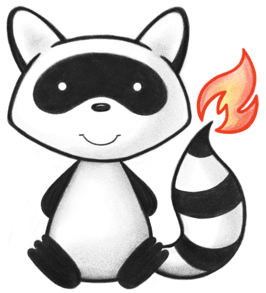
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.List; 034 035import org.hl7.fhir.exceptions.FHIRException; 036import org.hl7.fhir.instance.model.api.ICompositeType; 037import org.hl7.fhir.utilities.Utilities; 038 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.DatatypeDef; 041import ca.uhn.fhir.model.api.annotation.Description; 042 043/** 044 * Details for all kinds of technology mediated contact points for a person or 045 * organization, including telephone, email, etc. 046 */ 047@DatatypeDef(name = "ContactPoint") 048public class ContactPoint extends Type implements ICompositeType { 049 050 public enum ContactPointSystem { 051 /** 052 * The value is a telephone number used for voice calls. Use of full 053 * international numbers starting with + is recommended to enable automatic 054 * dialing support but not required. 055 */ 056 PHONE, 057 /** 058 * The value is a fax machine. Use of full international numbers starting with + 059 * is recommended to enable automatic dialing support but not required. 060 */ 061 FAX, 062 /** 063 * The value is an email address. 064 */ 065 EMAIL, 066 /** 067 * The value is a pager number. These may be local pager numbers that are only 068 * usable on a particular pager system. 069 */ 070 PAGER, 071 /** 072 * A contact that is not a phone, fax, pager or email address and is expressed 073 * as a URL. This is intended for various institutional or personal contacts 074 * including web sites, blogs, Skype, Twitter, Facebook, etc. Do not use for 075 * email addresses. 076 */ 077 URL, 078 /** 079 * A contact that can be used for sending an sms message (e.g. mobile phones, 080 * some landlines). 081 */ 082 SMS, 083 /** 084 * A contact that is not a phone, fax, page or email address and is not 085 * expressible as a URL. E.g. Internal mail address. This SHOULD NOT be used for 086 * contacts that are expressible as a URL (e.g. Skype, Twitter, Facebook, etc.) 087 * Extensions may be used to distinguish "other" contact types. 088 */ 089 OTHER, 090 /** 091 * added to help the parsers with the generic types 092 */ 093 NULL; 094 095 public static ContactPointSystem fromCode(String codeString) throws FHIRException { 096 if (codeString == null || "".equals(codeString)) 097 return null; 098 if ("phone".equals(codeString)) 099 return PHONE; 100 if ("fax".equals(codeString)) 101 return FAX; 102 if ("email".equals(codeString)) 103 return EMAIL; 104 if ("pager".equals(codeString)) 105 return PAGER; 106 if ("url".equals(codeString)) 107 return URL; 108 if ("sms".equals(codeString)) 109 return SMS; 110 if ("other".equals(codeString)) 111 return OTHER; 112 if (Configuration.isAcceptInvalidEnums()) 113 return null; 114 else 115 throw new FHIRException("Unknown ContactPointSystem code '" + codeString + "'"); 116 } 117 118 public String toCode() { 119 switch (this) { 120 case PHONE: 121 return "phone"; 122 case FAX: 123 return "fax"; 124 case EMAIL: 125 return "email"; 126 case PAGER: 127 return "pager"; 128 case URL: 129 return "url"; 130 case SMS: 131 return "sms"; 132 case OTHER: 133 return "other"; 134 case NULL: 135 return null; 136 default: 137 return "?"; 138 } 139 } 140 141 public String getSystem() { 142 switch (this) { 143 case PHONE: 144 return "http://hl7.org/fhir/contact-point-system"; 145 case FAX: 146 return "http://hl7.org/fhir/contact-point-system"; 147 case EMAIL: 148 return "http://hl7.org/fhir/contact-point-system"; 149 case PAGER: 150 return "http://hl7.org/fhir/contact-point-system"; 151 case URL: 152 return "http://hl7.org/fhir/contact-point-system"; 153 case SMS: 154 return "http://hl7.org/fhir/contact-point-system"; 155 case OTHER: 156 return "http://hl7.org/fhir/contact-point-system"; 157 case NULL: 158 return null; 159 default: 160 return "?"; 161 } 162 } 163 164 public String getDefinition() { 165 switch (this) { 166 case PHONE: 167 return "The value is a telephone number used for voice calls. Use of full international numbers starting with + is recommended to enable automatic dialing support but not required."; 168 case FAX: 169 return "The value is a fax machine. Use of full international numbers starting with + is recommended to