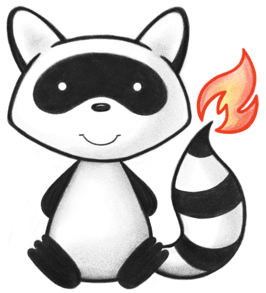
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * The CoverageEligibilityRequest provides patient and insurance coverage 049 * information to an insurer for them to respond, in the form of an 050 * CoverageEligibilityResponse, with information regarding whether the stated 051 * coverage is valid and in-force and optionally to provide the insurance 052 * details of the policy. 053 */ 054@ResourceDef(name = "CoverageEligibilityRequest", profile = "http://hl7.org/fhir/StructureDefinition/CoverageEligibilityRequest") 055public class CoverageEligibilityRequest extends DomainResource { 056 057 public enum EligibilityRequestStatus { 058 /** 059 * The instance is currently in-force. 060 */ 061 ACTIVE, 062 /** 063 * The instance is withdrawn, rescinded or reversed. 064 */ 065 CANCELLED, 066 /** 067 * A new instance the contents of which is not complete. 068 */ 069 DRAFT, 070 /** 071 * The instance was entered in error. 072 */ 073 ENTEREDINERROR, 074 /** 075 * added to help the parsers with the generic types 076 */ 077 NULL; 078 079 public static EligibilityRequestStatus fromCode(String codeString) throws FHIRException { 080 if (codeString == null || "".equals(codeString)) 081 return null; 082 if ("active".equals(codeString)) 083 return ACTIVE; 084 if ("cancelled".equals(codeString)) 085 return CANCELLED; 086 if ("draft".equals(codeString)) 087 return DRAFT; 088 if ("entered-in-error".equals(codeString)) 089 return ENTEREDINERROR; 090 if (Configuration.isAcceptInvalidEnums()) 091 return null; 092 else 093 throw new FHIRException("Unknown EligibilityRequestStatus code '" + codeString + "'"); 094 } 095 096 public String toCode() { 097 switch (this) { 098 case ACTIVE: 099 return "active"; 100 case CANCELLED: 101 return "cancelled"; 102 case DRAFT: 103 return "draft"; 104 case ENTEREDINERROR: 105 return "entered-in-error"; 106 case NULL: 107 return null; 108 default: 109 return "?"; 110 } 111 } 112 113 public String getSystem() { 114 switch (this) { 115 case ACTIVE: 116 return "http://hl7.org/fhir/fm-status"; 117 case CANCELLED: 118 return "http://hl7.org/fhir/fm-status"; 119 case DRAFT: 120 return "http://hl7.org/fhir/fm-status"; 121 case ENTEREDINERROR: 122 return "http://hl7.org/fhir/fm-status"; 123 case NULL: 124 return null; 125 default: 126 return "?"; 127 } 128 } 129 130 public String getDefinition() { 131 switch (this) { 132 case ACTIVE: 133 return "The instance is currently in-force."; 134 case CANCELLED: 135 return "The instance is withdrawn, rescinded or reversed."; 136 case DRAFT: 137 return "A new instance the contents of which is not complete."; 138 case ENTEREDINERROR: 139 return "The instance was entered in error."; 140 case NULL: 141 return null; 142 default: 143 return "?"; 144 } 145 } 146 147 public String getDisplay() { 148 switch (this) { 149 case ACTIVE: 150 return "Active"; 151 case CANCELLED: 152 return "Cancelled"; 153 case DRAFT: 154 return "Draft"; 155 case ENTEREDINERROR: 156 return "Entered in Error"; 157 case NULL: 158 return null; 159 default: 160 return "?"; 161 } 162 } 163 } 164 165 public static class EligibilityRequestStatusEnumFactory implements EnumFactory<EligibilityRequestStatus> { 166 public EligibilityRequestStatus fromCode(String codeString) throws IllegalArgumentException { 167 if (codeString == null || "".equals(codeString)) 168 if (codeString == null || "".equals(codeString)) 169 return null; 170 if ("active".equals(codeString)) 171 return EligibilityRequestStatus.ACTIVE; 172 if ("cancelled".equals(codeString)) 173 return EligibilityRequestStatus.CANCELLED; 174 if ("draft".equals(codeString)) 175 return EligibilityRequestStatus.DRAFT; 176 if ("entered-in-error".equals(codeString)) 177 return EligibilityRequestStatus.ENTEREDINERROR; 178 throw new IllegalArgumentException("Unknown EligibilityRequestStatus code '" + codeString + "'"); 179 } 180 181 public Enumeration<EligibilityRequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 182 if (code == null) 183 return null; 184 if (code.isEmpty()) 185 return new Enumeration<EligibilityRequestStatus>(this, EligibilityRequestStatus.NULL, code); 186 String codeString = code.asStringValue(); 187 if (codeString == null || "".equals(codeString)) 188 return new Enumeration<EligibilityRequestStatus>(this, EligibilityRequestStatus.NULL, code); 189 if ("active".equals(codeString)) 190 return new Enumeration<EligibilityRequestStatus>(this, EligibilityRequestStatus.ACTIVE, code); 191 if ("cancelled".equals(codeString)) 192 return new Enumeration<EligibilityRequestStatus>(this, EligibilityRequestStatus.CANCELLED, code); 193 if ("draft".equals(codeString)) 194 return new Enumeration<EligibilityRequestStatus>(this, EligibilityRequestStatus.DRAFT, code); 195 if ("entered-in-error".equals(codeString)) 196 return new Enumeration<EligibilityRequestStatus>(this, EligibilityRequestStatus.ENTEREDINERROR, code); 197 throw new FHIRException("Unknown EligibilityRequestStatus code '" + codeString + "'"); 198 } 199 200 public String toCode(EligibilityRequestStatus code) { 201 if (code == EligibilityRequestStatus.NULL) 202 return null; 203 if (code == EligibilityRequestStatus.ACTIVE) 204 return "active"; 205 if (code == EligibilityRequestStatus.CANCELLED) 206 return "cancelled"; 207 if (code == EligibilityRequestStatus.DRAFT) 208 return "draft"; 209 if (code == EligibilityRequestStatus.ENTEREDINERROR) 210 return "entered-in-error"; 211 return "?"; 212 } 213 214 public String toSystem(EligibilityRequestStatus code) { 215 return code.getSystem(); 216 } 217 } 218 219 public enum EligibilityRequestPurpose { 220 /** 221 * The prior authorization requirements for the listed, or discovered if 222 * specified, converages for the categories of service and/or specifed biling 223 * codes are requested. 224 */ 225 AUTHREQUIREMENTS, 226 /** 227 * The plan benefits and optionally benefits consumed for the listed, or 228 * discovered if specified, converages are requested. 229 */ 230 BENEFITS, 231 /** 232 * The insurer is requested to report on any coverages which they are aware of 233 * in addition to any specifed. 234 */ 235 DISCOVERY, 236 /** 237 * A check that the specified coverages are in-force is requested. 238 */ 239 VALIDATION, 240 /** 241 * added to help the parsers with the generic types 242 */ 243 NULL; 244 245 public static EligibilityRequestPurpose fromCode(String codeString) throws FHIRException { 246 if (codeString == null || "".equals(codeString)) 247 return null; 248 if ("auth-requirements".equals(codeString)) 249 return AUTHREQUIREMENTS; 250 if ("benefits".equals(codeString)) 251 return BENEFITS; 252 if ("discovery".equals(codeString)) 253 return DISCOVERY; 254 if ("validation".equals(codeString)) 255 return VALIDATION; 256 if (Configuration.isAcceptInvalidEnums()) 257 return null; 258 else 259 throw new FHIRException("Unknown EligibilityRequestPurpose code '" + codeString + "'"); 260 } 261 262 public String toCode() { 263 switch (this) { 264 case AUTHREQUIREMENTS: 265 return "auth-requirements"; 266 case BENEFITS: 267 return "benefits"; 268 case DISCOVERY: 269 return "discovery"; 270 case VALIDATION: 271 return "validation"; 272 case NULL: 273 return null; 274 default: 275 return "?"; 276 } 277 } 278 279 public String getSystem() { 280 switch (this) { 281 case AUTHREQUIREMENTS: 282 return "http://hl7.org/fhir/eligibilityrequest-purpose"; 283 case BENEFITS: 284 return "http://hl7.org/fhir/eligibilityrequest-purpose"; 285 case DISCOVERY: 286 return "http://hl7.org/fhir/eligibilityrequest-purpose"; 287 case VALIDATION: 288 return "http://hl7.org/fhir/eligibilityrequest-purpose"; 289 case NULL: 290 return null; 291 default: 292 return "?"; 293 } 294 } 295 296 public String getDefinition() { 297 switch (this) { 298 case AUTHREQUIREMENTS: 299 return "The prior authorization requirements for the listed, or discovered if specified, converages for the categories of service and/or specifed biling codes are requested."; 300 case BENEFITS: 301 return "The plan benefits and optionally benefits consumed for the listed, or discovered if specified, converages are requested."; 302 case DISCOVERY: 303 return "The insurer is requested to report on any coverages which they are aware of in addition to any specifed."; 304 case VALIDATION: 305 return "A check that the specified coverages are in-force is requested."; 306 case NULL: 307 return null; 308 default: 309 return "?"; 310 } 311 } 312 313 public String getDisplay() { 314 switch (this) { 315 case AUTHREQUIREMENTS: 316 return "Coverage auth-requirements"; 317 case BENEFITS: 318 return "Coverage benefits"; 319 case DISCOVERY: 320 return "Coverage Discovery"; 321 case VALIDATION: 322 return "Coverage Validation"; 323 case NULL: 324 return null; 325 default: 326 return "?"; 327 } 328 } 329 } 330 331 public static class EligibilityRequestPurposeEnumFactory implements EnumFactory<EligibilityRequestPurpose> { 332 public EligibilityRequestPurpose fromCode(String codeString) throws IllegalArgumentException { 333 if (codeString == null || "".equals(codeString)) 334 if (codeString == null || "".equals(codeString)) 335 return null; 336 if ("auth-requirements".equals(codeString)) 337 return EligibilityRequestPurpose.AUTHREQUIREMENTS; 338 if ("benefits".equals(codeString)) 339 return EligibilityRequestPurpose.BENEFITS; 340 if ("discovery".equals(codeString)) 341 return EligibilityRequestPurpose.DISCOVERY; 342 if ("validation".equals(codeString)) 343 return EligibilityRequestPurpose.VALIDATION; 344 throw new IllegalArgumentException("Unknown EligibilityRequestPurpose code '" + codeString + "'"); 345 } 346 347 public Enumeration<EligibilityRequestPurpose> fromType(PrimitiveType<?> code) throws FHIRException { 348 if (code == null) 349 return null; 350 if (code.isEmpty()) 351 return new Enumeration<EligibilityRequestPurpose>(this, EligibilityRequestPurpose.NULL, code); 352 String codeString = code.asStringValue(); 353 if (codeString == null || "".equals(codeString)) 354 return new Enumeration<EligibilityRequestPurpose>(this, EligibilityRequestPurpose.NULL, code); 355 if ("auth-requirements".equals(codeString)) 356 return new Enumeration<EligibilityRequestPurpose>(this, EligibilityRequestPurpose.AUTHREQUIREMENTS, code); 357 if ("benefits".equals(codeString)) 358 return new Enumeration<EligibilityRequestPurpose>(this, EligibilityRequestPurpose.BENEFITS, code); 359 if ("discovery".equals(codeString)) 360 return new Enumeration<EligibilityRequestPurpose>(this, EligibilityRequestPurpose.DISCOVERY, code); 361 if ("validation".equals(codeString)) 362 return new Enumeration<EligibilityRequestPurpose>(this, EligibilityRequestPurpose.VALIDATION, code); 363 throw new FHIRException("Unknown EligibilityRequestPurpose code '" + codeString + "'"); 364 } 365 366 public String toCode(EligibilityRequestPurpose code) { 367 if (code == EligibilityRequestPurpose.NULL) 368 return null; 369 if (code == EligibilityRequestPurpose.AUTHREQUIREMENTS) 370 return "auth-requirements"; 371 if (code == EligibilityRequestPurpose.BENEFITS) 372 return "benefits"; 373 if (code == EligibilityRequestPurpose.DISCOVERY) 374 return "discovery"; 375 if (code == EligibilityRequestPurpose.VALIDATION) 376 return "validation"; 377 return "?"; 378 } 379 380 public String toSystem(EligibilityRequestPurpose code) { 381 return code.getSystem(); 382 } 383 } 384 385 @Block() 386 public static class SupportingInformationComponent extends BackboneElement implements IBaseBackboneElement { 387 /** 388 * A number to uniquely identify supporting information entries. 389 */ 390 @Child(name = "sequence", type = { 391 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 392 @Description(shortDefinition = "Information instance identifier", formalDefinition = "A number to uniquely identify supporting information entries.") 393 protected PositiveIntType sequence; 394 395 /** 396 * Additional data or information such as resources, documents, images etc. 397 * including references to the data or the actual inclusion of the data. 398 */ 399 @Child(name = "information", type = { 400 Reference.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 401 @Description(shortDefinition = "Data to be provided", formalDefinition = "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.") 402 protected Reference information; 403 404 /** 405 * The actual object that is the target of the reference (Additional data or 406 * information such as resources, documents, images etc. including references to 407 * the data or the actual inclusion of the data.) 408 */ 409 protected Resource informationTarget; 410 411 /** 412 * The supporting materials are applicable for all detail items, product/servce 413 * categories and specific billing codes. 414 */ 415 @Child(name = "appliesToAll", type = { 416 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 417 @Description(shortDefinition = "Applies to all items", formalDefinition = "The supporting materials are applicable for all detail items, product/servce categories and specific billing codes.") 418 protected BooleanType appliesToAll; 419 420 private static final long serialVersionUID = 819254843L; 421 422 /** 423 * Constructor 424 */ 425 public SupportingInformationComponent() { 426 super(); 427 } 428 429 /** 430 * Constructor 431 */ 432 public SupportingInformationComponent(PositiveIntType sequence, Reference information) { 433 super(); 434 this.sequence = sequence; 435 this.information = information; 436 } 437 438 /** 439 * @return {@link #sequence} (A number to uniquely identify supporting 440 * information entries.). This is the underlying object with id, value 441 * and extensions. The accessor "getSequence" gives direct access to the 442 * value 443 */ 444 public PositiveIntType getSequenceElement() { 445 if (this.sequence == null) 446 if (Configuration.errorOnAutoCreate()) 447 throw new Error("Attempt to auto-create SupportingInformationComponent.sequence"); 448 else if (Configuration.doAutoCreate()) 449 this.sequence = new PositiveIntType(); // bb 450 return this.sequence; 451 } 452 453 public boolean hasSequenceElement() { 454 return this.sequence != null && !this.sequence.isEmpty(); 455 } 456 457 public boolean hasSequence() { 458 return this.sequence != null && !this.sequence.isEmpty(); 459 } 460 461 /** 462 * @param value {@link #sequence} (A number to uniquely identify supporting 463 * information entries.). This is the underlying object with id, 464 * value and extensions. The accessor "getSequence" gives direct 465 * access to the value 466 */ 467 public SupportingInformationComponent setSequenceElement(PositiveIntType value) { 468 this.sequence = value; 469 return this; 470 } 471 472 /** 473 * @return A number to uniquely identify supporting information entries. 474 */ 475 public int getSequence() { 476 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 477 } 478 479 /** 480 * @param value A number to uniquely identify supporting information entries. 481 */ 482 public SupportingInformationComponent setSequence(int value) { 483 if (this.sequence == null) 484 this.sequence = new PositiveIntType(); 485 this.sequence.setValue(value); 486 return this; 487 } 488 489 /** 490 * @return {@link #information} (Additional data or information such as 491 * resources, documents, images etc. including references to the data or 492 * the actual inclusion of the data.) 493 */ 494 public Reference getInformation() { 495 if (this.information == null) 496 if (Configuration.errorOnAutoCreate()) 497 throw new Error("Attempt to auto-create SupportingInformationComponent.information"); 498 else if (Configuration.doAutoCreate()) 499 this.information = new Reference(); // cc 500 return this.information; 501 } 502 503 public boolean hasInformation() { 504 return this.information != null && !this.information.isEmpty(); 505 } 506 507 /** 508 * @param value {@link #information} (Additional data or information such as 509 * resources, documents, images etc. including references to the 510 * data or the actual inclusion of the data.) 511 */ 512 public SupportingInformationComponent setInformation(Reference value) { 513 this.information = value; 514 return this; 515 } 516 517 /** 518 * @return {@link #information} The actual object that is the target of the 519 * reference. The reference library doesn't populate this, but you can 520 * use it to hold the resource if you resolve it. (Additional data or 521 * information such as resources, documents, images etc. including 522 * references to the data or the actual inclusion of the data.) 523 */ 524 public Resource getInformationTarget() { 525 return this.informationTarget; 526 } 527 528 /** 529 * @param value {@link #information} The actual object that is the target of the 530 * reference. The reference library doesn't use these, but you can 531 * use it to hold the resource if you resolve it. (Additional data 532 * or information such as resources, documents, images etc. 533 * including references to the data or the actual inclusion of the 534 * data.) 535 */ 536 public SupportingInformationComponent setInformationTarget(Resource value) { 537 this.informationTarget = value; 538 return this; 539 } 540 541 /** 542 * @return {@link #appliesToAll} (The supporting materials are applicable for 543 * all detail items, product/servce categories and specific billing 544 * codes.). This is the underlying object with id, value and extensions. 