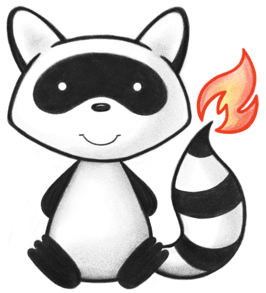
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * A request to convey information; e.g. the CDS system proposes that an alert 048 * be sent to a responsible provider, the CDS system proposes that the public 049 * health agency be notified about a reportable condition. 050 */ 051@ResourceDef(name = "CommunicationRequest", profile = "http://hl7.org/fhir/StructureDefinition/CommunicationRequest") 052public class CommunicationRequest extends DomainResource { 053 054 public enum CommunicationRequestStatus { 055 /** 056 * The request has been created but is not yet complete or ready for action. 057 */ 058 DRAFT, 059 /** 060 * The request is in force and ready to be acted upon. 061 */ 062 ACTIVE, 063 /** 064 * The request (and any implicit authorization to act) has been temporarily 065 * withdrawn but is expected to resume in the future. 066 */ 067 ONHOLD, 068 /** 069 * The request (and any implicit authorization to act) has been terminated prior 070 * to the known full completion of the intended actions. No further activity 071 * should occur. 072 */ 073 REVOKED, 074 /** 075 * The activity described by the request has been fully performed. No further 076 * activity will occur. 077 */ 078 COMPLETED, 079 /** 080 * This request should never have existed and should be considered 'void'. (It 081 * is possible that real-world decisions were based on it. If real-world 082 * activity has occurred, the status should be "revoked" rather than 083 * "entered-in-error".). 084 */ 085 ENTEREDINERROR, 086 /** 087 * The authoring/source system does not know which of the status values 088 * currently applies for this request. Note: This concept is not to be used for 089 * "other" - one of the listed statuses is presumed to apply, but the 090 * authoring/source system does not know which. 091 */ 092 UNKNOWN, 093 /** 094 * added to help the parsers with the generic types 095 */ 096 NULL; 097 098 public static CommunicationRequestStatus fromCode(String codeString) throws FHIRException { 099 if (codeString == null || "".equals(codeString)) 100 return null; 101 if ("draft".equals(codeString)) 102 return DRAFT; 103 if ("active".equals(codeString)) 104 return ACTIVE; 105 if ("on-hold".equals(codeString)) 106 return ONHOLD; 107 if ("revoked".equals(codeString)) 108 return REVOKED; 109 if ("completed".equals(codeString)) 110 return COMPLETED; 111 if ("entered-in-error".equals(codeString)) 112 return ENTEREDINERROR; 113 if ("unknown".equals(codeString)) 114 return UNKNOWN; 115 if (Configuration.isAcceptInvalidEnums()) 116 return null; 117 else 118 throw new FHIRException("Unknown CommunicationRequestStatus code '" + codeString + "'"); 119 } 120 121 public String toCode() { 122 switch (this) { 123 case DRAFT: 124 return "draft"; 125 case ACTIVE: 126 return "active"; 127 case ONHOLD: 128 return "on-hold"; 129 case REVOKED: 130 return "revoked"; 131 case COMPLETED: 132 return "completed"; 133 case ENTEREDINERROR: 134 return "entered-in-error"; 135 case UNKNOWN: 136 return "unknown"; 137 case NULL: 138 return null; 139 default: 140 return "?"; 141 } 142 } 143 144 public String getSystem() { 145 switch (this) { 146 case DRAFT: 147 return "http://hl7.org/fhir/request-status"; 148 case ACTIVE: 149 return "http://hl7.org/fhir/request-status"; 150 case ONHOLD: 151 return "http://hl7.org/fhir/request-status"; 152 case REVOKED: 153 return "http://hl7.org/fhir/request-status"; 154 case COMPLETED: 155 return "http://hl7.org/fhir/request-status"; 156 case ENTEREDINERROR: 157 return "http://hl7.org/fhir/request-status"; 158 case UNKNOWN: 159 return "http://hl7.org/fhir/request-status"; 160 case NULL: 161 return null; 162 default: 163 return "?"; 164 } 165 } 166 167 public String getDefinition() { 168 switch (this) { 169 case DRAFT: 170 return "The request has been created but is not yet complete or ready for action."; 171 case ACTIVE: 172 return "The request is in force and ready to be acted upon."; 173 case ONHOLD: 174 return "The request (and any implicit authorization to act) has been temporarily withdrawn but is expected to resume in the future."; 175 case REVOKED: 176 return "The request (and any implicit authorization to act) has been terminated prior to the known full completion of the intended actions. No further activity should occur."; 177 case COMPLETED: 178 return "The activity described by the request has been fully performed. No further activity will occur."; 179 case ENTEREDINERROR: 180 return "This request should never have existed and should be considered 'void'. (It is possible that real-world decisions were based on it. If real-world activity has occurred, the status should be \"revoked\" rather than \"entered-in-error\".)."; 181 case UNKNOWN: 182 return "The authoring/source system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 183 case NULL: 184 return null; 185 default: 186 return "?"; 187 } 188 } 189 190 public String getDisplay() { 191 switch (this) { 192 case DRAFT: 193 return "Draft"; 194 case ACTIVE: 195 return "Active"; 196 case ONHOLD: 197 return "On Hold"; 198 case REVOKED: 199 return "Revoked"; 200 case COMPLETED: 201 return "Completed"; 202 case ENTEREDINERROR: 203 return "Entered in Error"; 204 case UNKNOWN: 205 return "Unknown"; 206 case NULL: 207 return null; 208 default: 209 return "?"; 210 } 211 } 212 } 213 214 public static class CommunicationRequestStatusEnumFactory implements EnumFactory<CommunicationRequestStatus> { 215 public CommunicationRequestStatus fromCode(String codeString) throws IllegalArgumentException { 216 if (codeString == null || "".equals(codeString)) 217 if (codeString == null || "".equals(codeString)) 218 return null; 219 if ("draft".equals(codeString)) 220 return CommunicationRequestStatus.DRAFT; 221 if ("active".equals(codeString)) 222 return CommunicationRequestStatus.ACTIVE; 223 if ("on-hold".equals(codeString)) 224 return CommunicationRequestStatus.ONHOLD; 225 if ("revoked".equals(codeString)) 226 return CommunicationRequestStatus.REVOKED; 227 if ("completed".equals(codeString)) 228 return CommunicationRequestStatus.COMPLETED; 229 if ("entered-in-error".equals(codeString)) 230 return CommunicationRequestStatus.ENTEREDINERROR; 231 if ("unknown".equals(codeString)) 232 return CommunicationRequestStatus.UNKNOWN; 233 throw new IllegalArgumentException("Unknown CommunicationRequestStatus code '" + codeString + "'"); 234 } 235 236 public Enumeration<CommunicationRequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 237 if (code == null) 238 return null; 239 if (code.isEmpty()) 240 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.NULL, code); 241 String codeString = code.asStringValue(); 242 if (codeString == null || "".equals(codeString)) 243 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.NULL, code); 244 if ("draft".equals(codeString)) 245 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.DRAFT, code); 246 if ("active".equals(codeString)) 247 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.ACTIVE, code); 248 if ("on-hold".equals(codeString)) 249 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.ONHOLD, code); 250 if ("revoked".equals(codeString)) 251 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.REVOKED, code); 252 if ("completed".equals(codeString)) 253 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.COMPLETED, code); 254 if ("entered-in-error".equals(codeString)) 255 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.ENTEREDINERROR, code); 256 if ("unknown".equals(codeString)) 257 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.UNKNOWN, code); 258 throw new FHIRException("Unknown CommunicationRequestStatus code '" + codeString + "'"); 259 } 260 261 public String toCode(CommunicationRequestStatus code) { 262 if (code == CommunicationRequestStatus.DRAFT) 263 return "draft"; 264 if (code == CommunicationRequestStatus.ACTIVE) 265 return "active"; 266 if (code == CommunicationRequestStatus.ONHOLD) 267 return "on-hold"; 268 if (code == CommunicationRequestStatus.REVOKED) 269 return "revoked"; 270 if (code == CommunicationRequestStatus.COMPLETED) 271 return "completed"; 272 if (code == CommunicationRequestStatus.ENTEREDINERROR) 273 return "entered-in-error"; 274 if (code == CommunicationRequestStatus.UNKNOWN) 275 return "unknown"; 276 return "?"; 277 } 278 279 public String toSystem(CommunicationRequestStatus code) { 280 return code.getSystem(); 281 } 282 } 283 284 public enum CommunicationPriority { 285 /** 286 * The request has normal priority. 287 */ 288 ROUTINE, 289 /** 290 * The request should be actioned promptly - higher priority than routine. 291 */ 292 URGENT, 293 /** 294 * The request should be actioned as soon as possible - higher priority than 295 * urgent. 296 */ 297 ASAP, 298 /** 299 * The request should be actioned immediately - highest possible priority. E.g. 300 * an emergency. 301 */ 302 STAT, 303 /** 304 * added to help the parsers with the generic types 305 */ 306 NULL; 307 308 public static CommunicationPriority fromCode(String codeString) throws FHIRException { 309 if (codeString == null || "".equals(codeString)) 310 return null; 311 if ("routine".equals(codeString)) 312 return ROUTINE; 313 if ("urgent".equals(codeString)) 314 return URGENT; 315 if ("asap".equals(codeString)) 316 return ASAP; 317 if ("stat".equals(codeString)) 318 return STAT; 319 if (Configuration.isAcceptInvalidEnums()) 320 return null; 321 else 322 throw new FHIRException("Unknown CommunicationPriority code '" + codeString + "'"); 323 } 324 325 public String toCode() { 326 switch (this) { 327 case ROUTINE: 328 return "routine"; 329 case URGENT: 330 return "urgent"; 331 case ASAP: 332 return "asap"; 333 case STAT: 334 return "stat"; 335 case NULL: 336 return null; 337 default: 338 return "?"; 339 } 340 } 341 342 public String getSystem() { 343 switch (this) { 344 case ROUTINE: 345 return "http://hl7.org/fhir/request-priority"; 346 case URGENT: 347 return "http://hl7.org/fhir/request-priority"; 348 case ASAP: 349 return "http://hl7.org/fhir/request-priority"; 350 case STAT: 351 return "http://hl7.org/fhir/request-priority"; 352 case NULL: 353 return null; 354 default: 355 return "?"; 356 } 357 } 358 359 public String getDefinition() { 360 switch (this) { 361 case ROUTINE: 362 return "The request has normal priority."; 363 case URGENT: 364 return "The request should be actioned promptly - higher priority than routine."; 365 case ASAP: 366 return "The request should be actioned as soon as possible - higher priority than urgent."; 367 case STAT: 368 return "The request should be actioned immediately - highest possible priority. E.g. an emergency."; 369 case NULL: 370 return null; 371 default: 372 return "?"; 373 } 374 } 375 376 public String getDisplay() { 377 switch (this) { 378 case ROUTINE: 379 return "Routine"; 380 case URGENT: 381 return "Urgent"; 382 case ASAP: 383 return "ASAP"; 384 case STAT: 385 return "STAT"; 386 case NULL: 387 return null; 388 default: 389 return "?"; 390 } 391 } 392 } 393 394 public static class CommunicationPriorityEnumFactory implements EnumFactory<CommunicationPriority> { 395 public CommunicationPriority fromCode(String codeString) throws IllegalArgumentException { 396 if (codeString == null || "".equals(codeString)) 397 if (codeString == null || "".equals(codeString)) 398 return null; 399 if ("routine".equals(codeString)) 400 return CommunicationPriority.ROUTINE; 401 if ("urgent".equals(codeString)) 402 return CommunicationPriority.URGENT; 403 if ("asap".equals(codeString)) 404 return CommunicationPriority.ASAP; 405 if ("stat".equals(codeString)) 406 return CommunicationPriority.STAT; 407 throw new IllegalArgumentException("Unknown CommunicationPriority code '" + codeString + "'"); 408 } 409 410 public Enumeration<CommunicationPriority> fromType(PrimitiveType<?> code) throws FHIRException { 411 if (code == null) 412 return null; 413 if (code.isEmpty()) 414 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.NULL, code); 415 String codeString = code.asStringValue(); 416 if (codeString == null || "".equals(codeString)) 417 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.NULL, code); 418 if ("routine".equals(codeString)) 419 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.ROUTINE, code); 420 if ("urgent".equals(codeString)) 421 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.URGENT, code); 422 if ("asap".equals(codeString)) 423 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.ASAP, code); 424 if ("stat".equals(codeString)) 425 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.STAT, code); 426 throw new FHIRException("Unknown CommunicationPriority code '" + codeString + "'"); 427 } 428 429 public String toCode(CommunicationPriority code) { 430 if (code == CommunicationPriority.ROUTINE) 431 return "routine"; 432 if (code == CommunicationPriority.URGENT) 433 return "urgent"; 434 if (code == CommunicationPriority.ASAP) 435 return "asap"; 436 if (code == CommunicationPriority.STAT) 437 return "stat"; 438 return "?"; 439 } 440 441 public String toSystem(CommunicationPriority code) { 442 return code.getSystem(); 443 } 444 } 445 446 @Block() 447 public static class CommunicationRequestPayloadComponent extends BackboneElement implements IBaseBackboneElement { 448 /** 449 * The communicated content (or for multi-part communications, one portion of 450 * the communication). 451 */ 452 @Child(name = "content", type = { StringType.class, Attachment.class, 453 Reference.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 454 @Description(shortDefinition = "Message part content", formalDefinition = "The communicated content (or for multi-part communications, one portion of the communication).") 455 protected Type content; 456 457 private static final long serialVersionUID = -1763459053L; 458 459 /** 460 * Constructor 461 */ 462 public CommunicationRequestPayloadComponent() { 463 super(); 464 } 465 466 /** 467 * Constructor 468 */ 469 public CommunicationRequestPayloadComponent(Type content) { 470 super(); 471 this.content = content; 472 } 473 474 /** 475 * @return {@link #content} (The communicated content (or for multi-part 476 * communications, one portion of the communication).) 477 */ 478 public Type getContent() { 479 return this.content; 480 } 481 482 /** 483 * @return {@link #content} (The communicated content (or for multi-part 484 * communications, one portion of the communication).) 485 */ 486 public StringType getContentStringType() throws FHIRException { 487 if (this.content == null) 488 this.content = new StringType(); 489 if (!(this.content instanceof StringType)) 490 throw new FHIRException("Type mismatch: the type StringType was expected, but " 491 + this.content.getClass().getName() + " was encountered"); 492 return (StringType) this.content; 493 } 494 495 public boolean hasContentStringType() { 496 return this != null && this.content instanceof StringType; 497 } 498 499 /** 500 * @return {@link #content} (The communicated content (or for multi-part 501 * communications, one portion of the communication).) 502 */ 503 public Attachment getContentAttachment() throws FHIRException { 504 if (this.content == null) 505 this.content = new Attachment(); 506 if (!