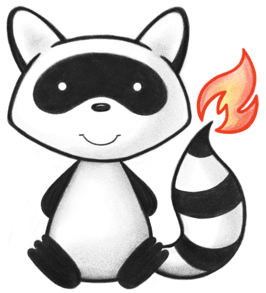
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * A record of a healthcare consumer?s choices, which permits or denies 049 * identified recipient(s) or recipient role(s) to perform one or more actions 050 * within a given policy context, for specific purposes and periods of time. 051 */ 052@ResourceDef(name = "Consent", profile = "http://hl7.org/fhir/StructureDefinition/Consent") 053public class Consent extends DomainResource { 054 055 public enum ConsentState { 056 /** 057 * The consent is in development or awaiting use but is not yet intended to be 058 * acted upon. 059 */ 060 DRAFT, 061 /** 062 * The consent has been proposed but not yet agreed to by all parties. The 063 * negotiation stage. 064 */ 065 PROPOSED, 066 /** 067 * The consent is to be followed and enforced. 068 */ 069 ACTIVE, 070 /** 071 * The consent has been rejected by one or more of the parties. 072 */ 073 REJECTED, 074 /** 075 * The consent is terminated or replaced. 076 */ 077 INACTIVE, 078 /** 079 * The consent was created wrongly (e.g. wrong patient) and should be ignored. 080 */ 081 ENTEREDINERROR, 082 /** 083 * added to help the parsers with the generic types 084 */ 085 NULL; 086 087 public static ConsentState fromCode(String codeString) throws FHIRException { 088 if (codeString == null || "".equals(codeString)) 089 return null; 090 if ("draft".equals(codeString)) 091 return DRAFT; 092 if ("proposed".equals(codeString)) 093 return PROPOSED; 094 if ("active".equals(codeString)) 095 return ACTIVE; 096 if ("rejected".equals(codeString)) 097 return REJECTED; 098 if ("inactive".equals(codeString)) 099 return INACTIVE; 100 if ("entered-in-error".equals(codeString)) 101 return ENTEREDINERROR; 102 if (Configuration.isAcceptInvalidEnums()) 103 return null; 104 else 105 throw new FHIRException("Unknown ConsentState code '" + codeString + "'"); 106 } 107 108 public String toCode() { 109 switch (this) { 110 case DRAFT: 111 return "draft"; 112 case PROPOSED: 113 return "proposed"; 114 case ACTIVE: 115 return "active"; 116 case REJECTED: 117 return "rejected"; 118 case INACTIVE: 119 return "inactive"; 120 case ENTEREDINERROR: 121 return "entered-in-error"; 122 case NULL: 123 return null; 124 default: 125 return "?"; 126 } 127 } 128 129 public String getSystem() { 130 switch (this) { 131 case DRAFT: 132 return "http://hl7.org/fhir/consent-state-codes"; 133 case PROPOSED: 134 return "http://hl7.org/fhir/consent-state-codes"; 135 case ACTIVE: 136 return "http://hl7.org/fhir/consent-state-codes"; 137 case REJECTED: 138 return "http://hl7.org/fhir/consent-state-codes"; 139 case INACTIVE: 140 return "http://hl7.org/fhir/consent-state-codes"; 141 case ENTEREDINERROR: 142 return "http://hl7.org/fhir/consent-state-codes"; 143 case NULL: 144 return null; 145 default: 146 return "?"; 147 } 148 } 149 150 public String getDefinition() { 151 switch (this) { 152 case DRAFT: 153 return "The consent is in development or awaiting use but is not yet intended to be acted upon."; 154 case PROPOSED: 155 return "The consent has been proposed but not yet agreed to by all parties. The negotiation stage."; 156 case ACTIVE: 157 return "The consent is to be followed and enforced."; 158 case REJECTED: 159 return "The consent has been rejected by one or more of the parties."; 160 case INACTIVE: 161 return "The consent is terminated or replaced."; 162 case ENTEREDINERROR: 163 return "The consent was created wrongly (e.g. wrong patient) and should be ignored."; 164 case NULL: 165 return null; 166 default: 167 return "?"; 168 } 169 } 170 171 public String getDisplay() { 172 switch (this) { 173 case DRAFT: 174 return "Pending"; 175 case PROPOSED: 176 return "Proposed"; 177 case ACTIVE: 178 return "Active"; 179 case REJECTED: 180 return "Rejected"; 181 case INACTIVE: 182 return "Inactive"; 183 case ENTEREDINERROR: 184 return "Entered in Error"; 185 case NULL: 186 return null; 187 default: 188 return "?"; 189 } 190 } 191 } 192 193 public static class ConsentStateEnumFactory implements EnumFactory<ConsentState> { 194 public ConsentState fromCode(String codeString) throws IllegalArgumentException { 195 if (codeString == null || "".equals(codeString)) 196 if (codeString == null || "".equals(codeString)) 197 return null; 198 if ("draft".equals(codeString)) 199 return ConsentState.DRAFT; 200 if ("proposed".equals(codeString)) 201 return ConsentState.PROPOSED; 202 if ("active".equals(codeString)) 203 return ConsentState.ACTIVE; 204 if ("rejected".equals(codeString)) 205 return ConsentState.REJECTED; 206 if ("inactive".equals(codeString)) 207 return ConsentState.INACTIVE; 208 if ("entered-in-error".equals(codeString)) 209 return ConsentState.ENTEREDINERROR; 210 throw new IllegalArgumentException("Unknown ConsentState code '" + codeString + "'"); 211 } 212 213 public Enumeration<ConsentState> fromType(PrimitiveType<?> code) throws FHIRException { 214 if (code == null) 215 return null; 216 if (code.isEmpty()) 217 return new Enumeration<ConsentState>(this, ConsentState.NULL, code); 218 String codeString = code.asStringValue(); 219 if (codeString == null || "".equals(codeString)) 220 return new Enumeration<ConsentState>(this, ConsentState.NULL, code); 221 if ("draft".equals(codeString)) 222 return new Enumeration<ConsentState>(this, ConsentState.DRAFT, code); 223 if ("proposed".equals(codeString)) 224 return new Enumeration<ConsentState>(this, ConsentState.PROPOSED, code); 225 if ("active".equals(codeString)) 226 return new Enumeration<ConsentState>(this, ConsentState.ACTIVE, code); 227 if ("rejected".equals(codeString)) 228 return new Enumeration<ConsentState>(this, ConsentState.REJECTED, code); 229 if ("inactive".equals(codeString)) 230 return new Enumeration<ConsentState>(this, ConsentState.INACTIVE, code); 231 if ("entered-in-error".equals(codeString)) 232 return new Enumeration<ConsentState>(this, ConsentState.ENTEREDINERROR, code); 233 throw new FHIRException("Unknown ConsentState code '" + codeString + "'"); 234 } 235 236 public String toCode(ConsentState code) { 237 if (code == ConsentState.NULL) 238 return null; 239 if (code == ConsentState.DRAFT) 240 return "draft"; 241 if (code == ConsentState.PROPOSED) 242 return "proposed"; 243 if (code == ConsentState.ACTIVE) 244 return "active"; 245 if (code == ConsentState.REJECTED) 246 return "rejected"; 247 if (code == ConsentState.INACTIVE) 248 return "inactive"; 249 if (code == ConsentState.ENTEREDINERROR) 250 return "entered-in-error"; 251 return "?"; 252 } 253 254 public String toSystem(ConsentState code) { 255 return code.getSystem(); 256 } 257 } 258 259 public enum ConsentProvisionType { 260 /** 261 * Consent is denied for actions meeting these rules. 262 */ 263 DENY, 264 /** 265 * Consent is provided for actions meeting these rules. 266 */ 267 PERMIT, 268 /** 269 * added to help the parsers with the generic types 270 */ 271 NULL; 272 273 public static ConsentProvisionType fromCode(String codeString) throws FHIRException { 274 if (codeString == null || "".equals(codeString)) 275 return null; 276 if ("deny".equals(codeString)) 277 return DENY; 278 if ("permit".equals(codeString)) 279 return PERMIT; 280 if (Configuration.isAcceptInvalidEnums()) 281 return null; 282 else 283 throw new FHIRException("Unknown ConsentProvisionType code '" + codeString + "'"); 284 } 285 286 public String toCode() { 287 switch (this) { 288 case DENY: 289 return "deny"; 290 case PERMIT: 291 return "permit"; 292 case NULL: 293 return null; 294 default: 295 return "?"; 296 } 297 } 298 299 public String getSystem() { 300 switch (this) { 301 case DENY: 302 return "http://hl7.org/fhir/consent-provision-type"; 303 case PERMIT: 304 return "http://hl7.org/fhir/consent-provision-type"; 305 case NULL: 306 return null; 307 default: 308 return "?"; 309 } 310 } 311 312 public String getDefinition() { 313 switch (this) { 314 case DENY: 315 return "Consent is denied for actions meeting these rules."; 316 case PERMIT: 317 return "Consent is provided for actions meeting these rules."; 318 case NULL: 319 return null; 320 default: 321 return "?"; 322 } 323 } 324 325 public String getDisplay() { 326 switch (this) { 327 case DENY: 328 return "Opt Out"; 329 case PERMIT: 330 return "Opt In"; 331 case NULL: 332 return null; 333 default: 334 return "?"; 335 } 336 } 337 } 338 339 public static class ConsentProvisionTypeEnumFactory implements EnumFactory<ConsentProvisionType> { 340 public ConsentProvisionType fromCode(String codeString) throws IllegalArgumentException { 341 if (codeString == null || "".equals(codeString)) 342 if (codeString == null || "".equals(codeString)) 343 return null; 344 if ("deny".equals(codeString)) 345 return ConsentProvisionType.DENY; 346 if ("permit".equals(codeString)) 347 return ConsentProvisionType.PERMIT; 348 throw new IllegalArgumentException("Unknown ConsentProvisionType code '" + codeString + "'"); 349 } 350 351 public Enumeration<ConsentProvisionType> fromType(PrimitiveType<?> code) throws FHIRException { 352 if (code == null) 353 return null; 354 if (code.isEmpty()) 355 return new Enumeration<ConsentProvisionType>(this, ConsentProvisionType.NULL, code); 356 String codeString = code.asStringValue(); 357 if (codeString == null || "".equals(codeString)) 358 return new Enumeration<ConsentProvisionType>(this, ConsentProvisionType.NULL, code); 359 if ("deny".equals(codeString)) 360 return new Enumeration<ConsentProvisionType>(this, ConsentProvisionType.DENY, code); 361 if ("permit".equals(codeString)) 362 return new Enumeration<ConsentProvisionType>(this, ConsentProvisionType.PERMIT, code); 363 throw new FHIRException("Unknown ConsentProvisionType code '" + codeString + "'"); 364 } 365 366 public String toCode(ConsentProvisionType code) { 367 if (code == ConsentProvisionType.NULL) 368 return null; 369 if (code == ConsentProvisionType.DENY) 370 return "deny"; 371 if (code == ConsentProvisionType.PERMIT) 372 return "permit"; 373 return "?"; 374 } 375 376 public String toSystem(ConsentProvisionType code) { 377 return code.getSystem(); 378 } 379 } 380 381 public enum ConsentDataMeaning { 382 /** 383 * The consent applies directly to the instance of the resource. 384 */ 385 INSTANCE, 386 /** 387 * The consent applies directly to the instance of the resource and instances it 388 * refers to. 389 */ 390 RELATED, 391 /** 392 * The consent applies directly to the instance of the resource and instances 393 * that refer to it. 394 */ 395 DEPENDENTS, 396 /** 397 * The consent applies to instances of resources that are authored by. 398 */ 399 AUTHOREDBY, 400 /** 401 * added to help the parsers with the generic types 402 */ 403 NULL; 404 405 public static ConsentDataMeaning fromCode(String codeString) throws FHIRException { 406 if (codeString == null || "".equals(codeString)) 407 return null; 408 if ("instance".equals(codeString)) 409 return INSTANCE; 410 if ("related".equals(codeString)) 411 return RELATED; 412 if ("dependents".equals(codeString)) 413 return DEPENDENTS; 414 if ("authoredby".equals(codeString)) 415 return AUTHOREDBY; 416 if (Configuration.isAcceptInvalidEnums()) 417 return null; 418 else 419 throw new FHIRException("Unknown ConsentDataMeaning code '" + codeString + "'"); 420 } 421 422 public String toCode() { 423 switch (this) { 424 case INSTANCE: 425 return "instance"; 426 case RELATED: 427 return "related"; 428 case DEPENDENTS: 429 return "dependents"; 430 case AUTHOREDBY: 431 return "authoredby"; 432 case NULL: 433 return null; 434 default: 435 return "?"; 436 } 437 } 438 439 public String getSystem() { 440 switch (this) { 441 case INSTANCE: 442 return "http://hl7.org/fhir/consent-data-meaning"; 443 case RELATED: 444 return "http://hl7.org/fhir/consent-data-meaning"; 445 case DEPENDENTS: 446 return "http://hl7.org/fhir/consent-data-meaning"; 447 case AUTHOREDBY: 448 return "http://hl7.org/fhir/consent-data-meaning"; 449 case NULL: 450 return null; 451 default: 452 return "?"; 453 } 454 } 455 456 public String getDefinition() { 457 switch (this) { 458 case INSTANCE: 459 return "The consent applies directly to the instance of the resource."; 460 case RELATED: 461 return "The consent applies directly to the instance of the resource and instances it refers to."; 462 case DEPENDENTS: 463 return "The consent applies directly to the instance of the resource and instances that refer to it."; 464 case AUTHOREDBY: 465 return "The consent applies to instances of resources that are authored by."; 466 case NULL: 467 return null; 468 default: 469 return "?"; 470 } 471 } 472 473 public String getDisplay() { 474 switch (this) { 475 case INSTANCE: 476 return "Instance"; 477 case RELATED: 478 return "Related"; 479 case DEPENDENTS: 480 return "Dependents"; 481 case AUTHOREDBY: 482 return "AuthoredBy"; 483 case NULL: 484 return null; 485 default: 486 return "?"; 487 } 488 } 489 } 490 491 public static class ConsentDataMeaningEnumFactory implements EnumFactory<ConsentDataMeaning> { 492 public ConsentDataMeaning fromCode(String codeString) throws IllegalArgumentException { 493 if (codeString == null || "".equals(codeString)) 494 if (codeString == null || "".equals(codeString)) 495 return null; 496 if ("instance".equals(codeString)) 497 return ConsentDataMeaning.INSTANCE; 498 if ("related".equals(codeString)) 499 return ConsentDataMeaning.RELATED; 500 if ("dependents".equals(codeString)) 501 return ConsentDataMeaning.DEPENDENTS; 502 if ("authoredby".equals(codeString)) 503 return ConsentDataMeaning.AUTHOREDBY; 504 throw new IllegalArgumentException("Unknown ConsentDataMeaning code '" + codeString + "'"); 505 } 506 507 public Enumeration<ConsentDataMeaning> fromType(PrimitiveType<?> code) throws FHIRException { 508 if (code == null) 509 return null; 510 if (code.isEmpty()) 511 return new Enumeration<ConsentDataMeaning>(this, ConsentDataMeaning.NULL, code); 512 String codeString = code.asStringValue(); 513 if (codeString == null || "".equals(codeString)) 514 return new Enumeration<ConsentDataMeaning>(this, ConsentDataMeaning.NULL, code); 515 if ("instance".equals(codeString)) 516 return new Enumeration<ConsentDataMeaning>(this, ConsentDataMeaning.INSTANCE, code); 517 if ("related".equals(codeString)) 518 return new Enumeration<ConsentDataMeaning>(this, ConsentDataMeaning.RELATED, code); 519 if ("dependents".equals(codeString)) 520 return new Enumeration<ConsentDataMeaning>(this, ConsentDataMeaning.DEPENDENTS, code); 521 if ("authoredby".equals(codeString)) 522 return new Enumeration<ConsentDataMeaning>(this, ConsentDataMeaning.AUTHOREDBY, code); 523 throw new FHIRException("Unknown ConsentDataMeaning code '" + codeString + "'"); 524 } 525 526 public String toCode(ConsentDataMeaning code) { 527 if (code == ConsentDataMeaning.NULL) 528 return null; 529 if (code == ConsentDataMeaning.INSTANCE) 530 return "instance"; 531 if (code == ConsentDataMeaning.RELATED) 532 return "related"; 533 if (code == ConsentDataMeaning.DEPENDENTS) 534 return "dependents"; 535 if (code == ConsentDataMeaning.AUTHOREDBY) 536 return "authoredby"; 537 return "?"; 538 } 539 540 public String toSystem(ConsentDataMeaning code) { 541 return code.getSystem(); 542 } 543 } 544 545 @Block() 546 public static class ConsentPolicyComponent extends BackboneElement implements IBaseBackboneElement { 547 /** 548 * Entity or Organization having regulatory jurisdiction or accountability for 549 * enforcing policies pertaining to Consent Directives. 550 */ 551 @Child(name = "authority", type = { UriType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 552 @Description(shortDefinition = "Enforcement source for policy", formalDefinition = "Entity or Organization having regulatory jurisdiction or accountability for enforcing policies pertaining to Consent Directives.") 553 protected UriType authority; 554 555 /** 556 * The references to the policies that are included in this consent scope. 557 * Policies may be organizational, but are often defined jurisdictionally, or in 558 * law. 559 */ 560 @Child(name = "uri", type = { UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 561 @Description(shortDefinition = "Specific policy covered by this consent", formalDefinition = "The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law.") 562 protected UriType uri; 563 564 private static final long serialVersionUID = 672275705L; 565 566 /** 567 * Constructor 568 */ 569 public ConsentPolicyComponent() { 570 super(); 571 } 572 573 /** 574 * @return {@link #authority} (Entity or Organization having regulatory 575 * jurisdiction or accountability for enforcing policies pertaining to 576 * Consent Directives.). This is the underlying object with id, value 577 * and extensions. The accessor "getAuthority" gives direct access to 578 * the value 579 */ 580 public UriType getAuthorityElement() { 581 if (this.authority == null) 582 if (Configuration.errorOnAutoCreate()) 583 throw new Error("Attempt to auto-create ConsentPolicyComponent.authority"); 584 else if (Configuration.doAutoCreate()) 585 this.authority = new UriType(); // bb 586 return this.authority; 587 } 588 589 public boolean hasAuthorityElement() { 590 return this.authority != null && !this.authority.isEmpty(); 591 } 592 593 public boolean hasAuthority() { 594 return this.authority != null && !this.authority.isEmpty(); 595 } 596 597 /** 598 * @param value {@link #authority} (Entity or Organization having regulatory 599 * jurisdiction or accountability for enforcing policies pertaining 600 * to Consent Directives.). This is the underlying object with id, 601 * value and extensions. The accessor "getAuthority" gives direct 602 * access to the value 603 */ 604 public ConsentPolicyComponent setAuthorityElement(UriType value) { 605 this.authority = value; 606 return this; 607 } 608 609 /** 610 * @return Entity or Organization having regulatory jurisdiction or 611 * accountability for enforcing policies pertaining to Consent 612 * Directives. 613 */ 614 public String getAuthority() { 615 return this.authority == null ? null : this.authority.getValue(); 616 } 617 618 /** 619 * @param value Entity or Organization having regulatory jurisdiction or 620 * accountability for enforcing policies pertaining to Consent 621 * Directives. 