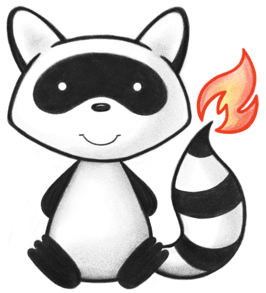
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * An occurrence of information being transmitted; e.g. an alert that was sent 048 * to a responsible provider, a public health agency that was notified about a 049 * reportable condition. 050 */ 051@ResourceDef(name = "Communication", profile = "http://hl7.org/fhir/StructureDefinition/Communication") 052public class Communication extends DomainResource { 053 054 public enum CommunicationStatus { 055 /** 056 * The core event has not started yet, but some staging activities have begun 057 * (e.g. surgical suite preparation). Preparation stages may be tracked for 058 * billing purposes. 059 */ 060 PREPARATION, 061 /** 062 * The event is currently occurring. 063 */ 064 INPROGRESS, 065 /** 066 * The event was terminated prior to any activity beyond preparation. I.e. The 067 * 'main' activity has not yet begun. The boundary between preparatory and the 068 * 'main' activity is context-specific. 069 */ 070 NOTDONE, 071 /** 072 * The event has been temporarily stopped but is expected to resume in the 073 * future. 074 */ 075 ONHOLD, 076 /** 077 * The event was terminated prior to the full completion of the intended 078 * activity but after at least some of the 'main' activity (beyond preparation) 079 * has occurred. 080 */ 081 STOPPED, 082 /** 083 * The event has now concluded. 084 */ 085 COMPLETED, 086 /** 087 * This electronic record should never have existed, though it is possible that 088 * real-world decisions were based on it. (If real-world activity has occurred, 089 * the status should be "stopped" rather than "entered-in-error".). 090 */ 091 ENTEREDINERROR, 092 /** 093 * The authoring/source system does not know which of the status values 094 * currently applies for this event. Note: This concept is not to be used for 095 * "other" - one of the listed statuses is presumed to apply, but the 096 * authoring/source system does not know which. 097 */ 098 UNKNOWN, 099 /** 100 * added to help the parsers with the generic types 101 */ 102 NULL; 103 104 public static CommunicationStatus fromCode(String codeString) throws FHIRException { 105 if (codeString == null || "".equals(codeString)) 106 return null; 107 if ("preparation".equals(codeString)) 108 return PREPARATION; 109 if ("in-progress".equals(codeString)) 110 return INPROGRESS; 111 if ("not-done".equals(codeString)) 112 return NOTDONE; 113 if ("on-hold".equals(codeString)) 114 return ONHOLD; 115 if ("stopped".equals(codeString)) 116 return STOPPED; 117 if ("completed".equals(codeString)) 118 return COMPLETED; 119 if ("entered-in-error".equals(codeString)) 120 return ENTEREDINERROR; 121 if ("unknown".equals(codeString)) 122 return UNKNOWN; 123 if (Configuration.isAcceptInvalidEnums()) 124 return null; 125 else 126 throw new FHIRException("Unknown CommunicationStatus code '" + codeString + "'"); 127 } 128 129 public String toCode() { 130 switch (this) { 131 case PREPARATION: 132 return "preparation"; 133 case INPROGRESS: 134 return "in-progress"; 135 case NOTDONE: 136 return "not-done"; 137 case ONHOLD: 138 return "on-hold"; 139 case STOPPED: 140 return "stopped"; 141 case COMPLETED: 142 return "completed"; 143 case ENTEREDINERROR: 144 return "entered-in-error"; 145 case UNKNOWN: 146 return "unknown"; 147 case NULL: 148 return null; 149 default: 150 return "?"; 151 } 152 } 153 154 public String getSystem() { 155 switch (this) { 156 case PREPARATION: 157 return "http://hl7.org/fhir/event-status"; 158 case INPROGRESS: 159 return "http://hl7.org/fhir/event-status"; 160 case NOTDONE: 161 return "http://hl7.org/fhir/event-status"; 162 case ONHOLD: 163 return "http://hl7.org/fhir/event-status"; 164 case STOPPED: 165 return "http://hl7.org/fhir/event-status"; 166 case COMPLETED: 167 return "http://hl7.org/fhir/event-status"; 168 case ENTEREDINERROR: 169 return "http://hl7.org/fhir/event-status"; 170 case UNKNOWN: 171 return "http://hl7.org/fhir/event-status"; 172 case NULL: 173 return null; 174 default: 175 return "?"; 176 } 177 } 178 179 public String getDefinition() { 180 switch (this) { 181 case PREPARATION: 182 return "The core event has not started yet, but some staging activities have begun (e.g. surgical suite preparation). Preparation stages may be tracked for billing purposes."; 183 case INPROGRESS: 184 return "The event is currently occurring."; 185 case NOTDONE: 186 return "The event was terminated prior to any activity beyond preparation. I.e. The 'main' activity has not yet begun. The boundary between preparatory and the 'main' activity is context-specific."; 187 case ONHOLD: 188 return "The event has been temporarily stopped but is expected to resume in the future."; 189 case STOPPED: 190 return "The event was terminated prior to the full completion of the intended activity but after at least some of the 'main' activity (beyond preparation) has occurred."; 191 case COMPLETED: 192 return "The event has now concluded."; 193 case ENTEREDINERROR: 194 return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"stopped\" rather than \"entered-in-error\".)."; 195 case UNKNOWN: 196 return "The authoring/source system does not know which of the status values currently applies for this event. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 197 case NULL: 198 return null; 199 default: 200 return "?"; 201 } 202 } 203 204 public String getDisplay() { 205 switch (this) { 206 case PREPARATION: 207 return "Preparation"; 208 case INPROGRESS: 209 return "In Progress"; 210 case NOTDONE: 211 return "Not Done"; 212 case ONHOLD: 213 return "On Hold"; 214 case STOPPED: 215 return "Stopped"; 216 case COMPLETED: 217 return "Completed"; 218 case ENTEREDINERROR: 219 return "Entered in Error"; 220 case UNKNOWN: 221 return "Unknown"; 222 case NULL: 223 return null; 224 default: 225 return "?"; 226 } 227 } 228 } 229 230 public static class CommunicationStatusEnumFactory implements EnumFactory<CommunicationStatus> { 231 public CommunicationStatus fromCode(String codeString) throws IllegalArgumentException { 232 if (codeString == null || "".equals(codeString)) 233 if (codeString == null || "".equals(codeString)) 234 return null; 235 if ("preparation".equals(codeString)) 236 return CommunicationStatus.PREPARATION; 237 if ("in-progress".equals(codeString)) 238 return CommunicationStatus.INPROGRESS; 239 if ("not-done".equals(codeString)) 240 return CommunicationStatus.NOTDONE; 241 if ("on-hold".equals(codeString)) 242 return CommunicationStatus.ONHOLD; 243 if ("stopped".equals(codeString)) 244 return CommunicationStatus.STOPPED; 245 if ("completed".equals(codeString)) 246 return CommunicationStatus.COMPLETED; 247 if ("entered-in-error".equals(codeString)) 248 return CommunicationStatus.ENTEREDINERROR; 249 if ("unknown".equals(codeString)) 250 return CommunicationStatus.UNKNOWN; 251 throw new IllegalArgumentException("Unknown CommunicationStatus code '" + codeString + "'"); 252 } 253 254 public Enumeration<CommunicationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 255 if (code == null) 256 return null; 257 if (code.isEmpty()) 258 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.NULL, code); 259 String codeString = code.asStringValue(); 260 if (codeString == null || "".equals(codeString)) 261 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.NULL, code); 262 if ("preparation".equals(codeString)) 263 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.PREPARATION, code); 264 if ("in-progress".equals(codeString)) 265 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.INPROGRESS, code); 266 if ("not-done".equals(codeString)) 267 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.NOTDONE, code); 268 if ("on-hold".equals(codeString)) 269 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.ONHOLD, code); 270 if ("stopped".equals(codeString)) 271 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.STOPPED, code); 272 if ("completed".equals(codeString)) 273 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.COMPLETED, code); 274 if ("entered-in-error".equals(codeString)) 275 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.ENTEREDINERROR, code); 276 if ("unknown".equals(codeString)) 277 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.UNKNOWN, code); 278 throw new FHIRException("Unknown CommunicationStatus code '" + codeString + "'"); 279 } 280 281 public String toCode(CommunicationStatus code) { 282 if (code == CommunicationStatus.NULL) 283 return null; 284 if (code == CommunicationStatus.PREPARATION) 285 return "preparation"; 286 if (code == CommunicationStatus.INPROGRESS) 287 return "in-progress"; 288 if (code == CommunicationStatus.NOTDONE) 289 return "not-done"; 290 if (code == CommunicationStatus.ONHOLD) 291 return "on-hold"; 292 if (code == CommunicationStatus.STOPPED) 293 return "stopped"; 294 if (code == CommunicationStatus.COMPLETED) 295 return "completed"; 296 if (code == CommunicationStatus.ENTEREDINERROR) 297 return "entered-in-error"; 298 if (code == CommunicationStatus.UNKNOWN) 299 return "unknown"; 300 return "?"; 301 } 302 303 public String toSystem(CommunicationStatus code) { 304 return code.getSystem(); 305 } 306 } 307 308 public enum CommunicationPriority { 309 /** 310 * The request has normal priority. 311 */ 312 ROUTINE, 313 /** 314 * The request should be actioned promptly - higher priority than routine. 315 */ 316 URGENT, 317 /** 318 * The request should be actioned as soon as possible - higher priority than 319 * urgent. 320 */ 321 ASAP, 322 /** 323 * The request should be actioned immediately - highest possible priority. E.g. 324 * an emergency. 325 */ 326 STAT, 327 /** 328 * added to help the parsers with the generic types 329 */ 330 NULL; 331 332 public static CommunicationPriority fromCode(String codeString) throws FHIRException { 333 if (codeString == null || "".equals(codeString)) 334 return null; 335 if ("routine".equals(codeString)) 336 return ROUTINE; 337 if ("urgent".equals(codeString)) 338 return URGENT; 339 if ("asap".equals(codeString)) 340 return ASAP; 341 if ("stat".equals(codeString)) 342 return STAT; 343 if (Configuration.isAcceptInvalidEnums()) 344 return null; 345 else 346 throw new FHIRException("Unknown CommunicationPriority code '" + codeString + "'"); 347 } 348 349 public String toCode() { 350 switch (this) { 351 case ROUTINE: 352 return "routine"; 353 case URGENT: 354 return "urgent"; 355 case ASAP: 356 return "asap"; 357 case STAT: 358 return "stat"; 359 case NULL: 360 return null; 361 default: 362 return "?"; 363 } 364 } 365 366 public String getSystem() { 367 switch (this) { 368 case ROUTINE: 369 return "http://hl7.org/fhir/request-priority"; 370 case URGENT: 371 return "http://hl7.org/fhir/request-priority"; 372 case ASAP: 373 return "http://hl7.org/fhir/request-priority"; 374 case STAT: 375 return "http://hl7.org/fhir/request-priority"; 376 case NULL: 377 return null; 378 default: 379 return "?"; 380 } 381 } 382 383 public String getDefinition() { 384 switch (this) { 385 case ROUTINE: 386 return "The request has normal priority."; 387 case URGENT: 388 return "The request should be actioned promptly - higher priority than routine."; 389 case ASAP: 390 return "The request should be actioned as soon as possible - higher priority than urgent."; 391 case STAT: 392 return "The request should be actioned immediately - highest possible priority. E.g. an emergency."; 393 case NULL: 394 return null; 395 default: 396 return "?"; 397 } 398 } 399 400 public String getDisplay() { 401 switch (this) { 402 case ROUTINE: 403 return "Routine"; 404 case URGENT: 405 return "Urgent"; 406 case ASAP: 407 return "ASAP"; 408 case STAT: 409 return "STAT"; 410 case NULL: 411 return null; 412 default: 413 return "?"; 414 } 415 } 416 } 417 418 public static class CommunicationPriorityEnumFactory implements EnumFactory<CommunicationPriority> { 419 public CommunicationPriority fromCode(String codeString) throws IllegalArgumentException { 420 if (codeString == null || "".equals(codeString)) 421 if (codeString == null || "".equals(codeString)) 422 return null; 423 if ("routine".equals(codeString)) 424 return CommunicationPriority.ROUTINE; 425 if ("urgent".equals(codeString)) 426 return CommunicationPriority.URGENT; 427 if ("asap".equals(codeString)) 428 return CommunicationPriority.ASAP; 429 if ("stat".equals(codeString)) 430 return CommunicationPriority.STAT; 431 throw new IllegalArgumentException("Unknown CommunicationPriority code '" + codeString + "'"); 432 } 433 434 public Enumeration<CommunicationPriority> fromType(PrimitiveType<?> code) throws FHIRException { 435 if (code == null) 436 return null; 437 if (code.isEmpty()) 438 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.NULL, code); 439 String codeString = code.asStringValue(); 440 if (codeString == null || "".equals(codeString)) 441 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.NULL, code); 442 if ("routine".equals(codeString)) 443 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.ROUTINE, code); 444 if ("urgent".equals(codeString)) 445 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.URGENT, code); 446 if ("asap".equals(codeString)) 447 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.ASAP, code); 448 if ("stat".equals(codeString)) 449 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.STAT, code); 450 throw new FHIRException("Unknown CommunicationPriority code '" + codeString + "'"); 451 } 452 453 public String toCode(CommunicationPriority code) { 454 if (code == CommunicationPriority.NULL) 455 return null; 456 if (code == CommunicationPriority.ROUTINE) 457 return "routine"; 458 if (code == CommunicationPriority.URGENT) 459 return "urgent"; 460 if (code == CommunicationPriority.ASAP) 461 return "asap"; 462 if (code == CommunicationPriority.STAT) 463 return "stat"; 464 return "?"; 465 } 466 467 public String toSystem(CommunicationPriority code) { 468 return code.getSystem(); 469 } 470 } 471 472 @Block() 473 public static class CommunicationPayloadComponent extends BackboneElement implements IBaseBackboneElement { 474 /** 475 * A communicated content (or for multi-part communications, one portion of the 476 * communication). 477 */ 478 @Child(name = "content", type = { StringType.class, Attachment.class, 479 Reference.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 480 @Description(shortDefinition = "Message part content", formalDefinition = "A communicated content (or for multi-part communications, one portion of the communication).") 481 protected Type content; 482 483 private static final long serialVersionUID = -1763459053L; 484 485 /** 486 * Constructor 487 */ 488 public CommunicationPayloadComponent() { 489 super(); 490 } 491 492 /** 493 * Constructor 494 */ 495 public CommunicationPayloadComponent(Type content) { 496 super(); 497 this.content = content; 498 } 499 500 /** 501 * @return {@link #content} (A communicated content (or for multi-part 502 * communications, one portion of the communication).) 503 */ 504 public Type getContent() { 505 return this.content; 506 } 507 508 /** 509 * @return {@link #content} (A communicated content (or for multi-part 510 * communications, one portion of the communication).) 511 */ 512 public StringType getContentStringType() throws FHIRException { 513 if (this.content == null) 514 this.content = new StringType(); 515 if (!(this.content instanceof StringType)) 516 throw new FHIRException("Type mismatch: the type StringType was expected, but " 517 + this.content.getClass().getName() + " was encountered"); 518 return (StringType) this.content; 519 } 520 521 public boolean hasContentStringType() { 522 return this != null && this.content instanceof StringType; 523 } 524 525 /** 526 * @return {@link #content} (A communicated content (or for multi-part 527 * communications, one portion of the communication).) 