545 * The accessor "getAppliesToAll" gives direct access to the value 546 */ 547 public BooleanType getAppliesToAllElement() { 548 if (this.appliesToAll == null) 549 if (Configuration.errorOnAutoCreate()) 550 throw new Error("Attempt to auto-create SupportingInformationComponent.appliesToAll"); 551 else if (Configuration.doAutoCreate()) 552 this.appliesToAll = new BooleanType(); // bb 553 return this.appliesToAll; 554 } 555 556 public boolean hasAppliesToAllElement() { 557 return this.appliesToAll != null && !this.appliesToAll.isEmpty(); 558 } 559 560 public boolean hasAppliesToAll() { 561 return this.appliesToAll != null && !this.appliesToAll.isEmpty(); 562 } 563 564 /** 565 * @param value {@link #appliesToAll} (The supporting materials are applicable 566 * for all detail items, product/servce categories and specific 567 * billing codes.). This is the underlying object with id, value 568 * and extensions. The accessor "getAppliesToAll" gives direct 569 * access to the value 570 */ 571 public SupportingInformationComponent setAppliesToAllElement(BooleanType value) { 572 this.appliesToAll = value; 573 return this; 574 } 575 576 /** 577 * @return The supporting materials are applicable for all detail items, 578 * product/servce categories and specific billing codes. 579 */ 580 public boolean getAppliesToAll() { 581 return this.appliesToAll == null || this.appliesToAll.isEmpty() ? false : this.appliesToAll.getValue(); 582 } 583 584 /** 585 * @param value The supporting materials are applicable for all detail items, 586 * product/servce categories and specific billing codes. 587 */ 588 public SupportingInformationComponent setAppliesToAll(boolean value) { 589 if (this.appliesToAll == null) 590 this.appliesToAll = new BooleanType(); 591 this.appliesToAll.setValue(value); 592 return this; 593 } 594 595 protected void listChildren(List<Property> children) { 596 super.listChildren(children); 597 children.add(new Property("sequence", "positiveInt", 598 "A number to uniquely identify supporting information entries.", 0, 1, sequence)); 599 children.add(new Property("information", "Reference(Any)", 600 "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 601 0, 1, information)); 602 children.add(new Property("appliesToAll", "boolean", 603 "The supporting materials are applicable for all detail items, product/servce categories and specific billing codes.", 604 0, 1, appliesToAll)); 605 } 606 607 @Override 608 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 609 switch (_hash) { 610 case 1349547969: 611 /* sequence */ return new Property("sequence", "positiveInt", 612 "A number to uniquely identify supporting information entries.", 0, 1, sequence); 613 case 1968600364: 614 /* information */ return new Property("information", "Reference(Any)", 615 "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 616 0, 1, information); 617 case -1096846342: 618 /* appliesToAll */ return new Property("appliesToAll", "boolean", 619 "The supporting materials are applicable for all detail items, product/servce categories and specific billing codes.", 620 0, 1, appliesToAll); 621 default: 622 return super.getNamedProperty(_hash, _name, _checkValid); 623 } 624 625 } 626 627 @Override 628 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 629 switch (hash) { 630 case 1349547969: 631 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 632 case 1968600364: 633 /* information */ return this.information == null ? new Base[0] : new Base[] { this.information }; // Reference 634 case -1096846342: 635 /* appliesToAll */ return this.appliesToAll == null ? new Base[0] : new Base[] { this.appliesToAll }; // BooleanType 636 default: 637 return super.getProperty(hash, name, checkValid); 638 } 639 640 } 641 642 @Override 643 public Base setProperty(int hash, String name, Base value) throws FHIRException { 644 switch (hash) { 645 case 1349547969: // sequence 646 this.sequence = castToPositiveInt(value); // PositiveIntType 647 return value; 648 case 1968600364: // information 649 this.information = castToReference(value); // Reference 650 return value; 651 case -1096846342: // appliesToAll 652 this.appliesToAll = castToBoolean(value); // BooleanType 653 return value; 654 default: 655 return super.setProperty(hash, name, value); 656 } 657 658 } 659 660 @Override 661 public Base setProperty(String name, Base value) throws FHIRException { 662 if (name.equals("sequence")) { 663 this.sequence = castToPositiveInt(value); // PositiveIntType 664 } else if (name.equals("information")) { 665 this.information = castToReference(value); // Reference 666 } else if (name.equals("appliesToAll")) { 667 this.appliesToAll = castToBoolean(value); // BooleanType 668 } else 669 return super.setProperty(name, value); 670 return value; 671 } 672 673 @Override 674 public void removeChild(String name, Base value) throws FHIRException { 675 if (name.equals("sequence")) { 676 this.sequence = null; 677 } else if (name.equals("information")) { 678 this.information = null; 679 } else if (name.equals("appliesToAll")) { 680 this.appliesToAll = null; 681 } else 682 super.removeChild(name, value); 683 684 } 685 686 @Override 687 public Base makeProperty(int hash, String name) throws FHIRException { 688 switch (hash) { 689 case 1349547969: 690 return getSequenceElement(); 691 case 1968600364: 692 return getInformation(); 693 case -1096846342: 694 return getAppliesToAllElement(); 695 default: 696 return super.makeProperty(hash, name); 697 } 698 699 } 700 701 @Override 702 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 703 switch (hash) { 704 case 1349547969: 705 /* sequence */ return new String[] { "positiveInt" }; 706 case 1968600364: 707 /* information */ return new String[] { "Reference" }; 708 case -1096846342: 709 /* appliesToAll */ return new String[] { "boolean" }; 710 default: 711 return super.getTypesForProperty(hash, name); 712 } 713 714 } 715 716 @Override 717 public Base addChild(String name) throws FHIRException { 718 if (name.equals("sequence")) { 719 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityRequest.sequence"); 720 } else if (name.equals("information")) { 721 this.information = new Reference(); 722 return this.information; 723 } else if (name.equals("appliesToAll")) { 724 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityRequest.appliesToAll"); 725 } else 726 return super.addChild(name); 727 } 728 729 public SupportingInformationComponent copy() { 730 SupportingInformationComponent dst = new SupportingInformationComponent(); 731 copyValues(dst); 732 return dst; 733 } 734 735 public void copyValues(SupportingInformationComponent dst) { 736 super.copyValues(dst); 737 dst.sequence = sequence == null ? null : sequence.copy(); 738 dst.information = information == null ? null : information.copy(); 739 dst.appliesToAll = appliesToAll == null ? null : appliesToAll.copy(); 740 } 741 742 @Override 743 public boolean equalsDeep(Base other_) { 744 if (!super.equalsDeep(other_)) 745 return false; 746 if (!(other_ instanceof SupportingInformationComponent)) 747 return false; 748 SupportingInformationComponent o = (SupportingInformationComponent) other_; 749 return compareDeep(sequence, o.sequence, true) && compareDeep(information, o.information, true) 750 && compareDeep(appliesToAll, o.appliesToAll, true); 751 } 752 753 @Override 754 public boolean equalsShallow(Base other_) { 755 if (!super.equalsShallow(other_)) 756 return false; 757 if (!(other_ instanceof SupportingInformationComponent)) 758 return false; 759 SupportingInformationComponent o = (SupportingInformationComponent) other_; 760 return compareValues(sequence, o.sequence, true) && compareValues(appliesToAll, o.appliesToAll, true); 761 } 762 763 public boolean isEmpty() { 764 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, information, appliesToAll); 765 } 766 767 public String fhirType() { 768 return "CoverageEligibilityRequest.supportingInfo"; 769 770 } 771 772 } 773 774 @Block() 775 public static class InsuranceComponent extends BackboneElement implements IBaseBackboneElement { 776 /** 777 * A flag to indicate that this Coverage is to be used for evaluation of this 778 * request when set to true. 779 */ 780 @Child(name = "focal", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 781 @Description(shortDefinition = "Applicable coverage", formalDefinition = "A flag to indicate that this Coverage is to be used for evaluation of this request when set to true.") 782 protected BooleanType focal; 783 784 /** 785 * Reference to the insurance card level information contained in the Coverage 786 * resource. The coverage issuing insurer will use these details to locate the 787 * patient's actual coverage within the insurer's information system. 788 */ 789 @Child(name = "coverage", type = { Coverage.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 790 @Description(shortDefinition = "Insurance information", formalDefinition = "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.") 791 protected Reference coverage; 792 793 /** 794 * The actual object that is the target of the reference (Reference to the 795 * insurance card level information contained in the Coverage resource. The 796 * coverage issuing insurer will use these details to locate the patient's 797 * actual coverage within the insurer's information system.) 798 */ 799 protected Coverage coverageTarget; 800 801 /** 802 * A business agreement number established between the provider and the insurer 803 * for special business processing purposes. 804 */ 805 @Child(name = "businessArrangement", type = { 806 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 807 @Description(shortDefinition = "Additional provider contract number", formalDefinition = "A business agreement number established between the provider and the insurer for special business processing purposes.") 808 protected StringType businessArrangement; 809 810 private static final long serialVersionUID = 692505842L; 811 812 /** 813 * Constructor 814 */ 815 public InsuranceComponent() { 816 super(); 817 } 818 819 /** 820 * Constructor 821 */ 822 public InsuranceComponent(Reference coverage) { 823 super(); 824 this.coverage = coverage; 825 } 826 827 /** 828 * @return {@link #focal} (A flag to indicate that this Coverage is to be used 829 * for evaluation of this request when set to true.). This is the 830 * underlying object with id, value and extensions. The accessor 831 * "getFocal" gives direct access to the value 832 */ 833 public BooleanType getFocalElement() { 834 if (this.focal == null) 835 if (Configuration.errorOnAutoCreate()) 836 throw new Error("Attempt to auto-create InsuranceComponent.focal"); 837 else if (Configuration.doAutoCreate()) 838 this.focal = new BooleanType(); // bb 839 return this.focal; 840 } 841 842 public boolean hasFocalElement() { 843 return this.focal != null && !this.focal.isEmpty(); 844 } 845 846 public boolean hasFocal() { 847 return this.focal != null && !this.focal.isEmpty(); 848 } 849 850 /** 851 * @param value {@link #focal} (A flag to indicate that this Coverage is to be 852 * used for evaluation of this request when set to true.). This is 853 * the underlying object with id, value and extensions. The 854 * accessor "getFocal" gives direct access to the value 855 */ 856 public InsuranceComponent setFocalElement(BooleanType value) { 857 this.focal = value; 858 return this; 859 } 860 861 /** 862 * @return A flag to indicate that this Coverage is to be used for evaluation of 863 * this request when set to true. 864 */ 865 public boolean getFocal() { 866 return this.focal == null || this.focal.isEmpty() ? false : this.focal.getValue(); 867 } 868 869 /** 870 * @param value A flag to indicate that this Coverage is to be used for 871 * evaluation of this request when set to true. 872 */ 873 public InsuranceComponent setFocal(boolean value) { 874 if (this.focal == null) 875 this.focal = new BooleanType(); 876 this.focal.setValue(value); 877 return this; 878 } 879 880 /** 881 * @return {@link #coverage} (Reference to the insurance card level information 882 * contained in the Coverage resource. The coverage issuing insurer will 883 * use these details to locate the patient's actual coverage within the 884 * insurer's information system.) 885 */ 886 public Reference getCoverage() { 887 if (this.coverage == null) 888 if (Configuration.errorOnAutoCreate()) 889 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 890 else if (Configuration.doAutoCreate()) 891 this.coverage = new Reference(); // cc 892 return this.coverage; 893 } 894 895 public boolean hasCoverage() { 896 return this.coverage != null && !this.coverage.isEmpty(); 897 } 898 899 /** 900 * @param value {@link #coverage} (Reference to the insurance card level 901 * information contained in the Coverage resource. The coverage 902 * issuing insurer will use these details to locate the patient's 903 * actual coverage within the insurer's information system.) 904 */ 905 public InsuranceComponent setCoverage(Reference value) { 906 this.coverage = value; 907 return this; 908 } 909 910 /** 911 * @return {@link #coverage} The actual object that is the target of the 912 * reference. The reference library doesn't populate this, but you can 913 * use it to hold the resource if you resolve it. (Reference to the 914 * insurance card level information contained in the Coverage resource. 915 * The coverage issuing insurer will use these details to locate the 916 * patient's actual coverage within the insurer's information system.) 917 */ 918 public Coverage getCoverageTarget() { 919 if (this.coverageTarget == null) 920 if (Configuration.errorOnAutoCreate()) 921 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 922 else if (Configuration.doAutoCreate()) 923 this.coverageTarget = new Coverage(); // aa 924 return this.coverageTarget; 925 } 926 927 /** 928 * @param value {@link #coverage} The actual object that is the target of the 929 * reference. The reference library doesn't use these, but you can 930 * use it to hold the resource if you resolve it. (Reference to the 931 * insurance card level information contained in the Coverage 932 * resource. The coverage issuing insurer will use these details to 933 * locate the patient's actual coverage within the insurer's 934 * information system.) 935 */ 936 public InsuranceComponent setCoverageTarget(Coverage value) { 937 this.coverageTarget = value; 938 return this; 939 } 940 941 /** 942 * @return {@link #businessArrangement} (A business agreement number established 943 * between the provider and the insurer for special business processing 944 * purposes.). This is the underlying object with id, value and 945 * extensions. The accessor "getBusinessArrangement" gives direct access 946 * to the value 947 */ 948 public StringType getBusinessArrangementElement() { 949 if (this.businessArrangement == null) 950 if (Configuration.errorOnAutoCreate()) 951 throw new Error("Attempt to auto-create InsuranceComponent.businessArrangement"); 952 else if (Configuration.doAutoCreate()) 953 this.businessArrangement = new StringType(); // bb 954 return this.businessArrangement; 955 } 956 957 public boolean hasBusinessArrangementElement() { 958 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 959 } 960 961 public boolean hasBusinessArrangement() { 962 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 963 } 964 965 /** 966 * @param value {@link #businessArrangement} (A business agreement number 967 * established between the provider and the insurer for special 968 * business processing purposes.). This is the underlying object 969 * with id, value and extensions. The accessor 970 * "getBusinessArrangement" gives direct access to the value 971 */ 972 public InsuranceComponent setBusinessArrangementElement(StringType value) { 973 this.businessArrangement = value; 974 return this; 975 } 976 977 /** 978 * @return A business agreement number established between the provider and the 979 * insurer for special business processing purposes. 980 */ 981 public String getBusinessArrangement() { 982 return this.businessArrangement == null ? null : this.businessArrangement.getValue(); 983 } 984 985 /** 986 * @param value A business agreement number established between the provider and 987 * the insurer for special business processing purposes. 