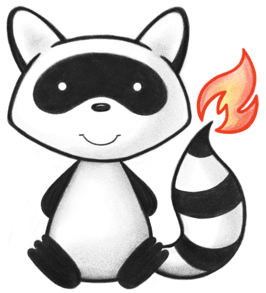
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * A record of a clinical assessment performed to determine what problem(s) may 049 * affect the patient and before planning the treatments or management 050 * strategies that are best to manage a patient's condition. Assessments are 051 * often 1:1 with a clinical consultation / encounter, but this varies greatly 052 * depending on the clinical workflow. This resource is called 053 * "ClinicalImpression" rather than "ClinicalAssessment" to avoid confusion with 054 * the recording of assessment tools such as Apgar score. 055 */ 056@ResourceDef(name = "ClinicalImpression", profile = "http://hl7.org/fhir/StructureDefinition/ClinicalImpression") 057public class ClinicalImpression extends DomainResource { 058 059 public enum ClinicalImpressionStatus { 060 /** 061 * null 062 */ 063 INPROGRESS, 064 /** 065 * null 066 */ 067 COMPLETED, 068 /** 069 * null 070 */ 071 ENTEREDINERROR, 072 /** 073 * added to help the parsers with the generic types 074 */ 075 NULL; 076 077 public static ClinicalImpressionStatus fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("in-progress".equals(codeString)) 081 return INPROGRESS; 082 if ("completed".equals(codeString)) 083 return COMPLETED; 084 if ("entered-in-error".equals(codeString)) 085 return ENTEREDINERROR; 086 if (Configuration.isAcceptInvalidEnums()) 087 return null; 088 else 089 throw new FHIRException("Unknown ClinicalImpressionStatus code '" + codeString + "'"); 090 } 091 092 public String toCode() { 093 switch (this) { 094 case INPROGRESS: 095 return "in-progress"; 096 case COMPLETED: 097 return "completed"; 098 case ENTEREDINERROR: 099 return "entered-in-error"; 100 case NULL: 101 return null; 102 default: 103 return "?"; 104 } 105 } 106 107 public String getSystem() { 108 switch (this) { 109 case INPROGRESS: 110 return "http://hl7.org/fhir/event-status"; 111 case COMPLETED: 112 return "http://hl7.org/fhir/event-status"; 113 case ENTEREDINERROR: 114 return "http://hl7.org/fhir/event-status"; 115 case NULL: 116 return null; 117 default: 118 return "?"; 119 } 120 } 121 122 public String getDefinition() { 123 switch (this) { 124 case INPROGRESS: 125 return ""; 126 case COMPLETED: 127 return ""; 128 case ENTEREDINERROR: 129 return ""; 130 case NULL: 131 return null; 132 default: 133 return "?"; 134 } 135 } 136 137 public String getDisplay() { 138 switch (this) { 139 case INPROGRESS: 140 return "in-progress"; 141 case COMPLETED: 142 return "completed"; 143 case ENTEREDINERROR: 144 return "entered-in-error"; 145 case NULL: 146 return null; 147 default: 148 return "?"; 149 } 150 } 151 } 152 153 public static class ClinicalImpressionStatusEnumFactory implements EnumFactory<ClinicalImpressionStatus> { 154 public ClinicalImpressionStatus fromCode(String codeString) throws IllegalArgumentException { 155 if (codeString == null || "".equals(codeString)) 156 if (codeString == null || "".equals(codeString)) 157 return null; 158 if ("in-progress".equals(codeString)) 159 return ClinicalImpressionStatus.INPROGRESS; 160 if ("completed".equals(codeString)) 161 return ClinicalImpressionStatus.COMPLETED; 162 if ("entered-in-error".equals(codeString)) 163 return ClinicalImpressionStatus.ENTEREDINERROR; 164 throw new IllegalArgumentException("Unknown ClinicalImpressionStatus code '" + codeString + "'"); 165 } 166 167 public Enumeration<ClinicalImpressionStatus> fromType(PrimitiveType<?> code) throws FHIRException { 168 if (code == null) 169 return null; 170 if (code.isEmpty()) 171 return new Enumeration<ClinicalImpressionStatus>(this, ClinicalImpressionStatus.NULL, code); 172 String codeString = code.asStringValue(); 173 if (codeString == null || "".equals(codeString)) 174 return new Enumeration<ClinicalImpressionStatus>(this, ClinicalImpressionStatus.NULL, code); 175 if ("in-progress".equals(codeString)) 176 return new Enumeration<ClinicalImpressionStatus>(this, ClinicalImpressionStatus.INPROGRESS, code); 177 if ("completed".equals(codeString)) 178 return new Enumeration<ClinicalImpressionStatus>(this, ClinicalImpressionStatus.COMPLETED, code); 179 if ("entered-in-error".equals(codeString)) 180 return new Enumeration<ClinicalImpressionStatus>(this, ClinicalImpressionStatus.ENTEREDINERROR, code); 181 throw new FHIRException("Unknown ClinicalImpressionStatus code '" + codeString + "'"); 182 } 183 184 public String toCode(ClinicalImpressionStatus code) { 185 if (code == ClinicalImpressionStatus.NULL) 186 return null; 187 if (code == ClinicalImpressionStatus.INPROGRESS) 188 return "in-progress"; 189 if (code == ClinicalImpressionStatus.COMPLETED) 190 return "completed"; 191 if (code == ClinicalImpressionStatus.ENTEREDINERROR) 192 return "entered-in-error"; 193 return "?"; 194 } 195 196 public String toSystem(ClinicalImpressionStatus code) { 197 return code.getSystem(); 198 } 199 } 200 201 @Block() 202 public static class ClinicalImpressionInvestigationComponent extends BackboneElement implements IBaseBackboneElement { 203 /** 204 * A name/code for the group ("set") of investigations. Typically, this will be 205 * something like "signs", "symptoms", "clinical", "diagnostic", but the list is 206 * not constrained, and others such groups such as 207 * (exposure|family|travel|nutritional) history may be used. 208 */ 209 @Child(name = "code", type = { 210 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 211 @Description(shortDefinition = "A name/code for the set", formalDefinition = "A name/code for the group (\"set\") of investigations. Typically, this will be something like \"signs\", \"symptoms\", \"clinical\", \"diagnostic\", but the list is not constrained, and others such groups such as (exposure|family|travel|nutritional) history may be used.") 212 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/investigation-sets") 213 protected CodeableConcept code; 214 215 /** 216 * A record of a specific investigation that was undertaken. 217 */ 218 @Child(name = "item", type = { Observation.class, QuestionnaireResponse.class, FamilyMemberHistory.class, 219 DiagnosticReport.class, RiskAssessment.class, ImagingStudy.class, 220 Media.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 221 @Description(shortDefinition = "Record of a specific investigation", formalDefinition = "A record of a specific investigation that was undertaken.") 222 protected List<Reference> item; 223 /** 224 * The actual objects that are the target of the reference (A record of a 225 * specific investigation that was undertaken.) 226 */ 227 protected List<Resource> itemTarget; 228 229 private static final long serialVersionUID = -301363326L; 230 231 /** 232 * Constructor 233 */ 234 public ClinicalImpressionInvestigationComponent() { 235 super(); 236 } 237 238 /** 239 * Constructor 240 */ 241 public ClinicalImpressionInvestigationComponent(CodeableConcept code) { 242 super(); 243 this.code = code; 244 } 245 246 /** 247 * @return {@link #code} (A name/code for the group ("set") of investigations. 248 * Typically, this will be something like "signs", "symptoms", 249 * "clinical", "diagnostic", but the list is not constrained, and others 250 * such groups such as (exposure|family|travel|nutritional) history may 251 * be used.) 252 */ 253 public CodeableConcept getCode() { 254 if (this.code == null) 255 if (Configuration.errorOnAutoCreate()) 256 throw new Error("Attempt to auto-create ClinicalImpressionInvestigationComponent.code"); 257 else if (Configuration.doAutoCreate()) 258 this.code = new CodeableConcept(); // cc 259 return this.code; 260 } 261 262 public boolean hasCode() { 263 return this.code != null && !this.code.isEmpty(); 264 } 265 266 /** 267 * @param value {@link #code} (A name/code for the group ("set") of 268 * investigations. Typically, this will be something like "signs", 269 * "symptoms", "clinical", "diagnostic", but the list is not 270 * constrained, and others such groups such as 271 * (exposure|family|travel|nutritional) history may be used.) 272 */ 273 public ClinicalImpressionInvestigationComponent setCode(CodeableConcept value) { 274 this.code = value; 275 return this; 276 } 277 278 /** 279 * @return {@link #item} (A record of a specific investigation that was 280 * undertaken.) 281 */ 282 public List<Reference> getItem() { 283 if (this.item == null) 284 this.item = new ArrayList<Reference>(); 285 return this.item; 286 } 287 288 /** 289 * @return Returns a reference to <code>this</code> for easy method chaining 290 */ 291 public ClinicalImpressionInvestigationComponent setItem(List<Reference> theItem) { 292 this.item = theItem; 293 return this; 294 } 295 296 public boolean hasItem() { 297 if (this.item == null) 298 return false; 299 for (Reference item : this.item) 300 if (!item.isEmpty()) 301 return true; 302 return false; 303 } 304 305 public Reference addItem() { // 3 306 Reference t = new Reference(); 307 if (this.item == null) 308 this.item = new ArrayList<Reference>(); 309 this.item.add(t); 310 return t; 311 } 312 313 public ClinicalImpressionInvestigationComponent addItem(Reference t) { // 3 314 if (t == null) 315 return this; 316 if (this.item == null) 317 this.item = new ArrayList<Reference>(); 318 this.item.add(t); 319 return this; 320 } 321 322 /** 323 * @return The first repetition of repeating field {@link #item}, creating it if 324 * it does not already exist 325 */ 326 public Reference getItemFirstRep() { 327 if (getItem().isEmpty()) { 328 addItem(); 329 } 330 return getItem().get(0); 331 } 332 333 /** 334 * @deprecated Use Reference#setResource(IBaseResource) instead 335 */ 336 @Deprecated 337 public List<Resource> getItemTarget() { 338 if (this.itemTarget == null) 339 this.itemTarget = new ArrayList<Resource>(); 340 return this.itemTarget; 341 } 342 343 protected void listChildren(List<Property> children) { 344 super.listChildren(children); 345 children.add(new Property("code", "CodeableConcept", 346 "A name/code for the group (\"set\") of investigations. Typically, this will be something like \"signs\", \"symptoms\", \"clinical\", \"diagnostic\", but the list is not constrained, and others such groups such as (exposure|family|travel|nutritional) history may be used.", 347 0, 1, code)); 348 children.add(new Property("item", 349 "Reference(Observation|QuestionnaireResponse|FamilyMemberHistory|DiagnosticReport|RiskAssessment|ImagingStudy|Media)", 350 "A record of a specific investigation that was undertaken.", 0, java.lang.Integer.MAX_VALUE, item)); 351 } 352 353 @Override 354 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 355 switch (_hash) { 356 case 3059181: 357 /* code */ return new Property("code", "CodeableConcept", 358 "A name/code for the group (\"set\") of investigations. Typically, this will be something like \"signs\", \"symptoms\", \"clinical\", \"diagnostic\", but the list is not constrained, and others such groups such as (exposure|family|travel|nutritional) history may be used.", 359 0, 1, code); 360 case 3242771: 361 /* item */ return new Property("item", 362 "Reference(Observation|QuestionnaireResponse|FamilyMemberHistory|DiagnosticReport|RiskAssessment|ImagingStudy|Media)", 363 "A record of a specific investigation that was undertaken.", 0, java.lang.Integer.MAX_VALUE, item); 364 default: 365 return super.getNamedProperty(_hash, _name, _checkValid); 366 } 367 368 } 369 370 @Override 371 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 372 switch (hash) { 373 case 3059181: 374 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 375 case 3242771: 376 /* item */ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // Reference 377 default: 378 return super.getProperty(hash, name, checkValid); 379 } 380 381 } 382 383 @Override 384 public Base setProperty(int hash, String name, Base value) throws FHIRException { 385 switch (hash) { 386 case 3059181: // code 387 this.code = castToCodeableConcept(value); // CodeableConcept 388 return value; 389 case 3242771: // item 390 this.getItem().add(castToReference(value)); // Reference 391 return value; 392 default: 393 return super.setProperty(hash, name, value); 394 } 395 396 } 397 398 @Override 399 public Base setProperty(String name, Base value) throws FHIRException { 400 if (name.equals("code")) { 401 this.code = castToCodeableConcept(value); // CodeableConcept 402 } else if (name.equals("item")) { 403 this.getItem().add(castToReference(value)); 404 } else 405 return super.setProperty(name, value); 406 return value; 407 } 408 409 @Override 410 public void removeChild(String name, Base value) throws FHIRException { 411 if (name.equals("code")) { 412 this.code = null; 413 } else if (name.equals("item")) { 414 this.getItem().remove(castToReference(value)); 415 } else 416 super.removeChild(name, value); 417 418 } 419 420 @Override 421 public Base makeProperty(int hash, String name) throws FHIRException { 422 switch (hash) { 423 case 3059181: 424 return getCode(); 425 case 3242771: 426 return addItem(); 427 default: 428 return super.makeProperty(hash, name); 429 } 430 431 } 432 433 @Override 434 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 435 switch (hash) { 436 case 3059181: 437 /* code */ return new String[] { "CodeableConcept" }; 438 case 3242771: 439 /* item */ return new String[] { "Reference" }; 440 default: 441 return super.getTypesForProperty(hash, name); 442 } 443 444 } 445 446 @Override 447 public Base addChild(String name) throws FHIRException { 448 if (name.equals("code")) { 449 this.code = new CodeableConcept(); 450 return this.code; 451 } else if (name.equals("item")) { 452 return addItem(); 453 } else 454 return super.addChild(name); 455 } 456 457 public ClinicalImpressionInvestigationComponent copy() { 458 ClinicalImpressionInvestigationComponent dst = new ClinicalImpressionInvestigationComponent(); 459 copyValues(dst); 460 return dst; 461 } 462 463 public void copyValues(ClinicalImpressionInvestigationComponent dst) { 464 super.copyValues(dst); 465 dst.code = code == null ? null : code.copy(); 466 if (item != null) { 467 dst.item = new ArrayList<Reference>(); 468 for (Reference i : item) 469 dst.item.add(i.copy()); 470 } 471 ; 472 } 473 474 @Override 475 public boolean equalsDeep(Base other_) { 476 if (!super.equalsDeep(other_)) 477 return false; 478 if (!(other_ instanceof ClinicalImpressionInvestigationComponent)) 479 return false; 480 ClinicalImpressionInvestigationComponent o = (ClinicalImpressionInvestigationComponent) other_; 481 return compareDeep(code, o.code, true) && compareDeep(item, o.item, true); 482 } 483 484 @Override 485 public boolean equalsShallow(Base other_) { 486 if (!super.equalsShallow(other_)) 487 return false; 488 if (!(other_ instanceof ClinicalImpressionInvestigationComponent)) 489 return false; 490 ClinicalImpressionInvestigationComponent o = (ClinicalImpressionInvestigationComponent) other_; 491 return true; 492 } 493 494 public boolean isEmpty() { 495 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, item); 496 } 497 498 public String fhirType() { 499 return "ClinicalImpression.investigation"; 500 501 } 502 503 } 504 505 @Block() 506 public static class ClinicalImpressionFindingComponent extends BackboneElement implements IBaseBackboneElement { 507 /** 508 * Specific text or code for finding or diagnosis, which may include ruled-out 509 * or resolved conditions. 