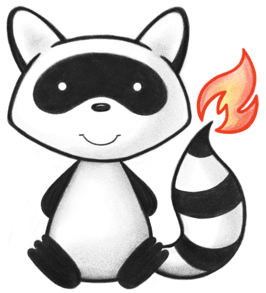
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * A set of healthcare-related information that is assembled together into a 049 * single logical package that provides a single coherent statement of meaning, 050 * establishes its own context and that has clinical attestation with regard to 051 * who is making the statement. A Composition defines the structure and 052 * narrative content necessary for a document. However, a Composition alone does 053 * not constitute a document. Rather, the Composition must be the first entry in 054 * a Bundle where Bundle.type=document, and any other resources referenced from 055 * Composition must be included as subsequent entries in the Bundle (for example 056 * Patient, Practitioner, Encounter, etc.). 057 */ 058@ResourceDef(name = "Composition", profile = "http://hl7.org/fhir/StructureDefinition/Composition") 059public class Composition extends DomainResource { 060 061 public enum CompositionStatus { 062 /** 063 * This is a preliminary composition or document (also known as initial or 064 * interim). The content may be incomplete or unverified. 065 */ 066 PRELIMINARY, 067 /** 068 * This version of the composition is complete and verified by an appropriate 069 * person and no further work is planned. Any subsequent updates would be on a 070 * new version of the composition. 071 */ 072 FINAL, 073 /** 074 * The composition content or the referenced resources have been modified 075 * (edited or added to) subsequent to being released as "final" and the 076 * composition is complete and verified by an authorized person. 077 */ 078 AMENDED, 079 /** 080 * The composition or document was originally created/issued in error, and this 081 * is an amendment that marks that the entire series should not be considered as 082 * valid. 083 */ 084 ENTEREDINERROR, 085 /** 086 * added to help the parsers with the generic types 087 */ 088 NULL; 089 090 public static CompositionStatus fromCode(String codeString) throws FHIRException { 091 if (codeString == null || "".equals(codeString)) 092 return null; 093 if ("preliminary".equals(codeString)) 094 return PRELIMINARY; 095 if ("final".equals(codeString)) 096 return FINAL; 097 if ("amended".equals(codeString)) 098 return AMENDED; 099 if ("entered-in-error".equals(codeString)) 100 return ENTEREDINERROR; 101 if (Configuration.isAcceptInvalidEnums()) 102 return null; 103 else 104 throw new FHIRException("Unknown CompositionStatus code '" + codeString + "'"); 105 } 106 107 public String toCode() { 108 switch (this) { 109 case PRELIMINARY: 110 return "preliminary"; 111 case FINAL: 112 return "final"; 113 case AMENDED: 114 return "amended"; 115 case ENTEREDINERROR: 116 return "entered-in-error"; 117 case NULL: 118 return null; 119 default: 120 return "?"; 121 } 122 } 123 124 public String getSystem() { 125 switch (this) { 126 case PRELIMINARY: 127 return "http://hl7.org/fhir/composition-status"; 128 case FINAL: 129 return "http://hl7.org/fhir/composition-status"; 130 case AMENDED: 131 return "http://hl7.org/fhir/composition-status"; 132 case ENTEREDINERROR: 133 return "http://hl7.org/fhir/composition-status"; 134 case NULL: 135 return null; 136 default: 137 return "?"; 138 } 139 } 140 141 public String getDefinition() { 142 switch (this) { 143 case PRELIMINARY: 144 return "This is a preliminary composition or document (also known as initial or interim). The content may be incomplete or unverified."; 145 case FINAL: 146 return "This version of the composition is complete and verified by an appropriate person and no further work is planned. Any subsequent updates would be on a new version of the composition."; 147 case AMENDED: 148 return "The composition content or the referenced resources have been modified (edited or added to) subsequent to being released as \"final\" and the composition is complete and verified by an authorized person."; 149 case ENTEREDINERROR: 150 return "The composition or document was originally created/issued in error, and this is an amendment that marks that the entire series should not be considered as valid."; 151 case NULL: 152 return null; 153 default: 154 return "?"; 155 } 156 } 157 158 public String getDisplay() { 159 switch (this) { 160 case PRELIMINARY: 161 return "Preliminary"; 162 case FINAL: 163 return "Final"; 164 case AMENDED: 165 return "Amended"; 166 case ENTEREDINERROR: 167 return "Entered in Error"; 168 case NULL: 169 return null; 170 default: 171 return "?"; 172 } 173 } 174 } 175 176 public static class CompositionStatusEnumFactory implements EnumFactory<CompositionStatus> { 177 public CompositionStatus fromCode(String codeString) throws IllegalArgumentException { 178 if (codeString == null || "".equals(codeString)) 179 if (codeString == null || "".equals(codeString)) 180 return null; 181 if ("preliminary".equals(codeString)) 182 return CompositionStatus.PRELIMINARY; 183 if ("final".equals(codeString)) 184 return CompositionStatus.FINAL; 185 if ("amended".equals(codeString)) 186 return CompositionStatus.AMENDED; 187 if ("entered-in-error".equals(codeString)) 188 return CompositionStatus.ENTEREDINERROR; 189 throw new IllegalArgumentException("Unknown CompositionStatus code '" + codeString + "'"); 190 } 191 192 public Enumeration<CompositionStatus> fromType(PrimitiveType<?> code) throws FHIRException { 193 if (code == null) 194 return null; 195 if (code.isEmpty()) 196 return new Enumeration<CompositionStatus>(this, CompositionStatus.NULL, code); 197 String codeString = code.asStringValue(); 198 if (codeString == null || "".equals(codeString)) 199 return new Enumeration<CompositionStatus>(this, CompositionStatus.NULL, code); 200 if ("preliminary".equals(codeString)) 201 return new Enumeration<CompositionStatus>(this, CompositionStatus.PRELIMINARY, code); 202 if ("final".equals(codeString)) 203 return new Enumeration<CompositionStatus>(this, CompositionStatus.FINAL, code); 204 if ("amended".equals(codeString)) 205 return new Enumeration<CompositionStatus>(this, CompositionStatus.AMENDED, code); 206 if ("entered-in-error".equals(codeString)) 207 return new Enumeration<CompositionStatus>(this, CompositionStatus.ENTEREDINERROR, code); 208 throw new FHIRException("Unknown CompositionStatus code '" + codeString + "'"); 209 } 210 211 public String toCode(CompositionStatus code) { 212 if (code == CompositionStatus.PRELIMINARY) 213 return "preliminary"; 214 if (code == CompositionStatus.FINAL) 215 return "final"; 216 if (code == CompositionStatus.AMENDED) 217 return "amended"; 218 if (code == CompositionStatus.ENTEREDINERROR) 219 return "entered-in-error"; 220 return "?"; 221 } 222 223 public String toSystem(CompositionStatus code) { 224 return code.getSystem(); 225 } 226 } 227 228 public enum DocumentConfidentiality { 229 /** 230 * null 231 */ 232 U, 233 /** 234 * null 235 */ 236 L, 237 /** 238 * null 239 */ 240 M, 241 /** 242 * null 243 */ 244 N, 245 /** 246 * null 247 */ 248 R, 249 /** 250 * null 251 */ 252 V, 253 /** 254 * added to help the parsers with the generic types 255 */ 256 NULL; 257 258 public static DocumentConfidentiality fromCode(String codeString) throws FHIRException { 259 if (codeString == null || "".equals(codeString)) 260 return null; 261 if ("U".equals(codeString)) 262 return U; 263 if ("L".equals(codeString)) 264 return L; 265 if ("M".equals(codeString)) 266 return M; 267 if ("N".equals(codeString)) 268 return N; 269 if ("R".equals(codeString)) 270 return R; 271 if ("V".equals(codeString)) 272 return V; 273 if (Configuration.isAcceptInvalidEnums()) 274 return null; 275 else 276 throw new FHIRException("Unknown DocumentConfidentiality code '" + codeString + "'"); 277 } 278 279 public String toCode() { 280 switch (this) { 281 case U: 282 return "U"; 283 case L: 284 return "L"; 285 case M: 286 return "M"; 287 case N: 288 return "N"; 289 case R: 290 return "R"; 291 case V: 292 return "V"; 293 case NULL: 294 return null; 295 default: 296 return "?"; 297 } 298 } 299 300 public String getSystem() { 301 switch (this) { 302 case U: 303 return "http://terminology.hl7.org/CodeSystem/v3-Confidentiality"; 304 case L: 305 return "http://terminology.hl7.org/CodeSystem/v3-Confidentiality"; 306 case M: 307 return "http://terminology.hl7.org/CodeSystem/v3-Confidentiality"; 308 case N: 309 return "http://terminology.hl7.org/CodeSystem/v3-Confidentiality"; 310 case R: 311 return "http://terminology.hl7.org/CodeSystem/v3-Confidentiality"; 312 case V: 313 return "http://terminology.hl7.org/CodeSystem/v3-Confidentiality"; 314 case NULL: 315 return null; 316 default: 317 return "?"; 318 } 319 } 320 321 public String getDefinition() { 322 switch (this) { 323 case U: 324 return ""; 325 case L: 326 return ""; 327 case M: 328 return ""; 329 case N: 330 return ""; 331 case R: 332 return ""; 333 case V: 334 return ""; 335 case NULL: 336 return null; 337 default: 338 return "?"; 339 } 340 } 341 342 public String getDisplay() { 343 switch (this) { 344 case U: 345 return "U"; 346 case L: 347 return "L"; 348 case M: 349 return "M"; 350 case N: 351 return "N"; 352 case R: 353 return "R"; 354 case V: 355 return "V"; 356 case NULL: 357 return null; 358 default: 359 return "?"; 360 } 361 } 362 } 363 364 public static class DocumentConfidentialityEnumFactory implements EnumFactory<DocumentConfidentiality> { 365 public DocumentConfidentiality fromCode(String codeString) throws IllegalArgumentException { 366 if (codeString == null || "".equals(codeString)) 367 if (codeString == null || "".equals(codeString)) 368 return null; 369 if ("U".equals(codeString)) 370 return DocumentConfidentiality.U; 371 if ("L".equals(codeString)) 372 return DocumentConfidentiality.L; 373 if ("M".equals(codeString)) 374 return DocumentConfidentiality.M; 375 if ("N".equals(codeString)) 376 return DocumentConfidentiality.N; 377 if ("R".equals(codeString)) 378 return DocumentConfidentiality.R; 379 if ("V".equals(codeString)) 380 return DocumentConfidentiality.V; 381 throw new IllegalArgumentException("Unknown DocumentConfidentiality code '" + codeString + "'"); 382 } 383 384 public Enumeration<DocumentConfidentiality> fromType(PrimitiveType<?> code) throws FHIRException { 385 if (code == null) 386 return null; 387 if (code.isEmpty()) 388 return new Enumeration<DocumentConfidentiality>(this, DocumentConfidentiality.NULL, code); 389 String codeString = code.asStringValue(); 390 if (codeString == null || "".equals(codeString)) 391 return new Enumeration<DocumentConfidentiality>(this, DocumentConfidentiality.NULL, code); 392 if ("U".equals(codeString)) 393 return new Enumeration<DocumentConfidentiality>(this, DocumentConfidentiality.U, code); 394 if ("L".equals(codeString)) 395 return new Enumeration<DocumentConfidentiality>(this, DocumentConfidentiality.L, code); 396 if ("M".equals(codeString)) 397 return new Enumeration<DocumentConfidentiality>(this, DocumentConfidentiality.M, code); 398 if ("N".equals(codeString)) 399 return new Enumeration<DocumentConfidentiality>(this, DocumentConfidentiality.N, code); 400 if ("R".equals(codeString)) 401 return new Enumeration<DocumentConfidentiality>(this, DocumentConfidentiality.R, code); 402 if ("V".equals(codeString)) 403 return new Enumeration<DocumentConfidentiality>(this, DocumentConfidentiality.V, code); 404 throw new FHIRException("Unknown DocumentConfidentiality code '" + codeString + "'"); 405 } 406 407 public String toCode(DocumentConfidentiality code) { 408 if (code == DocumentConfidentiality.U) 409 return "U"; 410 if (code == DocumentConfidentiality.L) 411 return "L"; 412 if (code == DocumentConfidentiality.M) 413 return "M"; 414 if (code == DocumentConfidentiality.N) 415 return "N"; 416 if (code == DocumentConfidentiality.R) 417 return "R"; 418 if (code == DocumentConfidentiality.V) 419 return "V"; 420 return "?"; 421 } 422 423 public String toSystem(DocumentConfidentiality code) { 424 return code.getSystem(); 425 } 426 } 427 428 public enum CompositionAttestationMode { 429 /** 430 * The person authenticated the content in their personal capacity. 431 */ 432 PERSONAL, 433 /** 434 * The person authenticated the content in their professional capacity. 435 */ 436 PROFESSIONAL, 437 /** 438 * The person authenticated the content and accepted legal responsibility for 439 * its content. 440 */ 441 LEGAL, 442 /** 443 * The organization authenticated the content as consistent with their policies 444 * and procedures. 445 */ 446 OFFICIAL, 447 /** 448 * added to help the parsers with the generic types 449 */ 450 NULL; 451 452 public static CompositionAttestationMode fromCode(String codeString) throws FHIRException { 453 if (codeString == null || "".equals(codeString)) 454 return null; 455 if ("personal".equals(codeString)) 456 return PERSONAL; 457 if ("professional".equals(codeString)) 458 return PROFESSIONAL; 459 if ("legal".equals(codeString)) 460 return LEGAL; 461 if ("official".equals(codeString)) 462 return OFFICIAL; 463 if (Configuration.isAcceptInvalidEnums()) 464 return null; 465 else 466 throw new FHIRException("Unknown CompositionAttestationMode code '" + codeString + "'"); 467 } 468 469 public String toCode() { 470 switch (this) { 471 case PERSONAL: 472 return "personal"; 473 case PROFESSIONAL: 474 return "professional"; 475 case LEGAL: 476 return "legal"; 477 case OFFICIAL: 478 return "official"; 479 case NULL: 480 return null; 481 default: 482 return "?"; 483 } 484 } 485 486 public String getSystem() { 487 switch (this) { 488 case PERSONAL: 489 return "http://hl7.org/fhir/composition-attestation-mode"; 490 case PROFESSIONAL: 491 return "http://hl7.org/fhir/composition-attestation-mode"; 492 case LEGAL: 493 return "http://hl7.org/fhir/composition-attestation-mode"; 494 case OFFICIAL: 495 return "http://hl7.org/fhir/composition-attestation-mode"; 496 case NULL: 497 return null; 498 default: 499 return "?"; 500 } 501 } 502 503 public String getDefinition() { 504 switch (this) { 505 case PERSONAL: 506 return "The person authenticated the content in their personal capacity."; 507 case PROFESSIONAL: 508 return "The person authenticated the content in their professional capacity."; 509 case LEGAL: 510 return "The person authenticated the content and accepted legal responsibility for its content."; 511 case OFFICIAL: 512 return "The organization authenticated the content as consistent with their policies and procedures."; 513 case NULL: 514 return null; 515 default: 516 return "?"; 517 } 518 } 519 520 public String getDisplay() { 521 switch (this) { 522 case PERSONAL: 523 return "Personal"; 524 case PROFESSIONAL: 525 return "Professional"; 526 case LEGAL: 527 return "Legal"; 528 case OFFICIAL: 529 return "Official"; 530 case NULL: 531 return null; 532 default: 533 return "?"; 534 } 535 } 536 } 537 538 public static class CompositionAttestationModeEnumFactory implements EnumFactory<CompositionAttestationMode> { 539 public CompositionAttestationMode fromCode(String codeString) throws IllegalArgumentException { 540 if (codeString == null || "".equals(codeString)) 541 if (codeString == null || "".equals(codeString)) 542 return null; 543 if ("personal".equals(codeString)) 544 return CompositionAttestationMode.PERSONAL; 545 if ("professional".equals(codeString)) 546 return CompositionAttestationMode.PROFESSIONAL; 547 if ("legal".equals(codeString)) 548 return CompositionAttestationMode.LEGAL; 549 if ("official".equals(codeString)) 550 return CompositionAttestationMode.OFFICIAL; 551 throw new IllegalArgumentException("Unknown CompositionAttestationMode code '" + codeString + "'"); 552 } 553 554 public Enumeration<CompositionAttestationMode> fromType(PrimitiveType<?> code) throws FHIRException { 555 if (code == null) 556 return null; 557 if (code.isEmpty()) 558 return new Enumeration<CompositionAttestationMode>(this, CompositionAttestationMode.NULL, code); 559 String codeString = code.asStringValue(); 560 if (codeString == null || "".equals(codeString)) 561 return new Enumeration<CompositionAttestationMode>(this, CompositionAttestationMode.NULL, code); 562 if ("personal".equals(codeString)) 563 return new Enumeration<CompositionAttestationMode>(this, CompositionAttestationMode.PERSONAL, code); 564 if ("professional".equals(codeString)) 565 return new Enumeration<CompositionAttestationMode>(this, CompositionAttestationMode.PROFESSIONAL, code); 566 if ("legal".equals(codeString)) 567 return new Enumeration<CompositionAttestationMode>(this, CompositionAttestationMode.LEGAL, code); 568 if ("official".equals(codeString)) 569 return new Enumeration<CompositionAttestationMode>(this, CompositionAttestationMode.OFFICIAL, code); 570 throw new FHIRException("Unknown CompositionAttestationMode code '" + codeString + "'"); 571 } 572 573 public String toCode(CompositionAttestationMode code) { 574 if (code == CompositionAttestationMode.PERSONAL) 575 return "personal"; 576 if (code == CompositionAttestationMode.PROFESSIONAL) 577 return "professional"; 578 if (code == CompositionAttestationMode.LEGAL) 579 return "legal"; 580 if (code == CompositionAttestationMode.OFFICIAL) 581 return "official"; 582 return "?"; 583 } 584 585 public String toSystem(CompositionAttestationMode code) { 586 return code.getSystem(); 587 } 588 } 589 590 public enum DocumentRelationshipType { 591 /** 592 * This document logically replaces or supersedes the target document. 593 */ 594 REPLACES, 595 /** 596 * This document was generated by transforming the target document (e.g. format 597 * or language conversion). 598 */ 599 TRANSFORMS, 600 /** 601 * This document is a signature of the target document. 602 */ 603 SIGNS, 604 /** 605 * This document adds additional information to the target document. 606 */ 607 APPENDS, 608 /** 609 * added to help the parsers with the generic types 610 */ 611 NULL; 612 613 public static DocumentRelationshipType fromCode(String codeString) throws FHIRException { 614 if (codeString == null || "".equals(codeString)) 615 return null; 616 if ("replaces".equals(codeString)) 617 return REPLACES; 618 if ("transforms".equals(codeString)) 619 return TRANSFORMS; 620 if ("signs".equals(codeString)) 621 return SIGNS; 622 if ("appends".equals(codeString)) 623 return APPENDS; 624 if (Configuration.isAcceptInvalidEnums()) 625 return null; 626 else 627 throw new FHIRException("Unknown DocumentRelationshipType code '" + codeString + "'"); 628 } 629 630 public String toCode() { 631 switch (this) { 632 case REPLACES: 633 return "replaces"; 634 case TRANSFORMS: 635 return "transforms"; 636 case SIGNS: 637 return "signs"; 638 case APPENDS: 639 return "appends"; 640 case NULL: 641 return null; 642 default: 643 return "?"; 644 } 645 } 646 647 public String getSystem() { 648 switch (this) { 649 case REPLACES: 650 return "http://hl7.org/fhir/document-relationship-type"; 651 case TRANSFORMS: 652 return "http://hl7.org/fhir/document-relationship-type"; 653 case SIGNS: 654 return "http://hl7.org/fhir/document-relationship-type"; 655 case APPENDS: 656 return "http://hl7.org/fhir/document-relationship-type"; 657 case NULL: 658 return null; 659 default: 660 return "?"; 661 } 662 } 663 664 public String getDefinition() { 665 switch (this) { 666 case REPLACES: 667 return "This document logically replaces or supersedes the target document."; 668 case TRANSFORMS: 669 return "This document was generated by transforming the target document (e.g. format or language conversion)."; 670 case SIGNS: 671 return "This document is a signature of the target document."; 672 case APPENDS: 673 return "This document adds additional information to the target document."; 674 case NULL: 675 return null; 676 default: 677 return "?"; 678 } 679 } 680 681 public String getDisplay() { 682 switch (this) { 683 case REPLACES: 684 return "Replaces"; 685 case TRANSFORMS: 686 return "Transforms"; 687 case SIGNS: 688 return "Signs"; 689 case APPENDS: 690 return "Appends"; 691 case NULL: 692 return null; 693 default: 694 return "?"; 695 } 696 } 697 } 698 699 public static class DocumentRelationshipTypeEnumFactory implements EnumFactory<DocumentRelationshipType> { 700 public DocumentRelationshipType fromCode(String codeString) throws IllegalArgumentException { 701 if (codeString == null || "".equals(codeString)) 702 if (codeString == null || "".equals(codeString)) 703 return null; 704 if ("replaces".equals(codeString)) 705 return DocumentRelationshipType.REPLACES; 706 if ("transforms".equals(codeString)) 707 return DocumentRelationshipType.TRANSFORMS; 708 if ("signs".equals(codeString)) 709 return DocumentRelationshipType.SIGNS; 710 if ("appends".equals(codeString)) 711 return DocumentRelationshipType.APPENDS; 712 throw new IllegalArgumentException("Unknown DocumentRelationshipType code '" + codeString + "'"); 713 } 714 715 public Enumeration<DocumentRelationshipType> fromType(PrimitiveType<?> code) throws FHIRException { 716 if (code == null) 717 return null; 718 if (code.isEmpty()) 719 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.NULL, code); 720 String codeString = code.asStringValue(); 721 if (codeString == null || "".equals(codeString)) 722 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.NULL, code); 723 if ("replaces".equals(codeString)) 724 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.REPLACES, code); 725 if ("transforms".equals(codeString)) 726 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.TRANSFORMS, code); 727 if ("signs".equals(codeString)) 728 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.SIGNS, code); 729 if ("appends".equals(codeString)) 730 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.APPENDS, code); 731 throw new FHIRException("Unknown DocumentRelationshipType code '" + codeString + "'"); 732 } 733 734 public String toCode(DocumentRelationshipType code) { 735 if (code == DocumentRelationshipType.REPLACES) 736 return "replaces"; 737 if (code == DocumentRelationshipType.TRANSFORMS) 738 return "transforms"; 739 if (code == DocumentRelationshipType.SIGNS) 740 return "signs"; 741 if (code == DocumentRelationshipType.APPENDS) 742 return "appends"; 743 return "?"; 744 } 745 746 public String toSystem(DocumentRelationshipType code) { 747 return code.getSystem(); 748 } 749 } 750 751 public enum SectionMode { 752 /** 753 * This list is the master list, maintained in an ongoing fashion with regular 754 * updates as the real world list it is tracking changes. 