enable automatic dialing support but not required."; 170 case EMAIL: 171 return "The value is an email address."; 172 case PAGER: 173 return "The value is a pager number. These may be local pager numbers that are only usable on a particular pager system."; 174 case URL: 175 return "A contact that is not a phone, fax, pager or email address and is expressed as a URL. This is intended for various institutional or personal contacts including web sites, blogs, Skype, Twitter, Facebook, etc. Do not use for email addresses."; 176 case SMS: 177 return "A contact that can be used for sending an sms message (e.g. mobile phones, some landlines)."; 178 case OTHER: 179 return "A contact that is not a phone, fax, page or email address and is not expressible as a URL. E.g. Internal mail address. This SHOULD NOT be used for contacts that are expressible as a URL (e.g. Skype, Twitter, Facebook, etc.) Extensions may be used to distinguish \"other\" contact types."; 180 case NULL: 181 return null; 182 default: 183 return "?"; 184 } 185 } 186 187 public String getDisplay() { 188 switch (this) { 189 case PHONE: 190 return "Phone"; 191 case FAX: 192 return "Fax"; 193 case EMAIL: 194 return "Email"; 195 case PAGER: 196 return "Pager"; 197 case URL: 198 return "URL"; 199 case SMS: 200 return "SMS"; 201 case OTHER: 202 return "Other"; 203 case NULL: 204 return null; 205 default: 206 return "?"; 207 } 208 } 209 } 210 211 public static class ContactPointSystemEnumFactory implements EnumFactory<ContactPointSystem> { 212 public ContactPointSystem fromCode(String codeString) throws IllegalArgumentException { 213 if (codeString == null || "".equals(codeString)) 214 if (codeString == null || "".equals(codeString)) 215 return null; 216 if ("phone".equals(codeString)) 217 return ContactPointSystem.PHONE; 218 if ("fax".equals(codeString)) 219 return ContactPointSystem.FAX; 220 if ("email".equals(codeString)) 221 return ContactPointSystem.EMAIL; 222 if ("pager".equals(codeString)) 223 return ContactPointSystem.PAGER; 224 if ("url".equals(codeString)) 225 return ContactPointSystem.URL; 226 if ("sms".equals(codeString)) 227 return ContactPointSystem.SMS; 228 if ("other".equals(codeString)) 229 return ContactPointSystem.OTHER; 230 throw new IllegalArgumentException("Unknown ContactPointSystem code '" + codeString + "'"); 231 } 232 233 public Enumeration<ContactPointSystem> fromType(PrimitiveType<?> code) throws FHIRException { 234 if (code == null) 235 return null; 236 if (code.isEmpty()) 237 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.NULL, code); 238 String codeString = code.asStringValue(); 239 if (codeString == null || "".equals(codeString)) 240 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.NULL, code); 241 if ("phone".equals(codeString)) 242 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.PHONE, code); 243 if ("fax".equals(codeString)) 244 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.FAX, code); 245 if ("email".equals(codeString)) 246 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.EMAIL, code); 247 if ("pager".equals(codeString)) 248 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.PAGER, code); 249 if ("url".equals(codeString)) 250 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.URL, code); 251 if ("sms".equals(codeString)) 252 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.SMS, code); 253 if ("other".equals(codeString)) 254 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.OTHER, code); 255 throw new FHIRException("Unknown ContactPointSystem code '" + codeString + "'"); 256 } 257 258 public String toCode(ContactPointSystem code) { 259 if (code == ContactPointSystem.NULL) 260 return null; 261 if (code == ContactPointSystem.PHONE) 262 return "phone"; 263 if (code == ContactPointSystem.FAX) 264 return "fax"; 265 if (code == ContactPointSystem.EMAIL) 266 return "email"; 267 if (code == ContactPointSystem.PAGER) 268 return "pager"; 269 if (code == ContactPointSystem.URL) 270 return "url"; 271 if (code == ContactPointSystem.SMS) 272 return "sms"; 273 if (code == ContactPointSystem.OTHER) 274 return "other"; 275 return "?"; 276 } 277 278 public String toSystem(ContactPointSystem code) { 279 return code.getSystem(); 280 } 281 } 282 283 public enum ContactPointUse { 284 /** 285 * A communication contact point at a home; attempted contacts for business 286 * purposes might intrude privacy and chances are one will contact family or 287 * other household members instead of the person one wishes to call. Typically 288 * used with urgent cases, or if no other contacts are available. 