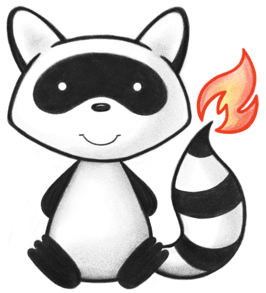
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * Financial instrument which may be used to reimburse or pay for health care 048 * products and services. Includes both insurance and self-payment. 049 */ 050@ResourceDef(name = "Coverage", profile = "http://hl7.org/fhir/StructureDefinition/Coverage") 051public class Coverage extends DomainResource { 052 053 public enum CoverageStatus { 054 /** 055 * The instance is currently in-force. 056 */ 057 ACTIVE, 058 /** 059 * The instance is withdrawn, rescinded or reversed. 060 */ 061 CANCELLED, 062 /** 063 * A new instance the contents of which is not complete. 064 */ 065 DRAFT, 066 /** 067 * The instance was entered in error. 068 */ 069 ENTEREDINERROR, 070 /** 071 * added to help the parsers with the generic types 072 */ 073 NULL; 074 075 public static CoverageStatus fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("active".equals(codeString)) 079 return ACTIVE; 080 if ("cancelled".equals(codeString)) 081 return CANCELLED; 082 if ("draft".equals(codeString)) 083 return DRAFT; 084 if ("entered-in-error".equals(codeString)) 085 return ENTEREDINERROR; 086 if (Configuration.isAcceptInvalidEnums()) 087 return null; 088 else 089 throw new FHIRException("Unknown CoverageStatus code '" + codeString + "'"); 090 } 091 092 public String toCode() { 093 switch (this) { 094 case ACTIVE: 095 return "active"; 096 case CANCELLED: 097 return "cancelled"; 098 case DRAFT: 099 return "draft"; 100 case ENTEREDINERROR: 101 return "entered-in-error"; 102 case NULL: 103 return null; 104 default: 105 return "?"; 106 } 107 } 108 109 public String getSystem() { 110 switch (this) { 111 case ACTIVE: 112 return "http://hl7.org/fhir/fm-status"; 113 case CANCELLED: 114 return "http://hl7.org/fhir/fm-status"; 115 case DRAFT: 116 return "http://hl7.org/fhir/fm-status"; 117 case ENTEREDINERROR: 118 return "http://hl7.org/fhir/fm-status"; 119 case NULL: 120 return null; 121 default: 122 return "?"; 123 } 124 } 125 126 public String getDefinition() { 127 switch (this) { 128 case ACTIVE: 129 return "The instance is currently in-force."; 130 case CANCELLED: 131 return "The instance is withdrawn, rescinded or reversed."; 132 case DRAFT: 133 return "A new instance the contents of which is not complete."; 134 case ENTEREDINERROR: 135 return "The instance was entered in error."; 136 case NULL: 137 return null; 138 default: 139 return "?"; 140 } 141 } 142 143 public String getDisplay() { 144 switch (this) { 145 case ACTIVE: 146 return "Active"; 147 case CANCELLED: 148 return "Cancelled"; 149 case DRAFT: 150 return "Draft"; 151 case ENTEREDINERROR: 152 return "Entered in Error"; 153 case NULL: 154 return null; 155 default: 156 return "?"; 157 } 158 } 159 } 160 161 public static class CoverageStatusEnumFactory implements EnumFactory<CoverageStatus> { 162 public CoverageStatus fromCode(String codeString) throws IllegalArgumentException { 163 if (codeString == null || "".equals(codeString)) 164 if (codeString == null || "".equals(codeString)) 165 return null; 166 if ("active".equals(codeString)) 167 return CoverageStatus.ACTIVE; 168 if ("cancelled".equals(codeString)) 169 return CoverageStatus.CANCELLED; 170 if ("draft".equals(codeString)) 171 return CoverageStatus.DRAFT; 172 if ("entered-in-error".equals(codeString)) 173 return CoverageStatus.ENTEREDINERROR; 174 throw new IllegalArgumentException("Unknown CoverageStatus code '" + codeString + "'"); 175 } 176 177 public Enumeration<CoverageStatus> fromType(PrimitiveType<?> code) throws FHIRException { 178 if (code == null) 179 return null; 180 if (code.isEmpty()) 181 return new Enumeration<CoverageStatus>(this, CoverageStatus.NULL, code); 182 String codeString = code.asStringValue(); 183 if (codeString == null || "".equals(codeString)) 184 return new Enumeration<CoverageStatus>(this, CoverageStatus.NULL, code); 185 if ("active".equals(codeString)) 186 return new Enumeration<CoverageStatus>(this, CoverageStatus.ACTIVE, code); 187 if ("cancelled".equals(codeString)) 188 return new Enumeration<CoverageStatus>(this, CoverageStatus.CANCELLED, code); 189 if ("draft".equals(codeString)) 190 return new Enumeration<CoverageStatus>(this, CoverageStatus.DRAFT, code); 191 if ("entered-in-error".equals(codeString)) 192 return new Enumeration<CoverageStatus>(this, CoverageStatus.ENTEREDINERROR, code); 193 throw new FHIRException("Unknown CoverageStatus code '" + codeString + "'"); 194 } 195 196 public String toCode(CoverageStatus code) { 197 if (code == CoverageStatus.ACTIVE) 198 return "active"; 199 if (code == CoverageStatus.CANCELLED) 200 return "cancelled"; 201 if (code == CoverageStatus.DRAFT) 202 return "draft"; 203 if (code == CoverageStatus.ENTEREDINERROR) 204 return "entered-in-error"; 205 return "?"; 206 } 207 208 public String toSystem(CoverageStatus code) { 209 return code.getSystem(); 210 } 211 } 212 213 @Block() 214 public static class ClassComponent extends BackboneElement implements IBaseBackboneElement { 215 /** 216 * The type of classification for which an insurer-specific class label or 217 * number and optional name is provided, for example may be used to identify a 218 * class of coverage or employer group, Policy, Plan. 219 */ 220 @Child(name = "type", type = { 221 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 222 @Description(shortDefinition = "Type of class such as 'group' or 'plan'", formalDefinition = "The type of classification for which an insurer-specific class label or number and optional name is provided, for example may be used to identify a class of coverage or employer group, Policy, Plan.") 223 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/coverage-class") 224 protected CodeableConcept type; 225 226 /** 227 * The alphanumeric string value associated with the insurer issued label. 228 */ 229 @Child(name = "value", type = { StringType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 230 @Description(shortDefinition = "Value associated with the type", formalDefinition = "The alphanumeric string value associated with the insurer issued label.") 231 protected StringType value; 232 233 /** 234 * A short description for the class. 235 */ 236 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 237 @Description(shortDefinition = "Human readable description of the type and value", formalDefinition = "A short description for the class.") 238 protected StringType name; 239 240 private static final long serialVersionUID = -1501519769L; 241 242 /** 243 * Constructor 244 */ 245 public ClassComponent() { 246 super(); 247 } 248 249 /** 250 * Constructor 251 */ 252 public ClassComponent(CodeableConcept type, StringType value) { 253 super(); 254 this.type = type; 255 this.value = value; 256 } 257 258 /** 259 * @return {@link #type} (The type of classification for which an 260 * insurer-specific class label or number and optional name is provided, 261 * for example may be used to identify a class of coverage or employer 262 * group, Policy, Plan.) 263 */ 264 public CodeableConcept getType() { 265 if (this.type == null) 266 if (Configuration.errorOnAutoCreate()) 267 throw new Error("Attempt to auto-create ClassComponent.type"); 268 else if (Configuration.doAutoCreate()) 269 this.type = new CodeableConcept(); // cc 270 return this.type; 271 } 272 273 public boolean hasType() { 274 return this.type != null && !this.type.isEmpty(); 275 } 276 277 /** 278 * @param value {@link #type} (The type of classification for which an 279 * insurer-specific class label or number and optional name is 280 * provided, for example may be used to identify a class of 281 * coverage or employer group, Policy, Plan.) 282 */ 283 public ClassComponent setType(CodeableConcept value) { 284 this.type = value; 285 return this; 286 } 287 288 /** 289 * @return {@link #value} (The alphanumeric string value associated with the 290 * insurer issued label.). This is the underlying object with id, value 291 * and extensions. The accessor "getValue" gives direct access to the 292 * value 293 */ 294 public StringType getValueElement() { 295 if (this.value == null) 296 if (Configuration.errorOnAutoCreate()) 297 throw new Error("Attempt to auto-create ClassComponent.value"); 298 else if (Configuration.doAutoCreate()) 299 this.value = new StringType(); // bb 300 return this.value; 301 } 302 303 public boolean hasValueElement() { 304 return this.value != null && !this.value.isEmpty(); 305 } 306 307 public boolean hasValue() { 308 return this.value != null && !this.value.isEmpty(); 309 } 310 311 /** 312 * @param value {@link #value} (The alphanumeric string value associated with 313 * the insurer issued label.). This is the underlying object with 314 * id, value and extensions. The accessor "getValue" gives direct 315 * access to the value 316 */ 317 public ClassComponent setValueElement(StringType value) { 318 this.value = value; 319 return this; 320 } 321 322 /** 323 * @return The alphanumeric string value associated with the insurer issued 324 * label. 325 */ 326 public String getValue() { 327 return this.value == null ? null : this.value.getValue(); 328 } 329 330 /** 331 * @param value The alphanumeric string value associated with the insurer issued 332 * label. 333 */ 334 public ClassComponent setValue(String value) { 335 if (this.value == null) 336 this.value = new StringType(); 337 this.value.setValue(value); 338 return this; 339 } 340 341 /** 342 * @return {@link #name} (A short description for the class.). This is the 343 * underlying object with id, value and extensions. The accessor 344 * "getName" gives direct access to the value 345 */ 346 public StringType getNameElement() { 347 if (this.name == null) 348 if (Configuration.errorOnAutoCreate()) 349 throw new Error("Attempt to auto-create ClassComponent.name"); 350 else if (Configuration.doAutoCreate()) 351 this.name = new StringType(); // bb 352 return this.name; 353 } 354 355 public boolean hasNameElement() { 356 return this.name != null && !this.name.isEmpty(); 357 } 358 359 public boolean hasName() { 360 return this.name != null && !this.name.isEmpty(); 361 } 362 363 /** 364 * @param value {@link #name} (A short description for the class.). This is the 365 * underlying object with id, value and extensions. The accessor 366 * "getName" gives direct access to the value 367 */ 368 public ClassComponent setNameElement(StringType value) { 369 this.name = value; 370 return this; 371 } 372 373 /** 374 * @return A short description for the class. 375 */ 376 public String getName() { 377 return this.name == null ? null : this.name.getValue(); 378 } 379 380 /** 381 * @param value A short description for the class. 382 */ 383 public ClassComponent setName(String value) { 384 if (Utilities.noString(value)) 385 this.name = null; 386 else { 387 if (this.name == null) 388 this.name = new StringType(); 389 this.name.setValue(value); 390 } 391 return this; 392 } 393 394 protected void listChildren(List<Property> children) { 395 super.listChildren(children); 396 children.add(new Property("type", "CodeableConcept", 397 "The type of classification for which an insurer-specific class label or number and optional name is provided, for example may be used to identify a class of coverage or employer group, Policy, Plan.", 398 0, 1, type)); 399 children.add(new Property("value", "string", 400 "The alphanumeric string value associated with the insurer issued label.", 0, 1, value)); 401 children.add(new Property("name", "string", "A short description for the class.", 0, 1, name)); 402 } 403 404 @Override 405 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 406 switch (_hash) { 407 case 3575610: 408 /* type */ return new Property("type", "CodeableConcept", 409 "The type of classification for which an insurer-specific class label or number and optional name is provided, for example may be used to identify a class of coverage or employer group, Policy, Plan.", 410 0, 1, type); 411 case 111972721: 412 /* value */ return new Property("value", "string", 413 "The alphanumeric string value associated with the insurer issued label.", 0, 1, value); 414 case 3373707: 415 /* name */ return new Property("name", "string", "A short description for the class.", 0, 1, name); 416 default: 417 return super.getNamedProperty(_hash, _name, _checkValid); 418 } 419 420 } 421 422 @Override 423 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 424 switch (hash) { 425 case 3575610: 426 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 427 case 111972721: 428 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // StringType 429 case 3373707: 430 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 431 default: 432 return super.getProperty(hash, name, checkValid); 433 } 434 435 } 436 437 @Override 438 public Base setProperty(int hash, String name, Base value) throws FHIRException { 439 switch (hash) { 440 case 3575610: // type 441 this.type = castToCodeableConcept(value); // CodeableConcept 442 return value; 443 case 111972721: // value 444 this.value = castToString(value); // StringType 445 return value; 446 case 3373707: // name 447 this.name = castToString(value); // StringType 448 return value; 449 default: 450 return super.setProperty(hash, name, value); 451 } 452 453 } 454 455 @Override 456 public Base setProperty(String name, Base value) throws FHIRException { 457 if (name.equals("type")) { 458 this.type = castToCodeableConcept(value); // CodeableConcept 459 } else if (name.equals("value")) { 460 this.value = castToString(value); // StringType 461 } else if (name.equals("name")) { 462 this.name = castToString(value); // StringType 463 } else 464 return super.setProperty(name, value); 465 return value; 466 } 467 468 @Override 469 public Base makeProperty(int hash, String name) throws FHIRException { 470 switch (hash) { 471 case 3575610: 472 return getType(); 473 case 111972721: 474 return getValueElement(); 475 case 3373707: 476 return getNameElement(); 477 default: 478 return super.makeProperty(hash, name); 479 } 480 481 } 482 483 @Override 484 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 485 switch (hash) { 486 case 3575610: 487 /* type */ return new String[] { "CodeableConcept" }; 488 case 111972721: 489 /* value */ return new String[] { "string" }; 490 case 3373707: 491 /* name */ return new String[] { "string" }; 492 default: 493 return super.getTypesForProperty(hash, name); 494 } 495 496 } 497 498 @Override 499 public Base addChild(String name) throws FHIRException { 500 if (name.equals("type")) { 501 this.type = new CodeableConcept(); 502 return this.type; 503 } else if (name.equals("value")) { 504 throw new FHIRException("Cannot call addChild on a singleton property Coverage.value"); 505 } else if (name.equals("name")) { 506 throw new FHIRException("Cannot call addChild on a singleton property Coverage.name"); 507 } else 508 return super.addChild(name); 509 } 510 511 public ClassComponent copy() { 512 ClassComponent dst = new ClassComponent(); 513 copyValues(dst); 514 return dst; 515 } 516 517 public void copyValues(ClassComponent dst) { 518 super.copyValues(dst); 519 dst.type = type == null ? null : type.copy(); 520 dst.value = value == null ? null : value.copy(); 521 dst.name = name == null ? null : name.copy(); 522 } 523 524 @Override 525 public boolean equalsDeep(Base other_) { 526 if (!super.equalsDeep(other_)) 527 return false; 528 if (!