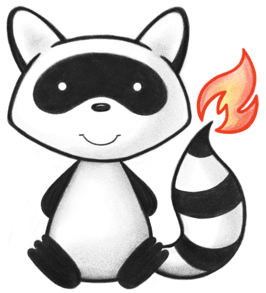
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * Describes a measurement, calculation or setting capability of a medical 048 * device. 049 */ 050@ResourceDef(name = "DeviceMetric", profile = "http://hl7.org/fhir/StructureDefinition/DeviceMetric") 051public class DeviceMetric extends DomainResource { 052 053 public enum DeviceMetricOperationalStatus { 054 /** 055 * The DeviceMetric is operating and will generate DeviceObservations. 056 */ 057 ON, 058 /** 059 * The DeviceMetric is not operating. 060 */ 061 OFF, 062 /** 063 * The DeviceMetric is operating, but will not generate any DeviceObservations. 064 */ 065 STANDBY, 066 /** 067 * The DeviceMetric was entered in error. 068 */ 069 ENTEREDINERROR, 070 /** 071 * added to help the parsers with the generic types 072 */ 073 NULL; 074 075 public static DeviceMetricOperationalStatus fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("on".equals(codeString)) 079 return ON; 080 if ("off".equals(codeString)) 081 return OFF; 082 if ("standby".equals(codeString)) 083 return STANDBY; 084 if ("entered-in-error".equals(codeString)) 085 return ENTEREDINERROR; 086 if (Configuration.isAcceptInvalidEnums()) 087 return null; 088 else 089 throw new FHIRException("Unknown DeviceMetricOperationalStatus code '" + codeString + "'"); 090 } 091 092 public String toCode() { 093 switch (this) { 094 case ON: 095 return "on"; 096 case OFF: 097 return "off"; 098 case STANDBY: 099 return "standby"; 100 case ENTEREDINERROR: 101 return "entered-in-error"; 102 case NULL: 103 return null; 104 default: 105 return "?"; 106 } 107 } 108 109 public String getSystem() { 110 switch (this) { 111 case ON: 112 return "http://hl7.org/fhir/metric-operational-status"; 113 case OFF: 114 return "http://hl7.org/fhir/metric-operational-status"; 115 case STANDBY: 116 return "http://hl7.org/fhir/metric-operational-status"; 117 case ENTEREDINERROR: 118 return "http://hl7.org/fhir/metric-operational-status"; 119 case NULL: 120 return null; 121 default: 122 return "?"; 123 } 124 } 125 126 public String getDefinition() { 127 switch (this) { 128 case ON: 129 return "The DeviceMetric is operating and will generate DeviceObservations."; 130 case OFF: 131 return "The DeviceMetric is not operating."; 132 case STANDBY: 133 return "The DeviceMetric is operating, but will not generate any DeviceObservations."; 134 case ENTEREDINERROR: 135 return "The DeviceMetric was entered in error."; 136 case NULL: 137 return null; 138 default: 139 return "?"; 140 } 141 } 142 143 public String getDisplay() { 144 switch (this) { 145 case ON: 146 return "On"; 147 case OFF: 148 return "Off"; 149 case STANDBY: 150 return "Standby"; 151 case ENTEREDINERROR: 152 return "Entered In Error"; 153 case NULL: 154 return null; 155 default: 156 return "?"; 157 } 158 } 159 } 160 161 public static class DeviceMetricOperationalStatusEnumFactory implements EnumFactory<DeviceMetricOperationalStatus> { 162 public DeviceMetricOperationalStatus fromCode(String codeString) throws IllegalArgumentException { 163 if (codeString == null || "".equals(codeString)) 164 if (codeString == null || "".equals(codeString)) 165 return null; 166 if ("on".equals(codeString)) 167 return DeviceMetricOperationalStatus.ON; 168 if ("off".equals(codeString)) 169 return DeviceMetricOperationalStatus.OFF; 170 if ("standby".equals(codeString)) 171 return DeviceMetricOperationalStatus.STANDBY; 172 if ("entered-in-error".equals(codeString)) 173 return DeviceMetricOperationalStatus.ENTEREDINERROR; 174 throw new IllegalArgumentException("Unknown DeviceMetricOperationalStatus code '" + codeString + "'"); 175 } 176 177 public Enumeration<DeviceMetricOperationalStatus> fromType(PrimitiveType<?> code) throws FHIRException { 178 if (code == null) 179 return null; 180 if (code.isEmpty()) 181 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.NULL, code); 182 String codeString = code.asStringValue(); 183 if (codeString == null || "".equals(codeString)) 184 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.NULL, code); 185 if ("on".equals(codeString)) 186 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.ON, code); 187 if ("off".equals(codeString)) 188 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.OFF, code); 189 if ("standby".equals(codeString)) 190 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.STANDBY, code); 191 if ("entered-in-error".equals(codeString)) 192 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.ENTEREDINERROR, code); 193 throw new FHIRException("Unknown DeviceMetricOperationalStatus code '" + codeString + "'"); 194 } 195 196 public String toCode(DeviceMetricOperationalStatus code) { 197 if (code == DeviceMetricOperationalStatus.ON) 198 return "on"; 199 if (code == DeviceMetricOperationalStatus.OFF) 200 return "off"; 201 if (code == DeviceMetricOperationalStatus.STANDBY) 202 return "standby"; 203 if (code == DeviceMetricOperationalStatus.ENTEREDINERROR) 204 return "entered-in-error"; 205 return "?"; 206 } 207 208 public String toSystem(DeviceMetricOperationalStatus code) { 209 return code.getSystem(); 210 } 211 } 212 213 public enum DeviceMetricColor { 214 /** 215 * Color for representation - black. 216 */ 217 BLACK, 218 /** 219 * Color for representation - red. 220 */ 221 RED, 222 /** 223 * Color for representation - green. 224 */ 225 GREEN, 226 /** 227 * Color for representation - yellow. 228 */ 229 YELLOW, 230 /** 231 * Color for representation - blue. 232 */ 233 BLUE, 234 /** 235 * Color for representation - magenta. 236 */ 237 MAGENTA, 238 /** 239 * Color for representation - cyan. 240 */ 241 CYAN, 242 /** 243 * Color for representation - white. 244 */ 245 WHITE, 246 /** 247 * added to help the parsers with the generic types 248 */ 249 NULL; 250 251 public static DeviceMetricColor fromCode(String codeString) throws FHIRException { 252 if (codeString == null || "".equals(codeString)) 253 return null; 254 if ("black".equals(codeString)) 255 return BLACK; 256 if ("red".equals(codeString)) 257 return RED; 258 if ("green".equals(codeString)) 259 return GREEN; 260 if ("yellow".equals(codeString)) 261 return YELLOW; 262 if ("blue".equals(codeString)) 263 return BLUE; 264 if ("magenta".equals(codeString)) 265 return MAGENTA; 266 if ("cyan".equals(codeString)) 267 return CYAN; 268 if ("white".equals(codeString)) 269 return WHITE; 270 if (Configuration.isAcceptInvalidEnums()) 271 return null; 272 else 273 throw new FHIRException("Unknown DeviceMetricColor code '" + codeString + "'"); 274 } 275 276 public String toCode() { 277 switch (this) { 278 case BLACK: 279 return "black"; 280 case RED: 281 return "red"; 282 case GREEN: 283 return "green"; 284 case YELLOW: 285 return "yellow"; 286 case BLUE: 287 return "blue"; 288 case MAGENTA: 289 return "magenta"; 290 case CYAN: 291 return "cyan"; 292 case WHITE: 293 return "white"; 294 case NULL: 295 return null; 296 default: 297 return "?"; 298 } 299 } 300 301 public String getSystem() { 302 switch (this) { 303 case BLACK: 304 return "http://hl7.org/fhir/metric-color"; 305 case RED: 306 return "http://hl7.org/fhir/metric-color"; 307 case GREEN: 308 return "http://hl7.org/fhir/metric-color"; 309 case YELLOW: 310 return "http://hl7.org/fhir/metric-color"; 311 case BLUE: 312 return "http://hl7.org/fhir/metric-color"; 313 case MAGENTA: 314 return "http://hl7.org/fhir/metric-color"; 315 case CYAN: 316 return "http://hl7.org/fhir/metric-color"; 317 case WHITE: 318 return "http://hl7.org/fhir/metric-color"; 319 case NULL: 320 return null; 321 default: 322 return "?"; 323 } 324 } 325 326 public String getDefinition() { 327 switch (this) { 328 case BLACK: 329 return "Color for representation - black."; 330 case RED: 331 return "Color for representation - red."; 332 case GREEN: 333 return "Color for representation - green."; 334 case YELLOW: 335 return "Color for representation - yellow."; 336 case BLUE: 337 return "Color for representation - blue."; 338 case MAGENTA: 339 return "Color for representation - magenta."; 340 case CYAN: 341 return "Color for representation - cyan."; 342 case WHITE: 343 return "Color for representation - white."; 344 case NULL: 345 return null; 346 default: 347 return "?"; 348 } 349 } 350 351 public String getDisplay() { 352 switch (this) { 353 case BLACK: 354 return "Color Black"; 355 case RED: 356 return "Color Red"; 357 case GREEN: 358 return "Color Green"; 359 case YELLOW: 360 return "Color Yellow"; 361 case BLUE: 362 return "Color Blue"; 363 case MAGENTA: 364 return "Color Magenta"; 365 case CYAN: 366 return "Color Cyan"; 367 case WHITE: 368 return "Color White"; 369 case NULL: 370 return null; 371 default: 372 return "?"; 373 } 374 } 375 } 376 377 public static class DeviceMetricColorEnumFactory implements EnumFactory<DeviceMetricColor> { 378 public DeviceMetricColor fromCode(String codeString) throws IllegalArgumentException { 379 if (codeString == null || "".equals(codeString)) 380 if (codeString == null || "".equals(codeString)) 381 return null; 382 if ("black".equals(codeString)) 383 return DeviceMetricColor.BLACK; 384 if ("red".equals(codeString)) 385 return DeviceMetricColor.RED; 386 if ("green".equals(codeString)) 387 return DeviceMetricColor.GREEN; 388 if ("yellow".equals(codeString)) 389 return DeviceMetricColor.YELLOW; 390 if ("blue".equals(codeString)) 391 return DeviceMetricColor.BLUE; 392 if ("magenta".equals(codeString)) 393 return DeviceMetricColor.MAGENTA; 394 if ("cyan".equals(codeString)) 395 return DeviceMetricColor.CYAN; 396 if ("white".equals(codeString)) 397 return DeviceMetricColor.WHITE; 398 throw new IllegalArgumentException("Unknown DeviceMetricColor code '" + codeString + "'"); 399 } 400 401 public Enumeration<DeviceMetricColor> fromType(PrimitiveType<?> code) throws FHIRException { 402 if (code == null) 403 return null; 404 if (code.isEmpty()) 405 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.NULL, code); 406 String codeString = code.asStringValue(); 407 if (codeString == null || "".equals(codeString)) 408 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.NULL, code); 409 if ("black".equals(codeString)) 410 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.BLACK, code); 411 if ("red".equals(codeString)) 412 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.RED, code); 413 if ("green".equals(codeString)) 414 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.GREEN, code); 415 if ("yellow".equals(codeString)) 416 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.YELLOW, code); 417 if ("blue".equals(codeString)) 418 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.BLUE, code); 419 if ("magenta".equals(codeString)) 420 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.MAGENTA, code); 421 if ("cyan".equals(codeString)) 422 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.CYAN, code); 423 if ("white".equals(codeString)) 424 return new Enumeration<DeviceMetricColor>(this, DeviceMetricColor.WHITE, code); 425 throw new FHIRException("Unknown DeviceMetricColor code '" + codeString + "'"); 426 } 427 428 public String toCode(DeviceMetricColor code) { 429 if (code == DeviceMetricColor.BLACK) 430 return "black"; 431 if (code == DeviceMetricColor.RED) 432 return "red"; 433 if (code == DeviceMetricColor.GREEN) 434 return "green"; 435 if (code == DeviceMetricColor.YELLOW) 436 return "yellow"; 437 if (code == DeviceMetricColor.BLUE) 438 return "blue"; 439 if (code == DeviceMetricColor.MAGENTA) 440 return "magenta"; 441 if (code == DeviceMetricColor.CYAN) 442 return "cyan"; 443 if (code == DeviceMetricColor.WHITE) 444 return "white"; 445 return "?"; 446 } 447 448 public String toSystem(DeviceMetricColor code) { 449 return code.getSystem(); 450 } 451 } 452 453 public enum DeviceMetricCategory { 454 /** 455 * DeviceObservations generated for this DeviceMetric are measured. 