289 */ 290 HOME, 291 /** 292 * An office contact point. First choice for business related contacts during 293 * business hours. 294 */ 295 WORK, 296 /** 297 * A temporary contact point. The period can provide more detailed information. 298 */ 299 TEMP, 300 /** 301 * This contact point is no longer in use (or was never correct, but retained 302 * for records). 303 */ 304 OLD, 305 /** 306 * A telecommunication device that moves and stays with its owner. May have 307 * characteristics of all other use codes, suitable for urgent matters, not the 308 * first choice for routine business. 309 */ 310 MOBILE, 311 /** 312 * added to help the parsers with the generic types 313 */ 314 NULL; 315 316 public static ContactPointUse fromCode(String codeString) throws FHIRException { 317 if (codeString == null || "".equals(codeString)) 318 return null; 319 if ("home".equals(codeString)) 320 return HOME; 321 if ("work".equals(codeString)) 322 return WORK; 323 if ("temp".equals(codeString)) 324 return TEMP; 325 if ("old".equals(codeString)) 326 return OLD; 327 if ("mobile".equals(codeString)) 328 return MOBILE; 329 if (Configuration.isAcceptInvalidEnums()) 330 return null; 331 else 332 throw new FHIRException("Unknown ContactPointUse code '" + codeString + "'"); 333 } 334 335 public String toCode() { 336 switch (this) { 337 case HOME: 338 return "home"; 339 case WORK: 340 return "work"; 341 case TEMP: 342 return "temp"; 343 case OLD: 344 return "old"; 345 case MOBILE: 346 return "mobile"; 347 case NULL: 348 return null; 349 default: 350 return "?"; 351 } 352 } 353 354 public String getSystem() { 355 switch (this) { 356 case HOME: 357 return "http://hl7.org/fhir/contact-point-use"; 358 case WORK: 359 return "http://hl7.org/fhir/contact-point-use"; 360 case TEMP: 361 return "http://hl7.org/fhir/contact-point-use"; 362 case OLD: 363 return "http://hl7.org/fhir/contact-point-use"; 364 case MOBILE: 365 return "http://hl7.org/fhir/contact-point-use"; 366 case NULL: 367 return null; 368 default: 369 return "?"; 370 } 371 } 372 373 public String getDefinition() { 374 switch (this) { 375 case HOME: 376 return "A communication contact point at a home; attempted contacts for business purposes might intrude privacy and chances are one will contact family or other household members instead of the person one wishes to call. Typically used with urgent cases, or if no other contacts are available."; 377 case WORK: 378 return "An office contact point. First choice for business related contacts during business hours."; 379 case TEMP: 380 return "A temporary contact point. The period can provide more detailed information."; 381 case OLD: 382 return "This contact point is no longer in use (or was never correct, but retained for records)."; 383 case MOBILE: 384 return "A telecommunication device that moves and stays with its owner. May have characteristics of all other use codes, suitable for urgent matters, not the first choice for routine business."; 385 case NULL: 386 return null; 387 default: 388 return "?"; 389 } 390 } 391 392 public String getDisplay() { 393 switch (this) { 394 case HOME: 395 return "Home"; 396 case WORK: 397 return "Work"; 398 case TEMP: 399 return "Temp"; 400 case OLD: 401 return "Old"; 402 case MOBILE: 403 return "Mobile"; 404 case NULL: 405 return null; 406 default: 407 return "?"; 408 } 409 } 410 } 411 412 public static class ContactPointUseEnumFactory implements EnumFactory<ContactPointUse> { 413 public ContactPointUse fromCode(String codeString) throws IllegalArgumentException { 414 if (codeString == null || "".equals(codeString)) 415 if (codeString == null || "".equals(codeString)) 416 return null; 417 if ("home".equals(codeString)) 418 return ContactPointUse.HOME; 419 if ("work".equals(codeString)) 420 return ContactPointUse.WORK; 421 if ("temp".equals(codeString)) 422 return ContactPointUse.TEMP; 423 if ("old".equals(codeString)) 424 return ContactPointUse.OLD; 425 if ("mobile".equals(codeString)) 426 return ContactPointUse.MOBILE; 427 throw new IllegalArgumentException("Unknown ContactPointUse code '" + codeString + "'"); 428 } 429 430 public Enumeration<ContactPointUse> fromType(PrimitiveType<?