528 */ 529 public Attachment getContentAttachment() throws FHIRException { 530 if (this.content == null) 531 this.content = new Attachment(); 532 if (!(this.content instanceof Attachment)) 533 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 534 + this.content.getClass().getName() + " was encountered"); 535 return (Attachment) this.content; 536 } 537 538 public boolean hasContentAttachment() { 539 return this != null && this.content instanceof Attachment; 540 } 541 542 /** 543 * @return {@link #content} (A communicated content (or for multi-part 544 * communications, one portion of the communication).) 545 */ 546 public Reference getContentReference() throws FHIRException { 547 if (this.content == null) 548 this.content = new Reference(); 549 if (!(this.content instanceof Reference)) 550 throw new FHIRException("Type mismatch: the type Reference was expected, but " 551 + this.content.getClass().getName() + " was encountered"); 552 return (Reference) this.content; 553 } 554 555 public boolean hasContentReference() { 556 return this != null && this.content instanceof Reference; 557 } 558 559 public boolean hasContent() { 560 return this.content != null && !this.content.isEmpty(); 561 } 562 563 /** 564 * @param value {@link #content} (A communicated content (or for multi-part 565 * communications, one portion of the communication).) 566 */ 567 public CommunicationPayloadComponent setContent(Type value) { 568 if (value != null && !(value instanceof StringType || value instanceof Attachment || value instanceof Reference)) 569 throw new Error("Not the right type for Communication.payload.content[x]: " + value.fhirType()); 570 this.content = value; 571 return this; 572 } 573 574 protected void listChildren(List<Property> children) { 575 super.listChildren(children); 576 children.add(new Property("content[x]", "string|Attachment|Reference(Any)", 577 "A communicated content (or for multi-part communications, one portion of the communication).", 0, 1, 578 content)); 579 } 580 581 @Override 582 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 583 switch (_hash) { 584 case 264548711: 585 /* content[x] */ return new Property("content[x]", "string|Attachment|Reference(Any)", 586 "A communicated content (or for multi-part communications, one portion of the communication).", 0, 1, 587 content); 588 case 951530617: 589 /* content */ return new Property("content[x]", "string|Attachment|Reference(Any)", 590 "A communicated content (or for multi-part communications, one portion of the communication).", 0, 1, 591 content); 592 case -326336022: 593 /* contentString */ return new Property("content[x]", "string|Attachment|Reference(Any)", 594 "A communicated content (or for multi-part communications, one portion of the communication).", 0, 1, 595 content); 596 case -702028164: 597 /* contentAttachment */ return new Property("content[x]", "string|Attachment|Reference(Any)", 598 "A communicated content (or for multi-part communications, one portion of the communication).", 0, 1, 599 content); 600 case 1193747154: 601 /* contentReference */ return new Property("content[x]", "string|Attachment|Reference(Any)", 602 "A communicated content (or for multi-part communications, one portion of the communication).", 0, 1, 603 content); 604 default: 605 return super.getNamedProperty(_hash, _name, _checkValid); 606 } 607 608 } 609 610 @Override 611 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 612 switch (hash) { 613 case 951530617: 614 /* content */ return this.content == null ? new Base[0] : new Base[] { this.content }; // Type 615 default: 616 return super.getProperty(hash, name, checkValid); 617 } 618 619 } 620 621 @Override 622 public Base setProperty(int hash, String name, Base value) throws FHIRException { 623 switch (hash) { 624 case 951530617: // content 625 this.content = castToType(value); // Type 626 return value; 627 default: 628 return super.setProperty(hash, name, value); 629 } 630 631 } 632 633 @Override 634 public Base setProperty(String name, Base value) throws FHIRException { 635 if (name.equals("content[x]")) { 636 this.content = castToType(value); // Type 637 } else 638 return super.setProperty(name, value); 639 return value; 640 } 641 642 @Override 643 public void removeChild(String name, Base value) throws FHIRException { 644 if (name.equals("content[x]")) { 645 this.content = null; 646 } else 647 super.removeChild(name, value); 648 649 } 650 651 @Override 652 public Base makeProperty(int hash, String name) throws FHIRException { 653 switch (hash) { 654 case 264548711: 655 return getContent(); 656 case 951530617: 657 return getContent(); 658 default: 659 return super.makeProperty(hash, name); 660 } 661 662 } 663 664 @Override 665 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 666 switch (hash) { 667 case 951530617: 668 /* content */ return new String[] { "string", "Attachment", "Reference" }; 669 default: 670 return super.getTypesForProperty(hash, name); 671 } 672 673 } 674 675 @Override 676 public Base addChild(String name) throws FHIRException { 677 if (name.equals("contentString")) { 678 this.content = new StringType(); 679 return this.content; 680 } else if (name.equals("contentAttachment")) { 681 this.content = new Attachment(); 682 return this.content; 683 } else if (name.equals("contentReference")) { 684 this.content = new Reference(); 685 return this.content; 686 } else 687 return super.addChild(name); 688 } 689 690 public CommunicationPayloadComponent copy() { 691 CommunicationPayloadComponent dst = new CommunicationPayloadComponent(); 692 copyValues(dst); 693 return dst; 694 } 695 696 public void copyValues(CommunicationPayloadComponent dst) { 697 super.copyValues(dst); 698 dst.content = content == null ? null : content.copy(); 699 } 700 701 @Override 702 public boolean equalsDeep(Base other_) { 703 if (!super.equalsDeep(other_)) 704 return false; 705 if (!(other_ instanceof CommunicationPayloadComponent)) 706 return false; 707 CommunicationPayloadComponent o = (CommunicationPayloadComponent) other_; 708 return compareDeep(content, o.content, true); 709 } 710 711 @Override 712 public boolean equalsShallow(Base other_) { 713 if (!super.equalsShallow(other_)) 714 return false; 715 if (!(other_ instanceof CommunicationPayloadComponent)) 716 return false; 717 CommunicationPayloadComponent o = (CommunicationPayloadComponent) other_; 718 return true; 719 } 720 721 public boolean isEmpty() { 722 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(content); 723 } 724 725 public String fhirType() { 726 return "Communication.payload"; 727 728 } 729 730 } 731 732 /** 733 * Business identifiers assigned to this communication by the performer or other 734 * systems which remain constant as the resource is updated and propagates from 735 * server to server. 736 */ 737 @Child(name = "identifier", type = { 738 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 739 @Description(shortDefinition = "Unique identifier", formalDefinition = "Business identifiers assigned to this communication by the performer or other systems which remain constant as the resource is updated and propagates from server to server.") 740 protected List<Identifier> identifier; 741 742 /** 743 * The URL pointing to a FHIR-defined protocol, guideline, orderset or other 744 * definition that is adhered to in whole or in part by this Communication. 745 */ 746 @Child(name = "instantiatesCanonical", type = { 747 CanonicalType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 748 @Description(shortDefinition = "Instantiates FHIR protocol or definition", formalDefinition = "The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Communication.") 749 protected List<CanonicalType> instantiatesCanonical; 750 751 /** 752 * The URL pointing to an externally maintained protocol, guideline, orderset or 753 * other definition that is adhered to in whole or in part by this 754 * Communication. 755 */ 756 @Child(name = "instantiatesUri", type = { 757 UriType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 758 @Description(shortDefinition = "Instantiates external protocol or definition", formalDefinition = "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Communication.") 759 protected List<UriType> instantiatesUri; 760 761 /** 762 * An order, proposal or plan fulfilled in whole or in part by this 763 * Communication. 764 */ 765 @Child(name = "basedOn", type = { 766 Reference.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 767 @Description(shortDefinition = "Request fulfilled by this communication", formalDefinition = "An order, proposal or plan fulfilled in whole or in part by this Communication.") 768 protected List<Reference> basedOn; 769 /** 770 * The actual objects that are the target of the reference (An order, proposal 771 * or plan fulfilled in whole or in part by this Communication.) 772 */ 773 protected List<Resource> basedOnTarget; 774 775 /** 776 * Part of this action. 777 */ 778 @Child(name = "partOf", type = { 779 Reference.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 780 @Description(shortDefinition = "Part of this action", formalDefinition = "Part of this action.") 781 protected List<Reference> partOf; 782 /** 783 * The actual objects that are the target of the reference (Part of this 784 * action.) 785 */ 786 protected List<Resource> partOfTarget; 787 788 /** 789 * Prior communication that this communication is in response to. 790 */ 791 @Child(name = "inResponseTo", type = { 792 Communication.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 793 @Description(shortDefinition = "Reply to", formalDefinition = "Prior communication that this communication is in response to.") 794 protected List<Reference> inResponseTo; 795 /** 796 * The actual objects that are the target of the reference (Prior communication 797 * that this communication is in response to.) 798 */ 799 protected List<Communication> inResponseToTarget; 800 801 /** 802 * The status of the transmission. 803 */ 804 @Child(name = "status", type = { CodeType.class }, order = 6, min = 1, max = 1, modifier = true, summary = true) 805 @Description(shortDefinition = "preparation | in-progress | not-done | on-hold | stopped | completed | entered-in-error | unknown", formalDefinition = "The status of the transmission.") 806 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/event-status") 807 protected Enumeration<CommunicationStatus> status; 808 809 /** 810 * Captures the reason for the current state of the Communication. 811 */ 812 @Child(name = "statusReason", type = { 813 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 814 @Description(shortDefinition = "Reason for current status", formalDefinition = "Captures the reason for the current state of the Communication.") 815 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/communication-not-done-reason") 816 protected CodeableConcept statusReason; 817 818 /** 819 * The type of message conveyed such as alert, notification, reminder, 820 * instruction, etc. 821 */ 822 @Child(name = "category", type = { 823 CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 824 @Description(shortDefinition = "Message category", formalDefinition = "The type of message conveyed such as alert, notification, reminder, instruction, etc.") 825 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/communication-category") 826 protected List<CodeableConcept> category; 827 828 /** 829 * Characterizes how quickly the planned or in progress communication must be 830 * addressed. Includes concepts such as stat, urgent, routine. 831 */ 832 @Child(name = "priority", type = { CodeType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 833 @Description(shortDefinition = "routine | urgent | asap | stat", formalDefinition = "Characterizes how quickly the planned or in progress communication must be addressed. Includes concepts such as stat, urgent, routine.") 834 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-priority") 835 protected Enumeration<CommunicationPriority> priority; 836 837 /** 838 * A channel that was used for this communication (e.g. email, fax). 839 */ 840 @Child(name = "medium", type = { 841 CodeableConcept.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 842 @Description(shortDefinition = "A channel of communication", formalDefinition = "A channel that was used for this communication (e.g. email, fax).") 843 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ParticipationMode") 844 protected List<CodeableConcept> medium; 845 846 /** 847 * The patient or group that was the focus of this communication. 848 */ 849 @Child(name = "subject", type = { Patient.class, 850 Group.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 851 @Description(shortDefinition = "Focus of message", formalDefinition = "The patient or group that was the focus of this communication.") 852 protected Reference subject; 853 854 /** 855 * The actual object that is the target of the reference (The patient or group 856 * that was the focus of this communication.) 857 */ 858 protected Resource subjectTarget; 859 860 /** 861 * Description of the purpose/content, similar to a subject line in an email. 862 */ 863 @Child(name = "topic", type = { 864 CodeableConcept.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 865 @Description(shortDefinition = "Description of the purpose/content", formalDefinition = "Description of the purpose/content, similar to a subject line in an email.") 866 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/communication-topic") 867 protected CodeableConcept topic; 868 869 /** 870 * Other resources that pertain to this communication and to which this 871 * communication should be associated. 872 */ 873 @Child(name = "about", type = { 874 Reference.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 875 @Description(shortDefinition = "Resources that pertain to this communication", formalDefinition = "Other resources that pertain to this communication and to which this communication should be associated.") 876 protected List<Reference> about; 877 /** 878 * The actual objects that are the target of the reference (Other resources that 879 * pertain to this communication and to which this communication should be 880 * associated.) 881 */ 882 protected List<Resource> aboutTarget; 883 884 /** 885 * The Encounter during which this Communication was created or to which the 886 * creation of this record is tightly associated. 887 */ 888 @Child(name = "encounter", type = { Encounter.class }, order = 14, min = 0, max = 1, modifier = false, summary = true) 889 @Description(shortDefinition = "Encounter created as part of", formalDefinition = "The Encounter during which this Communication was created or to which the creation of this record is tightly associated.") 890 protected Reference encounter; 891 892 /** 893 * The actual object that is the target of the reference (The Encounter during 894 * which this Communication was created or to which the creation of this record 895 * is tightly associated.) 896 */ 897 protected Encounter encounterTarget; 898 899 /** 900 * The time when this communication was sent. 901 */ 902 @Child(name = "sent", type = { DateTimeType.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 903 @Description(shortDefinition = "When sent", formalDefinition = "The time when this communication was sent.") 904 protected DateTimeType sent; 905 906 /** 907 * The time when this communication arrived at the destination. 908 */ 909 @Child(name = "received", type = { 910 DateTimeType.