456 */ 457 MEASUREMENT, 458 /** 459 * DeviceObservations generated for this DeviceMetric is a setting that will 460 * influence the behavior of the Device. 461 */ 462 SETTING, 463 /** 464 * DeviceObservations generated for this DeviceMetric are calculated. 465 */ 466 CALCULATION, 467 /** 468 * The category of this DeviceMetric is unspecified. 469 */ 470 UNSPECIFIED, 471 /** 472 * added to help the parsers with the generic types 473 */ 474 NULL; 475 476 public static DeviceMetricCategory fromCode(String codeString) throws FHIRException { 477 if (codeString == null || "".equals(codeString)) 478 return null; 479 if ("measurement".equals(codeString)) 480 return MEASUREMENT; 481 if ("setting".equals(codeString)) 482 return SETTING; 483 if ("calculation".equals(codeString)) 484 return CALCULATION; 485 if ("unspecified".equals(codeString)) 486 return UNSPECIFIED; 487 if (Configuration.isAcceptInvalidEnums()) 488 return null; 489 else 490 throw new FHIRException("Unknown DeviceMetricCategory code '" + codeString + "'"); 491 } 492 493 public String toCode() { 494 switch (this) { 495 case MEASUREMENT: 496 return "measurement"; 497 case SETTING: 498 return "setting"; 499 case CALCULATION: 500 return "calculation"; 501 case UNSPECIFIED: 502 return "unspecified"; 503 case NULL: 504 return null; 505 default: 506 return "?"; 507 } 508 } 509 510 public String getSystem() { 511 switch (this) { 512 case MEASUREMENT: 513 return "http://hl7.org/fhir/metric-category"; 514 case SETTING: 515 return "http://hl7.org/fhir/metric-category"; 516 case CALCULATION: 517 return "http://hl7.org/fhir/metric-category"; 518 case UNSPECIFIED: 519 return "http://hl7.org/fhir/metric-category"; 520 case NULL: 521 return null; 522 default: 523 return "?"; 524 } 525 } 526 527 public String getDefinition() { 528 switch (this) { 529 case MEASUREMENT: 530 return "DeviceObservations generated for this DeviceMetric are measured."; 531 case SETTING: 532 return "DeviceObservations generated for this DeviceMetric is a setting that will influence the behavior of the Device."; 533 case CALCULATION: 534 return "DeviceObservations generated for this DeviceMetric are calculated."; 535 case UNSPECIFIED: 536 return "The category of this DeviceMetric is unspecified."; 537 case NULL: 538 return null; 539 default: 540 return "?"; 541 } 542 } 543 544 public String getDisplay() { 545 switch (this) { 546 case MEASUREMENT: 547 return "Measurement"; 548 case SETTING: 549 return "Setting"; 550 case CALCULATION: 551 return "Calculation"; 552 case UNSPECIFIED: 553 return "Unspecified"; 554 case NULL: 555 return null; 556 default: 557 return "?"; 558 } 559 } 560 } 561 562 public static class DeviceMetricCategoryEnumFactory implements EnumFactory<DeviceMetricCategory> { 563 public DeviceMetricCategory fromCode(String codeString) throws IllegalArgumentException { 564 if (codeString == null || "".equals(codeString)) 565 if (codeString == null || "".equals(codeString)) 566 return null; 567 if ("measurement".equals(codeString)) 568 return DeviceMetricCategory.MEASUREMENT; 569 if ("setting".equals(codeString)) 570 return DeviceMetricCategory.SETTING; 571 if ("calculation".equals(codeString)) 572 return DeviceMetricCategory.CALCULATION; 573 if ("unspecified".equals(codeString)) 574 return DeviceMetricCategory.UNSPECIFIED; 575 throw new IllegalArgumentException("Unknown DeviceMetricCategory code '" + codeString + "'"); 576 } 577 578 public Enumeration<DeviceMetricCategory> fromType(PrimitiveType<?> code) throws FHIRException { 579 if (code == null) 580 return null; 581 if (code.isEmpty()) 582 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.NULL, code); 583 String codeString = code.asStringValue(); 584 if (codeString == null || "".equals(codeString)) 585 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.NULL, code); 586 if ("measurement".equals(codeString)) 587 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.MEASUREMENT, code); 588 if ("setting".equals(codeString)) 589 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.SETTING, code); 590 if ("calculation".equals(codeString)) 591 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.CALCULATION, code); 592 if ("unspecified".equals(codeString)) 593 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.UNSPECIFIED, code); 594 throw new FHIRException("Unknown DeviceMetricCategory code '" + codeString + "'"); 595 } 596 597 public String toCode(DeviceMetricCategory code) { 598 if (code == DeviceMetricCategory.MEASUREMENT) 599 return "measurement"; 600 if (code == DeviceMetricCategory.SETTING) 601 return "setting"; 602 if (code == DeviceMetricCategory.CALCULATION) 603 return "calculation"; 604 if (code == DeviceMetricCategory.UNSPECIFIED) 605 return "unspecified"; 606 return "?"; 607 } 608 609 public String toSystem(DeviceMetricCategory code) { 610 return code.getSystem(); 611 } 612 } 613 614 public enum DeviceMetricCalibrationType { 615 /** 616 * Metric calibration method has not been identified. 617 */ 618 UNSPECIFIED, 619 /** 620 * Offset metric calibration method. 621 */ 622 OFFSET, 623 /** 624 * Gain metric calibration method. 625 */ 626 GAIN, 627 /** 628 * Two-point metric calibration method. 629 */ 630 TWOPOINT, 631 /** 632 * added to help the parsers with the generic types 633 */ 634 NULL; 635 636 public static DeviceMetricCalibrationType fromCode(String codeString) throws FHIRException { 637 if (codeString == null || "".equals(codeString)) 638 return null; 639 if ("unspecified".equals(codeString)) 640 return UNSPECIFIED; 641 if ("offset".equals(codeString)) 642 return OFFSET; 643 if ("gain".equals(codeString)) 644 return GAIN; 645 if ("two-point".equals(codeString)) 646 return TWOPOINT; 647 if (Configuration.isAcceptInvalidEnums()) 648 return null; 649 else 650 throw new FHIRException("Unknown DeviceMetricCalibrationType code '" + codeString + "'"); 651 } 652 653 public String toCode() { 654 switch (this) { 655 case UNSPECIFIED: 656 return "unspecified"; 657 case OFFSET: 658 return "offset"; 659 case GAIN: 660 return "gain"; 661 case TWOPOINT: 662 return "two-point"; 663 case NULL: 664 return null; 665 default: 666 return "?"; 667 } 668 } 669 670 public String getSystem() { 671 switch (this) { 672 case UNSPECIFIED: 673 return "http://hl7.org/fhir/metric-calibration-type"; 674 case OFFSET: 675 return "http://hl7.org/fhir/metric-calibration-type"; 676 case GAIN: 677 return "http://hl7.org/fhir/metric-calibration-type"; 678 case TWOPOINT: 679 return "http://hl7.org/fhir/metric-calibration-type"; 680 case NULL: 681 return null; 682 default: 683 return "?"; 684 } 685 } 686 687 public String getDefinition() { 688 switch (this) { 689 case UNSPECIFIED: 690 return "Metric calibration method has not been identified."; 691 case OFFSET: 692 return "Offset metric calibration method."; 693 case GAIN: 694 return "Gain metric calibration method."; 695 case TWOPOINT: 696 return "Two-point metric calibration method."; 697 case NULL: 698 return null; 699 default: 700 return "?"; 701 } 702 } 703 704 public String getDisplay() { 705 switch (this) { 706 case UNSPECIFIED: 707 return "Unspecified"; 708 case OFFSET: 709 return "Offset"; 710 case GAIN: 711 return "Gain"; 712 case TWOPOINT: 713 return "Two Point"; 714 case NULL: 715 return null; 716 default: 717 return "?"; 718 } 719 } 720 } 721 722 public static class DeviceMetricCalibrationTypeEnumFactory implements EnumFactory<DeviceMetricCalibrationType> { 723 public DeviceMetricCalibrationType fromCode(String codeString) throws IllegalArgumentException { 724 if (codeString == null || "".equals(codeString)) 725 if (codeString == null || "".equals(codeString)) 726 return null; 727 if ("unspecified".equals(codeString)) 728 return DeviceMetricCalibrationType.UNSPECIFIED; 729 if ("offset".equals(codeString)) 730 return DeviceMetricCalibrationType.OFFSET; 731 if ("gain".equals(codeString)) 732 return DeviceMetricCalibrationType.GAIN; 733 if ("two-point".equals(codeString)) 734 return DeviceMetricCalibrationType.TWOPOINT; 735 throw new IllegalArgumentException("Unknown DeviceMetricCalibrationType code '" + codeString + "'"); 736 } 737 738 public Enumeration<DeviceMetricCalibrationType> fromType(PrimitiveType<?> code) throws FHIRException { 739 if (code == null) 740 return null; 741 if (code.isEmpty()) 742 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.NULL, code); 743 String codeString = code.asStringValue(); 744 if (codeString == null || "".equals(codeString)) 745 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.NULL, code); 746 if ("unspecified".equals(codeString)) 747 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.UNSPECIFIED, code); 748 if ("offset".equals(codeString)) 749 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.OFFSET, code); 750 if ("gain".equals(codeString)) 751 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.GAIN, code); 752 if ("two-point".equals(codeString)) 753 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.TWOPOINT, code); 754 throw new FHIRException("Unknown DeviceMetricCalibrationType code '" + codeString + "'"); 755 } 756 757 public String toCode(DeviceMetricCalibrationType code) { 758 if (code == DeviceMetricCalibrationType.UNSPECIFIED) 759 return "unspecified"; 760 if (code == DeviceMetricCalibrationType.OFFSET) 761 return "offset"; 762 if (code == DeviceMetricCalibrationType.GAIN) 763 return "gain"; 764 if (code == DeviceMetricCalibrationType.TWOPOINT) 765 return "two-point"; 766 return "?"; 767 } 768 769 public String toSystem(DeviceMetricCalibrationType code) { 770 return code.getSystem(); 771 } 772 } 773 774 public enum DeviceMetricCalibrationState { 775 /** 776 * The metric has not been calibrated. 777 */ 778 NOTCALIBRATED, 779 /** 780 * The metric needs to be calibrated. 781 */ 782 CALIBRATIONREQUIRED, 783 /** 784 * The metric has been calibrated. 785 */ 786 CALIBRATED, 787 /** 788 * The state of calibration of this metric is unspecified. 