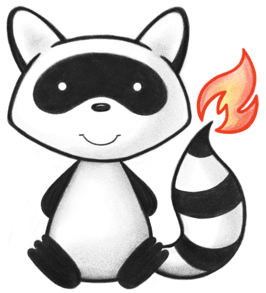
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048 049/** 050 * Legally enforceable, formally recorded unilateral or bilateral directive 051 * i.e., a policy or agreement. 052 */ 053@ResourceDef(name = "Contract", profile = "http://hl7.org/fhir/StructureDefinition/Contract") 054public class Contract extends DomainResource { 055 056 public enum ContractStatus { 057 /** 058 * Contract is augmented with additional information to correct errors in a 059 * predecessor or to updated values in a predecessor. Usage: Contract altered 060 * within effective time. Precedence Order = 9. Comparable FHIR and v.3 status 061 * codes: revised; replaced. 062 */ 063 AMENDED, 064 /** 065 * Contract is augmented with additional information that was missing from a 066 * predecessor Contract. Usage: Contract altered within effective time. 067 * Precedence Order = 9. Comparable FHIR and v.3 status codes: updated, 068 * replaced. 069 */ 070 APPENDED, 071 /** 072 * Contract is terminated due to failure of the Grantor and/or the Grantee to 073 * fulfil one or more contract provisions. Usage: Abnormal contract termination. 074 * Precedence Order = 10. Comparable FHIR and v.3 status codes: stopped; failed; 075 * aborted. 076 */ 077 CANCELLED, 078 /** 079 * Contract is pended to rectify failure of the Grantor or the Grantee to fulfil 080 * contract provision(s). E.g., Grantee complaint about Grantor's failure to 081 * comply with contract provisions. Usage: Contract pended. Precedence Order = 082 * 7. Comparable FHIR and v.3 status codes: on hold; pended; suspended. 083 */ 084 DISPUTED, 085 /** 086 * Contract was created in error. No Precedence Order. Status may be applied to 087 * a Contract with any status. 088 */ 089 ENTEREDINERROR, 090 /** 091 * Contract execution pending; may be executed when either the Grantor or the 092 * Grantee accepts the contract provisions by signing. I.e., where either the 093 * Grantor or the Grantee has signed, but not both. E.g., when an insurance 094 * applicant signs the insurers application, which references the policy. Usage: 095 * Optional first step of contract execution activity. May be skipped and 096 * contracting activity moves directly to executed state. Precedence Order = 3. 097 * Comparable FHIR and v.3 status codes: draft; preliminary; planned; intended; 098 * active. 099 */ 100 EXECUTABLE, 101 /** 102 * Contract is activated for period stipulated when both the Grantor and Grantee 103 * have signed it. Usage: Required state for normal completion of contracting 104 * activity. Precedence Order = 6. Comparable FHIR and v.3 status codes: 105 * accepted; completed. 106 */ 107 EXECUTED, 108 /** 109 * Contract execution is suspended while either or both the Grantor and Grantee 110 * propose and consider new or revised contract provisions. I.e., where the 111 * party which has not signed proposes changes to the terms. E .g., a life 112 * insurer declines to agree to the signed application because the life insurer 113 * has evidence that the applicant, who asserted to being younger or a 114 * non-smoker to get a lower premium rate - but offers instead to agree to a 115 * higher premium based on the applicants actual age or smoking status. Usage: 116 * Optional contract activity between executable and executed state. Precedence 117 * Order = 4. Comparable FHIR and v.3 status codes: in progress; review; held. 118 */ 119 NEGOTIABLE, 120 /** 121 * Contract is a proposal by either the Grantor or the Grantee. Aka - A Contract 122 * hard copy or electronic 'template', 'form' or 'application'. E.g., health 123 * insurance application; consent directive form. Usage: Beginning of contract 124 * negotiation, which may have been completed as a precondition because used for 125 * 0..* contracts. Precedence Order = 2. Comparable FHIR and v.3 status codes: 126 * requested; new. 127 */ 128 OFFERED, 129 /** 130 * Contract template is available as the basis for an application or offer by 131 * the Grantor or Grantee. E.g., health insurance policy; consent directive 132 * policy. Usage: Required initial contract activity, which may have been 133 * completed as a precondition because used for 0..* contracts. Precedence Order 134 * = 1. Comparable FHIR and v.3 status codes: proposed; intended. 135 */ 136 POLICY, 137 /** 138 * Execution of the Contract is not completed because either or both the Grantor 139 * and Grantee decline to accept some or all of the contract provisions. Usage: 140 * Optional contract activity between executable and abnormal termination. 141 * Precedence Order = 5. Comparable FHIR and v.3 status codes: stopped; 142 * cancelled. 143 */ 144 REJECTED, 145 /** 146 * Beginning of a successor Contract at the termination of predecessor Contract 147 * lifecycle. Usage: Follows termination of a preceding Contract that has 148 * reached its expiry date. Precedence Order = 13. Comparable FHIR and v.3 149 * status codes: superseded. 150 */ 151 RENEWED, 152 /** 153 * A Contract that is rescinded. May be required prior to replacing with an 154 * updated Contract. Comparable FHIR and v.3 status codes: nullified. 155 */ 156 REVOKED, 157 /** 158 * Contract is reactivated after being pended because of faulty execution. 159 * *E.g., competency of the signer(s), or where the policy is substantially 160 * different from and did not accompany the application/form so that the 161 * applicant could not compare them. Aka - ''reactivated''. Usage: Optional 162 * stage where a pended contract is reactivated. Precedence Order = 8. 163 * Comparable FHIR and v.3 status codes: reactivated. 164 */ 165 RESOLVED, 166 /** 167 * Contract reaches its expiry date. It might or might not be renewed or 168 * renegotiated. Usage: Normal end of contract period. Precedence Order = 12. 169 * Comparable FHIR and v.3 status codes: Obsoleted. 170 */ 171 TERMINATED, 172 /** 173 * added to help the parsers with the generic types 174 */ 175 NULL; 176 177 public static ContractStatus fromCode(String codeString) throws FHIRException { 178 if (codeString == null || "".equals(codeString)) 179 return null; 180 if ("amended".equals(codeString)) 181 return AMENDED; 182 if ("appended".equals(codeString)) 183 return APPENDED; 184 if ("cancelled".equals(codeString)) 185 return CANCELLED; 186 if ("disputed".equals(codeString)) 187 return DISPUTED; 188 if ("entered-in-error".equals(codeString)) 189 return ENTEREDINERROR; 190 if ("executable".equals(codeString)) 191 return EXECUTABLE; 192 if ("executed".equals(codeString)) 193 return EXECUTED; 194 if ("negotiable".equals(codeString)) 195 return NEGOTIABLE; 196 if ("offered".equals(codeString)) 197 return OFFERED; 198 if ("policy".equals(codeString)) 199 return POLICY; 200 if ("rejected".equals(codeString)) 201 return REJECTED; 202 if ("renewed".equals(codeString)) 203 return RENEWED; 204 if ("revoked".equals(codeString)) 205 return REVOKED; 206 if ("resolved".equals(codeString)) 207 return RESOLVED; 208 if ("terminated".equals(codeString)) 209 return TERMINATED; 210 if (Configuration.isAcceptInvalidEnums()) 211 return null; 212 else 213 throw new FHIRException("Unknown ContractStatus code '" + codeString + "'"); 214 } 215 216 public String toCode() { 217 switch (this) { 218 case AMENDED: 219 return "amended"; 220 case APPENDED: 221 return "appended"; 222 case CANCELLED: 223 return "cancelled"; 224 case DISPUTED: 225 return "disputed"; 226 case ENTEREDINERROR: 227 return "entered-in-error"; 228 case EXECUTABLE: 229 return "executable"; 230 case EXECUTED: 231 return "executed"; 232 case NEGOTIABLE: 233 return "negotiable"; 234 case OFFERED: 235 return "offered"; 236 case POLICY: 237 return "policy"; 238 case REJECTED: 239 return "rejected"; 240 case RENEWED: 241 return "renewed"; 242 case REVOKED: 243 return "revoked"; 244 case RESOLVED: 245 return "resolved"; 246 case TERMINATED: 247 return "terminated"; 248 case NULL: 249 return null; 250 default: 251 return "?"; 252 } 253 } 254 255 public String getSystem() { 256 switch (this) { 257 case AMENDED: 258 return "http://hl7.org/fhir/contract-status"; 259 case APPENDED: 260 return "http://hl7.org/fhir/contract-status"; 261 case CANCELLED: 262 return "http://hl7.org/fhir/contract-status"; 263 case DISPUTED: 264 return "http://hl7.org/fhir/contract-status"; 265 case ENTEREDINERROR: 266 return "http://hl7.org/fhir/contract-status"; 267 case EXECUTABLE: 268 return "http://hl7.org/fhir/contract-status"; 269 case EXECUTED: 270 return "http://hl7.org/fhir/contract-status"; 271 case NEGOTIABLE: 272 return "http://hl7.org/fhir/contract-status"; 273 case OFFERED: 274 return "http://hl7.org/fhir/contract-status"; 275 case POLICY: 276 return "http://hl7.org/fhir/contract-status"; 277 case REJECTED: 278 return "http://hl7.org/fhir/contract-status"; 279 case RENEWED: 280 return "http://hl7.org/fhir/contract-status"; 281 case REVOKED: 282 return "http://hl7.org/fhir/contract-status"; 283 case RESOLVED: 284 return "http://hl7.org/fhir/contract-status"; 285 case TERMINATED: 286 return "http://hl7.org/fhir/contract-status"; 287 case NULL: 288 return null; 289 default: 290 return "?"; 291 } 292 } 293 294 public String getDefinition() { 295 switch (this) { 296 case AMENDED: 297 return "Contract is augmented with additional information to correct errors in a predecessor or to updated values in a predecessor. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: revised; replaced."; 298 case APPENDED: 299 return "Contract is augmented with additional information that was missing from a predecessor Contract. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: updated, replaced."; 300 case CANCELLED: 301 return "Contract is terminated due to failure of the Grantor and/or the Grantee to fulfil one or more contract provisions. Usage: Abnormal contract termination. Precedence Order = 10. Comparable FHIR and v.3 status codes: stopped; failed; aborted."; 302 case DISPUTED: 303 return "Contract is pended to rectify failure of the Grantor or the Grantee to fulfil contract provision(s). E.g., Grantee complaint about Grantor's failure to comply with contract provisions. Usage: Contract pended. Precedence Order = 7. Comparable FHIR and v.3 status codes: on hold; pended; suspended."; 304 case ENTEREDINERROR: 305 return "Contract was created in error. No Precedence Order. Status may be applied to a Contract with any status."; 306 case EXECUTABLE: 307 return "Contract execution pending; may be executed when either the Grantor or the Grantee accepts the contract provisions by signing. I.e., where either the Grantor or the Grantee has signed, but not both. E.g., when an insurance applicant signs the insurers application, which references the policy. Usage: Optional first step of contract execution activity. May be skipped and contracting activity moves directly to executed state. Precedence Order = 3. Comparable FHIR and v.3 status codes: draft; preliminary; planned; intended; active."; 308 case EXECUTED: 309 return "Contract is activated for period stipulated when both the Grantor and Grantee have signed it. Usage: Required state for normal completion of contracting activity. Precedence Order = 6. Comparable FHIR and v.3 status codes: accepted; completed."; 310 case NEGOTIABLE: 311 return "Contract execution is suspended while either or both the Grantor and Grantee propose and consider new or revised contract provisions. I.e., where the party which has not signed proposes changes to the terms. E .g., a life insurer declines to agree to the signed application because the life insurer has evidence that the applicant, who asserted to being younger or a non-smoker to get a lower premium rate - but offers instead to agree to a higher premium based on the applicants actual age or smoking status. Usage: Optional contract activity between executable and executed state. Precedence Order = 4. Comparable FHIR and v.3 status codes: in progress; review; held."; 312 case OFFERED: 313 return "Contract is a proposal by either the Grantor or the Grantee. Aka - A Contract hard copy or electronic 'template', 'form' or 'application'. E.g., health insurance application; consent directive form. Usage: Beginning of contract negotiation, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 2. Comparable FHIR and v.3 status codes: requested; new."; 314 case POLICY: 315 return "Contract template is available as the basis for an application or offer by the Grantor or Grantee. E.g., health insurance policy; consent directive policy. Usage: Required initial contract activity, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 1. Comparable FHIR and v.3 status codes: proposed; intended."; 316 case REJECTED: 317 return " Execution of the Contract is not completed because either or both the Grantor and Grantee decline to accept some or all of the contract provisions. Usage: Optional contract activity between executable and abnormal termination. Precedence Order = 5. Comparable FHIR and v.3 status codes: stopped; cancelled."; 318 case RENEWED: 319 return "Beginning of a successor Contract at the termination of predecessor Contract lifecycle. Usage: Follows termination of a preceding Contract that has reached its expiry date. Precedence Order = 13. Comparable FHIR and v.3 status codes: superseded."; 320 case REVOKED: 321 return "A Contract that is rescinded. May be required prior to replacing with an updated Contract. Comparable FHIR and v.3 status codes: nullified."; 322 case RESOLVED: 323 return "Contract is reactivated after being pended because of faulty execution. *E.g., competency of the signer(s), or where the policy is substantially different from and did not accompany the application/form so that the applicant could not compare them. Aka - ''reactivated''. Usage: Optional stage where a pended contract is reactivated. Precedence Order = 8. Comparable FHIR and v.3 status codes: reactivated."; 324 case TERMINATED: 325 return "Contract reaches its expiry date. It might or might not be renewed or renegotiated. Usage: Normal end of contract period. Precedence Order = 12. Comparable FHIR and v.3 status codes: Obsoleted."; 326 case NULL: 327 return null; 328 default: 329 return "?"; 330 } 331 } 332 333 public String getDisplay() { 334 switch (this) { 335 case AMENDED: 336 return "Amended"; 337 case APPENDED: 338 return "Appended"; 339 case CANCELLED: 340 return "Cancelled"; 341 case DISPUTED: 342 return "Disputed"; 343 case ENTEREDINERROR: 344 return "Entered in Error"; 345 case EXECUTABLE: 346 return "Executable"; 347 case EXECUTED: 348 return "Executed"; 349 case NEGOTIABLE: 350 return "Negotiable"; 351 case OFFERED: 352 return "Offered"; 353 case POLICY: 354 return "Policy"; 355 case REJECTED: 356 return "Rejected"; 357 case RENEWED: 358 return "Renewed"; 359 case REVOKED: 360 return "Revoked"; 361 case RESOLVED: 362 return "Resolved"; 363 case TERMINATED: 364 return "Terminated"; 365 case NULL: 366 return null; 367 default: 368 return "?"; 369 } 370 } 371 } 372 373 public static class ContractStatusEnumFactory implements EnumFactory<ContractStatus> { 374 public ContractStatus fromCode(String codeString) throws IllegalArgumentException { 375 if (codeString == null || "".equals(codeString)) 376 if (codeString == null || "".equals(codeString)) 377 return null; 378 if ("amended".equals(codeString)) 379 return ContractStatus.AMENDED; 380 if ("appended".equals(codeString)) 381 return ContractStatus.APPENDED; 382 if ("cancelled".equals(codeString)) 383 return ContractStatus.CANCELLED; 384 if ("disputed".equals(codeString)) 385 return ContractStatus.DISPUTED; 386 if ("entered-in-error".equals(codeString)) 387 return ContractStatus.ENTEREDINERROR; 388 if ("executable".equals(codeString)) 389 return ContractStatus.EXECUTABLE; 390 if ("executed".equals(codeString)) 391 return ContractStatus.EXECUTED; 392 if ("negotiable".equals(codeString)) 393 return ContractStatus.NEGOTIABLE; 394 if ("offered".equals(codeString)) 395 return ContractStatus.OFFERED; 396 if ("policy".equals(codeString)) 397 return ContractStatus.POLICY; 398 if ("rejected".equals(codeString)) 399 return ContractStatus.REJECTED; 400 if ("renewed".equals(codeString)) 401 return ContractStatus.RENEWED; 402 if ("revoked".equals(codeString)) 403 return ContractStatus.REVOKED; 404 if ("resolved".equals(codeString)) 405 return ContractStatus.RESOLVED; 406 if ("terminated".equals(codeString)) 407 return ContractStatus.TERMINATED; 408 throw new IllegalArgumentException("Unknown ContractStatus code '" + codeString + "'"); 409 } 410 411 public Enumeration<ContractStatus> fromType(PrimitiveType<?> code) throws FHIRException { 412 if (code == null) 413 return null; 414 if (code.isEmpty()) 415 return new Enumeration<ContractStatus>(this, ContractStatus.NULL, code); 416 String codeString = code.asStringValue(); 417 if (codeString == null || "".equals(codeString)) 418 return new Enumeration<ContractStatus>(this, ContractStatus.NULL, code); 419 if ("amended".equals(codeString)) 420 return new Enumeration<ContractStatus>(this, ContractStatus.AMENDED, code); 421 if ("appended".equals(codeString)) 422 return new Enumeration<ContractStatus>(this, ContractStatus.APPENDED, code); 423 if ("cancelled".equals(codeString)) 424 return new Enumeration<ContractStatus>(this, ContractStatus.CANCELLED, code); 425 if ("disputed".equals(codeString)) 426 return new Enumeration<ContractStatus>(this, ContractStatus.DISPUTED, code); 427 if ("entered-in-error".equals(codeString)) 428 return new Enumeration<ContractStatus>(this, ContractStatus.ENTEREDINERROR, code); 429 if ("executable".equals(codeString)) 430 return new Enumeration<ContractStatus>(this, ContractStatus.EXECUTABLE, code); 431 if ("executed".equals(codeString)) 432 return new Enumeration<ContractStatus>(this, ContractStatus.EXECUTED, code); 433 if ("negotiable".equals(codeString)) 434 return new Enumeration<ContractStatus>(this, ContractStatus.NEGOTIABLE, code); 435 if ("offered".equals(codeString)) 436 return new Enumeration<ContractStatus>(this, ContractStatus.OFFERED, code); 437 if ("policy".equals(codeString)) 438 return new Enumeration<ContractStatus>(this, ContractStatus.POLICY, code); 439 if ("rejected".equals(codeString)) 440 return new Enumeration<ContractStatus>(this, ContractStatus.REJECTED, code); 441 if ("renewed".equals(codeString)) 442 return new Enumeration<ContractStatus>(this, ContractStatus.RENEWED, code); 443 if ("revoked".equals(codeString)) 444 return new Enumeration<ContractStatus>(this, ContractStatus.REVOKED, code); 445 if ("resolved".equals(codeString)) 446 return new Enumeration<ContractStatus>(this, ContractStatus.RESOLVED, code); 447 if ("terminated".equals(codeString)) 448 return new Enumeration<ContractStatus>(this, ContractStatus.TERMINATED, code); 449 throw new FHIRException("Unknown ContractStatus code '" + codeString + "'"); 450 } 451 452 public String toCode(ContractStatus code) { 453 if (code == ContractStatus.NULL) 454 return null; 455 if (code == ContractStatus.AMENDED) 456 return "amended"; 457 if (code == ContractStatus.APPENDED) 458 return "appended"; 459 if (code == ContractStatus.CANCELLED) 460 return "cancelled"; 461 if (code == ContractStatus.DISPUTED) 462 return "disputed"; 463 if (code == ContractStatus.ENTEREDINERROR) 464 return "entered-in-error"; 465 if (code == ContractStatus.EXECUTABLE) 466 return "executable"; 467 if (code == ContractStatus.EXECUTED) 468 return "executed"; 469 if (code == ContractStatus.NEGOTIABLE) 470 return "negotiable"; 471 if (code == ContractStatus.OFFERED) 472 return "offered"; 473 if (code == ContractStatus.POLICY) 474 return "policy"; 475 if (code == ContractStatus.REJECTED) 476 return "rejected"; 477 if (code == ContractStatus.RENEWED) 478 return "renewed"; 479 if (code == ContractStatus.REVOKED) 480 return "revoked"; 481 if (code == ContractStatus.RESOLVED) 482 return "resolved"; 483 if (code == ContractStatus.TERMINATED) 484 return "terminated"; 485 return "?"; 486 } 487 488 public String toSystem(ContractStatus code) { 489 return code.getSystem(); 490 } 491 } 492 493 public enum ContractPublicationStatus { 494 /** 495 * Contract is augmented with additional information to correct errors in a 496 * predecessor or to updated values in a predecessor. Usage: Contract altered 497 * within effective time. Precedence Order = 9. Comparable FHIR and v.3 status 498 * codes: revised; replaced. 499 */ 500 AMENDED, 501 /** 502 * Contract is augmented with additional information that was missing from a 503 * predecessor Contract. Usage: Contract altered within effective time. 504 * Precedence Order = 9. Comparable FHIR and v.3 status codes: updated, 505 * replaced. 506 */ 507 APPENDED, 508 /** 509 * Contract is terminated due to failure of the Grantor and/or the Grantee to 510 * fulfil one or more contract provisions. Usage: Abnormal contract termination. 511 * Precedence Order = 10. Comparable FHIR and v.3 status codes: stopped; failed; 512 * aborted. 513 */ 514 CANCELLED, 515 /** 516 * Contract is pended to rectify failure of the Grantor or the Grantee to fulfil 517 * contract provision(s). E.g., Grantee complaint about Grantor's failure to 518 * comply with contract provisions. Usage: Contract pended. Precedence Order = 519 * 7. Comparable FHIR and v.3 status codes: on hold; pended; suspended. 520 */ 521 DISPUTED, 522 /** 523 * Contract was created in error. No Precedence Order. Status may be applied to 524 * a Contract with any status. 525 */ 526 ENTEREDINERROR, 527 /** 528 * Contract execution pending; may be executed when either the Grantor or the 529 * Grantee accepts the contract provisions by signing. I.e., where either the 530 * Grantor or the Grantee has signed, but not both. E.g., when an insurance 531 * applicant signs the insurers application, which references the policy. Usage: 532 * Optional first step of contract execution activity. May be skipped and 533 * contracting activity moves directly to executed state. Precedence Order = 3. 534 * Comparable FHIR and v.3 status codes: draft; preliminary; planned; intended; 535 * active. 536 */ 537 EXECUTABLE, 538 /** 539 * Contract is activated for period stipulated when both the Grantor and Grantee 540 * have signed it. Usage: Required state for normal completion of contracting 541 * activity. Precedence Order = 6. Comparable FHIR and v.3 status codes: 542 * accepted; completed. 543 */ 544 EXECUTED, 545 /** 546 * Contract execution is suspended while either or both the Grantor and Grantee 547 * propose and consider new or revised contract provisions. I.e., where the 548 * party which has not signed proposes changes to the terms. E .g., a life 549 * insurer declines to agree to the signed application because the life insurer 550 * has evidence that the applicant, who asserted to being younger or a 551 * non-smoker to get a lower premium rate - but offers instead to agree to a 552 * higher premium based on the applicants actual age or smoking status. Usage: 553 * Optional contract activity between executable and executed state. Precedence 554 * Order = 4. Comparable FHIR and v.3 status codes: in progress; review; held. 555 */ 556 NEGOTIABLE, 557 /** 558 * Contract is a proposal by either the Grantor or the Grantee. Aka - A Contract 559 * hard copy or electronic 'template', 'form' or 'application'. E.g., health 560 * insurance application; consent directive form. Usage: Beginning of contract 561 * negotiation, which may have been completed as a precondition because used for 562 * 0..* contracts. Precedence Order = 2. Comparable FHIR and v.3 status codes: 563 * requested; new. 564 */ 565 OFFERED, 566 /** 567 * Contract template is available as the basis for an application or offer by 568 * the Grantor or Grantee. E.g., health insurance policy; consent directive 569 * policy. Usage: Required initial contract activity, which may have been 570 * completed as a precondition because used for 0..* contracts. Precedence Order 571 * = 1. Comparable FHIR and v.3 status codes: proposed; intended. 572 */ 573 POLICY, 574 /** 575 * Execution of the Contract is not completed because either or both the Grantor 576 * and Grantee decline to accept some or all of the contract provisions. Usage: 577 * Optional contract activity between executable and abnormal termination. 578 * Precedence Order = 5. Comparable FHIR and v.3 status codes: stopped; 579 * cancelled. 580 */ 581 REJECTED, 582 /** 583 * Beginning of a successor Contract at the termination of predecessor Contract 584 * lifecycle. Usage: Follows termination of a preceding Contract that has 585 * reached its expiry date. Precedence Order = 13. Comparable FHIR and v.3 586 * status codes: superseded. 587 */ 588 RENEWED, 589 /** 590 * A Contract that is rescinded. May be required prior to replacing with an 591 * updated Contract. Comparable FHIR and v.3 status codes: nullified. 592 */ 593 REVOKED, 594 /** 595 * Contract is reactivated after being pended because of faulty execution. 596 * *E.g., competency of the signer(s), or where the policy is substantially 597 * different from and did not accompany the application/form so that the 598 * applicant could not compare them. Aka - ''reactivated''. Usage: Optional 599 * stage where a pended contract is reactivated. Precedence Order = 8. 600 * Comparable FHIR and v.3 status codes: reactivated. 601 */ 602 RESOLVED, 603 /** 604 * Contract reaches its expiry date. It might or might not be renewed or 605 * renegotiated. Usage: Normal end of contract period. Precedence Order = 12. 606 * Comparable FHIR and v.3 status codes: Obsoleted. 607 */ 608 TERMINATED, 609 /** 610 * added to help the parsers with the generic types 611 */ 612 NULL; 613 614 public static ContractPublicationStatus fromCode(String codeString) throws FHIRException { 615 if (codeString == null || "".equals(codeString)) 616 return null; 617 if ("amended".equals(codeString)) 618 return AMENDED; 619 if ("appended".equals(codeString)) 620 return APPENDED; 621 if ("cancelled".equals(codeString)) 622 return CANCELLED; 623 if ("disputed".equals(codeString)) 624 return DISPUTED; 625 if ("entered-in-error".equals(codeString)) 626 return ENTEREDINERROR; 627 if ("executable".equals(codeString)) 628 return EXECUTABLE; 629 if ("executed".equals(codeString)) 630 return EXECUTED; 631 if ("negotiable".equals(codeString)) 632 return NEGOTIABLE; 633 if ("offered".equals(codeString)) 634 return OFFERED; 635 if ("policy".equals(codeString)) 636 return POLICY; 637 if ("rejected".equals(codeString)) 638 return REJECTED; 639 if ("renewed".equals(codeString)) 640 return RENEWED; 641 if ("revoked".equals(codeString)) 642 return REVOKED; 643 if ("resolved".equals(codeString)) 644 return RESOLVED; 645 if ("terminated".equals(codeString)) 646 return TERMINATED; 647 if (Configuration.isAcceptInvalidEnums()) 648 return null; 649 else 650 throw new FHIRException("Unknown ContractPublicationStatus code '" + codeString + "'"); 651 } 652 653 public String toCode() { 654 switch (this) { 655 case AMENDED: 656 return "amended"; 657 case APPENDED: 658 return "appended"; 659 case CANCELLED: 660 return "cancelled"; 661 case DISPUTED: 662 return "disputed"; 663 case ENTEREDINERROR: 664 return "entered-in-error"; 665 case EXECUTABLE: 666 return "executable"; 667 case EXECUTED: 668 return "executed"; 669 case NEGOTIABLE: 670 return "negotiable"; 671 case OFFERED: 672 return "offered"; 673 case POLICY: 674 return "policy"; 675 case REJECTED: 676 return "rejected"; 677 case RENEWED: 678 return "renewed"; 679 case REVOKED: 680 return "revoked"; 681 case RESOLVED: 682 return "resolved"; 683 case TERMINATED: 684 return "terminated"; 685 case NULL: 686 return null; 687 default: 688 return "?"; 689 } 690 } 691 692 public String getSystem() { 693 switch (this) { 694 case AMENDED: 695 return "http://hl7.org/fhir/contract-publicationstatus"; 696 case APPENDED: 697 return "http://hl7.org/fhir/contract-publicationstatus"; 698 case CANCELLED: 699 return "http://hl7.org/fhir/contract-publicationstatus"; 700 case DISPUTED: 701 return "http://hl7.org/fhir/contract-publicationstatus"; 702 case ENTEREDINERROR: 703 return "http://hl7.org/fhir/contract-publicationstatus"; 704 case EXECUTABLE: 705 return "http://hl7.org/fhir/contract-publicationstatus"; 706 case EXECUTED: 707 return "http://hl7.org/fhir/contract-publicationstatus"; 708 case NEGOTIABLE: 709 return "http://hl7.org/fhir/contract-publicationstatus"; 710 case OFFERED: 711 return "http://hl7.org/fhir/contract-publicationstatus"; 712 case POLICY: 713 return "http://hl7.org/fhir/contract-publicationstatus"; 714 case REJECTED: 715 return "http://hl7.org/fhir/contract-publicationstatus"; 716 case RENEWED: 717 return "http://hl7.org/fhir/contract-publicationstatus"; 718 case REVOKED: 719 return "http://hl7.org/fhir/contract-publicationstatus"; 720 case RESOLVED: 721 return "http://hl7.org/fhir/contract-publicationstatus"; 722 case TERMINATED: 723 return "http://hl7.org/fhir/contract-publicationstatus"; 724 case NULL: 725 return null; 726 default: 727 return "?"; 728 } 729 } 730 731 public String getDefinition() { 732 switch (this) { 733 case AMENDED: 734 return "Contract is augmented with additional information to correct errors in a predecessor or to updated values in a predecessor. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: revised; replaced."; 735 case APPENDED: 736 return "Contract is augmented with additional information that was missing from a predecessor Contract. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: updated, replaced."; 737 case CANCELLED: 738 return "Contract is terminated due to failure of the Grantor and/or the Grantee to fulfil one or more contract provisions. Usage: Abnormal contract termination. Precedence Order = 10. Comparable FHIR and v.3 status codes: stopped; failed; aborted."; 739 case DISPUTED: 740 return "Contract is pended to rectify failure of the Grantor or the Grantee to fulfil contract provision(s). E.g., Grantee complaint about Grantor's failure to comply with contract provisions. Usage: Contract pended. Precedence Order = 7. Comparable FHIR and v.3 status codes: on hold; pended; suspended."; 741 case ENTEREDINERROR: 742 return "Contract was created in error. No Precedence Order. Status may be applied to a Contract with any status."; 743 case EXECUTABLE: 744 return "Contract execution pending; may be executed when either the Grantor or the Grantee accepts the contract provisions by signing. I.e., where either the Grantor or the Grantee has signed, but not both. E.g., when an insurance applicant signs the insurers application, which references the policy. Usage: Optional first step of contract execution activity. May be skipped and contracting activity moves directly to executed state. Precedence Order = 3. Comparable FHIR and v.3 status codes: draft; preliminary; planned; intended; active."; 745 case EXECUTED: 746 return "Contract is activated for period stipulated when both the Grantor and Grantee have signed it. Usage: Required state for normal completion of contracting activity. Precedence Order = 6. Comparable FHIR and v.3 status codes: accepted; completed."; 747 case NEGOTIABLE: 748 return "Contract execution is suspended while either or both the Grantor and Grantee propose and consider new or revised contract provisions. I.e., where the party which has not signed proposes changes to the terms. E .g., a life insurer declines to agree to the signed application because the life insurer has evidence that the applicant, who asserted to being younger or a non-smoker to get a lower premium rate - but offers instead to agree to a higher premium based on the applicants actual age or smoking status. Usage: Optional contract activity between executable and executed state. Precedence Order = 4. Comparable FHIR and v.3 status codes: in progress; review; held."; 749 case OFFERED: 750 return "Contract is a proposal by either the Grantor or the Grantee. Aka - A Contract hard copy or electronic 'template', 'form' or 'application'. E.g., health insurance application; consent directive form. Usage: Beginning of contract negotiation, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 2. Comparable FHIR and v.3 status codes: requested; new."; 751 case POLICY: 752 return "Contract template is available as the basis for an application or offer by the Grantor or Grantee. E.g., health insurance policy; consent directive policy. Usage: Required initial contract activity, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 1. Comparable FHIR and v.3 status codes: proposed; intended."; 753 case REJECTED: 754 return " Execution of the Contract is not completed because either or both the Grantor and Grantee decline to accept some or all of the contract provisions. Usage: Optional contract activity between executable and abnormal termination. Precedence Order = 5. Comparable FHIR and v.3 status codes: stopped; cancelled."; 755 case RENEWED: 756 return "Beginning of a successor Contract at the termination of predecessor Contract lifecycle. Usage: Follows termination of a preceding Contract that has reached its expiry date. Precedence Order = 13. Comparable FHIR and v.3 status codes: superseded."; 757 case REVOKED: 758 return "A Contract that is rescinded. May be required prior to replacing with an updated Contract. Comparable FHIR and v.3 status codes: nullified."; 759 case RESOLVED: 760 return "Contract is reactivated after being pended because of faulty execution. *E.g., competency of the signer(s), or where the policy is substantially different from and did not accompany the application/form so that the applicant could not compare them. Aka - ''reactivated''. Usage: Optional stage where a pended contract is reactivated. Precedence Order = 8. Comparable FHIR and v.3 status codes: reactivated."; 761 case TERMINATED: 762 return "Contract reaches its expiry date. It might or might not be renewed or renegotiated. Usage: Normal end of contract period. Precedence Order = 12. Comparable FHIR and v.3 status codes: Obsoleted."; 763 case NULL: 764 return null; 765 default: 766 return "?"; 767 } 768 } 769 770 public String getDisplay() { 771 switch (this) { 772 case AMENDED: 773 return "Amended"; 774 case APPENDED: 775 return "Appended"; 776 case CANCELLED: 777 return "Cancelled"; 778 case DISPUTED: 779 return "Disputed"; 780 case ENTEREDINERROR: 781 return "Entered in Error"; 782 case EXECUTABLE: 783 return "Executable"; 784 case EXECUTED: 785 return "Executed"; 786 case NEGOTIABLE: 787 return "Negotiable"; 788 case OFFERED: 789 return "Offered"; 790 case POLICY: 791 return "Policy"; 792 case REJECTED: 793 return "Rejected"; 794 case RENEWED: 795 return "Renewed"; 796 case REVOKED: 797 return "Revoked"; 798 case RESOLVED: 799 return "Resolved"; 800 case TERMINATED: 801 return "Terminated"; 802 case NULL: 803 return null; 804 default: 805 return "?"; 806 } 807 } 808 } 809 810 public static class ContractPublicationStatusEnumFactory implements EnumFactory<ContractPublicationStatus> { 811 public ContractPublicationStatus fromCode(String codeString) throws IllegalArgumentException { 812 if (codeString == null || "".equals(codeString)) 813 if (codeString == null || "".equals(codeString)) 814 return null; 815 if ("amended".equals(codeString)) 816 return ContractPublicationStatus.AMENDED; 817 if ("appended".equals(codeString)) 818 return ContractPublicationStatus.APPENDED; 819 if ("cancelled".equals(codeString)) 820 return ContractPublicationStatus.CANCELLED; 821 if ("disputed".equals(codeString)) 822 return ContractPublicationStatus.DISPUTED; 823 if ("entered-in-error".equals(codeString)) 824 return ContractPublicationStatus.ENTEREDINERROR; 825 if ("executable".equals(codeString)) 826 return ContractPublicationStatus.EXECUTABLE; 827 if ("executed".equals(codeString)) 828 return ContractPublicationStatus.EXECUTED; 829 if ("negotiable".equals(codeString)) 830 return ContractPublicationStatus.NEGOTIABLE; 831 if ("offered".equals(codeString)) 832 return ContractPublicationStatus.OFFERED; 833 if ("policy".equals(codeString)) 834 return ContractPublicationStatus.POLICY; 835 if ("rejected".equals(codeString)) 836 return ContractPublicationStatus.REJECTED; 837 if ("renewed".equals(codeString)) 838 return ContractPublicationStatus.RENEWED; 839 if ("revoked".equals(codeString)) 840 return ContractPublicationStatus.REVOKED; 841 if ("resolved".equals(codeString)) 842 return ContractPublicationStatus.RESOLVED; 843 if ("terminated".equals(codeString)) 844 return ContractPublicationStatus.TERMINATED; 845 throw new IllegalArgumentException("Unknown ContractPublicationStatus code '" + codeString + "'"); 846 } 847 848 public Enumeration<ContractPublicationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 849 if (code == null) 850 return null; 851 if (code.isEmpty()) 852 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.NULL, code); 853 String codeString = code.asStringValue(); 854 if (codeString == null || "".equals(codeString)) 855 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.NULL, code); 856 if ("amended".equals(codeString)) 857 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.AMENDED, code); 858 if ("appended".equals(codeString)) 859 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.APPENDED, code); 860 if ("cancelled".equals(codeString)) 861 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.CANCELLED, code); 862 if ("disputed".equals(codeString)) 863 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.DISPUTED, code); 864 if ("entered-in-error".equals(codeString)) 865 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.ENTEREDINERROR, code); 866 if ("executable".equals(codeString)) 867 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.EXECUTABLE, code); 868 if ("executed".equals(codeString)) 869 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.EXECUTED, code); 870 if ("negotiable".equals(codeString)) 871 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.NEGOTIABLE, code); 872 if ("offered".equals(codeString)) 873 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.OFFERED, code); 874 if ("policy".equals(codeString)) 875 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.POLICY, code); 876 if ("rejected".equals(codeString)) 877 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.REJECTED, code); 878 if ("renewed".equals(codeString)) 879 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.RENEWED, code); 880 if ("revoked".equals(codeString)) 881 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.REVOKED, code); 882 if ("resolved".equals(codeString)) 883 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.RESOLVED, code); 884 if ("terminated".equals(codeString)) 885 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.TERMINATED, code); 886 throw new FHIRException("Unknown ContractPublicationStatus code '" + codeString + "'"); 887 } 888 889 public String toCode(ContractPublicationStatus code) { 890 if (code == ContractPublicationStatus.NULL) 891 return null; 892 if (code == ContractPublicationStatus.AMENDED) 893 return "amended"; 894 if (code == ContractPublicationStatus.APPENDED) 895 return "appended"; 896 if (code == ContractPublicationStatus.CANCELLED) 897 return "cancelled"; 898 if (code == ContractPublicationStatus.DISPUTED) 899 return "disputed"; 900 if (code == ContractPublicationStatus.ENTEREDINERROR) 901 return "entered-in-error"; 902 if (code == ContractPublicationStatus.EXECUTABLE) 903 return "executable"; 904 if (code == ContractPublicationStatus.EXECUTED) 905 return "executed"; 906 if (code == ContractPublicationStatus.NEGOTIABLE) 907 return "negotiable"; 908 if (code == ContractPublicationStatus.OFFERED) 909 return "offered"; 910 if (code == ContractPublicationStatus.POLICY) 911 return "policy"; 912 if (code == ContractPublicationStatus.REJECTED) 913 return "rejected"; 914 if (code == ContractPublicationStatus.RENEWED) 915 return "renewed"; 916 if (code == ContractPublicationStatus.REVOKED) 917 return "revoked"; 918 if (code == ContractPublicationStatus.RESOLVED) 919 return "resolved"; 920 if (code == ContractPublicationStatus.TERMINATED) 921 return "terminated"; 922 return "?"; 923 } 924 925 public String toSystem(ContractPublicationStatus code) { 926 return code.getSystem(); 927 } 928 } 929 930 @Block() 931 public static class ContentDefinitionComponent extends BackboneElement implements IBaseBackboneElement { 932 /** 933 * Precusory content structure and use, i.e., a boilerplate, template, 934 * application for a contract such as an insurance policy or benefits under a 935 * program, e.g., workers compensation. 936 */ 937 @Child(name = "type", type = { 938 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 939 @Description(shortDefinition = "Content structure and use", formalDefinition = "Precusory content structure and use, i.e., a boilerplate, template, application for a contract such as an insurance policy or benefits under a program, e.g., workers compensation.") 940 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-definition-type") 941 protected CodeableConcept type; 942 943 /** 944 * Detailed Precusory content type. 945 */ 946 @Child(name = "subType", type = { 947 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 948 @Description(shortDefinition = "Detailed Content Type Definition", formalDefinition = "Detailed Precusory content type.") 949 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-definition-subtype") 950 protected CodeableConcept subType; 951 952 /** 953 * The individual or organization that published the Contract precursor content. 954 */ 955 @Child(name = "publisher", type = { Practitioner.class, PractitionerRole.class, 956 Organization.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 957 @Description(shortDefinition = "Publisher Entity", formalDefinition = "The individual or organization that published the Contract precursor content.") 958 protected Reference publisher; 959 960 /** 961 * The actual object that is the target of the reference (The individual or 962 * organization that published the Contract precursor content.) 963 */ 964 protected Resource publisherTarget; 965 966 /** 967 * The date (and optionally time) when the contract was published. The date must 968 * change when the business version changes and it must change if the status 969 * code changes. In addition, it should change when the substantive content of 970 * the contract changes. 971 */ 972 @Child(name = "publicationDate", type = { 973 DateTimeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 974 @Description(shortDefinition = "When published", formalDefinition = "The date (and optionally time) when the contract was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the contract changes.") 975 protected DateTimeType publicationDate; 976 977 /** 978 * amended | appended | cancelled | disputed | entered-in-error | executable | 979 * executed | negotiable | offered | policy | rejected | renewed | revoked | 980 * resolved | terminated. 981 */ 982 @Child(name = "publicationStatus", type = { 983 CodeType.class }, order = 5, min = 1, max = 1, modifier = false, summary = false) 984 @Description(shortDefinition = "amended | appended | cancelled | disputed | entered-in-error | executable | executed | negotiable | offered | policy | rejected | renewed | revoked | resolved | terminated", formalDefinition = "amended | appended | cancelled | disputed | entered-in-error | executable | executed | negotiable | offered | policy | rejected | renewed | revoked | resolved | terminated.") 985 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-publicationstatus") 986 protected Enumeration<ContractPublicationStatus> publicationStatus; 987 988 /** 989 * A copyright statement relating to Contract precursor content. Copyright 990 * statements are generally legal restrictions on the use and publishing of the 991 * Contract precursor content. 992 */ 993 @Child(name = "copyright", type = { 994 MarkdownType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 995 @Description(shortDefinition = "Publication Ownership", formalDefinition = "A copyright statement relating to Contract precursor content. Copyright statements are generally legal restrictions on the use and publishing of the Contract precursor content.") 996 protected MarkdownType copyright; 997 998 private static final long serialVersionUID = -699592864L; 999 1000 /** 1001 * Constructor 1002 */ 1003 public ContentDefinitionComponent() { 1004 super(); 1005 } 1006 1007 /** 1008 * Constructor 1009 */ 1010 public ContentDefinitionComponent(CodeableConcept type, Enumeration<ContractPublicationStatus> publicationStatus) { 1011 super(); 1012 this.type = type; 1013 this.publicationStatus = publicationStatus; 1014 } 1015 1016 /** 1017 * @return {@link #type} (Precusory content structure and use, i.e., a 1018 * boilerplate, template, application for a contract such as an 1019 * insurance policy or benefits under a program, e.g., workers 1020 * compensation.) 1021 */ 1022 public CodeableConcept getType() { 1023 if (this.type == null) 1024 if (Configuration.errorOnAutoCreate()) 1025 throw new Error("Attempt to auto-create ContentDefinitionComponent.type"); 1026 else if (Configuration.doAutoCreate()) 1027 this.type = new CodeableConcept(); // cc 1028 return this.type; 1029 } 1030 1031 public boolean hasType() { 1032 return this.type != null && !this.type.isEmpty(); 1033 } 1034 1035 /** 1036 * @param value {@link #type} (Precusory content structure and use, i.e., a 1037 * boilerplate, template, application for a contract such as an 1038 * insurance policy or benefits under a program, e.g., workers 1039 * compensation.) 1040 */ 1041 public ContentDefinitionComponent setType(CodeableConcept value) { 1042 this.type = value; 1043 return this; 1044 } 1045 1046 /** 1047 * @return {@link #subType} (Detailed Precusory content type.) 1048 */ 1049 public CodeableConcept getSubType() { 1050 if (this.subType == null) 1051 if (Configuration.errorOnAutoCreate()) 1052 throw new Error("Attempt to auto-create ContentDefinitionComponent.subType"); 1053 else if (Configuration.doAutoCreate()) 1054 this.subType = new CodeableConcept(); // cc 1055 return this.subType; 1056 } 1057 1058 public boolean hasSubType() { 1059 return this.subType != null && !this.subType.isEmpty(); 1060 } 1061 1062 /** 1063 * @param value {@link #subType} (Detailed Precusory content type.) 1064 */ 1065 public ContentDefinitionComponent setSubType(CodeableConcept value) { 1066 this.subType = value; 1067 return this; 1068 } 1069 1070 /** 1071 * @return {@link #publisher} (The individual or organization that published the 1072 * Contract precursor content.) 1073 */ 1074 public Reference getPublisher() { 1075 if (this.publisher == null) 1076 if (Configuration.errorOnAutoCreate()) 1077 throw new Error("Attempt to auto-create ContentDefinitionComponent.publisher"); 1078 else if (Configuration.doAutoCreate()) 1079 this.publisher = new Reference(); // cc 1080 return this.publisher; 1081 } 1082 1083 public boolean hasPublisher() { 1084 return this.publisher != null && !this.publisher.isEmpty(); 1085 } 1086 1087 /** 1088 * @param value {@link #publisher} (The individual or organization that 1089 * published the Contract precursor content.) 1090 */ 1091 public ContentDefinitionComponent setPublisher(Reference value) { 1092 this.publisher = value; 1093 return this; 1094 } 1095 1096 /** 1097 * @return {@link #publisher} The actual object that is the target of the 1098 * reference. The reference library doesn't populate this, but you can 1099 * use it to hold the resource if you resolve it. (The individual or 1100 * organization that published the Contract precursor content.) 1101 */ 1102 public Resource getPublisherTarget() { 1103 return this.publisherTarget; 1104 } 1105 1106 /** 1107 * @param value {@link #publisher} The actual object that is the target of the 1108 * reference. The reference library doesn't use these, but you can 1109 * use it to hold the resource if you resolve it. (The individual 1110 * or organization that published the Contract precursor content.) 1111 */ 1112 public ContentDefinitionComponent setPublisherTarget(Resource value) { 1113 this.publisherTarget = value; 1114 return this; 1115 } 1116 1117 /** 1118 * @return {@link #publicationDate} (The date (and optionally time) when the 1119 * contract was published. The date must change when the business 1120 * version changes and it must change if the status code changes. In 1121 * addition, it should change when the substantive content of the 1122 * contract changes.). This is the underlying object with id, value and 1123 * extensions. The accessor "getPublicationDate" gives direct access to 1124 * the value 1125 */ 1126 public DateTimeType getPublicationDateElement() { 1127 if (this.publicationDate == null) 1128 if (Configuration.errorOnAutoCreate()) 1129 throw new Error("Attempt to auto-create ContentDefinitionComponent.publicationDate"); 1130 else if (Configuration.doAutoCreate()) 1131 this.publicationDate = new DateTimeType(); // bb 1132 return this.publicationDate; 1133 } 1134 1135 public boolean hasPublicationDateElement() { 1136 return this.publicationDate != null && !this.publicationDate.isEmpty(); 1137 } 1138 1139 public boolean hasPublicationDate() { 1140 return this.publicationDate != null && !this.publicationDate.isEmpty(); 1141 } 1142 1143 /** 1144 * @param value {@link #publicationDate} (The date (and optionally time) when 1145 * the contract was published. The date must change when the 1146 * business version changes and it must change if the status code 1147 * changes. In addition, it should change when the substantive 1148 * content of the contract changes.). This is the underlying object 1149 * with id, value and extensions. The accessor "getPublicationDate" 1150 * gives direct access to the value 1151 */ 1152 public ContentDefinitionComponent setPublicationDateElement(DateTimeType value) { 1153 this.publicationDate = value; 1154 return this; 1155 } 1156 1157 /** 1158 * @return The date (and optionally time) when the contract was published. The 1159 * date must change when the business version changes and it must change 1160 * if the status code changes. In addition, it should change when the 1161 * substantive content of the contract changes. 1162 */ 1163 public Date getPublicationDate() { 1164 return this.publicationDate == null ? null : this.publicationDate.getValue(); 1165 } 1166 1167 /** 1168 * @param value The date (and optionally time) when the contract was published. 1169 * The date must change when the business version changes and it 1170 * must change if the status code changes. In addition, it should 1171 * change when the substantive content of the contract changes. 1172 */ 1173 public ContentDefinitionComponent setPublicationDate(Date value) { 1174 if (value == null) 1175 this.publicationDate = null; 1176 else { 1177 if (this.publicationDate == null) 1178 this.publicationDate = new DateTimeType(); 1179 this.publicationDate.setValue(value); 1180 } 1181 return this; 1182 } 1183 1184 /** 1185 * @return {@link #publicationStatus} (amended | appended | cancelled | disputed 1186 * | entered-in-error | executable | executed | negotiable | offered | 1187 * policy | rejected | renewed | revoked | resolved | terminated.). This 1188 * is the underlying object with id, value and extensions. The accessor 1189 * "getPublicationStatus" gives direct access to the value 1190 */ 1191 public Enumeration<ContractPublicationStatus> getPublicationStatusElement() { 1192 if (this.publicationStatus == null) 1193 if (Configuration.errorOnAutoCreate()) 1194 throw new Error("Attempt to auto-create ContentDefinitionComponent.publicationStatus"); 1195 else if (Configuration.doAutoCreate()) 1196 this.publicationStatus = new Enumeration<ContractPublicationStatus>( 1197 new ContractPublicationStatusEnumFactory()); // bb 1198 return this.publicationStatus; 1199 } 1200 1201 public boolean hasPublicationStatusElement() { 1202 return this.publicationStatus != null && !this.publicationStatus.isEmpty(); 1203 } 1204 1205 public boolean hasPublicationStatus() { 1206 return this.publicationStatus != null && !this.publicationStatus.isEmpty(); 1207 } 1208 1209 /** 1210 * @param value {@link #publicationStatus} (amended | appended | cancelled | 1211 * disputed | entered-in-error | executable | executed | negotiable 1212 * | offered | policy | rejected | renewed | revoked | resolved | 1213 * terminated.). This is the underlying object with id, value and 1214 * extensions. The accessor "getPublicationStatus" gives direct 1215 * access to the value 1216 */ 1217 public ContentDefinitionComponent setPublicationStatusElement(Enumeration<ContractPublicationStatus> value) { 1218 this.publicationStatus = value; 1219 return this; 1220 } 1221 1222 /** 1223 * @return amended | appended | cancelled | disputed | entered-in-error | 1224 * executable | executed | negotiable | offered | policy | rejected | 1225 * renewed | revoked | resolved | terminated. 1226 */ 1227 public ContractPublicationStatus getPublicationStatus() { 1228 return this.publicationStatus == null ? null : this.publicationStatus.getValue(); 1229 } 1230 1231 /** 1232 * @param value amended | appended | cancelled | disputed | entered-in-error | 1233 * executable | executed | negotiable | offered | policy | rejected 1234 * | renewed | revoked | resolved | terminated. 1235 */ 1236 public ContentDefinitionComponent setPublicationStatus(ContractPublicationStatus value) { 1237 if (this.publicationStatus == null) 1238 this.publicationStatus = new Enumeration<ContractPublicationStatus>(new ContractPublicationStatusEnumFactory()); 1239 this.publicationStatus.setValue(value); 1240 return this; 1241 } 1242 1243 /** 1244 * @return {@link #copyright} (A copyright statement relating to Contract 1245 * precursor content. Copyright statements are generally legal 1246 * restrictions on the use and publishing of the Contract precursor 1247 * content.). This is the underlying object with id, value and 1248 * extensions. The accessor "getCopyright" gives direct access to the 1249 * value 1250 */ 1251 public MarkdownType getCopyrightElement() { 1252 if (this.copyright == null) 1253 if (Configuration.errorOnAutoCreate()) 1254 throw new Error("Attempt to auto-create ContentDefinitionComponent.copyright"); 1255 else if (Configuration.doAutoCreate()) 1256 this.copyright = new MarkdownType(); // bb 1257 return this.copyright; 1258 } 1259 1260 public boolean hasCopyrightElement() { 1261 return this.copyright != null && !this.copyright.isEmpty(); 1262 } 1263 1264 public boolean hasCopyright() { 1265 return this.copyright != null && !this.copyright.isEmpty(); 1266 } 1267 1268 /** 1269 * @param value {@link #copyright} (A copyright statement relating to Contract 1270 * precursor content. Copyright statements are generally legal 1271 * restrictions on the use and publishing of the Contract precursor 1272 * content.). This is the underlying object with id, value and 1273 * extensions. The accessor "getCopyright" gives direct access to 1274 * the value 1275 */ 1276 public ContentDefinitionComponent setCopyrightElement(MarkdownType value) { 1277 this.copyright = value; 1278 return this; 1279 } 1280 1281 /** 1282 * @return A copyright statement relating to Contract precursor content. 1283 * Copyright statements are generally legal restrictions on the use and 1284 * publishing of the Contract precursor content. 1285 */ 1286 public String getCopyright() { 1287 return this.copyright == null ? null : this.copyright.getValue(); 1288 } 1289 1290 /** 1291 * @param value A copyright statement relating to Contract precursor content. 1292 * Copyright statements are generally legal restrictions on the use 1293 * and publishing of the Contract precursor content. 1294 */ 1295 public ContentDefinitionComponent setCopyright(String value) { 1296 if (value == null) 1297 this.copyright = null; 1298 else { 1299 if (this.copyright == null) 1300 this.copyright = new MarkdownType(); 1301 this.copyright.setValue(value); 1302 } 1303 return this; 1304 } 1305 1306 protected void listChildren(List<Property> children) { 1307 super.listChildren(children); 1308 children.add(new Property("type", "CodeableConcept", 1309 "Precusory content structure and use, i.e., a boilerplate, template, application for a contract such as an insurance policy or benefits under a program, e.g., workers compensation.", 1310 0, 1, type)); 1311 children.add(new Property("subType", "CodeableConcept", "Detailed Precusory content type.", 0, 1, subType)); 1312 children.add(new Property("publisher", "Reference(Practitioner|PractitionerRole|Organization)", 1313 "The individual or organization that published the Contract precursor content.", 0, 1, publisher)); 1314 children.add(new Property("publicationDate", "dateTime", 1315 "The date (and optionally time) when the contract was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the contract changes.", 1316 0, 1, publicationDate)); 1317 children.add(new Property("publicationStatus", "code", 1318 "amended | appended | cancelled | disputed | entered-in-error | executable | executed | negotiable | offered | policy | rejected | renewed | revoked | resolved | terminated.", 1319 0, 1, publicationStatus)); 1320 children.add(new Property("copyright", "markdown", 1321 "A copyright statement relating to Contract precursor content. Copyright statements are generally legal restrictions on the use and publishing of the Contract precursor content.", 1322 0, 1, copyright)); 1323 } 1324 1325 @Override 1326 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1327 switch (_hash) { 1328 case 3575610: 1329 /* type */ return new Property("type", "CodeableConcept", 1330 "Precusory content structure and use, i.e., a boilerplate, template, application for a contract such as an insurance policy or benefits under a program, e.g., workers compensation.", 1331 0, 1, type); 1332 case -1868521062: 1333 /* subType */ return new Property("subType", "CodeableConcept", "Detailed Precusory content type.", 0, 1, 1334 subType); 1335 case 1447404028: 1336 /* publisher */ return new Property("publisher", "Reference(Practitioner|PractitionerRole|Organization)", 1337 "The individual or organization that published the Contract precursor content.", 0, 1, publisher); 1338 case 1470566394: 1339 /* publicationDate */ return new Property("publicationDate", "dateTime", 1340 "The date (and optionally time) when the contract was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the contract changes.", 1341 0, 1, publicationDate); 1342 case 616500542: 1343 /* publicationStatus */ return new Property("publicationStatus", "code", 1344 "amended | appended | cancelled | disputed | entered-in-error | executable | executed | negotiable | offered | policy | rejected | renewed | revoked | resolved | terminated.", 1345 0, 1, publicationStatus); 1346 case 1522889671: 1347 /* copyright */ return new Property("copyright", "markdown", 1348 "A copyright statement relating to Contract precursor content. Copyright statements are generally legal restrictions on the use and publishing of the Contract precursor content.", 1349 0, 1, copyright); 1350 default: 1351 return super.getNamedProperty(_hash, _name, _checkValid); 1352 } 1353 1354 } 1355 1356 @Override 1357 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1358 switch (hash) { 1359 case 3575610: 1360 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1361 case -1868521062: 1362 /* subType */ return this.subType == null ? new Base[0] : new Base[] { this.subType }; // CodeableConcept 1363 case 1447404028: 1364 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // Reference 1365 case 1470566394: 1366 /* publicationDate */ return this.publicationDate == null ? new Base[0] : new Base[] { this.publicationDate }; // DateTimeType 1367 case 616500542: 1368 /* publicationStatus */ return this.publicationStatus == null ? new Base[0] 1369 : new Base[] { this.publicationStatus }; // Enumeration<ContractPublicationStatus> 1370 case 1522889671: 1371 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 1372 default: 1373 return super.getProperty(hash, name, checkValid); 1374 } 1375 1376 } 1377 1378 @Override 1379 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1380 switch (hash) { 1381 case 3575610: // type 1382 this.type = castToCodeableConcept(value); // CodeableConcept 1383 return value; 1384 case -1868521062: // subType 1385 this.subType = castToCodeableConcept(value); // CodeableConcept 1386 return value; 1387 case 1447404028: // publisher 1388 this.publisher = castToReference(value); // Reference 1389 return value; 1390 case 1470566394: // publicationDate 1391 this.publicationDate = castToDateTime(value); // DateTimeType 1392 return value; 1393 case 616500542: // publicationStatus 1394 value = new ContractPublicationStatusEnumFactory().fromType(castToCode(value)); 1395 this.publicationStatus = (Enumeration) value; // Enumeration<ContractPublicationStatus> 1396 return value; 1397 case 1522889671: // copyright 1398 this.copyright = castToMarkdown(value); // MarkdownType 1399 return value; 1400 default: 1401 return super.setProperty(hash, name, value); 1402 } 1403 1404 } 1405 1406 @Override 1407 public Base setProperty(String name, Base value) throws FHIRException { 1408 if (name.equals("type")) { 1409 this.type = castToCodeableConcept(value); // CodeableConcept 1410 } else if (name.equals("subType")) { 1411 this.subType = castToCodeableConcept(value); // CodeableConcept 1412 } else if (name.equals("publisher")) { 1413 this.publisher = castToReference(value); // Reference 1414 } else if (name.equals("publicationDate")) { 1415 this.publicationDate = castToDateTime(value); // DateTimeType 1416 } else if (name.equals("publicationStatus")) { 1417 value = new ContractPublicationStatusEnumFactory().fromType(castToCode(value)); 1418 this.publicationStatus = (Enumeration) value; // Enumeration<ContractPublicationStatus> 1419 } else if (name.equals("copyright")) { 1420 this.copyright = castToMarkdown(value); // MarkdownType 1421 } else 1422 return super.setProperty(name, value); 1423 return value; 1424 } 1425 1426 @Override 1427 public void removeChild(String name, Base value) throws FHIRException { 1428 if (name.equals("type")) { 1429 this.type = null; 1430 } else if (name.equals("subType")) { 1431 this.subType = null; 1432 } else if (name.equals("publisher")) { 1433 this.publisher = null; 1434 } else if (name.equals("publicationDate")) { 1435 this.publicationDate = null; 1436 } else if (name.equals("publicationStatus")) { 1437 this.publicationStatus = null; 1438 } else if (name.equals("copyright")) { 1439 this.copyright = null; 1440 } else 1441 super.removeChild(name, value); 1442 1443 } 1444 1445 @Override 1446 public Base makeProperty(int hash, String name) throws FHIRException { 1447 switch (hash) { 1448 case 3575610: 1449 return getType(); 1450 case -1868521062: 1451 return getSubType(); 1452 case 1447404028: 1453 return getPublisher(); 1454 case 1470566394: 1455 return getPublicationDateElement(); 1456 case 616500542: 1457 return getPublicationStatusElement(); 1458 case 1522889671: 1459 return getCopyrightElement(); 1460 default: 1461 return super.makeProperty(hash, name); 1462 } 1463 1464 } 1465 1466 @Override 1467 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1468 switch (hash) { 1469 case 3575610: 1470 /* type */ return new String[] { "CodeableConcept" }; 1471 case -1868521062: 1472 /* subType */ return new String[] { "CodeableConcept" }; 1473 case 1447404028: 1474 /* publisher */ return new String[] { "Reference" }; 1475 case 1470566394: 1476 /* publicationDate */ return new String[] { "dateTime" }; 1477 case 616500542: 1478 /* publicationStatus */ return new String[] { "code" }; 1479 case 1522889671: 1480 /* copyright */ return new String[] { "markdown" }; 1481 default: 1482 return super.getTypesForProperty(hash, name); 1483 } 1484 1485 } 1486 1487 @Override 1488 public Base addChild(String name) throws FHIRException { 1489 if (name.equals("type")) { 1490 this.type = new CodeableConcept(); 1491 return this.type; 1492 } else if (name.equals("subType")) { 1493 this.subType = new CodeableConcept(); 1494 return this.subType; 1495 } else if (name.equals("publisher")) { 1496 this.publisher = new Reference(); 1497 return this.publisher; 1498 } else if (name.equals("publicationDate")) { 1499 throw new FHIRException("Cannot call addChild on a singleton property Contract.publicationDate"); 1500 } else if (name.equals("publicationStatus")) { 1501 throw new FHIRException("Cannot call addChild on a singleton property Contract.publicationStatus"); 1502 } else if (name.equals("copyright")) { 1503 throw new FHIRException("Cannot call addChild on a singleton property Contract.copyright"); 1504 } else 1505 return super.addChild(name); 1506 } 1507 1508 public ContentDefinitionComponent copy() { 1509 ContentDefinitionComponent dst = new ContentDefinitionComponent(); 1510 copyValues(dst); 1511 return dst; 1512 } 1513 1514 public void copyValues(ContentDefinitionComponent dst) { 1515 super.copyValues(dst); 1516 dst.type = type == null ? null : type.copy(); 1517 dst.subType = subType == null ? null : subType.copy(); 1518 dst.publisher = publisher == null ? null : publisher.copy(); 1519 dst.publicationDate = publicationDate == null ? null : publicationDate.copy(); 1520 dst.publicationStatus = publicationStatus == null ? null : publicationStatus.copy(); 1521 dst.copyright = copyright == null ? null : copyright.copy(); 1522 } 1523 1524 @Override 1525 public boolean equalsDeep(Base other_) { 1526 if (!super.equalsDeep(other_)) 1527 return false; 1528 if (!(other_ instanceof ContentDefinitionComponent)) 1529 return false; 1530 ContentDefinitionComponent o = (ContentDefinitionComponent) other_; 1531 return compareDeep(type, o.type, true) && compareDeep(subType, o.subType, true) 1532 && compareDeep(publisher, o.publisher, true) && compareDeep(publicationDate, o.publicationDate, true) 1533 && compareDeep(publicationStatus, o.publicationStatus, true) && compareDeep(copyright, o.copyright, true); 1534 } 1535 1536 @Override 1537 public boolean equalsShallow(Base other_) { 1538 if (!super.equalsShallow(other_)) 1539 return false; 1540 if (!(other_ instanceof ContentDefinitionComponent)) 1541 return false; 1542 ContentDefinitionComponent o = (ContentDefinitionComponent) other_; 1543 return compareValues(publicationDate, o.publicationDate, true) 1544 && compareValues(publicationStatus, o.publicationStatus, true) && compareValues(copyright, o.copyright, true); 1545 } 1546 1547 public boolean isEmpty() { 1548 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, subType, publisher, publicationDate, 1549 publicationStatus, copyright); 1550 } 1551 1552 public String fhirType() { 1553 return "Contract.contentDefinition"; 1554 1555 } 1556 1557 } 1558 1559 @Block() 1560 public static class TermComponent extends BackboneElement implements IBaseBackboneElement { 1561 /** 1562 * Unique identifier for this particular Contract Provision. 1563 */ 1564 @Child(name = "identifier", type = { 1565 Identifier.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1566 @Description(shortDefinition = "Contract Term Number", formalDefinition = "Unique identifier for this particular Contract Provision.") 1567 protected Identifier identifier; 1568 1569 /** 1570 * When this Contract Provision was issued. 1571 */ 1572 @Child(name = "issued", type = { 1573 DateTimeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1574 @Description(shortDefinition = "Contract Term Issue Date Time", formalDefinition = "When this Contract Provision was issued.") 1575 protected DateTimeType issued; 1576 1577 /** 1578 * Relevant time or time-period when this Contract Provision is applicable. 1579 */ 1580 @Child(name = "applies", type = { Period.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1581 @Description(shortDefinition = "Contract Term Effective Time", formalDefinition = "Relevant time or time-period when this Contract Provision is applicable.") 1582 protected Period applies; 1583 1584 /** 1585 * The entity that the term applies to. 1586 */ 1587 @Child(name = "topic", type = { CodeableConcept.class, 1588 Reference.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1589 @Description(shortDefinition = "Term Concern", formalDefinition = "The entity that the term applies to.") 1590 protected Type topic; 1591 1592 /** 1593 * A legal clause or condition contained within a contract that requires one or 1594 * both parties to perform a particular requirement by some specified time or 1595 * prevents one or both parties from performing a particular requirement by some 1596 * specified time. 1597 */ 1598 @Child(name = "type", type = { 1599 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1600 @Description(shortDefinition = "Contract Term Type or Form", formalDefinition = "A legal clause or condition contained within a contract that requires one or both parties to perform a particular requirement by some specified time or prevents one or both parties from performing a particular requirement by some specified time.") 1601 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-term-type") 1602 protected CodeableConcept type; 1603 1604 /** 1605 * A specialized legal clause or condition based on overarching contract type. 1606 */ 1607 @Child(name = "subType", type = { 1608 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1609 @Description(shortDefinition = "Contract Term Type specific classification", formalDefinition = "A specialized legal clause or condition based on overarching contract type.") 1610 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-term-subtype") 1611 protected CodeableConcept subType; 1612 1613 /** 1614 * Statement of a provision in a policy or a contract. 1615 */ 1616 @Child(name = "text", type = { StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1617 @Description(shortDefinition = "Term Statement", formalDefinition = "Statement of a provision in a policy or a contract.") 1618 protected StringType text; 1619 1620 /** 1621 * Security labels that protect the handling of information about the term and 1622 * its elements, which may be specifically identified.. 1623 */ 1624 @Child(name = "securityLabel", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1625 @Description(shortDefinition = "Protection for the Term", formalDefinition = "Security labels that protect the handling of information about the term and its elements, which may be specifically identified..") 1626 protected List<SecurityLabelComponent> securityLabel; 1627 1628 /** 1629 * The matter of concern in the context of this provision of the agrement. 1630 */ 1631 @Child(name = "offer", type = {}, order = 9, min = 1, max = 1, modifier = false, summary = false) 1632 @Description(shortDefinition = "Context of the Contract term", formalDefinition = "The matter of concern in the context of this provision of the agrement.") 1633 protected ContractOfferComponent offer; 1634 1635 /** 1636 * Contract Term Asset List. 1637 */ 1638 @Child(name = "asset", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1639 @Description(shortDefinition = "Contract Term Asset List", formalDefinition = "Contract Term Asset List.") 1640 protected List<ContractAssetComponent> asset; 1641 1642 /** 1643 * An actor taking a role in an activity for which it can be assigned some 1644 * degree of responsibility for the activity taking place. 