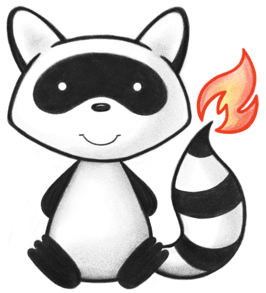
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence; 040import org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalenceEnumFactory; 041import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 042import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.ResourceDef; 050import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 051 052/** 053 * A statement of relationships from one set of concepts to one or more other 054 * concepts - either concepts in code systems, or data element/data element 055 * concepts, or classes in class models. 056 */ 057@ResourceDef(name = "ConceptMap", profile = "http://hl7.org/fhir/StructureDefinition/ConceptMap") 058@ChildOrder(names = { "url", "identifier", "version", "name", "title", "status", "experimental", "date", "publisher", 059 "contact", "description", "useContext", "jurisdiction", "purpose", "copyright", "source[x]", "target[x]", "group" }) 060public class ConceptMap extends MetadataResource { 061 062 public enum ConceptMapGroupUnmappedMode { 063 /** 064 * Use the code as provided in the $translate request. 065 */ 066 PROVIDED, 067 /** 068 * Use the code explicitly provided in the group.unmapped. 069 */ 070 FIXED, 071 /** 072 * Use the map identified by the canonical URL in the url element. 073 */ 074 OTHERMAP, 075 /** 076 * added to help the parsers with the generic types 077 */ 078 NULL; 079 080 public static ConceptMapGroupUnmappedMode fromCode(String codeString) throws FHIRException { 081 if (codeString == null || "".equals(codeString)) 082 return null; 083 if ("provided".equals(codeString)) 084 return PROVIDED; 085 if ("fixed".equals(codeString)) 086 return FIXED; 087 if ("other-map".equals(codeString)) 088 return OTHERMAP; 089 if (Configuration.isAcceptInvalidEnums()) 090 return null; 091 else 092 throw new FHIRException("Unknown ConceptMapGroupUnmappedMode code '" + codeString + "'"); 093 } 094 095 public String toCode() { 096 switch (this) { 097 case PROVIDED: 098 return "provided"; 099 case FIXED: 100 return "fixed"; 101 case OTHERMAP: 102 return "other-map"; 103 case NULL: 104 return null; 105 default: 106 return "?"; 107 } 108 } 109 110 public String getSystem() { 111 switch (this) { 112 case PROVIDED: 113 return "http://hl7.org/fhir/conceptmap-unmapped-mode"; 114 case FIXED: 115 return "http://hl7.org/fhir/conceptmap-unmapped-mode"; 116 case OTHERMAP: 117 return "http://hl7.org/fhir/conceptmap-unmapped-mode"; 118 case NULL: 119 return null; 120 default: 121 return "?"; 122 } 123 } 124 125 public String getDefinition() { 126 switch (this) { 127 case PROVIDED: 128 return "Use the code as provided in the $translate request."; 129 case FIXED: 130 return "Use the code explicitly provided in the group.unmapped."; 131 case OTHERMAP: 132 return "Use the map identified by the canonical URL in the url element."; 133 case NULL: 134 return null; 135 default: 136 return "?"; 137 } 138 } 139 140 public String getDisplay() { 141 switch (this) { 142 case PROVIDED: 143 return "Provided Code"; 144 case FIXED: 145 return "Fixed Code"; 146 case OTHERMAP: 147 return "Other Map"; 148 case NULL: 149 return null; 150 default: 151 return "?"; 152 } 153 } 154 } 155 156 public static class ConceptMapGroupUnmappedModeEnumFactory implements EnumFactory<ConceptMapGroupUnmappedMode> { 157 public ConceptMapGroupUnmappedMode fromCode(String codeString) throws IllegalArgumentException { 158 if (codeString == null || "".equals(codeString)) 159 if (codeString == null || "".equals(codeString)) 160 return null; 161 if ("provided".equals(codeString)) 162 return ConceptMapGroupUnmappedMode.PROVIDED; 163 if ("fixed".equals(codeString)) 164 return ConceptMapGroupUnmappedMode.FIXED; 165 if ("other-map".equals(codeString)) 166 return ConceptMapGroupUnmappedMode.OTHERMAP; 167 throw new IllegalArgumentException("Unknown ConceptMapGroupUnmappedMode code '" + codeString + "'"); 168 } 169 170 public Enumeration<ConceptMapGroupUnmappedMode> fromType(PrimitiveType<?> code) throws FHIRException { 171 if (code == null) 172 return null; 173 if (code.isEmpty()) 174 return new Enumeration<ConceptMapGroupUnmappedMode>(this, ConceptMapGroupUnmappedMode.NULL, code); 175 String codeString = code.asStringValue(); 176 if (codeString == null || "".equals(codeString)) 177 return new Enumeration<ConceptMapGroupUnmappedMode>(this, ConceptMapGroupUnmappedMode.NULL, code); 178 if ("provided".equals(codeString)) 179 return new Enumeration<ConceptMapGroupUnmappedMode>(this, ConceptMapGroupUnmappedMode.PROVIDED, code); 180 if ("fixed".equals(codeString)) 181 return new Enumeration<ConceptMapGroupUnmappedMode>(this, ConceptMapGroupUnmappedMode.FIXED, code); 182 if ("other-map".equals(codeString)) 183 return new Enumeration<ConceptMapGroupUnmappedMode>(this, ConceptMapGroupUnmappedMode.OTHERMAP, code); 184 throw new FHIRException("Unknown ConceptMapGroupUnmappedMode code '" + codeString + "'"); 185 } 186 187 public String toCode(ConceptMapGroupUnmappedMode code) { 188 if (code == ConceptMapGroupUnmappedMode.NULL) 189 return null; 190 if (code == ConceptMapGroupUnmappedMode.PROVIDED) 191 return "provided"; 192 if (code == ConceptMapGroupUnmappedMode.FIXED) 193 return "fixed"; 194 if (code == ConceptMapGroupUnmappedMode.OTHERMAP) 195 return "other-map"; 196 return "?"; 197 } 198 199 public String toSystem(ConceptMapGroupUnmappedMode code) { 200 return code.getSystem(); 201 } 202 } 203 204 @Block() 205 public static class ConceptMapGroupComponent extends BackboneElement implements IBaseBackboneElement { 206 /** 207 * An absolute URI that identifies the source system where the concepts to be 208 * mapped are defined. 209 */ 210 @Child(name = "source", type = { UriType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 211 @Description(shortDefinition = "Source system where concepts to be mapped are defined", formalDefinition = "An absolute URI that identifies the source system where the concepts to be mapped are defined.") 212 protected UriType source; 213 214 /** 215 * The specific version of the code system, as determined by the code system 216 * authority. 217 */ 218 @Child(name = "sourceVersion", type = { 219 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 220 @Description(shortDefinition = "Specific version of the code system", formalDefinition = "The specific version of the code system, as determined by the code system authority.") 221 protected StringType sourceVersion; 222 223 /** 224 * An absolute URI that identifies the target system that the concepts will be 225 * mapped to. 226 */ 227 @Child(name = "target", type = { UriType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 228 @Description(shortDefinition = "Target system that the concepts are to be mapped to", formalDefinition = "An absolute URI that identifies the target system that the concepts will be mapped to.") 229 protected UriType target; 230 231 /** 232 * The specific version of the code system, as determined by the code system 233 * authority. 234 */ 235 @Child(name = "targetVersion", type = { 236 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 237 @Description(shortDefinition = "Specific version of the code system", formalDefinition = "The specific version of the code system, as determined by the code system authority.") 238 protected StringType targetVersion; 239 240 /** 241 * Mappings for an individual concept in the source to one or more concepts in 242 * the target. 243 */ 244 @Child(name = "element", type = {}, order = 5, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 245 @Description(shortDefinition = "Mappings for a concept from the source set", formalDefinition = "Mappings for an individual concept in the source to one or more concepts in the target.") 246 protected List<SourceElementComponent> element; 247 248 /** 249 * What to do when there is no mapping for the source concept. "Unmapped" does 250 * not include codes that are unmatched, and the unmapped element is ignored in 251 * a code is specified to have equivalence = unmatched. 252 */ 253 @Child(name = "unmapped", type = {}, order = 6, min = 0, max = 1, modifier = false, summary = false) 254 @Description(shortDefinition = "What to do when there is no mapping for the source concept", formalDefinition = "What to do when there is no mapping for the source concept. \"Unmapped\" does not include codes that are unmatched, and the unmapped element is ignored in a code is specified to have equivalence = unmatched.") 255 protected ConceptMapGroupUnmappedComponent unmapped; 256 257 private static final long serialVersionUID = 1606357508L; 258 259 /** 260 * Constructor 261 */ 262 public ConceptMapGroupComponent() { 263 super(); 264 } 265 266 /** 267 * @return {@link #source} (An absolute URI that identifies the source system 268 * where the concepts to be mapped are defined.). This is the underlying 269 * object with id, value and extensions. The accessor "getSource" gives 270 * direct access to the value 271 */ 272 public UriType getSourceElement() { 273 if (this.source == null) 274 if (Configuration.errorOnAutoCreate()) 275 throw new Error("Attempt to auto-create ConceptMapGroupComponent.source"); 276 else if (Configuration.doAutoCreate()) 277 this.source = new UriType(); // bb 278 return this.source; 279 } 280 281 public boolean hasSourceElement() { 282 return this.source != null && !this.source.isEmpty(); 283 } 284 285 public boolean hasSource() { 286 return this.source != null && !this.source.isEmpty(); 287 } 288 289 /** 290 * @param value {@link #source} (An absolute URI that identifies the source 291 * system where the concepts to be mapped are defined.). This is 292 * the underlying object with id, value and extensions. The 293 * accessor "getSource" gives direct access to the value 294 */ 295 public ConceptMapGroupComponent setSourceElement(UriType value) { 296 this.source = value; 297 return this; 298 } 299 300 /** 301 * @return An absolute URI that identifies the source system where the concepts 302 * to be mapped are defined. 303 */ 304 public String getSource() { 305 return this.source == null ? null : this.source.getValue(); 306 } 307 308 /** 309 * @param value An absolute URI that identifies the source system where the 310 * concepts to be mapped are defined. 311 */ 312 public ConceptMapGroupComponent setSource(String value) { 313 if (Utilities.noString(value)) 314 this.source = null; 315 else { 316 if (this.source == null) 317 this.source = new UriType(); 318 this.source.setValue(value); 319 } 320 return this; 321 } 322 323 /** 324 * @return {@link #sourceVersion} (The specific version of the code system, as 325 * determined by the code system authority.). This is the underlying 326 * object with id, value and extensions. The accessor "getSourceVersion" 327 * gives direct access to the value 328 */ 329 public StringType getSourceVersionElement() { 330 if (this.sourceVersion == null) 331 if (Configuration.errorOnAutoCreate()) 332 throw new Error("Attempt to auto-create ConceptMapGroupComponent.sourceVersion"); 333 else if (Configuration.doAutoCreate()) 334 this.sourceVersion = new StringType(); // bb 335 return this.sourceVersion; 336 } 337 338 public boolean hasSourceVersionElement() { 339 return this.sourceVersion != null && !this.sourceVersion.isEmpty(); 340 } 341 342 public boolean hasSourceVersion() { 343 return this.sourceVersion != null && !this.sourceVersion.isEmpty(); 344 } 345 346 /** 347 * @param value {@link #sourceVersion} (The specific version of the code system, 348 * as determined by the code system authority.). This is the 349 * underlying object with id, value and extensions. The accessor 350 * "getSourceVersion" gives direct access to the value 351 */ 352 public ConceptMapGroupComponent setSourceVersionElement(StringType value) { 353 this.sourceVersion = value; 354 return this; 355 } 356 357 /** 358 * @return The specific version of the code system, as determined by the code 359 * system authority. 360 */ 361 public String getSourceVersion() { 362 return this.sourceVersion == null ? null : this.sourceVersion.getValue(); 363 } 364 365 /** 366 * @param value The specific version of the code system, as determined by the 367 * code system authority. 368 */ 369 public ConceptMapGroupComponent setSourceVersion(String value) { 370 if (Utilities.noString(value)) 371 this.sourceVersion = null; 372 else { 373 if (this.sourceVersion == null) 374 this.sourceVersion = new StringType(); 375 this.sourceVersion.setValue(value); 376 } 377 return this; 378 } 379 380 /** 381 * @return {@link #target} (An absolute URI that identifies the target system 382 * that the concepts will be mapped to.). This is the underlying object 383 * with id, value and extensions. The accessor "getTarget" gives direct 384 * access to the value 385 */ 386 public UriType getTargetElement() { 387 if (this.target == null) 388 if (Configuration.errorOnAutoCreate()) 389 throw new Error("Attempt to auto-create ConceptMapGroupComponent.target"); 390 else if (Configuration.doAutoCreate()) 391 this.target = new UriType(); // bb 392 return this.target; 393 } 394 395 public boolean hasTargetElement() { 396 return this.target != null && !this.target.isEmpty(); 397 } 398 399 public boolean hasTarget() { 400 return this.target != null && !this.target.isEmpty(); 401 } 402 403 /** 404 * @param value {@link #target} (An absolute URI that identifies the target 405 * system that the concepts will be mapped to.). This is the 406 * underlying object with id, value and extensions. The accessor 407 * "getTarget" gives direct access to the value 408 */ 409 public ConceptMapGroupComponent setTargetElement(UriType value) { 410 this.target = value; 411 return this; 412 } 413 414 /** 415 * @return An absolute URI that identifies the target system that the concepts 416 * will be mapped to. 417 */ 418 public String getTarget() { 419 return this.target == null ? null : this.target.getValue(); 420 } 421 422 /** 423 * @param value An absolute URI that identifies the target system that the 424 * concepts will be mapped to. 425 */ 426 public ConceptMapGroupComponent setTarget(String value) { 427 if (Utilities.noString(value)) 428 this.target = null; 429 else { 430 if (this.target == null) 431 this.target = new UriType(); 432 this.target.setValue(value); 433 } 434 return this; 435 } 436 437 /** 438 * @return {@link #targetVersion} (The specific version of the code system, as 439 * determined by the code system authority.). This is the underlying 440 * object with id, value and extensions. The accessor "getTargetVersion" 441 * gives direct access to the value 442 */ 443 public StringType getTargetVersionElement() { 444 if (this.targetVersion == null) 445 if (Configuration.errorOnAutoCreate()) 446 throw new Error("Attempt to auto-create ConceptMapGroupComponent.targetVersion"); 447 else if (Configuration.doAutoCreate()) 448 this.targetVersion = new StringType(); // bb 449 return this.targetVersion; 450 } 451 452 public boolean hasTargetVersionElement() { 453 return this.targetVersion != null && !this.targetVersion.isEmpty(); 454 } 455 456 public boolean hasTargetVersion() { 457 return this.targetVersion != null && !this.targetVersion.isEmpty(); 458 } 459 460 /** 461 * @param value {@link #targetVersion} (The specific version of the code system, 462 * as determined by the code system authority.). This is the 463 * underlying object with id, value and extensions. The accessor 464 * "getTargetVersion" gives direct access to the value 465 */ 466 public ConceptMapGroupComponent setTargetVersionElement(StringType value) { 467 this.targetVersion = value; 468 return this; 469 } 470 471 /** 472 * @return The specific version of the code system, as determined by the code 473 * system authority. 474 */ 475 public String getTargetVersion() { 476 return this.targetVersion == null ? null : this.targetVersion.getValue(); 477 } 478 479 /** 480 * @param value The specific version of the code system, as determined by the 481 * code system authority. 482 */ 483 public ConceptMapGroupComponent setTargetVersion(String value) { 484 if (Utilities.noString(value)) 485 this.targetVersion = null; 486 else { 487 if (this.targetVersion == null) 488 this.targetVersion = new StringType(); 489 this.targetVersion.setValue(value); 490 } 491 return this; 492 } 493 494 /** 495 * @return {@link #element} (Mappings for an individual concept in the source to 496 * one or more concepts in the target.) 497 */ 498 public List<SourceElementComponent> getElement() { 499 if (this.element == null) 500 this.element = new ArrayList<SourceElementComponent>(); 501 return this.element; 502 } 503 504 /** 505 * @return Returns a reference to <code>this</code> for easy method chaining 506 */ 507 public ConceptMapGroupComponent setElement(List<SourceElementComponent> theElement) { 508 this.element = theElement; 509 return this; 510 } 511 512 public boolean hasElement() { 513 if (this.element == null) 514 return false; 515 for (SourceElementComponent item : this.element) 516 if (!item.isEmpty()) 517 return true; 518 return false; 519 } 520 521 public SourceElementComponent addElement() { // 3 522 SourceElementComponent t = new SourceElementComponent(); 523 if (this.element == null) 524 this.element = new ArrayList<SourceElementComponent>(); 525 this.element.add(t); 526 return t; 527 } 528 529 public ConceptMapGroupComponent addElement(SourceElementComponent t) { // 3 530 if (t == null) 531 return this; 532 if (this.element == null) 533 this.element = new ArrayList<SourceElementComponent>(); 534 this.element.add(t); 535 return this; 536 } 537 538 /** 539 * @return The first repetition of repeating field {@link #element}, creating it 540 * if it does not already exist 541 */ 542 public SourceElementComponent getElementFirstRep() { 543 if (getElement().isEmpty()) { 544 addElement(); 545 } 546 return getElement().get(0); 547 } 548 549 /** 550 * @return {@link #unmapped} (What to do when there is no mapping for the source 551 * concept. "Unmapped" does not include codes that are unmatched, and 552 * the unmapped element is ignored in a code is specified to have 553 * equivalence = unmatched.) 554 */ 555 public ConceptMapGroupUnmappedComponent getUnmapped() { 556 if (this.unmapped == null) 557 if (Configuration.errorOnAutoCreate()) 558 throw new Error("Attempt to auto-create ConceptMapGroupComponent.unmapped"); 559 else if (Configuration.doAutoCreate()) 560 this.unmapped = new ConceptMapGroupUnmappedComponent(); // cc 561 return this.unmapped; 562 } 563 564 public boolean hasUnmapped() { 565 return this.unmapped != null && !this.unmapped.isEmpty(); 566 } 567 568 /** 569 * @param value {@link #unmapped} (What to do when there is no mapping for the 570 * source concept. "Unmapped" does not include codes that are 571 * unmatched, and the unmapped element is ignored in a code is 572 * specified to have equivalence = unmatched.) 573 */ 574 public ConceptMapGroupComponent setUnmapped(ConceptMapGroupUnmappedComponent value) { 575 this.unmapped = value; 576 return this; 577 } 578 579 protected void listChildren(List<Property> children) { 580 super.listChildren(children); 581 children.add(new Property("source", "uri", 582 "An absolute URI that identifies the source system where the concepts to be mapped are defined.", 0, 1, 583 source)); 584 children.add(new Property("sourceVersion", "string", 585 "The specific version of the code system, as determined by the code system authority.", 0, 1, sourceVersion)); 586 children.add(new Property("target", "uri", 587 "An absolute URI that identifies the target system that the concepts will be mapped to.", 0, 1, target)); 588 children.add(new Property("targetVersion", "string", 589 "The specific version of the code system, as determined by the code system authority.", 0, 1, targetVersion)); 590 children.add(new Property("element", "", 591 "Mappings for an individual concept in the source to one or more concepts in the target.", 0, 592 java.lang.Integer.MAX_VALUE, element)); 593 children.add(new Property("unmapped", "", 594 "What to do when there is no mapping for the source concept. \"Unmapped\" does not include codes that are unmatched, and the unmapped element is ignored in a code is specified to have equivalence = unmatched.", 595 0, 1, unmapped)); 596 } 597 598 @Override 599 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 600 switch (_hash) { 601 case -896505829: 602 /* source */ return new Property("source", "uri", 603 "An absolute URI that identifies the source system where the concepts to be mapped are defined.", 0, 1, 604 source); 605 case 446171197: 606 /* sourceVersion */ return new Property("sourceVersion", "string", 607 "The specific version of the code system, as determined by the code system authority.", 0, 1, 608 sourceVersion); 609 case -880905839: 610 /* target */ return new Property("target", "uri", 611 "An absolute URI that identifies the target system that the concepts will be mapped to.", 0, 1, target); 612 case -1639412217: 613 /* targetVersion */ return new Property("targetVersion", "string", 614 "The specific version of the code system, as determined by the code system authority.", 0, 1, 615 targetVersion); 616 case -1662836996: 617 /* element */ return new Property("element", "", 618 "Mappings for an individual concept in the source to one or more concepts in the target.", 0, 619 java.lang.Integer.MAX_VALUE, element); 620 case -194857460: 621 /* unmapped */ return new Property("unmapped", "", 622 "What to do when there is no mapping for the source concept. \"Unmapped\" does not include codes that are unmatched, and the unmapped element is ignored in a code is specified to have equivalence = unmatched.", 623 0, 1, unmapped); 624 default: 625 return super.getNamedProperty(_hash, _name, _checkValid); 626 } 627 628 } 629 630 @Override 631 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 632 switch (hash) { 633 case -896505829: 634 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // UriType 635 case 446171197: 636 /* sourceVersion */ return this.sourceVersion == null ? new Base[0] : new Base[] { this.sourceVersion }; // StringType 637 case -880905839: 638 /* target */ return this.target == null ? new Base[0] : new Base[] { this.target }; // UriType 639 case -1639412217: 640 /* targetVersion */ return this.targetVersion == null ? new Base[0] : new Base[] { this.targetVersion }; // StringType 641 case -1662836996: 642 /* element */ return this.element == null ? new Base[0] : this.element.toArray(new Base[this.element.size()]); // SourceElementComponent 643 case -194857460: 644 /* unmapped */ return this.unmapped == null ? new Base[0] : new Base[] { this.unmapped }; // ConceptMapGroupUnmappedComponent 645 default: 646 return super.getProperty(hash, name, checkValid); 647 } 648 649 } 650 651 @Override 652 public Base setProperty(int hash, String name, Base value) throws FHIRException { 653 switch (hash) { 654 case -896505829: // source 655 this.source = castToUri(value); // UriType 656 return value; 657 case 446171197: // sourceVersion 658 this.sourceVersion = castToString(value); // StringType 659 return value; 660 case -880905839: // target 661 this.target = castToUri(value); // UriType 662 return value; 663 case -1639412217: // targetVersion 664 this.targetVersion = castToString(value); // StringType 665 return value; 666 case -1662836996: // element 667 this.getElement().add((SourceElementComponent) value); // SourceElementComponent 668 return value; 669 case -194857460: // unmapped 670 this.unmapped = (ConceptMapGroupUnmappedComponent) value; // ConceptMapGroupUnmappedComponent 671 return value; 672 default: 673 return super.setProperty(hash, name, value); 674 } 675 676 } 677 678 @Override 679 public Base setProperty(String name, Base value) throws FHIRException { 680 if (name.equals("source")) { 681 this.source = castToUri(value); // UriType 682 } else if (name.equals("sourceVersion")) { 683 this.sourceVersion = castToString(value); // StringType 684 } else if (name.equals("target")) { 685 this.target = castToUri(value); // UriType 686 } else if (name.equals("targetVersion")) { 687 this.targetVersion = castToString(value); // StringType 688 } else if (name.equals("element")) { 689 this.getElement().add((SourceElementComponent) value); 690 } else if (name.equals("unmapped")) { 691 this.unmapped = (ConceptMapGroupUnmappedComponent) value; // ConceptMapGroupUnmappedComponent 692 } else 693 return super.setProperty(name, value); 694 return value; 695 } 696 697 @Override 698 public void removeChild(String name, Base value) throws FHIRException { 699 if (name.equals("source")) { 700 this.source = null; 701 } else if (name.equals("sourceVersion")) { 702 this.sourceVersion = null; 703 } else if (name.equals("target")) { 704 this.target = null; 705 } else if (name.equals("targetVersion")) { 706 this.targetVersion = null; 707 } else if (name.equals("element")) { 708 this.getElement().remove((SourceElementComponent) value); 709 } else if (name.equals("unmapped")) { 710 this.unmapped = (ConceptMapGroupUnmappedComponent) value; // ConceptMapGroupUnmappedComponent 711 } else 712 super.removeChild(name, value); 713 714 } 715 716 @Override 717 public Base makeProperty(int hash, String name) throws FHIRException { 718 switch (hash) { 719 case -896505829: 720 return getSourceElement(); 721 case 446171197: 722 return getSourceVersionElement(); 723 case -880905839: 724 return getTargetElement(); 725 case -1639412217: 726 return getTargetVersionElement(); 727 case -1662836996: 728 return addElement(); 729 case -194857460: 730 return getUnmapped(); 731 default: 732 return super.makeProperty(hash, name); 733 } 734 735 } 736 737 @Override 738 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 739 switch (hash) { 740 case -896505829: 741 /* source */ return new String[] { "uri" }; 742 case 446171197: 743 /* sourceVersion */ return new String[] { "string" }; 744 case -880905839: 745 /* target */ return new String[] { "uri" }; 746 case -1639412217: 747 /* targetVersion */ return new String[] { "string" }; 748 case -1662836996: 749 /* element */ return new String[] {}; 750 case -194857460: 751 /* unmapped */ return new String[] {}; 752 default: 753 return super.getTypesForProperty(hash, name); 754 } 755 756 } 757 758 @Override 759 public Base addChild(String name) throws FHIRException { 760 if (name.equals("source")) { 761 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.source"); 762 } else if (name.equals("sourceVersion")) { 763 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.sourceVersion"); 764 } else if (name.equals("target")) { 765 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.target"); 766 } else if (name.equals("targetVersion")) { 767 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.targetVersion"); 768 } else if (name.equals("element")) { 769 return addElement(); 770 } else if (name.equals("unmapped")) { 771 this.unmapped = new ConceptMapGroupUnmappedComponent(); 772 return this.unmapped; 773 } else 774 return super.addChild(name); 775 } 776 777 public ConceptMapGroupComponent copy() { 778 ConceptMapGroupComponent dst = new ConceptMapGroupComponent(); 779 copyValues(dst); 780 return dst; 781 } 782 783 public void copyValues(ConceptMapGroupComponent dst) { 784 super.copyValues(dst); 785 dst.source = source == null ? null : source.copy(); 786 dst.sourceVersion = sourceVersion == null ? null : sourceVersion.copy(); 787 dst.target = target == null ? null : target.copy(); 788 dst.targetVersion = targetVersion == null ? null : targetVersion.copy(); 789 if (element != null) { 790 dst.element = new ArrayList<SourceElementComponent>(); 791 for (SourceElementComponent i : element) 792 dst.element.add(i.copy()); 793 } 794 ; 795 dst.unmapped = unmapped == null ? null : unmapped.copy(); 796 } 797 798 @Override 799 public boolean equalsDeep(Base other_) { 800 if (!super.equalsDeep(other_)) 801 return false; 802 if (!