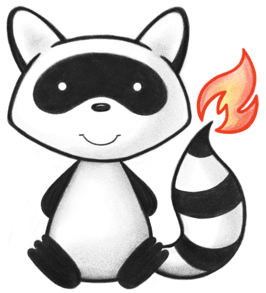
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence; 040import org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalenceEnumFactory; 041import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 042import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.ResourceDef; 050import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 051 052/** 053 * A statement of relationships from one set of concepts to one or more other 054 * concepts - either concepts in code systems, or data element/data element 055 * concepts, or classes in class models. 056 */ 057@ResourceDef(name = "ConceptMap", profile = "http://hl7.org/fhir/StructureDefinition/ConceptMap") 058@ChildOrder(names = { "url", "identifier", "version", "name", "title", "status", "experimental", "date", "publisher", 059 "contact", "description", "useContext", "jurisdiction", "purpose", "copyright", "source[x]", "target[x]", "group" }) 060public class ConceptMap extends MetadataResource { 061 062 public enum ConceptMapGroupUnmappedMode { 063 /** 064 * Use the code as provided in the $translate request. 065 */ 066 PROVIDED, 067 /** 068 * Use the code explicitly provided in the group.unmapped. 069 */ 070 FIXED, 071 /** 072 * Use the map identified by the canonical URL in the url element. 073 */ 074 OTHERMAP, 075 /** 076 * added to help the parsers with the generic types 077 */ 078 NULL; 079 080 public static ConceptMapGroupUnmappedMode fromCode(String codeString) throws FHIRException { 081 if (codeString == null || "".equals(codeString)) 082 return null; 083 if ("provided".equals(codeString)) 084 return PROVIDED; 085 if ("fixed".equals(codeString)) 086 return FIXED; 087 if ("other-map".equals(codeString)) 088 return OTHERMAP; 089 if (Configuration.isAcceptInvalidEnums()) 090 return null; 091 else 092 throw new FHIRException("Unknown ConceptMapGroupUnmappedMode code '" + codeString + "'"); 093 } 094 095 public String toCode() { 096 switch (this) { 097 case PROVIDED: 098 return "provided"; 099 case FIXED: 100 return "fixed"; 101 case OTHERMAP: 102 return "other-map"; 103 case NULL: 104 return null; 105 default: 106 return "?"; 107 } 108 } 109 110 public String getSystem() { 111 switch (this) { 112 case PROVIDED: 113 return "http://hl7.org/fhir/conceptmap-unmapped-mode"; 114 case FIXED: 115 return "http://hl7.org/fhir/conceptmap-unmapped-mode"; 116 case OTHERMAP: 117 return "http://hl7.org/fhir/conceptmap-unmapped-mode"; 118 case NULL: 119 return null; 120 default: 121 return "?"; 122 } 123 } 124 125 public String getDefinition() { 126 switch (this) { 127 case PROVIDED: 128 return "Use the code as provided in the $translate request."; 129 case FIXED: 130 return "Use the code explicitly provided in the group.unmapped."; 131 case OTHERMAP: 132 return "Use the map identified by the canonical URL in the url element."; 133 case NULL: 134 return null; 135 default: 136 return "?"; 137 } 138 } 139 140 public String getDisplay() { 141 switch (this) { 142 case PROVIDED: 143 return "Provided Code"; 144 case FIXED: 145 return "Fixed Code"; 146 case OTHERMAP: 147 return "Other Map"; 148 case NULL: 149 return null; 150 default: 151 return "?"; 152 } 153 } 154 } 155 156 public static class ConceptMapGroupUnmappedModeEnumFactory implements EnumFactory<ConceptMapGroupUnmappedMode> { 157 public ConceptMapGroupUnmappedMode fromCode(String codeString) throws IllegalArgumentException { 158 if (codeString == null || "".equals(codeString)) 159 if (codeString == null || "".equals(codeString)) 160 return null; 161 if ("provided".equals(codeString)) 162 return ConceptMapGroupUnmappedMode.PROVIDED; 163 if ("fixed".equals(codeString)) 164 return ConceptMapGroupUnmappedMode.FIXED; 165 if ("other-map".equals(codeString)) 166 return ConceptMapGroupUnmappedMode.OTHERMAP; 167 throw new IllegalArgumentException("Unknown ConceptMapGroupUnmappedMode code '" + codeString + "'"); 168 } 169 170 public Enumeration<ConceptMapGroupUnmappedMode> fromType(PrimitiveType<?> code) throws FHIRException { 171 if (code == null) 172 return null; 173 if (code.isEmpty()) 174 return new Enumeration<ConceptMapGroupUnmappedMode>(this, ConceptMapGroupUnmappedMode.NULL, code); 175 String codeString = code.asStringValue(); 176 if (codeString == null || "".equals(codeString)) 177 return new Enumeration<ConceptMapGroupUnmappedMode>(this, ConceptMapGroupUnmappedMode.NULL, code); 178 if ("provided".equals(codeString)) 179 return new Enumeration<ConceptMapGroupUnmappedMode>(this, ConceptMapGroupUnmappedMode.PROVIDED, code); 180 if ("fixed".equals(codeString)) 181 return new Enumeration<ConceptMapGroupUnmappedMode>(this, ConceptMapGroupUnmappedMode.FIXED, code); 182 if ("other-map".equals(codeString)) 183 return new Enumeration<ConceptMapGroupUnmappedMode>(this, ConceptMapGroupUnmappedMode.OTHERMAP, code); 184 throw new FHIRException("Unknown ConceptMapGroupUnmappedMode code '" + codeString + "'"); 185 } 186 187 public String toCode(ConceptMapGroupUnmappedMode code) { 188 if (code == ConceptMapGroupUnmappedMode.PROVIDED) 189 return "provided"; 190 if (code == ConceptMapGroupUnmappedMode.FIXED) 191 return "fixed"; 192 if (code == ConceptMapGroupUnmappedMode.OTHERMAP) 193 return "other-map"; 194 return "?"; 195 } 196 197 public String toSystem(ConceptMapGroupUnmappedMode code) { 198 return code.getSystem(); 199 } 200 } 201 202 @Block() 203 public static class ConceptMapGroupComponent extends BackboneElement implements IBaseBackboneElement { 204 /** 205 * An absolute URI that identifies the source system where the concepts to be 206 * mapped are defined. 207 */ 208 @Child(name = "source", type = { UriType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 209 @Description(shortDefinition = "Source system where concepts to be mapped are defined", formalDefinition = "An absolute URI that identifies the source system where the concepts to be mapped are defined.") 210 protected UriType source; 211 212 /** 213 * The specific version of the code system, as determined by the code system 214 * authority. 215 */ 216 @Child(name = "sourceVersion", type = { 217 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 218 @Description(shortDefinition = "Specific version of the code system", formalDefinition = "The specific version of the code system, as determined by the code system authority.") 219 protected StringType sourceVersion; 220 221 /** 222 * An absolute URI that identifies the target system that the concepts will be 223 * mapped to. 224 */ 225 @Child(name = "target", type = { UriType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 226 @Description(shortDefinition = "Target system that the concepts are to be mapped to", formalDefinition = "An absolute URI that identifies the target system that the concepts will be mapped to.") 227 protected UriType target; 228 229 /** 230 * The specific version of the code system, as determined by the code system 231 * authority. 232 */ 233 @Child(name = "targetVersion", type = { 234 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 235 @Description(shortDefinition = "Specific version of the code system", formalDefinition = "The specific version of the code system, as determined by the code system authority.") 236 protected StringType targetVersion; 237 238 /** 239 * Mappings for an individual concept in the source to one or more concepts in 240 * the target. 241 */ 242 @Child(name = "element", type = {}, order = 5, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 243 @Description(shortDefinition = "Mappings for a concept from the source set", formalDefinition = "Mappings for an individual concept in the source to one or more concepts in the target.") 244 protected List<SourceElementComponent> element; 245 246 /** 247 * What to do when there is no mapping for the source concept. "Unmapped" does 248 * not include codes that are unmatched, and the unmapped element is ignored in 249 * a code is specified to have equivalence = unmatched. 250 */ 251 @Child(name = "unmapped", type = {}, order = 6, min = 0, max = 1, modifier = false, summary = false) 252 @Description(shortDefinition = "What to do when there is no mapping for the source concept", formalDefinition = "What to do when there is no mapping for the source concept. \"Unmapped\" does not include codes that are unmatched, and the unmapped element is ignored in a code is specified to have equivalence = unmatched.") 253 protected ConceptMapGroupUnmappedComponent unmapped; 254 255 private static final long serialVersionUID = 1606357508L; 256 257 /** 258 * Constructor 259 */ 260 public ConceptMapGroupComponent() { 261 super(); 262 } 263 264 /** 265 * @return {@link #source} (An absolute URI that identifies the source system 266 * where the concepts to be mapped are defined.). This is the underlying 267 * object with id, value and extensions. The accessor "getSource" gives 268 * direct access to the value 269 */ 270 public UriType getSourceElement() { 271 if (this.source == null) 272 if (Configuration.errorOnAutoCreate()) 273 throw new Error("Attempt to auto-create ConceptMapGroupComponent.source"); 274 else if (Configuration.doAutoCreate()) 275 this.source = new UriType(); // bb 276 return this.source; 277 } 278 279 public boolean hasSourceElement() { 280 return this.source != null && !this.source.isEmpty(); 281 } 282 283 public boolean hasSource() { 284 return this.source != null && !this.source.isEmpty(); 285 } 286 287 /** 288 * @param value {@link #source} (An absolute URI that identifies the source 289 * system where the concepts to be mapped are defined.). This is 290 * the underlying object with id, value and extensions. The 291 * accessor "getSource" gives direct access to the value 292 */ 293 public ConceptMapGroupComponent setSourceElement(UriType value) { 294 this.source = value; 295 return this; 296 } 297 298 /** 299 * @return An absolute URI that identifies the source system where the concepts 300 * to be mapped are defined. 301 */ 302 public String getSource() { 303 return this.source == null ? null : this.source.getValue(); 304 } 305 306 /** 307 * @param value An absolute URI that identifies the source system where the 308 * concepts to be mapped are defined. 309 */ 310 public ConceptMapGroupComponent setSource(String value) { 311 if (Utilities.noString(value)) 312 this.source = null; 313 else { 314 if (this.source == null) 315 this.source = new UriType(); 316 this.source.setValue(value); 317 } 318 return this; 319 } 320 321 /** 322 * @return {@link #sourceVersion} (The specific version of the code system, as 323 * determined by the code system authority.). This is the underlying 324 * object with id, value and extensions. The accessor "getSourceVersion" 325 * gives direct access to the value 326 */ 327 public StringType getSourceVersionElement() { 328 if (this.sourceVersion == null) 329 if (Configuration.errorOnAutoCreate()) 330 throw new Error("Attempt to auto-create ConceptMapGroupComponent.sourceVersion"); 331 else if (Configuration.doAutoCreate()) 332 this.sourceVersion = new StringType(); // bb 333 return this.sourceVersion; 334 } 335 336 public boolean hasSourceVersionElement() { 337 return this.sourceVersion != null && !this.sourceVersion.isEmpty(); 338 } 339 340 public boolean hasSourceVersion() { 341 return this.sourceVersion != null && !this.sourceVersion.isEmpty(); 342 } 343 344 /** 345 * @param value {@link #sourceVersion} (The specific version of the code system, 346 * as determined by the code system authority.). This is the 347 * underlying object with id, value and extensions. The accessor 348 * "getSourceVersion" gives direct access to the value 349 */ 350 public ConceptMapGroupComponent setSourceVersionElement(StringType value) { 351 this.sourceVersion = value; 352 return this; 353 } 354 355 /** 356 * @return The specific version of the code system, as determined by the code 357 * system authority. 358 */ 359 public String getSourceVersion() { 360 return this.sourceVersion == null ? null : this.sourceVersion.getValue(); 361 } 362 363 /** 364 * @param value The specific version of the code system, as determined by the 365 * code system authority. 366 */ 367 public ConceptMapGroupComponent setSourceVersion(String value) { 368 if (Utilities.noString(value)) 369 this.sourceVersion = null; 370 else { 371 if (this.sourceVersion == null) 372 this.sourceVersion = new StringType(); 373 this.sourceVersion.setValue(value); 374 } 375 return this; 376 } 377 378 /** 379 * @return {@link #target} (An absolute URI that identifies the target system 380 * that the concepts will be mapped to.). This is the underlying object 381 * with id, value and extensions. The accessor "getTarget" gives direct 382 * access to the value 383 */ 384 public UriType getTargetElement() { 385 if (this.target == null) 386 if (Configuration.errorOnAutoCreate()) 387 throw new Error("Attempt to auto-create ConceptMapGroupComponent.target"); 388 else if (Configuration.doAutoCreate()) 389 this.target = new UriType(); // bb 390 return this.target; 391 } 392 393 public boolean hasTargetElement() { 394 return this.target != null && !this.target.isEmpty(); 395 } 396 397 public boolean hasTarget() { 398 return this.target != null && !this.target.isEmpty(); 399 } 400 401 /** 402 * @param value {@link #target} (An absolute URI that identifies the target 403 * system that the concepts will be mapped to.). This is the 404 * underlying object with id, value and extensions. The accessor 405 * "getTarget" gives direct access to the value 406 */ 407 public ConceptMapGroupComponent setTargetElement(UriType value) { 408 this.target = value; 409 return this; 410 } 411 412 /** 413 * @return An absolute URI that identifies the target system that the concepts 414 * will be mapped to. 415 */ 416 public String getTarget() { 417 return this.target == null ? null : this.target.getValue(); 418 } 419 420 /** 421 * @param value An absolute URI that identifies the target system that the 422 * concepts will be mapped to. 423 */ 424 public ConceptMapGroupComponent setTarget(String value) { 425 if (Utilities.noString(value)) 426 this.target = null; 427 else { 428 if (this.target == null) 429 this.target = new UriType(); 430 this.target.setValue(value); 431 } 432 return this; 433 } 434 435 /** 436 * @return {@link #targetVersion} (The specific version of the code system, as 437 * determined by the code system authority.). This is the underlying 438 * object with id, value and extensions. The accessor "getTargetVersion" 439 * gives direct access to the value 440 */ 441 public StringType getTargetVersionElement() { 442 if (this.targetVersion == null) 443 if (Configuration.errorOnAutoCreate()) 444 throw new Error("Attempt to auto-create ConceptMapGroupComponent.targetVersion"); 445 else if (Configuration.doAutoCreate()) 446 this.targetVersion = new StringType(); // bb 447 return this.targetVersion; 448 } 449 450 public boolean hasTargetVersionElement() { 451 return this.targetVersion != null && !this.targetVersion.isEmpty(); 452 } 453 454 public boolean hasTargetVersion() { 455 return this.targetVersion != null && !this.targetVersion.isEmpty(); 456 } 457 458 /** 459 * @param value {@link #targetVersion} (The specific version of the code system, 460 * as determined by the code system authority.). This is the 461 * underlying object with id, value and extensions. The accessor 462 * "getTargetVersion" gives direct access to the value 463 */ 464 public ConceptMapGroupComponent setTargetVersionElement(StringType value) { 465 this.targetVersion = value; 466 return this; 467 } 468 469 /** 470 * @return The specific version of the code system, as determined by the code 471 * system authority. 472 */ 473 public String getTargetVersion() { 474 return this.targetVersion == null ? null : this.targetVersion.getValue(); 475 } 476 477 /** 478 * @param value The specific version of the code system, as determined by the 479 * code system authority. 480 */ 481 public ConceptMapGroupComponent setTargetVersion(String value) { 482 if (Utilities.noString(value)) 483 this.targetVersion = null; 484 else { 485 if (this.targetVersion == null) 486 this.targetVersion = new StringType(); 487 this.targetVersion.setValue(value); 488 } 489 return this; 490 } 491 492 /** 493 * @return {@link #element} (Mappings for an individual concept in the source to 494 * one or more concepts in the target.) 495 */ 496 public List<SourceElementComponent> getElement() { 497 if (this.element == null) 498 this.element = new ArrayList<SourceElementComponent>(); 499 return this.element; 500 } 501 502 /** 503 * @return Returns a reference to <code>this</code> for easy method chaining 504 */ 505 public ConceptMapGroupComponent setElement(List<SourceElementComponent> theElement) { 506 this.element = theElement; 507 return this; 508 } 509 510 public boolean hasElement() { 511 if (this.element == null) 512 return false; 513 for (SourceElementComponent item : this.element) 514 if (!item.isEmpty()) 515 return true; 516 return false; 517 } 518 519 public SourceElementComponent addElement() { // 3 520 SourceElementComponent t = new SourceElementComponent(); 521 if (this.element == null) 522 this.element = new ArrayList<SourceElementComponent>(); 523 this.element.add(t); 524 return t; 525 } 526 527 public ConceptMapGroupComponent addElement(SourceElementComponent t) { // 3 528 if (t == null) 529 return this; 530 if (this.element == null) 531 this.element = new ArrayList<SourceElementComponent>(); 532 this.element.add(t); 533 return this; 534 } 535 536 /** 537 * @return The first repetition of repeating field {@link #element}, creating it 538 * if it does not already exist 539 */ 540 public SourceElementComponent getElementFirstRep() { 541 if (getElement().isEmpty()) { 542 addElement(); 543 } 544 return getElement().get(0); 545 } 546 547 /** 548 * @return {@link #unmapped} (What to do when there is no mapping for the source 549 * concept. "Unmapped" does not include codes that are unmatched, and 550 * the unmapped element is ignored in a code is specified to have 551 * equivalence = unmatched.) 552 */ 553 public ConceptMapGroupUnmappedComponent getUnmapped() { 554 if (this.unmapped == null) 555 if (Configuration.errorOnAutoCreate()) 556 throw new Error("Attempt to auto-create ConceptMapGroupComponent.unmapped"); 557 else if (Configuration.doAutoCreate()) 558 this.unmapped = new ConceptMapGroupUnmappedComponent(); // cc 559 return this.unmapped; 560 } 561 562 public boolean hasUnmapped() { 563 return this.unmapped != null && !this.unmapped.isEmpty(); 564 } 565 566 /** 567 * @param value {@link #unmapped} (What to do when there is no mapping for the 568 * source concept. "Unmapped" does not include codes that are 569 * unmatched, and the unmapped element is ignored in a code is 570 * specified to have equivalence = unmatched.) 571 */ 572 public ConceptMapGroupComponent setUnmapped(ConceptMapGroupUnmappedComponent value) { 573 this.unmapped = value; 574 return this; 575 } 576 577 protected void listChildren(List<Property> children) { 578 super.listChildren(children); 579 children.add(new Property("source", "uri", 580 "An absolute URI that identifies the source system where the concepts to be mapped are defined.", 0, 1, 581 source)); 582 children.add(new Property("sourceVersion", "string", 583 "The specific version of the code system, as determined by the code system authority.", 0, 1, sourceVersion)); 584 children.add(new Property("target", "uri", 585 "An absolute URI that identifies the target system that the concepts will be mapped to.", 0, 1, target)); 586 children.add(new Property("targetVersion", "string", 587 "The specific version of the code system, as determined by the code system authority.", 0, 1, targetVersion)); 588 children.add(new Property("element", "", 589 "Mappings for an individual concept in the source to one or more concepts in the target.", 0, 590 java.lang.Integer.MAX_VALUE, element)); 591 children.add(new Property("unmapped", "", 592 "What to do when there is no mapping for the source concept. \"Unmapped\" does not include codes that are unmatched, and the unmapped element is ignored in a code is specified to have equivalence = unmatched.", 593 0, 1, unmapped)); 594 } 595 596 @Override 597 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 598 switch (_hash) { 599 case -896505829: 600 /* source */ return new Property("source", "uri", 601 "An absolute URI that identifies the source system where the concepts to be mapped are defined.", 0, 1, 602 source); 603 case 446171197: 604 /* sourceVersion */ return new Property("sourceVersion", "string", 605 "The specific version of the code system, as determined by the code system authority.", 0, 1, 606 sourceVersion); 607 case -880905839: 608 /* target */ return new Property("target", "uri", 609 "An absolute URI that identifies the target system that the concepts will be mapped to.", 0, 1, target); 610 case -1639412217: 611 /* targetVersion */ return new Property("targetVersion", "string", 612 "The specific version of the code system, as determined by the code system authority.", 0, 1, 613 targetVersion); 614 case -1662836996: 615 /* element */ return new Property("element", "", 616 "Mappings for an individual concept in the source to one or more concepts in the target.", 0, 617 java.lang.Integer.MAX_VALUE, element); 618 case -194857460: 619 /* unmapped */ return new Property("unmapped", "", 620 "What to do when there is no mapping for the source concept. \"Unmapped\" does not include codes that are unmatched, and the unmapped element is ignored in a code is specified to have equivalence = unmatched.", 621 0, 1, unmapped); 622 default: 623 return super.getNamedProperty(_hash, _name, _checkValid); 624 } 625 626 } 627 628 @Override 629 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 630 switch (hash) { 631 case -896505829: 632 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // UriType 633 case 446171197: 634 /* sourceVersion */ return this.sourceVersion == null ? new Base[0] : new Base[] { this.sourceVersion }; // StringType 635 case -880905839: 636 /* target */ return this.target == null ? new Base[0] : new Base[] { this.target }; // UriType 637 case -1639412217: 638 /* targetVersion */ return this.targetVersion == null ? new Base[0] : new Base[] { this.targetVersion }; // StringType 639 case -1662836996: 640 /* element */ return this.element == null ? new Base[0] : this.element.toArray(new Base[this.element.size()]); // SourceElementComponent 641 case -194857460: 642 /* unmapped */ return this.unmapped == null ? new Base[0] : new Base[] { this.unmapped }; // ConceptMapGroupUnmappedComponent 643 default: 644 return super.getProperty(hash, name, checkValid); 645 } 646 647 } 648 649 @Override 650 public Base setProperty(int hash, String name, Base value) throws FHIRException { 651 switch (hash) { 652 case -896505829: // source 653 this.source = castToUri(value); // UriType 654 return value; 655 case 446171197: // sourceVersion 656 this.sourceVersion = castToString(value); // StringType 657 return value; 658 case -880905839: // target 659 this.target = castToUri(value); // UriType 660 return value; 661 case -1639412217: // targetVersion 662 this.targetVersion = castToString(value); // StringType 663 return value; 664 case -1662836996: // element 665 this.getElement().add((SourceElementComponent) value); // SourceElementComponent 666 return value; 667 case -194857460: // unmapped 668 this.unmapped = (ConceptMapGroupUnmappedComponent) value; // ConceptMapGroupUnmappedComponent 669 return value; 670 default: 671 return super.setProperty(hash, name, value); 672 } 673 674 } 675 676 @Override 677 public Base setProperty(String name, Base value) throws FHIRException { 678 if (name.equals("source")) { 679 this.source = castToUri(value); // UriType 680 } else if (name.equals("sourceVersion")) { 681 this.sourceVersion = castToString(value); // StringType 682 } else if (name.equals("target")) { 683 this.target = castToUri(value); // UriType 684 } else if (name.equals("targetVersion")) { 685 this.targetVersion = castToString(value); // StringType 686 } else if (name.equals("element")) { 687 this.getElement().add((SourceElementComponent) value); 688 } else if (name.equals("unmapped")) { 689 this.unmapped = (ConceptMapGroupUnmappedComponent) value; // ConceptMapGroupUnmappedComponent 690 } else 691 return super.setProperty(name, value); 692 return value; 693 } 694 695 @Override 696 public Base makeProperty(int hash, String name) throws FHIRException { 697 switch (hash) { 698 case -896505829: 699 return getSourceElement(); 700 case 446171197: 701 return getSourceVersionElement(); 702 case -880905839: 703 return getTargetElement(); 704 case -1639412217: 705 return getTargetVersionElement(); 706 case -1662836996: 707 return addElement(); 708 case -194857460: 709 return getUnmapped(); 710 default: 711 return super.makeProperty(hash, name); 712 } 713 714 } 715 716 @Override 717 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 718 switch (hash) { 719 case -896505829: 720 /* source */ return new String[] { "uri" }; 721 case 446171197: 722 /* sourceVersion */ return new String[] { "string" }; 723 case -880905839: 724 /* target */ return new String[] { "uri" }; 725 case -1639412217: 726 /* targetVersion */ return new String[] { "string" }; 727 case -1662836996: 728 /* element */ return new String[] {}; 729 case -194857460: 730 /* unmapped */ return new String[] {}; 731 default: 732 return super.getTypesForProperty(hash, name); 733 } 734 735 } 736 737 @Override 738 public Base addChild(String name) throws FHIRException { 739 if (name.equals("source")) { 740 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.source"); 741 } else if (name.equals("sourceVersion")) { 742 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.sourceVersion"); 743 } else if (name.equals("target")) { 744 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.target"); 745 } else if (name.equals("targetVersion")) { 746 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.targetVersion"); 747 } else if (name.equals("element")) { 748 return addElement(); 749 } else if (name.equals("unmapped")) { 750 this.unmapped = new ConceptMapGroupUnmappedComponent(); 751 return this.unmapped; 752 } else 753 return super.addChild(name); 754 } 755 756 public ConceptMapGroupComponent copy() { 757 ConceptMapGroupComponent dst = new ConceptMapGroupComponent(); 758 copyValues(dst); 759 return dst; 760 } 761 762 public void copyValues(ConceptMapGroupComponent dst) { 763 super.copyValues(dst); 764 dst.source = source == null ? null : source.copy(); 765 dst.sourceVersion = sourceVersion == null ? null : sourceVersion.copy(); 766 dst.target = target == null ? null : target.copy(); 767 dst.targetVersion = targetVersion == null ? null : targetVersion.copy(); 768 if (element != null) { 769 dst.element = new ArrayList<SourceElementComponent>(); 770 for (SourceElementComponent i : element) 771 dst.element.add(i.copy()); 772 } 773 ; 774 dst.unmapped = unmapped == null ? null : unmapped.copy(); 775 } 776 777 @Override 778 public boolean equalsDeep(Base other_) { 779 if (!super.equalsDeep(other_)) 780 return false; 781 if (!