789 */ 790 UNSPECIFIED, 791 /** 792 * added to help the parsers with the generic types 793 */ 794 NULL; 795 796 public static DeviceMetricCalibrationState fromCode(String codeString) throws FHIRException { 797 if (codeString == null || "".equals(codeString)) 798 return null; 799 if ("not-calibrated".equals(codeString)) 800 return NOTCALIBRATED; 801 if ("calibration-required".equals(codeString)) 802 return CALIBRATIONREQUIRED; 803 if ("calibrated".equals(codeString)) 804 return CALIBRATED; 805 if ("unspecified".equals(codeString)) 806 return UNSPECIFIED; 807 if (Configuration.isAcceptInvalidEnums()) 808 return null; 809 else 810 throw new FHIRException("Unknown DeviceMetricCalibrationState code '" + codeString + "'"); 811 } 812 813 public String toCode() { 814 switch (this) { 815 case NOTCALIBRATED: 816 return "not-calibrated"; 817 case CALIBRATIONREQUIRED: 818 return "calibration-required"; 819 case CALIBRATED: 820 return "calibrated"; 821 case UNSPECIFIED: 822 return "unspecified"; 823 case NULL: 824 return null; 825 default: 826 return "?"; 827 } 828 } 829 830 public String getSystem() { 831 switch (this) { 832 case NOTCALIBRATED: 833 return "http://hl7.org/fhir/metric-calibration-state"; 834 case CALIBRATIONREQUIRED: 835 return "http://hl7.org/fhir/metric-calibration-state"; 836 case CALIBRATED: 837 return "http://hl7.org/fhir/metric-calibration-state"; 838 case UNSPECIFIED: 839 return "http://hl7.org/fhir/metric-calibration-state"; 840 case NULL: 841 return null; 842 default: 843 return "?"; 844 } 845 } 846 847 public String getDefinition() { 848 switch (this) { 849 case NOTCALIBRATED: 850 return "The metric has not been calibrated."; 851 case CALIBRATIONREQUIRED: 852 return "The metric needs to be calibrated."; 853 case CALIBRATED: 854 return "The metric has been calibrated."; 855 case UNSPECIFIED: 856 return "The state of calibration of this metric is unspecified."; 857 case NULL: 858 return null; 859 default: 860 return "?"; 861 } 862 } 863 864 public String getDisplay() { 865 switch (this) { 866 case NOTCALIBRATED: 867 return "Not Calibrated"; 868 case CALIBRATIONREQUIRED: 869 return "Calibration Required"; 870 case CALIBRATED: 871 return "Calibrated"; 872 case UNSPECIFIED: 873 return "Unspecified"; 874 case NULL: 875 return null; 876 default: 877 return "?"; 878 } 879 } 880 } 881 882 public static class DeviceMetricCalibrationStateEnumFactory implements EnumFactory<DeviceMetricCalibrationState> { 883 public DeviceMetricCalibrationState fromCode(String codeString) throws IllegalArgumentException { 884 if (codeString == null || "".equals(codeString)) 885 if (codeString == null || "".equals(codeString)) 886 return null; 887 if ("not-calibrated".equals(codeString)) 888 return DeviceMetricCalibrationState.NOTCALIBRATED; 889 if ("calibration-required".equals(codeString)) 890 return DeviceMetricCalibrationState.CALIBRATIONREQUIRED; 891 if ("calibrated".equals(codeString)) 892 return DeviceMetricCalibrationState.CALIBRATED; 893 if ("unspecified".equals(codeString)) 894 return DeviceMetricCalibrationState.UNSPECIFIED; 895 throw new IllegalArgumentException("Unknown DeviceMetricCalibrationState code '" + codeString + "'"); 896 } 897 898 public Enumeration<DeviceMetricCalibrationState> fromType(PrimitiveType<?> code) throws FHIRException { 899 if (code == null) 900 return null; 901 if (code.isEmpty()) 902 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.NULL, code); 903 String codeString = code.asStringValue(); 904 if (codeString == null || "".equals(codeString)) 905 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.NULL, code); 906 if ("not-calibrated".equals(codeString)) 907 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.NOTCALIBRATED, code); 908 if ("calibration-required".equals(codeString)) 909 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.CALIBRATIONREQUIRED, 910 code); 911 if ("calibrated".equals(codeString)) 912 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.CALIBRATED, code); 913 if ("unspecified".equals(codeString)) 914 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.UNSPECIFIED, code); 915 throw new FHIRException("Unknown DeviceMetricCalibrationState code '" + codeString + "'"); 916 } 917 918 public String toCode(DeviceMetricCalibrationState code) { 919 if (code == DeviceMetricCalibrationState.NOTCALIBRATED) 920 return "not-calibrated"; 921 if (code == DeviceMetricCalibrationState.CALIBRATIONREQUIRED) 922 return "calibration-required"; 923 if (code == DeviceMetricCalibrationState.CALIBRATED) 924 return "calibrated"; 925 if (code == DeviceMetricCalibrationState.UNSPECIFIED) 926 return "unspecified"; 927 return "?"; 928 } 929 930 public String toSystem(DeviceMetricCalibrationState code) { 931 return code.getSystem(); 932 } 933 } 934 935 @Block() 936 public static class DeviceMetricCalibrationComponent extends BackboneElement implements IBaseBackboneElement { 937 /** 938 * Describes the type of the calibration method. 939 */ 940 @Child(name = "type", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 941 @Description(shortDefinition = "unspecified | offset | gain | two-point", formalDefinition = "Describes the type of the calibration method.") 942 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/metric-calibration-type") 943 protected Enumeration<DeviceMetricCalibrationType> type; 944 945 /** 946 * Describes the state of the calibration. 947 */ 948 @Child(name = "state", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 949 @Description(shortDefinition = "not-calibrated | calibration-required | calibrated | unspecified", formalDefinition = "Describes the state of the calibration.") 950 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/metric-calibration-state") 951 protected Enumeration<DeviceMetricCalibrationState> state; 952 953 /** 954 * Describes the time last calibration has been performed. 955 */ 956 @Child(name = "time", type = { InstantType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 957 @Description(shortDefinition = "Describes the time last calibration has been performed", formalDefinition = "Describes the time last calibration has been performed.") 958 protected InstantType time; 959 960 private static final long serialVersionUID = 1163986578L; 961 962 /** 963 * Constructor 964 */ 965 public DeviceMetricCalibrationComponent() { 966 super(); 967 } 968 969 /** 970 * @return {@link #type} (Describes the type of the calibration method.). This 971 * is the underlying object with id, value and extensions. The accessor 972 * "getType" gives direct access to the value 973 */ 974 public Enumeration<DeviceMetricCalibrationType> getTypeElement() { 975 if (this.type == null) 976 if (Configuration.errorOnAutoCreate()) 977 throw new Error("Attempt to auto-create DeviceMetricCalibrationComponent.type"); 978 else if (Configuration.doAutoCreate()) 979 this.type = new Enumeration<DeviceMetricCalibrationType>(new DeviceMetricCalibrationTypeEnumFactory()); // bb 980 return this.type; 981 } 982 983 public boolean hasTypeElement() { 984 return this.type != null && !this.type.isEmpty(); 985 } 986 987 public boolean hasType() { 988 return this.type != null && !this.type.isEmpty(); 989 } 990 991 /** 992 * @param value {@link #type} (Describes the type of the calibration method.). 993 * This is the underlying object with id, value and extensions. The 994 * accessor "getType" gives direct access to the value 995 */ 996 public DeviceMetricCalibrationComponent setTypeElement(Enumeration<DeviceMetricCalibrationType> value) { 997 this.type = value; 998 return this; 999 } 1000 1001 /** 1002 * @return Describes the type of the calibration method. 1003 */ 1004 public DeviceMetricCalibrationType getType() { 1005 return this.type == null ? null : this.type.getValue(); 1006 } 1007 1008 /** 1009 * @param value Describes the type of the calibration method. 1010 */ 1011 public DeviceMetricCalibrationComponent setType(DeviceMetricCalibrationType value) { 1012 if (value == null) 1013 this.type = null; 1014 else { 1015 if (this.type == null) 1016 this.type = new Enumeration<DeviceMetricCalibrationType>(new DeviceMetricCalibrationTypeEnumFactory()); 1017 this.type.setValue(value); 1018 } 1019 return this; 1020 } 1021 1022 /** 1023 * @return {@link #state} (Describes the state of the calibration.). This is the 1024 * underlying object with id, value and extensions. The accessor 1025 * "getState" gives direct access to the value 1026 */ 1027 public Enumeration<DeviceMetricCalibrationState> getStateElement() { 1028 if (this.state == null) 1029 if (Configuration.errorOnAutoCreate()) 1030 throw new Error("Attempt to auto-create DeviceMetricCalibrationComponent.state"); 1031 else if (Configuration.doAutoCreate()) 1032 this.state = new Enumeration<DeviceMetricCalibrationState>(new DeviceMetricCalibrationStateEnumFactory()); // bb 1033 return this.state; 1034 } 1035 1036 public boolean hasStateElement() { 1037 return this.state != null && !this.state.isEmpty(); 1038 } 1039 1040 public boolean hasState() { 1041 return this.state != null && !this.state.isEmpty(); 1042 } 1043 1044 /** 1045 * @param value {@link #state} (Describes the state of the calibration.). This 1046 * is the underlying object with id, value and extensions. The 1047 * accessor "getState" gives direct access to the value 1048 */ 1049 public DeviceMetricCalibrationComponent setStateElement(Enumeration<DeviceMetricCalibrationState> value) { 1050 this.state = value; 1051 return this; 1052 } 1053 1054 /** 1055 * @return Describes the state of the calibration. 1056 */ 1057 public DeviceMetricCalibrationState getState() { 1058 return this.state == null ? null : this.state.getValue(); 1059 } 1060 1061 /** 1062 * @param value Describes the state of the calibration. 1063 */ 1064 public DeviceMetricCalibrationComponent setState(DeviceMetricCalibrationState value) { 1065 if (value == null) 1066 this.state = null; 1067 else { 1068 if (this.state == null) 1069 this.state = new Enumeration<DeviceMetricCalibrationState>(new DeviceMetricCalibrationStateEnumFactory()); 1070 this.state.setValue(value); 1071 } 1072 return this; 1073 } 1074 1075 /** 1076 * @return {@link #time} (Describes the time last calibration has been 1077 * performed.). This is the underlying object with id, value and 1078 * extensions. The accessor "getTime" gives direct access to the value 1079 */ 1080 public InstantType getTimeElement() { 1081 if (this.time == null) 1082 if (Configuration.errorOnAutoCreate()) 1083 throw new Error("Attempt to auto-create DeviceMetricCalibrationComponent.time"); 1084 else if (Configuration.doAutoCreate()) 1085 this.time = new InstantType(); // bb 1086 return this.time; 1087 } 1088 1089 public boolean hasTimeElement() { 1090 return this.time != null && !this.time.isEmpty(); 1091 } 1092 1093 public boolean hasTime() { 1094 return this.time != null && !this.time.isEmpty(); 1095 } 1096 1097 /** 1098 * @param value {@link #time} (Describes the time last calibration has been 1099 * performed.). This is the underlying object with id, value and 1100 * extensions. The accessor "getTime" gives direct access to the 1101 * value 1102 */ 1103 public DeviceMetricCalibrationComponent setTimeElement(InstantType value) { 1104 this.time = value; 1105 return this; 1106 } 1107 1108 /** 1109 * @return Describes the time last calibration has been performed. 1110 */ 1111 public Date getTime() { 1112 return this.time == null ? null : this.time.getValue(); 1113 } 1114 1115 /** 1116 * @param value Describes the time last calibration has been performed. 