(other_ instanceof ConceptMapGroupComponent)) 803 return false; 804 ConceptMapGroupComponent o = (ConceptMapGroupComponent) other_; 805 return compareDeep(source, o.source, true) && compareDeep(sourceVersion, o.sourceVersion, true) 806 && compareDeep(target, o.target, true) && compareDeep(targetVersion, o.targetVersion, true) 807 && compareDeep(element, o.element, true) && compareDeep(unmapped, o.unmapped, true); 808 } 809 810 @Override 811 public boolean equalsShallow(Base other_) { 812 if (!super.equalsShallow(other_)) 813 return false; 814 if (!(other_ instanceof ConceptMapGroupComponent)) 815 return false; 816 ConceptMapGroupComponent o = (ConceptMapGroupComponent) other_; 817 return compareValues(source, o.source, true) && compareValues(sourceVersion, o.sourceVersion, true) 818 && compareValues(target, o.target, true) && compareValues(targetVersion, o.targetVersion, true); 819 } 820 821 public boolean isEmpty() { 822 return super.isEmpty() 823 && ca.uhn.fhir.util.ElementUtil.isEmpty(source, sourceVersion, target, targetVersion, element, unmapped); 824 } 825 826 public String fhirType() { 827 return "ConceptMap.group"; 828 829 } 830 831 } 832 833 @Block() 834 public static class SourceElementComponent extends BackboneElement implements IBaseBackboneElement { 835 /** 836 * Identity (code or path) or the element/item being mapped. 837 */ 838 @Child(name = "code", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 839 @Description(shortDefinition = "Identifies element being mapped", formalDefinition = "Identity (code or path) or the element/item being mapped.") 840 protected CodeType code; 841 842 /** 843 * The display for the code. The display is only provided to help editors when 844 * editing the concept map. 845 */ 846 @Child(name = "display", type = { 847 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 848 @Description(shortDefinition = "Display for the code", formalDefinition = "The display for the code. The display is only provided to help editors when editing the concept map.") 849 protected StringType display; 850 851 /** 852 * A concept from the target value set that this concept maps to. 853 */ 854 @Child(name = "target", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 855 @Description(shortDefinition = "Concept in target system for element", formalDefinition = "A concept from the target value set that this concept maps to.") 856 protected List<TargetElementComponent> target; 857 858 private static final long serialVersionUID = -1115258852L; 859 860 /** 861 * Constructor 862 */ 863 public SourceElementComponent() { 864 super(); 865 } 866 867 /** 868 * @return {@link #code} (Identity (code or path) or the element/item being 869 * mapped.). This is the underlying object with id, value and 870 * extensions. The accessor "getCode" gives direct access to the value 871 */ 872 public CodeType getCodeElement() { 873 if (this.code == null) 874 if (Configuration.errorOnAutoCreate()) 875 throw new Error("Attempt to auto-create SourceElementComponent.code"); 876 else if (Configuration.doAutoCreate()) 877 this.code = new CodeType(); // bb 878 return this.code; 879 } 880 881 public boolean hasCodeElement() { 882 return this.code != null && !this.code.isEmpty(); 883 } 884 885 public boolean hasCode() { 886 return this.code != null && !this.code.isEmpty(); 887 } 888 889 /** 890 * @param value {@link #code} (Identity (code or path) or the element/item being 891 * mapped.). This is the underlying object with id, value and 892 * extensions. The accessor "getCode" gives direct access to the 893 * value 894 */ 895 public SourceElementComponent setCodeElement(CodeType value) { 896 this.code = value; 897 return this; 898 } 899 900 /** 901 * @return Identity (code or path) or the element/item being mapped. 902 */ 903 public String getCode() { 904 return this.code == null ? null : this.code.getValue(); 905 } 906 907 /** 908 * @param value Identity (code or path) or the element/item being mapped. 909 */ 910 public SourceElementComponent setCode(String value) { 911 if (Utilities.noString(value)) 912 this.code = null; 913 else { 914 if (this.code == null) 915 this.code = new CodeType(); 916 this.code.setValue(value); 917 } 918 return this; 919 } 920 921 /** 922 * @return {@link #display} (The display for the code. The display is only 923 * provided to help editors when editing the concept map.). This is the 924 * underlying object with id, value and extensions. The accessor 925 * "getDisplay" gives direct access to the value 926 */ 927 public StringType getDisplayElement() { 928 if (this.display == null) 929 if (Configuration.errorOnAutoCreate()) 930 throw new Error("Attempt to auto-create SourceElementComponent.display"); 931 else if (Configuration.doAutoCreate()) 932 this.display = new StringType(); // bb 933 return this.display; 934 } 935 936 public boolean hasDisplayElement() { 937 return this.display != null && !this.display.isEmpty(); 938 } 939 940 public boolean hasDisplay() { 941 return this.display != null && !this.display.isEmpty(); 942 } 943 944 /** 945 * @param value {@link #display} (The display for the code. The display is only 946 * provided to help editors when editing the concept map.). This is 947 * the underlying object with id, value and extensions. The 948 * accessor "getDisplay" gives direct access to the value 949 */ 950 public SourceElementComponent setDisplayElement(StringType value) { 951 this.display = value; 952 return this; 953 } 954 955 /** 956 * @return The display for the code. The display is only provided to help 957 * editors when editing the concept map. 958 */ 959 public String getDisplay() { 960 return this.display == null ? null : this.display.getValue(); 961 } 962 963 /** 964 * @param value The display for the code. The display is only provided to help 965 * editors when editing the concept map. 966 */ 967 public SourceElementComponent setDisplay(String value) { 968 if (Utilities.noString(value)) 969 this.display = null; 970 else { 971 if (this.display == null) 972 this.display = new StringType(); 973 this.display.setValue(value); 974 } 975 return this; 976 } 977 978 /** 979 * @return {@link #target} (A concept from the target value set that this 980 * concept maps to.) 981 */ 982 public List<TargetElementComponent> getTarget() { 983 if (this.target == null) 984 this.target = new ArrayList<TargetElementComponent>(); 985 return this.target; 986 } 987 988 /** 989 * @return Returns a reference to <code>this</code> for easy method chaining 990 */ 991 public SourceElementComponent setTarget(List<TargetElementComponent> theTarget) { 992 this.target = theTarget; 993 return this; 994 } 995 996 public boolean hasTarget() { 997 if (this.target == null) 998 return false; 999 for (TargetElementComponent item : this.target) 1000 if (!item.isEmpty()) 1001 return true; 1002 return false; 1003 } 1004 1005 public TargetElementComponent addTarget() { // 3 1006 TargetElementComponent t = new TargetElementComponent(); 1007 if (this.target == null) 1008 this.target = new ArrayList<TargetElementComponent>(); 1009 this.target.add(t); 1010 return t; 1011 } 1012 1013 public SourceElementComponent addTarget(TargetElementComponent t) { // 3 1014 if (t == null) 1015 return this; 1016 if (this.target == null) 1017 this.target = new ArrayList<TargetElementComponent>(); 1018 this.target.add(t); 1019 return this; 1020 } 1021 1022 /** 1023 * @return The first repetition of repeating field {@link #target}, creating it 1024 * if it does not already exist 1025 */ 1026 public TargetElementComponent getTargetFirstRep() { 1027 if (getTarget().isEmpty()) { 1028 addTarget(); 1029 } 1030 return getTarget().get(0); 1031 } 1032 1033 protected void listChildren(List<Property> children) { 1034 super.listChildren(children); 1035 children 1036 .add(new Property("code", "code", "Identity (code or path) or the element/item being mapped.", 0, 1, code)); 1037 children.add(new Property("display", "string", 1038 "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 1, 1039 display)); 1040 children.add(new Property("target", "", "A concept from the target value set that this concept maps to.", 0, 1041 java.lang.Integer.MAX_VALUE, target)); 1042 } 1043 1044 @Override 1045 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1046 switch (_hash) { 1047 case 3059181: 1048 /* code */ return new Property("code", "code", "Identity (code or path) or the element/item being mapped.", 0, 1049 1, code); 1050 case 1671764162: 1051 /* display */ return new Property("display", "string", 1052 "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 1053 1, display); 1054 case -880905839: 1055 /* target */ return new Property("target", "", "A concept from the target value set that this concept maps to.", 1056 0, java.lang.Integer.MAX_VALUE, target); 1057 default: 1058 return super.getNamedProperty(_hash, _name, _checkValid); 1059 } 1060 1061 } 1062 1063 @Override 1064 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1065 switch (hash) { 1066 case 3059181: 1067 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeType 1068 case 1671764162: 1069 /* display */ return this.display == null ? new Base[0] : new Base[] { this.display }; // StringType 1070 case -880905839: 1071 /* target */ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // TargetElementComponent 1072 default: 1073 return super.getProperty(hash, name, checkValid); 1074 } 1075 1076 } 1077 1078 @Override 1079 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1080 switch (hash) { 1081 case 3059181: // code 1082 this.code = castToCode(value); // CodeType 1083 return value; 1084 case 1671764162: // display 1085 this.display = castToString(value); // StringType 1086 return value; 1087 case -880905839: // target 1088 this.getTarget().add((TargetElementComponent) value); // TargetElementComponent 1089 return value; 1090 default: 1091 return super.setProperty(hash, name, value); 1092 } 1093 1094 } 1095 1096 @Override 1097 public Base setProperty(String name, Base value) throws FHIRException { 1098 if (name.equals("code")) { 1099 this.code = castToCode(value); // CodeType 1100 } else if (name.equals("display")) { 1101 this.display = castToString(value); // StringType 1102 } else if (name.equals("target")) { 1103 this.getTarget().add((TargetElementComponent) value); 1104 } else 1105 return super.setProperty(name, value); 1106 return value; 1107 } 1108 1109 @Override 1110 public void removeChild(String name, Base value) throws FHIRException { 1111 if (name.equals("code")) { 1112 this.code = null; 1113 } else if (name.equals("display")) { 1114 this.display = null; 1115 } else if (name.equals("target")) { 1116 this.getTarget().remove((TargetElementComponent) value); 1117 } else 1118 super.removeChild(name, value); 1119 1120 } 1121 1122 @Override 1123 public Base makeProperty(int hash, String name) throws FHIRException { 1124 switch (hash) { 1125 case 3059181: 1126 return getCodeElement(); 1127 case 1671764162: 1128 return getDisplayElement(); 1129 case -880905839: 1130 return addTarget(); 1131 default: 1132 return super.makeProperty(hash, name); 1133 } 1134 1135 } 1136 1137 @Override 1138 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1139 switch (hash) { 1140 case 3059181: 1141 /* code */ return new String[] { "code" }; 1142 case 1671764162: 1143 /* display */ return new String[] { "string" }; 1144 case -880905839: 1145 /* target */ return new String[] {}; 1146 default: 1147 return super.getTypesForProperty(hash, name); 1148 } 1149 1150 } 1151 1152 @Override 1153 public Base addChild(String name) throws FHIRException { 1154 if (name.equals("code")) { 1155 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.code"); 1156 } else if (name.equals("display")) { 1157 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.display"); 1158 } else if (name.equals("target")) { 1159 return addTarget(); 1160 } else 1161 return super.addChild(name); 1162 } 1163 1164 public SourceElementComponent copy() { 1165 SourceElementComponent dst = new SourceElementComponent(); 1166 copyValues(dst); 1167 return dst; 1168 } 1169 1170 public void copyValues(SourceElementComponent dst) { 1171 super.copyValues(dst); 1172 dst.code = code == null ? null : code.copy(); 1173 dst.display = display == null ? null : display.copy(); 1174 if (target != null) { 1175 dst.target = new ArrayList<TargetElementComponent>(); 1176 for (TargetElementComponent i : target) 1177 dst.target.add(i.copy()); 1178 } 1179 ; 1180 } 1181 1182 @Override 1183 public boolean equalsDeep(Base other_) { 1184 if (!super.equalsDeep(other_)) 1185 return false; 1186 if (!(other_ instanceof SourceElementComponent)) 1187 return false; 1188 SourceElementComponent o = (SourceElementComponent) other_; 1189 return compareDeep(code, o.code, true) && compareDeep(display, o.display, true) 1190 && compareDeep(target, o.target, true); 1191 } 1192 1193 @Override 1194 public boolean equalsShallow(Base other_) { 1195 if (!super.equalsShallow(other_)) 1196 return false; 1197 if (!(other_ instanceof SourceElementComponent)) 1198 return false; 1199 SourceElementComponent o = (SourceElementComponent) other_; 1200 return compareValues(code, o.code, true) && compareValues(display, o.display, true); 1201 } 1202 1203 public boolean isEmpty() { 1204 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, display, target); 1205 } 1206 1207 public String fhirType() { 1208 return "ConceptMap.group.element"; 1209 1210 } 1211 1212 } 1213 1214 @Block() 1215 public static class TargetElementComponent extends BackboneElement implements IBaseBackboneElement { 1216 /** 1217 * Identity (code or path) or the element/item that the map refers to. 1218 */ 1219 @Child(name = "code", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1220 @Description(shortDefinition = "Code that identifies the target element", formalDefinition = "Identity (code or path) or the element/item that the map refers to.") 1221 protected CodeType code; 1222 1223 /** 1224 * The display for the code. The display is only provided to help editors when 1225 * editing the concept map. 1226 */ 1227 @Child(name = "display", type = { 1228 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1229 @Description(shortDefinition = "Display for the code", formalDefinition = "The display for the code. The display is only provided to help editors when editing the concept map.") 1230 protected StringType display; 1231 1232 /** 1233 * The equivalence between the source and target concepts (counting for the 1234 * dependencies and products). The equivalence is read from target to source 1235 * (e.g. the target is 'wider' than the source). 1236 */ 1237 @Child(name = "equivalence", type = { 1238 CodeType.class }, order = 3, min = 1, max = 1, modifier = true, summary = false) 1239 @Description(shortDefinition = "relatedto | equivalent | equal | wider | subsumes | narrower | specializes | inexact | unmatched | disjoint", formalDefinition = "The equivalence between the source and target concepts (counting for the dependencies and products). The equivalence is read from target to source (e.g. the target is 'wider' than the source).") 1240 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/concept-map-equivalence") 1241 protected Enumeration<ConceptMapEquivalence> equivalence; 1242 1243 /** 1244 * A description of status/issues in mapping that conveys additional information 1245 * not represented in the structured data. 1246 */ 1247 @Child(name = "comment", type = { 1248 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1249 @Description(shortDefinition = "Description of status/issues in mapping", formalDefinition = "A description of status/issues in mapping that conveys additional information not represented in the structured data.") 1250 protected StringType comment; 1251 1252 /** 1253 * A set of additional dependencies for this mapping to hold. This mapping is 1254 * only applicable if the specified element can be resolved, and it has the 1255 * specified value. 1256 */ 1257 @Child(name = "dependsOn", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1258 @Description(shortDefinition = "Other elements required for this mapping (from context)", formalDefinition = "A set of additional dependencies for this mapping to hold. This mapping is only applicable if the specified element can be resolved, and it has the specified value.") 1259 protected List<OtherElementComponent> dependsOn; 1260 1261 /** 1262 * A set of additional outcomes from this mapping to other elements. To properly 1263 * execute this mapping, the specified element must be mapped to some data 1264 * element or source that is in context. The mapping may still be useful without 1265 * a place for the additional data elements, but the equivalence cannot be 1266 * relied on. 1267 */ 1268 @Child(name = "product", type = { 1269 OtherElementComponent.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1270 @Description(shortDefinition = "Other concepts that this mapping also produces", formalDefinition = "A set of additional outcomes from this mapping to other elements. To properly execute this mapping, the specified element must be mapped to some data element or source that is in context. The mapping may still be useful without a place for the additional data elements, but the equivalence cannot be relied on.") 1271 protected List<OtherElementComponent> product; 1272 1273 private static final long serialVersionUID = -2008997477L; 1274 1275 /** 1276 * Constructor 1277 */ 1278 public TargetElementComponent() { 1279 super(); 1280 } 1281 1282 /** 1283 * Constructor 1284 */ 1285 public TargetElementComponent(Enumeration<ConceptMapEquivalence> equivalence) { 1286 super(); 1287 this.equivalence = equivalence; 1288 } 1289 1290 /** 1291 * @return {@link #code} (Identity (code or path) or the element/item that the 1292 * map refers to.). This is the underlying object with id, value and 1293 * extensions. The accessor "getCode" gives direct access to the value 1294 */ 1295 public CodeType getCodeElement() { 1296 if (this.code == null) 1297 if (Configuration.errorOnAutoCreate()) 1298 throw new Error("Attempt to auto-create TargetElementComponent.code"); 1299 else if (Configuration.doAutoCreate()) 1300 this.code = new CodeType(); // bb 1301 return this.code; 1302 } 1303 1304 public boolean hasCodeElement() { 1305 return this.code != null && !this.code.isEmpty(); 1306 } 1307 1308 public boolean hasCode() { 1309 return this.code != null && !this.code.isEmpty(); 1310 } 1311 1312 /** 1313 * @param value {@link #code} (Identity (code or path) or the element/item that 1314 * the map refers to.). This is the underlying object with id, 1315 * value and extensions. The accessor "getCode" gives direct access 1316 * to the value 1317 */ 1318 public TargetElementComponent setCodeElement(CodeType value) { 1319 this.code = value; 1320 return this; 1321 } 1322 1323 /** 1324 * @return Identity (code or path) or the element/item that the map refers to. 1325 */ 1326 public String getCode() { 1327 return this.code == null ? null : this.code.getValue(); 1328 } 1329 1330 /** 1331 * @param value Identity (code or path) or the element/item that the map refers 1332 * to. 1333 */ 1334 public TargetElementComponent setCode(String value) { 1335 if (Utilities.noString(value)) 1336 this.code = null; 1337 else { 1338 if (this.code == null) 1339 this.code = new CodeType(); 1340 this.code.setValue(value); 1341 } 1342 return this; 1343 } 1344 1345 /** 1346 * @return {@link #display} (The display for the code. The display is only 1347 * provided to help editors when editing the concept map.). This is the 1348 * underlying object with id, value and extensions. The accessor 1349 * "getDisplay" gives direct access to the value 1350 */ 1351 public StringType getDisplayElement() { 1352 if (this.display == null) 1353 if (Configuration.errorOnAutoCreate()) 1354 throw new Error("Attempt to auto-create TargetElementComponent.display"); 1355 else if (Configuration.doAutoCreate()) 1356 this.display = new StringType(); // bb 1357 return this.display; 1358 } 1359 1360 public boolean hasDisplayElement() { 1361 return this.display != null && !this.display.isEmpty(); 1362 } 1363 1364 public boolean hasDisplay() { 1365 return this.display != null && !this.display.isEmpty(); 1366 } 1367 1368 /** 1369 * @param value {@link #display} (The display for the code. The display is only 1370 * provided to help editors when editing the concept map.). This is 1371 * the underlying object with id, value and extensions. The 1372 * accessor "getDisplay" gives direct access to the value 1373 */ 1374 public TargetElementComponent setDisplayElement(StringType value) { 1375 this.display = value; 1376 return this; 1377 } 1378 1379 /** 1380 * @return The display for the code. The display is only provided to help 1381 * editors when editing the concept map. 1382 */ 1383 public String getDisplay() { 1384 return this.display == null ? null : this.display.getValue(); 1385 } 1386 1387 /** 1388 * @param value The display for the code. The display is only provided to help 1389 * editors when editing the concept map. 1390 */ 1391 public TargetElementComponent setDisplay(String value) { 1392 if (Utilities.noString(value)) 1393 this.display = null; 1394 else { 1395 if (this.display == null) 1396 this.display = new StringType(); 1397 this.display.setValue(value); 1398 } 1399 return this; 1400 } 1401 1402 /** 1403 * @return {@link #equivalence} (The equivalence between the source and target 1404 * concepts (counting for the dependencies and products). The 1405 * equivalence is read from target to source (e.g. the target is 'wider' 1406 * than the source).). This is the underlying object with id, value and 1407 * extensions. The accessor "getEquivalence" gives direct access to the 1408 * value 1409 */ 1410 public Enumeration<ConceptMapEquivalence> getEquivalenceElement() { 1411 if (this.equivalence == null) 1412 if (Configuration.errorOnAutoCreate()) 1413 throw new Error("Attempt to auto-create TargetElementComponent.equivalence"); 1414 else if (Configuration.doAutoCreate()) 1415 this.equivalence = new Enumeration<ConceptMapEquivalence>(new ConceptMapEquivalenceEnumFactory()); // bb 1416 return this.equivalence; 1417 } 1418 1419 public boolean hasEquivalenceElement() { 1420 return this.equivalence != null && !this.equivalence.isEmpty(); 1421 } 1422 1423 public boolean hasEquivalence() { 1424 return this.equivalence != null && !this.equivalence.isEmpty(); 1425 } 1426 1427 /** 1428 * @param value {@link #equivalence} (The equivalence between the source and 1429 * target concepts (counting for the dependencies and products). 