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 911 @Description(shortDefinition = "When received", formalDefinition = "The time when this communication arrived at the destination.") 912 protected DateTimeType received; 913 914 /** 915 * The entity (e.g. person, organization, clinical information system, care team 916 * or device) which was the target of the communication. If receipts need to be 917 * tracked by an individual, a separate resource instance will need to be 918 * created for each recipient. Multiple recipient communications are intended 919 * where either receipts are not tracked (e.g. a mass mail-out) or a receipt is 920 * captured in aggregate (all emails confirmed received by a particular time). 921 */ 922 @Child(name = "recipient", type = { Device.class, Organization.class, Patient.class, Practitioner.class, 923 PractitionerRole.class, RelatedPerson.class, Group.class, CareTeam.class, 924 HealthcareService.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 925 @Description(shortDefinition = "Message recipient", formalDefinition = "The entity (e.g. person, organization, clinical information system, care team or device) which was the target of the communication. If receipts need to be tracked by an individual, a separate resource instance will need to be created for each recipient. Multiple recipient communications are intended where either receipts are not tracked (e.g. a mass mail-out) or a receipt is captured in aggregate (all emails confirmed received by a particular time).") 926 protected List<Reference> recipient; 927 /** 928 * The actual objects that are the target of the reference (The entity (e.g. 929 * person, organization, clinical information system, care team or device) which 930 * was the target of the communication. If receipts need to be tracked by an 931 * individual, a separate resource instance will need to be created for each 932 * recipient. Multiple recipient communications are intended where either 933 * receipts are not tracked (e.g. a mass mail-out) or a receipt is captured in 934 * aggregate (all emails confirmed received by a particular time).) 935 */ 936 protected List<Resource> recipientTarget; 937 938 /** 939 * The entity (e.g. person, organization, clinical information system, or 940 * device) which was the source of the communication. 941 */ 942 @Child(name = "sender", type = { Device.class, Organization.class, Patient.class, Practitioner.class, 943 PractitionerRole.class, RelatedPerson.class, 944 HealthcareService.class }, order = 18, min = 0, max = 1, modifier = false, summary = false) 945 @Description(shortDefinition = "Message sender", formalDefinition = "The entity (e.g. person, organization, clinical information system, or device) which was the source of the communication.") 946 protected Reference sender; 947 948 /** 949 * The actual object that is the target of the reference (The entity (e.g. 950 * person, organization, clinical information system, or device) which was the 951 * source of the communication.) 952 */ 953 protected Resource senderTarget; 954 955 /** 956 * The reason or justification for the communication. 957 */ 958 @Child(name = "reasonCode", type = { 959 CodeableConcept.class }, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 960 @Description(shortDefinition = "Indication for message", formalDefinition = "The reason or justification for the communication.") 961 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/clinical-findings") 962 protected List<CodeableConcept> reasonCode; 963 964 /** 965 * Indicates another resource whose existence justifies this communication. 966 */ 967 @Child(name = "reasonReference", type = { Condition.class, Observation.class, DiagnosticReport.class, 968 DocumentReference.class }, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 969 @Description(shortDefinition = "Why was communication done?", formalDefinition = "Indicates another resource whose existence justifies this communication.") 970 protected List<Reference> reasonReference; 971 /** 972 * The actual objects that are the target of the reference (Indicates another 973 * resource whose existence justifies this communication.) 974 */ 975 protected List<Resource> reasonReferenceTarget; 976 977 /** 978 * Text, attachment(s), or resource(s) that was communicated to the recipient. 979 */ 980 @Child(name = "payload", type = {}, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 981 @Description(shortDefinition = "Message payload", formalDefinition = "Text, attachment(s), or resource(s) that was communicated to the recipient.") 982 protected List<CommunicationPayloadComponent> payload; 983 984 /** 985 * Additional notes or commentary about the communication by the sender, 986 * receiver or other interested parties. 987 */ 988 @Child(name = "note", type = { 989 Annotation.class }, order = 22, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 990 @Description(shortDefinition = "Comments made about the communication", formalDefinition = "Additional notes or commentary about the communication by the sender, receiver or other interested parties.") 991 protected List<Annotation> note; 992 993 private static final long serialVersionUID = 1325359310L; 994 995 /** 996 * Constructor 997 */ 998 public Communication() { 999 super(); 1000 } 1001 1002 /** 1003 * Constructor 1004 */ 1005 public Communication(Enumeration<CommunicationStatus> status) { 1006 super(); 1007 this.status = status; 1008 } 1009 1010 /** 1011 * @return {@link #identifier} (Business identifiers assigned to this 1012 * communication by the performer or other systems which remain constant 1013 * as the resource is updated and propagates from server to server.) 1014 */ 1015 public List<Identifier> getIdentifier() { 1016 if (this.identifier == null) 1017 this.identifier = new ArrayList<Identifier>(); 1018 return this.identifier; 1019 } 1020 1021 /** 1022 * @return Returns a reference to <code>this</code> for easy method chaining 1023 */ 1024 public Communication setIdentifier(List<Identifier> theIdentifier) { 1025 this.identifier = theIdentifier; 1026 return this; 1027 } 1028 1029 public boolean hasIdentifier() { 1030 if (this.identifier == null) 1031 return false; 1032 for (Identifier item : this.identifier) 1033 if (!item.isEmpty()) 1034 return true; 1035 return false; 1036 } 1037 1038 public Identifier addIdentifier() { // 3 1039 Identifier t = new Identifier(); 1040 if (this.identifier == null) 1041 this.identifier = new ArrayList<Identifier>(); 1042 this.identifier.add(t); 1043 return t; 1044 } 1045 1046 public Communication addIdentifier(Identifier t) { // 3 1047 if (t == null) 1048 return this; 1049 if (this.identifier == null) 1050 this.identifier = new ArrayList<Identifier>(); 1051 this.identifier.add(t); 1052 return this; 1053 } 1054 1055 /** 1056 * @return The first repetition of repeating field {@link #identifier}, creating 1057 * it if it does not already exist 1058 */ 1059 public Identifier getIdentifierFirstRep() { 1060 if (getIdentifier().isEmpty()) { 1061 addIdentifier(); 1062 } 1063 return getIdentifier().get(0); 1064 } 1065 1066 /** 1067 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined 1068 * protocol, guideline, orderset or other definition that is adhered to 1069 * in whole or in part by this Communication.) 1070 */ 1071 public List<CanonicalType> getInstantiatesCanonical() { 1072 if (this.instantiatesCanonical == null) 1073 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1074 return this.instantiatesCanonical; 1075 } 1076 1077 /** 1078 * @return Returns a reference to <code>this</code> for easy method chaining 1079 */ 1080 public Communication setInstantiatesCanonical(List<CanonicalType> theInstantiatesCanonical) { 1081 this.instantiatesCanonical = theInstantiatesCanonical; 1082 return this; 1083 } 1084 1085 public boolean hasInstantiatesCanonical() { 1086 if (this.instantiatesCanonical == null) 1087 return false; 1088 for (CanonicalType item : this.instantiatesCanonical) 1089 if (!item.isEmpty()) 1090 return true; 1091 return false; 1092 } 1093 1094 /** 1095 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined 1096 * protocol, guideline, orderset or other definition that is adhered to 1097 * in whole or in part by this Communication.) 1098 */ 1099 public CanonicalType addInstantiatesCanonicalElement() {// 2 1100 CanonicalType t = new CanonicalType(); 1101 if (this.instantiatesCanonical == null) 1102 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1103 this.instantiatesCanonical.add(t); 1104 return t; 1105 } 1106 1107 /** 1108 * @param value {@link #instantiatesCanonical} (The URL pointing to a 1109 * FHIR-defined protocol, guideline, orderset or other definition 1110 * that is adhered to in whole or in part by this Communication.) 1111 */ 1112 public Communication addInstantiatesCanonical(String value) { // 1 1113 CanonicalType t = new CanonicalType(); 1114 t.setValue(value); 1115 if (this.instantiatesCanonical == null) 1116 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1117 this.instantiatesCanonical.add(t); 1118 return this; 1119 } 1120 1121 /** 1122 * @param value {@link #instantiatesCanonical} (The URL pointing to a 1123 * FHIR-defined protocol, guideline, orderset or other definition 1124 * that is adhered to in whole or in part by this Communication.) 1125 */ 1126 public boolean hasInstantiatesCanonical(String value) { 1127 if (this.instantiatesCanonical == null) 1128 return false; 1129 for (CanonicalType v : this.instantiatesCanonical) 1130 if (v.getValue().equals(value)) // canonical(PlanDefinition|ActivityDefinition|Measure|OperationDefinition|Questionnaire) 1131 return true; 1132 return false; 1133 } 1134 1135 /** 1136 * @return {@link #instantiatesUri} (The URL pointing to an externally 1137 * maintained protocol, guideline, orderset or other definition that is 1138 * adhered to in whole or in part by this Communication.) 1139 */ 1140 public List<UriType> getInstantiatesUri() { 1141 if (this.instantiatesUri == null) 1142 this.instantiatesUri = new ArrayList<UriType>(); 1143 return this.instantiatesUri; 1144 } 1145 1146 /** 1147 * @return Returns a reference to <code>this</code> for easy method chaining 1148 */ 1149 public Communication setInstantiatesUri(List<UriType> theInstantiatesUri) { 1150 this.instantiatesUri = theInstantiatesUri; 1151 return this; 1152 } 1153 1154 public boolean hasInstantiatesUri() { 1155 if (this.instantiatesUri == null) 1156 return false; 1157 for (UriType item : this.instantiatesUri) 1158 if (!item.isEmpty()) 1159 return true; 1160 return false; 1161 } 1162 1163 /** 1164 * @return {@link #instantiatesUri} (The URL pointing to an externally 1165 * maintained protocol, guideline, orderset or other definition that is 1166 * adhered to in whole or in part by this Communication.) 1167 */ 1168 public UriType addInstantiatesUriElement() {// 2 1169 UriType t = new UriType(); 1170 if (this.instantiatesUri == null) 1171 this.instantiatesUri = new ArrayList<UriType>(); 1172 this.instantiatesUri.add(t); 1173 return t; 1174 } 1175 1176 /** 1177 * @param value {@link #instantiatesUri} (The URL pointing to an externally 1178 * maintained protocol, guideline, orderset or other definition 1179 * that is adhered to in whole or in part by this Communication.) 1180 */ 1181 public Communication addInstantiatesUri(String value) { // 1 1182 UriType t = new UriType(); 1183 t.setValue(value); 1184 if (this.instantiatesUri == null) 1185 this.instantiatesUri = new ArrayList<UriType>(); 1186 this.instantiatesUri.add(t); 1187 return this; 1188 } 1189 1190 /** 1191 * @param value {@link #instantiatesUri} (The URL pointing to an externally 1192 * maintained protocol, guideline, orderset or other definition 1193 * that is adhered to in whole or in part by this Communication.) 1194 */ 1195 public boolean hasInstantiatesUri(String value) { 1196 if (this.instantiatesUri == null) 1197 return false; 1198 for (UriType v : this.instantiatesUri) 1199 if (v.getValue().equals(value)) // uri 1200 return true; 1201 return false; 1202 } 1203 1204 /** 1205 * @return {@link #basedOn} (An order, proposal or plan fulfilled in whole or in 1206 * part by this Communication.) 1207 */ 1208 public List<Reference> getBasedOn() { 1209 if (this.basedOn == null) 1210 this.basedOn = new ArrayList<Reference>(); 1211 return this.basedOn; 1212 } 1213 1214 /** 1215 * @return Returns a reference to <code>this</code> for easy method chaining 1216 */ 1217 public Communication setBasedOn(List<Reference> theBasedOn) { 1218 this.basedOn = theBasedOn; 1219 return this; 1220 } 1221 1222 public boolean hasBasedOn() { 1223 if (this.basedOn == null) 1224 return false; 1225 for (Reference item : this.basedOn) 1226 if (!item.isEmpty()) 1227 return true; 1228 return false; 1229 } 1230 1231 public Reference addBasedOn() { // 3 1232 Reference t = new Reference(); 1233 if (this.basedOn == null) 1234 this.basedOn = new ArrayList<Reference>(); 1235 this.basedOn.add(t); 1236 return t; 1237 } 1238 1239 public Communication addBasedOn(Reference t) { // 3 1240 if (t == null) 1241 return this; 1242 if (this.basedOn == null) 1243 this.basedOn = new ArrayList<Reference>(); 1244 this.basedOn.add(t); 1245 return this; 1246 } 1247 1248 /** 1249 * @return The first repetition of repeating field {@link #basedOn}, creating it 1250 * if it does not already exist 1251 */ 1252 public Reference getBasedOnFirstRep() { 1253 if (getBasedOn().isEmpty()) { 1254 addBasedOn(); 1255 } 1256 return getBasedOn().get(0); 1257 } 1258 1259 /** 1260 * @deprecated Use Reference#setResource(IBaseResource) instead 1261 */ 1262 @Deprecated 1263 public List<Resource> getBasedOnTarget() { 1264 if (this.basedOnTarget == null) 1265 this.basedOnTarget = new ArrayList<Resource>(); 1266 return this.basedOnTarget; 1267 } 1268 1269 /** 1270 * @return {@link #partOf} (Part of this action.) 