(this.content instanceof Attachment)) 507 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 508 + this.content.getClass().getName() + " was encountered"); 509 return (Attachment) this.content; 510 } 511 512 public boolean hasContentAttachment() { 513 return this != null && this.content instanceof Attachment; 514 } 515 516 /** 517 * @return {@link #content} (The communicated content (or for multi-part 518 * communications, one portion of the communication).) 519 */ 520 public Reference getContentReference() throws FHIRException { 521 if (this.content == null) 522 this.content = new Reference(); 523 if (!(this.content instanceof Reference)) 524 throw new FHIRException("Type mismatch: the type Reference was expected, but " 525 + this.content.getClass().getName() + " was encountered"); 526 return (Reference) this.content; 527 } 528 529 public boolean hasContentReference() { 530 return this != null && this.content instanceof Reference; 531 } 532 533 public boolean hasContent() { 534 return this.content != null && !this.content.isEmpty(); 535 } 536 537 /** 538 * @param value {@link #content} (The communicated content (or for multi-part 539 * communications, one portion of the communication).) 540 */ 541 public CommunicationRequestPayloadComponent setContent(Type value) { 542 if (value != null && !(value instanceof StringType || value instanceof Attachment || value instanceof Reference)) 543 throw new Error("Not the right type for CommunicationRequest.payload.content[x]: " + value.fhirType()); 544 this.content = value; 545 return this; 546 } 547 548 protected void listChildren(List<Property> children) { 549 super.listChildren(children); 550 children.add(new Property("content[x]", "string|Attachment|Reference(Any)", 551 "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, 552 content)); 553 } 554 555 @Override 556 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 557 switch (_hash) { 558 case 264548711: 559 /* content[x] */ return new Property("content[x]", "string|Attachment|Reference(Any)", 560 "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, 561 content); 562 case 951530617: 563 /* content */ return new Property("content[x]", "string|Attachment|Reference(Any)", 564 "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, 565 content); 566 case -326336022: 567 /* contentString */ return new Property("content[x]", "string|Attachment|Reference(Any)", 568 "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, 569 content); 570 case -702028164: 571 /* contentAttachment */ return new Property("content[x]", "string|Attachment|Reference(Any)", 572 "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, 573 content); 574 case 1193747154: 575 /* contentReference */ return new Property("content[x]", "string|Attachment|Reference(Any)", 576 "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, 577 content); 578 default: 579 return super.getNamedProperty(_hash, _name, _checkValid); 580 } 581 582 } 583 584 @Override 585 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 586 switch (hash) { 587 case 951530617: 588 /* content */ return this.content == null ? new Base[0] : new Base[] { this.content }; // Type 589 default: 590 return super.getProperty(hash, name, checkValid); 591 } 592 593 } 594 595 @Override 596 public Base setProperty(int hash, String name, Base value) throws FHIRException { 597 switch (hash) { 598 case 951530617: // content 599 this.content = castToType(value); // Type 600 return value; 601 default: 602 return super.setProperty(hash, name, value); 603 } 604 605 } 606 607 @Override 608 public Base setProperty(String name, Base value) throws FHIRException { 609 if (name.equals("content[x]")) { 610 this.content = castToType(value); // Type 611 } else 612 return super.setProperty(name, value); 613 return value; 614 } 615 616 @Override 617 public void removeChild(String name, Base value) throws FHIRException { 618 if (name.equals("content[x]")) { 619 this.content = null; 620 } else 621 super.removeChild(name, value); 622 623 } 624 625 @Override 626 public Base makeProperty(int hash, String name) throws FHIRException { 627 switch (hash) { 628 case 264548711: 629 return getContent(); 630 case 951530617: 631 return getContent(); 632 default: 633 return super.makeProperty(hash, name); 634 } 635 636 } 637 638 @Override 639 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 640 switch (hash) { 641 case 951530617: 642 /* content */ return new String[] { "string", "Attachment", "Reference" }; 643 default: 644 return super.getTypesForProperty(hash, name); 645 } 646 647 } 648 649 @Override 650 public Base addChild(String name) throws FHIRException { 651 if (name.equals("contentString")) { 652 this.content = new StringType(); 653 return this.content; 654 } else if (name.equals("contentAttachment")) { 655 this.content = new Attachment(); 656 return this.content; 657 } else if (name.equals("contentReference")) { 658 this.content = new Reference(); 659 return this.content; 660 } else 661 return super.addChild(name); 662 } 663 664 public CommunicationRequestPayloadComponent copy() { 665 CommunicationRequestPayloadComponent dst = new CommunicationRequestPayloadComponent(); 666 copyValues(dst); 667 return dst; 668 } 669 670 public void copyValues(CommunicationRequestPayloadComponent dst) { 671 super.copyValues(dst); 672 dst.content = content == null ? null : content.copy(); 673 } 674 675 @Override 676 public boolean equalsDeep(Base other_) { 677 if (!super.equalsDeep(other_)) 678 return false; 679 if (!(other_ instanceof CommunicationRequestPayloadComponent)) 680 return false; 681 CommunicationRequestPayloadComponent o = (CommunicationRequestPayloadComponent) other_; 682 return compareDeep(content, o.content, true); 683 } 684 685 @Override 686 public boolean equalsShallow(Base other_) { 687 if (!super.equalsShallow(other_)) 688 return false; 689 if (!(other_ instanceof CommunicationRequestPayloadComponent)) 690 return false; 691 CommunicationRequestPayloadComponent o = (CommunicationRequestPayloadComponent) other_; 692 return true; 693 } 694 695 public boolean isEmpty() { 696 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(content); 697 } 698 699 public String fhirType() { 700 return "CommunicationRequest.payload"; 701 702 } 703 704 } 705 706 /** 707 * Business identifiers assigned to this communication request by the performer 708 * or other systems which remain constant as the resource is updated and 709 * propagates from server to server. 710 */ 711 @Child(name = "identifier", type = { 712 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 713 @Description(shortDefinition = "Unique identifier", formalDefinition = "Business identifiers assigned to this communication request by the performer or other systems which remain constant as the resource is updated and propagates from server to server.") 714 protected List<Identifier> identifier; 715 716 /** 717 * A plan or proposal that is fulfilled in whole or in part by this request. 718 */ 719 @Child(name = "basedOn", type = { 720 Reference.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 721 @Description(shortDefinition = "Fulfills plan or proposal", formalDefinition = "A plan or proposal that is fulfilled in whole or in part by this request.") 722 protected List<Reference> basedOn; 723 /** 724 * The actual objects that are the target of the reference (A plan or proposal 725 * that is fulfilled in whole or in part by this request.) 726 */ 727 protected List<Resource> basedOnTarget; 728 729 /** 730 * Completed or terminated request(s) whose function is taken by this new 731 * request. 732 */ 733 @Child(name = "replaces", type = { 734 CommunicationRequest.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 735 @Description(shortDefinition = "Request(s) replaced by this request", formalDefinition = "Completed or terminated request(s) whose function is taken by this new request.") 736 protected List<Reference> replaces; 737 /** 738 * The actual objects that are the target of the reference (Completed or 739 * terminated request(s) whose function is taken by this new request.) 740 */ 741 protected List<CommunicationRequest> replacesTarget; 742 743 /** 744 * A shared identifier common to all requests that were authorized more or less 745 * simultaneously by a single author, representing the identifier of the 746 * requisition, prescription or similar form. 747 */ 748 @Child(name = "groupIdentifier", type = { 749 Identifier.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 750 @Description(shortDefinition = "Composite request this is part of", formalDefinition = "A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.") 751 protected Identifier groupIdentifier; 752 753 /** 754 * The status of the proposal or order. 755 */ 756 @Child(name = "status", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = true, summary = true) 757 @Description(shortDefinition = "draft | active | on-hold | revoked | completed | entered-in-error | unknown", formalDefinition = "The status of the proposal or order.") 758 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-status") 759 protected Enumeration<CommunicationRequestStatus> status; 760 761 /** 762 * Captures the reason for the current state of the CommunicationRequest. 763 */ 764 @Child(name = "statusReason", type = { 765 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 766 @Description(shortDefinition = "Reason for current status", formalDefinition = "Captures the reason for the current state of the CommunicationRequest.") 767 protected CodeableConcept statusReason; 768 769 /** 770 * The type of message to be sent such as alert, notification, reminder, 771 * instruction, etc. 772 */ 773 @Child(name = "category", type = { 774 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 775 @Description(shortDefinition = "Message category", formalDefinition = "The type of message to be sent such as alert, notification, reminder, instruction, etc.") 776 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/communication-category") 777 protected List<CodeableConcept> category; 778 779 /** 780 * Characterizes how quickly the proposed act must be initiated. Includes 781 * concepts such as stat, urgent, routine. 782 */ 783 @Child(name = "priority", type = { CodeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 784 @Description(shortDefinition = "routine | urgent | asap | stat", formalDefinition = "Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine.") 785 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-priority") 786 protected Enumeration<CommunicationPriority> priority; 787 788 /** 789 * If true indicates that the CommunicationRequest is asking for the specified 790 * action to *not* occur. 791 */ 792 @Child(name = "doNotPerform", type = { 793 BooleanType.class }, order = 8, min = 0, max = 1, modifier = true, summary = true) 794 @Description(shortDefinition = "True if request is prohibiting action", formalDefinition = "If true indicates that the CommunicationRequest is asking for the specified action to *not* occur.") 795 protected BooleanType doNotPerform; 796 797 /** 798 * A channel that was used for this communication (e.g. email, fax). 799 */ 800 @Child(name = "medium", type = { 801 CodeableConcept.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 802 @Description(shortDefinition = "A channel of communication", formalDefinition = "A channel that was used for this communication (e.g. email, fax).") 803 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ParticipationMode") 804 protected List<CodeableConcept> medium; 805 806 /** 807 * The patient or group that is the focus of this communication request. 808 */ 809 @Child(name = "subject", type = { Patient.class, 810 Group.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 811 @Description(shortDefinition = "Focus of message", formalDefinition = "The patient or group that is the focus of this communication request.") 812 protected Reference subject; 813 814 /** 815 * The actual object that is the target of the reference (The patient or group 816 * that is the focus of this communication request.) 817 */ 818 protected Resource subjectTarget; 819 820 /** 821 * Other resources that pertain to this communication request and to which this 822 * communication request should be associated. 823 */ 824 @Child(name = "about", type = { 825 Reference.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 826 @Description(shortDefinition = "Resources that pertain to this communication request", formalDefinition = "Other resources that pertain to this communication request and to which this communication request should be associated.") 827 protected List<Reference> about; 828 /** 829 * The actual objects that are the target of the reference (Other resources that 830 * pertain to this communication request and to which this communication request 831 * should be associated.) 832 */ 833 protected List<Resource> aboutTarget; 834 835 /** 836 * The Encounter during which this CommunicationRequest was created or to which 837 * the creation of this record is tightly associated. 838 */ 839 @Child(name = "encounter", type = { Encounter.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 840 @Description(shortDefinition = "Encounter created as part of", formalDefinition = "The Encounter during which this CommunicationRequest was created or to which the creation of this record is tightly associated.") 841 protected Reference encounter; 842 843 /** 844 * The actual object that is the target of the reference (The Encounter during 845 * which this CommunicationRequest was created or to which the creation of this 846 * record is tightly associated.) 847 */ 848 protected Encounter encounterTarget; 849 850 /** 851 * Text, attachment(s), or resource(s) to be communicated to the recipient. 852 */ 853 @Child(name = "payload", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 854 @Description(shortDefinition = "Message payload", formalDefinition = "Text, attachment(s), or resource(s) to be communicated to the recipient.") 855 protected List<CommunicationRequestPayloadComponent> payload; 856 857 /** 858 * The time when this communication is to occur. 859 */ 860 @Child(name = "occurrence", type = { DateTimeType.class, 861 Period.class }, order = 14, min = 0, max = 1, modifier = false, summary = true) 862 @Description(shortDefinition = "When scheduled", formalDefinition = "The time when this communication is to occur.") 863 protected Type occurrence; 864 865 /** 866 * For draft requests, indicates the date of initial creation. For requests with 867 * other statuses, indicates the date of activation. 868 */ 869 @Child(name = "authoredOn", type = { 870 DateTimeType.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 871 @Description(shortDefinition = "When request transitioned to being actionable", formalDefinition = "For draft requests, indicates the date of initial creation. For requests with other statuses, indicates the date of activation.") 872 protected DateTimeType authoredOn; 873 874 /** 875 * The device, individual, or organization who initiated the request and has 876 * responsibility for its activation. 