510 */ 511 @Child(name = "itemCodeableConcept", type = { 512 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 513 @Description(shortDefinition = "What was found", formalDefinition = "Specific text or code for finding or diagnosis, which may include ruled-out or resolved conditions.") 514 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/condition-code") 515 protected CodeableConcept itemCodeableConcept; 516 517 /** 518 * Specific reference for finding or diagnosis, which may include ruled-out or 519 * resolved conditions. 520 */ 521 @Child(name = "itemReference", type = { Condition.class, Observation.class, 522 Media.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 523 @Description(shortDefinition = "What was found", formalDefinition = "Specific reference for finding or diagnosis, which may include ruled-out or resolved conditions.") 524 protected Reference itemReference; 525 526 /** 527 * The actual object that is the target of the reference (Specific reference for 528 * finding or diagnosis, which may include ruled-out or resolved conditions.) 529 */ 530 protected Resource itemReferenceTarget; 531 532 /** 533 * Which investigations support finding or diagnosis. 534 */ 535 @Child(name = "basis", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 536 @Description(shortDefinition = "Which investigations support finding", formalDefinition = "Which investigations support finding or diagnosis.") 537 protected StringType basis; 538 539 private static final long serialVersionUID = -1578446448L; 540 541 /** 542 * Constructor 543 */ 544 public ClinicalImpressionFindingComponent() { 545 super(); 546 } 547 548 /** 549 * @return {@link #itemCodeableConcept} (Specific text or code for finding or 550 * diagnosis, which may include ruled-out or resolved conditions.) 551 */ 552 public CodeableConcept getItemCodeableConcept() { 553 if (this.itemCodeableConcept == null) 554 if (Configuration.errorOnAutoCreate()) 555 throw new Error("Attempt to auto-create ClinicalImpressionFindingComponent.itemCodeableConcept"); 556 else if (Configuration.doAutoCreate()) 557 this.itemCodeableConcept = new CodeableConcept(); // cc 558 return this.itemCodeableConcept; 559 } 560 561 public boolean hasItemCodeableConcept() { 562 return this.itemCodeableConcept != null && !this.itemCodeableConcept.isEmpty(); 563 } 564 565 /** 566 * @param value {@link #itemCodeableConcept} (Specific text or code for finding 567 * or diagnosis, which may include ruled-out or resolved 568 * conditions.) 569 */ 570 public ClinicalImpressionFindingComponent setItemCodeableConcept(CodeableConcept value) { 571 this.itemCodeableConcept = value; 572 return this; 573 } 574 575 /** 576 * @return {@link #itemReference} (Specific reference for finding or diagnosis, 577 * which may include ruled-out or resolved conditions.) 578 */ 579 public Reference getItemReference() { 580 if (this.itemReference == null) 581 if (Configuration.errorOnAutoCreate()) 582 throw new Error("Attempt to auto-create ClinicalImpressionFindingComponent.itemReference"); 583 else if (Configuration.doAutoCreate()) 584 this.itemReference = new Reference(); // cc 585 return this.itemReference; 586 } 587 588 public boolean hasItemReference() { 589 return this.itemReference != null && !this.itemReference.isEmpty(); 590 } 591 592 /** 593 * @param value {@link #itemReference} (Specific reference for finding or 594 * diagnosis, which may include ruled-out or resolved conditions.) 595 */ 596 public ClinicalImpressionFindingComponent setItemReference(Reference value) { 597 this.itemReference = value; 598 return this; 599 } 600 601 /** 602 * @return {@link #itemReference} The actual object that is the target of the 603 * reference. The reference library doesn't populate this, but you can 604 * use it to hold the resource if you resolve it. (Specific reference 605 * for finding or diagnosis, which may include ruled-out or resolved 606 * conditions.) 607 */ 608 public Resource getItemReferenceTarget() { 609 return this.itemReferenceTarget; 610 } 611 612 /** 613 * @param value {@link #itemReference} The actual object that is the target of 614 * the reference. The reference library doesn't use these, but you 615 * can use it to hold the resource if you resolve it. (Specific 616 * reference for finding or diagnosis, which may include ruled-out 617 * or resolved conditions.) 618 */ 619 public ClinicalImpressionFindingComponent setItemReferenceTarget(Resource value) { 620 this.itemReferenceTarget = value; 621 return this; 622 } 623 624 /** 625 * @return {@link #basis} (Which investigations support finding or diagnosis.). 626 * This is the underlying object with id, value and extensions. The 627 * accessor "getBasis" gives direct access to the value 628 */ 629 public StringType getBasisElement() { 630 if (this.basis == null) 631 if (Configuration.errorOnAutoCreate()) 632 throw new Error("Attempt to auto-create ClinicalImpressionFindingComponent.basis"); 633 else if (Configuration.doAutoCreate()) 634 this.basis = new StringType(); // bb 635 return this.basis; 636 } 637 638 public boolean hasBasisElement() { 639 return this.basis != null && !this.basis.isEmpty(); 640 } 641 642 public boolean hasBasis() { 643 return this.basis != null && !this.basis.isEmpty(); 644 } 645 646 /** 647 * @param value {@link #basis} (Which investigations support finding or 648 * diagnosis.). This is the underlying object with id, value and 649 * extensions. The accessor "getBasis" gives direct access to the 650 * value 651 */ 652 public ClinicalImpressionFindingComponent setBasisElement(StringType value) { 653 this.basis = value; 654 return this; 655 } 656 657 /** 658 * @return Which investigations support finding or diagnosis. 659 */ 660 public String getBasis() { 661 return this.basis == null ? null : this.basis.getValue(); 662 } 663 664 /** 665 * @param value Which investigations support finding or diagnosis. 666 */ 667 public ClinicalImpressionFindingComponent setBasis(String value) { 668 if (Utilities.noString(value)) 669 this.basis = null; 670 else { 671 if (this.basis == null) 672 this.basis = new StringType(); 673 this.basis.setValue(value); 674 } 675 return this; 676 } 677 678 protected void listChildren(List<Property> children) { 679 super.listChildren(children); 680 children.add(new Property("itemCodeableConcept", "CodeableConcept", 681 "Specific text or code for finding or diagnosis, which may include ruled-out or resolved conditions.", 0, 1, 682 itemCodeableConcept)); 683 children.add(new Property("itemReference", "Reference(Condition|Observation|Media)", 684 "Specific reference for finding or diagnosis, which may include ruled-out or resolved conditions.", 0, 1, 685 itemReference)); 686 children.add(new Property("basis", "string", "Which investigations support finding or diagnosis.", 0, 1, basis)); 687 } 688 689 @Override 690 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 691 switch (_hash) { 692 case 106644494: 693 /* itemCodeableConcept */ return new Property("itemCodeableConcept", "CodeableConcept", 694 "Specific text or code for finding or diagnosis, which may include ruled-out or resolved conditions.", 0, 1, 695 itemCodeableConcept); 696 case 1376364920: 697 /* itemReference */ return new Property("itemReference", "Reference(Condition|Observation|Media)", 698 "Specific reference for finding or diagnosis, which may include ruled-out or resolved conditions.", 0, 1, 699 itemReference); 700 case 93508670: 701 /* basis */ return new Property("basis", "string", "Which investigations support finding or diagnosis.", 0, 1, 702 basis); 703 default: 704 return super.getNamedProperty(_hash, _name, _checkValid); 705 } 706 707 } 708 709 @Override 710 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 711 switch (hash) { 712 case 106644494: 713 /* itemCodeableConcept */ return this.itemCodeableConcept == null ? new Base[0] 714 : new Base[] { this.itemCodeableConcept }; // CodeableConcept 715 case 1376364920: 716 /* itemReference */ return this.itemReference == null ? new Base[0] : new Base[] { this.itemReference }; // Reference 717 case 93508670: 718 /* basis */ return this.basis == null ? new Base[0] : new Base[] { this.basis }; // StringType 719 default: 720 return super.getProperty(hash, name, checkValid); 721 } 722 723 } 724 725 @Override 726 public Base setProperty(int hash, String name, Base value) throws FHIRException { 727 switch (hash) { 728 case 106644494: // itemCodeableConcept 729 this.itemCodeableConcept = castToCodeableConcept(value); // CodeableConcept 730 return value; 731 case 1376364920: // itemReference 732 this.itemReference = castToReference(value); // Reference 733 return value; 734 case 93508670: // basis 735 this.basis = castToString(value); // StringType 736 return value; 737 default: 738 return super.setProperty(hash, name, value); 739 } 740 741 } 742 743 @Override 744 public Base setProperty(String name, Base value) throws FHIRException { 745 if (name.equals("itemCodeableConcept")) { 746 this.itemCodeableConcept = castToCodeableConcept(value); // CodeableConcept 747 } else if (name.equals("itemReference")) { 748 this.itemReference = castToReference(value); // Reference 749 } else if (name.equals("basis")) { 750 this.basis = castToString(value); // StringType 751 } else 752 return super.setProperty(name, value); 753 return value; 754 } 755 756 @Override 757 public void removeChild(String name, Base value) throws FHIRException { 758 if (name.equals("itemCodeableConcept")) { 759 this.itemCodeableConcept = null; 760 } else if (name.equals("itemReference")) { 761 this.itemReference = null; 762 } else if (name.equals("basis")) { 763 this.basis = null; 764 } else 765 super.removeChild(name, value); 766 767 } 768 769 @Override 770 public Base makeProperty(int hash, String name) throws FHIRException { 771 switch (hash) { 772 case 106644494: 773 return getItemCodeableConcept(); 774 case 1376364920: 775 return getItemReference(); 776 case 93508670: 777 return getBasisElement(); 778 default: 779 return super.makeProperty(hash, name); 780 } 781 782 } 783 784 @Override 785 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 786 switch (hash) { 787 case 106644494: 788 /* itemCodeableConcept */ return new String[] { "CodeableConcept" }; 789 case 1376364920: 790 /* itemReference */ return new String[] { "Reference" }; 791 case 93508670: 792 /* basis */ return new String[] { "string" }; 793 default: 794 return super.getTypesForProperty(hash, name); 795 } 796 797 } 798 799 @Override 800 public Base addChild(String name) throws FHIRException { 801 if (name.equals("itemCodeableConcept")) { 802 this.itemCodeableConcept = new CodeableConcept(); 803 return this.itemCodeableConcept; 804 } else if (name.equals("itemReference")) { 805 this.itemReference = new Reference(); 806 return this.itemReference; 807 } else if (name.equals("basis")) { 808 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.basis"); 809 } else 810 return super.addChild(name); 811 } 812 813 public ClinicalImpressionFindingComponent copy() { 814 ClinicalImpressionFindingComponent dst = new ClinicalImpressionFindingComponent(); 815 copyValues(dst); 816 return dst; 817 } 818 819 public void copyValues(ClinicalImpressionFindingComponent dst) { 820 super.copyValues(dst); 821 dst.itemCodeableConcept = itemCodeableConcept == null ? null : itemCodeableConcept.copy(); 822 dst.itemReference = itemReference == null ? null : itemReference.copy(); 823 dst.basis = basis == null ? null : basis.copy(); 824 } 825 826 @Override 827 public boolean equalsDeep(Base other_) { 828 if (!super.equalsDeep(other_)) 829 return false; 830 if (!(other_ instanceof ClinicalImpressionFindingComponent)) 831 return false; 832 ClinicalImpressionFindingComponent o = (ClinicalImpressionFindingComponent) other_; 833 return compareDeep(itemCodeableConcept, o.itemCodeableConcept, true) 834 && compareDeep(itemReference, o.itemReference, true) && compareDeep(basis, o.basis, true); 835 } 836 837 @Override 838 public boolean equalsShallow(Base other_) { 839 if (!super.equalsShallow(other_)) 840 return false; 841 if (!(other_ instanceof ClinicalImpressionFindingComponent)) 842 return false; 843 ClinicalImpressionFindingComponent o = (ClinicalImpressionFindingComponent) other_; 844 return compareValues(basis, o.basis, true); 845 } 846 847 public boolean isEmpty() { 848 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(itemCodeableConcept, itemReference, basis); 849 } 850 851 public String fhirType() { 852 return "ClinicalImpression.finding"; 853 854 } 855 856 } 857 858 /** 859 * Business identifiers assigned to this clinical impression by the performer or 860 * other systems which remain constant as the resource is updated and propagates 861 * from server to server. 862 */ 863 @Child(name = "identifier", type = { 864 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 865 @Description(shortDefinition = "Business identifier", formalDefinition = "Business identifiers assigned to this clinical impression by the performer or other systems which remain constant as the resource is updated and propagates from server to server.") 866 protected List<Identifier> identifier; 867 868 /** 869 * Identifies the workflow status of the assessment. 870 */ 871 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 872 @Description(shortDefinition = "in-progress | completed | entered-in-error", formalDefinition = "Identifies the workflow status of the assessment.") 873 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/clinicalimpression-status") 874 protected Enumeration<ClinicalImpressionStatus> status; 875 876 /** 877 * Captures the reason for the current state of the ClinicalImpression. 878 */ 879 @Child(name = "statusReason", type = { 880 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 881 @Description(shortDefinition = "Reason for current status", formalDefinition = "Captures the reason for the current state of the ClinicalImpression.") 882 protected CodeableConcept statusReason; 883 884 /** 885 * Categorizes the type of clinical assessment performed. 886 */ 887 @Child(name = "code", type = { CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 888 @Description(shortDefinition = "Kind of assessment performed", formalDefinition = "Categorizes the type of clinical assessment performed.") 889 protected CodeableConcept code; 890 891 /** 892 * A summary of the context and/or cause of the assessment - why / where it was 893 * performed, and what patient events/status prompted it. 894 */ 895 @Child(name = "description", type = { 896 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 897 @Description(shortDefinition = "Why/how the assessment was performed", formalDefinition = "A summary of the context and/or cause of the assessment - why / where it was performed, and what patient events/status prompted it.") 898 protected StringType description; 899 900 /** 901 * The patient or group of individuals assessed as part of this record. 