1271 */ 1272 public List<Reference> getPartOf() { 1273 if (this.partOf == null) 1274 this.partOf = new ArrayList<Reference>(); 1275 return this.partOf; 1276 } 1277 1278 /** 1279 * @return Returns a reference to <code>this</code> for easy method chaining 1280 */ 1281 public Communication setPartOf(List<Reference> thePartOf) { 1282 this.partOf = thePartOf; 1283 return this; 1284 } 1285 1286 public boolean hasPartOf() { 1287 if (this.partOf == null) 1288 return false; 1289 for (Reference item : this.partOf) 1290 if (!item.isEmpty()) 1291 return true; 1292 return false; 1293 } 1294 1295 public Reference addPartOf() { // 3 1296 Reference t = new Reference(); 1297 if (this.partOf == null) 1298 this.partOf = new ArrayList<Reference>(); 1299 this.partOf.add(t); 1300 return t; 1301 } 1302 1303 public Communication addPartOf(Reference t) { // 3 1304 if (t == null) 1305 return this; 1306 if (this.partOf == null) 1307 this.partOf = new ArrayList<Reference>(); 1308 this.partOf.add(t); 1309 return this; 1310 } 1311 1312 /** 1313 * @return The first repetition of repeating field {@link #partOf}, creating it 1314 * if it does not already exist 1315 */ 1316 public Reference getPartOfFirstRep() { 1317 if (getPartOf().isEmpty()) { 1318 addPartOf(); 1319 } 1320 return getPartOf().get(0); 1321 } 1322 1323 /** 1324 * @deprecated Use Reference#setResource(IBaseResource) instead 1325 */ 1326 @Deprecated 1327 public List<Resource> getPartOfTarget() { 1328 if (this.partOfTarget == null) 1329 this.partOfTarget = new ArrayList<Resource>(); 1330 return this.partOfTarget; 1331 } 1332 1333 /** 1334 * @return {@link #inResponseTo} (Prior communication that this communication is 1335 * in response to.) 1336 */ 1337 public List<Reference> getInResponseTo() { 1338 if (this.inResponseTo == null) 1339 this.inResponseTo = new ArrayList<Reference>(); 1340 return this.inResponseTo; 1341 } 1342 1343 /** 1344 * @return Returns a reference to <code>this</code> for easy method chaining 1345 */ 1346 public Communication setInResponseTo(List<Reference> theInResponseTo) { 1347 this.inResponseTo = theInResponseTo; 1348 return this; 1349 } 1350 1351 public boolean hasInResponseTo() { 1352 if (this.inResponseTo == null) 1353 return false; 1354 for (Reference item : this.inResponseTo) 1355 if (!item.isEmpty()) 1356 return true; 1357 return false; 1358 } 1359 1360 public Reference addInResponseTo() { // 3 1361 Reference t = new Reference(); 1362 if (this.inResponseTo == null) 1363 this.inResponseTo = new ArrayList<Reference>(); 1364 this.inResponseTo.add(t); 1365 return t; 1366 } 1367 1368 public Communication addInResponseTo(Reference t) { // 3 1369 if (t == null) 1370 return this; 1371 if (this.inResponseTo == null) 1372 this.inResponseTo = new ArrayList<Reference>(); 1373 this.inResponseTo.add(t); 1374 return this; 1375 } 1376 1377 /** 1378 * @return The first repetition of repeating field {@link #inResponseTo}, 1379 * creating it if it does not already exist 1380 */ 1381 public Reference getInResponseToFirstRep() { 1382 if (getInResponseTo().isEmpty()) { 1383 addInResponseTo(); 1384 } 1385 return getInResponseTo().get(0); 1386 } 1387 1388 /** 1389 * @deprecated Use Reference#setResource(IBaseResource) instead 1390 */ 1391 @Deprecated 1392 public List<Communication> getInResponseToTarget() { 1393 if (this.inResponseToTarget == null) 1394 this.inResponseToTarget = new ArrayList<Communication>(); 1395 return this.inResponseToTarget; 1396 } 1397 1398 /** 1399 * @deprecated Use Reference#setResource(IBaseResource) instead 1400 */ 1401 @Deprecated 1402 public Communication addInResponseToTarget() { 1403 Communication r = new Communication(); 1404 if (this.inResponseToTarget == null) 1405 this.inResponseToTarget = new ArrayList<Communication>(); 1406 this.inResponseToTarget.add(r); 1407 return r; 1408 } 1409 1410 /** 1411 * @return {@link #status} (The status of the transmission.). This is the 1412 * underlying object with id, value and extensions. The accessor 1413 * "getStatus" gives direct access to the value 1414 */ 1415 public Enumeration<CommunicationStatus> getStatusElement() { 1416 if (this.status == null) 1417 if (Configuration.errorOnAutoCreate()) 1418 throw new Error("Attempt to auto-create Communication.status"); 1419 else if (Configuration.doAutoCreate()) 1420 this.status = new Enumeration<CommunicationStatus>(new CommunicationStatusEnumFactory()); // bb 1421 return this.status; 1422 } 1423 1424 public boolean hasStatusElement() { 1425 return this.status != null && !this.status.isEmpty(); 1426 } 1427 1428 public boolean hasStatus() { 1429 return this.status != null && !this.status.isEmpty(); 1430 } 1431 1432 /** 1433 * @param value {@link #status} (The status of the transmission.). This is the 1434 * underlying object with id, value and extensions. The accessor 1435 * "getStatus" gives direct access to the value 1436 */ 1437 public Communication setStatusElement(Enumeration<CommunicationStatus> value) { 1438 this.status = value; 1439 return this; 1440 } 1441 1442 /** 1443 * @return The status of the transmission. 1444 */ 1445 public CommunicationStatus getStatus() { 1446 return this.status == null ? null : this.status.getValue(); 1447 } 1448 1449 /** 1450 * @param value The status of the transmission. 1451 */ 1452 public Communication setStatus(CommunicationStatus value) { 1453 if (this.status == null) 1454 this.status = new Enumeration<CommunicationStatus>(new CommunicationStatusEnumFactory()); 1455 this.status.setValue(value); 1456 return this; 1457 } 1458 1459 /** 1460 * @return {@link #statusReason} (Captures the reason for the current state of 1461 * the Communication.) 1462 */ 1463 public CodeableConcept getStatusReason() { 1464 if (this.statusReason == null) 1465 if (Configuration.errorOnAutoCreate()) 1466 throw new Error("Attempt to auto-create Communication.statusReason"); 1467 else if (Configuration.doAutoCreate()) 1468 this.statusReason = new CodeableConcept(); // cc 1469 return this.statusReason; 1470 } 1471 1472 public boolean hasStatusReason() { 1473 return this.statusReason != null && !this.statusReason.isEmpty(); 1474 } 1475 1476 /** 1477 * @param value {@link #statusReason} (Captures the reason for the current state 1478 * of the Communication.) 1479 */ 1480 public Communication setStatusReason(CodeableConcept value) { 1481 this.statusReason = value; 1482 return this; 1483 } 1484 1485 /** 1486 * @return {@link #category} (The type of message conveyed such as alert, 1487 * notification, reminder, instruction, etc.) 1488 */ 1489 public List<CodeableConcept> getCategory() { 1490 if (this.category == null) 1491 this.category = new ArrayList<CodeableConcept>(); 1492 return this.category; 1493 } 1494 1495 /** 1496 * @return Returns a reference to <code>this</code> for easy method chaining 1497 */ 1498 public Communication setCategory(List<CodeableConcept> theCategory) { 1499 this.category = theCategory; 1500 return this; 1501 } 1502 1503 public boolean hasCategory() { 1504 if (this.category == null) 1505 return false; 1506 for (CodeableConcept item : this.category) 1507 if (!item.isEmpty()) 1508 return true; 1509 return false; 1510 } 1511 1512 public CodeableConcept addCategory() { // 3 1513 CodeableConcept t = new CodeableConcept(); 1514 if (this.category == null) 1515 this.category = new ArrayList<CodeableConcept>(); 1516 this.category.add(t); 1517 return t; 1518 } 1519 1520 public Communication addCategory(CodeableConcept t) { // 3 1521 if (t == null) 1522 return this; 1523 if (this.category == null) 1524 this.category = new ArrayList<CodeableConcept>(); 1525 this.category.add(t); 1526 return this; 1527 } 1528 1529 /** 1530 * @return The first repetition of repeating field {@link #category}, creating 1531 * it if it does not already exist 1532 */ 1533 public CodeableConcept getCategoryFirstRep() { 1534 if (getCategory().isEmpty()) { 1535 addCategory(); 1536 } 1537 return getCategory().get(0); 1538 } 1539 1540 /** 1541 * @return {@link #priority} (Characterizes how quickly the planned or in 1542 * progress communication must be addressed. Includes concepts such as 1543 * stat, urgent, routine.). This is the underlying object with id, value 1544 * and extensions. The accessor "getPriority" gives direct access to the 1545 * value 1546 */ 1547 public Enumeration<CommunicationPriority> getPriorityElement() { 1548 if (this.priority == null) 1549 if (Configuration.errorOnAutoCreate()) 1550 throw new Error("Attempt to auto-create Communication.priority"); 1551 else if (Configuration.doAutoCreate()) 1552 this.priority = new Enumeration<CommunicationPriority>(new CommunicationPriorityEnumFactory()); // bb 1553 return this.priority; 1554 } 1555 1556 public boolean hasPriorityElement() { 1557 return this.priority != null && !this.priority.isEmpty(); 1558 } 1559 1560 public boolean hasPriority() { 1561 return this.priority != null && !this.priority.isEmpty(); 1562 } 1563 1564 /** 1565 * @param value {@link #priority} (Characterizes how quickly the planned or in 1566 * progress communication must be addressed. Includes concepts such 1567 * as stat, urgent, routine.). This is the underlying object with 1568 * id, value and extensions. The accessor "getPriority" gives 1569 * direct access to the value 1570 */ 1571 public Communication setPriorityElement(Enumeration<CommunicationPriority> value) { 1572 this.priority = value; 1573 return this; 1574 } 1575 1576 /** 1577 * @return Characterizes how quickly the planned or in progress communication 1578 * must be addressed. Includes concepts such as stat, urgent, routine. 1579 */ 1580 public CommunicationPriority getPriority() { 1581 return this.priority == null ? null : this.priority.getValue(); 1582 } 1583 1584 /** 1585 * @param value Characterizes how quickly the planned or in progress 1586 * communication must be addressed. Includes concepts such as stat, 1587 * urgent, routine. 1588 */ 1589 public Communication setPriority(CommunicationPriority value) { 1590 if (value == null) 1591 this.priority = null; 1592 else { 1593 if (this.priority == null) 1594 this.priority = new Enumeration<CommunicationPriority>(new CommunicationPriorityEnumFactory()); 1595 this.priority.setValue(value); 1596 } 1597 return this; 1598 } 1599 1600 /** 1601 * @return {@link #medium} (A channel that was used for this communication (e.g. 1602 * email, fax).) 1603 */ 1604 public List<CodeableConcept> getMedium() { 1605 if (this.medium == null) 1606 this.medium = new ArrayList<CodeableConcept>(); 1607 return this.medium; 1608 } 1609 1610 /** 1611 * @return Returns a reference to <code>this</code> for easy method chaining 1612 */ 1613 public Communication setMedium(List<CodeableConcept> theMedium) { 1614 this.medium = theMedium; 1615 return this; 1616 } 1617 1618 public boolean hasMedium() { 1619 if (this.medium == null) 1620 return false; 1621 for (CodeableConcept item : this.medium) 1622 if (!item.isEmpty()) 1623 return true; 1624 return false; 1625 } 1626 1627 public CodeableConcept addMedium() { // 3 1628 CodeableConcept t = new CodeableConcept(); 1629 if (this.medium == null) 1630 this.medium = new ArrayList<CodeableConcept>(); 1631 this.medium.add(t); 1632 return t; 1633 } 1634 1635 public Communication addMedium(CodeableConcept t) { // 3 1636 if (t == null) 1637 return this; 1638 if (this.medium == null) 1639 this.medium = new ArrayList<CodeableConcept>(); 1640 this.medium.add(t); 1641 return this; 1642 } 1643 1644 /** 1645 * @return The first repetition of repeating field {@link #medium}, creating it 1646 * if it does not already exist 1647 */ 1648 public CodeableConcept getMediumFirstRep() { 1649 if (getMedium().isEmpty()) { 1650 addMedium(); 1651 } 1652 return getMedium().get(0); 1653 } 1654 1655 /** 1656 * @return {@link #subject} (The patient or group that was the focus of this 1657 * communication.) 1658 */ 1659 public Reference getSubject() { 1660 if (this.subject == null) 1661 if (Configuration.errorOnAutoCreate()) 1662 throw new Error("Attempt to auto-create Communication.subject"); 1663 else if (Configuration.doAutoCreate()) 1664 this.subject = new Reference(); // cc 1665 return this.subject; 1666 } 1667 1668 public boolean hasSubject() { 1669 return this.subject != null && !this.subject.isEmpty(); 1670 } 1671 1672 /** 1673 * @param value {@link #subject} (The patient or group that was the focus of 1674 * this communication.) 1675 */ 1676 public Communication setSubject(Reference value) { 1677 this.subject = value; 1678 return this; 1679 } 1680 1681 /** 1682 * @return {@link #subject} The actual object that is the target of the 1683 * reference. The reference library doesn't populate this, but you can 1684 * use it to hold the resource if you resolve it. (The patient or group 1685 * that was the focus of this communication.) 1686 */ 1687 public Resource getSubjectTarget() { 1688 return this.subjectTarget; 1689 } 1690 1691 /** 1692 * @param value {@link #subject} The actual object that is the target of the 1693 * reference. The reference library doesn't use these, but you can 1694 * use it to hold the resource if you resolve it. (The patient or 1695 * group that was the focus of this communication.) 1696 */ 1697 public Communication setSubjectTarget(Resource value) { 1698 this.subjectTarget = value; 1699 return this; 1700 } 1701 1702 /** 1703 * @return {@link #topic} (Description of the purpose/content, similar to a 1704 * subject line in an email.) 1705 */ 1706 public CodeableConcept getTopic() { 1707 if (this.topic == null) 1708 if (Configuration.errorOnAutoCreate()) 1709 throw new Error("Attempt to auto-create Communication.topic"); 1710 else if (Configuration.doAutoCreate()) 1711 this.topic = new CodeableConcept(); // cc 1712 return this.topic; 1713 } 1714 1715 public boolean hasTopic() { 1716 return this.topic != null && !this.topic.isEmpty(); 1717 } 1718 1719 /** 1720 * @param value {@link #topic} (Description of the purpose/content, similar to a 1721 * subject line in an email.) 1722 */ 1723 public Communication setTopic(CodeableConcept value) { 1724 this.topic = value; 1725 return this; 1726 } 1727 1728 /** 1729 * @return {@link #about} (Other resources that pertain to this communication 1730 * and to which this communication should be associated.) 1731 */ 1732 public List<Reference> getAbout() { 1733 if (this.about == null) 1734 this.about = new ArrayList<Reference>(); 1735 return this.about; 1736 } 1737 1738 /** 1739 * @return Returns a reference to <code>this</code> for easy method chaining 1740 */ 1741 public Communication setAbout(List<Reference> theAbout) { 1742 this.about = theAbout; 1743 return this; 1744 } 1745 1746 public boolean hasAbout() { 1747 if (this.about == null) 1748 return false; 1749 for (Reference item : this.about) 1750 if (!item.isEmpty()) 1751 return true; 1752 return false; 1753 } 1754 1755 public Reference addAbout() { // 3 1756 Reference t = new Reference(); 1757 if (this.about == null) 1758 this.about = new ArrayList<Reference>(); 1759 this.about.add(t); 1760 return t; 1761 } 1762 1763 public Communication addAbout(Reference t) { // 3 1764 if (t == null) 1765 return this; 1766 if (this.about == null) 1767 this.about = new ArrayList<Reference>(); 1768 this.about.add(t); 1769 return this; 1770 } 1771 1772 /** 1773 * @return The first repetition of repeating field {@link #about}, creating it 1774 * if it does not already exist 1775 */ 1776 public Reference getAboutFirstRep() { 1777 if (getAbout().isEmpty()) { 1778 addAbout(); 1779 } 1780 return getAbout().get(0); 1781 } 1782 1783 /** 1784 * @deprecated Use Reference#setResource(IBaseResource) instead 1785 */ 1786 @Deprecated 1787 public List<Resource> getAboutTarget() { 1788 if (this.aboutTarget == null) 1789 this.aboutTarget = new ArrayList<Resource>(); 1790 return this.aboutTarget; 1791 } 1792 1793 /** 1794 * @return {@link #encounter} (The Encounter during which this Communication was 1795 * created or to which the creation of this record is tightly 1796 * associated.) 1797 */ 1798 public Reference getEncounter() { 1799 if (this.encounter == null) 1800 if (Configuration.errorOnAutoCreate()) 1801 throw new Error("Attempt to auto-create Communication.encounter"); 1802 else if (Configuration.doAutoCreate()) 1803 this.encounter = new Reference(); // cc 1804 return this.encounter; 1805 } 1806 1807 public boolean hasEncounter() { 1808 return this.encounter != null && !this.encounter.isEmpty(); 1809 } 1810 1811 /** 1812 * @param value {@link #encounter} (The Encounter during which this 1813 * Communication was created or to which the creation of this 1814 * record is tightly associated.) 1815 */ 1816 public Communication setEncounter(Reference value) { 1817 this.encounter = value; 1818 return this; 1819 } 1820 1821 /** 1822 * @return {@link #encounter} The actual object that is the target of the 1823 * reference. The reference library doesn't populate this, but you can 1824 * use it to hold the resource if you resolve it. (The Encounter during 1825 * which this Communication was created or to which the creation of this 1826 * record is tightly associated.) 1827 */ 1828 public Encounter getEncounterTarget() { 1829 if (this.encounterTarget == null) 1830 if (Configuration.errorOnAutoCreate()) 1831 throw new Error("Attempt to auto-create Communication.encounter"); 1832 else if (Configuration.doAutoCreate()) 1833 this.encounterTarget = new Encounter(); // aa 1834 return this.encounterTarget; 1835 } 1836 1837 /** 1838 * @param value {@link #encounter} The actual object that is the target of the 1839 * reference. The reference library doesn't use these, but you can 1840 * use it to hold the resource if you resolve it. (The Encounter 1841 * during which this Communication was created or to which the 1842 * creation of this record is tightly associated.) 