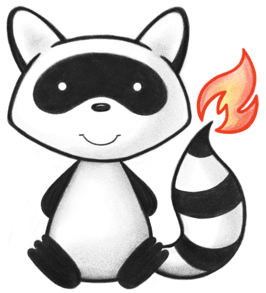
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * Represents a request for a patient to employ a medical device. The device may 048 * be an implantable device, or an external assistive device, such as a walker. 049 */ 050@ResourceDef(name = "DeviceRequest", profile = "http://hl7.org/fhir/StructureDefinition/DeviceRequest") 051public class DeviceRequest extends DomainResource { 052 053 public enum DeviceRequestStatus { 054 /** 055 * The request has been created but is not yet complete or ready for action. 056 */ 057 DRAFT, 058 /** 059 * The request is in force and ready to be acted upon. 060 */ 061 ACTIVE, 062 /** 063 * The request (and any implicit authorization to act) has been temporarily 064 * withdrawn but is expected to resume in the future. 065 */ 066 ONHOLD, 067 /** 068 * The request (and any implicit authorization to act) has been terminated prior 069 * to the known full completion of the intended actions. No further activity 070 * should occur. 071 */ 072 REVOKED, 073 /** 074 * The activity described by the request has been fully performed. No further 075 * activity will occur. 076 */ 077 COMPLETED, 078 /** 079 * This request should never have existed and should be considered 'void'. (It 080 * is possible that real-world decisions were based on it. If real-world 081 * activity has occurred, the status should be "revoked" rather than 082 * "entered-in-error".). 083 */ 084 ENTEREDINERROR, 085 /** 086 * The authoring/source system does not know which of the status values 087 * currently applies for this request. Note: This concept is not to be used for 088 * "other" - one of the listed statuses is presumed to apply, but the 089 * authoring/source system does not know which. 090 */ 091 UNKNOWN, 092 /** 093 * added to help the parsers with the generic types 094 */ 095 NULL; 096 097 public static DeviceRequestStatus fromCode(String codeString) throws FHIRException { 098 if (codeString == null || "".equals(codeString)) 099 return null; 100 if ("draft".equals(codeString)) 101 return DRAFT; 102 if ("active".equals(codeString)) 103 return ACTIVE; 104 if ("on-hold".equals(codeString)) 105 return ONHOLD; 106 if ("revoked".equals(codeString)) 107 return REVOKED; 108 if ("completed".equals(codeString)) 109 return COMPLETED; 110 if ("entered-in-error".equals(codeString)) 111 return ENTEREDINERROR; 112 if ("unknown".equals(codeString)) 113 return UNKNOWN; 114 if (Configuration.isAcceptInvalidEnums()) 115 return null; 116 else 117 throw new FHIRException("Unknown DeviceRequestStatus code '" + codeString + "'"); 118 } 119 120 public String toCode() { 121 switch (this) { 122 case DRAFT: 123 return "draft"; 124 case ACTIVE: 125 return "active"; 126 case ONHOLD: 127 return "on-hold"; 128 case REVOKED: 129 return "revoked"; 130 case COMPLETED: 131 return "completed"; 132 case ENTEREDINERROR: 133 return "entered-in-error"; 134 case UNKNOWN: 135 return "unknown"; 136 case NULL: 137 return null; 138 default: 139 return "?"; 140 } 141 } 142 143 public String getSystem() { 144 switch (this) { 145 case DRAFT: 146 return "http://hl7.org/fhir/request-status"; 147 case ACTIVE: 148 return "http://hl7.org/fhir/request-status"; 149 case ONHOLD: 150 return "http://hl7.org/fhir/request-status"; 151 case REVOKED: 152 return "http://hl7.org/fhir/request-status"; 153 case COMPLETED: 154 return "http://hl7.org/fhir/request-status"; 155 case ENTEREDINERROR: 156 return "http://hl7.org/fhir/request-status"; 157 case UNKNOWN: 158 return "http://hl7.org/fhir/request-status"; 159 case NULL: 160 return null; 161 default: 162 return "?"; 163 } 164 } 165 166 public String getDefinition() { 167 switch (this) { 168 case DRAFT: 169 return "The request has been created but is not yet complete or ready for action."; 170 case ACTIVE: 171 return "The request is in force and ready to be acted upon."; 172 case ONHOLD: 173 return "The request (and any implicit authorization to act) has been temporarily withdrawn but is expected to resume in the future."; 174 case REVOKED: 175 return "The request (and any implicit authorization to act) has been terminated prior to the known full completion of the intended actions. No further activity should occur."; 176 case COMPLETED: 177 return "The activity described by the request has been fully performed. No further activity will occur."; 178 case ENTEREDINERROR: 179 return "This request should never have existed and should be considered 'void'. (It is possible that real-world decisions were based on it. If real-world activity has occurred, the status should be \"revoked\" rather than \"entered-in-error\".)."; 180 case UNKNOWN: 181 return "The authoring/source system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 182 case NULL: 183 return null; 184 default: 185 return "?"; 186 } 187 } 188 189 public String getDisplay() { 190 switch (this) { 191 case DRAFT: 192 return "Draft"; 193 case ACTIVE: 194 return "Active"; 195 case ONHOLD: 196 return "On Hold"; 197 case REVOKED: 198 return "Revoked"; 199 case COMPLETED: 200 return "Completed"; 201 case ENTEREDINERROR: 202 return "Entered in Error"; 203 case UNKNOWN: 204 return "Unknown"; 205 case NULL: 206 return null; 207 default: 208 return "?"; 209 } 210 } 211 } 212 213 public static class DeviceRequestStatusEnumFactory implements EnumFactory<DeviceRequestStatus> { 214 public DeviceRequestStatus fromCode(String codeString) throws IllegalArgumentException { 215 if (codeString == null || "".equals(codeString)) 216 if (codeString == null || "".equals(codeString)) 217 return null; 218 if ("draft".equals(codeString)) 219 return DeviceRequestStatus.DRAFT; 220 if ("active".equals(codeString)) 221 return DeviceRequestStatus.ACTIVE; 222 if ("on-hold".equals(codeString)) 223 return DeviceRequestStatus.ONHOLD; 224 if ("revoked".equals(codeString)) 225 return DeviceRequestStatus.REVOKED; 226 if ("completed".equals(codeString)) 227 return DeviceRequestStatus.COMPLETED; 228 if ("entered-in-error".equals(codeString)) 229 return DeviceRequestStatus.ENTEREDINERROR; 230 if ("unknown".equals(codeString)) 231 return DeviceRequestStatus.UNKNOWN; 232 throw new IllegalArgumentException("Unknown DeviceRequestStatus code '" + codeString + "'"); 233 } 234 235 public Enumeration<DeviceRequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 236 if (code == null) 237 return null; 238 if (code.isEmpty()) 239 return new Enumeration<DeviceRequestStatus>(this, DeviceRequestStatus.NULL, code); 240 String codeString = code.asStringValue(); 241 if (codeString == null || "".equals(codeString)) 242 return new Enumeration<DeviceRequestStatus>(this, DeviceRequestStatus.NULL, code); 243 if ("draft".equals(codeString)) 244 return new Enumeration<DeviceRequestStatus>(this, DeviceRequestStatus.DRAFT, code); 245 if ("active".equals(codeString)) 246 return new Enumeration<DeviceRequestStatus>(this, DeviceRequestStatus.ACTIVE, code); 247 if ("on-hold".equals(codeString)) 248 return new Enumeration<DeviceRequestStatus>(this, DeviceRequestStatus.ONHOLD, code); 249 if ("revoked".equals(codeString)) 250 return new Enumeration<DeviceRequestStatus>(this, DeviceRequestStatus.REVOKED, code); 251 if ("completed".equals(codeString)) 252 return new Enumeration<DeviceRequestStatus>(this, DeviceRequestStatus.COMPLETED, code); 253 if ("entered-in-error".equals(codeString)) 254 return new Enumeration<DeviceRequestStatus>(this, DeviceRequestStatus.ENTEREDINERROR, code); 255 if ("unknown".equals(codeString)) 256 return new Enumeration<DeviceRequestStatus>(this, DeviceRequestStatus.UNKNOWN, code); 257 throw new FHIRException("Unknown DeviceRequestStatus code '" + codeString + "'"); 258 } 259 260 public String toCode(DeviceRequestStatus code) { 261 if (code == DeviceRequestStatus.NULL) 262 return null; 263 if (code == DeviceRequestStatus.DRAFT) 264 return "draft"; 265 if (code == DeviceRequestStatus.ACTIVE) 266 return "active"; 267 if (code == DeviceRequestStatus.ONHOLD) 268 return "on-hold"; 269 if (code == DeviceRequestStatus.REVOKED) 270 return "revoked"; 271 if (code == DeviceRequestStatus.COMPLETED) 272 return "completed"; 273 if (code == DeviceRequestStatus.ENTEREDINERROR) 274 return "entered-in-error"; 275 if (code == DeviceRequestStatus.UNKNOWN) 276 return "unknown"; 277 return "?"; 278 } 279 280 public String toSystem(DeviceRequestStatus code) { 281 return code.getSystem(); 282 } 283 } 284 285 public enum RequestIntent { 286 /** 287 * The request is a suggestion made by someone/something that does not have an 288 * intention to ensure it occurs and without providing an authorization to act. 289 */ 290 PROPOSAL, 291 /** 292 * The request represents an intention to ensure something occurs without 293 * providing an authorization for others to act. 294 */ 295 PLAN, 296 /** 297 * The request represents a legally binding instruction authored by a Patient or 298 * RelatedPerson. 299 */ 300 DIRECTIVE, 301 /** 302 * The request represents a request/demand and authorization for action by a 303 * Practitioner. 304 */ 305 ORDER, 306 /** 307 * The request represents an original authorization for action. 308 */ 309 ORIGINALORDER, 310 /** 311 * The request represents an automatically generated supplemental authorization 312 * for action based on a parent authorization together with initial results of 313 * the action taken against that parent authorization. 314 */ 315 REFLEXORDER, 316 /** 317 * The request represents the view of an authorization instantiated by a 318 * fulfilling system representing the details of the fulfiller's intention to 319 * act upon a submitted order. 320 */ 321 FILLERORDER, 322 /** 323 * An order created in fulfillment of a broader order that represents the 324 * authorization for a single activity occurrence. E.g. The administration of a 325 * single dose of a drug. 326 */ 327 INSTANCEORDER, 328 /** 329 * The request represents a component or option for a RequestGroup that 330 * establishes timing, conditionality and/or other constraints among a set of 331 * requests. Refer to [[[RequestGroup]]] for additional information on how this 332 * status is used. 333 */ 334 OPTION, 335 /** 336 * added to help the parsers with the generic types 337 */ 338 NULL; 339 340 public static RequestIntent fromCode(String codeString) throws FHIRException { 341 if (codeString == null || "".equals(codeString)) 342 return null; 343 if ("proposal".equals(codeString)) 344 return PROPOSAL; 345 if ("plan".equals(codeString)) 346 return PLAN; 347 if ("directive".equals(codeString)) 348 return DIRECTIVE; 349 if ("order".equals(codeString)) 350 return ORDER; 351 if ("original-order".equals(codeString)) 352 return ORIGINALORDER; 353 if ("reflex-order".equals(codeString)) 354 return REFLEXORDER; 355 if ("filler-order".equals(codeString)) 356 return FILLERORDER; 357 if ("instance-order".equals(codeString)) 358 return INSTANCEORDER; 359 if ("option".equals(codeString)) 360 return OPTION; 361 if (Configuration.isAcceptInvalidEnums()) 362 return null; 363 else 364 throw new FHIRException("Unknown RequestIntent code '" + codeString + "'"); 365 } 366 367 public String toCode() { 368 switch (this) { 369 case PROPOSAL: 370 return "proposal"; 371 case PLAN: 372 return "plan"; 373 case DIRECTIVE: 374 return "directive"; 375 case ORDER: 376 return "order"; 377 case ORIGINALORDER: 378 return "original-order"; 379 case REFLEXORDER: 380 return "reflex-order"; 381 case FILLERORDER: 382 return "filler-order"; 383 case INSTANCEORDER: 384 return "instance-order"; 385 case OPTION: 386 return "option"; 387 case NULL: 388 return null; 389 default: 390 return "?"; 391 } 392 } 393 394 public String getSystem() { 395 switch (this) { 396 case PROPOSAL: 397 return "http://hl7.org/fhir/request-intent"; 398 case PLAN: 399 return "http://hl7.org/fhir/request-intent"; 400 case DIRECTIVE: 401 return "http://hl7.org/fhir/request-intent"; 402 case ORDER: 403 return "http://hl7.org/fhir/request-intent"; 404 case ORIGINALORDER: 405 return "http://hl7.org/fhir/request-intent"; 406 case REFLEXORDER: 407 return "http://hl7.org/fhir/request-intent"; 408 case FILLERORDER: 409 return "http://hl7.org/fhir/request-intent"; 410 case INSTANCEORDER: 411 return "http://hl7.org/fhir/request-intent"; 412 case OPTION: 413 return "http://hl7.org/fhir/request-intent"; 414 case NULL: 415 return null; 416 default: 417 return "?"; 418 } 419 } 420 421 public String getDefinition() { 422 switch (this) { 423 case PROPOSAL: 424 return "The request is a suggestion made by someone/something that does not have an intention to ensure it occurs and without providing an authorization to act."; 425 case PLAN: 426 return "The request