622 */ 623 public ConsentPolicyComponent setAuthority(String value) { 624 if (Utilities.noString(value)) 625 this.authority = null; 626 else { 627 if (this.authority == null) 628 this.authority = new UriType(); 629 this.authority.setValue(value); 630 } 631 return this; 632 } 633 634 /** 635 * @return {@link #uri} (The references to the policies that are included in 636 * this consent scope. Policies may be organizational, but are often 637 * defined jurisdictionally, or in law.). This is the underlying object 638 * with id, value and extensions. The accessor "getUri" gives direct 639 * access to the value 640 */ 641 public UriType getUriElement() { 642 if (this.uri == null) 643 if (Configuration.errorOnAutoCreate()) 644 throw new Error("Attempt to auto-create ConsentPolicyComponent.uri"); 645 else if (Configuration.doAutoCreate()) 646 this.uri = new UriType(); // bb 647 return this.uri; 648 } 649 650 public boolean hasUriElement() { 651 return this.uri != null && !this.uri.isEmpty(); 652 } 653 654 public boolean hasUri() { 655 return this.uri != null && !this.uri.isEmpty(); 656 } 657 658 /** 659 * @param value {@link #uri} (The references to the policies that are included 660 * in this consent scope. Policies may be organizational, but are 661 * often defined jurisdictionally, or in law.). This is the 662 * underlying object with id, value and extensions. The accessor 663 * "getUri" gives direct access to the value 664 */ 665 public ConsentPolicyComponent setUriElement(UriType value) { 666 this.uri = value; 667 return this; 668 } 669 670 /** 671 * @return The references to the policies that are included in this consent 672 * scope. Policies may be organizational, but are often defined 673 * jurisdictionally, or in law. 674 */ 675 public String getUri() { 676 return this.uri == null ? null : this.uri.getValue(); 677 } 678 679 /** 680 * @param value The references to the policies that are included in this consent 681 * scope. Policies may be organizational, but are often defined 682 * jurisdictionally, or in law. 683 */ 684 public ConsentPolicyComponent setUri(String value) { 685 if (Utilities.noString(value)) 686 this.uri = null; 687 else { 688 if (this.uri == null) 689 this.uri = new UriType(); 690 this.uri.setValue(value); 691 } 692 return this; 693 } 694 695 protected void listChildren(List<Property> children) { 696 super.listChildren(children); 697 children.add(new Property("authority", "uri", 698 "Entity or Organization having regulatory jurisdiction or accountability for enforcing policies pertaining to Consent Directives.", 699 0, 1, authority)); 700 children.add(new Property("uri", "uri", 701 "The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law.", 702 0, 1, uri)); 703 } 704 705 @Override 706 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 707 switch (_hash) { 708 case 1475610435: 709 /* authority */ return new Property("authority", "uri", 710 "Entity or Organization having regulatory jurisdiction or accountability for enforcing policies pertaining to Consent Directives.", 711 0, 1, authority); 712 case 116076: 713 /* uri */ return new Property("uri", "uri", 714 "The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law.", 715 0, 1, uri); 716 default: 717 return super.getNamedProperty(_hash, _name, _checkValid); 718 } 719 720 } 721 722 @Override 723 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 724 switch (hash) { 725 case 1475610435: 726 /* authority */ return this.authority == null ? new Base[0] : new Base[] { this.authority }; // UriType 727 case 116076: 728 /* uri */ return this.uri == null ? new Base[0] : new Base[] { this.uri }; // UriType 729 default: 730 return super.getProperty(hash, name, checkValid); 731 } 732 733 } 734 735 @Override 736 public Base setProperty(int hash, String name, Base value) throws FHIRException { 737 switch (hash) { 738 case 1475610435: // authority 739 this.authority = castToUri(value); // UriType 740 return value; 741 case 116076: // uri 742 this.uri = castToUri(value); // UriType 743 return value; 744 default: 745 return super.setProperty(hash, name, value); 746 } 747 748 } 749 750 @Override 751 public Base setProperty(String name, Base value) throws FHIRException { 752 if (name.equals("authority")) { 753 this.authority = castToUri(value); // UriType 754 } else if (name.equals("uri")) { 755 this.uri = castToUri(value); // UriType 756 } else 757 return super.setProperty(name, value); 758 return value; 759 } 760 761 @Override 762 public void removeChild(String name, Base value) throws FHIRException { 763 if (name.equals("authority")) { 764 this.authority = null; 765 } else if (name.equals("uri")) { 766 this.uri = null; 767 } else 768 super.removeChild(name, value); 769 770 } 771 772 @Override 773 public Base makeProperty(int hash, String name) throws FHIRException { 774 switch (hash) { 775 case 1475610435: 776 return getAuthorityElement(); 777 case 116076: 778 return getUriElement(); 779 default: 780 return super.makeProperty(hash, name); 781 } 782 783 } 784 785 @Override 786 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 787 switch (hash) { 788 case 1475610435: 789 /* authority */ return new String[] { "uri" }; 790 case 116076: 791 /* uri */ return new String[] { "uri" }; 792 default: 793 return super.getTypesForProperty(hash, name); 794 } 795 796 } 797 798 @Override 799 public Base addChild(String name) throws FHIRException { 800 if (name.equals("authority")) { 801 throw new FHIRException("Cannot call addChild on a singleton property Consent.authority"); 802 } else if (name.equals("uri")) { 803 throw new FHIRException("Cannot call addChild on a singleton property Consent.uri"); 804 } else 805 return super.addChild(name); 806 } 807 808 public ConsentPolicyComponent copy() { 809 ConsentPolicyComponent dst = new ConsentPolicyComponent(); 810 copyValues(dst); 811 return dst; 812 } 813 814 public void copyValues(ConsentPolicyComponent dst) { 815 super.copyValues(dst); 816 dst.authority = authority == null ? null : authority.copy(); 817 dst.uri = uri == null ? null : uri.copy(); 818 } 819 820 @Override 821 public boolean equalsDeep(Base other_) { 822 if (!super.equalsDeep(other_)) 823 return false; 824 if (!(other_ instanceof ConsentPolicyComponent)) 825 return false; 826 ConsentPolicyComponent o = (ConsentPolicyComponent) other_; 827 return compareDeep(authority, o.authority, true) && compareDeep(uri, o.uri, true); 828 } 829 830 @Override 831 public boolean equalsShallow(Base other_) { 832 if (!super.equalsShallow(other_)) 833 return false; 834 if (!(other_ instanceof ConsentPolicyComponent)) 835 return false; 836 ConsentPolicyComponent o = (ConsentPolicyComponent) other_; 837 return compareValues(authority, o.authority, true) && compareValues(uri, o.uri, true); 838 } 839 840 public boolean isEmpty() { 841 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(authority, uri); 842 } 843 844 public String fhirType() { 845 return "Consent.policy"; 846 847 } 848 849 } 850 851 @Block() 852 public static class ConsentVerificationComponent extends BackboneElement implements IBaseBackboneElement { 853 /** 854 * Has the instruction been verified. 855 */ 856 @Child(name = "verified", type = { 857 BooleanType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 858 @Description(shortDefinition = "Has been verified", formalDefinition = "Has the instruction been verified.") 859 protected BooleanType verified; 860 861 /** 862 * Who verified the instruction (Patient, Relative or other Authorized Person). 863 */ 864 @Child(name = "verifiedWith", type = { Patient.class, 865 RelatedPerson.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 866 @Description(shortDefinition = "Person who verified", formalDefinition = "Who verified the instruction (Patient, Relative or other Authorized Person).") 867 protected Reference verifiedWith; 868 869 /** 870 * The actual object that is the target of the reference (Who verified the 871 * instruction (Patient, Relative or other Authorized Person).) 872 */ 873 protected Resource verifiedWithTarget; 874 875 /** 876 * Date verification was collected. 877 */ 878 @Child(name = "verificationDate", type = { 879 DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 880 @Description(shortDefinition = "When consent verified", formalDefinition = "Date verification was collected.") 881 protected DateTimeType verificationDate; 882 883 private static final long serialVersionUID = 1305161458L; 884 885 /** 886 * Constructor 887 */ 888 public ConsentVerificationComponent() { 889 super(); 890 } 891 892 /** 893 * Constructor 894 */ 895 public ConsentVerificationComponent(BooleanType verified) { 896 super(); 897 this.verified = verified; 898 } 899 900 /** 901 * @return {@link #verified} (Has the instruction been verified.). This is the 902 * underlying object with id, value and extensions. The accessor 903 * "getVerified" gives direct access to the value 904 */ 905 public BooleanType getVerifiedElement() { 906 if (this.verified == null) 907 if (Configuration.errorOnAutoCreate()) 908 throw new Error("Attempt to auto-create ConsentVerificationComponent.verified"); 909 else if (Configuration.doAutoCreate()) 910 this.verified = new BooleanType(); // bb 911 return this.verified; 912 } 913 914 public boolean hasVerifiedElement() { 915 return this.verified != null && !this.verified.isEmpty(); 916 } 917 918 public boolean hasVerified() { 919 return this.verified != null && !this.verified.isEmpty(); 920 } 921 922 /** 923 * @param value {@link #verified} (Has the instruction been verified.). This is 924 * the underlying object with id, value and extensions. The 925 * accessor "getVerified" gives direct access to the value 926 */ 927 public ConsentVerificationComponent setVerifiedElement(BooleanType value) { 928 this.verified = value; 929 return this; 930 } 931 932 /** 933 * @return Has the instruction been verified. 934 */ 935 public boolean getVerified() { 936 return this.verified == null || this.verified.isEmpty() ? false : this.verified.getValue(); 937 } 938 939 /** 940 * @param value Has the instruction been verified. 941 */ 942 public ConsentVerificationComponent setVerified(boolean value) { 943 if (this.verified == null) 944 this.verified = new BooleanType(); 945 this.verified.setValue(value); 946 return this; 947 } 948 949 /** 950 * @return {@link #verifiedWith} (Who verified the instruction (Patient, 951 * Relative or other Authorized Person).) 952 */ 953 public Reference getVerifiedWith() { 954 if (this.verifiedWith == null) 955 if (Configuration.errorOnAutoCreate()) 956 throw new Error("Attempt to auto-create ConsentVerificationComponent.verifiedWith"); 957 else if (Configuration.doAutoCreate()) 958 this.verifiedWith = new Reference(); // cc 959 return this.verifiedWith; 960 } 961 962 public boolean hasVerifiedWith() { 963 return this.verifiedWith != null && !this.verifiedWith.isEmpty(); 964 } 965 966 /** 967 * @param value {@link #verifiedWith} (Who verified the instruction (Patient, 968 * Relative or other Authorized Person).) 969 */ 970 public ConsentVerificationComponent setVerifiedWith(Reference value) { 971 this.verifiedWith = value; 972 return this; 973 } 974 975 /** 976 * @return {@link #verifiedWith} The actual object that is the target of the 977 * reference. The reference library doesn't populate this, but you can 978 * use it to hold the resource if you resolve it. (Who verified the 979 * instruction (Patient, Relative or other Authorized Person).) 980 */ 981 public Resource getVerifiedWithTarget() { 982 return this.verifiedWithTarget; 983 } 984 985 /** 986 * @param value {@link #verifiedWith} The actual object that is the target of 987 * the reference. The reference library doesn't use these, but you 988 * can use it to hold the resource if you resolve it. (Who verified 989 * the instruction (Patient, Relative or other Authorized Person).) 990 */ 991 public ConsentVerificationComponent setVerifiedWithTarget(Resource value) { 992 this.verifiedWithTarget = value; 993 return this; 994 } 995 996 /** 997 * @return {@link #verificationDate} (Date verification was collected.). This is 998 * the underlying object with id, value and extensions. The accessor 999 * "getVerificationDate" gives direct access to the value 1000 */ 1001 public DateTimeType getVerificationDateElement() { 1002 if (this.verificationDate == null) 1003 if (Configuration.errorOnAutoCreate()) 1004 throw new Error("Attempt to auto-create ConsentVerificationComponent.verificationDate"); 1005 else if (Configuration.doAutoCreate()) 1006 this.verificationDate = new DateTimeType(); // bb 1007 return this.verificationDate; 1008 } 1009 1010 public boolean hasVerificationDateElement() { 1011 return this.verificationDate != null && !this.verificationDate.isEmpty(); 1012 } 1013 1014 public boolean hasVerificationDate() { 1015 return this.verificationDate != null && !this.verificationDate.isEmpty(); 1016 } 1017 1018 /** 1019 * @param value {@link #verificationDate} (Date verification was collected.). 1020 * This is the underlying object with id, value and extensions. The 1021 * accessor "getVerificationDate" gives direct access to the value 1022 */ 1023 public ConsentVerificationComponent setVerificationDateElement(DateTimeType value) { 1024 this.verificationDate = value; 1025 return this; 1026 } 1027 1028 /** 1029 * @return Date verification was collected. 1030 */ 1031 public Date getVerificationDate() { 1032 return this.verificationDate == null ? null : this.verificationDate.getValue(); 1033 } 1034 1035 /** 1036 * @param value Date verification was collected. 1037 */ 1038 public ConsentVerificationComponent setVerificationDate(Date value) { 1039 if (value == null) 1040 this.verificationDate = null; 1041 else { 1042 if (this.verificationDate == null) 1043 this.verificationDate = new DateTimeType(); 1044 this.verificationDate.setValue(value); 1045 } 1046 return this; 1047 } 1048 1049 protected void listChildren(List<Property> children) { 1050 super.listChildren(children); 1051 children.add(new Property("verified", "boolean", "Has the instruction been verified.", 0, 1, verified)); 1052 children.add(new Property("verifiedWith", "Reference(Patient|RelatedPerson)", 1053 "Who verified the instruction (Patient, Relative or other Authorized Person).", 0, 1, verifiedWith)); 1054 children.add( 1055 new Property("verificationDate", "dateTime", "Date verification was collected.", 0, 1, verificationDate)); 1056 } 1057 1058 @Override 1059 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1060 switch (_hash) { 1061 case -1994383672: 1062 /* verified */ return new Property("verified", "boolean", "Has the instruction been verified.", 0, 1, verified); 1063 case -1425236050: 1064 /* verifiedWith */ return new Property("verifiedWith", "Reference(Patient|RelatedPerson)", 1065 "Who verified the instruction (Patient, Relative or other Authorized Person).", 0, 1, verifiedWith); 1066 case 642233449: 1067 /* verificationDate */ return new Property("verificationDate", "dateTime", "Date verification was collected.", 1068 0, 1, verificationDate); 1069 default: 1070 return super.getNamedProperty(_hash, _name, _checkValid); 1071 } 1072 1073 } 1074 1075 @Override 1076 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1077 switch (hash) { 1078 case -1994383672: 1079 /* verified */ return this.verified == null ? new Base[0] : new Base[] { this.verified }; // BooleanType 1080 case -1425236050: 1081 /* verifiedWith */ return this.verifiedWith == null ? new Base[0] : new Base[] { this.verifiedWith }; // Reference 1082 case 642233449: 1083 /* verificationDate */ return this.verificationDate == null ? new Base[0] 1084 : new Base[] { this.verificationDate }; // DateTimeType 1085 default: 1086 return super.getProperty(hash, name, checkValid); 1087 } 1088 1089 } 1090 1091 @Override 1092 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1093 switch (hash) { 1094 case -1994383672: // verified 1095 this.verified = castToBoolean(value); // BooleanType 1096 return value; 1097 case -1425236050: // verifiedWith 1098 this.verifiedWith = castToReference(value); // Reference 1099 return value; 1100 case 642233449: // verificationDate 1101 this.verificationDate = castToDateTime(value); // DateTimeType 1102 return value; 1103 default: 1104 return super.setProperty(hash, name, value); 1105 } 1106 1107 } 1108 1109 @Override 1110 public Base setProperty(String name, Base value) throws FHIRException { 1111 if (name.equals("verified")) { 1112 this.verified = castToBoolean(value); // BooleanType 1113 } else if (name.equals("verifiedWith")) { 1114 this.verifiedWith = castToReference(value); // Reference 1115 } else if (name.equals("verificationDate")) { 1116 this.verificationDate = castToDateTime(value); // DateTimeType 1117 } else 1118 return super.setProperty(name, value); 1119 return value; 1120 } 1121 1122 @Override 1123 public void removeChild(String name, Base value) throws FHIRException { 1124 if (name.equals("verified")) { 1125 this.verified = null; 1126 } else if (name.equals("verifiedWith")) { 1127 this.verifiedWith = null; 1128 } else if (name.equals("verificationDate")) { 1129 this.verificationDate = null; 1130 } else 1131 super.removeChild(name, value); 1132 1133 } 1134 1135 @Override 1136 public Base makeProperty(int hash, String name) throws FHIRException { 1137 switch (hash) { 1138 case -1994383672: 1139 return getVerifiedElement(); 1140 case -1425236050: 1141 return getVerifiedWith(); 1142 case 642233449: 1143 return getVerificationDateElement(); 1144 default: 1145 return super.makeProperty(hash, name); 1146 } 1147 1148 } 1149 1150 @Override 1151 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1152 switch (hash) { 1153 case -1994383672: 1154 /* verified */ return new String[] { "boolean" }; 1155 case -1425236050: 1156 /* verifiedWith */ return new String[] { "Reference" }; 1157 case 642233449: 1158 /* verificationDate */ return new String[] { "dateTime" }; 1159 default: 1160 return super.getTypesForProperty(hash, name); 1161 } 1162 1163 } 1164 1165 @Override 1166 public Base addChild(String name) throws FHIRException { 1167 if (name.equals("verified")) { 1168 throw new FHIRException("Cannot call addChild on a singleton property Consent.verified"); 1169 } else if (name.equals("verifiedWith")) { 1170 this.verifiedWith = new Reference(); 1171 return this.verifiedWith; 1172 } else if (name.equals("verificationDate")) { 1173 throw new FHIRException("Cannot call addChild on a singleton property Consent.verificationDate"); 1174 } else 1175 return super.addChild(name); 1176 } 1177 1178 public ConsentVerificationComponent copy() { 1179 ConsentVerificationComponent dst = new ConsentVerificationComponent(); 1180 copyValues(dst); 1181 return dst; 1182 } 1183 1184 public void copyValues(ConsentVerificationComponent dst) { 1185 super.copyValues(dst); 1186 dst.verified = verified == null ? null : verified.copy(); 1187 dst.verifiedWith = verifiedWith == null ? null : verifiedWith.copy(); 1188 dst.verificationDate = verificationDate == null ? null : verificationDate.copy(); 1189 } 1190 1191 @Override 1192 public boolean equalsDeep(Base other_) { 1193 if (!super.equalsDeep(other_)) 1194 return false; 1195 if (!(other_ instanceof ConsentVerificationComponent)) 1196 return false; 1197 ConsentVerificationComponent o = (ConsentVerificationComponent) other_; 1198 return compareDeep(verified, o.verified, true) && compareDeep(verifiedWith, o.verifiedWith, true) 1199 && compareDeep(verificationDate, o.verificationDate, true); 1200 } 1201 1202 @Override 1203 public boolean equalsShallow(Base other_) { 1204 if (!super.equalsShallow(other_)) 1205 return false; 1206 if (!(other_ instanceof ConsentVerificationComponent)) 1207 return false; 1208 ConsentVerificationComponent o = (ConsentVerificationComponent) other_; 1209 return compareValues(verified, o.verified, true) && compareValues(verificationDate, o.verificationDate, true); 1210 } 1211 1212 public boolean isEmpty() { 1213 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(verified, verifiedWith, verificationDate); 1214 } 1215 1216 public String fhirType() { 1217 return "Consent.verification"; 1218 1219 } 1220 1221 } 1222 1223 @Block() 1224 public static class ProvisionComponent extends BackboneElement implements IBaseBackboneElement { 1225 /** 1226 * Action to take - permit or deny - when the rule conditions are met. Not 1227 * permitted in root rule, required in all nested rules. 1228 */ 1229 @Child(name = "type", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1230 @Description(shortDefinition = "deny | permit", formalDefinition = "Action to take - permit or deny - when the rule conditions are met. Not permitted in root rule, required in all nested rules.") 1231 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/consent-provision-type") 1232 protected Enumeration<ConsentProvisionType> type; 1233 1234 /** 1235 * The timeframe in this rule is valid. 1236 */ 1237 @Child(name = "period", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1238 @Description(shortDefinition = "Timeframe for this rule", formalDefinition = "The timeframe in this rule is valid.") 1239 protected Period period; 1240 1241 /** 1242 * Who or what is controlled by this rule. Use group to identify a set of actors 1243 * by some property they share (e.g. 'admitting officers'). 1244 */ 1245 @Child(name = "actor", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1246 @Description(shortDefinition = "Who|what controlled by this rule (or group, by role)", formalDefinition = "Who or what is controlled by this rule. Use group to identify a set of actors by some property they share (e.g. 'admitting officers').") 1247 protected List<provisionActorComponent> actor; 1248 1249 /** 1250 * Actions controlled by this Rule. 1251 */ 1252 @Child(name = "action", type = { 1253 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1254 @Description(shortDefinition = "Actions controlled by this rule", formalDefinition = "Actions controlled by this Rule.") 1255 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/consent-action") 1256 protected List<CodeableConcept> action; 1257 1258 /** 1259 * A security label, comprised of 0..* security label fields (Privacy tags), 1260 * which define which resources are controlled by this exception. 1261 */ 1262 @Child(name = "securityLabel", type = { 1263 Coding.