1843 */ 1844 public Communication setEncounterTarget(Encounter value) { 1845 this.encounterTarget = value; 1846 return this; 1847 } 1848 1849 /** 1850 * @return {@link #sent} (The time when this communication was sent.). This is 1851 * the underlying object with id, value and extensions. The accessor 1852 * "getSent" gives direct access to the value 1853 */ 1854 public DateTimeType getSentElement() { 1855 if (this.sent == null) 1856 if (Configuration.errorOnAutoCreate()) 1857 throw new Error("Attempt to auto-create Communication.sent"); 1858 else if (Configuration.doAutoCreate()) 1859 this.sent = new DateTimeType(); // bb 1860 return this.sent; 1861 } 1862 1863 public boolean hasSentElement() { 1864 return this.sent != null && !this.sent.isEmpty(); 1865 } 1866 1867 public boolean hasSent() { 1868 return this.sent != null && !this.sent.isEmpty(); 1869 } 1870 1871 /** 1872 * @param value {@link #sent} (The time when this communication was sent.). This 1873 * is the underlying object with id, value and extensions. The 1874 * accessor "getSent" gives direct access to the value 1875 */ 1876 public Communication setSentElement(DateTimeType value) { 1877 this.sent = value; 1878 return this; 1879 } 1880 1881 /** 1882 * @return The time when this communication was sent. 1883 */ 1884 public Date getSent() { 1885 return this.sent == null ? null : this.sent.getValue(); 1886 } 1887 1888 /** 1889 * @param value The time when this communication was sent. 1890 */ 1891 public Communication setSent(Date value) { 1892 if (value == null) 1893 this.sent = null; 1894 else { 1895 if (this.sent == null) 1896 this.sent = new DateTimeType(); 1897 this.sent.setValue(value); 1898 } 1899 return this; 1900 } 1901 1902 /** 1903 * @return {@link #received} (The time when this communication arrived at the 1904 * destination.). This is the underlying object with id, value and 1905 * extensions. The accessor "getReceived" gives direct access to the 1906 * value 1907 */ 1908 public DateTimeType getReceivedElement() { 1909 if (this.received == null) 1910 if (Configuration.errorOnAutoCreate()) 1911 throw new Error("Attempt to auto-create Communication.received"); 1912 else if (Configuration.doAutoCreate()) 1913 this.received = new DateTimeType(); // bb 1914 return this.received; 1915 } 1916 1917 public boolean hasReceivedElement() { 1918 return this.received != null && !this.received.isEmpty(); 1919 } 1920 1921 public boolean hasReceived() { 1922 return this.received != null && !this.received.isEmpty(); 1923 } 1924 1925 /** 1926 * @param value {@link #received} (The time when this communication arrived at 1927 * the destination.). This is the underlying object with id, value 1928 * and extensions. The accessor "getReceived" gives direct access 1929 * to the value 1930 */ 1931 public Communication setReceivedElement(DateTimeType value) { 1932 this.received = value; 1933 return this; 1934 } 1935 1936 /** 1937 * @return The time when this communication arrived at the destination. 1938 */ 1939 public Date getReceived() { 1940 return this.received == null ? null : this.received.getValue(); 1941 } 1942 1943 /** 1944 * @param value The time when this communication arrived at the destination. 1945 */ 1946 public Communication setReceived(Date value) { 1947 if (value == null) 1948 this.received = null; 1949 else { 1950 if (this.received == null) 1951 this.received = new DateTimeType(); 1952 this.received.setValue(value); 1953 } 1954 return this; 1955 } 1956 1957 /** 1958 * @return {@link #recipient} (The entity (e.g. person, organization, clinical 1959 * information system, care team or device) which was the target of the 1960 * communication. If receipts need to be tracked by an individual, a 1961 * separate resource instance will need to be created for each 1962 * recipient. Multiple recipient communications are intended where 1963 * either receipts are not tracked (e.g. a mass mail-out) or a receipt 1964 * is captured in aggregate (all emails confirmed received by a 1965 * particular time).) 1966 */ 1967 public List<Reference> getRecipient() { 1968 if (this.recipient == null) 1969 this.recipient = new ArrayList<Reference>(); 1970 return this.recipient; 1971 } 1972 1973 /** 1974 * @return Returns a reference to <code>this</code> for easy method chaining 1975 */ 1976 public Communication setRecipient(List<Reference> theRecipient) { 1977 this.recipient = theRecipient; 1978 return this; 1979 } 1980 1981 public boolean hasRecipient() { 1982 if (this.recipient == null) 1983 return false; 1984 for (Reference item : this.recipient) 1985 if (!item.isEmpty()) 1986 return true; 1987 return false; 1988 } 1989 1990 public Reference addRecipient() { // 3 1991 Reference t = new Reference(); 1992 if (this.recipient == null) 1993 this.recipient = new ArrayList<Reference>(); 1994 this.recipient.add(t); 1995 return t; 1996 } 1997 1998 public Communication addRecipient(Reference t) { // 3 1999 if (t == null) 2000 return this; 2001 if (this.recipient == null) 2002 this.recipient = new ArrayList<Reference>(); 2003 this.recipient.add(t); 2004 return this; 2005 } 2006 2007 /** 2008 * @return The first repetition of repeating field {@link #recipient}, creating 2009 * it if it does not already exist 2010 */ 2011 public Reference getRecipientFirstRep() { 2012 if (getRecipient().isEmpty()) { 2013 addRecipient(); 2014 } 2015 return getRecipient().get(0); 2016 } 2017 2018 /** 2019 * @deprecated Use Reference#setResource(IBaseResource) instead 2020 */ 2021 @Deprecated 2022 public List<Resource> getRecipientTarget() { 2023 if (this.recipientTarget == null) 2024 this.recipientTarget = new ArrayList<Resource>(); 2025 return this.recipientTarget; 2026 } 2027 2028 /** 2029 * @return {@link #sender} (The entity (e.g. person, organization, clinical 2030 * information system, or device) which was the source of the 2031 * communication.) 2032 */ 2033 public Reference getSender() { 2034 if (this.sender == null) 2035 if (Configuration.errorOnAutoCreate()) 2036 throw new Error("Attempt to auto-create Communication.sender"); 2037 else if (Configuration.doAutoCreate()) 2038 this.sender = new Reference(); // cc 2039 return this.sender; 2040 } 2041 2042 public boolean hasSender() { 2043 return this.sender != null && !this.sender.isEmpty(); 2044 } 2045 2046 /** 2047 * @param value {@link #sender} (The entity (e.g. person, organization, clinical 2048 * information system, or device) which was the source of the 2049 * communication.) 2050 */ 2051 public Communication setSender(Reference value) { 2052 this.sender = value; 2053 return this; 2054 } 2055 2056 /** 2057 * @return {@link #sender} The actual object that is the target of the 2058 * reference. The reference library doesn't populate this, but you can 2059 * use it to hold the resource if you resolve it. (The entity (e.g. 2060 * person, organization, clinical information system, or device) which 2061 * was the source of the communication.) 2062 */ 2063 public Resource getSenderTarget() { 2064 return this.senderTarget; 2065 } 2066 2067 /** 2068 * @param value {@link #sender} The actual object that is the target of the 2069 * reference. The reference library doesn't use these, but you can 2070 * use it to hold the resource if you resolve it. (The entity (e.g. 2071 * person, organization, clinical information system, or device) 2072 * which was the source of the communication.) 2073 */ 2074 public Communication setSenderTarget(Resource value) { 2075 this.senderTarget = value; 2076 return this; 2077 } 2078 2079 /** 2080 * @return {@link #reasonCode} (The reason or justification for the 2081 * communication.) 2082 */ 2083 public List<CodeableConcept> getReasonCode() { 2084 if (this.reasonCode == null) 2085 this.reasonCode = new ArrayList<CodeableConcept>(); 2086 return this.reasonCode; 2087 } 2088 2089 /** 2090 * @return Returns a reference to <code>this</code> for easy method chaining 2091 */ 2092 public Communication setReasonCode(List<CodeableConcept> theReasonCode) { 2093 this.reasonCode = theReasonCode; 2094 return this; 2095 } 2096 2097 public boolean hasReasonCode() { 2098 if (this.reasonCode == null) 2099 return false; 2100 for (CodeableConcept item : this.reasonCode) 2101 if (!item.isEmpty()) 2102 return true; 2103 return false; 2104 } 2105 2106 public CodeableConcept addReasonCode() { // 3 2107 CodeableConcept t = new CodeableConcept(); 2108 if (this.reasonCode == null) 2109 this.reasonCode = new ArrayList<CodeableConcept>(); 2110 this.reasonCode.add(t); 2111 return t; 2112 } 2113 2114 public Communication addReasonCode(CodeableConcept t) { // 3 2115 if (t == null) 2116 return this; 2117 if (this.reasonCode == null) 2118 this.reasonCode = new ArrayList<CodeableConcept>(); 2119 this.reasonCode.add(t); 2120 return this; 2121 } 2122 2123 /** 2124 * @return The first repetition of repeating field {@link #reasonCode}, creating 2125 * it if it does not already exist 2126 */ 2127 public CodeableConcept getReasonCodeFirstRep() { 2128 if (getReasonCode().isEmpty()) { 2129 addReasonCode(); 2130 } 2131 return getReasonCode().get(0); 2132 } 2133 2134 /** 2135 * @return {@link #reasonReference} (Indicates another resource whose existence 2136 * justifies this communication.) 2137 */ 2138 public List<Reference> getReasonReference() { 2139 if (this.reasonReference == null) 2140 this.reasonReference = new ArrayList<Reference>(); 2141 return this.reasonReference; 2142 } 2143 2144 /** 2145 * @return Returns a reference to <code>this</code> for easy method chaining 2146 */ 2147 public Communication setReasonReference(List<Reference> theReasonReference) { 2148 this.reasonReference = theReasonReference; 2149 return this; 2150 } 2151 2152 public boolean hasReasonReference() { 2153 if (this.reasonReference == null) 2154 return false; 2155 for (Reference item : this.reasonReference) 2156 if (!item.isEmpty()) 2157 return true; 2158 return false; 2159 } 2160 2161 public Reference addReasonReference() { // 3 2162 Reference t = new Reference(); 2163 if (this.reasonReference == null) 2164 this.reasonReference = new ArrayList<Reference>(); 2165 this.reasonReference.add(t); 2166 return t; 2167 } 2168 2169 public Communication addReasonReference(Reference t) { // 3 2170 if (t == null) 2171 return this; 2172 if (this.reasonReference == null) 2173 this.reasonReference = new ArrayList<Reference>(); 2174 this.reasonReference.add(t); 2175 return this; 2176 } 2177 2178 /** 2179 * @return The first repetition of repeating field {@link #reasonReference}, 2180 * creating it if it does not already exist 2181 */ 2182 public Reference getReasonReferenceFirstRep() { 2183 if (getReasonReference().isEmpty()) { 2184 addReasonReference(); 2185 } 2186 return getReasonReference().get(0); 2187 } 2188 2189 /** 2190 * @deprecated Use Reference#setResource(IBaseResource) instead 2191 */ 2192 @Deprecated 2193 public List<Resource> getReasonReferenceTarget() { 2194 if (this.reasonReferenceTarget == null) 2195 this.reasonReferenceTarget = new ArrayList<Resource>(); 2196 return this.reasonReferenceTarget; 2197 } 2198 2199 /** 2200 * @return {@link #payload} (Text, attachment(s), or resource(s) that was 2201 * communicated to the recipient.) 2202 */ 2203 public List<CommunicationPayloadComponent> getPayload() { 2204 if (this.payload == null) 2205 this.payload = new ArrayList<CommunicationPayloadComponent>(); 2206 return this.payload; 2207 } 2208 2209 /** 2210 * @return Returns a reference to <code>this</code> for easy method chaining 2211 */ 2212 public Communication setPayload(List<CommunicationPayloadComponent> thePayload) { 2213 this.payload = thePayload; 2214 return this; 2215 } 2216 2217 public boolean hasPayload() { 2218 if (this.payload == null) 2219 return false; 2220 for (CommunicationPayloadComponent item : this.payload) 2221 if (!item.isEmpty()) 2222 return true; 2223 return false; 2224 } 2225 2226 public CommunicationPayloadComponent addPayload() { // 3 2227 CommunicationPayloadComponent t = new CommunicationPayloadComponent(); 2228 if (this.payload == null) 2229 this.payload = new ArrayList<CommunicationPayloadComponent>(); 2230 this.payload.add(t); 2231 return t; 2232 } 2233 2234 public Communication addPayload(CommunicationPayloadComponent t) { // 3 2235 if (t == null) 2236 return this; 2237 if (this.payload == null) 2238 this.payload = new ArrayList<CommunicationPayloadComponent>(); 2239 this.payload.add(t); 2240 return this; 2241 } 2242 2243 /** 2244 * @return The first repetition of repeating field {@link #payload}, creating it 2245 * if it does not already exist 2246 */ 2247 public CommunicationPayloadComponent getPayloadFirstRep() { 2248 if (getPayload().isEmpty()) { 2249 addPayload(); 2250 } 2251 return getPayload().get(0); 2252 } 2253 2254 /** 2255 * @return {@link #note} (Additional notes or commentary about the communication 2256 * by the sender, receiver or other interested parties.) 2257 */ 2258 public List<Annotation> getNote() { 2259 if (this.note == null) 2260 this.note = new ArrayList<Annotation>(); 2261 return this.note; 2262 } 2263 2264 /** 2265 * @return Returns a reference to <code>this</code> for easy method chaining 2266 */ 2267 public Communication setNote(List<Annotation> theNote) { 2268 this.note = theNote; 2269 return this; 2270 } 2271 2272 public boolean hasNote() { 2273 if (this.note == null) 2274 return false; 2275 for (Annotation item : this.note) 2276 if (!item.isEmpty()) 2277 return true; 2278 return false; 2279 } 2280 2281 public Annotation addNote() { // 3 2282 Annotation t = new Annotation(); 2283 if (this.note == null) 2284 this.note = new ArrayList<Annotation>(); 2285 this.note.add(t); 2286 return t; 2287 } 2288 2289 public Communication addNote(Annotation t) { // 3 2290 if (t == null) 2291 return this; 2292 if (this.note == null) 2293 this.note = new ArrayList<Annotation>(); 2294 this.note.add(t); 2295 return this; 2296 } 2297 2298 /** 2299 * @return The first repetition of repeating field {@link #note}, creating it if 2300 * it does not already exist 2301 */ 2302 public Annotation getNoteFirstRep() { 2303 if (getNote().isEmpty()) { 2304 addNote(); 2305 } 2306 return getNote().get(0); 2307 } 2308 2309 protected void listChildren(List<Property> children) { 2310 super.listChildren(children); 2311 children.add(new Property("identifier", "Identifier", 2312 "Business identifiers assigned to this communication by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 2313 0, java.lang.Integer.MAX_VALUE, identifier)); 2314 children.add(new Property("instantiatesCanonical", 2315 "canonical(PlanDefinition|ActivityDefinition|Measure|OperationDefinition|Questionnaire)", 2316 "The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Communication.", 2317 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical)); 2318 children.add(new Property("instantiatesUri", "uri", 2319 "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Communication.", 2320 0, java.lang.Integer.MAX_VALUE, instantiatesUri)); 2321 children.add(new Property("basedOn", "Reference(Any)", 2322 "An order, proposal or plan fulfilled in whole or in part by this Communication.", 0, 2323 java.lang.Integer.MAX_VALUE, basedOn)); 2324 children 2325 .add(new Property("partOf", "Reference(Any)", "Part of this action.", 0, java.lang.Integer.MAX_VALUE, partOf)); 2326 children.add(new Property("inResponseTo", "Reference(Communication)", 2327 "Prior communication that this communication is in response to.", 0, java.lang.Integer.MAX_VALUE, 2328 inResponseTo)); 2329 children.add(new Property("status", "code", "The status of the transmission.", 0, 1, status)); 2330 