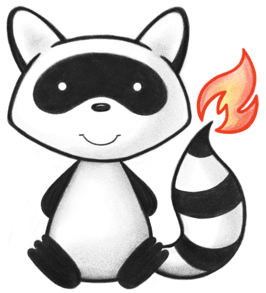
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.ICompositeType; 038 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.DatatypeDef; 041import ca.uhn.fhir.model.api.annotation.Description; 042 043/** 044 * A contributor to the content of a knowledge asset, including authors, 045 * editors, reviewers, and endorsers. 046 */ 047@DatatypeDef(name = "Contributor") 048public class Contributor extends Type implements ICompositeType { 049 050 public enum ContributorType { 051 /** 052 * An author of the content of the module. 053 */ 054 AUTHOR, 055 /** 056 * An editor of the content of the module. 057 */ 058 EDITOR, 059 /** 060 * A reviewer of the content of the module. 061 */ 062 REVIEWER, 063 /** 064 * An endorser of the content of the module. 065 */ 066 ENDORSER, 067 /** 068 * added to help the parsers with the generic types 069 */ 070 NULL; 071 072 public static ContributorType fromCode(String codeString) throws FHIRException { 073 if (codeString == null || "".equals(codeString)) 074 return null; 075 if ("author".equals(codeString)) 076 return AUTHOR; 077 if ("editor".equals(codeString)) 078 return EDITOR; 079 if ("reviewer".equals(codeString)) 080 return REVIEWER; 081 if ("endorser".equals(codeString)) 082 return ENDORSER; 083 if (Configuration.isAcceptInvalidEnums()) 084 return null; 085 else 086 throw new FHIRException("Unknown ContributorType code '" + codeString + "'"); 087 } 088 089 public String toCode() { 090 switch (this) { 091 case AUTHOR: 092 return "author"; 093 case EDITOR: 094 return "editor"; 095 case REVIEWER: 096 return "reviewer"; 097 case ENDORSER: 098 return "endorser"; 099 case NULL: 100 return null; 101 default: 102 return "?"; 103 } 104 } 105 106 public String getSystem() { 107 switch (this) { 108 case AUTHOR: 109 return "http://hl7.org/fhir/contributor-type"; 110 case EDITOR: 111 return "http://hl7.org/fhir/contributor-type"; 112 case REVIEWER: 113 return "http://hl7.org/fhir/contributor-type"; 114 case ENDORSER: 115 return "http://hl7.org/fhir/contributor-type"; 116 case NULL: 117 return null; 118 default: 119 return "?"; 120 } 121 } 122 123 public String getDefinition() { 124 switch (this) { 125 case AUTHOR: 126 return "An author of the content of the module."; 127 case EDITOR: 128 return "An editor of the content of the module."; 129 case REVIEWER: 130 return "A reviewer of the content of the module."; 131 case ENDORSER: 132 return "An endorser of the content of the module."; 133 case NULL: 134 return null; 135 default: 136 return "?"; 137 } 138 } 139 140 public String getDisplay() { 141 switch (this) { 142 case AUTHOR: 143 return "Author"; 144 case EDITOR: 145 return "Editor"; 146 case REVIEWER: 147 return "Reviewer"; 148 case ENDORSER: 149 return "Endorser"; 150 case NULL: 151 return null; 152 default: 153 return "?"; 154 } 155 } 156 } 157 158 public static class ContributorTypeEnumFactory implements EnumFactory<ContributorType> { 159 public ContributorType fromCode(String codeString) throws IllegalArgumentException { 160 if (codeString == null || "".equals(codeString)) 161 if (codeString == null || "".equals(codeString)) 162 return null; 163 if ("author".equals(codeString)) 164 return ContributorType.AUTHOR; 165 if ("editor".equals(codeString)) 166 return ContributorType.EDITOR; 167 if ("reviewer".equals(codeString)) 168 return ContributorType.REVIEWER; 169 if ("endorser".equals(codeString)) 170 return ContributorType.ENDORSER; 171 throw new IllegalArgumentException("Unknown ContributorType code '" + codeString + "'"); 172 } 173 174 public Enumeration<ContributorType> fromType(PrimitiveType<?