represents an intention to ensure something occurs without providing an authorization for others to act."; 427 case DIRECTIVE: 428 return "The request represents a legally binding instruction authored by a Patient or RelatedPerson."; 429 case ORDER: 430 return "The request represents a request/demand and authorization for action by a Practitioner."; 431 case ORIGINALORDER: 432 return "The request represents an original authorization for action."; 433 case REFLEXORDER: 434 return "The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization."; 435 case FILLERORDER: 436 return "The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order."; 437 case INSTANCEORDER: 438 return "An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug."; 439 case OPTION: 440 return "The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests. Refer to [[[RequestGroup]]] for additional information on how this status is used."; 441 case NULL: 442 return null; 443 default: 444 return "?"; 445 } 446 } 447 448 public String getDisplay() { 449 switch (this) { 450 case PROPOSAL: 451 return "Proposal"; 452 case PLAN: 453 return "Plan"; 454 case DIRECTIVE: 455 return "Directive"; 456 case ORDER: 457 return "Order"; 458 case ORIGINALORDER: 459 return "Original Order"; 460 case REFLEXORDER: 461 return "Reflex Order"; 462 case FILLERORDER: 463 return "Filler Order"; 464 case INSTANCEORDER: 465 return "Instance Order"; 466 case OPTION: 467 return "Option"; 468 case NULL: 469 return null; 470 default: 471 return "?"; 472 } 473 } 474 } 475 476 public static class RequestIntentEnumFactory implements EnumFactory<RequestIntent> { 477 public RequestIntent fromCode(String codeString) throws IllegalArgumentException { 478 if (codeString == null || "".equals(codeString)) 479 if (codeString == null || "".equals(codeString)) 480 return null; 481 if ("proposal".equals(codeString)) 482 return RequestIntent.PROPOSAL; 483 if ("plan".equals(codeString)) 484 return RequestIntent.PLAN; 485 if ("directive".equals(codeString)) 486 return RequestIntent.DIRECTIVE; 487 if ("order".equals(codeString)) 488 return RequestIntent.ORDER; 489 if ("original-order".equals(codeString)) 490 return RequestIntent.ORIGINALORDER; 491 if ("reflex-order".equals(codeString)) 492 return RequestIntent.REFLEXORDER; 493 if ("filler-order".equals(codeString)) 494 return RequestIntent.FILLERORDER; 495 if ("instance-order".equals(codeString)) 496 return RequestIntent.INSTANCEORDER; 497 if ("option".equals(codeString)) 498 return RequestIntent.OPTION; 499 throw new IllegalArgumentException("Unknown RequestIntent code '" + codeString + "'"); 500 } 501 502 public Enumeration<RequestIntent> fromType(PrimitiveType<?> code) throws FHIRException { 503 if (code == null) 504 return null; 505 if (code.isEmpty()) 506 return new Enumeration<RequestIntent>(this, RequestIntent.NULL, code); 507 String codeString = code.asStringValue(); 508 if (codeString == null || "".equals(codeString)) 509 return new Enumeration<RequestIntent>(this, RequestIntent.NULL, code); 510 if ("proposal".equals(codeString)) 511 return new Enumeration<RequestIntent>(this, RequestIntent.PROPOSAL, code); 512 if ("plan".equals(codeString)) 513 return new Enumeration<RequestIntent>(this, RequestIntent.PLAN, code); 514 if ("directive".equals(codeString)) 515 return new Enumeration<RequestIntent>(this, RequestIntent.DIRECTIVE, code); 516 if ("order".equals(codeString)) 517 return new Enumeration<RequestIntent>(this, RequestIntent.ORDER, code); 518 if ("original-order".equals(codeString)) 519 return new Enumeration<RequestIntent>(this, RequestIntent.ORIGINALORDER, code); 520 if ("reflex-order".equals(codeString)) 521 return new Enumeration<RequestIntent>(this, RequestIntent.REFLEXORDER, code); 522 if ("filler-order".equals(codeString)) 523 return new Enumeration<RequestIntent>(this, RequestIntent.FILLERORDER, code); 524 if ("instance-order".equals(codeString)) 525 return new Enumeration<RequestIntent>(this, RequestIntent.INSTANCEORDER, code); 526 if ("option".equals(codeString)) 527 return new Enumeration<RequestIntent>(this, RequestIntent.OPTION, code); 528 throw new FHIRException("Unknown RequestIntent code '" + codeString + "'"); 529 } 530 531 public String toCode(RequestIntent code) { 532 if (code == RequestIntent.NULL) 533 return null; 534 if (code == RequestIntent.PROPOSAL) 535 return "proposal"; 536 if (code == RequestIntent.PLAN) 537 return "plan"; 538 if (code == RequestIntent.DIRECTIVE) 539 return "directive"; 540 if (code == RequestIntent.ORDER) 541 return "order"; 542 if (code == RequestIntent.ORIGINALORDER) 543 return "original-order"; 544 if (code == RequestIntent.REFLEXORDER) 545 return "reflex-order"; 546 if (code == RequestIntent.FILLERORDER) 547 return "filler-order"; 548 if (code == RequestIntent.INSTANCEORDER) 549 return "instance-order"; 550 if (code == RequestIntent.OPTION) 551 return "option"; 552 return "?"; 553 } 554 555 public String toSystem(RequestIntent code) { 556 return code.getSystem(); 557 } 558 } 559 560 public enum RequestPriority { 561 /** 562 * The request has normal priority. 563 */ 564 ROUTINE, 565 /** 566 * The request should be actioned promptly - higher priority than routine. 567 */ 568 URGENT, 569 /** 570 * The request should be actioned as soon as possible - higher priority than 571 * urgent. 572 */ 573 ASAP, 574 /** 575 * The request should be actioned immediately - highest possible priority. E.g. 576 * an emergency. 577 */ 578 STAT, 579 /** 580 * added to help the parsers with the generic types 581 */ 582 NULL; 583 584 public static RequestPriority fromCode(String codeString) throws FHIRException { 585 if (codeString == null || "".equals(codeString)) 586 return null; 587 if ("routine".equals(codeString)) 588 return ROUTINE; 589 if ("urgent".equals(codeString)) 590 return URGENT; 591 if ("asap".equals(codeString)) 592 return ASAP; 593 if ("stat".equals(codeString)) 594 return STAT; 595 if (Configuration.isAcceptInvalidEnums()) 596 return null; 597 else 598 throw new FHIRException("Unknown RequestPriority code '" + codeString + "'"); 599 } 600 601 public String toCode() { 602 switch (this) { 603 case ROUTINE: 604 return "routine"; 605 case URGENT: 606 return "urgent"; 607 case ASAP: 608 return "asap"; 609 case STAT: 610 return "stat"; 611 case NULL: 612 return null; 613 default: 614 return "?"; 615 } 616 } 617 618 public String getSystem() { 619 switch (this) { 620 case ROUTINE: 621 return "http://hl7.org/fhir/request-priority"; 622 case URGENT: 623 return "http://hl7.org/fhir/request-priority"; 624 case ASAP: 625 return "http://hl7.org/fhir/request-priority"; 626 case STAT: 627 return "http://hl7.org/fhir/request-priority"; 628 case NULL: 629 return null; 630 default: 631 return "?"; 632 } 633 } 634 635 public String getDefinition() { 636 switch (this) { 637 case ROUTINE: 638 return "The request has normal priority."; 639 case URGENT: 640 return "The request should be actioned promptly - higher priority than routine."; 641 case ASAP: 642 return "The request should be actioned as soon as possible - higher priority than urgent."; 643 case STAT: 644 return "The request should be actioned immediately - highest possible priority. E.g. an emergency."; 645 case NULL: 646 return null; 647 default: 648 return "?"; 649 } 650 } 651 652 public String getDisplay() { 653 switch (this) { 654 case ROUTINE: 655 return "Routine"; 656 case URGENT: 657 return "Urgent"; 658 case ASAP: 659 return "ASAP"; 660 case STAT: 661 return "STAT"; 662 case NULL: 663 return null; 664 default: 665 return "?"; 666 } 667 } 668 } 669 670 public static class RequestPriorityEnumFactory implements EnumFactory<RequestPriority> { 671 public RequestPriority fromCode(String codeString) throws IllegalArgumentException { 672 if (codeString == null || "".equals(codeString)) 673 if (codeString == null || "".equals(codeString)) 674 return null; 675 if ("routine".equals(codeString)) 676 return RequestPriority.ROUTINE; 677 if ("urgent".equals(codeString)) 678 return RequestPriority.URGENT; 679 if ("asap".equals(codeString)) 680 return RequestPriority.ASAP; 681 if ("stat".equals(codeString)) 682 return RequestPriority.STAT; 683 throw new IllegalArgumentException("Unknown RequestPriority code '" + codeString + "'"); 684 } 685 686 public Enumeration<RequestPriority> fromType(PrimitiveType<?> code) throws FHIRException { 687 if (code == null) 688 return null; 689 if (code.isEmpty()) 690 return new Enumeration<RequestPriority>(this, RequestPriority.NULL, code); 691 String codeString = code.asStringValue(); 692 if (codeString == null || "".equals(codeString)) 693 return new Enumeration<RequestPriority>(this, RequestPriority.NULL, code); 694 if ("routine".equals(codeString)) 695 return new Enumeration<RequestPriority>(this, RequestPriority.ROUTINE, code); 696 if ("urgent".equals(codeString)) 697 return new Enumeration<RequestPriority>(this, RequestPriority.URGENT, code); 698 if ("asap".equals(codeString)) 699 return new Enumeration<RequestPriority>(this, RequestPriority.ASAP, code); 700 if ("stat".equals(codeString)) 701 return new Enumeration<RequestPriority>(this, RequestPriority.STAT, code); 702 throw new FHIRException("Unknown RequestPriority code '" + codeString + "'"); 703 } 704 705 public String toCode(RequestPriority code) { 706 if (code == RequestPriority.NULL) 707 return null; 708 if (code == RequestPriority.ROUTINE) 709 return "routine"; 710 if (code == RequestPriority.URGENT) 711 return "urgent"; 712 if (code == RequestPriority.ASAP) 713 return "asap"; 714 if (code == RequestPriority.STAT) 715 return "stat"; 716 return "?"; 717 } 718 719 public String toSystem(RequestPriority code) { 720 return code.getSystem(); 721 } 722 } 723 724 @Block() 725 public static class DeviceRequestParameterComponent extends BackboneElement implements IBaseBackboneElement { 726 /** 727 * A code or string that identifies the device detail being asserted. 728 */ 729 @Child(name = "code", type = { 730 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 731 @Description(shortDefinition = "Device detail", formalDefinition = "A code or string that identifies the device detail being asserted.") 732 protected CodeableConcept code; 733 734 /** 735 * The value of the device detail. 736 */ 737 @Child(name = "value", type = { CodeableConcept.class, Quantity.class, Range.class, 738 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 739 @Description(shortDefinition = "Value of detail", formalDefinition = "The value of the device detail.") 740 protected Type value; 741 742 private static final long serialVersionUID = 884525025L; 743 744 /** 745 * Constructor 746 */ 747 public DeviceRequestParameterComponent() { 748 super(); 749 } 750 751 /** 752 * @return {@link #code} (A code or string that identifies the device detail 753 * being asserted.) 754 */ 755 public CodeableConcept getCode() { 756 if (this.code == null) 757 if (Configuration.errorOnAutoCreate()) 758 throw new Error("Attempt to auto-create DeviceRequestParameterComponent.code"); 759 else if (Configuration.doAutoCreate()) 760 this.code = new CodeableConcept(); // cc 761 return this.code; 762 } 763 764 public boolean hasCode() { 765 return this.code != null && !this.code.isEmpty(); 766 } 767 768 /** 769 * @param value {@link #code} (A code or string that identifies the device 770 * detail being asserted.) 771 */ 772 public DeviceRequestParameterComponent setCode(CodeableConcept value) { 773 this.code = value; 774 return this; 775 } 776 777 /** 778 * @return {@link #value} (The value of the device detail.) 779 */ 780 public Type getValue() { 781 return this.value; 782 } 783 784 /** 785 * @return {@link #value} (The value of the device detail.) 786 */ 787 public CodeableConcept getValueCodeableConcept() throws FHIRException { 788 if (this.value == null) 789 this.value = new CodeableConcept(); 790 if (!(this.value instanceof CodeableConcept)) 791 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 792 + this.value.getClass().getName() + " was encountered"); 793 return (CodeableConcept) this.value; 794 } 795 796 public boolean hasValueCodeableConcept() { 797 return this != null && this.value instanceof CodeableConcept; 798 } 799 800 /** 801 * @return {@link #value} (The value of the device detail.) 802 */ 803 public Quantity getValueQuantity() throws FHIRException { 804 if (this.value == null) 805 this.value = new Quantity(); 806 if (!(this.value instanceof Quantity)) 807 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName() 808 + " was encountered"); 809 return (Quantity) this.value; 810 } 811 812 public boolean hasValueQuantity() { 813 return this != null && this.value instanceof Quantity; 814 } 815 816 /** 817 * @return {@link #value} (The value of the device detail.) 