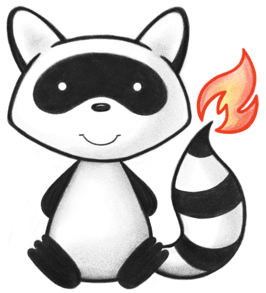
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * Indicates an actual or potential clinical issue with or between one or more 049 * active or proposed clinical actions for a patient; e.g. Drug-drug 050 * interaction, Ineffective treatment frequency, Procedure-condition conflict, 051 * etc. 052 */ 053@ResourceDef(name = "DetectedIssue", profile = "http://hl7.org/fhir/StructureDefinition/DetectedIssue") 054public class DetectedIssue extends DomainResource { 055 056 public enum DetectedIssueStatus { 057 /** 058 * The existence of the observation is registered, but there is no result yet 059 * available. 060 */ 061 REGISTERED, 062 /** 063 * This is an initial or interim observation: data may be incomplete or 064 * unverified. 065 */ 066 PRELIMINARY, 067 /** 068 * The observation is complete and there are no further actions needed. 069 * Additional information such "released", "signed", etc would be represented 070 * using [Provenance](provenance.html) which provides not only the act but also 071 * the actors and dates and other related data. These act states would be 072 * associated with an observation status of `preliminary` until they are all 073 * completed and then a status of `final` would be applied. 074 */ 075 FINAL, 076 /** 077 * Subsequent to being Final, the observation has been modified subsequent. This 078 * includes updates/new information and corrections. 079 */ 080 AMENDED, 081 /** 082 * Subsequent to being Final, the observation has been modified to correct an 083 * error in the test result. 084 */ 085 CORRECTED, 086 /** 087 * The observation is unavailable because the measurement was not started or not 088 * completed (also sometimes called "aborted"). 089 */ 090 CANCELLED, 091 /** 092 * The observation has been withdrawn following previous final release. This 093 * electronic record should never have existed, though it is possible that 094 * real-world decisions were based on it. (If real-world activity has occurred, 095 * the status should be "cancelled" rather than "entered-in-error".). 096 */ 097 ENTEREDINERROR, 098 /** 099 * The authoring/source system does not know which of the status values 100 * currently applies for this observation. Note: This concept is not to be used 101 * for "other" - one of the listed statuses is presumed to apply, but the 102 * authoring/source system does not know which. 103 */ 104 UNKNOWN, 105 /** 106 * added to help the parsers with the generic types 107 */ 108 NULL; 109 110 public static DetectedIssueStatus fromCode(String codeString) throws FHIRException { 111 if (codeString == null || "".equals(codeString)) 112 return null; 113 if ("registered".equals(codeString)) 114 return REGISTERED; 115 if ("preliminary".equals(codeString)) 116 return PRELIMINARY; 117 if ("final".equals(codeString)) 118 return FINAL; 119 if ("amended".equals(codeString)) 120 return AMENDED; 121 if ("corrected".equals(codeString)) 122 return CORRECTED; 123 if ("cancelled".equals(codeString)) 124 return CANCELLED; 125 if ("entered-in-error".equals(codeString)) 126 return ENTEREDINERROR; 127 if ("unknown".equals(codeString)) 128 return UNKNOWN; 129 if (Configuration.isAcceptInvalidEnums()) 130 return null; 131 else 132 throw new FHIRException("Unknown DetectedIssueStatus code '" + codeString + "'"); 133 } 134 135 public String toCode() { 136 switch (this) { 137 case REGISTERED: 138 return "registered"; 139 case PRELIMINARY: 140 return "preliminary"; 141 case FINAL: 142 return "final"; 143 case AMENDED: 144 return "amended"; 145 case CORRECTED: 146 return "corrected"; 147 case CANCELLED: 148 return "cancelled"; 149 case ENTEREDINERROR: 150 return "entered-in-error"; 151 case UNKNOWN: 152 return "unknown"; 153 case NULL: 154 return null; 155 default: 156 return "?"; 157 } 158 } 159 160 public String getSystem() { 161 switch (this) { 162 case REGISTERED: 163 return "http://hl7.org/fhir/observation-status"; 164 case PRELIMINARY: 165 return "http://hl7.org/fhir/observation-status"; 166 case FINAL: 167 return "http://hl7.org/fhir/observation-status"; 168 case AMENDED: 169 return "http://hl7.org/fhir/observation-status"; 170 case CORRECTED: 171 return "http://hl7.org/fhir/observation-status"; 172 case CANCELLED: 173 return "http://hl7.org/fhir/observation-status"; 174 case ENTEREDINERROR: 175 return "http://hl7.org/fhir/observation-status"; 176 case UNKNOWN: 177 return "http://hl7.org/fhir/observation-status"; 178 case NULL: 179 return null; 180 default: 181 return "?"; 182 } 183 } 184 185 public String getDefinition() { 186 switch (this) { 187 case REGISTERED: 188 return "The existence of the observation is registered, but there is no result yet available."; 189 case PRELIMINARY: 190 return "This is an initial or interim observation: data may be incomplete or unverified."; 191 case FINAL: 192 return "The observation is complete and there are no further actions needed. Additional information such \"released\", \"signed\", etc would be represented using [Provenance](provenance.html) which provides not only the act but also the actors and dates and other related data. These act states would be associated with an observation status of `preliminary` until they are all completed and then a status of `final` would be applied."; 193 case AMENDED: 194 return "Subsequent to being Final, the observation has been modified subsequent. This includes updates/new information and corrections."; 195 case CORRECTED: 196 return "Subsequent to being Final, the observation has been modified to correct an error in the test result."; 197 case CANCELLED: 198 return "The observation is unavailable because the measurement was not started or not completed (also sometimes called \"aborted\")."; 199 case ENTEREDINERROR: 200 return "The observation has been withdrawn following previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 201 case UNKNOWN: 202 return "The authoring/source system does not know which of the status values currently applies for this observation. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 203 case NULL: 204 return null; 205 default: 206 return "?"; 207 } 208 } 209 210 public String getDisplay() { 211 switch (this) { 212 case REGISTERED: 213 return "Registered"; 214 case PRELIMINARY: 215 return "Preliminary"; 216 case FINAL: 217 return "Final"; 218 case AMENDED: 219 return "Amended"; 220 case CORRECTED: 221 return "Corrected"; 222 case CANCELLED: 223 return "Cancelled"; 224 case ENTEREDINERROR: 225 return "Entered in Error"; 226 case UNKNOWN: 227 return "Unknown"; 228 case NULL: 229 return null; 230 default: 231 return "?"; 232 } 233 } 234 } 235 236 public static class DetectedIssueStatusEnumFactory implements EnumFactory<DetectedIssueStatus> { 237 public DetectedIssueStatus fromCode(String codeString) throws IllegalArgumentException { 238 if (codeString == null || "".equals(codeString)) 239 if (codeString == null || "".equals(codeString)) 240 return null; 241 if ("registered".equals(codeString)) 242 return DetectedIssueStatus.REGISTERED; 243 if ("preliminary".equals(codeString)) 244 return DetectedIssueStatus.PRELIMINARY; 245 if ("final".equals(codeString)) 246 return DetectedIssueStatus.FINAL; 247 if ("amended".equals(codeString)) 248 return DetectedIssueStatus.AMENDED; 249 if ("corrected".equals(codeString)) 250 return DetectedIssueStatus.CORRECTED; 251 if ("cancelled".equals(codeString)) 252 return DetectedIssueStatus.CANCELLED; 253 if ("entered-in-error".equals(codeString)) 254 return DetectedIssueStatus.ENTEREDINERROR; 255 if ("unknown".equals(codeString)) 256 return DetectedIssueStatus.UNKNOWN; 257 throw new IllegalArgumentException("Unknown DetectedIssueStatus code '" + codeString + "'"); 258 } 259 260 public Enumeration<DetectedIssueStatus> fromType(PrimitiveType<?> code) throws FHIRException { 261 if (code == null) 262 return null; 263 if (code.isEmpty()) 264 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.NULL, code); 265 String codeString = code.asStringValue(); 266 if (codeString == null || "".equals(codeString)) 267 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.NULL, code); 268 if ("registered".equals(codeString)) 269 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.REGISTERED, code); 270 if ("preliminary".equals(codeString)) 271 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.PRELIMINARY, code); 272 if ("final".equals(codeString)) 273 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.FINAL, code); 274 if ("amended".equals(codeString)) 275 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.AMENDED, code); 276 if ("corrected".equals(codeString)) 277 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.CORRECTED, code); 278 if ("cancelled".equals(codeString)) 279 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.CANCELLED, code); 280 if ("entered-in-error".equals(codeString)) 281 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.ENTEREDINERROR, code); 282 if ("unknown".equals(codeString)) 283 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.UNKNOWN, code); 284 throw new FHIRException("Unknown DetectedIssueStatus code '" + codeString + "'"); 285 } 286 287 public String toCode(DetectedIssueStatus code) { 288 if (code == DetectedIssueStatus.REGISTERED) 289 return "registered"; 290 if (code == DetectedIssueStatus.PRELIMINARY) 291 return "preliminary"; 292 if (code == DetectedIssueStatus.FINAL) 293 return "final"; 294 if (code == DetectedIssueStatus.AMENDED) 295 return "amended"; 296 if (code == DetectedIssueStatus.CORRECTED) 297 return "corrected"; 298 if (code == DetectedIssueStatus.CANCELLED) 299 return "cancelled"; 300 if (code == DetectedIssueStatus.ENTEREDINERROR) 301 return "entered-in-error"; 302 if (code == DetectedIssueStatus.UNKNOWN) 303 return "unknown"; 304 return "?"; 305 } 306 307 public String toSystem(DetectedIssueStatus code) { 308 return code.getSystem(); 309 } 310 } 311 312 public enum DetectedIssueSeverity { 313 /** 314 * Indicates the issue may be life-threatening or has the potential to cause 315 * permanent injury. 316 */ 317 HIGH, 318 /** 319 * Indicates the issue may result in noticeable adverse consequences but is 320 * unlikely to be life-threatening or cause permanent injury. 321 */ 322 MODERATE, 323 /** 324 * Indicates the issue may result in some adverse consequences but is unlikely 325 * to substantially affect the situation of the subject. 