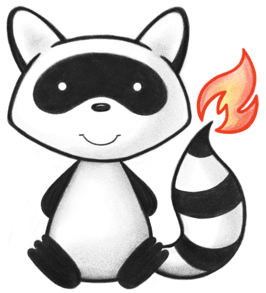
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * Describes a measurement, calculation or setting capability of a medical 048 * device. 049 */ 050@ResourceDef(name = "DeviceMetric", profile = "http://hl7.org/fhir/StructureDefinition/DeviceMetric") 051public class DeviceMetric extends DomainResource { 052 053 public enum DeviceMetricOperationalStatus { 054 /** 055 * The DeviceMetric is operating and will generate DeviceObservations. 056 */ 057 ON, 058 /** 059 * The DeviceMetric is not operating. 060 */ 061 OFF, 062 /** 063 * The DeviceMetric is operating, but will not generate any DeviceObservations. 064 */ 065 STANDBY, 066 /** 067 * The DeviceMetric was entered in error. 068 */ 069 ENTEREDINERROR, 070 /** 071 * added to help the parsers with the generic types 072 */ 073 NULL; 074 075 public static DeviceMetricOperationalStatus fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("on".equals(codeString)) 079 return ON; 080 if ("off".equals(codeString)) 081 return OFF; 082 if ("standby".equals(codeString)) 083 return STANDBY; 084 if ("entered-in-error".equals(codeString)) 085 return ENTEREDINERROR; 086 if (Configuration.isAcceptInvalidEnums()) 087 return null; 088 else 089 throw new FHIRException("Unknown DeviceMetricOperationalStatus code '" + codeString + "'"); 090 } 091 092 public String toCode() { 093 switch (this) { 094 case ON: 095 return "on"; 096 case OFF: 097 return "off"; 098 case STANDBY: 099 return "standby"; 100 case ENTEREDINERROR: 101 return "entered-in-error"; 102 case NULL: 103 return null; 104 default: 105 return "?"; 106 } 107 } 108 109 public String getSystem() { 110 switch (this) { 111 case ON: 112 return "http://hl7.org/fhir/metric-operational-status"; 113 case OFF: 114 return "http://hl7.org/fhir/metric-operational-status"; 115 case STANDBY: 116 return "http://hl7.org/fhir/metric-operational-status"; 117 case ENTEREDINERROR: 118 return "http://hl7.org/fhir/metric-operational-status"; 119 case NULL: 120 return null; 121 default: 122 return "?"; 123 } 124 } 125 126 public String getDefinition() { 127 switch (this) { 128 case ON: 129 return "The DeviceMetric is operating and will generate DeviceObservations."; 130 case OFF: 131 return "The DeviceMetric is not operating."; 132 case STANDBY: 133 return "The DeviceMetric is operating, but will not generate any DeviceObservations."; 134 case ENTEREDINERROR: 135 return "The DeviceMetric was entered in error."; 136 case NULL: 137 return null; 138 default: 139 return "?"; 140 } 141 } 142 143 public String getDisplay() { 144 switch (this) { 145 case ON: 146 return "On"; 147 case OFF: 148 return "Off"; 149 case STANDBY: 150 return "Standby"; 151 case ENTEREDINERROR: 152 return "Entered In Error"; 153 case NULL: 154 return null; 155 default: 156 return "?"; 157 } 158 } 159 } 160 161 public static class DeviceMetricOperationalStatusEnumFactory implements EnumFactory<DeviceMetricOperationalStatus> { 162 public DeviceMetricOperationalStatus fromCode(String codeString) throws IllegalArgumentException { 163 if (codeString == null || "".equals(codeString)) 164 if (codeString == null || "".equals(codeString)) 165 return null; 166 if ("on".equals(codeString)) 167 return DeviceMetricOperationalStatus.ON; 168 if ("off".equals(codeString)) 169 return DeviceMetricOperationalStatus.OFF; 170 if ("standby".equals(codeString)) 171 return DeviceMetricOperationalStatus.STANDBY; 172 if ("entered-in-error".equals(codeString)) 173 return DeviceMetricOperationalStatus.ENTEREDINERROR; 174 throw new IllegalArgumentException("Unknown DeviceMetricOperationalStatus code '" + codeString + "'"); 175 } 176 177 public Enumeration<DeviceMetricOperationalStatus> fromType(PrimitiveType<?> code) throws FHIRException { 178 if (code == null) 179 return null; 180 if (code.isEmpty()) 181 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.NULL, code); 182 String codeString = code.asStringValue(); 183 if (codeString == null || "".equals(codeString)) 184 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.NULL, code); 185 if ("on".equals(codeString)) 186 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.ON, code); 187 if ("off".equals(codeString)) 188 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.OFF, code); 189 if ("standby".equals(codeString)) 190 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.STANDBY, code); 191 if ("entered-in-error".equals(codeString)) 192 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.ENTEREDINERROR, code); 193 throw new FHIRException("Unknown DeviceMetricOperationalStatus code '" + codeString + "'"); 194 } 195 196 public String toCode(DeviceMetricOperationalStatus code) { 197 if (code == DeviceMetricOperationalStatus.NULL) 198 return null; 199 if (code == DeviceMetricOperationalStatus.ON) 200 return "on"; 201 if (code == DeviceMetricOperationalStatus.OFF) 202 return "off"; 203 if (code == DeviceMetricOperationalStatus.STANDBY) 204 return "standby"; 205 if (code == DeviceMetricOperationalStatus.ENTEREDINERROR) 206 return "entered-in-error"; 207 return "?"; 208 } 209 210 public String toSystem(DeviceMetricOperationalStatus code) { 211 return code.getSystem(); 212 } 213 } 214 215 public enum DeviceMetricColor { 216 /** 217 * Color for representation - black. 218 */ 219 BLACK, 220 /** 221 * Color for representation - red. 222 */ 223 RED, 224 /** 225 * Color for representation - green. 226 */ 227 GREEN, 228 /** 229 * Color for representation - yellow. 230 */ 231 YELLOW, 232 /** 233 * Color for representation - blue. 234 */ 235 BLUE, 236 /** 237 * Color for representation - magenta. 238 */ 239 MAGENTA, 240 /** 241 * Color for representation - cyan. 242 */ 243 CYAN, 244 /** 245 * Color for representation - white. 246 */ 247 WHITE, 248 /** 249 * added to help the parsers with the generic types 250 */ 251 NULL; 252 253 public static DeviceMetricColor fromCode(String codeString) throws FHIRException { 254 if (codeString == null || "".equals(codeString)) 255 return null; 256 if ("black".equals(codeString)) 257 return BLACK; 258 if ("red".equals(codeString)) 259 return RED; 260 if ("green".equals(codeString)) 261 return GREEN; 262 if ("yellow".equals(codeString)) 263 return YELLOW; 264 if ("blue".equals(codeString)) 265 return BLUE; 266 if ("magenta".equals(codeString)) 267 return MAGENTA; 268 if ("cyan".equals(codeString)) 269 return CYAN; 270 if ("white".equals(codeString)) 271 return WHITE; 272 if (Configuration.isAcceptInvalidEnums()) 273 return null; 274 else 275 throw new FHIRException("Unknown DeviceMetricColor code '" + codeString + "'"); 276 } 277 278 public String toCode() { 279 switch (this) { 280 case BLACK: 281 return "black"; 282 case RED: 283 return "red"; 284 case GREEN: 285 return "green"; 286 case YELLOW: 287 return "yellow"; 288 case BLUE: 289 return "blue"; 290 case MAGENTA: 291 return "magenta"; 292 case CYAN: 293 return "cyan"; 294 case WHITE: 295 return "white"; 296 case NULL: 297 return null; 298 default: 299 return "?"; 300 } 301 } 302 303 public String getSystem() { 304 switch (this) { 305 case BLACK: 306 return "http://hl7.org/fhir/metric-color"; 307 case RED: 308 return "http://hl7.org/fhir/metric-color"; 309 case GREEN: 310 return "http://hl7.org/fhir/metric-color"; 311 case YELLOW: 312 return "http://hl7.org/fhir/metric-color"; 313 case BLUE: 314 return "http://hl7.org/fhir/metric-color"; 315 case MAGENTA: 316 return "http://hl7.org/fhir/metric-color"; 317 case CYAN: 318 return "http://hl7.org/fhir/metric-color"; 319 case WHITE: 320 return "http://hl7.org/fhir/metric-color"; 321 case NULL: 322 return null; 323 default: 324 return "?"; 325 } 326 } 327 328 public String getDefinition() { 329 switch (this) { 330 case BLACK: 331 return "Color for representation - black."; 332 case RED: 333 return "Color for representation - red."; 334 case GREEN: 335 return "Color for representation - green."; 336 case YELLOW: 337 return "Color for representation - yellow."; 338 case BLUE: 339 return "Color for representation - blue."; 340 case MAGENTA: 341 return "Color for representation - magenta."; 342 case CYAN: 343 return "Color for representation - cyan."; 344 case WHITE: 345 return "Color for representation - white."; 346 case NULL: 347 return null; 348 default: 349 return "?"; 350 } 351 } 352 353 public String getDisplay() { 354 switch (this) { 355 case BLACK: 356 return "Color Black"; 357 case RED: 358 return "Color Red"; 359 case GREEN: 360 return "Color Green"; 361 case YELLOW: 362 return "Color Yellow"; 363 case BLUE: 364 return "Color Blue"; 365 case MAGENTA: 366 return "Color Magenta"; 367 case CYAN: 368 return "Color Cyan"; 369 case WHITE: 370 return "Color White"; 371 case NULL: 372 return null; 373 default: 374 return "?"; 375 } 376 } 377 } 378 379 public static class DeviceMetricColorEnumFactory implements EnumFactory<DeviceMetricColor> { 380 public DeviceMetricColor fromCode(String codeString) throws IllegalArgumentException { 381 if (codeString == null || "".equals(codeString)) 382 if (codeString == null || "".equals(codeString)) 383 return null; 384 if ("black".equals(codeString)) 385 return DeviceMetricColor.BLACK; 386 if ("red".equals(codeString)) 387 return DeviceMetricColor.RED; 388 if ("green".equals(codeString)) 389 return DeviceMetricColor.GREEN; 390 if ("yellow".equals(codeString)) 391 return DeviceMetricColor.YELLOW; 392 if ("blue".equals(codeString)) 393 return DeviceMetricColor.BLUE; 394 if ("magenta".equals(codeString)) 395 return DeviceMetricColor.MAGENTA; 396 if ("cyan".equals(codeString)) 397 return DeviceMetricColor.CYAN; 398 if ("white".equals(codeString)) 399 return DeviceMetricColor.WHITE; 400 throw new IllegalArgumentException("Unknown DeviceMetricColor code '" + codeString + "'"); 401 } 402 403 public Enumeration<DeviceMetricColor> fromType(PrimitiveType<?> code) throws FHIRException { 404 if (code == null) 405 return null; 406 if (code.isEmpty()) 407 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.NULL, code); 408 String codeString = code.asStringValue(); 409 if (codeString == null || "".equals(codeString)) 410 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.NULL, code); 411 if ("black".equals(codeString)) 412 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.BLACK, code); 413 if ("red".equals(codeString)) 414 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.RED, code); 415 if ("green".equals(codeString)) 416 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.GREEN, code); 417 if ("yellow".equals(codeString)) 418 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.YELLOW, code); 419 if ("blue".equals(codeString)) 420 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.BLUE, code); 421 if ("magenta".equals(codeString)) 422 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.MAGENTA, code); 423 if ("cyan".equals(codeString)) 424 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.CYAN, code); 425 if ("white".equals(codeString)) 426 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.WHITE, code); 427 throw new FHIRException("Unknown DeviceMetricColor code '" + codeString + "'"); 428 } 429 430 public String toCode(DeviceMetricColor code) { 431 if (code == DeviceMetricColor.NULL) 432 return null; 433 if (code == DeviceMetricColor.BLACK) 434 return "black"; 435 if (code == DeviceMetricColor.RED) 436 return "red"; 437 if (code == DeviceMetricColor.GREEN) 438 return "green"; 439 if (code == DeviceMetricColor.YELLOW) 440 return "yellow"; 441 if (code == DeviceMetricColor.BLUE) 442 return "blue"; 443 if (code == DeviceMetricColor.MAGENTA) 444 return "magenta"; 445 if (code == DeviceMetricColor.CYAN) 446 return "cyan"; 447 if (code == DeviceMetricColor.WHITE) 448 return "white"; 449 return "?"; 450 } 451 452 public String toSystem(DeviceMetricColor code) { 453 return code.getSystem(); 454 } 455 } 456 457 public enum DeviceMetricCategory { 458 /** 459 * DeviceObservations generated for this DeviceMetric are measured. 