818 */ 819 public Range getValueRange() throws FHIRException { 820 if (this.value == null) 821 this.value = new Range(); 822 if (!(this.value instanceof Range)) 823 throw new FHIRException( 824 "Type mismatch: the type Range was expected, but " + this.value.getClass().getName() + " was encountered"); 825 return (Range) this.value; 826 } 827 828 public boolean hasValueRange() { 829 return this != null && this.value instanceof Range; 830 } 831 832 /** 833 * @return {@link #value} (The value of the device detail.) 834 */ 835 public BooleanType getValueBooleanType() throws FHIRException { 836 if (this.value == null) 837 this.value = new BooleanType(); 838 if (!(this.value instanceof BooleanType)) 839 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 840 + this.value.getClass().getName() + " was encountered"); 841 return (BooleanType) this.value; 842 } 843 844 public boolean hasValueBooleanType() { 845 return this != null && this.value instanceof BooleanType; 846 } 847 848 public boolean hasValue() { 849 return this.value != null && !this.value.isEmpty(); 850 } 851 852 /** 853 * @param value {@link #value} (The value of the device detail.) 854 */ 855 public DeviceRequestParameterComponent setValue(Type value) { 856 if (value != null && !(value instanceof CodeableConcept || value instanceof Quantity || value instanceof Range 857 || value instanceof BooleanType)) 858 throw new Error("Not the right type for DeviceRequest.parameter.value[x]: " + value.fhirType()); 859 this.value = value; 860 return this; 861 } 862 863 protected void listChildren(List<Property> children) { 864 super.listChildren(children); 865 children.add(new Property("code", "CodeableConcept", 866 "A code or string that identifies the device detail being asserted.", 0, 1, code)); 867 children.add(new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", "The value of the device detail.", 868 0, 1, value)); 869 } 870 871 @Override 872 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 873 switch (_hash) { 874 case 3059181: 875 /* code */ return new Property("code", "CodeableConcept", 876 "A code or string that identifies the device detail being asserted.", 0, 1, code); 877 case -1410166417: 878 /* value[x] */ return new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", 879 "The value of the device detail.", 0, 1, value); 880 case 111972721: 881 /* value */ return new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", 882 "The value of the device detail.", 0, 1, value); 883 case 924902896: 884 /* valueCodeableConcept */ return new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", 885 "The value of the device detail.", 0, 1, value); 886 case -2029823716: 887 /* valueQuantity */ return new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", 888 "The value of the device detail.", 0, 1, value); 889 case 2030761548: 890 /* valueRange */ return new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", 891 "The value of the device detail.", 0, 1, value); 892 case 733421943: 893 /* valueBoolean */ return new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", 894 "The value of the device detail.", 0, 1, value); 895 default: 896 return super.getNamedProperty(_hash, _name, _checkValid); 897 } 898 899 } 900 901 @Override 902 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 903 switch (hash) { 904 case 3059181: 905 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 906 case 111972721: 907 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 908 default: 909 return super.getProperty(hash, name, checkValid); 910 } 911 912 } 913 914 @Override 915 public Base setProperty(int hash, String name, Base value) throws FHIRException { 916 switch (hash) { 917 case 3059181: // code 918 this.code = castToCodeableConcept(value); // CodeableConcept 919 return value; 920 case 111972721: // value 921 this.value = castToType(value); // Type 922 return value; 923 default: 924 return super.setProperty(hash, name, value); 925 } 926 927 } 928 929 @Override 930 public Base setProperty(String name, Base value) throws FHIRException { 931 if (name.equals("code")) { 932 this.code = castToCodeableConcept(value); // CodeableConcept 933 } else if (name.equals("value[x]")) { 934 this.value = castToType(value); // Type 935 } else 936 return super.setProperty(name, value); 937 return value; 938 } 939 940 @Override 941 public void removeChild(String name, Base value) throws FHIRException { 942 if (name.equals("code")) { 943 this.code = null; 944 } else if (name.equals("value[x]")) { 945 this.value = null; 946 } else 947 super.removeChild(name, value); 948 949 } 950 951 @Override 952 public Base makeProperty(int hash, String name) throws FHIRException { 953 switch (hash) { 954 case 3059181: 955 return getCode(); 956 case -1410166417: 957 return getValue(); 958 case 111972721: 959 return getValue(); 960 default: 961 return super.makeProperty(hash, name); 962 } 963 964 } 965 966 @Override 967 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 968 switch (hash) { 969 case 3059181: 970 /* code */ return new String[] { "CodeableConcept" }; 971 case 111972721: 972 /* value */ return new String[] { "CodeableConcept", "Quantity", "Range", "boolean" }; 973 default: 974 return super.getTypesForProperty(hash, name); 975 } 976 977 } 978 979 @Override 980 public Base addChild(String name) throws FHIRException { 981 if (name.equals("code")) { 982 this.code = new CodeableConcept(); 983 return this.code; 984 } else if (name.equals("valueCodeableConcept")) { 985 this.value = new CodeableConcept(); 986 return this.value; 987 } else if (name.equals("valueQuantity")) { 988 this.value = new Quantity(); 989 return this.value; 990 } else if (name.equals("valueRange")) { 991 this.value = new Range(); 992 return this.value; 993 } else if (name.equals("valueBoolean")) { 994 this.value = new BooleanType(); 995 return this.value; 996 } else 997 return super.addChild(name); 998 } 999 1000 public DeviceRequestParameterComponent copy() { 1001 DeviceRequestParameterComponent dst = new DeviceRequestParameterComponent(); 1002 copyValues(dst); 1003 return dst; 1004 } 1005 1006 public void copyValues(DeviceRequestParameterComponent dst) { 1007 super.copyValues(dst); 1008 dst.code = code == null ? null : code.copy(); 1009 dst.value = value == null ? null : value.copy(); 1010 } 1011 1012 @Override 1013 public boolean equalsDeep(Base other_) { 1014 if (!super.equalsDeep(other_)) 1015 return false; 1016 if (!(other_ instanceof DeviceRequestParameterComponent)) 1017 return false; 1018 DeviceRequestParameterComponent o = (DeviceRequestParameterComponent) other_; 1019 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true); 1020 } 1021 1022 @Override 1023 public boolean equalsShallow(Base other_) { 1024 if (!super.equalsShallow(other_)) 1025 return false; 1026 if (!(other_ instanceof DeviceRequestParameterComponent)) 1027 return false; 1028 DeviceRequestParameterComponent o = (DeviceRequestParameterComponent) other_; 1029 return true; 1030 } 1031 1032 public boolean isEmpty() { 1033 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value); 1034 } 1035 1036 public String fhirType() { 1037 return "DeviceRequest.parameter"; 1038 1039 } 1040 1041 } 1042 1043 /** 1044 * Identifiers assigned to this order by the orderer or by the receiver. 1045 */ 1046 @Child(name = "identifier", type = { 1047 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1048 @Description(shortDefinition = "External Request identifier", formalDefinition = "Identifiers assigned to this order by the orderer or by the receiver.") 1049 protected List<Identifier> identifier; 1050 1051 /** 1052 * The URL pointing to a FHIR-defined protocol, guideline, orderset or other 1053 * definition that is adhered to in whole or in part by this DeviceRequest. 1054 */ 1055 @Child(name = "instantiatesCanonical", type = { 1056 CanonicalType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1057 @Description(shortDefinition = "Instantiates FHIR protocol or definition", formalDefinition = "The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this DeviceRequest.") 1058 protected List<CanonicalType> instantiatesCanonical; 1059 1060 /** 1061 * The URL pointing to an externally maintained protocol, guideline, orderset or 1062 * other definition that is adhered to in whole or in part by this 1063 * DeviceRequest. 1064 */ 1065 @Child(name = "instantiatesUri", type = { 1066 UriType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1067 @Description(shortDefinition = "Instantiates external protocol or definition", formalDefinition = "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this DeviceRequest.") 1068 protected List<UriType> instantiatesUri; 1069 1070 /** 1071 * Plan/proposal/order fulfilled by this request. 1072 */ 1073 @Child(name = "basedOn", type = { 1074 Reference.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1075 @Description(shortDefinition = "What request fulfills", formalDefinition = "Plan/proposal/order fulfilled by this request.") 1076 protected List<Reference> basedOn; 1077 /** 1078 * The actual objects that are the target of the reference (Plan/proposal/order 1079 * fulfilled by this request.) 1080 */ 1081 protected List<Resource> basedOnTarget; 1082 1083 /** 1084 * The request takes the place of the referenced completed or terminated 1085 * request(s). 1086 */ 1087 @Child(name = "priorRequest", type = { 1088 Reference.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1089 @Description(shortDefinition = "What request replaces", formalDefinition = "The request takes the place of the referenced completed or terminated request(s).") 1090 protected List<Reference> priorRequest; 1091 /** 1092 * The actual objects that are the target of the reference (The request takes 1093 * the place of the referenced completed or terminated request(s).) 1094 */ 1095 protected List<Resource> priorRequestTarget; 1096 1097 /** 1098 * Composite request this is part of. 1099 */ 1100 @Child(name = "groupIdentifier", type = { 1101 Identifier.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1102 @Description(shortDefinition = "Identifier of composite request", formalDefinition = "Composite request this is part of.") 1103 protected Identifier groupIdentifier; 1104 1105 /** 1106 * The status of the request. 1107 */ 1108 @Child(name = "status", type = { CodeType.class }, order = 6, min = 0, max = 1, modifier = true, summary = true) 1109 @Description(shortDefinition = "draft | active | on-hold | revoked | completed | entered-in-error | unknown", formalDefinition = "The status of the request.") 1110 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-status") 1111 protected Enumeration<DeviceRequestStatus> status; 1112 1113 /** 1114 * Whether the request is a proposal, plan, an original order or a reflex order. 1115 */ 1116 @Child(name = "intent", type = { CodeType.class }, order = 7, min = 1, max = 1, modifier = true, summary = true) 1117 @Description(shortDefinition = "proposal | plan | directive | order | original-order | reflex-order | filler-order | instance-order | option", formalDefinition = "Whether the request is a proposal, plan, an original order or a reflex order.") 1118 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-intent") 1119 protected Enumeration<RequestIntent> intent; 1120 1121 /** 1122 * Indicates how quickly the {{title}} should be addressed with respect to other 1123 * requests. 1124 */ 1125 @Child(name = "priority", type = { CodeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1126 @Description(shortDefinition = "routine | urgent | asap | stat", formalDefinition = "Indicates how quickly the {{title}} should be addressed with respect to other requests.") 1127 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-priority") 1128 protected Enumeration<RequestPriority> priority; 1129 1130 /** 1131 * The details of the device to be used. 1132 */ 1133 @Child(name = "code", type = { Device.class, 1134 CodeableConcept.class }, order = 9, min = 1, max = 1, modifier = false, summary = true) 1135 @Description(shortDefinition = "Device requested", formalDefinition = "The details of the device to be used.") 1136 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/device-kind") 1137 protected Type code; 1138 1139 /** 1140 * Specific parameters for the ordered item. For example, the prism value for 1141 * lenses. 1142 */ 1143 @Child(name = "parameter", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1144 @Description(shortDefinition = "Device details", formalDefinition = "Specific parameters for the ordered item. For example, the prism value for lenses.") 