1645 */ 1646 @Child(name = "action", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1647 @Description(shortDefinition = "Entity being ascribed responsibility", formalDefinition = "An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.") 1648 protected List<ActionComponent> action; 1649 1650 /** 1651 * Nested group of Contract Provisions. 1652 */ 1653 @Child(name = "group", type = { 1654 TermComponent.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1655 @Description(shortDefinition = "Nested Contract Term Group", formalDefinition = "Nested group of Contract Provisions.") 1656 protected List<TermComponent> group; 1657 1658 private static final long serialVersionUID = -460907186L; 1659 1660 /** 1661 * Constructor 1662 */ 1663 public TermComponent() { 1664 super(); 1665 } 1666 1667 /** 1668 * Constructor 1669 */ 1670 public TermComponent(ContractOfferComponent offer) { 1671 super(); 1672 this.offer = offer; 1673 } 1674 1675 /** 1676 * @return {@link #identifier} (Unique identifier for this particular Contract 1677 * Provision.) 1678 */ 1679 public Identifier getIdentifier() { 1680 if (this.identifier == null) 1681 if (Configuration.errorOnAutoCreate()) 1682 throw new Error("Attempt to auto-create TermComponent.identifier"); 1683 else if (Configuration.doAutoCreate()) 1684 this.identifier = new Identifier(); // cc 1685 return this.identifier; 1686 } 1687 1688 public boolean hasIdentifier() { 1689 return this.identifier != null && !this.identifier.isEmpty(); 1690 } 1691 1692 /** 1693 * @param value {@link #identifier} (Unique identifier for this particular 1694 * Contract Provision.) 1695 */ 1696 public TermComponent setIdentifier(Identifier value) { 1697 this.identifier = value; 1698 return this; 1699 } 1700 1701 /** 1702 * @return {@link #issued} (When this Contract Provision was issued.). This is 1703 * the underlying object with id, value and extensions. The accessor 1704 * "getIssued" gives direct access to the value 1705 */ 1706 public DateTimeType getIssuedElement() { 1707 if (this.issued == null) 1708 if (Configuration.errorOnAutoCreate()) 1709 throw new Error("Attempt to auto-create TermComponent.issued"); 1710 else if (Configuration.doAutoCreate()) 1711 this.issued = new DateTimeType(); // bb 1712 return this.issued; 1713 } 1714 1715 public boolean hasIssuedElement() { 1716 return this.issued != null && !this.issued.isEmpty(); 1717 } 1718 1719 public boolean hasIssued() { 1720 return this.issued != null && !this.issued.isEmpty(); 1721 } 1722 1723 /** 1724 * @param value {@link #issued} (When this Contract Provision was issued.). This 1725 * is the underlying object with id, value and extensions. The 1726 * accessor "getIssued" gives direct access to the value 1727 */ 1728 public TermComponent setIssuedElement(DateTimeType value) { 1729 this.issued = value; 1730 return this; 1731 } 1732 1733 /** 1734 * @return When this Contract Provision was issued. 1735 */ 1736 public Date getIssued() { 1737 return this.issued == null ? null : this.issued.getValue(); 1738 } 1739 1740 /** 1741 * @param value When this Contract Provision was issued. 1742 */ 1743 public TermComponent setIssued(Date value) { 1744 if (value == null) 1745 this.issued = null; 1746 else { 1747 if (this.issued == null) 1748 this.issued = new DateTimeType(); 1749 this.issued.setValue(value); 1750 } 1751 return this; 1752 } 1753 1754 /** 1755 * @return {@link #applies} (Relevant time or time-period when this Contract 1756 * Provision is applicable.) 1757 */ 1758 public Period getApplies() { 1759 if (this.applies == null) 1760 if (Configuration.errorOnAutoCreate()) 1761 throw new Error("Attempt to auto-create TermComponent.applies"); 1762 else if (Configuration.doAutoCreate()) 1763 this.applies = new Period(); // cc 1764 return this.applies; 1765 } 1766 1767 public boolean hasApplies() { 1768 return this.applies != null && !this.applies.isEmpty(); 1769 } 1770 1771 /** 1772 * @param value {@link #applies} (Relevant time or time-period when this 1773 * Contract Provision is applicable.) 1774 */ 1775 public TermComponent setApplies(Period value) { 1776 this.applies = value; 1777 return this; 1778 } 1779 1780 /** 1781 * @return {@link #topic} (The entity that the term applies to.) 1782 */ 1783 public Type getTopic() { 1784 return this.topic; 1785 } 1786 1787 /** 1788 * @return {@link #topic} (The entity that the term applies to.) 1789 */ 1790 public CodeableConcept getTopicCodeableConcept() throws FHIRException { 1791 if (this.topic == null) 1792 this.topic = new CodeableConcept(); 1793 if (!(this.topic instanceof CodeableConcept)) 1794 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1795 + this.topic.getClass().getName() + " was encountered"); 1796 return (CodeableConcept) this.topic; 1797 } 1798 1799 public boolean hasTopicCodeableConcept() { 1800 return this != null && this.topic instanceof CodeableConcept; 1801 } 1802 1803 /** 1804 * @return {@link #topic} (The entity that the term applies to.) 1805 */ 1806 public Reference getTopicReference() throws FHIRException { 1807 if (this.topic == null) 1808 this.topic = new Reference(); 1809 if (!(this.topic instanceof Reference)) 1810 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.topic.getClass().getName() 1811 + " was encountered"); 1812 return (Reference) this.topic; 1813 } 1814 1815 public boolean hasTopicReference() { 1816 return this != null && this.topic instanceof Reference; 1817 } 1818 1819 public boolean hasTopic() { 1820 return this.topic != null && !this.topic.isEmpty(); 1821 } 1822 1823 /** 1824 * @param value {@link #topic} (The entity that the term applies to.) 1825 */ 1826 public TermComponent setTopic(Type value) { 1827 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1828 throw new Error("Not the right type for Contract.term.topic[x]: " + value.fhirType()); 1829 this.topic = value; 1830 return this; 1831 } 1832 1833 /** 1834 * @return {@link #type} (A legal clause or condition contained within a 1835 * contract that requires one or both parties to perform a particular 1836 * requirement by some specified time or prevents one or both parties 1837 * from performing a particular requirement by some specified time.) 1838 */ 1839 public CodeableConcept getType() { 1840 if (this.type == null) 1841 if (Configuration.errorOnAutoCreate()) 1842 throw new Error("Attempt to auto-create TermComponent.type"); 1843 else if (Configuration.doAutoCreate()) 1844 this.type = new CodeableConcept(); // cc 1845 return this.type; 1846 } 1847 1848 public boolean hasType() { 1849 return this.type != null && !this.type.isEmpty(); 1850 } 1851 1852 /** 1853 * @param value {@link #type} (A legal clause or condition contained within a 1854 * contract that requires one or both parties to perform a 1855 * particular requirement by some specified time or prevents one or 1856 * both parties from performing a particular requirement by some 1857 * specified time.) 1858 */ 1859 public TermComponent setType(CodeableConcept value) { 1860 this.type = value; 1861 return this; 1862 } 1863 1864 /** 1865 * @return {@link #subType} (A specialized legal clause or condition based on 1866 * overarching contract type.) 1867 */ 1868 public CodeableConcept getSubType() { 1869 if (this.subType == null) 1870 if (Configuration.errorOnAutoCreate()) 1871 throw new Error("Attempt to auto-create TermComponent.subType"); 1872 else if (Configuration.doAutoCreate()) 1873 this.subType = new CodeableConcept(); // cc 1874 return this.subType; 1875 } 1876 1877 public boolean hasSubType() { 1878 return this.subType != null && !this.subType.isEmpty(); 1879 } 1880 1881 /** 1882 * @param value {@link #subType} (A specialized legal clause or condition based 1883 * on overarching contract type.) 1884 */ 1885 public TermComponent setSubType(CodeableConcept value) { 1886 this.subType = value; 1887 return this; 1888 } 1889 1890 /** 1891 * @return {@link #text} (Statement of a provision in a policy or a contract.). 1892 * This is the underlying object with id, value and extensions. The 1893 * accessor "getText" gives direct access to the value 1894 */ 1895 public StringType getTextElement() { 1896 if (this.text == null) 1897 if (Configuration.errorOnAutoCreate()) 1898 throw new Error("Attempt to auto-create TermComponent.text"); 1899 else if (Configuration.doAutoCreate()) 1900 this.text = new StringType(); // bb 1901 return this.text; 1902 } 1903 1904 public boolean hasTextElement() { 1905 return this.text != null && !this.text.isEmpty(); 1906 } 1907 1908 public boolean hasText() { 1909 return this.text != null && !this.text.isEmpty(); 1910 } 1911 1912 /** 1913 * @param value {@link #text} (Statement of a provision in a policy or a 1914 * contract.). This is the underlying object with id, value and 1915 * extensions. The accessor "getText" gives direct access to the 1916 * value 1917 */ 1918 public TermComponent setTextElement(StringType value) { 1919 this.text = value; 1920 return this; 1921 } 1922 1923 /** 1924 * @return Statement of a provision in a policy or a contract. 1925 */ 1926 public String getText() { 1927 return this.text == null ? null : this.text.getValue(); 1928 } 1929 1930 /** 1931 * @param value Statement of a provision in a policy or a contract. 1932 */ 1933 public TermComponent setText(String value) { 1934 if (Utilities.noString(value)) 1935 this.text = null; 1936 else { 1937 if (this.text == null) 1938 this.text = new StringType(); 1939 this.text.setValue(value); 1940 } 1941 return this; 1942 } 1943 1944 /** 1945 * @return {@link #securityLabel} (Security labels that protect the handling of 1946 * information about the term and its elements, which may be 1947 * specifically identified..) 1948 */ 1949 public List<SecurityLabelComponent> getSecurityLabel() { 1950 if (this.securityLabel == null) 1951 this.securityLabel = new ArrayList<SecurityLabelComponent>(); 1952 return this.securityLabel; 1953 } 1954 1955 /** 1956 * @return Returns a reference to <code>this</code> for easy method chaining 1957 */ 1958 public TermComponent setSecurityLabel(List<SecurityLabelComponent> theSecurityLabel) { 1959 this.securityLabel = theSecurityLabel; 1960 return this; 1961 } 1962 1963 public boolean hasSecurityLabel() { 1964 if (this.securityLabel == null) 1965 return false; 1966 for (SecurityLabelComponent item : this.securityLabel) 1967 if (!item.isEmpty()) 1968 return true; 1969 return false; 1970 } 1971 1972 public SecurityLabelComponent addSecurityLabel() { // 3 1973 SecurityLabelComponent t = new SecurityLabelComponent(); 1974 if (this.securityLabel == null) 1975 this.securityLabel = new ArrayList<SecurityLabelComponent>(); 1976 this.securityLabel.add(t); 1977 return t; 1978 } 1979 1980 public TermComponent addSecurityLabel(SecurityLabelComponent t) { // 3 1981 if (t == null) 1982 return this; 1983 if (this.securityLabel == null) 1984 this.securityLabel = new ArrayList<SecurityLabelComponent>(); 1985 this.securityLabel.add(t); 1986 return this; 1987 } 1988 1989 /** 1990 * @return The first repetition of repeating field {@link #securityLabel}, 1991 * creating it if it does not already exist 1992 */ 1993 public SecurityLabelComponent getSecurityLabelFirstRep() { 1994 if (getSecurityLabel().isEmpty()) { 1995 addSecurityLabel(); 1996 } 1997 return getSecurityLabel().get(0); 1998 } 1999 2000 /** 2001 * @return {@link #offer} (The matter of concern in the context of this 2002 * provision of the agrement.) 2003 */ 2004 public ContractOfferComponent getOffer() { 2005 if (this.offer == null) 2006 if (Configuration.errorOnAutoCreate()) 2007 throw new Error("Attempt to auto-create TermComponent.offer"); 2008 else if (Configuration.doAutoCreate()) 2009 this.offer = new ContractOfferComponent(); // cc 2010 return this.offer; 2011 } 2012 2013 public boolean hasOffer() { 2014 return this.offer != null && !this.offer.isEmpty(); 2015 } 2016 2017 /** 2018 * @param value {@link #offer} (The matter of concern in the context of this 2019 * provision of the agrement.) 2020 */ 2021 public TermComponent setOffer(ContractOfferComponent value) { 2022 this.offer = value; 2023 return this; 2024 } 2025 2026 /** 2027 * @return {@link #asset} (Contract Term Asset List.) 2028 */ 2029 public List<ContractAssetComponent> getAsset() { 2030 if (this.asset == null) 2031 this.asset = new ArrayList<ContractAssetComponent>(); 2032 return this.asset; 2033 } 2034 2035 /** 2036 * @return Returns a reference to <code>this</code> for easy method chaining 2037 */ 2038 public TermComponent setAsset(List<ContractAssetComponent> theAsset) { 2039 this.asset = theAsset; 2040 return this; 2041 } 2042 2043 public boolean hasAsset() { 2044 if (this.asset == null) 2045 return false; 2046 for (ContractAssetComponent item : this.asset) 2047 if (!item.isEmpty()) 2048 return true; 2049 return false; 2050 } 2051 2052 public ContractAssetComponent addAsset() { // 3 2053 ContractAssetComponent t = new ContractAssetComponent(); 2054 if (this.asset == null) 2055 this.asset = new ArrayList<ContractAssetComponent>(); 2056 this.asset.add(t); 2057 return t; 2058 } 2059 2060 public TermComponent addAsset(ContractAssetComponent t) { // 3 2061 if (t == null) 2062 return this; 2063 if (this.asset == null) 2064 this.asset = new ArrayList<ContractAssetComponent>(); 2065 this.asset.add(t); 2066 return this; 2067 } 2068 2069 /** 2070 * @return The first repetition of repeating field {@link #asset}, creating it 2071 * if it does not already exist 2072 */ 2073 public ContractAssetComponent getAssetFirstRep() { 2074 if (getAsset().isEmpty()) { 2075 addAsset(); 2076 } 2077 return getAsset().get(0); 2078 } 2079 2080 /** 2081 * @return {@link #action} (An actor taking a role in an activity for which it 2082 * can be assigned some degree of responsibility for the activity taking 2083 * place.) 2084 */ 2085 public List<ActionComponent> getAction() { 2086 if (this.action == null) 2087 this.action = new ArrayList<ActionComponent>(); 2088 return this.action; 2089 } 2090 2091 /** 2092 * @return Returns a reference to <code>this</code> for easy method chaining 2093 */ 2094 public TermComponent setAction(List<ActionComponent> theAction) { 2095 this.action = theAction; 2096 return this; 2097 } 2098 2099 public boolean hasAction() { 2100 if (this.action == null) 2101 return false; 2102 for (ActionComponent item : this.action) 2103 if (!item.isEmpty()) 2104 return true; 2105 return false; 2106 } 2107 2108 public ActionComponent addAction() { // 3 2109 ActionComponent t = new ActionComponent(); 2110 if (this.action == null) 2111 this.action = new ArrayList<ActionComponent>(); 2112 this.action.add(t); 2113 return t; 2114 } 2115 2116 public TermComponent addAction(ActionComponent t) { // 3 2117 if (t == null) 2118 return this; 2119 if (this.action == null) 2120 this.action = new ArrayList<ActionComponent>(); 2121 this.action.add(t); 2122 return this; 2123 } 2124 2125 /** 2126 * @return The first repetition of repeating field {@link #action}, creating it 2127 * if it does not already exist 2128 */ 2129 public ActionComponent getActionFirstRep() { 2130 if (getAction().isEmpty()) { 2131 addAction(); 2132 } 2133 return getAction().get(0); 2134 } 2135 2136 /** 2137 * @return {@link #group} (Nested group of Contract Provisions.) 2138 */ 2139 public List<TermComponent> getGroup() { 2140 if (this.group == null) 2141 this.group = new ArrayList<TermComponent>(); 2142 return this.group; 2143 } 2144 2145 /** 2146 * @return Returns a reference to <code>this</code> for easy method chaining 2147 */ 2148 public TermComponent setGroup(List<TermComponent> theGroup) { 2149 this.group = theGroup; 2150 return this; 2151 } 2152 2153 public boolean hasGroup() { 2154 if (this.group == null) 2155 return false; 2156 for (TermComponent item : this.group) 2157 if (!item.isEmpty()) 2158 return true; 2159 return false; 2160 } 2161 2162 public TermComponent addGroup() { // 3 2163 TermComponent t = new TermComponent(); 2164 if (this.group == null) 2165 this.group = new ArrayList<TermComponent>(); 2166 this.group.add(t); 2167 return t; 2168 } 2169 2170 public TermComponent addGroup(TermComponent t) { // 3 2171 if (t == null) 2172 return this; 2173 if (this.group == null) 2174 this.group = new ArrayList<TermComponent>(); 2175 this.group.add(t); 2176 return this; 2177 } 2178 2179 /** 2180 * @return The first repetition of repeating field {@link #group}, creating it 2181 * if it does not already exist 2182 */ 2183 public TermComponent getGroupFirstRep() { 2184 if (getGroup().isEmpty()) { 2185 addGroup(); 2186 } 2187 return getGroup().get(0); 2188 } 2189 2190 protected void listChildren(List<Property> children) { 2191 super.listChildren(children); 2192 children.add(new Property("identifier", "Identifier", "Unique identifier for this particular Contract Provision.", 2193 0, 1, identifier)); 2194 children.add(new Property("issued", "dateTime", "When this Contract Provision was issued.", 0, 1, issued)); 2195 children.add(new Property("applies", "Period", 2196 "Relevant time or time-period when this Contract Provision is applicable.", 0, 1, applies)); 2197 children.add(new Property("topic[x]", "CodeableConcept|Reference(Any)", "The entity that the term applies to.", 0, 2198 1, topic)); 2199 children.add(new Property("type", "CodeableConcept", 2200 "A legal clause or condition contained within a contract that requires one or both parties to perform a particular requirement by some specified time or prevents one or both parties from performing a particular requirement by some specified time.", 2201 0, 1, type)); 2202 children.add(new Property("subType", "CodeableConcept", 2203 "A specialized legal clause or condition based on overarching contract type.", 0, 1, subType)); 2204 children.add(new Property("text", "string", "Statement of a provision in a policy or a contract.", 0, 1, text)); 2205 children.add(new Property("securityLabel", "", 2206 "Security labels that protect the handling of information about the term and its elements, which may be specifically identified..", 2207 0, java.lang.Integer.MAX_VALUE, securityLabel)); 2208 children.add(new Property("offer", "", "The matter of concern in the context of this provision of the agrement.", 2209 0, 1, offer)); 2210 children.add(new Property("asset", "", "Contract Term Asset List.", 0, java.lang.Integer.MAX_VALUE, asset)); 2211 children.add(new Property("action", "", 2212 "An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.", 2213 0, java.lang.Integer.MAX_VALUE, action)); 2214 children.add(new Property("group", "@Contract.term", "Nested group of Contract Provisions.", 0, 2215 java.lang.Integer.MAX_VALUE, group)); 2216 } 2217 2218 @Override 2219 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2220 switch (_hash) { 2221 case -1618432855: 2222 /* identifier */ return new Property("identifier", "Identifier", 2223 "Unique identifier for this particular Contract Provision.", 0, 1, identifier); 2224 case -1179159893: 2225 /* issued */ return new Property("issued", "dateTime", "When this Contract Provision was issued.", 0, 1, 2226 issued); 2227 case -793235316: 2228 /* applies */ return new Property("applies", "Period", 2229 "Relevant time or time-period when this Contract Provision is applicable.", 0, 1, applies); 2230 case -957295375: 2231 /* topic[x] */ return new Property("topic[x]", "CodeableConcept|Reference(Any)", 2232 "The entity that the term applies to.", 0, 1, topic); 2233 case 110546223: 2234 /* topic */ return new Property("topic[x]", "CodeableConcept|Reference(Any)", 2235 "The entity that the term applies to.", 0, 1, topic); 2236 case 777778802: 2237 /* topicCodeableConcept */ return new Property("topic[x]", "CodeableConcept|Reference(Any)", 2238 "The entity that the term applies to.", 0, 1, topic); 2239 case -343345444: 2240 /* topicReference */ return new Property("topic[x]", "CodeableConcept|Reference(Any)", 2241 "The entity that the term applies to.", 0, 1, topic); 2242 case 3575610: 2243 /* type */ return new Property("type", "CodeableConcept", 2244 "A legal clause or condition contained within a contract that requires one or both parties to perform a particular requirement by some specified time or prevents one or both parties from performing a particular requirement by some specified time.", 2245 0, 1, type); 2246 case -1868521062: 2247 /* subType */ return new Property("subType", "CodeableConcept", 2248 "A specialized legal clause or condition based on overarching contract type.", 0, 1, subType); 2249 case 3556653: 2250 /* text */ return new Property("text", "string", "Statement of a provision in a policy or a contract.", 0, 1, 2251 text); 2252 case -722296940: 2253 /* securityLabel */ return new Property("securityLabel", "", 2254 "Security labels that protect the handling of information about the term and its elements, which may be specifically identified..", 2255 0, java.lang.Integer.MAX_VALUE, securityLabel); 2256 case 105650780: 2257 /* offer */ return new Property("offer", "", 2258 "The matter of concern in the context of this provision of the agrement.", 0, 1, offer); 2259 case 93121264: 2260 /* asset */ return new Property("asset", "", "Contract Term Asset List.", 0, java.lang.Integer.MAX_VALUE, 2261 asset); 2262 case -1422950858: 2263 /* action */ return new Property("action", "", 2264 "An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.", 2265 0, java.lang.Integer.MAX_VALUE, action); 2266 case 98629247: 2267 /* group */ return new Property("group", "@Contract.term", "Nested group of Contract Provisions.", 0, 2268 java.lang.Integer.MAX_VALUE, group); 2269 default: 2270 return super.getNamedProperty(_hash, _name, _checkValid); 2271 } 2272 2273 } 2274 2275 @Override 2276 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2277 switch (hash) { 2278 case -1618432855: 2279 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 2280 case -1179159893: 2281 /* issued */ return this.issued == null ? new Base[0] : new Base[] { this.issued }; // DateTimeType 2282 case -793235316: 2283 /* applies */ return this.applies == null ? new Base[0] : new Base[] { this.applies }; // Period 2284 case 110546223: 2285 /* topic */ return this.topic == null ? new Base[0] : new Base[] { this.topic }; // Type 2286 case 3575610: 2287 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2288 case -1868521062: 2289 /* subType */ return this.subType == null ? new Base[0] : new Base[] { this.subType }; // CodeableConcept 2290 case 3556653: 2291 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType 2292 case -722296940: 2293 /* securityLabel */ return this.securityLabel == null ? new Base[0] 2294 : this.securityLabel.toArray(new Base[this.securityLabel.size()]); // SecurityLabelComponent 2295 case 105650780: 2296 /* offer */ return this.offer == null ? new Base[0] : new Base[] { this.offer }; // ContractOfferComponent 2297 case 93121264: 2298 /* asset */ return this.asset == null ? new Base[0] : this.asset.toArray(new Base[this.asset.size()]); // ContractAssetComponent 2299 case -1422950858: 2300 /* action */ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // ActionComponent 2301 case 98629247: 2302 /* group */ return this.group == null ? new Base[0] : this.group.toArray(new Base[this.group.size()]); // TermComponent 2303 default: 2304 return super.getProperty(hash, name, checkValid); 2305 } 2306 2307 } 2308 2309 @Override 2310 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2311 switch (hash) { 2312 case -1618432855: // identifier 2313 this.identifier = castToIdentifier(value); // Identifier 2314 return value; 2315 case -1179159893: // issued 2316 this.issued = castToDateTime(value); // DateTimeType 2317 return value; 2318 case -793235316: // applies 2319 this.applies = castToPeriod(value); // Period 2320 return value; 2321 case 110546223: // topic 2322 this.topic = castToType(value); // Type 2323 return value; 2324 case 3575610: // type 2325 this.type = castToCodeableConcept(value); // CodeableConcept 2326 return value; 2327 case -1868521062: // subType 2328 this.subType = castToCodeableConcept(value); // CodeableConcept 2329 return value; 2330 case 3556653: // text 2331 this.text = castToString(value); // StringType 2332 return value; 2333 case -722296940: // securityLabel 2334 this.getSecurityLabel().add((SecurityLabelComponent) value); // SecurityLabelComponent 2335 return value; 2336 case 105650780: // offer 2337 this.offer = (ContractOfferComponent) value; // ContractOfferComponent 2338 return value; 2339 case 93121264: // asset 2340 this.getAsset().add((ContractAssetComponent) value); // ContractAssetComponent 2341 return value; 2342 case -1422950858: // action 2343 this.getAction().add((ActionComponent) value); // ActionComponent 2344 return value; 2345 case 98629247: // group 2346 this.getGroup().add((TermComponent) value); // TermComponent 2347 return value; 2348 default: 2349 return super.setProperty(hash, name, value); 2350 } 2351 2352 } 2353 2354 @Override 2355 public Base setProperty(String name, Base value) throws FHIRException { 2356 if (name.equals("identifier")) { 2357 this.identifier = castToIdentifier(value); // Identifier 2358 } else if (name.equals("issued")) { 2359 this.issued = castToDateTime(value); // DateTimeType 2360 } else if (name.equals("applies")) { 2361 this.applies = castToPeriod(value); // Period 2362 } else if (name.equals("topic[x]")) { 2363 this.topic = castToType(value); // Type 2364 } else if (name.equals("type")) { 2365 this.type = castToCodeableConcept(value); // CodeableConcept 2366 } else if (name.equals("subType")) { 2367 this.subType = castToCodeableConcept(value); // CodeableConcept 2368 } else if (name.equals("text")) { 2369 this.text = castToString(value); // StringType 2370 } else if (name.equals("securityLabel")) { 2371 this.getSecurityLabel().add((SecurityLabelComponent) value); 2372 } else if (name.equals("offer")) { 2373 this.offer = (ContractOfferComponent) value; // ContractOfferComponent 2374 } else if (name.equals("asset")) { 2375 this.getAsset().add((ContractAssetComponent) value); 2376 } else if (name.equals("action")) { 2377 this.getAction().add((ActionComponent) value); 2378 } else if (name.equals("group")) { 2379 this.getGroup().add((TermComponent) value); 2380 } else 2381 return super.setProperty(name, value); 2382 return value; 2383 } 2384 2385 @Override 2386 public void removeChild(String name, Base value) throws FHIRException { 2387 if (name.equals("identifier")) { 2388 this.identifier = null; 2389 } else if (name.equals("issued")) { 2390 this.issued = null; 2391 } else if (name.equals("applies")) { 2392 this.applies = null; 2393 } else if (name.equals("topic[x]")) { 2394 this.topic = null; 2395 } else if (name.equals("type")) { 2396 this.type = null; 2397 } else if (name.equals("subType")) { 2398 this.subType = null; 2399 } else if (name.equals("text")) { 2400 this.text = null; 2401 } else if (name.equals("securityLabel")) { 2402 this.getSecurityLabel().remove((SecurityLabelComponent) value); 2403 } else if (name.equals("offer")) { 2404 this.offer = (ContractOfferComponent) value; // ContractOfferComponent 2405 } else if (name.equals("asset")) { 2406 this.getAsset().remove((ContractAssetComponent) value); 2407 } else if (name.equals("action")) { 2408 this.getAction().remove((ActionComponent) value); 2409 } else if (name.equals("group")) { 2410 this.getGroup().remove((TermComponent) value); 2411 } else 2412 super.removeChild(name, value); 2413 2414 } 2415 2416 @Override 2417 public Base makeProperty(int hash, String name) throws FHIRException { 2418 switch (hash) { 2419 case -1618432855: 2420 return getIdentifier(); 2421 case -1179159893: 2422 return getIssuedElement(); 2423 case -793235316: 2424 return getApplies(); 2425 case -957295375: 2426 return getTopic(); 2427 case 110546223: 2428 return getTopic(); 2429 case 3575610: 2430 return getType(); 2431 case -1868521062: 2432 return getSubType(); 2433 case 3556653: 2434 return getTextElement(); 2435 case -722296940: 2436 return addSecurityLabel(); 2437 case 105650780: 2438 return getOffer(); 2439 case 93121264: 2440 return addAsset(); 2441 case -1422950858: 2442 return addAction(); 2443 case 98629247: 2444 return addGroup(); 2445 default: 2446 return super.makeProperty(hash, name); 2447 } 2448 2449 } 2450 2451 @Override 2452 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2453 switch (hash) { 2454 case -1618432855: 2455 /* identifier */ return new String[] { "Identifier" }; 2456 case -1179159893: 2457 /* issued */ return new String[] { "dateTime" }; 2458 case -793235316: 2459 /* applies */ return new String[] { "Period" }; 2460 case 110546223: 2461 /* topic */ return new String[] { "CodeableConcept", "Reference" }; 2462 case 3575610: 2463 /* type */ return new String[] { "CodeableConcept" }; 2464 case -1868521062: 2465 /* subType */ return new String[] { "CodeableConcept" }; 2466 case 3556653: 2467 /* text */ return new String[] { "string" }; 2468 case -722296940: 2469 /* securityLabel */ return new String[] {}; 2470 case 105650780: 2471 /* offer */ return new String[] {}; 2472 case 93121264: 2473 /* asset */ return new String[] {}; 2474 case -1422950858: 2475 /* action */ return new String[] {}; 2476 case 98629247: 2477 /* group */ return new String[] { "@Contract.term" }; 2478 default: 2479 return super.getTypesForProperty(hash, name); 2480 } 2481 2482 } 2483 2484 @Override 2485 public Base addChild(String name) throws FHIRException { 2486 if (name.equals("identifier")) { 2487 this.identifier = new Identifier(); 2488 return this.identifier; 2489 } else if (name.equals("issued")) { 2490 throw new FHIRException("Cannot call addChild on a singleton property Contract.issued"); 2491 } else if (name.equals("applies")) { 2492 this.applies = new Period(); 2493 return this.applies; 2494 } else if (name.equals("topicCodeableConcept")) { 2495 this.topic = new CodeableConcept(); 2496 return this.topic; 2497 } else if (name.equals("topicReference")) { 2498 this.topic = new Reference(); 2499 return this.topic; 2500 } else if (name.equals("type")) { 2501 this.type = new CodeableConcept(); 2502 return this.type; 2503 } else if (name.equals("subType")) { 2504 this.subType = new CodeableConcept(); 2505 return this.subType; 2506 } else if (name.equals("text")) { 2507 throw new FHIRException("Cannot call addChild on a singleton property Contract.text"); 2508 } else if (name.equals("securityLabel")) { 2509 return addSecurityLabel(); 2510 } else if (name.equals("offer")) { 2511 this.offer = new ContractOfferComponent(); 2512 return this.offer; 2513 } else if (name.equals("asset")) { 2514 return addAsset(); 2515 } else if (name.equals("action")) { 2516 return addAction(); 2517 } else if (name.equals("group")) { 2518 return addGroup(); 2519 } else 2520 return super.addChild(name); 2521 } 2522 2523 public TermComponent copy() { 2524 TermComponent dst = new TermComponent(); 2525 copyValues(dst); 2526 return dst; 2527 } 2528 2529 public void copyValues(TermComponent dst) { 2530 super.copyValues(dst); 2531 dst.identifier = identifier == null ? null : identifier.copy(); 2532 dst.issued = issued == null ? null : issued.copy(); 2533 dst.applies = applies == null ? null : applies.copy(); 2534 dst.topic = topic == null ? null : topic.copy(); 2535 dst.type = type == null ? null : type.copy(); 2536 dst.subType = subType == null ? null : subType.copy(); 2537 dst.text = text == null ? null : text.copy(); 2538 if (securityLabel != null) { 2539 dst.securityLabel = new ArrayList<SecurityLabelComponent>(); 2540 for (SecurityLabelComponent i : securityLabel) 2541 dst.securityLabel.add(i.copy()); 2542 } 2543 ; 2544 dst.offer = offer == null ? null : offer.copy(); 2545 if (asset != null) { 2546 dst.asset = new ArrayList<ContractAssetComponent>(); 2547 for (ContractAssetComponent i : asset) 2548 dst.asset.add(i.copy()); 2549 } 2550 ; 2551 if (action != null) { 2552 dst.action = new ArrayList<ActionComponent>(); 2553 for (ActionComponent i : action) 2554 dst.action.add(i.copy()); 2555 } 2556 ; 2557 if (group != null) { 2558 dst.group = new ArrayList<TermComponent>(); 2559 for (TermComponent i : group) 2560 dst.group.add(i.copy()); 2561 } 2562 ; 2563 } 2564 2565 @Override 2566 public boolean equalsDeep(Base other_) { 2567 if (!super.equalsDeep(other_)) 2568 return false; 2569 if (!(other_ instanceof TermComponent)) 2570 return false; 2571 TermComponent o = (TermComponent) other_; 2572 return compareDeep(identifier, o.identifier, true) && compareDeep(issued, o.issued, true) 2573 && compareDeep(applies, o.applies, true) && compareDeep(topic, o.topic, true) 2574 && compareDeep(type, o.type, true) && compareDeep(subType, o.subType, true) && compareDeep(text, o.text, true) 2575 && compareDeep(securityLabel, o.securityLabel, true) && compareDeep(offer, o.offer, true) 2576 && compareDeep(asset, o.asset, true) && compareDeep(action, o.action, true) 2577 && compareDeep(group, o.group, true); 2578 } 2579 2580 @Override 2581 public boolean equalsShallow(Base other_) { 2582 if (!super.equalsShallow(other_)) 2583 return false; 2584 if (!(other_ instanceof TermComponent)) 2585 return false; 2586 TermComponent o = (TermComponent) other_; 2587 return compareValues(issued, o.issued, true) && compareValues(text, o.text, true); 2588 } 2589 2590 public boolean isEmpty() { 2591 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, issued, applies, topic, type, subType, 2592 text, securityLabel, offer, asset, action, group); 2593 } 2594 2595 public String fhirType() { 2596 return "Contract.term"; 2597 2598 } 2599 2600 } 2601 2602 @Block() 2603 public static class SecurityLabelComponent extends BackboneElement implements IBaseBackboneElement { 2604 /** 2605 * Number used to link this term or term element to the applicable Security 2606 * Label. 2607 */ 2608 @Child(name = "number", type = { 2609 UnsignedIntType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2610 @Description(shortDefinition = "Link to Security Labels", formalDefinition = "Number used to link this term or term element to the applicable Security Label.") 2611 protected List<UnsignedIntType> number; 2612 2613 /** 2614 * Security label privacy tag that species the level of confidentiality 2615 * protection required for this term and/or term elements. 2616 */ 2617 @Child(name = "classification", type = { 2618 Coding.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 2619 @Description(shortDefinition = "Confidentiality Protection", formalDefinition = "Security label privacy tag that species the level of confidentiality protection required for this term and/or term elements.") 2620 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-security-classification") 2621 protected Coding classification; 2622 2623 /** 2624 * Security label privacy tag that species the applicable privacy and security 2625 * policies governing this term and/or term elements. 2626 */ 2627 @Child(name = "category", type = { 2628 Coding.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2629 @Description(shortDefinition = "Applicable Policy", formalDefinition = "Security label privacy tag that species the applicable privacy and security policies governing this term and/or term elements.") 2630 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-security-category") 2631 protected List<Coding> category; 2632 2633 /** 2634 * Security label privacy tag that species the manner in which term and/or term 2635 * elements are to be protected. 2636 */ 2637 @Child(name = "control", type = { 2638 Coding.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2639 @Description(shortDefinition = "Handling Instructions", formalDefinition = "Security label privacy tag that species the manner in which term and/or term elements are to be protected.") 2640 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-security-control") 2641 protected List<Coding> control; 2642 2643 private static final long serialVersionUID = 788281758L; 2644 2645 /** 2646 * Constructor 2647 */ 2648 public SecurityLabelComponent() { 2649 super(); 2650 } 2651 2652 /** 2653 * Constructor 2654 */ 2655 public SecurityLabelComponent(Coding classification) { 2656 super(); 2657 this.classification = classification; 2658 } 2659 2660 /** 2661 * @return {@link #number} (Number used to link this term or term element to the 2662 * applicable Security Label.) 2663 */ 2664 public List<UnsignedIntType> getNumber() { 2665 if (this.number == null) 2666 this.number = new ArrayList<UnsignedIntType>(); 2667 return this.number; 2668 } 2669 2670 /** 2671 * @return Returns a reference to <code>this</code> for easy method chaining 2672 */ 2673 public SecurityLabelComponent setNumber(List<UnsignedIntType> theNumber) { 2674 this.number = theNumber; 2675 return this; 2676 } 2677 2678 public boolean hasNumber() { 2679 if (this.number == null) 2680 return false; 2681 for (UnsignedIntType item : this.number) 2682 if (!item.isEmpty()) 2683 return true; 2684 return false; 2685 } 2686 2687 /** 2688 * @return {@link #number} (Number used to link this term or term element to the 2689 * applicable Security Label.) 2690 */ 2691 public UnsignedIntType addNumberElement() {// 2 2692 UnsignedIntType t = new UnsignedIntType(); 2693 if (this.number == null) 2694 this.number = new ArrayList<UnsignedIntType>(); 2695 this.number.add(t); 2696 return t; 2697 } 2698 2699 /** 2700 * @param value {@link #number} (Number used to link this term or term element 2701 * to the applicable Security Label.) 2702 */ 2703 public SecurityLabelComponent addNumber(int value) { // 1 2704 UnsignedIntType t = new UnsignedIntType(); 2705 t.setValue(value); 2706 if (this.number == null) 2707 this.number = new ArrayList<UnsignedIntType>(); 2708 this.number.add(t); 2709 return this; 2710 } 2711 2712 /** 2713 * @param value {@link #number} (Number used to link this term or term element 2714 * to the applicable Security Label.) 2715 */ 2716 public boolean hasNumber(int value) { 2717 if (this.number == null) 2718 return false; 2719 for (UnsignedIntType v : this.number) 2720 if (v.getValue().equals(value)) // unsignedInt 2721 return true; 2722 return false; 2723 } 2724 2725 /** 2726 * @return {@link #classification} (Security label privacy tag that species the 2727 * level of confidentiality protection required for this term and/or 2728 * term elements.) 2729 */ 2730 public Coding getClassification() { 2731 if (this.classification == null) 2732 if (Configuration.errorOnAutoCreate()) 2733 throw new Error("Attempt to auto-create SecurityLabelComponent.classification"); 2734 else if (Configuration.doAutoCreate()) 2735 this.classification = new Coding(); // cc 2736 return this.classification; 2737 } 2738 2739 public boolean hasClassification() { 2740 return this.classification != null && !this.classification.isEmpty(); 2741 } 2742 2743 /** 2744 * @param value {@link #classification} (Security label privacy tag that species 2745 * the level of confidentiality protection required for this term 2746 * and/or term elements.) 2747 */ 2748 public SecurityLabelComponent setClassification(Coding value) { 2749 this.classification = value; 2750 return this; 2751 } 2752 2753 /** 2754 * @return {@link #category} (Security label privacy tag that species the 2755 * applicable privacy and security policies governing this term and/or 2756 * term elements.) 2757 */ 2758 public List<Coding> getCategory() { 2759 if (this.category == null) 2760 this.category = new ArrayList<Coding>(); 2761 return this.category; 2762 } 2763 2764 /** 2765 * @return Returns a reference to <code>this</code> for easy method chaining 2766 */ 2767 public SecurityLabelComponent setCategory(List<Coding> theCategory) { 2768 this.category = theCategory; 2769 return this; 2770 } 2771 2772 public boolean hasCategory() { 2773 if (this.category == null) 2774 return false; 2775 for (Coding item : this.category) 2776 if (!item.isEmpty()) 2777 return true; 2778 return false; 2779 } 2780 2781 public Coding addCategory() { // 3 2782 Coding t = new Coding(); 2783 if (this.category == null) 2784 this.category = new ArrayList<Coding>(); 2785 this.category.add(t); 2786 return t; 2787 } 2788 2789 public SecurityLabelComponent addCategory(Coding t) { // 3 2790 if (t == null) 2791 return this; 2792 if (this.category == null) 2793 this.category = new ArrayList<Coding>(); 2794 this.category.add(t); 2795 return this; 2796 } 2797 2798 /** 2799 * @return The first repetition of repeating field {@link #category}, creating 2800 * it if it does not already exist 2801 */ 2802 public Coding getCategoryFirstRep() { 2803 if (getCategory().isEmpty()) { 2804 addCategory(); 2805 } 2806 return getCategory().get(0); 2807 } 2808 2809 /** 2810 * @return {@link #control} (Security label privacy tag that species the manner 2811 * in which term and/or term elements are to be protected.) 2812 */ 2813 public List<Coding> getControl() { 2814 if (this.control == null) 2815 this.control = new ArrayList<Coding>(); 2816 return this.control; 2817 } 2818 2819 /** 2820 * @return Returns a reference to <code>this</code> for easy method chaining 2821 */ 2822 public SecurityLabelComponent setControl(List<Coding> theControl) { 2823 this.control = theControl; 2824 return this; 2825 } 2826 2827 public boolean hasControl() { 2828 if (this.control == null) 2829 return false; 2830 for (Coding item : this.control) 2831 if (!item.isEmpty()) 2832 return true; 2833 return false; 2834 } 2835 2836 public Coding addControl() { // 3 2837 Coding t = new Coding(); 2838 if (this.control == null) 2839 this.control = new ArrayList<Coding>(); 2840 this.control.add(t); 2841 return t; 2842 } 2843 2844 public SecurityLabelComponent addControl(Coding t) { // 3 2845 if (t == null) 2846 return this; 2847 if (this.control == null) 2848 this.control = new ArrayList<Coding>(); 2849 this.control.add(t); 2850 return this; 2851 } 2852 2853 /** 2854 * @return The first repetition of repeating field {@link #control}, creating it 2855 * if it does not already exist 2856 */ 2857 public Coding getControlFirstRep() { 2858 if (getControl().isEmpty()) { 2859 addControl(); 2860 } 2861 return getControl().get(0); 2862 } 2863 2864 protected void listChildren(List<Property> children) { 2865 super.listChildren(children); 2866 children.add(new Property("number", "unsignedInt", 2867 "Number used to link this term or term element to the applicable Security Label.", 0, 2868 java.lang.Integer.MAX_VALUE, number)); 2869 children.add(new Property("classification", "Coding", 2870 "Security label privacy tag that species the level of confidentiality protection required for this term and/or term elements.", 2871 0, 1, classification)); 2872 children.add(new Property("category", "Coding", 2873 "Security label privacy tag that species the applicable privacy and security policies governing this term and/or term elements.", 2874 0, java.lang.Integer.MAX_VALUE, category)); 2875 children.add(new Property("control", "Coding", 2876 "Security label privacy tag that species the manner in which term and/or term elements are to be protected.", 2877 0, java.lang.Integer.MAX_VALUE, control)); 2878 } 2879 2880 @Override 2881 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2882 switch (_hash) { 2883 case -1034364087: 2884 /* number */ return new Property("number", "unsignedInt", 2885 "Number used to link this term or term element to the applicable Security Label.", 0, 2886 java.lang.Integer.MAX_VALUE, number); 2887 case 382350310: 2888 /* classification */ return new Property("classification", "Coding", 2889 "Security label privacy tag that species the level of confidentiality protection required for this term and/or term elements.", 2890 0, 1, classification); 2891 case 50511102: 2892 /* category */ return new Property("category", "Coding", 2893 "Security label privacy tag that species the applicable privacy and security policies governing this term and/or term elements.", 2894 0, java.lang.Integer.MAX_VALUE, category); 2895 case 951543133: 2896 /* control */ return new Property("control", "Coding", 2897 "Security label privacy tag that species the manner in which term and/or term elements are to be protected.", 2898 0, java.lang.Integer.MAX_VALUE, control); 2899 default: 2900 return super.getNamedProperty(_hash, _name, _checkValid); 2901 } 2902 2903 } 2904 2905 @Override 2906 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2907 switch (hash) { 2908 case -1034364087: 2909 /* number */ return this.number == null ? new Base[0] : this.number.toArray(new Base[this.number.size()]); // UnsignedIntType 2910 case 382350310: 2911 /* classification */ return this.classification == null ? new Base[0] : new Base[] { this.classification }; // Coding 2912 case 50511102: 2913 /* category */ return this.category == null ? new Base[0] 2914 : this.category.toArray(new Base[this.category.size()]); // Coding 2915 case 951543133: 2916 /* control */ return this.control == null ? new Base[0] : this.control.toArray(new Base[this.control.size()]); // Coding 2917 default: 2918 return super.getProperty(hash, name, checkValid); 2919 } 2920 2921 } 2922 2923 @Override 2924 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2925 switch (hash) { 2926 case -1034364087: // number 2927 this.getNumber().add(castToUnsignedInt(value)); // UnsignedIntType 2928 return value; 2929 case 382350310: // classification 2930 this.classification = castToCoding(value); // Coding 2931 return value; 2932 case 50511102: // category 2933 this.getCategory().add(castToCoding(value)); // Coding 2934 return value; 2935 case 951543133: // control 2936 this.getControl().add(castToCoding(value)); // Coding 2937 return value; 2938 default: 2939 return super.setProperty(hash, name, value); 2940 } 2941 2942 } 2943 2944 @Override 2945 public Base setProperty(String name, Base value) throws FHIRException { 2946 if (name.equals("number")) { 2947 this.getNumber().add(castToUnsignedInt(value)); 2948 } else if (name.equals("classification")) { 2949 this.classification = castToCoding(value); // Coding 2950 } else if (name.equals("category")) { 2951 this.getCategory().add(castToCoding(value)); 2952 } else if (name.equals("control")) { 2953 this.getControl().add(castToCoding(value)); 2954 } else 2955 return super.setProperty(name, value); 2956 return value; 2957 } 2958 2959 @Override 2960 public void removeChild(String name, Base value) throws FHIRException { 2961 if (name.equals("number")) { 2962 this.getNumber().remove(castToUnsignedInt(value)); 2963 } else if (name.equals("classification")) { 2964 this.classification = null; 2965 } else if (name.equals("category")) { 2966 this.getCategory().remove(castToCoding(value)); 2967 } else if (name.equals("control")) { 2968 this.getControl().remove(castToCoding(value)); 2969 } else 2970 super.removeChild(name, value); 2971 2972 } 2973 2974 @Override 2975 public Base makeProperty(int hash, String name) throws FHIRException { 2976 switch (hash) { 2977 case -1034364087: 2978 return addNumberElement(); 2979 case 382350310: 2980 return getClassification(); 2981 case 50511102: 2982 return addCategory(); 2983 case 951543133: 2984 return addControl(); 2985 default: 2986 return super.makeProperty(hash, name); 2987 } 2988 2989 } 2990 2991 @Override 2992 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2993 switch (hash) { 2994 case -1034364087: 2995 /* number */ return new String[] { "unsignedInt" }; 2996 case 382350310: 2997 /* classification */ return new String[] { "Coding" }; 2998 case 50511102: 2999 /* category */ return new String[] { "Coding" }; 3000 case 951543133: 3001 /* control */ return new String[] { "Coding" }; 3002 default: 3003 return super.getTypesForProperty(hash, name); 3004 } 3005 3006 } 3007 3008 @Override 3009 public Base addChild(String name) throws FHIRException { 3010 if (name.equals("number")) { 3011 throw new FHIRException("Cannot call addChild on a singleton property Contract.number"); 3012 } else if (name.equals("classification")) { 3013 this.classification = new Coding(); 3014 return this.classification; 3015 } else if (name.equals("category")) { 3016 return addCategory(); 3017 } else if (name.equals("control")) { 3018 return addControl(); 3019 } else 3020 return super.addChild(name); 3021 } 3022 3023 public SecurityLabelComponent copy() { 3024 SecurityLabelComponent dst = new SecurityLabelComponent(); 3025 copyValues(dst); 3026 return dst; 3027 } 3028 3029 public void copyValues(SecurityLabelComponent dst) { 3030 super.copyValues(dst); 3031 if (number != null) { 3032 dst.number = new ArrayList<UnsignedIntType>(); 3033 for (UnsignedIntType i : number) 3034 dst.number.add(i.copy()); 3035 } 3036 ; 3037 dst.classification = classification == null ? null : classification.copy(); 3038 if (category != null) { 3039 dst.category = new ArrayList<Coding>(); 3040 for (Coding i : category) 3041 dst.category.add(i.copy()); 3042 } 3043 ; 3044 if (control != null) { 3045 dst.control = new ArrayList<Coding>(); 3046 for (Coding i : control) 3047 dst.control.add(i.copy()); 3048 } 3049 ; 3050 } 3051 3052 @Override 3053 public boolean equalsDeep(Base other_) { 3054 if (!super.equalsDeep(other_)) 3055 return false; 3056 if (!(other_ instanceof SecurityLabelComponent)) 3057 return false; 3058 SecurityLabelComponent o = (SecurityLabelComponent) other_; 3059 return compareDeep(number, o.number, true) && compareDeep(classification, o.classification, true) 3060 && compareDeep(category, o.category, true) && compareDeep(control, o.control, true); 3061 } 3062 3063 @Override 3064 public boolean equalsShallow(Base other_) { 3065 if (!super.equalsShallow(other_)) 3066 return false; 3067 if (!(other_ instanceof SecurityLabelComponent)) 3068 return false; 3069 SecurityLabelComponent o = (SecurityLabelComponent) other_; 3070 return compareValues(number, o.number, true); 3071 } 3072 3073 public boolean isEmpty() { 3074 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(number, classification, category, control); 3075 } 3076 3077 public String fhirType() { 3078 return "Contract.term.securityLabel"; 3079 3080 } 3081 3082 } 3083 3084 @Block() 3085 public static class ContractOfferComponent extends BackboneElement implements IBaseBackboneElement { 3086 /** 3087 * Unique identifier for this particular Contract Provision. 3088 */ 3089 @Child(name = "identifier", type = { 3090 Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3091 @Description(shortDefinition = "Offer business ID", formalDefinition = "Unique identifier for this particular Contract Provision.") 3092 protected List<Identifier> identifier; 3093 3094 /** 3095 * Offer Recipient. 3096 */ 3097 @Child(name = "party", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3098 @Description(shortDefinition = "Offer Recipient", formalDefinition = "Offer Recipient.") 3099 protected List<ContractPartyComponent> party; 3100 3101 /** 3102 * The owner of an asset has the residual control rights over the asset: the 3103 * right to decide all usages of the asset in any way not inconsistent with a 3104 * prior contract, custom, or law (Hart, 1995, p. 30). 3105 */ 3106 @Child(name = "topic", type = { Reference.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 3107 @Description(shortDefinition = "Negotiable offer asset", formalDefinition = "The owner of an asset has the residual control rights over the asset: the right to decide all usages of the asset in any way not inconsistent with a prior contract, custom, or law (Hart, 1995, p. 30).") 3108 protected Reference topic; 3109 3110 /** 3111 * The actual object that is the target of the reference (The owner of an asset 3112 * has the residual control rights over the asset: the right to decide all 3113 * usages of the asset in any way not inconsistent with a prior contract, 3114 * custom, or law (Hart, 1995, p. 30).) 3115 */ 3116 protected Resource topicTarget; 3117 3118 /** 3119 * Type of Contract Provision such as specific requirements, purposes for 3120 * actions, obligations, prohibitions, e.g. life time maximum benefit. 3121 */ 3122 @Child(name = "type", type = { 3123 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 3124 @Description(shortDefinition = "Contract Offer Type or Form", formalDefinition = "Type of Contract Provision such as specific requirements, purposes for actions, obligations, prohibitions, e.g. life time maximum benefit.") 3125 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-term-type") 3126 protected CodeableConcept type; 3127 3128 /** 3129 * Type of choice made by accepting party with respect to an offer made by an 3130 * offeror/ grantee. 3131 */ 3132 @Child(name = "decision", type = { 3133 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 3134 @Description(shortDefinition = "Accepting party choice", formalDefinition = "Type of choice made by accepting party with respect to an offer made by an offeror/ grantee.") 3135 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ActConsentDirective") 3136 protected CodeableConcept decision; 3137 3138 /** 3139 * How the decision about a Contract was conveyed. 3140 */ 3141 @Child(name = "decisionMode", type = { 3142 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3143 @Description(shortDefinition = "How decision is conveyed", formalDefinition = "How the decision about a Contract was conveyed.") 3144 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-decision-mode") 3145 protected List<CodeableConcept> decisionMode; 3146 3147 /** 3148 * Response to offer text. 3149 */ 3150 @Child(name = "answer", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3151 @Description(shortDefinition = "Response to offer text", formalDefinition = "Response to offer text.") 3152 protected List<AnswerComponent> answer; 3153 3154 /** 3155 * Human readable form of this Contract Offer. 3156 */ 3157 @Child(name = "text", type = { StringType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 3158 @Description(shortDefinition = "Human readable offer text", formalDefinition = "Human readable form of this Contract Offer.") 3159 protected StringType text; 3160 3161 /** 3162 * The id of the clause or question text of the offer in the referenced 3163 * questionnaire/response. 3164 */ 3165 @Child(name = "linkId", type = { 3166 StringType.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3167 @Description(shortDefinition = "Pointer to text", formalDefinition = "The id of the clause or question text of the offer in the referenced questionnaire/response.") 3168 protected List<StringType> linkId; 3169 3170 /** 3171 * Security labels that protects the offer. 3172 */ 3173 @Child(name = "securityLabelNumber", type = { 3174 UnsignedIntType.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3175 @Description(shortDefinition = "Offer restriction numbers", formalDefinition = "Security labels that protects the offer.") 3176 protected List<UnsignedIntType> securityLabelNumber; 3177 3178 private static final long serialVersionUID = -395674449L; 3179 3180 /** 3181 * Constructor 3182 */ 3183 public ContractOfferComponent() { 3184 super(); 3185 } 3186 3187 /** 3188 * @return {@link #identifier} (Unique identifier for this particular Contract 3189 * Provision.) 3190 */ 3191 public List<Identifier> getIdentifier() { 3192 if (this.identifier == null) 3193 this.identifier = new ArrayList<Identifier>(); 3194 return this.identifier; 3195 } 3196 3197 /** 3198 * @return Returns a reference to <code>this</code> for easy method chaining 3199 */ 3200 public ContractOfferComponent setIdentifier(List<Identifier> theIdentifier) { 3201 this.identifier = theIdentifier; 3202 return this; 3203 } 3204 3205 public boolean hasIdentifier() { 3206 if (this.identifier == null) 3207 return false; 3208 for (Identifier item : this.identifier) 3209 if (!item.isEmpty()) 3210 return true; 3211 return false; 3212 } 3213 3214 public Identifier addIdentifier() { // 3 3215 Identifier t = new Identifier(); 3216 if (this.identifier == null) 3217 this.identifier = new ArrayList<Identifier>(); 3218 this.identifier.add(t); 3219 return t; 3220 } 3221 3222 public ContractOfferComponent addIdentifier(Identifier t) { // 3 3223 if (t == null) 3224 return this; 3225 if (this.identifier == null) 3226 this.identifier = new ArrayList<Identifier>(); 3227 this.identifier.add(t); 3228 return this; 3229 } 3230 3231 /** 3232 * @return The first repetition of repeating field {@link #identifier}, creating 3233 * it if it does not already exist 3234 */ 3235 public Identifier getIdentifierFirstRep() { 3236 if (getIdentifier().isEmpty()) { 3237 addIdentifier(); 3238 } 3239 return getIdentifier().get(0); 3240 } 3241 3242 /** 3243 * @return {@link #party} (Offer Recipient.) 3244 */ 3245 public List<ContractPartyComponent> getParty() { 3246 if (this.party == null) 3247 this.party = new ArrayList<ContractPartyComponent>(); 3248 return this.party; 3249 } 3250 3251 /** 3252 * @return Returns a reference to <code>this</code> for easy method chaining 3253 */ 3254 public ContractOfferComponent setParty(List<ContractPartyComponent> theParty) { 3255 this.party = theParty; 3256 return this; 3257 } 3258 3259 public boolean hasParty() { 3260 if (this.party == null) 3261 return false; 3262 for (ContractPartyComponent item : this.party) 3263 if (!item.isEmpty()) 3264 return true; 3265 return false; 3266 } 3267 3268 public ContractPartyComponent addParty() { // 3 3269 ContractPartyComponent t = new ContractPartyComponent(); 3270 if (this.party == null) 3271 this.party = new ArrayList<ContractPartyComponent>(); 3272 this.party.add(t); 3273 return t; 3274 } 3275 3276 public ContractOfferComponent addParty(ContractPartyComponent t) { // 3 3277 if (t == null) 3278 return this; 3279 if (this.party == null) 3280 this.party = new ArrayList<ContractPartyComponent>(); 3281 this.party.add(t); 3282 return this; 3283 } 3284 3285 /** 3286 * @return The first repetition of repeating field {@link #party}, creating it 3287 * if it does not already exist 3288 */ 3289 public ContractPartyComponent getPartyFirstRep() { 3290 if (getParty().isEmpty()) { 3291 addParty(); 3292 } 3293 return getParty().get(0); 3294 } 3295 3296 /** 3297 * @return {@link #topic} (The owner of an asset has the residual control rights 3298 * over the asset: the right to decide all usages of the asset in any 3299 * way not inconsistent with a prior contract, custom, or law (Hart, 3300 * 1995, p. 30).) 3301 */ 3302 public Reference getTopic() { 3303 if (this.topic == null) 3304 if (Configuration.errorOnAutoCreate()) 3305 throw new Error("Attempt to auto-create ContractOfferComponent.topic"); 3306 else if (Configuration.doAutoCreate()) 3307 this.topic = new Reference(); // cc 3308 return this.topic; 3309 } 3310 3311 public boolean hasTopic() { 3312 return this.topic != null && !this.topic.isEmpty(); 3313 } 3314 3315 /** 3316 * @param value {@link #topic} (The owner of an asset has the residual control 3317 * rights over the asset: the right to decide all usages of the 3318 * asset in any way not inconsistent with a prior contract, custom, 3319 * or law (Hart, 1995, p. 30).) 3320 */ 3321 public ContractOfferComponent setTopic(Reference value) { 3322 this.topic = value; 3323 return this; 3324 } 3325 3326 /** 3327 * @return {@link #topic} The actual object that is the target of the reference. 3328 * The reference library doesn't populate this, but you can use it to 3329 * hold the resource if you resolve it. (The owner of an asset has the 3330 * residual control rights over the asset: the right to decide all 3331 * usages of the asset in any way not inconsistent with a prior 3332 * contract, custom, or law (Hart, 1995, p. 30).) 3333 */ 3334 public Resource getTopicTarget() { 3335 return this.topicTarget; 3336 } 3337 3338 /** 3339 * @param value {@link #topic} The actual object that is the target of the 3340 * reference. The reference library doesn't use these, but you can 3341 * use it to hold the resource if you resolve it. (The owner of an 3342 * asset has the residual control rights over the asset: the right 3343 * to decide all usages of the asset in any way not inconsistent 3344 * with a prior contract, custom, or law (Hart, 1995, p. 30).) 3345 */ 3346 public ContractOfferComponent setTopicTarget(Resource value) { 3347 this.topicTarget = value; 3348 return this; 3349 } 3350 3351 /** 3352 * @return {@link #type} (Type of Contract Provision such as specific 3353 * requirements, purposes for actions, obligations, prohibitions, e.g. 3354 * life time maximum benefit.) 3355 */ 3356 public CodeableConcept getType() { 3357 if (this.type == null) 3358 if (Configuration.errorOnAutoCreate()) 3359 throw new Error("Attempt to auto-create ContractOfferComponent.type"); 3360 else if (Configuration.doAutoCreate()) 3361 this.type = new CodeableConcept(); // cc 3362 return this.type; 3363 } 3364 3365 public boolean hasType() { 3366 return this.type != null && !this.type.isEmpty(); 3367 } 3368 3369 /** 3370 * @param value {@link #type} (Type of Contract Provision such as specific 3371 * requirements, purposes for actions, obligations, prohibitions, 3372 * e.g. life time maximum benefit.) 3373 */ 3374 public ContractOfferComponent setType(CodeableConcept value) { 3375 this.type = value; 3376 return this; 3377 } 3378 3379 /** 3380 * @return {@link #decision} (Type of choice made by accepting party with 3381 * respect to an offer made by an offeror/ grantee.) 3382 */ 3383 public CodeableConcept getDecision() { 3384 if (this.decision == null) 3385 if (Configuration.errorOnAutoCreate()) 3386 throw new Error("Attempt to auto-create ContractOfferComponent.decision"); 3387 else if (Configuration.doAutoCreate()) 3388 this.decision = new CodeableConcept(); // cc 3389 return this.decision; 3390 } 3391 3392 public boolean hasDecision() { 3393 return this.decision != null && !this.decision.isEmpty(); 3394 } 3395 3396 /** 3397 * @param value {@link #decision} (Type of choice made by accepting party with 3398 * respect to an offer made by an offeror/ grantee.) 3399 */ 3400 public ContractOfferComponent setDecision(CodeableConcept value) { 3401 this.decision = value; 3402 return this; 3403 } 3404 3405 /** 3406 * @return {@link #decisionMode} (How the decision about a Contract was 3407 * conveyed.) 3408 */ 3409 public List<CodeableConcept> getDecisionMode() { 3410 if (this.decisionMode == null) 3411 this.decisionMode = new ArrayList<CodeableConcept>(); 3412 return this.decisionMode; 3413 } 3414 3415 /** 3416 * @return Returns a reference to <code>this</code> for easy method chaining 3417 */ 3418 public ContractOfferComponent setDecisionMode(List<CodeableConcept> theDecisionMode) { 3419 this.decisionMode = theDecisionMode; 3420 return this; 3421 } 3422 3423 public boolean hasDecisionMode() { 3424 if (this.decisionMode == null) 3425 return false; 3426 for (CodeableConcept item : this.decisionMode) 3427 if (!item.isEmpty()) 3428 return true; 3429 return false; 3430 } 3431 3432 public CodeableConcept addDecisionMode() { // 3 3433 CodeableConcept t = new CodeableConcept(); 3434 if (this.decisionMode == null) 3435 this.decisionMode = new ArrayList<CodeableConcept>(); 3436 this.decisionMode.add(t); 3437 return t; 3438 } 3439 3440 public ContractOfferComponent addDecisionMode(CodeableConcept t) { // 3 3441 if (t == null) 3442 return this; 3443 if (this.decisionMode == null) 3444 this.decisionMode = new ArrayList<CodeableConcept>(); 3445 this.decisionMode.add(t); 3446 return this; 3447 } 3448 3449 /** 3450 * @return The first repetition of repeating field {@link #decisionMode}, 3451 * creating it if it does not already exist 3452 */ 3453 public CodeableConcept getDecisionModeFirstRep() { 3454 if (getDecisionMode().isEmpty()) { 3455 addDecisionMode(); 3456 } 3457 return getDecisionMode().get(0); 3458 } 3459 3460 /** 3461 * @return {@link #answer} (Response to offer text.) 3462 */ 3463 public List<AnswerComponent> getAnswer() { 3464 if (this.answer == null) 3465 this.answer = new ArrayList<AnswerComponent>(); 3466 return this.answer; 3467 } 3468 3469 /** 3470 * @return Returns a reference to <code>this</code> for easy method chaining 3471 */ 3472 public ContractOfferComponent setAnswer(List<AnswerComponent> theAnswer) { 3473 this.answer = theAnswer; 3474 return this; 3475 } 3476 3477 public boolean hasAnswer() { 3478 if (this.answer == null) 3479 return false; 3480 for (AnswerComponent item : this.answer) 3481 if (!item.isEmpty()) 3482 return true; 3483 return false; 3484 } 3485 3486 public AnswerComponent addAnswer() { // 3 3487 AnswerComponent t = new AnswerComponent(); 3488 if (this.answer == null) 3489 this.answer = new ArrayList<AnswerComponent>(); 3490 this.answer.add(t); 3491 return t; 3492 } 3493 3494 public ContractOfferComponent addAnswer(AnswerComponent t) { // 3 3495 if (t == null) 3496 return this; 3497 if (this.answer == null) 3498 this.answer = new ArrayList<AnswerComponent>(); 3499 this.answer.add(t); 3500 return this; 3501 } 3502 3503 /** 3504 * @return The first repetition of repeating field {@link #answer}, creating it 3505 * if it does not already exist 3506 */ 3507 public AnswerComponent getAnswerFirstRep() { 3508 if (getAnswer().isEmpty()) { 3509 addAnswer(); 3510 } 3511 return getAnswer().get(0); 3512 } 3513 3514 /** 3515 * @return {@link #text} (Human readable form of this Contract Offer.). This is 3516 * the underlying object with id, value and extensions. The accessor 3517 * "getText" gives direct access to the value 3518 */ 3519 public StringType getTextElement() { 3520 if (this.text == null) 3521 if (Configuration.errorOnAutoCreate()) 3522 throw new Error("Attempt to auto-create ContractOfferComponent.text"); 3523 else if (Configuration.doAutoCreate()) 3524 this.text = new StringType(); // bb 3525 return this.text; 3526 } 3527 3528 public boolean hasTextElement() { 3529 return this.text != null && !this.text.isEmpty(); 3530 } 3531 3532 public boolean hasText() { 3533 return this.text != null && !this.text.isEmpty(); 3534 } 3535 3536 /** 3537 * @param value {@link #text} (Human readable form of this Contract Offer.). 3538 * This is the underlying object with id, value and extensions. The 3539 * accessor "getText" gives direct access to the value 3540 */ 3541 public ContractOfferComponent setTextElement(StringType value) { 3542 this.text = value; 3543 return this; 3544 } 3545 3546 /** 3547 * @return Human readable form of this Contract Offer. 3548 */ 3549 public String getText() { 3550 return this.text == null ? null : this.text.getValue(); 3551 } 3552 3553 /** 3554 * @param value Human readable form of this Contract Offer. 3555 */ 3556 public ContractOfferComponent setText(String value) { 3557 if (Utilities.noString(value)) 3558 this.text = null; 3559 else { 3560 if (this.text == null) 3561 this.text = new StringType(); 3562 this.text.setValue(value); 3563 } 3564 return this; 3565 } 3566 3567 /** 3568 * @return {@link #linkId} (The id of the clause or question text of the offer 3569 * in the referenced questionnaire/response.) 3570 */ 3571 public List<StringType> getLinkId() { 3572 if (this.linkId == null) 3573 this.linkId = new ArrayList<StringType>(); 3574 return this.linkId; 3575 } 3576 3577 /** 3578 * @return Returns a reference to <code>this</code> for easy method chaining 3579 */ 3580 public ContractOfferComponent setLinkId(List<StringType> theLinkId) { 3581 this.linkId = theLinkId; 3582 return this; 3583 } 3584 3585 public boolean hasLinkId() { 3586 if (this.linkId == null) 3587 return false; 3588 for (StringType item : this.linkId) 3589 if (!item.isEmpty()) 3590 return true; 3591 return false; 3592 } 3593 3594 /** 3595 * @return {@link #linkId} (The id of the clause or question text of the offer 3596 * in the referenced questionnaire/response.) 3597 */ 3598 public StringType addLinkIdElement() {// 2 3599 StringType t = new StringType(); 3600 if (this.linkId == null) 3601 this.linkId = new ArrayList<StringType>(); 3602 this.linkId.add(t); 3603 return t; 3604 } 3605 3606 /** 3607 * @param value {@link #linkId} (The id of the clause or question text of the 3608 * offer in the referenced questionnaire/response.) 3609 */ 3610 public ContractOfferComponent addLinkId(String value) { // 1 3611 StringType t = new StringType(); 3612 t.setValue(value); 3613 if (this.linkId == null) 3614 this.linkId = new ArrayList<StringType>(); 3615 this.linkId.add(t); 3616 return this; 3617 } 3618 3619 /** 3620 * @param value {@link #linkId} (The id of the clause or question text of the 3621 * offer in the referenced questionnaire/response.) 3622 */ 3623 public boolean hasLinkId(String value) { 3624 if (this.linkId == null) 3625 return false; 3626 for (StringType v : this.linkId) 3627 if (v.getValue().equals(value)) // string 3628 return true; 3629 return false; 3630 } 3631 3632 /** 3633 * @return {@link #securityLabelNumber} (Security labels that protects the 3634 * offer.) 3635 */ 3636 public List<UnsignedIntType> getSecurityLabelNumber() { 3637 if (this.securityLabelNumber == null) 3638 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 3639 return this.securityLabelNumber; 3640 } 3641 3642 /** 3643 * @return Returns a reference to <code>this</code> for easy method chaining 3644 */ 3645 public ContractOfferComponent setSecurityLabelNumber(List<UnsignedIntType> theSecurityLabelNumber) { 3646 this.securityLabelNumber = theSecurityLabelNumber; 3647 return this; 3648 } 3649 3650 public boolean hasSecurityLabelNumber() { 3651 if (this.securityLabelNumber == null) 3652 return false; 3653 for (UnsignedIntType item : this.securityLabelNumber) 3654 if (!item.isEmpty()) 3655 return true; 3656 return false; 3657 } 3658 3659 /** 3660 * @return {@link #securityLabelNumber} (Security labels that protects the 3661 * offer.) 3662 */ 3663 public UnsignedIntType addSecurityLabelNumberElement() {// 2 3664 UnsignedIntType t = new UnsignedIntType(); 3665 if (this.securityLabelNumber == null) 3666 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 3667 this.securityLabelNumber.add(t); 3668 return t; 3669 } 3670 3671 /** 3672 * @param value {@link #securityLabelNumber} (Security labels that protects the 3673 * offer.) 3674 */ 3675 public ContractOfferComponent addSecurityLabelNumber(int value) { // 1 3676 UnsignedIntType t = new UnsignedIntType(); 3677 t.setValue(value); 3678 if (this.securityLabelNumber == null) 3679 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 3680 this.securityLabelNumber.add(t); 3681 return this; 3682 } 3683 3684 /** 3685 * @param value {@link #securityLabelNumber} (Security labels that protects the 3686 * offer.) 