> code) throws FHIRException { 175 if (code == null) 176 return null; 177 if (code.isEmpty()) 178 return new Enumeration<ContributorType>(this, ContributorType.NULL, code); 179 String codeString = code.asStringValue(); 180 if (codeString == null || "".equals(codeString)) 181 return new Enumeration<ContributorType>(this, ContributorType.NULL, code); 182 if ("author".equals(codeString)) 183 return new Enumeration<ContributorType>(this, ContributorType.AUTHOR, code); 184 if ("editor".equals(codeString)) 185 return new Enumeration<ContributorType>(this, ContributorType.EDITOR, code); 186 if ("reviewer".equals(codeString)) 187 return new Enumeration<ContributorType>(this, ContributorType.REVIEWER, code); 188 if ("endorser".equals(codeString)) 189 return new Enumeration<ContributorType>(this, ContributorType.ENDORSER, code); 190 throw new FHIRException("Unknown ContributorType code '" + codeString + "'"); 191 } 192 193 public String toCode(ContributorType code) { 194 if (code == ContributorType.NULL) 195 return null; 196 if (code == ContributorType.AUTHOR) 197 return "author"; 198 if (code == ContributorType.EDITOR) 199 return "editor"; 200 if (code == ContributorType.REVIEWER) 201 return "reviewer"; 202 if (code == ContributorType.ENDORSER) 203 return "endorser"; 204 return "?"; 205 } 206 207 public String toSystem(ContributorType code) { 208 return code.getSystem(); 209 } 210 } 211 212 /** 213 * The type of contributor. 214 */ 215 @Child(name = "type", type = { CodeType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 216 @Description(shortDefinition = "author | editor | reviewer | endorser", formalDefinition = "The type of contributor.") 217 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contributor-type") 218 protected Enumeration<ContributorType> type; 219 220 /** 221 * The name of the individual or organization responsible for the contribution. 222 */ 223 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 224 @Description(shortDefinition = "Who contributed the content", formalDefinition = "The name of the individual or organization responsible for the contribution.") 225 protected StringType name; 226 227 /** 228 * Contact details to assist a user in finding and communicating with the 229 * contributor. 230 */ 231 @Child(name = "contact", type = { 232 ContactDetail.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 233 @Description(shortDefinition = "Contact details of the contributor", formalDefinition = "Contact details to assist a user in finding and communicating with the contributor.") 234 protected List<ContactDetail> contact; 235 236 private static final long serialVersionUID = -609887113L; 237 238 /** 239 * Constructor 240 */ 241 public Contributor() { 242 super(); 243 } 244 245 /** 246 * Constructor 247 */ 248 public Contributor(Enumeration<ContributorType> type, StringType name) { 249 super(); 250 this.type = type; 251 this.name = name; 252 } 253 254 /** 255 * @return {@link #type} (The type of contributor.). This is the underlying 256 * object with id, value and extensions. The accessor "getType" gives 257 * direct access to the value 258 */ 259 public Enumeration<ContributorType> getTypeElement() { 260 if (this.type == null) 261 if (Configuration.errorOnAutoCreate()) 262 throw new Error("Attempt to auto-create Contributor.type"); 263 else if (Configuration.doAutoCreate()) 264 this.type = new Enumeration<ContributorType>(new ContributorTypeEnumFactory()); // bb 265 return this.type; 266 } 267 268 public boolean hasTypeElement() { 269 return this.type != null && !this.type.isEmpty(); 270 } 271 272 public boolean hasType() { 273 return this.type != null && !this.type.isEmpty(); 274 } 275 276 /** 277 * @param value {@link #type} (The type of contributor.). This is the underlying 278 * object with id, value and extensions. The accessor "getType" 279 * gives direct access to the value 280 */ 281 public Contributor setTypeElement(Enumeration<ContributorType> value) { 282 this.type = value; 283 return this; 284 } 285 286 /** 287 * @return The type of contributor. 288 */ 289 public ContributorType getType() { 290 return this.type == null ? null : this.type.getValue(); 291 } 292 293 /** 294 * @param value The type of contributor. 