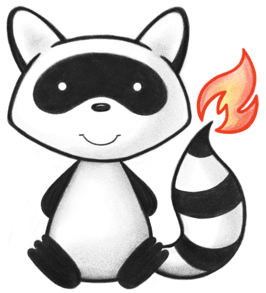
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * A set of healthcare-related information that is assembled together into a 049 * single logical package that provides a single coherent statement of meaning, 050 * establishes its own context and that has clinical attestation with regard to 051 * who is making the statement. A Composition defines the structure and 052 * narrative content necessary for a document. However, a Composition alone does 053 * not constitute a document. Rather, the Composition must be the first entry in 054 * a Bundle where Bundle.type=document, and any other resources referenced from 055 * Composition must be included as subsequent entries in the Bundle (for example 056 * Patient, Practitioner, Encounter, etc.). 057 */ 058@ResourceDef(name = "Composition", profile = "http://hl7.org/fhir/StructureDefinition/Composition") 059public class Composition extends DomainResource { 060 061 public enum CompositionStatus { 062 /** 063 * This is a preliminary composition or document (also known as initial or 064 * interim). The content may be incomplete or unverified. 065 */ 066 PRELIMINARY, 067 /** 068 * This version of the composition is complete and verified by an appropriate 069 * person and no further work is planned. Any subsequent updates would be on a 070 * new version of the composition. 071 */ 072 FINAL, 073 /** 074 * The composition content or the referenced resources have been modified 075 * (edited or added to) subsequent to being released as "final" and the 076 * composition is complete and verified by an authorized person. 077 */ 078 AMENDED, 079 /** 080 * The composition or document was originally created/issued in error, and this 081 * is an amendment that marks that the entire series should not be considered as 082 * valid. 083 */ 084 ENTEREDINERROR, 085 /** 086 * added to help the parsers with the generic types 087 */ 088 NULL; 089 090 public static CompositionStatus fromCode(String codeString) throws FHIRException { 091 if (codeString == null || "".equals(codeString)) 092 return null; 093 if ("preliminary".equals(codeString)) 094 return PRELIMINARY; 095 if ("final".equals(codeString)) 096 return FINAL; 097 if ("amended".equals(codeString)) 098 return AMENDED; 099 if ("entered-in-error".equals(codeString)) 100 return ENTEREDINERROR; 101 if (Configuration.isAcceptInvalidEnums()) 102 return null; 103 else 104 throw new FHIRException("Unknown CompositionStatus code '" + codeString + "'"); 105 } 106 107 public String toCode() { 108 switch (this) { 109 case PRELIMINARY: 110 return "preliminary"; 111 case FINAL: 112 return "final"; 113 case AMENDED: 114 return "amended"; 115 case ENTEREDINERROR: 116 return "entered-in-error"; 117 case NULL: 118 return null; 119 default: 120 return "?"; 121 } 122 } 123 124 public String getSystem() { 125 switch (this) { 126 case PRELIMINARY: 127 return "http://hl7.org/fhir/composition-status"; 128 case FINAL: 129 return "http://hl7.org/fhir/composition-status"; 130 case AMENDED: 131 return "http://hl7.org/fhir/composition-status"; 132 case ENTEREDINERROR: 133 return "http://hl7.org/fhir/composition-status"; 134 case NULL: 135 return null; 136 default: 137 return "?"; 138 } 139 } 140 141 public String getDefinition() { 142 switch (this) { 143 case PRELIMINARY: 144 return "This is a preliminary composition or document (also known as initial or interim). The content may be incomplete or unverified."; 145 case FINAL: 146 return "This version of the composition is complete and verified by an appropriate person and no further work is planned. Any subsequent updates would be on a new version of the composition."; 147 case AMENDED: 148 return "The composition content or the referenced resources have been modified (edited or added to) subsequent to being released as \"final\" and the composition is complete and verified by an authorized person."; 149 case ENTEREDINERROR: 150 return "The composition or document was originally created/issued in error, and this is an amendment that marks that the entire series should not be considered as valid."; 151 case NULL: 152 return null; 153 default: 154 return "?"; 155 } 156 } 157 158 public String getDisplay() { 159 switch (this) { 160 case PRELIMINARY: 161 return "Preliminary"; 162 case FINAL: 163 return "Final"; 164 case AMENDED: 165 return "Amended"; 166 case ENTEREDINERROR: 167 return "Entered in Error"; 168 case NULL: 169 return null; 170 default: 171 return "?"; 172 } 173 } 174 } 175 176 public static class CompositionStatusEnumFactory implements EnumFactory<CompositionStatus> { 177 public CompositionStatus fromCode(String codeString) throws IllegalArgumentException { 178 if (codeString == null || "".equals(codeString)) 179 if (codeString == null || "".equals(codeString)) 180 return null; 181 if ("preliminary".equals(codeString)) 182 return CompositionStatus.PRELIMINARY; 183 if ("final".equals(codeString)) 184 return CompositionStatus.FINAL; 185 if ("amended".equals(codeString)) 186 return CompositionStatus.AMENDED; 187 if ("entered-in-error".equals(codeString)) 188 return CompositionStatus.ENTEREDINERROR; 189 throw new IllegalArgumentException("Unknown CompositionStatus code '" + codeString + "'"); 190 } 191 192 public Enumeration<CompositionStatus> fromType(PrimitiveType<?> code) throws FHIRException { 193 if (code == null) 194 return null; 195 if (code.isEmpty()) 196 return new Enumeration<CompositionStatus>(this, CompositionStatus.NULL, code); 197 String codeString = code.asStringValue(); 198 if (codeString == null || "".equals(codeString)) 199 return new Enumeration<CompositionStatus>(this, CompositionStatus.NULL, code); 200 if ("preliminary".equals(codeString)) 201 return new Enumeration<CompositionStatus>(this, CompositionStatus.PRELIMINARY, code); 202 if ("final".equals(codeString)) 203 return new Enumeration<CompositionStatus>(this, CompositionStatus.FINAL, code); 204 if ("amended".equals(codeString)) 205 return new Enumeration<CompositionStatus>(this, CompositionStatus.AMENDED, code); 206 if ("entered-in-error".equals(codeString)) 207 return new Enumeration<CompositionStatus>(this, CompositionStatus.ENTEREDINERROR, code); 208 throw new FHIRException("Unknown CompositionStatus code '" + codeString + "'"); 209 } 210 211 public String toCode(CompositionStatus code) { 212 if (code == CompositionStatus.NULL) 213 return null; 214 if (code == CompositionStatus.PRELIMINARY) 215 return "preliminary"; 216 if (code == CompositionStatus.FINAL) 217 return "final"; 218 if (code == CompositionStatus.AMENDED) 219 return "amended"; 220 if (code == CompositionStatus.ENTEREDINERROR) 221 return "entered-in-error"; 222 return "?"; 223 } 224 225 public String toSystem(CompositionStatus code) { 226 return code.getSystem(); 227 } 228 } 229 230 public enum DocumentConfidentiality { 231 /** 232 * null 233 */ 234 U, 235 /** 236 * null 237 */ 238 L, 239 /** 240 * null 241 */ 242 M, 243 /** 244 * null 245 */ 246 N, 247 /** 248 * null 249 */ 250 R, 251 /** 252 * null 253 */ 254 V, 255 /** 256 * added to help the parsers with the generic types 257 */ 258 NULL; 259 260 public static DocumentConfidentiality fromCode(String codeString) throws FHIRException { 261 if (codeString == null || "".equals(codeString)) 262 return null; 263 if ("U".equals(codeString)) 264 return U; 265 if ("L".equals(codeString)) 266 return L; 267 if ("M".equals(codeString)) 268 return M; 269 if ("N".equals(codeString)) 270 return N; 271 if ("R".equals(codeString)) 272 return R; 273 if ("V".equals(codeString)) 274 return V; 275 if (Configuration.isAcceptInvalidEnums()) 276 return null; 277 else 278 throw new FHIRException("Unknown DocumentConfidentiality code '" + codeString + "'"); 279 } 280 281 public String toCode() { 282 switch (this) { 283 case U: 284 return "U"; 285 case L: 286 return "L"; 287 case M: 288 return "M"; 289 case N: 290 return "N"; 291 case R: 292 return "R"; 293 case V: 294 return "V"; 295 case NULL: 296 return null; 297 default: 298 return "?"; 299 } 300 } 301 302 public String getSystem() { 303 switch (this) { 304 case U: 305 return "http://terminology.hl7.org/CodeSystem/v3-Confidentiality"; 306 case L: 307 return "http://terminology.hl7.org/CodeSystem/v3-Confidentiality"; 308 case M: 309 return "http://terminology.hl7.org/CodeSystem/v3-Confidentiality"; 310 case N: 311 return "http://terminology.hl7.org/CodeSystem/v3-Confidentiality"; 312 case R: 313 return "http://terminology.hl7.org/CodeSystem/v3-Confidentiality"; 314 case V: 315 return "http://terminology.hl7.org/CodeSystem/v3-Confidentiality"; 316 case NULL: 317 return null; 318 default: 319 return "?"; 320 } 321 } 322 323 public String getDefinition() { 324 switch (this) { 325 case U: 326 return ""; 327 case L: 328 return ""; 329 case M: 330 return ""; 331 case N: 332 return ""; 333 case R: 334 return ""; 335 case V: 336 return ""; 337 case NULL: 338 return null; 339 default: 340 return "?"; 341 } 342 } 343 344 public String getDisplay() { 345 switch (this) { 346 case U: 347 return "U"; 348 case L: 349 return "L"; 350 case M: 351 return "M"; 352 case N: 353 return "N"; 354 case R: 355 return "R"; 356 case V: 357 return "V"; 358 case NULL: 359 return null; 360 default: 361 return "?"; 362 } 363 } 364 } 365 366 public static class DocumentConfidentialityEnumFactory implements EnumFactory<DocumentConfidentiality> { 367 public DocumentConfidentiality fromCode(String codeString) throws IllegalArgumentException { 368 if (codeString == null || "".equals(codeString)) 369 if (codeString == null || "".equals(codeString)) 370 return null; 371 if ("U".equals(codeString)) 372 return DocumentConfidentiality.U; 373 if ("L".equals(codeString)) 374 return DocumentConfidentiality.L; 375 if ("M".equals(codeString)) 376 return DocumentConfidentiality.M; 377 if ("N".equals(codeString)) 378 return DocumentConfidentiality.N; 379 if ("R".equals(codeString)) 380 return DocumentConfidentiality.R; 381 if ("V".equals(codeString)) 382 return DocumentConfidentiality.V; 383 throw new IllegalArgumentException("Unknown DocumentConfidentiality code '" + codeString + "'"); 384 } 385 386 public Enumeration<DocumentConfidentiality> fromType(PrimitiveType<?> code) throws FHIRException { 387 if (code == null) 388 return null; 389 if (code.isEmpty()) 390 return new Enumeration<DocumentConfidentiality>(this, DocumentConfidentiality.NULL, code); 391 String codeString = code.asStringValue(); 392 if (codeString == null || "".equals(codeString)) 393 return new Enumeration<DocumentConfidentiality>(this, DocumentConfidentiality.NULL, code); 394 if ("U".equals(codeString)) 395 return new Enumeration<DocumentConfidentiality>(this, DocumentConfidentiality.U, code); 396 if ("L".equals(codeString)) 397 return new Enumeration<DocumentConfidentiality>(this, DocumentConfidentiality.L, code); 398 if ("M".equals(codeString)) 399 return new Enumeration<DocumentConfidentiality>(this, DocumentConfidentiality.M, code); 400 if ("N".equals(codeString)) 401 return new Enumeration<DocumentConfidentiality>(this, DocumentConfidentiality.N, code); 402 if ("R".equals(codeString)) 403 return new Enumeration<DocumentConfidentiality>(this, DocumentConfidentiality.R, code); 404 if ("V".equals(codeString)) 405 return new Enumeration<DocumentConfidentiality>(this, DocumentConfidentiality.V, code); 406 throw new FHIRException("Unknown DocumentConfidentiality code '" + codeString + "'"); 407 } 408 409 public String toCode(DocumentConfidentiality code) { 410 if (code == DocumentConfidentiality.NULL) 411 return null; 412 if (code == DocumentConfidentiality.U) 413 return "U"; 414 if (code == DocumentConfidentiality.L) 415 return "L"; 416 if (code == DocumentConfidentiality.M) 417 return "M"; 418 if (code == DocumentConfidentiality.N) 419 return "N"; 420 if (code == DocumentConfidentiality.R) 421 return "R"; 422 if (code == DocumentConfidentiality.V) 423 return "V"; 424 return "?"; 425 } 426 427 public String toSystem(DocumentConfidentiality code) { 428 return code.getSystem(); 429 } 430 } 431 432 public enum CompositionAttestationMode { 433 /** 434 * The person authenticated the content in their personal capacity. 435 */ 436 PERSONAL, 437 /** 438 * The person authenticated the content in their professional capacity. 439 */ 440 PROFESSIONAL, 441 /** 442 * The person authenticated the content and accepted legal responsibility for 443 * its content. 444 */ 445 LEGAL, 446 /** 447 * The organization authenticated the content as consistent with their policies 448 * and procedures. 449 */ 450 OFFICIAL, 451 /** 452 * added to help the parsers with the generic types 453 */ 454 NULL; 455 456 public static CompositionAttestationMode fromCode(String codeString) throws FHIRException { 457 if (codeString == null || "".equals(codeString)) 458 return null; 459 if ("personal".equals(codeString)) 460 return PERSONAL; 461 if ("professional".equals(codeString)) 462 return PROFESSIONAL; 463 if ("legal".equals(codeString)) 464 return LEGAL; 465 if ("official".equals(codeString)) 466 return OFFICIAL; 467 if (Configuration.isAcceptInvalidEnums()) 468 return null; 469 else 470 throw new FHIRException("Unknown CompositionAttestationMode code '" + codeString + "'"); 471 } 472 473 public String toCode() { 474 switch (this) { 475 case PERSONAL: 476 return "personal"; 477 case PROFESSIONAL: 478 return "professional"; 479 case LEGAL: 480 return "legal"; 481 case OFFICIAL: 482 return "official"; 483 case NULL: 484 return null; 485 default: 486 return "?"; 487 } 488 } 489 490 public String getSystem() { 491 switch (this) { 492 case PERSONAL: 493 return "http://hl7.org/fhir/composition-attestation-mode"; 494 case PROFESSIONAL: 495 return "http://hl7.org/fhir/composition-attestation-mode"; 496 case LEGAL: 497 return "http://hl7.org/fhir/composition-attestation-mode"; 498 case OFFICIAL: 499 return "http://hl7.org/fhir/composition-attestation-mode"; 500 case NULL: 501 return null; 502 default: 503 return "?"; 504 } 505 } 506 507 public String getDefinition() { 508 switch (this) { 509 case PERSONAL: 510 return "The person authenticated the content in their personal capacity."; 511 case PROFESSIONAL: 512 return "The person authenticated the content in their professional capacity."; 513 case LEGAL: 514 return "The person authenticated the content and accepted legal responsibility for its content."; 515 case OFFICIAL: 516 return "The organization authenticated the content as consistent with their policies and procedures."; 517 case NULL: 518 return null; 519 default: 520 return "?"; 521 } 522 } 523 524 public String getDisplay() { 525 switch (this) { 526 case PERSONAL: 527 return "Personal"; 528 case PROFESSIONAL: 529 return "Professional"; 530 case LEGAL: 531 return "Legal"; 532 case OFFICIAL: 533 return "Official"; 534 case NULL: 535 return null; 536 default: 537 return "?"; 538 } 539 } 540 } 541 542 public static class CompositionAttestationModeEnumFactory implements EnumFactory<CompositionAttestationMode> { 543 public CompositionAttestationMode fromCode(String codeString) throws IllegalArgumentException { 544 if (codeString == null || "".equals(codeString)) 545 if (codeString == null || "".equals(codeString)) 546 return null; 547 if ("personal".equals(codeString)) 548 return CompositionAttestationMode.PERSONAL; 549 if ("professional".equals(codeString)) 550 return CompositionAttestationMode.PROFESSIONAL; 551 if ("legal".equals(codeString)) 552 return CompositionAttestationMode.LEGAL; 553 if ("official".equals(codeString)) 554 return CompositionAttestationMode.OFFICIAL; 555 throw new IllegalArgumentException("Unknown CompositionAttestationMode code '" + codeString + "'"); 556 } 557 558 public Enumeration<CompositionAttestationMode> fromType(PrimitiveType<?> code) throws FHIRException { 559 if (code == null) 560 return null; 561 if (code.isEmpty()) 562 return new Enumeration<CompositionAttestationMode>(this, CompositionAttestationMode.NULL, code); 563 String codeString = code.asStringValue(); 564 if (codeString == null || "".equals(codeString)) 565 return new Enumeration<CompositionAttestationMode>(this, CompositionAttestationMode.NULL, code); 566 if ("personal".equals(codeString)) 567 return new Enumeration<CompositionAttestationMode>(this, CompositionAttestationMode.PERSONAL, code); 568 if ("professional".equals(codeString)) 569 return new Enumeration<CompositionAttestationMode>(this, CompositionAttestationMode.PROFESSIONAL, code); 570 if ("legal".equals(codeString)) 571 return new Enumeration<CompositionAttestationMode>(this, CompositionAttestationMode.LEGAL, code); 572 if ("official".equals(codeString)) 573 return new Enumeration<CompositionAttestationMode>(this, CompositionAttestationMode.OFFICIAL, code); 574 throw new FHIRException("Unknown CompositionAttestationMode code '" + codeString + "'"); 575 } 576 577 public String toCode(CompositionAttestationMode code) { 578 if (code == CompositionAttestationMode.NULL) 579 return null; 580 if (code == CompositionAttestationMode.PERSONAL) 581 return "personal"; 582 if (code == CompositionAttestationMode.PROFESSIONAL) 583 return "professional"; 584 if (code == CompositionAttestationMode.LEGAL) 585 return "legal"; 586 if (code == CompositionAttestationMode.OFFICIAL) 587 return "official"; 588 return "?"; 589 } 590 591 public String toSystem(CompositionAttestationMode code) { 592 return code.getSystem(); 593 } 594 } 595 596 public enum DocumentRelationshipType { 597 /** 598 * This document logically replaces or supersedes the target document. 599 */ 600 REPLACES, 601 /** 602 * This document was generated by transforming the target document (e.g. format 603 * or language conversion). 604 */ 605 TRANSFORMS, 606 /** 607 * This document is a signature of the target document. 608 */ 609 SIGNS, 610 /** 611 * This document adds additional information to the target document. 612 */ 613 APPENDS, 614 /** 615 * added to help the parsers with the generic types 616 */ 617 NULL; 618 619 public static DocumentRelationshipType fromCode(String codeString) throws FHIRException { 620 if (codeString == null || "".equals(codeString)) 621 return null; 622 if ("replaces".equals(codeString)) 623 return REPLACES; 624 if ("transforms".equals(codeString)) 625 return TRANSFORMS; 626 if ("signs".equals(codeString)) 627 return SIGNS; 628 if ("appends".equals(codeString)) 629 return APPENDS; 630 if (Configuration.isAcceptInvalidEnums()) 631 return null; 632 else 633 throw new FHIRException("Unknown DocumentRelationshipType code '" + codeString + "'"); 634 } 635 636 public String toCode() { 637 switch (this) { 638 case REPLACES: 639 return "replaces"; 640 case TRANSFORMS: 641 return "transforms"; 642 case SIGNS: 643 return "signs"; 644 case APPENDS: 645 return "appends"; 646 case NULL: 647 return null; 648 default: 649 return "?"; 650 } 651 } 652 653 public String getSystem() { 654 switch (this) { 655 case REPLACES: 656 return "http://hl7.org/fhir/document-relationship-type"; 657 case TRANSFORMS: 658 return "http://hl7.org/fhir/document-relationship-type"; 659 case SIGNS: 660 return "http://hl7.org/fhir/document-relationship-type"; 661 case APPENDS: 662 return "http://hl7.org/fhir/document-relationship-type"; 663 case NULL: 664 return null; 665 default: 666 return "?"; 667 } 668 } 669 670 public String getDefinition() { 671 switch (this) { 672 case REPLACES: 673 return "This document logically replaces or supersedes the target document."; 674 case TRANSFORMS: 675 return "This document was generated by transforming the target document (e.g. format or language conversion)."; 676 case SIGNS: 677 return "This document is a signature of the target document."; 678 case APPENDS: 679 return "This document adds additional information to the target document."; 680 case NULL: 681 return null; 682 default: 683 return "?"; 684 } 685 } 686 687 public String getDisplay() { 688 switch (this) { 689 case REPLACES: 690 return "Replaces"; 691 case TRANSFORMS: 692 return "Transforms"; 693 case SIGNS: 694 return "Signs"; 695 case APPENDS: 696 return "Appends"; 697 case NULL: 698 return null; 699 default: 700 return "?"; 701 } 702 } 703 } 704 705 public static class DocumentRelationshipTypeEnumFactory implements EnumFactory<DocumentRelationshipType> { 706 public DocumentRelationshipType fromCode(String codeString) throws IllegalArgumentException { 707 if (codeString == null || "".equals(codeString)) 708 if (codeString == null || "".equals(codeString)) 709 return null; 710 if ("replaces".equals(codeString)) 711 return DocumentRelationshipType.REPLACES; 712 if ("transforms".equals(codeString)) 713 return DocumentRelationshipType.TRANSFORMS; 714 if ("signs".equals(codeString)) 715 return DocumentRelationshipType.SIGNS; 716 if ("appends".equals(codeString)) 717 return DocumentRelationshipType.APPENDS; 718 throw new IllegalArgumentException("Unknown DocumentRelationshipType code '" + codeString + "'"); 719 } 720 721 public Enumeration<DocumentRelationshipType> fromType(PrimitiveType<?> code) throws FHIRException { 722 if (code == null) 723 return null; 724 if (code.isEmpty()) 725 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.NULL, code); 726 String codeString = code.asStringValue(); 727 if (codeString == null || "".equals(codeString)) 728 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.NULL, code); 729 if ("replaces".equals(codeString)) 730 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.REPLACES, code); 731 if ("transforms".equals(codeString)) 732 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.TRANSFORMS, code); 733 if ("signs".equals(codeString)) 734 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.SIGNS, code); 735 if ("appends".equals(codeString)) 736 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.APPENDS, code); 737 throw new FHIRException("Unknown DocumentRelationshipType code '" + codeString + "'"); 738 } 739 740 public String toCode(DocumentRelationshipType code) { 741 if (code == DocumentRelationshipType.NULL) 742 return null; 743 if (code == DocumentRelationshipType.REPLACES) 744 return "replaces"; 745 if (code == DocumentRelationshipType.TRANSFORMS) 746 return "transforms"; 747 if (code == DocumentRelationshipType.SIGNS) 748 return "signs"; 749 if (code == DocumentRelationshipType.APPENDS) 750 return "appends"; 751 return "?"; 752 } 753 754 public String toSystem(DocumentRelationshipType code) { 755 return code.getSystem(); 756 } 757 } 758 759 public enum SectionMode { 760 /** 761 * This list is the master list, maintained in an ongoing fashion with regular 762 * updates as the real world list it is tracking changes. 