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1264 @Description(shortDefinition = "Security Labels that define affected resources", formalDefinition = "A security label, comprised of 0..* security label fields (Privacy tags), which define which resources are controlled by this exception.") 1265 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/security-labels") 1266 protected List<Coding> securityLabel; 1267 1268 /** 1269 * The context of the activities a user is taking - why the user is accessing 1270 * the data - that are controlled by this rule. 1271 */ 1272 @Child(name = "purpose", type = { 1273 Coding.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1274 @Description(shortDefinition = "Context of activities covered by this rule", formalDefinition = "The context of the activities a user is taking - why the user is accessing the data - that are controlled by this rule.") 1275 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-PurposeOfUse") 1276 protected List<Coding> purpose; 1277 1278 /** 1279 * The class of information covered by this rule. The type can be a FHIR 1280 * resource type, a profile on a type, or a CDA document, or some other type 1281 * that indicates what sort of information the consent relates to. 1282 */ 1283 @Child(name = "class", type = { 1284 Coding.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1285 @Description(shortDefinition = "e.g. Resource Type, Profile, CDA, etc.", formalDefinition = "The class of information covered by this rule. The type can be a FHIR resource type, a profile on a type, or a CDA document, or some other type that indicates what sort of information the consent relates to.") 1286 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/consent-content-class") 1287 protected List<Coding> class_; 1288 1289 /** 1290 * If this code is found in an instance, then the rule applies. 1291 */ 1292 @Child(name = "code", type = { 1293 CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1294 @Description(shortDefinition = "e.g. LOINC or SNOMED CT code, etc. in the content", formalDefinition = "If this code is found in an instance, then the rule applies.") 1295 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/consent-content-code") 1296 protected List<CodeableConcept> code; 1297 1298 /** 1299 * Clinical or Operational Relevant period of time that bounds the data 1300 * controlled by this rule. 1301 */ 1302 @Child(name = "dataPeriod", type = { Period.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 1303 @Description(shortDefinition = "Timeframe for data controlled by this rule", formalDefinition = "Clinical or Operational Relevant period of time that bounds the data controlled by this rule.") 1304 protected Period dataPeriod; 1305 1306 /** 1307 * The resources controlled by this rule if specific resources are referenced. 1308 */ 1309 @Child(name = "data", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1310 @Description(shortDefinition = "Data controlled by this rule", formalDefinition = "The resources controlled by this rule if specific resources are referenced.") 1311 protected List<provisionDataComponent> data; 1312 1313 /** 1314 * Rules which provide exceptions to the base rule or subrules. 1315 */ 1316 @Child(name = "provision", type = { 1317 ProvisionComponent.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1318 @Description(shortDefinition = "Nested Exception Rules", formalDefinition = "Rules which provide exceptions to the base rule or subrules.") 1319 protected List<ProvisionComponent> provision; 1320 1321 private static final long serialVersionUID = -1280172451L; 1322 1323 /** 1324 * Constructor 1325 */ 1326 public ProvisionComponent() { 1327 super(); 1328 } 1329 1330 /** 1331 * @return {@link #type} (Action to take - permit or deny - when the rule 1332 * conditions are met. Not permitted in root rule, required in all 1333 * nested rules.). This is the underlying object with id, value and 1334 * extensions. The accessor "getType" gives direct access to the value 1335 */ 1336 public Enumeration<ConsentProvisionType> getTypeElement() { 1337 if (this.type == null) 1338 if (Configuration.errorOnAutoCreate()) 1339 throw new Error("Attempt to auto-create provisionComponent.type"); 1340 else if (Configuration.doAutoCreate()) 1341 this.type = new Enumeration<ConsentProvisionType>(new ConsentProvisionTypeEnumFactory()); // bb 1342 return this.type; 1343 } 1344 1345 public boolean hasTypeElement() { 1346 return this.type != null && !this.type.isEmpty(); 1347 } 1348 1349 public boolean hasType() { 1350 return this.type != null && !this.type.isEmpty(); 1351 } 1352 1353 /** 1354 * @param value {@link #type} (Action to take - permit or deny - when the rule 1355 * conditions are met. Not permitted in root rule, required in all 1356 * nested rules.). This is the underlying object with id, value and 1357 * extensions. The accessor "getType" gives direct access to the 1358 * value 1359 */ 1360 public ProvisionComponent setTypeElement(Enumeration<ConsentProvisionType> value) { 1361 this.type = value; 1362 return this; 1363 } 1364 1365 /** 1366 * @return Action to take - permit or deny - when the rule conditions are met. 1367 * Not permitted in root rule, required in all nested rules. 1368 */ 1369 public ConsentProvisionType getType() { 1370 return this.type == null ? null : this.type.getValue(); 1371 } 1372 1373 /** 1374 * @param value Action to take - permit or deny - when the rule conditions are 1375 * met. Not permitted in root rule, required in all nested rules. 1376 */ 1377 public ProvisionComponent setType(ConsentProvisionType value) { 1378 if (value == null) 1379 this.type = null; 1380 else { 1381 if (this.type == null) 1382 this.type = new Enumeration<ConsentProvisionType>(new ConsentProvisionTypeEnumFactory()); 1383 this.type.setValue(value); 1384 } 1385 return this; 1386 } 1387 1388 /** 1389 * @return {@link #period} (The timeframe in this rule is valid.) 1390 */ 1391 public Period getPeriod() { 1392 if (this.period == null) 1393 if (Configuration.errorOnAutoCreate()) 1394 throw new Error("Attempt to auto-create provisionComponent.period"); 1395 else if (Configuration.doAutoCreate()) 1396 this.period = new Period(); // cc 1397 return this.period; 1398 } 1399 1400 public boolean hasPeriod() { 1401 return this.period != null && !this.period.isEmpty(); 1402 } 1403 1404 /** 1405 * @param value {@link #period} (The timeframe in this rule is valid.) 1406 */ 1407 public ProvisionComponent setPeriod(Period value) { 1408 this.period = value; 1409 return this; 1410 } 1411 1412 /** 1413 * @return {@link #actor} (Who or what is controlled by this rule. Use group to 1414 * identify a set of actors by some property they share (e.g. 'admitting 1415 * officers').) 1416 */ 1417 public List<provisionActorComponent> getActor() { 1418 if (this.actor == null) 1419 this.actor = new ArrayList<provisionActorComponent>(); 1420 return this.actor; 1421 } 1422 1423 /** 1424 * @return Returns a reference to <code>this</code> for easy method chaining 1425 */ 1426 public ProvisionComponent setActor(List<provisionActorComponent> theActor) { 1427 this.actor = theActor; 1428 return this; 1429 } 1430 1431 public boolean hasActor() { 1432 if (this.actor == null) 1433 return false; 1434 for (provisionActorComponent item : this.actor) 1435 if (!item.isEmpty()) 1436 return true; 1437 return false; 1438 } 1439 1440 public provisionActorComponent addActor() { // 3 1441 provisionActorComponent t = new provisionActorComponent(); 1442 if (this.actor == null) 1443 this.actor = new ArrayList<provisionActorComponent>(); 1444 this.actor.add(t); 1445 return t; 1446 } 1447 1448 public ProvisionComponent addActor(provisionActorComponent t) { // 3 1449 if (t == null) 1450 return this; 1451 if (this.actor == null) 1452 this.actor = new ArrayList<provisionActorComponent>(); 1453 this.actor.add(t); 1454 return this; 1455 } 1456 1457 /** 1458 * @return The first repetition of repeating field {@link #actor}, creating it 1459 * if it does not already exist 1460 */ 1461 public provisionActorComponent getActorFirstRep() { 1462 if (getActor().isEmpty()) { 1463 addActor(); 1464 } 1465 return getActor().get(0); 1466 } 1467 1468 /** 1469 * @return {@link #action} (Actions controlled by this Rule.) 1470 */ 1471 public List<CodeableConcept> getAction() { 1472 if (this.action == null) 1473 this.action = new ArrayList<CodeableConcept>(); 1474 return this.action; 1475 } 1476 1477 /** 1478 * @return Returns a reference to <code>this</code> for easy method chaining 1479 */ 1480 public ProvisionComponent setAction(List<CodeableConcept> theAction) { 1481 this.action = theAction; 1482 return this; 1483 } 1484 1485 public boolean hasAction() { 1486 if (this.action == null) 1487 return false; 1488 for (CodeableConcept item : this.action) 1489 if (!item.isEmpty()) 1490 return true; 1491 return false; 1492 } 1493 1494 public CodeableConcept addAction() { // 3 1495 CodeableConcept t = new CodeableConcept(); 1496 if (this.action == null) 1497 this.action = new ArrayList<CodeableConcept>(); 1498 this.action.add(t); 1499 return t; 1500 } 1501 1502 public ProvisionComponent addAction(CodeableConcept t) { // 3 1503 if (t == null) 1504 return this; 1505 if (this.action == null) 1506 this.action = new ArrayList<CodeableConcept>(); 1507 this.action.add(t); 1508 return this; 1509 } 1510 1511 /** 1512 * @return The first repetition of repeating field {@link #action}, creating it 1513 * if it does not already exist 1514 */ 1515 public CodeableConcept getActionFirstRep() { 1516 if (getAction().isEmpty()) { 1517 addAction(); 1518 } 1519 return getAction().get(0); 1520 } 1521 1522 /** 1523 * @return {@link #securityLabel} (A security label, comprised of 0..* security 1524 * label fields (Privacy tags), which define which resources are 1525 * controlled by this exception.) 1526 */ 1527 public List<Coding> getSecurityLabel() { 1528 if (this.securityLabel == null) 1529 this.securityLabel = new ArrayList<Coding>(); 1530 return this.securityLabel; 1531 } 1532 1533 /** 1534 * @return Returns a reference to <code>this</code> for easy method chaining 1535 */ 1536 public ProvisionComponent setSecurityLabel(List<Coding> theSecurityLabel) { 1537 this.securityLabel = theSecurityLabel; 1538 return this; 1539 } 1540 1541 public boolean hasSecurityLabel() { 1542 if (this.securityLabel == null) 1543 return false; 1544 for (Coding item : this.securityLabel) 1545 if (!item.isEmpty()) 1546 return true; 1547 return false; 1548 } 1549 1550 public Coding addSecurityLabel() { // 3 1551 Coding t = new Coding(); 1552 if (this.securityLabel == null) 1553 this.securityLabel = new ArrayList<Coding>(); 1554 this.securityLabel.add(t); 1555 return t; 1556 } 1557 1558 public ProvisionComponent addSecurityLabel(Coding t) { // 3 1559 if (t == null) 1560 return this; 1561 if (this.securityLabel == null) 1562 this.securityLabel = new ArrayList<Coding>(); 1563 this.securityLabel.add(t); 1564 return this; 1565 } 1566 1567 /** 1568 * @return The first repetition of repeating field {@link #securityLabel}, 1569 * creating it if it does not already exist 1570 */ 1571 public Coding getSecurityLabelFirstRep() { 1572 if (getSecurityLabel().isEmpty()) { 1573 addSecurityLabel(); 1574 } 1575 return getSecurityLabel().get(0); 1576 } 1577 1578 /** 1579 * @return {@link #purpose} (The context of the activities a user is taking - 1580 * why the user is accessing the data - that are controlled by this 1581 * rule.) 1582 */ 1583 public List<Coding> getPurpose() { 1584 if (this.purpose == null) 1585 this.purpose = new ArrayList<Coding>(); 1586 return this.purpose; 1587 } 1588 1589 /** 1590 * @return Returns a reference to <code>this</code> for easy method chaining 1591 */ 1592 public ProvisionComponent setPurpose(List<Coding> thePurpose) { 1593 this.purpose = thePurpose; 1594 return this; 1595 } 1596 1597 public boolean hasPurpose() { 1598 if (this.purpose == null) 1599 return false; 1600 for (Coding item : this.purpose) 1601 if (!item.isEmpty()) 1602 return true; 1603 return false; 1604 } 1605 1606 public Coding addPurpose() { // 3 1607 Coding t = new Coding(); 1608 if (this.purpose == null) 1609 this.purpose = new ArrayList<Coding>(); 1610 this.purpose.add(t); 1611 return t; 1612 } 1613 1614 public ProvisionComponent addPurpose(Coding t) { // 3 1615 if (t == null) 1616 return this; 1617 if (this.purpose == null) 1618 this.purpose = new ArrayList<Coding>(); 1619 this.purpose.add(t); 1620 return this; 1621 } 1622 1623 /** 1624 * @return The first repetition of repeating field {@link #purpose}, creating it 1625 * if it does not already exist 1626 */ 1627 public Coding getPurposeFirstRep() { 1628 if (getPurpose().isEmpty()) { 1629 addPurpose(); 1630 } 1631 return getPurpose().get(0); 1632 } 1633 1634 /** 1635 * @return {@link #class_} (The class of information covered by this rule. The 1636 * type can be a FHIR resource type, a profile on a type, or a CDA 1637 * document, or some other type that indicates what sort of information 1638 * the consent relates to.) 1639 */ 1640 public List<Coding> getClass_() { 1641 if (this.class_ == null) 1642 this.class_ = new ArrayList<Coding>(); 1643 return this.class_; 1644 } 1645 1646 /** 1647 * @return Returns a reference to <code>this</code> for easy method chaining 1648 */ 1649 public ProvisionComponent setClass_(List<Coding> theClass_) { 1650 this.class_ = theClass_; 1651 return this; 1652 } 1653 1654 public boolean hasClass_() { 1655 if (this.class_ == null) 1656 return false; 1657 for (Coding item : this.class_) 1658 if (!item.isEmpty()) 1659 return true; 1660 return false; 1661 } 1662 1663 public Coding addClass_() { // 3 1664 Coding t = new Coding(); 1665 if (this.class_ == null) 1666 this.class_ = new ArrayList<Coding>(); 1667 this.class_.add(t); 1668 return t; 1669 } 1670 1671 public ProvisionComponent addClass_(Coding t) { // 3 1672 if (t == null) 1673 return this; 1674 if (this.class_ == null) 1675 this.class_ = new ArrayList<Coding>(); 1676 this.class_.add(t); 1677 return this; 1678 } 1679 1680 /** 1681 * @return The first repetition of repeating field {@link #class_}, creating it 1682 * if it does not already exist 1683 */ 1684 public Coding getClass_FirstRep() { 1685 if (getClass_().isEmpty()) { 1686 addClass_(); 1687 } 1688 return getClass_().get(0); 1689 } 1690 1691 /** 1692 * @return {@link #code} (If this code is found in an instance, then the rule 1693 * applies.) 1694 */ 1695 public List<CodeableConcept> getCode() { 1696 if (this.code == null) 1697 this.code = new ArrayList<CodeableConcept>(); 1698 return this.code; 1699 } 1700 1701 /** 1702 * @return Returns a reference to <code>this</code> for easy method chaining 1703 */ 1704 public ProvisionComponent setCode(List<CodeableConcept> theCode) { 1705 this.code = theCode; 1706 return this; 1707 } 1708 1709 public boolean hasCode() { 1710 if (this.code == null) 1711 return false; 1712 for (CodeableConcept item : this.code) 1713 if (!item.isEmpty()) 1714 return true; 1715 return false; 1716 } 1717 1718 public CodeableConcept addCode() { // 3 1719 CodeableConcept t = new CodeableConcept(); 1720 if (this.code == null) 1721 this.code = new ArrayList<CodeableConcept>(); 1722 this.code.add(t); 1723 return t; 1724 } 1725 1726 public ProvisionComponent addCode(CodeableConcept t) { // 3 1727 if (t == null) 1728 return this; 1729 if (this.code == null) 1730 this.code = new ArrayList<CodeableConcept>(); 1731 this.code.add(t); 1732 return this; 1733 } 1734 1735 /** 1736 * @return The first repetition of repeating field {@link #code}, creating it if 1737 * it does not already exist 1738 */ 1739 public CodeableConcept getCodeFirstRep() { 1740 if (getCode().isEmpty()) { 1741 addCode(); 1742 } 1743 return getCode().get(0); 1744 } 1745 1746 /** 1747 * @return {@link #dataPeriod} (Clinical or Operational Relevant period of time 1748 * that bounds the data controlled by this rule.) 1749 */ 1750 public Period getDataPeriod() { 1751 if (this.dataPeriod == null) 1752 if (Configuration.errorOnAutoCreate()) 1753 throw new Error("Attempt to auto-create provisionComponent.dataPeriod"); 1754 else if (Configuration.doAutoCreate()) 1755 this.dataPeriod = new Period(); // cc 1756 return this.dataPeriod; 1757 } 1758 1759 public boolean hasDataPeriod() { 1760 return this.dataPeriod != null && !this.dataPeriod.isEmpty(); 1761 } 1762 1763 /** 1764 * @param value {@link #dataPeriod} (Clinical or Operational Relevant period of 1765 * time that bounds the data controlled by this rule.) 1766 */ 1767 public ProvisionComponent setDataPeriod(Period value) { 1768 this.dataPeriod = value; 1769 return this; 1770 } 1771 1772 /** 1773 * @return {@link #data} (The resources controlled by this rule if specific 1774 * resources are referenced.) 1775 */ 1776 public List<provisionDataComponent> getData() { 1777 if (this.data == null) 1778 this.data = new ArrayList<provisionDataComponent>(); 1779 return this.data; 1780 } 1781 1782 /** 1783 * @return Returns a reference to <code>this</code> for easy method chaining 1784 */ 1785 public ProvisionComponent setData(List<provisionDataComponent> theData) { 1786 this.data = theData; 1787 return this; 1788 } 1789 1790 public boolean hasData() { 1791 if (this.data == null) 1792 return false; 1793 for (provisionDataComponent item : this.data) 1794 if (!item.isEmpty()) 1795 return true; 1796 return false; 1797 } 1798 1799 public provisionDataComponent addData() { // 3 1800 provisionDataComponent t = new provisionDataComponent(); 1801 if (this.data == null) 1802 this.data = new ArrayList<provisionDataComponent>(); 1803 this.data.add(t); 1804 return t; 1805 } 1806 1807 public ProvisionComponent addData(provisionDataComponent t) { // 3 1808 if (t == null) 1809 return this; 1810 if (this.data == null) 1811 this.data = new ArrayList<provisionDataComponent>(); 1812 this.data.add(t); 1813 return this; 1814 } 1815 1816 /** 1817 * @return The first repetition of repeating field {@link #data}, creating it if 1818 * it does not already exist 1819 */ 1820 public provisionDataComponent getDataFirstRep() { 1821 if (getData().isEmpty()) { 1822 addData(); 1823 } 1824 return getData().get(0); 1825 } 1826 1827 /** 1828 * @return {@link #provision} (Rules which provide exceptions to the base rule 1829 * or subrules.) 1830 */ 1831 public List<ProvisionComponent> getProvision() { 1832 if (this.provision == null) 1833 this.provision = new ArrayList<ProvisionComponent>(); 1834 return this.provision; 1835 } 1836 1837 /** 1838 * @return Returns a reference to <code>this</code> for easy method chaining 1839 */ 1840 public ProvisionComponent setProvision(List<ProvisionComponent> theProvision) { 1841 this.provision = theProvision; 1842 return this; 1843 } 1844 1845 public boolean hasProvision() { 1846 if (this.provision == null) 1847 return false; 1848 for (ProvisionComponent item : this.provision) 1849 if (!item.isEmpty()) 1850 return true; 1851 return false; 1852 } 1853 1854 public ProvisionComponent addProvision() { // 3 1855 ProvisionComponent t = new ProvisionComponent(); 1856 if (this.provision == null) 1857 this.provision = new ArrayList<ProvisionComponent>(); 1858 this.provision.add(t); 1859 return t; 1860 } 1861 1862 public ProvisionComponent addProvision(ProvisionComponent t) { // 3 1863 if (t == null) 1864 return this; 1865 if (this.provision == null) 1866 this.provision = new ArrayList<ProvisionComponent>(); 1867 this.provision.add(t); 1868 return this; 1869 } 1870 1871 /** 1872 * @return The first repetition of repeating field {@link #provision}, creating 1873 * it if it does not already exist 1874 */ 1875 public ProvisionComponent getProvisionFirstRep() { 1876 if (getProvision().isEmpty()) { 1877 addProvision(); 1878 } 1879 return getProvision().get(0); 1880 } 1881 1882 protected void listChildren(List<Property> children) { 1883 super.listChildren(children); 1884 children.add(new Property("type", "code", 1885 "Action to take - permit or deny - when the rule conditions are met. Not permitted in root rule, required in all nested rules.", 1886 0, 1, type)); 1887 children.add(new Property("period", "Period", "The timeframe in this rule is valid.", 0, 1, period)); 1888 children.add(new Property("actor", "", 1889 "Who or what is controlled by this rule. Use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 1890 0, java.lang.Integer.MAX_VALUE, actor)); 1891 children.add(new Property("action", "CodeableConcept", "Actions controlled by this Rule.", 0, 1892 java.lang.Integer.MAX_VALUE, action)); 1893 children.add(new Property("securityLabel", "Coding", 1894 "A security label, comprised of 0..