902 */ 903 @Child(name = "subject", type = { Patient.class, 904 Group.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 905 @Description(shortDefinition = "Patient or group assessed", formalDefinition = "The patient or group of individuals assessed as part of this record.") 906 protected Reference subject; 907 908 /** 909 * The actual object that is the target of the reference (The patient or group 910 * of individuals assessed as part of this record.) 911 */ 912 protected Resource subjectTarget; 913 914 /** 915 * The Encounter during which this ClinicalImpression was created or to which 916 * the creation of this record is tightly associated. 917 */ 918 @Child(name = "encounter", type = { Encounter.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 919 @Description(shortDefinition = "Encounter created as part of", formalDefinition = "The Encounter during which this ClinicalImpression was created or to which the creation of this record is tightly associated.") 920 protected Reference encounter; 921 922 /** 923 * The actual object that is the target of the reference (The Encounter during 924 * which this ClinicalImpression was created or to which the creation of this 925 * record is tightly associated.) 926 */ 927 protected Encounter encounterTarget; 928 929 /** 930 * The point in time or period over which the subject was assessed. 931 */ 932 @Child(name = "effective", type = { DateTimeType.class, 933 Period.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 934 @Description(shortDefinition = "Time of assessment", formalDefinition = "The point in time or period over which the subject was assessed.") 935 protected Type effective; 936 937 /** 938 * Indicates when the documentation of the assessment was complete. 939 */ 940 @Child(name = "date", type = { DateTimeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 941 @Description(shortDefinition = "When the assessment was documented", formalDefinition = "Indicates when the documentation of the assessment was complete.") 942 protected DateTimeType date; 943 944 /** 945 * The clinician performing the assessment. 946 */ 947 @Child(name = "assessor", type = { Practitioner.class, 948 PractitionerRole.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 949 @Description(shortDefinition = "The clinician performing the assessment", formalDefinition = "The clinician performing the assessment.") 950 protected Reference assessor; 951 952 /** 953 * The actual object that is the target of the reference (The clinician 954 * performing the assessment.) 955 */ 956 protected Resource assessorTarget; 957 958 /** 959 * A reference to the last assessment that was conducted on this patient. 960 * Assessments are often/usually ongoing in nature; a care provider 961 * (practitioner or team) will make new assessments on an ongoing basis as new 962 * data arises or the patient's conditions changes. 963 */ 964 @Child(name = "previous", type = { 965 ClinicalImpression.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 966 @Description(shortDefinition = "Reference to last assessment", formalDefinition = "A reference to the last assessment that was conducted on this patient. Assessments are often/usually ongoing in nature; a care provider (practitioner or team) will make new assessments on an ongoing basis as new data arises or the patient's conditions changes.") 967 protected Reference previous; 968 969 /** 970 * The actual object that is the target of the reference (A reference to the 971 * last assessment that was conducted on this patient. Assessments are 972 * often/usually ongoing in nature; a care provider (practitioner or team) will 973 * make new assessments on an ongoing basis as new data arises or the patient's 974 * conditions changes.) 975 */ 976 protected ClinicalImpression previousTarget; 977 978 /** 979 * A list of the relevant problems/conditions for a patient. 980 */ 981 @Child(name = "problem", type = { Condition.class, 982 AllergyIntolerance.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 983 @Description(shortDefinition = "Relevant impressions of patient state", formalDefinition = "A list of the relevant problems/conditions for a patient.") 984 protected List<Reference> problem; 985 /** 986 * The actual objects that are the target of the reference (A list of the 987 * relevant problems/conditions for a patient.) 988 */ 989 protected List<Resource> problemTarget; 990 991 /** 992 * One or more sets of investigations (signs, symptoms, etc.). The actual 993 * grouping of investigations varies greatly depending on the type and context 994 * of the assessment. These investigations may include data generated during the 995 * assessment process, or data previously generated and recorded that is 996 * pertinent to the outcomes. 997 */ 998 @Child(name = "investigation", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 999 @Description(shortDefinition = "One or more sets of investigations (signs, symptoms, etc.)", formalDefinition = "One or more sets of investigations (signs, symptoms, etc.). The actual grouping of investigations varies greatly depending on the type and context of the assessment. These investigations may include data generated during the assessment process, or data previously generated and recorded that is pertinent to the outcomes.") 1000 protected List<ClinicalImpressionInvestigationComponent> investigation; 1001 1002 /** 1003 * Reference to a specific published clinical protocol that was followed during 1004 * this assessment, and/or that provides evidence in support of the diagnosis. 1005 */ 1006 @Child(name = "protocol", type = { 1007 UriType.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1008 @Description(shortDefinition = "Clinical Protocol followed", formalDefinition = "Reference to a specific published clinical protocol that was followed during this assessment, and/or that provides evidence in support of the diagnosis.") 1009 protected List<UriType> protocol; 1010 1011 /** 1012 * A text summary of the investigations and the diagnosis. 1013 */ 1014 @Child(name = "summary", type = { StringType.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 1015 @Description(shortDefinition = "Summary of the assessment", formalDefinition = "A text summary of the investigations and the diagnosis.") 1016 protected StringType summary; 1017 1018 /** 1019 * Specific findings or diagnoses that were considered likely or relevant to 1020 * ongoing treatment. 1021 */ 1022 @Child(name = "finding", type = {}, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1023 @Description(shortDefinition = "Possible or likely findings and diagnoses", formalDefinition = "Specific findings or diagnoses that were considered likely or relevant to ongoing treatment.") 1024 protected List<ClinicalImpressionFindingComponent> finding; 1025 1026 /** 1027 * Estimate of likely outcome. 1028 */ 1029 @Child(name = "prognosisCodeableConcept", type = { 1030 CodeableConcept.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1031 @Description(shortDefinition = "Estimate of likely outcome", formalDefinition = "Estimate of likely outcome.") 1032 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/clinicalimpression-prognosis") 1033 protected List<CodeableConcept> prognosisCodeableConcept; 1034 1035 /** 1036 * RiskAssessment expressing likely outcome. 1037 */ 1038 @Child(name = "prognosisReference", type = { 1039 RiskAssessment.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1040 @Description(shortDefinition = "RiskAssessment expressing likely outcome", formalDefinition = "RiskAssessment expressing likely outcome.") 1041 protected List<Reference> prognosisReference; 1042 /** 1043 * The actual objects that are the target of the reference (RiskAssessment 1044 * expressing likely outcome.) 1045 */ 1046 protected List<RiskAssessment> prognosisReferenceTarget; 1047 1048 /** 1049 * Information supporting the clinical impression. 1050 */ 1051 @Child(name = "supportingInfo", type = { 1052 Reference.class }, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1053 @Description(shortDefinition = "Information supporting the clinical impression", formalDefinition = "Information supporting the clinical impression.") 1054 protected List<Reference> supportingInfo; 1055 /** 1056 * The actual objects that are the target of the reference (Information 1057 * supporting the clinical impression.) 1058 */ 1059 protected List<Resource> supportingInfoTarget; 1060 1061 /** 1062 * Commentary about the impression, typically recorded after the impression 1063 * itself was made, though supplemental notes by the original author could also 1064 * appear. 1065 */ 1066 @Child(name = "note", type = { 1067 Annotation.class }, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1068 @Description(shortDefinition = "Comments made about the ClinicalImpression", formalDefinition = "Commentary about the impression, typically recorded after the impression itself was made, though supplemental notes by the original author could also appear.") 1069 protected List<Annotation> note; 1070 1071 private static final long serialVersionUID = 1158874575L; 1072 1073 /** 1074 * Constructor 1075 */ 1076 public ClinicalImpression() { 1077 super(); 1078 } 1079 1080 /** 1081 * Constructor 1082 */ 1083 public ClinicalImpression(Enumeration<ClinicalImpressionStatus> status, Reference subject) { 1084 super(); 1085 this.status = status; 1086 this.subject = subject; 1087 } 1088 1089 /** 1090 * @return {@link #identifier} (Business identifiers assigned to this clinical 1091 * impression by the performer or other systems which remain constant as 1092 * the resource is updated and propagates from server to server.) 1093 */ 1094 public List<Identifier> getIdentifier() { 1095 if (this.identifier == null) 1096 this.identifier = new ArrayList<Identifier>(); 1097 return this.identifier; 1098 } 1099 1100 /** 1101 * @return Returns a reference to <code>this</code> for easy method chaining 1102 */ 1103 public ClinicalImpression setIdentifier(List<Identifier> theIdentifier) { 1104 this.identifier = theIdentifier; 1105 return this; 1106 } 1107 1108 public boolean hasIdentifier() { 1109 if (this.identifier == null) 1110 return false; 1111 for (Identifier item : this.identifier) 1112 if (!item.isEmpty()) 1113 return true; 1114 return false; 1115 } 1116 1117 public Identifier addIdentifier() { // 3 1118 Identifier t = new Identifier(); 1119 if (this.identifier == null) 1120 this.identifier = new ArrayList<Identifier>(); 1121 this.identifier.add(t); 1122 return t; 1123 } 1124 1125 public ClinicalImpression addIdentifier(Identifier t) { // 3 1126 if (t == null) 1127 return this; 1128 if (this.identifier == null) 1129 this.identifier = new ArrayList<Identifier>(); 1130 this.identifier.add(t); 1131 return this; 1132 } 1133 1134 /** 1135 * @return The first repetition of repeating field {@link #identifier}, creating 1136 * it if it does not already exist 1137 */ 1138 public Identifier getIdentifierFirstRep() { 1139 if (getIdentifier().isEmpty()) { 1140 addIdentifier(); 1141 } 1142 return getIdentifier().get(0); 1143 } 1144 1145 /** 1146 * @return {@link #status} (Identifies the workflow status of the assessment.). 1147 * This is the underlying object with id, value and extensions. The 1148 * accessor "getStatus" gives direct access to the value 1149 */ 1150 public Enumeration<ClinicalImpressionStatus> getStatusElement() { 1151 if (this.status == null) 1152 if (Configuration.errorOnAutoCreate()) 1153 throw new Error("Attempt to auto-create ClinicalImpression.status"); 1154 else if (Configuration.doAutoCreate()) 1155 this.status = new Enumeration<ClinicalImpressionStatus>(new ClinicalImpressionStatusEnumFactory()); // bb 1156 return this.status; 1157 } 1158 1159 public boolean hasStatusElement() { 1160 return this.status != null && !this.status.isEmpty(); 1161 } 1162 1163 public boolean hasStatus() { 1164 return this.status != null && !this.status.isEmpty(); 1165 } 1166 1167 /** 1168 * @param value {@link #status} (Identifies the workflow status of the 1169 * assessment.). This is the underlying object with id, value and 1170 * extensions. The accessor "getStatus" gives direct access to the 1171 * value 1172 */ 1173 public ClinicalImpression setStatusElement(Enumeration<ClinicalImpressionStatus> value) { 1174 this.status = value; 1175 return this; 1176 } 1177 1178 /** 1179 * @return Identifies the workflow status of the assessment. 1180 */ 1181 public ClinicalImpressionStatus getStatus() { 1182 return this.status == null ? null : this.status.getValue(); 1183 } 1184 1185 /** 1186 * @param value Identifies the workflow status of the assessment. 1187 */ 1188 public ClinicalImpression setStatus(ClinicalImpressionStatus value) { 1189 if (this.status == null) 1190 this.status = new Enumeration<ClinicalImpressionStatus>(new ClinicalImpressionStatusEnumFactory()); 1191 this.status.setValue(value); 1192 return this; 1193 } 1194 1195 /** 1196 * @return {@link #statusReason} (Captures the reason for the current state of 1197 * the ClinicalImpression.) 1198 */ 1199 public CodeableConcept getStatusReason() { 1200 if (this.statusReason == null) 1201 if (Configuration.errorOnAutoCreate()) 1202 throw new Error("Attempt to auto-create ClinicalImpression.statusReason"); 1203 else if (Configuration.doAutoCreate()) 1204 this.statusReason = new CodeableConcept(); // cc 1205 return this.statusReason; 1206 } 1207 1208 public boolean hasStatusReason() { 1209 return this.statusReason != null && !this.statusReason.isEmpty(); 1210 } 1211 1212 /** 1213 * @param value {@link #statusReason} (Captures the reason for the current state 1214 * of the ClinicalImpression.) 1215 */ 1216 public ClinicalImpression setStatusReason(CodeableConcept value) { 1217 this.statusReason = value; 1218 return this; 1219 } 1220 1221 /** 1222 * @return {@link #code} (Categorizes the type of clinical assessment 1223 * performed.) 1224 */ 1225 public CodeableConcept getCode() { 1226 if (this.code == null) 1227 if (Configuration.errorOnAutoCreate()) 1228 throw new Error("Attempt to auto-create ClinicalImpression.code"); 1229 else if (Configuration.doAutoCreate()) 1230 this.code = new CodeableConcept(); // cc 1231 return this.code; 1232 } 1233 1234 public boolean hasCode() { 1235 return this.code != null && !this.code.isEmpty(); 1236 } 1237 1238 /** 1239 * @param value {@link #code} (Categorizes the type of clinical assessment 1240 * performed.) 