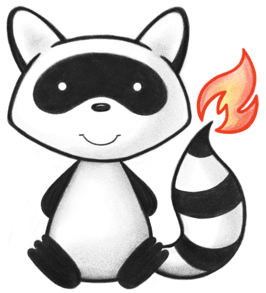
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * Indicates an actual or potential clinical issue with or between one or more 049 * active or proposed clinical actions for a patient; e.g. Drug-drug 050 * interaction, Ineffective treatment frequency, Procedure-condition conflict, 051 * etc. 052 */ 053@ResourceDef(name = "DetectedIssue", profile = "http://hl7.org/fhir/StructureDefinition/DetectedIssue") 054public class DetectedIssue extends DomainResource { 055 056 public enum DetectedIssueStatus { 057 /** 058 * The existence of the observation is registered, but there is no result yet 059 * available. 060 */ 061 REGISTERED, 062 /** 063 * This is an initial or interim observation: data may be incomplete or 064 * unverified. 065 */ 066 PRELIMINARY, 067 /** 068 * The observation is complete and there are no further actions needed. 069 * Additional information such "released", "signed", etc would be represented 070 * using [Provenance](provenance.html) which provides not only the act but also 071 * the actors and dates and other related data. These act states would be 072 * associated with an observation status of `preliminary` until they are all 073 * completed and then a status of `final` would be applied. 074 */ 075 FINAL, 076 /** 077 * Subsequent to being Final, the observation has been modified subsequent. This 078 * includes updates/new information and corrections. 079 */ 080 AMENDED, 081 /** 082 * Subsequent to being Final, the observation has been modified to correct an 083 * error in the test result. 084 */ 085 CORRECTED, 086 /** 087 * The observation is unavailable because the measurement was not started or not 088 * completed (also sometimes called "aborted"). 089 */ 090 CANCELLED, 091 /** 092 * The observation has been withdrawn following previous final release. This 093 * electronic record should never have existed, though it is possible that 094 * real-world decisions were based on it. (If real-world activity has occurred, 095 * the status should be "cancelled" rather than "entered-in-error".). 096 */ 097 ENTEREDINERROR, 098 /** 099 * The authoring/source system does not know which of the status values 100 * currently applies for this observation. Note: This concept is not to be used 101 * for "other" - one of the listed statuses is presumed to apply, but the 102 * authoring/source system does not know which. 103 */ 104 UNKNOWN, 105 /** 106 * added to help the parsers with the generic types 107 */ 108 NULL; 109 110 public static DetectedIssueStatus fromCode(String codeString) throws FHIRException { 111 if (codeString == null || "".equals(codeString)) 112 return null; 113 if ("registered".equals(codeString)) 114 return REGISTERED; 115 if ("preliminary".equals(codeString)) 116 return PRELIMINARY; 117 if ("final".equals(codeString)) 118 return FINAL; 119 if ("amended".equals(codeString)) 120 return AMENDED; 121 if ("corrected".equals(codeString)) 122 return CORRECTED; 123 if ("cancelled".equals(codeString)) 124 return CANCELLED; 125 if ("entered-in-error".equals(codeString)) 126 return ENTEREDINERROR; 127 if ("unknown".equals(codeString)) 128 return UNKNOWN; 129 if (Configuration.isAcceptInvalidEnums()) 130 return null; 131 else 132 throw new FHIRException("Unknown DetectedIssueStatus code '" + codeString + "'"); 133 } 134 135 public String toCode() { 136 switch (this) { 137 case REGISTERED: 138 return "registered"; 139 case PRELIMINARY: 140 return "preliminary"; 141 case FINAL: 142 return "final"; 143 case AMENDED: 144 return "amended"; 145 case CORRECTED: 146 return "corrected"; 147 case CANCELLED: 148 return "cancelled"; 149 case ENTEREDINERROR: 150 return "entered-in-error"; 151 case UNKNOWN: 152 return "unknown"; 153 case NULL: 154 return null; 155 default: 156 return "?"; 157 } 158 } 159 160 public String getSystem() { 161 switch (this) { 162 case REGISTERED: 163 return "http://hl7.org/fhir/observation-status"; 164 case PRELIMINARY: 165 return "http://hl7.org/fhir/observation-status"; 166 case FINAL: 167 return "http://hl7.org/fhir/observation-status"; 168 case AMENDED: 169 return "http://hl7.org/fhir/observation-status"; 170 case CORRECTED: 171 return "http://hl7.org/fhir/observation-status"; 172 case CANCELLED: 173 return "http://hl7.org/fhir/observation-status"; 174 case ENTEREDINERROR: 175 return "http://hl7.org/fhir/observation-status"; 176 case UNKNOWN: 177 return "http://hl7.org/fhir/observation-status"; 178 case NULL: 179 return null; 180 default: 181 return "?"; 182 } 183 } 184 185 public String getDefinition() { 186 switch (this) { 187 case REGISTERED: 188 return "The existence of the observation is registered, but there is no result yet available."; 189 case PRELIMINARY: 190 return "This is an initial or interim observation: data may be incomplete or unverified."; 191 case FINAL: 192 return "The observation is complete and there are no further actions needed. Additional information such \"released\", \"signed\", etc would be represented using [Provenance](provenance.html) which provides not only the act but also the actors and dates and other related data. These act states would be associated with an observation status of `preliminary` until they are all completed and then a status of `final` would be applied."; 193 case AMENDED: 194 return "Subsequent to being Final, the observation has been modified subsequent. This includes updates/new information and corrections."; 195 case CORRECTED: 196 return "Subsequent to being Final, the observation has been modified to correct an error in the test result."; 197 case CANCELLED: 198 return "The observation is unavailable because the measurement was not started or not completed (also sometimes called \"aborted\")."; 199 case ENTEREDINERROR: 200 return "The observation has been withdrawn following previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 201 case UNKNOWN: 202 return "The authoring/source system does not know which of the status values currently applies for this observation. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 203 case NULL: 204 return null; 205 default: 206 return "?"; 207 } 208 } 209 210 public String getDisplay() { 211 switch (this) { 212 case REGISTERED: 213 return "Registered"; 214 case PRELIMINARY: 215 return "Preliminary"; 216 case FINAL: 217 return "Final"; 218 case AMENDED: 219 return "Amended"; 220 case CORRECTED: 221 return "Corrected"; 222 case CANCELLED: 223 return "Cancelled"; 224 case ENTEREDINERROR: 225 return "Entered in Error"; 226 case UNKNOWN: 227 return "Unknown"; 228 case NULL: 229 return null; 230 default: 231 return "?"; 232 } 233 } 234 } 235 236 public static class DetectedIssueStatusEnumFactory implements EnumFactory<DetectedIssueStatus> { 237 public DetectedIssueStatus fromCode(String codeString) throws IllegalArgumentException { 238 if (codeString == null || "".equals(codeString)) 239 if (codeString == null || "".equals(codeString)) 240 return null; 241 if ("registered".equals(codeString)) 242 return DetectedIssueStatus.REGISTERED; 243 if ("preliminary".equals(codeString)) 244 return DetectedIssueStatus.PRELIMINARY; 245 if ("final".equals(codeString)) 246 return DetectedIssueStatus.FINAL; 247 if ("amended".equals(codeString)) 248 return DetectedIssueStatus.AMENDED; 249 if ("corrected".equals(codeString)) 250 return DetectedIssueStatus.CORRECTED; 251 if ("cancelled".equals(codeString)) 252 return DetectedIssueStatus.CANCELLED; 253 if ("entered-in-error".equals(codeString)) 254 return DetectedIssueStatus.ENTEREDINERROR; 255 if ("unknown".equals(codeString)) 256 return DetectedIssueStatus.UNKNOWN; 257 throw new IllegalArgumentException("Unknown DetectedIssueStatus code '" + codeString + "'"); 258 } 259 260 public Enumeration<DetectedIssueStatus> fromType(PrimitiveType<?> code) throws FHIRException { 261 if (code == null) 262 return null; 263 if (code.isEmpty()) 264 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.NULL, code); 265 String codeString = code.asStringValue(); 266 if (codeString == null || "".equals(codeString)) 267 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.NULL, code); 268 if ("registered".equals(codeString)) 269 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.REGISTERED, code); 270 if ("preliminary".equals(codeString)) 271 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.PRELIMINARY, code); 272 if ("final".equals(codeString)) 273 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.FINAL, code); 274 if ("amended".equals(codeString)) 275 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.AMENDED, code); 276 if ("corrected".equals(codeString)) 277 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.CORRECTED, code); 278 if ("cancelled".equals(codeString)) 279 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.CANCELLED, code); 280 if ("entered-in-error".equals(codeString)) 281 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.ENTEREDINERROR, code); 282 if ("unknown".equals(codeString)) 283 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.UNKNOWN, code); 284 throw new FHIRException("Unknown DetectedIssueStatus code '" + codeString + "'"); 285 } 286 287 public String toCode(DetectedIssueStatus code) { 288 if (code == DetectedIssueStatus.NULL) 289 return null; 290 if (code == DetectedIssueStatus.REGISTERED) 291 return "registered"; 292 if (code == DetectedIssueStatus.PRELIMINARY) 293 return "preliminary"; 294 if (code == DetectedIssueStatus.FINAL) 295 return "final"; 296 if (code == DetectedIssueStatus.AMENDED) 297 return "amended"; 298 if (code == DetectedIssueStatus.CORRECTED) 299 return "corrected"; 300 if (code == DetectedIssueStatus.CANCELLED) 301 return "cancelled"; 302 if (code == DetectedIssueStatus.ENTEREDINERROR) 303 return "entered-in-error"; 304 if (code == DetectedIssueStatus.UNKNOWN) 305 return "unknown"; 306 return "?"; 307 } 308 309 public String toSystem(DetectedIssueStatus code) { 310 return code.getSystem(); 311 } 312 } 313 314 public enum DetectedIssueSeverity { 315 /** 316 * Indicates the issue may be life-threatening or has the potential to cause 317 * permanent injury. 318 */ 319 HIGH, 320 /** 321 * Indicates the issue may result in noticeable adverse consequences but is 322 * unlikely to be life-threatening or cause permanent injury. 323 */ 324 MODERATE, 325 /** 326 * Indicates the issue may result in some adverse consequences but is unlikely 327 * to substantially affect the situation of the subject. 