* security label fields (Privacy tags), which define which resources are controlled by this exception.", 1895 0, java.lang.Integer.MAX_VALUE, securityLabel)); 1896 children.add(new Property("purpose", "Coding", 1897 "The context of the activities a user is taking - why the user is accessing the data - that are controlled by this rule.", 1898 0, java.lang.Integer.MAX_VALUE, purpose)); 1899 children.add(new Property("class", "Coding", 1900 "The class of information covered by this rule. The type can be a FHIR resource type, a profile on a type, or a CDA document, or some other type that indicates what sort of information the consent relates to.", 1901 0, java.lang.Integer.MAX_VALUE, class_)); 1902 children.add(new Property("code", "CodeableConcept", 1903 "If this code is found in an instance, then the rule applies.", 0, java.lang.Integer.MAX_VALUE, code)); 1904 children.add(new Property("dataPeriod", "Period", 1905 "Clinical or Operational Relevant period of time that bounds the data controlled by this rule.", 0, 1, 1906 dataPeriod)); 1907 children 1908 .add(new Property("data", "", "The resources controlled by this rule if specific resources are referenced.", 1909 0, java.lang.Integer.MAX_VALUE, data)); 1910 children.add(new Property("provision", "@Consent.provision", 1911 "Rules which provide exceptions to the base rule or subrules.", 0, java.lang.Integer.MAX_VALUE, provision)); 1912 } 1913 1914 @Override 1915 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1916 switch (_hash) { 1917 case 3575610: 1918 /* type */ return new Property("type", "code", 1919 "Action to take - permit or deny - when the rule conditions are met. Not permitted in root rule, required in all nested rules.", 1920 0, 1, type); 1921 case -991726143: 1922 /* period */ return new Property("period", "Period", "The timeframe in this rule is valid.", 0, 1, period); 1923 case 92645877: 1924 /* actor */ return new Property("actor", "", 1925 "Who or what is controlled by this rule. Use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 1926 0, java.lang.Integer.MAX_VALUE, actor); 1927 case -1422950858: 1928 /* action */ return new Property("action", "CodeableConcept", "Actions controlled by this Rule.", 0, 1929 java.lang.Integer.MAX_VALUE, action); 1930 case -722296940: 1931 /* securityLabel */ return new Property("securityLabel", "Coding", 1932 "A security label, comprised of 0..* security label fields (Privacy tags), which define which resources are controlled by this exception.", 1933 0, java.lang.Integer.MAX_VALUE, securityLabel); 1934 case -220463842: 1935 /* purpose */ return new Property("purpose", "Coding", 1936 "The context of the activities a user is taking - why the user is accessing the data - that are controlled by this rule.", 1937 0, java.lang.Integer.MAX_VALUE, purpose); 1938 case 94742904: 1939 /* class */ return new Property("class", "Coding", 1940 "The class of information covered by this rule. The type can be a FHIR resource type, a profile on a type, or a CDA document, or some other type that indicates what sort of information the consent relates to.", 1941 0, java.lang.Integer.MAX_VALUE, class_); 1942 case 3059181: 1943 /* code */ return new Property("code", "CodeableConcept", 1944 "If this code is found in an instance, then the rule applies.", 0, java.lang.Integer.MAX_VALUE, code); 1945 case 1177250315: 1946 /* dataPeriod */ return new Property("dataPeriod", "Period", 1947 "Clinical or Operational Relevant period of time that bounds the data controlled by this rule.", 0, 1, 1948 dataPeriod); 1949 case 3076010: 1950 /* data */ return new Property("data", "", 1951 "The resources controlled by this rule if specific resources are referenced.", 0, 1952 java.lang.Integer.MAX_VALUE, data); 1953 case -547120939: 1954 /* provision */ return new Property("provision", "@Consent.provision", 1955 "Rules which provide exceptions to the base rule or subrules.", 0, java.lang.Integer.MAX_VALUE, provision); 1956 default: 1957 return super.getNamedProperty(_hash, _name, _checkValid); 1958 } 1959 1960 } 1961 1962 @Override 1963 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1964 switch (hash) { 1965 case 3575610: 1966 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<ConsentProvisionType> 1967 case -991726143: 1968 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 1969 case 92645877: 1970 /* actor */ return this.actor == null ? new Base[0] : this.actor.toArray(new Base[this.actor.size()]); // provisionActorComponent 1971 case -1422950858: 1972 /* action */ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // CodeableConcept 1973 case -722296940: 1974 /* securityLabel */ return this.securityLabel == null ? new Base[0] 1975 : this.securityLabel.toArray(new Base[this.securityLabel.size()]); // Coding 1976 case -220463842: 1977 /* purpose */ return this.purpose == null ? new Base[0] : this.purpose.toArray(new Base[this.purpose.size()]); // Coding 1978 case 94742904: 1979 /* class */ return this.class_ == null ? new Base[0] : this.class_.toArray(new Base[this.class_.size()]); // Coding 1980 case 3059181: 1981 /* code */ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 1982 case 1177250315: 1983 /* dataPeriod */ return this.dataPeriod == null ? new Base[0] : new Base[] { this.dataPeriod }; // Period 1984 case 3076010: 1985 /* data */ return this.data == null ? new Base[0] : this.data.toArray(new Base[this.data.size()]); // provisionDataComponent 1986 case -547120939: 1987 /* provision */ return this.provision == null ? new Base[0] 1988 : this.provision.toArray(new Base[this.provision.size()]); // provisionComponent 1989 default: 1990 return super.getProperty(hash, name, checkValid); 1991 } 1992 1993 } 1994 1995 @Override 1996 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1997 switch (hash) { 1998 case 3575610: // type 1999 value = new ConsentProvisionTypeEnumFactory().fromType(castToCode(value)); 2000 this.type = (Enumeration) value; // Enumeration<ConsentProvisionType> 2001 return value; 2002 case -991726143: // period 2003 this.period = castToPeriod(value); // Period 2004 return value; 2005 case 92645877: // actor 2006 this.getActor().add((provisionActorComponent) value); // provisionActorComponent 2007 return value; 2008 case -1422950858: // action 2009 this.getAction().add(castToCodeableConcept(value)); // CodeableConcept 2010 return value; 2011 case -722296940: // securityLabel 2012 this.getSecurityLabel().add(castToCoding(value)); // Coding 2013 return value; 2014 case -220463842: // purpose 2015 this.getPurpose().add(castToCoding(value)); // Coding 2016 return value; 2017 case 94742904: // class 2018 this.getClass_().add(castToCoding(value)); // Coding 2019 return value; 2020 case 3059181: // code 2021 this.getCode().add(castToCodeableConcept(value)); // CodeableConcept 2022 return value; 2023 case 1177250315: // dataPeriod 2024 this.dataPeriod = castToPeriod(value); // Period 2025 return value; 2026 case 3076010: // data 2027 this.getData().add((provisionDataComponent) value); // provisionDataComponent 2028 return value; 2029 case -547120939: // provision 2030 this.getProvision().add((ProvisionComponent) value); // provisionComponent 2031 return value; 2032 default: 2033 return super.setProperty(hash, name, value); 2034 } 2035 2036 } 2037 2038 @Override 2039 public Base setProperty(String name, Base value) throws FHIRException { 2040 if (name.equals("type")) { 2041 value = new ConsentProvisionTypeEnumFactory().fromType(castToCode(value)); 2042 this.type = (Enumeration) value; // Enumeration<ConsentProvisionType> 2043 } else if (name.equals("period")) { 2044 this.period = castToPeriod(value); // Period 2045 } else if (name.equals("actor")) { 2046 this.getActor().add((provisionActorComponent) value); 2047 } else if (name.equals("action")) { 2048 this.getAction().add(castToCodeableConcept(value)); 2049 } else if (name.equals("securityLabel")) { 2050 this.getSecurityLabel().add(castToCoding(value)); 2051 } else if (name.equals("purpose")) { 2052 this.getPurpose().add(castToCoding(value)); 2053 } else if (name.equals("class")) { 2054 this.getClass_().add(castToCoding(value)); 2055 } else if (name.equals("code")) { 2056 this.getCode().add(castToCodeableConcept(value)); 2057 } else if (name.equals("dataPeriod")) { 2058 this.dataPeriod = castToPeriod(value); // Period 2059 } else if (name.equals("data")) { 2060 this.getData().add((provisionDataComponent) value); 2061 } else if (name.equals("provision")) { 2062 this.getProvision().add((ProvisionComponent) value); 2063 } else 2064 return super.setProperty(name, value); 2065 return value; 2066 } 2067 2068 @Override 2069 public void removeChild(String name, Base value) throws FHIRException { 2070 if (name.equals("type")) { 2071 this.type = null; 2072 } else if (name.equals("period")) { 2073 this.period = null; 2074 } else if (name.equals("actor")) { 2075 this.getActor().remove((provisionActorComponent) value); 2076 } else if (name.equals("action")) { 2077 this.getAction().remove(castToCodeableConcept(value)); 2078 } else if (name.equals("securityLabel")) { 2079 this.getSecurityLabel().remove(castToCoding(value)); 2080 } else if (name.equals("purpose")) { 2081 this.getPurpose().remove(castToCoding(value)); 2082 } else if (name.equals("class")) { 2083 this.getClass_().remove(castToCoding(value)); 2084 } else if (name.equals("code")) { 2085 this.getCode().remove(castToCodeableConcept(value)); 2086 } else if (name.equals("dataPeriod")) { 2087 this.dataPeriod = null; 2088 } else if (name.equals("data")) { 2089 this.getData().remove((provisionDataComponent) value); 2090 } else if (name.equals("provision")) { 2091 this.getProvision().remove((ProvisionComponent) value); 2092 } else 2093 super.removeChild(name, value); 2094 2095 } 2096 2097 @Override 2098 public Base makeProperty(int hash, String name) throws FHIRException { 2099 switch (hash) { 2100 case 3575610: 2101 return getTypeElement(); 2102 case -991726143: 2103 return getPeriod(); 2104 case 92645877: 2105 return addActor(); 2106 case -1422950858: 2107 return addAction(); 2108 case -722296940: 2109 return addSecurityLabel(); 2110 case -220463842: 2111 return addPurpose(); 2112 case 94742904: 2113 return addClass_(); 2114 case 3059181: 2115 return addCode(); 2116 case 1177250315: 2117 return getDataPeriod(); 2118 case 3076010: 2119 return addData(); 2120 case -547120939: 2121 return addProvision(); 2122 default: 2123 return super.makeProperty(hash, name); 2124 } 2125 2126 } 2127 2128 @Override 2129 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2130 switch (hash) { 2131 case 3575610: 2132 /* type */ return new String[] { "code" }; 2133 case -991726143: 2134 /* period */ return new String[] { "Period" }; 2135 case 92645877: 2136 /* actor */ return new String[] {}; 2137 case -1422950858: 2138 /* action */ return new String[] { "CodeableConcept" }; 2139 case -722296940: 2140 /* securityLabel */ return new String[] { "Coding" }; 2141 case -220463842: 2142 /* purpose */ return new String[] { "Coding" }; 2143 case 94742904: 2144 /* class */ return new String[] { "Coding" }; 2145 case 3059181: 2146 /* code */ return new String[] { "CodeableConcept" }; 2147 case 1177250315: 2148 /* dataPeriod */ return new String[] { "Period" }; 2149 case 3076010: 2150 /* data */ return new String[] {}; 2151 case -547120939: 2152 /* provision */ return new String[] { "@Consent.provision" }; 2153 default: 2154 return super.getTypesForProperty(hash, name); 2155 } 2156 2157 } 2158 2159 @Override 2160 public Base addChild(String name) throws FHIRException { 2161 if (name.equals("type")) { 2162 throw new FHIRException("Cannot call addChild on a singleton property Consent.type"); 2163 } else if (name.equals("period")) { 2164 this.period = new Period(); 2165 return this.period; 2166 } else if (name.equals("actor")) { 2167 return addActor(); 2168 } else if (name.equals("action")) { 2169 return addAction(); 2170 } else if (name.equals("securityLabel")) { 2171 return addSecurityLabel(); 2172 } else if (name.equals("purpose")) { 2173 return addPurpose(); 2174 } else if (name.equals("class")) { 2175 return addClass_(); 2176 } else if (name.equals("code")) { 2177 return addCode(); 2178 } else if (name.equals("dataPeriod")) { 2179 this.dataPeriod = new Period(); 2180 return this.dataPeriod; 2181 } else if (name.equals("data")) { 2182 return addData(); 2183 } else if (name.equals("provision")) { 2184 return addProvision(); 2185 } else 2186 return super.addChild(name); 2187 } 2188 2189 public ProvisionComponent copy() { 2190 ProvisionComponent dst = new ProvisionComponent(); 2191 copyValues(dst); 2192 return dst; 2193 } 2194 2195 public void copyValues(ProvisionComponent dst) { 2196 super.copyValues(dst); 2197 dst.type = type == null ? null : type.copy(); 2198 dst.period = period == null ? null : period.copy(); 2199 if (actor != null) { 2200 dst.actor = new ArrayList<provisionActorComponent>(); 2201 for (provisionActorComponent i : actor) 2202 dst.actor.add(i.copy()); 2203 } 2204 ; 2205 if (action != null) { 2206 dst.action = new ArrayList<CodeableConcept>(); 2207 for (CodeableConcept i : action) 2208 dst.action.add(i.copy()); 2209 } 2210 ; 2211 if (securityLabel != null) { 2212 dst.securityLabel = new ArrayList<Coding>(); 2213 for (Coding i : securityLabel) 2214 dst.securityLabel.add(i.copy()); 2215 } 2216 ; 2217 if (purpose != null) { 2218 dst.purpose = new ArrayList<Coding>(); 2219 for (Coding i : purpose) 2220 dst.purpose.add(i.copy()); 2221 } 2222 ; 2223 if (class_ != null) { 2224 dst.class_ = new ArrayList<Coding>(); 2225 for (Coding i : class_) 2226 dst.class_.add(i.copy()); 2227 } 2228 ; 2229 if (code != null) { 2230 dst.code = new ArrayList<CodeableConcept>(); 2231 for (CodeableConcept i : code) 2232 dst.code.add(i.copy()); 2233 } 2234 ; 2235 dst.dataPeriod = dataPeriod == null ? null : dataPeriod.copy(); 2236 if (data != null) { 2237 dst.data = new ArrayList<provisionDataComponent>(); 2238 for (provisionDataComponent i : data) 2239 dst.data.add(i.copy()); 2240 } 2241 ; 2242 if (provision != null) { 2243 dst.provision = new ArrayList<ProvisionComponent>(); 2244 for (ProvisionComponent i : provision) 2245 dst.provision.add(i.copy()); 2246 } 2247 ; 2248 } 2249 2250 @Override 2251 public boolean equalsDeep(Base other_) { 2252 if (!super.equalsDeep(other_)) 2253 return false; 2254 if (!(other_ instanceof ProvisionComponent)) 2255 return false; 2256 ProvisionComponent o = (ProvisionComponent) other_; 2257 return compareDeep(type, o.type, true) && compareDeep(period, o.period, true) && compareDeep(actor, o.actor, true) 2258 && compareDeep(action, o.action, true) && compareDeep(securityLabel, o.securityLabel, true) 2259 && compareDeep(purpose, o.purpose, true) && compareDeep(class_, o.class_, true) 2260 && compareDeep(code, o.code, true) && compareDeep(dataPeriod, o.dataPeriod, true) 2261 && compareDeep(data, o.data, true) && compareDeep(provision, o.provision, true); 2262 } 2263 2264 @Override 2265 public boolean equalsShallow(Base other_) { 2266 if (!super.equalsShallow(other_)) 2267 return false; 2268 if (!(other_ instanceof ProvisionComponent)) 2269 return false; 2270 ProvisionComponent o = (ProvisionComponent) other_; 2271 return compareValues(type, o.type, true); 2272 } 2273 2274 public boolean isEmpty() { 2275 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, period, actor, action, securityLabel, 2276 purpose, class_, code, dataPeriod, data, provision); 2277 } 2278 2279 public String fhirType() { 2280 return "Consent.provision"; 2281 2282 } 2283 2284 } 2285 2286 @Block() 2287 public static class provisionActorComponent extends BackboneElement implements IBaseBackboneElement { 2288 /** 2289 * How the individual is involved in the resources content that is described in 2290 * the exception. 2291 */ 2292 @Child(name = "role", type = { 2293 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2294 @Description(shortDefinition = "How the actor is involved", formalDefinition = "How the individual is involved in the resources content that is described in the exception.") 2295 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/security-role-type") 2296 protected CodeableConcept role; 2297 2298 /** 2299 * The resource that identifies the actor. To identify actors by type, use group 2300 * to identify a set of actors by some property they share (e.g. 'admitting 2301 * officers'). 2302 */ 2303 @Child(name = "reference", type = { Device.class, Group.class, CareTeam.class, Organization.class, Patient.class, 2304 Practitioner.class, RelatedPerson.class, 2305 PractitionerRole.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 2306 @Description(shortDefinition = "Resource for the actor (or group, by role)", formalDefinition = "The resource that identifies the actor. To identify actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').") 2307 protected Reference reference; 2308 2309 /** 2310 * The actual object that is the target of the reference (The resource that 2311 * identifies the actor. To identify actors by type, use group to identify a set 2312 * of actors by some property they share (e.g. 'admitting officers').) 2313 */ 2314 protected Resource referenceTarget; 2315 2316 private static final long serialVersionUID = 1152919415L; 2317 2318 /** 2319 * Constructor 2320 */ 2321 public provisionActorComponent() { 2322 super(); 2323 } 2324 2325 /** 2326 * Constructor 2327 */ 2328 public provisionActorComponent(CodeableConcept role, Reference reference) { 2329 super(); 2330 this.role = role; 2331 this.reference = reference; 2332 } 2333 2334 /** 2335 * @return {@link #role} (How the individual is involved in the resources 2336 * content that is described in the exception.) 2337 */ 2338 public CodeableConcept getRole() { 2339 if (this.role == null) 2340 if (Configuration.errorOnAutoCreate()) 2341 throw new Error("Attempt to auto-create provisionActorComponent.role"); 2342 else if (Configuration.doAutoCreate()) 2343 this.role = new CodeableConcept(); // cc 2344 return this.role; 2345 } 2346 2347 public boolean hasRole() { 2348 return this.role != null && !this.role.isEmpty(); 2349 } 2350 2351 /** 2352 * @param value {@link #role} (How the individual is involved in the resources 2353 * content that is described in the exception.) 2354 */ 2355 public provisionActorComponent setRole(CodeableConcept value) { 2356 this.role = value; 2357 return this; 2358 } 2359 2360 /** 2361 * @return {@link #reference} (The resource that identifies the actor. To 2362 * identify actors by type, use group to identify a set of actors by 2363 * some property they share (e.g. 'admitting officers').) 2364 */ 2365 public Reference getReference() { 2366 if (this.reference == null) 2367 if (Configuration.errorOnAutoCreate()) 2368 throw new Error("Attempt to auto-create provisionActorComponent.reference"); 2369 else if (Configuration.doAutoCreate()) 2370 this.reference = new Reference(); // cc 2371 return this.reference; 2372 } 2373 2374 public boolean hasReference() { 2375 return this.reference != null && !this.reference.isEmpty(); 2376 } 2377 2378 /** 2379 * @param value {@link #reference} (The resource that identifies the actor. To 2380 * identify actors by type, use group to identify a set of actors 2381 * by some property they share (e.g. 'admitting officers').) 2382 */ 2383 public provisionActorComponent setReference(Reference value) { 2384 this.reference = value; 2385 return this; 2386 } 2387 2388 /** 2389 * @return {@link #reference} The actual object that is the target of the 2390 * reference. The reference library doesn't populate this, but you can 2391 * use it to hold the resource if you resolve it. (The resource that 2392 * identifies the actor. To identify actors by type, use group to 2393 * identify a set of actors by some property they share (e.g. 'admitting 2394 * officers').) 