1145 protected List<DeviceRequestParameterComponent> parameter; 1146 1147 /** 1148 * The patient who will use the device. 1149 */ 1150 @Child(name = "subject", type = { Patient.class, Group.class, Location.class, 1151 Device.class }, order = 11, min = 1, max = 1, modifier = false, summary = true) 1152 @Description(shortDefinition = "Focus of request", formalDefinition = "The patient who will use the device.") 1153 protected Reference subject; 1154 1155 /** 1156 * The actual object that is the target of the reference (The patient who will 1157 * use the device.) 1158 */ 1159 protected Resource subjectTarget; 1160 1161 /** 1162 * An encounter that provides additional context in which this request is made. 1163 */ 1164 @Child(name = "encounter", type = { Encounter.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 1165 @Description(shortDefinition = "Encounter motivating request", formalDefinition = "An encounter that provides additional context in which this request is made.") 1166 protected Reference encounter; 1167 1168 /** 1169 * The actual object that is the target of the reference (An encounter that 1170 * provides additional context in which this request is made.) 1171 */ 1172 protected Encounter encounterTarget; 1173 1174 /** 1175 * The timing schedule for the use of the device. The Schedule data type allows 1176 * many different expressions, for example. "Every 8 hours"; "Three times a 1177 * day"; "1/2 an hour before breakfast for 10 days from 23-Dec 2011:"; "15 Oct 1178 * 2013, 17 Oct 2013 and 1 Nov 2013". 1179 */ 1180 @Child(name = "occurrence", type = { DateTimeType.class, Period.class, 1181 Timing.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 1182 @Description(shortDefinition = "Desired time or schedule for use", formalDefinition = "The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".") 1183 protected Type occurrence; 1184 1185 /** 1186 * When the request transitioned to being actionable. 1187 */ 1188 @Child(name = "authoredOn", type = { 1189 DateTimeType.class }, order = 14, min = 0, max = 1, modifier = false, summary = true) 1190 @Description(shortDefinition = "When recorded", formalDefinition = "When the request transitioned to being actionable.") 1191 protected DateTimeType authoredOn; 1192 1193 /** 1194 * The individual who initiated the request and has responsibility for its 1195 * activation. 1196 */ 1197 @Child(name = "requester", type = { Device.class, Practitioner.class, PractitionerRole.class, 1198 Organization.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 1199 @Description(shortDefinition = "Who/what is requesting diagnostics", formalDefinition = "The individual who initiated the request and has responsibility for its activation.") 1200 protected Reference requester; 1201 1202 /** 1203 * The actual object that is the target of the reference (The individual who 1204 * initiated the request and has responsibility for its activation.) 1205 */ 1206 protected Resource requesterTarget; 1207 1208 /** 1209 * Desired type of performer for doing the diagnostic testing. 1210 */ 1211 @Child(name = "performerType", type = { 1212 CodeableConcept.class }, order = 16, min = 0, max = 1, modifier = false, summary = true) 1213 @Description(shortDefinition = "Filler role", formalDefinition = "Desired type of performer for doing the diagnostic testing.") 1214 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/participant-role") 1215 protected CodeableConcept performerType; 1216 1217 /** 1218 * The desired performer for doing the diagnostic testing. 1219 */ 1220 @Child(name = "performer", type = { Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class, 1221 HealthcareService.class, Patient.class, Device.class, 1222 RelatedPerson.class }, order = 17, min = 0, max = 1, modifier = false, summary = true) 1223 @Description(shortDefinition = "Requested Filler", formalDefinition = "The desired performer for doing the diagnostic testing.") 1224 protected Reference performer; 1225 1226 /** 1227 * The actual object that is the target of the reference (The desired performer 1228 * for doing the diagnostic testing.) 1229 */ 1230 protected Resource performerTarget; 1231 1232 /** 1233 * Reason or justification for the use of this device. 1234 */ 1235 @Child(name = "reasonCode", type = { 1236 CodeableConcept.class }, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1237 @Description(shortDefinition = "Coded Reason for request", formalDefinition = "Reason or justification for the use of this device.") 1238 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/condition-code") 1239 protected List<CodeableConcept> reasonCode; 1240 1241 /** 1242 * Reason or justification for the use of this device. 1243 */ 1244 @Child(name = "reasonReference", type = { Condition.class, Observation.class, DiagnosticReport.class, 1245 DocumentReference.class }, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1246 @Description(shortDefinition = "Linked Reason for request", formalDefinition = "Reason or justification for the use of this device.") 1247 protected List<Reference> reasonReference; 1248 /** 1249 * The actual objects that are the target of the reference (Reason or 1250 * justification for the use of this device.) 1251 */ 1252 protected List<Resource> reasonReferenceTarget; 1253 1254 /** 1255 * Insurance plans, coverage extensions, pre-authorizations and/or 1256 * pre-determinations that may be required for delivering the requested service. 1257 */ 1258 @Child(name = "insurance", type = { Coverage.class, 1259 ClaimResponse.class }, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1260 @Description(shortDefinition = "Associated insurance coverage", formalDefinition = "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be required for delivering the requested service.") 1261 protected List<Reference> insurance; 1262 /** 1263 * The actual objects that are the target of the reference (Insurance plans, 1264 * coverage extensions, pre-authorizations and/or pre-determinations that may be 1265 * required for delivering the requested service.) 1266 */ 1267 protected List<Resource> insuranceTarget; 1268 1269 /** 1270 * Additional clinical information about the patient that may influence the 1271 * request fulfilment. For example, this may include where on the subject's body 1272 * the device will be used (i.e. the target site). 1273 */ 1274 @Child(name = "supportingInfo", type = { 1275 Reference.class }, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1276 @Description(shortDefinition = "Additional clinical information", formalDefinition = "Additional clinical information about the patient that may influence the request fulfilment. For example, this may include where on the subject's body the device will be used (i.e. the target site).") 1277 protected List<Reference> supportingInfo; 1278 /** 1279 * The actual objects that are the target of the reference (Additional clinical 1280 * information about the patient that may influence the request fulfilment. For 1281 * example, this may include where on the subject's body the device will be used 1282 * (i.e. the target site).) 1283 */ 1284 protected List<Resource> supportingInfoTarget; 1285 1286 /** 1287 * Details about this request that were not represented at all or sufficiently 1288 * in one of the attributes provided in a class. These may include for example a 1289 * comment, an instruction, or a note associated with the statement. 1290 */ 1291 @Child(name = "note", type = { 1292 Annotation.class }, order = 22, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1293 @Description(shortDefinition = "Notes or comments", formalDefinition = "Details about this request that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.") 1294 protected List<Annotation> note; 1295 1296 /** 1297 * Key events in the history of the request. 1298 */ 1299 @Child(name = "relevantHistory", type = { 1300 Provenance.class }, order = 23, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1301 @Description(shortDefinition = "Request provenance", formalDefinition = "Key events in the history of the request.") 1302 protected List<Reference> relevantHistory; 1303 /** 1304 * The actual objects that are the target of the reference (Key events in the 1305 * history of the request.) 1306 */ 1307 protected List<Provenance> relevantHistoryTarget; 1308 1309 private static final long serialVersionUID = 1484452423L; 1310 1311 /** 1312 * Constructor 1313 */ 1314 public DeviceRequest() { 1315 super(); 1316 } 1317 1318 /** 1319 * Constructor 1320 */ 1321 public DeviceRequest(Enumeration<RequestIntent> intent, Type code, Reference subject) { 1322 super(); 1323 this.intent = intent; 1324 this.code = code; 1325 this.subject = subject; 1326 } 1327 1328 /** 1329 * @return {@link #identifier} (Identifiers assigned to this order by the 1330 * orderer or by the receiver.) 1331 */ 1332 public List<Identifier> getIdentifier() { 1333 if (this.identifier == null) 1334 this.identifier = new ArrayList<Identifier>(); 1335 return this.identifier; 1336 } 1337 1338 /** 1339 * @return Returns a reference to <code>this</code> for easy method chaining 1340 */ 1341 public DeviceRequest setIdentifier(List<Identifier> theIdentifier) { 1342 this.identifier = theIdentifier; 1343 return this; 1344 } 1345 1346 public boolean hasIdentifier() { 1347 if (this.identifier == null) 1348 return false; 1349 for (Identifier item : this.identifier) 1350 if (!item.isEmpty()) 1351 return true; 1352 return false; 1353 } 1354 1355 public Identifier addIdentifier() { // 3 1356 Identifier t = new Identifier(); 1357 if (this.identifier == null) 1358 this.identifier = new ArrayList<Identifier>(); 1359 this.identifier.add(t); 1360 return t; 1361 } 1362 1363 public DeviceRequest addIdentifier(Identifier t) { // 3 1364 if (t == null) 1365 return this; 1366 if (this.identifier == null) 1367 this.identifier = new ArrayList<Identifier>(); 1368 this.identifier.add(t); 1369 return this; 1370 } 1371 1372 /** 1373 * @return The first repetition of repeating field {@link #identifier}, creating 1374 * it if it does not already exist 1375 */ 1376 public Identifier getIdentifierFirstRep() { 1377 if (getIdentifier().isEmpty()) { 1378 addIdentifier(); 1379 } 1380 return getIdentifier().get(0); 1381 } 1382 1383 /** 1384 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined 1385 * protocol, guideline, orderset or other definition that is adhered to 1386 * in whole or in part by this DeviceRequest.) 1387 */ 1388 public List<CanonicalType> getInstantiatesCanonical() { 1389 if (this.instantiatesCanonical == null) 1390 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1391 return this.instantiatesCanonical; 1392 } 1393 1394 /** 1395 * @return Returns a reference to <code>this</code> for easy method chaining 1396 */ 1397 public DeviceRequest setInstantiatesCanonical(List<CanonicalType> theInstantiatesCanonical) { 1398 this.instantiatesCanonical = theInstantiatesCanonical; 1399 return this; 1400 } 1401 1402 public boolean hasInstantiatesCanonical() { 1403 if (this.instantiatesCanonical == null) 1404 return false; 1405 for (CanonicalType item : this.instantiatesCanonical) 1406 if (!item.isEmpty()) 1407 return true; 1408 return false; 1409 } 1410 1411 /** 1412 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined 1413 * protocol, guideline, orderset or other definition that is adhered to 1414 * in whole or in part by this DeviceRequest.) 1415 */ 1416 public CanonicalType addInstantiatesCanonicalElement() {// 2 1417 CanonicalType t = new CanonicalType(); 1418 if (this.instantiatesCanonical == null) 1419 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1420 this.instantiatesCanonical.add(t); 1421 return t; 1422 } 1423 1424 /** 1425 * @param value {@link #instantiatesCanonical} (The URL pointing to a 1426 * FHIR-defined protocol, guideline, orderset or other definition 1427 * that is adhered to in whole or in part by this DeviceRequest.) 1428 */ 1429 public DeviceRequest addInstantiatesCanonical(String value) { // 1 1430 CanonicalType t = new CanonicalType(); 1431 t.setValue(value); 1432 if (this.instantiatesCanonical == null) 1433 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1434 this.instantiatesCanonical.add(t); 1435 return this; 1436 } 1437 1438 /** 1439 * @param value {@link #instantiatesCanonical} (The URL pointing to a 1440 * FHIR-defined protocol, guideline, orderset or other definition 1441 * that is adhered to in whole or in part by this DeviceRequest.) 