295 */ 296 public Contributor setType(ContributorType value) { 297 if (this.type == null) 298 this.type = new Enumeration<ContributorType>(new ContributorTypeEnumFactory()); 299 this.type.setValue(value); 300 return this; 301 } 302 303 /** 304 * @return {@link #name} (The name of the individual or organization responsible 305 * for the contribution.). This is the underlying object with id, value 306 * and extensions. The accessor "getName" gives direct access to the 307 * value 308 */ 309 public StringType getNameElement() { 310 if (this.name == null) 311 if (Configuration.errorOnAutoCreate()) 312 throw new Error("Attempt to auto-create Contributor.name"); 313 else if (Configuration.doAutoCreate()) 314 this.name = new StringType(); // bb 315 return this.name; 316 } 317 318 public boolean hasNameElement() { 319 return this.name != null && !this.name.isEmpty(); 320 } 321 322 public boolean hasName() { 323 return this.name != null && !this.name.isEmpty(); 324 } 325 326 /** 327 * @param value {@link #name} (The name of the individual or organization 328 * responsible for the contribution.). This is the underlying 329 * object with id, value and extensions. The accessor "getName" 330 * gives direct access to the value 331 */ 332 public Contributor setNameElement(StringType value) { 333 this.name = value; 334 return this; 335 } 336 337 /** 338 * @return The name of the individual or organization responsible for the 339 * contribution. 340 */ 341 public String getName() { 342 return this.name == null ? null : this.name.getValue(); 343 } 344 345 /** 346 * @param value The name of the individual or organization responsible for the 347 * contribution. 348 */ 349 public Contributor setName(String value) { 350 if (this.name == null) 351 this.name = new StringType(); 352 this.name.setValue(value); 353 return this; 354 } 355 356 /** 357 * @return {@link #contact} (Contact details to assist a user in finding and 358 * communicating with the contributor.) 359 */ 360 public List<ContactDetail> getContact() { 361 if (this.contact == null) 362 this.contact = new ArrayList<ContactDetail>(); 363 return this.contact; 364 } 365 366 /** 367 * @return Returns a reference to <code>this</code> for easy method chaining 368 */ 369 public Contributor setContact(List<ContactDetail> theContact) { 370 this.contact = theContact; 371 return this; 372 } 373 374 public boolean hasContact() { 375 if (this.contact == null) 376 return false; 377 for (ContactDetail item : this.contact) 378 if (!item.isEmpty()) 379 return true; 380 return false; 381 } 382 383 public ContactDetail addContact() { // 3 384 ContactDetail t = new ContactDetail(); 385 if (this.contact == null) 386 this.contact = new ArrayList<ContactDetail>(); 387 this.contact.add(t); 388 return t; 389 } 390 391 public Contributor addContact(ContactDetail t) { // 3 392 if (t == null) 393 return this; 394 if (this.contact == null) 395 this.contact = new ArrayList<ContactDetail>(); 396 this.contact.add(t); 397 return this; 398 } 399 400 /** 401 * @return The first repetition of repeating field {@link #contact}, creating it 402 * if it does not already exist 403 */ 404 public ContactDetail getContactFirstRep() { 405 if (getContact().isEmpty()) { 406 addContact(); 407 } 408 return getContact().get(0); 409 } 410 411 protected void listChildren(List<Property> children) { 412 super.listChildren(children); 413 children.add(new Property("type", "code", "The type of contributor.", 0, 1, type)); 414 children.add(new Property("name", "string", 415 "The name of the individual or organization responsible for the contribution.", 0, 1, name)); 416 children.add(new Property("contact", "ContactDetail", 417 "Contact details to assist a user in finding and communicating with the contributor.", 0, 418 java.lang.Integer.MAX_VALUE, contact)); 419 } 420 421 @Override 422 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 423 switch (_hash) { 424 case 3575610: 425 /* type */ return new Property("type", "code", "The type of contributor.", 0, 1, type); 426 case 3373707: 427 /* name */ return new Property("name", "string", 428 "The name of the individual or organization responsible for the contribution.", 0, 1, name); 429 case 951526432: 430 /* contact */ return new Property("contact", "ContactDetail", 431 "Contact details to assist a user in finding and communicating with the contributor.", 0, 432 java.lang.Integer.MAX_VALUE, contact); 433 default: 434 return super.getNamedProperty(_hash, _name, _checkValid); 435 } 436 437 } 438 439 @Override 440 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 441 switch (hash) { 442 case 3575610: 443 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<ContributorType> 444 case 3373707: 445 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 