(other_ instanceof ClassComponent)) 529 return false; 530 ClassComponent o = (ClassComponent) other_; 531 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true) && compareDeep(name, o.name, true); 532 } 533 534 @Override 535 public boolean equalsShallow(Base other_) { 536 if (!super.equalsShallow(other_)) 537 return false; 538 if (!(other_ instanceof ClassComponent)) 539 return false; 540 ClassComponent o = (ClassComponent) other_; 541 return compareValues(value, o.value, true) && compareValues(name, o.name, true); 542 } 543 544 public boolean isEmpty() { 545 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value, name); 546 } 547 548 public String fhirType() { 549 return "Coverage.class"; 550 551 } 552 553 } 554 555 @Block() 556 public static class CostToBeneficiaryComponent extends BackboneElement implements IBaseBackboneElement { 557 /** 558 * The category of patient centric costs associated with treatment. 559 */ 560 @Child(name = "type", type = { 561 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 562 @Description(shortDefinition = "Cost category", formalDefinition = "The category of patient centric costs associated with treatment.") 563 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/coverage-copay-type") 564 protected CodeableConcept type; 565 566 /** 567 * The amount due from the patient for the cost category. 568 */ 569 @Child(name = "value", type = { Quantity.class, 570 Money.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 571 @Description(shortDefinition = "The amount or percentage due from the beneficiary", formalDefinition = "The amount due from the patient for the cost category.") 572 protected Type value; 573 574 /** 575 * A suite of codes indicating exceptions or reductions to patient costs and 576 * their effective periods. 577 */ 578 @Child(name = "exception", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 579 @Description(shortDefinition = "Exceptions for patient payments", formalDefinition = "A suite of codes indicating exceptions or reductions to patient costs and their effective periods.") 580 protected List<ExemptionComponent> exception; 581 582 private static final long serialVersionUID = -1302829059L; 583 584 /** 585 * Constructor 586 */ 587 public CostToBeneficiaryComponent() { 588 super(); 589 } 590 591 /** 592 * Constructor 593 */ 594 public CostToBeneficiaryComponent(Type value) { 595 super(); 596 this.value = value; 597 } 598 599 /** 600 * @return {@link #type} (The category of patient centric costs associated with 601 * treatment.) 602 */ 603 public CodeableConcept getType() { 604 if (this.type == null) 605 if (Configuration.errorOnAutoCreate()) 606 throw new Error("Attempt to auto-create CostToBeneficiaryComponent.type"); 607 else if (Configuration.doAutoCreate()) 608 this.type = new CodeableConcept(); // cc 609 return this.type; 610 } 611 612 public boolean hasType() { 613 return this.type != null && !this.type.isEmpty(); 614 } 615 616 /** 617 * @param value {@link #type} (The category of patient centric costs associated 618 * with treatment.) 619 */ 620 public CostToBeneficiaryComponent setType(CodeableConcept value) { 621 this.type = value; 622 return this; 623 } 624 625 /** 626 * @return {@link #value} (The amount due from the patient for the cost 627 * category.) 628 */ 629 public Type getValue() { 630 return this.value; 631 } 632 633 /** 634 * @return {@link #value} (The amount due from the patient for the cost 635 * category.) 636 */ 637 public Quantity getValueQuantity() throws FHIRException { 638 if (this.value == null) 639 this.value = new Quantity(); 640 if (!(this.value instanceof Quantity)) 641 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName() 642 + " was encountered"); 643 return (Quantity) this.value; 644 } 645 646 public boolean hasValueQuantity() { 647 return this != null && this.value instanceof Quantity; 648 } 649 650 /** 651 * @return {@link #value} (The amount due from the patient for the cost 652 * category.) 653 */ 654 public Money getValueMoney() throws FHIRException { 655 if (this.value == null) 656 this.value = new Money(); 657 if (!(this.value instanceof Money)) 658 throw new FHIRException( 659 "Type mismatch: the type Money was expected, but " + this.value.getClass().getName() + " was encountered"); 660 return (Money) this.value; 661 } 662 663 public boolean hasValueMoney() { 664 return this != null && this.value instanceof Money; 665 } 666 667 public boolean hasValue() { 668 return this.value != null && !this.value.isEmpty(); 669 } 670 671 /** 672 * @param value {@link #value} (The amount due from the patient for the cost 673 * category.) 674 */ 675 public CostToBeneficiaryComponent setValue(Type value) { 676 if (value != null && !(value instanceof Quantity || value instanceof Money)) 677 throw new Error("Not the right type for Coverage.costToBeneficiary.value[x]: " + value.fhirType()); 678 this.value = value; 679 return this; 680 } 681 682 /** 683 * @return {@link #exception} (A suite of codes indicating exceptions or 684 * reductions to patient costs and their effective periods.) 685 */ 686 public List<ExemptionComponent> getException() { 687 if (this.exception == null) 688 this.exception = new ArrayList<ExemptionComponent>(); 689 return this.exception; 690 } 691 692 /** 693 * @return Returns a reference to <code>this</code> for easy method chaining 694 */ 695 public CostToBeneficiaryComponent setException(List<ExemptionComponent> theException) { 696 this.exception = theException; 697 return this; 698 } 699 700 public boolean hasException() { 701 if (this.exception == null) 702 return false; 703 for (ExemptionComponent item : this.exception) 704 if (!item.isEmpty()) 705 return true; 706 return false; 707 } 708 709 public ExemptionComponent addException() { // 3 710 ExemptionComponent t = new ExemptionComponent(); 711 if (this.exception == null) 712 this.exception = new ArrayList<ExemptionComponent>(); 713 this.exception.add(t); 714 return t; 715 } 716 717 public CostToBeneficiaryComponent addException(ExemptionComponent t) { // 3 718 if (t == null) 719 return this; 720 if (this.exception == null) 721 this.exception = new ArrayList<ExemptionComponent>(); 722 this.exception.add(t); 723 return this; 724 } 725 726 /** 727 * @return The first repetition of repeating field {@link #exception}, creating 728 * it if it does not already exist 729 */ 730 public ExemptionComponent getExceptionFirstRep() { 731 if (getException().isEmpty()) { 732 addException(); 733 } 734 return getException().get(0); 735 } 736 737 protected void listChildren(List<Property> children) { 738 super.listChildren(children); 739 children.add(new Property("type", "CodeableConcept", 740 "The category of patient centric costs associated with treatment.", 0, 1, type)); 741 children.add(new Property("value[x]", "SimpleQuantity|Money", 742 "The amount due from the patient for the cost category.", 0, 1, value)); 743 children.add(new Property("exception", "", 744 "A suite of codes indicating exceptions or reductions to patient costs and their effective periods.", 0, 745 java.lang.Integer.MAX_VALUE, exception)); 746 } 747 748 @Override 749 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 750 switch (_hash) { 751 case 3575610: 752 /* type */ return new Property("type", "CodeableConcept", 753 "The category of patient centric costs associated with treatment.", 0, 1, type); 754 case -1410166417: 755 /* value[x] */ return new Property("value[x]", "SimpleQuantity|Money", 756 "The amount due from the patient for the cost category.", 0, 1, value); 757 case 111972721: 758 /* value */ return new Property("value[x]", "SimpleQuantity|Money", 759 "The amount due from the patient for the cost category.", 0, 1, value); 760 case -2029823716: 761 /* valueQuantity */ return new Property("value[x]", "SimpleQuantity|Money", 762 "The amount due from the patient for the cost category.", 0, 1, value); 763 case 2026560975: 764 /* valueMoney */ return new Property("value[x]", "SimpleQuantity|Money", 765 "The amount due from the patient for the cost category.", 0, 1, value); 766 case 1481625679: 767 /* exception */ return new Property("exception", "", 768 "A suite of codes indicating exceptions or reductions to patient costs and their effective periods.", 0, 769 java.lang.Integer.MAX_VALUE, exception); 770 default: 771 return super.getNamedProperty(_hash, _name, _checkValid); 772 } 773 774 } 775 776 @Override 777 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 778 switch (hash) { 779 case 3575610: 780 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 781 case 111972721: 782 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 783 case 1481625679: 784 /* exception */ return this.exception == null ? new Base[0] 785 : this.exception.toArray(new Base[this.exception.size()]); // ExemptionComponent 786 default: 787 return super.getProperty(hash, name, checkValid); 788 } 789 790 } 791 792 @Override 793 public Base setProperty(int hash, String name, Base value) throws FHIRException { 794 switch (hash) { 795 case 3575610: // type 796 this.type = castToCodeableConcept(value); // CodeableConcept 797 return value; 798 case 111972721: // value 799 this.value = castToType(value); // Type 800 return value; 801 case 1481625679: // exception 802 this.getException().add((ExemptionComponent) value); // ExemptionComponent 803 return value; 804 default: 805 return super.setProperty(hash, name, value); 806 } 807 808 } 809 810 @Override 811 public Base setProperty(String name, Base value) throws FHIRException { 812 if (name.equals("type")) { 813 this.type = castToCodeableConcept(value); // CodeableConcept 814 } else if (name.equals("value[x]")) { 815 this.value = castToType(value); // Type 816 } else if (name.equals("exception")) { 817 this.getException().add((ExemptionComponent) value); 818 } else 819 return super.setProperty(name, value); 820 return value; 821 } 822 823 @Override 824 public Base makeProperty(int hash, String name) throws FHIRException { 825 switch (hash) { 826 case 3575610: 827 return getType(); 828 case -1410166417: 829 return getValue(); 830 case 111972721: 831 return getValue(); 832 case 1481625679: 833 return addException(); 834 default: 835 return super.makeProperty(hash, name); 836 } 837 838 } 839 840 @Override 841 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 842 switch (hash) { 843 case 3575610: 844 /* type */ return new String[] { "CodeableConcept" }; 845 case 111972721: 846 /* value */ return new String[] { "SimpleQuantity", "Money" }; 847 case 1481625679: 848 /* exception */ return new String[] {}; 849 default: 850 return super.getTypesForProperty(hash, name); 851 } 852 853 } 854 855 @Override 856 public Base addChild(String name) throws FHIRException { 857 if (name.equals("type")) { 858 this.type = new CodeableConcept(); 859 return this.type; 860 } else if (name.equals("valueQuantity")) { 861 this.value = new Quantity(); 862 return this.value; 863 } else if (name.equals("valueMoney")) { 864 this.value = new Money(); 865 return this.value; 866 } else if (name.equals("exception")) { 867 return addException(); 868 } else 869 return super.addChild(name); 870 } 871 872 public CostToBeneficiaryComponent copy() { 873 CostToBeneficiaryComponent dst = new CostToBeneficiaryComponent(); 874 copyValues(dst); 875 return dst; 876 } 877 878 public void copyValues(CostToBeneficiaryComponent dst) { 879 super.copyValues(dst); 880 dst.type = type == null ? null : type.copy(); 881 dst.value = value == null ? null : value.copy(); 882 if (exception != null) { 883 dst.exception = new ArrayList<ExemptionComponent>(); 884 for (ExemptionComponent i : exception) 885 dst.exception.add(i.copy()); 886 } 887 ; 888 } 889 890 @Override 891 public boolean equalsDeep(Base other_) { 892 if (!super.equalsDeep(other_)) 893 return false; 894 if (!