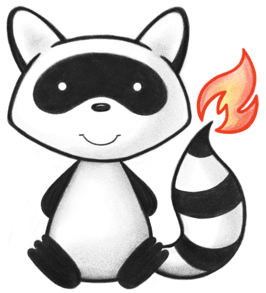
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * A type of a manufactured item that is used in the provision of healthcare 049 * without being substantially changed through that activity. The device may be 050 * a medical or non-medical device. 051 */ 052@ResourceDef(name = "Device", profile = "http://hl7.org/fhir/StructureDefinition/Device") 053public class Device extends DomainResource { 054 055 public enum UDIEntryType { 056 /** 057 * a barcodescanner captured the data from the device label. 058 */ 059 BARCODE, 060 /** 061 * An RFID chip reader captured the data from the device label. 062 */ 063 RFID, 064 /** 065 * The data was read from the label by a person and manually entered. (e.g. via 066 * a keyboard). 067 */ 068 MANUAL, 069 /** 070 * The data originated from a patient's implant card and was read by an 071 * operator. 072 */ 073 CARD, 074 /** 075 * The data originated from a patient source and was not directly scanned or 076 * read from a label or card. 077 */ 078 SELFREPORTED, 079 /** 080 * The method of data capture has not been determined. 081 */ 082 UNKNOWN, 083 /** 084 * added to help the parsers with the generic types 085 */ 086 NULL; 087 088 public static UDIEntryType fromCode(String codeString) throws FHIRException { 089 if (codeString == null || "".equals(codeString)) 090 return null; 091 if ("barcode".equals(codeString)) 092 return BARCODE; 093 if ("rfid".equals(codeString)) 094 return RFID; 095 if ("manual".equals(codeString)) 096 return MANUAL; 097 if ("card".equals(codeString)) 098 return CARD; 099 if ("self-reported".equals(codeString)) 100 return SELFREPORTED; 101 if ("unknown".equals(codeString)) 102 return UNKNOWN; 103 if (Configuration.isAcceptInvalidEnums()) 104 return null; 105 else 106 throw new FHIRException("Unknown UDIEntryType code '" + codeString + "'"); 107 } 108 109 public String toCode() { 110 switch (this) { 111 case BARCODE: 112 return "barcode"; 113 case RFID: 114 return "rfid"; 115 case MANUAL: 116 return "manual"; 117 case CARD: 118 return "card"; 119 case SELFREPORTED: 120 return "self-reported"; 121 case UNKNOWN: 122 return "unknown"; 123 case NULL: 124 return null; 125 default: 126 return "?"; 127 } 128 } 129 130 public String getSystem() { 131 switch (this) { 132 case BARCODE: 133 return "http://hl7.org/fhir/udi-entry-type"; 134 case RFID: 135 return "http://hl7.org/fhir/udi-entry-type"; 136 case MANUAL: 137 return "http://hl7.org/fhir/udi-entry-type"; 138 case CARD: 139 return "http://hl7.org/fhir/udi-entry-type"; 140 case SELFREPORTED: 141 return "http://hl7.org/fhir/udi-entry-type"; 142 case UNKNOWN: 143 return "http://hl7.org/fhir/udi-entry-type"; 144 case NULL: 145 return null; 146 default: 147 return "?"; 148 } 149 } 150 151 public String getDefinition() { 152 switch (this) { 153 case BARCODE: 154 return "a barcodescanner captured the data from the device label."; 155 case RFID: 156 return "An RFID chip reader captured the data from the device label."; 157 case MANUAL: 158 return "The data was read from the label by a person and manually entered. (e.g. via a keyboard)."; 159 case CARD: 160 return "The data originated from a patient's implant card and was read by an operator."; 161 case SELFREPORTED: 162 return "The data originated from a patient source and was not directly scanned or read from a label or card."; 163 case UNKNOWN: 164 return "The method of data capture has not been determined."; 165 case NULL: 166 return null; 167 default: 168 return "?"; 169 } 170 } 171 172 public String getDisplay() { 173 switch (this) { 174 case BARCODE: 175 return "Barcode"; 176 case RFID: 177 return "RFID"; 178 case MANUAL: 179 return "Manual"; 180 case CARD: 181 return "Card"; 182 case SELFREPORTED: 183 return "Self Reported"; 184 case UNKNOWN: 185 return "Unknown"; 186 case NULL: 187 return null; 188 default: 189 return "?"; 190 } 191 } 192 } 193 194 public static class UDIEntryTypeEnumFactory implements EnumFactory<UDIEntryType> { 195 public UDIEntryType fromCode(String codeString) throws IllegalArgumentException { 196 if (codeString == null || "".equals(codeString)) 197 if (codeString == null || "".equals(codeString)) 198 return null; 199 if ("barcode".equals(codeString)) 200 return UDIEntryType.BARCODE; 201 if ("rfid".equals(codeString)) 202 return UDIEntryType.RFID; 203 if ("manual".equals(codeString)) 204 return UDIEntryType.MANUAL; 205 if ("card".equals(codeString)) 206 return UDIEntryType.CARD; 207 if ("self-reported".equals(codeString)) 208 return UDIEntryType.SELFREPORTED; 209 if ("unknown".equals(codeString)) 210 return UDIEntryType.UNKNOWN; 211 throw new IllegalArgumentException("Unknown UDIEntryType code '" + codeString + "'"); 212 } 213 214 public Enumeration<UDIEntryType> fromType(PrimitiveType<?> code) throws FHIRException { 215 if (code == null) 216 return null; 217 if (code.isEmpty()) 218 return new Enumeration<UDIEntryType>(this, UDIEntryType.NULL, code); 219 String codeString = code.asStringValue(); 220 if (codeString == null || "".equals(codeString)) 221 return new Enumeration<UDIEntryType>(this, UDIEntryType.NULL, code); 222 if ("barcode".equals(codeString)) 223 return new Enumeration<UDIEntryType>(this, UDIEntryType.BARCODE, code); 224 if ("rfid".equals(codeString)) 225 return new Enumeration<UDIEntryType>(this, UDIEntryType.RFID, code); 226 if ("manual".equals(codeString)) 227 return new Enumeration<UDIEntryType>(this, UDIEntryType.MANUAL, code); 228 if ("card".equals(codeString)) 229 return new Enumeration<UDIEntryType>(this, UDIEntryType.CARD, code); 230 if ("self-reported".equals(codeString)) 231 return new Enumeration<UDIEntryType>(this, UDIEntryType.SELFREPORTED, code); 232 if ("unknown".equals(codeString)) 233 return new Enumeration<UDIEntryType>(this, UDIEntryType.UNKNOWN, code); 234 throw new FHIRException("Unknown UDIEntryType code '" + codeString + "'"); 235 } 236 237 public String toCode(UDIEntryType code) { 238 if (code == UDIEntryType.NULL) 239 return null; 240 if (code == UDIEntryType.BARCODE) 241 return "barcode"; 242 if (code == UDIEntryType.RFID) 243 return "rfid"; 244 if (code == UDIEntryType.MANUAL) 245 return "manual"; 246 if (code == UDIEntryType.CARD) 247 return "card"; 248 if (code == UDIEntryType.SELFREPORTED) 249 return "self-reported"; 250 if (code == UDIEntryType.UNKNOWN) 251 return "unknown"; 252 return "?"; 253 } 254 255 public String toSystem(UDIEntryType code) { 256 return code.getSystem(); 257 } 258 } 259 260 public enum FHIRDeviceStatus { 261 /** 262 * The device is available for use. Note: For *implanted devices* this means 263 * that the device is implanted in the patient. 264 */ 265 ACTIVE, 266 /** 267 * The device is no longer available for use (e.g. lost, expired, damaged). 268 * Note: For *implanted devices* this means that the device has been removed 269 * from the patient. 270 */ 271 INACTIVE, 272 /** 273 * The device was entered in error and voided. 274 */ 275 ENTEREDINERROR, 276 /** 277 * The status of the device has not been determined. 278 */ 279 UNKNOWN, 280 /** 281 * added to help the parsers with the generic types 282 */ 283 NULL; 284 285 public static FHIRDeviceStatus fromCode(String codeString) throws FHIRException { 286 if (codeString == null || "".equals(codeString)) 287 return null; 288 if ("active".equals(codeString)) 289 return ACTIVE; 290 if ("inactive".equals(codeString)) 291 return INACTIVE; 292 if ("entered-in-error".equals(codeString)) 293 return ENTEREDINERROR; 294 if ("unknown".equals(codeString)) 295 return UNKNOWN; 296 if (Configuration.isAcceptInvalidEnums()) 297 return null; 298 else 299 throw new FHIRException("Unknown FHIRDeviceStatus code '" + codeString + "'"); 300 } 301 302 public String toCode() { 303 switch (this) { 304 case ACTIVE: 305 return "active"; 306 case INACTIVE: 307 return "inactive"; 308 case ENTEREDINERROR: 309 return "entered-in-error"; 310 case UNKNOWN: 311 return "unknown"; 312 case NULL: 313 return null; 314 default: 315 return "?"; 316 } 317 } 318 319 public String getSystem() { 320 switch (this) { 321 case ACTIVE: 322 return "http://hl7.org/fhir/device-status"; 323 case INACTIVE: 324 return "http://hl7.org/fhir/device-status"; 325 case ENTEREDINERROR: 326 return "http://hl7.org/fhir/device-status"; 327 case UNKNOWN: 328 return "http://hl7.org/fhir/device-status"; 329 case NULL: 330 return null; 331 default: 332 return "?"; 333 } 334 } 335 336 public String getDefinition() { 337 switch (this) { 338 case ACTIVE: 339 return "The device is available for use. Note: For *implanted devices* this means that the device is implanted in the patient."; 340 case INACTIVE: 341 return "The device is no longer available for use (e.g. lost, expired, damaged). Note: For *implanted devices* this means that the device has been removed from the patient."; 342 case ENTEREDINERROR: 343 return "The device was entered in error and voided."; 344 case UNKNOWN: 345 return "The status of the device has not been determined."; 346 case NULL: 347 return null; 348 default: 349 return "?"; 350 } 351 } 352 353 public String getDisplay() { 354 switch (this) { 355 case ACTIVE: 356 return "Active"; 357 case INACTIVE: 358 return "Inactive"; 359 case ENTEREDINERROR: 360 return "Entered in Error"; 361 case UNKNOWN: 362 return "Unknown"; 363 case NULL: 364 return null; 365 default: 366 return "?"; 367 } 368 } 369 } 370 371 public static class FHIRDeviceStatusEnumFactory implements EnumFactory<FHIRDeviceStatus> { 372 public FHIRDeviceStatus fromCode(String codeString) throws IllegalArgumentException { 373 if (codeString == null || "".equals(codeString)) 374 if (codeString == null || "".equals(codeString)) 375 return null; 376 if ("active".equals(codeString)) 377 return FHIRDeviceStatus.ACTIVE; 378 if ("inactive".equals(codeString)) 379 return FHIRDeviceStatus.INACTIVE; 380 if ("entered-in-error".equals(codeString)) 381 return FHIRDeviceStatus.ENTEREDINERROR; 382 if ("unknown".equals(codeString)) 383 return FHIRDeviceStatus.UNKNOWN; 384 throw new IllegalArgumentException("Unknown FHIRDeviceStatus code '" + codeString + "'"); 385 } 386 387 public Enumeration<FHIRDeviceStatus> fromType(PrimitiveType<?> code) throws FHIRException { 388 if (code == null) 389 return null; 390 if (code.isEmpty()) 391 return new Enumeration<FHIRDeviceStatus>(this, FHIRDeviceStatus.NULL, code); 392 String codeString = code.asStringValue(); 393 if (codeString == null || "".equals(codeString)) 394 return new Enumeration<FHIRDeviceStatus>(this, FHIRDeviceStatus.NULL, code); 395 if ("active".equals(codeString)) 396 return new Enumeration<FHIRDeviceStatus>(this, FHIRDeviceStatus.ACTIVE, code); 397 if ("inactive".equals(codeString)) 398 return new Enumeration<FHIRDeviceStatus>(this, FHIRDeviceStatus.INACTIVE, code); 399 if ("entered-in-error".equals(codeString)) 400 return new Enumeration<FHIRDeviceStatus>(this, FHIRDeviceStatus.ENTEREDINERROR, code); 401 if ("unknown".equals(codeString)) 402 return new Enumeration<FHIRDeviceStatus>(this, FHIRDeviceStatus.UNKNOWN, code); 403 throw new FHIRException("Unknown FHIRDeviceStatus code '" + codeString + "'"); 404 } 405 406 public String toCode(FHIRDeviceStatus code) { 407 if (code == FHIRDeviceStatus.NULL) 408 return null; 409 if (code == FHIRDeviceStatus.ACTIVE) 410 return "active"; 411 if (code == FHIRDeviceStatus.INACTIVE) 412 return "inactive"; 413 if (code == FHIRDeviceStatus.ENTEREDINERROR) 414 return "entered-in-error"; 415 if (code == FHIRDeviceStatus.UNKNOWN) 416 return "unknown"; 417 return "?"; 418 } 419 420 public String toSystem(FHIRDeviceStatus code) { 421 return code.getSystem(); 422 } 423 } 424 425 public enum DeviceNameType { 426 /** 427 * UDI Label name. 428 */ 429 UDILABELNAME, 430 /** 431 * User Friendly name. 432 */ 433 USERFRIENDLYNAME, 434 /** 435 * Patient Reported name. 436 */ 437 PATIENTREPORTEDNAME, 438 /** 439 * Manufacturer name. 440 */ 441 MANUFACTURERNAME, 442 /** 443 * Model name. 444 */ 445 MODELNAME, 446 /** 447 * other. 448 */ 449 OTHER, 450 /** 451 * added to help the parsers with the generic types 452 */ 453 NULL; 454 455 public static DeviceNameType fromCode(String codeString) throws FHIRException { 456 if (codeString == null || "".equals(codeString)) 457 return null; 458 if ("udi-label-name".equals(codeString)) 459 return UDILABELNAME; 460 if ("user-friendly-name".equals(codeString)) 461 return USERFRIENDLYNAME; 462 if ("patient-reported-name".equals(codeString)) 463 return PATIENTREPORTEDNAME; 464 if ("manufacturer-name".equals(codeString)) 465 return MANUFACTURERNAME; 466 if ("model-name".equals(codeString)) 467 return MODELNAME; 468 if ("other".equals(codeString)) 469 return OTHER; 470 if (Configuration.isAcceptInvalidEnums()) 471 return null; 472 else 473 throw new FHIRException("Unknown DeviceNameType code '" + codeString + "'"); 474 } 475 476 public String toCode() { 477 switch (this) { 478 case UDILABELNAME: 479 return "udi-label-name"; 480 case USERFRIENDLYNAME: 481 return "user-friendly-name"; 482 case PATIENTREPORTEDNAME: 483 return "patient-reported-name"; 484 case MANUFACTURERNAME: 485 return "manufacturer-name"; 486 case MODELNAME: 487 return "model-name"; 488 case OTHER: 489 return "other"; 490 case NULL: 491 return null; 492 default: 493 return "?"; 494 } 495 } 496 497 public String getSystem() { 498 switch (this) { 499 case UDILABELNAME: 500 return "http://hl7.org/fhir/device-nametype"; 501 case USERFRIENDLYNAME: 502 return "http://hl7.org/fhir/device-nametype"; 503 case PATIENTREPORTEDNAME: 504 return "http://hl7.org/fhir/device-nametype"; 505 case MANUFACTURERNAME: 506 return "http://hl7.org/fhir/device-nametype"; 507 case MODELNAME: 508 return "http://hl7.org/fhir/device-nametype"; 509 case OTHER: 510 return "http://hl7.org/fhir/device-nametype"; 511 case NULL: 512 return null; 513 default: 514 return "?"; 515 } 516 } 517 518 public String getDefinition() { 519 switch (this) { 520 case UDILABELNAME: 521 return "UDI Label name."; 522 case USERFRIENDLYNAME: 523 return "User Friendly name."; 524 case PATIENTREPORTEDNAME: 525 return "Patient Reported name."; 526 case MANUFACTURERNAME: 527 return "Manufacturer name."; 528 case MODELNAME: 529 return "Model name."; 530 case OTHER: 531 return "other."; 532 case NULL: 533 return null; 534 default: 535 return "?"; 536 } 537 } 538 539 public String getDisplay() { 540 switch (this) { 541 case UDILABELNAME: 542 return "UDI Label name"; 543 case USERFRIENDLYNAME: 544 return "User Friendly name"; 545 case PATIENTREPORTEDNAME: 546 return "Patient Reported name"; 547 case MANUFACTURERNAME: 548 return "Manufacturer name"; 549 case MODELNAME: 550 return "Model name"; 551 case OTHER: 552 return "other"; 553 case NULL: 554 return null; 555 default: 556 return "?"; 557 } 558 } 559 } 560 561 public static class DeviceNameTypeEnumFactory implements EnumFactory<DeviceNameType> { 562 public DeviceNameType fromCode(String codeString) throws IllegalArgumentException { 563 if (codeString == null || "".equals(codeString)) 564 if (codeString == null || "".equals(codeString)) 565 return null; 566 if ("udi-label-name".equals(codeString)) 567 return DeviceNameType.UDILABELNAME; 568 if ("user-friendly-name".equals(codeString)) 569 return DeviceNameType.USERFRIENDLYNAME; 570 if ("patient-reported-name".equals(codeString)) 571 return DeviceNameType.PATIENTREPORTEDNAME; 572 if ("manufacturer-name".equals(codeString)) 573 return DeviceNameType.MANUFACTURERNAME; 574 if ("model-name".equals(codeString)) 575 return DeviceNameType.MODELNAME; 576 if ("other".equals(codeString)) 577 return DeviceNameType.OTHER; 578 throw new IllegalArgumentException("Unknown DeviceNameType code '" + codeString + "'"); 579 } 580 581 public Enumeration<DeviceNameType> fromType(PrimitiveType<?> code) throws FHIRException { 582 if (code == null) 583 return null; 584 if (code.isEmpty()) 585 return new Enumeration<DeviceNameType>(this, DeviceNameType.NULL, code); 586 String codeString = code.asStringValue(); 587 if (codeString == null || "".equals(codeString)) 588 return new Enumeration<DeviceNameType>(this, DeviceNameType.NULL, code); 589 if ("udi-label-name".equals(codeString)) 590 return new Enumeration<DeviceNameType>(this, DeviceNameType.UDILABELNAME, code); 591 if ("user-friendly-name".equals(codeString)) 592 return new Enumeration<DeviceNameType>(this, DeviceNameType.USERFRIENDLYNAME, code); 593 if ("patient-reported-name".equals(codeString)) 594 return new Enumeration<DeviceNameType>(this, DeviceNameType.PATIENTREPORTEDNAME, code); 595 if ("manufacturer-name".equals(codeString)) 596 return new Enumeration<DeviceNameType>(this, DeviceNameType.MANUFACTURERNAME, code); 597 if ("model-name".equals(codeString)) 598 return new Enumeration<DeviceNameType>(this, DeviceNameType.MODELNAME, code); 599 if ("other".equals(codeString)) 600 return new Enumeration<DeviceNameType>(this, DeviceNameType.OTHER, code); 601 throw new FHIRException("Unknown DeviceNameType code '" + codeString + "'"); 602 } 603 604 public String toCode(DeviceNameType code) { 605 if (code == DeviceNameType.NULL) 606 return null; 607 if (code == DeviceNameType.UDILABELNAME) 608 return "udi-label-name"; 609 if (code == DeviceNameType.USERFRIENDLYNAME) 610 return "user-friendly-name"; 611 if (code == DeviceNameType.PATIENTREPORTEDNAME) 612 return "patient-reported-name"; 613 if (code == DeviceNameType.MANUFACTURERNAME) 614 return "manufacturer-name"; 615 if (code == DeviceNameType.MODELNAME) 616 return "model-name"; 617 if (code == DeviceNameType.OTHER) 618 return "other"; 619 return "?"; 620 } 621 622 public String toSystem(DeviceNameType code) { 623 return code.getSystem(); 624 } 625 } 626 627 @Block() 628 public static class DeviceUdiCarrierComponent extends BackboneElement implements IBaseBackboneElement { 629 /** 630 * The device identifier (DI) is a mandatory, fixed portion of a UDI that 631 * identifies the labeler and the specific version or model of a device. 632 */ 633 @Child(name = "deviceIdentifier", type = { 634 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 635 @Description(shortDefinition = "Mandatory fixed portion of UDI", formalDefinition = "The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device.") 636 protected StringType deviceIdentifier; 637 638 /** 639 * Organization that is charged with issuing UDIs for devices. For example, the 640 * US FDA issuers include : 1) GS1: http://hl7.org/fhir/NamingSystem/gs1-di, 2) 641 * HIBCC: http://hl7.org/fhir/NamingSystem/hibcc-dI, 3) ICCBBA for blood 642 * containers: http://hl7.org/fhir/NamingSystem/iccbba-blood-di, 4) ICCBA for 643 * other devices: http://hl7.org/fhir/NamingSystem/iccbba-other-di. 644 */ 645 @Child(name = "issuer", type = { UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 646 @Description(shortDefinition = "UDI Issuing Organization", formalDefinition = "Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include :\n1) GS1: \nhttp://hl7.org/fhir/NamingSystem/gs1-di, \n2) HIBCC:\nhttp://hl7.org/fhir/NamingSystem/hibcc-dI, \n3) ICCBBA for blood containers:\nhttp://hl7.org/fhir/NamingSystem/iccbba-blood-di, \n4) ICCBA for other devices:\nhttp://hl7.org/fhir/NamingSystem/iccbba-other-di.") 647 protected UriType issuer; 648 649 /** 650 * The identity of the authoritative source for UDI generation within a 651 * jurisdiction. All UDIs are globally unique within a single namespace with the 652 * appropriate repository uri as the system. For example, UDIs of devices 653 * managed in the U.S. by the FDA, the value is 654 * http://hl7.org/fhir/NamingSystem/fda-udi. 655 */ 656 @Child(name = "jurisdiction", type = { 657 UriType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 658 @Description(shortDefinition = "Regional UDI authority", formalDefinition = "The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/fda-udi.") 659 protected UriType jurisdiction; 660 661 /** 662 * The full UDI carrier of the Automatic Identification and Data Capture (AIDC) 663 * technology representation of the barcode string as printed on the packaging 664 * of the device - e.g., a barcode or RFID. Because of limitations on character 665 * sets in XML and the need to round-trip JSON data through XML, AIDC Formats 666 * *SHALL* be base64 encoded. 667 */ 668 @Child(name = "carrierAIDC", type = { 669 Base64BinaryType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 670 @Description(shortDefinition = "UDI Machine Readable Barcode String", formalDefinition = "The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - e.g., a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded.") 671 protected Base64BinaryType carrierAIDC; 672 673 /** 674 * The full UDI carrier as the human readable form (HRF) representation of the 675 * barcode string as printed on the packaging of the device. 676 */ 677 @Child(name = "carrierHRF", type = { 678 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 679 @Description(shortDefinition = "UDI Human Readable Barcode String", formalDefinition = "The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device.") 680 protected StringType carrierHRF; 681 682 /** 683 * A coded entry to indicate how the data was entered. 684 */ 685 @Child(name = "entryType", type = { 686 CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 687 @Description(shortDefinition = "barcode | rfid | manual +", formalDefinition = "A coded entry to indicate how the data was entered.") 688 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/udi-entry-type") 689 protected Enumeration<UDIEntryType> entryType; 690 691 private static final long serialVersionUID = -191630425L; 692 693 /** 694 * Constructor 695 */ 696 public DeviceUdiCarrierComponent() { 697 super(); 698 } 699 700 /** 701 * @return {@link #deviceIdentifier} (The device identifier (DI) is a mandatory, 702 * fixed portion of a UDI that identifies the labeler and the specific 703 * version or model of a device.). This is the underlying object with 704 * id, value and extensions. The accessor "getDeviceIdentifier" gives 705 * direct access to the value 706 */ 707 public StringType getDeviceIdentifierElement() { 708 if (this.deviceIdentifier == null) 709 if (Configuration.errorOnAutoCreate()) 710 throw new Error("Attempt to auto-create DeviceUdiCarrierComponent.deviceIdentifier"); 711 else if (Configuration.doAutoCreate()) 712 this.deviceIdentifier = new StringType(); // bb 713 return this.deviceIdentifier; 714 } 715 716 public boolean hasDeviceIdentifierElement() { 717 return this.deviceIdentifier != null && !this.deviceIdentifier.isEmpty(); 718 } 719 720 public boolean hasDeviceIdentifier() { 721 return this.deviceIdentifier != null && !this.deviceIdentifier.isEmpty(); 722 } 723 724 /** 725 * @param value {@link #deviceIdentifier} (The device identifier (DI) is a 726 * mandatory, fixed portion of a UDI that identifies the labeler 727 * and the specific version or model of a device.). This is the 728 * underlying object with id, value and extensions. The accessor 729 * "getDeviceIdentifier" gives direct access to the value 730 */ 731 public DeviceUdiCarrierComponent setDeviceIdentifierElement(StringType value) { 732 this.deviceIdentifier = value; 733 return this; 734 } 735 736 /** 737 * @return The device identifier (DI) is a mandatory, fixed portion of a UDI 738 * that identifies the labeler and the specific version or model of a 739 * device. 740 */ 741 public String getDeviceIdentifier() { 742 return this.deviceIdentifier == null ? null : this.deviceIdentifier.getValue(); 743 } 744 745 /** 746 * @param value The device identifier (DI) is a mandatory, fixed portion of a 747 * UDI that identifies the labeler and the specific version or 748 * model of a device. 