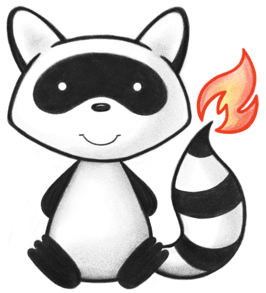
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049 050/** 051 * A compartment definition that defines how resources are accessed on a server. 052 */ 053@ResourceDef(name = "CompartmentDefinition", profile = "http://hl7.org/fhir/StructureDefinition/CompartmentDefinition") 054@ChildOrder(names = { "url", "version", "name", "status", "experimental", "date", "publisher", "contact", "description", 055 "useContext", "purpose", "code", "search", "resource" }) 056public class CompartmentDefinition extends MetadataResource { 057 058 public enum CompartmentType { 059 /** 060 * The compartment definition is for the patient compartment. 061 */ 062 PATIENT, 063 /** 064 * The compartment definition is for the encounter compartment. 065 */ 066 ENCOUNTER, 067 /** 068 * The compartment definition is for the related-person compartment. 069 */ 070 RELATEDPERSON, 071 /** 072 * The compartment definition is for the practitioner compartment. 073 */ 074 PRACTITIONER, 075 /** 076 * The compartment definition is for the device compartment. 077 */ 078 DEVICE, 079 /** 080 * added to help the parsers with the generic types 081 */ 082 NULL; 083 084 public static CompartmentType fromCode(String codeString) throws FHIRException { 085 if (codeString == null || "".equals(codeString)) 086 return null; 087 if ("Patient".equals(codeString)) 088 return PATIENT; 089 if ("Encounter".equals(codeString)) 090 return ENCOUNTER; 091 if ("RelatedPerson".equals(codeString)) 092 return RELATEDPERSON; 093 if ("Practitioner".equals(codeString)) 094 return PRACTITIONER; 095 if ("Device".equals(codeString)) 096 return DEVICE; 097 if (Configuration.isAcceptInvalidEnums()) 098 return null; 099 else 100 throw new FHIRException("Unknown CompartmentType code '" + codeString + "'"); 101 } 102 103 public String toCode() { 104 switch (this) { 105 case PATIENT: 106 return "Patient"; 107 case ENCOUNTER: 108 return "Encounter"; 109 case RELATEDPERSON: 110 return "RelatedPerson"; 111 case PRACTITIONER: 112 return "Practitioner"; 113 case DEVICE: 114 return "Device"; 115 case NULL: 116 return null; 117 default: 118 return "?"; 119 } 120 } 121 122 public String getSystem() { 123 switch (this) { 124 case PATIENT: 125 return "http://hl7.org/fhir/compartment-type"; 126 case ENCOUNTER: 127 return "http://hl7.org/fhir/compartment-type"; 128 case RELATEDPERSON: 129 return "http://hl7.org/fhir/compartment-type"; 130 case PRACTITIONER: 131 return "http://hl7.org/fhir/compartment-type"; 132 case DEVICE: 133 return "http://hl7.org/fhir/compartment-type"; 134 case NULL: 135 return null; 136 default: 137 return "?"; 138 } 139 } 140 141 public String getDefinition() { 142 switch (this) { 143 case PATIENT: 144 return "The compartment definition is for the patient compartment."; 145 case ENCOUNTER: 146 return "The compartment definition is for the encounter compartment."; 147 case RELATEDPERSON: 148 return "The compartment definition is for the related-person compartment."; 149 case PRACTITIONER: 150 return "The compartment definition is for the practitioner compartment."; 151 case DEVICE: 152 return "The compartment definition is for the device compartment."; 153 case NULL: 154 return null; 155 default: 156 return "?"; 157 } 158 } 159 160 public String getDisplay() { 161 switch (this) { 162 case PATIENT: 163 return "Patient"; 164 case ENCOUNTER: 165 return "Encounter"; 166 case RELATEDPERSON: 167 return "RelatedPerson"; 168 case PRACTITIONER: 169 return "Practitioner"; 170 case DEVICE: 171 return "Device"; 172 case NULL: 173 return null; 174 default: 175 return "?"; 176 } 177 } 178 } 179 180 public static class CompartmentTypeEnumFactory implements EnumFactory<CompartmentType> { 181 public CompartmentType fromCode(String codeString) throws IllegalArgumentException { 182 if (codeString == null || "".equals(codeString)) 183 if (codeString == null || "".equals(codeString)) 184 return null; 185 if ("Patient".equals(codeString)) 186 return CompartmentType.PATIENT; 187 if ("Encounter".equals(codeString)) 188 return CompartmentType.ENCOUNTER; 189 if ("RelatedPerson".equals(codeString)) 190 return CompartmentType.RELATEDPERSON; 191 if ("Practitioner".equals(codeString)) 192 return CompartmentType.PRACTITIONER; 193 if ("Device".equals(codeString)) 194 return CompartmentType.DEVICE; 195 throw new IllegalArgumentException("Unknown CompartmentType code '" + codeString + "'"); 196 } 197 198 public Enumeration<CompartmentType> fromType(PrimitiveType<?> code) throws FHIRException { 199 if (code == null) 200 return null; 201 if (code.isEmpty()) 202 return new Enumeration<CompartmentType>(this, CompartmentType.NULL, code); 203 String codeString = code.asStringValue(); 204 if (codeString == null || "".equals(codeString)) 205 return new Enumeration<CompartmentType>(this, CompartmentType.NULL, code); 206 if ("Patient".equals(codeString)) 207 return new Enumeration<CompartmentType>(this, CompartmentType.PATIENT, code); 208 if ("Encounter".equals(codeString)) 209 return new Enumeration<CompartmentType>(this, CompartmentType.ENCOUNTER, code); 210 if ("RelatedPerson".equals(codeString)) 211 return new Enumeration<CompartmentType>(this, CompartmentType.RELATEDPERSON, code); 212 if ("Practitioner".equals(codeString)) 213 return new Enumeration<CompartmentType>(this, CompartmentType.PRACTITIONER, code); 214 if ("Device".equals(codeString)) 215 return new Enumeration<CompartmentType>(this, CompartmentType.DEVICE, code); 216 throw new FHIRException("Unknown CompartmentType code '" + codeString + "'"); 217 } 218 219 public String toCode(CompartmentType code) { 220 if (code == CompartmentType.PATIENT) 221 return "Patient"; 222 if (code == CompartmentType.ENCOUNTER) 223 return "Encounter"; 224 if (code == CompartmentType.RELATEDPERSON) 225 return "RelatedPerson"; 226 if (code == CompartmentType.PRACTITIONER) 227 return "Practitioner"; 228 if (code == CompartmentType.DEVICE) 229 return "Device"; 230 return "?"; 231 } 232 233 public String toSystem(CompartmentType code) { 234 return code.getSystem(); 235 } 236 } 237 238 @Block() 239 public static class CompartmentDefinitionResourceComponent extends BackboneElement implements IBaseBackboneElement { 240 /** 241 * The name of a resource supported by the server. 242 */ 243 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 244 @Description(shortDefinition = "Name of resource type", formalDefinition = "The name of a resource supported by the server.") 245 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/resource-types") 246 protected CodeType code; 247 248 /** 249 * The name of a search parameter that represents the link to the compartment. 250 * More than one may be listed because a resource may be linked to a compartment 251 * in more than one way,. 252 */ 253 @Child(name = "param", type = { 254 StringType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 255 @Description(shortDefinition = "Search Parameter Name, or chained parameters", formalDefinition = "The name of a search parameter that represents the link to the compartment. More than one may be listed because a resource may be linked to a compartment in more than one way,.") 256 protected List<StringType> param; 257 258 /** 259 * Additional documentation about the resource and compartment. 260 */ 261 @Child(name = "documentation", type = { 262 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 263 @Description(shortDefinition = "Additional documentation about the resource and compartment", formalDefinition = "Additional documentation about the resource and compartment.") 264 protected StringType documentation; 265 266 private static final long serialVersionUID = 988080897L; 267 268 /** 269 * Constructor 270 */ 271 public CompartmentDefinitionResourceComponent() { 272 super(); 273 } 274 275 /** 276 * Constructor 277 */ 278 public CompartmentDefinitionResourceComponent(CodeType code) { 279 super(); 280 this.code = code; 281 } 282 283 /** 284 * @return {@link #code} (The name of a resource supported by the server.). This 285 * is the underlying object with id, value and extensions. The accessor 286 * "getCode" gives direct access to the value 287 */ 288 public CodeType getCodeElement() { 289 if (this.code == null) 290 if (Configuration.errorOnAutoCreate()) 291 throw new Error("Attempt to auto-create CompartmentDefinitionResourceComponent.code"); 292 else if (Configuration.doAutoCreate()) 293 this.code = new CodeType(); // bb 294 return this.code; 295 } 296 297 public boolean hasCodeElement() { 298 return this.code != null && !this.code.isEmpty(); 299 } 300 301 public boolean hasCode() { 302 return this.code != null && !this.code.isEmpty(); 303 } 304 305 /** 306 * @param value {@link #code} (The name of a resource supported by the server.). 307 * This is the underlying object with id, value and extensions. The 308 * accessor "getCode" gives direct access to the value 309 */ 310 public CompartmentDefinitionResourceComponent setCodeElement(CodeType value) { 311 this.code = value; 312 return this; 313 } 314 315 /** 316 * @return The name of a resource supported by the server. 317 */ 318 public String getCode() { 319 return this.code == null ? null : this.code.getValue(); 320 } 321 322 /** 323 * @param value The name of a resource supported by the server. 324 */ 325 public CompartmentDefinitionResourceComponent setCode(String value) { 326 if (this.code == null) 327 this.code = new CodeType(); 328 this.code.setValue(value); 329 return this; 330 } 331 332 /** 333 * @return {@link #param} (The name of a search parameter that represents the 334 * link to the compartment. More than one may be listed because a 335 * resource may be linked to a compartment in more than one way,.) 336 */ 337 public List<StringType> getParam() { 338 if (this.param == null) 339 this.param = new ArrayList<StringType>(); 340 return this.param; 341 } 342 343 /** 344 * @return Returns a reference to <code>this</code> for easy method chaining 345 */ 346 public CompartmentDefinitionResourceComponent setParam(List<StringType> theParam) { 347 this.param = theParam; 348 return this; 349 } 350 351 public boolean hasParam() { 352 if (this.param == null) 353 return false; 354 for (StringType item : this.param) 355 if (!item.isEmpty()) 356 return true; 357 return false; 358 } 359 360 /** 361 * @return {@link #param} (The name of a search parameter that represents the 362 * link to the compartment. More than one may be listed because a 363 * resource may be linked to a compartment in more than one way,.) 