763 */ 764 WORKING, 765 /** 766 * This list was prepared as a snapshot. It should not be assumed to be current. 767 */ 768 SNAPSHOT, 769 /** 770 * A point-in-time list that shows what changes have been made or recommended. 771 * E.g. a discharge medication list showing what was added and removed during an 772 * encounter. 773 */ 774 CHANGES, 775 /** 776 * added to help the parsers with the generic types 777 */ 778 NULL; 779 780 public static SectionMode fromCode(String codeString) throws FHIRException { 781 if (codeString == null || "".equals(codeString)) 782 return null; 783 if ("working".equals(codeString)) 784 return WORKING; 785 if ("snapshot".equals(codeString)) 786 return SNAPSHOT; 787 if ("changes".equals(codeString)) 788 return CHANGES; 789 if (Configuration.isAcceptInvalidEnums()) 790 return null; 791 else 792 throw new FHIRException("Unknown SectionMode code '" + codeString + "'"); 793 } 794 795 public String toCode() { 796 switch (this) { 797 case WORKING: 798 return "working"; 799 case SNAPSHOT: 800 return "snapshot"; 801 case CHANGES: 802 return "changes"; 803 case NULL: 804 return null; 805 default: 806 return "?"; 807 } 808 } 809 810 public String getSystem() { 811 switch (this) { 812 case WORKING: 813 return "http://hl7.org/fhir/list-mode"; 814 case SNAPSHOT: 815 return "http://hl7.org/fhir/list-mode"; 816 case CHANGES: 817 return "http://hl7.org/fhir/list-mode"; 818 case NULL: 819 return null; 820 default: 821 return "?"; 822 } 823 } 824 825 public String getDefinition() { 826 switch (this) { 827 case WORKING: 828 return "This list is the master list, maintained in an ongoing fashion with regular updates as the real world list it is tracking changes."; 829 case SNAPSHOT: 830 return "This list was prepared as a snapshot. It should not be assumed to be current."; 831 case CHANGES: 832 return "A point-in-time list that shows what changes have been made or recommended. E.g. a discharge medication list showing what was added and removed during an encounter."; 833 case NULL: 834 return null; 835 default: 836 return "?"; 837 } 838 } 839 840 public String getDisplay() { 841 switch (this) { 842 case WORKING: 843 return "Working List"; 844 case SNAPSHOT: 845 return "Snapshot List"; 846 case CHANGES: 847 return "Change List"; 848 case NULL: 849 return null; 850 default: 851 return "?"; 852 } 853 } 854 } 855 856 public static class SectionModeEnumFactory implements EnumFactory<SectionMode> { 857 public SectionMode fromCode(String codeString) throws IllegalArgumentException { 858 if (codeString == null || "".equals(codeString)) 859 if (codeString == null || "".equals(codeString)) 860 return null; 861 if ("working".equals(codeString)) 862 return SectionMode.WORKING; 863 if ("snapshot".equals(codeString)) 864 return SectionMode.SNAPSHOT; 865 if ("changes".equals(codeString)) 866 return SectionMode.CHANGES; 867 throw new IllegalArgumentException("Unknown SectionMode code '" + codeString + "'"); 868 } 869 870 public Enumeration<SectionMode> fromType(PrimitiveType<?> code) throws FHIRException { 871 if (code == null) 872 return null; 873 if (code.isEmpty()) 874 return new Enumeration<SectionMode>(this, SectionMode.NULL, code); 875 String codeString = code.asStringValue(); 876 if (codeString == null || "".equals(codeString)) 877 return new Enumeration<SectionMode>(this, SectionMode.NULL, code); 878 if ("working".equals(codeString)) 879 return new Enumeration<SectionMode>(this, SectionMode.WORKING, code); 880 if ("snapshot".equals(codeString)) 881 return new Enumeration<SectionMode>(this, SectionMode.SNAPSHOT, code); 882 if ("changes".equals(codeString)) 883 return new Enumeration<SectionMode>(this, SectionMode.CHANGES, code); 884 throw new FHIRException("Unknown SectionMode code '" + codeString + "'"); 885 } 886 887 public String toCode(SectionMode code) { 888 if (code == SectionMode.NULL) 889 return null; 890 if (code == SectionMode.WORKING) 891 return "working"; 892 if (code == SectionMode.SNAPSHOT) 893 return "snapshot"; 894 if (code == SectionMode.CHANGES) 895 return "changes"; 896 return "?"; 897 } 898 899 public String toSystem(SectionMode code) { 900 return code.getSystem(); 901 } 902 } 903 904 @Block() 905 public static class CompositionAttesterComponent extends BackboneElement implements IBaseBackboneElement { 906 /** 907 * The type of attestation the authenticator offers. 908 */ 909 @Child(name = "mode", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 910 @Description(shortDefinition = "personal | professional | legal | official", formalDefinition = "The type of attestation the authenticator offers.") 911 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/composition-attestation-mode") 912 protected Enumeration<CompositionAttestationMode> mode; 913 914 /** 915 * When the composition was attested by the party. 916 */ 917 @Child(name = "time", type = { DateTimeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 918 @Description(shortDefinition = "When the composition was attested", formalDefinition = "When the composition was attested by the party.") 919 protected DateTimeType time; 920 921 /** 922 * Who attested the composition in the specified way. 923 */ 924 @Child(name = "party", type = { Patient.class, RelatedPerson.class, Practitioner.class, PractitionerRole.class, 925 Organization.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 926 @Description(shortDefinition = "Who attested the composition", formalDefinition = "Who attested the composition in the specified way.") 927 protected Reference party; 928 929 /** 930 * The actual object that is the target of the reference (Who attested the 931 * composition in the specified way.) 932 */ 933 protected Resource partyTarget; 934 935 private static final long serialVersionUID = -1917768205L; 936 937 /** 938 * Constructor 939 */ 940 public CompositionAttesterComponent() { 941 super(); 942 } 943 944 /** 945 * Constructor 946 */ 947 public CompositionAttesterComponent(Enumeration<CompositionAttestationMode> mode) { 948 super(); 949 this.mode = mode; 950 } 951 952 /** 953 * @return {@link #mode} (The type of attestation the authenticator offers.). 954 * This is the underlying object with id, value and extensions. The 955 * accessor "getMode" gives direct access to the value 956 */ 957 public Enumeration<CompositionAttestationMode> getModeElement() { 958 if (this.mode == null) 959 if (Configuration.errorOnAutoCreate()) 960 throw new Error("Attempt to auto-create CompositionAttesterComponent.mode"); 961 else if (Configuration.doAutoCreate()) 962 this.mode = new Enumeration<CompositionAttestationMode>(new CompositionAttestationModeEnumFactory()); // bb 963 return this.mode; 964 } 965 966 public boolean hasModeElement() { 967 return this.mode != null && !this.mode.isEmpty(); 968 } 969 970 public boolean hasMode() { 971 return this.mode != null && !this.mode.isEmpty(); 972 } 973 974 /** 975 * @param value {@link #mode} (The type of attestation the authenticator 976 * offers.). This is the underlying object with id, value and 977 * extensions. The accessor "getMode" gives direct access to the 978 * value 979 */ 980 public CompositionAttesterComponent setModeElement(Enumeration<CompositionAttestationMode> value) { 981 this.mode = value; 982 return this; 983 } 984 985 /** 986 * @return The type of attestation the authenticator offers. 987 */ 988 public CompositionAttestationMode getMode() { 989 return this.mode == null ? null : this.mode.getValue(); 990 } 991 992 /** 993 * @param value The type of attestation the authenticator offers. 994 */ 995 public CompositionAttesterComponent setMode(CompositionAttestationMode value) { 996 if (this.mode == null) 997 this.mode = new Enumeration<CompositionAttestationMode>(new CompositionAttestationModeEnumFactory()); 998 this.mode.setValue(value); 999 return this; 1000 } 1001 1002 /** 1003 * @return {@link #time} (When the composition was attested by the party.). This 1004 * is the underlying object with id, value and extensions. The accessor 1005 * "getTime" gives direct access to the value 1006 */ 1007 public DateTimeType getTimeElement() { 1008 if (this.time == null) 1009 if (Configuration.errorOnAutoCreate()) 1010 throw new Error("Attempt to auto-create CompositionAttesterComponent.time"); 1011 else if (Configuration.doAutoCreate()) 1012 this.time = new DateTimeType(); // bb 1013 return this.time; 1014 } 1015 1016 public boolean hasTimeElement() { 1017 return this.time != null && !this.time.isEmpty(); 1018 } 1019 1020 public boolean hasTime() { 1021 return this.time != null && !this.time.isEmpty(); 1022 } 1023 1024 /** 1025 * @param value {@link #time} (When the composition was attested by the party.). 1026 * This is the underlying object with id, value and extensions. The 1027 * accessor "getTime" gives direct access to the value 1028 */ 1029 public CompositionAttesterComponent setTimeElement(DateTimeType value) { 1030 this.time = value; 1031 return this; 1032 } 1033 1034 /** 1035 * @return When the composition was attested by the party. 1036 */ 1037 public Date getTime() { 1038 return this.time == null ? null : this.time.getValue(); 1039 } 1040 1041 /** 1042 * @param value When the composition was attested by the party. 1043 */ 1044 public CompositionAttesterComponent setTime(Date value) { 1045 if (value == null) 1046 this.time = null; 1047 else { 1048 if (this.time == null) 1049 this.time = new DateTimeType(); 1050 this.time.setValue(value); 1051 } 1052 return this; 1053 } 1054 1055 /** 1056 * @return {@link #party} (Who attested the composition in the specified way.) 1057 */ 1058 public Reference getParty() { 1059 if (this.party == null) 1060 if (Configuration.errorOnAutoCreate()) 1061 throw new Error("Attempt to auto-create CompositionAttesterComponent.party"); 1062 else if (Configuration.doAutoCreate()) 1063 this.party = new Reference(); // cc 1064 return this.party; 1065 } 1066 1067 public boolean hasParty() { 1068 return this.party != null && !this.party.isEmpty(); 1069 } 1070 1071 /** 1072 * @param value {@link #party} (Who attested the composition in the specified 1073 * way.) 1074 */ 1075 public CompositionAttesterComponent setParty(Reference value) { 1076 this.party = value; 1077 return this; 1078 } 1079 1080 /** 1081 * @return {@link #party} The actual object that is the target of the reference. 1082 * The reference library doesn't populate this, but you can use it to 1083 * hold the resource if you resolve it. (Who attested the composition in 1084 * the specified way.) 1085 */ 1086 public Resource getPartyTarget() { 1087 return this.partyTarget; 1088 } 1089 1090 /** 1091 * @param value {@link #party} The actual object that is the target of the 1092 * reference. The reference library doesn't use these, but you can 1093 * use it to hold the resource if you resolve it. (Who attested the 1094 * composition in the specified way.) 1095 */ 1096 public CompositionAttesterComponent setPartyTarget(Resource value) { 1097 this.partyTarget = value; 1098 return this; 1099 } 1100 1101 protected void listChildren(List<Property> children) { 1102 super.listChildren(children); 1103 children.add(new Property("mode", "code", "The type of attestation the authenticator offers.", 0, 1, mode)); 1104 children.add(new Property("time", "dateTime", "When the composition was attested by the party.", 0, 1, time)); 1105 children.add(new Property("party", "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Organization)", 1106 "Who attested the composition in the specified way.", 0, 1, party)); 1107 } 1108 1109 @Override 1110 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1111 switch (_hash) { 1112 case 3357091: 1113 /* mode */ return new Property("mode", "code", "The type of attestation the authenticator offers.", 0, 1, mode); 1114 case 3560141: 1115 /* time */ return new Property("time", "dateTime", "When the composition was attested by the party.", 0, 1, 1116 time); 1117 case 106437350: 1118 /* party */ return new Property("party", 1119 "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Organization)", 1120 "Who attested the composition in the specified way.", 0, 1, party); 1121 default: 1122 return super.getNamedProperty(_hash, _name, _checkValid); 1123 } 1124 1125 } 1126 1127 @Override 1128 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1129 switch (hash) { 1130 case 3357091: 1131 /* mode */ return this.mode == null ? new Base[0] : new Base[] { this.mode }; // Enumeration<CompositionAttestationMode> 1132 case 3560141: 1133 /* time */ return this.time == null ? new Base[0] : new Base[] { this.time }; // DateTimeType 1134 case 106437350: 1135 /* party */ return this.party == null ? new Base[0] : new Base[] { this.party }; // Reference 1136 default: 1137 return super.getProperty(hash, name, checkValid); 1138 } 1139 1140 } 1141 1142 @Override 1143 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1144 switch (hash) { 1145 case 3357091: // mode 1146 value = new CompositionAttestationModeEnumFactory().fromType(castToCode(value)); 1147 this.mode = (Enumeration) value; // Enumeration<CompositionAttestationMode> 1148 return value; 1149 case 3560141: // time 1150 this.time = castToDateTime(value); // DateTimeType 1151 return value; 1152 case 106437350: // party 1153 this.party = castToReference(value); // Reference 1154 return value; 1155 default: 1156 return super.setProperty(hash, name, value); 1157 } 1158 1159 } 1160 1161 @Override 1162 public Base setProperty(String name, Base value) throws FHIRException { 1163 if (name.equals("mode")) { 1164 value = new CompositionAttestationModeEnumFactory().fromType(castToCode(value)); 1165 this.mode = (Enumeration) value; // Enumeration<CompositionAttestationMode> 1166 } else if (name.equals("time")) { 1167 this.time = castToDateTime(value); // DateTimeType 1168 } else if (name.equals("party")) { 1169 this.party = castToReference(value); // Reference 1170 } else 1171 return super.setProperty(name, value); 1172 return value; 1173 } 1174 1175 @Override 1176 public void removeChild(String name, Base value) throws FHIRException { 1177 if (name.equals("mode")) { 1178 this.mode = null; 1179 } else if (name.equals("time")) { 1180 this.time = null; 1181 } else if (name.equals("party")) { 1182 this.party = null; 1183 } else 1184 super.removeChild(name, value); 1185 1186 } 1187 1188 @Override 1189 public Base makeProperty(int hash, String name) throws FHIRException { 1190 switch (hash) { 1191 case 3357091: 1192 return getModeElement(); 1193 case 3560141: 1194 return getTimeElement(); 1195 case 106437350: 1196 return getParty(); 1197 default: 1198 return super.makeProperty(hash, name); 1199 } 1200 1201 } 1202 1203 @Override 1204 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1205 switch (hash) { 1206 case 3357091: 1207 /* mode */ return new String[] { "code" }; 1208 case 3560141: 1209 /* time */ return new String[] { "dateTime" }; 1210 case 106437350: 1211 /* party */ return new String[] { "Reference" }; 1212 default: 1213 return super.getTypesForProperty(hash, name); 1214 } 1215 1216 } 1217 1218 @Override 1219 public Base addChild(String name) throws FHIRException { 1220 if (name.equals("mode")) { 1221 throw new FHIRException("Cannot call addChild on a singleton property Composition.mode"); 1222 } else if (name.equals("time")) { 1223 throw new FHIRException("Cannot call addChild on a singleton property Composition.time"); 1224 } else if (name.equals("party")) { 1225 this.party = new Reference(); 1226 return this.party; 1227 } else 1228 return super.addChild(name); 1229 } 1230 1231 public CompositionAttesterComponent copy() { 1232 CompositionAttesterComponent dst = new CompositionAttesterComponent(); 1233 copyValues(dst); 1234 return dst; 1235 } 1236 1237 public void copyValues(CompositionAttesterComponent dst) { 1238 super.copyValues(dst); 1239 dst.mode = mode == null ? null : mode.copy(); 1240 dst.time = time == null ? null : time.copy(); 1241 dst.party = party == null ? null : party.copy(); 1242 } 1243 1244 @Override 1245 public boolean equalsDeep(Base other_) { 1246 if (!super.equalsDeep(other_)) 1247 return false; 1248 if (!(other_ instanceof CompositionAttesterComponent)) 1249 return false; 1250 CompositionAttesterComponent o = (CompositionAttesterComponent) other_; 1251 return compareDeep(mode, o.mode, true) && compareDeep(time, o.time, true) && compareDeep(party, o.party, true); 1252 } 1253 1254 @Override 1255 public boolean equalsShallow(Base other_) { 1256 if (!super.equalsShallow(other_)) 1257 return false; 1258 if (!(other_ instanceof CompositionAttesterComponent)) 1259 return false; 1260 CompositionAttesterComponent o = (CompositionAttesterComponent) other_; 1261 return compareValues(mode, o.mode, true) && compareValues(time, o.time, true); 1262 } 1263 1264 public boolean isEmpty() { 1265 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(mode, time, party); 1266 } 1267 1268 public String fhirType() { 1269 return "Composition.attester"; 1270 1271 } 1272 1273 } 1274 1275 @Block() 1276 public static class CompositionRelatesToComponent extends BackboneElement implements IBaseBackboneElement { 1277 /** 1278 * The type of relationship that this composition has with anther composition or 1279 * document. 1280 */ 1281 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1282 @Description(shortDefinition = "replaces | transforms | signs | appends", formalDefinition = "The type of relationship that this composition has with anther composition or document.") 1283 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/document-relationship-type") 1284 protected Enumeration<DocumentRelationshipType> code; 1285 1286 /** 1287 * The target composition/document of this relationship. 1288 */ 1289 @Child(name = "target", type = { Identifier.class, 1290 Composition.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 1291 @Description(shortDefinition = "Target of the relationship", formalDefinition = "The target composition/document of this relationship.") 1292 protected Type target; 1293 1294 private static final long serialVersionUID = 1536930280L; 1295 1296 /** 1297 * Constructor 1298 */ 1299 public CompositionRelatesToComponent() { 1300 super(); 1301 } 1302 1303 /** 1304 * Constructor 1305 */ 1306 public CompositionRelatesToComponent(Enumeration<DocumentRelationshipType> code, Type target) { 1307 super(); 1308 this.code = code; 1309 this.target = target; 1310 } 1311 1312 /** 1313 * @return {@link #code} (The type of relationship that this composition has 1314 * with anther composition or document.). This is the underlying object 1315 * with id, value and extensions. The accessor "getCode" gives direct 1316 * access to the value 1317 */ 1318 public Enumeration<DocumentRelationshipType> getCodeElement() { 1319 if (this.code == null) 1320 if (Configuration.errorOnAutoCreate()) 1321 throw new Error("Attempt to auto-create CompositionRelatesToComponent.code"); 1322 else if (Configuration.doAutoCreate()) 1323 this.code = new Enumeration<DocumentRelationshipType>(new DocumentRelationshipTypeEnumFactory()); // bb 1324 return this.code; 1325 } 1326 1327 public boolean hasCodeElement() { 1328 return this.code != null && !this.code.isEmpty(); 1329 } 1330 1331 public boolean hasCode() { 1332 return this.code != null && !this.code.isEmpty(); 1333 } 1334 1335 /** 1336 * @param value {@link #code} (The type of relationship that this composition 1337 * has with anther composition or document.). This is the 1338 * underlying object with id, value and extensions. The accessor 1339 * "getCode" gives direct access to the value 1340 */ 1341 public CompositionRelatesToComponent setCodeElement(Enumeration<DocumentRelationshipType> value) { 1342 this.code = value; 1343 return this; 1344 } 1345 1346 /** 1347 * @return The type of relationship that this composition has with anther 1348 * composition or document. 1349 */ 1350 public DocumentRelationshipType getCode() { 1351 return this.code == null ? null : this.code.getValue(); 1352 } 1353 1354 /** 1355 * @param value The type of relationship that this composition has with anther 1356 * composition or document. 1357 */ 1358 public CompositionRelatesToComponent setCode(DocumentRelationshipType value) { 1359 if (this.code == null) 1360 this.code = new Enumeration<DocumentRelationshipType>(new DocumentRelationshipTypeEnumFactory()); 1361 this.code.setValue(value); 1362 return this; 1363 } 1364 1365 /** 1366 * @return {@link #target} (The target composition/document of this 1367 * relationship.) 1368 */ 1369 public Type getTarget() { 1370 return this.target; 1371 } 1372 1373 /** 1374 * @return {@link #target} (The target composition/document of this 1375 * relationship.) 1376 */ 1377 public Identifier getTargetIdentifier() throws FHIRException { 1378 if (this.target == null) 1379 this.target = new Identifier(); 1380 if (!(this.target instanceof Identifier)) 1381 throw new FHIRException("Type mismatch: the type Identifier was expected, but " 1382 + this.target.getClass().getName() + " was encountered"); 1383 return (Identifier) this.target; 1384 } 1385 1386 public boolean hasTargetIdentifier() { 1387 return this != null && this.target instanceof Identifier; 1388 } 1389 1390 /** 1391 * @return {@link #target} (The target composition/document of this 1392 * relationship.) 1393 */ 1394 public Reference getTargetReference() throws FHIRException { 1395 if (this.target == null) 1396 this.target = new Reference(); 1397 if (!(this.target instanceof Reference)) 1398 throw new FHIRException("Type mismatch: the type Reference was expected, but " 1399 + this.target.getClass().getName() + " was encountered"); 1400 return (Reference) this.target; 1401 } 1402 1403 public boolean hasTargetReference() { 1404 return this != null && this.target instanceof Reference; 1405 } 1406 1407 public boolean hasTarget() { 1408 return this.target != null && !this.target.isEmpty(); 1409 } 1410 1411 /** 1412 * @param value {@link #target} (The target composition/document of this 1413 * relationship.) 1414 */ 1415 public CompositionRelatesToComponent setTarget(Type value) { 1416 if (value != null && !