460 */ 461 MEASUREMENT, 462 /** 463 * DeviceObservations generated for this DeviceMetric is a setting that will 464 * influence the behavior of the Device. 465 */ 466 SETTING, 467 /** 468 * DeviceObservations generated for this DeviceMetric are calculated. 469 */ 470 CALCULATION, 471 /** 472 * The category of this DeviceMetric is unspecified. 473 */ 474 UNSPECIFIED, 475 /** 476 * added to help the parsers with the generic types 477 */ 478 NULL; 479 480 public static DeviceMetricCategory fromCode(String codeString) throws FHIRException { 481 if (codeString == null || "".equals(codeString)) 482 return null; 483 if ("measurement".equals(codeString)) 484 return MEASUREMENT; 485 if ("setting".equals(codeString)) 486 return SETTING; 487 if ("calculation".equals(codeString)) 488 return CALCULATION; 489 if ("unspecified".equals(codeString)) 490 return UNSPECIFIED; 491 if (Configuration.isAcceptInvalidEnums()) 492 return null; 493 else 494 throw new FHIRException("Unknown DeviceMetricCategory code '" + codeString + "'"); 495 } 496 497 public String toCode() { 498 switch (this) { 499 case MEASUREMENT: 500 return "measurement"; 501 case SETTING: 502 return "setting"; 503 case CALCULATION: 504 return "calculation"; 505 case UNSPECIFIED: 506 return "unspecified"; 507 case NULL: 508 return null; 509 default: 510 return "?"; 511 } 512 } 513 514 public String getSystem() { 515 switch (this) { 516 case MEASUREMENT: 517 return "http://hl7.org/fhir/metric-category"; 518 case SETTING: 519 return "http://hl7.org/fhir/metric-category"; 520 case CALCULATION: 521 return "http://hl7.org/fhir/metric-category"; 522 case UNSPECIFIED: 523 return "http://hl7.org/fhir/metric-category"; 524 case NULL: 525 return null; 526 default: 527 return "?"; 528 } 529 } 530 531 public String getDefinition() { 532 switch (this) { 533 case MEASUREMENT: 534 return "DeviceObservations generated for this DeviceMetric are measured."; 535 case SETTING: 536 return "DeviceObservations generated for this DeviceMetric is a setting that will influence the behavior of the Device."; 537 case CALCULATION: 538 return "DeviceObservations generated for this DeviceMetric are calculated."; 539 case UNSPECIFIED: 540 return "The category of this DeviceMetric is unspecified."; 541 case NULL: 542 return null; 543 default: 544 return "?"; 545 } 546 } 547 548 public String getDisplay() { 549 switch (this) { 550 case MEASUREMENT: 551 return "Measurement"; 552 case SETTING: 553 return "Setting"; 554 case CALCULATION: 555 return "Calculation"; 556 case UNSPECIFIED: 557 return "Unspecified"; 558 case NULL: 559 return null; 560 default: 561 return "?"; 562 } 563 } 564 } 565 566 public static class DeviceMetricCategoryEnumFactory implements EnumFactory<DeviceMetricCategory> { 567 public DeviceMetricCategory fromCode(String codeString) throws IllegalArgumentException { 568 if (codeString == null || "".equals(codeString)) 569 if (codeString == null || "".equals(codeString)) 570 return null; 571 if ("measurement".equals(codeString)) 572 return DeviceMetricCategory.MEASUREMENT; 573 if ("setting".equals(codeString)) 574 return DeviceMetricCategory.SETTING; 575 if ("calculation".equals(codeString)) 576 return DeviceMetricCategory.CALCULATION; 577 if ("unspecified".equals(codeString)) 578 return DeviceMetricCategory.UNSPECIFIED; 579 throw new IllegalArgumentException("Unknown DeviceMetricCategory code '" + codeString + "'"); 580 } 581 582 public Enumeration<DeviceMetricCategory> fromType(PrimitiveType<?> code) throws FHIRException { 583 if (code == null) 584 return null; 585 if (code.isEmpty()) 586 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.NULL, code); 587 String codeString = code.asStringValue(); 588 if (codeString == null || "".equals(codeString)) 589 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.NULL, code); 590 if ("measurement".equals(codeString)) 591 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.MEASUREMENT, code); 592 if ("setting".equals(codeString)) 593 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.SETTING, code); 594 if ("calculation".equals(codeString)) 595 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.CALCULATION, code); 596 if ("unspecified".equals(codeString)) 597 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.UNSPECIFIED, code); 598 throw new FHIRException("Unknown DeviceMetricCategory code '" + codeString + "'"); 599 } 600 601 public String toCode(DeviceMetricCategory code) { 602 if (code == DeviceMetricCategory.NULL) 603 return null; 604 if (code == DeviceMetricCategory.MEASUREMENT) 605 return "measurement"; 606 if (code == DeviceMetricCategory.SETTING) 607 return "setting"; 608 if (code == DeviceMetricCategory.CALCULATION) 609 return "calculation"; 610 if (code == DeviceMetricCategory.UNSPECIFIED) 611 return "unspecified"; 612 return "?"; 613 } 614 615 public String toSystem(DeviceMetricCategory code) { 616 return code.getSystem(); 617 } 618 } 619 620 public enum DeviceMetricCalibrationType { 621 /** 622 * Metric calibration method has not been identified. 623 */ 624 UNSPECIFIED, 625 /** 626 * Offset metric calibration method. 627 */ 628 OFFSET, 629 /** 630 * Gain metric calibration method. 631 */ 632 GAIN, 633 /** 634 * Two-point metric calibration method. 635 */ 636 TWOPOINT, 637 /** 638 * added to help the parsers with the generic types 639 */ 640 NULL; 641 642 public static DeviceMetricCalibrationType fromCode(String codeString) throws FHIRException { 643 if (codeString == null || "".equals(codeString)) 644 return null; 645 if ("unspecified".equals(codeString)) 646 return UNSPECIFIED; 647 if ("offset".equals(codeString)) 648 return OFFSET; 649 if ("gain".equals(codeString)) 650 return GAIN; 651 if ("two-point".equals(codeString)) 652 return TWOPOINT; 653 if (Configuration.isAcceptInvalidEnums()) 654 return null; 655 else 656 throw new FHIRException("Unknown DeviceMetricCalibrationType code '" + codeString + "'"); 657 } 658 659 public String toCode() { 660 switch (this) { 661 case UNSPECIFIED: 662 return "unspecified"; 663 case OFFSET: 664 return "offset"; 665 case GAIN: 666 return "gain"; 667 case TWOPOINT: 668 return "two-point"; 669 case NULL: 670 return null; 671 default: 672 return "?"; 673 } 674 } 675 676 public String getSystem() { 677 switch (this) { 678 case UNSPECIFIED: 679 return "http://hl7.org/fhir/metric-calibration-type"; 680 case OFFSET: 681 return "http://hl7.org/fhir/metric-calibration-type"; 682 case GAIN: 683 return "http://hl7.org/fhir/metric-calibration-type"; 684 case TWOPOINT: 685 return "http://hl7.org/fhir/metric-calibration-type"; 686 case NULL: 687 return null; 688 default: 689 return "?"; 690 } 691 } 692 693 public String getDefinition() { 694 switch (this) { 695 case UNSPECIFIED: 696 return "Metric calibration method has not been identified."; 697 case OFFSET: 698 return "Offset metric calibration method."; 699 case GAIN: 700 return "Gain metric calibration method."; 701 case TWOPOINT: 702 return "Two-point metric calibration method."; 703 case NULL: 704 return null; 705 default: 706 return "?"; 707 } 708 } 709 710 public String getDisplay() { 711 switch (this) { 712 case UNSPECIFIED: 713 return "Unspecified"; 714 case OFFSET: 715 return "Offset"; 716 case GAIN: 717 return "Gain"; 718 case TWOPOINT: 719 return "Two Point"; 720 case NULL: 721 return null; 722 default: 723 return "?"; 724 } 725 } 726 } 727 728 public static class DeviceMetricCalibrationTypeEnumFactory implements EnumFactory<DeviceMetricCalibrationType> { 729 public DeviceMetricCalibrationType fromCode(String codeString) throws IllegalArgumentException { 730 if (codeString == null || "".equals(codeString)) 731 if (codeString == null || "".equals(codeString)) 732 return null; 733 if ("unspecified".equals(codeString)) 734 return DeviceMetricCalibrationType.UNSPECIFIED; 735 if ("offset".equals(codeString)) 736 return DeviceMetricCalibrationType.OFFSET; 737 if ("gain".equals(codeString)) 738 return DeviceMetricCalibrationType.GAIN; 739 if ("two-point".equals(codeString)) 740 return DeviceMetricCalibrationType.TWOPOINT; 741 throw new IllegalArgumentException("Unknown DeviceMetricCalibrationType code '" + codeString + "'"); 742 } 743 744 public Enumeration<DeviceMetricCalibrationType> fromType(PrimitiveType<?> code) throws FHIRException { 745 if (code == null) 746 return null; 747 if (code.isEmpty()) 748 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.NULL, code); 749 String codeString = code.asStringValue(); 750 if (codeString == null || "".equals(codeString)) 751 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.NULL, code); 752 if ("unspecified".equals(codeString)) 753 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.UNSPECIFIED, code); 754 if ("offset".equals(codeString)) 755 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.OFFSET, code); 756 if ("gain".equals(codeString)) 757 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.GAIN, code); 758 if ("two-point".equals(codeString)) 759 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.TWOPOINT, code); 760 throw new FHIRException("Unknown DeviceMetricCalibrationType code '" + codeString + "'"); 761 } 762 763 public String toCode(DeviceMetricCalibrationType code) { 764 if (code == DeviceMetricCalibrationType.NULL) 765 return null; 766 if (code == DeviceMetricCalibrationType.UNSPECIFIED) 767 return "unspecified"; 768 if (code == DeviceMetricCalibrationType.OFFSET) 769 return "offset"; 770 if (code == DeviceMetricCalibrationType.GAIN) 771 return "gain"; 772 if (code == DeviceMetricCalibrationType.TWOPOINT) 773 return "two-point"; 774 return "?"; 775 } 776 777 public String toSystem(DeviceMetricCalibrationType code) { 778 return code.getSystem(); 779 } 780 } 781 782 public enum DeviceMetricCalibrationState { 783 /** 784 * The metric has not been calibrated. 785 */ 786 NOTCALIBRATED, 787 /** 788 * The metric needs to be calibrated. 789 */ 790 CALIBRATIONREQUIRED, 791 /** 792 * The metric has been calibrated. 793 */ 794 CALIBRATED, 795 /** 796 * The state of calibration of this metric is unspecified. 