3687 */ 3688 public boolean hasSecurityLabelNumber(int value) { 3689 if (this.securityLabelNumber == null) 3690 return false; 3691 for (UnsignedIntType v : this.securityLabelNumber) 3692 if (v.getValue().equals(value)) // unsignedInt 3693 return true; 3694 return false; 3695 } 3696 3697 protected void listChildren(List<Property> children) { 3698 super.listChildren(children); 3699 children.add(new Property("identifier", "Identifier", "Unique identifier for this particular Contract Provision.", 3700 0, java.lang.Integer.MAX_VALUE, identifier)); 3701 children.add(new Property("party", "", "Offer Recipient.", 0, java.lang.Integer.MAX_VALUE, party)); 3702 children.add(new Property("topic", "Reference(Any)", 3703 "The owner of an asset has the residual control rights over the asset: the right to decide all usages of the asset in any way not inconsistent with a prior contract, custom, or law (Hart, 1995, p. 30).", 3704 0, 1, topic)); 3705 children.add(new Property("type", "CodeableConcept", 3706 "Type of Contract Provision such as specific requirements, purposes for actions, obligations, prohibitions, e.g. life time maximum benefit.", 3707 0, 1, type)); 3708 children.add(new Property("decision", "CodeableConcept", 3709 "Type of choice made by accepting party with respect to an offer made by an offeror/ grantee.", 0, 1, 3710 decision)); 3711 children.add(new Property("decisionMode", "CodeableConcept", "How the decision about a Contract was conveyed.", 0, 3712 java.lang.Integer.MAX_VALUE, decisionMode)); 3713 children.add(new Property("answer", "", "Response to offer text.", 0, java.lang.Integer.MAX_VALUE, answer)); 3714 children.add(new Property("text", "string", "Human readable form of this Contract Offer.", 0, 1, text)); 3715 children.add(new Property("linkId", "string", 3716 "The id of the clause or question text of the offer in the referenced questionnaire/response.", 0, 3717 java.lang.Integer.MAX_VALUE, linkId)); 3718 children.add(new Property("securityLabelNumber", "unsignedInt", "Security labels that protects the offer.", 0, 3719 java.lang.Integer.MAX_VALUE, securityLabelNumber)); 3720 } 3721 3722 @Override 3723 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3724 switch (_hash) { 3725 case -1618432855: 3726 /* identifier */ return new Property("identifier", "Identifier", 3727 "Unique identifier for this particular Contract Provision.", 0, java.lang.Integer.MAX_VALUE, identifier); 3728 case 106437350: 3729 /* party */ return new Property("party", "", "Offer Recipient.", 0, java.lang.Integer.MAX_VALUE, party); 3730 case 110546223: 3731 /* topic */ return new Property("topic", "Reference(Any)", 3732 "The owner of an asset has the residual control rights over the asset: the right to decide all usages of the asset in any way not inconsistent with a prior contract, custom, or law (Hart, 1995, p. 30).", 3733 0, 1, topic); 3734 case 3575610: 3735 /* type */ return new Property("type", "CodeableConcept", 3736 "Type of Contract Provision such as specific requirements, purposes for actions, obligations, prohibitions, e.g. life time maximum benefit.", 3737 0, 1, type); 3738 case 565719004: 3739 /* decision */ return new Property("decision", "CodeableConcept", 3740 "Type of choice made by accepting party with respect to an offer made by an offeror/ grantee.", 0, 1, 3741 decision); 3742 case 675909535: 3743 /* decisionMode */ return new Property("decisionMode", "CodeableConcept", 3744 "How the decision about a Contract was conveyed.", 0, java.lang.Integer.MAX_VALUE, decisionMode); 3745 case -1412808770: 3746 /* answer */ return new Property("answer", "", "Response to offer text.", 0, java.lang.Integer.MAX_VALUE, 3747 answer); 3748 case 3556653: 3749 /* text */ return new Property("text", "string", "Human readable form of this Contract Offer.", 0, 1, text); 3750 case -1102667083: 3751 /* linkId */ return new Property("linkId", "string", 3752 "The id of the clause or question text of the offer in the referenced questionnaire/response.", 0, 3753 java.lang.Integer.MAX_VALUE, linkId); 3754 case -149460995: 3755 /* securityLabelNumber */ return new Property("securityLabelNumber", "unsignedInt", 3756 "Security labels that protects the offer.", 0, java.lang.Integer.MAX_VALUE, securityLabelNumber); 3757 default: 3758 return super.getNamedProperty(_hash, _name, _checkValid); 3759 } 3760 3761 } 3762 3763 @Override 3764 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3765 switch (hash) { 3766 case -1618432855: 3767 /* identifier */ return this.identifier == null ? new Base[0] 3768 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3769 case 106437350: 3770 /* party */ return this.party == null ? new Base[0] : this.party.toArray(new Base[this.party.size()]); // ContractPartyComponent 3771 case 110546223: 3772 /* topic */ return this.topic == null ? new Base[0] : new Base[] { this.topic }; // Reference 3773 case 3575610: 3774 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 3775 case 565719004: 3776 /* decision */ return this.decision == null ? new Base[0] : new Base[] { this.decision }; // CodeableConcept 3777 case 675909535: 3778 /* decisionMode */ return this.decisionMode == null ? new Base[0] 3779 : this.decisionMode.toArray(new Base[this.decisionMode.size()]); // CodeableConcept 3780 case -1412808770: 3781 /* answer */ return this.answer == null ? new Base[0] : this.answer.toArray(new Base[this.answer.size()]); // AnswerComponent 3782 case 3556653: 3783 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType 3784 case -1102667083: 3785 /* linkId */ return this.linkId == null ? new Base[0] : this.linkId.toArray(new Base[this.linkId.size()]); // StringType 3786 case -149460995: 3787 /* securityLabelNumber */ return this.securityLabelNumber == null ? new Base[0] 3788 : this.securityLabelNumber.toArray(new Base[this.securityLabelNumber.size()]); // UnsignedIntType 3789 default: 3790 return super.getProperty(hash, name, checkValid); 3791 } 3792 3793 } 3794 3795 @Override 3796 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3797 switch (hash) { 3798 case -1618432855: // identifier 3799 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3800 return value; 3801 case 106437350: // party 3802 this.getParty().add((ContractPartyComponent) value); // ContractPartyComponent 3803 return value; 3804 case 110546223: // topic 3805 this.topic = castToReference(value); // Reference 3806 return value; 3807 case 3575610: // type 3808 this.type = castToCodeableConcept(value); // CodeableConcept 3809 return value; 3810 case 565719004: // decision 3811 this.decision = castToCodeableConcept(value); // CodeableConcept 3812 return value; 3813 case 675909535: // decisionMode 3814 this.getDecisionMode().add(castToCodeableConcept(value)); // CodeableConcept 3815 return value; 3816 case -1412808770: // answer 3817 this.getAnswer().add((AnswerComponent) value); // AnswerComponent 3818 return value; 3819 case 3556653: // text 3820 this.text = castToString(value); // StringType 3821 return value; 3822 case -1102667083: // linkId 3823 this.getLinkId().add(castToString(value)); // StringType 3824 return value; 3825 case -149460995: // securityLabelNumber 3826 this.getSecurityLabelNumber().add(castToUnsignedInt(value)); // UnsignedIntType 3827 return value; 3828 default: 3829 return super.setProperty(hash, name, value); 3830 } 3831 3832 } 3833 3834 @Override 3835 public Base setProperty(String name, Base value) throws FHIRException { 3836 if (name.equals("identifier")) { 3837 this.getIdentifier().add(castToIdentifier(value)); 3838 } else if (name.equals("party")) { 3839 this.getParty().add((ContractPartyComponent) value); 3840 } else if (name.equals("topic")) { 3841 this.topic = castToReference(value); // Reference 3842 } else if (name.equals("type")) { 3843 this.type = castToCodeableConcept(value); // CodeableConcept 3844 } else if (name.equals("decision")) { 3845 this.decision = castToCodeableConcept(value); // CodeableConcept 3846 } else if (name.equals("decisionMode")) { 3847 this.getDecisionMode().add(castToCodeableConcept(value)); 3848 } else if (name.equals("answer")) { 3849 this.getAnswer().add((AnswerComponent) value); 3850 } else if (name.equals("text")) { 3851 this.text = castToString(value); // StringType 3852 } else if (name.equals("linkId")) { 3853 this.getLinkId().add(castToString(value)); 3854 } else if (name.equals("securityLabelNumber")) { 3855 this.getSecurityLabelNumber().add(castToUnsignedInt(value)); 3856 } else 3857 return super.setProperty(name, value); 3858 return value; 3859 } 3860 3861 @Override 3862 public void removeChild(String name, Base value) throws FHIRException { 3863 if (name.equals("identifier")) { 3864 this.getIdentifier().remove(castToIdentifier(value)); 3865 } else if (name.equals("party")) { 3866 this.getParty().remove((ContractPartyComponent) value); 3867 } else if (name.equals("topic")) { 3868 this.topic = null; 3869 } else if (name.equals("type")) { 3870 this.type = null; 3871 } else if (name.equals("decision")) { 3872 this.decision = null; 3873 } else if (name.equals("decisionMode")) { 3874 this.getDecisionMode().remove(castToCodeableConcept(value)); 3875 } else if (name.equals("answer")) { 3876 this.getAnswer().remove((AnswerComponent) value); 3877 } else if (name.equals("text")) { 3878 this.text = null; 3879 } else if (name.equals("linkId")) { 3880 this.getLinkId().remove(castToString(value)); 3881 } else if (name.equals("securityLabelNumber")) { 3882 this.getSecurityLabelNumber().remove(castToUnsignedInt(value)); 3883 } else 3884 super.removeChild(name, value); 3885 3886 } 3887 3888 @Override 3889 public Base makeProperty(int hash, String name) throws FHIRException { 3890 switch (hash) { 3891 case -1618432855: 3892 return addIdentifier(); 3893 case 106437350: 3894 return addParty(); 3895 case 110546223: 3896 return getTopic(); 3897 case 3575610: 3898 return getType(); 3899 case 565719004: 3900 return getDecision(); 3901 case 675909535: 3902 return addDecisionMode(); 3903 case -1412808770: 3904 return addAnswer(); 3905 case 3556653: 3906 return getTextElement(); 3907 case -1102667083: 3908 return addLinkIdElement(); 3909 case -149460995: 3910 return addSecurityLabelNumberElement(); 3911 default: 3912 return super.makeProperty(hash, name); 3913 } 3914 3915 } 3916 3917 @Override 3918 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3919 switch (hash) { 3920 case -1618432855: 3921 /* identifier */ return new String[] { "Identifier" }; 3922 case 106437350: 3923 /* party */ return new String[] {}; 3924 case 110546223: 3925 /* topic */ return new String[] { "Reference" }; 3926 case 3575610: 3927 /* type */ return new String[] { "CodeableConcept" }; 3928 case 565719004: 3929 /* decision */ return new String[] { "CodeableConcept" }; 3930 case 675909535: 3931 /* decisionMode */ return new String[] { "CodeableConcept" }; 3932 case -1412808770: 3933 /* answer */ return new String[] {}; 3934 case 3556653: 3935 /* text */ return new String[] { "string" }; 3936 case -1102667083: 3937 /* linkId */ return new String[] { "string" }; 3938 case -149460995: 3939 /* securityLabelNumber */ return new String[] { "unsignedInt" }; 3940 default: 3941 return super.getTypesForProperty(hash, name); 3942 } 3943 3944 } 3945 3946 @Override 3947 public Base addChild(String name) throws FHIRException { 3948 if (name.equals("identifier")) { 3949 return addIdentifier(); 3950 } else if (name.equals("party")) { 3951 return addParty(); 3952 } else if (name.equals("topic")) { 3953 this.topic = new Reference(); 3954 return this.topic; 3955 } else if (name.equals("type")) { 3956 this.type = new CodeableConcept(); 3957 return this.type; 3958 } else if (name.equals("decision")) { 3959 this.decision = new CodeableConcept(); 3960 return this.decision; 3961 } else if (name.equals("decisionMode")) { 3962 return addDecisionMode(); 3963 } else if (name.equals("answer")) { 3964 return addAnswer(); 3965 } else if (name.equals("text")) { 3966 throw new FHIRException("Cannot call addChild on a singleton property Contract.text"); 3967 } else if (name.equals("linkId")) { 3968 throw new FHIRException("Cannot call addChild on a singleton property Contract.linkId"); 3969 } else if (name.equals("securityLabelNumber")) { 3970 throw new FHIRException("Cannot call addChild on a singleton property Contract.securityLabelNumber"); 3971 } else 3972 return super.addChild(name); 3973 } 3974 3975 public ContractOfferComponent copy() { 3976 ContractOfferComponent dst = new ContractOfferComponent(); 3977 copyValues(dst); 3978 return dst; 3979 } 3980 3981 public void copyValues(ContractOfferComponent dst) { 3982 super.copyValues(dst); 3983 if (identifier != null) { 3984 dst.identifier = new ArrayList<Identifier>(); 3985 for (Identifier i : identifier) 3986 dst.identifier.add(i.copy()); 3987 } 3988 ; 3989 if (party != null) { 3990 dst.party = new ArrayList<ContractPartyComponent>(); 3991 for (ContractPartyComponent i : party) 3992 dst.party.add(i.copy()); 3993 } 3994 ; 3995 dst.topic = topic == null ? null : topic.copy(); 3996 dst.type = type == null ? null : type.copy(); 3997 dst.decision = decision == null ? null : decision.copy(); 3998 if (decisionMode != null) { 3999 dst.decisionMode = new ArrayList<CodeableConcept>(); 4000 for (CodeableConcept i : decisionMode) 4001 dst.decisionMode.add(i.copy()); 4002 } 4003 ; 4004 if (answer != null) { 4005 dst.answer = new ArrayList<AnswerComponent>(); 4006 for (AnswerComponent i : answer) 4007 dst.answer.add(i.copy()); 4008 } 4009 ; 4010 dst.text = text == null ? null : text.copy(); 4011 if (linkId != null) { 4012 dst.linkId = new ArrayList<StringType>(); 4013 for (StringType i : linkId) 4014 dst.linkId.add(i.copy()); 4015 } 4016 ; 4017 if (securityLabelNumber != null) { 4018 dst.securityLabelNumber = new ArrayList<UnsignedIntType>(); 4019 for (UnsignedIntType i : securityLabelNumber) 4020 dst.securityLabelNumber.add(i.copy()); 4021 } 4022 ; 4023 } 4024 4025 @Override 4026 public boolean equalsDeep(Base other_) { 4027 if (!super.equalsDeep(other_)) 4028 return false; 4029 if (!(other_ instanceof ContractOfferComponent)) 4030 return false; 4031 ContractOfferComponent o = (ContractOfferComponent) other_; 4032 return compareDeep(identifier, o.identifier, true) && compareDeep(party, o.party, true) 4033 && compareDeep(topic, o.topic, true) && compareDeep(type, o.type, true) 4034 && compareDeep(decision, o.decision, true) && compareDeep(decisionMode, o.decisionMode, true) 4035 && compareDeep(answer, o.answer, true) && compareDeep(text, o.text, true) 4036 && compareDeep(linkId, o.linkId, true) && compareDeep(securityLabelNumber, o.securityLabelNumber, true); 4037 } 4038 4039 @Override 4040 public boolean equalsShallow(Base other_) { 4041 if (!super.equalsShallow(other_)) 4042 return false; 4043 if (!(other_ instanceof ContractOfferComponent)) 4044 return false; 4045 ContractOfferComponent o = (ContractOfferComponent) other_; 4046 return compareValues(text, o.text, true) && compareValues(linkId, o.linkId, true) 4047 && compareValues(securityLabelNumber, o.securityLabelNumber, true); 4048 } 4049 4050 public boolean isEmpty() { 4051 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, party, topic, type, decision, 4052 decisionMode, answer, text, linkId, securityLabelNumber); 4053 } 4054 4055 public String fhirType() { 4056 return "Contract.term.offer"; 4057 4058 } 4059 4060 } 4061 4062 @Block() 4063 public static class ContractPartyComponent extends BackboneElement implements IBaseBackboneElement { 4064 /** 4065 * Participant in the offer. 4066 */ 4067 @Child(name = "reference", type = { Patient.class, RelatedPerson.class, Practitioner.class, PractitionerRole.class, 4068 Device.class, Group.class, 4069 Organization.class }, order = 1, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4070 @Description(shortDefinition = "Referenced entity", formalDefinition = "Participant in the offer.") 4071 protected List<Reference> reference; 4072 /** 4073 * The actual objects that are the target of the reference (Participant in the 4074 * offer.) 4075 */ 4076 protected List<Resource> referenceTarget; 4077 4078 /** 4079 * How the party participates in the offer. 4080 */ 4081 @Child(name = "role", type = { 4082 CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 4083 @Description(shortDefinition = "Participant engagement type", formalDefinition = "How the party participates in the offer.") 4084 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-party-role") 4085 protected CodeableConcept role; 4086 4087 private static final long serialVersionUID = 128949255L; 4088 4089 /** 4090 * Constructor 4091 */ 4092 public ContractPartyComponent() { 4093 super(); 4094 } 4095 4096 /** 4097 * Constructor 4098 */ 4099 public ContractPartyComponent(CodeableConcept role) { 4100 super(); 4101 this.role = role; 4102 } 4103 4104 /** 4105 * @return {@link #reference} (Participant in the offer.) 4106 */ 4107 public List<Reference> getReference() { 4108 if (this.reference == null) 4109 this.reference = new ArrayList<Reference>(); 4110 return this.reference; 4111 } 4112 4113 /** 4114 * @return Returns a reference to <code>this</code> for easy method chaining 4115 */ 4116 public ContractPartyComponent setReference(List<Reference> theReference) { 4117 this.reference = theReference; 4118 return this; 4119 } 4120 4121 public boolean hasReference() { 4122 if (this.reference == null) 4123 return false; 4124 for (Reference item : this.reference) 4125 if (!item.isEmpty()) 4126 return true; 4127 return false; 4128 } 4129 4130 public Reference addReference() { // 3 4131 Reference t = new Reference(); 4132 if (this.reference == null) 4133 this.reference = new ArrayList<Reference>(); 4134 this.reference.add(t); 4135 return t; 4136 } 4137 4138 public ContractPartyComponent addReference(Reference t) { // 3 4139 if (t == null) 4140 return this; 4141 if (this.reference == null) 4142 this.reference = new ArrayList<Reference>(); 4143 this.reference.add(t); 4144 return this; 4145 } 4146 4147 /** 4148 * @return The first repetition of repeating field {@link #reference}, creating 4149 * it if it does not already exist 4150 */ 4151 public Reference getReferenceFirstRep() { 4152 if (getReference().isEmpty()) { 4153 addReference(); 4154 } 4155 return getReference().get(0); 4156 } 4157 4158 /** 4159 * @deprecated Use Reference#setResource(IBaseResource) instead 4160 */ 4161 @Deprecated 4162 public List<Resource> getReferenceTarget() { 4163 if (this.referenceTarget == null) 4164 this.referenceTarget = new ArrayList<Resource>(); 4165 return this.referenceTarget; 4166 } 4167 4168 /** 4169 * @return {@link #role} (How the party participates in the offer.) 4170 */ 4171 public CodeableConcept getRole() { 4172 if (this.role == null) 4173 if (Configuration.errorOnAutoCreate()) 4174 throw new Error("Attempt to auto-create ContractPartyComponent.role"); 4175 else if (Configuration.doAutoCreate()) 4176 this.role = new CodeableConcept(); // cc 4177 return this.role; 4178 } 4179 4180 public boolean hasRole() { 4181 return this.role != null && !this.role.isEmpty(); 4182 } 4183 4184 /** 4185 * @param value {@link #role} (How the party participates in the offer.) 4186 */ 4187 public ContractPartyComponent setRole(CodeableConcept value) { 4188 this.role = value; 4189 return this; 4190 } 4191 4192 protected void listChildren(List<Property> children) { 4193 super.listChildren(children); 4194 children.add(new Property("reference", 4195 "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Device|Group|Organization)", 4196 "Participant in the offer.", 0, java.lang.Integer.MAX_VALUE, reference)); 4197 children.add(new Property("role", "CodeableConcept", "How the party participates in the offer.", 0, 1, role)); 4198 } 4199 4200 @Override 4201 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4202 switch (_hash) { 4203 case -925155509: 4204 /* reference */ return new Property("reference", 4205 "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Device|Group|Organization)", 4206 "Participant in the offer.", 0, java.lang.Integer.MAX_VALUE, reference); 4207 case 3506294: 4208 /* role */ return new Property("role", "CodeableConcept", "How the party participates in the offer.", 0, 1, 4209 role); 4210 default: 4211 return super.getNamedProperty(_hash, _name, _checkValid); 4212 } 4213 4214 } 4215 4216 @Override 4217 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4218 switch (hash) { 4219 case -925155509: 4220 /* reference */ return this.reference == null ? new Base[0] 4221 : this.reference.toArray(new Base[this.reference.size()]); // Reference 4222 case 3506294: 4223 /* role */ return this.role == null ? new Base[0] : new Base[] { this.role }; // CodeableConcept 4224 default: 4225 return super.getProperty(hash, name, checkValid); 4226 } 4227 4228 } 4229 4230 @Override 4231 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4232 switch (hash) { 4233 case -925155509: // reference 4234 this.getReference().add(castToReference(value)); // Reference 4235 return value; 4236 case 3506294: // role 4237 this.role = castToCodeableConcept(value); // CodeableConcept 4238 return value; 4239 default: 4240 return super.setProperty(hash, name, value); 4241 } 4242 4243 } 4244 4245 @Override 4246 public Base setProperty(String name, Base value) throws FHIRException { 4247 if (name.equals("reference")) { 4248 this.getReference().add(castToReference(value)); 4249 } else if (name.equals("role")) { 4250 this.role = castToCodeableConcept(value); // CodeableConcept 4251 } else 4252 return super.setProperty(name, value); 4253 return value; 4254 } 4255 4256 @Override 4257 public void removeChild(String name, Base value) throws FHIRException { 4258 if (name.equals("reference")) { 4259 this.getReference().remove(castToReference(value)); 4260 } else if (name.equals("role")) { 4261 this.role = null; 4262 } else 4263 super.removeChild(name, value); 4264 4265 } 4266 4267 @Override 4268 public Base makeProperty(int hash, String name) throws FHIRException { 4269 switch (hash) { 4270 case -925155509: 4271 return addReference(); 4272 case 3506294: 4273 return getRole(); 4274 default: 4275 return super.makeProperty(hash, name); 4276 } 4277 4278 } 4279 4280 @Override 4281 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4282 switch (hash) { 4283 case -925155509: 4284 /* reference */ return new String[] { "Reference" }; 4285 case 3506294: 4286 /* role */ return new String[] { "CodeableConcept" }; 4287 default: 4288 return super.getTypesForProperty(hash, name); 4289 } 4290 4291 } 4292 4293 @Override 4294 public Base addChild(String name) throws FHIRException { 4295 if (name.equals("reference")) { 4296 return addReference(); 4297 } else if (name.equals("role")) { 4298 this.role = new CodeableConcept(); 4299 return this.role; 4300 } else 4301 return super.addChild(name); 4302 } 4303 4304 public ContractPartyComponent copy() { 4305 ContractPartyComponent dst = new ContractPartyComponent(); 4306 copyValues(dst); 4307 return dst; 4308 } 4309 4310 public void copyValues(ContractPartyComponent dst) { 4311 super.copyValues(dst); 4312 if (reference != null) { 4313 dst.reference = new ArrayList<Reference>(); 4314 for (Reference i : reference) 4315 dst.reference.add(i.copy()); 4316 } 4317 ; 4318 dst.role = role == null ? null : role.copy(); 4319 } 4320 4321 @Override 4322 public boolean equalsDeep(Base other_) { 4323 if (!super.equalsDeep(other_)) 4324 return false; 4325 if (!(other_ instanceof ContractPartyComponent)) 4326 return false; 4327 ContractPartyComponent o = (ContractPartyComponent) other_; 4328 return compareDeep(reference, o.reference, true) && compareDeep(role, o.role, true); 4329 } 4330 4331 @Override 4332 public boolean equalsShallow(Base other_) { 4333 if (!super.equalsShallow(other_)) 4334 return false; 4335 if (!(other_ instanceof ContractPartyComponent)) 4336 return false; 4337 ContractPartyComponent o = (ContractPartyComponent) other_; 4338 return true; 4339 } 4340 4341 public boolean isEmpty() { 4342 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(reference, role); 4343 } 4344 4345 public String fhirType() { 4346 return "Contract.term.offer.party"; 4347 4348 } 4349 4350 } 4351 4352 @Block() 4353 public static class AnswerComponent extends BackboneElement implements IBaseBackboneElement { 4354 /** 4355 * Response to an offer clause or question text, which enables selection of 4356 * values to be agreed to, e.g., the period of participation, the date of 4357 * occupancy of a rental, warrently duration, or whether biospecimen may be used 4358 * for further research. 4359 */ 4360 @Child(name = "value", type = { BooleanType.class, DecimalType.class, IntegerType.class, DateType.class, 4361 DateTimeType.class, TimeType.class, StringType.class, UriType.class, Attachment.class, Coding.class, 4362 Quantity.class, Reference.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 4363 @Description(shortDefinition = "The actual answer response", formalDefinition = "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.") 4364 protected Type value; 4365 4366 private static final long serialVersionUID = -732981989L; 4367 4368 /** 4369 * Constructor 4370 */ 4371 public AnswerComponent() { 4372 super(); 4373 } 4374 4375 /** 4376 * Constructor 4377 */ 4378 public AnswerComponent(Type value) { 4379 super(); 4380 this.value = value; 4381 } 4382 4383 /** 4384 * @return {@link #value} (Response to an offer clause or question text, which 4385 * enables selection of values to be agreed to, e.g., the period of 4386 * participation, the date of occupancy of a rental, warrently duration, 4387 * or whether biospecimen may be used for further research.) 4388 */ 4389 public Type getValue() { 4390 return this.value; 4391 } 4392 4393 /** 4394 * @return {@link #value} (Response to an offer clause or question text, which 4395 * enables selection of values to be agreed to, e.g., the period of 4396 * participation, the date of occupancy of a rental, warrently duration, 4397 * or whether biospecimen may be used for further research.) 4398 */ 4399 public BooleanType getValueBooleanType() throws FHIRException { 4400 if (this.value == null) 4401 this.value = new BooleanType(); 4402 if (!(this.value instanceof BooleanType)) 4403 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 4404 + this.value.getClass().getName() + " was encountered"); 4405 return (BooleanType) this.value; 4406 } 4407 4408 public boolean hasValueBooleanType() { 4409 return this != null && this.value instanceof BooleanType; 4410 } 4411 4412 /** 4413 * @return {@link #value} (Response to an offer clause or question text, which 4414 * enables selection of values to be agreed to, e.g., the period of 4415 * participation, the date of occupancy of a rental, warrently duration, 4416 * or whether biospecimen may be used for further research.) 4417 */ 4418 public DecimalType getValueDecimalType() throws FHIRException { 4419 if (this.value == null) 4420 this.value = new DecimalType(); 4421 if (!(this.value instanceof DecimalType)) 4422 throw new FHIRException("Type mismatch: the type DecimalType was expected, but " 4423 + this.value.getClass().getName() + " was encountered"); 4424 return (DecimalType) this.value; 4425 } 4426 4427 public boolean hasValueDecimalType() { 4428 return this != null && this.value instanceof DecimalType; 4429 } 4430 4431 /** 4432 * @return {@link #value} (Response to an offer clause or question text, which 4433 * enables selection of values to be agreed to, e.g., the period of 4434 * participation, the date of occupancy of a rental, warrently duration, 4435 * or whether biospecimen may be used for further research.) 4436 */ 4437 public IntegerType getValueIntegerType() throws FHIRException { 4438 if (this.value == null) 4439 this.value = new IntegerType(); 4440 if (!(this.value instanceof IntegerType)) 4441 throw new FHIRException("Type mismatch: the type IntegerType was expected, but " 4442 + this.value.getClass().getName() + " was encountered"); 4443 return (IntegerType) this.value; 4444 } 4445 4446 public boolean hasValueIntegerType() { 4447 return this != null && this.value instanceof IntegerType; 4448 } 4449 4450 /** 4451 * @return {@link #value} (Response to an offer clause or question text, which 4452 * enables selection of values to be agreed to, e.g., the period of 4453 * participation, the date of occupancy of a rental, warrently duration, 4454 * or whether biospecimen may be used for further research.) 4455 */ 4456 public DateType getValueDateType() throws FHIRException { 4457 if (this.value == null) 4458 this.value = new DateType(); 4459 if (!(this.value instanceof DateType)) 4460 throw new FHIRException("Type mismatch: the type DateType was expected, but " + this.value.getClass().getName() 4461 + " was encountered"); 4462 return (DateType) this.value; 4463 } 4464 4465 public boolean hasValueDateType() { 4466 return this != null && this.value instanceof DateType; 4467 } 4468 4469 /** 4470 * @return {@link #value} (Response to an offer clause or question text, which 4471 * enables selection of values to be agreed to, e.g., the period of 4472 * participation, the date of occupancy of a rental, warrently duration, 4473 * or whether biospecimen may be used for further research.) 4474 */ 4475 public DateTimeType getValueDateTimeType() throws FHIRException { 4476 if (this.value == null) 4477 this.value = new DateTimeType(); 4478 if (!(this.value instanceof DateTimeType)) 4479 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 4480 + this.value.getClass().getName() + " was encountered"); 4481 return (DateTimeType) this.value; 4482 } 4483 4484 public boolean hasValueDateTimeType() { 4485 return this != null && this.value instanceof DateTimeType; 4486 } 4487 4488 /** 4489 * @return {@link #value} (Response to an offer clause or question text, which 4490 * enables selection of values to be agreed to, e.g., the period of 4491 * participation, the date of occupancy of a rental, warrently duration, 4492 * or whether biospecimen may be used for further research.) 4493 */ 4494 public TimeType getValueTimeType() throws FHIRException { 4495 if (this.value == null) 4496 this.value = new TimeType(); 4497 if (!(this.value instanceof TimeType)) 4498 throw new FHIRException("Type mismatch: the type TimeType was expected, but " + this.value.getClass().getName() 4499 + " was encountered"); 4500 return (TimeType) this.value; 4501 } 4502 4503 public boolean hasValueTimeType() { 4504 return this != null && this.value instanceof TimeType; 4505 } 4506 4507 /** 4508 * @return {@link #value} (Response to an offer clause or question text, which 4509 * enables selection of values to be agreed to, e.g., the period of 4510 * participation, the date of occupancy of a rental, warrently duration, 4511 * or whether biospecimen may be used for further research.) 4512 */ 4513 public StringType getValueStringType() throws FHIRException { 4514 if (this.value == null) 4515 this.value = new StringType(); 4516 if (!(this.value instanceof StringType)) 4517 throw new FHIRException("Type mismatch: the type StringType was expected, but " 4518 + this.value.getClass().getName() + " was encountered"); 4519 return (StringType) this.value; 4520 } 4521 4522 public boolean hasValueStringType() { 4523 return this != null && this.value instanceof StringType; 4524 } 4525 4526 /** 4527 * @return {@link #value} (Response to an offer clause or question text, which 4528 * enables selection of values to be agreed to, e.g., the period of 4529 * participation, the date of occupancy of a rental, warrently duration, 4530 * or whether biospecimen may be used for further research.) 4531 */ 4532 public UriType getValueUriType() throws FHIRException { 4533 if (this.value == null) 4534 this.value = new UriType(); 4535 if (!(this.value instanceof UriType)) 4536 throw new FHIRException("Type mismatch: the type UriType was expected, but " + this.value.getClass().getName() 4537 + " was encountered"); 4538 return (UriType) this.value; 4539 } 4540 4541 public boolean hasValueUriType() { 4542 return this != null && this.value instanceof UriType; 4543 } 4544 4545 /** 4546 * @return {@link #value} (Response to an offer clause or question text, which 4547 * enables selection of values to be agreed to, e.g., the period of 4548 * participation, the date of occupancy of a rental, warrently duration, 4549 * or whether biospecimen may be used for further research.) 4550 */ 4551 public Attachment getValueAttachment() throws FHIRException { 4552 if (this.value == null) 4553 this.value = new Attachment(); 4554 if (!(this.value instanceof Attachment)) 4555 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 4556 + this.value.getClass().getName() + " was encountered"); 4557 return (Attachment) this.value; 4558 } 4559 4560 public boolean hasValueAttachment() { 4561 return this != null && this.value instanceof Attachment; 4562 } 4563 4564 /** 4565 * @return {@link #value} (Response to an offer clause or question text, which 4566 * enables selection of values to be agreed to, e.g., the period of 4567 * participation, the date of occupancy of a rental, warrently duration, 4568 * or whether biospecimen may be used for further research.) 4569 */ 4570 public Coding getValueCoding() throws FHIRException { 4571 if (this.value == null) 4572 this.value = new Coding(); 4573 if (!(this.value instanceof Coding)) 4574 throw new FHIRException( 4575 "Type mismatch: the type Coding was expected, but " + this.value.getClass().getName() + " was encountered"); 4576 return (Coding) this.value; 4577 } 4578 4579 public boolean hasValueCoding() { 4580 return this != null && this.value instanceof Coding; 4581 } 4582 4583 /** 4584 * @return {@link #value} (Response to an offer clause or question text, which 4585 * enables selection of values to be agreed to, e.g., the period of 4586 * participation, the date of occupancy of a rental, warrently duration, 4587 * or whether biospecimen may be used for further research.) 4588 */ 4589 public Quantity getValueQuantity() throws FHIRException { 4590 if (this.value == null) 4591 this.value = new Quantity(); 4592 if (!(this.value instanceof Quantity)) 4593 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName() 4594 + " was encountered"); 4595 return (Quantity) this.value; 4596 } 4597 4598 public boolean hasValueQuantity() { 4599 return this != null && this.value instanceof Quantity; 4600 } 4601 4602 /** 4603 * @return {@link #value} (Response to an offer clause or question text, which 4604 * enables selection of values to be agreed to, e.g., the period of 4605 * participation, the date of occupancy of a rental, warrently duration, 4606 * or whether biospecimen may be used for further research.) 4607 */ 4608 public Reference getValueReference() throws FHIRException { 4609 if (this.value == null) 4610 this.value = new Reference(); 4611 if (!(this.value instanceof Reference)) 4612 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.value.getClass().getName() 4613 + " was encountered"); 4614 return (Reference) this.value; 4615 } 4616 4617 public boolean hasValueReference() { 4618 return this != null && this.value instanceof Reference; 4619 } 4620 4621 public boolean hasValue() { 4622 return this.value != null && !this.value.isEmpty(); 4623 } 4624 4625 /** 4626 * @param value {@link #value} (Response to an offer clause or question text, 4627 * which enables selection of values to be agreed to, e.g., the 4628 * period of participation, the date of occupancy of a rental, 4629 * warrently duration, or whether biospecimen may be used for 4630 * further research.) 4631 */ 4632 public AnswerComponent setValue(Type value) { 4633 if (value != null 4634 && !(value instanceof BooleanType || value instanceof DecimalType || value instanceof IntegerType 4635 || value instanceof DateType || value instanceof DateTimeType || value instanceof TimeType 4636 || value instanceof StringType || value instanceof UriType || value instanceof Attachment 4637 || value instanceof Coding || value instanceof Quantity || value instanceof Reference)) 4638 throw new Error("Not the right type for Contract.term.offer.answer.value[x]: " + value.fhirType()); 4639 this.value = value; 4640 return this; 4641 } 4642 4643 protected void listChildren(List<Property> children) { 4644 super.listChildren(children); 4645 children.add(new Property("value[x]", 4646 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4647 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4648 0, 1, value)); 4649 } 4650 4651 @Override 4652 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4653 switch (_hash) { 4654 case -1410166417: 4655 /* value[x] */ return new Property("value[x]", 4656 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4657 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4658 0, 1, value); 4659 case 111972721: 4660 /* value */ return new Property("value[x]", 4661 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4662 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4663 0, 1, value); 4664 case 733421943: 4665 /* valueBoolean */ return new Property("value[x]", 4666 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4667 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4668 0, 1, value); 4669 case -2083993440: 4670 /* valueDecimal */ return new Property("value[x]", 4671 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4672 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4673 0, 1, value); 4674 case -1668204915: 4675 /* valueInteger */ return new Property("value[x]", 4676 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4677 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4678 0, 1, value); 4679 case -766192449: 4680 /* valueDate */ return new Property("value[x]", 4681 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4682 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4683 0, 1, value); 4684 case 1047929900: 4685 /* valueDateTime */ return new Property("value[x]", 4686 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4687 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4688 0, 1, value); 4689 case -765708322: 4690 /* valueTime */ return new Property("value[x]", 4691 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4692 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4693 0, 1, value); 4694 case -1424603934: 4695 /* valueString */ return new Property("value[x]", 4696 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4697 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4698 0, 1, value); 4699 case -1410172357: 4700 /* valueUri */ return new Property("value[x]", 4701 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4702 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4703 0, 1, value); 4704 case -475566732: 4705 /* valueAttachment */ return new Property("value[x]", 4706 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4707 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4708 0, 1, value); 4709 case -1887705029: 4710 /* valueCoding */ return new Property("value[x]", 4711 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4712 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4713 0, 1, value); 4714 case -2029823716: 4715 /* valueQuantity */ return new Property("value[x]", 4716 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4717 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4718 0, 1, value); 4719 case 1755241690: 4720 /* valueReference */ return new Property("value[x]", 4721 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4722 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4723 0, 1, value); 4724 default: 4725 return super.getNamedProperty(_hash, _name, _checkValid); 4726 } 4727 4728 } 4729 4730 @Override 4731 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4732 switch (hash) { 4733 case 111972721: 4734 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 4735 default: 4736 return super.getProperty(hash, name, checkValid); 4737 } 4738 4739 } 4740 4741 @Override 4742 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4743 switch (hash) { 4744 case 111972721: // value 4745 this.value = castToType(value); // Type 4746 return value; 4747 default: 4748 return super.setProperty(hash, name, value); 4749 } 4750 4751 } 4752 4753 @Override 4754 public Base setProperty(String name, Base value) throws FHIRException { 4755 if (name.equals("value[x]")) { 4756 this.value = castToType(value); // Type 4757 } else 4758 return super.setProperty(name, value); 4759 return value; 4760 } 4761 4762 @Override 4763 public void removeChild(String name, Base value) throws FHIRException { 4764 if (name.equals("value[x]")) { 4765 this.value = null; 4766 } else 4767 super.removeChild(name, value); 4768 4769 } 4770 4771 @Override 4772 public Base makeProperty(int hash, String name) throws FHIRException { 4773 switch (hash) { 4774 case -1410166417: 4775 return getValue(); 4776 case 111972721: 4777 return getValue(); 4778 default: 4779 return super.makeProperty(hash, name); 4780 } 4781 4782 } 4783 4784 @Override 4785 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4786 switch (hash) { 4787 case 111972721: 4788 /* value */ return new String[] { "boolean", "decimal", "integer", "date", "dateTime", "time", "string", "uri", 4789 "Attachment", "Coding", "Quantity", "Reference" }; 4790 default: 4791 return super.getTypesForProperty(hash, name); 4792 } 4793 4794 } 4795 4796 @Override 4797 public Base addChild(String name) throws FHIRException { 4798 if (name.equals("valueBoolean")) { 4799 this.value = new BooleanType(); 4800 return this.value; 4801 } else if (name.equals("valueDecimal")) { 4802 this.value = new DecimalType(); 4803 return this.value; 4804 } else if (name.equals("valueInteger")) { 4805 this.value = new IntegerType(); 4806 return this.value; 4807 } else if (name.equals("valueDate")) { 4808 this.value = new DateType(); 4809 return this.value; 4810 } else if (name.equals("valueDateTime")) { 4811 this.value = new DateTimeType(); 4812 return this.value; 4813 } else if (name.equals("valueTime")) { 4814 this.value = new TimeType(); 4815 return this.value; 4816 } else if (name.equals("valueString")) { 4817 this.value = new StringType(); 4818 return this.value; 4819 } else if (name.equals("valueUri")) { 4820 this.value = new UriType(); 4821 return this.value; 4822 } else if (name.equals("valueAttachment")) { 4823 this.value = new Attachment(); 4824 return this.value; 4825 } else if (name.equals("valueCoding")) { 4826 this.value = new Coding(); 4827 return this.value; 4828 } else if (name.equals("valueQuantity")) { 4829 this.value = new Quantity(); 4830 return this.value; 4831 } else if (name.equals("valueReference")) { 4832 this.value = new Reference(); 4833 return this.value; 4834 } else 4835 return super.addChild(name); 4836 } 4837 4838 public AnswerComponent copy() { 4839 AnswerComponent dst = new AnswerComponent(); 4840 copyValues(dst); 4841 return dst; 4842 } 4843 4844 public void copyValues(AnswerComponent dst) { 4845 super.copyValues(dst); 4846 dst.value = value == null ? null : value.copy(); 4847 } 4848 4849 @Override 4850 public boolean equalsDeep(Base other_) { 4851 if (!super.equalsDeep(other_)) 4852 return false; 4853 if (!(other_ instanceof AnswerComponent)) 4854 return false; 4855 AnswerComponent o = (AnswerComponent) other_; 4856 return compareDeep(value, o.value, true); 4857 } 4858 4859 @Override 4860 public boolean equalsShallow(Base other_) { 4861 if (!super.equalsShallow(other_)) 4862 return false; 4863 if (!(other_ instanceof AnswerComponent)) 4864 return false; 4865 AnswerComponent o = (AnswerComponent) other_; 4866 return true; 4867 } 4868 4869 public boolean isEmpty() { 4870 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value); 4871 } 4872 4873 public String fhirType() { 4874 return "Contract.term.offer.answer"; 4875 4876 } 4877 4878 } 4879 4880 @Block() 4881 public static class ContractAssetComponent extends BackboneElement implements IBaseBackboneElement { 4882 /** 4883 * Differentiates the kind of the asset . 4884 */ 4885 @Child(name = "scope", type = { 4886 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 4887 @Description(shortDefinition = "Range of asset", formalDefinition = "Differentiates the kind of the asset .") 4888 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-assetscope") 4889 protected CodeableConcept scope; 4890 4891 /** 4892 * Target entity type about which the term may be concerned. 4893 */ 4894 @Child(name = "type", type = { 4895 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4896 @Description(shortDefinition = "Asset category", formalDefinition = "Target entity type about which the term may be concerned.") 4897 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-assettype") 4898 protected List<CodeableConcept> type; 4899 4900 /** 4901 * Associated entities. 4902 */ 4903 @Child(name = "typeReference", type = { 4904 Reference.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4905 @Description(shortDefinition = "Associated entities", formalDefinition = "Associated entities.") 4906 protected List<Reference> typeReference; 4907 /** 4908 * The actual objects that are the target of the reference (Associated 4909 * entities.) 4910 */ 4911 protected List<Resource> typeReferenceTarget; 4912 4913 /** 4914 * May be a subtype or part of an offered asset. 4915 */ 4916 @Child(name = "subtype", type = { 4917 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4918 @Description(shortDefinition = "Asset sub-category", formalDefinition = "May be a subtype or part of an offered asset.") 4919 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-assetsubtype") 4920 protected List<CodeableConcept> subtype; 4921 4922 /** 4923 * Specifies the applicability of the term to an asset resource instance, and 4924 * instances it refers to orinstances that refer to it, and/or are owned by the 4925 * offeree. 4926 */ 4927 @Child(name = "relationship", type = { 4928 Coding.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 4929 @Description(shortDefinition = "Kinship of the asset", formalDefinition = "Specifies the applicability of the term to an asset resource instance, and instances it refers to orinstances that refer to it, and/or are owned by the offeree.") 4930 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/consent-content-class") 4931 protected Coding relationship; 4932 4933 /** 4934 * Circumstance of the asset. 4935 */ 4936 @Child(name = "context", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4937 @Description(shortDefinition = "Circumstance of the asset", formalDefinition = "Circumstance of the asset.") 4938 protected List<AssetContextComponent> context; 4939 4940 /** 4941 * Description of the quality and completeness of the asset that imay be a 4942 * factor in its valuation. 4943 */ 4944 @Child(name = "condition", type = { 4945 StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 4946 @Description(shortDefinition = "Quality desctiption of asset", formalDefinition = "Description of the quality and completeness of the asset that imay be a factor in its valuation.") 4947 protected StringType condition; 4948 4949 /** 4950 * Type of Asset availability for use or ownership. 4951 */ 4952 @Child(name = "periodType", type = { 4953 CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4954 @Description(shortDefinition = "Asset availability types", formalDefinition = "Type of Asset availability for use or ownership.") 4955 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/asset-availability") 4956 protected List<CodeableConcept> periodType; 4957 4958 /** 4959 * Asset relevant contractual time period. 4960 */ 4961 @Child(name = "period", type = { 4962 Period.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4963 @Description(shortDefinition = "Time period of the asset", formalDefinition = "Asset relevant contractual time period.") 4964 protected List<Period> period; 4965 4966 /** 4967 * Time period of asset use. 4968 */ 4969 @Child(name = "usePeriod", type = { 4970 Period.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4971 @Description(shortDefinition = "Time period", formalDefinition = "Time period of asset use.") 4972 protected List<Period> usePeriod; 4973 4974 /** 4975 * Clause or question text (Prose Object) concerning the asset in a linked form, 4976 * such as a QuestionnaireResponse used in the formation of the contract. 4977 */ 4978 @Child(name = "text", type = { StringType.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 4979 @Description(shortDefinition = "Asset clause or question text", formalDefinition = "Clause or question text (Prose Object) concerning the asset in a linked form, such as a QuestionnaireResponse used in the formation of the contract.") 4980 protected StringType text; 4981 4982 /** 4983 * Id [identifier??] of the clause or question text about the asset in the 4984 * referenced form or QuestionnaireResponse. 4985 */ 4986 @Child(name = "linkId", type = { 4987 StringType.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4988 @Description(shortDefinition = "Pointer to asset text", formalDefinition = "Id [identifier??] of the clause or question text about the asset in the referenced form or QuestionnaireResponse.") 4989 protected List<StringType> linkId; 4990 4991 /** 4992 * Response to assets. 4993 */ 4994 @Child(name = "answer", type = { 4995 AnswerComponent.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4996 @Description(shortDefinition = "Response to assets", formalDefinition = "Response to assets.") 4997 protected List<AnswerComponent> answer; 4998 4999 /** 5000 * Security labels that protects the asset. 5001 */ 5002 @Child(name = "securityLabelNumber", type = { 5003 UnsignedIntType.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5004 @Description(shortDefinition = "Asset restriction numbers", formalDefinition = "Security labels that protects the asset.") 5005 protected List<UnsignedIntType> securityLabelNumber; 5006 5007 /** 5008 * Contract Valued Item List. 5009 */ 5010 @Child(name = "valuedItem", type = {}, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5011 @Description(shortDefinition = "Contract Valued Item List", formalDefinition = "Contract Valued Item List.") 5012 protected List<ValuedItemComponent> valuedItem; 5013 5014 private static final long serialVersionUID = -1080398792L; 5015 5016 /** 5017 * Constructor 5018 */ 5019 public ContractAssetComponent() { 5020 super(); 5021 } 5022 5023 /** 5024 * @return {@link #scope} (Differentiates the kind of the asset .) 5025 */ 5026 public CodeableConcept getScope() { 5027 if (this.scope == null) 5028 if (Configuration.errorOnAutoCreate()) 5029 throw new Error("Attempt to auto-create ContractAssetComponent.scope"); 5030 else if (Configuration.doAutoCreate()) 5031 this.scope = new CodeableConcept(); // cc 5032 return this.scope; 5033 } 5034 5035 public boolean hasScope() { 5036 return this.scope != null && !this.scope.isEmpty(); 5037 } 5038 5039 /** 5040 * @param value {@link #scope} (Differentiates the kind of the asset .) 5041 */ 5042 public ContractAssetComponent setScope(CodeableConcept value) { 5043 this.scope = value; 5044 return this; 5045 } 5046 5047 /** 5048 * @return {@link #type} (Target entity type about which the term may be 5049 * concerned.) 5050 */ 5051 public List<CodeableConcept> getType() { 5052 if (this.type == null) 5053 this.type = new ArrayList<CodeableConcept>(); 5054 return this.type; 5055 } 5056 5057 /** 5058 * @return Returns a reference to <code>this</code> for easy method chaining 5059 */ 5060 public ContractAssetComponent setType(List<CodeableConcept> theType) { 5061 this.type = theType; 5062 return this; 5063 } 5064 5065 public boolean hasType() { 5066 if (this.type == null) 5067 return false; 5068 for (CodeableConcept item : this.type) 5069 if (!item.isEmpty()) 5070 return true; 5071 return false; 5072 } 5073 5074 public CodeableConcept addType() { // 3 5075 CodeableConcept t = new CodeableConcept(); 5076 if (this.type == null) 5077 this.type = new ArrayList<CodeableConcept>(); 5078 this.type.add(t); 5079 return t; 5080 } 5081 5082 public ContractAssetComponent addType(CodeableConcept t) { // 3 5083 if (t == null) 5084 return this; 5085 if (this.type == null) 5086 this.type = new ArrayList<CodeableConcept>(); 5087 this.type.add(t); 5088 return this; 5089 } 5090 5091 /** 5092 * @return The first repetition of repeating field {@link #type}, creating it if 5093 * it does not already exist 5094 */ 5095 public CodeableConcept getTypeFirstRep() { 5096 if (getType().isEmpty()) { 5097 addType(); 5098 } 5099 return getType().get(0); 5100 } 5101 5102 /** 5103 * @return {@link #typeReference} (Associated entities.) 5104 */ 5105 public List<Reference> getTypeReference() { 5106 if (this.typeReference == null) 5107 this.typeReference = new ArrayList<Reference>(); 5108 return this.typeReference; 5109 } 5110 5111 /** 5112 * @return Returns a reference to <code>this</code> for easy method chaining 5113 */ 5114 public ContractAssetComponent setTypeReference(List<Reference> theTypeReference) { 5115 this.typeReference = theTypeReference; 5116 return this; 5117 } 5118 5119 public boolean hasTypeReference() { 5120 if (this.typeReference == null) 5121 return false; 5122 for (Reference item : this.typeReference) 5123 if (!item.isEmpty()) 5124 return true; 5125 return false; 5126 } 5127 5128 public Reference addTypeReference() { // 3 5129 Reference t = new Reference(); 5130 if (this.typeReference == null) 5131 this.typeReference = new ArrayList<Reference>(); 5132 this.typeReference.add(t); 5133 return t; 5134 } 5135 5136 public ContractAssetComponent addTypeReference(Reference t) { // 3 5137 if (t == null) 5138 return this; 5139 if (this.typeReference == null) 5140 this.typeReference = new ArrayList<Reference>(); 5141 this.typeReference.add(t); 5142 return this; 5143 } 5144 5145 /** 5146 * @return The first repetition of repeating field {@link #typeReference}, 5147 * creating it if it does not already exist 5148 */ 5149 public Reference getTypeReferenceFirstRep() { 5150 if (getTypeReference().isEmpty()) { 5151 addTypeReference(); 5152 } 5153 return getTypeReference().get(0); 5154 } 5155 5156 /** 5157 * @deprecated Use Reference#setResource(IBaseResource) instead 5158 */ 5159 @Deprecated 5160 public List<Resource> getTypeReferenceTarget() { 5161 if (this.typeReferenceTarget == null) 5162 this.typeReferenceTarget = new ArrayList<Resource>(); 5163 return this.typeReferenceTarget; 5164 } 5165 5166 /** 5167 * @return {@link #subtype} (May be a subtype or part of an offered asset.) 5168 */ 5169 public List<CodeableConcept> getSubtype() { 5170 if (this.subtype == null) 5171 this.subtype = new ArrayList<CodeableConcept>(); 5172 return this.subtype; 5173 } 5174 5175 /** 5176 * @return Returns a reference to <code>this</code> for easy method chaining 5177 */ 5178 public ContractAssetComponent setSubtype(List<CodeableConcept> theSubtype) { 5179 this.subtype = theSubtype; 5180 return this; 5181 } 5182 5183 public boolean hasSubtype() { 5184 if (this.subtype == null) 5185 return false; 5186 for (CodeableConcept item : this.subtype) 5187 if (!item.isEmpty()) 5188 return true; 5189 return false; 5190 } 5191 5192 public CodeableConcept addSubtype() { // 3 5193 CodeableConcept t = new CodeableConcept(); 5194 if (this.subtype == null) 5195 this.subtype = new ArrayList<CodeableConcept>(); 5196 this.subtype.add(t); 5197 return t; 5198 } 5199 5200 public ContractAssetComponent addSubtype(CodeableConcept t) { // 3 5201 if (t == null) 5202 return this; 5203 if (this.subtype == null) 5204 this.subtype = new ArrayList<CodeableConcept>(); 5205 this.subtype.add(t); 5206 return this; 5207 } 5208 5209 /** 5210 * @return The first repetition of repeating field {@link #subtype}, creating it 5211 * if it does not already exist 5212 */ 5213 public CodeableConcept getSubtypeFirstRep() { 5214 if (getSubtype().isEmpty()) { 5215 addSubtype(); 5216 } 5217 return getSubtype().get(0); 5218 } 5219 5220 /** 5221 * @return {@link #relationship} (Specifies the applicability of the term to an 5222 * asset resource instance, and instances it refers to orinstances that 5223 * refer to it, and/or are owned by the offeree.) 5224 */ 5225 public Coding getRelationship() { 5226 if (this.relationship == null) 5227 if (Configuration.errorOnAutoCreate()) 5228 throw new Error("Attempt to auto-create ContractAssetComponent.relationship"); 5229 else if (Configuration.doAutoCreate()) 5230 this.relationship = new Coding(); // cc 5231 return this.relationship; 5232 } 5233 5234 public boolean hasRelationship() { 5235 return this.relationship != null && !this.relationship.isEmpty(); 5236 } 5237 5238 /** 5239 * @param value {@link #relationship} (Specifies the applicability of the term 5240 * to an asset resource instance, and instances it refers to 5241 * orinstances that refer to it, and/or are owned by the offeree.) 5242 */ 5243 public ContractAssetComponent setRelationship(Coding value) { 5244 this.relationship = value; 5245 return this; 5246 } 5247 5248 /** 5249 * @return {@link #context} (Circumstance of the asset.) 5250 */ 5251 public List<AssetContextComponent> getContext() { 5252 if (this.context == null) 5253 this.context = new ArrayList<AssetContextComponent>(); 5254 return this.context; 5255 } 5256 5257 /** 5258 * @return Returns a reference to <code>this</code> for easy method chaining 5259 */ 5260 public ContractAssetComponent setContext(List<AssetContextComponent> theContext) { 5261 this.context = theContext; 5262 return this; 5263 } 5264 5265 public boolean hasContext() { 5266 if (this.context == null) 5267 return false; 5268 for (AssetContextComponent item : this.context) 5269 if (!item.isEmpty()) 5270 return true; 5271 return false; 5272 } 5273 5274 public AssetContextComponent addContext() { // 3 5275 AssetContextComponent t = new AssetContextComponent(); 5276 if (this.context == null) 5277 this.context = new ArrayList<AssetContextComponent>(); 5278 this.context.add(t); 5279 return t; 5280 } 5281 5282 public ContractAssetComponent addContext(AssetContextComponent t) { // 3 5283 if (t == null) 5284 return this; 5285 if (this.context == null) 5286 this.context = new ArrayList<AssetContextComponent>(); 5287 this.context.add(t); 5288 return this; 5289 } 5290 5291 /** 5292 * @return The first repetition of repeating field {@link #context}, creating it 5293 * if it does not already exist 5294 */ 5295 public AssetContextComponent getContextFirstRep() { 5296 if (getContext().isEmpty()) { 5297 addContext(); 5298 } 5299 return getContext().get(0); 5300 } 5301 5302 /** 5303 * @return {@link #condition} (Description of the quality and completeness of 5304 * the asset that imay be a factor in its valuation.). This is the 5305 * underlying object with id, value and extensions. The accessor 5306 * "getCondition" gives direct access to the value 5307 */ 5308 public StringType getConditionElement() { 5309 if (this.condition == null) 5310 if (Configuration.errorOnAutoCreate()) 5311 throw new Error("Attempt to auto-create ContractAssetComponent.condition"); 5312 else if (Configuration.doAutoCreate()) 5313 this.condition = new StringType(); // bb 5314 return this.condition; 5315 } 5316 5317 public boolean hasConditionElement() { 5318 return this.condition != null && !this.condition.isEmpty(); 5319 } 5320 5321 public boolean hasCondition() { 5322 return this.condition != null && !this.condition.isEmpty(); 5323 } 5324 5325 /** 5326 * @param value {@link #condition} (Description of the quality and completeness 5327 * of the asset that imay be a factor in its valuation.). This is 5328 * the underlying object with id, value and extensions. The 5329 * accessor "getCondition" gives direct access to the value 5330 */ 5331 public ContractAssetComponent setConditionElement(StringType value) { 5332 this.condition = value; 5333 return this; 5334 } 5335 5336 /** 5337 * @return Description of the quality and completeness of the asset that imay be 5338 * a factor in its valuation. 5339 */ 5340 public String getCondition() { 5341 return this.condition == null ? null : this.condition.getValue(); 5342 } 5343 5344 /** 5345 * @param value Description of the quality and completeness of the asset that 5346 * imay be a factor in its valuation. 5347 */ 5348 public ContractAssetComponent setCondition(String value) { 5349 if (Utilities.noString(value)) 5350 this.condition = null; 5351 else { 5352 if (this.condition == null) 5353 this.condition = new StringType(); 5354 this.condition.setValue(value); 5355 } 5356 return this; 5357 } 5358 5359 /** 5360 * @return {@link #periodType} (Type of Asset availability for use or 5361 * ownership.) 5362 */ 5363 public List<CodeableConcept> getPeriodType() { 5364 if (this.periodType == null) 5365 this.periodType = new ArrayList<CodeableConcept>(); 5366 return this.periodType; 5367 } 5368 5369 /** 5370 * @return Returns a reference to <code>this</code> for easy method chaining 5371 */ 5372 public ContractAssetComponent setPeriodType(List<CodeableConcept> thePeriodType) { 5373 this.periodType = thePeriodType; 5374 return this; 5375 } 5376 5377 public boolean hasPeriodType() { 5378 if (this.periodType == null) 5379 return false; 5380 for (CodeableConcept item : this.periodType) 5381 if (!item.isEmpty()) 5382 return true; 5383 return false; 5384 } 5385 5386 public CodeableConcept addPeriodType() { // 3 5387 CodeableConcept t = new CodeableConcept(); 5388 if (this.periodType == null) 5389 this.periodType = new ArrayList<CodeableConcept>(); 5390 this.periodType.add(t); 5391 return t; 5392 } 5393 5394 public ContractAssetComponent addPeriodType(CodeableConcept t) { // 3 5395 if (t == null) 5396 return this; 5397 if (this.periodType == null) 5398 this.periodType = new ArrayList<CodeableConcept>(); 5399 this.periodType.add(t); 5400 return this; 5401 } 5402 5403 /** 5404 * @return The first repetition of repeating field {@link #periodType}, creating 5405 * it if it does not already exist 5406 */ 5407 public CodeableConcept getPeriodTypeFirstRep() { 5408 if (getPeriodType().isEmpty()) { 5409 addPeriodType(); 5410 } 5411 return getPeriodType().get(0); 5412 } 5413 5414 /** 5415 * @return {@link #period} (Asset relevant contractual time period.) 5416 */ 5417 public List<Period> getPeriod() { 5418 if (this.period == null) 5419 this.period = new ArrayList<Period>(); 5420 return this.period; 5421 } 5422 5423 /** 5424 * @return Returns a reference to <code>this</code> for easy method chaining 5425 */ 5426 public ContractAssetComponent setPeriod(List<Period> thePeriod) { 5427 this.period = thePeriod; 5428 return this; 5429 } 5430 5431 public boolean hasPeriod() { 5432 if (this.period == null) 5433 return false; 5434 for (Period item : this.period) 5435 if (!item.isEmpty()) 5436 return true; 5437 return false; 5438 } 5439 5440 public Period addPeriod() { // 3 5441 Period t = new Period(); 5442 if (this.period == null) 5443 this.period = new ArrayList<Period>(); 5444 this.period.add(t); 5445 return t; 5446 } 5447 5448 public ContractAssetComponent addPeriod(Period t) { // 3 5449 if (t == null) 5450 return this; 5451 if (this.period == null) 5452 this.period = new ArrayList<Period>(); 5453 this.period.add(t); 5454 return this; 5455 } 5456 5457 /** 5458 * @return The first repetition of repeating field {@link #period}, creating it 5459 * if it does not already exist 5460 */ 5461 public Period getPeriodFirstRep() { 5462 if (getPeriod().isEmpty()) { 5463 addPeriod(); 5464 } 5465 return getPeriod().get(0); 5466 } 5467 5468 /** 5469 * @return {@link #usePeriod} (Time period of asset use.) 5470 */ 5471 public List<Period> getUsePeriod() { 5472 if (this.usePeriod == null) 5473 this.usePeriod = new ArrayList<Period>(); 5474 return this.usePeriod; 5475 } 5476 5477 /** 5478 * @return Returns a reference to <code>this</code> for easy method chaining 5479 */ 5480 public ContractAssetComponent setUsePeriod(List<Period> theUsePeriod) { 5481 this.usePeriod = theUsePeriod; 5482 return this; 5483 } 5484 5485 public boolean hasUsePeriod() { 5486 if (this.usePeriod == null) 5487 return false; 5488 for (Period item : this.usePeriod) 5489 if (!item.isEmpty()) 5490 return true; 5491 return false; 5492 } 5493 5494 public Period addUsePeriod() { // 3 5495 Period t = new Period(); 5496 if (this.usePeriod == null) 5497 this.usePeriod = new ArrayList<Period>(); 5498 this.usePeriod.add(t); 5499 return t; 5500 } 5501 5502 public ContractAssetComponent addUsePeriod(Period t) { // 3 5503 if (t == null) 5504 return this; 5505 if (this.usePeriod == null) 5506 this.usePeriod = new ArrayList<Period>(); 5507 this.usePeriod.add(t); 5508 return this; 5509 } 5510 5511 /** 5512 * @return The first repetition of repeating field {@link #usePeriod}, creating 5513 * it if it does not already exist 5514 */ 5515 public Period getUsePeriodFirstRep() { 5516 if (getUsePeriod().isEmpty()) { 5517 addUsePeriod(); 5518 } 5519 return getUsePeriod().get(0); 5520 } 5521 5522 /** 5523 * @return {@link #text} (Clause or question text (Prose Object) concerning the 5524 * asset in a linked form, such as a QuestionnaireResponse used in the 5525 * formation of the contract.). This is the underlying object with id, 5526 * value and extensions. The accessor "getText" gives direct access to 5527 * the value 5528 */ 5529 public StringType getTextElement() { 5530 if (this.text == null) 5531 if (Configuration.errorOnAutoCreate()) 5532 throw new Error("Attempt to auto-create ContractAssetComponent.text"); 5533 else if (Configuration.doAutoCreate()) 5534 this.text = new StringType(); // bb 5535 return this.text; 5536 } 5537 5538 public boolean hasTextElement() { 5539 return this.text != null && !this.text.isEmpty(); 5540 } 5541 5542 public boolean hasText() { 5543 return this.text != null && !this.text.isEmpty(); 5544 } 5545 5546 /** 5547 * @param value {@link #text} (Clause or question text (Prose Object) concerning 5548 * the asset in a linked form, such as a QuestionnaireResponse used 5549 * in the formation of the contract.). This is the underlying 5550 * object with id, value and extensions. The accessor "getText" 5551 * gives direct access to the value 5552 */ 5553 public ContractAssetComponent setTextElement(StringType value) { 5554 this.text = value; 5555 return this; 5556 } 5557 5558 /** 5559 * @return Clause or question text (Prose Object) concerning the asset in a 5560 * linked form, such as a QuestionnaireResponse used in the formation of 5561 * the contract. 5562 */ 5563 public String getText() { 5564 return this.text == null ? null : this.text.getValue(); 5565 } 5566 5567 /** 5568 * @param value Clause or question text (Prose Object) concerning the asset in a 5569 * linked form, such as a QuestionnaireResponse used in the 5570 * formation of the contract. 5571 */ 5572 public ContractAssetComponent setText(String value) { 5573 if (Utilities.noString(value)) 5574 this.text = null; 5575 else { 5576 if (this.text == null) 5577 this.text = new StringType(); 5578 this.text.setValue(value); 5579 } 5580 return this; 5581 } 5582 5583 /** 5584 * @return {@link #linkId} (Id [identifier??] of the clause or question text 5585 * about the asset in the referenced form or QuestionnaireResponse.) 5586 */ 5587 public List<StringType> getLinkId() { 5588 if (this.linkId == null) 5589 this.linkId = new ArrayList<StringType>(); 5590 return this.linkId; 5591 } 5592 5593 /** 5594 * @return Returns a reference to <code>this</code> for easy method chaining 5595 */ 5596 public ContractAssetComponent setLinkId(List<StringType> theLinkId) { 5597 this.linkId = theLinkId; 5598 return this; 5599 } 5600 5601 public boolean hasLinkId() { 5602 if (this.linkId == null) 5603 return false; 5604 for (StringType item : this.linkId) 5605 if (!item.isEmpty()) 5606 return true; 5607 return false; 5608 } 5609 5610 /** 5611 * @return {@link #linkId} (Id [identifier??] of the clause or question text 5612 * about the asset in the referenced form or QuestionnaireResponse.) 5613 */ 5614 public StringType addLinkIdElement() {// 2 5615 StringType t = new StringType(); 5616 if (this.linkId == null) 5617 this.linkId = new ArrayList<StringType>(); 5618 this.linkId.add(t); 5619 return t; 5620 } 5621 5622 /** 5623 * @param value {@link #linkId} (Id [identifier??] of the clause or question 5624 * text about the asset in the referenced form or 5625 * QuestionnaireResponse.) 5626 */ 5627 public ContractAssetComponent addLinkId(String value) { // 1 5628 StringType t = new StringType(); 5629 t.setValue(value); 5630 if (this.linkId == null) 5631 this.linkId = new ArrayList<StringType>(); 5632 this.linkId.add(t); 5633 return this; 5634 } 5635 5636 /** 5637 * @param value {@link #linkId} (Id [identifier??] of the clause or question 5638 * text about the asset in the referenced form or 5639 * QuestionnaireResponse.) 5640 */ 5641 public boolean hasLinkId(String value) { 5642 if (this.linkId == null) 5643 return false; 5644 for (StringType v : this.linkId) 5645 if (v.getValue().equals(value)) // string 5646 return true; 5647 return false; 5648 } 5649 5650 /** 5651 * @return {@link #answer} (Response to assets.) 5652 */ 5653 public List<AnswerComponent> getAnswer() { 5654 if (this.answer == null) 5655 this.answer = new ArrayList<AnswerComponent>(); 5656 return this.answer; 5657 } 5658 5659 /** 5660 * @return Returns a reference to <code>this</code> for easy method chaining 5661 */ 5662 public ContractAssetComponent setAnswer(List<AnswerComponent> theAnswer) { 5663 this.answer = theAnswer; 5664 return this; 5665 } 5666 5667 public boolean hasAnswer() { 5668 if (this.answer == null) 5669 return false; 5670 for (AnswerComponent item : this.answer) 5671 if (!item.isEmpty()) 5672 return true; 5673 return false; 5674 } 5675 5676 public AnswerComponent addAnswer() { // 3 5677 AnswerComponent t = new AnswerComponent(); 5678 if (this.answer == null) 5679 this.answer = new ArrayList<AnswerComponent>(); 5680 this.answer.add(t); 5681 return t; 5682 } 5683 5684 public ContractAssetComponent addAnswer(AnswerComponent t) { // 3 5685 if (t == null) 5686 return this; 5687 if (this.answer == null) 5688 this.answer = new ArrayList<AnswerComponent>(); 5689 this.answer.add(t); 5690 return this; 5691 } 5692 5693 /** 5694 * @return The first repetition of repeating field {@link #answer}, creating it 5695 * if it does not already exist 5696 */ 5697 public AnswerComponent getAnswerFirstRep() { 5698 if (getAnswer().isEmpty()) { 5699 addAnswer(); 5700 } 5701 return getAnswer().get(0); 5702 } 5703 5704 /** 5705 * @return {@link #securityLabelNumber} (Security labels that protects the 5706 * asset.) 5707 */ 5708 public List<UnsignedIntType> getSecurityLabelNumber() { 5709 if (this.securityLabelNumber == null) 5710 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 5711 return this.securityLabelNumber; 5712 } 5713 5714 /** 5715 * @return Returns a reference to <code>this</code> for easy method chaining 5716 */ 5717 public ContractAssetComponent setSecurityLabelNumber(List<UnsignedIntType> theSecurityLabelNumber) { 5718 this.securityLabelNumber = theSecurityLabelNumber; 5719 return this; 5720 } 5721 5722 public boolean hasSecurityLabelNumber() { 5723 if (this.securityLabelNumber == null) 5724 return false; 5725 for (UnsignedIntType item : this.securityLabelNumber) 5726 if (!item.isEmpty()) 5727 return true; 5728 return false; 5729 } 5730 5731 /** 5732 * @return {@link #securityLabelNumber} (Security labels that protects the 5733 * asset.) 5734 */ 5735 public UnsignedIntType addSecurityLabelNumberElement() {// 2 5736 UnsignedIntType t = new UnsignedIntType(); 5737 if (this.securityLabelNumber == null) 5738 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 5739 this.securityLabelNumber.add(t); 5740 return t; 5741 } 5742 5743 /** 5744 * @param value {@link #securityLabelNumber} (Security labels that protects the 5745 * asset.) 5746 */ 5747 public ContractAssetComponent addSecurityLabelNumber(int value) { // 1 5748 UnsignedIntType t = new UnsignedIntType(); 5749 t.setValue(value); 5750 if (this.securityLabelNumber == null) 5751 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 5752 this.securityLabelNumber.add(t); 5753 return this; 5754 } 5755 5756 /** 5757 * @param value {@link #securityLabelNumber} (Security labels that protects the 5758 * asset.) 