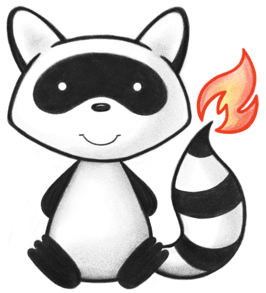
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * A request to convey information; e.g. the CDS system proposes that an alert 048 * be sent to a responsible provider, the CDS system proposes that the public 049 * health agency be notified about a reportable condition. 050 */ 051@ResourceDef(name = "CommunicationRequest", profile = "http://hl7.org/fhir/StructureDefinition/CommunicationRequest") 052public class CommunicationRequest extends DomainResource { 053 054 public enum CommunicationRequestStatus { 055 /** 056 * The request has been created but is not yet complete or ready for action. 057 */ 058 DRAFT, 059 /** 060 * The request is in force and ready to be acted upon. 061 */ 062 ACTIVE, 063 /** 064 * The request (and any implicit authorization to act) has been temporarily 065 * withdrawn but is expected to resume in the future. 066 */ 067 ONHOLD, 068 /** 069 * The request (and any implicit authorization to act) has been terminated prior 070 * to the known full completion of the intended actions. No further activity 071 * should occur. 072 */ 073 REVOKED, 074 /** 075 * The activity described by the request has been fully performed. No further 076 * activity will occur. 077 */ 078 COMPLETED, 079 /** 080 * This request should never have existed and should be considered 'void'. (It 081 * is possible that real-world decisions were based on it. If real-world 082 * activity has occurred, the status should be "revoked" rather than 083 * "entered-in-error".). 084 */ 085 ENTEREDINERROR, 086 /** 087 * The authoring/source system does not know which of the status values 088 * currently applies for this request. Note: This concept is not to be used for 089 * "other" - one of the listed statuses is presumed to apply, but the 090 * authoring/source system does not know which. 091 */ 092 UNKNOWN, 093 /** 094 * added to help the parsers with the generic types 095 */ 096 NULL; 097 098 public static CommunicationRequestStatus fromCode(String codeString) throws FHIRException { 099 if (codeString == null || "".equals(codeString)) 100 return null; 101 if ("draft".equals(codeString)) 102 return DRAFT; 103 if ("active".equals(codeString)) 104 return ACTIVE; 105 if ("on-hold".equals(codeString)) 106 return ONHOLD; 107 if ("revoked".equals(codeString)) 108 return REVOKED; 109 if ("completed".equals(codeString)) 110 return COMPLETED; 111 if ("entered-in-error".equals(codeString)) 112 return ENTEREDINERROR; 113 if ("unknown".equals(codeString)) 114 return UNKNOWN; 115 if (Configuration.isAcceptInvalidEnums()) 116 return null; 117 else 118 throw new FHIRException("Unknown CommunicationRequestStatus code '" + codeString + "'"); 119 } 120 121 public String toCode() { 122 switch (this) { 123 case DRAFT: 124 return "draft"; 125 case ACTIVE: 126 return "active"; 127 case ONHOLD: 128 return "on-hold"; 129 case REVOKED: 130 return "revoked"; 131 case COMPLETED: 132 return "completed"; 133 case ENTEREDINERROR: 134 return "entered-in-error"; 135 case UNKNOWN: 136 return "unknown"; 137 case NULL: 138 return null; 139 default: 140 return "?"; 141 } 142 } 143 144 public String getSystem() { 145 switch (this) { 146 case DRAFT: 147 return "http://hl7.org/fhir/request-status"; 148 case ACTIVE: 149 return "http://hl7.org/fhir/request-status"; 150 case ONHOLD: 151 return "http://hl7.org/fhir/request-status"; 152 case REVOKED: 153 return "http://hl7.org/fhir/request-status"; 154 case COMPLETED: 155 return "http://hl7.org/fhir/request-status"; 156 case ENTEREDINERROR: 157 return "http://hl7.org/fhir/request-status"; 158 case UNKNOWN: 159 return "http://hl7.org/fhir/request-status"; 160 case NULL: 161 return null; 162 default: 163 return "?"; 164 } 165 } 166 167 public String getDefinition() { 168 switch (this) { 169 case DRAFT: 170 return "The request has been created but is not yet complete or ready for action."; 171 case ACTIVE: 172 return "The request is in force and ready to be acted upon."; 173 case ONHOLD: 174 return "The request (and any implicit authorization to act) has been temporarily withdrawn but is expected to resume in the future."; 175 case REVOKED: 176 return "The request (and any implicit authorization to act) has been terminated prior to the known full completion of the intended actions. No further activity should occur."; 177 case COMPLETED: 178 return "The activity described by the request has been fully performed. No further activity will occur."; 179 case ENTEREDINERROR: 180 return "This request should never have existed and should be considered 'void'. (It is possible that real-world decisions were based on it. If real-world activity has occurred, the status should be \"revoked\" rather than \"entered-in-error\".)."; 181 case UNKNOWN: 182 return "The authoring/source system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 183 case NULL: 184 return null; 185 default: 186 return "?"; 187 } 188 } 189 190 public String getDisplay() { 191 switch (this) { 192 case DRAFT: 193 return "Draft"; 194 case ACTIVE: 195 return "Active"; 196 case ONHOLD: 197 return "On Hold"; 198 case REVOKED: 199 return "Revoked"; 200 case COMPLETED: 201 return "Completed"; 202 case ENTEREDINERROR: 203 return "Entered in Error"; 204 case UNKNOWN: 205 return "Unknown"; 206 case NULL: 207 return null; 208 default: 209 return "?"; 210 } 211 } 212 } 213 214 public static class CommunicationRequestStatusEnumFactory implements EnumFactory<CommunicationRequestStatus> { 215 public CommunicationRequestStatus fromCode(String codeString) throws IllegalArgumentException { 216 if (codeString == null || "".equals(codeString)) 217 if (codeString == null || "".equals(codeString)) 218 return null; 219 if ("draft".equals(codeString)) 220 return CommunicationRequestStatus.DRAFT; 221 if ("active".equals(codeString)) 222 return CommunicationRequestStatus.ACTIVE; 223 if ("on-hold".equals(codeString)) 224 return CommunicationRequestStatus.ONHOLD; 225 if ("revoked".equals(codeString)) 226 return CommunicationRequestStatus.REVOKED; 227 if ("completed".equals(codeString)) 228 return CommunicationRequestStatus.COMPLETED; 229 if ("entered-in-error".equals(codeString)) 230 return CommunicationRequestStatus.ENTEREDINERROR; 231 if ("unknown".equals(codeString)) 232 return CommunicationRequestStatus.UNKNOWN; 233 throw new IllegalArgumentException("Unknown CommunicationRequestStatus code '" + codeString + "'"); 234 } 235 236 public Enumeration<CommunicationRequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 237 if (code == null) 238 return null; 239 if (code.isEmpty()) 240 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.NULL, code); 241 String codeString = code.asStringValue(); 242 if (codeString == null || "".equals(codeString)) 243 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.NULL, code); 244 if ("draft".equals(codeString)) 245 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.DRAFT, code); 246 if ("active".equals(codeString)) 247 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.ACTIVE, code); 248 if ("on-hold".equals(codeString)) 249 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.ONHOLD, code); 250 if ("revoked".equals(codeString)) 251 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.REVOKED, code); 252 if ("completed".equals(codeString)) 253 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.COMPLETED, code); 254 if ("entered-in-error".equals(codeString)) 255 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.ENTEREDINERROR, code); 256 if ("unknown".equals(codeString)) 257 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.UNKNOWN, code); 258 throw new FHIRException("Unknown CommunicationRequestStatus code '" + codeString + "'"); 259 } 260 261 public String toCode(CommunicationRequestStatus code) { 262 if (code == CommunicationRequestStatus.NULL) 263 return null; 264 if (code == CommunicationRequestStatus.DRAFT) 265 return "draft"; 266 if (code == CommunicationRequestStatus.ACTIVE) 267 return "active"; 268 if (code == CommunicationRequestStatus.ONHOLD) 269 return "on-hold"; 270 if (code == CommunicationRequestStatus.REVOKED) 271 return "revoked"; 272 if (code == CommunicationRequestStatus.COMPLETED) 273 return "completed"; 274 if (code == CommunicationRequestStatus.ENTEREDINERROR) 275 return "entered-in-error"; 276 if (code == CommunicationRequestStatus.UNKNOWN) 277 return "unknown"; 278 return "?"; 279 } 280 281 public String toSystem(CommunicationRequestStatus code) { 282 return code.getSystem(); 283 } 284 } 285 286 public enum CommunicationPriority { 287 /** 288 * The request has normal priority. 289 */ 290 ROUTINE, 291 /** 292 * The request should be actioned promptly - higher priority than routine. 293 */ 294 URGENT, 295 /** 296 * The request should be actioned as soon as possible - higher priority than 297 * urgent. 298 */ 299 ASAP, 300 /** 301 * The request should be actioned immediately - highest possible priority. E.g. 302 * an emergency. 303 */ 304 STAT, 305 /** 306 * added to help the parsers with the generic types 307 */ 308 NULL; 309 310 public static CommunicationPriority fromCode(String codeString) throws FHIRException { 311 if (codeString == null || "".equals(codeString)) 312 return null; 313 if ("routine".equals(codeString)) 314 return ROUTINE; 315 if ("urgent".equals(codeString)) 316 return URGENT; 317 if ("asap".equals(codeString)) 318 return ASAP; 319 if ("stat".equals(codeString)) 320 return STAT; 321 if (Configuration.isAcceptInvalidEnums()) 322 return null; 323 else 324 throw new FHIRException("Unknown CommunicationPriority code '" + codeString + "'"); 325 } 326 327 public String toCode() { 328 switch (this) { 329 case ROUTINE: 330 return "routine"; 331 case URGENT: 332 return "urgent"; 333 case ASAP: 334 return "asap"; 335 case STAT: 336 return "stat"; 337 case NULL: 338 return null; 339 default: 340 return "?"; 341 } 342 } 343 344 public String getSystem() { 345 switch (this) { 346 case ROUTINE: 347 return "http://hl7.org/fhir/request-priority"; 348 case URGENT: 349 return "http://hl7.org/fhir/request-priority"; 350 case ASAP: 351 return "http://hl7.org/fhir/request-priority"; 352 case STAT: 353 return "http://hl7.org/fhir/request-priority"; 354 case NULL: 355 return null; 356 default: 357 return "?"; 358 } 359 } 360 361 public String getDefinition() { 362 switch (this) { 363 case ROUTINE: 364 return "The request has normal priority."; 365 case URGENT: 366 return "The request should be actioned promptly - higher priority than routine."; 367 case ASAP: 368 return "The request should be actioned as soon as possible - higher priority than urgent."; 369 case STAT: 370 return "The request should be actioned immediately - highest possible priority. E.g. an emergency."; 371 case NULL: 372 return null; 373 default: 374 return "?"; 375 } 376 } 377 378 public String getDisplay() { 379 switch (this) { 380 case ROUTINE: 381 return "Routine"; 382 case URGENT: 383 return "Urgent"; 384 case ASAP: 385 return "ASAP"; 386 case STAT: 387 return "STAT"; 388 case NULL: 389 return null; 390 default: 391 return "?"; 392 } 393 } 394 } 395 396 public static class CommunicationPriorityEnumFactory implements EnumFactory<CommunicationPriority> { 397 public CommunicationPriority fromCode(String codeString) throws IllegalArgumentException { 398 if (codeString == null || "".equals(codeString)) 399 if (codeString == null || "".equals(codeString)) 400 return null; 401 if ("routine".equals(codeString)) 402 return CommunicationPriority.ROUTINE; 403 if ("urgent".equals(codeString)) 404 return CommunicationPriority.URGENT; 405 if ("asap".equals(codeString)) 406 return CommunicationPriority.ASAP; 407 if ("stat".equals(codeString)) 408 return CommunicationPriority.STAT; 409 throw new IllegalArgumentException("Unknown CommunicationPriority code '" + codeString + "'"); 410 } 411 412 public Enumeration<CommunicationPriority> fromType(PrimitiveType<?> code) throws FHIRException { 413 if (code == null) 414 return null; 415 if (code.isEmpty()) 416 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.NULL, code); 417 String codeString = code.asStringValue(); 418 if (codeString == null || "".equals(codeString)) 419 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.NULL, code); 420 if ("routine".equals(codeString)) 421 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.ROUTINE, code); 422 if ("urgent".equals(codeString)) 423 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.URGENT, code); 424 if ("asap".equals(codeString)) 425 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.ASAP, code); 426 if ("stat".equals(codeString)) 427 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.STAT, code); 428 throw new FHIRException("Unknown CommunicationPriority code '" + codeString + "'"); 429 } 430 431 public String toCode(CommunicationPriority code) { 432 if (code == CommunicationPriority.NULL) 433 return null; 434 if (code == CommunicationPriority.ROUTINE) 435 return "routine"; 436 if (code == CommunicationPriority.URGENT) 437 return "urgent"; 438 if (code == CommunicationPriority.ASAP) 439 return "asap"; 440 if (code == CommunicationPriority.STAT) 441 return "stat"; 442 return "?"; 443 } 444 445 public String toSystem(CommunicationPriority code) { 446 return code.getSystem(); 447 } 448 } 449 450 @Block() 451 public static class CommunicationRequestPayloadComponent extends BackboneElement implements IBaseBackboneElement { 452 /** 453 * The communicated content (or for multi-part communications, one portion of 454 * the communication). 455 */ 456 @Child(name = "content", type = { StringType.class, Attachment.class, 457 Reference.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 458 @Description(shortDefinition = "Message part content", formalDefinition = "The communicated content (or for multi-part communications, one portion of the communication).") 459 protected Type content; 460 461 private static final long serialVersionUID = -1763459053L; 462 463 /** 464 * Constructor 465 */ 466 public CommunicationRequestPayloadComponent() { 467 super(); 468 } 469 470 /** 471 * Constructor 472 */ 473 public CommunicationRequestPayloadComponent(Type content) { 474 super(); 475 this.content = content; 476 } 477 478 /** 479 * @return {@link #content} (The communicated content (or for multi-part 480 * communications, one portion of the communication).) 481 */ 482 public Type getContent() { 483 return this.content; 484 } 485 486 /** 487 * @return {@link #content} (The communicated content (or for multi-part 488 * communications, one portion of the communication).) 489 */ 490 public StringType getContentStringType() throws FHIRException { 491 if (this.content == null) 492 this.content = new StringType(); 493 if (!(this.content instanceof StringType)) 494 throw new FHIRException("Type mismatch: the type StringType was expected, but " 495 + this.content.getClass().getName() + " was encountered"); 496 return (StringType) this.content; 497 } 498 499 public boolean hasContentStringType() { 500 return this.content instanceof StringType; 501 } 502 503 /** 504 * @return {@link #content} (The communicated content (or for multi-part 505 * communications, one portion of the communication).) 506 */ 507 public Attachment getContentAttachment() throws FHIRException { 508 if (this.content == null) 509 this.content = new Attachment(); 510 if (!