797 */ 798 UNSPECIFIED, 799 /** 800 * added to help the parsers with the generic types 801 */ 802 NULL; 803 804 public static DeviceMetricCalibrationState fromCode(String codeString) throws FHIRException { 805 if (codeString == null || "".equals(codeString)) 806 return null; 807 if ("not-calibrated".equals(codeString)) 808 return NOTCALIBRATED; 809 if ("calibration-required".equals(codeString)) 810 return CALIBRATIONREQUIRED; 811 if ("calibrated".equals(codeString)) 812 return CALIBRATED; 813 if ("unspecified".equals(codeString)) 814 return UNSPECIFIED; 815 if (Configuration.isAcceptInvalidEnums()) 816 return null; 817 else 818 throw new FHIRException("Unknown DeviceMetricCalibrationState code '" + codeString + "'"); 819 } 820 821 public String toCode() { 822 switch (this) { 823 case NOTCALIBRATED: 824 return "not-calibrated"; 825 case CALIBRATIONREQUIRED: 826 return "calibration-required"; 827 case CALIBRATED: 828 return "calibrated"; 829 case UNSPECIFIED: 830 return "unspecified"; 831 case NULL: 832 return null; 833 default: 834 return "?"; 835 } 836 } 837 838 public String getSystem() { 839 switch (this) { 840 case NOTCALIBRATED: 841 return "http://hl7.org/fhir/metric-calibration-state"; 842 case CALIBRATIONREQUIRED: 843 return "http://hl7.org/fhir/metric-calibration-state"; 844 case CALIBRATED: 845 return "http://hl7.org/fhir/metric-calibration-state"; 846 case UNSPECIFIED: 847 return "http://hl7.org/fhir/metric-calibration-state"; 848 case NULL: 849 return null; 850 default: 851 return "?"; 852 } 853 } 854 855 public String getDefinition() { 856 switch (this) { 857 case NOTCALIBRATED: 858 return "The metric has not been calibrated."; 859 case CALIBRATIONREQUIRED: 860 return "The metric needs to be calibrated."; 861 case CALIBRATED: 862 return "The metric has been calibrated."; 863 case UNSPECIFIED: 864 return "The state of calibration of this metric is unspecified."; 865 case NULL: 866 return null; 867 default: 868 return "?"; 869 } 870 } 871 872 public String getDisplay() { 873 switch (this) { 874 case NOTCALIBRATED: 875 return "Not Calibrated"; 876 case CALIBRATIONREQUIRED: 877 return "Calibration Required"; 878 case CALIBRATED: 879 return "Calibrated"; 880 case UNSPECIFIED: 881 return "Unspecified"; 882 case NULL: 883 return null; 884 default: 885 return "?"; 886 } 887 } 888 } 889 890 public static class DeviceMetricCalibrationStateEnumFactory implements EnumFactory<DeviceMetricCalibrationState> { 891 public DeviceMetricCalibrationState fromCode(String codeString) throws IllegalArgumentException { 892 if (codeString == null || "".equals(codeString)) 893 if (codeString == null || "".equals(codeString)) 894 return null; 895 if ("not-calibrated".equals(codeString)) 896 return DeviceMetricCalibrationState.NOTCALIBRATED; 897 if ("calibration-required".equals(codeString)) 898 return DeviceMetricCalibrationState.CALIBRATIONREQUIRED; 899 if ("calibrated".equals(codeString)) 900 return DeviceMetricCalibrationState.CALIBRATED; 901 if ("unspecified".equals(codeString)) 902 return DeviceMetricCalibrationState.UNSPECIFIED; 903 throw new IllegalArgumentException("Unknown DeviceMetricCalibrationState code '" + codeString + "'"); 904 } 905 906 public Enumeration<DeviceMetricCalibrationState> fromType(PrimitiveType<?> code) throws FHIRException { 907 if (code == null) 908 return null; 909 if (code.isEmpty()) 910 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.NULL, code); 911 String codeString = code.asStringValue(); 912 if (codeString == null || "".equals(codeString)) 913 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.NULL, code); 914 if ("not-calibrated".equals(codeString)) 915 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.NOTCALIBRATED, code); 916 if ("calibration-required".equals(codeString)) 917 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.CALIBRATIONREQUIRED, 918 code); 919 if ("calibrated".equals(codeString)) 920 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.CALIBRATED, code); 921 if ("unspecified".equals(codeString)) 922 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.UNSPECIFIED, code); 923 throw new FHIRException("Unknown DeviceMetricCalibrationState code '" + codeString + "'"); 924 } 925 926 public String toCode(DeviceMetricCalibrationState code) { 927 if (code == DeviceMetricCalibrationState.NULL) 928 return null; 929 if (code == DeviceMetricCalibrationState.NOTCALIBRATED) 930 return "not-calibrated"; 931 if (code == DeviceMetricCalibrationState.CALIBRATIONREQUIRED) 932 return "calibration-required"; 933 if (code == DeviceMetricCalibrationState.CALIBRATED) 934 return "calibrated"; 935 if (code == DeviceMetricCalibrationState.UNSPECIFIED) 936 return "unspecified"; 937 return "?"; 938 } 939 940 public String toSystem(DeviceMetricCalibrationState code) { 941 return code.getSystem(); 942 } 943 } 944 945 @Block() 946 public static class DeviceMetricCalibrationComponent extends BackboneElement implements IBaseBackboneElement { 947 /** 948 * Describes the type of the calibration method. 949 */ 950 @Child(name = "type", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 951 @Description(shortDefinition = "unspecified | offset | gain | two-point", formalDefinition = "Describes the type of the calibration method.") 952 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/metric-calibration-type") 953 protected Enumeration<DeviceMetricCalibrationType> type; 954 955 /** 956 * Describes the state of the calibration. 957 */ 958 @Child(name = "state", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 959 @Description(shortDefinition = "not-calibrated | calibration-required | calibrated | unspecified", formalDefinition = "Describes the state of the calibration.") 960 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/metric-calibration-state") 961 protected Enumeration<DeviceMetricCalibrationState> state; 962 963 /** 964 * Describes the time last calibration has been performed. 965 */ 966 @Child(name = "time", type = { InstantType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 967 @Description(shortDefinition = "Describes the time last calibration has been performed", formalDefinition = "Describes the time last calibration has been performed.") 968 protected InstantType time; 969 970 private static final long serialVersionUID = 1163986578L; 971 972 /** 973 * Constructor 974 */ 975 public DeviceMetricCalibrationComponent() { 976 super(); 977 } 978 979 /** 980 * @return {@link #type} (Describes the type of the calibration method.). This 981 * is the underlying object with id, value and extensions. The accessor 982 * "getType" gives direct access to the value 983 */ 984 public Enumeration<DeviceMetricCalibrationType> getTypeElement() { 985 if (this.type == null) 986 if (Configuration.errorOnAutoCreate()) 987 throw new Error("Attempt to auto-create DeviceMetricCalibrationComponent.type"); 988 else if (Configuration.doAutoCreate()) 989 this.type = new Enumeration<DeviceMetricCalibrationType>(new DeviceMetricCalibrationTypeEnumFactory()); // bb 990 return this.type; 991 } 992 993 public boolean hasTypeElement() { 994 return this.type != null && !this.type.isEmpty(); 995 } 996 997 public boolean hasType() { 998 return this.type != null && !this.type.isEmpty(); 999 } 1000 1001 /** 1002 * @param value {@link #type} (Describes the type of the calibration method.). 1003 * This is the underlying object with id, value and extensions. The 1004 * accessor "getType" gives direct access to the value 1005 */ 1006 public DeviceMetricCalibrationComponent setTypeElement(Enumeration<DeviceMetricCalibrationType> value) { 1007 this.type = value; 1008 return this; 1009 } 1010 1011 /** 1012 * @return Describes the type of the calibration method. 1013 */ 1014 public DeviceMetricCalibrationType getType() { 1015 return this.type == null ? null : this.type.getValue(); 1016 } 1017 1018 /** 1019 * @param value Describes the type of the calibration method. 1020 */ 1021 public DeviceMetricCalibrationComponent setType(DeviceMetricCalibrationType value) { 1022 if (value == null) 1023 this.type = null; 1024 else { 1025 if (this.type == null) 1026 this.type = new Enumeration<DeviceMetricCalibrationType>(new DeviceMetricCalibrationTypeEnumFactory()); 1027 this.type.setValue(value); 1028 } 1029 return this; 1030 } 1031 1032 /** 1033 * @return {@link #state} (Describes the state of the calibration.). This is the 1034 * underlying object with id, value and extensions. The accessor 1035 * "getState" gives direct access to the value 1036 */ 1037 public Enumeration<DeviceMetricCalibrationState> getStateElement() { 1038 if (this.state == null) 1039 if (Configuration.errorOnAutoCreate()) 1040 throw new Error("Attempt to auto-create DeviceMetricCalibrationComponent.state"); 1041 else if (Configuration.doAutoCreate()) 1042 this.state = new Enumeration<DeviceMetricCalibrationState>(new DeviceMetricCalibrationStateEnumFactory()); // bb 1043 return this.state; 1044 } 1045 1046 public boolean hasStateElement() { 1047 return this.state != null && !this.state.isEmpty(); 1048 } 1049 1050 public boolean hasState() { 1051 return this.state != null && !this.state.isEmpty(); 1052 } 1053 1054 /** 1055 * @param value {@link #state} (Describes the state of the calibration.). This 1056 * is the underlying object with id, value and extensions. The 1057 * accessor "getState" gives direct access to the value 1058 */ 1059 public DeviceMetricCalibrationComponent setStateElement(Enumeration<DeviceMetricCalibrationState> value) { 1060 this.state = value; 1061 return this; 1062 } 1063 1064 /** 1065 * @return Describes the state of the calibration. 1066 */ 1067 public DeviceMetricCalibrationState getState() { 1068 return this.state == null ? null : this.state.getValue(); 1069 } 1070 1071 /** 1072 * @param value Describes the state of the calibration. 1073 */ 1074 public DeviceMetricCalibrationComponent setState(DeviceMetricCalibrationState value) { 1075 if (value == null) 1076 this.state = null; 1077 else { 1078 if (this.state == null) 1079 this.state = new Enumeration<DeviceMetricCalibrationState>(new DeviceMetricCalibrationStateEnumFactory()); 1080 this.state.setValue(value); 1081 } 1082 return this; 1083 } 1084 1085 /** 1086 * @return {@link #time} (Describes the time last calibration has been 1087 * performed.). This is the underlying object with id, value and 1088 * extensions. The accessor "getTime" gives direct access to the value 1089 */ 1090 public InstantType getTimeElement() { 1091 if (this.time == null) 1092 if (Configuration.errorOnAutoCreate()) 1093 throw new Error("Attempt to auto-create DeviceMetricCalibrationComponent.time"); 1094 else if (Configuration.doAutoCreate()) 1095 this.time = new InstantType(); // bb 1096 return this.time; 1097 } 1098 1099 public boolean hasTimeElement() { 1100 return this.time != null && !this.time.isEmpty(); 1101 } 1102 1103 public boolean hasTime() { 1104 return this.time != null && !this.time.isEmpty(); 1105 } 1106 1107 /** 1108 * @param value {@link #time} (Describes the time last calibration has been 1109 * performed.). This is the underlying object with id, value and 1110 * extensions. The accessor "getTime" gives direct access to the 1111 * value 1112 */ 1113 public DeviceMetricCalibrationComponent setTimeElement(InstantType value) { 1114 this.time = value; 1115 return this; 1116 } 1117 1118 /** 1119 * @return Describes the time last calibration has been performed. 1120 */ 1121 public Date getTime() { 1122 return this.time == null ? null : this.time.getValue(); 1123 } 1124 1125 /** 1126 * @param value Describes the time last calibration has been performed. 