1442 */ 1443 public boolean hasInstantiatesCanonical(String value) { 1444 if (this.instantiatesCanonical == null) 1445 return false; 1446 for (CanonicalType v : this.instantiatesCanonical) 1447 if (v.getValue().equals(value)) // canonical(ActivityDefinition|PlanDefinition) 1448 return true; 1449 return false; 1450 } 1451 1452 /** 1453 * @return {@link #instantiatesUri} (The URL pointing to an externally 1454 * maintained protocol, guideline, orderset or other definition that is 1455 * adhered to in whole or in part by this DeviceRequest.) 1456 */ 1457 public List<UriType> getInstantiatesUri() { 1458 if (this.instantiatesUri == null) 1459 this.instantiatesUri = new ArrayList<UriType>(); 1460 return this.instantiatesUri; 1461 } 1462 1463 /** 1464 * @return Returns a reference to <code>this</code> for easy method chaining 1465 */ 1466 public DeviceRequest setInstantiatesUri(List<UriType> theInstantiatesUri) { 1467 this.instantiatesUri = theInstantiatesUri; 1468 return this; 1469 } 1470 1471 public boolean hasInstantiatesUri() { 1472 if (this.instantiatesUri == null) 1473 return false; 1474 for (UriType item : this.instantiatesUri) 1475 if (!item.isEmpty()) 1476 return true; 1477 return false; 1478 } 1479 1480 /** 1481 * @return {@link #instantiatesUri} (The URL pointing to an externally 1482 * maintained protocol, guideline, orderset or other definition that is 1483 * adhered to in whole or in part by this DeviceRequest.) 1484 */ 1485 public UriType addInstantiatesUriElement() {// 2 1486 UriType t = new UriType(); 1487 if (this.instantiatesUri == null) 1488 this.instantiatesUri = new ArrayList<UriType>(); 1489 this.instantiatesUri.add(t); 1490 return t; 1491 } 1492 1493 /** 1494 * @param value {@link #instantiatesUri} (The URL pointing to an externally 1495 * maintained protocol, guideline, orderset or other definition 1496 * that is adhered to in whole or in part by this DeviceRequest.) 1497 */ 1498 public DeviceRequest addInstantiatesUri(String value) { // 1 1499 UriType t = new UriType(); 1500 t.setValue(value); 1501 if (this.instantiatesUri == null) 1502 this.instantiatesUri = new ArrayList<UriType>(); 1503 this.instantiatesUri.add(t); 1504 return this; 1505 } 1506 1507 /** 1508 * @param value {@link #instantiatesUri} (The URL pointing to an externally 1509 * maintained protocol, guideline, orderset or other definition 1510 * that is adhered to in whole or in part by this DeviceRequest.) 1511 */ 1512 public boolean hasInstantiatesUri(String value) { 1513 if (this.instantiatesUri == null) 1514 return false; 1515 for (UriType v : this.instantiatesUri) 1516 if (v.getValue().equals(value)) // uri 1517 return true; 1518 return false; 1519 } 1520 1521 /** 1522 * @return {@link #basedOn} (Plan/proposal/order fulfilled by this request.) 1523 */ 1524 public List<Reference> getBasedOn() { 1525 if (this.basedOn == null) 1526 this.basedOn = new ArrayList<Reference>(); 1527 return this.basedOn; 1528 } 1529 1530 /** 1531 * @return Returns a reference to <code>this</code> for easy method chaining 1532 */ 1533 public DeviceRequest setBasedOn(List<Reference> theBasedOn) { 1534 this.basedOn = theBasedOn; 1535 return this; 1536 } 1537 1538 public boolean hasBasedOn() { 1539 if (this.basedOn == null) 1540 return false; 1541 for (Reference item : this.basedOn) 1542 if (!item.isEmpty()) 1543 return true; 1544 return false; 1545 } 1546 1547 public Reference addBasedOn() { // 3 1548 Reference t = new Reference(); 1549 if (this.basedOn == null) 1550 this.basedOn = new ArrayList<Reference>(); 1551 this.basedOn.add(t); 1552 return t; 1553 } 1554 1555 public DeviceRequest addBasedOn(Reference t) { // 3 1556 if (t == null) 1557 return this; 1558 if (this.basedOn == null) 1559 this.basedOn = new ArrayList<Reference>(); 1560 this.basedOn.add(t); 1561 return this; 1562 } 1563 1564 /** 1565 * @return The first repetition of repeating field {@link #basedOn}, creating it 1566 * if it does not already exist 1567 */ 1568 public Reference getBasedOnFirstRep() { 1569 if (getBasedOn().isEmpty()) { 1570 addBasedOn(); 1571 } 1572 return getBasedOn().get(0); 1573 } 1574 1575 /** 1576 * @deprecated Use Reference#setResource(IBaseResource) instead 1577 */ 1578 @Deprecated 1579 public List<Resource> getBasedOnTarget() { 1580 if (this.basedOnTarget == null) 1581 this.basedOnTarget = new ArrayList<Resource>(); 1582 return this.basedOnTarget; 1583 } 1584 1585 /** 1586 * @return {@link #priorRequest} (The request takes the place of the referenced 1587 * completed or terminated request(s).) 1588 */ 1589 public List<Reference> getPriorRequest() { 1590 if (this.priorRequest == null) 1591 this.priorRequest = new ArrayList<Reference>(); 1592 return this.priorRequest; 1593 } 1594 1595 /** 1596 * @return Returns a reference to <code>this</code> for easy method chaining 1597 */ 1598 public DeviceRequest setPriorRequest(List<Reference> thePriorRequest) { 1599 this.priorRequest = thePriorRequest; 1600 return this; 1601 } 1602 1603 public boolean hasPriorRequest() { 1604 if (this.priorRequest == null) 1605 return false; 1606 for (Reference item : this.priorRequest) 1607 if (!item.isEmpty()) 1608 return true; 1609 return false; 1610 } 1611 1612 public Reference addPriorRequest() { // 3 1613 Reference t = new Reference(); 1614 if (this.priorRequest == null) 1615 this.priorRequest = new ArrayList<Reference>(); 1616 this.priorRequest.add(t); 1617 return t; 1618 } 1619 1620 public DeviceRequest addPriorRequest(Reference t) { // 3 1621 if (t == null) 1622 return this; 1623 if (this.priorRequest == null) 1624 this.priorRequest = new ArrayList<Reference>(); 1625 this.priorRequest.add(t); 1626 return this; 1627 } 1628 1629 /** 1630 * @return The first repetition of repeating field {@link #priorRequest}, 1631 * creating it if it does not already exist 1632 */ 1633 public Reference getPriorRequestFirstRep() { 1634 if (getPriorRequest().isEmpty()) { 1635 addPriorRequest(); 1636 } 1637 return getPriorRequest().get(0); 1638 } 1639 1640 /** 1641 * @deprecated Use Reference#setResource(IBaseResource) instead 1642 */ 1643 @Deprecated 1644 public List<Resource> getPriorRequestTarget() { 1645 if (this.priorRequestTarget == null) 1646 this.priorRequestTarget = new ArrayList<Resource>(); 1647 return this.priorRequestTarget; 1648 } 1649 1650 /** 1651 * @return {@link #groupIdentifier} (Composite request this is part of.) 1652 */ 1653 public Identifier getGroupIdentifier() { 1654 if (this.groupIdentifier == null) 1655 if (Configuration.errorOnAutoCreate()) 1656 throw new Error("Attempt to auto-create DeviceRequest.groupIdentifier"); 1657 else if (Configuration.doAutoCreate()) 1658 this.groupIdentifier = new Identifier(); // cc 1659 return this.groupIdentifier; 1660 } 1661 1662 public boolean hasGroupIdentifier() { 1663 return this.groupIdentifier != null && !this.groupIdentifier.isEmpty(); 1664 } 1665 1666 /** 1667 * @param value {@link #groupIdentifier} (Composite request this is part of.) 1668 */ 1669 public DeviceRequest setGroupIdentifier(Identifier value) { 1670 this.groupIdentifier = value; 1671 return this; 1672 } 1673 1674 /** 1675 * @return {@link #status} (The status of the request.). This is the underlying 1676 * object with id, value and extensions. The accessor "getStatus" gives 1677 * direct access to the value 1678 */ 1679 public Enumeration<DeviceRequestStatus> getStatusElement() { 1680 if (this.status == null) 1681 if (Configuration.errorOnAutoCreate()) 1682 throw new Error("Attempt to auto-create DeviceRequest.status"); 1683 else if (Configuration.doAutoCreate()) 1684 this.status = new Enumeration<DeviceRequestStatus>(new DeviceRequestStatusEnumFactory()); // bb 1685 return this.status; 1686 } 1687 1688 public boolean hasStatusElement() { 1689 return this.status != null && !this.status.isEmpty(); 1690 } 1691 1692 public boolean hasStatus() { 1693 return this.status != null && !this.status.isEmpty(); 1694 } 1695 1696 /** 1697 * @param value {@link #status} (The status of the request.). This is the 1698 * underlying object with id, value and extensions. The accessor 1699 * "getStatus" gives direct access to the value 1700 */ 1701 public DeviceRequest setStatusElement(Enumeration<DeviceRequestStatus> value) { 1702 this.status = value; 1703 return this; 1704 } 1705 1706 /** 1707 * @return The status of the request. 1708 */ 1709 public DeviceRequestStatus getStatus() { 1710 return this.status == null ? null : this.status.getValue(); 1711 } 1712 1713 /** 1714 * @param value The status of the request. 1715 */ 1716 public DeviceRequest setStatus(DeviceRequestStatus value) { 1717 if (value == null) 1718 this.status = null; 1719 else { 1720 if (this.status == null) 1721 this.status = new Enumeration<DeviceRequestStatus>(new DeviceRequestStatusEnumFactory()); 1722 this.status.setValue(value); 1723 } 1724 return this; 1725 } 1726 1727 /** 1728 * @return {@link #intent} (Whether the request is a proposal, plan, an original 1729 * order or a reflex order.). This is the underlying object with id, 1730 * value and extensions. The accessor "getIntent" gives direct access to 1731 * the value 1732 */ 1733 public Enumeration<RequestIntent> getIntentElement() { 1734 if (this.intent == null) 1735 if (Configuration.errorOnAutoCreate()) 1736 throw new Error("Attempt to auto-create DeviceRequest.intent"); 1737 else if (Configuration.doAutoCreate()) 1738 this.intent = new Enumeration<RequestIntent>(new RequestIntentEnumFactory()); // bb 1739 return this.intent; 1740 } 1741 1742 public boolean hasIntentElement() { 1743 return this.intent != null && !this.intent.isEmpty(); 1744 } 1745 1746 public boolean hasIntent() { 1747 return this.intent != null && !this.intent.isEmpty(); 1748 } 1749 1750 /** 1751 * @param value {@link #intent} (Whether the request is a proposal, plan, an 1752 * original order or a reflex order.). This is the underlying 1753 * object with id, value and extensions. The accessor "getIntent" 1754 * gives direct access to the value 1755 */ 1756 public DeviceRequest setIntentElement(Enumeration<RequestIntent> value) { 1757 this.intent = value; 1758 return this; 1759 } 1760 1761 /** 1762 * @return Whether the request is a proposal, plan, an original order or a 1763 * reflex order. 1764 */ 1765 public RequestIntent getIntent() { 1766 return this.intent == null ? null : this.intent.getValue(); 1767 } 1768 1769 /** 1770 * @param value Whether the request is a proposal, plan, an original order or a 1771 * reflex order. 1772 */ 1773 public DeviceRequest setIntent(RequestIntent value) { 1774 if (this.intent == null) 1775 this.intent = new Enumeration<RequestIntent>(new RequestIntentEnumFactory()); 1776 this.intent.setValue(value); 1777 return this; 1778 } 1779 1780 /** 1781 * @return {@link #priority} (Indicates how quickly the {{title}} should be 1782 * addressed with respect to other requests.). This is the underlying 1783 * object with id, value and extensions. The accessor "getPriority" 1784 * gives direct access to the value 1785 */ 1786 public Enumeration<RequestPriority> getPriorityElement() { 1787 if (this.priority == null) 1788 if (Configuration.errorOnAutoCreate()) 1789 throw new Error("Attempt to auto-create DeviceRequest.priority"); 1790 else if (Configuration.doAutoCreate()) 1791 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 1792 return this.priority; 1793 } 1794 1795 public boolean hasPriorityElement() { 1796 return this.priority != null && !this.priority.isEmpty(); 1797 } 1798 1799 public boolean hasPriority() { 1800 return this.priority != null && !this.priority.isEmpty(); 1801 } 1802 1803 /** 1804 * @param value {@link #priority} (Indicates how quickly the {{title}} should be 1805 * addressed with respect to other requests.). This is the 1806 * underlying object with id, value and extensions. The accessor 1807 * "getPriority" gives direct access to the value 1808 */ 1809 public DeviceRequest setPriorityElement(Enumeration<RequestPriority> value) { 1810 this.priority = value; 1811 return this; 1812 } 1813 1814 /** 1815 * @return Indicates how quickly the {{title}} should be addressed with respect 1816 * to other requests. 1817 */ 1818 public RequestPriority getPriority() { 1819 return this.priority == null ? null : this.priority.getValue(); 1820 } 1821 1822 /** 1823 * @param value Indicates how quickly the {{title}} should be addressed with 1824 * respect to other requests. 