328 */ 329 LOW, 330 /** 331 * added to help the parsers with the generic types 332 */ 333 NULL; 334 335 public static DetectedIssueSeverity fromCode(String codeString) throws FHIRException { 336 if (codeString == null || "".equals(codeString)) 337 return null; 338 if ("high".equals(codeString)) 339 return HIGH; 340 if ("moderate".equals(codeString)) 341 return MODERATE; 342 if ("low".equals(codeString)) 343 return LOW; 344 if (Configuration.isAcceptInvalidEnums()) 345 return null; 346 else 347 throw new FHIRException("Unknown DetectedIssueSeverity code '" + codeString + "'"); 348 } 349 350 public String toCode() { 351 switch (this) { 352 case HIGH: 353 return "high"; 354 case MODERATE: 355 return "moderate"; 356 case LOW: 357 return "low"; 358 case NULL: 359 return null; 360 default: 361 return "?"; 362 } 363 } 364 365 public String getSystem() { 366 switch (this) { 367 case HIGH: 368 return "http://hl7.org/fhir/detectedissue-severity"; 369 case MODERATE: 370 return "http://hl7.org/fhir/detectedissue-severity"; 371 case LOW: 372 return "http://hl7.org/fhir/detectedissue-severity"; 373 case NULL: 374 return null; 375 default: 376 return "?"; 377 } 378 } 379 380 public String getDefinition() { 381 switch (this) { 382 case HIGH: 383 return "Indicates the issue may be life-threatening or has the potential to cause permanent injury."; 384 case MODERATE: 385 return "Indicates the issue may result in noticeable adverse consequences but is unlikely to be life-threatening or cause permanent injury."; 386 case LOW: 387 return "Indicates the issue may result in some adverse consequences but is unlikely to substantially affect the situation of the subject."; 388 case NULL: 389 return null; 390 default: 391 return "?"; 392 } 393 } 394 395 public String getDisplay() { 396 switch (this) { 397 case HIGH: 398 return "High"; 399 case MODERATE: 400 return "Moderate"; 401 case LOW: 402 return "Low"; 403 case NULL: 404 return null; 405 default: 406 return "?"; 407 } 408 } 409 } 410 411 public static class DetectedIssueSeverityEnumFactory implements EnumFactory<DetectedIssueSeverity> { 412 public DetectedIssueSeverity fromCode(String codeString) throws IllegalArgumentException { 413 if (codeString == null || "".equals(codeString)) 414 if (codeString == null || "".equals(codeString)) 415 return null; 416 if ("high".equals(codeString)) 417 return DetectedIssueSeverity.HIGH; 418 if ("moderate".equals(codeString)) 419 return DetectedIssueSeverity.MODERATE; 420 if ("low".equals(codeString)) 421 return DetectedIssueSeverity.LOW; 422 throw new IllegalArgumentException("Unknown DetectedIssueSeverity code '" + codeString + "'"); 423 } 424 425 public Enumeration<DetectedIssueSeverity> fromType(PrimitiveType<?> code) throws FHIRException { 426 if (code == null) 427 return null; 428 if (code.isEmpty()) 429 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.NULL, code); 430 String codeString = code.asStringValue(); 431 if (codeString == null || "".equals(codeString)) 432 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.NULL, code); 433 if ("high".equals(codeString)) 434 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.HIGH, code); 435 if ("moderate".equals(codeString)) 436 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.MODERATE, code); 437 if ("low".equals(codeString)) 438 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.LOW, code); 439 throw new FHIRException("Unknown DetectedIssueSeverity code '" + codeString + "'"); 440 } 441 442 public String toCode(DetectedIssueSeverity code) { 443 if (code == DetectedIssueSeverity.NULL) 444 return null; 445 if (code == DetectedIssueSeverity.HIGH) 446 return "high"; 447 if (code == DetectedIssueSeverity.MODERATE) 448 return "moderate"; 449 if (code == DetectedIssueSeverity.LOW) 450 return "low"; 451 return "?"; 452 } 453 454 public String toSystem(DetectedIssueSeverity code) { 455 return code.getSystem(); 456 } 457 } 458 459 @Block() 460 public static class DetectedIssueEvidenceComponent extends BackboneElement implements IBaseBackboneElement { 461 /** 462 * A manifestation that led to the recording of this detected issue. 463 */ 464 @Child(name = "code", type = { 465 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 466 @Description(shortDefinition = "Manifestation", formalDefinition = "A manifestation that led to the recording of this detected issue.") 467 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/manifestation-or-symptom") 468 protected List<CodeableConcept> code; 469 470 /** 471 * Links to resources that constitute evidence for the detected issue such as a 472 * GuidanceResponse or MeasureReport. 473 */ 474 @Child(name = "detail", type = { 475 Reference.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 476 @Description(shortDefinition = "Supporting information", formalDefinition = "Links to resources that constitute evidence for the detected issue such as a GuidanceResponse or MeasureReport.") 477 protected List<Reference> detail; 478 /** 479 * The actual objects that are the target of the reference (Links to resources 480 * that constitute evidence for the detected issue such as a GuidanceResponse or 481 * MeasureReport.) 482 */ 483 protected List<Resource> detailTarget; 484 485 private static final long serialVersionUID = 1135831276L; 486 487 /** 488 * Constructor 489 */ 490 public DetectedIssueEvidenceComponent() { 491 super(); 492 } 493 494 /** 495 * @return {@link #code} (A manifestation that led to the recording of this 496 * detected issue.) 497 */ 498 public List<CodeableConcept> getCode() { 499 if (this.code == null) 500 this.code = new ArrayList<CodeableConcept>(); 501 return this.code; 502 } 503 504 /** 505 * @return Returns a reference to <code>this</code> for easy method chaining 506 */ 507 public DetectedIssueEvidenceComponent setCode(List<CodeableConcept> theCode) { 508 this.code = theCode; 509 return this; 510 } 511 512 public boolean hasCode() { 513 if (this.code == null) 514 return false; 515 for (CodeableConcept item : this.code) 516 if (!item.isEmpty()) 517 return true; 518 return false; 519 } 520 521 public CodeableConcept addCode() { // 3 522 CodeableConcept t = new CodeableConcept(); 523 if (this.code == null) 524 this.code = new ArrayList<CodeableConcept>(); 525 this.code.add(t); 526 return t; 527 } 528 529 public DetectedIssueEvidenceComponent addCode(CodeableConcept t) { // 3 530 if (t == null) 531 return this; 532 if (this.code == null) 533 this.code = new ArrayList<CodeableConcept>(); 534 this.code.add(t); 535 return this; 536 } 537 538 /** 539 * @return The first repetition of repeating field {@link #code}, creating it if 540 * it does not already exist 541 */ 542 public CodeableConcept getCodeFirstRep() { 543 if (getCode().isEmpty()) { 544 addCode(); 545 } 546 return getCode().get(0); 547 } 548 549 /** 550 * @return {@link #detail} (Links to resources that constitute evidence for the 551 * detected issue such as a GuidanceResponse or MeasureReport.) 552 */ 553 public List<Reference> getDetail() { 554 if (this.detail == null) 555 this.detail = new ArrayList<Reference>(); 556 return this.detail; 557 } 558 559 /** 560 * @return Returns a reference to <code>this</code> for easy method chaining 561 */ 562 public DetectedIssueEvidenceComponent setDetail(List<Reference> theDetail) { 563 this.detail = theDetail; 564 return this; 565 } 566 567 public boolean hasDetail() { 568 if (this.detail == null) 569 return false; 570 for (Reference item : this.detail) 571 if (!item.isEmpty()) 572 return true; 573 return false; 574 } 575 576 public Reference addDetail() { // 3 577 Reference t = new Reference(); 578 if (this.detail == null) 579 this.detail = new ArrayList<Reference>(); 580 this.detail.add(t); 581 return t; 582 } 583 584 public DetectedIssueEvidenceComponent addDetail(Reference t) { // 3 585 if (t == null) 586 return this; 587 if (this.detail == null) 588 this.detail = new ArrayList<Reference>(); 589 this.detail.add(t); 590 return this; 591 } 592 593 /** 594 * @return The first repetition of repeating field {@link #detail}, creating it 595 * if it does not already exist 596 */ 597 public Reference getDetailFirstRep() { 598 if (getDetail().isEmpty()) { 599 addDetail(); 600 } 601 return getDetail().get(0); 602 } 603 604 /** 605 * @deprecated Use Reference#setResource(IBaseResource) instead 606 */ 607 @Deprecated 608 public List<Resource> getDetailTarget() { 609 if (this.detailTarget == null) 610 this.detailTarget = new ArrayList<Resource>(); 611 return this.detailTarget; 612 } 613 614 protected void listChildren(List<Property> children) { 615 super.listChildren(children); 616 children.add(new Property("code", "CodeableConcept", 617 "A manifestation that led to the recording of this detected issue.", 0, java.lang.Integer.MAX_VALUE, code)); 618 children.add(new Property("detail", "Reference(Any)", 619 "Links to resources that constitute evidence for the detected issue such as a GuidanceResponse or MeasureReport.", 620 0, java.lang.Integer.MAX_VALUE, detail)); 621 } 622 623 @Override 624 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 625 switch (_hash) { 626 case 3059181: 627 /* code */ return new Property("code", "CodeableConcept", 628 "A manifestation that led to the recording of this detected issue.", 0, java.lang.Integer.MAX_VALUE, code); 629 case -1335224239: 630 /* detail */ return new Property("detail", "Reference(Any)", 631 "Links to resources that constitute evidence for the detected issue such as a GuidanceResponse or MeasureReport.", 632 0, java.lang.Integer.MAX_VALUE, detail); 633 default: 634 return super.getNamedProperty(_hash, _name, _checkValid); 635 } 636 637 } 638 639 @Override 640 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 641 switch (hash) { 642 case 3059181: 643 /* code */ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 644 case -1335224239: 645 /* detail */ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // Reference 646 default: 647 return super.getProperty(hash, name, checkValid); 648 } 649 650 } 651 652 @Override 653 public Base setProperty(int hash, String name, Base value) throws FHIRException { 654 switch (hash) { 655 case 3059181: // code 656 this.getCode().add(castToCodeableConcept(value)); // CodeableConcept 657 return value; 658 case -1335224239: // detail 659 this.getDetail().add(castToReference(value)); // Reference 660 return value; 661 default: 662 return super.setProperty(hash, name, value); 663 } 664 665 } 666 667 @Override 668 public Base setProperty(String name, Base value) throws FHIRException { 669 if (name.equals("code")) { 670 this.getCode().add(castToCodeableConcept(value)); 671 } else if (name.equals("detail")) { 672 this.getDetail().add(castToReference(value)); 673 } else 674 return super.setProperty(name, value); 675 return value; 676 } 677 678 @Override 679 public void removeChild(String name, Base value) throws FHIRException { 680 if (name.equals("code")) { 681 this.getCode().remove(castToCodeableConcept(value)); 682 } else if (name.equals("detail")) { 683 this.getDetail().remove(castToReference(value)); 684 } else 685 super.removeChild(name, value); 686 687 } 688 689 @Override 690 public Base makeProperty(int hash, String name) throws FHIRException { 691 switch (hash) { 692 case 3059181: 693 return addCode(); 694 case -1335224239: 695 return addDetail(); 696 default: 697 return super.makeProperty(hash, name); 698 } 699 700 } 701 702 @Override 703 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 704 switch (hash) { 705 case 3059181: 706 /* code */ return new String[] { "CodeableConcept" }; 707 case -1335224239: 708 /* detail */ return new String[] { "Reference" }; 709 default: 710 return super.getTypesForProperty(hash, name); 711 } 712 713 } 714 715 @Override 716 public Base addChild(String name) throws FHIRException { 717 if (name.equals("code")) { 718 return addCode(); 719 } else if (name.equals("detail")) { 720 return addDetail(); 721 } else 722 return super.addChild(name); 723 } 724 725 public DetectedIssueEvidenceComponent copy() { 726 DetectedIssueEvidenceComponent dst = new DetectedIssueEvidenceComponent(); 727 copyValues(dst); 728 return dst; 729 } 730 731 public void copyValues(DetectedIssueEvidenceComponent dst) { 732 super.copyValues(dst); 733 if (code != null) { 734 dst.code = new ArrayList<CodeableConcept>(); 735 for (CodeableConcept i : code) 736 dst.code.add(i.copy()); 737 } 738 ; 739 if (detail != null) { 740 dst.detail = new ArrayList<Reference>(); 741 for (Reference i : detail) 742 dst.detail.add(i.copy()); 743 } 744 ; 745 } 746 747 @Override 748 public boolean equalsDeep(Base other_) { 749 if (!super.equalsDeep(other_)) 750 return false; 751 if (!