(other_ instanceof CostToBeneficiaryComponent)) 895 return false; 896 CostToBeneficiaryComponent o = (CostToBeneficiaryComponent) other_; 897 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true) 898 && compareDeep(exception, o.exception, true); 899 } 900 901 @Override 902 public boolean equalsShallow(Base other_) { 903 if (!super.equalsShallow(other_)) 904 return false; 905 if (!(other_ instanceof CostToBeneficiaryComponent)) 906 return false; 907 CostToBeneficiaryComponent o = (CostToBeneficiaryComponent) other_; 908 return true; 909 } 910 911 public boolean isEmpty() { 912 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value, exception); 913 } 914 915 public String fhirType() { 916 return "Coverage.costToBeneficiary"; 917 918 } 919 920 } 921 922 @Block() 923 public static class ExemptionComponent extends BackboneElement implements IBaseBackboneElement { 924 /** 925 * The code for the specific exception. 926 */ 927 @Child(name = "type", type = { 928 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 929 @Description(shortDefinition = "Exception category", formalDefinition = "The code for the specific exception.") 930 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/coverage-financial-exception") 931 protected CodeableConcept type; 932 933 /** 934 * The timeframe during when the exception is in force. 935 */ 936 @Child(name = "period", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 937 @Description(shortDefinition = "The effective period of the exception", formalDefinition = "The timeframe during when the exception is in force.") 938 protected Period period; 939 940 private static final long serialVersionUID = 523191991L; 941 942 /** 943 * Constructor 944 */ 945 public ExemptionComponent() { 946 super(); 947 } 948 949 /** 950 * Constructor 951 */ 952 public ExemptionComponent(CodeableConcept type) { 953 super(); 954 this.type = type; 955 } 956 957 /** 958 * @return {@link #type} (The code for the specific exception.) 959 */ 960 public CodeableConcept getType() { 961 if (this.type == null) 962 if (Configuration.errorOnAutoCreate()) 963 throw new Error("Attempt to auto-create ExemptionComponent.type"); 964 else if (Configuration.doAutoCreate()) 965 this.type = new CodeableConcept(); // cc 966 return this.type; 967 } 968 969 public boolean hasType() { 970 return this.type != null && !this.type.isEmpty(); 971 } 972 973 /** 974 * @param value {@link #type} (The code for the specific exception.) 975 */ 976 public ExemptionComponent setType(CodeableConcept value) { 977 this.type = value; 978 return this; 979 } 980 981 /** 982 * @return {@link #period} (The timeframe during when the exception is in 983 * force.) 984 */ 985 public Period getPeriod() { 986 if (this.period == null) 987 if (Configuration.errorOnAutoCreate()) 988 throw new Error("Attempt to auto-create ExemptionComponent.period"); 989 else if (Configuration.doAutoCreate()) 990 this.period = new Period(); // cc 991 return this.period; 992 } 993 994 public boolean hasPeriod() { 995 return this.period != null && !this.period.isEmpty(); 996 } 997 998 /** 999 * @param value {@link #period} (The timeframe during when the exception is in 1000 * force.) 1001 */ 1002 public ExemptionComponent setPeriod(Period value) { 1003 this.period = value; 1004 return this; 1005 } 1006 1007 protected void listChildren(List<Property> children) { 1008 super.listChildren(children); 1009 children.add(new Property("type", "CodeableConcept", "The code for the specific exception.", 0, 1, type)); 1010 children 1011 .add(new Property("period", "Period", "The timeframe during when the exception is in force.", 0, 1, period)); 1012 } 1013 1014 @Override 1015 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1016 switch (_hash) { 1017 case 3575610: 1018 /* type */ return new Property("type", "CodeableConcept", "The code for the specific exception.", 0, 1, type); 1019 case -991726143: 1020 /* period */ return new Property("period", "Period", "The timeframe during when the exception is in force.", 0, 1021 1, period); 1022 default: 1023 return super.getNamedProperty(_hash, _name, _checkValid); 1024 } 1025 1026 } 1027 1028 @Override 1029 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1030 switch (hash) { 1031 case 3575610: 1032 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1033 case -991726143: 1034 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 1035 default: 1036 return super.getProperty(hash, name, checkValid); 1037 } 1038 1039 } 1040 1041 @Override 1042 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1043 switch (hash) { 1044 case 3575610: // type 1045 this.type = castToCodeableConcept(value); // CodeableConcept 1046 return value; 1047 case -991726143: // period 1048 this.period = castToPeriod(value); // Period 1049 return value; 1050 default: 1051 return super.setProperty(hash, name, value); 1052 } 1053 1054 } 1055 1056 @Override 1057 public Base setProperty(String name, Base value) throws FHIRException { 1058 if (name.equals("type")) { 1059 this.type = castToCodeableConcept(value); // CodeableConcept 1060 } else if (name.equals("period")) { 1061 this.period = castToPeriod(value); // Period 1062 } else 1063 return super.setProperty(name, value); 1064 return value; 1065 } 1066 1067 @Override 1068 public Base makeProperty(int hash, String name) throws FHIRException { 1069 switch (hash) { 1070 case 3575610: 1071 return getType(); 1072 case -991726143: 1073 return getPeriod(); 1074 default: 1075 return super.makeProperty(hash, name); 1076 } 1077 1078 } 1079 1080 @Override 1081 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1082 switch (hash) { 1083 case 3575610: 1084 /* type */ return new String[] { "CodeableConcept" }; 1085 case -991726143: 1086 /* period */ return new String[] { "Period" }; 1087 default: 1088 return super.getTypesForProperty(hash, name); 1089 } 1090 1091 } 1092 1093 @Override 1094 public Base addChild(String name) throws FHIRException { 1095 if (name.equals("type")) { 1096 this.type = new CodeableConcept(); 1097 return this.type; 1098 } else if (name.equals("period")) { 1099 this.period = new Period(); 1100 return this.period; 1101 } else 1102 return super.addChild(name); 1103 } 1104 1105 public ExemptionComponent copy() { 1106 ExemptionComponent dst = new ExemptionComponent(); 1107 copyValues(dst); 1108 return dst; 1109 } 1110 1111 public void copyValues(ExemptionComponent dst) { 1112 super.copyValues(dst); 1113 dst.type = type == null ? null : type.copy(); 1114 dst.period = period == null ? null : period.copy(); 1115 } 1116 1117 @Override 1118 public boolean equalsDeep(Base other_) { 1119 if (!super.equalsDeep(other_)) 1120 return false; 1121 if (!(other_ instanceof ExemptionComponent)) 1122 return false; 1123 ExemptionComponent o = (ExemptionComponent) other_; 1124 return compareDeep(type, o.type, true) && compareDeep(period, o.period, true); 1125 } 1126 1127 @Override 1128 public boolean equalsShallow(Base other_) { 1129 if (!super.equalsShallow(other_)) 1130 return false; 1131 if (!(other_ instanceof ExemptionComponent)) 1132 return false; 1133 ExemptionComponent o = (ExemptionComponent) other_; 1134 return true; 1135 } 1136 1137 public boolean isEmpty() { 1138 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, period); 1139 } 1140 1141 public String fhirType() { 1142 return "Coverage.costToBeneficiary.exception"; 1143 1144 } 1145 1146 } 1147 1148 /** 1149 * A unique identifier assigned to this coverage. 1150 */ 1151 @Child(name = "identifier", type = { 1152 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1153 @Description(shortDefinition = "Business Identifier for the coverage", formalDefinition = "A unique identifier assigned to this coverage.") 1154 protected List<Identifier> identifier; 1155 1156 /** 1157 * The status of the resource instance. 1158 */ 1159 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 1160 @Description(shortDefinition = "active | cancelled | draft | entered-in-error", formalDefinition = "The status of the resource instance.") 1161 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/fm-status") 1162 protected Enumeration<CoverageStatus> status; 1163 1164 /** 1165 * The type of coverage: social program, medical plan, accident coverage 1166 * (workers compensation, auto), group health or payment by an individual or 1167 * organization. 1168 */ 1169 @Child(name = "type", type = { CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1170 @Description(shortDefinition = "Coverage category such as medical or accident", formalDefinition = "The type of coverage: social program, medical plan, accident coverage (workers compensation, auto), group health or payment by an individual or organization.") 1171 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/coverage-type") 1172 protected CodeableConcept type; 1173 1174 /** 1175 * The party who 'owns' the insurance policy. 1176 */ 1177 @Child(name = "policyHolder", type = { Patient.class, RelatedPerson.class, 1178 Organization.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1179 @Description(shortDefinition = "Owner of the policy", formalDefinition = "The party who 'owns' the insurance policy.") 1180 protected Reference policyHolder; 1181 1182 /** 1183 * The actual object that is the target of the reference (The party who 'owns' 1184 * the insurance policy.) 1185 */ 1186 protected Resource policyHolderTarget; 1187 1188 /** 1189 * The party who has signed-up for or 'owns' the contractual relationship to the 1190 * policy or to whom the benefit of the policy for services rendered to them or 1191 * their family is due. 1192 */ 1193 @Child(name = "subscriber", type = { Patient.class, 1194 RelatedPerson.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1195 @Description(shortDefinition = "Subscriber to the policy", formalDefinition = "The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due.") 1196 protected Reference subscriber; 1197 1198 /** 1199 * The actual object that is the target of the reference (The party who has 1200 * signed-up for or 'owns' the contractual relationship to the policy or to whom 1201 * the benefit of the policy for services rendered to them or their family is 1202 * due.) 1203 */ 1204 protected Resource subscriberTarget; 1205 1206 /** 1207 * The insurer assigned ID for the Subscriber. 1208 */ 1209 @Child(name = "subscriberId", type = { 1210 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1211 @Description(shortDefinition = "ID assigned to the subscriber", formalDefinition = "The insurer assigned ID for the Subscriber.") 1212 protected StringType subscriberId; 1213 1214 /** 1215 * The party who benefits from the insurance coverage; the patient when products 1216 * and/or services are provided. 1217 */ 1218 @Child(name = "beneficiary", type = { Patient.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 1219 @Description(shortDefinition = "Plan beneficiary", formalDefinition = "The party who benefits from the insurance coverage; the patient when products and/or services are provided.") 1220 protected Reference beneficiary; 1221 1222 /** 1223 * The actual object that is the target of the reference (The party who benefits 1224 * from the insurance coverage; the patient when products and/or services are 1225 * provided.) 1226 */ 1227 protected Patient beneficiaryTarget; 1228 1229 /** 1230 * A unique identifier for a dependent under the coverage. 1231 */ 1232 @Child(name = "dependent", type = { StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1233 @Description(shortDefinition = "Dependent number", formalDefinition = "A unique identifier for a dependent under the coverage.") 1234 protected StringType dependent; 1235 1236 /** 1237 * The relationship of beneficiary (patient) to the subscriber. 1238 */ 1239 @Child(name = "relationship", type = { 1240 CodeableConcept.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1241 @Description(shortDefinition = "Beneficiary relationship to the subscriber", formalDefinition = "The relationship of beneficiary (patient) to the subscriber.") 1242 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/subscriber-relationship") 1243 protected CodeableConcept relationship; 1244 1245 /** 1246 * Time period during which the coverage is in force. A missing start date 1247 * indicates the start date isn't known, a missing end date means the coverage 1248 * is continuing to be in force. 1249 */ 1250 @Child(name = "period", type = { Period.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 1251 @Description(shortDefinition = "Coverage start and end dates", formalDefinition = "Time period during which the coverage is in force. A missing start date indicates the start date isn't known, a missing end date means the coverage is continuing to be in force.") 1252 protected Period period; 1253 1254 /** 1255 * The program or plan underwriter or payor including both insurance and 1256 * non-insurance agreements, such as patient-pay agreements. 1257 */ 1258 @Child(name = "payor", type = { Organization.class, Patient.class, 1259 RelatedPerson.class }, order = 10, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1260 @Description(shortDefinition = "Issuer of the policy", formalDefinition = "The program or plan underwriter or payor including both insurance and non-insurance agreements, such as patient-pay agreements.") 