1117 */ 1118 public DeviceMetricCalibrationComponent setTime(Date value) { 1119 if (value == null) 1120 this.time = null; 1121 else { 1122 if (this.time == null) 1123 this.time = new InstantType(); 1124 this.time.setValue(value); 1125 } 1126 return this; 1127 } 1128 1129 protected void listChildren(List<Property> children) { 1130 super.listChildren(children); 1131 children.add(new Property("type", "code", "Describes the type of the calibration method.", 0, 1, type)); 1132 children.add(new Property("state", "code", "Describes the state of the calibration.", 0, 1, state)); 1133 children 1134 .add(new Property("time", "instant", "Describes the time last calibration has been performed.", 0, 1, time)); 1135 } 1136 1137 @Override 1138 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1139 switch (_hash) { 1140 case 3575610: 1141 /* type */ return new Property("type", "code", "Describes the type of the calibration method.", 0, 1, type); 1142 case 109757585: 1143 /* state */ return new Property("state", "code", "Describes the state of the calibration.", 0, 1, state); 1144 case 3560141: 1145 /* time */ return new Property("time", "instant", "Describes the time last calibration has been performed.", 0, 1146 1, time); 1147 default: 1148 return super.getNamedProperty(_hash, _name, _checkValid); 1149 } 1150 1151 } 1152 1153 @Override 1154 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1155 switch (hash) { 1156 case 3575610: 1157 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<DeviceMetricCalibrationType> 1158 case 109757585: 1159 /* state */ return this.state == null ? new Base[0] : new Base[] { this.state }; // Enumeration<DeviceMetricCalibrationState> 1160 case 3560141: 1161 /* time */ return this.time == null ? new Base[0] : new Base[] { this.time }; // InstantType 1162 default: 1163 return super.getProperty(hash, name, checkValid); 1164 } 1165 1166 } 1167 1168 @Override 1169 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1170 switch (hash) { 1171 case 3575610: // type 1172 value = new DeviceMetricCalibrationTypeEnumFactory().fromType(castToCode(value)); 1173 this.type = (Enumeration) value; // Enumeration<DeviceMetricCalibrationType> 1174 return value; 1175 case 109757585: // state 1176 value = new DeviceMetricCalibrationStateEnumFactory().fromType(castToCode(value)); 1177 this.state = (Enumeration) value; // Enumeration<DeviceMetricCalibrationState> 1178 return value; 1179 case 3560141: // time 1180 this.time = castToInstant(value); // InstantType 1181 return value; 1182 default: 1183 return super.setProperty(hash, name, value); 1184 } 1185 1186 } 1187 1188 @Override 1189 public Base setProperty(String name, Base value) throws FHIRException { 1190 if (name.equals("type")) { 1191 value = new DeviceMetricCalibrationTypeEnumFactory().fromType(castToCode(value)); 1192 this.type = (Enumeration) value; // Enumeration<DeviceMetricCalibrationType> 1193 } else if (name.equals("state")) { 1194 value = new DeviceMetricCalibrationStateEnumFactory().fromType(castToCode(value)); 1195 this.state = (Enumeration) value; // Enumeration<DeviceMetricCalibrationState> 1196 } else if (name.equals("time")) { 1197 this.time = castToInstant(value); // InstantType 1198 } else 1199 return super.setProperty(name, value); 1200 return value; 1201 } 1202 1203 @Override 1204 public void removeChild(String name, Base value) throws FHIRException { 1205 if (name.equals("type")) { 1206 this.type = null; 1207 } else if (name.equals("state")) { 1208 this.state = null; 1209 } else if (name.equals("time")) { 1210 this.time = null; 1211 } else 1212 super.removeChild(name, value); 1213 1214 } 1215 1216 @Override 1217 public Base makeProperty(int hash, String name) throws FHIRException { 1218 switch (hash) { 1219 case 3575610: 1220 return getTypeElement(); 1221 case 109757585: 1222 return getStateElement(); 1223 case 3560141: 1224 return getTimeElement(); 1225 default: 1226 return super.makeProperty(hash, name); 1227 } 1228 1229 } 1230 1231 @Override 1232 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1233 switch (hash) { 1234 case 3575610: 1235 /* type */ return new String[] { "code" }; 1236 case 109757585: 1237 /* state */ return new String[] { "code" }; 1238 case 3560141: 1239 /* time */ return new String[] { "instant" }; 1240 default: 1241 return super.getTypesForProperty(hash, name); 1242 } 1243 1244 } 1245 1246 @Override 1247 public Base addChild(String name) throws FHIRException { 1248 if (name.equals("type")) { 1249 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.type"); 1250 } else if (name.equals("state")) { 1251 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.state"); 1252 } else if (name.equals("time")) { 1253 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.time"); 1254 } else 1255 return super.addChild(name); 1256 } 1257 1258 public DeviceMetricCalibrationComponent copy() { 1259 DeviceMetricCalibrationComponent dst = new DeviceMetricCalibrationComponent(); 1260 copyValues(dst); 1261 return dst; 1262 } 1263 1264 public void copyValues(DeviceMetricCalibrationComponent dst) { 1265 super.copyValues(dst); 1266 dst.type = type == null ? null : type.copy(); 1267 dst.state = state == null ? null : state.copy(); 1268 dst.time = time == null ? null : time.copy(); 1269 } 1270 1271 @Override 1272 public boolean equalsDeep(Base other_) { 1273 if (!super.equalsDeep(other_)) 1274 return false; 1275 if (!(other_ instanceof DeviceMetricCalibrationComponent)) 1276 return false; 1277 DeviceMetricCalibrationComponent o = (DeviceMetricCalibrationComponent) other_; 1278 return compareDeep(type, o.type, true) && compareDeep(state, o.state, true) && compareDeep(time, o.time, true); 1279 } 1280 1281 @Override 1282 public boolean equalsShallow(Base other_) { 1283 if (!super.equalsShallow(other_)) 1284 return false; 1285 if (!(other_ instanceof DeviceMetricCalibrationComponent)) 1286 return false; 1287 DeviceMetricCalibrationComponent o = (DeviceMetricCalibrationComponent) other_; 1288 return compareValues(type, o.type, true) && compareValues(state, o.state, true) 1289 && compareValues(time, o.time, true); 1290 } 1291 1292 public boolean isEmpty() { 1293 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, state, time); 1294 } 1295 1296 public String fhirType() { 1297 return "DeviceMetric.calibration"; 1298 1299 } 1300 1301 } 1302 1303 /** 1304 * Unique instance identifiers assigned to a device by the device or gateway 1305 * software, manufacturers, other organizations or owners. For example: handle 1306 * ID. 1307 */ 1308 @Child(name = "identifier", type = { 1309 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1310 @Description(shortDefinition = "Instance identifier", formalDefinition = "Unique instance identifiers assigned to a device by the device or gateway software, manufacturers, other organizations or owners. For example: handle ID.") 1311 protected List<Identifier> identifier; 1312 1313 /** 1314 * Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc. 1315 */ 1316 @Child(name = "type", type = { CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1317 @Description(shortDefinition = "Identity of metric, for example Heart Rate or PEEP Setting", formalDefinition = "Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc.") 1318 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/devicemetric-type") 1319 protected CodeableConcept type; 1320 1321 /** 1322 * Describes the unit that an observed value determined for this metric will 1323 * have. For example: Percent, Seconds, etc. 1324 */ 1325 @Child(name = "unit", type = { CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1326 @Description(shortDefinition = "Unit of Measure for the Metric", formalDefinition = "Describes the unit that an observed value determined for this metric will have. For example: Percent, Seconds, etc.") 1327 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/devicemetric-type") 1328 protected CodeableConcept unit; 1329 1330 /** 1331 * Describes the link to the Device that this DeviceMetric belongs to and that 1332 * contains administrative device information such as manufacturer, serial 1333 * number, etc. 1334 */ 1335 @Child(name = "source", type = { Device.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1336 @Description(shortDefinition = "Describes the link to the source Device", formalDefinition = "Describes the link to the Device that this DeviceMetric belongs to and that contains administrative device information such as manufacturer, serial number, etc.") 1337 protected Reference source; 1338 1339 /** 1340 * The actual object that is the target of the reference (Describes the link to 1341 * the Device that this DeviceMetric belongs to and that contains administrative 1342 * device information such as manufacturer, serial number, etc.) 1343 */ 1344 protected Device sourceTarget; 1345 1346 /** 1347 * Describes the link to the Device that this DeviceMetric belongs to and that 1348 * provide information about the location of this DeviceMetric in the 1349 * containment structure of the parent Device. An example would be a Device that 1350 * represents a Channel. This reference can be used by a client application to 1351 * distinguish DeviceMetrics that have the same type, but should be interpreted 1352 * based on their containment location. 1353 */ 1354 @Child(name = "parent", type = { Device.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1355 @Description(shortDefinition = "Describes the link to the parent Device", formalDefinition = "Describes the link to the Device that this DeviceMetric belongs to and that provide information about the location of this DeviceMetric in the containment structure of the parent Device. An example would be a Device that represents a Channel. This reference can be used by a client application to distinguish DeviceMetrics that have the same type, but should be interpreted based on their containment location.") 1356 protected Reference parent; 1357 1358 /** 1359 * The actual object that is the target of the reference (Describes the link to 1360 * the Device that this DeviceMetric belongs to and that provide information 1361 * about the location of this DeviceMetric in the containment structure of the 1362 * parent Device. An example would be a Device that represents a Channel. This 1363 * reference can be used by a client application to distinguish DeviceMetrics 1364 * that have the same type, but should be interpreted based on their containment 1365 * location.) 1366 */ 1367 protected Device parentTarget; 1368 1369 /** 1370 * Indicates current operational state of the device. For example: On, Off, 1371 * Standby, etc. 1372 */ 1373 @Child(name = "operationalStatus", type = { 1374 CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1375 @Description(shortDefinition = "on | off | standby | entered-in-error", formalDefinition = "Indicates current operational state of the device. For example: On, Off, Standby, etc.") 1376 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/metric-operational-status") 1377 protected Enumeration<DeviceMetricOperationalStatus> operationalStatus; 1378 1379 /** 1380 * Describes the color representation for the metric. This is often used to aid 1381 * clinicians to track and identify parameter types by color. In practice, 1382 * consider a Patient Monitor that has ECG/HR and Pleth for example; the 1383 * parameters are displayed in different characteristic colors, such as HR-blue, 1384 * BP-green, and PR and SpO2- magenta. 1385 */ 1386 @Child(name = "color", type = { CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1387 @Description(shortDefinition = "black | red | green | yellow | blue | magenta | cyan | white", formalDefinition = "Describes the color representation for the metric. This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth for example; the parameters are displayed in different characteristic colors, such as HR-blue, BP-green, and PR and SpO2- magenta.") 