1430 * The equivalence is read from target to source (e.g. the target 1431 * is 'wider' than the source).). This is the underlying object 1432 * with id, value and extensions. The accessor "getEquivalence" 1433 * gives direct access to the value 1434 */ 1435 public TargetElementComponent setEquivalenceElement(Enumeration<ConceptMapEquivalence> value) { 1436 this.equivalence = value; 1437 return this; 1438 } 1439 1440 /** 1441 * @return The equivalence between the source and target concepts (counting for 1442 * the dependencies and products). The equivalence is read from target 1443 * to source (e.g. the target is 'wider' than the source). 1444 */ 1445 public ConceptMapEquivalence getEquivalence() { 1446 return this.equivalence == null ? null : this.equivalence.getValue(); 1447 } 1448 1449 /** 1450 * @param value The equivalence between the source and target concepts (counting 1451 * for the dependencies and products). The equivalence is read from 1452 * target to source (e.g. the target is 'wider' than the source). 1453 */ 1454 public TargetElementComponent setEquivalence(ConceptMapEquivalence value) { 1455 if (this.equivalence == null) 1456 this.equivalence = new Enumeration<ConceptMapEquivalence>(new ConceptMapEquivalenceEnumFactory()); 1457 this.equivalence.setValue(value); 1458 return this; 1459 } 1460 1461 /** 1462 * @return {@link #comment} (A description of status/issues in mapping that 1463 * conveys additional information not represented in the structured 1464 * data.). This is the underlying object with id, value and extensions. 1465 * The accessor "getComment" gives direct access to the value 1466 */ 1467 public StringType getCommentElement() { 1468 if (this.comment == null) 1469 if (Configuration.errorOnAutoCreate()) 1470 throw new Error("Attempt to auto-create TargetElementComponent.comment"); 1471 else if (Configuration.doAutoCreate()) 1472 this.comment = new StringType(); // bb 1473 return this.comment; 1474 } 1475 1476 public boolean hasCommentElement() { 1477 return this.comment != null && !this.comment.isEmpty(); 1478 } 1479 1480 public boolean hasComment() { 1481 return this.comment != null && !this.comment.isEmpty(); 1482 } 1483 1484 /** 1485 * @param value {@link #comment} (A description of status/issues in mapping that 1486 * conveys additional information not represented in the structured 1487 * data.). This is the underlying object with id, value and 1488 * extensions. The accessor "getComment" gives direct access to the 1489 * value 1490 */ 1491 public TargetElementComponent setCommentElement(StringType value) { 1492 this.comment = value; 1493 return this; 1494 } 1495 1496 /** 1497 * @return A description of status/issues in mapping that conveys additional 1498 * information not represented in the structured data. 1499 */ 1500 public String getComment() { 1501 return this.comment == null ? null : this.comment.getValue(); 1502 } 1503 1504 /** 1505 * @param value A description of status/issues in mapping that conveys 1506 * additional information not represented in the structured data. 1507 */ 1508 public TargetElementComponent setComment(String value) { 1509 if (Utilities.noString(value)) 1510 this.comment = null; 1511 else { 1512 if (this.comment == null) 1513 this.comment = new StringType(); 1514 this.comment.setValue(value); 1515 } 1516 return this; 1517 } 1518 1519 /** 1520 * @return {@link #dependsOn} (A set of additional dependencies for this mapping 1521 * to hold. This mapping is only applicable if the specified element can 1522 * be resolved, and it has the specified value.) 1523 */ 1524 public List<OtherElementComponent> getDependsOn() { 1525 if (this.dependsOn == null) 1526 this.dependsOn = new ArrayList<OtherElementComponent>(); 1527 return this.dependsOn; 1528 } 1529 1530 /** 1531 * @return Returns a reference to <code>this</code> for easy method chaining 1532 */ 1533 public TargetElementComponent setDependsOn(List<OtherElementComponent> theDependsOn) { 1534 this.dependsOn = theDependsOn; 1535 return this; 1536 } 1537 1538 public boolean hasDependsOn() { 1539 if (this.dependsOn == null) 1540 return false; 1541 for (OtherElementComponent item : this.dependsOn) 1542 if (!item.isEmpty()) 1543 return true; 1544 return false; 1545 } 1546 1547 public OtherElementComponent addDependsOn() { // 3 1548 OtherElementComponent t = new OtherElementComponent(); 1549 if (this.dependsOn == null) 1550 this.dependsOn = new ArrayList<OtherElementComponent>(); 1551 this.dependsOn.add(t); 1552 return t; 1553 } 1554 1555 public TargetElementComponent addDependsOn(OtherElementComponent t) { // 3 1556 if (t == null) 1557 return this; 1558 if (this.dependsOn == null) 1559 this.dependsOn = new ArrayList<OtherElementComponent>(); 1560 this.dependsOn.add(t); 1561 return this; 1562 } 1563 1564 /** 1565 * @return The first repetition of repeating field {@link #dependsOn}, creating 1566 * it if it does not already exist 1567 */ 1568 public OtherElementComponent getDependsOnFirstRep() { 1569 if (getDependsOn().isEmpty()) { 1570 addDependsOn(); 1571 } 1572 return getDependsOn().get(0); 1573 } 1574 1575 /** 1576 * @return {@link #product} (A set of additional outcomes from this mapping to 1577 * other elements. To properly execute this mapping, the specified 1578 * element must be mapped to some data element or source that is in 1579 * context. The mapping may still be useful without a place for the 1580 * additional data elements, but the equivalence cannot be relied on.) 1581 */ 1582 public List<OtherElementComponent> getProduct() { 1583 if (this.product == null) 1584 this.product = new ArrayList<OtherElementComponent>(); 1585 return this.product; 1586 } 1587 1588 /** 1589 * @return Returns a reference to <code>this</code> for easy method chaining 1590 */ 1591 public TargetElementComponent setProduct(List<OtherElementComponent> theProduct) { 1592 this.product = theProduct; 1593 return this; 1594 } 1595 1596 public boolean hasProduct() { 1597 if (this.product == null) 1598 return false; 1599 for (OtherElementComponent item : this.product) 1600 if (!item.isEmpty()) 1601 return true; 1602 return false; 1603 } 1604 1605 public OtherElementComponent addProduct() { // 3 1606 OtherElementComponent t = new OtherElementComponent(); 1607 if (this.product == null) 1608 this.product = new ArrayList<OtherElementComponent>(); 1609 this.product.add(t); 1610 return t; 1611 } 1612 1613 public TargetElementComponent addProduct(OtherElementComponent t) { // 3 1614 if (t == null) 1615 return this; 1616 if (this.product == null) 1617 this.product = new ArrayList<OtherElementComponent>(); 1618 this.product.add(t); 1619 return this; 1620 } 1621 1622 /** 1623 * @return The first repetition of repeating field {@link #product}, creating it 1624 * if it does not already exist 1625 */ 1626 public OtherElementComponent getProductFirstRep() { 1627 if (getProduct().isEmpty()) { 1628 addProduct(); 1629 } 1630 return getProduct().get(0); 1631 } 1632 1633 protected void listChildren(List<Property> children) { 1634 super.listChildren(children); 1635 children.add(new Property("code", "code", "Identity (code or path) or the element/item that the map refers to.", 1636 0, 1, code)); 1637 children.add(new Property("display", "string", 1638 "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 1, 1639 display)); 1640 children.add(new Property("equivalence", "code", 1641 "The equivalence between the source and target concepts (counting for the dependencies and products). The equivalence is read from target to source (e.g. the target is 'wider' than the source).", 1642 0, 1, equivalence)); 1643 children.add(new Property("comment", "string", 1644 "A description of status/issues in mapping that conveys additional information not represented in the structured data.", 1645 0, 1, comment)); 1646 children.add(new Property("dependsOn", "", 1647 "A set of additional dependencies for this mapping to hold. This mapping is only applicable if the specified element can be resolved, and it has the specified value.", 1648 0, java.lang.Integer.MAX_VALUE, dependsOn)); 1649 children.add(new Property("product", "@ConceptMap.group.element.target.dependsOn", 1650 "A set of additional outcomes from this mapping to other elements. To properly execute this mapping, the specified element must be mapped to some data element or source that is in context. The mapping may still be useful without a place for the additional data elements, but the equivalence cannot be relied on.", 1651 0, java.lang.Integer.MAX_VALUE, product)); 1652 } 1653 1654 @Override 1655 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1656 switch (_hash) { 1657 case 3059181: 1658 /* code */ return new Property("code", "code", 1659 "Identity (code or path) or the element/item that the map refers to.", 0, 1, code); 1660 case 1671764162: 1661 /* display */ return new Property("display", "string", 1662 "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 1663 1, display); 1664 case -15828692: 1665 /* equivalence */ return new Property("equivalence", "code", 1666 "The equivalence between the source and target concepts (counting for the dependencies and products). The equivalence is read from target to source (e.g. the target is 'wider' than the source).", 1667 0, 1, equivalence); 1668 case 950398559: 1669 /* comment */ return new Property("comment", "string", 1670 "A description of status/issues in mapping that conveys additional information not represented in the structured data.", 1671 0, 1, comment); 1672 case -1109214266: 1673 /* dependsOn */ return new Property("dependsOn", "", 1674 "A set of additional dependencies for this mapping to hold. This mapping is only applicable if the specified element can be resolved, and it has the specified value.", 1675 0, java.lang.Integer.MAX_VALUE, dependsOn); 1676 case -309474065: 1677 /* product */ return new Property("product", "@ConceptMap.group.element.target.dependsOn", 1678 "A set of additional outcomes from this mapping to other elements. To properly execute this mapping, the specified element must be mapped to some data element or source that is in context. The mapping may still be useful without a place for the additional data elements, but the equivalence cannot be relied on.", 1679 0, java.lang.Integer.MAX_VALUE, product); 1680 default: 1681 return super.getNamedProperty(_hash, _name, _checkValid); 1682 } 1683 1684 } 1685 1686 @Override 1687 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1688 switch (hash) { 1689 case 3059181: 1690 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeType 1691 case 1671764162: 1692 /* display */ return this.display == null ? new Base[0] : new Base[] { this.display }; // StringType 1693 case -15828692: 1694 /* equivalence */ return this.equivalence == null ? new Base[0] : new Base[] { this.equivalence }; // Enumeration<ConceptMapEquivalence> 1695 case 950398559: 1696 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // StringType 1697 case -1109214266: 1698 /* dependsOn */ return this.dependsOn == null ? new Base[0] 1699 : this.dependsOn.toArray(new Base[this.dependsOn.size()]); // OtherElementComponent 1700 case -309474065: 1701 /* product */ return this.product == null ? new Base[0] : this.product.toArray(new Base[this.product.size()]); // OtherElementComponent 1702 default: 1703 return super.getProperty(hash, name, checkValid); 1704 } 1705 1706 } 1707 1708 @Override 1709 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1710 switch (hash) { 1711 case 3059181: // code 1712 this.code = castToCode(value); // CodeType 1713 return value; 1714 case 1671764162: // display 1715 this.display = castToString(value); // StringType 1716 return value; 1717 case -15828692: // equivalence 1718 value = new ConceptMapEquivalenceEnumFactory().fromType(castToCode(value)); 1719 this.equivalence = (Enumeration) value; // Enumeration<ConceptMapEquivalence> 1720 return value; 1721 case 950398559: // comment 1722 this.comment = castToString(value); // StringType 1723 return value; 1724 case -1109214266: // dependsOn 1725 this.getDependsOn().add((OtherElementComponent) value); // OtherElementComponent 1726 return value; 1727 case -309474065: // product 1728 this.getProduct().add((OtherElementComponent) value); // OtherElementComponent 1729 return value; 1730 default: 1731 return super.setProperty(hash, name, value); 1732 } 1733 1734 } 1735 1736 @Override 1737 public Base setProperty(String name, Base value) throws FHIRException { 1738 if (name.equals("code")) { 1739 this.code = castToCode(value); // CodeType 1740 } else if (name.equals("display")) { 1741 this.display = castToString(value); // StringType 1742 } else if (name.equals("equivalence")) { 1743 value = new ConceptMapEquivalenceEnumFactory().fromType(castToCode(value)); 1744 this.equivalence = (Enumeration) value; // Enumeration<ConceptMapEquivalence> 1745 } else if (name.equals("comment")) { 1746 this.comment = castToString(value); // StringType 1747 } else if (name.equals("dependsOn")) { 1748 this.getDependsOn().add((OtherElementComponent) value); 1749 } else if (name.equals("product")) { 1750 this.getProduct().add((OtherElementComponent) value); 1751 } else 1752 return super.setProperty(name, value); 1753 return value; 1754 } 1755 1756 @Override 1757 public void removeChild(String name, Base value) throws FHIRException { 1758 if (name.equals("code")) { 1759 this.code = null; 1760 } else if (name.equals("display")) { 1761 this.display = null; 1762 } else if (name.equals("equivalence")) { 1763 this.equivalence = null; 1764 } else if (name.equals("comment")) { 1765 this.comment = null; 1766 } else if (name.equals("dependsOn")) { 1767 this.getDependsOn().remove((OtherElementComponent) value); 1768 } else if (name.equals("product")) { 1769 this.getProduct().remove((OtherElementComponent) value); 1770 } else 1771 super.removeChild(name, value); 1772 1773 } 1774 1775 @Override 1776 public Base makeProperty(int hash, String name) throws FHIRException { 1777 switch (hash) { 1778 case 3059181: 1779 return getCodeElement(); 1780 case 1671764162: 1781 return getDisplayElement(); 1782 case -15828692: 1783 return getEquivalenceElement(); 1784 case 950398559: 1785 return getCommentElement(); 1786 case -1109214266: 1787 return addDependsOn(); 1788 case -309474065: 1789 return addProduct(); 1790 default: 1791 return super.makeProperty(hash, name); 1792 } 1793 1794 } 1795 1796 @Override 1797 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1798 switch (hash) { 1799 case 3059181: 1800 /* code */ return new String[] { "code" }; 1801 case 1671764162: 1802 /* display */ return new String[] { "string" }; 1803 case -15828692: 1804 /* equivalence */ return new String[] { "code" }; 1805 case 950398559: 1806 /* comment */ return new String[] { "string" }; 1807 case -1109214266: 1808 /* dependsOn */ return new String[] {}; 1809 case -309474065: 1810 /* product */ return new String[] { "@ConceptMap.group.element.target.dependsOn" }; 1811 default: 1812 return super.getTypesForProperty(hash, name); 1813 } 1814 1815 } 1816 1817 @Override 1818 public Base addChild(String name) throws FHIRException { 1819 if (name.equals("code")) { 1820 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.code"); 1821 } else if (name.equals("display")) { 1822 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.display"); 1823 } else if (name.equals("equivalence")) { 1824 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.equivalence"); 1825 } else if (name.equals("comment")) { 1826 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.comment"); 1827 } else if (name.equals("dependsOn")) { 1828 return addDependsOn(); 1829 } else if (name.equals("product")) { 1830 return addProduct(); 1831 } else 1832 return super.addChild(name); 1833 } 1834 1835 public TargetElementComponent copy() { 1836 TargetElementComponent dst = new TargetElementComponent(); 1837 copyValues(dst); 1838 return dst; 1839 } 1840 1841 public void copyValues(TargetElementComponent dst) { 1842 super.copyValues(dst); 1843 dst.code = code == null ? null : code.copy(); 1844 dst.display = display == null ? null : display.copy(); 1845 dst.equivalence = equivalence == null ? null : equivalence.copy(); 1846 dst.comment = comment == null ? null : comment.copy(); 1847 if (dependsOn != null) { 1848 dst.dependsOn = new ArrayList<OtherElementComponent>(); 1849 for (OtherElementComponent i : dependsOn) 1850 dst.dependsOn.add(i.copy()); 1851 } 1852 ; 1853 if (product != null) { 1854 dst.product = new ArrayList<OtherElementComponent>(); 1855 for (OtherElementComponent i : product) 1856 dst.product.add(i.copy()); 1857 } 1858 ; 1859 } 1860 1861 @Override 1862 public boolean equalsDeep(Base other_) { 1863 if (!super.equalsDeep(other_)) 1864 return false; 1865 if (!(other_ instanceof TargetElementComponent)) 1866 return false; 1867 TargetElementComponent o = (TargetElementComponent) other_; 1868 return compareDeep(code, o.code, true) && compareDeep(display, o.display, true) 1869 && compareDeep(equivalence, o.equivalence, true) && compareDeep(comment, o.comment, true) 1870 && compareDeep(dependsOn, o.dependsOn, true) && compareDeep(product, o.product, true); 1871 } 1872 1873 @Override 1874 public boolean equalsShallow(Base other_) { 1875 if (!super.equalsShallow(other_)) 1876 return false; 1877 if (!(other_ instanceof TargetElementComponent)) 1878 return false; 1879 TargetElementComponent o = (TargetElementComponent) other_; 1880 return compareValues(code, o.code, true) && compareValues(display, o.display, true) 1881 && compareValues(equivalence, o.equivalence, true) && compareValues(comment, o.comment, true); 1882 } 1883 1884 public boolean isEmpty() { 1885 return super.isEmpty() 1886 && ca.uhn.fhir.util.ElementUtil.isEmpty(code, display, equivalence, comment, dependsOn, product); 1887 } 1888 1889 public String fhirType() { 1890 return "ConceptMap.group.element.target"; 1891 1892 } 1893 1894 } 1895 1896 @Block() 1897 public static class OtherElementComponent extends BackboneElement implements IBaseBackboneElement { 1898 /** 1899 * A reference to an element that holds a coded value that corresponds to a code 1900 * system property. The idea is that the information model carries an element 1901 * somewhere that is labeled to correspond with a code system property. 1902 */ 1903 @Child(name = "property", type = { UriType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1904 @Description(shortDefinition = "Reference to property mapping depends on", formalDefinition = "A reference to an element that holds a coded value that corresponds to a code system property. The idea is that the information model carries an element somewhere that is labeled to correspond with a code system property.") 1905 protected UriType property; 1906 1907 /** 1908 * An absolute URI that identifies the code system of the dependency code (if 1909 * the source/dependency is a value set that crosses code systems). 1910 */ 1911 @Child(name = "system", type = { 1912 CanonicalType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1913 @Description(shortDefinition = "Code System (if necessary)", formalDefinition = "An absolute URI that identifies the code system of the dependency code (if the source/dependency is a value set that crosses code systems).") 1914 protected CanonicalType system; 1915 1916 /** 1917 * Identity (code or path) or the element/item/ValueSet/text that the map 1918 * depends on / refers to. 1919 */ 1920 @Child(name = "value", type = { StringType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 1921 @Description(shortDefinition = "Value of the referenced element", formalDefinition = "Identity (code or path) or the element/item/ValueSet/text that the map depends on / refers to.") 1922 protected StringType value; 1923 1924 /** 1925 * The display for the code. The display is only provided to help editors when 1926 * editing the concept map. 1927 */ 1928 @Child(name = "display", type = { 1929 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1930 @Description(shortDefinition = "Display for the code (if value is a code)", formalDefinition = "The display for the code. The display is only provided to help editors when editing the concept map.") 1931 protected StringType display; 1932 1933 private static final long serialVersionUID = -1836341923L; 1934 1935 /** 1936 * Constructor 1937 */ 1938 public OtherElementComponent() { 1939 super(); 1940 } 1941 1942 /** 1943 * Constructor 1944 */ 1945 public OtherElementComponent(UriType property, StringType value) { 1946 super(); 1947 this.property = property; 1948 this.value = value; 1949 } 1950 1951 /** 1952 * @return {@link #property} (A reference to an element that holds a coded value 1953 * that corresponds to a code system property. The idea is that the 1954 * information model carries an element somewhere that is labeled to 1955 * correspond with a code system property.). This is the underlying 1956 * object with id, value and extensions. The accessor "getProperty" 1957 * gives direct access to the value 1958 */ 1959 public UriType getPropertyElement() { 1960 if (this.property == null) 1961 if (Configuration.errorOnAutoCreate()) 1962 throw new Error("Attempt to auto-create OtherElementComponent.property"); 1963 else if (Configuration.doAutoCreate()) 1964 this.property = new UriType(); // bb 1965 return this.property; 1966 } 1967 1968 public boolean hasPropertyElement() { 1969 return this.property != null && !this.property.isEmpty(); 1970 } 1971 1972 public boolean hasProperty() { 1973 return this.property != null && !this.property.isEmpty(); 1974 } 1975 1976 /** 1977 * @param value {@link #property} (A reference to an element that holds a coded 1978 * value that corresponds to a code system property. The idea is 1979 * that the information model carries an element somewhere that is 1980 * labeled to correspond with a code system property.). This is the 1981 * underlying object with id, value and extensions. The accessor 1982 * "getProperty" gives direct access to the value 1983 */ 1984 public OtherElementComponent setPropertyElement(UriType value) { 1985 this.property = value; 1986 return this; 1987 } 1988 1989 /** 1990 * @return A reference to an element that holds a coded value that corresponds 1991 * to a code system property. The idea is that the information model 1992 * carries an element somewhere that is labeled to correspond with a 1993 * code system property. 1994 */ 1995 public String getProperty() { 1996 return this.property == null ? null : this.property.getValue(); 1997 } 1998 1999 /** 2000 * @param value A reference to an element that holds a coded value that 2001 * corresponds to a code system property. The idea is that the 2002 * information model carries an element somewhere that is labeled 2003 * to correspond with a code system property. 2004 */ 2005 public OtherElementComponent setProperty(String value) { 2006 if (this.property == null) 2007 this.property = new UriType(); 2008 this.property.setValue(value); 2009 return this; 2010 } 2011 2012 /** 2013 * @return {@link #system} (An absolute URI that identifies the code system of 2014 * the dependency code (if the source/dependency is a value set that 2015 * crosses code systems).). This is the underlying object with id, value 2016 * and extensions. The accessor "getSystem" gives direct access to the 2017 * value 2018 */ 2019 public CanonicalType getSystemElement() { 2020 if (this.system == null) 2021 if (Configuration.errorOnAutoCreate()) 2022 throw new Error("Attempt to auto-create OtherElementComponent.system"); 2023 else if (Configuration.doAutoCreate()) 2024 this.system = new CanonicalType(); // bb 2025 return this.system; 2026 } 2027 2028 public boolean hasSystemElement() { 2029 return this.system != null && !this.system.isEmpty(); 2030 } 2031 2032 public boolean hasSystem() { 2033 return this.system != null && !this.system.isEmpty(); 2034 } 2035 2036 /** 2037 * @param value {@link #system} (An absolute URI that identifies the code system 2038 * of the dependency code (if the source/dependency is a value set 2039 * that crosses code systems).). This is the underlying object with 2040 * id, value and extensions. The accessor "getSystem" gives direct 2041 * access to the value 2042 */ 2043 public OtherElementComponent setSystemElement(CanonicalType value) { 2044 this.system = value; 2045 return this; 2046 } 2047 2048 /** 2049 * @return An absolute URI that identifies the code system of the dependency 2050 * code (if the source/dependency is a value set that crosses code 2051 * systems). 