2395 */ 2396 public Resource getReferenceTarget() { 2397 return this.referenceTarget; 2398 } 2399 2400 /** 2401 * @param value {@link #reference} The actual object that is the target of the 2402 * reference. The reference library doesn't use these, but you can 2403 * use it to hold the resource if you resolve it. (The resource 2404 * that identifies the actor. To identify actors by type, use group 2405 * to identify a set of actors by some property they share (e.g. 2406 * 'admitting officers').) 2407 */ 2408 public provisionActorComponent setReferenceTarget(Resource value) { 2409 this.referenceTarget = value; 2410 return this; 2411 } 2412 2413 protected void listChildren(List<Property> children) { 2414 super.listChildren(children); 2415 children.add(new Property("role", "CodeableConcept", 2416 "How the individual is involved in the resources content that is described in the exception.", 0, 1, role)); 2417 children.add(new Property("reference", 2418 "Reference(Device|Group|CareTeam|Organization|Patient|Practitioner|RelatedPerson|PractitionerRole)", 2419 "The resource that identifies the actor. To identify actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 2420 0, 1, reference)); 2421 } 2422 2423 @Override 2424 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2425 switch (_hash) { 2426 case 3506294: 2427 /* role */ return new Property("role", "CodeableConcept", 2428 "How the individual is involved in the resources content that is described in the exception.", 0, 1, role); 2429 case -925155509: 2430 /* reference */ return new Property("reference", 2431 "Reference(Device|Group|CareTeam|Organization|Patient|Practitioner|RelatedPerson|PractitionerRole)", 2432 "The resource that identifies the actor. To identify actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 2433 0, 1, reference); 2434 default: 2435 return super.getNamedProperty(_hash, _name, _checkValid); 2436 } 2437 2438 } 2439 2440 @Override 2441 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2442 switch (hash) { 2443 case 3506294: 2444 /* role */ return this.role == null ? new Base[0] : new Base[] { this.role }; // CodeableConcept 2445 case -925155509: 2446 /* reference */ return this.reference == null ? new Base[0] : new Base[] { this.reference }; // Reference 2447 default: 2448 return super.getProperty(hash, name, checkValid); 2449 } 2450 2451 } 2452 2453 @Override 2454 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2455 switch (hash) { 2456 case 3506294: // role 2457 this.role = castToCodeableConcept(value); // CodeableConcept 2458 return value; 2459 case -925155509: // reference 2460 this.reference = castToReference(value); // Reference 2461 return value; 2462 default: 2463 return super.setProperty(hash, name, value); 2464 } 2465 2466 } 2467 2468 @Override 2469 public Base setProperty(String name, Base value) throws FHIRException { 2470 if (name.equals("role")) { 2471 this.role = castToCodeableConcept(value); // CodeableConcept 2472 } else if (name.equals("reference")) { 2473 this.reference = castToReference(value); // Reference 2474 } else 2475 return super.setProperty(name, value); 2476 return value; 2477 } 2478 2479 @Override 2480 public void removeChild(String name, Base value) throws FHIRException { 2481 if (name.equals("role")) { 2482 this.role = null; 2483 } else if (name.equals("reference")) { 2484 this.reference = null; 2485 } else 2486 super.removeChild(name, value); 2487 2488 } 2489 2490 @Override 2491 public Base makeProperty(int hash, String name) throws FHIRException { 2492 switch (hash) { 2493 case 3506294: 2494 return getRole(); 2495 case -925155509: 2496 return getReference(); 2497 default: 2498 return super.makeProperty(hash, name); 2499 } 2500 2501 } 2502 2503 @Override 2504 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2505 switch (hash) { 2506 case 3506294: 2507 /* role */ return new String[] { "CodeableConcept" }; 2508 case -925155509: 2509 /* reference */ return new String[] { "Reference" }; 2510 default: 2511 return super.getTypesForProperty(hash, name); 2512 } 2513 2514 } 2515 2516 @Override 2517 public Base addChild(String name) throws FHIRException { 2518 if (name.equals("role")) { 2519 this.role = new CodeableConcept(); 2520 return this.role; 2521 } else if (name.equals("reference")) { 2522 this.reference = new Reference(); 2523 return this.reference; 2524 } else 2525 return super.addChild(name); 2526 } 2527 2528 public provisionActorComponent copy() { 2529 provisionActorComponent dst = new provisionActorComponent(); 2530 copyValues(dst); 2531 return dst; 2532 } 2533 2534 public void copyValues(provisionActorComponent dst) { 2535 super.copyValues(dst); 2536 dst.role = role == null ? null : role.copy(); 2537 dst.reference = reference == null ? null : reference.copy(); 2538 } 2539 2540 @Override 2541 public boolean equalsDeep(Base other_) { 2542 if (!super.equalsDeep(other_)) 2543 return false; 2544 if (!(other_ instanceof provisionActorComponent)) 2545 return false; 2546 provisionActorComponent o = (provisionActorComponent) other_; 2547 return compareDeep(role, o.role, true) && compareDeep(reference, o.reference, true); 2548 } 2549 2550 @Override 2551 public boolean equalsShallow(Base other_) { 2552 if (!super.equalsShallow(other_)) 2553 return false; 2554 if (!(other_ instanceof provisionActorComponent)) 2555 return false; 2556 provisionActorComponent o = (provisionActorComponent) other_; 2557 return true; 2558 } 2559 2560 public boolean isEmpty() { 2561 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, reference); 2562 } 2563 2564 public String fhirType() { 2565 return "Consent.provision.actor"; 2566 2567 } 2568 2569 } 2570 2571 @Block() 2572 public static class provisionDataComponent extends BackboneElement implements IBaseBackboneElement { 2573 /** 2574 * How the resource reference is interpreted when testing consent restrictions. 2575 */ 2576 @Child(name = "meaning", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2577 @Description(shortDefinition = "instance | related | dependents | authoredby", formalDefinition = "How the resource reference is interpreted when testing consent restrictions.") 2578 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/consent-data-meaning") 2579 protected Enumeration<ConsentDataMeaning> meaning; 2580 2581 /** 2582 * A reference to a specific resource that defines which resources are covered 2583 * by this consent. 2584 */ 2585 @Child(name = "reference", type = { 2586 Reference.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 2587 @Description(shortDefinition = "The actual data reference", formalDefinition = "A reference to a specific resource that defines which resources are covered by this consent.") 2588 protected Reference reference; 2589 2590 /** 2591 * The actual object that is the target of the reference (A reference to a 2592 * specific resource that defines which resources are covered by this consent.) 2593 */ 2594 protected Resource referenceTarget; 2595 2596 private static final long serialVersionUID = -424898645L; 2597 2598 /** 2599 * Constructor 2600 */ 2601 public provisionDataComponent() { 2602 super(); 2603 } 2604 2605 /** 2606 * Constructor 2607 */ 2608 public provisionDataComponent(Enumeration<ConsentDataMeaning> meaning, Reference reference) { 2609 super(); 2610 this.meaning = meaning; 2611 this.reference = reference; 2612 } 2613 2614 /** 2615 * @return {@link #meaning} (How the resource reference is interpreted when 2616 * testing consent restrictions.). This is the underlying object with 2617 * id, value and extensions. The accessor "getMeaning" gives direct 2618 * access to the value 2619 */ 2620 public Enumeration<ConsentDataMeaning> getMeaningElement() { 2621 if (this.meaning == null) 2622 if (Configuration.errorOnAutoCreate()) 2623 throw new Error("Attempt to auto-create provisionDataComponent.meaning"); 2624 else if (Configuration.doAutoCreate()) 2625 this.meaning = new Enumeration<ConsentDataMeaning>(new ConsentDataMeaningEnumFactory()); // bb 2626 return this.meaning; 2627 } 2628 2629 public boolean hasMeaningElement() { 2630 return this.meaning != null && !this.meaning.isEmpty(); 2631 } 2632 2633 public boolean hasMeaning() { 2634 return this.meaning != null && !this.meaning.isEmpty(); 2635 } 2636 2637 /** 2638 * @param value {@link #meaning} (How the resource reference is interpreted when 2639 * testing consent restrictions.). This is the underlying object 2640 * with id, value and extensions. The accessor "getMeaning" gives 2641 * direct access to the value 2642 */ 2643 public provisionDataComponent setMeaningElement(Enumeration<ConsentDataMeaning> value) { 2644 this.meaning = value; 2645 return this; 2646 } 2647 2648 /** 2649 * @return How the resource reference is interpreted when testing consent 2650 * restrictions. 2651 */ 2652 public ConsentDataMeaning getMeaning() { 2653 return this.meaning == null ? null : this.meaning.getValue(); 2654 } 2655 2656 /** 2657 * @param value How the resource reference is interpreted when testing consent 2658 * restrictions. 2659 */ 2660 public provisionDataComponent setMeaning(ConsentDataMeaning value) { 2661 if (this.meaning == null) 2662 this.meaning = new Enumeration<ConsentDataMeaning>(new ConsentDataMeaningEnumFactory()); 2663 this.meaning.setValue(value); 2664 return this; 2665 } 2666 2667 /** 2668 * @return {@link #reference} (A reference to a specific resource that defines 2669 * which resources are covered by this consent.) 2670 */ 2671 public Reference getReference() { 2672 if (this.reference == null) 2673 if (Configuration.errorOnAutoCreate()) 2674 throw new Error("Attempt to auto-create provisionDataComponent.reference"); 2675 else if (Configuration.doAutoCreate()) 2676 this.reference = new Reference(); // cc 2677 return this.reference; 2678 } 2679 2680 public boolean hasReference() { 2681 return this.reference != null && !this.reference.isEmpty(); 2682 } 2683 2684 /** 2685 * @param value {@link #reference} (A reference to a specific resource that 2686 * defines which resources are covered by this consent.) 2687 */ 2688 public provisionDataComponent setReference(Reference value) { 2689 this.reference = value; 2690 return this; 2691 } 2692 2693 /** 2694 * @return {@link #reference} The actual object that is the target of the 2695 * reference. The reference library doesn't populate this, but you can 2696 * use it to hold the resource if you resolve it. (A reference to a 2697 * specific resource that defines which resources are covered by this 2698 * consent.) 2699 */ 2700 public Resource getReferenceTarget() { 2701 return this.referenceTarget; 2702 } 2703 2704 /** 2705 * @param value {@link #reference} The actual object that is the target of the 2706 * reference. The reference library doesn't use these, but you can 2707 * use it to hold the resource if you resolve it. (A reference to a 2708 * specific resource that defines which resources are covered by 2709 * this consent.) 2710 */ 2711 public provisionDataComponent setReferenceTarget(Resource value) { 2712 this.referenceTarget = value; 2713 return this; 2714 } 2715 2716 protected void listChildren(List<Property> children) { 2717 super.listChildren(children); 2718 children.add(new Property("meaning", "code", 2719 "How the resource reference is interpreted when testing consent restrictions.", 0, 1, meaning)); 2720 children.add(new Property("reference", "Reference(Any)", 2721 "A reference to a specific resource that defines which resources are covered by this consent.", 0, 1, 2722 reference)); 2723 } 2724 2725 @Override 2726 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2727 switch (_hash) { 2728 case 938160637: 2729 /* meaning */ return new Property("meaning", "code", 2730 "How the resource reference is interpreted when testing consent restrictions.", 0, 1, meaning); 2731 case -925155509: 2732 /* reference */ return new Property("reference", "Reference(Any)", 2733 "A reference to a specific resource that defines which resources are covered by this consent.", 0, 1, 2734 reference); 2735 default: 2736 return super.getNamedProperty(_hash, _name, _checkValid); 2737 } 2738 2739 } 2740 2741 @Override 2742 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2743 switch (hash) { 2744 case 938160637: 2745 /* meaning */ return this.meaning == null ? new Base[0] : new Base[] { this.meaning }; // Enumeration<ConsentDataMeaning> 2746 case -925155509: 2747 /* reference */ return this.reference == null ? new Base[0] : new Base[] { this.reference }; // Reference 2748 default: 2749 return super.getProperty(hash, name, checkValid); 2750 } 2751 2752 } 2753 2754 @Override 2755 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2756 switch (hash) { 2757 case 938160637: // meaning 2758 value = new ConsentDataMeaningEnumFactory().fromType(castToCode(value)); 2759 this.meaning = (Enumeration) value; // Enumeration<ConsentDataMeaning> 2760 return value; 2761 case -925155509: // reference 2762 this.reference = castToReference(value); // Reference 2763 return value; 2764 default: 2765 return super.setProperty(hash, name, value); 2766 } 2767 2768 } 2769 2770 @Override 2771 public Base setProperty(String name, Base value) throws FHIRException { 2772 if (name.equals("meaning")) { 2773 value = new ConsentDataMeaningEnumFactory().fromType(castToCode(value)); 2774 this.meaning = (Enumeration) value; // Enumeration<ConsentDataMeaning> 2775 } else if (name.equals("reference")) { 2776 this.reference = castToReference(value); // Reference 2777 } else 2778 return super.setProperty(name, value); 2779 return value; 2780 } 2781 2782 @Override 2783 public void removeChild(String name, Base value) throws FHIRException { 2784 if (name.equals("meaning")) { 2785 this.meaning = null; 2786 } else if (name.equals("reference")) { 2787 this.reference = null; 2788 } else 2789 super.removeChild(name, value); 2790 2791 } 2792 2793 @Override 2794 public Base makeProperty(int hash, String name) throws FHIRException { 2795 switch (hash) { 2796 case 938160637: 2797 return getMeaningElement(); 2798 case -925155509: 2799 return getReference(); 2800 default: 2801 return super.makeProperty(hash, name); 2802 } 2803 2804 } 2805 2806 @Override 2807 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2808 switch (hash) { 2809 case 938160637: 2810 /* meaning */ return new String[] { "code" }; 2811 case -925155509: 2812 /* reference */ return new String[] { "Reference" }; 2813 default: 2814 return super.getTypesForProperty(hash, name); 2815 } 2816 2817 } 2818 2819 @Override 2820 public Base addChild(String name) throws FHIRException { 2821 if (name.equals("meaning")) { 2822 throw new FHIRException("Cannot call addChild on a singleton property Consent.meaning"); 2823 } else if (name.equals("reference")) { 2824 this.reference = new Reference(); 2825 return this.reference; 2826 } else 2827 return super.addChild(name); 2828 } 2829 2830 public provisionDataComponent copy() { 2831 provisionDataComponent dst = new provisionDataComponent(); 2832 copyValues(dst); 2833 return dst; 2834 } 2835 2836 public void copyValues(provisionDataComponent dst) { 2837 super.copyValues(dst); 2838 dst.meaning = meaning == null ? null : meaning.copy(); 2839 dst.reference = reference == null ? null : reference.copy(); 2840 } 2841 2842 @Override 2843 public boolean equalsDeep(Base other_) { 2844 if (!super.equalsDeep(other_)) 2845 return false; 2846 if (!(other_ instanceof provisionDataComponent)) 2847 return false; 2848 provisionDataComponent o = (provisionDataComponent) other_; 2849 return compareDeep(meaning, o.meaning, true) && compareDeep(reference, o.reference, true); 2850 } 2851 2852 @Override 2853 public boolean equalsShallow(Base other_) { 2854 if (!super.equalsShallow(other_)) 2855 return false; 2856 if (!(other_ instanceof provisionDataComponent)) 2857 return false; 2858 provisionDataComponent o = (provisionDataComponent) other_; 2859 return compareValues(meaning, o.meaning, true); 2860 } 2861 2862 public boolean isEmpty() { 2863 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(meaning, reference); 2864 } 2865 2866 public String fhirType() { 2867 return "Consent.provision.data"; 2868 2869 } 2870 2871 } 2872 2873 /** 2874 * Unique identifier for this copy of the Consent Statement. 2875 */ 2876 @Child(name = "identifier", type = { 2877 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2878 @Description(shortDefinition = "Identifier for this record (external references)", formalDefinition = "Unique identifier for this copy of the Consent Statement.") 2879 protected List<Identifier> identifier; 2880 2881 /** 2882 * Indicates the current state of this consent. 2883 */ 2884 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 2885 @Description(shortDefinition = "draft | proposed | active | rejected | inactive | entered-in-error", formalDefinition = "Indicates the current state of this consent.") 2886 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/consent-state-codes") 2887 protected Enumeration<ConsentState> status; 2888 2889 /** 2890 * A selector of the type of consent being presented: ADR, Privacy, Treatment, 2891 * Research. This list is now extensible. 2892 */ 2893 @Child(name = "scope", type = { CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = true, summary = true) 2894 @Description(shortDefinition = "Which of the four areas this resource covers (extensible)", formalDefinition = "A selector of the type of consent being presented: ADR, Privacy, Treatment, Research. This list is now extensible.") 2895 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/consent-scope") 2896 protected CodeableConcept scope; 2897 2898 /** 2899 * A classification of the type of consents found in the statement. This element 2900 * supports indexing and retrieval of consent statements. 2901 */ 2902 @Child(name = "category", type = { 2903 CodeableConcept.class }, order = 3, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2904 @Description(shortDefinition = "Classification of the consent statement - for indexing/retrieval", formalDefinition = "A classification of the type of consents found in the statement. This element supports indexing and retrieval of consent statements.") 2905 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/consent-category") 2906 protected List<CodeableConcept> category; 2907 2908 /** 2909 * The patient/healthcare consumer to whom this consent applies. 2910 */ 2911 @Child(name = "patient", type = { Patient.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 2912 @Description(shortDefinition = "Who the consent applies to", formalDefinition = "The patient/healthcare consumer to whom this consent applies.") 2913 protected Reference patient; 2914 2915 /** 2916 * The actual object that is the target of the reference (The patient/healthcare 2917 * consumer to whom this consent applies.) 2918 */ 2919 protected Patient patientTarget; 2920 2921 /** 2922 * When this Consent was issued / created / indexed. 2923 */ 2924 @Child(name = "dateTime", type = { 2925 DateTimeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 2926 @Description(shortDefinition = "When this Consent was created or indexed", formalDefinition = "When this Consent was issued / created / indexed.") 2927 protected DateTimeType dateTime; 2928 2929 /** 2930 * Either the Grantor, which is the entity responsible for granting the rights 2931 * listed in a Consent Directive or the Grantee, which is the entity responsible 2932 * for complying with the Consent Directive, including any obligations or 2933 * limitations on authorizations and enforcement of prohibitions. 2934 */ 2935 @Child(name = "performer", type = { Organization.class, Patient.class, Practitioner.class, RelatedPerson.class, 2936 PractitionerRole.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2937 @Description(shortDefinition = "Who is agreeing to the policy and rules", formalDefinition = "Either the Grantor, which is the entity responsible for granting the rights listed in a Consent Directive or the Grantee, which is the entity responsible for complying with the Consent Directive, including any obligations or limitations on authorizations and enforcement of prohibitions.") 