364 */ 365 public StringType addParamElement() {// 2 366 StringType t = new StringType(); 367 if (this.param == null) 368 this.param = new ArrayList<StringType>(); 369 this.param.add(t); 370 return t; 371 } 372 373 /** 374 * @param value {@link #param} (The name of a search parameter that represents 375 * the link to the compartment. More than one may be listed because 376 * a resource may be linked to a compartment in more than one 377 * way,.) 378 */ 379 public CompartmentDefinitionResourceComponent addParam(String value) { // 1 380 StringType t = new StringType(); 381 t.setValue(value); 382 if (this.param == null) 383 this.param = new ArrayList<StringType>(); 384 this.param.add(t); 385 return this; 386 } 387 388 /** 389 * @param value {@link #param} (The name of a search parameter that represents 390 * the link to the compartment. More than one may be listed because 391 * a resource may be linked to a compartment in more than one 392 * way,.) 393 */ 394 public boolean hasParam(String value) { 395 if (this.param == null) 396 return false; 397 for (StringType v : this.param) 398 if (v.getValue().equals(value)) // string 399 return true; 400 return false; 401 } 402 403 /** 404 * @return {@link #documentation} (Additional documentation about the resource 405 * and compartment.). This is the underlying object with id, value and 406 * extensions. The accessor "getDocumentation" gives direct access to 407 * the value 408 */ 409 public StringType getDocumentationElement() { 410 if (this.documentation == null) 411 if (Configuration.errorOnAutoCreate()) 412 throw new Error("Attempt to auto-create CompartmentDefinitionResourceComponent.documentation"); 413 else if (Configuration.doAutoCreate()) 414 this.documentation = new StringType(); // bb 415 return this.documentation; 416 } 417 418 public boolean hasDocumentationElement() { 419 return this.documentation != null && !this.documentation.isEmpty(); 420 } 421 422 public boolean hasDocumentation() { 423 return this.documentation != null && !this.documentation.isEmpty(); 424 } 425 426 /** 427 * @param value {@link #documentation} (Additional documentation about the 428 * resource and compartment.). This is the underlying object with 429 * id, value and extensions. The accessor "getDocumentation" gives 430 * direct access to the value 431 */ 432 public CompartmentDefinitionResourceComponent setDocumentationElement(StringType value) { 433 this.documentation = value; 434 return this; 435 } 436 437 /** 438 * @return Additional documentation about the resource and compartment. 439 */ 440 public String getDocumentation() { 441 return this.documentation == null ? null : this.documentation.getValue(); 442 } 443 444 /** 445 * @param value Additional documentation about the resource and compartment. 446 */ 447 public CompartmentDefinitionResourceComponent setDocumentation(String value) { 448 if (Utilities.noString(value)) 449 this.documentation = null; 450 else { 451 if (this.documentation == null) 452 this.documentation = new StringType(); 453 this.documentation.setValue(value); 454 } 455 return this; 456 } 457 458 protected void listChildren(List<Property> children) { 459 super.listChildren(children); 460 children.add(new Property("code", "code", "The name of a resource supported by the server.", 0, 1, code)); 461 children.add(new Property("param", "string", 462 "The name of a search parameter that represents the link to the compartment. More than one may be listed because a resource may be linked to a compartment in more than one way,.", 463 0, java.lang.Integer.MAX_VALUE, param)); 464 children.add(new Property("documentation", "string", 465 "Additional documentation about the resource and compartment.", 0, 1, documentation)); 466 } 467 468 @Override 469 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 470 switch (_hash) { 471 case 3059181: 472 /* code */ return new Property("code", "code", "The name of a resource supported by the server.", 0, 1, code); 473 case 106436749: 474 /* param */ return new Property("param", "string", 475 "The name of a search parameter that represents the link to the compartment. More than one may be listed because a resource may be linked to a compartment in more than one way,.", 476 0, java.lang.Integer.MAX_VALUE, param); 477 case 1587405498: 478 /* documentation */ return new Property("documentation", "string", 479 "Additional documentation about the resource and compartment.", 0, 1, documentation); 480 default: 481 return super.getNamedProperty(_hash, _name, _checkValid); 482 } 483 484 } 485 486 @Override 487 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 488 switch (hash) { 489 case 3059181: 490 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeType 491 case 106436749: 492 /* param */ return this.param == null ? new Base[0] : this.param.toArray(new Base[this.param.size()]); // StringType 493 case 1587405498: 494 /* documentation */ return this.documentation == null ? new Base[0] : new Base[] { this.documentation }; // StringType 495 default: 496 return super.getProperty(hash, name, checkValid); 497 } 498 499 } 500 501 @Override 502 public Base setProperty(int hash, String name, Base value) throws FHIRException { 503 switch (hash) { 504 case 3059181: // code 505 this.code = castToCode(value); // CodeType 506 return value; 507 case 106436749: // param 508 this.getParam().add(castToString(value)); // StringType 509 return value; 510 case 1587405498: // documentation 511 this.documentation = castToString(value); // StringType 512 return value; 513 default: 514 return super.setProperty(hash, name, value); 515 } 516 517 } 518 519 @Override 520 public Base setProperty(String name, Base value) throws FHIRException { 521 if (name.equals("code")) { 522 this.code = castToCode(value); // CodeType 523 } else if (name.equals("param")) { 524 this.getParam().add(castToString(value)); 525 } else if (name.equals("documentation")) { 526 this.documentation = castToString(value); // StringType 527 } else 528 return super.setProperty(name, value); 529 return value; 530 } 531 532 @Override 533 public void removeChild(String name, Base value) throws FHIRException { 534 if (name.equals("code")) { 535 this.code = null; 536 } else if (name.equals("param")) { 537 this.getParam().remove(castToString(value)); 538 } else if (name.equals("documentation")) { 539 this.documentation = null; 540 } else 541 super.removeChild(name, value); 542 543 } 544 545 @Override 546 public Base makeProperty(int hash, String name) throws FHIRException { 547 switch (hash) { 548 case 3059181: 549 return getCodeElement(); 550 case 106436749: 551 return addParamElement(); 552 case 1587405498: 553 return getDocumentationElement(); 554 default: 555 return super.makeProperty(hash, name); 556 } 557 558 } 559 560 @Override 561 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 562 switch (hash) { 563 case 3059181: 564 /* code */ return new String[] { "code" }; 565 case 106436749: 566 /* param */ return new String[] { "string" }; 567 case 1587405498: 568 /* documentation */ return new String[] { "string" }; 569 default: 570 return super.getTypesForProperty(hash, name); 571 } 572 573 } 574 575 @Override 576 public Base addChild(String name) throws FHIRException { 577 if (name.equals("code")) { 578 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.code"); 579 } else if (name.equals("param")) { 580 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.param"); 581 } else if (name.equals("documentation")) { 582 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.documentation"); 583 } else 584 return super.addChild(name); 585 } 586 587 public CompartmentDefinitionResourceComponent copy() { 588 CompartmentDefinitionResourceComponent dst = new CompartmentDefinitionResourceComponent(); 589 copyValues(dst); 590 return dst; 591 } 592 593 public void copyValues(CompartmentDefinitionResourceComponent dst) { 594 super.copyValues(dst); 595 dst.code = code == null ? null : code.copy(); 596 if (param != null) { 597 dst.param = new ArrayList<StringType>(); 598 for (StringType i : param) 599 dst.param.add(i.copy()); 600 } 601 ; 602 dst.documentation = documentation == null ? null : documentation.copy(); 603 } 604 605 @Override 606 public boolean equalsDeep(Base other_) { 607 if (!super.equalsDeep(other_)) 608 return false; 609 if (!(other_ instanceof CompartmentDefinitionResourceComponent)) 610 return false; 611 CompartmentDefinitionResourceComponent o = (CompartmentDefinitionResourceComponent) other_; 612 return compareDeep(code, o.code, true) && compareDeep(param, o.param, true) 613 && compareDeep(documentation, o.documentation, true); 614 } 615 616 @Override 617 public boolean equalsShallow(Base other_) { 618 if (!super.equalsShallow(other_)) 619 return false; 620 if (!(other_ instanceof CompartmentDefinitionResourceComponent)) 621 return false; 622 CompartmentDefinitionResourceComponent o = (CompartmentDefinitionResourceComponent) other_; 623 return compareValues(code, o.code, true) && compareValues(param, o.param, true) 624 && compareValues(documentation, o.documentation, true); 625 } 626 627 public boolean isEmpty() { 628 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, param, documentation); 629 } 630 631 public String fhirType() { 632 return "CompartmentDefinition.resource"; 633 634 } 635 636 } 637 638 /** 639 * Explanation of why this compartment definition is needed and why it has been 640 * designed as it has. 641 */ 642 @Child(name = "purpose", type = { 643 MarkdownType.class }, order = 0, min = 0, max = 1, modifier = false, summary = false) 644 @Description(shortDefinition = "Why this compartment definition is defined", formalDefinition = "Explanation of why this compartment definition is needed and why it has been designed as it has.") 645 protected MarkdownType purpose; 646 647 /** 648 * Which compartment this definition describes. 649 */ 650 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 651 @Description(shortDefinition = "Patient | Encounter | RelatedPerson | Practitioner | Device", formalDefinition = "Which compartment this definition describes.") 652 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/compartment-type") 653 protected Enumeration<CompartmentType> code; 654 655 /** 656 * Whether the search syntax is supported,. 