2052 */ 2053 public String getSystem() { 2054 return this.system == null ? null : this.system.getValue(); 2055 } 2056 2057 /** 2058 * @param value An absolute URI that identifies the code system of the 2059 * dependency code (if the source/dependency is a value set that 2060 * crosses code systems). 2061 */ 2062 public OtherElementComponent setSystem(String value) { 2063 if (Utilities.noString(value)) 2064 this.system = null; 2065 else { 2066 if (this.system == null) 2067 this.system = new CanonicalType(); 2068 this.system.setValue(value); 2069 } 2070 return this; 2071 } 2072 2073 /** 2074 * @return {@link #value} (Identity (code or path) or the 2075 * element/item/ValueSet/text that the map depends on / refers to.). 2076 * This is the underlying object with id, value and extensions. The 2077 * accessor "getValue" gives direct access to the value 2078 */ 2079 public StringType getValueElement() { 2080 if (this.value == null) 2081 if (Configuration.errorOnAutoCreate()) 2082 throw new Error("Attempt to auto-create OtherElementComponent.value"); 2083 else if (Configuration.doAutoCreate()) 2084 this.value = new StringType(); // bb 2085 return this.value; 2086 } 2087 2088 public boolean hasValueElement() { 2089 return this.value != null && !this.value.isEmpty(); 2090 } 2091 2092 public boolean hasValue() { 2093 return this.value != null && !this.value.isEmpty(); 2094 } 2095 2096 /** 2097 * @param value {@link #value} (Identity (code or path) or the 2098 * element/item/ValueSet/text that the map depends on / refers 2099 * to.). This is the underlying object with id, value and 2100 * extensions. The accessor "getValue" gives direct access to the 2101 * value 2102 */ 2103 public OtherElementComponent setValueElement(StringType value) { 2104 this.value = value; 2105 return this; 2106 } 2107 2108 /** 2109 * @return Identity (code or path) or the element/item/ValueSet/text that the 2110 * map depends on / refers to. 2111 */ 2112 public String getValue() { 2113 return this.value == null ? null : this.value.getValue(); 2114 } 2115 2116 /** 2117 * @param value Identity (code or path) or the element/item/ValueSet/text that 2118 * the map depends on / refers to. 2119 */ 2120 public OtherElementComponent setValue(String value) { 2121 if (this.value == null) 2122 this.value = new StringType(); 2123 this.value.setValue(value); 2124 return this; 2125 } 2126 2127 /** 2128 * @return {@link #display} (The display for the code. The display is only 2129 * provided to help editors when editing the concept map.). This is the 2130 * underlying object with id, value and extensions. The accessor 2131 * "getDisplay" gives direct access to the value 2132 */ 2133 public StringType getDisplayElement() { 2134 if (this.display == null) 2135 if (Configuration.errorOnAutoCreate()) 2136 throw new Error("Attempt to auto-create OtherElementComponent.display"); 2137 else if (Configuration.doAutoCreate()) 2138 this.display = new StringType(); // bb 2139 return this.display; 2140 } 2141 2142 public boolean hasDisplayElement() { 2143 return this.display != null && !this.display.isEmpty(); 2144 } 2145 2146 public boolean hasDisplay() { 2147 return this.display != null && !this.display.isEmpty(); 2148 } 2149 2150 /** 2151 * @param value {@link #display} (The display for the code. The display is only 2152 * provided to help editors when editing the concept map.). This is 2153 * the underlying object with id, value and extensions. The 2154 * accessor "getDisplay" gives direct access to the value 2155 */ 2156 public OtherElementComponent setDisplayElement(StringType value) { 2157 this.display = value; 2158 return this; 2159 } 2160 2161 /** 2162 * @return The display for the code. The display is only provided to help 2163 * editors when editing the concept map. 2164 */ 2165 public String getDisplay() { 2166 return this.display == null ? null : this.display.getValue(); 2167 } 2168 2169 /** 2170 * @param value The display for the code. The display is only provided to help 2171 * editors when editing the concept map. 2172 */ 2173 public OtherElementComponent setDisplay(String value) { 2174 if (Utilities.noString(value)) 2175 this.display = null; 2176 else { 2177 if (this.display == null) 2178 this.display = new StringType(); 2179 this.display.setValue(value); 2180 } 2181 return this; 2182 } 2183 2184 protected void listChildren(List<Property> children) { 2185 super.listChildren(children); 2186 children.add(new Property("property", "uri", 2187 "A reference to an element that holds a coded value that corresponds to a code system property. The idea is that the information model carries an element somewhere that is labeled to correspond with a code system property.", 2188 0, 1, property)); 2189 children.add(new Property("system", "canonical(CodeSystem)", 2190 "An absolute URI that identifies the code system of the dependency code (if the source/dependency is a value set that crosses code systems).", 2191 0, 1, system)); 2192 children.add(new Property("value", "string", 2193 "Identity (code or path) or the element/item/ValueSet/text that the map depends on / refers to.", 0, 1, 2194 value)); 2195 children.add(new Property("display", "string", 2196 "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 1, 2197 display)); 2198 } 2199 2200 @Override 2201 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2202 switch (_hash) { 2203 case -993141291: 2204 /* property */ return new Property("property", "uri", 2205 "A reference to an element that holds a coded value that corresponds to a code system property. The idea is that the information model carries an element somewhere that is labeled to correspond with a code system property.", 2206 0, 1, property); 2207 case -887328209: 2208 /* system */ return new Property("system", "canonical(CodeSystem)", 2209 "An absolute URI that identifies the code system of the dependency code (if the source/dependency is a value set that crosses code systems).", 2210 0, 1, system); 2211 case 111972721: 2212 /* value */ return new Property("value", "string", 2213 "Identity (code or path) or the element/item/ValueSet/text that the map depends on / refers to.", 0, 1, 2214 value); 2215 case 1671764162: 2216 /* display */ return new Property("display", "string", 2217 "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 2218 1, display); 2219 default: 2220 return super.getNamedProperty(_hash, _name, _checkValid); 2221 } 2222 2223 } 2224 2225 @Override 2226 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2227 switch (hash) { 2228 case -993141291: 2229 /* property */ return this.property == null ? new Base[0] : new Base[] { this.property }; // UriType 2230 case -887328209: 2231 /* system */ return this.system == null ? new Base[0] : new Base[] { this.system }; // CanonicalType 2232 case 111972721: 2233 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // StringType 2234 case 1671764162: 2235 /* display */ return this.display == null ? new Base[0] : new Base[] { this.display }; // StringType 2236 default: 2237 return super.getProperty(hash, name, checkValid); 2238 } 2239 2240 } 2241 2242 @Override 2243 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2244 switch (hash) { 2245 case -993141291: // property 2246 this.property = castToUri(value); // UriType 2247 return value; 2248 case -887328209: // system 2249 this.system = castToCanonical(value); // CanonicalType 2250 return value; 2251 case 111972721: // value 2252 this.value = castToString(value); // StringType 2253 return value; 2254 case 1671764162: // display 2255 this.display = castToString(value); // StringType 2256 return value; 2257 default: 2258 return super.setProperty(hash, name, value); 2259 } 2260 2261 } 2262 2263 @Override 2264 public Base setProperty(String name, Base value) throws FHIRException { 2265 if (name.equals("property")) { 2266 this.property = castToUri(value); // UriType 2267 } else if (name.equals("system")) { 2268 this.system = castToCanonical(value); // CanonicalType 2269 } else if (name.equals("value")) { 2270 this.value = castToString(value); // StringType 2271 } else if (name.equals("display")) { 2272 this.display = castToString(value); // StringType 2273 } else 2274 return super.setProperty(name, value); 2275 return value; 2276 } 2277 2278 @Override 2279 public void removeChild(String name, Base value) throws FHIRException { 2280 if (name.equals("property")) { 2281 this.property = null; 2282 } else if (name.equals("system")) { 2283 this.system = null; 2284 } else if (name.equals("value")) { 2285 this.value = null; 2286 } else if (name.equals("display")) { 2287 this.display = null; 2288 } else 2289 super.removeChild(name, value); 2290 2291 } 2292 2293 @Override 2294 public Base makeProperty(int hash, String name) throws FHIRException { 2295 switch (hash) { 2296 case -993141291: 2297 return getPropertyElement(); 2298 case -887328209: 2299 return getSystemElement(); 2300 case 111972721: 2301 return getValueElement(); 2302 case 1671764162: 2303 return getDisplayElement(); 2304 default: 2305 return super.makeProperty(hash, name); 2306 } 2307 2308 } 2309 2310 @Override 2311 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2312 switch (hash) { 2313 case -993141291: 2314 /* property */ return new String[] { "uri" }; 2315 case -887328209: 2316 /* system */ return new String[] { "canonical" }; 2317 case 111972721: 2318 /* value */ return new String[] { "string" }; 2319 case 1671764162: 2320 /* display */ return new String[] { "string" }; 2321 default: 2322 return super.getTypesForProperty(hash, name); 2323 } 2324 2325 } 2326 2327 @Override 2328 public Base addChild(String name) throws FHIRException { 2329 if (name.equals("property")) { 2330 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.property"); 2331 } else if (name.equals("system")) { 2332 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.system"); 2333 } else if (name.equals("value")) { 2334 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.value"); 2335 } else if (name.equals("display")) { 2336 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.display"); 2337 } else 2338 return super.addChild(name); 2339 } 2340 2341 public OtherElementComponent copy() { 2342 OtherElementComponent dst = new OtherElementComponent(); 2343 copyValues(dst); 2344 return dst; 2345 } 2346 2347 public void copyValues(OtherElementComponent dst) { 2348 super.copyValues(dst); 2349 dst.property = property == null ? null : property.copy(); 2350 dst.system = system == null ? null : system.copy(); 2351 dst.value = value == null ? null : value.copy(); 2352 dst.display = display == null ? null : display.copy(); 2353 } 2354 2355 @Override 2356 public boolean equalsDeep(Base other_) { 2357 if (!super.equalsDeep(other_)) 2358 return false; 2359 if (!(other_ instanceof OtherElementComponent)) 2360 return false; 2361 OtherElementComponent o = (OtherElementComponent) other_; 2362 return compareDeep(property, o.property, true) && compareDeep(system, o.system, true) 2363 && compareDeep(value, o.value, true) && compareDeep(display, o.display, true); 2364 } 2365 2366 @Override 2367 public boolean equalsShallow(Base other_) { 2368 if (!super.equalsShallow(other_)) 2369 return false; 2370 if (!(other_ instanceof OtherElementComponent)) 2371 return false; 2372 OtherElementComponent o = (OtherElementComponent) other_; 2373 return compareValues(property, o.property, true) && compareValues(value, o.value, true) 2374 && compareValues(display, o.display, true); 2375 } 2376 2377 public boolean isEmpty() { 2378 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(property, system, value, display); 2379 } 2380 2381 public String fhirType() { 2382 return "ConceptMap.group.element.target.dependsOn"; 2383 2384 } 2385 2386 } 2387 2388 @Block() 2389 public static class ConceptMapGroupUnmappedComponent extends BackboneElement implements IBaseBackboneElement { 2390 /** 2391 * Defines which action to take if there is no match for the source concept in 2392 * the target system designated for the group. One of 3 actions are possible: 2393 * use the unmapped code (this is useful when doing a mapping between versions, 2394 * and only a few codes have changed), use a fixed code (a default code), or 2395 * alternatively, a reference to a different concept map can be provided (by 2396 * canonical URL). 2397 */ 2398 @Child(name = "mode", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2399 @Description(shortDefinition = "provided | fixed | other-map", formalDefinition = "Defines which action to take if there is no match for the source concept in the target system designated for the group. One of 3 actions are possible: use the unmapped code (this is useful when doing a mapping between versions, and only a few codes have changed), use a fixed code (a default code), or alternatively, a reference to a different concept map can be provided (by canonical URL).") 2400 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/conceptmap-unmapped-mode") 2401 protected Enumeration<ConceptMapGroupUnmappedMode> mode; 2402 2403 /** 2404 * The fixed code to use when the mode = 'fixed' - all unmapped codes are mapped 2405 * to a single fixed code. 2406 */ 2407 @Child(name = "code", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2408 @Description(shortDefinition = "Fixed code when mode = fixed", formalDefinition = "The fixed code to use when the mode = 'fixed' - all unmapped codes are mapped to a single fixed code.") 2409 protected CodeType code; 2410 2411 /** 2412 * The display for the code. The display is only provided to help editors when 2413 * editing the concept map. 2414 */ 2415 @Child(name = "display", type = { 2416 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2417 @Description(shortDefinition = "Display for the code", formalDefinition = "The display for the code. The display is only provided to help editors when editing the concept map.") 2418 protected StringType display; 2419 2420 /** 2421 * The canonical reference to an additional ConceptMap resource instance to use 2422 * for mapping if this ConceptMap resource contains no matching mapping for the 2423 * source concept. 2424 */ 2425 @Child(name = "url", type = { CanonicalType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2426 @Description(shortDefinition = "canonical reference to an additional ConceptMap to use for mapping if the source concept is unmapped", formalDefinition = "The canonical reference to an additional ConceptMap resource instance to use for mapping if this ConceptMap resource contains no matching mapping for the source concept.") 2427 protected CanonicalType url; 2428 2429 private static final long serialVersionUID = 1261364354L; 2430 2431 /** 2432 * Constructor 2433 */ 2434 public ConceptMapGroupUnmappedComponent() { 2435 super(); 2436 } 2437 2438 /** 2439 * Constructor 2440 */ 2441 public ConceptMapGroupUnmappedComponent(Enumeration<ConceptMapGroupUnmappedMode> mode) { 2442 super(); 2443 this.mode = mode; 2444 } 2445 2446 /** 2447 * @return {@link #mode} (Defines which action to take if there is no match for 2448 * the source concept in the target system designated for the group. One 2449 * of 3 actions are possible: use the unmapped code (this is useful when 2450 * doing a mapping between versions, and only a few codes have changed), 2451 * use a fixed code (a default code), or alternatively, a reference to a 2452 * different concept map can be provided (by canonical URL).). This is 2453 * the underlying object with id, value and extensions. The accessor 2454 * "getMode" gives direct access to the value 2455 */ 2456 public Enumeration<ConceptMapGroupUnmappedMode> getModeElement() { 2457 if (this.mode == null) 2458 if (Configuration.errorOnAutoCreate()) 2459 throw new Error("Attempt to auto-create ConceptMapGroupUnmappedComponent.mode"); 2460 else if (Configuration.doAutoCreate()) 2461 this.mode = new Enumeration<ConceptMapGroupUnmappedMode>(new ConceptMapGroupUnmappedModeEnumFactory()); // bb 2462 return this.mode; 2463 } 2464 2465 public boolean hasModeElement() { 2466 return this.mode != null && !this.mode.isEmpty(); 2467 } 2468 2469 public boolean hasMode() { 2470 return this.mode != null && !this.mode.isEmpty(); 2471 } 2472 2473 /** 2474 * @param value {@link #mode} (Defines which action to take if there is no match 2475 * for the source concept in the target system designated for the 2476 * group. One of 3 actions are possible: use the unmapped code 2477 * (this is useful when doing a mapping between versions, and only 2478 * a few codes have changed), use a fixed code (a default code), or 2479 * alternatively, a reference to a different concept map can be 2480 * provided (by canonical URL).). This is the underlying object 2481 * with id, value and extensions. The accessor "getMode" gives 2482 * direct access to the value 2483 */ 2484 public ConceptMapGroupUnmappedComponent setModeElement(Enumeration<ConceptMapGroupUnmappedMode> value) { 2485 this.mode = value; 2486 return this; 2487 } 2488 2489 /** 2490 * @return Defines which action to take if there is no match for the source 2491 * concept in the target system designated for the group. One of 3 2492 * actions are possible: use the unmapped code (this is useful when 2493 * doing a mapping between versions, and only a few codes have changed), 2494 * use a fixed code (a default code), or alternatively, a reference to a 2495 * different concept map can be provided (by canonical URL). 2496 */ 2497 public ConceptMapGroupUnmappedMode getMode() { 2498 return this.mode == null ? null : this.mode.getValue(); 2499 } 2500 2501 /** 2502 * @param value Defines which action to take if there is no match for the source 2503 * concept in the target system designated for the group. One of 3 2504 * actions are possible: use the unmapped code (this is useful when 2505 * doing a mapping between versions, and only a few codes have 2506 * changed), use a fixed code (a default code), or alternatively, a 2507 * reference to a different concept map can be provided (by 2508 * canonical URL). 2509 */ 2510 public ConceptMapGroupUnmappedComponent setMode(ConceptMapGroupUnmappedMode value) { 2511 if (this.mode == null) 2512 this.mode = new Enumeration<ConceptMapGroupUnmappedMode>(new ConceptMapGroupUnmappedModeEnumFactory()); 2513 this.mode.setValue(value); 2514 return this; 2515 } 2516 2517 /** 2518 * @return {@link #code} (The fixed code to use when the mode = 'fixed' - all 2519 * unmapped codes are mapped to a single fixed code.). This is the 2520 * underlying object with id, value and extensions. The accessor 2521 * "getCode" gives direct access to the value 2522 */ 2523 public CodeType getCodeElement() { 2524 if (this.code == null) 2525 if (Configuration.errorOnAutoCreate()) 2526 throw new Error("Attempt to auto-create ConceptMapGroupUnmappedComponent.code"); 2527 else if (Configuration.doAutoCreate()) 2528 this.code = new CodeType(); // bb 2529 return this.code; 2530 } 2531 2532 public boolean hasCodeElement() { 2533 return this.code != null && !this.code.isEmpty(); 2534 } 2535 2536 public boolean hasCode() { 2537 return this.code != null && !this.code.isEmpty(); 2538 } 2539 2540 /** 2541 * @param value {@link #code} (The fixed code to use when the mode = 'fixed' - 2542 * all unmapped codes are mapped to a single fixed code.). This is 2543 * the underlying object with id, value and extensions. The 2544 * accessor "getCode" gives direct access to the value 2545 */ 2546 public ConceptMapGroupUnmappedComponent setCodeElement(CodeType value) { 2547 this.code = value; 2548 return this; 2549 } 2550 2551 /** 2552 * @return The fixed code to use when the mode = 'fixed' - all unmapped codes 2553 * are mapped to a single fixed code. 2554 */ 2555 public String getCode() { 2556 return this.code == null ? null : this.code.getValue(); 2557 } 2558 2559 /** 2560 * @param value The fixed code to use when the mode = 'fixed' - all unmapped 2561 * codes are mapped to a single fixed code. 2562 */ 2563 public ConceptMapGroupUnmappedComponent setCode(String value) { 2564 if (Utilities.noString(value)) 2565 this.code = null; 2566 else { 2567 if (this.code == null) 2568 this.code = new CodeType(); 2569 this.code.setValue(value); 2570 } 2571 return this; 2572 } 2573 2574 /** 2575 * @return {@link #display} (The display for the code. The display is only 2576 * provided to help editors when editing the concept map.). This is the 2577 * underlying object with id, value and extensions. The accessor 2578 * "getDisplay" gives direct access to the value 2579 */ 2580 public StringType getDisplayElement() { 2581 if (this.display == null) 2582 if (Configuration.errorOnAutoCreate()) 2583 throw new Error("Attempt to auto-create ConceptMapGroupUnmappedComponent.display"); 2584 else if (Configuration.doAutoCreate()) 2585 this.display = new StringType(); // bb 2586 return this.display; 2587 } 2588 2589 public boolean hasDisplayElement() { 2590 return this.display != null && !this.display.isEmpty(); 2591 } 2592 2593 public boolean hasDisplay() { 2594 return this.display != null && !this.display.isEmpty(); 2595 } 2596 2597 /** 2598 * @param value {@link #display} (The display for the code. The display is only 2599 * provided to help editors when editing the concept map.). This is 2600 * the underlying object with id, value and extensions. The 2601 * accessor "getDisplay" gives direct access to the value 2602 */ 2603 public ConceptMapGroupUnmappedComponent setDisplayElement(StringType value) { 2604 this.display = value; 2605 return this; 2606 } 2607 2608 /** 2609 * @return The display for the code. The display is only provided to help 2610 * editors when editing the concept map. 2611 */ 2612 public String getDisplay() { 2613 return this.display == null ? null : this.display.getValue(); 2614 } 2615 2616 /** 2617 * @param value The display for the code. The display is only provided to help 2618 * editors when editing the concept map. 2619 */ 2620 public ConceptMapGroupUnmappedComponent setDisplay(String value) { 2621 if (Utilities.noString(value)) 2622 this.display = null; 2623 else { 2624 if (this.display == null) 2625 this.display = new StringType(); 2626 this.display.setValue(value); 2627 } 2628 return this; 2629 } 2630 2631 /** 2632 * @return {@link #url} (The canonical reference to an additional ConceptMap 2633 * resource instance to use for mapping if this ConceptMap resource 2634 * contains no matching mapping for the source concept.). This is the 2635 * underlying object with id, value and extensions. The accessor 2636 * "getUrl" gives direct access to the value 2637 */ 2638 public CanonicalType getUrlElement() { 2639 if (this.url == null) 2640 if (Configuration.errorOnAutoCreate()) 2641 throw new Error("Attempt to auto-create ConceptMapGroupUnmappedComponent.url"); 2642 else if (Configuration.doAutoCreate()) 2643 this.url = new CanonicalType(); // bb 2644 return this.url; 2645 } 2646 2647 public boolean hasUrlElement() { 2648 return this.url != null && !this.url.isEmpty(); 2649 } 2650 2651 public boolean hasUrl() { 2652 return this.url != null && !this.url.isEmpty(); 2653 } 2654 2655 /** 2656 * @param value {@link #url} (The canonical reference to an additional 2657 * ConceptMap resource instance to use for mapping if this 2658 * ConceptMap resource contains no matching mapping for the source 2659 * concept.). This is the underlying object with id, value and 2660 * extensions. The accessor "getUrl" gives direct access to the 2661 * value 2662 */ 2663 public ConceptMapGroupUnmappedComponent setUrlElement(CanonicalType value) { 2664 this.url = value; 2665 return this; 2666 } 2667 2668 /** 2669 * @return The canonical reference to an additional ConceptMap resource instance 2670 * to use for mapping if this ConceptMap resource contains no matching 2671 * mapping for the source concept. 2672 */ 2673 public String getUrl() { 2674 return this.url == null ? null : this.url.getValue(); 2675 } 2676 2677 /** 2678 * @param value The canonical reference to an additional ConceptMap resource 2679 * instance to use for mapping if this ConceptMap resource contains 2680 * no matching mapping for the source concept. 