(other_ instanceof ConceptMapGroupComponent)) 782 return false; 783 ConceptMapGroupComponent o = (ConceptMapGroupComponent) other_; 784 return compareDeep(source, o.source, true) && compareDeep(sourceVersion, o.sourceVersion, true) 785 && compareDeep(target, o.target, true) && compareDeep(targetVersion, o.targetVersion, true) 786 && compareDeep(element, o.element, true) && compareDeep(unmapped, o.unmapped, true); 787 } 788 789 @Override 790 public boolean equalsShallow(Base other_) { 791 if (!super.equalsShallow(other_)) 792 return false; 793 if (!(other_ instanceof ConceptMapGroupComponent)) 794 return false; 795 ConceptMapGroupComponent o = (ConceptMapGroupComponent) other_; 796 return compareValues(source, o.source, true) && compareValues(sourceVersion, o.sourceVersion, true) 797 && compareValues(target, o.target, true) && compareValues(targetVersion, o.targetVersion, true); 798 } 799 800 public boolean isEmpty() { 801 return super.isEmpty() 802 && ca.uhn.fhir.util.ElementUtil.isEmpty(source, sourceVersion, target, targetVersion, element, unmapped); 803 } 804 805 public String fhirType() { 806 return "ConceptMap.group"; 807 808 } 809 810 } 811 812 @Block() 813 public static class SourceElementComponent extends BackboneElement implements IBaseBackboneElement { 814 /** 815 * Identity (code or path) or the element/item being mapped. 816 */ 817 @Child(name = "code", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 818 @Description(shortDefinition = "Identifies element being mapped", formalDefinition = "Identity (code or path) or the element/item being mapped.") 819 protected CodeType code; 820 821 /** 822 * The display for the code. The display is only provided to help editors when 823 * editing the concept map. 824 */ 825 @Child(name = "display", type = { 826 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 827 @Description(shortDefinition = "Display for the code", formalDefinition = "The display for the code. The display is only provided to help editors when editing the concept map.") 828 protected StringType display; 829 830 /** 831 * A concept from the target value set that this concept maps to. 832 */ 833 @Child(name = "target", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 834 @Description(shortDefinition = "Concept in target system for element", formalDefinition = "A concept from the target value set that this concept maps to.") 835 protected List<TargetElementComponent> target; 836 837 private static final long serialVersionUID = -1115258852L; 838 839 /** 840 * Constructor 841 */ 842 public SourceElementComponent() { 843 super(); 844 } 845 846 /** 847 * @return {@link #code} (Identity (code or path) or the element/item being 848 * mapped.). This is the underlying object with id, value and 849 * extensions. The accessor "getCode" gives direct access to the value 850 */ 851 public CodeType getCodeElement() { 852 if (this.code == null) 853 if (Configuration.errorOnAutoCreate()) 854 throw new Error("Attempt to auto-create SourceElementComponent.code"); 855 else if (Configuration.doAutoCreate()) 856 this.code = new CodeType(); // bb 857 return this.code; 858 } 859 860 public boolean hasCodeElement() { 861 return this.code != null && !this.code.isEmpty(); 862 } 863 864 public boolean hasCode() { 865 return this.code != null && !this.code.isEmpty(); 866 } 867 868 /** 869 * @param value {@link #code} (Identity (code or path) or the element/item being 870 * mapped.). This is the underlying object with id, value and 871 * extensions. The accessor "getCode" gives direct access to the 872 * value 873 */ 874 public SourceElementComponent setCodeElement(CodeType value) { 875 this.code = value; 876 return this; 877 } 878 879 /** 880 * @return Identity (code or path) or the element/item being mapped. 881 */ 882 public String getCode() { 883 return this.code == null ? null : this.code.getValue(); 884 } 885 886 /** 887 * @param value Identity (code or path) or the element/item being mapped. 888 */ 889 public SourceElementComponent setCode(String value) { 890 if (Utilities.noString(value)) 891 this.code = null; 892 else { 893 if (this.code == null) 894 this.code = new CodeType(); 895 this.code.setValue(value); 896 } 897 return this; 898 } 899 900 /** 901 * @return {@link #display} (The display for the code. The display is only 902 * provided to help editors when editing the concept map.). This is the 903 * underlying object with id, value and extensions. The accessor 904 * "getDisplay" gives direct access to the value 905 */ 906 public StringType getDisplayElement() { 907 if (this.display == null) 908 if (Configuration.errorOnAutoCreate()) 909 throw new Error("Attempt to auto-create SourceElementComponent.display"); 910 else if (Configuration.doAutoCreate()) 911 this.display = new StringType(); // bb 912 return this.display; 913 } 914 915 public boolean hasDisplayElement() { 916 return this.display != null && !this.display.isEmpty(); 917 } 918 919 public boolean hasDisplay() { 920 return this.display != null && !this.display.isEmpty(); 921 } 922 923 /** 924 * @param value {@link #display} (The display for the code. The display is only 925 * provided to help editors when editing the concept map.). This is 926 * the underlying object with id, value and extensions. The 927 * accessor "getDisplay" gives direct access to the value 928 */ 929 public SourceElementComponent setDisplayElement(StringType value) { 930 this.display = value; 931 return this; 932 } 933 934 /** 935 * @return The display for the code. The display is only provided to help 936 * editors when editing the concept map. 937 */ 938 public String getDisplay() { 939 return this.display == null ? null : this.display.getValue(); 940 } 941 942 /** 943 * @param value The display for the code. The display is only provided to help 944 * editors when editing the concept map. 945 */ 946 public SourceElementComponent setDisplay(String value) { 947 if (Utilities.noString(value)) 948 this.display = null; 949 else { 950 if (this.display == null) 951 this.display = new StringType(); 952 this.display.setValue(value); 953 } 954 return this; 955 } 956 957 /** 958 * @return {@link #target} (A concept from the target value set that this 959 * concept maps to.) 960 */ 961 public List<TargetElementComponent> getTarget() { 962 if (this.target == null) 963 this.target = new ArrayList<TargetElementComponent>(); 964 return this.target; 965 } 966 967 /** 968 * @return Returns a reference to <code>this</code> for easy method chaining 969 */ 970 public SourceElementComponent setTarget(List<TargetElementComponent> theTarget) { 971 this.target = theTarget; 972 return this; 973 } 974 975 public boolean hasTarget() { 976 if (this.target == null) 977 return false; 978 for (TargetElementComponent item : this.target) 979 if (!item.isEmpty()) 980 return true; 981 return false; 982 } 983 984 public TargetElementComponent addTarget() { // 3 985 TargetElementComponent t = new TargetElementComponent(); 986 if (this.target == null) 987 this.target = new ArrayList<TargetElementComponent>(); 988 this.target.add(t); 989 return t; 990 } 991 992 public SourceElementComponent addTarget(TargetElementComponent t) { // 3 993 if (t == null) 994 return this; 995 if (this.target == null) 996 this.target = new ArrayList<TargetElementComponent>(); 997 this.target.add(t); 998 return this; 999 } 1000 1001 /** 1002 * @return The first repetition of repeating field {@link #target}, creating it 1003 * if it does not already exist 1004 */ 1005 public TargetElementComponent getTargetFirstRep() { 1006 if (getTarget().isEmpty()) { 1007 addTarget(); 1008 } 1009 return getTarget().get(0); 1010 } 1011 1012 protected void listChildren(List<Property> children) { 1013 super.listChildren(children); 1014 children 1015 .add(new Property("code", "code", "Identity (code or path) or the element/item being mapped.", 0, 1, code)); 1016 children.add(new Property("display", "string", 1017 "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 1, 1018 display)); 1019 children.add(new Property("target", "", "A concept from the target value set that this concept maps to.", 0, 1020 java.lang.Integer.MAX_VALUE, target)); 1021 } 1022 1023 @Override 1024 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1025 switch (_hash) { 1026 case 3059181: 1027 /* code */ return new Property("code", "code", "Identity (code or path) or the element/item being mapped.", 0, 1028 1, code); 1029 case 1671764162: 1030 /* display */ return new Property("display", "string", 1031 "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 1032 1, display); 1033 case -880905839: 1034 /* target */ return new Property("target", "", "A concept from the target value set that this concept maps to.", 1035 0, java.lang.Integer.MAX_VALUE, target); 1036 default: 1037 return super.getNamedProperty(_hash, _name, _checkValid); 1038 } 1039 1040 } 1041 1042 @Override 1043 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1044 switch (hash) { 1045 case 3059181: 1046 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeType 1047 case 1671764162: 1048 /* display */ return this.display == null ? new Base[0] : new Base[] { this.display }; // StringType 1049 case -880905839: 1050 /* target */ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // TargetElementComponent 1051 default: 1052 return super.getProperty(hash, name, checkValid); 1053 } 1054 1055 } 1056 1057 @Override 1058 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1059 switch (hash) { 1060 case 3059181: // code 1061 this.code = castToCode(value); // CodeType 1062 return value; 1063 case 1671764162: // display 1064 this.display = castToString(value); // StringType 1065 return value; 1066 case -880905839: // target 1067 this.getTarget().add((TargetElementComponent) value); // TargetElementComponent 1068 return value; 1069 default: 1070 return super.setProperty(hash, name, value); 1071 } 1072 1073 } 1074 1075 @Override 1076 public Base setProperty(String name, Base value) throws FHIRException { 1077 if (name.equals("code")) { 1078 this.code = castToCode(value); // CodeType 1079 } else if (name.equals("display")) { 1080 this.display = castToString(value); // StringType 1081 } else if (name.equals("target")) { 1082 this.getTarget().add((TargetElementComponent) value); 1083 } else 1084 return super.setProperty(name, value); 1085 return value; 1086 } 1087 1088 @Override 1089 public Base makeProperty(int hash, String name) throws FHIRException { 1090 switch (hash) { 1091 case 3059181: 1092 return getCodeElement(); 1093 case 1671764162: 1094 return getDisplayElement(); 1095 case -880905839: 1096 return addTarget(); 1097 default: 1098 return super.makeProperty(hash, name); 1099 } 1100 1101 } 1102 1103 @Override 1104 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1105 switch (hash) { 1106 case 3059181: 1107 /* code */ return new String[] { "code" }; 1108 case 1671764162: 1109 /* display */ return new String[] { "string" }; 1110 case -880905839: 1111 /* target */ return new String[] {}; 1112 default: 1113 return super.getTypesForProperty(hash, name); 1114 } 1115 1116 } 1117 1118 @Override 1119 public Base addChild(String name) throws FHIRException { 1120 if (name.equals("code")) { 1121 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.code"); 1122 } else if (name.equals("display")) { 1123 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.display"); 1124 } else if (name.equals("target")) { 1125 return addTarget(); 1126 } else 1127 return super.addChild(name); 1128 } 1129 1130 public SourceElementComponent copy() { 1131 SourceElementComponent dst = new SourceElementComponent(); 1132 copyValues(dst); 1133 return dst; 1134 } 1135 1136 public void copyValues(SourceElementComponent dst) { 1137 super.copyValues(dst); 1138 dst.code = code == null ? null : code.copy(); 1139 dst.display = display == null ? null : display.copy(); 1140 if (target != null) { 1141 dst.target = new ArrayList<TargetElementComponent>(); 1142 for (TargetElementComponent i : target) 1143 dst.target.add(i.copy()); 1144 } 1145 ; 1146 } 1147 1148 @Override 1149 public boolean equalsDeep(Base other_) { 1150 if (!super.equalsDeep(other_)) 1151 return false; 1152 if (!(other_ instanceof SourceElementComponent)) 1153 return false; 1154 SourceElementComponent o = (SourceElementComponent) other_; 1155 return compareDeep(code, o.code, true) && compareDeep(display, o.display, true) 1156 && compareDeep(target, o.target, true); 1157 } 1158 1159 @Override 1160 public boolean equalsShallow(Base other_) { 1161 if (!super.equalsShallow(other_)) 1162 return false; 1163 if (!(other_ instanceof SourceElementComponent)) 1164 return false; 1165 SourceElementComponent o = (SourceElementComponent) other_; 1166 return compareValues(code, o.code, true) && compareValues(display, o.display, true); 1167 } 1168 1169 public boolean isEmpty() { 1170 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, display, target); 1171 } 1172 1173 public String fhirType() { 1174 return "ConceptMap.group.element"; 1175 1176 } 1177 1178 } 1179 1180 @Block() 1181 public static class TargetElementComponent extends BackboneElement implements IBaseBackboneElement { 1182 /** 1183 * Identity (code or path) or the element/item that the map refers to. 1184 */ 1185 @Child(name = "code", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1186 @Description(shortDefinition = "Code that identifies the target element", formalDefinition = "Identity (code or path) or the element/item that the map refers to.") 1187 protected CodeType code; 1188 1189 /** 1190 * The display for the code. The display is only provided to help editors when 1191 * editing the concept map. 1192 */ 1193 @Child(name = "display", type = { 1194 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1195 @Description(shortDefinition = "Display for the code", formalDefinition = "The display for the code. The display is only provided to help editors when editing the concept map.") 1196 protected StringType display; 1197 1198 /** 1199 * The equivalence between the source and target concepts (counting for the 1200 * dependencies and products). The equivalence is read from target to source 1201 * (e.g. the target is 'wider' than the source). 1202 */ 1203 @Child(name = "equivalence", type = { 1204 CodeType.class }, order = 3, min = 1, max = 1, modifier = true, summary = false) 1205 @Description(shortDefinition = "relatedto | equivalent | equal | wider | subsumes | narrower | specializes | inexact | unmatched | disjoint", formalDefinition = "The equivalence between the source and target concepts (counting for the dependencies and products). The equivalence is read from target to source (e.g. the target is 'wider' than the source).") 1206 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/concept-map-equivalence") 1207 protected Enumeration<ConceptMapEquivalence> equivalence; 1208 1209 /** 1210 * A description of status/issues in mapping that conveys additional information 1211 * not represented in the structured data. 1212 */ 1213 @Child(name = "comment", type = { 1214 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1215 @Description(shortDefinition = "Description of status/issues in mapping", formalDefinition = "A description of status/issues in mapping that conveys additional information not represented in the structured data.") 1216 protected StringType comment; 1217 1218 /** 1219 * A set of additional dependencies for this mapping to hold. This mapping is 1220 * only applicable if the specified element can be resolved, and it has the 1221 * specified value. 1222 */ 1223 @Child(name = "dependsOn", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1224 @Description(shortDefinition = "Other elements required for this mapping (from context)", formalDefinition = "A set of additional dependencies for this mapping to hold. This mapping is only applicable if the specified element can be resolved, and it has the specified value.") 1225 protected List<OtherElementComponent> dependsOn; 1226 1227 /** 1228 * A set of additional outcomes from this mapping to other elements. To properly 1229 * execute this mapping, the specified element must be mapped to some data 1230 * element or source that is in context. The mapping may still be useful without 1231 * a place for the additional data elements, but the equivalence cannot be 1232 * relied on. 1233 */ 1234 @Child(name = "product", type = { 1235 OtherElementComponent.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1236 @Description(shortDefinition = "Other concepts that this mapping also produces", formalDefinition = "A set of additional outcomes from this mapping to other elements. To properly execute this mapping, the specified element must be mapped to some data element or source that is in context. The mapping may still be useful without a place for the additional data elements, but the equivalence cannot be relied on.") 1237 protected List<OtherElementComponent> product; 1238 1239 private static final long serialVersionUID = -2008997477L; 1240 1241 /** 1242 * Constructor 1243 */ 1244 public TargetElementComponent() { 1245 super(); 1246 } 1247 1248 /** 1249 * Constructor 1250 */ 1251 public TargetElementComponent(Enumeration<ConceptMapEquivalence> equivalence) { 1252 super(); 1253 this.equivalence = equivalence; 1254 } 1255 1256 /** 1257 * @return {@link #code} (Identity (code or path) or the element/item that the 1258 * map refers to.). This is the underlying object with id, value and 1259 * extensions. The accessor "getCode" gives direct access to the value 1260 */ 1261 public CodeType getCodeElement() { 1262 if (this.code == null) 1263 if (Configuration.errorOnAutoCreate()) 1264 throw new Error("Attempt to auto-create TargetElementComponent.code"); 1265 else if (Configuration.doAutoCreate()) 1266 this.code = new CodeType(); // bb 1267 return this.code; 1268 } 1269 1270 public boolean hasCodeElement() { 1271 return this.code != null && !this.code.isEmpty(); 1272 } 1273 1274 public boolean hasCode() { 1275 return this.code != null && !this.code.isEmpty(); 1276 } 1277 1278 /** 1279 * @param value {@link #code} (Identity (code or path) or the element/item that 1280 * the map refers to.). This is the underlying object with id, 1281 * value and extensions. The accessor "getCode" gives direct access 1282 * to the value 1283 */ 1284 public TargetElementComponent setCodeElement(CodeType value) { 1285 this.code = value; 1286 return this; 1287 } 1288 1289 /** 1290 * @return Identity (code or path) or the element/item that the map refers to. 1291 */ 1292 public String getCode() { 1293 return this.code == null ? null : this.code.getValue(); 1294 } 1295 1296 /** 1297 * @param value Identity (code or path) or the element/item that the map refers 1298 * to. 1299 */ 1300 public TargetElementComponent setCode(String value) { 1301 if (Utilities.noString(value)) 1302 this.code = null; 1303 else { 1304 if (this.code == null) 1305 this.code = new CodeType(); 1306 this.code.setValue(value); 1307 } 1308 return this; 1309 } 1310 1311 /** 1312 * @return {@link #display} (The display for the code. The display is only 1313 * provided to help editors when editing the concept map.). This is the 1314 * underlying object with id, value and extensions. The accessor 1315 * "getDisplay" gives direct access to the value 1316 */ 1317 public StringType getDisplayElement() { 1318 if (this.display == null) 1319 if (Configuration.errorOnAutoCreate()) 1320 throw new Error("Attempt to auto-create TargetElementComponent.display"); 1321 else if (Configuration.doAutoCreate()) 1322 this.display = new StringType(); // bb 1323 return this.display; 1324 } 1325 1326 public boolean hasDisplayElement() { 1327 return this.display != null && !this.display.isEmpty(); 1328 } 1329 1330 public boolean hasDisplay() { 1331 return this.display != null && !this.display.isEmpty(); 1332 } 1333 1334 /** 1335 * @param value {@link #display} (The display for the code. The display is only 1336 * provided to help editors when editing the concept map.). This is 1337 * the underlying object with id, value and extensions. The 1338 * accessor "getDisplay" gives direct access to the value 1339 */ 1340 public TargetElementComponent setDisplayElement(StringType value) { 1341 this.display = value; 1342 return this; 1343 } 1344 1345 /** 1346 * @return The display for the code. The display is only provided to help 1347 * editors when editing the concept map. 1348 */ 1349 public String getDisplay() { 1350 return this.display == null ? null : this.display.getValue(); 1351 } 1352 1353 /** 1354 * @param value The display for the code. The display is only provided to help 1355 * editors when editing the concept map. 1356 */ 1357 public TargetElementComponent setDisplay(String value) { 1358 if (Utilities.noString(value)) 1359 this.display = null; 1360 else { 1361 if (this.display == null) 1362 this.display = new StringType(); 1363 this.display.setValue(value); 1364 } 1365 return this; 1366 } 1367 1368 /** 1369 * @return {@link #equivalence} (The equivalence between the source and target 1370 * concepts (counting for the dependencies and products). The 1371 * equivalence is read from target to source (e.g. the target is 'wider' 1372 * than the source).). This is the underlying object with id, value and 1373 * extensions. The accessor "getEquivalence" gives direct access to the 1374 * value 1375 */ 1376 public Enumeration<ConceptMapEquivalence> getEquivalenceElement() { 1377 if (this.equivalence == null) 1378 if (Configuration.errorOnAutoCreate()) 1379 throw new Error("Attempt to auto-create TargetElementComponent.equivalence"); 1380 else if (Configuration.doAutoCreate()) 1381 this.equivalence = new Enumeration<ConceptMapEquivalence>(new ConceptMapEquivalenceEnumFactory()); // bb 1382 return this.equivalence; 1383 } 1384 1385 public boolean hasEquivalenceElement() { 1386 return this.equivalence != null && !this.equivalence.isEmpty(); 1387 } 1388 1389 public boolean hasEquivalence() { 1390 return this.equivalence != null && !this.equivalence.isEmpty(); 1391 } 1392 1393 /** 1394 * @param value {@link #equivalence} (The equivalence between the source and 1395 * target concepts (counting for the dependencies and products). 