1261 protected List<Reference> payor; 1262 /** 1263 * The actual objects that are the target of the reference (The program or plan 1264 * underwriter or payor including both insurance and non-insurance agreements, 1265 * such as patient-pay agreements.) 1266 */ 1267 protected List<Resource> payorTarget; 1268 1269 /** 1270 * A suite of underwriter specific classifiers. 1271 */ 1272 @Child(name = "class", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1273 @Description(shortDefinition = "Additional coverage classifications", formalDefinition = "A suite of underwriter specific classifiers.") 1274 protected List<ClassComponent> class_; 1275 1276 /** 1277 * The order of applicability of this coverage relative to other coverages which 1278 * are currently in force. Note, there may be gaps in the numbering and this 1279 * does not imply primary, secondary etc. as the specific positioning of 1280 * coverages depends upon the episode of care. 1281 */ 1282 @Child(name = "order", type = { 1283 PositiveIntType.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 1284 @Description(shortDefinition = "Relative order of the coverage", formalDefinition = "The order of applicability of this coverage relative to other coverages which are currently in force. Note, there may be gaps in the numbering and this does not imply primary, secondary etc. as the specific positioning of coverages depends upon the episode of care.") 1285 protected PositiveIntType order; 1286 1287 /** 1288 * The insurer-specific identifier for the insurer-defined network of providers 1289 * to which the beneficiary may seek treatment which will be covered at the 1290 * 'in-network' rate, otherwise 'out of network' terms and conditions apply. 1291 */ 1292 @Child(name = "network", type = { StringType.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 1293 @Description(shortDefinition = "Insurer network", formalDefinition = "The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply.") 1294 protected StringType network; 1295 1296 /** 1297 * A suite of codes indicating the cost category and associated amount which 1298 * have been detailed in the policy and may have been included on the health 1299 * card. 1300 */ 1301 @Child(name = "costToBeneficiary", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1302 @Description(shortDefinition = "Patient payments for services/products", formalDefinition = "A suite of codes indicating the cost category and associated amount which have been detailed in the policy and may have been included on the health card.") 1303 protected List<CostToBeneficiaryComponent> costToBeneficiary; 1304 1305 /** 1306 * When 'subrogation=true' this insurance instance has been included not for 1307 * adjudication but to provide insurers with the details to recover costs. 1308 */ 1309 @Child(name = "subrogation", type = { 1310 BooleanType.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 1311 @Description(shortDefinition = "Reimbursement to insurer", formalDefinition = "When 'subrogation=true' this insurance instance has been included not for adjudication but to provide insurers with the details to recover costs.") 1312 protected BooleanType subrogation; 1313 1314 /** 1315 * The policy(s) which constitute this insurance coverage. 1316 */ 1317 @Child(name = "contract", type = { 1318 Contract.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1319 @Description(shortDefinition = "Contract details", formalDefinition = "The policy(s) which constitute this insurance coverage.") 1320 protected List<Reference> contract; 1321 /** 1322 * The actual objects that are the target of the reference (The policy(s) which 1323 * constitute this insurance coverage.) 1324 */ 1325 protected List<Contract> contractTarget; 1326 1327 private static final long serialVersionUID = 212315315L; 1328 1329 /** 1330 * Constructor 1331 */ 1332 public Coverage() { 1333 super(); 1334 } 1335 1336 /** 1337 * Constructor 1338 */ 1339 public Coverage(Enumeration<CoverageStatus> status, Reference beneficiary) { 1340 super(); 1341 this.status = status; 1342 this.beneficiary = beneficiary; 1343 } 1344 1345 /** 1346 * @return {@link #identifier} (A unique identifier assigned to this coverage.) 1347 */ 1348 public List<Identifier> getIdentifier() { 1349 if (this.identifier == null) 1350 this.identifier = new ArrayList<Identifier>(); 1351 return this.identifier; 1352 } 1353 1354 /** 1355 * @return Returns a reference to <code>this</code> for easy method chaining 1356 */ 1357 public Coverage setIdentifier(List<Identifier> theIdentifier) { 1358 this.identifier = theIdentifier; 1359 return this; 1360 } 1361 1362 public boolean hasIdentifier() { 1363 if (this.identifier == null) 1364 return false; 1365 for (Identifier item : this.identifier) 1366 if (!item.isEmpty()) 1367 return true; 1368 return false; 1369 } 1370 1371 public Identifier addIdentifier() { // 3 1372 Identifier t = new Identifier(); 1373 if (this.identifier == null) 1374 this.identifier = new ArrayList<Identifier>(); 1375 this.identifier.add(t); 1376 return t; 1377 } 1378 1379 public Coverage addIdentifier(Identifier t) { // 3 1380 if (t == null) 1381 return this; 1382 if (this.identifier == null) 1383 this.identifier = new ArrayList<Identifier>(); 1384 this.identifier.add(t); 1385 return this; 1386 } 1387 1388 /** 1389 * @return The first repetition of repeating field {@link #identifier}, creating 1390 * it if it does not already exist 1391 */ 1392 public Identifier getIdentifierFirstRep() { 1393 if (getIdentifier().isEmpty()) { 1394 addIdentifier(); 1395 } 1396 return getIdentifier().get(0); 1397 } 1398 1399 /** 1400 * @return {@link #status} (The status of the resource instance.). This is the 1401 * underlying object with id, value and extensions. The accessor 1402 * "getStatus" gives direct access to the value 1403 */ 1404 public Enumeration<CoverageStatus> getStatusElement() { 1405 if (this.status == null) 1406 if (Configuration.errorOnAutoCreate()) 1407 throw new Error("Attempt to auto-create Coverage.status"); 1408 else if (Configuration.doAutoCreate()) 1409 this.status = new Enumeration<CoverageStatus>(new CoverageStatusEnumFactory()); // bb 1410 return this.status; 1411 } 1412 1413 public boolean hasStatusElement() { 1414 return this.status != null && !this.status.isEmpty(); 1415 } 1416 1417 public boolean hasStatus() { 1418 return this.status != null && !this.status.isEmpty(); 1419 } 1420 1421 /** 1422 * @param value {@link #status} (The status of the resource instance.). This is 1423 * the underlying object with id, value and extensions. The 1424 * accessor "getStatus" gives direct access to the value 1425 */ 1426 public Coverage setStatusElement(Enumeration<CoverageStatus> value) { 1427 this.status = value; 1428 return this; 1429 } 1430 1431 /** 1432 * @return The status of the resource instance. 1433 */ 1434 public CoverageStatus getStatus() { 1435 return this.status == null ? null : this.status.getValue(); 1436 } 1437 1438 /** 1439 * @param value The status of the resource instance. 1440 */ 1441 public Coverage setStatus(CoverageStatus value) { 1442 if (this.status == null) 1443 this.status = new Enumeration<CoverageStatus>(new CoverageStatusEnumFactory()); 1444 this.status.setValue(value); 1445 return this; 1446 } 1447 1448 /** 1449 * @return {@link #type} (The type of coverage: social program, medical plan, 1450 * accident coverage (workers compensation, auto), group health or 1451 * payment by an individual or organization.) 1452 */ 1453 public CodeableConcept getType() { 1454 if (this.type == null) 1455 if (Configuration.errorOnAutoCreate()) 1456 throw new Error("Attempt to auto-create Coverage.type"); 1457 else if (Configuration.doAutoCreate()) 1458 this.type = new CodeableConcept(); // cc 1459 return this.type; 1460 } 1461 1462 public boolean hasType() { 1463 return this.type != null && !this.type.isEmpty(); 1464 } 1465 1466 /** 1467 * @param value {@link #type} (The type of coverage: social program, medical 1468 * plan, accident coverage (workers compensation, auto), group 1469 * health or payment by an individual or organization.) 1470 */ 1471 public Coverage setType(CodeableConcept value) { 1472 this.type = value; 1473 return this; 1474 } 1475 1476 /** 1477 * @return {@link #policyHolder} (The party who 'owns' the insurance policy.) 1478 */ 1479 public Reference getPolicyHolder() { 1480 if (this.policyHolder == null) 1481 if (Configuration.errorOnAutoCreate()) 1482 throw new Error("Attempt to auto-create Coverage.policyHolder"); 1483 else if (Configuration.doAutoCreate()) 1484 this.policyHolder = new Reference(); // cc 1485 return this.policyHolder; 1486 } 1487 1488 public boolean hasPolicyHolder() { 1489 return this.policyHolder != null && !this.policyHolder.isEmpty(); 1490 } 1491 1492 /** 1493 * @param value {@link #policyHolder} (The party who 'owns' the insurance 1494 * policy.) 1495 */ 1496 public Coverage setPolicyHolder(Reference value) { 1497 this.policyHolder = value; 1498 return this; 1499 } 1500 1501 /** 1502 * @return {@link #policyHolder} The actual object that is the target of the 1503 * reference. The reference library doesn't populate this, but you can 1504 * use it to hold the resource if you resolve it. (The party who 'owns' 1505 * the insurance policy.) 1506 */ 1507 public Resource getPolicyHolderTarget() { 1508 return this.policyHolderTarget; 1509 } 1510 1511 /** 1512 * @param value {@link #policyHolder} The actual object that is the target of 1513 * the reference. The reference library doesn't use these, but you 1514 * can use it to hold the resource if you resolve it. (The party 1515 * who 'owns' the insurance policy.) 1516 */ 1517 public Coverage setPolicyHolderTarget(Resource value) { 1518 this.policyHolderTarget = value; 1519 return this; 1520 } 1521 1522 /** 1523 * @return {@link #subscriber} (The party who has signed-up for or 'owns' the 1524 * contractual relationship to the policy or to whom the benefit of the 1525 * policy for services rendered to them or their family is due.) 1526 */ 1527 public Reference getSubscriber() { 1528 if (this.subscriber == null) 1529 if (Configuration.errorOnAutoCreate()) 1530 throw new Error("Attempt to auto-create Coverage.subscriber"); 1531 else if (Configuration.doAutoCreate()) 1532 this.subscriber = new Reference(); // cc 1533 return this.subscriber; 1534 } 1535 1536 public boolean hasSubscriber() { 1537 return this.subscriber != null && !this.subscriber.isEmpty(); 1538 } 1539 1540 /** 1541 * @param value {@link #subscriber} (The party who has signed-up for or 'owns' 1542 * the contractual relationship to the policy or to whom the 1543 * benefit of the policy for services rendered to them or their 1544 * family is due.) 1545 */ 1546 public Coverage setSubscriber(Reference value) { 1547 this.subscriber = value; 1548 return this; 1549 } 1550 1551 /** 1552 * @return {@link #subscriber} The actual object that is the target of the 1553 * reference. The reference library doesn't populate this, but you can 1554 * use it to hold the resource if you resolve it. (The party who has 1555 * signed-up for or 'owns' the contractual relationship to the policy or 1556 * to whom the benefit of the policy for services rendered to them or 1557 * their family is due.) 1558 */ 1559 public Resource getSubscriberTarget() { 1560 return this.subscriberTarget; 1561 } 1562 1563 /** 1564 * @param value {@link #subscriber} The actual object that is the target of the 1565 * reference. The reference library doesn't use these, but you can 1566 * use it to hold the resource if you resolve it. (The party who 1567 * has signed-up for or 'owns' the contractual relationship to the 1568 * policy or to whom the benefit of the policy for services 1569 * rendered to them or their family is due.) 1570 */ 1571 public Coverage setSubscriberTarget(Resource value) { 1572 this.subscriberTarget = value; 1573 return this; 1574 } 1575 1576 /** 1577 * @return {@link #subscriberId} (The insurer assigned ID for the Subscriber.). 1578 * This is the underlying object with id, value and extensions. The 1579 * accessor "getSubscriberId" gives direct access to the value 1580 */ 1581 public StringType getSubscriberIdElement() { 1582 if (this.subscriberId == null) 1583 if (Configuration.errorOnAutoCreate()) 1584 throw new Error("Attempt to auto-create Coverage.subscriberId"); 1585 else if (Configuration.doAutoCreate()) 1586 this.subscriberId = new StringType(); // bb 1587 return this.subscriberId; 1588 } 1589 1590 public boolean hasSubscriberIdElement() { 1591 return this.subscriberId != null && !this.subscriberId.isEmpty(); 1592 } 1593 1594 public boolean hasSubscriberId() { 1595 return this.subscriberId != null && !this.subscriberId.isEmpty(); 1596 } 1597 1598 /** 1599 * @param value {@link #subscriberId} (The insurer assigned ID for the 1600 * Subscriber.). This is the underlying object with id, value and 1601 * extensions. The accessor "getSubscriberId" gives direct access 1602 * to the value 1603 */ 1604 public Coverage setSubscriberIdElement(StringType value) { 1605 this.subscriberId = value; 1606 return this; 1607 } 1608 1609 /** 1610 * @return The insurer assigned ID for the Subscriber. 1611 */ 1612 public String getSubscriberId() { 1613 return this.subscriberId == null ? null : this.subscriberId.getValue(); 1614 } 1615 1616 /** 1617 * @param value The insurer assigned ID for the Subscriber. 