1825 */ 1826 public DeviceRequest setPriority(RequestPriority value) { 1827 if (value == null) 1828 this.priority = null; 1829 else { 1830 if (this.priority == null) 1831 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 1832 this.priority.setValue(value); 1833 } 1834 return this; 1835 } 1836 1837 /** 1838 * @return {@link #code} (The details of the device to be used.) 1839 */ 1840 public Type getCode() { 1841 return this.code; 1842 } 1843 1844 /** 1845 * @return {@link #code} (The details of the device to be used.) 1846 */ 1847 public Reference getCodeReference() throws FHIRException { 1848 if (this.code == null) 1849 this.code = new Reference(); 1850 if (!(this.code instanceof Reference)) 1851 throw new FHIRException( 1852 "Type mismatch: the type Reference was expected, but " + this.code.getClass().getName() + " was encountered"); 1853 return (Reference) this.code; 1854 } 1855 1856 public boolean hasCodeReference() { 1857 return this != null && this.code instanceof Reference; 1858 } 1859 1860 /** 1861 * @return {@link #code} (The details of the device to be used.) 1862 */ 1863 public CodeableConcept getCodeCodeableConcept() throws FHIRException { 1864 if (this.code == null) 1865 this.code = new CodeableConcept(); 1866 if (!(this.code instanceof CodeableConcept)) 1867 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1868 + this.code.getClass().getName() + " was encountered"); 1869 return (CodeableConcept) this.code; 1870 } 1871 1872 public boolean hasCodeCodeableConcept() { 1873 return this != null && this.code instanceof CodeableConcept; 1874 } 1875 1876 public boolean hasCode() { 1877 return this.code != null && !this.code.isEmpty(); 1878 } 1879 1880 /** 1881 * @param value {@link #code} (The details of the device to be used.) 1882 */ 1883 public DeviceRequest setCode(Type value) { 1884 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept)) 1885 throw new Error("Not the right type for DeviceRequest.code[x]: " + value.fhirType()); 1886 this.code = value; 1887 return this; 1888 } 1889 1890 /** 1891 * @return {@link #parameter} (Specific parameters for the ordered item. For 1892 * example, the prism value for lenses.) 1893 */ 1894 public List<DeviceRequestParameterComponent> getParameter() { 1895 if (this.parameter == null) 1896 this.parameter = new ArrayList<DeviceRequestParameterComponent>(); 1897 return this.parameter; 1898 } 1899 1900 /** 1901 * @return Returns a reference to <code>this</code> for easy method chaining 1902 */ 1903 public DeviceRequest setParameter(List<DeviceRequestParameterComponent> theParameter) { 1904 this.parameter = theParameter; 1905 return this; 1906 } 1907 1908 public boolean hasParameter() { 1909 if (this.parameter == null) 1910 return false; 1911 for (DeviceRequestParameterComponent item : this.parameter) 1912 if (!item.isEmpty()) 1913 return true; 1914 return false; 1915 } 1916 1917 public DeviceRequestParameterComponent addParameter() { // 3 1918 DeviceRequestParameterComponent t = new DeviceRequestParameterComponent(); 1919 if (this.parameter == null) 1920 this.parameter = new ArrayList<DeviceRequestParameterComponent>(); 1921 this.parameter.add(t); 1922 return t; 1923 } 1924 1925 public DeviceRequest addParameter(DeviceRequestParameterComponent t) { // 3 1926 if (t == null) 1927 return this; 1928 if (this.parameter == null) 1929 this.parameter = new ArrayList<DeviceRequestParameterComponent>(); 1930 this.parameter.add(t); 1931 return this; 1932 } 1933 1934 /** 1935 * @return The first repetition of repeating field {@link #parameter}, creating 1936 * it if it does not already exist 1937 */ 1938 public DeviceRequestParameterComponent getParameterFirstRep() { 1939 if (getParameter().isEmpty()) { 1940 addParameter(); 1941 } 1942 return getParameter().get(0); 1943 } 1944 1945 /** 1946 * @return {@link #subject} (The patient who will use the device.) 1947 */ 1948 public Reference getSubject() { 1949 if (this.subject == null) 1950 if (Configuration.errorOnAutoCreate()) 1951 throw new Error("Attempt to auto-create DeviceRequest.subject"); 1952 else if (Configuration.doAutoCreate()) 1953 this.subject = new Reference(); // cc 1954 return this.subject; 1955 } 1956 1957 public boolean hasSubject() { 1958 return this.subject != null && !this.subject.isEmpty(); 1959 } 1960 1961 /** 1962 * @param value {@link #subject} (The patient who will use the device.) 1963 */ 1964 public DeviceRequest setSubject(Reference value) { 1965 this.subject = value; 1966 return this; 1967 } 1968 1969 /** 1970 * @return {@link #subject} The actual object that is the target of the 1971 * reference. The reference library doesn't populate this, but you can 1972 * use it to hold the resource if you resolve it. (The patient who will 1973 * use the device.) 1974 */ 1975 public Resource getSubjectTarget() { 1976 return this.subjectTarget; 1977 } 1978 1979 /** 1980 * @param value {@link #subject} The actual object that is the target of the 1981 * reference. The reference library doesn't use these, but you can 1982 * use it to hold the resource if you resolve it. (The patient who 1983 * will use the device.) 1984 */ 1985 public DeviceRequest setSubjectTarget(Resource value) { 1986 this.subjectTarget = value; 1987 return this; 1988 } 1989 1990 /** 1991 * @return {@link #encounter} (An encounter that provides additional context in 1992 * which this request is made.) 1993 */ 1994 public Reference getEncounter() { 1995 if (this.encounter == null) 1996 if (Configuration.errorOnAutoCreate()) 1997 throw new Error("Attempt to auto-create DeviceRequest.encounter"); 1998 else if (Configuration.doAutoCreate()) 1999 this.encounter = new Reference(); // cc 2000 return this.encounter; 2001 } 2002 2003 public boolean hasEncounter() { 2004 return this.encounter != null && !this.encounter.isEmpty(); 2005 } 2006 2007 /** 2008 * @param value {@link #encounter} (An encounter that provides additional 2009 * context in which this request is made.) 2010 */ 2011 public DeviceRequest setEncounter(Reference value) { 2012 this.encounter = value; 2013 return this; 2014 } 2015 2016 /** 2017 * @return {@link #encounter} The actual object that is the target of the 2018 * reference. The reference library doesn't populate this, but you can 2019 * use it to hold the resource if you resolve it. (An encounter that 2020 * provides additional context in which this request is made.) 2021 */ 2022 public Encounter getEncounterTarget() { 2023 if (this.encounterTarget == null) 2024 if (Configuration.errorOnAutoCreate()) 2025 throw new Error("Attempt to auto-create DeviceRequest.encounter"); 2026 else if (Configuration.doAutoCreate()) 2027 this.encounterTarget = new Encounter(); // aa 2028 return this.encounterTarget; 2029 } 2030 2031 /** 2032 * @param value {@link #encounter} The actual object that is the target of the 2033 * reference. The reference library doesn't use these, but you can 2034 * use it to hold the resource if you resolve it. (An encounter 2035 * that provides additional context in which this request is made.) 2036 */ 2037 public DeviceRequest setEncounterTarget(Encounter value) { 2038 this.encounterTarget = value; 2039 return this; 2040 } 2041 2042 /** 2043 * @return {@link #occurrence} (The timing schedule for the use of the device. 2044 * The Schedule data type allows many different expressions, for 2045 * example. "Every 8 hours"; "Three times a day"; "1/2 an hour before 2046 * breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 2047 * and 1 Nov 2013".) 2048 */ 2049 public Type getOccurrence() { 2050 return this.occurrence; 2051 } 2052 2053 /** 2054 * @return {@link #occurrence} (The timing schedule for the use of the device. 2055 * The Schedule data type allows many different expressions, for 2056 * example. "Every 8 hours"; "Three times a day"; "1/2 an hour before 2057 * breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 2058 * and 1 Nov 2013".) 2059 */ 2060 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 2061 if (this.occurrence == null) 2062 this.occurrence = new DateTimeType(); 2063 if (!(this.occurrence instanceof DateTimeType)) 2064 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 2065 + this.occurrence.getClass().getName() + " was encountered"); 2066 return (DateTimeType) this.occurrence; 2067 } 2068 2069 public boolean hasOccurrenceDateTimeType() { 2070 return this != null && this.occurrence instanceof DateTimeType; 2071 } 2072 2073 /** 2074 * @return {@link #occurrence} (The timing schedule for the use of the device. 2075 * The Schedule data type allows many different expressions, for 2076 * example. "Every 8 hours"; "Three times a day"; "1/2 an hour before 2077 * breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 2078 * and 1 Nov 2013".) 2079 */ 2080 public Period getOccurrencePeriod() throws FHIRException { 2081 if (this.occurrence == null) 2082 this.occurrence = new Period(); 2083 if (!(this.occurrence instanceof Period)) 2084 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.occurrence.getClass().getName() 2085 + " was encountered"); 2086 return (Period) this.occurrence; 2087 } 2088 2089 public boolean hasOccurrencePeriod() { 2090 return this != null && this.occurrence instanceof Period; 2091 } 2092 2093 /** 2094 * @return {@link #occurrence} (The timing schedule for the use of the device. 2095 * The Schedule data type allows many different expressions, for 2096 * example. "Every 8 hours"; "Three times a day"; "1/2 an hour before 2097 * breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 2098 * and 1 Nov 2013".) 2099 */ 2100 public Timing getOccurrenceTiming() throws FHIRException { 2101 if (this.occurrence == null) 2102 this.occurrence = new Timing(); 2103 if (!(this.occurrence instanceof Timing)) 2104 throw new FHIRException("Type mismatch: the type Timing was expected, but " + this.occurrence.getClass().getName() 2105 + " was encountered"); 2106 return (Timing) this.occurrence; 2107 } 2108 2109 public boolean hasOccurrenceTiming() { 2110 return this != null && this.occurrence instanceof Timing; 2111 } 2112 2113 public boolean hasOccurrence() { 2114 return this.occurrence != null && !this.occurrence.isEmpty(); 2115 } 2116 2117 /** 2118 * @param value {@link #occurrence} (The timing schedule for the use of the 2119 * device. The Schedule data type allows many different 2120 * expressions, for example. "Every 8 hours"; "Three times a day"; 2121 * "1/2 an hour before breakfast for 10 days from 23-Dec 2011:"; 2122 * "15 Oct 2013, 17 Oct 2013 and 1 Nov 2013".) 2123 */ 2124 public DeviceRequest setOccurrence(Type value) { 2125 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 2126 throw new Error("Not the right type for DeviceRequest.occurrence[x]: " + value.fhirType()); 2127 this.occurrence = value; 2128 return this; 2129 } 2130 2131 /** 2132 * @return {@link #authoredOn} (When the request transitioned to being 2133 * actionable.). This is the underlying object with id, value and 2134 * extensions. The accessor "getAuthoredOn" gives direct access to the 2135 * value 2136 */ 2137 public DateTimeType getAuthoredOnElement() { 2138 if (this.authoredOn == null) 2139 if (Configuration.errorOnAutoCreate()) 2140 throw new Error("Attempt to auto-create DeviceRequest.authoredOn"); 2141 else if (Configuration.doAutoCreate()) 2142 this.authoredOn = new DateTimeType(); // bb 2143 return this.authoredOn; 2144 } 2145 2146 public boolean hasAuthoredOnElement() { 2147 return this.authoredOn != null && !this.authoredOn.isEmpty(); 2148 } 2149 2150 public boolean hasAuthoredOn() { 2151 return this.authoredOn != null && !this.authoredOn.isEmpty(); 2152 } 2153 2154 /** 2155 * @param value {@link #authoredOn} (When the request transitioned to being 2156 * actionable.). This is the underlying object with id, value and 2157 * extensions. The accessor "getAuthoredOn" gives direct access to 2158 * the value 2159 */ 2160 public DeviceRequest setAuthoredOnElement(DateTimeType value) { 2161 this.authoredOn = value; 2162 return this; 2163 } 2164 2165 /** 2166 * @return When the request transitioned to being actionable. 2167 */ 2168 public Date getAuthoredOn() { 2169 return this.authoredOn == null ? null : this.authoredOn.getValue(); 2170 } 2171 2172 /** 2173 * @param value When the request transitioned to being actionable. 2174 */ 2175 public DeviceRequest setAuthoredOn(Date value) { 2176 if (value == null) 2177 this.authoredOn = null; 2178 else { 2179 if (this.authoredOn == null) 2180 this.authoredOn = new DateTimeType(); 2181 this.authoredOn.setValue(value); 2182 } 2183 return this; 2184 } 2185 2186 /** 2187 * @return {@link #requester} (The individual who initiated the request and has 2188 * responsibility for its activation.) 