2938 protected List<Reference> performer; 2939 /** 2940 * The actual objects that are the target of the reference (Either the Grantor, 2941 * which is the entity responsible for granting the rights listed in a Consent 2942 * Directive or the Grantee, which is the entity responsible for complying with 2943 * the Consent Directive, including any obligations or limitations on 2944 * authorizations and enforcement of prohibitions.) 2945 */ 2946 protected List<Resource> performerTarget; 2947 2948 /** 2949 * The organization that manages the consent, and the framework within which it 2950 * is executed. 2951 */ 2952 @Child(name = "organization", type = { 2953 Organization.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2954 @Description(shortDefinition = "Custodian of the consent", formalDefinition = "The organization that manages the consent, and the framework within which it is executed.") 2955 protected List<Reference> organization; 2956 /** 2957 * The actual objects that are the target of the reference (The organization 2958 * that manages the consent, and the framework within which it is executed.) 2959 */ 2960 protected List<Organization> organizationTarget; 2961 2962 /** 2963 * The source on which this consent statement is based. The source might be a 2964 * scanned original paper form, or a reference to a consent that links back to 2965 * such a source, a reference to a document repository (e.g. XDS) that stores 2966 * the original consent document. 2967 */ 2968 @Child(name = "source", type = { Attachment.class, Consent.class, DocumentReference.class, Contract.class, 2969 QuestionnaireResponse.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 2970 @Description(shortDefinition = "Source from which this consent is taken", formalDefinition = "The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.") 2971 protected Type source; 2972 2973 /** 2974 * The references to the policies that are included in this consent scope. 2975 * Policies may be organizational, but are often defined jurisdictionally, or in 2976 * law. 2977 */ 2978 @Child(name = "policy", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2979 @Description(shortDefinition = "Policies covered by this consent", formalDefinition = "The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law.") 2980 protected List<ConsentPolicyComponent> policy; 2981 2982 /** 2983 * A reference to the specific base computable regulation or policy. 2984 */ 2985 @Child(name = "policyRule", type = { 2986 CodeableConcept.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 2987 @Description(shortDefinition = "Regulation that this consents to", formalDefinition = "A reference to the specific base computable regulation or policy.") 2988 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/consent-policy") 2989 protected CodeableConcept policyRule; 2990 2991 /** 2992 * Whether a treatment instruction (e.g. artificial respiration yes or no) was 2993 * verified with the patient, his/her family or another authorized person. 2994 */ 2995 @Child(name = "verification", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2996 @Description(shortDefinition = "Consent Verified by patient or family", formalDefinition = "Whether a treatment instruction (e.g. artificial respiration yes or no) was verified with the patient, his/her family or another authorized person.") 2997 protected List<ConsentVerificationComponent> verification; 2998 2999 /** 3000 * An exception to the base policy of this consent. An exception can be an 3001 * addition or removal of access permissions. 3002 */ 3003 @Child(name = "provision", type = {}, order = 12, min = 0, max = 1, modifier = false, summary = true) 3004 @Description(shortDefinition = "Constraints to the base Consent.policyRule", formalDefinition = "An exception to the base policy of this consent. An exception can be an addition or removal of access permissions.") 3005 protected ProvisionComponent provision; 3006 3007 private static final long serialVersionUID = 206528051L; 3008 3009 /** 3010 * Constructor 3011 */ 3012 public Consent() { 3013 super(); 3014 } 3015 3016 /** 3017 * Constructor 3018 */ 3019 public Consent(Enumeration<ConsentState> status, CodeableConcept scope) { 3020 super(); 3021 this.status = status; 3022 this.scope = scope; 3023 } 3024 3025 /** 3026 * @return {@link #identifier} (Unique identifier for this copy of the Consent 3027 * Statement.) 3028 */ 3029 public List<Identifier> getIdentifier() { 3030 if (this.identifier == null) 3031 this.identifier = new ArrayList<Identifier>(); 3032 return this.identifier; 3033 } 3034 3035 /** 3036 * @return Returns a reference to <code>this</code> for easy method chaining 3037 */ 3038 public Consent setIdentifier(List<Identifier> theIdentifier) { 3039 this.identifier = theIdentifier; 3040 return this; 3041 } 3042 3043 public boolean hasIdentifier() { 3044 if (this.identifier == null) 3045 return false; 3046 for (Identifier item : this.identifier) 3047 if (!item.isEmpty()) 3048 return true; 3049 return false; 3050 } 3051 3052 public Identifier addIdentifier() { // 3 3053 Identifier t = new Identifier(); 3054 if (this.identifier == null) 3055 this.identifier = new ArrayList<Identifier>(); 3056 this.identifier.add(t); 3057 return t; 3058 } 3059 3060 public Consent addIdentifier(Identifier t) { // 3 3061 if (t == null) 3062 return this; 3063 if (this.identifier == null) 3064 this.identifier = new ArrayList<Identifier>(); 3065 this.identifier.add(t); 3066 return this; 3067 } 3068 3069 /** 3070 * @return The first repetition of repeating field {@link #identifier}, creating 3071 * it if it does not already exist 3072 */ 3073 public Identifier getIdentifierFirstRep() { 3074 if (getIdentifier().isEmpty()) { 3075 addIdentifier(); 3076 } 3077 return getIdentifier().get(0); 3078 } 3079 3080 /** 3081 * @return {@link #status} (Indicates the current state of this consent.). This 3082 * is the underlying object with id, value and extensions. The accessor 3083 * "getStatus" gives direct access to the value 3084 */ 3085 public Enumeration<ConsentState> getStatusElement() { 3086 if (this.status == null) 3087 if (Configuration.errorOnAutoCreate()) 3088 throw new Error("Attempt to auto-create Consent.status"); 3089 else if (Configuration.doAutoCreate()) 3090 this.status = new Enumeration<ConsentState>(new ConsentStateEnumFactory()); // bb 3091 return this.status; 3092 } 3093 3094 public boolean hasStatusElement() { 3095 return this.status != null && !this.status.isEmpty(); 3096 } 3097 3098 public boolean hasStatus() { 3099 return this.status != null && !this.status.isEmpty(); 3100 } 3101 3102 /** 3103 * @param value {@link #status} (Indicates the current state of this consent.). 3104 * This is the underlying object with id, value and extensions. The 3105 * accessor "getStatus" gives direct access to the value 3106 */ 3107 public Consent setStatusElement(Enumeration<ConsentState> value) { 3108 this.status = value; 3109 return this; 3110 } 3111 3112 /** 3113 * @return Indicates the current state of this consent. 3114 */ 3115 public ConsentState getStatus() { 3116 return this.status == null ? null : this.status.getValue(); 3117 } 3118 3119 /** 3120 * @param value Indicates the current state of this consent. 3121 */ 3122 public Consent setStatus(ConsentState value) { 3123 if (this.status == null) 3124 this.status = new Enumeration<ConsentState>(new ConsentStateEnumFactory()); 3125 this.status.setValue(value); 3126 return this; 3127 } 3128 3129 /** 3130 * @return {@link #scope} (A selector of the type of consent being presented: 3131 * ADR, Privacy, Treatment, Research. This list is now extensible.) 3132 */ 3133 public CodeableConcept getScope() { 3134 if (this.scope == null) 3135 if (Configuration.errorOnAutoCreate()) 3136 throw new Error("Attempt to auto-create Consent.scope"); 3137 else if (Configuration.doAutoCreate()) 3138 this.scope = new CodeableConcept(); // cc 3139 return this.scope; 3140 } 3141 3142 public boolean hasScope() { 3143 return this.scope != null && !this.scope.isEmpty(); 3144 } 3145 3146 /** 3147 * @param value {@link #scope} (A selector of the type of consent being 3148 * presented: ADR, Privacy, Treatment, Research. This list is now 3149 * extensible.) 3150 */ 3151 public Consent setScope(CodeableConcept value) { 3152 this.scope = value; 3153 return this; 3154 } 3155 3156 /** 3157 * @return {@link #category} (A classification of the type of consents found in 3158 * the statement. This element supports indexing and retrieval of 3159 * consent statements.) 3160 */ 3161 public List<CodeableConcept> getCategory() { 3162 if (this.category == null) 3163 this.category = new ArrayList<CodeableConcept>(); 3164 return this.category; 3165 } 3166 3167 /** 3168 * @return Returns a reference to <code>this</code> for easy method chaining 3169 */ 3170 public Consent setCategory(List<CodeableConcept> theCategory) { 3171 this.category = theCategory; 3172 return this; 3173 } 3174 3175 public boolean hasCategory() { 3176 if (this.category == null) 3177 return false; 3178 for (CodeableConcept item : this.category) 3179 if (!item.isEmpty()) 3180 return true; 3181 return false; 3182 } 3183 3184 public CodeableConcept addCategory() { // 3 3185 CodeableConcept t = new CodeableConcept(); 3186 if (this.category == null) 3187 this.category = new ArrayList<CodeableConcept>(); 3188 this.category.add(t); 3189 return t; 3190 } 3191 3192 public Consent addCategory(CodeableConcept t) { // 3 3193 if (t == null) 3194 return this; 3195 if (this.category == null) 3196 this.category = new ArrayList<CodeableConcept>(); 3197 this.category.add(t); 3198 return this; 3199 } 3200 3201 /** 3202 * @return The first repetition of repeating field {@link #category}, creating 3203 * it if it does not already exist 3204 */ 3205 public CodeableConcept getCategoryFirstRep() { 3206 if (getCategory().isEmpty()) { 3207 addCategory(); 3208 } 3209 return getCategory().get(0); 3210 } 3211 3212 /** 3213 * @return {@link #patient} (The patient/healthcare consumer to whom this 3214 * consent applies.) 3215 */ 3216 public Reference getPatient() { 3217 if (this.patient == null) 3218 if (Configuration.errorOnAutoCreate()) 3219 throw new Error("Attempt to auto-create Consent.patient"); 3220 else if (Configuration.doAutoCreate()) 3221 this.patient = new Reference(); // cc 3222 return this.patient; 3223 } 3224 3225 public boolean hasPatient() { 3226 return this.patient != null && !this.patient.isEmpty(); 3227 } 3228 3229 /** 3230 * @param value {@link #patient} (The patient/healthcare consumer to whom this 3231 * consent applies.) 3232 */ 3233 public Consent setPatient(Reference value) { 3234 this.patient = value; 3235 return this; 3236 } 3237 3238 /** 3239 * @return {@link #patient} The actual object that is the target of the 3240 * reference. The reference library doesn't populate this, but you can 3241 * use it to hold the resource if you resolve it. (The 3242 * patient/healthcare consumer to whom this consent applies.) 3243 */ 3244 public Patient getPatientTarget() { 3245 if (this.patientTarget == null) 3246 if (Configuration.errorOnAutoCreate()) 3247 throw new Error("Attempt to auto-create Consent.patient"); 3248 else if (Configuration.doAutoCreate()) 3249 this.patientTarget = new Patient(); // aa 3250 return this.patientTarget; 3251 } 3252 3253 /** 3254 * @param value {@link #patient} The actual object that is the target of the 3255 * reference. The reference library doesn't use these, but you can 3256 * use it to hold the resource if you resolve it. (The 3257 * patient/healthcare consumer to whom this consent applies.) 3258 */ 3259 public Consent setPatientTarget(Patient value) { 3260 this.patientTarget = value; 3261 return this; 3262 } 3263 3264 /** 3265 * @return {@link #dateTime} (When this Consent was issued / created / 3266 * indexed.). This is the underlying object with id, value and 3267 * extensions. The accessor "getDateTime" gives direct access to the 3268 * value 3269 */ 3270 public DateTimeType getDateTimeElement() { 3271 if (this.dateTime == null) 3272 if (Configuration.errorOnAutoCreate()) 3273 throw new Error("Attempt to auto-create Consent.dateTime"); 3274 else if (Configuration.doAutoCreate()) 3275 this.dateTime = new DateTimeType(); // bb 3276 return this.dateTime; 3277 } 3278 3279 public boolean hasDateTimeElement() { 3280 return this.dateTime != null && !this.dateTime.isEmpty(); 3281 } 3282 3283 public boolean hasDateTime() { 3284 return this.dateTime != null && !this.dateTime.isEmpty(); 3285 } 3286 3287 /** 3288 * @param value {@link #dateTime} (When this Consent was issued / created / 3289 * indexed.). This is the underlying object with id, value and 3290 * extensions. The accessor "getDateTime" gives direct access to 3291 * the value 3292 */ 3293 public Consent setDateTimeElement(DateTimeType value) { 3294 this.dateTime = value; 3295 return this; 3296 } 3297 3298 /** 3299 * @return When this Consent was issued / created / indexed. 3300 */ 3301 public Date getDateTime() { 3302 return this.dateTime == null ? null : this.dateTime.getValue(); 3303 } 3304 3305 /** 3306 * @param value When this Consent was issued / created / indexed. 3307 */ 3308 public Consent setDateTime(Date value) { 3309 if (value == null) 3310 this.dateTime = null; 3311 else { 3312 if (this.dateTime == null) 3313 this.dateTime = new DateTimeType(); 3314 this.dateTime.setValue(value); 3315 } 3316 return this; 3317 } 3318 3319 /** 3320 * @return {@link #performer} (Either the Grantor, which is the entity 3321 * responsible for granting the rights listed in a Consent Directive or 3322 * the Grantee, which is the entity responsible for complying with the 3323 * Consent Directive, including any obligations or limitations on 3324 * authorizations and enforcement of prohibitions.) 3325 */ 3326 public List<Reference> getPerformer() { 3327 if (this.performer == null) 3328 this.performer = new ArrayList<Reference>(); 3329 return this.performer; 3330 } 3331 3332 /** 3333 * @return Returns a reference to <code>this</code> for easy method chaining 3334 */ 3335 public Consent setPerformer(List<Reference> thePerformer) { 3336 this.performer = thePerformer; 3337 return this; 3338 } 3339 3340 public boolean hasPerformer() { 3341 if (this.performer == null) 3342 return false; 3343 for (Reference item : this.performer) 3344 if (!item.isEmpty()) 3345 return true; 3346 return false; 3347 } 3348 3349 public Reference addPerformer() { // 3 3350 Reference t = new Reference(); 3351 if (this.performer == null) 3352 this.performer = new ArrayList<Reference>(); 3353 this.performer.add(t); 3354 return t; 3355 } 3356 3357 public Consent addPerformer(Reference t) { // 3 3358 if (t == null) 3359 return this; 3360 if (this.performer == null) 3361 this.performer = new ArrayList<Reference>(); 3362 this.performer.add(t); 3363 return this; 3364 } 3365 3366 /** 3367 * @return The first repetition of repeating field {@link #performer}, creating 3368 * it if it does not already exist 3369 */ 3370 public Reference getPerformerFirstRep() { 3371 if (getPerformer().isEmpty()) { 3372 addPerformer(); 3373 } 3374 return getPerformer().get(0); 3375 } 3376 3377 /** 3378 * @deprecated Use Reference#setResource(IBaseResource) instead 3379 */ 3380 @Deprecated 3381 public List<Resource> getPerformerTarget() { 3382 if (this.performerTarget == null) 3383 this.performerTarget = new ArrayList<Resource>(); 3384 return this.performerTarget; 3385 } 3386 3387 /** 3388 * @return {@link #organization} (The organization that manages the consent, and 3389 * the framework within which it is executed.) 3390 */ 3391 public List<Reference> getOrganization() { 3392 if (this.organization == null) 3393 this.organization = new ArrayList<Reference>(); 3394 return this.organization; 3395 } 3396 3397 /** 3398 * @return Returns a reference to <code>this</code> for easy method chaining 3399 */ 3400 public Consent setOrganization(List<Reference> theOrganization) { 3401 this.organization = theOrganization; 3402 return this; 3403 } 3404 3405 public boolean hasOrganization() { 3406 if (this.organization == null) 3407 return false; 3408 for (Reference item : this.organization) 3409 if (!item.isEmpty()) 3410 return true; 3411 return false; 3412 } 3413 3414 public Reference addOrganization() { // 3 3415 Reference t = new Reference(); 3416 if (this.organization == null) 3417 this.organization = new ArrayList<Reference>(); 3418 this.organization.add(t); 3419 return t; 3420 } 3421 3422 public Consent addOrganization(Reference t) { // 3 3423 if (t == null) 3424 return this; 3425 if (this.organization == null) 3426 this.organization = new ArrayList<Reference>(); 3427 this.organization.add(t); 3428 return this; 3429 } 3430 3431 /** 3432 * @return The first repetition of repeating field {@link #organization}, 3433 * creating it if it does not already exist 3434 */ 3435 public Reference getOrganizationFirstRep() { 3436 if (getOrganization().isEmpty()) { 3437 addOrganization(); 3438 } 3439 return getOrganization().get(0); 3440 } 3441 3442 /** 3443 * @deprecated Use Reference#setResource(IBaseResource) instead 3444 */ 3445 @Deprecated 3446 public List<Organization> getOrganizationTarget() { 3447 if (this.organizationTarget == null) 3448 this.organizationTarget = new ArrayList<Organization>(); 3449 return this.organizationTarget; 3450 } 3451 3452 /** 3453 * @deprecated Use Reference#setResource(IBaseResource) instead 3454 */ 3455 @Deprecated 3456 public Organization addOrganizationTarget() { 3457 Organization r = new Organization(); 3458 if (this.organizationTarget == null) 3459 this.organizationTarget = new ArrayList<Organization>(); 3460 this.organizationTarget.add(r); 3461 return r; 3462 } 3463 3464 /** 3465 * @return {@link #source} (The source on which this consent statement is based. 3466 * The source might be a scanned original paper form, or a reference to 3467 * a consent that links back to such a source, a reference to a document 3468 * repository (e.g. XDS) that stores the original consent document.) 3469 */ 3470 public Type getSource() { 3471 return this.source; 3472 } 3473 3474 /** 3475 * @return {@link #source} (The source on which this consent statement is based. 3476 * The source might be a scanned original paper form, or a reference to 3477 * a consent that links back to such a source, a reference to a document 3478 * repository (e.g. XDS) that stores the original consent document.) 3479 */ 3480 public Attachment getSourceAttachment() throws FHIRException { 3481 if (this.source == null) 3482 this.source = new Attachment(); 3483 if (!(this.source instanceof Attachment)) 3484 throw new FHIRException("Type mismatch: the type Attachment was expected, but " + this.source.getClass().getName() 3485 + " was encountered"); 3486 return (Attachment) this.source; 3487 } 3488 3489 public boolean hasSourceAttachment() { 3490 return this != null && this.source instanceof Attachment; 3491 } 3492 3493 /** 3494 * @return {@link #source} (The source on which this consent statement is based. 3495 * The source might be a scanned original paper form, or a reference to 3496 * a consent that links back to such a source, a reference to a document 3497 * repository (e.g. XDS) that stores the original consent document.) 3498 */ 3499 public Reference getSourceReference() throws FHIRException { 3500 if (this.source == null) 3501 this.source = new Reference(); 3502 if (!(this.source instanceof Reference)) 3503 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.source.getClass().getName() 3504 + " was encountered"); 3505 return (Reference) this.source; 3506 } 3507 3508 public boolean hasSourceReference() { 3509 return this != null && this.source instanceof Reference; 3510 } 3511 3512 public boolean hasSource() { 3513 return this.source != null && !this.source.isEmpty(); 3514 } 3515 3516 /** 3517 * @param value {@link #source} (The source on which this consent statement is 3518 * based. The source might be a scanned original paper form, or a 3519 * reference to a consent that links back to such a source, a 3520 * reference to a document repository (e.g. XDS) that stores the 3521 * original consent document.) 3522 */ 3523 public Consent setSource(Type value) { 3524 if (value != null && !