326 */ 327 LOW, 328 /** 329 * added to help the parsers with the generic types 330 */ 331 NULL; 332 333 public static DetectedIssueSeverity fromCode(String codeString) throws FHIRException { 334 if (codeString == null || "".equals(codeString)) 335 return null; 336 if ("high".equals(codeString)) 337 return HIGH; 338 if ("moderate".equals(codeString)) 339 return MODERATE; 340 if ("low".equals(codeString)) 341 return LOW; 342 if (Configuration.isAcceptInvalidEnums()) 343 return null; 344 else 345 throw new FHIRException("Unknown DetectedIssueSeverity code '" + codeString + "'"); 346 } 347 348 public String toCode() { 349 switch (this) { 350 case HIGH: 351 return "high"; 352 case MODERATE: 353 return "moderate"; 354 case LOW: 355 return "low"; 356 case NULL: 357 return null; 358 default: 359 return "?"; 360 } 361 } 362 363 public String getSystem() { 364 switch (this) { 365 case HIGH: 366 return "http://hl7.org/fhir/detectedissue-severity"; 367 case MODERATE: 368 return "http://hl7.org/fhir/detectedissue-severity"; 369 case LOW: 370 return "http://hl7.org/fhir/detectedissue-severity"; 371 case NULL: 372 return null; 373 default: 374 return "?"; 375 } 376 } 377 378 public String getDefinition() { 379 switch (this) { 380 case HIGH: 381 return "Indicates the issue may be life-threatening or has the potential to cause permanent injury."; 382 case MODERATE: 383 return "Indicates the issue may result in noticeable adverse consequences but is unlikely to be life-threatening or cause permanent injury."; 384 case LOW: 385 return "Indicates the issue may result in some adverse consequences but is unlikely to substantially affect the situation of the subject."; 386 case NULL: 387 return null; 388 default: 389 return "?"; 390 } 391 } 392 393 public String getDisplay() { 394 switch (this) { 395 case HIGH: 396 return "High"; 397 case MODERATE: 398 return "Moderate"; 399 case LOW: 400 return "Low"; 401 case NULL: 402 return null; 403 default: 404 return "?"; 405 } 406 } 407 } 408 409 public static class DetectedIssueSeverityEnumFactory implements EnumFactory<DetectedIssueSeverity> { 410 public DetectedIssueSeverity fromCode(String codeString) throws IllegalArgumentException { 411 if (codeString == null || "".equals(codeString)) 412 if (codeString == null || "".equals(codeString)) 413 return null; 414 if ("high".equals(codeString)) 415 return DetectedIssueSeverity.HIGH; 416 if ("moderate".equals(codeString)) 417 return DetectedIssueSeverity.MODERATE; 418 if ("low".equals(codeString)) 419 return DetectedIssueSeverity.LOW; 420 throw new IllegalArgumentException("Unknown DetectedIssueSeverity code '" + codeString + "'"); 421 } 422 423 public Enumeration<DetectedIssueSeverity> fromType(PrimitiveType<?> code) throws FHIRException { 424 if (code == null) 425 return null; 426 if (code.isEmpty()) 427 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.NULL, code); 428 String codeString = code.asStringValue(); 429 if (codeString == null || "".equals(codeString)) 430 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.NULL, code); 431 if ("high".equals(codeString)) 432 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.HIGH, code); 433 if ("moderate".equals(codeString)) 434 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.MODERATE, code); 435 if ("low".equals(codeString)) 436 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.LOW, code); 437 throw new FHIRException("Unknown DetectedIssueSeverity code '" + codeString + "'"); 438 } 439 440 public String toCode(DetectedIssueSeverity code) { 441 if (code == DetectedIssueSeverity.HIGH) 442 return "high"; 443 if (code == DetectedIssueSeverity.MODERATE) 444 return "moderate"; 445 if (code == DetectedIssueSeverity.LOW) 446 return "low"; 447 return "?"; 448 } 449 450 public String toSystem(DetectedIssueSeverity code) { 451 return code.getSystem(); 452 } 453 } 454 455 @Block() 456 public static class DetectedIssueEvidenceComponent extends BackboneElement implements IBaseBackboneElement { 457 /** 458 * A manifestation that led to the recording of this detected issue. 459 */ 460 @Child(name = "code", type = { 461 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 462 @Description(shortDefinition = "Manifestation", formalDefinition = "A manifestation that led to the recording of this detected issue.") 463 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/manifestation-or-symptom") 464 protected List<CodeableConcept> code; 465 466 /** 467 * Links to resources that constitute evidence for the detected issue such as a 468 * GuidanceResponse or MeasureReport. 469 */ 470 @Child(name = "detail", type = { 471 Reference.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 472 @Description(shortDefinition = "Supporting information", formalDefinition = "Links to resources that constitute evidence for the detected issue such as a GuidanceResponse or MeasureReport.") 473 protected List<Reference> detail; 474 /** 475 * The actual objects that are the target of the reference (Links to resources 476 * that constitute evidence for the detected issue such as a GuidanceResponse or 477 * MeasureReport.) 478 */ 479 protected List<Resource> detailTarget; 480 481 private static final long serialVersionUID = 1135831276L; 482 483 /** 484 * Constructor 485 */ 486 public DetectedIssueEvidenceComponent() { 487 super(); 488 } 489 490 /** 491 * @return {@link #code} (A manifestation that led to the recording of this 492 * detected issue.) 493 */ 494 public List<CodeableConcept> getCode() { 495 if (this.code == null) 496 this.code = new ArrayList<CodeableConcept>(); 497 return this.code; 498 } 499 500 /** 501 * @return Returns a reference to <code>this</code> for easy method chaining 502 */ 503 public DetectedIssueEvidenceComponent setCode(List<CodeableConcept> theCode) { 504 this.code = theCode; 505 return this; 506 } 507 508 public boolean hasCode() { 509 if (this.code == null) 510 return false; 511 for (CodeableConcept item : this.code) 512 if (!item.isEmpty()) 513 return true; 514 return false; 515 } 516 517 public CodeableConcept addCode() { // 3 518 CodeableConcept t = new CodeableConcept(); 519 if (this.code == null) 520 this.code = new ArrayList<CodeableConcept>(); 521 this.code.add(t); 522 return t; 523 } 524 525 public DetectedIssueEvidenceComponent addCode(CodeableConcept t) { // 3 526 if (t == null) 527 return this; 528 if (this.code == null) 529 this.code = new ArrayList<CodeableConcept>(); 530 this.code.add(t); 531 return this; 532 } 533 534 /** 535 * @return The first repetition of repeating field {@link #code}, creating it if 536 * it does not already exist 537 */ 538 public CodeableConcept getCodeFirstRep() { 539 if (getCode().isEmpty()) { 540 addCode(); 541 } 542 return getCode().get(0); 543 } 544 545 /** 546 * @return {@link #detail} (Links to resources that constitute evidence for the 547 * detected issue such as a GuidanceResponse or MeasureReport.) 548 */ 549 public List<Reference> getDetail() { 550 if (this.detail == null) 551 this.detail = new ArrayList<Reference>(); 552 return this.detail; 553 } 554 555 /** 556 * @return Returns a reference to <code>this</code> for easy method chaining 557 */ 558 public DetectedIssueEvidenceComponent setDetail(List<Reference> theDetail) { 559 this.detail = theDetail; 560 return this; 561 } 562 563 public boolean hasDetail() { 564 if (this.detail == null) 565 return false; 566 for (Reference item : this.detail) 567 if (!item.isEmpty()) 568 return true; 569 return false; 570 } 571 572 public Reference addDetail() { // 3 573 Reference t = new Reference(); 574 if (this.detail == null) 575 this.detail = new ArrayList<Reference>(); 576 this.detail.add(t); 577 return t; 578 } 579 580 public DetectedIssueEvidenceComponent addDetail(Reference t) { // 3 581 if (t == null) 582 return this; 583 if (this.detail == null) 584 this.detail = new ArrayList<Reference>(); 585 this.detail.add(t); 586 return this; 587 } 588 589 /** 590 * @return The first repetition of repeating field {@link #detail}, creating it 591 * if it does not already exist 592 */ 593 public Reference getDetailFirstRep() { 594 if (getDetail().isEmpty()) { 595 addDetail(); 596 } 597 return getDetail().get(0); 598 } 599 600 /** 601 * @deprecated Use Reference#setResource(IBaseResource) instead 602 */ 603 @Deprecated 604 public List<Resource> getDetailTarget() { 605 if (this.detailTarget == null) 606 this.detailTarget = new ArrayList<Resource>(); 607 return this.detailTarget; 608 } 609 610 protected void listChildren(List<Property> children) { 611 super.listChildren(children); 612 children.add(new Property("code", "CodeableConcept", 613 "A manifestation that led to the recording of this detected issue.", 0, java.lang.Integer.MAX_VALUE, code)); 614 children.add(new Property("detail", "Reference(Any)", 615 "Links to resources that constitute evidence for the detected issue such as a GuidanceResponse or MeasureReport.", 616 0, java.lang.Integer.MAX_VALUE, detail)); 617 } 618 619 @Override 620 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 621 switch (_hash) { 622 case 3059181: 623 /* code */ return new Property("code", "CodeableConcept", 624 "A manifestation that led to the recording of this detected issue.", 0, java.lang.Integer.MAX_VALUE, code); 625 case -1335224239: 626 /* detail */ return new Property("detail", "Reference(Any)", 627 "Links to resources that constitute evidence for the detected issue such as a GuidanceResponse or MeasureReport.", 628 0, java.lang.Integer.MAX_VALUE, detail); 629 default: 630 return super.getNamedProperty(_hash, _name, _checkValid); 631 } 632 633 } 634 635 @Override 636 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 637 switch (hash) { 638 case 3059181: 639 /* code */ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 640 case -1335224239: 641 /* detail */ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // Reference 642 default: 643 return super.getProperty(hash, name, checkValid); 644 } 645 646 } 647 648 @Override 649 public Base setProperty(int hash, String name, Base value) throws FHIRException { 650 switch (hash) { 651 case 3059181: // code 652 this.getCode().add(castToCodeableConcept(value)); // CodeableConcept 653 return value; 654 case -1335224239: // detail 655 this.getDetail().add(castToReference(value)); // Reference 656 return value; 657 default: 658 return super.setProperty(hash, name, value); 659 } 660 661 } 662 663 @Override 664 public Base setProperty(String name, Base value) throws FHIRException { 665 if (name.equals("code")) { 666 this.getCode().add(castToCodeableConcept(value)); 667 } else if (name.equals("detail")) { 668 this.getDetail().add(castToReference(value)); 669 } else 670 return super.setProperty(name, value); 671 return value; 672 } 673 674 @Override 675 public void removeChild(String name, Base value) throws FHIRException { 676 if (name.equals("code")) { 677 this.getCode().remove(castToCodeableConcept(value)); 678 } else if (name.equals("detail")) { 679 this.getDetail().remove(castToReference(value)); 680 } else 681 super.removeChild(name, value); 682 683 } 684 685 @Override 686 public Base makeProperty(int hash, String name) throws FHIRException { 687 switch (hash) { 688 case 3059181: 689 return addCode(); 690 case -1335224239: 691 return addDetail(); 692 default: 693 return super.makeProperty(hash, name); 694 } 695 696 } 697 698 @Override 699 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 700 switch (hash) { 701 case 3059181: 702 /* code */ return new String[] { "CodeableConcept" }; 703 case -1335224239: 704 /* detail */ return new String[] { "Reference" }; 705 default: 706 return super.getTypesForProperty(hash, name); 707 } 708 709 } 710 711 @Override 712 public Base addChild(String name) throws FHIRException { 713 if (name.equals("code")) { 714 return addCode(); 715 } else if (name.equals("detail")) { 716 return addDetail(); 717 } else 718 return super.addChild(name); 719 } 720 721 public DetectedIssueEvidenceComponent copy() { 722 DetectedIssueEvidenceComponent dst = new DetectedIssueEvidenceComponent(); 723 copyValues(dst); 724 return dst; 725 } 726 727 public void copyValues(DetectedIssueEvidenceComponent dst) { 728 super.copyValues(dst); 729 if (code != null) { 730 dst.code = new ArrayList<CodeableConcept>(); 731 for (CodeableConcept i : code) 732 dst.code.add(i.copy()); 733 } 734 ; 735 if (detail != null) { 736 dst.detail = new ArrayList<Reference>(); 737 for (Reference i : detail) 738 dst.detail.add(i.copy()); 739 } 740 ; 741 } 742 743 @Override 744 public boolean equalsDeep(Base other_) { 745 if (!super.equalsDeep(other_)) 746 return false; 747 if (!