1618 */ 1619 public Coverage setSubscriberId(String value) { 1620 if (Utilities.noString(value)) 1621 this.subscriberId = null; 1622 else { 1623 if (this.subscriberId == null) 1624 this.subscriberId = new StringType(); 1625 this.subscriberId.setValue(value); 1626 } 1627 return this; 1628 } 1629 1630 /** 1631 * @return {@link #beneficiary} (The party who benefits from the insurance 1632 * coverage; the patient when products and/or services are provided.) 1633 */ 1634 public Reference getBeneficiary() { 1635 if (this.beneficiary == null) 1636 if (Configuration.errorOnAutoCreate()) 1637 throw new Error("Attempt to auto-create Coverage.beneficiary"); 1638 else if (Configuration.doAutoCreate()) 1639 this.beneficiary = new Reference(); // cc 1640 return this.beneficiary; 1641 } 1642 1643 public boolean hasBeneficiary() { 1644 return this.beneficiary != null && !this.beneficiary.isEmpty(); 1645 } 1646 1647 /** 1648 * @param value {@link #beneficiary} (The party who benefits from the insurance 1649 * coverage; the patient when products and/or services are 1650 * provided.) 1651 */ 1652 public Coverage setBeneficiary(Reference value) { 1653 this.beneficiary = value; 1654 return this; 1655 } 1656 1657 /** 1658 * @return {@link #beneficiary} The actual object that is the target of the 1659 * reference. The reference library doesn't populate this, but you can 1660 * use it to hold the resource if you resolve it. (The party who 1661 * benefits from the insurance coverage; the patient when products 1662 * and/or services are provided.) 1663 */ 1664 public Patient getBeneficiaryTarget() { 1665 if (this.beneficiaryTarget == null) 1666 if (Configuration.errorOnAutoCreate()) 1667 throw new Error("Attempt to auto-create Coverage.beneficiary"); 1668 else if (Configuration.doAutoCreate()) 1669 this.beneficiaryTarget = new Patient(); // aa 1670 return this.beneficiaryTarget; 1671 } 1672 1673 /** 1674 * @param value {@link #beneficiary} The actual object that is the target of the 1675 * reference. The reference library doesn't use these, but you can 1676 * use it to hold the resource if you resolve it. (The party who 1677 * benefits from the insurance coverage; the patient when products 1678 * and/or services are provided.) 1679 */ 1680 public Coverage setBeneficiaryTarget(Patient value) { 1681 this.beneficiaryTarget = value; 1682 return this; 1683 } 1684 1685 /** 1686 * @return {@link #dependent} (A unique identifier for a dependent under the 1687 * coverage.). This is the underlying object with id, value and 1688 * extensions. The accessor "getDependent" gives direct access to the 1689 * value 1690 */ 1691 public StringType getDependentElement() { 1692 if (this.dependent == null) 1693 if (Configuration.errorOnAutoCreate()) 1694 throw new Error("Attempt to auto-create Coverage.dependent"); 1695 else if (Configuration.doAutoCreate()) 1696 this.dependent = new StringType(); // bb 1697 return this.dependent; 1698 } 1699 1700 public boolean hasDependentElement() { 1701 return this.dependent != null && !this.dependent.isEmpty(); 1702 } 1703 1704 public boolean hasDependent() { 1705 return this.dependent != null && !this.dependent.isEmpty(); 1706 } 1707 1708 /** 1709 * @param value {@link #dependent} (A unique identifier for a dependent under 1710 * the coverage.). This is the underlying object with id, value and 1711 * extensions. The accessor "getDependent" gives direct access to 1712 * the value 1713 */ 1714 public Coverage setDependentElement(StringType value) { 1715 this.dependent = value; 1716 return this; 1717 } 1718 1719 /** 1720 * @return A unique identifier for a dependent under the coverage. 1721 */ 1722 public String getDependent() { 1723 return this.dependent == null ? null : this.dependent.getValue(); 1724 } 1725 1726 /** 1727 * @param value A unique identifier for a dependent under the coverage. 1728 */ 1729 public Coverage setDependent(String value) { 1730 if (Utilities.noString(value)) 1731 this.dependent = null; 1732 else { 1733 if (this.dependent == null) 1734 this.dependent = new StringType(); 1735 this.dependent.setValue(value); 1736 } 1737 return this; 1738 } 1739 1740 /** 1741 * @return {@link #relationship} (The relationship of beneficiary (patient) to 1742 * the subscriber.) 1743 */ 1744 public CodeableConcept getRelationship() { 1745 if (this.relationship == null) 1746 if (Configuration.errorOnAutoCreate()) 1747 throw new Error("Attempt to auto-create Coverage.relationship"); 1748 else if (Configuration.doAutoCreate()) 1749 this.relationship = new CodeableConcept(); // cc 1750 return this.relationship; 1751 } 1752 1753 public boolean hasRelationship() { 1754 return this.relationship != null && !this.relationship.isEmpty(); 1755 } 1756 1757 /** 1758 * @param value {@link #relationship} (The relationship of beneficiary (patient) 1759 * to the subscriber.) 1760 */ 1761 public Coverage setRelationship(CodeableConcept value) { 1762 this.relationship = value; 1763 return this; 1764 } 1765 1766 /** 1767 * @return {@link #period} (Time period during which the coverage is in force. A 1768 * missing start date indicates the start date isn't known, a missing 1769 * end date means the coverage is continuing to be in force.) 1770 */ 1771 public Period getPeriod() { 1772 if (this.period == null) 1773 if (Configuration.errorOnAutoCreate()) 1774 throw new Error("Attempt to auto-create Coverage.period"); 1775 else if (Configuration.doAutoCreate()) 1776 this.period = new Period(); // cc 1777 return this.period; 1778 } 1779 1780 public boolean hasPeriod() { 1781 return this.period != null && !this.period.isEmpty(); 1782 } 1783 1784 /** 1785 * @param value {@link #period} (Time period during which the coverage is in 1786 * force. A missing start date indicates the start date isn't 1787 * known, a missing end date means the coverage is continuing to be 1788 * in force.) 1789 */ 1790 public Coverage setPeriod(Period value) { 1791 this.period = value; 1792 return this; 1793 } 1794 1795 /** 1796 * @return {@link #payor} (The program or plan underwriter or payor including 1797 * both insurance and non-insurance agreements, such as patient-pay 1798 * agreements.) 1799 */ 1800 public List<Reference> getPayor() { 1801 if (this.payor == null) 1802 this.payor = new ArrayList<Reference>(); 1803 return this.payor; 1804 } 1805 1806 /** 1807 * @return Returns a reference to <code>this</code> for easy method chaining 1808 */ 1809 public Coverage setPayor(List<Reference> thePayor) { 1810 this.payor = thePayor; 1811 return this; 1812 } 1813 1814 public boolean hasPayor() { 1815 if (this.payor == null) 1816 return false; 1817 for (Reference item : this.payor) 1818 if (!item.isEmpty()) 1819 return true; 1820 return false; 1821 } 1822 1823 public Reference addPayor() { // 3 1824 Reference t = new Reference(); 1825 if (this.payor == null) 1826 this.payor = new ArrayList<Reference>(); 1827 this.payor.add(t); 1828 return t; 1829 } 1830 1831 public Coverage addPayor(Reference t) { // 3 1832 if (t == null) 1833 return this; 1834 if (this.payor == null) 1835 this.payor = new ArrayList<Reference>(); 1836 this.payor.add(t); 1837 return this; 1838 } 1839 1840 /** 1841 * @return The first repetition of repeating field {@link #payor}, creating it 1842 * if it does not already exist 1843 */ 1844 public Reference getPayorFirstRep() { 1845 if (getPayor().isEmpty()) { 1846 addPayor(); 1847 } 1848 return getPayor().get(0); 1849 } 1850 1851 /** 1852 * @deprecated Use Reference#setResource(IBaseResource) instead 1853 */ 1854 @Deprecated 1855 public List<Resource> getPayorTarget() { 1856 if (this.payorTarget == null) 1857 this.payorTarget = new ArrayList<Resource>(); 1858 return this.payorTarget; 1859 } 1860 1861 /** 1862 * @return {@link #class_} (A suite of underwriter specific classifiers.) 1863 */ 1864 public List<ClassComponent> getClass_() { 1865 if (this.class_ == null) 1866 this.class_ = new ArrayList<ClassComponent>(); 1867 return this.class_; 1868 } 1869 1870 /** 1871 * @return Returns a reference to <code>this</code> for easy method chaining 1872 */ 1873 public Coverage setClass_(List<ClassComponent> theClass_) { 1874 this.class_ = theClass_; 1875 return this; 1876 } 1877 1878 public boolean hasClass_() { 1879 if (this.class_ == null) 1880 return false; 1881 for (ClassComponent item : this.class_) 1882 if (!item.isEmpty()) 1883 return true; 1884 return false; 1885 } 1886 1887 public ClassComponent addClass_() { // 3 1888 ClassComponent t = new ClassComponent(); 1889 if (this.class_ == null) 1890 this.class_ = new ArrayList<ClassComponent>(); 1891 this.class_.add(t); 1892 return t; 1893 } 1894 1895 public Coverage addClass_(ClassComponent t) { // 3 1896 if (t == null) 1897 return this; 1898 if (this.class_ == null) 1899 this.class_ = new ArrayList<ClassComponent>(); 1900 this.class_.add(t); 1901 return this; 1902 } 1903 1904 /** 1905 * @return The first repetition of repeating field {@link #class_}, creating it 1906 * if it does not already exist 1907 */ 1908 public ClassComponent getClass_FirstRep() { 1909 if (getClass_().isEmpty()) { 1910 addClass_(); 1911 } 1912 return getClass_().get(0); 1913 } 1914 1915 /** 1916 * @return {@link #order} (The order of applicability of this coverage relative 1917 * to other coverages which are currently in force. Note, there may be 1918 * gaps in the numbering and this does not imply primary, secondary etc. 1919 * as the specific positioning of coverages depends upon the episode of 1920 * care.). This is the underlying object with id, value and extensions. 1921 * The accessor "getOrder" gives direct access to the value 1922 */ 1923 public PositiveIntType getOrderElement() { 1924 if (this.order == null) 1925 if (Configuration.errorOnAutoCreate()) 1926 throw new Error("Attempt to auto-create Coverage.order"); 1927 else if (Configuration.doAutoCreate()) 1928 this.order = new PositiveIntType(); // bb 1929 return this.order; 1930 } 1931 1932 public boolean hasOrderElement() { 1933 return this.order != null && !this.order.isEmpty(); 1934 } 1935 1936 public boolean hasOrder() { 1937 return this.order != null && !this.order.isEmpty(); 1938 } 1939 1940 /** 1941 * @param value {@link #order} (The order of applicability of this coverage 1942 * relative to other coverages which are currently in force. Note, 1943 * there may be gaps in the numbering and this does not imply 1944 * primary, secondary etc. as the specific positioning of coverages 1945 * depends upon the episode of care.). This is the underlying 1946 * object with id, value and extensions. The accessor "getOrder" 1947 * gives direct access to the value 1948 */ 1949 public Coverage setOrderElement(PositiveIntType value) { 1950 this.order = value; 1951 return this; 1952 } 1953 1954 /** 1955 * @return The order of applicability of this coverage relative to other 1956 * coverages which are currently in force. Note, there may be gaps in 1957 * the numbering and this does not imply primary, secondary etc. as the 1958 * specific positioning of coverages depends upon the episode of care. 1959 */ 1960 public int getOrder() { 1961 return this.order == null || this.order.isEmpty() ? 0 : this.order.getValue(); 1962 } 1963 1964 /** 1965 * @param value The order of applicability of this coverage relative to other 1966 * coverages which are currently in force. Note, there may be gaps 1967 * in the numbering and this does not imply primary, secondary etc. 1968 * as the specific positioning of coverages depends upon the 1969 * episode of care. 1970 */ 1971 public Coverage setOrder(int value) { 1972 if (this.order == null) 1973 this.order = new PositiveIntType(); 1974 this.order.setValue(value); 1975 return this; 1976 } 1977 1978 /** 1979 * @return {@link #network} (The insurer-specific identifier for the 1980 * insurer-defined network of providers to which the beneficiary may 1981 * seek treatment which will be covered at the 'in-network' rate, 1982 * otherwise 'out of network' terms and conditions apply.). This is the 1983 * underlying object with id, value and extensions. The accessor 1984 * "getNetwork" gives direct access to the value 1985 */ 1986 public StringType getNetworkElement() { 1987 if (this.network == null) 1988 if (Configuration.errorOnAutoCreate()) 1989 throw new Error("Attempt to auto-create Coverage.network"); 1990 else if (Configuration.doAutoCreate()) 1991 this.network = new StringType(); // bb 1992 return this.network; 1993 } 1994 1995 public boolean hasNetworkElement() { 1996 return this.network != null && !this.network.isEmpty(); 1997 } 1998 1999 public boolean hasNetwork() { 2000 return this.network != null && !this.network.isEmpty(); 2001 } 2002 2003 /** 2004 * @param value {@link #network} (The insurer-specific identifier for the 2005 * insurer-defined network of providers to which the beneficiary 2006 * may seek treatment which will be covered at the 'in-network' 2007 * rate, otherwise 'out of network' terms and conditions apply.). 2008 * This is the underlying object with id, value and extensions. The 2009 * accessor "getNetwork" gives direct access to the value 2010 */ 2011 public Coverage setNetworkElement(StringType value) { 2012 this.network = value; 2013 return this; 2014 } 2015 2016 /** 2017 * @return The insurer-specific identifier for the insurer-defined network of 2018 * providers to which the beneficiary may seek treatment which will be 2019 * covered at the 'in-network' rate, otherwise 'out of network' terms 2020 * and conditions apply. 