755 */ 756 WORKING, 757 /** 758 * This list was prepared as a snapshot. It should not be assumed to be current. 759 */ 760 SNAPSHOT, 761 /** 762 * A point-in-time list that shows what changes have been made or recommended. 763 * E.g. a discharge medication list showing what was added and removed during an 764 * encounter. 765 */ 766 CHANGES, 767 /** 768 * added to help the parsers with the generic types 769 */ 770 NULL; 771 772 public static SectionMode fromCode(String codeString) throws FHIRException { 773 if (codeString == null || "".equals(codeString)) 774 return null; 775 if ("working".equals(codeString)) 776 return WORKING; 777 if ("snapshot".equals(codeString)) 778 return SNAPSHOT; 779 if ("changes".equals(codeString)) 780 return CHANGES; 781 if (Configuration.isAcceptInvalidEnums()) 782 return null; 783 else 784 throw new FHIRException("Unknown SectionMode code '" + codeString + "'"); 785 } 786 787 public String toCode() { 788 switch (this) { 789 case WORKING: 790 return "working"; 791 case SNAPSHOT: 792 return "snapshot"; 793 case CHANGES: 794 return "changes"; 795 case NULL: 796 return null; 797 default: 798 return "?"; 799 } 800 } 801 802 public String getSystem() { 803 switch (this) { 804 case WORKING: 805 return "http://hl7.org/fhir/list-mode"; 806 case SNAPSHOT: 807 return "http://hl7.org/fhir/list-mode"; 808 case CHANGES: 809 return "http://hl7.org/fhir/list-mode"; 810 case NULL: 811 return null; 812 default: 813 return "?"; 814 } 815 } 816 817 public String getDefinition() { 818 switch (this) { 819 case WORKING: 820 return "This list is the master list, maintained in an ongoing fashion with regular updates as the real world list it is tracking changes."; 821 case SNAPSHOT: 822 return "This list was prepared as a snapshot. It should not be assumed to be current."; 823 case CHANGES: 824 return "A point-in-time list that shows what changes have been made or recommended. E.g. a discharge medication list showing what was added and removed during an encounter."; 825 case NULL: 826 return null; 827 default: 828 return "?"; 829 } 830 } 831 832 public String getDisplay() { 833 switch (this) { 834 case WORKING: 835 return "Working List"; 836 case SNAPSHOT: 837 return "Snapshot List"; 838 case CHANGES: 839 return "Change List"; 840 case NULL: 841 return null; 842 default: 843 return "?"; 844 } 845 } 846 } 847 848 public static class SectionModeEnumFactory implements EnumFactory<SectionMode> { 849 public SectionMode fromCode(String codeString) throws IllegalArgumentException { 850 if (codeString == null || "".equals(codeString)) 851 if (codeString == null || "".equals(codeString)) 852 return null; 853 if ("working".equals(codeString)) 854 return SectionMode.WORKING; 855 if ("snapshot".equals(codeString)) 856 return SectionMode.SNAPSHOT; 857 if ("changes".equals(codeString)) 858 return SectionMode.CHANGES; 859 throw new IllegalArgumentException("Unknown SectionMode code '" + codeString + "'"); 860 } 861 862 public Enumeration<SectionMode> fromType(PrimitiveType<?> code) throws FHIRException { 863 if (code == null) 864 return null; 865 if (code.isEmpty()) 866 return new Enumeration<SectionMode>(this, SectionMode.NULL, code); 867 String codeString = code.asStringValue(); 868 if (codeString == null || "".equals(codeString)) 869 return new Enumeration<SectionMode>(this, SectionMode.NULL, code); 870 if ("working".equals(codeString)) 871 return new Enumeration<SectionMode>(this, SectionMode.WORKING, code); 872 if ("snapshot".equals(codeString)) 873 return new Enumeration<SectionMode>(this, SectionMode.SNAPSHOT, code); 874 if ("changes".equals(codeString)) 875 return new Enumeration<SectionMode>(this, SectionMode.CHANGES, code); 876 throw new FHIRException("Unknown SectionMode code '" + codeString + "'"); 877 } 878 879 public String toCode(SectionMode code) { 880 if (code == SectionMode.WORKING) 881 return "working"; 882 if (code == SectionMode.SNAPSHOT) 883 return "snapshot"; 884 if (code == SectionMode.CHANGES) 885 return "changes"; 886 return "?"; 887 } 888 889 public String toSystem(SectionMode code) { 890 return code.getSystem(); 891 } 892 } 893 894 @Block() 895 public static class CompositionAttesterComponent extends BackboneElement implements IBaseBackboneElement { 896 /** 897 * The type of attestation the authenticator offers. 898 */ 899 @Child(name = "mode", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 900 @Description(shortDefinition = "personal | professional | legal | official", formalDefinition = "The type of attestation the authenticator offers.") 901 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/composition-attestation-mode") 902 protected Enumeration<CompositionAttestationMode> mode; 903 904 /** 905 * When the composition was attested by the party. 906 */ 907 @Child(name = "time", type = { DateTimeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 908 @Description(shortDefinition = "When the composition was attested", formalDefinition = "When the composition was attested by the party.") 909 protected DateTimeType time; 910 911 /** 912 * Who attested the composition in the specified way. 913 */ 914 @Child(name = "party", type = { Patient.class, RelatedPerson.class, Practitioner.class, PractitionerRole.class, 915 Organization.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 916 @Description(shortDefinition = "Who attested the composition", formalDefinition = "Who attested the composition in the specified way.") 917 protected Reference party; 918 919 /** 920 * The actual object that is the target of the reference (Who attested the 921 * composition in the specified way.) 922 */ 923 protected Resource partyTarget; 924 925 private static final long serialVersionUID = -1917768205L; 926 927 /** 928 * Constructor 929 */ 930 public CompositionAttesterComponent() { 931 super(); 932 } 933 934 /** 935 * Constructor 936 */ 937 public CompositionAttesterComponent(Enumeration<CompositionAttestationMode> mode) { 938 super(); 939 this.mode = mode; 940 } 941 942 /** 943 * @return {@link #mode} (The type of attestation the authenticator offers.). 944 * This is the underlying object with id, value and extensions. The 945 * accessor "getMode" gives direct access to the value 946 */ 947 public Enumeration<CompositionAttestationMode> getModeElement() { 948 if (this.mode == null) 949 if (Configuration.errorOnAutoCreate()) 950 throw new Error("Attempt to auto-create CompositionAttesterComponent.mode"); 951 else if (Configuration.doAutoCreate()) 952 this.mode = new Enumeration<CompositionAttestationMode>(new CompositionAttestationModeEnumFactory()); // bb 953 return this.mode; 954 } 955 956 public boolean hasModeElement() { 957 return this.mode != null && !this.mode.isEmpty(); 958 } 959 960 public boolean hasMode() { 961 return this.mode != null && !this.mode.isEmpty(); 962 } 963 964 /** 965 * @param value {@link #mode} (The type of attestation the authenticator 966 * offers.). This is the underlying object with id, value and 967 * extensions. The accessor "getMode" gives direct access to the 968 * value 969 */ 970 public CompositionAttesterComponent setModeElement(Enumeration<CompositionAttestationMode> value) { 971 this.mode = value; 972 return this; 973 } 974 975 /** 976 * @return The type of attestation the authenticator offers. 977 */ 978 public CompositionAttestationMode getMode() { 979 return this.mode == null ? null : this.mode.getValue(); 980 } 981 982 /** 983 * @param value The type of attestation the authenticator offers. 984 */ 985 public CompositionAttesterComponent setMode(CompositionAttestationMode value) { 986 if (this.mode == null) 987 this.mode = new Enumeration<CompositionAttestationMode>(new CompositionAttestationModeEnumFactory()); 988 this.mode.setValue(value); 989 return this; 990 } 991 992 /** 993 * @return {@link #time} (When the composition was attested by the party.). This 994 * is the underlying object with id, value and extensions. The accessor 995 * "getTime" gives direct access to the value 996 */ 997 public DateTimeType getTimeElement() { 998 if (this.time == null) 999 if (Configuration.errorOnAutoCreate()) 1000 throw new Error("Attempt to auto-create CompositionAttesterComponent.time"); 1001 else if (Configuration.doAutoCreate()) 1002 this.time = new DateTimeType(); // bb 1003 return this.time; 1004 } 1005 1006 public boolean hasTimeElement() { 1007 return this.time != null && !this.time.isEmpty(); 1008 } 1009 1010 public boolean hasTime() { 1011 return this.time != null && !this.time.isEmpty(); 1012 } 1013 1014 /** 1015 * @param value {@link #time} (When the composition was attested by the party.). 1016 * This is the underlying object with id, value and extensions. The 1017 * accessor "getTime" gives direct access to the value 1018 */ 1019 public CompositionAttesterComponent setTimeElement(DateTimeType value) { 1020 this.time = value; 1021 return this; 1022 } 1023 1024 /** 1025 * @return When the composition was attested by the party. 1026 */ 1027 public Date getTime() { 1028 return this.time == null ? null : this.time.getValue(); 1029 } 1030 1031 /** 1032 * @param value When the composition was attested by the party. 1033 */ 1034 public CompositionAttesterComponent setTime(Date value) { 1035 if (value == null) 1036 this.time = null; 1037 else { 1038 if (this.time == null) 1039 this.time = new DateTimeType(); 1040 this.time.setValue(value); 1041 } 1042 return this; 1043 } 1044 1045 /** 1046 * @return {@link #party} (Who attested the composition in the specified way.) 1047 */ 1048 public Reference getParty() { 1049 if (this.party == null) 1050 if (Configuration.errorOnAutoCreate()) 1051 throw new Error("Attempt to auto-create CompositionAttesterComponent.party"); 1052 else if (Configuration.doAutoCreate()) 1053 this.party = new Reference(); // cc 1054 return this.party; 1055 } 1056 1057 public boolean hasParty() { 1058 return this.party != null && !this.party.isEmpty(); 1059 } 1060 1061 /** 1062 * @param value {@link #party} (Who attested the composition in the specified 1063 * way.) 1064 */ 1065 public CompositionAttesterComponent setParty(Reference value) { 1066 this.party = value; 1067 return this; 1068 } 1069 1070 /** 1071 * @return {@link #party} The actual object that is the target of the reference. 1072 * The reference library doesn't populate this, but you can use it to 1073 * hold the resource if you resolve it. (Who attested the composition in 1074 * the specified way.) 1075 */ 1076 public Resource getPartyTarget() { 1077 return this.partyTarget; 1078 } 1079 1080 /** 1081 * @param value {@link #party} The actual object that is the target of the 1082 * reference. The reference library doesn't use these, but you can 1083 * use it to hold the resource if you resolve it. (Who attested the 1084 * composition in the specified way.) 1085 */ 1086 public CompositionAttesterComponent setPartyTarget(Resource value) { 1087 this.partyTarget = value; 1088 return this; 1089 } 1090 1091 protected void listChildren(List<Property> children) { 1092 super.listChildren(children); 1093 children.add(new Property("mode", "code", "The type of attestation the authenticator offers.", 0, 1, mode)); 1094 children.add(new Property("time", "dateTime", "When the composition was attested by the party.", 0, 1, time)); 1095 children.add(new Property("party", "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Organization)", 1096 "Who attested the composition in the specified way.", 0, 1, party)); 1097 } 1098 1099 @Override 1100 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1101 switch (_hash) { 1102 case 3357091: 1103 /* mode */ return new Property("mode", "code", "The type of attestation the authenticator offers.", 0, 1, mode); 1104 case 3560141: 1105 /* time */ return new Property("time", "dateTime", "When the composition was attested by the party.", 0, 1, 1106 time); 1107 case 106437350: 1108 /* party */ return new Property("party", 1109 "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Organization)", 1110 "Who attested the composition in the specified way.", 0, 1, party); 1111 default: 1112 return super.getNamedProperty(_hash, _name, _checkValid); 1113 } 1114 1115 } 1116 1117 @Override 1118 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1119 switch (hash) { 1120 case 3357091: 1121 /* mode */ return this.mode == null ? new Base[0] : new Base[] { this.mode }; // Enumeration<CompositionAttestationMode> 1122 case 3560141: 1123 /* time */ return this.time == null ? new Base[0] : new Base[] { this.time }; // DateTimeType 1124 case 106437350: 1125 /* party */ return this.party == null ? new Base[0] : new Base[] { this.party }; // Reference 1126 default: 1127 return super.getProperty(hash, name, checkValid); 1128 } 1129 1130 } 1131 1132 @Override 1133 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1134 switch (hash) { 1135 case 3357091: // mode 1136 value = new CompositionAttestationModeEnumFactory().fromType(castToCode(value)); 1137 this.mode = (Enumeration) value; // Enumeration<CompositionAttestationMode> 1138 return value; 1139 case 3560141: // time 1140 this.time = castToDateTime(value); // DateTimeType 1141 return value; 1142 case 106437350: // party 1143 this.party = castToReference(value); // Reference 1144 return value; 1145 default: 1146 return super.setProperty(hash, name, value); 1147 } 1148 1149 } 1150 1151 @Override 1152 public Base setProperty(String name, Base value) throws FHIRException { 1153 if (name.equals("mode")) { 1154 value = new CompositionAttestationModeEnumFactory().fromType(castToCode(value)); 1155 this.mode = (Enumeration) value; // Enumeration<CompositionAttestationMode> 1156 } else if (name.equals("time")) { 1157 this.time = castToDateTime(value); // DateTimeType 1158 } else if (name.equals("party")) { 1159 this.party = castToReference(value); // Reference 1160 } else 1161 return super.setProperty(name, value); 1162 return value; 1163 } 1164 1165 @Override 1166 public void removeChild(String name, Base value) throws FHIRException { 1167 if (name.equals("mode")) { 1168 this.mode = null; 1169 } else if (name.equals("time")) { 1170 this.time = null; 1171 } else if (name.equals("party")) { 1172 this.party = null; 1173 } else 1174 super.removeChild(name, value); 1175 1176 } 1177 1178 @Override 1179 public Base makeProperty(int hash, String name) throws FHIRException { 1180 switch (hash) { 1181 case 3357091: 1182 return getModeElement(); 1183 case 3560141: 1184 return getTimeElement(); 1185 case 106437350: 1186 return getParty(); 1187 default: 1188 return super.makeProperty(hash, name); 1189 } 1190 1191 } 1192 1193 @Override 1194 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1195 switch (hash) { 1196 case 3357091: 1197 /* mode */ return new String[] { "code" }; 1198 case 3560141: 1199 /* time */ return new String[] { "dateTime" }; 1200 case 106437350: 1201 /* party */ return new String[] { "Reference" }; 1202 default: 1203 return super.getTypesForProperty(hash, name); 1204 } 1205 1206 } 1207 1208 @Override 1209 public Base addChild(String name) throws FHIRException { 1210 if (name.equals("mode")) { 1211 throw new FHIRException("Cannot call addChild on a singleton property Composition.mode"); 1212 } else if (name.equals("time")) { 1213 throw new FHIRException("Cannot call addChild on a singleton property Composition.time"); 1214 } else if (name.equals("party")) { 1215 this.party = new Reference(); 1216 return this.party; 1217 } else 1218 return super.addChild(name); 1219 } 1220 1221 public CompositionAttesterComponent copy() { 1222 CompositionAttesterComponent dst = new CompositionAttesterComponent(); 1223 copyValues(dst); 1224 return dst; 1225 } 1226 1227 public void copyValues(CompositionAttesterComponent dst) { 1228 super.copyValues(dst); 1229 dst.mode = mode == null ? null : mode.copy(); 1230 dst.time = time == null ? null : time.copy(); 1231 dst.party = party == null ? null : party.copy(); 1232 } 1233 1234 @Override 1235 public boolean equalsDeep(Base other_) { 1236 if (!super.equalsDeep(other_)) 1237 return false; 1238 if (!(other_ instanceof CompositionAttesterComponent)) 1239 return false; 1240 CompositionAttesterComponent o = (CompositionAttesterComponent) other_; 1241 return compareDeep(mode, o.mode, true) && compareDeep(time, o.time, true) && compareDeep(party, o.party, true); 1242 } 1243 1244 @Override 1245 public boolean equalsShallow(Base other_) { 1246 if (!super.equalsShallow(other_)) 1247 return false; 1248 if (!(other_ instanceof CompositionAttesterComponent)) 1249 return false; 1250 CompositionAttesterComponent o = (CompositionAttesterComponent) other_; 1251 return compareValues(mode, o.mode, true) && compareValues(time, o.time, true); 1252 } 1253 1254 public boolean isEmpty() { 1255 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(mode, time, party); 1256 } 1257 1258 public String fhirType() { 1259 return "Composition.attester"; 1260 1261 } 1262 1263 } 1264 1265 @Block() 1266 public static class CompositionRelatesToComponent extends BackboneElement implements IBaseBackboneElement { 1267 /** 1268 * The type of relationship that this composition has with anther composition or 1269 * document. 1270 */ 1271 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1272 @Description(shortDefinition = "replaces | transforms | signs | appends", formalDefinition = "The type of relationship that this composition has with anther composition or document.") 1273 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/document-relationship-type") 1274 protected Enumeration<DocumentRelationshipType> code; 1275 1276 /** 1277 * The target composition/document of this relationship. 1278 */ 1279 @Child(name = "target", type = { Identifier.class, 1280 Composition.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 1281 @Description(shortDefinition = "Target of the relationship", formalDefinition = "The target composition/document of this relationship.") 1282 protected Type target; 1283 1284 private static final long serialVersionUID = 1536930280L; 1285 1286 /** 1287 * Constructor 1288 */ 1289 public CompositionRelatesToComponent() { 1290 super(); 1291 } 1292 1293 /** 1294 * Constructor 1295 */ 1296 public CompositionRelatesToComponent(Enumeration<DocumentRelationshipType> code, Type target) { 1297 super(); 1298 this.code = code; 1299 this.target = target; 1300 } 1301 1302 /** 1303 * @return {@link #code} (The type of relationship that this composition has 1304 * with anther composition or document.). This is the underlying object 1305 * with id, value and extensions. The accessor "getCode" gives direct 1306 * access to the value 1307 */ 1308 public Enumeration<DocumentRelationshipType> getCodeElement() { 1309 if (this.code == null) 1310 if (Configuration.errorOnAutoCreate()) 1311 throw new Error("Attempt to auto-create CompositionRelatesToComponent.code"); 1312 else if (Configuration.doAutoCreate()) 1313 this.code = new Enumeration<DocumentRelationshipType>(new DocumentRelationshipTypeEnumFactory()); // bb 1314 return this.code; 1315 } 1316 1317 public boolean hasCodeElement() { 1318 return this.code != null && !this.code.isEmpty(); 1319 } 1320 1321 public boolean hasCode() { 1322 return this.code != null && !this.code.isEmpty(); 1323 } 1324 1325 /** 1326 * @param value {@link #code} (The type of relationship that this composition 1327 * has with anther composition or document.). This is the 1328 * underlying object with id, value and extensions. The accessor 1329 * "getCode" gives direct access to the value 1330 */ 1331 public CompositionRelatesToComponent setCodeElement(Enumeration<DocumentRelationshipType> value) { 1332 this.code = value; 1333 return this; 1334 } 1335 1336 /** 1337 * @return The type of relationship that this composition has with anther 1338 * composition or document. 1339 */ 1340 public DocumentRelationshipType getCode() { 1341 return this.code == null ? null : this.code.getValue(); 1342 } 1343 1344 /** 1345 * @param value The type of relationship that this composition has with anther 1346 * composition or document. 1347 */ 1348 public CompositionRelatesToComponent setCode(DocumentRelationshipType value) { 1349 if (this.code == null) 1350 this.code = new Enumeration<DocumentRelationshipType>(new DocumentRelationshipTypeEnumFactory()); 1351 this.code.setValue(value); 1352 return this; 1353 } 1354 1355 /** 1356 * @return {@link #target} (The target composition/document of this 1357 * relationship.) 1358 */ 1359 public Type getTarget() { 1360 return this.target; 1361 } 1362 1363 /** 1364 * @return {@link #target} (The target composition/document of this 1365 * relationship.) 1366 */ 1367 public Identifier getTargetIdentifier() throws FHIRException { 1368 if (this.target == null) 1369 this.target = new Identifier(); 1370 if (!(this.target instanceof Identifier)) 1371 throw new FHIRException("Type mismatch: the type Identifier was expected, but " 1372 + this.target.getClass().getName() + " was encountered"); 1373 return (Identifier) this.target; 1374 } 1375 1376 public boolean hasTargetIdentifier() { 1377 return this != null && this.target instanceof Identifier; 1378 } 1379 1380 /** 1381 * @return {@link #target} (The target composition/document of this 1382 * relationship.) 1383 */ 1384 public Reference getTargetReference() throws FHIRException { 1385 if (this.target == null) 1386 this.target = new Reference(); 1387 if (!(this.target instanceof Reference)) 1388 throw new FHIRException("Type mismatch: the type Reference was expected, but " 1389 + this.target.getClass().getName() + " was encountered"); 1390 return (Reference) this.target; 1391 } 1392 1393 public boolean hasTargetReference() { 1394 return this != null && this.target instanceof Reference; 1395 } 1396 1397 public boolean hasTarget() { 1398 return this.target != null && !this.target.isEmpty(); 1399 } 1400 1401 /** 1402 * @param value {@link #target} (The target composition/document of this 1403 * relationship.) 1404 */ 1405 public CompositionRelatesToComponent setTarget(Type value) { 1406 if (value != null && !