(other_ instanceof DetectedIssueEvidenceComponent)) 748 return false; 749 DetectedIssueEvidenceComponent o = (DetectedIssueEvidenceComponent) other_; 750 return compareDeep(code, o.code, true) && compareDeep(detail, o.detail, true); 751 } 752 753 @Override 754 public boolean equalsShallow(Base other_) { 755 if (!super.equalsShallow(other_)) 756 return false; 757 if (!(other_ instanceof DetectedIssueEvidenceComponent)) 758 return false; 759 DetectedIssueEvidenceComponent o = (DetectedIssueEvidenceComponent) other_; 760 return true; 761 } 762 763 public boolean isEmpty() { 764 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, detail); 765 } 766 767 public String fhirType() { 768 return "DetectedIssue.evidence"; 769 770 } 771 772 } 773 774 @Block() 775 public static class DetectedIssueMitigationComponent extends BackboneElement implements IBaseBackboneElement { 776 /** 777 * Describes the action that was taken or the observation that was made that 778 * reduces/eliminates the risk associated with the identified issue. 779 */ 780 @Child(name = "action", type = { 781 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 782 @Description(shortDefinition = "What mitigation?", formalDefinition = "Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue.") 783 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/detectedissue-mitigation-action") 784 protected CodeableConcept action; 785 786 /** 787 * Indicates when the mitigating action was documented. 788 */ 789 @Child(name = "date", type = { DateTimeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 790 @Description(shortDefinition = "Date committed", formalDefinition = "Indicates when the mitigating action was documented.") 791 protected DateTimeType date; 792 793 /** 794 * Identifies the practitioner who determined the mitigation and takes 795 * responsibility for the mitigation step occurring. 796 */ 797 @Child(name = "author", type = { Practitioner.class, 798 PractitionerRole.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 799 @Description(shortDefinition = "Who is committing?", formalDefinition = "Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.") 800 protected Reference author; 801 802 /** 803 * The actual object that is the target of the reference (Identifies the 804 * practitioner who determined the mitigation and takes responsibility for the 805 * mitigation step occurring.) 806 */ 807 protected Resource authorTarget; 808 809 private static final long serialVersionUID = -1928864832L; 810 811 /** 812 * Constructor 813 */ 814 public DetectedIssueMitigationComponent() { 815 super(); 816 } 817 818 /** 819 * Constructor 820 */ 821 public DetectedIssueMitigationComponent(CodeableConcept action) { 822 super(); 823 this.action = action; 824 } 825 826 /** 827 * @return {@link #action} (Describes the action that was taken or the 828 * observation that was made that reduces/eliminates the risk associated 829 * with the identified issue.) 830 */ 831 public CodeableConcept getAction() { 832 if (this.action == null) 833 if (Configuration.errorOnAutoCreate()) 834 throw new Error("Attempt to auto-create DetectedIssueMitigationComponent.action"); 835 else if (Configuration.doAutoCreate()) 836 this.action = new CodeableConcept(); // cc 837 return this.action; 838 } 839 840 public boolean hasAction() { 841 return this.action != null && !this.action.isEmpty(); 842 } 843 844 /** 845 * @param value {@link #action} (Describes the action that was taken or the 846 * observation that was made that reduces/eliminates the risk 847 * associated with the identified issue.) 848 */ 849 public DetectedIssueMitigationComponent setAction(CodeableConcept value) { 850 this.action = value; 851 return this; 852 } 853 854 /** 855 * @return {@link #date} (Indicates when the mitigating action was documented.). 856 * This is the underlying object with id, value and extensions. The 857 * accessor "getDate" gives direct access to the value 858 */ 859 public DateTimeType getDateElement() { 860 if (this.date == null) 861 if (Configuration.errorOnAutoCreate()) 862 throw new Error("Attempt to auto-create DetectedIssueMitigationComponent.date"); 863 else if (Configuration.doAutoCreate()) 864 this.date = new DateTimeType(); // bb 865 return this.date; 866 } 867 868 public boolean hasDateElement() { 869 return this.date != null && !this.date.isEmpty(); 870 } 871 872 public boolean hasDate() { 873 return this.date != null && !this.date.isEmpty(); 874 } 875 876 /** 877 * @param value {@link #date} (Indicates when the mitigating action was 878 * documented.). This is the underlying object with id, value and 879 * extensions. The accessor "getDate" gives direct access to the 880 * value 881 */ 882 public DetectedIssueMitigationComponent setDateElement(DateTimeType value) { 883 this.date = value; 884 return this; 885 } 886 887 /** 888 * @return Indicates when the mitigating action was documented. 889 */ 890 public Date getDate() { 891 return this.date == null ? null : this.date.getValue(); 892 } 893 894 /** 895 * @param value Indicates when the mitigating action was documented. 896 */ 897 public DetectedIssueMitigationComponent setDate(Date value) { 898 if (value == null) 899 this.date = null; 900 else { 901 if (this.date == null) 902 this.date = new DateTimeType(); 903 this.date.setValue(value); 904 } 905 return this; 906 } 907 908 /** 909 * @return {@link #author} (Identifies the practitioner who determined the 910 * mitigation and takes responsibility for the mitigation step 911 * occurring.) 912 */ 913 public Reference getAuthor() { 914 if (this.author == null) 915 if (Configuration.errorOnAutoCreate()) 916 throw new Error("Attempt to auto-create DetectedIssueMitigationComponent.author"); 917 else if (Configuration.doAutoCreate()) 918 this.author = new Reference(); // cc 919 return this.author; 920 } 921 922 public boolean hasAuthor() { 923 return this.author != null && !this.author.isEmpty(); 924 } 925 926 /** 927 * @param value {@link #author} (Identifies the practitioner who determined the 928 * mitigation and takes responsibility for the mitigation step 929 * occurring.) 930 */ 931 public DetectedIssueMitigationComponent setAuthor(Reference value) { 932 this.author = value; 933 return this; 934 } 935 936 /** 937 * @return {@link #author} The actual object that is the target of the 938 * reference. The reference library doesn't populate this, but you can 939 * use it to hold the resource if you resolve it. (Identifies the 940 * practitioner who determined the mitigation and takes responsibility 941 * for the mitigation step occurring.) 942 */ 943 public Resource getAuthorTarget() { 944 return this.authorTarget; 945 } 946 947 /** 948 * @param value {@link #author} The actual object that is the target of the 949 * reference. The reference library doesn't use these, but you can 950 * use it to hold the resource if you resolve it. (Identifies the 951 * practitioner who determined the mitigation and takes 952 * responsibility for the mitigation step occurring.) 953 */ 954 public DetectedIssueMitigationComponent setAuthorTarget(Resource value) { 955 this.authorTarget = value; 956 return this; 957 } 958 959 protected void listChildren(List<Property> children) { 960 super.listChildren(children); 961 children.add(new Property("action", "CodeableConcept", 962 "Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue.", 963 0, 1, action)); 964 children 965 .add(new Property("date", "dateTime", "Indicates when the mitigating action was documented.", 0, 1, date)); 966 children.add(new Property("author", "Reference(Practitioner|PractitionerRole)", 967 "Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.", 968 0, 1, author)); 969 } 970 971 @Override 972 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 973 switch (_hash) { 974 case -1422950858: 975 /* action */ return new Property("action", "CodeableConcept", 976 "Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue.", 977 0, 1, action); 978 case 3076014: 979 /* date */ return new Property("date", "dateTime", "Indicates when the mitigating action was documented.", 0, 1, 980 date); 981 case -1406328437: 982 /* author */ return new Property("author", "Reference(Practitioner|PractitionerRole)", 983 "Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.", 984 0, 1, author); 985 default: 986 return super.getNamedProperty(_hash, _name, _checkValid); 987 } 988 989 } 990 991 @Override 992 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 993 switch (hash) { 994 case -1422950858: 995 /* action */ return this.action == null ? new Base[0] : new Base[] { this.action }; // CodeableConcept 996 case 3076014: 997 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 998 case -1406328437: 999 /* author */ return this.author == null ? new Base[0] : new Base[] { this.author }; // Reference 1000 default: 1001 return super.getProperty(hash, name, checkValid); 1002 } 1003 1004 } 1005 1006 @Override 1007 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1008 switch (hash) { 1009 case -1422950858: // action 1010 this.action = castToCodeableConcept(value); // CodeableConcept 1011 return value; 1012 case 3076014: // date 1013 this.date = castToDateTime(value); // DateTimeType 1014 return value; 1015 case -1406328437: // author 1016 this.author = castToReference(value); // Reference 1017 return value; 1018 default: 1019 return super.setProperty(hash, name, value); 1020 } 1021 1022 } 1023 1024 @Override 1025 public Base setProperty(String name, Base value) throws FHIRException { 1026 if (name.equals("action")) { 1027 this.action = castToCodeableConcept(value); // CodeableConcept 1028 } else if (name.equals("date")) { 1029 this.date = castToDateTime(value); // DateTimeType 1030 } else if (name.equals("author")) { 1031 this.author = castToReference(value); // Reference 1032 } else 1033 return super.setProperty(name, value); 1034 return value; 1035 } 1036 1037 @Override 1038 