1388 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/metric-color") 1389 protected Enumeration<DeviceMetricColor> color; 1390 1391 /** 1392 * Indicates the category of the observation generation process. A DeviceMetric 1393 * can be for example a setting, measurement, or calculation. 1394 */ 1395 @Child(name = "category", type = { CodeType.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 1396 @Description(shortDefinition = "measurement | setting | calculation | unspecified", formalDefinition = "Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation.") 1397 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/metric-category") 1398 protected Enumeration<DeviceMetricCategory> category; 1399 1400 /** 1401 * Describes the measurement repetition time. This is not necessarily the same 1402 * as the update period. The measurement repetition time can range from 1403 * milliseconds up to hours. An example for a measurement repetition time in the 1404 * range of milliseconds is the sampling rate of an ECG. An example for a 1405 * measurement repetition time in the range of hours is a NIBP that is triggered 1406 * automatically every hour. The update period may be different than the 1407 * measurement repetition time, if the device does not update the published 1408 * observed value with the same frequency as it was measured. 1409 */ 1410 @Child(name = "measurementPeriod", type = { 1411 Timing.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1412 @Description(shortDefinition = "Describes the measurement repetition time", formalDefinition = "Describes the measurement repetition time. This is not necessarily the same as the update period. The measurement repetition time can range from milliseconds up to hours. An example for a measurement repetition time in the range of milliseconds is the sampling rate of an ECG. An example for a measurement repetition time in the range of hours is a NIBP that is triggered automatically every hour. The update period may be different than the measurement repetition time, if the device does not update the published observed value with the same frequency as it was measured.") 1413 protected Timing measurementPeriod; 1414 1415 /** 1416 * Describes the calibrations that have been performed or that are required to 1417 * be performed. 1418 */ 1419 @Child(name = "calibration", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1420 @Description(shortDefinition = "Describes the calibrations that have been performed or that are required to be performed", formalDefinition = "Describes the calibrations that have been performed or that are required to be performed.") 1421 protected List<DeviceMetricCalibrationComponent> calibration; 1422 1423 private static final long serialVersionUID = 1309955219L; 1424 1425 /** 1426 * Constructor 1427 */ 1428 public DeviceMetric() { 1429 super(); 1430 } 1431 1432 /** 1433 * Constructor 1434 */ 1435 public DeviceMetric(CodeableConcept type, Enumeration<DeviceMetricCategory> category) { 1436 super(); 1437 this.type = type; 1438 this.category = category; 1439 } 1440 1441 /** 1442 * @return {@link #identifier} (Unique instance identifiers assigned to a device 1443 * by the device or gateway software, manufacturers, other organizations 1444 * or owners. For example: handle ID.) 1445 */ 1446 public List<Identifier> getIdentifier() { 1447 if (this.identifier == null) 1448 this.identifier = new ArrayList<Identifier>(); 1449 return this.identifier; 1450 } 1451 1452 /** 1453 * @return Returns a reference to <code>this</code> for easy method chaining 1454 */ 1455 public DeviceMetric setIdentifier(List<Identifier> theIdentifier) { 1456 this.identifier = theIdentifier; 1457 return this; 1458 } 1459 1460 public boolean hasIdentifier() { 1461 if (this.identifier == null) 1462 return false; 1463 for (Identifier item : this.identifier) 1464 if (!item.isEmpty()) 1465 return true; 1466 return false; 1467 } 1468 1469 public Identifier addIdentifier() { // 3 1470 Identifier t = new Identifier(); 1471 if (this.identifier == null) 1472 this.identifier = new ArrayList<Identifier>(); 1473 this.identifier.add(t); 1474 return t; 1475 } 1476 1477 public DeviceMetric addIdentifier(Identifier t) { // 3 1478 if (t == null) 1479 return this; 1480 if (this.identifier == null) 1481 this.identifier = new ArrayList<Identifier>(); 1482 this.identifier.add(t); 1483 return this; 1484 } 1485 1486 /** 1487 * @return The first repetition of repeating field {@link #identifier}, creating 1488 * it if it does not already exist 1489 */ 1490 public Identifier getIdentifierFirstRep() { 1491 if (getIdentifier().isEmpty()) { 1492 addIdentifier(); 1493 } 1494 return getIdentifier().get(0); 1495 } 1496 1497 /** 1498 * @return {@link #type} (Describes the type of the metric. For example: Heart 1499 * Rate, PEEP Setting, etc.) 1500 */ 1501 public CodeableConcept getType() { 1502 if (this.type == null) 1503 if (Configuration.errorOnAutoCreate()) 1504 throw new Error("Attempt to auto-create DeviceMetric.type"); 1505 else if (Configuration.doAutoCreate()) 1506 this.type = new CodeableConcept(); // cc 1507 return this.type; 1508 } 1509 1510 public boolean hasType() { 1511 return this.type != null && !this.type.isEmpty(); 1512 } 1513 1514 /** 1515 * @param value {@link #type} (Describes the type of the metric. For example: 1516 * Heart Rate, PEEP Setting, etc.) 1517 */ 1518 public DeviceMetric setType(CodeableConcept value) { 1519 this.type = value; 1520 return this; 1521 } 1522 1523 /** 1524 * @return {@link #unit} (Describes the unit that an observed value determined 1525 * for this metric will have. For example: Percent, Seconds, etc.) 1526 */ 1527 public CodeableConcept getUnit() { 1528 if (this.unit == null) 1529 if (Configuration.errorOnAutoCreate()) 1530 throw new Error("Attempt to auto-create DeviceMetric.unit"); 1531 else if (Configuration.doAutoCreate()) 1532 this.unit = new CodeableConcept(); // cc 1533 return this.unit; 1534 } 1535 1536 public boolean hasUnit() { 1537 return this.unit != null && !this.unit.isEmpty(); 1538 } 1539 1540 /** 1541 * @param value {@link #unit} (Describes the unit that an observed value 1542 * determined for this metric will have. For example: Percent, 1543 * Seconds, etc.) 1544 */ 1545 public DeviceMetric setUnit(CodeableConcept value) { 1546 this.unit = value; 1547 return this; 1548 } 1549 1550 /** 1551 * @return {@link #source} (Describes the link to the Device that this 1552 * DeviceMetric belongs to and that contains administrative device 1553 * information such as manufacturer, serial number, etc.) 1554 */ 1555 public Reference getSource() { 1556 if (this.source == null) 1557 if (Configuration.errorOnAutoCreate()) 1558 throw new Error("Attempt to auto-create DeviceMetric.source"); 1559 else if (Configuration.doAutoCreate()) 1560 this.source = new Reference(); // cc 1561 return this.source; 1562 } 1563 1564 public boolean hasSource() { 1565 return this.source != null && !this.source.isEmpty(); 1566 } 1567 1568 /** 1569 * @param value {@link #source} (Describes the link to the Device that this 1570 * DeviceMetric belongs to and that contains administrative device 1571 * information such as manufacturer, serial number, etc.) 1572 */ 1573 public DeviceMetric setSource(Reference value) { 1574 this.source = value; 1575 return this; 1576 } 1577 1578 /** 1579 * @return {@link #source} The actual object that is the target of the 1580 * reference. The reference library doesn't populate this, but you can 1581 * use it to hold the resource if you resolve it. (Describes the link to 1582 * the Device that this DeviceMetric belongs to and that contains 1583 * administrative device information such as manufacturer, serial 1584 * number, etc.) 1585 */ 1586 public Device getSourceTarget() { 1587 if (this.sourceTarget == null) 1588 if (Configuration.errorOnAutoCreate()) 1589 throw new Error("Attempt to auto-create DeviceMetric.source"); 1590 else if (Configuration.doAutoCreate()) 1591 this.sourceTarget = new Device(); // aa 1592 return this.sourceTarget; 1593 } 1594 1595 /** 1596 * @param value {@link #source} The actual object that is the target of the 1597 * reference. The reference library doesn't use these, but you can 1598 * use it to hold the resource if you resolve it. (Describes the 1599 * link to the Device that this DeviceMetric belongs to and that 1600 * contains administrative device information such as manufacturer, 1601 * serial number, etc.) 1602 */ 1603 public DeviceMetric setSourceTarget(Device value) { 1604 this.sourceTarget = value; 1605 return this; 1606 } 1607 1608 /** 1609 * @return {@link #parent} (Describes the link to the Device that this 1610 * DeviceMetric belongs to and that provide information about the 1611 * location of this DeviceMetric in the containment structure of the 1612 * parent Device. An example would be a Device that represents a 1613 * Channel. This reference can be used by a client application to 1614 * distinguish DeviceMetrics that have the same type, but should be 1615 * interpreted based on their containment location.) 1616 */ 1617 public Reference getParent() { 1618 if (this.parent == null) 1619 if (Configuration.errorOnAutoCreate()) 1620 throw new Error("Attempt to auto-create DeviceMetric.parent"); 1621 else if (Configuration.doAutoCreate()) 1622 this.parent = new Reference(); // cc 1623 return this.parent; 1624 } 1625 1626 public boolean hasParent() { 1627 return this.parent != null && !this.parent.isEmpty(); 1628 } 1629 1630 /** 1631 * @param value {@link #parent} (Describes the link to the Device that this 1632 * DeviceMetric belongs to and that provide information about the 1633 * location of this DeviceMetric in the containment structure of 1634 * the parent Device. An example would be a Device that represents 1635 * a Channel. This reference can be used by a client application to 1636 * distinguish DeviceMetrics that have the same type, but should be 1637 * interpreted based on their containment location.) 1638 */ 1639 public DeviceMetric setParent(Reference value) { 1640 this.parent = value; 1641 return this; 1642 } 1643 1644 /** 1645 * @return {@link #parent} The actual object that is the target of the 1646 * reference. The reference library doesn't populate this, but you can 1647 * use it to hold the resource if you resolve it. (Describes the link to 1648 * the Device that this DeviceMetric belongs to and that provide 1649 * information about the location of this DeviceMetric in the 1650 * containment structure of the parent Device. An example would be a 1651 * Device that represents a Channel. This reference can be used by a 1652 * client application to distinguish DeviceMetrics that have the same 1653 * type, but should be interpreted based on their containment location.) 1654 */ 1655 public Device getParentTarget() { 1656 if (this.parentTarget == null) 1657 if (Configuration.errorOnAutoCreate()) 1658 throw new Error("Attempt to auto-create DeviceMetric.parent"); 1659 else if (Configuration.doAutoCreate()) 1660 this.parentTarget = new Device(); // aa 1661 return this.parentTarget; 1662 } 1663 1664 /** 1665 * @param value {@link #parent} The actual object that is the target of the 1666 * reference. The reference library doesn't use these, but you can 1667 * use it to hold the resource if you resolve it. (Describes the 1668 * link to the Device that this DeviceMetric belongs to and that 1669 * provide information about the location of this DeviceMetric in 1670 * the containment structure of the parent Device. An example would 1671 * be a Device that represents a Channel. This reference can be 1672 * used by a client application to distinguish DeviceMetrics that 1673 * have the same type, but should be interpreted based on their 1674 * containment location.) 