1241 */ 1242 public ClinicalImpression setCode(CodeableConcept value) { 1243 this.code = value; 1244 return this; 1245 } 1246 1247 /** 1248 * @return {@link #description} (A summary of the context and/or cause of the 1249 * assessment - why / where it was performed, and what patient 1250 * events/status prompted it.). This is the underlying object with id, 1251 * value and extensions. The accessor "getDescription" gives direct 1252 * access to the value 1253 */ 1254 public StringType getDescriptionElement() { 1255 if (this.description == null) 1256 if (Configuration.errorOnAutoCreate()) 1257 throw new Error("Attempt to auto-create ClinicalImpression.description"); 1258 else if (Configuration.doAutoCreate()) 1259 this.description = new StringType(); // bb 1260 return this.description; 1261 } 1262 1263 public boolean hasDescriptionElement() { 1264 return this.description != null && !this.description.isEmpty(); 1265 } 1266 1267 public boolean hasDescription() { 1268 return this.description != null && !this.description.isEmpty(); 1269 } 1270 1271 /** 1272 * @param value {@link #description} (A summary of the context and/or cause of 1273 * the assessment - why / where it was performed, and what patient 1274 * events/status prompted it.). This is the underlying object with 1275 * id, value and extensions. The accessor "getDescription" gives 1276 * direct access to the value 1277 */ 1278 public ClinicalImpression setDescriptionElement(StringType value) { 1279 this.description = value; 1280 return this; 1281 } 1282 1283 /** 1284 * @return A summary of the context and/or cause of the assessment - why / where 1285 * it was performed, and what patient events/status prompted it. 1286 */ 1287 public String getDescription() { 1288 return this.description == null ? null : this.description.getValue(); 1289 } 1290 1291 /** 1292 * @param value A summary of the context and/or cause of the assessment - why / 1293 * where it was performed, and what patient events/status prompted 1294 * it. 1295 */ 1296 public ClinicalImpression setDescription(String value) { 1297 if (Utilities.noString(value)) 1298 this.description = null; 1299 else { 1300 if (this.description == null) 1301 this.description = new StringType(); 1302 this.description.setValue(value); 1303 } 1304 return this; 1305 } 1306 1307 /** 1308 * @return {@link #subject} (The patient or group of individuals assessed as 1309 * part of this record.) 1310 */ 1311 public Reference getSubject() { 1312 if (this.subject == null) 1313 if (Configuration.errorOnAutoCreate()) 1314 throw new Error("Attempt to auto-create ClinicalImpression.subject"); 1315 else if (Configuration.doAutoCreate()) 1316 this.subject = new Reference(); // cc 1317 return this.subject; 1318 } 1319 1320 public boolean hasSubject() { 1321 return this.subject != null && !this.subject.isEmpty(); 1322 } 1323 1324 /** 1325 * @param value {@link #subject} (The patient or group of individuals assessed 1326 * as part of this record.) 1327 */ 1328 public ClinicalImpression setSubject(Reference value) { 1329 this.subject = value; 1330 return this; 1331 } 1332 1333 /** 1334 * @return {@link #subject} The actual object that is the target of the 1335 * reference. The reference library doesn't populate this, but you can 1336 * use it to hold the resource if you resolve it. (The patient or group 1337 * of individuals assessed as part of this record.) 1338 */ 1339 public Resource getSubjectTarget() { 1340 return this.subjectTarget; 1341 } 1342 1343 /** 1344 * @param value {@link #subject} The actual object that is the target of the 1345 * reference. The reference library doesn't use these, but you can 1346 * use it to hold the resource if you resolve it. (The patient or 1347 * group of individuals assessed as part of this record.) 1348 */ 1349 public ClinicalImpression setSubjectTarget(Resource value) { 1350 this.subjectTarget = value; 1351 return this; 1352 } 1353 1354 /** 1355 * @return {@link #encounter} (The Encounter during which this 1356 * ClinicalImpression was created or to which the creation of this 1357 * record is tightly associated.) 1358 */ 1359 public Reference getEncounter() { 1360 if (this.encounter == null) 1361 if (Configuration.errorOnAutoCreate()) 1362 throw new Error("Attempt to auto-create ClinicalImpression.encounter"); 1363 else if (Configuration.doAutoCreate()) 1364 this.encounter = new Reference(); // cc 1365 return this.encounter; 1366 } 1367 1368 public boolean hasEncounter() { 1369 return this.encounter != null && !this.encounter.isEmpty(); 1370 } 1371 1372 /** 1373 * @param value {@link #encounter} (The Encounter during which this 1374 * ClinicalImpression was created or to which the creation of this 1375 * record is tightly associated.) 1376 */ 1377 public ClinicalImpression setEncounter(Reference value) { 1378 this.encounter = value; 1379 return this; 1380 } 1381 1382 /** 1383 * @return {@link #encounter} The actual object that is the target of the 1384 * reference. The reference library doesn't populate this, but you can 1385 * use it to hold the resource if you resolve it. (The Encounter during 1386 * which this ClinicalImpression was created or to which the creation of 1387 * this record is tightly associated.) 1388 */ 1389 public Encounter getEncounterTarget() { 1390 if (this.encounterTarget == null) 1391 if (Configuration.errorOnAutoCreate()) 1392 throw new Error("Attempt to auto-create ClinicalImpression.encounter"); 1393 else if (Configuration.doAutoCreate()) 1394 this.encounterTarget = new Encounter(); // aa 1395 return this.encounterTarget; 1396 } 1397 1398 /** 1399 * @param value {@link #encounter} The actual object that is the target of the 1400 * reference. The reference library doesn't use these, but you can 1401 * use it to hold the resource if you resolve it. (The Encounter 1402 * during which this ClinicalImpression was created or to which the 1403 * creation of this record is tightly associated.) 1404 */ 1405 public ClinicalImpression setEncounterTarget(Encounter value) { 1406 this.encounterTarget = value; 1407 return this; 1408 } 1409 1410 /** 1411 * @return {@link #effective} (The point in time or period over which the 1412 * subject was assessed.) 1413 */ 1414 public Type getEffective() { 1415 return this.effective; 1416 } 1417 1418 /** 1419 * @return {@link #effective} (The point in time or period over which the 1420 * subject was assessed.) 1421 */ 1422 public DateTimeType getEffectiveDateTimeType() throws FHIRException { 1423 if (this.effective == null) 1424 this.effective = new DateTimeType(); 1425 if (!(this.effective instanceof DateTimeType)) 1426 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1427 + this.effective.getClass().getName() + " was encountered"); 1428 return (DateTimeType) this.effective; 1429 } 1430 1431 public boolean hasEffectiveDateTimeType() { 1432 return this != null && this.effective instanceof DateTimeType; 1433 } 1434 1435 /** 1436 * @return {@link #effective} (The point in time or period over which the 1437 * subject was assessed.) 1438 */ 1439 public Period getEffectivePeriod() throws FHIRException { 1440 if (this.effective == null) 1441 this.effective = new Period(); 1442 if (!(this.effective instanceof Period)) 1443 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.effective.getClass().getName() 1444 + " was encountered"); 1445 return (Period) this.effective; 1446 } 1447 1448 public boolean hasEffectivePeriod() { 1449 return this != null && this.effective instanceof Period; 1450 } 1451 1452 public boolean hasEffective() { 1453 return this.effective != null && !this.effective.isEmpty(); 1454 } 1455 1456 /** 1457 * @param value {@link #effective} (The point in time or period over which the 1458 * subject was assessed.) 1459 */ 1460 public ClinicalImpression setEffective(Type value) { 1461 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1462 throw new Error("Not the right type for ClinicalImpression.effective[x]: " + value.fhirType()); 1463 this.effective = value; 1464 return this; 1465 } 1466 1467 /** 1468 * @return {@link #date} (Indicates when the documentation of the assessment was 1469 * complete.). This is the underlying object with id, value and 1470 * extensions. The accessor "getDate" gives direct access to the value 1471 */ 1472 public DateTimeType getDateElement() { 1473 if (this.date == null) 1474 if (Configuration.errorOnAutoCreate()) 1475 throw new Error("Attempt to auto-create ClinicalImpression.date"); 1476 else if (Configuration.doAutoCreate()) 1477 this.date = new DateTimeType(); // bb 1478 return this.date; 1479 } 1480 1481 public boolean hasDateElement() { 1482 return this.date != null && !this.date.isEmpty(); 1483 } 1484 1485 public boolean hasDate() { 1486 return this.date != null && !this.date.isEmpty(); 1487 } 1488 1489 /** 1490 * @param value {@link #date} (Indicates when the documentation of the 1491 * assessment was complete.). This is the underlying object with 1492 * id, value and extensions. The accessor "getDate" gives direct 1493 * access to the value 1494 */ 1495 public ClinicalImpression setDateElement(DateTimeType value) { 1496 this.date = value; 1497 return this; 1498 } 1499 1500 /** 1501 * @return Indicates when the documentation of the assessment was complete. 1502 */ 1503 public Date getDate() { 1504 return this.date == null ? null : this.date.getValue(); 1505 } 1506 1507 /** 1508 * @param value Indicates when the documentation of the assessment was complete. 1509 */ 1510 public ClinicalImpression setDate(Date value) { 1511 if (value == null) 1512 this.date = null; 1513 else { 1514 if (this.date == null) 1515 this.date = new DateTimeType(); 1516 this.date.setValue(value); 1517 } 1518 return this; 1519 } 1520 1521 /** 1522 * @return {@link #assessor} (The clinician performing the assessment.) 1523 */ 1524 public Reference getAssessor() { 1525 if (this.assessor == null) 1526 if (Configuration.errorOnAutoCreate()) 1527 throw new Error("Attempt to auto-create ClinicalImpression.assessor"); 1528 else if (Configuration.doAutoCreate()) 1529 this.assessor = new Reference(); // cc 1530 return this.assessor; 1531 } 1532 1533 public boolean hasAssessor() { 1534 return this.assessor != null && !this.assessor.isEmpty(); 1535 } 1536 1537 /** 1538 * @param value {@link #assessor} (The clinician performing the assessment.) 1539 */ 1540 public ClinicalImpression setAssessor(Reference value) { 1541 this.assessor = value; 1542 return this; 1543 } 1544 1545 /** 1546 * @return {@link #assessor} The actual object that is the target of the 1547 * reference. The reference library doesn't populate this, but you can 1548 * use it to hold the resource if you resolve it. (The clinician 1549 * performing the assessment.) 1550 */ 1551 public Resource getAssessorTarget() { 1552 return this.assessorTarget; 1553 } 1554 1555 /** 1556 * @param value {@link #assessor} The actual object that is the target of the 1557 * reference. The reference library doesn't use these, but you can 1558 * use it to hold the resource if you resolve it. (The clinician 1559 * performing the assessment.) 1560 */ 1561 public ClinicalImpression setAssessorTarget(Resource value) { 1562 this.assessorTarget = value; 1563 return this; 1564 } 1565 1566 /** 1567 * @return {@link #previous} (A reference to the last assessment that was 1568 * conducted on this patient. Assessments are often/usually ongoing in 1569 * nature; a care provider (practitioner or team) will make new 1570 * assessments on an ongoing basis as new data arises or the patient's 1571 * conditions changes.) 1572 */ 1573 public Reference getPrevious() { 1574 if (this.previous == null) 1575 if (Configuration.errorOnAutoCreate()) 1576 throw new Error("Attempt to auto-create ClinicalImpression.previous"); 1577 else if (Configuration.doAutoCreate()) 1578 this.previous = new Reference(); // cc 1579 return this.previous; 1580 } 1581 1582 public boolean hasPrevious() { 1583 return this.previous != null && !this.previous.isEmpty(); 1584 } 1585 1586 /** 1587 * @param value {@link #previous} (A reference to the last assessment that was 1588 * conducted on this patient. Assessments are often/usually ongoing 1589 * in nature; a care provider (practitioner or team) will make new 1590 * assessments on an ongoing basis as new data arises or the 1591 * patient's conditions changes.) 1592 */ 1593 public ClinicalImpression setPrevious(Reference value) { 1594 this.previous = value; 1595 return this; 1596 } 1597 1598 /** 1599 * @return {@link #previous} The actual object that is the target of the 1600 * reference. The reference library doesn't populate this, but you can 1601 * use it to hold the resource if you resolve it. (A reference to the 1602 * last assessment that was conducted on this patient. Assessments are 1603 * often/usually ongoing in nature; a care provider (practitioner or 1604 * team) will make new assessments on an ongoing basis as new data 1605 * arises or the patient's conditions changes.) 1606 */ 1607 public ClinicalImpression getPreviousTarget() { 1608 if (this.previousTarget == null) 1609 if (Configuration.errorOnAutoCreate()) 1610 throw new Error("Attempt to auto-create ClinicalImpression.previous"); 1611 else if (Configuration.doAutoCreate()) 1612 this.previousTarget = new ClinicalImpression(); // aa 1613 return this.previousTarget; 1614 } 1615 1616 /** 1617 * @param value {@link #previous} The actual object that is the target of the 1618 * reference. The reference library doesn't use these, but you can 1619 * use it to hold the resource if you resolve it. (A reference to 1620 * the last assessment that was conducted on this patient. 1621 * Assessments are often/usually ongoing in nature; a care provider 1622 * (practitioner or team) will make new assessments on an ongoing 1623 * basis as new data arises or the patient's conditions changes.) 1624 */ 1625 public ClinicalImpression setPreviousTarget(ClinicalImpression value) { 1626 this.previousTarget = value; 1627 return this; 1628 } 1629 1630 /** 1631 * @return {@link #problem} (A list of the relevant problems/conditions for a 1632 * patient.) 1633 */ 1634 public List<Reference> getProblem() { 1635 if (this.problem == null) 1636 this.problem = new ArrayList<Reference>(); 1637 return this.problem; 1638 } 1639 1640 /** 1641 * @return Returns a reference to <code>this</code> for easy method chaining 1642 */ 1643 public ClinicalImpression setProblem(List<Reference> theProblem) { 1644 this.problem = theProblem; 1645 return this; 1646 } 1647 1648 public boolean hasProblem() { 1649 if (this.problem == null) 1650 return false; 1651 for (Reference item : this.problem) 1652 if (!item.isEmpty()) 1653 return true; 1654 return false; 1655 } 1656 1657 public Reference addProblem() { // 3 1658 Reference t = new Reference(); 1659 if (this.problem == null) 1660 this.problem = new ArrayList<Reference>(); 1661 this.problem.add(t); 1662 return t; 1663 } 1664 1665 public ClinicalImpression addProblem(Reference t) { // 3 1666 if (t == null) 1667 return this; 1668 if (this.problem == null) 1669 this.problem = new ArrayList<Reference>(); 1670 this.problem.add(t); 1671 return this; 1672 } 1673 1674 /** 1675 * @return The first repetition of repeating field {@link #problem}, creating it 1676 * if it does not already exist 1677 */ 1678 public Reference getProblemFirstRep() { 1679 if (getProblem().isEmpty()) { 1680 addProblem(); 1681 } 1682 return getProblem().get(0); 1683 } 1684 1685 /** 1686 * @deprecated Use Reference#setResource(IBaseResource) instead 1687 */ 1688 @Deprecated 1689 public List<Resource> getProblemTarget() { 1690 if (this.problemTarget == null) 1691 this.problemTarget = new ArrayList<Resource>(); 1692 return this.problemTarget; 1693 } 1694 1695 /** 1696 * @return {@link #investigation} (One or more sets of investigations (signs, 1697 * symptoms, etc.). The actual grouping of investigations varies greatly 1698 * depending on the type and context of the assessment. These 1699 * investigations may include data generated during the assessment 1700 * process, or data previously generated and recorded that is pertinent 1701 * to the outcomes.) 