5759 */ 5760 public boolean hasSecurityLabelNumber(int value) { 5761 if (this.securityLabelNumber == null) 5762 return false; 5763 for (UnsignedIntType v : this.securityLabelNumber) 5764 if (v.getValue().equals(value)) // unsignedInt 5765 return true; 5766 return false; 5767 } 5768 5769 /** 5770 * @return {@link #valuedItem} (Contract Valued Item List.) 5771 */ 5772 public List<ValuedItemComponent> getValuedItem() { 5773 if (this.valuedItem == null) 5774 this.valuedItem = new ArrayList<ValuedItemComponent>(); 5775 return this.valuedItem; 5776 } 5777 5778 /** 5779 * @return Returns a reference to <code>this</code> for easy method chaining 5780 */ 5781 public ContractAssetComponent setValuedItem(List<ValuedItemComponent> theValuedItem) { 5782 this.valuedItem = theValuedItem; 5783 return this; 5784 } 5785 5786 public boolean hasValuedItem() { 5787 if (this.valuedItem == null) 5788 return false; 5789 for (ValuedItemComponent item : this.valuedItem) 5790 if (!item.isEmpty()) 5791 return true; 5792 return false; 5793 } 5794 5795 public ValuedItemComponent addValuedItem() { // 3 5796 ValuedItemComponent t = new ValuedItemComponent(); 5797 if (this.valuedItem == null) 5798 this.valuedItem = new ArrayList<ValuedItemComponent>(); 5799 this.valuedItem.add(t); 5800 return t; 5801 } 5802 5803 public ContractAssetComponent addValuedItem(ValuedItemComponent t) { // 3 5804 if (t == null) 5805 return this; 5806 if (this.valuedItem == null) 5807 this.valuedItem = new ArrayList<ValuedItemComponent>(); 5808 this.valuedItem.add(t); 5809 return this; 5810 } 5811 5812 /** 5813 * @return The first repetition of repeating field {@link #valuedItem}, creating 5814 * it if it does not already exist 5815 */ 5816 public ValuedItemComponent getValuedItemFirstRep() { 5817 if (getValuedItem().isEmpty()) { 5818 addValuedItem(); 5819 } 5820 return getValuedItem().get(0); 5821 } 5822 5823 protected void listChildren(List<Property> children) { 5824 super.listChildren(children); 5825 children.add(new Property("scope", "CodeableConcept", "Differentiates the kind of the asset .", 0, 1, scope)); 5826 children.add(new Property("type", "CodeableConcept", "Target entity type about which the term may be concerned.", 5827 0, java.lang.Integer.MAX_VALUE, type)); 5828 children.add(new Property("typeReference", "Reference(Any)", "Associated entities.", 0, 5829 java.lang.Integer.MAX_VALUE, typeReference)); 5830 children.add(new Property("subtype", "CodeableConcept", "May be a subtype or part of an offered asset.", 0, 5831 java.lang.Integer.MAX_VALUE, subtype)); 5832 children.add(new Property("relationship", "Coding", 5833 "Specifies the applicability of the term to an asset resource instance, and instances it refers to orinstances that refer to it, and/or are owned by the offeree.", 5834 0, 1, relationship)); 5835 children.add(new Property("context", "", "Circumstance of the asset.", 0, java.lang.Integer.MAX_VALUE, context)); 5836 children.add(new Property("condition", "string", 5837 "Description of the quality and completeness of the asset that imay be a factor in its valuation.", 0, 1, 5838 condition)); 5839 children.add(new Property("periodType", "CodeableConcept", "Type of Asset availability for use or ownership.", 0, 5840 java.lang.Integer.MAX_VALUE, periodType)); 5841 children.add(new Property("period", "Period", "Asset relevant contractual time period.", 0, 5842 java.lang.Integer.MAX_VALUE, period)); 5843 children.add( 5844 new Property("usePeriod", "Period", "Time period of asset use.", 0, java.lang.Integer.MAX_VALUE, usePeriod)); 5845 children.add(new Property("text", "string", 5846 "Clause or question text (Prose Object) concerning the asset in a linked form, such as a QuestionnaireResponse used in the formation of the contract.", 5847 0, 1, text)); 5848 children.add(new Property("linkId", "string", 5849 "Id [identifier??] of the clause or question text about the asset in the referenced form or QuestionnaireResponse.", 5850 0, java.lang.Integer.MAX_VALUE, linkId)); 5851 children.add(new Property("answer", "@Contract.term.offer.answer", "Response to assets.", 0, 5852 java.lang.Integer.MAX_VALUE, answer)); 5853 children.add(new Property("securityLabelNumber", "unsignedInt", "Security labels that protects the asset.", 0, 5854 java.lang.Integer.MAX_VALUE, securityLabelNumber)); 5855 children.add( 5856 new Property("valuedItem", "", "Contract Valued Item List.", 0, java.lang.Integer.MAX_VALUE, valuedItem)); 5857 } 5858 5859 @Override 5860 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5861 switch (_hash) { 5862 case 109264468: 5863 /* scope */ return new Property("scope", "CodeableConcept", "Differentiates the kind of the asset .", 0, 1, 5864 scope); 5865 case 3575610: 5866 /* type */ return new Property("type", "CodeableConcept", 5867 "Target entity type about which the term may be concerned.", 0, java.lang.Integer.MAX_VALUE, type); 5868 case 2074825009: 5869 /* typeReference */ return new Property("typeReference", "Reference(Any)", "Associated entities.", 0, 5870 java.lang.Integer.MAX_VALUE, typeReference); 5871 case -1867567750: 5872 /* subtype */ return new Property("subtype", "CodeableConcept", "May be a subtype or part of an offered asset.", 5873 0, java.lang.Integer.MAX_VALUE, subtype); 5874 case -261851592: 5875 /* relationship */ return new Property("relationship", "Coding", 5876 "Specifies the applicability of the term to an asset resource instance, and instances it refers to orinstances that refer to it, and/or are owned by the offeree.", 5877 0, 1, relationship); 5878 case 951530927: 5879 /* context */ return new Property("context", "", "Circumstance of the asset.", 0, java.lang.Integer.MAX_VALUE, 5880 context); 5881 case -861311717: 5882 /* condition */ return new Property("condition", "string", 5883 "Description of the quality and completeness of the asset that imay be a factor in its valuation.", 0, 1, 5884 condition); 5885 case 384348315: 5886 /* periodType */ return new Property("periodType", "CodeableConcept", 5887 "Type of Asset availability for use or ownership.", 0, java.lang.Integer.MAX_VALUE, periodType); 5888 case -991726143: 5889 /* period */ return new Property("period", "Period", "Asset relevant contractual time period.", 0, 5890 java.lang.Integer.MAX_VALUE, period); 5891 case -628382168: 5892 /* usePeriod */ return new Property("usePeriod", "Period", "Time period of asset use.", 0, 5893 java.lang.Integer.MAX_VALUE, usePeriod); 5894 case 3556653: 5895 /* text */ return new Property("text", "string", 5896 "Clause or question text (Prose Object) concerning the asset in a linked form, such as a QuestionnaireResponse used in the formation of the contract.", 5897 0, 1, text); 5898 case -1102667083: 5899 /* linkId */ return new Property("linkId", "string", 5900 "Id [identifier??] of the clause or question text about the asset in the referenced form or QuestionnaireResponse.", 5901 0, java.lang.Integer.MAX_VALUE, linkId); 5902 case -1412808770: 5903 /* answer */ return new Property("answer", "@Contract.term.offer.answer", "Response to assets.", 0, 5904 java.lang.Integer.MAX_VALUE, answer); 5905 case -149460995: 5906 /* securityLabelNumber */ return new Property("securityLabelNumber", "unsignedInt", 5907 "Security labels that protects the asset.", 0, java.lang.Integer.MAX_VALUE, securityLabelNumber); 5908 case 2046675654: 5909 /* valuedItem */ return new Property("valuedItem", "", "Contract Valued Item List.", 0, 5910 java.lang.Integer.MAX_VALUE, valuedItem); 5911 default: 5912 return super.getNamedProperty(_hash, _name, _checkValid); 5913 } 5914 5915 } 5916 5917 @Override 5918 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5919 switch (hash) { 5920 case 109264468: 5921 /* scope */ return this.scope == null ? new Base[0] : new Base[] { this.scope }; // CodeableConcept 5922 case 3575610: 5923 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 5924 case 2074825009: 5925 /* typeReference */ return this.typeReference == null ? new Base[0] 5926 : this.typeReference.toArray(new Base[this.typeReference.size()]); // Reference 5927 case -1867567750: 5928 /* subtype */ return this.subtype == null ? new Base[0] : this.subtype.toArray(new Base[this.subtype.size()]); // CodeableConcept 5929 case -261851592: 5930 /* relationship */ return this.relationship == null ? new Base[0] : new Base[] { this.relationship }; // Coding 5931 case 951530927: 5932 /* context */ return this.context == null ? new Base[0] : this.context.toArray(new Base[this.context.size()]); // AssetContextComponent 5933 case -861311717: 5934 /* condition */ return this.condition == null ? new Base[0] : new Base[] { this.condition }; // StringType 5935 case 384348315: 5936 /* periodType */ return this.periodType == null ? new Base[0] 5937 : this.periodType.toArray(new Base[this.periodType.size()]); // CodeableConcept 5938 case -991726143: 5939 /* period */ return this.period == null ? new Base[0] : this.period.toArray(new Base[this.period.size()]); // Period 5940 case -628382168: 5941 /* usePeriod */ return this.usePeriod == null ? new Base[0] 5942 : this.usePeriod.toArray(new Base[this.usePeriod.size()]); // Period 5943 case 3556653: 5944 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType 5945 case -1102667083: 5946 /* linkId */ return this.linkId == null ? new Base[0] : this.linkId.toArray(new Base[this.linkId.size()]); // StringType 5947 case -1412808770: 5948 /* answer */ return this.answer == null ? new Base[0] : this.answer.toArray(new Base[this.answer.size()]); // AnswerComponent 5949 case -149460995: 5950 /* securityLabelNumber */ return this.securityLabelNumber == null ? new Base[0] 5951 : this.securityLabelNumber.toArray(new Base[this.securityLabelNumber.size()]); // UnsignedIntType 5952 case 2046675654: 5953 /* valuedItem */ return this.valuedItem == null ? new Base[0] 5954 : this.valuedItem.toArray(new Base[this.valuedItem.size()]); // ValuedItemComponent 5955 default: 5956 return super.getProperty(hash, name, checkValid); 5957 } 5958 5959 } 5960 5961 @Override 5962 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5963 switch (hash) { 5964 case 109264468: // scope 5965 this.scope = castToCodeableConcept(value); // CodeableConcept 5966 return value; 5967 case 3575610: // type 5968 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 5969 return value; 5970 case 2074825009: // typeReference 5971 this.getTypeReference().add(castToReference(value)); // Reference 5972 return value; 5973 case -1867567750: // subtype 5974 this.getSubtype().add(castToCodeableConcept(value)); // CodeableConcept 5975 return value; 5976 case -261851592: // relationship 5977 this.relationship = castToCoding(value); // Coding 5978 return value; 5979 case 951530927: // context 5980 this.getContext().add((AssetContextComponent) value); // AssetContextComponent 5981 return value; 5982 case -861311717: // condition 5983 this.condition = castToString(value); // StringType 5984 return value; 5985 case 384348315: // periodType 5986 this.getPeriodType().add(castToCodeableConcept(value)); // CodeableConcept 5987 return value; 5988 case -991726143: // period 5989 this.getPeriod().add(castToPeriod(value)); // Period 5990 return value; 5991 case -628382168: // usePeriod 5992 this.getUsePeriod().add(castToPeriod(value)); // Period 5993 return value; 5994 case 3556653: // text 5995 this.text = castToString(value); // StringType 5996 return value; 5997 case -1102667083: // linkId 5998 this.getLinkId().add(castToString(value)); // StringType 5999 return value; 6000 case -1412808770: // answer 6001 this.getAnswer().add((AnswerComponent) value); // AnswerComponent 6002 return value; 6003 case -149460995: // securityLabelNumber 6004 this.getSecurityLabelNumber().add(castToUnsignedInt(value)); // UnsignedIntType 6005 return value; 6006 case 2046675654: // valuedItem 6007 this.getValuedItem().add((ValuedItemComponent) value); // ValuedItemComponent 6008 return value; 6009 default: 6010 return super.setProperty(hash, name, value); 6011 } 6012 6013 } 6014 6015 @Override 6016 public Base setProperty(String name, Base value) throws FHIRException { 6017 if (name.equals("scope")) { 6018 this.scope = castToCodeableConcept(value); // CodeableConcept 6019 } else if (name.equals("type")) { 6020 this.getType().add(castToCodeableConcept(value)); 6021 } else if (name.equals("typeReference")) { 6022 this.getTypeReference().add(castToReference(value)); 6023 } else if (name.equals("subtype")) { 6024 this.getSubtype().add(castToCodeableConcept(value)); 6025 } else if (name.equals("relationship")) { 6026 this.relationship = castToCoding(value); // Coding 6027 } else if (name.equals("context")) { 6028 this.getContext().add((AssetContextComponent) value); 6029 } else if (name.equals("condition")) { 6030 this.condition = castToString(value); // StringType 6031 } else if (name.equals("periodType")) { 6032 this.getPeriodType().add(castToCodeableConcept(value)); 6033 } else if (name.equals("period")) { 6034 this.getPeriod().add(castToPeriod(value)); 6035 } else if (name.equals("usePeriod")) { 6036 this.getUsePeriod().add(castToPeriod(value)); 6037 } else if (name.equals("text")) { 6038 this.text = castToString(value); // StringType 6039 } else if (name.equals("linkId")) { 6040 this.getLinkId().add(castToString(value)); 6041 } else if (name.equals("answer")) { 6042 this.getAnswer().add((AnswerComponent) value); 6043 } else if (name.equals("securityLabelNumber")) { 6044 this.getSecurityLabelNumber().add(castToUnsignedInt(value)); 6045 } else if (name.equals("valuedItem")) { 6046 this.getValuedItem().add((ValuedItemComponent) value); 6047 } else 6048 return super.setProperty(name, value); 6049 return value; 6050 } 6051 6052 @Override 6053 public void removeChild(String name, Base value) throws FHIRException { 6054 if (name.equals("scope")) { 6055 this.scope = null; 6056 } else if (name.equals("type")) { 6057 this.getType().remove(castToCodeableConcept(value)); 6058 } else if (name.equals("typeReference")) { 6059 this.getTypeReference().remove(castToReference(value)); 6060 } else if (name.equals("subtype")) { 6061 this.getSubtype().remove(castToCodeableConcept(value)); 6062 } else if (name.equals("relationship")) { 6063 this.relationship = null; 6064 } else if (name.equals("context")) { 6065 this.getContext().remove((AssetContextComponent) value); 6066 } else if (name.equals("condition")) { 6067 this.condition = null; 6068 } else if (name.equals("periodType")) { 6069 this.getPeriodType().remove(castToCodeableConcept(value)); 6070 } else if (name.equals("period")) { 6071 this.getPeriod().remove(castToPeriod(value)); 6072 } else if (name.equals("usePeriod")) { 6073 this.getUsePeriod().remove(castToPeriod(value)); 6074 } else if (name.equals("text")) { 6075 this.text = null; 6076 } else if (name.equals("linkId")) { 6077 this.getLinkId().remove(castToString(value)); 6078 } else if (name.equals("answer")) { 6079 this.getAnswer().remove((AnswerComponent) value); 6080 } else if (name.equals("securityLabelNumber")) { 6081 this.getSecurityLabelNumber().remove(castToUnsignedInt(value)); 6082 } else if (name.equals("valuedItem")) { 6083 this.getValuedItem().remove((ValuedItemComponent) value); 6084 } else 6085 super.removeChild(name, value); 6086 6087 } 6088 6089 @Override 6090 public Base makeProperty(int hash, String name) throws FHIRException { 6091 switch (hash) { 6092 case 109264468: 6093 return getScope(); 6094 case 3575610: 6095 return addType(); 6096 case 2074825009: 6097 return addTypeReference(); 6098 case -1867567750: 6099 return addSubtype(); 6100 case -261851592: 6101 return getRelationship(); 6102 case 951530927: 6103 return addContext(); 6104 case -861311717: 6105 return getConditionElement(); 6106 case 384348315: 6107 return addPeriodType(); 6108 case -991726143: 6109 return addPeriod(); 6110 case -628382168: 6111 return addUsePeriod(); 6112 case 3556653: 6113 return getTextElement(); 6114 case -1102667083: 6115 return addLinkIdElement(); 6116 case -1412808770: 6117 return addAnswer(); 6118 case -149460995: 6119 return addSecurityLabelNumberElement(); 6120 case 2046675654: 6121 return addValuedItem(); 6122 default: 6123 return super.makeProperty(hash, name); 6124 } 6125 6126 } 6127 6128 @Override 6129 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6130 switch (hash) { 6131 case 109264468: 6132 /* scope */ return new String[] { "CodeableConcept" }; 6133 case 3575610: 6134 /* type */ return new String[] { "CodeableConcept" }; 6135 case 2074825009: 6136 /* typeReference */ return new String[] { "Reference" }; 6137 case -1867567750: 6138 /* subtype */ return new String[] { "CodeableConcept" }; 6139 case -261851592: 6140 /* relationship */ return new String[] { "Coding" }; 6141 case 951530927: 6142 /* context */ return new String[] {}; 6143 case -861311717: 6144 /* condition */ return new String[] { "string" }; 6145 case 384348315: 6146 /* periodType */ return new String[] { "CodeableConcept" }; 6147 case -991726143: 6148 /* period */ return new String[] { "Period" }; 6149 case -628382168: 6150 /* usePeriod */ return new String[] { "Period" }; 6151 case 3556653: 6152 /* text */ return new String[] { "string" }; 6153 case -1102667083: 6154 /* linkId */ return new String[] { "string" }; 6155 case -1412808770: 6156 /* answer */ return new String[] { "@Contract.term.offer.answer" }; 6157 case -149460995: 6158 /* securityLabelNumber */ return new String[] { "unsignedInt" }; 6159 case 2046675654: 6160 /* valuedItem */ return new String[] {}; 6161 default: 6162 return super.getTypesForProperty(hash, name); 6163 } 6164 6165 } 6166 6167 @Override 6168 public Base addChild(String name) throws FHIRException { 6169 if (name.equals("scope")) { 6170 this.scope = new CodeableConcept(); 6171 return this.scope; 6172 } else if (name.equals("type")) { 6173 return addType(); 6174 } else if (name.equals("typeReference")) { 6175 return addTypeReference(); 6176 } else if (name.equals("subtype")) { 6177 return addSubtype(); 6178 } else if (name.equals("relationship")) { 6179 this.relationship = new Coding(); 6180 return this.relationship; 6181 } else if (name.equals("context")) { 6182 return addContext(); 6183 } else if (name.equals("condition")) { 6184 throw new FHIRException("Cannot call addChild on a singleton property Contract.condition"); 6185 } else if (name.equals("periodType")) { 6186 return addPeriodType(); 6187 } else if (name.equals("period")) { 6188 return addPeriod(); 6189 } else if (name.equals("usePeriod")) { 6190 return addUsePeriod(); 6191 } else if (name.equals("text")) { 6192 throw new FHIRException("Cannot call addChild on a singleton property Contract.text"); 6193 } else if (name.equals("linkId")) { 6194 throw new FHIRException("Cannot call addChild on a singleton property Contract.linkId"); 6195 } else if (name.equals("answer")) { 6196 return addAnswer(); 6197 } else if (name.equals("securityLabelNumber")) { 6198 throw new FHIRException("Cannot call addChild on a singleton property Contract.securityLabelNumber"); 6199 } else if (name.equals("valuedItem")) { 6200 return addValuedItem(); 6201 } else 6202 return super.addChild(name); 6203 } 6204 6205 public ContractAssetComponent copy() { 6206 ContractAssetComponent dst = new ContractAssetComponent(); 6207 copyValues(dst); 6208 return dst; 6209 } 6210 6211 public void copyValues(ContractAssetComponent dst) { 6212 super.copyValues(dst); 6213 dst.scope = scope == null ? null : scope.copy(); 6214 if (type != null) { 6215 dst.type = new ArrayList<CodeableConcept>(); 6216 for (CodeableConcept i : type) 6217 dst.type.add(i.copy()); 6218 } 6219 ; 6220 if (typeReference != null) { 6221 dst.typeReference = new ArrayList<Reference>(); 6222 for (Reference i : typeReference) 6223 dst.typeReference.add(i.copy()); 6224 } 6225 ; 6226 if (subtype != null) { 6227 dst.subtype = new ArrayList<CodeableConcept>(); 6228 for (CodeableConcept i : subtype) 6229 dst.subtype.add(i.copy()); 6230 } 6231 ; 6232 dst.relationship = relationship == null ? null : relationship.copy(); 6233 if (context != null) { 6234 dst.context = new ArrayList<AssetContextComponent>(); 6235 for (AssetContextComponent i : context) 6236 dst.context.add(i.copy()); 6237 } 6238 ; 6239 dst.condition = condition == null ? null : condition.copy(); 6240 if (periodType != null) { 6241 dst.periodType = new ArrayList<CodeableConcept>(); 6242 for (CodeableConcept i : periodType) 6243 dst.periodType.add(i.copy()); 6244 } 6245 ; 6246 if (period != null) { 6247 dst.period = new ArrayList<Period>(); 6248 for (Period i : period) 6249 dst.period.add(i.copy()); 6250 } 6251 ; 6252 if (usePeriod != null) { 6253 dst.usePeriod = new ArrayList<Period>(); 6254 for (Period i : usePeriod) 6255 dst.usePeriod.add(i.copy()); 6256 } 6257 ; 6258 dst.text = text == null ? null : text.copy(); 6259 if (linkId != null) { 6260 dst.linkId = new ArrayList<StringType>(); 6261 for (StringType i : linkId) 6262 dst.linkId.add(i.copy()); 6263 } 6264 ; 6265 if (answer != null) { 6266 dst.answer = new ArrayList<AnswerComponent>(); 6267 for (AnswerComponent i : answer) 6268 dst.answer.add(i.copy()); 6269 } 6270 ; 6271 if (securityLabelNumber != null) { 6272 dst.securityLabelNumber = new ArrayList<UnsignedIntType>(); 6273 for (UnsignedIntType i : securityLabelNumber) 6274 dst.securityLabelNumber.add(i.copy()); 6275 } 6276 ; 6277 if (valuedItem != null) { 6278 dst.valuedItem = new ArrayList<ValuedItemComponent>(); 6279 for (ValuedItemComponent i : valuedItem) 6280 dst.valuedItem.add(i.copy()); 6281 } 6282 ; 6283 } 6284 6285 @Override 6286 public boolean equalsDeep(Base other_) { 6287 if (!super.equalsDeep(other_)) 6288 return false; 6289 if (!(other_ instanceof ContractAssetComponent)) 6290 return false; 6291 ContractAssetComponent o = (ContractAssetComponent) other_; 6292 return compareDeep(scope, o.scope, true) && compareDeep(type, o.type, true) 6293 && compareDeep(typeReference, o.typeReference, true) && compareDeep(subtype, o.subtype, true) 6294 && compareDeep(relationship, o.relationship, true) && compareDeep(context, o.context, true) 6295 && compareDeep(condition, o.condition, true) && compareDeep(periodType, o.periodType, true) 6296 && compareDeep(period, o.period, true) && compareDeep(usePeriod, o.usePeriod, true) 6297 && compareDeep(text, o.text, true) && compareDeep(linkId, o.linkId, true) 6298 && compareDeep(answer, o.answer, true) && compareDeep(securityLabelNumber, o.securityLabelNumber, true) 6299 && compareDeep(valuedItem, o.valuedItem, true); 6300 } 6301 6302 @Override 6303 public boolean equalsShallow(Base other_) { 6304 if (!super.equalsShallow(other_)) 6305 return false; 6306 if (!(other_ instanceof ContractAssetComponent)) 6307 return false; 6308 ContractAssetComponent o = (ContractAssetComponent) other_; 6309 return compareValues(condition, o.condition, true) && compareValues(text, o.text, true) 6310 && compareValues(linkId, o.linkId, true) && compareValues(securityLabelNumber, o.securityLabelNumber, true); 6311 } 6312 6313 public boolean isEmpty() { 6314 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(scope, type, typeReference, subtype, relationship, 6315 context, condition, periodType, period, usePeriod, text, linkId, answer, securityLabelNumber, valuedItem); 6316 } 6317 6318 public String fhirType() { 6319 return "Contract.term.asset"; 6320 6321 } 6322 6323 } 6324 6325 @Block() 6326 public static class AssetContextComponent extends BackboneElement implements IBaseBackboneElement { 6327 /** 6328 * Asset context reference may include the creator, custodian, or owning Person 6329 * or Organization (e.g., bank, repository), location held, e.g., building, 6330 * jurisdiction. 6331 */ 6332 @Child(name = "reference", type = { 6333 Reference.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 6334 @Description(shortDefinition = "Creator,custodian or owner", formalDefinition = "Asset context reference may include the creator, custodian, or owning Person or Organization (e.g., bank, repository), location held, e.g., building, jurisdiction.") 6335 protected Reference reference; 6336 6337 /** 6338 * The actual object that is the target of the reference (Asset context 6339 * reference may include the creator, custodian, or owning Person or 6340 * Organization (e.g., bank, repository), location held, e.g., building, 6341 * jurisdiction.) 6342 */ 6343 protected Resource referenceTarget; 6344 6345 /** 6346 * Coded representation of the context generally or of the Referenced entity, 6347 * such as the asset holder type or location. 6348 */ 6349 @Child(name = "code", type = { 6350 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6351 @Description(shortDefinition = "Codeable asset context", formalDefinition = "Coded representation of the context generally or of the Referenced entity, such as the asset holder type or location.") 6352 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-assetcontext") 6353 protected List<CodeableConcept> code; 6354 6355 /** 6356 * Context description. 6357 */ 6358 @Child(name = "text", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 6359 @Description(shortDefinition = "Context description", formalDefinition = "Context description.") 6360 protected StringType text; 6361 6362 private static final long serialVersionUID = -634115628L; 6363 6364 /** 6365 * Constructor 6366 */ 6367 public AssetContextComponent() { 6368 super(); 6369 } 6370 6371 /** 6372 * @return {@link #reference} (Asset context reference may include the creator, 6373 * custodian, or owning Person or Organization (e.g., bank, repository), 6374 * location held, e.g., building, jurisdiction.) 6375 */ 6376 public Reference getReference() { 6377 if (this.reference == null) 6378 if (Configuration.errorOnAutoCreate()) 6379 throw new Error("Attempt to auto-create AssetContextComponent.reference"); 6380 else if (Configuration.doAutoCreate()) 6381 this.reference = new Reference(); // cc 6382 return this.reference; 6383 } 6384 6385 public boolean hasReference() { 6386 return this.reference != null && !this.reference.isEmpty(); 6387 } 6388 6389 /** 6390 * @param value {@link #reference} (Asset context reference may include the 6391 * creator, custodian, or owning Person or Organization (e.g., 6392 * bank, repository), location held, e.g., building, jurisdiction.) 6393 */ 6394 public AssetContextComponent setReference(Reference value) { 6395 this.reference = value; 6396 return this; 6397 } 6398 6399 /** 6400 * @return {@link #reference} The actual object that is the target of the 6401 * reference. The reference library doesn't populate this, but you can 6402 * use it to hold the resource if you resolve it. (Asset context 6403 * reference may include the creator, custodian, or owning Person or 6404 * Organization (e.g., bank, repository), location held, e.g., building, 6405 * jurisdiction.) 6406 */ 6407 public Resource getReferenceTarget() { 6408 return this.referenceTarget; 6409 } 6410 6411 /** 6412 * @param value {@link #reference} The actual object that is the target of the 6413 * reference. The reference library doesn't use these, but you can 6414 * use it to hold the resource if you resolve it. (Asset context 6415 * reference may include the creator, custodian, or owning Person 6416 * or Organization (e.g., bank, repository), location held, e.g., 6417 * building, jurisdiction.) 6418 */ 6419 public AssetContextComponent setReferenceTarget(Resource value) { 6420 this.referenceTarget = value; 6421 return this; 6422 } 6423 6424 /** 6425 * @return {@link #code} (Coded representation of the context generally or of 6426 * the Referenced entity, such as the asset holder type or location.) 6427 */ 6428 public List<CodeableConcept> getCode() { 6429 if (this.code == null) 6430 this.code = new ArrayList<CodeableConcept>(); 6431 return this.code; 6432 } 6433 6434 /** 6435 * @return Returns a reference to <code>this</code> for easy method chaining 6436 */ 6437 public AssetContextComponent setCode(List<CodeableConcept> theCode) { 6438 this.code = theCode; 6439 return this; 6440 } 6441 6442 public boolean hasCode() { 6443 if (this.code == null) 6444 return false; 6445 for (CodeableConcept item : this.code) 6446 if (!item.isEmpty()) 6447 return true; 6448 return false; 6449 } 6450 6451 public CodeableConcept addCode() { // 3 6452 CodeableConcept t = new CodeableConcept(); 6453 if (this.code == null) 6454 this.code = new ArrayList<CodeableConcept>(); 6455 this.code.add(t); 6456 return t; 6457 } 6458 6459 public AssetContextComponent addCode(CodeableConcept t) { // 3 6460 if (t == null) 6461 return this; 6462 if (this.code == null) 6463 this.code = new ArrayList<CodeableConcept>(); 6464 this.code.add(t); 6465 return this; 6466 } 6467 6468 /** 6469 * @return The first repetition of repeating field {@link #code}, creating it if 6470 * it does not already exist 6471 */ 6472 public CodeableConcept getCodeFirstRep() { 6473 if (getCode().isEmpty()) { 6474 addCode(); 6475 } 6476 return getCode().get(0); 6477 } 6478 6479 /** 6480 * @return {@link #text} (Context description.). This is the underlying object 6481 * with id, value and extensions. The accessor "getText" gives direct 6482 * access to the value 6483 */ 6484 public StringType getTextElement() { 6485 if (this.text == null) 6486 if (Configuration.errorOnAutoCreate()) 6487 throw new Error("Attempt to auto-create AssetContextComponent.text"); 6488 else if (Configuration.doAutoCreate()) 6489 this.text = new StringType(); // bb 6490 return this.text; 6491 } 6492 6493 public boolean hasTextElement() { 6494 return this.text != null && !this.text.isEmpty(); 6495 } 6496 6497 public boolean hasText() { 6498 return this.text != null && !this.text.isEmpty(); 6499 } 6500 6501 /** 6502 * @param value {@link #text} (Context description.). This is the underlying 6503 * object with id, value and extensions. The accessor "getText" 6504 * gives direct access to the value 6505 */ 6506 public AssetContextComponent setTextElement(StringType value) { 6507 this.text = value; 6508 return this; 6509 } 6510 6511 /** 6512 * @return Context description. 6513 */ 6514 public String getText() { 6515 return this.text == null ? null : this.text.getValue(); 6516 } 6517 6518 /** 6519 * @param value Context description. 6520 */ 6521 public AssetContextComponent setText(String value) { 6522 if (Utilities.noString(value)) 6523 this.text = null; 6524 else { 6525 if (this.text == null) 6526 this.text = new StringType(); 6527 this.text.setValue(value); 6528 } 6529 return this; 6530 } 6531 6532 protected void listChildren(List<Property> children) { 6533 super.listChildren(children); 6534 children.add(new Property("reference", "Reference(Any)", 6535 "Asset context reference may include the creator, custodian, or owning Person or Organization (e.g., bank, repository), location held, e.g., building, jurisdiction.", 6536 0, 1, reference)); 6537 children.add(new Property("code", "CodeableConcept", 6538 "Coded representation of the context generally or of the Referenced entity, such as the asset holder type or location.", 6539 0, java.lang.Integer.MAX_VALUE, code)); 6540 children.add(new Property("text", "string", "Context description.", 0, 1, text)); 6541 } 6542 6543 @Override 6544 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6545 switch (_hash) { 6546 case -925155509: 6547 /* reference */ return new Property("reference", "Reference(Any)", 6548 "Asset context reference may include the creator, custodian, or owning Person or Organization (e.g., bank, repository), location held, e.g., building, jurisdiction.", 6549 0, 1, reference); 6550 case 3059181: 6551 /* code */ return new Property("code", "CodeableConcept", 6552 "Coded representation of the context generally or of the Referenced entity, such as the asset holder type or location.", 6553 0, java.lang.Integer.MAX_VALUE, code); 6554 case 3556653: 6555 /* text */ return new Property("text", "string", "Context description.", 0, 1, text); 6556 default: 6557 return super.getNamedProperty(_hash, _name, _checkValid); 6558 } 6559 6560 } 6561 6562 @Override 6563 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6564 switch (hash) { 6565 case -925155509: 6566 /* reference */ return this.reference == null ? new Base[0] : new Base[] { this.reference }; // Reference 6567 case 3059181: 6568 /* code */ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 6569 case 3556653: 6570 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType 6571 default: 6572 return super.getProperty(hash, name, checkValid); 6573 } 6574 6575 } 6576 6577 @Override 6578 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6579 switch (hash) { 6580 case -925155509: // reference 6581 this.reference = castToReference(value); // Reference 6582 return value; 6583 case 3059181: // code 6584 this.getCode().add(castToCodeableConcept(value)); // CodeableConcept 6585 return value; 6586 case 3556653: // text 6587 this.text = castToString(value); // StringType 6588 return value; 6589 default: 6590 return super.setProperty(hash, name, value); 6591 } 6592 6593 } 6594 6595 @Override 6596 public Base setProperty(String name, Base value) throws FHIRException { 6597 if (name.equals("reference")) { 6598 this.reference = castToReference(value); // Reference 6599 } else if (name.equals("code")) { 6600 this.getCode().add(castToCodeableConcept(value)); 6601 } else if (name.equals("text")) { 6602 this.text = castToString(value); // StringType 6603 } else 6604 return super.setProperty(name, value); 6605 return value; 6606 } 6607 6608 @Override 6609 public void removeChild(String name, Base value) throws FHIRException { 6610 if (name.equals("reference")) { 6611 this.reference = null; 6612 } else if (name.equals("code")) { 6613 this.getCode().remove(castToCodeableConcept(value)); 6614 } else if (name.equals("text")) { 6615 this.text = null; 6616 } else 6617 super.removeChild(name, value); 6618 6619 } 6620 6621 @Override 6622 public Base makeProperty(int hash, String name) throws FHIRException { 6623 switch (hash) { 6624 case -925155509: 6625 return getReference(); 6626 case 3059181: 6627 return addCode(); 6628 case 3556653: 6629 return getTextElement(); 6630 default: 6631 return super.makeProperty(hash, name); 6632 } 6633 6634 } 6635 6636 @Override 6637 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6638 switch (hash) { 6639 case -925155509: 6640 /* reference */ return new String[] { "Reference" }; 6641 case 3059181: 6642 /* code */ return new String[] { "CodeableConcept" }; 6643 case 3556653: 6644 /* text */ return new String[] { "string" }; 6645 default: 6646 return super.getTypesForProperty(hash, name); 6647 } 6648 6649 } 6650 6651 @Override 6652 public Base addChild(String name) throws FHIRException { 6653 if (name.equals("reference")) { 6654 this.reference = new Reference(); 6655 return this.reference; 6656 } else if (name.equals("code")) { 6657 return addCode(); 6658 } else if (name.equals("text")) { 6659 throw new FHIRException("Cannot call addChild on a singleton property Contract.text"); 6660 } else 6661 return super.addChild(name); 6662 } 6663 6664 public AssetContextComponent copy() { 6665 AssetContextComponent dst = new AssetContextComponent(); 6666 copyValues(dst); 6667 return dst; 6668 } 6669 6670 public void copyValues(AssetContextComponent dst) { 6671 super.copyValues(dst); 6672 dst.reference = reference == null ? null : reference.copy(); 6673 if (code != null) { 6674 dst.code = new ArrayList<CodeableConcept>(); 6675 for (CodeableConcept i : code) 6676 dst.code.add(i.copy()); 6677 } 6678 ; 6679 dst.text = text == null ? null : text.copy(); 6680 } 6681 6682 @Override 6683 public boolean equalsDeep(Base other_) { 6684 if (!super.equalsDeep(other_)) 6685 return false; 6686 if (!(other_ instanceof AssetContextComponent)) 6687 return false; 6688 AssetContextComponent o = (AssetContextComponent) other_; 6689 return compareDeep(reference, o.reference, true) && compareDeep(code, o.code, true) 6690 && compareDeep(text, o.text, true); 6691 } 6692 6693 @Override 6694 public boolean equalsShallow(Base other_) { 6695 if (!super.equalsShallow(other_)) 6696 return false; 6697 if (!(other_ instanceof AssetContextComponent)) 6698 return false; 6699 AssetContextComponent o = (AssetContextComponent) other_; 6700 return compareValues(text, o.text, true); 6701 } 6702 6703 public boolean isEmpty() { 6704 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(reference, code, text); 6705 } 6706 6707 public String fhirType() { 6708 return "Contract.term.asset.context"; 6709 6710 } 6711 6712 } 6713 6714 @Block() 6715 public static class ValuedItemComponent extends BackboneElement implements IBaseBackboneElement { 6716 /** 6717 * Specific type of Contract Valued Item that may be priced. 6718 */ 6719 @Child(name = "entity", type = { CodeableConcept.class, 6720 Reference.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 6721 @Description(shortDefinition = "Contract Valued Item Type", formalDefinition = "Specific type of Contract Valued Item that may be priced.") 6722 protected Type entity; 6723 6724 /** 6725 * Identifies a Contract Valued Item instance. 6726 */ 6727 @Child(name = "identifier", type = { 6728 Identifier.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 6729 @Description(shortDefinition = "Contract Valued Item Number", formalDefinition = "Identifies a Contract Valued Item instance.") 6730 protected Identifier identifier; 6731 6732 /** 6733 * Indicates the time during which this Contract ValuedItem information is 6734 * effective. 6735 */ 6736 @Child(name = "effectiveTime", type = { 6737 DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 6738 @Description(shortDefinition = "Contract Valued Item Effective Tiem", formalDefinition = "Indicates the time during which this Contract ValuedItem information is effective.") 6739 protected DateTimeType effectiveTime; 6740 6741 /** 6742 * Specifies the units by which the Contract Valued Item is measured or counted, 6743 * and quantifies the countable or measurable Contract Valued Item instances. 6744 */ 6745 @Child(name = "quantity", type = { Quantity.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 6746 @Description(shortDefinition = "Count of Contract Valued Items", formalDefinition = "Specifies the units by which the Contract Valued Item is measured or counted, and quantifies the countable or measurable Contract Valued Item instances.") 6747 protected Quantity quantity; 6748 6749 /** 6750 * A Contract Valued Item unit valuation measure. 6751 */ 6752 @Child(name = "unitPrice", type = { Money.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 6753 @Description(shortDefinition = "Contract Valued Item fee, charge, or cost", formalDefinition = "A Contract Valued Item unit valuation measure.") 6754 protected Money unitPrice; 6755 6756 /** 6757 * A real number that represents a multiplier used in determining the overall 6758 * value of the Contract Valued Item delivered. The concept of a Factor allows 6759 * for a discount or surcharge multiplier to be applied to a monetary amount. 6760 */ 6761 @Child(name = "factor", type = { 6762 DecimalType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 6763 @Description(shortDefinition = "Contract Valued Item Price Scaling Factor", formalDefinition = "A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.") 6764 protected DecimalType factor; 6765 6766 /** 6767 * An amount that expresses the weighting (based on difficulty, cost and/or 6768 * resource intensiveness) associated with the Contract Valued Item delivered. 6769 * The concept of Points allows for assignment of point values for a Contract 6770 * Valued Item, such that a monetary amount can be assigned to each point. 6771 */ 6772 @Child(name = "points", type = { 6773 DecimalType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 6774 @Description(shortDefinition = "Contract Valued Item Difficulty Scaling Factor", formalDefinition = "An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point.") 6775 protected DecimalType points; 6776 6777 /** 6778 * Expresses the product of the Contract Valued Item unitQuantity and the 6779 * unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per 6780 * Point) * factor Number * points = net Amount. Quantity, factor and points are 6781 * assumed to be 1 if not supplied. 6782 */ 6783 @Child(name = "net", type = { Money.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 6784 @Description(shortDefinition = "Total Contract Valued Item Value", formalDefinition = "Expresses the product of the Contract Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.") 6785 protected Money net; 6786 6787 /** 6788 * Terms of valuation. 6789 */ 6790 @Child(name = "payment", type = { 6791 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 6792 @Description(shortDefinition = "Terms of valuation", formalDefinition = "Terms of valuation.") 6793 protected StringType payment; 6794 6795 /** 6796 * When payment is due. 6797 */ 6798 @Child(name = "paymentDate", type = { 6799 DateTimeType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 6800 @Description(shortDefinition = "When payment is due", formalDefinition = "When payment is due.") 6801 protected DateTimeType paymentDate; 6802 6803 /** 6804 * Who will make payment. 6805 */ 6806 @Child(name = "responsible", type = { Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, 6807 RelatedPerson.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 6808 @Description(shortDefinition = "Who will make payment", formalDefinition = "Who will make payment.") 6809 protected Reference responsible; 6810 6811 /** 6812 * The actual object that is the target of the reference (Who will make 6813 * payment.) 6814 */ 6815 protected Resource responsibleTarget; 6816 6817 /** 6818 * Who will receive payment. 6819 */ 6820 @Child(name = "recipient", type = { Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, 6821 RelatedPerson.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 6822 @Description(shortDefinition = "Who will receive payment", formalDefinition = "Who will receive payment.") 6823 protected Reference recipient; 6824 6825 /** 6826 * The actual object that is the target of the reference (Who will receive 6827 * payment.) 6828 */ 6829 protected Resource recipientTarget; 6830 6831 /** 6832 * Id of the clause or question text related to the context of this valuedItem 6833 * in the referenced form or QuestionnaireResponse. 6834 */ 6835 @Child(name = "linkId", type = { 6836 StringType.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6837 @Description(shortDefinition = "Pointer to specific item", formalDefinition = "Id of the clause or question text related to the context of this valuedItem in the referenced form or QuestionnaireResponse.") 6838 protected List<StringType> linkId; 6839 6840 /** 6841 * A set of security labels that define which terms are controlled by this 6842 * condition. 6843 */ 6844 @Child(name = "securityLabelNumber", type = { 6845 UnsignedIntType.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6846 @Description(shortDefinition = "Security Labels that define affected terms", formalDefinition = "A set of security labels that define which terms are controlled by this condition.") 6847 protected List<UnsignedIntType> securityLabelNumber; 6848 6849 private static final long serialVersionUID = 1894951601L; 6850 6851 /** 6852 * Constructor 6853 */ 6854 public ValuedItemComponent() { 6855 super(); 6856 } 6857 6858 /** 6859 * @return {@link #entity} (Specific type of Contract Valued Item that may be 6860 * priced.) 6861 */ 6862 public Type getEntity() { 6863 return this.entity; 6864 } 6865 6866 /** 6867 * @return {@link #entity} (Specific type of Contract Valued Item that may be 6868 * priced.) 6869 */ 6870 public CodeableConcept getEntityCodeableConcept() throws FHIRException { 6871 if (this.entity == null) 6872 this.entity = new CodeableConcept(); 6873 if (!(this.entity instanceof CodeableConcept)) 6874 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 6875 + this.entity.getClass().getName() + " was encountered"); 6876 return (CodeableConcept) this.entity; 6877 } 6878 6879 public boolean hasEntityCodeableConcept() { 6880 return this != null && this.entity instanceof CodeableConcept; 6881 } 6882 6883 /** 6884 * @return {@link #entity} (Specific type of Contract Valued Item that may be 6885 * priced.) 6886 */ 6887 public Reference getEntityReference() throws FHIRException { 6888 if (this.entity == null) 6889 this.entity = new Reference(); 6890 if (!(this.entity instanceof Reference)) 6891 throw new FHIRException("Type mismatch: the type Reference was expected, but " 6892 + this.entity.getClass().getName() + " was encountered"); 6893 return (Reference) this.entity; 6894 } 6895 6896 public boolean hasEntityReference() { 6897 return this != null && this.entity instanceof Reference; 6898 } 6899 6900 public boolean hasEntity() { 6901 return this.entity != null && !this.entity.isEmpty(); 6902 } 6903 6904 /** 6905 * @param value {@link #entity} (Specific type of Contract Valued Item that may 6906 * be priced.) 6907 */ 6908 public ValuedItemComponent setEntity(Type value) { 6909 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 6910 throw new Error("Not the right type for Contract.term.asset.valuedItem.entity[x]: " + value.fhirType()); 6911 this.entity = value; 6912 return this; 6913 } 6914 6915 /** 6916 * @return {@link #identifier} (Identifies a Contract Valued Item instance.) 6917 */ 6918 public Identifier getIdentifier() { 6919 if (this.identifier == null) 6920 if (Configuration.errorOnAutoCreate()) 6921 throw new Error("Attempt to auto-create ValuedItemComponent.identifier"); 6922 else if (Configuration.doAutoCreate()) 6923 this.identifier = new Identifier(); // cc 6924 return this.identifier; 6925 } 6926 6927 public boolean hasIdentifier() { 6928 return this.identifier != null && !this.identifier.isEmpty(); 6929 } 6930 6931 /** 6932 * @param value {@link #identifier} (Identifies a Contract Valued Item 6933 * instance.) 6934 */ 6935 public ValuedItemComponent setIdentifier(Identifier value) { 6936 this.identifier = value; 6937 return this; 6938 } 6939 6940 /** 6941 * @return {@link #effectiveTime} (Indicates the time during which this Contract 6942 * ValuedItem information is effective.). This is the underlying object 6943 * with id, value and extensions. The accessor "getEffectiveTime" gives 6944 * direct access to the value 6945 */ 6946 public DateTimeType getEffectiveTimeElement() { 6947 if (this.effectiveTime == null) 6948 if (Configuration.errorOnAutoCreate()) 6949 throw new Error("Attempt to auto-create ValuedItemComponent.effectiveTime"); 6950 else if (Configuration.doAutoCreate()) 6951 this.effectiveTime = new DateTimeType(); // bb 6952 return this.effectiveTime; 6953 } 6954 6955 public boolean hasEffectiveTimeElement() { 6956 return this.effectiveTime != null && !this.effectiveTime.isEmpty(); 6957 } 6958 6959 public boolean hasEffectiveTime() { 6960 return this.effectiveTime != null && !this.effectiveTime.isEmpty(); 6961 } 6962 6963 /** 6964 * @param value {@link #effectiveTime} (Indicates the time during which this 6965 * Contract ValuedItem information is effective.). This is the 6966 * underlying object with id, value and extensions. The accessor 6967 * "getEffectiveTime" gives direct access to the value 6968 */ 6969 public ValuedItemComponent setEffectiveTimeElement(DateTimeType value) { 6970 this.effectiveTime = value; 6971 return this; 6972 } 6973 6974 /** 6975 * @return Indicates the time during which this Contract ValuedItem information 6976 * is effective. 6977 */ 6978 public Date getEffectiveTime() { 6979 return this.effectiveTime == null ? null : this.effectiveTime.getValue(); 6980 } 6981 6982 /** 6983 * @param value Indicates the time during which this Contract ValuedItem 6984 * information is effective. 6985 */ 6986 public ValuedItemComponent setEffectiveTime(Date value) { 6987 if (value == null) 6988 this.effectiveTime = null; 6989 else { 6990 if (this.effectiveTime == null) 6991 this.effectiveTime = new DateTimeType(); 6992 this.effectiveTime.setValue(value); 6993 } 6994 return this; 6995 } 6996 6997 /** 6998 * @return {@link #quantity} (Specifies the units by which the Contract Valued 6999 * Item is measured or counted, and quantifies the countable or 7000 * measurable Contract Valued Item instances.) 7001 */ 7002 public Quantity getQuantity() { 7003 if (this.quantity == null) 7004 if (Configuration.errorOnAutoCreate()) 7005 throw new Error("Attempt to auto-create ValuedItemComponent.quantity"); 7006 else if (Configuration.doAutoCreate()) 7007 this.quantity = new Quantity(); // cc 7008 return this.quantity; 7009 } 7010 7011 public boolean hasQuantity() { 7012 return this.quantity != null && !this.quantity.isEmpty(); 7013 } 7014 7015 /** 7016 * @param value {@link #quantity} (Specifies the units by which the Contract 7017 * Valued Item is measured or counted, and quantifies the countable 7018 * or measurable Contract Valued Item instances.) 7019 */ 7020 public ValuedItemComponent setQuantity(Quantity value) { 7021 this.quantity = value; 7022 return this; 7023 } 7024 7025 /** 7026 * @return {@link #unitPrice} (A Contract Valued Item unit valuation measure.) 7027 */ 7028 public Money getUnitPrice() { 7029 if (this.unitPrice == null) 7030 if (Configuration.errorOnAutoCreate()) 7031 throw new Error("Attempt to auto-create ValuedItemComponent.unitPrice"); 7032 else if (Configuration.doAutoCreate()) 7033 this.unitPrice = new Money(); // cc 7034 return this.unitPrice; 7035 } 7036 7037 public boolean hasUnitPrice() { 7038 return this.unitPrice != null && !this.unitPrice.isEmpty(); 7039 } 7040 7041 /** 7042 * @param value {@link #unitPrice} (A Contract Valued Item unit valuation 7043 * measure.) 7044 */ 7045 public ValuedItemComponent setUnitPrice(Money value) { 7046 this.unitPrice = value; 7047 return this; 7048 } 7049 7050 /** 7051 * @return {@link #factor} (A real number that represents a multiplier used in 7052 * determining the overall value of the Contract Valued Item delivered. 7053 * The concept of a Factor allows for a discount or surcharge multiplier 7054 * to be applied to a monetary amount.). This is the underlying object 7055 * with id, value and extensions. The accessor "getFactor" gives direct 7056 * access to the value 7057 */ 7058 public DecimalType getFactorElement() { 7059 if (this.factor == null) 7060 if (Configuration.errorOnAutoCreate()) 7061 throw new Error("Attempt to auto-create ValuedItemComponent.factor"); 7062 else if (Configuration.doAutoCreate()) 7063 this.factor = new DecimalType(); // bb 7064 return this.factor; 7065 } 7066 7067 public boolean hasFactorElement() { 7068 return this.factor != null && !this.factor.isEmpty(); 7069 } 7070 7071 public boolean hasFactor() { 7072 return this.factor != null && !this.factor.isEmpty(); 7073 } 7074 7075 /** 7076 * @param value {@link #factor} (A real number that represents a multiplier used 7077 * in determining the overall value of the Contract Valued Item 7078 * delivered. The concept of a Factor allows for a discount or 7079 * surcharge multiplier to be applied to a monetary amount.). This 7080 * is the underlying object with id, value and extensions. The 7081 * accessor "getFactor" gives direct access to the value 7082 */ 7083 public ValuedItemComponent setFactorElement(DecimalType value) { 7084 this.factor = value; 7085 return this; 7086 } 7087 7088 /** 7089 * @return A real number that represents a multiplier used in determining the 7090 * overall value of the Contract Valued Item delivered. The concept of a 7091 * Factor allows for a discount or surcharge multiplier to be applied to 7092 * a monetary amount. 7093 */ 7094 public BigDecimal getFactor() { 7095 return this.factor == null ? null : this.factor.getValue(); 7096 } 7097 7098 /** 7099 * @param value A real number that represents a multiplier used in determining 7100 * the overall value of the Contract Valued Item delivered. The 7101 * concept of a Factor allows for a discount or surcharge 7102 * multiplier to be applied to a monetary amount. 7103 */ 7104 public ValuedItemComponent setFactor(BigDecimal value) { 7105 if (value == null) 7106 this.factor = null; 7107 else { 7108 if (this.factor == null) 7109 this.factor = new DecimalType(); 7110 this.factor.setValue(value); 7111 } 7112 return this; 7113 } 7114 7115 /** 7116 * @param value A real number that represents a multiplier used in determining 7117 * the overall value of the Contract Valued Item delivered. The 7118 * concept of a Factor allows for a discount or surcharge 7119 * multiplier to be applied to a monetary amount. 7120 */ 7121 public ValuedItemComponent setFactor(long value) { 7122 this.factor = new DecimalType(); 7123 this.factor.setValue(value); 7124 return this; 7125 } 7126 7127 /** 7128 * @param value A real number that represents a multiplier used in determining 7129 * the overall value of the Contract Valued Item delivered. The 7130 * concept of a Factor allows for a discount or surcharge 7131 * multiplier to be applied to a monetary amount. 7132 */ 7133 public ValuedItemComponent setFactor(double value) { 7134 this.factor = new DecimalType(); 7135 this.factor.setValue(value); 7136 return this; 7137 } 7138 7139 /** 7140 * @return {@link #points} (An amount that expresses the weighting (based on 7141 * difficulty, cost and/or resource intensiveness) associated with the 7142 * Contract Valued Item delivered. The concept of Points allows for 7143 * assignment of point values for a Contract Valued Item, such that a 7144 * monetary amount can be assigned to each point.). This is the 7145 * underlying object with id, value and extensions. The accessor 7146 * "getPoints" gives direct access to the value 7147 */ 7148 public DecimalType getPointsElement() { 7149 if (this.points == null) 7150 if (Configuration.errorOnAutoCreate()) 7151 throw new Error("Attempt to auto-create ValuedItemComponent.points"); 7152 else if (Configuration.doAutoCreate()) 7153 this.points = new DecimalType(); // bb 7154 return this.points; 7155 } 7156 7157 public boolean hasPointsElement() { 7158 return this.points != null && !this.points.isEmpty(); 7159 } 7160 7161 public boolean hasPoints() { 7162 return this.points != null && !this.points.isEmpty(); 7163 } 7164 7165 /** 7166 * @param value {@link #points} (An amount that expresses the weighting (based 7167 * on difficulty, cost and/or resource intensiveness) associated 7168 * with the Contract Valued Item delivered. The concept of Points 7169 * allows for assignment of point values for a Contract Valued 7170 * Item, such that a monetary amount can be assigned to each 7171 * point.). This is the underlying object with id, value and 7172 * extensions. The accessor "getPoints" gives direct access to the 7173 * value 7174 */ 7175 public ValuedItemComponent setPointsElement(DecimalType value) { 7176 this.points = value; 7177 return this; 7178 } 7179 7180 /** 7181 * @return An amount that expresses the weighting (based on difficulty, cost 7182 * and/or resource intensiveness) associated with the Contract Valued 7183 * Item delivered. The concept of Points allows for assignment of point 7184 * values for a Contract Valued Item, such that a monetary amount can be 7185 * assigned to each point. 7186 */ 7187 public BigDecimal getPoints() { 7188 return this.points == null ? null : this.points.getValue(); 7189 } 7190 7191 /** 7192 * @param value An amount that expresses the weighting (based on difficulty, 7193 * cost and/or resource intensiveness) associated with the Contract 7194 * Valued Item delivered. The concept of Points allows for 7195 * assignment of point values for a Contract Valued Item, such that 7196 * a monetary amount can be assigned to each point. 7197 */ 7198 public ValuedItemComponent setPoints(BigDecimal value) { 7199 if (value == null) 7200 this.points = null; 7201 else { 7202 if (this.points == null) 7203 this.points = new DecimalType(); 7204 this.points.setValue(value); 7205 } 7206 return this; 7207 } 7208 7209 /** 7210 * @param value An amount that expresses the weighting (based on difficulty, 7211 * cost and/or resource intensiveness) associated with the Contract 7212 * Valued Item delivered. The concept of Points allows for 7213 * assignment of point values for a Contract Valued Item, such that 7214 * a monetary amount can be assigned to each point. 7215 */ 7216 public ValuedItemComponent setPoints(long value) { 7217 this.points = new DecimalType(); 7218 this.points.setValue(value); 7219 return this; 7220 } 7221 7222 /** 7223 * @param value An amount that expresses the weighting (based on difficulty, 7224 * cost and/or resource intensiveness) associated with the Contract 7225 * Valued Item delivered. The concept of Points allows for 7226 * assignment of point values for a Contract Valued Item, such that 7227 * a monetary amount can be assigned to each point. 7228 */ 7229 public ValuedItemComponent setPoints(double value) { 7230 this.points = new DecimalType(); 7231 this.points.setValue(value); 7232 return this; 7233 } 7234 7235 /** 7236 * @return {@link #net} (Expresses the product of the Contract Valued Item 7237 * unitQuantity and the unitPriceAmt. For example, the formula: unit 7238 * Quantity * unit Price (Cost per Point) * factor Number * points = net 7239 * Amount. Quantity, factor and points are assumed to be 1 if not 7240 * supplied.) 7241 */ 7242 public Money getNet() { 7243 if (this.net == null) 7244 if (Configuration.errorOnAutoCreate()) 7245 throw new Error("Attempt to auto-create ValuedItemComponent.net"); 7246 else if (Configuration.doAutoCreate()) 7247 this.net = new Money(); // cc 7248 return this.net; 7249 } 7250 7251 public boolean hasNet() { 7252 return this.net != null && !this.net.isEmpty(); 7253 } 7254 7255 /** 7256 * @param value {@link #net} (Expresses the product of the Contract Valued Item 7257 * unitQuantity and the unitPriceAmt. For example, the formula: 7258 * unit Quantity * unit Price (Cost per Point) * factor Number * 7259 * points = net Amount. Quantity, factor and points are assumed to 7260 * be 1 if not supplied.) 7261 */ 7262 public ValuedItemComponent setNet(Money value) { 7263 this.net = value; 7264 return this; 7265 } 7266 7267 /** 7268 * @return {@link #payment} (Terms of valuation.). This is the underlying object 7269 * with id, value and extensions. The accessor "getPayment" gives direct 7270 * access to the value 7271 */ 7272 public StringType getPaymentElement() { 7273 if (this.payment == null) 7274 if (Configuration.errorOnAutoCreate()) 7275 throw new Error("Attempt to auto-create ValuedItemComponent.payment"); 7276 else if (Configuration.doAutoCreate()) 7277 this.payment = new StringType(); // bb 7278 return this.payment; 7279 } 7280 7281 public boolean hasPaymentElement() { 7282 return this.payment != null && !this.payment.isEmpty(); 7283 } 7284 7285 public boolean hasPayment() { 7286 return this.payment != null && !this.payment.isEmpty(); 7287 } 7288 7289 /** 7290 * @param value {@link #payment} (Terms of valuation.). This is the underlying 7291 * object with id, value and extensions. The accessor "getPayment" 7292 * gives direct access to the value 7293 */ 7294 public ValuedItemComponent setPaymentElement(StringType value) { 7295 this.payment = value; 7296 return this; 7297 } 7298 7299 /** 7300 * @return Terms of valuation. 7301 */ 7302 public String getPayment() { 7303 return this.payment == null ? null : this.payment.getValue(); 7304 } 7305 7306 /** 7307 * @param value Terms of valuation. 7308 */ 7309 public ValuedItemComponent setPayment(String value) { 7310 if (Utilities.noString(value)) 7311 this.payment = null; 7312 else { 7313 if (this.payment == null) 7314 this.payment = new StringType(); 7315 this.payment.setValue(value); 7316 } 7317 return this; 7318 } 7319 7320 /** 7321 * @return {@link #paymentDate} (When payment is due.). This is the underlying 7322 * object with id, value and extensions. The accessor "getPaymentDate" 7323 * gives direct access to the value 7324 */ 7325 public DateTimeType getPaymentDateElement() { 7326 if (this.paymentDate == null) 7327 if (Configuration.errorOnAutoCreate()) 7328 throw new Error("Attempt to auto-create ValuedItemComponent.paymentDate"); 7329 else if (Configuration.doAutoCreate()) 7330 this.paymentDate = new DateTimeType(); // bb 7331 return this.paymentDate; 7332 } 7333 7334 public boolean hasPaymentDateElement() { 7335 return this.paymentDate != null && !this.paymentDate.isEmpty(); 7336 } 7337 7338 public boolean hasPaymentDate() { 7339 return this.paymentDate != null && !this.paymentDate.isEmpty(); 7340 } 7341 7342 /** 7343 * @param value {@link #paymentDate} (When payment is due.). This is the 7344 * underlying object with id, value and extensions. The accessor 7345 * "getPaymentDate" gives direct access to the value 7346 */ 7347 public ValuedItemComponent setPaymentDateElement(DateTimeType value) { 7348 this.paymentDate = value; 7349 return this; 7350 } 7351 7352 /** 7353 * @return When payment is due. 7354 */ 7355 public Date getPaymentDate() { 7356 return this.paymentDate == null ? null : this.paymentDate.getValue(); 7357 } 7358 7359 /** 7360 * @param value When payment is due. 7361 */ 7362 public ValuedItemComponent setPaymentDate(Date value) { 7363 if (value == null) 7364 this.paymentDate = null; 7365 else { 7366 if (this.paymentDate == null) 7367 this.paymentDate = new DateTimeType(); 7368 this.paymentDate.setValue(value); 7369 } 7370 return this; 7371 } 7372 7373 /** 7374 * @return {@link #responsible} (Who will make payment.) 7375 */ 7376 public Reference getResponsible() { 7377 if (this.responsible == null) 7378 if (Configuration.errorOnAutoCreate()) 7379 throw new Error("Attempt to auto-create ValuedItemComponent.responsible"); 7380 else if (Configuration.doAutoCreate()) 7381 this.responsible = new Reference(); // cc 7382 return this.responsible; 7383 } 7384 7385 public boolean hasResponsible() { 7386 return this.responsible != null && !this.responsible.isEmpty(); 7387 } 7388 7389 /** 7390 * @param value {@link #responsible} (Who will make payment.) 7391 */ 7392 public ValuedItemComponent setResponsible(Reference value) { 7393 this.responsible = value; 7394 return this; 7395 } 7396 7397 /** 7398 * @return {@link #responsible} The actual object that is the target of the 7399 * reference. The reference library doesn't populate this, but you can 7400 * use it to hold the resource if you resolve it. (Who will make 7401 * payment.) 7402 */ 7403 public Resource getResponsibleTarget() { 7404 return this.responsibleTarget; 7405 } 7406 7407 /** 7408 * @param value {@link #responsible} The actual object that is the target of the 7409 * reference. The reference library doesn't use these, but you can 7410 * use it to hold the resource if you resolve it. (Who will make 7411 * payment.) 7412 */ 7413 public ValuedItemComponent setResponsibleTarget(Resource value) { 7414 this.responsibleTarget = value; 7415 return this; 7416 } 7417 7418 /** 7419 * @return {@link #recipient} (Who will receive payment.) 7420 */ 7421 public Reference getRecipient() { 7422 if (this.recipient == null) 7423 if (Configuration.errorOnAutoCreate()) 7424 throw new Error("Attempt to auto-create ValuedItemComponent.recipient"); 7425 else if (Configuration.doAutoCreate()) 7426 this.recipient = new Reference(); // cc 7427 return this.recipient; 7428 } 7429 7430 public boolean hasRecipient() { 7431 return this.recipient != null && !this.recipient.isEmpty(); 7432 } 7433 7434 /** 7435 * @param value {@link #recipient} (Who will receive payment.) 7436 */ 7437 public ValuedItemComponent setRecipient(Reference value) { 7438 this.recipient = value; 7439 return this; 7440 } 7441 7442 /** 7443 * @return {@link #recipient} The actual object that is the target of the 7444 * reference. The reference library doesn't populate this, but you can 7445 * use it to hold the resource if you resolve it. (Who will receive 7446 * payment.) 7447 */ 7448 public Resource getRecipientTarget() { 7449 return this.recipientTarget; 7450 } 7451 7452 /** 7453 * @param value {@link #recipient} The actual object that is the target of the 7454 * reference. The reference library doesn't use these, but you can 7455 * use it to hold the resource if you resolve it. (Who will receive 7456 * payment.) 7457 */ 7458 public ValuedItemComponent setRecipientTarget(Resource value) { 7459 this.recipientTarget = value; 7460 return this; 7461 } 7462 7463 /** 7464 * @return {@link #linkId} (Id of the clause or question text related to the 7465 * context of this valuedItem in the referenced form or 7466 * QuestionnaireResponse.) 7467 */ 7468 public List<StringType> getLinkId() { 7469 if (this.linkId == null) 7470 this.linkId = new ArrayList<StringType>(); 7471 return this.linkId; 7472 } 7473 7474 /** 7475 * @return Returns a reference to <code>this</code> for easy method chaining 7476 */ 7477 public ValuedItemComponent setLinkId(List<StringType> theLinkId) { 7478 this.linkId = theLinkId; 7479 return this; 7480 } 7481 7482 public boolean hasLinkId() { 7483 if (this.linkId == null) 7484 return false; 7485 for (StringType item : this.linkId) 7486 if (!item.isEmpty()) 7487 return true; 7488 return false; 7489 } 7490 7491 /** 7492 * @return {@link #linkId} (Id of the clause or question text related to the 7493 * context of this valuedItem in the referenced form or 7494 * QuestionnaireResponse.) 7495 */ 7496 public StringType addLinkIdElement() {// 2 7497 StringType t = new StringType(); 7498 if (this.linkId == null) 7499 this.linkId = new ArrayList<StringType>(); 7500 this.linkId.add(t); 7501 return t; 7502 } 7503 7504 /** 7505 * @param value {@link #linkId} (Id of the clause or question text related to 7506 * the context of this valuedItem in the referenced form or 7507 * QuestionnaireResponse.) 7508 */ 7509 public ValuedItemComponent addLinkId(String value) { // 1 7510 StringType t = new StringType(); 7511 t.setValue(value); 7512 if (this.linkId == null) 7513 this.linkId = new ArrayList<StringType>(); 7514 this.linkId.add(t); 7515 return this; 7516 } 7517 7518 /** 7519 * @param value {@link #linkId} (Id of the clause or question text related to 7520 * the context of this valuedItem in the referenced form or 7521 * QuestionnaireResponse.) 7522 */ 7523 public boolean hasLinkId(String value) { 7524 if (this.linkId == null) 7525 return false; 7526 for (StringType v : this.linkId) 7527 if (v.getValue().equals(value)) // string 7528 return true; 7529 return false; 7530 } 7531 7532 /** 7533 * @return {@link #securityLabelNumber} (A set of security labels that define 7534 * which terms are controlled by this condition.) 7535 */ 7536 public List<UnsignedIntType> getSecurityLabelNumber() { 7537 if (this.securityLabelNumber == null) 7538 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 7539 return this.securityLabelNumber; 7540 } 7541 7542 /** 7543 * @return Returns a reference to <code>this</code> for easy method chaining 7544 */ 7545 public ValuedItemComponent setSecurityLabelNumber(List<UnsignedIntType> theSecurityLabelNumber) { 7546 this.securityLabelNumber = theSecurityLabelNumber; 7547 return this; 7548 } 7549 7550 public boolean hasSecurityLabelNumber() { 7551 if (this.securityLabelNumber == null) 7552 return false; 7553 for (UnsignedIntType item : this.securityLabelNumber) 7554 if (!item.isEmpty()) 7555 return true; 7556 return false; 7557 } 7558 7559 /** 7560 * @return {@link #securityLabelNumber} (A set of security labels that define 7561 * which terms are controlled by this condition.) 7562 */ 7563 public UnsignedIntType addSecurityLabelNumberElement() {// 2 7564 UnsignedIntType t = new UnsignedIntType(); 7565 if (this.securityLabelNumber == null) 7566 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 7567 this.securityLabelNumber.add(t); 7568 return t; 7569 } 7570 7571 /** 7572 * @param value {@link #securityLabelNumber} (A set of security labels that 7573 * define which terms are controlled by this condition.) 7574 */ 7575 public ValuedItemComponent addSecurityLabelNumber(int value) { // 1 7576 UnsignedIntType t = new UnsignedIntType(); 7577 t.setValue(value); 7578 if (this.securityLabelNumber == null) 7579 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 7580 this.securityLabelNumber.add(t); 7581 return this; 7582 } 7583 7584 /** 7585 * @param value {@link #securityLabelNumber} (A set of security labels that 7586 * define which terms are controlled by this condition.) 