> code) throws FHIRException { 431 if (code == null) 432 return null; 433 if (code.isEmpty()) 434 return new Enumeration<ContactPointUse>(this, ContactPointUse.NULL, code); 435 String codeString = code.asStringValue(); 436 if (codeString == null || "".equals(codeString)) 437 return new Enumeration<ContactPointUse>(this, ContactPointUse.NULL, code); 438 if ("home".equals(codeString)) 439 return new Enumeration<ContactPointUse>(this, ContactPointUse.HOME, code); 440 if ("work".equals(codeString)) 441 return new Enumeration<ContactPointUse>(this, ContactPointUse.WORK, code); 442 if ("temp".equals(codeString)) 443 return new Enumeration<ContactPointUse>(this, ContactPointUse.TEMP, code); 444 if ("old".equals(codeString)) 445 return new Enumeration<ContactPointUse>(this, ContactPointUse.OLD, code); 446 if ("mobile".equals(codeString)) 447 return new Enumeration<ContactPointUse>(this, ContactPointUse.MOBILE, code); 448 throw new FHIRException("Unknown ContactPointUse code '" + codeString + "'"); 449 } 450 451 public String toCode(ContactPointUse code) { 452 if (code == ContactPointUse.NULL) 453 return null; 454 if (code == ContactPointUse.HOME) 455 return "home"; 456 if (code == ContactPointUse.WORK) 457 return "work"; 458 if (code == ContactPointUse.TEMP) 459 return "temp"; 460 if (code == ContactPointUse.OLD) 461 return "old"; 462 if (code == ContactPointUse.MOBILE) 463 return "mobile"; 464 return "?"; 465 } 466 467 public String toSystem(ContactPointUse code) { 468 return code.getSystem(); 469 } 470 } 471 472 /** 473 * Telecommunications form for contact point - what communications system is 474 * required to make use of the contact. 475 */ 476 @Child(name = "system", type = { CodeType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 477 @Description(shortDefinition = "phone | fax | email | pager | url | sms | other", formalDefinition = "Telecommunications form for contact point - what communications system is required to make use of the contact.") 478 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contact-point-system") 479 protected Enumeration<ContactPointSystem> system; 480 481 /** 482 * The actual contact point details, in a form that is meaningful to the 483 * designated communication system (i.e. phone number or email address). 484 */ 485 @Child(name = "value", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 486 @Description(shortDefinition = "The actual contact point details", formalDefinition = "The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address).") 487 protected StringType value; 488 489 /** 490 * Identifies the purpose for the contact point. 491 */ 492 @Child(name = "use", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = true, summary = true) 493 @Description(shortDefinition = "home | work | temp | old | mobile - purpose of this contact point", formalDefinition = "Identifies the purpose for the contact point.") 494 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contact-point-use") 495 protected Enumeration<ContactPointUse> use; 496 497 /** 498 * Specifies a preferred order in which to use a set of contacts. ContactPoints 499 * with lower rank values are more preferred than those with higher rank values. 500 */ 501 @Child(name = "rank", type = { PositiveIntType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 502 @Description(shortDefinition = "Specify preferred order of use (1 = highest)", formalDefinition = "Specifies a preferred order in which to use a set of contacts. ContactPoints with lower rank values are more preferred than those with higher rank values.") 