public void removeChild(String name, Base value) throws FHIRException { 1039 if (name.equals("action")) { 1040 this.action = null; 1041 } else if (name.equals("date")) { 1042 this.date = null; 1043 } else if (name.equals("author")) { 1044 this.author = null; 1045 } else 1046 super.removeChild(name, value); 1047 1048 } 1049 1050 @Override 1051 public Base makeProperty(int hash, String name) throws FHIRException { 1052 switch (hash) { 1053 case -1422950858: 1054 return getAction(); 1055 case 3076014: 1056 return getDateElement(); 1057 case -1406328437: 1058 return getAuthor(); 1059 default: 1060 return super.makeProperty(hash, name); 1061 } 1062 1063 } 1064 1065 @Override 1066 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1067 switch (hash) { 1068 case -1422950858: 1069 /* action */ return new String[] { "CodeableConcept" }; 1070 case 3076014: 1071 /* date */ return new String[] { "dateTime" }; 1072 case -1406328437: 1073 /* author */ return new String[] { "Reference" }; 1074 default: 1075 return super.getTypesForProperty(hash, name); 1076 } 1077 1078 } 1079 1080 @Override 1081 public Base addChild(String name) throws FHIRException { 1082 if (name.equals("action")) { 1083 this.action = new CodeableConcept(); 1084 return this.action; 1085 } else if (name.equals("date")) { 1086 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.date"); 1087 } else if (name.equals("author")) { 1088 this.author = new Reference(); 1089 return this.author; 1090 } else 1091 return super.addChild(name); 1092 } 1093 1094 public DetectedIssueMitigationComponent copy() { 1095 DetectedIssueMitigationComponent dst = new DetectedIssueMitigationComponent(); 1096 copyValues(dst); 1097 return dst; 1098 } 1099 1100 public void copyValues(DetectedIssueMitigationComponent dst) { 1101 super.copyValues(dst); 1102 dst.action = action == null ? null : action.copy(); 1103 dst.date = date == null ? null : date.copy(); 1104 dst.author = author == null ? null : author.copy(); 1105 } 1106 1107 @Override 1108 public boolean equalsDeep(Base other_) { 1109 if (!super.equalsDeep(other_)) 1110 return false; 1111 if (!(other_ instanceof DetectedIssueMitigationComponent)) 1112 return false; 1113 DetectedIssueMitigationComponent o = (DetectedIssueMitigationComponent) other_; 1114 return compareDeep(action, o.action, true) && compareDeep(date, o.date, true) 1115 && compareDeep(author, o.author, true); 1116 } 1117 1118 @Override 1119 public boolean equalsShallow(Base other_) { 1120 if (!super.equalsShallow(other_)) 1121 return false; 1122 if (!(other_ instanceof DetectedIssueMitigationComponent)) 1123 return false; 1124 DetectedIssueMitigationComponent o = (DetectedIssueMitigationComponent) other_; 1125 return compareValues(date, o.date, true); 1126 } 1127 1128 public boolean isEmpty() { 1129 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(action, date, author); 1130 } 1131 1132 public String fhirType() { 1133 return "DetectedIssue.mitigation"; 1134 1135 } 1136 1137 } 1138 1139 /** 1140 * Business identifier associated with the detected issue record. 1141 */ 1142 @Child(name = "identifier", type = { 1143 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1144 @Description(shortDefinition = "Unique id for the detected issue", formalDefinition = "Business identifier associated with the detected issue record.") 1145 protected List<Identifier> identifier; 1146 1147 /** 1148 * Indicates the status of the detected issue. 1149 */ 1150 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 1151 @Description(shortDefinition = "registered | preliminary | final | amended +", formalDefinition = "Indicates the status of the detected issue.") 1152 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-status") 1153 protected Enumeration<DetectedIssueStatus> status; 1154 1155 /** 1156 * Identifies the general type of issue identified. 1157 */ 1158 @Child(name = "code", type = { CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1159 @Description(shortDefinition = "Issue Category, e.g. drug-drug, duplicate therapy, etc.", formalDefinition = "Identifies the general type of issue identified.") 1160 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/detectedissue-category") 1161 protected CodeableConcept code; 1162 1163 /** 1164 * Indicates the degree of importance associated with the identified issue based 1165 * on the potential impact on the patient. 1166 */ 1167 @Child(name = "severity", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1168 @Description(shortDefinition = "high | moderate | low", formalDefinition = "Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.") 1169 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/detectedissue-severity") 1170 protected Enumeration<DetectedIssueSeverity> severity; 1171 1172 /** 1173 * Indicates the patient whose record the detected issue is associated with. 1174 */ 1175 @Child(name = "patient", type = { Patient.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1176 @Description(shortDefinition = "Associated patient", formalDefinition = "Indicates the patient whose record the detected issue is associated with.") 1177 protected Reference patient; 1178 1179 /** 1180 * The actual object that is the target of the reference (Indicates the patient 1181 * whose record the detected issue is associated with.) 1182 */ 1183 protected Patient patientTarget; 1184 1185 /** 1186 * The date or period when the detected issue was initially identified. 1187 */ 1188 @Child(name = "identified", type = { DateTimeType.class, 1189 Period.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1190 @Description(shortDefinition = "When identified", formalDefinition = "The date or period when the detected issue was initially identified.") 1191 protected Type identified; 1192 1193 /** 1194 * Individual or device responsible for the issue being raised. For example, a 1195 * decision support application or a pharmacist conducting a medication review. 1196 */ 1197 @Child(name = "author", type = { Practitioner.class, PractitionerRole.class, 1198 Device.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1199 @Description(shortDefinition = "The provider or device that identified the issue", formalDefinition = "Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.") 1200 protected Reference author; 1201 1202 /** 1203 * The actual object that is the target of the reference (Individual or device 1204 * responsible for the issue being raised. For example, a decision support 1205 * application or a pharmacist conducting a medication review.) 1206 */ 1207 protected Resource authorTarget; 1208 1209 /** 1210 * Indicates the resource representing the current activity or proposed activity 1211 * that is potentially problematic. 1212 */ 1213 @Child(name = "implicated", type = { 1214 Reference.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1215 @Description(shortDefinition = "Problem resource", formalDefinition = "Indicates the resource representing the current activity or proposed activity that is potentially problematic.") 1216 protected List<Reference> implicated; 1217 /** 1218 * The actual objects that are the target of the reference (Indicates the 1219 * resource representing the current activity or proposed activity that is 1220 * potentially problematic.) 1221 */ 1222 protected List<Resource> implicatedTarget; 1223 1224 /** 1225 * Supporting evidence or manifestations that provide the basis for identifying 1226 * the detected issue such as a GuidanceResponse or MeasureReport. 1227 */ 1228 @Child(name = "evidence", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1229 @Description(shortDefinition = "Supporting evidence", formalDefinition = "Supporting evidence or manifestations that provide the basis for identifying the detected issue such as a GuidanceResponse or MeasureReport.") 1230 protected List<DetectedIssueEvidenceComponent> evidence; 1231 1232 /** 1233 * A textual explanation of the detected issue. 1234 */ 1235 @Child(name = "detail", type = { StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1236 @Description(shortDefinition = "Description and context", formalDefinition = "A textual explanation of the detected issue.") 1237 protected StringType detail; 1238 1239 /** 1240 * The literature, knowledge-base or similar reference that describes the 1241 * propensity for the detected issue identified. 1242 */ 1243 @Child(name = "reference", type = { UriType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 1244 @Description(shortDefinition = "Authority for issue", formalDefinition = "The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.") 1245 protected UriType reference; 1246 1247 /** 1248 * Indicates an action that has been taken or is committed to reduce or 1249 * eliminate the likelihood of the risk identified by the detected issue from 1250 * manifesting. Can also reflect an observation of known mitigating factors that 1251 * may reduce/eliminate the need for any action. 1252 */ 1253 @Child(name = "mitigation", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1254 @Description(shortDefinition = "Step taken to address", formalDefinition = "Indicates an action that has been taken or is committed to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action.") 1255 protected List<DetectedIssueMitigationComponent> mitigation; 1256 1257 private static final long serialVersionUID = 1404426283L; 1258 1259 /** 1260 * Constructor 1261 */ 1262 public DetectedIssue() { 1263 super(); 1264 } 1265 1266 /** 1267 * Constructor 1268 */ 1269 public DetectedIssue(Enumeration<DetectedIssueStatus> status) { 1270 super(); 1271 this.status = status; 1272 } 1273 1274 /** 1275 * @return {@link #identifier} (Business identifier associated with the detected 1276 * issue record.) 1277 */ 1278 public List<Identifier> getIdentifier() { 1279 if (this.identifier == null) 1280 this.identifier = new ArrayList<Identifier>(); 1281 return this.identifier; 1282 } 1283 1284 /** 1285 * @return Returns a reference to <code>this</code> for easy method chaining 1286 */ 1287 public DetectedIssue setIdentifier(List<Identifier> theIdentifier) { 1288 this.identifier = theIdentifier; 1289 return this; 1290 } 1291 1292 public boolean hasIdentifier() { 1293 if (this.identifier == null) 1294 return false; 1295 for (Identifier item : this.identifier) 1296 if (!item.isEmpty()) 1297 return true; 1298 return false; 1299 } 1300 1301 public Identifier addIdentifier() { // 3 1302 Identifier t = new Identifier(); 1303 if (this.identifier == null) 1304 this.identifier = new ArrayList<Identifier>(); 1305 this.identifier.add(t); 1306 return t; 1307 } 1308 1309 public DetectedIssue addIdentifier(Identifier t) { // 3 1310 if (t == null) 1311 return this; 1312 if (this.identifier == null) 1313 this.identifier = new ArrayList<Identifier>(); 1314 this.identifier.add(t); 1315 return this; 1316 } 1317 1318 /** 1319 * @return The first repetition of repeating field {@link #identifier}, creating 1320 * it if it does not already exist 1321 */ 1322 public Identifier getIdentifierFirstRep() { 1323 if (getIdentifier().isEmpty()) { 1324 addIdentifier(); 1325 } 1326 return getIdentifier().get(0); 1327 } 1328 1329 /** 1330 * @return {@link #status} (Indicates the status of the detected issue.). This 1331 * is the underlying object with id, value and extensions. The accessor 1332 * "getStatus" gives direct access to the value 1333 */ 1334 public Enumeration<DetectedIssueStatus> getStatusElement() { 1335 if (this.status == null) 1336 if (Configuration.errorOnAutoCreate()) 1337 throw new Error("Attempt to auto-create DetectedIssue.status"); 1338 else if (Configuration.doAutoCreate()) 1339 this.status = new Enumeration<DetectedIssueStatus>(new DetectedIssueStatusEnumFactory()); // bb 1340 return this.status; 1341 } 1342 1343 public boolean hasStatusElement() { 1344 return this.status != null && !this.status.isEmpty(); 1345 } 1346 1347 public boolean hasStatus() { 1348 return this.status != null && !this.status.isEmpty(); 1349 } 1350 1351 /** 1352 * @param value {@link #status} (Indicates the status of the detected issue.). 