2021 */ 2022 public String getNetwork() { 2023 return this.network == null ? null : this.network.getValue(); 2024 } 2025 2026 /** 2027 * @param value The insurer-specific identifier for the insurer-defined network 2028 * of providers to which the beneficiary may seek treatment which 2029 * will be covered at the 'in-network' rate, otherwise 'out of 2030 * network' terms and conditions apply. 2031 */ 2032 public Coverage setNetwork(String value) { 2033 if (Utilities.noString(value)) 2034 this.network = null; 2035 else { 2036 if (this.network == null) 2037 this.network = new StringType(); 2038 this.network.setValue(value); 2039 } 2040 return this; 2041 } 2042 2043 /** 2044 * @return {@link #costToBeneficiary} (A suite of codes indicating the cost 2045 * category and associated amount which have been detailed in the policy 2046 * and may have been included on the health card.) 2047 */ 2048 public List<CostToBeneficiaryComponent> getCostToBeneficiary() { 2049 if (this.costToBeneficiary == null) 2050 this.costToBeneficiary = new ArrayList<CostToBeneficiaryComponent>(); 2051 return this.costToBeneficiary; 2052 } 2053 2054 /** 2055 * @return Returns a reference to <code>this</code> for easy method chaining 2056 */ 2057 public Coverage setCostToBeneficiary(List<CostToBeneficiaryComponent> theCostToBeneficiary) { 2058 this.costToBeneficiary = theCostToBeneficiary; 2059 return this; 2060 } 2061 2062 public boolean hasCostToBeneficiary() { 2063 if (this.costToBeneficiary == null) 2064 return false; 2065 for (CostToBeneficiaryComponent item : this.costToBeneficiary) 2066 if (!item.isEmpty()) 2067 return true; 2068 return false; 2069 } 2070 2071 public CostToBeneficiaryComponent addCostToBeneficiary() { // 3 2072 CostToBeneficiaryComponent t = new CostToBeneficiaryComponent(); 2073 if (this.costToBeneficiary == null) 2074 this.costToBeneficiary = new ArrayList<CostToBeneficiaryComponent>(); 2075 this.costToBeneficiary.add(t); 2076 return t; 2077 } 2078 2079 public Coverage addCostToBeneficiary(CostToBeneficiaryComponent t) { // 3 2080 if (t == null) 2081 return this; 2082 if (this.costToBeneficiary == null) 2083 this.costToBeneficiary = new ArrayList<CostToBeneficiaryComponent>(); 2084 this.costToBeneficiary.add(t); 2085 return this; 2086 } 2087 2088 /** 2089 * @return The first repetition of repeating field {@link #costToBeneficiary}, 2090 * creating it if it does not already exist 2091 */ 2092 public CostToBeneficiaryComponent getCostToBeneficiaryFirstRep() { 2093 if (getCostToBeneficiary().isEmpty()) { 2094 addCostToBeneficiary(); 2095 } 2096 return getCostToBeneficiary().get(0); 2097 } 2098 2099 /** 2100 * @return {@link #subrogation} (When 'subrogation=true' this insurance instance 2101 * has been included not for adjudication but to provide insurers with 2102 * the details to recover costs.). This is the underlying object with 2103 * id, value and extensions. The accessor "getSubrogation" gives direct 2104 * access to the value 2105 */ 2106 public BooleanType getSubrogationElement() { 2107 if (this.subrogation == null) 2108 if (Configuration.errorOnAutoCreate()) 2109 throw new Error("Attempt to auto-create Coverage.subrogation"); 2110 else if (Configuration.doAutoCreate()) 2111 this.subrogation = new BooleanType(); // bb 2112 return this.subrogation; 2113 } 2114 2115 public boolean hasSubrogationElement() { 2116 return this.subrogation != null && !this.subrogation.isEmpty(); 2117 } 2118 2119 public boolean hasSubrogation() { 2120 return this.subrogation != null && !this.subrogation.isEmpty(); 2121 } 2122 2123 /** 2124 * @param value {@link #subrogation} (When 'subrogation=true' this insurance 2125 * instance has been included not for adjudication but to provide 2126 * insurers with the details to recover costs.). This is the 2127 * underlying object with id, value and extensions. The accessor 2128 * "getSubrogation" gives direct access to the value 2129 */ 2130 public Coverage setSubrogationElement(BooleanType value) { 2131 this.subrogation = value; 2132 return this; 2133 } 2134 2135 /** 2136 * @return When 'subrogation=true' this insurance instance has been included not 2137 * for adjudication but to provide insurers with the details to recover 2138 * costs. 2139 */ 2140 public boolean getSubrogation() { 2141 return this.subrogation == null || this.subrogation.isEmpty() ? false : this.subrogation.getValue(); 2142 } 2143 2144 /** 2145 * @param value When 'subrogation=true' this insurance instance has been 2146 * included not for adjudication but to provide insurers with the 2147 * details to recover costs. 2148 */ 2149 public Coverage setSubrogation(boolean value) { 2150 if (this.subrogation == null) 2151 this.subrogation = new BooleanType(); 2152 this.subrogation.setValue(value); 2153 return this; 2154 } 2155 2156 /** 2157 * @return {@link #contract} (The policy(s) which constitute this insurance 2158 * coverage.) 2159 */ 2160 public List<Reference> getContract() { 2161 if (this.contract == null) 2162 this.contract = new ArrayList<Reference>(); 2163 return this.contract; 2164 } 2165 2166 /** 2167 * @return Returns a reference to <code>this</code> for easy method chaining 2168 */ 2169 public Coverage setContract(List<Reference> theContract) { 2170 this.contract = theContract; 2171 return this; 2172 } 2173 2174 public boolean hasContract() { 2175 if (this.contract == null) 2176 return false; 2177 for (Reference item : this.contract) 2178 if (!item.isEmpty()) 2179 return true; 2180 return false; 2181 } 2182 2183 public Reference addContract() { // 3 2184 Reference t = new Reference(); 2185 if (this.contract == null) 2186 this.contract = new ArrayList<Reference>(); 2187 this.contract.add(t); 2188 return t; 2189 } 2190 2191 public Coverage addContract(Reference t) { // 3 2192 if (t == null) 2193 return this; 2194 if (this.contract == null) 2195 this.contract = new ArrayList<Reference>(); 2196 this.contract.add(t); 2197 return this; 2198 } 2199 2200 /** 2201 * @return The first repetition of repeating field {@link #contract}, creating 2202 * it if it does not already exist 2203 */ 2204 public Reference getContractFirstRep() { 2205 if (getContract().isEmpty()) { 2206 addContract(); 2207 } 2208 return getContract().get(0); 2209 } 2210 2211 /** 2212 * @deprecated Use Reference#setResource(IBaseResource) instead 2213 */ 2214 @Deprecated 2215 public List<Contract> getContractTarget() { 2216 if (this.contractTarget == null) 2217 this.contractTarget = new ArrayList<Contract>(); 2218 return this.contractTarget; 2219 } 2220 2221 /** 2222 * @deprecated Use Reference#setResource(IBaseResource) instead 2223 */ 2224 @Deprecated 2225 public Contract addContractTarget() { 2226 Contract r = new Contract(); 2227 if (this.contractTarget == null) 2228 this.contractTarget = new ArrayList<Contract>(); 2229 this.contractTarget.add(r); 2230 return r; 2231 } 2232 2233 protected void listChildren(List<Property> children) { 2234 super.listChildren(children); 2235 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this coverage.", 0, 2236 java.lang.Integer.MAX_VALUE, identifier)); 2237 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 2238 children.add(new Property("type", "CodeableConcept", 2239 "The type of coverage: social program, medical plan, accident coverage (workers compensation, auto), group health or payment by an individual or organization.", 2240 0, 1, type)); 2241 children.add(new Property("policyHolder", "Reference(Patient|RelatedPerson|Organization)", 2242 "The party who 'owns' the insurance policy.", 0, 1, policyHolder)); 2243 children.add(new Property("subscriber", "Reference(Patient|RelatedPerson)", 2244 "The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due.", 2245 0, 1, subscriber)); 2246 children 2247 .add(new Property("subscriberId", "string", "The insurer assigned ID for the Subscriber.", 0, 1, subscriberId)); 2248 children.add(new Property("beneficiary", "Reference(Patient)", 2249 "The party who benefits from the insurance coverage; the patient when products and/or services are provided.", 2250 0, 1, beneficiary)); 2251 children.add(new Property("dependent", "string", "A unique identifier for a dependent under the coverage.", 0, 1, 2252 dependent)); 2253 children.add(new Property("relationship", "CodeableConcept", 2254 "The relationship of beneficiary (patient) to the subscriber.", 0, 1, relationship)); 2255 children.add(new Property("period", "Period", 2256 "Time period during which the coverage is in force. A missing start date indicates the start date isn't known, a missing end date means the coverage is continuing to be in force.", 2257 0, 1, period)); 2258 children.add(new Property("payor", "Reference(Organization|Patient|RelatedPerson)", 2259 "The program or plan underwriter or payor including both insurance and non-insurance agreements, such as patient-pay agreements.", 2260 0, java.lang.Integer.MAX_VALUE, payor)); 2261 children.add(new Property("class", "", "A suite of underwriter specific classifiers.", 0, 2262 java.lang.Integer.MAX_VALUE, class_)); 2263 children.add(new Property("order", "positiveInt", 2264 "The order of applicability of this coverage relative to other coverages which are currently in force. Note, there may be gaps in the numbering and this does not imply primary, secondary etc. as the specific positioning of coverages depends upon the episode of care.", 2265 0, 1, order)); 2266 children.add(new Property("network", "string", 2267 "The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply.", 2268 0, 1, network)); 2269 children.add(new Property("costToBeneficiary", "", 2270 "A suite of codes indicating the cost category and associated amount which have been detailed in the policy and may have been included on the health card.", 2271 0, java.lang.Integer.MAX_VALUE, costToBeneficiary)); 2272 children.add(new Property("subrogation", "boolean", 2273 "When 'subrogation=true' this insurance instance has been included not for adjudication but to provide insurers with the details to recover costs.", 2274 0, 1, subrogation)); 2275 children.add(new Property("contract", "Reference(Contract)", 2276 "The policy(s) which constitute this insurance coverage.", 0, java.lang.Integer.MAX_VALUE, contract)); 2277 } 2278 2279 @Override 2280 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2281 switch (_hash) { 2282 case -1618432855: 2283 /* identifier */ return new Property("identifier", "Identifier", "A unique identifier assigned to this coverage.", 2284 0, java.lang.Integer.MAX_VALUE, identifier); 2285 case -892481550: 2286 /* status */ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 2287 case 3575610: 2288 /* type */ return new Property("type", "CodeableConcept", 2289 "The type of coverage: social program, medical plan, accident coverage (workers compensation, auto), group health or payment by an individual or organization.", 2290 0, 1, type); 2291 case 2046898558: 2292 /* policyHolder */ return new Property("policyHolder", "Reference(Patient|RelatedPerson|Organization)", 2293 "The party who 'owns' the insurance policy.", 0, 1, policyHolder); 2294 case -1219769240: 2295 /* subscriber */ return new Property("subscriber", "Reference(Patient|RelatedPerson)", 2296 "The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due.", 2297 0, 1, subscriber); 2298 case 327834531: 2299 /* subscriberId */ return new Property("subscriberId", "string", "The insurer assigned ID for the Subscriber.", 0, 2300 1, subscriberId); 2301 case -565102875: 2302 /* beneficiary */ return new Property("beneficiary", "Reference(Patient)", 2303 "The party who benefits from the insurance coverage; the patient when products and/or services are provided.", 2304 0, 1, beneficiary); 2305 case -1109226753: 2306 /* dependent */ return new Property("dependent", "string", 2307 "A unique identifier for a dependent under the coverage.", 0, 1, dependent); 2308 case -261851592: 2309 /* relationship */ return new Property("relationship", "CodeableConcept", 2310 "The relationship of beneficiary (patient) to the subscriber.", 0, 1, relationship); 2311 case -991726143: 2312 /* period */ return new Property("period", "Period", 2313 "Time period during which the coverage is in force. A missing start date indicates the start date isn't known, a missing end date means the coverage is continuing to be in force.", 2314 0, 1, period); 2315 case 106443915: 2316 /* payor */ return new Property("payor", "Reference(Organization|Patient|RelatedPerson)", 2317 "The program or plan underwriter or payor including both insurance and non-insurance agreements, such as patient-pay agreements.", 2318 0, java.lang.Integer.MAX_VALUE, payor); 2319 case 94742904: 2320 /* class */ return new Property("class", "", "A suite of underwriter specific classifiers.", 0, 2321 java.lang.Integer.MAX_VALUE, class_); 2322 case 106006350: 2323 /* order */ return new Property("order", "positiveInt", 2324 "The order of applicability of this coverage relative to other coverages which are currently in force. Note, there may be gaps in the numbering and this does not imply primary, secondary etc. as the specific positioning of coverages depends upon the episode of care.", 2325 0, 1, order); 2326 case 1843485230: 2327 /* network */ return new Property("network", "string", 2328 "The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply.", 2329 0, 1, network); 2330 case -1866474851: 2331 /* costToBeneficiary */ return new Property("costToBeneficiary", "", 2332 "A suite of codes indicating the cost category and associated amount which have been detailed in the policy and may have been included on the health card.", 2333 0, java.lang.Integer.MAX_VALUE, costToBeneficiary); 