877 */ 878 @Child(name = "requester", type = { Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, 879 RelatedPerson.class, Device.class }, order = 16, min = 0, max = 1, modifier = false, summary = true) 880 @Description(shortDefinition = "Who/what is requesting service", formalDefinition = "The device, individual, or organization who initiated the request and has responsibility for its activation.") 881 protected Reference requester; 882 883 /** 884 * The actual object that is the target of the reference (The device, 885 * individual, or organization who initiated the request and has responsibility 886 * for its activation.) 887 */ 888 protected Resource requesterTarget; 889 890 /** 891 * The entity (e.g. person, organization, clinical information system, device, 892 * group, or care team) which is the intended target of the communication. 893 */ 894 @Child(name = "recipient", type = { Device.class, Organization.class, Patient.class, Practitioner.class, 895 PractitionerRole.class, RelatedPerson.class, Group.class, CareTeam.class, 896 HealthcareService.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 897 @Description(shortDefinition = "Message recipient", formalDefinition = "The entity (e.g. person, organization, clinical information system, device, group, or care team) which is the intended target of the communication.") 898 protected List<Reference> recipient; 899 /** 900 * The actual objects that are the target of the reference (The entity (e.g. 901 * person, organization, clinical information system, device, group, or care 902 * team) which is the intended target of the communication.) 903 */ 904 protected List<Resource> recipientTarget; 905 906 /** 907 * The entity (e.g. person, organization, clinical information system, or 908 * device) which is to be the source of the communication. 909 */ 910 @Child(name = "sender", type = { Device.class, Organization.class, Patient.class, Practitioner.class, 911 PractitionerRole.class, RelatedPerson.class, 912 HealthcareService.class }, order = 18, min = 0, max = 1, modifier = false, summary = true) 913 @Description(shortDefinition = "Message sender", formalDefinition = "The entity (e.g. person, organization, clinical information system, or device) which is to be the source of the communication.") 914 protected Reference sender; 915 916 /** 917 * The actual object that is the target of the reference (The entity (e.g. 918 * person, organization, clinical information system, or device) which is to be 919 * the source of the communication.) 920 */ 921 protected Resource senderTarget; 922 923 /** 924 * Describes why the request is being made in coded or textual form. 925 */ 926 @Child(name = "reasonCode", type = { 927 CodeableConcept.class }, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 928 @Description(shortDefinition = "Why is communication needed?", formalDefinition = "Describes why the request is being made in coded or textual form.") 929 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ActReason") 930 protected List<CodeableConcept> reasonCode; 931 932 /** 933 * Indicates another resource whose existence justifies this request. 934 */ 935 @Child(name = "reasonReference", type = { Condition.class, Observation.class, DiagnosticReport.class, 936 DocumentReference.class }, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 937 @Description(shortDefinition = "Why is communication needed?", formalDefinition = "Indicates another resource whose existence justifies this request.") 938 protected List<Reference> reasonReference; 939 /** 940 * The actual objects that are the target of the reference (Indicates another 941 * resource whose existence justifies this request.) 942 */ 943 protected List<Resource> reasonReferenceTarget; 944 945 /** 946 * Comments made about the request by the requester, sender, recipient, subject 947 * or other participants. 948 */ 949 @Child(name = "note", type = { 950 Annotation.class }, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 951 @Description(shortDefinition = "Comments made about communication request", formalDefinition = "Comments made about the request by the requester, sender, recipient, subject or other participants.") 952 protected List<Annotation> note; 953 954 private static final long serialVersionUID = 2131096857L; 955 956 /** 957 * Constructor 958 */ 959 public CommunicationRequest() { 960 super(); 961 } 962 963 /** 964 * Constructor 965 */ 966 public CommunicationRequest(Enumeration<CommunicationRequestStatus> status) { 967 super(); 968 this.status = status; 969 } 970 971 /** 972 * @return {@link #identifier} (Business identifiers assigned to this 973 * communication request by the performer or other systems which remain 974 * constant as the resource is updated and propagates from server to 975 * server.) 976 */ 977 public List<Identifier> getIdentifier() { 978 if (this.identifier == null) 979 this.identifier = new ArrayList<Identifier>(); 980 return this.identifier; 981 } 982 983 /** 984 * @return Returns a reference to <code>this</code> for easy method chaining 985 */ 986 public CommunicationRequest setIdentifier(List<Identifier> theIdentifier) { 987 this.identifier = theIdentifier; 988 return this; 989 } 990 991 public boolean hasIdentifier() { 992 if (this.identifier == null) 993 return false; 994 for (Identifier item : this.identifier) 995 if (!item.isEmpty()) 996 return true; 997 return false; 998 } 999 1000 public Identifier addIdentifier() { // 3 1001 Identifier t = new Identifier(); 1002 if (this.identifier == null) 1003 this.identifier = new ArrayList<Identifier>(); 1004 this.identifier.add(t); 1005 return t; 1006 } 1007 1008 public CommunicationRequest addIdentifier(Identifier t) { // 3 1009 if (t == null) 1010 return this; 1011 if (this.identifier == null) 1012 this.identifier = new ArrayList<Identifier>(); 1013 this.identifier.add(t); 1014 return this; 1015 } 1016 1017 /** 1018 * @return The first repetition of repeating field {@link #identifier}, creating 1019 * it if it does not already exist 1020 */ 1021 public Identifier getIdentifierFirstRep() { 1022 if (getIdentifier().isEmpty()) { 1023 addIdentifier(); 1024 } 1025 return getIdentifier().get(0); 1026 } 1027 1028 /** 1029 * @return {@link #basedOn} (A plan or proposal that is fulfilled in whole or in 1030 * part by this request.) 1031 */ 1032 public List<Reference> getBasedOn() { 1033 if (this.basedOn == null) 1034 this.basedOn = new ArrayList<Reference>(); 1035 return this.basedOn; 1036 } 1037 1038 /** 1039 * @return Returns a reference to <code>this</code> for easy method chaining 1040 */ 1041 public CommunicationRequest setBasedOn(List<Reference> theBasedOn) { 1042 this.basedOn = theBasedOn; 1043 return this; 1044 } 1045 1046 public boolean hasBasedOn() { 1047 if (this.basedOn == null) 1048 return false; 1049 for (Reference item : this.basedOn) 1050 if (!item.isEmpty()) 1051 return true; 1052 return false; 1053 } 1054 1055 public Reference addBasedOn() { // 3 1056 Reference t = new Reference(); 1057 if (this.basedOn == null) 1058 this.basedOn = new ArrayList<Reference>(); 1059 this.basedOn.add(t); 1060 return t; 1061 } 1062 1063 public CommunicationRequest addBasedOn(Reference t) { // 3 1064 if (t == null) 1065 return this; 1066 if (this.basedOn == null) 1067 this.basedOn = new ArrayList<Reference>(); 1068 this.basedOn.add(t); 1069 return this; 1070 } 1071 1072 /** 1073 * @return The first repetition of repeating field {@link #basedOn}, creating it 1074 * if it does not already exist 1075 */ 1076 public Reference getBasedOnFirstRep() { 1077 if (getBasedOn().isEmpty()) { 1078 addBasedOn(); 1079 } 1080 return getBasedOn().get(0); 1081 } 1082 1083 /** 1084 * @deprecated Use Reference#setResource(IBaseResource) instead 1085 */ 1086 @Deprecated 1087 public List<Resource> getBasedOnTarget() { 1088 if (this.basedOnTarget == null) 1089 this.basedOnTarget = new ArrayList<Resource>(); 1090 return this.basedOnTarget; 1091 } 1092 1093 /** 1094 * @return {@link #replaces} (Completed or terminated request(s) whose function 1095 * is taken by this new request.) 1096 */ 1097 public List<Reference> getReplaces() { 1098 if (this.replaces == null) 1099 this.replaces = new ArrayList<Reference>(); 1100 return this.replaces; 1101 } 1102 1103 /** 1104 * @return Returns a reference to <code>this</code> for easy method chaining 1105 */ 1106 public CommunicationRequest setReplaces(List<Reference> theReplaces) { 1107 this.replaces = theReplaces; 1108 return this; 1109 } 1110 1111 public boolean hasReplaces() { 1112 if (this.replaces == null) 1113 return false; 1114 for (Reference item : this.replaces) 1115 if (!item.isEmpty()) 1116 return true; 1117 return false; 1118 } 1119 1120 public Reference addReplaces() { // 3 1121 Reference t = new Reference(); 1122 if (this.replaces == null) 1123 this.replaces = new ArrayList<Reference>(); 1124 this.replaces.add(t); 1125 return t; 1126 } 1127 1128 public CommunicationRequest addReplaces(Reference t) { // 3 1129 if (t == null) 1130 return this; 1131 if (this.replaces == null) 1132 this.replaces = new ArrayList<Reference>(); 1133 this.replaces.add(t); 1134 return this; 1135 } 1136 1137 /** 1138 * @return The first repetition of repeating field {@link #replaces}, creating 1139 * it if it does not already exist 1140 */ 1141 public Reference getReplacesFirstRep() { 1142 if (getReplaces().isEmpty()) { 1143 addReplaces(); 1144 } 1145 return getReplaces().get(0); 1146 } 1147 1148 /** 1149 * @deprecated Use Reference#setResource(IBaseResource) instead 1150 */ 1151 @Deprecated 1152 public List<CommunicationRequest> getReplacesTarget() { 1153 if (this.replacesTarget == null) 1154 this.replacesTarget = new ArrayList<CommunicationRequest>(); 1155 return this.replacesTarget; 1156 } 1157 1158 /** 1159 * @deprecated Use Reference#setResource(IBaseResource) instead 1160 */ 1161 @Deprecated 1162 public CommunicationRequest addReplacesTarget() { 1163 CommunicationRequest r = new CommunicationRequest(); 1164 if (this.replacesTarget == null) 1165 this.replacesTarget = new ArrayList<CommunicationRequest>(); 1166 this.replacesTarget.add(r); 1167 return r; 1168 } 1169 1170 /** 1171 * @return {@link #groupIdentifier} (A shared identifier common to all requests 1172 * that were authorized more or less simultaneously by a single author, 1173 * representing the identifier of the requisition, prescription or 1174 * similar form.) 1175 */ 1176 public Identifier getGroupIdentifier() { 1177 if (this.groupIdentifier == null) 1178 if (Configuration.errorOnAutoCreate()) 1179 throw new Error("Attempt to auto-create CommunicationRequest.groupIdentifier"); 1180 else if (Configuration.doAutoCreate()) 1181 this.groupIdentifier = new Identifier(); // cc 1182 return this.groupIdentifier; 1183 } 1184 1185 public boolean hasGroupIdentifier() { 1186 return this.groupIdentifier != null && !this.groupIdentifier.isEmpty(); 1187 } 1188 1189 /** 1190 * @param value {@link #groupIdentifier} (A shared identifier common to all 1191 * requests that were authorized more or less simultaneously by a 1192 * single author, representing the identifier of the requisition, 1193 * prescription or similar form.) 1194 */ 1195 public CommunicationRequest setGroupIdentifier(Identifier value) { 1196 this.groupIdentifier = value; 1197 return this; 1198 } 1199 1200 /** 1201 * @return {@link #status} (The status of the proposal or order.). This is the 1202 * underlying object with id, value and extensions. The accessor 1203 * "getStatus" gives direct access to the value 1204 */ 1205 public Enumeration<CommunicationRequestStatus> getStatusElement() { 1206 if (this.status == null) 1207 if (Configuration.errorOnAutoCreate()) 1208 throw new Error("Attempt to auto-create CommunicationRequest.status"); 1209 else if (Configuration.doAutoCreate()) 1210 this.status = new Enumeration<CommunicationRequestStatus>(new CommunicationRequestStatusEnumFactory()); // bb 1211 return this.status; 1212 } 1213 1214 public boolean hasStatusElement() { 1215 return this.status != null && !this.status.isEmpty(); 1216 } 1217 1218 public boolean hasStatus() { 1219 return this.status != null && !this.status.isEmpty(); 1220 } 1221 1222 /** 1223 * @param value {@link #status} (The status of the proposal or order.). This is 1224 * the underlying object with id, value and extensions. The 1225 * accessor "getStatus" gives direct access to the value 1226 */ 1227 public CommunicationRequest setStatusElement(Enumeration<CommunicationRequestStatus> value) { 1228 this.status = value; 1229 return this; 1230 } 1231 1232 /** 1233 * @return The status of the proposal or order. 1234 */ 1235 public CommunicationRequestStatus getStatus() { 1236 return this.status == null ? null : this.status.getValue(); 1237 } 1238 1239 /** 1240 * @param value The status of the proposal or order. 1241 */ 1242 public CommunicationRequest setStatus(CommunicationRequestStatus value) { 1243 if (this.status == null) 1244 this.status = new Enumeration<CommunicationRequestStatus>(new CommunicationRequestStatusEnumFactory()); 1245 this.status.setValue(value); 1246 return this; 1247 } 1248 1249 /** 1250 * @return {@link #statusReason} (Captures the reason for the current state of 1251 * the CommunicationRequest.) 1252 */ 1253 public CodeableConcept getStatusReason() { 1254 if (this.statusReason == null) 1255 if (Configuration.errorOnAutoCreate()) 1256 throw new Error("Attempt to auto-create CommunicationRequest.statusReason"); 1257 else if (Configuration.doAutoCreate()) 1258 this.statusReason = new CodeableConcept(); // cc 1259 return this.statusReason; 1260 } 1261 1262 public boolean hasStatusReason() { 1263 return this.statusReason != null && !this.statusReason.isEmpty(); 1264 } 1265 1266 /** 1267 * @param value {@link #statusReason} (Captures the reason for the current state 1268 * of the CommunicationRequest.) 