1702 */ 1703 public List<ClinicalImpressionInvestigationComponent> getInvestigation() { 1704 if (this.investigation == null) 1705 this.investigation = new ArrayList<ClinicalImpressionInvestigationComponent>(); 1706 return this.investigation; 1707 } 1708 1709 /** 1710 * @return Returns a reference to <code>this</code> for easy method chaining 1711 */ 1712 public ClinicalImpression setInvestigation(List<ClinicalImpressionInvestigationComponent> theInvestigation) { 1713 this.investigation = theInvestigation; 1714 return this; 1715 } 1716 1717 public boolean hasInvestigation() { 1718 if (this.investigation == null) 1719 return false; 1720 for (ClinicalImpressionInvestigationComponent item : this.investigation) 1721 if (!item.isEmpty()) 1722 return true; 1723 return false; 1724 } 1725 1726 public ClinicalImpressionInvestigationComponent addInvestigation() { // 3 1727 ClinicalImpressionInvestigationComponent t = new ClinicalImpressionInvestigationComponent(); 1728 if (this.investigation == null) 1729 this.investigation = new ArrayList<ClinicalImpressionInvestigationComponent>(); 1730 this.investigation.add(t); 1731 return t; 1732 } 1733 1734 public ClinicalImpression addInvestigation(ClinicalImpressionInvestigationComponent t) { // 3 1735 if (t == null) 1736 return this; 1737 if (this.investigation == null) 1738 this.investigation = new ArrayList<ClinicalImpressionInvestigationComponent>(); 1739 this.investigation.add(t); 1740 return this; 1741 } 1742 1743 /** 1744 * @return The first repetition of repeating field {@link #investigation}, 1745 * creating it if it does not already exist 1746 */ 1747 public ClinicalImpressionInvestigationComponent getInvestigationFirstRep() { 1748 if (getInvestigation().isEmpty()) { 1749 addInvestigation(); 1750 } 1751 return getInvestigation().get(0); 1752 } 1753 1754 /** 1755 * @return {@link #protocol} (Reference to a specific published clinical 1756 * protocol that was followed during this assessment, and/or that 1757 * provides evidence in support of the diagnosis.) 1758 */ 1759 public List<UriType> getProtocol() { 1760 if (this.protocol == null) 1761 this.protocol = new ArrayList<UriType>(); 1762 return this.protocol; 1763 } 1764 1765 /** 1766 * @return Returns a reference to <code>this</code> for easy method chaining 1767 */ 1768 public ClinicalImpression setProtocol(List<UriType> theProtocol) { 1769 this.protocol = theProtocol; 1770 return this; 1771 } 1772 1773 public boolean hasProtocol() { 1774 if (this.protocol == null) 1775 return false; 1776 for (UriType item : this.protocol) 1777 if (!item.isEmpty()) 1778 return true; 1779 return false; 1780 } 1781 1782 /** 1783 * @return {@link #protocol} (Reference to a specific published clinical 1784 * protocol that was followed during this assessment, and/or that 1785 * provides evidence in support of the diagnosis.) 1786 */ 1787 public UriType addProtocolElement() {// 2 1788 UriType t = new UriType(); 1789 if (this.protocol == null) 1790 this.protocol = new ArrayList<UriType>(); 1791 this.protocol.add(t); 1792 return t; 1793 } 1794 1795 /** 1796 * @param value {@link #protocol} (Reference to a specific published clinical 1797 * protocol that was followed during this assessment, and/or that 1798 * provides evidence in support of the diagnosis.) 1799 */ 1800 public ClinicalImpression addProtocol(String value) { // 1 1801 UriType t = new UriType(); 1802 t.setValue(value); 1803 if (this.protocol == null) 1804 this.protocol = new ArrayList<UriType>(); 1805 this.protocol.add(t); 1806 return this; 1807 } 1808 1809 /** 1810 * @param value {@link #protocol} (Reference to a specific published clinical 1811 * protocol that was followed during this assessment, and/or that 1812 * provides evidence in support of the diagnosis.) 1813 */ 1814 public boolean hasProtocol(String value) { 1815 if (this.protocol == null) 1816 return false; 1817 for (UriType v : this.protocol) 1818 if (v.getValue().equals(value)) // uri 1819 return true; 1820 return false; 1821 } 1822 1823 /** 1824 * @return {@link #summary} (A text summary of the investigations and the 1825 * diagnosis.). This is the underlying object with id, value and 1826 * extensions. The accessor "getSummary" gives direct access to the 1827 * value 1828 */ 1829 public StringType getSummaryElement() { 1830 if (this.summary == null) 1831 if (Configuration.errorOnAutoCreate()) 1832 throw new Error("Attempt to auto-create ClinicalImpression.summary"); 1833 else if (Configuration.doAutoCreate()) 1834 this.summary = new StringType(); // bb 1835 return this.summary; 1836 } 1837 1838 public boolean hasSummaryElement() { 1839 return this.summary != null && !this.summary.isEmpty(); 1840 } 1841 1842 public boolean hasSummary() { 1843 return this.summary != null && !this.summary.isEmpty(); 1844 } 1845 1846 /** 1847 * @param value {@link #summary} (A text summary of the investigations and the 1848 * diagnosis.). This is the underlying object with id, value and 1849 * extensions. The accessor "getSummary" gives direct access to the 1850 * value 1851 */ 1852 public ClinicalImpression setSummaryElement(StringType value) { 1853 this.summary = value; 1854 return this; 1855 } 1856 1857 /** 1858 * @return A text summary of the investigations and the diagnosis. 1859 */ 1860 public String getSummary() { 1861 return this.summary == null ? null : this.summary.getValue(); 1862 } 1863 1864 /** 1865 * @param value A text summary of the investigations and the diagnosis. 1866 */ 1867 public ClinicalImpression setSummary(String value) { 1868 if (Utilities.noString(value)) 1869 this.summary = null; 1870 else { 1871 if (this.summary == null) 1872 this.summary = new StringType(); 1873 this.summary.setValue(value); 1874 } 1875 return this; 1876 } 1877 1878 /** 1879 * @return {@link #finding} (Specific findings or diagnoses that were considered 1880 * likely or relevant to ongoing treatment.) 1881 */ 1882 public List<ClinicalImpressionFindingComponent> getFinding() { 1883 if (this.finding == null) 1884 this.finding = new ArrayList<ClinicalImpressionFindingComponent>(); 1885 return this.finding; 1886 } 1887 1888 /** 1889 * @return Returns a reference to <code>this</code> for easy method chaining 1890 */ 1891 public ClinicalImpression setFinding(List<ClinicalImpressionFindingComponent> theFinding) { 1892 this.finding = theFinding; 1893 return this; 1894 } 1895 1896 public boolean hasFinding() { 1897 if (this.finding == null) 1898 return false; 1899 for (ClinicalImpressionFindingComponent item : this.finding) 1900 if (!item.isEmpty()) 1901 return true; 1902 return false; 1903 } 1904 1905 public ClinicalImpressionFindingComponent addFinding() { // 3 1906 ClinicalImpressionFindingComponent t = new ClinicalImpressionFindingComponent(); 1907 if (this.finding == null) 1908 this.finding = new ArrayList<ClinicalImpressionFindingComponent>(); 1909 this.finding.add(t); 1910 return t; 1911 } 1912 1913 public ClinicalImpression addFinding(ClinicalImpressionFindingComponent t) { // 3 1914 if (t == null) 1915 return this; 1916 if (this.finding == null) 1917 this.finding = new ArrayList<ClinicalImpressionFindingComponent>(); 1918 this.finding.add(t); 1919 return this; 1920 } 1921 1922 /** 1923 * @return The first repetition of repeating field {@link #finding}, creating it 1924 * if it does not already exist 1925 */ 1926 public ClinicalImpressionFindingComponent getFindingFirstRep() { 1927 if (getFinding().isEmpty()) { 1928 addFinding(); 1929 } 1930 return getFinding().get(0); 1931 } 1932 1933 /** 1934 * @return {@link #prognosisCodeableConcept} (Estimate of likely outcome.) 1935 */ 1936 public List<CodeableConcept> getPrognosisCodeableConcept() { 1937 if (this.prognosisCodeableConcept == null) 1938 this.prognosisCodeableConcept = new ArrayList<CodeableConcept>(); 1939 return this.prognosisCodeableConcept; 1940 } 1941 1942 /** 1943 * @return Returns a reference to <code>this</code> for easy method chaining 1944 */ 1945 public ClinicalImpression setPrognosisCodeableConcept(List<CodeableConcept> thePrognosisCodeableConcept) { 1946 this.prognosisCodeableConcept = thePrognosisCodeableConcept; 1947 return this; 1948 } 1949 1950 public boolean hasPrognosisCodeableConcept() { 1951 if (this.prognosisCodeableConcept == null) 1952 return false; 1953 for (CodeableConcept item : this.prognosisCodeableConcept) 1954 if (!item.isEmpty()) 1955 return true; 1956 return false; 1957 } 1958 1959 public CodeableConcept addPrognosisCodeableConcept() { // 3 1960 CodeableConcept t = new CodeableConcept(); 1961 if (this.prognosisCodeableConcept == null) 1962 this.prognosisCodeableConcept = new ArrayList<CodeableConcept>(); 1963 this.prognosisCodeableConcept.add(t); 1964 return t; 1965 } 1966 1967 public ClinicalImpression addPrognosisCodeableConcept(CodeableConcept t) { // 3 1968 if (t == null) 1969 return this; 1970 if (this.prognosisCodeableConcept == null) 1971 this.prognosisCodeableConcept = new ArrayList<CodeableConcept>(); 1972 this.prognosisCodeableConcept.add(t); 1973 return this; 1974 } 1975 1976 /** 1977 * @return The first repetition of repeating field 1978 * {@link #prognosisCodeableConcept}, creating it if it does not already 1979 * exist 1980 */ 1981 public CodeableConcept getPrognosisCodeableConceptFirstRep() { 1982 if (getPrognosisCodeableConcept().isEmpty()) { 1983 addPrognosisCodeableConcept(); 1984 } 1985 return getPrognosisCodeableConcept().get(0); 1986 } 1987 1988 /** 1989 * @return {@link #prognosisReference} (RiskAssessment expressing likely 1990 * outcome.) 1991 */ 1992 public List<Reference> getPrognosisReference() { 1993 if (this.prognosisReference == null) 1994 this.prognosisReference = new ArrayList<Reference>(); 1995 return this.prognosisReference; 1996 } 1997 1998 /** 1999 * @return Returns a reference to <code>this</code> for easy method chaining 2000 */ 2001 public ClinicalImpression setPrognosisReference(List<Reference> thePrognosisReference) { 2002 this.prognosisReference = thePrognosisReference; 2003 return this; 2004 } 2005 2006 public boolean hasPrognosisReference() { 2007 if (this.prognosisReference == null) 2008 return false; 2009 for (Reference item : this.prognosisReference) 2010 if (!item.isEmpty()) 2011 return true; 2012 return false; 2013 } 2014 2015 public Reference addPrognosisReference() { // 3 2016 Reference t = new Reference(); 2017 if (this.prognosisReference == null) 2018 this.prognosisReference = new ArrayList<Reference>(); 2019 this.prognosisReference.add(t); 2020 return t; 2021 } 2022 2023 public ClinicalImpression addPrognosisReference(Reference t) { // 3 2024 if (t == null) 2025 return this; 2026 if (this.prognosisReference == null) 2027 this.prognosisReference = new ArrayList<Reference>(); 2028 this.prognosisReference.add(t); 2029 return this; 2030 } 2031 2032 /** 2033 * @return The first repetition of repeating field {@link #prognosisReference}, 2034 * creating it if it does not already exist 2035 */ 2036 public Reference getPrognosisReferenceFirstRep() { 2037 if (getPrognosisReference().isEmpty()) { 2038 addPrognosisReference(); 2039 } 2040 return getPrognosisReference().get(0); 2041 } 2042 2043 /** 2044 * @deprecated Use Reference#setResource(IBaseResource) instead 2045 */ 2046 @Deprecated 2047 public List<RiskAssessment> getPrognosisReferenceTarget() { 2048 if (this.prognosisReferenceTarget == null) 2049 this.prognosisReferenceTarget = new ArrayList<RiskAssessment>(); 2050 return this.prognosisReferenceTarget; 2051 } 2052 2053 /** 2054 * @deprecated Use Reference#setResource(IBaseResource) instead 2055 */ 2056 @Deprecated 2057 public RiskAssessment addPrognosisReferenceTarget() { 2058 RiskAssessment r = new RiskAssessment(); 2059 if (this.prognosisReferenceTarget == null) 2060 this.prognosisReferenceTarget = new ArrayList<RiskAssessment>(); 2061 this.prognosisReferenceTarget.add(r); 2062 return r; 2063 } 2064 2065 /** 2066 * @return {@link #supportingInfo} (Information supporting the clinical 2067 * impression.) 2068 */ 2069 public List<Reference> getSupportingInfo() { 2070 if (this.supportingInfo == null) 2071 this.supportingInfo = new ArrayList<Reference>(); 2072 return this.supportingInfo; 2073 } 2074 2075 /** 2076 * @return Returns a reference to <code>this</code> for easy method chaining 2077 */ 2078 public ClinicalImpression setSupportingInfo(List<Reference> theSupportingInfo) { 2079 this.supportingInfo = theSupportingInfo; 2080 return this; 2081 } 2082 2083 public boolean hasSupportingInfo() { 2084 if (this.supportingInfo == null) 2085 return false; 2086 for (Reference item : this.supportingInfo) 2087 if (!item.isEmpty()) 2088 return true; 2089 return false; 2090 } 2091 2092 public Reference addSupportingInfo() { // 3 2093 Reference t = new Reference(); 2094 if (this.supportingInfo == null) 2095 this.supportingInfo = new ArrayList<Reference>(); 2096 this.supportingInfo.add(t); 2097 return t; 2098 } 2099 2100 public ClinicalImpression addSupportingInfo(Reference t) { // 3 2101 if (t == null) 2102 return this; 2103 if (this.supportingInfo == null) 2104 this.supportingInfo = new ArrayList<Reference>(); 2105 this.supportingInfo.add(t); 2106 return this; 2107 } 2108 2109 /** 2110 * @return The first repetition of repeating field {@link #supportingInfo}, 2111 * creating it if it does not already exist 2112 */ 2113 public Reference getSupportingInfoFirstRep() { 2114 if (getSupportingInfo().isEmpty()) { 2115 addSupportingInfo(); 2116 } 2117 return getSupportingInfo().get(0); 2118 } 2119 2120 /** 2121 * @deprecated Use Reference#setResource(IBaseResource) instead 2122 */ 2123 @Deprecated 2124 public List<Resource> getSupportingInfoTarget() { 2125 if (this.supportingInfoTarget == null) 2126 this.supportingInfoTarget = new ArrayList<Resource>(); 2127 return this.supportingInfoTarget; 2128 } 2129 2130 /** 2131 * @return {@link #note} (Commentary about the impression, typically recorded 2132 * after the impression itself was made, though supplemental notes by 2133 * the original author could also appear.) 