7587 */ 7588 public boolean hasSecurityLabelNumber(int value) { 7589 if (this.securityLabelNumber == null) 7590 return false; 7591 for (UnsignedIntType v : this.securityLabelNumber) 7592 if (v.getValue().equals(value)) // unsignedInt 7593 return true; 7594 return false; 7595 } 7596 7597 protected void listChildren(List<Property> children) { 7598 super.listChildren(children); 7599 children.add(new Property("entity[x]", "CodeableConcept|Reference(Any)", 7600 "Specific type of Contract Valued Item that may be priced.", 0, 1, entity)); 7601 children.add( 7602 new Property("identifier", "Identifier", "Identifies a Contract Valued Item instance.", 0, 1, identifier)); 7603 children.add(new Property("effectiveTime", "dateTime", 7604 "Indicates the time during which this Contract ValuedItem information is effective.", 0, 1, effectiveTime)); 7605 children.add(new Property("quantity", "SimpleQuantity", 7606 "Specifies the units by which the Contract Valued Item is measured or counted, and quantifies the countable or measurable Contract Valued Item instances.", 7607 0, 1, quantity)); 7608 children 7609 .add(new Property("unitPrice", "Money", "A Contract Valued Item unit valuation measure.", 0, 1, unitPrice)); 7610 children.add(new Property("factor", "decimal", 7611 "A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 7612 0, 1, factor)); 7613 children.add(new Property("points", "decimal", 7614 "An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point.", 7615 0, 1, points)); 7616 children.add(new Property("net", "Money", 7617 "Expresses the product of the Contract Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 7618 0, 1, net)); 7619 children.add(new Property("payment", "string", "Terms of valuation.", 0, 1, payment)); 7620 children.add(new Property("paymentDate", "dateTime", "When payment is due.", 0, 1, paymentDate)); 7621 children.add( 7622 new Property("responsible", "Reference(Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", 7623 "Who will make payment.", 0, 1, responsible)); 7624 children 7625 .add(new Property("recipient", "Reference(Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", 7626 "Who will receive payment.", 0, 1, recipient)); 7627 children.add(new Property("linkId", "string", 7628 "Id of the clause or question text related to the context of this valuedItem in the referenced form or QuestionnaireResponse.", 7629 0, java.lang.Integer.MAX_VALUE, linkId)); 7630 children.add(new Property("securityLabelNumber", "unsignedInt", 7631 "A set of security labels that define which terms are controlled by this condition.", 0, 7632 java.lang.Integer.MAX_VALUE, securityLabelNumber)); 7633 } 7634 7635 @Override 7636 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7637 switch (_hash) { 7638 case -740568643: 7639 /* entity[x] */ return new Property("entity[x]", "CodeableConcept|Reference(Any)", 7640 "Specific type of Contract Valued Item that may be priced.", 0, 1, entity); 7641 case -1298275357: 7642 /* entity */ return new Property("entity[x]", "CodeableConcept|Reference(Any)", 7643 "Specific type of Contract Valued Item that may be priced.", 0, 1, entity); 7644 case 924197182: 7645 /* entityCodeableConcept */ return new Property("entity[x]", "CodeableConcept|Reference(Any)", 7646 "Specific type of Contract Valued Item that may be priced.", 0, 1, entity); 7647 case -356635992: 7648 /* entityReference */ return new Property("entity[x]", "CodeableConcept|Reference(Any)", 7649 "Specific type of Contract Valued Item that may be priced.", 0, 1, entity); 7650 case -1618432855: 7651 /* identifier */ return new Property("identifier", "Identifier", "Identifies a Contract Valued Item instance.", 7652 0, 1, identifier); 7653 case -929905388: 7654 /* effectiveTime */ return new Property("effectiveTime", "dateTime", 7655 "Indicates the time during which this Contract ValuedItem information is effective.", 0, 1, effectiveTime); 7656 case -1285004149: 7657 /* quantity */ return new Property("quantity", "SimpleQuantity", 7658 "Specifies the units by which the Contract Valued Item is measured or counted, and quantifies the countable or measurable Contract Valued Item instances.", 7659 0, 1, quantity); 7660 case -486196699: 7661 /* unitPrice */ return new Property("unitPrice", "Money", "A Contract Valued Item unit valuation measure.", 0, 7662 1, unitPrice); 7663 case -1282148017: 7664 /* factor */ return new Property("factor", "decimal", 7665 "A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 7666 0, 1, factor); 7667 case -982754077: 7668 /* points */ return new Property("points", "decimal", 7669 "An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point.", 7670 0, 1, points); 7671 case 108957: 7672 /* net */ return new Property("net", "Money", 7673 "Expresses the product of the Contract Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 7674 0, 1, net); 7675 case -786681338: 7676 /* payment */ return new Property("payment", "string", "Terms of valuation.", 0, 1, payment); 7677 case -1540873516: 7678 /* paymentDate */ return new Property("paymentDate", "dateTime", "When payment is due.", 0, 1, paymentDate); 7679 case 1847674614: 7680 /* responsible */ return new Property("responsible", 7681 "Reference(Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "Who will make payment.", 0, 7682 1, responsible); 7683 case 820081177: 7684 /* recipient */ return new Property("recipient", 7685 "Reference(Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "Who will receive payment.", 7686 0, 1, recipient); 7687 case -1102667083: 7688 /* linkId */ return new Property("linkId", "string", 7689 "Id of the clause or question text related to the context of this valuedItem in the referenced form or QuestionnaireResponse.", 7690 0, java.lang.Integer.MAX_VALUE, linkId); 7691 case -149460995: 7692 /* securityLabelNumber */ return new Property("securityLabelNumber", "unsignedInt", 7693 "A set of security labels that define which terms are controlled by this condition.", 0, 7694 java.lang.Integer.MAX_VALUE, securityLabelNumber); 7695 default: 7696 return super.getNamedProperty(_hash, _name, _checkValid); 7697 } 7698 7699 } 7700 7701 @Override 7702 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7703 switch (hash) { 7704 case -1298275357: 7705 /* entity */ return this.entity == null ? new Base[0] : new Base[] { this.entity }; // Type 7706 case -1618432855: 7707 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 7708 case -929905388: 7709 /* effectiveTime */ return this.effectiveTime == null ? new Base[0] : new Base[] { this.effectiveTime }; // DateTimeType 7710 case -1285004149: 7711 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 7712 case -486196699: 7713 /* unitPrice */ return this.unitPrice == null ? new Base[0] : new Base[] { this.unitPrice }; // Money 7714 case -1282148017: 7715 /* factor */ return this.factor == null ? new Base[0] : new Base[] { this.factor }; // DecimalType 7716 case -982754077: 7717 /* points */ return this.points == null ? new Base[0] : new Base[] { this.points }; // DecimalType 7718 case 108957: 7719 /* net */ return this.net == null ? new Base[0] : new Base[] { this.net }; // Money 7720 case -786681338: 7721 /* payment */ return this.payment == null ? new Base[0] : new Base[] { this.payment }; // StringType 7722 case -1540873516: 7723 /* paymentDate */ return this.paymentDate == null ? new Base[0] : new Base[] { this.paymentDate }; // DateTimeType 7724 case 1847674614: 7725 /* responsible */ return this.responsible == null ? new Base[0] : new Base[] { this.responsible }; // Reference 7726 case 820081177: 7727 /* recipient */ return this.recipient == null ? new Base[0] : new Base[] { this.recipient }; // Reference 7728 case -1102667083: 7729 /* linkId */ return this.linkId == null ? new Base[0] : this.linkId.toArray(new Base[this.linkId.size()]); // StringType 7730 case -149460995: 7731 /* securityLabelNumber */ return this.securityLabelNumber == null ? new Base[0] 7732 : this.securityLabelNumber.toArray(new Base[this.securityLabelNumber.size()]); // UnsignedIntType 7733 default: 7734 return super.getProperty(hash, name, checkValid); 7735 } 7736 7737 } 7738 7739 @Override 7740 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7741 switch (hash) { 7742 case -1298275357: // entity 7743 this.entity = castToType(value); // Type 7744 return value; 7745 case -1618432855: // identifier 7746 this.identifier = castToIdentifier(value); // Identifier 7747 return value; 7748 case -929905388: // effectiveTime 7749 this.effectiveTime = castToDateTime(value); // DateTimeType 7750 return value; 7751 case -1285004149: // quantity 7752 this.quantity = castToQuantity(value); // Quantity 7753 return value; 7754 case -486196699: // unitPrice 7755 this.unitPrice = castToMoney(value); // Money 7756 return value; 7757 case -1282148017: // factor 7758 this.factor = castToDecimal(value); // DecimalType 7759 return value; 7760 case -982754077: // points 7761 this.points = castToDecimal(value); // DecimalType 7762 return value; 7763 case 108957: // net 7764 this.net = castToMoney(value); // Money 7765 return value; 7766 case -786681338: // payment 7767 this.payment = castToString(value); // StringType 7768 return value; 7769 case -1540873516: // paymentDate 7770 this.paymentDate = castToDateTime(value); // DateTimeType 7771 return value; 7772 case 1847674614: // responsible 7773 this.responsible = castToReference(value); // Reference 7774 return value; 7775 case 820081177: // recipient 7776 this.recipient = castToReference(value); // Reference 7777 return value; 7778 case -1102667083: // linkId 7779 this.getLinkId().add(castToString(value)); // StringType 7780 return value; 7781 case -149460995: // securityLabelNumber 7782 this.getSecurityLabelNumber().add(castToUnsignedInt(value)); // UnsignedIntType 7783 return value; 7784 default: 7785 return super.setProperty(hash, name, value); 7786 } 7787 7788 } 7789 7790 @Override 7791 public Base setProperty(String name, Base value) throws FHIRException { 7792 if (name.equals("entity[x]")) { 7793 this.entity = castToType(value); // Type 7794 } else if (name.equals("identifier")) { 7795 this.identifier = castToIdentifier(value); // Identifier 7796 } else if (name.equals("effectiveTime")) { 7797 this.effectiveTime = castToDateTime(value); // DateTimeType 7798 } else if (name.equals("quantity")) { 7799 this.quantity = castToQuantity(value); // Quantity 7800 } else if (name.equals("unitPrice")) { 7801 this.unitPrice = castToMoney(value); // Money 7802 } else if (name.equals("factor")) { 7803 this.factor = castToDecimal(value); // DecimalType 7804 } else if (name.equals("points")) { 7805 this.points = castToDecimal(value); // DecimalType 7806 } else if (name.equals("net")) { 7807 this.net = castToMoney(value); // Money 7808 } else if (name.equals("payment")) { 7809 this.payment = castToString(value); // StringType 7810 } else if (name.equals("paymentDate")) { 7811 this.paymentDate = castToDateTime(value); // DateTimeType 7812 } else if (name.equals("responsible")) { 7813 this.responsible = castToReference(value); // Reference 7814 } else if (name.equals("recipient")) { 7815 this.recipient = castToReference(value); // Reference 7816 } else if (name.equals("linkId")) { 7817 this.getLinkId().add(castToString(value)); 7818 } else if (name.equals("securityLabelNumber")) { 7819 this.getSecurityLabelNumber().add(castToUnsignedInt(value)); 7820 } else 7821 return super.setProperty(name, value); 7822 return value; 7823 } 7824 7825 @Override 7826 public void removeChild(String name, Base value) throws FHIRException { 7827 if (name.equals("entity[x]")) { 7828 this.entity = null; 7829 } else if (name.equals("identifier")) { 7830 this.identifier = null; 7831 } else if (name.equals("effectiveTime")) { 7832 this.effectiveTime = null; 7833 } else if (name.equals("quantity")) { 7834 this.quantity = null; 7835 } else if (name.equals("unitPrice")) { 7836 this.unitPrice = null; 7837 } else if (name.equals("factor")) { 7838 this.factor = null; 7839 } else if (name.equals("points")) { 7840 this.points = null; 7841 } else if (name.equals("net")) { 7842 this.net = null; 7843 } else if (name.equals("payment")) { 7844 this.payment = null; 7845 } else if (name.equals("paymentDate")) { 7846 this.paymentDate = null; 7847 } else if (name.equals("responsible")) { 7848 this.responsible = null; 7849 } else if (name.equals("recipient")) { 7850 this.recipient = null; 7851 } else if (name.equals("linkId")) { 7852 this.getLinkId().remove(castToString(value)); 7853 } else if (name.equals("securityLabelNumber")) { 7854 this.getSecurityLabelNumber().remove(castToUnsignedInt(value)); 7855 } else 7856 super.removeChild(name, value); 7857 7858 } 7859 7860 @Override 7861 public Base makeProperty(int hash, String name) throws FHIRException { 7862 switch (hash) { 7863 case -740568643: 7864 return getEntity(); 7865 case -1298275357: 7866 return getEntity(); 7867 case -1618432855: 7868 return getIdentifier(); 7869 case -929905388: 7870 return getEffectiveTimeElement(); 7871 case -1285004149: 7872 return getQuantity(); 7873 case -486196699: 7874 return getUnitPrice(); 7875 case -1282148017: 7876 return getFactorElement(); 7877 case -982754077: 7878 return getPointsElement(); 7879 case 108957: 7880 return getNet(); 7881 case -786681338: 7882 return getPaymentElement(); 7883 case -1540873516: 7884 return getPaymentDateElement(); 7885 case 1847674614: 7886 return getResponsible(); 7887 case 820081177: 7888 return getRecipient(); 7889 case -1102667083: 7890 return addLinkIdElement(); 7891 case -149460995: 7892 return addSecurityLabelNumberElement(); 7893 default: 7894 return super.makeProperty(hash, name); 7895 } 7896 7897 } 7898 7899 @Override 7900 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7901 switch (hash) { 7902 case -1298275357: 7903 /* entity */ return new String[] { "CodeableConcept", "Reference" }; 7904 case -1618432855: 7905 /* identifier */ return new String[] { "Identifier" }; 7906 case -929905388: 7907 /* effectiveTime */ return new String[] { "dateTime" }; 7908 case -1285004149: 7909 /* quantity */ return new String[] { "SimpleQuantity" }; 7910 case -486196699: 7911 /* unitPrice */ return new String[] { "Money" }; 7912 case -1282148017: 7913 /* factor */ return new String[] { "decimal" }; 7914 case -982754077: 7915 /* points */ return new String[] { "decimal" }; 7916 case 108957: 7917 /* net */ return new String[] { "Money" }; 7918 case -786681338: 7919 /* payment */ return new String[] { "string" }; 7920 case -1540873516: 7921 /* paymentDate */ return new String[] { "dateTime" }; 7922 case 1847674614: 7923 /* responsible */ return new String[] { "Reference" }; 7924 case 820081177: 7925 /* recipient */ return new String[] { "Reference" }; 7926 case -1102667083: 7927 /* linkId */ return new String[] { "string" }; 7928 case -149460995: 7929 /* securityLabelNumber */ return new String[] { "unsignedInt" }; 7930 default: 7931 return super.getTypesForProperty(hash, name); 7932 } 7933 7934 } 7935 7936 @Override 7937 public Base addChild(String name) throws FHIRException { 7938 if (name.equals("entityCodeableConcept")) { 7939 this.entity = new CodeableConcept(); 7940 return this.entity; 7941 } else if (name.equals("entityReference")) { 7942 this.entity = new Reference(); 7943 return this.entity; 7944 } else if (name.equals("identifier")) { 7945 this.identifier = new Identifier(); 7946 return this.identifier; 7947 } else if (name.equals("effectiveTime")) { 7948 throw new FHIRException("Cannot call addChild on a singleton property Contract.effectiveTime"); 7949 } else if (name.equals("quantity")) { 7950 this.quantity = new Quantity(); 7951 return this.quantity; 7952 } else if (name.equals("unitPrice")) { 7953 this.unitPrice = new Money(); 7954 return this.unitPrice; 7955 } else if (name.equals("factor")) { 7956 throw new FHIRException("Cannot call addChild on a singleton property Contract.factor"); 7957 } else if (name.equals("points")) { 7958 throw new FHIRException("Cannot call addChild on a singleton property Contract.points"); 7959 } else if (name.equals("net")) { 7960 this.net = new Money(); 7961 return this.net; 7962 } else if (name.equals("payment")) { 7963 throw new FHIRException("Cannot call addChild on a singleton property Contract.payment"); 7964 } else if (name.equals("paymentDate")) { 7965 throw new FHIRException("Cannot call addChild on a singleton property Contract.paymentDate"); 7966 } else if (name.equals("responsible")) { 7967 this.responsible = new Reference(); 7968 return this.responsible; 7969 } else if (name.equals("recipient")) { 7970 this.recipient = new Reference(); 7971 return this.recipient; 7972 } else if (name.equals("linkId")) { 7973 throw new FHIRException("Cannot call addChild on a singleton property Contract.linkId"); 7974 } else if (name.equals("securityLabelNumber")) { 7975 throw new FHIRException("Cannot call addChild on a singleton property Contract.securityLabelNumber"); 7976 } else 7977 return super.addChild(name); 7978 } 7979 7980 public ValuedItemComponent copy() { 7981 ValuedItemComponent dst = new ValuedItemComponent(); 7982 copyValues(dst); 7983 return dst; 7984 } 7985 7986 public void copyValues(ValuedItemComponent dst) { 7987 super.copyValues(dst); 7988 dst.entity = entity == null ? null : entity.copy(); 7989 dst.identifier = identifier == null ? null : identifier.copy(); 7990 dst.effectiveTime = effectiveTime == null ? null : effectiveTime.copy(); 7991 dst.quantity = quantity == null ? null : quantity.copy(); 7992 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 7993 dst.factor = factor == null ? null : factor.copy(); 7994 dst.points = points == null ? null : points.copy(); 7995 dst.net = net == null ? null : net.copy(); 7996 dst.payment = payment == null ? null : payment.copy(); 7997 dst.paymentDate = paymentDate == null ? null : paymentDate.copy(); 7998 dst.responsible = responsible == null ? null : responsible.copy(); 7999 dst.recipient = recipient == null ? null : recipient.copy(); 8000 if (linkId != null) { 8001 dst.linkId = new ArrayList<StringType>(); 8002 for (StringType i : linkId) 8003 dst.linkId.add(i.copy()); 8004 } 8005 ; 8006 if (securityLabelNumber != null) { 8007 dst.securityLabelNumber = new ArrayList<UnsignedIntType>(); 8008 for (UnsignedIntType i : securityLabelNumber) 8009 dst.securityLabelNumber.add(i.copy()); 8010 } 8011 ; 8012 } 8013 8014 @Override 8015 public boolean equalsDeep(Base other_) { 8016 if (!super.equalsDeep(other_)) 8017 return false; 8018 if (!(other_ instanceof ValuedItemComponent)) 8019 return false; 8020 ValuedItemComponent o = (ValuedItemComponent) other_; 8021 return compareDeep(entity, o.entity, true) && compareDeep(identifier, o.identifier, true) 8022 && compareDeep(effectiveTime, o.effectiveTime, true) && compareDeep(quantity, o.quantity, true) 8023 && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 8024 && compareDeep(points, o.points, true) && compareDeep(net, o.net, true) 8025 && compareDeep(payment, o.payment, true) && compareDeep(paymentDate, o.paymentDate, true) 8026 && compareDeep(responsible, o.responsible, true) && compareDeep(recipient, o.recipient, true) 8027 && compareDeep(linkId, o.linkId, true) && compareDeep(securityLabelNumber, o.securityLabelNumber, true); 8028 } 8029 8030 @Override 8031 public boolean equalsShallow(Base other_) { 8032 if (!super.equalsShallow(other_)) 8033 return false; 8034 if (!(other_ instanceof ValuedItemComponent)) 8035 return false; 8036 ValuedItemComponent o = (ValuedItemComponent) other_; 8037 return compareValues(effectiveTime, o.effectiveTime, true) && compareValues(factor, o.factor, true) 8038 && compareValues(points, o.points, true) && compareValues(payment, o.payment, true) 8039 && compareValues(paymentDate, o.paymentDate, true) && compareValues(linkId, o.linkId, true) 8040 && compareValues(securityLabelNumber, o.securityLabelNumber, true); 8041 } 8042 8043 public boolean isEmpty() { 8044 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(entity, identifier, effectiveTime, quantity, 8045 unitPrice, factor, points, net, payment, paymentDate, responsible, recipient, linkId, securityLabelNumber); 8046 } 8047 8048 public String fhirType() { 8049 return "Contract.term.asset.valuedItem"; 8050 8051 } 8052 8053 } 8054 8055 @Block() 8056 public static class ActionComponent extends BackboneElement implements IBaseBackboneElement { 8057 /** 8058 * True if the term prohibits the action. 8059 */ 8060 @Child(name = "doNotPerform", type = { 8061 BooleanType.class }, order = 1, min = 0, max = 1, modifier = true, summary = false) 8062 @Description(shortDefinition = "True if the term prohibits the action", formalDefinition = "True if the term prohibits the action.") 8063 protected BooleanType doNotPerform; 8064 8065 /** 8066 * Activity or service obligation to be done or not done, performed or not 8067 * performed, effectuated or not by this Contract term. 8068 */ 8069 @Child(name = "type", type = { 8070 CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 8071 @Description(shortDefinition = "Type or form of the action", formalDefinition = "Activity or service obligation to be done or not done, performed or not performed, effectuated or not by this Contract term.") 8072 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-action") 8073 protected CodeableConcept type; 8074 8075 /** 8076 * Entity of the action. 8077 */ 8078 @Child(name = "subject", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8079 @Description(shortDefinition = "Entity of the action", formalDefinition = "Entity of the action.") 8080 protected List<ActionSubjectComponent> subject; 8081 8082 /** 8083 * Reason or purpose for the action stipulated by this Contract Provision. 8084 */ 8085 @Child(name = "intent", type = { 8086 CodeableConcept.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 8087 @Description(shortDefinition = "Purpose for the Contract Term Action", formalDefinition = "Reason or purpose for the action stipulated by this Contract Provision.") 8088 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-PurposeOfUse") 8089 protected CodeableConcept intent; 8090 8091 /** 8092 * Id [identifier??] of the clause or question text related to this action in 8093 * the referenced form or QuestionnaireResponse. 8094 */ 8095 @Child(name = "linkId", type = { 8096 StringType.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8097 @Description(shortDefinition = "Pointer to specific item", formalDefinition = "Id [identifier??] of the clause or question text related to this action in the referenced form or QuestionnaireResponse.") 8098 protected List<StringType> linkId; 8099 8100 /** 8101 * Current state of the term action. 8102 */ 8103 @Child(name = "status", type = { 8104 CodeableConcept.class }, order = 6, min = 1, max = 1, modifier = false, summary = false) 8105 @Description(shortDefinition = "State of the action", formalDefinition = "Current state of the term action.") 8106 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-actionstatus") 8107 protected CodeableConcept status; 8108 8109 /** 8110 * Encounter or Episode with primary association to specified term activity. 8111 */ 8112 @Child(name = "context", type = { Encounter.class, 8113 EpisodeOfCare.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 8114 @Description(shortDefinition = "Episode associated with action", formalDefinition = "Encounter or Episode with primary association to specified term activity.") 8115 protected Reference context; 8116 8117 /** 8118 * The actual object that is the target of the reference (Encounter or Episode 8119 * with primary association to specified term activity.) 8120 */ 8121 protected Resource contextTarget; 8122 8123 /** 8124 * Id [identifier??] of the clause or question text related to the requester of 8125 * this action in the referenced form or QuestionnaireResponse. 8126 */ 8127 @Child(name = "contextLinkId", type = { 8128 StringType.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8129 @Description(shortDefinition = "Pointer to specific item", formalDefinition = "Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse.") 8130 protected List<StringType> contextLinkId; 8131 8132 /** 8133 * When action happens. 8134 */ 8135 @Child(name = "occurrence", type = { DateTimeType.class, Period.class, 8136 Timing.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 8137 @Description(shortDefinition = "When action happens", formalDefinition = "When action happens.") 8138 protected Type occurrence; 8139 8140 /** 8141 * Who or what initiated the action and has responsibility for its activation. 8142 */ 8143 @Child(name = "requester", type = { Patient.class, RelatedPerson.class, Practitioner.class, PractitionerRole.class, 8144 Device.class, Group.class, 8145 Organization.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8146 @Description(shortDefinition = "Who asked for action", formalDefinition = "Who or what initiated the action and has responsibility for its activation.") 8147 protected List<Reference> requester; 8148 /** 8149 * The actual objects that are the target of the reference (Who or what 8150 * initiated the action and has responsibility for its activation.) 8151 */ 8152 protected List<Resource> requesterTarget; 8153 8154 /** 8155 * Id [identifier??] of the clause or question text related to the requester of 8156 * this action in the referenced form or QuestionnaireResponse. 8157 */ 8158 @Child(name = "requesterLinkId", type = { 8159 StringType.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8160 @Description(shortDefinition = "Pointer to specific item", formalDefinition = "Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse.") 8161 protected List<StringType> requesterLinkId; 8162 8163 /** 8164 * The type of individual that is desired or required to perform or not perform 8165 * the action. 8166 */ 8167 @Child(name = "performerType", type = { 8168 CodeableConcept.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8169 @Description(shortDefinition = "Kind of service performer", formalDefinition = "The type of individual that is desired or required to perform or not perform the action.") 8170 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/provenance-agent-type") 8171 protected List<CodeableConcept> performerType; 8172 8173 /** 8174 * The type of role or competency of an individual desired or required to 8175 * perform or not perform the action. 8176 */ 8177 @Child(name = "performerRole", type = { 8178 CodeableConcept.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 8179 @Description(shortDefinition = "Competency of the performer", formalDefinition = "The type of role or competency of an individual desired or required to perform or not perform the action.") 8180 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/provenance-agent-role") 8181 protected CodeableConcept performerRole; 8182 8183 /** 8184 * Indicates who or what is being asked to perform (or not perform) the ction. 8185 */ 8186 @Child(name = "performer", type = { RelatedPerson.class, Patient.class, Practitioner.class, PractitionerRole.class, 8187 CareTeam.class, Device.class, Substance.class, Organization.class, 8188 Location.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 8189 @Description(shortDefinition = "Actor that wil execute (or not) the action", formalDefinition = "Indicates who or what is being asked to perform (or not perform) the ction.") 8190 protected Reference performer; 8191 8192 /** 8193 * The actual object that is the target of the reference (Indicates who or what 8194 * is being asked to perform (or not perform) the ction.) 8195 */ 8196 protected Resource performerTarget; 8197 8198 /** 8199 * Id [identifier??] of the clause or question text related to the reason type 8200 * or reference of this action in the referenced form or QuestionnaireResponse. 8201 */ 8202 @Child(name = "performerLinkId", type = { 8203 StringType.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8204 @Description(shortDefinition = "Pointer to specific item", formalDefinition = "Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse.") 8205 protected List<StringType> performerLinkId; 8206 8207 /** 8208 * Rationale for the action to be performed or not performed. Describes why the 8209 * action is permitted or prohibited. 8210 */ 8211 @Child(name = "reasonCode", type = { 8212 CodeableConcept.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8213 @Description(shortDefinition = "Why is action (not) needed?", formalDefinition = "Rationale for the action to be performed or not performed. Describes why the action is permitted or prohibited.") 8214 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-PurposeOfUse") 8215 protected List<CodeableConcept> reasonCode; 8216 8217 /** 8218 * Indicates another resource whose existence justifies permitting or not 8219 * permitting this action. 8220 */ 8221 @Child(name = "reasonReference", type = { Condition.class, Observation.class, DiagnosticReport.class, 8222 DocumentReference.class, Questionnaire.class, 8223 QuestionnaireResponse.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8224 @Description(shortDefinition = "Why is action (not) needed?", formalDefinition = "Indicates another resource whose existence justifies permitting or not permitting this action.") 8225 protected List<Reference> reasonReference; 8226 /** 8227 * The actual objects that are the target of the reference (Indicates another 8228 * resource whose existence justifies permitting or not permitting this action.) 8229 */ 8230 protected List<Resource> reasonReferenceTarget; 8231 8232 /** 8233 * Describes why the action is to be performed or not performed in textual form. 8234 */ 8235 @Child(name = "reason", type = { 8236 StringType.class }, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8237 @Description(shortDefinition = "Why action is to be performed", formalDefinition = "Describes why the action is to be performed or not performed in textual form.") 8238 protected List<StringType> reason; 8239 8240 /** 8241 * Id [identifier??] of the clause or question text related to the reason type 8242 * or reference of this action in the referenced form or QuestionnaireResponse. 8243 */ 8244 @Child(name = "reasonLinkId", type = { 8245 StringType.class }, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8246 @Description(shortDefinition = "Pointer to specific item", formalDefinition = "Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse.") 8247 protected List<StringType> reasonLinkId; 8248 8249 /** 8250 * Comments made about the term action made by the requester, performer, subject 8251 * or other participants. 8252 */ 8253 @Child(name = "note", type = { 8254 Annotation.class }, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8255 @Description(shortDefinition = "Comments about the action", formalDefinition = "Comments made about the term action made by the requester, performer, subject or other participants.") 8256 protected List<Annotation> note; 8257 8258 /** 8259 * Security labels that protects the action. 8260 */ 8261 @Child(name = "securityLabelNumber", type = { 8262 UnsignedIntType.class }, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8263 @Description(shortDefinition = "Action restriction numbers", formalDefinition = "Security labels that protects the action.") 8264 protected List<UnsignedIntType> securityLabelNumber; 8265 8266 private static final long serialVersionUID = -178728180L; 8267 8268 /** 8269 * Constructor 8270 */ 8271 public ActionComponent() { 8272 super(); 8273 } 8274 8275 /** 8276 * Constructor 8277 */ 8278 public ActionComponent(CodeableConcept type, CodeableConcept intent, CodeableConcept status) { 8279 super(); 8280 this.type = type; 8281 this.intent = intent; 8282 this.status = status; 8283 } 8284 8285 /** 8286 * @return {@link #doNotPerform} (True if the term prohibits the action.). This 8287 * is the underlying object with id, value and extensions. The accessor 8288 * "getDoNotPerform" gives direct access to the value 8289 */ 8290 public BooleanType getDoNotPerformElement() { 8291 if (this.doNotPerform == null) 8292 if (Configuration.errorOnAutoCreate()) 8293 throw new Error("Attempt to auto-create ActionComponent.doNotPerform"); 8294 else if (Configuration.doAutoCreate()) 8295 this.doNotPerform = new BooleanType(); // bb 8296 return this.doNotPerform; 8297 } 8298 8299 public boolean hasDoNotPerformElement() { 8300 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 8301 } 8302 8303 public boolean hasDoNotPerform() { 8304 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 8305 } 8306 8307 /** 8308 * @param value {@link #doNotPerform} (True if the term prohibits the action.). 8309 * This is the underlying object with id, value and extensions. The 8310 * accessor "getDoNotPerform" gives direct access to the value 8311 */ 8312 public ActionComponent setDoNotPerformElement(BooleanType value) { 8313 this.doNotPerform = value; 8314 return this; 8315 } 8316 8317 /** 8318 * @return True if the term prohibits the action. 8319 */ 8320 public boolean getDoNotPerform() { 8321 return this.doNotPerform == null || this.doNotPerform.isEmpty() ? false : this.doNotPerform.getValue(); 8322 } 8323 8324 /** 8325 * @param value True if the term prohibits the action. 8326 */ 8327 public ActionComponent setDoNotPerform(boolean value) { 8328 if (this.doNotPerform == null) 8329 this.doNotPerform = new BooleanType(); 8330 this.doNotPerform.setValue(value); 8331 return this; 8332 } 8333 8334 /** 8335 * @return {@link #type} (Activity or service obligation to be done or not done, 8336 * performed or not performed, effectuated or not by this Contract 8337 * term.) 8338 */ 8339 public CodeableConcept getType() { 8340 if (this.type == null) 8341 if (Configuration.errorOnAutoCreate()) 8342 throw new Error("Attempt to auto-create ActionComponent.type"); 8343 else if (Configuration.doAutoCreate()) 8344 this.type = new CodeableConcept(); // cc 8345 return this.type; 8346 } 8347 8348 public boolean hasType() { 8349 return this.type != null && !this.type.isEmpty(); 8350 } 8351 8352 /** 8353 * @param value {@link #type} (Activity or service obligation to be done or not 8354 * done, performed or not performed, effectuated or not by this 8355 * Contract term.) 8356 */ 8357 public ActionComponent setType(CodeableConcept value) { 8358 this.type = value; 8359 return this; 8360 } 8361 8362 /** 8363 * @return {@link #subject} (Entity of the action.) 8364 */ 8365 public List<ActionSubjectComponent> getSubject() { 8366 if (this.subject == null) 8367 this.subject = new ArrayList<ActionSubjectComponent>(); 8368 return this.subject; 8369 } 8370 8371 /** 8372 * @return Returns a reference to <code>this</code> for easy method chaining 8373 */ 8374 public ActionComponent setSubject(List<ActionSubjectComponent> theSubject) { 8375 this.subject = theSubject; 8376 return this; 8377 } 8378 8379 public boolean hasSubject() { 8380 if (this.subject == null) 8381 return false; 8382 for (ActionSubjectComponent item : this.subject) 8383 if (!item.isEmpty()) 8384 return true; 8385 return false; 8386 } 8387 8388 public ActionSubjectComponent addSubject() { // 3 8389 ActionSubjectComponent t = new ActionSubjectComponent(); 8390 if (this.subject == null) 8391 this.subject = new ArrayList<ActionSubjectComponent>(); 8392 this.subject.add(t); 8393 return t; 8394 } 8395 8396 public ActionComponent addSubject(ActionSubjectComponent t) { // 3 8397 if (t == null) 8398 return this; 8399 if (this.subject == null) 8400 this.subject = new ArrayList<ActionSubjectComponent>(); 8401 this.subject.add(t); 8402 return this; 8403 } 8404 8405 /** 8406 * @return The first repetition of repeating field {@link #subject}, creating it 8407 * if it does not already exist 8408 */ 8409 public ActionSubjectComponent getSubjectFirstRep() { 8410 if (getSubject().isEmpty()) { 8411 addSubject(); 8412 } 8413 return getSubject().get(0); 8414 } 8415 8416 /** 8417 * @return {@link #intent} (Reason or purpose for the action stipulated by this 8418 * Contract Provision.) 8419 */ 8420 public CodeableConcept getIntent() { 8421 if (this.intent == null) 8422 if (Configuration.errorOnAutoCreate()) 8423 throw new Error("Attempt to auto-create ActionComponent.intent"); 8424 else if (Configuration.doAutoCreate()) 8425 this.intent = new CodeableConcept(); // cc 8426 return this.intent; 8427 } 8428 8429 public boolean hasIntent() { 8430 return this.intent != null && !this.intent.isEmpty(); 8431 } 8432 8433 /** 8434 * @param value {@link #intent} (Reason or purpose for the action stipulated by 8435 * this Contract Provision.) 8436 */ 8437 public ActionComponent setIntent(CodeableConcept value) { 8438 this.intent = value; 8439 return this; 8440 } 8441 8442 /** 8443 * @return {@link #linkId} (Id [identifier??] of the clause or question text 8444 * related to this action in the referenced form or 8445 * QuestionnaireResponse.) 8446 */ 8447 public List<StringType> getLinkId() { 8448 if (this.linkId == null) 8449 this.linkId = new ArrayList<StringType>(); 8450 return this.linkId; 8451 } 8452 8453 /** 8454 * @return Returns a reference to <code>this</code> for easy method chaining 8455 */ 8456 public ActionComponent setLinkId(List<StringType> theLinkId) { 8457 this.linkId = theLinkId; 8458 return this; 8459 } 8460 8461 public boolean hasLinkId() { 8462 if (this.linkId == null) 8463 return false; 8464 for (StringType item : this.linkId) 8465 if (!item.isEmpty()) 8466 return true; 8467 return false; 8468 } 8469 8470 /** 8471 * @return {@link #linkId} (Id [identifier??] of the clause or question text 8472 * related to this action in the referenced form or 8473 * QuestionnaireResponse.) 8474 */ 8475 public StringType addLinkIdElement() {// 2 8476 StringType t = new StringType(); 8477 if (this.linkId == null) 8478 this.linkId = new ArrayList<StringType>(); 8479 this.linkId.add(t); 8480 return t; 8481 } 8482 8483 /** 8484 * @param value {@link #linkId} (Id [identifier??] of the clause or question 8485 * text related to this action in the referenced form or 8486 * QuestionnaireResponse.) 8487 */ 8488 public ActionComponent addLinkId(String value) { // 1 8489 StringType t = new StringType(); 8490 t.setValue(value); 8491 if (this.linkId == null) 8492 this.linkId = new ArrayList<StringType>(); 8493 this.linkId.add(t); 8494 return this; 8495 } 8496 8497 /** 8498 * @param value {@link #linkId} (Id [identifier??] of the clause or question 8499 * text related to this action in the referenced form or 8500 * QuestionnaireResponse.) 8501 */ 8502 public boolean hasLinkId(String value) { 8503 if (this.linkId == null) 8504 return false; 8505 for (StringType v : this.linkId) 8506 if (v.getValue().equals(value)) // string 8507 return true; 8508 return false; 8509 } 8510 8511 /** 8512 * @return {@link #status} (Current state of the term action.) 8513 */ 8514 public CodeableConcept getStatus() { 8515 if (this.status == null) 8516 if (Configuration.errorOnAutoCreate()) 8517 throw new Error("Attempt to auto-create ActionComponent.status"); 8518 else if (Configuration.doAutoCreate()) 8519 this.status = new CodeableConcept(); // cc 8520 return this.status; 8521 } 8522 8523 public boolean hasStatus() { 8524 return this.status != null && !this.status.isEmpty(); 8525 } 8526 8527 /** 8528 * @param value {@link #status} (Current state of the term action.) 8529 */ 8530 public ActionComponent setStatus(CodeableConcept value) { 8531 this.status = value; 8532 return this; 8533 } 8534 8535 /** 8536 * @return {@link #context} (Encounter or Episode with primary association to 8537 * specified term activity.) 8538 */ 8539 public Reference getContext() { 8540 if (this.context == null) 8541 if (Configuration.errorOnAutoCreate()) 8542 throw new Error("Attempt to auto-create ActionComponent.context"); 8543 else if (Configuration.doAutoCreate()) 8544 this.context = new Reference(); // cc 8545 return this.context; 8546 } 8547 8548 public boolean hasContext() { 8549 return this.context != null && !this.context.isEmpty(); 8550 } 8551 8552 /** 8553 * @param value {@link #context} (Encounter or Episode with primary association 8554 * to specified term activity.) 8555 */ 8556 public ActionComponent setContext(Reference value) { 8557 this.context = value; 8558 return this; 8559 } 8560 8561 /** 8562 * @return {@link #context} The actual object that is the target of the 8563 * reference. The reference library doesn't populate this, but you can 8564 * use it to hold the resource if you resolve it. (Encounter or Episode 8565 * with primary association to specified term activity.) 8566 */ 8567 public Resource getContextTarget() { 8568 return this.contextTarget; 8569 } 8570 8571 /** 8572 * @param value {@link #context} The actual object that is the target of the 8573 * reference. The reference library doesn't use these, but you can 8574 * use it to hold the resource if you resolve it. (Encounter or 8575 * Episode with primary association to specified term activity.) 8576 */ 8577 public ActionComponent setContextTarget(Resource value) { 8578 this.contextTarget = value; 8579 return this; 8580 } 8581 8582 /** 8583 * @return {@link #contextLinkId} (Id [identifier??] of the clause or question 8584 * text related to the requester of this action in the referenced form 8585 * or QuestionnaireResponse.) 8586 */ 8587 public List<StringType> getContextLinkId() { 8588 if (this.contextLinkId == null) 8589 this.contextLinkId = new ArrayList<StringType>(); 8590 return this.contextLinkId; 8591 } 8592 8593 /** 8594 * @return Returns a reference to <code>this</code> for easy method chaining 8595 */ 8596 public ActionComponent setContextLinkId(List<StringType> theContextLinkId) { 8597 this.contextLinkId = theContextLinkId; 8598 return this; 8599 } 8600 8601 public boolean hasContextLinkId() { 8602 if (this.contextLinkId == null) 8603 return false; 8604 for (StringType item : this.contextLinkId) 8605 if (!item.isEmpty()) 8606 return true; 8607 return false; 8608 } 8609 8610 /** 8611 * @return {@link #contextLinkId} (Id [identifier??] of the clause or question 8612 * text related to the requester of this action in the referenced form 8613 * or QuestionnaireResponse.) 8614 */ 8615 public StringType addContextLinkIdElement() {// 2 8616 StringType t = new StringType(); 8617 if (this.contextLinkId == null) 8618 this.contextLinkId = new ArrayList<StringType>(); 8619 this.contextLinkId.add(t); 8620 return t; 8621 } 8622 8623 /** 8624 * @param value {@link #contextLinkId} (Id [identifier??] of the clause or 8625 * question text related to the requester of this action in the 8626 * referenced form or QuestionnaireResponse.) 8627 */ 8628 public ActionComponent addContextLinkId(String value) { // 1 8629 StringType t = new StringType(); 8630 t.setValue(value); 8631 if (this.contextLinkId == null) 8632 this.contextLinkId = new ArrayList<StringType>(); 8633 this.contextLinkId.add(t); 8634 return this; 8635 } 8636 8637 /** 8638 * @param value {@link #contextLinkId} (Id [identifier??] of the clause or 8639 * question text related to the requester of this action in the 8640 * referenced form or QuestionnaireResponse.) 8641 */ 8642 public boolean hasContextLinkId(String value) { 8643 if (this.contextLinkId == null) 8644 return false; 8645 for (StringType v : this.contextLinkId) 8646 if (v.getValue().equals(value)) // string 8647 return true; 8648 return false; 8649 } 8650 8651 /** 8652 * @return {@link #occurrence} (When action happens.) 8653 */ 8654 public Type getOccurrence() { 8655 return this.occurrence; 8656 } 8657 8658 /** 8659 * @return {@link #occurrence} (When action happens.) 8660 */ 8661 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 8662 if (this.occurrence == null) 8663 this.occurrence = new DateTimeType(); 8664 if (!(this.occurrence instanceof DateTimeType)) 8665 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 8666 + this.occurrence.getClass().getName() + " was encountered"); 8667 return (DateTimeType) this.occurrence; 8668 } 8669 8670 public boolean hasOccurrenceDateTimeType() { 8671 return this != null && this.occurrence instanceof DateTimeType; 8672 } 8673 8674 /** 8675 * @return {@link #occurrence} (When action happens.) 8676 */ 8677 public Period getOccurrencePeriod() throws FHIRException { 8678 if (this.occurrence == null) 8679 this.occurrence = new Period(); 8680 if (!(this.occurrence instanceof Period)) 8681 throw new FHIRException("Type mismatch: the type Period was expected, but " 8682 + this.occurrence.getClass().getName() + " was encountered"); 8683 return (Period) this.occurrence; 8684 } 8685 8686 public boolean hasOccurrencePeriod() { 8687 return this != null && this.occurrence instanceof Period; 8688 } 8689 8690 /** 8691 * @return {@link #occurrence} (When action happens.) 8692 */ 8693 public Timing getOccurrenceTiming() throws FHIRException { 8694 if (this.occurrence == null) 8695 this.occurrence = new Timing(); 8696 if (!(this.occurrence instanceof Timing)) 8697 throw new FHIRException("Type mismatch: the type Timing was expected, but " 8698 + this.occurrence.getClass().getName() + " was encountered"); 8699 return (Timing) this.occurrence; 8700 } 8701 8702 public boolean hasOccurrenceTiming() { 8703 return this != null && this.occurrence instanceof Timing; 8704 } 8705 8706 public boolean hasOccurrence() { 8707 return this.occurrence != null && !this.occurrence.isEmpty(); 8708 } 8709 8710 /** 8711 * @param value {@link #occurrence} (When action happens.) 8712 */ 8713 public ActionComponent setOccurrence(Type value) { 8714 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 8715 throw new Error("Not the right type for Contract.term.action.occurrence[x]: " + value.fhirType()); 8716 this.occurrence = value; 8717 return this; 8718 } 8719 8720 /** 8721 * @return {@link #requester} (Who or what initiated the action and has 8722 * responsibility for its activation.) 8723 */ 8724 public List<Reference> getRequester() { 8725 if (this.requester == null) 8726 this.requester = new ArrayList<Reference>(); 8727 return this.requester; 8728 } 8729 8730 /** 8731 * @return Returns a reference to <code>this</code> for easy method chaining 8732 */ 8733 public ActionComponent setRequester(List<Reference> theRequester) { 8734 this.requester = theRequester; 8735 return this; 8736 } 8737 8738 public boolean hasRequester() { 8739 if (this.requester == null) 8740 return false; 8741 for (Reference item : this.requester) 8742 if (!item.isEmpty()) 8743 return true; 8744 return false; 8745 } 8746 8747 public Reference addRequester() { // 3 8748 Reference t = new Reference(); 8749 if (this.requester == null) 8750 this.requester = new ArrayList<Reference>(); 8751 this.requester.add(t); 8752 return t; 8753 } 8754 8755 public ActionComponent addRequester(Reference t) { // 3 8756 if (t == null) 8757 return this; 8758 if (this.requester == null) 8759 this.requester = new ArrayList<Reference>(); 8760 this.requester.add(t); 8761 return this; 8762 } 8763 8764 /** 8765 * @return The first repetition of repeating field {@link #requester}, creating 8766 * it if it does not already exist 8767 */ 8768 public Reference getRequesterFirstRep() { 8769 if (getRequester().isEmpty()) { 8770 addRequester(); 8771 } 8772 return getRequester().get(0); 8773 } 8774 8775 /** 8776 * @deprecated Use Reference#setResource(IBaseResource) instead 8777 */ 8778 @Deprecated 8779 public List<Resource> getRequesterTarget() { 8780 if (this.requesterTarget == null) 8781 this.requesterTarget = new ArrayList<Resource>(); 8782 return this.requesterTarget; 8783 } 8784 8785 /** 8786 * @return {@link #requesterLinkId} (Id [identifier??] of the clause or question 8787 * text related to the requester of this action in the referenced form 8788 * or QuestionnaireResponse.) 8789 */ 8790 public List<StringType> getRequesterLinkId() { 8791 if (this.requesterLinkId == null) 8792 this.requesterLinkId = new ArrayList<StringType>(); 8793 return this.requesterLinkId; 8794 } 8795 8796 /** 8797 * @return Returns a reference to <code>this</code> for easy method chaining 8798 */ 8799 public ActionComponent setRequesterLinkId(List<StringType> theRequesterLinkId) { 8800 this.requesterLinkId = theRequesterLinkId; 8801 return this; 8802 } 8803 8804 public boolean hasRequesterLinkId() { 8805 if (this.requesterLinkId == null) 8806 return false; 8807 for (StringType item : this.requesterLinkId) 8808 if (!item.isEmpty()) 8809 return true; 8810 return false; 8811 } 8812 8813 /** 8814 * @return {@link #requesterLinkId} (Id [identifier??] of the clause or question 8815 * text related to the requester of this action in the referenced form 8816 * or QuestionnaireResponse.) 8817 */ 8818 public StringType addRequesterLinkIdElement() {// 2 8819 StringType t = new StringType(); 8820 if (this.requesterLinkId == null) 8821 this.requesterLinkId = new ArrayList<StringType>(); 8822 this.requesterLinkId.add(t); 8823 return t; 8824 } 8825 8826 /** 8827 * @param value {@link #requesterLinkId} (Id [identifier??] of the clause or 8828 * question text related to the requester of this action in the 8829 * referenced form or QuestionnaireResponse.) 8830 */ 8831 public ActionComponent addRequesterLinkId(String value) { // 1 8832 StringType t = new StringType(); 8833 t.setValue(value); 8834 if (this.requesterLinkId == null) 8835 this.requesterLinkId = new ArrayList<StringType>(); 8836 this.requesterLinkId.add(t); 8837 return this; 8838 } 8839 8840 /** 8841 * @param value {@link #requesterLinkId} (Id [identifier??] of the clause or 8842 * question text related to the requester of this action in the 8843 * referenced form or QuestionnaireResponse.) 8844 */ 8845 public boolean hasRequesterLinkId(String value) { 8846 if (this.requesterLinkId == null) 8847 return false; 8848 for (StringType v : this.requesterLinkId) 8849 if (v.getValue().equals(value)) // string 8850 return true; 8851 return false; 8852 } 8853 8854 /** 8855 * @return {@link #performerType} (The type of individual that is desired or 8856 * required to perform or not perform the action.) 8857 */ 8858 public List<CodeableConcept> getPerformerType() { 8859 if (this.performerType == null) 8860 this.performerType = new ArrayList<CodeableConcept>(); 8861 return this.performerType; 8862 } 8863 8864 /** 8865 * @return Returns a reference to <code>this</code> for easy method chaining 8866 */ 8867 public ActionComponent setPerformerType(List<CodeableConcept> thePerformerType) { 8868 this.performerType = thePerformerType; 8869 return this; 8870 } 8871 8872 public boolean hasPerformerType() { 8873 if (this.performerType == null) 8874 return false; 8875 for (CodeableConcept item : this.performerType) 8876 if (!item.isEmpty()) 8877 return true; 8878 return false; 8879 } 8880 8881 public CodeableConcept addPerformerType() { // 3 8882 CodeableConcept t = new CodeableConcept(); 8883 if (this.performerType == null) 8884 this.performerType = new ArrayList<CodeableConcept>(); 8885 this.performerType.add(t); 8886 return t; 8887 } 8888 8889 public ActionComponent addPerformerType(CodeableConcept t) { // 3 8890 if (t == null) 8891 return this; 8892 if (this.performerType == null) 8893 this.performerType = new ArrayList<CodeableConcept>(); 8894 this.performerType.add(t); 8895 return this; 8896 } 8897 8898 /** 8899 * @return The first repetition of repeating field {@link #performerType}, 8900 * creating it if it does not already exist 8901 */ 8902 public CodeableConcept getPerformerTypeFirstRep() { 8903 if (getPerformerType().isEmpty()) { 8904 addPerformerType(); 8905 } 8906 return getPerformerType().get(0); 8907 } 8908 8909 /** 8910 * @return {@link #performerRole} (The type of role or competency of an 8911 * individual desired or required to perform or not perform the action.) 8912 */ 8913 public CodeableConcept getPerformerRole() { 8914 if (this.performerRole == null) 8915 if (Configuration.errorOnAutoCreate()) 8916 throw new Error("Attempt to auto-create ActionComponent.performerRole"); 8917 else if (Configuration.doAutoCreate()) 8918 this.performerRole = new CodeableConcept(); // cc 8919 return this.performerRole; 8920 } 8921 8922 public boolean hasPerformerRole() { 8923 return this.performerRole != null && !this.performerRole.isEmpty(); 8924 } 8925 8926 /** 8927 * @param value {@link #performerRole} (The type of role or competency of an 8928 * individual desired or required to perform or not perform the 8929 * action.) 8930 */ 8931 public ActionComponent setPerformerRole(CodeableConcept value) { 8932 this.performerRole = value; 8933 return this; 8934 } 8935 8936 /** 8937 * @return {@link #performer} (Indicates who or what is being asked to perform 8938 * (or not perform) the ction.) 8939 */ 8940 public Reference getPerformer() { 8941 if (this.performer == null) 8942 if (Configuration.errorOnAutoCreate()) 8943 throw new Error("Attempt to auto-create ActionComponent.performer"); 8944 else if (Configuration.doAutoCreate()) 8945 this.performer = new Reference(); // cc 8946 return this.performer; 8947 } 8948 8949 public boolean hasPerformer() { 8950 return this.performer != null && !this.performer.isEmpty(); 8951 } 8952 8953 /** 8954 * @param value {@link #performer} (Indicates who or what is being asked to 8955 * perform (or not perform) the ction.) 8956 */ 8957 public ActionComponent setPerformer(Reference value) { 8958 this.performer = value; 8959 return this; 8960 } 8961 8962 /** 8963 * @return {@link #performer} The actual object that is the target of the 8964 * reference. The reference library doesn't populate this, but you can 8965 * use it to hold the resource if you resolve it. (Indicates who or what 8966 * is being asked to perform (or not perform) the ction.) 8967 */ 8968 public Resource getPerformerTarget() { 8969 return this.performerTarget; 8970 } 8971 8972 /** 8973 * @param value {@link #performer} The actual object that is the target of the 8974 * reference. The reference library doesn't use these, but you can 8975 * use it to hold the resource if you resolve it. (Indicates who or 8976 * what is being asked to perform (or not perform) the ction.) 8977 */ 8978 public ActionComponent setPerformerTarget(Resource value) { 8979 this.performerTarget = value; 8980 return this; 8981 } 8982 8983 /** 8984 * @return {@link #performerLinkId} (Id [identifier??] of the clause or question 8985 * text related to the reason type or reference of this action in the 8986 * referenced form or QuestionnaireResponse.) 8987 */ 8988 public List<StringType> getPerformerLinkId() { 8989 if (this.performerLinkId == null) 8990 this.performerLinkId = new ArrayList<StringType>(); 8991 return this.performerLinkId; 8992 } 8993 8994 /** 8995 * @return Returns a reference to <code>this</code> for easy method chaining 8996 */ 8997 public ActionComponent setPerformerLinkId(List<StringType> thePerformerLinkId) { 8998 this.performerLinkId = thePerformerLinkId; 8999 return this; 9000 } 9001 9002 public boolean hasPerformerLinkId() { 9003 if (this.performerLinkId == null) 9004 return false; 9005 for (StringType item : this.performerLinkId) 9006 if (!item.isEmpty()) 9007 return true; 9008 return false; 9009 } 9010 9011 /** 9012 * @return {@link #performerLinkId} (Id [identifier??] of the clause or question 9013 * text related to the reason type or reference of this action in the 9014 * referenced form or QuestionnaireResponse.) 9015 */ 9016 public StringType addPerformerLinkIdElement() {// 2 9017 StringType t = new StringType(); 9018 if (this.performerLinkId == null) 9019 this.performerLinkId = new ArrayList<StringType>(); 9020 this.performerLinkId.add(t); 9021 return t; 9022 } 9023 9024 /** 9025 * @param value {@link #performerLinkId} (Id [identifier??] of the clause or 9026 * question text related to the reason type or reference of this 9027 * action in the referenced form or QuestionnaireResponse.) 9028 */ 9029 public ActionComponent addPerformerLinkId(String value) { // 1 9030 StringType t = new StringType(); 9031 t.setValue(value); 9032 if (this.performerLinkId == null) 9033 this.performerLinkId = new ArrayList<StringType>(); 9034 this.performerLinkId.add(t); 9035 return this; 9036 } 9037 9038 /** 9039 * @param value {@link #performerLinkId} (Id [identifier??] of the clause or 9040 * question text related to the reason type or reference of this 9041 * action in the referenced form or QuestionnaireResponse.) 9042 */ 9043 public boolean hasPerformerLinkId(String value) { 9044 if (this.performerLinkId == null) 9045 return false; 9046 for (StringType v : this.performerLinkId) 9047 if (v.getValue().equals(value)) // string 9048 return true; 9049 return false; 9050 } 9051 9052 /** 9053 * @return {@link #reasonCode} (Rationale for the action to be performed or not 9054 * performed. Describes why the action is permitted or prohibited.) 9055 */ 9056 public List<CodeableConcept> getReasonCode() { 9057 if (this.reasonCode == null) 9058 this.reasonCode = new ArrayList<CodeableConcept>(); 9059 return this.reasonCode; 9060 } 9061 9062 /** 9063 * @return Returns a reference to <code>this</code> for easy method chaining 9064 */ 9065 public ActionComponent setReasonCode(List<CodeableConcept> theReasonCode) { 9066 this.reasonCode = theReasonCode; 9067 return this; 9068 } 9069 9070 public boolean hasReasonCode() { 9071 if (this.reasonCode == null) 9072 return false; 9073 for (CodeableConcept item : this.reasonCode) 9074 if (!item.isEmpty()) 9075 return true; 9076 return false; 9077 } 9078 9079 public CodeableConcept addReasonCode() { // 3 9080 CodeableConcept t = new CodeableConcept(); 9081 if (this.reasonCode == null) 9082 this.reasonCode = new ArrayList<CodeableConcept>(); 9083 this.reasonCode.add(t); 9084 return t; 9085 } 9086 9087 public ActionComponent addReasonCode(CodeableConcept t) { // 3 9088 if (t == null) 9089 return this; 9090 if (this.reasonCode == null) 9091 this.reasonCode = new ArrayList<CodeableConcept>(); 9092 this.reasonCode.add(t); 9093 return this; 9094 } 9095 9096 /** 9097 * @return The first repetition of repeating field {@link #reasonCode}, creating 9098 * it if it does not already exist 9099 */ 9100 public CodeableConcept getReasonCodeFirstRep() { 9101 if (getReasonCode().isEmpty()) { 9102 addReasonCode(); 9103 } 9104 return getReasonCode().get(0); 9105 } 9106 9107 /** 9108 * @return {@link #reasonReference} (Indicates another resource whose existence 9109 * justifies permitting or not permitting this action.) 9110 */ 9111 public List<Reference> getReasonReference() { 9112 if (this.reasonReference == null) 9113 this.reasonReference = new ArrayList<Reference>(); 9114 return this.reasonReference; 9115 } 9116 9117 /** 9118 * @return Returns a reference to <code>this</code> for easy method chaining 9119 */ 9120 public ActionComponent setReasonReference(List<Reference> theReasonReference) { 9121 this.reasonReference = theReasonReference; 9122 return this; 9123 } 9124 9125 public boolean hasReasonReference() { 9126 if (this.reasonReference == null) 9127 return false; 9128 for (Reference item : this.reasonReference) 9129 if (!item.isEmpty()) 9130 return true; 9131 return false; 9132 } 9133 9134 public Reference addReasonReference() { // 3 9135 Reference t = new Reference(); 9136 if (this.reasonReference == null) 9137 this.reasonReference = new ArrayList<Reference>(); 9138 this.reasonReference.add(t); 9139 return t; 9140 } 9141 9142 public ActionComponent addReasonReference(Reference t) { // 3 9143 if (t == null) 9144 return this; 9145 if (this.reasonReference == null) 9146 this.reasonReference = new ArrayList<Reference>(); 9147 this.reasonReference.add(t); 9148 return this; 9149 } 9150 9151 /** 9152 * @return The first repetition of repeating field {@link #reasonReference}, 9153 * creating it if it does not already exist 9154 */ 9155 public Reference getReasonReferenceFirstRep() { 9156 if (getReasonReference().isEmpty()) { 9157 addReasonReference(); 9158 } 9159 return getReasonReference().get(0); 9160 } 9161 9162 /** 9163 * @deprecated Use Reference#setResource(IBaseResource) instead 9164 */ 9165 @Deprecated 9166 public List<Resource> getReasonReferenceTarget() { 9167 if (this.reasonReferenceTarget == null) 9168 this.reasonReferenceTarget = new ArrayList<Resource>(); 9169 return this.reasonReferenceTarget; 9170 } 9171 9172 /** 9173 * @return {@link #reason} (Describes why the action is to be performed or not 9174 * performed in textual form.) 9175 */ 9176 public List<StringType> getReason() { 9177 if (this.reason == null) 9178 this.reason = new ArrayList<StringType>(); 9179 return this.reason; 9180 } 9181 9182 /** 9183 * @return Returns a reference to <code>this</code> for easy method chaining 9184 */ 9185 public ActionComponent setReason(List<StringType> theReason) { 9186 this.reason = theReason; 9187 return this; 9188 } 9189 9190 public boolean hasReason() { 9191 if (this.reason == null) 9192 return false; 9193 for (StringType item : this.reason) 9194 if (!item.isEmpty()) 9195 return true; 9196 return false; 9197 } 9198 9199 /** 9200 * @return {@link #reason} (Describes why the action is to be performed or not 9201 * performed in textual form.) 9202 */ 9203 public StringType addReasonElement() {// 2 9204 StringType t = new StringType(); 9205 if (this.reason == null) 9206 this.reason = new ArrayList<StringType>(); 9207 this.reason.add(t); 9208 return t; 9209 } 9210 9211 /** 9212 * @param value {@link #reason} (Describes why the action is to be performed or 9213 * not performed in textual form.) 9214 */ 9215 public ActionComponent addReason(String value) { // 1 9216 StringType t = new StringType(); 9217 t.setValue(value); 9218 if (this.reason == null) 9219 this.reason = new ArrayList<StringType>(); 9220 this.reason.add(t); 9221 return this; 9222 } 9223 9224 /** 9225 * @param value {@link #reason} (Describes why the action is to be performed or 9226 * not performed in textual form.) 9227 */ 9228 public boolean hasReason(String value) { 9229 if (this.reason == null) 9230 return false; 9231 for (StringType v : this.reason) 9232 if (v.getValue().equals(value)) // string 9233 return true; 9234 return false; 9235 } 9236 9237 /** 9238 * @return {@link #reasonLinkId} (Id [identifier??] of the clause or question 9239 * text related to the reason type or reference of this action in the 9240 * referenced form or QuestionnaireResponse.) 9241 */ 9242 public List<StringType> getReasonLinkId() { 9243 if (this.reasonLinkId == null) 9244 this.reasonLinkId = new ArrayList<StringType>(); 9245 return this.reasonLinkId; 9246 } 9247 9248 /** 9249 * @return Returns a reference to <code>this</code> for easy method chaining 9250 */ 9251 public ActionComponent setReasonLinkId(List<StringType> theReasonLinkId) { 9252 this.reasonLinkId = theReasonLinkId; 9253 return this; 9254 } 9255 9256 public boolean hasReasonLinkId() { 9257 if (this.reasonLinkId == null) 9258 return false; 9259 for (StringType item : this.reasonLinkId) 9260 if (!item.isEmpty()) 9261 return true; 9262 return false; 9263 } 9264 9265 /** 9266 * @return {@link #reasonLinkId} (Id [identifier??] of the clause or question 9267 * text related to the reason type or reference of this action in the 9268 * referenced form or QuestionnaireResponse.) 9269 */ 9270 public StringType addReasonLinkIdElement() {// 2 9271 StringType t = new StringType(); 9272 if (this.reasonLinkId == null) 9273 this.reasonLinkId = new ArrayList<StringType>(); 9274 this.reasonLinkId.add(t); 9275 return t; 9276 } 9277 9278 /** 9279 * @param value {@link #reasonLinkId} (Id [identifier??] of the clause or 9280 * question text related to the reason type or reference of this 9281 * action in the referenced form or QuestionnaireResponse.) 9282 */ 9283 public ActionComponent addReasonLinkId(String value) { // 1 9284 StringType t = new StringType(); 9285 t.setValue(value); 9286 if (this.reasonLinkId == null) 9287 this.reasonLinkId = new ArrayList<StringType>(); 9288 this.reasonLinkId.add(t); 9289 return this; 9290 } 9291 9292 /** 9293 * @param value {@link #reasonLinkId} (Id [identifier??] of the clause or 9294 * question text related to the reason type or reference of this 9295 * action in the referenced form or QuestionnaireResponse.) 9296 */ 9297 public boolean hasReasonLinkId(String value) { 9298 if (this.reasonLinkId == null) 9299 return false; 9300 for (StringType v : this.reasonLinkId) 9301 if (v.getValue().equals(value)) // string 9302 return true; 9303 return false; 9304 } 9305 9306 /** 9307 * @return {@link #note} (Comments made about the term action made by the 9308 * requester, performer, subject or other participants.) 9309 */ 9310 public List<Annotation> getNote() { 9311 if (this.note == null) 9312 this.note = new ArrayList<Annotation>(); 9313 return this.note; 9314 } 9315 9316 /** 9317 * @return Returns a reference to <code>this</code> for easy method chaining 9318 */ 9319 public ActionComponent setNote(List<Annotation> theNote) { 9320 this.note = theNote; 9321 return this; 9322 } 9323 9324 public boolean hasNote() { 9325 if (this.note == null) 9326 return false; 9327 for (Annotation item : this.note) 9328 if (!item.isEmpty()) 9329 return true; 9330 return false; 9331 } 9332 9333 public Annotation addNote() { // 3 9334 Annotation t = new Annotation(); 9335 if (this.note == null) 9336 this.note = new ArrayList<Annotation>(); 9337 this.note.add(t); 9338 return t; 9339 } 9340 9341 public ActionComponent addNote(Annotation t) { // 3 9342 if (t == null) 9343 return this; 9344 if (this.note == null) 9345 this.note = new ArrayList<Annotation>(); 9346 this.note.add(t); 9347 return this; 9348 } 9349 9350 /** 9351 * @return The first repetition of repeating field {@link #note}, creating it if 9352 * it does not already exist 9353 */ 9354 public Annotation getNoteFirstRep() { 9355 if (getNote().isEmpty()) { 9356 addNote(); 9357 } 9358 return getNote().get(0); 9359 } 9360 9361 /** 9362 * @return {@link #securityLabelNumber} (Security labels that protects the 9363 * action.) 9364 */ 9365 public List<UnsignedIntType> getSecurityLabelNumber() { 9366 if (this.securityLabelNumber == null) 9367 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 9368 return this.securityLabelNumber; 9369 } 9370 9371 /** 9372 * @return Returns a reference to <code>this</code> for easy method chaining 9373 */ 9374 public ActionComponent setSecurityLabelNumber(List<UnsignedIntType> theSecurityLabelNumber) { 9375 this.securityLabelNumber = theSecurityLabelNumber; 9376 return this; 9377 } 9378 9379 public boolean hasSecurityLabelNumber() { 9380 if (this.securityLabelNumber == null) 9381 return false; 9382 for (UnsignedIntType item : this.securityLabelNumber) 9383 if (!item.isEmpty()) 9384 return true; 9385 return false; 9386 } 9387 9388 /** 9389 * @return {@link #securityLabelNumber} (Security labels that protects the 9390 * action.) 9391 */ 9392 public UnsignedIntType addSecurityLabelNumberElement() {// 2 9393 UnsignedIntType t = new UnsignedIntType(); 9394 if (this.securityLabelNumber == null) 9395 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 9396 this.securityLabelNumber.add(t); 9397 return t; 9398 } 9399 9400 /** 9401 * @param value {@link #securityLabelNumber} (Security labels that protects the 9402 * action.) 9403 */ 9404 public ActionComponent addSecurityLabelNumber(int value) { // 1 9405 UnsignedIntType t = new UnsignedIntType(); 9406 t.setValue(value); 9407 if (this.securityLabelNumber == null) 9408 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 9409 this.securityLabelNumber.add(t); 9410 return this; 9411 } 9412 9413 /** 9414 * @param value {@link #securityLabelNumber} (Security labels that protects the 9415 * action.) 