(value instanceof Identifier || value instanceof Reference)) 1407 throw new Error("Not the right type for Composition.relatesTo.target[x]: " + value.fhirType()); 1408 this.target = value; 1409 return this; 1410 } 1411 1412 protected void listChildren(List<Property> children) { 1413 super.listChildren(children); 1414 children.add(new Property("code", "code", 1415 "The type of relationship that this composition has with anther composition or document.", 0, 1, code)); 1416 children.add(new Property("target[x]", "Identifier|Reference(Composition)", 1417 "The target composition/document of this relationship.", 0, 1, target)); 1418 } 1419 1420 @Override 1421 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1422 switch (_hash) { 1423 case 3059181: 1424 /* code */ return new Property("code", "code", 1425 "The type of relationship that this composition has with anther composition or document.", 0, 1, code); 1426 case -815579825: 1427 /* target[x] */ return new Property("target[x]", "Identifier|Reference(Composition)", 1428 "The target composition/document of this relationship.", 0, 1, target); 1429 case -880905839: 1430 /* target */ return new Property("target[x]", "Identifier|Reference(Composition)", 1431 "The target composition/document of this relationship.", 0, 1, target); 1432 case 1690892570: 1433 /* targetIdentifier */ return new Property("target[x]", "Identifier|Reference(Composition)", 1434 "The target composition/document of this relationship.", 0, 1, target); 1435 case 1259806906: 1436 /* targetReference */ return new Property("target[x]", "Identifier|Reference(Composition)", 1437 "The target composition/document of this relationship.", 0, 1, target); 1438 default: 1439 return super.getNamedProperty(_hash, _name, _checkValid); 1440 } 1441 1442 } 1443 1444 @Override 1445 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1446 switch (hash) { 1447 case 3059181: 1448 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // Enumeration<DocumentRelationshipType> 1449 case -880905839: 1450 /* target */ return this.target == null ? new Base[0] : new Base[] { this.target }; // Type 1451 default: 1452 return super.getProperty(hash, name, checkValid); 1453 } 1454 1455 } 1456 1457 @Override 1458 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1459 switch (hash) { 1460 case 3059181: // code 1461 value = new DocumentRelationshipTypeEnumFactory().fromType(castToCode(value)); 1462 this.code = (Enumeration) value; // Enumeration<DocumentRelationshipType> 1463 return value; 1464 case -880905839: // target 1465 this.target = castToType(value); // Type 1466 return value; 1467 default: 1468 return super.setProperty(hash, name, value); 1469 } 1470 1471 } 1472 1473 @Override 1474 public Base setProperty(String name, Base value) throws FHIRException { 1475 if (name.equals("code")) { 1476 value = new DocumentRelationshipTypeEnumFactory().fromType(castToCode(value)); 1477 this.code = (Enumeration) value; // Enumeration<DocumentRelationshipType> 1478 } else if (name.equals("target[x]")) { 1479 this.target = castToType(value); // Type 1480 } else 1481 return super.setProperty(name, value); 1482 return value; 1483 } 1484 1485 @Override 1486 public void removeChild(String name, Base value) throws FHIRException { 1487 if (name.equals("code")) { 1488 this.code = null; 1489 } else if (name.equals("target[x]")) { 1490 this.target = null; 1491 } else 1492 super.removeChild(name, value); 1493 1494 } 1495 1496 @Override 1497 public Base makeProperty(int hash, String name) throws FHIRException { 1498 switch (hash) { 1499 case 3059181: 1500 return getCodeElement(); 1501 case -815579825: 1502 return getTarget(); 1503 case -880905839: 1504 return getTarget(); 1505 default: 1506 return super.makeProperty(hash, name); 1507 } 1508 1509 } 1510 1511 @Override 1512 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1513 switch (hash) { 1514 case 3059181: 1515 /* code */ return new String[] { "code" }; 1516 case -880905839: 1517 /* target */ return new String[] { "Identifier", "Reference" }; 1518 default: 1519 return super.getTypesForProperty(hash, name); 1520 } 1521 1522 } 1523 1524 @Override 1525 public Base addChild(String name) throws FHIRException { 1526 if (name.equals("code")) { 1527 throw new FHIRException("Cannot call addChild on a singleton property Composition.code"); 1528 } else if (name.equals("targetIdentifier")) { 1529 this.target = new Identifier(); 1530 return this.target; 1531 } else if (name.equals("targetReference")) { 1532 this.target = new Reference(); 1533 return this.target; 1534 } else 1535 return super.addChild(name); 1536 } 1537 1538 public CompositionRelatesToComponent copy() { 1539 CompositionRelatesToComponent dst = new CompositionRelatesToComponent(); 1540 copyValues(dst); 1541 return dst; 1542 } 1543 1544 public void copyValues(CompositionRelatesToComponent dst) { 1545 super.copyValues(dst); 1546 dst.code = code == null ? null : code.copy(); 1547 dst.target = target == null ? null : target.copy(); 1548 } 1549 1550 @Override 1551 public boolean equalsDeep(Base other_) { 1552 if (!super.equalsDeep(other_)) 1553 return false; 1554 if (!(other_ instanceof CompositionRelatesToComponent)) 1555 return false; 1556 CompositionRelatesToComponent o = (CompositionRelatesToComponent) other_; 1557 return compareDeep(code, o.code, true) && compareDeep(target, o.target, true); 1558 } 1559 1560 @Override 1561 public boolean equalsShallow(Base other_) { 1562 if (!super.equalsShallow(other_)) 1563 return false; 1564 if (!(other_ instanceof CompositionRelatesToComponent)) 1565 return false; 1566 CompositionRelatesToComponent o = (CompositionRelatesToComponent) other_; 1567 return compareValues(code, o.code, true); 1568 } 1569 1570 public boolean isEmpty() { 1571 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, target); 1572 } 1573 1574 public String fhirType() { 1575 return "Composition.relatesTo"; 1576 1577 } 1578 1579 } 1580 1581 @Block() 1582 public static class CompositionEventComponent extends BackboneElement implements IBaseBackboneElement { 1583 /** 1584 * This list of codes represents the main clinical acts, such as a colonoscopy 1585 * or an appendectomy, being documented. In some cases, the event is inherent in 1586 * the typeCode, such as a "History and Physical Report" in which the procedure 1587 * being documented is necessarily a "History and Physical" act. 1588 */ 1589 @Child(name = "code", type = { 1590 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1591 @Description(shortDefinition = "Code(s) that apply to the event being documented", formalDefinition = "This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the typeCode, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act.") 1592 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ActCode") 1593 protected List<CodeableConcept> code; 1594 1595 /** 1596 * The period of time covered by the documentation. There is no assertion that 1597 * the documentation is a complete representation for this period, only that it 1598 * documents events during this time. 1599 */ 1600 @Child(name = "period", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1601 @Description(shortDefinition = "The period covered by the documentation", formalDefinition = "The period of time covered by the documentation. There is no assertion that the documentation is a complete representation for this period, only that it documents events during this time.") 1602 protected Period period; 1603 1604 /** 1605 * The description and/or reference of the event(s) being documented. For 1606 * example, this could be used to document such a colonoscopy or an 1607 * appendectomy. 1608 */ 1609 @Child(name = "detail", type = { 1610 Reference.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1611 @Description(shortDefinition = "The event(s) being documented", formalDefinition = "The description and/or reference of the event(s) being documented. For example, this could be used to document such a colonoscopy or an appendectomy.") 1612 protected List<Reference> detail; 1613 /** 1614 * The actual objects that are the target of the reference (The description 1615 * and/or reference of the event(s) being documented. For example, this could be 1616 * used to document such a colonoscopy or an appendectomy.) 1617 */ 1618 protected List<Resource> detailTarget; 1619 1620 private static final long serialVersionUID = -1581379774L; 1621 1622 /** 1623 * Constructor 1624 */ 1625 public CompositionEventComponent() { 1626 super(); 1627 } 1628 1629 /** 1630 * @return {@link #code} (This list of codes represents the main clinical acts, 1631 * such as a colonoscopy or an appendectomy, being documented. In some 1632 * cases, the event is inherent in the typeCode, such as a "History and 1633 * Physical Report" in which the procedure being documented is 1634 * necessarily a "History and Physical" act.) 1635 */ 1636 public List<CodeableConcept> getCode() { 1637 if (this.code == null) 1638 this.code = new ArrayList<CodeableConcept>(); 1639 return this.code; 1640 } 1641 1642 /** 1643 * @return Returns a reference to <code>this</code> for easy method chaining 1644 */ 1645 public CompositionEventComponent setCode(List<CodeableConcept> theCode) { 1646 this.code = theCode; 1647 return this; 1648 } 1649 1650 public boolean hasCode() { 1651 if (this.code == null) 1652 return false; 1653 for (CodeableConcept item : this.code) 1654 if (!item.isEmpty()) 1655 return true; 1656 return false; 1657 } 1658 1659 public CodeableConcept addCode() { // 3 1660 CodeableConcept t = new CodeableConcept(); 1661 if (this.code == null) 1662 this.code = new ArrayList<CodeableConcept>(); 1663 this.code.add(t); 1664 return t; 1665 } 1666 1667 public CompositionEventComponent addCode(CodeableConcept t) { // 3 1668 if (t == null) 1669 return this; 1670 if (this.code == null) 1671 this.code = new ArrayList<CodeableConcept>(); 1672 this.code.add(t); 1673 return this; 1674 } 1675 1676 /** 1677 * @return The first repetition of repeating field {@link #code}, creating it if 1678 * it does not already exist 1679 */ 1680 public CodeableConcept getCodeFirstRep() { 1681 if (getCode().isEmpty()) { 1682 addCode(); 1683 } 1684 return getCode().get(0); 1685 } 1686 1687 /** 1688 * @return {@link #period} (The period of time covered by the documentation. 1689 * There is no assertion that the documentation is a complete 1690 * representation for this period, only that it documents events during 1691 * this time.) 1692 */ 1693 public Period getPeriod() { 1694 if (this.period == null) 1695 if (Configuration.errorOnAutoCreate()) 1696 throw new Error("Attempt to auto-create CompositionEventComponent.period"); 1697 else if (Configuration.doAutoCreate()) 1698 this.period = new Period(); // cc 1699 return this.period; 1700 } 1701 1702 public boolean hasPeriod() { 1703 return this.period != null && !this.period.isEmpty(); 1704 } 1705 1706 /** 1707 * @param value {@link #period} (The period of time covered by the 1708 * documentation. There is no assertion that the documentation is a 1709 * complete representation for this period, only that it documents 1710 * events during this time.) 1711 */ 1712 public CompositionEventComponent setPeriod(Period value) { 1713 this.period = value; 1714 return this; 1715 } 1716 1717 /** 1718 * @return {@link #detail} (The description and/or reference of the event(s) 1719 * being documented. For example, this could be used to document such a 1720 * colonoscopy or an appendectomy.) 1721 */ 1722 public List<Reference> getDetail() { 1723 if (this.detail == null) 1724 this.detail = new ArrayList<Reference>(); 1725 return this.detail; 1726 } 1727 1728 /** 1729 * @return Returns a reference to <code>this</code> for easy method chaining 1730 */ 1731 public CompositionEventComponent setDetail(List<Reference> theDetail) { 1732 this.detail = theDetail; 1733 return this; 1734 } 1735 1736 public boolean hasDetail() { 1737 if (this.detail == null) 1738 return false; 1739 for (Reference item : this.detail) 1740 if (!item.isEmpty()) 1741 return true; 1742 return false; 1743 } 1744 1745 public Reference addDetail() { // 3 1746 Reference t = new Reference(); 1747 if (this.detail == null) 1748 this.detail = new ArrayList<Reference>(); 1749 this.detail.add(t); 1750 return t; 1751 } 1752 1753 public CompositionEventComponent addDetail(Reference t) { // 3 1754 if (t == null) 1755 return this; 1756 if (this.detail == null) 1757 this.detail = new ArrayList<Reference>(); 1758 this.detail.add(t); 1759 return this; 1760 } 1761 1762 /** 1763 * @return The first repetition of repeating field {@link #detail}, creating it 1764 * if it does not already exist 1765 */ 1766 public Reference getDetailFirstRep() { 1767 if (getDetail().isEmpty()) { 1768 addDetail(); 1769 } 1770 return getDetail().get(0); 1771 } 1772 1773 /** 1774 * @deprecated Use Reference#setResource(IBaseResource) instead 1775 */ 1776 @Deprecated 1777 public List<Resource> getDetailTarget() { 1778 if (this.detailTarget == null) 1779 this.detailTarget = new ArrayList<Resource>(); 1780 return this.detailTarget; 1781 } 1782 1783 protected void listChildren(List<Property> children) { 1784 super.listChildren(children); 1785 children.add(new Property("code", "CodeableConcept", 1786 "This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the typeCode, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act.", 1787 0, java.lang.Integer.MAX_VALUE, code)); 1788 children.add(new Property("period", "Period", 1789 "The period of time covered by the documentation. There is no assertion that the documentation is a complete representation for this period, only that it documents events during this time.", 1790 0, 1, period)); 1791 children.add(new Property("detail", "Reference(Any)", 1792 "The description and/or reference of the event(s) being documented. For example, this could be used to document such a colonoscopy or an appendectomy.", 1793 0, java.lang.Integer.MAX_VALUE, detail)); 1794 } 1795 1796 @Override 1797 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1798 switch (_hash) { 1799 case 3059181: 1800 /* code */ return new Property("code", "CodeableConcept", 1801 "This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the typeCode, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act.", 1802 0, java.lang.Integer.MAX_VALUE, code); 1803 case -991726143: 1804 /* period */ return new Property("period", "Period", 1805 "The period of time covered by the documentation. There is no assertion that the documentation is a complete representation for this period, only that it documents events during this time.", 1806 0, 1, period); 1807 case -1335224239: 1808 /* detail */ return new Property("detail", "Reference(Any)", 1809 "The description and/or reference of the event(s) being documented. For example, this could be used to document such a colonoscopy or an appendectomy.", 1810 0, java.lang.Integer.MAX_VALUE, detail); 1811 default: 1812 return super.getNamedProperty(_hash, _name, _checkValid); 1813 } 1814 1815 } 1816 1817 @Override 1818 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1819 switch (hash) { 1820 case 3059181: 1821 /* code */ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 1822 case -991726143: 1823 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 1824 case -1335224239: 1825 /* detail */ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // Reference 1826 default: 1827 return super.getProperty(hash, name, checkValid); 1828 } 1829 1830 } 1831 1832 @Override 1833 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1834 switch (hash) { 1835 case 3059181: // code 1836 this.getCode().add(castToCodeableConcept(value)); // CodeableConcept 1837 return value; 1838 case -991726143: // period 1839 this.period = castToPeriod(value); // Period 1840 return value; 1841 case -1335224239: // detail 1842 this.getDetail().add(castToReference(value)); // Reference 1843 return value; 1844 default: 1845 return super.setProperty(hash, name, value); 1846 } 1847 1848 } 1849 1850 @Override 1851 public Base setProperty(String name, Base value) throws FHIRException { 1852 if (name.equals("code")) { 1853 this.getCode().add(castToCodeableConcept(value)); 1854 } else if (name.equals("period")) { 1855 this.period = castToPeriod(value); // Period 1856 } else if (name.equals("detail")) { 1857 this.getDetail().add(castToReference(value)); 1858 } else 1859 return super.setProperty(name, value); 1860 return value; 1861 } 1862 1863 @Override 1864 public void removeChild(String name, Base value) throws FHIRException { 1865 if (name.equals("code")) { 1866 this.getCode().remove(castToCodeableConcept(value)); 1867 } else if (name.equals("period")) { 1868 this.period = null; 1869 } else if (name.equals("detail")) { 1870 this.getDetail().remove(castToReference(value)); 1871 } else 1872 super.removeChild(name, value); 1873 1874 } 1875 1876 @Override 1877 public Base makeProperty(int hash, String name) throws FHIRException { 1878 switch (hash) { 1879 case 3059181: 1880 return addCode(); 1881 case -991726143: 1882 return getPeriod(); 1883 case -1335224239: 1884 return addDetail(); 1885 default: 1886 return super.makeProperty(hash, name); 1887 } 1888 1889 } 1890 1891 @Override 1892 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1893 switch (hash) { 1894 case 3059181: 1895 /* code */ return new String[] { "CodeableConcept" }; 1896 case -991726143: 1897 /* period */ return new String[] { "Period" }; 1898 case -1335224239: 1899 /* detail */ return new String[] { "Reference" }; 1900 default: 1901 return super.getTypesForProperty(hash, name); 1902 } 1903 1904 } 1905 1906 @Override 1907 public Base addChild(String name) throws FHIRException { 1908 if (name.equals("code")) { 1909 return addCode(); 1910 } else if (name.equals("period")) { 1911 this.period = new Period(); 1912 return this.period; 1913 } else if (name.equals("detail")) { 1914 return addDetail(); 1915 } else 1916 return super.addChild(name); 1917 } 1918 1919 public CompositionEventComponent copy() { 1920 CompositionEventComponent dst = new CompositionEventComponent(); 1921 copyValues(dst); 1922 return dst; 1923 } 1924 1925 public void copyValues(CompositionEventComponent dst) { 1926 super.copyValues(dst); 1927 if (code != null) { 1928 dst.code = new ArrayList<CodeableConcept>(); 1929 for (CodeableConcept i : code) 1930 dst.code.add(i.copy()); 1931 } 1932 ; 1933 dst.period = period == null ? null : period.copy(); 1934 if (detail != null) { 1935 dst.detail = new ArrayList<Reference>(); 1936 for (Reference i : detail) 1937 dst.detail.add(i.copy()); 1938 } 1939 ; 1940 } 1941 1942 @Override 1943 public boolean equalsDeep(Base other_) { 1944 if (!super.equalsDeep(other_)) 1945 return false; 1946 if (!(other_ instanceof CompositionEventComponent)) 1947 return false; 1948 CompositionEventComponent o = (CompositionEventComponent) other_; 1949 return compareDeep(code, o.code, true) && compareDeep(period, o.period, true) 1950 && compareDeep(detail, o.detail, true); 1951 } 1952 1953 @Override 1954 public boolean equalsShallow(Base other_) { 1955 if (!super.equalsShallow(other_)) 1956 return false; 1957 if (!(other_ instanceof CompositionEventComponent)) 1958 return false; 1959 CompositionEventComponent o = (CompositionEventComponent) other_; 1960 return true; 1961 } 1962 1963 public boolean isEmpty() { 1964 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, period, detail); 1965 } 1966 1967 public String fhirType() { 1968 return "Composition.event"; 1969 1970 } 1971 1972 } 1973 1974 @Block() 1975 public static class SectionComponent extends BackboneElement implements IBaseBackboneElement { 1976 /** 1977 * The label for this particular section. This will be part of the rendered 1978 * content for the document, and is often used to build a table of contents. 1979 */ 1980 @Child(name = "title", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1981 @Description(shortDefinition = "Label for section (e.g. for ToC)", formalDefinition = "The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents.") 1982 protected StringType title; 1983 1984 /** 1985 * A code identifying the kind of content contained within the section. This 1986 * must be consistent with the section title. 1987 */ 1988 @Child(name = "code", type = { 1989 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1990 @Description(shortDefinition = "Classification of section (recommended)", formalDefinition = "A code identifying the kind of content contained within the section. This must be consistent with the section title.") 1991 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/doc-section-codes") 1992 protected CodeableConcept code; 1993 1994 /** 1995 * Identifies who is responsible for the information in this section, not 1996 * necessarily who typed it in. 1997 */ 1998 @Child(name = "author", type = { Practitioner.class, PractitionerRole.class, Device.class, Patient.class, 1999 RelatedPerson.class, 2000 Organization.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2001 @Description(shortDefinition = "Who and/or what authored the section", formalDefinition = "Identifies who is responsible for the information in this section, not necessarily who typed it in.") 2002 protected List<Reference> author; 2003 /** 2004 * The actual objects that are the target of the reference (Identifies who is 2005 * responsible for the information in this section, not necessarily who typed it 2006 * in.) 2007 */ 2008 protected List<Resource> authorTarget; 2009 2010 /** 2011 * The actual focus of the section when it is not the subject of the 2012 * composition, but instead represents something or someone associated with the 2013 * subject such as (for a patient subject) a spouse, parent, fetus, or donor. If 2014 * not focus is specified, the focus is assumed to be focus of the parent 2015 * section, or, for a section in the Composition itself, the subject of the 2016 * composition. Sections with a focus SHALL only include resources where the 2017 * logical subject (patient, subject, focus, etc.) matches the section focus, or 2018 * the resources have no logical subject (few resources). 2019 */ 2020 @Child(name = "focus", type = { Reference.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2021 @Description(shortDefinition = "Who/what the section is about, when it is not about the subject of composition", formalDefinition = "The actual focus of the section when it is not the subject of the composition, but instead represents something or someone associated with the subject such as (for a patient subject) a spouse, parent, fetus, or donor. If not focus is specified, the focus is assumed to be focus of the parent section, or, for a section in the Composition itself, the subject of the composition. Sections with a focus SHALL only include resources where the logical subject (patient, subject, focus, etc.) matches the section focus, or the resources have no logical subject (few resources).") 