2134 */ 2135 public List<Annotation> getNote() { 2136 if (this.note == null) 2137 this.note = new ArrayList<Annotation>(); 2138 return this.note; 2139 } 2140 2141 /** 2142 * @return Returns a reference to <code>this</code> for easy method chaining 2143 */ 2144 public ClinicalImpression setNote(List<Annotation> theNote) { 2145 this.note = theNote; 2146 return this; 2147 } 2148 2149 public boolean hasNote() { 2150 if (this.note == null) 2151 return false; 2152 for (Annotation item : this.note) 2153 if (!item.isEmpty()) 2154 return true; 2155 return false; 2156 } 2157 2158 public Annotation addNote() { // 3 2159 Annotation t = new Annotation(); 2160 if (this.note == null) 2161 this.note = new ArrayList<Annotation>(); 2162 this.note.add(t); 2163 return t; 2164 } 2165 2166 public ClinicalImpression addNote(Annotation t) { // 3 2167 if (t == null) 2168 return this; 2169 if (this.note == null) 2170 this.note = new ArrayList<Annotation>(); 2171 this.note.add(t); 2172 return this; 2173 } 2174 2175 /** 2176 * @return The first repetition of repeating field {@link #note}, creating it if 2177 * it does not already exist 2178 */ 2179 public Annotation getNoteFirstRep() { 2180 if (getNote().isEmpty()) { 2181 addNote(); 2182 } 2183 return getNote().get(0); 2184 } 2185 2186 protected void listChildren(List<Property> children) { 2187 super.listChildren(children); 2188 children.add(new Property("identifier", "Identifier", 2189 "Business identifiers assigned to this clinical impression by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 2190 0, java.lang.Integer.MAX_VALUE, identifier)); 2191 children.add(new Property("status", "code", "Identifies the workflow status of the assessment.", 0, 1, status)); 2192 children.add(new Property("statusReason", "CodeableConcept", 2193 "Captures the reason for the current state of the ClinicalImpression.", 0, 1, statusReason)); 2194 children.add( 2195 new Property("code", "CodeableConcept", "Categorizes the type of clinical assessment performed.", 0, 1, code)); 2196 children.add(new Property("description", "string", 2197 "A summary of the context and/or cause of the assessment - why / where it was performed, and what patient events/status prompted it.", 2198 0, 1, description)); 2199 children.add(new Property("subject", "Reference(Patient|Group)", 2200 "The patient or group of individuals assessed as part of this record.", 0, 1, subject)); 2201 children.add(new Property("encounter", "Reference(Encounter)", 2202 "The Encounter during which this ClinicalImpression was created or to which the creation of this record is tightly associated.", 2203 0, 1, encounter)); 2204 children.add(new Property("effective[x]", "dateTime|Period", 2205 "The point in time or period over which the subject was assessed.", 0, 1, effective)); 2206 children.add(new Property("date", "dateTime", "Indicates when the documentation of the assessment was complete.", 0, 2207 1, date)); 2208 children.add(new Property("assessor", "Reference(Practitioner|PractitionerRole)", 2209 "The clinician performing the assessment.", 0, 1, assessor)); 2210 children.add(new Property("previous", "Reference(ClinicalImpression)", 2211 "A reference to the last assessment that was conducted on this patient. Assessments are often/usually ongoing in nature; a care provider (practitioner or team) will make new assessments on an ongoing basis as new data arises or the patient's conditions changes.", 2212 0, 1, previous)); 2213 children.add(new Property("problem", "Reference(Condition|AllergyIntolerance)", 2214 "A list of the relevant problems/conditions for a patient.", 0, java.lang.Integer.MAX_VALUE, problem)); 2215 children.add(new Property("investigation", "", 2216 "One or more sets of investigations (signs, symptoms, etc.). The actual grouping of investigations varies greatly depending on the type and context of the assessment. These investigations may include data generated during the assessment process, or data previously generated and recorded that is pertinent to the outcomes.", 2217 0, java.lang.Integer.MAX_VALUE, investigation)); 2218 children.add(new Property("protocol", "uri", 2219 "Reference to a specific published clinical protocol that was followed during this assessment, and/or that provides evidence in support of the diagnosis.", 2220 0, java.lang.Integer.MAX_VALUE, protocol)); 2221 children.add( 2222 new Property("summary", "string", "A text summary of the investigations and the diagnosis.", 0, 1, summary)); 2223 children.add(new Property("finding", "", 2224 "Specific findings or diagnoses that were considered likely or relevant to ongoing treatment.", 0, 2225 java.lang.Integer.MAX_VALUE, finding)); 2226 children.add(new Property("prognosisCodeableConcept", "CodeableConcept", "Estimate of likely outcome.", 0, 2227 java.lang.Integer.MAX_VALUE, prognosisCodeableConcept)); 2228 children.add(new Property("prognosisReference", "Reference(RiskAssessment)", 2229 "RiskAssessment expressing likely outcome.", 0, java.lang.Integer.MAX_VALUE, prognosisReference)); 2230 children.add(new Property("supportingInfo", "Reference(Any)", "Information supporting the clinical impression.", 0, 2231 java.lang.Integer.MAX_VALUE, supportingInfo)); 2232 children.add(new Property("note", "Annotation", 2233 "Commentary about the impression, typically recorded after the impression itself was made, though supplemental notes by the original author could also appear.", 2234 0, java.lang.Integer.MAX_VALUE, note)); 2235 } 2236 2237 @Override 2238 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2239 switch (_hash) { 2240 case -1618432855: 2241 /* identifier */ return new Property("identifier", "Identifier", 2242 "Business identifiers assigned to this clinical impression by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 2243 0, java.lang.Integer.MAX_VALUE, identifier); 2244 case -892481550: 2245 /* status */ return new Property("status", "code", "Identifies the workflow status of the assessment.", 0, 1, 2246 status); 2247 case 2051346646: 2248 /* statusReason */ return new Property("statusReason", "CodeableConcept", 2249 "Captures the reason for the current state of the ClinicalImpression.", 0, 1, statusReason); 2250 case 3059181: 2251 /* code */ return new Property("code", "CodeableConcept", 2252 "Categorizes the type of clinical assessment performed.", 0, 1, code); 2253 case -1724546052: 2254 /* description */ return new Property("description", "string", 2255 "A summary of the context and/or cause of the assessment - why / where it was performed, and what patient events/status prompted it.", 2256 0, 1, description); 2257 case -1867885268: 2258 /* subject */ return new Property("subject", "Reference(Patient|Group)", 2259 "The patient or group of individuals assessed as part of this record.", 0, 1, subject); 2260 case 1524132147: 2261 /* encounter */ return new Property("encounter", "Reference(Encounter)", 2262 "The Encounter during which this ClinicalImpression was created or to which the creation of this record is tightly associated.", 2263 0, 1, encounter); 2264 case 247104889: 2265 /* effective[x] */ return new Property("effective[x]", "dateTime|Period", 2266 "The point in time or period over which the subject was assessed.", 0, 1, effective); 2267 case -1468651097: 2268 /* effective */ return new Property("effective[x]", "dateTime|Period", 2269 "The point in time or period over which the subject was assessed.", 0, 1, effective); 2270 case -275306910: 2271 /* effectiveDateTime */ return new Property("effective[x]", "dateTime|Period", 2272 "The point in time or period over which the subject was assessed.", 0, 1, effective); 2273 case -403934648: 2274 /* effectivePeriod */ return new Property("effective[x]", "dateTime|Period", 2275 "The point in time or period over which the subject was assessed.", 0, 1, effective); 2276 case 3076014: 2277 /* date */ return new Property("date", "dateTime", 2278 "Indicates when the documentation of the assessment was complete.", 0, 1, date); 2279 case -373213113: 2280 /* assessor */ return new Property("assessor", "Reference(Practitioner|PractitionerRole)", 2281 "The clinician performing the assessment.", 0, 1, assessor); 2282 case -1273775369: 2283 /* previous */ return new Property("previous", "Reference(ClinicalImpression)", 2284 "A reference to the last assessment that was conducted on this patient. Assessments are often/usually ongoing in nature; a care provider (practitioner or team) will make new assessments on an ongoing basis as new data arises or the patient's conditions changes.", 2285 0, 1, previous); 2286 case -309542241: 2287 /* problem */ return new Property("problem", "Reference(Condition|AllergyIntolerance)", 2288 "A list of the relevant problems/conditions for a patient.", 0, java.lang.Integer.MAX_VALUE, problem); 2289 case 956015362: 2290 /* investigation */ return new Property("investigation", "", 2291 "One or more sets of investigations (signs, symptoms, etc.). The actual grouping of investigations varies greatly depending on the type and context of the assessment. These investigations may include data generated during the assessment process, or data previously generated and recorded that is pertinent to the outcomes.", 2292 0, java.lang.Integer.MAX_VALUE, investigation); 2293 case -989163880: 2294 /* protocol */ return new Property("protocol", "uri", 2295 "Reference to a specific published clinical protocol that was followed during this assessment, and/or that provides evidence in support of the diagnosis.", 2296 0, java.lang.Integer.MAX_VALUE, protocol); 2297 case -1857640538: 2298 /* summary */ return new Property("summary", "string", "A text summary of the investigations and the diagnosis.", 2299 0, 1, summary); 2300 case -853173367: 2301 /* finding */ return new Property("finding", "", 2302 "Specific findings or diagnoses that were considered likely or relevant to ongoing treatment.", 0, 2303 java.lang.Integer.MAX_VALUE, finding); 2304 case -676337953: 2305 /* prognosisCodeableConcept */ return new Property("prognosisCodeableConcept", "CodeableConcept", 2306 "Estimate of likely outcome.", 0, java.lang.Integer.MAX_VALUE, prognosisCodeableConcept); 2307 case -587137783: 2308 /* prognosisReference */ return new Property("prognosisReference", "Reference(RiskAssessment)", 2309 "RiskAssessment expressing likely outcome.", 0, java.lang.Integer.MAX_VALUE, prognosisReference); 2310 case 1922406657: 2311 /* supportingInfo */ return new Property("supportingInfo", "Reference(Any)", 2312 "Information supporting the clinical impression.", 0, java.lang.Integer.MAX_VALUE, supportingInfo); 2313 case 3387378: 2314 /* note */ return new Property("note", "Annotation", 2315 "Commentary about the impression, typically recorded after the impression itself was made, though supplemental notes by the original author could also appear.", 2316 0, java.lang.Integer.MAX_VALUE, note); 2317 default: 2318 return super.getNamedProperty(_hash, _name, _checkValid); 2319 } 2320 2321 } 2322 2323 @Override 2324 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2325 switch (hash) { 2326 case -1618432855: 2327 /* identifier */ return this.identifier == null ? new Base[0] 2328 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2329 case -892481550: 2330 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<ClinicalImpressionStatus> 2331 case 2051346646: 2332 /* statusReason */ return this.statusReason == null ? new Base[0] : new Base[] { this.statusReason }; // CodeableConcept 2333 case 3059181: 2334 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 2335 case -1724546052: 2336 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 2337 case -1867885268: 2338 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2339 case 1524132147: 2340 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 2341 case -1468651097: 2342 /* effective */ return this.effective == null ? new Base[0] : new Base[] { this.effective }; // Type 2343 case 3076014: 2344 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 2345 case -373213113: 2346 /* assessor */ return this.assessor == null ? new Base[0] : new Base[] { this.assessor }; // Reference 2347 case -1273775369: 2348 /* previous */ return this.previous == null ? new Base[0] : new Base[] { this.previous }; // Reference 2349 case -309542241: 2350 /* problem */ return this.problem == null ? new Base[0] : this.problem.toArray(new Base[this.problem.size()]); // Reference 2351 case 956015362: 2352 /* investigation */ return this.investigation == null ? new Base[0] 2353 : this.investigation.toArray(new Base[this.investigation.size()]); // ClinicalImpressionInvestigationComponent 2354 case -989163880: 2355 /* protocol */ return this.protocol == null ? new Base[0] : this.protocol.toArray(new Base[this.protocol.size()]); // UriType 2356 case -1857640538: 2357 /* summary */ return this.summary == null ? new Base[0] : new Base[] { this.summary }; // StringType 2358 case -853173367: 2359 /* finding */ return this.finding == null ? new Base[0] : this.finding.toArray(new Base[this.finding.size()]); // ClinicalImpressionFindingComponent 2360 case -676337953: 2361 /* prognosisCodeableConcept */ return this.prognosisCodeableConcept == null ? new Base[0] 2362 : this.prognosisCodeableConcept.toArray(new Base[this.prognosisCodeableConcept.size()]); // CodeableConcept 2363 case -587137783: 2364 /* prognosisReference */ return this.prognosisReference == null ? new Base[0] 2365 : this.prognosisReference.toArray(new Base[this.prognosisReference.size()]); // Reference 2366 case 1922406657: 2367 /* supportingInfo */ return this.supportingInfo == null ? new Base[0] 2368 : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // Reference 2369 case 3387378: 2370 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2371 default: 2372 return super.getProperty(hash, name, checkValid); 2373 } 2374 2375 } 2376 2377 @Override 2378 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2379 switch (hash) { 2380 case -1618432855: // identifier 2381 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2382 return value; 2383 case -892481550: // status 2384 value = new ClinicalImpressionStatusEnumFactory().fromType(castToCode(value)); 2385 this.status = (Enumeration) value; // Enumeration<ClinicalImpressionStatus> 2386 return value; 2387 case 2051346646: // statusReason 2388 this.statusReason = castToCodeableConcept(value); // CodeableConcept 2389 return value; 2390 case 3059181: // code 2391 this.code = castToCodeableConcept(value); // CodeableConcept 2392 return value; 2393 case -1724546052: // description 2394 this.description = castToString(value); // StringType 2395 return value; 2396 case -1867885268: // subject 2397 this.subject = castToReference(value); // Reference 2398 return value; 2399 case 1524132147: // encounter 2400 this.encounter = castToReference(value); // Reference 2401 return value; 2402 case -1468651097: // effective 2403 this.effective = castToType(value); // Type 2404 return value; 2405 case 3076014: // date 2406 this.date = castToDateTime(value); // DateTimeType 2407 return value; 2408 case -373213113: // assessor 2409 this.assessor = castToReference(value); // Reference 2410 return value; 2411 case -1273775369: // previous 2412 this.previous = castToReference(value); // Reference 2413 return value; 