503 protected PositiveIntType rank; 504 505 /** 506 * Time period when the contact point was/is in use. 507 */ 508 @Child(name = "period", type = { Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 509 @Description(shortDefinition = "Time period when the contact point was/is in use", formalDefinition = "Time period when the contact point was/is in use.") 510 protected Period period; 511 512 private static final long serialVersionUID = 1509610874L; 513 514 /** 515 * Constructor 516 */ 517 public ContactPoint() { 518 super(); 519 } 520 521 /** 522 * @return {@link #system} (Telecommunications form for contact point - what 523 * communications system is required to make use of the contact.). This 524 * is the underlying object with id, value and extensions. The accessor 525 * "getSystem" gives direct access to the value 526 */ 527 public Enumeration<ContactPointSystem> getSystemElement() { 528 if (this.system == null) 529 if (Configuration.errorOnAutoCreate()) 530 throw new Error("Attempt to auto-create ContactPoint.system"); 531 else if (Configuration.doAutoCreate()) 532 this.system = new Enumeration<ContactPointSystem>(new ContactPointSystemEnumFactory()); // bb 533 return this.system; 534 } 535 536 public boolean hasSystemElement() { 537 return this.system != null && !this.system.isEmpty(); 538 } 539 540 public boolean hasSystem() { 541 return this.system != null && !this.system.isEmpty(); 542 } 543 544 /** 545 * @param value {@link #system} (Telecommunications form for contact point - 546 * what communications system is required to make use of the 547 * contact.). This is the underlying object with id, value and 548 * extensions. The accessor "getSystem" gives direct access to the 549 * value 550 */ 551 public ContactPoint setSystemElement(Enumeration<ContactPointSystem> value) { 552 this.system = value; 553 return this; 554 } 555 556 /** 557 * @return Telecommunications form for contact point - what communications 558 * system is required to make use of the contact. 559 */ 560 public ContactPointSystem getSystem() { 561 return this.system == null ? null : this.system.getValue(); 562 } 563 564 /** 565 * @param value Telecommunications form for contact point - what communications 566 * system is required to make use of the contact. 567 */ 568 public ContactPoint setSystem(ContactPointSystem value) { 569 if (value == null) 570 this.system = null; 571 else { 572 if (this.system == null) 573 this.system = new Enumeration<ContactPointSystem>(new ContactPointSystemEnumFactory()); 574 this.system.setValue(value); 575 } 576 return this; 577 } 578 579 /** 580 * @return {@link #value} (The actual contact point details, in a form that is 581 * meaningful to the designated communication system (i.e. phone number 582 * or email address).). This is the underlying object with id, value and 583 * extensions. The accessor "getValue" gives direct access to the value 584 */ 585 public StringType getValueElement() { 586 if (this.value == null) 587 if (Configuration.errorOnAutoCreate()) 588 throw new Error("Attempt to auto-create ContactPoint.value"); 589 else if (Configuration.doAutoCreate()) 590 this.value = new StringType(); // bb 591 return this.value; 592 } 593 594 public boolean hasValueElement() { 595 return this.value != null && !this.value.isEmpty(); 596 } 597 598 public boolean hasValue() { 599 return this.value != null && !this.value.isEmpty(); 600 } 601 602 /** 603 * @param value {@link #value} (The actual contact point details, in a form that 604 * is meaningful to the designated communication system (i.e. phone 605 * number or email address).). This is the underlying object with 606 * id, value and extensions. The accessor "getValue" gives direct 607 * access to the value 608 */ 609 public ContactPoint setValueElement(StringType value) { 610 this.value = value; 611 return this; 612 } 613 614 /** 615 * @return The actual contact point details, in a form that is meaningful to the 616 * designated communication system (i.e. phone number or email address). 617 */ 618 public String getValue() { 619 return this.value == null ? null : this.value.getValue(); 620 } 621 622 /** 623 * @param value The actual contact point details, in a form that is meaningful 624 * to the designated communication system (i.e. phone number or 625 * email address). 