(other_ instanceof DetectedIssueEvidenceComponent)) 752 return false; 753 DetectedIssueEvidenceComponent o = (DetectedIssueEvidenceComponent) other_; 754 return compareDeep(code, o.code, true) && compareDeep(detail, o.detail, true); 755 } 756 757 @Override 758 public boolean equalsShallow(Base other_) { 759 if (!super.equalsShallow(other_)) 760 return false; 761 if (!(other_ instanceof DetectedIssueEvidenceComponent)) 762 return false; 763 DetectedIssueEvidenceComponent o = (DetectedIssueEvidenceComponent) other_; 764 return true; 765 } 766 767 public boolean isEmpty() { 768 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, detail); 769 } 770 771 public String fhirType() { 772 return "DetectedIssue.evidence"; 773 774 } 775 776 } 777 778 @Block() 779 public static class DetectedIssueMitigationComponent extends BackboneElement implements IBaseBackboneElement { 780 /** 781 * Describes the action that was taken or the observation that was made that 782 * reduces/eliminates the risk associated with the identified issue. 783 */ 784 @Child(name = "action", type = { 785 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 786 @Description(shortDefinition = "What mitigation?", formalDefinition = "Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue.") 787 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/detectedissue-mitigation-action") 788 protected CodeableConcept action; 789 790 /** 791 * Indicates when the mitigating action was documented. 792 */ 793 @Child(name = "date", type = { DateTimeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 794 @Description(shortDefinition = "Date committed", formalDefinition = "Indicates when the mitigating action was documented.") 795 protected DateTimeType date; 796 797 /** 798 * Identifies the practitioner who determined the mitigation and takes 799 * responsibility for the mitigation step occurring. 800 */ 801 @Child(name = "author", type = { Practitioner.class, 802 PractitionerRole.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 803 @Description(shortDefinition = "Who is committing?", formalDefinition = "Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.") 804 protected Reference author; 805 806 /** 807 * The actual object that is the target of the reference (Identifies the 808 * practitioner who determined the mitigation and takes responsibility for the 809 * mitigation step occurring.) 810 */ 811 protected Resource authorTarget; 812 813 private static final long serialVersionUID = -1928864832L; 814 815 /** 816 * Constructor 817 */ 818 public DetectedIssueMitigationComponent() { 819 super(); 820 } 821 822 /** 823 * Constructor 824 */ 825 public DetectedIssueMitigationComponent(CodeableConcept action) { 826 super(); 827 this.action = action; 828 } 829 830 /** 831 * @return {@link #action} (Describes the action that was taken or the 832 * observation that was made that reduces/eliminates the risk associated 833 * with the identified issue.) 834 */ 835 public CodeableConcept getAction() { 836 if (this.action == null) 837 if (Configuration.errorOnAutoCreate()) 838 throw new Error("Attempt to auto-create DetectedIssueMitigationComponent.action"); 839 else if (Configuration.doAutoCreate()) 840 this.action = new CodeableConcept(); // cc 841 return this.action; 842 } 843 844 public boolean hasAction() { 845 return this.action != null && !this.action.isEmpty(); 846 } 847 848 /** 849 * @param value {@link #action} (Describes the action that was taken or the 850 * observation that was made that reduces/eliminates the risk 851 * associated with the identified issue.) 852 */ 853 public DetectedIssueMitigationComponent setAction(CodeableConcept value) { 854 this.action = value; 855 return this; 856 } 857 858 /** 859 * @return {@link #date} (Indicates when the mitigating action was documented.). 860 * This is the underlying object with id, value and extensions. The 861 * accessor "getDate" gives direct access to the value 862 */ 863 public DateTimeType getDateElement() { 864 if (this.date == null) 865 if (Configuration.errorOnAutoCreate()) 866 throw new Error("Attempt to auto-create DetectedIssueMitigationComponent.date"); 867 else if (Configuration.doAutoCreate()) 868 this.date = new DateTimeType(); // bb 869 return this.date; 870 } 871 872 public boolean hasDateElement() { 873 return this.date != null && !this.date.isEmpty(); 874 } 875 876 public boolean hasDate() { 877 return this.date != null && !this.date.isEmpty(); 878 } 879 880 /** 881 * @param value {@link #date} (Indicates when the mitigating action was 882 * documented.). This is the underlying object with id, value and 883 * extensions. The accessor "getDate" gives direct access to the 884 * value 885 */ 886 public DetectedIssueMitigationComponent setDateElement(DateTimeType value) { 887 this.date = value; 888 return this; 889 } 890 891 /** 892 * @return Indicates when the mitigating action was documented. 893 */ 894 public Date getDate() { 895 return this.date == null ? null : this.date.getValue(); 896 } 897 898 /** 899 * @param value Indicates when the mitigating action was documented. 900 */ 901 public DetectedIssueMitigationComponent setDate(Date value) { 902 if (value == null) 903 this.date = null; 904 else { 905 if (this.date == null) 906 this.date = new DateTimeType(); 907 this.date.setValue(value); 908 } 909 return this; 910 } 911 912 /** 913 * @return {@link #author} (Identifies the practitioner who determined the 914 * mitigation and takes responsibility for the mitigation step 915 * occurring.) 916 */ 917 public Reference getAuthor() { 918 if (this.author == null) 919 if (Configuration.errorOnAutoCreate()) 920 throw new Error("Attempt to auto-create DetectedIssueMitigationComponent.author"); 921 else if (Configuration.doAutoCreate()) 922 this.author = new Reference(); // cc 923 return this.author; 924 } 925 926 public boolean hasAuthor() { 927 return this.author != null && !this.author.isEmpty(); 928 } 929 930 /** 931 * @param value {@link #author} (Identifies the practitioner who determined the 932 * mitigation and takes responsibility for the mitigation step 933 * occurring.) 934 */ 935 public DetectedIssueMitigationComponent setAuthor(Reference value) { 936 this.author = value; 937 return this; 938 } 939 940 /** 941 * @return {@link #author} The actual object that is the target of the 942 * reference. The reference library doesn't populate this, but you can 943 * use it to hold the resource if you resolve it. (Identifies the 944 * practitioner who determined the mitigation and takes responsibility 945 * for the mitigation step occurring.) 946 */ 947 public Resource getAuthorTarget() { 948 return this.authorTarget; 949 } 950 951 /** 952 * @param value {@link #author} The actual object that is the target of the 953 * reference. The reference library doesn't use these, but you can 954 * use it to hold the resource if you resolve it. (Identifies the 955 * practitioner who determined the mitigation and takes 956 * responsibility for the mitigation step occurring.) 957 */ 958 public DetectedIssueMitigationComponent setAuthorTarget(Resource value) { 959 this.authorTarget = value; 960 return this; 961 } 962 963 protected void listChildren(List<Property> children) { 964 super.listChildren(children); 965 children.add(new Property("action", "CodeableConcept", 966 "Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue.", 967 0, 1, action)); 968 children 969 .add(new Property("date", "dateTime", "Indicates when the mitigating action was documented.", 0, 1, date)); 970 children.add(new Property("author", "Reference(Practitioner|PractitionerRole)", 971 "Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.", 972 0, 1, author)); 973 } 974 975 @Override 976 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 977 switch (_hash) { 978 case -1422950858: 979 /* action */ return new Property("action", "CodeableConcept", 980 "Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue.", 981 0, 1, action); 982 case 3076014: 983 /* date */ return new Property("date", "dateTime", "Indicates when the mitigating action was documented.", 0, 1, 984 date); 985 case -1406328437: 986 /* author */ return new Property("author", "Reference(Practitioner|PractitionerRole)", 987 "Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.", 988 0, 1, author); 989 default: 990 return super.getNamedProperty(_hash, _name, _checkValid); 991 } 992 993 } 994 995 @Override 996 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 997 switch (hash) { 998 case -1422950858: 999 /* action */ return this.action == null ? new Base[0] : new Base[] { this.action }; // CodeableConcept 1000 case 3076014: 1001 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 1002 case -1406328437: 1003 /* author */ return this.author == null ? new Base[0] : new Base[] { this.author }; // Reference 1004 default: 1005 return super.getProperty(hash, name, checkValid); 1006 } 1007 1008 } 1009 1010 @Override 1011 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1012 switch (hash) { 1013 case -1422950858: // action 1014 this.action = castToCodeableConcept(value); // CodeableConcept 1015 return value; 1016 case 3076014: // date 1017 this.date = castToDateTime(value); // DateTimeType 1018 return value; 1019 case -1406328437: // author 1020 this.author = castToReference(value); // Reference 1021 return value; 1022 default: 1023 return super.setProperty(hash, name, value); 1024 } 1025 1026 } 1027 1028 @Override 1029 public Base setProperty(String name, Base value) throws FHIRException { 1030 if (name.equals("action")) { 1031 this.action = castToCodeableConcept(value); // CodeableConcept 1032 } else if (name.equals("date")) { 1033 this.date = castToDateTime(value); // DateTimeType 1034 } else if (name.equals("author")) { 1035 this.author = castToReference(value); // Reference 1036 } else 1037 return