1127 */ 1128 public DeviceMetricCalibrationComponent setTime(Date value) { 1129 if (value == null) 1130 this.time = null; 1131 else { 1132 if (this.time == null) 1133 this.time = new InstantType(); 1134 this.time.setValue(value); 1135 } 1136 return this; 1137 } 1138 1139 protected void listChildren(List<Property> children) { 1140 super.listChildren(children); 1141 children.add(new Property("type", "code", "Describes the type of the calibration method.", 0, 1, type)); 1142 children.add(new Property("state", "code", "Describes the state of the calibration.", 0, 1, state)); 1143 children 1144 .add(new Property("time", "instant", "Describes the time last calibration has been performed.", 0, 1, time)); 1145 } 1146 1147 @Override 1148 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1149 switch (_hash) { 1150 case 3575610: 1151 /* type */ return new Property("type", "code", "Describes the type of the calibration method.", 0, 1, type); 1152 case 109757585: 1153 /* state */ return new Property("state", "code", "Describes the state of the calibration.", 0, 1, state); 1154 case 3560141: 1155 /* time */ return new Property("time", "instant", "Describes the time last calibration has been performed.", 0, 1156 1, time); 1157 default: 1158 return super.getNamedProperty(_hash, _name, _checkValid); 1159 } 1160 1161 } 1162 1163 @Override 1164 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1165 switch (hash) { 1166 case 3575610: 1167 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<DeviceMetricCalibrationType> 1168 case 109757585: 1169 /* state */ return this.state == null ? new Base[0] : new Base[] { this.state }; // Enumeration<DeviceMetricCalibrationState> 1170 case 3560141: 1171 /* time */ return this.time == null ? new Base[0] : new Base[] { this.time }; // InstantType 1172 default: 1173 return super.getProperty(hash, name, checkValid); 1174 } 1175 1176 } 1177 1178 @Override 1179 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1180 switch (hash) { 1181 case 3575610: // type 1182 value = new DeviceMetricCalibrationTypeEnumFactory().fromType(castToCode(value)); 1183 this.type = (Enumeration) value; // Enumeration<DeviceMetricCalibrationType> 1184 return value; 1185 case 109757585: // state 1186 value = new DeviceMetricCalibrationStateEnumFactory().fromType(castToCode(value)); 1187 this.state = (Enumeration) value; // Enumeration<DeviceMetricCalibrationState> 1188 return value; 1189 case 3560141: // time 1190 this.time = castToInstant(value); // InstantType 1191 return value; 1192 default: 1193 return super.setProperty(hash, name, value); 1194 } 1195 1196 } 1197 1198 @Override 1199 public Base setProperty(String name, Base value) throws FHIRException { 1200 if (name.equals("type")) { 1201 value = new DeviceMetricCalibrationTypeEnumFactory().fromType(castToCode(value)); 1202 this.type = (Enumeration) value; // Enumeration<DeviceMetricCalibrationType> 1203 } else if (name.equals("state")) { 1204 value = new DeviceMetricCalibrationStateEnumFactory().fromType(castToCode(value)); 1205 this.state = (Enumeration) value; // Enumeration<DeviceMetricCalibrationState> 1206 } else if (name.equals("time")) { 1207 this.time = castToInstant(value); // InstantType 1208 } else 1209 return super.setProperty(name, value); 1210 return value; 1211 } 1212 1213 @Override 1214 public void removeChild(String name, Base value) throws FHIRException { 1215 if (name.equals("type")) { 1216 this.type = null; 1217 } else if (name.equals("state")) { 1218 this.state = null; 1219 } else if (name.equals("time")) { 1220 this.time = null; 1221 } else 1222 super.removeChild(name, value); 1223 1224 } 1225 1226 @Override 1227 public Base makeProperty(int hash, String name) throws FHIRException { 1228 switch (hash) { 1229 case 3575610: 1230 return getTypeElement(); 1231 case 109757585: 1232 return getStateElement(); 1233 case 3560141: 1234 return getTimeElement(); 1235 default: 1236 return super.makeProperty(hash, name); 1237 } 1238 1239 } 1240 1241 @Override 1242 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1243 switch (hash) { 1244 case 3575610: 1245 /* type */ return new String[] { "code" }; 1246 case 109757585: 1247 /* state */ return new String[] { "code" }; 1248 case 3560141: 1249 /* time */ return new String[] { "instant" }; 1250 default: 1251 return super.getTypesForProperty(hash, name); 1252 } 1253 1254 } 1255 1256 @Override 1257 public Base addChild(String name) throws FHIRException { 1258 if (name.equals("type")) { 1259 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.type"); 1260 } else if (name.equals("state")) { 1261 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.state"); 1262 } else if (name.equals("time")) { 1263 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.time"); 1264 } else 1265 return super.addChild(name); 1266 } 1267 1268 public DeviceMetricCalibrationComponent copy() { 1269 DeviceMetricCalibrationComponent dst = new DeviceMetricCalibrationComponent(); 1270 copyValues(dst); 1271 return dst; 1272 } 1273 1274 public void copyValues(DeviceMetricCalibrationComponent dst) { 1275 super.copyValues(dst); 1276 dst.type = type == null ? null : type.copy(); 1277 dst.state = state == null ? null : state.copy(); 1278 dst.time = time == null ? null : time.copy(); 1279 } 1280 1281 @Override 1282 public boolean equalsDeep(Base other_) { 1283 if (!super.equalsDeep(other_)) 1284 return false; 1285 if (!(other_ instanceof DeviceMetricCalibrationComponent)) 1286 return false; 1287 DeviceMetricCalibrationComponent o = (DeviceMetricCalibrationComponent) other_; 1288 return compareDeep(type, o.type, true) && compareDeep(state, o.state, true) && compareDeep(time, o.time, true); 1289 } 1290 1291 @Override 1292 public boolean equalsShallow(Base other_) { 1293 if (!super.equalsShallow(other_)) 1294 return false; 1295 if (!(other_ instanceof DeviceMetricCalibrationComponent)) 1296 return false; 1297 DeviceMetricCalibrationComponent o = (DeviceMetricCalibrationComponent) other_; 1298 return compareValues(type, o.type, true) && compareValues(state, o.state, true) 1299 && compareValues(time, o.time, true); 1300 } 1301 1302 public boolean isEmpty() { 1303 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, state, time); 1304 } 1305 1306 public String fhirType() { 1307 return "DeviceMetric.calibration"; 1308 1309 } 1310 1311 } 1312 1313 /** 1314 * Unique instance identifiers assigned to a device by the device or gateway 1315 * software, manufacturers, other organizations or owners. For example: handle 1316 * ID. 1317 */ 1318 @Child(name = "identifier", type = { 1319 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1320 @Description(shortDefinition = "Instance identifier", formalDefinition = "Unique instance identifiers assigned to a device by the device or gateway software, manufacturers, other organizations or owners. For example: handle ID.") 1321 protected List<Identifier> identifier; 1322 1323 /** 1324 * Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc. 1325 */ 1326 @Child(name = "type", type = { CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1327 @Description(shortDefinition = "Identity of metric, for example Heart Rate or PEEP Setting", formalDefinition = "Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc.") 1328 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/devicemetric-type") 1329 protected CodeableConcept type; 1330 1331 /** 1332 * Describes the unit that an observed value determined for this metric will 1333 * have. For example: Percent, Seconds, etc. 1334 */ 1335 @Child(name = "unit", type = { CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1336 @Description(shortDefinition = "Unit of Measure for the Metric", formalDefinition = "Describes the unit that an observed value determined for this metric will have. For example: Percent, Seconds, etc.") 1337 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/devicemetric-type") 1338 protected CodeableConcept unit; 1339 1340 /** 1341 * Describes the link to the Device that this DeviceMetric belongs to and that 1342 * contains administrative device information such as manufacturer, serial 1343 * number, etc. 1344 */ 1345 @Child(name = "source", type = { Device.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1346 @Description(shortDefinition = "Describes the link to the source Device", formalDefinition = "Describes the link to the Device that this DeviceMetric belongs to and that contains administrative device information such as manufacturer, serial number, etc.") 1347 protected Reference source; 1348 1349 /** 1350 * The actual object that is the target of the reference (Describes the link to 1351 * the Device that this DeviceMetric belongs to and that contains administrative 1352 * device information such as manufacturer, serial number, etc.) 1353 */ 1354 protected Device sourceTarget; 1355 1356 /** 1357 * Describes the link to the Device that this DeviceMetric belongs to and that 1358 * provide information about the location of this DeviceMetric in the 1359 * containment structure of the parent Device. An example would be a Device that 1360 * represents a Channel. This reference can be used by a client application to 1361 * distinguish DeviceMetrics that have the same type, but should be interpreted 1362 * based on their containment location. 1363 */ 1364 @Child(name = "parent", type = { Device.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1365 @Description(shortDefinition = "Describes the link to the parent Device", formalDefinition = "Describes the link to the Device that this DeviceMetric belongs to and that provide information about the location of this DeviceMetric in the containment structure of the parent Device. An example would be a Device that represents a Channel. This reference can be used by a client application to distinguish DeviceMetrics that have the same type, but should be interpreted based on their containment location.") 1366 protected Reference parent; 1367 1368 /** 1369 * The actual object that is the target of the reference (Describes the link to 1370 * the Device that this DeviceMetric belongs to and that provide information 1371 * about the location of this DeviceMetric in the containment structure of the 1372 * parent Device. An example would be a Device that represents a Channel. This 1373 * reference can be used by a client application to distinguish DeviceMetrics 1374 * that have the same type, but should be interpreted based on their containment 1375 * location.) 1376 */ 1377 protected Device parentTarget; 1378 1379 /** 1380 * Indicates current operational state of the device. For example: On, Off, 1381 * Standby, etc. 1382 */ 1383 @Child(name = "operationalStatus", type = { 1384 CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1385 @Description(shortDefinition = "on | off | standby | entered-in-error", formalDefinition = "Indicates current operational state of the device. For example: On, Off, Standby, etc.") 1386 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/metric-operational-status") 1387 protected Enumeration<DeviceMetricOperationalStatus> operationalStatus; 1388 1389 /** 1390 * Describes the color representation for the metric. This is often used to aid 1391 * clinicians to track and identify parameter types by color. In practice, 1392 * consider a Patient Monitor that has ECG/HR and Pleth for example; the 1393 * parameters are displayed in different characteristic colors, such as HR-blue, 1394 * BP-green, and PR and SpO2- magenta. 1395 */ 1396 @Child(name = "color", type = { CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1397 @Description(shortDefinition = "black | red | green | yellow | blue | magenta | cyan | white", formalDefinition = "Describes the color representation for the metric. This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth for example; the parameters are displayed in different characteristic colors, such as HR-blue, BP-green, and PR and SpO2- magenta.") 