2189 */ 2190 public Reference getRequester() { 2191 if (this.requester == null) 2192 if (Configuration.errorOnAutoCreate()) 2193 throw new Error("Attempt to auto-create DeviceRequest.requester"); 2194 else if (Configuration.doAutoCreate()) 2195 this.requester = new Reference(); // cc 2196 return this.requester; 2197 } 2198 2199 public boolean hasRequester() { 2200 return this.requester != null && !this.requester.isEmpty(); 2201 } 2202 2203 /** 2204 * @param value {@link #requester} (The individual who initiated the request and 2205 * has responsibility for its activation.) 2206 */ 2207 public DeviceRequest setRequester(Reference value) { 2208 this.requester = value; 2209 return this; 2210 } 2211 2212 /** 2213 * @return {@link #requester} The actual object that is the target of the 2214 * reference. The reference library doesn't populate this, but you can 2215 * use it to hold the resource if you resolve it. (The individual who 2216 * initiated the request and has responsibility for its activation.) 2217 */ 2218 public Resource getRequesterTarget() { 2219 return this.requesterTarget; 2220 } 2221 2222 /** 2223 * @param value {@link #requester} The actual object that is the target of the 2224 * reference. The reference library doesn't use these, but you can 2225 * use it to hold the resource if you resolve it. (The individual 2226 * who initiated the request and has responsibility for its 2227 * activation.) 2228 */ 2229 public DeviceRequest setRequesterTarget(Resource value) { 2230 this.requesterTarget = value; 2231 return this; 2232 } 2233 2234 /** 2235 * @return {@link #performerType} (Desired type of performer for doing the 2236 * diagnostic testing.) 2237 */ 2238 public CodeableConcept getPerformerType() { 2239 if (this.performerType == null) 2240 if (Configuration.errorOnAutoCreate()) 2241 throw new Error("Attempt to auto-create DeviceRequest.performerType"); 2242 else if (Configuration.doAutoCreate()) 2243 this.performerType = new CodeableConcept(); // cc 2244 return this.performerType; 2245 } 2246 2247 public boolean hasPerformerType() { 2248 return this.performerType != null && !this.performerType.isEmpty(); 2249 } 2250 2251 /** 2252 * @param value {@link #performerType} (Desired type of performer for doing the 2253 * diagnostic testing.) 2254 */ 2255 public DeviceRequest setPerformerType(CodeableConcept value) { 2256 this.performerType = value; 2257 return this; 2258 } 2259 2260 /** 2261 * @return {@link #performer} (The desired performer for doing the diagnostic 2262 * testing.) 2263 */ 2264 public Reference getPerformer() { 2265 if (this.performer == null) 2266 if (Configuration.errorOnAutoCreate()) 2267 throw new Error("Attempt to auto-create DeviceRequest.performer"); 2268 else if (Configuration.doAutoCreate()) 2269 this.performer = new Reference(); // cc 2270 return this.performer; 2271 } 2272 2273 public boolean hasPerformer() { 2274 return this.performer != null && !this.performer.isEmpty(); 2275 } 2276 2277 /** 2278 * @param value {@link #performer} (The desired performer for doing the 2279 * diagnostic testing.) 2280 */ 2281 public DeviceRequest setPerformer(Reference value) { 2282 this.performer = value; 2283 return this; 2284 } 2285 2286 /** 2287 * @return {@link #performer} The actual object that is the target of the 2288 * reference. The reference library doesn't populate this, but you can 2289 * use it to hold the resource if you resolve it. (The desired performer 2290 * for doing the diagnostic testing.) 2291 */ 2292 public Resource getPerformerTarget() { 2293 return this.performerTarget; 2294 } 2295 2296 /** 2297 * @param value {@link #performer} The actual object that is the target of the 2298 * reference. The reference library doesn't use these, but you can 2299 * use it to hold the resource if you resolve it. (The desired 2300 * performer for doing the diagnostic testing.) 2301 */ 2302 public DeviceRequest setPerformerTarget(Resource value) { 2303 this.performerTarget = value; 2304 return this; 2305 } 2306 2307 /** 2308 * @return {@link #reasonCode} (Reason or justification for the use of this 2309 * device.) 2310 */ 2311 public List<CodeableConcept> getReasonCode() { 2312 if (this.reasonCode == null) 2313 this.reasonCode = new ArrayList<CodeableConcept>(); 2314 return this.reasonCode; 2315 } 2316 2317 /** 2318 * @return Returns a reference to <code>this</code> for easy method chaining 2319 */ 2320 public DeviceRequest setReasonCode(List<CodeableConcept> theReasonCode) { 2321 this.reasonCode = theReasonCode; 2322 return this; 2323 } 2324 2325 public boolean hasReasonCode() { 2326 if (this.reasonCode == null) 2327 return false; 2328 for (CodeableConcept item : this.reasonCode) 2329 if (!item.isEmpty()) 2330 return true; 2331 return false; 2332 } 2333 2334 public CodeableConcept addReasonCode() { // 3 2335 CodeableConcept t = new CodeableConcept(); 2336 if (this.reasonCode == null) 2337 this.reasonCode = new ArrayList<CodeableConcept>(); 2338 this.reasonCode.add(t); 2339 return t; 2340 } 2341 2342 public DeviceRequest addReasonCode(CodeableConcept t) { // 3 2343 if (t == null) 2344 return this; 2345 if (this.reasonCode == null) 2346 this.reasonCode = new ArrayList<CodeableConcept>(); 2347 this.reasonCode.add(t); 2348 return this; 2349 } 2350 2351 /** 2352 * @return The first repetition of repeating field {@link #reasonCode}, creating 2353 * it if it does not already exist 2354 */ 2355 public CodeableConcept getReasonCodeFirstRep() { 2356 if (getReasonCode().isEmpty()) { 2357 addReasonCode(); 2358 } 2359 return getReasonCode().get(0); 2360 } 2361 2362 /** 2363 * @return {@link #reasonReference} (Reason or justification for the use of this 2364 * device.) 2365 */ 2366 public List<Reference> getReasonReference() { 2367 if (this.reasonReference == null) 2368 this.reasonReference = new ArrayList<Reference>(); 2369 return this.reasonReference; 2370 } 2371 2372 /** 2373 * @return Returns a reference to <code>this</code> for easy method chaining 2374 */ 2375 public DeviceRequest setReasonReference(List<Reference> theReasonReference) { 2376 this.reasonReference = theReasonReference; 2377 return this; 2378 } 2379 2380 public boolean hasReasonReference() { 2381 if (this.reasonReference == null) 2382 return false; 2383 for (Reference item : this.reasonReference) 2384 if (!item.isEmpty()) 2385 return true; 2386 return false; 2387 } 2388 2389 public Reference addReasonReference() { // 3 2390 Reference t = new Reference(); 2391 if (this.reasonReference == null) 2392 this.reasonReference = new ArrayList<Reference>(); 2393 this.reasonReference.add(t); 2394 return t; 2395 } 2396 2397 public DeviceRequest addReasonReference(Reference t) { // 3 2398 if (t == null) 2399 return this; 2400 if (this.reasonReference == null) 2401 this.reasonReference = new ArrayList<Reference>(); 2402 this.reasonReference.add(t); 2403 return this; 2404 } 2405 2406 /** 2407 * @return The first repetition of repeating field {@link #reasonReference}, 2408 * creating it if it does not already exist 2409 */ 2410 public Reference getReasonReferenceFirstRep() { 2411 if (getReasonReference().isEmpty()) { 2412 addReasonReference(); 2413 } 2414 return getReasonReference().get(0); 2415 } 2416 2417 /** 2418 * @deprecated Use Reference#setResource(IBaseResource) instead 2419 */ 2420 @Deprecated 2421 public List<Resource> getReasonReferenceTarget() { 2422 if (this.reasonReferenceTarget == null) 2423 this.reasonReferenceTarget = new ArrayList<Resource>(); 2424 return this.reasonReferenceTarget; 2425 } 2426 2427 /** 2428 * @return {@link #insurance} (Insurance plans, coverage extensions, 2429 * pre-authorizations and/or pre-determinations that may be required for 2430 * delivering the requested service.) 2431 */ 2432 public List<Reference> getInsurance() { 2433 if (this.insurance == null) 2434 this.insurance = new ArrayList<Reference>(); 2435 return this.insurance; 2436 } 2437 2438 /** 2439 * @return Returns a reference to <code>this</code> for easy method chaining 2440 */ 2441 public DeviceRequest setInsurance(List<Reference> theInsurance) { 2442 this.insurance = theInsurance; 2443 return this; 2444 } 2445 2446 public boolean hasInsurance() { 2447 if (this.insurance == null) 2448 return false; 2449 for (Reference item : this.insurance) 2450 if (!item.isEmpty()) 2451 return true; 2452 return false; 2453 } 2454 2455 public Reference addInsurance() { // 3 2456 Reference t = new Reference(); 2457 if (this.insurance == null) 2458 this.insurance = new ArrayList<Reference>(); 2459 this.insurance.add(t); 2460 return t; 2461 } 2462 2463 public DeviceRequest addInsurance(Reference t) { // 3 2464 if (t == null) 2465 return this; 2466 if (this.insurance == null) 2467 this.insurance = new ArrayList<Reference>(); 2468 this.insurance.add(t); 2469 return this; 2470 } 2471 2472 /** 2473 * @return The first repetition of repeating field {@link #insurance}, creating 2474 * it if it does not already exist 2475 */ 2476 public Reference getInsuranceFirstRep() { 2477 if (getInsurance().isEmpty()) { 2478 addInsurance(); 2479 } 2480 return getInsurance().get(0); 2481 } 2482 2483 /** 2484 * @deprecated Use Reference#setResource(IBaseResource) instead 2485 */ 2486 @Deprecated 2487 public List<Resource> getInsuranceTarget() { 2488 if (this.insuranceTarget == null) 2489 this.insuranceTarget = new ArrayList<Resource>(); 2490 return this.insuranceTarget; 2491 } 2492 2493 /** 2494 * @return {@link #supportingInfo} (Additional clinical information about the 2495 * patient that may influence the request fulfilment. For example, this 2496 * may include where on the subject's body the device will be used (i.e. 2497 * the target site).) 2498 */ 2499 public List<Reference> getSupportingInfo() { 2500 if (this.supportingInfo == null) 2501 this.supportingInfo = new ArrayList<Reference>(); 2502 return this.supportingInfo; 2503 } 2504 2505 /** 2506 * @return Returns a reference to <code>this</code> for easy method chaining 2507 */ 2508 public DeviceRequest setSupportingInfo(List<Reference> theSupportingInfo) { 2509 this.supportingInfo = theSupportingInfo; 2510 return this; 2511 } 2512 2513 public boolean hasSupportingInfo() { 2514 if (this.supportingInfo == null) 2515 return false; 2516 for (Reference item : this.supportingInfo) 2517 if (!item.isEmpty()) 2518 return true; 2519 return false; 2520 } 2521 2522 public Reference addSupportingInfo() { // 3 2523 Reference t = new Reference(); 2524 if (this.supportingInfo == null) 2525 this.supportingInfo = new ArrayList<Reference>(); 2526 this.supportingInfo.add(t); 2527 return t; 2528 } 2529 2530 public DeviceRequest addSupportingInfo(Reference t) { // 3 2531 if (t == null) 2532 return this; 2533 if (this.supportingInfo == null) 2534 this.supportingInfo = new ArrayList<Reference>(); 2535 this.supportingInfo.add(t); 2536 return this; 2537 } 2538 2539 /** 2540 * @return The first repetition of repeating field {@link #supportingInfo}, 2541 * creating it if it does not already exist 2542 */ 2543 public Reference getSupportingInfoFirstRep() { 2544 if (getSupportingInfo().isEmpty()) { 2545 addSupportingInfo(); 2546 } 2547 return getSupportingInfo().get(0); 2548 } 2549 2550 /** 2551 * @deprecated Use Reference#setResource(IBaseResource) instead 2552 */ 2553 @Deprecated 2554 public List<Resource> getSupportingInfoTarget() { 2555 if (this.supportingInfoTarget == null) 2556 this.supportingInfoTarget = new ArrayList<Resource>(); 2557 return this.supportingInfoTarget; 2558 } 2559 2560 /** 2561 * @return {@link #note} (Details about this request that were not represented 2562 * at all or sufficiently in one of the attributes provided in a class. 2563 * These may include for example a comment, an instruction, or a note 2564 * associated with the statement.) 2565 */ 2566 public List<Annotation> getNote() { 2567 if (this.note == null) 2568 this.note = new ArrayList<Annotation>(); 2569 return this.note; 2570 } 2571 2572 /** 2573 * @return Returns a reference to <code>this</code> for easy method chaining 2574 */ 2575 public DeviceRequest setNote(List<Annotation> theNote) { 2576 this.note = theNote; 2577 return this; 2578 } 2579 2580 public boolean hasNote() { 2581 if (this.note == null) 2582 return false; 2583 for (Annotation item : this.note) 2584 if (!item.isEmpty()) 2585 return true; 2586 return false; 2587 } 2588 2589 public Annotation addNote() { // 3 2590 Annotation t = new Annotation(); 2591 if (this.note == null) 2592 this.note = new ArrayList<Annotation>(); 2593 this.note.add(t); 2594 return t; 2595 } 2596 2597 public DeviceRequest addNote(Annotation t) { // 3 2598 if (t == null) 2599 return this; 2600 if (this.note == null) 2601 this.note = new ArrayList<Annotation>(); 2602 this.note.add(t); 2603 return this; 2604 } 2605 2606 /** 2607 * @return The first repetition of repeating field {@link #note}, creating it if 2608 * it does not already exist 2609 */ 2610 public Annotation getNoteFirstRep() { 2611 if (getNote().isEmpty()) { 2612 addNote(); 2613 } 2614 return getNote().get(0); 2615 } 2616 2617 /** 2618 * @return {@link #relevantHistory} (Key events in the history of the request.) 