(this.content instanceof Attachment)) 511 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 512 + this.content.getClass().getName() + " was encountered"); 513 return (Attachment) this.content; 514 } 515 516 public boolean hasContentAttachment() { 517 return this.content instanceof Attachment; 518 } 519 520 /** 521 * @return {@link #content} (The communicated content (or for multi-part 522 * communications, one portion of the communication).) 523 */ 524 public Reference getContentReference() throws FHIRException { 525 if (this.content == null) 526 this.content = new Reference(); 527 if (!(this.content instanceof Reference)) 528 throw new FHIRException("Type mismatch: the type Reference was expected, but " 529 + this.content.getClass().getName() + " was encountered"); 530 return (Reference) this.content; 531 } 532 533 public boolean hasContentReference() { 534 return this.content instanceof Reference; 535 } 536 537 public boolean hasContent() { 538 return this.content != null && !this.content.isEmpty(); 539 } 540 541 /** 542 * @param value {@link #content} (The communicated content (or for multi-part 543 * communications, one portion of the communication).) 544 */ 545 public CommunicationRequestPayloadComponent setContent(Type value) { 546 if (value != null && !(value instanceof StringType || value instanceof Attachment || value instanceof Reference)) 547 throw new Error("Not the right type for CommunicationRequest.payload.content[x]: " + value.fhirType()); 548 this.content = value; 549 return this; 550 } 551 552 protected void listChildren(List<Property> children) { 553 super.listChildren(children); 554 children.add(new Property("content[x]", "string|Attachment|Reference(Any)", 555 "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, 556 content)); 557 } 558 559 @Override 560 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 561 switch (_hash) { 562 case 264548711: 563 /* content[x] */ return new Property("content[x]", "string|Attachment|Reference(Any)", 564 "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, 565 content); 566 case 951530617: 567 /* content */ return new Property("content[x]", "string|Attachment|Reference(Any)", 568 "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, 569 content); 570 case -326336022: 571 /* contentString */ return new Property("content[x]", "string|Attachment|Reference(Any)", 572 "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, 573 content); 574 case -702028164: 575 /* contentAttachment */ return new Property("content[x]", "string|Attachment|Reference(Any)", 576 "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, 577 content); 578 case 1193747154: 579 /* contentReference */ return new Property("content[x]", "string|Attachment|Reference(Any)", 580 "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, 581 content); 582 default: 583 return super.getNamedProperty(_hash, _name, _checkValid); 584 } 585 586 } 587 588 @Override 589 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 590 switch (hash) { 591 case 951530617: 592 /* content */ return this.content == null ? new Base[0] : new Base[] { this.content }; // Type 593 default: 594 return super.getProperty(hash, name, checkValid); 595 } 596 597 } 598 599 @Override 600 public Base setProperty(int hash, String name, Base value) throws FHIRException { 601 switch (hash) { 602 case 951530617: // content 603 this.content = castToType(value); // Type 604 return value; 605 default: 606 return super.setProperty(hash, name, value); 607 } 608 609 } 610 611 @Override 612 public Base setProperty(String name, Base value) throws FHIRException { 613 if (name.equals("content[x]")) { 614 this.content = castToType(value); // Type 615 } else 616 return super.setProperty(name, value); 617 return value; 618 } 619 620 @Override 621 public void removeChild(String name, Base value) throws FHIRException { 622 if (name.equals("content[x]")) { 623 this.content = null; 624 } else 625 super.removeChild(name, value); 626 627 } 628 629 @Override 630 public Base makeProperty(int hash, String name) throws FHIRException { 631 switch (hash) { 632 case 264548711: 633 return getContent(); 634 case 951530617: 635 return getContent(); 636 default: 637 return super.makeProperty(hash, name); 638 } 639 640 } 641 642 @Override 643 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 644 switch (hash) { 645 case 951530617: 646 /* content */ return new String[] { "string", "Attachment", "Reference" }; 647 default: 648 return super.getTypesForProperty(hash, name); 649 } 650 651 } 652 653 @Override 654 public Base addChild(String name) throws FHIRException { 655 if (name.equals("contentString")) { 656 this.content = new StringType(); 657 return this.content; 658 } else if (name.equals("contentAttachment")) { 659 this.content = new Attachment(); 660 return this.content; 661 } else if (name.equals("contentReference")) { 662 this.content = new Reference(); 663 return this.content; 664 } else 665 return super.addChild(name); 666 } 667 668 public CommunicationRequestPayloadComponent copy() { 669 CommunicationRequestPayloadComponent dst = new CommunicationRequestPayloadComponent(); 670 copyValues(dst); 671 return dst; 672 } 673 674 public void copyValues(CommunicationRequestPayloadComponent dst) { 675 super.copyValues(dst); 676 dst.content = content == null ? null : content.copy(); 677 } 678 679 @Override 680 public boolean equalsDeep(Base other_) { 681 if (!super.equalsDeep(other_)) 682 return false; 683 if (!(other_ instanceof CommunicationRequestPayloadComponent)) 684 return false; 685 CommunicationRequestPayloadComponent o = (CommunicationRequestPayloadComponent) other_; 686 return compareDeep(content, o.content, true); 687 } 688 689 @Override 690 public boolean equalsShallow(Base other_) { 691 if (!super.equalsShallow(other_)) 692 return false; 693 if (!(other_ instanceof CommunicationRequestPayloadComponent)) 694 return false; 695 CommunicationRequestPayloadComponent o = (CommunicationRequestPayloadComponent) other_; 696 return true; 697 } 698 699 public boolean isEmpty() { 700 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(content); 701 } 702 703 public String fhirType() { 704 return "CommunicationRequest.payload"; 705 706 } 707 708 } 709 710 /** 711 * Business identifiers assigned to this communication request by the performer 712 * or other systems which remain constant as the resource is updated and 713 * propagates from server to server. 714 */ 715 @Child(name = "identifier", type = { 716 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 717 @Description(shortDefinition = "Unique identifier", formalDefinition = "Business identifiers assigned to this communication request by the performer or other systems which remain constant as the resource is updated and propagates from server to server.") 718 protected List<Identifier> identifier; 719 720 /** 721 * A plan or proposal that is fulfilled in whole or in part by this request. 722 */ 723 @Child(name = "basedOn", type = { 724 Reference.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 725 @Description(shortDefinition = "Fulfills plan or proposal", formalDefinition = "A plan or proposal that is fulfilled in whole or in part by this request.") 726 protected List<Reference> basedOn; 727 /** 728 * The actual objects that are the target of the reference (A plan or proposal 729 * that is fulfilled in whole or in part by this request.) 730 */ 731 protected List<Resource> basedOnTarget; 732 733 /** 734 * Completed or terminated request(s) whose function is taken by this new 735 * request. 736 */ 737 @Child(name = "replaces", type = { 738 CommunicationRequest.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 739 @Description(shortDefinition = "Request(s) replaced by this request", formalDefinition = "Completed or terminated request(s) whose function is taken by this new request.") 740 protected List<Reference> replaces; 741 /** 742 * The actual objects that are the target of the reference (Completed or 743 * terminated request(s) whose function is taken by this new request.) 744 */ 745 protected List<CommunicationRequest> replacesTarget; 746 747 /** 748 * A shared identifier common to all requests that were authorized more or less 749 * simultaneously by a single author, representing the identifier of the 750 * requisition, prescription or similar form. 751 */ 752 @Child(name = "groupIdentifier", type = { 753 Identifier.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 754 @Description(shortDefinition = "Composite request this is part of", formalDefinition = "A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.") 755 protected Identifier groupIdentifier; 756 757 /** 758 * The status of the proposal or order. 759 */ 760 @Child(name = "status", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = true, summary = true) 761 @Description(shortDefinition = "draft | active | on-hold | revoked | completed | entered-in-error | unknown", formalDefinition = "The status of the proposal or order.") 762 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-status") 763 protected Enumeration<CommunicationRequestStatus> status; 764 765 /** 766 * Captures the reason for the current state of the CommunicationRequest. 767 */ 768 @Child(name = "statusReason", type = { 769 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 770 @Description(shortDefinition = "Reason for current status", formalDefinition = "Captures the reason for the current state of the CommunicationRequest.") 771 protected CodeableConcept statusReason; 772 773 /** 774 * The type of message to be sent such as alert, notification, reminder, 775 * instruction, etc. 776 */ 777 @Child(name = "category", type = { 778 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 779 @Description(shortDefinition = "Message category", formalDefinition = "The type of message to be sent such as alert, notification, reminder, instruction, etc.") 780 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/communication-category") 781 protected List<CodeableConcept> category; 782 783 /** 784 * Characterizes how quickly the proposed act must be initiated. Includes 785 * concepts such as stat, urgent, routine. 786 */ 787 @Child(name = "priority", type = { CodeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 788 @Description(shortDefinition = "routine | urgent | asap | stat", formalDefinition = "Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine.") 789 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-priority") 790 protected Enumeration<CommunicationPriority> priority; 791 792 /** 793 * If true indicates that the CommunicationRequest is asking for the specified 794 * action to *not* occur. 795 */ 796 @Child(name = "doNotPerform", type = { 797 BooleanType.class }, order = 8, min = 0, max = 1, modifier = true, summary = true) 798 @Description(shortDefinition = "True if request is prohibiting action", formalDefinition = "If true indicates that the CommunicationRequest is asking for the specified action to *not* occur.") 799 protected BooleanType doNotPerform; 800 801 /** 802 * A channel that was used for this communication (e.g. email, fax). 803 */ 804 @Child(name = "medium", type = { 805 CodeableConcept.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 806 @Description(shortDefinition = "A channel of communication", formalDefinition = "A channel that was used for this communication (e.g. email, fax).") 807 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ParticipationMode") 808 protected List<CodeableConcept> medium; 809 810 /** 811 * The patient or group that is the focus of this communication request. 812 */ 813 @Child(name = "subject", type = { Patient.class, 814 Group.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 815 @Description(shortDefinition = "Focus of message", formalDefinition = "The patient or group that is the focus of this communication request.") 816 protected Reference subject; 817 818 /** 819 * The actual object that is the target of the reference (The patient or group 820 * that is the focus of this communication request.) 821 */ 822 protected Resource subjectTarget; 823 824 /** 825 * Other resources that pertain to this communication request and to which this 826 * communication request should be associated. 827 */ 828 @Child(name = "about", type = { 829 Reference.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 830 @Description(shortDefinition = "Resources that pertain to this communication request", formalDefinition = "Other resources that pertain to this communication request and to which this communication request should be associated.") 831 protected List<Reference> about; 832 /** 833 * The actual objects that are the target of the reference (Other resources that 834 * pertain to this communication request and to which this communication request 835 * should be associated.) 836 */ 837 protected List<Resource> aboutTarget; 838 839 /** 840 * The Encounter during which this CommunicationRequest was created or to which 841 * the creation of this record is tightly associated. 842 */ 843 @Child(name = "encounter", type = { Encounter.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 844 @Description(shortDefinition = "Encounter created as part of", formalDefinition = "The Encounter during which this CommunicationRequest was created or to which the creation of this record is tightly associated.") 845 protected Reference encounter; 846 847 /** 848 * The actual object that is the target of the reference (The Encounter during 849 * which this CommunicationRequest was created or to which the creation of this 850 * record is tightly associated.) 851 */ 852 protected Encounter encounterTarget; 853 854 /** 855 * Text, attachment(s), or resource(s) to be communicated to the recipient. 856 */ 857 @Child(name = "payload", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 858 @Description(shortDefinition = "Message payload", formalDefinition = "Text, attachment(s), or resource(s) to be communicated to the recipient.") 859 protected List<CommunicationRequestPayloadComponent> payload; 860 861 /** 862 * The time when this communication is to occur. 863 */ 864 @Child(name = "occurrence", type = { DateTimeType.class, 865 Period.class }, order = 14, min = 0, max = 1, modifier = false, summary = true) 866 @Description(shortDefinition = "When scheduled", formalDefinition = "The time when this communication is to occur.") 867 protected Type occurrence; 868 869 /** 870 * For draft requests, indicates the date of initial creation. For requests with 871 * other statuses, indicates the date of activation. 872 */ 873 @Child(name = "authoredOn", type = { 874 DateTimeType.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 875 @Description(shortDefinition = "When request transitioned to being actionable", formalDefinition = "For draft requests, indicates the date of initial creation. For requests with other statuses, indicates the date of activation.") 876 protected DateTimeType authoredOn; 877 878 /** 879 * The device, individual, or organization who initiated the request and has 880 * responsibility for its activation. 