2681 */ 2682 public ConceptMapGroupUnmappedComponent setUrl(String value) { 2683 if (Utilities.noString(value)) 2684 this.url = null; 2685 else { 2686 if (this.url == null) 2687 this.url = new CanonicalType(); 2688 this.url.setValue(value); 2689 } 2690 return this; 2691 } 2692 2693 protected void listChildren(List<Property> children) { 2694 super.listChildren(children); 2695 children.add(new Property("mode", "code", 2696 "Defines which action to take if there is no match for the source concept in the target system designated for the group. One of 3 actions are possible: use the unmapped code (this is useful when doing a mapping between versions, and only a few codes have changed), use a fixed code (a default code), or alternatively, a reference to a different concept map can be provided (by canonical URL).", 2697 0, 1, mode)); 2698 children.add(new Property("code", "code", 2699 "The fixed code to use when the mode = 'fixed' - all unmapped codes are mapped to a single fixed code.", 0, 2700 1, code)); 2701 children.add(new Property("display", "string", 2702 "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 1, 2703 display)); 2704 children.add(new Property("url", "canonical(ConceptMap)", 2705 "The canonical reference to an additional ConceptMap resource instance to use for mapping if this ConceptMap resource contains no matching mapping for the source concept.", 2706 0, 1, url)); 2707 } 2708 2709 @Override 2710 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2711 switch (_hash) { 2712 case 3357091: 2713 /* mode */ return new Property("mode", "code", 2714 "Defines which action to take if there is no match for the source concept in the target system designated for the group. One of 3 actions are possible: use the unmapped code (this is useful when doing a mapping between versions, and only a few codes have changed), use a fixed code (a default code), or alternatively, a reference to a different concept map can be provided (by canonical URL).", 2715 0, 1, mode); 2716 case 3059181: 2717 /* code */ return new Property("code", "code", 2718 "The fixed code to use when the mode = 'fixed' - all unmapped codes are mapped to a single fixed code.", 0, 2719 1, code); 2720 case 1671764162: 2721 /* display */ return new Property("display", "string", 2722 "The display for the code. The display is only provided to help editors when editing the concept map.", 0, 2723 1, display); 2724 case 116079: 2725 /* url */ return new Property("url", "canonical(ConceptMap)", 2726 "The canonical reference to an additional ConceptMap resource instance to use for mapping if this ConceptMap resource contains no matching mapping for the source concept.", 2727 0, 1, url); 2728 default: 2729 return super.getNamedProperty(_hash, _name, _checkValid); 2730 } 2731 2732 } 2733 2734 @Override 2735 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2736 switch (hash) { 2737 case 3357091: 2738 /* mode */ return this.mode == null ? new Base[0] : new Base[] { this.mode }; // Enumeration<ConceptMapGroupUnmappedMode> 2739 case 3059181: 2740 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeType 2741 case 1671764162: 2742 /* display */ return this.display == null ? new Base[0] : new Base[] { this.display }; // StringType 2743 case 116079: 2744 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // CanonicalType 2745 default: 2746 return super.getProperty(hash, name, checkValid); 2747 } 2748 2749 } 2750 2751 @Override 2752 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2753 switch (hash) { 2754 case 3357091: // mode 2755 value = new ConceptMapGroupUnmappedModeEnumFactory().fromType(castToCode(value)); 2756 this.mode = (Enumeration) value; // Enumeration<ConceptMapGroupUnmappedMode> 2757 return value; 2758 case 3059181: // code 2759 this.code = castToCode(value); // CodeType 2760 return value; 2761 case 1671764162: // display 2762 this.display = castToString(value); // StringType 2763 return value; 2764 case 116079: // url 2765 this.url = castToCanonical(value); // CanonicalType 2766 return value; 2767 default: 2768 return super.setProperty(hash, name, value); 2769 } 2770 2771 } 2772 2773 @Override 2774 public Base setProperty(String name, Base value) throws FHIRException { 2775 if (name.equals("mode")) { 2776 value = new ConceptMapGroupUnmappedModeEnumFactory().fromType(castToCode(value)); 2777 this.mode = (Enumeration) value; // Enumeration<ConceptMapGroupUnmappedMode> 2778 } else if (name.equals("code")) { 2779 this.code = castToCode(value); // CodeType 2780 } else if (name.equals("display")) { 2781 this.display = castToString(value); // StringType 2782 } else if (name.equals("url")) { 2783 this.url = castToCanonical(value); // CanonicalType 2784 } else 2785 return super.setProperty(name, value); 2786 return value; 2787 } 2788 2789 @Override 2790 public void removeChild(String name, Base value) throws FHIRException { 2791 if (name.equals("mode")) { 2792 this.mode = null; 2793 } else if (name.equals("code")) { 2794 this.code = null; 2795 } else if (name.equals("display")) { 2796 this.display = null; 2797 } else if (name.equals("url")) { 2798 this.url = null; 2799 } else 2800 super.removeChild(name, value); 2801 2802 } 2803 2804 @Override 2805 public Base makeProperty(int hash, String name) throws FHIRException { 2806 switch (hash) { 2807 case 3357091: 2808 return getModeElement(); 2809 case 3059181: 2810 return getCodeElement(); 2811 case 1671764162: 2812 return getDisplayElement(); 2813 case 116079: 2814 return getUrlElement(); 2815 default: 2816 return super.makeProperty(hash, name); 2817 } 2818 2819 } 2820 2821 @Override 2822 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2823 switch (hash) { 2824 case 3357091: 2825 /* mode */ return new String[] { "code" }; 2826 case 3059181: 2827 /* code */ return new String[] { "code" }; 2828 case 1671764162: 2829 /* display */ return new String[] { "string" }; 2830 case 116079: 2831 /* url */ return new String[] { "canonical" }; 2832 default: 2833 return super.getTypesForProperty(hash, name); 2834 } 2835 2836 } 2837 2838 @Override 2839 public Base addChild(String name) throws FHIRException { 2840 if (name.equals("mode")) { 2841 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.mode"); 2842 } else if (name.equals("code")) { 2843 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.code"); 2844 } else if (name.equals("display")) { 2845 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.display"); 2846 } else if (name.equals("url")) { 2847 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.url"); 2848 } else 2849 return super.addChild(name); 2850 } 2851 2852 public ConceptMapGroupUnmappedComponent copy() { 2853 ConceptMapGroupUnmappedComponent dst = new ConceptMapGroupUnmappedComponent(); 2854 copyValues(dst); 2855 return dst; 2856 } 2857 2858 public void copyValues(ConceptMapGroupUnmappedComponent dst) { 2859 super.copyValues(dst); 2860 dst.mode = mode == null ? null : mode.copy(); 2861 dst.code = code == null ? null : code.copy(); 2862 dst.display = display == null ? null : display.copy(); 2863 dst.url = url == null ? null : url.copy(); 2864 } 2865 2866 @Override 2867 public boolean equalsDeep(Base other_) { 2868 if (!super.equalsDeep(other_)) 2869 return false; 2870 if (!(other_ instanceof ConceptMapGroupUnmappedComponent)) 2871 return false; 2872 ConceptMapGroupUnmappedComponent o = (ConceptMapGroupUnmappedComponent) other_; 2873 return compareDeep(mode, o.mode, true) && compareDeep(code, o.code, true) && compareDeep(display, o.display, true) 2874 && compareDeep(url, o.url, true); 2875 } 2876 2877 @Override 2878 public boolean equalsShallow(Base other_) { 2879 if (!super.equalsShallow(other_)) 2880 return false; 2881 if (!(other_ instanceof ConceptMapGroupUnmappedComponent)) 2882 return false; 2883 ConceptMapGroupUnmappedComponent o = (ConceptMapGroupUnmappedComponent) other_; 2884 return compareValues(mode, o.mode, true) && compareValues(code, o.code, true) 2885 && compareValues(display, o.display, true); 2886 } 2887 2888 public boolean isEmpty() { 2889 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(mode, code, display, url); 2890 } 2891 2892 public String fhirType() { 2893 return "ConceptMap.group.unmapped"; 2894 2895 } 2896 2897 } 2898 2899 /** 2900 * A formal identifier that is used to identify this concept map when it is 2901 * represented in other formats, or referenced in a specification, model, design 2902 * or an instance. 2903 */ 2904 @Child(name = "identifier", type = { 2905 Identifier.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 2906 @Description(shortDefinition = "Additional identifier for the concept map", formalDefinition = "A formal identifier that is used to identify this concept map when it is represented in other formats, or referenced in a specification, model, design or an instance.") 2907 protected Identifier identifier; 2908 2909 /** 2910 * Explanation of why this concept map is needed and why it has been designed as 2911 * it has. 2912 */ 2913 @Child(name = "purpose", type = { 2914 MarkdownType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2915 @Description(shortDefinition = "Why this concept map is defined", formalDefinition = "Explanation of why this concept map is needed and why it has been designed as it has.") 2916 protected MarkdownType purpose; 2917 2918 /** 2919 * A copyright statement relating to the concept map and/or its contents. 2920 * Copyright statements are generally legal restrictions on the use and 2921 * publishing of the concept map. 2922 */ 2923 @Child(name = "copyright", type = { 2924 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2925 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the concept map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the concept map.") 2926 protected MarkdownType copyright; 2927 2928 /** 2929 * Identifier for the source value set that contains the concepts that are being 2930 * mapped and provides context for the mappings. 2931 */ 2932 @Child(name = "source", type = { UriType.class, 2933 CanonicalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 2934 @Description(shortDefinition = "The source value set that contains the concepts that are being mapped", formalDefinition = "Identifier for the source value set that contains the concepts that are being mapped and provides context for the mappings.") 2935 protected Type source; 2936 2937 /** 2938 * The target value set provides context for the mappings. Note that the mapping 2939 * is made between concepts, not between value sets, but the value set provides 2940 * important context about how the concept mapping choices are made. 2941 */ 2942 @Child(name = "target", type = { UriType.class, 2943 CanonicalType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 2944 @Description(shortDefinition = "The target value set which provides context for the mappings", formalDefinition = "The target value set provides context for the mappings. Note that the mapping is made between concepts, not between value sets, but the value set provides important context about how the concept mapping choices are made.") 2945 protected Type target; 2946 2947 /** 2948 * A group of mappings that all have the same source and target system. 2949 */ 2950 @Child(name = "group", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2951 @Description(shortDefinition = "Same source and target systems", formalDefinition = "A group of mappings that all have the same source and target system.") 2952 protected List<ConceptMapGroupComponent> group; 2953 2954 private static final long serialVersionUID = -2081872580L; 2955 2956 /** 2957 * Constructor 2958 */ 2959 public ConceptMap() { 2960 super(); 2961 } 2962 2963 /** 2964 * Constructor 2965 */ 2966 public ConceptMap(Enumeration<PublicationStatus> status) { 2967 super(); 2968 this.status = status; 2969 } 2970 2971 /** 2972 * @return {@link #url} (An absolute URI that is used to identify this concept 2973 * map when it is referenced in a specification, model, design or an 2974 * instance; also called its canonical identifier. This SHOULD be 2975 * globally unique and SHOULD be a literal address at which at which an 2976 * authoritative instance of this concept map is (or will be) published. 2977 * This URL can be the target of a canonical reference. It SHALL remain 2978 * the same when the concept map is stored on different servers.). This 2979 * is the underlying object with id, value and extensions. The accessor 2980 * "getUrl" gives direct access to the value 2981 */ 2982 public UriType getUrlElement() { 2983 if (this.url == null) 2984 if (Configuration.errorOnAutoCreate()) 2985 throw new Error("Attempt to auto-create ConceptMap.url"); 2986 else if (Configuration.doAutoCreate()) 2987 this.url = new UriType(); // bb 2988 return this.url; 2989 } 2990 2991 public boolean hasUrlElement() { 2992 return this.url != null && !this.url.isEmpty(); 2993 } 2994 2995 public boolean hasUrl() { 2996 return this.url != null && !this.url.isEmpty(); 2997 } 2998 2999 /** 3000 * @param value {@link #url} (An absolute URI that is used to identify this 3001 * concept map when it is referenced in a specification, model, 3002 * design or an instance; also called its canonical identifier. 3003 * This SHOULD be globally unique and SHOULD be a literal address 3004 * at which at which an authoritative instance of this concept map 3005 * is (or will be) published. This URL can be the target of a 3006 * canonical reference. It SHALL remain the same when the concept 3007 * map is stored on different servers.). This is the underlying 3008 * object with id, value and extensions. The accessor "getUrl" 3009 * gives direct access to the value 3010 */ 3011 public ConceptMap setUrlElement(UriType value) { 3012 this.url = value; 3013 return this; 3014 } 3015 3016 /** 3017 * @return An absolute URI that is used to identify this concept map when it is 3018 * referenced in a specification, model, design or an instance; also 3019 * called its canonical identifier. This SHOULD be globally unique and 3020 * SHOULD be a literal address at which at which an authoritative 3021 * instance of this concept map is (or will be) published. This URL can 3022 * be the target of a canonical reference. It SHALL remain the same when 3023 * the concept map is stored on different servers. 3024 */ 3025 public String getUrl() { 3026 return this.url == null ? null : this.url.getValue(); 3027 } 3028 3029 /** 3030 * @param value An absolute URI that is used to identify this concept map when 3031 * it is referenced in a specification, model, design or an 3032 * instance; also called its canonical identifier. This SHOULD be 3033 * globally unique and SHOULD be a literal address at which at 3034 * which an authoritative instance of this concept map is (or will 3035 * be) published. This URL can be the target of a canonical 3036 * reference. It SHALL remain the same when the concept map is 3037 * stored on different servers. 3038 */ 3039 public ConceptMap setUrl(String value) { 3040 if (Utilities.noString(value)) 3041 this.url = null; 3042 else { 3043 if (this.url == null) 3044 this.url = new UriType(); 3045 this.url.setValue(value); 3046 } 3047 return this; 3048 } 3049 3050 /** 3051 * @return {@link #identifier} (A formal identifier that is used to identify 3052 * this concept map when it is represented in other formats, or 3053 * referenced in a specification, model, design or an instance.) 3054 */ 3055 public Identifier getIdentifier() { 3056 if (this.identifier == null) 3057 if (Configuration.errorOnAutoCreate()) 3058 throw new Error("Attempt to auto-create ConceptMap.identifier"); 3059 else if (Configuration.doAutoCreate()) 3060 this.identifier = new Identifier(); // cc 3061 return this.identifier; 3062 } 3063 3064 public boolean hasIdentifier() { 3065 return this.identifier != null && !this.identifier.isEmpty(); 3066 } 3067 3068 /** 3069 * @param value {@link #identifier} (A formal identifier that is used to 3070 * identify this concept map when it is represented in other 3071 * formats, or referenced in a specification, model, design or an 3072 * instance.) 3073 */ 3074 public ConceptMap setIdentifier(Identifier value) { 3075 this.identifier = value; 3076 return this; 3077 } 3078 3079 /** 3080 * @return {@link #version} (The identifier that is used to identify this 3081 * version of the concept map when it is referenced in a specification, 3082 * model, design or instance. This is an arbitrary value managed by the 3083 * concept map author and is not expected to be globally unique. For 3084 * example, it might be a timestamp (e.g. yyyymmdd) if a managed version 3085 * is not available. There is also no expectation that versions can be 3086 * placed in a lexicographical sequence.). This is the underlying object 3087 * with id, value and extensions. The accessor "getVersion" gives direct 3088 * access to the value 3089 */ 3090 public StringType getVersionElement() { 3091 if (this.version == null) 3092 if (Configuration.errorOnAutoCreate()) 3093 throw new Error("Attempt to auto-create ConceptMap.version"); 3094 else if (Configuration.doAutoCreate()) 3095 this.version = new StringType(); // bb 3096 return this.version; 3097 } 3098 3099 public boolean hasVersionElement() { 3100 return this.version != null && !this.version.isEmpty(); 3101 } 3102 3103 public boolean hasVersion() { 3104 return this.version != null && !this.version.isEmpty(); 3105 } 3106 3107 /** 3108 * @param value {@link #version} (The identifier that is used to identify this 3109 * version of the concept map when it is referenced in a 3110 * specification, model, design or instance. This is an arbitrary 3111 * value managed by the concept map author and is not expected to 3112 * be globally unique. For example, it might be a timestamp (e.g. 3113 * yyyymmdd) if a managed version is not available. There is also 3114 * no expectation that versions can be placed in a lexicographical 3115 * sequence.). This is the underlying object with id, value and 3116 * extensions. The accessor "getVersion" gives direct access to the 3117 * value 3118 */ 3119 public ConceptMap setVersionElement(StringType value) { 3120 this.version = value; 3121 return this; 3122 } 3123 3124 /** 3125 * @return The identifier that is used to identify this version of the concept 3126 * map when it is referenced in a specification, model, design or 3127 * instance. This is an arbitrary value managed by the concept map 3128 * author and is not expected to be globally unique. For example, it 3129 * might be a timestamp (e.g. yyyymmdd) if a managed version is not 3130 * available. There is also no expectation that versions can be placed 3131 * in a lexicographical sequence. 3132 */ 3133 public String getVersion() { 3134 return this.version == null ? null : this.version.getValue(); 3135 } 3136 3137 /** 3138 * @param value The identifier that is used to identify this version of the 3139 * concept map when it is referenced in a specification, model, 3140 * design or instance. This is an arbitrary value managed by the 3141 * concept map author and is not expected to be globally unique. 3142 * For example, it might be a timestamp (e.g. yyyymmdd) if a 3143 * managed version is not available. There is also no expectation 3144 * that versions can be placed in a lexicographical sequence. 3145 */ 3146 public ConceptMap setVersion(String value) { 3147 if (Utilities.noString(value)) 3148 this.version = null; 3149 else { 3150 if (this.version == null) 3151 this.version = new StringType(); 3152 this.version.setValue(value); 3153 } 3154 return this; 3155 } 3156 3157 /** 3158 * @return {@link #name} (A natural language name identifying the concept map. 3159 * This name should be usable as an identifier for the module by machine 3160 * processing applications such as code generation.). This is the 3161 * underlying object with id, value and extensions. The accessor 3162 * "getName" gives direct access to the value 3163 */ 3164 public StringType getNameElement() { 3165 if (this.name == null) 3166 if (Configuration.errorOnAutoCreate()) 3167 throw new Error("Attempt to auto-create ConceptMap.name"); 3168 else if (Configuration.doAutoCreate()) 3169 this.name = new StringType(); // bb 3170 return this.name; 3171 } 3172 3173 public boolean hasNameElement() { 3174 return this.name != null && !this.name.isEmpty(); 3175 } 3176 3177 public boolean hasName() { 3178 return this.name != null && !this.name.isEmpty(); 3179 } 3180 3181 /** 3182 * @param value {@link #name} (A natural language name identifying the concept 3183 * map. This name should be usable as an identifier for the module 3184 * by machine processing applications such as code generation.). 3185 * This is the underlying object with id, value and extensions. The 3186 * accessor "getName" gives direct access to the value 3187 */ 3188 public ConceptMap setNameElement(StringType value) { 3189 this.name = value; 3190 return this; 3191 } 3192 3193 /** 3194 * @return A natural language name identifying the concept map. This name should 3195 * be usable as an identifier for the module by machine processing 3196 * applications such as code generation. 3197 */ 3198 public String getName() { 3199 return this.name == null ? null : this.name.getValue(); 3200 } 3201 3202 /** 3203 * @param value A natural language name identifying the concept map. This name 3204 * should be usable as an identifier for the module by machine 3205 * processing applications such as code generation. 3206 */ 3207 public ConceptMap setName(String value) { 3208 if (Utilities.noString(value)) 3209 this.name = null; 3210 else { 3211 if (this.name == null) 3212 this.name = new StringType(); 3213 this.name.setValue(value); 3214 } 3215 return this; 3216 } 3217 3218 /** 3219 * @return {@link #title} (A short, descriptive, user-friendly title for the 3220 * concept map.). This is the underlying object with id, value and 3221 * extensions. The accessor "getTitle" gives direct access to the value 3222 */ 3223 public StringType getTitleElement() { 3224 if (this.title == null) 3225 if (Configuration.errorOnAutoCreate()) 3226 throw new Error("Attempt to auto-create ConceptMap.title"); 3227 else if (Configuration.doAutoCreate()) 3228 this.title = new StringType(); // bb 3229 return this.title; 3230 } 3231 3232 public boolean hasTitleElement() { 3233 return this.title != null && !this.title.isEmpty(); 3234 } 3235 3236 public boolean hasTitle() { 3237 return this.title != null && !this.title.isEmpty(); 3238 } 3239 3240 /** 3241 * @param value {@link #title} (A short, descriptive, user-friendly title for 3242 * the concept map.). This is the underlying object with id, value 3243 * and extensions. The accessor "getTitle" gives direct access to 3244 * the value 3245 */ 3246 public ConceptMap setTitleElement(StringType value) { 3247 this.title = value; 3248 return this; 3249 } 3250 3251 /** 3252 * @return A short, descriptive, user-friendly title for the concept map. 3253 */ 3254 public String getTitle() { 3255 return this.title == null ? null : this.title.getValue(); 3256 } 3257 3258 /** 3259 * @param value A short, descriptive, user-friendly title for the concept map. 3260 */ 3261 public ConceptMap setTitle(String value) { 3262 if (Utilities.noString(value)) 3263 this.title = null; 3264 else { 3265 if (this.title == null) 3266 this.title = new StringType(); 3267 this.title.setValue(value); 3268 } 3269 return this; 3270 } 3271 3272 /** 3273 * @return {@link #status} (The status of this concept map. Enables tracking the 3274 * life-cycle of the content.). This is the underlying object with id, 3275 * value and extensions. The accessor "getStatus" gives direct access to 3276 * the value 3277 */ 3278 public Enumeration<PublicationStatus> getStatusElement() { 3279 if (this.status == null) 3280 if (Configuration.errorOnAutoCreate()) 3281 throw new Error("Attempt to auto-create ConceptMap.status"); 3282 else if (Configuration.doAutoCreate()) 3283 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 3284 return this.status; 3285 } 3286 3287 public boolean hasStatusElement() { 3288 return this.status != null && !this.status.isEmpty(); 3289 } 3290 3291 public boolean hasStatus() { 3292 return this.status != null && !this.status.isEmpty(); 3293 } 3294 3295 /** 3296 * @param value {@link #status} (The status of this concept map. Enables 3297 * tracking the life-cycle of the content.). This is the underlying 3298 * object with id, value and extensions. The accessor "getStatus" 3299 * gives direct access to the value 3300 */ 3301 public ConceptMap setStatusElement(Enumeration<PublicationStatus> value) { 3302 this.status = value; 3303 return this; 3304 } 3305 3306 /** 3307 * @return The status of this concept map. Enables tracking the life-cycle of 3308 * the content. 