1353 * This is the underlying object with id, value and extensions. The 1354 * accessor "getStatus" gives direct access to the value 1355 */ 1356 public DetectedIssue setStatusElement(Enumeration<DetectedIssueStatus> value) { 1357 this.status = value; 1358 return this; 1359 } 1360 1361 /** 1362 * @return Indicates the status of the detected issue. 1363 */ 1364 public DetectedIssueStatus getStatus() { 1365 return this.status == null ? null : this.status.getValue(); 1366 } 1367 1368 /** 1369 * @param value Indicates the status of the detected issue. 1370 */ 1371 public DetectedIssue setStatus(DetectedIssueStatus value) { 1372 if (this.status == null) 1373 this.status = new Enumeration<DetectedIssueStatus>(new DetectedIssueStatusEnumFactory()); 1374 this.status.setValue(value); 1375 return this; 1376 } 1377 1378 /** 1379 * @return {@link #code} (Identifies the general type of issue identified.) 1380 */ 1381 public CodeableConcept getCode() { 1382 if (this.code == null) 1383 if (Configuration.errorOnAutoCreate()) 1384 throw new Error("Attempt to auto-create DetectedIssue.code"); 1385 else if (Configuration.doAutoCreate()) 1386 this.code = new CodeableConcept(); // cc 1387 return this.code; 1388 } 1389 1390 public boolean hasCode() { 1391 return this.code != null && !this.code.isEmpty(); 1392 } 1393 1394 /** 1395 * @param value {@link #code} (Identifies the general type of issue identified.) 1396 */ 1397 public DetectedIssue setCode(CodeableConcept value) { 1398 this.code = value; 1399 return this; 1400 } 1401 1402 /** 1403 * @return {@link #severity} (Indicates the degree of importance associated with 1404 * the identified issue based on the potential impact on the patient.). 1405 * This is the underlying object with id, value and extensions. The 1406 * accessor "getSeverity" gives direct access to the value 1407 */ 1408 public Enumeration<DetectedIssueSeverity> getSeverityElement() { 1409 if (this.severity == null) 1410 if (Configuration.errorOnAutoCreate()) 1411 throw new Error("Attempt to auto-create DetectedIssue.severity"); 1412 else if (Configuration.doAutoCreate()) 1413 this.severity = new Enumeration<DetectedIssueSeverity>(new DetectedIssueSeverityEnumFactory()); // bb 1414 return this.severity; 1415 } 1416 1417 public boolean hasSeverityElement() { 1418 return this.severity != null && !this.severity.isEmpty(); 1419 } 1420 1421 public boolean hasSeverity() { 1422 return this.severity != null && !this.severity.isEmpty(); 1423 } 1424 1425 /** 1426 * @param value {@link #severity} (Indicates the degree of importance associated 1427 * with the identified issue based on the potential impact on the 1428 * patient.). This is the underlying object with id, value and 1429 * extensions. The accessor "getSeverity" gives direct access to 1430 * the value 1431 */ 1432 public DetectedIssue setSeverityElement(Enumeration<DetectedIssueSeverity> value) { 1433 this.severity = value; 1434 return this; 1435 } 1436 1437 /** 1438 * @return Indicates the degree of importance associated with the identified 1439 * issue based on the potential impact on the patient. 1440 */ 1441 public DetectedIssueSeverity getSeverity() { 1442 return this.severity == null ? null : this.severity.getValue(); 1443 } 1444 1445 /** 1446 * @param value Indicates the degree of importance associated with the 1447 * identified issue based on the potential impact on the patient. 1448 */ 1449 public DetectedIssue setSeverity(DetectedIssueSeverity value) { 1450 if (value == null) 1451 this.severity = null; 1452 else { 1453 if (this.severity == null) 1454 this.severity = new Enumeration<DetectedIssueSeverity>(new DetectedIssueSeverityEnumFactory()); 1455 this.severity.setValue(value); 1456 } 1457 return this; 1458 } 1459 1460 /** 1461 * @return {@link #patient} (Indicates the patient whose record the detected 1462 * issue is associated with.) 1463 */ 1464 public Reference getPatient() { 1465 if (this.patient == null) 1466 if (Configuration.errorOnAutoCreate()) 1467 throw new Error("Attempt to auto-create DetectedIssue.patient"); 1468 else if (Configuration.doAutoCreate()) 1469 this.patient = new Reference(); // cc 1470 return this.patient; 1471 } 1472 1473 public boolean hasPatient() { 1474 return this.patient != null && !this.patient.isEmpty(); 1475 } 1476 1477 /** 1478 * @param value {@link #patient} (Indicates the patient whose record the 1479 * detected issue is associated with.) 1480 */ 1481 public DetectedIssue setPatient(Reference value) { 1482 this.patient = value; 1483 return this; 1484 } 1485 1486 /** 1487 * @return {@link #patient} The actual object that is the target of the 1488 * reference. The reference library doesn't populate this, but you can 1489 * use it to hold the resource if you resolve it. (Indicates the patient 1490 * whose record the detected issue is associated with.) 1491 */ 1492 public Patient getPatientTarget() { 1493 if (this.patientTarget == null) 1494 if (Configuration.errorOnAutoCreate()) 1495 throw new Error("Attempt to auto-create DetectedIssue.patient"); 1496 else if (Configuration.doAutoCreate()) 1497 this.patientTarget = new Patient(); // aa 1498 return this.patientTarget; 1499 } 1500 1501 /** 1502 * @param value {@link #patient} The actual object that is the target of the 1503 * reference. The reference library doesn't use these, but you can 1504 * use it to hold the resource if you resolve it. (Indicates the 1505 * patient whose record the detected issue is associated with.) 1506 */ 1507 public DetectedIssue setPatientTarget(Patient value) { 1508 this.patientTarget = value; 1509 return this; 1510 } 1511 1512 /** 1513 * @return {@link #identified} (The date or period when the detected issue was 1514 * initially identified.) 1515 */ 1516 public Type getIdentified() { 1517 return this.identified; 1518 } 1519 1520 /** 1521 * @return {@link #identified} (The date or period when the detected issue was 1522 * initially identified.) 1523 */ 1524 public DateTimeType getIdentifiedDateTimeType() throws FHIRException { 1525 if (this.identified == null) 1526 this.identified = new DateTimeType(); 1527 if (!(this.identified instanceof DateTimeType)) 1528 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1529 + this.identified.getClass().getName() + " was encountered"); 1530 return (DateTimeType) this.identified; 1531 } 1532 1533 public boolean hasIdentifiedDateTimeType() { 1534 return this != null && this.identified instanceof DateTimeType; 1535 } 1536 1537 /** 1538 * @return {@link #identified} (The date or period when the detected issue was 1539 * initially identified.) 1540 */ 1541 public Period getIdentifiedPeriod() throws FHIRException { 1542 if (this.identified == null) 1543 this.identified = new Period(); 1544 if (!(this.identified instanceof Period)) 1545 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.identified.getClass().getName() 1546 + " was encountered"); 1547 return (Period) this.identified; 1548 } 1549 1550 public boolean hasIdentifiedPeriod() { 1551 return this != null && this.identified instanceof Period; 1552 } 1553 1554 public boolean hasIdentified() { 1555 return this.identified != null && !this.identified.isEmpty(); 1556 } 1557 1558 /** 1559 * @param value {@link #identified} (The date or period when the detected issue 1560 * was initially identified.) 1561 */ 1562 public DetectedIssue setIdentified(Type value) { 1563 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1564 throw new Error("Not the right type for DetectedIssue.identified[x]: " + value.fhirType()); 1565 this.identified = value; 1566 return this; 1567 } 1568 1569 /** 1570 * @return {@link #author} (Individual or device responsible for the issue being 1571 * raised. For example, a decision support application or a pharmacist 1572 * conducting a medication review.) 1573 */ 1574 public Reference getAuthor() { 1575 if (this.author == null) 1576 if (Configuration.errorOnAutoCreate()) 1577 throw new Error("Attempt to auto-create DetectedIssue.author"); 1578 else if (Configuration.doAutoCreate()) 1579 this.author = new Reference(); // cc 1580 return this.author; 1581 } 1582 1583 public boolean hasAuthor() { 1584 return this.author != null && !this.author.isEmpty(); 1585 } 1586 1587 /** 1588 * @param value {@link #author} (Individual or device responsible for the issue 1589 * being raised. For example, a decision support application or a 1590 * pharmacist conducting a medication review.) 1591 */ 1592 public DetectedIssue setAuthor(Reference value) { 1593 this.author = value; 1594 return this; 1595 } 1596 1597 /** 1598 * @return {@link #author} The actual object that is the target of the 1599 * reference. The reference library doesn't populate this, but you can 1600 * use it to hold the resource if you resolve it. (Individual or device 1601 * responsible for the issue being raised. For example, a decision 1602 * support application or a pharmacist conducting a medication review.) 1603 */ 1604 public Resource getAuthorTarget() { 1605 return this.authorTarget; 1606 } 1607 1608 /** 1609 * @param value {@link #author} The actual object that is the target of the 1610 * reference. The reference library doesn't use these, but you can 1611 * use it to hold the resource if you resolve it. (Individual or 1612 * device responsible for the issue being raised. For example, a 1613 * decision support application or a pharmacist conducting a 1614 * medication review.) 1615 */ 1616 public DetectedIssue setAuthorTarget(Resource value) { 1617 this.authorTarget = value; 1618 return this; 1619 } 1620 1621 /** 1622 * @return {@link #implicated} (Indicates the resource representing the current 1623 * activity or proposed activity that is potentially problematic.) 