749 */ 750 public DeviceUdiCarrierComponent setDeviceIdentifier(String value) { 751 if (Utilities.noString(value)) 752 this.deviceIdentifier = null; 753 else { 754 if (this.deviceIdentifier == null) 755 this.deviceIdentifier = new StringType(); 756 this.deviceIdentifier.setValue(value); 757 } 758 return this; 759 } 760 761 /** 762 * @return {@link #issuer} (Organization that is charged with issuing UDIs for 763 * devices. For example, the US FDA issuers include : 1) GS1: 764 * http://hl7.org/fhir/NamingSystem/gs1-di, 2) HIBCC: 765 * http://hl7.org/fhir/NamingSystem/hibcc-dI, 3) ICCBBA for blood 766 * containers: http://hl7.org/fhir/NamingSystem/iccbba-blood-di, 4) 767 * ICCBA for other devices: 768 * http://hl7.org/fhir/NamingSystem/iccbba-other-di.). This is the 769 * underlying object with id, value and extensions. The accessor 770 * "getIssuer" gives direct access to the value 771 */ 772 public UriType getIssuerElement() { 773 if (this.issuer == null) 774 if (Configuration.errorOnAutoCreate()) 775 throw new Error("Attempt to auto-create DeviceUdiCarrierComponent.issuer"); 776 else if (Configuration.doAutoCreate()) 777 this.issuer = new UriType(); // bb 778 return this.issuer; 779 } 780 781 public boolean hasIssuerElement() { 782 return this.issuer != null && !this.issuer.isEmpty(); 783 } 784 785 public boolean hasIssuer() { 786 return this.issuer != null && !this.issuer.isEmpty(); 787 } 788 789 /** 790 * @param value {@link #issuer} (Organization that is charged with issuing UDIs 791 * for devices. For example, the US FDA issuers include : 1) GS1: 792 * http://hl7.org/fhir/NamingSystem/gs1-di, 2) HIBCC: 793 * http://hl7.org/fhir/NamingSystem/hibcc-dI, 3) ICCBBA for blood 794 * containers: http://hl7.org/fhir/NamingSystem/iccbba-blood-di, 4) 795 * ICCBA for other devices: 796 * http://hl7.org/fhir/NamingSystem/iccbba-other-di.). This is the 797 * underlying object with id, value and extensions. The accessor 798 * "getIssuer" gives direct access to the value 799 */ 800 public DeviceUdiCarrierComponent setIssuerElement(UriType value) { 801 this.issuer = value; 802 return this; 803 } 804 805 /** 806 * @return Organization that is charged with issuing UDIs for devices. For 807 * example, the US FDA issuers include : 1) GS1: 808 * http://hl7.org/fhir/NamingSystem/gs1-di, 2) HIBCC: 809 * http://hl7.org/fhir/NamingSystem/hibcc-dI, 3) ICCBBA for blood 810 * containers: http://hl7.org/fhir/NamingSystem/iccbba-blood-di, 4) 811 * ICCBA for other devices: 812 * http://hl7.org/fhir/NamingSystem/iccbba-other-di. 813 */ 814 public String getIssuer() { 815 return this.issuer == null ? null : this.issuer.getValue(); 816 } 817 818 /** 819 * @param value Organization that is charged with issuing UDIs for devices. For 820 * example, the US FDA issuers include : 1) GS1: 821 * http://hl7.org/fhir/NamingSystem/gs1-di, 2) HIBCC: 822 * http://hl7.org/fhir/NamingSystem/hibcc-dI, 3) ICCBBA for blood 823 * containers: http://hl7.org/fhir/NamingSystem/iccbba-blood-di, 4) 824 * ICCBA for other devices: 825 * http://hl7.org/fhir/NamingSystem/iccbba-other-di. 826 */ 827 public DeviceUdiCarrierComponent setIssuer(String value) { 828 if (Utilities.noString(value)) 829 this.issuer = null; 830 else { 831 if (this.issuer == null) 832 this.issuer = new UriType(); 833 this.issuer.setValue(value); 834 } 835 return this; 836 } 837 838 /** 839 * @return {@link #jurisdiction} (The identity of the authoritative source for 840 * UDI generation within a jurisdiction. All UDIs are globally unique 841 * within a single namespace with the appropriate repository uri as the 842 * system. For example, UDIs of devices managed in the U.S. by the FDA, 843 * the value is http://hl7.org/fhir/NamingSystem/fda-udi.). This is the 844 * underlying object with id, value and extensions. The accessor 845 * "getJurisdiction" gives direct access to the value 846 */ 847 public UriType getJurisdictionElement() { 848 if (this.jurisdiction == null) 849 if (Configuration.errorOnAutoCreate()) 850 throw new Error("Attempt to auto-create DeviceUdiCarrierComponent.jurisdiction"); 851 else if (Configuration.doAutoCreate()) 852 this.jurisdiction = new UriType(); // bb 853 return this.jurisdiction; 854 } 855 856 public boolean hasJurisdictionElement() { 857 return this.jurisdiction != null && !this.jurisdiction.isEmpty(); 858 } 859 860 public boolean hasJurisdiction() { 861 return this.jurisdiction != null && !this.jurisdiction.isEmpty(); 862 } 863 864 /** 865 * @param value {@link #jurisdiction} (The identity of the authoritative source 866 * for UDI generation within a jurisdiction. All UDIs are globally 867 * unique within a single namespace with the appropriate repository 868 * uri as the system. For example, UDIs of devices managed in the 869 * U.S. by the FDA, the value is 870 * http://hl7.org/fhir/NamingSystem/fda-udi.). This is the 871 * underlying object with id, value and extensions. The accessor 872 * "getJurisdiction" gives direct access to the value 873 */ 874 public DeviceUdiCarrierComponent setJurisdictionElement(UriType value) { 875 this.jurisdiction = value; 876 return this; 877 } 878 879 /** 880 * @return The identity of the authoritative source for UDI generation within a 881 * jurisdiction. All UDIs are globally unique within a single namespace 882 * with the appropriate repository uri as the system. For example, UDIs 883 * of devices managed in the U.S. by the FDA, the value is 884 * http://hl7.org/fhir/NamingSystem/fda-udi. 885 */ 886 public String getJurisdiction() { 887 return this.jurisdiction == null ? null : this.jurisdiction.getValue(); 888 } 889 890 /** 891 * @param value The identity of the authoritative source for UDI generation 892 * within a jurisdiction. All UDIs are globally unique within a 893 * single namespace with the appropriate repository uri as the 894 * system. For example, UDIs of devices managed in the U.S. by the 895 * FDA, the value is http://hl7.org/fhir/NamingSystem/fda-udi. 896 */ 897 public DeviceUdiCarrierComponent setJurisdiction(String value) { 898 if (Utilities.noString(value)) 899 this.jurisdiction = null; 900 else { 901 if (this.jurisdiction == null) 902 this.jurisdiction = new UriType(); 903 this.jurisdiction.setValue(value); 904 } 905 return this; 906 } 907 908 /** 909 * @return {@link #carrierAIDC} (The full UDI carrier of the Automatic 910 * Identification and Data Capture (AIDC) technology representation of 911 * the barcode string as printed on the packaging of the device - e.g., 912 * a barcode or RFID. Because of limitations on character sets in XML 913 * and the need to round-trip JSON data through XML, AIDC Formats 914 * *SHALL* be base64 encoded.). This is the underlying object with id, 915 * value and extensions. The accessor "getCarrierAIDC" gives direct 916 * access to the value 917 */ 918 public Base64BinaryType getCarrierAIDCElement() { 919 if (this.carrierAIDC == null) 920 if (Configuration.errorOnAutoCreate()) 921 throw new Error("Attempt to auto-create DeviceUdiCarrierComponent.carrierAIDC"); 922 else if (Configuration.doAutoCreate()) 923 this.carrierAIDC = new Base64BinaryType(); // bb 924 return this.carrierAIDC; 925 } 926 927 public boolean hasCarrierAIDCElement() { 928 return this.carrierAIDC != null && !this.carrierAIDC.isEmpty(); 929 } 930 931 public boolean hasCarrierAIDC() { 932 return this.carrierAIDC != null && !this.carrierAIDC.isEmpty(); 933 } 934 935 /** 936 * @param value {@link #carrierAIDC} (The full UDI carrier of the Automatic 937 * Identification and Data Capture (AIDC) technology representation 938 * of the barcode string as printed on the packaging of the device 939 * - e.g., a barcode or RFID. Because of limitations on character 940 * sets in XML and the need to round-trip JSON data through XML, 941 * AIDC Formats *SHALL* be base64 encoded.). This is the underlying 942 * object with id, value and extensions. The accessor 943 * "getCarrierAIDC" gives direct access to the value 944 */ 945 public DeviceUdiCarrierComponent setCarrierAIDCElement(Base64BinaryType value) { 946 this.carrierAIDC = value; 947 return this; 948 } 949 950 /** 951 * @return The full UDI carrier of the Automatic Identification and Data Capture 952 * (AIDC) technology representation of the barcode string as printed on 953 * the packaging of the device - e.g., a barcode or RFID. Because of 954 * limitations on character sets in XML and the need to round-trip JSON 955 * data through XML, AIDC Formats *SHALL* be base64 encoded. 956 */ 957 public byte[] getCarrierAIDC() { 958 return this.carrierAIDC == null ? null : this.carrierAIDC.getValue(); 959 } 960 961 /** 962 * @param value The full UDI carrier of the Automatic Identification and Data 963 * Capture (AIDC) technology representation of the barcode string 964 * as printed on the packaging of the device - e.g., a barcode or 965 * RFID. Because of limitations on character sets in XML and the 966 * need to round-trip JSON data through XML, AIDC Formats *SHALL* 967 * be base64 encoded. 968 */ 969 public DeviceUdiCarrierComponent setCarrierAIDC(byte[] value) { 970 if (value == null) 971 this.carrierAIDC = null; 972 else { 973 if (this.carrierAIDC == null) 974 this.carrierAIDC = new Base64BinaryType(); 975 this.carrierAIDC.setValue(value); 976 } 977 return this; 978 } 979 980 /** 981 * @return {@link #carrierHRF} (The full UDI carrier as the human readable form 982 * (HRF) representation of the barcode string as printed on the 983 * packaging of the device.). This is the underlying object with id, 984 * value and extensions. The accessor "getCarrierHRF" gives direct 985 * access to the value 986 */ 987 public StringType getCarrierHRFElement() { 988 if (this.carrierHRF == null) 989 if (Configuration.errorOnAutoCreate()) 990 throw new Error("Attempt to auto-create DeviceUdiCarrierComponent.carrierHRF"); 991 else if (Configuration.doAutoCreate()) 992 this.carrierHRF = new StringType(); // bb 993 return this.carrierHRF; 994 } 995 996 public boolean hasCarrierHRFElement() { 997 return this.carrierHRF != null && !this.carrierHRF.isEmpty(); 998 } 999 1000 public boolean hasCarrierHRF() { 1001 return this.carrierHRF != null && !this.carrierHRF.isEmpty(); 1002 } 1003 1004 /** 1005 * @param value {@link #carrierHRF} (The full UDI carrier as the human readable 1006 * form (HRF) representation of the barcode string as printed on 1007 * the packaging of the device.). This is the underlying object 1008 * with id, value and extensions. The accessor "getCarrierHRF" 1009 * gives direct access to the value 1010 */ 1011 public DeviceUdiCarrierComponent setCarrierHRFElement(StringType value) { 1012 this.carrierHRF = value; 1013 return this; 1014 } 1015 1016 /** 1017 * @return The full UDI carrier as the human readable form (HRF) representation 1018 * of the barcode string as printed on the packaging of the device. 1019 */ 1020 public String getCarrierHRF() { 1021 return this.carrierHRF == null ? null : this.carrierHRF.getValue(); 1022 } 1023 1024 /** 1025 * @param value The full UDI carrier as the human readable form (HRF) 1026 * representation of the barcode string as printed on the packaging 1027 * of the device. 1028 */ 1029 public DeviceUdiCarrierComponent setCarrierHRF(String value) { 1030 if (Utilities.noString(value)) 1031 this.carrierHRF = null; 1032 else { 1033 if (this.carrierHRF == null) 1034 this.carrierHRF = new StringType(); 1035 this.carrierHRF.setValue(value); 1036 } 1037 return this; 1038 } 1039 1040 /** 1041 * @return {@link #entryType} (A coded entry to indicate how the data was 1042 * entered.). This is the underlying object with id, value and 1043 * extensions. The accessor "getEntryType" gives direct access to the 1044 * value 1045 */ 1046 public Enumeration<UDIEntryType> getEntryTypeElement() { 1047 if (this.entryType == null) 1048 if (Configuration.errorOnAutoCreate()) 1049 throw new Error("Attempt to auto-create DeviceUdiCarrierComponent.entryType"); 1050 else if (Configuration.doAutoCreate()) 1051 this.entryType = new Enumeration<UDIEntryType>(new UDIEntryTypeEnumFactory()); // bb 1052 return this.entryType; 1053 } 1054 1055 public boolean hasEntryTypeElement() { 1056 return this.entryType != null && !this.entryType.isEmpty(); 1057 } 1058 1059 public boolean hasEntryType() { 1060 return this.entryType != null && !this.entryType.isEmpty(); 1061 } 1062 1063 /** 1064 * @param value {@link #entryType} (A coded entry to indicate how the data was 1065 * entered.). This is the underlying object with id, value and 1066 * extensions. The accessor "getEntryType" gives direct access to 1067 * the value 1068 */ 1069 public DeviceUdiCarrierComponent setEntryTypeElement(Enumeration<UDIEntryType> value) { 1070 this.entryType = value; 1071 return this; 1072 } 1073 1074 /** 1075 * @return A coded entry to indicate how the data was entered. 1076 */ 1077 public UDIEntryType getEntryType() { 1078 return this.entryType == null ? null : this.entryType.getValue(); 1079 } 1080 1081 /** 1082 * @param value A coded entry to indicate how the data was entered. 1083 */ 1084 public DeviceUdiCarrierComponent setEntryType(UDIEntryType value) { 1085 if (value == null) 1086 this.entryType = null; 1087 else { 1088 if (this.entryType == null) 1089 this.entryType = new Enumeration<UDIEntryType>(new UDIEntryTypeEnumFactory()); 1090 this.entryType.setValue(value); 1091 } 1092 return this; 1093 } 1094 1095 protected void listChildren(List<Property> children) { 1096 super.listChildren(children); 1097 children.add(new Property("deviceIdentifier", "string", 1098 "The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device.", 1099 0, 1, deviceIdentifier)); 1100 children.add(new Property("issuer", "uri", 1101 "Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include :\n1) GS1: \nhttp://hl7.org/fhir/NamingSystem/gs1-di, \n2) HIBCC:\nhttp://hl7.org/fhir/NamingSystem/hibcc-dI, \n3) ICCBBA for blood containers:\nhttp://hl7.org/fhir/NamingSystem/iccbba-blood-di, \n4) ICCBA for other devices:\nhttp://hl7.org/fhir/NamingSystem/iccbba-other-di.", 1102 0, 1, issuer)); 1103 children.add(new Property("jurisdiction", "uri", 1104 "The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/fda-udi.", 1105 0, 1, jurisdiction)); 1106 children.add(new Property("carrierAIDC", "base64Binary", 1107 "The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - e.g., a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded.", 1108 0, 1, carrierAIDC)); 1109 children.add(new Property("carrierHRF", "string", 1110 "The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device.", 1111 0, 1, carrierHRF)); 1112 children.add( 1113 new Property("entryType", "code", "A coded entry to indicate how the data was entered.", 0, 1, entryType)); 1114 } 1115 1116 @Override 1117 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1118 switch (_hash) { 1119 case 1322005407: 1120 /* deviceIdentifier */ return new Property("deviceIdentifier", "string", 1121 "The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device.", 1122 0, 1, deviceIdentifier); 1123 case -1179159879: 1124 /* issuer */ return new Property("issuer", "uri", 1125 "Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include :\n1) GS1: \nhttp://hl7.org/fhir/NamingSystem/gs1-di, \n2) HIBCC:\nhttp://hl7.org/fhir/NamingSystem/hibcc-dI, \n3) ICCBBA for blood containers:\nhttp://hl7.org/fhir/NamingSystem/iccbba-blood-di, \n4) ICCBA for other devices:\nhttp://hl7.org/fhir/NamingSystem/iccbba-other-di.", 1126 0, 1, issuer); 1127 case -507075711: 1128 /* jurisdiction */ return new Property("jurisdiction", "uri", 1129 "The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/fda-udi.", 1130 0, 1, jurisdiction); 1131 case -768521825: 1132 /* carrierAIDC */ return new Property("carrierAIDC", "base64Binary", 1133 "The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - e.g., a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded.", 1134 0, 1, carrierAIDC); 1135 case 806499972: 1136 /* carrierHRF */ return new Property("carrierHRF", "string", 1137 "The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device.", 1138 0, 1, carrierHRF); 1139 case -479362356: 1140 /* entryType */ return new Property("entryType", "code", "A coded entry to indicate how the data was entered.", 1141 0, 1, entryType); 1142 default: 1143 return super.getNamedProperty(_hash, _name, _checkValid); 1144 } 1145 1146 } 1147 1148 @Override 1149 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1150 switch (hash) { 1151 case 1322005407: 1152 /* deviceIdentifier */ return this.deviceIdentifier == null ? new Base[0] 1153 : new Base[] { this.deviceIdentifier }; // StringType 1154 case -1179159879: 1155 /* issuer */ return this.issuer == null ? new Base[0] : new Base[] { this.issuer }; // UriType 1156 case -507075711: 1157 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] : new Base[] { this.jurisdiction }; // UriType 1158 case -768521825: 1159 /* carrierAIDC */ return this.carrierAIDC == null ? new Base[0] : new Base[] { this.carrierAIDC }; // Base64BinaryType 1160 case 806499972: 1161 /* carrierHRF */ return this.carrierHRF == null ? new Base[0] : new Base[] { this.carrierHRF }; // StringType 1162 case -479362356: 1163 /* entryType */ return this.entryType == null ? new Base[0] : new Base[] { this.entryType }; // Enumeration<UDIEntryType> 1164 default: 1165 return super.getProperty(hash, name, checkValid); 1166 } 1167 1168 } 1169 1170 @Override 1171 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1172 switch (hash) { 1173 case 1322005407: // deviceIdentifier 1174 this.deviceIdentifier = castToString(value); // StringType 1175 return value; 1176 case -1179159879: // issuer 1177 this.issuer = castToUri(value); // UriType 1178 return value; 1179 case -507075711: // jurisdiction 1180 this.jurisdiction = castToUri(value); // UriType 1181 return value; 1182 case -768521825: // carrierAIDC 1183 this.carrierAIDC = castToBase64Binary(value); // Base64BinaryType 1184 return value; 1185 case 806499972: // carrierHRF 1186 this.carrierHRF = castToString(value); // StringType 1187 return value; 1188 case -479362356: // entryType 1189 value = new UDIEntryTypeEnumFactory().fromType(castToCode(value)); 1190 this.entryType = (Enumeration) value; // Enumeration<UDIEntryType> 1191 return value; 1192 default: 1193 return super.setProperty(hash, name, value); 1194 } 1195 1196 } 1197 1198 @Override 1199 public Base setProperty(String name, Base value) throws FHIRException { 1200 if (name.equals("deviceIdentifier")) { 1201 this.deviceIdentifier = castToString(value); // StringType 1202 } else if (name.equals("issuer")) { 1203 this.issuer = castToUri(value); // UriType 1204 } else if (name.equals("jurisdiction")) { 1205 this.jurisdiction = castToUri(value); // UriType 1206 } else if (name.equals("carrierAIDC")) { 1207 this.carrierAIDC = castToBase64Binary(value); // Base64BinaryType 1208 } else if (name.equals("carrierHRF")) { 1209 this.carrierHRF = castToString(value); // StringType 1210 } else if (name.equals("entryType")) { 1211 value = new UDIEntryTypeEnumFactory().fromType(castToCode(value)); 1212 this.entryType = (Enumeration) value; // Enumeration<UDIEntryType> 1213 } else 1214 return super.setProperty(name, value); 1215 return value; 1216 } 1217 1218 @Override 1219 public void removeChild(String name, Base value) throws FHIRException { 1220 if (name.equals("deviceIdentifier")) { 1221 this.deviceIdentifier = null; 1222 } else if (name.equals("issuer")) { 1223 this.issuer = null; 1224 } else if (name.equals("jurisdiction")) { 1225 this.jurisdiction = null; 1226 } else if (name.equals("carrierAIDC")) { 1227 this.carrierAIDC = null; 1228 } else if (name.equals("carrierHRF")) { 1229 this.carrierHRF = null; 1230 } else if (name.equals("entryType")) { 1231 this.entryType = null; 1232 } else 1233 super.removeChild(name, value); 1234 1235 } 1236 1237 @Override 1238 public Base makeProperty(int hash, String name) throws FHIRException { 1239 switch (hash) { 1240 case 1322005407: 1241 return getDeviceIdentifierElement(); 1242 case -1179159879: 1243 return getIssuerElement(); 1244 case -507075711: 1245 return getJurisdictionElement(); 1246 case -768521825: 1247 return getCarrierAIDCElement(); 1248 case 806499972: 1249 return getCarrierHRFElement(); 1250 case -479362356: 1251 return getEntryTypeElement(); 1252 default: 1253 return super.makeProperty(hash, name); 1254 } 1255 1256 } 1257 1258 @Override 1259 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1260 switch (hash) { 1261 case 1322005407: 1262 /* deviceIdentifier */ return new String[] { "string" }; 1263 case -1179159879: 1264 /* issuer */ return new String[] { "uri" }; 1265 case -507075711: 1266 /* jurisdiction */ return new String[] { "uri" }; 1267 case -768521825: 1268 /* carrierAIDC */ return new String[] { "base64Binary" }; 1269 case 806499972: 1270 /* carrierHRF */ return new String[] { "string" }; 1271 case -479362356: 1272 /* entryType */ return new String[] { "code" }; 1273 default: 1274 return super.getTypesForProperty(hash, name); 1275 } 1276 1277 } 1278 1279 @Override 1280 public Base addChild(String name) throws FHIRException { 1281 if (name.equals("deviceIdentifier")) { 1282 throw new FHIRException("Cannot call addChild on a singleton property Device.deviceIdentifier"); 1283 } else if (name.equals("issuer")) { 1284 throw new FHIRException("Cannot call addChild on a singleton property Device.issuer"); 1285 } else if (name.equals("jurisdiction")) { 1286 throw new FHIRException("Cannot call addChild on a singleton property Device.jurisdiction"); 1287 } else if (name.equals("carrierAIDC")) { 1288 throw new FHIRException("Cannot call addChild on a singleton property Device.carrierAIDC"); 1289 } else if (name.equals("carrierHRF")) { 1290 throw new FHIRException("Cannot call addChild on a singleton property Device.carrierHRF"); 1291 } else if (name.equals("entryType")) { 1292 throw new FHIRException("Cannot call addChild on a singleton property Device.entryType"); 1293 } else 1294 return super.addChild(name); 1295 } 1296 1297 public DeviceUdiCarrierComponent copy() { 1298 DeviceUdiCarrierComponent dst = new DeviceUdiCarrierComponent(); 1299 copyValues(dst); 1300 return dst; 1301 } 1302 1303 public void copyValues(DeviceUdiCarrierComponent dst) { 1304 super.copyValues(dst); 1305 dst.deviceIdentifier = deviceIdentifier == null ? null : deviceIdentifier.copy(); 1306 dst.issuer = issuer == null ? null : issuer.copy(); 1307 dst.jurisdiction = jurisdiction == null ? null : jurisdiction.copy(); 1308 dst.carrierAIDC = carrierAIDC == null ? null : carrierAIDC.copy(); 1309 dst.carrierHRF = carrierHRF == null ? null : carrierHRF.copy(); 1310 dst.entryType = entryType == null ? null : entryType.copy(); 1311 } 1312 1313 @Override 1314 public boolean equalsDeep(Base other_) { 1315 if (!super.equalsDeep(other_)) 1316 return false; 1317 if (!(other_ instanceof DeviceUdiCarrierComponent)) 1318 return false; 1319 DeviceUdiCarrierComponent o = (DeviceUdiCarrierComponent) other_; 1320 return compareDeep(deviceIdentifier, o.deviceIdentifier, true) && compareDeep(issuer, o.issuer, true) 1321 && compareDeep(jurisdiction, o.jurisdiction, true) && compareDeep(carrierAIDC, o.carrierAIDC, true) 1322 && compareDeep(carrierHRF, o.carrierHRF, true) && compareDeep(entryType, o.entryType, true); 1323 } 1324 1325 @Override 1326 public boolean equalsShallow(Base other_) { 1327 if (!super.equalsShallow(other_)) 1328 return false; 1329 if (!(other_ instanceof DeviceUdiCarrierComponent)) 1330 return false; 1331 DeviceUdiCarrierComponent o = (DeviceUdiCarrierComponent) other_; 1332 return compareValues(deviceIdentifier, o.deviceIdentifier, true) && compareValues(issuer, o.issuer, true) 1333 && compareValues(jurisdiction, o.jurisdiction, true) && compareValues(carrierAIDC, o.carrierAIDC, true) 1334 && compareValues(carrierHRF, o.carrierHRF, true) && compareValues(entryType, o.entryType, true); 1335 } 1336 1337 public boolean isEmpty() { 1338 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(deviceIdentifier, issuer, jurisdiction, 1339 carrierAIDC, carrierHRF, entryType); 1340 } 1341 1342 public String fhirType() { 1343 return "Device.udiCarrier"; 1344 1345 } 1346 1347 } 1348 1349 @Block() 1350 public static class DeviceDeviceNameComponent extends BackboneElement implements IBaseBackboneElement { 1351 /** 1352 * The name of the device. 