super.setProperty(name, value); 1038 return value; 1039 } 1040 1041 @Override 1042 public void removeChild(String name, Base value) throws FHIRException { 1043 if (name.equals("action")) { 1044 this.action = null; 1045 } else if (name.equals("date")) { 1046 this.date = null; 1047 } else if (name.equals("author")) { 1048 this.author = null; 1049 } else 1050 super.removeChild(name, value); 1051 1052 } 1053 1054 @Override 1055 public Base makeProperty(int hash, String name) throws FHIRException { 1056 switch (hash) { 1057 case -1422950858: 1058 return getAction(); 1059 case 3076014: 1060 return getDateElement(); 1061 case -1406328437: 1062 return getAuthor(); 1063 default: 1064 return super.makeProperty(hash, name); 1065 } 1066 1067 } 1068 1069 @Override 1070 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1071 switch (hash) { 1072 case -1422950858: 1073 /* action */ return new String[] { "CodeableConcept" }; 1074 case 3076014: 1075 /* date */ return new String[] { "dateTime" }; 1076 case -1406328437: 1077 /* author */ return new String[] { "Reference" }; 1078 default: 1079 return super.getTypesForProperty(hash, name); 1080 } 1081 1082 } 1083 1084 @Override 1085 public Base addChild(String name) throws FHIRException { 1086 if (name.equals("action")) { 1087 this.action = new CodeableConcept(); 1088 return this.action; 1089 } else if (name.equals("date")) { 1090 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.date"); 1091 } else if (name.equals("author")) { 1092 this.author = new Reference(); 1093 return this.author; 1094 } else 1095 return super.addChild(name); 1096 } 1097 1098 public DetectedIssueMitigationComponent copy() { 1099 DetectedIssueMitigationComponent dst = new DetectedIssueMitigationComponent(); 1100 copyValues(dst); 1101 return dst; 1102 } 1103 1104 public void copyValues(DetectedIssueMitigationComponent dst) { 1105 super.copyValues(dst); 1106 dst.action = action == null ? null : action.copy(); 1107 dst.date = date == null ? null : date.copy(); 1108 dst.author = author == null ? null : author.copy(); 1109 } 1110 1111 @Override 1112 public boolean equalsDeep(Base other_) { 1113 if (!super.equalsDeep(other_)) 1114 return false; 1115 if (!(other_ instanceof DetectedIssueMitigationComponent)) 1116 return false; 1117 DetectedIssueMitigationComponent o = (DetectedIssueMitigationComponent) other_; 1118 return compareDeep(action, o.action, true) && compareDeep(date, o.date, true) 1119 && compareDeep(author, o.author, true); 1120 } 1121 1122 @Override 1123 public boolean equalsShallow(Base other_) { 1124 if (!super.equalsShallow(other_)) 1125 return false; 1126 if (!(other_ instanceof DetectedIssueMitigationComponent)) 1127 return false; 1128 DetectedIssueMitigationComponent o = (DetectedIssueMitigationComponent) other_; 1129 return compareValues(date, o.date, true); 1130 } 1131 1132 public boolean isEmpty() { 1133 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(action, date, author); 1134 } 1135 1136 public String fhirType() { 1137 return "DetectedIssue.mitigation"; 1138 1139 } 1140 1141 } 1142 1143 /** 1144 * Business identifier associated with the detected issue record. 1145 */ 1146 @Child(name = "identifier", type = { 1147 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1148 @Description(shortDefinition = "Unique id for the detected issue", formalDefinition = "Business identifier associated with the detected issue record.") 1149 protected List<Identifier> identifier; 1150 1151 /** 1152 * Indicates the status of the detected issue. 1153 */ 1154 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 1155 @Description(shortDefinition = "registered | preliminary | final | amended +", formalDefinition = "Indicates the status of the detected issue.") 1156 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-status") 1157 protected Enumeration<DetectedIssueStatus> status; 1158 1159 /** 1160 * Identifies the general type of issue identified. 1161 */ 1162 @Child(name = "code", type = { CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1163 @Description(shortDefinition = "Issue Category, e.g. drug-drug, duplicate therapy, etc.", formalDefinition = "Identifies the general type of issue identified.") 1164 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/detectedissue-category") 1165 protected CodeableConcept code; 1166 1167 /** 1168 * Indicates the degree of importance associated with the identified issue based 1169 * on the potential impact on the patient. 1170 */ 1171 @Child(name = "severity", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1172 @Description(shortDefinition = "high | moderate | low", formalDefinition = "Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.") 1173 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/detectedissue-severity") 1174 protected Enumeration<DetectedIssueSeverity> severity; 1175 1176 /** 1177 * Indicates the patient whose record the detected issue is associated with. 1178 */ 1179 @Child(name = "patient", type = { Patient.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1180 @Description(shortDefinition = "Associated patient", formalDefinition = "Indicates the patient whose record the detected issue is associated with.") 1181 protected Reference patient; 1182 1183 /** 1184 * The actual object that is the target of the reference (Indicates the patient 1185 * whose record the detected issue is associated with.) 1186 */ 1187 protected Patient patientTarget; 1188 1189 /** 1190 * The date or period when the detected issue was initially identified. 1191 */ 1192 @Child(name = "identified", type = { DateTimeType.class, 1193 Period.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1194 @Description(shortDefinition = "When identified", formalDefinition = "The date or period when the detected issue was initially identified.") 1195 protected Type identified; 1196 1197 /** 1198 * Individual or device responsible for the issue being raised. For example, a 1199 * decision support application or a pharmacist conducting a medication review. 1200 */ 1201 @Child(name = "author", type = { Practitioner.class, PractitionerRole.class, 1202 Device.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1203 @Description(shortDefinition = "The provider or device that identified the issue", formalDefinition = "Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.") 1204 protected Reference author; 1205 1206 /** 1207 * The actual object that is the target of the reference (Individual or device 1208 * responsible for the issue being raised. For example, a decision support 1209 * application or a pharmacist conducting a medication review.) 1210 */ 1211 protected Resource authorTarget; 1212 1213 /** 1214 * Indicates the resource representing the current activity or proposed activity 1215 * that is potentially problematic. 1216 */ 1217 @Child(name = "implicated", type = { 1218 Reference.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1219 @Description(shortDefinition = "Problem resource", formalDefinition = "Indicates the resource representing the current activity or proposed activity that is potentially problematic.") 1220 protected List<Reference> implicated; 1221 /** 1222 * The actual objects that are the target of the reference (Indicates the 1223 * resource representing the current activity or proposed activity that is 1224 * potentially problematic.) 1225 */ 1226 protected List<Resource> implicatedTarget; 1227 1228 /** 1229 * Supporting evidence or manifestations that provide the basis for identifying 1230 * the detected issue such as a GuidanceResponse or MeasureReport. 1231 */ 1232 @Child(name = "evidence", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1233 @Description(shortDefinition = "Supporting evidence", formalDefinition = "Supporting evidence or manifestations that provide the basis for identifying the detected issue such as a GuidanceResponse or MeasureReport.") 1234 protected List<DetectedIssueEvidenceComponent> evidence; 1235 1236 /** 1237 * A textual explanation of the detected issue. 1238 */ 1239 @Child(name = "detail", type = { StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1240 @Description(shortDefinition = "Description and context", formalDefinition = "A textual explanation of the detected issue.") 1241 protected StringType detail; 1242 1243 /** 1244 * The literature, knowledge-base or similar reference that describes the 1245 * propensity for the detected issue identified. 1246 */ 1247 @Child(name = "reference", type = { UriType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 1248 @Description(shortDefinition = "Authority for issue", formalDefinition = "The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.") 1249 protected UriType reference; 1250 1251 /** 1252 * Indicates an action that has been taken or is committed to reduce or 1253 * eliminate the likelihood of the risk identified by the detected issue from 1254 * manifesting. Can also reflect an observation of known mitigating factors that 1255 * may reduce/eliminate the need for any action. 1256 */ 1257 @Child(name = "mitigation", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1258 @Description(shortDefinition = "Step taken to address", formalDefinition = "Indicates an action that has been taken or is committed to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action.") 1259 protected List<DetectedIssueMitigationComponent> mitigation; 1260 1261 private static final long serialVersionUID = 1404426283L; 1262 1263 /** 1264 * Constructor 1265 */ 1266 public DetectedIssue() { 1267 super(); 1268 } 1269 1270 /** 1271 * Constructor 1272 */ 1273 public DetectedIssue(Enumeration<DetectedIssueStatus> status) { 1274 super(); 1275 this.status = status; 1276 } 1277 1278 /** 1279 * @return {@link #identifier} (Business identifier associated with the detected 1280 * issue record.) 1281 */ 1282 public List<Identifier> getIdentifier() { 1283 if (this.identifier == null) 1284 this.identifier = new ArrayList<Identifier>(); 1285 return this.identifier; 1286 } 1287 1288 /** 1289 * @return Returns a reference to <code>this</code> for easy method chaining 1290 */ 1291 public DetectedIssue setIdentifier(List<Identifier> theIdentifier) { 1292 this.identifier = theIdentifier; 1293 return this; 1294 } 1295 1296 public boolean hasIdentifier() { 1297 if (this.identifier == null) 1298 return false; 1299 for (Identifier item : this.identifier) 1300 if (!item.isEmpty()) 1301 return true; 1302 return false; 1303 } 1304 1305 public Identifier addIdentifier() { // 3 1306 Identifier t = new Identifier(); 1307 if (this.identifier == null) 1308 this.identifier = new ArrayList<Identifier>(); 1309 this.identifier.add(t); 1310 return t; 1311 } 1312 1313 public DetectedIssue addIdentifier(Identifier t) { // 3 1314 if (t == null) 1315 return this; 1316 if (this.identifier == null) 1317 this.identifier = new ArrayList<Identifier>(); 1318 this.identifier.add(t); 1319 return this; 1320 } 1321 1322 /** 1323 * @return The first repetition of repeating field {@link #identifier}, creating 1324 * it if it does not already exist 1325 */ 1326 public Identifier getIdentifierFirstRep() { 1327 if (getIdentifier().isEmpty()) { 1328 addIdentifier(); 1329 } 1330 return getIdentifier().get(0); 1331 } 1332 1333 /** 1334 * @return {@link #status} (Indicates the status of the detected issue.). This 1335 * is the underlying object with id, value and extensions. The accessor 1336 * "getStatus" gives direct access to the value 1337 */ 1338 public Enumeration<DetectedIssueStatus> getStatusElement() { 1339 if (this.status == null) 1340 if (Configuration.errorOnAutoCreate()) 1341 throw new Error("Attempt to auto-create DetectedIssue.status"); 1342 else if (Configuration.doAutoCreate()) 1343 this.status = new Enumeration<DetectedIssueStatus>(new DetectedIssueStatusEnumFactory()); // bb 1344 return this.status; 1345 } 1346 1347 public boolean hasStatusElement() { 1348 return this.status != null && !this.status.isEmpty(); 1349 } 1350 1351 public boolean hasStatus() { 1352 return this.status != null && !this.status.isEmpty(); 1353 } 1354 1355 /** 1356 * @param value {@link #status} (Indicates the status of the detected issue.). 