1269 */ 1270 public CommunicationRequest setStatusReason(CodeableConcept value) { 1271 this.statusReason = value; 1272 return this; 1273 } 1274 1275 /** 1276 * @return {@link #category} (The type of message to be sent such as alert, 1277 * notification, reminder, instruction, etc.) 1278 */ 1279 public List<CodeableConcept> getCategory() { 1280 if (this.category == null) 1281 this.category = new ArrayList<CodeableConcept>(); 1282 return this.category; 1283 } 1284 1285 /** 1286 * @return Returns a reference to <code>this</code> for easy method chaining 1287 */ 1288 public CommunicationRequest setCategory(List<CodeableConcept> theCategory) { 1289 this.category = theCategory; 1290 return this; 1291 } 1292 1293 public boolean hasCategory() { 1294 if (this.category == null) 1295 return false; 1296 for (CodeableConcept item : this.category) 1297 if (!item.isEmpty()) 1298 return true; 1299 return false; 1300 } 1301 1302 public CodeableConcept addCategory() { // 3 1303 CodeableConcept t = new CodeableConcept(); 1304 if (this.category == null) 1305 this.category = new ArrayList<CodeableConcept>(); 1306 this.category.add(t); 1307 return t; 1308 } 1309 1310 public CommunicationRequest addCategory(CodeableConcept t) { // 3 1311 if (t == null) 1312 return this; 1313 if (this.category == null) 1314 this.category = new ArrayList<CodeableConcept>(); 1315 this.category.add(t); 1316 return this; 1317 } 1318 1319 /** 1320 * @return The first repetition of repeating field {@link #category}, creating 1321 * it if it does not already exist 1322 */ 1323 public CodeableConcept getCategoryFirstRep() { 1324 if (getCategory().isEmpty()) { 1325 addCategory(); 1326 } 1327 return getCategory().get(0); 1328 } 1329 1330 /** 1331 * @return {@link #priority} (Characterizes how quickly the proposed act must be 1332 * initiated. Includes concepts such as stat, urgent, routine.). This is 1333 * the underlying object with id, value and extensions. The accessor 1334 * "getPriority" gives direct access to the value 1335 */ 1336 public Enumeration<CommunicationPriority> getPriorityElement() { 1337 if (this.priority == null) 1338 if (Configuration.errorOnAutoCreate()) 1339 throw new Error("Attempt to auto-create CommunicationRequest.priority"); 1340 else if (Configuration.doAutoCreate()) 1341 this.priority = new Enumeration<CommunicationPriority>(new CommunicationPriorityEnumFactory()); // bb 1342 return this.priority; 1343 } 1344 1345 public boolean hasPriorityElement() { 1346 return this.priority != null && !this.priority.isEmpty(); 1347 } 1348 1349 public boolean hasPriority() { 1350 return this.priority != null && !this.priority.isEmpty(); 1351 } 1352 1353 /** 1354 * @param value {@link #priority} (Characterizes how quickly the proposed act 1355 * must be initiated. Includes concepts such as stat, urgent, 1356 * routine.). This is the underlying object with id, value and 1357 * extensions. The accessor "getPriority" gives direct access to 1358 * the value 1359 */ 1360 public CommunicationRequest setPriorityElement(Enumeration<CommunicationPriority> value) { 1361 this.priority = value; 1362 return this; 1363 } 1364 1365 /** 1366 * @return Characterizes how quickly the proposed act must be initiated. 1367 * Includes concepts such as stat, urgent, routine. 1368 */ 1369 public CommunicationPriority getPriority() { 1370 return this.priority == null ? null : this.priority.getValue(); 1371 } 1372 1373 /** 1374 * @param value Characterizes how quickly the proposed act must be initiated. 1375 * Includes concepts such as stat, urgent, routine. 1376 */ 1377 public CommunicationRequest setPriority(CommunicationPriority value) { 1378 if (value == null) 1379 this.priority = null; 1380 else { 1381 if (this.priority == null) 1382 this.priority = new Enumeration<CommunicationPriority>(new CommunicationPriorityEnumFactory()); 1383 this.priority.setValue(value); 1384 } 1385 return this; 1386 } 1387 1388 /** 1389 * @return {@link #doNotPerform} (If true indicates that the 1390 * CommunicationRequest is asking for the specified action to *not* 1391 * occur.). This is the underlying object with id, value and extensions. 1392 * The accessor "getDoNotPerform" gives direct access to the value 1393 */ 1394 public BooleanType getDoNotPerformElement() { 1395 if (this.doNotPerform == null) 1396 if (Configuration.errorOnAutoCreate()) 1397 throw new Error("Attempt to auto-create CommunicationRequest.doNotPerform"); 1398 else if (Configuration.doAutoCreate()) 1399 this.doNotPerform = new BooleanType(); // bb 1400 return this.doNotPerform; 1401 } 1402 1403 public boolean hasDoNotPerformElement() { 1404 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 1405 } 1406 1407 public boolean hasDoNotPerform() { 1408 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 1409 } 1410 1411 /** 1412 * @param value {@link #doNotPerform} (If true indicates that the 1413 * CommunicationRequest is asking for the specified action to *not* 1414 * occur.). This is the underlying object with id, value and 1415 * extensions. The accessor "getDoNotPerform" gives direct access 1416 * to the value 1417 */ 1418 public CommunicationRequest setDoNotPerformElement(BooleanType value) { 1419 this.doNotPerform = value; 1420 return this; 1421 } 1422 1423 /** 1424 * @return If true indicates that the CommunicationRequest is asking for the 1425 * specified action to *not* occur. 1426 */ 1427 public boolean getDoNotPerform() { 1428 return this.doNotPerform == null || this.doNotPerform.isEmpty() ? false : this.doNotPerform.getValue(); 1429 } 1430 1431 /** 1432 * @param value If true indicates that the CommunicationRequest is asking for 1433 * the specified action to *not* occur. 1434 */ 1435 public CommunicationRequest setDoNotPerform(boolean value) { 1436 if (this.doNotPerform == null) 1437 this.doNotPerform = new BooleanType(); 1438 this.doNotPerform.setValue(value); 1439 return this; 1440 } 1441 1442 /** 1443 * @return {@link #medium} (A channel that was used for this communication (e.g. 1444 * email, fax).) 1445 */ 1446 public List<CodeableConcept> getMedium() { 1447 if (this.medium == null) 1448 this.medium = new ArrayList<CodeableConcept>(); 1449 return this.medium; 1450 } 1451 1452 /** 1453 * @return Returns a reference to <code>this</code> for easy method chaining 1454 */ 1455 public CommunicationRequest setMedium(List<CodeableConcept> theMedium) { 1456 this.medium = theMedium; 1457 return this; 1458 } 1459 1460 public boolean hasMedium() { 1461 if (this.medium == null) 1462 return false; 1463 for (CodeableConcept item : this.medium) 1464 if (!item.isEmpty()) 1465 return true; 1466 return false; 1467 } 1468 1469 public CodeableConcept addMedium() { // 3 1470 CodeableConcept t = new CodeableConcept(); 1471 if (this.medium == null) 1472 this.medium = new ArrayList<CodeableConcept>(); 1473 this.medium.add(t); 1474 return t; 1475 } 1476 1477 public CommunicationRequest addMedium(CodeableConcept t) { // 3 1478 if (t == null) 1479 return this; 1480 if (this.medium == null) 1481 this.medium = new ArrayList<CodeableConcept>(); 1482 this.medium.add(t); 1483 return this; 1484 } 1485 1486 /** 1487 * @return The first repetition of repeating field {@link #medium}, creating it 1488 * if it does not already exist 1489 */ 1490 public CodeableConcept getMediumFirstRep() { 1491 if (getMedium().isEmpty()) { 1492 addMedium(); 1493 } 1494 return getMedium().get(0); 1495 } 1496 1497 /** 1498 * @return {@link #subject} (The patient or group that is the focus of this 1499 * communication request.) 1500 */ 1501 public Reference getSubject() { 1502 if (this.subject == null) 1503 if (Configuration.errorOnAutoCreate()) 1504 throw new Error("Attempt to auto-create CommunicationRequest.subject"); 1505 else if (Configuration.doAutoCreate()) 1506 this.subject = new Reference(); // cc 1507 return this.subject; 1508 } 1509 1510 public boolean hasSubject() { 1511 return this.subject != null && !this.subject.isEmpty(); 1512 } 1513 1514 /** 1515 * @param value {@link #subject} (The patient or group that is the focus of this 1516 * communication request.) 1517 */ 1518 public CommunicationRequest setSubject(Reference value) { 1519 this.subject = value; 1520 return this; 1521 } 1522 1523 /** 1524 * @return {@link #subject} The actual object that is the target of the 1525 * reference. The reference library doesn't populate this, but you can 1526 * use it to hold the resource if you resolve it. (The patient or group 1527 * that is the focus of this communication request.) 1528 */ 1529 public Resource getSubjectTarget() { 1530 return this.subjectTarget; 1531 } 1532 1533 /** 1534 * @param value {@link #subject} The actual object that is the target of the 1535 * reference. The reference library doesn't use these, but you can 1536 * use it to hold the resource if you resolve it. (The patient or 1537 * group that is the focus of this communication request.) 1538 */ 1539 public CommunicationRequest setSubjectTarget(Resource value) { 1540 this.subjectTarget = value; 1541 return this; 1542 } 1543 1544 /** 1545 * @return {@link #about} (Other resources that pertain to this communication 1546 * request and to which this communication request should be 1547 * associated.) 1548 */ 1549 public List<Reference> getAbout() { 1550 if (this.about == null) 1551 this.about = new ArrayList<Reference>(); 1552 return this.about; 1553 } 1554 1555 /** 1556 * @return Returns a reference to <code>this</code> for easy method chaining 1557 */ 1558 public CommunicationRequest setAbout(List<Reference> theAbout) { 1559 this.about = theAbout; 1560 return this; 1561 } 1562 1563 public boolean hasAbout() { 1564 if (this.about == null) 1565 return false; 1566 for (Reference item : this.about) 1567 if (!item.isEmpty()) 1568 return true; 1569 return false; 1570 } 1571 1572 public Reference addAbout() { // 3 1573 Reference t = new Reference(); 1574 if (this.about == null) 1575 this.about = new ArrayList<Reference>(); 1576 this.about.add(t); 1577 return t; 1578 } 1579 1580 public CommunicationRequest addAbout(Reference t) { // 3 1581 if (t == null) 1582 return this; 1583 if (this.about == null) 1584 this.about = new ArrayList<Reference>(); 1585 this.about.add(t); 1586 return this; 1587 } 1588 1589 /** 1590 * @return The first repetition of repeating field {@link #about}, creating it 1591 * if it does not already exist 1592 */ 1593 public Reference getAboutFirstRep() { 1594 if (getAbout().isEmpty()) { 1595 addAbout(); 1596 } 1597 return getAbout().get(0); 1598 } 1599 1600 /** 1601 * @deprecated Use Reference#setResource(IBaseResource) instead 1602 */ 1603 @Deprecated 1604 public List<Resource> getAboutTarget() { 1605 if (this.aboutTarget == null) 1606 this.aboutTarget = new ArrayList<Resource>(); 1607 return this.aboutTarget; 1608 } 1609 1610 /** 1611 * @return {@link #encounter} (The Encounter during which this 1612 * CommunicationRequest was created or to which the creation of this 1613 * record is tightly associated.) 1614 */ 1615 public Reference getEncounter() { 1616 if (this.encounter == null) 1617 if (Configuration.errorOnAutoCreate()) 1618 throw new Error("Attempt to auto-create CommunicationRequest.encounter"); 1619 else if (Configuration.doAutoCreate()) 1620 this.encounter = new Reference(); // cc 1621 return this.encounter; 1622 } 1623 1624 public boolean hasEncounter() { 1625 return this.encounter != null && !this.encounter.isEmpty(); 1626 } 1627 1628 /** 1629 * @param value {@link #encounter} (The Encounter during which this 1630 * CommunicationRequest was created or to which the creation of 1631 * this record is tightly associated.) 1632 */ 1633 public CommunicationRequest setEncounter(Reference value) { 1634 this.encounter = value; 1635 return this; 1636 } 1637 1638 /** 1639 * @return {@link #encounter} The actual object that is the target of the 1640 * reference. The reference library doesn't populate this, but you can 1641 * use it to hold the resource if you resolve it. (The Encounter during 1642 * which this CommunicationRequest was created or to which the creation 1643 * of this record is tightly associated.) 1644 */ 1645 public Encounter getEncounterTarget() { 1646 if (this.encounterTarget == null) 1647 if (Configuration.errorOnAutoCreate()) 1648 throw new Error("Attempt to auto-create CommunicationRequest.encounter"); 1649 else if (Configuration.doAutoCreate()) 1650 this.encounterTarget = new Encounter(); // aa 1651 return this.encounterTarget; 1652 } 1653 1654 /** 1655 * @param value {@link #encounter} The actual object that is the target of the 1656 * reference. The reference library doesn't use these, but you can 1657 * use it to hold the resource if you resolve it. (The Encounter 1658 * during which this CommunicationRequest was created or to which 1659 * the creation of this record is tightly associated.) 1660 */ 1661 public CommunicationRequest setEncounterTarget(Encounter value) { 1662 this.encounterTarget = value; 1663 return this; 1664 } 1665 1666 /** 1667 * @return {@link #payload} (Text, attachment(s), or resource(s) to be 1668 * communicated to the recipient.) 1669 */ 1670 public List<CommunicationRequestPayloadComponent> getPayload() { 1671 if (this.payload == null) 1672 this.payload = new ArrayList<CommunicationRequestPayloadComponent>(); 1673 return this.payload; 1674 } 1675 1676 /** 1677 * @return Returns a reference to <code>this</code> for easy method chaining 1678 */ 1679 public CommunicationRequest setPayload(List<CommunicationRequestPayloadComponent> thePayload) { 1680 this.payload = thePayload; 1681 return this; 1682 } 1683 1684 public boolean hasPayload() { 1685 if (this.payload == null) 1686 return false; 1687 for (CommunicationRequestPayloadComponent item : this.payload) 1688 if (!item.isEmpty()) 1689 return true; 1690 return false; 1691 } 1692 1693 public CommunicationRequestPayloadComponent addPayload() { // 3 1694 CommunicationRequestPayloadComponent t = new CommunicationRequestPayloadComponent(); 1695 if (this.payload == null) 1696 this.payload = new ArrayList<CommunicationRequestPayloadComponent>(); 1697 this.payload.add(t); 1698 return t; 1699 } 1700 1701 public CommunicationRequest addPayload(CommunicationRequestPayloadComponent t) { // 3 1702 if (t == null) 1703 return this; 1704 if (this.payload == null) 1705 this.payload = new ArrayList<CommunicationRequestPayloadComponent>(); 1706 this.payload.add(t); 1707 return this; 1708 } 1709 1710 /** 1711 * @return The first repetition of repeating field {@link #payload}, creating it 1712 * if it does not already exist 1713 */ 1714 public CommunicationRequestPayloadComponent getPayloadFirstRep() { 1715 if (getPayload().isEmpty()) { 1716 addPayload(); 1717 } 1718 return getPayload().get(0); 1719 } 1720 1721 /** 1722 * @return {@link #occurrence} (The time when this communication is to occur.) 1723 */ 1724 public Type getOccurrence() { 1725 return this.occurrence; 1726 } 1727 1728 /** 1729 * @return {@link #occurrence} (The time when this communication is to occur.) 1730 */ 1731 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 1732 if (this.occurrence == null) 1733 this.occurrence = new DateTimeType(); 1734 if (!(this.occurrence instanceof DateTimeType)) 1735 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1736 + this.occurrence.getClass().getName() + " was encountered"); 1737 return (DateTimeType) this.occurrence; 1738 } 1739 1740 public boolean hasOccurrenceDateTimeType() { 1741 return this != null && this.occurrence instanceof DateTimeType; 1742 } 1743 1744 /** 1745 * @return {@link #occurrence} (The time when this communication is to occur.) 1746 */ 1747 public Period getOccurrencePeriod() throws FHIRException { 1748 if (this.occurrence == null) 1749 this.occurrence = new Period(); 1750 if (!(this.occurrence instanceof Period)) 1751 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.occurrence.getClass().getName() 1752 + " was encountered"); 1753 return (Period) this.occurrence; 1754 } 1755 1756 public boolean hasOccurrencePeriod() { 1757 return this != null && this.occurrence instanceof Period; 1758 } 1759 1760 public boolean hasOccurrence() { 1761 return this.occurrence != null && !this.occurrence.isEmpty(); 1762 } 1763 1764 /** 1765 * @param value {@link #occurrence} (The time when this communication is to 1766 * occur.) 