657 */ 658 @Child(name = "search", type = { BooleanType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 659 @Description(shortDefinition = "Whether the search syntax is supported", formalDefinition = "Whether the search syntax is supported,.") 660 protected BooleanType search; 661 662 /** 663 * Information about how a resource is related to the compartment. 664 */ 665 @Child(name = "resource", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 666 @Description(shortDefinition = "How a resource is related to the compartment", formalDefinition = "Information about how a resource is related to the compartment.") 667 protected List<CompartmentDefinitionResourceComponent> resource; 668 669 private static final long serialVersionUID = -1159172945L; 670 671 /** 672 * Constructor 673 */ 674 public CompartmentDefinition() { 675 super(); 676 } 677 678 /** 679 * Constructor 680 */ 681 public CompartmentDefinition(UriType url, StringType name, Enumeration<PublicationStatus> status, 682 Enumeration<CompartmentType> code, BooleanType search) { 683 super(); 684 this.url = url; 685 this.name = name; 686 this.status = status; 687 this.code = code; 688 this.search = search; 689 } 690 691 /** 692 * @return {@link #url} (An absolute URI that is used to identify this 693 * compartment definition when it is referenced in a specification, 694 * model, design or an instance; also called its canonical identifier. 695 * This SHOULD be globally unique and SHOULD be a literal address at 696 * which at which an authoritative instance of this compartment 697 * definition is (or will be) published. This URL can be the target of a 698 * canonical reference. It SHALL remain the same when the compartment 699 * definition is stored on different servers.). This is the underlying 700 * object with id, value and extensions. The accessor "getUrl" gives 701 * direct access to the value 702 */ 703 public UriType getUrlElement() { 704 if (this.url == null) 705 if (Configuration.errorOnAutoCreate()) 706 throw new Error("Attempt to auto-create CompartmentDefinition.url"); 707 else if (Configuration.doAutoCreate()) 708 this.url = new UriType(); // bb 709 return this.url; 710 } 711 712 public boolean hasUrlElement() { 713 return this.url != null && !this.url.isEmpty(); 714 } 715 716 public boolean hasUrl() { 717 return this.url != null && !this.url.isEmpty(); 718 } 719 720 /** 721 * @param value {@link #url} (An absolute URI that is used to identify this 722 * compartment definition when it is referenced in a specification, 723 * model, design or an instance; also called its canonical 724 * identifier. This SHOULD be globally unique and SHOULD be a 725 * literal address at which at which an authoritative instance of 726 * this compartment definition is (or will be) published. This URL 727 * can be the target of a canonical reference. It SHALL remain the 728 * same when the compartment definition is stored on different 729 * servers.). This is the underlying object with id, value and 730 * extensions. The accessor "getUrl" gives direct access to the 731 * value 732 */ 733 public CompartmentDefinition setUrlElement(UriType value) { 734 this.url = value; 735 return this; 736 } 737 738 /** 739 * @return An absolute URI that is used to identify this compartment definition 740 * when it is referenced in a specification, model, design or an 741 * instance; also called its canonical identifier. This SHOULD be 742 * globally unique and SHOULD be a literal address at which at which an 743 * authoritative instance of this compartment definition is (or will be) 744 * published. This URL can be the target of a canonical reference. It 745 * SHALL remain the same when the compartment definition is stored on 746 * different servers. 747 */ 748 public String getUrl() { 749 return this.url == null ? null : this.url.getValue(); 750 } 751 752 /** 753 * @param value An absolute URI that is used to identify this compartment 754 * definition when it is referenced in a specification, model, 755 * design or an instance; also called its canonical identifier. 756 * This SHOULD be globally unique and SHOULD be a literal address 757 * at which at which an authoritative instance of this compartment 758 * definition is (or will be) published. This URL can be the target 759 * of a canonical reference. It SHALL remain the same when the 760 * compartment definition is stored on different servers. 761 */ 762 public CompartmentDefinition setUrl(String value) { 763 if (this.url == null) 764 this.url = new UriType(); 765 this.url.setValue(value); 766 return this; 767 } 768 769 /** 770 * @return {@link #version} (The identifier that is used to identify this 771 * version of the compartment definition when it is referenced in a 772 * specification, model, design or instance. This is an arbitrary value 773 * managed by the compartment definition author and is not expected to 774 * be globally unique. For example, it might be a timestamp (e.g. 775 * yyyymmdd) if a managed version is not available. There is also no 776 * expectation that versions can be placed in a lexicographical 777 * sequence.). This is the underlying object with id, value and 778 * extensions. The accessor "getVersion" gives direct access to the 779 * value 780 */ 781 public StringType getVersionElement() { 782 if (this.version == null) 783 if (Configuration.errorOnAutoCreate()) 784 throw new Error("Attempt to auto-create CompartmentDefinition.version"); 785 else if (Configuration.doAutoCreate()) 786 this.version = new StringType(); // bb 787 return this.version; 788 } 789 790 public boolean hasVersionElement() { 791 return this.version != null && !this.version.isEmpty(); 792 } 793 794 public boolean hasVersion() { 795 return this.version != null && !this.version.isEmpty(); 796 } 797 798 /** 799 * @param value {@link #version} (The identifier that is used to identify this 800 * version of the compartment definition when it is referenced in a 801 * specification, model, design or instance. This is an arbitrary 802 * value managed by the compartment definition author and is not 803 * expected to be globally unique. For example, it might be a 804 * timestamp (e.g. yyyymmdd) if a managed version is not available. 805 * There is also no expectation that versions can be placed in a 806 * lexicographical sequence.). This is the underlying object with 807 * id, value and extensions. The accessor "getVersion" gives direct 808 * access to the value 809 */ 810 public CompartmentDefinition setVersionElement(StringType value) { 811 this.version = value; 812 return this; 813 } 814 815 /** 816 * @return The identifier that is used to identify this version of the 817 * compartment definition when it is referenced in a specification, 818 * model, design or instance. This is an arbitrary value managed by the 819 * compartment definition author and is not expected to be globally 820 * unique. For example, it might be a timestamp (e.g. yyyymmdd) if a 821 * managed version is not available. There is also no expectation that 822 * versions can be placed in a lexicographical sequence. 823 */ 824 public String getVersion() { 825 return this.version == null ? null : this.version.getValue(); 826 } 827 828 /** 829 * @param value The identifier that is used to identify this version of the 830 * compartment definition when it is referenced in a specification, 831 * model, design or instance. This is an arbitrary value managed by 832 * the compartment definition author and is not expected to be 833 * globally unique. For example, it might be a timestamp (e.g. 834 * yyyymmdd) if a managed version is not available. There is also 835 * no expectation that versions can be placed in a lexicographical 836 * sequence. 837 */ 838 public CompartmentDefinition setVersion(String value) { 839 if (Utilities.noString(value)) 840 this.version = null; 841 else { 842 if (this.version == null) 843 this.version = new StringType(); 844 this.version.setValue(value); 845 } 846 return this; 847 } 848 849 /** 850 * @return {@link #name} (A natural language name identifying the compartment 851 * definition. This name should be usable as an identifier for the 852 * module by machine processing applications such as code generation.). 853 * This is the underlying object with id, value and extensions. The 854 * accessor "getName" gives direct access to the value 855 */ 856 public StringType getNameElement() { 857 if (this.name == null) 858 if (Configuration.errorOnAutoCreate()) 859 throw new Error("Attempt to auto-create CompartmentDefinition.name"); 860 else if (Configuration.doAutoCreate()) 861 this.name = new StringType(); // bb 862 return this.name; 863 } 864 865 public boolean hasNameElement() { 866 return this.name != null && !this.name.isEmpty(); 867 } 868 869 public boolean hasName() { 870 return this.name != null && !this.name.isEmpty(); 871 } 872 873 /** 874 * @param value {@link #name} (A natural language name identifying the 875 * compartment definition. This name should be usable as an 876 * identifier for the module by machine processing applications 877 * such as code generation.). This is the underlying object with 878 * id, value and extensions. The accessor "getName" gives direct 879 * access to the value 880 */ 881 public CompartmentDefinition setNameElement(StringType value) { 882 this.name = value; 883 return this; 884 } 885 886 /** 887 * @return A natural language name identifying the compartment definition. This 888 * name should be usable as an identifier for the module by machine 889 * processing applications such as code generation. 890 */ 891 public String getName() { 892 return this.name == null ? null : this.name.getValue(); 893 } 894 895 /** 896 * @param value A natural language name identifying the compartment definition. 897 * This name should be usable as an identifier for the module by 898 * machine processing applications such as code generation. 899 */ 900 public CompartmentDefinition setName(String value) { 901 if (this.name == null) 902 this.name = new StringType(); 903 this.name.setValue(value); 904 return this; 905 } 906 907 /** 908 * @return {@link #status} (The status of this compartment definition. Enables 909 * tracking the life-cycle of the content.). This is the underlying 910 * object with id, value and extensions. The accessor "getStatus" gives 911 * direct access to the value 912 */ 913 public Enumeration<PublicationStatus> getStatusElement() { 914 if (this.status == null) 915 if (Configuration.errorOnAutoCreate()) 916 throw new Error("Attempt to auto-create CompartmentDefinition.status"); 917 else if (Configuration.doAutoCreate()) 918 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 919 return this.status; 920 } 921 922 public boolean hasStatusElement() { 923 return this.status != null && !this.status.isEmpty(); 924 } 925 926 public boolean hasStatus() { 927 return this.status != null && !this.status.isEmpty(); 928 } 929 930 /** 931 * @param value {@link #status} (The status of this compartment definition. 