988 */ 989 public InsuranceComponent setBusinessArrangement(String value) { 990 if (Utilities.noString(value)) 991 this.businessArrangement = null; 992 else { 993 if (this.businessArrangement == null) 994 this.businessArrangement = new StringType(); 995 this.businessArrangement.setValue(value); 996 } 997 return this; 998 } 999 1000 protected void listChildren(List<Property> children) { 1001 super.listChildren(children); 1002 children.add(new Property("focal", "boolean", 1003 "A flag to indicate that this Coverage is to be used for evaluation of this request when set to true.", 0, 1, 1004 focal)); 1005 children.add(new Property("coverage", "Reference(Coverage)", 1006 "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 1007 0, 1, coverage)); 1008 children.add(new Property("businessArrangement", "string", 1009 "A business agreement number established between the provider and the insurer for special business processing purposes.", 1010 0, 1, businessArrangement)); 1011 } 1012 1013 @Override 1014 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1015 switch (_hash) { 1016 case 97604197: 1017 /* focal */ return new Property("focal", "boolean", 1018 "A flag to indicate that this Coverage is to be used for evaluation of this request when set to true.", 0, 1019 1, focal); 1020 case -351767064: 1021 /* coverage */ return new Property("coverage", "Reference(Coverage)", 1022 "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 1023 0, 1, coverage); 1024 case 259920682: 1025 /* businessArrangement */ return new Property("businessArrangement", "string", 1026 "A business agreement number established between the provider and the insurer for special business processing purposes.", 1027 0, 1, businessArrangement); 1028 default: 1029 return super.getNamedProperty(_hash, _name, _checkValid); 1030 } 1031 1032 } 1033 1034 @Override 1035 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1036 switch (hash) { 1037 case 97604197: 1038 /* focal */ return this.focal == null ? new Base[0] : new Base[] { this.focal }; // BooleanType 1039 case -351767064: 1040 /* coverage */ return this.coverage == null ? new Base[0] : new Base[] { this.coverage }; // Reference 1041 case 259920682: 1042 /* businessArrangement */ return this.businessArrangement == null ? new Base[0] 1043 : new Base[] { this.businessArrangement }; // StringType 1044 default: 1045 return super.getProperty(hash, name, checkValid); 1046 } 1047 1048 } 1049 1050 @Override 1051 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1052 switch (hash) { 1053 case 97604197: // focal 1054 this.focal = castToBoolean(value); // BooleanType 1055 return value; 1056 case -351767064: // coverage 1057 this.coverage = castToReference(value); // Reference 1058 return value; 1059 case 259920682: // businessArrangement 1060 this.businessArrangement = castToString(value); // StringType 1061 return value; 1062 default: 1063 return super.setProperty(hash, name, value); 1064 } 1065 1066 } 1067 1068 @Override 1069 public Base setProperty(String name, Base value) throws FHIRException { 1070 if (name.equals("focal")) { 1071 this.focal = castToBoolean(value); // BooleanType 1072 } else if (name.equals("coverage")) { 1073 this.coverage = castToReference(value); // Reference 1074 } else if (name.equals("businessArrangement")) { 1075 this.businessArrangement = castToString(value); // StringType 1076 } else 1077 return super.setProperty(name, value); 1078 return value; 1079 } 1080 1081 @Override 1082 public void removeChild(String name, Base value) throws FHIRException { 1083 if (name.equals("focal")) { 1084 this.focal = null; 1085 } else if (name.equals("coverage")) { 1086 this.coverage = null; 1087 } else if (name.equals("businessArrangement")) { 1088 this.businessArrangement = null; 1089 } else 1090 super.removeChild(name, value); 1091 1092 } 1093 1094 @Override 1095 public Base makeProperty(int hash, String name) throws FHIRException { 1096 switch (hash) { 1097 case 97604197: 1098 return getFocalElement(); 1099 case -351767064: 1100 return getCoverage(); 1101 case 259920682: 1102 return getBusinessArrangementElement(); 1103 default: 1104 return super.makeProperty(hash, name); 1105 } 1106 1107 } 1108 1109 @Override 1110 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1111 switch (hash) { 1112 case 97604197: 1113 /* focal */ return new String[] { "boolean" }; 1114 case -351767064: 1115 /* coverage */ return new String[] { "Reference" }; 1116 case 259920682: 1117 /* businessArrangement */ return new String[] { "string" }; 1118 default: 1119 return super.getTypesForProperty(hash, name); 1120 } 1121 1122 } 1123 1124 @Override 1125 public Base addChild(String name) throws FHIRException { 1126 if (name.equals("focal")) { 1127 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityRequest.focal"); 1128 } else if (name.equals("coverage")) { 1129 this.coverage = new Reference(); 1130 return this.coverage; 1131 } else if (name.equals("businessArrangement")) { 1132 throw new FHIRException( 1133 "Cannot call addChild on a singleton property CoverageEligibilityRequest.businessArrangement"); 1134 } else 1135 return super.addChild(name); 1136 } 1137 1138 public InsuranceComponent copy() { 1139 InsuranceComponent dst = new InsuranceComponent(); 1140 copyValues(dst); 1141 return dst; 1142 } 1143 1144 public void copyValues(InsuranceComponent dst) { 1145 super.copyValues(dst); 1146 dst.focal = focal == null ? null : focal.copy(); 1147 dst.coverage = coverage == null ? null : coverage.copy(); 1148 dst.businessArrangement = businessArrangement == null ? null : businessArrangement.copy(); 1149 } 1150 1151 @Override 1152 public boolean equalsDeep(Base other_) { 1153 if (!super.equalsDeep(other_)) 1154 return false; 1155 if (!(other_ instanceof InsuranceComponent)) 1156 return false; 1157 InsuranceComponent o = (InsuranceComponent) other_; 1158 return compareDeep(focal, o.focal, true) && compareDeep(coverage, o.coverage, true) 1159 && compareDeep(businessArrangement, o.businessArrangement, true); 1160 } 1161 1162 @Override 1163 public boolean equalsShallow(Base other_) { 1164 if (!super.equalsShallow(other_)) 1165 return false; 1166 if (!(other_ instanceof InsuranceComponent)) 1167 return false; 1168 InsuranceComponent o = (InsuranceComponent) other_; 1169 return compareValues(focal, o.focal, true) && compareValues(businessArrangement, o.businessArrangement, true); 1170 } 1171 1172 public boolean isEmpty() { 1173 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(focal, coverage, businessArrangement); 1174 } 1175 1176 public String fhirType() { 1177 return "CoverageEligibilityRequest.insurance"; 1178 1179 } 1180 1181 } 1182 1183 @Block() 1184 public static class DetailsComponent extends BackboneElement implements IBaseBackboneElement { 1185 /** 1186 * Exceptions, special conditions and supporting information applicable for this 1187 * service or product line. 1188 */ 1189 @Child(name = "supportingInfoSequence", type = { 1190 PositiveIntType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1191 @Description(shortDefinition = "Applicable exception or supporting information", formalDefinition = "Exceptions, special conditions and supporting information applicable for this service or product line.") 1192 protected List<PositiveIntType> supportingInfoSequence; 1193 1194 /** 1195 * Code to identify the general type of benefits under which products and 1196 * services are provided. 1197 */ 1198 @Child(name = "category", type = { 1199 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1200 @Description(shortDefinition = "Benefit classification", formalDefinition = "Code to identify the general type of benefits under which products and services are provided.") 1201 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-benefitcategory") 1202 protected CodeableConcept category; 1203 1204 /** 1205 * This contains the product, service, drug or other billing code for the item. 1206 */ 1207 @Child(name = "productOrService", type = { 1208 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1209 @Description(shortDefinition = "Billing, service, product, or drug code", formalDefinition = "This contains the product, service, drug or other billing code for the item.") 1210 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-uscls") 1211 protected CodeableConcept productOrService; 1212 1213 /** 1214 * Item typification or modifiers codes to convey additional context for the 1215 * product or service. 1216 */ 1217 @Child(name = "modifier", type = { 1218 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1219 @Description(shortDefinition = "Product or service billing modifiers", formalDefinition = "Item typification or modifiers codes to convey additional context for the product or service.") 1220 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-modifiers") 1221 protected List<CodeableConcept> modifier; 1222 1223 /** 1224 * The practitioner who is responsible for the product or service to be rendered 1225 * to the patient. 1226 */ 1227 @Child(name = "provider", type = { Practitioner.class, 1228 PractitionerRole.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1229 @Description(shortDefinition = "Perfoming practitioner", formalDefinition = "The practitioner who is responsible for the product or service to be rendered to the patient.") 1230 protected Reference provider; 1231 1232 /** 1233 * The actual object that is the target of the reference (The practitioner who 1234 * is responsible for the product or service to be rendered to the patient.) 1235 */ 1236 protected Resource providerTarget; 1237 1238 /** 1239 * The number of repetitions of a service or product. 1240 */ 1241 @Child(name = "quantity", type = { Quantity.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1242 @Description(shortDefinition = "Count of products or services", formalDefinition = "The number of repetitions of a service or product.") 1243 protected Quantity quantity; 1244 1245 /** 1246 * The amount charged to the patient by the provider for a single unit. 1247 */ 1248 @Child(name = "unitPrice", type = { Money.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 1249 @Description(shortDefinition = "Fee, charge or cost per item", formalDefinition = "The amount charged to the patient by the provider for a single unit.") 1250 protected Money unitPrice; 1251 1252 /** 1253 * Facility where the services will be provided. 1254 */ 1255 @Child(name = "facility", type = { Location.class, 1256 Organization.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1257 @Description(shortDefinition = "Servicing facility", formalDefinition = "Facility where the services will be provided.") 1258 protected Reference facility; 1259 1260 /** 1261 * The actual object that is the target of the reference (Facility where the 1262 * services will be provided.) 1263 */ 1264 protected Resource facilityTarget; 1265 1266 /** 1267 * Patient diagnosis for which care is sought. 1268 */ 1269 @Child(name = "diagnosis", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1270 @Description(shortDefinition = "Applicable diagnosis", formalDefinition = "Patient diagnosis for which care is sought.") 1271 protected List<DiagnosisComponent> diagnosis; 1272 1273 /** 1274 * The plan/proposal/order describing the proposed service in detail. 1275 */ 1276 @Child(name = "detail", type = { 1277 Reference.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1278 @Description(shortDefinition = "Product or service details", formalDefinition = "The plan/proposal/order describing the proposed service in detail.") 1279 protected List<Reference> detail; 1280 /** 1281 * The actual objects that are the target of the reference (The 1282 * plan/proposal/order describing the proposed service in detail.) 1283 */ 1284 protected List<Resource> detailTarget; 1285 1286 private static final long serialVersionUID = 389110539L; 1287 1288 /** 1289 * Constructor 1290 */ 1291 public DetailsComponent() { 1292 super(); 1293 } 1294 1295 /** 1296 * @return {@link #supportingInfoSequence} (Exceptions, special conditions and 1297 * supporting information applicable for this service or product line.) 1298 */ 1299 public List<PositiveIntType> getSupportingInfoSequence() { 1300 if (this.supportingInfoSequence == null) 1301 this.supportingInfoSequence = new ArrayList<PositiveIntType>(); 1302 return this.supportingInfoSequence; 1303 } 1304 1305 /** 1306 * @return Returns a reference to <code>this</code> for easy method chaining 1307 */ 1308 public DetailsComponent setSupportingInfoSequence(List<PositiveIntType> theSupportingInfoSequence) { 1309 this.supportingInfoSequence = theSupportingInfoSequence; 1310 return this; 1311 } 1312 1313 public boolean hasSupportingInfoSequence() { 1314 if (this.supportingInfoSequence == null) 1315 return false; 1316 for (PositiveIntType item : this.supportingInfoSequence) 1317 if (!item.isEmpty()) 1318 return true; 1319 return false; 1320 } 1321 1322 /** 1323 * @return {@link #supportingInfoSequence} (Exceptions, special conditions and 1324 * supporting information applicable for this service or product line.) 1325 */ 1326 public PositiveIntType addSupportingInfoSequenceElement() {// 2 1327 PositiveIntType t = new PositiveIntType(); 1328 if (this.supportingInfoSequence == null) 1329 this.supportingInfoSequence = new ArrayList<PositiveIntType>(); 1330 this.supportingInfoSequence.add(t); 1331 return t; 1332 } 1333 1334 /** 1335 * @param value {@link #supportingInfoSequence} (Exceptions, special conditions 1336 * and supporting information applicable for this service or 1337 * product line.) 1338 */ 1339 public DetailsComponent addSupportingInfoSequence(int value) { // 1 1340 PositiveIntType t = new PositiveIntType(); 1341 t.setValue(value); 1342 if (this.supportingInfoSequence == null) 1343 this.supportingInfoSequence = new ArrayList<PositiveIntType>(); 1344 this.supportingInfoSequence.add(t); 1345 return this; 1346 } 1347 1348 /** 1349 * @param value {@link #supportingInfoSequence} (Exceptions, special conditions 1350 * and supporting information applicable for this service or 1351 * product line.) 1352 */ 1353 public boolean hasSupportingInfoSequence(int value) { 1354 if (this.supportingInfoSequence == null) 1355 return false; 1356 for (PositiveIntType v : this.supportingInfoSequence) 1357 if (v.getValue().equals(value)) // positiveInt 1358 return true; 1359 return false; 1360 } 1361 1362 /** 1363 * @return {@link #category} (Code to identify the general type of benefits 1364 * under which products and services are provided.) 1365 */ 1366 public CodeableConcept getCategory() { 1367 if (this.category == null) 1368 if (Configuration.errorOnAutoCreate()) 1369 throw new Error("Attempt to auto-create DetailsComponent.category"); 1370 else if (Configuration.doAutoCreate()) 1371 this.category = new CodeableConcept(); // cc 1372 return this.category; 1373 } 1374 1375 public boolean hasCategory() { 1376 return this.category != null && !this.category.isEmpty(); 1377 } 1378 1379 /** 1380 * @param value {@link #category} (Code to identify the general type of benefits 1381 * under which products and services are provided.) 1382 */ 1383 public DetailsComponent setCategory(CodeableConcept value) { 1384 this.category = value; 1385 return this; 1386 } 1387 1388 /** 1389 * @return {@link #productOrService} (This contains the product, service, drug 1390 * or other billing code for the item.) 1391 */ 1392 public CodeableConcept getProductOrService() { 1393 if (this.productOrService == null) 1394 if (Configuration.errorOnAutoCreate()) 1395 throw new Error("Attempt to auto-create DetailsComponent.productOrService"); 1396 else if (Configuration.doAutoCreate()) 1397 this.productOrService = new CodeableConcept(); // cc 1398 return this.productOrService; 1399 } 1400 1401 public boolean hasProductOrService() { 1402 return this.productOrService != null && !this.productOrService.isEmpty(); 1403 } 1404 1405 /** 1406 * @param value {@link #productOrService} (This contains the product, service, 1407 * drug or other billing code for the item.) 1408 */ 1409 public DetailsComponent setProductOrService(CodeableConcept value) { 1410 this.productOrService = value; 1411 return this; 1412 } 1413 1414 /** 1415 * @return {@link #modifier} (Item typification or modifiers codes to convey 1416 * additional context for the product or service.) 1417 */ 1418 public List<CodeableConcept> getModifier() { 1419 if (this.modifier == null) 1420 this.modifier = new ArrayList<CodeableConcept>(); 1421 return this.modifier; 1422 } 1423 1424 /** 1425 * @return Returns a reference to <code>this</code> for easy method chaining 1426 */ 1427 public DetailsComponent setModifier(List<CodeableConcept> theModifier) { 1428 this.modifier = theModifier; 1429 return this; 1430 } 1431 1432 public boolean hasModifier() { 1433 if (this.modifier == null) 1434 return false; 1435 for (CodeableConcept item : this.modifier) 1436 if (!item.isEmpty()) 1437 return true; 1438 return false; 1439 } 1440 1441 public CodeableConcept addModifier() { // 3 1442 CodeableConcept t = new CodeableConcept(); 1443 if (this.modifier == null) 1444 this.modifier = new ArrayList<CodeableConcept>(); 1445 this.modifier.add(t); 1446 return t; 1447 } 1448 1449 public DetailsComponent addModifier(CodeableConcept t) { // 3 1450 if (t == null) 1451 return this; 1452 if (this.modifier == null) 1453 this.modifier = new ArrayList<CodeableConcept>(); 1454 this.modifier.add(t); 1455 return this; 1456 } 1457 1458 /** 1459 * @return The first repetition of repeating field {@link #modifier}, creating 1460 * it if it does not already exist 1461 */ 1462 public CodeableConcept getModifierFirstRep() { 1463 if (getModifier().isEmpty()) { 1464 addModifier(); 1465 } 1466 return getModifier().get(0); 1467 } 1468 1469 /** 1470 * @return {@link #provider} (The practitioner who is responsible for the 1471 * product or service to be rendered to the patient.) 1472 */ 1473 public Reference getProvider() { 1474 if (this.provider == null) 1475 if (Configuration.errorOnAutoCreate()) 1476 throw new Error("Attempt to auto-create DetailsComponent.provider"); 1477 else if (Configuration.doAutoCreate()) 1478 this.provider = new Reference(); // cc 1479 return this.provider; 1480 } 1481 1482 public boolean hasProvider() { 1483 return this.provider != null && !this.provider.isEmpty(); 1484 } 1485 1486 /** 1487 * @param value {@link #provider} (The practitioner who is responsible for the 1488 * product or service to be rendered to the patient.) 1489 */ 1490 public DetailsComponent setProvider(Reference value) { 1491 this.provider = value; 1492 return this; 1493 } 1494 1495 /** 1496 * @return {@link #provider} The actual object that is the target of the 1497 * reference. The reference library doesn't populate this, but you can 1498 * use it to hold the resource if you resolve it. (The practitioner who 1499 * is responsible for the product or service to be rendered to the 1500 * patient.) 1501 */ 1502 public Resource getProviderTarget() { 1503 return this.providerTarget; 1504 } 1505 1506 /** 1507 * @param value {@link #provider} The actual object that is the target of the 1508 * reference. The reference library doesn't use these, but you can 1509 * use it to hold the resource if you resolve it. (The practitioner 1510 * who is responsible for the product or service to be rendered to 1511 * the patient.) 1512 */ 1513 public DetailsComponent setProviderTarget(Resource value) { 1514 this.providerTarget = value; 1515 return this; 1516 } 1517 1518 /** 1519 * @return {@link #quantity} (The number of repetitions of a service or 1520 * product.) 1521 */ 1522 public Quantity getQuantity() { 1523 if (this.quantity == null) 1524 if (Configuration.errorOnAutoCreate()) 1525 throw new Error("Attempt to auto-create DetailsComponent.quantity"); 1526 else if (Configuration.doAutoCreate()) 1527 this.quantity = new Quantity(); // cc 1528 return this.quantity; 1529 } 1530 1531 public boolean hasQuantity() { 1532 return this.quantity != null && !this.quantity.isEmpty(); 1533 } 1534 1535 /** 1536 * @param value {@link #quantity} (The number of repetitions of a service or 1537 * product.) 