1398 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/metric-color") 1399 protected Enumeration<DeviceMetricColor> color; 1400 1401 /** 1402 * Indicates the category of the observation generation process. A DeviceMetric 1403 * can be for example a setting, measurement, or calculation. 1404 */ 1405 @Child(name = "category", type = { CodeType.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 1406 @Description(shortDefinition = "measurement | setting | calculation | unspecified", formalDefinition = "Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation.") 1407 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/metric-category") 1408 protected Enumeration<DeviceMetricCategory> category; 1409 1410 /** 1411 * Describes the measurement repetition time. This is not necessarily the same 1412 * as the update period. The measurement repetition time can range from 1413 * milliseconds up to hours. An example for a measurement repetition time in the 1414 * range of milliseconds is the sampling rate of an ECG. An example for a 1415 * measurement repetition time in the range of hours is a NIBP that is triggered 1416 * automatically every hour. The update period may be different than the 1417 * measurement repetition time, if the device does not update the published 1418 * observed value with the same frequency as it was measured. 1419 */ 1420 @Child(name = "measurementPeriod", type = { 1421 Timing.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1422 @Description(shortDefinition = "Describes the measurement repetition time", formalDefinition = "Describes the measurement repetition time. This is not necessarily the same as the update period. The measurement repetition time can range from milliseconds up to hours. An example for a measurement repetition time in the range of milliseconds is the sampling rate of an ECG. An example for a measurement repetition time in the range of hours is a NIBP that is triggered automatically every hour. The update period may be different than the measurement repetition time, if the device does not update the published observed value with the same frequency as it was measured.") 1423 protected Timing measurementPeriod; 1424 1425 /** 1426 * Describes the calibrations that have been performed or that are required to 1427 * be performed. 1428 */ 1429 @Child(name = "calibration", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1430 @Description(shortDefinition = "Describes the calibrations that have been performed or that are required to be performed", formalDefinition = "Describes the calibrations that have been performed or that are required to be performed.") 1431 protected List<DeviceMetricCalibrationComponent> calibration; 1432 1433 private static final long serialVersionUID = 1309955219L; 1434 1435 /** 1436 * Constructor 1437 */ 1438 public DeviceMetric() { 1439 super(); 1440 } 1441 1442 /** 1443 * Constructor 1444 */ 1445 public DeviceMetric(CodeableConcept type, Enumeration<DeviceMetricCategory> category) { 1446 super(); 1447 this.type = type; 1448 this.category = category; 1449 } 1450 1451 /** 1452 * @return {@link #identifier} (Unique instance identifiers assigned to a device 1453 * by the device or gateway software, manufacturers, other organizations 1454 * or owners. For example: handle ID.) 1455 */ 1456 public List<Identifier> getIdentifier() { 1457 if (this.identifier == null) 1458 this.identifier = new ArrayList<Identifier>(); 1459 return this.identifier; 1460 } 1461 1462 /** 1463 * @return Returns a reference to <code>this</code> for easy method chaining 1464 */ 1465 public DeviceMetric setIdentifier(List<Identifier> theIdentifier) { 1466 this.identifier = theIdentifier; 1467 return this; 1468 } 1469 1470 public boolean hasIdentifier() { 1471 if (this.identifier == null) 1472 return false; 1473 for (Identifier item : this.identifier) 1474 if (!item.isEmpty()) 1475 return true; 1476 return false; 1477 } 1478 1479 public Identifier addIdentifier() { // 3 1480 Identifier t = new Identifier(); 1481 if (this.identifier == null) 1482 this.identifier = new ArrayList<Identifier>(); 1483 this.identifier.add(t); 1484 return t; 1485 } 1486 1487 public DeviceMetric addIdentifier(Identifier t) { // 3 1488 if (t == null) 1489 return this; 1490 if (this.identifier == null) 1491 this.identifier = new ArrayList<Identifier>(); 1492 this.identifier.add(t); 1493 return this; 1494 } 1495 1496 /** 1497 * @return The first repetition of repeating field {@link #identifier}, creating 1498 * it if it does not already exist 1499 */ 1500 public Identifier getIdentifierFirstRep() { 1501 if (getIdentifier().isEmpty()) { 1502 addIdentifier(); 1503 } 1504 return getIdentifier().get(0); 1505 } 1506 1507 /** 1508 * @return {@link #type} (Describes the type of the metric. For example: Heart 1509 * Rate, PEEP Setting, etc.) 1510 */ 1511 public CodeableConcept getType() { 1512 if (this.type == null) 1513 if (Configuration.errorOnAutoCreate()) 1514 throw new Error("Attempt to auto-create DeviceMetric.type"); 1515 else if (Configuration.doAutoCreate()) 1516 this.type = new CodeableConcept(); // cc 1517 return this.type; 1518 } 1519 1520 public boolean hasType() { 1521 return this.type != null && !this.type.isEmpty(); 1522 } 1523 1524 /** 1525 * @param value {@link #type} (Describes the type of the metric. For example: 1526 * Heart Rate, PEEP Setting, etc.) 1527 */ 1528 public DeviceMetric setType(CodeableConcept value) { 1529 this.type = value; 1530 return this; 1531 } 1532 1533 /** 1534 * @return {@link #unit} (Describes the unit that an observed value determined 1535 * for this metric will have. For example: Percent, Seconds, etc.) 1536 */ 1537 public CodeableConcept getUnit() { 1538 if (this.unit == null) 1539 if (Configuration.errorOnAutoCreate()) 1540 throw new Error("Attempt to auto-create DeviceMetric.unit"); 1541 else if (Configuration.doAutoCreate()) 1542 this.unit = new CodeableConcept(); // cc 1543 return this.unit; 1544 } 1545 1546 public boolean hasUnit() { 1547 return this.unit != null && !this.unit.isEmpty(); 1548 } 1549 1550 /** 1551 * @param value {@link #unit} (Describes the unit that an observed value 1552 * determined for this metric will have. For example: Percent, 1553 * Seconds, etc.) 1554 */ 1555 public DeviceMetric setUnit(CodeableConcept value) { 1556 this.unit = value; 1557 return this; 1558 } 1559 1560 /** 1561 * @return {@link #source} (Describes the link to the Device that this 1562 * DeviceMetric belongs to and that contains administrative device 1563 * information such as manufacturer, serial number, etc.) 1564 */ 1565 public Reference getSource() { 1566 if (this.source == null) 1567 if (Configuration.errorOnAutoCreate()) 1568 throw new Error("Attempt to auto-create DeviceMetric.source"); 1569 else if (Configuration.doAutoCreate()) 1570 this.source = new Reference(); // cc 1571 return this.source; 1572 } 1573 1574 public boolean hasSource() { 1575 return this.source != null && !this.source.isEmpty(); 1576 } 1577 1578 /** 1579 * @param value {@link #source} (Describes the link to the Device that this 1580 * DeviceMetric belongs to and that contains administrative device 1581 * information such as manufacturer, serial number, etc.) 1582 */ 1583 public DeviceMetric setSource(Reference value) { 1584 this.source = value; 1585 return this; 1586 } 1587 1588 /** 1589 * @return {@link #source} The actual object that is the target of the 1590 * reference. The reference library doesn't populate this, but you can 1591 * use it to hold the resource if you resolve it. (Describes the link to 1592 * the Device that this DeviceMetric belongs to and that contains 1593 * administrative device information such as manufacturer, serial 1594 * number, etc.) 1595 */ 1596 public Device getSourceTarget() { 1597 if (this.sourceTarget == null) 1598 if (Configuration.errorOnAutoCreate()) 1599 throw new Error("Attempt to auto-create DeviceMetric.source"); 1600 else if (Configuration.doAutoCreate()) 1601 this.sourceTarget = new Device(); // aa 1602 return this.sourceTarget; 1603 } 1604 1605 /** 1606 * @param value {@link #source} The actual object that is the target of the 1607 * reference. The reference library doesn't use these, but you can 1608 * use it to hold the resource if you resolve it. (Describes the 1609 * link to the Device that this DeviceMetric belongs to and that 1610 * contains administrative device information such as manufacturer, 1611 * serial number, etc.) 1612 */ 1613 public DeviceMetric setSourceTarget(Device value) { 1614 this.sourceTarget = value; 1615 return this; 1616 } 1617 1618 /** 1619 * @return {@link #parent} (Describes the link to the Device that this 1620 * DeviceMetric belongs to and that provide information about the 1621 * location of this DeviceMetric in the containment structure of the 1622 * parent Device. An example would be a Device that represents a 1623 * Channel. This reference can be used by a client application to 1624 * distinguish DeviceMetrics that have the same type, but should be 1625 * interpreted based on their containment location.) 1626 */ 1627 public Reference getParent() { 1628 if (this.parent == null) 1629 if (Configuration.errorOnAutoCreate()) 1630 throw new Error("Attempt to auto-create DeviceMetric.parent"); 1631 else if (Configuration.doAutoCreate()) 1632 this.parent = new Reference(); // cc 1633 return this.parent; 1634 } 1635 1636 public boolean hasParent() { 1637 return this.parent != null && !this.parent.isEmpty(); 1638 } 1639 1640 /** 1641 * @param value {@link #parent} (Describes the link to the Device that this 1642 * DeviceMetric belongs to and that provide information about the 1643 * location of this DeviceMetric in the containment structure of 1644 * the parent Device. An example would be a Device that represents 1645 * a Channel. This reference can be used by a client application to 1646 * distinguish DeviceMetrics that have the same type, but should be 1647 * interpreted based on their containment location.) 1648 */ 1649 public DeviceMetric setParent(Reference value) { 1650 this.parent = value; 1651 return this; 1652 } 1653 1654 /** 1655 * @return {@link #parent} The actual object that is the target of the 1656 * reference. The reference library doesn't populate this, but you can 1657 * use it to hold the resource if you resolve it. (Describes the link to 1658 * the Device that this DeviceMetric belongs to and that provide 1659 * information about the location of this DeviceMetric in the 1660 * containment structure of the parent Device. An example would be a 1661 * Device that represents a Channel. This reference can be used by a 1662 * client application to distinguish DeviceMetrics that have the same 1663 * type, but should be interpreted based on their containment location.) 