children.add(new Property("statusReason", "CodeableConcept", 2331 "Captures the reason for the current state of the Communication.", 0, 1, statusReason)); 2332 children.add(new Property("category", "CodeableConcept", 2333 "The type of message conveyed such as alert, notification, reminder, instruction, etc.", 0, 2334 java.lang.Integer.MAX_VALUE, category)); 2335 children.add(new Property("priority", "code", 2336 "Characterizes how quickly the planned or in progress communication must be addressed. Includes concepts such as stat, urgent, routine.", 2337 0, 1, priority)); 2338 children.add(new Property("medium", "CodeableConcept", 2339 "A channel that was used for this communication (e.g. email, fax).", 0, java.lang.Integer.MAX_VALUE, medium)); 2340 children.add(new Property("subject", "Reference(Patient|Group)", 2341 "The patient or group that was the focus of this communication.", 0, 1, subject)); 2342 children.add(new Property("topic", "CodeableConcept", 2343 "Description of the purpose/content, similar to a subject line in an email.", 0, 1, topic)); 2344 children.add(new Property("about", "Reference(Any)", 2345 "Other resources that pertain to this communication and to which this communication should be associated.", 0, 2346 java.lang.Integer.MAX_VALUE, about)); 2347 children.add(new Property("encounter", "Reference(Encounter)", 2348 "The Encounter during which this Communication was created or to which the creation of this record is tightly associated.", 2349 0, 1, encounter)); 2350 children.add(new Property("sent", "dateTime", "The time when this communication was sent.", 0, 1, sent)); 2351 children.add(new Property("received", "dateTime", "The time when this communication arrived at the destination.", 0, 2352 1, received)); 2353 children.add(new Property("recipient", 2354 "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson|Group|CareTeam|HealthcareService)", 2355 "The entity (e.g. person, organization, clinical information system, care team or device) which was the target of the communication. If receipts need to be tracked by an individual, a separate resource instance will need to be created for each recipient. Multiple recipient communications are intended where either receipts are not tracked (e.g. a mass mail-out) or a receipt is captured in aggregate (all emails confirmed received by a particular time).", 2356 0, java.lang.Integer.MAX_VALUE, recipient)); 2357 children.add(new Property("sender", 2358 "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson|HealthcareService)", 2359 "The entity (e.g. person, organization, clinical information system, or device) which was the source of the communication.", 2360 0, 1, sender)); 2361 children.add(new Property("reasonCode", "CodeableConcept", "The reason or justification for the communication.", 0, 2362 java.lang.Integer.MAX_VALUE, reasonCode)); 2363 children.add(new Property("reasonReference", "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 2364 "Indicates another resource whose existence justifies this communication.", 0, java.lang.Integer.MAX_VALUE, 2365 reasonReference)); 2366 children 2367 .add(new Property("payload", "", "Text, attachment(s), or resource(s) that was communicated to the recipient.", 2368 0, java.lang.Integer.MAX_VALUE, payload)); 2369 children.add(new Property("note", "Annotation", 2370 "Additional notes or commentary about the communication by the sender, receiver or other interested parties.", 2371 0, java.lang.Integer.MAX_VALUE, note)); 2372 } 2373 2374 @Override 2375 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2376 switch (_hash) { 2377 case -1618432855: 2378 /* identifier */ return new Property("identifier", "Identifier", 2379 "Business identifiers assigned to this communication by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 2380 0, java.lang.Integer.MAX_VALUE, identifier); 2381 case 8911915: 2382 /* instantiatesCanonical */ return new Property("instantiatesCanonical", 2383 "canonical(PlanDefinition|ActivityDefinition|Measure|OperationDefinition|Questionnaire)", 2384 "The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Communication.", 2385 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical); 2386 case -1926393373: 2387 /* instantiatesUri */ return new Property("instantiatesUri", "uri", 2388 "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Communication.", 2389 0, java.lang.Integer.MAX_VALUE, instantiatesUri); 2390 case -332612366: 2391 /* basedOn */ return new Property("basedOn", "Reference(Any)", 2392 "An order, proposal or plan fulfilled in whole or in part by this Communication.", 0, 2393 java.lang.Integer.MAX_VALUE, basedOn); 2394 case -995410646: 2395 /* partOf */ return new Property("partOf", "Reference(Any)", "Part of this action.", 0, 2396 java.lang.Integer.MAX_VALUE, partOf); 2397 case 1932956065: 2398 /* inResponseTo */ return new Property("inResponseTo", "Reference(Communication)", 2399 "Prior communication that this communication is in response to.", 0, java.lang.Integer.MAX_VALUE, 2400 inResponseTo); 2401 case -892481550: 2402 /* status */ return new Property("status", "code", "The status of the transmission.", 0, 1, status); 2403 case 2051346646: 2404 /* statusReason */ return new Property("statusReason", "CodeableConcept", 2405 "Captures the reason for the current state of the Communication.", 0, 1, statusReason); 2406 case 50511102: 2407 /* category */ return new Property("category", "CodeableConcept", 2408 "The type of message conveyed such as alert, notification, reminder, instruction, etc.", 0, 2409 java.lang.Integer.MAX_VALUE, category); 2410 case -1165461084: 2411 /* priority */ return new Property("priority", "code", 2412 "Characterizes how quickly the planned or in progress communication must be addressed. Includes concepts such as stat, urgent, routine.", 2413 0, 1, priority); 2414 case -1078030475: 2415 /* medium */ return new Property("medium", "CodeableConcept", 2416 "A channel that was used for this communication (e.g. email, fax).", 0, java.lang.Integer.MAX_VALUE, medium); 2417 case -1867885268: 2418 /* subject */ return new Property("subject", "Reference(Patient|Group)", 2419 "The patient or group that was the focus of this communication.", 0, 1, subject); 2420 case 110546223: 2421 /* topic */ return new Property("topic", "CodeableConcept", 2422 "Description of the purpose/content, similar to a subject line in an email.", 0, 1, topic); 2423 case 92611469: 2424 /* about */ return new Property("about", "Reference(Any)", 2425 "Other resources that pertain to this communication and to which this communication should be associated.", 0, 2426 java.lang.Integer.MAX_VALUE, about); 2427 case 1524132147: 2428 /* encounter */ return new Property("encounter", "Reference(Encounter)", 2429 "The Encounter during which this Communication was created or to which the creation of this record is tightly associated.", 2430 0, 1, encounter); 2431 case 3526552: 2432 /* sent */ return new Property("sent", "dateTime", "The time when this communication was sent.", 0, 1, sent); 2433 case -808719903: 2434 /* received */ return new Property("received", "dateTime", 2435 "The time when this communication arrived at the destination.", 0, 1, received); 2436 case 820081177: 2437 /* recipient */ return new Property("recipient", 2438 "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson|Group|CareTeam|HealthcareService)", 2439 "The entity (e.g. person, organization, clinical information system, care team or device) which was the target of the communication. If receipts need to be tracked by an individual, a separate resource instance will need to be created for each recipient. Multiple recipient communications are intended where either receipts are not tracked (e.g. a mass mail-out) or a receipt is captured in aggregate (all emails confirmed received by a particular time).", 2440 0, java.lang.Integer.MAX_VALUE, recipient); 2441 case -905962955: 2442 /* sender */ return new Property("sender", 2443 "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson|HealthcareService)", 2444 "The entity (e.g. person, organization, clinical information system, or device) which was the source of the communication.", 2445 0, 1, sender); 2446 case 722137681: 2447 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 2448 "The reason or justification for the communication.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 2449 case -1146218137: 2450 /* reasonReference */ return new Property("reasonReference", 2451 "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 2452 "Indicates another resource whose existence justifies this communication.", 0, java.lang.Integer.MAX_VALUE, 2453 reasonReference); 2454 case -786701938: 2455 /* payload */ return new Property("payload", "", 2456 "Text, attachment(s), or resource(s) that was communicated to the recipient.", 0, java.lang.Integer.MAX_VALUE, 2457 payload); 2458 case 3387378: 2459 /* note */ return new Property("note", "Annotation", 2460 "Additional notes or commentary about the communication by the sender, receiver or other interested parties.", 2461 0, java.lang.Integer.MAX_VALUE, note); 2462 default: 2463 return super.getNamedProperty(_hash, _name, _checkValid); 2464 } 2465 2466 } 2467 2468 @Override 2469 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2470 switch (hash) { 2471 case -1618432855: 2472 /* identifier */ return this.identifier == null ? new Base[0] 2473 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2474 case 8911915: 2475 /* instantiatesCanonical */ return this.instantiatesCanonical == null ? new Base[0] 2476 : this.instantiatesCanonical.toArray(new Base[this.instantiatesCanonical.size()]); // CanonicalType 2477 case -1926393373: 2478 /* instantiatesUri */ return this.instantiatesUri == null ? new Base[0] 2479 : this.instantiatesUri.toArray(new Base[this.instantiatesUri.size()]); // UriType 2480 case -332612366: 2481 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2482 case -995410646: 2483 /* partOf */ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 2484 case 1932956065: 2485 /* inResponseTo */ return this.inResponseTo == null ? new Base[0] 2486 : this.inResponseTo.toArray(new Base[this.inResponseTo.size()]); // Reference 2487 case -892481550: 2488 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<CommunicationStatus> 2489 case 2051346646: 2490 /* statusReason */ return this.statusReason == null ? new Base[0] : new Base[] { this.statusReason }; // CodeableConcept 2491 case 50511102: 2492 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2493 case -1165461084: 2494 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // Enumeration<CommunicationPriority> 2495 case -1078030475: 2496 /* medium */ return this.medium == null ? new Base[0] : this.medium.toArray(new Base[this.medium.size()]); // CodeableConcept 2497 case -1867885268: 2498 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2499 case 110546223: 2500 /* topic */ return this.topic == null ? new Base[0] : new Base[] { this.topic }; // CodeableConcept 2501 case 92611469: 2502 /* about */ return this.about == null ? new Base[0] : this.about.toArray(new Base[this.about.size()]); // Reference 2503 case 1524132147: 2504 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 2505 case 3526552: 2506 /* sent */ return this.sent == null ? new Base[0] : new Base[] { this.sent }; // DateTimeType 2507 case -808719903: 2508 /* received */ return this.received == null ? new Base[0] : new Base[] { this.received }; // DateTimeType 2509 case 820081177: 2510 /* recipient */ return this.recipient == null ? new Base[0] 2511 : this.recipient.toArray(new Base[this.recipient.size()]); // Reference 2512 case -905962955: 2513 /* sender */ return this.sender == null ? new Base[0] : new Base[] { this.sender }; // Reference 2514 case 722137681: 2515 /* reasonCode */ return this.reasonCode == null ? new Base[0] 2516 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 2517 case -1146218137: 2518 /* reasonReference */ return this.reasonReference == null ? new Base[0] 2519 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 2520 case -786701938: 2521 /* payload */ return this.payload == null ? new Base[0] : this.payload.toArray(new Base[this.payload.size()]); // CommunicationPayloadComponent 2522 case 3387378: 2523 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2524 default: 2525 return super.getProperty(hash, name, checkValid); 2526 } 2527 2528 } 2529 2530 @Override 2531 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2532 switch (hash) { 2533 case -1618432855: // identifier 2534 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2535 return value; 2536 case 8911915: // instantiatesCanonical 2537 this.getInstantiatesCanonical().add(castToCanonical(value)); // CanonicalType 2538 return value; 2539 case -1926393373: // instantiatesUri 2540 this.getInstantiatesUri().add(castToUri(value)); // UriType 2541 return value; 2542 case -332612366: // basedOn 2543 this.getBasedOn().add(castToReference(value)); // Reference 2544 return value; 2545 case -995410646: // partOf 2546 this.getPartOf().add(castToReference(value)); // Reference 2547 return value; 2548 case 1932956065: // inResponseTo 2549 this.getInResponseTo().add(castToReference(value)); // Reference 2550 return value; 2551 case -892481550: // status 2552 value = new CommunicationStatusEnumFactory().fromType(castToCode(value)); 2553 this.status = (Enumeration) value; // Enumeration<CommunicationStatus> 2554 return value; 2555 case 2051346646: // statusReason 2556 this.statusReason = castToCodeableConcept(value); // CodeableConcept 2557 return value; 2558 case 50511102: // category 2559 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 2560 return value; 2561 case -1165461084: // priority 2562 value = new CommunicationPriorityEnumFactory().fromType(castToCode(value)); 2563 this.priority = (Enumeration) value; // Enumeration<CommunicationPriority> 2564 return value; 2565 case -1078030475: // medium 2566 this.getMedium().add(castToCodeableConcept(value)); // CodeableConcept 2567 return value; 2568 case -1867885268: // subject 2569 this.subject = castToReference(value); // Reference 2570 return value; 2571 case 110546223: // topic 2572 this.topic = castToCodeableConcept(value); // CodeableConcept 2573 return value; 2574 case 92611469: // about 2575 this.getAbout().add(castToReference(value)); // Reference 2576 return value; 2577 case 1524132147: // encounter 2578 this.encounter = castToReference(value); // Reference 2579 return value; 2580 case 3526552: // sent 2581 this.sent = castToDateTime(value); // DateTimeType 2582 return value; 2583 case -808719903: // received 2584 this.received = castToDateTime(value); // DateTimeType 2585 return value; 2586 case 820081177: // recipient 2587 this.getRecipient().add(castToReference(value)); // Reference 2588 return value; 2589 case -905962955: // sender 2590 this.sender = castToReference(value); // Reference 2591 return value; 2592 case 722137681: // reasonCode 2593 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 2594 return value; 2595 case -1146218137: // reasonReference 2596 this.getReasonReference().add(castToReference(value)); // Reference 2597 return value; 2598 case -786701938: // payload 2599 this.getPayload().add((CommunicationPayloadComponent) value); // CommunicationPayloadComponent 2600 return value; 2601 case 3387378: // note 2602 this.getNote().add(castToAnnotation(value)); // Annotation 2603 return value; 2604 default: 2605 return super.setProperty(hash, name, value); 2606 } 2607 2608 } 2609 2610 @Override 2611 public Base setProperty(String name, Base value) throws FHIRException { 2612 if (name.equals("identifier")) { 2613 