446 case 951526432: 447 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 448 default: 449 return super.getProperty(hash, name, checkValid); 450 } 451 452 } 453 454 @Override 455 public Base setProperty(int hash, String name, Base value) throws FHIRException { 456 switch (hash) { 457 case 3575610: // type 458 value = new ContributorTypeEnumFactory().fromType(castToCode(value)); 459 this.type = (Enumeration) value; // Enumeration<ContributorType> 460 return value; 461 case 3373707: // name 462 this.name = castToString(value); // StringType 463 return value; 464 case 951526432: // contact 465 this.getContact().add(castToContactDetail(value)); // ContactDetail 466 return value; 467 default: 468 return super.setProperty(hash, name, value); 469 } 470 471 } 472 473 @Override 474 public Base setProperty(String name, Base value) throws FHIRException { 475 if (name.equals("type")) { 476 value = new ContributorTypeEnumFactory().fromType(castToCode(value)); 477 this.type = (Enumeration) value; // Enumeration<ContributorType> 478 } else if (name.equals("name")) { 479 this.name = castToString(value); // StringType 480 } else if (name.equals("contact")) { 481 this.getContact().add(castToContactDetail(value)); 482 } else 483 return super.setProperty(name, value); 484 return value; 485 } 486 487 @Override 488 public void removeChild(String name, Base value) throws FHIRException { 489 if (name.equals("type")) { 490 this.type = null; 491 } else if (name.equals("name")) { 492 this.name = null; 493 } else if (name.equals("contact")) { 494 this.getContact().remove(castToContactDetail(value)); 495 } else 496 super.removeChild(name, value); 497 498 } 499 500 @Override 501 public Base makeProperty(int hash, String name) throws FHIRException { 502 switch (hash) { 503 case 3575610: 504 return getTypeElement(); 505 case 3373707: 506 return getNameElement(); 507 case 951526432: 508 return addContact(); 509 default: 510 return super.makeProperty(hash, name); 511 } 512 513 } 514 515 @Override 516 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 517 switch (hash) { 518 case 3575610: 519 /* type */ return new String[] { "code" }; 520 case 3373707: 521 /* name */ return new String[] { "string" }; 522 case 951526432: 523 /* contact */ return new String[] { "ContactDetail" }; 524 default: 525 return super.getTypesForProperty(hash, name); 526 } 527 528 } 529 530 @Override 531 public Base addChild(String name) throws FHIRException { 532 if (name.equals("type")) { 533 throw new FHIRException("Cannot call addChild on a singleton property Contributor.type"); 534 } else if (name.equals("name")) { 535 throw new FHIRException("Cannot call addChild on a singleton property Contributor.name"); 536 } else if (name.equals("contact")) { 537 return addContact(); 538 } else 539 return super.addChild(name); 540 } 541 542 public String fhirType() { 543 return "Contributor"; 544 545 } 546 547 public Contributor copy() { 548 Contributor dst = new Contributor(); 549 copyValues(dst); 550 return dst; 551 } 552 553 public void copyValues(Contributor dst) { 554 super.copyValues(dst); 555 dst.type = type == null ? null : type.copy(); 556 dst.name = name == null ? null : name.copy(); 557 if (contact != null) { 558 dst.contact = new ArrayList<ContactDetail>(); 559 for (ContactDetail i : contact) 560 dst.contact.add(i.copy()); 561 } 562 ; 563 } 564 565 protected Contributor typedCopy() { 566 return copy(); 567 } 568 569 @Override 570 public boolean equalsDeep(Base other_) { 571 if (!super.equalsDeep(other_)) 572 return false; 573 if (!(other_ instanceof Contributor)) 574 return false; 575 Contributor o = (Contributor) other_; 576 return compareDeep(type, o.type, true) && compareDeep(name, o.name, true) && compareDeep(contact, o.contact, true); 577 } 578 579 @Override 580 public boolean equalsShallow(Base other_) { 581 if (!super.equalsShallow(other_)) 582 return false; 583 if (!(other_ instanceof Contributor)) 584 return false; 585 Contributor o = (Contributor) other_; 586 return compareValues(type, o.type, true) && compareValues(name, o.name, true); 587 } 588 589 public boolean isEmpty() { 590 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, name, contact); 591 } 592 593}