626 */ 627 public ContactPoint setValue(String value) { 628 if (Utilities.noString(value)) 629 this.value = null; 630 else { 631 if (this.value == null) 632 this.value = new StringType(); 633 this.value.setValue(value); 634 } 635 return this; 636 } 637 638 /** 639 * @return {@link #use} (Identifies the purpose for the contact point.). This is 640 * the underlying object with id, value and extensions. The accessor 641 * "getUse" gives direct access to the value 642 */ 643 public Enumeration<ContactPointUse> getUseElement() { 644 if (this.use == null) 645 if (Configuration.errorOnAutoCreate()) 646 throw new Error("Attempt to auto-create ContactPoint.use"); 647 else if (Configuration.doAutoCreate()) 648 this.use = new Enumeration<ContactPointUse>(new ContactPointUseEnumFactory()); // bb 649 return this.use; 650 } 651 652 public boolean hasUseElement() { 653 return this.use != null && !this.use.isEmpty(); 654 } 655 656 public boolean hasUse() { 657 return this.use != null && !this.use.isEmpty(); 658 } 659 660 /** 661 * @param value {@link #use} (Identifies the purpose for the contact point.). 662 * This is the underlying object with id, value and extensions. The 663 * accessor "getUse" gives direct access to the value 664 */ 665 public ContactPoint setUseElement(Enumeration<ContactPointUse> value) { 666 this.use = value; 667 return this; 668 } 669 670 /** 671 * @return Identifies the purpose for the contact point. 672 */ 673 public ContactPointUse getUse() { 674 return this.use == null ? null : this.use.getValue(); 675 } 676 677 /** 678 * @param value Identifies the purpose for the contact point. 679 */ 680 public ContactPoint setUse(ContactPointUse value) { 681 if (value == null) 682 this.use = null; 683 else { 684 if (this.use == null) 685 this.use = new Enumeration<ContactPointUse>(new ContactPointUseEnumFactory()); 686 this.use.setValue(value); 687 } 688 return this; 689 } 690 691 /** 692 * @return {@link #rank} (Specifies a preferred order in which to use a set of 693 * contacts. ContactPoints with lower rank values are more preferred 694 * than those with higher rank values.). This is the underlying object 695 * with id, value and extensions. The accessor "getRank" gives direct 696 * access to the value 697 */ 698 public PositiveIntType getRankElement() { 699 if (this.rank == null) 700 if (Configuration.errorOnAutoCreate()) 701 throw new Error("Attempt to auto-create ContactPoint.rank"); 702 else if (Configuration.doAutoCreate()) 703 this.rank = new PositiveIntType(); // bb 704 return this.rank; 705 } 706 707 public boolean hasRankElement() { 708 return this.rank != null && !this.rank.isEmpty(); 709 } 710 711 public boolean hasRank() { 712 return this.rank != null && !this.rank.isEmpty(); 713 } 714 715 /** 716 * @param value {@link #rank} (Specifies a preferred order in which to use a set 717 * of contacts. ContactPoints with lower rank values are more 718 * preferred than those with higher rank values.). This is the 719 * underlying object with id, value and extensions. The accessor 720 * "getRank" gives direct access to the value 721 */ 722 public ContactPoint setRankElement(PositiveIntType value) { 723 this.rank = value; 724 return this; 725 } 726 727 /** 728 * @return Specifies a preferred order in which to use a set of contacts. 729 * ContactPoints with lower rank values are more preferred than those 730 * with higher rank values. 731 */ 732 public int getRank() { 733 return this.rank == null || this.rank.isEmpty() ? 0 : this.rank.getValue(); 734 } 735 736 /** 737 * @param value Specifies a preferred order in which to use a set of contacts. 738 * ContactPoints with lower rank values are more preferred than 739 * those with higher rank values. 