1675 */ 1676 public DeviceMetric setParentTarget(Device value) { 1677 this.parentTarget = value; 1678 return this; 1679 } 1680 1681 /** 1682 * @return {@link #operationalStatus} (Indicates current operational state of 1683 * the device. For example: On, Off, Standby, etc.). This is the 1684 * underlying object with id, value and extensions. The accessor 1685 * "getOperationalStatus" gives direct access to the value 1686 */ 1687 public Enumeration<DeviceMetricOperationalStatus> getOperationalStatusElement() { 1688 if (this.operationalStatus == null) 1689 if (Configuration.errorOnAutoCreate()) 1690 throw new Error("Attempt to auto-create DeviceMetric.operationalStatus"); 1691 else if (Configuration.doAutoCreate()) 1692 this.operationalStatus = new Enumeration<DeviceMetricOperationalStatus>( 1693 new DeviceMetricOperationalStatusEnumFactory()); // bb 1694 return this.operationalStatus; 1695 } 1696 1697 public boolean hasOperationalStatusElement() { 1698 return this.operationalStatus != null && !this.operationalStatus.isEmpty(); 1699 } 1700 1701 public boolean hasOperationalStatus() { 1702 return this.operationalStatus != null && !this.operationalStatus.isEmpty(); 1703 } 1704 1705 /** 1706 * @param value {@link #operationalStatus} (Indicates current operational state 1707 * of the device. For example: On, Off, Standby, etc.). This is the 1708 * underlying object with id, value and extensions. The accessor 1709 * "getOperationalStatus" gives direct access to the value 1710 */ 1711 public DeviceMetric setOperationalStatusElement(Enumeration<DeviceMetricOperationalStatus> value) { 1712 this.operationalStatus = value; 1713 return this; 1714 } 1715 1716 /** 1717 * @return Indicates current operational state of the device. For example: On, 1718 * Off, Standby, etc. 1719 */ 1720 public DeviceMetricOperationalStatus getOperationalStatus() { 1721 return this.operationalStatus == null ? null : this.operationalStatus.getValue(); 1722 } 1723 1724 /** 1725 * @param value Indicates current operational state of the device. For example: 1726 * On, Off, Standby, etc. 1727 */ 1728 public DeviceMetric setOperationalStatus(DeviceMetricOperationalStatus value) { 1729 if (value == null) 1730 this.operationalStatus = null; 1731 else { 1732 if (this.operationalStatus == null) 1733 this.operationalStatus = new Enumeration<DeviceMetricOperationalStatus>( 1734 new DeviceMetricOperationalStatusEnumFactory()); 1735 this.operationalStatus.setValue(value); 1736 } 1737 return this; 1738 } 1739 1740 /** 1741 * @return {@link #color} (Describes the color representation for the metric. 1742 * This is often used to aid clinicians to track and identify parameter 1743 * types by color. In practice, consider a Patient Monitor that has 1744 * ECG/HR and Pleth for example; the parameters are displayed in 1745 * different characteristic colors, such as HR-blue, BP-green, and PR 1746 * and SpO2- magenta.). This is the underlying object with id, value and 1747 * extensions. The accessor "getColor" gives direct access to the value 1748 */ 1749 public Enumeration<DeviceMetricColor> getColorElement() { 1750 if (this.color == null) 1751 if (Configuration.errorOnAutoCreate()) 1752 throw new Error("Attempt to auto-create DeviceMetric.color"); 1753 else if (Configuration.doAutoCreate()) 1754 this.color = new Enumeration<DeviceMetricColor>(new DeviceMetricColorEnumFactory()); // bb 1755 return this.color; 1756 } 1757 1758 public boolean hasColorElement() { 1759 return this.color != null && !this.color.isEmpty(); 1760 } 1761 1762 public boolean hasColor() { 1763 return this.color != null && !this.color.isEmpty(); 1764 } 1765 1766 /** 1767 * @param value {@link #color} (Describes the color representation for the 1768 * metric. This is often used to aid clinicians to track and 1769 * identify parameter types by color. In practice, consider a 1770 * Patient Monitor that has ECG/HR and Pleth for example; the 1771 * parameters are displayed in different characteristic colors, 1772 * such as HR-blue, BP-green, and PR and SpO2- magenta.). This is 1773 * the underlying object with id, value and extensions. The 1774 * accessor "getColor" gives direct access to the value 1775 */ 1776 public DeviceMetric setColorElement(Enumeration<DeviceMetricColor> value) { 1777 this.color = value; 1778 return this; 1779 } 1780 1781 /** 1782 * @return Describes the color representation for the metric. This is often used 1783 * to aid clinicians to track and identify parameter types by color. In 1784 * practice, consider a Patient Monitor that has ECG/HR and Pleth for 1785 * example; the parameters are displayed in different characteristic 1786 * colors, such as HR-blue, BP-green, and PR and SpO2- magenta. 1787 */ 1788 public DeviceMetricColor getColor() { 1789 return this.color == null ? null : this.color.getValue(); 1790 } 1791 1792 /** 1793 * @param value Describes the color representation for the metric. This is often 1794 * used to aid clinicians to track and identify parameter types by 1795 * color. In practice, consider a Patient Monitor that has ECG/HR 1796 * and Pleth for example; the parameters are displayed in different 1797 * characteristic colors, such as HR-blue, BP-green, and PR and 1798 * SpO2- magenta. 1799 */ 1800 public DeviceMetric setColor(DeviceMetricColor value) { 1801 if (value == null) 1802 this.color = null; 1803 else { 1804 if (this.color == null) 1805 this.color = new Enumeration<DeviceMetricColor>(new DeviceMetricColorEnumFactory()); 1806 this.color.setValue(value); 1807 } 1808 return this; 1809 } 1810 1811 /** 1812 * @return {@link #category} (Indicates the category of the observation 1813 * generation process. A DeviceMetric can be for example a setting, 1814 * measurement, or calculation.). This is the underlying object with id, 1815 * value and extensions. The accessor "getCategory" gives direct access 1816 * to the value 1817 */ 1818 public Enumeration<DeviceMetricCategory> getCategoryElement() { 1819 if (this.category == null) 1820 if (Configuration.errorOnAutoCreate()) 1821 throw new Error("Attempt to auto-create DeviceMetric.category"); 1822 else if (Configuration.doAutoCreate()) 1823 this.category = new Enumeration<DeviceMetricCategory>(new DeviceMetricCategoryEnumFactory()); // bb 1824 return this.category; 1825 } 1826 1827 public boolean hasCategoryElement() { 1828 return this.category != null && !this.category.isEmpty(); 1829 } 1830 1831 public boolean hasCategory() { 1832 return this.category != null && !this.category.isEmpty(); 1833 } 1834 1835 /** 1836 * @param value {@link #category} (Indicates the category of the observation 1837 * generation process. A DeviceMetric can be for example a setting, 1838 * measurement, or calculation.). This is the underlying object 1839 * with id, value and extensions. The accessor "getCategory" gives 1840 * direct access to the value 1841 */ 1842 public DeviceMetric setCategoryElement(Enumeration<DeviceMetricCategory> value) { 1843 this.category = value; 1844 return this; 1845 } 1846 1847 /** 1848 * @return Indicates the category of the observation generation process. A 1849 * DeviceMetric can be for example a setting, measurement, or 1850 * calculation. 1851 */ 1852 public DeviceMetricCategory getCategory() { 1853 return this.category == null ? null : this.category.getValue(); 1854 } 1855 1856 /** 1857 * @param value Indicates the category of the observation generation process. A 1858 * DeviceMetric can be for example a setting, measurement, or 1859 * calculation. 1860 */ 1861 public DeviceMetric setCategory(DeviceMetricCategory value) { 1862 if (this.category == null) 1863 this.category = new Enumeration<DeviceMetricCategory>(new DeviceMetricCategoryEnumFactory()); 1864 this.category.setValue(value); 1865 return this; 1866 } 1867 1868 /** 1869 * @return {@link #measurementPeriod} (Describes the measurement repetition 1870 * time. This is not necessarily the same as the update period. The 1871 * measurement repetition time can range from milliseconds up to hours. 1872 * An example for a measurement repetition time in the range of 1873 * milliseconds is the sampling rate of an ECG. An example for a 1874 * measurement repetition time in the range of hours is a NIBP that is 1875 * triggered automatically every hour. The update period may be 1876 * different than the measurement repetition time, if the device does 1877 * not update the published observed value with the same frequency as it 1878 * was measured.) 1879 */ 1880 public Timing getMeasurementPeriod() { 1881 if (this.measurementPeriod == null) 1882 if (Configuration.errorOnAutoCreate()) 1883 throw new Error("Attempt to auto-create DeviceMetric.measurementPeriod"); 1884 else if (Configuration.doAutoCreate()) 1885 this.measurementPeriod = new Timing(); // cc 1886 return this.measurementPeriod; 1887 } 1888 1889 public boolean hasMeasurementPeriod() { 1890 return this.measurementPeriod != null && !this.measurementPeriod.isEmpty(); 1891 } 1892 1893 /** 1894 * @param value {@link #measurementPeriod} (Describes the measurement repetition 1895 * time. This is not necessarily the same as the update period. The 1896 * measurement repetition time can range from milliseconds up to 1897 * hours. An example for a measurement repetition time in the range 1898 * of milliseconds is the sampling rate of an ECG. An example for a 1899 * measurement repetition time in the range of hours is a NIBP that 1900 * is triggered automatically every hour. The update period may be 1901 * different than the measurement repetition time, if the device 1902 * does not update the published observed value with the same 1903 * frequency as it was measured.) 1904 */ 1905 public DeviceMetric setMeasurementPeriod(Timing value) { 1906 this.measurementPeriod = value; 1907 return this; 1908 } 1909 1910 /** 1911 * @return {@link #calibration} (Describes the calibrations that have been 1912 * performed or that are required to be performed.) 