1664 */ 1665 public Device getParentTarget() { 1666 if (this.parentTarget == null) 1667 if (Configuration.errorOnAutoCreate()) 1668 throw new Error("Attempt to auto-create DeviceMetric.parent"); 1669 else if (Configuration.doAutoCreate()) 1670 this.parentTarget = new Device(); // aa 1671 return this.parentTarget; 1672 } 1673 1674 /** 1675 * @param value {@link #parent} The actual object that is the target of the 1676 * reference. The reference library doesn't use these, but you can 1677 * use it to hold the resource if you resolve it. (Describes the 1678 * link to the Device that this DeviceMetric belongs to and that 1679 * provide information about the location of this DeviceMetric in 1680 * the containment structure of the parent Device. An example would 1681 * be a Device that represents a Channel. This reference can be 1682 * used by a client application to distinguish DeviceMetrics that 1683 * have the same type, but should be interpreted based on their 1684 * containment location.) 1685 */ 1686 public DeviceMetric setParentTarget(Device value) { 1687 this.parentTarget = value; 1688 return this; 1689 } 1690 1691 /** 1692 * @return {@link #operationalStatus} (Indicates current operational state of 1693 * the device. For example: On, Off, Standby, etc.). This is the 1694 * underlying object with id, value and extensions. The accessor 1695 * "getOperationalStatus" gives direct access to the value 1696 */ 1697 public Enumeration<DeviceMetricOperationalStatus> getOperationalStatusElement() { 1698 if (this.operationalStatus == null) 1699 if (Configuration.errorOnAutoCreate()) 1700 throw new Error("Attempt to auto-create DeviceMetric.operationalStatus"); 1701 else if (Configuration.doAutoCreate()) 1702 this.operationalStatus = new Enumeration<DeviceMetricOperationalStatus>( 1703 new DeviceMetricOperationalStatusEnumFactory()); // bb 1704 return this.operationalStatus; 1705 } 1706 1707 public boolean hasOperationalStatusElement() { 1708 return this.operationalStatus != null && !this.operationalStatus.isEmpty(); 1709 } 1710 1711 public boolean hasOperationalStatus() { 1712 return this.operationalStatus != null && !this.operationalStatus.isEmpty(); 1713 } 1714 1715 /** 1716 * @param value {@link #operationalStatus} (Indicates current operational state 1717 * of the device. For example: On, Off, Standby, etc.). This is the 1718 * underlying object with id, value and extensions. The accessor 1719 * "getOperationalStatus" gives direct access to the value 1720 */ 1721 public DeviceMetric setOperationalStatusElement(Enumeration<DeviceMetricOperationalStatus> value) { 1722 this.operationalStatus = value; 1723 return this; 1724 } 1725 1726 /** 1727 * @return Indicates current operational state of the device. For example: On, 1728 * Off, Standby, etc. 1729 */ 1730 public DeviceMetricOperationalStatus getOperationalStatus() { 1731 return this.operationalStatus == null ? null : this.operationalStatus.getValue(); 1732 } 1733 1734 /** 1735 * @param value Indicates current operational state of the device. For example: 1736 * On, Off, Standby, etc. 1737 */ 1738 public DeviceMetric setOperationalStatus(DeviceMetricOperationalStatus value) { 1739 if (value == null) 1740 this.operationalStatus = null; 1741 else { 1742 if (this.operationalStatus == null) 1743 this.operationalStatus = new Enumeration<DeviceMetricOperationalStatus>( 1744 new DeviceMetricOperationalStatusEnumFactory()); 1745 this.operationalStatus.setValue(value); 1746 } 1747 return this; 1748 } 1749 1750 /** 1751 * @return {@link #color} (Describes the color representation for the metric. 1752 * This is often used to aid clinicians to track and identify parameter 1753 * types by color. In practice, consider a Patient Monitor that has 1754 * ECG/HR and Pleth for example; the parameters are displayed in 1755 * different characteristic colors, such as HR-blue, BP-green, and PR 1756 * and SpO2- magenta.). This is the underlying object with id, value and 1757 * extensions. The accessor "getColor" gives direct access to the value 1758 */ 1759 public Enumeration<DeviceMetricColor> getColorElement() { 1760 if (this.color == null) 1761 if (Configuration.errorOnAutoCreate()) 1762 throw new Error("Attempt to auto-create DeviceMetric.color"); 1763 else if (Configuration.doAutoCreate()) 1764 this.color = new Enumeration<DeviceMetricColor>(new DeviceMetricColorEnumFactory()); // bb 1765 return this.color; 1766 } 1767 1768 public boolean hasColorElement() { 1769 return this.color != null && !this.color.isEmpty(); 1770 } 1771 1772 public boolean hasColor() { 1773 return this.color != null && !this.color.isEmpty(); 1774 } 1775 1776 /** 1777 * @param value {@link #color} (Describes the color representation for the 1778 * metric. This is often used to aid clinicians to track and 1779 * identify parameter types by color. In practice, consider a 1780 * Patient Monitor that has ECG/HR and Pleth for example; the 1781 * parameters are displayed in different characteristic colors, 1782 * such as HR-blue, BP-green, and PR and SpO2- magenta.). This is 1783 * the underlying object with id, value and extensions. The 1784 * accessor "getColor" gives direct access to the value 1785 */ 1786 public DeviceMetric setColorElement(Enumeration<DeviceMetricColor> value) { 1787 this.color = value; 1788 return this; 1789 } 1790 1791 /** 1792 * @return Describes the color representation for the metric. This is often used 1793 * to aid clinicians to track and identify parameter types by color. In 1794 * practice, consider a Patient Monitor that has ECG/HR and Pleth for 1795 * example; the parameters are displayed in different characteristic 1796 * colors, such as HR-blue, BP-green, and PR and SpO2- magenta. 1797 */ 1798 public DeviceMetricColor getColor() { 1799 return this.color == null ? null : this.color.getValue(); 1800 } 1801 1802 /** 1803 * @param value Describes the color representation for the metric. This is often 1804 * used to aid clinicians to track and identify parameter types by 1805 * color. In practice, consider a Patient Monitor that has ECG/HR 1806 * and Pleth for example; the parameters are displayed in different 1807 * characteristic colors, such as HR-blue, BP-green, and PR and 1808 * SpO2- magenta. 1809 */ 1810 public DeviceMetric setColor(DeviceMetricColor value) { 1811 if (value == null) 1812 this.color = null; 1813 else { 1814 if (this.color == null) 1815 this.color = new Enumeration<DeviceMetricColor>(new DeviceMetricColorEnumFactory()); 1816 this.color.setValue(value); 1817 } 1818 return this; 1819 } 1820 1821 /** 1822 * @return {@link #category} (Indicates the category of the observation 1823 * generation process. A DeviceMetric can be for example a setting, 1824 * measurement, or calculation.). This is the underlying object with id, 1825 * value and extensions. The accessor "getCategory" gives direct access 1826 * to the value 1827 */ 1828 public Enumeration<DeviceMetricCategory> getCategoryElement() { 1829 if (this.category == null) 1830 if (Configuration.errorOnAutoCreate()) 1831 throw new Error("Attempt to auto-create DeviceMetric.category"); 1832 else if (Configuration.doAutoCreate()) 1833 this.category = new Enumeration<DeviceMetricCategory>(new DeviceMetricCategoryEnumFactory()); // bb 1834 return this.category; 1835 } 1836 1837 public boolean hasCategoryElement() { 1838 return this.category != null && !this.category.isEmpty(); 1839 } 1840 1841 public boolean hasCategory() { 1842 return this.category != null && !this.category.isEmpty(); 1843 } 1844 1845 /** 1846 * @param value {@link #category} (Indicates the category of the observation 1847 * generation process. A DeviceMetric can be for example a setting, 1848 * measurement, or calculation.). This is the underlying object 1849 * with id, value and extensions. The accessor "getCategory" gives 1850 * direct access to the value 1851 */ 1852 public DeviceMetric setCategoryElement(Enumeration<DeviceMetricCategory> value) { 1853 this.category = value; 1854 return this; 1855 } 1856 1857 /** 1858 * @return Indicates the category of the observation generation process. A 1859 * DeviceMetric can be for example a setting, measurement, or 1860 * calculation. 1861 */ 1862 public DeviceMetricCategory getCategory() { 1863 return this.category == null ? null : this.category.getValue(); 1864 } 1865 1866 /** 1867 * @param value Indicates the category of the observation generation process. A 1868 * DeviceMetric can be for example a setting, measurement, or 1869 * calculation. 1870 */ 1871 public DeviceMetric setCategory(DeviceMetricCategory value) { 1872 if (this.category == null) 1873 this.category = new Enumeration<DeviceMetricCategory>(new DeviceMetricCategoryEnumFactory()); 1874 this.category.setValue(value); 1875 return this; 1876 } 1877 1878 /** 1879 * @return {@link #measurementPeriod} (Describes the measurement repetition 1880 * time. This is not necessarily the same as the update period. The 1881 * measurement repetition time can range from milliseconds up to hours. 1882 * An example for a measurement repetition time in the range of 1883 * milliseconds is the sampling rate of an ECG. An example for a 1884 * measurement repetition time in the range of hours is a NIBP that is 1885 * triggered automatically every hour. The update period may be 1886 * different than the measurement repetition time, if the device does 1887 * not update the published observed value with the same frequency as it 1888 * was measured.) 1889 */ 1890 public Timing getMeasurementPeriod() { 1891 if (this.measurementPeriod == null) 1892 if (Configuration.errorOnAutoCreate()) 1893 throw new Error("Attempt to auto-create DeviceMetric.measurementPeriod"); 1894 else if (Configuration.doAutoCreate()) 1895 this.measurementPeriod = new Timing(); // cc 1896 return this.measurementPeriod; 1897 } 1898 1899 public boolean hasMeasurementPeriod() { 1900 return this.measurementPeriod != null && !this.measurementPeriod.isEmpty(); 1901 } 1902 1903 /** 1904 * @param value {@link #measurementPeriod} (Describes the measurement repetition 1905 * time. This is not necessarily the same as the update period. The 1906 * measurement repetition time can range from milliseconds up to 1907 * hours. An example for a measurement repetition time in the range 1908 * of milliseconds is the sampling rate of an ECG. An example for a 1909 * measurement repetition time in the range of hours is a NIBP that 1910 * is triggered automatically every hour. The update period may be 1911 * different than the measurement repetition time, if the device 1912 * does not update the published observed value with the same 1913 * frequency as it was measured.) 1914 */ 1915 public DeviceMetric setMeasurementPeriod(Timing value) { 1916 this.measurementPeriod = value; 1917 return this; 1918 } 1919 1920 /** 1921 * @return {@link #calibration} (Describes the calibrations that have been 1922 * performed or that are required to be performed.) 