2022 protected Reference focus; 2023 2024 /** 2025 * The actual object that is the target of the reference (The actual focus of 2026 * the section when it is not the subject of the composition, but instead 2027 * represents something or someone associated with the subject such as (for a 2028 * patient subject) a spouse, parent, fetus, or donor. If not focus is 2029 * specified, the focus is assumed to be focus of the parent section, or, for a 2030 * section in the Composition itself, the subject of the composition. Sections 2031 * with a focus SHALL only include resources where the logical subject (patient, 2032 * subject, focus, etc.) matches the section focus, or the resources have no 2033 * logical subject (few resources).) 2034 */ 2035 protected Resource focusTarget; 2036 2037 /** 2038 * A human-readable narrative that contains the attested content of the section, 2039 * used to represent the content of the resource to a human. The narrative need 2040 * not encode all the structured data, but is required to contain sufficient 2041 * detail to make it "clinically safe" for a human to just read the narrative. 2042 */ 2043 @Child(name = "text", type = { Narrative.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 2044 @Description(shortDefinition = "Text summary of the section, for human interpretation", formalDefinition = "A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative.") 2045 protected Narrative text; 2046 2047 /** 2048 * How the entry list was prepared - whether it is a working list that is 2049 * suitable for being maintained on an ongoing basis, or if it represents a 2050 * snapshot of a list of items from another source, or whether it is a prepared 2051 * list where items may be marked as added, modified or deleted. 2052 */ 2053 @Child(name = "mode", type = { CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 2054 @Description(shortDefinition = "working | snapshot | changes", formalDefinition = "How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.") 2055 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/list-mode") 2056 protected Enumeration<SectionMode> mode; 2057 2058 /** 2059 * Specifies the order applied to the items in the section entries. 2060 */ 2061 @Child(name = "orderedBy", type = { 2062 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 2063 @Description(shortDefinition = "Order of section entries", formalDefinition = "Specifies the order applied to the items in the section entries.") 2064 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/list-order") 2065 protected CodeableConcept orderedBy; 2066 2067 /** 2068 * A reference to the actual resource from which the narrative in the section is 2069 * derived. 2070 */ 2071 @Child(name = "entry", type = { 2072 Reference.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2073 @Description(shortDefinition = "A reference to data that supports this section", formalDefinition = "A reference to the actual resource from which the narrative in the section is derived.") 2074 protected List<Reference> entry; 2075 /** 2076 * The actual objects that are the target of the reference (A reference to the 2077 * actual resource from which the narrative in the section is derived.) 2078 */ 2079 protected List<Resource> entryTarget; 2080 2081 /** 2082 * If the section is empty, why the list is empty. An empty section typically 2083 * has some text explaining the empty reason. 2084 */ 2085 @Child(name = "emptyReason", type = { 2086 CodeableConcept.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 2087 @Description(shortDefinition = "Why the section is empty", formalDefinition = "If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason.") 2088 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/list-empty-reason") 2089 protected CodeableConcept emptyReason; 2090 2091 /** 2092 * A nested sub-section within this section. 2093 */ 2094 @Child(name = "section", type = { 2095 SectionComponent.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2096 @Description(shortDefinition = "Nested Section", formalDefinition = "A nested sub-section within this section.") 2097 protected List<SectionComponent> section; 2098 2099 private static final long serialVersionUID = -797396954L; 2100 2101 /** 2102 * Constructor 2103 */ 2104 public SectionComponent() { 2105 super(); 2106 } 2107 2108 /** 2109 * @return {@link #title} (The label for this particular section. This will be 2110 * part of the rendered content for the document, and is often used to 2111 * build a table of contents.). This is the underlying object with id, 2112 * value and extensions. The accessor "getTitle" gives direct access to 2113 * the value 2114 */ 2115 public StringType getTitleElement() { 2116 if (this.title == null) 2117 if (Configuration.errorOnAutoCreate()) 2118 throw new Error("Attempt to auto-create SectionComponent.title"); 2119 else if (Configuration.doAutoCreate()) 2120 this.title = new StringType(); // bb 2121 return this.title; 2122 } 2123 2124 public boolean hasTitleElement() { 2125 return this.title != null && !this.title.isEmpty(); 2126 } 2127 2128 public boolean hasTitle() { 2129 return this.title != null && !this.title.isEmpty(); 2130 } 2131 2132 /** 2133 * @param value {@link #title} (The label for this particular section. This will 2134 * be part of the rendered content for the document, and is often 2135 * used to build a table of contents.). This is the underlying 2136 * object with id, value and extensions. The accessor "getTitle" 2137 * gives direct access to the value 2138 */ 2139 public SectionComponent setTitleElement(StringType value) { 2140 this.title = value; 2141 return this; 2142 } 2143 2144 /** 2145 * @return The label for this particular section. This will be part of the 2146 * rendered content for the document, and is often used to build a table 2147 * of contents. 2148 */ 2149 public String getTitle() { 2150 return this.title == null ? null : this.title.getValue(); 2151 } 2152 2153 /** 2154 * @param value The label for this particular section. This will be part of the 2155 * rendered content for the document, and is often used to build a 2156 * table of contents. 2157 */ 2158 public SectionComponent setTitle(String value) { 2159 if (Utilities.noString(value)) 2160 this.title = null; 2161 else { 2162 if (this.title == null) 2163 this.title = new StringType(); 2164 this.title.setValue(value); 2165 } 2166 return this; 2167 } 2168 2169 /** 2170 * @return {@link #code} (A code identifying the kind of content contained 2171 * within the section. This must be consistent with the section title.) 2172 */ 2173 public CodeableConcept getCode() { 2174 if (this.code == null) 2175 if (Configuration.errorOnAutoCreate()) 2176 throw new Error("Attempt to auto-create SectionComponent.code"); 2177 else if (Configuration.doAutoCreate()) 2178 this.code = new CodeableConcept(); // cc 2179 return this.code; 2180 } 2181 2182 public boolean hasCode() { 2183 return this.code != null && !this.code.isEmpty(); 2184 } 2185 2186 /** 2187 * @param value {@link #code} (A code identifying the kind of content contained 2188 * within the section. This must be consistent with the section 2189 * title.) 2190 */ 2191 public SectionComponent setCode(CodeableConcept value) { 2192 this.code = value; 2193 return this; 2194 } 2195 2196 /** 2197 * @return {@link #author} (Identifies who is responsible for the information in 2198 * this section, not necessarily who typed it in.) 2199 */ 2200 public List<Reference> getAuthor() { 2201 if (this.author == null) 2202 this.author = new ArrayList<Reference>(); 2203 return this.author; 2204 } 2205 2206 /** 2207 * @return Returns a reference to <code>this</code> for easy method chaining 2208 */ 2209 public SectionComponent setAuthor(List<Reference> theAuthor) { 2210 this.author = theAuthor; 2211 return this; 2212 } 2213 2214 public boolean hasAuthor() { 2215 if (this.author == null) 2216 return false; 2217 for (Reference item : this.author) 2218 if (!item.isEmpty()) 2219 return true; 2220 return false; 2221 } 2222 2223 public Reference addAuthor() { // 3 2224 Reference t = new Reference(); 2225 if (this.author == null) 2226 this.author = new ArrayList<Reference>(); 2227 this.author.add(t); 2228 return t; 2229 } 2230 2231 public SectionComponent addAuthor(Reference t) { // 3 2232 if (t == null) 2233 return this; 2234 if (this.author == null) 2235 this.author = new ArrayList<Reference>(); 2236 this.author.add(t); 2237 return this; 2238 } 2239 2240 /** 2241 * @return The first repetition of repeating field {@link #author}, creating it 2242 * if it does not already exist 2243 */ 2244 public Reference getAuthorFirstRep() { 2245 if (getAuthor().isEmpty()) { 2246 addAuthor(); 2247 } 2248 return getAuthor().get(0); 2249 } 2250 2251 /** 2252 * @deprecated Use Reference#setResource(IBaseResource) instead 2253 */ 2254 @Deprecated 2255 public List<Resource> getAuthorTarget() { 2256 if (this.authorTarget == null) 2257 this.authorTarget = new ArrayList<Resource>(); 2258 return this.authorTarget; 2259 } 2260 2261 /** 2262 * @return {@link #focus} (The actual focus of the section when it is not the 2263 * subject of the composition, but instead represents something or 2264 * someone associated with the subject such as (for a patient subject) a 2265 * spouse, parent, fetus, or donor. If not focus is specified, the focus 2266 * is assumed to be focus of the parent section, or, for a section in 2267 * the Composition itself, the subject of the composition. Sections with 2268 * a focus SHALL only include resources where the logical subject 2269 * (patient, subject, focus, etc.) matches the section focus, or the 2270 * resources have no logical subject (few resources).) 2271 */ 2272 public Reference getFocus() { 2273 if (this.focus == null) 2274 if (Configuration.errorOnAutoCreate()) 2275 throw new Error("Attempt to auto-create SectionComponent.focus"); 2276 else if (Configuration.doAutoCreate()) 2277 this.focus = new Reference(); // cc 2278 return this.focus; 2279 } 2280 2281 public boolean hasFocus() { 2282 return this.focus != null && !this.focus.isEmpty(); 2283 } 2284 2285 /** 2286 * @param value {@link #focus} (The actual focus of the section when it is not 2287 * the subject of the composition, but instead represents something 2288 * or someone associated with the subject such as (for a patient 2289 * subject) a spouse, parent, fetus, or donor. If not focus is 2290 * specified, the focus is assumed to be focus of the parent 2291 * section, or, for a section in the Composition itself, the 2292 * subject of the composition. Sections with a focus SHALL only 2293 * include resources where the logical subject (patient, subject, 2294 * focus, etc.) matches the section focus, or the resources have no 2295 * logical subject (few resources).) 2296 */ 2297 public SectionComponent setFocus(Reference value) { 2298 this.focus = value; 2299 return this; 2300 } 2301 2302 /** 2303 * @return {@link #focus} The actual object that is the target of the reference. 2304 * The reference library doesn't populate this, but you can use it to 2305 * hold the resource if you resolve it. (The actual focus of the section 2306 * when it is not the subject of the composition, but instead represents 2307 * something or someone associated with the subject such as (for a 2308 * patient subject) a spouse, parent, fetus, or donor. If not focus is 2309 * specified, the focus is assumed to be focus of the parent section, 2310 * or, for a section in the Composition itself, the subject of the 2311 * composition. Sections with a focus SHALL only include resources where 2312 * the logical subject (patient, subject, focus, etc.) matches the 2313 * section focus, or the resources have no logical subject (few 2314 * resources).) 2315 */ 2316 public Resource getFocusTarget() { 2317 return this.focusTarget; 2318 } 2319 2320 /** 2321 * @param value {@link #focus} The actual object that is the target of the 2322 * reference. The reference library doesn't use these, but you can 2323 * use it to hold the resource if you resolve it. (The actual focus 2324 * of the section when it is not the subject of the composition, 2325 * but instead represents something or someone associated with the 2326 * subject such as (for a patient subject) a spouse, parent, fetus, 2327 * or donor. If not focus is specified, the focus is assumed to be 2328 * focus of the parent section, or, for a section in the 2329 * Composition itself, the subject of the composition. Sections 2330 * with a focus SHALL only include resources where the logical 2331 * subject (patient, subject, focus, etc.) matches the section 2332 * focus, or the resources have no logical subject (few 2333 * resources).) 2334 */ 2335 public SectionComponent setFocusTarget(Resource value) { 2336 this.focusTarget = value; 2337 return this; 2338 } 2339 2340 /** 2341 * @return {@link #text} (A human-readable narrative that contains the attested 2342 * content of the section, used to represent the content of the resource 2343 * to a human. The narrative need not encode all the structured data, 2344 * but is required to contain sufficient detail to make it "clinically 2345 * safe" for a human to just read the narrative.) 2346 */ 2347 public Narrative getText() { 2348 if (this.text == null) 2349 if (Configuration.errorOnAutoCreate()) 2350 throw new Error("Attempt to auto-create SectionComponent.text"); 2351 else if (Configuration.doAutoCreate()) 2352 this.text = new Narrative(); // cc 2353 return this.text; 2354 } 2355 2356 public boolean hasText() { 2357 return this.text != null && !this.text.isEmpty(); 2358 } 2359 2360 /** 2361 * @param value {@link #text} (A human-readable narrative that contains the 2362 * attested content of the section, used to represent the content 2363 * of the resource to a human. The narrative need not encode all 2364 * the structured data, but is required to contain sufficient 2365 * detail to make it "clinically safe" for a human to just read the 2366 * narrative.) 2367 */ 2368 public SectionComponent setText(Narrative value) { 2369 this.text = value; 2370 return this; 2371 } 2372 2373 /** 2374 * @return {@link #mode} (How the entry list was prepared - whether it is a 2375 * working list that is suitable for being maintained on an ongoing 2376 * basis, or if it represents a snapshot of a list of items from another 2377 * source, or whether it is a prepared list where items may be marked as 2378 * added, modified or deleted.). This is the underlying object with id, 2379 * value and extensions. The accessor "getMode" gives direct access to 2380 * the value 2381 */ 2382 public Enumeration<SectionMode> getModeElement() { 2383 if (this.mode == null) 2384 if (Configuration.errorOnAutoCreate()) 2385 throw new Error("Attempt to auto-create SectionComponent.mode"); 2386 else if (Configuration.doAutoCreate()) 2387 this.mode = new Enumeration<SectionMode>(new SectionModeEnumFactory()); // bb 2388 return this.mode; 2389 } 2390 2391 public boolean hasModeElement() { 2392 return this.mode != null && !this.mode.isEmpty(); 2393 } 2394 2395 public boolean hasMode() { 2396 return this.mode != null && !this.mode.isEmpty(); 2397 } 2398 2399 /** 2400 * @param value {@link #mode} (How the entry list was prepared - whether it is a 2401 * working list that is suitable for being maintained on an ongoing 2402 * basis, or if it represents a snapshot of a list of items from 2403 * another source, or whether it is a prepared list where items may 2404 * be marked as added, modified or deleted.). This is the 2405 * underlying object with id, value and extensions. The accessor 2406 * "getMode" gives direct access to the value 2407 */ 2408 public SectionComponent setModeElement(Enumeration<SectionMode> value) { 2409 this.mode = value; 2410 return this; 2411 } 2412 2413 /** 2414 * @return How the entry list was prepared - whether it is a working list that 2415 * is suitable for being maintained on an ongoing basis, or if it 2416 * represents a snapshot of a list of items from another source, or 2417 * whether it is a prepared list where items may be marked as added, 2418 * modified or deleted. 2419 */ 2420 public SectionMode getMode() { 2421 return this.mode == null ? null : this.mode.getValue(); 2422 } 2423 2424 /** 2425 * @param value How the entry list was prepared - whether it is a working list 2426 * that is suitable for being maintained on an ongoing basis, or if 2427 * it represents a snapshot of a list of items from another source, 2428 * or whether it is a prepared list where items may be marked as 2429 * added, modified or deleted. 2430 */ 2431 public SectionComponent setMode(SectionMode value) { 2432 if (value == null) 2433 this.mode = null; 2434 else { 2435 if (this.mode == null) 2436 this.mode = new Enumeration<SectionMode>(new SectionModeEnumFactory()); 2437 this.mode.setValue(value); 2438 } 2439 return this; 2440 } 2441 2442 /** 2443 * @return {@link #orderedBy} (Specifies the order applied to the items in the 2444 * section entries.) 2445 */ 2446 public CodeableConcept getOrderedBy() { 2447 if (this.orderedBy == null) 2448 if (Configuration.errorOnAutoCreate()) 2449 throw new Error("Attempt to auto-create SectionComponent.orderedBy"); 2450 else if (Configuration.doAutoCreate()) 2451 this.orderedBy = new CodeableConcept(); // cc 2452 return this.orderedBy; 2453 } 2454 2455 public boolean hasOrderedBy() { 2456 return this.orderedBy != null && !this.orderedBy.isEmpty(); 2457 } 2458 2459 /** 2460 * @param value {@link #orderedBy} (Specifies the order applied to the items in 2461 * the section entries.) 2462 */ 2463 public SectionComponent setOrderedBy(CodeableConcept value) { 2464 this.orderedBy = value; 2465 return this; 2466 } 2467 2468 /** 2469 * @return {@link #entry} (A reference to the actual resource from which the 2470 * narrative in the section is derived.) 2471 */ 2472 public List<Reference> getEntry() { 2473 if (this.entry == null) 2474 this.entry = new ArrayList<Reference>(); 2475 return this.entry; 2476 } 2477 2478 /** 2479 * @return Returns a reference to <code>this</code> for easy method chaining 2480 */ 2481 public SectionComponent setEntry(List<Reference> theEntry) { 2482 this.entry = theEntry; 2483 return this; 2484 } 2485 2486 public boolean hasEntry() { 2487 if (this.entry == null) 2488 return false; 2489 for (Reference item : this.entry) 2490 if (!item.isEmpty()) 2491 return true; 2492 return false; 2493 } 2494 2495 public Reference addEntry() { // 3 2496 Reference t = new Reference(); 2497 if (this.entry == null) 2498 this.entry = new ArrayList<Reference>(); 2499 this.entry.add(t); 2500 return t; 2501 } 2502 2503 public SectionComponent addEntry(Reference t) { // 3 2504 if (t == null) 2505 return this; 2506 if (this.entry == null) 2507 this.entry = new ArrayList<Reference>(); 2508 this.entry.add(t); 2509 return this; 2510 } 2511 2512 /** 2513 * @return The first repetition of repeating field {@link #entry}, creating it 2514 * if it does not already exist 2515 */ 2516 public Reference getEntryFirstRep() { 2517 if (getEntry().isEmpty()) { 2518 addEntry(); 2519 } 2520 return getEntry().get(0); 2521 } 2522 2523 /** 2524 * @deprecated Use Reference#setResource(IBaseResource) instead 2525 */ 2526 @Deprecated 2527 public List<Resource> getEntryTarget() { 2528 if (this.entryTarget == null) 2529 this.entryTarget = new ArrayList<Resource>(); 2530 return this.entryTarget; 2531 } 2532 2533 /** 2534 * @return {@link #emptyReason} (If the section is empty, why the list is empty. 2535 * An empty section typically has some text explaining the empty 2536 * reason.) 2537 */ 2538 public CodeableConcept getEmptyReason() { 2539 if (this.emptyReason == null) 2540 if (Configuration.errorOnAutoCreate()) 2541 throw new Error("Attempt to auto-create SectionComponent.emptyReason"); 2542 else if (Configuration.doAutoCreate()) 2543 this.emptyReason = new CodeableConcept(); // cc 2544 return this.emptyReason; 2545 } 2546 2547 public boolean hasEmptyReason() { 2548 return this.emptyReason != null && !this.emptyReason.isEmpty(); 2549 } 2550 2551 /** 2552 * @param value {@link #emptyReason} (If the section is empty, why the list is 2553 * empty. An empty section typically has some text explaining the 2554 * empty reason.) 2555 */ 2556 public SectionComponent setEmptyReason(CodeableConcept value) { 2557 this.emptyReason = value; 2558 return this; 2559 } 2560 2561 /** 2562 * @return {@link #section} (A nested sub-section within this section.) 