1357 * This is the underlying object with id, value and extensions. The 1358 * accessor "getStatus" gives direct access to the value 1359 */ 1360 public DetectedIssue setStatusElement(Enumeration<DetectedIssueStatus> value) { 1361 this.status = value; 1362 return this; 1363 } 1364 1365 /** 1366 * @return Indicates the status of the detected issue. 1367 */ 1368 public DetectedIssueStatus getStatus() { 1369 return this.status == null ? null : this.status.getValue(); 1370 } 1371 1372 /** 1373 * @param value Indicates the status of the detected issue. 1374 */ 1375 public DetectedIssue setStatus(DetectedIssueStatus value) { 1376 if (this.status == null) 1377 this.status = new Enumeration<DetectedIssueStatus>(new DetectedIssueStatusEnumFactory()); 1378 this.status.setValue(value); 1379 return this; 1380 } 1381 1382 /** 1383 * @return {@link #code} (Identifies the general type of issue identified.) 1384 */ 1385 public CodeableConcept getCode() { 1386 if (this.code == null) 1387 if (Configuration.errorOnAutoCreate()) 1388 throw new Error("Attempt to auto-create DetectedIssue.code"); 1389 else if (Configuration.doAutoCreate()) 1390 this.code = new CodeableConcept(); // cc 1391 return this.code; 1392 } 1393 1394 public boolean hasCode() { 1395 return this.code != null && !this.code.isEmpty(); 1396 } 1397 1398 /** 1399 * @param value {@link #code} (Identifies the general type of issue identified.) 1400 */ 1401 public DetectedIssue setCode(CodeableConcept value) { 1402 this.code = value; 1403 return this; 1404 } 1405 1406 /** 1407 * @return {@link #severity} (Indicates the degree of importance associated with 1408 * the identified issue based on the potential impact on the patient.). 1409 * This is the underlying object with id, value and extensions. The 1410 * accessor "getSeverity" gives direct access to the value 1411 */ 1412 public Enumeration<DetectedIssueSeverity> getSeverityElement() { 1413 if (this.severity == null) 1414 if (Configuration.errorOnAutoCreate()) 1415 throw new Error("Attempt to auto-create DetectedIssue.severity"); 1416 else if (Configuration.doAutoCreate()) 1417 this.severity = new Enumeration<DetectedIssueSeverity>(new DetectedIssueSeverityEnumFactory()); // bb 1418 return this.severity; 1419 } 1420 1421 public boolean hasSeverityElement() { 1422 return this.severity != null && !this.severity.isEmpty(); 1423 } 1424 1425 public boolean hasSeverity() { 1426 return this.severity != null && !this.severity.isEmpty(); 1427 } 1428 1429 /** 1430 * @param value {@link #severity} (Indicates the degree of importance associated 1431 * with the identified issue based on the potential impact on the 1432 * patient.). This is the underlying object with id, value and 1433 * extensions. The accessor "getSeverity" gives direct access to 1434 * the value 1435 */ 1436 public DetectedIssue setSeverityElement(Enumeration<DetectedIssueSeverity> value) { 1437 this.severity = value; 1438 return this; 1439 } 1440 1441 /** 1442 * @return Indicates the degree of importance associated with the identified 1443 * issue based on the potential impact on the patient. 1444 */ 1445 public DetectedIssueSeverity getSeverity() { 1446 return this.severity == null ? null : this.severity.getValue(); 1447 } 1448 1449 /** 1450 * @param value Indicates the degree of importance associated with the 1451 * identified issue based on the potential impact on the patient. 1452 */ 1453 public DetectedIssue setSeverity(DetectedIssueSeverity value) { 1454 if (value == null) 1455 this.severity = null; 1456 else { 1457 if (this.severity == null) 1458 this.severity = new Enumeration<DetectedIssueSeverity>(new DetectedIssueSeverityEnumFactory()); 1459 this.severity.setValue(value); 1460 } 1461 return this; 1462 } 1463 1464 /** 1465 * @return {@link #patient} (Indicates the patient whose record the detected 1466 * issue is associated with.) 1467 */ 1468 public Reference getPatient() { 1469 if (this.patient == null) 1470 if (Configuration.errorOnAutoCreate()) 1471 throw new Error("Attempt to auto-create DetectedIssue.patient"); 1472 else if (Configuration.doAutoCreate()) 1473 this.patient = new Reference(); // cc 1474 return this.patient; 1475 } 1476 1477 public boolean hasPatient() { 1478 return this.patient != null && !this.patient.isEmpty(); 1479 } 1480 1481 /** 1482 * @param value {@link #patient} (Indicates the patient whose record the 1483 * detected issue is associated with.) 1484 */ 1485 public DetectedIssue setPatient(Reference value) { 1486 this.patient = value; 1487 return this; 1488 } 1489 1490 /** 1491 * @return {@link #patient} The actual object that is the target of the 1492 * reference. The reference library doesn't populate this, but you can 1493 * use it to hold the resource if you resolve it. (Indicates the patient 1494 * whose record the detected issue is associated with.) 1495 */ 1496 public Patient getPatientTarget() { 1497 if (this.patientTarget == null) 1498 if (Configuration.errorOnAutoCreate()) 1499 throw new Error("Attempt to auto-create DetectedIssue.patient"); 1500 else if (Configuration.doAutoCreate()) 1501 this.patientTarget = new Patient(); // aa 1502 return this.patientTarget; 1503 } 1504 1505 /** 1506 * @param value {@link #patient} The actual object that is the target of the 1507 * reference. The reference library doesn't use these, but you can 1508 * use it to hold the resource if you resolve it. (Indicates the 1509 * patient whose record the detected issue is associated with.) 1510 */ 1511 public DetectedIssue setPatientTarget(Patient value) { 1512 this.patientTarget = value; 1513 return this; 1514 } 1515 1516 /** 1517 * @return {@link #identified} (The date or period when the detected issue was 1518 * initially identified.) 1519 */ 1520 public Type getIdentified() { 1521 return this.identified; 1522 } 1523 1524 /** 1525 * @return {@link #identified} (The date or period when the detected issue was 1526 * initially identified.) 1527 */ 1528 public DateTimeType getIdentifiedDateTimeType() throws FHIRException { 1529 if (this.identified == null) 1530 this.identified = new DateTimeType(); 1531 if (!(this.identified instanceof DateTimeType)) 1532 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1533 + this.identified.getClass().getName() + " was encountered"); 1534 return (DateTimeType) this.identified; 1535 } 1536 1537 public boolean hasIdentifiedDateTimeType() { 1538 return this != null && this.identified instanceof DateTimeType; 1539 } 1540 1541 /** 1542 * @return {@link #identified} (The date or period when the detected issue was 1543 * initially identified.) 1544 */ 1545 public Period getIdentifiedPeriod() throws FHIRException { 1546 if (this.identified == null) 1547 this.identified = new Period(); 1548 if (!(this.identified instanceof Period)) 1549 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.identified.getClass().getName() 1550 + " was encountered"); 1551 return (Period) this.identified; 1552 } 1553 1554 public boolean hasIdentifiedPeriod() { 1555 return this != null && this.identified instanceof Period; 1556 } 1557 1558 public boolean hasIdentified() { 1559 return this.identified != null && !this.identified.isEmpty(); 1560 } 1561 1562 /** 1563 * @param value {@link #identified} (The date or period when the detected issue 1564 * was initially identified.) 1565 */ 1566 public DetectedIssue setIdentified(Type value) { 1567 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1568 throw new Error("Not the right type for DetectedIssue.identified[x]: " + value.fhirType()); 1569 this.identified = value; 1570 return this; 1571 } 1572 1573 /** 1574 * @return {@link #author} (Individual or device responsible for the issue being 1575 * raised. For example, a decision support application or a pharmacist 1576 * conducting a medication review.) 1577 */ 1578 public Reference getAuthor() { 1579 if (this.author == null) 1580 if (Configuration.errorOnAutoCreate()) 1581 throw new Error("Attempt to auto-create DetectedIssue.author"); 1582 else if (Configuration.doAutoCreate()) 1583 this.author = new Reference(); // cc 1584 return this.author; 1585 } 1586 1587 public boolean hasAuthor() { 1588 return this.author != null && !this.author.isEmpty(); 1589 } 1590 1591 /** 1592 * @param value {@link #author} (Individual or device responsible for the issue 1593 * being raised. For example, a decision support application or a 1594 * pharmacist conducting a medication review.) 1595 */ 1596 public DetectedIssue setAuthor(Reference value) { 1597 this.author = value; 1598 return this; 1599 } 1600 1601 /** 1602 * @return {@link #author} The actual object that is the target of the 1603 * reference. The reference library doesn't populate this, but you can 1604 * use it to hold the resource if you resolve it. (Individual or device 1605 * responsible for the issue being raised. For example, a decision 1606 * support application or a pharmacist conducting a medication review.) 1607 */ 1608 public Resource getAuthorTarget() { 1609 return this.authorTarget; 1610 } 1611 1612 /** 1613 * @param value {@link #author} The actual object that is the target of the 1614 * reference. The reference library doesn't use these, but you can 1615 * use it to hold the resource if you resolve it. (Individual or 1616 * device responsible for the issue being raised. For example, a 1617 * decision support application or a pharmacist conducting a 1618 * medication review.) 1619 */ 1620 public DetectedIssue setAuthorTarget(Resource value) { 1621 this.authorTarget = value; 1622 return this; 1623 } 1624 1625 /** 1626 * @return {@link #implicated} (Indicates the resource representing the current 1627 * activity or proposed activity that is potentially problematic.) 