1913 */ 1914 public List<DeviceMetricCalibrationComponent> getCalibration() { 1915 if (this.calibration == null) 1916 this.calibration = new ArrayList<DeviceMetricCalibrationComponent>(); 1917 return this.calibration; 1918 } 1919 1920 /** 1921 * @return Returns a reference to <code>this</code> for easy method chaining 1922 */ 1923 public DeviceMetric setCalibration(List<DeviceMetricCalibrationComponent> theCalibration) { 1924 this.calibration = theCalibration; 1925 return this; 1926 } 1927 1928 public boolean hasCalibration() { 1929 if (this.calibration == null) 1930 return false; 1931 for (DeviceMetricCalibrationComponent item : this.calibration) 1932 if (!item.isEmpty()) 1933 return true; 1934 return false; 1935 } 1936 1937 public DeviceMetricCalibrationComponent addCalibration() { // 3 1938 DeviceMetricCalibrationComponent t = new DeviceMetricCalibrationComponent(); 1939 if (this.calibration == null) 1940 this.calibration = new ArrayList<DeviceMetricCalibrationComponent>(); 1941 this.calibration.add(t); 1942 return t; 1943 } 1944 1945 public DeviceMetric addCalibration(DeviceMetricCalibrationComponent t) { // 3 1946 if (t == null) 1947 return this; 1948 if (this.calibration == null) 1949 this.calibration = new ArrayList<DeviceMetricCalibrationComponent>(); 1950 this.calibration.add(t); 1951 return this; 1952 } 1953 1954 /** 1955 * @return The first repetition of repeating field {@link #calibration}, 1956 * creating it if it does not already exist 1957 */ 1958 public DeviceMetricCalibrationComponent getCalibrationFirstRep() { 1959 if (getCalibration().isEmpty()) { 1960 addCalibration(); 1961 } 1962 return getCalibration().get(0); 1963 } 1964 1965 protected void listChildren(List<Property> children) { 1966 super.listChildren(children); 1967 children.add(new Property("identifier", "Identifier", 1968 "Unique instance identifiers assigned to a device by the device or gateway software, manufacturers, other organizations or owners. For example: handle ID.", 1969 0, java.lang.Integer.MAX_VALUE, identifier)); 1970 children.add(new Property("type", "CodeableConcept", 1971 "Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc.", 0, 1, type)); 1972 children.add(new Property("unit", "CodeableConcept", 1973 "Describes the unit that an observed value determined for this metric will have. For example: Percent, Seconds, etc.", 1974 0, 1, unit)); 1975 children.add(new Property("source", "Reference(Device)", 1976 "Describes the link to the Device that this DeviceMetric belongs to and that contains administrative device information such as manufacturer, serial number, etc.", 1977 0, 1, source)); 1978 children.add(new Property("parent", "Reference(Device)", 1979 "Describes the link to the Device that this DeviceMetric belongs to and that provide information about the location of this DeviceMetric in the containment structure of the parent Device. An example would be a Device that represents a Channel. This reference can be used by a client application to distinguish DeviceMetrics that have the same type, but should be interpreted based on their containment location.", 1980 0, 1, parent)); 1981 children.add(new Property("operationalStatus", "code", 1982 "Indicates current operational state of the device. For example: On, Off, Standby, etc.", 0, 1, 1983 operationalStatus)); 1984 children.add(new Property("color", "code", 1985 "Describes the color representation for the metric. This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth for example; the parameters are displayed in different characteristic colors, such as HR-blue, BP-green, and PR and SpO2- magenta.", 1986 0, 1, color)); 1987 children.add(new Property("category", "code", 1988 "Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation.", 1989 0, 1, category)); 1990 children.add(new Property("measurementPeriod", "Timing", 1991 "Describes the measurement repetition time. This is not necessarily the same as the update period. The measurement repetition time can range from milliseconds up to hours. An example for a measurement repetition time in the range of milliseconds is the sampling rate of an ECG. An example for a measurement repetition time in the range of hours is a NIBP that is triggered automatically every hour. The update period may be different than the measurement repetition time, if the device does not update the published observed value with the same frequency as it was measured.", 1992 0, 1, measurementPeriod)); 1993 children.add(new Property("calibration", "", 1994 "Describes the calibrations that have been performed or that are required to be performed.", 0, 1995 java.lang.Integer.MAX_VALUE, calibration)); 1996 } 1997 1998 @Override 1999 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2000 switch (_hash) { 2001 case -1618432855: 2002 /* identifier */ return new Property("identifier", "Identifier", 2003 "Unique instance identifiers assigned to a device by the device or gateway software, manufacturers, other organizations or owners. For example: handle ID.", 2004 0, java.lang.Integer.MAX_VALUE, identifier); 2005 case 3575610: 2006 /* type */ return new Property("type", "CodeableConcept", 2007 "Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc.", 0, 1, type); 2008 case 3594628: 2009 /* unit */ return new Property("unit", "CodeableConcept", 2010 "Describes the unit that an observed value determined for this metric will have. For example: Percent, Seconds, etc.", 2011 0, 1, unit); 2012 case -896505829: 2013 /* source */ return new Property("source", "Reference(Device)", 2014 "Describes the link to the Device that this DeviceMetric belongs to and that contains administrative device information such as manufacturer, serial number, etc.", 2015 0, 1, source); 2016 case -995424086: 2017 /* parent */ return new Property("parent", "Reference(Device)", 2018 "Describes the link to the Device that this DeviceMetric belongs to and that provide information about the location of this DeviceMetric in the containment structure of the parent Device. An example would be a Device that represents a Channel. This reference can be used by a client application to distinguish DeviceMetrics that have the same type, but should be interpreted based on their containment location.", 2019 0, 1, parent); 2020 case -2103166364: 2021 /* operationalStatus */ return new Property("operationalStatus", "code", 2022 "Indicates current operational state of the device. For example: On, Off, Standby, etc.", 0, 1, 2023 operationalStatus); 2024 case 94842723: 2025 /* color */ return new Property("color", "code", 2026 "Describes the color representation for the metric. This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth for example; the parameters are displayed in different characteristic colors, such as HR-blue, BP-green, and PR and SpO2- magenta.", 2027 0, 1, color); 2028 case 50511102: 2029 /* category */ return new Property("category", "code", 2030 "Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation.", 2031 0, 1, category); 2032 case -1300332387: 2033 /* measurementPeriod */ return new Property("measurementPeriod", "Timing", 2034 "Describes the measurement repetition time. This is not necessarily the same as the update period. The measurement repetition time can range from milliseconds up to hours. An example for a measurement repetition time in the range of milliseconds is the sampling rate of an ECG. An example for a measurement repetition time in the range of hours is a NIBP that is triggered automatically every hour. The update period may be different than the measurement repetition time, if the device does not update the published observed value with the same frequency as it was measured.", 2035 0, 1, measurementPeriod); 2036 case 1421318634: 2037 /* calibration */ return new Property("calibration", "", 2038 "Describes the calibrations that have been performed or that are required to be performed.", 0, 2039 java.lang.Integer.MAX_VALUE, calibration); 2040 default: 2041 return super.getNamedProperty(_hash, _name, _checkValid); 2042 } 2043 2044 } 2045 2046 @Override 2047 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2048 switch (hash) { 2049 case -1618432855: 2050 /* identifier */ return this.identifier == null ? new Base[0] 2051 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2052 case 3575610: 2053 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2054 case 3594628: 2055 /* unit */ return this.unit == null ? new Base[0] : new Base[] { this.unit }; // CodeableConcept 2056 case -896505829: 2057 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // Reference 2058 case -995424086: 2059 /* parent */ return this.parent == null ? new Base[0] : new Base[] { this.parent }; // Reference 2060 case -2103166364: 2061 /* operationalStatus */ return this.operationalStatus == null ? new Base[0] 2062 : new Base[] { this.operationalStatus }; // Enumeration<DeviceMetricOperationalStatus> 2063 case 94842723: 2064 /* color */ return this.color == null ? new Base[0] : new Base[] { this.color }; // Enumeration<DeviceMetricColor> 2065 case 50511102: 2066 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // Enumeration<DeviceMetricCategory> 2067 case -1300332387: 2068 /* measurementPeriod */ return this.measurementPeriod == null ? new Base[0] 2069 : new Base[] { this.measurementPeriod }; // Timing 2070 case 1421318634: 2071 /* calibration */ return this.calibration == null ? new Base[0] 2072 : this.calibration.toArray(new Base[this.calibration.size()]); // DeviceMetricCalibrationComponent 2073 default: 2074 return super.getProperty(hash, name, checkValid); 2075 } 2076 2077 } 2078 2079 @Override 2080 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2081 switch (hash) { 2082 case -1618432855: // identifier 2083 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2084 return value; 2085 case 3575610: // type 2086 this.type = castToCodeableConcept(value); // CodeableConcept 2087 return value; 2088 case 3594628: // unit 2089 this.unit = castToCodeableConcept(value); // CodeableConcept 2090 return value; 2091 case -896505829: // source 2092 this.source = castToReference(value); // Reference 2093 return value; 2094 case -995424086: // parent 2095 this.parent = castToReference(value); // Reference 2096 return value; 2097 case -2103166364: // operationalStatus 2098 value = new DeviceMetricOperationalStatusEnumFactory().fromType(castToCode(value)); 2099 this.operationalStatus = (Enumeration) value; // Enumeration<DeviceMetricOperationalStatus> 2100 return value; 2101 case 94842723: // color 2102 value = new DeviceMetricColorEnumFactory().fromType(castToCode(value)); 2103 this.color = (Enumeration) value; // Enumeration<DeviceMetricColor> 2104 return value; 2105 case 50511102: // category 2106 value = new DeviceMetricCategoryEnumFactory().fromType(castToCode(value)); 2107 this.category = (Enumeration) value; // Enumeration<DeviceMetricCategory> 2108 return value; 2109 case -1300332387: // measurementPeriod 2110 this.measurementPeriod = castToTiming(value); // Timing 2111 return value; 2112 case 1421318634: // calibration 2113 this.getCalibration().add((DeviceMetricCalibrationComponent) value); // DeviceMetricCalibrationComponent 2114 return value; 2115 default: 2116 return super.setProperty(hash, name, value); 2117 } 2118 2119 } 2120 2121 @Override 2122 public Base setProperty(String name, Base value) throws FHIRException { 2123 if (name.equals("identifier")) { 2124 this.getIdentifier().add(castToIdentifier(value)); 2125 } else if (name.equals("type")) { 2126 this.type = castToCodeableConcept(value); // CodeableConcept 2127 } else if (name.equals("unit")) { 2128 this.unit = castToCodeableConcept(value); // CodeableConcept 2129 } else if (name.equals("source")) { 2130 this.source = castToReference(value); // Reference 2131 } else if (name.equals("parent")) { 2132 this.parent = castToReference(value); // Reference 2133 } else if (name.equals("operationalStatus")) { 2134 value = new DeviceMetricOperationalStatusEnumFactory().fromType(castToCode(value)); 2135 this.operationalStatus = (Enumeration) value; // Enumeration<DeviceMetricOperationalStatus> 2136 } else if (name.equals("color")) { 2137 value = new DeviceMetricColorEnumFactory().fromType(castToCode(value)); 