2563 */ 2564 public List<SectionComponent> getSection() { 2565 if (this.section == null) 2566 this.section = new ArrayList<SectionComponent>(); 2567 return this.section; 2568 } 2569 2570 /** 2571 * @return Returns a reference to <code>this</code> for easy method chaining 2572 */ 2573 public SectionComponent setSection(List<SectionComponent> theSection) { 2574 this.section = theSection; 2575 return this; 2576 } 2577 2578 public boolean hasSection() { 2579 if (this.section == null) 2580 return false; 2581 for (SectionComponent item : this.section) 2582 if (!item.isEmpty()) 2583 return true; 2584 return false; 2585 } 2586 2587 public SectionComponent addSection() { // 3 2588 SectionComponent t = new SectionComponent(); 2589 if (this.section == null) 2590 this.section = new ArrayList<SectionComponent>(); 2591 this.section.add(t); 2592 return t; 2593 } 2594 2595 public SectionComponent addSection(SectionComponent t) { // 3 2596 if (t == null) 2597 return this; 2598 if (this.section == null) 2599 this.section = new ArrayList<SectionComponent>(); 2600 this.section.add(t); 2601 return this; 2602 } 2603 2604 /** 2605 * @return The first repetition of repeating field {@link #section}, creating it 2606 * if it does not already exist 2607 */ 2608 public SectionComponent getSectionFirstRep() { 2609 if (getSection().isEmpty()) { 2610 addSection(); 2611 } 2612 return getSection().get(0); 2613 } 2614 2615 protected void listChildren(List<Property> children) { 2616 super.listChildren(children); 2617 children.add(new Property("title", "string", 2618 "The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents.", 2619 0, 1, title)); 2620 children.add(new Property("code", "CodeableConcept", 2621 "A code identifying the kind of content contained within the section. This must be consistent with the section title.", 2622 0, 1, code)); 2623 children.add( 2624 new Property("author", "Reference(Practitioner|PractitionerRole|Device|Patient|RelatedPerson|Organization)", 2625 "Identifies who is responsible for the information in this section, not necessarily who typed it in.", 0, 2626 java.lang.Integer.MAX_VALUE, author)); 2627 children.add(new Property("focus", "Reference(Any)", 2628 "The actual focus of the section when it is not the subject of the composition, but instead represents something or someone associated with the subject such as (for a patient subject) a spouse, parent, fetus, or donor. If not focus is specified, the focus is assumed to be focus of the parent section, or, for a section in the Composition itself, the subject of the composition. Sections with a focus SHALL only include resources where the logical subject (patient, subject, focus, etc.) matches the section focus, or the resources have no logical subject (few resources).", 2629 0, 1, focus)); 2630 children.add(new Property("text", "Narrative", 2631 "A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative.", 2632 0, 1, text)); 2633 children.add(new Property("mode", "code", 2634 "How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.", 2635 0, 1, mode)); 2636 children.add(new Property("orderedBy", "CodeableConcept", 2637 "Specifies the order applied to the items in the section entries.", 0, 1, orderedBy)); 2638 children.add(new Property("entry", "Reference(Any)", 2639 "A reference to the actual resource from which the narrative in the section is derived.", 0, 2640 java.lang.Integer.MAX_VALUE, entry)); 2641 children.add(new Property("emptyReason", "CodeableConcept", 2642 "If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason.", 2643 0, 1, emptyReason)); 2644 children.add(new Property("section", "@Composition.section", "A nested sub-section within this section.", 0, 2645 java.lang.Integer.MAX_VALUE, section)); 2646 } 2647 2648 @Override 2649 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2650 switch (_hash) { 2651 case 110371416: 2652 /* title */ return new Property("title", "string", 2653 "The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents.", 2654 0, 1, title); 2655 case 3059181: 2656 /* code */ return new Property("code", "CodeableConcept", 2657 "A code identifying the kind of content contained within the section. This must be consistent with the section title.", 2658 0, 1, code); 2659 case -1406328437: 2660 /* author */ return new Property("author", 2661 "Reference(Practitioner|PractitionerRole|Device|Patient|RelatedPerson|Organization)", 2662 "Identifies who is responsible for the information in this section, not necessarily who typed it in.", 0, 2663 java.lang.Integer.MAX_VALUE, author); 2664 case 97604824: 2665 /* focus */ return new Property("focus", "Reference(Any)", 2666 "The actual focus of the section when it is not the subject of the composition, but instead represents something or someone associated with the subject such as (for a patient subject) a spouse, parent, fetus, or donor. If not focus is specified, the focus is assumed to be focus of the parent section, or, for a section in the Composition itself, the subject of the composition. Sections with a focus SHALL only include resources where the logical subject (patient, subject, focus, etc.) matches the section focus, or the resources have no logical subject (few resources).", 2667 0, 1, focus); 2668 case 3556653: 2669 /* text */ return new Property("text", "Narrative", 2670 "A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative.", 2671 0, 1, text); 2672 case 3357091: 2673 /* mode */ return new Property("mode", "code", 2674 "How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.", 2675 0, 1, mode); 2676 case -391079516: 2677 /* orderedBy */ return new Property("orderedBy", "CodeableConcept", 2678 "Specifies the order applied to the items in the section entries.", 0, 1, orderedBy); 2679 case 96667762: 2680 /* entry */ return new Property("entry", "Reference(Any)", 2681 "A reference to the actual resource from which the narrative in the section is derived.", 0, 2682 java.lang.Integer.MAX_VALUE, entry); 2683 case 1140135409: 2684 /* emptyReason */ return new Property("emptyReason", "CodeableConcept", 2685 "If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason.", 2686 0, 1, emptyReason); 2687 case 1970241253: 2688 /* section */ return new Property("section", "@Composition.section", 2689 "A nested sub-section within this section.", 0, java.lang.Integer.MAX_VALUE, section); 2690 default: 2691 return super.getNamedProperty(_hash, _name, _checkValid); 2692 } 2693 2694 } 2695 2696 @Override 2697 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2698 switch (hash) { 2699 case 110371416: 2700 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 2701 case 3059181: 2702 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 2703 case -1406328437: 2704 /* author */ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // Reference 2705 case 97604824: 2706 /* focus */ return this.focus == null ? new Base[0] : new Base[] { this.focus }; // Reference 2707 case 3556653: 2708 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // Narrative 2709 case 3357091: 2710 /* mode */ return this.mode == null ? new Base[0] : new Base[] { this.mode }; // Enumeration<SectionMode> 2711 case -391079516: 2712 /* orderedBy */ return this.orderedBy == null ? new Base[0] : new Base[] { this.orderedBy }; // CodeableConcept 2713 case 96667762: 2714 /* entry */ return this.entry == null ? new Base[0] : this.entry.toArray(new Base[this.entry.size()]); // Reference 2715 case 1140135409: 2716 /* emptyReason */ return this.emptyReason == null ? new Base[0] : new Base[] { this.emptyReason }; // CodeableConcept 2717 case 1970241253: 2718 /* section */ return this.section == null ? new Base[0] : this.section.toArray(new Base[this.section.size()]); // SectionComponent 2719 default: 2720 return super.getProperty(hash, name, checkValid); 2721 } 2722 2723 } 2724 2725 @Override 2726 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2727 switch (hash) { 2728 case 110371416: // title 2729 this.title = castToString(value); // StringType 2730 return value; 2731 case 3059181: // code 2732 this.code = castToCodeableConcept(value); // CodeableConcept 2733 return value; 2734 case -1406328437: // author 2735 this.getAuthor().add(castToReference(value)); // Reference 2736 return value; 2737 case 97604824: // focus 2738 this.focus = castToReference(value); // Reference 2739 return value; 2740 case 3556653: // text 2741 this.text = castToNarrative(value); // Narrative 2742 return value; 2743 case 3357091: // mode 2744 value = new SectionModeEnumFactory().fromType(castToCode(value)); 2745 this.mode = (Enumeration) value; // Enumeration<SectionMode> 2746 return value; 2747 case -391079516: // orderedBy 2748 this.orderedBy = castToCodeableConcept(value); // CodeableConcept 2749 return value; 2750 case 96667762: // entry 2751 this.getEntry().add(castToReference(value)); // Reference 2752 return value; 2753 case 1140135409: // emptyReason 2754 this.emptyReason = castToCodeableConcept(value); // CodeableConcept 2755 return value; 2756 case 1970241253: // section 2757 this.getSection().add((SectionComponent) value); // SectionComponent 2758 return value; 2759 default: 2760 return super.setProperty(hash, name, value); 2761 } 2762 2763 } 2764 2765 @Override 2766 public Base setProperty(String name, Base value) throws FHIRException { 2767 if (name.equals("title")) { 2768 this.title = castToString(value); // StringType 2769 } else if (name.equals("code")) { 2770 this.code = castToCodeableConcept(value); // CodeableConcept 2771 } else if (name.equals("author")) { 2772 this.getAuthor().add(castToReference(value)); 2773 } else if (name.equals("focus")) { 2774 this.focus = castToReference(value); // Reference 2775 } else if (name.equals("text")) { 2776 this.text = castToNarrative(value); // Narrative 2777 } else if (name.equals("mode")) { 2778 value = new SectionModeEnumFactory().fromType(castToCode(value)); 2779 this.mode = (Enumeration) value; // Enumeration<SectionMode> 2780 } else if (name.equals("orderedBy")) { 2781 this.orderedBy = castToCodeableConcept(value); // CodeableConcept 2782 } else if (name.equals("entry")) { 2783 this.getEntry().add(castToReference(value)); 2784 } else if (name.equals("emptyReason")) { 2785 this.emptyReason = castToCodeableConcept(value); // CodeableConcept 2786 } else if (name.equals("section")) { 2787 this.getSection().add((SectionComponent) value); 2788 } else 2789 return super.setProperty(name, value); 2790 return value; 2791 } 2792 2793 @Override 2794 public void removeChild(String name, Base value) throws FHIRException { 2795 if (name.equals("title")) { 2796 this.title = null; 2797 } else if (name.equals("code")) { 2798 this.code = null; 2799 } else if (name.equals("author")) { 2800 this.getAuthor().remove(castToReference(value)); 2801 } else if (name.equals("focus")) { 2802 this.focus = null; 2803 } else if (name.equals("text")) { 2804 this.text = null; 2805 } else if (name.equals("mode")) { 2806 this.mode = null; 2807 } else if (name.equals("orderedBy")) { 2808 this.orderedBy = null; 2809 } else if (name.equals("entry")) { 2810 this.getEntry().remove(castToReference(value)); 2811 } else if (name.equals("emptyReason")) { 2812 this.emptyReason = null; 2813 } else if (name.equals("section")) { 2814 this.getSection().remove((SectionComponent) value); 2815 } else 2816 super.removeChild(name, value); 2817 2818 } 2819 2820 @Override 2821 public Base makeProperty(int hash, String name) throws FHIRException { 2822 switch (hash) { 2823 case 110371416: 2824 return getTitleElement(); 2825 case 3059181: 2826 return getCode(); 2827 case -1406328437: 2828 return addAuthor(); 2829 case 97604824: 2830 return getFocus(); 2831 case 3556653: 2832 return getText(); 2833 case 3357091: 2834 return getModeElement(); 2835 case -391079516: 2836 return getOrderedBy(); 2837 case 96667762: 2838 return addEntry(); 2839 case 1140135409: 2840 return getEmptyReason(); 2841 case 1970241253: 2842 return addSection(); 2843 default: 2844 return super.makeProperty(hash, name); 2845 } 2846 2847 } 2848 2849 @Override 2850 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2851 switch (hash) { 2852 case 110371416: 2853 /* title */ return new String[] { "string" }; 2854 case 3059181: 2855 /* code */ return new String[] { "CodeableConcept" }; 2856 case -1406328437: 2857 /* author */ return new String[] { "Reference" }; 2858 case 97604824: 2859 /* focus */ return new String[] { "Reference" }; 2860 case 3556653: 2861 /* text */ return new String[] { "Narrative" }; 2862 case 3357091: 2863 /* mode */ return new String[] { "code" }; 2864 case -391079516: 2865 /* orderedBy */ return new String[] { "CodeableConcept" }; 2866 case 96667762: 2867 /* entry */ return new String[] { "Reference" }; 2868 case 1140135409: 2869 /* emptyReason */ return new String[] { "CodeableConcept" }; 2870 case 1970241253: 2871 /* section */ return new String[] { "@Composition.section" }; 2872 default: 2873 return super.getTypesForProperty(hash, name); 2874 } 2875 2876 } 2877 2878 @Override 2879 public Base addChild(String name) throws FHIRException { 2880 if (name.equals("title")) { 2881 throw new FHIRException("Cannot call addChild on a singleton property Composition.title"); 2882 } else if (name.equals("code")) { 2883 this.code = new CodeableConcept(); 2884 return this.code; 2885 } else if (name.equals("author")) { 2886 return addAuthor(); 2887 } else if (name.equals("focus")) { 2888 this.focus = new Reference(); 2889 return this.focus; 2890 } else if (name.equals("text")) { 2891 this.text = new Narrative(); 2892 return this.text; 2893 } else if (name.equals("mode")) { 2894 throw new FHIRException("Cannot call addChild on a singleton property Composition.mode"); 2895 } else if (name.equals("orderedBy")) { 2896 this.orderedBy = new CodeableConcept(); 2897 return this.orderedBy; 2898 } else if (name.equals("entry")) { 2899 return addEntry(); 2900 } else if (name.equals("emptyReason")) { 2901 this.emptyReason = new CodeableConcept(); 2902 return this.emptyReason; 2903 } else if (name.equals("section")) { 2904 return addSection(); 2905 } else 2906 return super.addChild(name); 2907 } 2908 2909 public SectionComponent copy() { 2910 SectionComponent dst = new SectionComponent(); 2911 copyValues(dst); 2912 return dst; 2913 } 2914 2915 public void copyValues(SectionComponent dst) { 2916 super.copyValues(dst); 2917 dst.title = title == null ? null : title.copy(); 2918 dst.code = code == null ? null : code.copy(); 2919 if (author != null) { 2920 dst.author = new ArrayList<Reference>(); 2921 for (Reference i : author) 2922 dst.author.add(i.copy()); 2923 } 2924 ; 2925 dst.focus = focus == null ? null : focus.copy(); 2926 dst.text = text == null ? null : text.copy(); 2927 dst.mode = mode == null ? null : mode.copy(); 2928 dst.orderedBy = orderedBy == null ? null : orderedBy.copy(); 2929 if (entry != null) { 2930 dst.entry = new ArrayList<Reference>(); 2931 for (Reference i : entry) 2932 dst.entry.add(i.copy()); 2933 } 2934 ; 2935 dst.emptyReason = emptyReason == null ? null : emptyReason.copy(); 2936 if (section != null) { 2937 dst.section = new ArrayList<SectionComponent>(); 2938 for (SectionComponent i : section) 2939 dst.section.add(i.copy()); 2940 } 2941 ; 2942 } 2943 2944 @Override 2945 public boolean equalsDeep(Base other_) { 2946 if (!super.equalsDeep(other_)) 2947 return false; 2948 if (!(other_ instanceof SectionComponent)) 2949 return false; 2950 SectionComponent o = (SectionComponent) other_; 2951 return compareDeep(title, o.title, true) && compareDeep(code, o.code, true) && compareDeep(author, o.author, true) 2952 && compareDeep(focus, o.focus, true) && compareDeep(text, o.text, true) && compareDeep(mode, o.mode, true) 2953 && compareDeep(orderedBy, o.orderedBy, true) && compareDeep(entry, o.entry, true) 2954 && compareDeep(emptyReason, o.emptyReason, true) && compareDeep(section, o.section, true); 2955 } 2956 2957 @Override 2958 public boolean equalsShallow(Base other_) { 2959 if (!super.equalsShallow(other_)) 2960 return false; 2961 if (!(other_ instanceof SectionComponent)) 2962 return false; 2963 SectionComponent o = (SectionComponent) other_; 2964 return compareValues(title, o.title, true) && compareValues(mode, o.mode, true); 2965 } 2966 2967 public boolean isEmpty() { 2968 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(title, code, author, focus, text, mode, orderedBy, 2969 entry, emptyReason, section); 2970 } 2971 2972 public String fhirType() { 2973 return "Composition.section"; 2974 2975 } 2976 2977 } 2978 2979 /** 2980 * A version-independent identifier for the Composition. This identifier stays 2981 * constant as the composition is changed over time. 2982 */ 2983 @Child(name = "identifier", type = { 2984 Identifier.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 2985 @Description(shortDefinition = "Version-independent identifier for the Composition", formalDefinition = "A version-independent identifier for the Composition. This identifier stays constant as the composition is changed over time.") 2986 protected Identifier identifier; 2987 2988 /** 2989 * The workflow/clinical status of this composition. The status is a marker for 2990 * the clinical standing of the document. 2991 */ 2992 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 2993 @Description(shortDefinition = "preliminary | final | amended | entered-in-error", formalDefinition = "The workflow/clinical status of this composition. The status is a marker for the clinical standing of the document.") 2994 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/composition-status") 2995 protected Enumeration<CompositionStatus> status; 2996 2997 /** 2998 * Specifies the particular kind of composition (e.g. History and Physical, 2999 * Discharge Summary, Progress Note). This usually equates to the purpose of 3000 * making the composition. 3001 */ 3002 @Child(name = "type", type = { CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 3003 @Description(shortDefinition = "Kind of composition (LOINC if possible)", formalDefinition = "Specifies the particular kind of composition (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the composition.") 3004 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/doc-typecodes") 3005 protected CodeableConcept type; 3006 3007 /** 3008 * A categorization for the type of the composition - helps for indexing and 3009 * searching. This may be implied by or derived from the code specified in the 3010 * Composition Type. 3011 */ 3012 @Child(name = "category", type = { 3013 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3014 @Description(shortDefinition = "Categorization of Composition", formalDefinition = "A categorization for the type of the composition - helps for indexing and searching. This may be implied by or derived from the code specified in the Composition Type.") 3015 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/document-classcodes") 3016 protected List<CodeableConcept> category; 3017 3018 /** 3019 * Who or what the composition is about. The composition can be about a person, 3020 * (patient or healthcare practitioner), a device (e.g. a machine) or even a 3021 * group of subjects (such as a document about a herd of livestock, or a set of 3022 * patients that share a common exposure). 3023 */ 3024 @Child(name = "subject", type = { Reference.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 3025 @Description(shortDefinition = "Who and/or what the composition is about", formalDefinition = "Who or what the composition is about. The composition can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of livestock, or a set of patients that share a common exposure).") 3026 protected Reference subject; 3027 3028 /** 3029 * The actual object that is the target of the reference (Who or what the 3030 * composition is about. The composition can be about a person, (patient or 3031 * healthcare practitioner), a device (e.g. a machine) or even a group of 3032 * subjects (such as a document about a herd of livestock, or a set of patients 3033 * that share a common exposure).) 3034 */ 3035 protected Resource subjectTarget; 3036 3037 /** 3038 * Describes the clinical encounter or type of care this documentation is 3039 * associated with. 3040 */ 3041 @Child(name = "encounter", type = { Encounter.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 3042 @Description(shortDefinition = "Context of the Composition", formalDefinition = "Describes the clinical encounter or type of care this documentation is associated with.") 3043 protected Reference encounter; 3044 3045 /** 3046 * The actual object that is the target of the reference (Describes the clinical 3047 * encounter or type of care this documentation is associated with.) 3048 */ 3049 protected Encounter encounterTarget; 3050 3051 /** 3052 * The composition editing time, when the composition was last logically changed 3053 * by the author. 3054 */ 3055 @Child(name = "date", type = { DateTimeType.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 3056 @Description(shortDefinition = "Composition editing time", formalDefinition = "The composition editing time, when the composition was last logically changed by the author.") 3057 protected DateTimeType date; 3058 3059 /** 3060 * Identifies who is responsible for the information in the composition, not 3061 * necessarily who typed it in. 3062 */ 3063 @Child(name = "author", type = { Practitioner.class, PractitionerRole.class, Device.class, Patient.class, 3064 RelatedPerson.class, 3065 Organization.class }, order = 7, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3066 @Description(shortDefinition = "Who and/or what authored the composition", formalDefinition = "Identifies who is responsible for the information in the composition, not necessarily who typed it in.") 3067 protected List<Reference> author; 3068 /** 3069 * The actual objects that are the target of the reference (Identifies who is 3070 * responsible for the information in the composition, not necessarily who typed 3071 * it in.) 3072 */ 3073 protected List<Resource> authorTarget; 3074 3075 /** 3076 * Official human-readable label for the composition. 3077 */ 3078 @Child(name = "title", type = { StringType.class }, order = 8, min = 1, max = 1, modifier = false, summary = true) 3079 @Description(shortDefinition = "Human Readable name/title", formalDefinition = "Official human-readable label for the composition.") 3080 protected StringType title; 3081 3082 /** 3083 * The code specifying the level of confidentiality of the Composition. 3084 */ 3085 @Child(name = "confidentiality", type = { 3086 CodeType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 3087 @Description(shortDefinition = "As defined by affinity domain", formalDefinition = "The code specifying the level of confidentiality of the Composition.") 3088 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ConfidentialityClassification") 3089 protected Enumeration<DocumentConfidentiality> confidentiality; 3090 3091 /** 3092 * A participant who has attested to the accuracy of the composition/document. 3093 */ 3094 @Child(name = "attester", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3095 @Description(shortDefinition = "Attests to accuracy of composition", formalDefinition = "A participant who has attested to the accuracy of the composition/document.") 3096 protected List<CompositionAttesterComponent> attester; 3097 3098 /** 3099 * Identifies the organization or group who is responsible for ongoing 3100 * maintenance of and access to the composition/document information. 3101 */ 3102 @Child(name = "custodian", type = { 3103 Organization.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 3104 @Description(shortDefinition = "Organization which maintains the composition", formalDefinition = "Identifies the organization or group who is responsible for ongoing maintenance of and access to the composition/document information.") 