1628 */ 1629 public List<Reference> getImplicated() { 1630 if (this.implicated == null) 1631 this.implicated = new ArrayList<Reference>(); 1632 return this.implicated; 1633 } 1634 1635 /** 1636 * @return Returns a reference to <code>this</code> for easy method chaining 1637 */ 1638 public DetectedIssue setImplicated(List<Reference> theImplicated) { 1639 this.implicated = theImplicated; 1640 return this; 1641 } 1642 1643 public boolean hasImplicated() { 1644 if (this.implicated == null) 1645 return false; 1646 for (Reference item : this.implicated) 1647 if (!item.isEmpty()) 1648 return true; 1649 return false; 1650 } 1651 1652 public Reference addImplicated() { // 3 1653 Reference t = new Reference(); 1654 if (this.implicated == null) 1655 this.implicated = new ArrayList<Reference>(); 1656 this.implicated.add(t); 1657 return t; 1658 } 1659 1660 public DetectedIssue addImplicated(Reference t) { // 3 1661 if (t == null) 1662 return this; 1663 if (this.implicated == null) 1664 this.implicated = new ArrayList<Reference>(); 1665 this.implicated.add(t); 1666 return this; 1667 } 1668 1669 /** 1670 * @return The first repetition of repeating field {@link #implicated}, creating 1671 * it if it does not already exist 1672 */ 1673 public Reference getImplicatedFirstRep() { 1674 if (getImplicated().isEmpty()) { 1675 addImplicated(); 1676 } 1677 return getImplicated().get(0); 1678 } 1679 1680 /** 1681 * @deprecated Use Reference#setResource(IBaseResource) instead 1682 */ 1683 @Deprecated 1684 public List<Resource> getImplicatedTarget() { 1685 if (this.implicatedTarget == null) 1686 this.implicatedTarget = new ArrayList<Resource>(); 1687 return this.implicatedTarget; 1688 } 1689 1690 /** 1691 * @return {@link #evidence} (Supporting evidence or manifestations that provide 1692 * the basis for identifying the detected issue such as a 1693 * GuidanceResponse or MeasureReport.) 1694 */ 1695 public List<DetectedIssueEvidenceComponent> getEvidence() { 1696 if (this.evidence == null) 1697 this.evidence = new ArrayList<DetectedIssueEvidenceComponent>(); 1698 return this.evidence; 1699 } 1700 1701 /** 1702 * @return Returns a reference to <code>this</code> for easy method chaining 1703 */ 1704 public DetectedIssue setEvidence(List<DetectedIssueEvidenceComponent> theEvidence) { 1705 this.evidence = theEvidence; 1706 return this; 1707 } 1708 1709 public boolean hasEvidence() { 1710 if (this.evidence == null) 1711 return false; 1712 for (DetectedIssueEvidenceComponent item : this.evidence) 1713 if (!item.isEmpty()) 1714 return true; 1715 return false; 1716 } 1717 1718 public DetectedIssueEvidenceComponent addEvidence() { // 3 1719 DetectedIssueEvidenceComponent t = new DetectedIssueEvidenceComponent(); 1720 if (this.evidence == null) 1721 this.evidence = new ArrayList<DetectedIssueEvidenceComponent>(); 1722 this.evidence.add(t); 1723 return t; 1724 } 1725 1726 public DetectedIssue addEvidence(DetectedIssueEvidenceComponent t) { // 3 1727 if (t == null) 1728 return this; 1729 if (this.evidence == null) 1730 this.evidence = new ArrayList<DetectedIssueEvidenceComponent>(); 1731 this.evidence.add(t); 1732 return this; 1733 } 1734 1735 /** 1736 * @return The first repetition of repeating field {@link #evidence}, creating 1737 * it if it does not already exist 1738 */ 1739 public DetectedIssueEvidenceComponent getEvidenceFirstRep() { 1740 if (getEvidence().isEmpty()) { 1741 addEvidence(); 1742 } 1743 return getEvidence().get(0); 1744 } 1745 1746 /** 1747 * @return {@link #detail} (A textual explanation of the detected issue.). This 1748 * is the underlying object with id, value and extensions. The accessor 1749 * "getDetail" gives direct access to the value 1750 */ 1751 public StringType getDetailElement() { 1752 if (this.detail == null) 1753 if (Configuration.errorOnAutoCreate()) 1754 throw new Error("Attempt to auto-create DetectedIssue.detail"); 1755 else if (Configuration.doAutoCreate()) 1756 this.detail = new StringType(); // bb 1757 return this.detail; 1758 } 1759 1760 public boolean hasDetailElement() { 1761 return this.detail != null && !this.detail.isEmpty(); 1762 } 1763 1764 public boolean hasDetail() { 1765 return this.detail != null && !this.detail.isEmpty(); 1766 } 1767 1768 /** 1769 * @param value {@link #detail} (A textual explanation of the detected issue.). 1770 * This is the underlying object with id, value and extensions. The 1771 * accessor "getDetail" gives direct access to the value 1772 */ 1773 public DetectedIssue setDetailElement(StringType value) { 1774 this.detail = value; 1775 return this; 1776 } 1777 1778 /** 1779 * @return A textual explanation of the detected issue. 1780 */ 1781 public String getDetail() { 1782 return this.detail == null ? null : this.detail.getValue(); 1783 } 1784 1785 /** 1786 * @param value A textual explanation of the detected issue. 1787 */ 1788 public DetectedIssue setDetail(String value) { 1789 if (Utilities.noString(value)) 1790 this.detail = null; 1791 else { 1792 if (this.detail == null) 1793 this.detail = new StringType(); 1794 this.detail.setValue(value); 1795 } 1796 return this; 1797 } 1798 1799 /** 1800 * @return {@link #reference} (The literature, knowledge-base or similar 1801 * reference that describes the propensity for the detected issue 1802 * identified.). This is the underlying object with id, value and 1803 * extensions. The accessor "getReference" gives direct access to the 1804 * value 1805 */ 1806 public UriType getReferenceElement() { 1807 if (this.reference == null) 1808 if (Configuration.errorOnAutoCreate()) 1809 throw new Error("Attempt to auto-create DetectedIssue.reference"); 1810 else if (Configuration.doAutoCreate()) 1811 this.reference = new UriType(); // bb 1812 return this.reference; 1813 } 1814 1815 public boolean hasReferenceElement() { 1816 return this.reference != null && !this.reference.isEmpty(); 1817 } 1818 1819 public boolean hasReference() { 1820 return this.reference != null && !this.reference.isEmpty(); 1821 } 1822 1823 /** 1824 * @param value {@link #reference} (The literature, knowledge-base or similar 1825 * reference that describes the propensity for the detected issue 1826 * identified.). This is the underlying object with id, value and 1827 * extensions. The accessor "getReference" gives direct access to 1828 * the value 1829 */ 1830 public DetectedIssue setReferenceElement(UriType value) { 1831 this.reference = value; 1832 return this; 1833 } 1834 1835 /** 1836 * @return The literature, knowledge-base or similar reference that describes 1837 * the propensity for the detected issue identified. 1838 */ 1839 public String getReference() { 1840 return this.reference == null ? null : this.reference.getValue(); 1841 } 1842 1843 /** 1844 * @param value The literature, knowledge-base or similar reference that 1845 * describes the propensity for the detected issue identified. 1846 */ 1847 public DetectedIssue setReference(String value) { 1848 if (Utilities.noString(value)) 1849 this.reference = null; 1850 else { 1851 if (this.reference == null) 1852 this.reference = new UriType(); 1853 this.reference.setValue(value); 1854 } 1855 return this; 1856 } 1857 1858 /** 1859 * @return {@link #mitigation} (Indicates an action that has been taken or is 1860 * committed to reduce or eliminate the likelihood of the risk 1861 * identified by the detected issue from manifesting. Can also reflect 1862 * an observation of known mitigating factors that may reduce/eliminate 1863 * the need for any action.) 1864 */ 1865 public List<DetectedIssueMitigationComponent> getMitigation() { 1866 if (this.mitigation == null) 1867 this.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 1868 return this.mitigation; 1869 } 1870 1871 /** 1872 * @return Returns a reference to <code>this</code> for easy method chaining 1873 */ 1874 public DetectedIssue setMitigation(List<DetectedIssueMitigationComponent> theMitigation) { 1875 this.mitigation = theMitigation; 1876 return this; 1877 } 1878 1879 public boolean hasMitigation() { 1880 if (this.mitigation == null) 1881 return false; 1882 for (DetectedIssueMitigationComponent item : this.mitigation) 1883 if (!item.isEmpty()) 1884 return true; 1885 return false; 1886 } 1887 1888 public DetectedIssueMitigationComponent addMitigation() { // 3 1889 DetectedIssueMitigationComponent t = new DetectedIssueMitigationComponent(); 1890 if (this.mitigation == null) 1891 this.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 1892 this.mitigation.add(t); 1893 return t; 1894 } 1895 1896 public DetectedIssue addMitigation(DetectedIssueMitigationComponent t) { // 3 1897 if (t == null) 1898 return this; 1899 if (this.mitigation == null) 1900 this.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 1901 this.mitigation.add(t); 1902 return this; 1903 } 1904 1905 /** 1906 * @return The first repetition of repeating field {@link #mitigation}, creating 1907 * it if it does not already exist 1908 */ 1909 public DetectedIssueMitigationComponent getMitigationFirstRep() { 1910 if (getMitigation().isEmpty()) { 1911 addMitigation(); 1912 } 1913 return getMitigation().get(0); 1914 } 1915 1916 protected void listChildren(List<Property> children) { 1917 super.listChildren(children); 1918 children.add(new Property("identifier", "Identifier", 1919 "Business identifier associated with the detected issue record.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1920 children.add(new Property("status", "code", "Indicates the status of the detected issue.", 0, 1, status)); 1921 children 1922 .add(new Property("code", "CodeableConcept", "Identifies the general type of issue identified.", 0, 1, code)); 1923 children.add(new Property("severity", "code", 1924 "Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.", 1925 0, 1, severity)); 1926 children.add(new Property("patient", "Reference(Patient)", 1927 "Indicates the patient whose record the detected issue is associated with.", 0, 1, patient)); 1928 children.add(new Property("identified[x]", "dateTime|Period", 1929 "The date or period when the detected issue was initially identified.", 0, 1, identified)); 1930 children.add(new Property("author", "Reference(Practitioner|PractitionerRole|Device)", 1931 "Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.", 1932 0, 1, author)); 1933 children.add(new Property("implicated", "Reference(Any)", 1934 "Indicates the resource representing the current activity or proposed activity that is potentially problematic.", 1935 0, java.lang.Integer.MAX_VALUE, implicated)); 1936 children.add(new Property("evidence", "", 1937 "Supporting evidence or manifestations that provide the basis for identifying the detected issue such as a GuidanceResponse or MeasureReport.", 1938 0, java.lang.Integer.MAX_VALUE, evidence)); 1939 children.add(new Property("detail", "string", "A textual explanation of the detected issue.", 0, 1, detail)); 1940 children.add(new Property("reference", "uri", 1941 "The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.", 1942 0, 1, reference)); 1943 children.add(new Property("mitigation", "", 1944 "Indicates an action that has been taken or is