(value instanceof Identifier || value instanceof Reference)) 1417 throw new Error("Not the right type for Composition.relatesTo.target[x]: " + value.fhirType()); 1418 this.target = value; 1419 return this; 1420 } 1421 1422 protected void listChildren(List<Property> children) { 1423 super.listChildren(children); 1424 children.add(new Property("code", "code", 1425 "The type of relationship that this composition has with anther composition or document.", 0, 1, code)); 1426 children.add(new Property("target[x]", "Identifier|Reference(Composition)", 1427 "The target composition/document of this relationship.", 0, 1, target)); 1428 } 1429 1430 @Override 1431 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1432 switch (_hash) { 1433 case 3059181: 1434 /* code */ return new Property("code", "code", 1435 "The type of relationship that this composition has with anther composition or document.", 0, 1, code); 1436 case -815579825: 1437 /* target[x] */ return new Property("target[x]", "Identifier|Reference(Composition)", 1438 "The target composition/document of this relationship.", 0, 1, target); 1439 case -880905839: 1440 /* target */ return new Property("target[x]", "Identifier|Reference(Composition)", 1441 "The target composition/document of this relationship.", 0, 1, target); 1442 case 1690892570: 1443 /* targetIdentifier */ return new Property("target[x]", "Identifier|Reference(Composition)", 1444 "The target composition/document of this relationship.", 0, 1, target); 1445 case 1259806906: 1446 /* targetReference */ return new Property("target[x]", "Identifier|Reference(Composition)", 1447 "The target composition/document of this relationship.", 0, 1, target); 1448 default: 1449 return super.getNamedProperty(_hash, _name, _checkValid); 1450 } 1451 1452 } 1453 1454 @Override 1455 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1456 switch (hash) { 1457 case 3059181: 1458 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // Enumeration<DocumentRelationshipType> 1459 case -880905839: 1460 /* target */ return this.target == null ? new Base[0] : new Base[] { this.target }; // Type 1461 default: 1462 return super.getProperty(hash, name, checkValid); 1463 } 1464 1465 } 1466 1467 @Override 1468 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1469 switch (hash) { 1470 case 3059181: // code 1471 value = new DocumentRelationshipTypeEnumFactory().fromType(castToCode(value)); 1472 this.code = (Enumeration) value; // Enumeration<DocumentRelationshipType> 1473 return value; 1474 case -880905839: // target 1475 this.target = castToType(value); // Type 1476 return value; 1477 default: 1478 return super.setProperty(hash, name, value); 1479 } 1480 1481 } 1482 1483 @Override 1484 public Base setProperty(String name, Base value) throws FHIRException { 1485 if (name.equals("code")) { 1486 value = new DocumentRelationshipTypeEnumFactory().fromType(castToCode(value)); 1487 this.code = (Enumeration) value; // Enumeration<DocumentRelationshipType> 1488 } else if (name.equals("target[x]")) { 1489 this.target = castToType(value); // Type 1490 } else 1491 return super.setProperty(name, value); 1492 return value; 1493 } 1494 1495 @Override 1496 public void removeChild(String name, Base value) throws FHIRException { 1497 if (name.equals("code")) { 1498 this.code = null; 1499 } else if (name.equals("target[x]")) { 1500 this.target = null; 1501 } else 1502 super.removeChild(name, value); 1503 1504 } 1505 1506 @Override 1507 public Base makeProperty(int hash, String name) throws FHIRException { 1508 switch (hash) { 1509 case 3059181: 1510 return getCodeElement(); 1511 case -815579825: 1512 return getTarget(); 1513 case -880905839: 1514 return getTarget(); 1515 default: 1516 return super.makeProperty(hash, name); 1517 } 1518 1519 } 1520 1521 @Override 1522 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1523 switch (hash) { 1524 case 3059181: 1525 /* code */ return new String[] { "code" }; 1526 case -880905839: 1527 /* target */ return new String[] { "Identifier", "Reference" }; 1528 default: 1529 return super.getTypesForProperty(hash, name); 1530 } 1531 1532 } 1533 1534 @Override 1535 public Base addChild(String name) throws FHIRException { 1536 if (name.equals("code")) { 1537 throw new FHIRException("Cannot call addChild on a singleton property Composition.code"); 1538 } else if (name.equals("targetIdentifier")) { 1539 this.target = new Identifier(); 1540 return this.target; 1541 } else if (name.equals("targetReference")) { 1542 this.target = new Reference(); 1543 return this.target; 1544 } else 1545 return super.addChild(name); 1546 } 1547 1548 public CompositionRelatesToComponent copy() { 1549 CompositionRelatesToComponent dst = new CompositionRelatesToComponent(); 1550 copyValues(dst); 1551 return dst; 1552 } 1553 1554 public void copyValues(CompositionRelatesToComponent dst) { 1555 super.copyValues(dst); 1556 dst.code = code == null ? null : code.copy(); 1557 dst.target = target == null ? null : target.copy(); 1558 } 1559 1560 @Override 1561 public boolean equalsDeep(Base other_) { 1562 if (!super.equalsDeep(other_)) 1563 return false; 1564 if (!(other_ instanceof CompositionRelatesToComponent)) 1565 return false; 1566 CompositionRelatesToComponent o = (CompositionRelatesToComponent) other_; 1567 return compareDeep(code, o.code, true) && compareDeep(target, o.target, true); 1568 } 1569 1570 @Override 1571 public boolean equalsShallow(Base other_) { 1572 if (!super.equalsShallow(other_)) 1573 return false; 1574 if (!(other_ instanceof CompositionRelatesToComponent)) 1575 return false; 1576 CompositionRelatesToComponent o = (CompositionRelatesToComponent) other_; 1577 return compareValues(code, o.code, true); 1578 } 1579 1580 public boolean isEmpty() { 1581 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, target); 1582 } 1583 1584 public String fhirType() { 1585 return "Composition.relatesTo"; 1586 1587 } 1588 1589 } 1590 1591 @Block() 1592 public static class CompositionEventComponent extends BackboneElement implements IBaseBackboneElement { 1593 /** 1594 * This list of codes represents the main clinical acts, such as a colonoscopy 1595 * or an appendectomy, being documented. In some cases, the event is inherent in 1596 * the typeCode, such as a "History and Physical Report" in which the procedure 1597 * being documented is necessarily a "History and Physical" act. 1598 */ 1599 @Child(name = "code", type = { 1600 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1601 @Description(shortDefinition = "Code(s) that apply to the event being documented", formalDefinition = "This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the typeCode, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act.") 1602 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ActCode") 1603 protected List<CodeableConcept> code; 1604 1605 /** 1606 * The period of time covered by the documentation. There is no assertion that 1607 * the documentation is a complete representation for this period, only that it 1608 * documents events during this time. 1609 */ 1610 @Child(name = "period", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1611 @Description(shortDefinition = "The period covered by the documentation", formalDefinition = "The period of time covered by the documentation. There is no assertion that the documentation is a complete representation for this period, only that it documents events during this time.") 1612 protected Period period; 1613 1614 /** 1615 * The description and/or reference of the event(s) being documented. For 1616 * example, this could be used to document such a colonoscopy or an 1617 * appendectomy. 1618 */ 1619 @Child(name = "detail", type = { 1620 Reference.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1621 @Description(shortDefinition = "The event(s) being documented", formalDefinition = "The description and/or reference of the event(s) being documented. For example, this could be used to document such a colonoscopy or an appendectomy.") 1622 protected List<Reference> detail; 1623 /** 1624 * The actual objects that are the target of the reference (The description 1625 * and/or reference of the event(s) being documented. For example, this could be 1626 * used to document such a colonoscopy or an appendectomy.) 1627 */ 1628 protected List<Resource> detailTarget; 1629 1630 private static final long serialVersionUID = -1581379774L; 1631 1632 /** 1633 * Constructor 1634 */ 1635 public CompositionEventComponent() { 1636 super(); 1637 } 1638 1639 /** 1640 * @return {@link #code} (This list of codes represents the main clinical acts, 1641 * such as a colonoscopy or an appendectomy, being documented. In some 1642 * cases, the event is inherent in the typeCode, such as a "History and 1643 * Physical Report" in which the procedure being documented is 1644 * necessarily a "History and Physical" act.) 1645 */ 1646 public List<CodeableConcept> getCode() { 1647 if (this.code == null) 1648 this.code = new ArrayList<CodeableConcept>(); 1649 return this.code; 1650 } 1651 1652 /** 1653 * @return Returns a reference to <code>this</code> for easy method chaining 1654 */ 1655 public CompositionEventComponent setCode(List<CodeableConcept> theCode) { 1656 this.code = theCode; 1657 return this; 1658 } 1659 1660 public boolean hasCode() { 1661 if (this.code == null) 1662 return false; 1663 for (CodeableConcept item : this.code) 1664 if (!item.isEmpty()) 1665 return true; 1666 return false; 1667 } 1668 1669 public CodeableConcept addCode() { // 3 1670 CodeableConcept t = new CodeableConcept(); 1671 if (this.code == null) 1672 this.code = new ArrayList<CodeableConcept>(); 1673 this.code.add(t); 1674 return t; 1675 } 1676 1677 public CompositionEventComponent addCode(CodeableConcept t) { // 3 1678 if (t == null) 1679 return this; 1680 if (this.code == null) 1681 this.code = new ArrayList<CodeableConcept>(); 1682 this.code.add(t); 1683 return this; 1684 } 1685 1686 /** 1687 * @return The first repetition of repeating field {@link #code}, creating it if 1688 * it does not already exist 1689 */ 1690 public CodeableConcept getCodeFirstRep() { 1691 if (getCode().isEmpty()) { 1692 addCode(); 1693 } 1694 return getCode().get(0); 1695 } 1696 1697 /** 1698 * @return {@link #period} (The period of time covered by the documentation. 1699 * There is no assertion that the documentation is a complete 1700 * representation for this period, only that it documents events during 1701 * this time.) 1702 */ 1703 public Period getPeriod() { 1704 if (this.period == null) 1705 if (Configuration.errorOnAutoCreate()) 1706 throw new Error("Attempt to auto-create CompositionEventComponent.period"); 1707 else if (Configuration.doAutoCreate()) 1708 this.period = new Period(); // cc 1709 return this.period; 1710 } 1711 1712 public boolean hasPeriod() { 1713 return this.period != null && !this.period.isEmpty(); 1714 } 1715 1716 /** 1717 * @param value {@link #period} (The period of time covered by the 1718 * documentation. There is no assertion that the documentation is a 1719 * complete representation for this period, only that it documents 1720 * events during this time.) 1721 */ 1722 public CompositionEventComponent setPeriod(Period value) { 1723 this.period = value; 1724 return this; 1725 } 1726 1727 /** 1728 * @return {@link #detail} (The description and/or reference of the event(s) 1729 * being documented. For example, this could be used to document such a 1730 * colonoscopy or an appendectomy.) 1731 */ 1732 public List<Reference> getDetail() { 1733 if (this.detail == null) 1734 this.detail = new ArrayList<Reference>(); 1735 return this.detail; 1736 } 1737 1738 /** 1739 * @return Returns a reference to <code>this</code> for easy method chaining 1740 */ 1741 public CompositionEventComponent setDetail(List<Reference> theDetail) { 1742 this.detail = theDetail; 1743 return this; 1744 } 1745 1746 public boolean hasDetail() { 1747 if (this.detail == null) 1748 return false; 1749 for (Reference item : this.detail) 1750 if (!item.isEmpty()) 1751 return true; 1752 return false; 1753 } 1754 1755 public Reference addDetail() { // 3 1756 Reference t = new Reference(); 1757 if (this.detail == null) 1758 this.detail = new ArrayList<Reference>(); 1759 this.detail.add(t); 1760 return t; 1761 } 1762 1763 public CompositionEventComponent addDetail(Reference t) { // 3 1764 if (t == null) 1765 return this; 1766 if (this.detail == null) 1767 this.detail = new ArrayList<Reference>(); 1768 this.detail.add(t); 1769 return this; 1770 } 1771 1772 /** 1773 * @return The first repetition of repeating field {@link #detail}, creating it 1774 * if it does not already exist 1775 */ 1776 public Reference getDetailFirstRep() { 1777 if (getDetail().isEmpty()) { 1778 addDetail(); 1779 } 1780 return getDetail().get(0); 1781 } 1782 1783 /** 1784 * @deprecated Use Reference#setResource(IBaseResource) instead 1785 */ 1786 @Deprecated 1787 public List<Resource> getDetailTarget() { 1788 if (this.detailTarget == null) 1789 this.detailTarget = new ArrayList<Resource>(); 1790 return this.detailTarget; 1791 } 1792 1793 protected void listChildren(List<Property> children) { 1794 super.listChildren(children); 1795 children.add(new Property("code", "CodeableConcept", 1796 "This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the typeCode, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act.", 1797 0, java.lang.Integer.MAX_VALUE, code)); 1798 children.add(new Property("period", "Period", 1799 "The period of time covered by the documentation. There is no assertion that the documentation is a complete representation for this period, only that it documents events during this time.", 1800 0, 1, period)); 1801 children.add(new Property("detail", "Reference(Any)", 1802 "The description and/or reference of the event(s) being documented. For example, this could be used to document such a colonoscopy or an appendectomy.", 1803 0, java.lang.Integer.MAX_VALUE, detail)); 1804 } 1805 1806 @Override 1807 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1808 switch (_hash) { 1809 case 3059181: 1810 /* code */ return new Property("code", "CodeableConcept", 1811 "This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the typeCode, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act.", 1812 0, java.lang.Integer.MAX_VALUE, code); 1813 case -991726143: 1814 /* period */ return new Property("period", "Period", 1815 "The period of time covered by the documentation. There is no assertion that the documentation is a complete representation for this period, only that it documents events during this time.", 1816 0, 1, period); 1817 case -1335224239: 1818 /* detail */ return new Property("detail", "Reference(Any)", 1819 "The description and/or reference of the event(s) being documented. For example, this could be used to document such a colonoscopy or an appendectomy.", 1820 0, java.lang.Integer.MAX_VALUE, detail); 1821 default: 1822 return super.getNamedProperty(_hash, _name, _checkValid); 1823 } 1824 1825 } 1826 1827 @Override 1828 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1829 switch (hash) { 1830 case 3059181: 1831 /* code */ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 1832 case -991726143: 1833 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 1834 case -1335224239: 1835 /* detail */ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // Reference 1836 default: 1837 return super.getProperty(hash, name, checkValid); 1838 } 1839 1840 } 1841 1842 @Override 1843 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1844 switch (hash) { 1845 case 3059181: // code 1846 this.getCode().add(castToCodeableConcept(value)); // CodeableConcept 1847 return value; 1848 case -991726143: // period 1849 this.period = castToPeriod(value); // Period 1850 return value; 1851 case -1335224239: // detail 1852 this.getDetail().add(castToReference(value)); // Reference 1853 return value; 1854 default: 1855 return super.setProperty(hash, name, value); 1856 } 1857 1858 } 1859 1860 @Override 1861 public Base setProperty(String name, Base value) throws FHIRException { 1862 if (name.equals("code")) { 1863 this.getCode().add(castToCodeableConcept(value)); 1864 } else if (name.equals("period")) { 1865 this.period = castToPeriod(value); // Period 1866 } else if (name.equals("detail")) { 1867 this.getDetail().add(castToReference(value)); 1868 } else 1869 return super.setProperty(name, value); 1870 return value; 1871 } 1872 1873 @Override 1874 public void removeChild(String name, Base value) throws FHIRException { 1875 if (name.equals("code")) { 1876 this.getCode().remove(castToCodeableConcept(value)); 1877 } else if (name.equals("period")) { 1878 this.period = null; 1879 } else if (name.equals("detail")) { 1880 this.getDetail().remove(castToReference(value)); 1881 } else 1882 super.removeChild(name, value); 1883 1884 } 1885 1886 @Override 1887 public Base makeProperty(int hash, String name) throws FHIRException { 1888 switch (hash) { 1889 case 3059181: 1890 return addCode(); 1891 case -991726143: 1892 return getPeriod(); 1893 case -1335224239: 1894 return addDetail(); 1895 default: 1896 return super.makeProperty(hash, name); 1897 } 1898 1899 } 1900 1901 @Override 1902 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1903 switch (hash) { 1904 case 3059181: 1905 /* code */ return new String[] { "CodeableConcept" }; 1906 case -991726143: 1907 /* period */ return new String[] { "Period" }; 1908 case -1335224239: 1909 /* detail */ return new String[] { "Reference" }; 1910 default: 1911 return super.getTypesForProperty(hash, name); 1912 } 1913 1914 } 1915 1916 @Override 1917 public Base addChild(String name) throws FHIRException { 1918 if (name.equals("code")) { 1919 return addCode(); 1920 } else if (name.equals("period")) { 1921 this.period = new Period(); 1922 return this.period; 1923 } else if (name.equals("detail")) { 1924 return addDetail(); 1925 } else 1926 return super.addChild(name); 1927 } 1928 1929 public CompositionEventComponent copy() { 1930 CompositionEventComponent dst = new CompositionEventComponent(); 1931 copyValues(dst); 1932 return dst; 1933 } 1934 1935 public void copyValues(CompositionEventComponent dst) { 1936 super.copyValues(dst); 1937 if (code != null) { 1938 dst.code = new ArrayList<CodeableConcept>(); 1939 for (CodeableConcept i : code) 1940 dst.code.add(i.copy()); 1941 } 1942 ; 1943 dst.period = period == null ? null : period.copy(); 1944 if (detail != null) { 1945 dst.detail = new ArrayList<Reference>(); 1946 for (Reference i : detail) 1947 dst.detail.add(i.copy()); 1948 } 1949 ; 1950 } 1951 1952 @Override 1953 public boolean equalsDeep(Base other_) { 1954 if (!super.equalsDeep(other_)) 1955 return false; 1956 if (!(other_ instanceof CompositionEventComponent)) 1957 return false; 1958 CompositionEventComponent o = (CompositionEventComponent) other_; 1959 return compareDeep(code, o.code, true) && compareDeep(period, o.period, true) 1960 && compareDeep(detail, o.detail, true); 1961 } 1962 1963 @Override 1964 public boolean equalsShallow(Base other_) { 1965 if (!super.equalsShallow(other_)) 1966 return false; 1967 if (!(other_ instanceof CompositionEventComponent)) 1968 return false; 1969 CompositionEventComponent o = (CompositionEventComponent) other_; 1970 return true; 1971 } 1972 1973 public boolean isEmpty() { 1974 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, period, detail); 1975 } 1976 1977 public String fhirType() { 1978 return "Composition.event"; 1979 1980 } 1981 1982 } 1983 1984 @Block() 1985 public static class SectionComponent extends BackboneElement implements IBaseBackboneElement { 1986 /** 1987 * The label for this particular section. This will be part of the rendered 1988 * content for the document, and is often used to build a table of contents. 1989 */ 1990 @Child(name = "title", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1991 @Description(shortDefinition = "Label for section (e.g. for ToC)", formalDefinition = "The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents.") 1992 protected StringType title; 1993 1994 /** 1995 * A code identifying the kind of content contained within the section. This 1996 * must be consistent with the section title. 1997 */ 1998 @Child(name = "code", type = { 1999 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2000 @Description(shortDefinition = "Classification of section (recommended)", formalDefinition = "A code identifying the kind of content contained within the section. This must be consistent with the section title.") 2001 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/doc-section-codes") 2002 protected CodeableConcept code; 2003 2004 /** 2005 * Identifies who is responsible for the information in this section, not 2006 * necessarily who typed it in. 2007 */ 2008 @Child(name = "author", type = { Practitioner.class, PractitionerRole.class, Device.class, Patient.class, 2009 RelatedPerson.class, 2010 Organization.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2011 @Description(shortDefinition = "Who and/or what authored the section", formalDefinition = "Identifies who is responsible for the information in this section, not necessarily who typed it in.") 2012 protected List<Reference> author; 2013 /** 2014 * The actual objects that are the target of the reference (Identifies who is 2015 * responsible for the information in this section, not necessarily who typed it 2016 * in.) 2017 */ 2018 protected List<Resource> authorTarget; 2019 2020 /** 2021 * The actual focus of the section when it is not the subject of the 2022 * composition, but instead represents something or someone associated with the 2023 * subject such as (for a patient subject) a spouse, parent, fetus, or donor. If 2024 * not focus is specified, the focus is assumed to be focus of the parent 2025 * section, or, for a section in the Composition itself, the subject of the 2026 * composition. Sections with a focus SHALL only include resources where the 2027 * logical subject (patient, subject, focus, etc.) matches the section focus, or 2028 * the resources have no logical subject (few resources). 2029 */ 2030 @Child(name = "focus", type = { Reference.