this.getIdentifier().add(castToIdentifier(value)); 2614 } else if (name.equals("instantiatesCanonical")) { 2615 this.getInstantiatesCanonical().add(castToCanonical(value)); 2616 } else if (name.equals("instantiatesUri")) { 2617 this.getInstantiatesUri().add(castToUri(value)); 2618 } else if (name.equals("basedOn")) { 2619 this.getBasedOn().add(castToReference(value)); 2620 } else if (name.equals("partOf")) { 2621 this.getPartOf().add(castToReference(value)); 2622 } else if (name.equals("inResponseTo")) { 2623 this.getInResponseTo().add(castToReference(value)); 2624 } else if (name.equals("status")) { 2625 value = new CommunicationStatusEnumFactory().fromType(castToCode(value)); 2626 this.status = (Enumeration) value; // Enumeration<CommunicationStatus> 2627 } else if (name.equals("statusReason")) { 2628 this.statusReason = castToCodeableConcept(value); // CodeableConcept 2629 } else if (name.equals("category")) { 2630 this.getCategory().add(castToCodeableConcept(value)); 2631 } else if (name.equals("priority")) { 2632 value = new CommunicationPriorityEnumFactory().fromType(castToCode(value)); 2633 this.priority = (Enumeration) value; // Enumeration<CommunicationPriority> 2634 } else if (name.equals("medium")) { 2635 this.getMedium().add(castToCodeableConcept(value)); 2636 } else if (name.equals("subject")) { 2637 this.subject = castToReference(value); // Reference 2638 } else if (name.equals("topic")) { 2639 this.topic = castToCodeableConcept(value); // CodeableConcept 2640 } else if (name.equals("about")) { 2641 this.getAbout().add(castToReference(value)); 2642 } else if (name.equals("encounter")) { 2643 this.encounter = castToReference(value); // Reference 2644 } else if (name.equals("sent")) { 2645 this.sent = castToDateTime(value); // DateTimeType 2646 } else if (name.equals("received")) { 2647 this.received = castToDateTime(value); // DateTimeType 2648 } else if (name.equals("recipient")) { 2649 this.getRecipient().add(castToReference(value)); 2650 } else if (name.equals("sender")) { 2651 this.sender = castToReference(value); // Reference 2652 } else if (name.equals("reasonCode")) { 2653 this.getReasonCode().add(castToCodeableConcept(value)); 2654 } else if (name.equals("reasonReference")) { 2655 this.getReasonReference().add(castToReference(value)); 2656 } else if (name.equals("payload")) { 2657 this.getPayload().add((CommunicationPayloadComponent) value); 2658 } else if (name.equals("note")) { 2659 this.getNote().add(castToAnnotation(value)); 2660 } else 2661 return super.setProperty(name, value); 2662 return value; 2663 } 2664 2665 @Override 2666 public void removeChild(String name, Base value) throws FHIRException { 2667 if (name.equals("identifier")) { 2668 this.getIdentifier().remove(castToIdentifier(value)); 2669 } else if (name.equals("instantiatesCanonical")) { 2670 this.getInstantiatesCanonical().remove(castToCanonical(value)); 2671 } else if (name.equals("instantiatesUri")) { 2672 this.getInstantiatesUri().remove(castToUri(value)); 2673 } else if (name.equals("basedOn")) { 2674 this.getBasedOn().remove(castToReference(value)); 2675 } else if (name.equals("partOf")) { 2676 this.getPartOf().remove(castToReference(value)); 2677 } else if (name.equals("inResponseTo")) { 2678 this.getInResponseTo().remove(castToReference(value)); 2679 } else if (name.equals("status")) { 2680 this.status = null; 2681 } else if (name.equals("statusReason")) { 2682 this.statusReason = null; 2683 } else if (name.equals("category")) { 2684 this.getCategory().remove(castToCodeableConcept(value)); 2685 } else if (name.equals("priority")) { 2686 this.priority = null; 2687 } else if (name.equals("medium")) { 2688 this.getMedium().remove(castToCodeableConcept(value)); 2689 } else if (name.equals("subject")) { 2690 this.subject = null; 2691 } else if (name.equals("topic")) { 2692 this.topic = null; 2693 } else if (name.equals("about")) { 2694 this.getAbout().remove(castToReference(value)); 2695 } else if (name.equals("encounter")) { 2696 this.encounter = null; 2697 } else if (name.equals("sent")) { 2698 this.sent = null; 2699 } else if (name.equals("received")) { 2700 this.received = null; 2701 } else if (name.equals("recipient")) { 2702 this.getRecipient().remove(castToReference(value)); 2703 } else if (name.equals("sender")) { 2704 this.sender = null; 2705 } else if (name.equals("reasonCode")) { 2706 this.getReasonCode().remove(castToCodeableConcept(value)); 2707 } else if (name.equals("reasonReference")) { 2708 this.getReasonReference().remove(castToReference(value)); 2709 } else if (name.equals("payload")) { 2710 this.getPayload().remove((CommunicationPayloadComponent) value); 2711 } else if (name.equals("note")) { 2712 this.getNote().remove(castToAnnotation(value)); 2713 } else 2714 super.removeChild(name, value); 2715 2716 } 2717 2718 @Override 2719 public Base makeProperty(int hash, String name) throws FHIRException { 2720 switch (hash) { 2721 case -1618432855: 2722 return addIdentifier(); 2723 case 8911915: 2724 return addInstantiatesCanonicalElement(); 2725 case -1926393373: 2726 return addInstantiatesUriElement(); 2727 case -332612366: 2728 return addBasedOn(); 2729 case -995410646: 2730 return addPartOf(); 2731 case 1932956065: 2732 return addInResponseTo(); 2733 case -892481550: 2734 return getStatusElement(); 2735 case 2051346646: 2736 return getStatusReason(); 2737 case 50511102: 2738 return addCategory(); 2739 case -1165461084: 2740 return getPriorityElement(); 2741 case -1078030475: 2742 return addMedium(); 2743 case -1867885268: 2744 return getSubject(); 2745 case 110546223: 2746 return getTopic(); 2747 case 92611469: 2748 return addAbout(); 2749 case 1524132147: 2750 return getEncounter(); 2751 case 3526552: 2752 return getSentElement(); 2753 case -808719903: 2754 return getReceivedElement(); 2755 case 820081177: 2756 return addRecipient(); 2757 case -905962955: 2758 return getSender(); 2759 case 722137681: 2760 return addReasonCode(); 2761 case -1146218137: 2762 return addReasonReference(); 2763 case -786701938: 2764 return addPayload(); 2765 case 3387378: 2766 return addNote(); 2767 default: 2768 return super.makeProperty(hash, name); 2769 } 2770 2771 } 2772 2773 @Override 2774 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2775 switch (hash) { 2776 case -1618432855: 2777 /* identifier */ return new String[] { "Identifier" }; 2778 case 8911915: 2779 /* instantiatesCanonical */ return new String[] { "canonical" }; 2780 case -1926393373: 2781 /* instantiatesUri */ return new String[] { "uri" }; 2782 case -332612366: 2783 /* basedOn */ return new String[] { "Reference" }; 2784 case -995410646: 2785 /* partOf */ return new String[] { "Reference" }; 2786 case 1932956065: 2787 /* inResponseTo */ return new String[] { "Reference" }; 2788 case -892481550: 2789 /* status */ return new String[] { "code" }; 2790 case 2051346646: 2791 /* statusReason */ return new String[] { "CodeableConcept" }; 2792 case 50511102: 2793 /* category */ return new String[] { "CodeableConcept" }; 2794 case -1165461084: 2795 /* priority */ return new String[] { "code" }; 2796 case -1078030475: 2797 /* medium */ return new String[] { "CodeableConcept" }; 2798 case -1867885268: 2799 /* subject */ return new String[] { "Reference" }; 2800 case 110546223: 2801 /* topic */ return new String[] { "CodeableConcept" }; 2802 case 92611469: 2803 /* about */ return new String[] { "Reference" }; 2804 case 1524132147: 2805 /* encounter */ return new String[] { "Reference" }; 2806 case 3526552: 2807 /* sent */ return new String[] { "dateTime" }; 2808 case -808719903: 2809 /* received */ return new String[] { "dateTime" }; 2810 case 820081177: 2811 /* recipient */ return new String[] { "Reference" }; 2812 case -905962955: 2813 /* sender */ return new String[] { "Reference" }; 2814 case 722137681: 2815 /* reasonCode */ return new String[] { "CodeableConcept" }; 2816 case -1146218137: 2817 /* reasonReference */ return new String[] { "Reference" }; 2818 case -786701938: 2819 /* payload */ return new String[] {}; 2820 case 3387378: 2821 /* note */ return new String[] { "Annotation" }; 2822 default: 2823 return super.getTypesForProperty(hash, name); 2824 } 2825 2826 } 2827 2828 @Override 2829 public Base addChild(String name) throws FHIRException { 2830 if (name.equals("identifier")) { 2831 return addIdentifier(); 2832 } else if (name.equals("instantiatesCanonical")) { 2833 throw new FHIRException("Cannot call addChild on a singleton property Communication.instantiatesCanonical"); 2834 } else if (name.equals("instantiatesUri")) { 2835 throw new FHIRException("Cannot call addChild on a singleton property Communication.instantiatesUri"); 2836 } else if (name.equals("basedOn")) { 2837 return addBasedOn(); 2838 } else if (name.equals("partOf")) { 2839 return addPartOf(); 2840 } else if (name.equals("inResponseTo")) { 2841 return addInResponseTo(); 2842 } else if (name.equals("status")) { 2843 throw new FHIRException("Cannot call addChild on a singleton property Communication.status"); 2844 } else if (name.equals("statusReason")) { 2845 this.statusReason = new CodeableConcept(); 2846 return this.statusReason; 2847 } else if (name.equals("category")) { 2848 return addCategory(); 2849 } else if (name.equals("priority")) { 2850 throw new FHIRException("Cannot call addChild on a singleton property Communication.priority"); 2851 } else if (name.equals("medium")) { 2852 return addMedium(); 2853 } else if (name.equals("subject")) { 2854 this.subject = new Reference(); 2855 return this.subject; 2856 } else if (name.equals("topic")) { 2857 this.topic = new CodeableConcept(); 2858 return this.topic; 2859 } else if (name.equals("about")) { 2860 return addAbout(); 2861 } else if (name.equals("encounter")) { 2862 this.encounter = new Reference(); 2863 return this.encounter; 2864 } else if (name.equals("sent")) { 2865 throw new FHIRException("Cannot call addChild on a singleton property Communication.sent"); 2866 } else if (name.equals("received")) { 2867 throw new FHIRException("Cannot call addChild on a singleton property Communication.received"); 2868 } else if (name.equals("recipient")) { 2869 return addRecipient(); 2870 } else if (name.equals("sender")) { 2871 this.sender = new Reference(); 2872 return this.sender; 2873 } else if (name.equals("reasonCode")) { 2874 return addReasonCode(); 2875 } else if (name.equals("reasonReference")) { 2876 return addReasonReference(); 2877 } else if (name.equals("payload")) { 2878 return addPayload(); 2879 } else if (name.equals("note")) { 2880 return addNote(); 2881 } else 2882 return super.addChild(name); 2883 } 2884 2885 public String fhirType() { 2886 return "Communication"; 2887 2888 } 2889 2890 public Communication copy() { 2891 Communication dst = new Communication(); 2892 copyValues(dst); 2893 return dst; 2894 } 2895 2896 public void copyValues(Communication dst) { 2897 super.copyValues(dst); 2898 if (identifier != null) { 2899 dst.identifier = new ArrayList<Identifier>(); 2900 for (Identifier i : identifier) 2901 dst.identifier.add(i.copy()); 2902 } 2903 ; 2904 if (instantiatesCanonical != null) { 2905 dst.instantiatesCanonical = new ArrayList<CanonicalType>(); 2906 for (CanonicalType i : instantiatesCanonical) 2907 dst.instantiatesCanonical.add(i.copy()); 2908 } 2909 ; 2910 if (instantiatesUri != null) { 2911 dst.instantiatesUri = new ArrayList<UriType>(); 2912 for (UriType i : instantiatesUri) 2913 dst.instantiatesUri.add(i.copy()); 2914 } 2915 ; 2916 if (basedOn != null) { 2917 dst.basedOn = new ArrayList<Reference>(); 2918 for (Reference i : basedOn) 2919 dst.basedOn.add(i.copy()); 2920 } 2921 ; 2922 if (partOf != null) { 2923 dst.partOf = new ArrayList<Reference>(); 2924 for (Reference i : partOf) 2925 dst.partOf.add(i.copy()); 2926 } 2927 ; 2928 if (inResponseTo != null) { 2929 dst.inResponseTo = new ArrayList<Reference>(); 2930 for (Reference i : inResponseTo) 2931 dst.inResponseTo.add(i.copy()); 2932 } 2933 ; 2934 dst.status = status == null ? null : status.copy(); 2935 dst.statusReason = statusReason == null ? null : statusReason.copy(); 2936 if (category != null) { 2937 dst.category = new ArrayList<CodeableConcept>(); 2938 for (CodeableConcept i : category) 2939 dst.category.add(i.copy()); 2940 } 2941 ; 2942 dst.priority = priority == null ? null : priority.copy(); 2943 if (medium != null) { 2944 dst.medium = new ArrayList<CodeableConcept>(); 2945 for (CodeableConcept i : medium) 2946 dst.medium.add(i.copy()); 2947 } 2948 ; 2949 dst.subject = subject == null ? null : subject.copy(); 2950 dst.topic = topic == null ? null : topic.copy(); 2951 if (about != null) { 2952 dst.about = new ArrayList<Reference>(); 2953 for (Reference i : about) 2954 dst.about.add(i.copy()); 2955 } 2956 ; 2957 dst.encounter = encounter == null ? null : encounter.copy(); 2958 dst.sent = sent == null ? null : sent.copy(); 2959 dst.received = received == null ? null : received.copy(); 2960 if (recipient != null) { 2961 dst.recipient = new ArrayList<Reference>(); 2962 for (Reference i : recipient) 2963 dst.recipient.add(i.copy()); 2964 } 2965 ; 2966 dst.sender = sender == null ? null : sender.copy(); 2967 if (reasonCode != null) { 2968 dst.reasonCode = new ArrayList<CodeableConcept>(); 2969 for (CodeableConcept i : reasonCode) 2970 dst.reasonCode.add(i.copy()); 2971 } 2972 ; 2973 if (reasonReference != null) { 2974 dst.reasonReference = new ArrayList<Reference>(); 2975 for (Reference i : reasonReference) 2976 dst.reasonReference.add(i.copy()); 2977 } 2978 ; 2979 if (payload != null) { 2980 dst.payload = new ArrayList<CommunicationPayloadComponent>(); 2981 for (CommunicationPayloadComponent i : payload) 2982 dst.payload.add(i.copy()); 2983 } 2984 ; 2985 if (note != null) { 2986 dst.note = new ArrayList<Annotation>(); 2987 for (Annotation i : note) 2988 dst.note.add(i.copy()); 2989 } 2990 ; 2991 } 2992 2993 protected Communication typedCopy() { 2994 return copy(); 2995 } 2996 2997 @Override 2998 public boolean equalsDeep(Base other_) { 2999 if (!super.equalsDeep(other_)) 3000 return false; 3001 if (!(other_ instanceof Communication)) 3002 return false; 3003 Communication o = (Communication) other_; 3004 return compareDeep(identifier, o.identifier, true) 3005 && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 3006 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(basedOn, o.basedOn, true) 3007 && compareDeep(partOf, o.partOf, true) && compareDeep(inResponseTo, o.inResponseTo, true) 3008 && compareDeep(status, o.status, true) && compareDeep(statusReason, o.statusReason, true) 3009 && compareDeep(category, o.category, true) && compareDeep(priority, o.priority, true) 3010 && compareDeep(medium, o.medium, true) && compareDeep(subject, o.subject, true) 3011 && compareDeep(topic, o.topic, true) && compareDeep(about, o.about, true) 3012 && compareDeep(encounter, o.encounter, true) && compareDeep(sent, o.sent, true) 3013 && compareDeep(received, o.received, true) && compareDeep(recipient, o.recipient, true) 3014 && compareDeep(sender, o.sender, true) && compareDeep(reasonCode, o.reasonCode, true) 3015 && compareDeep(reasonReference, o.reasonReference, true) && compareDeep(payload, o.payload, true) 3016 && compareDeep(note, o.note, true); 3017 } 3018 3019 @Override 3020 public boolean equalsShallow(Base other_) { 3021 if (!super.equalsShallow(other_)) 3022 return false; 3023 if (!