committed to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action.", 1945 0, java.lang.Integer.MAX_VALUE, mitigation)); 1946 } 1947 1948 @Override 1949 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1950 switch (_hash) { 1951 case -1618432855: 1952 /* identifier */ return new Property("identifier", "Identifier", 1953 "Business identifier associated with the detected issue record.", 0, java.lang.Integer.MAX_VALUE, identifier); 1954 case -892481550: 1955 /* status */ return new Property("status", "code", "Indicates the status of the detected issue.", 0, 1, status); 1956 case 3059181: 1957 /* code */ return new Property("code", "CodeableConcept", "Identifies the general type of issue identified.", 0, 1958 1, code); 1959 case 1478300413: 1960 /* severity */ return new Property("severity", "code", 1961 "Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.", 1962 0, 1, severity); 1963 case -791418107: 1964 /* patient */ return new Property("patient", "Reference(Patient)", 1965 "Indicates the patient whose record the detected issue is associated with.", 0, 1, patient); 1966 case 569355781: 1967 /* identified[x] */ return new Property("identified[x]", "dateTime|Period", 1968 "The date or period when the detected issue was initially identified.", 0, 1, identified); 1969 case -1618432869: 1970 /* identified */ return new Property("identified[x]", "dateTime|Period", 1971 "The date or period when the detected issue was initially identified.", 0, 1, identified); 1972 case -968788650: 1973 /* identifiedDateTime */ return new Property("identified[x]", "dateTime|Period", 1974 "The date or period when the detected issue was initially identified.", 0, 1, identified); 1975 case 520482364: 1976 /* identifiedPeriod */ return new Property("identified[x]", "dateTime|Period", 1977 "The date or period when the detected issue was initially identified.", 0, 1, identified); 1978 case -1406328437: 1979 /* author */ return new Property("author", "Reference(Practitioner|PractitionerRole|Device)", 1980 "Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.", 1981 0, 1, author); 1982 case -810216884: 1983 /* implicated */ return new Property("implicated", "Reference(Any)", 1984 "Indicates the resource representing the current activity or proposed activity that is potentially problematic.", 1985 0, java.lang.Integer.MAX_VALUE, implicated); 1986 case 382967383: 1987 /* evidence */ return new Property("evidence", "", 1988 "Supporting evidence or manifestations that provide the basis for identifying the detected issue such as a GuidanceResponse or MeasureReport.", 1989 0, java.lang.Integer.MAX_VALUE, evidence); 1990 case -1335224239: 1991 /* detail */ return new Property("detail", "string", "A textual explanation of the detected issue.", 0, 1, 1992 detail); 1993 case -925155509: 1994 /* reference */ return new Property("reference", "uri", 1995 "The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.", 1996 0, 1, reference); 1997 case 1293793087: 1998 /* mitigation */ return new Property("mitigation", "", 1999 "Indicates an action that has been taken or is committed to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action.", 2000 0, java.lang.Integer.MAX_VALUE, mitigation); 2001 default: 2002 return super.getNamedProperty(_hash, _name, _checkValid); 2003 } 2004 2005 } 2006 2007 @Override 2008 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2009 switch (hash) { 2010 case -1618432855: 2011 /* identifier */ return this.identifier == null ? new Base[0] 2012 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2013 case -892481550: 2014 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<DetectedIssueStatus> 2015 case 3059181: 2016 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 2017 case 1478300413: 2018 /* severity */ return this.severity == null ? new Base[0] : new Base[] { this.severity }; // Enumeration<DetectedIssueSeverity> 2019 case -791418107: 2020 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 2021 case -1618432869: 2022 /* identified */ return this.identified == null ? new Base[0] : new Base[] { this.identified }; // Type 2023 case -1406328437: 2024 /* author */ return this.author == null ? new Base[0] : new Base[] { this.author }; // Reference 2025 case -810216884: 2026 /* implicated */ return this.implicated == null ? new Base[0] 2027 : this.implicated.toArray(new Base[this.implicated.size()]); // Reference 2028 case 382967383: 2029 /* evidence */ return this.evidence == null ? new Base[0] : this.evidence.toArray(new Base[this.evidence.size()]); // DetectedIssueEvidenceComponent 2030 case -1335224239: 2031 /* detail */ return this.detail == null ? new Base[0] : new Base[] { this.detail }; // StringType 2032 case -925155509: 2033 /* reference */ return this.reference == null ? new Base[0] : new Base[] { this.reference }; // UriType 2034 case 1293793087: 2035 /* mitigation */ return this.mitigation == null ? new Base[0] 2036 : this.mitigation.toArray(new Base[this.mitigation.size()]); // DetectedIssueMitigationComponent 2037 default: 2038 return super.getProperty(hash, name, checkValid); 2039 } 2040 2041 } 2042 2043 @Override 2044 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2045 switch (hash) { 2046 case -1618432855: // identifier 2047 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2048 return value; 2049 case -892481550: // status 2050 value = new DetectedIssueStatusEnumFactory().fromType(castToCode(value)); 2051 this.status = (Enumeration) value; // Enumeration<DetectedIssueStatus> 2052 return value; 2053 case 3059181: // code 2054 this.code = castToCodeableConcept(value); // CodeableConcept 2055 return value; 2056 case 1478300413: // severity 2057 value = new DetectedIssueSeverityEnumFactory().fromType(castToCode(value)); 2058 this.severity = (Enumeration) value; // Enumeration<DetectedIssueSeverity> 2059 return value; 2060 case -791418107: // patient 2061 this.patient = castToReference(value); // Reference 2062 return value; 2063 case -1618432869: // identified 2064 this.identified = castToType(value); // Type 2065 return value; 2066 case -1406328437: // author 2067 this.author = castToReference(value); // Reference 2068 return value; 2069 case -810216884: // implicated 2070 this.getImplicated().add(castToReference(value)); // Reference 2071 return value; 2072 case 382967383: // evidence 2073 this.getEvidence().add((DetectedIssueEvidenceComponent) value); // DetectedIssueEvidenceComponent 2074 return value; 2075 case -1335224239: // detail 2076 this.detail = castToString(value); // StringType 2077 return value; 2078 case -925155509: // reference 2079 this.reference = castToUri(value); // UriType 2080 return value; 2081 case 1293793087: // mitigation 2082 this.getMitigation().add((DetectedIssueMitigationComponent) value); // DetectedIssueMitigationComponent 2083 return value; 2084 default: 2085 return super.setProperty(hash, name, value); 2086 } 2087 2088 } 2089 2090 @Override 2091 public Base setProperty(String name, Base value) throws FHIRException { 2092 if (name.equals("identifier")) { 2093 this.getIdentifier().add(castToIdentifier(value)); 2094 } else if (name.equals("status")) { 2095 value = new DetectedIssueStatusEnumFactory().fromType(castToCode(value)); 2096 this.status = (Enumeration) value; // Enumeration<DetectedIssueStatus> 2097 } else if (name.equals("code")) { 2098 this.code = castToCodeableConcept(value); // CodeableConcept 2099 } else if (name.equals("severity")) { 2100 value = new DetectedIssueSeverityEnumFactory().fromType(castToCode(value)); 2101 this.severity = (Enumeration) value; // Enumeration<DetectedIssueSeverity> 2102 } else if (name.equals("patient")) { 2103 this.patient = castToReference(value); // Reference 2104 } else if (name.equals("identified[x]")) { 2105 this.identified = castToType(value); // Type 2106 } else if (name.equals("author")) { 2107 this.author = castToReference(value); // Reference 2108 } else if (name.equals("implicated")) { 2109 this.getImplicated().add(castToReference(value)); 2110 } else if (name.equals("evidence")) { 2111 this.getEvidence().add((DetectedIssueEvidenceComponent) value); 2112 } else if (name.equals("detail")) { 2113 this.detail = castToString(value); // StringType 2114 } else if (name.equals("reference")) { 2115 this.reference = castToUri(value); // UriType 2116 } else if (name.equals("mitigation")) { 2117 this.getMitigation().add((DetectedIssueMitigationComponent) value); 2118 } else 2119 return super.setProperty(name, value); 2120 return value; 2121 } 2122 2123 @Override 2124 public void removeChild(String name, Base value) throws FHIRException { 2125 if (name.equals("identifier")) { 2126 this.getIdentifier().remove(castToIdentifier(value)); 2127 } else if (name.equals("status")) { 2128 this.status = null; 2129 } else if (name.equals("code")) { 2130 this.code = null; 2131 } else if (name.equals("severity")) { 2132 this.severity = null; 2133 } else if (name.equals("patient")) { 2134 this.patient = null; 2135 } else if (name.equals("identified[x]")) { 2136 this.identified = null; 2137 } else if (name.equals("author")) { 2138 this.author = null; 2139 } else if (name.equals("implicated")) { 2140 this.getImplicated().remove(castToReference(value)); 2141 } else if (name.equals("evidence")) { 2142 this.getEvidence().remove((DetectedIssueEvidenceComponent) value); 2143 } else if (name.equals("detail")) { 2144 this.detail = null; 2145 } else if (name.equals("reference")) { 2146 this.reference = null; 2147 } else if (name.equals("mitigation")) { 2148 this.getMitigation().remove((DetectedIssueMitigationComponent) value); 2149 } else 2150 super.removeChild(name, value); 2151 2152 } 2153 2154 @Override 2155 public Base makeProperty(int hash, String name) throws FHIRException { 2156 switch (hash) { 2157 case -1618432855: 2158 return addIdentifier(); 2159 case -892481550: 2160 return getStatusElement(); 2161 case 3059181: 2162 return getCode(); 2163 case 1478300413: 2164 return getSeverityElement(); 2165 case -791418107: 2166 return getPatient(); 2167 case 569355781: 2168 return getIdentified(); 2169 case -1618432869: 2170 return getIdentified(); 2171 case -1406328437: 2172 return getAuthor(); 2173 case -810216884: 2174 return addImplicated(); 2175 case 382967383: 2176 return addEvidence(); 2177 case -1335224239: 2178 return getDetailElement(); 2179 case -925155509: 2180 return getReferenceElement(); 2181 case 1293793087: 2182 return addMitigation(); 2183 default: 2184 return super.makeProperty(hash, name); 2185 } 2186 2187 } 2188 2189 @Override 2190 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2191 switch (hash) { 2192 case -1618432855: 2193 /* identifier */ return new String[] { "Identifier" }; 2194 case -892481550: 2195 /* status */ return new String[] { "code" }; 2196 case 3059181: 2197 /* code */ return new String[] { "CodeableConcept" }; 2198 case 1478300413: 2199 /* severity */ return new String[] { "code" }; 2200 case -791418107: 2201 /* patient */ return new String[] { "Reference" }; 