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2031 @Description(shortDefinition = "Who/what the section is about, when it is not about the subject of composition", formalDefinition = "The actual focus of the section when it is not the subject of the composition, but instead represents something or someone associated with the subject such as (for a patient subject) a spouse, parent, fetus, or donor. If not focus is specified, the focus is assumed to be focus of the parent section, or, for a section in the Composition itself, the subject of the composition. Sections with a focus SHALL only include resources where the logical subject (patient, subject, focus, etc.) matches the section focus, or the resources have no logical subject (few resources).") 2032 protected Reference focus; 2033 2034 /** 2035 * The actual object that is the target of the reference (The actual focus of 2036 * the section when it is not the subject of the composition, but instead 2037 * represents something or someone associated with the subject such as (for a 2038 * patient subject) a spouse, parent, fetus, or donor. If not focus is 2039 * specified, the focus is assumed to be focus of the parent section, or, for a 2040 * section in the Composition itself, the subject of the composition. Sections 2041 * with a focus SHALL only include resources where the logical subject (patient, 2042 * subject, focus, etc.) matches the section focus, or the resources have no 2043 * logical subject (few resources).) 2044 */ 2045 protected Resource focusTarget; 2046 2047 /** 2048 * A human-readable narrative that contains the attested content of the section, 2049 * used to represent the content of the resource to a human. The narrative need 2050 * not encode all the structured data, but is required to contain sufficient 2051 * detail to make it "clinically safe" for a human to just read the narrative. 2052 */ 2053 @Child(name = "text", type = { Narrative.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 2054 @Description(shortDefinition = "Text summary of the section, for human interpretation", formalDefinition = "A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative.") 2055 protected Narrative text; 2056 2057 /** 2058 * How the entry list was prepared - whether it is a working list that is 2059 * suitable for being maintained on an ongoing basis, or if it represents a 2060 * snapshot of a list of items from another source, or whether it is a prepared 2061 * list where items may be marked as added, modified or deleted. 2062 */ 2063 @Child(name = "mode", type = { CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 2064 @Description(shortDefinition = "working | snapshot | changes", formalDefinition = "How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.") 2065 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/list-mode") 2066 protected Enumeration<SectionMode> mode; 2067 2068 /** 2069 * Specifies the order applied to the items in the section entries. 2070 */ 2071 @Child(name = "orderedBy", type = { 2072 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 2073 @Description(shortDefinition = "Order of section entries", formalDefinition = "Specifies the order applied to the items in the section entries.") 2074 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/list-order") 2075 protected CodeableConcept orderedBy; 2076 2077 /** 2078 * A reference to the actual resource from which the narrative in the section is 2079 * derived. 2080 */ 2081 @Child(name = "entry", type = { 2082 Reference.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2083 @Description(shortDefinition = "A reference to data that supports this section", formalDefinition = "A reference to the actual resource from which the narrative in the section is derived.") 2084 protected List<Reference> entry; 2085 /** 2086 * The actual objects that are the target of the reference (A reference to the 2087 * actual resource from which the narrative in the section is derived.) 2088 */ 2089 protected List<Resource> entryTarget; 2090 2091 /** 2092 * If the section is empty, why the list is empty. An empty section typically 2093 * has some text explaining the empty reason. 2094 */ 2095 @Child(name = "emptyReason", type = { 2096 CodeableConcept.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 2097 @Description(shortDefinition = "Why the section is empty", formalDefinition = "If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason.") 2098 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/list-empty-reason") 2099 protected CodeableConcept emptyReason; 2100 2101 /** 2102 * A nested sub-section within this section. 2103 */ 2104 @Child(name = "section", type = { 2105 SectionComponent.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2106 @Description(shortDefinition = "Nested Section", formalDefinition = "A nested sub-section within this section.") 2107 protected List<SectionComponent> section; 2108 2109 private static final long serialVersionUID = -797396954L; 2110 2111 /** 2112 * Constructor 2113 */ 2114 public SectionComponent() { 2115 super(); 2116 } 2117 2118 /** 2119 * @return {@link #title} (The label for this particular section. This will be 2120 * part of the rendered content for the document, and is often used to 2121 * build a table of contents.). This is the underlying object with id, 2122 * value and extensions. The accessor "getTitle" gives direct access to 2123 * the value 2124 */ 2125 public StringType getTitleElement() { 2126 if (this.title == null) 2127 if (Configuration.errorOnAutoCreate()) 2128 throw new Error("Attempt to auto-create SectionComponent.title"); 2129 else if (Configuration.doAutoCreate()) 2130 this.title = new StringType(); // bb 2131 return this.title; 2132 } 2133 2134 public boolean hasTitleElement() { 2135 return this.title != null && !this.title.isEmpty(); 2136 } 2137 2138 public boolean hasTitle() { 2139 return this.title != null && !this.title.isEmpty(); 2140 } 2141 2142 /** 2143 * @param value {@link #title} (The label for this particular section. This will 2144 * be part of the rendered content for the document, and is often 2145 * used to build a table of contents.). This is the underlying 2146 * object with id, value and extensions. The accessor "getTitle" 2147 * gives direct access to the value 2148 */ 2149 public SectionComponent setTitleElement(StringType value) { 2150 this.title = value; 2151 return this; 2152 } 2153 2154 /** 2155 * @return The label for this particular section. This will be part of the 2156 * rendered content for the document, and is often used to build a table 2157 * of contents. 2158 */ 2159 public String getTitle() { 2160 return this.title == null ? null : this.title.getValue(); 2161 } 2162 2163 /** 2164 * @param value The label for this particular section. This will be part of the 2165 * rendered content for the document, and is often used to build a 2166 * table of contents. 2167 */ 2168 public SectionComponent setTitle(String value) { 2169 if (Utilities.noString(value)) 2170 this.title = null; 2171 else { 2172 if (this.title == null) 2173 this.title = new StringType(); 2174 this.title.setValue(value); 2175 } 2176 return this; 2177 } 2178 2179 /** 2180 * @return {@link #code} (A code identifying the kind of content contained 2181 * within the section. This must be consistent with the section title.) 2182 */ 2183 public CodeableConcept getCode() { 2184 if (this.code == null) 2185 if (Configuration.errorOnAutoCreate()) 2186 throw new Error("Attempt to auto-create SectionComponent.code"); 2187 else if (Configuration.doAutoCreate()) 2188 this.code = new CodeableConcept(); // cc 2189 return this.code; 2190 } 2191 2192 public boolean hasCode() { 2193 return this.code != null && !this.code.isEmpty(); 2194 } 2195 2196 /** 2197 * @param value {@link #code} (A code identifying the kind of content contained 2198 * within the section. This must be consistent with the section 2199 * title.) 2200 */ 2201 public SectionComponent setCode(CodeableConcept value) { 2202 this.code = value; 2203 return this; 2204 } 2205 2206 /** 2207 * @return {@link #author} (Identifies who is responsible for the information in 2208 * this section, not necessarily who typed it in.) 2209 */ 2210 public List<Reference> getAuthor() { 2211 if (this.author == null) 2212 this.author = new ArrayList<Reference>(); 2213 return this.author; 2214 } 2215 2216 /** 2217 * @return Returns a reference to <code>this</code> for easy method chaining 2218 */ 2219 public SectionComponent setAuthor(List<Reference> theAuthor) { 2220 this.author = theAuthor; 2221 return this; 2222 } 2223 2224 public boolean hasAuthor() { 2225 if (this.author == null) 2226 return false; 2227 for (Reference item : this.author) 2228 if (!item.isEmpty()) 2229 return true; 2230 return false; 2231 } 2232 2233 public Reference addAuthor() { // 3 2234 Reference t = new Reference(); 2235 if (this.author == null) 2236 this.author = new ArrayList<Reference>(); 2237 this.author.add(t); 2238 return t; 2239 } 2240 2241 public SectionComponent addAuthor(Reference t) { // 3 2242 if (t == null) 2243 return this; 2244 if (this.author == null) 2245 this.author = new ArrayList<Reference>(); 2246 this.author.add(t); 2247 return this; 2248 } 2249 2250 /** 2251 * @return The first repetition of repeating field {@link #author}, creating it 2252 * if it does not already exist 2253 */ 2254 public Reference getAuthorFirstRep() { 2255 if (getAuthor().isEmpty()) { 2256 addAuthor(); 2257 } 2258 return getAuthor().get(0); 2259 } 2260 2261 /** 2262 * @deprecated Use Reference#setResource(IBaseResource) instead 2263 */ 2264 @Deprecated 2265 public List<Resource> getAuthorTarget() { 2266 if (this.authorTarget == null) 2267 this.authorTarget = new ArrayList<Resource>(); 2268 return this.authorTarget; 2269 } 2270 2271 /** 2272 * @return {@link #focus} (The actual focus of the section when it is not the 2273 * subject of the composition, but instead represents something or 2274 * someone associated with the subject such as (for a patient subject) a 2275 * spouse, parent, fetus, or donor. If not focus is specified, the focus 2276 * is assumed to be focus of the parent section, or, for a section in 2277 * the Composition itself, the subject of the composition. Sections with 2278 * a focus SHALL only include resources where the logical subject 2279 * (patient, subject, focus, etc.) matches the section focus, or the 2280 * resources have no logical subject (few resources).) 2281 */ 2282 public Reference getFocus() { 2283 if (this.focus == null) 2284 if (Configuration.errorOnAutoCreate()) 2285 throw new Error("Attempt to auto-create SectionComponent.focus"); 2286 else if (Configuration.doAutoCreate()) 2287 this.focus = new Reference(); // cc 2288 return this.focus; 2289 } 2290 2291 public boolean hasFocus() { 2292 return this.focus != null && !this.focus.isEmpty(); 2293 } 2294 2295 /** 2296 * @param value {@link #focus} (The actual focus of the section when it is not 2297 * the subject of the composition, but instead represents something 2298 * or someone associated with the subject such as (for a patient 2299 * subject) a spouse, parent, fetus, or donor. If not focus is 2300 * specified, the focus is assumed to be focus of the parent 2301 * section, or, for a section in the Composition itself, the 2302 * subject of the composition. Sections with a focus SHALL only 2303 * include resources where the logical subject (patient, subject, 2304 * focus, etc.) matches the section focus, or the resources have no 2305 * logical subject (few resources).) 2306 */ 2307 public SectionComponent setFocus(Reference value) { 2308 this.focus = value; 2309 return this; 2310 } 2311 2312 /** 2313 * @return {@link #focus} The actual object that is the target of the reference. 2314 * The reference library doesn't populate this, but you can use it to 2315 * hold the resource if you resolve it. (The actual focus of the section 2316 * when it is not the subject of the composition, but instead represents 2317 * something or someone associated with the subject such as (for a 2318 * patient subject) a spouse, parent, fetus, or donor. If not focus is 2319 * specified, the focus is assumed to be focus of the parent section, 2320 * or, for a section in the Composition itself, the subject of the 2321 * composition. Sections with a focus SHALL only include resources where 2322 * the logical subject (patient, subject, focus, etc.) matches the 2323 * section focus, or the resources have no logical subject (few 2324 * resources).) 2325 */ 2326 public Resource getFocusTarget() { 2327 return this.focusTarget; 2328 } 2329 2330 /** 2331 * @param value {@link #focus} The actual object that is the target of the 2332 * reference. The reference library doesn't use these, but you can 2333 * use it to hold the resource if you resolve it. (The actual focus 2334 * of the section when it is not the subject of the composition, 2335 * but instead represents something or someone associated with the 2336 * subject such as (for a patient subject) a spouse, parent, fetus, 2337 * or donor. If not focus is specified, the focus is assumed to be 2338 * focus of the parent section, or, for a section in the 2339 * Composition itself, the subject of the composition. Sections 2340 * with a focus SHALL only include resources where the logical 2341 * subject (patient, subject, focus, etc.) matches the section 2342 * focus, or the resources have no logical subject (few 2343 * resources).) 2344 */ 2345 public SectionComponent setFocusTarget(Resource value) { 2346 this.focusTarget = value; 2347 return this; 2348 } 2349 2350 /** 2351 * @return {@link #text} (A human-readable narrative that contains the attested 2352 * content of the section, used to represent the content of the resource 2353 * to a human. The narrative need not encode all the structured data, 2354 * but is required to contain sufficient detail to make it "clinically 2355 * safe" for a human to just read the narrative.) 2356 */ 2357 public Narrative getText() { 2358 if (this.text == null) 2359 if (Configuration.errorOnAutoCreate()) 2360 throw new Error("Attempt to auto-create SectionComponent.text"); 2361 else if (Configuration.doAutoCreate()) 2362 this.text = new Narrative(); // cc 2363 return this.text; 2364 } 2365 2366 public boolean hasText() { 2367 return this.text != null && !this.text.isEmpty(); 2368 } 2369 2370 /** 2371 * @param value {@link #text} (A human-readable narrative that contains the 2372 * attested content of the section, used to represent the content 2373 * of the resource to a human. The narrative need not encode all 2374 * the structured data, but is required to contain sufficient 2375 * detail to make it "clinically safe" for a human to just read the 2376 * narrative.) 2377 */ 2378 public SectionComponent setText(Narrative value) { 2379 this.text = value; 2380 return this; 2381 } 2382 2383 /** 2384 * @return {@link #mode} (How the entry list was prepared - whether it is a 2385 * working list that is suitable for being maintained on an ongoing 2386 * basis, or if it represents a snapshot of a list of items from another 2387 * source, or whether it is a prepared list where items may be marked as 2388 * added, modified or deleted.). This is the underlying object with id, 2389 * value and extensions. The accessor "getMode" gives direct access to 2390 * the value 2391 */ 2392 public Enumeration<SectionMode> getModeElement() { 2393 if (this.mode == null) 2394 if (Configuration.errorOnAutoCreate()) 2395 throw new Error("Attempt to auto-create SectionComponent.mode"); 2396 else if (Configuration.doAutoCreate()) 2397 this.mode = new Enumeration<SectionMode>(new SectionModeEnumFactory()); // bb 2398 return this.mode; 2399 } 2400 2401 public boolean hasModeElement() { 2402 return this.mode != null && !this.mode.isEmpty(); 2403 } 2404 2405 public boolean hasMode() { 2406 return this.mode != null && !this.mode.isEmpty(); 2407 } 2408 2409 /** 2410 * @param value {@link #mode} (How the entry list was prepared - whether it is a 2411 * working list that is suitable for being maintained on an ongoing 2412 * basis, or if it represents a snapshot of a list of items from 2413 * another source, or whether it is a prepared list where items may 2414 * be marked as added, modified or deleted.). This is the 2415 * underlying object with id, value and extensions. The accessor 2416 * "getMode" gives direct access to the value 2417 */ 2418 public SectionComponent setModeElement(Enumeration<SectionMode> value) { 2419 this.mode = value; 2420 return this; 2421 } 2422 2423 /** 2424 * @return How the entry list was prepared - whether it is a working list that 2425 * is suitable for being maintained on an ongoing basis, or if it 2426 * represents a snapshot of a list of items from another source, or 2427 * whether it is a prepared list where items may be marked as added, 2428 * modified or deleted. 2429 */ 2430 public SectionMode getMode() { 2431 return this.mode == null ? null : this.mode.getValue(); 2432 } 2433 2434 /** 2435 * @param value How the entry list was prepared - whether it is a working list 2436 * that is suitable for being maintained on an ongoing basis, or if 2437 * it represents a snapshot of a list of items from another source, 2438 * or whether it is a prepared list where items may be marked as 2439 * added, modified or deleted. 2440 */ 2441 public SectionComponent setMode(SectionMode value) { 2442 if (value == null) 2443 this.mode = null; 2444 else { 2445 if (this.mode == null) 2446 this.mode = new Enumeration<SectionMode>(new SectionModeEnumFactory()); 2447 this.mode.setValue(value); 2448 } 2449 return this; 2450 } 2451 2452 /** 2453 * @return {@link #orderedBy} (Specifies the order applied to the items in the 2454 * section entries.) 2455 */ 2456 public CodeableConcept getOrderedBy() { 2457 if (this.orderedBy == null) 2458 if (Configuration.errorOnAutoCreate()) 2459 throw new Error("Attempt to auto-create SectionComponent.orderedBy"); 2460 else if (Configuration.doAutoCreate()) 2461 this.orderedBy = new CodeableConcept(); // cc 2462 return this.orderedBy; 2463 } 2464 2465 public boolean hasOrderedBy() { 2466 return this.orderedBy != null && !this.orderedBy.isEmpty(); 2467 } 2468 2469 /** 2470 * @param value {@link #orderedBy} (Specifies the order applied to the items in 2471 * the section entries.) 2472 */ 2473 public SectionComponent setOrderedBy(CodeableConcept value) { 2474 this.orderedBy = value; 2475 return this; 2476 } 2477 2478 /** 2479 * @return {@link #entry} (A reference to the actual resource from which the 2480 * narrative in the section is derived.) 2481 */ 2482 public List<Reference> getEntry() { 2483 if (this.entry == null) 2484 this.entry = new ArrayList<Reference>(); 2485 return this.entry; 2486 } 2487 2488 /** 2489 * @return Returns a reference to <code>this</code> for easy method chaining 2490 */ 2491 public SectionComponent setEntry(List<Reference> theEntry) { 2492 this.entry = theEntry; 2493 return this; 2494 } 2495 2496 public boolean hasEntry() { 2497 if (this.entry == null) 2498 return false; 2499 for (Reference item : this.entry) 2500 if (!item.isEmpty()) 2501 return true; 2502 return false; 2503 } 2504 2505 public Reference addEntry() { // 3 2506 Reference t = new Reference(); 2507 if (this.entry == null) 2508 this.entry = new ArrayList<Reference>(); 2509 this.entry.add(t); 2510 return t; 2511 } 2512 2513 public SectionComponent addEntry(Reference t) { // 3 2514 if (t == null) 2515 return this; 2516 if (this.entry == null) 2517 this.entry = new ArrayList<Reference>(); 2518 this.entry.add(t); 2519 return this; 2520 } 2521 2522 /** 2523 * @return The first repetition of repeating field {@link #entry}, creating it 2524 * if it does not already exist 2525 */ 2526 public Reference getEntryFirstRep() { 2527 if (getEntry().isEmpty()) { 2528 addEntry(); 2529 } 2530 return getEntry().get(0); 2531 } 2532 2533 /** 2534 * @deprecated Use Reference#setResource(IBaseResource) instead 2535 */ 2536 @Deprecated 2537 public List<Resource> getEntryTarget() { 2538 if (this.entryTarget == null) 2539 this.entryTarget = new ArrayList<Resource>(); 2540 return this.entryTarget; 2541 } 2542 2543 /** 2544 * @return {@link #emptyReason} (If the section is empty, why the list is empty. 2545 * An empty section typically has some text explaining the empty 2546 * reason.) 2547 */ 2548 public CodeableConcept getEmptyReason() { 2549 if (this.emptyReason == null) 2550 if (Configuration.errorOnAutoCreate()) 2551 throw new Error("Attempt to auto-create SectionComponent.emptyReason"); 2552 else if (Configuration.doAutoCreate()) 2553 this.emptyReason = new CodeableConcept(); // cc 2554 return this.emptyReason; 2555 } 2556 2557 public boolean hasEmptyReason() { 2558 return this.emptyReason != null && !this.emptyReason.isEmpty(); 2559 } 2560 2561 /** 2562 * @param value {@link #emptyReason} (If the section is empty, why the list is 2563 * empty. An empty section typically has some text explaining the 2564 * empty reason.) 2565 */ 2566 public SectionComponent setEmptyReason(CodeableConcept value) { 2567 this.emptyReason = value; 2568 return this; 2569 } 2570 2571 /** 2572 * @return {@link #section} (A nested sub-section within this section.) 