932 * Enables tracking the life-cycle of the content.). This is the 933 * underlying object with id, value and extensions. The accessor 934 * "getStatus" gives direct access to the value 935 */ 936 public CompartmentDefinition setStatusElement(Enumeration<PublicationStatus> value) { 937 this.status = value; 938 return this; 939 } 940 941 /** 942 * @return The status of this compartment definition. Enables tracking the 943 * life-cycle of the content. 944 */ 945 public PublicationStatus getStatus() { 946 return this.status == null ? null : this.status.getValue(); 947 } 948 949 /** 950 * @param value The status of this compartment definition. Enables tracking the 951 * life-cycle of the content. 952 */ 953 public CompartmentDefinition setStatus(PublicationStatus value) { 954 if (this.status == null) 955 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 956 this.status.setValue(value); 957 return this; 958 } 959 960 /** 961 * @return {@link #experimental} (A Boolean value to indicate that this 962 * compartment definition is authored for testing purposes (or 963 * education/evaluation/marketing) and is not intended to be used for 964 * genuine usage.). This is the underlying object with id, value and 965 * extensions. The accessor "getExperimental" gives direct access to the 966 * value 967 */ 968 public BooleanType getExperimentalElement() { 969 if (this.experimental == null) 970 if (Configuration.errorOnAutoCreate()) 971 throw new Error("Attempt to auto-create CompartmentDefinition.experimental"); 972 else if (Configuration.doAutoCreate()) 973 this.experimental = new BooleanType(); // bb 974 return this.experimental; 975 } 976 977 public boolean hasExperimentalElement() { 978 return this.experimental != null && !this.experimental.isEmpty(); 979 } 980 981 public boolean hasExperimental() { 982 return this.experimental != null && !this.experimental.isEmpty(); 983 } 984 985 /** 986 * @param value {@link #experimental} (A Boolean value to indicate that this 987 * compartment definition is authored for testing purposes (or 988 * education/evaluation/marketing) and is not intended to be used 989 * for genuine usage.). This is the underlying object with id, 990 * value and extensions. The accessor "getExperimental" gives 991 * direct access to the value 992 */ 993 public CompartmentDefinition setExperimentalElement(BooleanType value) { 994 this.experimental = value; 995 return this; 996 } 997 998 /** 999 * @return A Boolean value to indicate that this compartment definition is 1000 * authored for testing purposes (or education/evaluation/marketing) and 1001 * is not intended to be used for genuine usage. 1002 */ 1003 public boolean getExperimental() { 1004 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1005 } 1006 1007 /** 1008 * @param value A Boolean value to indicate that this compartment definition is 1009 * authored for testing purposes (or 1010 * education/evaluation/marketing) and is not intended to be used 1011 * for genuine usage. 1012 */ 1013 public CompartmentDefinition setExperimental(boolean value) { 1014 if (this.experimental == null) 1015 this.experimental = new BooleanType(); 1016 this.experimental.setValue(value); 1017 return this; 1018 } 1019 1020 /** 1021 * @return {@link #date} (The date (and optionally time) when the compartment 1022 * definition was published. The date must change when the business 1023 * version changes and it must change if the status code changes. In 1024 * addition, it should change when the substantive content of the 1025 * compartment definition changes.). This is the underlying object with 1026 * id, value and extensions. The accessor "getDate" gives direct access 1027 * to the value 1028 */ 1029 public DateTimeType getDateElement() { 1030 if (this.date == null) 1031 if (Configuration.errorOnAutoCreate()) 1032 throw new Error("Attempt to auto-create CompartmentDefinition.date"); 1033 else if (Configuration.doAutoCreate()) 1034 this.date = new DateTimeType(); // bb 1035 return this.date; 1036 } 1037 1038 public boolean hasDateElement() { 1039 return this.date != null && !this.date.isEmpty(); 1040 } 1041 1042 public boolean hasDate() { 1043 return this.date != null && !this.date.isEmpty(); 1044 } 1045 1046 /** 1047 * @param value {@link #date} (The date (and optionally time) when the 1048 * compartment definition was published. The date must change when 1049 * the business version changes and it must change if the status 1050 * code changes. In addition, it should change when the substantive 1051 * content of the compartment definition changes.). This is the 1052 * underlying object with id, value and extensions. The accessor 1053 * "getDate" gives direct access to the value 1054 */ 1055 public CompartmentDefinition setDateElement(DateTimeType value) { 1056 this.date = value; 1057 return this; 1058 } 1059 1060 /** 1061 * @return The date (and optionally time) when the compartment definition was 1062 * published. The date must change when the business version changes and 1063 * it must change if the status code changes. In addition, it should 1064 * change when the substantive content of the compartment definition 1065 * changes. 1066 */ 1067 public Date getDate() { 1068 return this.date == null ? null : this.date.getValue(); 1069 } 1070 1071 /** 1072 * @param value The date (and optionally time) when the compartment definition 1073 * was published. The date must change when the business version 1074 * changes and it must change if the status code changes. In 1075 * addition, it should change when the substantive content of the 1076 * compartment definition changes. 1077 */ 1078 public CompartmentDefinition setDate(Date value) { 1079 if (value == null) 1080 this.date = null; 1081 else { 1082 if (this.date == null) 1083 this.date = new DateTimeType(); 1084 this.date.setValue(value); 1085 } 1086 return this; 1087 } 1088 1089 /** 1090 * @return {@link #publisher} (The name of the organization or individual that 1091 * published the compartment definition.). This is the underlying object 1092 * with id, value and extensions. The accessor "getPublisher" gives 1093 * direct access to the value 1094 */ 1095 public StringType getPublisherElement() { 1096 if (this.publisher == null) 1097 if (Configuration.errorOnAutoCreate()) 1098 throw new Error("Attempt to auto-create CompartmentDefinition.publisher"); 1099 else if (Configuration.doAutoCreate()) 1100 this.publisher = new StringType(); // bb 1101 return this.publisher; 1102 } 1103 1104 public boolean hasPublisherElement() { 1105 return this.publisher != null && !this.publisher.isEmpty(); 1106 } 1107 1108 public boolean hasPublisher() { 1109 return this.publisher != null && !this.publisher.isEmpty(); 1110 } 1111 1112 /** 1113 * @param value {@link #publisher} (The name of the organization or individual 1114 * that published the compartment definition.). This is the 1115 * underlying object with id, value and extensions. The accessor 1116 * "getPublisher" gives direct access to the value 1117 */ 1118 public CompartmentDefinition setPublisherElement(StringType value) { 1119 this.publisher = value; 1120 return this; 1121 } 1122 1123 /** 1124 * @return The name of the organization or individual that published the 1125 * compartment definition. 1126 */ 1127 public String getPublisher() { 1128 return this.publisher == null ? null : this.publisher.getValue(); 1129 } 1130 1131 /** 1132 * @param value The name of the organization or individual that published the 1133 * compartment definition. 1134 */ 1135 public CompartmentDefinition setPublisher(String value) { 1136 if (Utilities.noString(value)) 1137 this.publisher = null; 1138 else { 1139 if (this.publisher == null) 1140 this.publisher = new StringType(); 1141 this.publisher.setValue(value); 1142 } 1143 return this; 1144 } 1145 1146 /** 1147 * @return {@link #contact} (Contact details to assist a user in finding and 1148 * communicating with the publisher.) 1149 */ 1150 public List<ContactDetail> getContact() { 1151 if (this.contact == null) 1152 this.contact = new ArrayList<ContactDetail>(); 1153 return this.contact; 1154 } 1155 1156 /** 1157 * @return Returns a reference to <code>this</code> for easy method chaining 1158 */ 1159 public CompartmentDefinition setContact(List<ContactDetail> theContact) { 1160 this.contact = theContact; 1161 return this; 1162 } 1163 1164 public boolean hasContact() { 1165 if (this.contact == null) 1166 return false; 1167 for (ContactDetail item : this.contact) 1168 if (!item.isEmpty()) 1169 return true; 1170 return false; 1171 } 1172 1173 public ContactDetail addContact() { // 3 1174 ContactDetail t = new ContactDetail(); 1175 if (this.contact == null) 1176 this.contact = new ArrayList<ContactDetail>(); 1177 this.contact.add(t); 1178 return t; 1179 } 1180 1181 public CompartmentDefinition addContact(ContactDetail t) { // 3 1182 if (t == null) 1183 return this; 1184 if (this.contact == null) 1185 this.contact = new ArrayList<ContactDetail>(); 1186 this.contact.add(t); 1187 return this; 1188 } 1189 1190 /** 1191 * @return The first repetition of repeating field {@link #contact}, creating it 1192 * if it does not already exist 1193 */ 1194 public ContactDetail getContactFirstRep() { 1195 if (getContact().isEmpty()) { 1196 addContact(); 1197 } 1198 return getContact().get(0); 1199 } 1200 1201 /** 1202 * @return {@link #description} (A free text natural language description of the 1203 * compartment definition from a consumer's perspective.). This is the 1204 * underlying object with id, value and extensions. The accessor 1205 * "getDescription" gives direct access to the value 1206 */ 1207 public MarkdownType getDescriptionElement() { 1208 if (this.description == null) 1209 if (Configuration.errorOnAutoCreate()) 1210 throw new Error("Attempt to auto-create CompartmentDefinition.description"); 1211 else if (Configuration.doAutoCreate()) 1212 this.description = new MarkdownType(); // bb 1213 return this.description; 1214 } 1215 1216 public boolean hasDescriptionElement() { 1217 return this.description != null && !this.description.isEmpty(); 1218 } 1219 1220 public boolean hasDescription() { 1221 return this.description != null && !this.description.isEmpty(); 1222 } 1223 1224 /** 1225 * @param value {@link #description} (A free text natural language description 1226 * of the compartment definition from a consumer's perspective.). 