1624 */ 1625 public List<Reference> getImplicated() { 1626 if (this.implicated == null) 1627 this.implicated = new ArrayList<Reference>(); 1628 return this.implicated; 1629 } 1630 1631 /** 1632 * @return Returns a reference to <code>this</code> for easy method chaining 1633 */ 1634 public DetectedIssue setImplicated(List<Reference> theImplicated) { 1635 this.implicated = theImplicated; 1636 return this; 1637 } 1638 1639 public boolean hasImplicated() { 1640 if (this.implicated == null) 1641 return false; 1642 for (Reference item : this.implicated) 1643 if (!item.isEmpty()) 1644 return true; 1645 return false; 1646 } 1647 1648 public Reference addImplicated() { // 3 1649 Reference t = new Reference(); 1650 if (this.implicated == null) 1651 this.implicated = new ArrayList<Reference>(); 1652 this.implicated.add(t); 1653 return t; 1654 } 1655 1656 public DetectedIssue addImplicated(Reference t) { // 3 1657 if (t == null) 1658 return this; 1659 if (this.implicated == null) 1660 this.implicated = new ArrayList<Reference>(); 1661 this.implicated.add(t); 1662 return this; 1663 } 1664 1665 /** 1666 * @return The first repetition of repeating field {@link #implicated}, creating 1667 * it if it does not already exist 1668 */ 1669 public Reference getImplicatedFirstRep() { 1670 if (getImplicated().isEmpty()) { 1671 addImplicated(); 1672 } 1673 return getImplicated().get(0); 1674 } 1675 1676 /** 1677 * @deprecated Use Reference#setResource(IBaseResource) instead 1678 */ 1679 @Deprecated 1680 public List<Resource> getImplicatedTarget() { 1681 if (this.implicatedTarget == null) 1682 this.implicatedTarget = new ArrayList<Resource>(); 1683 return this.implicatedTarget; 1684 } 1685 1686 /** 1687 * @return {@link #evidence} (Supporting evidence or manifestations that provide 1688 * the basis for identifying the detected issue such as a 1689 * GuidanceResponse or MeasureReport.) 1690 */ 1691 public List<DetectedIssueEvidenceComponent> getEvidence() { 1692 if (this.evidence == null) 1693 this.evidence = new ArrayList<DetectedIssueEvidenceComponent>(); 1694 return this.evidence; 1695 } 1696 1697 /** 1698 * @return Returns a reference to <code>this</code> for easy method chaining 1699 */ 1700 public DetectedIssue setEvidence(List<DetectedIssueEvidenceComponent> theEvidence) { 1701 this.evidence = theEvidence; 1702 return this; 1703 } 1704 1705 public boolean hasEvidence() { 1706 if (this.evidence == null) 1707 return false; 1708 for (DetectedIssueEvidenceComponent item : this.evidence) 1709 if (!item.isEmpty()) 1710 return true; 1711 return false; 1712 } 1713 1714 public DetectedIssueEvidenceComponent addEvidence() { // 3 1715 DetectedIssueEvidenceComponent t = new DetectedIssueEvidenceComponent(); 1716 if (this.evidence == null) 1717 this.evidence = new ArrayList<DetectedIssueEvidenceComponent>(); 1718 this.evidence.add(t); 1719 return t; 1720 } 1721 1722 public DetectedIssue addEvidence(DetectedIssueEvidenceComponent t) { // 3 1723 if (t == null) 1724 return this; 1725 if (this.evidence == null) 1726 this.evidence = new ArrayList<DetectedIssueEvidenceComponent>(); 1727 this.evidence.add(t); 1728 return this; 1729 } 1730 1731 /** 1732 * @return The first repetition of repeating field {@link #evidence}, creating 1733 * it if it does not already exist 1734 */ 1735 public DetectedIssueEvidenceComponent getEvidenceFirstRep() { 1736 if (getEvidence().isEmpty()) { 1737 addEvidence(); 1738 } 1739 return getEvidence().get(0); 1740 } 1741 1742 /** 1743 * @return {@link #detail} (A textual explanation of the detected issue.). This 1744 * is the underlying object with id, value and extensions. The accessor 1745 * "getDetail" gives direct access to the value 1746 */ 1747 public StringType getDetailElement() { 1748 if (this.detail == null) 1749 if (Configuration.errorOnAutoCreate()) 1750 throw new Error("Attempt to auto-create DetectedIssue.detail"); 1751 else if (Configuration.doAutoCreate()) 1752 this.detail = new StringType(); // bb 1753 return this.detail; 1754 } 1755 1756 public boolean hasDetailElement() { 1757 return this.detail != null && !this.detail.isEmpty(); 1758 } 1759 1760 public boolean hasDetail() { 1761 return this.detail != null && !this.detail.isEmpty(); 1762 } 1763 1764 /** 1765 * @param value {@link #detail} (A textual explanation of the detected issue.). 1766 * This is the underlying object with id, value and extensions. The 1767 * accessor "getDetail" gives direct access to the value 1768 */ 1769 public DetectedIssue setDetailElement(StringType value) { 1770 this.detail = value; 1771 return this; 1772 } 1773 1774 /** 1775 * @return A textual explanation of the detected issue. 1776 */ 1777 public String getDetail() { 1778 return this.detail == null ? null : this.detail.getValue(); 1779 } 1780 1781 /** 1782 * @param value A textual explanation of the detected issue. 1783 */ 1784 public DetectedIssue setDetail(String value) { 1785 if (Utilities.noString(value)) 1786 this.detail = null; 1787 else { 1788 if (this.detail == null) 1789 this.detail = new StringType(); 1790 this.detail.setValue(value); 1791 } 1792 return this; 1793 } 1794 1795 /** 1796 * @return {@link #reference} (The literature, knowledge-base or similar 1797 * reference that describes the propensity for the detected issue 1798 * identified.). This is the underlying object with id, value and 1799 * extensions. The accessor "getReference" gives direct access to the 1800 * value 1801 */ 1802 public UriType getReferenceElement() { 1803 if (this.reference == null) 1804 if (Configuration.errorOnAutoCreate()) 1805 throw new Error("Attempt to auto-create DetectedIssue.reference"); 1806 else if (Configuration.doAutoCreate()) 1807 this.reference = new UriType(); // bb 1808 return this.reference; 1809 } 1810 1811 public boolean hasReferenceElement() { 1812 return this.reference != null && !this.reference.isEmpty(); 1813 } 1814 1815 public boolean hasReference() { 1816 return this.reference != null && !this.reference.isEmpty(); 1817 } 1818 1819 /** 1820 * @param value {@link #reference} (The literature, knowledge-base or similar 1821 * reference that describes the propensity for the detected issue 1822 * identified.). This is the underlying object with id, value and 1823 * extensions. The accessor "getReference" gives direct access to 1824 * the value 1825 */ 1826 public DetectedIssue setReferenceElement(UriType value) { 1827 this.reference = value; 1828 return this; 1829 } 1830 1831 /** 1832 * @return The literature, knowledge-base or similar reference that describes 1833 * the propensity for the detected issue identified. 1834 */ 1835 public String getReference() { 1836 return this.reference == null ? null : this.reference.getValue(); 1837 } 1838 1839 /** 1840 * @param value The literature, knowledge-base or similar reference that 1841 * describes the propensity for the detected issue identified. 1842 */ 1843 public DetectedIssue setReference(String value) { 1844 if (Utilities.noString(value)) 1845 this.reference = null; 1846 else { 1847 if (this.reference == null) 1848 this.reference = new UriType(); 1849 this.reference.setValue(value); 1850 } 1851 return this; 1852 } 1853 1854 /** 1855 * @return {@link #mitigation} (Indicates an action that has been taken or is 1856 * committed to reduce or eliminate the likelihood of the risk 1857 * identified by the detected issue from manifesting. Can also reflect 1858 * an observation of known mitigating factors that may reduce/eliminate 1859 * the need for any action.) 1860 */ 1861 public List<DetectedIssueMitigationComponent> getMitigation() { 1862 if (this.mitigation == null) 1863 this.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 1864 return this.mitigation; 1865 } 1866 1867 /** 1868 * @return Returns a reference to <code>this</code> for easy method chaining 1869 */ 1870 public DetectedIssue setMitigation(List<DetectedIssueMitigationComponent> theMitigation) { 1871 this.mitigation = theMitigation; 1872 return this; 1873 } 1874 1875 public boolean hasMitigation() { 1876 if (this.mitigation == null) 1877 return false; 1878 for (DetectedIssueMitigationComponent item : this.mitigation) 1879 if (!item.isEmpty()) 1880 return true; 1881 return false; 1882 } 1883 1884 public DetectedIssueMitigationComponent addMitigation() { // 3 1885 DetectedIssueMitigationComponent t = new DetectedIssueMitigationComponent(); 1886 if (this.mitigation == null) 1887 this.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 1888 this.mitigation.add(t); 1889 return t; 1890 } 1891 1892 public DetectedIssue addMitigation(DetectedIssueMitigationComponent t) { // 3 1893 if (t == null) 1894 return this; 1895 if (this.mitigation == null) 1896 this.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 1897 this.mitigation.add(t); 1898 return this; 1899 } 1900 1901 /** 1902 * @return The first repetition of repeating field {@link #mitigation}, creating 1903 * it if it does not already exist 1904 */ 1905 public DetectedIssueMitigationComponent getMitigationFirstRep() { 1906 if (getMitigation().isEmpty()) { 1907 addMitigation(); 1908 } 1909 return getMitigation().get(0); 1910 } 1911 1912 protected void listChildren(List<Property> children) { 1913 super.listChildren(children); 1914 children.add(new Property("identifier", "Identifier", 1915 "Business identifier associated with the detected issue record.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1916 children.add(new Property("status", "code", "Indicates the status of the detected issue.", 0, 1, status)); 1917 children 1918 .add(new Property("code", "CodeableConcept", "Identifies the general type of issue identified.", 0, 1, code)); 1919 children.add(new Property("severity", "code", 1920 "Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.", 1921 0, 1, severity)); 1922 children.add(new Property("patient", "Reference(Patient)", 1923 "Indicates the patient whose record the detected issue is associated with.", 0, 1, patient)); 1924 children.add(new Property("identified[x]", "dateTime|Period", 1925 "The date or period when the detected issue was initially identified.", 0, 1, identified)); 1926 children.add(new Property("author", "Reference(Practitioner|PractitionerRole|Device)", 1927 "Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.", 1928 0, 1, author)); 1929 children.add(new Property("implicated", "Reference(Any)", 1930 "Indicates the resource representing the current activity or proposed activity that is potentially problematic.", 1931 0, java.lang.Integer.MAX_VALUE, implicated)); 1932 children.add(new Property("evidence", "", 1933 "Supporting evidence or manifestations that provide the basis for identifying the detected issue such as a GuidanceResponse or MeasureReport.", 1934 0, java.lang.Integer.MAX_VALUE, evidence)); 1935 children.add(new Property("detail", "string", "A textual explanation of the detected issue.", 0, 1, detail)); 1936 children.add(new Property("reference", "uri", 1937 "The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.", 1938 0, 1, reference)); 1939 children.add(new Property("mitigation", "", 1940 "Indicates an action that has been taken or is committed