2573 */ 2574 public List<SectionComponent> getSection() { 2575 if (this.section == null) 2576 this.section = new ArrayList<SectionComponent>(); 2577 return this.section; 2578 } 2579 2580 /** 2581 * @return Returns a reference to <code>this</code> for easy method chaining 2582 */ 2583 public SectionComponent setSection(List<SectionComponent> theSection) { 2584 this.section = theSection; 2585 return this; 2586 } 2587 2588 public boolean hasSection() { 2589 if (this.section == null) 2590 return false; 2591 for (SectionComponent item : this.section) 2592 if (!item.isEmpty()) 2593 return true; 2594 return false; 2595 } 2596 2597 public SectionComponent addSection() { // 3 2598 SectionComponent t = new SectionComponent(); 2599 if (this.section == null) 2600 this.section = new ArrayList<SectionComponent>(); 2601 this.section.add(t); 2602 return t; 2603 } 2604 2605 public SectionComponent addSection(SectionComponent t) { // 3 2606 if (t == null) 2607 return this; 2608 if (this.section == null) 2609 this.section = new ArrayList<SectionComponent>(); 2610 this.section.add(t); 2611 return this; 2612 } 2613 2614 /** 2615 * @return The first repetition of repeating field {@link #section}, creating it 2616 * if it does not already exist 2617 */ 2618 public SectionComponent getSectionFirstRep() { 2619 if (getSection().isEmpty()) { 2620 addSection(); 2621 } 2622 return getSection().get(0); 2623 } 2624 2625 protected void listChildren(List<Property> children) { 2626 super.listChildren(children); 2627 children.add(new Property("title", "string", 2628 "The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents.", 2629 0, 1, title)); 2630 children.add(new Property("code", "CodeableConcept", 2631 "A code identifying the kind of content contained within the section. This must be consistent with the section title.", 2632 0, 1, code)); 2633 children.add( 2634 new Property("author", "Reference(Practitioner|PractitionerRole|Device|Patient|RelatedPerson|Organization)", 2635 "Identifies who is responsible for the information in this section, not necessarily who typed it in.", 0, 2636 java.lang.Integer.MAX_VALUE, author)); 2637 children.add(new Property("focus", "Reference(Any)", 2638 "The actual focus of the section when it is not the subject of the composition, but instead represents something or someone associated with the subject such as (for a patient subject) a spouse, parent, fetus, or donor. If not focus is specified, the focus is assumed to be focus of the parent section, or, for a section in the Composition itself, the subject of the composition. Sections with a focus SHALL only include resources where the logical subject (patient, subject, focus, etc.) matches the section focus, or the resources have no logical subject (few resources).", 2639 0, 1, focus)); 2640 children.add(new Property("text", "Narrative", 2641 "A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative.", 2642 0, 1, text)); 2643 children.add(new Property("mode", "code", 2644 "How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.", 2645 0, 1, mode)); 2646 children.add(new Property("orderedBy", "CodeableConcept", 2647 "Specifies the order applied to the items in the section entries.", 0, 1, orderedBy)); 2648 children.add(new Property("entry", "Reference(Any)", 2649 "A reference to the actual resource from which the narrative in the section is derived.", 0, 2650 java.lang.Integer.MAX_VALUE, entry)); 2651 children.add(new Property("emptyReason", "CodeableConcept", 2652 "If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason.", 2653 0, 1, emptyReason)); 2654 children.add(new Property("section", "@Composition.section", "A nested sub-section within this section.", 0, 2655 java.lang.Integer.MAX_VALUE, section)); 2656 } 2657 2658 @Override 2659 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2660 switch (_hash) { 2661 case 110371416: 2662 /* title */ return new Property("title", "string", 2663 "The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents.", 2664 0, 1, title); 2665 case 3059181: 2666 /* code */ return new Property("code", "CodeableConcept", 2667 "A code identifying the kind of content contained within the section. This must be consistent with the section title.", 2668 0, 1, code); 2669 case -1406328437: 2670 /* author */ return new Property("author", 2671 "Reference(Practitioner|PractitionerRole|Device|Patient|RelatedPerson|Organization)", 2672 "Identifies who is responsible for the information in this section, not necessarily who typed it in.", 0, 2673 java.lang.Integer.MAX_VALUE, author); 2674 case 97604824: 2675 /* focus */ return new Property("focus", "Reference(Any)", 2676 "The actual focus of the section when it is not the subject of the composition, but instead represents something or someone associated with the subject such as (for a patient subject) a spouse, parent, fetus, or donor. If not focus is specified, the focus is assumed to be focus of the parent section, or, for a section in the Composition itself, the subject of the composition. Sections with a focus SHALL only include resources where the logical subject (patient, subject, focus, etc.) matches the section focus, or the resources have no logical subject (few resources).", 2677 0, 1, focus); 2678 case 3556653: 2679 /* text */ return new Property("text", "Narrative", 2680 "A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative.", 2681 0, 1, text); 2682 case 3357091: 2683 /* mode */ return new Property("mode", "code", 2684 "How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.", 2685 0, 1, mode); 2686 case -391079516: 2687 /* orderedBy */ return new Property("orderedBy", "CodeableConcept", 2688 "Specifies the order applied to the items in the section entries.", 0, 1, orderedBy); 2689 case 96667762: 2690 /* entry */ return new Property("entry", "Reference(Any)", 2691 "A reference to the actual resource from which the narrative in the section is derived.", 0, 2692 java.lang.Integer.MAX_VALUE, entry); 2693 case 1140135409: 2694 /* emptyReason */ return new Property("emptyReason", "CodeableConcept", 2695 "If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason.", 2696 0, 1, emptyReason); 2697 case 1970241253: 2698 /* section */ return new Property("section", "@Composition.section", 2699 "A nested sub-section within this section.", 0, java.lang.Integer.MAX_VALUE, section); 2700 default: 2701 return super.getNamedProperty(_hash, _name, _checkValid); 2702 } 2703 2704 } 2705 2706 @Override 2707 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2708 switch (hash) { 2709 case 110371416: 2710 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 2711 case 3059181: 2712 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 2713 case -1406328437: 2714 /* author */ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // Reference 2715 case 97604824: 2716 /* focus */ return this.focus == null ? new Base[0] : new Base[] { this.focus }; // Reference 2717 case 3556653: 2718 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // Narrative 2719 case 3357091: 2720 /* mode */ return this.mode == null ? new Base[0] : new Base[] { this.mode }; // Enumeration<SectionMode> 2721 case -391079516: 2722 /* orderedBy */ return this.orderedBy == null ? new Base[0] : new Base[] { this.orderedBy }; // CodeableConcept 2723 case 96667762: 2724 /* entry */ return this.entry == null ? new Base[0] : this.entry.toArray(new Base[this.entry.size()]); // Reference 2725 case 1140135409: 2726 /* emptyReason */ return this.emptyReason == null ? new Base[0] : new Base[] { this.emptyReason }; // CodeableConcept 2727 case 1970241253: 2728 /* section */ return this.section == null ? new Base[0] : this.section.toArray(new Base[this.section.size()]); // SectionComponent 2729 default: 2730 return super.getProperty(hash, name, checkValid); 2731 } 2732 2733 } 2734 2735 @Override 2736 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2737 switch (hash) { 2738 case 110371416: // title 2739 this.title = castToString(value); // StringType 2740 return value; 2741 case 3059181: // code 2742 this.code = castToCodeableConcept(value); // CodeableConcept 2743 return value; 2744 case -1406328437: // author 2745 this.getAuthor().add(castToReference(value)); // Reference 2746 return value; 2747 case 97604824: // focus 2748 this.focus = castToReference(value); // Reference 2749 return value; 2750 case 3556653: // text 2751 this.text = castToNarrative(value); // Narrative 2752 return value; 2753 case 3357091: // mode 2754 value = new SectionModeEnumFactory().fromType(castToCode(value)); 2755 this.mode = (Enumeration) value; // Enumeration<SectionMode> 2756 return value; 2757 case -391079516: // orderedBy 2758 this.orderedBy = castToCodeableConcept(value); // CodeableConcept 2759 return value; 2760 case 96667762: // entry 2761 this.getEntry().add(castToReference(value)); // Reference 2762 return value; 2763 case 1140135409: // emptyReason 2764 this.emptyReason = castToCodeableConcept(value); // CodeableConcept 2765 return value; 2766 case 1970241253: // section 2767 this.getSection().add((SectionComponent) value); // SectionComponent 2768 return value; 2769 default: 2770 return super.setProperty(hash, name, value); 2771 } 2772 2773 } 2774 2775 @Override 2776 public Base setProperty(String name, Base value) throws FHIRException { 2777 if (name.equals("title")) { 2778 this.title = castToString(value); // StringType 2779 } else if (name.equals("code")) { 2780 this.code = castToCodeableConcept(value); // CodeableConcept 2781 } else if (name.equals("author")) { 2782 this.getAuthor().add(castToReference(value)); 2783 } else if (name.equals("focus")) { 2784 this.focus = castToReference(value); // Reference 2785 } else if (name.equals("text")) { 2786 this.text = castToNarrative(value); // Narrative 2787 } else if (name.equals("mode")) { 2788 value = new SectionModeEnumFactory().fromType(castToCode(value)); 2789 this.mode = (Enumeration) value; // Enumeration<SectionMode> 2790 } else if (name.equals("orderedBy")) { 2791 this.orderedBy = castToCodeableConcept(value); // CodeableConcept 2792 } else if (name.equals("entry")) { 2793 this.getEntry().add(castToReference(value)); 2794 } else if (name.equals("emptyReason")) { 2795 this.emptyReason = castToCodeableConcept(value); // CodeableConcept 2796 } else if (name.equals("section")) { 2797 this.getSection().add((SectionComponent) value); 2798 } else 2799 return super.setProperty(name, value); 2800 return value; 2801 } 2802 2803 @Override 2804 public void removeChild(String name, Base value) throws FHIRException { 2805 if (name.equals("title")) { 2806 this.title = null; 2807 } else if (name.equals("code")) { 2808 this.code = null; 2809 } else if (name.equals("author")) { 2810 this.getAuthor().remove(castToReference(value)); 2811 } else if (name.equals("focus")) { 2812 this.focus = null; 2813 } else if (name.equals("text")) { 2814 this.text = null; 2815 } else if (name.equals("mode")) { 2816 this.mode = null; 2817 } else if (name.equals("orderedBy")) { 2818 this.orderedBy = null; 2819 } else if (name.equals("entry")) { 2820 this.getEntry().remove(castToReference(value)); 2821 } else if (name.equals("emptyReason")) { 2822 this.emptyReason = null; 2823 } else if (name.equals("section")) { 2824 this.getSection().remove((SectionComponent) value); 2825 } else 2826 super.removeChild(name, value); 2827 2828 } 2829 2830 @Override 2831 public Base makeProperty(int hash, String name) throws FHIRException { 2832 switch (hash) { 2833 case 110371416: 2834 return getTitleElement(); 2835 case 3059181: 2836 return getCode(); 2837 case -1406328437: 2838 return addAuthor(); 2839 case 97604824: 2840 return getFocus(); 2841 case 3556653: 2842 return getText(); 2843 case 3357091: 2844 return getModeElement(); 2845 case -391079516: 2846 return getOrderedBy(); 2847 case 96667762: 2848 return addEntry(); 2849 case 1140135409: 2850 return getEmptyReason(); 2851 case 1970241253: 2852 return addSection(); 2853 default: 2854 return super.makeProperty(hash, name); 2855 } 2856 2857 } 2858 2859 @Override 2860 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2861 switch (hash) { 2862 case 110371416: 2863 /* title */ return new String[] { "string" }; 2864 case 3059181: 2865 /* code */ return new String[] { "CodeableConcept" }; 2866 case -1406328437: 2867 /* author */ return new String[] { "Reference" }; 2868 case 97604824: 2869 /* focus */ return new String[] { "Reference" }; 2870 case 3556653: 2871 /* text */ return new String[] { "Narrative" }; 2872 case 3357091: 2873 /* mode */ return new String[] { "code" }; 2874 case -391079516: 2875 /* orderedBy */ return new String[] { "CodeableConcept" }; 2876 case 96667762: 2877 /* entry */ return new String[] { "Reference" }; 2878 case 1140135409: 2879 /* emptyReason */ return new String[] { "CodeableConcept" }; 2880 case 1970241253: 2881 /* section */ return new String[] { "@Composition.section" }; 2882 default: 2883 return super.getTypesForProperty(hash, name); 2884 } 2885 2886 } 2887 2888 @Override 2889 public Base addChild(String name) throws FHIRException { 2890 if (name.equals("title")) { 2891 throw new FHIRException("Cannot call addChild on a singleton property Composition.title"); 2892 } else if (name.equals("code")) { 2893 this.code = new CodeableConcept(); 2894 return this.code; 2895 } else if (name.equals("author")) { 2896 return addAuthor(); 2897 } else if (name.equals("focus")) { 2898 this.focus = new Reference(); 2899 return this.focus; 2900 } else if (name.equals("text")) { 2901 this.text = new Narrative(); 2902 return this.text; 2903 } else if (name.equals("mode")) { 2904 throw new FHIRException("Cannot call addChild on a singleton property Composition.mode"); 2905 } else if (name.equals("orderedBy")) { 2906 this.orderedBy = new CodeableConcept(); 2907 return this.orderedBy; 2908 } else if (name.equals("entry")) { 2909 return addEntry(); 2910 } else if (name.equals("emptyReason")) { 2911 this.emptyReason = new CodeableConcept(); 2912 return this.emptyReason; 2913 } else if (name.equals("section")) { 2914 return addSection(); 2915 } else 2916 return super.addChild(name); 2917 } 2918 2919 public SectionComponent copy() { 2920 SectionComponent dst = new SectionComponent(); 2921 copyValues(dst); 2922 return dst; 2923 } 2924 2925 public void copyValues(SectionComponent dst) { 2926 super.copyValues(dst); 2927 dst.title = title == null ? null : title.copy(); 2928 dst.code = code == null ? null : code.copy(); 2929 if (author != null) { 2930 dst.author = new ArrayList<Reference>(); 2931 for (Reference i : author) 2932 dst.author.add(i.copy()); 2933 } 2934 ; 2935 dst.focus = focus == null ? null : focus.copy(); 2936 dst.text = text == null ? null : text.copy(); 2937 dst.mode = mode == null ? null : mode.copy(); 2938 dst.orderedBy = orderedBy == null ? null : orderedBy.copy(); 2939 if (entry != null) { 2940 dst.entry = new ArrayList<Reference>(); 2941 for (Reference i : entry) 2942 dst.entry.add(i.copy()); 2943 } 2944 ; 2945 dst.emptyReason = emptyReason == null ? null : emptyReason.copy(); 2946 if (section != null) { 2947 dst.section = new ArrayList<SectionComponent>(); 2948 for (SectionComponent i : section) 2949 dst.section.add(i.copy()); 2950 } 2951 ; 2952 } 2953 2954 @Override 2955 public boolean equalsDeep(Base other_) { 2956 if (!super.equalsDeep(other_)) 2957 return false; 2958 if (!(other_ instanceof SectionComponent)) 2959 return false; 2960 SectionComponent o = (SectionComponent) other_; 2961 return compareDeep(title, o.title, true) && compareDeep(code, o.code, true) && compareDeep(author, o.author, true) 2962 && compareDeep(focus, o.focus, true) && compareDeep(text, o.text, true) && compareDeep(mode, o.mode, true) 2963 && compareDeep(orderedBy, o.orderedBy, true) && compareDeep(entry, o.entry, true) 2964 && compareDeep(emptyReason, o.emptyReason, true) && compareDeep(section, o.section, true); 2965 } 2966 2967 @Override 2968 public boolean equalsShallow(Base other_) { 2969 if (!super.equalsShallow(other_)) 2970 return false; 2971 if (!(other_ instanceof SectionComponent)) 2972 return false; 2973 SectionComponent o = (SectionComponent) other_; 2974 return compareValues(title, o.title, true) && compareValues(mode, o.mode, true); 2975 } 2976 2977 public boolean isEmpty() { 2978 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(title, code, author, focus, text, mode, orderedBy, 2979 entry, emptyReason, section); 2980 } 2981 2982 public String fhirType() { 2983 return "Composition.section"; 2984 2985 } 2986 2987 } 2988 2989 /** 2990 * A version-independent identifier for the Composition. This identifier stays 2991 * constant as the composition is changed over time. 2992 */ 2993 @Child(name = "identifier", type = { 2994 Identifier.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 2995 @Description(shortDefinition = "Version-independent identifier for the Composition", formalDefinition = "A version-independent identifier for the Composition. This identifier stays constant as the composition is changed over time.") 2996 protected Identifier identifier; 2997 2998 /** 2999 * The workflow/clinical status of this composition. The status is a marker for 3000 * the clinical standing of the document. 3001 */ 3002 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 3003 @Description(shortDefinition = "preliminary | final | amended | entered-in-error", formalDefinition = "The workflow/clinical status of this composition. The status is a marker for the clinical standing of the document.") 3004 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/composition-status") 3005 protected Enumeration<CompositionStatus> status; 3006 3007 /** 3008 * Specifies the particular kind of composition (e.g. History and Physical, 3009 * Discharge Summary, Progress Note). This usually equates to the purpose of 3010 * making the composition. 3011 */ 3012 @Child(name = "type", type = { CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 3013 @Description(shortDefinition = "Kind of composition (LOINC if possible)", formalDefinition = "Specifies the particular kind of composition (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the composition.") 3014 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/doc-typecodes") 3015 protected CodeableConcept type; 3016 3017 /** 3018 * A categorization for the type of the composition - helps for indexing and 3019 * searching. This may be implied by or derived from the code specified in the 3020 * Composition Type. 3021 */ 3022 @Child(name = "category", type = { 3023 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3024 @Description(shortDefinition = "Categorization of Composition", formalDefinition = "A categorization for the type of the composition - helps for indexing and searching. This may be implied by or derived from the code specified in the Composition Type.") 3025 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/document-classcodes") 3026 protected List<CodeableConcept> category; 3027 3028 /** 3029 * Who or what the composition is about. The composition can be about a person, 3030 * (patient or healthcare practitioner), a device (e.g. a machine) or even a 3031 * group of subjects (such as a document about a herd of livestock, or a set of 3032 * patients that share a common exposure). 3033 */ 3034 @Child(name = "subject", type = { Reference.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 3035 @Description(shortDefinition = "Who and/or what the composition is about", formalDefinition = "Who or what the composition is about. The composition can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of livestock, or a set of patients that share a common exposure).") 3036 protected Reference subject; 3037 3038 /** 3039 * The actual object that is the target of the reference (Who or what the 3040 * composition is about. The composition can be about a person, (patient or 3041 * healthcare practitioner), a device (e.g. a machine) or even a group of 3042 * subjects (such as a document about a herd of livestock, or a set of patients 3043 * that share a common exposure).) 3044 */ 3045 protected Resource subjectTarget; 3046 3047 /** 3048 * Describes the clinical encounter or type of care this documentation is 3049 * associated with. 3050 */ 3051 @Child(name = "encounter", type = { Encounter.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 3052 @Description(shortDefinition = "Context of the Composition", formalDefinition = "Describes the clinical encounter or type of care this documentation is associated with.") 3053 protected Reference encounter; 3054 3055 /** 3056 * The actual object that is the target of the reference (Describes the clinical 3057 * encounter or type of care this documentation is associated with.) 3058 */ 3059 protected Encounter encounterTarget; 3060 3061 /** 3062 * The composition editing time, when the composition was last logically changed 3063 * by the author. 3064 */ 3065 @Child(name = "date", type = { DateTimeType.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 3066 @Description(shortDefinition = "Composition editing time", formalDefinition = "The composition editing time, when the composition was last logically changed by the author.") 3067 protected DateTimeType date; 3068 3069 /** 3070 * Identifies who is responsible for the information in the composition, not 3071 * necessarily who typed it in. 3072 */ 3073 @Child(name = "author", type = { Practitioner.class, PractitionerRole.class, Device.class, Patient.class, 3074 RelatedPerson.class, 3075 Organization.class }, order = 7, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3076 @Description(shortDefinition = "Who and/or what authored the composition", formalDefinition = "Identifies who is responsible for the information in the composition, not necessarily who typed it in.") 3077 protected List<Reference> author; 3078 /** 3079 * The actual objects that are the target of the reference (Identifies who is 3080 * responsible for the information in the composition, not necessarily who typed 3081 * it in.) 3082 */ 3083 protected List<Resource> authorTarget; 3084 3085 /** 3086 * Official human-readable label for the composition. 3087 */ 3088 @Child(name = "title", type = { StringType.class }, order = 8, min = 1, max = 1, modifier = false, summary = true) 3089 @Description(shortDefinition = "Human Readable name/title", formalDefinition = "Official human-readable label for the composition.") 3090 protected StringType title; 3091 3092 /** 3093 * The code specifying the level of confidentiality of the Composition. 3094 */ 3095 @Child(name = "confidentiality", type = { 3096 CodeType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 3097 @Description(shortDefinition = "As defined by affinity domain", formalDefinition = "The code specifying the level of confidentiality of the Composition.") 3098 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ConfidentialityClassification") 3099 protected Enumeration<DocumentConfidentiality> confidentiality; 3100 3101 /** 3102 * A participant who has attested to the accuracy of the composition/document. 3103 */ 3104 @Child(name = "attester", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3105 @Description(shortDefinition = "Attests to accuracy of composition", formalDefinition = "A participant who has attested to the accuracy of the composition/document.") 3106 protected List<CompositionAttesterComponent> attester; 3107 3108 /** 3109 * Identifies the organization or group who is responsible for ongoing 3110 * maintenance of and access to the composition/document information. 3111 */ 3112 @Child(name = "custodian", type = { 3113 Organization.