881 */ 882 @Child(name = "requester", type = { Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, 883 RelatedPerson.class, Device.class }, order = 16, min = 0, max = 1, modifier = false, summary = true) 884 @Description(shortDefinition = "Who/what is requesting service", formalDefinition = "The device, individual, or organization who initiated the request and has responsibility for its activation.") 885 protected Reference requester; 886 887 /** 888 * The actual object that is the target of the reference (The device, 889 * individual, or organization who initiated the request and has responsibility 890 * for its activation.) 891 */ 892 protected Resource requesterTarget; 893 894 /** 895 * The entity (e.g. person, organization, clinical information system, device, 896 * group, or care team) which is the intended target of the communication. 897 */ 898 @Child(name = "recipient", type = { Device.class, Organization.class, Patient.class, Practitioner.class, 899 PractitionerRole.class, RelatedPerson.class, Group.class, CareTeam.class, 900 HealthcareService.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 901 @Description(shortDefinition = "Message recipient", formalDefinition = "The entity (e.g. person, organization, clinical information system, device, group, or care team) which is the intended target of the communication.") 902 protected List<Reference> recipient; 903 /** 904 * The actual objects that are the target of the reference (The entity (e.g. 905 * person, organization, clinical information system, device, group, or care 906 * team) which is the intended target of the communication.) 907 */ 908 protected List<Resource> recipientTarget; 909 910 /** 911 * The entity (e.g. person, organization, clinical information system, or 912 * device) which is to be the source of the communication. 913 */ 914 @Child(name = "sender", type = { Device.class, Organization.class, Patient.class, Practitioner.class, 915 PractitionerRole.class, RelatedPerson.class, 916 HealthcareService.class }, order = 18, min = 0, max = 1, modifier = false, summary = true) 917 @Description(shortDefinition = "Message sender", formalDefinition = "The entity (e.g. person, organization, clinical information system, or device) which is to be the source of the communication.") 918 protected Reference sender; 919 920 /** 921 * The actual object that is the target of the reference (The entity (e.g. 922 * person, organization, clinical information system, or device) which is to be 923 * the source of the communication.) 924 */ 925 protected Resource senderTarget; 926 927 /** 928 * Describes why the request is being made in coded or textual form. 929 */ 930 @Child(name = "reasonCode", type = { 931 CodeableConcept.class }, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 932 @Description(shortDefinition = "Why is communication needed?", formalDefinition = "Describes why the request is being made in coded or textual form.") 933 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ActReason") 934 protected List<CodeableConcept> reasonCode; 935 936 /** 937 * Indicates another resource whose existence justifies this request. 938 */ 939 @Child(name = "reasonReference", type = { Condition.class, Observation.class, DiagnosticReport.class, 940 DocumentReference.class }, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 941 @Description(shortDefinition = "Why is communication needed?", formalDefinition = "Indicates another resource whose existence justifies this request.") 942 protected List<Reference> reasonReference; 943 /** 944 * The actual objects that are the target of the reference (Indicates another 945 * resource whose existence justifies this request.) 946 */ 947 protected List<Resource> reasonReferenceTarget; 948 949 /** 950 * Comments made about the request by the requester, sender, recipient, subject 951 * or other participants. 952 */ 953 @Child(name = "note", type = { 954 Annotation.class }, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 955 @Description(shortDefinition = "Comments made about communication request", formalDefinition = "Comments made about the request by the requester, sender, recipient, subject or other participants.") 956 protected List<Annotation> note; 957 958 private static final long serialVersionUID = 2131096857L; 959 960 /** 961 * Constructor 962 */ 963 public CommunicationRequest() { 964 super(); 965 } 966 967 /** 968 * Constructor 969 */ 970 public CommunicationRequest(Enumeration<CommunicationRequestStatus> status) { 971 super(); 972 this.status = status; 973 } 974 975 /** 976 * @return {@link #identifier} (Business identifiers assigned to this 977 * communication request by the performer or other systems which remain 978 * constant as the resource is updated and propagates from server to 979 * server.) 980 */ 981 public List<Identifier> getIdentifier() { 982 if (this.identifier == null) 983 this.identifier = new ArrayList<Identifier>(); 984 return this.identifier; 985 } 986 987 /** 988 * @return Returns a reference to <code>this</code> for easy method chaining 989 */ 990 public CommunicationRequest setIdentifier(List<Identifier> theIdentifier) { 991 this.identifier = theIdentifier; 992 return this; 993 } 994 995 public boolean hasIdentifier() { 996 if (this.identifier == null) 997 return false; 998 for (Identifier item : this.identifier) 999 if (!item.isEmpty()) 1000 return true; 1001 return false; 1002 } 1003 1004 public Identifier addIdentifier() { // 3 1005 Identifier t = new Identifier(); 1006 if (this.identifier == null) 1007 this.identifier = new ArrayList<Identifier>(); 1008 this.identifier.add(t); 1009 return t; 1010 } 1011 1012 public CommunicationRequest addIdentifier(Identifier t) { // 3 1013 if (t == null) 1014 return this; 1015 if (this.identifier == null) 1016 this.identifier = new ArrayList<Identifier>(); 1017 this.identifier.add(t); 1018 return this; 1019 } 1020 1021 /** 1022 * @return The first repetition of repeating field {@link #identifier}, creating 1023 * it if it does not already exist 1024 */ 1025 public Identifier getIdentifierFirstRep() { 1026 if (getIdentifier().isEmpty()) { 1027 addIdentifier(); 1028 } 1029 return getIdentifier().get(0); 1030 } 1031 1032 /** 1033 * @return {@link #basedOn} (A plan or proposal that is fulfilled in whole or in 1034 * part by this request.) 1035 */ 1036 public List<Reference> getBasedOn() { 1037 if (this.basedOn == null) 1038 this.basedOn = new ArrayList<Reference>(); 1039 return this.basedOn; 1040 } 1041 1042 /** 1043 * @return Returns a reference to <code>this</code> for easy method chaining 1044 */ 1045 public CommunicationRequest setBasedOn(List<Reference> theBasedOn) { 1046 this.basedOn = theBasedOn; 1047 return this; 1048 } 1049 1050 public boolean hasBasedOn() { 1051 if (this.basedOn == null) 1052 return false; 1053 for (Reference item : this.basedOn) 1054 if (!item.isEmpty()) 1055 return true; 1056 return false; 1057 } 1058 1059 public Reference addBasedOn() { // 3 1060 Reference t = new Reference(); 1061 if (this.basedOn == null) 1062 this.basedOn = new ArrayList<Reference>(); 1063 this.basedOn.add(t); 1064 return t; 1065 } 1066 1067 public CommunicationRequest addBasedOn(Reference t) { // 3 1068 if (t == null) 1069 return this; 1070 if (this.basedOn == null) 1071 this.basedOn = new ArrayList<Reference>(); 1072 this.basedOn.add(t); 1073 return this; 1074 } 1075 1076 /** 1077 * @return The first repetition of repeating field {@link #basedOn}, creating it 1078 * if it does not already exist 1079 */ 1080 public Reference getBasedOnFirstRep() { 1081 if (getBasedOn().isEmpty()) { 1082 addBasedOn(); 1083 } 1084 return getBasedOn().get(0); 1085 } 1086 1087 /** 1088 * @deprecated Use Reference#setResource(IBaseResource) instead 1089 */ 1090 @Deprecated 1091 public List<Resource> getBasedOnTarget() { 1092 if (this.basedOnTarget == null) 1093 this.basedOnTarget = new ArrayList<Resource>(); 1094 return this.basedOnTarget; 1095 } 1096 1097 /** 1098 * @return {@link #replaces} (Completed or terminated request(s) whose function 1099 * is taken by this new request.) 1100 */ 1101 public List<Reference> getReplaces() { 1102 if (this.replaces == null) 1103 this.replaces = new ArrayList<Reference>(); 1104 return this.replaces; 1105 } 1106 1107 /** 1108 * @return Returns a reference to <code>this</code> for easy method chaining 1109 */ 1110 public CommunicationRequest setReplaces(List<Reference> theReplaces) { 1111 this.replaces = theReplaces; 1112 return this; 1113 } 1114 1115 public boolean hasReplaces() { 1116 if (this.replaces == null) 1117 return false; 1118 for (Reference item : this.replaces) 1119 if (!item.isEmpty()) 1120 return true; 1121 return false; 1122 } 1123 1124 public Reference addReplaces() { // 3 1125 Reference t = new Reference(); 1126 if (this.replaces == null) 1127 this.replaces = new ArrayList<Reference>(); 1128 this.replaces.add(t); 1129 return t; 1130 } 1131 1132 public CommunicationRequest addReplaces(Reference t) { // 3 1133 if (t == null) 1134 return this; 1135 if (this.replaces == null) 1136 this.replaces = new ArrayList<Reference>(); 1137 this.replaces.add(t); 1138 return this; 1139 } 1140 1141 /** 1142 * @return The first repetition of repeating field {@link #replaces}, creating 1143 * it if it does not already exist 1144 */ 1145 public Reference getReplacesFirstRep() { 1146 if (getReplaces().isEmpty()) { 1147 addReplaces(); 1148 } 1149 return getReplaces().get(0); 1150 } 1151 1152 /** 1153 * @deprecated Use Reference#setResource(IBaseResource) instead 1154 */ 1155 @Deprecated 1156 public List<CommunicationRequest> getReplacesTarget() { 1157 if (this.replacesTarget == null) 1158 this.replacesTarget = new ArrayList<CommunicationRequest>(); 1159 return this.replacesTarget; 1160 } 1161 1162 /** 1163 * @deprecated Use Reference#setResource(IBaseResource) instead 1164 */ 1165 @Deprecated 1166 public CommunicationRequest addReplacesTarget() { 1167 CommunicationRequest r = new CommunicationRequest(); 1168 if (this.replacesTarget == null) 1169 this.replacesTarget = new ArrayList<CommunicationRequest>(); 1170 this.replacesTarget.add(r); 1171 return r; 1172 } 1173 1174 /** 1175 * @return {@link #groupIdentifier} (A shared identifier common to all requests 1176 * that were authorized more or less simultaneously by a single author, 1177 * representing the identifier of the requisition, prescription or 1178 * similar form.) 1179 */ 1180 public Identifier getGroupIdentifier() { 1181 if (this.groupIdentifier == null) 1182 if (Configuration.errorOnAutoCreate()) 1183 throw new Error("Attempt to auto-create CommunicationRequest.groupIdentifier"); 1184 else if (Configuration.doAutoCreate()) 1185 this.groupIdentifier = new Identifier(); // cc 1186 return this.groupIdentifier; 1187 } 1188 1189 public boolean hasGroupIdentifier() { 1190 return this.groupIdentifier != null && !this.groupIdentifier.isEmpty(); 1191 } 1192 1193 /** 1194 * @param value {@link #groupIdentifier} (A shared identifier common to all 1195 * requests that were authorized more or less simultaneously by a 1196 * single author, representing the identifier of the requisition, 1197 * prescription or similar form.) 1198 */ 1199 public CommunicationRequest setGroupIdentifier(Identifier value) { 1200 this.groupIdentifier = value; 1201 return this; 1202 } 1203 1204 /** 1205 * @return {@link #status} (The status of the proposal or order.). This is the 1206 * underlying object with id, value and extensions. The accessor 1207 * "getStatus" gives direct access to the value 1208 */ 1209 public Enumeration<CommunicationRequestStatus> getStatusElement() { 1210 if (this.status == null) 1211 if (Configuration.errorOnAutoCreate()) 1212 throw new Error("Attempt to auto-create CommunicationRequest.status"); 1213 else if (Configuration.doAutoCreate()) 1214 this.status = new Enumeration<CommunicationRequestStatus>(new CommunicationRequestStatusEnumFactory()); // bb 1215 return this.status; 1216 } 1217 1218 public boolean hasStatusElement() { 1219 return this.status != null && !this.status.isEmpty(); 1220 } 1221 1222 public boolean hasStatus() { 1223 return this.status != null && !this.status.isEmpty(); 1224 } 1225 1226 /** 1227 * @param value {@link #status} (The status of the proposal or order.). This is 1228 * the underlying object with id, value and extensions. The 1229 * accessor "getStatus" gives direct access to the value 1230 */ 1231 public CommunicationRequest setStatusElement(Enumeration<CommunicationRequestStatus> value) { 1232 this.status = value; 1233 return this; 1234 } 1235 1236 /** 1237 * @return The status of the proposal or order. 1238 */ 1239 public CommunicationRequestStatus getStatus() { 1240 return this.status == null ? null : this.status.getValue(); 1241 } 1242 1243 /** 1244 * @param value The status of the proposal or order. 1245 */ 1246 public CommunicationRequest setStatus(CommunicationRequestStatus value) { 1247 if (this.status == null) 1248 this.status = new Enumeration<CommunicationRequestStatus>(new CommunicationRequestStatusEnumFactory()); 1249 this.status.setValue(value); 1250 return this; 1251 } 1252 1253 /** 1254 * @return {@link #statusReason} (Captures the reason for the current state of 1255 * the CommunicationRequest.) 1256 */ 1257 public CodeableConcept getStatusReason() { 1258 if (this.statusReason == null) 1259 if (Configuration.errorOnAutoCreate()) 1260 throw new Error("Attempt to auto-create CommunicationRequest.statusReason"); 1261 else if (Configuration.doAutoCreate()) 1262 this.statusReason = new CodeableConcept(); // cc 1263 return this.statusReason; 1264 } 1265 1266 public boolean hasStatusReason() { 1267 return this.statusReason != null && !this.statusReason.isEmpty(); 1268 } 1269 1270 /** 1271 * @param value {@link #statusReason} (Captures the reason for the current state 1272 * of the CommunicationRequest.) 