1353 */ 1354 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1355 @Description(shortDefinition = "The name of the device", formalDefinition = "The name of the device.") 1356 protected StringType name; 1357 1358 /** 1359 * The type of deviceName. UDILabelName | UserFriendlyName | PatientReportedName 1360 * | ManufactureDeviceName | ModelName. 1361 */ 1362 @Child(name = "type", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 1363 @Description(shortDefinition = "udi-label-name | user-friendly-name | patient-reported-name | manufacturer-name | model-name | other", formalDefinition = "The type of deviceName.\nUDILabelName | UserFriendlyName | PatientReportedName | ManufactureDeviceName | ModelName.") 1364 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/device-nametype") 1365 protected Enumeration<DeviceNameType> type; 1366 1367 private static final long serialVersionUID = 918983440L; 1368 1369 /** 1370 * Constructor 1371 */ 1372 public DeviceDeviceNameComponent() { 1373 super(); 1374 } 1375 1376 /** 1377 * Constructor 1378 */ 1379 public DeviceDeviceNameComponent(StringType name, Enumeration<DeviceNameType> type) { 1380 super(); 1381 this.name = name; 1382 this.type = type; 1383 } 1384 1385 /** 1386 * @return {@link #name} (The name of the device.). This is the underlying 1387 * object with id, value and extensions. The accessor "getName" gives 1388 * direct access to the value 1389 */ 1390 public StringType getNameElement() { 1391 if (this.name == null) 1392 if (Configuration.errorOnAutoCreate()) 1393 throw new Error("Attempt to auto-create DeviceDeviceNameComponent.name"); 1394 else if (Configuration.doAutoCreate()) 1395 this.name = new StringType(); // bb 1396 return this.name; 1397 } 1398 1399 public boolean hasNameElement() { 1400 return this.name != null && !this.name.isEmpty(); 1401 } 1402 1403 public boolean hasName() { 1404 return this.name != null && !this.name.isEmpty(); 1405 } 1406 1407 /** 1408 * @param value {@link #name} (The name of the device.). This is the underlying 1409 * object with id, value and extensions. The accessor "getName" 1410 * gives direct access to the value 1411 */ 1412 public DeviceDeviceNameComponent setNameElement(StringType value) { 1413 this.name = value; 1414 return this; 1415 } 1416 1417 /** 1418 * @return The name of the device. 1419 */ 1420 public String getName() { 1421 return this.name == null ? null : this.name.getValue(); 1422 } 1423 1424 /** 1425 * @param value The name of the device. 1426 */ 1427 public DeviceDeviceNameComponent setName(String value) { 1428 if (this.name == null) 1429 this.name = new StringType(); 1430 this.name.setValue(value); 1431 return this; 1432 } 1433 1434 /** 1435 * @return {@link #type} (The type of deviceName. UDILabelName | 1436 * UserFriendlyName | PatientReportedName | ManufactureDeviceName | 1437 * ModelName.). This is the underlying object with id, value and 1438 * extensions. The accessor "getType" gives direct access to the value 1439 */ 1440 public Enumeration<DeviceNameType> getTypeElement() { 1441 if (this.type == null) 1442 if (Configuration.errorOnAutoCreate()) 1443 throw new Error("Attempt to auto-create DeviceDeviceNameComponent.type"); 1444 else if (Configuration.doAutoCreate()) 1445 this.type = new Enumeration<DeviceNameType>(new DeviceNameTypeEnumFactory()); // bb 1446 return this.type; 1447 } 1448 1449 public boolean hasTypeElement() { 1450 return this.type != null && !this.type.isEmpty(); 1451 } 1452 1453 public boolean hasType() { 1454 return this.type != null && !this.type.isEmpty(); 1455 } 1456 1457 /** 1458 * @param value {@link #type} (The type of deviceName. UDILabelName | 1459 * UserFriendlyName | PatientReportedName | ManufactureDeviceName | 1460 * ModelName.). This is the underlying object with id, value and 1461 * extensions. The accessor "getType" gives direct access to the 1462 * value 1463 */ 1464 public DeviceDeviceNameComponent setTypeElement(Enumeration<DeviceNameType> value) { 1465 this.type = value; 1466 return this; 1467 } 1468 1469 /** 1470 * @return The type of deviceName. UDILabelName | UserFriendlyName | 1471 * PatientReportedName | ManufactureDeviceName | ModelName. 1472 */ 1473 public DeviceNameType getType() { 1474 return this.type == null ? null : this.type.getValue(); 1475 } 1476 1477 /** 1478 * @param value The type of deviceName. UDILabelName | UserFriendlyName | 1479 * PatientReportedName | ManufactureDeviceName | ModelName. 1480 */ 1481 public DeviceDeviceNameComponent setType(DeviceNameType value) { 1482 if (this.type == null) 1483 this.type = new Enumeration<DeviceNameType>(new DeviceNameTypeEnumFactory()); 1484 this.type.setValue(value); 1485 return this; 1486 } 1487 1488 protected void listChildren(List<Property> children) { 1489 super.listChildren(children); 1490 children.add(new Property("name", "string", "The name of the device.", 0, 1, name)); 1491 children.add(new Property("type", "code", 1492 "The type of deviceName.\nUDILabelName | UserFriendlyName | PatientReportedName | ManufactureDeviceName | ModelName.", 1493 0, 1, type)); 1494 } 1495 1496 @Override 1497 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1498 switch (_hash) { 1499 case 3373707: 1500 /* name */ return new Property("name", "string", "The name of the device.", 0, 1, name); 1501 case 3575610: 1502 /* type */ return new Property("type", "code", 1503 "The type of deviceName.\nUDILabelName | UserFriendlyName | PatientReportedName | ManufactureDeviceName | ModelName.", 1504 0, 1, type); 1505 default: 1506 return super.getNamedProperty(_hash, _name, _checkValid); 1507 } 1508 1509 } 1510 1511 @Override 1512 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1513 switch (hash) { 1514 case 3373707: 1515 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 1516 case 3575610: 1517 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<DeviceNameType> 1518 default: 1519 return super.getProperty(hash, name, checkValid); 1520 } 1521 1522 } 1523 1524 @Override 1525 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1526 switch (hash) { 1527 case 3373707: // name 1528 this.name = castToString(value); // StringType 1529 return value; 1530 case 3575610: // type 1531 value = new DeviceNameTypeEnumFactory().fromType(castToCode(value)); 1532 this.type = (Enumeration) value; // Enumeration<DeviceNameType> 1533 return value; 1534 default: 1535 return super.setProperty(hash, name, value); 1536 } 1537 1538 } 1539 1540 @Override 1541 public Base setProperty(String name, Base value) throws FHIRException { 1542 if (name.equals("name")) { 1543 this.name = castToString(value); // StringType 1544 } else if (name.equals("type")) { 1545 value = new DeviceNameTypeEnumFactory().fromType(castToCode(value)); 1546 this.type = (Enumeration) value; // Enumeration<DeviceNameType> 1547 } else 1548 return super.setProperty(name, value); 1549 return value; 1550 } 1551 1552 @Override 1553 public void removeChild(String name, Base value) throws FHIRException { 1554 if (name.equals("name")) { 1555 this.name = null; 1556 } else if (name.equals("type")) { 1557 this.type = null; 1558 } else 1559 super.removeChild(name, value); 1560 1561 } 1562 1563 @Override 1564 public Base makeProperty(int hash, String name) throws FHIRException { 1565 switch (hash) { 1566 case 3373707: 1567 return getNameElement(); 1568 case 3575610: 1569 return getTypeElement(); 1570 default: 1571 return super.makeProperty(hash, name); 1572 } 1573 1574 } 1575 1576 @Override 1577 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1578 switch (hash) { 1579 case 3373707: 1580 /* name */ return new String[] { "string" }; 1581 case 3575610: 1582 /* type */ return new String[] { "code" }; 1583 default: 1584 return super.getTypesForProperty(hash, name); 1585 } 1586 1587 } 1588 1589 @Override 1590 public Base addChild(String name) throws FHIRException { 1591 if (name.equals("name")) { 1592 throw new FHIRException("Cannot call addChild on a singleton property Device.name"); 1593 } else if (name.equals("type")) { 1594 throw new FHIRException("Cannot call addChild on a singleton property Device.type"); 1595 } else 1596 return super.addChild(name); 1597 } 1598 1599 public DeviceDeviceNameComponent copy() { 1600 DeviceDeviceNameComponent dst = new DeviceDeviceNameComponent(); 1601 copyValues(dst); 1602 return dst; 1603 } 1604 1605 public void copyValues(DeviceDeviceNameComponent dst) { 1606 super.copyValues(dst); 1607 dst.name = name == null ? null : name.copy(); 1608 dst.type = type == null ? null : type.copy(); 1609 } 1610 1611 @Override 1612 public boolean equalsDeep(Base other_) { 1613 if (!super.equalsDeep(other_)) 1614 return false; 1615 if (!(other_ instanceof DeviceDeviceNameComponent)) 1616 return false; 1617 DeviceDeviceNameComponent o = (DeviceDeviceNameComponent) other_; 1618 return compareDeep(name, o.name, true) && compareDeep(type, o.type, true); 1619 } 1620 1621 @Override 1622 public boolean equalsShallow(Base other_) { 1623 if (!super.equalsShallow(other_)) 1624 return false; 1625 if (!(other_ instanceof DeviceDeviceNameComponent)) 1626 return false; 1627 DeviceDeviceNameComponent o = (DeviceDeviceNameComponent) other_; 1628 return compareValues(name, o.name, true) && compareValues(type, o.type, true); 1629 } 1630 1631 public boolean isEmpty() { 1632 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, type); 1633 } 1634 1635 public String fhirType() { 1636 return "Device.deviceName"; 1637 1638 } 1639 1640 } 1641 1642 @Block() 1643 public static class DeviceSpecializationComponent extends BackboneElement implements IBaseBackboneElement { 1644 /** 1645 * The standard that is used to operate and communicate. 1646 */ 1647 @Child(name = "systemType", type = { 1648 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1649 @Description(shortDefinition = "The standard that is used to operate and communicate", formalDefinition = "The standard that is used to operate and communicate.") 1650 protected CodeableConcept systemType; 1651 1652 /** 1653 * The version of the standard that is used to operate and communicate. 1654 */ 1655 @Child(name = "version", type = { 1656 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1657 @Description(shortDefinition = "The version of the standard that is used to operate and communicate", formalDefinition = "The version of the standard that is used to operate and communicate.") 1658 protected StringType version; 1659 1660 private static final long serialVersionUID = 1557342629L; 1661 1662 /** 1663 * Constructor 1664 */ 1665 public DeviceSpecializationComponent() { 1666 super(); 1667 } 1668 1669 /** 1670 * Constructor 1671 */ 1672 public DeviceSpecializationComponent(CodeableConcept systemType) { 1673 super(); 1674 this.systemType = systemType; 1675 } 1676 1677 /** 1678 * @return {@link #systemType} (The standard that is used to operate and 1679 * communicate.) 1680 */ 1681 public CodeableConcept getSystemType() { 1682 if (this.systemType == null) 1683 if (Configuration.errorOnAutoCreate()) 1684 throw new Error("Attempt to auto-create DeviceSpecializationComponent.systemType"); 1685 else if (Configuration.doAutoCreate()) 1686 this.systemType = new CodeableConcept(); // cc 1687 return this.systemType; 1688 } 1689 1690 public boolean hasSystemType() { 1691 return this.systemType != null && !this.systemType.isEmpty(); 1692 } 1693 1694 /** 1695 * @param value {@link #systemType} (The standard that is used to operate and 1696 * communicate.) 1697 */ 1698 public DeviceSpecializationComponent setSystemType(CodeableConcept value) { 1699 this.systemType = value; 1700 return this; 1701 } 1702 1703 /** 1704 * @return {@link #version} (The version of the standard that is used to operate 1705 * and communicate.). This is the underlying object with id, value and 1706 * extensions. The accessor "getVersion" gives direct access to the 1707 * value 1708 */ 1709 public StringType getVersionElement() { 1710 if (this.version == null) 1711 if (Configuration.errorOnAutoCreate()) 1712 throw new Error("Attempt to auto-create DeviceSpecializationComponent.version"); 1713 else if (Configuration.doAutoCreate()) 1714 this.version = new StringType(); // bb 1715 return this.version; 1716 } 1717 1718 public boolean hasVersionElement() { 1719 return this.version != null && !this.version.isEmpty(); 1720 } 1721 1722 public boolean hasVersion() { 1723 return this.version != null && !this.version.isEmpty(); 1724 } 1725 1726 /** 1727 * @param value {@link #version} (The version of the standard that is used to 1728 * operate and communicate.). This is the underlying object with 1729 * id, value and extensions. The accessor "getVersion" gives direct 1730 * access to the value 1731 */ 1732 public DeviceSpecializationComponent setVersionElement(StringType value) { 1733 this.version = value; 1734 return this; 1735 } 1736 1737 /** 1738 * @return The version of the standard that is used to operate and communicate. 1739 */ 1740 public String getVersion() { 1741 return this.version == null ? null : this.version.getValue(); 1742 } 1743 1744 /** 1745 * @param value The version of the standard that is used to operate and 1746 * communicate. 1747 */ 1748 public DeviceSpecializationComponent setVersion(String value) { 1749 if (Utilities.noString(value)) 1750 this.version = null; 1751 else { 1752 if (this.version == null) 1753 this.version = new StringType(); 1754 this.version.setValue(value); 1755 } 1756 return this; 1757 } 1758 1759 protected void listChildren(List<Property> children) { 1760 super.listChildren(children); 1761 children.add(new Property("systemType", "CodeableConcept", 1762 "The standard that is used to operate and communicate.", 0, 1, systemType)); 1763 children.add(new Property("version", "string", 1764 "The version of the standard that is used to operate and communicate.", 0, 1, version)); 1765 } 1766 1767 @Override 1768 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1769 switch (_hash) { 1770 case 642893321: 1771 /* systemType */ return new Property("systemType", "CodeableConcept", 1772 "The standard that is used to operate and communicate.", 0, 1, systemType); 1773 case 351608024: 1774 /* version */ return new Property("version", "string", 1775 "The version of the standard that is used to operate and communicate.", 0, 1, version); 1776 default: 1777 return super.getNamedProperty(_hash, _name, _checkValid); 1778 } 1779 1780 } 1781 1782 @Override 1783 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1784 switch (hash) { 1785 case 642893321: 1786 /* systemType */ return this.systemType == null ? new Base[0] : new Base[] { this.systemType }; // CodeableConcept 1787 case 351608024: 1788 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 1789 default: 1790 return super.getProperty(hash, name, checkValid); 1791 } 1792 1793 } 1794 1795 @Override 1796 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1797 switch (hash) { 1798 case 642893321: // systemType 1799 this.systemType = castToCodeableConcept(value); // CodeableConcept 1800 return value; 1801 case 351608024: // version 1802 this.version = castToString(value); // StringType 1803 return value; 1804 default: 1805 return super.setProperty(hash, name, value); 1806 } 1807 1808 } 1809 1810 @Override 1811 public Base setProperty(String name, Base value) throws FHIRException { 1812 if (name.equals("systemType")) { 1813 this.systemType = castToCodeableConcept(value); // CodeableConcept 1814 } else if (name.equals("version")) { 1815 this.version = castToString(value); // StringType 1816 } else 1817 return super.setProperty(name, value); 1818 return value; 1819 } 1820 1821 @Override 1822 public void removeChild(String name, Base value) throws FHIRException { 1823 if (name.equals("systemType")) { 1824 this.systemType = null; 1825 } else if (name.equals("version")) { 1826 this.version = null; 1827 } else 1828 super.removeChild(name, value); 1829 1830 } 1831 1832 @Override 1833 public Base makeProperty(int hash, String name) throws FHIRException { 1834 switch (hash) { 1835 case 642893321: 1836 return getSystemType(); 1837 case 351608024: 1838 return getVersionElement(); 1839 default: 1840 return super.makeProperty(hash, name); 1841 } 1842 1843 } 1844 1845 @Override 1846 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1847 switch (hash) { 1848 case 642893321: 1849 /* systemType */ return new String[] { "CodeableConcept" }; 1850 case 351608024: 1851 /* version */ return new String[] { "string" }; 1852 default: 1853 return super.getTypesForProperty(hash, name); 1854 } 1855 1856 } 1857 1858 @Override 1859 public Base addChild(String name) throws FHIRException { 1860 if (name.equals("systemType")) { 1861 this.systemType = new CodeableConcept(); 1862 return this.systemType; 1863 } else if (name.equals("version")) { 1864 throw new FHIRException("Cannot call addChild on a singleton property Device.version"); 1865 } else 1866 return super.addChild(name); 1867 } 1868 1869 public DeviceSpecializationComponent copy() { 1870 DeviceSpecializationComponent dst = new DeviceSpecializationComponent(); 1871 copyValues(dst); 1872 return dst; 1873 } 1874 1875 public void copyValues(DeviceSpecializationComponent dst) { 1876 super.copyValues(dst); 1877 dst.systemType = systemType == null ? null : systemType.copy(); 1878 dst.version = version == null ? null : version.copy(); 1879 } 1880 1881 @Override 1882 public boolean equalsDeep(Base other_) { 1883 if (!super.equalsDeep(other_)) 1884 return false; 1885 if (!(other_ instanceof DeviceSpecializationComponent)) 1886 return false; 1887 DeviceSpecializationComponent o = (DeviceSpecializationComponent) other_; 1888 return compareDeep(systemType, o.systemType, true) && compareDeep(version, o.version, true); 1889 } 1890 1891 @Override 1892 public boolean equalsShallow(Base other_) { 1893 if (!super.equalsShallow(other_)) 1894 return false; 1895 if (!(other_ instanceof DeviceSpecializationComponent)) 1896 return false; 1897 DeviceSpecializationComponent o = (DeviceSpecializationComponent) other_; 1898 return compareValues(version, o.version, true); 1899 } 1900 1901 public boolean isEmpty() { 1902 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(systemType, version); 1903 } 1904 1905 public String fhirType() { 1906 return "Device.specialization"; 1907 1908 } 1909 1910 } 1911 1912 @Block() 1913 public static class DeviceVersionComponent extends BackboneElement implements IBaseBackboneElement { 1914 /** 1915 * The type of the device version. 1916 */ 1917 @Child(name = "type", type = { 1918 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1919 @Description(shortDefinition = "The type of the device version", formalDefinition = "The type of the device version.") 1920 protected CodeableConcept type; 1921 1922 /** 1923 * A single component of the device version. 1924 */ 1925 @Child(name = "component", type = { 1926 Identifier.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1927 @Description(shortDefinition = "A single component of the device version", formalDefinition = "A single component of the device version.") 1928 protected Identifier component; 1929 1930 /** 1931 * The version text. 1932 */ 1933 @Child(name = "value", type = { StringType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 1934 @Description(shortDefinition = "The version text", formalDefinition = "The version text.") 1935 protected StringType value; 1936 1937 private static final long serialVersionUID = 645214295L; 1938 1939 /** 1940 * Constructor 1941 */ 1942 public DeviceVersionComponent() { 1943 super(); 1944 } 1945 1946 /** 1947 * Constructor 1948 */ 1949 public DeviceVersionComponent(StringType value) { 1950 super(); 1951 this.value = value; 1952 } 1953 1954 /** 1955 * @return {@link #type} (The type of the device version.) 1956 */ 1957 public CodeableConcept getType() { 1958 if (this.type == null) 1959 if (Configuration.errorOnAutoCreate()) 1960 throw new Error("Attempt to auto-create DeviceVersionComponent.type"); 1961 else if (Configuration.doAutoCreate()) 1962 this.type = new CodeableConcept(); // cc 1963 return this.type; 1964 } 1965 1966 public boolean hasType() { 1967 return this.type != null && !this.type.isEmpty(); 1968 } 1969 1970 /** 1971 * @param value {@link #type} (The type of the device version.) 1972 */ 1973 public DeviceVersionComponent setType(CodeableConcept value) { 1974 this.type = value; 1975 return this; 1976 } 1977 1978 /** 1979 * @return {@link #component} (A single component of the device version.) 1980 */ 1981 public Identifier getComponent() { 1982 if (this.component == null) 1983 if (Configuration.errorOnAutoCreate()) 1984 throw new Error("Attempt to auto-create DeviceVersionComponent.component"); 1985 else if (Configuration.doAutoCreate()) 1986 this.component = new Identifier(); // cc 1987 return this.component; 1988 } 1989 1990 public boolean hasComponent() { 1991 return this.component != null && !this.component.isEmpty(); 1992 } 1993 1994 /** 1995 * @param value {@link #component} (A single component of the device version.) 