1767 */ 1768 public CommunicationRequest setOccurrence(Type value) { 1769 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1770 throw new Error("Not the right type for CommunicationRequest.occurrence[x]: " + value.fhirType()); 1771 this.occurrence = value; 1772 return this; 1773 } 1774 1775 /** 1776 * @return {@link #authoredOn} (For draft requests, indicates the date of 1777 * initial creation. For requests with other statuses, indicates the 1778 * date of activation.). This is the underlying object with id, value 1779 * and extensions. The accessor "getAuthoredOn" gives direct access to 1780 * the value 1781 */ 1782 public DateTimeType getAuthoredOnElement() { 1783 if (this.authoredOn == null) 1784 if (Configuration.errorOnAutoCreate()) 1785 throw new Error("Attempt to auto-create CommunicationRequest.authoredOn"); 1786 else if (Configuration.doAutoCreate()) 1787 this.authoredOn = new DateTimeType(); // bb 1788 return this.authoredOn; 1789 } 1790 1791 public boolean hasAuthoredOnElement() { 1792 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1793 } 1794 1795 public boolean hasAuthoredOn() { 1796 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1797 } 1798 1799 /** 1800 * @param value {@link #authoredOn} (For draft requests, indicates the date of 1801 * initial creation. For requests with other statuses, indicates 1802 * the date of activation.). This is the underlying object with id, 1803 * value and extensions. The accessor "getAuthoredOn" gives direct 1804 * access to the value 1805 */ 1806 public CommunicationRequest setAuthoredOnElement(DateTimeType value) { 1807 this.authoredOn = value; 1808 return this; 1809 } 1810 1811 /** 1812 * @return For draft requests, indicates the date of initial creation. For 1813 * requests with other statuses, indicates the date of activation. 1814 */ 1815 public Date getAuthoredOn() { 1816 return this.authoredOn == null ? null : this.authoredOn.getValue(); 1817 } 1818 1819 /** 1820 * @param value For draft requests, indicates the date of initial creation. For 1821 * requests with other statuses, indicates the date of activation. 1822 */ 1823 public CommunicationRequest setAuthoredOn(Date value) { 1824 if (value == null) 1825 this.authoredOn = null; 1826 else { 1827 if (this.authoredOn == null) 1828 this.authoredOn = new DateTimeType(); 1829 this.authoredOn.setValue(value); 1830 } 1831 return this; 1832 } 1833 1834 /** 1835 * @return {@link #requester} (The device, individual, or organization who 1836 * initiated the request and has responsibility for its activation.) 1837 */ 1838 public Reference getRequester() { 1839 if (this.requester == null) 1840 if (Configuration.errorOnAutoCreate()) 1841 throw new Error("Attempt to auto-create CommunicationRequest.requester"); 1842 else if (Configuration.doAutoCreate()) 1843 this.requester = new Reference(); // cc 1844 return this.requester; 1845 } 1846 1847 public boolean hasRequester() { 1848 return this.requester != null && !this.requester.isEmpty(); 1849 } 1850 1851 /** 1852 * @param value {@link #requester} (The device, individual, or organization who 1853 * initiated the request and has responsibility for its 1854 * activation.) 1855 */ 1856 public CommunicationRequest setRequester(Reference value) { 1857 this.requester = value; 1858 return this; 1859 } 1860 1861 /** 1862 * @return {@link #requester} The actual object that is the target of the 1863 * reference. The reference library doesn't populate this, but you can 1864 * use it to hold the resource if you resolve it. (The device, 1865 * individual, or organization who initiated the request and has 1866 * responsibility for its activation.) 1867 */ 1868 public Resource getRequesterTarget() { 1869 return this.requesterTarget; 1870 } 1871 1872 /** 1873 * @param value {@link #requester} The actual object that is the target of the 1874 * reference. The reference library doesn't use these, but you can 1875 * use it to hold the resource if you resolve it. (The device, 1876 * individual, or organization who initiated the request and has 1877 * responsibility for its activation.) 1878 */ 1879 public CommunicationRequest setRequesterTarget(Resource value) { 1880 this.requesterTarget = value; 1881 return this; 1882 } 1883 1884 /** 1885 * @return {@link #recipient} (The entity (e.g. person, organization, clinical 1886 * information system, device, group, or care team) which is the 1887 * intended target of the communication.) 1888 */ 1889 public List<Reference> getRecipient() { 1890 if (this.recipient == null) 1891 this.recipient = new ArrayList<Reference>(); 1892 return this.recipient; 1893 } 1894 1895 /** 1896 * @return Returns a reference to <code>this</code> for easy method chaining 1897 */ 1898 public CommunicationRequest setRecipient(List<Reference> theRecipient) { 1899 this.recipient = theRecipient; 1900 return this; 1901 } 1902 1903 public boolean hasRecipient() { 1904 if (this.recipient == null) 1905 return false; 1906 for (Reference item : this.recipient) 1907 if (!item.isEmpty()) 1908 return true; 1909 return false; 1910 } 1911 1912 public Reference addRecipient() { // 3 1913 Reference t = new Reference(); 1914 if (this.recipient == null) 1915 this.recipient = new ArrayList<Reference>(); 1916 this.recipient.add(t); 1917 return t; 1918 } 1919 1920 public CommunicationRequest addRecipient(Reference t) { // 3 1921 if (t == null) 1922 return this; 1923 if (this.recipient == null) 1924 this.recipient = new ArrayList<Reference>(); 1925 this.recipient.add(t); 1926 return this; 1927 } 1928 1929 /** 1930 * @return The first repetition of repeating field {@link #recipient}, creating 1931 * it if it does not already exist 1932 */ 1933 public Reference getRecipientFirstRep() { 1934 if (getRecipient().isEmpty()) { 1935 addRecipient(); 1936 } 1937 return getRecipient().get(0); 1938 } 1939 1940 /** 1941 * @deprecated Use Reference#setResource(IBaseResource) instead 1942 */ 1943 @Deprecated 1944 public List<Resource> getRecipientTarget() { 1945 if (this.recipientTarget == null) 1946 this.recipientTarget = new ArrayList<Resource>(); 1947 return this.recipientTarget; 1948 } 1949 1950 /** 1951 * @return {@link #sender} (The entity (e.g. person, organization, clinical 1952 * information system, or device) which is to be the source of the 1953 * communication.) 1954 */ 1955 public Reference getSender() { 1956 if (this.sender == null) 1957 if (Configuration.errorOnAutoCreate()) 1958 throw new Error("Attempt to auto-create CommunicationRequest.sender"); 1959 else if (Configuration.doAutoCreate()) 1960 this.sender = new Reference(); // cc 1961 return this.sender; 1962 } 1963 1964 public boolean hasSender() { 1965 return this.sender != null && !this.sender.isEmpty(); 1966 } 1967 1968 /** 1969 * @param value {@link #sender} (The entity (e.g. person, organization, clinical 1970 * information system, or device) which is to be the source of the 1971 * communication.) 1972 */ 1973 public CommunicationRequest setSender(Reference value) { 1974 this.sender = value; 1975 return this; 1976 } 1977 1978 /** 1979 * @return {@link #sender} The actual object that is the target of the 1980 * reference. The reference library doesn't populate this, but you can 1981 * use it to hold the resource if you resolve it. (The entity (e.g. 1982 * person, organization, clinical information system, or device) which 1983 * is to be the source of the communication.) 1984 */ 1985 public Resource getSenderTarget() { 1986 return this.senderTarget; 1987 } 1988 1989 /** 1990 * @param value {@link #sender} The actual object that is the target of the 1991 * reference. The reference library doesn't use these, but you can 1992 * use it to hold the resource if you resolve it. (The entity (e.g. 1993 * person, organization, clinical information system, or device) 1994 * which is to be the source of the communication.) 1995 */ 1996 public CommunicationRequest setSenderTarget(Resource value) { 1997 this.senderTarget = value; 1998 return this; 1999 } 2000 2001 /** 2002 * @return {@link #reasonCode} (Describes why the request is being made in coded 2003 * or textual form.) 2004 */ 2005 public List<CodeableConcept> getReasonCode() { 2006 if (this.reasonCode == null) 2007 this.reasonCode = new ArrayList<CodeableConcept>(); 2008 return this.reasonCode; 2009 } 2010 2011 /** 2012 * @return Returns a reference to <code>this</code> for easy method chaining 2013 */ 2014 public CommunicationRequest setReasonCode(List<CodeableConcept> theReasonCode) { 2015 this.reasonCode = theReasonCode; 2016 return this; 2017 } 2018 2019 public boolean hasReasonCode() { 2020 if (this.reasonCode == null) 2021 return false; 2022 for (CodeableConcept item : this.reasonCode) 2023 if (!item.isEmpty()) 2024 return true; 2025 return false; 2026 } 2027 2028 public CodeableConcept addReasonCode() { // 3 2029 CodeableConcept t = new CodeableConcept(); 2030 if (this.reasonCode == null) 2031 this.reasonCode = new ArrayList<CodeableConcept>(); 2032 this.reasonCode.add(t); 2033 return t; 2034 } 2035 2036 public CommunicationRequest addReasonCode(CodeableConcept t) { // 3 2037 if (t == null) 2038 return this; 2039 if (this.reasonCode == null) 2040 this.reasonCode = new ArrayList<CodeableConcept>(); 2041 this.reasonCode.add(t); 2042 return this; 2043 } 2044 2045 /** 2046 * @return The first repetition of repeating field {@link #reasonCode}, creating 2047 * it if it does not already exist 2048 */ 2049 public CodeableConcept getReasonCodeFirstRep() { 2050 if (getReasonCode().isEmpty()) { 2051 addReasonCode(); 2052 } 2053 return getReasonCode().get(0); 2054 } 2055 2056 /** 2057 * @return {@link #reasonReference} (Indicates another resource whose existence 2058 * justifies this request.) 2059 */ 2060 public List<Reference> getReasonReference() { 2061 if (this.reasonReference == null) 2062 this.reasonReference = new ArrayList<Reference>(); 2063 return this.reasonReference; 2064 } 2065 2066 /** 2067 * @return Returns a reference to <code>this</code> for easy method chaining 2068 */ 2069 public CommunicationRequest setReasonReference(List<Reference> theReasonReference) { 2070 this.reasonReference = theReasonReference; 2071 return this; 2072 } 2073 2074 public boolean hasReasonReference() { 2075 if (this.reasonReference == null) 2076 return false; 2077 for (Reference item : this.reasonReference) 2078 if (!item.isEmpty()) 2079 return true; 2080 return false; 2081 } 2082 2083 public Reference addReasonReference() { // 3 2084 Reference t = new Reference(); 2085 if (this.reasonReference == null) 2086 this.reasonReference = new ArrayList<Reference>(); 2087 this.reasonReference.add(t); 2088 return t; 2089 } 2090 2091 public CommunicationRequest addReasonReference(Reference t) { // 3 2092 if (t == null) 2093 return this; 2094 if (this.reasonReference == null) 2095 this.reasonReference = new ArrayList<Reference>(); 2096 this.reasonReference.add(t); 2097 return this; 2098 } 2099 2100 /** 2101 * @return The first repetition of repeating field {@link #reasonReference}, 2102 * creating it if it does not already exist 2103 */ 2104 public Reference getReasonReferenceFirstRep() { 2105 if (getReasonReference().isEmpty()) { 2106 addReasonReference(); 2107 } 2108 return getReasonReference().get(0); 2109 } 2110 2111 /** 2112 * @deprecated Use Reference#setResource(IBaseResource) instead 2113 */ 2114 @Deprecated 2115 public List<Resource> getReasonReferenceTarget() { 2116 if (this.reasonReferenceTarget == null) 2117 this.reasonReferenceTarget = new ArrayList<Resource>(); 2118 return this.reasonReferenceTarget; 2119 } 2120 2121 /** 2122 * @return {@link #note} (Comments made about the request by the requester, 2123 * sender, recipient, subject or other participants.) 2124 */ 2125 public List<Annotation> getNote() { 2126 if (this.note == null) 2127 this.note = new ArrayList<Annotation>(); 2128 return this.note; 2129 } 2130 2131 /** 2132 * @return Returns a reference to <code>this</code> for easy method chaining 2133 */ 2134 public CommunicationRequest setNote(List<Annotation> theNote) { 2135 this.note = theNote; 2136 return this; 2137 } 2138 2139 public boolean hasNote() { 2140 if (this.note == null) 2141 return false; 2142 for (Annotation item : this.note) 2143 if (!item.isEmpty()) 2144 return true; 2145 return false; 2146 } 2147 2148 public Annotation addNote() { // 3 2149 Annotation t = new Annotation(); 2150 if (this.note == null) 2151 this.note = new ArrayList<Annotation>(); 2152 this.note.add(t); 2153 return t; 2154 } 2155 2156 public CommunicationRequest addNote(Annotation t) { // 3 2157 if (t == null) 2158 return this; 2159 if (this.note == null) 2160 this.note = new ArrayList<Annotation>(); 2161 this.note.add(t); 2162 return this; 2163 } 2164 2165 /** 2166 * @return The first repetition of repeating field {@link #note}, creating it if 2167 * it does not already exist 2168 */ 2169 public Annotation getNoteFirstRep() { 2170 if (getNote().isEmpty()) { 2171 addNote(); 2172 } 2173 return getNote().get(0); 2174 } 2175 2176 protected void listChildren(List<Property> children) { 2177 super.listChildren(children); 2178 children.add(new Property("identifier", "Identifier", 2179 "Business identifiers assigned to this communication request by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 2180 0, java.lang.Integer.MAX_VALUE, identifier)); 2181 children.add(new Property("basedOn", "Reference(Any)", 2182 "A plan or proposal that is fulfilled in whole or in part by this request.", 0, java.lang.Integer.MAX_VALUE, 2183 basedOn)); 2184 children.add(new Property("replaces", "Reference(CommunicationRequest)", 2185 "Completed or terminated request(s) whose function is taken by this new request.", 0, 2186 java.lang.Integer.MAX_VALUE, replaces)); 2187 children.add(new Property("groupIdentifier", "Identifier", 2188 "A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.", 2189 0, 1, groupIdentifier)); 2190 children.add(new Property("status", "code", "The status of the proposal or order.", 0, 1, status)); 2191 children.add(new Property("statusReason", "CodeableConcept", 2192 "Captures the reason for the current state of the CommunicationRequest.", 0, 1, statusReason)); 2193 children.add(new Property("category", "CodeableConcept", 2194 "The type of message to be sent such as alert, notification, reminder, instruction, etc.", 0, 2195 java.lang.Integer.MAX_VALUE, category)); 2196 children.add(new Property("priority", "code", 2197 "Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine.", 2198 0, 1, priority)); 2199 children.add(new Property("doNotPerform", "boolean", 2200 "If true indicates that the CommunicationRequest is asking for the specified action to *not* occur.", 0, 1, 2201 doNotPerform)); 2202 children.add(new Property("medium", "CodeableConcept", 2203 "A channel that was used for this communication (e.g. email, fax).", 0, java.lang.Integer.MAX_VALUE, medium)); 2204 children.add(new Property("subject", "Reference(Patient|Group)", 2205 "The patient or group that is the focus of this communication request.", 0, 1, subject)); 2206 children.add(new Property("about", "Reference(Any)", 2207 "Other resources that pertain to this communication request and to which this communication request should be associated.", 2208 0, java.lang.Integer.MAX_VALUE, about)); 2209 children.add(new Property("encounter", "Reference(Encounter)", 2210 "The Encounter during which this CommunicationRequest was created or to which the creation of this record is tightly associated.", 2211 0, 1, encounter)); 2212 children.add(new Property("payload", "", "Text, attachment(s), or resource(s) to be communicated to the recipient.", 2213 0, java.lang.Integer.MAX_VALUE, payload)); 2214 children.add(new Property("occurrence[x]", "dateTime|Period", "The time when this communication is to occur.", 0, 1, 2215 occurrence)); 2216 children.add(new Property("authoredOn", "dateTime", 