1273 */ 1274 public CommunicationRequest setStatusReason(CodeableConcept value) { 1275 this.statusReason = value; 1276 return this; 1277 } 1278 1279 /** 1280 * @return {@link #category} (The type of message to be sent such as alert, 1281 * notification, reminder, instruction, etc.) 1282 */ 1283 public List<CodeableConcept> getCategory() { 1284 if (this.category == null) 1285 this.category = new ArrayList<CodeableConcept>(); 1286 return this.category; 1287 } 1288 1289 /** 1290 * @return Returns a reference to <code>this</code> for easy method chaining 1291 */ 1292 public CommunicationRequest setCategory(List<CodeableConcept> theCategory) { 1293 this.category = theCategory; 1294 return this; 1295 } 1296 1297 public boolean hasCategory() { 1298 if (this.category == null) 1299 return false; 1300 for (CodeableConcept item : this.category) 1301 if (!item.isEmpty()) 1302 return true; 1303 return false; 1304 } 1305 1306 public CodeableConcept addCategory() { // 3 1307 CodeableConcept t = new CodeableConcept(); 1308 if (this.category == null) 1309 this.category = new ArrayList<CodeableConcept>(); 1310 this.category.add(t); 1311 return t; 1312 } 1313 1314 public CommunicationRequest addCategory(CodeableConcept t) { // 3 1315 if (t == null) 1316 return this; 1317 if (this.category == null) 1318 this.category = new ArrayList<CodeableConcept>(); 1319 this.category.add(t); 1320 return this; 1321 } 1322 1323 /** 1324 * @return The first repetition of repeating field {@link #category}, creating 1325 * it if it does not already exist 1326 */ 1327 public CodeableConcept getCategoryFirstRep() { 1328 if (getCategory().isEmpty()) { 1329 addCategory(); 1330 } 1331 return getCategory().get(0); 1332 } 1333 1334 /** 1335 * @return {@link #priority} (Characterizes how quickly the proposed act must be 1336 * initiated. Includes concepts such as stat, urgent, routine.). This is 1337 * the underlying object with id, value and extensions. The accessor 1338 * "getPriority" gives direct access to the value 1339 */ 1340 public Enumeration<CommunicationPriority> getPriorityElement() { 1341 if (this.priority == null) 1342 if (Configuration.errorOnAutoCreate()) 1343 throw new Error("Attempt to auto-create CommunicationRequest.priority"); 1344 else if (Configuration.doAutoCreate()) 1345 this.priority = new Enumeration<CommunicationPriority>(new CommunicationPriorityEnumFactory()); // bb 1346 return this.priority; 1347 } 1348 1349 public boolean hasPriorityElement() { 1350 return this.priority != null && !this.priority.isEmpty(); 1351 } 1352 1353 public boolean hasPriority() { 1354 return this.priority != null && !this.priority.isEmpty(); 1355 } 1356 1357 /** 1358 * @param value {@link #priority} (Characterizes how quickly the proposed act 1359 * must be initiated. Includes concepts such as stat, urgent, 1360 * routine.). This is the underlying object with id, value and 1361 * extensions. The accessor "getPriority" gives direct access to 1362 * the value 1363 */ 1364 public CommunicationRequest setPriorityElement(Enumeration<CommunicationPriority> value) { 1365 this.priority = value; 1366 return this; 1367 } 1368 1369 /** 1370 * @return Characterizes how quickly the proposed act must be initiated. 1371 * Includes concepts such as stat, urgent, routine. 1372 */ 1373 public CommunicationPriority getPriority() { 1374 return this.priority == null ? null : this.priority.getValue(); 1375 } 1376 1377 /** 1378 * @param value Characterizes how quickly the proposed act must be initiated. 1379 * Includes concepts such as stat, urgent, routine. 1380 */ 1381 public CommunicationRequest setPriority(CommunicationPriority value) { 1382 if (value == null) 1383 this.priority = null; 1384 else { 1385 if (this.priority == null) 1386 this.priority = new Enumeration<CommunicationPriority>(new CommunicationPriorityEnumFactory()); 1387 this.priority.setValue(value); 1388 } 1389 return this; 1390 } 1391 1392 /** 1393 * @return {@link #doNotPerform} (If true indicates that the 1394 * CommunicationRequest is asking for the specified action to *not* 1395 * occur.). This is the underlying object with id, value and extensions. 1396 * The accessor "getDoNotPerform" gives direct access to the value 1397 */ 1398 public BooleanType getDoNotPerformElement() { 1399 if (this.doNotPerform == null) 1400 if (Configuration.errorOnAutoCreate()) 1401 throw new Error("Attempt to auto-create CommunicationRequest.doNotPerform"); 1402 else if (Configuration.doAutoCreate()) 1403 this.doNotPerform = new BooleanType(); // bb 1404 return this.doNotPerform; 1405 } 1406 1407 public boolean hasDoNotPerformElement() { 1408 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 1409 } 1410 1411 public boolean hasDoNotPerform() { 1412 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 1413 } 1414 1415 /** 1416 * @param value {@link #doNotPerform} (If true indicates that the 1417 * CommunicationRequest is asking for the specified action to *not* 1418 * occur.). This is the underlying object with id, value and 1419 * extensions. The accessor "getDoNotPerform" gives direct access 1420 * to the value 1421 */ 1422 public CommunicationRequest setDoNotPerformElement(BooleanType value) { 1423 this.doNotPerform = value; 1424 return this; 1425 } 1426 1427 /** 1428 * @return If true indicates that the CommunicationRequest is asking for the 1429 * specified action to *not* occur. 1430 */ 1431 public boolean getDoNotPerform() { 1432 return this.doNotPerform == null || this.doNotPerform.isEmpty() ? false : this.doNotPerform.getValue(); 1433 } 1434 1435 /** 1436 * @param value If true indicates that the CommunicationRequest is asking for 1437 * the specified action to *not* occur. 1438 */ 1439 public CommunicationRequest setDoNotPerform(boolean value) { 1440 if (this.doNotPerform == null) 1441 this.doNotPerform = new BooleanType(); 1442 this.doNotPerform.setValue(value); 1443 return this; 1444 } 1445 1446 /** 1447 * @return {@link #medium} (A channel that was used for this communication (e.g. 1448 * email, fax).) 1449 */ 1450 public List<CodeableConcept> getMedium() { 1451 if (this.medium == null) 1452 this.medium = new ArrayList<CodeableConcept>(); 1453 return this.medium; 1454 } 1455 1456 /** 1457 * @return Returns a reference to <code>this</code> for easy method chaining 1458 */ 1459 public CommunicationRequest setMedium(List<CodeableConcept> theMedium) { 1460 this.medium = theMedium; 1461 return this; 1462 } 1463 1464 public boolean hasMedium() { 1465 if (this.medium == null) 1466 return false; 1467 for (CodeableConcept item : this.medium) 1468 if (!item.isEmpty()) 1469 return true; 1470 return false; 1471 } 1472 1473 public CodeableConcept addMedium() { // 3 1474 CodeableConcept t = new CodeableConcept(); 1475 if (this.medium == null) 1476 this.medium = new ArrayList<CodeableConcept>(); 1477 this.medium.add(t); 1478 return t; 1479 } 1480 1481 public CommunicationRequest addMedium(CodeableConcept t) { // 3 1482 if (t == null) 1483 return this; 1484 if (this.medium == null) 1485 this.medium = new ArrayList<CodeableConcept>(); 1486 this.medium.add(t); 1487 return this; 1488 } 1489 1490 /** 1491 * @return The first repetition of repeating field {@link #medium}, creating it 1492 * if it does not already exist 1493 */ 1494 public CodeableConcept getMediumFirstRep() { 1495 if (getMedium().isEmpty()) { 1496 addMedium(); 1497 } 1498 return getMedium().get(0); 1499 } 1500 1501 /** 1502 * @return {@link #subject} (The patient or group that is the focus of this 1503 * communication request.) 1504 */ 1505 public Reference getSubject() { 1506 if (this.subject == null) 1507 if (Configuration.errorOnAutoCreate()) 1508 throw new Error("Attempt to auto-create CommunicationRequest.subject"); 1509 else if (Configuration.doAutoCreate()) 1510 this.subject = new Reference(); // cc 1511 return this.subject; 1512 } 1513 1514 public boolean hasSubject() { 1515 return this.subject != null && !this.subject.isEmpty(); 1516 } 1517 1518 /** 1519 * @param value {@link #subject} (The patient or group that is the focus of this 1520 * communication request.) 1521 */ 1522 public CommunicationRequest setSubject(Reference value) { 1523 this.subject = value; 1524 return this; 1525 } 1526 1527 /** 1528 * @return {@link #subject} The actual object that is the target of the 1529 * reference. The reference library doesn't populate this, but you can 1530 * use it to hold the resource if you resolve it. (The patient or group 1531 * that is the focus of this communication request.) 1532 */ 1533 public Resource getSubjectTarget() { 1534 return this.subjectTarget; 1535 } 1536 1537 /** 1538 * @param value {@link #subject} The actual object that is the target of the 1539 * reference. The reference library doesn't use these, but you can 1540 * use it to hold the resource if you resolve it. (The patient or 1541 * group that is the focus of this communication request.) 1542 */ 1543 public CommunicationRequest setSubjectTarget(Resource value) { 1544 this.subjectTarget = value; 1545 return this; 1546 } 1547 1548 /** 1549 * @return {@link #about} (Other resources that pertain to this communication 1550 * request and to which this communication request should be 1551 * associated.) 1552 */ 1553 public List<Reference> getAbout() { 1554 if (this.about == null) 1555 this.about = new ArrayList<Reference>(); 1556 return this.about; 1557 } 1558 1559 /** 1560 * @return Returns a reference to <code>this</code> for easy method chaining 1561 */ 1562 public CommunicationRequest setAbout(List<Reference> theAbout) { 1563 this.about = theAbout; 1564 return this; 1565 } 1566 1567 public boolean hasAbout() { 1568 if (this.about == null) 1569 return false; 1570 for (Reference item : this.about) 1571 if (!item.isEmpty()) 1572 return true; 1573 return false; 1574 } 1575 1576 public Reference addAbout() { // 3 1577 Reference t = new Reference(); 1578 if (this.about == null) 1579 this.about = new ArrayList<Reference>(); 1580 this.about.add(t); 1581 return t; 1582 } 1583 1584 public CommunicationRequest addAbout(Reference t) { // 3 1585 if (t == null) 1586 return this; 1587 if (this.about == null) 1588 this.about = new ArrayList<Reference>(); 1589 this.about.add(t); 1590 return this; 1591 } 1592 1593 /** 1594 * @return The first repetition of repeating field {@link #about}, creating it 1595 * if it does not already exist 1596 */ 1597 public Reference getAboutFirstRep() { 1598 if (getAbout().isEmpty()) { 1599 addAbout(); 1600 } 1601 return getAbout().get(0); 1602 } 1603 1604 /** 1605 * @deprecated Use Reference#setResource(IBaseResource) instead 1606 */ 1607 @Deprecated 1608 public List<Resource> getAboutTarget() { 1609 if (this.aboutTarget == null) 1610 this.aboutTarget = new ArrayList<Resource>(); 1611 return this.aboutTarget; 1612 } 1613 1614 /** 1615 * @return {@link #encounter} (The Encounter during which this 1616 * CommunicationRequest was created or to which the creation of this 1617 * record is tightly associated.) 1618 */ 1619 public Reference getEncounter() { 1620 if (this.encounter == null) 1621 if (Configuration.errorOnAutoCreate()) 1622 throw new Error("Attempt to auto-create CommunicationRequest.encounter"); 1623 else if (Configuration.doAutoCreate()) 1624 this.encounter = new Reference(); // cc 1625 return this.encounter; 1626 } 1627 1628 public boolean hasEncounter() { 1629 return this.encounter != null && !this.encounter.isEmpty(); 1630 } 1631 1632 /** 1633 * @param value {@link #encounter} (The Encounter during which this 1634 * CommunicationRequest was created or to which the creation of 1635 * this record is tightly associated.) 1636 */ 1637 public CommunicationRequest setEncounter(Reference value) { 1638 this.encounter = value; 1639 return this; 1640 } 1641 1642 /** 1643 * @return {@link #encounter} The actual object that is the target of the 1644 * reference. The reference library doesn't populate this, but you can 1645 * use it to hold the resource if you resolve it. (The Encounter during 1646 * which this CommunicationRequest was created or to which the creation 1647 * of this record is tightly associated.) 1648 */ 1649 public Encounter getEncounterTarget() { 1650 if (this.encounterTarget == null) 1651 if (Configuration.errorOnAutoCreate()) 1652 throw new Error("Attempt to auto-create CommunicationRequest.encounter"); 1653 else if (Configuration.doAutoCreate()) 1654 this.encounterTarget = new Encounter(); // aa 1655 return this.encounterTarget; 1656 } 1657 1658 /** 1659 * @param value {@link #encounter} The actual object that is the target of the 1660 * reference. The reference library doesn't use these, but you can 1661 * use it to hold the resource if you resolve it. (The Encounter 1662 * during which this CommunicationRequest was created or to which 1663 * the creation of this record is tightly associated.) 1664 */ 1665 public CommunicationRequest setEncounterTarget(Encounter value) { 1666 this.encounterTarget = value; 1667 return this; 1668 } 1669 1670 /** 1671 * @return {@link #payload} (Text, attachment(s), or resource(s) to be 1672 * communicated to the recipient.) 1673 */ 1674 public List<CommunicationRequestPayloadComponent> getPayload() { 1675 if (this.payload == null) 1676 this.payload = new ArrayList<CommunicationRequestPayloadComponent>(); 1677 return this.payload; 1678 } 1679 1680 /** 1681 * @return Returns a reference to <code>this</code> for easy method chaining 1682 */ 1683 public CommunicationRequest setPayload(List<CommunicationRequestPayloadComponent> thePayload) { 1684 this.payload = thePayload; 1685 return this; 1686 } 1687 1688 public boolean hasPayload() { 1689 if (this.payload == null) 1690 return false; 1691 for (CommunicationRequestPayloadComponent item : this.payload) 1692 if (!item.isEmpty()) 1693 return true; 1694 return false; 1695 } 1696 1697 public CommunicationRequestPayloadComponent addPayload() { // 3 1698 CommunicationRequestPayloadComponent t = new CommunicationRequestPayloadComponent(); 1699 if (this.payload == null) 1700 this.payload = new ArrayList<CommunicationRequestPayloadComponent>(); 1701 this.payload.add(t); 1702 return t; 1703 } 1704 1705 public CommunicationRequest addPayload(CommunicationRequestPayloadComponent t) { // 3 1706 if (t == null) 1707 return this; 1708 if (this.payload == null) 1709 this.payload = new ArrayList<CommunicationRequestPayloadComponent>(); 1710 this.payload.add(t); 1711 return this; 1712 } 1713 1714 /** 1715 * @return The first repetition of repeating field {@link #payload}, creating it 1716 * if it does not already exist 1717 */ 1718 public CommunicationRequestPayloadComponent getPayloadFirstRep() { 1719 if (getPayload().isEmpty()) { 1720 addPayload(); 1721 } 1722 return getPayload().get(0); 1723 } 1724 1725 /** 1726 * @return {@link #occurrence} (The time when this communication is to occur.) 1727 */ 1728 public Type getOccurrence() { 1729 return this.occurrence; 1730 } 1731 1732 /** 1733 * @return {@link #occurrence} (The time when this communication is to occur.) 1734 */ 1735 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 1736 if (this.occurrence == null) 1737 this.occurrence = new DateTimeType(); 1738 if (!(this.occurrence instanceof DateTimeType)) 1739 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1740 + this.occurrence.getClass().getName() + " was encountered"); 1741 return (DateTimeType) this.occurrence; 1742 } 1743 1744 public boolean hasOccurrenceDateTimeType() { 1745 return this.occurrence instanceof DateTimeType; 1746 } 1747 1748 /** 1749 * @return {@link #occurrence} (The time when this communication is to occur.) 1750 */ 1751 public Period getOccurrencePeriod() throws FHIRException { 1752 if (this.occurrence == null) 1753 this.occurrence = new Period(); 1754 if (!(this.occurrence instanceof Period)) 1755 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.occurrence.getClass().getName() 1756 + " was encountered"); 1757 return (Period) this.occurrence; 1758 } 1759 1760 public boolean hasOccurrencePeriod() { 1761 return this.occurrence instanceof Period; 1762 } 1763 1764 public boolean hasOccurrence() { 1765 return this.occurrence != null && !this.occurrence.isEmpty(); 1766 } 1767 1768 /** 1769 * @param value {@link #occurrence} (The time when this communication is to 1770 * occur.) 