1538 */ 1539 public DetailsComponent setQuantity(Quantity value) { 1540 this.quantity = value; 1541 return this; 1542 } 1543 1544 /** 1545 * @return {@link #unitPrice} (The amount charged to the patient by the provider 1546 * for a single unit.) 1547 */ 1548 public Money getUnitPrice() { 1549 if (this.unitPrice == null) 1550 if (Configuration.errorOnAutoCreate()) 1551 throw new Error("Attempt to auto-create DetailsComponent.unitPrice"); 1552 else if (Configuration.doAutoCreate()) 1553 this.unitPrice = new Money(); // cc 1554 return this.unitPrice; 1555 } 1556 1557 public boolean hasUnitPrice() { 1558 return this.unitPrice != null && !this.unitPrice.isEmpty(); 1559 } 1560 1561 /** 1562 * @param value {@link #unitPrice} (The amount charged to the patient by the 1563 * provider for a single unit.) 1564 */ 1565 public DetailsComponent setUnitPrice(Money value) { 1566 this.unitPrice = value; 1567 return this; 1568 } 1569 1570 /** 1571 * @return {@link #facility} (Facility where the services will be provided.) 1572 */ 1573 public Reference getFacility() { 1574 if (this.facility == null) 1575 if (Configuration.errorOnAutoCreate()) 1576 throw new Error("Attempt to auto-create DetailsComponent.facility"); 1577 else if (Configuration.doAutoCreate()) 1578 this.facility = new Reference(); // cc 1579 return this.facility; 1580 } 1581 1582 public boolean hasFacility() { 1583 return this.facility != null && !this.facility.isEmpty(); 1584 } 1585 1586 /** 1587 * @param value {@link #facility} (Facility where the services will be 1588 * provided.) 1589 */ 1590 public DetailsComponent setFacility(Reference value) { 1591 this.facility = value; 1592 return this; 1593 } 1594 1595 /** 1596 * @return {@link #facility} The actual object that is the target of the 1597 * reference. The reference library doesn't populate this, but you can 1598 * use it to hold the resource if you resolve it. (Facility where the 1599 * services will be provided.) 1600 */ 1601 public Resource getFacilityTarget() { 1602 return this.facilityTarget; 1603 } 1604 1605 /** 1606 * @param value {@link #facility} The actual object that is the target of the 1607 * reference. The reference library doesn't use these, but you can 1608 * use it to hold the resource if you resolve it. (Facility where 1609 * the services will be provided.) 1610 */ 1611 public DetailsComponent setFacilityTarget(Resource value) { 1612 this.facilityTarget = value; 1613 return this; 1614 } 1615 1616 /** 1617 * @return {@link #diagnosis} (Patient diagnosis for which care is sought.) 1618 */ 1619 public List<DiagnosisComponent> getDiagnosis() { 1620 if (this.diagnosis == null) 1621 this.diagnosis = new ArrayList<DiagnosisComponent>(); 1622 return this.diagnosis; 1623 } 1624 1625 /** 1626 * @return Returns a reference to <code>this</code> for easy method chaining 1627 */ 1628 public DetailsComponent setDiagnosis(List<DiagnosisComponent> theDiagnosis) { 1629 this.diagnosis = theDiagnosis; 1630 return this; 1631 } 1632 1633 public boolean hasDiagnosis() { 1634 if (this.diagnosis == null) 1635 return false; 1636 for (DiagnosisComponent item : this.diagnosis) 1637 if (!item.isEmpty()) 1638 return true; 1639 return false; 1640 } 1641 1642 public DiagnosisComponent addDiagnosis() { // 3 1643 DiagnosisComponent t = new DiagnosisComponent(); 1644 if (this.diagnosis == null) 1645 this.diagnosis = new ArrayList<DiagnosisComponent>(); 1646 this.diagnosis.add(t); 1647 return t; 1648 } 1649 1650 public DetailsComponent addDiagnosis(DiagnosisComponent t) { // 3 1651 if (t == null) 1652 return this; 1653 if (this.diagnosis == null) 1654 this.diagnosis = new ArrayList<DiagnosisComponent>(); 1655 this.diagnosis.add(t); 1656 return this; 1657 } 1658 1659 /** 1660 * @return The first repetition of repeating field {@link #diagnosis}, creating 1661 * it if it does not already exist 1662 */ 1663 public DiagnosisComponent getDiagnosisFirstRep() { 1664 if (getDiagnosis().isEmpty()) { 1665 addDiagnosis(); 1666 } 1667 return getDiagnosis().get(0); 1668 } 1669 1670 /** 1671 * @return {@link #detail} (The plan/proposal/order describing the proposed 1672 * service in detail.) 1673 */ 1674 public List<Reference> getDetail() { 1675 if (this.detail == null) 1676 this.detail = new ArrayList<Reference>(); 1677 return this.detail; 1678 } 1679 1680 /** 1681 * @return Returns a reference to <code>this</code> for easy method chaining 1682 */ 1683 public DetailsComponent setDetail(List<Reference> theDetail) { 1684 this.detail = theDetail; 1685 return this; 1686 } 1687 1688 public boolean hasDetail() { 1689 if (this.detail == null) 1690 return false; 1691 for (Reference item : this.detail) 1692 if (!item.isEmpty()) 1693 return true; 1694 return false; 1695 } 1696 1697 public Reference addDetail() { // 3 1698 Reference t = new Reference(); 1699 if (this.detail == null) 1700 this.detail = new ArrayList<Reference>(); 1701 this.detail.add(t); 1702 return t; 1703 } 1704 1705 public DetailsComponent addDetail(Reference t) { // 3 1706 if (t == null) 1707 return this; 1708 if (this.detail == null) 1709 this.detail = new ArrayList<Reference>(); 1710 this.detail.add(t); 1711 return this; 1712 } 1713 1714 /** 1715 * @return The first repetition of repeating field {@link #detail}, creating it 1716 * if it does not already exist 1717 */ 1718 public Reference getDetailFirstRep() { 1719 if (getDetail().isEmpty()) { 1720 addDetail(); 1721 } 1722 return getDetail().get(0); 1723 } 1724 1725 /** 1726 * @deprecated Use Reference#setResource(IBaseResource) instead 1727 */ 1728 @Deprecated 1729 public List<Resource> getDetailTarget() { 1730 if (this.detailTarget == null) 1731 this.detailTarget = new ArrayList<Resource>(); 1732 return this.detailTarget; 1733 } 1734 1735 protected void listChildren(List<Property> children) { 1736 super.listChildren(children); 1737 children.add(new Property("supportingInfoSequence", "positiveInt", 1738 "Exceptions, special conditions and supporting information applicable for this service or product line.", 0, 1739 java.lang.Integer.MAX_VALUE, supportingInfoSequence)); 1740 children.add(new Property("category", "CodeableConcept", 1741 "Code to identify the general type of benefits under which products and services are provided.", 0, 1, 1742 category)); 1743 children.add(new Property("productOrService", "CodeableConcept", 1744 "This contains the product, service, drug or other billing code for the item.", 0, 1, productOrService)); 1745 children.add(new Property("modifier", "CodeableConcept", 1746 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 1747 java.lang.Integer.MAX_VALUE, modifier)); 1748 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole)", 1749 "The practitioner who is responsible for the product or service to be rendered to the patient.", 0, 1, 1750 provider)); 1751 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 1752 1, quantity)); 1753 children.add(new Property("unitPrice", "Money", 1754 "The amount charged to the patient by the provider for a single unit.", 0, 1, unitPrice)); 1755 children.add(new Property("facility", "Reference(Location|Organization)", 1756 "Facility where the services will be provided.", 0, 1, facility)); 1757 children.add(new Property("diagnosis", "", "Patient diagnosis for which care is sought.", 0, 1758 java.lang.Integer.MAX_VALUE, diagnosis)); 1759 children.add( 1760 new Property("detail", "Reference(Any)", "The plan/proposal/order describing the proposed service in detail.", 1761 0, java.lang.Integer.MAX_VALUE, detail)); 1762 } 1763 1764 @Override 1765 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1766 switch (_hash) { 1767 case -595860510: 1768 /* supportingInfoSequence */ return new Property("supportingInfoSequence", "positiveInt", 1769 "Exceptions, special conditions and supporting information applicable for this service or product line.", 0, 1770 java.lang.Integer.MAX_VALUE, supportingInfoSequence); 1771 case 50511102: 1772 /* category */ return new Property("category", "CodeableConcept", 1773 "Code to identify the general type of benefits under which products and services are provided.", 0, 1, 1774 category); 1775 case 1957227299: 1776 /* productOrService */ return new Property("productOrService", "CodeableConcept", 1777 "This contains the product, service, drug or other billing code for the item.", 0, 1, productOrService); 1778 case -615513385: 1779 /* modifier */ return new Property("modifier", "CodeableConcept", 1780 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 1781 java.lang.Integer.MAX_VALUE, modifier); 1782 case -987494927: 1783 /* provider */ return new Property("provider", "Reference(Practitioner|PractitionerRole)", 1784 "The practitioner who is responsible for the product or service to be rendered to the patient.", 0, 1, 1785 provider); 1786 case -1285004149: 1787 /* quantity */ return new Property("quantity", "SimpleQuantity", 1788 "The number of repetitions of a service or product.", 0, 1, quantity); 1789 case -486196699: 1790 /* unitPrice */ return new Property("unitPrice", "Money", 1791 "The amount charged to the patient by the provider for a single unit.", 0, 1, unitPrice); 1792 case 501116579: 1793 /* facility */ return new Property("facility", "Reference(Location|Organization)", 1794 "Facility where the services will be provided.", 0, 1, facility); 1795 case 1196993265: 1796 /* diagnosis */ return new Property("diagnosis", "", "Patient diagnosis for which care is sought.", 0, 1797 java.lang.Integer.MAX_VALUE, diagnosis); 1798 case -1335224239: 1799 /* detail */ return new Property("detail", "Reference(Any)", 1800 "The plan/proposal/order describing the proposed service in detail.", 0, java.lang.Integer.MAX_VALUE, 1801 detail); 1802 default: 1803 return super.getNamedProperty(_hash, _name, _checkValid); 1804 } 1805 1806 } 1807 1808 @Override 1809 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1810 switch (hash) { 1811 case -595860510: 1812 /* supportingInfoSequence */ return this.supportingInfoSequence == null ? new Base[0] 1813 : this.supportingInfoSequence.toArray(new Base[this.supportingInfoSequence.size()]); // PositiveIntType 1814 case 50511102: 1815 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 1816 case 1957227299: 1817 /* productOrService */ return this.productOrService == null ? new Base[0] 1818 : new Base[] { this.productOrService }; // CodeableConcept 1819 case -615513385: 1820 /* modifier */ return this.modifier == null ? new Base[0] 1821 : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 1822 case -987494927: 1823 /* provider */ return this.provider == null ? new Base[0] : new Base[] { this.provider }; // Reference 1824 case -1285004149: 1825 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 1826 case -486196699: 1827 /* unitPrice */ return this.unitPrice == null ? new Base[0] : new Base[] { this.unitPrice }; // Money 1828 case 501116579: 1829 /* facility */ return this.facility == null ? new Base[0] : new Base[] { this.facility }; // Reference 1830 case 1196993265: 1831 /* diagnosis */ return this.diagnosis == null ? new Base[0] 1832 : this.diagnosis.toArray(new Base[this.diagnosis.size()]); // DiagnosisComponent 1833 case -1335224239: 1834 /* detail */ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // Reference 1835 default: 1836 return super.getProperty(hash, name, checkValid); 1837 } 1838 1839 } 1840 1841 @Override 1842 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1843 switch (hash) { 1844 case -595860510: // supportingInfoSequence 1845 this.getSupportingInfoSequence().add(castToPositiveInt(value)); // PositiveIntType 1846 return value; 1847 case 50511102: // category 1848 this.category = castToCodeableConcept(value); // CodeableConcept 1849 return value; 1850 case 1957227299: // productOrService 1851 this.productOrService = castToCodeableConcept(value); // CodeableConcept 1852 return value; 1853 case -615513385: // modifier 1854 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 1855 return value; 1856 case -987494927: // provider 1857 this.provider = castToReference(value); // Reference 1858 return value; 1859 case -1285004149: // quantity 1860 this.quantity = castToQuantity(value); // Quantity 1861 return value; 1862 case -486196699: // unitPrice 1863 this.unitPrice = castToMoney(value); // Money 1864 return value; 1865 case 501116579: // facility 1866 this.facility = castToReference(value); // Reference 1867 return value; 1868 case 1196993265: // diagnosis 1869 this.getDiagnosis().add((DiagnosisComponent) value); // DiagnosisComponent 1870 return value; 1871 case -1335224239: // detail 1872 this.getDetail().add(castToReference(value)); // Reference 1873 return value; 1874 default: 1875 return super.setProperty(hash, name, value); 1876 } 1877 1878 } 1879 1880 @Override 1881 public Base setProperty(String name, Base value) throws FHIRException { 1882 if (name.equals("supportingInfoSequence")) { 1883 this.getSupportingInfoSequence().add(castToPositiveInt(value)); 1884 } else if (name.equals("category")) { 1885 this.category = castToCodeableConcept(value); // CodeableConcept 1886 } else if (name.equals("productOrService")) { 1887 this.productOrService = castToCodeableConcept(value); // CodeableConcept 1888 } else if (name.equals("modifier")) { 1889 this.getModifier().add(castToCodeableConcept(value)); 1890 } else if (name.equals("provider")) { 1891 this.provider = castToReference(value); // Reference 1892 } else if (name.equals("quantity")) { 1893 this.quantity = castToQuantity(value); // Quantity 1894 } else if (name.equals("unitPrice")) { 1895 this.unitPrice = castToMoney(value); // Money 1896 } else if (name.equals("facility")) { 1897 this.facility = castToReference(value); // Reference 1898 } else if (name.equals("diagnosis")) { 1899 this.getDiagnosis().add((DiagnosisComponent) value); 1900 } else if (name.equals("detail")) { 1901 this.getDetail().add(castToReference(value)); 1902 } else 1903 return super.setProperty(name, value); 1904 return value; 1905 } 1906 1907 @Override 1908 public void removeChild(String name, Base value) throws FHIRException { 1909 if (name.equals("supportingInfoSequence")) { 1910 this.getSupportingInfoSequence().remove(castToPositiveInt(value)); 1911 } else if (name.equals("category")) { 1912 this.category = null; 1913 } else if (name.equals("productOrService")) { 1914 this.productOrService = null; 1915 } else if (name.equals("modifier")) { 1916 this.getModifier().remove(castToCodeableConcept(value)); 1917 } else if (name.equals("provider")) { 1918 this.provider = null; 1919 } else if (name.equals("quantity")) { 1920 this.quantity = null; 1921 } else if (name.equals("unitPrice")) { 1922 this.unitPrice = null; 1923 } else if (name.equals("facility")) { 1924 this.facility = null; 1925 } else if (name.equals("diagnosis")) { 1926 this.getDiagnosis().remove((DiagnosisComponent) value); 1927 } else if (name.equals("detail")) { 1928 this.getDetail().remove(castToReference(value)); 1929 } else 1930 super.removeChild(name, value); 1931 1932 } 1933 1934 @Override 1935 public Base makeProperty(int hash, String name) throws FHIRException { 1936 switch (hash) { 1937 case -595860510: 1938 return addSupportingInfoSequenceElement(); 1939 case 50511102: 1940 return getCategory(); 1941 case 1957227299: 1942 return getProductOrService(); 1943 case -615513385: 1944 return addModifier(); 1945 case -987494927: 1946 return getProvider(); 1947 case -1285004149: 1948 return getQuantity(); 1949 case -486196699: 1950 return getUnitPrice(); 1951 case 501116579: 1952 return getFacility(); 1953 case 1196993265: 1954 return addDiagnosis(); 1955 case -1335224239: 1956 return addDetail(); 1957 default: 1958 return super.makeProperty(hash, name); 1959 } 1960 1961 } 1962 1963 @Override 1964 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1965 switch (hash) { 1966 case -595860510: 1967 /* supportingInfoSequence */ return new String[] { "positiveInt" }; 1968 case 50511102: 1969 /* category */ return new String[] { "CodeableConcept" }; 1970 case 1957227299: 1971 /* productOrService */ return new String[] { "CodeableConcept" }; 1972 case -615513385: 1973 /* modifier */ return new String[] { "CodeableConcept" }; 1974 case -987494927: 1975 /* provider */ return new String[] { "Reference" }; 1976 case -1285004149: 1977 /* quantity */ return new String[] { "SimpleQuantity" }; 1978 case -486196699: 1979 /* unitPrice */ return new String[] { "Money" }; 1980 case 501116579: 1981 /* facility */ return new String[] { "Reference" }; 1982 case 1196993265: 1983 /* diagnosis */ return new String[] {}; 1984 case -1335224239: 1985 /* detail */ return new String[] { "Reference" }; 1986 default: 1987 return super.getTypesForProperty(hash, name); 1988 } 1989 1990 } 1991 1992 @Override 1993 public Base addChild(String name) throws FHIRException { 1994 if (name.equals("supportingInfoSequence")) { 1995 throw new FHIRException( 1996 "Cannot call addChild on a singleton property CoverageEligibilityRequest.supportingInfoSequence"); 1997 } else if (name.equals("category")) { 1998 this.category = new CodeableConcept(); 1999 return this.category; 2000 } else if (name.equals("productOrService")) { 2001 this.productOrService = new CodeableConcept(); 2002 return this.productOrService; 2003 } else if (name.equals("modifier")) { 2004 return addModifier(); 2005 } else if (name.equals("provider")) { 2006 this.provider = new Reference(); 2007 return this.provider; 2008 } else if (name.equals("quantity")) { 2009 this.quantity = new Quantity(); 2010 return this.quantity; 2011 } else if (name.equals("unitPrice")) { 2012 this.unitPrice = new Money(); 2013 return this.unitPrice; 2014 } else if (name.equals("facility")) { 2015 this.facility = new Reference(); 2016 return this.facility; 2017 } else if (name.equals("diagnosis")) { 2018 return addDiagnosis(); 2019 } else if (name.equals("detail")) { 2020 return addDetail(); 2021 } else 2022 return super.addChild(name); 2023 } 2024 2025 public DetailsComponent copy() { 2026 DetailsComponent dst = new DetailsComponent(); 2027 copyValues(dst); 2028 return dst; 2029 } 2030 2031 public void copyValues(DetailsComponent dst) { 2032 super.copyValues(dst); 2033 if (supportingInfoSequence != null) { 2034 dst.supportingInfoSequence = new ArrayList<PositiveIntType>(); 2035 for (PositiveIntType i : supportingInfoSequence) 2036 dst.supportingInfoSequence.add(i.copy()); 2037 } 2038 ; 2039 dst.category = category == null ? null : category.copy(); 2040 dst.productOrService = productOrService == null ? null : productOrService.copy(); 2041 if (modifier != null) { 2042 dst.modifier = new ArrayList<CodeableConcept>(); 2043 for (CodeableConcept i : modifier) 2044 dst.modifier.add(i.copy()); 2045 } 2046 ; 2047 dst.provider = provider == null ? null : provider.copy(); 2048 dst.quantity = quantity == null ? null : quantity.copy(); 2049 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 2050 dst.facility = facility == null ? null : facility.copy(); 2051 if (diagnosis != null) { 2052 dst.diagnosis = new ArrayList<DiagnosisComponent>(); 2053 for (DiagnosisComponent i : diagnosis) 2054 dst.diagnosis.add(i.copy()); 2055 } 2056 ; 2057 if (detail != null) { 2058 dst.detail = new ArrayList<Reference>(); 2059 for (Reference i : detail) 2060 dst.detail.add(i.copy()); 2061 } 2062 ; 2063 } 2064 2065 @Override 2066 public boolean equalsDeep(Base other_) { 2067 if (!super.equalsDeep(other_)) 2068 return false; 2069 if (!