2334 case 837389739: 2335 /* subrogation */ return new Property("subrogation", "boolean", 2336 "When 'subrogation=true' this insurance instance has been included not for adjudication but to provide insurers with the details to recover costs.", 2337 0, 1, subrogation); 2338 case -566947566: 2339 /* contract */ return new Property("contract", "Reference(Contract)", 2340 "The policy(s) which constitute this insurance coverage.", 0, java.lang.Integer.MAX_VALUE, contract); 2341 default: 2342 return super.getNamedProperty(_hash, _name, _checkValid); 2343 } 2344 2345 } 2346 2347 @Override 2348 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2349 switch (hash) { 2350 case -1618432855: 2351 /* identifier */ return this.identifier == null ? new Base[0] 2352 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2353 case -892481550: 2354 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<CoverageStatus> 2355 case 3575610: 2356 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2357 case 2046898558: 2358 /* policyHolder */ return this.policyHolder == null ? new Base[0] : new Base[] { this.policyHolder }; // Reference 2359 case -1219769240: 2360 /* subscriber */ return this.subscriber == null ? new Base[0] : new Base[] { this.subscriber }; // Reference 2361 case 327834531: 2362 /* subscriberId */ return this.subscriberId == null ? new Base[0] : new Base[] { this.subscriberId }; // StringType 2363 case -565102875: 2364 /* beneficiary */ return this.beneficiary == null ? new Base[0] : new Base[] { this.beneficiary }; // Reference 2365 case -1109226753: 2366 /* dependent */ return this.dependent == null ? new Base[0] : new Base[] { this.dependent }; // StringType 2367 case -261851592: 2368 /* relationship */ return this.relationship == null ? new Base[0] : new Base[] { this.relationship }; // CodeableConcept 2369 case -991726143: 2370 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 2371 case 106443915: 2372 /* payor */ return this.payor == null ? new Base[0] : this.payor.toArray(new Base[this.payor.size()]); // Reference 2373 case 94742904: 2374 /* class */ return this.class_ == null ? new Base[0] : this.class_.toArray(new Base[this.class_.size()]); // ClassComponent 2375 case 106006350: 2376 /* order */ return this.order == null ? new Base[0] : new Base[] { this.order }; // PositiveIntType 2377 case 1843485230: 2378 /* network */ return this.network == null ? new Base[0] : new Base[] { this.network }; // StringType 2379 case -1866474851: 2380 /* costToBeneficiary */ return this.costToBeneficiary == null ? new Base[0] 2381 : this.costToBeneficiary.toArray(new Base[this.costToBeneficiary.size()]); // CostToBeneficiaryComponent 2382 case 837389739: 2383 /* subrogation */ return this.subrogation == null ? new Base[0] : new Base[] { this.subrogation }; // BooleanType 2384 case -566947566: 2385 /* contract */ return this.contract == null ? new Base[0] : this.contract.toArray(new Base[this.contract.size()]); // Reference 2386 default: 2387 return super.getProperty(hash, name, checkValid); 2388 } 2389 2390 } 2391 2392 @Override 2393 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2394 switch (hash) { 2395 case -1618432855: // identifier 2396 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2397 return value; 2398 case -892481550: // status 2399 value = new CoverageStatusEnumFactory().fromType(castToCode(value)); 2400 this.status = (Enumeration) value; // Enumeration<CoverageStatus> 2401 return value; 2402 case 3575610: // type 2403 this.type = castToCodeableConcept(value); // CodeableConcept 2404 return value; 2405 case 2046898558: // policyHolder 2406 this.policyHolder = castToReference(value); // Reference 2407 return value; 2408 case -1219769240: // subscriber 2409 this.subscriber = castToReference(value); // Reference 2410 return value; 2411 case 327834531: // subscriberId 2412 this.subscriberId = castToString(value); // StringType 2413 return value; 2414 case -565102875: // beneficiary 2415 this.beneficiary = castToReference(value); // Reference 2416 return value; 2417 case -1109226753: // dependent 2418 this.dependent = castToString(value); // StringType 2419 return value; 2420 case -261851592: // relationship 2421 this.relationship = castToCodeableConcept(value); // CodeableConcept 2422 return value; 2423 case -991726143: // period 2424 this.period = castToPeriod(value); // Period 2425 return value; 2426 case 106443915: // payor 2427 this.getPayor().add(castToReference(value)); // Reference 2428 return value; 2429 case 94742904: // class 2430 this.getClass_().add((ClassComponent) value); // ClassComponent 2431 return value; 2432 case 106006350: // order 2433 this.order = castToPositiveInt(value); // PositiveIntType 2434 return value; 2435 case 1843485230: // network 2436 this.network = castToString(value); // StringType 2437 return value; 2438 case -1866474851: // costToBeneficiary 2439 this.getCostToBeneficiary().add((CostToBeneficiaryComponent) value); // CostToBeneficiaryComponent 2440 return value; 2441 case 837389739: // subrogation 2442 this.subrogation = castToBoolean(value); // BooleanType 2443 return value; 2444 case -566947566: // contract 2445 this.getContract().add(castToReference(value)); // Reference 2446 return value; 2447 default: 2448 return super.setProperty(hash, name, value); 2449 } 2450 2451 } 2452 2453 @Override 2454 public Base setProperty(String name, Base value) throws FHIRException { 2455 if (name.equals("identifier")) { 2456 this.getIdentifier().add(castToIdentifier(value)); 2457 } else if (name.equals("status")) { 2458 value = new CoverageStatusEnumFactory().fromType(castToCode(value)); 2459 this.status = (Enumeration) value; // Enumeration<CoverageStatus> 2460 } else if (name.equals("type")) { 2461 this.type = castToCodeableConcept(value); // CodeableConcept 2462 } else if (name.equals("policyHolder")) { 2463 this.policyHolder = castToReference(value); // Reference 2464 } else if (name.equals("subscriber")) { 2465 this.subscriber = castToReference(value); // Reference 2466 } else if (name.equals("subscriberId")) { 2467 this.subscriberId = castToString(value); // StringType 2468 } else if (name.equals("beneficiary")) { 2469 this.beneficiary = castToReference(value); // Reference 2470 } else if (name.equals("dependent")) { 2471 this.dependent = castToString(value); // StringType 2472 } else if (name.equals("relationship")) { 2473 this.relationship = castToCodeableConcept(value); // CodeableConcept 2474 } else if (name.equals("period")) { 2475 this.period = castToPeriod(value); // Period 2476 } else if (name.equals("payor")) { 2477 this.getPayor().add(castToReference(value)); 2478 } else if (name.equals("class")) { 2479 this.getClass_().add((ClassComponent) value); 2480 } else if (name.equals("order")) { 2481 this.order = castToPositiveInt(value); // PositiveIntType 2482 } else if (name.equals("network")) { 2483 this.network = castToString(value); // StringType 2484 } else if (name.equals("costToBeneficiary")) { 2485 this.getCostToBeneficiary().add((CostToBeneficiaryComponent) value); 2486 } else if (name.equals("subrogation")) { 2487 this.subrogation = castToBoolean(value); // BooleanType 2488 } else if (name.equals("contract")) { 2489 this.getContract().add(castToReference(value)); 2490 } else 2491 return super.setProperty(name, value); 2492 return value; 2493 } 2494 2495 @Override 2496 public Base makeProperty(int hash, String name) throws FHIRException { 2497 switch (hash) { 2498 case -1618432855: 2499 return addIdentifier(); 2500 case -892481550: 2501 return getStatusElement(); 2502 case 3575610: 2503 return getType(); 2504 case 2046898558: 2505 return getPolicyHolder(); 2506 case -1219769240: 2507 return getSubscriber(); 2508 case 327834531: 2509 return getSubscriberIdElement(); 2510 case -565102875: 2511 return getBeneficiary(); 2512 case -1109226753: 2513 return getDependentElement(); 2514 case -261851592: 2515 return getRelationship(); 2516 case -991726143: 2517 return getPeriod(); 2518 case 106443915: 2519 return addPayor(); 2520 case 94742904: 2521 return addClass_(); 2522 case 106006350: 2523 return getOrderElement(); 2524 case 1843485230: 2525 return getNetworkElement(); 2526 case -1866474851: 2527 return addCostToBeneficiary(); 2528 case 837389739: 2529 return getSubrogationElement(); 2530 case -566947566: 2531 return addContract(); 2532 default: 2533 return super.makeProperty(hash, name); 2534 } 2535 2536 } 2537 2538 @Override 2539 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2540 switch (hash) { 2541 case -1618432855: 2542 /* identifier */ return new String[] { "Identifier" }; 2543 case -892481550: 2544 /* status */ return new String[] { "code" }; 2545 case 3575610: 2546 /* type */ return new String[] { "CodeableConcept" }; 2547 case 2046898558: 2548 /* policyHolder */ return new String[] { "Reference" }; 2549 case -1219769240: 2550 /* subscriber */ return new String[] { "Reference" }; 2551 case 327834531: 2552 /* subscriberId */ return new String[] { "string" }; 2553 case -565102875: 2554 /* beneficiary */ return new String[] { "Reference" }; 2555 case -1109226753: 2556 /* dependent */ return new String[] { "string" }; 2557 case -261851592: 2558 /* relationship */ return new String[] { "CodeableConcept" }; 2559 case -991726143: 2560 /* period */ return new String[] { "Period" }; 2561 case 106443915: 2562 /* payor */ return new String[] { "Reference" }; 2563 case 94742904: 2564 /* class */ return new String[] {}; 2565 case 106006350: 2566 /* order */ return new String[] { "positiveInt" }; 2567 case 1843485230: 2568 /* network */ return new String[] { "string" }; 2569 case -1866474851: 2570 /* costToBeneficiary */ return new String[] {}; 2571 case 837389739: 2572 /* subrogation */ return new String[] { "boolean" }; 2573 case -566947566: 2574 /* contract */ return new String[] { "Reference" }; 2575 default: 2576 return super.getTypesForProperty(hash, name); 2577 } 2578 2579 } 2580 2581 @Override 2582 public Base addChild(String name) throws FHIRException { 2583 if (name.equals("identifier")) { 2584 return addIdentifier(); 2585 } else if (name.equals("status")) { 2586 throw new FHIRException("Cannot call addChild on a singleton property Coverage.status"); 2587 } else if (name.equals("type")) { 2588 this.type = new CodeableConcept(); 2589 return this.type; 2590 } else if (name.equals("policyHolder")) { 2591 this.policyHolder = new Reference(); 2592 return this.policyHolder; 2593 } else if (name.equals("subscriber")) { 2594 this.subscriber = new Reference(); 2595 return this.subscriber; 2596 } else if (name.equals("subscriberId")) { 2597 throw new FHIRException("Cannot call addChild on a singleton property Coverage.subscriberId"); 2598 } else if (name.equals("beneficiary")) { 2599 this.beneficiary = new Reference(); 2600 return this.beneficiary; 2601 } else if (name.equals("dependent")) { 2602 throw new FHIRException("Cannot call addChild on a singleton property Coverage.dependent"); 2603 } else if (name.equals("relationship")) { 2604 this.relationship = new CodeableConcept(); 2605 return this.relationship; 2606 } else if (name.equals("period")) { 2607 this.period = new Period(); 2608 return this.period; 2609 } else if (name.equals("payor")) { 2610 return addPayor(); 2611 } else if (name.equals("class")) { 2612 return addClass_(); 2613 } else if (name.equals("order")) { 2614 throw new FHIRException("Cannot call addChild on a singleton property Coverage.order"); 2615 } else if (name.equals("network")) { 2616 throw new FHIRException("Cannot call addChild on a singleton property Coverage.network"); 2617 } else if (name.equals("costToBeneficiary")) { 2618 return addCostToBeneficiary(); 2619 } else if (name.equals("subrogation")) { 2620 throw new FHIRException("Cannot call addChild on a singleton property Coverage.subrogation"); 2621 } else if (name.equals("contract")) { 2622 return addContract(); 2623 } else 2624 return super.addChild(name); 2625 } 2626 2627 public String fhirType() { 2628 return "Coverage"; 2629 2630 } 2631 2632 public Coverage copy() { 2633 Coverage dst = new Coverage(); 2634 copyValues(dst); 2635 return dst; 2636 } 2637 2638 public void copyValues(Coverage dst) { 2639 super.copyValues(dst); 2640 if (identifier != null) { 2641 dst.identifier = new ArrayList<Identifier>(); 2642 for (Identifier i : identifier) 2643 dst.identifier.add(i.copy()); 2644 } 2645 ; 2646 dst.status = status == null ? null : status.copy(); 2647 dst.type = type == null ? null : type.copy(); 2648 dst.policyHolder = policyHolder == null ? null : policyHolder.copy(); 2649 dst.subscriber = subscriber == null ? null : subscriber.copy(); 2650 dst.subscriberId = subscriberId == null ? null : subscriberId.copy(); 2651 dst.beneficiary = beneficiary == null ? null : beneficiary.copy(); 2652 dst.dependent = dependent == null ? null : dependent.copy(); 2653 dst.relationship = relationship == null ? null : relationship.copy(); 2654 dst.period = period == null ? null : period.copy(); 2655 if (payor != null) { 2656 dst.payor = new ArrayList<Reference>(); 2657 for (Reference i : payor) 2658 dst.payor.add(i.copy()); 2659 } 2660 ; 2661 if (class_ != null) { 2662 dst.class_ = new ArrayList<ClassComponent>(); 2663 for (ClassComponent i : class_) 2664 dst.class_.add(i.copy()); 2665 } 2666 ; 2667 dst.order = order == null ? null : order.copy(); 2668 dst.network = network == null ? null : network.copy(); 2669 if (costToBeneficiary != null) { 2670 dst.costToBeneficiary = new ArrayList<CostToBeneficiaryComponent>(); 2671 for (CostToBeneficiaryComponent i : costToBeneficiary) 2672 dst.costToBeneficiary.add(i.copy()); 2673 } 2674 ; 2675 dst.subrogation = subrogation == null ? null : subrogation.copy(); 2676 if (contract != null) { 2677 dst.contract = new ArrayList<Reference>(); 2678 for (Reference i : contract) 2679 dst.contract.add(i.copy()); 2680 } 2681 ; 2682 } 2683 2684 protected Coverage typedCopy() { 2685 return copy(); 2686 } 2687 2688 @Override 2689 public boolean equalsDeep(Base other_) { 2690 if (!super.equalsDeep(other_)) 2691 return false; 2692 if (!