740 */ 741 public ContactPoint setRank(int value) { 742 if (this.rank == null) 743 this.rank = new PositiveIntType(); 744 this.rank.setValue(value); 745 return this; 746 } 747 748 /** 749 * @return {@link #period} (Time period when the contact point was/is in use.) 750 */ 751 public Period getPeriod() { 752 if (this.period == null) 753 if (Configuration.errorOnAutoCreate()) 754 throw new Error("Attempt to auto-create ContactPoint.period"); 755 else if (Configuration.doAutoCreate()) 756 this.period = new Period(); // cc 757 return this.period; 758 } 759 760 public boolean hasPeriod() { 761 return this.period != null && !this.period.isEmpty(); 762 } 763 764 /** 765 * @param value {@link #period} (Time period when the contact point was/is in 766 * use.) 767 */ 768 public ContactPoint setPeriod(Period value) { 769 this.period = value; 770 return this; 771 } 772 773 protected void listChildren(List<Property> children) { 774 super.listChildren(children); 775 children.add(new Property("system", "code", 776 "Telecommunications form for contact point - what communications system is required to make use of the contact.", 777 0, 1, system)); 778 children.add(new Property("value", "string", 779 "The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address).", 780 0, 1, value)); 781 children.add(new Property("use", "code", "Identifies the purpose for the contact point.", 0, 1, use)); 782 children.add(new Property("rank", "positiveInt", 783 "Specifies a preferred order in which to use a set of contacts. ContactPoints with lower rank values are more preferred than those with higher rank values.", 784 0, 1, rank)); 785 children.add(new Property("period", "Period", "Time period when the contact point was/is in use.", 0, 1, period)); 786 } 787 788 @Override 789 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 790 switch (_hash) { 791 case -887328209: 792 /* system */ return new Property("system", "code", 793 "Telecommunications form for contact point - what communications system is required to make use of the contact.", 794 0, 1, system); 795 case 111972721: 796 /* value */ return new Property("value", "string", 797 "The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address).", 798 0, 1, value); 799 case 116103: 800 /* use */ return new Property("use", "code", "Identifies the purpose for the contact point.", 0, 1, use); 801 case 3492908: 802 /* rank */ return new Property("rank", "positiveInt", 803 "Specifies a preferred order in which to use a set of contacts. ContactPoints with lower rank values are more preferred than those with higher rank values.", 804 0, 1, rank); 805 case -991726143: 806 /* period */ return new Property("period", "Period", "Time period when the contact point was/is in use.", 0, 1, 807 period); 808 default: 809 return super.getNamedProperty(_hash, _name, _checkValid); 810 } 811 812 } 813 814 @Override 815 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 816 switch (hash) { 817 case -887328209: 818 /* system */ return this.system == null ? new Base[0] : new Base[] { this.system }; // Enumeration<ContactPointSystem> 819 case 111972721: 820 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // StringType 821 case 116103: 822 /* use */ return this.use == null ? new Base[0] : new Base[] { this.use }; // Enumeration<ContactPointUse> 823 case 3492908: 824 /* rank */ return this.rank == null ? new Base[0] : new Base[] { this.rank }; // PositiveIntType 825 case -991726143: 826 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 827 default: 828 return super.getProperty(hash, name, checkValid); 829 } 830 831 } 832 833 @Override 834 public Base setProperty(int hash, String name, Base value) throws FHIRException { 835 switch (hash) { 836 case -887328209: // system 837 value = new ContactPointSystemEnumFactory().fromType(castToCode(value)); 838 this.system = (Enumeration) value; // Enumeration<ContactPointSystem> 839 return value; 840 case 111972721: // value 841 this.value = castToString(value); // StringType 842 return value; 843 case 116103: // use 844 value = new ContactPointUseEnumFactory().fromType(castToCode(value)); 845 this.use = (Enumeration) value; // Enumeration<ContactPointUse> 846 return value; 847 case 3492908: // rank 848 this.rank = castToPositiveInt(value); // PositiveIntType 849 return value; 850 case -991726143: // period 851 this.period = castToPeriod(value); // Period 852 return value; 853 default: 854 return super.setProperty(hash, name, value); 855 } 856 857 } 858 859 @Override 860 public Base setProperty(String name, Base value) throws FHIRException { 861 if (name.equals("system")) { 862 value = new ContactPointSystemEnumFactory().fromType(castToCode(value)); 863 this.system = (Enumeration) value; // Enumeration<ContactPointSystem> 864 } else if (name.equals("value")) { 865 this.value = castToString(value); // StringType 866 } else if (name.equals("use")) { 867 value = new ContactPointUseEnumFactory().fromType(castToCode(value)); 868 this.use = (Enumeration) value; // Enumeration<ContactPointUse> 869 } else if (name.equals("rank")) { 870 this.rank = castToPositiveInt(value); // PositiveIntType 871 } else if (name.equals("period")) { 872 this.period = castToPeriod(value); // Period 873 } else 874 return super.setProperty(name, value); 875 return value; 876 } 877 878 @Override 879 public void removeChild(String name, Base value) throws FHIRException { 880 if (name.equals("system")) { 881 this.system = null; 882 } else if (name.equals("value")) { 883 this.value = null; 884 } else if (name.equals("use")) { 885 this.use = null; 886 } else if (name.equals("rank")) { 887 this.rank = null; 888 } else if (name.equals("period")) { 889 this.period = null; 890 } else 891 super.removeChild(name, value); 892 893 } 894 895 @Override 896 public Base makeProperty(int hash, String name) throws FHIRException { 897 switch (hash) { 898 case -887328209: 899 return getSystemElement(); 900 case 111972721: 901 return getValueElement(); 902 case 116103: 903 return getUseElement(); 904 case 3492908: 905 return getRankElement(); 906 case -991726143: 907 return getPeriod(); 908 default: 909 return super.makeProperty(hash, name); 910 } 911 912 } 913 914 @Override 915 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 916 switch (hash) { 917 case -887328209: 918 /* system */ return new String[] { "code" }; 919 case 111972721: 920 /* value */ return new String[] { "string" }; 921 case 116103: 922 /* use */ return new String[] { "code" }; 923 case 3492908: 924 /* rank */ return new String[] { "positiveInt" }; 925 case -991726143: 926 /* period */ return new String[] { "Period" }; 927 default: 928 return super.getTypesForProperty(hash, name); 929 } 930 931 } 932 933 @Override 934 public Base addChild(String name) throws FHIRException { 935 if (name.equals("system")) { 936 throw new FHIRException("Cannot call addChild on a singleton property ContactPoint.system"); 937 } else if (name.equals("value")) { 938 throw new FHIRException("Cannot call addChild on a singleton property ContactPoint.value"); 939 } else if (name.equals("use")) { 940 throw new FHIRException("Cannot call addChild on a singleton property ContactPoint.use"); 941 } else if (name.equals("rank")) { 942 throw new FHIRException("Cannot call addChild on a singleton property ContactPoint.rank"); 943 } else if (name.equals("period")) { 944 this.period = new Period(); 945 return this.period; 946 } else 947 return super.addChild(name); 948 } 949 950 public String fhirType() { 951 return "ContactPoint"; 952 953 } 954 955 public ContactPoint copy() { 956 ContactPoint dst = new ContactPoint(); 957 copyValues(dst); 958 return dst; 959 } 960 961 public void copyValues(ContactPoint dst) { 962 super.copyValues(dst); 963 dst.system = system == null ? null : system.copy(); 964 dst.value = value == null ? null : value.copy(); 965 dst.use = use == null ? null : use.copy(); 966 dst.rank = rank == null ? null : rank.copy(); 967 dst.period = period == null ? null : period.copy(); 968 } 969 970 protected ContactPoint typedCopy() { 971 return copy(); 972 } 973 974 @Override 975 public boolean equalsDeep(Base other_) { 976 if (!super.equalsDeep(other_)) 977 return false; 978 if (!(other_ instanceof ContactPoint)) 979 return false; 980 ContactPoint o = (ContactPoint) other_; 981 return compareDeep(system, o.system, true) && compareDeep(value, o.value, true) && compareDeep(use, o.use, true) 982 && compareDeep(rank, o.rank, true) && compareDeep(period, o.period, true); 983 } 984 985 @Override 986 public boolean equalsShallow(Base other_) { 987 if (!super.equalsShallow(other_)) 988 return false; 989 if (!(other_ instanceof ContactPoint)) 990 return false; 991 ContactPoint o = (ContactPoint) other_; 992 return compareValues(system, o.system, true) && compareValues(value, o.value, true) 993 && compareValues(use, o.use, true) && compareValues(rank, o.rank, true); 994 } 995 996 public boolean isEmpty() { 997 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(system, value, use, rank, period); 998 } 999 1000}