1996 */ 1997 public DeviceVersionComponent setComponent(Identifier value) { 1998 this.component = value; 1999 return this; 2000 } 2001 2002 /** 2003 * @return {@link #value} (The version text.). This is the underlying object 2004 * with id, value and extensions. The accessor "getValue" gives direct 2005 * access to the value 2006 */ 2007 public StringType getValueElement() { 2008 if (this.value == null) 2009 if (Configuration.errorOnAutoCreate()) 2010 throw new Error("Attempt to auto-create DeviceVersionComponent.value"); 2011 else if (Configuration.doAutoCreate()) 2012 this.value = new StringType(); // bb 2013 return this.value; 2014 } 2015 2016 public boolean hasValueElement() { 2017 return this.value != null && !this.value.isEmpty(); 2018 } 2019 2020 public boolean hasValue() { 2021 return this.value != null && !this.value.isEmpty(); 2022 } 2023 2024 /** 2025 * @param value {@link #value} (The version text.). This is the underlying 2026 * object with id, value and extensions. The accessor "getValue" 2027 * gives direct access to the value 2028 */ 2029 public DeviceVersionComponent setValueElement(StringType value) { 2030 this.value = value; 2031 return this; 2032 } 2033 2034 /** 2035 * @return The version text. 2036 */ 2037 public String getValue() { 2038 return this.value == null ? null : this.value.getValue(); 2039 } 2040 2041 /** 2042 * @param value The version text. 2043 */ 2044 public DeviceVersionComponent setValue(String value) { 2045 if (this.value == null) 2046 this.value = new StringType(); 2047 this.value.setValue(value); 2048 return this; 2049 } 2050 2051 protected void listChildren(List<Property> children) { 2052 super.listChildren(children); 2053 children.add(new Property("type", "CodeableConcept", "The type of the device version.", 0, 1, type)); 2054 children 2055 .add(new Property("component", "Identifier", "A single component of the device version.", 0, 1, component)); 2056 children.add(new Property("value", "string", "The version text.", 0, 1, value)); 2057 } 2058 2059 @Override 2060 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2061 switch (_hash) { 2062 case 3575610: 2063 /* type */ return new Property("type", "CodeableConcept", "The type of the device version.", 0, 1, type); 2064 case -1399907075: 2065 /* component */ return new Property("component", "Identifier", "A single component of the device version.", 0, 2066 1, component); 2067 case 111972721: 2068 /* value */ return new Property("value", "string", "The version text.", 0, 1, value); 2069 default: 2070 return super.getNamedProperty(_hash, _name, _checkValid); 2071 } 2072 2073 } 2074 2075 @Override 2076 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2077 switch (hash) { 2078 case 3575610: 2079 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2080 case -1399907075: 2081 /* component */ return this.component == null ? new Base[0] : new Base[] { this.component }; // Identifier 2082 case 111972721: 2083 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // StringType 2084 default: 2085 return super.getProperty(hash, name, checkValid); 2086 } 2087 2088 } 2089 2090 @Override 2091 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2092 switch (hash) { 2093 case 3575610: // type 2094 this.type = castToCodeableConcept(value); // CodeableConcept 2095 return value; 2096 case -1399907075: // component 2097 this.component = castToIdentifier(value); // Identifier 2098 return value; 2099 case 111972721: // value 2100 this.value = castToString(value); // StringType 2101 return value; 2102 default: 2103 return super.setProperty(hash, name, value); 2104 } 2105 2106 } 2107 2108 @Override 2109 public Base setProperty(String name, Base value) throws FHIRException { 2110 if (name.equals("type")) { 2111 this.type = castToCodeableConcept(value); // CodeableConcept 2112 } else if (name.equals("component")) { 2113 this.component = castToIdentifier(value); // Identifier 2114 } else if (name.equals("value")) { 2115 this.value = castToString(value); // StringType 2116 } else 2117 return super.setProperty(name, value); 2118 return value; 2119 } 2120 2121 @Override 2122 public void removeChild(String name, Base value) throws FHIRException { 2123 if (name.equals("type")) { 2124 this.type = null; 2125 } else if (name.equals("component")) { 2126 this.component = null; 2127 } else if (name.equals("value")) { 2128 this.value = null; 2129 } else 2130 super.removeChild(name, value); 2131 2132 } 2133 2134 @Override 2135 public Base makeProperty(int hash, String name) throws FHIRException { 2136 switch (hash) { 2137 case 3575610: 2138 return getType(); 2139 case -1399907075: 2140 return getComponent(); 2141 case 111972721: 2142 return getValueElement(); 2143 default: 2144 return super.makeProperty(hash, name); 2145 } 2146 2147 } 2148 2149 @Override 2150 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2151 switch (hash) { 2152 case 3575610: 2153 /* type */ return new String[] { "CodeableConcept" }; 2154 case -1399907075: 2155 /* component */ return new String[] { "Identifier" }; 2156 case 111972721: 2157 /* value */ return new String[] { "string" }; 2158 default: 2159 return super.getTypesForProperty(hash, name); 2160 } 2161 2162 } 2163 2164 @Override 2165 public Base addChild(String name) throws FHIRException { 2166 if (name.equals("type")) { 2167 this.type = new CodeableConcept(); 2168 return this.type; 2169 } else if (name.equals("component")) { 2170 this.component = new Identifier(); 2171 return this.component; 2172 } else if (name.equals("value")) { 2173 throw new FHIRException("Cannot call addChild on a singleton property Device.value"); 2174 } else 2175 return super.addChild(name); 2176 } 2177 2178 public DeviceVersionComponent copy() { 2179 DeviceVersionComponent dst = new DeviceVersionComponent(); 2180 copyValues(dst); 2181 return dst; 2182 } 2183 2184 public void copyValues(DeviceVersionComponent dst) { 2185 super.copyValues(dst); 2186 dst.type = type == null ? null : type.copy(); 2187 dst.component = component == null ? null : component.copy(); 2188 dst.value = value == null ? null : value.copy(); 2189 } 2190 2191 @Override 2192 public boolean equalsDeep(Base other_) { 2193 if (!super.equalsDeep(other_)) 2194 return false; 2195 if (!(other_ instanceof DeviceVersionComponent)) 2196 return false; 2197 DeviceVersionComponent o = (DeviceVersionComponent) other_; 2198 return compareDeep(type, o.type, true) && compareDeep(component, o.component, true) 2199 && compareDeep(value, o.value, true); 2200 } 2201 2202 @Override 2203 public boolean equalsShallow(Base other_) { 2204 if (!super.equalsShallow(other_)) 2205 return false; 2206 if (!(other_ instanceof DeviceVersionComponent)) 2207 return false; 2208 DeviceVersionComponent o = (DeviceVersionComponent) other_; 2209 return compareValues(value, o.value, true); 2210 } 2211 2212 public boolean isEmpty() { 2213 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, component, value); 2214 } 2215 2216 public String fhirType() { 2217 return "Device.version"; 2218 2219 } 2220 2221 } 2222 2223 @Block() 2224 public static class DevicePropertyComponent extends BackboneElement implements IBaseBackboneElement { 2225 /** 2226 * Code that specifies the property DeviceDefinitionPropetyCode (Extensible). 2227 */ 2228 @Child(name = "type", type = { 2229 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2230 @Description(shortDefinition = "Code that specifies the property DeviceDefinitionPropetyCode (Extensible)", formalDefinition = "Code that specifies the property DeviceDefinitionPropetyCode (Extensible).") 2231 protected CodeableConcept type; 2232 2233 /** 2234 * Property value as a quantity. 2235 */ 2236 @Child(name = "valueQuantity", type = { 2237 Quantity.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2238 @Description(shortDefinition = "Property value as a quantity", formalDefinition = "Property value as a quantity.") 2239 protected List<Quantity> valueQuantity; 2240 2241 /** 2242 * Property value as a code, e.g., NTP4 (synced to NTP). 2243 */ 2244 @Child(name = "valueCode", type = { 2245 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2246 @Description(shortDefinition = "Property value as a code, e.g., NTP4 (synced to NTP)", formalDefinition = "Property value as a code, e.g., NTP4 (synced to NTP).") 2247 protected List<CodeableConcept> valueCode; 2248 2249 private static final long serialVersionUID = 1512172633L; 2250 2251 /** 2252 * Constructor 2253 */ 2254 public DevicePropertyComponent() { 2255 super(); 2256 } 2257 2258 /** 2259 * Constructor 2260 */ 2261 public DevicePropertyComponent(CodeableConcept type) { 2262 super(); 2263 this.type = type; 2264 } 2265 2266 /** 2267 * @return {@link #type} (Code that specifies the property 2268 * DeviceDefinitionPropetyCode (Extensible).) 2269 */ 2270 public CodeableConcept getType() { 2271 if (this.type == null) 2272 if (Configuration.errorOnAutoCreate()) 2273 throw new Error("Attempt to auto-create DevicePropertyComponent.type"); 2274 else if (Configuration.doAutoCreate()) 2275 this.type = new CodeableConcept(); // cc 2276 return this.type; 2277 } 2278 2279 public boolean hasType() { 2280 return this.type != null && !this.type.isEmpty(); 2281 } 2282 2283 /** 2284 * @param value {@link #type} (Code that specifies the property 2285 * DeviceDefinitionPropetyCode (Extensible).) 2286 */ 2287 public DevicePropertyComponent setType(CodeableConcept value) { 2288 this.type = value; 2289 return this; 2290 } 2291 2292 /** 2293 * @return {@link #valueQuantity} (Property value as a quantity.) 2294 */ 2295 public List<Quantity> getValueQuantity() { 2296 if (this.valueQuantity == null) 2297 this.valueQuantity = new ArrayList<Quantity>(); 2298 return this.valueQuantity; 2299 } 2300 2301 /** 2302 * @return Returns a reference to <code>this</code> for easy method chaining 2303 */ 2304 public DevicePropertyComponent setValueQuantity(List<Quantity> theValueQuantity) { 2305 this.valueQuantity = theValueQuantity; 2306 return this; 2307 } 2308 2309 public boolean hasValueQuantity() { 2310 if (this.valueQuantity == null) 2311 return false; 2312 for (Quantity item : this.valueQuantity) 2313 if (!item.isEmpty()) 2314 return true; 2315 return false; 2316 } 2317 2318 public Quantity addValueQuantity() { // 3 2319 Quantity t = new Quantity(); 2320 if (this.valueQuantity == null) 2321 this.valueQuantity = new ArrayList<Quantity>(); 2322 this.valueQuantity.add(t); 2323 return t; 2324 } 2325 2326 public DevicePropertyComponent addValueQuantity(Quantity t) { // 3 2327 if (t == null) 2328 return this; 2329 if (this.valueQuantity == null) 2330 this.valueQuantity = new ArrayList<Quantity>(); 2331 this.valueQuantity.add(t); 2332 return this; 2333 } 2334 2335 /** 2336 * @return The first repetition of repeating field {@link #valueQuantity}, 2337 * creating it if it does not already exist 2338 */ 2339 public Quantity getValueQuantityFirstRep() { 2340 if (getValueQuantity().isEmpty()) { 2341 addValueQuantity(); 2342 } 2343 return getValueQuantity().get(0); 2344 } 2345 2346 /** 2347 * @return {@link #valueCode} (Property value as a code, e.g., NTP4 (synced to 2348 * NTP).) 2349 */ 2350 public List<CodeableConcept> getValueCode() { 2351 if (this.valueCode == null) 2352 this.valueCode = new ArrayList<CodeableConcept>(); 2353 return this.valueCode; 2354 } 2355 2356 /** 2357 * @return Returns a reference to <code>this</code> for easy method chaining 2358 */ 2359 public DevicePropertyComponent setValueCode(List<CodeableConcept> theValueCode) { 2360 this.valueCode = theValueCode; 2361 return this; 2362 } 2363 2364 public boolean hasValueCode() { 2365 if (this.valueCode == null) 2366 return false; 2367 for (CodeableConcept item : this.valueCode) 2368 if (!item.isEmpty()) 2369 return true; 2370 return false; 2371 } 2372 2373 public CodeableConcept addValueCode() { // 3 2374 CodeableConcept t = new CodeableConcept(); 2375 if (this.valueCode == null) 2376 this.valueCode = new ArrayList<CodeableConcept>(); 2377 this.valueCode.add(t); 2378 return t; 2379 } 2380 2381 public DevicePropertyComponent addValueCode(CodeableConcept t) { // 3 2382 if (t == null) 2383 return this; 2384 if (this.valueCode == null) 2385 this.valueCode = new ArrayList<CodeableConcept>(); 2386 this.valueCode.add(t); 2387 return this; 2388 } 2389 2390 /** 2391 * @return The first repetition of repeating field {@link #valueCode}, creating 2392 * it if it does not already exist 2393 */ 2394 public CodeableConcept getValueCodeFirstRep() { 2395 if (getValueCode().isEmpty()) { 2396 addValueCode(); 2397 } 2398 return getValueCode().get(0); 2399 } 2400 2401 protected void listChildren(List<Property> children) { 2402 super.listChildren(children); 2403 children.add(new Property("type", "CodeableConcept", 2404 "Code that specifies the property DeviceDefinitionPropetyCode (Extensible).", 0, 1, type)); 2405 children.add(new Property("valueQuantity", "Quantity", "Property value as a quantity.", 0, 2406 java.lang.Integer.MAX_VALUE, valueQuantity)); 2407 children.add(new Property("valueCode", "CodeableConcept", "Property value as a code, e.g., NTP4 (synced to NTP).", 2408 0, java.lang.Integer.MAX_VALUE, valueCode)); 2409 } 2410 2411 @Override 2412 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2413 switch (_hash) { 2414 case 3575610: 2415 /* type */ return new Property("type", "CodeableConcept", 2416 "Code that specifies the property DeviceDefinitionPropetyCode (Extensible).", 0, 1, type); 2417 case -2029823716: 2418 /* valueQuantity */ return new Property("valueQuantity", "Quantity", "Property value as a quantity.", 0, 2419 java.lang.Integer.MAX_VALUE, valueQuantity); 2420 case -766209282: 2421 /* valueCode */ return new Property("valueCode", "CodeableConcept", 2422 "Property value as a code, e.g., NTP4 (synced to NTP).", 0, java.lang.Integer.MAX_VALUE, valueCode); 2423 default: 2424 return super.getNamedProperty(_hash, _name, _checkValid); 2425 } 2426 2427 } 2428 2429 @Override 2430 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2431 switch (hash) { 2432 case 3575610: 2433 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2434 case -2029823716: 2435 /* valueQuantity */ return this.valueQuantity == null ? new Base[0] 2436 : this.valueQuantity.toArray(new Base[this.valueQuantity.size()]); // Quantity 2437 case -766209282: 2438 /* valueCode */ return this.valueCode == null ? new Base[0] 2439 : this.valueCode.toArray(new Base[this.valueCode.size()]); // CodeableConcept 2440 default: 2441 return super.getProperty(hash, name, checkValid); 2442 } 2443 2444 } 2445 2446 @Override 2447 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2448 switch (hash) { 2449 case 3575610: // type 2450 this.type = castToCodeableConcept(value); // CodeableConcept 2451 return value; 2452 case -2029823716: // valueQuantity 2453 this.getValueQuantity().add(castToQuantity(value)); // Quantity 2454 return value; 2455 case -766209282: // valueCode 2456 this.getValueCode().add(castToCodeableConcept(value)); // CodeableConcept 2457 return value; 2458 default: 2459 return super.setProperty(hash, name, value); 2460 } 2461 2462 } 2463 2464 @Override 2465 public Base setProperty(String name, Base value) throws FHIRException { 2466 if (name.equals("type")) { 2467 this.type = castToCodeableConcept(value); // CodeableConcept 2468 } else if (name.equals("valueQuantity")) { 2469 this.getValueQuantity().add(castToQuantity(value)); 2470 } else if (name.equals("valueCode")) { 2471 this.getValueCode().add(castToCodeableConcept(value)); 2472 } else 2473 return super.setProperty(name, value); 2474 return value; 2475 } 2476 2477 @Override 2478 public void removeChild(String name, Base value) throws FHIRException { 2479 if (name.equals("type")) { 2480 this.type = null; 2481 } else if (name.equals("valueQuantity")) { 2482 this.getValueQuantity().remove(castToQuantity(value)); 2483 } else if (name.equals("valueCode")) { 2484 this.getValueCode().remove(castToCodeableConcept(value)); 2485 } else 2486 super.removeChild(name, value); 2487 2488 } 2489 2490 @Override 2491 public Base makeProperty(int hash, String name) throws FHIRException { 2492 switch (hash) { 2493 case 3575610: 2494 return getType(); 2495 case -2029823716: 2496 return addValueQuantity(); 2497 case -766209282: 2498 return addValueCode(); 2499 default: 2500 return super.makeProperty(hash, name); 2501 } 2502 2503 } 2504 2505 @Override 2506 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2507 switch (hash) { 2508 case 3575610: 2509 /* type */ return new String[] { "CodeableConcept" }; 2510 case -2029823716: 2511 /* valueQuantity */ return new String[] { "Quantity" }; 2512 case -766209282: 2513 /* valueCode */ return new String[] { "CodeableConcept" }; 2514 default: 2515 return super.getTypesForProperty(hash, name); 2516 } 2517 2518 } 2519 2520 @Override 2521 public Base addChild(String name) throws FHIRException { 2522 if (name.equals("type")) { 2523 this.type = new CodeableConcept(); 2524 return this.type; 2525 } else if (name.equals("valueQuantity")) { 2526 return addValueQuantity(); 2527 } else if (name.equals("valueCode")) { 2528 return addValueCode(); 2529 } else 2530 return super.addChild(name); 2531 } 2532 2533 public DevicePropertyComponent copy() { 2534 DevicePropertyComponent dst = new DevicePropertyComponent(); 2535 copyValues(dst); 2536 return dst; 2537 } 2538 2539 public void copyValues(DevicePropertyComponent dst) { 2540 super.copyValues(dst); 2541 dst.type = type == null ? null : type.copy(); 2542 if (valueQuantity != null) { 2543 dst.valueQuantity = new ArrayList<Quantity>(); 2544 for (Quantity i : valueQuantity) 2545 dst.valueQuantity.add(i.copy()); 2546 } 2547 ; 2548 if (valueCode != null) { 2549 dst.valueCode = new ArrayList<CodeableConcept>(); 2550 for (CodeableConcept i : valueCode) 2551 dst.valueCode.add(i.copy()); 2552 } 2553 ; 2554 } 2555 2556 @Override 2557 public boolean equalsDeep(Base other_) { 2558 if (!super.equalsDeep(other_)) 2559 return false; 2560 if (!(other_ instanceof DevicePropertyComponent)) 2561 return false; 2562 DevicePropertyComponent o = (DevicePropertyComponent) other_; 2563 return compareDeep(type, o.type, true) && compareDeep(valueQuantity, o.valueQuantity, true) 2564 && compareDeep(valueCode, o.valueCode, true); 2565 } 2566 2567 @Override 2568 public boolean equalsShallow(Base other_) { 2569 if (!super.equalsShallow(other_)) 2570 return false; 2571 if (!(other_ instanceof DevicePropertyComponent)) 2572 return false; 2573 DevicePropertyComponent o = (DevicePropertyComponent) other_; 2574 return true; 2575 } 2576 2577 public boolean isEmpty() { 2578 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, valueQuantity, valueCode); 2579 } 2580 2581 public String fhirType() { 2582 return "Device.property"; 2583 2584 } 2585 2586 } 2587 2588 /** 2589 * Unique instance identifiers assigned to a device by manufacturers other 2590 * organizations or owners. 2591 */ 2592 @Child(name = "identifier", type = { 2593 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2594 @Description(shortDefinition = "Instance identifier", formalDefinition = "Unique instance identifiers assigned to a device by manufacturers other organizations or owners.") 2595 protected List<Identifier> identifier; 2596 2597 /** 2598 * The reference to the definition for the device. 2599 */ 2600 @Child(name = "definition", type = { 2601 DeviceDefinition.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2602 @Description(shortDefinition = "The reference to the definition for the device", formalDefinition = "The reference to the definition for the device.") 2603 protected Reference definition; 2604 2605 /** 2606 * The actual object that is the target of the reference (The reference to the 2607 * definition for the device.) 2608 */ 2609 protected DeviceDefinition definitionTarget; 2610 2611 /** 2612 * Unique device identifier (UDI) assigned to device label or package. Note that 2613 * the Device may include multiple udiCarriers as it either may include just the 2614 * udiCarrier for the jurisdiction it is sold, or for multiple jurisdictions it 2615 * could have been sold. 2616 */ 2617 @Child(name = "udiCarrier", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2618 @Description(shortDefinition = "Unique Device Identifier (UDI) Barcode string", formalDefinition = "Unique device identifier (UDI) assigned to device label or package. Note that the Device may include multiple udiCarriers as it either may include just the udiCarrier for the jurisdiction it is sold, or for multiple jurisdictions it could have been sold.") 2619 protected List<DeviceUdiCarrierComponent> udiCarrier; 2620 2621 /** 2622 * Status of the Device availability. 2623 */ 2624 @Child(name = "status", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = true, summary = true) 2625 @Description(shortDefinition = "active | inactive | entered-in-error | unknown", formalDefinition = "Status of the Device availability.") 2626 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/device-status") 2627 protected Enumeration<FHIRDeviceStatus> status; 2628 2629 /** 2630 * Reason for the dtatus of the Device availability. 2631 */ 2632 @Child(name = "statusReason", type = { 2633 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2634 @Description(shortDefinition = "online | paused | standby | offline | not-ready | transduc-discon | hw-discon | off", formalDefinition = "Reason for the dtatus of the Device availability.") 2635 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/device-status-reason") 2636 protected List<CodeableConcept> statusReason; 2637 2638 /** 2639 * The distinct identification string as required by regulation for a human 2640 * cell, tissue, or cellular and tissue-based product. 2641 */ 2642 @Child(name = "distinctIdentifier", type = { 2643 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 2644 @Description(shortDefinition = "The distinct identification string", formalDefinition = "The distinct identification string as required by regulation for a human cell, tissue, or cellular and tissue-based product.") 2645 protected StringType distinctIdentifier; 2646 2647 /** 2648 * A name of the manufacturer. 2649 */ 2650 @Child(name = "manufacturer", type = { 2651 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 2652 @Description(shortDefinition = "Name of device manufacturer", formalDefinition = "A name of the manufacturer.") 2653 protected StringType manufacturer; 2654 2655 /** 2656 * The date and time when the device was manufactured. 2657 */ 2658 @Child(name = "manufactureDate", type = { 2659 DateTimeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 2660 @Description(shortDefinition = "Date when the device was made", formalDefinition = "The date and time when the device was manufactured.") 2661 protected DateTimeType manufactureDate; 2662 2663 /** 2664 * The date and time beyond which this device is no longer valid or should not 2665 * be used (if applicable). 2666 */ 2667 @Child(name = "expirationDate", type = { 2668 DateTimeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 2669 @Description(shortDefinition = "Date and time of expiry of this device (if applicable)", formalDefinition = "The date and time beyond which this device is no longer valid or should not be used (if applicable).") 2670 protected DateTimeType expirationDate; 2671 2672 /** 2673 * Lot number assigned by the manufacturer. 2674 */ 2675 @Child(name = "lotNumber", type = { 2676 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 2677 @Description(shortDefinition = "Lot number of manufacture", formalDefinition = "Lot number assigned by the manufacturer.") 2678 protected StringType lotNumber; 2679 2680 /** 2681 * The serial number assigned by the organization when the device was 2682 * manufactured. 2683 */ 2684 @Child(name = "serialNumber", type = { 2685 StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 2686 @Description(shortDefinition = "Serial number assigned by the manufacturer", formalDefinition = "The serial number assigned by the organization when the device was manufactured.") 2687 protected StringType serialNumber; 2688 2689 /** 2690 * This represents the manufacturer's name of the device as provided by the 2691 * device, from a UDI label, or by a person describing the Device. This 2692 * typically would be used when a person provides the name(s) or when the device 2693 * represents one of the names available from DeviceDefinition. 2694 */ 2695 @Child(name = "deviceName", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2696 @Description(shortDefinition = "The name of the device as given by the manufacturer", formalDefinition = "This represents the manufacturer's name of the device as provided by the device, from a UDI label, or by a person describing the Device. This typically would be used when a person provides the name(s) or when the device represents one of the names available from DeviceDefinition.") 2697 protected List<DeviceDeviceNameComponent> deviceName; 2698 2699 /** 2700 * The model number for the device. 2701 */ 2702 @Child(name = "modelNumber", type = { 2703 StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 2704 @Description(shortDefinition = "The model number for the device", formalDefinition = "The model number for the device.") 2705 protected StringType modelNumber; 2706 2707 /** 2708 * The part number of the device. 2709 */ 2710 @Child(name = "partNumber", type = { 2711 StringType.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 2712 @Description(shortDefinition = "The part number of the device", formalDefinition = "The part number of the device.") 2713 protected StringType partNumber; 2714 2715 /** 2716 * The kind or type of device. 2717 */ 2718 @Child(name = "type", type = { 2719 CodeableConcept.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 2720 @Description(shortDefinition = "The kind or type of device", formalDefinition = "The kind or type of device.") 2721 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/device-type") 2722 protected CodeableConcept type; 2723 2724 /** 2725 * The capabilities supported on a device, the standards to which the device 2726 * conforms for a particular purpose, and used for the communication. 2727 */ 2728 @Child(name = "specialization", type = {}, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2729 @Description(shortDefinition = "The capabilities supported on a device, the standards to which the device conforms for a particular purpose, and used for the communication", formalDefinition = "The capabilities supported on a device, the standards to which the device conforms for a particular purpose, and used for the communication.") 2730 protected List<DeviceSpecializationComponent> specialization; 2731 2732 /** 2733 * The actual design of the device or software version running on the device. 