2217 "For draft requests, indicates the date of initial creation. For requests with other statuses, indicates the date of activation.", 2218 0, 1, authoredOn)); 2219 children.add(new Property("requester", 2220 "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device)", 2221 "The device, individual, or organization who initiated the request and has responsibility for its activation.", 2222 0, 1, requester)); 2223 children.add(new Property("recipient", 2224 "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson|Group|CareTeam|HealthcareService)", 2225 "The entity (e.g. person, organization, clinical information system, device, group, or care team) which is the intended target of the communication.", 2226 0, java.lang.Integer.MAX_VALUE, recipient)); 2227 children.add(new Property("sender", 2228 "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson|HealthcareService)", 2229 "The entity (e.g. person, organization, clinical information system, or device) which is to be the source of the communication.", 2230 0, 1, sender)); 2231 children.add(new Property("reasonCode", "CodeableConcept", 2232 "Describes why the request is being made in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, 2233 reasonCode)); 2234 children.add(new Property("reasonReference", "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 2235 "Indicates another resource whose existence justifies this request.", 0, java.lang.Integer.MAX_VALUE, 2236 reasonReference)); 2237 children.add(new Property("note", "Annotation", 2238 "Comments made about the request by the requester, sender, recipient, subject or other participants.", 0, 2239 java.lang.Integer.MAX_VALUE, note)); 2240 } 2241 2242 @Override 2243 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2244 switch (_hash) { 2245 case -1618432855: 2246 /* identifier */ return new Property("identifier", "Identifier", 2247 "Business identifiers assigned to this communication request by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 2248 0, java.lang.Integer.MAX_VALUE, identifier); 2249 case -332612366: 2250 /* basedOn */ return new Property("basedOn", "Reference(Any)", 2251 "A plan or proposal that is fulfilled in whole or in part by this request.", 0, java.lang.Integer.MAX_VALUE, 2252 basedOn); 2253 case -430332865: 2254 /* replaces */ return new Property("replaces", "Reference(CommunicationRequest)", 2255 "Completed or terminated request(s) whose function is taken by this new request.", 0, 2256 java.lang.Integer.MAX_VALUE, replaces); 2257 case -445338488: 2258 /* groupIdentifier */ return new Property("groupIdentifier", "Identifier", 2259 "A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.", 2260 0, 1, groupIdentifier); 2261 case -892481550: 2262 /* status */ return new Property("status", "code", "The status of the proposal or order.", 0, 1, status); 2263 case 2051346646: 2264 /* statusReason */ return new Property("statusReason", "CodeableConcept", 2265 "Captures the reason for the current state of the CommunicationRequest.", 0, 1, statusReason); 2266 case 50511102: 2267 /* category */ return new Property("category", "CodeableConcept", 2268 "The type of message to be sent such as alert, notification, reminder, instruction, etc.", 0, 2269 java.lang.Integer.MAX_VALUE, category); 2270 case -1165461084: 2271 /* priority */ return new Property("priority", "code", 2272 "Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine.", 2273 0, 1, priority); 2274 case -1788508167: 2275 /* doNotPerform */ return new Property("doNotPerform", "boolean", 2276 "If true indicates that the CommunicationRequest is asking for the specified action to *not* occur.", 0, 1, 2277 doNotPerform); 2278 case -1078030475: 2279 /* medium */ return new Property("medium", "CodeableConcept", 2280 "A channel that was used for this communication (e.g. email, fax).", 0, java.lang.Integer.MAX_VALUE, medium); 2281 case -1867885268: 2282 /* subject */ return new Property("subject", "Reference(Patient|Group)", 2283 "The patient or group that is the focus of this communication request.", 0, 1, subject); 2284 case 92611469: 2285 /* about */ return new Property("about", "Reference(Any)", 2286 "Other resources that pertain to this communication request and to which this communication request should be associated.", 2287 0, java.lang.Integer.MAX_VALUE, about); 2288 case 1524132147: 2289 /* encounter */ return new Property("encounter", "Reference(Encounter)", 2290 "The Encounter during which this CommunicationRequest was created or to which the creation of this record is tightly associated.", 2291 0, 1, encounter); 2292 case -786701938: 2293 /* payload */ return new Property("payload", "", 2294 "Text, attachment(s), or resource(s) to be communicated to the recipient.", 0, java.lang.Integer.MAX_VALUE, 2295 payload); 2296 case -2022646513: 2297 /* occurrence[x] */ return new Property("occurrence[x]", "dateTime|Period", 2298 "The time when this communication is to occur.", 0, 1, occurrence); 2299 case 1687874001: 2300 /* occurrence */ return new Property("occurrence[x]", "dateTime|Period", 2301 "The time when this communication is to occur.", 0, 1, occurrence); 2302 case -298443636: 2303 /* occurrenceDateTime */ return new Property("occurrence[x]", "dateTime|Period", 2304 "The time when this communication is to occur.", 0, 1, occurrence); 2305 case 1397156594: 2306 /* occurrencePeriod */ return new Property("occurrence[x]", "dateTime|Period", 2307 "The time when this communication is to occur.", 0, 1, occurrence); 2308 case -1500852503: 2309 /* authoredOn */ return new Property("authoredOn", "dateTime", 2310 "For draft requests, indicates the date of initial creation. For requests with other statuses, indicates the date of activation.", 2311 0, 1, authoredOn); 2312 case 693933948: 2313 /* requester */ return new Property("requester", 2314 "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device)", 2315 "The device, individual, or organization who initiated the request and has responsibility for its activation.", 2316 0, 1, requester); 2317 case 820081177: 2318 /* recipient */ return new Property("recipient", 2319 "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson|Group|CareTeam|HealthcareService)", 2320 "The entity (e.g. person, organization, clinical information system, device, group, or care team) which is the intended target of the communication.", 2321 0, java.lang.Integer.MAX_VALUE, recipient); 2322 case -905962955: 2323 /* sender */ return new Property("sender", 2324 "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson|HealthcareService)", 2325 "The entity (e.g. person, organization, clinical information system, or device) which is to be the source of the communication.", 2326 0, 1, sender); 2327 case 722137681: 2328 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 2329 "Describes why the request is being made in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, 2330 reasonCode); 2331 case -1146218137: 2332 /* reasonReference */ return new Property("reasonReference", 2333 "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 2334 "Indicates another resource whose existence justifies this request.", 0, java.lang.Integer.MAX_VALUE, 2335 reasonReference); 2336 case 3387378: 2337 /* note */ return new Property("note", "Annotation", 2338 "Comments made about the request by the requester, sender, recipient, subject or other participants.", 0, 2339 java.lang.Integer.MAX_VALUE, note); 2340 default: 2341 return super.getNamedProperty(_hash, _name, _checkValid); 2342 } 2343 2344 } 2345 2346 @Override 2347 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2348 switch (hash) { 2349 case -1618432855: 2350 /* identifier */ return this.identifier == null ? new Base[0] 2351 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2352 case -332612366: 2353 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2354 case -430332865: 2355 /* replaces */ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // Reference 2356 case -445338488: 2357 /* groupIdentifier */ return this.groupIdentifier == null ? new Base[0] : new Base[] { this.groupIdentifier }; // Identifier 2358 case -892481550: 2359 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<CommunicationRequestStatus> 2360 case 2051346646: 2361 /* statusReason */ return this.statusReason == null ? new Base[0] : new Base[] { this.statusReason }; // CodeableConcept 2362 case 50511102: 2363 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2364 case -1165461084: 2365 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // Enumeration<CommunicationPriority> 2366 case -1788508167: 2367 /* doNotPerform */ return this.doNotPerform == null ? new Base[0] : new Base[] { this.doNotPerform }; // BooleanType 2368 case -1078030475: 2369 /* medium */ return this.medium == null ? new Base[0] : this.medium.toArray(new Base[this.medium.size()]); // CodeableConcept 2370 case -1867885268: 2371 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2372 case 92611469: 2373 /* about */ return this.about == null ? new Base[0] : this.about.toArray(new Base[this.about.size()]); // Reference 2374 case 1524132147: 2375 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 2376 case -786701938: 2377 /* payload */ return this.payload == null ? new Base[0] : this.payload.toArray(new Base[this.payload.size()]); // CommunicationRequestPayloadComponent 2378 case 1687874001: 2379 /* occurrence */ return this.occurrence == null ? new Base[0] : new Base[] { this.occurrence }; // Type 2380 case -1500852503: 2381 /* authoredOn */ return this.authoredOn == null ? new Base[0] : new Base[] { this.authoredOn }; // DateTimeType 2382 case 693933948: 2383 /* requester */ return this.requester == null ? new Base[0] : new Base[] { this.requester }; // Reference 2384 case 820081177: 2385 /* recipient */ return this.recipient == null ? new Base[0] 2386 : this.recipient.toArray(new Base[this.recipient.size()]); // Reference 2387 case -905962955: 2388 /* sender */ return this.sender == null ? new Base[0] : new Base[] { this.sender }; // Reference 2389 case 722137681: 2390 /* reasonCode */ return this.reasonCode == null ? new Base[0] 2391 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 2392 case -1146218137: 2393 /* reasonReference */ return this.reasonReference == null ? new Base[0] 2394 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 2395 case 3387378: 2396 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2397 default: 2398 return super.getProperty(hash, name, checkValid); 2399 } 2400 2401 } 2402 2403 @Override 2404 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2405 switch (hash) { 2406 case -1618432855: // identifier 2407 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2408 return value; 2409 case -332612366: // basedOn 2410 this.getBasedOn().add(castToReference(value)); // Reference 2411 return value; 2412 case -430332865: // replaces 2413 this.getReplaces().add(castToReference(value)); // Reference 2414 return value; 2415 case -445338488: // groupIdentifier 2416 this.groupIdentifier = castToIdentifier(value); // Identifier 2417 return value; 2418 case -892481550: // status 2419 value = new CommunicationRequestStatusEnumFactory().fromType(castToCode(value)); 2420 this.status = (Enumeration) value; // Enumeration<CommunicationRequestStatus> 2421 return value; 2422 case 2051346646: // statusReason 2423 this.statusReason = castToCodeableConcept(value); // CodeableConcept 2424 return value; 2425 case 50511102: // category 2426 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 2427 return value; 2428 case -1165461084: // priority 2429 value = new CommunicationPriorityEnumFactory().fromType(castToCode(value)); 2430 this.priority = (Enumeration) value; // Enumeration<CommunicationPriority> 2431 return value; 2432 case -1788508167: // doNotPerform 2433 this.doNotPerform = castToBoolean(value); // BooleanType 2434 return value; 2435 case -1078030475: // medium 2436 this.getMedium().add(castToCodeableConcept(value)); // CodeableConcept 2437 return value; 2438 case -1867885268: // subject 2439 this.subject = castToReference(value); // Reference 2440 return value; 2441 case 92611469: // about 2442 this.getAbout().add(castToReference(value)); // Reference 2443 return value; 2444 case 1524132147: // encounter 2445 this.encounter = castToReference(value); // Reference 2446 return value; 2447 case -786701938: // payload 2448 this.getPayload().add((CommunicationRequestPayloadComponent) value); // CommunicationRequestPayloadComponent 2449 return value; 2450 case 1687874001: // occurrence 2451 this.occurrence = castToType(value); // Type 2452 return value; 2453 case -1500852503: // authoredOn 2454 this.authoredOn = castToDateTime(value); // DateTimeType 2455 return value; 2456 case 693933948: // requester 2457 this.requester = castToReference(value); // Reference 2458 return value; 2459 case 820081177: // recipient 2460 this.getRecipient().add(castToReference(value)); // Reference 2461 return value; 2462 case -905962955: // sender 2463 this.sender = castToReference(value); // Reference 2464 return value; 2465 case 722137681: // reasonCode 2466 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 2467 return value; 2468 case -1146218137: // reasonReference 2469 this.getReasonReference().add(castToReference(value)); // Reference 2470 return value; 2471 case 3387378: // note 2472 this.getNote().add(castToAnnotation(value)); // Annotation 2473 return value; 2474 default: 2475 return super.setProperty(hash, name, value); 2476 } 2477 2478 } 2479 2480 @Override 2481 public Base setProperty(String name, Base value) throws FHIRException { 2482 if (name.equals("identifier")) { 2483 this.getIdentifier().add(castToIdentifier(value)); 2484 } else if (name.equals("basedOn")) { 2485 this.getBasedOn().add(castToReference(value)); 2486 } else if (name.equals("replaces")) { 2487 this.getReplaces().add(castToReference(value)); 2488 } else if (name.equals("groupIdentifier")) { 2489 this.groupIdentifier = castToIdentifier(value); // Identifier 2490 } else if (name.equals("status")) { 2491 value = new CommunicationRequestStatusEnumFactory().fromType(castToCode(value)); 2492 this.status = (Enumeration) value; // Enumeration<CommunicationRequestStatus> 2493 } else if (name.equals("statusReason")) { 2494 this.statusReason = castToCodeableConcept(value); // CodeableConcept 2495 } else if (name.equals("category")) { 2496 this.getCategory().add(castToCodeableConcept(value)); 2497 } else if (name.equals("priority")) { 2498 value = new CommunicationPriorityEnumFactory().fromType(castToCode(value)); 2499 this.priority = (Enumeration) value; // Enumeration<CommunicationPriority> 2500 } else if (name.equals("doNotPerform")) { 2501 this.doNotPerform = castToBoolean(value); // BooleanType 2502 } else if (name.equals("medium")) { 2503 this.getMedium().add(castToCodeableConcept(value)); 2504 } else if (name.equals("subject")) { 2505 this.subject = castToReference(value); // Reference 2506 } else if (name.equals("about")) { 2507 this.getAbout().add(castToReference(value)); 2508 } else if (name.equals("encounter")) { 2509 this.encounter = castToReference(value); // Reference 2510 } else if (name.equals("payload")) { 2511 this.getPayload().add((CommunicationRequestPayloadComponent) value); 