1771 */ 1772 public CommunicationRequest setOccurrence(Type value) { 1773 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1774 throw new Error("Not the right type for CommunicationRequest.occurrence[x]: " + value.fhirType()); 1775 this.occurrence = value; 1776 return this; 1777 } 1778 1779 /** 1780 * @return {@link #authoredOn} (For draft requests, indicates the date of 1781 * initial creation. For requests with other statuses, indicates the 1782 * date of activation.). This is the underlying object with id, value 1783 * and extensions. The accessor "getAuthoredOn" gives direct access to 1784 * the value 1785 */ 1786 public DateTimeType getAuthoredOnElement() { 1787 if (this.authoredOn == null) 1788 if (Configuration.errorOnAutoCreate()) 1789 throw new Error("Attempt to auto-create CommunicationRequest.authoredOn"); 1790 else if (Configuration.doAutoCreate()) 1791 this.authoredOn = new DateTimeType(); // bb 1792 return this.authoredOn; 1793 } 1794 1795 public boolean hasAuthoredOnElement() { 1796 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1797 } 1798 1799 public boolean hasAuthoredOn() { 1800 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1801 } 1802 1803 /** 1804 * @param value {@link #authoredOn} (For draft requests, indicates the date of 1805 * initial creation. For requests with other statuses, indicates 1806 * the date of activation.). This is the underlying object with id, 1807 * value and extensions. The accessor "getAuthoredOn" gives direct 1808 * access to the value 1809 */ 1810 public CommunicationRequest setAuthoredOnElement(DateTimeType value) { 1811 this.authoredOn = value; 1812 return this; 1813 } 1814 1815 /** 1816 * @return For draft requests, indicates the date of initial creation. For 1817 * requests with other statuses, indicates the date of activation. 1818 */ 1819 public Date getAuthoredOn() { 1820 return this.authoredOn == null ? null : this.authoredOn.getValue(); 1821 } 1822 1823 /** 1824 * @param value For draft requests, indicates the date of initial creation. For 1825 * requests with other statuses, indicates the date of activation. 1826 */ 1827 public CommunicationRequest setAuthoredOn(Date value) { 1828 if (value == null) 1829 this.authoredOn = null; 1830 else { 1831 if (this.authoredOn == null) 1832 this.authoredOn = new DateTimeType(); 1833 this.authoredOn.setValue(value); 1834 } 1835 return this; 1836 } 1837 1838 /** 1839 * @return {@link #requester} (The device, individual, or organization who 1840 * initiated the request and has responsibility for its activation.) 1841 */ 1842 public Reference getRequester() { 1843 if (this.requester == null) 1844 if (Configuration.errorOnAutoCreate()) 1845 throw new Error("Attempt to auto-create CommunicationRequest.requester"); 1846 else if (Configuration.doAutoCreate()) 1847 this.requester = new Reference(); // cc 1848 return this.requester; 1849 } 1850 1851 public boolean hasRequester() { 1852 return this.requester != null && !this.requester.isEmpty(); 1853 } 1854 1855 /** 1856 * @param value {@link #requester} (The device, individual, or organization who 1857 * initiated the request and has responsibility for its 1858 * activation.) 1859 */ 1860 public CommunicationRequest setRequester(Reference value) { 1861 this.requester = value; 1862 return this; 1863 } 1864 1865 /** 1866 * @return {@link #requester} The actual object that is the target of the 1867 * reference. The reference library doesn't populate this, but you can 1868 * use it to hold the resource if you resolve it. (The device, 1869 * individual, or organization who initiated the request and has 1870 * responsibility for its activation.) 1871 */ 1872 public Resource getRequesterTarget() { 1873 return this.requesterTarget; 1874 } 1875 1876 /** 1877 * @param value {@link #requester} The actual object that is the target of the 1878 * reference. The reference library doesn't use these, but you can 1879 * use it to hold the resource if you resolve it. (The device, 1880 * individual, or organization who initiated the request and has 1881 * responsibility for its activation.) 1882 */ 1883 public CommunicationRequest setRequesterTarget(Resource value) { 1884 this.requesterTarget = value; 1885 return this; 1886 } 1887 1888 /** 1889 * @return {@link #recipient} (The entity (e.g. person, organization, clinical 1890 * information system, device, group, or care team) which is the 1891 * intended target of the communication.) 1892 */ 1893 public List<Reference> getRecipient() { 1894 if (this.recipient == null) 1895 this.recipient = new ArrayList<Reference>(); 1896 return this.recipient; 1897 } 1898 1899 /** 1900 * @return Returns a reference to <code>this</code> for easy method chaining 1901 */ 1902 public CommunicationRequest setRecipient(List<Reference> theRecipient) { 1903 this.recipient = theRecipient; 1904 return this; 1905 } 1906 1907 public boolean hasRecipient() { 1908 if (this.recipient == null) 1909 return false; 1910 for (Reference item : this.recipient) 1911 if (!item.isEmpty()) 1912 return true; 1913 return false; 1914 } 1915 1916 public Reference addRecipient() { // 3 1917 Reference t = new Reference(); 1918 if (this.recipient == null) 1919 this.recipient = new ArrayList<Reference>(); 1920 this.recipient.add(t); 1921 return t; 1922 } 1923 1924 public CommunicationRequest addRecipient(Reference t) { // 3 1925 if (t == null) 1926 return this; 1927 if (this.recipient == null) 1928 this.recipient = new ArrayList<Reference>(); 1929 this.recipient.add(t); 1930 return this; 1931 } 1932 1933 /** 1934 * @return The first repetition of repeating field {@link #recipient}, creating 1935 * it if it does not already exist 1936 */ 1937 public Reference getRecipientFirstRep() { 1938 if (getRecipient().isEmpty()) { 1939 addRecipient(); 1940 } 1941 return getRecipient().get(0); 1942 } 1943 1944 /** 1945 * @deprecated Use Reference#setResource(IBaseResource) instead 1946 */ 1947 @Deprecated 1948 public List<Resource> getRecipientTarget() { 1949 if (this.recipientTarget == null) 1950 this.recipientTarget = new ArrayList<Resource>(); 1951 return this.recipientTarget; 1952 } 1953 1954 /** 1955 * @return {@link #sender} (The entity (e.g. person, organization, clinical 1956 * information system, or device) which is to be the source of the 1957 * communication.) 1958 */ 1959 public Reference getSender() { 1960 if (this.sender == null) 1961 if (Configuration.errorOnAutoCreate()) 1962 throw new Error("Attempt to auto-create CommunicationRequest.sender"); 1963 else if (Configuration.doAutoCreate()) 1964 this.sender = new Reference(); // cc 1965 return this.sender; 1966 } 1967 1968 public boolean hasSender() { 1969 return this.sender != null && !this.sender.isEmpty(); 1970 } 1971 1972 /** 1973 * @param value {@link #sender} (The entity (e.g. person, organization, clinical 1974 * information system, or device) which is to be the source of the 1975 * communication.) 1976 */ 1977 public CommunicationRequest setSender(Reference value) { 1978 this.sender = value; 1979 return this; 1980 } 1981 1982 /** 1983 * @return {@link #sender} The actual object that is the target of the 1984 * reference. The reference library doesn't populate this, but you can 1985 * use it to hold the resource if you resolve it. (The entity (e.g. 1986 * person, organization, clinical information system, or device) which 1987 * is to be the source of the communication.) 1988 */ 1989 public Resource getSenderTarget() { 1990 return this.senderTarget; 1991 } 1992 1993 /** 1994 * @param value {@link #sender} The actual object that is the target of the 1995 * reference. The reference library doesn't use these, but you can 1996 * use it to hold the resource if you resolve it. (The entity (e.g. 1997 * person, organization, clinical information system, or device) 1998 * which is to be the source of the communication.) 1999 */ 2000 public CommunicationRequest setSenderTarget(Resource value) { 2001 this.senderTarget = value; 2002 return this; 2003 } 2004 2005 /** 2006 * @return {@link #reasonCode} (Describes why the request is being made in coded 2007 * or textual form.) 2008 */ 2009 public List<CodeableConcept> getReasonCode() { 2010 if (this.reasonCode == null) 2011 this.reasonCode = new ArrayList<CodeableConcept>(); 2012 return this.reasonCode; 2013 } 2014 2015 /** 2016 * @return Returns a reference to <code>this</code> for easy method chaining 2017 */ 2018 public CommunicationRequest setReasonCode(List<CodeableConcept> theReasonCode) { 2019 this.reasonCode = theReasonCode; 2020 return this; 2021 } 2022 2023 public boolean hasReasonCode() { 2024 if (this.reasonCode == null) 2025 return false; 2026 for (CodeableConcept item : this.reasonCode) 2027 if (!item.isEmpty()) 2028 return true; 2029 return false; 2030 } 2031 2032 public CodeableConcept addReasonCode() { // 3 2033 CodeableConcept t = new CodeableConcept(); 2034 if (this.reasonCode == null) 2035 this.reasonCode = new ArrayList<CodeableConcept>(); 2036 this.reasonCode.add(t); 2037 return t; 2038 } 2039 2040 public CommunicationRequest addReasonCode(CodeableConcept t) { // 3 2041 if (t == null) 2042 return this; 2043 if (this.reasonCode == null) 2044 this.reasonCode = new ArrayList<CodeableConcept>(); 2045 this.reasonCode.add(t); 2046 return this; 2047 } 2048 2049 /** 2050 * @return The first repetition of repeating field {@link #reasonCode}, creating 2051 * it if it does not already exist 2052 */ 2053 public CodeableConcept getReasonCodeFirstRep() { 2054 if (getReasonCode().isEmpty()) { 2055 addReasonCode(); 2056 } 2057 return getReasonCode().get(0); 2058 } 2059 2060 /** 2061 * @return {@link #reasonReference} (Indicates another resource whose existence 2062 * justifies this request.) 2063 */ 2064 public List<Reference> getReasonReference() { 2065 if (this.reasonReference == null) 2066 this.reasonReference = new ArrayList<Reference>(); 2067 return this.reasonReference; 2068 } 2069 2070 /** 2071 * @return Returns a reference to <code>this</code> for easy method chaining 2072 */ 2073 public CommunicationRequest setReasonReference(List<Reference> theReasonReference) { 2074 this.reasonReference = theReasonReference; 2075 return this; 2076 } 2077 2078 public boolean hasReasonReference() { 2079 if (this.reasonReference == null) 2080 return false; 2081 for (Reference item : this.reasonReference) 2082 if (!item.isEmpty()) 2083 return true; 2084 return false; 2085 } 2086 2087 public Reference addReasonReference() { // 3 2088 Reference t = new Reference(); 2089 if (this.reasonReference == null) 2090 this.reasonReference = new ArrayList<Reference>(); 2091 this.reasonReference.add(t); 2092 return t; 2093 } 2094 2095 public CommunicationRequest addReasonReference(Reference t) { // 3 2096 if (t == null) 2097 return this; 2098 if (this.reasonReference == null) 2099 this.reasonReference = new ArrayList<Reference>(); 2100 this.reasonReference.add(t); 2101 return this; 2102 } 2103 2104 /** 2105 * @return The first repetition of repeating field {@link #reasonReference}, 2106 * creating it if it does not already exist 2107 */ 2108 public Reference getReasonReferenceFirstRep() { 2109 if (getReasonReference().isEmpty()) { 2110 addReasonReference(); 2111 } 2112 return getReasonReference().get(0); 2113 } 2114 2115 /** 2116 * @deprecated Use Reference#setResource(IBaseResource) instead 2117 */ 2118 @Deprecated 2119 public List<Resource> getReasonReferenceTarget() { 2120 if (this.reasonReferenceTarget == null) 2121 this.reasonReferenceTarget = new ArrayList<Resource>(); 2122 return this.reasonReferenceTarget; 2123 } 2124 2125 /** 2126 * @return {@link #note} (Comments made about the request by the requester, 2127 * sender, recipient, subject or other participants.) 2128 */ 2129 public List<Annotation> getNote() { 2130 if (this.note == null) 2131 this.note = new ArrayList<Annotation>(); 2132 return this.note; 2133 } 2134 2135 /** 2136 * @return Returns a reference to <code>this</code> for easy method chaining 2137 */ 2138 public CommunicationRequest setNote(List<Annotation> theNote) { 2139 this.note = theNote; 2140 return this; 2141 } 2142 2143 public boolean hasNote() { 2144 if (this.note == null) 2145 return false; 2146 for (Annotation item : this.note) 2147 if (!item.isEmpty()) 2148 return true; 2149 return false; 2150 } 2151 2152 public Annotation addNote() { // 3 2153 Annotation t = new Annotation(); 2154 if (this.note == null) 2155 this.note = new ArrayList<Annotation>(); 2156 this.note.add(t); 2157 return t; 2158 } 2159 2160 public CommunicationRequest addNote(Annotation t) { // 3 2161 if (t == null) 2162 return this; 2163 if (this.note == null) 2164 this.note = new ArrayList<Annotation>(); 2165 this.note.add(t); 2166 return this; 2167 } 2168 2169 /** 2170 * @return The first repetition of repeating field {@link #note}, creating it if 2171 * it does not already exist 2172 */ 2173 public Annotation getNoteFirstRep() { 2174 if (getNote().isEmpty()) { 2175 addNote(); 2176 } 2177 return getNote().get(0); 2178 } 2179 2180 protected void listChildren(List<Property> children) { 2181 super.listChildren(children); 2182 children.add(new Property("identifier", "Identifier", 2183 "Business identifiers assigned to this communication request by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 2184 0, java.lang.Integer.MAX_VALUE, identifier)); 2185 children.add(new Property("basedOn", "Reference(Any)", 2186 "A plan or proposal that is fulfilled in whole or in part by this request.", 0, java.lang.Integer.MAX_VALUE, 2187 basedOn)); 2188 children.add(new Property("replaces", "Reference(CommunicationRequest)", 2189 "Completed or terminated request(s) whose function is taken by this new request.", 0, 2190 java.lang.Integer.MAX_VALUE, replaces)); 2191 children.add(new Property("groupIdentifier", "Identifier", 2192 "A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.", 2193 0, 1, groupIdentifier)); 2194 children.add(new Property("status", "code", "The status of the proposal or order.", 0, 1, status)); 2195 children.add(new Property("statusReason", "CodeableConcept", 2196 "Captures the reason for the current state of the CommunicationRequest.", 0, 1, statusReason)); 2197 children.add(new Property("category", "CodeableConcept", 2198 "The type of message to be sent such as alert, notification, reminder, instruction, etc.", 0, 2199 java.lang.Integer.MAX_VALUE, category)); 2200 children.add(new Property("priority", "code", 2201 "Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine.", 2202 0, 1, priority)); 2203 children.add(new Property("doNotPerform", "boolean", 2204 "If true indicates that the CommunicationRequest is asking for the specified action to *not* occur.", 0, 1, 2205 doNotPerform)); 2206 children.add(new Property("medium", "CodeableConcept", 2207 "A channel that was used for this communication (e.g. email, fax).", 0, java.lang.Integer.MAX_VALUE, medium)); 2208 children.add(new Property("subject", "Reference(Patient|Group)", 2209 "The patient or group that is the focus of this communication request.", 0, 1, subject)); 2210 children.add(new Property("about", "Reference(Any)", 2211 "Other resources that pertain to this communication request and to which this communication request should be associated.", 2212 0, java.lang.Integer.MAX_VALUE, about)); 2213 children.add(new Property("encounter", "Reference(Encounter)", 2214 "The Encounter during which this CommunicationRequest was created or to which the creation of this record is tightly associated.", 2215 0, 1, encounter)); 2216 children.add(new Property("payload", "", "Text, attachment(s), or resource(s) to be communicated to the recipient.", 2217 0, java.lang.Integer.MAX_VALUE, payload)); 2218 children.add(new Property("occurrence[x]", "dateTime|Period", "The time when this communication is to occur.", 0, 1, 2219 occurrence)); 2220 children.add(new