1227 * This is the underlying object with id, value and extensions. The 1228 * accessor "getDescription" gives direct access to the value 1229 */ 1230 public CompartmentDefinition setDescriptionElement(MarkdownType value) { 1231 this.description = value; 1232 return this; 1233 } 1234 1235 /** 1236 * @return A free text natural language description of the compartment 1237 * definition from a consumer's perspective. 1238 */ 1239 public String getDescription() { 1240 return this.description == null ? null : this.description.getValue(); 1241 } 1242 1243 /** 1244 * @param value A free text natural language description of the compartment 1245 * definition from a consumer's perspective. 1246 */ 1247 public CompartmentDefinition setDescription(String value) { 1248 if (value == null) 1249 this.description = null; 1250 else { 1251 if (this.description == null) 1252 this.description = new MarkdownType(); 1253 this.description.setValue(value); 1254 } 1255 return this; 1256 } 1257 1258 /** 1259 * @return {@link #useContext} (The content was developed with a focus and 1260 * intent of supporting the contexts that are listed. These contexts may 1261 * be general categories (gender, age, ...) or may be references to 1262 * specific programs (insurance plans, studies, ...) and may be used to 1263 * assist with indexing and searching for appropriate compartment 1264 * definition instances.) 1265 */ 1266 public List<UsageContext> getUseContext() { 1267 if (this.useContext == null) 1268 this.useContext = new ArrayList<UsageContext>(); 1269 return this.useContext; 1270 } 1271 1272 /** 1273 * @return Returns a reference to <code>this</code> for easy method chaining 1274 */ 1275 public CompartmentDefinition setUseContext(List<UsageContext> theUseContext) { 1276 this.useContext = theUseContext; 1277 return this; 1278 } 1279 1280 public boolean hasUseContext() { 1281 if (this.useContext == null) 1282 return false; 1283 for (UsageContext item : this.useContext) 1284 if (!item.isEmpty()) 1285 return true; 1286 return false; 1287 } 1288 1289 public UsageContext addUseContext() { // 3 1290 UsageContext t = new UsageContext(); 1291 if (this.useContext == null) 1292 this.useContext = new ArrayList<UsageContext>(); 1293 this.useContext.add(t); 1294 return t; 1295 } 1296 1297 public CompartmentDefinition addUseContext(UsageContext t) { // 3 1298 if (t == null) 1299 return this; 1300 if (this.useContext == null) 1301 this.useContext = new ArrayList<UsageContext>(); 1302 this.useContext.add(t); 1303 return this; 1304 } 1305 1306 /** 1307 * @return The first repetition of repeating field {@link #useContext}, creating 1308 * it if it does not already exist 1309 */ 1310 public UsageContext getUseContextFirstRep() { 1311 if (getUseContext().isEmpty()) { 1312 addUseContext(); 1313 } 1314 return getUseContext().get(0); 1315 } 1316 1317 /** 1318 * @return {@link #purpose} (Explanation of why this compartment definition is 1319 * needed and why it has been designed as it has.). This is the 1320 * underlying object with id, value and extensions. The accessor 1321 * "getPurpose" gives direct access to the value 1322 */ 1323 public MarkdownType getPurposeElement() { 1324 if (this.purpose == null) 1325 if (Configuration.errorOnAutoCreate()) 1326 throw new Error("Attempt to auto-create CompartmentDefinition.purpose"); 1327 else if (Configuration.doAutoCreate()) 1328 this.purpose = new MarkdownType(); // bb 1329 return this.purpose; 1330 } 1331 1332 public boolean hasPurposeElement() { 1333 return this.purpose != null && !this.purpose.isEmpty(); 1334 } 1335 1336 public boolean hasPurpose() { 1337 return this.purpose != null && !this.purpose.isEmpty(); 1338 } 1339 1340 /** 1341 * @param value {@link #purpose} (Explanation of why this compartment definition 1342 * is needed and why it has been designed as it has.). This is the 1343 * underlying object with id, value and extensions. The accessor 1344 * "getPurpose" gives direct access to the value 1345 */ 1346 public CompartmentDefinition setPurposeElement(MarkdownType value) { 1347 this.purpose = value; 1348 return this; 1349 } 1350 1351 /** 1352 * @return Explanation of why this compartment definition is needed and why it 1353 * has been designed as it has. 1354 */ 1355 public String getPurpose() { 1356 return this.purpose == null ? null : this.purpose.getValue(); 1357 } 1358 1359 /** 1360 * @param value Explanation of why this compartment definition is needed and why 1361 * it has been designed as it has. 1362 */ 1363 public CompartmentDefinition setPurpose(String value) { 1364 if (value == null) 1365 this.purpose = null; 1366 else { 1367 if (this.purpose == null) 1368 this.purpose = new MarkdownType(); 1369 this.purpose.setValue(value); 1370 } 1371 return this; 1372 } 1373 1374 /** 1375 * @return {@link #code} (Which compartment this definition describes.). This is 1376 * the underlying object with id, value and extensions. The accessor 1377 * "getCode" gives direct access to the value 1378 */ 1379 public Enumeration<CompartmentType> getCodeElement() { 1380 if (this.code == null) 1381 if (Configuration.errorOnAutoCreate()) 1382 throw new Error("Attempt to auto-create CompartmentDefinition.code"); 1383 else if (Configuration.doAutoCreate()) 1384 this.code = new Enumeration<CompartmentType>(new CompartmentTypeEnumFactory()); // bb 1385 return this.code; 1386 } 1387 1388 public boolean hasCodeElement() { 1389 return this.code != null && !this.code.isEmpty(); 1390 } 1391 1392 public boolean hasCode() { 1393 return this.code != null && !this.code.isEmpty(); 1394 } 1395 1396 /** 1397 * @param value {@link #code} (Which compartment this definition describes.). 1398 * This is the underlying object with id, value and extensions. The 1399 * accessor "getCode" gives direct access to the value 1400 */ 1401 public CompartmentDefinition setCodeElement(Enumeration<CompartmentType> value) { 1402 this.code = value; 1403 return this; 1404 } 1405 1406 /** 1407 * @return Which compartment this definition describes. 1408 */ 1409 public CompartmentType getCode() { 1410 return this.code == null ? null : this.code.getValue(); 1411 } 1412 1413 /** 1414 * @param value Which compartment this definition describes. 1415 */ 1416 public CompartmentDefinition setCode(CompartmentType value) { 1417 if (this.code == null) 1418 this.code = new Enumeration<CompartmentType>(new CompartmentTypeEnumFactory()); 1419 this.code.setValue(value); 1420 return this; 1421 } 1422 1423 /** 1424 * @return {@link #search} (Whether the search syntax is supported,.). This is 1425 * the underlying object with id, value and extensions. The accessor 1426 * "getSearch" gives direct access to the value 1427 */ 1428 public BooleanType getSearchElement() { 1429 if (this.search == null) 1430 if (Configuration.errorOnAutoCreate()) 1431 throw new Error("Attempt to auto-create CompartmentDefinition.search"); 1432 else if (Configuration.doAutoCreate()) 1433 this.search = new BooleanType(); // bb 1434 return this.search; 1435 } 1436 1437 public boolean hasSearchElement() { 1438 return this.search != null && !this.search.isEmpty(); 1439 } 1440 1441 public boolean hasSearch() { 1442 return this.search != null && !this.search.isEmpty(); 1443 } 1444 1445 /** 1446 * @param value {@link #search} (Whether the search syntax is supported,.). This 1447 * is the underlying object with id, value and extensions. The 1448 * accessor "getSearch" gives direct access to the value 1449 */ 1450 public CompartmentDefinition setSearchElement(BooleanType value) { 1451 this.search = value; 1452 return this; 1453 } 1454 1455 /** 1456 * @return Whether the search syntax is supported,. 1457 */ 1458 public boolean getSearch() { 1459 return this.search == null || this.search.isEmpty() ? false : this.search.getValue(); 1460 } 1461 1462 /** 1463 * @param value Whether the search syntax is supported,. 1464 */ 1465 public CompartmentDefinition setSearch(boolean value) { 1466 if (this.search == null) 1467 this.search = new BooleanType(); 1468 this.search.setValue(value); 1469 return this; 1470 } 1471 1472 /** 1473 * @return {@link #resource} (Information about how a resource is related to the 1474 * compartment.) 1475 */ 1476 public List<CompartmentDefinitionResourceComponent> getResource() { 1477 if (this.resource == null) 1478 this.resource = new ArrayList<CompartmentDefinitionResourceComponent>(); 1479 return this.resource; 1480 } 1481 1482 /** 1483 * @return Returns a reference to <code>this</code> for easy method chaining 1484 */ 1485 public CompartmentDefinition setResource(List<CompartmentDefinitionResourceComponent> theResource) { 1486 this.resource = theResource; 1487 return this; 1488 } 1489 1490 public boolean hasResource() { 1491 if (this.resource == null) 1492 return false; 1493 for (CompartmentDefinitionResourceComponent item : this.resource) 1494 if (!item.isEmpty()) 1495 return true; 1496 return false; 1497 } 1498 1499 public CompartmentDefinitionResourceComponent addResource() { // 3 1500 CompartmentDefinitionResourceComponent t = new CompartmentDefinitionResourceComponent(); 1501 if (this.resource == null) 1502 this.resource = new ArrayList<CompartmentDefinitionResourceComponent>(); 1503 this.resource.add(t); 1504 return t; 1505 } 1506 1507 public CompartmentDefinition addResource(CompartmentDefinitionResourceComponent t) { // 3 1508 if (t == null) 1509 return this; 1510 if (this.resource == null) 1511 this.resource = new ArrayList<CompartmentDefinitionResourceComponent>(); 1512 this.resource.add(t); 1513 return this; 1514 } 1515 1516 /** 1517 * @return The first repetition of repeating field {@link #resource}, creating 1518 * it if it does not already exist 1519 */ 1520 public CompartmentDefinitionResourceComponent getResourceFirstRep() { 1521 if (getResource().isEmpty()) { 1522 addResource(); 1523 } 1524 return getResource().get(0); 1525 } 1526 1527 protected void listChildren(List<Property> children) { 