(other_ instanceof DetailsComponent)) 2070 return false; 2071 DetailsComponent o = (DetailsComponent) other_; 2072 return compareDeep(supportingInfoSequence, o.supportingInfoSequence, true) 2073 && compareDeep(category, o.category, true) && compareDeep(productOrService, o.productOrService, true) 2074 && compareDeep(modifier, o.modifier, true) && compareDeep(provider, o.provider, true) 2075 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 2076 && compareDeep(facility, o.facility, true) && compareDeep(diagnosis, o.diagnosis, true) 2077 && compareDeep(detail, o.detail, true); 2078 } 2079 2080 @Override 2081 public boolean equalsShallow(Base other_) { 2082 if (!super.equalsShallow(other_)) 2083 return false; 2084 if (!(other_ instanceof DetailsComponent)) 2085 return false; 2086 DetailsComponent o = (DetailsComponent) other_; 2087 return compareValues(supportingInfoSequence, o.supportingInfoSequence, true); 2088 } 2089 2090 public boolean isEmpty() { 2091 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(supportingInfoSequence, category, productOrService, 2092 modifier, provider, quantity, unitPrice, facility, diagnosis, detail); 2093 } 2094 2095 public String fhirType() { 2096 return "CoverageEligibilityRequest.item"; 2097 2098 } 2099 2100 } 2101 2102 @Block() 2103 public static class DiagnosisComponent extends BackboneElement implements IBaseBackboneElement { 2104 /** 2105 * The nature of illness or problem in a coded form or as a reference to an 2106 * external defined Condition. 2107 */ 2108 @Child(name = "diagnosis", type = { CodeableConcept.class, 2109 Condition.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2110 @Description(shortDefinition = "Nature of illness or problem", formalDefinition = "The nature of illness or problem in a coded form or as a reference to an external defined Condition.") 2111 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/icd-10") 2112 protected Type diagnosis; 2113 2114 private static final long serialVersionUID = -454532709L; 2115 2116 /** 2117 * Constructor 2118 */ 2119 public DiagnosisComponent() { 2120 super(); 2121 } 2122 2123 /** 2124 * @return {@link #diagnosis} (The nature of illness or problem in a coded form 2125 * or as a reference to an external defined Condition.) 2126 */ 2127 public Type getDiagnosis() { 2128 return this.diagnosis; 2129 } 2130 2131 /** 2132 * @return {@link #diagnosis} (The nature of illness or problem in a coded form 2133 * or as a reference to an external defined Condition.) 2134 */ 2135 public CodeableConcept getDiagnosisCodeableConcept() throws FHIRException { 2136 if (this.diagnosis == null) 2137 this.diagnosis = new CodeableConcept(); 2138 if (!(this.diagnosis instanceof CodeableConcept)) 2139 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2140 + this.diagnosis.getClass().getName() + " was encountered"); 2141 return (CodeableConcept) this.diagnosis; 2142 } 2143 2144 public boolean hasDiagnosisCodeableConcept() { 2145 return this != null && this.diagnosis instanceof CodeableConcept; 2146 } 2147 2148 /** 2149 * @return {@link #diagnosis} (The nature of illness or problem in a coded form 2150 * or as a reference to an external defined Condition.) 2151 */ 2152 public Reference getDiagnosisReference() throws FHIRException { 2153 if (this.diagnosis == null) 2154 this.diagnosis = new Reference(); 2155 if (!(this.diagnosis instanceof Reference)) 2156 throw new FHIRException("Type mismatch: the type Reference was expected, but " 2157 + this.diagnosis.getClass().getName() + " was encountered"); 2158 return (Reference) this.diagnosis; 2159 } 2160 2161 public boolean hasDiagnosisReference() { 2162 return this != null && this.diagnosis instanceof Reference; 2163 } 2164 2165 public boolean hasDiagnosis() { 2166 return this.diagnosis != null && !this.diagnosis.isEmpty(); 2167 } 2168 2169 /** 2170 * @param value {@link #diagnosis} (The nature of illness or problem in a coded 2171 * form or as a reference to an external defined Condition.) 2172 */ 2173 public DiagnosisComponent setDiagnosis(Type value) { 2174 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2175 throw new Error( 2176 "Not the right type for CoverageEligibilityRequest.item.diagnosis.diagnosis[x]: " + value.fhirType()); 2177 this.diagnosis = value; 2178 return this; 2179 } 2180 2181 protected void listChildren(List<Property> children) { 2182 super.listChildren(children); 2183 children.add(new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", 2184 "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, 2185 diagnosis)); 2186 } 2187 2188 @Override 2189 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2190 switch (_hash) { 2191 case -1487009809: 2192 /* diagnosis[x] */ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", 2193 "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 2194 1, diagnosis); 2195 case 1196993265: 2196 /* diagnosis */ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", 2197 "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 2198 1, diagnosis); 2199 case 277781616: 2200 /* diagnosisCodeableConcept */ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", 2201 "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 2202 1, diagnosis); 2203 case 2050454362: 2204 /* diagnosisReference */ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", 2205 "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 2206 1, diagnosis); 2207 default: 2208 return super.getNamedProperty(_hash, _name, _checkValid); 2209 } 2210 2211 } 2212 2213 @Override 2214 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2215 switch (hash) { 2216 case 1196993265: 2217 /* diagnosis */ return this.diagnosis == null ? new Base[0] : new Base[] { this.diagnosis }; // Type 2218 default: 2219 return super.getProperty(hash, name, checkValid); 2220 } 2221 2222 } 2223 2224 @Override 2225 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2226 switch (hash) { 2227 case 1196993265: // diagnosis 2228 this.diagnosis = castToType(value); // Type 2229 return value; 2230 default: 2231 return super.setProperty(hash, name, value); 2232 } 2233 2234 } 2235 2236 @Override 2237 public Base setProperty(String name, Base value) throws FHIRException { 2238 if (name.equals("diagnosis[x]")) { 2239 this.diagnosis = castToType(value); // Type 2240 } else 2241 return super.setProperty(name, value); 2242 return value; 2243 } 2244 2245 @Override 2246 public void removeChild(String name, Base value) throws FHIRException { 2247 if (name.equals("diagnosis[x]")) { 2248 this.diagnosis = null; 2249 } else 2250 super.removeChild(name, value); 2251 2252 } 2253 2254 @Override 2255 public Base makeProperty(int hash, String name) throws FHIRException { 2256 switch (hash) { 2257 case -1487009809: 2258 return getDiagnosis(); 2259 case 1196993265: 2260 return getDiagnosis(); 2261 default: 2262 return super.makeProperty(hash, name); 2263 } 2264 2265 } 2266 2267 @Override 2268 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2269 switch (hash) { 2270 case 1196993265: 2271 /* diagnosis */ return new String[] { "CodeableConcept", "Reference" }; 2272 default: 2273 return super.getTypesForProperty(hash, name); 2274 } 2275 2276 } 2277 2278 @Override 2279 public Base addChild(String name) throws FHIRException { 2280 if (name.equals("diagnosisCodeableConcept")) { 2281 this.diagnosis = new CodeableConcept(); 2282 return this.diagnosis; 2283 } else if (name.equals("diagnosisReference")) { 2284 this.diagnosis = new Reference(); 2285 return this.diagnosis; 2286 } else 2287 return super.addChild(name); 2288 } 2289 2290 public DiagnosisComponent copy() { 2291 DiagnosisComponent dst = new DiagnosisComponent(); 2292 copyValues(dst); 2293 return dst; 2294 } 2295 2296 public void copyValues(DiagnosisComponent dst) { 2297 super.copyValues(dst); 2298 dst.diagnosis = diagnosis == null ? null : diagnosis.copy(); 2299 } 2300 2301 @Override 2302 public boolean equalsDeep(Base other_) { 2303 if (!super.equalsDeep(other_)) 2304 return false; 2305 if (!(other_ instanceof DiagnosisComponent)) 2306 return false; 2307 DiagnosisComponent o = (DiagnosisComponent) other_; 2308 return compareDeep(diagnosis, o.diagnosis, true); 2309 } 2310 2311 @Override 2312 public boolean equalsShallow(Base other_) { 2313 if (!super.equalsShallow(other_)) 2314 return false; 2315 if (!(other_ instanceof DiagnosisComponent)) 2316 return false; 2317 DiagnosisComponent o = (DiagnosisComponent) other_; 2318 return true; 2319 } 2320 2321 public boolean isEmpty() { 2322 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(diagnosis); 2323 } 2324 2325 public String fhirType() { 2326 return "CoverageEligibilityRequest.item.diagnosis"; 2327 2328 } 2329 2330 } 2331 2332 /** 2333 * A unique identifier assigned to this coverage eligiblity request. 2334 */ 2335 @Child(name = "identifier", type = { 2336 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2337 @Description(shortDefinition = "Business Identifier for coverage eligiblity request", formalDefinition = "A unique identifier assigned to this coverage eligiblity request.") 2338 protected List<Identifier> identifier; 2339 2340 /** 2341 * The status of the resource instance. 2342 */ 2343 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 2344 @Description(shortDefinition = "active | cancelled | draft | entered-in-error", formalDefinition = "The status of the resource instance.") 2345 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/fm-status") 2346 protected Enumeration<EligibilityRequestStatus> status; 2347 2348 /** 2349 * When the requestor expects the processor to complete processing. 2350 */ 2351 @Child(name = "priority", type = { 2352 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2353 @Description(shortDefinition = "Desired processing priority", formalDefinition = "When the requestor expects the processor to complete processing.") 2354 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/process-priority") 2355 protected CodeableConcept priority; 2356 2357 /** 2358 * Code to specify whether requesting: prior authorization requirements for some 2359 * service categories or billing codes; benefits for coverages specified or 2360 * discovered; discovery and return of coverages for the patient; and/or 2361 * validation that the specified coverage is in-force at the date/period 2362 * specified or 'now' if not specified. 2363 */ 2364 @Child(name = "purpose", type = { 2365 CodeType.class }, order = 3, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2366 @Description(shortDefinition = "auth-requirements | benefits | discovery | validation", formalDefinition = "Code to specify whether requesting: prior authorization requirements for some service categories or billing codes; benefits for coverages specified or discovered; discovery and return of coverages for the patient; and/or validation that the specified coverage is in-force at the date/period specified or 'now' if not specified.") 2367 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/eligibilityrequest-purpose") 2368 protected List<Enumeration<EligibilityRequestPurpose>> purpose; 2369 2370 /** 2371 * The party who is the beneficiary of the supplied coverage and for whom 2372 * eligibility is sought. 2373 */ 2374 @Child(name = "patient", type = { Patient.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 2375 @Description(shortDefinition = "Intended recipient of products and services", formalDefinition = "The party who is the beneficiary of the supplied coverage and for whom eligibility is sought.") 2376 protected Reference patient; 2377 2378 /** 2379 * The actual object that is the target of the reference (The party who is the 2380 * beneficiary of the supplied coverage and for whom eligibility is sought.) 2381 */ 2382 protected Patient patientTarget; 2383 2384 /** 2385 * The date or dates when the enclosed suite of services were performed or 2386 * completed. 2387 */ 2388 @Child(name = "serviced", type = { DateType.class, 2389 Period.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 2390 @Description(shortDefinition = "Estimated date or dates of service", formalDefinition = "The date or dates when the enclosed suite of services were performed or completed.") 2391 protected Type serviced; 2392 2393 /** 2394 * The date when this resource was created. 2395 */ 2396 @Child(name = "created", type = { DateTimeType.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 2397 @Description(shortDefinition = "Creation date", formalDefinition = "The date when this resource was created.") 2398 protected DateTimeType created; 2399 2400 /** 2401 * Person who created the request. 2402 */ 2403 @Child(name = "enterer", type = { Practitioner.class, 2404 PractitionerRole.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 2405 @Description(shortDefinition = "Author", formalDefinition = "Person who created the request.") 2406 protected Reference enterer; 2407 2408 /** 2409 * The actual object that is the target of the reference (Person who created the 2410 * request.) 2411 */ 2412 protected Resource entererTarget; 2413 2414 /** 2415 * The provider which is responsible for the request. 2416 */ 2417 @Child(name = "provider", type = { Practitioner.class, PractitionerRole.class, 2418 Organization.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 2419 @Description(shortDefinition = "Party responsible for the request", formalDefinition = "The provider which is responsible for the request.") 2420 protected Reference provider; 2421 2422 /** 2423 * The actual object that is the target of the reference (The provider which is 2424 * responsible for the request.) 2425 */ 2426 protected Resource providerTarget; 2427 2428 /** 2429 * The Insurer who issued the coverage in question and is the recipient of the 2430 * request. 2431 */ 2432 @Child(name = "insurer", type = { Organization.class }, order = 9, min = 1, max = 1, modifier = false, summary = true) 2433 @Description(shortDefinition = "Coverage issuer", formalDefinition = "The Insurer who issued the coverage in question and is the recipient of the request.") 2434 protected Reference insurer; 2435 2436 /** 2437 * The actual object that is the target of the reference (The Insurer who issued 2438 * the coverage in question and is the recipient of the request.) 2439 */ 2440 protected Organization insurerTarget; 2441 2442 /** 2443 * Facility where the services are intended to be provided. 2444 */ 2445 @Child(name = "facility", type = { Location.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 2446 @Description(shortDefinition = "Servicing facility", formalDefinition = "Facility where the services are intended to be provided.") 2447 protected Reference facility; 2448 2449 /** 2450 * The actual object that is the target of the reference (Facility where the 2451 * services are intended to be provided.) 2452 */ 2453 protected Location facilityTarget; 2454 2455 /** 2456 * Additional information codes regarding exceptions, special considerations, 2457 * the condition, situation, prior or concurrent issues. 2458 */ 2459 @Child(name = "supportingInfo", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2460 @Description(shortDefinition = "Supporting information", formalDefinition = "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.") 2461 protected List<SupportingInformationComponent> supportingInfo; 2462 2463 /** 2464 * Financial instruments for reimbursement for the health care products and 2465 * services. 2466 */ 2467 @Child(name = "insurance", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2468 @Description(shortDefinition = "Patient insurance information", formalDefinition = "Financial instruments for reimbursement for the health care products and services.") 2469 protected List<InsuranceComponent> insurance; 2470 2471 /** 2472 * Service categories or billable services for which benefit details and/or an 2473 * authorization prior to service delivery may be required by the payor. 2474 */ 2475 @Child(name = "item", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2476 @Description(shortDefinition = "Item to be evaluated for eligibiity", formalDefinition = "Service categories or billable services for which benefit details and/or an authorization prior to service delivery may be required by the payor.") 