1396 * The equivalence is read from target to source (e.g. the target 1397 * is 'wider' than the source).). This is the underlying object 1398 * with id, value and extensions. The accessor "getEquivalence" 1399 * gives direct access to the value 1400 */ 1401 public TargetElementComponent setEquivalenceElement(Enumeration<ConceptMapEquivalence> value) { 1402 this.equivalence = value; 1403 return this; 1404 } 1405 1406 /** 1407 * @return The equivalence between the source and target concepts (counting for 1408 * the dependencies and products). The equivalence is read from target 1409 * to source (e.g. the target is 'wider' than the source). 1410 */ 1411 public ConceptMapEquivalence getEquivalence() { 1412 return this.equivalence == null ? null : this.equivalence.getValue(); 1413 } 1414 1415 /** 1416 * @param value The equivalence between the source and target concepts (counting 1417 * for the dependencies and products). The equivalence is read from 1418 * target to source (e.g. the target is 'wider' than the source). 1419 */ 1420 public TargetElementComponent setEquivalence(ConceptMapEquivalence value) { 1421 if (this.equivalence == null) 1422 this.equivalence = new Enumeration<ConceptMapEquivalence>(new ConceptMapEquivalenceEnumFactory()); 1423 this.equivalence.setValue(value); 1424 return this; 1425 } 1426 1427 /** 1428 * @return {@link #comment} (A description of status/issues in mapping that 1429 * conveys additional information not represented in the structured 1430 * data.). This is the underlying object with id, value and extensions. 1431 * The accessor "getComment" gives direct access to the value 1432 */ 1433 public StringType getCommentElement() { 1434 if (this.comment == null) 1435 if (Configuration.errorOnAutoCreate()) 1436 throw new Error("Attempt to auto-create TargetElementComponent.comment"); 1437 else if (Configuration.doAutoCreate()) 1438 this.comment = new StringType(); // bb 1439 return this.comment; 1440 } 1441 1442 public boolean hasCommentElement() { 1443 return this.comment != null && !this.comment.isEmpty(); 1444 } 1445 1446 public boolean hasComment() { 1447 return this.comment != null && !this.comment.isEmpty(); 1448 } 1449 1450 /** 1451 * @param value {@link #comment} (A description of status/issues in mapping that 1452 * conveys additional information not represented in the structured 1453 * data.). This is the underlying object with id, value and 1454 * extensions. The accessor "getComment" gives direct access to the 1455 * value 1456 */ 1457 public TargetElementComponent setCommentElement(StringType value) { 1458 this.comment = value; 1459 return this; 1460 } 1461 1462 /** 1463 * @return A description of status/issues in mapping that conveys additional 1464 * information not represented in the structured data. 1465 */ 1466 public String getComment() { 1467 return this.comment == null ? null : this.comment.getValue(); 1468 } 1469 1470 /** 1471 * @param value A description of status/issues in mapping that conveys 1472 * additional information not represented in the structured data. 1473 */ 1474 public TargetElementComponent setComment(String value) { 1475 if (Utilities.noString(value)) 1476 this.comment = null; 1477 else { 1478 if (this.comment == null) 1479 this.comment = new StringType(); 1480 this.comment.setValue(value); 1481 } 1482 return this; 1483 } 1484 1485 /** 1486 * @return {@link #dependsOn} (A set of additional dependencies for this mapping 1487 * to hold. This mapping is only applicable if the specified element can 1488 * be resolved, and it has the specified value.) 1489 */ 1490 public List<OtherElementComponent> getDependsOn() { 1491 if (this.dependsOn == null) 1492 this.dependsOn = new ArrayList<OtherElementComponent>(); 1493 return this.dependsOn; 1494 } 1495 1496 /** 1497 * @return Returns a reference to <code>this</code> for easy method chaining 1498 */ 1499 public TargetElementComponent setDependsOn(List<OtherElementComponent> theDependsOn) { 1500 this.dependsOn = theDependsOn; 1501 return this; 1502 } 1503 1504 public boolean hasDependsOn() { 1505 if (this.dependsOn == null) 1506 return false; 1507 for (OtherElementComponent item : this.dependsOn) 1508 if (!item.isEmpty()) 1509 return true; 1510 return false; 1511 } 1512 1513 public OtherElementComponent addDependsOn() { // 3 1514 OtherElementComponent t = new OtherElementComponent(); 1515 if (this.dependsOn == null) 1516 this.dependsOn = new ArrayList<OtherElementComponent>(); 1517 this.dependsOn.add(t); 1518 return t; 1519 } 1520 1521 public TargetElementComponent addDependsOn(OtherElementComponent t) { // 3 1522 if (t == null) 1523 return this; 1524 if (this.dependsOn == null) 1525 this.dependsOn = new ArrayList<OtherElementComponent>(); 1526 this.dependsOn.add(t); 1527 return this; 1528 } 1529 1530 /** 1531 * @return The first repetition of repeating field {@link #dependsOn}, creating 1532 * it if it does not already exist 1533 */ 1534 public OtherElementComponent getDependsOnFirstRep() { 1535 if (getDependsOn().isEmpty()) { 1536 addDependsOn(); 1537 } 1538 return getDependsOn().get(0); 1539 } 1540 1541 /** 1542 * @return {@link #product} (A set of additional outcomes from this mapping to 1543 * other elements. To properly execute this mapping, the specified 1544 * element must be mapped to some data element or source that is in 1545 * context. The mapping may still be useful without a place for the 1546 * additional data elements, but the equivalence cannot be relied on.) 1547 */ 1548 public List<OtherElementComponent> getProduct() { 1549 if (this.product == null) 1550 this.product = new ArrayList<OtherElementComponent>(); 1551 return this.product; 1552 } 1553 1554 /** 1555 * @return Returns a reference to <code>this</code> for easy method chaining 1556 */ 1557 public TargetElementComponent setProduct(List<OtherElementComponent> theProduct) { 1558 this.product = theProduct; 1559 return this; 1560 } 1561 1562 public boolean hasProduct() { 1563 if (this.product == null) 1564 return false; 1565 for (OtherElementComponent item : this.product) 1566 if (!item.isEmpty()) 1567 return true; 1568 return false; 1569 } 1570 1571 public OtherElementComponent addProduct() { // 3 1572 OtherElementComponent t = new OtherElementComponent(); 1573 if (this.product == null) 1574 this.product = new ArrayList<OtherElementComponent>(); 1575 this.product.add(t); 1576 return t; 1577 } 1578 1579 public TargetElementComponent addProduct(OtherElementComponent t) { // 3 1580 if (t == null) 1581 return this; 1582 if (this.product == null) 1583 this.product = new ArrayList<OtherElementComponent>(); 1584 this.product.add(t); 1585 return this; 1586 } 1587 1588 /** 1589 * @return The first repetition of repeating field {@link #product}, creating it 1590 * if it does not already exist 1591 */ 1592 public OtherElementComponent getProductFirstRep() { 1593 if (getProduct().isEmpty()) { 1594 addProduct(); 1595 } 1596 return getProduct().get(0); 1597 } 1598 1599 protected void listChildren(List<Property> children) { 1600 super.listChildren(children); 1601 children.add(new Property("code", "code", "Identity (code or path) or the element/item that the map refers to.", 1602 0, 1, code)); 1603 children.add(new Property("display", "string", 1604 "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 1, 1605 display)); 1606 children.add(new Property("equivalence", "code", 1607 "The equivalence between the source and target concepts (counting for the dependencies and products). The equivalence is read from target to source (e.g. the target is 'wider' than the source).", 1608 0, 1, equivalence)); 1609 children.add(new Property("comment", "string", 1610 "A description of status/issues in mapping that conveys additional information not represented in the structured data.", 1611 0, 1, comment)); 1612 children.add(new Property("dependsOn", "", 1613 "A set of additional dependencies for this mapping to hold. This mapping is only applicable if the specified element can be resolved, and it has the specified value.", 1614 0, java.lang.Integer.MAX_VALUE, dependsOn)); 1615 children.add(new Property("product", "@ConceptMap.group.element.target.dependsOn", 1616 "A set of additional outcomes from this mapping to other elements. To properly execute this mapping, the specified element must be mapped to some data element or source that is in context. The mapping may still be useful without a place for the additional data elements, but the equivalence cannot be relied on.", 1617 0, java.lang.Integer.MAX_VALUE, product)); 1618 } 1619 1620 @Override 1621 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1622 switch (_hash) { 1623 case 3059181: 1624 /* code */ return new Property("code", "code", 1625 "Identity (code or path) or the element/item that the map refers to.", 0, 1, code); 1626 case 1671764162: 1627 /* display */ return new Property("display", "string", 1628 "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 1629 1, display); 1630 case -15828692: 1631 /* equivalence */ return new Property("equivalence", "code", 1632 "The equivalence between the source and target concepts (counting for the dependencies and products). The equivalence is read from target to source (e.g. the target is 'wider' than the source).", 1633 0, 1, equivalence); 1634 case 950398559: 1635 /* comment */ return new Property("comment", "string", 1636 "A description of status/issues in mapping that conveys additional information not represented in the structured data.", 1637 0, 1, comment); 1638 case -1109214266: 1639 /* dependsOn */ return new Property("dependsOn", "", 1640 "A set of additional dependencies for this mapping to hold. This mapping is only applicable if the specified element can be resolved, and it has the specified value.", 1641 0, java.lang.Integer.MAX_VALUE, dependsOn); 1642 case -309474065: 1643 /* product */ return new Property("product", "@ConceptMap.group.element.target.dependsOn", 1644 "A set of additional outcomes from this mapping to other elements. To properly execute this mapping, the specified element must be mapped to some data element or source that is in context. The mapping may still be useful without a place for the additional data elements, but the equivalence cannot be relied on.", 1645 0, java.lang.Integer.MAX_VALUE, product); 1646 default: 1647 return super.getNamedProperty(_hash, _name, _checkValid); 1648 } 1649 1650 } 1651 1652 @Override 1653 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1654 switch (hash) { 1655 case 3059181: 1656 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeType 1657 case 1671764162: 1658 /* display */ return this.display == null ? new Base[0] : new Base[] { this.display }; // StringType 1659 case -15828692: 1660 /* equivalence */ return this.equivalence == null ? new Base[0] : new Base[] { this.equivalence }; // Enumeration<ConceptMapEquivalence> 1661 case 950398559: 1662 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // StringType 1663 case -1109214266: 1664 /* dependsOn */ return this.dependsOn == null ? new Base[0] 1665 : this.dependsOn.toArray(new Base[this.dependsOn.size()]); // OtherElementComponent 1666 case -309474065: 1667 /* product */ return this.product == null ? new Base[0] : this.product.toArray(new Base[this.product.size()]); // OtherElementComponent 1668 default: 1669 return super.getProperty(hash, name, checkValid); 1670 } 1671 1672 } 1673 1674 @Override 1675 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1676 switch (hash) { 1677 case 3059181: // code 1678 this.code = castToCode(value); // CodeType 1679 return value; 1680 case 1671764162: // display 1681 this.display = castToString(value); // StringType 1682 return value; 1683 case -15828692: // equivalence 1684 value = new ConceptMapEquivalenceEnumFactory().fromType(castToCode(value)); 1685 this.equivalence = (Enumeration) value; // Enumeration<ConceptMapEquivalence> 1686 return value; 1687 case 950398559: // comment 1688 this.comment = castToString(value); // StringType 1689 return value; 1690 case -1109214266: // dependsOn 1691 this.getDependsOn().add((OtherElementComponent) value); // OtherElementComponent 1692 return value; 1693 case -309474065: // product 1694 this.getProduct().add((OtherElementComponent) value); // OtherElementComponent 1695 return value; 1696 default: 1697 return super.setProperty(hash, name, value); 1698 } 1699 1700 } 1701 1702 @Override 1703 public Base setProperty(String name, Base value) throws FHIRException { 1704 if (name.equals("code")) { 1705 this.code = castToCode(value); // CodeType 1706 } else if (name.equals("display")) { 1707 this.display = castToString(value); // StringType 1708 } else if (name.equals("equivalence")) { 1709 value = new ConceptMapEquivalenceEnumFactory().fromType(castToCode(value)); 1710 this.equivalence = (Enumeration) value; // Enumeration<ConceptMapEquivalence> 1711 } else if (name.equals("comment")) { 1712 this.comment = castToString(value); // StringType 1713 } else if (name.equals("dependsOn")) { 1714 this.getDependsOn().add((OtherElementComponent) value); 1715 } else if (name.equals("product")) { 1716 this.getProduct().add((OtherElementComponent) value); 1717 } else 1718 return super.setProperty(name, value); 1719 return value; 1720 } 1721 1722 @Override 1723 public Base makeProperty(int hash, String name) throws FHIRException { 1724 switch (hash) { 1725 case 3059181: 1726 return getCodeElement(); 1727 case 1671764162: 1728 return getDisplayElement(); 1729 case -15828692: 1730 return getEquivalenceElement(); 1731 case 950398559: 1732 return getCommentElement(); 1733 case -1109214266: 1734 return addDependsOn(); 1735 case -309474065: 1736 return addProduct(); 1737 default: 1738 return super.makeProperty(hash, name); 1739 } 1740 1741 } 1742 1743 @Override 1744 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1745 switch (hash) { 1746 case 3059181: 1747 /* code */ return new String[] { "code" }; 1748 case 1671764162: 1749 /* display */ return new String[] { "string" }; 1750 case -15828692: 1751 /* equivalence */ return new String[] { "code" }; 1752 case 950398559: 1753 /* comment */ return new String[] { "string" }; 1754 case -1109214266: 1755 /* dependsOn */ return new String[] {}; 1756 case -309474065: 1757 /* product */ return new String[] { "@ConceptMap.group.element.target.dependsOn" }; 1758 default: 1759 return super.getTypesForProperty(hash, name); 1760 } 1761 1762 } 1763 1764 @Override 1765 public Base addChild(String name) throws FHIRException { 1766 if (name.equals("code")) { 1767 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.code"); 1768 } else if (name.equals("display")) { 1769 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.display"); 1770 } else if (name.equals("equivalence")) { 1771 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.equivalence"); 1772 } else if (name.equals("comment")) { 1773 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.comment"); 1774 } else if (name.equals("dependsOn")) { 1775 return addDependsOn(); 1776 } else if (name.equals("product")) { 1777 return addProduct(); 1778 } else 1779 return super.addChild(name); 1780 } 1781 1782 public TargetElementComponent copy() { 1783 TargetElementComponent dst = new TargetElementComponent(); 1784 copyValues(dst); 1785 return dst; 1786 } 1787 1788 public void copyValues(TargetElementComponent dst) { 1789 super.copyValues(dst); 1790 dst.code = code == null ? null : code.copy(); 1791 dst.display = display == null ? null : display.copy(); 1792 dst.equivalence = equivalence == null ? null : equivalence.copy(); 1793 dst.comment = comment == null ? null : comment.copy(); 1794 if (dependsOn != null) { 1795 dst.dependsOn = new ArrayList<OtherElementComponent>(); 1796 for (OtherElementComponent i : dependsOn) 1797 dst.dependsOn.add(i.copy()); 1798 } 1799 ; 1800 if (product != null) { 1801 dst.product = new ArrayList<OtherElementComponent>(); 1802 for (OtherElementComponent i : product) 1803 dst.product.add(i.copy()); 1804 } 1805 ; 1806 } 1807 1808 @Override 1809 public boolean equalsDeep(Base other_) { 1810 if (!super.equalsDeep(other_)) 1811 return false; 1812 if (!(other_ instanceof TargetElementComponent)) 1813 return false; 1814 TargetElementComponent o = (TargetElementComponent) other_; 1815 return compareDeep(code, o.code, true) && compareDeep(display, o.display, true) 1816 && compareDeep(equivalence, o.equivalence, true) && compareDeep(comment, o.comment, true) 1817 && compareDeep(dependsOn, o.dependsOn, true) && compareDeep(product, o.product, true); 1818 } 1819 1820 @Override 1821 public boolean equalsShallow(Base other_) { 1822 if (!super.equalsShallow(other_)) 1823 return false; 1824 if (!(other_ instanceof TargetElementComponent)) 1825 return false; 1826 TargetElementComponent o = (TargetElementComponent) other_; 1827 return compareValues(code, o.code, true) && compareValues(display, o.display, true) 1828 && compareValues(equivalence, o.equivalence, true) && compareValues(comment, o.comment, true); 1829 } 1830 1831 public boolean isEmpty() { 1832 return super.isEmpty() 1833 && ca.uhn.fhir.util.ElementUtil.isEmpty(code, display, equivalence, comment, dependsOn, product); 1834 } 1835 1836 public String fhirType() { 1837 return "ConceptMap.group.element.target"; 1838 1839 } 1840 1841 } 1842 1843 @Block() 1844 public static class OtherElementComponent extends BackboneElement implements IBaseBackboneElement { 1845 /** 1846 * A reference to an element that holds a coded value that corresponds to a code 1847 * system property. The idea is that the information model carries an element 1848 * somewhere that is labeled to correspond with a code system property. 1849 */ 1850 @Child(name = "property", type = { UriType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1851 @Description(shortDefinition = "Reference to property mapping depends on", formalDefinition = "A reference to an element that holds a coded value that corresponds to a code system property. The idea is that the information model carries an element somewhere that is labeled to correspond with a code system property.") 1852 protected UriType property; 1853 1854 /** 1855 * An absolute URI that identifies the code system of the dependency code (if 1856 * the source/dependency is a value set that crosses code systems). 1857 */ 1858 @Child(name = "system", type = { 1859 CanonicalType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1860 @Description(shortDefinition = "Code System (if necessary)", formalDefinition = "An absolute URI that identifies the code system of the dependency code (if the source/dependency is a value set that crosses code systems).") 1861 protected CanonicalType system; 1862 1863 /** 1864 * Identity (code or path) or the element/item/ValueSet/text that the map 1865 * depends on / refers to. 1866 */ 1867 @Child(name = "value", type = { StringType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 1868 @Description(shortDefinition = "Value of the referenced element", formalDefinition = "Identity (code or path) or the element/item/ValueSet/text that the map depends on / refers to.") 1869 protected StringType value; 1870 1871 /** 1872 * The display for the code. The display is only provided to help editors when 1873 * editing the concept map. 1874 */ 1875 @Child(name = "display", type = { 1876 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1877 @Description(shortDefinition = "Display for the code (if value is a code)", formalDefinition = "The display for the code. The display is only provided to help editors when editing the concept map.") 1878 protected StringType display; 1879 1880 private static final long serialVersionUID = -1836341923L; 1881 1882 /** 1883 * Constructor 1884 */ 1885 public OtherElementComponent() { 1886 super(); 1887 } 1888 1889 /** 1890 * Constructor 1891 */ 1892 public OtherElementComponent(UriType property, StringType value) { 1893 super(); 1894 this.property = property; 1895 this.value = value; 1896 } 1897 1898 /** 1899 * @return {@link #property} (A reference to an element that holds a coded value 1900 * that corresponds to a code system property. The idea is that the 1901 * information model carries an element somewhere that is labeled to 1902 * correspond with a code system property.). This is the underlying 1903 * object with id, value and extensions. The accessor "getProperty" 1904 * gives direct access to the value 1905 */ 1906 public UriType getPropertyElement() { 1907 if (this.property == null) 1908 if (Configuration.errorOnAutoCreate()) 1909 throw new Error("Attempt to auto-create OtherElementComponent.property"); 1910 else if (Configuration.doAutoCreate()) 1911 this.property = new UriType(); // bb 1912 return this.property; 1913 } 1914 1915 public boolean hasPropertyElement() { 1916 return this.property != null && !this.property.isEmpty(); 1917 } 1918 1919 public boolean hasProperty() { 1920 return this.property != null && !this.property.isEmpty(); 1921 } 1922 1923 /** 1924 * @param value {@link #property} (A reference to an element that holds a coded 1925 * value that corresponds to a code system property. The idea is 1926 * that the information model carries an element somewhere that is 1927 * labeled to correspond with a code system property.). This is the 1928 * underlying object with id, value and extensions. The accessor 1929 * "getProperty" gives direct access to the value 1930 */ 1931 public OtherElementComponent setPropertyElement(UriType value) { 1932 this.property = value; 1933 return this; 1934 } 1935 1936 /** 1937 * @return A reference to an element that holds a coded value that corresponds 1938 * to a code system property. The idea is that the information model 1939 * carries an element somewhere that is labeled to correspond with a 1940 * code system property. 1941 */ 1942 public String getProperty() { 1943 return this.property == null ? null : this.property.getValue(); 1944 } 1945 1946 /** 1947 * @param value A reference to an element that holds a coded value that 1948 * corresponds to a code system property. The idea is that the 1949 * information model carries an element somewhere that is labeled 1950 * to correspond with a code system property. 1951 */ 1952 public OtherElementComponent setProperty(String value) { 1953 if (this.property == null) 1954 this.property = new UriType(); 1955 this.property.setValue(value); 1956 return this; 1957 } 1958 1959 /** 1960 * @return {@link #system} (An absolute URI that identifies the code system of 1961 * the dependency code (if the source/dependency is a value set that 1962 * crosses code systems).). This is the underlying object with id, value 1963 * and extensions. The accessor "getSystem" gives direct access to the 1964 * value 1965 */ 1966 public CanonicalType getSystemElement() { 1967 if (this.system == null) 1968 if (Configuration.errorOnAutoCreate()) 1969 throw new Error("Attempt to auto-create OtherElementComponent.system"); 1970 else if (Configuration.doAutoCreate()) 1971 this.system = new CanonicalType(); // bb 1972 return this.system; 1973 } 1974 1975 public boolean hasSystemElement() { 1976 return this.system != null && !this.system.isEmpty(); 1977 } 1978 1979 public boolean hasSystem() { 1980 return this.system != null && !this.system.isEmpty(); 1981 } 1982 1983 /** 1984 * @param value {@link #system} (An absolute URI that identifies the code system 1985 * of the dependency code (if the source/dependency is a value set 1986 * that crosses code systems).). This is the underlying object with 1987 * id, value and extensions. The accessor "getSystem" gives direct 1988 * access to the value 1989 */ 1990 public OtherElementComponent setSystemElement(CanonicalType value) { 1991 this.system = value; 1992 return this; 1993 } 1994 1995 /** 1996 * @return An absolute URI that identifies the code system of the dependency 1997 * code (if the source/dependency is a value set that crosses code 1998 * systems). 