9416 */ 9417 public boolean hasSecurityLabelNumber(int value) { 9418 if (this.securityLabelNumber == null) 9419 return false; 9420 for (UnsignedIntType v : this.securityLabelNumber) 9421 if (v.getValue().equals(value)) // unsignedInt 9422 return true; 9423 return false; 9424 } 9425 9426 protected void listChildren(List<Property> children) { 9427 super.listChildren(children); 9428 children 9429 .add(new Property("doNotPerform", "boolean", "True if the term prohibits the action.", 0, 1, doNotPerform)); 9430 children.add(new Property("type", "CodeableConcept", 9431 "Activity or service obligation to be done or not done, performed or not performed, effectuated or not by this Contract term.", 9432 0, 1, type)); 9433 children.add(new Property("subject", "", "Entity of the action.", 0, java.lang.Integer.MAX_VALUE, subject)); 9434 children.add(new Property("intent", "CodeableConcept", 9435 "Reason or purpose for the action stipulated by this Contract Provision.", 0, 1, intent)); 9436 children.add(new Property("linkId", "string", 9437 "Id [identifier??] of the clause or question text related to this action in the referenced form or QuestionnaireResponse.", 9438 0, java.lang.Integer.MAX_VALUE, linkId)); 9439 children.add(new Property("status", "CodeableConcept", "Current state of the term action.", 0, 1, status)); 9440 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", 9441 "Encounter or Episode with primary association to specified term activity.", 0, 1, context)); 9442 children.add(new Property("contextLinkId", "string", 9443 "Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse.", 9444 0, java.lang.Integer.MAX_VALUE, contextLinkId)); 9445 children.add(new Property("occurrence[x]", "dateTime|Period|Timing", "When action happens.", 0, 1, occurrence)); 9446 children.add(new Property("requester", 9447 "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Device|Group|Organization)", 9448 "Who or what initiated the action and has responsibility for its activation.", 0, java.lang.Integer.MAX_VALUE, 9449 requester)); 9450 children.add(new Property("requesterLinkId", "string", 9451 "Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse.", 9452 0, java.lang.Integer.MAX_VALUE, requesterLinkId)); 9453 children.add(new Property("performerType", "CodeableConcept", 9454 "The type of individual that is desired or required to perform or not perform the action.", 0, 9455 java.lang.Integer.MAX_VALUE, performerType)); 9456 children.add(new Property("performerRole", "CodeableConcept", 9457 "The type of role or competency of an individual desired or required to perform or not perform the action.", 9458 0, 1, performerRole)); 9459 children.add(new Property("performer", 9460 "Reference(RelatedPerson|Patient|Practitioner|PractitionerRole|CareTeam|Device|Substance|Organization|Location)", 9461 "Indicates who or what is being asked to perform (or not perform) the ction.", 0, 1, performer)); 9462 children.add(new Property("performerLinkId", "string", 9463 "Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse.", 9464 0, java.lang.Integer.MAX_VALUE, performerLinkId)); 9465 children.add(new Property("reasonCode", "CodeableConcept", 9466 "Rationale for the action to be performed or not performed. Describes why the action is permitted or prohibited.", 9467 0, java.lang.Integer.MAX_VALUE, reasonCode)); 9468 children.add(new Property("reasonReference", 9469 "Reference(Condition|Observation|DiagnosticReport|DocumentReference|Questionnaire|QuestionnaireResponse)", 9470 "Indicates another resource whose existence justifies permitting or not permitting this action.", 0, 9471 java.lang.Integer.MAX_VALUE, reasonReference)); 9472 children.add(new Property("reason", "string", 9473 "Describes why the action is to be performed or not performed in textual form.", 0, 9474 java.lang.Integer.MAX_VALUE, reason)); 9475 children.add(new Property("reasonLinkId", "string", 9476 "Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse.", 9477 0, java.lang.Integer.MAX_VALUE, reasonLinkId)); 9478 children.add(new Property("note", "Annotation", 9479 "Comments made about the term action made by the requester, performer, subject or other participants.", 0, 9480 java.lang.Integer.MAX_VALUE, note)); 9481 children.add(new Property("securityLabelNumber", "unsignedInt", "Security labels that protects the action.", 0, 9482 java.lang.Integer.MAX_VALUE, securityLabelNumber)); 9483 } 9484 9485 @Override 9486 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 9487 switch (_hash) { 9488 case -1788508167: 9489 /* doNotPerform */ return new Property("doNotPerform", "boolean", "True if the term prohibits the action.", 0, 9490 1, doNotPerform); 9491 case 3575610: 9492 /* type */ return new Property("type", "CodeableConcept", 9493 "Activity or service obligation to be done or not done, performed or not performed, effectuated or not by this Contract term.", 9494 0, 1, type); 9495 case -1867885268: 9496 /* subject */ return new Property("subject", "", "Entity of the action.", 0, java.lang.Integer.MAX_VALUE, 9497 subject); 9498 case -1183762788: 9499 /* intent */ return new Property("intent", "CodeableConcept", 9500 "Reason or purpose for the action stipulated by this Contract Provision.", 0, 1, intent); 9501 case -1102667083: 9502 /* linkId */ return new Property("linkId", "string", 9503 "Id [identifier??] of the clause or question text related to this action in the referenced form or QuestionnaireResponse.", 9504 0, java.lang.Integer.MAX_VALUE, linkId); 9505 case -892481550: 9506 /* status */ return new Property("status", "CodeableConcept", "Current state of the term action.", 0, 1, 9507 status); 9508 case 951530927: 9509 /* context */ return new Property("context", "Reference(Encounter|EpisodeOfCare)", 9510 "Encounter or Episode with primary association to specified term activity.", 0, 1, context); 9511 case -288783036: 9512 /* contextLinkId */ return new Property("contextLinkId", "string", 9513 "Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse.", 9514 0, java.lang.Integer.MAX_VALUE, contextLinkId); 9515 case -2022646513: 9516 /* occurrence[x] */ return new Property("occurrence[x]", "dateTime|Period|Timing", "When action happens.", 0, 1, 9517 occurrence); 9518 case 1687874001: 9519 /* occurrence */ return new Property("occurrence[x]", "dateTime|Period|Timing", "When action happens.", 0, 1, 9520 occurrence); 9521 case -298443636: 9522 /* occurrenceDateTime */ return new Property("occurrence[x]", "dateTime|Period|Timing", "When action happens.", 9523 0, 1, occurrence); 9524 case 1397156594: 9525 /* occurrencePeriod */ return new Property("occurrence[x]", "dateTime|Period|Timing", "When action happens.", 0, 9526 1, occurrence); 9527 case 1515218299: 9528 /* occurrenceTiming */ return new Property("occurrence[x]", "dateTime|Period|Timing", "When action happens.", 0, 9529 1, occurrence); 9530 case 693933948: 9531 /* requester */ return new Property("requester", 9532 "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Device|Group|Organization)", 9533 "Who or what initiated the action and has responsibility for its activation.", 0, 9534 java.lang.Integer.MAX_VALUE, requester); 9535 case -1468032687: 9536 /* requesterLinkId */ return new Property("requesterLinkId", "string", 9537 "Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse.", 9538 0, java.lang.Integer.MAX_VALUE, requesterLinkId); 9539 case -901444568: 9540 /* performerType */ return new Property("performerType", "CodeableConcept", 9541 "The type of individual that is desired or required to perform or not perform the action.", 0, 9542 java.lang.Integer.MAX_VALUE, performerType); 9543 case -901513884: 9544 /* performerRole */ return new Property("performerRole", "CodeableConcept", 9545 "The type of role or competency of an individual desired or required to perform or not perform the action.", 9546 0, 1, performerRole); 9547 case 481140686: 9548 /* performer */ return new Property("performer", 9549 "Reference(RelatedPerson|Patient|Practitioner|PractitionerRole|CareTeam|Device|Substance|Organization|Location)", 9550 "Indicates who or what is being asked to perform (or not perform) the ction.", 0, 1, performer); 9551 case 1051302947: 9552 /* performerLinkId */ return new Property("performerLinkId", "string", 9553 "Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse.", 9554 0, java.lang.Integer.MAX_VALUE, performerLinkId); 9555 case 722137681: 9556 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 9557 "Rationale for the action to be performed or not performed. Describes why the action is permitted or prohibited.", 9558 0, java.lang.Integer.MAX_VALUE, reasonCode); 9559 case -1146218137: 9560 /* reasonReference */ return new Property("reasonReference", 9561 "Reference(Condition|Observation|DiagnosticReport|DocumentReference|Questionnaire|QuestionnaireResponse)", 9562 "Indicates another resource whose existence justifies permitting or not permitting this action.", 0, 9563 java.lang.Integer.MAX_VALUE, reasonReference); 9564 case -934964668: 9565 /* reason */ return new Property("reason", "string", 9566 "Describes why the action is to be performed or not performed in textual form.", 0, 9567 java.lang.Integer.MAX_VALUE, reason); 9568 case -1557963239: 9569 /* reasonLinkId */ return new Property("reasonLinkId", "string", 9570 "Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse.", 9571 0, java.lang.Integer.MAX_VALUE, reasonLinkId); 9572 case 3387378: 9573 /* note */ return new Property("note", "Annotation", 9574 "Comments made about the term action made by the requester, performer, subject or other participants.", 0, 9575 java.lang.Integer.MAX_VALUE, note); 9576 case -149460995: 9577 /* securityLabelNumber */ return new Property("securityLabelNumber", "unsignedInt", 9578 "Security labels that protects the action.", 0, java.lang.Integer.MAX_VALUE, securityLabelNumber); 9579 default: 9580 return super.getNamedProperty(_hash, _name, _checkValid); 9581 } 9582 9583 } 9584 9585 @Override 9586 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 9587 switch (hash) { 9588 case -1788508167: 9589 /* doNotPerform */ return this.doNotPerform == null ? new Base[0] : new Base[] { this.doNotPerform }; // BooleanType 9590 case 3575610: 9591 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 9592 case -1867885268: 9593 /* subject */ return this.subject == null ? new Base[0] : this.subject.toArray(new Base[this.subject.size()]); // ActionSubjectComponent 9594 case -1183762788: 9595 /* intent */ return this.intent == null ? new Base[0] : new Base[] { this.intent }; // CodeableConcept 9596 case -1102667083: 9597 /* linkId */ return this.linkId == null ? new Base[0] : this.linkId.toArray(new Base[this.linkId.size()]); // StringType 9598 case -892481550: 9599 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // CodeableConcept 9600 case 951530927: 9601 /* context */ return this.context == null ? new Base[0] : new Base[] { this.context }; // Reference 9602 case -288783036: 9603 /* contextLinkId */ return this.contextLinkId == null ? new Base[0] 9604 : this.contextLinkId.toArray(new Base[this.contextLinkId.size()]); // StringType 9605 case 1687874001: 9606 /* occurrence */ return this.occurrence == null ? new Base[0] : new Base[] { this.occurrence }; // Type 9607 case 693933948: 9608 /* requester */ return this.requester == null ? new Base[0] 9609 : this.requester.toArray(new Base[this.requester.size()]); // Reference 9610 case -1468032687: 9611 /* requesterLinkId */ return this.requesterLinkId == null ? new Base[0] 9612 : this.requesterLinkId.toArray(new Base[this.requesterLinkId.size()]); // StringType 9613 case -901444568: 9614 /* performerType */ return this.performerType == null ? new Base[0] 9615 : this.performerType.toArray(new Base[this.performerType.size()]); // CodeableConcept 9616 case -901513884: 9617 /* performerRole */ return this.performerRole == null ? new Base[0] : new Base[] { this.performerRole }; // CodeableConcept 9618 case 481140686: 9619 /* performer */ return this.performer == null ? new Base[0] : new Base[] { this.performer }; // Reference 9620 case 1051302947: 9621 /* performerLinkId */ return this.performerLinkId == null ? new Base[0] 9622 : this.performerLinkId.toArray(new Base[this.performerLinkId.size()]); // StringType 9623 case 722137681: 9624 /* reasonCode */ return this.reasonCode == null ? new Base[0] 9625 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 9626 case -1146218137: 9627 /* reasonReference */ return this.reasonReference == null ? new Base[0] 9628 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 9629 case -934964668: 9630 /* reason */ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // StringType 9631 case -1557963239: 9632 /* reasonLinkId */ return this.reasonLinkId == null ? new Base[0] 9633 : this.reasonLinkId.toArray(new Base[this.reasonLinkId.size()]); // StringType 9634 case 3387378: 9635 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 9636 case -149460995: 9637 /* securityLabelNumber */ return this.securityLabelNumber == null ? new Base[0] 9638 : this.securityLabelNumber.toArray(new Base[this.securityLabelNumber.size()]); // UnsignedIntType 9639 default: 9640 return super.getProperty(hash, name, checkValid); 9641 } 9642 9643 } 9644 9645 @Override 9646 public Base setProperty(int hash, String name, Base value) throws FHIRException { 9647 switch (hash) { 9648 case -1788508167: // doNotPerform 9649 this.doNotPerform = castToBoolean(value); // BooleanType 9650 return value; 9651 case 3575610: // type 9652 this.type = castToCodeableConcept(value); // CodeableConcept 9653 return value; 9654 case -1867885268: // subject 9655 this.getSubject().add((ActionSubjectComponent) value); // ActionSubjectComponent 9656 return value; 9657 case -1183762788: // intent 9658 this.intent = castToCodeableConcept(value); // CodeableConcept 9659 return value; 9660 case -1102667083: // linkId 9661 this.getLinkId().add(castToString(value)); // StringType 9662 return value; 9663 case -892481550: // status 9664 this.status = castToCodeableConcept(value); // CodeableConcept 9665 return value; 9666 case 951530927: // context 9667 this.context = castToReference(value); // Reference 9668 return value; 9669 case -288783036: // contextLinkId 9670 this.getContextLinkId().add(castToString(value)); // StringType 9671 return value; 9672 case 1687874001: // occurrence 9673 this.occurrence = castToType(value); // Type 9674 return value; 9675 case 693933948: // requester 9676 this.getRequester().add(castToReference(value)); // Reference 9677 return value; 9678 case -1468032687: // requesterLinkId 9679 this.getRequesterLinkId().add(castToString(value)); // StringType 9680 return value; 9681 case -901444568: // performerType 9682 this.getPerformerType().add(castToCodeableConcept(value)); // CodeableConcept 9683 return value; 9684 case -901513884: // performerRole 9685 this.performerRole = castToCodeableConcept(value); // CodeableConcept 9686 return value; 9687 case 481140686: // performer 9688 this.performer = castToReference(value); // Reference 9689 return value; 9690 case 1051302947: // performerLinkId 9691 this.getPerformerLinkId().add(castToString(value)); // StringType 9692 return value; 9693 case 722137681: // reasonCode 9694 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 9695 return value; 9696 case -1146218137: // reasonReference 9697 this.getReasonReference().add(castToReference(value)); // Reference 9698 return value; 9699 case -934964668: // reason 9700 this.getReason().add(castToString(value)); // StringType 9701 return value; 9702 case -1557963239: // reasonLinkId 9703 this.getReasonLinkId().add(castToString(value)); // StringType 9704 return value; 9705 case 3387378: // note 9706 this.getNote().add(castToAnnotation(value)); // Annotation 9707 return value; 9708 case -149460995: // securityLabelNumber 9709 this.getSecurityLabelNumber().add(castToUnsignedInt(value)); // UnsignedIntType 9710 return value; 9711 default: 9712 return super.setProperty(hash, name, value); 9713 } 9714 9715 } 9716 9717 @Override 9718 public Base setProperty(String name, Base value) throws FHIRException { 9719 if (name.equals("doNotPerform")) { 9720 this.doNotPerform = castToBoolean(value); // BooleanType 9721 } else if (name.equals("type")) { 9722 this.type = castToCodeableConcept(value); // CodeableConcept 9723 } else if (name.equals("subject")) { 9724 this.getSubject().add((ActionSubjectComponent) value); 9725 } else if (name.equals("intent")) { 9726 this.intent = castToCodeableConcept(value); // CodeableConcept 9727 } else if (name.equals("linkId")) { 9728 this.getLinkId().add(castToString(value)); 9729 } else if (name.equals("status")) { 9730 this.status = castToCodeableConcept(value); // CodeableConcept 9731 } else if (name.equals("context")) { 9732 this.context = castToReference(value); // Reference 9733 } else if (name.equals("contextLinkId")) { 9734 this.getContextLinkId().add(castToString(value)); 9735 } else if (name.equals("occurrence[x]")) { 9736 this.occurrence = castToType(value); // Type 9737 } else if (name.equals("requester")) { 9738 this.getRequester().add(castToReference(value)); 9739 } else if (name.equals("requesterLinkId")) { 9740 this.getRequesterLinkId().add(castToString(value)); 9741 } else if (name.equals("performerType")) { 9742 this.getPerformerType().add(castToCodeableConcept(value)); 9743 } else if (name.equals("performerRole")) { 9744 this.performerRole = castToCodeableConcept(value); // CodeableConcept 9745 } else if (name.equals("performer")) { 9746 this.performer = castToReference(value); // Reference 9747 } else if (name.equals("performerLinkId")) { 9748 this.getPerformerLinkId().add(castToString(value)); 9749 } else if (name.equals("reasonCode")) { 9750 this.getReasonCode().add(castToCodeableConcept(value)); 9751 } else if (name.equals("reasonReference")) { 9752 this.getReasonReference().add(castToReference(value)); 9753 } else if (name.equals("reason")) { 9754 this.getReason().add(castToString(value)); 9755 } else if (name.equals("reasonLinkId")) { 9756 this.getReasonLinkId().add(castToString(value)); 9757 } else if (name.equals("note")) { 9758 this.getNote().add(castToAnnotation(value)); 9759 } else if (name.equals("securityLabelNumber")) { 9760 this.getSecurityLabelNumber().add(castToUnsignedInt(value)); 9761 } else 9762 return super.setProperty(name, value); 9763 return value; 9764 } 9765 9766 @Override 9767 public void removeChild(String name, Base value) throws FHIRException { 9768 if (name.equals("doNotPerform")) { 9769 this.doNotPerform = null; 9770 } else if (name.equals("type")) { 9771 this.type = null; 9772 } else if (name.equals("subject")) { 9773 this.getSubject().remove((ActionSubjectComponent) value); 9774 } else if (name.equals("intent")) { 9775 this.intent = null; 9776 } else if (name.equals("linkId")) { 9777 this.getLinkId().remove(castToString(value)); 9778 } else if (name.equals("status")) { 9779 this.status = null; 9780 } else if (name.equals("context")) { 9781 this.context = null; 9782 } else if (name.equals("contextLinkId")) { 9783 this.getContextLinkId().remove(castToString(value)); 9784 } else if (name.equals("occurrence[x]")) { 9785 this.occurrence = null; 9786 } else if (name.equals("requester")) { 9787 this.getRequester().remove(castToReference(value)); 9788 } else if (name.equals("requesterLinkId")) { 9789 this.getRequesterLinkId().remove(castToString(value)); 9790 } else if (name.equals("performerType")) { 9791 this.getPerformerType().remove(castToCodeableConcept(value)); 9792 } else if (name.equals("performerRole")) { 9793 this.performerRole = null; 9794 } else if (name.equals("performer")) { 9795 this.performer = null; 9796 } else if (name.equals("performerLinkId")) { 9797 this.getPerformerLinkId().remove(castToString(value)); 9798 } else if (name.equals("reasonCode")) { 9799 this.getReasonCode().remove(castToCodeableConcept(value)); 9800 } else if (name.equals("reasonReference")) { 9801 this.getReasonReference().remove(castToReference(value)); 9802 } else if (name.equals("reason")) { 9803 this.getReason().remove(castToString(value)); 9804 } else if (name.equals("reasonLinkId")) { 9805 this.getReasonLinkId().remove(castToString(value)); 9806 } else if (name.equals("note")) { 9807 this.getNote().remove(castToAnnotation(value)); 9808 } else if (name.equals("securityLabelNumber")) { 9809 this.getSecurityLabelNumber().remove(castToUnsignedInt(value)); 9810 } else 9811 super.removeChild(name, value); 9812 9813 } 9814 9815 @Override 9816 public Base makeProperty(int hash, String name) throws FHIRException { 9817 switch (hash) { 9818 case -1788508167: 9819 return getDoNotPerformElement(); 9820 case 3575610: 9821 return getType(); 9822 case -1867885268: 9823 return addSubject(); 9824 case -1183762788: 9825 return getIntent(); 9826 case -1102667083: 9827 return addLinkIdElement(); 9828 case -892481550: 9829 return getStatus(); 9830 case 951530927: 9831 return getContext(); 9832 case -288783036: 9833 return addContextLinkIdElement(); 9834 case -2022646513: 9835 return getOccurrence(); 9836 case 1687874001: 9837 return getOccurrence(); 9838 case 693933948: 9839 return addRequester(); 9840 case -1468032687: 9841 return addRequesterLinkIdElement(); 9842 case -901444568: 9843 return addPerformerType(); 9844 case -901513884: 9845 return getPerformerRole(); 9846 case 481140686: 9847 return getPerformer(); 9848 case 1051302947: 9849 return addPerformerLinkIdElement(); 9850 case 722137681: 9851 return addReasonCode(); 9852 case -1146218137: 9853 return addReasonReference(); 9854 case -934964668: 9855 return addReasonElement(); 9856 case -1557963239: 9857 return addReasonLinkIdElement(); 9858 case 3387378: 9859 return addNote(); 9860 case -149460995: 9861 return addSecurityLabelNumberElement(); 9862 default: 9863 return super.makeProperty(hash, name); 9864 } 9865 9866 } 9867 9868 @Override 9869 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9870 switch (hash) { 9871 case -1788508167: 9872 /* doNotPerform */ return new String[] { "boolean" }; 9873 case 3575610: 9874 /* type */ return new String[] { "CodeableConcept" }; 9875 case -1867885268: 9876 /* subject */ return new String[] {}; 9877 case -1183762788: 9878 /* intent */ return new String[] { "CodeableConcept" }; 9879 case -1102667083: 9880 /* linkId */ return new String[] { "string" }; 9881 case -892481550: 9882 /* status */ return new String[] { "CodeableConcept" }; 9883 case 951530927: 9884 /* context */ return new String[] { "Reference" }; 9885 case -288783036: 9886 /* contextLinkId */ return new String[] { "string" }; 9887 case 1687874001: 9888 /* occurrence */ return new String[] { "dateTime", "Period", "Timing" }; 9889 case 693933948: 9890 /* requester */ return new String[] { "Reference" }; 9891 case -1468032687: 9892 /* requesterLinkId */ return new String[] { "string" }; 9893 case -901444568: 9894 /* performerType */ return new String[] { "CodeableConcept" }; 9895 case -901513884: 9896 /* performerRole */ return new String[] { "CodeableConcept" }; 9897 case 481140686: 9898 /* performer */ return new String[] { "Reference" }; 9899 case 1051302947: 9900 /* performerLinkId */ return new String[] { "string" }; 9901 case 722137681: 9902 /* reasonCode */ return new String[] { "CodeableConcept" }; 9903 case -1146218137: 9904 /* reasonReference */ return new String[] { "Reference" }; 9905 case -934964668: 9906 /* reason */ return new String[] { "string" }; 9907 case -1557963239: 9908 /* reasonLinkId */ return new String[] { "string" }; 9909 case 3387378: 9910 /* note */ return new String[] { "Annotation" }; 9911 case -149460995: 9912 /* securityLabelNumber */ return new String[] { "unsignedInt" }; 9913 default: 9914 return super.getTypesForProperty(hash, name); 9915 } 9916 9917 } 9918 9919 @Override 9920 public Base addChild(String name) throws FHIRException { 9921 if (name.equals("doNotPerform")) { 9922 throw new FHIRException("Cannot call addChild on a singleton property Contract.doNotPerform"); 9923 } else if (name.equals("type")) { 9924 this.type = new CodeableConcept(); 9925 return this.type; 9926 } else if (name.equals("subject")) { 9927 return addSubject(); 9928 } else if (name.equals("intent")) { 9929 this.intent = new CodeableConcept(); 9930 return this.intent; 9931 } else if (name.equals("linkId")) { 9932 throw new FHIRException("Cannot call addChild on a singleton property Contract.linkId"); 9933 } else if (name.equals("status")) { 9934 this.status = new CodeableConcept(); 9935 return this.status; 9936 } else if (name.equals("context")) { 9937 this.context = new Reference(); 9938 return this.context; 9939 } else if (name.equals("contextLinkId")) { 9940 throw new FHIRException("Cannot call addChild on a singleton property Contract.contextLinkId"); 9941 } else if (name.equals("occurrenceDateTime")) { 9942 this.occurrence = new DateTimeType(); 9943 return this.occurrence; 9944 } else if (name.equals("occurrencePeriod")) { 9945 this.occurrence = new Period(); 9946 return this.occurrence; 9947 } else if (name.equals("occurrenceTiming")) { 9948 this.occurrence = new Timing(); 9949 return this.occurrence; 9950 } else if (name.equals("requester")) { 9951 return addRequester(); 9952 } else if (name.equals("requesterLinkId")) { 9953 throw new FHIRException("Cannot call addChild on a singleton property Contract.requesterLinkId"); 9954 } else if (name.equals("performerType")) { 9955 return addPerformerType(); 9956 } else if (name.equals("performerRole")) { 9957 this.performerRole = new CodeableConcept(); 9958 return this.performerRole; 9959 } else if (name.equals("performer")) { 9960 this.performer = new Reference(); 9961 return this.performer; 9962 } else if (name.equals("performerLinkId")) { 9963 throw new FHIRException("Cannot call addChild on a singleton property Contract.performerLinkId"); 9964 } else if (name.equals("reasonCode")) { 9965 return addReasonCode(); 9966 } else if (name.equals("reasonReference")) { 9967 return addReasonReference(); 9968 } else if (name.equals("reason")) { 9969 throw new FHIRException("Cannot call addChild on a singleton property Contract.reason"); 9970 } else if (name.equals("reasonLinkId")) { 9971 throw new FHIRException("Cannot call addChild on a singleton property Contract.reasonLinkId"); 9972 } else if (name.equals("note")) { 9973 return addNote(); 9974 } else if (name.equals("securityLabelNumber")) { 9975 throw new FHIRException("Cannot call addChild on a singleton property Contract.securityLabelNumber"); 9976 } else 9977 return super.addChild(name); 9978 } 9979 9980 public ActionComponent copy() { 9981 ActionComponent dst = new ActionComponent(); 9982 copyValues(dst); 9983 return dst; 9984 } 9985 9986 public void copyValues(ActionComponent dst) { 9987 super.copyValues(dst); 9988 dst.doNotPerform = doNotPerform == null ? null : doNotPerform.copy(); 9989 dst.type = type == null ? null : type.copy(); 9990 if (subject != null) { 9991 dst.subject = new ArrayList<ActionSubjectComponent>(); 9992 for (ActionSubjectComponent i : subject) 9993 dst.subject.add(i.copy()); 9994 } 9995 ; 9996 dst.intent = intent == null ? null : intent.copy(); 9997 if (linkId != null) { 9998 dst.linkId = new ArrayList<StringType>(); 9999 for (StringType i : linkId) 10000 dst.linkId.add(i.copy()); 10001 } 10002 ; 10003 dst.status = status == null ? null : status.copy(); 10004 dst.context = context == null ? null : context.copy(); 10005 if (contextLinkId != null) { 10006 dst.contextLinkId = new ArrayList<StringType>(); 10007 for (StringType i : contextLinkId) 10008 dst.contextLinkId.add(i.copy()); 10009 } 10010 ; 10011 dst.occurrence = occurrence == null ? null : occurrence.copy(); 10012 if (requester != null) { 10013 dst.requester = new ArrayList<Reference>(); 10014 for (Reference i : requester) 10015 dst.requester.add(i.copy()); 10016 } 10017 ; 10018 if (requesterLinkId != null) { 10019 dst.requesterLinkId = new ArrayList<StringType>(); 10020 for (StringType i : requesterLinkId) 10021 dst.requesterLinkId.add(i.copy()); 10022 } 10023 ; 10024 if (performerType != null) { 10025 dst.performerType = new ArrayList<CodeableConcept>(); 10026 for (CodeableConcept i : performerType) 10027 dst.performerType.add(i.copy()); 10028 } 10029 ; 10030 dst.performerRole = performerRole == null ? null : performerRole.copy(); 10031 dst.performer = performer == null ? null : performer.copy(); 10032 if (performerLinkId != null) { 10033 dst.performerLinkId = new ArrayList<StringType>(); 10034 for (StringType i : performerLinkId) 10035 dst.performerLinkId.add(i.copy()); 10036 } 10037 ; 10038 if (reasonCode != null) { 10039 dst.reasonCode = new ArrayList<CodeableConcept>(); 10040 for (CodeableConcept i : reasonCode) 10041 dst.reasonCode.add(i.copy()); 10042 } 10043 ; 10044 if (reasonReference != null) { 10045 dst.reasonReference = new ArrayList<Reference>(); 10046 for (Reference i : reasonReference) 10047 dst.reasonReference.add(i.copy()); 10048 } 10049 ; 10050 if (reason != null) { 10051 dst.reason = new ArrayList<StringType>(); 10052 for (StringType i : reason) 10053 dst.reason.add(i.copy()); 10054 } 10055 ; 10056 if (reasonLinkId != null) { 10057 dst.reasonLinkId = new ArrayList<StringType>(); 10058 for (StringType i : reasonLinkId) 10059 dst.reasonLinkId.add(i.copy()); 10060 } 10061 ; 10062 if (note != null) { 10063 dst.note = new ArrayList<Annotation>(); 10064 for (Annotation i : note) 10065 dst.note.add(i.copy()); 10066 } 10067 ; 10068 if (securityLabelNumber != null) { 10069 dst.securityLabelNumber = new ArrayList<UnsignedIntType>(); 10070 for (UnsignedIntType i : securityLabelNumber) 10071 dst.securityLabelNumber.add(i.copy()); 10072 } 10073 ; 10074 } 10075 10076 @Override 10077 public boolean equalsDeep(Base other_) { 10078 if (!super.equalsDeep(other_)) 10079 return false; 10080 if (!(other_ instanceof ActionComponent)) 10081 return false; 10082 ActionComponent o = (ActionComponent) other_; 10083 return compareDeep(doNotPerform, o.doNotPerform, true) && compareDeep(type, o.type, true) 10084 && compareDeep(subject, o.subject, true) && compareDeep(intent, o.intent, true) 10085 && compareDeep(linkId, o.linkId, true) && compareDeep(status, o.status, true) 10086 && compareDeep(context, o.context, true) && compareDeep(contextLinkId, o.contextLinkId, true) 10087 && compareDeep(occurrence, o.occurrence, true) && compareDeep(requester, o.requester, true) 10088 && compareDeep(requesterLinkId, o.requesterLinkId, true) && compareDeep(performerType, o.performerType, true) 10089 && compareDeep(performerRole, o.performerRole, true) && compareDeep(performer, o.performer, true) 10090 && compareDeep(performerLinkId, o.performerLinkId, true) && compareDeep(reasonCode, o.reasonCode, true) 10091 && compareDeep(reasonReference, o.reasonReference, true) && compareDeep(reason, o.reason, true) 10092 && compareDeep(reasonLinkId, o.reasonLinkId, true) && compareDeep(note, o.note, true) 10093 && compareDeep(securityLabelNumber, o.securityLabelNumber, true); 10094 } 10095 10096 @Override 10097 public boolean equalsShallow(Base other_) { 10098 if (!super.equalsShallow(other_)) 10099 return false; 10100 if (!(other_ instanceof ActionComponent)) 10101 return false; 10102 ActionComponent o = (ActionComponent) other_; 10103 return compareValues(doNotPerform, o.doNotPerform, true) && compareValues(linkId, o.linkId, true) 10104 && compareValues(contextLinkId, o.contextLinkId, true) 10105 && compareValues(requesterLinkId, o.requesterLinkId, true) 10106 && compareValues(performerLinkId, o.performerLinkId, true) && compareValues(reason, o.reason, true) 10107 && compareValues(reasonLinkId, o.reasonLinkId, true) 10108 && compareValues(securityLabelNumber, o.securityLabelNumber, true); 10109 } 10110 10111 public boolean isEmpty() { 10112 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(doNotPerform, type, subject, intent, linkId, 10113 status, context, contextLinkId, occurrence, requester, requesterLinkId, performerType, performerRole, 10114 performer, performerLinkId, reasonCode, reasonReference, reason, reasonLinkId, note, securityLabelNumber); 10115 } 10116 10117 public String fhirType() { 10118 return "Contract.term.action"; 10119 10120 } 10121 10122 } 10123 10124 @Block() 10125 public static class ActionSubjectComponent extends BackboneElement implements IBaseBackboneElement { 10126 /** 10127 * The entity the action is performed or not performed on or for. 10128 */ 10129 @Child(name = "reference", type = { Patient.class, RelatedPerson.class, Practitioner.class, PractitionerRole.class, 10130 Device.class, Group.class, 10131 Organization.class }, order = 1, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 10132 @Description(shortDefinition = "Entity of the action", formalDefinition = "The entity the action is performed or not performed on or for.") 10133 protected List<Reference> reference; 10134 /** 10135 * The actual objects that are the target of the reference (The entity the 10136 * action is performed or not performed on or for.) 10137 */ 10138 protected List<Resource> referenceTarget; 10139 10140 /** 10141 * Role type of agent assigned roles in this Contract. 10142 */ 10143 @Child(name = "role", type = { 10144 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 10145 @Description(shortDefinition = "Role type of the agent", formalDefinition = "Role type of agent assigned roles in this Contract.") 10146 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-actorrole") 10147 protected CodeableConcept role; 10148 10149 private static final long serialVersionUID = 128949255L; 10150 10151 /** 10152 * Constructor 10153 */ 10154 public ActionSubjectComponent() { 10155 super(); 10156 } 10157 10158 /** 10159 * @return {@link #reference} (The entity the action is performed or not 10160 * performed on or for.) 10161 */ 10162 public List<Reference> getReference() { 10163 if (this.reference == null) 10164 this.reference = new ArrayList<Reference>(); 10165 return this.reference; 10166 } 10167 10168 /** 10169 * @return Returns a reference to <code>this</code> for easy method chaining 10170 */ 10171 public ActionSubjectComponent setReference(List<Reference> theReference) { 10172 this.reference = theReference; 10173 return this; 10174 } 10175 10176 public boolean hasReference() { 10177 if (this.reference == null) 10178 return false; 10179 for (Reference item : this.reference) 10180 if (!item.isEmpty()) 10181 return true; 10182 return false; 10183 } 10184 10185 public Reference addReference() { // 3 10186 Reference t = new Reference(); 10187 if (this.reference == null) 10188 this.reference = new ArrayList<Reference>(); 10189 this.reference.add(t); 10190 return t; 10191 } 10192 10193 public ActionSubjectComponent addReference(Reference t) { // 3 10194 if (t == null) 10195 return this; 10196 if (this.reference == null) 10197 this.reference = new ArrayList<Reference>(); 10198 this.reference.add(t); 10199 return this; 10200 } 10201 10202 /** 10203 * @return The first repetition of repeating field {@link #reference}, creating 10204 * it if it does not already exist 10205 */ 10206 public Reference getReferenceFirstRep() { 10207 if (getReference().isEmpty()) { 10208 addReference(); 10209 } 10210 return getReference().get(0); 10211 } 10212 10213 /** 10214 * @deprecated Use Reference#setResource(IBaseResource) instead 10215 */ 10216 @Deprecated 10217 public List<Resource> getReferenceTarget() { 10218 if (this.referenceTarget == null) 10219 this.referenceTarget = new ArrayList<Resource>(); 10220 return this.referenceTarget; 10221 } 10222 10223 /** 10224 * @return {@link #role} (Role type of agent assigned roles in this Contract.) 10225 */ 10226 public CodeableConcept getRole() { 10227 if (this.role == null) 10228 if (Configuration.errorOnAutoCreate()) 10229 throw new Error("Attempt to auto-create ActionSubjectComponent.role"); 10230 else if (Configuration.doAutoCreate()) 10231 this.role = new CodeableConcept(); // cc 10232 return this.role; 10233 } 10234 10235 public boolean hasRole() { 10236 return this.role != null && !this.role.isEmpty(); 10237 } 10238 10239 /** 10240 * @param value {@link #role} (Role type of agent assigned roles in this 10241 * Contract.) 10242 */ 10243 public ActionSubjectComponent setRole(CodeableConcept value) { 10244 this.role = value; 10245 return this; 10246 } 10247 10248 protected void listChildren(List<Property> children) { 10249 super.listChildren(children); 10250 children.add(new Property("reference", 10251 "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Device|Group|Organization)", 10252 "The entity the action is performed or not performed on or for.", 0, java.lang.Integer.MAX_VALUE, reference)); 10253 children.add( 10254 new Property("role", "CodeableConcept", "Role type of agent assigned roles in this Contract.", 0, 1, role)); 10255 } 10256 10257 @Override 10258 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 10259 switch (_hash) { 10260 case -925155509: 10261 /* reference */ return new Property("reference", 10262 "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Device|Group|Organization)", 10263 "The entity the action is performed or not performed on or for.", 0, java.lang.Integer.MAX_VALUE, 10264 reference); 10265 case 3506294: 10266 /* role */ return new Property("role", "CodeableConcept", "Role type of agent assigned roles in this Contract.", 10267 0, 1, role); 10268 default: 10269 return super.getNamedProperty(_hash, _name, _checkValid); 10270 } 10271 10272 } 10273 10274 @Override 10275 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 10276 switch (hash) { 10277 case -925155509: 10278 /* reference */ return this.reference == null ? new Base[0] 10279 : this.reference.toArray(new Base[this.reference.size()]); // Reference 10280 case 3506294: 10281 /* role */ return this.role == null ? new Base[0] : new Base[] { this.role }; // CodeableConcept 10282 default: 10283 return super.getProperty(hash, name, checkValid); 10284 } 10285 10286 } 10287 10288 @Override 10289 public Base setProperty(int hash, String name, Base value) throws FHIRException { 10290 switch (hash) { 10291 case -925155509: // reference 10292 this.getReference().add(castToReference(value)); // Reference 10293 return value; 10294 case 3506294: // role 10295 this.role = castToCodeableConcept(value); // CodeableConcept 10296 return value; 10297 default: 10298 return super.setProperty(hash, name, value); 10299 } 10300 10301 } 10302 10303 @Override 10304 public Base setProperty(String name, Base value) throws FHIRException { 10305 if (name.equals("reference")) { 10306 this.getReference().add(castToReference(value)); 10307 } else if (name.equals("role")) { 10308 this.role = castToCodeableConcept(value); // CodeableConcept 10309 } else 10310 return super.setProperty(name, value); 10311 return value; 10312 } 10313 10314 @Override 10315 public void removeChild(String name, Base value) throws FHIRException { 10316 if (name.equals("reference")) { 10317 this.getReference().remove(castToReference(value)); 10318 } else if (name.equals("role")) { 10319 this.role = null; 10320 } else 10321 super.removeChild(name, value); 10322 10323 } 10324 10325 @Override 10326 public Base makeProperty(int hash, String name) throws FHIRException { 10327 switch (hash) { 10328 case -925155509: 10329 return addReference(); 10330 case 3506294: 10331 return getRole(); 10332 default: 10333 return super.makeProperty(hash, name); 10334 } 10335 10336 } 10337 10338 @Override 10339 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 10340 switch (hash) { 10341 case -925155509: 10342 /* reference */ return new String[] { "Reference" }; 10343 case 3506294: 10344 /* role */ return new String[] { "CodeableConcept" }; 10345 default: 10346 return super.getTypesForProperty(hash, name); 10347 } 10348 10349 } 10350 10351 @Override 10352 public Base addChild(String name) throws FHIRException { 10353 if (name.equals("reference")) { 10354 return addReference(); 10355 } else if (name.equals("role")) { 10356 this.role = new CodeableConcept(); 10357 return this.role; 10358 } else 10359 return super.addChild(name); 10360 } 10361 10362 public ActionSubjectComponent copy() { 10363 ActionSubjectComponent dst = new ActionSubjectComponent(); 10364 copyValues(dst); 10365 return dst; 10366 } 10367 10368 public void copyValues(ActionSubjectComponent dst) { 10369 super.copyValues(dst); 10370 if (reference != null) { 10371 dst.reference = new ArrayList<Reference>(); 10372 for (Reference i : reference) 10373 dst.reference.add(i.copy()); 10374 } 10375 ; 10376 dst.role = role == null ? null : role.copy(); 10377 } 10378 10379 @Override 10380 public boolean equalsDeep(Base other_) { 10381 if (!super.equalsDeep(other_)) 10382 return false; 10383 if (!(other_ instanceof ActionSubjectComponent)) 10384 return false; 10385 ActionSubjectComponent o = (ActionSubjectComponent) other_; 10386 return compareDeep(reference, o.reference, true) && compareDeep(role, o.role, true); 10387 } 10388 10389 @Override 10390 public boolean equalsShallow(Base other_) { 10391 if (!super.equalsShallow(other_)) 10392 return false; 10393 if (!(other_ instanceof ActionSubjectComponent)) 10394 return false; 10395 ActionSubjectComponent o = (ActionSubjectComponent) other_; 10396 return true; 10397 } 10398 10399 public boolean isEmpty() { 10400 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(reference, role); 10401 } 10402 10403 public String fhirType() { 10404 return "Contract.term.action.subject"; 10405 10406 } 10407 10408 } 10409 10410 @Block() 10411 public static class SignatoryComponent extends BackboneElement implements IBaseBackboneElement { 10412 /** 10413 * Role of this Contract signer, e.g. notary, grantee. 10414 */ 10415 @Child(name = "type", type = { Coding.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 10416 @Description(shortDefinition = "Contract Signatory Role", formalDefinition = "Role of this Contract signer, e.g. notary, grantee.") 10417 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-signer-type") 10418 protected Coding type; 10419 10420 /** 10421 * Party which is a signator to this Contract. 10422 */ 10423 @Child(name = "party", type = { Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, 10424 RelatedPerson.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 10425 @Description(shortDefinition = "Contract Signatory Party", formalDefinition = "Party which is a signator to this Contract.") 10426 protected Reference party; 10427 10428 /** 10429 * The actual object that is the target of the reference (Party which is a 10430 * signator to this Contract.) 10431 */ 10432 protected Resource partyTarget; 10433 10434 /** 10435 * Legally binding Contract DSIG signature contents in Base64. 10436 */ 10437 @Child(name = "signature", type = { 10438 Signature.class }, order = 3, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 10439 @Description(shortDefinition = "Contract Documentation Signature", formalDefinition = "Legally binding Contract DSIG signature contents in Base64.") 10440 protected List<Signature> signature; 10441 10442 private static final long serialVersionUID = 1948139228L; 10443 10444 /** 10445 * Constructor 10446 */ 10447 public SignatoryComponent() { 10448 super(); 10449 } 10450 10451 /** 10452 * Constructor 10453 */ 10454 public SignatoryComponent(Coding type, Reference party) { 10455 super(); 10456 this.type = type; 10457 this.party = party; 10458 } 10459 10460 /** 10461 * @return {@link #type} (Role of this Contract signer, e.g. notary, grantee.) 10462 */ 10463 public Coding getType() { 10464 if (this.type == null) 10465 if (Configuration.errorOnAutoCreate()) 10466 throw new Error("Attempt to auto-create SignatoryComponent.type"); 10467 else if (Configuration.doAutoCreate()) 10468 this.type = new Coding(); // cc 10469 return this.type; 10470 } 10471 10472 public boolean hasType() { 10473 return this.type != null && !this.type.isEmpty(); 10474 } 10475 10476 /** 10477 * @param value {@link #type} (Role of this Contract signer, e.g. notary, 10478 * grantee.) 10479 */ 10480 public SignatoryComponent setType(Coding value) { 10481 this.type = value; 10482 return this; 10483 } 10484 10485 /** 10486 * @return {@link #party} (Party which is a signator to this Contract.) 10487 */ 10488 public Reference getParty() { 10489 if (this.party == null) 10490 if (Configuration.errorOnAutoCreate()) 10491 throw new Error("Attempt to auto-create SignatoryComponent.party"); 10492 else if (Configuration.doAutoCreate()) 10493 this.party = new Reference(); // cc 10494 return this.party; 10495 } 10496 10497 public boolean hasParty() { 10498 return this.party != null && !this.party.isEmpty(); 10499 } 10500 10501 /** 10502 * @param value {@link #party} (Party which is a signator to this Contract.) 10503 */ 10504 public SignatoryComponent setParty(Reference value) { 10505 this.party = value; 10506 return this; 10507 } 10508 10509 /** 10510 * @return {@link #party} The actual object that is the target of the reference. 10511 * The reference library doesn't populate this, but you can use it to 10512 * hold the resource if you resolve it. (Party which is a signator to 10513 * this Contract.) 10514 */ 10515 public Resource getPartyTarget() { 10516 return this.partyTarget; 10517 } 10518 10519 /** 10520 * @param value {@link #party} The actual object that is the target of the 10521 * reference. The reference library doesn't use these, but you can 10522 * use it to hold the resource if you resolve it. (Party which is a 10523 * signator to this Contract.) 10524 */ 10525 public SignatoryComponent setPartyTarget(Resource value) { 10526 this.partyTarget = value; 10527 return this; 10528 } 10529 10530 /** 10531 * @return {@link #signature} (Legally binding Contract DSIG signature contents 10532 * in Base64.) 10533 */ 10534 public List<Signature> getSignature() { 10535 if (this.signature == null) 10536 this.signature = new ArrayList<Signature>(); 10537 return this.signature; 10538 } 10539 10540 /** 10541 * @return Returns a reference to <code>this</code> for easy method chaining 10542 */ 10543 public SignatoryComponent setSignature(List<Signature> theSignature) { 10544 this.signature = theSignature; 10545 return this; 10546 } 10547 10548 public boolean hasSignature() { 10549 if (this.signature == null) 10550 return false; 10551 for (Signature item : this.signature) 10552 if (!item.isEmpty()) 10553 return true; 10554 return false; 10555 } 10556 10557 public Signature addSignature() { // 3 10558 Signature t = new Signature(); 10559 if (this.signature == null) 10560 this.signature = new ArrayList<Signature>(); 10561 this.signature.add(t); 10562 return t; 10563 } 10564 10565 public SignatoryComponent addSignature(Signature t) { // 3 10566 if (t == null) 10567 return this; 10568 if (this.signature == null) 10569 this.signature = new ArrayList<Signature>(); 10570 this.signature.add(t); 10571 return this; 10572 } 10573 10574 /** 10575 * @return The first repetition of repeating field {@link #signature}, creating 10576 * it if it does not already exist 10577 */ 10578 public Signature getSignatureFirstRep() { 10579 if (getSignature().isEmpty()) { 10580 addSignature(); 10581 } 10582 return getSignature().get(0); 10583 } 10584 10585 protected void listChildren(List<Property> children) { 10586 super.listChildren(children); 10587 children.add(new Property("type", "Coding", "Role of this Contract signer, e.g. notary, grantee.", 0, 1, type)); 10588 children.add(new Property("party", "Reference(Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", 10589 "Party which is a signator to this Contract.", 0, 1, party)); 10590 children.add(new Property("signature", "Signature", "Legally binding Contract DSIG signature contents in Base64.", 10591 0, java.lang.Integer.MAX_VALUE, signature)); 10592 } 10593 10594 @Override 10595 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 10596 switch (_hash) { 10597 case 3575610: 10598 /* type */ return new Property("type", "Coding", "Role of this Contract signer, e.g. notary, grantee.", 0, 1, 10599 type); 10600 case 106437350: 10601 /* party */ return new Property("party", 10602 "Reference(Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", 10603 "Party which is a signator to this Contract.", 0, 1, party); 10604 case 1073584312: 10605 /* signature */ return new Property("signature", "Signature", 10606 "Legally binding Contract DSIG signature contents in Base64.", 0, java.lang.Integer.MAX_VALUE, signature); 10607 default: 10608 return super.getNamedProperty(_hash, _name, _checkValid); 10609 } 10610 10611 } 10612 10613 @Override 10614 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 10615 switch (hash) { 10616 case 3575610: 10617 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Coding 10618 case 106437350: 10619 /* party */ return this.party == null ? new Base[0] : new Base[] { this.party }; // Reference 10620 case 1073584312: 10621 /* signature */ return this.signature == null ? new Base[0] 10622 : this.signature.toArray(new Base[this.signature.size()]); // Signature 10623 default: 10624 return super.getProperty(hash, name, checkValid); 10625 } 10626 10627 } 10628 10629 @Override 10630 public Base setProperty(int hash, String name, Base value) throws FHIRException { 10631 switch (hash) { 10632 case 3575610: // type 10633 this.type = castToCoding(value); // Coding 10634 return value; 10635 case 106437350: // party 10636 this.party = castToReference(value); // Reference 10637 return value; 10638 case 1073584312: // signature 10639 this.getSignature().add(castToSignature(value)); // Signature 10640 return value; 10641 default: 10642 return super.setProperty(hash, name, value); 10643 } 10644 10645 } 10646 10647 @Override 10648 public Base setProperty(String name, Base value) throws FHIRException { 10649 if (name.equals("type")) { 10650 this.type = castToCoding(value); // Coding 10651 } else if (name.equals("party")) { 10652 this.party = castToReference(value); // Reference 10653 } else if (name.equals("signature")) { 10654 this.getSignature().add(castToSignature(value)); 10655 } else 10656 return super.setProperty(name, value); 10657 return value; 10658 } 10659 10660 @Override 10661 public void removeChild(String name, Base value) throws FHIRException { 10662 if (name.equals("type")) { 10663 this.type = null; 10664 } else if (name.equals("party")) { 10665 this.party = null; 10666 } else if (name.equals("signature")) { 10667 this.getSignature().remove(castToSignature(value)); 10668 } else 10669 super.removeChild(name, value); 10670 10671 } 10672 10673 @Override 10674 public Base makeProperty(int hash, String name) throws FHIRException { 10675 switch (hash) { 10676 case 3575610: 10677 return getType(); 10678 case 106437350: 10679 return getParty(); 10680 case 1073584312: 10681 return addSignature(); 10682 default: 10683 return super.makeProperty(hash, name); 10684 } 10685 10686 } 10687 10688 @Override 10689 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 10690 switch (hash) { 10691 case 3575610: 10692 /* type */ return new String[] { "Coding" }; 10693 case 106437350: 10694 /* party */ return new String[] { "Reference" }; 10695 case 1073584312: 10696 /* signature */ return new String[] { "Signature" }; 10697 default: 10698 return super.getTypesForProperty(hash, name); 10699 } 10700 10701 } 10702 10703 @Override 10704 public Base addChild(String name) throws FHIRException { 10705 if (name.equals("type")) { 10706 this.type = new Coding(); 10707 return this.type; 10708 } else if (name.equals("party")) { 10709 this.party = new Reference(); 10710 return this.party; 10711 } else if (name.equals("signature")) { 10712 return addSignature(); 10713 } else 10714 return super.addChild(name); 10715 } 10716 10717 public SignatoryComponent copy() { 10718 SignatoryComponent dst = new SignatoryComponent(); 10719 copyValues(dst); 10720 return dst; 10721 } 10722 10723 public void copyValues(SignatoryComponent dst) { 10724 super.copyValues(dst); 10725 dst.type = type == null ? null : type.copy(); 10726 dst.party = party == null ? null : party.copy(); 10727 if (signature != null) { 10728 dst.signature = new ArrayList<Signature>(); 10729 for (Signature i : signature) 10730 dst.signature.add(i.copy()); 10731 } 10732 ; 10733 } 10734 10735 @Override 10736 public boolean equalsDeep(Base other_) { 10737 if (!super.equalsDeep(other_)) 10738 return false; 10739 if (!(other_ instanceof SignatoryComponent)) 10740 return false; 10741 SignatoryComponent o = (SignatoryComponent) other_; 10742 return compareDeep(type, o.type, true) && compareDeep(party, o.party, true) 10743 && compareDeep(signature, o.signature, true); 10744 } 10745 10746 @Override 10747 public boolean equalsShallow(Base other_) { 10748 if (!super.equalsShallow(other_)) 10749 return false; 10750 if (!(other_ instanceof SignatoryComponent)) 10751 return false; 10752 SignatoryComponent o = (SignatoryComponent) other_; 10753 return true; 10754 } 10755 10756 public boolean isEmpty() { 10757 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, party, signature); 10758 } 10759 10760 public String fhirType() { 10761 return "Contract.signer"; 10762 10763 } 10764 10765 } 10766 10767 @Block() 10768 public static class FriendlyLanguageComponent extends BackboneElement implements IBaseBackboneElement { 10769 /** 10770 * Human readable rendering of this Contract in a format and representation 10771 * intended to enhance comprehension and ensure understandability. 10772 */ 10773 @Child(name = "content", type = { Attachment.class, Composition.class, DocumentReference.class, 10774 QuestionnaireResponse.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 10775 @Description(shortDefinition = "Easily comprehended representation of this Contract", formalDefinition = "Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.") 10776 protected Type content; 10777 10778 private static final long serialVersionUID = -1763459053L; 10779 10780 /** 10781 * Constructor 10782 */ 10783 public FriendlyLanguageComponent() { 10784 super(); 10785 } 10786 10787 /** 10788 * Constructor 10789 */ 10790 public FriendlyLanguageComponent(Type content) { 10791 super(); 10792 this.content = content; 10793 } 10794 10795 /** 10796 * @return {@link #content} (Human readable rendering of this Contract in a 10797 * format and representation intended to enhance comprehension and 10798 * ensure understandability.) 10799 */ 10800 public Type getContent() { 10801 return this.content; 10802 } 10803 10804 /** 10805 * @return {@link #content} (Human readable rendering of this Contract in a 10806 * format and representation intended to enhance comprehension and 10807 * ensure understandability.) 10808 */ 10809 public Attachment getContentAttachment() throws FHIRException { 10810 if (this.content == null) 10811 this.content = new Attachment(); 10812 if (!(this.content instanceof Attachment)) 10813 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 10814 + this.content.getClass().getName() + " was encountered"); 10815 return (Attachment) this.content; 10816 } 10817 10818 public boolean hasContentAttachment() { 10819 return this != null && this.content instanceof Attachment; 10820 } 10821 10822 /** 10823 * @return {@link #content} (Human readable rendering of this Contract in a 10824 * format and representation intended to enhance comprehension and 10825 * ensure understandability.) 10826 */ 10827 public Reference getContentReference() throws FHIRException { 10828 if (this.content == null) 10829 this.content = new Reference(); 10830 if (!(this.content instanceof Reference)) 10831 throw new FHIRException("Type mismatch: the type Reference was expected, but " 10832 + this.content.getClass().getName() + " was encountered"); 10833 return (Reference) this.content; 10834 } 10835 10836 public boolean hasContentReference() { 10837 return this != null && this.content instanceof Reference; 10838 } 10839 10840 public boolean hasContent() { 10841 return this.content != null && !this.content.isEmpty(); 10842 } 10843 10844 /** 10845 * @param value {@link #content} (Human readable rendering of this Contract in a 10846 * format and representation intended to enhance comprehension and 10847 * ensure understandability.) 10848 */ 10849 public FriendlyLanguageComponent setContent(Type value) { 10850 if (value != null && !(value instanceof Attachment || value instanceof Reference)) 10851 throw new Error("Not the right type for Contract.friendly.content[x]: " + value.fhirType()); 10852 this.content = value; 10853 return this; 10854 } 10855 10856 protected void listChildren(List<Property> children) { 10857 super.listChildren(children); 10858 children.add(new Property("content[x]", 10859 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", 10860 "Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.", 10861 0, 1, content)); 10862 } 10863 10864 @Override 10865 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 10866 switch (_hash) { 10867 case 264548711: 10868 /* content[x] */ return new Property("content[x]", 10869 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", 10870 "Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.", 10871 0, 1, content); 10872 case 951530617: 10873 /* content */ return new Property("content[x]", 10874 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", 10875 "Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.", 10876 0, 1, content); 10877 case -702028164: 10878 /* contentAttachment */ return new Property("content[x]", 10879 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", 10880 "Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.", 10881 0, 1, content); 10882 case 1193747154: 10883 /* contentReference */ return new Property("content[x]", 10884 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", 10885 "Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.", 10886 0, 1, content); 10887 default: 10888 return super.getNamedProperty(_hash, _name, _checkValid); 10889 } 10890 10891 } 10892 10893 @Override 10894 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 10895 switch (hash) { 10896 case 951530617: 10897 /* content */ return this.content == null ? new Base[0] : new Base[] { this.content }; // Type 10898 default: 10899 return super.getProperty(hash, name, checkValid); 10900 } 10901 10902 } 10903 10904 @Override 10905 public Base setProperty(int hash, String name, Base value) throws FHIRException { 10906 switch (hash) { 10907 case 951530617: // content 10908 this.content = castToType(value); // Type 10909 return value; 10910 default: 10911 return super.setProperty(hash, name, value); 10912 } 10913 10914 } 10915 10916 @Override 10917 public Base setProperty(String name, Base value) throws FHIRException { 10918 if (name.equals("content[x]")) { 10919 this.content = castToType(value); // Type 10920 } else 10921 return super.setProperty(name, value); 10922 return value; 10923 } 10924 10925 @Override 10926 public void removeChild(String name, Base value) throws FHIRException { 10927 if (name.equals("content[x]")) { 10928 this.content = null; 10929 } else 10930 super.removeChild(name, value); 10931 10932 } 10933 10934 @Override 10935 public Base makeProperty(int hash, String name) throws FHIRException { 10936 switch (hash) { 10937 case 264548711: 10938 return getContent(); 10939 case 951530617: 10940 return getContent(); 10941 default: 10942 return super.makeProperty(hash, name); 10943 } 10944 10945 } 10946 10947 @Override 10948 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 10949 switch (hash) { 10950 case 951530617: 10951 /* content */ return new String[] { "Attachment", "Reference" }; 10952 default: 10953 return super.getTypesForProperty(hash, name); 10954 } 10955 10956 } 10957 10958 @Override 10959 public Base addChild(String name) throws FHIRException { 10960 if (name.equals("contentAttachment")) { 10961 this.content = new Attachment(); 10962 return this.content; 10963 } else if (name.equals("contentReference")) { 10964 this.content = new Reference(); 10965 return this.content; 10966 } else 10967 return super.addChild(name); 10968 } 10969 10970 public FriendlyLanguageComponent copy() { 10971 FriendlyLanguageComponent dst = new FriendlyLanguageComponent(); 10972 copyValues(dst); 10973 return dst; 10974 } 10975 10976 public void copyValues(FriendlyLanguageComponent dst) { 10977 super.copyValues(dst); 10978 dst.content = content == null ? null : content.copy(); 10979 } 10980 10981 @Override 10982 public boolean equalsDeep(Base other_) { 10983 if (!super.equalsDeep(other_)) 10984 return false; 10985 if (!(other_ instanceof FriendlyLanguageComponent)) 10986 return false; 10987 FriendlyLanguageComponent o = (FriendlyLanguageComponent) other_; 10988 return compareDeep(content, o.content, true); 10989 } 10990 10991 @Override 10992 public boolean equalsShallow(Base other_) { 10993 if (!super.equalsShallow(other_)) 10994 return false; 10995 if (!(other_ instanceof FriendlyLanguageComponent)) 10996 return false; 10997 FriendlyLanguageComponent o = (FriendlyLanguageComponent) other_; 10998 return true; 10999 } 11000 11001 public boolean isEmpty() { 11002 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(content); 11003 } 11004 11005 public String fhirType() { 11006 return "Contract.friendly"; 11007 11008 } 11009 11010 } 11011 11012 @Block() 11013 public static class LegalLanguageComponent extends BackboneElement implements IBaseBackboneElement { 11014 /** 11015 * Contract legal text in human renderable form. 11016 */ 11017 @Child(name = "content", type = { Attachment.class, Composition.class, DocumentReference.class, 11018 QuestionnaireResponse.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 11019 @Description(shortDefinition = "Contract Legal Text", formalDefinition = "Contract legal text in human renderable form.") 11020 protected Type content; 11021 11022 private static final long serialVersionUID = -1763459053L; 11023 11024 /** 11025 * Constructor 11026 */ 11027 public LegalLanguageComponent() { 11028 super(); 11029 } 11030 11031 /** 11032 * Constructor 11033 */ 11034 public LegalLanguageComponent(Type content) { 11035 super(); 11036 this.content = content; 11037 } 11038 11039 /** 11040 * @return {@link #content} (Contract legal text in human renderable form.) 11041 */ 11042 public Type getContent() { 11043 return this.content; 11044 } 11045 11046 /** 11047 * @return {@link #content} (Contract legal text in human renderable form.) 11048 */ 11049 public Attachment getContentAttachment() throws FHIRException { 11050 if (this.content == null) 11051 this.content = new Attachment(); 11052 if (!(this.content instanceof Attachment)) 11053 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 11054 + this.content.getClass().getName() + " was encountered"); 11055 return (Attachment) this.content; 11056 } 11057 11058 public boolean hasContentAttachment() { 11059 return this != null && this.content instanceof Attachment; 11060 } 11061 11062 /** 11063 * @return {@link #content} (Contract legal text in human renderable form.) 11064 */ 11065 public Reference getContentReference() throws FHIRException { 11066 if (this.content == null) 11067 this.content = new Reference(); 11068 if (!(this.content instanceof Reference)) 11069 throw new FHIRException("Type mismatch: the type Reference was expected, but " 11070 + this.content.getClass().getName() + " was encountered"); 11071 return (Reference) this.content; 11072 } 11073 11074 public boolean hasContentReference() { 11075 return this != null && this.content instanceof Reference; 11076 } 11077 11078 public boolean hasContent() { 11079 return this.content != null && !this.content.isEmpty(); 11080 } 11081 11082 /** 11083 * @param value {@link #content} (Contract legal text in human renderable form.) 11084 */ 11085 public LegalLanguageComponent setContent(Type value) { 11086 if (value != null && !(value instanceof Attachment || value instanceof Reference)) 11087 throw new Error("Not the right type for Contract.legal.content[x]: " + value.fhirType()); 11088 this.content = value; 11089 return this; 11090 } 11091 11092 protected void listChildren(List<Property> children) { 11093 super.listChildren(children); 11094 children 11095 .add(new Property("content[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", 11096 "Contract legal text in human renderable form.", 0, 1, content)); 11097 } 11098 11099 @Override 11100 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 11101 switch (_hash) { 11102 case 264548711: 11103 /* content[x] */ return new Property("content[x]", 11104 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", 11105 "Contract legal text in human renderable form.", 0, 1, content); 11106 case 951530617: 11107 /* content */ return new Property("content[x]", 11108 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", 11109 "Contract legal text in human renderable form.", 0, 1, content); 11110 case -702028164: 11111 /* contentAttachment */ return new Property("content[x]", 11112 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", 11113 "Contract legal text in human renderable form.", 0, 1, content); 11114 case 1193747154: 11115 /* contentReference */ return new Property("content[x]", 11116 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", 11117 "Contract legal text in human renderable form.", 0, 1, content); 11118 default: 11119 return super.getNamedProperty(_hash, _name, _checkValid); 11120 } 11121 11122 } 11123 11124 @Override 11125 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 11126 switch (hash) { 11127 case 951530617: 11128 /* content */ return this.content == null ? new Base[0] : new Base[] { this.content }; // Type 11129 default: 11130 return super.getProperty(hash, name, checkValid); 11131 } 11132 11133 } 11134 11135 @Override 11136 public Base setProperty(int hash, String name, Base value) throws FHIRException { 11137 switch (hash) { 11138 case 951530617: // content 11139 this.content = castToType(value); // Type 11140 return value; 11141 default: 11142 return super.setProperty(hash, name, value); 11143 } 11144 11145 } 11146 11147 @Override 11148 public Base setProperty(String name, Base value) throws FHIRException { 11149 if (name.equals("content[x]")) { 11150 this.content = castToType(value); // Type 11151 } else 11152 return super.setProperty(name, value); 11153 return value; 11154 } 11155 11156 @Override 11157 public void removeChild(String name, Base value) throws FHIRException { 11158 if (name.equals("content[x]")) { 11159 this.content = null; 11160 } else 11161 super.removeChild(name, value); 11162 11163 } 11164 11165 @Override 11166 public Base makeProperty(int hash, String name) throws FHIRException { 11167 switch (hash) { 11168 case 264548711: 11169 return getContent(); 11170 case 951530617: 11171 return getContent(); 11172 default: 11173 return super.makeProperty(hash, name); 11174 } 11175 11176 } 11177 11178 @Override 11179 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 11180 switch (hash) { 11181 case 951530617: 11182 /* content */ return new String[] { "Attachment", "Reference" }; 11183 default: 11184 return super.getTypesForProperty(hash, name); 11185 } 11186 11187 } 11188 11189 @Override 11190 public Base addChild(String name) throws FHIRException { 11191 if (name.equals("contentAttachment")) { 11192 this.content = new Attachment(); 11193 return this.content; 11194 } else if (name.equals("contentReference")) { 11195 this.content = new Reference(); 11196 return this.content; 11197 } else 11198 return super.addChild(name); 11199 } 11200 11201 public LegalLanguageComponent copy() { 11202 LegalLanguageComponent dst = new LegalLanguageComponent(); 11203 copyValues(dst); 11204 return dst; 11205 } 11206 11207 public void copyValues(LegalLanguageComponent dst) { 11208 super.copyValues(dst); 11209 dst.content = content == null ? null : content.copy(); 11210 } 11211 11212 @Override 11213 public boolean equalsDeep(Base other_) { 11214 if (!super.equalsDeep(other_)) 11215 return false; 11216 if (!(other_ instanceof LegalLanguageComponent)) 11217 return false; 11218 LegalLanguageComponent o = (LegalLanguageComponent) other_; 11219 return compareDeep(content, o.content, true); 11220 } 11221 11222 @Override 11223 public boolean equalsShallow(Base other_) { 11224 if (!super.equalsShallow(other_)) 11225 return false; 11226 if (!(other_ instanceof LegalLanguageComponent)) 11227 return false; 11228 LegalLanguageComponent o = (LegalLanguageComponent) other_; 11229 return true; 11230 } 11231 11232 public boolean isEmpty() { 11233 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(content); 11234 } 11235 11236 public String fhirType() { 11237 return "Contract.legal"; 11238 11239 } 11240 11241 } 11242 11243 @Block() 11244 public static class ComputableLanguageComponent extends BackboneElement implements IBaseBackboneElement { 11245 /** 11246 * Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, 11247 * SecPal). 11248 */ 11249 @Child(name = "content", type = { Attachment.class, 11250 DocumentReference.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 11251 @Description(shortDefinition = "Computable Contract Rules", formalDefinition = "Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).") 11252 protected Type content; 11253 11254 private static final long serialVersionUID = -1763459053L; 11255 11256 /** 11257 * Constructor 11258 */ 11259 public ComputableLanguageComponent() { 11260 super(); 11261 } 11262 11263 /** 11264 * Constructor 11265 */ 11266 public ComputableLanguageComponent(Type content) { 11267 super(); 11268 this.content = content; 11269 } 11270 11271 /** 11272 * @return {@link #content} (Computable Contract conveyed using a policy rule 11273 * language (e.g. XACML, DKAL, SecPal).) 11274 */ 11275 public Type getContent() { 11276 return this.content; 11277 } 11278 11279 /** 11280 * @return {@link #content} (Computable Contract conveyed using a policy rule 11281 * language (e.g. XACML, DKAL, SecPal).) 11282 */ 11283 public Attachment getContentAttachment() throws FHIRException { 11284 if (this.content == null) 11285 this.content = new Attachment(); 11286 if (!(this.content instanceof Attachment)) 11287 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 11288 + this.content.getClass().getName() + " was encountered"); 11289 return (Attachment) this.content; 11290 } 11291 11292 public boolean hasContentAttachment() { 11293 return this != null && this.content instanceof Attachment; 11294 } 11295 11296 /** 11297 * @return {@link #content} (Computable Contract conveyed using a policy rule 11298 * language (e.g. XACML, DKAL, SecPal).) 11299 */ 11300 public Reference getContentReference() throws FHIRException { 11301 if (this.content == null) 11302 this.content = new Reference(); 11303 if (!(this.content instanceof Reference)) 11304 throw new FHIRException("Type mismatch: the type Reference was expected, but " 11305 + this.content.getClass().getName() + " was encountered"); 11306 return (Reference) this.content; 11307 } 11308 11309 public boolean hasContentReference() { 11310 return this != null && this.content instanceof Reference; 11311 } 11312 11313 public boolean hasContent() { 11314 return this.content != null && !this.content.isEmpty(); 11315 } 11316 11317 /** 11318 * @param value {@link #content} (Computable Contract conveyed using a policy 11319 * rule language (e.g. XACML, DKAL, SecPal).) 11320 */ 11321 public ComputableLanguageComponent setContent(Type value) { 11322 if (value != null && !(value instanceof Attachment || value instanceof Reference)) 11323 throw new Error("Not the right type for Contract.rule.content[x]: " + value.fhirType()); 11324 this.content = value; 11325 return this; 11326 } 11327 11328 protected void listChildren(List<Property> children) { 11329 super.listChildren(children); 11330 children.add(new Property("content[x]", "Attachment|Reference(DocumentReference)", 11331 "Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).", 0, 1, content)); 11332 } 11333 11334 @Override 11335 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 11336 switch (_hash) { 11337 case 264548711: 11338 /* content[x] */ return new Property("content[x]", "Attachment|Reference(DocumentReference)", 11339 "Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).", 0, 1, content); 11340 case 951530617: 11341 /* content */ return new Property("content[x]", "Attachment|Reference(DocumentReference)", 11342 "Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).", 0, 1, content); 11343 case -702028164: 11344 /* contentAttachment */ return new Property("content[x]", "Attachment|Reference(DocumentReference)", 11345 "Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).", 0, 1, content); 11346 case 1193747154: 11347 /* contentReference */ return new Property("content[x]", "Attachment|Reference(DocumentReference)", 11348 "Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).", 0, 1, content); 11349 default: 11350 return super.getNamedProperty(_hash, _name, _checkValid); 11351 } 11352 11353 } 11354 11355 @Override 11356 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 11357 switch (hash) { 11358 case 951530617: 11359 /* content */ return this.content == null ? new Base[0] : new Base[] { this.content }; // Type 11360 default: 11361 return super.getProperty(hash, name, checkValid); 11362 } 11363 11364 } 11365 11366 @Override 11367 public Base setProperty(int hash, String name, Base value) throws FHIRException { 11368 switch (hash) { 11369 case 951530617: // content 11370 this.content = castToType(value); // Type 11371 return value; 11372 default: 11373 return super.setProperty(hash, name, value); 11374 } 11375 11376 } 11377 11378 @Override 11379 public Base setProperty(String name, Base value) throws FHIRException { 11380 if (name.equals("content[x]")) { 11381 this.content = castToType(value); // Type 11382 } else 11383 return super.setProperty(name, value); 11384 return value; 11385 } 11386 11387 @Override 11388 public void removeChild(String name, Base value) throws FHIRException { 11389 if (name.equals("content[x]")) { 11390 this.content = null; 11391 } else 11392 super.removeChild(name, value); 11393 11394 } 11395 11396 @Override 11397 public Base makeProperty(int hash, String name) throws FHIRException { 11398 switch (hash) { 11399 case 264548711: 11400 return getContent(); 11401 case 951530617: 11402 return getContent(); 11403 default: 11404 return super.makeProperty(hash, name); 11405 } 11406 11407 } 11408 11409 @Override 11410 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 11411 switch (hash) { 11412 case 951530617: 11413 /* content */ return new String[] { "Attachment", "Reference" }; 11414 default: 11415 return super.getTypesForProperty(hash, name); 11416 } 11417 11418 } 11419 11420 @Override 11421 public Base addChild(String name) throws FHIRException { 11422 if (name.equals("contentAttachment")) { 11423 this.content = new Attachment(); 11424 return this.content; 11425 } else if (name.equals("contentReference")) { 11426 this.content = new Reference(); 11427 return this.content; 11428 } else 11429 return super.addChild(name); 11430 } 11431 11432 public ComputableLanguageComponent copy() { 11433 ComputableLanguageComponent dst = new ComputableLanguageComponent(); 11434 copyValues(dst); 11435 return dst; 11436 } 11437 11438 public void copyValues(ComputableLanguageComponent dst) { 11439 super.copyValues(dst); 11440 dst.content = content == null ? null : content.copy(); 11441 } 11442 11443 @Override 11444 public boolean equalsDeep(Base other_) { 11445 if (!super.equalsDeep(other_)) 11446 return false; 11447 if (!(other_ instanceof ComputableLanguageComponent)) 11448 return false; 11449 ComputableLanguageComponent o = (ComputableLanguageComponent) other_; 11450 return compareDeep(content, o.content, true); 11451 } 11452 11453 @Override 11454 public boolean equalsShallow(Base other_) { 11455 if (!super.equalsShallow(other_)) 11456 return false; 11457 if (!(other_ instanceof ComputableLanguageComponent)) 11458 return false; 11459 ComputableLanguageComponent o = (ComputableLanguageComponent) other_; 11460 return true; 11461 } 11462 11463 public boolean isEmpty() { 11464 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(content); 11465 } 11466 11467 public String fhirType() { 11468 return "Contract.rule"; 11469 11470 } 11471 11472 } 11473 11474 /** 11475 * Unique identifier for this Contract or a derivative that references a Source 11476 * Contract. 11477 */ 11478 @Child(name = "identifier", type = { 11479 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 11480 @Description(shortDefinition = "Contract number", formalDefinition = "Unique identifier for this Contract or a derivative that references a Source Contract.") 11481 protected List<Identifier> identifier; 11482 11483 /** 11484 * Canonical identifier for this contract, represented as a URI (globally 11485 * unique). 11486 */ 11487 @Child(name = "url", type = { UriType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 11488 @Description(shortDefinition = "Basal definition", formalDefinition = "Canonical identifier for this contract, represented as a URI (globally unique).") 11489 protected UriType url; 11490 11491 /** 11492 * An edition identifier used for business purposes to label business 11493 * significant variants. 11494 */ 11495 @Child(name = "version", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 11496 @Description(shortDefinition = "Business edition", formalDefinition = "An edition identifier used for business purposes to label business significant variants.") 11497 protected StringType version; 11498 11499 /** 11500 * The status of the resource instance. 11501 */ 11502 @Child(name = "status", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = true, summary = true) 11503 @Description(shortDefinition = "amended | appended | cancelled | disputed | entered-in-error | executable | executed | negotiable | offered | policy | rejected | renewed | revoked | resolved | terminated", formalDefinition = "The status of the resource instance.") 11504 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-status") 11505 protected Enumeration<ContractStatus> status; 11506 11507 /** 11508 * Legal states of the formation of a legal instrument, which is a formally 11509 * executed written document that can be formally attributed to its author, 11510 * records and formally expresses a legally enforceable act, process, or 11511 * contractual duty, obligation, or right, and therefore evidences that act, 11512 * process, or agreement. 11513 */ 11514 @Child(name = "legalState", type = { 11515 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 11516 @Description(shortDefinition = "Negotiation status", formalDefinition = "Legal states of the formation of a legal instrument, which is a formally executed written document that can be formally attributed to its author, records and formally expresses a legally enforceable act, process, or contractual duty, obligation, or right, and therefore evidences that act, process, or agreement.") 