2138 this.color = (Enumeration) value; // Enumeration<DeviceMetricColor> 2139 } else if (name.equals("category")) { 2140 value = new DeviceMetricCategoryEnumFactory().fromType(castToCode(value)); 2141 this.category = (Enumeration) value; // Enumeration<DeviceMetricCategory> 2142 } else if (name.equals("measurementPeriod")) { 2143 this.measurementPeriod = castToTiming(value); // Timing 2144 } else if (name.equals("calibration")) { 2145 this.getCalibration().add((DeviceMetricCalibrationComponent) value); 2146 } else 2147 return super.setProperty(name, value); 2148 return value; 2149 } 2150 2151 @Override 2152 public void removeChild(String name, Base value) throws FHIRException { 2153 if (name.equals("identifier")) { 2154 this.getIdentifier().remove(castToIdentifier(value)); 2155 } else if (name.equals("type")) { 2156 this.type = null; 2157 } else if (name.equals("unit")) { 2158 this.unit = null; 2159 } else if (name.equals("source")) { 2160 this.source = null; 2161 } else if (name.equals("parent")) { 2162 this.parent = null; 2163 } else if (name.equals("operationalStatus")) { 2164 this.operationalStatus = null; 2165 } else if (name.equals("color")) { 2166 this.color = null; 2167 } else if (name.equals("category")) { 2168 this.category = null; 2169 } else if (name.equals("measurementPeriod")) { 2170 this.measurementPeriod = null; 2171 } else if (name.equals("calibration")) { 2172 this.getCalibration().remove((DeviceMetricCalibrationComponent) value); 2173 } else 2174 super.removeChild(name, value); 2175 2176 } 2177 2178 @Override 2179 public Base makeProperty(int hash, String name) throws FHIRException { 2180 switch (hash) { 2181 case -1618432855: 2182 return addIdentifier(); 2183 case 3575610: 2184 return getType(); 2185 case 3594628: 2186 return getUnit(); 2187 case -896505829: 2188 return getSource(); 2189 case -995424086: 2190 return getParent(); 2191 case -2103166364: 2192 return getOperationalStatusElement(); 2193 case 94842723: 2194 return getColorElement(); 2195 case 50511102: 2196 return getCategoryElement(); 2197 case -1300332387: 2198 return getMeasurementPeriod(); 2199 case 1421318634: 2200 return addCalibration(); 2201 default: 2202 return super.makeProperty(hash, name); 2203 } 2204 2205 } 2206 2207 @Override 2208 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2209 switch (hash) { 2210 case -1618432855: 2211 /* identifier */ return new String[] { "Identifier" }; 2212 case 3575610: 2213 /* type */ return new String[] { "CodeableConcept" }; 2214 case 3594628: 2215 /* unit */ return new String[] { "CodeableConcept" }; 2216 case -896505829: 2217 /* source */ return new String[] { "Reference" }; 2218 case -995424086: 2219 /* parent */ return new String[] { "Reference" }; 2220 case -2103166364: 2221 /* operationalStatus */ return new String[] { "code" }; 2222 case 94842723: 2223 /* color */ return new String[] { "code" }; 2224 case 50511102: 2225 /* category */ return new String[] { "code" }; 2226 case -1300332387: 2227 /* measurementPeriod */ return new String[] { "Timing" }; 2228 case 1421318634: 2229 /* calibration */ return new String[] {}; 2230 default: 2231 return super.getTypesForProperty(hash, name); 2232 } 2233 2234 } 2235 2236 @Override 2237 public Base addChild(String name) throws FHIRException { 2238 if (name.equals("identifier")) { 2239 return addIdentifier(); 2240 } else if (name.equals("type")) { 2241 this.type = new CodeableConcept(); 2242 return this.type; 2243 } else if (name.equals("unit")) { 2244 this.unit = new CodeableConcept(); 2245 return this.unit; 2246 } else if (name.equals("source")) { 2247 this.source = new Reference(); 2248 return this.source; 2249 } else if (name.equals("parent")) { 2250 this.parent = new Reference(); 2251 return this.parent; 2252 } else if (name.equals("operationalStatus")) { 2253 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.operationalStatus"); 2254 } else if (name.equals("color")) { 2255 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.color"); 2256 } else if (name.equals("category")) { 2257 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.category"); 2258 } else if (name.equals("measurementPeriod")) { 2259 this.measurementPeriod = new Timing(); 2260 return this.measurementPeriod; 2261 } else if (name.equals("calibration")) { 2262 return addCalibration(); 2263 } else 2264 return super.addChild(name); 2265 } 2266 2267 public String fhirType() { 2268 return "DeviceMetric"; 2269 2270 } 2271 2272 public DeviceMetric copy() { 2273 DeviceMetric dst = new DeviceMetric(); 2274 copyValues(dst); 2275 return dst; 2276 } 2277 2278 public void copyValues(DeviceMetric dst) { 2279 super.copyValues(dst); 2280 if (identifier != null) { 2281 dst.identifier = new ArrayList<Identifier>(); 2282 for (Identifier i : identifier) 2283 dst.identifier.add(i.copy()); 2284 } 2285 ; 2286 dst.type = type == null ? null : type.copy(); 2287 dst.unit = unit == null ? null : unit.copy(); 2288 dst.source = source == null ? null : source.copy(); 2289 dst.parent = parent == null ? null : parent.copy(); 2290 dst.operationalStatus = operationalStatus == null ? null : operationalStatus.copy(); 2291 dst.color = color == null ? null : color.copy(); 2292 dst.category = category == null ? null : category.copy(); 2293 dst.measurementPeriod = measurementPeriod == null ? null : measurementPeriod.copy(); 2294 if (calibration != null) { 2295 dst.calibration = new ArrayList<DeviceMetricCalibrationComponent>(); 2296 for (DeviceMetricCalibrationComponent i : calibration) 2297 dst.calibration.add(i.copy()); 2298 } 2299 ; 2300 } 2301 2302 protected DeviceMetric typedCopy() { 2303 return copy(); 2304 } 2305 2306 @Override 2307 public boolean equalsDeep(Base other_) { 2308 if (!super.equalsDeep(other_)) 2309 return false; 2310 if (!(other_ instanceof DeviceMetric)) 2311 return false; 2312 DeviceMetric o = (DeviceMetric) other_; 2313 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) 2314 && compareDeep(unit, o.unit, true) && compareDeep(source, o.source, true) && compareDeep(parent, o.parent, true) 2315 && compareDeep(operationalStatus, o.operationalStatus, true) && compareDeep(color, o.color, true) 2316 && compareDeep(category, o.category, true) && compareDeep(measurementPeriod, o.measurementPeriod, true) 2317 && compareDeep(calibration, o.calibration, true); 2318 } 2319 2320 @Override 2321 public boolean equalsShallow(Base other_) { 2322 if (!super.equalsShallow(other_)) 2323 return false; 2324 if (!(other_ instanceof DeviceMetric)) 2325 return false; 2326 DeviceMetric o = (DeviceMetric) other_; 2327 return compareValues(operationalStatus, o.operationalStatus, true) && compareValues(color, o.color, true) 2328 && compareValues(category, o.category, true); 2329 } 2330 2331 public boolean isEmpty() { 2332 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, unit, source, parent, 2333 operationalStatus, color, category, measurementPeriod, calibration); 2334 } 2335 2336 @Override 2337 public ResourceType getResourceType() { 2338 return ResourceType.DeviceMetric; 2339 } 2340 2341 /** 2342 * Search parameter: <b>parent</b> 2343 * <p> 2344 * Description: <b>The parent DeviceMetric resource</b><br> 2345 * Type: <b>reference</b><br> 2346 * Path: <b>DeviceMetric.parent</b><br> 2347 * </p> 2348 */ 2349 @SearchParamDefinition(name = "parent", path = "DeviceMetric.parent", description = "The parent DeviceMetric resource", type = "reference", target = { 2350 Device.class }) 2351 public static final String SP_PARENT = "parent"; 2352 /** 2353 * <b>Fluent Client</b> search parameter constant for <b>parent</b> 2354 * <p> 2355 * Description: <b>The parent DeviceMetric resource</b><br> 2356 * Type: <b>reference</b><br> 2357 * Path: <b>DeviceMetric.parent</b><br> 2358 * </p> 2359 */ 2360 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2361 SP_PARENT); 2362 2363 /** 2364 * Constant for fluent queries to be used to add include statements. Specifies 2365 * the path value of "<b>DeviceMetric:parent</b>". 2366 */ 2367 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARENT = new ca.uhn.fhir.model.api.Include( 2368 "DeviceMetric:parent").toLocked(); 2369 2370 /** 2371 * Search parameter: <b>identifier</b> 2372 * <p> 2373 * Description: <b>The identifier of the metric</b><br> 2374 * Type: <b>token</b><br> 2375 * Path: <b>DeviceMetric.identifier</b><br> 2376 * </p> 2377 */ 2378 @SearchParamDefinition(name = "identifier", path = "DeviceMetric.identifier", description = "The identifier of the metric", type = "token") 2379 public static final String SP_IDENTIFIER = "identifier"; 2380 /** 2381 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2382 * <p> 2383 * Description: <b>The identifier of the metric</b><br> 2384 * Type: <b>token</b><br> 2385 * Path: <b>DeviceMetric.identifier</b><br> 2386 * </p> 2387 */ 2388 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2389 SP_IDENTIFIER); 2390 2391 /** 2392 * Search parameter: <b>source</b> 2393 * <p> 2394 * Description: <b>The device resource</b><br> 2395 * Type: <b>reference</b><br> 2396 * Path: <b>DeviceMetric.source</b><br> 2397 * </p> 2398 */ 2399 @SearchParamDefinition(name = "source", path = "DeviceMetric.source", description = "The device resource", type = "reference", target = { 2400 Device.class }) 2401 public static final String SP_SOURCE = "source"; 2402 /** 2403 * <b>Fluent Client</b> search parameter constant for <b>source</b> 2404 * <p> 2405 * Description: <b>The device resource</b><br> 2406 * Type: <b>reference</b><br> 2407 * Path: <b>DeviceMetric.source</b><br> 2408 * </p> 2409 */ 2410 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2411 SP_SOURCE); 2412 2413 /** 2414 * Constant for fluent queries to be used to add include statements. Specifies 2415 * the path value of "<b>DeviceMetric:source</b>". 2416 */ 2417 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include( 2418 "DeviceMetric:source").toLocked(); 2419 2420 /** 2421 * Search parameter: <b>type</b> 2422 * <p> 2423 * Description: <b>The component type</b><br> 2424 * Type: <b>token</b><br> 2425 * Path: <b>DeviceMetric.type</b><br> 2426 * </p> 2427 */ 2428 @SearchParamDefinition(name = "type", path = "DeviceMetric.type", description = "The component type", type = "token") 2429 public static final String SP_TYPE = "type"; 2430 /** 2431 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2432 * <p> 2433 * Description: <b>The component type</b><br> 2434 * Type: <b>token</b><br> 2435 * Path: <b>DeviceMetric.type</b><br> 2436 * </p> 2437 */ 2438 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2439 SP_TYPE); 2440 2441 /** 2442 * Search parameter: <b>category</b> 2443 * <p> 2444 * Description: <b>The category of the metric</b><br> 2445 * Type: <b>token</b><br> 2446 * Path: <b>DeviceMetric.category</b><br> 2447 * </p> 2448 */ 2449 @SearchParamDefinition(name = "category", path = "DeviceMetric.category", description = "The category of the metric", type = "token") 2450 public static final String SP_CATEGORY = "category"; 2451 /** 2452 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2453 * <p> 2454 * Description: <b>The category of the metric</b><br> 2455 * Type: <b>token</b><br> 2456 * Path: <b>DeviceMetric.category</b><br> 2457 * </p> 2458 */ 2459 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2460 SP_CATEGORY); 2461 2462}