3105 protected Reference custodian; 3106 3107 /** 3108 * The actual object that is the target of the reference (Identifies the 3109 * organization or group who is responsible for ongoing maintenance of and 3110 * access to the composition/document information.) 3111 */ 3112 protected Organization custodianTarget; 3113 3114 /** 3115 * Relationships that this composition has with other compositions or documents 3116 * that already exist. 3117 */ 3118 @Child(name = "relatesTo", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3119 @Description(shortDefinition = "Relationships to other compositions/documents", formalDefinition = "Relationships that this composition has with other compositions or documents that already exist.") 3120 protected List<CompositionRelatesToComponent> relatesTo; 3121 3122 /** 3123 * The clinical service, such as a colonoscopy or an appendectomy, being 3124 * documented. 3125 */ 3126 @Child(name = "event", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3127 @Description(shortDefinition = "The clinical service(s) being documented", formalDefinition = "The clinical service, such as a colonoscopy or an appendectomy, being documented.") 3128 protected List<CompositionEventComponent> event; 3129 3130 /** 3131 * The root of the sections that make up the composition. 3132 */ 3133 @Child(name = "section", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3134 @Description(shortDefinition = "Composition is broken into sections", formalDefinition = "The root of the sections that make up the composition.") 3135 protected List<SectionComponent> section; 3136 3137 private static final long serialVersionUID = -1490206663L; 3138 3139 /** 3140 * Constructor 3141 */ 3142 public Composition() { 3143 super(); 3144 } 3145 3146 /** 3147 * Constructor 3148 */ 3149 public Composition(Enumeration<CompositionStatus> status, CodeableConcept type, DateTimeType date, StringType title) { 3150 super(); 3151 this.status = status; 3152 this.type = type; 3153 this.date = date; 3154 this.title = title; 3155 } 3156 3157 /** 3158 * @return {@link #identifier} (A version-independent identifier for the 3159 * Composition. This identifier stays constant as the composition is 3160 * changed over time.) 3161 */ 3162 public Identifier getIdentifier() { 3163 if (this.identifier == null) 3164 if (Configuration.errorOnAutoCreate()) 3165 throw new Error("Attempt to auto-create Composition.identifier"); 3166 else if (Configuration.doAutoCreate()) 3167 this.identifier = new Identifier(); // cc 3168 return this.identifier; 3169 } 3170 3171 public boolean hasIdentifier() { 3172 return this.identifier != null && !this.identifier.isEmpty(); 3173 } 3174 3175 /** 3176 * @param value {@link #identifier} (A version-independent identifier for the 3177 * Composition. This identifier stays constant as the composition 3178 * is changed over time.) 3179 */ 3180 public Composition setIdentifier(Identifier value) { 3181 this.identifier = value; 3182 return this; 3183 } 3184 3185 /** 3186 * @return {@link #status} (The workflow/clinical status of this composition. 3187 * The status is a marker for the clinical standing of the document.). 3188 * This is the underlying object with id, value and extensions. The 3189 * accessor "getStatus" gives direct access to the value 3190 */ 3191 public Enumeration<CompositionStatus> getStatusElement() { 3192 if (this.status == null) 3193 if (Configuration.errorOnAutoCreate()) 3194 throw new Error("Attempt to auto-create Composition.status"); 3195 else if (Configuration.doAutoCreate()) 3196 this.status = new Enumeration<CompositionStatus>(new CompositionStatusEnumFactory()); // bb 3197 return this.status; 3198 } 3199 3200 public boolean hasStatusElement() { 3201 return this.status != null && !this.status.isEmpty(); 3202 } 3203 3204 public boolean hasStatus() { 3205 return this.status != null && !this.status.isEmpty(); 3206 } 3207 3208 /** 3209 * @param value {@link #status} (The workflow/clinical status of this 3210 * composition. The status is a marker for the clinical standing of 3211 * the document.). This is the underlying object with id, value and 3212 * extensions. The accessor "getStatus" gives direct access to the 3213 * value 3214 */ 3215 public Composition setStatusElement(Enumeration<CompositionStatus> value) { 3216 this.status = value; 3217 return this; 3218 } 3219 3220 /** 3221 * @return The workflow/clinical status of this composition. The status is a 3222 * marker for the clinical standing of the document. 3223 */ 3224 public CompositionStatus getStatus() { 3225 return this.status == null ? null : this.status.getValue(); 3226 } 3227 3228 /** 3229 * @param value The workflow/clinical status of this composition. The status is 3230 * a marker for the clinical standing of the document. 3231 */ 3232 public Composition setStatus(CompositionStatus value) { 3233 if (this.status == null) 3234 this.status = new Enumeration<CompositionStatus>(new CompositionStatusEnumFactory()); 3235 this.status.setValue(value); 3236 return this; 3237 } 3238 3239 /** 3240 * @return {@link #type} (Specifies the particular kind of composition (e.g. 3241 * History and Physical, Discharge Summary, Progress Note). This usually 3242 * equates to the purpose of making the composition.) 3243 */ 3244 public CodeableConcept getType() { 3245 if (this.type == null) 3246 if (Configuration.errorOnAutoCreate()) 3247 throw new Error("Attempt to auto-create Composition.type"); 3248 else if (Configuration.doAutoCreate()) 3249 this.type = new CodeableConcept(); // cc 3250 return this.type; 3251 } 3252 3253 public boolean hasType() { 3254 return this.type != null && !this.type.isEmpty(); 3255 } 3256 3257 /** 3258 * @param value {@link #type} (Specifies the particular kind of composition 3259 * (e.g. History and Physical, Discharge Summary, Progress Note). 3260 * This usually equates to the purpose of making the composition.) 3261 */ 3262 public Composition setType(CodeableConcept value) { 3263 this.type = value; 3264 return this; 3265 } 3266 3267 /** 3268 * @return {@link #category} (A categorization for the type of the composition - 3269 * helps for indexing and searching. This may be implied by or derived 3270 * from the code specified in the Composition Type.) 3271 */ 3272 public List<CodeableConcept> getCategory() { 3273 if (this.category == null) 3274 this.category = new ArrayList<CodeableConcept>(); 3275 return this.category; 3276 } 3277 3278 /** 3279 * @return Returns a reference to <code>this</code> for easy method chaining 3280 */ 3281 public Composition setCategory(List<CodeableConcept> theCategory) { 3282 this.category = theCategory; 3283 return this; 3284 } 3285 3286 public boolean hasCategory() { 3287 if (this.category == null) 3288 return false; 3289 for (CodeableConcept item : this.category) 3290 if (!item.isEmpty()) 3291 return true; 3292 return false; 3293 } 3294 3295 public CodeableConcept addCategory() { // 3 3296 CodeableConcept t = new CodeableConcept(); 3297 if (this.category == null) 3298 this.category = new ArrayList<CodeableConcept>(); 3299 this.category.add(t); 3300 return t; 3301 } 3302 3303 public Composition addCategory(CodeableConcept t) { // 3 3304 if (t == null) 3305 return this; 3306 if (this.category == null) 3307 this.category = new ArrayList<CodeableConcept>(); 3308 this.category.add(t); 3309 return this; 3310 } 3311 3312 /** 3313 * @return The first repetition of repeating field {@link #category}, creating 3314 * it if it does not already exist 3315 */ 3316 public CodeableConcept getCategoryFirstRep() { 3317 if (getCategory().isEmpty()) { 3318 addCategory(); 3319 } 3320 return getCategory().get(0); 3321 } 3322 3323 /** 3324 * @return {@link #subject} (Who or what the composition is about. The 3325 * composition can be about a person, (patient or healthcare 3326 * practitioner), a device (e.g. a machine) or even a group of subjects 3327 * (such as a document about a herd of livestock, or a set of patients 3328 * that share a common exposure).) 3329 */ 3330 public Reference getSubject() { 3331 if (this.subject == null) 3332 if (Configuration.errorOnAutoCreate()) 3333 throw new Error("Attempt to auto-create Composition.subject"); 3334 else if (Configuration.doAutoCreate()) 3335 this.subject = new Reference(); // cc 3336 return this.subject; 3337 } 3338 3339 public boolean hasSubject() { 3340 return this.subject != null && !this.subject.isEmpty(); 3341 } 3342 3343 /** 3344 * @param value {@link #subject} (Who or what the composition is about. The 3345 * composition can be about a person, (patient or healthcare 3346 * practitioner), a device (e.g. a machine) or even a group of 3347 * subjects (such as a document about a herd of livestock, or a set 3348 * of patients that share a common exposure).) 3349 */ 3350 public Composition setSubject(Reference value) { 3351 this.subject = value; 3352 return this; 3353 } 3354 3355 /** 3356 * @return {@link #subject} The actual object that is the target of the 3357 * reference. The reference library doesn't populate this, but you can 3358 * use it to hold the resource if you resolve it. (Who or what the 3359 * composition is about. The composition can be about a person, (patient 3360 * or healthcare practitioner), a device (e.g. a machine) or even a 3361 * group of subjects (such as a document about a herd of livestock, or a 3362 * set of patients that share a common exposure).) 3363 */ 3364 public Resource getSubjectTarget() { 3365 return this.subjectTarget; 3366 } 3367 3368 /** 3369 * @param value {@link #subject} The actual object that is the target of the 3370 * reference. The reference library doesn't use these, but you can 3371 * use it to hold the resource if you resolve it. (Who or what the 3372 * composition is about. The composition can be about a person, 3373 * (patient or healthcare practitioner), a device (e.g. a machine) 3374 * or even a group of subjects (such as a document about a herd of 3375 * livestock, or a set of patients that share a common exposure).) 3376 */ 3377 public Composition setSubjectTarget(Resource value) { 3378 this.subjectTarget = value; 3379 return this; 3380 } 3381 3382 /** 3383 * @return {@link #encounter} (Describes the clinical encounter or type of care 3384 * this documentation is associated with.) 3385 */ 3386 public Reference getEncounter() { 3387 if (this.encounter == null) 3388 if (Configuration.errorOnAutoCreate()) 3389 throw new Error("Attempt to auto-create Composition.encounter"); 3390 else if (Configuration.doAutoCreate()) 3391 this.encounter = new Reference(); // cc 3392 return this.encounter; 3393 } 3394 3395 public boolean hasEncounter() { 3396 return this.encounter != null && !this.encounter.isEmpty(); 3397 } 3398 3399 /** 3400 * @param value {@link #encounter} (Describes the clinical encounter or type of 3401 * care this documentation is associated with.) 3402 */ 3403 public Composition setEncounter(Reference value) { 3404 this.encounter = value; 3405 return this; 3406 } 3407 3408 /** 3409 * @return {@link #encounter} The actual object that is the target of the 3410 * reference. The reference library doesn't populate this, but you can 3411 * use it to hold the resource if you resolve it. (Describes the 3412 * clinical encounter or type of care this documentation is associated 3413 * with.) 3414 */ 3415 public Encounter getEncounterTarget() { 3416 if (this.encounterTarget == null) 3417 if (Configuration.errorOnAutoCreate()) 3418 throw new Error("Attempt to auto-create Composition.encounter"); 3419 else if (Configuration.doAutoCreate()) 3420 this.encounterTarget = new Encounter(); // aa 3421 return this.encounterTarget; 3422 } 3423 3424 /** 3425 * @param value {@link #encounter} The actual object that is the target of the 3426 * reference. The reference library doesn't use these, but you can 3427 * use it to hold the resource if you resolve it. (Describes the 3428 * clinical encounter or type of care this documentation is 3429 * associated with.) 3430 */ 3431 public Composition setEncounterTarget(Encounter value) { 3432 this.encounterTarget = value; 3433 return this; 3434 } 3435 3436 /** 3437 * @return {@link #date} (The composition editing time, when the composition was 3438 * last logically changed by the author.). This is the underlying object 3439 * with id, value and extensions. The accessor "getDate" gives direct 3440 * access to the value 3441 */ 3442 public DateTimeType getDateElement() { 3443 if (this.date == null) 3444 if (Configuration.errorOnAutoCreate()) 3445 throw new Error("Attempt to auto-create Composition.date"); 3446 else if (Configuration.doAutoCreate()) 3447 this.date = new DateTimeType(); // bb 3448 return this.date; 3449 } 3450 3451 public boolean hasDateElement() { 3452 return this.date != null && !this.date.isEmpty(); 3453 } 3454 3455 public boolean hasDate() { 3456 return this.date != null && !this.date.isEmpty(); 3457 } 3458 3459 /** 3460 * @param value {@link #date} (The composition editing time, when the 3461 * composition was last logically changed by the author.). This is 3462 * the underlying object with id, value and extensions. The 3463 * accessor "getDate" gives direct access to the value 3464 */ 3465 public Composition setDateElement(DateTimeType value) { 3466 this.date = value; 3467 return this; 3468 } 3469 3470 /** 3471 * @return The composition editing time, when the composition was last logically 3472 * changed by the author. 3473 */ 3474 public Date getDate() { 3475 return this.date == null ? null : this.date.getValue(); 3476 } 3477 3478 /** 3479 * @param value The composition editing time, when the composition was last 3480 * logically changed by the author. 3481 */ 3482 public Composition setDate(Date value) { 3483 if (this.date == null) 3484 this.date = new DateTimeType(); 3485 this.date.setValue(value); 3486 return this; 3487 } 3488 3489 /** 3490 * @return {@link #author} (Identifies who is responsible for the information in 3491 * the composition, not necessarily who typed it in.) 3492 */ 3493 public List<Reference> getAuthor() { 3494 if (this.author == null) 3495 this.author = new ArrayList<Reference>(); 3496 return this.author; 3497 } 3498 3499 /** 3500 * @return Returns a reference to <code>this</code> for easy method chaining 3501 */ 3502 public Composition setAuthor(List<Reference> theAuthor) { 3503 this.author = theAuthor; 3504 return this; 3505 } 3506 3507 public boolean hasAuthor() { 3508 if (this.author == null) 3509 return false; 3510 for (Reference item : this.author) 3511 if (!item.isEmpty()) 3512 return true; 3513 return false; 3514 } 3515 3516 public Reference addAuthor() { // 3 3517 Reference t = new Reference(); 3518 if (this.author == null) 3519 this.author = new ArrayList<Reference>(); 3520 this.author.add(t); 3521 return t; 3522 } 3523 3524 public Composition addAuthor(Reference t) { // 3 3525 if (t == null) 3526 return this; 3527 if (this.author == null) 3528 this.author = new ArrayList<Reference>(); 3529 this.author.add(t); 3530 return this; 3531 } 3532 3533 /** 3534 * @return The first repetition of repeating field {@link #author}, creating it 3535 * if it does not already exist 3536 */ 3537 public Reference getAuthorFirstRep() { 3538 if (getAuthor().isEmpty()) { 3539 addAuthor(); 3540 } 3541 return getAuthor().get(0); 3542 } 3543 3544 /** 3545 * @deprecated Use Reference#setResource(IBaseResource) instead 3546 */ 3547 @Deprecated 3548 public List<Resource> getAuthorTarget() { 3549 if (this.authorTarget == null) 3550 this.authorTarget = new ArrayList<Resource>(); 3551 return this.authorTarget; 3552 } 3553 3554 /** 3555 * @return {@link #title} (Official human-readable label for the composition.). 3556 * This is the underlying object with id, value and extensions. The 3557 * accessor "getTitle" gives direct access to the value 3558 */ 3559 public StringType getTitleElement() { 3560 if (this.title == null) 3561 if (Configuration.errorOnAutoCreate()) 3562 throw new Error("Attempt to auto-create Composition.title"); 3563 else if (Configuration.doAutoCreate()) 3564 this.title = new StringType(); // bb 3565 return this.title; 3566 } 3567 3568 public boolean hasTitleElement() { 3569 return this.title != null && !this.title.isEmpty(); 3570 } 3571 3572 public boolean hasTitle() { 3573 return this.title != null && !this.title.isEmpty(); 3574 } 3575 3576 /** 3577 * @param value {@link #title} (Official human-readable label for the 3578 * composition.). This is the underlying object with id, value and 3579 * extensions. The accessor "getTitle" gives direct access to the 3580 * value 3581 */ 3582 public Composition setTitleElement(StringType value) { 3583 this.title = value; 3584 return this; 3585 } 3586 3587 /** 3588 * @return Official human-readable label for the composition. 3589 */ 3590 public String getTitle() { 3591 return this.title == null ? null : this.title.getValue(); 3592 } 3593 3594 /** 3595 * @param value Official human-readable label for the composition. 3596 */ 3597 public Composition setTitle(String value) { 3598 if (this.title == null) 3599 this.title = new StringType(); 3600 this.title.setValue(value); 3601 return this; 3602 } 3603 3604 /** 3605 * @return {@link #confidentiality} (The code specifying the level of 3606 * confidentiality of the Composition.). This is the underlying object 3607 * with id, value and extensions. The accessor "getConfidentiality" 3608 * gives direct access to the value 3609 */ 3610 public Enumeration<DocumentConfidentiality> getConfidentialityElement() { 3611 if (this.confidentiality == null) 3612 if (Configuration.errorOnAutoCreate()) 3613 throw new Error("Attempt to auto-create Composition.confidentiality"); 3614 else if (Configuration.doAutoCreate()) 3615 this.confidentiality = new Enumeration<DocumentConfidentiality>(new DocumentConfidentialityEnumFactory()); // bb 3616 return this.confidentiality; 3617 } 3618 3619 public boolean hasConfidentialityElement() { 3620 return this.confidentiality != null && !this.confidentiality.isEmpty(); 3621 } 3622 3623 public boolean hasConfidentiality() { 3624 return this.confidentiality != null && !this.confidentiality.isEmpty(); 3625 } 3626 3627 /** 3628 * @param value {@link #confidentiality} (The code specifying the level of 3629 * confidentiality of the Composition.). This is the underlying 3630 * object with id, value and extensions. The accessor 3631 * "getConfidentiality" gives direct access to the value 3632 */ 3633 public Composition setConfidentialityElement(Enumeration<DocumentConfidentiality> value) { 3634 this.confidentiality = value; 3635 return this; 3636 } 3637 3638 /** 3639 * @return The code specifying the level of confidentiality of the Composition. 3640 */ 3641 public DocumentConfidentiality getConfidentiality() { 3642 return this.confidentiality == null ? null : this.confidentiality.getValue(); 3643 } 3644 3645 /** 3646 * @param value The code specifying the level of confidentiality of the 3647 * Composition. 3648 */ 3649 public Composition setConfidentiality(DocumentConfidentiality value) { 3650 if (value == null) 3651 this.confidentiality = null; 3652 else { 3653 if (this.confidentiality == null) 3654 this.confidentiality = new Enumeration<DocumentConfidentiality>(new DocumentConfidentialityEnumFactory()); 3655 this.confidentiality.setValue(value); 3656 } 3657 return this; 3658 } 3659 3660 /** 3661 * @return {@link #attester} (A participant who has attested to the accuracy of 3662 * the composition/document.) 3663 */ 3664 public List<CompositionAttesterComponent> getAttester() { 3665 if (this.attester == null) 3666 this.attester = new ArrayList<CompositionAttesterComponent>(); 3667 return this.attester; 3668 } 3669 3670 /** 3671 * @return Returns a reference to <code>this</code> for easy method chaining 3672 */ 3673 public Composition setAttester(List<CompositionAttesterComponent> theAttester) { 3674 this.attester = theAttester; 3675 return this; 3676 } 3677 3678 public boolean hasAttester() { 3679 if (this.attester == null) 3680 return false; 3681 for (CompositionAttesterComponent item : this.attester) 3682 if (!item.isEmpty()) 3683 return true; 3684 return false; 3685 } 3686 3687 public CompositionAttesterComponent addAttester() { // 3 3688 CompositionAttesterComponent t = new CompositionAttesterComponent(); 3689 if (this.attester == null) 3690 this.attester = new ArrayList<CompositionAttesterComponent>(); 3691 this.attester.add(t); 3692 return t; 3693 } 3694 3695 public Composition addAttester(CompositionAttesterComponent t) { // 3 3696 if (t == null) 3697 return this; 3698 if (this.attester == null) 3699 this.attester = new ArrayList<CompositionAttesterComponent>(); 3700 this.attester.add(t); 3701 return this; 3702 } 3703 3704 /** 3705 * @return The first repetition of repeating field {@link #attester}, creating 3706 * it if it does not already exist 3707 */ 3708 public CompositionAttesterComponent getAttesterFirstRep() { 3709 if (getAttester().isEmpty()) { 3710 addAttester(); 3711 } 3712 return getAttester().get(0); 3713 } 3714 3715 /** 3716 * @return {@link #custodian} (Identifies the organization or group who is 3717 * responsible for ongoing maintenance of and access to the 3718 * composition/document information.) 3719 */ 3720 public Reference getCustodian() { 3721 if (this.custodian == null) 3722 if (Configuration.errorOnAutoCreate()) 3723 throw new Error("Attempt to auto-create Composition.custodian"); 3724 else if (Configuration.doAutoCreate()) 3725 this.custodian = new Reference(); // cc 3726 return this.custodian; 3727 } 3728 3729 public boolean hasCustodian() { 3730 return this.custodian != null && !this.custodian.isEmpty(); 3731 } 3732 3733 /** 3734 * @param value {@link #custodian} (Identifies the organization or group who is 3735 * responsible for ongoing maintenance of and access to the 3736 * composition/document information.) 3737 */ 3738 public Composition setCustodian(Reference value) { 3739 this.custodian = value; 3740 return this; 3741 } 3742 3743 /** 3744 * @return {@link #custodian} The actual object that is the target of the 3745 * reference. The reference library doesn't populate this, but you can 3746 * use it to hold the resource if you resolve it. (Identifies the 3747 * organization or group who is responsible for ongoing maintenance of 3748 * and access to the composition/document information.) 