11517 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-legalstate") 11518 protected CodeableConcept legalState; 11519 11520 /** 11521 * The URL pointing to a FHIR-defined Contract Definition that is adhered to in 11522 * whole or part by this Contract. 11523 */ 11524 @Child(name = "instantiatesCanonical", type = { 11525 Contract.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 11526 @Description(shortDefinition = "Source Contract Definition", formalDefinition = "The URL pointing to a FHIR-defined Contract Definition that is adhered to in whole or part by this Contract.") 11527 protected Reference instantiatesCanonical; 11528 11529 /** 11530 * The actual object that is the target of the reference (The URL pointing to a 11531 * FHIR-defined Contract Definition that is adhered to in whole or part by this 11532 * Contract.) 11533 */ 11534 protected Contract instantiatesCanonicalTarget; 11535 11536 /** 11537 * The URL pointing to an externally maintained definition that is adhered to in 11538 * whole or in part by this Contract. 11539 */ 11540 @Child(name = "instantiatesUri", type = { 11541 UriType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 11542 @Description(shortDefinition = "External Contract Definition", formalDefinition = "The URL pointing to an externally maintained definition that is adhered to in whole or in part by this Contract.") 11543 protected UriType instantiatesUri; 11544 11545 /** 11546 * The minimal content derived from the basal information source at a specific 11547 * stage in its lifecycle. 11548 */ 11549 @Child(name = "contentDerivative", type = { 11550 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 11551 @Description(shortDefinition = "Content derived from the basal information", formalDefinition = "The minimal content derived from the basal information source at a specific stage in its lifecycle.") 11552 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-content-derivative") 11553 protected CodeableConcept contentDerivative; 11554 11555 /** 11556 * When this Contract was issued. 11557 */ 11558 @Child(name = "issued", type = { DateTimeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 11559 @Description(shortDefinition = "When this Contract was issued", formalDefinition = "When this Contract was issued.") 11560 protected DateTimeType issued; 11561 11562 /** 11563 * Relevant time or time-period when this Contract is applicable. 11564 */ 11565 @Child(name = "applies", type = { Period.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 11566 @Description(shortDefinition = "Effective time", formalDefinition = "Relevant time or time-period when this Contract is applicable.") 11567 protected Period applies; 11568 11569 /** 11570 * Event resulting in discontinuation or termination of this Contract instance 11571 * by one or more parties to the contract. 11572 */ 11573 @Child(name = "expirationType", type = { 11574 CodeableConcept.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 11575 @Description(shortDefinition = "Contract cessation cause", formalDefinition = "Event resulting in discontinuation or termination of this Contract instance by one or more parties to the contract.") 11576 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-expiration-type") 11577 protected CodeableConcept expirationType; 11578 11579 /** 11580 * The target entity impacted by or of interest to parties to the agreement. 11581 */ 11582 @Child(name = "subject", type = { 11583 Reference.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 11584 @Description(shortDefinition = "Contract Target Entity", formalDefinition = "The target entity impacted by or of interest to parties to the agreement.") 11585 protected List<Reference> subject; 11586 /** 11587 * The actual objects that are the target of the reference (The target entity 11588 * impacted by or of interest to parties to the agreement.) 11589 */ 11590 protected List<Resource> subjectTarget; 11591 11592 /** 11593 * A formally or informally recognized grouping of people, principals, 11594 * organizations, or jurisdictions formed for the purpose of achieving some form 11595 * of collective action such as the promulgation, administration and enforcement 11596 * of contracts and policies. 11597 */ 11598 @Child(name = "authority", type = { 11599 Organization.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11600 @Description(shortDefinition = "Authority under which this Contract has standing", formalDefinition = "A formally or informally recognized grouping of people, principals, organizations, or jurisdictions formed for the purpose of achieving some form of collective action such as the promulgation, administration and enforcement of contracts and policies.") 11601 protected List<Reference> authority; 11602 /** 11603 * The actual objects that are the target of the reference (A formally or 11604 * informally recognized grouping of people, principals, organizations, or 11605 * jurisdictions formed for the purpose of achieving some form of collective 11606 * action such as the promulgation, administration and enforcement of contracts 11607 * and policies.) 11608 */ 11609 protected List<Organization> authorityTarget; 11610 11611 /** 11612 * Recognized governance framework or system operating with a circumscribed 11613 * scope in accordance with specified principles, policies, processes or 11614 * procedures for managing rights, actions, or behaviors of parties or 11615 * principals relative to resources. 11616 */ 11617 @Child(name = "domain", type = { 11618 Location.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11619 @Description(shortDefinition = "A sphere of control governed by an authoritative jurisdiction, organization, or person", formalDefinition = "Recognized governance framework or system operating with a circumscribed scope in accordance with specified principles, policies, processes or procedures for managing rights, actions, or behaviors of parties or principals relative to resources.") 11620 protected List<Reference> domain; 11621 /** 11622 * The actual objects that are the target of the reference (Recognized 11623 * governance framework or system operating with a circumscribed scope in 11624 * accordance with specified principles, policies, processes or procedures for 11625 * managing rights, actions, or behaviors of parties or principals relative to 11626 * resources.) 11627 */ 11628 protected List<Location> domainTarget; 11629 11630 /** 11631 * Sites in which the contract is complied with, exercised, or in force. 11632 */ 11633 @Child(name = "site", type = { 11634 Location.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11635 @Description(shortDefinition = "Specific Location", formalDefinition = "Sites in which the contract is complied with, exercised, or in force.") 11636 protected List<Reference> site; 11637 /** 11638 * The actual objects that are the target of the reference (Sites in which the 11639 * contract is complied with, exercised, or in force.) 11640 */ 11641 protected List<Location> siteTarget; 11642 11643 /** 11644 * A natural language name identifying this Contract definition, derivative, or 11645 * instance in any legal state. Provides additional information about its 11646 * content. This name should be usable as an identifier for the module by 11647 * machine processing applications such as code generation. 11648 */ 11649 @Child(name = "name", type = { StringType.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 11650 @Description(shortDefinition = "Computer friendly designation", formalDefinition = "A natural language name identifying this Contract definition, derivative, or instance in any legal state. Provides additional information about its content. This name should be usable as an identifier for the module by machine processing applications such as code generation.") 11651 protected StringType name; 11652 11653 /** 11654 * A short, descriptive, user-friendly title for this Contract definition, 11655 * derivative, or instance in any legal state.t giving additional information 11656 * about its content. 11657 */ 11658 @Child(name = "title", type = { StringType.class }, order = 16, min = 0, max = 1, modifier = false, summary = true) 11659 @Description(shortDefinition = "Human Friendly name", formalDefinition = "A short, descriptive, user-friendly title for this Contract definition, derivative, or instance in any legal state.t giving additional information about its content.") 11660 protected StringType title; 11661 11662 /** 11663 * An explanatory or alternate user-friendly title for this Contract definition, 11664 * derivative, or instance in any legal state.t giving additional information 11665 * about its content. 11666 */ 11667 @Child(name = "subtitle", type = { 11668 StringType.class }, order = 17, min = 0, max = 1, modifier = false, summary = false) 11669 @Description(shortDefinition = "Subordinate Friendly name", formalDefinition = "An explanatory or alternate user-friendly title for this Contract definition, derivative, or instance in any legal state.t giving additional information about its content.") 11670 protected StringType subtitle; 11671 11672 /** 11673 * Alternative representation of the title for this Contract definition, 11674 * derivative, or instance in any legal state., e.g., a domain specific contract 11675 * number related to legislation. 11676 */ 11677 @Child(name = "alias", type = { 11678 StringType.class }, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11679 @Description(shortDefinition = "Acronym or short name", formalDefinition = "Alternative representation of the title for this Contract definition, derivative, or instance in any legal state., e.g., a domain specific contract number related to legislation.") 11680 protected List<StringType> alias; 11681 11682 /** 11683 * The individual or organization that authored the Contract definition, 11684 * derivative, or instance in any legal state. 11685 */ 11686 @Child(name = "author", type = { Patient.class, Practitioner.class, PractitionerRole.class, 11687 Organization.class }, order = 19, min = 0, max = 1, modifier = false, summary = false) 11688 @Description(shortDefinition = "Source of Contract", formalDefinition = "The individual or organization that authored the Contract definition, derivative, or instance in any legal state.") 11689 protected Reference author; 11690 11691 /** 11692 * The actual object that is the target of the reference (The individual or 11693 * organization that authored the Contract definition, derivative, or instance 11694 * in any legal state.) 11695 */ 11696 protected Resource authorTarget; 11697 11698 /** 11699 * A selector of legal concerns for this Contract definition, derivative, or 11700 * instance in any legal state. 11701 */ 11702 @Child(name = "scope", type = { 11703 CodeableConcept.class }, order = 20, min = 0, max = 1, modifier = false, summary = false) 11704 @Description(shortDefinition = "Range of Legal Concerns", formalDefinition = "A selector of legal concerns for this Contract definition, derivative, or instance in any legal state.") 11705 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-scope") 11706 protected CodeableConcept scope; 11707 11708 /** 11709 * Narrows the range of legal concerns to focus on the achievement of specific 11710 * contractual objectives. 11711 */ 11712 @Child(name = "topic", type = { CodeableConcept.class, 11713 Reference.class }, order = 21, min = 0, max = 1, modifier = false, summary = false) 11714 @Description(shortDefinition = "Focus of contract interest", formalDefinition = "Narrows the range of legal concerns to focus on the achievement of specific contractual objectives.") 11715 protected Type topic; 11716 11717 /** 11718 * A high-level category for the legal instrument, whether constructed as a 11719 * Contract definition, derivative, or instance in any legal state. Provides 11720 * additional information about its content within the context of the Contract's 11721 * scope to distinguish the kinds of systems that would be interested in the 11722 * contract. 11723 */ 11724 @Child(name = "type", type = { 11725 CodeableConcept.class }, order = 22, min = 0, max = 1, modifier = false, summary = true) 11726 @Description(shortDefinition = "Legal instrument category", formalDefinition = "A high-level category for the legal instrument, whether constructed as a Contract definition, derivative, or instance in any legal state. Provides additional information about its content within the context of the Contract's scope to distinguish the kinds of systems that would be interested in the contract.") 11727 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-type") 11728 protected CodeableConcept type; 11729 11730 /** 11731 * Sub-category for the Contract that distinguishes the kinds of systems that 11732 * would be interested in the Contract within the context of the Contract's 11733 * scope. 11734 */ 11735 @Child(name = "subType", type = { 11736 CodeableConcept.class }, order = 23, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 11737 @Description(shortDefinition = "Subtype within the context of type", formalDefinition = "Sub-category for the Contract that distinguishes the kinds of systems that would be interested in the Contract within the context of the Contract's scope.") 11738 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-subtype") 11739 protected List<CodeableConcept> subType; 11740 11741 /** 11742 * Precusory content developed with a focus and intent of supporting the 11743 * formation a Contract instance, which may be associated with and transformable 11744 * into a Contract. 11745 */ 11746 @Child(name = "contentDefinition", type = {}, order = 24, min = 0, max = 1, modifier = false, summary = false) 11747 @Description(shortDefinition = "Contract precursor content", formalDefinition = "Precusory content developed with a focus and intent of supporting the formation a Contract instance, which may be associated with and transformable into a Contract.") 11748 protected ContentDefinitionComponent contentDefinition; 11749 11750 /** 11751 * One or more Contract Provisions, which may be related and conveyed as a 11752 * group, and may contain nested groups. 11753 */ 11754 @Child(name = "term", type = {}, order = 25, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11755 @Description(shortDefinition = "Contract Term List", formalDefinition = "One or more Contract Provisions, which may be related and conveyed as a group, and may contain nested groups.") 11756 protected List<TermComponent> term; 11757 11758 /** 11759 * Information that may be needed by/relevant to the performer in their 11760 * execution of this term action. 11761 */ 11762 @Child(name = "supportingInfo", type = { 11763 Reference.class }, order = 26, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11764 @Description(shortDefinition = "Extra Information", formalDefinition = "Information that may be needed by/relevant to the performer in their execution of this term action.") 11765 protected List<Reference> supportingInfo; 11766 /** 11767 * The actual objects that are the target of the reference (Information that may 11768 * be needed by/relevant to the performer in their execution of this term 11769 * action.) 11770 */ 11771 protected List<Resource> supportingInfoTarget; 11772 11773 /** 11774 * Links to Provenance records for past versions of this Contract definition, 11775 * derivative, or instance, which identify key state transitions or updates that 11776 * are likely to be relevant to a user looking at the current version of the 11777 * Contract. The Provence.entity indicates the target that was changed in the 11778 * update. http://build.fhir.org/provenance-definitions.html#Provenance.entity. 11779 */ 11780 @Child(name = "relevantHistory", type = { 11781 Provenance.class }, order = 27, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11782 @Description(shortDefinition = "Key event in Contract History", formalDefinition = "Links to Provenance records for past versions of this Contract definition, derivative, or instance, which identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the Contract. The Provence.entity indicates the target that was changed in the update. http://build.fhir.org/provenance-definitions.html#Provenance.entity.") 11783 protected List<Reference> relevantHistory; 11784 /** 11785 * The actual objects that are the target of the reference (Links to Provenance 11786 * records for past versions of this Contract definition, derivative, or 11787 * instance, which identify key state transitions or updates that are likely to 11788 * be relevant to a user looking at the current version of the Contract. The 11789 * Provence.entity indicates the target that was changed in the update. 11790 * http://build.fhir.org/provenance-definitions.html#Provenance.entity.) 11791 */ 11792 protected List<Provenance> relevantHistoryTarget; 11793 11794 /** 11795 * Parties with legal standing in the Contract, including the principal parties, 11796 * the grantor(s) and grantee(s), which are any person or organization bound by 11797 * the contract, and any ancillary parties, which facilitate the execution of 11798 * the contract such as a notary or witness. 11799 */ 11800 @Child(name = "signer", type = {}, order = 28, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11801 @Description(shortDefinition = "Contract Signatory", formalDefinition = "Parties with legal standing in the Contract, including the principal parties, the grantor(s) and grantee(s), which are any person or organization bound by the contract, and any ancillary parties, which facilitate the execution of the contract such as a notary or witness.") 11802 protected List<SignatoryComponent> signer; 11803 11804 /** 11805 * The "patient friendly language" versionof the Contract in whole or in parts. 11806 * "Patient friendly language" means the representation of the Contract and 11807 * Contract Provisions in a manner that is readily accessible and understandable 11808 * by a layperson in accordance with best practices for communication styles 11809 * that ensure that those agreeing to or signing the Contract understand the 11810 * roles, actions, obligations, responsibilities, and implication of the 11811 * agreement. 11812 */ 11813 @Child(name = "friendly", type = {}, order = 29, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11814 @Description(shortDefinition = "Contract Friendly Language", formalDefinition = "The \"patient friendly language\" versionof the Contract in whole or in parts. \"Patient friendly language\" means the representation of the Contract and Contract Provisions in a manner that is readily accessible and understandable by a layperson in accordance with best practices for communication styles that ensure that those agreeing to or signing the Contract understand the roles, actions, obligations, responsibilities, and implication of the agreement.") 11815 protected List<FriendlyLanguageComponent> friendly; 11816 11817 /** 11818 * List of Legal expressions or representations of this Contract. 11819 */ 11820 @Child(name = "legal", type = {}, order = 30, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11821 @Description(shortDefinition = "Contract Legal Language", formalDefinition = "List of Legal expressions or representations of this Contract.") 11822 protected List<LegalLanguageComponent> legal; 11823 11824 /** 11825 * List of Computable Policy Rule Language Representations of this Contract. 11826 */ 11827 @Child(name = "rule", type = {}, order = 31, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11828 @Description(shortDefinition = "Computable Contract Language", formalDefinition = "List of Computable Policy Rule Language Representations of this Contract.") 11829 protected List<ComputableLanguageComponent> rule; 11830 11831 /** 11832 * Legally binding Contract: This is the signed and legally recognized 11833 * representation of the Contract, which is considered the "source of truth" and 11834 * which would be the basis for legal action related to enforcement of this 11835 * Contract. 11836 */ 11837 @Child(name = "legallyBinding", type = { Attachment.class, Composition.class, DocumentReference.class, 11838 QuestionnaireResponse.class, Contract.class }, order = 32, min = 0, max = 1, modifier = false, summary = false) 11839 @Description(shortDefinition = "Binding Contract", formalDefinition = "Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the \"source of truth\" and which would be the basis for legal action related to enforcement of this Contract.") 11840 protected Type legallyBinding; 11841 11842 private static final long serialVersionUID = -1388892487L; 11843 11844 /** 11845 * Constructor 11846 */ 11847 public Contract() { 11848 super(); 11849 } 11850 11851 /** 11852 * @return {@link #identifier} (Unique identifier for this Contract or a 11853 * derivative that references a Source Contract.) 11854 */ 11855 public List<Identifier> getIdentifier() { 11856 if (this.identifier == null) 11857 this.identifier = new ArrayList<Identifier>(); 11858 return this.identifier; 11859 } 11860 11861 /** 11862 * @return Returns a reference to <code>this</code> for easy method chaining 11863 */ 11864 public Contract setIdentifier(List<Identifier> theIdentifier) { 11865 this.identifier = theIdentifier; 11866 return this; 11867 } 11868 11869 public boolean hasIdentifier() { 11870 if (this.identifier == null) 11871 return false; 11872 for (Identifier item : this.identifier) 11873 if (!item.isEmpty()) 11874 return true; 11875 return false; 11876 } 11877 11878 public Identifier addIdentifier() { // 3 11879 Identifier t = new Identifier(); 11880 if (this.identifier == null) 11881 this.identifier = new ArrayList<Identifier>(); 11882 this.identifier.add(t); 11883 return t; 11884 } 11885 11886 public Contract addIdentifier(Identifier t) { // 3 11887 if (t == null) 11888 return this; 11889 if (this.identifier == null) 11890 this.identifier = new ArrayList<Identifier>(); 11891 this.identifier.add(t); 11892 return this; 11893 } 11894 11895 /** 11896 * @return The first repetition of repeating field {@link #identifier}, creating 11897 * it if it does not already exist 11898 */ 11899 public Identifier getIdentifierFirstRep() { 11900 if (getIdentifier().isEmpty()) { 11901 addIdentifier(); 11902 } 11903 return getIdentifier().get(0); 11904 } 11905 11906 /** 11907 * @return {@link #url} (Canonical identifier for this contract, represented as 11908 * a URI (globally unique).). This is the underlying object with id, 11909 * value and extensions. The accessor "getUrl" gives direct access to 11910 * the value 11911 */ 11912 public UriType getUrlElement() { 11913 if (this.url == null) 11914 if (Configuration.errorOnAutoCreate()) 11915 throw new Error("Attempt to auto-create Contract.url"); 11916 else if (Configuration.doAutoCreate()) 11917 this.url = new UriType(); // bb 11918 return this.url; 11919 } 11920 11921 public boolean hasUrlElement() { 11922 return this.url != null && !this.url.isEmpty(); 11923 } 11924 11925 public boolean hasUrl() { 11926 return this.url != null && !this.url.isEmpty(); 11927 } 11928 11929 /** 11930 * @param value {@link #url} (Canonical identifier for this contract, 11931 * represented as a URI (globally unique).). This is the underlying 11932 * object with id, value and extensions. The accessor "getUrl" 11933 * gives direct access to the value 11934 */ 11935 public Contract setUrlElement(UriType value) { 11936 this.url = value; 11937 return this; 11938 } 11939 11940 /** 11941 * @return Canonical identifier for this contract, represented as a URI 11942 * (globally unique). 11943 */ 11944 public String getUrl() { 11945 return this.url == null ? null : this.url.getValue(); 11946 } 11947 11948 /** 11949 * @param value Canonical identifier for this contract, represented as a URI 11950 * (globally unique). 11951 */ 11952 public Contract setUrl(String value) { 11953 if (Utilities.noString(value)) 11954 this.url = null; 11955 else { 11956 if (this.url == null) 11957 this.url = new UriType(); 11958 this.url.setValue(value); 11959 } 11960 return this; 11961 } 11962 11963 /** 11964 * @return {@link #version} (An edition identifier used for business purposes to 11965 * label business significant variants.). This is the underlying object 11966 * with id, value and extensions. The accessor "getVersion" gives direct 11967 * access to the value 11968 */ 11969 public StringType getVersionElement() { 11970 if (this.version == null) 11971 if (Configuration.errorOnAutoCreate()) 11972 throw new Error("Attempt to auto-create Contract.version"); 11973 else if (Configuration.doAutoCreate()) 11974 this.version = new StringType(); // bb 11975 return this.version; 11976 } 11977 11978 public boolean hasVersionElement() { 11979 return this.version != null && !this.version.isEmpty(); 11980 } 11981 11982 public boolean hasVersion() { 11983 return this.version != null && !this.version.isEmpty(); 11984 } 11985 11986 /** 11987 * @param value {@link #version} (An edition identifier used for business 11988 * purposes to label business significant variants.). This is the 11989 * underlying object with id, value and extensions. The accessor 11990 * "getVersion" gives direct access to the value 11991 */ 11992 public Contract setVersionElement(StringType value) { 11993 this.version = value; 11994 return this; 11995 } 11996 11997 /** 11998 * @return An edition identifier used for business purposes to label business 11999 * significant variants. 12000 */ 12001 public String getVersion() { 12002 return this.version == null ? null : this.version.getValue(); 12003 } 12004 12005 /** 12006 * @param value An edition identifier used for business purposes to label 12007 * business significant variants. 12008 */ 12009 public Contract setVersion(String value) { 12010 if (Utilities.noString(value)) 12011 this.version = null; 12012 else { 12013 if (this.version == null) 12014 this.version = new StringType(); 12015 this.version.setValue(value); 12016 } 12017 return this; 12018 } 12019 12020 /** 12021 * @return {@link #status} (The status of the resource instance.). This is the 12022 * underlying object with id, value and extensions. The accessor 12023 * "getStatus" gives direct access to the value 12024 */ 12025 public Enumeration<ContractStatus> getStatusElement() { 12026 if (this.status == null) 12027 if (Configuration.errorOnAutoCreate()) 12028 throw new Error("Attempt to auto-create Contract.status"); 12029 else if (Configuration.doAutoCreate()) 12030 this.status = new Enumeration<ContractStatus>(new ContractStatusEnumFactory()); // bb 12031 return this.status; 12032 } 12033 12034 public boolean hasStatusElement() { 12035 return this.status != null && !this.status.isEmpty(); 12036 } 12037 12038 public boolean hasStatus() { 12039 return this.status != null && !this.status.isEmpty(); 12040 } 12041 12042 /** 12043 * @param value {@link #status} (The status of the resource instance.). This is 12044 * the underlying object with id, value and extensions. The 12045 * accessor "getStatus" gives direct access to the value 12046 */ 12047 public Contract setStatusElement(Enumeration<ContractStatus> value) { 12048 this.status = value; 12049 return this; 12050 } 12051 12052 /** 12053 * @return The status of the resource instance. 12054 */ 12055 public ContractStatus getStatus() { 12056 return this.status == null ? null : this.status.getValue(); 12057 } 12058 12059 /** 12060 * @param value The status of the resource instance. 12061 */ 12062 public Contract setStatus(ContractStatus value) { 12063 if (value == null) 12064 this.status = null; 12065 else { 12066 if (this.status == null) 12067 this.status = new Enumeration<ContractStatus>(new ContractStatusEnumFactory()); 12068 this.status.setValue(value); 12069 } 12070 return this; 12071 } 12072 12073 /** 12074 * @return {@link #legalState} (Legal states of the formation of a legal 12075 * instrument, which is a formally executed written document that can be 12076 * formally attributed to its author, records and formally expresses a 12077 * legally enforceable act, process, or contractual duty, obligation, or 12078 * right, and therefore evidences that act, process, or agreement.) 12079 */ 12080 public CodeableConcept getLegalState() { 12081 if (this.legalState == null) 12082 if (Configuration.errorOnAutoCreate()) 12083 throw new Error("Attempt to auto-create Contract.legalState"); 12084 else if (Configuration.doAutoCreate()) 12085 this.legalState = new CodeableConcept(); // cc 12086 return this.legalState; 12087 } 12088 12089 public boolean hasLegalState() { 12090 return this.legalState != null && !this.legalState.isEmpty(); 12091 } 12092 12093 /** 12094 * @param value {@link #legalState} (Legal states of the formation of a legal 12095 * instrument, which is a formally executed written document that 12096 * can be formally attributed to its author, records and formally 12097 * expresses a legally enforceable act, process, or contractual 12098 * duty, obligation, or right, and therefore evidences that act, 12099 * process, or agreement.) 12100 */ 12101 public Contract setLegalState(CodeableConcept value) { 12102 this.legalState = value; 12103 return this; 12104 } 12105 12106 /** 12107 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined 12108 * Contract Definition that is adhered to in whole or part by this 12109 * Contract.) 12110 */ 12111 public Reference getInstantiatesCanonical() { 12112 if (this.instantiatesCanonical == null) 12113 if (Configuration.errorOnAutoCreate()) 12114 throw new Error("Attempt to auto-create Contract.instantiatesCanonical"); 12115 else if (Configuration.doAutoCreate()) 12116 this.instantiatesCanonical = new Reference(); // cc 12117 return this.instantiatesCanonical; 12118 } 12119 12120 public boolean hasInstantiatesCanonical() { 12121 return this.instantiatesCanonical != null && !this.instantiatesCanonical.isEmpty(); 12122 } 12123 12124 /** 12125 * @param value {@link #instantiatesCanonical} (The URL pointing to a 12126 * FHIR-defined Contract Definition that is adhered to in whole or 12127 * part by this Contract.) 12128 */ 12129 public Contract setInstantiatesCanonical(Reference value) { 12130 this.instantiatesCanonical = value; 12131 return this; 12132 } 12133 12134 /** 12135 * @return {@link #instantiatesCanonical} The actual object that is the target 12136 * of the reference. The reference library doesn't populate this, but 12137 * you can use it to hold the resource if you resolve it. (The URL 12138 * pointing to a FHIR-defined Contract Definition that is adhered to in 12139 * whole or part by this Contract.) 12140 */ 12141 public Contract getInstantiatesCanonicalTarget() { 12142 if (this.instantiatesCanonicalTarget == null) 12143 if (Configuration.errorOnAutoCreate()) 12144 throw new Error("Attempt to auto-create Contract.instantiatesCanonical"); 12145 else if (Configuration.doAutoCreate()) 12146 this.instantiatesCanonicalTarget = new Contract(); // aa 12147 return this.instantiatesCanonicalTarget; 12148 } 12149 12150 /** 12151 * @param value {@link #instantiatesCanonical} The actual object that is the 12152 * target of the reference. The reference library doesn't use 12153 * these, but you can use it to hold the resource if you resolve 12154 * it. (The URL pointing to a FHIR-defined Contract Definition that 12155 * is adhered to in whole or part by this Contract.) 12156 */ 12157 public Contract setInstantiatesCanonicalTarget(Contract value) { 12158 this.instantiatesCanonicalTarget = value; 12159 return this; 12160 } 12161 12162 /** 12163 * @return {@link #instantiatesUri} (The URL pointing to an externally 12164 * maintained definition that is adhered to in whole or in part by this 12165 * Contract.). This is the underlying object with id, value and 12166 * extensions. The accessor "getInstantiatesUri" gives direct access to 12167 * the value 12168 */ 12169 public UriType getInstantiatesUriElement() { 12170 if (this.instantiatesUri == null) 12171 if (Configuration.errorOnAutoCreate()) 12172 throw new Error("Attempt to auto-create Contract.instantiatesUri"); 12173 else if (Configuration.doAutoCreate()) 12174 this.instantiatesUri = new UriType(); // bb 12175 return this.instantiatesUri; 12176 } 12177 12178 public boolean hasInstantiatesUriElement() { 12179 return this.instantiatesUri != null && !this.instantiatesUri.isEmpty(); 12180 } 12181 12182 public boolean hasInstantiatesUri() { 12183 return this.instantiatesUri != null && !this.instantiatesUri.isEmpty(); 12184 } 12185 12186 /** 12187 * @param value {@link #instantiatesUri} (The URL pointing to an externally 12188 * maintained definition that is adhered to in whole or in part by 12189 * this Contract.). This is the underlying object with id, value 12190 * and extensions. The accessor "getInstantiatesUri" gives direct 12191 * access to the value 12192 */ 12193 public Contract setInstantiatesUriElement(UriType value) { 12194 this.instantiatesUri = value; 12195 return this; 12196 } 12197 12198 /** 12199 * @return The URL pointing to an externally maintained definition that is 12200 * adhered to in whole or in part by this Contract. 12201 */ 12202 public String getInstantiatesUri() { 12203 return this.instantiatesUri == null ? null : this.instantiatesUri.getValue(); 12204 } 12205 12206 /** 12207 * @param value The URL pointing to an externally maintained definition that is 12208 * adhered to in whole or in part by this Contract. 12209 */ 12210 public Contract setInstantiatesUri(String value) { 12211 if (Utilities.noString(value)) 12212 this.instantiatesUri = null; 12213 else { 12214 if (this.instantiatesUri == null) 12215 this.instantiatesUri = new UriType(); 12216 this.instantiatesUri.setValue(value); 12217 } 12218 return this; 12219 } 12220 12221 /** 12222 * @return {@link #contentDerivative} (The minimal content derived from the 12223 * basal information source at a specific stage in its lifecycle.) 12224 */ 12225 public CodeableConcept getContentDerivative() { 12226 if (this.contentDerivative == null) 12227 if (Configuration.errorOnAutoCreate()) 12228 throw new Error("Attempt to auto-create Contract.contentDerivative"); 12229 else if (Configuration.doAutoCreate()) 12230 this.contentDerivative = new CodeableConcept(); // cc 12231 return this.contentDerivative; 12232 } 12233 12234 public boolean hasContentDerivative() { 12235 return this.contentDerivative != null && !this.contentDerivative.isEmpty(); 12236 } 12237 12238 /** 12239 * @param value {@link #contentDerivative} (The minimal content derived from the 12240 * basal information source at a specific stage in its lifecycle.) 12241 */ 12242 public Contract setContentDerivative(CodeableConcept value) { 12243 this.contentDerivative = value; 12244 return this; 12245 } 12246 12247 /** 12248 * @return {@link #issued} (When this Contract was issued.). This is the 12249 * underlying object with id, value and extensions. The accessor 12250 * "getIssued" gives direct access to the value 12251 */ 12252 public DateTimeType getIssuedElement() { 12253 if (this.issued == null) 12254 if (Configuration.errorOnAutoCreate()) 12255 throw new Error("Attempt to auto-create Contract.issued"); 12256 else if (Configuration.doAutoCreate()) 12257 this.issued = new DateTimeType(); // bb 12258 return this.issued; 12259 } 12260 12261 public boolean hasIssuedElement() { 12262 return this.issued != null && !this.issued.isEmpty(); 12263 } 12264 12265 public boolean hasIssued() { 12266 return this.issued != null && !this.issued.isEmpty(); 12267 } 12268 12269 /** 12270 * @param value {@link #issued} (When this Contract was issued.). This is the 12271 * underlying object with id, value and extensions. The accessor 12272 * "getIssued" gives direct access to the value 12273 */ 12274 public Contract setIssuedElement(DateTimeType value) { 12275 this.issued = value; 12276 return this; 12277 } 12278 12279 /** 12280 * @return When this Contract was issued. 12281 */ 12282 public Date getIssued() { 12283 return this.issued == null ? null : this.issued.getValue(); 12284 } 12285 12286 /** 12287 * @param value When this Contract was issued. 12288 */ 12289 public Contract setIssued(Date value) { 12290 if (value == null) 12291 this.issued = null; 12292 else { 12293 if (this.issued == null) 12294 this.issued = new DateTimeType(); 12295 this.issued.setValue(value); 12296 } 12297 return this; 12298 } 12299 12300 /** 12301 * @return {@link #applies} (Relevant time or time-period when this Contract is 12302 * applicable.) 12303 */ 12304 public Period getApplies() { 12305 if (this.applies == null) 12306 if (Configuration.errorOnAutoCreate()) 12307 throw new Error("Attempt to auto-create Contract.applies"); 12308 else if (Configuration.doAutoCreate()) 12309 this.applies = new Period(); // cc 12310 return this.applies; 12311 } 12312 12313 public boolean hasApplies() { 12314 return this.applies != null && !this.applies.isEmpty(); 12315 } 12316 12317 /** 12318 * @param value {@link #applies} (Relevant time or time-period when this 12319 * Contract is applicable.) 12320 */ 12321 public Contract setApplies(Period value) { 12322 this.applies = value; 12323 return this; 12324 } 12325 12326 /** 12327 * @return {@link #expirationType} (Event resulting in discontinuation or 12328 * termination of this Contract instance by one or more parties to the 12329 * contract.) 12330 */ 12331 public CodeableConcept getExpirationType() { 12332 if (this.expirationType == null) 12333 if (Configuration.errorOnAutoCreate()) 12334 throw new Error("Attempt to auto-create Contract.expirationType"); 12335 else if (Configuration.doAutoCreate()) 12336 this.expirationType = new CodeableConcept(); // cc 12337 return this.expirationType; 12338 } 12339 12340 public boolean hasExpirationType() { 12341 return this.expirationType != null && !this.expirationType.isEmpty(); 12342 } 12343 12344 /** 12345 * @param value {@link #expirationType} (Event resulting in discontinuation or 12346 * termination of this Contract instance by one or more parties to 12347 * the contract.) 12348 */ 12349 public Contract setExpirationType(CodeableConcept value) { 12350 this.expirationType = value; 12351 return this; 12352 } 12353 12354 /** 12355 * @return {@link #subject} (The target entity impacted by or of interest to 12356 * parties to the agreement.) 12357 */ 12358 public List<Reference> getSubject() { 12359 if (this.subject == null) 12360 this.subject = new ArrayList<Reference>(); 12361 return this.subject; 12362 } 12363 12364 /** 12365 * @return Returns a reference to <code>this</code> for easy method chaining 12366 */ 12367 public Contract setSubject(List<Reference> theSubject) { 12368 this.subject = theSubject; 12369 return this; 12370 } 12371 12372 public boolean hasSubject() { 12373 if (this.subject == null) 12374 return false; 12375 for (Reference item : this.subject) 12376 if (!item.isEmpty()) 12377 return true; 12378 return false; 12379 } 12380 12381 public Reference addSubject() { // 3 12382 Reference t = new Reference(); 12383 if (this.subject == null) 12384 this.subject = new ArrayList<Reference>(); 12385 this.subject.add(t); 12386 return t; 12387 } 12388 12389 public Contract addSubject(Reference t) { // 3 12390 if (t == null) 12391 return this; 12392 if (this.subject == null) 12393 this.subject = new ArrayList<Reference>(); 12394 this.subject.add(t); 12395 return this; 12396 } 12397 12398 /** 12399 * @return The first repetition of repeating field {@link #subject}, creating it 12400 * if it does not already exist 12401 */ 12402 public Reference getSubjectFirstRep() { 12403 if (getSubject().isEmpty()) { 12404 addSubject(); 12405 } 12406 return getSubject().get(0); 12407 } 12408 12409 /** 12410 * @deprecated Use Reference#setResource(IBaseResource) instead 12411 */ 12412 @Deprecated 12413 public List<Resource> getSubjectTarget() { 12414 if (this.subjectTarget == null) 12415 this.subjectTarget = new ArrayList<Resource>(); 12416 return this.subjectTarget; 12417 } 12418 12419 /** 12420 * @return {@link #authority} (A formally or informally recognized grouping of 12421 * people, principals, organizations, or jurisdictions formed for the 12422 * purpose of achieving some form of collective action such as the 12423 * promulgation, administration and enforcement of contracts and 12424 * policies.) 12425 */ 12426 public List<Reference> getAuthority() { 12427 if (this.authority == null) 12428 this.authority = new ArrayList<Reference>(); 12429 return this.authority; 12430 } 12431 12432 /** 12433 * @return Returns a reference to <code>this</code> for easy method chaining 12434 */ 12435 public Contract setAuthority(List<Reference> theAuthority) { 12436 this.authority = theAuthority; 12437 return this; 12438 } 12439 12440 public boolean hasAuthority() { 12441 if (this.authority == null) 12442 return false; 12443 for (Reference item : this.authority) 12444 if (!item.isEmpty()) 12445 return true; 12446 return false; 12447 } 12448 12449 public Reference addAuthority() { // 3 12450 Reference t = new Reference(); 12451 if (this.authority == null) 12452 this.authority = new ArrayList<Reference>(); 12453 this.authority.add(t); 12454 return t; 12455 } 12456 12457 public Contract addAuthority(Reference t) { // 3 12458 if (t == null) 12459 return this; 12460 if (this.authority == null) 12461 this.authority = new ArrayList<Reference>(); 12462 this.authority.add(t); 12463 return this; 12464 } 12465 12466 /** 12467 * @return The first repetition of repeating field {@link #authority}, creating 12468 * it if it does not already exist 12469 */ 12470 public Reference getAuthorityFirstRep() { 12471 if (getAuthority().isEmpty()) { 12472 addAuthority(); 12473 } 12474 return getAuthority().get(0); 12475 } 12476 12477 /** 12478 * @deprecated Use Reference#setResource(IBaseResource) instead 12479 */ 12480 @Deprecated 12481 public List<Organization> getAuthorityTarget() { 12482 if (this.authorityTarget == null) 12483 this.authorityTarget = new ArrayList<Organization>(); 12484 return this.authorityTarget; 12485 } 12486 12487 /** 12488 * @deprecated Use Reference#setResource(IBaseResource) instead 12489 */ 12490 @Deprecated 12491 public Organization addAuthorityTarget() { 12492 Organization r = new Organization(); 12493 if (this.authorityTarget == null) 12494 this.authorityTarget = new ArrayList<Organization>(); 12495 this.authorityTarget.add(r); 12496 return r; 12497 } 12498 12499 /** 12500 * @return {@link #domain} (Recognized governance framework or system operating 12501 * with a circumscribed scope in accordance with specified principles, 12502 * policies, processes or procedures for managing rights, actions, or 12503 * behaviors of parties or principals relative to resources.) 12504 */ 12505 public List<Reference> getDomain() { 12506 if (this.domain == null) 12507 this.domain = new ArrayList<Reference>(); 12508 return this.domain; 12509 } 12510 12511 /** 12512 * @return Returns a reference to <code>this</code> for easy method chaining 12513 */ 12514 public Contract setDomain(List<Reference> theDomain) { 12515 this.domain = theDomain; 12516 return this; 12517 } 12518 12519 public boolean hasDomain() { 12520 if (this.domain == null) 12521 return false; 12522 for (Reference item : this.domain) 12523 if (!item.isEmpty()) 12524 return true; 12525 return false; 12526 } 12527 12528 public Reference addDomain() { // 3 12529 Reference t = new Reference(); 12530 if (this.domain == null) 12531 this.domain = new ArrayList<Reference>(); 12532 this.domain.add(t); 12533 return t; 12534 } 12535 12536 public Contract addDomain(Reference t) { // 3 12537 if (t == null) 12538 return this; 12539 if (this.domain == null) 12540 this.domain = new ArrayList<Reference>(); 12541 this.domain.add(t); 12542 return this; 12543 } 12544 12545 /** 12546 * @return The first repetition of repeating field {@link #domain}, creating it 12547 * if it does not already exist 12548 */ 12549 public Reference getDomainFirstRep() { 12550 if (getDomain().isEmpty()) { 12551 addDomain(); 12552 } 12553 return getDomain().get(0); 12554 } 12555 12556 /** 12557 * @deprecated Use Reference#setResource(IBaseResource) instead 12558 */ 12559 @Deprecated 12560 public List<Location> getDomainTarget() { 12561 if (this.domainTarget == null) 12562 this.domainTarget = new ArrayList<Location>(); 12563 return this.domainTarget; 12564 } 12565 12566 /** 12567 * @deprecated Use Reference#setResource(IBaseResource) instead 12568 */ 12569 @Deprecated 12570 public Location addDomainTarget() { 12571 Location r = new Location(); 12572 if (this.domainTarget == null) 12573 this.domainTarget = new ArrayList<Location>(); 12574 this.domainTarget.add(r); 12575 return r; 12576 } 12577 12578 /** 12579 * @return {@link #site} (Sites in which the contract is complied with, 12580 * exercised, or in force.) 12581 */ 12582 public List<Reference> getSite() { 12583 if (this.site == null) 12584 this.site = new ArrayList<Reference>(); 12585 return this.site; 12586 } 12587 12588 /** 12589 * @return Returns a reference to <code>this</code> for easy method chaining 12590 */ 12591 public Contract setSite(List<Reference> theSite) { 12592 this.site = theSite; 12593 return this; 12594 } 12595 12596 public boolean hasSite() { 12597 if (this.site == null) 12598 return false; 12599 for (Reference item : this.site) 12600 if (!item.isEmpty()) 12601 return true; 12602 return false; 12603 } 12604 12605 public Reference addSite() { // 3 12606 Reference t = new Reference(); 12607 if (this.site == null) 12608 this.site = new ArrayList<Reference>(); 12609 this.site.add(t); 12610 return t; 12611 } 12612 12613 public Contract addSite(Reference t) { // 3 12614 if (t == null) 12615 return this; 12616 if (this.site == null) 12617 this.site = new ArrayList<Reference>(); 12618 this.site.add(t); 12619 return this; 12620 } 12621 12622 /** 12623 * @return The first repetition of repeating field {@link #site}, creating it if 12624 * it does not already exist 12625 */ 12626 public Reference getSiteFirstRep() { 12627 if (getSite().isEmpty()) { 12628 addSite(); 12629 } 12630 return getSite().get(0); 12631 } 12632 12633 /** 12634 * @deprecated Use Reference#setResource(IBaseResource) instead 12635 */ 12636 @Deprecated 12637 public List<Location> getSiteTarget() { 12638 if (this.siteTarget == null) 12639 this.siteTarget = new ArrayList<Location>(); 12640 return this.siteTarget; 12641 } 12642 12643 /** 12644 * @deprecated Use Reference#setResource(IBaseResource) instead 12645 */ 12646 @Deprecated 12647 public Location addSiteTarget() { 12648 Location r = new Location(); 12649 if (this.siteTarget == null) 12650 this.siteTarget = new ArrayList<Location>(); 12651 this.siteTarget.add(r); 12652 return r; 12653 } 12654 12655 /** 12656 * @return {@link #name} (A natural language name identifying this Contract 12657 * definition, derivative, or instance in any legal state. Provides 12658 * additional information about its content. This name should be usable 12659 * as an identifier for the module by machine processing applications 12660 * such as code generation.). This is the underlying object with id, 12661 * value and extensions. The accessor "getName" gives direct access to 12662 * the value 12663 */ 12664 public StringType getNameElement() { 12665 if (this.name == null) 12666 if (Configuration.errorOnAutoCreate()) 12667 throw new Error("Attempt to auto-create Contract.name"); 12668 else if (Configuration.doAutoCreate()) 12669 this.name = new StringType(); // bb 12670 return this.name; 12671 } 12672 12673 public boolean hasNameElement() { 12674 return this.name != null && !this.name.isEmpty(); 12675 } 12676 12677 public boolean hasName() { 12678 return this.name != null && !this.name.isEmpty(); 12679 } 12680 12681 /** 12682 * @param value {@link #name} (A natural language name identifying this Contract 12683 * definition, derivative, or instance in any legal state. Provides 12684 * additional information about its content. This name should be 12685 * usable as an identifier for the module by machine processing 12686 * applications such as code generation.). This is the underlying 12687 * object with id, value and extensions. The accessor "getName" 12688 * gives direct access to the value 12689 */ 12690 public Contract setNameElement(StringType value) { 12691 this.name = value; 12692 return this; 12693 } 12694 12695 /** 12696 * @return A natural language name identifying this Contract definition, 12697 * derivative, or instance in any legal state. Provides additional 12698 * information about its content. This name should be usable as an 12699 * identifier for the module by machine processing applications such as 12700 * code generation. 12701 */ 12702 public String getName() { 12703 return this.name == null ? null : this.name.getValue(); 12704 } 12705 12706 /** 12707 * @param value A natural language name identifying this Contract definition, 12708 * derivative, or instance in any legal state. Provides additional 12709 * information about its content. This name should be usable as an 12710 * identifier for the module by machine processing applications 12711 * such as code generation. 12712 */ 12713 public Contract setName(String value) { 12714 if (Utilities.noString(value)) 12715 this.name = null; 12716 else { 12717 if (this.name == null) 12718 this.name = new StringType(); 12719 this.name.setValue(value); 12720 } 12721 return this; 12722 } 12723 12724 /** 12725 * @return {@link #title} (A short, descriptive, user-friendly title for this 12726 * Contract definition, derivative, or instance in any legal state.t 12727 * giving additional information about its content.). This is the 12728 * underlying object with id, value and extensions. The accessor 12729 * "getTitle" gives direct access to the value 12730 */ 12731 public StringType getTitleElement() { 12732 if (this.title == null) 12733 if (Configuration.errorOnAutoCreate()) 12734 throw new Error("Attempt to auto-create Contract.title"); 12735 else if (Configuration.doAutoCreate()) 12736 this.title = new StringType(); // bb 12737 return this.title; 12738 } 12739 12740 public boolean hasTitleElement() { 12741 return this.title != null && !this.title.isEmpty(); 12742 } 12743 12744 public boolean hasTitle() { 12745 return this.title != null && !this.title.isEmpty(); 12746 } 12747 12748 /** 12749 * @param value {@link #title} (A short, descriptive, user-friendly title for 12750 * this Contract definition, derivative, or instance in any legal 12751 * state.t giving additional information about its content.). This 12752 * is the underlying object with id, value and extensions. The 12753 * accessor "getTitle" gives direct access to the value 12754 */ 12755 public Contract setTitleElement(StringType value) { 12756 this.title = value; 12757 return this; 12758 } 12759 12760 /** 12761 * @return A short, descriptive, user-friendly title for this Contract 12762 * definition, derivative, or instance in any legal state.t giving 12763 * additional information about its content. 12764 */ 12765 public String getTitle() { 12766 return this.title == null ? null : this.title.getValue(); 12767 } 12768 12769 /** 12770 * @param value A short, descriptive, user-friendly title for this Contract 12771 * definition, derivative, or instance in any legal state.t giving 12772 * additional information about its content. 12773 */ 12774 public Contract setTitle(String value) { 12775 if (Utilities.noString(value)) 12776 this.title = null; 12777 else { 12778 if (this.title == null) 12779 this.title = new StringType(); 12780 this.title.setValue(value); 12781 } 12782 return this; 12783 } 12784 12785 /** 12786 * @return {@link #subtitle} (An explanatory or alternate user-friendly title 12787 * for this Contract definition, derivative, or instance in any legal 12788 * state.t giving additional information about its content.). This is 12789 * the underlying object with id, value and extensions. The accessor 12790 * "getSubtitle" gives direct access to the value 12791 */ 12792 public StringType getSubtitleElement() { 12793 if (this.subtitle == null) 12794 if (Configuration.errorOnAutoCreate()) 12795 throw new Error("Attempt to auto-create Contract.subtitle"); 12796 else if (Configuration.doAutoCreate()) 12797 this.subtitle = new StringType(); // bb 12798 return this.subtitle; 12799 } 12800 12801 public boolean hasSubtitleElement() { 12802 return this.subtitle != null && !this.subtitle.isEmpty(); 12803 } 12804 12805 public boolean hasSubtitle() { 12806 return this.subtitle != null && !this.subtitle.isEmpty(); 12807 } 12808 12809 /** 12810 * @param value {@link #subtitle} (An explanatory or alternate user-friendly 12811 * title for this Contract definition, derivative, or instance in 12812 * any legal state.t giving additional information about its 12813 * content.). This is the underlying object with id, value and 12814 * extensions. The accessor "getSubtitle" gives direct access to 12815 * the value 12816 */ 12817 public Contract setSubtitleElement(StringType value) { 12818 this.subtitle = value; 12819 return this; 12820 } 12821 12822 /** 12823 * @return An explanatory or alternate user-friendly title for this Contract 12824 * definition, derivative, or instance in any legal state.t giving 12825 * additional information about its content. 12826 */ 12827 public String getSubtitle() { 12828 return this.subtitle == null ? null : this.subtitle.getValue(); 12829 } 12830 12831 /** 12832 * @param value An explanatory or alternate user-friendly title for this 12833 * Contract definition, derivative, or instance in any legal 12834 * state.t giving additional information about its content. 12835 */ 12836 public Contract setSubtitle(String value) { 12837 if (Utilities.noString(value)) 12838 this.subtitle = null; 12839 else { 12840 if (this.subtitle == null) 12841 this.subtitle = new StringType(); 12842 this.subtitle.setValue(value); 12843 } 12844 return this; 12845 } 12846 12847 /** 12848 * @return {@link #alias} (Alternative representation of the title for this 12849 * Contract definition, derivative, or instance in any legal state., 12850 * e.g., a domain specific contract number related to legislation.) 12851 */ 12852 public List<StringType> getAlias() { 12853 if (this.alias == null) 12854 this.alias = new ArrayList<StringType>(); 12855 return this.alias; 12856 } 12857 12858 /** 12859 * @return Returns a reference to <code>this</code> for easy method chaining 12860 */ 12861 public Contract setAlias(List<StringType> theAlias) { 12862 this.alias = theAlias; 12863 return this; 12864 } 12865 12866 public boolean hasAlias() { 12867 if (this.alias == null) 12868 return false; 12869 for (StringType item : this.alias) 12870 if (!item.isEmpty()) 12871 return true; 12872 return false; 12873 } 12874 12875 /** 12876 * @return {@link #alias} (Alternative representation of the title for this 12877 * Contract definition, derivative, or instance in any legal state., 12878 * e.g., a domain specific contract number related to legislation.) 12879 */ 12880 public StringType addAliasElement() {// 2 12881 StringType t = new StringType(); 12882 if (this.alias == null) 12883 this.alias = new ArrayList<StringType>(); 12884 this.alias.add(t); 12885 return t; 12886 } 12887 12888 /** 12889 * @param value {@link #alias} (Alternative representation of the title for this 12890 * Contract definition, derivative, or instance in any legal 12891 * state., e.g., a domain specific contract number related to 12892 * legislation.) 12893 */ 12894 public Contract addAlias(String value) { // 1 12895 StringType t = new StringType(); 12896 t.setValue(value); 12897 if (this.alias == null) 12898 this.alias = new ArrayList<StringType>(); 12899 this.alias.add(t); 12900 return this; 12901 } 12902 12903 /** 12904 * @param value {@link #alias} (Alternative representation of the title for this 12905 * Contract definition, derivative, or instance in any legal 12906 * state., e.g., a domain specific contract number related to 12907 * legislation.) 12908 */ 12909 public boolean hasAlias(String value) { 12910 if (this.alias == null) 12911 return false; 12912 for (StringType v : this.alias) 12913 if (v.getValue().equals(value)) // string 12914 return true; 12915 return false; 12916 } 12917 12918 /** 12919 * @return {@link #author} (The individual or organization that authored the 12920 * Contract definition, derivative, or instance in any legal state.) 12921 */ 12922 public Reference getAuthor() { 12923 if (this.author == null) 12924 if (Configuration.errorOnAutoCreate()) 12925 throw new Error("Attempt to auto-create Contract.author"); 12926 else if (Configuration.doAutoCreate()) 12927 this.author = new Reference(); // cc 12928 return this.author; 12929 } 12930 12931 public boolean hasAuthor() { 12932 return this.author != null && !this.author.isEmpty(); 12933 } 12934 12935 /** 12936 * @param value {@link #author} (The individual or organization that authored 12937 * the Contract definition, derivative, or instance in any legal 12938 * state.) 12939 */ 12940 public Contract setAuthor(Reference value) { 12941 this.author = value; 12942 return this; 12943 } 12944 12945 /** 12946 * @return {@link #author} The actual object that is the target of the 12947 * reference. The reference library doesn't populate this, but you can 12948 * use it to hold the resource if you resolve it. (The individual or 12949 * organization that authored the Contract definition, derivative, or 12950 * instance in any legal state.) 12951 */ 12952 public Resource getAuthorTarget() { 12953 return this.authorTarget; 12954 } 12955 12956 /** 12957 * @param value {@link #author} The actual object that is the target of the 12958 * reference. The reference library doesn't use these, but you can 12959 * use it to hold the resource if you resolve it. (The individual 12960 * or organization that authored the Contract definition, 12961 * derivative, or instance in any legal state.) 12962 */ 12963 public Contract setAuthorTarget(Resource value) { 12964 this.authorTarget = value; 12965 return this; 12966 } 12967 12968 /** 12969 * @return {@link #scope} (A selector of legal concerns for this Contract 12970 * definition, derivative, or instance in any legal state.) 12971 */ 12972 public CodeableConcept getScope() { 12973 if (this.scope == null) 12974 if (Configuration.errorOnAutoCreate()) 12975 throw new Error("Attempt to auto-create Contract.scope"); 12976 else if (Configuration.doAutoCreate()) 12977 this.scope = new CodeableConcept(); // cc 12978 return this.scope; 12979 } 12980 12981 public boolean hasScope() { 12982 return this.scope != null && !this.scope.isEmpty(); 12983 } 12984 12985 /** 12986 * @param value {@link #scope} (A selector of legal concerns for this Contract 12987 * definition, derivative, or instance in any legal state.) 12988 */ 12989 public Contract setScope(CodeableConcept value) { 12990 this.scope = value; 12991 return this; 12992 } 12993 12994 /** 12995 * @return {@link #topic} (Narrows the range of legal concerns to focus on the 12996 * achievement of specific contractual objectives.) 12997 */ 12998 public Type getTopic() { 12999 return this.topic; 13000 } 13001 13002 /** 13003 * @return {@link #topic} (Narrows the range of legal concerns to focus on the 13004 * achievement of specific contractual objectives.) 13005 */ 13006 public CodeableConcept getTopicCodeableConcept() throws FHIRException { 13007 if (this.topic == null) 13008 this.topic = new CodeableConcept(); 13009 if (!(this.topic instanceof CodeableConcept)) 13010 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 13011 + this.topic.getClass().getName() + " was encountered"); 13012 return (CodeableConcept) this.topic; 13013 } 13014 13015 public boolean hasTopicCodeableConcept() { 13016 return this != null && this.topic instanceof CodeableConcept; 13017 } 13018 13019 /** 13020 * @return {@link #topic} (Narrows the range of legal concerns to focus on the 13021 * achievement of specific contractual objectives.) 13022 */ 13023 public Reference getTopicReference() throws FHIRException { 13024 if (this.topic == null) 13025 this.topic = new Reference(); 13026 if (!(this.topic instanceof Reference)) 13027 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.topic.getClass().getName() 13028 + " was encountered"); 13029 return (Reference) this.topic; 13030 } 13031 13032 public boolean hasTopicReference() { 13033 return this != null && this.topic instanceof Reference; 13034 } 13035 13036 public boolean hasTopic() { 13037 return this.topic != null && !this.topic.isEmpty(); 13038 } 13039 13040 /** 13041 * @param value {@link #topic} (Narrows the range of legal concerns to focus on 13042 * the achievement of specific contractual objectives.) 13043 */ 13044 public Contract setTopic(Type value) { 13045 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 13046 throw new Error("Not the right type for Contract.topic[x]: " + value.fhirType()); 13047 this.topic = value; 13048 return this; 13049 } 13050 13051 /** 13052 * @return {@link #type} (A high-level category for the legal instrument, 13053 * whether constructed as a Contract definition, derivative, or instance 13054 * in any legal state. Provides additional information about its content 13055 * within the context of the Contract's scope to distinguish the kinds 13056 * of systems that would be interested in the contract.) 13057 */ 13058 public CodeableConcept getType() { 13059 if (this.type == null) 13060 if (Configuration.errorOnAutoCreate()) 13061 throw new Error("Attempt to auto-create Contract.type"); 13062 else if (Configuration.doAutoCreate()) 13063 this.type = new CodeableConcept(); // cc 13064 return this.type; 13065 } 13066 13067 public boolean hasType() { 13068 return this.type != null && !this.type.isEmpty(); 13069 } 13070 13071 /** 13072 * @param value {@link #type} (A high-level category for the legal instrument, 13073 * whether constructed as a Contract definition, derivative, or 13074 * instance in any legal state. Provides additional information 13075 * about its content within the context of the Contract's scope to 13076 * distinguish the kinds of systems that would be interested in the 13077 * contract.) 13078 */ 13079 public Contract setType(CodeableConcept value) { 13080 this.type = value; 13081 return this; 13082 } 13083 13084 /** 13085 * @return {@link #subType} (Sub-category for the Contract that distinguishes 13086 * the kinds of systems that would be interested in the Contract within 13087 * the context of the Contract's scope.) 13088 */ 13089 public List<CodeableConcept> getSubType() { 13090 if (this.subType == null) 13091 this.subType = new ArrayList<CodeableConcept>(); 13092 return this.subType; 13093 } 13094 13095 /** 13096 * @return Returns a reference to <code>this</code> for easy method chaining 13097 */ 13098 public Contract setSubType(List<CodeableConcept> theSubType) { 13099 this.subType = theSubType; 13100 return this; 13101 } 13102 13103 public boolean hasSubType() { 13104 if (this.subType == null) 13105 return false; 13106 for (CodeableConcept item : this.subType) 13107 if (!item.isEmpty()) 13108 return true; 13109 return false; 13110 } 13111 13112 public CodeableConcept addSubType() { // 3 13113 CodeableConcept t = new CodeableConcept(); 13114 if (this.subType == null) 13115 this.subType = new ArrayList<CodeableConcept>(); 13116 this.subType.add(t); 13117 return t; 13118 } 13119 13120 public Contract addSubType(CodeableConcept t) { // 3 13121 if (t == null) 13122 return this; 13123 if (this.subType == null) 13124 this.subType = new ArrayList<CodeableConcept>(); 13125 this.subType.add(t); 13126 return this; 13127 } 13128 13129 /** 13130 * @return The first repetition of repeating field {@link #subType}, creating it 13131 * if it does not already exist 13132 */ 13133 public CodeableConcept getSubTypeFirstRep() { 13134 if (getSubType().isEmpty()) { 13135 addSubType(); 13136 } 13137 return getSubType().get(0); 13138 } 13139 13140 /** 13141 * @return {@link #contentDefinition} (Precusory content developed with a focus 13142 * and intent of supporting the formation a Contract instance, which may 13143 * be associated with and transformable into a Contract.) 13144 */ 13145 public ContentDefinitionComponent getContentDefinition() { 13146 if (this.contentDefinition == null) 13147 if (Configuration.errorOnAutoCreate()) 13148 throw new Error("Attempt to auto-create Contract.contentDefinition"); 13149 else if (Configuration.doAutoCreate()) 13150 this.contentDefinition = new ContentDefinitionComponent(); // cc 13151 return this.contentDefinition; 13152 } 13153 13154 public boolean hasContentDefinition() { 13155 return this.contentDefinition != null && !this.contentDefinition.isEmpty(); 13156 } 13157 13158 /** 13159 * @param value {@link #contentDefinition} (Precusory content developed with a 13160 * focus and intent of supporting the formation a Contract 13161 * instance, which may be associated with and transformable into a 13162 * Contract.) 13163 */ 13164 public Contract setContentDefinition(ContentDefinitionComponent value) { 13165 this.contentDefinition = value; 13166 return this; 13167 } 13168 13169 /** 13170 * @return {@link #term} (One or more Contract Provisions, which may be related 13171 * and conveyed as a group, and may contain nested groups.) 13172 */ 13173 public List<TermComponent> getTerm() { 13174 if (this.term == null) 13175 this.term = new ArrayList<TermComponent>(); 13176 return this.term; 13177 } 13178 13179 /** 13180 * @return Returns a reference to <code>this</code> for easy method chaining 13181 */ 13182 public Contract setTerm(List<TermComponent> theTerm) { 13183 this.term = theTerm; 13184 return this; 13185 } 13186 13187 public boolean hasTerm() { 13188 if (this.term == null) 13189 return false; 13190 for (TermComponent item : this.term) 13191 if (!item.isEmpty()) 13192 return true; 13193 return false; 13194 } 13195 13196 public TermComponent addTerm() { // 3 13197 TermComponent t = new TermComponent(); 13198 if (this.term == null) 13199 this.term = new ArrayList<TermComponent>(); 13200 this.term.add(t); 13201 return t; 13202 } 13203 13204 public Contract addTerm(TermComponent t) { // 3 13205 if (t == null) 13206 return this; 13207 if (this.term == null) 13208 this.term = new ArrayList<TermComponent>(); 13209 this.term.add(t); 13210 return this; 13211 } 13212 13213 /** 13214 * @return The first repetition of repeating field {@link #term}, creating it if 13215 * it does not already exist 13216 */ 13217 public TermComponent getTermFirstRep() { 13218 if (getTerm().isEmpty()) { 13219 addTerm(); 13220 } 13221 return getTerm().get(0); 13222 } 13223 13224 /** 13225 * @return {@link #supportingInfo} (Information that may be needed by/relevant 13226 * to the performer in their execution of this term action.) 13227 */ 13228 public List<Reference> getSupportingInfo() { 13229 if (this.supportingInfo == null) 13230 this.supportingInfo = new ArrayList<Reference>(); 13231 return this.supportingInfo; 13232 } 13233 13234 /** 13235 * @return Returns a reference to <code>this</code> for easy method chaining 13236 */ 13237 public Contract setSupportingInfo(List<Reference> theSupportingInfo) { 13238 this.supportingInfo = theSupportingInfo; 13239 return this; 13240 } 13241 13242 public boolean hasSupportingInfo() { 13243 if (this.supportingInfo == null) 13244 return false; 13245 for (Reference item : this.supportingInfo) 13246 if (!item.isEmpty()) 13247 return true; 13248 return false; 13249 } 13250 13251 public Reference addSupportingInfo() { // 3 13252 Reference t = new Reference(); 13253 if (this.supportingInfo == null) 13254 this.supportingInfo = new ArrayList<Reference>(); 13255 this.supportingInfo.add(t); 13256 return t; 13257 } 13258 13259 public Contract addSupportingInfo(Reference t) { // 3 13260 if (t == null) 13261 return this; 13262 if (this.supportingInfo == null) 13263 this.supportingInfo = new ArrayList<Reference>(); 13264 this.supportingInfo.add(t); 13265 return this; 13266 } 13267 13268 /** 13269 * @return The first repetition of repeating field {@link #supportingInfo}, 13270 * creating it if it does not already exist 13271 */ 13272 public Reference getSupportingInfoFirstRep() { 13273 if (getSupportingInfo().isEmpty()) { 13274 addSupportingInfo(); 13275 } 13276 return getSupportingInfo().get(0); 13277 } 13278 13279 /** 13280 * @deprecated Use Reference#setResource(IBaseResource) instead 13281 */ 13282 @Deprecated 13283 public List<Resource> getSupportingInfoTarget() { 13284 if (this.supportingInfoTarget == null) 13285 this.supportingInfoTarget = new ArrayList<Resource>(); 13286 return this.supportingInfoTarget; 13287 } 13288 13289 /** 13290 * @return {@link #relevantHistory} (Links to Provenance records for past 13291 * versions of this Contract definition, derivative, or instance, which 13292 * identify key state transitions or updates that are likely to be 13293 * relevant to a user looking at the current version of the Contract. 13294 * The Provence.entity indicates the target that was changed in the 13295 * update. 13296 * http://build.fhir.org/provenance-definitions.html#Provenance.entity.) 13297 */ 13298 public List<Reference> getRelevantHistory() { 13299 if (this.relevantHistory == null) 13300 this.relevantHistory = new ArrayList<Reference>(); 13301 return this.relevantHistory; 13302 } 13303 13304 /** 13305 * @return Returns a reference to <code>this</code> for easy method chaining 13306 */ 13307 public Contract setRelevantHistory(List<Reference> theRelevantHistory) { 13308 this.relevantHistory = theRelevantHistory; 13309 return this; 13310 } 13311 13312 public boolean hasRelevantHistory() { 13313 if (this.relevantHistory == null) 13314 return false; 13315 for (Reference item : this.relevantHistory) 13316 if (!item.isEmpty()) 13317 return true; 13318 return false; 13319 } 13320 13321 public Reference addRelevantHistory() { // 3 13322 Reference t = new Reference(); 13323 if (this.relevantHistory == null) 13324 this.relevantHistory = new ArrayList<Reference>(); 13325 this.relevantHistory.add(t); 13326 return t; 13327 } 13328 13329 public Contract addRelevantHistory(Reference t) { // 3 13330 if (t == null) 13331 return this; 13332 if (this.relevantHistory == null) 13333 this.relevantHistory = new ArrayList<Reference>(); 13334 this.relevantHistory.add(t); 13335 return this; 13336 } 13337 13338 /** 13339 * @return The first repetition of repeating field {@link #relevantHistory}, 13340 * creating it if it does not already exist 13341 */ 13342 public Reference getRelevantHistoryFirstRep() { 13343 if (getRelevantHistory().isEmpty()) { 13344 addRelevantHistory(); 13345 } 13346 return getRelevantHistory().get(0); 13347 } 13348 13349 /** 13350 * @deprecated Use Reference#setResource(IBaseResource) instead 13351 */ 13352 @Deprecated 13353 public List<Provenance> getRelevantHistoryTarget() { 13354 if (this.relevantHistoryTarget == null) 13355 this.relevantHistoryTarget = new ArrayList<Provenance>(); 13356 return this.relevantHistoryTarget; 13357 } 13358 13359 /** 13360 * @deprecated Use Reference#setResource(IBaseResource) instead 13361 */ 13362 @Deprecated 13363 public Provenance addRelevantHistoryTarget() { 13364 Provenance r = new Provenance(); 13365 if (this.relevantHistoryTarget == null) 13366 this.relevantHistoryTarget = new ArrayList<Provenance>(); 13367 this.relevantHistoryTarget.add(r); 13368 return r; 13369 } 13370 13371 /** 13372 * @return {@link #signer} (Parties with legal standing in the Contract, 13373 * including the principal parties, the grantor(s) and grantee(s), which 13374 * are any person or organization bound by the contract, and any 13375 * ancillary parties, which facilitate the execution of the contract 13376 * such as a notary or witness.) 