2477 protected List<DetailsComponent> item; 2478 2479 private static final long serialVersionUID = 1371127108L; 2480 2481 /** 2482 * Constructor 2483 */ 2484 public CoverageEligibilityRequest() { 2485 super(); 2486 } 2487 2488 /** 2489 * Constructor 2490 */ 2491 public CoverageEligibilityRequest(Enumeration<EligibilityRequestStatus> status, Reference patient, 2492 DateTimeType created, Reference insurer) { 2493 super(); 2494 this.status = status; 2495 this.patient = patient; 2496 this.created = created; 2497 this.insurer = insurer; 2498 } 2499 2500 /** 2501 * @return {@link #identifier} (A unique identifier assigned to this coverage 2502 * eligiblity request.) 2503 */ 2504 public List<Identifier> getIdentifier() { 2505 if (this.identifier == null) 2506 this.identifier = new ArrayList<Identifier>(); 2507 return this.identifier; 2508 } 2509 2510 /** 2511 * @return Returns a reference to <code>this</code> for easy method chaining 2512 */ 2513 public CoverageEligibilityRequest setIdentifier(List<Identifier> theIdentifier) { 2514 this.identifier = theIdentifier; 2515 return this; 2516 } 2517 2518 public boolean hasIdentifier() { 2519 if (this.identifier == null) 2520 return false; 2521 for (Identifier item : this.identifier) 2522 if (!item.isEmpty()) 2523 return true; 2524 return false; 2525 } 2526 2527 public Identifier addIdentifier() { // 3 2528 Identifier t = new Identifier(); 2529 if (this.identifier == null) 2530 this.identifier = new ArrayList<Identifier>(); 2531 this.identifier.add(t); 2532 return t; 2533 } 2534 2535 public CoverageEligibilityRequest addIdentifier(Identifier t) { // 3 2536 if (t == null) 2537 return this; 2538 if (this.identifier == null) 2539 this.identifier = new ArrayList<Identifier>(); 2540 this.identifier.add(t); 2541 return this; 2542 } 2543 2544 /** 2545 * @return The first repetition of repeating field {@link #identifier}, creating 2546 * it if it does not already exist 2547 */ 2548 public Identifier getIdentifierFirstRep() { 2549 if (getIdentifier().isEmpty()) { 2550 addIdentifier(); 2551 } 2552 return getIdentifier().get(0); 2553 } 2554 2555 /** 2556 * @return {@link #status} (The status of the resource instance.). This is the 2557 * underlying object with id, value and extensions. The accessor 2558 * "getStatus" gives direct access to the value 2559 */ 2560 public Enumeration<EligibilityRequestStatus> getStatusElement() { 2561 if (this.status == null) 2562 if (Configuration.errorOnAutoCreate()) 2563 throw new Error("Attempt to auto-create CoverageEligibilityRequest.status"); 2564 else if (Configuration.doAutoCreate()) 2565 this.status = new Enumeration<EligibilityRequestStatus>(new EligibilityRequestStatusEnumFactory()); // bb 2566 return this.status; 2567 } 2568 2569 public boolean hasStatusElement() { 2570 return this.status != null && !this.status.isEmpty(); 2571 } 2572 2573 public boolean hasStatus() { 2574 return this.status != null && !this.status.isEmpty(); 2575 } 2576 2577 /** 2578 * @param value {@link #status} (The status of the resource instance.). This is 2579 * the underlying object with id, value and extensions. The 2580 * accessor "getStatus" gives direct access to the value 2581 */ 2582 public CoverageEligibilityRequest setStatusElement(Enumeration<EligibilityRequestStatus> value) { 2583 this.status = value; 2584 return this; 2585 } 2586 2587 /** 2588 * @return The status of the resource instance. 2589 */ 2590 public EligibilityRequestStatus getStatus() { 2591 return this.status == null ? null : this.status.getValue(); 2592 } 2593 2594 /** 2595 * @param value The status of the resource instance. 2596 */ 2597 public CoverageEligibilityRequest setStatus(EligibilityRequestStatus value) { 2598 if (this.status == null) 2599 this.status = new Enumeration<EligibilityRequestStatus>(new EligibilityRequestStatusEnumFactory()); 2600 this.status.setValue(value); 2601 return this; 2602 } 2603 2604 /** 2605 * @return {@link #priority} (When the requestor expects the processor to 2606 * complete processing.) 2607 */ 2608 public CodeableConcept getPriority() { 2609 if (this.priority == null) 2610 if (Configuration.errorOnAutoCreate()) 2611 throw new Error("Attempt to auto-create CoverageEligibilityRequest.priority"); 2612 else if (Configuration.doAutoCreate()) 2613 this.priority = new CodeableConcept(); // cc 2614 return this.priority; 2615 } 2616 2617 public boolean hasPriority() { 2618 return this.priority != null && !this.priority.isEmpty(); 2619 } 2620 2621 /** 2622 * @param value {@link #priority} (When the requestor expects the processor to 2623 * complete processing.) 2624 */ 2625 public CoverageEligibilityRequest setPriority(CodeableConcept value) { 2626 this.priority = value; 2627 return this; 2628 } 2629 2630 /** 2631 * @return {@link #purpose} (Code to specify whether requesting: prior 2632 * authorization requirements for some service categories or billing 2633 * codes; benefits for coverages specified or discovered; discovery and 2634 * return of coverages for the patient; and/or validation that the 2635 * specified coverage is in-force at the date/period specified or 'now' 2636 * if not specified.) 2637 */ 2638 public List<Enumeration<EligibilityRequestPurpose>> getPurpose() { 2639 if (this.purpose == null) 2640 this.purpose = new ArrayList<Enumeration<EligibilityRequestPurpose>>(); 2641 return this.purpose; 2642 } 2643 2644 /** 2645 * @return Returns a reference to <code>this</code> for easy method chaining 2646 */ 2647 public CoverageEligibilityRequest setPurpose(List<Enumeration<EligibilityRequestPurpose>> thePurpose) { 2648 this.purpose = thePurpose; 2649 return this; 2650 } 2651 2652 public boolean hasPurpose() { 2653 if (this.purpose == null) 2654 return false; 2655 for (Enumeration<EligibilityRequestPurpose> item : this.purpose) 2656 if (!item.isEmpty()) 2657 return true; 2658 return false; 2659 } 2660 2661 /** 2662 * @return {@link #purpose} (Code to specify whether requesting: prior 2663 * authorization requirements for some service categories or billing 2664 * codes; benefits for coverages specified or discovered; discovery and 2665 * return of coverages for the patient; and/or validation that the 2666 * specified coverage is in-force at the date/period specified or 'now' 2667 * if not specified.) 2668 */ 2669 public Enumeration<EligibilityRequestPurpose> addPurposeElement() {// 2 2670 Enumeration<EligibilityRequestPurpose> t = new Enumeration<EligibilityRequestPurpose>( 2671 new EligibilityRequestPurposeEnumFactory()); 2672 if (this.purpose == null) 2673 this.purpose = new ArrayList<Enumeration<EligibilityRequestPurpose>>(); 2674 this.purpose.add(t); 2675 return t; 2676 } 2677 2678 /** 2679 * @param value {@link #purpose} (Code to specify whether requesting: prior 2680 * authorization requirements for some service categories or 2681 * billing codes; benefits for coverages specified or discovered; 2682 * discovery and return of coverages for the patient; and/or 2683 * validation that the specified coverage is in-force at the 2684 * date/period specified or 'now' if not specified.) 2685 */ 2686 public CoverageEligibilityRequest addPurpose(EligibilityRequestPurpose value) { // 1 2687 Enumeration<EligibilityRequestPurpose> t = new Enumeration<EligibilityRequestPurpose>( 2688 new EligibilityRequestPurposeEnumFactory()); 2689 t.setValue(value); 2690 if (this.purpose == null) 2691 this.purpose = new ArrayList<Enumeration<EligibilityRequestPurpose>>(); 2692 this.purpose.add(t); 2693 return this; 2694 } 2695 2696 /** 2697 * @param value {@link #purpose} (Code to specify whether requesting: prior 2698 * authorization requirements for some service categories or 2699 * billing codes; benefits for coverages specified or discovered; 2700 * discovery and return of coverages for the patient; and/or 2701 * validation that the specified coverage is in-force at the 2702 * date/period specified or 'now' if not specified.) 2703 */ 2704 public boolean hasPurpose(EligibilityRequestPurpose value) { 2705 if (this.purpose == null) 2706 return false; 2707 for (Enumeration<EligibilityRequestPurpose> v : this.purpose) 2708 if (v.getValue().equals(value)) // code 2709 return true; 2710 return false; 2711 } 2712 2713 /** 2714 * @return {@link #patient} (The party who is the beneficiary of the supplied 2715 * coverage and for whom eligibility is sought.) 2716 */ 2717 public Reference getPatient() { 2718 if (this.patient == null) 2719 if (Configuration.errorOnAutoCreate()) 2720 throw new Error("Attempt to auto-create CoverageEligibilityRequest.patient"); 2721 else if (Configuration.doAutoCreate()) 2722 this.patient = new Reference(); // cc 2723 return this.patient; 2724 } 2725 2726 public boolean hasPatient() { 2727 return this.patient != null && !this.patient.isEmpty(); 2728 } 2729 2730 /** 2731 * @param value {@link #patient} (The party who is the beneficiary of the 2732 * supplied coverage and for whom eligibility is sought.) 2733 */ 2734 public CoverageEligibilityRequest setPatient(Reference value) { 2735 this.patient = value; 2736 return this; 2737 } 2738 2739 /** 2740 * @return {@link #patient} The actual object that is the target of the 2741 * reference. The reference library doesn't populate this, but you can 2742 * use it to hold the resource if you resolve it. (The party who is the 2743 * beneficiary of the supplied coverage and for whom eligibility is 2744 * sought.) 2745 */ 2746 public Patient getPatientTarget() { 2747 if (this.patientTarget == null) 2748 if (Configuration.errorOnAutoCreate()) 2749 throw new Error("Attempt to auto-create CoverageEligibilityRequest.patient"); 2750 else if (Configuration.doAutoCreate()) 2751 this.patientTarget = new Patient(); // aa 2752 return this.patientTarget; 2753 } 2754 2755 /** 2756 * @param value {@link #patient} The actual object that is the target of the 2757 * reference. The reference library doesn't use these, but you can 2758 * use it to hold the resource if you resolve it. (The party who is 2759 * the beneficiary of the supplied coverage and for whom 2760 * eligibility is sought.) 2761 */ 2762 public CoverageEligibilityRequest setPatientTarget(Patient value) { 2763 this.patientTarget = value; 2764 return this; 2765 } 2766 2767 /** 2768 * @return {@link #serviced} (The date or dates when the enclosed suite of 2769 * services were performed or completed.) 2770 */ 2771 public Type getServiced() { 2772 return this.serviced; 2773 } 2774 2775 /** 2776 * @return {@link #serviced} (The date or dates when the enclosed suite of 2777 * services were performed or completed.) 2778 */ 2779 public DateType getServicedDateType() throws FHIRException { 2780 if (this.serviced == null) 2781 this.serviced = new DateType(); 2782 if (!(this.serviced instanceof DateType)) 2783 throw new FHIRException("Type mismatch: the type DateType was expected, but " + this.serviced.getClass().getName() 2784 + " was encountered"); 2785 return (DateType) this.serviced; 2786 } 2787 2788 public boolean hasServicedDateType() { 2789 return this != null && this.serviced instanceof DateType; 2790 } 2791 2792 /** 2793 * @return {@link #serviced} (The date or dates when the enclosed suite of 2794 * services were performed or completed.) 2795 */ 2796 public Period getServicedPeriod() throws FHIRException { 2797 if (this.serviced == null) 2798 this.serviced = new Period(); 2799 if (!(this.serviced instanceof Period)) 2800 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.serviced.getClass().getName() 2801 + " was encountered"); 2802 return (Period) this.serviced; 2803 } 2804 2805 public boolean hasServicedPeriod() { 2806 return this != null && this.serviced instanceof Period; 2807 } 2808 2809 public boolean hasServiced() { 2810 return this.serviced != null && !this.serviced.isEmpty(); 2811 } 2812 2813 /** 2814 * @param value {@link #serviced} (The date or dates when the enclosed suite of 2815 * services were performed or completed.) 2816 */ 2817 public CoverageEligibilityRequest setServiced(Type value) { 2818 if (value != null && !(value instanceof DateType || value instanceof Period)) 2819 throw new Error("Not the right type for CoverageEligibilityRequest.serviced[x]: " + value.fhirType()); 2820 this.serviced = value; 2821 return this; 2822 } 2823 2824 /** 2825 * @return {@link #created} (The date when this resource was created.). This is 2826 * the underlying object with id, value and extensions. The accessor 2827 * "getCreated" gives direct access to the value 2828 */ 2829 public DateTimeType getCreatedElement() { 2830 if (this.created == null) 2831 if (Configuration.errorOnAutoCreate()) 2832 throw new Error("Attempt to auto-create CoverageEligibilityRequest.created"); 2833 else if (Configuration.doAutoCreate()) 2834 this.created = new DateTimeType(); // bb 2835 return this.created; 2836 } 2837 2838 public boolean hasCreatedElement() { 2839 return this.created != null && !this.created.isEmpty(); 2840 } 2841 2842 public boolean hasCreated() { 2843 return this.created != null && !this.created.isEmpty(); 2844 } 2845 2846 /** 2847 * @param value {@link #created} (The date when this resource was created.). 2848 * This is the underlying object with id, value and extensions. The 2849 * accessor "getCreated" gives direct access to the value 2850 */ 2851 public CoverageEligibilityRequest setCreatedElement(DateTimeType value) { 2852 this.created = value; 2853 return this; 2854 } 2855 2856 /** 2857 * @return The date when this resource was created. 2858 */ 2859 public Date getCreated() { 2860 return this.created == null ? null : this.created.getValue(); 2861 } 2862 2863 /** 2864 * @param value The date when this resource was created. 2865 */ 2866 public CoverageEligibilityRequest setCreated(Date value) { 2867 if (this.created == null) 2868 this.created = new DateTimeType(); 2869 this.created.setValue(value); 2870 return this; 2871 } 2872 2873 /** 2874 * @return {@link #enterer} (Person who created the request.) 2875 */ 2876 public Reference getEnterer() { 2877 if (this.enterer == null) 2878 if (Configuration.errorOnAutoCreate()) 2879 throw new Error("Attempt to auto-create CoverageEligibilityRequest.enterer"); 2880 else if (Configuration.doAutoCreate()) 2881 this.enterer = new Reference(); // cc 2882 return this.enterer; 2883 } 2884 2885 public boolean hasEnterer() { 2886 return this.enterer != null && !this.enterer.isEmpty(); 2887 } 2888 2889 /** 2890 * @param value {@link #enterer} (Person who created the request.) 2891 */ 2892 public CoverageEligibilityRequest setEnterer(Reference value) { 2893 this.enterer = value; 2894 return this; 2895 } 2896 2897 /** 2898 * @return {@link #enterer} The actual object that is the target of the 2899 * reference. The reference library doesn't populate this, but you can 2900 * use it to hold the resource if you resolve it. (Person who created 2901 * the request.) 2902 */ 2903 public Resource getEntererTarget() { 2904 return this.entererTarget; 2905 } 2906 2907 /** 2908 * @param value {@link #enterer} The actual object that is the target of the 2909 * reference. The reference library doesn't use these, but you can 2910 * use it to hold the resource if you resolve it. (Person who 2911 * created the request.) 2912 */ 2913 public CoverageEligibilityRequest setEntererTarget(Resource value) { 2914 this.entererTarget = value; 2915 return this; 2916 } 2917 2918 /** 2919 * @return {@link #provider} (The provider which is responsible for the 2920 * request.) 2921 */ 2922 public Reference getProvider() { 2923 if (this.provider == null) 2924 if (Configuration.errorOnAutoCreate()) 2925 throw new Error("Attempt to auto-create CoverageEligibilityRequest.provider"); 2926 else if (Configuration.doAutoCreate()) 2927 this.provider = new Reference(); // cc 2928 return this.provider; 2929 } 2930 2931 public boolean hasProvider() { 2932 return this.provider != null && !this.provider.isEmpty(); 2933 } 2934 2935 /** 2936 * @param value {@link #provider} (The provider which is responsible for the 2937 * request.) 2938 */ 2939 public CoverageEligibilityRequest setProvider(Reference value) { 2940 this.provider = value; 2941 return this; 2942 } 2943 2944 /** 2945 * @return {@link #provider} The actual object that is the target of the 2946 * reference. The reference library doesn't populate this, but you can 2947 * use it to hold the resource if you resolve it. (The provider which is 2948 * responsible for the request.) 2949 */ 2950 public Resource getProviderTarget() { 2951 return this.providerTarget; 2952 } 2953 2954 /** 2955 * @param value {@link #provider} The actual object that is the target of the 2956 * reference. The reference library doesn't use these, but you can 2957 * use it to hold the resource if you resolve it. (The provider 2958 * which is responsible for the request.) 2959 */ 2960 public CoverageEligibilityRequest setProviderTarget(Resource value) { 2961 this.providerTarget = value; 2962 return this; 2963 } 2964 2965 /** 2966 * @return {@link #insurer} (The Insurer who issued the coverage in question and 2967 * is the recipient of the request.) 2968 */ 2969 public Reference getInsurer() { 2970 if (this.insurer == null) 2971 if (Configuration.errorOnAutoCreate()) 2972 throw new Error("Attempt to auto-create CoverageEligibilityRequest.insurer"); 2973 else if (Configuration.doAutoCreate()) 2974 this.insurer = new Reference(); // cc 2975 return this.insurer; 2976 } 2977 2978 public boolean hasInsurer() { 2979 return this.insurer != null && !this.insurer.isEmpty(); 2980 } 2981 2982 /** 2983 * @param value {@link #insurer} (The Insurer who issued the coverage in 2984 * question and is the recipient of the request.) 2985 */ 2986 public CoverageEligibilityRequest setInsurer(Reference value) { 2987 this.insurer = value; 2988 return this; 2989 } 2990 2991 /** 2992 * @return {@link #insurer} The actual object that is the target of the 2993 * reference. The reference library doesn't populate this, but you can 2994 * use it to hold the resource if you resolve it. (The Insurer who 2995 * issued the coverage in question and is the recipient of the request.) 