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 3114 @Description(shortDefinition = "Organization which maintains the composition", formalDefinition = "Identifies the organization or group who is responsible for ongoing maintenance of and access to the composition/document information.") 3115 protected Reference custodian; 3116 3117 /** 3118 * The actual object that is the target of the reference (Identifies the 3119 * organization or group who is responsible for ongoing maintenance of and 3120 * access to the composition/document information.) 3121 */ 3122 protected Organization custodianTarget; 3123 3124 /** 3125 * Relationships that this composition has with other compositions or documents 3126 * that already exist. 3127 */ 3128 @Child(name = "relatesTo", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3129 @Description(shortDefinition = "Relationships to other compositions/documents", formalDefinition = "Relationships that this composition has with other compositions or documents that already exist.") 3130 protected List<CompositionRelatesToComponent> relatesTo; 3131 3132 /** 3133 * The clinical service, such as a colonoscopy or an appendectomy, being 3134 * documented. 3135 */ 3136 @Child(name = "event", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3137 @Description(shortDefinition = "The clinical service(s) being documented", formalDefinition = "The clinical service, such as a colonoscopy or an appendectomy, being documented.") 3138 protected List<CompositionEventComponent> event; 3139 3140 /** 3141 * The root of the sections that make up the composition. 3142 */ 3143 @Child(name = "section", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3144 @Description(shortDefinition = "Composition is broken into sections", formalDefinition = "The root of the sections that make up the composition.") 3145 protected List<SectionComponent> section; 3146 3147 private static final long serialVersionUID = -1490206663L; 3148 3149 /** 3150 * Constructor 3151 */ 3152 public Composition() { 3153 super(); 3154 } 3155 3156 /** 3157 * Constructor 3158 */ 3159 public Composition(Enumeration<CompositionStatus> status, CodeableConcept type, DateTimeType date, StringType title) { 3160 super(); 3161 this.status = status; 3162 this.type = type; 3163 this.date = date; 3164 this.title = title; 3165 } 3166 3167 /** 3168 * @return {@link #identifier} (A version-independent identifier for the 3169 * Composition. This identifier stays constant as the composition is 3170 * changed over time.) 3171 */ 3172 public Identifier getIdentifier() { 3173 if (this.identifier == null) 3174 if (Configuration.errorOnAutoCreate()) 3175 throw new Error("Attempt to auto-create Composition.identifier"); 3176 else if (Configuration.doAutoCreate()) 3177 this.identifier = new Identifier(); // cc 3178 return this.identifier; 3179 } 3180 3181 public boolean hasIdentifier() { 3182 return this.identifier != null && !this.identifier.isEmpty(); 3183 } 3184 3185 /** 3186 * @param value {@link #identifier} (A version-independent identifier for the 3187 * Composition. This identifier stays constant as the composition 3188 * is changed over time.) 3189 */ 3190 public Composition setIdentifier(Identifier value) { 3191 this.identifier = value; 3192 return this; 3193 } 3194 3195 /** 3196 * @return {@link #status} (The workflow/clinical status of this composition. 3197 * The status is a marker for the clinical standing of the document.). 3198 * This is the underlying object with id, value and extensions. The 3199 * accessor "getStatus" gives direct access to the value 3200 */ 3201 public Enumeration<CompositionStatus> getStatusElement() { 3202 if (this.status == null) 3203 if (Configuration.errorOnAutoCreate()) 3204 throw new Error("Attempt to auto-create Composition.status"); 3205 else if (Configuration.doAutoCreate()) 3206 this.status = new Enumeration<CompositionStatus>(new CompositionStatusEnumFactory()); // bb 3207 return this.status; 3208 } 3209 3210 public boolean hasStatusElement() { 3211 return this.status != null && !this.status.isEmpty(); 3212 } 3213 3214 public boolean hasStatus() { 3215 return this.status != null && !this.status.isEmpty(); 3216 } 3217 3218 /** 3219 * @param value {@link #status} (The workflow/clinical status of this 3220 * composition. The status is a marker for the clinical standing of 3221 * the document.). This is the underlying object with id, value and 3222 * extensions. The accessor "getStatus" gives direct access to the 3223 * value 3224 */ 3225 public Composition setStatusElement(Enumeration<CompositionStatus> value) { 3226 this.status = value; 3227 return this; 3228 } 3229 3230 /** 3231 * @return The workflow/clinical status of this composition. The status is a 3232 * marker for the clinical standing of the document. 3233 */ 3234 public CompositionStatus getStatus() { 3235 return this.status == null ? null : this.status.getValue(); 3236 } 3237 3238 /** 3239 * @param value The workflow/clinical status of this composition. The status is 3240 * a marker for the clinical standing of the document. 3241 */ 3242 public Composition setStatus(CompositionStatus value) { 3243 if (this.status == null) 3244 this.status = new Enumeration<CompositionStatus>(new CompositionStatusEnumFactory()); 3245 this.status.setValue(value); 3246 return this; 3247 } 3248 3249 /** 3250 * @return {@link #type} (Specifies the particular kind of composition (e.g. 3251 * History and Physical, Discharge Summary, Progress Note). This usually 3252 * equates to the purpose of making the composition.) 3253 */ 3254 public CodeableConcept getType() { 3255 if (this.type == null) 3256 if (Configuration.errorOnAutoCreate()) 3257 throw new Error("Attempt to auto-create Composition.type"); 3258 else if (Configuration.doAutoCreate()) 3259 this.type = new CodeableConcept(); // cc 3260 return this.type; 3261 } 3262 3263 public boolean hasType() { 3264 return this.type != null && !this.type.isEmpty(); 3265 } 3266 3267 /** 3268 * @param value {@link #type} (Specifies the particular kind of composition 3269 * (e.g. History and Physical, Discharge Summary, Progress Note). 3270 * This usually equates to the purpose of making the composition.) 3271 */ 3272 public Composition setType(CodeableConcept value) { 3273 this.type = value; 3274 return this; 3275 } 3276 3277 /** 3278 * @return {@link #category} (A categorization for the type of the composition - 3279 * helps for indexing and searching. This may be implied by or derived 3280 * from the code specified in the Composition Type.) 3281 */ 3282 public List<CodeableConcept> getCategory() { 3283 if (this.category == null) 3284 this.category = new ArrayList<CodeableConcept>(); 3285 return this.category; 3286 } 3287 3288 /** 3289 * @return Returns a reference to <code>this</code> for easy method chaining 3290 */ 3291 public Composition setCategory(List<CodeableConcept> theCategory) { 3292 this.category = theCategory; 3293 return this; 3294 } 3295 3296 public boolean hasCategory() { 3297 if (this.category == null) 3298 return false; 3299 for (CodeableConcept item : this.category) 3300 if (!item.isEmpty()) 3301 return true; 3302 return false; 3303 } 3304 3305 public CodeableConcept addCategory() { // 3 3306 CodeableConcept t = new CodeableConcept(); 3307 if (this.category == null) 3308 this.category = new ArrayList<CodeableConcept>(); 3309 this.category.add(t); 3310 return t; 3311 } 3312 3313 public Composition addCategory(CodeableConcept t) { // 3 3314 if (t == null) 3315 return this; 3316 if (this.category == null) 3317 this.category = new ArrayList<CodeableConcept>(); 3318 this.category.add(t); 3319 return this; 3320 } 3321 3322 /** 3323 * @return The first repetition of repeating field {@link #category}, creating 3324 * it if it does not already exist 3325 */ 3326 public CodeableConcept getCategoryFirstRep() { 3327 if (getCategory().isEmpty()) { 3328 addCategory(); 3329 } 3330 return getCategory().get(0); 3331 } 3332 3333 /** 3334 * @return {@link #subject} (Who or what the composition is about. The 3335 * composition can be about a person, (patient or healthcare 3336 * practitioner), a device (e.g. a machine) or even a group of subjects 3337 * (such as a document about a herd of livestock, or a set of patients 3338 * that share a common exposure).) 3339 */ 3340 public Reference getSubject() { 3341 if (this.subject == null) 3342 if (Configuration.errorOnAutoCreate()) 3343 throw new Error("Attempt to auto-create Composition.subject"); 3344 else if (Configuration.doAutoCreate()) 3345 this.subject = new Reference(); // cc 3346 return this.subject; 3347 } 3348 3349 public boolean hasSubject() { 3350 return this.subject != null && !this.subject.isEmpty(); 3351 } 3352 3353 /** 3354 * @param value {@link #subject} (Who or what the composition is about. The 3355 * composition can be about a person, (patient or healthcare 3356 * practitioner), a device (e.g. a machine) or even a group of 3357 * subjects (such as a document about a herd of livestock, or a set 3358 * of patients that share a common exposure).) 3359 */ 3360 public Composition setSubject(Reference value) { 3361 this.subject = value; 3362 return this; 3363 } 3364 3365 /** 3366 * @return {@link #subject} The actual object that is the target of the 3367 * reference. The reference library doesn't populate this, but you can 3368 * use it to hold the resource if you resolve it. (Who or what the 3369 * composition is about. The composition can be about a person, (patient 3370 * or healthcare practitioner), a device (e.g. a machine) or even a 3371 * group of subjects (such as a document about a herd of livestock, or a 3372 * set of patients that share a common exposure).) 3373 */ 3374 public Resource getSubjectTarget() { 3375 return this.subjectTarget; 3376 } 3377 3378 /** 3379 * @param value {@link #subject} The actual object that is the target of the 3380 * reference. The reference library doesn't use these, but you can 3381 * use it to hold the resource if you resolve it. (Who or what the 3382 * composition is about. The composition can be about a person, 3383 * (patient or healthcare practitioner), a device (e.g. a machine) 3384 * or even a group of subjects (such as a document about a herd of 3385 * livestock, or a set of patients that share a common exposure).) 3386 */ 3387 public Composition setSubjectTarget(Resource value) { 3388 this.subjectTarget = value; 3389 return this; 3390 } 3391 3392 /** 3393 * @return {@link #encounter} (Describes the clinical encounter or type of care 3394 * this documentation is associated with.) 3395 */ 3396 public Reference getEncounter() { 3397 if (this.encounter == null) 3398 if (Configuration.errorOnAutoCreate()) 3399 throw new Error("Attempt to auto-create Composition.encounter"); 3400 else if (Configuration.doAutoCreate()) 3401 this.encounter = new Reference(); // cc 3402 return this.encounter; 3403 } 3404 3405 public boolean hasEncounter() { 3406 return this.encounter != null && !this.encounter.isEmpty(); 3407 } 3408 3409 /** 3410 * @param value {@link #encounter} (Describes the clinical encounter or type of 3411 * care this documentation is associated with.) 3412 */ 3413 public Composition setEncounter(Reference value) { 3414 this.encounter = value; 3415 return this; 3416 } 3417 3418 /** 3419 * @return {@link #encounter} The actual object that is the target of the 3420 * reference. The reference library doesn't populate this, but you can 3421 * use it to hold the resource if you resolve it. (Describes the 3422 * clinical encounter or type of care this documentation is associated 3423 * with.) 3424 */ 3425 public Encounter getEncounterTarget() { 3426 if (this.encounterTarget == null) 3427 if (Configuration.errorOnAutoCreate()) 3428 throw new Error("Attempt to auto-create Composition.encounter"); 3429 else if (Configuration.doAutoCreate()) 3430 this.encounterTarget = new Encounter(); // aa 3431 return this.encounterTarget; 3432 } 3433 3434 /** 3435 * @param value {@link #encounter} The actual object that is the target of the 3436 * reference. The reference library doesn't use these, but you can 3437 * use it to hold the resource if you resolve it. (Describes the 3438 * clinical encounter or type of care this documentation is 3439 * associated with.) 3440 */ 3441 public Composition setEncounterTarget(Encounter value) { 3442 this.encounterTarget = value; 3443 return this; 3444 } 3445 3446 /** 3447 * @return {@link #date} (The composition editing time, when the composition was 3448 * last logically changed by the author.). This is the underlying object 3449 * with id, value and extensions. The accessor "getDate" gives direct 3450 * access to the value 3451 */ 3452 public DateTimeType getDateElement() { 3453 if (this.date == null) 3454 if (Configuration.errorOnAutoCreate()) 3455 throw new Error("Attempt to auto-create Composition.date"); 3456 else if (Configuration.doAutoCreate()) 3457 this.date = new DateTimeType(); // bb 3458 return this.date; 3459 } 3460 3461 public boolean hasDateElement() { 3462 return this.date != null && !this.date.isEmpty(); 3463 } 3464 3465 public boolean hasDate() { 3466 return this.date != null && !this.date.isEmpty(); 3467 } 3468 3469 /** 3470 * @param value {@link #date} (The composition editing time, when the 3471 * composition was last logically changed by the author.). This is 3472 * the underlying object with id, value and extensions. The 3473 * accessor "getDate" gives direct access to the value 3474 */ 3475 public Composition setDateElement(DateTimeType value) { 3476 this.date = value; 3477 return this; 3478 } 3479 3480 /** 3481 * @return The composition editing time, when the composition was last logically 3482 * changed by the author. 3483 */ 3484 public Date getDate() { 3485 return this.date == null ? null : this.date.getValue(); 3486 } 3487 3488 /** 3489 * @param value The composition editing time, when the composition was last 3490 * logically changed by the author. 3491 */ 3492 public Composition setDate(Date value) { 3493 if (this.date == null) 3494 this.date = new DateTimeType(); 3495 this.date.setValue(value); 3496 return this; 3497 } 3498 3499 /** 3500 * @return {@link #author} (Identifies who is responsible for the information in 3501 * the composition, not necessarily who typed it in.) 3502 */ 3503 public List<Reference> getAuthor() { 3504 if (this.author == null) 3505 this.author = new ArrayList<Reference>(); 3506 return this.author; 3507 } 3508 3509 /** 3510 * @return Returns a reference to <code>this</code> for easy method chaining 3511 */ 3512 public Composition setAuthor(List<Reference> theAuthor) { 3513 this.author = theAuthor; 3514 return this; 3515 } 3516 3517 public boolean hasAuthor() { 3518 if (this.author == null) 3519 return false; 3520 for (Reference item : this.author) 3521 if (!item.isEmpty()) 3522 return true; 3523 return false; 3524 } 3525 3526 public Reference addAuthor() { // 3 3527 Reference t = new Reference(); 3528 if (this.author == null) 3529 this.author = new ArrayList<Reference>(); 3530 this.author.add(t); 3531 return t; 3532 } 3533 3534 public Composition addAuthor(Reference t) { // 3 3535 if (t == null) 3536 return this; 3537 if (this.author == null) 3538 this.author = new ArrayList<Reference>(); 3539 this.author.add(t); 3540 return this; 3541 } 3542 3543 /** 3544 * @return The first repetition of repeating field {@link #author}, creating it 3545 * if it does not already exist 3546 */ 3547 public Reference getAuthorFirstRep() { 3548 if (getAuthor().isEmpty()) { 3549 addAuthor(); 3550 } 3551 return getAuthor().get(0); 3552 } 3553 3554 /** 3555 * @deprecated Use Reference#setResource(IBaseResource) instead 3556 */ 3557 @Deprecated 3558 public List<Resource> getAuthorTarget() { 3559 if (this.authorTarget == null) 3560 this.authorTarget = new ArrayList<Resource>(); 3561 return this.authorTarget; 3562 } 3563 3564 /** 3565 * @return {@link #title} (Official human-readable label for the composition.). 3566 * This is the underlying object with id, value and extensions. The 3567 * accessor "getTitle" gives direct access to the value 3568 */ 3569 public StringType getTitleElement() { 3570 if (this.title == null) 3571 if (Configuration.errorOnAutoCreate()) 3572 throw new Error("Attempt to auto-create Composition.title"); 3573 else if (Configuration.doAutoCreate()) 3574 this.title = new StringType(); // bb 3575 return this.title; 3576 } 3577 3578 public boolean hasTitleElement() { 3579 return this.title != null && !this.title.isEmpty(); 3580 } 3581 3582 public boolean hasTitle() { 3583 return this.title != null && !this.title.isEmpty(); 3584 } 3585 3586 /** 3587 * @param value {@link #title} (Official human-readable label for the 3588 * composition.). This is the underlying object with id, value and 3589 * extensions. The accessor "getTitle" gives direct access to the 3590 * value 3591 */ 3592 public Composition setTitleElement(StringType value) { 3593 this.title = value; 3594 return this; 3595 } 3596 3597 /** 3598 * @return Official human-readable label for the composition. 3599 */ 3600 public String getTitle() { 3601 return this.title == null ? null : this.title.getValue(); 3602 } 3603 3604 /** 3605 * @param value Official human-readable label for the composition. 3606 */ 3607 public Composition setTitle(String value) { 3608 if (this.title == null) 3609 this.title = new StringType(); 3610 this.title.setValue(value); 3611 return this; 3612 } 3613 3614 /** 3615 * @return {@link #confidentiality} (The code specifying the level of 3616 * confidentiality of the Composition.). This is the underlying object 3617 * with id, value and extensions. The accessor "getConfidentiality" 3618 * gives direct access to the value 3619 */ 3620 public Enumeration<DocumentConfidentiality> getConfidentialityElement() { 3621 if (this.confidentiality == null) 3622 if (Configuration.errorOnAutoCreate()) 3623 throw new Error("Attempt to auto-create Composition.confidentiality"); 3624 else if (Configuration.doAutoCreate()) 3625 this.confidentiality = new Enumeration<DocumentConfidentiality>(new DocumentConfidentialityEnumFactory()); // bb 3626 return this.confidentiality; 3627 } 3628 3629 public boolean hasConfidentialityElement() { 3630 return this.confidentiality != null && !this.confidentiality.isEmpty(); 3631 } 3632 3633 public boolean hasConfidentiality() { 3634 return this.confidentiality != null && !this.confidentiality.isEmpty(); 3635 } 3636 3637 /** 3638 * @param value {@link #confidentiality} (The code specifying the level of 3639 * confidentiality of the Composition.). This is the underlying 3640 * object with id, value and extensions. The accessor 3641 * "getConfidentiality" gives direct access to the value 3642 */ 3643 public Composition setConfidentialityElement(Enumeration<DocumentConfidentiality> value) { 3644 this.confidentiality = value; 3645 return this; 3646 } 3647 3648 /** 3649 * @return The code specifying the level of confidentiality of the Composition. 3650 */ 3651 public DocumentConfidentiality getConfidentiality() { 3652 return this.confidentiality == null ? null : this.confidentiality.getValue(); 3653 } 3654 3655 /** 3656 * @param value The code specifying the level of confidentiality of the 3657 * Composition. 3658 */ 3659 public Composition setConfidentiality(DocumentConfidentiality value) { 3660 if (value == null) 3661 this.confidentiality = null; 3662 else { 3663 if (this.confidentiality == null) 3664 this.confidentiality = new Enumeration<DocumentConfidentiality>(new DocumentConfidentialityEnumFactory()); 3665 this.confidentiality.setValue(value); 3666 } 3667 return this; 3668 } 3669 3670 /** 3671 * @return {@link #attester} (A participant who has attested to the accuracy of 3672 * the composition/document.) 3673 */ 3674 public List<CompositionAttesterComponent> getAttester() { 3675 if (this.attester == null) 3676 this.attester = new ArrayList<CompositionAttesterComponent>(); 3677 return this.attester; 3678 } 3679 3680 /** 3681 * @return Returns a reference to <code>this</code> for easy method chaining 3682 */ 3683 public Composition setAttester(List<CompositionAttesterComponent> theAttester) { 3684 this.attester = theAttester; 3685 return this; 3686 } 3687 3688 public boolean hasAttester() { 3689 if (this.attester == null) 3690 return false; 3691 for (CompositionAttesterComponent item : this.attester) 3692 if (!item.isEmpty()) 3693 return true; 3694 return false; 3695 } 3696 3697 public CompositionAttesterComponent addAttester() { // 3 3698 CompositionAttesterComponent t = new CompositionAttesterComponent(); 3699 if (this.attester == null) 3700 this.attester = new ArrayList<CompositionAttesterComponent>(); 3701 this.attester.add(t); 3702 return t; 3703 } 3704 3705 public Composition addAttester(CompositionAttesterComponent t) { // 3 3706 if (t == null) 3707 return this; 3708 if (this.attester == null) 3709 this.attester = new ArrayList<CompositionAttesterComponent>(); 3710 this.attester.add(t); 3711 return this; 3712 } 3713 3714 /** 3715 * @return The first repetition of repeating field {@link #attester}, creating 3716 * it if it does not already exist 3717 */ 3718 public CompositionAttesterComponent getAttesterFirstRep() { 3719 if (getAttester().isEmpty()) { 3720 addAttester(); 3721 } 3722 return getAttester().get(0); 3723 } 3724 3725 /** 3726 * @return {@link #custodian} (Identifies the organization or group who is 3727 * responsible for ongoing maintenance of and access to the 3728 * composition/document information.) 3729 */ 3730 public Reference getCustodian() { 3731 if (this.custodian == null) 3732 if (Configuration.errorOnAutoCreate()) 3733 throw new Error("Attempt to auto-create Composition.custodian"); 3734 else if (Configuration.doAutoCreate()) 3735 this.custodian = new Reference(); // cc 3736 return this.custodian; 3737 } 3738 3739 public boolean hasCustodian() { 3740 return this.custodian != null && !this.custodian.isEmpty(); 3741 } 3742 3743 /** 3744 * @param value {@link #custodian} (Identifies the organization or group who is 3745 * responsible for ongoing maintenance of and access to the 3746 * composition/document information.) 3747 */ 3748 public Composition setCustodian(Reference value) { 3749 this.custodian = value; 3750 return this; 3751 } 3752 3753 /** 3754 * @return {@link #custodian} The actual object that is the target of the 3755 * reference. The reference library doesn't populate this, but you can 3756 * use it to hold the resource if you resolve it. (Identifies the 3757 * organization or group who is responsible for ongoing maintenance of 3758 * and access to the composition/document information.) 