3309 */ 3310 public PublicationStatus getStatus() { 3311 return this.status == null ? null : this.status.getValue(); 3312 } 3313 3314 /** 3315 * @param value The status of this concept map. Enables tracking the life-cycle 3316 * of the content. 3317 */ 3318 public ConceptMap setStatus(PublicationStatus value) { 3319 if (this.status == null) 3320 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 3321 this.status.setValue(value); 3322 return this; 3323 } 3324 3325 /** 3326 * @return {@link #experimental} (A Boolean value to indicate that this concept 3327 * map is authored for testing purposes (or 3328 * education/evaluation/marketing) and is not intended to be used for 3329 * genuine usage.). This is the underlying object with id, value and 3330 * extensions. The accessor "getExperimental" gives direct access to the 3331 * value 3332 */ 3333 public BooleanType getExperimentalElement() { 3334 if (this.experimental == null) 3335 if (Configuration.errorOnAutoCreate()) 3336 throw new Error("Attempt to auto-create ConceptMap.experimental"); 3337 else if (Configuration.doAutoCreate()) 3338 this.experimental = new BooleanType(); // bb 3339 return this.experimental; 3340 } 3341 3342 public boolean hasExperimentalElement() { 3343 return this.experimental != null && !this.experimental.isEmpty(); 3344 } 3345 3346 public boolean hasExperimental() { 3347 return this.experimental != null && !this.experimental.isEmpty(); 3348 } 3349 3350 /** 3351 * @param value {@link #experimental} (A Boolean value to indicate that this 3352 * concept map is authored for testing purposes (or 3353 * education/evaluation/marketing) and is not intended to be used 3354 * for genuine usage.). This is the underlying object with id, 3355 * value and extensions. The accessor "getExperimental" gives 3356 * direct access to the value 3357 */ 3358 public ConceptMap setExperimentalElement(BooleanType value) { 3359 this.experimental = value; 3360 return this; 3361 } 3362 3363 /** 3364 * @return A Boolean value to indicate that this concept map is authored for 3365 * testing purposes (or education/evaluation/marketing) and is not 3366 * intended to be used for genuine usage. 3367 */ 3368 public boolean getExperimental() { 3369 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 3370 } 3371 3372 /** 3373 * @param value A Boolean value to indicate that this concept map is authored 3374 * for testing purposes (or education/evaluation/marketing) and is 3375 * not intended to be used for genuine usage. 3376 */ 3377 public ConceptMap setExperimental(boolean value) { 3378 if (this.experimental == null) 3379 this.experimental = new BooleanType(); 3380 this.experimental.setValue(value); 3381 return this; 3382 } 3383 3384 /** 3385 * @return {@link #date} (The date (and optionally time) when the concept map 3386 * was published. The date must change when the business version changes 3387 * and it must change if the status code changes. In addition, it should 3388 * change when the substantive content of the concept map changes.). 3389 * This is the underlying object with id, value and extensions. The 3390 * accessor "getDate" gives direct access to the value 3391 */ 3392 public DateTimeType getDateElement() { 3393 if (this.date == null) 3394 if (Configuration.errorOnAutoCreate()) 3395 throw new Error("Attempt to auto-create ConceptMap.date"); 3396 else if (Configuration.doAutoCreate()) 3397 this.date = new DateTimeType(); // bb 3398 return this.date; 3399 } 3400 3401 public boolean hasDateElement() { 3402 return this.date != null && !this.date.isEmpty(); 3403 } 3404 3405 public boolean hasDate() { 3406 return this.date != null && !this.date.isEmpty(); 3407 } 3408 3409 /** 3410 * @param value {@link #date} (The date (and optionally time) when the concept 3411 * map was published. The date must change when the business 3412 * version changes and it must change if the status code changes. 3413 * In addition, it should change when the substantive content of 3414 * the concept map changes.). This is the underlying object with 3415 * id, value and extensions. The accessor "getDate" gives direct 3416 * access to the value 3417 */ 3418 public ConceptMap setDateElement(DateTimeType value) { 3419 this.date = value; 3420 return this; 3421 } 3422 3423 /** 3424 * @return The date (and optionally time) when the concept map was published. 3425 * The date must change when the business version changes and it must 3426 * change if the status code changes. In addition, it should change when 3427 * the substantive content of the concept map changes. 3428 */ 3429 public Date getDate() { 3430 return this.date == null ? null : this.date.getValue(); 3431 } 3432 3433 /** 3434 * @param value The date (and optionally time) when the concept map was 3435 * published. The date must change when the business version 3436 * changes and it must change if the status code changes. In 3437 * addition, it should change when the substantive content of the 3438 * concept map changes. 3439 */ 3440 public ConceptMap setDate(Date value) { 3441 if (value == null) 3442 this.date = null; 3443 else { 3444 if (this.date == null) 3445 this.date = new DateTimeType(); 3446 this.date.setValue(value); 3447 } 3448 return this; 3449 } 3450 3451 /** 3452 * @return {@link #publisher} (The name of the organization or individual that 3453 * published the concept map.). This is the underlying object with id, 3454 * value and extensions. The accessor "getPublisher" gives direct access 3455 * to the value 3456 */ 3457 public StringType getPublisherElement() { 3458 if (this.publisher == null) 3459 if (Configuration.errorOnAutoCreate()) 3460 throw new Error("Attempt to auto-create ConceptMap.publisher"); 3461 else if (Configuration.doAutoCreate()) 3462 this.publisher = new StringType(); // bb 3463 return this.publisher; 3464 } 3465 3466 public boolean hasPublisherElement() { 3467 return this.publisher != null && !this.publisher.isEmpty(); 3468 } 3469 3470 public boolean hasPublisher() { 3471 return this.publisher != null && !this.publisher.isEmpty(); 3472 } 3473 3474 /** 3475 * @param value {@link #publisher} (The name of the organization or individual 3476 * that published the concept map.). This is the underlying object 3477 * with id, value and extensions. The accessor "getPublisher" gives 3478 * direct access to the value 3479 */ 3480 public ConceptMap setPublisherElement(StringType value) { 3481 this.publisher = value; 3482 return this; 3483 } 3484 3485 /** 3486 * @return The name of the organization or individual that published the concept 3487 * map. 3488 */ 3489 public String getPublisher() { 3490 return this.publisher == null ? null : this.publisher.getValue(); 3491 } 3492 3493 /** 3494 * @param value The name of the organization or individual that published the 3495 * concept map. 3496 */ 3497 public ConceptMap setPublisher(String value) { 3498 if (Utilities.noString(value)) 3499 this.publisher = null; 3500 else { 3501 if (this.publisher == null) 3502 this.publisher = new StringType(); 3503 this.publisher.setValue(value); 3504 } 3505 return this; 3506 } 3507 3508 /** 3509 * @return {@link #contact} (Contact details to assist a user in finding and 3510 * communicating with the publisher.) 3511 */ 3512 public List<ContactDetail> getContact() { 3513 if (this.contact == null) 3514 this.contact = new ArrayList<ContactDetail>(); 3515 return this.contact; 3516 } 3517 3518 /** 3519 * @return Returns a reference to <code>this</code> for easy method chaining 3520 */ 3521 public ConceptMap setContact(List<ContactDetail> theContact) { 3522 this.contact = theContact; 3523 return this; 3524 } 3525 3526 public boolean hasContact() { 3527 if (this.contact == null) 3528 return false; 3529 for (ContactDetail item : this.contact) 3530 if (!item.isEmpty()) 3531 return true; 3532 return false; 3533 } 3534 3535 public ContactDetail addContact() { // 3 3536 ContactDetail t = new ContactDetail(); 3537 if (this.contact == null) 3538 this.contact = new ArrayList<ContactDetail>(); 3539 this.contact.add(t); 3540 return t; 3541 } 3542 3543 public ConceptMap addContact(ContactDetail t) { // 3 3544 if (t == null) 3545 return this; 3546 if (this.contact == null) 3547 this.contact = new ArrayList<ContactDetail>(); 3548 this.contact.add(t); 3549 return this; 3550 } 3551 3552 /** 3553 * @return The first repetition of repeating field {@link #contact}, creating it 3554 * if it does not already exist 3555 */ 3556 public ContactDetail getContactFirstRep() { 3557 if (getContact().isEmpty()) { 3558 addContact(); 3559 } 3560 return getContact().get(0); 3561 } 3562 3563 /** 3564 * @return {@link #description} (A free text natural language description of the 3565 * concept map from a consumer's perspective.). This is the underlying 3566 * object with id, value and extensions. The accessor "getDescription" 3567 * gives direct access to the value 3568 */ 3569 public MarkdownType getDescriptionElement() { 3570 if (this.description == null) 3571 if (Configuration.errorOnAutoCreate()) 3572 throw new Error("Attempt to auto-create ConceptMap.description"); 3573 else if (Configuration.doAutoCreate()) 3574 this.description = new MarkdownType(); // bb 3575 return this.description; 3576 } 3577 3578 public boolean hasDescriptionElement() { 3579 return this.description != null && !this.description.isEmpty(); 3580 } 3581 3582 public boolean hasDescription() { 3583 return this.description != null && !this.description.isEmpty(); 3584 } 3585 3586 /** 3587 * @param value {@link #description} (A free text natural language description 3588 * of the concept map from a consumer's perspective.). This is the 3589 * underlying object with id, value and extensions. The accessor 3590 * "getDescription" gives direct access to the value 3591 */ 3592 public ConceptMap setDescriptionElement(MarkdownType value) { 3593 this.description = value; 3594 return this; 3595 } 3596 3597 /** 3598 * @return A free text natural language description of the concept map from a 3599 * consumer's perspective. 3600 */ 3601 public String getDescription() { 3602 return this.description == null ? null : this.description.getValue(); 3603 } 3604 3605 /** 3606 * @param value A free text natural language description of the concept map from 3607 * a consumer's perspective. 3608 */ 3609 public ConceptMap setDescription(String value) { 3610 if (value == null) 3611 this.description = null; 3612 else { 3613 if (this.description == null) 3614 this.description = new MarkdownType(); 3615 this.description.setValue(value); 3616 } 3617 return this; 3618 } 3619 3620 /** 3621 * @return {@link #useContext} (The content was developed with a focus and 3622 * intent of supporting the contexts that are listed. These contexts may 3623 * be general categories (gender, age, ...) or may be references to 3624 * specific programs (insurance plans, studies, ...) and may be used to 3625 * assist with indexing and searching for appropriate concept map 3626 * instances.) 3627 */ 3628 public List<UsageContext> getUseContext() { 3629 if (this.useContext == null) 3630 this.useContext = new ArrayList<UsageContext>(); 3631 return this.useContext; 3632 } 3633 3634 /** 3635 * @return Returns a reference to <code>this</code> for easy method chaining 3636 */ 3637 public ConceptMap setUseContext(List<UsageContext> theUseContext) { 3638 this.useContext = theUseContext; 3639 return this; 3640 } 3641 3642 public boolean hasUseContext() { 3643 if (this.useContext == null) 3644 return false; 3645 for (UsageContext item : this.useContext) 3646 if (!item.isEmpty()) 3647 return true; 3648 return false; 3649 } 3650 3651 public UsageContext addUseContext() { // 3 3652 UsageContext t = new UsageContext(); 3653 if (this.useContext == null) 3654 this.useContext = new ArrayList<UsageContext>(); 3655 this.useContext.add(t); 3656 return t; 3657 } 3658 3659 public ConceptMap addUseContext(UsageContext t) { // 3 3660 if (t == null) 3661 return this; 3662 if (this.useContext == null) 3663 this.useContext = new ArrayList<UsageContext>(); 3664 this.useContext.add(t); 3665 return this; 3666 } 3667 3668 /** 3669 * @return The first repetition of repeating field {@link #useContext}, creating 3670 * it if it does not already exist 3671 */ 3672 public UsageContext getUseContextFirstRep() { 3673 if (getUseContext().isEmpty()) { 3674 addUseContext(); 3675 } 3676 return getUseContext().get(0); 3677 } 3678 3679 /** 3680 * @return {@link #jurisdiction} (A legal or geographic region in which the 3681 * concept map is intended to be used.) 3682 */ 3683 public List<CodeableConcept> getJurisdiction() { 3684 if (this.jurisdiction == null) 3685 this.jurisdiction = new ArrayList<CodeableConcept>(); 3686 return this.jurisdiction; 3687 } 3688 3689 /** 3690 * @return Returns a reference to <code>this</code> for easy method chaining 3691 */ 3692 public ConceptMap setJurisdiction(List<CodeableConcept> theJurisdiction) { 3693 this.jurisdiction = theJurisdiction; 3694 return this; 3695 } 3696 3697 public boolean hasJurisdiction() { 3698 if (this.jurisdiction == null) 3699 return false; 3700 for (CodeableConcept item : this.jurisdiction) 3701 if (!item.isEmpty()) 3702 return true; 3703 return false; 3704 } 3705 3706 public CodeableConcept addJurisdiction() { // 3 3707 CodeableConcept t = new CodeableConcept(); 3708 if (this.jurisdiction == null) 3709 this.jurisdiction = new ArrayList<CodeableConcept>(); 3710 this.jurisdiction.add(t); 3711 return t; 3712 } 3713 3714 public ConceptMap addJurisdiction(CodeableConcept t) { // 3 3715 if (t == null) 3716 return this; 3717 if (this.jurisdiction == null) 3718 this.jurisdiction = new ArrayList<CodeableConcept>(); 3719 this.jurisdiction.add(t); 3720 return this; 3721 } 3722 3723 /** 3724 * @return The first repetition of repeating field {@link #jurisdiction}, 3725 * creating it if it does not already exist 3726 */ 3727 public CodeableConcept getJurisdictionFirstRep() { 3728 if (getJurisdiction().isEmpty()) { 3729 addJurisdiction(); 3730 } 3731 return getJurisdiction().get(0); 3732 } 3733 3734 /** 3735 * @return {@link #purpose} (Explanation of why this concept map is needed and 3736 * why it has been designed as it has.). This is the underlying object 3737 * with id, value and extensions. The accessor "getPurpose" gives direct 3738 * access to the value 3739 */ 3740 public MarkdownType getPurposeElement() { 3741 if (this.purpose == null) 3742 if (Configuration.errorOnAutoCreate()) 3743 throw new Error("Attempt to auto-create ConceptMap.purpose"); 3744 else if (Configuration.doAutoCreate()) 3745 this.purpose = new MarkdownType(); // bb 3746 return this.purpose; 3747 } 3748 3749 public boolean hasPurposeElement() { 3750 return this.purpose != null && !this.purpose.isEmpty(); 3751 } 3752 3753 public boolean hasPurpose() { 3754 return this.purpose != null && !this.purpose.isEmpty(); 3755 } 3756 3757 /** 3758 * @param value {@link #purpose} (Explanation of why this concept map is needed 3759 * and why it has been designed as it has.). This is the underlying 3760 * object with id, value and extensions. The accessor "getPurpose" 3761 * gives direct access to the value 3762 */ 3763 public ConceptMap setPurposeElement(MarkdownType value) { 3764 this.purpose = value; 3765 return this; 3766 } 3767 3768 /** 3769 * @return Explanation of why this concept map is needed and why it has been 3770 * designed as it has. 3771 */ 3772 public String getPurpose() { 3773 return this.purpose == null ? null : this.purpose.getValue(); 3774 } 3775 3776 /** 3777 * @param value Explanation of why this concept map is needed and why it has 3778 * been designed as it has. 3779 */ 3780 public ConceptMap setPurpose(String value) { 3781 if (value == null) 3782 this.purpose = null; 3783 else { 3784 if (this.purpose == null) 3785 this.purpose = new MarkdownType(); 3786 this.purpose.setValue(value); 3787 } 3788 return this; 3789 } 3790 3791 /** 3792 * @return {@link #copyright} (A copyright statement relating to the concept map 3793 * and/or its contents. Copyright statements are generally legal 3794 * restrictions on the use and publishing of the concept map.). This is 3795 * the underlying object with id, value and extensions. The accessor 3796 * "getCopyright" gives direct access to the value 3797 */ 3798 public MarkdownType getCopyrightElement() { 3799 if (this.copyright == null) 3800 if (Configuration.errorOnAutoCreate()) 3801 throw new Error("Attempt to auto-create ConceptMap.copyright"); 3802 else if (Configuration.doAutoCreate()) 3803 this.copyright = new MarkdownType(); // bb 3804 return this.copyright; 3805 } 3806 3807 public boolean hasCopyrightElement() { 3808 return this.copyright != null && !this.copyright.isEmpty(); 3809 } 3810 3811 public boolean hasCopyright() { 3812 return this.copyright != null && !this.copyright.isEmpty(); 3813 } 3814 3815 /** 3816 * @param value {@link #copyright} (A copyright statement relating to the 3817 * concept map and/or its contents. Copyright statements are 3818 * generally legal restrictions on the use and publishing of the 3819 * concept map.). This is the underlying object with id, value and 3820 * extensions. The accessor "getCopyright" gives direct access to 3821 * the value 3822 */ 3823 public ConceptMap setCopyrightElement(MarkdownType value) { 3824 this.copyright = value; 3825 return this; 3826 } 3827 3828 /** 3829 * @return A copyright statement relating to the concept map and/or its 3830 * contents. Copyright statements are generally legal restrictions on 3831 * the use and publishing of the concept map. 3832 */ 3833 public String getCopyright() { 3834 return this.copyright == null ? null : this.copyright.getValue(); 3835 } 3836 3837 /** 3838 * @param value A copyright statement relating to the concept map and/or its 3839 * contents. Copyright statements are generally legal restrictions 3840 * on the use and publishing of the concept map. 3841 */ 3842 public ConceptMap setCopyright(String value) { 3843 if (value == null) 3844 this.copyright = null; 3845 else { 3846 if (this.copyright == null) 3847 this.copyright = new MarkdownType(); 3848 this.copyright.setValue(value); 3849 } 3850 return this; 3851 } 3852 3853 /** 3854 * @return {@link #source} (Identifier for the source value set that contains 3855 * the concepts that are being mapped and provides context for the 3856 * mappings.) 3857 */ 3858 public Type getSource() { 3859 return this.source; 3860 } 3861 3862 /** 3863 * @return {@link #source} (Identifier for the source value set that contains 3864 * the concepts that are being mapped and provides context for the 3865 * mappings.) 3866 */ 3867 public UriType getSourceUriType() throws FHIRException { 3868 if (this.source == null) 3869 this.source = new UriType(); 3870 if (!(this.source instanceof UriType)) 3871 throw new FHIRException( 3872 "Type mismatch: the type UriType was expected, but " + this.source.getClass().getName() + " was encountered"); 3873 return (UriType) this.source; 3874 } 3875 3876 public boolean hasSourceUriType() { 3877 return this != null && this.source instanceof UriType; 3878 } 3879 3880 /** 3881 * @return {@link #source} (Identifier for the source value set that contains 3882 * the concepts that are being mapped and provides context for the 3883 * mappings.) 3884 */ 3885 public CanonicalType getSourceCanonicalType() throws FHIRException { 3886 if (this.source == null) 3887 this.source = new CanonicalType(); 3888 if (!(this.source instanceof CanonicalType)) 3889 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but " 3890 + this.source.getClass().getName() + " was encountered"); 3891 return (CanonicalType) this.source; 3892 } 3893 3894 public boolean hasSourceCanonicalType() { 3895 return this != null && this.source instanceof CanonicalType; 3896 } 3897 3898 public boolean hasSource() { 3899 return this.source != null && !this.source.isEmpty(); 3900 } 3901 3902 /** 3903 * @param value {@link #source} (Identifier for the source value set that 3904 * contains the concepts that are being mapped and provides context 3905 * for the mappings.) 3906 */ 3907 public ConceptMap setSource(Type value) { 3908 if (value != null && !(value instanceof UriType || value instanceof CanonicalType)) 3909 throw new Error("Not the right type for ConceptMap.source[x]: " + value.fhirType()); 3910 this.source = value; 3911 return this; 3912 } 3913 3914 /** 3915 * @return {@link #target} (The target value set provides context for the 3916 * mappings. Note that the mapping is made between concepts, not between 3917 * value sets, but the value set provides important context about how 3918 * the concept mapping choices are made.) 3919 */ 3920 public Type getTarget() { 3921 return this.target; 3922 } 3923 3924 /** 3925 * @return {@link #target} (The target value set provides context for the 3926 * mappings. Note that the mapping is made between concepts, not between 3927 * value sets, but the value set provides important context about how 3928 * the concept mapping choices are made.) 3929 */ 3930 public UriType getTargetUriType() throws FHIRException { 3931 if (this.target == null) 3932 this.target = new UriType(); 3933 if (!(this.target instanceof UriType)) 3934 throw new FHIRException( 3935 "Type mismatch: the type UriType was expected, but " + this.target.getClass().getName() + " was encountered"); 3936 return (UriType) this.target; 3937 } 3938 3939 public boolean hasTargetUriType() { 3940 return this != null && this.target instanceof UriType; 3941 } 3942 3943 /** 3944 * @return {@link #target} (The target value set provides context for the 3945 * mappings. Note that the mapping is made between concepts, not between 3946 * value sets, but the value set provides important context about how 3947 * the concept mapping choices are made.) 3948 */ 3949 public CanonicalType getTargetCanonicalType() throws FHIRException { 3950 if (this.target == null) 3951 this.target = new CanonicalType(); 3952 if (!(this.target instanceof CanonicalType)) 3953 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but " 3954 + this.target.getClass().getName() + " was encountered"); 3955 return (CanonicalType) this.target; 3956 } 3957 3958 public boolean hasTargetCanonicalType() { 3959 return this != null && this.target instanceof CanonicalType; 3960 } 3961 3962 public boolean hasTarget() { 3963 return this.target != null && !this.target.isEmpty(); 3964 } 3965 3966 /** 3967 * @param value {@link #target} (The target value set provides context for the 3968 * mappings. Note that the mapping is made between concepts, not 3969 * between value sets, but the value set provides important context 3970 * about how the concept mapping choices are made.) 3971 */ 3972 public ConceptMap setTarget(Type value) { 3973 if (value != null && !(value instanceof UriType || value instanceof CanonicalType)) 3974 throw new Error("Not the right type for ConceptMap.target[x]: " + value.fhirType()); 3975 this.target = value; 3976 return this; 3977 } 3978 3979 /** 3980 * @return {@link #group} (A group of mappings that all have the same source and 3981 * target system.) 3982 */ 3983 public List<ConceptMapGroupComponent> getGroup() { 3984 if (this.group == null) 3985 this.group = new ArrayList<ConceptMapGroupComponent>(); 3986 return this.group; 3987 } 3988 3989 /** 3990 * @return Returns a reference to <code>this</code> for easy method chaining 3991 */ 3992 public ConceptMap setGroup(List<ConceptMapGroupComponent> theGroup) { 3993 this.group = theGroup; 3994 return this; 3995 } 3996 3997 public boolean hasGroup() { 3998 if (this.group == null) 3999 return false; 4000 for (ConceptMapGroupComponent item : this.group) 4001 if (!item.isEmpty()) 4002 return true; 4003 return false; 4004 } 4005 4006 public ConceptMapGroupComponent addGroup() { // 3 4007 ConceptMapGroupComponent t = new ConceptMapGroupComponent(); 4008 if (this.group == null) 4009 this.group = new ArrayList<ConceptMapGroupComponent>(); 4010 this.group.add(t); 4011 return t; 4012 } 4013 4014 public ConceptMap addGroup(ConceptMapGroupComponent t) { // 3 4015 if (t == null) 4016 return this; 4017 if (this.group == null) 4018 this.group = new ArrayList<ConceptMapGroupComponent>(); 4019 this.group.add(t); 4020 return this; 4021 } 4022 4023 /** 4024 * @return The first repetition of repeating field {@link #group}, creating it 4025 * if it does not already exist 4026 */ 4027 public ConceptMapGroupComponent getGroupFirstRep() { 4028 if (getGroup().isEmpty()) { 4029 addGroup(); 4030 } 4031 return getGroup().get(0); 4032 } 4033 4034 protected void listChildren(List<Property> children) { 4035 super.listChildren(children); 4036 children.add(new Property("url", "uri", 4037 "An absolute URI that is used to identify this concept map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this concept map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the concept map is stored on different servers.", 4038 0, 1, url)); 4039 children.add(new Property("identifier", "Identifier", 4040 "A formal identifier that is used to identify this concept map when it is represented in other formats, or referenced in a specification, model, design or an instance.", 4041 0, 1, identifier)); 4042 children.add(new Property("version", "string", 4043 "The identifier that is used to identify this version of the concept map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the concept map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 4044 0, 1, version)); 4045 children.add(new Property("name", "string", 4046 "A natural language name identifying the concept map. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 4047 0, 1, name)); 4048 children.add( 4049 new Property("title", "string", "A short, descriptive, user-friendly title for the concept map.", 0, 1, title)); 4050 children.add(new Property("status", "code", 4051 "The status of this concept map. Enables tracking the life-cycle of the content.", 0, 1, status)); 4052 children.add(new Property("experimental", "boolean", 4053 "A Boolean value to indicate that this concept map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 4054 0, 1, experimental)); 4055 children.add(new Property("date", "dateTime", 4056 "The date (and optionally time) when the concept map was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the concept map changes.", 4057 0, 1, date)); 4058 children.add(new Property("publisher", "string", 4059 "The name of the organization or individual that published the concept map.", 0, 1, publisher)); 4060 children.add(new Property("contact", "ContactDetail", 4061 "Contact details to assist a user in finding and communicating with the publisher.", 0, 4062 java.lang.Integer.MAX_VALUE, contact)); 4063 children.add(new Property("description", "markdown", 4064 "A free text natural language description of the concept map from a consumer's perspective.", 0, 1, 4065 description)); 4066 children.add(new Property("useContext", "UsageContext", 4067 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate concept map instances.", 4068 0, java.lang.Integer.MAX_VALUE, useContext)); 4069 children.add(new Property("jurisdiction", "CodeableConcept", 4070 "A legal or geographic region in which the concept map is intended to be used.", 0, java.lang.Integer.MAX_VALUE, 4071 jurisdiction)); 4072 children.add(new Property("purpose", "markdown", 4073 "Explanation of why this concept map is needed and why it has been designed as it has.", 0, 1, purpose)); 4074 children.add(new Property("copyright", "markdown", 4075 "A copyright statement relating to the concept map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the concept map.", 4076 0, 1, copyright)); 4077 children.add(new Property("source[x]", "uri|canonical(ValueSet)", 4078 "Identifier for the source value set that contains the concepts that are being mapped and provides context for the mappings.", 4079 0, 1, source)); 4080 children.add(new Property("target[x]", "uri|canonical(ValueSet)", 4081 "The target value set provides context for the mappings. Note that the mapping is made between concepts, not between value sets, but the value set provides important context about how the concept mapping choices are made.", 4082 0, 1, target)); 4083 children.add(new Property("group", "", "A group of mappings that all have the same source and target system.", 0, 4084 java.lang.Integer.MAX_VALUE, group)); 4085 } 4086 4087 @Override 4088 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4089 switch (_hash) { 4090 case 116079: 4091 /* url */ return new Property("url", "uri", 4092 "An absolute URI that is used to identify this concept map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this concept map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the concept map is stored on different servers.", 4093 0, 1, url); 4094 case -1618432855: 4095 /* identifier */ return new Property("identifier", "Identifier", 4096 "A formal identifier that is used to identify this concept map when it is represented in other formats, or referenced in a specification, model, design or an instance.", 4097 0, 1, identifier); 4098 case 351608024: 4099 /* version */ return new Property("version", "string", 4100 "The identifier that is used to identify this version of the concept map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the concept map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 4101 0, 1, version); 4102 case 3373707: 4103 /* name */ return new Property("name", "string", 4104 "A natural language name identifying the concept map. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 4105 0, 1, name); 4106 case 110371416: 4107 /* title */ return new Property("title", "string", 4108 "A short, descriptive, user-friendly title for the concept map.", 0, 1, title); 4109 case -892481550: 4110 /* status */ return new Property("status", "code", 4111 "The status of this concept map. Enables tracking the life-cycle of the content.", 0, 1, status); 4112 case -404562712: 4113 /* experimental */ return new Property("experimental", "boolean", 4114 "A Boolean value to indicate that this concept map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 4115 0, 1, experimental); 4116 case 3076014: 4117 /* date */ return new Property("date", "dateTime", 4118 "The date (and optionally time) when the concept map was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the concept map changes.", 4119 0, 1, date); 4120 case 1447404028: 4121 /* publisher */ return new Property("publisher", "string", 4122 "The name of the organization or individual that published the concept map.", 0, 1, publisher); 4123 case 951526432: 4124 /* contact */ return new Property("contact", "ContactDetail", 4125 "Contact details to assist a user in finding and communicating with the publisher.", 0, 4126 java.lang.Integer.MAX_VALUE, contact); 4127 case -1724546052: 4128 /* description */ return new Property("description", "markdown", 4129 "A free text natural language description of the concept map from a consumer's perspective.", 0, 1, 4130 description); 4131 case -669707736: 4132 /* useContext */ return new Property("useContext", "UsageContext", 4133 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate concept map instances.", 4134 0, java.lang.Integer.MAX_VALUE, useContext); 4135 case -507075711: 4136 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 4137 "A legal or geographic region in which the concept map is intended to be used.", 0, 4138 java.lang.Integer.MAX_VALUE, jurisdiction); 4139 case -220463842: 4140 /* purpose */ return new Property("purpose", "markdown", 4141 "Explanation of why this concept map is needed and why it has been designed as it has.", 0, 1, purpose); 4142 case 1522889671: 4143 /* copyright */ return new Property("copyright", "markdown", 4144 "A copyright statement relating to the concept map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the concept map.", 4145 0, 1, copyright); 4146 case -1698413947: 4147 /* source[x] */ return new Property("source[x]", "uri|canonical(ValueSet)", 4148 "Identifier for the source value set that contains the concepts that are being mapped and provides context for the mappings.", 4149 0, 1, source); 4150 case -896505829: 4151 /* source */ return new Property("source[x]", "uri|canonical(ValueSet)", 4152 "Identifier for the source value set that contains the concepts that are being mapped and provides context for the mappings.", 4153 0, 1, source); 4154 case -1698419887: 4155 /* sourceUri */ return new Property("source[x]", "uri|canonical(ValueSet)", 4156 "Identifier for the source value set that contains the concepts that are being mapped and provides context for the mappings.", 4157 0, 1, source); 4158 case 1509247769: 4159 /* sourceCanonical */ return new Property("source[x]", "uri|canonical(ValueSet)", 4160 "Identifier for the source value set that contains the concepts that are being mapped and provides context for the mappings.", 4161 0, 1, source); 4162 case -815579825: 4163 /* target[x] */ return new Property("target[x]", "uri|canonical(ValueSet)", 4164 "The target value set provides context for the mappings. Note that the mapping is made between concepts, not between value sets, but the value set provides important context about how the concept mapping choices are made.", 4165 0, 1, target); 4166 case -880905839: 4167 /* target */ return new Property("target[x]", "uri|canonical(ValueSet)", 4168 "The target value set provides context for the mappings. Note that the mapping is made between concepts, not between value sets, but the value set provides important context about how the concept mapping choices are made.", 4169 0, 1, target); 4170 case -815585765: 4171 /* targetUri */ return new Property("target[x]", "uri|canonical(ValueSet)", 4172 "The target value set provides context for the mappings. Note that the mapping is made between concepts, not between value sets, but the value set provides important context about how the concept mapping choices are made.", 4173 0, 1, target); 4174 case -1281653149: 4175 /* targetCanonical */ return new Property("target[x]", "uri|canonical(ValueSet)", 4176 "The target value set provides context for the mappings. Note that the mapping is made between concepts, not between value sets, but the value set provides important context about how the concept mapping choices are made.", 4177 0, 1, target); 4178 case 98629247: 4179 /* group */ return new Property("group", "", 4180 "A group of mappings that all have the same source and target system.", 0, java.lang.Integer.MAX_VALUE, 4181 group); 4182 default: 4183 return super.getNamedProperty(_hash, _name, _checkValid); 4184 } 4185 4186 } 4187 4188 @Override 4189 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4190 switch (hash) { 4191 case 116079: 4192 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 4193 case -1618432855: 4194 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 4195 case 351608024: 4196 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 4197 case 3373707: 4198 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 4199 case 110371416: 4200 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 4201 case -892481550: 4202 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 4203 case -404562712: 4204 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 4205 case 3076014: 4206 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 4207 case 1447404028: 4208 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 4209 case 951526432: 4210 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 4211 case -1724546052: 4212 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 4213 case -669707736: 4214 /* useContext */ return this.useContext == null ? new Base[0] 4215 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 4216 case -507075711: 4217 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 4218 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 4219 case -220463842: 4220 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 4221 case 1522889671: 4222 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 4223 case -896505829: 4224 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // Type 4225 case -880905839: 4226 /* target */ return this.target == null ? new Base[0] : new Base[] { this.target }; // Type 4227 case 98629247: 4228 /* group */ return this.group == null ? new Base[0] : this.group.toArray(new Base[this.group.size()]); // ConceptMapGroupComponent 4229 default: 4230 return super.getProperty(hash, name, checkValid); 4231 } 4232 4233 } 4234 4235 @Override 4236 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4237 switch (hash) { 4238 case 116079: // url 4239 this.url = castToUri(value); // UriType 4240 return value; 4241 case -1618432855: // identifier 4242 this.identifier = castToIdentifier(value); // Identifier 4243 return value; 4244 case 351608024: // version 4245 this.version = castToString(value); // StringType 4246 return value; 4247 case 3373707: // name 4248 this.name = castToString(value); // StringType 4249 return value; 4250 case 110371416: // title 4251 this.title = castToString(value); // StringType 4252 return value; 4253 case -892481550: // status 4254 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 4255 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4256 return value; 4257 case -404562712: // experimental 4258 this.experimental = castToBoolean(value); // BooleanType 4259 return value; 4260 case 3076014: // date 4261 this.date = castToDateTime(value); // DateTimeType 4262 return value; 4263 case 1447404028: // publisher 4264 this.publisher = castToString(value); // StringType 4265 return value; 4266 case 951526432: // contact 4267 this.getContact().add(castToContactDetail(value)); // ContactDetail 4268 return value; 4269 case -1724546052: // description 4270 this.description = castToMarkdown(value); // MarkdownType 4271 return value; 4272 case -669707736: // useContext 4273 this.getUseContext().add(castToUsageContext(value)); // UsageContext 4274 return value; 4275 case -507075711: // jurisdiction 4276 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 4277 return value; 4278 case -220463842: // purpose 4279 this.purpose = castToMarkdown(value); // MarkdownType 4280 return value; 4281 case 1522889671: // copyright 4282 this.copyright = castToMarkdown(value); // MarkdownType 4283 return value; 4284 case -896505829: // source 4285 this.source = castToType(value); // Type 4286 return value; 4287 case -880905839: // target 4288 this.target = castToType(value); // Type 4289 return value; 4290 case 98629247: // group 4291 this.getGroup().add((ConceptMapGroupComponent) value); // ConceptMapGroupComponent 4292 return value; 4293 default: 4294 return super.setProperty(hash, name, value); 4295 } 4296 4297 } 4298 4299 @Override 4300 public Base setProperty(String name, Base value) throws FHIRException { 4301 if (name.equals("url")) { 4302 this.url = castToUri(value); // UriType 4303 } else if (name.equals("identifier")) { 4304 this.identifier = castToIdentifier(value); // Identifier 4305 } else if (name.equals("version")) { 4306 this.version = castToString(value); // StringType 4307 } else if (name.equals("name")) { 4308 this.name = castToString(value); // StringType 4309 } else if (name.equals("title")) { 4310 this.title = castToString(value); // StringType 4311 } else if (name.equals("status")) { 4312 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 4313 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4314 } else if (name.equals("experimental")) { 4315 this.experimental = castToBoolean(value); // BooleanType 4316 } else if (name.equals("date")) { 4317 this.date = castToDateTime(value); // DateTimeType 4318 } else if (name.equals("publisher")) { 4319 this.publisher = castToString(value); // StringType 4320 } else if (name.equals("contact")) { 4321 this.getContact().add(castToContactDetail(value)); 4322 } else if (name.equals("description")) { 4323 this.description = castToMarkdown(value); // MarkdownType 4324 } else if (name.equals("useContext")) { 4325 this.getUseContext().add(castToUsageContext(value)); 4326 } else if (name.equals("jurisdiction")) { 4327 this.getJurisdiction().add(castToCodeableConcept(value)); 4328 } else if (name.equals("purpose")) { 4329 this.purpose = castToMarkdown(value); // MarkdownType 4330 } else if (name.equals("copyright")) { 4331 this.copyright = castToMarkdown(value); // MarkdownType 4332 } else if (name.equals("source[x]")) { 4333 this.source = castToType(value); // Type 4334 } else if (name.equals("target[x]")) { 4335 this.target = castToType(value); // Type 4336 } else if (name.equals("group")) { 4337 this.getGroup().add((ConceptMapGroupComponent) value); 4338 } else 4339 return super.setProperty(name, value); 4340 return value; 4341 } 4342 4343 @Override 4344 public void removeChild(String name, Base value) throws FHIRException { 4345 if (name.equals("url")) { 4346 this.url = null; 4347 } else if (name.equals("identifier")) { 4348 this.identifier = null; 4349 } else if (name.equals("version")) { 4350 this.version = null; 4351 } else if (name.equals("name")) { 4352 this.name = null; 4353 } else if (name.equals("title")) { 4354 this.title = null; 4355 } else if (name.equals("status")) { 4356 this.status = null; 4357 } else if (name.equals("experimental")) { 4358 this.experimental = null; 4359 } else if (name.equals("date")) { 4360 this.date = null; 4361 } else if (name.equals("publisher")) { 4362 this.publisher = null; 4363 } else if (name.equals("contact")) { 4364 this.getContact().remove(castToContactDetail(value)); 4365 } else if (name.equals("description")) { 4366 this.description = null; 4367 } else if (name.equals("useContext")) { 4368 this.getUseContext().remove(castToUsageContext(value)); 4369 } else if (name.equals("jurisdiction")) { 4370 this.getJurisdiction().remove(castToCodeableConcept(value)); 4371 } else if (name.equals("purpose")) { 4372 this.purpose = null; 4373 } else if (name.equals("copyright")) { 4374 this.copyright = null; 4375 } else if (name.equals("source[x]")) { 4376 this.source = null; 4377 } else if (name.equals("target[x]")) { 4378 this.target = null; 4379 } else if (name.equals("group")) { 4380 this.getGroup().remove((ConceptMapGroupComponent) value); 4381 } else 4382 super.removeChild(name, value); 4383 4384 } 4385 4386 @Override 4387 public Base makeProperty(int hash, String name) throws FHIRException { 4388 switch (hash) { 4389 case 116079: 4390 return getUrlElement(); 4391 case -1618432855: 4392 return getIdentifier(); 4393 case 351608024: 4394 return getVersionElement(); 4395 case 3373707: 4396 return getNameElement(); 4397 case 110371416: 4398 return getTitleElement(); 4399 case -892481550: 4400 return getStatusElement(); 4401 case -404562712: 4402 return getExperimentalElement(); 4403 case 3076014: 4404 return getDateElement(); 4405 case 1447404028: 4406 return getPublisherElement(); 4407 case 951526432: 4408 return addContact(); 4409 case -1724546052: 4410 return getDescriptionElement(); 4411 case -669707736: 4412 return addUseContext(); 4413 case -507075711: 4414 return addJurisdiction(); 4415 case -220463842: 4416 return getPurposeElement(); 4417 case 1522889671: 4418 return getCopyrightElement(); 4419 case -1698413947: 4420 return getSource(); 4421 case -896505829: 4422 return getSource(); 4423 case -815579825: 4424 return getTarget(); 4425 case -880905839: 4426 return getTarget(); 4427 case 98629247: 4428 return addGroup(); 4429 default: 4430 return super.makeProperty(hash, name); 4431 } 4432 4433 } 4434 4435 @Override 4436 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4437 switch (hash) { 4438 case 116079: 4439 /* url */ return new String[] { "uri" }; 4440 case -1618432855: 4441 /* identifier */ return new String[] { "Identifier" }; 4442 case 351608024: 4443 /* version */ return new String[] { "string" }; 4444 case 3373707: 4445 /* name */ return new String[] { "string" }; 4446 case 110371416: 4447 /* title */ return new String[] { "string" }; 4448 case -892481550: 4449 /* status */ return new String[] { "code" }; 4450 case -404562712: 4451 /* experimental */ return new String[] { "boolean" }; 4452 case 3076014: 4453 /* date */ return new String[] { "dateTime" }; 4454 case 1447404028: 4455 /* publisher */ return new String[] { "string" }; 4456 case 951526432: 4457 /* contact */ return new String[] { "ContactDetail" }; 4458 case -1724546052: 4459 /* description */ return new String[] { "markdown" }; 4460 case -669707736: 4461 /* useContext */ return new String[] { "UsageContext" }; 4462 case -507075711: 4463 /* jurisdiction */ return new String[] { "CodeableConcept" }; 4464 case -220463842: 4465 /* purpose */ return new String[] { "markdown" }; 4466 case 1522889671: 4467 /* copyright */ return new String[] { "markdown" }; 4468 case -896505829: 4469 /* source */ return new String[] { "uri", "canonical" }; 4470 case -880905839: 4471 /* target */ return new String[] { "uri", "canonical" }; 4472 case 98629247: 4473 /* group */ return new String[] {}; 4474 default: 4475 return super.getTypesForProperty(hash, name); 4476 } 4477 4478 } 4479 4480 @Override 4481 public Base addChild(String name) throws FHIRException { 4482 if (name.equals("url")) { 4483 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.url"); 4484 } else if (name.equals("identifier")) { 4485 this.identifier = new Identifier(); 4486 return this.identifier; 4487 } else if (name.equals("version")) { 4488 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.version"); 4489 } else if (name.equals("name")) { 4490 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.name"); 4491 } else if (name.equals("title")) { 4492 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.title"); 4493 } else if (name.equals("status")) { 4494 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.status"); 4495 } else if (name.equals("experimental")) { 4496 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.experimental"); 4497 } else if (name.equals("date")) { 4498 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.date"); 4499 } else if (name.equals("publisher")) { 4500 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.publisher"); 4501 } else if (name.equals("contact")) { 4502 return addContact(); 4503 } else if (name.equals("description")) { 4504 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.description"); 4505 } else if (name.equals("useContext")) { 4506 return addUseContext(); 4507 } else if (name.equals("jurisdiction")) { 4508 return addJurisdiction(); 4509 } else if (name.equals("purpose")) { 4510 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.purpose"); 4511 } else if (name.equals("copyright")) { 4512 throw new FHIRException("Cannot call addChild on a singleton property ConceptMap.copyright"); 4513 } else if (name.equals("sourceUri")) { 4514 this.source = new UriType(); 4515 return this.source; 4516 } else if (name.equals("sourceCanonical")) { 4517 this.source = new CanonicalType(); 4518 return this.source; 4519 } else if (name.equals("targetUri")) { 4520 this.target = new UriType(); 4521 return this.target; 4522 } else if (name.equals("targetCanonical")) { 4523 this.target = new CanonicalType(); 4524 return this.target; 4525 } else if (name.equals("group")) { 4526 return addGroup(); 4527 } else 4528 return super.addChild(name); 4529 } 4530 4531 public String fhirType() { 4532 return "ConceptMap"; 4533 4534 } 4535 4536 public ConceptMap copy() { 4537 ConceptMap dst = new ConceptMap(); 4538 copyValues(dst); 4539 return dst; 4540 } 4541 4542 public void copyValues(ConceptMap dst) { 4543 super.copyValues(dst); 4544 dst.url = url == null ? null : url.copy(); 4545 dst.identifier = identifier == null ? null : identifier.copy(); 4546 dst.version = version == null ? null : version.copy(); 4547 dst.name = name == null ? null : name.copy(); 4548 dst.title = title == null ? null : title.copy(); 4549 dst.status = status == null ? null : status.copy(); 4550 dst.experimental = experimental == null ? null : experimental.copy(); 4551 dst.date = date == null ? null : date.copy(); 4552 dst.publisher = publisher == null ? null : publisher.copy(); 4553 if (contact != null) { 4554 dst.contact = new ArrayList<ContactDetail>(); 4555 for (ContactDetail i : contact) 4556 dst.contact.add(i.copy()); 4557 } 4558 ; 4559 dst.description = description == null ? null : description.copy(); 4560 if (useContext != null) { 4561 dst.useContext = new ArrayList<UsageContext>(); 4562 for (UsageContext i : useContext) 4563 dst.useContext.add(i.copy()); 4564 } 4565 ; 4566 if (jurisdiction != null) { 4567 dst.jurisdiction = new ArrayList<CodeableConcept>(); 4568 for (CodeableConcept i : jurisdiction) 4569 dst.jurisdiction.add(i.copy()); 4570 } 4571 ; 4572 dst.purpose = purpose == null ? null : purpose.copy(); 4573 dst.copyright = copyright == null ? null : copyright.copy(); 4574 dst.source = source == null ? null : source.copy(); 4575 dst.target = target == null ? null : target.copy(); 4576 if (group != null) { 4577 dst.group = new ArrayList<ConceptMapGroupComponent>(); 4578 for (ConceptMapGroupComponent i : group) 4579 dst.group.add(i.copy()); 4580 } 4581 ; 4582 } 4583 4584 protected ConceptMap typedCopy() { 4585 return copy(); 4586 } 4587 4588 @Override 4589 public boolean equalsDeep(Base other_) { 4590 if (!super.equalsDeep(other_)) 4591 return false; 4592 if (!(other_ instanceof ConceptMap)) 4593 return false; 4594 ConceptMap o = (ConceptMap) other_; 4595 return compareDeep(identifier, o.identifier, true) && compareDeep(purpose, o.purpose, true) 4596 && compareDeep(copyright, o.copyright, true) && compareDeep(source, o.source, true) 4597 && compareDeep(target, o.target, true) && compareDeep(group, o.group, true); 4598 } 4599 4600 @Override 4601 public boolean equalsShallow(Base other_) { 4602 if (!super.equalsShallow(other_)) 4603 return false; 4604 if (!