(value instanceof Attachment || value instanceof Reference)) 3525 throw new Error("Not the right type for Consent.source[x]: " + value.fhirType()); 3526 this.source = value; 3527 return this; 3528 } 3529 3530 /** 3531 * @return {@link #policy} (The references to the policies that are included in 3532 * this consent scope. Policies may be organizational, but are often 3533 * defined jurisdictionally, or in law.) 3534 */ 3535 public List<ConsentPolicyComponent> getPolicy() { 3536 if (this.policy == null) 3537 this.policy = new ArrayList<ConsentPolicyComponent>(); 3538 return this.policy; 3539 } 3540 3541 /** 3542 * @return Returns a reference to <code>this</code> for easy method chaining 3543 */ 3544 public Consent setPolicy(List<ConsentPolicyComponent> thePolicy) { 3545 this.policy = thePolicy; 3546 return this; 3547 } 3548 3549 public boolean hasPolicy() { 3550 if (this.policy == null) 3551 return false; 3552 for (ConsentPolicyComponent item : this.policy) 3553 if (!item.isEmpty()) 3554 return true; 3555 return false; 3556 } 3557 3558 public ConsentPolicyComponent addPolicy() { // 3 3559 ConsentPolicyComponent t = new ConsentPolicyComponent(); 3560 if (this.policy == null) 3561 this.policy = new ArrayList<ConsentPolicyComponent>(); 3562 this.policy.add(t); 3563 return t; 3564 } 3565 3566 public Consent addPolicy(ConsentPolicyComponent t) { // 3 3567 if (t == null) 3568 return this; 3569 if (this.policy == null) 3570 this.policy = new ArrayList<ConsentPolicyComponent>(); 3571 this.policy.add(t); 3572 return this; 3573 } 3574 3575 /** 3576 * @return The first repetition of repeating field {@link #policy}, creating it 3577 * if it does not already exist 3578 */ 3579 public ConsentPolicyComponent getPolicyFirstRep() { 3580 if (getPolicy().isEmpty()) { 3581 addPolicy(); 3582 } 3583 return getPolicy().get(0); 3584 } 3585 3586 /** 3587 * @return {@link #policyRule} (A reference to the specific base computable 3588 * regulation or policy.) 3589 */ 3590 public CodeableConcept getPolicyRule() { 3591 if (this.policyRule == null) 3592 if (Configuration.errorOnAutoCreate()) 3593 throw new Error("Attempt to auto-create Consent.policyRule"); 3594 else if (Configuration.doAutoCreate()) 3595 this.policyRule = new CodeableConcept(); // cc 3596 return this.policyRule; 3597 } 3598 3599 public boolean hasPolicyRule() { 3600 return this.policyRule != null && !this.policyRule.isEmpty(); 3601 } 3602 3603 /** 3604 * @param value {@link #policyRule} (A reference to the specific base computable 3605 * regulation or policy.) 3606 */ 3607 public Consent setPolicyRule(CodeableConcept value) { 3608 this.policyRule = value; 3609 return this; 3610 } 3611 3612 /** 3613 * @return {@link #verification} (Whether a treatment instruction (e.g. 3614 * artificial respiration yes or no) was verified with the patient, 3615 * his/her family or another authorized person.) 3616 */ 3617 public List<ConsentVerificationComponent> getVerification() { 3618 if (this.verification == null) 3619 this.verification = new ArrayList<ConsentVerificationComponent>(); 3620 return this.verification; 3621 } 3622 3623 /** 3624 * @return Returns a reference to <code>this</code> for easy method chaining 3625 */ 3626 public Consent setVerification(List<ConsentVerificationComponent> theVerification) { 3627 this.verification = theVerification; 3628 return this; 3629 } 3630 3631 public boolean hasVerification() { 3632 if (this.verification == null) 3633 return false; 3634 for (ConsentVerificationComponent item : this.verification) 3635 if (!item.isEmpty()) 3636 return true; 3637 return false; 3638 } 3639 3640 public ConsentVerificationComponent addVerification() { // 3 3641 ConsentVerificationComponent t = new ConsentVerificationComponent(); 3642 if (this.verification == null) 3643 this.verification = new ArrayList<ConsentVerificationComponent>(); 3644 this.verification.add(t); 3645 return t; 3646 } 3647 3648 public Consent addVerification(ConsentVerificationComponent t) { // 3 3649 if (t == null) 3650 return this; 3651 if (this.verification == null) 3652 this.verification = new ArrayList<ConsentVerificationComponent>(); 3653 this.verification.add(t); 3654 return this; 3655 } 3656 3657 /** 3658 * @return The first repetition of repeating field {@link #verification}, 3659 * creating it if it does not already exist 3660 */ 3661 public ConsentVerificationComponent getVerificationFirstRep() { 3662 if (getVerification().isEmpty()) { 3663 addVerification(); 3664 } 3665 return getVerification().get(0); 3666 } 3667 3668 /** 3669 * @return {@link #provision} (An exception to the base policy of this consent. 3670 * An exception can be an addition or removal of access permissions.) 3671 */ 3672 public ProvisionComponent getProvision() { 3673 if (this.provision == null) 3674 if (Configuration.errorOnAutoCreate()) 3675 throw new Error("Attempt to auto-create Consent.provision"); 3676 else if (Configuration.doAutoCreate()) 3677 this.provision = new ProvisionComponent(); // cc 3678 return this.provision; 3679 } 3680 3681 public boolean hasProvision() { 3682 return this.provision != null && !this.provision.isEmpty(); 3683 } 3684 3685 /** 3686 * @param value {@link #provision} (An exception to the base policy of this 3687 * consent. An exception can be an addition or removal of access 3688 * permissions.) 3689 */ 3690 public Consent setProvision(ProvisionComponent value) { 3691 this.provision = value; 3692 return this; 3693 } 3694 3695 protected void listChildren(List<Property> children) { 3696 super.listChildren(children); 3697 children.add(new Property("identifier", "Identifier", "Unique identifier for this copy of the Consent Statement.", 3698 0, java.lang.Integer.MAX_VALUE, identifier)); 3699 children.add(new Property("status", "code", "Indicates the current state of this consent.", 0, 1, status)); 3700 children.add(new Property("scope", "CodeableConcept", 3701 "A selector of the type of consent being presented: ADR, Privacy, Treatment, Research. This list is now extensible.", 3702 0, 1, scope)); 3703 children.add(new Property("category", "CodeableConcept", 3704 "A classification of the type of consents found in the statement. This element supports indexing and retrieval of consent statements.", 3705 0, java.lang.Integer.MAX_VALUE, category)); 3706 children.add(new Property("patient", "Reference(Patient)", 3707 "The patient/healthcare consumer to whom this consent applies.", 0, 1, patient)); 3708 children.add( 3709 new Property("dateTime", "dateTime", "When this Consent was issued / created / indexed.", 0, 1, dateTime)); 3710 children.add(new Property("performer", 3711 "Reference(Organization|Patient|Practitioner|RelatedPerson|PractitionerRole)", 3712 "Either the Grantor, which is the entity responsible for granting the rights listed in a Consent Directive or the Grantee, which is the entity responsible for complying with the Consent Directive, including any obligations or limitations on authorizations and enforcement of prohibitions.", 3713 0, java.lang.Integer.MAX_VALUE, performer)); 3714 children.add(new Property("organization", "Reference(Organization)", 3715 "The organization that manages the consent, and the framework within which it is executed.", 0, 3716 java.lang.Integer.MAX_VALUE, organization)); 3717 children.add(new Property("source[x]", 3718 "Attachment|Reference(Consent|DocumentReference|Contract|QuestionnaireResponse)", 3719 "The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.", 3720 0, 1, source)); 3721 children.add(new Property("policy", "", 3722 "The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law.", 3723 0, java.lang.Integer.MAX_VALUE, policy)); 3724 children.add(new Property("policyRule", "CodeableConcept", 3725 "A reference to the specific base computable regulation or policy.", 0, 1, policyRule)); 3726 children.add(new Property("verification", "", 3727 "Whether a treatment instruction (e.g. artificial respiration yes or no) was verified with the patient, his/her family or another authorized person.", 3728 0, java.lang.Integer.MAX_VALUE, verification)); 3729 children.add(new Property("provision", "", 3730 "An exception to the base policy of this consent. An exception can be an addition or removal of access permissions.", 3731 0, 1, provision)); 3732 } 3733 3734 @Override 3735 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3736 switch (_hash) { 3737 case -1618432855: 3738 /* identifier */ return new Property("identifier", "Identifier", 3739 "Unique identifier for this copy of the Consent Statement.", 0, java.lang.Integer.MAX_VALUE, identifier); 3740 case -892481550: 3741 /* status */ return new Property("status", "code", "Indicates the current state of this consent.", 0, 1, status); 3742 case 109264468: 3743 /* scope */ return new Property("scope", "CodeableConcept", 3744 "A selector of the type of consent being presented: ADR, Privacy, Treatment, Research. This list is now extensible.", 3745 0, 1, scope); 3746 case 50511102: 3747 /* category */ return new Property("category", "CodeableConcept", 3748 "A classification of the type of consents found in the statement. This element supports indexing and retrieval of consent statements.", 3749 0, java.lang.Integer.MAX_VALUE, category); 3750 case -791418107: 3751 /* patient */ return new Property("patient", "Reference(Patient)", 3752 "The patient/healthcare consumer to whom this consent applies.", 0, 1, patient); 3753 case 1792749467: 3754 /* dateTime */ return new Property("dateTime", "dateTime", "When this Consent was issued / created / indexed.", 3755 0, 1, dateTime); 3756 case 481140686: 3757 /* performer */ return new Property("performer", 3758 "Reference(Organization|Patient|Practitioner|RelatedPerson|PractitionerRole)", 3759 "Either the Grantor, which is the entity responsible for granting the rights listed in a Consent Directive or the Grantee, which is the entity responsible for complying with the Consent Directive, including any obligations or limitations on authorizations and enforcement of prohibitions.", 3760 0, java.lang.Integer.MAX_VALUE, performer); 3761 case 1178922291: 3762 /* organization */ return new Property("organization", "Reference(Organization)", 3763 "The organization that manages the consent, and the framework within which it is executed.", 0, 3764 java.lang.Integer.MAX_VALUE, organization); 3765 case -1698413947: 3766 /* source[x] */ return new Property("source[x]", 3767 "Attachment|Reference(Consent|DocumentReference|Contract|QuestionnaireResponse)", 3768 "The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.", 3769 0, 1, source); 3770 case -896505829: 3771 /* source */ return new Property("source[x]", 3772 "Attachment|Reference(Consent|DocumentReference|Contract|QuestionnaireResponse)", 3773 "The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.", 3774 0, 1, source); 3775 case 1964406686: 3776 /* sourceAttachment */ return new Property("source[x]", 3777 "Attachment|Reference(Consent|DocumentReference|Contract|QuestionnaireResponse)", 3778 "The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.", 3779 0, 1, source); 3780 case -244259472: 3781 /* sourceReference */ return new Property("source[x]", 3782 "Attachment|Reference(Consent|DocumentReference|Contract|QuestionnaireResponse)", 3783 "The source on which this consent statement is based. The source might be a scanned original paper form, or a reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.", 3784 0, 1, source); 3785 case -982670030: 3786 /* policy */ return new Property("policy", "", 3787 "The references to the policies that are included in this consent scope. Policies may be organizational, but are often defined jurisdictionally, or in law.", 3788 0, java.lang.Integer.MAX_VALUE, policy); 3789 case 1593493326: 3790 /* policyRule */ return new Property("policyRule", "CodeableConcept", 3791 "A reference to the specific base computable regulation or policy.", 0, 1, policyRule); 3792 case -1484401125: 3793 /* verification */ return new Property("verification", "", 3794 "Whether a treatment instruction (e.g. artificial respiration yes or no) was verified with the patient, his/her family or another authorized person.", 3795 0, java.lang.Integer.MAX_VALUE, verification); 3796 case -547120939: 3797 /* provision */ return new Property("provision", "", 3798 "An exception to the base policy of this consent. An exception can be an addition or removal of access permissions.", 3799 0, 1, provision); 3800 default: 3801 return super.getNamedProperty(_hash, _name, _checkValid); 3802 } 3803 3804 } 3805 3806 @Override 3807 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3808 switch (hash) { 3809 case -1618432855: 3810 /* identifier */ return this.identifier == null ? new Base[0] 3811 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3812 case -892481550: 3813 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<ConsentState> 3814 case 109264468: 3815 /* scope */ return this.scope == null ? new Base[0] : new Base[] { this.scope }; // CodeableConcept 3816 case 50511102: 3817 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 3818 case -791418107: 3819 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 3820 case 1792749467: 3821 /* dateTime */ return this.dateTime == null ? new Base[0] : new Base[] { this.dateTime }; // DateTimeType 3822 case 481140686: 3823 /* performer */ return this.performer == null ? new Base[0] 3824 : this.performer.toArray(new Base[this.performer.size()]); // Reference 3825 case 1178922291: 3826 /* organization */ return this.organization == null ? new Base[0] 3827 : this.organization.toArray(new Base[this.organization.size()]); // Reference 3828 case -896505829: 3829 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // Type 3830 case -982670030: 3831 /* policy */ return this.policy == null ? new Base[0] : this.policy.toArray(new Base[this.policy.size()]); // ConsentPolicyComponent 3832 case 1593493326: 3833 /* policyRule */ return this.policyRule == null ? new Base[0] : new Base[] { this.policyRule }; // CodeableConcept 3834 case -1484401125: 3835 /* verification */ return this.verification == null ? new Base[0] 3836 : this.verification.toArray(new Base[this.verification.size()]); // ConsentVerificationComponent 3837 case -547120939: 3838 /* provision */ return this.provision == null ? new Base[0] : new Base[] { this.provision }; // provisionComponent 3839 default: 3840 return super.getProperty(hash, name, checkValid); 3841 } 3842 3843 } 3844 3845 @Override 3846 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3847 switch (hash) { 3848 case -1618432855: // identifier 3849 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3850 return value; 3851 case -892481550: // status 3852 value = new ConsentStateEnumFactory().fromType(castToCode(value)); 3853 this.status = (Enumeration) value; // Enumeration<ConsentState> 3854 return value; 3855 case 109264468: // scope 3856 this.scope = castToCodeableConcept(value); // CodeableConcept 3857 return value; 3858 case 50511102: // category 3859 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 3860 return value; 3861 case -791418107: // patient 3862 this.patient = castToReference(value); // Reference 3863 return value; 3864 case 1792749467: // dateTime 3865 this.dateTime = castToDateTime(value); // DateTimeType 3866 return value; 3867 case 481140686: // performer 3868 this.getPerformer().add(castToReference(value)); // Reference 3869 return value; 3870 case 1178922291: // organization 3871 this.getOrganization().add(castToReference(value)); // Reference 3872 return value; 3873 case -896505829: // source 3874 this.source = castToType(value); // Type 3875 return value; 3876 case -982670030: // policy 3877 this.getPolicy().add((ConsentPolicyComponent) value); // ConsentPolicyComponent 3878 return value; 3879 case 1593493326: // policyRule 3880 this.policyRule = castToCodeableConcept(value); // CodeableConcept 3881 return value; 3882 case -1484401125: // verification 3883 this.getVerification().add((ConsentVerificationComponent) value); // ConsentVerificationComponent 3884 return value; 3885 case -547120939: // provision 3886 this.provision = (ProvisionComponent) value; // provisionComponent 3887 return value; 3888 default: 3889 return super.setProperty(hash, name, value); 3890 } 3891 3892 } 3893 3894 @Override 3895 public Base setProperty(String name, Base value) throws FHIRException { 3896 if (name.equals("identifier")) { 3897 this.getIdentifier().add(castToIdentifier(value)); 3898 } else if (name.equals("status")) { 3899 value = new ConsentStateEnumFactory().fromType(castToCode(value)); 3900 this.status = (Enumeration) value; // Enumeration<ConsentState> 3901 } else if (name.equals("scope")) { 3902 this.scope = castToCodeableConcept(value); // CodeableConcept 3903 } else if (name.equals("category")) { 3904 this.getCategory().add(castToCodeableConcept(value)); 3905 } else if (name.equals("patient")) { 3906 this.patient = castToReference(value); // Reference 3907 } else if (name.equals("dateTime")) { 3908 this.dateTime = castToDateTime(value); // DateTimeType 3909 } else if (name.equals("performer")) { 3910 this.getPerformer().add(castToReference(value)); 3911 } else if (name.equals("organization")) { 3912 this.getOrganization().add(castToReference(value)); 3913 } else if (name.equals("source[x]")) { 3914 this.source = castToType(value); // Type 3915 } else if (name.equals("policy")) { 3916 this.getPolicy().add((ConsentPolicyComponent) value); 3917 } else if (name.equals("policyRule")) { 3918 this.policyRule = castToCodeableConcept(value); // CodeableConcept 3919 } else if (name.equals("verification")) { 3920 this.getVerification().add((ConsentVerificationComponent) value); 3921 } else if (name.equals("provision")) { 3922 this.provision = (ProvisionComponent) value; // provisionComponent 3923 } else 3924 return super.setProperty(name, value); 3925 return value; 3926 } 3927 3928 @Override 3929 public void removeChild(String name, Base value) throws FHIRException { 3930 if (name.equals("identifier")) { 3931 this.getIdentifier().remove(castToIdentifier(value)); 3932 } else if (name.equals("status")) { 3933 this.status = null; 3934 } else if (name.equals("scope")) { 3935 this.scope = null; 3936 } else if (name.equals("category")) { 3937 this.getCategory().remove(castToCodeableConcept(value)); 3938 } else if (name.equals("patient")) { 3939 this.patient = null; 3940 } else if (name.equals("dateTime")) { 3941 this.dateTime = null; 3942 } else if (name.equals("performer")) { 3943 this.getPerformer().remove(castToReference(value)); 3944 } else if (name.equals("organization")) { 3945 this.getOrganization().remove(castToReference(value)); 3946 } else if (name.equals("source[x]")) { 3947 this.source = null; 3948 } else if (name.equals("policy")) { 3949 this.getPolicy().remove((ConsentPolicyComponent) value); 3950 } else if (name.equals("policyRule")) { 3951 this.policyRule = null; 3952 } else if (name.equals("verification")) { 3953 this.getVerification().remove((ConsentVerificationComponent) value); 3954 } else if (name.equals("provision")) { 3955 this.provision = (ProvisionComponent) value; // provisionComponent 3956 } else 3957 super.removeChild(name, value); 3958 3959 } 3960 3961 @Override 3962 public Base makeProperty(int hash, String name) throws FHIRException { 3963 switch (hash) { 3964 case -1618432855: 3965 return addIdentifier(); 3966 case -892481550: 3967 return getStatusElement(); 3968 case 109264468: 3969 return getScope(); 3970 case 50511102: 3971 return addCategory(); 3972 case -791418107: 3973 return getPatient(); 3974 case 1792749467: 3975 return getDateTimeElement(); 3976 case 481140686: 3977 return addPerformer(); 3978 case 1178922291: 3979 return addOrganization(); 3980 case -1698413947: 3981 return getSource(); 3982 case -896505829: 3983 return getSource(); 3984 case -982670030: 3985 return addPolicy(); 3986 case 1593493326: 3987 return getPolicyRule(); 3988 case -1484401125: 3989 return addVerification(); 3990 case -547120939: 3991 return getProvision(); 3992 default: 3993 return super.makeProperty(hash, name); 3994 } 3995 3996 } 3997 3998 @Override 3999 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4000 switch (hash) { 4001 case -1618432855: 4002 /* identifier */ return new String[] { "Identifier" }; 4003 case -892481550: 4004 /* status */ return new