2734 */ 2735 @Child(name = "version", type = {}, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2736 @Description(shortDefinition = "The actual design of the device or software version running on the device", formalDefinition = "The actual design of the device or software version running on the device.") 2737 protected List<DeviceVersionComponent> version; 2738 2739 /** 2740 * The actual configuration settings of a device as it actually operates, e.g., 2741 * regulation status, time properties. 2742 */ 2743 @Child(name = "property", type = {}, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2744 @Description(shortDefinition = "The actual configuration settings of a device as it actually operates, e.g., regulation status, time properties", formalDefinition = "The actual configuration settings of a device as it actually operates, e.g., regulation status, time properties.") 2745 protected List<DevicePropertyComponent> property; 2746 2747 /** 2748 * Patient information, If the device is affixed to a person. 2749 */ 2750 @Child(name = "patient", type = { Patient.class }, order = 18, min = 0, max = 1, modifier = false, summary = false) 2751 @Description(shortDefinition = "Patient to whom Device is affixed", formalDefinition = "Patient information, If the device is affixed to a person.") 2752 protected Reference patient; 2753 2754 /** 2755 * The actual object that is the target of the reference (Patient information, 2756 * If the device is affixed to a person.) 2757 */ 2758 protected Patient patientTarget; 2759 2760 /** 2761 * An organization that is responsible for the provision and ongoing maintenance 2762 * of the device. 2763 */ 2764 @Child(name = "owner", type = { Organization.class }, order = 19, min = 0, max = 1, modifier = false, summary = false) 2765 @Description(shortDefinition = "Organization responsible for device", formalDefinition = "An organization that is responsible for the provision and ongoing maintenance of the device.") 2766 protected Reference owner; 2767 2768 /** 2769 * The actual object that is the target of the reference (An organization that 2770 * is responsible for the provision and ongoing maintenance of the device.) 2771 */ 2772 protected Organization ownerTarget; 2773 2774 /** 2775 * Contact details for an organization or a particular human that is responsible 2776 * for the device. 2777 */ 2778 @Child(name = "contact", type = { 2779 ContactPoint.class }, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2780 @Description(shortDefinition = "Details for human/organization for support", formalDefinition = "Contact details for an organization or a particular human that is responsible for the device.") 2781 protected List<ContactPoint> contact; 2782 2783 /** 2784 * The place where the device can be found. 2785 */ 2786 @Child(name = "location", type = { Location.class }, order = 21, min = 0, max = 1, modifier = false, summary = false) 2787 @Description(shortDefinition = "Where the device is found", formalDefinition = "The place where the device can be found.") 2788 protected Reference location; 2789 2790 /** 2791 * The actual object that is the target of the reference (The place where the 2792 * device can be found.) 2793 */ 2794 protected Location locationTarget; 2795 2796 /** 2797 * A network address on which the device may be contacted directly. 2798 */ 2799 @Child(name = "url", type = { UriType.class }, order = 22, min = 0, max = 1, modifier = false, summary = false) 2800 @Description(shortDefinition = "Network address to contact device", formalDefinition = "A network address on which the device may be contacted directly.") 2801 protected UriType url; 2802 2803 /** 2804 * Descriptive information, usage information or implantation information that 2805 * is not captured in an existing element. 2806 */ 2807 @Child(name = "note", type = { 2808 Annotation.class }, order = 23, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2809 @Description(shortDefinition = "Device notes and comments", formalDefinition = "Descriptive information, usage information or implantation information that is not captured in an existing element.") 2810 protected List<Annotation> note; 2811 2812 /** 2813 * Provides additional safety characteristics about a medical device. For 2814 * example devices containing latex. 2815 */ 2816 @Child(name = "safety", type = { 2817 CodeableConcept.class }, order = 24, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2818 @Description(shortDefinition = "Safety Characteristics of Device", formalDefinition = "Provides additional safety characteristics about a medical device. For example devices containing latex.") 2819 protected List<CodeableConcept> safety; 2820 2821 /** 2822 * The parent device. 2823 */ 2824 @Child(name = "parent", type = { Device.class }, order = 25, min = 0, max = 1, modifier = false, summary = false) 2825 @Description(shortDefinition = "The parent device", formalDefinition = "The parent device.") 2826 protected Reference parent; 2827 2828 /** 2829 * The actual object that is the target of the reference (The parent device.) 2830 */ 2831 protected Device parentTarget; 2832 2833 private static final long serialVersionUID = -298380419L; 2834 2835 /** 2836 * Constructor 2837 */ 2838 public Device() { 2839 super(); 2840 } 2841 2842 /** 2843 * @return {@link #identifier} (Unique instance identifiers assigned to a device 2844 * by manufacturers other organizations or owners.) 2845 */ 2846 public List<Identifier> getIdentifier() { 2847 if (this.identifier == null) 2848 this.identifier = new ArrayList<Identifier>(); 2849 return this.identifier; 2850 } 2851 2852 /** 2853 * @return Returns a reference to <code>this</code> for easy method chaining 2854 */ 2855 public Device setIdentifier(List<Identifier> theIdentifier) { 2856 this.identifier = theIdentifier; 2857 return this; 2858 } 2859 2860 public boolean hasIdentifier() { 2861 if (this.identifier == null) 2862 return false; 2863 for (Identifier item : this.identifier) 2864 if (!item.isEmpty()) 2865 return true; 2866 return false; 2867 } 2868 2869 public Identifier addIdentifier() { // 3 2870 Identifier t = new Identifier(); 2871 if (this.identifier == null) 2872 this.identifier = new ArrayList<Identifier>(); 2873 this.identifier.add(t); 2874 return t; 2875 } 2876 2877 public Device addIdentifier(Identifier t) { // 3 2878 if (t == null) 2879 return this; 2880 if (this.identifier == null) 2881 this.identifier = new ArrayList<Identifier>(); 2882 this.identifier.add(t); 2883 return this; 2884 } 2885 2886 /** 2887 * @return The first repetition of repeating field {@link #identifier}, creating 2888 * it if it does not already exist 2889 */ 2890 public Identifier getIdentifierFirstRep() { 2891 if (getIdentifier().isEmpty()) { 2892 addIdentifier(); 2893 } 2894 return getIdentifier().get(0); 2895 } 2896 2897 /** 2898 * @return {@link #definition} (The reference to the definition for the device.) 2899 */ 2900 public Reference getDefinition() { 2901 if (this.definition == null) 2902 if (Configuration.errorOnAutoCreate()) 2903 throw new Error("Attempt to auto-create Device.definition"); 2904 else if (Configuration.doAutoCreate()) 2905 this.definition = new Reference(); // cc 2906 return this.definition; 2907 } 2908 2909 public boolean hasDefinition() { 2910 return this.definition != null && !this.definition.isEmpty(); 2911 } 2912 2913 /** 2914 * @param value {@link #definition} (The reference to the definition for the 2915 * device.) 2916 */ 2917 public Device setDefinition(Reference value) { 2918 this.definition = value; 2919 return this; 2920 } 2921 2922 /** 2923 * @return {@link #definition} The actual object that is the target of the 2924 * reference. The reference library doesn't populate this, but you can 2925 * use it to hold the resource if you resolve it. (The reference to the 2926 * definition for the device.) 2927 */ 2928 public DeviceDefinition getDefinitionTarget() { 2929 if (this.definitionTarget == null) 2930 if (Configuration.errorOnAutoCreate()) 2931 throw new Error("Attempt to auto-create Device.definition"); 2932 else if (Configuration.doAutoCreate()) 2933 this.definitionTarget = new DeviceDefinition(); // aa 2934 return this.definitionTarget; 2935 } 2936 2937 /** 2938 * @param value {@link #definition} The actual object that is the target of the 2939 * reference. The reference library doesn't use these, but you can 2940 * use it to hold the resource if you resolve it. (The reference to 2941 * the definition for the device.) 2942 */ 2943 public Device setDefinitionTarget(DeviceDefinition value) { 2944 this.definitionTarget = value; 2945 return this; 2946 } 2947 2948 /** 2949 * @return {@link #udiCarrier} (Unique device identifier (UDI) assigned to 2950 * device label or package. Note that the Device may include multiple 2951 * udiCarriers as it either may include just the udiCarrier for the 2952 * jurisdiction it is sold, or for multiple jurisdictions it could have 2953 * been sold.) 2954 */ 2955 public List<DeviceUdiCarrierComponent> getUdiCarrier() { 2956 if (this.udiCarrier == null) 2957 this.udiCarrier = new ArrayList<DeviceUdiCarrierComponent>(); 2958 return this.udiCarrier; 2959 } 2960 2961 /** 2962 * @return Returns a reference to <code>this</code> for easy method chaining 2963 */ 2964 public Device setUdiCarrier(List<DeviceUdiCarrierComponent> theUdiCarrier) { 2965 this.udiCarrier = theUdiCarrier; 2966 return this; 2967 } 2968 2969 public boolean hasUdiCarrier() { 2970 if (this.udiCarrier == null) 2971 return false; 2972 for (DeviceUdiCarrierComponent item : this.udiCarrier) 2973 if (!item.isEmpty()) 2974 return true; 2975 return false; 2976 } 2977 2978 public DeviceUdiCarrierComponent addUdiCarrier() { // 3 2979 DeviceUdiCarrierComponent t = new DeviceUdiCarrierComponent(); 2980 if (this.udiCarrier == null) 2981 this.udiCarrier = new ArrayList<DeviceUdiCarrierComponent>(); 2982 this.udiCarrier.add(t); 2983 return t; 2984 } 2985 2986 public Device addUdiCarrier(DeviceUdiCarrierComponent t) { // 3 2987 if (t == null) 2988 return this; 2989 if (this.udiCarrier == null) 2990 this.udiCarrier = new ArrayList<DeviceUdiCarrierComponent>(); 2991 this.udiCarrier.add(t); 2992 return this; 2993 } 2994 2995 /** 2996 * @return The first repetition of repeating field {@link #udiCarrier}, creating 2997 * it if it does not already exist 2998 */ 2999 public DeviceUdiCarrierComponent getUdiCarrierFirstRep() { 3000 if (getUdiCarrier().isEmpty()) { 3001 addUdiCarrier(); 3002 } 3003 return getUdiCarrier().get(0); 3004 } 3005 3006 /** 3007 * @return {@link #status} (Status of the Device availability.). This is the 3008 * underlying object with id, value and extensions. The accessor 3009 * "getStatus" gives direct access to the value 3010 */ 3011 public Enumeration<FHIRDeviceStatus> getStatusElement() { 3012 if (this.status == null) 3013 if (Configuration.errorOnAutoCreate()) 3014 throw new Error("Attempt to auto-create Device.status"); 3015 else if (Configuration.doAutoCreate()) 3016 this.status = new Enumeration<FHIRDeviceStatus>(new FHIRDeviceStatusEnumFactory()); // bb 3017 return this.status; 3018 } 3019 3020 public boolean hasStatusElement() { 3021 return this.status != null && !this.status.isEmpty(); 3022 } 3023 3024 public boolean hasStatus() { 3025 return this.status != null && !this.status.isEmpty(); 3026 } 3027 3028 /** 3029 * @param value {@link #status} (Status of the Device availability.). This is 3030 * the underlying object with id, value and extensions. The 3031 * accessor "getStatus" gives direct access to the value 3032 */ 3033 public Device setStatusElement(Enumeration<FHIRDeviceStatus> value) { 3034 this.status = value; 3035 return this; 3036 } 3037 3038 /** 3039 * @return Status of the Device availability. 3040 */ 3041 public FHIRDeviceStatus getStatus() { 3042 return this.status == null ? null : this.status.getValue(); 3043 } 3044 3045 /** 3046 * @param value Status of the Device availability. 3047 */ 3048 public Device setStatus(FHIRDeviceStatus value) { 3049 if (value == null) 3050 this.status = null; 3051 else { 3052 if (this.status == null) 3053 this.status = new Enumeration<FHIRDeviceStatus>(new FHIRDeviceStatusEnumFactory()); 3054 this.status.setValue(value); 3055 } 3056 return this; 3057 } 3058 3059 /** 3060 * @return {@link #statusReason} (Reason for the dtatus of the Device 3061 * availability.) 3062 */ 3063 public List<CodeableConcept> getStatusReason() { 3064 if (this.statusReason == null) 3065 this.statusReason = new ArrayList<CodeableConcept>(); 3066 return this.statusReason; 3067 } 3068 3069 /** 3070 * @return Returns a reference to <code>this</code> for easy method chaining 3071 */ 3072 public Device setStatusReason(List<CodeableConcept> theStatusReason) { 3073 this.statusReason = theStatusReason; 3074 return this; 3075 } 3076 3077 public boolean hasStatusReason() { 3078 if (this.statusReason == null) 3079 return false; 3080 for (CodeableConcept item : this.statusReason) 3081 if (!item.isEmpty()) 3082 return true; 3083 return false; 3084 } 3085 3086 public CodeableConcept addStatusReason() { // 3 3087 CodeableConcept t = new CodeableConcept(); 3088 if (this.statusReason == null) 3089 this.statusReason = new ArrayList<CodeableConcept>(); 3090 this.statusReason.add(t); 3091 return t; 3092 } 3093 3094 public Device addStatusReason(CodeableConcept t) { // 3 3095 if (t == null) 3096 return this; 3097 if (this.statusReason == null) 3098 this.statusReason = new ArrayList<CodeableConcept>(); 3099 this.statusReason.add(t); 3100 return this; 3101 } 3102 3103 /** 3104 * @return The first repetition of repeating field {@link #statusReason}, 3105 * creating it if it does not already exist 3106 */ 3107 public CodeableConcept getStatusReasonFirstRep() { 3108 if (getStatusReason().isEmpty()) { 3109 addStatusReason(); 3110 } 3111 return getStatusReason().get(0); 3112 } 3113 3114 /** 3115 * @return {@link #distinctIdentifier} (The distinct identification string as 3116 * required by regulation for a human cell, tissue, or cellular and 3117 * tissue-based product.). This is the underlying object with id, value 3118 * and extensions. The accessor "getDistinctIdentifier" gives direct 3119 * access to the value 3120 */ 3121 public StringType getDistinctIdentifierElement() { 3122 if (this.distinctIdentifier == null) 3123 if (Configuration.errorOnAutoCreate()) 3124 throw new Error("Attempt to auto-create Device.distinctIdentifier"); 3125 else if (Configuration.doAutoCreate()) 3126 this.distinctIdentifier = new StringType(); // bb 3127 return this.distinctIdentifier; 3128 } 3129 3130 public boolean hasDistinctIdentifierElement() { 3131 return this.distinctIdentifier != null && !this.distinctIdentifier.isEmpty(); 3132 } 3133 3134 public boolean hasDistinctIdentifier() { 3135 return this.distinctIdentifier != null && !this.distinctIdentifier.isEmpty(); 3136 } 3137 3138 /** 3139 * @param value {@link #distinctIdentifier} (The distinct identification string 3140 * as required by regulation for a human cell, tissue, or cellular 3141 * and tissue-based product.). This is the underlying object with 3142 * id, value and extensions. The accessor "getDistinctIdentifier" 3143 * gives direct access to the value 3144 */ 3145 public Device setDistinctIdentifierElement(StringType value) { 3146 this.distinctIdentifier = value; 3147 return this; 3148 } 3149 3150 /** 3151 * @return The distinct identification string as required by regulation for a 3152 * human cell, tissue, or cellular and tissue-based product. 3153 */ 3154 public String getDistinctIdentifier() { 3155 return this.distinctIdentifier == null ? null : this.distinctIdentifier.getValue(); 3156 } 3157 3158 /** 3159 * @param value The distinct identification string as required by regulation for 3160 * a human cell, tissue, or cellular and tissue-based product. 3161 */ 3162 public Device setDistinctIdentifier(String value) { 3163 if (Utilities.noString(value)) 3164 this.distinctIdentifier = null; 3165 else { 3166 if (this.distinctIdentifier == null) 3167 this.distinctIdentifier = new StringType(); 3168 this.distinctIdentifier.setValue(value); 3169 } 3170 return this; 3171 } 3172 3173 /** 3174 * @return {@link #manufacturer} (A name of the manufacturer.). This is the 3175 * underlying object with id, value and extensions. The accessor 3176 * "getManufacturer" gives direct access to the value 3177 */ 3178 public StringType getManufacturerElement() { 3179 if (this.manufacturer == null) 3180 if (Configuration.errorOnAutoCreate()) 3181 throw new Error("Attempt to auto-create Device.manufacturer"); 3182 else if (Configuration.doAutoCreate()) 3183 this.manufacturer = new StringType(); // bb 3184 return this.manufacturer; 3185 } 3186 3187 public boolean hasManufacturerElement() { 3188 return this.manufacturer != null && !this.manufacturer.isEmpty(); 3189 } 3190 3191 public boolean hasManufacturer() { 3192 return this.manufacturer != null && !this.manufacturer.isEmpty(); 3193 } 3194 3195 /** 3196 * @param value {@link #manufacturer} (A name of the manufacturer.). This is the 3197 * underlying object with id, value and extensions. The accessor 3198 * "getManufacturer" gives direct access to the value 3199 */ 3200 public Device setManufacturerElement(StringType value) { 3201 this.manufacturer = value; 3202 return this; 3203 } 3204 3205 /** 3206 * @return A name of the manufacturer. 3207 */ 3208 public String getManufacturer() { 3209 return this.manufacturer == null ? null : this.manufacturer.getValue(); 3210 } 3211 3212 /** 3213 * @param value A name of the manufacturer. 3214 */ 3215 public Device setManufacturer(String value) { 3216 if (Utilities.noString(value)) 3217 this.manufacturer = null; 3218 else { 3219 if (this.manufacturer == null) 3220 this.manufacturer = new StringType(); 3221 this.manufacturer.setValue(value); 3222 } 3223 return this; 3224 } 3225 3226 /** 3227 * @return {@link #manufactureDate} (The date and time when the device was 3228 * manufactured.). This is the underlying object with id, value and 3229 * extensions. The accessor "getManufactureDate" gives direct access to 3230 * the value 3231 */ 3232 public DateTimeType getManufactureDateElement() { 3233 if (this.manufactureDate == null) 3234 if (Configuration.errorOnAutoCreate()) 3235 throw new Error("Attempt to auto-create Device.manufactureDate"); 3236 else if (Configuration.doAutoCreate()) 3237 this.manufactureDate = new DateTimeType(); // bb 3238 return this.manufactureDate; 3239 } 3240 3241 public boolean hasManufactureDateElement() { 3242 return this.manufactureDate != null && !this.manufactureDate.isEmpty(); 3243 } 3244 3245 public boolean hasManufactureDate() { 3246 return this.manufactureDate != null && !this.manufactureDate.isEmpty(); 3247 } 3248 3249 /** 3250 * @param value {@link #manufactureDate} (The date and time when the device was 3251 * manufactured.). This is the underlying object with id, value and 3252 * extensions. The accessor "getManufactureDate" gives direct 3253 * access to the value 3254 */ 3255 public Device setManufactureDateElement(DateTimeType value) { 3256 this.manufactureDate = value; 3257 return this; 3258 } 3259 3260 /** 3261 * @return The date and time when the device was manufactured. 3262 */ 3263 public Date getManufactureDate() { 3264 return this.manufactureDate == null ? null : this.manufactureDate.getValue(); 3265 } 3266 3267 /** 3268 * @param value The date and time when the device was manufactured. 3269 */ 3270 public Device setManufactureDate(Date value) { 3271 if (value == null) 3272 this.manufactureDate = null; 3273 else { 3274 if (this.manufactureDate == null) 3275 this.manufactureDate = new DateTimeType(); 3276 this.manufactureDate.setValue(value); 3277 } 3278 return this; 3279 } 3280 3281 /** 3282 * @return {@link #expirationDate} (The date and time beyond which this device 3283 * is no longer valid or should not be used (if applicable).). This is 3284 * the underlying object with id, value and extensions. The accessor 3285 * "getExpirationDate" gives direct access to the value 3286 */ 3287 public DateTimeType getExpirationDateElement() { 3288 if (this.expirationDate == null) 3289 if (Configuration.errorOnAutoCreate()) 3290 throw new Error("Attempt to auto-create Device.expirationDate"); 3291 else if (Configuration.doAutoCreate()) 3292 this.expirationDate = new DateTimeType(); // bb 3293 return this.expirationDate; 3294 } 3295 3296 public boolean hasExpirationDateElement() { 3297 return this.expirationDate != null && !this.expirationDate.isEmpty(); 3298 } 3299 3300 public boolean hasExpirationDate() { 3301 return this.expirationDate != null && !this.expirationDate.isEmpty(); 3302 } 3303 3304 /** 3305 * @param value {@link #expirationDate} (The date and time beyond which this 3306 * device is no longer valid or should not be used (if 3307 * applicable).). This is the underlying object with id, value and 3308 * extensions. The accessor "getExpirationDate" gives direct access 3309 * to the value 3310 */ 3311 public Device setExpirationDateElement(DateTimeType value) { 3312 this.expirationDate = value; 3313 return this; 3314 } 3315 3316 /** 3317 * @return The date and time beyond which this device is no longer valid or 3318 * should not be used (if applicable). 3319 */ 3320 public Date getExpirationDate() { 3321 return this.expirationDate == null ? null : this.expirationDate.getValue(); 3322 } 3323 3324 /** 3325 * @param value The date and time beyond which this device is no longer valid or 3326 * should not be used (if applicable). 3327 */ 3328 public Device setExpirationDate(Date value) { 3329 if (value == null) 3330 this.expirationDate = null; 3331 else { 3332 if (this.expirationDate == null) 3333 this.expirationDate = new DateTimeType(); 3334 this.expirationDate.setValue(value); 3335 } 3336 return this; 3337 } 3338 3339 /** 3340 * @return {@link #lotNumber} (Lot number assigned by the manufacturer.). This 3341 * is the underlying object with id, value and extensions. The accessor 3342 * "getLotNumber" gives direct access to the value 3343 */ 3344 public StringType getLotNumberElement() { 3345 if (this.lotNumber == null) 3346 if (Configuration.errorOnAutoCreate()) 3347 throw new Error("Attempt to auto-create Device.lotNumber"); 3348 else if (Configuration.doAutoCreate()) 3349 this.lotNumber = new StringType(); // bb 3350 return this.lotNumber; 3351 } 3352 3353 public boolean hasLotNumberElement() { 3354 return this.lotNumber != null && !this.lotNumber.isEmpty(); 3355 } 3356 3357 public boolean hasLotNumber() { 3358 return this.lotNumber != null && !this.lotNumber.isEmpty(); 3359 } 3360 3361 /** 3362 * @param value {@link #lotNumber} (Lot number assigned by the manufacturer.). 3363 * This is the underlying object with id, value and extensions. The 3364 * accessor "getLotNumber" gives direct access to the value 3365 */ 3366 public Device setLotNumberElement(StringType value) { 3367 this.lotNumber = value; 3368 return this; 3369 } 3370 3371 /** 3372 * @return Lot number assigned by the manufacturer. 3373 */ 3374 public String getLotNumber() { 3375 return this.lotNumber == null ? null : this.lotNumber.getValue(); 3376 } 3377 3378 /** 3379 * @param value Lot number assigned by the manufacturer. 