1528 super.listChildren(children); 1529 children.add(new Property("url", "uri", 1530 "An absolute URI that is used to identify this compartment definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this compartment definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the compartment definition is stored on different servers.", 1531 0, 1, url)); 1532 children.add(new Property("version", "string", 1533 "The identifier that is used to identify this version of the compartment definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the compartment definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 1534 0, 1, version)); 1535 children.add(new Property("name", "string", 1536 "A natural language name identifying the compartment definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 1537 0, 1, name)); 1538 children.add(new Property("status", "code", 1539 "The status of this compartment definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 1540 children.add(new Property("experimental", "boolean", 1541 "A Boolean value to indicate that this compartment definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 1542 0, 1, experimental)); 1543 children.add(new Property("date", "dateTime", 1544 "The date (and optionally time) when the compartment definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the compartment definition changes.", 1545 0, 1, date)); 1546 children.add(new Property("publisher", "string", 1547 "The name of the organization or individual that published the compartment definition.", 0, 1, publisher)); 1548 children.add(new Property("contact", "ContactDetail", 1549 "Contact details to assist a user in finding and communicating with the publisher.", 0, 1550 java.lang.Integer.MAX_VALUE, contact)); 1551 children.add(new Property("description", "markdown", 1552 "A free text natural language description of the compartment definition from a consumer's perspective.", 0, 1, 1553 description)); 1554 children.add(new Property("useContext", "UsageContext", 1555 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate compartment definition instances.", 1556 0, java.lang.Integer.MAX_VALUE, useContext)); 1557 children.add(new Property("purpose", "markdown", 1558 "Explanation of why this compartment definition is needed and why it has been designed as it has.", 0, 1, 1559 purpose)); 1560 children.add(new Property("code", "code", "Which compartment this definition describes.", 0, 1, code)); 1561 children.add(new Property("search", "boolean", "Whether the search syntax is supported,.", 0, 1, search)); 1562 children.add(new Property("resource", "", "Information about how a resource is related to the compartment.", 0, 1563 java.lang.Integer.MAX_VALUE, resource)); 1564 } 1565 1566 @Override 1567 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1568 switch (_hash) { 1569 case 116079: 1570 /* url */ return new Property("url", "uri", 1571 "An absolute URI that is used to identify this compartment definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this compartment definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the compartment definition is stored on different servers.", 1572 0, 1, url); 1573 case 351608024: 1574 /* version */ return new Property("version", "string", 1575 "The identifier that is used to identify this version of the compartment definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the compartment definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 1576 0, 1, version); 1577 case 3373707: 1578 /* name */ return new Property("name", "string", 1579 "A natural language name identifying the compartment definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 1580 0, 1, name); 1581 case -892481550: 1582 /* status */ return new Property("status", "code", 1583 "The status of this compartment definition. Enables tracking the life-cycle of the content.", 0, 1, status); 1584 case -404562712: 1585 /* experimental */ return new Property("experimental", "boolean", 1586 "A Boolean value to indicate that this compartment definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 1587 0, 1, experimental); 1588 case 3076014: 1589 /* date */ return new Property("date", "dateTime", 1590 "The date (and optionally time) when the compartment definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the compartment definition changes.", 1591 0, 1, date); 1592 case 1447404028: 1593 /* publisher */ return new Property("publisher", "string", 1594 "The name of the organization or individual that published the compartment definition.", 0, 1, publisher); 1595 case 951526432: 1596 /* contact */ return new Property("contact", "ContactDetail", 1597 "Contact details to assist a user in finding and communicating with the publisher.", 0, 1598 java.lang.Integer.MAX_VALUE, contact); 1599 case -1724546052: 1600 /* description */ return new Property("description", "markdown", 1601 "A free text natural language description of the compartment definition from a consumer's perspective.", 0, 1, 1602 description); 1603 case -669707736: 1604 /* useContext */ return new Property("useContext", "UsageContext", 1605 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate compartment definition instances.", 1606 0, java.lang.Integer.MAX_VALUE, useContext); 1607 case -220463842: 1608 /* purpose */ return new Property("purpose", "markdown", 1609 "Explanation of why this compartment definition is needed and why it has been designed as it has.", 0, 1, 1610 purpose); 1611 case 3059181: 1612 /* code */ return new Property("code", "code", "Which compartment this definition describes.", 0, 1, code); 1613 case -906336856: 1614 /* search */ return new Property("search", "boolean", "Whether the search syntax is supported,.", 0, 1, search); 1615 case -341064690: 1616 /* resource */ return new Property("resource", "", 1617 "Information about how a resource is related to the compartment.", 0, java.lang.Integer.MAX_VALUE, resource); 1618 default: 1619 return super.getNamedProperty(_hash, _name, _checkValid); 1620 } 1621 1622 } 1623 1624 @Override 1625 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1626 switch (hash) { 1627 case 116079: 1628 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 1629 case 351608024: 1630 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 1631 case 3373707: 1632 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 1633 case -892481550: 1634 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 1635 case -404562712: 1636 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 1637 case 3076014: 1638 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 1639 case 1447404028: 1640 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 1641 case 951526432: 1642 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 1643 case -1724546052: 1644 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 1645 case -669707736: 1646 /* useContext */ return this.useContext == null ? new Base[0] 1647 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 1648 case -220463842: 1649 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 1650 case 3059181: 1651 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // Enumeration<CompartmentType> 1652 case -906336856: 1653 /* search */ return this.search == null ? new Base[0] : new Base[] { this.search }; // BooleanType 1654 case -341064690: 1655 /* resource */ return this.resource == null ? new Base[0] : this.resource.toArray(new Base[this.resource.size()]); // CompartmentDefinitionResourceComponent 1656 default: 1657 return super.getProperty(hash, name, checkValid); 1658 } 1659 1660 } 1661 1662 @Override 1663 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1664 switch (hash) { 1665 case 116079: // url 1666 this.url = castToUri(value); // UriType 1667 return value; 1668 case 351608024: // version 1669 this.version = castToString(value); // StringType 1670 return value; 1671 case 3373707: // name 1672 this.name = castToString(value); // StringType 1673 return value; 1674 case -892481550: // status 1675 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 1676 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1677 return value; 1678 case -404562712: // experimental 1679 this.experimental = castToBoolean(value); // BooleanType 1680 return value; 1681 case 3076014: // date 1682 this.date = castToDateTime(value); // DateTimeType 1683 return value; 1684 case 1447404028: // publisher 1685 this.publisher = castToString(value); // StringType 1686 return value; 1687 case 951526432: // contact 1688 this.getContact().add(castToContactDetail(value)); // ContactDetail 1689 return value; 1690 case -1724546052: // description 1691 this.description = castToMarkdown(value); // MarkdownType 1692 return value; 1693 case -669707736: // useContext 1694 this.getUseContext().add(castToUsageContext(value)); // UsageContext 1695 return value; 1696 case -220463842: // purpose 1697 this.purpose = castToMarkdown(value); // MarkdownType 1698 return value; 1699 case 3059181: // code 1700 value = new CompartmentTypeEnumFactory().fromType(castToCode(value)); 1701 this.code = (Enumeration) value; // Enumeration<CompartmentType> 1702 return value; 1703 case -906336856: // search 1704 this.search = castToBoolean(value); // BooleanType 1705 return value; 1706 case -341064690: // resource 1707 this.getResource().add((CompartmentDefinitionResourceComponent) value); // CompartmentDefinitionResourceComponent 1708 return value; 1709 default: 1710 return super.setProperty(hash, name, value); 1711 } 1712 1713 } 1714 1715 @Override 1716 public Base setProperty(String name, Base value) throws FHIRException { 1717 if (name.equals("url")) { 1718 this.url = castToUri(value); // UriType 1719 } else if (name.equals("version")) { 1720 this.version = castToString(value); // StringType 1721 } else if (name.equals("name")) { 1722 this.name = castToString(value); // StringType 1723 } else if (name.equals("status")) { 1724 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 1725 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1726 } else if (name.equals("experimental")) { 1727 this.experimental = castToBoolean(value); // BooleanType 1728 } else if (name.equals("date")) { 1729 this.date = castToDateTime(value); // DateTimeType 1730 } else if (name.equals("publisher")) { 1731 this.publisher = castToString(value); // StringType 1732 } else if (name.equals("contact")) { 1733 