3749 */ 3750 public Organization getCustodianTarget() { 3751 if (this.custodianTarget == null) 3752 if (Configuration.errorOnAutoCreate()) 3753 throw new Error("Attempt to auto-create Composition.custodian"); 3754 else if (Configuration.doAutoCreate()) 3755 this.custodianTarget = new Organization(); // aa 3756 return this.custodianTarget; 3757 } 3758 3759 /** 3760 * @param value {@link #custodian} The actual object that is the target of the 3761 * reference. The reference library doesn't use these, but you can 3762 * use it to hold the resource if you resolve it. (Identifies the 3763 * organization or group who is responsible for ongoing maintenance 3764 * of and access to the composition/document information.) 3765 */ 3766 public Composition setCustodianTarget(Organization value) { 3767 this.custodianTarget = value; 3768 return this; 3769 } 3770 3771 /** 3772 * @return {@link #relatesTo} (Relationships that this composition has with 3773 * other compositions or documents that already exist.) 3774 */ 3775 public List<CompositionRelatesToComponent> getRelatesTo() { 3776 if (this.relatesTo == null) 3777 this.relatesTo = new ArrayList<CompositionRelatesToComponent>(); 3778 return this.relatesTo; 3779 } 3780 3781 /** 3782 * @return Returns a reference to <code>this</code> for easy method chaining 3783 */ 3784 public Composition setRelatesTo(List<CompositionRelatesToComponent> theRelatesTo) { 3785 this.relatesTo = theRelatesTo; 3786 return this; 3787 } 3788 3789 public boolean hasRelatesTo() { 3790 if (this.relatesTo == null) 3791 return false; 3792 for (CompositionRelatesToComponent item : this.relatesTo) 3793 if (!item.isEmpty()) 3794 return true; 3795 return false; 3796 } 3797 3798 public CompositionRelatesToComponent addRelatesTo() { // 3 3799 CompositionRelatesToComponent t = new CompositionRelatesToComponent(); 3800 if (this.relatesTo == null) 3801 this.relatesTo = new ArrayList<CompositionRelatesToComponent>(); 3802 this.relatesTo.add(t); 3803 return t; 3804 } 3805 3806 public Composition addRelatesTo(CompositionRelatesToComponent t) { // 3 3807 if (t == null) 3808 return this; 3809 if (this.relatesTo == null) 3810 this.relatesTo = new ArrayList<CompositionRelatesToComponent>(); 3811 this.relatesTo.add(t); 3812 return this; 3813 } 3814 3815 /** 3816 * @return The first repetition of repeating field {@link #relatesTo}, creating 3817 * it if it does not already exist 3818 */ 3819 public CompositionRelatesToComponent getRelatesToFirstRep() { 3820 if (getRelatesTo().isEmpty()) { 3821 addRelatesTo(); 3822 } 3823 return getRelatesTo().get(0); 3824 } 3825 3826 /** 3827 * @return {@link #event} (The clinical service, such as a colonoscopy or an 3828 * appendectomy, being documented.) 3829 */ 3830 public List<CompositionEventComponent> getEvent() { 3831 if (this.event == null) 3832 this.event = new ArrayList<CompositionEventComponent>(); 3833 return this.event; 3834 } 3835 3836 /** 3837 * @return Returns a reference to <code>this</code> for easy method chaining 3838 */ 3839 public Composition setEvent(List<CompositionEventComponent> theEvent) { 3840 this.event = theEvent; 3841 return this; 3842 } 3843 3844 public boolean hasEvent() { 3845 if (this.event == null) 3846 return false; 3847 for (CompositionEventComponent item : this.event) 3848 if (!item.isEmpty()) 3849 return true; 3850 return false; 3851 } 3852 3853 public CompositionEventComponent addEvent() { // 3 3854 CompositionEventComponent t = new CompositionEventComponent(); 3855 if (this.event == null) 3856 this.event = new ArrayList<CompositionEventComponent>(); 3857 this.event.add(t); 3858 return t; 3859 } 3860 3861 public Composition addEvent(CompositionEventComponent t) { // 3 3862 if (t == null) 3863 return this; 3864 if (this.event == null) 3865 this.event = new ArrayList<CompositionEventComponent>(); 3866 this.event.add(t); 3867 return this; 3868 } 3869 3870 /** 3871 * @return The first repetition of repeating field {@link #event}, creating it 3872 * if it does not already exist 3873 */ 3874 public CompositionEventComponent getEventFirstRep() { 3875 if (getEvent().isEmpty()) { 3876 addEvent(); 3877 } 3878 return getEvent().get(0); 3879 } 3880 3881 /** 3882 * @return {@link #section} (The root of the sections that make up the 3883 * composition.) 3884 */ 3885 public List<SectionComponent> getSection() { 3886 if (this.section == null) 3887 this.section = new ArrayList<SectionComponent>(); 3888 return this.section; 3889 } 3890 3891 /** 3892 * @return Returns a reference to <code>this</code> for easy method chaining 3893 */ 3894 public Composition setSection(List<SectionComponent> theSection) { 3895 this.section = theSection; 3896 return this; 3897 } 3898 3899 public boolean hasSection() { 3900 if (this.section == null) 3901 return false; 3902 for (SectionComponent item : this.section) 3903 if (!item.isEmpty()) 3904 return true; 3905 return false; 3906 } 3907 3908 public SectionComponent addSection() { // 3 3909 SectionComponent t = new SectionComponent(); 3910 if (this.section == null) 3911 this.section = new ArrayList<SectionComponent>(); 3912 this.section.add(t); 3913 return t; 3914 } 3915 3916 public Composition addSection(SectionComponent t) { // 3 3917 if (t == null) 3918 return this; 3919 if (this.section == null) 3920 this.section = new ArrayList<SectionComponent>(); 3921 this.section.add(t); 3922 return this; 3923 } 3924 3925 /** 3926 * @return The first repetition of repeating field {@link #section}, creating it 3927 * if it does not already exist 3928 */ 3929 public SectionComponent getSectionFirstRep() { 3930 if (getSection().isEmpty()) { 3931 addSection(); 3932 } 3933 return getSection().get(0); 3934 } 3935 3936 protected void listChildren(List<Property> children) { 3937 super.listChildren(children); 3938 children.add(new Property("identifier", "Identifier", 3939 "A version-independent identifier for the Composition. This identifier stays constant as the composition is changed over time.", 3940 0, 1, identifier)); 3941 children.add(new Property("status", "code", 3942 "The workflow/clinical status of this composition. The status is a marker for the clinical standing of the document.", 3943 0, 1, status)); 3944 children.add(new Property("type", "CodeableConcept", 3945 "Specifies the particular kind of composition (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the composition.", 3946 0, 1, type)); 3947 children.add(new Property("category", "CodeableConcept", 3948 "A categorization for the type of the composition - helps for indexing and searching. This may be implied by or derived from the code specified in the Composition Type.", 3949 0, java.lang.Integer.MAX_VALUE, category)); 3950 children.add(new Property("subject", "Reference(Any)", 3951 "Who or what the composition is about. The composition can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of livestock, or a set of patients that share a common exposure).", 3952 0, 1, subject)); 3953 children.add(new Property("encounter", "Reference(Encounter)", 3954 "Describes the clinical encounter or type of care this documentation is associated with.", 0, 1, encounter)); 3955 children.add(new Property("date", "dateTime", 3956 "The composition editing time, when the composition was last logically changed by the author.", 0, 1, date)); 3957 children.add( 3958 new Property("author", "Reference(Practitioner|PractitionerRole|Device|Patient|RelatedPerson|Organization)", 3959 "Identifies who is responsible for the information in the composition, not necessarily who typed it in.", 0, 3960 java.lang.Integer.MAX_VALUE, author)); 3961 children.add(new Property("title", "string", "Official human-readable label for the composition.", 0, 1, title)); 3962 children.add(new Property("confidentiality", "code", 3963 "The code specifying the level of confidentiality of the Composition.", 0, 1, confidentiality)); 3964 children 3965 .add(new Property("attester", "", "A participant who has attested to the accuracy of the composition/document.", 3966 0, java.lang.Integer.MAX_VALUE, attester)); 3967 children.add(new Property("custodian", "Reference(Organization)", 3968 "Identifies the organization or group who is responsible for ongoing maintenance of and access to the composition/document information.", 3969 0, 1, custodian)); 3970 children.add(new Property("relatesTo", "", 3971 "Relationships that this composition has with other compositions or documents that already exist.", 0, 3972 java.lang.Integer.MAX_VALUE, relatesTo)); 3973 children.add( 3974 new Property("event", "", "The clinical service, such as a colonoscopy or an appendectomy, being documented.", 3975 0, java.lang.Integer.MAX_VALUE, event)); 3976 children.add(new Property("section", "", "The root of the sections that make up the composition.", 0, 3977 java.lang.Integer.MAX_VALUE, section)); 3978 } 3979 3980 @Override 3981 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3982 switch (_hash) { 3983 case -1618432855: 3984 /* identifier */ return new Property("identifier", "Identifier", 3985 "A version-independent identifier for the Composition. This identifier stays constant as the composition is changed over time.", 3986 0, 1, identifier); 3987 case -892481550: 3988 /* status */ return new Property("status", "code", 3989 "The workflow/clinical status of this composition. The status is a marker for the clinical standing of the document.", 3990 0, 1, status); 3991 case 3575610: 3992 /* type */ return new Property("type", "CodeableConcept", 3993 "Specifies the particular kind of composition (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the composition.", 3994 0, 1, type); 3995 case 50511102: 3996 /* category */ return new Property("category", "CodeableConcept", 3997 "A categorization for the type of the composition - helps for indexing and searching. This may be implied by or derived from the code specified in the Composition Type.", 3998 0, java.lang.Integer.MAX_VALUE, category); 3999 case -1867885268: 4000 /* subject */ return new Property("subject", "Reference(Any)", 4001 "Who or what the composition is about. The composition can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of livestock, or a set of patients that share a common exposure).", 4002 0, 1, subject); 4003 case 1524132147: 4004 /* encounter */ return new Property("encounter", "Reference(Encounter)", 4005 "Describes the clinical encounter or type of care this documentation is associated with.", 0, 1, encounter); 4006 case 3076014: 4007 /* date */ return new Property("date", "dateTime", 4008 "The composition editing time, when the composition was last logically changed by the author.", 0, 1, date); 4009 case -1406328437: 4010 /* author */ return new Property("author", 4011 "Reference(Practitioner|PractitionerRole|Device|Patient|RelatedPerson|Organization)", 4012 "Identifies who is responsible for the information in the composition, not necessarily who typed it in.", 0, 4013 java.lang.Integer.MAX_VALUE, author); 4014 case 110371416: 4015 /* title */ return new Property("title", "string", "Official human-readable label for the composition.", 0, 1, 4016 title); 4017 case -1923018202: 4018 /* confidentiality */ return new Property("confidentiality", "code", 4019 "The code specifying the level of confidentiality of the Composition.", 0, 1, confidentiality); 4020 case 542920370: 4021 /* attester */ return new Property("attester", "", 4022 "A participant who has attested to the accuracy of the composition/document.", 0, java.lang.Integer.MAX_VALUE, 4023 attester); 4024 case 1611297262: 4025 /* custodian */ return new Property("custodian", "Reference(Organization)", 4026 "Identifies the organization or group who is responsible for ongoing maintenance of and access to the composition/document information.", 4027 0, 1, custodian); 4028 case -7765931: 4029 /* relatesTo */ return new Property("relatesTo", "", 4030 "Relationships that this composition has with other compositions or documents that already exist.", 0, 4031 java.lang.Integer.MAX_VALUE, relatesTo); 4032 case 96891546: 4033 /* event */ return new Property("event", "", 4034 "The clinical service, such as a colonoscopy or an appendectomy, being documented.", 0, 4035 java.lang.Integer.MAX_VALUE, event); 4036 case 1970241253: 4037 /* section */ return new Property("section", "", "The root of the sections that make up the composition.", 0, 4038 java.lang.Integer.MAX_VALUE, section); 4039 default: 4040 return super.getNamedProperty(_hash, _name, _checkValid); 4041 } 4042 4043 } 4044 4045 @Override 4046 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4047 switch (hash) { 4048 case -1618432855: 4049 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 4050 case -892481550: 4051 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<CompositionStatus> 4052 case 3575610: 4053 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 4054 case 50511102: 4055 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 4056 case -1867885268: 4057 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 4058 case 1524132147: 4059 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 4060 case 3076014: 4061 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 4062 case -1406328437: 4063 /* author */ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // Reference 4064 case 110371416: 4065 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 4066 case -1923018202: 4067 /* confidentiality */ return this.confidentiality == null ? new Base[0] : new Base[] { this.confidentiality }; // Enumeration<DocumentConfidentiality> 4068 case 542920370: 4069 /* attester */ return this.attester == null ? new Base[0] : this.attester.toArray(new Base[this.attester.size()]); // CompositionAttesterComponent 4070 case 1611297262: 4071 /* custodian */ return this.custodian == null ? new Base[0] : new Base[] { this.custodian }; // Reference 4072 case -7765931: 4073 /* relatesTo */ return this.relatesTo == null ? new Base[0] 4074 : this.relatesTo.toArray(new Base[this.relatesTo.size()]); // CompositionRelatesToComponent 4075 case 96891546: 4076 /* event */ return this.event == null ? new Base[0] : this.event.toArray(new Base[this.event.size()]); // CompositionEventComponent 4077 case 1970241253: 4078 /* section */ return this.section == null ? new Base[0] : this.section.toArray(new Base[this.section.size()]); // SectionComponent 4079 default: 4080 return super.getProperty(hash, name, checkValid); 4081 } 4082 4083 } 4084 4085 @Override 4086 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4087 switch (hash) { 4088 case -1618432855: // identifier 4089 this.identifier = castToIdentifier(value); // Identifier 4090 return value; 4091 case -892481550: // status 4092 value = new CompositionStatusEnumFactory().fromType(castToCode(value)); 4093 this.status = (Enumeration) value; // Enumeration<CompositionStatus> 4094 return value; 4095 case 3575610: // type 4096 this.type = castToCodeableConcept(value); // CodeableConcept 4097 return value; 4098 case 50511102: // category 4099 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 4100 return value; 4101 case -1867885268: // subject 4102 this.subject = castToReference(value); // Reference 4103 return value; 4104 case 1524132147: // encounter 4105 this.encounter = castToReference(value); // Reference 4106 return value; 4107 case 3076014: // date 4108 this.date = castToDateTime(value); // DateTimeType 4109 return value; 4110 case -1406328437: // author 4111 this.getAuthor().add(castToReference(value)); // Reference 4112 return value; 4113 case 110371416: // title 4114 this.title = castToString(value); // StringType 4115 return value; 4116 case -1923018202: // confidentiality 4117 value = new DocumentConfidentialityEnumFactory().fromType(castToCode(value)); 4118 this.confidentiality = (Enumeration) value; // Enumeration<DocumentConfidentiality> 4119 return value; 4120 case 542920370: // attester 4121 this.getAttester().add((CompositionAttesterComponent) value); // CompositionAttesterComponent 4122 return value; 4123 case 1611297262: // custodian 4124 this.custodian = castToReference(value); // Reference 4125 return value; 4126 case -7765931: // relatesTo 4127 this.getRelatesTo().add((CompositionRelatesToComponent) value); // CompositionRelatesToComponent 4128 return value; 4129 case 96891546: // event 4130 this.getEvent().add((CompositionEventComponent) value); // CompositionEventComponent 4131 return value; 4132 case 1970241253: // section 4133 this.getSection().add((SectionComponent) value); // SectionComponent 4134 return value; 4135 default: 4136 return super.setProperty(hash, name, value); 4137 } 4138 4139 } 4140 4141 @Override 4142 public Base setProperty(String name, Base value) throws FHIRException { 4143 if (name.equals("identifier")) { 4144 this.identifier = castToIdentifier(value); // Identifier 4145 } else if (name.equals("status")) { 4146 value = new CompositionStatusEnumFactory().fromType(castToCode(value)); 4147 this.status = (Enumeration) value; // Enumeration<CompositionStatus> 4148 } else if (name.equals("type")) { 4149 this.type = castToCodeableConcept(value); // CodeableConcept 4150 } else if (name.equals("category")) { 4151 this.getCategory().add(castToCodeableConcept(value)); 4152 } else if (name.equals("subject")) { 4153 this.subject = castToReference(value); // Reference 4154 } else if (name.equals("encounter")) { 4155 this.encounter = castToReference(value); // Reference 4156 } else if (name.equals("date")) { 4157 this.date = castToDateTime(value); // DateTimeType 4158 } else if (name.equals("author")) { 4159 this.getAuthor().add(castToReference(value)); 4160 } else if (name.equals("title")) { 4161 this.title = castToString(value); // StringType 4162 } else if (name.equals("confidentiality")) { 4163 value = new DocumentConfidentialityEnumFactory().fromType(castToCode(value)); 4164 this.confidentiality = (Enumeration) value; // Enumeration<DocumentConfidentiality> 4165 } else if (name.equals("attester")) { 4166 this.getAttester().add((CompositionAttesterComponent) value); 4167 } else if (name.equals("custodian")) { 4168 this.custodian = castToReference(value); // Reference 4169 } else if (name.equals("relatesTo")) { 4170 this.getRelatesTo().add((CompositionRelatesToComponent) value); 4171 } else if (name.equals("event")) { 4172 this.getEvent().add((CompositionEventComponent) value); 4173 } else if (name.equals("section")) { 4174 this.getSection().add((SectionComponent) value); 4175 } else 4176 return super.setProperty(name, value); 4177 return value; 4178 } 4179 4180 @Override 4181 public void removeChild(String name, Base value) throws FHIRException { 4182 if (name.equals("identifier")) { 4183 this.identifier = null; 4184 } else if (name.equals("status")) { 4185 this.status = null; 4186 } else if (name.equals("type")) { 4187 this.type = null; 4188 } else if (name.equals("category")) { 4189 this.getCategory().remove(castToCodeableConcept(value)); 4190 } else if (name.equals("subject")) { 4191 this.subject = null; 4192 } else if (name.equals("encounter")) { 4193 this.encounter = null; 4194 } else if (name.equals("date")) { 4195 this.date = null; 4196 } else if (name.equals("author")) { 4197 this.getAuthor().remove(castToReference(value)); 4198 } else if (name.equals("title")) { 4199 this.title = null; 4200 } else if (name.equals("confidentiality")) { 4201 this.confidentiality = null; 4202 } else if (name.equals("attester")) { 4203 this.getAttester().remove((CompositionAttesterComponent) value); 4204 } else if (name.equals("custodian")) { 4205 this.custodian = null; 4206 } else if (name.equals("relatesTo")) { 4207 this.getRelatesTo().remove((CompositionRelatesToComponent) value); 4208 } else if (name.equals("event")) { 4209 this.getEvent().remove((CompositionEventComponent) value); 4210 } else if (name.equals("section")) { 4211 this.getSection().remove((SectionComponent) value); 4212 } else 4213 super.removeChild(name, value); 4214 4215 } 4216 4217 @Override 4218 public Base makeProperty(int hash, String name) throws FHIRException { 4219 switch (hash) { 4220 case -1618432855: 4221 return getIdentifier(); 4222 case -892481550: 4223 return getStatusElement(); 4224 case 3575610: 4225 return getType(); 4226 case 50511102: 4227 return addCategory(); 4228 case -1867885268: 4229 return getSubject(); 4230 case 1524132147: 4231 return getEncounter(); 4232 case 3076014: 4233 return getDateElement(); 4234 case -1406328437: 4235 return addAuthor(); 4236 case 110371416: 4237 return getTitleElement(); 4238 case -1923018202: 4239 return getConfidentialityElement(); 4240 case 542920370: 4241 return addAttester(); 4242 case 1611297262: 4243 return getCustodian(); 4244 case -7765931: 4245 return addRelatesTo(); 4246 case 96891546: 4247 return addEvent(); 4248 case 1970241253: 4249 return addSection(); 4250 default: 4251 return super.makeProperty(hash, name); 4252 } 4253 4254 } 4255 4256 @Override 4257 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4258 switch (hash) { 4259 case -1618432855: 4260 /* identifier */ return new String[] { "Identifier" }; 4261 case -892481550: 4262 /* status */ return new String[] { "code" }; 4263 case 3575610: 4264 /* type */ return new String[] { "CodeableConcept" }; 4265 case 50511102: 4266 /* category */ return new String[] { "CodeableConcept" }; 4267 case -1867885268: 4268 /* subject */ return new String[] { "Reference" }; 4269 case 1524132147: 4270 /* encounter */ return new String[] { "Reference" }; 4271 case 3076014: 4272 /* date */ return new String[] { "dateTime" }; 4273 case -1406328437: 4274 /* author */ return new String[] { "Reference" }; 4275 case 110371416: 4276 /* title */ return new String[] { "string" }; 4277 case -1923018202: 4278 /* confidentiality */ return new String[] { "code" }; 4279 case 542920370: 4280 /* attester */ return new String[] {}; 4281 case 1611297262: 4282 /* custodian */ return new String[] { "Reference" }; 4283 case -7765931: 4284 /* relatesTo */ return new String[] {}; 4285 case 96891546: 4286 /* event */ return new String[] {}; 4287 case 1970241253: 4288 /* section */ return new String[] {}; 4289 default: 4290 return super.getTypesForProperty(hash, name); 4291 } 4292 4293 } 4294 4295 @Override 4296 public Base addChild(String name) throws