1999 */ 2000 public String getSystem() { 2001 return this.system == null ? null : this.system.getValue(); 2002 } 2003 2004 /** 2005 * @param value An absolute URI that identifies the code system of the 2006 * dependency code (if the source/dependency is a value set that 2007 * crosses code systems). 2008 */ 2009 public OtherElementComponent setSystem(String value) { 2010 if (Utilities.noString(value)) 2011 this.system = null; 2012 else { 2013 if (this.system == null) 2014 this.system = new CanonicalType(); 2015 this.system.setValue(value); 2016 } 2017 return this; 2018 } 2019 2020 /** 2021 * @return {@link #value} (Identity (code or path) or the 2022 * element/item/ValueSet/text that the map depends on / refers to.). 2023 * This is the underlying object with id, value and extensions. The 2024 * accessor "getValue" gives direct access to the value 2025 */ 2026 public StringType getValueElement() { 2027 if (this.value == null) 2028 if (Configuration.errorOnAutoCreate()) 2029 throw new Error("Attempt to auto-create OtherElementComponent.value"); 2030 else if (Configuration.doAutoCreate()) 2031 this.value = new StringType(); // bb 2032 return this.value; 2033 } 2034 2035 public boolean hasValueElement() { 2036 return this.value != null && !this.value.isEmpty(); 2037 } 2038 2039 public boolean hasValue() { 2040 return this.value != null && !this.value.isEmpty(); 2041 } 2042 2043 /** 2044 * @param value {@link #value} (Identity (code or path) or the 2045 * element/item/ValueSet/text that the map depends on / refers 2046 * to.). This is the underlying object with id, value and 2047 * extensions. The accessor "getValue" gives direct access to the 2048 * value 2049 */ 2050 public OtherElementComponent setValueElement(StringType value) { 2051 this.value = value; 2052 return this; 2053 } 2054 2055 /** 2056 * @return Identity (code or path) or the element/item/ValueSet/text that the 2057 * map depends on / refers to. 2058 */ 2059 public String getValue() { 2060 return this.value == null ? null : this.value.getValue(); 2061 } 2062 2063 /** 2064 * @param value Identity (code or path) or the element/item/ValueSet/text that 2065 * the map depends on / refers to. 2066 */ 2067 public OtherElementComponent setValue(String value) { 2068 if (this.value == null) 2069 this.value = new StringType(); 2070 this.value.setValue(value); 2071 return this; 2072 } 2073 2074 /** 2075 * @return {@link #display} (The display for the code. The display is only 2076 * provided to help editors when editing the concept map.). This is the 2077 * underlying object with id, value and extensions. The accessor 2078 * "getDisplay" gives direct access to the value 2079 */ 2080 public StringType getDisplayElement() { 2081 if (this.display == null) 2082 if (Configuration.errorOnAutoCreate()) 2083 throw new Error("Attempt to auto-create OtherElementComponent.display"); 2084 else if (Configuration.doAutoCreate()) 2085 this.display = new StringType(); // bb 2086 return this.display; 2087 } 2088 2089 public boolean hasDisplayElement() { 2090 return this.display != null && !this.display.isEmpty(); 2091 } 2092 2093 public boolean hasDisplay() { 2094 return this.display != null && !this.display.isEmpty(); 2095 } 2096 2097 /** 2098 * @param value {@link #display} (The display for the code. The display is only 2099 * provided to help editors when editing the concept map.). This is 2100 * the underlying object with id, value and extensions. The 2101 * accessor "getDisplay" gives direct access to the value 2102 */ 2103 public OtherElementComponent setDisplayElement(StringType value) { 2104 this.display = value; 2105 return this; 2106 } 2107 2108 /** 2109 * @return The display for the code. The display is only provided to help 2110 * editors when editing the concept map. 2111 */ 2112 public String getDisplay() { 2113 return this.display == null ? null : this.display.getValue(); 2114 } 2115 2116 /** 2117 * @param value The display for the code. The display is only provided to help 2118 * editors when editing the concept map. 2119 */ 2120 public OtherElementComponent setDisplay(String value) { 2121 if (Utilities.noString(value)) 2122 this.display = null; 2123 else { 2124 if (this.display == null) 2125 this.display = new StringType(); 2126 this.display.setValue(value); 2127 } 2128 return this; 2129 } 2130 2131 protected void listChildren(List<Property> children) { 2132 super.listChildren(children); 2133 children.add(new Property("property", "uri", 2134 "A reference to an element that holds a coded value that corresponds to a code system property. The idea is that the information model carries an element somewhere that is labeled to correspond with a code system property.", 2135 0, 1, property)); 2136 children.add(new Property("system", "canonical(CodeSystem)", 2137 "An absolute URI that identifies the code system of the dependency code (if the source/dependency is a value set that crosses code systems).", 2138 0, 1, system)); 2139 children.add(new Property("value", "string", 2140 "Identity (code or path) or the element/item/ValueSet/text that the map depends on / refers to.", 0, 1, 2141 value)); 2142 children.add(new Property("display", "string", 2143 "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 1, 2144 display)); 2145 } 2146 2147 @Override 2148 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2149 switch (_hash) { 2150 case -993141291: 2151 /* property */ return new Property("property", "uri", 2152 "A reference to an element that holds a coded value that corresponds to a code system property. The idea is that the information model carries an element somewhere that is labeled to correspond with a code system property.", 2153 0, 1, property); 2154 case -887328209: 2155 /* system */ return new Property("system", "canonical(CodeSystem)", 2156 "An absolute URI that identifies the code system of the dependency code (if the source/dependency is a value set that crosses code systems).", 2157 0, 1, system); 2158 case 111972721: 2159 /* value */ return new Property("value", "string", 2160 "Identity (code or path) or the element/item/ValueSet/text that the map depends on / refers to.", 0, 1, 2161 value); 2162 case 1671764162: 2163 /* display */ return new Property("display", "string", 2164 "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 2165 1, display); 2166 default: 2167 return super.getNamedProperty(_hash, _name, _checkValid); 2168 } 2169 2170 } 2171 2172 @Override 2173 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2174 switch (hash) { 2175 case -993141291: 2176 /* property */ return this.property == null ? new Base[0] : new Base[] { this.property }; // UriType 2177 case -887328209: 2178 /* system */ return this.system == null ? new Base[0] : new Base[] { this.system }; // CanonicalType 2179 case 111972721: 2180 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // StringType 2181 case 1671764162: 2182 /* display */ return this.display == null ? new Base[0] : new Base[] { this.display }; // StringType 2183 default: 2184 return super.getProperty(hash, name, checkValid); 2185 } 2186 2187 } 2188 2189 @Override 2190 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2191 switch (hash) { 2192 case -993141291: // property 2193 this.property = castToUri(value); // UriType 2194 return value; 2195 case -887328209: // system 2196 this.system = castToCanonical(value); // CanonicalType 2197 return value; 2198 case 111972721: // value 2199 this.value = castToString(value); // StringType 2200 return value; 2201 case 1671764162: // display 2202 this.display = castToString(value); // StringType 2203 return value; 2204 default: 2205 return super.setProperty(hash, name, value); 2206 } 2207 2208 } 2209 2210 @Override 2211 public Base setProperty(String name, Base value) throws FHIRException { 2212 if (name.equals("property")) { 2213 this.property = castToUri(value); // UriType 2214 } else if (name.equals("system")) { 2215 this.system = castToCanonical(value); // CanonicalType 2216 } else if (name.equals("value")) { 2217 this.value = castToString(value); // StringType 2218 } else if (name.equals("display")) { 2219 this.display = castToString(value); // StringType 2220 } else 2221 return super.setProperty(name, value); 2222 return value; 2223 } 2224 2225 @Override 2226 public Base makeProperty(int hash, String name) throws FHIRException { 2227 switch (hash) { 2228 case -993141291: 2229 return getPropertyElement(); 2230 case -887328209: 2231 return getSystemElement(); 2232 case 111972721: 2233 return getValueElement(); 2234 case 1671764162: 2235 return getDisplayElement(); 2236 default: 2237 return super.makeProperty(hash, name); 2238 } 2239 2240 } 2241 2242 @Override 2243 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2244 switch (hash) { 2245 case -993141291: 2246 /* property */ return new String[] { "uri" }; 2247 case -887328209: 2248 /* system */ return new String[] { "canonical" }; 2249 case 111972721: 2250 /* value */ return new String[] { "string" }; 2251 case 1671764162: 2252 /* display */ return new String[] { "string" }; 2253 default: 2254 return super.getTypesForProperty(hash, name); 2255 } 2256 2257 } 2258 2259 @Override 2260 public Base addChild(String name) throws FHIRException { 2261 if (name.equals("property")) { 2262 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.property"); 2263 } else if (name.equals("system")) { 2264 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.system"); 2265 } else if (name.equals("value")) { 2266 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.value"); 2267 } else if (name.equals("display")) { 2268 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.display"); 2269 } else 2270 return super.addChild(name); 2271 } 2272 2273 public OtherElementComponent copy() { 2274 OtherElementComponent dst = new OtherElementComponent(); 2275 copyValues(dst); 2276 return dst; 2277 } 2278 2279 public void copyValues(OtherElementComponent dst) { 2280 super.copyValues(dst); 2281 dst.property = property == null ? null : property.copy(); 2282 dst.system = system == null ? null : system.copy(); 2283 dst.value = value == null ? null : value.copy(); 2284 dst.display = display == null ? null : display.copy(); 2285 } 2286 2287 @Override 2288 public boolean equalsDeep(Base other_) { 2289 if (!super.equalsDeep(other_)) 2290 return false; 2291 if (!(other_ instanceof OtherElementComponent)) 2292 return false; 2293 OtherElementComponent o = (OtherElementComponent) other_; 2294 return compareDeep(property, o.property, true) && compareDeep(system, o.system, true) 2295 && compareDeep(value, o.value, true) && compareDeep(display, o.display, true); 2296 } 2297 2298 @Override 2299 public boolean equalsShallow(Base other_) { 2300 if (!super.equalsShallow(other_)) 2301 return false; 2302 if (!(other_ instanceof OtherElementComponent)) 2303 return false; 2304 OtherElementComponent o = (OtherElementComponent) other_; 2305 return compareValues(property, o.property, true) && compareValues(value, o.value, true) 2306 && compareValues(display, o.display, true); 2307 } 2308 2309 public boolean isEmpty() { 2310 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(property, system, value, display); 2311 } 2312 2313 public String fhirType() { 2314 return "ConceptMap.group.element.target.dependsOn"; 2315 2316 } 2317 2318 } 2319 2320 @Block() 2321 public static class ConceptMapGroupUnmappedComponent extends BackboneElement implements IBaseBackboneElement { 2322 /** 2323 * Defines which action to take if there is no match for the source concept in 2324 * the target system designated for the group. One of 3 actions are possible: 2325 * use the unmapped code (this is useful when doing a mapping between versions, 2326 * and only a few codes have changed), use a fixed code (a default code), or 2327 * alternatively, a reference to a different concept map can be provided (by 2328 * canonical URL). 2329 */ 2330 @Child(name = "mode", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2331 @Description(shortDefinition = "provided | fixed | other-map", formalDefinition = "Defines which action to take if there is no match for the source concept in the target system designated for the group. One of 3 actions are possible: use the unmapped code (this is useful when doing a mapping between versions, and only a few codes have changed), use a fixed code (a default code), or alternatively, a reference to a different concept map can be provided (by canonical URL).") 2332 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/conceptmap-unmapped-mode") 2333 protected Enumeration<ConceptMapGroupUnmappedMode> mode; 2334 2335 /** 2336 * The fixed code to use when the mode = 'fixed' - all unmapped codes are mapped 2337 * to a single fixed code. 2338 */ 2339 @Child(name = "code", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2340 @Description(shortDefinition = "Fixed code when mode = fixed", formalDefinition = "The fixed code to use when the mode = 'fixed' - all unmapped codes are mapped to a single fixed code.") 2341 protected CodeType code; 2342 2343 /** 2344 * The display for the code. The display is only provided to help editors when 2345 * editing the concept map. 2346 */ 2347 @Child(name = "display", type = { 2348 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2349 @Description(shortDefinition = "Display for the code", formalDefinition = "The display for the code. The display is only provided to help editors when editing the concept map.") 2350 protected StringType display; 2351 2352 /** 2353 * The canonical reference to an additional ConceptMap resource instance to use 2354 * for mapping if this ConceptMap resource contains no matching mapping for the 2355 * source concept. 2356 */ 2357 @Child(name = "url", type = { CanonicalType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2358 @Description(shortDefinition = "canonical reference to an additional ConceptMap to use for mapping if the source concept is unmapped", formalDefinition = "The canonical reference to an additional ConceptMap resource instance to use for mapping if this ConceptMap resource contains no matching mapping for the source concept.") 2359 protected CanonicalType url; 2360 2361 private static final long serialVersionUID = 1261364354L; 2362 2363 /** 2364 * Constructor 2365 */ 2366 public ConceptMapGroupUnmappedComponent() { 2367 super(); 2368 } 2369 2370 /** 2371 * Constructor 2372 */ 2373 public ConceptMapGroupUnmappedComponent(Enumeration<ConceptMapGroupUnmappedMode> mode) { 2374 super(); 2375 this.mode = mode; 2376 } 2377 2378 /** 2379 * @return {@link #mode} (Defines which action to take if there is no match for 2380 * the source concept in the target system designated for the group. One 2381 * of 3 actions are possible: use the unmapped code (this is useful when 2382 * doing a mapping between versions, and only a few codes have changed), 2383 * use a fixed code (a default code), or alternatively, a reference to a 2384 * different concept map can be provided (by canonical URL).). This is 2385 * the underlying object with id, value and extensions. The accessor 2386 * "getMode" gives direct access to the value 2387 */ 2388 public Enumeration<ConceptMapGroupUnmappedMode> getModeElement() { 2389 if (this.mode == null) 2390 if (Configuration.errorOnAutoCreate()) 2391 throw new Error("Attempt to auto-create ConceptMapGroupUnmappedComponent.mode"); 2392 else if (Configuration.doAutoCreate()) 2393 this.mode = new Enumeration<ConceptMapGroupUnmappedMode>(new ConceptMapGroupUnmappedModeEnumFactory()); // bb 2394 return this.mode; 2395 } 2396 2397 public boolean hasModeElement() { 2398 return this.mode != null && !this.mode.isEmpty(); 2399 } 2400 2401 public boolean hasMode() { 2402 return this.mode != null && !this.mode.isEmpty(); 2403 } 2404 2405 /** 2406 * @param value {@link #mode} (Defines which action to take if there is no match 2407 * for the source concept in the target system designated for the 2408 * group. One of 3 actions are possible: use the unmapped code 2409 * (this is useful when doing a mapping between versions, and only 2410 * a few codes have changed), use a fixed code (a default code), or 2411 * alternatively, a reference to a different concept map can be 2412 * provided (by canonical URL).). This is the underlying object 2413 * with id, value and extensions. The accessor "getMode" gives 2414 * direct access to the value 2415 */ 2416 public ConceptMapGroupUnmappedComponent setModeElement(Enumeration<ConceptMapGroupUnmappedMode> value) { 2417 this.mode = value; 2418 return this; 2419 } 2420 2421 /** 2422 * @return Defines which action to take if there is no match for the source 2423 * concept in the target system designated for the group. One of 3 2424 * actions are possible: use the unmapped code (this is useful when 2425 * doing a mapping between versions, and only a few codes have changed), 2426 * use a fixed code (a default code), or alternatively, a reference to a 2427 * different concept map can be provided (by canonical URL). 2428 */ 2429 public ConceptMapGroupUnmappedMode getMode() { 2430 return this.mode == null ? null : this.mode.getValue(); 2431 } 2432 2433 /** 2434 * @param value Defines which action to take if there is no match for the source 2435 * concept in the target system designated for the group. One of 3 2436 * actions are possible: use the unmapped code (this is useful when 2437 * doing a mapping between versions, and only a few codes have 2438 * changed), use a fixed code (a default code), or alternatively, a 2439 * reference to a different concept map can be provided (by 2440 * canonical URL). 2441 */ 2442 public ConceptMapGroupUnmappedComponent setMode(ConceptMapGroupUnmappedMode value) { 2443 if (this.mode == null) 2444 this.mode = new Enumeration<ConceptMapGroupUnmappedMode>(new ConceptMapGroupUnmappedModeEnumFactory()); 2445 this.mode.setValue(value); 2446 return this; 2447 } 2448 2449 /** 2450 * @return {@link #code} (The fixed code to use when the mode = 'fixed' - all 2451 * unmapped codes are mapped to a single fixed code.). This is the 2452 * underlying object with id, value and extensions. The accessor 2453 * "getCode" gives direct access to the value 2454 */ 2455 public CodeType getCodeElement() { 2456 if (this.code == null) 2457 if (Configuration.errorOnAutoCreate()) 2458 throw new Error("Attempt to auto-create ConceptMapGroupUnmappedComponent.code"); 2459 else if (Configuration.doAutoCreate()) 2460 this.code = new CodeType(); // bb 2461 return this.code; 2462 } 2463 2464 public boolean hasCodeElement() { 2465 return this.code != null && !this.code.isEmpty(); 2466 } 2467 2468 public boolean hasCode() { 2469 return this.code != null && !this.code.isEmpty(); 2470 } 2471 2472 /** 2473 * @param value {@link #code} (The fixed code to use when the mode = 'fixed' - 2474 * all unmapped codes are mapped to a single fixed code.). This is 2475 * the underlying object with id, value and extensions. The 2476 * accessor "getCode" gives direct access to the value 2477 */ 2478 public ConceptMapGroupUnmappedComponent setCodeElement(CodeType value) { 2479 this.code = value; 2480 return this; 2481 } 2482 2483 /** 2484 * @return The fixed code to use when the mode = 'fixed' - all unmapped codes 2485 * are mapped to a single fixed code. 2486 */ 2487 public String getCode() { 2488 return this.code == null ? null : this.code.getValue(); 2489 } 2490 2491 /** 2492 * @param value The fixed code to use when the mode = 'fixed' - all unmapped 2493 * codes are mapped to a single fixed code. 2494 */ 2495 public ConceptMapGroupUnmappedComponent setCode(String value) { 2496 if (Utilities.noString(value)) 2497 this.code = null; 2498 else { 2499 if (this.code == null) 2500 this.code = new CodeType(); 2501 this.code.setValue(value); 2502 } 2503 return this; 2504 } 2505 2506 /** 2507 * @return {@link #display} (The display for the code. The display is only 2508 * provided to help editors when editing the concept map.). This is the 2509 * underlying object with id, value and extensions. The accessor 2510 * "getDisplay" gives direct access to the value 2511 */ 2512 public StringType getDisplayElement() { 2513 if (this.display == null) 2514 if (Configuration.errorOnAutoCreate()) 2515 throw new Error("Attempt to auto-create ConceptMapGroupUnmappedComponent.display"); 2516 else if (Configuration.doAutoCreate()) 2517 this.display = new StringType(); // bb 2518 return this.display; 2519 } 2520 2521 public boolean hasDisplayElement() { 2522 return this.display != null && !this.display.isEmpty(); 2523 } 2524 2525 public boolean hasDisplay() { 2526 return this.display != null && !this.display.isEmpty(); 2527 } 2528 2529 /** 2530 * @param value {@link #display} (The display for the code. The display is only 2531 * provided to help editors when editing the concept map.). This is 2532 * the underlying object with id, value and extensions. The 2533 * accessor "getDisplay" gives direct access to the value 2534 */ 2535 public ConceptMapGroupUnmappedComponent setDisplayElement(StringType value) { 2536 this.display = value; 2537 return this; 2538 } 2539 2540 /** 2541 * @return The display for the code. The display is only provided to help 2542 * editors when editing the concept map. 2543 */ 2544 public String getDisplay() { 2545 return this.display == null ? null : this.display.getValue(); 2546 } 2547 2548 /** 2549 * @param value The display for the code. The display is only provided to help 2550 * editors when editing the concept map. 2551 */ 2552 public ConceptMapGroupUnmappedComponent setDisplay(String value) { 2553 if (Utilities.noString(value)) 2554 this.display = null; 2555 else { 2556 if (this.display == null) 2557 this.display = new StringType(); 2558 this.display.setValue(value); 2559 } 2560 return this; 2561 } 2562 2563 /** 2564 * @return {@link #url} (The canonical reference to an additional ConceptMap 2565 * resource instance to use for mapping if this ConceptMap resource 2566 * contains no matching mapping for the source concept.). This is the 2567 * underlying object with id, value and extensions. The accessor 2568 * "getUrl" gives direct access to the value 2569 */ 2570 public CanonicalType getUrlElement() { 2571 if (this.url == null) 2572 if (Configuration.errorOnAutoCreate()) 2573 throw new Error("Attempt to auto-create ConceptMapGroupUnmappedComponent.url"); 2574 else if (Configuration.doAutoCreate()) 2575 this.url = new CanonicalType(); // bb 2576 return this.url; 2577 } 2578 2579 public boolean hasUrlElement() { 2580 return this.url != null && !this.url.isEmpty(); 2581 } 2582 2583 public boolean hasUrl() { 2584 return this.url != null && !this.url.isEmpty(); 2585 } 2586 2587 /** 2588 * @param value {@link #url} (The canonical reference to an additional 2589 * ConceptMap resource instance to use for mapping if this 2590 * ConceptMap resource contains no matching mapping for the source 2591 * concept.). This is the underlying object with id, value and 2592 * extensions. The accessor "getUrl" gives direct access to the 2593 * value 2594 */ 2595 public ConceptMapGroupUnmappedComponent setUrlElement(CanonicalType value) { 2596 this.url = value; 2597 return this; 2598 } 2599 2600 /** 2601 * @return The canonical reference to an additional ConceptMap resource instance 2602 * to use for mapping if this ConceptMap resource contains no matching 2603 * mapping for the source concept. 2604 */ 2605 public String getUrl() { 2606 return this.url == null ? null : this.url.getValue(); 2607 } 2608 2609 /** 2610 * @param value The canonical reference to an additional ConceptMap resource 2611 * instance to use for mapping if this ConceptMap resource contains 2612 * no matching mapping for the source concept. 