2512 } else if (name.equals("occurrence[x]")) { 2513 this.occurrence = castToType(value); // Type 2514 } else if (name.equals("authoredOn")) { 2515 this.authoredOn = castToDateTime(value); // DateTimeType 2516 } else if (name.equals("requester")) { 2517 this.requester = castToReference(value); // Reference 2518 } else if (name.equals("recipient")) { 2519 this.getRecipient().add(castToReference(value)); 2520 } else if (name.equals("sender")) { 2521 this.sender = castToReference(value); // Reference 2522 } else if (name.equals("reasonCode")) { 2523 this.getReasonCode().add(castToCodeableConcept(value)); 2524 } else if (name.equals("reasonReference")) { 2525 this.getReasonReference().add(castToReference(value)); 2526 } else if (name.equals("note")) { 2527 this.getNote().add(castToAnnotation(value)); 2528 } else 2529 return super.setProperty(name, value); 2530 return value; 2531 } 2532 2533 @Override 2534 public void removeChild(String name, Base value) throws FHIRException { 2535 if (name.equals("identifier")) { 2536 this.getIdentifier().remove(castToIdentifier(value)); 2537 } else if (name.equals("basedOn")) { 2538 this.getBasedOn().remove(castToReference(value)); 2539 } else if (name.equals("replaces")) { 2540 this.getReplaces().remove(castToReference(value)); 2541 } else if (name.equals("groupIdentifier")) { 2542 this.groupIdentifier = null; 2543 } else if (name.equals("status")) { 2544 this.status = null; 2545 } else if (name.equals("statusReason")) { 2546 this.statusReason = null; 2547 } else if (name.equals("category")) { 2548 this.getCategory().remove(castToCodeableConcept(value)); 2549 } else if (name.equals("priority")) { 2550 this.priority = null; 2551 } else if (name.equals("doNotPerform")) { 2552 this.doNotPerform = null; 2553 } else if (name.equals("medium")) { 2554 this.getMedium().remove(castToCodeableConcept(value)); 2555 } else if (name.equals("subject")) { 2556 this.subject = null; 2557 } else if (name.equals("about")) { 2558 this.getAbout().remove(castToReference(value)); 2559 } else if (name.equals("encounter")) { 2560 this.encounter = null; 2561 } else if (name.equals("payload")) { 2562 this.getPayload().remove((CommunicationRequestPayloadComponent) value); 2563 } else if (name.equals("occurrence[x]")) { 2564 this.occurrence = null; 2565 } else if (name.equals("authoredOn")) { 2566 this.authoredOn = null; 2567 } else if (name.equals("requester")) { 2568 this.requester = null; 2569 } else if (name.equals("recipient")) { 2570 this.getRecipient().remove(castToReference(value)); 2571 } else if (name.equals("sender")) { 2572 this.sender = null; 2573 } else if (name.equals("reasonCode")) { 2574 this.getReasonCode().remove(castToCodeableConcept(value)); 2575 } else if (name.equals("reasonReference")) { 2576 this.getReasonReference().remove(castToReference(value)); 2577 } else if (name.equals("note")) { 2578 this.getNote().remove(castToAnnotation(value)); 2579 } else 2580 super.removeChild(name, value); 2581 2582 } 2583 2584 @Override 2585 public Base makeProperty(int hash, String name) throws FHIRException { 2586 switch (hash) { 2587 case -1618432855: 2588 return addIdentifier(); 2589 case -332612366: 2590 return addBasedOn(); 2591 case -430332865: 2592 return addReplaces(); 2593 case -445338488: 2594 return getGroupIdentifier(); 2595 case -892481550: 2596 return getStatusElement(); 2597 case 2051346646: 2598 return getStatusReason(); 2599 case 50511102: 2600 return addCategory(); 2601 case -1165461084: 2602 return getPriorityElement(); 2603 case -1788508167: 2604 return getDoNotPerformElement(); 2605 case -1078030475: 2606 return addMedium(); 2607 case -1867885268: 2608 return getSubject(); 2609 case 92611469: 2610 return addAbout(); 2611 case 1524132147: 2612 return getEncounter(); 2613 case -786701938: 2614 return addPayload(); 2615 case -2022646513: 2616 return getOccurrence(); 2617 case 1687874001: 2618 return getOccurrence(); 2619 case -1500852503: 2620 return getAuthoredOnElement(); 2621 case 693933948: 2622 return getRequester(); 2623 case 820081177: 2624 return addRecipient(); 2625 case -905962955: 2626 return getSender(); 2627 case 722137681: 2628 return addReasonCode(); 2629 case -1146218137: 2630 return addReasonReference(); 2631 case 3387378: 2632 return addNote(); 2633 default: 2634 return super.makeProperty(hash, name); 2635 } 2636 2637 } 2638 2639 @Override 2640 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2641 switch (hash) { 2642 case -1618432855: 2643 /* identifier */ return new String[] { "Identifier" }; 2644 case -332612366: 2645 /* basedOn */ return new String[] { "Reference" }; 2646 case -430332865: 2647 /* replaces */ return new String[] { "Reference" }; 2648 case -445338488: 2649 /* groupIdentifier */ return new String[] { "Identifier" }; 2650 case -892481550: 2651 /* status */ return new String[] { "code" }; 2652 case 2051346646: 2653 /* statusReason */ return new String[] { "CodeableConcept" }; 2654 case 50511102: 2655 /* category */ return new String[] { "CodeableConcept" }; 2656 case -1165461084: 2657 /* priority */ return new String[] { "code" }; 2658 case -1788508167: 2659 /* doNotPerform */ return new String[] { "boolean" }; 2660 case -1078030475: 2661 /* medium */ return new String[] { "CodeableConcept" }; 2662 case -1867885268: 2663 /* subject */ return new String[] { "Reference" }; 2664 case 92611469: 2665 /* about */ return new String[] { "Reference" }; 2666 case 1524132147: 2667 /* encounter */ return new String[] { "Reference" }; 2668 case -786701938: 2669 /* payload */ return new String[] {}; 2670 case 1687874001: 2671 /* occurrence */ return new String[] { "dateTime", "Period" }; 2672 case -1500852503: 2673 /* authoredOn */ return new String[] { "dateTime" }; 2674 case 693933948: 2675 /* requester */ return new String[] { "Reference" }; 2676 case 820081177: 2677 /* recipient */ return new String[] { "Reference" }; 2678 case -905962955: 2679 /* sender */ return new String[] { "Reference" }; 2680 case 722137681: 2681 /* reasonCode */ return new String[] { "CodeableConcept" }; 2682 case -1146218137: 2683 /* reasonReference */ return new String[] { "Reference" }; 2684 case 3387378: 2685 /* note */ return new String[] { "Annotation" }; 2686 default: 2687 return super.getTypesForProperty(hash, name); 2688 } 2689 2690 } 2691 2692 @Override 2693 public Base addChild(String name) throws FHIRException { 2694 if (name.equals("identifier")) { 2695 return addIdentifier(); 2696 } else if (name.equals("basedOn")) { 2697 return addBasedOn(); 2698 } else if (name.equals("replaces")) { 2699 return addReplaces(); 2700 } else if (name.equals("groupIdentifier")) { 2701 this.groupIdentifier = new Identifier(); 2702 return this.groupIdentifier; 2703 } else if (name.equals("status")) { 2704 throw new FHIRException("Cannot call addChild on a singleton property CommunicationRequest.status"); 2705 } else if (name.equals("statusReason")) { 2706 this.statusReason = new CodeableConcept(); 2707 return this.statusReason; 2708 } else if (name.equals("category")) { 2709 return addCategory(); 2710 } else if (name.equals("priority")) { 2711 throw new FHIRException("Cannot call addChild on a singleton property CommunicationRequest.priority"); 2712 } else if (name.equals("doNotPerform")) { 2713 throw new FHIRException("Cannot call addChild on a singleton property CommunicationRequest.doNotPerform"); 2714 } else if (name.equals("medium")) { 2715 return addMedium(); 2716 } else if (name.equals("subject")) { 2717 this.subject = new Reference(); 2718 return this.subject; 2719 } else if (name.equals("about")) { 2720 return addAbout(); 2721 } else if (name.equals("encounter")) { 2722 this.encounter = new Reference(); 2723 return this.encounter; 2724 } else if (name.equals("payload")) { 2725 return addPayload(); 2726 } else if (name.equals("occurrenceDateTime")) { 2727 this.occurrence = new DateTimeType(); 2728 return this.occurrence; 2729 } else if (name.equals("occurrencePeriod")) { 2730 this.occurrence = new Period(); 2731 return this.occurrence; 2732 } else if (name.equals("authoredOn")) { 2733 throw new FHIRException("Cannot call addChild on a singleton property CommunicationRequest.authoredOn"); 2734 } else if (name.equals("requester")) { 2735 this.requester = new Reference(); 2736 return this.requester; 2737 } else if (name.equals("recipient")) { 2738 return addRecipient(); 2739 } else if (name.equals("sender")) { 2740 this.sender = new Reference(); 2741 return this.sender; 2742 } else if (name.equals("reasonCode")) { 2743 return addReasonCode(); 2744 } else if (name.equals("reasonReference")) { 2745 return addReasonReference(); 2746 } else if (name.equals("note")) { 2747 return addNote(); 2748 } else 2749 return super.addChild(name); 2750 } 2751 2752 public String fhirType() { 2753 return "CommunicationRequest"; 2754 2755 } 2756 2757 public CommunicationRequest copy() { 2758 CommunicationRequest dst = new CommunicationRequest(); 2759 copyValues(dst); 2760 return dst; 2761 } 2762 2763 public void copyValues(CommunicationRequest dst) { 2764 super.copyValues(dst); 2765 if (identifier != null) { 2766 dst.identifier = new ArrayList<Identifier>(); 2767 for (Identifier i : identifier) 2768 dst.identifier.add(i.copy()); 2769 } 2770 ; 2771 if (basedOn != null) { 2772 dst.basedOn = new ArrayList<Reference>(); 2773 for (Reference i : basedOn) 2774 dst.basedOn.add(i.copy()); 2775 } 2776 ; 2777 if (replaces != null) { 2778 dst.replaces = new ArrayList<Reference>(); 2779 for (Reference i : replaces) 2780 dst.replaces.add(i.copy()); 2781 } 2782 ; 2783 dst.groupIdentifier = groupIdentifier == null ? null : groupIdentifier.copy(); 2784 dst.status = status == null ? null : status.copy(); 2785 dst.statusReason = statusReason == null ? null : statusReason.copy(); 2786 if (category != null) { 2787 dst.category = new ArrayList<CodeableConcept>(); 2788 for (CodeableConcept i : category) 2789 dst.category.add(i.copy()); 2790 } 2791 ; 2792 dst.priority = priority == null ? null : priority.copy(); 2793 dst.doNotPerform = doNotPerform == null ? null : doNotPerform.copy(); 2794 if (medium != null) { 2795 dst.medium = new ArrayList<CodeableConcept>(); 2796 for (CodeableConcept i : medium) 2797 dst.medium.add(i.copy()); 2798 } 2799 ; 2800 dst.subject = subject == null ? null : subject.copy(); 2801 if (about != null) { 2802 dst.about = new ArrayList<Reference>(); 2803 for (Reference i : about) 2804 dst.about.add(i.copy()); 2805 } 2806 ; 2807 dst.encounter = encounter == null ? null : encounter.copy(); 2808 if (payload != null) { 2809 dst.payload = new ArrayList<CommunicationRequestPayloadComponent>(); 2810 for (CommunicationRequestPayloadComponent i : payload) 2811 dst.payload.add(i.copy()); 2812 } 2813 ; 2814 dst.occurrence = occurrence == null ? null : occurrence.copy(); 2815 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 2816 dst.requester = requester == null ? null : requester.copy(); 2817 if (recipient != null) { 2818 dst.recipient = new ArrayList<Reference>(); 2819 for (Reference i : recipient) 2820 dst.recipient.add(i.copy()); 2821 } 2822 ; 2823 dst.sender = sender == null ? null : sender.copy(); 2824 if (reasonCode != null) { 2825 dst.reasonCode = new ArrayList<CodeableConcept>(); 2826 for (CodeableConcept i : reasonCode) 2827 dst.reasonCode.add(i.copy()); 2828 } 2829 ; 2830 if (reasonReference != null) { 2831 dst.reasonReference = new ArrayList<Reference>(); 2832 for (Reference i : reasonReference) 2833 dst.reasonReference.add(i.copy()); 2834 } 2835 ; 2836 if (note != null) { 2837 dst.note = new ArrayList<Annotation>(); 2838 for (Annotation i : note) 2839 dst.note.add(i.copy()); 2840 } 2841 ; 2842 } 2843 2844 protected CommunicationRequest typedCopy() { 2845 return copy(); 2846 } 2847 2848 @Override 2849 public boolean equalsDeep(Base other_) { 2850 if (!super.equalsDeep(other_)) 2851 return false; 2852 if (!(other_ instanceof CommunicationRequest)) 2853 return false; 2854 CommunicationRequest o = (CommunicationRequest) other_; 2855 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) 2856 && compareDeep(replaces, o.replaces, true) && compareDeep(groupIdentifier, o.groupIdentifier, true) 2857 && compareDeep(status, o.status, true) && compareDeep(statusReason, o.statusReason, true) 2858 && compareDeep(category, o.category, true) && compareDeep(priority, o.priority, true) 2859 && compareDeep(doNotPerform, o.doNotPerform, true) && compareDeep(medium, o.medium, true) 2860 && compareDeep(subject, o.subject, true) && compareDeep(about, o.about, true) 2861 && compareDeep(encounter, o.encounter, true) && compareDeep(payload, o.payload, true) 2862 && compareDeep(occurrence, o.occurrence, true) && compareDeep(authoredOn, o.authoredOn, true) 2863 && compareDeep(requester, o.requester, true) && compareDeep(recipient, o.recipient, true) 2864 && compareDeep(sender, o.sender, true) && compareDeep(reasonCode, o.reasonCode, true) 2865 && compareDeep(reasonReference, o.reasonReference, true) && compareDeep(note, o.note, true); 2866 } 2867 2868 @Override 2869 public boolean equalsShallow(Base other_) { 2870 if (!super.equalsShallow(other_)) 2871 return false; 2872 if (!(other_ instanceof CommunicationRequest)) 2873 return false; 2874 CommunicationRequest o = (CommunicationRequest) other_; 2875 return compareValues(status, o.status, true) && compareValues(priority, o.priority, true) 2876 && compareValues(doNotPerform, o.doNotPerform, true) && compareValues(authoredOn, o.authoredOn, true); 2877 } 2878 2879 public boolean isEmpty() { 2880 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, replaces, groupIdentifier, 2881 status, statusReason, category, priority, doNotPerform, medium, subject, about, encounter, payload, occurrence, 2882 authoredOn, requester, recipient, sender, reasonCode, reasonReference, note); 2883 } 2884 2885 @Override 2886 public ResourceType getResourceType() { 2887 return ResourceType.CommunicationRequest; 2888 } 2889 2890 /** 2891 * Search parameter: <b>requester</b> 2892 * <p> 2893 * Description: <b>Who/what is requesting service</b><br> 2894 * Type: <b>reference</b><br> 2895 * Path: <b>CommunicationRequest.requester</b><br> 2896 * </p> 2897 */ 2898 @SearchParamDefinition(name = "requester", path = "CommunicationRequest.requester", description = "Who/what is requesting service", type = "reference", providesMembershipIn = { 2899 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 2900 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 2901 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, 2902 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 2903 public static final String SP_REQUESTER = "requester"; 2904 /** 2905 * <b>Fluent Client</b> search parameter constant for <b>requester</b> 2906 * <p> 2907 * Description: <b>Who/what is requesting service</b><br> 2908 * Type: <b>reference</b><br> 2909 * Path: <b>CommunicationRequest.requester</b><br> 2910 * </p> 2911 */ 2912 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2913 SP_REQUESTER); 2914 2915 /** 2916 * Constant for fluent queries to be used to add include statements. Specifies 2917 * the path value of "<b>CommunicationRequest:requester</b>". 