this.getContact().add(castToContactDetail(value)); 1734 } else if (name.equals("description")) { 1735 this.description = castToMarkdown(value); // MarkdownType 1736 } else if (name.equals("useContext")) { 1737 this.getUseContext().add(castToUsageContext(value)); 1738 } else if (name.equals("purpose")) { 1739 this.purpose = castToMarkdown(value); // MarkdownType 1740 } else if (name.equals("code")) { 1741 value = new CompartmentTypeEnumFactory().fromType(castToCode(value)); 1742 this.code = (Enumeration) value; // Enumeration<CompartmentType> 1743 } else if (name.equals("search")) { 1744 this.search = castToBoolean(value); // BooleanType 1745 } else if (name.equals("resource")) { 1746 this.getResource().add((CompartmentDefinitionResourceComponent) value); 1747 } else 1748 return super.setProperty(name, value); 1749 return value; 1750 } 1751 1752 @Override 1753 public void removeChild(String name, Base value) throws FHIRException { 1754 if (name.equals("url")) { 1755 this.url = null; 1756 } else if (name.equals("version")) { 1757 this.version = null; 1758 } else if (name.equals("name")) { 1759 this.name = null; 1760 } else if (name.equals("status")) { 1761 this.status = null; 1762 } else if (name.equals("experimental")) { 1763 this.experimental = null; 1764 } else if (name.equals("date")) { 1765 this.date = null; 1766 } else if (name.equals("publisher")) { 1767 this.publisher = null; 1768 } else if (name.equals("contact")) { 1769 this.getContact().remove(castToContactDetail(value)); 1770 } else if (name.equals("description")) { 1771 this.description = null; 1772 } else if (name.equals("useContext")) { 1773 this.getUseContext().remove(castToUsageContext(value)); 1774 } else if (name.equals("purpose")) { 1775 this.purpose = null; 1776 } else if (name.equals("code")) { 1777 this.code = null; 1778 } else if (name.equals("search")) { 1779 this.search = null; 1780 } else if (name.equals("resource")) { 1781 this.getResource().remove((CompartmentDefinitionResourceComponent) value); 1782 } else 1783 super.removeChild(name, value); 1784 1785 } 1786 1787 @Override 1788 public Base makeProperty(int hash, String name) throws FHIRException { 1789 switch (hash) { 1790 case 116079: 1791 return getUrlElement(); 1792 case 351608024: 1793 return getVersionElement(); 1794 case 3373707: 1795 return getNameElement(); 1796 case -892481550: 1797 return getStatusElement(); 1798 case -404562712: 1799 return getExperimentalElement(); 1800 case 3076014: 1801 return getDateElement(); 1802 case 1447404028: 1803 return getPublisherElement(); 1804 case 951526432: 1805 return addContact(); 1806 case -1724546052: 1807 return getDescriptionElement(); 1808 case -669707736: 1809 return addUseContext(); 1810 case -220463842: 1811 return getPurposeElement(); 1812 case 3059181: 1813 return getCodeElement(); 1814 case -906336856: 1815 return getSearchElement(); 1816 case -341064690: 1817 return addResource(); 1818 default: 1819 return super.makeProperty(hash, name); 1820 } 1821 1822 } 1823 1824 @Override 1825 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1826 switch (hash) { 1827 case 116079: 1828 /* url */ return new String[] { "uri" }; 1829 case 351608024: 1830 /* version */ return new String[] { "string" }; 1831 case 3373707: 1832 /* name */ return new String[] { "string" }; 1833 case -892481550: 1834 /* status */ return new String[] { "code" }; 1835 case -404562712: 1836 /* experimental */ return new String[] { "boolean" }; 1837 case 3076014: 1838 /* date */ return new String[] { "dateTime" }; 1839 case 1447404028: 1840 /* publisher */ return new String[] { "string" }; 1841 case 951526432: 1842 /* contact */ return new String[] { "ContactDetail" }; 1843 case -1724546052: 1844 /* description */ return new String[] { "markdown" }; 1845 case -669707736: 1846 /* useContext */ return new String[] { "UsageContext" }; 1847 case -220463842: 1848 /* purpose */ return new String[] { "markdown" }; 1849 case 3059181: 1850 /* code */ return new String[] { "code" }; 1851 case -906336856: 1852 /* search */ return new String[] { "boolean" }; 1853 case -341064690: 1854 /* resource */ return new String[] {}; 1855 default: 1856 return super.getTypesForProperty(hash, name); 1857 } 1858 1859 } 1860 1861 @Override 1862 public Base addChild(String name) throws FHIRException { 1863 if (name.equals("url")) { 1864 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.url"); 1865 } else if (name.equals("version")) { 1866 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.version"); 1867 } else if (name.equals("name")) { 1868 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.name"); 1869 } else if (name.equals("status")) { 1870 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.status"); 1871 } else if (name.equals("experimental")) { 1872 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.experimental"); 1873 } else if (name.equals("date")) { 1874 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.date"); 1875 } else if (name.equals("publisher")) { 1876 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.publisher"); 1877 } else if (name.equals("contact")) { 1878 return addContact(); 1879 } else if (name.equals("description")) { 1880 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.description"); 1881 } else if (name.equals("useContext")) { 1882 return addUseContext(); 1883 } else if (name.equals("purpose")) { 1884 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.purpose"); 1885 } else if (name.equals("code")) { 1886 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.code"); 1887 } else if (name.equals("search")) { 1888 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.search"); 1889 } else if (name.equals("resource")) { 1890 return addResource(); 1891 } else 1892 return super.addChild(name); 1893 } 1894 1895 public String fhirType() { 1896 return "CompartmentDefinition"; 1897 1898 } 1899 1900 public CompartmentDefinition copy() { 1901 CompartmentDefinition dst = new CompartmentDefinition(); 1902 copyValues(dst); 1903 return dst; 1904 } 1905 1906 public void copyValues(CompartmentDefinition dst) { 1907 super.copyValues(dst); 1908 dst.url = url == null ? null : url.copy(); 1909 dst.version = version == null ? null : version.copy(); 1910 dst.name = name == null ? null : name.copy(); 1911 dst.status = status == null ? null : status.copy(); 1912 dst.experimental = experimental == null ? null : experimental.copy(); 1913 dst.date = date == null ? null : date.copy(); 1914 dst.publisher = publisher == null ? null : publisher.copy(); 1915 if (contact != null) { 1916 dst.contact = new ArrayList<ContactDetail>(); 1917 for (ContactDetail i : contact) 1918 dst.contact.add(i.copy()); 1919 } 1920 ; 1921 dst.description = description == null ? null : description.copy(); 1922 if (useContext != null) { 1923 dst.useContext = new ArrayList<UsageContext>(); 1924 for (UsageContext i : useContext) 1925 dst.useContext.add(i.copy()); 1926 } 1927 ; 1928 dst.purpose = purpose == null ? null : purpose.copy(); 1929 dst.code = code == null ? null : code.copy(); 1930 dst.search = search == null ? null : search.copy(); 1931 if (resource != null) { 1932 dst.resource = new ArrayList<CompartmentDefinitionResourceComponent>(); 1933 for (CompartmentDefinitionResourceComponent i : resource) 1934 dst.resource.add(i.copy()); 1935 } 1936 ; 1937 } 1938 1939 protected CompartmentDefinition typedCopy() { 1940 return copy(); 1941 } 1942 1943 @Override 1944 public boolean equalsDeep(Base other_) { 1945 if (!super.equalsDeep(other_)) 1946 return false; 1947 if (!(other_ instanceof CompartmentDefinition)) 1948 return false; 1949 CompartmentDefinition o = (CompartmentDefinition) other_; 1950 return compareDeep(purpose, o.purpose, true) && compareDeep(code, o.code, true) 1951 && compareDeep(search, o.search, true) && compareDeep(resource, o.resource, true); 1952 } 1953 1954 @Override 1955 public boolean equalsShallow(Base other_) { 1956 if (!super.equalsShallow(other_)) 1957 return false; 1958 if (!(other_ instanceof CompartmentDefinition)) 1959 return false; 1960 CompartmentDefinition o = (CompartmentDefinition) other_; 1961 return compareValues(purpose, o.purpose, true) && compareValues(code, o.code, true) 1962 && compareValues(search, o.search, true); 1963 } 1964 1965 public boolean isEmpty() { 1966 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(purpose, code, search, resource); 1967 } 1968 1969 @Override 1970 public ResourceType getResourceType() { 1971 return ResourceType.CompartmentDefinition; 1972 } 1973 1974 /** 1975 * Search parameter: <b>date</b> 1976 * <p> 1977 * Description: <b>The compartment definition publication date</b><br> 1978 * Type: <b>date</b><br> 1979 * Path: <b>CompartmentDefinition.date</b><br> 1980 * </p> 1981 */ 1982 @SearchParamDefinition(name = "date", path = "CompartmentDefinition.date", description = "The compartment definition publication date", type = "date") 1983 public static final String SP_DATE = "date"; 1984 /** 1985 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1986 * <p> 1987 * Description: <b>The compartment definition publication date</b><br> 1988 * Type: <b>date</b><br> 1989 * Path: <b>CompartmentDefinition.date</b><br> 1990 * </p> 1991 */ 1992 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 1993 SP_DATE); 1994 1995 /** 1996 * Search parameter: <b>code</b> 1997 * <p> 1998 * Description: <b>Patient | Encounter | RelatedPerson | Practitioner | 1999 * Device</b><br> 2000 * Type: <b>token</b><br> 2001 * Path: <b>CompartmentDefinition.code</b><br> 2002 * </p> 2003 */ 2004 @SearchParamDefinition(name = "code", path = "CompartmentDefinition.code", description = "Patient | Encounter | RelatedPerson | Practitioner | Device", type = "token") 2005 public static final String SP_CODE = "code"; 2006 /** 2007 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2008 * <p> 2009 * Description: <b>Patient | Encounter | RelatedPerson | Practitioner | 2010 * Device</b><br> 2011 * Type: <b>token</b><br> 2012 * Path: <b>CompartmentDefinition.code</b><br> 2013 * </p> 2014 */ 2015 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2016 SP_CODE); 2017 2018 /** 2019 * Search parameter: <b>context-type-value</b> 2020 * <p> 2021 * Description: <b>A use context type and value assigned to the compartment 2022 * definition</b><br> 2023 * Type: <b>composite</b><br> 2024 * Path: <b></b><br> 2025 * </p> 2026 */ 2027 @SearchParamDefinition(name = "context-type-value", path = "CompartmentDefinition.useContext", description = "A use context type and value assigned to the compartment definition", type = "composite", compositeOf = { 2028 "context-type", "context" }) 2029 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 2030 /** 2031 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 2032 * <p> 2033 * Description: <b>A use context type and value assigned to the compartment 2034 * definition</b><br> 2035 * Type: <b>composite</b><br> 2036 * Path: <b></b><br> 2037 * </p> 2038 */ 2039 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 2040 SP_CONTEXT_TYPE_VALUE); 2041 2042 /** 2043 * Search parameter: <b>resource</b> 2044 * <p> 2045 * Description: <b>Name of resource type</b><br> 2046 * Type: <b>token</b><br> 2047 * Path: <b>CompartmentDefinition.resource.code</b><br> 2048 * </p> 2049 */ 2050 @SearchParamDefinition(name = "resource", path = "CompartmentDefinition.resource.code", description = "Name of resource type", type = "token") 2051 public static final String SP_RESOURCE = "resource"; 2052 /** 2053 * <b>Fluent Client</b> search parameter constant for <b>resource</b> 2054 * <p> 2055 * Description: <b>Name of resource type</b><br> 2056 * Type: <b>token</b><br> 2057 * Path: <b>CompartmentDefinition.resource.code</b><br> 2058 * </p> 2059 */ 2060 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RESOURCE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2061 SP_RESOURCE); 2062 2063 /** 2064 * Search parameter: <b>description</b> 2065 * <p> 2066 * Description: <b>The description of the compartment definition</b><br> 2067 * Type: <b>string</b><br> 2068 * Path: <b>CompartmentDefinition.description</b><br> 2069 * </p> 2070 */ 2071 @SearchParamDefinition(name = "description", path = "CompartmentDefinition.description", description = "The description of the compartment definition", type = "string") 2072 public static final String SP_DESCRIPTION = "description"; 2073 /** 2074 * <b>Fluent Client</b> search parameter constant for <b>description</b> 2075 * <p> 2076 * Description: <b>The description of the compartment definition</b><br> 2077 * Type: <b>string</b><br> 2078 * Path: <b>CompartmentDefinition.description</b><br> 2079 * </p> 2080 */ 2081 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 2082 SP_DESCRIPTION); 2083 2084 /** 2085 * Search parameter: <b>context-type</b> 2086 * <p> 2087 * Description: <b>A type of use context assigned to the compartment 2088 * definition</b><br> 2089 * Type: <b>token</b><br> 2090 * Path: <b>CompartmentDefinition.useContext.code</b><br> 2091 * </p> 2092 */ 2093 @SearchParamDefinition(name = "context-type", path = "CompartmentDefinition.useContext.code", description = "A type of use context assigned to the compartment definition", type = "token") 2094 public static final String SP_CONTEXT_TYPE = "context-type"; 2095 /** 2096 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 2097 * <p> 2098 * Description: <b>A type of use context assigned to the compartment 2099 * definition</b><br> 2100 * Type: <b>token</b><br> 2101 * Path: <b>CompartmentDefinition.useContext.code</b><br> 2102 * </p> 2103 */ 2104 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2105 SP_CONTEXT_TYPE); 2106 2107 /** 2108 * Search parameter: <b>version</b> 2109 * <p> 2110 * Description: <b>The business version of the compartment definition</b><br> 2111 * Type: <b>token</b><br> 2112 * Path: <b>CompartmentDefinition.version</b><br> 2113 * </p> 2114 */ 2115 @SearchParamDefinition(name = "version", path = "CompartmentDefinition.version", description = "The business version of the compartment definition", type = "token") 2116 public static final String SP_VERSION = "version"; 2117 /** 2118 * <b>Fluent Client</b> search parameter constant for <b>version</b> 2119 * <p> 2120 * Description: <b>The business version of the compartment definition</b><br> 2121 * Type: <b>token</b><br> 2122 * Path: <b>CompartmentDefinition.version</b><br> 2123 * </p> 2124 */ 2125 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2126 SP_VERSION); 2127 2128 /** 2129 * Search parameter: <b>url</b> 2130 * <p> 2131 * Description: <b>The uri that identifies the compartment definition</b><br> 2132 * Type: <b>uri</b><br> 2133 * Path: <b>CompartmentDefinition.url</b><br> 2134 * </p> 2135 */ 2136 @SearchParamDefinition(name = "url", path = "CompartmentDefinition.url", description = "The uri that identifies the compartment definition", type = "uri") 2137 public static final String SP_URL = "url"; 2138 /** 2139 * <b>Fluent Client</b> search parameter constant for <b>url</b> 2140 * <p> 2141 * Description: <b>The uri that identifies the compartment definition</b><br> 2142 * Type: <b>uri</b><br> 2143 * Path: <b>CompartmentDefinition.url</b><br> 2144 * </p> 2145 */ 2146 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 2147 2148 /** 2149 * Search parameter: <b>context-quantity</b> 2150 * <p> 2151 * Description: <b>A quantity- or range-valued use context assigned to the 2152 * compartment definition</b><br> 2153 * Type: <b>quantity</b><br> 2154 * Path: <b>CompartmentDefinition.useContext.valueQuantity, 2155 * CompartmentDefinition.useContext.valueRange</b><br> 2156 * </p> 2157 */ 2158 @SearchParamDefinition(name = "context-quantity", path = "(CompartmentDefinition.useContext.value as Quantity) | (CompartmentDefinition.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the compartment definition", type = "quantity") 2159 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 2160 /** 2161 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 2162 * <p> 2163 * Description: <b>A quantity- or range-valued use context assigned to the 2164 * compartment definition</b><br> 2165 * Type: <b>quantity</b><br> 2166 * Path: <b>CompartmentDefinition.useContext.valueQuantity, 2167 * CompartmentDefinition.useContext.valueRange</b><br> 2168 * </p> 2169 */ 2170 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 2171 SP_CONTEXT_QUANTITY); 2172 2173 /** 2174 * Search parameter: <b>name</b> 2175 * <p> 2176 * Description: <b>Computationally friendly name of the compartment 2177 * definition</b><br> 2178 * Type: <b>string</b><br> 2179 * Path: <b>CompartmentDefinition.name</b><br> 2180 * </p> 2181 */ 2182 @SearchParamDefinition(name = "name", path = "CompartmentDefinition.name", description = "Computationally friendly name of the compartment definition", type = "string") 2183 public static final String SP_NAME = "name"; 2184 /** 2185 * <b>Fluent Client</b> search parameter constant for <b>name</b> 2186 * <p> 2187 * Description: <b>Computationally friendly name of the compartment 2188 * definition</b><br> 2189 * Type: <b>string</b><br> 2190 * Path: <b>CompartmentDefinition.name</b><br> 2191 * </p> 2192 */ 2193 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 2194 SP_NAME); 2195 2196 /** 2197 * Search parameter: <b>context</b> 2198 * <p> 2199 * Description: <b>A use context assigned to the compartment definition</b><br> 2200 * Type: <b>token</b><br> 2201 * Path: <b>CompartmentDefinition.useContext.valueCodeableConcept</b><br> 2202 * </p> 2203 */ 2204 @SearchParamDefinition(name = "context", path = "(CompartmentDefinition.useContext.value as CodeableConcept)", description = "A use context assigned to the compartment definition", type = "token") 2205 public static final String SP_CONTEXT = "context"; 2206 /** 2207 * <b>Fluent Client</b> search parameter constant for <b>context</b> 2208 * <p> 2209 * Description: <b>A use context assigned to the compartment definition</b><br> 2210 * Type: <b>token</b><br> 2211 * Path: <b>CompartmentDefinition.useContext.valueCodeableConcept</b><br> 2212 * </p> 2213 */ 2214 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2215 SP_CONTEXT); 2216 2217 /** 2218 * Search parameter: <b>publisher</b> 2219 * <p> 2220 * Description: <b>Name of the publisher of the compartment definition</b><br> 2221 * Type: <b>string</b><br> 2222 * Path: <b>CompartmentDefinition.publisher</b><br> 2223 * </p> 2224 */ 2225 @SearchParamDefinition(name = "publisher", path = "CompartmentDefinition.publisher", description = "Name of the publisher of the compartment definition", type = "string") 2226 public static final String SP_PUBLISHER = "publisher"; 2227 /** 2228 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 2229 * <p> 2230 * Description: <b>Name of the publisher of the compartment definition</b><br> 2231 * Type: <b>string</b><br> 2232 * Path: <b>CompartmentDefinition.publisher</b><br> 2233 * </p> 2234 */ 2235 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 2236 SP_PUBLISHER); 2237 2238 /** 2239 * Search parameter: <b>context-type-quantity</b> 2240 * <p> 2241 * Description: <b>A use context type and quantity- or range-based value 2242 * assigned to the compartment definition</b><br> 2243 * Type: <b>composite</b><br> 2244 * Path: <b></b><br> 2245 * </p> 2246 */ 2247 @SearchParamDefinition(name = "context-type-quantity", path = "CompartmentDefinition.useContext", description = "A use context type and quantity- or range-based value assigned to the compartment definition", type = "composite", compositeOf = { 2248 "context-type", "context-quantity" }) 2249 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 2250 /** 2251 * <b>Fluent Client</b> search parameter constant for 2252 * <b>context-type-quantity</b> 2253 * <p> 2254 * Description: <b>A use context type and quantity- or range-based value 2255 * assigned to the compartment definition</b><br> 2256 * Type: <b>composite</b><br> 2257 * Path: <b></b><br> 2258 * </p> 2259 */ 2260 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 2261 SP_CONTEXT_TYPE_QUANTITY); 2262 2263 /** 2264 * Search parameter: <b>status</b> 2265 * <p> 2266 * Description: <b>The current status of the compartment definition</b><br> 2267 * Type: <b>token</b><br> 2268 * Path: <b>CompartmentDefinition.status</b><br> 2269 * </p> 2270 */ 2271 @SearchParamDefinition(name = "status", path = "CompartmentDefinition.status", description = "The current status of the compartment definition", type = "token") 2272 public static final String SP_STATUS = "status"; 2273 /** 2274 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2275 * <p> 2276 * Description: <b>The current status of the compartment definition</b><br> 2277 * Type: <b>token</b><br> 2278 * Path: <b>CompartmentDefinition.status</b><br> 2279 * </p> 2280 */ 2281 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2282 SP_STATUS); 2283 2284}