2613 */ 2614 public ConceptMapGroupUnmappedComponent setUrl(String value) { 2615 if (Utilities.noString(value)) 2616 this.url = null; 2617 else { 2618 if (this.url == null) 2619 this.url = new CanonicalType(); 2620 this.url.setValue(value); 2621 } 2622 return this; 2623 } 2624 2625 protected void listChildren(List<Property> children) { 2626 super.listChildren(children); 2627 children.add(new Property("mode", "code", 2628 "Defines which action to take if there is no match for the source concept in the target system designated for the group. One of 3 actions are possible: use the unmapped code (this is useful when doing a mapping between versions, and only a few codes have changed), use a fixed code (a default code), or alternatively, a reference to a different concept map can be provided (by canonical URL).", 2629 0, 1, mode)); 2630 children.add(new Property("code", "code", 2631 "The fixed code to use when the mode = 'fixed' - all unmapped codes are mapped to a single fixed code.", 0, 2632 1, code)); 2633 children.add(new Property("display", "string", 2634 "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 1, 2635 display)); 2636 children.add(new Property("url", "canonical(ConceptMap)", 2637 "The canonical reference to an additional ConceptMap resource instance to use for mapping if this ConceptMap resource contains no matching mapping for the source concept.", 2638 0, 1, url)); 2639 } 2640 2641 @Override 2642 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2643 switch (_hash) { 2644 case 3357091: 2645 /* mode */ return new Property("mode", "code", 2646 "Defines which action to take if there is no match for the source concept in the target system designated for the group. One of 3 actions are possible: use the unmapped code (this is useful when doing a mapping between versions, and only a few codes have changed), use a fixed code (a default code), or alternatively, a reference to a different concept map can be provided (by canonical URL).", 2647 0, 1, mode); 2648 case 3059181: 2649 /* code */ return new Property("code", "code", 2650 "The fixed code to use when the mode = 'fixed' - all unmapped codes are mapped to a single fixed code.", 0, 2651 1, code); 2652 case 1671764162: 2653 /* display */ return new Property("display", "string", 2654 "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 2655 1, display); 2656 case 116079: 2657 /* url */ return new Property("url", "canonical(ConceptMap)", 2658 "The canonical reference to an additional ConceptMap resource instance to use for mapping if this ConceptMap resource contains no matching mapping for the source concept.", 2659 0, 1, url); 2660 default: 2661 return super.getNamedProperty(_hash, _name, _checkValid); 2662 } 2663 2664 } 2665 2666 @Override 2667 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2668 switch (hash) { 2669 case 3357091: 2670 /* mode */ return this.mode == null ? new Base[0] : new Base[] { this.mode }; // Enumeration<ConceptMapGroupUnmappedMode> 2671 case 3059181: 2672 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeType 2673 case 1671764162: 2674 /* display */ return this.display == null ? new Base[0] : new Base[] { this.display }; // StringType 2675 case 116079: 2676 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // CanonicalType 2677 default: 2678 return super.getProperty(hash, name, checkValid); 2679 } 2680 2681 } 2682 2683 @Override 2684 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2685 switch (hash) { 2686 case 3357091: // mode 2687 value = new ConceptMapGroupUnmappedModeEnumFactory().fromType(castToCode(value)); 2688 this.mode = (Enumeration) value; // Enumeration<ConceptMapGroupUnmappedMode> 2689 return value; 2690 case 3059181: // code 2691 this.code = castToCode(value); // CodeType 2692 return value; 2693 case 1671764162: // display 2694 this.display = castToString(value); // StringType 2695 return value; 2696 case 116079: // url 2697 this.url = castToCanonical(value); // CanonicalType 2698 return value; 2699 default: 2700 return super.setProperty(hash, name, value); 2701 } 2702 2703 } 2704 2705 @Override 2706 public Base setProperty(String name, Base value) throws FHIRException { 2707 if (name.equals("mode")) { 2708 value = new ConceptMapGroupUnmappedModeEnumFactory().fromType(castToCode(value)); 2709 this.mode = (Enumeration) value; // Enumeration<ConceptMapGroupUnmappedMode> 2710 } else if (name.equals("code")) { 2711 this.code = castToCode(value); // CodeType 2712 } else if (name.equals("display")) { 2713 this.display = castToString(value); // StringType 2714 } else if (name.equals("url")) { 2715 this.url = castToCanonical(value); // CanonicalType 2716 } else 2717 return super.setProperty(name, value); 2718 return value; 2719 } 2720 2721 @Override 2722 public Base makeProperty(int hash, String name) throws FHIRException { 2723 switch (hash) { 2724 case 3357091: 2725 return getModeElement(); 2726 case 3059181: 2727 return getCodeElement(); 2728 case 1671764162: 2729 return getDisplayElement(); 2730 case 116079: 2731 return getUrlElement(); 2732 default: 2733 return super.makeProperty(hash, name); 2734 } 2735 2736 } 2737 2738 @Override 2739 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2740 switch (hash) { 2741 case 3357091: 2742 /* mode */ return new String[] { "code" }; 2743 case 3059181: 2744 /* code */ return new String[] { "code" }; 2745 case 1671764162: 2746 /* display */ return new String[] { "string" }; 2747 case 116079: 2748 /* url */ return new String[] { "canonical" }; 2749 default: 2750 return super.getTypesForProperty(hash, name); 2751 } 2752 2753 } 2754 2755 @Override 2756 public Base addChild(String name) throws FHIRException { 2757 if (name.equals("mode")) { 2758 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.mode"); 2759 } else if (name.equals("code")) { 2760 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.code"); 2761 } else if (name.equals("display")) { 2762 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.display"); 2763 } else if (name.equals("url")) { 2764 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.url"); 2765 } else 2766 return super.addChild(name); 2767 } 2768 2769 public ConceptMapGroupUnmappedComponent copy() { 2770 ConceptMapGroupUnmappedComponent dst = new ConceptMapGroupUnmappedComponent(); 2771 copyValues(dst); 2772 return dst; 2773 } 2774 2775 public void copyValues(ConceptMapGroupUnmappedComponent dst) { 2776 super.copyValues(dst); 2777 dst.mode = mode == null ? null : mode.copy(); 2778 dst.code = code == null ? null : code.copy(); 2779 dst.display = display == null ? null : display.copy(); 2780 dst.url = url == null ? null : url.copy(); 2781 } 2782 2783 @Override 2784 public boolean equalsDeep(Base other_) { 2785 if (!super.equalsDeep(other_)) 2786 return false; 2787 if (!(other_ instanceof ConceptMapGroupUnmappedComponent)) 2788 return false; 2789 ConceptMapGroupUnmappedComponent o = (ConceptMapGroupUnmappedComponent) other_; 2790 return compareDeep(mode, o.mode, true) && compareDeep(code, o.code, true) && compareDeep(display, o.display, true) 2791 && compareDeep(url, o.url, true); 2792 } 2793 2794 @Override 2795 public boolean equalsShallow(Base other_) { 2796 if (!super.equalsShallow(other_)) 2797 return false; 2798 if (!(other_ instanceof ConceptMapGroupUnmappedComponent)) 2799 return false; 2800 ConceptMapGroupUnmappedComponent o = (ConceptMapGroupUnmappedComponent) other_; 2801 return compareValues(mode, o.mode, true) && compareValues(code, o.code, true) 2802 && compareValues(display, o.display, true); 2803 } 2804 2805 public boolean isEmpty() { 2806 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(mode, code, display, url); 2807 } 2808 2809 public String fhirType() { 2810 return "ConceptMap.group.unmapped"; 2811 2812 } 2813 2814 } 2815 2816 /** 2817 * A formal identifier that is used to identify this concept map when it is 2818 * represented in other formats, or referenced in a specification, model, design 2819 * or an instance. 2820 */ 2821 @Child(name = "identifier", type = { 2822 Identifier.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 2823 @Description(shortDefinition = "Additional identifier for the concept map", formalDefinition = "A formal identifier that is used to identify this concept map when it is represented in other formats, or referenced in a specification, model, design or an instance.") 2824 protected Identifier identifier; 2825 2826 /** 2827 * Explanation of why this concept map is needed and why it has been designed as 2828 * it has. 2829 */ 2830 @Child(name = "purpose", type = { 2831 MarkdownType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2832 @Description(shortDefinition = "Why this concept map is defined", formalDefinition = "Explanation of why this concept map is needed and why it has been designed as it has.") 2833 protected MarkdownType purpose; 2834 2835 /** 2836 * A copyright statement relating to the concept map and/or its contents. 2837 * Copyright statements are generally legal restrictions on the use and 2838 * publishing of the concept map. 2839 */ 2840 @Child(name = "copyright", type = { 2841 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2842 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the concept map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the concept map.") 2843 protected MarkdownType copyright; 2844 2845 /** 2846 * Identifier for the source value set that contains the concepts that are being 2847 * mapped and provides context for the mappings. 2848 */ 2849 @Child(name = "source", type = { UriType.class, 2850 CanonicalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 2851 @Description(shortDefinition = "The source value set that contains the concepts that are being mapped", formalDefinition = "Identifier for the source value set that contains the concepts that are being mapped and provides context for the mappings.") 2852 protected Type source; 2853 2854 /** 2855 * The target value set provides context for the mappings. Note that the mapping 2856 * is made between concepts, not between value sets, but the value set provides 2857 * important context about how the concept mapping choices are made. 2858 */ 2859 @Child(name = "target", type = { UriType.class, 2860 CanonicalType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 2861 @Description(shortDefinition = "The target value set which provides context for the mappings", formalDefinition = "The target value set provides context for the mappings. Note that the mapping is made between concepts, not between value sets, but the value set provides important context about how the concept mapping choices are made.") 2862 protected Type target; 2863 2864 /** 2865 * A group of mappings that all have the same source and target system. 2866 */ 2867 @Child(name = "group", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2868 @Description(shortDefinition = "Same source and target systems", formalDefinition = "A group of mappings that all have the same source and target system.") 2869 protected List<ConceptMapGroupComponent> group; 2870 2871 private static final long serialVersionUID = -2081872580L; 2872 2873 /** 2874 * Constructor 2875 */ 2876 public ConceptMap() { 2877 super(); 2878 } 2879 2880 /** 2881 * Constructor 2882 */ 2883 public ConceptMap(Enumeration<PublicationStatus> status) { 2884 super(); 2885 this.status = status; 2886 } 2887 2888 /** 2889 * @return {@link #url} (An absolute URI that is used to identify this concept 2890 * map when it is referenced in a specification, model, design or an 2891 * instance; also called its canonical identifier. This SHOULD be 2892 * globally unique and SHOULD be a literal address at which at which an 2893 * authoritative instance of this concept map is (or will be) published. 2894 * This URL can be the target of a canonical reference. It SHALL remain 2895 * the same when the concept map is stored on different servers.). This 2896 * is the underlying object with id, value and extensions. The accessor 2897 * "getUrl" gives direct access to the value 2898 */ 2899 public UriType getUrlElement() { 2900 if (this.url == null) 2901 if (Configuration.errorOnAutoCreate()) 2902 throw new Error("Attempt to auto-create ConceptMap.url"); 2903 else if (Configuration.doAutoCreate()) 2904 this.url = new UriType(); // bb 2905 return this.url; 2906 } 2907 2908 public boolean hasUrlElement() { 2909 return this.url != null && !this.url.isEmpty(); 2910 } 2911 2912 public boolean hasUrl() { 2913 return this.url != null && !this.url.isEmpty(); 2914 } 2915 2916 /** 2917 * @param value {@link #url} (An absolute URI that is used to identify this 2918 * concept map when it is referenced in a specification, model, 2919 * design or an instance; also called its canonical identifier. 2920 * This SHOULD be globally unique and SHOULD be a literal address 2921 * at which at which an authoritative instance of this concept map 2922 * is (or will be) published. This URL can be the target of a 2923 * canonical reference. It SHALL remain the same when the concept 2924 * map is stored on different servers.). This is the underlying 2925 * object with id, value and extensions. The accessor "getUrl" 2926 * gives direct access to the value 2927 */ 2928 public ConceptMap setUrlElement(UriType value) { 2929 this.url = value; 2930 return this; 2931 } 2932 2933 /** 2934 * @return An absolute URI that is used to identify this concept map when it is 2935 * referenced in a specification, model, design or an instance; also 2936 * called its canonical identifier. This SHOULD be globally unique and 2937 * SHOULD be a literal address at which at which an authoritative 2938 * instance of this concept map is (or will be) published. This URL can 2939 * be the target of a canonical reference. It SHALL remain the same when 2940 * the concept map is stored on different servers. 2941 */ 2942 public String getUrl() { 2943 return this.url == null ? null : this.url.getValue(); 2944 } 2945 2946 /** 2947 * @param value An absolute URI that is used to identify this concept map when 2948 * it is referenced in a specification, model, design or an 2949 * instance; also called its canonical identifier. This SHOULD be 2950 * globally unique and SHOULD be a literal address at which at 2951 * which an authoritative instance of this concept map is (or will 2952 * be) published. This URL can be the target of a canonical 2953 * reference. It SHALL remain the same when the concept map is 2954 * stored on different servers. 2955 */ 2956 public ConceptMap setUrl(String value) { 2957 if (Utilities.noString(value)) 2958 this.url = null; 2959 else { 2960 if (this.url == null) 2961 this.url = new UriType(); 2962 this.url.setValue(value); 2963 } 2964 return this; 2965 } 2966 2967 /** 2968 * @return {@link #identifier} (A formal identifier that is used to identify 2969 * this concept map when it is represented in other formats, or 2970 * referenced in a specification, model, design or an instance.) 2971 */ 2972 public Identifier getIdentifier() { 2973 if (this.identifier == null) 2974 if (Configuration.errorOnAutoCreate()) 2975 throw new Error("Attempt to auto-create ConceptMap.identifier"); 2976 else if (Configuration.doAutoCreate()) 2977 this.identifier = new Identifier(); // cc 2978 return this.identifier; 2979 } 2980 2981 public boolean hasIdentifier() { 2982 return this.identifier != null && !this.identifier.isEmpty(); 2983 } 2984 2985 /** 2986 * @param value {@link #identifier} (A formal identifier that is used to 2987 * identify this concept map when it is represented in other 2988 * formats, or referenced in a specification, model, design or an 2989 * instance.) 2990 */ 2991 public ConceptMap setIdentifier(Identifier value) { 2992 this.identifier = value; 2993 return this; 2994 } 2995 2996 /** 2997 * @return {@link #version} (The identifier that is used to identify this 2998 * version of the concept map when it is referenced in a specification, 2999 * model, design or instance. This is an arbitrary value managed by the 3000 * concept map author and is not expected to be globally unique. For 3001 * example, it might be a timestamp (e.g. yyyymmdd) if a managed version 3002 * is not available. There is also no expectation that versions can be 3003 * placed in a lexicographical sequence.). This is the underlying object 3004 * with id, value and extensions. The accessor "getVersion" gives direct 3005 * access to the value 3006 */ 3007 public StringType getVersionElement() { 3008 if (this.version == null) 3009 if (Configuration.errorOnAutoCreate()) 3010 throw new Error("Attempt to auto-create ConceptMap.version"); 3011 else if (Configuration.doAutoCreate()) 3012 this.version = new StringType(); // bb 3013 return this.version; 3014 } 3015 3016 public boolean hasVersionElement() { 3017 return this.version != null && !this.version.isEmpty(); 3018 } 3019 3020 public boolean hasVersion() { 3021 return this.version != null && !this.version.isEmpty(); 3022 } 3023 3024 /** 3025 * @param value {@link #version} (The identifier that is used to identify this 3026 * version of the concept map when it is referenced in a 3027 * specification, model, design or instance. This is an arbitrary 3028 * value managed by the concept map author and is not expected to 3029 * be globally unique. For example, it might be a timestamp (e.g. 3030 * yyyymmdd) if a managed version is not available. There is also 3031 * no expectation that versions can be placed in a lexicographical 3032 * sequence.). This is the underlying object with id, value and 3033 * extensions. The accessor "getVersion" gives direct access to the 3034 * value 3035 */ 3036 public ConceptMap setVersionElement(StringType value) { 3037 this.version = value; 3038 return this; 3039 } 3040 3041 /** 3042 * @return The identifier that is used to identify this version of the concept 3043 * map when it is referenced in a specification, model, design or 3044 * instance. This is an arbitrary value managed by the concept map 3045 * author and is not expected to be globally unique. For example, it 3046 * might be a timestamp (e.g. yyyymmdd) if a managed version is not 3047 * available. There is also no expectation that versions can be placed 3048 * in a lexicographical sequence. 3049 */ 3050 public String getVersion() { 3051 return this.version == null ? null : this.version.getValue(); 3052 } 3053 3054 /** 3055 * @param value The identifier that is used to identify this version of the 3056 * concept map when it is referenced in a specification, model, 3057 * design or instance. This is an arbitrary value managed by the 3058 * concept map author and is not expected to be globally unique. 3059 * For example, it might be a timestamp (e.g. yyyymmdd) if a 3060 * managed version is not available. There is also no expectation 3061 * that versions can be placed in a lexicographical sequence. 3062 */ 3063 public ConceptMap setVersion(String value) { 3064 if (Utilities.noString(value)) 3065 this.version = null; 3066 else { 3067 if (this.version == null) 3068 this.version = new StringType(); 3069 this.version.setValue(value); 3070 } 3071 return this; 3072 } 3073 3074 /** 3075 * @return {@link #name} (A natural language name identifying the concept map. 3076 * This name should be usable as an identifier for the module by machine 3077 * processing applications such as code generation.). This is the 3078 * underlying object with id, value and extensions. The accessor 3079 * "getName" gives direct access to the value 3080 */ 3081 public StringType getNameElement() { 3082 if (this.name == null) 3083 if (Configuration.errorOnAutoCreate()) 3084 throw new Error("Attempt to auto-create ConceptMap.name"); 3085 else if (Configuration.doAutoCreate()) 3086 this.name = new StringType(); // bb 3087 return this.name; 3088 } 3089 3090 public boolean hasNameElement() { 3091 return this.name != null && !this.name.isEmpty(); 3092 } 3093 3094 public boolean hasName() { 3095 return this.name != null && !this.name.isEmpty(); 3096 } 3097 3098 /** 3099 * @param value {@link #name} (A natural language name identifying the concept 3100 * map. This name should be usable as an identifier for the module 3101 * by machine processing applications such as code generation.). 3102 * This is the underlying object with id, value and extensions. The 3103 * accessor "getName" gives direct access to the value 3104 */ 3105 public ConceptMap setNameElement(StringType value) { 3106 this.name = value; 3107 return this; 3108 } 3109 3110 /** 3111 * @return A natural language name identifying the concept map. This name should 3112 * be usable as an identifier for the module by machine processing 3113 * applications such as code generation. 3114 */ 3115 public String getName() { 3116 return this.name == null ? null : this.name.getValue(); 3117 } 3118 3119 /** 3120 * @param value A natural language name identifying the concept map. This name 3121 * should be usable as an identifier for the module by machine 3122 * processing applications such as code generation. 3123 */ 3124 public ConceptMap setName(String value) { 3125 if (Utilities.noString(value)) 3126 this.name = null; 3127 else { 3128 if (this.name == null) 3129 this.name = new StringType(); 3130 this.name.setValue(value); 3131 } 3132 return this; 3133 } 3134 3135 /** 3136 * @return {@link #title} (A short, descriptive, user-friendly title for the 3137 * concept map.). This is the underlying object with id, value and 3138 * extensions. The accessor "getTitle" gives direct access to the value 3139 */ 3140 public StringType getTitleElement() { 3141 if (this.title == null) 3142 if (Configuration.errorOnAutoCreate()) 3143 throw new Error("Attempt to auto-create ConceptMap.title"); 3144 else if (Configuration.doAutoCreate()) 3145 this.title = new StringType(); // bb 3146 return this.title; 3147 } 3148 3149 public boolean hasTitleElement() { 3150 return this.title != null && !this.title.isEmpty(); 3151 } 3152 3153 public boolean hasTitle() { 3154 return this.title != null && !this.title.isEmpty(); 3155 } 3156 3157 /** 3158 * @param value {@link #title} (A short, descriptive, user-friendly title for 3159 * the concept map.). This is the underlying object with id, value 3160 * and extensions. The accessor "getTitle" gives direct access to 3161 * the value 3162 */ 3163 public ConceptMap setTitleElement(StringType value) { 3164 this.title = value; 3165 return this; 3166 } 3167 3168 /** 3169 * @return A short, descriptive, user-friendly title for the concept map. 3170 */ 3171 public String getTitle() { 3172 return this.title == null ? null : this.title.getValue(); 3173 } 3174 3175 /** 3176 * @param value A short, descriptive, user-friendly title for the concept map. 3177 */ 3178 public ConceptMap setTitle(String value) { 3179 if (Utilities.noString(value)) 3180 this.title = null; 3181 else { 3182 if (this.title == null) 3183 this.title = new StringType(); 3184 this.title.setValue(value); 3185 } 3186 return this; 3187 } 3188 3189 /** 3190 * @return {@link #status} (The status of this concept map. Enables tracking the 3191 * life-cycle of the content.). This is the underlying object with id, 3192 * value and extensions. The accessor "getStatus" gives direct access to 3193 * the value 3194 */ 3195 public Enumeration<PublicationStatus> getStatusElement() { 3196 if (this.status == null) 3197 if (Configuration.errorOnAutoCreate()) 3198 throw new Error("Attempt to auto-create ConceptMap.status"); 3199 else if (Configuration.doAutoCreate()) 3200 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 3201 return this.status; 3202 } 3203 3204 public boolean hasStatusElement() { 3205 return this.status != null && !this.status.isEmpty(); 3206 } 3207 3208 public boolean hasStatus() { 3209 return this.status != null && !this.status.isEmpty(); 3210 } 3211 3212 /** 3213 * @param value {@link #status} (The status of this concept map. Enables 3214 * tracking the life-cycle of the content.). This is the underlying 3215 * object with id, value and extensions. The accessor "getStatus" 3216 * gives direct access to the value 3217 */ 3218 public ConceptMap setStatusElement(Enumeration<PublicationStatus> value) { 3219 this.status = value; 3220 return this; 3221 } 3222 3223 /** 3224 * @return The status of this concept map. Enables tracking the life-cycle of 3225 * the content. 