2918 */ 2919 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTER = new ca.uhn.fhir.model.api.Include( 2920 "CommunicationRequest:requester").toLocked(); 2921 2922 /** 2923 * Search parameter: <b>authored</b> 2924 * <p> 2925 * Description: <b>When request transitioned to being actionable</b><br> 2926 * Type: <b>date</b><br> 2927 * Path: <b>CommunicationRequest.authoredOn</b><br> 2928 * </p> 2929 */ 2930 @SearchParamDefinition(name = "authored", path = "CommunicationRequest.authoredOn", description = "When request transitioned to being actionable", type = "date") 2931 public static final String SP_AUTHORED = "authored"; 2932 /** 2933 * <b>Fluent Client</b> search parameter constant for <b>authored</b> 2934 * <p> 2935 * Description: <b>When request transitioned to being actionable</b><br> 2936 * Type: <b>date</b><br> 2937 * Path: <b>CommunicationRequest.authoredOn</b><br> 2938 * </p> 2939 */ 2940 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED = new ca.uhn.fhir.rest.gclient.DateClientParam( 2941 SP_AUTHORED); 2942 2943 /** 2944 * Search parameter: <b>identifier</b> 2945 * <p> 2946 * Description: <b>Unique identifier</b><br> 2947 * Type: <b>token</b><br> 2948 * Path: <b>CommunicationRequest.identifier</b><br> 2949 * </p> 2950 */ 2951 @SearchParamDefinition(name = "identifier", path = "CommunicationRequest.identifier", description = "Unique identifier", type = "token") 2952 public static final String SP_IDENTIFIER = "identifier"; 2953 /** 2954 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2955 * <p> 2956 * Description: <b>Unique identifier</b><br> 2957 * Type: <b>token</b><br> 2958 * Path: <b>CommunicationRequest.identifier</b><br> 2959 * </p> 2960 */ 2961 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2962 SP_IDENTIFIER); 2963 2964 /** 2965 * Search parameter: <b>subject</b> 2966 * <p> 2967 * Description: <b>Focus of message</b><br> 2968 * Type: <b>reference</b><br> 2969 * Path: <b>CommunicationRequest.subject</b><br> 2970 * </p> 2971 */ 2972 @SearchParamDefinition(name = "subject", path = "CommunicationRequest.subject", description = "Focus of message", type = "reference", providesMembershipIn = { 2973 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Group.class, Patient.class }) 2974 public static final String SP_SUBJECT = "subject"; 2975 /** 2976 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2977 * <p> 2978 * Description: <b>Focus of message</b><br> 2979 * Type: <b>reference</b><br> 2980 * Path: <b>CommunicationRequest.subject</b><br> 2981 * </p> 2982 */ 2983 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2984 SP_SUBJECT); 2985 2986 /** 2987 * Constant for fluent queries to be used to add include statements. Specifies 2988 * the path value of "<b>CommunicationRequest:subject</b>". 2989 */ 2990 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 2991 "CommunicationRequest:subject").toLocked(); 2992 2993 /** 2994 * Search parameter: <b>replaces</b> 2995 * <p> 2996 * Description: <b>Request(s) replaced by this request</b><br> 2997 * Type: <b>reference</b><br> 2998 * Path: <b>CommunicationRequest.replaces</b><br> 2999 * </p> 3000 */ 3001 @SearchParamDefinition(name = "replaces", path = "CommunicationRequest.replaces", description = "Request(s) replaced by this request", type = "reference", target = { 3002 CommunicationRequest.class }) 3003 public static final String SP_REPLACES = "replaces"; 3004 /** 3005 * <b>Fluent Client</b> search parameter constant for <b>replaces</b> 3006 * <p> 3007 * Description: <b>Request(s) replaced by this request</b><br> 3008 * Type: <b>reference</b><br> 3009 * Path: <b>CommunicationRequest.replaces</b><br> 3010 * </p> 3011 */ 3012 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REPLACES = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3013 SP_REPLACES); 3014 3015 /** 3016 * Constant for fluent queries to be used to add include statements. Specifies 3017 * the path value of "<b>CommunicationRequest:replaces</b>". 3018 */ 3019 public static final ca.uhn.fhir.model.api.Include INCLUDE_REPLACES = new ca.uhn.fhir.model.api.Include( 3020 "CommunicationRequest:replaces").toLocked(); 3021 3022 /** 3023 * Search parameter: <b>medium</b> 3024 * <p> 3025 * Description: <b>A channel of communication</b><br> 3026 * Type: <b>token</b><br> 3027 * Path: <b>CommunicationRequest.medium</b><br> 3028 * </p> 3029 */ 3030 @SearchParamDefinition(name = "medium", path = "CommunicationRequest.medium", description = "A channel of communication", type = "token") 3031 public static final String SP_MEDIUM = "medium"; 3032 /** 3033 * <b>Fluent Client</b> search parameter constant for <b>medium</b> 3034 * <p> 3035 * Description: <b>A channel of communication</b><br> 3036 * Type: <b>token</b><br> 3037 * Path: <b>CommunicationRequest.medium</b><br> 3038 * </p> 3039 */ 3040 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MEDIUM = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3041 SP_MEDIUM); 3042 3043 /** 3044 * Search parameter: <b>encounter</b> 3045 * <p> 3046 * Description: <b>Encounter created as part of</b><br> 3047 * Type: <b>reference</b><br> 3048 * Path: <b>CommunicationRequest.encounter</b><br> 3049 * </p> 3050 */ 3051 @SearchParamDefinition(name = "encounter", path = "CommunicationRequest.encounter", description = "Encounter created as part of", type = "reference", providesMembershipIn = { 3052 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 3053 public static final String SP_ENCOUNTER = "encounter"; 3054 /** 3055 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 3056 * <p> 3057 * Description: <b>Encounter created as part of</b><br> 3058 * Type: <b>reference</b><br> 3059 * Path: <b>CommunicationRequest.encounter</b><br> 3060 * </p> 3061 */ 3062 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3063 SP_ENCOUNTER); 3064 3065 /** 3066 * Constant for fluent queries to be used to add include statements. Specifies 3067 * the path value of "<b>CommunicationRequest:encounter</b>". 3068 */ 3069 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 3070 "CommunicationRequest:encounter").toLocked(); 3071 3072 /** 3073 * Search parameter: <b>occurrence</b> 3074 * <p> 3075 * Description: <b>When scheduled</b><br> 3076 * Type: <b>date</b><br> 3077 * Path: <b>CommunicationRequest.occurrenceDateTime</b><br> 3078 * </p> 3079 */ 3080 @SearchParamDefinition(name = "occurrence", path = "(CommunicationRequest.occurrence as dateTime)", description = "When scheduled", type = "date") 3081 public static final String SP_OCCURRENCE = "occurrence"; 3082 /** 3083 * <b>Fluent Client</b> search parameter constant for <b>occurrence</b> 3084 * <p> 3085 * Description: <b>When scheduled</b><br> 3086 * Type: <b>date</b><br> 3087 * Path: <b>CommunicationRequest.occurrenceDateTime</b><br> 3088 * </p> 3089 */ 3090 public static final ca.uhn.fhir.rest.gclient.DateClientParam OCCURRENCE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3091 SP_OCCURRENCE); 3092 3093 /** 3094 * Search parameter: <b>priority</b> 3095 * <p> 3096 * Description: <b>routine | urgent | asap | stat</b><br> 3097 * Type: <b>token</b><br> 3098 * Path: <b>CommunicationRequest.priority</b><br> 3099 * </p> 3100 */ 3101 @SearchParamDefinition(name = "priority", path = "CommunicationRequest.priority", description = "routine | urgent | asap | stat", type = "token") 3102 public static final String SP_PRIORITY = "priority"; 3103 /** 3104 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 3105 * <p> 3106 * Description: <b>routine | urgent | asap | stat</b><br> 3107 * Type: <b>token</b><br> 3108 * Path: <b>CommunicationRequest.priority</b><br> 3109 * </p> 3110 */ 3111 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3112 SP_PRIORITY); 3113 3114 /** 3115 * Search parameter: <b>group-identifier</b> 3116 * <p> 3117 * Description: <b>Composite request this is part of</b><br> 3118 * Type: <b>token</b><br> 3119 * Path: <b>CommunicationRequest.groupIdentifier</b><br> 3120 * </p> 3121 */ 3122 @SearchParamDefinition(name = "group-identifier", path = "CommunicationRequest.groupIdentifier", description = "Composite request this is part of", type = "token") 3123 public static final String SP_GROUP_IDENTIFIER = "group-identifier"; 3124 /** 3125 * <b>Fluent Client</b> search parameter constant for <b>group-identifier</b> 3126 * <p> 3127 * Description: <b>Composite request this is part of</b><br> 3128 * Type: <b>token</b><br> 3129 * Path: <b>CommunicationRequest.groupIdentifier</b><br> 3130 * </p> 3131 */ 3132 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GROUP_IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3133 SP_GROUP_IDENTIFIER); 3134 3135 /** 3136 * Search parameter: <b>based-on</b> 3137 * <p> 3138 * Description: <b>Fulfills plan or proposal</b><br> 3139 * Type: <b>reference</b><br> 3140 * Path: <b>CommunicationRequest.basedOn</b><br> 3141 * </p> 3142 */ 3143 @SearchParamDefinition(name = "based-on", path = "CommunicationRequest.basedOn", description = "Fulfills plan or proposal", type = "reference") 3144 public static final String SP_BASED_ON = "based-on"; 3145 /** 3146 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 3147 * <p> 3148 * Description: <b>Fulfills plan or proposal</b><br> 3149 * Type: <b>reference</b><br> 3150 * Path: <b>CommunicationRequest.basedOn</b><br> 3151 * </p> 3152 */ 3153 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3154 SP_BASED_ON); 3155 3156 /** 3157 * Constant for fluent queries to be used to add include statements. Specifies 3158 * the path value of "<b>CommunicationRequest:based-on</b>". 3159 */ 3160 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include( 3161 "CommunicationRequest:based-on").toLocked(); 3162 3163 /** 3164 * Search parameter: <b>sender</b> 3165 * <p> 3166 * Description: <b>Message sender</b><br> 3167 * Type: <b>reference</b><br> 3168 * Path: <b>CommunicationRequest.sender</b><br> 3169 * </p> 3170 */ 3171 @SearchParamDefinition(name = "sender", path = "CommunicationRequest.sender", description = "Message sender", type = "reference", providesMembershipIn = { 3172 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 3173 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3174 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 3175 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, 3176 HealthcareService.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, 3177 RelatedPerson.class }) 3178 public static final String SP_SENDER = "sender"; 3179 /** 3180 * <b>Fluent Client</b> search parameter constant for <b>sender</b> 3181 * <p> 3182 * Description: <b>Message sender</b><br> 3183 * Type: <b>reference</b><br> 3184 * Path: <b>CommunicationRequest.sender</b><br> 3185 * </p> 3186 */ 3187 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SENDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3188 SP_SENDER); 3189 3190 /** 3191 * Constant for fluent queries to be used to add include statements. Specifies 3192 * the path value of "<b>CommunicationRequest:sender</b>". 3193 */ 3194 public static final ca.uhn.fhir.model.api.Include INCLUDE_SENDER = new ca.uhn.fhir.model.api.Include( 3195 "CommunicationRequest:sender").toLocked(); 3196 3197 /** 3198 * Search parameter: <b>patient</b> 3199 * <p> 3200 * Description: <b>Focus of message</b><br> 3201 * Type: <b>reference</b><br> 3202 * Path: <b>CommunicationRequest.subject</b><br> 3203 * </p> 3204 */ 3205 @SearchParamDefinition(name = "patient", path = "CommunicationRequest.subject.where(resolve() is Patient)", description = "Focus of message", type = "reference", target = { 3206 Patient.class }) 3207 public static final String SP_PATIENT = "patient"; 3208 /** 3209 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3210 * <p> 3211 * Description: <b>Focus of message</b><br> 3212 * Type: <b>reference</b><br> 3213 * Path: <b>CommunicationRequest.subject</b><br> 3214 * </p> 3215 */ 3216 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3217 SP_PATIENT); 3218 3219 /** 3220 * Constant for fluent queries to be used to add include statements. Specifies 3221 * the path value of "<b>CommunicationRequest:patient</b>". 3222 */ 3223 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 3224 "CommunicationRequest:patient").toLocked(); 3225 3226 /** 3227 * Search parameter: <b>recipient</b> 3228 * <p> 3229 * Description: <b>Message recipient</b><br> 3230 * Type: <b>reference</b><br> 3231 * Path: <b>CommunicationRequest.recipient</b><br> 3232 * </p> 3233 */ 3234 @SearchParamDefinition(name = "recipient", path = "CommunicationRequest.recipient", description = "Message recipient", type = "reference", providesMembershipIn = { 3235 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 3236 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3237 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 3238 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { CareTeam.class, Device.class, 3239 Group.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, 3240 PractitionerRole.class, RelatedPerson.class }) 3241 public static final String SP_RECIPIENT = "recipient"; 3242 /** 3243 * <b>Fluent Client</b> search parameter constant for <b>recipient</b> 3244 * <p> 3245 * Description: <b>Message recipient</b><br> 3246 * Type: <b>reference</b><br> 3247 * Path: <b>CommunicationRequest.recipient</b><br> 3248 * </p> 3249 */ 3250 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECIPIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3251 SP_RECIPIENT); 3252 3253 /** 3254 * Constant for fluent queries to be used to add include statements. Specifies 3255 * the path value of "<b>CommunicationRequest:recipient</b>". 3256 */ 3257 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECIPIENT = new ca.uhn.fhir.model.api.Include( 3258 "CommunicationRequest:recipient").toLocked(); 3259 3260 /** 3261 * Search parameter: <b>category</b> 3262 * <p> 3263 * Description: <b>Message category</b><br> 3264 * Type: <b>token</b><br> 3265 * Path: <b>CommunicationRequest.category</b><br> 3266 * </p> 3267 */ 3268 @SearchParamDefinition(name = "category", path = "CommunicationRequest.category", description = "Message category", type = "token") 3269 public static final String SP_CATEGORY = "category"; 3270 /** 3271 * <b>Fluent Client</b> search parameter constant for <b>category</b> 3272 * <p> 3273 * Description: <b>Message category</b><br> 3274 * Type: <b>token</b><br> 3275 * Path: <b>CommunicationRequest.category</b><br> 3276 * </p> 3277 */ 3278 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3279 SP_CATEGORY); 3280 3281 /** 3282 * Search parameter: <b>status</b> 3283 * <p> 3284 * Description: <b>draft | active | on-hold | revoked | completed | 3285 * entered-in-error | unknown</b><br> 3286 * Type: <b>token</b><br> 3287 * Path: <b>CommunicationRequest.status</b><br> 3288 * </p> 3289 */ 3290 @SearchParamDefinition(name = "status", path = "CommunicationRequest.status", description = "draft | active | on-hold | revoked | completed | entered-in-error | unknown", type = "token") 3291 public static final String SP_STATUS = "status"; 3292 /** 3293 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3294 * <p> 3295 * Description: <b>draft | active | on-hold | revoked | completed | 3296 * entered-in-error | unknown</b><br> 3297 * Type: <b>token</b><br> 3298 * Path: <b>CommunicationRequest.status</b><br> 3299 * </p> 3300 */ 3301 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3302 SP_STATUS); 3303 3304}