2996 */ 2997 public Organization getInsurerTarget() { 2998 if (this.insurerTarget == null) 2999 if (Configuration.errorOnAutoCreate()) 3000 throw new Error("Attempt to auto-create CoverageEligibilityRequest.insurer"); 3001 else if (Configuration.doAutoCreate()) 3002 this.insurerTarget = new Organization(); // aa 3003 return this.insurerTarget; 3004 } 3005 3006 /** 3007 * @param value {@link #insurer} The actual object that is the target of the 3008 * reference. The reference library doesn't use these, but you can 3009 * use it to hold the resource if you resolve it. (The Insurer who 3010 * issued the coverage in question and is the recipient of the 3011 * request.) 3012 */ 3013 public CoverageEligibilityRequest setInsurerTarget(Organization value) { 3014 this.insurerTarget = value; 3015 return this; 3016 } 3017 3018 /** 3019 * @return {@link #facility} (Facility where the services are intended to be 3020 * provided.) 3021 */ 3022 public Reference getFacility() { 3023 if (this.facility == null) 3024 if (Configuration.errorOnAutoCreate()) 3025 throw new Error("Attempt to auto-create CoverageEligibilityRequest.facility"); 3026 else if (Configuration.doAutoCreate()) 3027 this.facility = new Reference(); // cc 3028 return this.facility; 3029 } 3030 3031 public boolean hasFacility() { 3032 return this.facility != null && !this.facility.isEmpty(); 3033 } 3034 3035 /** 3036 * @param value {@link #facility} (Facility where the services are intended to 3037 * be provided.) 3038 */ 3039 public CoverageEligibilityRequest setFacility(Reference value) { 3040 this.facility = value; 3041 return this; 3042 } 3043 3044 /** 3045 * @return {@link #facility} The actual object that is the target of the 3046 * reference. The reference library doesn't populate this, but you can 3047 * use it to hold the resource if you resolve it. (Facility where the 3048 * services are intended to be provided.) 3049 */ 3050 public Location getFacilityTarget() { 3051 if (this.facilityTarget == null) 3052 if (Configuration.errorOnAutoCreate()) 3053 throw new Error("Attempt to auto-create CoverageEligibilityRequest.facility"); 3054 else if (Configuration.doAutoCreate()) 3055 this.facilityTarget = new Location(); // aa 3056 return this.facilityTarget; 3057 } 3058 3059 /** 3060 * @param value {@link #facility} The actual object that is the target of the 3061 * reference. The reference library doesn't use these, but you can 3062 * use it to hold the resource if you resolve it. (Facility where 3063 * the services are intended to be provided.) 3064 */ 3065 public CoverageEligibilityRequest setFacilityTarget(Location value) { 3066 this.facilityTarget = value; 3067 return this; 3068 } 3069 3070 /** 3071 * @return {@link #supportingInfo} (Additional information codes regarding 3072 * exceptions, special considerations, the condition, situation, prior 3073 * or concurrent issues.) 3074 */ 3075 public List<SupportingInformationComponent> getSupportingInfo() { 3076 if (this.supportingInfo == null) 3077 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 3078 return this.supportingInfo; 3079 } 3080 3081 /** 3082 * @return Returns a reference to <code>this</code> for easy method chaining 3083 */ 3084 public CoverageEligibilityRequest setSupportingInfo(List<SupportingInformationComponent> theSupportingInfo) { 3085 this.supportingInfo = theSupportingInfo; 3086 return this; 3087 } 3088 3089 public boolean hasSupportingInfo() { 3090 if (this.supportingInfo == null) 3091 return false; 3092 for (SupportingInformationComponent item : this.supportingInfo) 3093 if (!item.isEmpty()) 3094 return true; 3095 return false; 3096 } 3097 3098 public SupportingInformationComponent addSupportingInfo() { // 3 3099 SupportingInformationComponent t = new SupportingInformationComponent(); 3100 if (this.supportingInfo == null) 3101 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 3102 this.supportingInfo.add(t); 3103 return t; 3104 } 3105 3106 public CoverageEligibilityRequest addSupportingInfo(SupportingInformationComponent t) { // 3 3107 if (t == null) 3108 return this; 3109 if (this.supportingInfo == null) 3110 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 3111 this.supportingInfo.add(t); 3112 return this; 3113 } 3114 3115 /** 3116 * @return The first repetition of repeating field {@link #supportingInfo}, 3117 * creating it if it does not already exist 3118 */ 3119 public SupportingInformationComponent getSupportingInfoFirstRep() { 3120 if (getSupportingInfo().isEmpty()) { 3121 addSupportingInfo(); 3122 } 3123 return getSupportingInfo().get(0); 3124 } 3125 3126 /** 3127 * @return {@link #insurance} (Financial instruments for reimbursement for the 3128 * health care products and services.) 3129 */ 3130 public List<InsuranceComponent> getInsurance() { 3131 if (this.insurance == null) 3132 this.insurance = new ArrayList<InsuranceComponent>(); 3133 return this.insurance; 3134 } 3135 3136 /** 3137 * @return Returns a reference to <code>this</code> for easy method chaining 3138 */ 3139 public CoverageEligibilityRequest setInsurance(List<InsuranceComponent> theInsurance) { 3140 this.insurance = theInsurance; 3141 return this; 3142 } 3143 3144 public boolean hasInsurance() { 3145 if (this.insurance == null) 3146 return false; 3147 for (InsuranceComponent item : this.insurance) 3148 if (!item.isEmpty()) 3149 return true; 3150 return false; 3151 } 3152 3153 public InsuranceComponent addInsurance() { // 3 3154 InsuranceComponent t = new InsuranceComponent(); 3155 if (this.insurance == null) 3156 this.insurance = new ArrayList<InsuranceComponent>(); 3157 this.insurance.add(t); 3158 return t; 3159 } 3160 3161 public CoverageEligibilityRequest addInsurance(InsuranceComponent t) { // 3 3162 if (t == null) 3163 return this; 3164 if (this.insurance == null) 3165 this.insurance = new ArrayList<InsuranceComponent>(); 3166 this.insurance.add(t); 3167 return this; 3168 } 3169 3170 /** 3171 * @return The first repetition of repeating field {@link #insurance}, creating 3172 * it if it does not already exist 3173 */ 3174 public InsuranceComponent getInsuranceFirstRep() { 3175 if (getInsurance().isEmpty()) { 3176 addInsurance(); 3177 } 3178 return getInsurance().get(0); 3179 } 3180 3181 /** 3182 * @return {@link #item} (Service categories or billable services for which 3183 * benefit details and/or an authorization prior to service delivery may 3184 * be required by the payor.) 3185 */ 3186 public List<DetailsComponent> getItem() { 3187 if (this.item == null) 3188 this.item = new ArrayList<DetailsComponent>(); 3189 return this.item; 3190 } 3191 3192 /** 3193 * @return Returns a reference to <code>this</code> for easy method chaining 3194 */ 3195 public CoverageEligibilityRequest setItem(List<DetailsComponent> theItem) { 3196 this.item = theItem; 3197 return this; 3198 } 3199 3200 public boolean hasItem() { 3201 if (this.item == null) 3202 return false; 3203 for (DetailsComponent item : this.item) 3204 if (!item.isEmpty()) 3205 return true; 3206 return false; 3207 } 3208 3209 public DetailsComponent addItem() { // 3 3210 DetailsComponent t = new DetailsComponent(); 3211 if (this.item == null) 3212 this.item = new ArrayList<DetailsComponent>(); 3213 this.item.add(t); 3214 return t; 3215 } 3216 3217 public CoverageEligibilityRequest addItem(DetailsComponent t) { // 3 3218 if (t == null) 3219 return this; 3220 if (this.item == null) 3221 this.item = new ArrayList<DetailsComponent>(); 3222 this.item.add(t); 3223 return this; 3224 } 3225 3226 /** 3227 * @return The first repetition of repeating field {@link #item}, creating it if 3228 * it does not already exist 3229 */ 3230 public DetailsComponent getItemFirstRep() { 3231 if (getItem().isEmpty()) { 3232 addItem(); 3233 } 3234 return getItem().get(0); 3235 } 3236 3237 protected void listChildren(List<Property> children) { 3238 super.listChildren(children); 3239 children.add( 3240 new Property("identifier", "Identifier", "A unique identifier assigned to this coverage eligiblity request.", 0, 3241 java.lang.Integer.MAX_VALUE, identifier)); 3242 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 3243 children.add(new Property("priority", "CodeableConcept", 3244 "When the requestor expects the processor to complete processing.", 0, 1, priority)); 3245 children.add(new Property("purpose", "code", 3246 "Code to specify whether requesting: prior authorization requirements for some service categories or billing codes; benefits for coverages specified or discovered; discovery and return of coverages for the patient; and/or validation that the specified coverage is in-force at the date/period specified or 'now' if not specified.", 3247 0, java.lang.Integer.MAX_VALUE, purpose)); 3248 children.add(new Property("patient", "Reference(Patient)", 3249 "The party who is the beneficiary of the supplied coverage and for whom eligibility is sought.", 0, 1, 3250 patient)); 3251 children.add(new Property("serviced[x]", "date|Period", 3252 "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced)); 3253 children.add(new Property("created", "dateTime", "The date when this resource was created.", 0, 1, created)); 3254 children.add(new Property("enterer", "Reference(Practitioner|PractitionerRole)", "Person who created the request.", 3255 0, 1, enterer)); 3256 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", 3257 "The provider which is responsible for the request.", 0, 1, provider)); 3258 children.add(new Property("insurer", "Reference(Organization)", 3259 "The Insurer who issued the coverage in question and is the recipient of the request.", 0, 1, insurer)); 3260 children.add(new Property("facility", "Reference(Location)", 3261 "Facility where the services are intended to be provided.", 0, 1, facility)); 3262 children.add(new Property("supportingInfo", "", 3263 "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.", 3264 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 3265 children.add(new Property("insurance", "", 3266 "Financial instruments for reimbursement for the health care products and services.", 0, 3267 java.lang.Integer.MAX_VALUE, insurance)); 3268 children.add(new Property("item", "", 3269 "Service categories or billable services for which benefit details and/or an authorization prior to service delivery may be required by the payor.", 3270 0, java.lang.Integer.MAX_VALUE, item)); 3271 } 3272 3273 @Override 3274 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3275 switch (_hash) { 3276 case -1618432855: 3277 /* identifier */ return new Property("identifier", "Identifier", 3278 "A unique identifier assigned to this coverage eligiblity request.", 0, java.lang.Integer.MAX_VALUE, 3279 identifier); 3280 case -892481550: 3281 /* status */ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 3282 case -1165461084: 3283 /* priority */ return new Property("priority", "CodeableConcept", 3284 "When the requestor expects the processor to complete processing.", 0, 1, priority); 3285 case -220463842: 3286 /* purpose */ return new Property("purpose", "code", 3287 "Code to specify whether requesting: prior authorization requirements for some service categories or billing codes; benefits for coverages specified or discovered; discovery and return of coverages for the patient; and/or validation that the specified coverage is in-force at the date/period specified or 'now' if not specified.", 3288 0, java.lang.Integer.MAX_VALUE, purpose); 3289 case -791418107: 3290 /* patient */ return new Property("patient", "Reference(Patient)", 3291 "The party who is the beneficiary of the supplied coverage and for whom eligibility is sought.", 0, 1, 3292 patient); 3293 case -1927922223: 3294 /* serviced[x] */ return new Property("serviced[x]", "date|Period", 3295 "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 3296 case 1379209295: 3297 /* serviced */ return new Property("serviced[x]", "date|Period", 3298 "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 3299 case 363246749: 3300 /* servicedDate */ return new Property("serviced[x]", "date|Period", 3301 "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 3302 case 1534966512: 3303 /* servicedPeriod */ return new Property("serviced[x]", "date|Period", 3304 "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 3305 case 1028554472: 3306 /* created */ return new Property("created", "dateTime", "The date when this resource was created.", 0, 1, 3307 created); 3308 case -1591951995: 3309 /* enterer */ return new Property("enterer", "Reference(Practitioner|PractitionerRole)", 3310 "Person who created the request.", 0, 1, enterer); 3311 case -987494927: 3312 /* provider */ return new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", 3313 "The provider which is responsible for the request.", 0, 1, provider); 3314 case 1957615864: 3315 /* insurer */ return new Property("insurer", "Reference(Organization)", 3316 "The Insurer who issued the coverage in question and is the recipient of the request.", 0, 1, insurer); 3317 case 501116579: 3318 /* facility */ return new Property("facility", "Reference(Location)", 3319 "Facility where the services are intended to be provided.", 0, 1, facility); 3320 case 1922406657: 3321 /* supportingInfo */ return new Property("supportingInfo", "", 3322 "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.", 3323 0, java.lang.Integer.MAX_VALUE, supportingInfo); 3324 case 73049818: 3325 /* insurance */ return new Property("insurance", "", 3326 "Financial instruments for reimbursement for the health care products and services.", 0, 3327 java.lang.Integer.MAX_VALUE, insurance); 3328 case 3242771: 3329 /* item */ return new Property("item", "", 3330 "Service categories or billable services for which benefit details and/or an authorization prior to service delivery may be required by the payor.", 3331 0, java.lang.Integer.MAX_VALUE, item); 3332 default: 3333 return super.getNamedProperty(_hash, _name, _checkValid); 3334 } 3335 3336 } 3337 3338 @Override 3339 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3340 switch (hash) { 3341 case -1618432855: 3342 /* identifier */ return this.identifier == null ? new Base[0] 3343 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3344 case -892481550: 3345 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<EligibilityRequestStatus> 3346 case -1165461084: 3347 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // CodeableConcept 3348 case -220463842: 3349 /* purpose */ return this.purpose == null ? new Base[0] : this.purpose.toArray(new Base[this.purpose.size()]); // Enumeration<EligibilityRequestPurpose> 3350 case -791418107: 3351 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 3352 case 1379209295: 3353 /* serviced */ return this.serviced == null ? new Base[0] : new Base[] { this.serviced }; // Type 3354 case 1028554472: 3355 /* created */ return this.created == null ? new Base[0] : new Base[] { this.created }; // DateTimeType 3356 case -1591951995: 3357 /* enterer */ return this.enterer == null ? new Base[0] : new Base[] { this.enterer }; // Reference 3358 case -987494927: 3359 /* provider */ return this.provider == null ? new Base[0] : new Base[] { this.provider }; // Reference 3360 case 1957615864: 3361 /* insurer */ return this.insurer == null ? new Base[0] : new Base[] { this.insurer }; // Reference 3362 case 501116579: 3363 /* facility */ return this.facility == null ? new Base[0] : new Base[] { this.facility }; // Reference 3364 case 1922406657: 3365 /* supportingInfo */ return this.supportingInfo == null ? new Base[0] 3366 : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // SupportingInformationComponent 3367 case 73049818: 3368 /* insurance */ return this.insurance == null ? new Base[0] 3369 : this.insurance.toArray(new Base[this.insurance.size()]); // InsuranceComponent 3370 case 3242771: 3371 /* item */ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // DetailsComponent 3372 default: 3373 return super.getProperty(hash, name, checkValid); 3374 } 3375 3376 } 3377 3378 @Override 3379 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3380 switch (hash) { 3381 case -1618432855: // identifier 3382 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3383 return value; 3384 case -892481550: // status 3385 value = new EligibilityRequestStatusEnumFactory().fromType(castToCode(value)); 3386 this.status = (Enumeration) value; // Enumeration<EligibilityRequestStatus> 3387 return value; 3388 case -1165461084: // priority 3389 this.priority = castToCodeableConcept(value); // CodeableConcept 3390 return value; 3391 case -220463842: // purpose 3392 value = new EligibilityRequestPurposeEnumFactory().fromType(castToCode(value)); 3393 this.getPurpose().add((Enumeration) value); // Enumeration<EligibilityRequestPurpose> 3394 return value; 3395 case -791418107: // patient 3396 this.patient = castToReference(value); // Reference 3397 return value; 3398 case 1379209295: // serviced 3399 this.serviced = castToType(value); // Type 3400 return value; 3401 case 1028554472: // created 3402 this.created = castToDateTime(value); // DateTimeType 3403 return value; 3404 case -1591951995: // enterer 3405 this.enterer = castToReference(value); // Reference 3406 return value; 3407 case -987494927: // provider 3408 this.provider = castToReference(value); // Reference 3409 return value; 3410 case 1957615864: // insurer 3411 this.insurer = castToReference(value); // Reference 3412 return value; 3413 case 501116579: // facility 3414 this.facility = castToReference(value); // Reference 3415 return value; 3416 case 1922406657: // supportingInfo 3417 this.getSupportingInfo().add((SupportingInformationComponent) value); // SupportingInformationComponent 3418 return value; 3419 case 73049818: // insurance 3420 this.getInsurance().add((InsuranceComponent) value); // InsuranceComponent 3421 return value; 3422 case 3242771: // item 3423 this.getItem().add((DetailsComponent) value); // DetailsComponent 3424 return value; 3425 default: 3426 return super.setProperty(hash, name, value); 3427 } 3428 3429 } 3430 3431 @Override 3432 public Base setProperty(String name, Base