3226 */ 3227 public PublicationStatus getStatus() { 3228 return this.status == null ? null : this.status.getValue(); 3229 } 3230 3231 /** 3232 * @param value The status of this concept map. Enables tracking the life-cycle 3233 * of the content. 3234 */ 3235 public ConceptMap setStatus(PublicationStatus value) { 3236 if (this.status == null) 3237 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 3238 this.status.setValue(value); 3239 return this; 3240 } 3241 3242 /** 3243 * @return {@link #experimental} (A Boolean value to indicate that this concept 3244 * map is authored for testing purposes (or 3245 * education/evaluation/marketing) and is not intended to be used for 3246 * genuine usage.). This is the underlying object with id, value and 3247 * extensions. The accessor "getExperimental" gives direct access to the 3248 * value 3249 */ 3250 public BooleanType getExperimentalElement() { 3251 if (this.experimental == null) 3252 if (Configuration.errorOnAutoCreate()) 3253 throw new Error("Attempt to auto-create ConceptMap.experimental"); 3254 else if (Configuration.doAutoCreate()) 3255 this.experimental = new BooleanType(); // bb 3256 return this.experimental; 3257 } 3258 3259 public boolean hasExperimentalElement() { 3260 return this.experimental != null && !this.experimental.isEmpty(); 3261 } 3262 3263 public boolean hasExperimental() { 3264 return this.experimental != null && !this.experimental.isEmpty(); 3265 } 3266 3267 /** 3268 * @param value {@link #experimental} (A Boolean value to indicate that this 3269 * concept map is authored for testing purposes (or 3270 * education/evaluation/marketing) and is not intended to be used 3271 * for genuine usage.). This is the underlying object with id, 3272 * value and extensions. The accessor "getExperimental" gives 3273 * direct access to the value 3274 */ 3275 public ConceptMap setExperimentalElement(BooleanType value) { 3276 this.experimental = value; 3277 return this; 3278 } 3279 3280 /** 3281 * @return A Boolean value to indicate that this concept map is authored for 3282 * testing purposes (or education/evaluation/marketing) and is not 3283 * intended to be used for genuine usage. 3284 */ 3285 public boolean getExperimental() { 3286 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 3287 } 3288 3289 /** 3290 * @param value A Boolean value to indicate that this concept map is authored 3291 * for testing purposes (or education/evaluation/marketing) and is 3292 * not intended to be used for genuine usage. 3293 */ 3294 public ConceptMap setExperimental(boolean value) { 3295 if (this.experimental == null) 3296 this.experimental = new BooleanType(); 3297 this.experimental.setValue(value); 3298 return this; 3299 } 3300 3301 /** 3302 * @return {@link #date} (The date (and optionally time) when the concept map 3303 * was published. The date must change when the business version changes 3304 * and it must change if the status code changes. In addition, it should 3305 * change when the substantive content of the concept map changes.). 3306 * This is the underlying object with id, value and extensions. The 3307 * accessor "getDate" gives direct access to the value 3308 */ 3309 public DateTimeType getDateElement() { 3310 if (this.date == null) 3311 if (Configuration.errorOnAutoCreate()) 3312 throw new Error("Attempt to auto-create ConceptMap.date"); 3313 else if (Configuration.doAutoCreate()) 3314 this.date = new DateTimeType(); // bb 3315 return this.date; 3316 } 3317 3318 public boolean hasDateElement() { 3319 return this.date != null && !this.date.isEmpty(); 3320 } 3321 3322 public boolean hasDate() { 3323 return this.date != null && !this.date.isEmpty(); 3324 } 3325 3326 /** 3327 * @param value {@link #date} (The date (and optionally time) when the concept 3328 * map was published. The date must change when the business 3329 * version changes and it must change if the status code changes. 3330 * In addition, it should change when the substantive content of 3331 * the concept map changes.). This is the underlying object with 3332 * id, value and extensions. The accessor "getDate" gives direct 3333 * access to the value 3334 */ 3335 public ConceptMap setDateElement(DateTimeType value) { 3336 this.date = value; 3337 return this; 3338 } 3339 3340 /** 3341 * @return The date (and optionally time) when the concept map was published. 3342 * The date must change when the business version changes and it must 3343 * change if the status code changes. In addition, it should change when 3344 * the substantive content of the concept map changes. 3345 */ 3346 public Date getDate() { 3347 return this.date == null ? null : this.date.getValue(); 3348 } 3349 3350 /** 3351 * @param value The date (and optionally time) when the concept map was 3352 * published. The date must change when the business version 3353 * changes and it must change if the status code changes. In 3354 * addition, it should change when the substantive content of the 3355 * concept map changes. 3356 */ 3357 public ConceptMap setDate(Date value) { 3358 if (value == null) 3359 this.date = null; 3360 else { 3361 if (this.date == null) 3362 this.date = new DateTimeType(); 3363 this.date.setValue(value); 3364 } 3365 return this; 3366 } 3367 3368 /** 3369 * @return {@link #publisher} (The name of the organization or individual that 3370 * published the concept map.). This is the underlying object with id, 3371 * value and extensions. The accessor "getPublisher" gives direct access 3372 * to the value 3373 */ 3374 public StringType getPublisherElement() { 3375 if (this.publisher == null) 3376 if (Configuration.errorOnAutoCreate()) 3377 throw new Error("Attempt to auto-create ConceptMap.publisher"); 3378 else if (Configuration.doAutoCreate()) 3379 this.publisher = new StringType(); // bb 3380 return this.publisher; 3381 } 3382 3383 public boolean hasPublisherElement() { 3384 return this.publisher != null && !this.publisher.isEmpty(); 3385 } 3386 3387 public boolean hasPublisher() { 3388 return this.publisher != null && !this.publisher.isEmpty(); 3389 } 3390 3391 /** 3392 * @param value {@link #publisher} (The name of the organization or individual 3393 * that published the concept map.). This is the underlying object 3394 * with id, value and extensions. The accessor "getPublisher" gives 3395 * direct access to the value 3396 */ 3397 public ConceptMap setPublisherElement(StringType value) { 3398 this.publisher = value; 3399 return this; 3400 } 3401 3402 /** 3403 * @return The name of the organization or individual that published the concept 3404 * map. 3405 */ 3406 public String getPublisher() { 3407 return this.publisher == null ? null : this.publisher.getValue(); 3408 } 3409 3410 /** 3411 * @param value The name of the organization or individual that published the 3412 * concept map. 3413 */ 3414 public ConceptMap setPublisher(String value) { 3415 if (Utilities.noString(value)) 3416 this.publisher = null; 3417 else { 3418 if (this.publisher == null) 3419 this.publisher = new StringType(); 3420 this.publisher.setValue(value); 3421 } 3422 return this; 3423 } 3424 3425 /** 3426 * @return {@link #contact} (Contact details to assist a user in finding and 3427 * communicating with the publisher.) 3428 */ 3429 public List<ContactDetail> getContact() { 3430 if (this.contact == null) 3431 this.contact = new ArrayList<ContactDetail>(); 3432 return this.contact; 3433 } 3434 3435 /** 3436 * @return Returns a reference to <code>this</code> for easy method chaining 3437 */ 3438 public ConceptMap setContact(List<ContactDetail> theContact) { 3439 this.contact = theContact; 3440 return this; 3441 } 3442 3443 public boolean hasContact() { 3444 if (this.contact == null) 3445 return false; 3446 for (ContactDetail item : this.contact) 3447 if (!item.isEmpty()) 3448 return true; 3449 return false; 3450 } 3451 3452 public ContactDetail addContact() { // 3 3453 ContactDetail t = new ContactDetail(); 3454 if (this.contact == null) 3455 this.contact = new ArrayList<ContactDetail>(); 3456 this.contact.add(t); 3457 return t; 3458 } 3459 3460 public ConceptMap addContact(ContactDetail t) { // 3 3461 if (t == null) 3462 return this; 3463 if (this.contact == null) 3464 this.contact = new ArrayList<ContactDetail>(); 3465 this.contact.add(t); 3466 return this; 3467 } 3468 3469 /** 3470 * @return The first repetition of repeating field {@link #contact}, creating it 3471 * if it does not already exist 3472 */ 3473 public ContactDetail getContactFirstRep() { 3474 if (getContact().isEmpty()) { 3475 addContact(); 3476 } 3477 return getContact().get(0); 3478 } 3479 3480 /** 3481 * @return {@link #description} (A free text natural language description of the 3482 * concept map from a consumer's perspective.). This is the underlying 3483 * object with id, value and extensions. The accessor "getDescription" 3484 * gives direct access to the value 3485 */ 3486 public MarkdownType getDescriptionElement() { 3487 if (this.description == null) 3488 if (Configuration.errorOnAutoCreate()) 3489 throw new Error("Attempt to auto-create ConceptMap.description"); 3490 else if (Configuration.doAutoCreate()) 3491 this.description = new MarkdownType(); // bb 3492 return this.description; 3493 } 3494 3495 public boolean hasDescriptionElement() { 3496 return this.description != null && !this.description.isEmpty(); 3497 } 3498 3499 public boolean hasDescription() { 3500 return this.description != null && !this.description.isEmpty(); 3501 } 3502 3503 /** 3504 * @param value {@link #description} (A free text natural language description 3505 * of the concept map from a consumer's perspective.). This is the 3506 * underlying object with id, value and extensions. The accessor 3507 * "getDescription" gives direct access to the value 3508 */ 3509 public ConceptMap setDescriptionElement(MarkdownType value) { 3510 this.description = value; 3511 return this; 3512 } 3513 3514 /** 3515 * @return A free text natural language description of the concept map from a 3516 * consumer's perspective. 3517 */ 3518 public String getDescription() { 3519 return this.description == null ? null : this.description.getValue(); 3520 } 3521 3522 /** 3523 * @param value A free text natural language description of the concept map from 3524 * a consumer's perspective. 3525 */ 3526 public ConceptMap setDescription(String value) { 3527 if (value == null) 3528 this.description = null; 3529 else { 3530 if (this.description == null) 3531 this.description = new MarkdownType(); 3532 this.description.setValue(value); 3533 } 3534 return this; 3535 } 3536 3537 /** 3538 * @return {@link #useContext} (The content was developed with a focus and 3539 * intent of supporting the contexts that are listed. These contexts may 3540 * be general categories (gender, age, ...) or may be references to 3541 * specific programs (insurance plans, studies, ...) and may be used to 3542 * assist with indexing and searching for appropriate concept map 3543 * instances.) 3544 */ 3545 public List<UsageContext> getUseContext() { 3546 if (this.useContext == null) 3547 this.useContext = new ArrayList<UsageContext>(); 3548 return this.useContext; 3549 } 3550 3551 /** 3552 * @return Returns a reference to <code>this</code> for easy method chaining 3553 */ 3554 public ConceptMap setUseContext(List<UsageContext> theUseContext) { 3555 this.useContext = theUseContext; 3556 return this; 3557 } 3558 3559 public boolean hasUseContext() { 3560 if (this.useContext == null) 3561 return false; 3562 for (UsageContext item : this.useContext) 3563 if (!item.isEmpty()) 3564 return true; 3565 return false; 3566 } 3567 3568 public UsageContext addUseContext() { // 3 3569 UsageContext t = new UsageContext(); 3570 if (this.useContext == null) 3571 this.useContext = new ArrayList<UsageContext>(); 3572 this.useContext.add(t); 3573 return t; 3574 } 3575 3576 public ConceptMap addUseContext(UsageContext t) { // 3 3577 if (t == null) 3578 return this; 3579 if (this.useContext == null) 3580 this.useContext = new ArrayList<UsageContext>(); 3581 this.useContext.add(t); 3582 return this; 3583 } 3584 3585 /** 3586 * @return The first repetition of repeating field {@link #useContext}, creating 3587 * it if it does not already exist 3588 */ 3589 public UsageContext getUseContextFirstRep() { 3590 if (getUseContext().isEmpty()) { 3591 addUseContext(); 3592 } 3593 return getUseContext().get(0); 3594 } 3595 3596 /** 3597 * @return {@link #jurisdiction} (A legal or geographic region in which the 3598 * concept map is intended to be used.) 3599 */ 3600 public List<CodeableConcept> getJurisdiction() { 3601 if (this.jurisdiction == null) 3602 this.jurisdiction = new ArrayList<CodeableConcept>(); 3603 return this.jurisdiction; 3604 } 3605 3606 /** 3607 * @return Returns a reference to <code>this</code> for easy method chaining 3608 */ 3609 public ConceptMap setJurisdiction(List<CodeableConcept> theJurisdiction) { 3610 this.jurisdiction = theJurisdiction; 3611 return this; 3612 } 3613 3614 public boolean hasJurisdiction() { 3615 if (this.jurisdiction == null) 3616 return false; 3617 for (CodeableConcept item : this.jurisdiction) 3618 if (!item.isEmpty()) 3619 return true; 3620 return false; 3621 } 3622 3623 public CodeableConcept addJurisdiction() { // 3 3624 CodeableConcept t = new CodeableConcept(); 3625 if (this.jurisdiction == null) 3626 this.jurisdiction = new ArrayList<CodeableConcept>(); 3627 this.jurisdiction.add(t); 3628 return t; 3629 } 3630 3631 public ConceptMap addJurisdiction(CodeableConcept t) { // 3 3632 if (t == null) 3633 return this; 3634 if (this.jurisdiction == null) 3635 this.jurisdiction = new ArrayList<CodeableConcept>(); 3636 this.jurisdiction.add(t); 3637 return this; 3638 } 3639 3640 /** 3641 * @return The first repetition of repeating field {@link #jurisdiction}, 3642 * creating it if it does not already exist 3643 */ 3644 public CodeableConcept getJurisdictionFirstRep() { 3645 if (getJurisdiction().isEmpty()) { 3646 addJurisdiction(); 3647 } 3648 return getJurisdiction().get(0); 3649 } 3650 3651 /** 3652 * @return {@link #purpose} (Explanation of why this concept map is needed and 3653 * why it has been designed as it has.). This is the underlying object 3654 * with id, value and extensions. The accessor "getPurpose" gives direct 3655 * access to the value 3656 */ 3657 public MarkdownType getPurposeElement() { 3658 if (this.purpose == null) 3659 if (Configuration.errorOnAutoCreate()) 3660 throw new Error("Attempt to auto-create ConceptMap.purpose"); 3661 else if (Configuration.doAutoCreate()) 3662 this.purpose = new MarkdownType(); // bb 3663 return this.purpose; 3664 } 3665 3666 public boolean hasPurposeElement() { 3667 return this.purpose != null && !this.purpose.isEmpty(); 3668 } 3669 3670 public boolean hasPurpose() { 3671 return this.purpose != null && !this.purpose.isEmpty(); 3672 } 3673 3674 /** 3675 * @param value {@link #purpose} (Explanation of why this concept map is needed 3676 * and why it has been designed as it has.). This is the underlying 3677 * object with id, value and extensions. The accessor "getPurpose" 3678 * gives direct access to the value 3679 */ 3680 public ConceptMap setPurposeElement(MarkdownType value) { 3681 this.purpose = value; 3682 return this; 3683 } 3684 3685 /** 3686 * @return Explanation of why this concept map is needed and why it has been 3687 * designed as it has. 3688 */ 3689 public String getPurpose() { 3690 return this.purpose == null ? null : this.purpose.getValue(); 3691 } 3692 3693 /** 3694 * @param value Explanation of why this concept map is needed and why it has 3695 * been designed as it has. 3696 */ 3697 public ConceptMap setPurpose(String value) { 3698 if (value == null) 3699 this.purpose = null; 3700 else { 3701 if (this.purpose == null) 3702 this.purpose = new MarkdownType(); 3703 this.purpose.setValue(value); 3704 } 3705 return this; 3706 } 3707 3708 /** 3709 * @return {@link #copyright} (A copyright statement relating to the concept map 3710 * and/or its contents. Copyright statements are generally legal 3711 * restrictions on the use and publishing of the concept map.). This is 3712 * the underlying object with id, value and extensions. The accessor 3713 * "getCopyright" gives direct access to the value 3714 */ 3715 public MarkdownType getCopyrightElement() { 3716 if (this.copyright == null) 3717 if (Configuration.errorOnAutoCreate()) 3718 throw new Error("Attempt to auto-create ConceptMap.copyright"); 3719 else if (Configuration.doAutoCreate()) 3720 this.copyright = new MarkdownType(); // bb 3721 return this.copyright; 3722 } 3723 3724 public boolean hasCopyrightElement() { 3725 return this.copyright != null && !this.copyright.isEmpty(); 3726 } 3727 3728 public boolean hasCopyright() { 3729 return this.copyright != null && !this.copyright.isEmpty(); 3730 } 3731 3732 /** 3733 * @param value {@link #copyright} (A copyright statement relating to the 3734 * concept map and/or its contents. Copyright statements are 3735 * generally legal restrictions on the use and publishing of the 3736 * concept map.). This is the underlying object with id, value and 3737 * extensions. The accessor "getCopyright" gives direct access to 3738 * the value 3739 */ 3740 public ConceptMap setCopyrightElement(MarkdownType value) { 3741 this.copyright = value; 3742 return this; 3743 } 3744 3745 /** 3746 * @return A copyright statement relating to the concept map and/or its 3747 * contents. Copyright statements are generally legal restrictions on 3748 * the use and publishing of the concept map. 3749 */ 3750 public String getCopyright() { 3751 return this.copyright == null ? null : this.copyright.getValue(); 3752 } 3753 3754 /** 3755 * @param value A copyright statement relating to the concept map and/or its 3756 * contents. Copyright statements are generally legal restrictions 3757 * on the use and publishing of the concept map. 3758 */ 3759 public ConceptMap setCopyright(String value) { 3760 if (value == null) 3761 this.copyright = null; 3762 else { 3763 if (this.copyright == null) 3764 this.copyright = new MarkdownType(); 3765 this.copyright.setValue(value); 3766 } 3767 return this; 3768 } 3769 3770 /** 3771 * @return {@link #source} (Identifier for the source value set that contains 3772 * the concepts that are being mapped and provides context for the 3773 * mappings.) 3774 */ 3775 public Type getSource() { 3776 return this.source; 3777 } 3778 3779 /** 3780 * @return {@link #source} (Identifier for the source value set that contains 3781 * the concepts that are being mapped and provides context for the 3782 * mappings.) 3783 */ 3784 public UriType getSourceUriType() throws FHIRException { 3785 if (this.source == null) 3786 this.source = new UriType(); 3787 if (!(this.source instanceof UriType)) 3788 throw new FHIRException( 3789 "Type mismatch: the type UriType was expected, but " + this.source.getClass().getName() + " was encountered"); 3790 return (UriType) this.source; 3791 } 3792 3793 public boolean hasSourceUriType() { 3794 return this != null && this.source instanceof UriType; 3795 } 3796 3797 /** 3798 * @return {@link #source} (Identifier for the source value set that contains 3799 * the concepts that are being mapped and provides context for the 3800 * mappings.) 3801 */ 3802 public CanonicalType getSourceCanonicalType() throws FHIRException { 3803 if (this.source == null) 3804 this.source = new CanonicalType(); 3805 if (!(this.source instanceof CanonicalType)) 3806 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but " 3807 + this.source.getClass().getName() + " was encountered"); 3808 return (CanonicalType) this.source; 3809 } 3810 3811 public boolean hasSourceCanonicalType() { 3812 return this != null && this.source instanceof CanonicalType; 3813 } 3814 3815 public boolean hasSource() { 3816 return this.source != null && !this.source.isEmpty(); 3817 } 3818 3819 /** 3820 * @param value {@link #source} (Identifier for the source value set that 3821 * contains the concepts that are being mapped and provides context 3822 * for the mappings.) 3823 */ 3824 public ConceptMap setSource(Type value) { 3825 if (value != null && !(value instanceof UriType || value instanceof CanonicalType)) 3826 throw new Error("Not the right type for ConceptMap.source[x]: " + value.fhirType()); 3827 this.source = value; 3828 return this; 3829 } 3830 3831 /** 3832 * @return {@link #target} (The target value set provides context for the 3833 * mappings. Note that the mapping is made between concepts, not between 3834 * value sets, but the value set provides important context about how 3835 * the concept mapping choices are made.) 3836 */ 3837 public Type getTarget() { 3838 return this.target; 3839 } 3840 3841 /** 3842 * @return {@link #target} (The target value set provides context for the 3843 * mappings. Note that the mapping is made between concepts, not between 3844 * value sets, but the value set provides important context about how 3845 * the concept mapping choices are made.) 3846 */ 3847 public UriType getTargetUriType() throws FHIRException { 3848 if (this.target == null) 3849 this.target = new UriType(); 3850 if (!(this.target instanceof UriType)) 3851 throw new FHIRException( 3852 "Type mismatch: the type UriType was expected, but " + this.target.getClass().getName() + " was encountered"); 3853 return (UriType) this.target; 3854 } 3855 3856 public boolean hasTargetUriType() { 3857 return this != null && this.target instanceof UriType; 3858 } 3859 3860 /** 3861 * @return {@link #target} (The target value set provides context for the 3862 * mappings. Note that the mapping is made between concepts, not between 3863 * value sets, but the value set provides important context about how 3864 * the concept mapping choices are made.) 3865 */ 3866 public CanonicalType getTargetCanonicalType() throws FHIRException { 3867 if (this.target == null) 3868 this.target = new CanonicalType(); 3869 if (!(this.target instanceof CanonicalType)) 3870 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but " 3871 + this.target.getClass().getName() + " was encountered"); 3872 return (CanonicalType) this.target; 3873 } 3874 3875 public boolean hasTargetCanonicalType() { 3876 return this != null && this.target instanceof CanonicalType; 3877 } 3878 3879 public boolean hasTarget() { 3880 return this.target != null && !this.target.isEmpty(); 3881 } 3882 3883 /** 3884 * @param value {@link #target} (The target value set provides context for the 3885 * mappings. Note that the mapping is made between concepts, not 3886 * between value sets, but the value set provides important context 3887 * about how the concept mapping choices are made.) 3888 */ 3889 public ConceptMap setTarget(Type value) { 3890 if (value != null && !(value instanceof UriType || value instanceof CanonicalType)) 3891 throw new Error("Not the right type for ConceptMap.target[x]: " + value.fhirType()); 3892 this.target = value; 3893 return this; 3894 } 3895 3896 /** 3897 * @return {@link #group} (A group of mappings that all have the same source and 3898 * target system.) 3899 */ 3900 public List<ConceptMapGroupComponent> getGroup() { 3901 if (this.group == null) 3902 this.group = new ArrayList<ConceptMapGroupComponent>(); 3903 return this.group; 3904 } 3905 3906 /** 3907 * @return Returns a reference to <code>this</code> for easy method chaining 3908 */ 3909 public ConceptMap setGroup(List<ConceptMapGroupComponent> theGroup) { 3910 this.group = theGroup; 3911 return this; 3912 } 3913 3914 public boolean hasGroup() { 3915 if (this.group == null) 3916 return false; 3917 for (ConceptMapGroupComponent item : this.group) 3918 if (!item.isEmpty()) 3919 return true; 3920 return false; 3921 } 3922 3923 public ConceptMapGroupComponent addGroup() { // 3 3924 ConceptMapGroupComponent t = new ConceptMapGroupComponent(); 3925 if (this.group == null) 3926 this.group = new ArrayList<ConceptMapGroupComponent>(); 3927 this.group.add(t); 3928 return t; 3929 } 3930 3931 public ConceptMap addGroup(ConceptMapGroupComponent t) { // 3 3932 if (t == null) 3933 return this; 3934 if (this.group == null) 3935 this.group = new ArrayList<ConceptMapGroupComponent>(); 3936 this.group.add(t); 3937 return this; 3938 } 3939 3940 /** 3941 * @return The first repetition of repeating field {@link #group}, creating it 3942 * if it does not already exist 3943 */ 3944 public ConceptMapGroupComponent getGroupFirstRep() { 3945 if (getGroup().isEmpty()) { 3946 addGroup(); 3947 } 3948 return getGroup().get(0); 3949 } 3950 3951 protected void listChildren(List<Property> children) { 3952 super.listChildren(children); 3953 children.add(new Property("url", "uri", 3954 "An absolute URI that is used to identify this concept map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this concept map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the concept map is stored on different servers.", 3955 0, 1, url)); 3956 children.add(new Property("identifier", "Identifier", 3957 "A formal identifier that is used to identify this concept map when it is represented in other formats, or referenced in a specification, model, design or an instance.", 3958 0, 1, identifier)); 3959 children.add(new Property("version", "string", 3960 "The identifier that is used to identify this version of the concept map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the concept map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 3961 0, 1, version)); 3962 children.add(new Property("name", "string", 3963 "A natural language name identifying the concept map. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 3964 0, 1, name)); 3965 children.add( 3966 new Property("title", "string", "A short, descriptive, user-friendly title for the concept map.", 0, 1, title)); 3967 children.add(new Property("status", "code", 3968 "The status of this concept map. Enables tracking the life-cycle of the content.", 0, 1, status)); 3969 children.add(new Property("experimental", "boolean", 3970 "A Boolean value to indicate that this concept map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 3971 0, 1, experimental)); 3972 children.add(new Property("date", "dateTime", 3973 "The date (and optionally time) when the concept map was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the concept map changes.", 3974 0, 1, date)); 3975 children.add(new Property("publisher", "string", 3976 "The name of the organization or individual that published the concept map.", 0, 1, publisher)); 3977 children.add(new Property("contact", "ContactDetail", 3978 "Contact details to assist a user in finding and communicating with the publisher.", 0, 3979 java.lang.Integer.MAX_VALUE, contact)); 3980 children.add(new Property("description", "markdown", 3981 "A free text natural language description of the concept map from a consumer's perspective.", 0, 1, 3982 description)); 3983 children.add(new Property("useContext", "UsageContext", 3984 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate concept map instances.", 3985 0, java.lang.Integer.MAX_VALUE, useContext)); 3986 children.add(new Property("jurisdiction", "CodeableConcept", 3987 "A legal or geographic region in which the concept map is intended to be used.", 0, java.lang.Integer.MAX_VALUE, 3988 jurisdiction)); 3989 children.add(new Property("purpose", "markdown", 3990 "Explanation of why this concept map is needed and why it has been designed as it has.", 0, 1, purpose)); 3991 children.add(new Property("copyright", "markdown", 3992 "A copyright statement relating to the concept map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the concept map.", 3993 0, 1, copyright)); 3994 children.add(new Property("source[x]", "uri|canonical(ValueSet)", 3995 "Identifier for the source value set that contains the concepts that are being mapped and provides context for the mappings.", 3996 0, 1, source)); 3997 children.add(new Property("target[x]", "uri|canonical(ValueSet)", 3998 "The target value set provides context for the mappings. Note that the mapping is made between concepts, not between value sets, but the value set provides important context about how the concept mapping choices are made.", 3999 0, 1, target)); 4000 children.add(new Property("group", "", "A group of mappings that all have the same source and target system.", 0, 4001 java.lang.Integer.MAX_VALUE, group)); 4002 } 4003 4004 @Override 4005 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4006 switch (_hash) { 4007 case 116079: 4008 /* url */ return new Property("url", "uri", 4009 "An absolute URI that is used to identify this concept map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this concept map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the concept map is stored on different servers.", 4010 0, 1, url); 4011 case -1618432855: 4012 /* identifier */ return new Property("identifier", "Identifier", 4013 "A formal identifier that is used to identify this concept map when it is represented in other formats, or referenced in a specification, model, design or an instance.", 4014 0, 1, identifier); 4015 case 351608024: 4016 /* version */ return new Property("version", "string", 4017 "The identifier that is used to identify this version of the concept map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the concept map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 4018 0, 1, version); 4019 case 3373707: 4020 /* name */ return new Property("name", "string", 4021 "A natural language name identifying the concept map. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 4022 0, 1, name); 4023 case 110371416: 4024 /* title */ return new Property("title", "string", 4025 "A short, descriptive, user-friendly title for the concept map.", 0, 1, title); 4026 case -892481550: 4027 /* status */ return new Property("status", "code", 4028 "The status of this concept map. Enables tracking the life-cycle of the content.", 0, 1, status); 4029 case -404562712: 4030 /* experimental */ return new Property("experimental", "boolean", 4031 "A Boolean value to indicate that this concept map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 4032 0, 1, experimental); 4033 case 3076014: 4034 /* date */ return new Property("date", "dateTime", 4035 "The date (and optionally time) when the concept map was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the concept map changes.", 4036 0, 1, date); 4037 case 1447404028: 4038 /* publisher */ return new Property("publisher", "string", 4039 "The name of the organization or individual that published the concept map.", 0, 1, publisher); 4040 case 951526432: 4041 /* contact */ return new Property("contact", "ContactDetail", 4042 "Contact details to assist a user in finding and communicating with the publisher.", 0, 4043 java.lang.Integer.MAX_VALUE, contact); 4044 case -1724546052: 4045 /* description */ return new Property("description", "markdown", 4046 "A free text natural language description of the concept map from a consumer's perspective.", 0, 1, 4047 description); 4048 case -669707736: 4049 /* useContext */ return new Property("useContext", "UsageContext", 4050 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate concept map instances.", 4051 0, java.lang.Integer.MAX_VALUE, useContext); 4052 case -507075711: 4053 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 4054 "A legal or geographic region in which the concept map is intended to be used.", 0, 4055 java.lang.Integer.MAX_VALUE, jurisdiction); 4056 case -220463842: 4057 /* purpose */ return new Property("purpose", "markdown", 4058 "Explanation of why this concept map is needed and why it has been designed as it has.", 0, 1, purpose); 4059 case 1522889671: 4060 /* copyright */ return new Property("copyright", "markdown", 4061 "A copyright statement relating to the concept map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the concept map.", 4062 0, 1, copyright); 4063 case -1698413947: 4064 /* source[x] */ return new Property("source[x]", "uri|canonical(ValueSet)", 4065 "Identifier for the source value set that contains the concepts that are being mapped and provides context for the mappings.", 4066 0, 1, source); 4067 case -896505829: 4068 /* source */ return new Property("source[x]", "uri|canonical(ValueSet)", 4069 "Identifier for the source value set that contains the concepts that are being mapped and provides context for the mappings.", 4070 0, 1, source); 4071 case -1698419887: 4072 /* sourceUri */ return new Property("source[x]", "uri|canonical(ValueSet)", 4073 "Identifier for the source value set that contains the concepts that are being mapped and provides context for the mappings.", 4074 0, 1, source); 4075 case 1509247769: 4076 /* sourceCanonical */ return new Property("source[x]", "uri|canonical(ValueSet)", 4077 "Identifier for the source value set that contains the concepts that are being mapped and provides context for the mappings.", 4078 0, 1, source); 4079 case -815579825: 4080 /* target[x] */ return new Property("target[x]", "uri|canonical(ValueSet)", 4081 "The target value set provides context for the mappings. Note that the mapping is made between concepts, not between value sets, but the value set provides important context about how the concept mapping choices are made.", 4082 0, 1, target); 4083 case -880905839: 4084 /* target */ return new Property("target[x]", "uri|canonical(ValueSet)", 4085 "The target value set provides context for the mappings. Note that the mapping is made between concepts, not between value sets, but the value set provides important context about how the concept mapping choices are made.", 4086 0, 1, target); 4087 case -815585765: 4088 /* targetUri */ return new Property("target[x]", "uri|canonical(ValueSet)", 4089 "The target value set provides context for the mappings. Note that the mapping is made between concepts, not between value sets, but the value set provides important context about how the concept mapping choices are made.", 4090 0, 1, target); 4091 case -1281653149: 4092 /* targetCanonical */ return new Property("target[x]", "uri|canonical(ValueSet)", 4093 "The target value set provides context for the mappings. Note that the mapping is made between concepts, not between value sets, but the value set provides important context about how the concept mapping choices are made.", 4094 0, 1, target); 4095 case 98629247: 4096 /* group */ return new Property("group", "", 4097 "A group of mappings that all have the same source and target system.", 0, java.lang.Integer.MAX_VALUE, 4098 group); 4099 default: 4100 return super.getNamedProperty(_hash, _name, _checkValid); 4101 } 4102 4103 } 4104 4105 @Override 4106 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4107 switch (hash) { 4108 case 116079: 4109 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 4110 case -1618432855: 4111 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 4112 case 351608024: 4113 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 4114 case 3373707: 4115 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 4116 case 110371416: 4117 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 4118 case -892481550: 4119 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 4120 case -404562712: 4121 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 4122 case 3076014: 4123 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 4124 case 1447404028: 4125 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 4126 case 951526432: 4127 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 4128 case -1724546052: 4129 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 4130 case -669707736: 4131 /* useContext */ return this.useContext == null ? new Base[0] 4132 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 4133 case -507075711: 4134 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 4135 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 4136 case -220463842: 4137 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 4138 case 1522889671: 4139 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 4140 case -896505829: 4141 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // Type 4142 case -880905839: 4143 /* target */ return this.target == null ? new Base[0] : new Base[] { this.target }; // Type 4144 case 98629247: 4145 /* group */ return this.group == null ? new Base[0] : this.group.toArray(new Base[this.group.size()]); // ConceptMapGroupComponent 4146 default: 4147 return super.getProperty(hash, name, checkValid); 4148 } 4149 4150 } 4151 4152 @Override 4153 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4154 switch (hash) { 4155 case 116079: // url 4156 this.url = castToUri(value); // UriType 4157 return value; 4158 case -1618432855: // identifier 4159 this.identifier = castToIdentifier(value); // Identifier 4160 return value; 4161 case 351608024: // version 4162 this.version = castToString(value); // StringType 4163 return value; 4164 case 3373707: // name 4165 this.name = castToString(value); // StringType 4166 return value; 4167 case 110371416: // title 4168 this.title = castToString(value); // StringType 4169 return value; 4170 case -892481550: // status 4171 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 4172 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4173 return value; 4174 case -404562712: // experimental 4175 this.experimental = castToBoolean(value); // BooleanType 4176 return value; 4177 case 3076014: // date 4178 this.date = castToDateTime(value); // DateTimeType 4179 return value; 4180 case 1447404028: // publisher 4181 this.publisher = castToString(value); // StringType 4182 return value; 4183 case 951526432: // contact 4184 this.getContact().add(castToContactDetail(value)); // ContactDetail 4185 return value; 4186 case -1724546052: // description 4187 this.description = castToMarkdown(value); // MarkdownType 4188 return value; 4189 case -669707736: // useContext 4190 this.getUseContext().add(castToUsageContext(value)); // UsageContext 4191 return value; 4192 case -507075711: // jurisdiction 4193 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 4194 return value; 4195 case -220463842: // purpose 4196 this.purpose = castToMarkdown(value); // MarkdownType 4197 return value; 4198 case 1522889671: // copyright 4199 this.copyright = castToMarkdown(value); // MarkdownType 4200 return value; 4201 case -896505829: // source 4202 this.source = castToType(value); // Type 4203 return value; 4204 case -880905839: // target 4205 this.target = castToType(value); // Type 4206 return value; 4207 case 98629247: // group 4208 this.getGroup().add((ConceptMapGroupComponent) value); // ConceptMapGroupComponent 4209 return value; 4210 default: 4211 return super.setProperty(hash, name, value); 4212 } 4213 4214 } 4215 4216 @Override 4217 public Base setProperty(String name, Base value) throws FHIRException { 4218 if (name.equals("url")) { 4219 this.url = castToUri(value); // UriType 4220 } else if (name.equals("identifier")) { 4221 this.identifier = castToIdentifier(value); // Identifier 4222 } else if (name.equals("version")) { 4223 this.version = castToString(value); // StringType 4224 } else if (name.equals("name")) { 4225 this.name = castToString(value); // StringType 4226 } else if (name.equals("title")) { 4227 this.title = castToString(value); // StringType 4228 } else if (name.equals("status")) { 4229 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 4230 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4231 } else if (name.equals("experimental")) { 4232 this.experimental = castToBoolean(value); // BooleanType 4233 } else if (name.equals("date")) { 4234 this.date = castToDateTime(value); // DateTimeType 4235 } else if (name.equals("publisher")) { 4236 this.publisher = castToString(value); // StringType 4237 } else if (name.equals("contact")) { 4238 this.getContact().add(castToContactDetail(value)); 4239 } else if (name.equals("description")) { 4240 this.description = castToMarkdown(value); // MarkdownType 4241 } else if (name.equals("useContext")) { 4242 this.getUseContext().add(castToUsageContext(value)); 4243 } else if (name.equals("jurisdiction")) { 4244 this.getJurisdiction().add(castToCodeableConcept(value)); 4245 } else if (name.equals("purpose")) { 4246 this.purpose = castToMarkdown(value); // MarkdownType 4247 } else if (name.equals("copyright")) { 4248 this.copyright = castToMarkdown(value); // MarkdownType 4249 } else if (name.equals("source[x]")) { 4250 this.source = castToType(value); // Type 4251 } else if (name.equals("target[x]")) { 4252 this.target = castToType(value); // Type 4253 } else if (name.equals("group")) { 4254 this.getGroup().add((ConceptMapGroupComponent) value); 4255 } else 4256 return super.setProperty(name, value); 4257 return value; 4258 } 4259 4260 @Override 4261 public Base makeProperty(int hash, String name) throws FHIRException { 4262 switch (hash) { 4263 case 116079: 4264 return getUrlElement(); 4265 case -1618432855: 4266 return getIdentifier(); 4267 case 351608024: 4268 return getVersionElement(); 4269 case 3373707: 4270 return getNameElement(); 4271 case 110371416: 4272 return getTitleElement(); 4273 case -892481550: 4274 return getStatusElement(); 4275 case -404562712: 4276 return getExperimentalElement(); 4277 case 3076014: 4278 return getDateElement(); 4279 case 1447404028: 4280 return getPublisherElement(); 4281 case 951526432: 4282 return addContact(); 4283 case -1724546052: 4284 return getDescriptionElement(); 4285 case -669707736: 4286 return addUseContext(); 4287 case -507075711: 4288 return addJurisdiction(); 4289 case -220463842: 4290 return getPurposeElement(); 4291 case 1522889671: 4292 return getCopyrightElement(); 4293 case -1698413947: 4294 return getSource(); 4295 case -896505829: 4296 return getSource(); 4297 case -815579825: 4298 return getTarget(); 4299 case -880905839: 4300 return getTarget(); 4301 case 98629247: 4302 return addGroup(); 4303 default: 4304 return super.makeProperty(hash, name); 4305 } 4306 4307 } 4308 4309 @Override 4310 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4311 switch (hash) { 4312 case 116079: 4313 /* url */ return new String[] { "uri" }; 4314 case -1618432855: 4315 /* identifier */ return new String[] { "Identifier" }; 4316 case 351608024: 4317 /* version */ return new String[] { "string" }; 4318 case 3373707: 4319 /* name */ return new String[] { "string" }; 4320 case 110371416: 4321 /* title */ return new String[] { "string" }; 4322 case -892481550: 4323 /* status */ return new String[] { "code" }; 4324 case -404562712: 4325 /* experimental */ return new String[] { "boolean" }; 4326 case 3076014: 4327 /* date */ return new String[] { "dateTime" }; 4328 case 1447404028: 4329 /* publisher */ return new String[] { "string" }; 4330 case 951526432: 4331 /* contact */ return new String[] { "ContactDetail" }; 4332 case -1724546052: 4333 /* description */ return new String[] { "markdown" }; 4334 case -669707736: 4335 /* useContext */ return new String[] { "UsageContext" }; 4336 case -507075711: 4337 /* jurisdiction */ return new String[] { "CodeableConcept" }; 4338 case -220463842: 4339 /* purpose */ return new String[] { "markdown" }; 4340 case 1522889671: 4341 /* copyright */ return new String[] { "markdown" }; 4342 case -896505829: 4343 /* source */ return new String[] { "uri", "canonical" }; 4344 case -880905839: 4345 /* target */ return new String[] { "uri", "canonical" }; 4346 case 98629247: 4347 /* group */ return new String[] {}; 4348 default: 4349 return super.getTypesForProperty(hash, name); 4350 } 4351 4352 } 4353 4354 @Override 4355 public Base addChild(String name) throws FHIRException { 4356 if (name.equals("url")) { 4357 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.url"); 4358 } else if (name.equals("identifier")) { 4359 this.identifier = new Identifier(); 4360 return this.identifier; 4361 } else if (name.equals("version")) { 4362 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.version"); 4363 } else if (name.equals("name")) { 4364 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.name"); 4365 } else if (name.equals("title")) { 4366 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.title"); 4367 } else if (name.equals("status")) { 4368 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.status"); 4369 } else if (name.equals("experimental")) { 4370 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.experimental"); 4371 } else if (name.equals("date")) { 4372 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.date"); 4373 } else if (name.equals("publisher")) { 4374 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.publisher"); 4375 } else if (name.equals("contact")) { 4376 return addContact(); 4377 } else if (name.equals("description")) { 4378 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.description"); 4379 } else if (name.equals("useContext")) { 4380 return addUseContext(); 4381 } else if (name.equals("jurisdiction")) { 4382 return addJurisdiction(); 4383 } else if (name.equals("purpose")) { 4384 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.purpose"); 4385 } else if (name.equals("copyright")) { 4386 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.copyright"); 4387 } else if (name.equals("sourceUri")) { 4388 this.source = new UriType(); 4389 return this.source; 4390 } else if (name.equals("sourceCanonical")) { 4391 this.source = new CanonicalType(); 4392 return this.source; 4393 } else if (name.equals("targetUri")) { 4394 this.target = new UriType(); 4395 return this.target; 4396 } else if (name.equals("targetCanonical")) { 4397 this.target = new CanonicalType(); 4398 return this.target; 4399 } else if (name.equals("group")) { 4400 return addGroup(); 4401 } else 4402 return super.addChild(name); 4403 } 4404 4405 public String fhirType() { 4406 return "ConceptMap"; 4407 4408 } 4409 4410 public ConceptMap copy() { 4411 ConceptMap dst = new ConceptMap(); 4412 copyValues(dst); 4413 return dst; 4414 } 4415 4416 public void copyValues(ConceptMap dst) { 4417 super.copyValues(dst); 4418 dst.url = url == null ? null : url.copy(); 4419 dst.identifier = identifier == null ? null : identifier.copy(); 4420 dst.version = version == null ? null : version.copy(); 4421 dst.name = name == null ? null : name.copy(); 4422 dst.title = title == null ? null : title.copy(); 4423 dst.status = status == null ? null : status.copy(); 4424 dst.experimental = experimental == null ? null : experimental.copy(); 4425 dst.date = date == null ? null : date.copy(); 4426 dst.publisher = publisher == null ? null : publisher.copy(); 4427 if (contact != null) { 4428 dst.contact = new ArrayList<ContactDetail>(); 4429 for (ContactDetail i : contact) 4430 dst.contact.add(i.copy()); 4431 } 4432 ; 4433 dst.description = description == null ? null : description.copy(); 4434 if (useContext != null) { 4435 dst.useContext = new ArrayList<UsageContext>(); 4436 for (UsageContext i : useContext) 4437 dst.useContext.add(i.copy()); 4438 } 4439 ; 4440 if (jurisdiction != null) { 4441 dst.jurisdiction = new ArrayList<CodeableConcept>(); 4442 for (CodeableConcept i : jurisdiction) 4443 dst.jurisdiction.add(i.copy()); 4444 } 4445 ; 4446 dst.purpose = purpose == null ? null : purpose.copy(); 4447 dst.copyright = copyright == null ? null : copyright.copy(); 4448 dst.source = source == null ? null : source.copy(); 4449 dst.target = target == null ? null : target.copy(); 4450 if (group != null) { 4451 dst.group = new ArrayList<ConceptMapGroupComponent>(); 4452 for (ConceptMapGroupComponent i : group) 4453 dst.group.add(i.copy()); 4454 } 4455 ; 4456 } 4457 4458 protected ConceptMap typedCopy() { 4459 return copy(); 4460 } 4461 4462 @Override 4463 public boolean equalsDeep(Base other_) { 4464 if (!super.equalsDeep(other_)) 4465 return false; 4466 if (!(other_ instanceof ConceptMap)) 4467 return false; 4468 ConceptMap o = (ConceptMap) other_; 4469 return compareDeep(identifier, o.identifier, true) && compareDeep(purpose, o.purpose, true) 4470 && compareDeep(copyright, o.copyright, true) && compareDeep(source, o.source, true) 4471 && compareDeep(target, o.target, true) && compareDeep(group, o.group, true); 4472 } 4473 4474 @Override 4475 public boolean equalsShallow(Base other_) { 4476 if (!super.equalsShallow(other_)) 4477 return false; 4478 if (!