Property("authoredOn", "dateTime", 2221 "For draft requests, indicates the date of initial creation. For requests with other statuses, indicates the date of activation.", 2222 0, 1, authoredOn)); 2223 children.add(new Property("requester", 2224 "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device)", 2225 "The device, individual, or organization who initiated the request and has responsibility for its activation.", 2226 0, 1, requester)); 2227 children.add(new Property("recipient", 2228 "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson|Group|CareTeam|HealthcareService)", 2229 "The entity (e.g. person, organization, clinical information system, device, group, or care team) which is the intended target of the communication.", 2230 0, java.lang.Integer.MAX_VALUE, recipient)); 2231 children.add(new Property("sender", 2232 "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson|HealthcareService)", 2233 "The entity (e.g. person, organization, clinical information system, or device) which is to be the source of the communication.", 2234 0, 1, sender)); 2235 children.add(new Property("reasonCode", "CodeableConcept", 2236 "Describes why the request is being made in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, 2237 reasonCode)); 2238 children.add(new Property("reasonReference", "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 2239 "Indicates another resource whose existence justifies this request.", 0, java.lang.Integer.MAX_VALUE, 2240 reasonReference)); 2241 children.add(new Property("note", "Annotation", 2242 "Comments made about the request by the requester, sender, recipient, subject or other participants.", 0, 2243 java.lang.Integer.MAX_VALUE, note)); 2244 } 2245 2246 @Override 2247 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2248 switch (_hash) { 2249 case -1618432855: 2250 /* identifier */ return new Property("identifier", "Identifier", 2251 "Business identifiers assigned to this communication request by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 2252 0, java.lang.Integer.MAX_VALUE, identifier); 2253 case -332612366: 2254 /* basedOn */ return new Property("basedOn", "Reference(Any)", 2255 "A plan or proposal that is fulfilled in whole or in part by this request.", 0, java.lang.Integer.MAX_VALUE, 2256 basedOn); 2257 case -430332865: 2258 /* replaces */ return new Property("replaces", "Reference(CommunicationRequest)", 2259 "Completed or terminated request(s) whose function is taken by this new request.", 0, 2260 java.lang.Integer.MAX_VALUE, replaces); 2261 case -445338488: 2262 /* groupIdentifier */ return new Property("groupIdentifier", "Identifier", 2263 "A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.", 2264 0, 1, groupIdentifier); 2265 case -892481550: 2266 /* status */ return new Property("status", "code", "The status of the proposal or order.", 0, 1, status); 2267 case 2051346646: 2268 /* statusReason */ return new Property("statusReason", "CodeableConcept", 2269 "Captures the reason for the current state of the CommunicationRequest.", 0, 1, statusReason); 2270 case 50511102: 2271 /* category */ return new Property("category", "CodeableConcept", 2272 "The type of message to be sent such as alert, notification, reminder, instruction, etc.", 0, 2273 java.lang.Integer.MAX_VALUE, category); 2274 case -1165461084: 2275 /* priority */ return new Property("priority", "code", 2276 "Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine.", 2277 0, 1, priority); 2278 case -1788508167: 2279 /* doNotPerform */ return new Property("doNotPerform", "boolean", 2280 "If true indicates that the CommunicationRequest is asking for the specified action to *not* occur.", 0, 1, 2281 doNotPerform); 2282 case -1078030475: 2283 /* medium */ return new Property("medium", "CodeableConcept", 2284 "A channel that was used for this communication (e.g. email, fax).", 0, java.lang.Integer.MAX_VALUE, medium); 2285 case -1867885268: 2286 /* subject */ return new Property("subject", "Reference(Patient|Group)", 2287 "The patient or group that is the focus of this communication request.", 0, 1, subject); 2288 case 92611469: 2289 /* about */ return new Property("about", "Reference(Any)", 2290 "Other resources that pertain to this communication request and to which this communication request should be associated.", 2291 0, java.lang.Integer.MAX_VALUE, about); 2292 case 1524132147: 2293 /* encounter */ return new Property("encounter", "Reference(Encounter)", 2294 "The Encounter during which this CommunicationRequest was created or to which the creation of this record is tightly associated.", 2295 0, 1, encounter); 2296 case -786701938: 2297 /* payload */ return new Property("payload", "", 2298 "Text, attachment(s), or resource(s) to be communicated to the recipient.", 0, java.lang.Integer.MAX_VALUE, 2299 payload); 2300 case -2022646513: 2301 /* occurrence[x] */ return new Property("occurrence[x]", "dateTime|Period", 2302 "The time when this communication is to occur.", 0, 1, occurrence); 2303 case 1687874001: 2304 /* occurrence */ return new Property("occurrence[x]", "dateTime|Period", 2305 "The time when this communication is to occur.", 0, 1, occurrence); 2306 case -298443636: 2307 /* occurrenceDateTime */ return new Property("occurrence[x]", "dateTime|Period", 2308 "The time when this communication is to occur.", 0, 1, occurrence); 2309 case 1397156594: 2310 /* occurrencePeriod */ return new Property("occurrence[x]", "dateTime|Period", 2311 "The time when this communication is to occur.", 0, 1, occurrence); 2312 case -1500852503: 2313 /* authoredOn */ return new Property("authoredOn", "dateTime", 2314 "For draft requests, indicates the date of initial creation. For requests with other statuses, indicates the date of activation.", 2315 0, 1, authoredOn); 2316 case 693933948: 2317 /* requester */ return new Property("requester", 2318 "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device)", 2319 "The device, individual, or organization who initiated the request and has responsibility for its activation.", 2320 0, 1, requester); 2321 case 820081177: 2322 /* recipient */ return new Property("recipient", 2323 "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson|Group|CareTeam|HealthcareService)", 2324 "The entity (e.g. person, organization, clinical information system, device, group, or care team) which is the intended target of the communication.", 2325 0, java.lang.Integer.MAX_VALUE, recipient); 2326 case -905962955: 2327 /* sender */ return new Property("sender", 2328 "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson|HealthcareService)", 2329 "The entity (e.g. person, organization, clinical information system, or device) which is to be the source of the communication.", 2330 0, 1, sender); 2331 case 722137681: 2332 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 2333 "Describes why the request is being made in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, 2334 reasonCode); 2335 case -1146218137: 2336 /* reasonReference */ return new Property("reasonReference", 2337 "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 2338 "Indicates another resource whose existence justifies this request.", 0, java.lang.Integer.MAX_VALUE, 2339 reasonReference); 2340 case 3387378: 2341 /* note */ return new Property("note", "Annotation", 2342 "Comments made about the request by the requester, sender, recipient, subject or other participants.", 0, 2343 java.lang.Integer.MAX_VALUE, note); 2344 default: 2345 return super.getNamedProperty(_hash, _name, _checkValid); 2346 } 2347 2348 } 2349 2350 @Override 2351 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2352 switch (hash) { 2353 case -1618432855: 2354 /* identifier */ return this.identifier == null ? new Base[0] 2355 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2356 case -332612366: 2357 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2358 case -430332865: 2359 /* replaces */ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // Reference 2360 case -445338488: 2361 /* groupIdentifier */ return this.groupIdentifier == null ? new Base[0] : new Base[] { this.groupIdentifier }; // Identifier 2362 case -892481550: 2363 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<CommunicationRequestStatus> 2364 case 2051346646: 2365 /* statusReason */ return this.statusReason == null ? new Base[0] : new Base[] { this.statusReason }; // CodeableConcept 2366 case 50511102: 2367 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2368 case -1165461084: 2369 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // Enumeration<CommunicationPriority> 2370 case -1788508167: 2371 /* doNotPerform */ return this.doNotPerform == null ? new Base[0] : new Base[] { this.doNotPerform }; // BooleanType 2372 case -1078030475: 2373 /* medium */ return this.medium == null ? new Base[0] : this.medium.toArray(new Base[this.medium.size()]); // CodeableConcept 2374 case -1867885268: 2375 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2376 case 92611469: 2377 /* about */ return this.about == null ? new Base[0] : this.about.toArray(new Base[this.about.size()]); // Reference 2378 case 1524132147: 2379 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 2380 case -786701938: 2381 /* payload */ return this.payload == null ? new Base[0] : this.payload.toArray(new Base[this.payload.size()]); // CommunicationRequestPayloadComponent 2382 case 1687874001: 2383 /* occurrence */ return this.occurrence == null ? new Base[0] : new Base[] { this.occurrence }; // Type 2384 case -1500852503: 2385 /* authoredOn */ return this.authoredOn == null ? new Base[0] : new Base[] { this.authoredOn }; // DateTimeType 2386 case 693933948: 2387 /* requester */ return this.requester == null ? new Base[0] : new Base[] { this.requester }; // Reference 2388 case 820081177: 2389 /* recipient */ return this.recipient == null ? new Base[0] 2390 : this.recipient.toArray(new Base[this.recipient.size()]); // Reference 2391 case -905962955: 2392 /* sender */ return this.sender == null ? new Base[0] : new Base[] { this.sender }; // Reference 2393 case 722137681: 2394 /* reasonCode */ return this.reasonCode == null ? new Base[0] 2395 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 2396 case -1146218137: 2397 /* reasonReference */ return this.reasonReference == null ? new Base[0] 2398 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 2399 case 3387378: 2400 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2401 default: 2402 return super.getProperty(hash, name, checkValid); 2403 } 2404 2405 } 2406 2407 @Override 2408 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2409 switch (hash) { 2410 case -1618432855: // identifier 2411 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2412 return value; 2413 case -332612366: // basedOn 2414 this.getBasedOn().add(castToReference(value)); // Reference 2415 return value; 2416 case -430332865: // replaces 2417 this.getReplaces().add(castToReference(value)); // Reference 2418 return value; 2419 case -445338488: // groupIdentifier 2420 this.groupIdentifier = castToIdentifier(value); // Identifier 2421 return value; 2422 case -892481550: // status 2423 value = new CommunicationRequestStatusEnumFactory().fromType(castToCode(value)); 2424 this.status = (Enumeration) value; // Enumeration<CommunicationRequestStatus> 2425 return value; 2426 case 2051346646: // statusReason 2427 this.statusReason = castToCodeableConcept(value); // CodeableConcept 2428 return value; 2429 case 50511102: // category 2430 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 2431 return value; 2432 case -1165461084: // priority 2433 value = new CommunicationPriorityEnumFactory().fromType(castToCode(value)); 2434 this.priority = (Enumeration) value; // Enumeration<CommunicationPriority> 2435 return value; 2436 case -1788508167: // doNotPerform 2437 this.doNotPerform = castToBoolean(value); // BooleanType 2438 return value; 2439 case -1078030475: // medium 2440 this.getMedium().add(castToCodeableConcept(value)); // CodeableConcept 2441 return value; 2442 case -1867885268: // subject 2443 this.subject = castToReference(value); // Reference 2444 return value; 2445 case 92611469: // about 2446 this.getAbout().add(castToReference(value)); // Reference 2447 return value; 2448 case 1524132147: // encounter 2449 this.encounter = castToReference(value); // Reference 2450 return value; 2451 case -786701938: // payload 2452 this.getPayload().add((CommunicationRequestPayloadComponent) value); // CommunicationRequestPayloadComponent 2453 return value; 2454 case 1687874001: // occurrence 2455 this.occurrence = castToType(value); // Type 2456 return value; 2457 case -1500852503: // authoredOn 2458 this.authoredOn = castToDateTime(value); // DateTimeType 2459 return value; 2460 case 693933948: // requester 2461 this.requester = castToReference(value); // Reference 2462 return value; 2463 case 820081177: // recipient 2464 this.getRecipient().add(castToReference(value)); // Reference 2465 return value; 2466 case -905962955: // sender 2467 this.sender = castToReference(value); // Reference 2468 return value; 2469 case 722137681: // reasonCode 2470 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 2471 return value; 2472 case -1146218137: // reasonReference 2473 this.getReasonReference().add(castToReference(value)); // Reference 2474 return value; 2475 case 3387378: // note 2476 this.getNote().add(castToAnnotation(value)); // Annotation 2477 return value; 2478 default: 2479 return super.setProperty(hash, name, value); 2480 } 2481 2482 } 2483 2484 @Override 2485 public Base setProperty(String name, Base value) throws FHIRException { 2486 if (name.equals("identifier")) { 2487 this.getIdentifier().add(castToIdentifier(value)); 2488 } else if (name.equals("basedOn")) { 2489 this.getBasedOn().add(castToReference(value)); 2490 } else if (name.equals("replaces")) { 2491 this.getReplaces().add(castToReference(value)); 2492 } else if (name.equals("groupIdentifier")) { 2493 this.groupIdentifier = castToIdentifier(value); // Identifier 2494 } else if (name.equals("status")) { 2495 value = new CommunicationRequestStatusEnumFactory().fromType(castToCode(value)); 2496 this.status = (Enumeration) value; // Enumeration<CommunicationRequestStatus> 2497 } else if (name.equals("statusReason")) { 2498 this.statusReason = castToCodeableConcept(value); // CodeableConcept 2499 } else if (name.equals("category")) { 2500 this.getCategory().add(castToCodeableConcept(value)); 2501 } else if (name.equals("priority")) { 2502 value = new CommunicationPriorityEnumFactory().fromType(castToCode(value)); 2503 this.priority = (Enumeration) value; // Enumeration<CommunicationPriority> 2504 } else if (name.equals("doNotPerform")) { 2505 this.doNotPerform = castToBoolean(value); // BooleanType 2506 } else if (name.equals("medium")) { 2507 this.getMedium().add(castToCodeableConcept(value)); 2508 } else if (name.equals("subject")) { 2509 this.subject = castToReference(value); // Reference 2510 } else if (name.equals("about")) { 2511 this.getAbout().add(castToReference(value)); 2512 } else if (name.equals("encounter")) { 2513 this.encounter = castToReference(value); // Reference 2514 } else if (name.equals("payload")) { 2515 this.getPayload().add((CommunicationRequestPayloadComponent) value); 