value) throws FHIRException { 3433 if (name.equals("identifier")) { 3434 this.getIdentifier().add(castToIdentifier(value)); 3435 } else if (name.equals("status")) { 3436 value = new EligibilityRequestStatusEnumFactory().fromType(castToCode(value)); 3437 this.status = (Enumeration) value; // Enumeration<EligibilityRequestStatus> 3438 } else if (name.equals("priority")) { 3439 this.priority = castToCodeableConcept(value); // CodeableConcept 3440 } else if (name.equals("purpose")) { 3441 value = new EligibilityRequestPurposeEnumFactory().fromType(castToCode(value)); 3442 this.getPurpose().add((Enumeration) value); 3443 } else if (name.equals("patient")) { 3444 this.patient = castToReference(value); // Reference 3445 } else if (name.equals("serviced[x]")) { 3446 this.serviced = castToType(value); // Type 3447 } else if (name.equals("created")) { 3448 this.created = castToDateTime(value); // DateTimeType 3449 } else if (name.equals("enterer")) { 3450 this.enterer = castToReference(value); // Reference 3451 } else if (name.equals("provider")) { 3452 this.provider = castToReference(value); // Reference 3453 } else if (name.equals("insurer")) { 3454 this.insurer = castToReference(value); // Reference 3455 } else if (name.equals("facility")) { 3456 this.facility = castToReference(value); // Reference 3457 } else if (name.equals("supportingInfo")) { 3458 this.getSupportingInfo().add((SupportingInformationComponent) value); 3459 } else if (name.equals("insurance")) { 3460 this.getInsurance().add((InsuranceComponent) value); 3461 } else if (name.equals("item")) { 3462 this.getItem().add((DetailsComponent) value); 3463 } else 3464 return super.setProperty(name, value); 3465 return value; 3466 } 3467 3468 @Override 3469 public void removeChild(String name, Base value) throws FHIRException { 3470 if (name.equals("identifier")) { 3471 this.getIdentifier().remove(castToIdentifier(value)); 3472 } else if (name.equals("status")) { 3473 this.status = null; 3474 } else if (name.equals("priority")) { 3475 this.priority = null; 3476 } else if (name.equals("purpose")) { 3477 this.getPurpose().remove((Enumeration) value); 3478 } else if (name.equals("patient")) { 3479 this.patient = null; 3480 } else if (name.equals("serviced[x]")) { 3481 this.serviced = null; 3482 } else if (name.equals("created")) { 3483 this.created = null; 3484 } else if (name.equals("enterer")) { 3485 this.enterer = null; 3486 } else if (name.equals("provider")) { 3487 this.provider = null; 3488 } else if (name.equals("insurer")) { 3489 this.insurer = null; 3490 } else if (name.equals("facility")) { 3491 this.facility = null; 3492 } else if (name.equals("supportingInfo")) { 3493 this.getSupportingInfo().remove((SupportingInformationComponent) value); 3494 } else if (name.equals("insurance")) { 3495 this.getInsurance().remove((InsuranceComponent) value); 3496 } else if (name.equals("item")) { 3497 this.getItem().remove((DetailsComponent) value); 3498 } else 3499 super.removeChild(name, value); 3500 3501 } 3502 3503 @Override 3504 public Base makeProperty(int hash, String name) throws FHIRException { 3505 switch (hash) { 3506 case -1618432855: 3507 return addIdentifier(); 3508 case -892481550: 3509 return getStatusElement(); 3510 case -1165461084: 3511 return getPriority(); 3512 case -220463842: 3513 return addPurposeElement(); 3514 case -791418107: 3515 return getPatient(); 3516 case -1927922223: 3517 return getServiced(); 3518 case 1379209295: 3519 return getServiced(); 3520 case 1028554472: 3521 return getCreatedElement(); 3522 case -1591951995: 3523 return getEnterer(); 3524 case -987494927: 3525 return getProvider(); 3526 case 1957615864: 3527 return getInsurer(); 3528 case 501116579: 3529 return getFacility(); 3530 case 1922406657: 3531 return addSupportingInfo(); 3532 case 73049818: 3533 return addInsurance(); 3534 case 3242771: 3535 return addItem(); 3536 default: 3537 return super.makeProperty(hash, name); 3538 } 3539 3540 } 3541 3542 @Override 3543 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3544 switch (hash) { 3545 case -1618432855: 3546 /* identifier */ return new String[] { "Identifier" }; 3547 case -892481550: 3548 /* status */ return new String[] { "code" }; 3549 case -1165461084: 3550 /* priority */ return new String[] { "CodeableConcept" }; 3551 case -220463842: 3552 /* purpose */ return new String[] { "code" }; 3553 case -791418107: 3554 /* patient */ return new String[] { "Reference" }; 3555 case 1379209295: 3556 /* serviced */ return new String[] { "date", "Period" }; 3557 case 1028554472: 3558 /* created */ return new String[] { "dateTime" }; 3559 case -1591951995: 3560 /* enterer */ return new String[] { "Reference" }; 3561 case -987494927: 3562 /* provider */ return new String[] { "Reference" }; 3563 case 1957615864: 3564 /* insurer */ return new String[] { "Reference" }; 3565 case 501116579: 3566 /* facility */ return new String[] { "Reference" }; 3567 case 1922406657: 3568 /* supportingInfo */ return new String[] {}; 3569 case 73049818: 3570 /* insurance */ return new String[] {}; 3571 case 3242771: 3572 /* item */ return new String[] {}; 3573 default: 3574 return super.getTypesForProperty(hash, name); 3575 } 3576 3577 } 3578 3579 @Override 3580 public Base addChild(String name) throws FHIRException { 3581 if (name.equals("identifier")) { 3582 return addIdentifier(); 3583 } else if (name.equals("status")) { 3584 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityRequest.status"); 3585 } else if (name.equals("priority")) { 3586 this.priority = new CodeableConcept(); 3587 return this.priority; 3588 } else if (name.equals("purpose")) { 3589 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityRequest.purpose"); 3590 } else if (name.equals("patient")) { 3591 this.patient = new Reference(); 3592 return this.patient; 3593 } else if (name.equals("servicedDate")) { 3594 this.serviced = new DateType(); 3595 return this.serviced; 3596 } else if (name.equals("servicedPeriod")) { 3597 this.serviced = new Period(); 3598 return this.serviced; 3599 } else if (name.equals("created")) { 3600 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityRequest.created"); 3601 } else if (name.equals("enterer")) { 3602 this.enterer = new Reference(); 3603 return this.enterer; 3604 } else if (name.equals("provider")) { 3605 this.provider = new Reference(); 3606 return this.provider; 3607 } else if (name.equals("insurer")) { 3608 this.insurer = new Reference(); 3609 return this.insurer; 3610 } else if (name.equals("facility")) { 3611 this.facility = new Reference(); 3612 return this.facility; 3613 } else if (name.equals("supportingInfo")) { 3614 return addSupportingInfo(); 3615 } else if (name.equals("insurance")) { 3616 return addInsurance(); 3617 } else if (name.equals("item")) { 3618 return addItem(); 3619 } else 3620 return super.addChild(name); 3621 } 3622 3623 public String fhirType() { 3624 return "CoverageEligibilityRequest"; 3625 3626 } 3627 3628 public CoverageEligibilityRequest copy() { 3629 CoverageEligibilityRequest dst = new CoverageEligibilityRequest(); 3630 copyValues(dst); 3631 return dst; 3632 } 3633 3634 public void copyValues(CoverageEligibilityRequest dst) { 3635 super.copyValues(dst); 3636 if (identifier != null) { 3637 dst.identifier = new ArrayList<Identifier>(); 3638 for (Identifier i : identifier) 3639 dst.identifier.add(i.copy()); 3640 } 3641 ; 3642 dst.status = status == null ? null : status.copy(); 3643 dst.priority = priority == null ? null : priority.copy(); 3644 if (purpose != null) { 3645 dst.purpose = new ArrayList<Enumeration<EligibilityRequestPurpose>>(); 3646 for (Enumeration<EligibilityRequestPurpose> i : purpose) 3647 dst.purpose.add(i.copy()); 3648 } 3649 ; 3650 dst.patient = patient == null ? null : patient.copy(); 3651 dst.serviced = serviced == null ? null : serviced.copy(); 3652 dst.created = created == null ? null : created.copy(); 3653 dst.enterer = enterer == null ? null : enterer.copy(); 3654 dst.provider = provider == null ? null : provider.copy(); 3655 dst.insurer = insurer == null ? null : insurer.copy(); 3656 dst.facility = facility == null ? null : facility.copy(); 3657 if (supportingInfo != null) { 3658 dst.supportingInfo = new ArrayList<SupportingInformationComponent>(); 3659 for (SupportingInformationComponent i : supportingInfo) 3660 dst.supportingInfo.add(i.copy()); 3661 } 3662 ; 3663 if (insurance != null) { 3664 dst.insurance = new ArrayList<InsuranceComponent>(); 3665 for (InsuranceComponent i : insurance) 3666 dst.insurance.add(i.copy()); 3667 } 3668 ; 3669 if (item != null) { 3670 dst.item = new ArrayList<DetailsComponent>(); 3671 for (DetailsComponent i : item) 3672 dst.item.add(i.copy()); 3673 } 3674 ; 3675 } 3676 3677 protected CoverageEligibilityRequest typedCopy() { 3678 return copy(); 3679 } 3680 3681 @Override 3682 public boolean equalsDeep(Base other_) { 3683 if (!super.equalsDeep(other_)) 3684 return false; 3685 if (!(other_ instanceof CoverageEligibilityRequest)) 3686 return false; 3687 CoverageEligibilityRequest o = (CoverageEligibilityRequest) other_; 3688 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 3689 && compareDeep(priority, o.priority, true) && compareDeep(purpose, o.purpose, true) 3690 && compareDeep(patient, o.patient, true) && compareDeep(serviced, o.serviced, true) 3691 && compareDeep(created, o.created, true) && compareDeep(enterer, o.enterer, true) 3692 && compareDeep(provider, o.provider, true) && compareDeep(insurer, o.insurer, true) 3693 && compareDeep(facility, o.facility, true) && compareDeep(supportingInfo, o.supportingInfo, true) 3694 && compareDeep(insurance, o.insurance, true) && compareDeep(item, o.item, true); 3695 } 3696 3697 @Override 3698 public boolean equalsShallow(Base other_) { 3699 if (!super.equalsShallow(other_)) 3700 return false; 3701 if (!(other_ instanceof CoverageEligibilityRequest)) 3702 return false; 3703 CoverageEligibilityRequest o = (CoverageEligibilityRequest) other_; 3704 return compareValues(status, o.status, true) && compareValues(purpose, o.purpose, true) 3705 && compareValues(created, o.created, true); 3706 } 3707 3708 public boolean isEmpty() { 3709 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, priority, purpose, patient, 3710 serviced, created, enterer, provider, insurer, facility, supportingInfo, insurance, item); 3711 } 3712 3713 @Override 3714 public ResourceType getResourceType() { 3715 return ResourceType.CoverageEligibilityRequest; 3716 } 3717 3718 /** 3719 * Search parameter: <b>identifier</b> 3720 * <p> 3721 * Description: <b>The business identifier of the Eligibility</b><br> 3722 * Type: <b>token</b><br> 3723 * Path: <b>CoverageEligibilityRequest.identifier</b><br> 3724 * </p> 3725 */ 3726 @SearchParamDefinition(name = "identifier", path = "CoverageEligibilityRequest.identifier", description = "The business identifier of the Eligibility", type = "token") 3727 public static final String SP_IDENTIFIER = "identifier"; 3728 /** 3729 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3730 * <p> 3731 * Description: <b>The business identifier of the Eligibility</b><br> 3732 * Type: <b>token</b><br> 3733 * Path: <b>CoverageEligibilityRequest.identifier</b><br> 3734 * </p> 3735 */ 3736 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3737 SP_IDENTIFIER); 3738 3739 /** 3740 * Search parameter: <b>provider</b> 3741 * <p> 3742 * Description: <b>The reference to the provider</b><br> 3743 * Type: <b>reference</b><br> 3744 * Path: <b>CoverageEligibilityRequest.provider</b><br> 3745 * </p> 3746 */ 3747 @SearchParamDefinition(name = "provider", path = "CoverageEligibilityRequest.provider", description = "The reference to the provider", type = "reference", providesMembershipIn = { 3748 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 3749 Practitioner.class, PractitionerRole.class }) 3750 public static final String SP_PROVIDER = "provider"; 3751 /** 3752 * <b>Fluent Client</b> search parameter constant for <b>provider</b> 3753 * <p> 3754 * Description: <b>The reference to the provider</b><br> 3755 * Type: <b>reference</b><br> 3756 * Path: <b>CoverageEligibilityRequest.provider</b><br> 3757 * </p> 3758 */ 3759 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3760 SP_PROVIDER); 3761 3762 /** 3763 * Constant for fluent queries to be used to add include statements. Specifies 3764 * the path value of "<b>CoverageEligibilityRequest:provider</b>". 3765 */ 3766 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROVIDER = new ca.uhn.fhir.model.api.Include( 3767 "CoverageEligibilityRequest:provider").toLocked(); 3768 3769 /** 3770 * Search parameter: <b>patient</b> 3771 * <p> 3772 * Description: <b>The reference to the patient</b><br> 3773 * Type: <b>reference</b><br> 3774 * Path: <b>CoverageEligibilityRequest.patient</b><br> 3775 * </p> 3776 */ 3777 @SearchParamDefinition(name = "patient", path = "CoverageEligibilityRequest.patient", description = "The reference to the patient", type = "reference", providesMembershipIn = { 3778 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 3779 public static final String SP_PATIENT = "patient"; 3780 /** 3781 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3782 * <p> 3783 * Description: <b>The reference to the patient</b><br> 3784 * Type: <b>reference</b><br> 3785 * Path: <b>CoverageEligibilityRequest.patient</b><br> 3786 * </p> 3787 */ 3788 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3789 SP_PATIENT); 3790 3791 /** 3792 * Constant for fluent queries to be used to add include statements. Specifies 3793 * the path value of "<b>CoverageEligibilityRequest:patient</b>". 3794 */ 3795 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 3796 "CoverageEligibilityRequest:patient").toLocked(); 3797 3798 /** 3799 * Search parameter: <b>created</b> 3800 * <p> 3801 * Description: <b>The creation date for the EOB</b><br> 3802 * Type: <b>date</b><br> 3803 * Path: <b>CoverageEligibilityRequest.created</b><br> 3804 * </p> 3805 */ 3806 @SearchParamDefinition(name = "created", path = "CoverageEligibilityRequest.created", description = "The creation date for the EOB", type = "date") 3807 public static final String SP_CREATED = "created"; 3808 /** 3809 * <b>Fluent Client</b> search parameter constant for <b>created</b> 3810 * <p> 3811 * Description: <b>The creation date for the EOB</b><br> 3812 * Type: <b>date</b><br> 3813 * Path: <b>CoverageEligibilityRequest.created</b><br> 3814 * </p> 3815 */ 3816 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam( 3817 SP_CREATED); 3818 3819 /** 3820 * Search parameter: <b>enterer</b> 3821 * <p> 3822 * Description: <b>The party who is responsible for the request</b><br> 3823 * Type: <b>reference</b><br> 3824 * Path: <b>CoverageEligibilityRequest.enterer</b><br> 3825 * </p> 3826 */ 3827 @SearchParamDefinition(name = "enterer", path = "CoverageEligibilityRequest.enterer", description = "The party who is responsible for the request", type = "reference", providesMembershipIn = { 3828 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Practitioner.class, 3829 PractitionerRole.class }) 3830 public static final String SP_ENTERER = "enterer"; 3831 /** 3832 * <b>Fluent Client</b> search parameter constant for <b>enterer</b> 3833 * <p> 3834 * Description: <b>The party who is responsible for the request</b><br> 3835 * Type: <b>reference</b><br> 3836 * Path: <b>CoverageEligibilityRequest.enterer</b><br> 3837 * </p> 3838 */ 3839 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTERER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3840 SP_ENTERER); 3841 3842 /** 3843 * Constant for fluent queries to be used to add include statements. Specifies 3844 * the path value of "<b>CoverageEligibilityRequest:enterer</b>". 3845 */ 3846 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTERER = new ca.uhn.fhir.model.api.Include( 3847 "CoverageEligibilityRequest:enterer").toLocked(); 3848 3849 /** 3850 * Search parameter: <b>facility</b> 3851 * <p> 3852 * Description: <b>Facility responsible for the goods and services</b><br> 3853 * Type: <b>reference</b><br> 3854 * Path: <b>CoverageEligibilityRequest.facility</b><br> 3855 * </p> 3856 */ 3857 @SearchParamDefinition(name = "facility", path = "CoverageEligibilityRequest.facility", description = "Facility responsible for the goods and services", type = "reference", target = { 3858 Location.class }) 3859 public static final String SP_FACILITY = "facility"; 3860 /** 3861 * <b>Fluent Client</b> search parameter constant for <b>facility</b> 3862 * <p> 3863 * Description: <b>Facility responsible for the goods and services</b><br> 3864 * Type: <b>reference</b><br> 3865 * Path: <b>CoverageEligibilityRequest.facility</b><br> 3866 * </p> 3867 */ 3868 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FACILITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3869 SP_FACILITY); 3870 3871 /** 3872 * Constant for fluent queries to be used to add include statements. Specifies 3873 * the path value of "<b>CoverageEligibilityRequest:facility</b>". 3874 */ 3875 public static final ca.uhn.fhir.model.api.Include INCLUDE_FACILITY = new ca.uhn.fhir.model.api.Include( 3876 "CoverageEligibilityRequest:facility").toLocked(); 3877 3878 /** 3879 * Search parameter: <b>status</b> 3880 * <p> 3881 * Description: <b>The status of the EligibilityRequest</b><br> 3882 * Type: <b>token</b><br> 3883 * Path: <b>CoverageEligibilityRequest.status</b><br> 3884 * </p> 3885 */ 3886 @SearchParamDefinition(name = "status", path = "CoverageEligibilityRequest.status", description = "The status of the EligibilityRequest", type = "token") 3887 public static final String SP_STATUS = "status"; 3888 /** 3889 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3890 * <p> 3891 * Description: <b>The status of the EligibilityRequest</b><br> 3892 * Type: <b>token</b><br> 3893 * Path: <b>CoverageEligibilityRequest.status</b><br> 3894 * </p> 3895 */ 3896 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3897 SP_STATUS); 3898 3899}