2414 case -309542241: // problem 2415 this.getProblem().add(castToReference(value)); // Reference 2416 return value; 2417 case 956015362: // investigation 2418 this.getInvestigation().add((ClinicalImpressionInvestigationComponent) value); // ClinicalImpressionInvestigationComponent 2419 return value; 2420 case -989163880: // protocol 2421 this.getProtocol().add(castToUri(value)); // UriType 2422 return value; 2423 case -1857640538: // summary 2424 this.summary = castToString(value); // StringType 2425 return value; 2426 case -853173367: // finding 2427 this.getFinding().add((ClinicalImpressionFindingComponent) value); // ClinicalImpressionFindingComponent 2428 return value; 2429 case -676337953: // prognosisCodeableConcept 2430 this.getPrognosisCodeableConcept().add(castToCodeableConcept(value)); // CodeableConcept 2431 return value; 2432 case -587137783: // prognosisReference 2433 this.getPrognosisReference().add(castToReference(value)); // Reference 2434 return value; 2435 case 1922406657: // supportingInfo 2436 this.getSupportingInfo().add(castToReference(value)); // Reference 2437 return value; 2438 case 3387378: // note 2439 this.getNote().add(castToAnnotation(value)); // Annotation 2440 return value; 2441 default: 2442 return super.setProperty(hash, name, value); 2443 } 2444 2445 } 2446 2447 @Override 2448 public Base setProperty(String name, Base value) throws FHIRException { 2449 if (name.equals("identifier")) { 2450 this.getIdentifier().add(castToIdentifier(value)); 2451 } else if (name.equals("status")) { 2452 value = new ClinicalImpressionStatusEnumFactory().fromType(castToCode(value)); 2453 this.status = (Enumeration) value; // Enumeration<ClinicalImpressionStatus> 2454 } else if (name.equals("statusReason")) { 2455 this.statusReason = castToCodeableConcept(value); // CodeableConcept 2456 } else if (name.equals("code")) { 2457 this.code = castToCodeableConcept(value); // CodeableConcept 2458 } else if (name.equals("description")) { 2459 this.description = castToString(value); // StringType 2460 } else if (name.equals("subject")) { 2461 this.subject = castToReference(value); // Reference 2462 } else if (name.equals("encounter")) { 2463 this.encounter = castToReference(value); // Reference 2464 } else if (name.equals("effective[x]")) { 2465 this.effective = castToType(value); // Type 2466 } else if (name.equals("date")) { 2467 this.date = castToDateTime(value); // DateTimeType 2468 } else if (name.equals("assessor")) { 2469 this.assessor = castToReference(value); // Reference 2470 } else if (name.equals("previous")) { 2471 this.previous = castToReference(value); // Reference 2472 } else if (name.equals("problem")) { 2473 this.getProblem().add(castToReference(value)); 2474 } else if (name.equals("investigation")) { 2475 this.getInvestigation().add((ClinicalImpressionInvestigationComponent) value); 2476 } else if (name.equals("protocol")) { 2477 this.getProtocol().add(castToUri(value)); 2478 } else if (name.equals("summary")) { 2479 this.summary = castToString(value); // StringType 2480 } else if (name.equals("finding")) { 2481 this.getFinding().add((ClinicalImpressionFindingComponent) value); 2482 } else if (name.equals("prognosisCodeableConcept")) { 2483 this.getPrognosisCodeableConcept().add(castToCodeableConcept(value)); 2484 } else if (name.equals("prognosisReference")) { 2485 this.getPrognosisReference().add(castToReference(value)); 2486 } else if (name.equals("supportingInfo")) { 2487 this.getSupportingInfo().add(castToReference(value)); 2488 } else if (name.equals("note")) { 2489 this.getNote().add(castToAnnotation(value)); 2490 } else 2491 return super.setProperty(name, value); 2492 return value; 2493 } 2494 2495 @Override 2496 public void removeChild(String name, Base value) throws FHIRException { 2497 if (name.equals("identifier")) { 2498 this.getIdentifier().remove(castToIdentifier(value)); 2499 } else if (name.equals("status")) { 2500 this.status = null; 2501 } else if (name.equals("statusReason")) { 2502 this.statusReason = null; 2503 } else if (name.equals("code")) { 2504 this.code = null; 2505 } else if (name.equals("description")) { 2506 this.description = null; 2507 } else if (name.equals("subject")) { 2508 this.subject = null; 2509 } else if (name.equals("encounter")) { 2510 this.encounter = null; 2511 } else if (name.equals("effective[x]")) { 2512 this.effective = null; 2513 } else if (name.equals("date")) { 2514 this.date = null; 2515 } else if (name.equals("assessor")) { 2516 this.assessor = null; 2517 } else if (name.equals("previous")) { 2518 this.previous = null; 2519 } else if (name.equals("problem")) { 2520 this.getProblem().remove(castToReference(value)); 2521 } else if (name.equals("investigation")) { 2522 this.getInvestigation().remove((ClinicalImpressionInvestigationComponent) value); 2523 } else if (name.equals("protocol")) { 2524 this.getProtocol().remove(castToUri(value)); 2525 } else if (name.equals("summary")) { 2526 this.summary = null; 2527 } else if (name.equals("finding")) { 2528 this.getFinding().remove((ClinicalImpressionFindingComponent) value); 2529 } else if (name.equals("prognosisCodeableConcept")) { 2530 this.getPrognosisCodeableConcept().remove(castToCodeableConcept(value)); 2531 } else if (name.equals("prognosisReference")) { 2532 this.getPrognosisReference().remove(castToReference(value)); 2533 } else if (name.equals("supportingInfo")) { 2534 this.getSupportingInfo().remove(castToReference(value)); 2535 } else if (name.equals("note")) { 2536 this.getNote().remove(castToAnnotation(value)); 2537 } else 2538 super.removeChild(name, value); 2539 2540 } 2541 2542 @Override 2543 public Base makeProperty(int hash, String name) throws FHIRException { 2544 switch (hash) { 2545 case -1618432855: 2546 return addIdentifier(); 2547 case -892481550: 2548 return getStatusElement(); 2549 case 2051346646: 2550 return getStatusReason(); 2551 case 3059181: 2552 return getCode(); 2553 case -1724546052: 2554 return getDescriptionElement(); 2555 case -1867885268: 2556 return getSubject(); 2557 case 1524132147: 2558 return getEncounter(); 2559 case 247104889: 2560 return getEffective(); 2561 case -1468651097: 2562 return getEffective(); 2563 case 3076014: 2564 return getDateElement(); 2565 case -373213113: 2566 return getAssessor(); 2567 case -1273775369: 2568 return getPrevious(); 2569 case -309542241: 2570 return addProblem(); 2571 case 956015362: 2572 return addInvestigation(); 2573 case -989163880: 2574 return addProtocolElement(); 2575 case -1857640538: 2576 return getSummaryElement(); 2577 case -853173367: 2578 return addFinding(); 2579 case -676337953: 2580 return addPrognosisCodeableConcept(); 2581 case -587137783: 2582 return addPrognosisReference(); 2583 case 1922406657: 2584 return addSupportingInfo(); 2585 case 3387378: 2586 return addNote(); 2587 default: 2588 return super.makeProperty(hash, name); 2589 } 2590 2591 } 2592 2593 @Override 2594 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2595 switch (hash) { 2596 case -1618432855: 2597 /* identifier */ return new String[] { "Identifier" }; 2598 case -892481550: 2599 /* status */ return new String[] { "code" }; 2600 case 2051346646: 2601 /* statusReason */ return new String[] { "CodeableConcept" }; 2602 case 3059181: 2603 /* code */ return new String[] { "CodeableConcept" }; 2604 case -1724546052: 2605 /* description */ return new String[] { "string" }; 2606 case -1867885268: 2607 /* subject */ return new String[] { "Reference" }; 2608 case 1524132147: 2609 /* encounter */ return new String[] { "Reference" }; 2610 case -1468651097: 2611 /* effective */ return new String[] { "dateTime", "Period" }; 2612 case 3076014: 2613 /* date */ return new String[] { "dateTime" }; 2614 case -373213113: 2615 /* assessor */ return new String[] { "Reference" }; 2616 case -1273775369: 2617 /* previous */ return new String[] { "Reference" }; 2618 case -309542241: 2619 /* problem */ return new String[] { "Reference" }; 2620 case 956015362: 2621 /* investigation */ return new String[] {}; 2622 case -989163880: 2623 /* protocol */ return new String[] { "uri" }; 2624 case -1857640538: 2625 /* summary */ return new String[] { "string" }; 2626 case -853173367: 2627 /* finding */ return new String[] {}; 2628 case -676337953: 2629 /* prognosisCodeableConcept */ return new String[] { "CodeableConcept" }; 2630 case -587137783: 2631 /* prognosisReference */ return new String[] { "Reference" }; 2632 case 1922406657: 2633 /* supportingInfo */ return new String[] { "Reference" }; 2634 case 3387378: 2635 /* note */ return new String[] { "Annotation" }; 2636 default: 2637 return super.getTypesForProperty(hash, name); 2638 } 2639 2640 } 2641 2642 @Override 2643 public Base addChild(String name) throws FHIRException { 2644 if (name.equals("identifier")) { 2645 return addIdentifier(); 2646 } else if (name.equals("status")) { 2647 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.status"); 2648 } else if (name.equals("statusReason")) { 2649 this.statusReason = new CodeableConcept(); 2650 return this.statusReason; 2651 } else if (name.equals("code")) { 2652 this.code = new CodeableConcept(); 2653 return this.code; 2654 } else if (name.equals("description")) { 2655 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.description"); 2656 } else if (name.equals("subject")) { 2657 this.subject = new Reference(); 2658 return this.subject; 2659 } else if (name.equals("encounter")) { 2660 this.encounter = new Reference(); 2661 return this.encounter; 2662 } else if (name.equals("effectiveDateTime")) { 2663 this.effective = new DateTimeType(); 2664 return this.effective; 2665 } else if (name.equals("effectivePeriod")) { 2666 this.effective = new Period(); 2667 return this.effective; 2668 } else if (name.equals("date")) { 2669 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.date"); 2670 } else if (name.equals("assessor")) { 2671 this.assessor = new Reference(); 2672 return this.assessor; 2673 } else if (name.equals("previous")) { 2674 this.previous = new Reference(); 2675 return this.previous; 2676 } else if (name.equals("problem")) { 2677 return addProblem(); 2678 } else if (name.equals("investigation")) { 2679 return addInvestigation(); 2680 } else if (name.equals("protocol")) { 2681 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.protocol"); 2682 } else if (name.equals("summary")) { 2683 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.summary"); 2684 } else if (name.equals("finding")) { 2685 return addFinding(); 2686 } else if (name.equals("prognosisCodeableConcept")) { 2687 return addPrognosisCodeableConcept(); 2688 } else if (name.equals("prognosisReference")) { 2689 return addPrognosisReference(); 2690 } else if (name.equals("supportingInfo")) { 2691 return addSupportingInfo(); 2692 } else if (name.equals("note")) { 2693 return addNote(); 2694 } else 2695 return super.addChild(name); 2696 } 2697 2698 public String fhirType() { 2699 return "ClinicalImpression"; 2700 2701 } 2702 2703 public ClinicalImpression copy() { 2704 ClinicalImpression dst = new ClinicalImpression(); 2705 copyValues(dst); 2706 return dst; 2707 } 2708 2709 public void copyValues(ClinicalImpression dst) { 2710 super.copyValues(dst); 2711 if (identifier != null) { 2712 dst.identifier = new ArrayList<Identifier>(); 2713 for (Identifier i : identifier) 2714 dst.identifier.add(i.copy()); 2715 } 2716 ; 2717 dst.status = status == null ? null : status.copy(); 2718 dst.statusReason = statusReason == null ? null : statusReason.copy(); 2719 dst.code = code == null ? null : code.copy(); 2720 dst.description = description == null ? null : description.copy(); 2721 dst.subject = subject == null ? null : subject.copy(); 2722 dst.encounter = encounter == null ? null : encounter.copy(); 2723 dst.effective = effective == null ? null : effective.copy(); 2724 dst.date = date == null ? null : date.copy(); 2725 dst.assessor = assessor == null ? null : assessor.copy(); 2726 dst.previous = previous == null ? null : previous.copy(); 2727 if (problem != null) { 2728 dst.problem = new ArrayList<Reference>(); 2729 for (Reference i : problem) 2730 dst.problem.add(i.copy()); 2731 } 2732 ; 2733 if (investigation != null) { 2734 dst.investigation = new ArrayList<ClinicalImpressionInvestigationComponent>(); 2735 for (ClinicalImpressionInvestigationComponent i : investigation) 2736 dst.investigation.add(i.copy()); 2737 } 2738 ; 2739 if (protocol != null) { 2740 dst.protocol = new ArrayList<UriType>(); 2741 for (UriType i : protocol) 2742 dst.protocol.add(i.copy()); 2743 } 2744 ; 2745 dst.summary = summary == null ? null : summary.copy(); 2746 if (finding != null) { 2747 dst.finding = new ArrayList<ClinicalImpressionFindingComponent>(); 2748 for (ClinicalImpressionFindingComponent i : finding) 2749 dst.finding.add(i.copy()); 2750 } 2751 ; 2752 if (prognosisCodeableConcept != null) { 2753 dst.prognosisCodeableConcept = new ArrayList<CodeableConcept>(); 2754 for (CodeableConcept i : prognosisCodeableConcept) 2755 dst.prognosisCodeableConcept.add(i.copy()); 2756 } 2757 ; 2758 if (prognosisReference != null) { 2759 dst.prognosisReference = new ArrayList<Reference>(); 2760 for (Reference i : prognosisReference) 2761 dst.prognosisReference.add(i.copy()); 2762 } 2763 ; 2764 if (supportingInfo != null) { 2765 dst.supportingInfo = new ArrayList<Reference>(); 2766 for (Reference i : supportingInfo) 2767 dst.supportingInfo.add(i.copy()); 2768 } 2769 ; 2770 if (note != null) { 2771 dst.note = new ArrayList<Annotation>(); 2772 for (Annotation i : note) 2773 dst.note.add(i.copy()); 2774 } 2775 ; 2776 } 2777 2778 protected ClinicalImpression typedCopy() { 2779 return copy(); 2780 } 2781 2782 @Override 2783 public boolean equalsDeep(Base other_) { 2784 if (!super.equalsDeep(other_)) 2785 return false; 2786 if (!(other_ instanceof ClinicalImpression)) 2787 return false; 2788 ClinicalImpression o = (ClinicalImpression) other_; 2789 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 2790 && compareDeep(statusReason, o.statusReason, true) && compareDeep(code, o.code, true) 2791 && compareDeep(description, o.description, true) && compareDeep(subject, o.subject, true) 2792 && compareDeep(encounter, o.encounter, true) && compareDeep(effective, o.effective, true) 2793 && compareDeep(date, o.date, true) && compareDeep(assessor, o.assessor, true) 2794 && compareDeep(previous, o.previous, true) && compareDeep(problem, o.problem, true) 2795 && compareDeep(investigation, o.investigation, true) && compareDeep(protocol, o.protocol, true) 2796 && compareDeep(summary, o.summary, true) && compareDeep(finding, o.finding, true) 2797 && compareDeep(prognosisCodeableConcept, o.prognosisCodeableConcept, true) 2798 && compareDeep(prognosisReference, o.prognosisReference, true) 2799 && compareDeep(supportingInfo, o.supportingInfo, true) && compareDeep(note, o.note, true); 2800 } 2801 2802 @Override 2803 public boolean equalsShallow(Base other_) { 2804 if (!super.equalsShallow(other_)) 2805 return false; 2806 if (!