2516 } else if (name.equals("occurrence[x]")) { 2517 this.occurrence = castToType(value); // Type 2518 } else if (name.equals("authoredOn")) { 2519 this.authoredOn = castToDateTime(value); // DateTimeType 2520 } else if (name.equals("requester")) { 2521 this.requester = castToReference(value); // Reference 2522 } else if (name.equals("recipient")) { 2523 this.getRecipient().add(castToReference(value)); 2524 } else if (name.equals("sender")) { 2525 this.sender = castToReference(value); // Reference 2526 } else if (name.equals("reasonCode")) { 2527 this.getReasonCode().add(castToCodeableConcept(value)); 2528 } else if (name.equals("reasonReference")) { 2529 this.getReasonReference().add(castToReference(value)); 2530 } else if (name.equals("note")) { 2531 this.getNote().add(castToAnnotation(value)); 2532 } else 2533 return super.setProperty(name, value); 2534 return value; 2535 } 2536 2537 @Override 2538 public void removeChild(String name, Base value) throws FHIRException { 2539 if (name.equals("identifier")) { 2540 this.getIdentifier().remove(castToIdentifier(value)); 2541 } else if (name.equals("basedOn")) { 2542 this.getBasedOn().remove(castToReference(value)); 2543 } else if (name.equals("replaces")) { 2544 this.getReplaces().remove(castToReference(value)); 2545 } else if (name.equals("groupIdentifier")) { 2546 this.groupIdentifier = null; 2547 } else if (name.equals("status")) { 2548 this.status = null; 2549 } else if (name.equals("statusReason")) { 2550 this.statusReason = null; 2551 } else if (name.equals("category")) { 2552 this.getCategory().remove(castToCodeableConcept(value)); 2553 } else if (name.equals("priority")) { 2554 this.priority = null; 2555 } else if (name.equals("doNotPerform")) { 2556 this.doNotPerform = null; 2557 } else if (name.equals("medium")) { 2558 this.getMedium().remove(castToCodeableConcept(value)); 2559 } else if (name.equals("subject")) { 2560 this.subject = null; 2561 } else if (name.equals("about")) { 2562 this.getAbout().remove(castToReference(value)); 2563 } else if (name.equals("encounter")) { 2564 this.encounter = null; 2565 } else if (name.equals("payload")) { 2566 this.getPayload().remove((CommunicationRequestPayloadComponent) value); 2567 } else if (name.equals("occurrence[x]")) { 2568 this.occurrence = null; 2569 } else if (name.equals("authoredOn")) { 2570 this.authoredOn = null; 2571 } else if (name.equals("requester")) { 2572 this.requester = null; 2573 } else if (name.equals("recipient")) { 2574 this.getRecipient().remove(castToReference(value)); 2575 } else if (name.equals("sender")) { 2576 this.sender = null; 2577 } else if (name.equals("reasonCode")) { 2578 this.getReasonCode().remove(castToCodeableConcept(value)); 2579 } else if (name.equals("reasonReference")) { 2580 this.getReasonReference().remove(castToReference(value)); 2581 } else if (name.equals("note")) { 2582 this.getNote().remove(castToAnnotation(value)); 2583 } else 2584 super.removeChild(name, value); 2585 2586 } 2587 2588 @Override 2589 public Base makeProperty(int hash, String name) throws FHIRException { 2590 switch (hash) { 2591 case -1618432855: 2592 return addIdentifier(); 2593 case -332612366: 2594 return addBasedOn(); 2595 case -430332865: 2596 return addReplaces(); 2597 case -445338488: 2598 return getGroupIdentifier(); 2599 case -892481550: 2600 return getStatusElement(); 2601 case 2051346646: 2602 return getStatusReason(); 2603 case 50511102: 2604 return addCategory(); 2605 case -1165461084: 2606 return getPriorityElement(); 2607 case -1788508167: 2608 return getDoNotPerformElement(); 2609 case -1078030475: 2610 return addMedium(); 2611 case -1867885268: 2612 return getSubject(); 2613 case 92611469: 2614 return addAbout(); 2615 case 1524132147: 2616 return getEncounter(); 2617 case -786701938: 2618 return addPayload(); 2619 case -2022646513: 2620 return getOccurrence(); 2621 case 1687874001: 2622 return getOccurrence(); 2623 case -1500852503: 2624 return getAuthoredOnElement(); 2625 case 693933948: 2626 return getRequester(); 2627 case 820081177: 2628 return addRecipient(); 2629 case -905962955: 2630 return getSender(); 2631 case 722137681: 2632 return addReasonCode(); 2633 case -1146218137: 2634 return addReasonReference(); 2635 case 3387378: 2636 return addNote(); 2637 default: 2638 return super.makeProperty(hash, name); 2639 } 2640 2641 } 2642 2643 @Override 2644 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2645 switch (hash) { 2646 case -1618432855: 2647 /* identifier */ return new String[] { "Identifier" }; 2648 case -332612366: 2649 /* basedOn */ return new String[] { "Reference" }; 2650 case -430332865: 2651 /* replaces */ return new String[] { "Reference" }; 2652 case -445338488: 2653 /* groupIdentifier */ return new String[] { "Identifier" }; 2654 case -892481550: 2655 /* status */ return new String[] { "code" }; 2656 case 2051346646: 2657 /* statusReason */ return new String[] { "CodeableConcept" }; 2658 case 50511102: 2659 /* category */ return new String[] { "CodeableConcept" }; 2660 case -1165461084: 2661 /* priority */ return new String[] { "code" }; 2662 case -1788508167: 2663 /* doNotPerform */ return new String[] { "boolean" }; 2664 case -1078030475: 2665 /* medium */ return new String[] { "CodeableConcept" }; 2666 case -1867885268: 2667 /* subject */ return new String[] { "Reference" }; 2668 case 92611469: 2669 /* about */ return new String[] { "Reference" }; 2670 case 1524132147: 2671 /* encounter */ return new String[] { "Reference" }; 2672 case -786701938: 2673 /* payload */ return new String[] {}; 2674 case 1687874001: 2675 /* occurrence */ return new String[] { "dateTime", "Period" }; 2676 case -1500852503: 2677 /* authoredOn */ return new String[] { "dateTime" }; 2678 case 693933948: 2679 /* requester */ return new String[] { "Reference" }; 2680 case 820081177: 2681 /* recipient */ return new String[] { "Reference" }; 2682 case -905962955: 2683 /* sender */ return new String[] { "Reference" }; 2684 case 722137681: 2685 /* reasonCode */ return new String[] { "CodeableConcept" }; 2686 case -1146218137: 2687 /* reasonReference */ return new String[] { "Reference" }; 2688 case 3387378: 2689 /* note */ return new String[] { "Annotation" }; 2690 default: 2691 return super.getTypesForProperty(hash, name); 2692 } 2693 2694 } 2695 2696 @Override 2697 public Base addChild(String name) throws FHIRException { 2698 if (name.equals("identifier")) { 2699 return addIdentifier(); 2700 } else if (name.equals("basedOn")) { 2701 return addBasedOn(); 2702 } else if (name.equals("replaces")) { 2703 return addReplaces(); 2704 } else if (name.equals("groupIdentifier")) { 2705 this.groupIdentifier = new Identifier(); 2706 return this.groupIdentifier; 2707 } else if (name.equals("status")) { 2708 throw new FHIRException("Cannot call addChild on a singleton property CommunicationRequest.status"); 2709 } else if (name.equals("statusReason")) { 2710 this.statusReason = new CodeableConcept(); 2711 return this.statusReason; 2712 } else if (name.equals("category")) { 2713 return addCategory(); 2714 } else if (name.equals("priority")) { 2715 throw new FHIRException("Cannot call addChild on a singleton property CommunicationRequest.priority"); 2716 } else if (name.equals("doNotPerform")) { 2717 throw new FHIRException("Cannot call addChild on a singleton property CommunicationRequest.doNotPerform"); 2718 } else if (name.equals("medium")) { 2719 return addMedium(); 2720 } else if (name.equals("subject")) { 2721 this.subject = new Reference(); 2722 return this.subject; 2723 } else if (name.equals("about")) { 2724 return addAbout(); 2725 } else if (name.equals("encounter")) { 2726 this.encounter = new Reference(); 2727 return this.encounter; 2728 } else if (name.equals("payload")) { 2729 return addPayload(); 2730 } else if (name.equals("occurrenceDateTime")) { 2731 this.occurrence = new DateTimeType(); 2732 return this.occurrence; 2733 } else if (name.equals("occurrencePeriod")) { 2734 this.occurrence = new Period(); 2735 return this.occurrence; 2736 } else if (name.equals("authoredOn")) { 2737 throw new FHIRException("Cannot call addChild on a singleton property CommunicationRequest.authoredOn"); 2738 } else if (name.equals("requester")) { 2739 this.requester = new Reference(); 2740 return this.requester; 2741 } else if (name.equals("recipient")) { 2742 return addRecipient(); 2743 } else if (name.equals("sender")) { 2744 this.sender = new Reference(); 2745 return this.sender; 2746 } else if (name.equals("reasonCode")) { 2747 return addReasonCode(); 2748 } else if (name.equals("reasonReference")) { 2749 return addReasonReference(); 2750 } else if (name.equals("note")) { 2751 return addNote(); 2752 } else 2753 return super.addChild(name); 2754 } 2755 2756 public String fhirType() { 2757 return "CommunicationRequest"; 2758 2759 } 2760 2761 public CommunicationRequest copy() { 2762 CommunicationRequest dst = new CommunicationRequest(); 2763 copyValues(dst); 2764 return dst; 2765 } 2766 2767 public void copyValues(CommunicationRequest dst) { 2768 super.copyValues(dst); 2769 if (identifier != null) { 2770 dst.identifier = new ArrayList<Identifier>(); 2771 for (Identifier i : identifier) 2772 dst.identifier.add(i.copy()); 2773 } 2774 ; 2775 if (basedOn != null) { 2776 dst.basedOn = new ArrayList<Reference>(); 2777 for (Reference i : basedOn) 2778 dst.basedOn.add(i.copy()); 2779 } 2780 ; 2781 if (replaces != null) { 2782 dst.replaces = new ArrayList<Reference>(); 2783 for (Reference i : replaces) 2784 dst.replaces.add(i.copy()); 2785 } 2786 ; 2787 dst.groupIdentifier = groupIdentifier == null ? null : groupIdentifier.copy(); 2788 dst.status = status == null ? null : status.copy(); 2789 dst.statusReason = statusReason == null ? null : statusReason.copy(); 2790 if (category != null) { 2791 dst.category = new ArrayList<CodeableConcept>(); 2792 for (CodeableConcept i : category) 2793 dst.category.add(i.copy()); 2794 } 2795 ; 2796 dst.priority = priority == null ? null : priority.copy(); 2797 dst.doNotPerform = doNotPerform == null ? null : doNotPerform.copy(); 2798 if (medium != null) { 2799 dst.medium = new ArrayList<CodeableConcept>(); 2800 for (CodeableConcept i : medium) 2801 dst.medium.add(i.copy()); 2802 } 2803 ; 2804 dst.subject = subject == null ? null : subject.copy(); 2805 if (about != null) { 2806 dst.about = new ArrayList<Reference>(); 2807 for (Reference i : about) 2808 dst.about.add(i.copy()); 2809 } 2810 ; 2811 dst.encounter = encounter == null ? null : encounter.copy(); 2812 if (payload != null) { 2813 dst.payload = new ArrayList<CommunicationRequestPayloadComponent>(); 2814 for (CommunicationRequestPayloadComponent i : payload) 2815 dst.payload.add(i.copy()); 2816 } 2817 ; 2818 dst.occurrence = occurrence == null ? null : occurrence.copy(); 2819 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 2820 dst.requester = requester == null ? null : requester.copy(); 2821 if (recipient != null) { 2822 dst.recipient = new ArrayList<Reference>(); 2823 for (Reference i : recipient) 2824 dst.recipient.add(i.copy()); 2825 } 2826 ; 2827 dst.sender = sender == null ? null : sender.copy(); 2828 if (reasonCode != null) { 2829 dst.reasonCode = new ArrayList<CodeableConcept>(); 2830 for (CodeableConcept i : reasonCode) 2831 dst.reasonCode.add(i.copy()); 2832 } 2833 ; 2834 if (reasonReference != null) { 2835 dst.reasonReference = new ArrayList<Reference>(); 2836 for (Reference i : reasonReference) 2837 dst.reasonReference.add(i.copy()); 2838 } 2839 ; 2840 if (note != null) { 2841 dst.note = new ArrayList<Annotation>(); 2842 for (Annotation i : note) 2843 dst.note.add(i.copy()); 2844 } 2845 ; 2846 } 2847 2848 protected CommunicationRequest typedCopy() { 2849 return copy(); 2850 } 2851 2852 @Override 2853 public boolean equalsDeep(Base other_) { 2854 if (!super.equalsDeep(other_)) 2855 return false; 2856 if (!(other_ instanceof CommunicationRequest)) 2857 return false; 2858 CommunicationRequest o = (CommunicationRequest) other_; 2859 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) 2860 && compareDeep(replaces, o.replaces, true) && compareDeep(groupIdentifier, o.groupIdentifier, true) 2861 && compareDeep(status, o.status, true) && compareDeep(statusReason, o.statusReason, true) 2862 && compareDeep(category, o.category, true) && compareDeep(priority, o.priority, true) 2863 && compareDeep(doNotPerform, o.doNotPerform, true) && compareDeep(medium, o.medium, true) 2864 && compareDeep(subject, o.subject, true) && compareDeep(about, o.about, true) 2865 && compareDeep(encounter, o.encounter, true) && compareDeep(payload, o.payload, true) 2866 && compareDeep(occurrence, o.occurrence, true) && compareDeep(authoredOn, o.authoredOn, true) 2867 && compareDeep(requester, o.requester, true) && compareDeep(recipient, o.recipient, true) 2868 && compareDeep(sender, o.sender, true) && compareDeep(reasonCode, o.reasonCode, true) 2869 && compareDeep(reasonReference, o.reasonReference, true) && compareDeep(note, o.note, true); 2870 } 2871 2872 @Override 2873 public boolean equalsShallow(Base other_) { 2874 if (!super.equalsShallow(other_)) 2875 return false; 2876 if (!(other_ instanceof CommunicationRequest)) 2877 return false; 2878 CommunicationRequest o = (CommunicationRequest) other_; 2879 return compareValues(status, o.status, true) && compareValues(priority, o.priority, true) 2880 && compareValues(doNotPerform, o.doNotPerform, true) && compareValues(authoredOn, o.authoredOn, true); 2881 } 2882 2883 public boolean isEmpty() { 2884 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, replaces, groupIdentifier, 2885 status, statusReason, category, priority, doNotPerform, medium, subject, about, encounter, payload, occurrence, 2886 authoredOn, requester, recipient, sender, reasonCode, reasonReference, note); 2887 } 2888 2889 @Override 2890 public ResourceType getResourceType() { 2891 return ResourceType.CommunicationRequest; 2892 } 2893 2894 /** 2895 * Search parameter: <b>requester</b> 2896 * <p> 2897 * Description: <b>Who/what is requesting service</b><br> 2898 * Type: <b>reference</b><br> 2899 * Path: <b>CommunicationRequest.requester</b><br> 2900 * </p> 2901 */ 2902 @SearchParamDefinition(name = "requester", path = "CommunicationRequest.requester", description = "Who/what is requesting service", type = "reference", providesMembershipIn = { 2903 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 2904 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 2905 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, 2906 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 2907 public static final String SP_REQUESTER = "requester"; 2908 /** 2909 * <b>Fluent Client</b> search parameter constant for <b>requester</b> 2910 * <p> 2911 * Description: <b>Who/what is requesting service</b><br> 2912 * Type: <b>reference</b><br> 2913 * Path: <b>CommunicationRequest.requester</b><br> 2914 * </p> 2915 */ 2916 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2917 SP_REQUESTER); 2918 2919 /** 2920 * Constant for fluent queries to be used to add include statements. Specifies 2921 * the path value of "<b>CommunicationRequest:requester</b>". 