1923 */ 1924 public List<DeviceMetricCalibrationComponent> getCalibration() { 1925 if (this.calibration == null) 1926 this.calibration = new ArrayList<DeviceMetricCalibrationComponent>(); 1927 return this.calibration; 1928 } 1929 1930 /** 1931 * @return Returns a reference to <code>this</code> for easy method chaining 1932 */ 1933 public DeviceMetric setCalibration(List<DeviceMetricCalibrationComponent> theCalibration) { 1934 this.calibration = theCalibration; 1935 return this; 1936 } 1937 1938 public boolean hasCalibration() { 1939 if (this.calibration == null) 1940 return false; 1941 for (DeviceMetricCalibrationComponent item : this.calibration) 1942 if (!item.isEmpty()) 1943 return true; 1944 return false; 1945 } 1946 1947 public DeviceMetricCalibrationComponent addCalibration() { // 3 1948 DeviceMetricCalibrationComponent t = new DeviceMetricCalibrationComponent(); 1949 if (this.calibration == null) 1950 this.calibration = new ArrayList<DeviceMetricCalibrationComponent>(); 1951 this.calibration.add(t); 1952 return t; 1953 } 1954 1955 public DeviceMetric addCalibration(DeviceMetricCalibrationComponent t) { // 3 1956 if (t == null) 1957 return this; 1958 if (this.calibration == null) 1959 this.calibration = new ArrayList<DeviceMetricCalibrationComponent>(); 1960 this.calibration.add(t); 1961 return this; 1962 } 1963 1964 /** 1965 * @return The first repetition of repeating field {@link #calibration}, 1966 * creating it if it does not already exist 1967 */ 1968 public DeviceMetricCalibrationComponent getCalibrationFirstRep() { 1969 if (getCalibration().isEmpty()) { 1970 addCalibration(); 1971 } 1972 return getCalibration().get(0); 1973 } 1974 1975 protected void listChildren(List<Property> children) { 1976 super.listChildren(children); 1977 children.add(new Property("identifier", "Identifier", 1978 "Unique instance identifiers assigned to a device by the device or gateway software, manufacturers, other organizations or owners. For example: handle ID.", 1979 0, java.lang.Integer.MAX_VALUE, identifier)); 1980 children.add(new Property("type", "CodeableConcept", 1981 "Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc.", 0, 1, type)); 1982 children.add(new Property("unit", "CodeableConcept", 1983 "Describes the unit that an observed value determined for this metric will have. For example: Percent, Seconds, etc.", 1984 0, 1, unit)); 1985 children.add(new Property("source", "Reference(Device)", 1986 "Describes the link to the Device that this DeviceMetric belongs to and that contains administrative device information such as manufacturer, serial number, etc.", 1987 0, 1, source)); 1988 children.add(new Property("parent", "Reference(Device)", 1989 "Describes the link to the Device that this DeviceMetric belongs to and that provide information about the location of this DeviceMetric in the containment structure of the parent Device. An example would be a Device that represents a Channel. This reference can be used by a client application to distinguish DeviceMetrics that have the same type, but should be interpreted based on their containment location.", 1990 0, 1, parent)); 1991 children.add(new Property("operationalStatus", "code", 1992 "Indicates current operational state of the device. For example: On, Off, Standby, etc.", 0, 1, 1993 operationalStatus)); 1994 children.add(new Property("color", "code", 1995 "Describes the color representation for the metric. This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth for example; the parameters are displayed in different characteristic colors, such as HR-blue, BP-green, and PR and SpO2- magenta.", 1996 0, 1, color)); 1997 children.add(new Property("category", "code", 1998 "Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation.", 1999 0, 1, category)); 2000 children.add(new Property("measurementPeriod", "Timing", 2001 "Describes the measurement repetition time. This is not necessarily the same as the update period. The measurement repetition time can range from milliseconds up to hours. An example for a measurement repetition time in the range of milliseconds is the sampling rate of an ECG. An example for a measurement repetition time in the range of hours is a NIBP that is triggered automatically every hour. The update period may be different than the measurement repetition time, if the device does not update the published observed value with the same frequency as it was measured.", 2002 0, 1, measurementPeriod)); 2003 children.add(new Property("calibration", "", 2004 "Describes the calibrations that have been performed or that are required to be performed.", 0, 2005 java.lang.Integer.MAX_VALUE, calibration)); 2006 } 2007 2008 @Override 2009 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2010 switch (_hash) { 2011 case -1618432855: 2012 /* identifier */ return new Property("identifier", "Identifier", 2013 "Unique instance identifiers assigned to a device by the device or gateway software, manufacturers, other organizations or owners. For example: handle ID.", 2014 0, java.lang.Integer.MAX_VALUE, identifier); 2015 case 3575610: 2016 /* type */ return new Property("type", "CodeableConcept", 2017 "Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc.", 0, 1, type); 2018 case 3594628: 2019 /* unit */ return new Property("unit", "CodeableConcept", 2020 "Describes the unit that an observed value determined for this metric will have. For example: Percent, Seconds, etc.", 2021 0, 1, unit); 2022 case -896505829: 2023 /* source */ return new Property("source", "Reference(Device)", 2024 "Describes the link to the Device that this DeviceMetric belongs to and that contains administrative device information such as manufacturer, serial number, etc.", 2025 0, 1, source); 2026 case -995424086: 2027 /* parent */ return new Property("parent", "Reference(Device)", 2028 "Describes the link to the Device that this DeviceMetric belongs to and that provide information about the location of this DeviceMetric in the containment structure of the parent Device. An example would be a Device that represents a Channel. This reference can be used by a client application to distinguish DeviceMetrics that have the same type, but should be interpreted based on their containment location.", 2029 0, 1, parent); 2030 case -2103166364: 2031 /* operationalStatus */ return new Property("operationalStatus", "code", 2032 "Indicates current operational state of the device. For example: On, Off, Standby, etc.", 0, 1, 2033 operationalStatus); 2034 case 94842723: 2035 /* color */ return new Property("color", "code", 2036 "Describes the color representation for the metric. This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth for example; the parameters are displayed in different characteristic colors, such as HR-blue, BP-green, and PR and SpO2- magenta.", 2037 0, 1, color); 2038 case 50511102: 2039 /* category */ return new Property("category", "code", 2040 "Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation.", 2041 0, 1, category); 2042 case -1300332387: 2043 /* measurementPeriod */ return new Property("measurementPeriod", "Timing", 2044 "Describes the measurement repetition time. This is not necessarily the same as the update period. The measurement repetition time can range from milliseconds up to hours. An example for a measurement repetition time in the range of milliseconds is the sampling rate of an ECG. An example for a measurement repetition time in the range of hours is a NIBP that is triggered automatically every hour. The update period may be different than the measurement repetition time, if the device does not update the published observed value with the same frequency as it was measured.", 2045 0, 1, measurementPeriod); 2046 case 1421318634: 2047 /* calibration */ return new Property("calibration", "", 2048 "Describes the calibrations that have been performed or that are required to be performed.", 0, 2049 java.lang.Integer.MAX_VALUE, calibration); 2050 default: 2051 return super.getNamedProperty(_hash, _name, _checkValid); 2052 } 2053 2054 } 2055 2056 @Override 2057 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2058 switch (hash) { 2059 case -1618432855: 2060 /* identifier */ return this.identifier == null ? new Base[0] 2061 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2062 case 3575610: 2063 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2064 case 3594628: 2065 /* unit */ return this.unit == null ? new Base[0] : new Base[] { this.unit }; // CodeableConcept 2066 case -896505829: 2067 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // Reference 2068 case -995424086: 2069 /* parent */ return this.parent == null ? new Base[0] : new Base[] { this.parent }; // Reference 2070 case -2103166364: 2071 /* operationalStatus */ return this.operationalStatus == null ? new Base[0] 2072 : new Base[] { this.operationalStatus }; // Enumeration<DeviceMetricOperationalStatus> 2073 case 94842723: 2074 /* color */ return this.color == null ? new Base[0] : new Base[] { this.color }; // Enumeration<DeviceMetricColor> 2075 case 50511102: 2076 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // Enumeration<DeviceMetricCategory> 2077 case -1300332387: 2078 /* measurementPeriod */ return this.measurementPeriod == null ? new Base[0] 2079 : new Base[] { this.measurementPeriod }; // Timing 2080 case 1421318634: 2081 /* calibration */ return this.calibration == null ? new Base[0] 2082 : this.calibration.toArray(new Base[this.calibration.size()]); // DeviceMetricCalibrationComponent 2083 default: 2084 return super.getProperty(hash, name, checkValid); 2085 } 2086 2087 } 2088 2089 @Override 2090 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2091 switch (hash) { 2092 case -1618432855: // identifier 2093 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2094 return value; 2095 case 3575610: // type 2096 this.type = castToCodeableConcept(value); // CodeableConcept 2097 return value; 2098 case 3594628: // unit 2099 this.unit = castToCodeableConcept(value); // CodeableConcept 2100 return value; 2101 case -896505829: // source 2102 this.source = castToReference(value); // Reference 2103 return value; 2104 case -995424086: // parent 2105 this.parent = castToReference(value); // Reference 2106 return value; 2107 case -2103166364: // operationalStatus 2108 value = new DeviceMetricOperationalStatusEnumFactory().fromType(castToCode(value)); 2109 this.operationalStatus = (Enumeration) value; // Enumeration<DeviceMetricOperationalStatus> 2110 return value; 2111 case 94842723: // color 2112 value = new DeviceMetricColorEnumFactory().fromType(castToCode(value)); 2113 this.color = (Enumeration) value; // Enumeration<DeviceMetricColor> 2114 return value; 2115 case 50511102: // category 2116 value = new DeviceMetricCategoryEnumFactory().fromType(castToCode(value)); 2117 this.category = (Enumeration) value; // Enumeration<DeviceMetricCategory> 2118 return value; 2119 case -1300332387: // measurementPeriod 2120 this.measurementPeriod = castToTiming(value); // Timing 2121 return value; 2122 case 1421318634: // calibration 2123 this.getCalibration().add((DeviceMetricCalibrationComponent) value); // DeviceMetricCalibrationComponent 2124 return value; 2125 default: 2126 return super.setProperty(hash, name, value); 2127 } 2128 2129 } 2130 2131 @Override 2132 public Base setProperty(String name, Base value) throws FHIRException { 2133 if (name.equals("identifier")) { 2134 this.getIdentifier().add(castToIdentifier(value)); 2135 } else if (name.equals("type")) { 2136 this.type = castToCodeableConcept(value); // CodeableConcept 2137 } else if (name.equals("unit")) { 2138 this.unit = castToCodeableConcept(value); // CodeableConcept 2139 } else if (name.equals("source")) { 2140 this.source = castToReference(value); // Reference 2141 } else if (name.equals("parent")) { 2142 this.parent = castToReference(value); // Reference 2143 } else if (name.equals("operationalStatus")) { 2144 value = new DeviceMetricOperationalStatusEnumFactory().fromType(castToCode(value)); 2145 this.operationalStatus = (Enumeration) value; // Enumeration<DeviceMetricOperationalStatus> 2146 } else if (name.equals("color")) { 2147 value = new