to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action.", 1941 0, java.lang.Integer.MAX_VALUE, mitigation)); 1942 } 1943 1944 @Override 1945 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1946 switch (_hash) { 1947 case -1618432855: 1948 /* identifier */ return new Property("identifier", "Identifier", 1949 "Business identifier associated with the detected issue record.", 0, java.lang.Integer.MAX_VALUE, identifier); 1950 case -892481550: 1951 /* status */ return new Property("status", "code", "Indicates the status of the detected issue.", 0, 1, status); 1952 case 3059181: 1953 /* code */ return new Property("code", "CodeableConcept", "Identifies the general type of issue identified.", 0, 1954 1, code); 1955 case 1478300413: 1956 /* severity */ return new Property("severity", "code", 1957 "Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.", 1958 0, 1, severity); 1959 case -791418107: 1960 /* patient */ return new Property("patient", "Reference(Patient)", 1961 "Indicates the patient whose record the detected issue is associated with.", 0, 1, patient); 1962 case 569355781: 1963 /* identified[x] */ return new Property("identified[x]", "dateTime|Period", 1964 "The date or period when the detected issue was initially identified.", 0, 1, identified); 1965 case -1618432869: 1966 /* identified */ return new Property("identified[x]", "dateTime|Period", 1967 "The date or period when the detected issue was initially identified.", 0, 1, identified); 1968 case -968788650: 1969 /* identifiedDateTime */ return new Property("identified[x]", "dateTime|Period", 1970 "The date or period when the detected issue was initially identified.", 0, 1, identified); 1971 case 520482364: 1972 /* identifiedPeriod */ return new Property("identified[x]", "dateTime|Period", 1973 "The date or period when the detected issue was initially identified.", 0, 1, identified); 1974 case -1406328437: 1975 /* author */ return new Property("author", "Reference(Practitioner|PractitionerRole|Device)", 1976 "Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.", 1977 0, 1, author); 1978 case -810216884: 1979 /* implicated */ return new Property("implicated", "Reference(Any)", 1980 "Indicates the resource representing the current activity or proposed activity that is potentially problematic.", 1981 0, java.lang.Integer.MAX_VALUE, implicated); 1982 case 382967383: 1983 /* evidence */ return new Property("evidence", "", 1984 "Supporting evidence or manifestations that provide the basis for identifying the detected issue such as a GuidanceResponse or MeasureReport.", 1985 0, java.lang.Integer.MAX_VALUE, evidence); 1986 case -1335224239: 1987 /* detail */ return new Property("detail", "string", "A textual explanation of the detected issue.", 0, 1, 1988 detail); 1989 case -925155509: 1990 /* reference */ return new Property("reference", "uri", 1991 "The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.", 1992 0, 1, reference); 1993 case 1293793087: 1994 /* mitigation */ return new Property("mitigation", "", 1995 "Indicates an action that has been taken or is committed to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action.", 1996 0, java.lang.Integer.MAX_VALUE, mitigation); 1997 default: 1998 return super.getNamedProperty(_hash, _name, _checkValid); 1999 } 2000 2001 } 2002 2003 @Override 2004 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2005 switch (hash) { 2006 case -1618432855: 2007 /* identifier */ return this.identifier == null ? new Base[0] 2008 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2009 case -892481550: 2010 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<DetectedIssueStatus> 2011 case 3059181: 2012 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 2013 case 1478300413: 2014 /* severity */ return this.severity == null ? new Base[0] : new Base[] { this.severity }; // Enumeration<DetectedIssueSeverity> 2015 case -791418107: 2016 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 2017 case -1618432869: 2018 /* identified */ return this.identified == null ? new Base[0] : new Base[] { this.identified }; // Type 2019 case -1406328437: 2020 /* author */ return this.author == null ? new Base[0] : new Base[] { this.author }; // Reference 2021 case -810216884: 2022 /* implicated */ return this.implicated == null ? new Base[0] 2023 : this.implicated.toArray(new Base[this.implicated.size()]); // Reference 2024 case 382967383: 2025 /* evidence */ return this.evidence == null ? new Base[0] : this.evidence.toArray(new Base[this.evidence.size()]); // DetectedIssueEvidenceComponent 2026 case -1335224239: 2027 /* detail */ return this.detail == null ? new Base[0] : new Base[] { this.detail }; // StringType 2028 case -925155509: 2029 /* reference */ return this.reference == null ? new Base[0] : new Base[] { this.reference }; // UriType 2030 case 1293793087: 2031 /* mitigation */ return this.mitigation == null ? new Base[0] 2032 : this.mitigation.toArray(new Base[this.mitigation.size()]); // DetectedIssueMitigationComponent 2033 default: 2034 return super.getProperty(hash, name, checkValid); 2035 } 2036 2037 } 2038 2039 @Override 2040 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2041 switch (hash) { 2042 case -1618432855: // identifier 2043 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2044 return value; 2045 case -892481550: // status 2046 value = new DetectedIssueStatusEnumFactory().fromType(castToCode(value)); 2047 this.status = (Enumeration) value; // Enumeration<DetectedIssueStatus> 2048 return value; 2049 case 3059181: // code 2050 this.code = castToCodeableConcept(value); // CodeableConcept 2051 return value; 2052 case 1478300413: // severity 2053 value = new DetectedIssueSeverityEnumFactory().fromType(castToCode(value)); 2054 this.severity = (Enumeration) value; // Enumeration<DetectedIssueSeverity> 2055 return value; 2056 case -791418107: // patient 2057 this.patient = castToReference(value); // Reference 2058 return value; 2059 case -1618432869: // identified 2060 this.identified = castToType(value); // Type 2061 return value; 2062 case -1406328437: // author 2063 this.author = castToReference(value); // Reference 2064 return value; 2065 case -810216884: // implicated 2066 this.getImplicated().add(castToReference(value)); // Reference 2067 return value; 2068 case 382967383: // evidence 2069 this.getEvidence().add((DetectedIssueEvidenceComponent) value); // DetectedIssueEvidenceComponent 2070 return value; 2071 case -1335224239: // detail 2072 this.detail = castToString(value); // StringType 2073 return value; 2074 case -925155509: // reference 2075 this.reference = castToUri(value); // UriType 2076 return value; 2077 case 1293793087: // mitigation 2078 this.getMitigation().add((DetectedIssueMitigationComponent) value); // DetectedIssueMitigationComponent 2079 return value; 2080 default: 2081 return super.setProperty(hash, name, value); 2082 } 2083 2084 } 2085 2086 @Override 2087 public Base setProperty(String name, Base value) throws FHIRException { 2088 if (name.equals("identifier")) { 2089 this.getIdentifier().add(castToIdentifier(value)); 2090 } else if (name.equals("status")) { 2091 value = new DetectedIssueStatusEnumFactory().fromType(castToCode(value)); 2092 this.status = (Enumeration) value; // Enumeration<DetectedIssueStatus> 2093 } else if (name.equals("code")) { 2094 this.code = castToCodeableConcept(value); // CodeableConcept 2095 } else if (name.equals("severity")) { 2096 value = new DetectedIssueSeverityEnumFactory().fromType(castToCode(value)); 2097 this.severity = (Enumeration) value; // Enumeration<DetectedIssueSeverity> 2098 } else if (name.equals("patient")) { 2099 this.patient = castToReference(value); // Reference 2100 } else if (name.equals("identified[x]")) { 2101 this.identified = castToType(value); // Type 2102 } else if (name.equals("author")) { 2103 this.author = castToReference(value); // Reference 2104 } else if (name.equals("implicated")) { 2105 this.getImplicated().add(castToReference(value)); 2106 } else if (name.equals("evidence")) { 2107 this.getEvidence().add((DetectedIssueEvidenceComponent) value); 2108 } else if (name.equals("detail")) { 2109 this.detail = castToString(value); // StringType 2110 } else if (name.equals("reference")) { 2111 this.reference = castToUri(value); // UriType 2112 } else if (name.equals("mitigation")) { 2113 this.getMitigation().add((DetectedIssueMitigationComponent) value); 2114 } else 2115 return super.setProperty(name, value); 2116 return value; 2117 } 2118 2119 @Override 2120 public void removeChild(String name, Base value) throws FHIRException { 2121 if (name.equals("identifier")) { 2122 this.getIdentifier().remove(castToIdentifier(value)); 2123 } else if (name.equals("status")) { 2124 this.status = null; 2125 } else if (name.equals("code")) { 2126 this.code = null; 2127 } else if (name.equals("severity")) { 2128 this.severity = null; 2129 } else if (name.equals("patient")) { 2130 this.patient = null; 2131 } else if (name.equals("identified[x]")) { 2132 this.identified = null; 2133 } else if (name.equals("author")) { 2134 this.author = null; 2135 } else if (name.equals("implicated")) { 2136 this.getImplicated().remove(castToReference(value)); 2137 } else if (name.equals("evidence")) { 2138 this.getEvidence().remove((DetectedIssueEvidenceComponent) value); 2139 } else if (name.equals("detail")) { 2140 this.detail = null; 2141 } else if (name.equals("reference")) { 2142 this.reference = null; 2143 } else if (name.equals("mitigation")) { 2144 this.getMitigation().remove((DetectedIssueMitigationComponent) value); 2145 } else 2146 super.removeChild(name, value); 2147 2148 } 2149 2150 @Override 2151 public Base makeProperty(int hash, String name) throws FHIRException { 2152 switch (hash) { 2153 case -1618432855: 2154 return addIdentifier(); 2155 case -892481550: 2156 return getStatusElement(); 2157 case 3059181: 2158 return getCode(); 2159 case 1478300413: 2160 return getSeverityElement(); 2161 case -791418107: 2162 return getPatient(); 2163 case 569355781: 2164 return getIdentified(); 2165 case -1618432869: 2166 return getIdentified(); 2167 case -1406328437: 2168 return getAuthor(); 2169 case -810216884: 2170 return addImplicated(); 2171 case 382967383: 2172 return addEvidence(); 2173 case -1335224239: 2174 return getDetailElement(); 2175 case -925155509: 2176 return getReferenceElement(); 2177 case 1293793087: 2178 return addMitigation(); 2179 default: 2180 return super.makeProperty(hash, name); 2181 } 2182 2183 } 2184 2185 @Override 2186 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2187 switch (hash) { 2188 case -1618432855: 2189 /* identifier */ return new String[] { "Identifier" }; 2190 case -892481550: 2191 /* status */ return new String[] { "code" }; 2192 case 3059181: 2193 /* code */ return new String[] { "CodeableConcept" }; 2194 case 1478300413: 2195 /* severity */ return new String[] { "code" }; 2196 case -791418107: 2197 /* patient */ return new String[] { "Reference" }; 2198 case -1618432869: 2199 /* identified */ return new String[] { "dateTime", "Period" }; 2200 case -1406328437: 2201 /* author */ return new String[] { "Reference" }; 2202 case -810216884: 2203 /* implicated */ return new String[] { "Reference" }; 2204 case 382967383: 2205 /* evidence */ return new String[] {}; 2206 case -1335224239: 2207 /* detail */ return new String[] { "string" }; 2208 case -925155509: 2209 /* reference */ return new String[] { "uri" }; 2210 case 1293793087: 2211 /* mitigation */ return new String[] {}; 2212 default: 2213 return super.getTypesForProperty(hash, name); 2214 } 2215 2216 } 2217 2218 @Override 2219 public Base addChild(String name) throws FHIRException { 2220 if (name.equals("identifier")) { 2221 return addIdentifier(); 2222 } else if (name.equals("status")) { 2223 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.status"); 2224 } else if (name.equals("code")) { 2225 this.code = new CodeableConcept(); 2226 return this.code; 2227 } else if (name.equals("severity")) { 2228 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.severity"); 2229 } else if (name.equals("patient")) { 2230 this.patient = new Reference(); 2231 return this.patient; 2232 } else if (name.equals("identifiedDateTime")) { 2233 this.identified = new DateTimeType(); 2234 return this.identified; 2235 } else if (name.equals("identifiedPeriod")) { 2236 this.identified = new Period(); 2237 return this.identified; 2238 } else if (name.equals("author")) { 2239 this.author = new Reference(); 2240 return this.author; 2241 } else if (name.equals("implicated")) { 2242 return addImplicated(); 2243 } else if (name.equals("evidence")) { 2244 return addEvidence(); 2245 } else if (name.equals("detail")) { 2246 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.detail"); 2247 } else if (name.equals("reference")) { 2248 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.reference"); 2249 } else if (name.equals("mitigation")) { 2250 return addMitigation(); 2251 } else 2252 return super.addChild(name); 2253 } 2254 2255 public String fhirType() { 2256 return "DetectedIssue"; 2257 2258 } 2259 2260 public DetectedIssue copy() { 2261 DetectedIssue dst = new DetectedIssue(); 2262 copyValues(dst); 2263 return dst; 2264 } 2265 2266 public void copyValues(DetectedIssue dst) { 2267 super.copyValues(dst); 2268 if (identifier != null) { 2269 dst.identifier = new ArrayList<Identifier>(); 2270 for (Identifier i : identifier) 2271 dst.identifier.add(i.copy()); 2272 } 2273 ; 2274 dst.status = status == null ? null : status.copy(); 2275 dst.code = code == null ? null : code.copy(); 2276 dst.severity = severity == null ? null : severity.copy(); 2277 dst.patient = patient == null ? null : patient.copy(); 2278 dst.identified = identified == null ? null : identified.copy(); 2279 dst.author = author == null ? null : author.copy(); 2280 if (implicated != null) { 2281 dst.implicated = new ArrayList<Reference>(); 2282 for (Reference i : implicated) 2283 dst.implicated.add(i.copy()); 2284 } 2285 ; 2286 if (evidence != null) { 2287 dst.evidence = new ArrayList<DetectedIssueEvidenceComponent>(); 2288 for (DetectedIssueEvidenceComponent i : evidence) 2289 dst.evidence.add(i.copy()); 2290 } 2291 ; 2292 dst.detail = detail == null ? null : detail.copy(); 2293 dst.reference = reference == null ? null : reference.copy(); 2294 if (mitigation != null) { 2295 dst.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 2296 for (DetectedIssueMitigationComponent i : mitigation) 2297 dst.mitigation.add(i.copy()); 2298 } 2299 ; 2300 } 2301 2302 protected DetectedIssue typedCopy() { 2303 return copy(); 2304 } 2305 2306 @Override 2307 public boolean equalsDeep(Base other_) { 2308 if (!super.equalsDeep(other_)) 2309 return false; 2310 if (!(other_ instanceof DetectedIssue)) 2311 return false; 2312 DetectedIssue o = (DetectedIssue) other_; 2313 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 2314 && compareDeep(code, o.code, true) && compareDeep(severity, o.severity, true) 2315 && compareDeep(patient, o.patient, true) && compareDeep(identified, o.identified, true) 2316 && compareDeep(author, o.author, true) && compareDeep(implicated, o.implicated, true) 2317 && compareDeep(evidence, o.evidence, true) && compareDeep(detail, o.detail, true) 2318 && compareDeep(reference, o.reference, true) && compareDeep(mitigation, o.mitigation, true); 2319 } 2320 2321 @Override 2322 public boolean equalsShallow(Base other_) { 2323 if (!super.equalsShallow(other_)) 2324 return false; 2325 if (!(other_ instanceof DetectedIssue)) 2326 return false; 2327 DetectedIssue o = (DetectedIssue) other_; 2328 return compareValues(status, o.status, true) && compareValues(severity, o.severity, true) 2329 && compareValues(detail, o.detail, true) && compareValues(reference, o.reference, true); 2330 } 2331 2332 public boolean isEmpty() { 2333 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, code, severity, patient, 2334 identified, author, implicated, evidence, detail, reference, mitigation); 2335 } 2336 2337 @Override 2338 public ResourceType getResourceType() { 2339 return ResourceType.DetectedIssue; 2340 } 2341 2342 /** 2343 * Search parameter: <b>identifier</b> 2344 * <p> 2345 * Description: <b>Unique id for the detected issue</b><br> 2346 * Type: <b>token</b><br> 2347 * Path: <b>DetectedIssue.identifier</b><br> 2348 * </p> 2349 */ 2350 @SearchParamDefinition(name = "identifier", path = "DetectedIssue.identifier", description = "Unique id for the detected issue", type = "token") 2351 public static final String SP_IDENTIFIER = "identifier"; 2352 /** 2353 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2354 * <p> 2355 * Description: <b>Unique id for the detected issue</b><br> 2356 * Type: <b>token</b><br> 2357 * Path: <b>DetectedIssue.identifier</b><br> 2358 * </p> 2359 */ 2360 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2361 SP_IDENTIFIER); 2362 2363 /** 2364 * Search parameter: <b>code</b> 2365 * <p> 2366 * Description: <b>Issue Category, e.g. drug-drug, duplicate therapy, 2367 * etc.</b><br> 2368 * Type: <b>token</b><br> 2369 * Path: <b>DetectedIssue.code</b><br> 2370 * </p> 2371 */ 2372 @SearchParamDefinition(name = "code", path = "DetectedIssue.code", description = "Issue Category, e.g. drug-drug, duplicate therapy, etc.", type = "token") 2373 public static final String SP_CODE = "code"; 2374 /** 2375 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2376 * <p> 2377 * Description: <b>Issue Category, e.g. drug-drug, duplicate therapy, 2378 * etc.</b><br> 2379 * Type: <b>token</b><br> 2380 * Path: <b>DetectedIssue.code</b><br> 2381 * </p> 2382 */ 2383 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2384 SP_CODE); 2385 2386 /** 2387 * Search parameter: <b>identified</b> 2388 * <p> 2389 * Description: <b>When identified</b><br> 2390 * Type: <b>date</b><br> 2391 * Path: <b>DetectedIssue.identified[x]</b><br> 2392 * </p> 2393 */ 2394 @SearchParamDefinition(name = "identified", path = "DetectedIssue.identified", description = "When identified", type = "date") 2395 public static final String SP_IDENTIFIED = "identified"; 2396 /** 2397 * <b>Fluent Client</b> search parameter constant for <b>identified</b> 2398 * <p> 2399 * Description: <b>When identified</b><br> 2400 * Type: <b>date</b><br> 2401 * Path: <b>DetectedIssue.identified[x]</b><br> 2402 * </p> 2403 */ 2404 public static final ca.uhn.fhir.rest.gclient.DateClientParam IDENTIFIED = new ca.uhn.fhir.rest.gclient.DateClientParam( 2405 SP_IDENTIFIED); 2406 2407 /** 2408 * Search parameter: <b>patient</b> 2409 * <p> 2410 * Description: <b>Associated patient</b><br> 2411 * Type: <b>reference</b><br> 2412 * Path: <b>DetectedIssue.patient</b><br> 2413 * </p> 2414 */ 2415 @SearchParamDefinition(name = "patient", path = "DetectedIssue.patient", description = "Associated patient", type = "reference", providesMembershipIn = { 2416 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 2417 public static final String SP_PATIENT = "patient"; 2418 /** 2419 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2420 * <p> 2421 * Description: <b>Associated patient</b><br> 2422 * Type: <b>reference</b><br> 2423 * Path: <b>DetectedIssue.patient</b><br> 2424 * </p> 2425 */ 2426 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2427 SP_PATIENT); 2428 2429 /** 2430 * Constant for fluent queries to be used to add include statements. Specifies 2431 * the path value of "<b>DetectedIssue:patient</b>". 2432 */ 2433 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 2434 "DetectedIssue:patient").toLocked(); 2435 2436 /** 2437 * Search parameter: <b>author</b> 2438 * <p> 2439 * Description: <b>The provider or device that identified the issue</b><br> 2440 * Type: <b>reference</b><br> 2441 * Path: <b>DetectedIssue.author</b><br> 2442 * </p> 2443 */ 2444 @SearchParamDefinition(name = "author", path = "DetectedIssue.author", description = "The provider or device that identified the issue", type = "reference", providesMembershipIn = { 2445 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 2446 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Device.class, 2447 Practitioner.class, PractitionerRole.class }) 2448 public static final String SP_AUTHOR = "author"; 2449 /** 2450 * <b>Fluent Client</b> search parameter constant for <b>author</b> 2451 * <p> 2452 * Description: <b>The provider or device that identified the issue</b><br> 2453 * Type: <b>reference</b><br> 2454 * Path: <b>DetectedIssue.author</b><br> 2455 * </p> 2456 */ 2457 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2458 SP_AUTHOR); 2459 2460 /** 2461 * Constant for fluent queries to be used to add include statements. Specifies 2462 * the path value of "<b>DetectedIssue:author</b>". 2463 */ 2464 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include( 2465 "DetectedIssue:author").toLocked(); 2466 2467 /** 2468 * Search parameter: <b>implicated</b> 2469 * <p> 2470 * Description: <b>Problem resource</b><br> 2471 * Type: <b>reference</b><br> 2472 * Path: <b>DetectedIssue.implicated</b><br> 2473 * </p> 2474 */ 2475 @SearchParamDefinition(name = "implicated", path = "DetectedIssue.implicated", description = "Problem resource", type = "reference") 2476 public static final String SP_IMPLICATED = "implicated"; 2477 /** 2478 * <b>Fluent Client</b> search parameter constant for <b>implicated</b> 2479 * <p> 2480 * Description: <b>Problem resource</b><br> 2481 * Type: <b>reference</b><br> 2482 * Path: <b>DetectedIssue.implicated</b><br> 2483 * </p> 2484 */ 2485 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam IMPLICATED = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2486 SP_IMPLICATED); 2487 2488 /** 2489 * Constant for fluent queries to be used to add include statements. Specifies 2490 * the path value of "<b>DetectedIssue:implicated</b>". 2491 */ 2492 public static final ca.uhn.fhir.model.api.Include INCLUDE_IMPLICATED = new ca.uhn.fhir.model.api.Include( 2493 "DetectedIssue:implicated").toLocked(); 2494 2495}