3380 */ 3381 public Device setLotNumber(String value) { 3382 if (Utilities.noString(value)) 3383 this.lotNumber = null; 3384 else { 3385 if (this.lotNumber == null) 3386 this.lotNumber = new StringType(); 3387 this.lotNumber.setValue(value); 3388 } 3389 return this; 3390 } 3391 3392 /** 3393 * @return {@link #serialNumber} (The serial number assigned by the organization 3394 * when the device was manufactured.). This is the underlying object 3395 * with id, value and extensions. The accessor "getSerialNumber" gives 3396 * direct access to the value 3397 */ 3398 public StringType getSerialNumberElement() { 3399 if (this.serialNumber == null) 3400 if (Configuration.errorOnAutoCreate()) 3401 throw new Error("Attempt to auto-create Device.serialNumber"); 3402 else if (Configuration.doAutoCreate()) 3403 this.serialNumber = new StringType(); // bb 3404 return this.serialNumber; 3405 } 3406 3407 public boolean hasSerialNumberElement() { 3408 return this.serialNumber != null && !this.serialNumber.isEmpty(); 3409 } 3410 3411 public boolean hasSerialNumber() { 3412 return this.serialNumber != null && !this.serialNumber.isEmpty(); 3413 } 3414 3415 /** 3416 * @param value {@link #serialNumber} (The serial number assigned by the 3417 * organization when the device was manufactured.). This is the 3418 * underlying object with id, value and extensions. The accessor 3419 * "getSerialNumber" gives direct access to the value 3420 */ 3421 public Device setSerialNumberElement(StringType value) { 3422 this.serialNumber = value; 3423 return this; 3424 } 3425 3426 /** 3427 * @return The serial number assigned by the organization when the device was 3428 * manufactured. 3429 */ 3430 public String getSerialNumber() { 3431 return this.serialNumber == null ? null : this.serialNumber.getValue(); 3432 } 3433 3434 /** 3435 * @param value The serial number assigned by the organization when the device 3436 * was manufactured. 3437 */ 3438 public Device setSerialNumber(String value) { 3439 if (Utilities.noString(value)) 3440 this.serialNumber = null; 3441 else { 3442 if (this.serialNumber == null) 3443 this.serialNumber = new StringType(); 3444 this.serialNumber.setValue(value); 3445 } 3446 return this; 3447 } 3448 3449 /** 3450 * @return {@link #deviceName} (This represents the manufacturer's name of the 3451 * device as provided by the device, from a UDI label, or by a person 3452 * describing the Device. This typically would be used when a person 3453 * provides the name(s) or when the device represents one of the names 3454 * available from DeviceDefinition.) 3455 */ 3456 public List<DeviceDeviceNameComponent> getDeviceName() { 3457 if (this.deviceName == null) 3458 this.deviceName = new ArrayList<DeviceDeviceNameComponent>(); 3459 return this.deviceName; 3460 } 3461 3462 /** 3463 * @return Returns a reference to <code>this</code> for easy method chaining 3464 */ 3465 public Device setDeviceName(List<DeviceDeviceNameComponent> theDeviceName) { 3466 this.deviceName = theDeviceName; 3467 return this; 3468 } 3469 3470 public boolean hasDeviceName() { 3471 if (this.deviceName == null) 3472 return false; 3473 for (DeviceDeviceNameComponent item : this.deviceName) 3474 if (!item.isEmpty()) 3475 return true; 3476 return false; 3477 } 3478 3479 public DeviceDeviceNameComponent addDeviceName() { // 3 3480 DeviceDeviceNameComponent t = new DeviceDeviceNameComponent(); 3481 if (this.deviceName == null) 3482 this.deviceName = new ArrayList<DeviceDeviceNameComponent>(); 3483 this.deviceName.add(t); 3484 return t; 3485 } 3486 3487 public Device addDeviceName(DeviceDeviceNameComponent t) { // 3 3488 if (t == null) 3489 return this; 3490 if (this.deviceName == null) 3491 this.deviceName = new ArrayList<DeviceDeviceNameComponent>(); 3492 this.deviceName.add(t); 3493 return this; 3494 } 3495 3496 /** 3497 * @return The first repetition of repeating field {@link #deviceName}, creating 3498 * it if it does not already exist 3499 */ 3500 public DeviceDeviceNameComponent getDeviceNameFirstRep() { 3501 if (getDeviceName().isEmpty()) { 3502 addDeviceName(); 3503 } 3504 return getDeviceName().get(0); 3505 } 3506 3507 /** 3508 * @return {@link #modelNumber} (The model number for the device.). This is the 3509 * underlying object with id, value and extensions. The accessor 3510 * "getModelNumber" gives direct access to the value 3511 */ 3512 public StringType getModelNumberElement() { 3513 if (this.modelNumber == null) 3514 if (Configuration.errorOnAutoCreate()) 3515 throw new Error("Attempt to auto-create Device.modelNumber"); 3516 else if (Configuration.doAutoCreate()) 3517 this.modelNumber = new StringType(); // bb 3518 return this.modelNumber; 3519 } 3520 3521 public boolean hasModelNumberElement() { 3522 return this.modelNumber != null && !this.modelNumber.isEmpty(); 3523 } 3524 3525 public boolean hasModelNumber() { 3526 return this.modelNumber != null && !this.modelNumber.isEmpty(); 3527 } 3528 3529 /** 3530 * @param value {@link #modelNumber} (The model number for the device.). This is 3531 * the underlying object with id, value and extensions. The 3532 * accessor "getModelNumber" gives direct access to the value 3533 */ 3534 public Device setModelNumberElement(StringType value) { 3535 this.modelNumber = value; 3536 return this; 3537 } 3538 3539 /** 3540 * @return The model number for the device. 3541 */ 3542 public String getModelNumber() { 3543 return this.modelNumber == null ? null : this.modelNumber.getValue(); 3544 } 3545 3546 /** 3547 * @param value The model number for the device. 3548 */ 3549 public Device setModelNumber(String value) { 3550 if (Utilities.noString(value)) 3551 this.modelNumber = null; 3552 else { 3553 if (this.modelNumber == null) 3554 this.modelNumber = new StringType(); 3555 this.modelNumber.setValue(value); 3556 } 3557 return this; 3558 } 3559 3560 /** 3561 * @return {@link #partNumber} (The part number of the device.). This is the 3562 * underlying object with id, value and extensions. The accessor 3563 * "getPartNumber" gives direct access to the value 3564 */ 3565 public StringType getPartNumberElement() { 3566 if (this.partNumber == null) 3567 if (Configuration.errorOnAutoCreate()) 3568 throw new Error("Attempt to auto-create Device.partNumber"); 3569 else if (Configuration.doAutoCreate()) 3570 this.partNumber = new StringType(); // bb 3571 return this.partNumber; 3572 } 3573 3574 public boolean hasPartNumberElement() { 3575 return this.partNumber != null && !this.partNumber.isEmpty(); 3576 } 3577 3578 public boolean hasPartNumber() { 3579 return this.partNumber != null && !this.partNumber.isEmpty(); 3580 } 3581 3582 /** 3583 * @param value {@link #partNumber} (The part number of the device.). This is 3584 * the underlying object with id, value and extensions. The 3585 * accessor "getPartNumber" gives direct access to the value 3586 */ 3587 public Device setPartNumberElement(StringType value) { 3588 this.partNumber = value; 3589 return this; 3590 } 3591 3592 /** 3593 * @return The part number of the device. 3594 */ 3595 public String getPartNumber() { 3596 return this.partNumber == null ? null : this.partNumber.getValue(); 3597 } 3598 3599 /** 3600 * @param value The part number of the device. 3601 */ 3602 public Device setPartNumber(String value) { 3603 if (Utilities.noString(value)) 3604 this.partNumber = null; 3605 else { 3606 if (this.partNumber == null) 3607 this.partNumber = new StringType(); 3608 this.partNumber.setValue(value); 3609 } 3610 return this; 3611 } 3612 3613 /** 3614 * @return {@link #type} (The kind or type of device.) 3615 */ 3616 public CodeableConcept getType() { 3617 if (this.type == null) 3618 if (Configuration.errorOnAutoCreate()) 3619 throw new Error("Attempt to auto-create Device.type"); 3620 else if (Configuration.doAutoCreate()) 3621 this.type = new CodeableConcept(); // cc 3622 return this.type; 3623 } 3624 3625 public boolean hasType() { 3626 return this.type != null && !this.type.isEmpty(); 3627 } 3628 3629 /** 3630 * @param value {@link #type} (The kind or type of device.) 3631 */ 3632 public Device setType(CodeableConcept value) { 3633 this.type = value; 3634 return this; 3635 } 3636 3637 /** 3638 * @return {@link #specialization} (The capabilities supported on a device, the 3639 * standards to which the device conforms for a particular purpose, and 3640 * used for the communication.) 3641 */ 3642 public List<DeviceSpecializationComponent> getSpecialization() { 3643 if (this.specialization == null) 3644 this.specialization = new ArrayList<DeviceSpecializationComponent>(); 3645 return this.specialization; 3646 } 3647 3648 /** 3649 * @return Returns a reference to <code>this</code> for easy method chaining 3650 */ 3651 public Device setSpecialization(List<DeviceSpecializationComponent> theSpecialization) { 3652 this.specialization = theSpecialization; 3653 return this; 3654 } 3655 3656 public boolean hasSpecialization() { 3657 if (this.specialization == null) 3658 return false; 3659 for (DeviceSpecializationComponent item : this.specialization) 3660 if (!item.isEmpty()) 3661 return true; 3662 return false; 3663 } 3664 3665 public DeviceSpecializationComponent addSpecialization() { // 3 3666 DeviceSpecializationComponent t = new DeviceSpecializationComponent(); 3667 if (this.specialization == null) 3668 this.specialization = new ArrayList<DeviceSpecializationComponent>(); 3669 this.specialization.add(t); 3670 return t; 3671 } 3672 3673 public Device addSpecialization(DeviceSpecializationComponent t) { // 3 3674 if (t == null) 3675 return this; 3676 if (this.specialization == null) 3677 this.specialization = new ArrayList<DeviceSpecializationComponent>(); 3678 this.specialization.add(t); 3679 return this; 3680 } 3681 3682 /** 3683 * @return The first repetition of repeating field {@link #specialization}, 3684 * creating it if it does not already exist 3685 */ 3686 public DeviceSpecializationComponent getSpecializationFirstRep() { 3687 if (getSpecialization().isEmpty()) { 3688 addSpecialization(); 3689 } 3690 return getSpecialization().get(0); 3691 } 3692 3693 /** 3694 * @return {@link #version} (The actual design of the device or software version 3695 * running on the device.) 3696 */ 3697 public List<DeviceVersionComponent> getVersion() { 3698 if (this.version == null) 3699 this.version = new ArrayList<DeviceVersionComponent>(); 3700 return this.version; 3701 } 3702 3703 /** 3704 * @return Returns a reference to <code>this</code> for easy method chaining 3705 */ 3706 public Device setVersion(List<DeviceVersionComponent> theVersion) { 3707 this.version = theVersion; 3708 return this; 3709 } 3710 3711 public boolean hasVersion() { 3712 if (this.version == null) 3713 return false; 3714 for (DeviceVersionComponent item : this.version) 3715 if (!item.isEmpty()) 3716 return true; 3717 return false; 3718 } 3719 3720 public DeviceVersionComponent addVersion() { // 3 3721 DeviceVersionComponent t = new DeviceVersionComponent(); 3722 if (this.version == null) 3723 this.version = new ArrayList<DeviceVersionComponent>(); 3724 this.version.add(t); 3725 return t; 3726 } 3727 3728 public Device addVersion(DeviceVersionComponent t) { // 3 3729 if (t == null) 3730 return this; 3731 if (this.version == null) 3732 this.version = new ArrayList<DeviceVersionComponent>(); 3733 this.version.add(t); 3734 return this; 3735 } 3736 3737 /** 3738 * @return The first repetition of repeating field {@link #version}, creating it 3739 * if it does not already exist 3740 */ 3741 public DeviceVersionComponent getVersionFirstRep() { 3742 if (getVersion().isEmpty()) { 3743 addVersion(); 3744 } 3745 return getVersion().get(0); 3746 } 3747 3748 /** 3749 * @return {@link #property} (The actual configuration settings of a device as 3750 * it actually operates, e.g., regulation status, time properties.) 3751 */ 3752 public List<DevicePropertyComponent> getProperty() { 3753 if (this.property == null) 3754 this.property = new ArrayList<DevicePropertyComponent>(); 3755 return this.property; 3756 } 3757 3758 /** 3759 * @return Returns a reference to <code>this</code> for easy method chaining 3760 */ 3761 public Device setProperty(List<DevicePropertyComponent> theProperty) { 3762 this.property = theProperty; 3763 return this; 3764 } 3765 3766 public boolean hasProperty() { 3767 if (this.property == null) 3768 return false; 3769 for (DevicePropertyComponent item : this.property) 3770 if (!item.isEmpty()) 3771 return true; 3772 return false; 3773 } 3774 3775 public DevicePropertyComponent addProperty() { // 3 3776 DevicePropertyComponent t = new DevicePropertyComponent(); 3777 if (this.property == null) 3778 this.property = new ArrayList<DevicePropertyComponent>(); 3779 this.property.add(t); 3780 return t; 3781 } 3782 3783 public Device addProperty(DevicePropertyComponent t) { // 3 3784 if (t == null) 3785 return this; 3786 if (this.property == null) 3787 this.property = new ArrayList<DevicePropertyComponent>(); 3788 this.property.add(t); 3789 return this; 3790 } 3791 3792 /** 3793 * @return The first repetition of repeating field {@link #property}, creating 3794 * it if it does not already exist 3795 */ 3796 public DevicePropertyComponent getPropertyFirstRep() { 3797 if (getProperty().isEmpty()) { 3798 addProperty(); 3799 } 3800 return getProperty().get(0); 3801 } 3802 3803 /** 3804 * @return {@link #patient} (Patient information, If the device is affixed to a 3805 * person.) 3806 */ 3807 public Reference getPatient() { 3808 if (this.patient == null) 3809 if (Configuration.errorOnAutoCreate()) 3810 throw new Error("Attempt to auto-create Device.patient"); 3811 else if (Configuration.doAutoCreate()) 3812 this.patient = new Reference(); // cc 3813 return this.patient; 3814 } 3815 3816 public boolean hasPatient() { 3817 return this.patient != null && !this.patient.isEmpty(); 3818 } 3819 3820 /** 3821 * @param value {@link #patient} (Patient information, If the device is affixed 3822 * to a person.) 3823 */ 3824 public Device setPatient(Reference value) { 3825 this.patient = value; 3826 return this; 3827 } 3828 3829 /** 3830 * @return {@link #patient} The actual object that is the target of the 3831 * reference. The reference library doesn't populate this, but you can 3832 * use it to hold the resource if you resolve it. (Patient information, 3833 * If the device is affixed to a person.) 3834 */ 3835 public Patient getPatientTarget() { 3836 if (this.patientTarget == null) 3837 if (Configuration.errorOnAutoCreate()) 3838 throw new Error("Attempt to auto-create Device.patient"); 3839 else if (Configuration.doAutoCreate()) 3840 this.patientTarget = new Patient(); // aa 3841 return this.patientTarget; 3842 } 3843 3844 /** 3845 * @param value {@link #patient} The actual object that is the target of the 3846 * reference. The reference library doesn't use these, but you can 3847 * use it to hold the resource if you resolve it. (Patient 3848 * information, If the device is affixed to a person.) 3849 */ 3850 public Device setPatientTarget(Patient value) { 3851 this.patientTarget = value; 3852 return this; 3853 } 3854 3855 /** 3856 * @return {@link #owner} (An organization that is responsible for the provision 3857 * and ongoing maintenance of the device.) 3858 */ 3859 public Reference getOwner() { 3860 if (this.owner == null) 3861 if (Configuration.errorOnAutoCreate()) 3862 throw new Error("Attempt to auto-create Device.owner"); 3863 else if (Configuration.doAutoCreate()) 3864 this.owner = new Reference(); // cc 3865 return this.owner; 3866 } 3867 3868 public boolean hasOwner() { 3869 return this.owner != null && !this.owner.isEmpty(); 3870 } 3871 3872 /** 3873 * @param value {@link #owner} (An organization that is responsible for the 3874 * provision and ongoing maintenance of the device.) 3875 */ 3876 public Device setOwner(Reference value) { 3877 this.owner = value; 3878 return this; 3879 } 3880 3881 /** 3882 * @return {@link #owner} The actual object that is the target of the reference. 3883 * The reference library doesn't populate this, but you can use it to 3884 * hold the resource if you resolve it. (An organization that is 3885 * responsible for the provision and ongoing maintenance of the device.) 3886 */ 3887 public Organization getOwnerTarget() { 3888 if (this.ownerTarget == null) 3889 if (Configuration.errorOnAutoCreate()) 3890 throw new Error("Attempt to auto-create Device.owner"); 3891 else if (Configuration.doAutoCreate()) 3892 this.ownerTarget = new Organization(); // aa 3893 return this.ownerTarget; 3894 } 3895 3896 /** 3897 * @param value {@link #owner} The actual object that is the target of the 3898 * reference. The reference library doesn't use these, but you can 3899 * use it to hold the resource if you resolve it. (An organization 3900 * that is responsible for the provision and ongoing maintenance of 3901 * the device.) 3902 */ 3903 public Device setOwnerTarget(Organization value) { 3904 this.ownerTarget = value; 3905 return this; 3906 } 3907 3908 /** 3909 * @return {@link #contact} (Contact details for an organization or a particular 3910 * human that is responsible for the device.) 3911 */ 3912 public List<ContactPoint> getContact() { 3913 if (this.contact == null) 3914 this.contact = new ArrayList<ContactPoint>(); 3915 return this.contact; 3916 } 3917 3918 /** 3919 * @return Returns a reference to <code>this</code> for easy method chaining 3920 */ 3921 public Device setContact(List<ContactPoint> theContact) { 3922 this.contact = theContact; 3923 return this; 3924 } 3925 3926 public boolean hasContact() { 3927 if (this.contact == null) 3928 return false; 3929 for (ContactPoint item : this.contact) 3930 if (!item.isEmpty()) 3931 return true; 3932 return false; 3933 } 3934 3935 public ContactPoint addContact() { // 3 3936 ContactPoint t = new ContactPoint(); 3937 if (this.contact == null) 3938 this.contact = new ArrayList<ContactPoint>(); 3939 this.contact.add(t); 3940 return t; 3941 } 3942 3943 public Device addContact(ContactPoint t) { // 3 3944 if (t == null) 3945 return this; 3946 if (this.contact == null) 3947 this.contact = new ArrayList<ContactPoint>(); 3948 this.contact.add(t); 3949 return this; 3950 } 3951 3952 /** 3953 * @return The first repetition of repeating field {@link #contact}, creating it 3954 * if it does not already exist 3955 */ 3956 public ContactPoint getContactFirstRep() { 3957 if (getContact().isEmpty()) { 3958 addContact(); 3959 } 3960 return getContact().get(0); 3961 } 3962 3963 /** 3964 * @return {@link #location} (The place where the device can be found.) 3965 */ 3966 public Reference getLocation() { 3967 if (this.location == null) 3968 if (Configuration.errorOnAutoCreate()) 3969 throw new Error("Attempt to auto-create Device.location"); 3970 else if (Configuration.doAutoCreate()) 3971 this.location = new Reference(); // cc 3972 return this.location; 3973 } 3974 3975 public boolean hasLocation() { 3976 return this.location != null && !this.location.isEmpty(); 3977 } 3978 3979 /** 3980 * @param value {@link #location} (The place where the device can be found.) 3981 */ 3982 public Device setLocation(Reference value) { 3983 this.location = value; 3984 return this; 3985 } 3986 3987 /** 3988 * @return {@link #location} The actual object that is the target of the 3989 * reference. The reference library doesn't populate this, but you can 3990 * use it to hold the resource if you resolve it. (The place where the 3991 * device can be found.) 3992 */ 3993 public Location getLocationTarget() { 3994 if (this.locationTarget == null) 3995 if (Configuration.errorOnAutoCreate()) 3996 throw new Error("Attempt to auto-create Device.location"); 3997 else if (Configuration.doAutoCreate()) 3998 this.locationTarget = new Location(); // aa 3999 return this.locationTarget; 4000 } 4001 4002 /** 4003 * @param value {@link #location} The actual object that is the target of the 4004 * reference. The reference library doesn't use these, but you can 4005 * use it to hold the resource if you resolve it. (The place where 4006 * the device can be found.) 4007 */ 4008 public Device setLocationTarget(Location value) { 4009 this.locationTarget = value; 4010 return this; 4011 } 4012 4013 /** 4014 * @return {@link #url} (A network address on which the device may be contacted 4015 * directly.). This is the underlying object with id, value and 4016 * extensions. The accessor "getUrl" gives direct access to the value 4017 */ 4018 public UriType getUrlElement() { 4019 if (this.url == null) 4020 if (Configuration.errorOnAutoCreate()) 4021 throw new Error("Attempt to auto-create Device.url"); 4022 else if (Configuration.doAutoCreate()) 4023 this.url = new UriType(); // bb 4024 return this.url; 4025 } 4026 4027 public boolean hasUrlElement() { 4028 return this.url != null && !this.url.isEmpty(); 4029 } 4030 4031 public boolean hasUrl() { 4032 return this.url != null && !this.url.isEmpty(); 4033 } 4034 4035 /** 4036 * @param value {@link #url} (A network address on which the device may be 4037 * contacted directly.). This is the underlying object with id, 4038 * value and extensions. The accessor "getUrl" gives direct access 4039 * to the value 4040 */ 4041 public Device setUrlElement(UriType value) { 4042 this.url = value; 4043 return this; 4044 } 4045 4046 /** 4047 * @return A network address on which the device may be contacted directly. 4048 */ 4049 public String getUrl() { 4050 return this.url == null ? null : this.url.getValue(); 4051 } 4052 4053 /** 4054 * @param value A network address on which the device may be contacted directly. 4055 */ 4056 public Device setUrl(String value) { 4057 if (Utilities.noString(value)) 4058 this.url = null; 4059 else { 4060 if (this.url == null) 4061 this.url = new UriType(); 4062 this.url.setValue(value); 4063 } 4064 return this; 4065 } 4066 4067 /** 4068 * @return {@link #note} (Descriptive information, usage information or 4069 * implantation information that is not captured in an existing 4070 * element.) 4071 */ 4072 public List<Annotation> getNote() { 4073 if (this.note == null) 4074 this.note = new ArrayList<Annotation>(); 4075 return this.note; 4076 } 4077 4078 /** 4079 * @return Returns a reference to <code>this</code> for easy method chaining 4080 */ 4081 public Device setNote(List<Annotation> theNote) { 4082 this.note = theNote; 4083 return this; 4084 } 4085 4086 public boolean hasNote() { 4087 if (this.note == null) 4088 return false; 4089 for (Annotation item : this.note) 4090 if (!item.isEmpty()) 4091 return true; 4092 return false; 4093 } 4094 4095 public Annotation addNote() { // 3 4096 Annotation t = new Annotation(); 4097 if (this.note == null) 4098 this.note = new ArrayList<Annotation>(); 4099 this.note.add(t); 4100 return t; 4101 } 4102 4103 public Device addNote(Annotation t) { // 3 4104 if (t == null) 4105 return this; 4106 if (this.note == null) 4107 this.note = new ArrayList<Annotation>(); 4108 this.note.add(t); 4109 return this; 4110 } 4111 4112 /** 4113 * @return The first repetition of repeating field {@link #note}, creating it if 4114 * it does not already exist 4115 */ 4116 public Annotation getNoteFirstRep() { 4117 if (getNote().isEmpty()) { 4118 addNote(); 4119 } 4120 return getNote().get(0); 4121 } 4122 4123 /** 4124 * @return {@link #safety} (Provides additional safety characteristics about a 4125 * medical device. For example devices containing latex.) 4126 */ 4127 public List<CodeableConcept> getSafety() { 4128 if (this.safety == null) 4129 this.safety = new ArrayList<CodeableConcept>(); 4130 return this.safety; 4131 } 4132 4133 /** 4134 * @return Returns a reference to <code>this</code> for easy method chaining 4135 */ 4136 public Device setSafety(List<CodeableConcept> theSafety) { 4137 this.safety = theSafety; 4138 return this; 4139 } 4140 4141 public boolean hasSafety() { 4142 if (this.safety == null) 4143 return false; 4144 for (CodeableConcept item : this.safety) 4145 if (!item.isEmpty()) 4146 return true; 4147 return false; 4148 } 4149 4150 public CodeableConcept addSafety() { // 3 4151 CodeableConcept t = new CodeableConcept(); 4152 if (this.safety == null) 4153 this.safety = new ArrayList<CodeableConcept>(); 4154 this.safety.add(t); 4155 return t; 4156 } 4157 4158 public Device addSafety(CodeableConcept t) { // 3 4159 if (t == null) 4160 return this; 4161 if (this.safety == null) 4162 this.safety = new ArrayList<CodeableConcept>(); 4163 this.safety.add(t); 4164 return this; 4165 } 4166 4167 /** 4168 * @return The first repetition of repeating field {@link #safety}, creating it 4169 * if it does not already exist 4170 */ 4171 public CodeableConcept getSafetyFirstRep() { 4172 if (getSafety().isEmpty()) { 4173 addSafety(); 4174 } 4175 return getSafety().get(0); 4176 } 4177 4178 /** 4179 * @return {@link #parent} (The parent device.) 