13377 */ 13378 public List<SignatoryComponent> getSigner() { 13379 if (this.signer == null) 13380 this.signer = new ArrayList<SignatoryComponent>(); 13381 return this.signer; 13382 } 13383 13384 /** 13385 * @return Returns a reference to <code>this</code> for easy method chaining 13386 */ 13387 public Contract setSigner(List<SignatoryComponent> theSigner) { 13388 this.signer = theSigner; 13389 return this; 13390 } 13391 13392 public boolean hasSigner() { 13393 if (this.signer == null) 13394 return false; 13395 for (SignatoryComponent item : this.signer) 13396 if (!item.isEmpty()) 13397 return true; 13398 return false; 13399 } 13400 13401 public SignatoryComponent addSigner() { // 3 13402 SignatoryComponent t = new SignatoryComponent(); 13403 if (this.signer == null) 13404 this.signer = new ArrayList<SignatoryComponent>(); 13405 this.signer.add(t); 13406 return t; 13407 } 13408 13409 public Contract addSigner(SignatoryComponent t) { // 3 13410 if (t == null) 13411 return this; 13412 if (this.signer == null) 13413 this.signer = new ArrayList<SignatoryComponent>(); 13414 this.signer.add(t); 13415 return this; 13416 } 13417 13418 /** 13419 * @return The first repetition of repeating field {@link #signer}, creating it 13420 * if it does not already exist 13421 */ 13422 public SignatoryComponent getSignerFirstRep() { 13423 if (getSigner().isEmpty()) { 13424 addSigner(); 13425 } 13426 return getSigner().get(0); 13427 } 13428 13429 /** 13430 * @return {@link #friendly} (The "patient friendly language" versionof the 13431 * Contract in whole or in parts. "Patient friendly language" means the 13432 * representation of the Contract and Contract Provisions in a manner 13433 * that is readily accessible and understandable by a layperson in 13434 * accordance with best practices for communication styles that ensure 13435 * that those agreeing to or signing the Contract understand the roles, 13436 * actions, obligations, responsibilities, and implication of the 13437 * agreement.) 13438 */ 13439 public List<FriendlyLanguageComponent> getFriendly() { 13440 if (this.friendly == null) 13441 this.friendly = new ArrayList<FriendlyLanguageComponent>(); 13442 return this.friendly; 13443 } 13444 13445 /** 13446 * @return Returns a reference to <code>this</code> for easy method chaining 13447 */ 13448 public Contract setFriendly(List<FriendlyLanguageComponent> theFriendly) { 13449 this.friendly = theFriendly; 13450 return this; 13451 } 13452 13453 public boolean hasFriendly() { 13454 if (this.friendly == null) 13455 return false; 13456 for (FriendlyLanguageComponent item : this.friendly) 13457 if (!item.isEmpty()) 13458 return true; 13459 return false; 13460 } 13461 13462 public FriendlyLanguageComponent addFriendly() { // 3 13463 FriendlyLanguageComponent t = new FriendlyLanguageComponent(); 13464 if (this.friendly == null) 13465 this.friendly = new ArrayList<FriendlyLanguageComponent>(); 13466 this.friendly.add(t); 13467 return t; 13468 } 13469 13470 public Contract addFriendly(FriendlyLanguageComponent t) { // 3 13471 if (t == null) 13472 return this; 13473 if (this.friendly == null) 13474 this.friendly = new ArrayList<FriendlyLanguageComponent>(); 13475 this.friendly.add(t); 13476 return this; 13477 } 13478 13479 /** 13480 * @return The first repetition of repeating field {@link #friendly}, creating 13481 * it if it does not already exist 13482 */ 13483 public FriendlyLanguageComponent getFriendlyFirstRep() { 13484 if (getFriendly().isEmpty()) { 13485 addFriendly(); 13486 } 13487 return getFriendly().get(0); 13488 } 13489 13490 /** 13491 * @return {@link #legal} (List of Legal expressions or representations of this 13492 * Contract.) 13493 */ 13494 public List<LegalLanguageComponent> getLegal() { 13495 if (this.legal == null) 13496 this.legal = new ArrayList<LegalLanguageComponent>(); 13497 return this.legal; 13498 } 13499 13500 /** 13501 * @return Returns a reference to <code>this</code> for easy method chaining 13502 */ 13503 public Contract setLegal(List<LegalLanguageComponent> theLegal) { 13504 this.legal = theLegal; 13505 return this; 13506 } 13507 13508 public boolean hasLegal() { 13509 if (this.legal == null) 13510 return false; 13511 for (LegalLanguageComponent item : this.legal) 13512 if (!item.isEmpty()) 13513 return true; 13514 return false; 13515 } 13516 13517 public LegalLanguageComponent addLegal() { // 3 13518 LegalLanguageComponent t = new LegalLanguageComponent(); 13519 if (this.legal == null) 13520 this.legal = new ArrayList<LegalLanguageComponent>(); 13521 this.legal.add(t); 13522 return t; 13523 } 13524 13525 public Contract addLegal(LegalLanguageComponent t) { // 3 13526 if (t == null) 13527 return this; 13528 if (this.legal == null) 13529 this.legal = new ArrayList<LegalLanguageComponent>(); 13530 this.legal.add(t); 13531 return this; 13532 } 13533 13534 /** 13535 * @return The first repetition of repeating field {@link #legal}, creating it 13536 * if it does not already exist 13537 */ 13538 public LegalLanguageComponent getLegalFirstRep() { 13539 if (getLegal().isEmpty()) { 13540 addLegal(); 13541 } 13542 return getLegal().get(0); 13543 } 13544 13545 /** 13546 * @return {@link #rule} (List of Computable Policy Rule Language 13547 * Representations of this Contract.) 13548 */ 13549 public List<ComputableLanguageComponent> getRule() { 13550 if (this.rule == null) 13551 this.rule = new ArrayList<ComputableLanguageComponent>(); 13552 return this.rule; 13553 } 13554 13555 /** 13556 * @return Returns a reference to <code>this</code> for easy method chaining 13557 */ 13558 public Contract setRule(List<ComputableLanguageComponent> theRule) { 13559 this.rule = theRule; 13560 return this; 13561 } 13562 13563 public boolean hasRule() { 13564 if (this.rule == null) 13565 return false; 13566 for (ComputableLanguageComponent item : this.rule) 13567 if (!item.isEmpty()) 13568 return true; 13569 return false; 13570 } 13571 13572 public ComputableLanguageComponent addRule() { // 3 13573 ComputableLanguageComponent t = new ComputableLanguageComponent(); 13574 if (this.rule == null) 13575 this.rule = new ArrayList<ComputableLanguageComponent>(); 13576 this.rule.add(t); 13577 return t; 13578 } 13579 13580 public Contract addRule(ComputableLanguageComponent t) { // 3 13581 if (t == null) 13582 return this; 13583 if (this.rule == null) 13584 this.rule = new ArrayList<ComputableLanguageComponent>(); 13585 this.rule.add(t); 13586 return this; 13587 } 13588 13589 /** 13590 * @return The first repetition of repeating field {@link #rule}, creating it if 13591 * it does not already exist 13592 */ 13593 public ComputableLanguageComponent getRuleFirstRep() { 13594 if (getRule().isEmpty()) { 13595 addRule(); 13596 } 13597 return getRule().get(0); 13598 } 13599 13600 /** 13601 * @return {@link #legallyBinding} (Legally binding Contract: This is the signed 13602 * and legally recognized representation of the Contract, which is 13603 * considered the "source of truth" and which would be the basis for 13604 * legal action related to enforcement of this Contract.) 13605 */ 13606 public Type getLegallyBinding() { 13607 return this.legallyBinding; 13608 } 13609 13610 /** 13611 * @return {@link #legallyBinding} (Legally binding Contract: This is the signed 13612 * and legally recognized representation of the Contract, which is 13613 * considered the "source of truth" and which would be the basis for 13614 * legal action related to enforcement of this Contract.) 13615 */ 13616 public Attachment getLegallyBindingAttachment() throws FHIRException { 13617 if (this.legallyBinding == null) 13618 this.legallyBinding = new Attachment(); 13619 if (!(this.legallyBinding instanceof Attachment)) 13620 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 13621 + this.legallyBinding.getClass().getName() + " was encountered"); 13622 return (Attachment) this.legallyBinding; 13623 } 13624 13625 public boolean hasLegallyBindingAttachment() { 13626 return this != null && this.legallyBinding instanceof Attachment; 13627 } 13628 13629 /** 13630 * @return {@link #legallyBinding} (Legally binding Contract: This is the signed 13631 * and legally recognized representation of the Contract, which is 13632 * considered the "source of truth" and which would be the basis for 13633 * legal action related to enforcement of this Contract.) 13634 */ 13635 public Reference getLegallyBindingReference() throws FHIRException { 13636 if (this.legallyBinding == null) 13637 this.legallyBinding = new Reference(); 13638 if (!(this.legallyBinding instanceof Reference)) 13639 throw new FHIRException("Type mismatch: the type Reference was expected, but " 13640 + this.legallyBinding.getClass().getName() + " was encountered"); 13641 return (Reference) this.legallyBinding; 13642 } 13643 13644 public boolean hasLegallyBindingReference() { 13645 return this != null && this.legallyBinding instanceof Reference; 13646 } 13647 13648 public boolean hasLegallyBinding() { 13649 return this.legallyBinding != null && !this.legallyBinding.isEmpty(); 13650 } 13651 13652 /** 13653 * @param value {@link #legallyBinding} (Legally binding Contract: This is the 13654 * signed and legally recognized representation of the Contract, 13655 * which is considered the "source of truth" and which would be the 13656 * basis for legal action related to enforcement of this Contract.) 13657 */ 13658 public Contract setLegallyBinding(Type value) { 13659 if (value != null && !(value instanceof Attachment || value instanceof Reference)) 13660 throw new Error("Not the right type for Contract.legallyBinding[x]: " + value.fhirType()); 13661 this.legallyBinding = value; 13662 return this; 13663 } 13664 13665 protected void listChildren(List<Property> children) { 13666 super.listChildren(children); 13667 children.add(new Property("identifier", "Identifier", 13668 "Unique identifier for this Contract or a derivative that references a Source Contract.", 0, 13669 java.lang.Integer.MAX_VALUE, identifier)); 13670 children.add(new Property("url", "uri", 13671 "Canonical identifier for this contract, represented as a URI (globally unique).", 0, 1, url)); 13672 children.add(new Property("version", "string", 13673 "An edition identifier used for business purposes to label business significant variants.", 0, 1, version)); 13674 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 13675 children.add(new Property("legalState", "CodeableConcept", 13676 "Legal states of the formation of a legal instrument, which is a formally executed written document that can be formally attributed to its author, records and formally expresses a legally enforceable act, process, or contractual duty, obligation, or right, and therefore evidences that act, process, or agreement.", 13677 0, 1, legalState)); 13678 children.add(new Property("instantiatesCanonical", "Reference(Contract)", 13679 "The URL pointing to a FHIR-defined Contract Definition that is adhered to in whole or part by this Contract.", 13680 0, 1, instantiatesCanonical)); 13681 children.add(new Property("instantiatesUri", "uri", 13682 "The URL pointing to an externally maintained definition that is adhered to in whole or in part by this Contract.", 13683 0, 1, instantiatesUri)); 13684 children.add(new Property("contentDerivative", "CodeableConcept", 13685 "The minimal content derived from the basal information source at a specific stage in its lifecycle.", 0, 1, 13686 contentDerivative)); 13687 children.add(new Property("issued", "dateTime", "When this Contract was issued.", 0, 1, issued)); 13688 children.add(new Property("applies", "Period", "Relevant time or time-period when this Contract is applicable.", 0, 13689 1, applies)); 13690 children.add(new Property("expirationType", "CodeableConcept", 13691 "Event resulting in discontinuation or termination of this Contract instance by one or more parties to the contract.", 13692 0, 1, expirationType)); 13693 children.add(new Property("subject", "Reference(Any)", 13694 "The target entity impacted by or of interest to parties to the agreement.", 0, java.lang.Integer.MAX_VALUE, 13695 subject)); 13696 children.add(new Property("authority", "Reference(Organization)", 13697 "A formally or informally recognized grouping of people, principals, organizations, or jurisdictions formed for the purpose of achieving some form of collective action such as the promulgation, administration and enforcement of contracts and policies.", 13698 0, java.lang.Integer.MAX_VALUE, authority)); 13699 children.add(new Property("domain", "Reference(Location)", 13700 "Recognized governance framework or system operating with a circumscribed scope in accordance with specified principles, policies, processes or procedures for managing rights, actions, or behaviors of parties or principals relative to resources.", 13701 0, java.lang.Integer.MAX_VALUE, domain)); 13702 children.add(new Property("site", "Reference(Location)", 13703 "Sites in which the contract is complied with, exercised, or in force.", 0, java.lang.Integer.MAX_VALUE, 13704 site)); 13705 children.add(new Property("name", "string", 13706 "A natural language name identifying this Contract definition, derivative, or instance in any legal state. Provides additional information about its content. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 13707 0, 1, name)); 13708 children.add(new Property("title", "string", 13709 "A short, descriptive, user-friendly title for this Contract definition, derivative, or instance in any legal state.t giving additional information about its content.", 13710 0, 1, title)); 13711 children.add(new Property("subtitle", "string", 13712 "An explanatory or alternate user-friendly title for this Contract definition, derivative, or instance in any legal state.t giving additional information about its content.", 13713 0, 1, subtitle)); 13714 children.add(new Property("alias", "string", 13715 "Alternative representation of the title for this Contract definition, derivative, or instance in any legal state., e.g., a domain specific contract number related to legislation.", 13716 0, java.lang.Integer.MAX_VALUE, alias)); 13717 children.add(new Property("author", "Reference(Patient|Practitioner|PractitionerRole|Organization)", 13718 "The individual or organization that authored the Contract definition, derivative, or instance in any legal state.", 13719 0, 1, author)); 13720 children.add(new Property("scope", "CodeableConcept", 13721 "A selector of legal concerns for this Contract definition, derivative, or instance in any legal state.", 0, 1, 13722 scope)); 13723 children.add(new Property("topic[x]", "CodeableConcept|Reference(Any)", 13724 "Narrows the range of legal concerns to focus on the achievement of specific contractual objectives.", 0, 1, 13725 topic)); 13726 children.add(new Property("type", "CodeableConcept", 13727 "A high-level category for the legal instrument, whether constructed as a Contract definition, derivative, or instance in any legal state. Provides additional information about its content within the context of the Contract's scope to distinguish the kinds of systems that would be interested in the contract.", 13728 0, 1, type)); 13729 children.add(new Property("subType", "CodeableConcept", 13730 "Sub-category for the Contract that distinguishes the kinds of systems that would be interested in the Contract within the context of the Contract's scope.", 13731 0, java.lang.Integer.MAX_VALUE, subType)); 13732 children.add(new Property("contentDefinition", "", 13733 "Precusory content developed with a focus and intent of supporting the formation a Contract instance, which may be associated with and transformable into a Contract.", 13734 0, 1, contentDefinition)); 13735 children.add(new Property("term", "", 13736 "One or more Contract Provisions, which may be related and conveyed as a group, and may contain nested groups.", 13737 0, java.lang.Integer.MAX_VALUE, term)); 13738 children.add(new Property("supportingInfo", "Reference(Any)", 13739 "Information that may be needed by/relevant to the performer in their execution of this term action.", 0, 13740 java.lang.Integer.MAX_VALUE, supportingInfo)); 13741 children.add(new Property("relevantHistory", "Reference(Provenance)", 13742 "Links to Provenance records for past versions of this Contract definition, derivative, or instance, which identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the Contract. The Provence.entity indicates the target that was changed in the update. http://build.fhir.org/provenance-definitions.html#Provenance.entity.", 13743 0, java.lang.Integer.MAX_VALUE, relevantHistory)); 13744 children.add(new Property("signer", "", 13745 "Parties with legal standing in the Contract, including the principal parties, the grantor(s) and grantee(s), which are any person or organization bound by the contract, and any ancillary parties, which facilitate the execution of the contract such as a notary or witness.", 13746 0, java.lang.Integer.MAX_VALUE, signer)); 13747 children.add(new Property("friendly", "", 13748 "The \"patient friendly language\" versionof the Contract in whole or in parts. \"Patient friendly language\" means the representation of the Contract and Contract Provisions in a manner that is readily accessible and understandable by a layperson in accordance with best practices for communication styles that ensure that those agreeing to or signing the Contract understand the roles, actions, obligations, responsibilities, and implication of the agreement.", 13749 0, java.lang.Integer.MAX_VALUE, friendly)); 13750 children.add(new Property("legal", "", "List of Legal expressions or representations of this Contract.", 0, 13751 java.lang.Integer.MAX_VALUE, legal)); 13752 children.add(new Property("rule", "", "List of Computable Policy Rule Language Representations of this Contract.", 13753 0, java.lang.Integer.MAX_VALUE, rule)); 13754 children.add(new Property("legallyBinding[x]", 13755 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse|Contract)", 13756 "Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the \"source of truth\" and which would be the basis for legal action related to enforcement of this Contract.", 13757 0, 1, legallyBinding)); 13758 } 13759 13760 @Override 13761 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 13762 switch (_hash) { 13763 case -1618432855: 13764 /* identifier */ return new Property("identifier", "Identifier", 13765 "Unique identifier for this Contract or a derivative that references a Source Contract.", 0, 13766 java.lang.Integer.MAX_VALUE, identifier); 13767 case 116079: 13768 /* url */ return new Property("url", "uri", 13769 "Canonical identifier for this contract, represented as a URI (globally unique).", 0, 1, url); 13770 case 351608024: 13771 /* version */ return new Property("version", "string", 13772 "An edition identifier used for business purposes to label business significant variants.", 0, 1, version); 13773 case -892481550: 13774 /* status */ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 13775 case 568606040: 13776 /* legalState */ return new Property("legalState", "CodeableConcept", 13777 "Legal states of the formation of a legal instrument, which is a formally executed written document that can be formally attributed to its author, records and formally expresses a legally enforceable act, process, or contractual duty, obligation, or right, and therefore evidences that act, process, or agreement.", 13778 0, 1, legalState); 13779 case 8911915: 13780 /* instantiatesCanonical */ return new Property("instantiatesCanonical", "Reference(Contract)", 13781 "The URL pointing to a FHIR-defined Contract Definition that is adhered to in whole or part by this Contract.", 13782 0, 1, instantiatesCanonical); 13783 case -1926393373: 13784 /* instantiatesUri */ return new Property("instantiatesUri", "uri", 13785 "The URL pointing to an externally maintained definition that is adhered to in whole or in part by this Contract.", 13786 0, 1, instantiatesUri); 13787 case -92412192: 13788 /* contentDerivative */ return new Property("contentDerivative", "CodeableConcept", 13789 "The minimal content derived from the basal information source at a specific stage in its lifecycle.", 0, 1, 13790 contentDerivative); 13791 case -1179159893: 13792 /* issued */ return new Property("issued", "dateTime", "When this Contract was issued.", 0, 1, issued); 13793 case -793235316: 13794 /* applies */ return new Property("applies", "Period", 13795 "Relevant time or time-period when this Contract is applicable.", 0, 1, applies); 13796 case -668311927: 13797 /* expirationType */ return new Property("expirationType", "CodeableConcept", 13798 "Event resulting in discontinuation or termination of this Contract instance by one or more parties to the contract.", 13799 0, 1, expirationType); 13800 case -1867885268: 13801 /* subject */ return new Property("subject", "Reference(Any)", 13802 "The target entity impacted by or of interest to parties to the agreement.", 0, java.lang.Integer.MAX_VALUE, 13803 subject); 13804 case 1475610435: 13805 /* authority */ return new Property("authority", "Reference(Organization)", 13806 "A formally or informally recognized grouping of people, principals, organizations, or jurisdictions formed for the purpose of achieving some form of collective action such as the promulgation, administration and enforcement of contracts and policies.", 13807 0, java.lang.Integer.MAX_VALUE, authority); 13808 case -1326197564: 13809 /* domain */ return new Property("domain", "Reference(Location)", 13810 "Recognized governance framework or system operating with a circumscribed scope in accordance with specified principles, policies, processes or procedures for managing rights, actions, or behaviors of parties or principals relative to resources.", 13811 0, java.lang.Integer.MAX_VALUE, domain); 13812 case 3530567: 13813 /* site */ return new Property("site", "Reference(Location)", 13814 "Sites in which the contract is complied with, exercised, or in force.", 0, java.lang.Integer.MAX_VALUE, 13815 site); 13816 case 3373707: 13817 /* name */ return new Property("name", "string", 13818 "A natural language name identifying this Contract definition, derivative, or instance in any legal state. Provides additional information about its content. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 13819 0, 1, name); 13820 case 110371416: 13821 /* title */ return new Property("title", "string", 13822 "A short, descriptive, user-friendly title for this Contract definition, derivative, or instance in any legal state.t giving additional information about its content.", 13823 0, 1, title); 13824 case -2060497896: 13825 /* subtitle */ return new Property("subtitle", "string", 13826 "An explanatory or alternate user-friendly title for this Contract definition, derivative, or instance in any legal state.t giving additional information about its content.", 13827 0, 1, subtitle); 13828 case 92902992: 13829 /* alias */ return new Property("alias", "string", 13830 "Alternative representation of the title for this Contract definition, derivative, or instance in any legal state., e.g., a domain specific contract number related to legislation.", 13831 0, java.lang.Integer.MAX_VALUE, alias); 13832 case -1406328437: 13833 /* author */ return new Property("author", "Reference(Patient|Practitioner|PractitionerRole|Organization)", 13834 "The individual or organization that authored the Contract definition, derivative, or instance in any legal state.", 13835 0, 1, author); 13836 case 109264468: 13837 /* scope */ return new Property("scope", "CodeableConcept", 13838 "A selector of legal concerns for this Contract definition, derivative, or instance in any legal state.", 0, 13839 1, scope); 13840 case -957295375: 13841 /* topic[x] */ return new Property("topic[x]", "CodeableConcept|Reference(Any)", 13842 "Narrows the range of legal concerns to focus on the achievement of specific contractual objectives.", 0, 1, 13843 topic); 13844 case 110546223: 13845 /* topic */ return new Property("topic[x]", "CodeableConcept|Reference(Any)", 13846 "Narrows the range of legal concerns to focus on the achievement of specific contractual objectives.", 0, 1, 13847 topic); 13848 case 777778802: 13849 /* topicCodeableConcept */ return new Property("topic[x]", "CodeableConcept|Reference(Any)", 13850 "Narrows the range of legal concerns to focus on the achievement of specific contractual objectives.", 0, 1, 13851 topic); 13852 case -343345444: 13853 /* topicReference */ return new Property("topic[x]", "CodeableConcept|Reference(Any)", 13854 "Narrows the range of legal concerns to focus on the achievement of specific contractual objectives.", 0, 1, 13855 topic); 13856 case 3575610: 13857 /* type */ return new Property("type", "CodeableConcept", 13858 "A high-level category for the legal instrument, whether constructed as a Contract definition, derivative, or instance in any legal state. Provides additional information about its content within the context of the Contract's scope to distinguish the kinds of systems that would be interested in the contract.", 13859 0, 1, type); 13860 case -1868521062: 13861 /* subType */ return new Property("subType", "CodeableConcept", 13862 "Sub-category for the Contract that distinguishes the kinds of systems that would be interested in the Contract within the context of the Contract's scope.", 13863 0, java.lang.Integer.MAX_VALUE, subType); 13864 case 247055020: 13865 /* contentDefinition */ return new Property("contentDefinition", "", 13866 "Precusory content developed with a focus and intent of supporting the formation a Contract instance, which may be associated with and transformable into a Contract.", 13867 0, 1, contentDefinition); 13868 case 3556460: 13869 /* term */ return new Property("term", "", 13870 "One or more Contract Provisions, which may be related and conveyed as a group, and may contain nested groups.", 13871 0, java.lang.Integer.MAX_VALUE, term); 13872 case 1922406657: 13873 /* supportingInfo */ return new Property("supportingInfo", "Reference(Any)", 13874 "Information that may be needed by/relevant to the performer in their execution of this term action.", 0, 13875 java.lang.Integer.MAX_VALUE, supportingInfo); 13876 case 1538891575: 13877 /* relevantHistory */ return new Property("relevantHistory", "Reference(Provenance)", 13878 "Links to Provenance records for past versions of this Contract definition, derivative, or instance, which identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the Contract. The Provence.entity indicates the target that was changed in the update. http://build.fhir.org/provenance-definitions.html#Provenance.entity.", 13879 0, java.lang.Integer.MAX_VALUE, relevantHistory); 13880 case -902467798: 13881 /* signer */ return new Property("signer", "", 13882 "Parties with legal standing in the Contract, including the principal parties, the grantor(s) and grantee(s), which are any person or organization bound by the contract, and any ancillary parties, which facilitate the execution of the contract such as a notary or witness.", 13883 0, java.lang.Integer.MAX_VALUE, signer); 13884 case -1423054677: 13885 /* friendly */ return new Property("friendly", "", 13886 "The \"patient friendly language\" versionof the Contract in whole or in parts. \"Patient friendly language\" means the representation of the Contract and Contract Provisions in a manner that is readily accessible and understandable by a layperson in accordance with best practices for communication styles that ensure that those agreeing to or signing the Contract understand the roles, actions, obligations, responsibilities, and implication of the agreement.", 13887 0, java.lang.Integer.MAX_VALUE, friendly); 13888 case 102851257: 13889 /* legal */ return new Property("legal", "", "List of Legal expressions or representations of this Contract.", 0, 13890 java.lang.Integer.MAX_VALUE, legal); 13891 case 3512060: 13892 /* rule */ return new Property("rule", "", 13893 "List of Computable Policy Rule Language Representations of this Contract.", 0, java.lang.Integer.MAX_VALUE, 13894 rule); 13895 case -772497791: 13896 /* legallyBinding[x] */ return new Property("legallyBinding[x]", 13897 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse|Contract)", 13898 "Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the \"source of truth\" and which would be the basis for legal action related to enforcement of this Contract.", 13899 0, 1, legallyBinding); 13900 case -126751329: 13901 /* legallyBinding */ return new Property("legallyBinding[x]", 13902 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse|Contract)", 13903 "Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the \"source of truth\" and which would be the basis for legal action related to enforcement of this Contract.", 13904 0, 1, legallyBinding); 13905 case 344057890: 13906 /* legallyBindingAttachment */ return new Property("legallyBinding[x]", 13907 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse|Contract)", 13908 "Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the \"source of truth\" and which would be the basis for legal action related to enforcement of this Contract.", 13909 0, 1, legallyBinding); 13910 case -296528788: 13911 /* legallyBindingReference */ return new Property("legallyBinding[x]", 13912 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse|Contract)", 13913 "Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the \"source of truth\" and which would be the basis for legal action related to enforcement of this Contract.", 13914 0, 1, legallyBinding); 13915 default: 13916 return super.getNamedProperty(_hash, _name, _checkValid); 13917 } 13918 13919 } 13920 13921 @Override 13922 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 13923 switch (hash) { 13924 case -1618432855: 13925 /* identifier */ return this.identifier == null ? new Base[0] 13926 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 13927 case 116079: 13928 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 13929 case 351608024: 13930 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 13931 case -892481550: 13932 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<ContractStatus> 13933 case 568606040: 13934 /* legalState */ return this.legalState == null ? new Base[0] : new Base[] { this.legalState }; // CodeableConcept 13935 case 8911915: 13936 /* instantiatesCanonical */ return this.instantiatesCanonical == null ? new Base[0] 13937 : new Base[] { this.instantiatesCanonical }; // Reference 13938 case -1926393373: 13939 /* instantiatesUri */ return this.instantiatesUri == null ? new Base[0] : new Base[] { this.instantiatesUri }; // UriType 13940 case -92412192: 13941 /* contentDerivative */ return this.contentDerivative == null ? new Base[0] 13942 : new Base[] { this.contentDerivative }; // CodeableConcept 13943 case -1179159893: 13944 /* issued */ return this.issued == null ? new Base[0] : new Base[] { this.issued }; // DateTimeType 13945 case -793235316: 13946 /* applies */ return this.applies == null ? new Base[0] : new Base[] { this.applies }; // Period 13947 case -668311927: 13948 /* expirationType */ return this.expirationType == null ? new Base[0] : new Base[] { this.expirationType }; // CodeableConcept 13949 case -1867885268: 13950 /* subject */ return this.subject == null ? new Base[0] : this.subject.toArray(new Base[this.subject.size()]); // Reference 13951 case 1475610435: 13952 /* authority */ return this.authority == null ? new Base[0] 13953 : this.authority.toArray(new Base[this.authority.size()]); // Reference 13954 case -1326197564: 13955 /* domain */ return this.domain == null ? new Base[0] : this.domain.toArray(new Base[this.domain.size()]); // Reference 13956 case 3530567: 13957 /* site */ return this.site == null ? new Base[0] : this.site.toArray(new Base[this.site.size()]); // Reference 13958 case 3373707: 13959 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 13960 case 110371416: 13961 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 13962 case -2060497896: 13963 /* subtitle */ return this.subtitle == null ? new Base[0] : new Base[] { this.subtitle }; // StringType 13964 case 92902992: 13965 /* alias */ return this.alias == null ? new Base[0] : this.alias.toArray(new Base[this.alias.size()]); // StringType 13966 case -1406328437: 13967 /* author */ return this.author == null ? new Base[0] : new Base[] { this.author }; // Reference 13968 case 109264468: 13969 /* scope */ return this.scope == null ? new Base[0] : new Base[] { this.scope }; // CodeableConcept 13970 case 110546223: 13971 /* topic */ return this.topic == null ? new Base[0] : new Base[] { this.topic }; // Type 13972 case 3575610: 13973 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 13974 case -1868521062: 13975 /* subType */ return this.subType == null ? new Base[0] : this.subType.toArray(new Base[this.subType.size()]); // CodeableConcept 13976 case 247055020: 13977 /* contentDefinition */ return this.contentDefinition == null ? new Base[0] 13978 : new Base[] { this.contentDefinition }; // ContentDefinitionComponent 13979 case 3556460: 13980 /* term */ return this.term == null ? new Base[0] : this.term.toArray(new Base[this.term.size()]); // TermComponent 13981 case 1922406657: 13982 /* supportingInfo */ return this.supportingInfo == null ? new Base[0] 13983 : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // Reference 13984 case 1538891575: 13985 /* relevantHistory */ return this.relevantHistory == null ? new Base[0] 13986 : this.relevantHistory.toArray(new Base[this.relevantHistory.size()]); // Reference 13987 case -902467798: 13988 /* signer */ return this.signer == null ? new Base[0] : this.signer.toArray(new Base[this.signer.size()]); // SignatoryComponent 13989 case -1423054677: 13990 /* friendly */ return this.friendly == null ? new Base[0] : this.friendly.toArray(new Base[this.friendly.size()]); // FriendlyLanguageComponent 13991 case 102851257: 13992 /* legal */ return this.legal == null ? new Base[0] : this.legal.toArray(new Base[this.legal.size()]); // LegalLanguageComponent 13993 case 3512060: 13994 /* rule */ return this.rule == null ? new Base[0] : this.rule.toArray(new Base[this.rule.size()]); // ComputableLanguageComponent 13995 case -126751329: 13996 /* legallyBinding */ return this.legallyBinding == null ? new Base[0] : new Base[] { this.legallyBinding }; // Type 13997 default: 13998 return super.getProperty(hash, name, checkValid); 13999 } 14000 14001 } 14002 14003 @Override 14004 public Base setProperty(int hash, String name, Base value) throws FHIRException { 14005 switch (hash) { 14006 case -1618432855: // identifier 14007 this.getIdentifier().add(castToIdentifier(value)); // Identifier 14008 return value; 14009 case 116079: // url 14010 this.url = castToUri(value); // UriType 14011 return value; 14012 case 351608024: // version 14013 this.version = castToString(value); // StringType 14014 return value; 14015 case -892481550: // status 14016 value = new ContractStatusEnumFactory().fromType(castToCode(value)); 14017 this.status = (Enumeration) value; // Enumeration<ContractStatus> 14018 return value; 14019 case 568606040: // legalState 14020 this.legalState = castToCodeableConcept(value); // CodeableConcept 14021 return value; 14022 case 8911915: // instantiatesCanonical 14023 this.instantiatesCanonical = castToReference(value); // Reference 14024 return value; 14025 case -1926393373: // instantiatesUri 14026 this.instantiatesUri = castToUri(value); // UriType 14027 return value; 14028 case -92412192: // contentDerivative 14029 this.contentDerivative = castToCodeableConcept(value); // CodeableConcept 14030 return value; 14031 case -1179159893: // issued 14032 this.issued = castToDateTime(value); // DateTimeType 14033 return value; 14034 case -793235316: // applies 14035 this.applies = castToPeriod(value); // Period 14036 return value; 14037 case -668311927: // expirationType 14038 this.expirationType = castToCodeableConcept(value); // CodeableConcept 14039 return value; 14040 case -1867885268: // subject 14041 this.getSubject().add(castToReference(value)); // Reference 14042 return value; 14043 case 1475610435: // authority 14044 this.getAuthority().add(castToReference(value)); // Reference 14045 return value; 14046 case -1326197564: // domain 14047 this.getDomain().add(castToReference(value)); // Reference 14048 return value; 14049 case 3530567: // site 14050 this.getSite().add(castToReference(value)); // Reference 14051 return value; 14052 case 3373707: // name 14053 this.name = castToString(value); // StringType 14054 return value; 14055 case 110371416: // title 14056 this.title = castToString(value); // StringType 14057 return value; 14058 case -2060497896: // subtitle 14059 this.subtitle = castToString(value); // StringType 14060 return value; 14061 case 92902992: // alias 14062 this.getAlias().add(castToString(value)); // StringType 14063 return value; 14064 case -1406328437: // author 14065 this.author = castToReference(value); // Reference 14066 return value; 14067 case 109264468: // scope 14068 this.scope = castToCodeableConcept(value); // CodeableConcept 14069 return value; 14070 case 110546223: // topic 14071 this.topic = castToType(value); // Type 14072 return value; 14073 case 3575610: // type 14074 this.type = castToCodeableConcept(value); // CodeableConcept 14075 return value; 14076 case -1868521062: // subType 14077 this.getSubType().add(castToCodeableConcept(value)); // CodeableConcept 14078 return value; 14079 case 247055020: // contentDefinition 14080 this.contentDefinition = (ContentDefinitionComponent) value; // ContentDefinitionComponent 14081 return value; 14082 case 3556460: // term 14083 this.getTerm().add((TermComponent) value); // TermComponent 14084 return value; 14085 case 1922406657: // supportingInfo 14086 this.getSupportingInfo().add(castToReference(value)); // Reference 14087 return value; 14088 case 1538891575: // relevantHistory 14089 this.getRelevantHistory().add(castToReference(value)); // Reference 14090 return value; 14091 case -902467798: // signer 14092 this.getSigner().add((SignatoryComponent) value); // SignatoryComponent 14093 return value; 14094 case -1423054677: // friendly 14095 this.getFriendly().add((FriendlyLanguageComponent) value); // FriendlyLanguageComponent 14096 return value; 14097 case 102851257: // legal 14098 this.getLegal().add((LegalLanguageComponent) value); // LegalLanguageComponent 14099 return value; 14100 case 3512060: // rule 14101 this.getRule().add((ComputableLanguageComponent) value); // ComputableLanguageComponent 14102 return value; 14103 case -126751329: // legallyBinding 14104 this.legallyBinding = castToType(value); // Type 14105 return value; 14106 default: 14107 return super.setProperty(hash, name, value); 14108 } 14109 14110 } 14111 14112 @Override 14113 public Base setProperty(String name, Base value) throws FHIRException { 14114 if (name.equals("identifier")) { 14115 this.getIdentifier().add(castToIdentifier(value)); 14116 } else if (name.equals("url")) { 14117 this.url = castToUri(value); // UriType 14118 } else if (name.equals("version")) { 14119 this.version = castToString(value); // StringType 14120 } else if (name.equals("status")) { 14121 value = new ContractStatusEnumFactory().fromType(castToCode(value)); 14122 this.status = (Enumeration) value; // Enumeration<ContractStatus> 14123 } else if (name.equals("legalState")) { 14124 this.legalState = castToCodeableConcept(value); // CodeableConcept 14125 } else if (name.equals("instantiatesCanonical")) { 14126 this.instantiatesCanonical = castToReference(value); // Reference 14127 } else if (name.equals("instantiatesUri")) { 14128 this.instantiatesUri = castToUri(value); // UriType 14129 } else if (name.equals("contentDerivative")) { 14130 this.contentDerivative = castToCodeableConcept(value); // CodeableConcept 14131 } else if (name.equals("issued")) { 14132 this.issued = castToDateTime(value); // DateTimeType 14133 } else if (name.equals("applies")) { 14134 this.applies = castToPeriod(value); // Period 14135 } else if (name.equals("expirationType")) { 14136 this.expirationType = castToCodeableConcept(value); // CodeableConcept 14137 } else if (name.equals("subject")) { 14138 this.getSubject().add(castToReference(value)); 14139 } else if (name.equals("authority")) { 14140 this.getAuthority().add(castToReference(value)); 14141 } else if (name.equals("domain")) { 14142 this.getDomain().add(castToReference(value)); 14143 } else if (name.equals("site")) { 14144 this.getSite().add(castToReference(value)); 14145 } else if (name.equals("name")) { 14146 this.name = castToString(value); // StringType 14147 } else if (name.equals("title")) { 14148 this.title = castToString(value); // StringType 14149 } else if (name.equals("subtitle")) { 14150 this.subtitle = castToString(value); // StringType 14151 } else if (name.equals("alias")) { 14152 this.getAlias().add(castToString(value)); 14153 } else if (name.equals("author")) { 14154 this.author = castToReference(value); // Reference 14155 } else if (name.equals("scope")) { 14156 this.scope = castToCodeableConcept(value); // CodeableConcept 14157 } else if (name.equals("topic[x]")) { 14158 this.topic = castToType(value); // Type 14159 } else if (name.equals("type")) { 14160 this.type = castToCodeableConcept(value); // CodeableConcept 14161 } else if (name.equals("subType")) { 14162 this.getSubType().add(castToCodeableConcept(value)); 14163 } else if (name.equals("contentDefinition")) { 14164 this.contentDefinition = (ContentDefinitionComponent) value; // ContentDefinitionComponent 14165 } else if (name.equals("term")) { 14166 this.getTerm().add((TermComponent) value); 14167 } else if (name.equals("supportingInfo")) { 14168 this.getSupportingInfo().add(castToReference(value)); 14169 } else if (name.equals("relevantHistory")) { 14170 this.getRelevantHistory().add(castToReference(value)); 14171 } else if (name.equals("signer")) { 14172 this.getSigner().add((SignatoryComponent) value); 14173 } else if (name.equals("friendly")) { 14174 this.getFriendly().add((FriendlyLanguageComponent) value); 14175 } else if (name.equals("legal")) { 14176 this.getLegal().add((LegalLanguageComponent) value); 14177 } else if (name.equals("rule")) { 14178 this.getRule().add((ComputableLanguageComponent) value); 14179 } else if (name.equals("legallyBinding[x]")) { 14180 this.legallyBinding = castToType(value); // Type 14181 } else 14182 return super.setProperty(name, value); 14183 return value; 14184 } 14185 14186 @Override 14187 public void removeChild(String name, Base value) throws FHIRException { 14188 if (name.equals("identifier")) { 14189 this.getIdentifier().remove(castToIdentifier(value)); 14190 } else if (name.equals("url")) { 14191 this.url = null; 14192 } else if (name.equals("version")) { 14193 this.version = null; 14194 } else if (name.equals("status")) { 14195 this.status = null; 14196 } else if (name.equals("legalState")) { 14197 this.legalState = null; 14198 } else if (name.equals("instantiatesCanonical")) { 14199 this.instantiatesCanonical = null; 14200 } else if (name.equals("instantiatesUri")) { 14201 this.instantiatesUri = null; 14202 } else if (name.equals("contentDerivative")) { 14203 this.contentDerivative = null; 14204 } else if (name.equals("issued")) { 14205 this.issued = null; 14206 } else if (name.equals("applies")) { 14207 this.applies = null; 14208 } else if (name.equals("expirationType")) { 14209 this.expirationType = null; 14210 } else if (name.equals("subject")) { 14211 this.getSubject().remove(castToReference(value)); 14212 } else if (name.equals("authority")) { 14213 this.getAuthority().remove(castToReference(value)); 14214 } else if (name.equals("domain")) { 14215 this.getDomain().remove(castToReference(value)); 14216 } else if (name.equals("site")) { 14217 this.getSite().remove(castToReference(value)); 14218 } else if (name.equals("name")) { 14219 this.name = null; 14220 } else if (name.equals("title")) { 14221 this.title = null; 14222 } else if (name.equals("subtitle")) { 14223 this.subtitle = null; 14224 } else if (name.equals("alias")) { 14225 this.getAlias().remove(castToString(value)); 14226 } else if (name.equals("author")) { 14227 this.author = null; 14228 } else if (name.equals("scope")) { 14229 this.scope = null; 14230 } else if (name.equals("topic[x]")) { 14231 this.topic = null; 14232 } else if (name.equals("type")) { 14233 this.type = null; 14234 } else if (name.equals("subType")) { 14235 this.getSubType().remove(castToCodeableConcept(value)); 14236 } else if (name.equals("contentDefinition")) { 14237 this.contentDefinition = (ContentDefinitionComponent) value; // ContentDefinitionComponent 14238 } else if (name.equals("term")) { 14239 this.getTerm().remove((TermComponent) value); 14240 } else if (name.equals("supportingInfo")) { 14241 this.getSupportingInfo().remove(castToReference(value)); 14242 } else if (name.equals("relevantHistory")) { 14243 this.getRelevantHistory().remove(castToReference(value)); 14244 } else if (name.equals("signer")) { 14245 this.getSigner().remove((SignatoryComponent) value); 14246 } else if (name.equals("friendly")) { 14247 this.getFriendly().remove((FriendlyLanguageComponent) value); 14248 } else if (name.equals("legal")) { 14249 this.getLegal().remove((LegalLanguageComponent) value); 14250 } else if (name.equals("rule")) { 14251 this.getRule().remove((ComputableLanguageComponent) value); 14252 } else if (name.equals("legallyBinding[x]")) { 14253 this.legallyBinding = null; 14254 } else 14255 super.removeChild(name, value); 14256 14257 } 14258 14259 @Override 14260 public Base makeProperty(int hash, String name) throws FHIRException { 14261 switch (hash) { 14262 case -1618432855: 14263 return addIdentifier(); 14264 case 116079: 14265 return getUrlElement(); 14266 case 351608024: 14267 return getVersionElement(); 14268 case -892481550: 14269 return getStatusElement(); 14270 case 568606040: 14271 return getLegalState(); 14272 case 8911915: 14273 return getInstantiatesCanonical(); 14274 case -1926393373: 14275 return getInstantiatesUriElement(); 14276 case -92412192: 14277 return getContentDerivative(); 14278 case -1179159893: 14279 return getIssuedElement(); 14280 case -793235316: 14281 return getApplies(); 14282 case -668311927: 14283 return getExpirationType(); 14284 case -1867885268: 14285 return addSubject(); 14286 case 1475610435: 14287 return addAuthority(); 14288 case -1326197564: 14289 return addDomain(); 14290 case 3530567: 14291 return addSite(); 14292 case 3373707: 14293 return getNameElement(); 14294 case 110371416: 14295 return getTitleElement(); 14296 case -2060497896: 14297 return getSubtitleElement(); 14298 case 92902992: 14299 return addAliasElement(); 14300 case -1406328437: 14301 return getAuthor(); 14302 case 109264468: 14303 return getScope(); 14304 case -957295375: 14305 return getTopic(); 14306 case 110546223: 14307 return getTopic(); 14308 case 3575610: 14309 return getType(); 14310 case -1868521062: 14311 return addSubType(); 14312 case 247055020: 14313 return getContentDefinition(); 14314 case 3556460: 14315 return addTerm(); 14316 case 1922406657: 14317 return addSupportingInfo(); 14318 case 1538891575: 14319 return addRelevantHistory(); 14320 case -902467798: 14321 return addSigner(); 14322 case -1423054677: 14323 return addFriendly(); 14324 case 102851257: 14325 return addLegal(); 14326 case 3512060: 14327 return addRule(); 14328 case -772497791: 14329 return getLegallyBinding(); 14330 case -126751329: 14331 return getLegallyBinding(); 14332 default: 14333 return super.makeProperty(hash, name); 14334 } 14335 14336 } 14337 14338 @Override 14339 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 14340 switch (hash) { 14341 case -1618432855: 14342 /* identifier */ return new String[] { "Identifier" }; 14343 case 116079: 14344 /* url */ return new String[] { "uri" }; 14345 case 351608024: 14346 /* version */ return new String[] { "string" }; 14347 case -892481550: 14348 /* status */ return new String[] { "code" }; 14349 case 568606040: 14350 /* legalState */ return new String[] { "CodeableConcept" }; 14351 case 8911915: 14352 /* instantiatesCanonical */ return new String[] { "Reference" }; 14353 case -1926393373: 14354 /* instantiatesUri */ return new String[] { "uri" }; 14355 case -92412192: 14356 /* contentDerivative */ return new String[] { "CodeableConcept" }; 14357 case -1179159893: 14358 /* issued */ return new String[] { "dateTime" }; 14359 case -793235316: 14360 /* applies */ return new String[] { "Period" }; 14361 case -668311927: 14362 /* expirationType */ return new String[] { "CodeableConcept" }; 14363 case -1867885268: 14364 /* subject */ return new String[] { "Reference" }; 14365 case 1475610435: 14366 /* authority */ return new String[] { "Reference" }; 14367 case -1326197564: 14368 /* domain */ return new String[] { "Reference" }; 14369 case 3530567: 14370 /* site */ return new String[] { "Reference" }; 14371 case 3373707: 14372 /* name */ return new String[] { "string" }; 14373 case 110371416: 14374 /* title */ return new String[] { "string" }; 14375 case -2060497896: 14376 /* subtitle */ return new String[] { "string" }; 14377 case 92902992: 14378 /* alias */ return new String[] { "string" }; 14379 case -1406328437: 14380 /* author */ return new String[] { "Reference" }; 14381 case 109264468: 14382 /* scope */ return new String[] { "CodeableConcept" }; 14383 case 110546223: 14384 /* topic */ return new String[] { "CodeableConcept", "Reference" }; 14385 case 3575610: 14386 /* type */ return new String[] { "CodeableConcept" }; 14387 case -1868521062: 14388 /* subType */ return new String[] { "CodeableConcept" }; 14389 case 247055020: 14390 /* contentDefinition */ return new String[] {}; 14391 case 3556460: 14392 /* term */ return new String[] {}; 14393 case 1922406657: 14394 /* supportingInfo */ return new String[] { "Reference" }; 14395 case 1538891575: 14396 /* relevantHistory */ return new String[] { "Reference" }; 14397 case -902467798: 14398 /* signer */ return new String[] {}; 14399 case -1423054677: 14400 /* friendly */ return new String[] {}; 14401 case 102851257: 14402 /* legal */ return new String[] {}; 14403 case 3512060: 14404 /* rule */ return new String[] {}; 14405 case -126751329: 14406 /* legallyBinding */ return new String[] { "Attachment", "Reference" }; 14407 default: 14408 return super.getTypesForProperty(hash, name); 14409 } 14410 14411 } 14412 14413 @Override 14414 public Base addChild(String name) throws FHIRException { 14415 if (name.equals("identifier")) { 14416 return addIdentifier(); 14417 } else if (name.equals("url")) { 14418 throw new FHIRException("Cannot call addChild on a singleton property Contract.url"); 14419 } else if (name.equals("version")) { 14420 throw new FHIRException("Cannot call addChild on a singleton property Contract.version"); 14421 } else if (name.equals("status")) { 14422 throw new FHIRException("Cannot call addChild on a singleton property Contract.status"); 14423 } else if (name.equals("legalState")) { 14424 this.legalState = new CodeableConcept(); 14425 return this.legalState; 14426 } else if (name.equals("instantiatesCanonical")) { 14427 this.instantiatesCanonical = new Reference(); 14428 return this.instantiatesCanonical; 14429 } else if (name.equals("instantiatesUri")) { 14430 throw new FHIRException("Cannot call addChild on a singleton property Contract.instantiatesUri"); 14431 } else if (name.equals("contentDerivative")) { 14432 this.contentDerivative = new CodeableConcept(); 14433 return this.contentDerivative; 14434 } else if (name.equals("issued")) { 14435 throw new FHIRException("Cannot call addChild on a singleton property Contract.issued"); 14436 } else if (name.equals("applies")) { 14437 this.applies = new Period(); 14438 return this.applies; 14439 } else if (name.equals("expirationType")) { 14440 this.expirationType = new CodeableConcept(); 14441 return this.expirationType; 14442 } else if (name.equals("subject")) { 14443 return addSubject(); 14444 } else if (name.equals("authority")) { 14445 return addAuthority(); 14446 } else if (name.equals("domain")) { 14447 return addDomain(); 14448 } else if (name.equals("site")) { 14449 return addSite(); 14450 } else if (name.equals("name")) { 14451 throw new FHIRException("Cannot call addChild on a singleton property Contract.name"); 14452 } else if (name.equals("title")) { 14453 throw new FHIRException("Cannot call addChild on a singleton property Contract.title"); 14454 } else if (name.equals("subtitle")) { 14455 throw new FHIRException("Cannot call addChild on a singleton property Contract.subtitle"); 14456 } else if (name.equals("alias")) { 14457 throw new FHIRException("Cannot call addChild on a singleton property Contract.alias"); 14458 } else if (name.equals("author")) { 14459 this.author = new Reference(); 14460 return this.author; 14461 } else if (name.equals("scope")) { 14462 this.scope = new CodeableConcept(); 14463 return this.scope; 14464 } else if (name.equals("topicCodeableConcept")) { 14465 this.topic = new CodeableConcept(); 14466 return this.topic; 14467 } else if (name.equals("topicReference")) { 14468 this.topic = new Reference(); 14469 return this.topic; 14470 } else if (name.equals("type")) { 14471 this.type = new CodeableConcept(); 14472 return this.type; 14473 } else if (name.equals("subType")) { 14474 return addSubType(); 14475 } else if (name.equals("contentDefinition")) { 14476 this.contentDefinition = new ContentDefinitionComponent(); 14477 return this.contentDefinition; 14478 } else if (name.equals("term")) { 14479 return addTerm(); 14480 } else if (name.equals("supportingInfo")) { 14481 return addSupportingInfo(); 14482 } else if (name.equals("relevantHistory")) { 14483 return addRelevantHistory(); 14484 } else if (name.equals("signer")) { 14485 return addSigner(); 14486 } else if (name.equals("friendly")) { 14487 return addFriendly(); 14488 } else if (name.equals("legal")) { 14489 return addLegal(); 14490 } else if (name.equals("rule")) { 14491 return addRule(); 14492 } else if (name.equals("legallyBindingAttachment")) { 14493 this.legallyBinding = new Attachment(); 14494 return this.legallyBinding; 14495 } else if (name.equals("legallyBindingReference")) { 14496 this.legallyBinding = new Reference(); 14497 return this.legallyBinding; 14498 } else 14499 return super.addChild(name); 14500 } 14501 14502 public String fhirType() { 14503 return "Contract"; 14504 14505 } 14506 14507 public Contract copy() { 14508 Contract dst = new Contract(); 14509 copyValues(dst); 14510 return dst; 14511 } 14512 14513 public void copyValues(Contract dst) { 14514 super.copyValues(dst); 14515 if (identifier != null) { 14516 dst.identifier = new ArrayList<Identifier>(); 14517 for (Identifier i : identifier) 14518 dst.identifier.add(i.copy()); 14519 } 14520 ; 14521 dst.url = url == null ? null : url.copy(); 14522 dst.version = version == null ? null : version.copy(); 14523 dst.status = status == null ? null : status.copy(); 14524 dst.legalState = legalState == null ? null : legalState.copy(); 14525 dst.instantiatesCanonical = instantiatesCanonical == null ? null : instantiatesCanonical.copy(); 14526 dst.instantiatesUri = instantiatesUri == null ? null : instantiatesUri.copy(); 14527 dst.contentDerivative = contentDerivative == null ? null : contentDerivative.copy(); 14528 dst.issued = issued == null ? null : issued.copy(); 14529 dst.applies = applies == null ? null : applies.copy(); 14530 dst.expirationType = expirationType == null ? null : expirationType.copy(); 14531 if (subject != null) { 14532 dst.subject = new ArrayList<Reference>(); 14533 for (Reference i : subject) 14534 dst.subject.add(i.copy()); 14535 } 14536 ; 14537 if (authority != null) { 14538 dst.authority = new ArrayList<Reference>(); 14539 for (Reference i : authority) 14540 dst.authority.add(i.copy()); 14541 } 14542 ; 14543 if (domain != null) { 14544 dst.domain = new ArrayList<Reference>(); 14545 for (Reference i : domain) 14546 dst.domain.add(i.copy()); 14547 } 14548 ; 14549 if (site != null) { 14550 dst.site = new ArrayList<Reference>(); 14551 for (Reference i : site) 14552 dst.site.add(i.copy()); 14553 } 14554 ; 14555 dst.name = name == null ? null : name.copy(); 14556 dst.title = title == null ? null : title.copy(); 14557 dst.subtitle = subtitle == null ? null : subtitle.copy(); 14558 if (alias != null) { 14559 dst.alias = new ArrayList<StringType>(); 14560 for (StringType i : alias) 14561 dst.alias.add(i.copy()); 14562 } 14563 ; 14564 dst.author = author == null ? null : author.copy(); 14565 dst.scope = scope == null ? null : scope.copy(); 14566 dst.topic = topic == null ? null : topic.copy(); 14567 dst.type = type == null ? null : type.copy(); 14568 if (subType != null) { 14569 dst.subType = new ArrayList<CodeableConcept>(); 14570 for (CodeableConcept i : subType) 14571 dst.subType.add(i.copy()); 14572 } 14573 ; 14574 dst.contentDefinition = contentDefinition == null ? null : contentDefinition.copy(); 14575 if (term != null) { 14576 dst.term = new ArrayList<TermComponent>(); 14577 for (TermComponent i : term) 14578 dst.term.add(i.copy()); 14579 } 14580 ; 14581 if (supportingInfo != null) { 14582 dst.supportingInfo = new ArrayList<Reference>(); 14583 for (Reference i : supportingInfo) 14584 dst.supportingInfo.add(i.copy()); 14585 } 14586 ; 14587 if (relevantHistory != null) { 14588 dst.relevantHistory = new ArrayList<Reference>(); 14589 for (Reference i : relevantHistory) 14590 dst.relevantHistory.add(i.copy()); 14591 } 14592 ; 14593 if (signer != null) { 14594 dst.signer = new ArrayList<SignatoryComponent>(); 14595 for (SignatoryComponent i : signer) 14596 dst.signer.add(i.copy()); 14597 } 14598 ; 14599 if (friendly != null) { 14600 dst.friendly = new ArrayList<FriendlyLanguageComponent>(); 14601 for (FriendlyLanguageComponent i : friendly) 14602 dst.friendly.add(i.copy()); 14603 } 14604 ; 14605 if (legal != null) { 14606 dst.legal = new ArrayList<LegalLanguageComponent>(); 14607 for (LegalLanguageComponent i : legal) 14608 dst.legal.add(i.copy()); 14609 } 14610 ; 14611 if (rule != null) { 14612 dst.rule = new ArrayList<ComputableLanguageComponent>(); 14613 for (ComputableLanguageComponent i : rule) 14614 dst.rule.add(i.copy()); 14615 } 14616 ; 14617 dst.legallyBinding = legallyBinding == null ? null : legallyBinding.copy(); 14618 } 14619 14620 protected Contract typedCopy() { 14621 return copy(); 14622 } 14623 14624 @Override 14625 public boolean equalsDeep(Base other_) { 14626 if (!super.equalsDeep(other_)) 14627 return false; 14628 if (!(other_ instanceof Contract)) 14629 return false; 14630 Contract o = (Contract) other_; 14631 return compareDeep(identifier, o.identifier, true) && compareDeep(url, o.url, true) 14632 && compareDeep(version, o.version, true) && compareDeep(status, o.status, true) 14633 && compareDeep(legalState, o.legalState, true) 14634 && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 14635 && compareDeep(instantiatesUri, o.instantiatesUri, true) 14636 && compareDeep(contentDerivative, o.contentDerivative, true) && compareDeep(issued, o.issued, true) 14637 && compareDeep(applies, o.applies, true) && compareDeep(expirationType, o.expirationType, true) 14638 && compareDeep(subject, o.subject, true) && compareDeep(authority, o.authority, true) 14639 && compareDeep(domain, o.domain, true) && compareDeep(site, o.site, true) && compareDeep(name, o.name, true) 14640 && compareDeep(title, o.title, true) && compareDeep(subtitle, o.subtitle, true) 14641 && compareDeep(alias, o.alias, true) && compareDeep(author, o.author, true) && compareDeep(scope, o.scope, true) 14642 && compareDeep(topic, o.topic, true) && compareDeep(type, o.type, true) && compareDeep(subType, o.subType, true) 14643 && compareDeep(contentDefinition, o.contentDefinition, true) && compareDeep(term, o.term, true) 14644 && compareDeep(supportingInfo, o.supportingInfo, true) && compareDeep(relevantHistory, o.relevantHistory, true) 14645 && compareDeep(signer, o.signer, true) && compareDeep(friendly, o.friendly, true) 14646 && compareDeep(legal, o.legal, true) && compareDeep(rule, o.rule, true) 14647 && compareDeep(legallyBinding, o.legallyBinding, true); 14648 } 14649 14650 @Override 14651 public boolean equalsShallow(Base other_) { 14652 if (!super.equalsShallow(other_)) 14653 return false; 14654 if (!(other_ instanceof Contract)) 14655 return false; 14656 Contract o = (Contract) other_; 14657 return compareValues(url, o.url, true) && compareValues(version, o.version, true) 14658 && compareValues(status, o.status, true) && compareValues(instantiatesUri, o.instantiatesUri, true) 14659 && compareValues(issued, o.issued, true) && compareValues(name, o.name, true) 14660 && compareValues(title, o.title, true) && compareValues(subtitle, o.subtitle, true) 14661 && compareValues(alias, o.alias, true); 14662 } 14663 14664 public boolean isEmpty() { 14665 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, url, version, status, legalState, 14666 instantiatesCanonical, instantiatesUri, contentDerivative, issued, applies, expirationType, subject, authority, 14667 domain, site, name, title, subtitle, alias, author, scope, topic, type, subType, contentDefinition, term, 14668 supportingInfo, relevantHistory, signer, friendly, legal, rule, legallyBinding); 14669 } 14670 14671 @Override 14672 public ResourceType getResourceType() { 14673 return ResourceType.Contract; 14674 } 14675 14676 /** 14677 * Search parameter: <b>identifier</b> 14678 * <p> 14679 * Description: <b>The identity of the contract</b><br> 14680 * Type: <b>token</b><br> 14681 * Path: <b>Contract.identifier</b><br> 14682 * </p> 14683 */ 14684 @SearchParamDefinition(name = "identifier", path = "Contract.identifier", description = "The identity of the contract", type = "token") 14685 public static final String SP_IDENTIFIER = "identifier"; 14686 /** 14687 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 14688 * <p> 14689 * Description: <b>The identity of the contract</b><br> 14690 * Type: <b>token</b><br> 14691 * Path: <b>Contract.identifier</b><br> 14692 * </p> 14693 */ 14694 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 14695 SP_IDENTIFIER); 14696 14697 /** 14698 * Search parameter: <b>instantiates</b> 14699 * <p> 14700 * Description: <b>A source definition of the contract</b><br> 14701 * Type: <b>uri</b><br> 14702 * Path: <b>Contract.instantiatesUri</b><br> 14703 * </p> 14704 */ 14705 @SearchParamDefinition(name = "instantiates", path = "Contract.instantiatesUri", description = "A source definition of the contract", type = "uri") 14706 public static final String SP_INSTANTIATES = "instantiates"; 14707 /** 14708 * <b>Fluent Client</b> search parameter constant for <b>instantiates</b> 14709 * <p> 14710 * Description: <b>A source definition of the contract</b><br> 14711 * Type: <b>uri</b><br> 14712 * Path: <b>Contract.instantiatesUri</b><br> 14713 * </p> 14714 */ 14715 public static final ca.uhn.fhir.rest.gclient.UriClientParam INSTANTIATES = new ca.uhn.fhir.rest.gclient.UriClientParam( 14716 SP_INSTANTIATES); 14717 14718 /** 14719 * Search parameter: <b>patient</b> 14720 * <p> 14721 * Description: <b>The identity of the subject of the contract (if a 14722 * patient)</b><br> 14723 * Type: <b>reference</b><br> 14724 * Path: <b>Contract.subject</b><br> 14725 * </p> 14726 */ 14727 @SearchParamDefinition(name = "patient", path = "Contract.subject.where(resolve() is Patient)", description = "The identity of the subject of the contract (if a patient)", type = "reference", target = { 14728 Patient.class }) 14729 public static final String SP_PATIENT = "patient"; 14730 /** 14731 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 14732 * <p> 14733 * Description: <b>The identity of the subject of the contract (if a 14734 * patient)</b><br> 14735 * Type: <b>reference</b><br> 14736 * Path: <b>Contract.subject</b><br> 14737 * </p> 14738 */ 14739 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 14740 SP_PATIENT); 14741 14742 /** 14743 * Constant for fluent queries to be used to add include statements. Specifies 14744 * the path value of "<b>Contract:patient</b>". 14745 */ 14746 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 14747 "Contract:patient").toLocked(); 14748 14749 /** 14750 * Search parameter: <b>subject</b> 14751 * <p> 14752 * Description: <b>The identity of the subject of the contract</b><br> 14753 * Type: <b>reference</b><br> 14754 * Path: <b>Contract.subject</b><br> 14755 * </p> 14756 */ 14757 @SearchParamDefinition(name = "subject", path = "Contract.subject", description = "The identity of the subject of the contract", type = "reference") 14758 public static final String SP_SUBJECT = "subject"; 14759 /** 14760 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 14761 * <p> 14762 * Description: <b>The identity of the subject of the contract</b><br> 14763 * Type: <b>reference</b><br> 14764 * Path: <b>Contract.subject</b><br> 14765 * </p> 14766 */ 14767 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 14768 SP_SUBJECT); 14769 14770 /** 14771 * Constant for fluent queries to be used to add include statements. Specifies 14772 * the path value of "<b>Contract:subject</b>". 14773 */ 14774 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 14775 "Contract:subject").toLocked(); 14776 14777 /** 14778 * Search parameter: <b>authority</b> 14779 * <p> 14780 * Description: <b>The authority of the contract</b><br> 14781 * Type: <b>reference</b><br> 14782 * Path: <b>Contract.authority</b><br> 14783 * </p> 14784 */ 14785 @SearchParamDefinition(name = "authority", path = "Contract.authority", description = "The authority of the contract", type = "reference", target = { 14786 Organization.class }) 14787 public static final String SP_AUTHORITY = "authority"; 14788 /** 14789 * <b>Fluent Client</b> search parameter constant for <b>authority</b> 14790 * <p> 14791 * Description: <b>The authority of the contract</b><br> 14792 * Type: <b>reference</b><br> 14793 * Path: <b>Contract.authority</b><br> 14794 * </p> 14795 */ 14796 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHORITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 14797 SP_AUTHORITY); 14798 14799 /** 14800 * Constant for fluent queries to be used to add include statements. Specifies 14801 * the path value of "<b>Contract:authority</b>". 14802 */ 14803 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHORITY = new ca.uhn.fhir.model.api.Include( 14804 "Contract:authority").toLocked(); 14805 14806 /** 14807 * Search parameter: <b>domain</b> 14808 * <p> 14809 * Description: <b>The domain of the contract</b><br> 14810 * Type: <b>reference</b><br> 14811 * Path: <b>Contract.domain</b><br> 14812 * </p> 14813 */ 14814 @SearchParamDefinition(name = "domain", path = "Contract.domain", description = "The domain of the contract", type = "reference", target = { 14815 Location.class }) 14816 public static final String SP_DOMAIN = "domain"; 14817 /** 14818 * <b>Fluent Client</b> search parameter constant for <b>domain</b> 14819 * <p> 14820 * Description: <b>The domain of the contract</b><br> 14821 * Type: <b>reference</b><br> 14822 * Path: <b>Contract.domain</b><br> 14823 * </p> 14824 */ 14825 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DOMAIN = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 14826 SP_DOMAIN); 14827 14828 /** 14829 * Constant for fluent queries to be used to add include statements. Specifies 14830 * the path value of "<b>Contract:domain</b>". 14831 */ 14832 public static final ca.uhn.fhir.model.api.Include INCLUDE_DOMAIN = new ca.uhn.fhir.model.api.Include( 14833 "Contract:domain").toLocked(); 14834 14835 /** 14836 * Search parameter: <b>issued</b> 14837 * <p> 14838 * Description: <b>The date/time the contract was issued</b><br> 14839 * Type: <b>date</b><br> 14840 * Path: <b>Contract.issued</b><br> 14841 * </p> 14842 */ 14843 @SearchParamDefinition(name = "issued", path = "Contract.issued", description = "The date/time the contract was issued", type = "date") 14844 public static final String SP_ISSUED = "issued"; 14845 /** 14846 * <b>Fluent Client</b> search parameter constant for <b>issued</b> 14847 * <p> 14848 * Description: <b>The date/time the contract was issued</b><br> 14849 * Type: <b>date</b><br> 14850 * Path: <b>Contract.issued</b><br> 14851 * </p> 14852 */ 14853 public static final ca.uhn.fhir.rest.gclient.DateClientParam ISSUED = new ca.uhn.fhir.rest.gclient.DateClientParam( 14854 SP_ISSUED); 14855 14856 /** 14857 * Search parameter: <b>url</b> 14858 * <p> 14859 * Description: <b>The basal contract definition</b><br> 14860 * Type: <b>uri</b><br> 14861 * Path: <b>Contract.url</b><br> 14862 * </p> 14863 */ 14864 @SearchParamDefinition(name = "url", path = "Contract.url", description = "The basal contract definition", type = "uri") 14865 public static final String SP_URL = "url"; 14866 /** 14867 * <b>Fluent Client</b> search parameter constant for <b>url</b> 14868 * <p> 14869 * Description: <b>The basal contract definition</b><br> 14870 * Type: <b>uri</b><br> 14871 * Path: <b>Contract.url</b><br> 14872 * </p> 14873 */ 14874 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 14875 14876 /** 14877 * Search parameter: <b>signer</b> 14878 * <p> 14879 * Description: <b>Contract Signatory Party</b><br> 14880 * Type: <b>reference</b><br> 14881 * Path: <b>Contract.signer.party</b><br> 14882 * </p> 14883 */ 14884 @SearchParamDefinition(name = "signer", path = "Contract.signer.party", description = "Contract Signatory Party", type = "reference", target = { 14885 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 14886 public static final String SP_SIGNER = "signer"; 14887 /** 14888 * <b>Fluent Client</b> search parameter constant for <b>signer</b> 14889 * <p> 14890 * Description: <b>Contract Signatory Party</b><br> 14891 * Type: <b>reference</b><br> 14892 * Path: <b>Contract.signer.party</b><br> 14893 * </p> 14894 */ 14895 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SIGNER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 14896 SP_SIGNER); 14897 14898 /** 14899 * Constant for fluent queries to be used to add include statements. Specifies 14900 * the path value of "<b>Contract:signer</b>". 14901 */ 14902 public static final ca.uhn.fhir.model.api.Include INCLUDE_SIGNER = new ca.uhn.fhir.model.api.Include( 14903 "Contract:signer").toLocked(); 14904 14905 /** 14906 * Search parameter: <b>status</b> 14907 * <p> 14908 * Description: <b>The status of the contract</b><br> 14909 * Type: <b>token</b><br> 14910 * Path: <b>Contract.status</b><br> 14911 * </p> 14912 */ 14913 @SearchParamDefinition(name = "status", path = "Contract.status", description = "The status of the contract", type = "token") 14914 public static final String SP_STATUS = "status"; 14915 /** 14916 * <b>Fluent Client</b> search parameter constant for <b>status</b> 14917 * <p> 14918 * Description: <b>The status of the contract</b><br> 14919 * Type: <b>token</b><br> 14920 * Path: <b>Contract.status</b><br> 14921 * </p> 14922 */ 14923 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 14924 SP_STATUS); 14925 14926}