DeviceMetricColorEnumFactory().fromType(castToCode(value)); 2148 this.color = (Enumeration) value; // Enumeration<DeviceMetricColor> 2149 } else if (name.equals("category")) { 2150 value = new DeviceMetricCategoryEnumFactory().fromType(castToCode(value)); 2151 this.category = (Enumeration) value; // Enumeration<DeviceMetricCategory> 2152 } else if (name.equals("measurementPeriod")) { 2153 this.measurementPeriod = castToTiming(value); // Timing 2154 } else if (name.equals("calibration")) { 2155 this.getCalibration().add((DeviceMetricCalibrationComponent) value); 2156 } else 2157 return super.setProperty(name, value); 2158 return value; 2159 } 2160 2161 @Override 2162 public void removeChild(String name, Base value) throws FHIRException { 2163 if (name.equals("identifier")) { 2164 this.getIdentifier().remove(castToIdentifier(value)); 2165 } else if (name.equals("type")) { 2166 this.type = null; 2167 } else if (name.equals("unit")) { 2168 this.unit = null; 2169 } else if (name.equals("source")) { 2170 this.source = null; 2171 } else if (name.equals("parent")) { 2172 this.parent = null; 2173 } else if (name.equals("operationalStatus")) { 2174 this.operationalStatus = null; 2175 } else if (name.equals("color")) { 2176 this.color = null; 2177 } else if (name.equals("category")) { 2178 this.category = null; 2179 } else if (name.equals("measurementPeriod")) { 2180 this.measurementPeriod = null; 2181 } else if (name.equals("calibration")) { 2182 this.getCalibration().remove((DeviceMetricCalibrationComponent) value); 2183 } else 2184 super.removeChild(name, value); 2185 2186 } 2187 2188 @Override 2189 public Base makeProperty(int hash, String name) throws FHIRException { 2190 switch (hash) { 2191 case -1618432855: 2192 return addIdentifier(); 2193 case 3575610: 2194 return getType(); 2195 case 3594628: 2196 return getUnit(); 2197 case -896505829: 2198 return getSource(); 2199 case -995424086: 2200 return getParent(); 2201 case -2103166364: 2202 return getOperationalStatusElement(); 2203 case 94842723: 2204 return getColorElement(); 2205 case 50511102: 2206 return getCategoryElement(); 2207 case -1300332387: 2208 return getMeasurementPeriod(); 2209 case 1421318634: 2210 return addCalibration(); 2211 default: 2212 return super.makeProperty(hash, name); 2213 } 2214 2215 } 2216 2217 @Override 2218 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2219 switch (hash) { 2220 case -1618432855: 2221 /* identifier */ return new String[] { "Identifier" }; 2222 case 3575610: 2223 /* type */ return new String[] { "CodeableConcept" }; 2224 case 3594628: 2225 /* unit */ return new String[] { "CodeableConcept" }; 2226 case -896505829: 2227 /* source */ return new String[] { "Reference" }; 2228 case -995424086: 2229 /* parent */ return new String[] { "Reference" }; 2230 case -2103166364: 2231 /* operationalStatus */ return new String[] { "code" }; 2232 case 94842723: 2233 /* color */ return new String[] { "code" }; 2234 case 50511102: 2235 /* category */ return new String[] { "code" }; 2236 case -1300332387: 2237 /* measurementPeriod */ return new String[] { "Timing" }; 2238 case 1421318634: 2239 /* calibration */ return new String[] {}; 2240 default: 2241 return super.getTypesForProperty(hash, name); 2242 } 2243 2244 } 2245 2246 @Override 2247 public Base addChild(String name) throws FHIRException { 2248 if (name.equals("identifier")) { 2249 return addIdentifier(); 2250 } else if (name.equals("type")) { 2251 this.type = new CodeableConcept(); 2252 return this.type; 2253 } else if (name.equals("unit")) { 2254 this.unit = new CodeableConcept(); 2255 return this.unit; 2256 } else if (name.equals("source")) { 2257 this.source = new Reference(); 2258 return this.source; 2259 } else if (name.equals("parent")) { 2260 this.parent = new Reference(); 2261 return this.parent; 2262 } else if (name.equals("operationalStatus")) { 2263 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.operationalStatus"); 2264 } else if (name.equals("color")) { 2265 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.color"); 2266 } else if (name.equals("category")) { 2267 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.category"); 2268 } else if (name.equals("measurementPeriod")) { 2269 this.measurementPeriod = new Timing(); 2270 return this.measurementPeriod; 2271 } else if (name.equals("calibration")) { 2272 return addCalibration(); 2273 } else 2274 return super.addChild(name); 2275 } 2276 2277 public String fhirType() { 2278 return "DeviceMetric"; 2279 2280 } 2281 2282 public DeviceMetric copy() { 2283 DeviceMetric dst = new DeviceMetric(); 2284 copyValues(dst); 2285 return dst; 2286 } 2287 2288 public void copyValues(DeviceMetric dst) { 2289 super.copyValues(dst); 2290 if (identifier != null) { 2291 dst.identifier = new ArrayList<Identifier>(); 2292 for (Identifier i : identifier) 2293 dst.identifier.add(i.copy()); 2294 } 2295 ; 2296 dst.type = type == null ? null : type.copy(); 2297 dst.unit = unit == null ? null : unit.copy(); 2298 dst.source = source == null ? null : source.copy(); 2299 dst.parent = parent == null ? null : parent.copy(); 2300 dst.operationalStatus = operationalStatus == null ? null : operationalStatus.copy(); 2301 dst.color = color == null ? null : color.copy(); 2302 dst.category = category == null ? null : category.copy(); 2303 dst.measurementPeriod = measurementPeriod == null ? null : measurementPeriod.copy(); 2304 if (calibration != null) { 2305 dst.calibration = new ArrayList<DeviceMetricCalibrationComponent>(); 2306 for (DeviceMetricCalibrationComponent i : calibration) 2307 dst.calibration.add(i.copy()); 2308 } 2309 ; 2310 } 2311 2312 protected DeviceMetric typedCopy() { 2313 return copy(); 2314 } 2315 2316 @Override 2317 public boolean equalsDeep(Base other_) { 2318 if (!super.equalsDeep(other_)) 2319 return false; 2320 if (!(other_ instanceof DeviceMetric)) 2321 return false; 2322 DeviceMetric o = (DeviceMetric) other_; 2323 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) 2324 && compareDeep(unit, o.unit, true) && compareDeep(source, o.source, true) && compareDeep(parent, o.parent, true) 2325 && compareDeep(operationalStatus, o.operationalStatus, true) && compareDeep(color, o.color, true) 2326 && compareDeep(category, o.category, true) && compareDeep(measurementPeriod, o.measurementPeriod, true) 2327 && compareDeep(calibration, o.calibration, true); 2328 } 2329 2330 @Override 2331 public boolean equalsShallow(Base other_) { 2332 if (!super.equalsShallow(other_)) 2333 return false; 2334 if (!(other_ instanceof DeviceMetric)) 2335 return false; 2336 DeviceMetric o = (DeviceMetric) other_; 2337 return compareValues(operationalStatus, o.operationalStatus, true) && compareValues(color, o.color, true) 2338 && compareValues(category, o.category, true); 2339 } 2340 2341 public boolean isEmpty() { 2342 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, unit, source, parent, 2343 operationalStatus, color, category, measurementPeriod, calibration); 2344 } 2345 2346 @Override 2347 public ResourceType getResourceType() { 2348 return ResourceType.DeviceMetric; 2349 } 2350 2351 /** 2352 * Search parameter: <b>parent</b> 2353 * <p> 2354 * Description: <b>The parent DeviceMetric resource</b><br> 2355 * Type: <b>reference</b><br> 2356 * Path: <b>DeviceMetric.parent</b><br> 2357 * </p> 2358 */ 2359 @SearchParamDefinition(name = "parent", path = "DeviceMetric.parent", description = "The parent DeviceMetric resource", type = "reference", target = { 2360 Device.class }) 2361 public static final String SP_PARENT = "parent"; 2362 /** 2363 * <b>Fluent Client</b> search parameter constant for <b>parent</b> 2364 * <p> 2365 * Description: <b>The parent DeviceMetric resource</b><br> 2366 * Type: <b>reference</b><br> 2367 * Path: <b>DeviceMetric.parent</b><br> 2368 * </p> 2369 */ 2370 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2371 SP_PARENT); 2372 2373 /** 2374 * Constant for fluent queries to be used to add include statements. Specifies 2375 * the path value of "<b>DeviceMetric:parent</b>". 2376 */ 2377 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARENT = new ca.uhn.fhir.model.api.Include( 2378 "DeviceMetric:parent").toLocked(); 2379 2380 /** 2381 * Search parameter: <b>identifier</b> 2382 * <p> 2383 * Description: <b>The identifier of the metric</b><br> 2384 * Type: <b>token</b><br> 2385 * Path: <b>DeviceMetric.identifier</b><br> 2386 * </p> 2387 */ 2388 @SearchParamDefinition(name = "identifier", path = "DeviceMetric.identifier", description = "The identifier of the metric", type = "token") 2389 public static final String SP_IDENTIFIER = "identifier"; 2390 /** 2391 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2392 * <p> 2393 * Description: <b>The identifier of the metric</b><br> 2394 * Type: <b>token</b><br> 2395 * Path: <b>DeviceMetric.identifier</b><br> 2396 * </p> 2397 */ 2398 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2399 SP_IDENTIFIER); 2400 2401 /** 2402 * Search parameter: <b>source</b> 2403 * <p> 2404 * Description: <b>The device resource</b><br> 2405 * Type: <b>reference</b><br> 2406 * Path: <b>DeviceMetric.source</b><br> 2407 * </p> 2408 */ 2409 @SearchParamDefinition(name = "source", path = "DeviceMetric.source", description = "The device resource", type = "reference", target = { 2410 Device.class }) 2411 public static final String SP_SOURCE = "source"; 2412 /** 2413 * <b>Fluent Client</b> search parameter constant for <b>source</b> 2414 * <p> 2415 * Description: <b>The device resource</b><br> 2416 * Type: <b>reference</b><br> 2417 * Path: <b>DeviceMetric.source</b><br> 2418 * </p> 2419 */ 2420 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2421 SP_SOURCE); 2422 2423 /** 2424 * Constant for fluent queries to be used to add include statements. Specifies 2425 * the path value of "<b>DeviceMetric:source</b>". 2426 */ 2427 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include( 2428 "DeviceMetric:source").toLocked(); 2429 2430 /** 2431 * Search parameter: <b>type</b> 2432 * <p> 2433 * Description: <b>The component type</b><br> 2434 * Type: <b>token</b><br> 2435 * Path: <b>DeviceMetric.type</b><br> 2436 * </p> 2437 */ 2438 @SearchParamDefinition(name = "type", path = "DeviceMetric.type", description = "The component type", type = "token") 2439 public static final String SP_TYPE = "type"; 2440 /** 2441 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2442 * <p> 2443 * Description: <b>The component type</b><br> 2444 * Type: <b>token</b><br> 2445 * Path: <b>DeviceMetric.type</b><br> 2446 * </p> 2447 */ 2448 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2449 SP_TYPE); 2450 2451 /** 2452 * Search parameter: <b>category</b> 2453 * <p> 2454 * Description: <b>The category of the metric</b><br> 2455 * Type: <b>token</b><br> 2456 * Path: <b>DeviceMetric.category</b><br> 2457 * </p> 2458 */ 2459 @SearchParamDefinition(name = "category", path = "DeviceMetric.category", description = "The category of the metric", type = "token") 2460 public static final String SP_CATEGORY = "category"; 2461 /** 2462 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2463 * <p> 2464 * Description: <b>The category of the metric</b><br> 2465 * Type: <b>token</b><br> 2466 * Path: <b>DeviceMetric.category</b><br> 2467 * </p> 2468 */ 2469 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2470 SP_CATEGORY); 2471 2472}