4180 */ 4181 public Reference getParent() { 4182 if (this.parent == null) 4183 if (Configuration.errorOnAutoCreate()) 4184 throw new Error("Attempt to auto-create Device.parent"); 4185 else if (Configuration.doAutoCreate()) 4186 this.parent = new Reference(); // cc 4187 return this.parent; 4188 } 4189 4190 public boolean hasParent() { 4191 return this.parent != null && !this.parent.isEmpty(); 4192 } 4193 4194 /** 4195 * @param value {@link #parent} (The parent device.) 4196 */ 4197 public Device setParent(Reference value) { 4198 this.parent = value; 4199 return this; 4200 } 4201 4202 /** 4203 * @return {@link #parent} The actual object that is the target of the 4204 * reference. The reference library doesn't populate this, but you can 4205 * use it to hold the resource if you resolve it. (The parent device.) 4206 */ 4207 public Device getParentTarget() { 4208 if (this.parentTarget == null) 4209 if (Configuration.errorOnAutoCreate()) 4210 throw new Error("Attempt to auto-create Device.parent"); 4211 else if (Configuration.doAutoCreate()) 4212 this.parentTarget = new Device(); // aa 4213 return this.parentTarget; 4214 } 4215 4216 /** 4217 * @param value {@link #parent} The actual object that is the target of the 4218 * reference. The reference library doesn't use these, but you can 4219 * use it to hold the resource if you resolve it. (The parent 4220 * device.) 4221 */ 4222 public Device setParentTarget(Device value) { 4223 this.parentTarget = value; 4224 return this; 4225 } 4226 4227 protected void listChildren(List<Property> children) { 4228 super.listChildren(children); 4229 children.add(new Property("identifier", "Identifier", 4230 "Unique instance identifiers assigned to a device by manufacturers other organizations or owners.", 0, 4231 java.lang.Integer.MAX_VALUE, identifier)); 4232 children.add(new Property("definition", "Reference(DeviceDefinition)", 4233 "The reference to the definition for the device.", 0, 1, definition)); 4234 children.add(new Property("udiCarrier", "", 4235 "Unique device identifier (UDI) assigned to device label or package. Note that the Device may include multiple udiCarriers as it either may include just the udiCarrier for the jurisdiction it is sold, or for multiple jurisdictions it could have been sold.", 4236 0, java.lang.Integer.MAX_VALUE, udiCarrier)); 4237 children.add(new Property("status", "code", "Status of the Device availability.", 0, 1, status)); 4238 children.add(new Property("statusReason", "CodeableConcept", "Reason for the dtatus of the Device availability.", 0, 4239 java.lang.Integer.MAX_VALUE, statusReason)); 4240 children.add(new Property("distinctIdentifier", "string", 4241 "The distinct identification string as required by regulation for a human cell, tissue, or cellular and tissue-based product.", 4242 0, 1, distinctIdentifier)); 4243 children.add(new Property("manufacturer", "string", "A name of the manufacturer.", 0, 1, manufacturer)); 4244 children.add(new Property("manufactureDate", "dateTime", "The date and time when the device was manufactured.", 0, 4245 1, manufactureDate)); 4246 children.add(new Property("expirationDate", "dateTime", 4247 "The date and time beyond which this device is no longer valid or should not be used (if applicable).", 0, 1, 4248 expirationDate)); 4249 children.add(new Property("lotNumber", "string", "Lot number assigned by the manufacturer.", 0, 1, lotNumber)); 4250 children.add(new Property("serialNumber", "string", 4251 "The serial number assigned by the organization when the device was manufactured.", 0, 1, serialNumber)); 4252 children.add(new Property("deviceName", "", 4253 "This represents the manufacturer's name of the device as provided by the device, from a UDI label, or by a person describing the Device. This typically would be used when a person provides the name(s) or when the device represents one of the names available from DeviceDefinition.", 4254 0, java.lang.Integer.MAX_VALUE, deviceName)); 4255 children.add(new Property("modelNumber", "string", "The model number for the device.", 0, 1, modelNumber)); 4256 children.add(new Property("partNumber", "string", "The part number of the device.", 0, 1, partNumber)); 4257 children.add(new Property("type", "CodeableConcept", "The kind or type of device.", 0, 1, type)); 4258 children.add(new Property("specialization", "", 4259 "The capabilities supported on a device, the standards to which the device conforms for a particular purpose, and used for the communication.", 4260 0, java.lang.Integer.MAX_VALUE, specialization)); 4261 children 4262 .add(new Property("version", "", "The actual design of the device or software version running on the device.", 4263 0, java.lang.Integer.MAX_VALUE, version)); 4264 children.add(new Property("property", "", 4265 "The actual configuration settings of a device as it actually operates, e.g., regulation status, time properties.", 4266 0, java.lang.Integer.MAX_VALUE, property)); 4267 children.add(new Property("patient", "Reference(Patient)", 4268 "Patient information, If the device is affixed to a person.", 0, 1, patient)); 4269 children.add(new Property("owner", "Reference(Organization)", 4270 "An organization that is responsible for the provision and ongoing maintenance of the device.", 0, 1, owner)); 4271 children.add(new Property("contact", "ContactPoint", 4272 "Contact details for an organization or a particular human that is responsible for the device.", 0, 4273 java.lang.Integer.MAX_VALUE, contact)); 4274 children.add( 4275 new Property("location", "Reference(Location)", "The place where the device can be found.", 0, 1, location)); 4276 children 4277 .add(new Property("url", "uri", "A network address on which the device may be contacted directly.", 0, 1, url)); 4278 children.add(new Property("note", "Annotation", 4279 "Descriptive information, usage information or implantation information that is not captured in an existing element.", 4280 0, java.lang.Integer.MAX_VALUE, note)); 4281 children.add(new Property("safety", "CodeableConcept", 4282 "Provides additional safety characteristics about a medical device. For example devices containing latex.", 0, 4283 java.lang.Integer.MAX_VALUE, safety)); 4284 children.add(new Property("parent", "Reference(Device)", "The parent device.", 0, 1, parent)); 4285 } 4286 4287 @Override 4288 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4289 switch (_hash) { 4290 case -1618432855: 4291 /* identifier */ return new Property("identifier", "Identifier", 4292 "Unique instance identifiers assigned to a device by manufacturers other organizations or owners.", 0, 4293 java.lang.Integer.MAX_VALUE, identifier); 4294 case -1014418093: 4295 /* definition */ return new Property("definition", "Reference(DeviceDefinition)", 4296 "The reference to the definition for the device.", 0, 1, definition); 4297 case -1343558178: 4298 /* udiCarrier */ return new Property("udiCarrier", "", 4299 "Unique device identifier (UDI) assigned to device label or package. Note that the Device may include multiple udiCarriers as it either may include just the udiCarrier for the jurisdiction it is sold, or for multiple jurisdictions it could have been sold.", 4300 0, java.lang.Integer.MAX_VALUE, udiCarrier); 4301 case -892481550: 4302 /* status */ return new Property("status", "code", "Status of the Device availability.", 0, 1, status); 4303 case 2051346646: 4304 /* statusReason */ return new Property("statusReason", "CodeableConcept", 4305 "Reason for the dtatus of the Device availability.", 0, java.lang.Integer.MAX_VALUE, statusReason); 4306 case -1836176187: 4307 /* distinctIdentifier */ return new Property("distinctIdentifier", "string", 4308 "The distinct identification string as required by regulation for a human cell, tissue, or cellular and tissue-based product.", 4309 0, 1, distinctIdentifier); 4310 case -1969347631: 4311 /* manufacturer */ return new Property("manufacturer", "string", "A name of the manufacturer.", 0, 1, 4312 manufacturer); 4313 case 416714767: 4314 /* manufactureDate */ return new Property("manufactureDate", "dateTime", 4315 "The date and time when the device was manufactured.", 0, 1, manufactureDate); 4316 case -668811523: 4317 /* expirationDate */ return new Property("expirationDate", "dateTime", 4318 "The date and time beyond which this device is no longer valid or should not be used (if applicable).", 0, 1, 4319 expirationDate); 4320 case 462547450: 4321 /* lotNumber */ return new Property("lotNumber", "string", "Lot number assigned by the manufacturer.", 0, 1, 4322 lotNumber); 4323 case 83787357: 4324 /* serialNumber */ return new Property("serialNumber", "string", 4325 "The serial number assigned by the organization when the device was manufactured.", 0, 1, serialNumber); 4326 case 780988929: 4327 /* deviceName */ return new Property("deviceName", "", 4328 "This represents the manufacturer's name of the device as provided by the device, from a UDI label, or by a person describing the Device. This typically would be used when a person provides the name(s) or when the device represents one of the names available from DeviceDefinition.", 4329 0, java.lang.Integer.MAX_VALUE, deviceName); 4330 case 346619858: 4331 /* modelNumber */ return new Property("modelNumber", "string", "The model number for the device.", 0, 1, 4332 modelNumber); 4333 case -731502308: 4334 /* partNumber */ return new Property("partNumber", "string", "The part number of the device.", 0, 1, partNumber); 4335 case 3575610: 4336 /* type */ return new Property("type", "CodeableConcept", "The kind or type of device.", 0, 1, type); 4337 case 682815883: 4338 /* specialization */ return new Property("specialization", "", 4339 "The capabilities supported on a device, the standards to which the device conforms for a particular purpose, and used for the communication.", 4340 0, java.lang.Integer.MAX_VALUE, specialization); 4341 case 351608024: 4342 /* version */ return new Property("version", "", 4343 "The actual design of the device or software version running on the device.", 0, java.lang.Integer.MAX_VALUE, 4344 version); 4345 case -993141291: 4346 /* property */ return new Property("property", "", 4347 "The actual configuration settings of a device as it actually operates, e.g., regulation status, time properties.", 4348 0, java.lang.Integer.MAX_VALUE, property); 4349 case -791418107: 4350 /* patient */ return new Property("patient", "Reference(Patient)", 4351 "Patient information, If the device is affixed to a person.", 0, 1, patient); 4352 case 106164915: 4353 /* owner */ return new Property("owner", "Reference(Organization)", 4354 "An organization that is responsible for the provision and ongoing maintenance of the device.", 0, 1, owner); 4355 case 951526432: 4356 /* contact */ return new Property("contact", "ContactPoint", 4357 "Contact details for an organization or a particular human that is responsible for the device.", 0, 4358 java.lang.Integer.MAX_VALUE, contact); 4359 case 1901043637: 4360 /* location */ return new Property("location", "Reference(Location)", "The place where the device can be found.", 4361 0, 1, location); 4362 case 116079: 4363 /* url */ return new Property("url", "uri", "A network address on which the device may be contacted directly.", 0, 4364 1, url); 4365 case 3387378: 4366 /* note */ return new Property("note", "Annotation", 4367 "Descriptive information, usage information or implantation information that is not captured in an existing element.", 4368 0, java.lang.Integer.MAX_VALUE, note); 4369 case -909893934: 4370 /* safety */ return new Property("safety", "CodeableConcept", 4371 "Provides additional safety characteristics about a medical device. For example devices containing latex.", 4372 0, java.lang.Integer.MAX_VALUE, safety); 4373 case -995424086: 4374 /* parent */ return new Property("parent", "Reference(Device)", "The parent device.", 0, 1, parent); 4375 default: 4376 return super.getNamedProperty(_hash, _name, _checkValid); 4377 } 4378 4379 } 4380 4381 @Override 4382 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4383 switch (hash) { 4384 case -1618432855: 4385 /* identifier */ return this.identifier == null ? new Base[0] 4386 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4387 case -1014418093: 4388 /* definition */ return this.definition == null ? new Base[0] : new Base[] { this.definition }; // Reference 4389 case -1343558178: 4390 /* udiCarrier */ return this.udiCarrier == null ? new Base[0] 4391 : this.udiCarrier.toArray(new Base[this.udiCarrier.size()]); // DeviceUdiCarrierComponent 4392 case -892481550: 4393 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<FHIRDeviceStatus> 4394 case 2051346646: 4395 /* statusReason */ return this.statusReason == null ? new Base[0] 4396 : this.statusReason.toArray(new Base[this.statusReason.size()]); // CodeableConcept 4397 case -1836176187: 4398 /* distinctIdentifier */ return this.distinctIdentifier == null ? new Base[0] 4399 : new Base[] { this.distinctIdentifier }; // StringType 4400 case -1969347631: 4401 /* manufacturer */ return this.manufacturer == null ? new Base[0] : new Base[] { this.manufacturer }; // StringType 4402 case 416714767: 4403 /* manufactureDate */ return this.manufactureDate == null ? new Base[0] : new Base[] { this.manufactureDate }; // DateTimeType 4404 case -668811523: 4405 /* expirationDate */ return this.expirationDate == null ? new Base[0] : new Base[] { this.expirationDate }; // DateTimeType 4406 case 462547450: 4407 /* lotNumber */ return this.lotNumber == null ? new Base[0] : new Base[] { this.lotNumber }; // StringType 4408 case 83787357: 4409 /* serialNumber */ return this.serialNumber == null ? new Base[0] : new Base[] { this.serialNumber }; // StringType 4410 case 780988929: 4411 /* deviceName */ return this.deviceName == null ? new Base[0] 4412 : this.deviceName.toArray(new Base[this.deviceName.size()]); // DeviceDeviceNameComponent 4413 case 346619858: 4414 /* modelNumber */ return this.modelNumber == null ? new Base[0] : new Base[] { this.modelNumber }; // StringType 4415 case -731502308: 4416 /* partNumber */ return this.partNumber == null ? new Base[0] : new Base[] { this.partNumber }; // StringType 4417 case 3575610: 4418 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 4419 case 682815883: 4420 /* specialization */ return this.specialization == null ? new Base[0] 4421 : this.specialization.toArray(new Base[this.specialization.size()]); // DeviceSpecializationComponent 4422 case 351608024: 4423 /* version */ return this.version == null ? new Base[0] : this.version.toArray(new Base[this.version.size()]); // DeviceVersionComponent 4424 case -993141291: 4425 /* property */ return this.property == null ? new Base[0] : this.property.toArray(new Base[this.property.size()]); // DevicePropertyComponent 4426 case -791418107: 4427 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 4428 case 106164915: 4429 /* owner */ return this.owner == null ? new Base[0] : new Base[] { this.owner }; // Reference 4430 case 951526432: 4431 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactPoint 4432 case 1901043637: 4433 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Reference 4434 case 116079: 4435 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 4436 case 3387378: 4437 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 4438 case -909893934: 4439 /* safety */ return this.safety == null ? new Base[0] : this.safety.toArray(new Base[this.safety.size()]); // CodeableConcept 4440 case -995424086: 4441 /* parent */ return this.parent == null ? new Base[0] : new Base[] { this.parent }; // Reference 4442 default: 4443 return super.getProperty(hash, name, checkValid); 4444 } 4445 4446 } 4447 4448 @Override 4449 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4450 switch (hash) { 4451 case -1618432855: // identifier 4452 this.getIdentifier().add(castToIdentifier(value)); // Identifier 4453 return value; 4454 case -1014418093: // definition 4455 this.definition = castToReference(value); // Reference 4456 return value; 4457 case -1343558178: // udiCarrier 4458 this.getUdiCarrier().add((DeviceUdiCarrierComponent) value); // DeviceUdiCarrierComponent 4459 return value; 4460 case -892481550: // status 4461 value = new FHIRDeviceStatusEnumFactory().fromType(castToCode(value)); 4462 this.status = (Enumeration) value; // Enumeration<FHIRDeviceStatus> 4463 return value; 4464 case 2051346646: // statusReason 4465 this.getStatusReason().add(castToCodeableConcept(value)); // CodeableConcept 4466 return value; 4467 case -1836176187: // distinctIdentifier 4468 this.distinctIdentifier = castToString(value); // StringType 4469 return value; 4470 case -1969347631: // manufacturer 4471 this.manufacturer = castToString(value); // StringType 4472 return value; 4473 case 416714767: // manufactureDate 4474 this.manufactureDate = castToDateTime(value); // DateTimeType 4475 return value; 4476 case -668811523: // expirationDate 4477 this.expirationDate = castToDateTime(value); // DateTimeType 4478 return value; 4479 case 462547450: // lotNumber 4480 this.lotNumber = castToString(value); // StringType 4481 return value; 4482 case 83787357: // serialNumber 4483 this.serialNumber = castToString(value); // StringType 4484 return value; 4485 case 780988929: // deviceName 4486 this.getDeviceName().add((DeviceDeviceNameComponent) value); // DeviceDeviceNameComponent 4487 return value; 4488 case 346619858: // modelNumber 4489 this.modelNumber = castToString(value); // StringType 4490 return value; 4491 case -731502308: // partNumber 4492 this.partNumber = castToString(value); // StringType 4493 return value; 4494 case 3575610: // type 4495 this.type = castToCodeableConcept(value); // CodeableConcept 4496 return value; 4497 case 682815883: // specialization 4498 this.getSpecialization().add((DeviceSpecializationComponent) value); // DeviceSpecializationComponent 4499 return value; 4500 case 351608024: // version 4501 this.getVersion().add((DeviceVersionComponent) value); // DeviceVersionComponent 4502 return value; 4503 case -993141291: // property 4504 this.getProperty().add((DevicePropertyComponent) value); // DevicePropertyComponent 4505 return value; 4506 case -791418107: // patient 4507 this.patient = castToReference(value); // Reference 4508 return value; 4509 case 106164915: // owner 4510 this.owner = castToReference(value); // Reference 4511 return value; 4512 case 951526432: // contact 4513 this.getContact().add(castToContactPoint(value)); // ContactPoint 4514 return value; 4515 case 1901043637: // location 4516 this.location = castToReference(value); // Reference 4517 return value; 4518 case 116079: // url 4519 this.url = castToUri(value); // UriType 4520 return value; 4521 case 3387378: // note 4522 this.getNote().add(castToAnnotation(value)); // Annotation 4523 return value; 4524 case -909893934: // safety 4525 this.getSafety().add(castToCodeableConcept(value)); // CodeableConcept 4526 return value; 4527 case -995424086: // parent 4528 this.parent = castToReference(value); // Reference 4529 return value; 4530 default: 4531 return super.setProperty(hash, name, value); 4532 } 4533 4534 } 4535 4536 @Override 4537 public Base setProperty(String name, Base value) throws FHIRException { 4538 if (name.equals("identifier")) { 4539 this.getIdentifier().add(castToIdentifier(value)); 4540 } else if (name.equals("definition")) { 4541 this.definition = castToReference(value); // Reference 4542 } else if (name.equals("udiCarrier")) { 4543 this.getUdiCarrier().add((DeviceUdiCarrierComponent) value); 4544 } else if (name.equals("status")) { 4545 value = new FHIRDeviceStatusEnumFactory().fromType(castToCode(value)); 4546 this.status = (Enumeration) value; // Enumeration<FHIRDeviceStatus> 4547 } else if (name.equals("statusReason")) { 4548 this.getStatusReason().add(castToCodeableConcept(value)); 4549 } else if (name.equals("distinctIdentifier")) { 4550 this.distinctIdentifier = castToString(value); // StringType 4551 } else if (name.equals("manufacturer")) { 4552 this.manufacturer = castToString(value); // StringType 4553 } else if (name.equals("manufactureDate")) { 4554 this.manufactureDate = castToDateTime(value); // DateTimeType 4555 } else if (name.equals("expirationDate")) { 4556 this.expirationDate = castToDateTime(value); // DateTimeType 4557 } else if (name.equals("lotNumber")) { 4558 this.lotNumber = castToString(value); // StringType 4559 } else if (name.equals("serialNumber")) { 4560 this.serialNumber = castToString(value); // StringType 4561 } else if (name.equals("deviceName")) { 4562 this.getDeviceName().add((DeviceDeviceNameComponent) value); 4563 } else if (name.equals("modelNumber")) { 4564 this.modelNumber = castToString(value); // StringType 4565 } else if (name.equals("partNumber")) { 4566 this.partNumber = castToString(value); // StringType 4567 } else if (name.equals("type")) { 4568 this.type = castToCodeableConcept(value); // CodeableConcept 4569 } else if (name.equals("specialization")) { 4570 this.getSpecialization().add((DeviceSpecializationComponent) value); 4571 } else if (name.equals("version")) { 4572 this.getVersion().add((DeviceVersionComponent) value); 4573 } else if (name.equals("property")) { 4574 this.getProperty().add((DevicePropertyComponent) value); 4575 } else if (name.equals("patient")) { 4576 this.patient = castToReference(value); // Reference 4577 } else if (name.equals("owner")) { 4578 this.owner = castToReference(value); // Reference 4579 } else if (name.equals("contact")) { 4580 this.getContact().add(castToContactPoint(value)); 4581 } else if (name.equals("location")) { 4582 this.location = castToReference(value); // Reference 4583 } else if (name.equals("url")) { 4584 this.url = castToUri(value); // UriType 4585 } else if (name.equals("note")) { 4586 this.getNote().add(castToAnnotation(value)); 4587 } else if (name.equals("safety")) { 4588 this.getSafety().add(castToCodeableConcept(value)); 4589 } else if (name.equals("parent")) { 4590 this.parent = castToReference(value); // Reference 4591 } else 4592 return super.setProperty(name, value); 4593 return value; 4594 } 4595 4596 @Override 4597 public void removeChild(String name, Base value) throws FHIRException { 4598 if (name.equals("identifier")) { 4599 this.getIdentifier().remove(castToIdentifier(value)); 4600 } else if (name.equals("definition")) { 4601 this.definition = null; 4602 } else if (name.equals("udiCarrier")) { 4603 this.getUdiCarrier().remove((DeviceUdiCarrierComponent) value); 4604 } else if (name.equals("status")) { 4605 this.status = null; 4606 } else if (name.equals("statusReason")) { 4607 this.getStatusReason().remove(castToCodeableConcept(value)); 4608 } else if (name.equals("distinctIdentifier")) { 4609 this.distinctIdentifier = null; 4610 } else if (name.equals("manufacturer")) { 4611 this.manufacturer = null; 4612 } else if (name.equals("manufactureDate")) { 4613 this.manufactureDate = null; 4614 } else if (name.equals("expirationDate")) { 4615 this.expirationDate = null; 4616 } else if (name.equals("lotNumber")) { 4617 this.lotNumber = null; 4618 } else if (name.equals("serialNumber")) { 4619 this.serialNumber = null; 4620 } else if (name.equals("deviceName")) { 4621 this.getDeviceName().remove((DeviceDeviceNameComponent) value); 4622 } else if (name.equals("modelNumber")) { 4623 this.modelNumber = null; 4624 } else if (name.equals("partNumber")) { 4625 this.partNumber = null; 4626 } else if (name.equals("type")) { 4627 this.type = null; 4628 } else if (name.equals("specialization")) { 4629 this.getSpecialization().remove((DeviceSpecializationComponent) value); 4630 } else if (name.equals("version")) { 4631 this.getVersion().remove((DeviceVersionComponent) value); 4632 } else if (name.equals("property")) { 4633 this.getProperty().remove((DevicePropertyComponent) value); 4634 } else if (name.equals("patient")) { 4635 this.patient = null; 4636 } else if (name.equals("owner")) { 4637 this.owner = null; 4638 } else if (name.equals("contact")) { 4639 this.getContact().remove(castToContactPoint(value)); 4640 } else if (name.equals("location")) { 4641 this.location = null; 4642 } else if (name.equals("url")) { 4643 this.url = null; 4644 } else if (name.equals("note")) { 4645 this.getNote().remove(castToAnnotation(value)); 4646 } else if (name.equals("safety")) { 4647 this.getSafety().remove(castToCodeableConcept(value)); 4648 } else if (name.equals("parent")) { 4649 this.parent = null; 4650 } else 4651 super.removeChild(name, value); 4652 4653 } 4654 4655 @Override 4656 public Base makeProperty(int hash, String name) throws FHIRException { 4657 switch (hash) { 4658 case -1618432855: 4659 return addIdentifier(); 4660 case -1014418093: 4661 return getDefinition(); 4662 case -1343558178: 4663 return addUdiCarrier(); 4664 case -892481550: 4665 return getStatusElement(); 4666 case 2051346646: 4667 return addStatusReason(); 4668 case -1836176187: 4669 return getDistinctIdentifierElement(); 4670 case -1969347631: 4671 return getManufacturerElement(); 4672 case 416714767: 4673 return getManufactureDateElement(); 4674 case -668811523: 4675 return getExpirationDateElement(); 4676 case 462547450: 4677 return getLotNumberElement(); 4678 case 83787357: 4679 return getSerialNumberElement(); 4680 case 780988929: 4681 return addDeviceName(); 4682 case 346619858: 4683 return getModelNumberElement(); 4684 case -731502308: 4685 return getPartNumberElement(); 4686 case 3575610: 4687 return getType(); 4688 case 682815883: 4689 return addSpecialization(); 4690 case 351608024: 4691 return addVersion(); 4692 case -993141291: 4693 return addProperty(); 4694 case -791418107: 4695 return getPatient(); 4696 case 106164915: 4697 return getOwner(); 4698 case 951526432: 4699 return addContact(); 4700 case 1901043637: 4701 return getLocation(); 4702 case 116079: 4703 return getUrlElement(); 4704 case 3387378: 4705 return addNote(); 4706 case -909893934: 4707 return addSafety(); 4708 case -995424086: 4709 return getParent(); 4710 default: 4711 return super.makeProperty(hash, name); 4712 } 4713 4714 } 4715 4716 @Override 4717 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4718 switch (hash) { 4719 case -1618432855: 4720 /* identifier */ return new String[] { "Identifier" }; 4721 case -1014418093: 4722 /* definition */ return new String[] { "Reference" }; 4723 case -1343558178: 4724 /* udiCarrier */ return new String[] {}; 4725 case -892481550: 4726 /* status */ return new String[] { "code" }; 4727 case 2051346646: 4728 /* statusReason */ return new String[] { "CodeableConcept" }; 4729 case -1836176187: 4730 /* distinctIdentifier */ return new String[] { "string" }; 4731 case -1969347631: 4732 /* manufacturer */ return new String[] { "string" }; 4733 case 416714767: 4734 /* manufactureDate */ return new String[] { "dateTime" }; 4735 case -668811523: 4736 /* expirationDate */ return new String[] { "dateTime" }; 4737 case 462547450: 4738 /* lotNumber */ return new String[] { "string" }; 4739 case 83787357: 4740 /* serialNumber */ return new String[] { "string" }; 4741 case 780988929: 4742 /* deviceName */ return new String[] {}; 4743 case 346619858: 4744 /* modelNumber */ return new String[] { "string" }; 4745 case -731502308: 4746 /* partNumber */ return new String[] { "string" }; 4747 case 3575610: 4748 /* type */ return new String[] { "CodeableConcept" }; 4749 case 682815883: 4750 /* specialization */ return new String[] {}; 4751 case 351608024: 4752 /* version */ return new String[] {}; 4753 case -993141291: 4754 /* property */ return new String[] {}; 4755 case -791418107: 4756 /* patient */ return new String[] { "Reference" }; 4757 case 106164915: 4758 /* owner */ return new String[] { "Reference" }; 4759 case 951526432: 4760 /* contact */ return new String[] { "ContactPoint" }; 4761 case 1901043637: 4762 /* location */ return new String[] { "Reference" }; 4763 case 116079: 4764 /* url */ return new String[] { "uri" }; 4765 case 3387378: 4766 /* note */ return new String[] { "Annotation" }; 4767 case -909893934: 4768 /* safety */ return new String[] { "CodeableConcept" }; 4769 case -995424086: 4770 /* parent */ return new String[] { "Reference" }; 4771 default: 4772 return super.getTypesForProperty(hash, name); 4773 } 4774 4775 } 4776 4777 @Override 4778 public Base addChild(String name) throws FHIRException { 4779 if (name.equals("identifier")) { 4780 return addIdentifier(); 4781 } else if (name.equals("definition")) { 4782 this.definition = new Reference(); 4783 return this.definition; 4784 } else if (name.equals("udiCarrier")) { 4785 return addUdiCarrier(); 4786 } else if (name.equals("status")) { 4787 throw new FHIRException("Cannot call addChild on a singleton property Device.status"); 4788 } else if (name.equals("statusReason")) { 4789 return addStatusReason(); 4790 } else if (name.equals("distinctIdentifier")) { 4791 throw new FHIRException("Cannot call addChild on a singleton property Device.distinctIdentifier"); 4792 } else if (name.equals("manufacturer")) { 4793 throw new FHIRException("Cannot call addChild on a singleton property Device.manufacturer"); 4794 } else if (name.equals("manufactureDate")) { 4795 throw new FHIRException("Cannot call addChild on a singleton property Device.manufactureDate"); 4796 } else if (name.equals("expirationDate")) { 4797 throw new FHIRException("Cannot call addChild on a singleton property Device.expirationDate"); 4798 } else if (name.equals("lotNumber")) { 4799 throw new FHIRException("Cannot call addChild on a singleton property Device.lotNumber"); 4800 } else if (name.equals("serialNumber")) { 4801 throw new FHIRException("Cannot call addChild on a singleton property Device.serialNumber"); 4802 } else if (name.equals("deviceName")) { 4803 return addDeviceName(); 4804 } else if (name.equals("modelNumber")) { 4805 throw new FHIRException("Cannot call addChild on a singleton property Device.modelNumber"); 4806 } else if (name.equals("partNumber")) { 4807 throw new FHIRException("Cannot call addChild on a singleton property Device.partNumber"); 4808 } else if (name.equals("type")) { 4809 this.type = new CodeableConcept(); 4810 return this.type; 4811 } else if (name.equals("specialization")) { 4812 return addSpecialization(); 4813 } else if (name.equals("version")) { 4814 return addVersion(); 4815 } else if (name.equals("property")) { 4816 return addProperty(); 4817 } else if (name.equals("patient")) { 4818 this.patient = new Reference(); 4819 return this.patient; 4820 } else if (name.equals("owner")) { 4821 this.owner = new Reference(); 4822 return this.owner; 4823 } else if (name.equals("contact")) { 4824 return addContact(); 4825 } else if (name.equals("location")) { 4826 this.location = new Reference(); 4827 return this.location; 4828 } else if (name.equals("url")) { 4829 throw new FHIRException("Cannot call addChild on a singleton property Device.url"); 4830 } else if (name.equals("note")) { 4831 return addNote(); 4832 } else if (name.equals("safety")) { 4833 return addSafety(); 4834 } else if (name.equals("parent")) { 4835 this.parent = new Reference(); 4836 return this.parent; 4837 } else 4838 return super.addChild(name); 4839 } 4840 4841 public String fhirType() { 4842 return "Device"; 4843 4844 } 4845 4846 public Device copy() { 4847 Device dst = new Device(); 4848 copyValues(dst); 4849 return dst; 4850 } 4851 4852 public void copyValues(Device dst) { 4853 super.copyValues(dst); 4854 if (identifier != null) { 4855 dst.identifier = new ArrayList<Identifier>(); 4856 for (Identifier i : identifier) 4857 dst.identifier.add(i.copy()); 4858 } 4859 ; 4860 dst.definition = definition == null ? null : definition.copy(); 4861 if (udiCarrier != null) { 4862 dst.udiCarrier = new ArrayList<DeviceUdiCarrierComponent>(); 4863 for (DeviceUdiCarrierComponent i : udiCarrier) 4864 dst.udiCarrier.add(i.copy()); 4865 } 4866 ; 4867 dst.status = status == null ? null : status.copy(); 4868 if (statusReason != null) { 4869 dst.statusReason = new ArrayList<CodeableConcept>(); 4870 for (CodeableConcept i : statusReason) 4871 dst.statusReason.add(i.copy()); 4872 } 4873 ; 4874 dst.distinctIdentifier = distinctIdentifier == null ? null : distinctIdentifier.copy(); 4875 dst.manufacturer = manufacturer == null ? null : manufacturer.copy(); 4876 dst.manufactureDate = manufactureDate == null ? null : manufactureDate.copy(); 4877 dst.expirationDate = expirationDate == null ? null : expirationDate.copy(); 4878 dst.lotNumber = lotNumber == null ? null : lotNumber.copy(); 4879 dst.serialNumber = serialNumber == null ? null : serialNumber.copy(); 4880 if (deviceName != null) { 4881 dst.deviceName = new ArrayList<DeviceDeviceNameComponent>(); 4882 for (DeviceDeviceNameComponent i : deviceName) 4883 dst.deviceName.add(i.copy()); 4884 } 4885 ; 4886 dst.modelNumber = modelNumber == null ? null : modelNumber.copy(); 4887 dst.partNumber = partNumber == null ? null : partNumber.copy(); 4888 dst.type = type == null ? null : type.copy(); 4889 if (specialization != null) { 4890 dst.specialization = new ArrayList<DeviceSpecializationComponent>(); 4891 for (DeviceSpecializationComponent i : specialization) 4892 dst.specialization.add(i.copy()); 4893 } 4894 ; 4895 if (version != null) { 4896 dst.version = new ArrayList<DeviceVersionComponent>(); 4897 for (DeviceVersionComponent i : version) 4898 dst.version.add(i.copy()); 4899 } 4900 ; 4901 if (property != null) { 4902 dst.property = new ArrayList<DevicePropertyComponent>(); 4903 for (DevicePropertyComponent i : property) 4904 dst.property.add(i.copy()); 4905 } 4906 ; 4907 dst.patient = patient == null ? null : patient.copy(); 4908 dst.owner = owner == null ? null : owner.copy(); 4909 if (contact != null) { 4910 dst.contact = new ArrayList<ContactPoint>(); 4911 for (ContactPoint i : contact) 4912 dst.contact.add(i.copy()); 4913 } 4914 ; 4915 dst.location = location == null ? null : location.copy(); 4916 dst.url = url == null ? null : url.copy(); 4917 if (note != null) { 4918 dst.note = new ArrayList<Annotation>(); 4919 for (Annotation i : note) 4920 dst.note.add(i.copy()); 4921 } 4922 ; 4923 if (safety != null) { 4924 dst.safety = new ArrayList<CodeableConcept>(); 4925 for (CodeableConcept i : safety) 4926 dst.safety.add(i.copy()); 4927 } 4928 ; 4929 dst.parent = parent == null ? null : parent.copy(); 4930 } 4931 4932 protected Device typedCopy() { 4933 return copy(); 4934 } 4935 4936 @Override 4937 public boolean equalsDeep(Base other_) { 4938 if (!super.equalsDeep(other_)) 4939 return false; 4940 if (!(other_ instanceof Device)) 4941 return false; 4942 Device o = (Device) other_; 4943 return compareDeep(identifier, o.identifier, true) && compareDeep(definition, o.definition, true) 4944 && compareDeep(udiCarrier, o.udiCarrier, true) && compareDeep(status, o.status, true) 4945 && compareDeep(statusReason, o.statusReason, true) 4946 && compareDeep(distinctIdentifier, o.distinctIdentifier, true) 4947 && compareDeep(manufacturer, o.manufacturer, true) && compareDeep(manufactureDate, o.manufactureDate, true) 4948 && compareDeep(expirationDate, o.expirationDate, true) && compareDeep(lotNumber, o.lotNumber, true) 4949 && compareDeep(serialNumber, o.serialNumber, true) && compareDeep(deviceName, o.deviceName, true) 4950 && compareDeep(modelNumber, o.modelNumber, true) && compareDeep(partNumber, o.partNumber, true) 4951 && compareDeep(type, o.type, true) && compareDeep(specialization, o.specialization, true) 4952 && compareDeep(version, o.version, true) && compareDeep(property, o.property, true) 4953 && compareDeep(patient, o.patient, true) && compareDeep(owner, o.owner, true) 4954 && compareDeep(contact, o.contact, true) && compareDeep(location, o.location, true) 4955 && compareDeep(url, o.url, true) && compareDeep(note, o.note, true) && compareDeep(safety, o.safety, true) 4956 && compareDeep(parent, o.parent, true); 4957 } 4958 4959 @Override 4960 public boolean equalsShallow(Base other_) { 4961 if (!super.equalsShallow(other_)) 4962 return false; 4963 if (!(other_ instanceof Device)) 4964 return false; 4965 Device o = (Device) other_; 4966 return compareValues(status, o.status, true) && compareValues(distinctIdentifier, o.distinctIdentifier, true) 4967 && compareValues(manufacturer, o.manufacturer, true) && compareValues(manufactureDate, o.manufactureDate, true) 4968 && compareValues(expirationDate, o.expirationDate, true) && compareValues(lotNumber, o.lotNumber, true) 4969 && compareValues(serialNumber, o.serialNumber, true) && compareValues(modelNumber, o.modelNumber, true) 4970 && compareValues(partNumber, o.partNumber, true) && compareValues(url, o.url, true); 4971 } 4972 4973 public boolean isEmpty() { 4974 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, definition, udiCarrier, status, 4975 statusReason, distinctIdentifier, manufacturer, manufactureDate, expirationDate, lotNumber, serialNumber, 4976 deviceName, modelNumber, partNumber, type, specialization, version, property, patient, owner, contact, location, 4977 url, note, safety, parent); 4978 } 4979 4980 @Override 4981 public ResourceType getResourceType() { 4982 return ResourceType.Device; 4983 } 4984 4985 /** 4986 * Search parameter: <b>udi-di</b> 4987 * <p> 4988 * Description: <b>The udi Device Identifier (DI)</b><br> 4989 * Type: <b>string</b><br> 4990 * Path: <b>Device.udiCarrier</b><br> 4991 * </p> 4992 */ 4993 @SearchParamDefinition(name = "udi-di", path = "Device.udiCarrier.deviceIdentifier", description = "The udi Device Identifier (DI)", type = "string") 4994 public static final String SP_UDI_DI = "udi-di"; 4995 /** 4996 * <b>Fluent Client</b> search parameter constant for <b>udi-di</b> 4997 * <p> 4998 * Description: <b>The udi Device Identifier (DI)</b><br> 4999 * Type: <b>string</b><br> 5000 * Path: <b>Device.udiCarrier</b><br> 5001 * </p> 5002 */ 5003 public static final ca.uhn.fhir.rest.gclient.StringClientParam UDI_DI = new ca.uhn.fhir.rest.gclient.StringClientParam( 5004 SP_UDI_DI); 5005 5006 /** 5007 * Search parameter: <b>identifier</b> 5008 * <p> 5009 * Description: <b>Instance id from manufacturer, owner, and others</b><br> 5010 * Type: <b>token</b><br> 5011 * Path: <b>Device.identifier</b><br> 5012 * </p> 5013 */ 5014 @SearchParamDefinition(name = "identifier", path = "Device.identifier", description = "Instance id from manufacturer, owner, and others", type = "token") 5015 public static final String SP_IDENTIFIER = "identifier"; 5016 /** 5017 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5018 * <p> 5019 * Description: <b>Instance id from manufacturer, owner, and others</b><br> 5020 * Type: <b>token</b><br> 5021 * Path: <b>Device.identifier</b><br> 5022 * </p> 5023 */ 5024 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5025 SP_IDENTIFIER); 5026 5027 /** 5028 * Search parameter: <b>udi-carrier</b> 5029 * <p> 5030 * Description: <b>UDI Barcode (RFID or other technology) string in *HRF* 5031 * format.</b><br> 5032 * Type: <b>string</b><br> 5033 * Path: <b>Device.udiCarrier</b><br> 5034 * </p> 5035 */ 5036 @SearchParamDefinition(name = "udi-carrier", path = "Device.udiCarrier.carrierHRF", description = "UDI Barcode (RFID or other technology) string in *HRF* format.", type = "string") 5037 public static final String SP_UDI_CARRIER = "udi-carrier"; 5038 /** 5039 * <b>Fluent Client</b> search parameter constant for <b>udi-carrier</b> 5040 * <p> 5041 * Description: <b>UDI Barcode (RFID or other technology) string in *HRF* 5042 * format.</b><br> 5043 * Type: <b>string</b><br> 5044 * Path: <b>Device.udiCarrier</b><br> 5045 * </p> 5046 */ 5047 public static final ca.uhn.fhir.rest.gclient.StringClientParam UDI_CARRIER = new ca.uhn.fhir.rest.gclient.StringClientParam( 5048 SP_UDI_CARRIER); 5049 5050 /** 5051 * Search parameter: <b>device-name</b> 5052 * <p> 5053 * Description: <b>A server defined search that may match any of the string 5054 * fields in Device.deviceName or Device.type.</b><br> 5055 * Type: <b>string</b><br> 5056 * Path: <b>Device.deviceName</b><br> 5057 * </p> 5058 */ 5059 @SearchParamDefinition(name = "device-name", path = "Device.deviceName.name | Device.type.coding.display | Device.type.text", description = "A server defined search that may match any of the string fields in Device.deviceName or Device.type.", type = "string") 5060 public static final String SP_DEVICE_NAME = "device-name"; 5061 /** 5062 * <b>Fluent Client</b> search parameter constant for <b>device-name</b> 5063 * <p> 5064 * Description: <b>A server defined search that may match any of the string 5065 * fields in Device.deviceName or Device.type.</b><br> 5066 * Type: <b>string</b><br> 5067 * Path: <b>Device.deviceName</b><br> 5068 * </p> 5069 */ 5070 public static final ca.uhn.fhir.rest.gclient.StringClientParam DEVICE_NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 5071 SP_DEVICE_NAME); 5072 5073 /** 5074 * Search parameter: <b>patient</b> 5075 * <p> 5076 * Description: <b>Patient information, if the resource is affixed to a 5077 * person</b><br> 5078 * Type: <b>reference</b><br> 5079 * Path: <b>Device.patient</b><br> 5080 * </p> 5081 */ 5082 @SearchParamDefinition(name = "patient", path = "Device.patient", description = "Patient information, if the resource is affixed to a person", type = "reference", target = { 5083 Patient.class }) 5084 public static final String SP_PATIENT = "patient"; 5085 /** 5086 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 5087 * <p> 5088 * Description: <b>Patient information, if the resource is affixed to a 5089 * person</b><br> 5090 * Type: <b>reference</b><br> 5091 * Path: <b>Device.patient</b><br> 5092 * </p> 5093 */ 5094 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5095 SP_PATIENT); 5096 5097 /** 5098 * Constant for fluent queries to be used to add include statements. Specifies 5099 * the path value of "<b>Device:patient</b>". 5100 */ 5101 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 5102 "Device:patient").toLocked(); 5103 5104 /** 5105 * Search parameter: <b>organization</b> 5106 * <p> 5107 * Description: <b>The organization responsible for the device</b><br> 5108 * Type: <b>reference</b><br> 5109 * Path: <b>Device.owner</b><br> 5110 * </p> 5111 */ 5112 @SearchParamDefinition(name = "organization", path = "Device.owner", description = "The organization responsible for the device", type = "reference", target = { 5113 Organization.class }) 5114 public static final String SP_ORGANIZATION = "organization"; 5115 /** 5116 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 5117 * <p> 5118 * Description: <b>The organization responsible for the device</b><br> 5119 * Type: <b>reference</b><br> 5120 * Path: <b>Device.owner</b><br> 5121 * </p> 5122 */ 5123 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5124 SP_ORGANIZATION); 5125 5126 /** 5127 * Constant for fluent queries to be used to add include statements. Specifies 5128 * the path value of "<b>Device:organization</b>". 5129 */ 5130 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include( 5131 "Device:organization").toLocked(); 5132 5133 /** 5134 * Search parameter: <b>model</b> 5135 * <p> 5136 * Description: <b>The model of the device</b><br> 5137 * Type: <b>string</b><br> 5138 * Path: <b>Device.modelNumber</b><br> 5139 * </p> 5140 */ 5141 @SearchParamDefinition(name = "model", path = "Device.modelNumber", description = "The model of the device", type = "string") 5142 public static final String SP_MODEL = "model"; 5143 /** 5144 * <b>Fluent Client</b> search parameter constant for <b>model</b> 5145 * <p> 5146 * Description: <b>The model of the device</b><br> 5147 * Type: <b>string</b><br> 5148 * Path: <b>Device.modelNumber</b><br> 5149 * </p> 5150 */ 5151 public static final ca.uhn.fhir.rest.gclient.StringClientParam MODEL = new ca.uhn.fhir.rest.gclient.StringClientParam( 5152 SP_MODEL); 5153 5154 /** 5155 * Search parameter: <b>location</b> 5156 * <p> 5157 * Description: <b>A location, where the resource is found</b><br> 5158 * Type: <b>reference</b><br> 5159 * Path: <b>Device.location</b><br> 5160 * </p> 5161 */ 5162 @SearchParamDefinition(name = "location", path = "Device.location", description = "A location, where the resource is found", type = "reference", target = { 5163 Location.class }) 5164 public static final String SP_LOCATION = "location"; 5165 /** 5166 * <b>Fluent Client</b> search parameter constant for <b>location</b> 5167 * <p> 5168 * Description: <b>A location, where the resource is found</b><br> 5169 * Type: <b>reference</b><br> 5170 * Path: <b>Device.location</b><br> 5171 * </p> 5172 */ 5173 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5174 SP_LOCATION); 5175 5176 /** 5177 * Constant for fluent queries to be used to add include statements. Specifies 5178 * the path value of "<b>Device:location</b>". 5179 */ 5180 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include( 5181 "Device:location").toLocked(); 5182 5183 /** 5184 * Search parameter: <b>type</b> 5185 * <p> 5186 * Description: <b>The type of the device</b><br> 5187 * Type: <b>token</b><br> 5188 * Path: <b>Device.type</b><br> 5189 * </p> 5190 */ 5191 @SearchParamDefinition(name = "type", path = "Device.type", description = "The type of the device", type = "token") 5192 public static final String SP_TYPE = "type"; 5193 /** 5194 * <b>Fluent Client</b> search parameter constant for <b>type</b> 5195 * <p> 5196 * Description: <b>The type of the device</b><br> 5197 * Type: <b>token</b><br> 5198 * Path: <b>Device.type</b><br> 5199 * </p> 5200 */ 5201 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5202 SP_TYPE); 5203 5204 /** 5205 * Search parameter: <b>url</b> 5206 * <p> 5207 * Description: <b>Network address to contact device</b><br> 5208 * Type: <b>uri</b><br> 5209 * Path: <b>Device.url</b><br> 5210 * </p> 5211 */ 5212 @SearchParamDefinition(name = "url", path = "Device.url", description = "Network address to contact device", type = "uri") 5213 public static final String SP_URL = "url"; 5214 /** 5215 * <b>Fluent Client</b> search parameter constant for <b>url</b> 5216 * <p> 5217 * Description: <b>Network address to contact device</b><br> 5218 * Type: <b>uri</b><br> 5219 * Path: <b>Device.url</b><br> 5220 * </p> 5221 */ 5222 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 5223 5224 /** 5225 * Search parameter: <b>manufacturer</b> 5226 * <p> 5227 * Description: <b>The manufacturer of the device</b><br> 5228 * Type: <b>string</b><br> 5229 * Path: <b>Device.manufacturer</b><br> 5230 * </p> 5231 */ 5232 @SearchParamDefinition(name = "manufacturer", path = "Device.manufacturer", description = "The manufacturer of the device", type = "string") 5233 public static final String SP_MANUFACTURER = "manufacturer"; 5234 /** 5235 * <b>Fluent Client</b> search parameter constant for <b>manufacturer</b> 5236 * <p> 5237 * Description: <b>The manufacturer of the device</b><br> 5238 * Type: <b>string</b><br> 5239 * Path: <b>Device.manufacturer</b><br> 5240 * </p> 5241 */ 5242 public static final ca.uhn.fhir.rest.gclient.StringClientParam MANUFACTURER = new ca.uhn.fhir.rest.gclient.StringClientParam( 5243 SP_MANUFACTURER); 5244 5245 /** 5246 * Search parameter: <b>status</b> 5247 * <p> 5248 * Description: <b>active | inactive | entered-in-error | unknown</b><br> 5249 * Type: <b>token</b><br> 5250 * Path: <b>Device.status</b><br> 5251 * </p> 5252 */ 5253 @SearchParamDefinition(name = "status", path = "Device.status", description = "active | inactive | entered-in-error | unknown", type = "token") 5254 public static final String SP_STATUS = "status"; 5255 /** 5256 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5257 * <p> 5258 * Description: <b>active | inactive | entered-in-error | unknown</b><br> 5259 * Type: <b>token</b><br> 5260 * Path: <b>Device.status</b><br> 5261 * </p> 5262 */ 5263 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5264 SP_STATUS); 5265 5266}