(other_ instanceof Communication)) 3024 return false; 3025 Communication o = (Communication) other_; 3026 return compareValues(instantiatesUri, o.instantiatesUri, true) && compareValues(status, o.status, true) 3027 && compareValues(priority, o.priority, true) && compareValues(sent, o.sent, true) 3028 && compareValues(received, o.received, true); 3029 } 3030 3031 public boolean isEmpty() { 3032 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiatesCanonical, instantiatesUri, 3033 basedOn, partOf, inResponseTo, status, statusReason, category, priority, medium, subject, topic, about, 3034 encounter, sent, received, recipient, sender, reasonCode, reasonReference, payload, note); 3035 } 3036 3037 @Override 3038 public ResourceType getResourceType() { 3039 return ResourceType.Communication; 3040 } 3041 3042 /** 3043 * Search parameter: <b>identifier</b> 3044 * <p> 3045 * Description: <b>Unique identifier</b><br> 3046 * Type: <b>token</b><br> 3047 * Path: <b>Communication.identifier</b><br> 3048 * </p> 3049 */ 3050 @SearchParamDefinition(name = "identifier", path = "Communication.identifier", description = "Unique identifier", type = "token") 3051 public static final String SP_IDENTIFIER = "identifier"; 3052 /** 3053 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3054 * <p> 3055 * Description: <b>Unique identifier</b><br> 3056 * Type: <b>token</b><br> 3057 * Path: <b>Communication.identifier</b><br> 3058 * </p> 3059 */ 3060 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3061 SP_IDENTIFIER); 3062 3063 /** 3064 * Search parameter: <b>subject</b> 3065 * <p> 3066 * Description: <b>Focus of message</b><br> 3067 * Type: <b>reference</b><br> 3068 * Path: <b>Communication.subject</b><br> 3069 * </p> 3070 */ 3071 @SearchParamDefinition(name = "subject", path = "Communication.subject", description = "Focus of message", type = "reference", providesMembershipIn = { 3072 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Group.class, Patient.class }) 3073 public static final String SP_SUBJECT = "subject"; 3074 /** 3075 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3076 * <p> 3077 * Description: <b>Focus of message</b><br> 3078 * Type: <b>reference</b><br> 3079 * Path: <b>Communication.subject</b><br> 3080 * </p> 3081 */ 3082 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3083 SP_SUBJECT); 3084 3085 /** 3086 * Constant for fluent queries to be used to add include statements. Specifies 3087 * the path value of "<b>Communication:subject</b>". 3088 */ 3089 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 3090 "Communication:subject").toLocked(); 3091 3092 /** 3093 * Search parameter: <b>instantiates-canonical</b> 3094 * <p> 3095 * Description: <b>Instantiates FHIR protocol or definition</b><br> 3096 * Type: <b>reference</b><br> 3097 * Path: <b>Communication.instantiatesCanonical</b><br> 3098 * </p> 3099 */ 3100 @SearchParamDefinition(name = "instantiates-canonical", path = "Communication.instantiatesCanonical", description = "Instantiates FHIR protocol or definition", type = "reference", target = { 3101 ActivityDefinition.class, Measure.class, OperationDefinition.class, PlanDefinition.class, Questionnaire.class }) 3102 public static final String SP_INSTANTIATES_CANONICAL = "instantiates-canonical"; 3103 /** 3104 * <b>Fluent Client</b> search parameter constant for 3105 * <b>instantiates-canonical</b> 3106 * <p> 3107 * Description: <b>Instantiates FHIR protocol or definition</b><br> 3108 * Type: <b>reference</b><br> 3109 * Path: <b>Communication.instantiatesCanonical</b><br> 3110 * </p> 3111 */ 3112 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSTANTIATES_CANONICAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3113 SP_INSTANTIATES_CANONICAL); 3114 3115 /** 3116 * Constant for fluent queries to be used to add include statements. Specifies 3117 * the path value of "<b>Communication:instantiates-canonical</b>". 3118 */ 3119 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSTANTIATES_CANONICAL = new ca.uhn.fhir.model.api.Include( 3120 "Communication:instantiates-canonical").toLocked(); 3121 3122 /** 3123 * Search parameter: <b>received</b> 3124 * <p> 3125 * Description: <b>When received</b><br> 3126 * Type: <b>date</b><br> 3127 * Path: <b>Communication.received</b><br> 3128 * </p> 3129 */ 3130 @SearchParamDefinition(name = "received", path = "Communication.received", description = "When received", type = "date") 3131 public static final String SP_RECEIVED = "received"; 3132 /** 3133 * <b>Fluent Client</b> search parameter constant for <b>received</b> 3134 * <p> 3135 * Description: <b>When received</b><br> 3136 * Type: <b>date</b><br> 3137 * Path: <b>Communication.received</b><br> 3138 * </p> 3139 */ 3140 public static final ca.uhn.fhir.rest.gclient.DateClientParam RECEIVED = new ca.uhn.fhir.rest.gclient.DateClientParam( 3141 SP_RECEIVED); 3142 3143 /** 3144 * Search parameter: <b>part-of</b> 3145 * <p> 3146 * Description: <b>Part of this action</b><br> 3147 * Type: <b>reference</b><br> 3148 * Path: <b>Communication.partOf</b><br> 3149 * </p> 3150 */ 3151 @SearchParamDefinition(name = "part-of", path = "Communication.partOf", description = "Part of this action", type = "reference") 3152 public static final String SP_PART_OF = "part-of"; 3153 /** 3154 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 3155 * <p> 3156 * Description: <b>Part of this action</b><br> 3157 * Type: <b>reference</b><br> 3158 * Path: <b>Communication.partOf</b><br> 3159 * </p> 3160 */ 3161 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3162 SP_PART_OF); 3163 3164 /** 3165 * Constant for fluent queries to be used to add include statements. Specifies 3166 * the path value of "<b>Communication:part-of</b>". 3167 */ 3168 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include( 3169 "Communication:part-of").toLocked(); 3170 3171 /** 3172 * Search parameter: <b>medium</b> 3173 * <p> 3174 * Description: <b>A channel of communication</b><br> 3175 * Type: <b>token</b><br> 3176 * Path: <b>Communication.medium</b><br> 3177 * </p> 3178 */ 3179 @SearchParamDefinition(name = "medium", path = "Communication.medium", description = "A channel of communication", type = "token") 3180 public static final String SP_MEDIUM = "medium"; 3181 /** 3182 * <b>Fluent Client</b> search parameter constant for <b>medium</b> 3183 * <p> 3184 * Description: <b>A channel of communication</b><br> 3185 * Type: <b>token</b><br> 3186 * Path: <b>Communication.medium</b><br> 3187 * </p> 3188 */ 3189 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MEDIUM = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3190 SP_MEDIUM); 3191 3192 /** 3193 * Search parameter: <b>encounter</b> 3194 * <p> 3195 * Description: <b>Encounter created as part of</b><br> 3196 * Type: <b>reference</b><br> 3197 * Path: <b>Communication.encounter</b><br> 3198 * </p> 3199 */ 3200 @SearchParamDefinition(name = "encounter", path = "Communication.encounter", description = "Encounter created as part of", type = "reference", providesMembershipIn = { 3201 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 3202 public static final String SP_ENCOUNTER = "encounter"; 3203 /** 3204 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 3205 * <p> 3206 * Description: <b>Encounter created as part of</b><br> 3207 * Type: <b>reference</b><br> 3208 * Path: <b>Communication.encounter</b><br> 3209 * </p> 3210 */ 3211 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3212 SP_ENCOUNTER); 3213 3214 /** 3215 * Constant for fluent queries to be used to add include statements. Specifies 3216 * the path value of "<b>Communication:encounter</b>". 3217 */ 3218 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 3219 "Communication:encounter").toLocked(); 3220 3221 /** 3222 * Search parameter: <b>sent</b> 3223 * <p> 3224 * Description: <b>When sent</b><br> 3225 * Type: <b>date</b><br> 3226 * Path: <b>Communication.sent</b><br> 3227 * </p> 3228 */ 3229 @SearchParamDefinition(name = "sent", path = "Communication.sent", description = "When sent", type = "date") 3230 public static final String SP_SENT = "sent"; 3231 /** 3232 * <b>Fluent Client</b> search parameter constant for <b>sent</b> 3233 * <p> 3234 * Description: <b>When sent</b><br> 3235 * Type: <b>date</b><br> 3236 * Path: <b>Communication.sent</b><br> 3237 * </p> 3238 */ 3239 public static final ca.uhn.fhir.rest.gclient.DateClientParam SENT = new ca.uhn.fhir.rest.gclient.DateClientParam( 3240 SP_SENT); 3241 3242 /** 3243 * Search parameter: <b>based-on</b> 3244 * <p> 3245 * Description: <b>Request fulfilled by this communication</b><br> 3246 * Type: <b>reference</b><br> 3247 * Path: <b>Communication.basedOn</b><br> 3248 * </p> 3249 */ 3250 @SearchParamDefinition(name = "based-on", path = "Communication.basedOn", description = "Request fulfilled by this communication", type = "reference") 3251 public static final String SP_BASED_ON = "based-on"; 3252 /** 3253 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 3254 * <p> 3255 * Description: <b>Request fulfilled by this communication</b><br> 3256 * Type: <b>reference</b><br> 3257 * Path: <b>Communication.basedOn</b><br> 3258 * </p> 3259 */ 3260 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3261 SP_BASED_ON); 3262 3263 /** 3264 * Constant for fluent queries to be used to add include statements. Specifies 3265 * the path value of "<b>Communication:based-on</b>". 3266 */ 3267 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include( 3268 "Communication:based-on").toLocked(); 3269 3270 /** 3271 * Search parameter: <b>sender</b> 3272 * <p> 3273 * Description: <b>Message sender</b><br> 3274 * Type: <b>reference</b><br> 3275 * Path: <b>Communication.sender</b><br> 3276 * </p> 3277 */ 3278 @SearchParamDefinition(name = "sender", path = "Communication.sender", description = "Message sender", type = "reference", providesMembershipIn = { 3279 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 3280 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3281 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 3282 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, 3283 HealthcareService.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, 3284 RelatedPerson.class }) 3285 public static final String SP_SENDER = "sender"; 3286 /** 3287 * <b>Fluent Client</b> search parameter constant for <b>sender</b> 3288 * <p> 3289 * Description: <b>Message sender</b><br> 3290 * Type: <b>reference</b><br> 3291 * Path: <b>Communication.sender</b><br> 3292 * </p> 3293 */ 3294 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SENDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3295 SP_SENDER); 3296 3297 /** 3298 * Constant for fluent queries to be used to add include statements. Specifies 3299 * the path value of "<b>Communication:sender</b>". 3300 */ 3301 public static final ca.uhn.fhir.model.api.Include INCLUDE_SENDER = new ca.uhn.fhir.model.api.Include( 3302 "Communication:sender").toLocked(); 3303 3304 /** 3305 * Search parameter: <b>patient</b> 3306 * <p> 3307 * Description: <b>Focus of message</b><br> 3308 * Type: <b>reference</b><br> 3309 * Path: <b>Communication.subject</b><br> 3310 * </p> 3311 */ 3312 @SearchParamDefinition(name = "patient", path = "Communication.subject.where(resolve() is Patient)", description = "Focus of message", type = "reference", target = { 3313 Patient.class }) 3314 public static final String SP_PATIENT = "patient"; 3315 /** 3316 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3317 * <p> 3318 * Description: <b>Focus of message</b><br> 3319 * Type: <b>reference</b><br> 3320 * Path: <b>Communication.subject</b><br> 3321 * </p> 3322 */ 3323 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3324 SP_PATIENT); 3325 3326 /** 3327 * Constant for fluent queries to be used to add include statements. Specifies 3328 * the path value of "<b>Communication:patient</b>". 3329 */ 3330 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 3331 "Communication:patient").toLocked(); 3332 3333 /** 3334 * Search parameter: <b>recipient</b> 3335 * <p> 3336 * Description: <b>Message recipient</b><br> 3337 * Type: <b>reference</b><br> 3338 * Path: <b>Communication.recipient</b><br> 3339 * </p> 3340 */ 3341 @SearchParamDefinition(name = "recipient", path = "Communication.recipient", description = "Message recipient", type = "reference", providesMembershipIn = { 3342 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 3343 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3344 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 3345 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { CareTeam.class, Device.class, 3346 Group.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, 3347 PractitionerRole.class, RelatedPerson.class }) 3348 public static final String SP_RECIPIENT = "recipient"; 3349 /** 3350 * <b>Fluent Client</b> search parameter constant for <b>recipient</b> 3351 * <p> 3352 * Description: <b>Message recipient</b><br> 3353 * Type: <b>reference</b><br> 3354 * Path: <b>Communication.recipient</b><br> 3355 * </p> 3356 */ 3357 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECIPIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3358 SP_RECIPIENT); 3359 3360 /** 3361 * Constant for fluent queries to be used to add include statements. Specifies 3362 * the path value of "<b>Communication:recipient</b>". 3363 */ 3364 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECIPIENT = new ca.uhn.fhir.model.api.Include( 3365 "Communication:recipient").toLocked(); 3366 3367 /** 3368 * Search parameter: <b>instantiates-uri</b> 3369 * <p> 3370 * Description: <b>Instantiates external protocol or definition</b><br> 3371 * Type: <b>uri</b><br> 3372 * Path: <b>Communication.instantiatesUri</b><br> 3373 * </p> 3374 */ 3375 @SearchParamDefinition(name = "instantiates-uri", path = "Communication.instantiatesUri", description = "Instantiates external protocol or definition", type = "uri") 3376 public static final String SP_INSTANTIATES_URI = "instantiates-uri"; 3377 /** 3378 * <b>Fluent Client</b> search parameter constant for <b>instantiates-uri</b> 3379 * <p> 3380 * Description: <b>Instantiates external protocol or definition</b><br> 3381 * Type: <b>uri</b><br> 3382 * Path: <b>Communication.instantiatesUri</b><br> 3383 * </p> 3384 */ 3385 public static final ca.uhn.fhir.rest.gclient.UriClientParam INSTANTIATES_URI = new ca.uhn.fhir.rest.gclient.UriClientParam( 3386 SP_INSTANTIATES_URI); 3387 3388 /** 3389 * Search parameter: <b>category</b> 3390 * <p> 3391 * Description: <b>Message category</b><br> 3392 * Type: <b>token</b><br> 3393 * Path: <b>Communication.category</b><br> 3394 * </p> 3395 */ 3396 @SearchParamDefinition(name = "category", path = "Communication.category", description = "Message category", type = "token") 3397 public static final String SP_CATEGORY = "category"; 3398 /** 3399 * <b>Fluent Client</b> search parameter constant for <b>category</b> 3400 * <p> 3401 * Description: <b>Message category</b><br> 3402 * Type: <b>token</b><br> 3403 * Path: <b>Communication.category</b><br> 3404 * </p> 3405 */ 3406 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3407 SP_CATEGORY); 3408 3409 /** 3410 * Search parameter: <b>status</b> 3411 * <p> 3412 * Description: <b>preparation | in-progress | not-done | on-hold | stopped | 3413 * completed | entered-in-error | unknown</b><br> 3414 * Type: <b>token</b><br> 3415 * Path: <b>Communication.status</b><br> 3416 * </p> 3417 */ 3418 @SearchParamDefinition(name = "status", path = "Communication.status", description = "preparation | in-progress | not-done | on-hold | stopped | completed | entered-in-error | unknown", type = "token") 3419 public static final String SP_STATUS = "status"; 3420 /** 3421 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3422 * <p> 3423 * Description: <b>preparation | in-progress | not-done | on-hold | stopped | 3424 * completed | entered-in-error | unknown</b><br> 3425 * Type: <b>token</b><br> 3426 * Path: <b>Communication.status</b><br> 3427 * </p> 3428 */ 3429 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3430 SP_STATUS); 3431 3432}