String[] { "code" }; 4005 case 109264468: 4006 /* scope */ return new String[] { "CodeableConcept" }; 4007 case 50511102: 4008 /* category */ return new String[] { "CodeableConcept" }; 4009 case -791418107: 4010 /* patient */ return new String[] { "Reference" }; 4011 case 1792749467: 4012 /* dateTime */ return new String[] { "dateTime" }; 4013 case 481140686: 4014 /* performer */ return new String[] { "Reference" }; 4015 case 1178922291: 4016 /* organization */ return new String[] { "Reference" }; 4017 case -896505829: 4018 /* source */ return new String[] { "Attachment", "Reference" }; 4019 case -982670030: 4020 /* policy */ return new String[] {}; 4021 case 1593493326: 4022 /* policyRule */ return new String[] { "CodeableConcept" }; 4023 case -1484401125: 4024 /* verification */ return new String[] {}; 4025 case -547120939: 4026 /* provision */ return new String[] {}; 4027 default: 4028 return super.getTypesForProperty(hash, name); 4029 } 4030 4031 } 4032 4033 @Override 4034 public Base addChild(String name) throws FHIRException { 4035 if (name.equals("identifier")) { 4036 return addIdentifier(); 4037 } else if (name.equals("status")) { 4038 throw new FHIRException("Cannot call addChild on a singleton property Consent.status"); 4039 } else if (name.equals("scope")) { 4040 this.scope = new CodeableConcept(); 4041 return this.scope; 4042 } else if (name.equals("category")) { 4043 return addCategory(); 4044 } else if (name.equals("patient")) { 4045 this.patient = new Reference(); 4046 return this.patient; 4047 } else if (name.equals("dateTime")) { 4048 throw new FHIRException("Cannot call addChild on a singleton property Consent.dateTime"); 4049 } else if (name.equals("performer")) { 4050 return addPerformer(); 4051 } else if (name.equals("organization")) { 4052 return addOrganization(); 4053 } else if (name.equals("sourceAttachment")) { 4054 this.source = new Attachment(); 4055 return this.source; 4056 } else if (name.equals("sourceReference")) { 4057 this.source = new Reference(); 4058 return this.source; 4059 } else if (name.equals("policy")) { 4060 return addPolicy(); 4061 } else if (name.equals("policyRule")) { 4062 this.policyRule = new CodeableConcept(); 4063 return this.policyRule; 4064 } else if (name.equals("verification")) { 4065 return addVerification(); 4066 } else if (name.equals("provision")) { 4067 this.provision = new ProvisionComponent(); 4068 return this.provision; 4069 } else 4070 return super.addChild(name); 4071 } 4072 4073 public String fhirType() { 4074 return "Consent"; 4075 4076 } 4077 4078 public Consent copy() { 4079 Consent dst = new Consent(); 4080 copyValues(dst); 4081 return dst; 4082 } 4083 4084 public void copyValues(Consent dst) { 4085 super.copyValues(dst); 4086 if (identifier != null) { 4087 dst.identifier = new ArrayList<Identifier>(); 4088 for (Identifier i : identifier) 4089 dst.identifier.add(i.copy()); 4090 } 4091 ; 4092 dst.status = status == null ? null : status.copy(); 4093 dst.scope = scope == null ? null : scope.copy(); 4094 if (category != null) { 4095 dst.category = new ArrayList<CodeableConcept>(); 4096 for (CodeableConcept i : category) 4097 dst.category.add(i.copy()); 4098 } 4099 ; 4100 dst.patient = patient == null ? null : patient.copy(); 4101 dst.dateTime = dateTime == null ? null : dateTime.copy(); 4102 if (performer != null) { 4103 dst.performer = new ArrayList<Reference>(); 4104 for (Reference i : performer) 4105 dst.performer.add(i.copy()); 4106 } 4107 ; 4108 if (organization != null) { 4109 dst.organization = new ArrayList<Reference>(); 4110 for (Reference i : organization) 4111 dst.organization.add(i.copy()); 4112 } 4113 ; 4114 dst.source = source == null ? null : source.copy(); 4115 if (policy != null) { 4116 dst.policy = new ArrayList<ConsentPolicyComponent>(); 4117 for (ConsentPolicyComponent i : policy) 4118 dst.policy.add(i.copy()); 4119 } 4120 ; 4121 dst.policyRule = policyRule == null ? null : policyRule.copy(); 4122 if (verification != null) { 4123 dst.verification = new ArrayList<ConsentVerificationComponent>(); 4124 for (ConsentVerificationComponent i : verification) 4125 dst.verification.add(i.copy()); 4126 } 4127 ; 4128 dst.provision = provision == null ? null : provision.copy(); 4129 } 4130 4131 protected Consent typedCopy() { 4132 return copy(); 4133 } 4134 4135 @Override 4136 public boolean equalsDeep(Base other_) { 4137 if (!super.equalsDeep(other_)) 4138 return false; 4139 if (!(other_ instanceof Consent)) 4140 return false; 4141 Consent o = (Consent) other_; 4142 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 4143 && compareDeep(scope, o.scope, true) && compareDeep(category, o.category, true) 4144 && compareDeep(patient, o.patient, true) && compareDeep(dateTime, o.dateTime, true) 4145 && compareDeep(performer, o.performer, true) && compareDeep(organization, o.organization, true) 4146 && compareDeep(source, o.source, true) && compareDeep(policy, o.policy, true) 4147 && compareDeep(policyRule, o.policyRule, true) && compareDeep(verification, o.verification, true) 4148 && compareDeep(provision, o.provision, true); 4149 } 4150 4151 @Override 4152 public boolean equalsShallow(Base other_) { 4153 if (!super.equalsShallow(other_)) 4154 return false; 4155 if (!(other_ instanceof Consent)) 4156 return false; 4157 Consent o = (Consent) other_; 4158 return compareValues(status, o.status, true) && compareValues(dateTime, o.dateTime, true); 4159 } 4160 4161 public boolean isEmpty() { 4162 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, scope, category, patient, 4163 dateTime, performer, organization, source, policy, policyRule, verification, provision); 4164 } 4165 4166 @Override 4167 public ResourceType getResourceType() { 4168 return ResourceType.Consent; 4169 } 4170 4171 /** 4172 * Search parameter: <b>date</b> 4173 * <p> 4174 * Description: <b>When this Consent was created or indexed</b><br> 4175 * Type: <b>date</b><br> 4176 * Path: <b>Consent.dateTime</b><br> 4177 * </p> 4178 */ 4179 @SearchParamDefinition(name = "date", path = "Consent.dateTime", description = "When this Consent was created or indexed", type = "date") 4180 public static final String SP_DATE = "date"; 4181 /** 4182 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4183 * <p> 4184 * Description: <b>When this Consent was created or indexed</b><br> 4185 * Type: <b>date</b><br> 4186 * Path: <b>Consent.dateTime</b><br> 4187 * </p> 4188 */ 4189 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 4190 SP_DATE); 4191 4192 /** 4193 * Search parameter: <b>identifier</b> 4194 * <p> 4195 * Description: <b>Identifier for this record (external references)</b><br> 4196 * Type: <b>token</b><br> 4197 * Path: <b>Consent.identifier</b><br> 4198 * </p> 4199 */ 4200 @SearchParamDefinition(name = "identifier", path = "Consent.identifier", description = "Identifier for this record (external references)", type = "token") 4201 public static final String SP_IDENTIFIER = "identifier"; 4202 /** 4203 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4204 * <p> 4205 * Description: <b>Identifier for this record (external references)</b><br> 4206 * Type: <b>token</b><br> 4207 * Path: <b>Consent.identifier</b><br> 4208 * </p> 4209 */ 4210 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4211 SP_IDENTIFIER); 4212 4213 /** 4214 * Search parameter: <b>period</b> 4215 * <p> 4216 * Description: <b>Timeframe for this rule</b><br> 4217 * Type: <b>date</b><br> 4218 * Path: <b>Consent.provision.period</b><br> 4219 * </p> 4220 */ 4221 @SearchParamDefinition(name = "period", path = "Consent.provision.period", description = "Timeframe for this rule", type = "date") 4222 public static final String SP_PERIOD = "period"; 4223 /** 4224 * <b>Fluent Client</b> search parameter constant for <b>period</b> 4225 * <p> 4226 * Description: <b>Timeframe for this rule</b><br> 4227 * Type: <b>date</b><br> 4228 * Path: <b>Consent.provision.period</b><br> 4229 * </p> 4230 */ 4231 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam( 4232 SP_PERIOD); 4233 4234 /** 4235 * Search parameter: <b>data</b> 4236 * <p> 4237 * Description: <b>The actual data reference</b><br> 4238 * Type: <b>reference</b><br> 4239 * Path: <b>Consent.provision.data.reference</b><br> 4240 * </p> 4241 */ 4242 @SearchParamDefinition(name = "data", path = "Consent.provision.data.reference", description = "The actual data reference", type = "reference") 4243 public static final String SP_DATA = "data"; 4244 /** 4245 * <b>Fluent Client</b> search parameter constant for <b>data</b> 4246 * <p> 4247 * Description: <b>The actual data reference</b><br> 4248 * Type: <b>reference</b><br> 4249 * Path: <b>Consent.provision.data.reference</b><br> 4250 * </p> 4251 */ 4252 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DATA = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4253 SP_DATA); 4254 4255 /** 4256 * Constant for fluent queries to be used to add include statements. Specifies 4257 * the path value of "<b>Consent:data</b>". 4258 */ 4259 public static final ca.uhn.fhir.model.api.Include INCLUDE_DATA = new ca.uhn.fhir.model.api.Include("Consent:data") 4260 .toLocked(); 4261 4262 /** 4263 * Search parameter: <b>purpose</b> 4264 * <p> 4265 * Description: <b>Context of activities covered by this rule</b><br> 4266 * Type: <b>token</b><br> 4267 * Path: <b>Consent.provision.purpose</b><br> 4268 * </p> 4269 */ 4270 @SearchParamDefinition(name = "purpose", path = "Consent.provision.purpose", description = "Context of activities covered by this rule", type = "token") 4271 public static final String SP_PURPOSE = "purpose"; 4272 /** 4273 * <b>Fluent Client</b> search parameter constant for <b>purpose</b> 4274 * <p> 4275 * Description: <b>Context of activities covered by this rule</b><br> 4276 * Type: <b>token</b><br> 4277 * Path: <b>Consent.provision.purpose</b><br> 4278 * </p> 4279 */ 4280 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PURPOSE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4281 SP_PURPOSE); 4282 4283 /** 4284 * Search parameter: <b>source-reference</b> 4285 * <p> 4286 * Description: <b>Search by reference to a Consent, DocumentReference, Contract 4287 * or QuestionnaireResponse</b><br> 4288 * Type: <b>reference</b><br> 4289 * Path: <b>Consent.source[x]</b><br> 4290 * </p> 4291 */ 4292 @SearchParamDefinition(name = "source-reference", path = "Consent.source", description = "Search by reference to a Consent, DocumentReference, Contract or QuestionnaireResponse", type = "reference", target = { 4293 Consent.class, Contract.class, DocumentReference.class, QuestionnaireResponse.class }) 4294 public static final String SP_SOURCE_REFERENCE = "source-reference"; 4295 /** 4296 * <b>Fluent Client</b> search parameter constant for <b>source-reference</b> 4297 * <p> 4298 * Description: <b>Search by reference to a Consent, DocumentReference, Contract 4299 * or QuestionnaireResponse</b><br> 4300 * Type: <b>reference</b><br> 4301 * Path: <b>Consent.source[x]</b><br> 4302 * </p> 4303 */ 4304 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4305 SP_SOURCE_REFERENCE); 4306 4307 /** 4308 * Constant for fluent queries to be used to add include statements. Specifies 4309 * the path value of "<b>Consent:source-reference</b>". 4310 */ 4311 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE_REFERENCE = new ca.uhn.fhir.model.api.Include( 4312 "Consent:source-reference").toLocked(); 4313 4314 /** 4315 * Search parameter: <b>actor</b> 4316 * <p> 4317 * Description: <b>Resource for the actor (or group, by role)</b><br> 4318 * Type: <b>reference</b><br> 4319 * Path: <b>Consent.provision.actor.reference</b><br> 4320 * </p> 4321 */ 4322 @SearchParamDefinition(name = "actor", path = "Consent.provision.actor.reference", description = "Resource for the actor (or group, by role)", type = "reference", target = { 4323 CareTeam.class, Device.class, Group.class, Organization.class, Patient.class, Practitioner.class, 4324 PractitionerRole.class, RelatedPerson.class }) 4325 public static final String SP_ACTOR = "actor"; 4326 /** 4327 * <b>Fluent Client</b> search parameter constant for <b>actor</b> 4328 * <p> 4329 * Description: <b>Resource for the actor (or group, by role)</b><br> 4330 * Type: <b>reference</b><br> 4331 * Path: <b>Consent.provision.actor.reference</b><br> 4332 * </p> 4333 */ 4334 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4335 SP_ACTOR); 4336 4337 /** 4338 * Constant for fluent queries to be used to add include statements. Specifies 4339 * the path value of "<b>Consent:actor</b>". 4340 */ 4341 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACTOR = new ca.uhn.fhir.model.api.Include("Consent:actor") 4342 .toLocked(); 4343 4344 /** 4345 * Search parameter: <b>security-label</b> 4346 * <p> 4347 * Description: <b>Security Labels that define affected resources</b><br> 4348 * Type: <b>token</b><br> 4349 * Path: <b>Consent.provision.securityLabel</b><br> 4350 * </p> 4351 */ 4352 @SearchParamDefinition(name = "security-label", path = "Consent.provision.securityLabel", description = "Security Labels that define affected resources", type = "token") 4353 public static final String SP_SECURITY_LABEL = "security-label"; 4354 /** 4355 * <b>Fluent Client</b> search parameter constant for <b>security-label</b> 4356 * <p> 4357 * Description: <b>Security Labels that define affected resources</b><br> 4358 * Type: <b>token</b><br> 4359 * Path: <b>Consent.provision.securityLabel</b><br> 4360 * </p> 4361 */ 4362 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SECURITY_LABEL = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4363 SP_SECURITY_LABEL); 4364 4365 /** 4366 * Search parameter: <b>patient</b> 4367 * <p> 4368 * Description: <b>Who the consent applies to</b><br> 4369 * Type: <b>reference</b><br> 4370 * Path: <b>Consent.patient</b><br> 4371 * </p> 4372 */ 4373 @SearchParamDefinition(name = "patient", path = "Consent.patient", description = "Who the consent applies to", type = "reference", providesMembershipIn = { 4374 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 4375 public static final String SP_PATIENT = "patient"; 4376 /** 4377 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4378 * <p> 4379 * Description: <b>Who the consent applies to</b><br> 4380 * Type: <b>reference</b><br> 4381 * Path: <b>Consent.patient</b><br> 4382 * </p> 4383 */ 4384 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4385 SP_PATIENT); 4386 4387 /** 4388 * Constant for fluent queries to be used to add include statements. Specifies 4389 * the path value of "<b>Consent:patient</b>". 4390 */ 4391 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 4392 "Consent:patient").toLocked(); 4393 4394 /** 4395 * Search parameter: <b>organization</b> 4396 * <p> 4397 * Description: <b>Custodian of the consent</b><br> 4398 * Type: <b>reference</b><br> 4399 * Path: <b>Consent.organization</b><br> 4400 * </p> 4401 */ 4402 @SearchParamDefinition(name = "organization", path = "Consent.organization", description = "Custodian of the consent", type = "reference", target = { 4403 Organization.class }) 4404 public static final String SP_ORGANIZATION = "organization"; 4405 /** 4406 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 4407 * <p> 4408 * Description: <b>Custodian of the consent</b><br> 4409 * Type: <b>reference</b><br> 4410 * Path: <b>Consent.organization</b><br> 4411 * </p> 4412 */ 4413 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4414 SP_ORGANIZATION); 4415 4416 /** 4417 * Constant for fluent queries to be used to add include statements. Specifies 4418 * the path value of "<b>Consent:organization</b>". 4419 */ 4420 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include( 4421 "Consent:organization").toLocked(); 4422 4423 /** 4424 * Search parameter: <b>scope</b> 4425 * <p> 4426 * Description: <b>Which of the four areas this resource covers 4427 * (extensible)</b><br> 4428 * Type: <b>token</b><br> 4429 * Path: <b>Consent.scope</b><br> 4430 * </p> 4431 */ 4432 @SearchParamDefinition(name = "scope", path = "Consent.scope", description = "Which of the four areas this resource covers (extensible)", type = "token") 4433 public static final String SP_SCOPE = "scope"; 4434 /** 4435 * <b>Fluent Client</b> search parameter constant for <b>scope</b> 4436 * <p> 4437 * Description: <b>Which of the four areas this resource covers 4438 * (extensible)</b><br> 4439 * Type: <b>token</b><br> 4440 * Path: <b>Consent.scope</b><br> 4441 * </p> 4442 */ 4443 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SCOPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4444 SP_SCOPE); 4445 4446 /** 4447 * Search parameter: <b>action</b> 4448 * <p> 4449 * Description: <b>Actions controlled by this rule</b><br> 4450 * Type: <b>token</b><br> 4451 * Path: <b>Consent.provision.action</b><br> 4452 * </p> 4453 */ 4454 @SearchParamDefinition(name = "action", path = "Consent.provision.action", description = "Actions controlled by this rule", type = "token") 4455 public static final String SP_ACTION = "action"; 4456 /** 4457 * <b>Fluent Client</b> search parameter constant for <b>action</b> 4458 * <p> 4459 * Description: <b>Actions controlled by this rule</b><br> 4460 * Type: <b>token</b><br> 4461 * Path: <b>Consent.provision.action</b><br> 4462 * </p> 4463 */ 4464 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4465 SP_ACTION); 4466 4467 /** 4468 * Search parameter: <b>consentor</b> 4469 * <p> 4470 * Description: <b>Who is agreeing to the policy and rules</b><br> 4471 * Type: <b>reference</b><br> 4472 * Path: <b>Consent.performer</b><br> 4473 * </p> 4474 */ 4475 @SearchParamDefinition(name = "consentor", path = "Consent.performer", description = "Who is agreeing to the policy and rules", type = "reference", target = { 4476 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 4477 public static final String SP_CONSENTOR = "consentor"; 4478 /** 4479 * <b>Fluent Client</b> search parameter constant for <b>consentor</b> 4480 * <p> 4481 * Description: <b>Who is agreeing to the policy and rules</b><br> 4482 * Type: <b>reference</b><br> 4483 * Path: <b>Consent.performer</b><br> 4484 * </p> 4485 */ 4486 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONSENTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4487 SP_CONSENTOR); 4488 4489 /** 4490 * Constant for fluent queries to be used to add include statements. Specifies 4491 * the path value of "<b>Consent:consentor</b>". 4492 */ 4493 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONSENTOR = new ca.uhn.fhir.model.api.Include( 4494 "Consent:consentor").toLocked(); 4495 4496 /** 4497 * Search parameter: <b>category</b> 4498 * <p> 4499 * Description: <b>Classification of the consent statement - for 4500 * indexing/retrieval</b><br> 4501 * Type: <b>token</b><br> 4502 * Path: <b>Consent.category</b><br> 4503 * </p> 4504 */ 4505 @SearchParamDefinition(name = "category", path = "Consent.category", description = "Classification of the consent statement - for indexing/retrieval", type = "token") 4506 public static final String SP_CATEGORY = "category"; 4507 /** 4508 * <b>Fluent Client</b> search parameter constant for <b>category</b> 4509 * <p> 4510 * Description: <b>Classification of the consent statement - for 4511 * indexing/retrieval</b><br> 4512 * Type: <b>token</b><br> 4513 * Path: <b>Consent.category</b><br> 4514 * </p> 4515 */ 4516 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4517 SP_CATEGORY); 4518 4519 /** 4520 * Search parameter: <b>status</b> 4521 * <p> 4522 * Description: <b>draft | proposed | active | rejected | inactive | 4523 * entered-in-error</b><br> 4524 * Type: <b>token</b><br> 4525 * Path: <b>Consent.status</b><br> 4526 * </p> 4527 */ 4528 @SearchParamDefinition(name = "status", path = "Consent.status", description = "draft | proposed | active | rejected | inactive | entered-in-error", type = "token") 4529 public static final String SP_STATUS = "status"; 4530 /** 4531 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4532 * <p> 4533 * Description: <b>draft | proposed | active | rejected | inactive | 4534 * entered-in-error</b><br> 4535 * Type: <b>token</b><br> 4536 * Path: <b>Consent.status</b><br> 4537 * </p> 4538 */ 4539 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4540 SP_STATUS); 4541 4542}