2922 */ 2923 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTER = new ca.uhn.fhir.model.api.Include( 2924 "CommunicationRequest:requester").toLocked(); 2925 2926 /** 2927 * Search parameter: <b>authored</b> 2928 * <p> 2929 * Description: <b>When request transitioned to being actionable</b><br> 2930 * Type: <b>date</b><br> 2931 * Path: <b>CommunicationRequest.authoredOn</b><br> 2932 * </p> 2933 */ 2934 @SearchParamDefinition(name = "authored", path = "CommunicationRequest.authoredOn", description = "When request transitioned to being actionable", type = "date") 2935 public static final String SP_AUTHORED = "authored"; 2936 /** 2937 * <b>Fluent Client</b> search parameter constant for <b>authored</b> 2938 * <p> 2939 * Description: <b>When request transitioned to being actionable</b><br> 2940 * Type: <b>date</b><br> 2941 * Path: <b>CommunicationRequest.authoredOn</b><br> 2942 * </p> 2943 */ 2944 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED = new ca.uhn.fhir.rest.gclient.DateClientParam( 2945 SP_AUTHORED); 2946 2947 /** 2948 * Search parameter: <b>identifier</b> 2949 * <p> 2950 * Description: <b>Unique identifier</b><br> 2951 * Type: <b>token</b><br> 2952 * Path: <b>CommunicationRequest.identifier</b><br> 2953 * </p> 2954 */ 2955 @SearchParamDefinition(name = "identifier", path = "CommunicationRequest.identifier", description = "Unique identifier", type = "token") 2956 public static final String SP_IDENTIFIER = "identifier"; 2957 /** 2958 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2959 * <p> 2960 * Description: <b>Unique identifier</b><br> 2961 * Type: <b>token</b><br> 2962 * Path: <b>CommunicationRequest.identifier</b><br> 2963 * </p> 2964 */ 2965 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2966 SP_IDENTIFIER); 2967 2968 /** 2969 * Search parameter: <b>subject</b> 2970 * <p> 2971 * Description: <b>Focus of message</b><br> 2972 * Type: <b>reference</b><br> 2973 * Path: <b>CommunicationRequest.subject</b><br> 2974 * </p> 2975 */ 2976 @SearchParamDefinition(name = "subject", path = "CommunicationRequest.subject", description = "Focus of message", type = "reference", providesMembershipIn = { 2977 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Group.class, Patient.class }) 2978 public static final String SP_SUBJECT = "subject"; 2979 /** 2980 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2981 * <p> 2982 * Description: <b>Focus of message</b><br> 2983 * Type: <b>reference</b><br> 2984 * Path: <b>CommunicationRequest.subject</b><br> 2985 * </p> 2986 */ 2987 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2988 SP_SUBJECT); 2989 2990 /** 2991 * Constant for fluent queries to be used to add include statements. Specifies 2992 * the path value of "<b>CommunicationRequest:subject</b>". 2993 */ 2994 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 2995 "CommunicationRequest:subject").toLocked(); 2996 2997 /** 2998 * Search parameter: <b>replaces</b> 2999 * <p> 3000 * Description: <b>Request(s) replaced by this request</b><br> 3001 * Type: <b>reference</b><br> 3002 * Path: <b>CommunicationRequest.replaces</b><br> 3003 * </p> 3004 */ 3005 @SearchParamDefinition(name = "replaces", path = "CommunicationRequest.replaces", description = "Request(s) replaced by this request", type = "reference", target = { 3006 CommunicationRequest.class }) 3007 public static final String SP_REPLACES = "replaces"; 3008 /** 3009 * <b>Fluent Client</b> search parameter constant for <b>replaces</b> 3010 * <p> 3011 * Description: <b>Request(s) replaced by this request</b><br> 3012 * Type: <b>reference</b><br> 3013 * Path: <b>CommunicationRequest.replaces</b><br> 3014 * </p> 3015 */ 3016 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REPLACES = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3017 SP_REPLACES); 3018 3019 /** 3020 * Constant for fluent queries to be used to add include statements. Specifies 3021 * the path value of "<b>CommunicationRequest:replaces</b>". 3022 */ 3023 public static final ca.uhn.fhir.model.api.Include INCLUDE_REPLACES = new ca.uhn.fhir.model.api.Include( 3024 "CommunicationRequest:replaces").toLocked(); 3025 3026 /** 3027 * Search parameter: <b>medium</b> 3028 * <p> 3029 * Description: <b>A channel of communication</b><br> 3030 * Type: <b>token</b><br> 3031 * Path: <b>CommunicationRequest.medium</b><br> 3032 * </p> 3033 */ 3034 @SearchParamDefinition(name = "medium", path = "CommunicationRequest.medium", description = "A channel of communication", type = "token") 3035 public static final String SP_MEDIUM = "medium"; 3036 /** 3037 * <b>Fluent Client</b> search parameter constant for <b>medium</b> 3038 * <p> 3039 * Description: <b>A channel of communication</b><br> 3040 * Type: <b>token</b><br> 3041 * Path: <b>CommunicationRequest.medium</b><br> 3042 * </p> 3043 */ 3044 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MEDIUM = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3045 SP_MEDIUM); 3046 3047 /** 3048 * Search parameter: <b>encounter</b> 3049 * <p> 3050 * Description: <b>Encounter created as part of</b><br> 3051 * Type: <b>reference</b><br> 3052 * Path: <b>CommunicationRequest.encounter</b><br> 3053 * </p> 3054 */ 3055 @SearchParamDefinition(name = "encounter", path = "CommunicationRequest.encounter", description = "Encounter created as part of", type = "reference", providesMembershipIn = { 3056 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 3057 public static final String SP_ENCOUNTER = "encounter"; 3058 /** 3059 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 3060 * <p> 3061 * Description: <b>Encounter created as part of</b><br> 3062 * Type: <b>reference</b><br> 3063 * Path: <b>CommunicationRequest.encounter</b><br> 3064 * </p> 3065 */ 3066 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3067 SP_ENCOUNTER); 3068 3069 /** 3070 * Constant for fluent queries to be used to add include statements. Specifies 3071 * the path value of "<b>CommunicationRequest:encounter</b>". 3072 */ 3073 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 3074 "CommunicationRequest:encounter").toLocked(); 3075 3076 /** 3077 * Search parameter: <b>occurrence</b> 3078 * <p> 3079 * Description: <b>When scheduled</b><br> 3080 * Type: <b>date</b><br> 3081 * Path: <b>CommunicationRequest.occurrenceDateTime</b><br> 3082 * </p> 3083 */ 3084 @SearchParamDefinition(name = "occurrence", path = "(CommunicationRequest.occurrence as dateTime)", description = "When scheduled", type = "date") 3085 public static final String SP_OCCURRENCE = "occurrence"; 3086 /** 3087 * <b>Fluent Client</b> search parameter constant for <b>occurrence</b> 3088 * <p> 3089 * Description: <b>When scheduled</b><br> 3090 * Type: <b>date</b><br> 3091 * Path: <b>CommunicationRequest.occurrenceDateTime</b><br> 3092 * </p> 3093 */ 3094 public static final ca.uhn.fhir.rest.gclient.DateClientParam OCCURRENCE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3095 SP_OCCURRENCE); 3096 3097 /** 3098 * Search parameter: <b>priority</b> 3099 * <p> 3100 * Description: <b>routine | urgent | asap | stat</b><br> 3101 * Type: <b>token</b><br> 3102 * Path: <b>CommunicationRequest.priority</b><br> 3103 * </p> 3104 */ 3105 @SearchParamDefinition(name = "priority", path = "CommunicationRequest.priority", description = "routine | urgent | asap | stat", type = "token") 3106 public static final String SP_PRIORITY = "priority"; 3107 /** 3108 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 3109 * <p> 3110 * Description: <b>routine | urgent | asap | stat</b><br> 3111 * Type: <b>token</b><br> 3112 * Path: <b>CommunicationRequest.priority</b><br> 3113 * </p> 3114 */ 3115 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3116 SP_PRIORITY); 3117 3118 /** 3119 * Search parameter: <b>group-identifier</b> 3120 * <p> 3121 * Description: <b>Composite request this is part of</b><br> 3122 * Type: <b>token</b><br> 3123 * Path: <b>CommunicationRequest.groupIdentifier</b><br> 3124 * </p> 3125 */ 3126 @SearchParamDefinition(name = "group-identifier", path = "CommunicationRequest.groupIdentifier", description = "Composite request this is part of", type = "token") 3127 public static final String SP_GROUP_IDENTIFIER = "group-identifier"; 3128 /** 3129 * <b>Fluent Client</b> search parameter constant for <b>group-identifier</b> 3130 * <p> 3131 * Description: <b>Composite request this is part of</b><br> 3132 * Type: <b>token</b><br> 3133 * Path: <b>CommunicationRequest.groupIdentifier</b><br> 3134 * </p> 3135 */ 3136 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GROUP_IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3137 SP_GROUP_IDENTIFIER); 3138 3139 /** 3140 * Search parameter: <b>based-on</b> 3141 * <p> 3142 * Description: <b>Fulfills plan or proposal</b><br> 3143 * Type: <b>reference</b><br> 3144 * Path: <b>CommunicationRequest.basedOn</b><br> 3145 * </p> 3146 */ 3147 @SearchParamDefinition(name = "based-on", path = "CommunicationRequest.basedOn", description = "Fulfills plan or proposal", type = "reference") 3148 public static final String SP_BASED_ON = "based-on"; 3149 /** 3150 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 3151 * <p> 3152 * Description: <b>Fulfills plan or proposal</b><br> 3153 * Type: <b>reference</b><br> 3154 * Path: <b>CommunicationRequest.basedOn</b><br> 3155 * </p> 3156 */ 3157 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3158 SP_BASED_ON); 3159 3160 /** 3161 * Constant for fluent queries to be used to add include statements. Specifies 3162 * the path value of "<b>CommunicationRequest:based-on</b>". 3163 */ 3164 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include( 3165 "CommunicationRequest:based-on").toLocked(); 3166 3167 /** 3168 * Search parameter: <b>sender</b> 3169 * <p> 3170 * Description: <b>Message sender</b><br> 3171 * Type: <b>reference</b><br> 3172 * Path: <b>CommunicationRequest.sender</b><br> 3173 * </p> 3174 */ 3175 @SearchParamDefinition(name = "sender", path = "CommunicationRequest.sender", description = "Message sender", type = "reference", providesMembershipIn = { 3176 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 3177 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3178 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 3179 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, 3180 HealthcareService.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, 3181 RelatedPerson.class }) 3182 public static final String SP_SENDER = "sender"; 3183 /** 3184 * <b>Fluent Client</b> search parameter constant for <b>sender</b> 3185 * <p> 3186 * Description: <b>Message sender</b><br> 3187 * Type: <b>reference</b><br> 3188 * Path: <b>CommunicationRequest.sender</b><br> 3189 * </p> 3190 */ 3191 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SENDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3192 SP_SENDER); 3193 3194 /** 3195 * Constant for fluent queries to be used to add include statements. Specifies 3196 * the path value of "<b>CommunicationRequest:sender</b>". 3197 */ 3198 public static final ca.uhn.fhir.model.api.Include INCLUDE_SENDER = new ca.uhn.fhir.model.api.Include( 3199 "CommunicationRequest:sender").toLocked(); 3200 3201 /** 3202 * Search parameter: <b>patient</b> 3203 * <p> 3204 * Description: <b>Focus of message</b><br> 3205 * Type: <b>reference</b><br> 3206 * Path: <b>CommunicationRequest.subject</b><br> 3207 * </p> 3208 */ 3209 @SearchParamDefinition(name = "patient", path = "CommunicationRequest.subject.where(resolve() is Patient)", description = "Focus of message", type = "reference", target = { 3210 Patient.class }) 3211 public static final String SP_PATIENT = "patient"; 3212 /** 3213 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3214 * <p> 3215 * Description: <b>Focus of message</b><br> 3216 * Type: <b>reference</b><br> 3217 * Path: <b>CommunicationRequest.subject</b><br> 3218 * </p> 3219 */ 3220 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3221 SP_PATIENT); 3222 3223 /** 3224 * Constant for fluent queries to be used to add include statements. Specifies 3225 * the path value of "<b>CommunicationRequest:patient</b>". 3226 */ 3227 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 3228 "CommunicationRequest:patient").toLocked(); 3229 3230 /** 3231 * Search parameter: <b>recipient</b> 3232 * <p> 3233 * Description: <b>Message recipient</b><br> 3234 * Type: <b>reference</b><br> 3235 * Path: <b>CommunicationRequest.recipient</b><br> 3236 * </p> 3237 */ 3238 @SearchParamDefinition(name = "recipient", path = "CommunicationRequest.recipient", description = "Message recipient", type = "reference", providesMembershipIn = { 3239 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 3240 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3241 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 3242 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { CareTeam.class, Device.class, 3243 Group.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, 3244 PractitionerRole.class, RelatedPerson.class }) 3245 public static final String SP_RECIPIENT = "recipient"; 3246 /** 3247 * <b>Fluent Client</b> search parameter constant for <b>recipient</b> 3248 * <p> 3249 * Description: <b>Message recipient</b><br> 3250 * Type: <b>reference</b><br> 3251 * Path: <b>CommunicationRequest.recipient</b><br> 3252 * </p> 3253 */ 3254 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECIPIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3255 SP_RECIPIENT); 3256 3257 /** 3258 * Constant for fluent queries to be used to add include statements. Specifies 3259 * the path value of "<b>CommunicationRequest:recipient</b>". 3260 */ 3261 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECIPIENT = new ca.uhn.fhir.model.api.Include( 3262 "CommunicationRequest:recipient").toLocked(); 3263 3264 /** 3265 * Search parameter: <b>category</b> 3266 * <p> 3267 * Description: <b>Message category</b><br> 3268 * Type: <b>token</b><br> 3269 * Path: <b>CommunicationRequest.category</b><br> 3270 * </p> 3271 */ 3272 @SearchParamDefinition(name = "category", path = "CommunicationRequest.category", description = "Message category", type = "token") 3273 public static final String SP_CATEGORY = "category"; 3274 /** 3275 * <b>Fluent Client</b> search parameter constant for <b>category</b> 3276 * <p> 3277 * Description: <b>Message category</b><br> 3278 * Type: <b>token</b><br> 3279 * Path: <b>CommunicationRequest.category</b><br> 3280 * </p> 3281 */ 3282 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3283 SP_CATEGORY); 3284 3285 /** 3286 * Search parameter: <b>status</b> 3287 * <p> 3288 * Description: <b>draft | active | on-hold | revoked | completed | 3289 * entered-in-error | unknown</b><br> 3290 * Type: <b>token</b><br> 3291 * Path: <b>CommunicationRequest.status</b><br> 3292 * </p> 3293 */ 3294 @SearchParamDefinition(name = "status", path = "CommunicationRequest.status", description = "draft | active | on-hold | revoked | completed | entered-in-error | unknown", type = "token") 3295 public static final String SP_STATUS = "status"; 3296 /** 3297 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3298 * <p> 3299 * Description: <b>draft | active | on-hold | revoked | completed | 3300 * entered-in-error | unknown</b><br> 3301 * Type: <b>token</b><br> 3302 * Path: <b>CommunicationRequest.status</b><br> 3303 * </p> 3304 */ 3305 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3306 SP_STATUS); 3307 3308}