2202 case -1618432869: 2203 /* identified */ return new String[] { "dateTime", "Period" }; 2204 case -1406328437: 2205 /* author */ return new String[] { "Reference" }; 2206 case -810216884: 2207 /* implicated */ return new String[] { "Reference" }; 2208 case 382967383: 2209 /* evidence */ return new String[] {}; 2210 case -1335224239: 2211 /* detail */ return new String[] { "string" }; 2212 case -925155509: 2213 /* reference */ return new String[] { "uri" }; 2214 case 1293793087: 2215 /* mitigation */ return new String[] {}; 2216 default: 2217 return super.getTypesForProperty(hash, name); 2218 } 2219 2220 } 2221 2222 @Override 2223 public Base addChild(String name) throws FHIRException { 2224 if (name.equals("identifier")) { 2225 return addIdentifier(); 2226 } else if (name.equals("status")) { 2227 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.status"); 2228 } else if (name.equals("code")) { 2229 this.code = new CodeableConcept(); 2230 return this.code; 2231 } else if (name.equals("severity")) { 2232 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.severity"); 2233 } else if (name.equals("patient")) { 2234 this.patient = new Reference(); 2235 return this.patient; 2236 } else if (name.equals("identifiedDateTime")) { 2237 this.identified = new DateTimeType(); 2238 return this.identified; 2239 } else if (name.equals("identifiedPeriod")) { 2240 this.identified = new Period(); 2241 return this.identified; 2242 } else if (name.equals("author")) { 2243 this.author = new Reference(); 2244 return this.author; 2245 } else if (name.equals("implicated")) { 2246 return addImplicated(); 2247 } else if (name.equals("evidence")) { 2248 return addEvidence(); 2249 } else if (name.equals("detail")) { 2250 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.detail"); 2251 } else if (name.equals("reference")) { 2252 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.reference"); 2253 } else if (name.equals("mitigation")) { 2254 return addMitigation(); 2255 } else 2256 return super.addChild(name); 2257 } 2258 2259 public String fhirType() { 2260 return "DetectedIssue"; 2261 2262 } 2263 2264 public DetectedIssue copy() { 2265 DetectedIssue dst = new DetectedIssue(); 2266 copyValues(dst); 2267 return dst; 2268 } 2269 2270 public void copyValues(DetectedIssue dst) { 2271 super.copyValues(dst); 2272 if (identifier != null) { 2273 dst.identifier = new ArrayList<Identifier>(); 2274 for (Identifier i : identifier) 2275 dst.identifier.add(i.copy()); 2276 } 2277 ; 2278 dst.status = status == null ? null : status.copy(); 2279 dst.code = code == null ? null : code.copy(); 2280 dst.severity = severity == null ? null : severity.copy(); 2281 dst.patient = patient == null ? null : patient.copy(); 2282 dst.identified = identified == null ? null : identified.copy(); 2283 dst.author = author == null ? null : author.copy(); 2284 if (implicated != null) { 2285 dst.implicated = new ArrayList<Reference>(); 2286 for (Reference i : implicated) 2287 dst.implicated.add(i.copy()); 2288 } 2289 ; 2290 if (evidence != null) { 2291 dst.evidence = new ArrayList<DetectedIssueEvidenceComponent>(); 2292 for (DetectedIssueEvidenceComponent i : evidence) 2293 dst.evidence.add(i.copy()); 2294 } 2295 ; 2296 dst.detail = detail == null ? null : detail.copy(); 2297 dst.reference = reference == null ? null : reference.copy(); 2298 if (mitigation != null) { 2299 dst.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 2300 for (DetectedIssueMitigationComponent i : mitigation) 2301 dst.mitigation.add(i.copy()); 2302 } 2303 ; 2304 } 2305 2306 protected DetectedIssue typedCopy() { 2307 return copy(); 2308 } 2309 2310 @Override 2311 public boolean equalsDeep(Base other_) { 2312 if (!super.equalsDeep(other_)) 2313 return false; 2314 if (!(other_ instanceof DetectedIssue)) 2315 return false; 2316 DetectedIssue o = (DetectedIssue) other_; 2317 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 2318 && compareDeep(code, o.code, true) && compareDeep(severity, o.severity, true) 2319 && compareDeep(patient, o.patient, true) && compareDeep(identified, o.identified, true) 2320 && compareDeep(author, o.author, true) && compareDeep(implicated, o.implicated, true) 2321 && compareDeep(evidence, o.evidence, true) && compareDeep(detail, o.detail, true) 2322 && compareDeep(reference, o.reference, true) && compareDeep(mitigation, o.mitigation, true); 2323 } 2324 2325 @Override 2326 public boolean equalsShallow(Base other_) { 2327 if (!super.equalsShallow(other_)) 2328 return false; 2329 if (!(other_ instanceof DetectedIssue)) 2330 return false; 2331 DetectedIssue o = (DetectedIssue) other_; 2332 return compareValues(status, o.status, true) && compareValues(severity, o.severity, true) 2333 && compareValues(detail, o.detail, true) && compareValues(reference, o.reference, true); 2334 } 2335 2336 public boolean isEmpty() { 2337 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, code, severity, patient, 2338 identified, author, implicated, evidence, detail, reference, mitigation); 2339 } 2340 2341 @Override 2342 public ResourceType getResourceType() { 2343 return ResourceType.DetectedIssue; 2344 } 2345 2346 /** 2347 * Search parameter: <b>identifier</b> 2348 * <p> 2349 * Description: <b>Unique id for the detected issue</b><br> 2350 * Type: <b>token</b><br> 2351 * Path: <b>DetectedIssue.identifier</b><br> 2352 * </p> 2353 */ 2354 @SearchParamDefinition(name = "identifier", path = "DetectedIssue.identifier", description = "Unique id for the detected issue", type = "token") 2355 public static final String SP_IDENTIFIER = "identifier"; 2356 /** 2357 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2358 * <p> 2359 * Description: <b>Unique id for the detected issue</b><br> 2360 * Type: <b>token</b><br> 2361 * Path: <b>DetectedIssue.identifier</b><br> 2362 * </p> 2363 */ 2364 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2365 SP_IDENTIFIER); 2366 2367 /** 2368 * Search parameter: <b>code</b> 2369 * <p> 2370 * Description: <b>Issue Category, e.g. drug-drug, duplicate therapy, 2371 * etc.</b><br> 2372 * Type: <b>token</b><br> 2373 * Path: <b>DetectedIssue.code</b><br> 2374 * </p> 2375 */ 2376 @SearchParamDefinition(name = "code", path = "DetectedIssue.code", description = "Issue Category, e.g. drug-drug, duplicate therapy, etc.", type = "token") 2377 public static final String SP_CODE = "code"; 2378 /** 2379 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2380 * <p> 2381 * Description: <b>Issue Category, e.g. drug-drug, duplicate therapy, 2382 * etc.</b><br> 2383 * Type: <b>token</b><br> 2384 * Path: <b>DetectedIssue.code</b><br> 2385 * </p> 2386 */ 2387 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2388 SP_CODE); 2389 2390 /** 2391 * Search parameter: <b>identified</b> 2392 * <p> 2393 * Description: <b>When identified</b><br> 2394 * Type: <b>date</b><br> 2395 * Path: <b>DetectedIssue.identified[x]</b><br> 2396 * </p> 2397 */ 2398 @SearchParamDefinition(name = "identified", path = "DetectedIssue.identified", description = "When identified", type = "date") 2399 public static final String SP_IDENTIFIED = "identified"; 2400 /** 2401 * <b>Fluent Client</b> search parameter constant for <b>identified</b> 2402 * <p> 2403 * Description: <b>When identified</b><br> 2404 * Type: <b>date</b><br> 2405 * Path: <b>DetectedIssue.identified[x]</b><br> 2406 * </p> 2407 */ 2408 public static final ca.uhn.fhir.rest.gclient.DateClientParam IDENTIFIED = new ca.uhn.fhir.rest.gclient.DateClientParam( 2409 SP_IDENTIFIED); 2410 2411 /** 2412 * Search parameter: <b>patient</b> 2413 * <p> 2414 * Description: <b>Associated patient</b><br> 2415 * Type: <b>reference</b><br> 2416 * Path: <b>DetectedIssue.patient</b><br> 2417 * </p> 2418 */ 2419 @SearchParamDefinition(name = "patient", path = "DetectedIssue.patient", description = "Associated patient", type = "reference", providesMembershipIn = { 2420 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 2421 public static final String SP_PATIENT = "patient"; 2422 /** 2423 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2424 * <p> 2425 * Description: <b>Associated patient</b><br> 2426 * Type: <b>reference</b><br> 2427 * Path: <b>DetectedIssue.patient</b><br> 2428 * </p> 2429 */ 2430 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2431 SP_PATIENT); 2432 2433 /** 2434 * Constant for fluent queries to be used to add include statements. Specifies 2435 * the path value of "<b>DetectedIssue:patient</b>". 2436 */ 2437 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 2438 "DetectedIssue:patient").toLocked(); 2439 2440 /** 2441 * Search parameter: <b>author</b> 2442 * <p> 2443 * Description: <b>The provider or device that identified the issue</b><br> 2444 * Type: <b>reference</b><br> 2445 * Path: <b>DetectedIssue.author</b><br> 2446 * </p> 2447 */ 2448 @SearchParamDefinition(name = "author", path = "DetectedIssue.author", description = "The provider or device that identified the issue", type = "reference", providesMembershipIn = { 2449 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 2450 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Device.class, 2451 Practitioner.class, PractitionerRole.class }) 2452 public static final String SP_AUTHOR = "author"; 2453 /** 2454 * <b>Fluent Client</b> search parameter constant for <b>author</b> 2455 * <p> 2456 * Description: <b>The provider or device that identified the issue</b><br> 2457 * Type: <b>reference</b><br> 2458 * Path: <b>DetectedIssue.author</b><br> 2459 * </p> 2460 */ 2461 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2462 SP_AUTHOR); 2463 2464 /** 2465 * Constant for fluent queries to be used to add include statements. Specifies 2466 * the path value of "<b>DetectedIssue:author</b>". 2467 */ 2468 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include( 2469 "DetectedIssue:author").toLocked(); 2470 2471 /** 2472 * Search parameter: <b>implicated</b> 2473 * <p> 2474 * Description: <b>Problem resource</b><br> 2475 * Type: <b>reference</b><br> 2476 * Path: <b>DetectedIssue.implicated</b><br> 2477 * </p> 2478 */ 2479 @SearchParamDefinition(name = "implicated", path = "DetectedIssue.implicated", description = "Problem resource", type = "reference") 2480 public static final String SP_IMPLICATED = "implicated"; 2481 /** 2482 * <b>Fluent Client</b> search parameter constant for <b>implicated</b> 2483 * <p> 2484 * Description: <b>Problem resource</b><br> 2485 * Type: <b>reference</b><br> 2486 * Path: <b>DetectedIssue.implicated</b><br> 2487 * </p> 2488 */ 2489 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam IMPLICATED = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2490 SP_IMPLICATED); 2491 2492 /** 2493 * Constant for fluent queries to be used to add include statements. Specifies 2494 * the path value of "<b>DetectedIssue:implicated</b>". 2495 */ 2496 public static final ca.uhn.fhir.model.api.Include INCLUDE_IMPLICATED = new ca.uhn.fhir.model.api.Include( 2497 "DetectedIssue:implicated").toLocked(); 2498 2499}