(other_ instanceof Coverage)) 2693 return false; 2694 Coverage o = (Coverage) other_; 2695 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 2696 && compareDeep(type, o.type, true) && compareDeep(policyHolder, o.policyHolder, true) 2697 && compareDeep(subscriber, o.subscriber, true) && compareDeep(subscriberId, o.subscriberId, true) 2698 && compareDeep(beneficiary, o.beneficiary, true) && compareDeep(dependent, o.dependent, true) 2699 && compareDeep(relationship, o.relationship, true) && compareDeep(period, o.period, true) 2700 && compareDeep(payor, o.payor, true) && compareDeep(class_, o.class_, true) && compareDeep(order, o.order, true) 2701 && compareDeep(network, o.network, true) && compareDeep(costToBeneficiary, o.costToBeneficiary, true) 2702 && compareDeep(subrogation, o.subrogation, true) && compareDeep(contract, o.contract, true); 2703 } 2704 2705 @Override 2706 public boolean equalsShallow(Base other_) { 2707 if (!super.equalsShallow(other_)) 2708 return false; 2709 if (!(other_ instanceof Coverage)) 2710 return false; 2711 Coverage o = (Coverage) other_; 2712 return compareValues(status, o.status, true) && compareValues(subscriberId, o.subscriberId, true) 2713 && compareValues(dependent, o.dependent, true) && compareValues(order, o.order, true) 2714 && compareValues(network, o.network, true) && compareValues(subrogation, o.subrogation, true); 2715 } 2716 2717 public boolean isEmpty() { 2718 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, type, policyHolder, subscriber, 2719 subscriberId, beneficiary, dependent, relationship, period, payor, class_, order, network, costToBeneficiary, 2720 subrogation, contract); 2721 } 2722 2723 @Override 2724 public ResourceType getResourceType() { 2725 return ResourceType.Coverage; 2726 } 2727 2728 /** 2729 * Search parameter: <b>identifier</b> 2730 * <p> 2731 * Description: <b>The primary identifier of the insured and the 2732 * coverage</b><br> 2733 * Type: <b>token</b><br> 2734 * Path: <b>Coverage.identifier</b><br> 2735 * </p> 2736 */ 2737 @SearchParamDefinition(name = "identifier", path = "Coverage.identifier", description = "The primary identifier of the insured and the coverage", type = "token") 2738 public static final String SP_IDENTIFIER = "identifier"; 2739 /** 2740 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2741 * <p> 2742 * Description: <b>The primary identifier of the insured and the 2743 * coverage</b><br> 2744 * Type: <b>token</b><br> 2745 * Path: <b>Coverage.identifier</b><br> 2746 * </p> 2747 */ 2748 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2749 SP_IDENTIFIER); 2750 2751 /** 2752 * Search parameter: <b>payor</b> 2753 * <p> 2754 * Description: <b>The identity of the insurer or party paying for 2755 * services</b><br> 2756 * Type: <b>reference</b><br> 2757 * Path: <b>Coverage.payor</b><br> 2758 * </p> 2759 */ 2760 @SearchParamDefinition(name = "payor", path = "Coverage.payor", description = "The identity of the insurer or party paying for services", type = "reference", providesMembershipIn = { 2761 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 2762 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Organization.class, 2763 Patient.class, RelatedPerson.class }) 2764 public static final String SP_PAYOR = "payor"; 2765 /** 2766 * <b>Fluent Client</b> search parameter constant for <b>payor</b> 2767 * <p> 2768 * Description: <b>The identity of the insurer or party paying for 2769 * services</b><br> 2770 * Type: <b>reference</b><br> 2771 * Path: <b>Coverage.payor</b><br> 2772 * </p> 2773 */ 2774 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PAYOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2775 SP_PAYOR); 2776 2777 /** 2778 * Constant for fluent queries to be used to add include statements. Specifies 2779 * the path value of "<b>Coverage:payor</b>". 2780 */ 2781 public static final ca.uhn.fhir.model.api.Include INCLUDE_PAYOR = new ca.uhn.fhir.model.api.Include("Coverage:payor") 2782 .toLocked(); 2783 2784 /** 2785 * Search parameter: <b>subscriber</b> 2786 * <p> 2787 * Description: <b>Reference to the subscriber</b><br> 2788 * Type: <b>reference</b><br> 2789 * Path: <b>Coverage.subscriber</b><br> 2790 * </p> 2791 */ 2792 @SearchParamDefinition(name = "subscriber", path = "Coverage.subscriber", description = "Reference to the subscriber", type = "reference", providesMembershipIn = { 2793 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 2794 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Patient.class, 2795 RelatedPerson.class }) 2796 public static final String SP_SUBSCRIBER = "subscriber"; 2797 /** 2798 * <b>Fluent Client</b> search parameter constant for <b>subscriber</b> 2799 * <p> 2800 * Description: <b>Reference to the subscriber</b><br> 2801 * Type: <b>reference</b><br> 2802 * Path: <b>Coverage.subscriber</b><br> 2803 * </p> 2804 */ 2805 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBSCRIBER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2806 SP_SUBSCRIBER); 2807 2808 /** 2809 * Constant for fluent queries to be used to add include statements. Specifies 2810 * the path value of "<b>Coverage:subscriber</b>". 2811 */ 2812 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBSCRIBER = new ca.uhn.fhir.model.api.Include( 2813 "Coverage:subscriber").toLocked(); 2814 2815 /** 2816 * Search parameter: <b>beneficiary</b> 2817 * <p> 2818 * Description: <b>Covered party</b><br> 2819 * Type: <b>reference</b><br> 2820 * Path: <b>Coverage.beneficiary</b><br> 2821 * </p> 2822 */ 2823 @SearchParamDefinition(name = "beneficiary", path = "Coverage.beneficiary", description = "Covered party", type = "reference", providesMembershipIn = { 2824 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 2825 public static final String SP_BENEFICIARY = "beneficiary"; 2826 /** 2827 * <b>Fluent Client</b> search parameter constant for <b>beneficiary</b> 2828 * <p> 2829 * Description: <b>Covered party</b><br> 2830 * Type: <b>reference</b><br> 2831 * Path: <b>Coverage.beneficiary</b><br> 2832 * </p> 2833 */ 2834 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BENEFICIARY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2835 SP_BENEFICIARY); 2836 2837 /** 2838 * Constant for fluent queries to be used to add include statements. Specifies 2839 * the path value of "<b>Coverage:beneficiary</b>". 2840 */ 2841 public static final ca.uhn.fhir.model.api.Include INCLUDE_BENEFICIARY = new ca.uhn.fhir.model.api.Include( 2842 "Coverage:beneficiary").toLocked(); 2843 2844 /** 2845 * Search parameter: <b>patient</b> 2846 * <p> 2847 * Description: <b>Retrieve coverages for a patient</b><br> 2848 * Type: <b>reference</b><br> 2849 * Path: <b>Coverage.beneficiary</b><br> 2850 * </p> 2851 */ 2852 @SearchParamDefinition(name = "patient", path = "Coverage.beneficiary", description = "Retrieve coverages for a patient", type = "reference", target = { 2853 Patient.class }) 2854 public static final String SP_PATIENT = "patient"; 2855 /** 2856 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2857 * <p> 2858 * Description: <b>Retrieve coverages for a patient</b><br> 2859 * Type: <b>reference</b><br> 2860 * Path: <b>Coverage.beneficiary</b><br> 2861 * </p> 2862 */ 2863 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2864 SP_PATIENT); 2865 2866 /** 2867 * Constant for fluent queries to be used to add include statements. Specifies 2868 * the path value of "<b>Coverage:patient</b>". 2869 */ 2870 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 2871 "Coverage:patient").toLocked(); 2872 2873 /** 2874 * Search parameter: <b>class-value</b> 2875 * <p> 2876 * Description: <b>Value of the class (eg. Plan number, group number)</b><br> 2877 * Type: <b>string</b><br> 2878 * Path: <b>Coverage.class.value</b><br> 2879 * </p> 2880 */ 2881 @SearchParamDefinition(name = "class-value", path = "Coverage.class.value", description = "Value of the class (eg. Plan number, group number)", type = "string") 2882 public static final String SP_CLASS_VALUE = "class-value"; 2883 /** 2884 * <b>Fluent Client</b> search parameter constant for <b>class-value</b> 2885 * <p> 2886 * Description: <b>Value of the class (eg. Plan number, group number)</b><br> 2887 * Type: <b>string</b><br> 2888 * Path: <b>Coverage.class.value</b><br> 2889 * </p> 2890 */ 2891 public static final ca.uhn.fhir.rest.gclient.StringClientParam CLASS_VALUE = new ca.uhn.fhir.rest.gclient.StringClientParam( 2892 SP_CLASS_VALUE); 2893 2894 /** 2895 * Search parameter: <b>type</b> 2896 * <p> 2897 * Description: <b>The kind of coverage (health plan, auto, Workers 2898 * Compensation)</b><br> 2899 * Type: <b>token</b><br> 2900 * Path: <b>Coverage.type</b><br> 2901 * </p> 2902 */ 2903 @SearchParamDefinition(name = "type", path = "Coverage.type", description = "The kind of coverage (health plan, auto, Workers Compensation)", type = "token") 2904 public static final String SP_TYPE = "type"; 2905 /** 2906 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2907 * <p> 2908 * Description: <b>The kind of coverage (health plan, auto, Workers 2909 * Compensation)</b><br> 2910 * Type: <b>token</b><br> 2911 * Path: <b>Coverage.type</b><br> 2912 * </p> 2913 */ 2914 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2915 SP_TYPE); 2916 2917 /** 2918 * Search parameter: <b>dependent</b> 2919 * <p> 2920 * Description: <b>Dependent number</b><br> 2921 * Type: <b>string</b><br> 2922 * Path: <b>Coverage.dependent</b><br> 2923 * </p> 2924 */ 2925 @SearchParamDefinition(name = "dependent", path = "Coverage.dependent", description = "Dependent number", type = "string") 2926 public static final String SP_DEPENDENT = "dependent"; 2927 /** 2928 * <b>Fluent Client</b> search parameter constant for <b>dependent</b> 2929 * <p> 2930 * Description: <b>Dependent number</b><br> 2931 * Type: <b>string</b><br> 2932 * Path: <b>Coverage.dependent</b><br> 2933 * </p> 2934 */ 2935 public static final ca.uhn.fhir.rest.gclient.StringClientParam DEPENDENT = new ca.uhn.fhir.rest.gclient.StringClientParam( 2936 SP_DEPENDENT); 2937 2938 /** 2939 * Search parameter: <b>class-type</b> 2940 * <p> 2941 * Description: <b>Coverage class (eg. plan, group)</b><br> 2942 * Type: <b>token</b><br> 2943 * Path: <b>Coverage.class.type</b><br> 2944 * </p> 2945 */ 2946 @SearchParamDefinition(name = "class-type", path = "Coverage.class.type", description = "Coverage class (eg. plan, group)", type = "token") 2947 public static final String SP_CLASS_TYPE = "class-type"; 2948 /** 2949 * <b>Fluent Client</b> search parameter constant for <b>class-type</b> 2950 * <p> 2951 * Description: <b>Coverage class (eg. plan, group)</b><br> 2952 * Type: <b>token</b><br> 2953 * Path: <b>Coverage.class.type</b><br> 2954 * </p> 2955 */ 2956 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLASS_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2957 SP_CLASS_TYPE); 2958 2959 /** 2960 * Search parameter: <b>policy-holder</b> 2961 * <p> 2962 * Description: <b>Reference to the policyholder</b><br> 2963 * Type: <b>reference</b><br> 2964 * Path: <b>Coverage.policyHolder</b><br> 2965 * </p> 2966 */ 2967 @SearchParamDefinition(name = "policy-holder", path = "Coverage.policyHolder", description = "Reference to the policyholder", type = "reference", providesMembershipIn = { 2968 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 2969 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Organization.class, 2970 Patient.class, RelatedPerson.class }) 2971 public static final String SP_POLICY_HOLDER = "policy-holder"; 2972 /** 2973 * <b>Fluent Client</b> search parameter constant for <b>policy-holder</b> 2974 * <p> 2975 * Description: <b>Reference to the policyholder</b><br> 2976 * Type: <b>reference</b><br> 2977 * Path: <b>Coverage.policyHolder</b><br> 2978 * </p> 2979 */ 2980 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam POLICY_HOLDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2981 SP_POLICY_HOLDER); 2982 2983 /** 2984 * Constant for fluent queries to be used to add include statements. Specifies 2985 * the path value of "<b>Coverage:policy-holder</b>". 2986 */ 2987 public static final ca.uhn.fhir.model.api.Include INCLUDE_POLICY_HOLDER = new ca.uhn.fhir.model.api.Include( 2988 "Coverage:policy-holder").toLocked(); 2989 2990 /** 2991 * Search parameter: <b>status</b> 2992 * <p> 2993 * Description: <b>The status of the Coverage</b><br> 2994 * Type: <b>token</b><br> 2995 * Path: <b>Coverage.status</b><br> 2996 * </p> 2997 */ 2998 @SearchParamDefinition(name = "status", path = "Coverage.status", description = "The status of the Coverage", type = "token") 2999 public static final String SP_STATUS = "status"; 3000 /** 3001 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3002 * <p> 3003 * Description: <b>The status of the Coverage</b><br> 3004 * Type: <b>token</b><br> 3005 * Path: <b>Coverage.status</b><br> 3006 * </p> 3007 */ 3008 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3009 SP_STATUS); 3010 3011}