(other_ instanceof ClinicalImpression)) 2807 return false; 2808 ClinicalImpression o = (ClinicalImpression) other_; 2809 return compareValues(status, o.status, true) && compareValues(description, o.description, true) 2810 && compareValues(date, o.date, true) && compareValues(protocol, o.protocol, true) 2811 && compareValues(summary, o.summary, true); 2812 } 2813 2814 public boolean isEmpty() { 2815 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, statusReason, code, description, 2816 subject, encounter, effective, date, assessor, previous, problem, investigation, protocol, summary, finding, 2817 prognosisCodeableConcept, prognosisReference, supportingInfo, note); 2818 } 2819 2820 @Override 2821 public ResourceType getResourceType() { 2822 return ResourceType.ClinicalImpression; 2823 } 2824 2825 /** 2826 * Search parameter: <b>date</b> 2827 * <p> 2828 * Description: <b>When the assessment was documented</b><br> 2829 * Type: <b>date</b><br> 2830 * Path: <b>ClinicalImpression.date</b><br> 2831 * </p> 2832 */ 2833 @SearchParamDefinition(name = "date", path = "ClinicalImpression.date", description = "When the assessment was documented", type = "date") 2834 public static final String SP_DATE = "date"; 2835 /** 2836 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2837 * <p> 2838 * Description: <b>When the assessment was documented</b><br> 2839 * Type: <b>date</b><br> 2840 * Path: <b>ClinicalImpression.date</b><br> 2841 * </p> 2842 */ 2843 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2844 SP_DATE); 2845 2846 /** 2847 * Search parameter: <b>identifier</b> 2848 * <p> 2849 * Description: <b>Business identifier</b><br> 2850 * Type: <b>token</b><br> 2851 * Path: <b>ClinicalImpression.identifier</b><br> 2852 * </p> 2853 */ 2854 @SearchParamDefinition(name = "identifier", path = "ClinicalImpression.identifier", description = "Business identifier", type = "token") 2855 public static final String SP_IDENTIFIER = "identifier"; 2856 /** 2857 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2858 * <p> 2859 * Description: <b>Business identifier</b><br> 2860 * Type: <b>token</b><br> 2861 * Path: <b>ClinicalImpression.identifier</b><br> 2862 * </p> 2863 */ 2864 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2865 SP_IDENTIFIER); 2866 2867 /** 2868 * Search parameter: <b>previous</b> 2869 * <p> 2870 * Description: <b>Reference to last assessment</b><br> 2871 * Type: <b>reference</b><br> 2872 * Path: <b>ClinicalImpression.previous</b><br> 2873 * </p> 2874 */ 2875 @SearchParamDefinition(name = "previous", path = "ClinicalImpression.previous", description = "Reference to last assessment", type = "reference", target = { 2876 ClinicalImpression.class }) 2877 public static final String SP_PREVIOUS = "previous"; 2878 /** 2879 * <b>Fluent Client</b> search parameter constant for <b>previous</b> 2880 * <p> 2881 * Description: <b>Reference to last assessment</b><br> 2882 * Type: <b>reference</b><br> 2883 * Path: <b>ClinicalImpression.previous</b><br> 2884 * </p> 2885 */ 2886 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREVIOUS = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2887 SP_PREVIOUS); 2888 2889 /** 2890 * Constant for fluent queries to be used to add include statements. Specifies 2891 * the path value of "<b>ClinicalImpression:previous</b>". 2892 */ 2893 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREVIOUS = new ca.uhn.fhir.model.api.Include( 2894 "ClinicalImpression:previous").toLocked(); 2895 2896 /** 2897 * Search parameter: <b>finding-code</b> 2898 * <p> 2899 * Description: <b>What was found</b><br> 2900 * Type: <b>token</b><br> 2901 * Path: <b>ClinicalImpression.finding.itemCodeableConcept</b><br> 2902 * </p> 2903 */ 2904 @SearchParamDefinition(name = "finding-code", path = "ClinicalImpression.finding.itemCodeableConcept", description = "What was found", type = "token") 2905 public static final String SP_FINDING_CODE = "finding-code"; 2906 /** 2907 * <b>Fluent Client</b> search parameter constant for <b>finding-code</b> 2908 * <p> 2909 * Description: <b>What was found</b><br> 2910 * Type: <b>token</b><br> 2911 * Path: <b>ClinicalImpression.finding.itemCodeableConcept</b><br> 2912 * </p> 2913 */ 2914 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FINDING_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2915 SP_FINDING_CODE); 2916 2917 /** 2918 * Search parameter: <b>assessor</b> 2919 * <p> 2920 * Description: <b>The clinician performing the assessment</b><br> 2921 * Type: <b>reference</b><br> 2922 * Path: <b>ClinicalImpression.assessor</b><br> 2923 * </p> 2924 */ 2925 @SearchParamDefinition(name = "assessor", path = "ClinicalImpression.assessor", description = "The clinician performing the assessment", type = "reference", providesMembershipIn = { 2926 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Practitioner.class, 2927 PractitionerRole.class }) 2928 public static final String SP_ASSESSOR = "assessor"; 2929 /** 2930 * <b>Fluent Client</b> search parameter constant for <b>assessor</b> 2931 * <p> 2932 * Description: <b>The clinician performing the assessment</b><br> 2933 * Type: <b>reference</b><br> 2934 * Path: <b>ClinicalImpression.assessor</b><br> 2935 * </p> 2936 */ 2937 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ASSESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2938 SP_ASSESSOR); 2939 2940 /** 2941 * Constant for fluent queries to be used to add include statements. Specifies 2942 * the path value of "<b>ClinicalImpression:assessor</b>". 2943 */ 2944 public static final ca.uhn.fhir.model.api.Include INCLUDE_ASSESSOR = new ca.uhn.fhir.model.api.Include( 2945 "ClinicalImpression:assessor").toLocked(); 2946 2947 /** 2948 * Search parameter: <b>subject</b> 2949 * <p> 2950 * Description: <b>Patient or group assessed</b><br> 2951 * Type: <b>reference</b><br> 2952 * Path: <b>ClinicalImpression.subject</b><br> 2953 * </p> 2954 */ 2955 @SearchParamDefinition(name = "subject", path = "ClinicalImpression.subject", description = "Patient or group assessed", type = "reference", providesMembershipIn = { 2956 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Group.class, Patient.class }) 2957 public static final String SP_SUBJECT = "subject"; 2958 /** 2959 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2960 * <p> 2961 * Description: <b>Patient or group assessed</b><br> 2962 * Type: <b>reference</b><br> 2963 * Path: <b>ClinicalImpression.subject</b><br> 2964 * </p> 2965 */ 2966 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2967 SP_SUBJECT); 2968 2969 /** 2970 * Constant for fluent queries to be used to add include statements. Specifies 2971 * the path value of "<b>ClinicalImpression:subject</b>". 2972 */ 2973 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 2974 "ClinicalImpression:subject").toLocked(); 2975 2976 /** 2977 * Search parameter: <b>encounter</b> 2978 * <p> 2979 * Description: <b>Encounter created as part of</b><br> 2980 * Type: <b>reference</b><br> 2981 * Path: <b>ClinicalImpression.encounter</b><br> 2982 * </p> 2983 */ 2984 @SearchParamDefinition(name = "encounter", path = "ClinicalImpression.encounter", description = "Encounter created as part of", type = "reference", providesMembershipIn = { 2985 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 2986 public static final String SP_ENCOUNTER = "encounter"; 2987 /** 2988 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2989 * <p> 2990 * Description: <b>Encounter created as part of</b><br> 2991 * Type: <b>reference</b><br> 2992 * Path: <b>ClinicalImpression.encounter</b><br> 2993 * </p> 2994 */ 2995 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2996 SP_ENCOUNTER); 2997 2998 /** 2999 * Constant for fluent queries to be used to add include statements. Specifies 3000 * the path value of "<b>ClinicalImpression:encounter</b>". 3001 */ 3002 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 3003 "ClinicalImpression:encounter").toLocked(); 3004 3005 /** 3006 * Search parameter: <b>finding-ref</b> 3007 * <p> 3008 * Description: <b>What was found</b><br> 3009 * Type: <b>reference</b><br> 3010 * Path: <b>ClinicalImpression.finding.itemReference</b><br> 3011 * </p> 3012 */ 3013 @SearchParamDefinition(name = "finding-ref", path = "ClinicalImpression.finding.itemReference", description = "What was found", type = "reference", target = { 3014 Condition.class, Media.class, Observation.class }) 3015 public static final String SP_FINDING_REF = "finding-ref"; 3016 /** 3017 * <b>Fluent Client</b> search parameter constant for <b>finding-ref</b> 3018 * <p> 3019 * Description: <b>What was found</b><br> 3020 * Type: <b>reference</b><br> 3021 * Path: <b>ClinicalImpression.finding.itemReference</b><br> 3022 * </p> 3023 */ 3024 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FINDING_REF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3025 SP_FINDING_REF); 3026 3027 /** 3028 * Constant for fluent queries to be used to add include statements. Specifies 3029 * the path value of "<b>ClinicalImpression:finding-ref</b>". 3030 */ 3031 public static final ca.uhn.fhir.model.api.Include INCLUDE_FINDING_REF = new ca.uhn.fhir.model.api.Include( 3032 "ClinicalImpression:finding-ref").toLocked(); 3033 3034 /** 3035 * Search parameter: <b>problem</b> 3036 * <p> 3037 * Description: <b>Relevant impressions of patient state</b><br> 3038 * Type: <b>reference</b><br> 3039 * Path: <b>ClinicalImpression.problem</b><br> 3040 * </p> 3041 */ 3042 @SearchParamDefinition(name = "problem", path = "ClinicalImpression.problem", description = "Relevant impressions of patient state", type = "reference", target = { 3043 AllergyIntolerance.class, Condition.class }) 3044 public static final String SP_PROBLEM = "problem"; 3045 /** 3046 * <b>Fluent Client</b> search parameter constant for <b>problem</b> 3047 * <p> 3048 * Description: <b>Relevant impressions of patient state</b><br> 3049 * Type: <b>reference</b><br> 3050 * Path: <b>ClinicalImpression.problem</b><br> 3051 * </p> 3052 */ 3053 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROBLEM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3054 SP_PROBLEM); 3055 3056 /** 3057 * Constant for fluent queries to be used to add include statements. Specifies 3058 * the path value of "<b>ClinicalImpression:problem</b>". 3059 */ 3060 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROBLEM = new ca.uhn.fhir.model.api.Include( 3061 "ClinicalImpression:problem").toLocked(); 3062 3063 /** 3064 * Search parameter: <b>patient</b> 3065 * <p> 3066 * Description: <b>Patient or group assessed</b><br> 3067 * Type: <b>reference</b><br> 3068 * Path: <b>ClinicalImpression.subject</b><br> 3069 * </p> 3070 */ 3071 @SearchParamDefinition(name = "patient", path = "ClinicalImpression.subject.where(resolve() is Patient)", description = "Patient or group assessed", type = "reference", target = { 3072 Patient.class }) 3073 public static final String SP_PATIENT = "patient"; 3074 /** 3075 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3076 * <p> 3077 * Description: <b>Patient or group assessed</b><br> 3078 * Type: <b>reference</b><br> 3079 * Path: <b>ClinicalImpression.subject</b><br> 3080 * </p> 3081 */ 3082 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3083 SP_PATIENT); 3084 3085 /** 3086 * Constant for fluent queries to be used to add include statements. Specifies 3087 * the path value of "<b>ClinicalImpression:patient</b>". 3088 */ 3089 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 3090 "ClinicalImpression:patient").toLocked(); 3091 3092 /** 3093 * Search parameter: <b>supporting-info</b> 3094 * <p> 3095 * Description: <b>Information supporting the clinical impression</b><br> 3096 * Type: <b>reference</b><br> 3097 * Path: <b>ClinicalImpression.supportingInfo</b><br> 3098 * </p> 3099 */ 3100 @SearchParamDefinition(name = "supporting-info", path = "ClinicalImpression.supportingInfo", description = "Information supporting the clinical impression", type = "reference") 3101 public static final String SP_SUPPORTING_INFO = "supporting-info"; 3102 /** 3103 * <b>Fluent Client</b> search parameter constant for <b>supporting-info</b> 3104 * <p> 3105 * Description: <b>Information supporting the clinical impression</b><br> 3106 * Type: <b>reference</b><br> 3107 * Path: <b>ClinicalImpression.supportingInfo</b><br> 3108 * </p> 3109 */ 3110 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUPPORTING_INFO = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3111 SP_SUPPORTING_INFO); 3112 3113 /** 3114 * Constant for fluent queries to be used to add include statements. Specifies 3115 * the path value of "<b>ClinicalImpression:supporting-info</b>". 3116 */ 3117 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUPPORTING_INFO = new ca.uhn.fhir.model.api.Include( 3118 "ClinicalImpression:supporting-info").toLocked(); 3119 3120 /** 3121 * Search parameter: <b>investigation</b> 3122 * <p> 3123 * Description: <b>Record of a specific investigation</b><br> 3124 * Type: <b>reference</b><br> 3125 * Path: <b>ClinicalImpression.investigation.item</b><br> 3126 * </p> 3127 */ 3128 @SearchParamDefinition(name = "investigation", path = "ClinicalImpression.investigation.item", description = "Record of a specific investigation", type = "reference", target = { 3129 DiagnosticReport.class, FamilyMemberHistory.class, ImagingStudy.class, Media.class, Observation.class, 3130 QuestionnaireResponse.class, RiskAssessment.class }) 3131 public static final String SP_INVESTIGATION = "investigation"; 3132 /** 3133 * <b>Fluent Client</b> search parameter constant for <b>investigation</b> 3134 * <p> 3135 * Description: <b>Record of a specific investigation</b><br> 3136 * Type: <b>reference</b><br> 3137 * Path: <b>ClinicalImpression.investigation.item</b><br> 3138 * </p> 3139 */ 3140 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INVESTIGATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3141 SP_INVESTIGATION); 3142 3143 /** 3144 * Constant for fluent queries to be used to add include statements. Specifies 3145 * the path value of "<b>ClinicalImpression:investigation</b>". 3146 */ 3147 public static final ca.uhn.fhir.model.api.Include INCLUDE_INVESTIGATION = new ca.uhn.fhir.model.api.Include( 3148 "ClinicalImpression:investigation").toLocked(); 3149 3150 /** 3151 * Search parameter: <b>status</b> 3152 * <p> 3153 * Description: <b>in-progress | completed | entered-in-error</b><br> 3154 * Type: <b>token</b><br> 3155 * Path: <b>ClinicalImpression.status</b><br> 3156 * </p> 3157 */ 3158 @SearchParamDefinition(name = "status", path = "ClinicalImpression.status", description = "in-progress | completed | entered-in-error", type = "token") 3159 public static final String SP_STATUS = "status"; 3160 /** 3161 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3162 * <p> 3163 * Description: <b>in-progress | completed | entered-in-error</b><br> 3164 * Type: <b>token</b><br> 3165 * Path: <b>ClinicalImpression.status</b><br> 3166 * </p> 3167 */ 3168 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3169 SP_STATUS); 3170 3171}