3759 */ 3760 public Organization getCustodianTarget() { 3761 if (this.custodianTarget == null) 3762 if (Configuration.errorOnAutoCreate()) 3763 throw new Error("Attempt to auto-create Composition.custodian"); 3764 else if (Configuration.doAutoCreate()) 3765 this.custodianTarget = new Organization(); // aa 3766 return this.custodianTarget; 3767 } 3768 3769 /** 3770 * @param value {@link #custodian} The actual object that is the target of the 3771 * reference. The reference library doesn't use these, but you can 3772 * use it to hold the resource if you resolve it. (Identifies the 3773 * organization or group who is responsible for ongoing maintenance 3774 * of and access to the composition/document information.) 3775 */ 3776 public Composition setCustodianTarget(Organization value) { 3777 this.custodianTarget = value; 3778 return this; 3779 } 3780 3781 /** 3782 * @return {@link #relatesTo} (Relationships that this composition has with 3783 * other compositions or documents that already exist.) 3784 */ 3785 public List<CompositionRelatesToComponent> getRelatesTo() { 3786 if (this.relatesTo == null) 3787 this.relatesTo = new ArrayList<CompositionRelatesToComponent>(); 3788 return this.relatesTo; 3789 } 3790 3791 /** 3792 * @return Returns a reference to <code>this</code> for easy method chaining 3793 */ 3794 public Composition setRelatesTo(List<CompositionRelatesToComponent> theRelatesTo) { 3795 this.relatesTo = theRelatesTo; 3796 return this; 3797 } 3798 3799 public boolean hasRelatesTo() { 3800 if (this.relatesTo == null) 3801 return false; 3802 for (CompositionRelatesToComponent item : this.relatesTo) 3803 if (!item.isEmpty()) 3804 return true; 3805 return false; 3806 } 3807 3808 public CompositionRelatesToComponent addRelatesTo() { // 3 3809 CompositionRelatesToComponent t = new CompositionRelatesToComponent(); 3810 if (this.relatesTo == null) 3811 this.relatesTo = new ArrayList<CompositionRelatesToComponent>(); 3812 this.relatesTo.add(t); 3813 return t; 3814 } 3815 3816 public Composition addRelatesTo(CompositionRelatesToComponent t) { // 3 3817 if (t == null) 3818 return this; 3819 if (this.relatesTo == null) 3820 this.relatesTo = new ArrayList<CompositionRelatesToComponent>(); 3821 this.relatesTo.add(t); 3822 return this; 3823 } 3824 3825 /** 3826 * @return The first repetition of repeating field {@link #relatesTo}, creating 3827 * it if it does not already exist 3828 */ 3829 public CompositionRelatesToComponent getRelatesToFirstRep() { 3830 if (getRelatesTo().isEmpty()) { 3831 addRelatesTo(); 3832 } 3833 return getRelatesTo().get(0); 3834 } 3835 3836 /** 3837 * @return {@link #event} (The clinical service, such as a colonoscopy or an 3838 * appendectomy, being documented.) 3839 */ 3840 public List<CompositionEventComponent> getEvent() { 3841 if (this.event == null) 3842 this.event = new ArrayList<CompositionEventComponent>(); 3843 return this.event; 3844 } 3845 3846 /** 3847 * @return Returns a reference to <code>this</code> for easy method chaining 3848 */ 3849 public Composition setEvent(List<CompositionEventComponent> theEvent) { 3850 this.event = theEvent; 3851 return this; 3852 } 3853 3854 public boolean hasEvent() { 3855 if (this.event == null) 3856 return false; 3857 for (CompositionEventComponent item : this.event) 3858 if (!item.isEmpty()) 3859 return true; 3860 return false; 3861 } 3862 3863 public CompositionEventComponent addEvent() { // 3 3864 CompositionEventComponent t = new CompositionEventComponent(); 3865 if (this.event == null) 3866 this.event = new ArrayList<CompositionEventComponent>(); 3867 this.event.add(t); 3868 return t; 3869 } 3870 3871 public Composition addEvent(CompositionEventComponent t) { // 3 3872 if (t == null) 3873 return this; 3874 if (this.event == null) 3875 this.event = new ArrayList<CompositionEventComponent>(); 3876 this.event.add(t); 3877 return this; 3878 } 3879 3880 /** 3881 * @return The first repetition of repeating field {@link #event}, creating it 3882 * if it does not already exist 3883 */ 3884 public CompositionEventComponent getEventFirstRep() { 3885 if (getEvent().isEmpty()) { 3886 addEvent(); 3887 } 3888 return getEvent().get(0); 3889 } 3890 3891 /** 3892 * @return {@link #section} (The root of the sections that make up the 3893 * composition.) 3894 */ 3895 public List<SectionComponent> getSection() { 3896 if (this.section == null) 3897 this.section = new ArrayList<SectionComponent>(); 3898 return this.section; 3899 } 3900 3901 /** 3902 * @return Returns a reference to <code>this</code> for easy method chaining 3903 */ 3904 public Composition setSection(List<SectionComponent> theSection) { 3905 this.section = theSection; 3906 return this; 3907 } 3908 3909 public boolean hasSection() { 3910 if (this.section == null) 3911 return false; 3912 for (SectionComponent item : this.section) 3913 if (!item.isEmpty()) 3914 return true; 3915 return false; 3916 } 3917 3918 public SectionComponent addSection() { // 3 3919 SectionComponent t = new SectionComponent(); 3920 if (this.section == null) 3921 this.section = new ArrayList<SectionComponent>(); 3922 this.section.add(t); 3923 return t; 3924 } 3925 3926 public Composition addSection(SectionComponent t) { // 3 3927 if (t == null) 3928 return this; 3929 if (this.section == null) 3930 this.section = new ArrayList<SectionComponent>(); 3931 this.section.add(t); 3932 return this; 3933 } 3934 3935 /** 3936 * @return The first repetition of repeating field {@link #section}, creating it 3937 * if it does not already exist 3938 */ 3939 public SectionComponent getSectionFirstRep() { 3940 if (getSection().isEmpty()) { 3941 addSection(); 3942 } 3943 return getSection().get(0); 3944 } 3945 3946 protected void listChildren(List<Property> children) { 3947 super.listChildren(children); 3948 children.add(new Property("identifier", "Identifier", 3949 "A version-independent identifier for the Composition. This identifier stays constant as the composition is changed over time.", 3950 0, 1, identifier)); 3951 children.add(new Property("status", "code", 3952 "The workflow/clinical status of this composition. The status is a marker for the clinical standing of the document.", 3953 0, 1, status)); 3954 children.add(new Property("type", "CodeableConcept", 3955 "Specifies the particular kind of composition (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the composition.", 3956 0, 1, type)); 3957 children.add(new Property("category", "CodeableConcept", 3958 "A categorization for the type of the composition - helps for indexing and searching. This may be implied by or derived from the code specified in the Composition Type.", 3959 0, java.lang.Integer.MAX_VALUE, category)); 3960 children.add(new Property("subject", "Reference(Any)", 3961 "Who or what the composition is about. The composition can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of livestock, or a set of patients that share a common exposure).", 3962 0, 1, subject)); 3963 children.add(new Property("encounter", "Reference(Encounter)", 3964 "Describes the clinical encounter or type of care this documentation is associated with.", 0, 1, encounter)); 3965 children.add(new Property("date", "dateTime", 3966 "The composition editing time, when the composition was last logically changed by the author.", 0, 1, date)); 3967 children.add( 3968 new Property("author", "Reference(Practitioner|PractitionerRole|Device|Patient|RelatedPerson|Organization)", 3969 "Identifies who is responsible for the information in the composition, not necessarily who typed it in.", 0, 3970 java.lang.Integer.MAX_VALUE, author)); 3971 children.add(new Property("title", "string", "Official human-readable label for the composition.", 0, 1, title)); 3972 children.add(new Property("confidentiality", "code", 3973 "The code specifying the level of confidentiality of the Composition.", 0, 1, confidentiality)); 3974 children 3975 .add(new Property("attester", "", "A participant who has attested to the accuracy of the composition/document.", 3976 0, java.lang.Integer.MAX_VALUE, attester)); 3977 children.add(new Property("custodian", "Reference(Organization)", 3978 "Identifies the organization or group who is responsible for ongoing maintenance of and access to the composition/document information.", 3979 0, 1, custodian)); 3980 children.add(new Property("relatesTo", "", 3981 "Relationships that this composition has with other compositions or documents that already exist.", 0, 3982 java.lang.Integer.MAX_VALUE, relatesTo)); 3983 children.add( 3984 new Property("event", "", "The clinical service, such as a colonoscopy or an appendectomy, being documented.", 3985 0, java.lang.Integer.MAX_VALUE, event)); 3986 children.add(new Property("section", "", "The root of the sections that make up the composition.", 0, 3987 java.lang.Integer.MAX_VALUE, section)); 3988 } 3989 3990 @Override 3991 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3992 switch (_hash) { 3993 case -1618432855: 3994 /* identifier */ return new Property("identifier", "Identifier", 3995 "A version-independent identifier for the Composition. This identifier stays constant as the composition is changed over time.", 3996 0, 1, identifier); 3997 case -892481550: 3998 /* status */ return new Property("status", "code", 3999 "The workflow/clinical status of this composition. The status is a marker for the clinical standing of the document.", 4000 0, 1, status); 4001 case 3575610: 4002 /* type */ return new Property("type", "CodeableConcept", 4003 "Specifies the particular kind of composition (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the composition.", 4004 0, 1, type); 4005 case 50511102: 4006 /* category */ return new Property("category", "CodeableConcept", 4007 "A categorization for the type of the composition - helps for indexing and searching. This may be implied by or derived from the code specified in the Composition Type.", 4008 0, java.lang.Integer.MAX_VALUE, category); 4009 case -1867885268: 4010 /* subject */ return new Property("subject", "Reference(Any)", 4011 "Who or what the composition is about. The composition can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of livestock, or a set of patients that share a common exposure).", 4012 0, 1, subject); 4013 case 1524132147: 4014 /* encounter */ return new Property("encounter", "Reference(Encounter)", 4015 "Describes the clinical encounter or type of care this documentation is associated with.", 0, 1, encounter); 4016 case 3076014: 4017 /* date */ return new Property("date", "dateTime", 4018 "The composition editing time, when the composition was last logically changed by the author.", 0, 1, date); 4019 case -1406328437: 4020 /* author */ return new Property("author", 4021 "Reference(Practitioner|PractitionerRole|Device|Patient|RelatedPerson|Organization)", 4022 "Identifies who is responsible for the information in the composition, not necessarily who typed it in.", 0, 4023 java.lang.Integer.MAX_VALUE, author); 4024 case 110371416: 4025 /* title */ return new Property("title", "string", "Official human-readable label for the composition.", 0, 1, 4026 title); 4027 case -1923018202: 4028 /* confidentiality */ return new Property("confidentiality", "code", 4029 "The code specifying the level of confidentiality of the Composition.", 0, 1, confidentiality); 4030 case 542920370: 4031 /* attester */ return new Property("attester", "", 4032 "A participant who has attested to the accuracy of the composition/document.", 0, java.lang.Integer.MAX_VALUE, 4033 attester); 4034 case 1611297262: 4035 /* custodian */ return new Property("custodian", "Reference(Organization)", 4036 "Identifies the organization or group who is responsible for ongoing maintenance of and access to the composition/document information.", 4037 0, 1, custodian); 4038 case -7765931: 4039 /* relatesTo */ return new Property("relatesTo", "", 4040 "Relationships that this composition has with other compositions or documents that already exist.", 0, 4041 java.lang.Integer.MAX_VALUE, relatesTo); 4042 case 96891546: 4043 /* event */ return new Property("event", "", 4044 "The clinical service, such as a colonoscopy or an appendectomy, being documented.", 0, 4045 java.lang.Integer.MAX_VALUE, event); 4046 case 1970241253: 4047 /* section */ return new Property("section", "", "The root of the sections that make up the composition.", 0, 4048 java.lang.Integer.MAX_VALUE, section); 4049 default: 4050 return super.getNamedProperty(_hash, _name, _checkValid); 4051 } 4052 4053 } 4054 4055 @Override 4056 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4057 switch (hash) { 4058 case -1618432855: 4059 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 4060 case -892481550: 4061 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<CompositionStatus> 4062 case 3575610: 4063 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 4064 case 50511102: 4065 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 4066 case -1867885268: 4067 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 4068 case 1524132147: 4069 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 4070 case 3076014: 4071 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 4072 case -1406328437: 4073 /* author */ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // Reference 4074 case 110371416: 4075 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 4076 case -1923018202: 4077 /* confidentiality */ return this.confidentiality == null ? new Base[0] : new Base[] { this.confidentiality }; // Enumeration<DocumentConfidentiality> 4078 case 542920370: 4079 /* attester */ return this.attester == null ? new Base[0] : this.attester.toArray(new Base[this.attester.size()]); // CompositionAttesterComponent 4080 case 1611297262: 4081 /* custodian */ return this.custodian == null ? new Base[0] : new Base[] { this.custodian }; // Reference 4082 case -7765931: 4083 /* relatesTo */ return this.relatesTo == null ? new Base[0] 4084 : this.relatesTo.toArray(new Base[this.relatesTo.size()]); // CompositionRelatesToComponent 4085 case 96891546: 4086 /* event */ return this.event == null ? new Base[0] : this.event.toArray(new Base[this.event.size()]); // CompositionEventComponent 4087 case 1970241253: 4088 /* section */ return this.section == null ? new Base[0] : this.section.toArray(new Base[this.section.size()]); // SectionComponent 4089 default: 4090 return super.getProperty(hash, name, checkValid); 4091 } 4092 4093 } 4094 4095 @Override 4096 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4097 switch (hash) { 4098 case -1618432855: // identifier 4099 this.identifier = castToIdentifier(value); // Identifier 4100 return value; 4101 case -892481550: // status 4102 value = new CompositionStatusEnumFactory().fromType(castToCode(value)); 4103 this.status = (Enumeration) value; // Enumeration<CompositionStatus> 4104 return value; 4105 case 3575610: // type 4106 this.type = castToCodeableConcept(value); // CodeableConcept 4107 return value; 4108 case 50511102: // category 4109 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 4110 return value; 4111 case -1867885268: // subject 4112 this.subject = castToReference(value); // Reference 4113 return value; 4114 case 1524132147: // encounter 4115 this.encounter = castToReference(value); // Reference 4116 return value; 4117 case 3076014: // date 4118 this.date = castToDateTime(value); // DateTimeType 4119 return value; 4120 case -1406328437: // author 4121 this.getAuthor().add(castToReference(value)); // Reference 4122 return value; 4123 case 110371416: // title 4124 this.title = castToString(value); // StringType 4125 return value; 4126 case -1923018202: // confidentiality 4127 value = new DocumentConfidentialityEnumFactory().fromType(castToCode(value)); 4128 this.confidentiality = (Enumeration) value; // Enumeration<DocumentConfidentiality> 4129 return value; 4130 case 542920370: // attester 4131 this.getAttester().add((CompositionAttesterComponent) value); // CompositionAttesterComponent 4132 return value; 4133 case 1611297262: // custodian 4134 this.custodian = castToReference(value); // Reference 4135 return value; 4136 case -7765931: // relatesTo 4137 this.getRelatesTo().add((CompositionRelatesToComponent) value); // CompositionRelatesToComponent 4138 return value; 4139 case 96891546: // event 4140 this.getEvent().add((CompositionEventComponent) value); // CompositionEventComponent 4141 return value; 4142 case 1970241253: // section 4143 this.getSection().add((SectionComponent) value); // SectionComponent 4144 return value; 4145 default: 4146 return super.setProperty(hash, name, value); 4147 } 4148 4149 } 4150 4151 @Override 4152 public Base setProperty(String name, Base value) throws FHIRException { 4153 if (name.equals("identifier")) { 4154 this.identifier = castToIdentifier(value); // Identifier 4155 } else if (name.equals("status")) { 4156 value = new CompositionStatusEnumFactory().fromType(castToCode(value)); 4157 this.status = (Enumeration) value; // Enumeration<CompositionStatus> 4158 } else if (name.equals("type")) { 4159 this.type = castToCodeableConcept(value); // CodeableConcept 4160 } else if (name.equals("category")) { 4161 this.getCategory().add(castToCodeableConcept(value)); 4162 } else if (name.equals("subject")) { 4163 this.subject = castToReference(value); // Reference 4164 } else if (name.equals("encounter")) { 4165 this.encounter = castToReference(value); // Reference 4166 } else if (name.equals("date")) { 4167 this.date = castToDateTime(value); // DateTimeType 4168 } else if (name.equals("author")) { 4169 this.getAuthor().add(castToReference(value)); 4170 } else if (name.equals("title")) { 4171 this.title = castToString(value); // StringType 4172 } else if (name.equals("confidentiality")) { 4173 value = new DocumentConfidentialityEnumFactory().fromType(castToCode(value)); 4174 this.confidentiality = (Enumeration) value; // Enumeration<DocumentConfidentiality> 4175 } else if (name.equals("attester")) { 4176 this.getAttester().add((CompositionAttesterComponent) value); 4177 } else if (name.equals("custodian")) { 4178 this.custodian = castToReference(value); // Reference 4179 } else if (name.equals("relatesTo")) { 4180 this.getRelatesTo().add((CompositionRelatesToComponent) value); 4181 } else if (name.equals("event")) { 4182 this.getEvent().add((CompositionEventComponent) value); 4183 } else if (name.equals("section")) { 4184 this.getSection().add((SectionComponent) value); 4185 } else 4186 return super.setProperty(name, value); 4187 return value; 4188 } 4189 4190 @Override 4191 public void removeChild(String name, Base value) throws FHIRException { 4192 if (name.equals("identifier")) { 4193 this.identifier = null; 4194 } else if (name.equals("status")) { 4195 this.status = null; 4196 } else if (name.equals("type")) { 4197 this.type = null; 4198 } else if (name.equals("category")) { 4199 this.getCategory().remove(castToCodeableConcept(value)); 4200 } else if (name.equals("subject")) { 4201 this.subject = null; 4202 } else if (name.equals("encounter")) { 4203 this.encounter = null; 4204 } else if (name.equals("date")) { 4205 this.date = null; 4206 } else if (name.equals("author")) { 4207 this.getAuthor().remove(castToReference(value)); 4208 } else if (name.equals("title")) { 4209 this.title = null; 4210 } else if (name.equals("confidentiality")) { 4211 this.confidentiality = null; 4212 } else if (name.equals("attester")) { 4213 this.getAttester().remove((CompositionAttesterComponent) value); 4214 } else if (name.equals("custodian")) { 4215 this.custodian = null; 4216 } else if (name.equals("relatesTo")) { 4217 this.getRelatesTo().remove((CompositionRelatesToComponent) value); 4218 } else if (name.equals("event")) { 4219 this.getEvent().remove((CompositionEventComponent) value); 4220 } else if (name.equals("section")) { 4221 this.getSection().remove((SectionComponent) value); 4222 } else 4223 super.removeChild(name, value); 4224 4225 } 4226 4227 @Override 4228 public Base makeProperty(int hash, String name) throws FHIRException { 4229 switch (hash) { 4230 case -1618432855: 4231 return getIdentifier(); 4232 case -892481550: 4233 return getStatusElement(); 4234 case 3575610: 4235 return getType(); 4236 case 50511102: 4237 return addCategory(); 4238 case -1867885268: 4239 return getSubject(); 4240 case 1524132147: 4241 return getEncounter(); 4242 case 3076014: 4243 return getDateElement(); 4244 case -1406328437: 4245 return addAuthor(); 4246 case 110371416: 4247 return getTitleElement(); 4248 case -1923018202: 4249 return getConfidentialityElement(); 4250 case 542920370: 4251 return addAttester(); 4252 case 1611297262: 4253 return getCustodian(); 4254 case -7765931: 4255 return addRelatesTo(); 4256 case 96891546: 4257 return addEvent(); 4258 case 1970241253: 4259 return addSection(); 4260 default: 4261 return super.makeProperty(hash, name); 4262 } 4263 4264 } 4265 4266 @Override 4267 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4268 switch (hash) { 4269 case -1618432855: 4270 /* identifier */ return new String[] { "Identifier" }; 4271 case -892481550: 4272 /* status */ return new String[] { "code" }; 4273 case 3575610: 4274 /* type */ return new String[] { "CodeableConcept" }; 4275 case 50511102: 4276 /* category */ return new String[] { "CodeableConcept" }; 4277 case -1867885268: 4278 /* subject */ return new String[] { "Reference" }; 4279 case 1524132147: 4280 /* encounter */ return new String[] { "Reference" }; 4281 case 3076014: 4282 /* date */ return new String[] { "dateTime" }; 4283 case -1406328437: 4284 /* author */ return new String[] { "Reference" }; 4285 case 110371416: 4286 /* title */ return new String[] { "string" }; 4287 case -1923018202: 4288 /* confidentiality */ return new String[] { "code" }; 4289 case 542920370: 4290 /* attester */ return new String[] {}; 4291 case 1611297262: 4292 /* custodian */ return new String[] { "Reference" }; 4293 case -7765931: 4294 /* relatesTo */ return new String[] {}; 4295 case 96891546: 4296 /* event */ return new String[] {}; 4297 case 1970241253: 4298 /* section */ return new String[] {}; 4299 default: 4300 return super.getTypesForProperty(hash, name); 4301 } 4302 4303 } 4304 4305 @Override 4306 