(other_ instanceof ConceptMap)) 4479 return false; 4480 ConceptMap o = (ConceptMap) other_; 4481 return compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true); 4482 } 4483 4484 public boolean isEmpty() { 4485 return super.isEmpty() 4486 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, purpose, copyright, source, target, group); 4487 } 4488 4489 @Override 4490 public ResourceType getResourceType() { 4491 return ResourceType.ConceptMap; 4492 } 4493 4494 /** 4495 * Search parameter: <b>date</b> 4496 * <p> 4497 * Description: <b>The concept map publication date</b><br> 4498 * Type: <b>date</b><br> 4499 * Path: <b>ConceptMap.date</b><br> 4500 * </p> 4501 */ 4502 @SearchParamDefinition(name = "date", path = "ConceptMap.date", description = "The concept map publication date", type = "date") 4503 public static final String SP_DATE = "date"; 4504 /** 4505 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4506 * <p> 4507 * Description: <b>The concept map publication date</b><br> 4508 * Type: <b>date</b><br> 4509 * Path: <b>ConceptMap.date</b><br> 4510 * </p> 4511 */ 4512 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 4513 SP_DATE); 4514 4515 /** 4516 * Search parameter: <b>other</b> 4517 * <p> 4518 * Description: <b>canonical reference to an additional ConceptMap to use for 4519 * mapping if the source concept is unmapped</b><br> 4520 * Type: <b>reference</b><br> 4521 * Path: <b>ConceptMap.group.unmapped.url</b><br> 4522 * </p> 4523 */ 4524 @SearchParamDefinition(name = "other", path = "ConceptMap.group.unmapped.url", description = "canonical reference to an additional ConceptMap to use for mapping if the source concept is unmapped", type = "reference", target = { 4525 ConceptMap.class }) 4526 public static final String SP_OTHER = "other"; 4527 /** 4528 * <b>Fluent Client</b> search parameter constant for <b>other</b> 4529 * <p> 4530 * Description: <b>canonical reference to an additional ConceptMap to use for 4531 * mapping if the source concept is unmapped</b><br> 4532 * Type: <b>reference</b><br> 4533 * Path: <b>ConceptMap.group.unmapped.url</b><br> 4534 * </p> 4535 */ 4536 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam OTHER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4537 SP_OTHER); 4538 4539 /** 4540 * Constant for fluent queries to be used to add include statements. Specifies 4541 * the path value of "<b>ConceptMap:other</b>". 4542 */ 4543 public static final ca.uhn.fhir.model.api.Include INCLUDE_OTHER = new ca.uhn.fhir.model.api.Include( 4544 "ConceptMap:other").toLocked(); 4545 4546 /** 4547 * Search parameter: <b>context-type-value</b> 4548 * <p> 4549 * Description: <b>A use context type and value assigned to the concept 4550 * map</b><br> 4551 * Type: <b>composite</b><br> 4552 * Path: <b></b><br> 4553 * </p> 4554 */ 4555 @SearchParamDefinition(name = "context-type-value", path = "ConceptMap.useContext", description = "A use context type and value assigned to the concept map", type = "composite", compositeOf = { 4556 "context-type", "context" }) 4557 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 4558 /** 4559 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 4560 * <p> 4561 * Description: <b>A use context type and value assigned to the concept 4562 * map</b><br> 4563 * Type: <b>composite</b><br> 4564 * Path: <b></b><br> 4565 * </p> 4566 */ 4567 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 4568 SP_CONTEXT_TYPE_VALUE); 4569 4570 /** 4571 * Search parameter: <b>target-system</b> 4572 * <p> 4573 * Description: <b>Target system that the concepts are to be mapped to</b><br> 4574 * Type: <b>uri</b><br> 4575 * Path: <b>ConceptMap.group.target</b><br> 4576 * </p> 4577 */ 4578 @SearchParamDefinition(name = "target-system", path = "ConceptMap.group.target", description = "Target system that the concepts are to be mapped to", type = "uri") 4579 public static final String SP_TARGET_SYSTEM = "target-system"; 4580 /** 4581 * <b>Fluent Client</b> search parameter constant for <b>target-system</b> 4582 * <p> 4583 * Description: <b>Target system that the concepts are to be mapped to</b><br> 4584 * Type: <b>uri</b><br> 4585 * Path: <b>ConceptMap.group.target</b><br> 4586 * </p> 4587 */ 4588 public static final ca.uhn.fhir.rest.gclient.UriClientParam TARGET_SYSTEM = new ca.uhn.fhir.rest.gclient.UriClientParam( 4589 SP_TARGET_SYSTEM); 4590 4591 /** 4592 * Search parameter: <b>dependson</b> 4593 * <p> 4594 * Description: <b>Reference to property mapping depends on</b><br> 4595 * Type: <b>uri</b><br> 4596 * Path: <b>ConceptMap.group.element.target.dependsOn.property</b><br> 4597 * </p> 4598 */ 4599 @SearchParamDefinition(name = "dependson", path = "ConceptMap.group.element.target.dependsOn.property", description = "Reference to property mapping depends on", type = "uri") 4600 public static final String SP_DEPENDSON = "dependson"; 4601 /** 4602 * <b>Fluent Client</b> search parameter constant for <b>dependson</b> 4603 * <p> 4604 * Description: <b>Reference to property mapping depends on</b><br> 4605 * Type: <b>uri</b><br> 4606 * Path: <b>ConceptMap.group.element.target.dependsOn.property</b><br> 4607 * </p> 4608 */ 4609 public static final ca.uhn.fhir.rest.gclient.UriClientParam DEPENDSON = new ca.uhn.fhir.rest.gclient.UriClientParam( 4610 SP_DEPENDSON); 4611 4612 /** 4613 * Search parameter: <b>jurisdiction</b> 4614 * <p> 4615 * Description: <b>Intended jurisdiction for the concept map</b><br> 4616 * Type: <b>token</b><br> 4617 * Path: <b>ConceptMap.jurisdiction</b><br> 4618 * </p> 4619 */ 4620 @SearchParamDefinition(name = "jurisdiction", path = "ConceptMap.jurisdiction", description = "Intended jurisdiction for the concept map", type = "token") 4621 public static final String SP_JURISDICTION = "jurisdiction"; 4622 /** 4623 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 4624 * <p> 4625 * Description: <b>Intended jurisdiction for the concept map</b><br> 4626 * Type: <b>token</b><br> 4627 * Path: <b>ConceptMap.jurisdiction</b><br> 4628 * </p> 4629 */ 4630 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4631 SP_JURISDICTION); 4632 4633 /** 4634 * Search parameter: <b>description</b> 4635 * <p> 4636 * Description: <b>The description of the concept map</b><br> 4637 * Type: <b>string</b><br> 4638 * Path: <b>ConceptMap.description</b><br> 4639 * </p> 4640 */ 4641 @SearchParamDefinition(name = "description", path = "ConceptMap.description", description = "The description of the concept map", type = "string") 4642 public static final String SP_DESCRIPTION = "description"; 4643 /** 4644 * <b>Fluent Client</b> search parameter constant for <b>description</b> 4645 * <p> 4646 * Description: <b>The description of the concept map</b><br> 4647 * Type: <b>string</b><br> 4648 * Path: <b>ConceptMap.description</b><br> 4649 * </p> 4650 */ 4651 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 4652 SP_DESCRIPTION); 4653 4654 /** 4655 * Search parameter: <b>context-type</b> 4656 * <p> 4657 * Description: <b>A type of use context assigned to the concept map</b><br> 4658 * Type: <b>token</b><br> 4659 * Path: <b>ConceptMap.useContext.code</b><br> 4660 * </p> 4661 */ 4662 @SearchParamDefinition(name = "context-type", path = "ConceptMap.useContext.code", description = "A type of use context assigned to the concept map", type = "token") 4663 public static final String SP_CONTEXT_TYPE = "context-type"; 4664 /** 4665 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 4666 * <p> 4667 * Description: <b>A type of use context assigned to the concept map</b><br> 4668 * Type: <b>token</b><br> 4669 * Path: <b>ConceptMap.useContext.code</b><br> 4670 * </p> 4671 */ 4672 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4673 SP_CONTEXT_TYPE); 4674 4675 /** 4676 * Search parameter: <b>source</b> 4677 * <p> 4678 * Description: <b>The source value set that contains the concepts that are 4679 * being mapped</b><br> 4680 * Type: <b>reference</b><br> 4681 * Path: <b>ConceptMap.sourceCanonical</b><br> 4682 * </p> 4683 */ 4684 @SearchParamDefinition(name = "source", path = "(ConceptMap.source as canonical)", description = "The source value set that contains the concepts that are being mapped", type = "reference", target = { 4685 ValueSet.class }) 4686 public static final String SP_SOURCE = "source"; 4687 /** 4688 * <b>Fluent Client</b> search parameter constant for <b>source</b> 4689 * <p> 4690 * Description: <b>The source value set that contains the concepts that are 4691 * being mapped</b><br> 4692 * Type: <b>reference</b><br> 4693 * Path: <b>ConceptMap.sourceCanonical</b><br> 4694 * </p> 4695 */ 4696 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4697 SP_SOURCE); 4698 4699 /** 4700 * Constant for fluent queries to be used to add include statements. Specifies 4701 * the path value of "<b>ConceptMap:source</b>". 4702 */ 4703 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include( 4704 "ConceptMap:source").toLocked(); 4705 4706 /** 4707 * Search parameter: <b>title</b> 4708 * <p> 4709 * Description: <b>The human-friendly name of the concept map</b><br> 4710 * Type: <b>string</b><br> 4711 * Path: <b>ConceptMap.title</b><br> 4712 * </p> 4713 */ 4714 @SearchParamDefinition(name = "title", path = "ConceptMap.title", description = "The human-friendly name of the concept map", type = "string") 4715 public static final String SP_TITLE = "title"; 4716 /** 4717 * <b>Fluent Client</b> search parameter constant for <b>title</b> 4718 * <p> 4719 * Description: <b>The human-friendly name of the concept map</b><br> 4720 * Type: <b>string</b><br> 4721 * Path: <b>ConceptMap.title</b><br> 4722 * </p> 4723 */ 4724 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 4725 SP_TITLE); 4726 4727 /** 4728 * Search parameter: <b>context-quantity</b> 4729 * <p> 4730 * Description: <b>A quantity- or range-valued use context assigned to the 4731 * concept map</b><br> 4732 * Type: <b>quantity</b><br> 4733 * Path: <b>ConceptMap.useContext.valueQuantity, 4734 * ConceptMap.useContext.valueRange</b><br> 4735 * </p> 4736 */ 4737 @SearchParamDefinition(name = "context-quantity", path = "(ConceptMap.useContext.value as Quantity) | (ConceptMap.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the concept map", type = "quantity") 4738 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 4739 /** 4740 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 4741 * <p> 4742 * Description: <b>A quantity- or range-valued use context assigned to the 4743 * concept map</b><br> 4744 * Type: <b>quantity</b><br> 4745 * Path: <b>ConceptMap.useContext.valueQuantity, 4746 * ConceptMap.useContext.valueRange</b><br> 4747 * </p> 4748 */ 4749 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 4750 SP_CONTEXT_QUANTITY); 4751 4752 /** 4753 * Search parameter: <b>source-uri</b> 4754 * <p> 4755 * Description: <b>The source value set that contains the concepts that are 4756 * being mapped</b><br> 4757 * Type: <b>reference</b><br> 4758 * Path: <b>ConceptMap.sourceUri</b><br> 4759 * </p> 4760 */ 4761 @SearchParamDefinition(name = "source-uri", path = "(ConceptMap.source as uri)", description = "The source value set that contains the concepts that are being mapped", type = "reference", target = { 4762 ValueSet.class }) 4763 public static final String SP_SOURCE_URI = "source-uri"; 4764 /** 4765 * <b>Fluent Client</b> search parameter constant for <b>source-uri</b> 4766 * <p> 4767 * Description: <b>The source value set that contains the concepts that are 4768 * being mapped</b><br> 4769 * Type: <b>reference</b><br> 4770 * Path: <b>ConceptMap.sourceUri</b><br> 4771 * </p> 4772 */ 4773 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE_URI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4774 SP_SOURCE_URI); 4775 4776 /** 4777 * Constant for fluent queries to be used to add include statements. Specifies 4778 * the path value of "<b>ConceptMap:source-uri</b>". 4779 */ 4780 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE_URI = new ca.uhn.fhir.model.api.Include( 4781 "ConceptMap:source-uri").toLocked(); 4782 4783 /** 4784 * Search parameter: <b>context</b> 4785 * <p> 4786 * Description: <b>A use context assigned to the concept map</b><br> 4787 * Type: <b>token</b><br> 4788 * Path: <b>ConceptMap.useContext.valueCodeableConcept</b><br> 4789 * </p> 4790 */ 4791 @SearchParamDefinition(name = "context", path = "(ConceptMap.useContext.value as CodeableConcept)", description = "A use context assigned to the concept map", type = "token") 4792 public static final String SP_CONTEXT = "context"; 4793 /** 4794 * <b>Fluent Client</b> search parameter constant for <b>context</b> 4795 * <p> 4796 * Description: <b>A use context assigned to the concept map</b><br> 4797 * Type: <b>token</b><br> 4798 * Path: <b>ConceptMap.useContext.valueCodeableConcept</b><br> 4799 * </p> 4800 */ 4801 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4802 SP_CONTEXT); 4803 4804 /** 4805 * Search parameter: <b>context-type-quantity</b> 4806 * <p> 4807 * Description: <b>A use context type and quantity- or range-based value 4808 * assigned to the concept map</b><br> 4809 * Type: <b>composite</b><br> 4810 * Path: <b></b><br> 4811 * </p> 4812 */ 4813 @SearchParamDefinition(name = "context-type-quantity", path = "ConceptMap.useContext", description = "A use context type and quantity- or range-based value assigned to the concept map", type = "composite", compositeOf = { 4814 "context-type", "context-quantity" }) 4815 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 4816 /** 4817 * <b>Fluent Client</b> search parameter constant for 4818 * <b>context-type-quantity</b> 4819 * <p> 4820 * Description: <b>A use context type and quantity- or range-based value 4821 * assigned to the concept map</b><br> 4822 * Type: <b>composite</b><br> 4823 * Path: <b></b><br> 4824 * </p> 4825 */ 4826 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 4827 SP_CONTEXT_TYPE_QUANTITY); 4828 4829 /** 4830 * Search parameter: <b>source-system</b> 4831 * <p> 4832 * Description: <b>Source system where concepts to be mapped are defined</b><br> 4833 * Type: <b>uri</b><br> 4834 * Path: <b>ConceptMap.group.source</b><br> 4835 * </p> 4836 */ 4837 @SearchParamDefinition(name = "source-system", path = "ConceptMap.group.source", description = "Source system where concepts to be mapped are defined", type = "uri") 4838 public static final String SP_SOURCE_SYSTEM = "source-system"; 4839 /** 4840 * <b>Fluent Client</b> search parameter constant for <b>source-system</b> 4841 * <p> 4842 * Description: <b>Source system where concepts to be mapped are defined</b><br> 4843 * Type: <b>uri</b><br> 4844 * Path: <b>ConceptMap.group.source</b><br> 4845 * </p> 4846 */ 4847 public static final ca.uhn.fhir.rest.gclient.UriClientParam SOURCE_SYSTEM = new ca.uhn.fhir.rest.gclient.UriClientParam( 4848 SP_SOURCE_SYSTEM); 4849 4850 /** 4851 * Search parameter: <b>target-code</b> 4852 * <p> 4853 * Description: <b>Code that identifies the target element</b><br> 4854 * Type: <b>token</b><br> 4855 * Path: <b>ConceptMap.group.element.target.code</b><br> 4856 * </p> 4857 */ 4858 @SearchParamDefinition(name = "target-code", path = "ConceptMap.group.element.target.code", description = "Code that identifies the target element", type = "token") 4859 public static final String SP_TARGET_CODE = "target-code"; 4860 /** 4861 * <b>Fluent Client</b> search parameter constant for <b>target-code</b> 4862 * <p> 4863 * Description: <b>Code that identifies the target element</b><br> 4864 * Type: <b>token</b><br> 4865 * Path: <b>ConceptMap.group.element.target.code</b><br> 4866 * </p> 4867 */ 4868 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TARGET_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4869 SP_TARGET_CODE); 4870 4871 /** 4872 * Search parameter: <b>target-uri</b> 4873 * <p> 4874 * Description: <b>The target value set which provides context for the 4875 * mappings</b><br> 4876 * Type: <b>reference</b><br> 4877 * Path: <b>ConceptMap.targetUri</b><br> 4878 * </p> 4879 */ 4880 @SearchParamDefinition(name = "target-uri", path = "(ConceptMap.target as uri)", description = "The target value set which provides context for the mappings", type = "reference", target = { 4881 ValueSet.class }) 4882 public static final String SP_TARGET_URI = "target-uri"; 4883 /** 4884 * <b>Fluent Client</b> search parameter constant for <b>target-uri</b> 4885 * <p> 4886 * Description: <b>The target value set which provides context for the 4887 * mappings</b><br> 4888 * Type: <b>reference</b><br> 4889 * Path: <b>ConceptMap.targetUri</b><br> 4890 * </p> 4891 */ 4892 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam TARGET_URI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4893 SP_TARGET_URI); 4894 4895 /** 4896 * Constant for fluent queries to be used to add include statements. Specifies 4897 * the path value of "<b>ConceptMap:target-uri</b>". 4898 */ 4899 public static final ca.uhn.fhir.model.api.Include INCLUDE_TARGET_URI = new ca.uhn.fhir.model.api.Include( 4900 "ConceptMap:target-uri").toLocked(); 4901 4902 /** 4903 * Search parameter: <b>identifier</b> 4904 * <p> 4905 * Description: <b>External identifier for the concept map</b><br> 4906 * Type: <b>token</b><br> 4907 * Path: <b>ConceptMap.identifier</b><br> 4908 * </p> 4909 */ 4910 @SearchParamDefinition(name = "identifier", path = "ConceptMap.identifier", description = "External identifier for the concept map", type = "token") 4911 public static final String SP_IDENTIFIER = "identifier"; 4912 /** 4913 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4914 * <p> 4915 * Description: <b>External identifier for the concept map</b><br> 4916 * Type: <b>token</b><br> 4917 * Path: <b>ConceptMap.identifier</b><br> 4918 * </p> 4919 */ 4920 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4921 SP_IDENTIFIER); 4922 4923 /** 4924 * Search parameter: <b>product</b> 4925 * <p> 4926 * Description: <b>Reference to property mapping depends on</b><br> 4927 * Type: <b>uri</b><br> 4928 * Path: <b>ConceptMap.group.element.target.product.property</b><br> 4929 * </p> 4930 */ 4931 @SearchParamDefinition(name = "product", path = "ConceptMap.group.element.target.product.property", description = "Reference to property mapping depends on", type = "uri") 4932 public static final String SP_PRODUCT = "product"; 4933 /** 4934 * <b>Fluent Client</b> search parameter constant for <b>product</b> 4935 * <p> 4936 * Description: <b>Reference to property mapping depends on</b><br> 4937 * Type: <b>uri</b><br> 4938 * Path: <b>ConceptMap.group.element.target.product.property</b><br> 4939 * </p> 4940 */ 4941 public static final ca.uhn.fhir.rest.gclient.UriClientParam PRODUCT = new ca.uhn.fhir.rest.gclient.UriClientParam( 4942 SP_PRODUCT); 4943 4944 /** 4945 * Search parameter: <b>version</b> 4946 * <p> 4947 * Description: <b>The business version of the concept map</b><br> 4948 * Type: <b>token</b><br> 4949 * Path: <b>ConceptMap.version</b><br> 4950 * </p> 4951 */ 4952 @SearchParamDefinition(name = "version", path = "ConceptMap.version", description = "The business version of the concept map", type = "token") 4953 public static final String SP_VERSION = "version"; 4954 /** 4955 * <b>Fluent Client</b> search parameter constant for <b>version</b> 4956 * <p> 4957 * Description: <b>The business version of the concept map</b><br> 4958 * Type: <b>token</b><br> 4959 * Path: <b>ConceptMap.version</b><br> 4960 * </p> 4961 */ 4962 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4963 SP_VERSION); 4964 4965 /** 4966 * Search parameter: <b>url</b> 4967 * <p> 4968 * Description: <b>The uri that identifies the concept map</b><br> 4969 * Type: <b>uri</b><br> 4970 * Path: <b>ConceptMap.url</b><br> 4971 * </p> 4972 */ 4973 @SearchParamDefinition(name = "url", path = "ConceptMap.url", description = "The uri that identifies the concept map", type = "uri") 4974 public static final String SP_URL = "url"; 4975 /** 4976 * <b>Fluent Client</b> search parameter constant for <b>url</b> 4977 * <p> 4978 * Description: <b>The uri that identifies the concept map</b><br> 4979 * Type: <b>uri</b><br> 4980 * Path: <b>ConceptMap.url</b><br> 4981 * </p> 4982 */ 4983 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 4984 4985 /** 4986 * Search parameter: <b>target</b> 4987 * <p> 4988 * Description: <b>The target value set which provides context for the 4989 * mappings</b><br> 4990 * Type: <b>reference</b><br> 4991 * Path: <b>ConceptMap.targetCanonical</b><br> 4992 * </p> 4993 */ 4994 @SearchParamDefinition(name = "target", path = "(ConceptMap.target as canonical)", description = "The target value set which provides context for the mappings", type = "reference", target = { 4995 ValueSet.class }) 4996 public static final String SP_TARGET = "target"; 4997 /** 4998 * <b>Fluent Client</b> search parameter constant for <b>target</b> 4999 * <p> 5000 * Description: <b>The target value set which provides context for the 5001 * mappings</b><br> 5002 * Type: <b>reference</b><br> 5003 * Path: <b>ConceptMap.targetCanonical</b><br> 5004 * </p> 5005 */ 5006 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam TARGET = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5007 SP_TARGET); 5008 5009 /** 5010 * Constant for fluent queries to be used to add include statements. Specifies 5011 * the path value of "<b>ConceptMap:target</b>". 5012 */ 5013 public static final ca.uhn.fhir.model.api.Include INCLUDE_TARGET = new ca.uhn.fhir.model.api.Include( 5014 "ConceptMap:target").toLocked(); 5015 5016 /** 5017 * Search parameter: <b>source-code</b> 5018 * <p> 5019 * Description: <b>Identifies element being mapped</b><br> 5020 * Type: <b>token</b><br> 5021 * Path: <b>ConceptMap.group.element.code</b><br> 5022 * </p> 5023 */ 5024 @SearchParamDefinition(name = "source-code", path = "ConceptMap.group.element.code", description = "Identifies element being mapped", type = "token") 5025 public static final String SP_SOURCE_CODE = "source-code"; 5026 /** 5027 * <b>Fluent Client</b> search parameter constant for <b>source-code</b> 5028 * <p> 5029 * Description: <b>Identifies element being mapped</b><br> 5030 * Type: <b>token</b><br> 5031 * Path: <b>ConceptMap.group.element.code</b><br> 5032 * </p> 5033 */ 5034 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SOURCE_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5035 SP_SOURCE_CODE); 5036 5037 /** 5038 * Search parameter: <b>name</b> 5039 * <p> 5040 * Description: <b>Computationally friendly name of the concept map</b><br> 5041 * Type: <b>string</b><br> 5042 * Path: <b>ConceptMap.name</b><br> 5043 * </p> 5044 */ 5045 @SearchParamDefinition(name = "name", path = "ConceptMap.name", description = "Computationally friendly name of the concept map", type = "string") 5046 public static final String SP_NAME = "name"; 5047 /** 5048 * <b>Fluent Client</b> search parameter constant for <b>name</b> 5049 * <p> 5050 * Description: <b>Computationally friendly name of the concept map</b><br> 5051 * Type: <b>string</b><br> 5052 * Path: <b>ConceptMap.name</b><br> 5053 * </p> 5054 */ 5055 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 5056 SP_NAME); 5057 5058 /** 5059 * Search parameter: <b>publisher</b> 5060 * <p> 5061 * Description: <b>Name of the publisher of the concept map</b><br> 5062 * Type: <b>string</b><br> 5063 * Path: <b>ConceptMap.publisher</b><br> 5064 * </p> 5065 */ 5066 @SearchParamDefinition(name = "publisher", path = "ConceptMap.publisher", description = "Name of the publisher of the concept map", type = "string") 5067 public static final String SP_PUBLISHER = "publisher"; 5068 /** 5069 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 5070 * <p> 5071 * Description: <b>Name of the publisher of the concept map</b><br> 5072 * Type: <b>string</b><br> 5073 * Path: <b>ConceptMap.publisher</b><br> 5074 * </p> 5075 */ 5076 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 5077 SP_PUBLISHER); 5078 5079 /** 5080 * Search parameter: <b>status</b> 5081 * <p> 5082 * Description: <b>The current status of the concept map</b><br> 5083 * Type: <b>token</b><br> 5084 * Path: <b>ConceptMap.status</b><br> 5085 * </p> 5086 */ 5087 @SearchParamDefinition(name = "status", path = "ConceptMap.status", description = "The current status of the concept map", type = "token") 5088 public static final String SP_STATUS = "status"; 5089 /** 5090 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5091 * <p> 5092 * Description: <b>The current status of the concept map</b><br> 5093 * Type: <b>token</b><br> 5094 * Path: <b>ConceptMap.status</b><br> 5095 * </p> 5096 */ 5097 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5098 SP_STATUS); 5099 5100}