(other_ instanceof ConceptMap)) 4605 return false; 4606 ConceptMap o = (ConceptMap) other_; 4607 return compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true); 4608 } 4609 4610 public boolean isEmpty() { 4611 return super.isEmpty() 4612 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, purpose, copyright, source, target, group); 4613 } 4614 4615 @Override 4616 public ResourceType getResourceType() { 4617 return ResourceType.ConceptMap; 4618 } 4619 4620 /** 4621 * Search parameter: <b>date</b> 4622 * <p> 4623 * Description: <b>The concept map publication date</b><br> 4624 * Type: <b>date</b><br> 4625 * Path: <b>ConceptMap.date</b><br> 4626 * </p> 4627 */ 4628 @SearchParamDefinition(name = "date", path = "ConceptMap.date", description = "The concept map publication date", type = "date") 4629 public static final String SP_DATE = "date"; 4630 /** 4631 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4632 * <p> 4633 * Description: <b>The concept map publication date</b><br> 4634 * Type: <b>date</b><br> 4635 * Path: <b>ConceptMap.date</b><br> 4636 * </p> 4637 */ 4638 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 4639 SP_DATE); 4640 4641 /** 4642 * Search parameter: <b>other</b> 4643 * <p> 4644 * Description: <b>canonical reference to an additional ConceptMap to use for 4645 * mapping if the source concept is unmapped</b><br> 4646 * Type: <b>reference</b><br> 4647 * Path: <b>ConceptMap.group.unmapped.url</b><br> 4648 * </p> 4649 */ 4650 @SearchParamDefinition(name = "other", path = "ConceptMap.group.unmapped.url", description = "canonical reference to an additional ConceptMap to use for mapping if the source concept is unmapped", type = "reference", target = { 4651 ConceptMap.class }) 4652 public static final String SP_OTHER = "other"; 4653 /** 4654 * <b>Fluent Client</b> search parameter constant for <b>other</b> 4655 * <p> 4656 * Description: <b>canonical reference to an additional ConceptMap to use for 4657 * mapping if the source concept is unmapped</b><br> 4658 * Type: <b>reference</b><br> 4659 * Path: <b>ConceptMap.group.unmapped.url</b><br> 4660 * </p> 4661 */ 4662 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam OTHER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4663 SP_OTHER); 4664 4665 /** 4666 * Constant for fluent queries to be used to add include statements. Specifies 4667 * the path value of "<b>ConceptMap:other</b>". 4668 */ 4669 public static final ca.uhn.fhir.model.api.Include INCLUDE_OTHER = new ca.uhn.fhir.model.api.Include( 4670 "ConceptMap:other").toLocked(); 4671 4672 /** 4673 * Search parameter: <b>context-type-value</b> 4674 * <p> 4675 * Description: <b>A use context type and value assigned to the concept 4676 * map</b><br> 4677 * Type: <b>composite</b><br> 4678 * Path: <b></b><br> 4679 * </p> 4680 */ 4681 @SearchParamDefinition(name = "context-type-value", path = "ConceptMap.useContext", description = "A use context type and value assigned to the concept map", type = "composite", compositeOf = { 4682 "context-type", "context" }) 4683 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 4684 /** 4685 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 4686 * <p> 4687 * Description: <b>A use context type and value assigned to the concept 4688 * map</b><br> 4689 * Type: <b>composite</b><br> 4690 * Path: <b></b><br> 4691 * </p> 4692 */ 4693 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 4694 SP_CONTEXT_TYPE_VALUE); 4695 4696 /** 4697 * Search parameter: <b>target-system</b> 4698 * <p> 4699 * Description: <b>Target system that the concepts are to be mapped to</b><br> 4700 * Type: <b>uri</b><br> 4701 * Path: <b>ConceptMap.group.target</b><br> 4702 * </p> 4703 */ 4704 @SearchParamDefinition(name = "target-system", path = "ConceptMap.group.target", description = "Target system that the concepts are to be mapped to", type = "uri") 4705 public static final String SP_TARGET_SYSTEM = "target-system"; 4706 /** 4707 * <b>Fluent Client</b> search parameter constant for <b>target-system</b> 4708 * <p> 4709 * Description: <b>Target system that the concepts are to be mapped to</b><br> 4710 * Type: <b>uri</b><br> 4711 * Path: <b>ConceptMap.group.target</b><br> 4712 * </p> 4713 */ 4714 public static final ca.uhn.fhir.rest.gclient.UriClientParam TARGET_SYSTEM = new ca.uhn.fhir.rest.gclient.UriClientParam( 4715 SP_TARGET_SYSTEM); 4716 4717 /** 4718 * Search parameter: <b>dependson</b> 4719 * <p> 4720 * Description: <b>Reference to property mapping depends on</b><br> 4721 * Type: <b>uri</b><br> 4722 * Path: <b>ConceptMap.group.element.target.dependsOn.property</b><br> 4723 * </p> 4724 */ 4725 @SearchParamDefinition(name = "dependson", path = "ConceptMap.group.element.target.dependsOn.property", description = "Reference to property mapping depends on", type = "uri") 4726 public static final String SP_DEPENDSON = "dependson"; 4727 /** 4728 * <b>Fluent Client</b> search parameter constant for <b>dependson</b> 4729 * <p> 4730 * Description: <b>Reference to property mapping depends on</b><br> 4731 * Type: <b>uri</b><br> 4732 * Path: <b>ConceptMap.group.element.target.dependsOn.property</b><br> 4733 * </p> 4734 */ 4735 public static final ca.uhn.fhir.rest.gclient.UriClientParam DEPENDSON = new ca.uhn.fhir.rest.gclient.UriClientParam( 4736 SP_DEPENDSON); 4737 4738 /** 4739 * Search parameter: <b>jurisdiction</b> 4740 * <p> 4741 * Description: <b>Intended jurisdiction for the concept map</b><br> 4742 * Type: <b>token</b><br> 4743 * Path: <b>ConceptMap.jurisdiction</b><br> 4744 * </p> 4745 */ 4746 @SearchParamDefinition(name = "jurisdiction", path = "ConceptMap.jurisdiction", description = "Intended jurisdiction for the concept map", type = "token") 4747 public static final String SP_JURISDICTION = "jurisdiction"; 4748 /** 4749 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 4750 * <p> 4751 * Description: <b>Intended jurisdiction for the concept map</b><br> 4752 * Type: <b>token</b><br> 4753 * Path: <b>ConceptMap.jurisdiction</b><br> 4754 * </p> 4755 */ 4756 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4757 SP_JURISDICTION); 4758 4759 /** 4760 * Search parameter: <b>description</b> 4761 * <p> 4762 * Description: <b>The description of the concept map</b><br> 4763 * Type: <b>string</b><br> 4764 * Path: <b>ConceptMap.description</b><br> 4765 * </p> 4766 */ 4767 @SearchParamDefinition(name = "description", path = "ConceptMap.description", description = "The description of the concept map", type = "string") 4768 public static final String SP_DESCRIPTION = "description"; 4769 /** 4770 * <b>Fluent Client</b> search parameter constant for <b>description</b> 4771 * <p> 4772 * Description: <b>The description of the concept map</b><br> 4773 * Type: <b>string</b><br> 4774 * Path: <b>ConceptMap.description</b><br> 4775 * </p> 4776 */ 4777 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 4778 SP_DESCRIPTION); 4779 4780 /** 4781 * Search parameter: <b>context-type</b> 4782 * <p> 4783 * Description: <b>A type of use context assigned to the concept map</b><br> 4784 * Type: <b>token</b><br> 4785 * Path: <b>ConceptMap.useContext.code</b><br> 4786 * </p> 4787 */ 4788 @SearchParamDefinition(name = "context-type", path = "ConceptMap.useContext.code", description = "A type of use context assigned to the concept map", type = "token") 4789 public static final String SP_CONTEXT_TYPE = "context-type"; 4790 /** 4791 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 4792 * <p> 4793 * Description: <b>A type of use context assigned to the concept map</b><br> 4794 * Type: <b>token</b><br> 4795 * Path: <b>ConceptMap.useContext.code</b><br> 4796 * </p> 4797 */ 4798 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4799 SP_CONTEXT_TYPE); 4800 4801 /** 4802 * Search parameter: <b>source</b> 4803 * <p> 4804 * Description: <b>The source value set that contains the concepts that are 4805 * being mapped</b><br> 4806 * Type: <b>reference</b><br> 4807 * Path: <b>ConceptMap.sourceCanonical</b><br> 4808 * </p> 4809 */ 4810 @SearchParamDefinition(name = "source", path = "(ConceptMap.source as canonical)", description = "The source value set that contains the concepts that are being mapped", type = "reference", target = { 4811 ValueSet.class }) 4812 public static final String SP_SOURCE = "source"; 4813 /** 4814 * <b>Fluent Client</b> search parameter constant for <b>source</b> 4815 * <p> 4816 * Description: <b>The source value set that contains the concepts that are 4817 * being mapped</b><br> 4818 * Type: <b>reference</b><br> 4819 * Path: <b>ConceptMap.sourceCanonical</b><br> 4820 * </p> 4821 */ 4822 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4823 SP_SOURCE); 4824 4825 /** 4826 * Constant for fluent queries to be used to add include statements. Specifies 4827 * the path value of "<b>ConceptMap:source</b>". 4828 */ 4829 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include( 4830 "ConceptMap:source").toLocked(); 4831 4832 /** 4833 * Search parameter: <b>title</b> 4834 * <p> 4835 * Description: <b>The human-friendly name of the concept map</b><br> 4836 * Type: <b>string</b><br> 4837 * Path: <b>ConceptMap.title</b><br> 4838 * </p> 4839 */ 4840 @SearchParamDefinition(name = "title", path = "ConceptMap.title", description = "The human-friendly name of the concept map", type = "string") 4841 public static final String SP_TITLE = "title"; 4842 /** 4843 * <b>Fluent Client</b> search parameter constant for <b>title</b> 4844 * <p> 4845 * Description: <b>The human-friendly name of the concept map</b><br> 4846 * Type: <b>string</b><br> 4847 * Path: <b>ConceptMap.title</b><br> 4848 * </p> 4849 */ 4850 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 4851 SP_TITLE); 4852 4853 /** 4854 * Search parameter: <b>context-quantity</b> 4855 * <p> 4856 * Description: <b>A quantity- or range-valued use context assigned to the 4857 * concept map</b><br> 4858 * Type: <b>quantity</b><br> 4859 * Path: <b>ConceptMap.useContext.valueQuantity, 4860 * ConceptMap.useContext.valueRange</b><br> 4861 * </p> 4862 */ 4863 @SearchParamDefinition(name = "context-quantity", path = "(ConceptMap.useContext.value as Quantity) | (ConceptMap.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the concept map", type = "quantity") 4864 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 4865 /** 4866 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 4867 * <p> 4868 * Description: <b>A quantity- or range-valued use context assigned to the 4869 * concept map</b><br> 4870 * Type: <b>quantity</b><br> 4871 * Path: <b>ConceptMap.useContext.valueQuantity, 4872 * ConceptMap.useContext.valueRange</b><br> 4873 * </p> 4874 */ 4875 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 4876 SP_CONTEXT_QUANTITY); 4877 4878 /** 4879 * Search parameter: <b>source-uri</b> 4880 * <p> 4881 * Description: <b>The source value set that contains the concepts that are 4882 * being mapped</b><br> 4883 * Type: <b>reference</b><br> 4884 * Path: <b>ConceptMap.sourceUri</b><br> 4885 * </p> 4886 */ 4887 @SearchParamDefinition(name = "source-uri", path = "(ConceptMap.source as uri)", description = "The source value set that contains the concepts that are being mapped", type = "reference", target = { 4888 ValueSet.class }) 4889 public static final String SP_SOURCE_URI = "source-uri"; 4890 /** 4891 * <b>Fluent Client</b> search parameter constant for <b>source-uri</b> 4892 * <p> 4893 * Description: <b>The source value set that contains the concepts that are 4894 * being mapped</b><br> 4895 * Type: <b>reference</b><br> 4896 * Path: <b>ConceptMap.sourceUri</b><br> 4897 * </p> 4898 */ 4899 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE_URI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4900 SP_SOURCE_URI); 4901 4902 /** 4903 * Constant for fluent queries to be used to add include statements. Specifies 4904 * the path value of "<b>ConceptMap:source-uri</b>". 4905 */ 4906 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE_URI = new ca.uhn.fhir.model.api.Include( 4907 "ConceptMap:source-uri").toLocked(); 4908 4909 /** 4910 * Search parameter: <b>context</b> 4911 * <p> 4912 * Description: <b>A use context assigned to the concept map</b><br> 4913 * Type: <b>token</b><br> 4914 * Path: <b>ConceptMap.useContext.valueCodeableConcept</b><br> 4915 * </p> 4916 */ 4917 @SearchParamDefinition(name = "context", path = "(ConceptMap.useContext.value as CodeableConcept)", description = "A use context assigned to the concept map", type = "token") 4918 public static final String SP_CONTEXT = "context"; 4919 /** 4920 * <b>Fluent Client</b> search parameter constant for <b>context</b> 4921 * <p> 4922 * Description: <b>A use context assigned to the concept map</b><br> 4923 * Type: <b>token</b><br> 4924 * Path: <b>ConceptMap.useContext.valueCodeableConcept</b><br> 4925 * </p> 4926 */ 4927 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4928 SP_CONTEXT); 4929 4930 /** 4931 * Search parameter: <b>context-type-quantity</b> 4932 * <p> 4933 * Description: <b>A use context type and quantity- or range-based value 4934 * assigned to the concept map</b><br> 4935 * Type: <b>composite</b><br> 4936 * Path: <b></b><br> 4937 * </p> 4938 */ 4939 @SearchParamDefinition(name = "context-type-quantity", path = "ConceptMap.useContext", description = "A use context type and quantity- or range-based value assigned to the concept map", type = "composite", compositeOf = { 4940 "context-type", "context-quantity" }) 4941 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 4942 /** 4943 * <b>Fluent Client</b> search parameter constant for 4944 * <b>context-type-quantity</b> 4945 * <p> 4946 * Description: <b>A use context type and quantity- or range-based value 4947 * assigned to the concept map</b><br> 4948 * Type: <b>composite</b><br> 4949 * Path: <b></b><br> 4950 * </p> 4951 */ 4952 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 4953 SP_CONTEXT_TYPE_QUANTITY); 4954 4955 /** 4956 * Search parameter: <b>source-system</b> 4957 * <p> 4958 * Description: <b>Source system where concepts to be mapped are defined</b><br> 4959 * Type: <b>uri</b><br> 4960 * Path: <b>ConceptMap.group.source</b><br> 4961 * </p> 4962 */ 4963 @SearchParamDefinition(name = "source-system", path = "ConceptMap.group.source", description = "Source system where concepts to be mapped are defined", type = "uri") 4964 public static final String SP_SOURCE_SYSTEM = "source-system"; 4965 /** 4966 * <b>Fluent Client</b> search parameter constant for <b>source-system</b> 4967 * <p> 4968 * Description: <b>Source system where concepts to be mapped are defined</b><br> 4969 * Type: <b>uri</b><br> 4970 * Path: <b>ConceptMap.group.source</b><br> 4971 * </p> 4972 */ 4973 public static final ca.uhn.fhir.rest.gclient.UriClientParam SOURCE_SYSTEM = new ca.uhn.fhir.rest.gclient.UriClientParam( 4974 SP_SOURCE_SYSTEM); 4975 4976 /** 4977 * Search parameter: <b>target-code</b> 4978 * <p> 4979 * Description: <b>Code that identifies the target element</b><br> 4980 * Type: <b>token</b><br> 4981 * Path: <b>ConceptMap.group.element.target.code</b><br> 4982 * </p> 4983 */ 4984 @SearchParamDefinition(name = "target-code", path = "ConceptMap.group.element.target.code", description = "Code that identifies the target element", type = "token") 4985 public static final String SP_TARGET_CODE = "target-code"; 4986 /** 4987 * <b>Fluent Client</b> search parameter constant for <b>target-code</b> 4988 * <p> 4989 * Description: <b>Code that identifies the target element</b><br> 4990 * Type: <b>token</b><br> 4991 * Path: <b>ConceptMap.group.element.target.code</b><br> 4992 * </p> 4993 */ 4994 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TARGET_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4995 SP_TARGET_CODE); 4996 4997 /** 4998 * Search parameter: <b>target-uri</b> 4999 * <p> 5000 * Description: <b>The target value set which provides context for the 5001 * mappings</b><br> 5002 * Type: <b>reference</b><br> 5003 * Path: <b>ConceptMap.targetUri</b><br> 5004 * </p> 5005 */ 5006 @SearchParamDefinition(name = "target-uri", path = "(ConceptMap.target as uri)", description = "The target value set which provides context for the mappings", type = "reference", target = { 5007 ValueSet.class }) 5008 public static final String SP_TARGET_URI = "target-uri"; 5009 /** 5010 * <b>Fluent Client</b> search parameter constant for <b>target-uri</b> 5011 * <p> 5012 * Description: <b>The target value set which provides context for the 5013 * mappings</b><br> 5014 * Type: <b>reference</b><br> 5015 * Path: <b>ConceptMap.targetUri</b><br> 5016 * </p> 5017 */ 5018 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam TARGET_URI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5019 SP_TARGET_URI); 5020 5021 /** 5022 * Constant for fluent queries to be used to add include statements. Specifies 5023 * the path value of "<b>ConceptMap:target-uri</b>". 5024 */ 5025 public static final ca.uhn.fhir.model.api.Include INCLUDE_TARGET_URI = new ca.uhn.fhir.model.api.Include( 5026 "ConceptMap:target-uri").toLocked(); 5027 5028 /** 5029 * Search parameter: <b>identifier</b> 5030 * <p> 5031 * Description: <b>External identifier for the concept map</b><br> 5032 * Type: <b>token</b><br> 5033 * Path: <b>ConceptMap.identifier</b><br> 5034 * </p> 5035 */ 5036 @SearchParamDefinition(name = "identifier", path = "ConceptMap.identifier", description = "External identifier for the concept map", type = "token") 5037 public static final String SP_IDENTIFIER = "identifier"; 5038 /** 5039 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5040 * <p> 5041 * Description: <b>External identifier for the concept map</b><br> 5042 * Type: <b>token</b><br> 5043 * Path: <b>ConceptMap.identifier</b><br> 5044 * </p> 5045 */ 5046 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5047 SP_IDENTIFIER); 5048 5049 /** 5050 * Search parameter: <b>product</b> 5051 * <p> 5052 * Description: <b>Reference to property mapping depends on</b><br> 5053 * Type: <b>uri</b><br> 5054 * Path: <b>ConceptMap.group.element.target.product.property</b><br> 5055 * </p> 5056 */ 5057 @SearchParamDefinition(name = "product", path = "ConceptMap.group.element.target.product.property", description = "Reference to property mapping depends on", type = "uri") 5058 public static final String SP_PRODUCT = "product"; 5059 /** 5060 * <b>Fluent Client</b> search parameter constant for <b>product</b> 5061 * <p> 5062 * Description: <b>Reference to property mapping depends on</b><br> 5063 * Type: <b>uri</b><br> 5064 * Path: <b>ConceptMap.group.element.target.product.property</b><br> 5065 * </p> 5066 */ 5067 public static final ca.uhn.fhir.rest.gclient.UriClientParam PRODUCT = new ca.uhn.fhir.rest.gclient.UriClientParam( 5068 SP_PRODUCT); 5069 5070 /** 5071 * Search parameter: <b>version</b> 5072 * <p> 5073 * Description: <b>The business version of the concept map</b><br> 5074 * Type: <b>token</b><br> 5075 * Path: <b>ConceptMap.version</b><br> 5076 * </p> 5077 */ 5078 @SearchParamDefinition(name = "version", path = "ConceptMap.version", description = "The business version of the concept map", type = "token") 5079 public static final String SP_VERSION = "version"; 5080 /** 5081 * <b>Fluent Client</b> search parameter constant for <b>version</b> 5082 * <p> 5083 * Description: <b>The business version of the concept map</b><br> 5084 * Type: <b>token</b><br> 5085 * Path: <b>ConceptMap.version</b><br> 5086 * </p> 5087 */ 5088 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5089 SP_VERSION); 5090 5091 /** 5092 * Search parameter: <b>url</b> 5093 * <p> 5094 * Description: <b>The uri that identifies the concept map</b><br> 5095 * Type: <b>uri</b><br> 5096 * Path: <b>ConceptMap.url</b><br> 5097 * </p> 5098 */ 5099 @SearchParamDefinition(name = "url", path = "ConceptMap.url", description = "The uri that identifies the concept map", type = "uri") 5100 public static final String SP_URL = "url"; 5101 /** 5102 * <b>Fluent Client</b> search parameter constant for <b>url</b> 5103 * <p> 5104 * Description: <b>The uri that identifies the concept map</b><br> 5105 * Type: <b>uri</b><br> 5106 * Path: <b>ConceptMap.url</b><br> 5107 * </p> 5108 */ 5109 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 5110 5111 /** 5112 * Search parameter: <b>target</b> 5113 * <p> 5114 * Description: <b>The target value set which provides context for the 5115 * mappings</b><br> 5116 * Type: <b>reference</b><br> 5117 * Path: <b>ConceptMap.targetCanonical</b><br> 5118 * </p> 5119 */ 5120 @SearchParamDefinition(name = "target", path = "(ConceptMap.target as canonical)", description = "The target value set which provides context for the mappings", type = "reference", target = { 5121 ValueSet.class }) 5122 public static final String SP_TARGET = "target"; 5123 /** 5124 * <b>Fluent Client</b> search parameter constant for <b>target</b> 5125 * <p> 5126 * Description: <b>The target value set which provides context for the 5127 * mappings</b><br> 5128 * Type: <b>reference</b><br> 5129 * Path: <b>ConceptMap.targetCanonical</b><br> 5130 * </p> 5131 */ 5132 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam TARGET = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5133 SP_TARGET); 5134 5135 /** 5136 * Constant for fluent queries to be used to add include statements. Specifies 5137 * the path value of "<b>ConceptMap:target</b>". 5138 */ 5139 public static final ca.uhn.fhir.model.api.Include INCLUDE_TARGET = new ca.uhn.fhir.model.api.Include( 5140 "ConceptMap:target").toLocked(); 5141 5142 /** 5143 * Search parameter: <b>source-code</b> 5144 * <p> 5145 * Description: <b>Identifies element being mapped</b><br> 5146 * Type: <b>token</b><br> 5147 * Path: <b>ConceptMap.group.element.code</b><br> 5148 * </p> 5149 */ 5150 @SearchParamDefinition(name = "source-code", path = "ConceptMap.group.element.code", description = "Identifies element being mapped", type = "token") 5151 public static final String SP_SOURCE_CODE = "source-code"; 5152 /** 5153 * <b>Fluent Client</b> search parameter constant for <b>source-code</b> 5154 * <p> 5155 * Description: <b>Identifies element being mapped</b><br> 5156 * Type: <b>token</b><br> 5157 * Path: <b>ConceptMap.group.element.code</b><br> 5158 * </p> 5159 */ 5160 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SOURCE_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5161 SP_SOURCE_CODE); 5162 5163 /** 5164 * Search parameter: <b>name</b> 5165 * <p> 5166 * Description: <b>Computationally friendly name of the concept map</b><br> 5167 * Type: <b>string</b><br> 5168 * Path: <b>ConceptMap.name</b><br> 5169 * </p> 5170 */ 5171 @SearchParamDefinition(name = "name", path = "ConceptMap.name", description = "Computationally friendly name of the concept map", type = "string") 5172 public static final String SP_NAME = "name"; 5173 /** 5174 * <b>Fluent Client</b> search parameter constant for <b>name</b> 5175 * <p> 5176 * Description: <b>Computationally friendly name of the concept map</b><br> 5177 * Type: <b>string</b><br> 5178 * Path: <b>ConceptMap.name</b><br> 5179 * </p> 5180 */ 5181 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 5182 SP_NAME); 5183 5184 /** 5185 * Search parameter: <b>publisher</b> 5186 * <p> 5187 * Description: <b>Name of the publisher of the concept map</b><br> 5188 * Type: <b>string</b><br> 5189 * Path: <b>ConceptMap.publisher</b><br> 5190 * </p> 5191 */ 5192 @SearchParamDefinition(name = "publisher", path = "ConceptMap.publisher", description = "Name of the publisher of the concept map", type = "string") 5193 public static final String SP_PUBLISHER = "publisher"; 5194 /** 5195 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 5196 * <p> 5197 * Description: <b>Name of the publisher of the concept map</b><br> 5198 * Type: <b>string</b><br> 5199 * Path: <b>ConceptMap.publisher</b><br> 5200 * </p> 5201 */ 5202 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 5203 SP_PUBLISHER); 5204 5205 /** 5206 * Search parameter: <b>status</b> 5207 * <p> 5208 * Description: <b>The current status of the concept map</b><br> 5209 * Type: <b>token</b><br> 5210 * Path: <b>ConceptMap.status</b><br> 5211 * </p> 5212 */ 5213 @SearchParamDefinition(name = "status", path = "ConceptMap.status", description = "The current status of the concept map", type = "token") 5214 public static final String SP_STATUS = "status"; 5215 /** 5216 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5217 * <p> 5218 * Description: <b>The current status of the concept map</b><br> 5219 * Type: <b>token</b><br> 5220 * Path: <b>ConceptMap.status</b><br> 5221 * </p> 5222 */ 5223 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5224 SP_STATUS); 5225 5226}