public Base addChild(String name) throws FHIRException { 4307 if (name.equals("identifier")) { 4308 this.identifier = new Identifier(); 4309 return this.identifier; 4310 } else if (name.equals("status")) { 4311 throw new FHIRException("Cannot call addChild on a singleton property Composition.status"); 4312 } else if (name.equals("type")) { 4313 this.type = new CodeableConcept(); 4314 return this.type; 4315 } else if (name.equals("category")) { 4316 return addCategory(); 4317 } else if (name.equals("subject")) { 4318 this.subject = new Reference(); 4319 return this.subject; 4320 } else if (name.equals("encounter")) { 4321 this.encounter = new Reference(); 4322 return this.encounter; 4323 } else if (name.equals("date")) { 4324 throw new FHIRException("Cannot call addChild on a singleton property Composition.date"); 4325 } else if (name.equals("author")) { 4326 return addAuthor(); 4327 } else if (name.equals("title")) { 4328 throw new FHIRException("Cannot call addChild on a singleton property Composition.title"); 4329 } else if (name.equals("confidentiality")) { 4330 throw new FHIRException("Cannot call addChild on a singleton property Composition.confidentiality"); 4331 } else if (name.equals("attester")) { 4332 return addAttester(); 4333 } else if (name.equals("custodian")) { 4334 this.custodian = new Reference(); 4335 return this.custodian; 4336 } else if (name.equals("relatesTo")) { 4337 return addRelatesTo(); 4338 } else if (name.equals("event")) { 4339 return addEvent(); 4340 } else if (name.equals("section")) { 4341 return addSection(); 4342 } else 4343 return super.addChild(name); 4344 } 4345 4346 public String fhirType() { 4347 return "Composition"; 4348 4349 } 4350 4351 public Composition copy() { 4352 Composition dst = new Composition(); 4353 copyValues(dst); 4354 return dst; 4355 } 4356 4357 public void copyValues(Composition dst) { 4358 super.copyValues(dst); 4359 dst.identifier = identifier == null ? null : identifier.copy(); 4360 dst.status = status == null ? null : status.copy(); 4361 dst.type = type == null ? null : type.copy(); 4362 if (category != null) { 4363 dst.category = new ArrayList<CodeableConcept>(); 4364 for (CodeableConcept i : category) 4365 dst.category.add(i.copy()); 4366 } 4367 ; 4368 dst.subject = subject == null ? null : subject.copy(); 4369 dst.encounter = encounter == null ? null : encounter.copy(); 4370 dst.date = date == null ? null : date.copy(); 4371 if (author != null) { 4372 dst.author = new ArrayList<Reference>(); 4373 for (Reference i : author) 4374 dst.author.add(i.copy()); 4375 } 4376 ; 4377 dst.title = title == null ? null : title.copy(); 4378 dst.confidentiality = confidentiality == null ? null : confidentiality.copy(); 4379 if (attester != null) { 4380 dst.attester = new ArrayList<CompositionAttesterComponent>(); 4381 for (CompositionAttesterComponent i : attester) 4382 dst.attester.add(i.copy()); 4383 } 4384 ; 4385 dst.custodian = custodian == null ? null : custodian.copy(); 4386 if (relatesTo != null) { 4387 dst.relatesTo = new ArrayList<CompositionRelatesToComponent>(); 4388 for (CompositionRelatesToComponent i : relatesTo) 4389 dst.relatesTo.add(i.copy()); 4390 } 4391 ; 4392 if (event != null) { 4393 dst.event = new ArrayList<CompositionEventComponent>(); 4394 for (CompositionEventComponent i : event) 4395 dst.event.add(i.copy()); 4396 } 4397 ; 4398 if (section != null) { 4399 dst.section = new ArrayList<SectionComponent>(); 4400 for (SectionComponent i : section) 4401 dst.section.add(i.copy()); 4402 } 4403 ; 4404 } 4405 4406 protected Composition typedCopy() { 4407 return copy(); 4408 } 4409 4410 @Override 4411 public boolean equalsDeep(Base other_) { 4412 if (!super.equalsDeep(other_)) 4413 return false; 4414 if (!(other_ instanceof Composition)) 4415 return false; 4416 Composition o = (Composition) other_; 4417 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 4418 && compareDeep(type, o.type, true) && compareDeep(category, o.category, true) 4419 && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 4420 && compareDeep(date, o.date, true) && compareDeep(author, o.author, true) && compareDeep(title, o.title, true) 4421 && compareDeep(confidentiality, o.confidentiality, true) && compareDeep(attester, o.attester, true) 4422 && compareDeep(custodian, o.custodian, true) && compareDeep(relatesTo, o.relatesTo, true) 4423 && compareDeep(event, o.event, true) && compareDeep(section, o.section, true); 4424 } 4425 4426 @Override 4427 public boolean equalsShallow(Base other_) { 4428 if (!super.equalsShallow(other_)) 4429 return false; 4430 if (!(other_ instanceof Composition)) 4431 return false; 4432 Composition o = (Composition) other_; 4433 return compareValues(status, o.status, true) && compareValues(date, o.date, true) 4434 && compareValues(title, o.title, true) && compareValues(confidentiality, o.confidentiality, true); 4435 } 4436 4437 public boolean isEmpty() { 4438 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, type, category, subject, 4439 encounter, date, author, title, confidentiality, attester, custodian, relatesTo, event, section); 4440 } 4441 4442 @Override 4443 public ResourceType getResourceType() { 4444 return ResourceType.Composition; 4445 } 4446 4447 /** 4448 * Search parameter: <b>date</b> 4449 * <p> 4450 * Description: <b>Composition editing time</b><br> 4451 * Type: <b>date</b><br> 4452 * Path: <b>Composition.date</b><br> 4453 * </p> 4454 */ 4455 @SearchParamDefinition(name = "date", path = "Composition.date", description = "Composition editing time", type = "date") 4456 public static final String SP_DATE = "date"; 4457 /** 4458 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4459 * <p> 4460 * Description: <b>Composition editing time</b><br> 4461 * Type: <b>date</b><br> 4462 * Path: <b>Composition.date</b><br> 4463 * </p> 4464 */ 4465 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 4466 SP_DATE); 4467 4468 /** 4469 * Search parameter: <b>identifier</b> 4470 * <p> 4471 * Description: <b>Version-independent identifier for the Composition</b><br> 4472 * Type: <b>token</b><br> 4473 * Path: <b>Composition.identifier</b><br> 4474 * </p> 4475 */ 4476 @SearchParamDefinition(name = "identifier", path = "Composition.identifier", description = "Version-independent identifier for the Composition", type = "token") 4477 public static final String SP_IDENTIFIER = "identifier"; 4478 /** 4479 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4480 * <p> 4481 * Description: <b>Version-independent identifier for the Composition</b><br> 4482 * Type: <b>token</b><br> 4483 * Path: <b>Composition.identifier</b><br> 4484 * </p> 4485 */ 4486 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4487 SP_IDENTIFIER); 4488 4489 /** 4490 * Search parameter: <b>period</b> 4491 * <p> 4492 * Description: <b>The period covered by the documentation</b><br> 4493 * Type: <b>date</b><br> 4494 * Path: <b>Composition.event.period</b><br> 4495 * </p> 4496 */ 4497 @SearchParamDefinition(name = "period", path = "Composition.event.period", description = "The period covered by the documentation", type = "date") 4498 public static final String SP_PERIOD = "period"; 4499 /** 4500 * <b>Fluent Client</b> search parameter constant for <b>period</b> 4501 * <p> 4502 * Description: <b>The period covered by the documentation</b><br> 4503 * Type: <b>date</b><br> 4504 * Path: <b>Composition.event.period</b><br> 4505 * </p> 4506 */ 4507 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam( 4508 SP_PERIOD); 4509 4510 /** 4511 * Search parameter: <b>related-id</b> 4512 * <p> 4513 * Description: <b>Target of the relationship</b><br> 4514 * Type: <b>token</b><br> 4515 * Path: <b>Composition.relatesTo.targetIdentifier</b><br> 4516 * </p> 4517 */ 4518 @SearchParamDefinition(name = "related-id", path = "(Composition.relatesTo.target as Identifier)", description = "Target of the relationship", type = "token") 4519 public static final String SP_RELATED_ID = "related-id"; 4520 /** 4521 * <b>Fluent Client</b> search parameter constant for <b>related-id</b> 4522 * <p> 4523 * Description: <b>Target of the relationship</b><br> 4524 * Type: <b>token</b><br> 4525 * Path: <b>Composition.relatesTo.targetIdentifier</b><br> 4526 * </p> 4527 */ 4528 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RELATED_ID = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4529 SP_RELATED_ID); 4530 4531 /** 4532 * Search parameter: <b>subject</b> 4533 * <p> 4534 * Description: <b>Who and/or what the composition is about</b><br> 4535 * Type: <b>reference</b><br> 4536 * Path: <b>Composition.subject</b><br> 4537 * </p> 4538 */ 4539 @SearchParamDefinition(name = "subject", path = "Composition.subject", description = "Who and/or what the composition is about", type = "reference", providesMembershipIn = { 4540 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 4541 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }) 4542 public static final String SP_SUBJECT = "subject"; 4543 /** 4544 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 4545 * <p> 4546 * Description: <b>Who and/or what the composition is about</b><br> 4547 * Type: <b>reference</b><br> 4548 * Path: <b>Composition.subject</b><br> 4549 * </p> 4550 */ 4551 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4552 SP_SUBJECT); 4553 4554 /** 4555 * Constant for fluent queries to be used to add include statements. Specifies 4556 * the path value of "<b>Composition:subject</b>". 4557 */ 4558 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 4559 "Composition:subject").toLocked(); 4560 4561 /** 4562 * Search parameter: <b>author</b> 4563 * <p> 4564 * Description: <b>Who and/or what authored the composition</b><br> 4565 * Type: <b>reference</b><br> 4566 * Path: <b>Composition.author</b><br> 4567 * </p> 4568 */ 4569 @SearchParamDefinition(name = "author", path = "Composition.author", description = "Who and/or what authored the composition", type = "reference", providesMembershipIn = { 4570 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 4571 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 4572 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 4573 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, 4574 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 4575 public static final String SP_AUTHOR = "author"; 4576 /** 4577 * <b>Fluent Client</b> search parameter constant for <b>author</b> 4578 * <p> 4579 * Description: <b>Who and/or what authored the composition</b><br> 4580 * Type: <b>reference</b><br> 4581 * Path: <b>Composition.author</b><br> 4582 * </p> 4583 */ 4584 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4585 SP_AUTHOR); 4586 4587 /** 4588 * Constant for fluent queries to be used to add include statements. Specifies 4589 * the path value of "<b>Composition:author</b>". 4590 */ 4591 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include( 4592 "Composition:author").toLocked(); 4593 4594 /** 4595 * Search parameter: <b>confidentiality</b> 4596 * <p> 4597 * Description: <b>As defined by affinity domain</b><br> 4598 * Type: <b>token</b><br> 4599 * Path: <b>Composition.confidentiality</b><br> 4600 * </p> 4601 */ 4602 @SearchParamDefinition(name = "confidentiality", path = "Composition.confidentiality", description = "As defined by affinity domain", type = "token") 4603 public static final String SP_CONFIDENTIALITY = "confidentiality"; 4604 /** 4605 * <b>Fluent Client</b> search parameter constant for <b>confidentiality</b> 4606 * <p> 4607 * Description: <b>As defined by affinity domain</b><br> 4608 * Type: <b>token</b><br> 4609 * Path: <b>Composition.confidentiality</b><br> 4610 * </p> 4611 */ 4612 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONFIDENTIALITY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4613 SP_CONFIDENTIALITY); 4614 4615 /** 4616 * Search parameter: <b>section</b> 4617 * <p> 4618 * Description: <b>Classification of section (recommended)</b><br> 4619 * Type: <b>token</b><br> 4620 * Path: <b>Composition.section.code</b><br> 4621 * </p> 4622 */ 4623 @SearchParamDefinition(name = "section", path = "Composition.section.code", description = "Classification of section (recommended)", type = "token") 4624 public static final String SP_SECTION = "section"; 4625 /** 4626 * <b>Fluent Client</b> search parameter constant for <b>section</b> 4627 * <p> 4628 * Description: <b>Classification of section (recommended)</b><br> 4629 * Type: <b>token</b><br> 4630 * Path: <b>Composition.section.code</b><br> 4631 * </p> 4632 */ 4633 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SECTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4634 SP_SECTION); 4635 4636 /** 4637 * Search parameter: <b>encounter</b> 4638 * <p> 4639 * Description: <b>Context of the Composition</b><br> 4640 * Type: <b>reference</b><br> 4641 * Path: <b>Composition.encounter</b><br> 4642 * </p> 4643 */ 4644 @SearchParamDefinition(name = "encounter", path = "Composition.encounter", description = "Context of the Composition", type = "reference", providesMembershipIn = { 4645 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 4646 public static final String SP_ENCOUNTER = "encounter"; 4647 /** 4648 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 4649 * <p> 4650 * Description: <b>Context of the Composition</b><br> 4651 * Type: <b>reference</b><br> 4652 * Path: <b>Composition.encounter</b><br> 4653 * </p> 4654 */ 4655 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4656 SP_ENCOUNTER); 4657 4658 /** 4659 * Constant for fluent queries to be used to add include statements. Specifies 4660 * the path value of "<b>Composition:encounter</b>". 4661 */ 4662 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 4663 "Composition:encounter").toLocked(); 4664 4665 /** 4666 * Search parameter: <b>type</b> 4667 * <p> 4668 * Description: <b>Kind of composition (LOINC if possible)</b><br> 4669 * Type: <b>token</b><br> 4670 * Path: <b>Composition.type</b><br> 4671 * </p> 4672 */ 4673 @SearchParamDefinition(name = "type", path = "Composition.type", description = "Kind of composition (LOINC if possible)", type = "token") 4674 public static final String SP_TYPE = "type"; 4675 /** 4676 * <b>Fluent Client</b> search parameter constant for <b>type</b> 4677 * <p> 4678 * Description: <b>Kind of composition (LOINC if possible)</b><br> 4679 * Type: <b>token</b><br> 4680 * Path: <b>Composition.type</b><br> 4681 * </p> 4682 */ 4683 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4684 SP_TYPE); 4685 4686 /** 4687 * Search parameter: <b>title</b> 4688 * <p> 4689 * Description: <b>Human Readable name/title</b><br> 4690 * Type: <b>string</b><br> 4691 * Path: <b>Composition.title</b><br> 4692 * </p> 4693 */ 4694 @SearchParamDefinition(name = "title", path = "Composition.title", description = "Human Readable name/title", type = "string") 4695 public static final String SP_TITLE = "title"; 4696 /** 4697 * <b>Fluent Client</b> search parameter constant for <b>title</b> 4698 * <p> 4699 * Description: <b>Human Readable name/title</b><br> 4700 * Type: <b>string</b><br> 4701 * Path: <b>Composition.title</b><br> 4702 * </p> 4703 */ 4704 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 4705 SP_TITLE); 4706 4707 /** 4708 * Search parameter: <b>attester</b> 4709 * <p> 4710 * Description: <b>Who attested the composition</b><br> 4711 * Type: <b>reference</b><br> 4712 * Path: <b>Composition.attester.party</b><br> 4713 * </p> 4714 */ 4715 @SearchParamDefinition(name = "attester", path = "Composition.attester.party", description = "Who attested the composition", type = "reference", providesMembershipIn = { 4716 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 4717 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 4718 Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 4719 public static final String SP_ATTESTER = "attester"; 4720 /** 4721 * <b>Fluent Client</b> search parameter constant for <b>attester</b> 4722 * <p> 4723 * Description: <b>Who attested the composition</b><br> 4724 * Type: <b>reference</b><br> 4725 * Path: <b>Composition.attester.party</b><br> 4726 * </p> 4727 */ 4728 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ATTESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4729 SP_ATTESTER); 4730 4731 /** 4732 * Constant for fluent queries to be used to add include statements. Specifies 4733 * the path value of "<b>Composition:attester</b>". 4734 */ 4735 public static final ca.uhn.fhir.model.api.Include INCLUDE_ATTESTER = new ca.uhn.fhir.model.api.Include( 4736 "Composition:attester").toLocked(); 4737 4738 /** 4739 * Search parameter: <b>entry</b> 4740 * <p> 4741 * Description: <b>A reference to data that supports this section</b><br> 4742 * Type: <b>reference</b><br> 4743 * Path: <b>Composition.section.entry</b><br> 4744 * </p> 4745 */ 4746 @SearchParamDefinition(name = "entry", path = "Composition.section.entry", description = "A reference to data that supports this section", type = "reference") 4747 public static final String SP_ENTRY = "entry"; 4748 /** 4749 * <b>Fluent Client</b> search parameter constant for <b>entry</b> 4750 * <p> 4751 * Description: <b>A reference to data that supports this section</b><br> 4752 * Type: <b>reference</b><br> 4753 * Path: <b>Composition.section.entry</b><br> 4754 * </p> 4755 */ 4756 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTRY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4757 SP_ENTRY); 4758 4759 /** 4760 * Constant for fluent queries to be used to add include statements. Specifies 4761 * the path value of "<b>Composition:entry</b>". 4762 */ 4763 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTRY = new ca.uhn.fhir.model.api.Include( 4764 "Composition:entry").toLocked(); 4765 4766 /** 4767 * Search parameter: <b>related-ref</b> 4768 * <p> 4769 * Description: <b>Target of the relationship</b><br> 4770 * Type: <b>reference</b><br> 4771 * Path: <b>Composition.relatesTo.targetReference</b><br> 4772 * </p> 4773 */ 4774 @SearchParamDefinition(name = "related-ref", path = "(Composition.relatesTo.target as Reference)", description = "Target of the relationship", type = "reference", target = { 4775 Composition.class }) 4776 public static final String SP_RELATED_REF = "related-ref"; 4777 /** 4778 * <b>Fluent Client</b> search parameter constant for <b>related-ref</b> 4779 * <p> 4780 * Description: <b>Target of the relationship</b><br> 4781 * Type: <b>reference</b><br> 4782 * Path: <b>Composition.relatesTo.targetReference</b><br> 4783 * </p> 4784 */ 4785 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RELATED_REF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4786 SP_RELATED_REF); 4787 4788 /** 4789 * Constant for fluent queries to be used to add include statements. Specifies 4790 * the path value of "<b>Composition:related-ref</b>". 4791 */ 4792 public static final ca.uhn.fhir.model.api.Include INCLUDE_RELATED_REF = new ca.uhn.fhir.model.api.Include( 4793 "Composition:related-ref").toLocked(); 4794 4795 /** 4796 * Search parameter: <b>patient</b> 4797 * <p> 4798 * Description: <b>Who and/or what the composition is about</b><br> 4799 * Type: <b>reference</b><br> 4800 * Path: <b>Composition.subject</b><br> 4801 * </p> 4802 */ 4803 @SearchParamDefinition(name = "patient", path = "Composition.subject.where(resolve() is Patient)", description = "Who and/or what the composition is about", type = "reference", target = { 4804 Patient.class }) 4805 public static final String SP_PATIENT = "patient"; 4806 /** 4807 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4808 * <p> 4809 * Description: <b>Who and/or what the composition is about</b><br> 4810 * Type: <b>reference</b><br> 4811 * Path: <b>Composition.subject</b><br> 4812 * </p> 4813 */ 4814 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4815 SP_PATIENT); 4816 4817 /** 4818 * Constant for fluent queries to be used to add include statements. Specifies 4819 * the path value of "<b>Composition:patient</b>". 4820 */ 4821 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 4822 "Composition:patient").toLocked(); 4823 4824 /** 4825 * Search parameter: <b>context</b> 4826 * <p> 4827 * Description: <b>Code(s) that apply to the event being documented</b><br> 4828 * Type: <b>token</b><br> 4829 * Path: <b>Composition.event.code</b><br> 4830 * </p> 4831 */ 4832 @SearchParamDefinition(name = "context", path = "Composition.event.code", description = "Code(s) that apply to the event being documented", type = "token") 4833 public static final String SP_CONTEXT = "context"; 4834 /** 4835 * <b>Fluent Client</b> search parameter constant for <b>context</b> 4836 * <p> 4837 * Description: <b>Code(s) that apply to the event being documented</b><br> 4838 * Type: <b>token</b><br> 4839 * Path: <b>Composition.event.code</b><br> 4840 * </p> 4841 */ 4842 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4843 SP_CONTEXT); 4844 4845 /** 4846 * Search parameter: <b>category</b> 4847 * <p> 4848 * Description: <b>Categorization of Composition</b><br> 4849 * Type: <b>token</b><br> 4850 * Path: <b>Composition.category</b><br> 4851 * </p> 4852 */ 4853 @SearchParamDefinition(name = "category", path = "Composition.category", description = "Categorization of Composition", type = "token") 4854 public static final String SP_CATEGORY = "category"; 4855 /** 4856 * <b>Fluent Client</b> search parameter constant for <b>category</b> 4857 * <p> 4858 * Description: <b>Categorization of Composition</b><br> 4859 * Type: <b>token</b><br> 4860 * Path: <b>Composition.category</b><br> 4861 * </p> 4862 */ 4863 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4864 SP_CATEGORY); 4865 4866 /** 4867 * Search parameter: <b>status</b> 4868 * <p> 4869 * Description: <b>preliminary | final | amended | entered-in-error</b><br> 4870 * Type: <b>token</b><br> 4871 * Path: <b>Composition.status</b><br> 4872 * </p> 4873 */ 4874 @SearchParamDefinition(name = "status", path = "Composition.status", description = "preliminary | final | amended | entered-in-error", type = "token") 4875 public static final String SP_STATUS = "status"; 4876 /** 4877 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4878 * <p> 4879 * Description: <b>preliminary | final | amended | entered-in-error</b><br> 4880 * Type: <b>token</b><br> 4881 * Path: <b>Composition.status</b><br> 4882 * </p> 4883 */ 4884 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4885 SP_STATUS); 4886 4887}