FHIRException { 4297 if (name.equals("identifier")) { 4298 this.identifier = new Identifier(); 4299 return this.identifier; 4300 } else if (name.equals("status")) { 4301 throw new FHIRException("Cannot call addChild on a singleton property Composition.status"); 4302 } else if (name.equals("type")) { 4303 this.type = new CodeableConcept(); 4304 return this.type; 4305 } else if (name.equals("category")) { 4306 return addCategory(); 4307 } else if (name.equals("subject")) { 4308 this.subject = new Reference(); 4309 return this.subject; 4310 } else if (name.equals("encounter")) { 4311 this.encounter = new Reference(); 4312 return this.encounter; 4313 } else if (name.equals("date")) { 4314 throw new FHIRException("Cannot call addChild on a singleton property Composition.date"); 4315 } else if (name.equals("author")) { 4316 return addAuthor(); 4317 } else if (name.equals("title")) { 4318 throw new FHIRException("Cannot call addChild on a singleton property Composition.title"); 4319 } else if (name.equals("confidentiality")) { 4320 throw new FHIRException("Cannot call addChild on a singleton property Composition.confidentiality"); 4321 } else if (name.equals("attester")) { 4322 return addAttester(); 4323 } else if (name.equals("custodian")) { 4324 this.custodian = new Reference(); 4325 return this.custodian; 4326 } else if (name.equals("relatesTo")) { 4327 return addRelatesTo(); 4328 } else if (name.equals("event")) { 4329 return addEvent(); 4330 } else if (name.equals("section")) { 4331 return addSection(); 4332 } else 4333 return super.addChild(name); 4334 } 4335 4336 public String fhirType() { 4337 return "Composition"; 4338 4339 } 4340 4341 public Composition copy() { 4342 Composition dst = new Composition(); 4343 copyValues(dst); 4344 return dst; 4345 } 4346 4347 public void copyValues(Composition dst) { 4348 super.copyValues(dst); 4349 dst.identifier = identifier == null ? null : identifier.copy(); 4350 dst.status = status == null ? null : status.copy(); 4351 dst.type = type == null ? null : type.copy(); 4352 if (category != null) { 4353 dst.category = new ArrayList<CodeableConcept>(); 4354 for (CodeableConcept i : category) 4355 dst.category.add(i.copy()); 4356 } 4357 ; 4358 dst.subject = subject == null ? null : subject.copy(); 4359 dst.encounter = encounter == null ? null : encounter.copy(); 4360 dst.date = date == null ? null : date.copy(); 4361 if (author != null) { 4362 dst.author = new ArrayList<Reference>(); 4363 for (Reference i : author) 4364 dst.author.add(i.copy()); 4365 } 4366 ; 4367 dst.title = title == null ? null : title.copy(); 4368 dst.confidentiality = confidentiality == null ? null : confidentiality.copy(); 4369 if (attester != null) { 4370 dst.attester = new ArrayList<CompositionAttesterComponent>(); 4371 for (CompositionAttesterComponent i : attester) 4372 dst.attester.add(i.copy()); 4373 } 4374 ; 4375 dst.custodian = custodian == null ? null : custodian.copy(); 4376 if (relatesTo != null) { 4377 dst.relatesTo = new ArrayList<CompositionRelatesToComponent>(); 4378 for (CompositionRelatesToComponent i : relatesTo) 4379 dst.relatesTo.add(i.copy()); 4380 } 4381 ; 4382 if (event != null) { 4383 dst.event = new ArrayList<CompositionEventComponent>(); 4384 for (CompositionEventComponent i : event) 4385 dst.event.add(i.copy()); 4386 } 4387 ; 4388 if (section != null) { 4389 dst.section = new ArrayList<SectionComponent>(); 4390 for (SectionComponent i : section) 4391 dst.section.add(i.copy()); 4392 } 4393 ; 4394 } 4395 4396 protected Composition typedCopy() { 4397 return copy(); 4398 } 4399 4400 @Override 4401 public boolean equalsDeep(Base other_) { 4402 if (!super.equalsDeep(other_)) 4403 return false; 4404 if (!(other_ instanceof Composition)) 4405 return false; 4406 Composition o = (Composition) other_; 4407 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 4408 && compareDeep(type, o.type, true) && compareDeep(category, o.category, true) 4409 && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 4410 && compareDeep(date, o.date, true) && compareDeep(author, o.author, true) && compareDeep(title, o.title, true) 4411 && compareDeep(confidentiality, o.confidentiality, true) && compareDeep(attester, o.attester, true) 4412 && compareDeep(custodian, o.custodian, true) && compareDeep(relatesTo, o.relatesTo, true) 4413 && compareDeep(event, o.event, true) && compareDeep(section, o.section, true); 4414 } 4415 4416 @Override 4417 public boolean equalsShallow(Base other_) { 4418 if (!super.equalsShallow(other_)) 4419 return false; 4420 if (!(other_ instanceof Composition)) 4421 return false; 4422 Composition o = (Composition) other_; 4423 return compareValues(status, o.status, true) && compareValues(date, o.date, true) 4424 && compareValues(title, o.title, true) && compareValues(confidentiality, o.confidentiality, true); 4425 } 4426 4427 public boolean isEmpty() { 4428 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, type, category, subject, 4429 encounter, date, author, title, confidentiality, attester, custodian, relatesTo, event, section); 4430 } 4431 4432 @Override 4433 public ResourceType getResourceType() { 4434 return ResourceType.Composition; 4435 } 4436 4437 /** 4438 * Search parameter: <b>date</b> 4439 * <p> 4440 * Description: <b>Composition editing time</b><br> 4441 * Type: <b>date</b><br> 4442 * Path: <b>Composition.date</b><br> 4443 * </p> 4444 */ 4445 @SearchParamDefinition(name = "date", path = "Composition.date", description = "Composition editing time", type = "date") 4446 public static final String SP_DATE = "date"; 4447 /** 4448 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4449 * <p> 4450 * Description: <b>Composition editing time</b><br> 4451 * Type: <b>date</b><br> 4452 * Path: <b>Composition.date</b><br> 4453 * </p> 4454 */ 4455 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 4456 SP_DATE); 4457 4458 /** 4459 * Search parameter: <b>identifier</b> 4460 * <p> 4461 * Description: <b>Version-independent identifier for the Composition</b><br> 4462 * Type: <b>token</b><br> 4463 * Path: <b>Composition.identifier</b><br> 4464 * </p> 4465 */ 4466 @SearchParamDefinition(name = "identifier", path = "Composition.identifier", description = "Version-independent identifier for the Composition", type = "token") 4467 public static final String SP_IDENTIFIER = "identifier"; 4468 /** 4469 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4470 * <p> 4471 * Description: <b>Version-independent identifier for the Composition</b><br> 4472 * Type: <b>token</b><br> 4473 * Path: <b>Composition.identifier</b><br> 4474 * </p> 4475 */ 4476 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4477 SP_IDENTIFIER); 4478 4479 /** 4480 * Search parameter: <b>period</b> 4481 * <p> 4482 * Description: <b>The period covered by the documentation</b><br> 4483 * Type: <b>date</b><br> 4484 * Path: <b>Composition.event.period</b><br> 4485 * </p> 4486 */ 4487 @SearchParamDefinition(name = "period", path = "Composition.event.period", description = "The period covered by the documentation", type = "date") 4488 public static final String SP_PERIOD = "period"; 4489 /** 4490 * <b>Fluent Client</b> search parameter constant for <b>period</b> 4491 * <p> 4492 * Description: <b>The period covered by the documentation</b><br> 4493 * Type: <b>date</b><br> 4494 * Path: <b>Composition.event.period</b><br> 4495 * </p> 4496 */ 4497 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam( 4498 SP_PERIOD); 4499 4500 /** 4501 * Search parameter: <b>related-id</b> 4502 * <p> 4503 * Description: <b>Target of the relationship</b><br> 4504 * Type: <b>token</b><br> 4505 * Path: <b>Composition.relatesTo.targetIdentifier</b><br> 4506 * </p> 4507 */ 4508 @SearchParamDefinition(name = "related-id", path = "(Composition.relatesTo.target as Identifier)", description = "Target of the relationship", type = "token") 4509 public static final String SP_RELATED_ID = "related-id"; 4510 /** 4511 * <b>Fluent Client</b> search parameter constant for <b>related-id</b> 4512 * <p> 4513 * Description: <b>Target of the relationship</b><br> 4514 * Type: <b>token</b><br> 4515 * Path: <b>Composition.relatesTo.targetIdentifier</b><br> 4516 * </p> 4517 */ 4518 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RELATED_ID = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4519 SP_RELATED_ID); 4520 4521 /** 4522 * Search parameter: <b>subject</b> 4523 * <p> 4524 * Description: <b>Who and/or what the composition is about</b><br> 4525 * Type: <b>reference</b><br> 4526 * Path: <b>Composition.subject</b><br> 4527 * </p> 4528 */ 4529 @SearchParamDefinition(name = "subject", path = "Composition.subject", description = "Who and/or what the composition is about", type = "reference", providesMembershipIn = { 4530 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 4531 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }) 4532 public static final String SP_SUBJECT = "subject"; 4533 /** 4534 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 4535 * <p> 4536 * Description: <b>Who and/or what the composition is about</b><br> 4537 * Type: <b>reference</b><br> 4538 * Path: <b>Composition.subject</b><br> 4539 * </p> 4540 */ 4541 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4542 SP_SUBJECT); 4543 4544 /** 4545 * Constant for fluent queries to be used to add include statements. Specifies 4546 * the path value of "<b>Composition:subject</b>". 4547 */ 4548 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 4549 "Composition:subject").toLocked(); 4550 4551 /** 4552 * Search parameter: <b>author</b> 4553 * <p> 4554 * Description: <b>Who and/or what authored the composition</b><br> 4555 * Type: <b>reference</b><br> 4556 * Path: <b>Composition.author</b><br> 4557 * </p> 4558 */ 4559 @SearchParamDefinition(name = "author", path = "Composition.author", description = "Who and/or what authored the composition", type = "reference", providesMembershipIn = { 4560 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 4561 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 4562 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 4563 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, 4564 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 4565 public static final String SP_AUTHOR = "author"; 4566 /** 4567 * <b>Fluent Client</b> search parameter constant for <b>author</b> 4568 * <p> 4569 * Description: <b>Who and/or what authored the composition</b><br> 4570 * Type: <b>reference</b><br> 4571 * Path: <b>Composition.author</b><br> 4572 * </p> 4573 */ 4574 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4575 SP_AUTHOR); 4576 4577 /** 4578 * Constant for fluent queries to be used to add include statements. Specifies 4579 * the path value of "<b>Composition:author</b>". 4580 */ 4581 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include( 4582 "Composition:author").toLocked(); 4583 4584 /** 4585 * Search parameter: <b>confidentiality</b> 4586 * <p> 4587 * Description: <b>As defined by affinity domain</b><br> 4588 * Type: <b>token</b><br> 4589 * Path: <b>Composition.confidentiality</b><br> 4590 * </p> 4591 */ 4592 @SearchParamDefinition(name = "confidentiality", path = "Composition.confidentiality", description = "As defined by affinity domain", type = "token") 4593 public static final String SP_CONFIDENTIALITY = "confidentiality"; 4594 /** 4595 * <b>Fluent Client</b> search parameter constant for <b>confidentiality</b> 4596 * <p> 4597 * Description: <b>As defined by affinity domain</b><br> 4598 * Type: <b>token</b><br> 4599 * Path: <b>Composition.confidentiality</b><br> 4600 * </p> 4601 */ 4602 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONFIDENTIALITY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4603 SP_CONFIDENTIALITY); 4604 4605 /** 4606 * Search parameter: <b>section</b> 4607 * <p> 4608 * Description: <b>Classification of section (recommended)</b><br> 4609 * Type: <b>token</b><br> 4610 * Path: <b>Composition.section.code</b><br> 4611 * </p> 4612 */ 4613 @SearchParamDefinition(name = "section", path = "Composition.section.code", description = "Classification of section (recommended)", type = "token") 4614 public static final String SP_SECTION = "section"; 4615 /** 4616 * <b>Fluent Client</b> search parameter constant for <b>section</b> 4617 * <p> 4618 * Description: <b>Classification of section (recommended)</b><br> 4619 * Type: <b>token</b><br> 4620 * Path: <b>Composition.section.code</b><br> 4621 * </p> 4622 */ 4623 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SECTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4624 SP_SECTION); 4625 4626 /** 4627 * Search parameter: <b>encounter</b> 4628 * <p> 4629 * Description: <b>Context of the Composition</b><br> 4630 * Type: <b>reference</b><br> 4631 * Path: <b>Composition.encounter</b><br> 4632 * </p> 4633 */ 4634 @SearchParamDefinition(name = "encounter", path = "Composition.encounter", description = "Context of the Composition", type = "reference", providesMembershipIn = { 4635 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 4636 public static final String SP_ENCOUNTER = "encounter"; 4637 /** 4638 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 4639 * <p> 4640 * Description: <b>Context of the Composition</b><br> 4641 * Type: <b>reference</b><br> 4642 * Path: <b>Composition.encounter</b><br> 4643 * </p> 4644 */ 4645 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4646 SP_ENCOUNTER); 4647 4648 /** 4649 * Constant for fluent queries to be used to add include statements. Specifies 4650 * the path value of "<b>Composition:encounter</b>". 4651 */ 4652 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 4653 "Composition:encounter").toLocked(); 4654 4655 /** 4656 * Search parameter: <b>type</b> 4657 * <p> 4658 * Description: <b>Kind of composition (LOINC if possible)</b><br> 4659 * Type: <b>token</b><br> 4660 * Path: <b>Composition.type</b><br> 4661 * </p> 4662 */ 4663 @SearchParamDefinition(name = "type", path = "Composition.type", description = "Kind of composition (LOINC if possible)", type = "token") 4664 public static final String SP_TYPE = "type"; 4665 /** 4666 * <b>Fluent Client</b> search parameter constant for <b>type</b> 4667 * <p> 4668 * Description: <b>Kind of composition (LOINC if possible)</b><br> 4669 * Type: <b>token</b><br> 4670 * Path: <b>Composition.type</b><br> 4671 * </p> 4672 */ 4673 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4674 SP_TYPE); 4675 4676 /** 4677 * Search parameter: <b>title</b> 4678 * <p> 4679 * Description: <b>Human Readable name/title</b><br> 4680 * Type: <b>string</b><br> 4681 * Path: <b>Composition.title</b><br> 4682 * </p> 4683 */ 4684 @SearchParamDefinition(name = "title", path = "Composition.title", description = "Human Readable name/title", type = "string") 4685 public static final String SP_TITLE = "title"; 4686 /** 4687 * <b>Fluent Client</b> search parameter constant for <b>title</b> 4688 * <p> 4689 * Description: <b>Human Readable name/title</b><br> 4690 * Type: <b>string</b><br> 4691 * Path: <b>Composition.title</b><br> 4692 * </p> 4693 */ 4694 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 4695 SP_TITLE); 4696 4697 /** 4698 * Search parameter: <b>attester</b> 4699 * <p> 4700 * Description: <b>Who attested the composition</b><br> 4701 * Type: <b>reference</b><br> 4702 * Path: <b>Composition.attester.party</b><br> 4703 * </p> 4704 */ 4705 @SearchParamDefinition(name = "attester", path = "Composition.attester.party", description = "Who attested the composition", type = "reference", providesMembershipIn = { 4706 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 4707 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 4708 Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 4709 public static final String SP_ATTESTER = "attester"; 4710 /** 4711 * <b>Fluent Client</b> search parameter constant for <b>attester</b> 4712 * <p> 4713 * Description: <b>Who attested the composition</b><br> 4714 * Type: <b>reference</b><br> 4715 * Path: <b>Composition.attester.party</b><br> 4716 * </p> 4717 */ 4718 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ATTESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4719 SP_ATTESTER); 4720 4721 /** 4722 * Constant for fluent queries to be used to add include statements. Specifies 4723 * the path value of "<b>Composition:attester</b>". 4724 */ 4725 public static final ca.uhn.fhir.model.api.Include INCLUDE_ATTESTER = new ca.uhn.fhir.model.api.Include( 4726 "Composition:attester").toLocked(); 4727 4728 /** 4729 * Search parameter: <b>entry</b> 4730 * <p> 4731 * Description: <b>A reference to data that supports this section</b><br> 4732 * Type: <b>reference</b><br> 4733 * Path: <b>Composition.section.entry</b><br> 4734 * </p> 4735 */ 4736 @SearchParamDefinition(name = "entry", path = "Composition.section.entry", description = "A reference to data that supports this section", type = "reference") 4737 public static final String SP_ENTRY = "entry"; 4738 /** 4739 * <b>Fluent Client</b> search parameter constant for <b>entry</b> 4740 * <p> 4741 * Description: <b>A reference to data that supports this section</b><br> 4742 * Type: <b>reference</b><br> 4743 * Path: <b>Composition.section.entry</b><br> 4744 * </p> 4745 */ 4746 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTRY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4747 SP_ENTRY); 4748 4749 /** 4750 * Constant for fluent queries to be used to add include statements. Specifies 4751 * the path value of "<b>Composition:entry</b>". 4752 */ 4753 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTRY = new ca.uhn.fhir.model.api.Include( 4754 "Composition:entry").toLocked(); 4755 4756 /** 4757 * Search parameter: <b>related-ref</b> 4758 * <p> 4759 * Description: <b>Target of the relationship</b><br> 4760 * Type: <b>reference</b><br> 4761 * Path: <b>Composition.relatesTo.targetReference</b><br> 4762 * </p> 4763 */ 4764 @SearchParamDefinition(name = "related-ref", path = "(Composition.relatesTo.target as Reference)", description = "Target of the relationship", type = "reference", target = { 4765 Composition.class }) 4766 public static final String SP_RELATED_REF = "related-ref"; 4767 /** 4768 * <b>Fluent Client</b> search parameter constant for <b>related-ref</b> 4769 * <p> 4770 * Description: <b>Target of the relationship</b><br> 4771 * Type: <b>reference</b><br> 4772 * Path: <b>Composition.relatesTo.targetReference</b><br> 4773 * </p> 4774 */ 4775 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RELATED_REF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4776 SP_RELATED_REF); 4777 4778 /** 4779 * Constant for fluent queries to be used to add include statements. Specifies 4780 * the path value of "<b>Composition:related-ref</b>". 4781 */ 4782 public static final ca.uhn.fhir.model.api.Include INCLUDE_RELATED_REF = new ca.uhn.fhir.model.api.Include( 4783 "Composition:related-ref").toLocked(); 4784 4785 /** 4786 * Search parameter: <b>patient</b> 4787 * <p> 4788 * Description: <b>Who and/or what the composition is about</b><br> 4789 * Type: <b>reference</b><br> 4790 * Path: <b>Composition.subject</b><br> 4791 * </p> 4792 */ 4793 @SearchParamDefinition(name = "patient", path = "Composition.subject.where(resolve() is Patient)", description = "Who and/or what the composition is about", type = "reference", target = { 4794 Patient.class }) 4795 public static final String SP_PATIENT = "patient"; 4796 /** 4797 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4798 * <p> 4799 * Description: <b>Who and/or what the composition is about</b><br> 4800 * Type: <b>reference</b><br> 4801 * Path: <b>Composition.subject</b><br> 4802 * </p> 4803 */ 4804 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4805 SP_PATIENT); 4806 4807 /** 4808 * Constant for fluent queries to be used to add include statements. Specifies 4809 * the path value of "<b>Composition:patient</b>". 4810 */ 4811 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 4812 "Composition:patient").toLocked(); 4813 4814 /** 4815 * Search parameter: <b>context</b> 4816 * <p> 4817 * Description: <b>Code(s) that apply to the event being documented</b><br> 4818 * Type: <b>token</b><br> 4819 * Path: <b>Composition.event.code</b><br> 4820 * </p> 4821 */ 4822 @SearchParamDefinition(name = "context", path = "Composition.event.code", description = "Code(s) that apply to the event being documented", type = "token") 4823 public static final String SP_CONTEXT = "context"; 4824 /** 4825 * <b>Fluent Client</b> search parameter constant for <b>context</b> 4826 * <p> 4827 * Description: <b>Code(s) that apply to the event being documented</b><br> 4828 * Type: <b>token</b><br> 4829 * Path: <b>Composition.event.code</b><br> 4830 * </p> 4831 */ 4832 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4833 SP_CONTEXT); 4834 4835 /** 4836 * Search parameter: <b>category</b> 4837 * <p> 4838 * Description: <b>Categorization of Composition</b><br> 4839 * Type: <b>token</b><br> 4840 * Path: <b>Composition.category</b><br> 4841 * </p> 4842 */ 4843 @SearchParamDefinition(name = "category", path = "Composition.category", description = "Categorization of Composition", type = "token") 4844 public static final String SP_CATEGORY = "category"; 4845 /** 4846 * <b>Fluent Client</b> search parameter constant for <b>category</b> 4847 * <p> 4848 * Description: <b>Categorization of Composition</b><br> 4849 * Type: <b>token</b><br> 4850 * Path: <b>Composition.category</b><br> 4851 * </p> 4852 */ 4853 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4854 SP_CATEGORY); 4855 4856 /** 4857 * Search parameter: <b>status</b> 4858 * <p> 4859 * Description: <b>preliminary | final | amended | entered-in-error</b><br> 4860 * Type: <b>token</b><br> 4861 * Path: <b>Composition.status</b><br> 4862 * </p> 4863 */ 4864 @SearchParamDefinition(name = "status", path = "Composition.status", description = "preliminary | final | amended | entered-in-error", type = "token") 4865 public static final String SP_STATUS = "status"; 4866 /** 4867 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4868 * <p> 4869 * Description: <b>preliminary | final | amended | entered-in-error</b><br> 4870 * Type: <b>token</b><br> 4871 * Path: <b>Composition.status</b><br> 4872 * </p> 4873 */ 4874 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4875 SP_STATUS); 4876 4877}