2619 */ 2620 public List<Reference> getRelevantHistory() { 2621 if (this.relevantHistory == null) 2622 this.relevantHistory = new ArrayList<Reference>(); 2623 return this.relevantHistory; 2624 } 2625 2626 /** 2627 * @return Returns a reference to <code>this</code> for easy method chaining 2628 */ 2629 public DeviceRequest setRelevantHistory(List<Reference> theRelevantHistory) { 2630 this.relevantHistory = theRelevantHistory; 2631 return this; 2632 } 2633 2634 public boolean hasRelevantHistory() { 2635 if (this.relevantHistory == null) 2636 return false; 2637 for (Reference item : this.relevantHistory) 2638 if (!item.isEmpty()) 2639 return true; 2640 return false; 2641 } 2642 2643 public Reference addRelevantHistory() { // 3 2644 Reference t = new Reference(); 2645 if (this.relevantHistory == null) 2646 this.relevantHistory = new ArrayList<Reference>(); 2647 this.relevantHistory.add(t); 2648 return t; 2649 } 2650 2651 public DeviceRequest addRelevantHistory(Reference t) { // 3 2652 if (t == null) 2653 return this; 2654 if (this.relevantHistory == null) 2655 this.relevantHistory = new ArrayList<Reference>(); 2656 this.relevantHistory.add(t); 2657 return this; 2658 } 2659 2660 /** 2661 * @return The first repetition of repeating field {@link #relevantHistory}, 2662 * creating it if it does not already exist 2663 */ 2664 public Reference getRelevantHistoryFirstRep() { 2665 if (getRelevantHistory().isEmpty()) { 2666 addRelevantHistory(); 2667 } 2668 return getRelevantHistory().get(0); 2669 } 2670 2671 /** 2672 * @deprecated Use Reference#setResource(IBaseResource) instead 2673 */ 2674 @Deprecated 2675 public List<Provenance> getRelevantHistoryTarget() { 2676 if (this.relevantHistoryTarget == null) 2677 this.relevantHistoryTarget = new ArrayList<Provenance>(); 2678 return this.relevantHistoryTarget; 2679 } 2680 2681 /** 2682 * @deprecated Use Reference#setResource(IBaseResource) instead 2683 */ 2684 @Deprecated 2685 public Provenance addRelevantHistoryTarget() { 2686 Provenance r = new Provenance(); 2687 if (this.relevantHistoryTarget == null) 2688 this.relevantHistoryTarget = new ArrayList<Provenance>(); 2689 this.relevantHistoryTarget.add(r); 2690 return r; 2691 } 2692 2693 protected void listChildren(List<Property> children) { 2694 super.listChildren(children); 2695 children.add(new Property("identifier", "Identifier", 2696 "Identifiers assigned to this order by the orderer or by the receiver.", 0, java.lang.Integer.MAX_VALUE, 2697 identifier)); 2698 children.add(new Property("instantiatesCanonical", "canonical(ActivityDefinition|PlanDefinition)", 2699 "The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this DeviceRequest.", 2700 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical)); 2701 children.add(new Property("instantiatesUri", "uri", 2702 "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this DeviceRequest.", 2703 0, java.lang.Integer.MAX_VALUE, instantiatesUri)); 2704 children.add(new Property("basedOn", "Reference(Any)", "Plan/proposal/order fulfilled by this request.", 0, 2705 java.lang.Integer.MAX_VALUE, basedOn)); 2706 children.add(new Property("priorRequest", "Reference(Any)", 2707 "The request takes the place of the referenced completed or terminated request(s).", 0, 2708 java.lang.Integer.MAX_VALUE, priorRequest)); 2709 children.add( 2710 new Property("groupIdentifier", "Identifier", "Composite request this is part of.", 0, 1, groupIdentifier)); 2711 children.add(new Property("status", "code", "The status of the request.", 0, 1, status)); 2712 children.add(new Property("intent", "code", 2713 "Whether the request is a proposal, plan, an original order or a reflex order.", 0, 1, intent)); 2714 children.add(new Property("priority", "code", 2715 "Indicates how quickly the {{title}} should be addressed with respect to other requests.", 0, 1, priority)); 2716 children.add(new Property("code[x]", "Reference(Device)|CodeableConcept", "The details of the device to be used.", 2717 0, 1, code)); 2718 children.add(new Property("parameter", "", 2719 "Specific parameters for the ordered item. For example, the prism value for lenses.", 0, 2720 java.lang.Integer.MAX_VALUE, parameter)); 2721 children.add(new Property("subject", "Reference(Patient|Group|Location|Device)", 2722 "The patient who will use the device.", 0, 1, subject)); 2723 children.add(new Property("encounter", "Reference(Encounter)", 2724 "An encounter that provides additional context in which this request is made.", 0, 1, encounter)); 2725 children.add(new Property("occurrence[x]", "dateTime|Period|Timing", 2726 "The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 2727 0, 1, occurrence)); 2728 children.add( 2729 new Property("authoredOn", "dateTime", "When the request transitioned to being actionable.", 0, 1, authoredOn)); 2730 children.add(new Property("requester", "Reference(Device|Practitioner|PractitionerRole|Organization)", 2731 "The individual who initiated the request and has responsibility for its activation.", 0, 1, requester)); 2732 children.add(new Property("performerType", "CodeableConcept", 2733 "Desired type of performer for doing the diagnostic testing.", 0, 1, performerType)); 2734 children.add(new Property("performer", 2735 "Reference(Practitioner|PractitionerRole|Organization|CareTeam|HealthcareService|Patient|Device|RelatedPerson)", 2736 "The desired performer for doing the diagnostic testing.", 0, 1, performer)); 2737 children.add(new Property("reasonCode", "CodeableConcept", "Reason or justification for the use of this device.", 0, 2738 java.lang.Integer.MAX_VALUE, reasonCode)); 2739 children.add(new Property("reasonReference", "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 2740 "Reason or justification for the use of this device.", 0, java.lang.Integer.MAX_VALUE, reasonReference)); 2741 children.add(new Property("insurance", "Reference(Coverage|ClaimResponse)", 2742 "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be required for delivering the requested service.", 2743 0, java.lang.Integer.MAX_VALUE, insurance)); 2744 children.add(new Property("supportingInfo", "Reference(Any)", 2745 "Additional clinical information about the patient that may influence the request fulfilment. For example, this may include where on the subject's body the device will be used (i.e. the target site).", 2746 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 2747 children.add(new Property("note", "Annotation", 2748 "Details about this request that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.", 2749 0, java.lang.Integer.MAX_VALUE, note)); 2750 children.add(new Property("relevantHistory", "Reference(Provenance)", "Key events in the history of the request.", 2751 0, java.lang.Integer.MAX_VALUE, relevantHistory)); 2752 } 2753 2754 @Override 2755 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2756 switch (_hash) { 2757 case -1618432855: 2758 /* identifier */ return new Property("identifier", "Identifier", 2759 "Identifiers assigned to this order by the orderer or by the receiver.", 0, java.lang.Integer.MAX_VALUE, 2760 identifier); 2761 case 8911915: 2762 /* instantiatesCanonical */ return new Property("instantiatesCanonical", 2763 "canonical(ActivityDefinition|PlanDefinition)", 2764 "The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this DeviceRequest.", 2765 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical); 2766 case -1926393373: 2767 /* instantiatesUri */ return new Property("instantiatesUri", "uri", 2768 "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this DeviceRequest.", 2769 0, java.lang.Integer.MAX_VALUE, instantiatesUri); 2770 case -332612366: 2771 /* basedOn */ return new Property("basedOn", "Reference(Any)", "Plan/proposal/order fulfilled by this request.", 2772 0, java.lang.Integer.MAX_VALUE, basedOn); 2773 case 237568101: 2774 /* priorRequest */ return new Property("priorRequest", "Reference(Any)", 2775 "The request takes the place of the referenced completed or terminated request(s).", 0, 2776 java.lang.Integer.MAX_VALUE, priorRequest); 2777 case -445338488: 2778 /* groupIdentifier */ return new Property("groupIdentifier", "Identifier", "Composite request this is part of.", 2779 0, 1, groupIdentifier); 2780 case -892481550: 2781 /* status */ return new Property("status", "code", "The status of the request.", 0, 1, status); 2782 case -1183762788: 2783 /* intent */ return new Property("intent", "code", 2784 "Whether the request is a proposal, plan, an original order or a reflex order.", 0, 1, intent); 2785 case -1165461084: 2786 /* priority */ return new Property("priority", "code", 2787 "Indicates how quickly the {{title}} should be addressed with respect to other requests.", 0, 1, priority); 2788 case 941839219: 2789 /* code[x] */ return new Property("code[x]", "Reference(Device)|CodeableConcept", 2790 "The details of the device to be used.", 0, 1, code); 2791 case 3059181: 2792 /* code */ return new Property("code[x]", "Reference(Device)|CodeableConcept", 2793 "The details of the device to be used.", 0, 1, code); 2794 case 1565461470: 2795 /* codeReference */ return new Property("code[x]", "Reference(Device)|CodeableConcept", 2796 "The details of the device to be used.", 0, 1, code); 2797 case 4899316: 2798 /* codeCodeableConcept */ return new Property("code[x]", "Reference(Device)|CodeableConcept", 2799 "The details of the device to be used.", 0, 1, code); 2800 case 1954460585: 2801 /* parameter */ return new Property("parameter", "", 2802 "Specific parameters for the ordered item. For example, the prism value for lenses.", 0, 2803 java.lang.Integer.MAX_VALUE, parameter); 2804 case -1867885268: 2805 /* subject */ return new Property("subject", "Reference(Patient|Group|Location|Device)", 2806 "The patient who will use the device.", 0, 1, subject); 2807 case 1524132147: 2808 /* encounter */ return new Property("encounter", "Reference(Encounter)", 2809 "An encounter that provides additional context in which this request is made.", 0, 1, encounter); 2810 case -2022646513: 2811 /* occurrence[x] */ return new Property("occurrence[x]", "dateTime|Period|Timing", 2812 "The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 2813 0, 1, occurrence); 2814 case 1687874001: 2815 /* occurrence */ return new Property("occurrence[x]", "dateTime|Period|Timing", 2816 "The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 2817 0, 1, occurrence); 2818 case -298443636: 2819 /* occurrenceDateTime */ return new Property("occurrence[x]", "dateTime|Period|Timing", 2820 "The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 2821 0, 1, occurrence); 2822 case 1397156594: 2823 /* occurrencePeriod */ return new Property("occurrence[x]", "dateTime|Period|Timing", 2824 "The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 2825 0, 1, occurrence); 2826 case 1515218299: 2827 /* occurrenceTiming */ return new Property("occurrence[x]", "dateTime|Period|Timing", 2828 "The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 2829 0, 1, occurrence); 2830 case -1500852503: 2831 /* authoredOn */ return new Property("authoredOn", "dateTime", 2832 "When the request transitioned to being actionable.", 0, 1, authoredOn); 2833 case 693933948: 2834 /* requester */ return new Property("requester", "Reference(Device|Practitioner|PractitionerRole|Organization)", 2835 "The individual who initiated the request and has responsibility for its activation.", 0, 1, requester); 2836 case -901444568: 2837 /* performerType */ return new Property("performerType", "CodeableConcept", 2838 "Desired type of performer for doing the diagnostic testing.", 0, 1, performerType); 2839 case 481140686: 2840 /* performer */ return new Property("performer", 2841 "Reference(Practitioner|PractitionerRole|Organization|CareTeam|HealthcareService|Patient|Device|RelatedPerson)", 2842 "The desired performer for doing the diagnostic testing.", 0, 1, performer); 2843 case 722137681: 2844 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 2845 "Reason or justification for the use of this device.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 2846 case -1146218137: 2847 /* reasonReference */ return new Property("reasonReference", 2848 "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 2849 "Reason or justification for the use of this device.", 0, java.lang.Integer.MAX_VALUE, reasonReference); 2850 case 73049818: 2851 /* insurance */ return new Property("insurance", "Reference(Coverage|ClaimResponse)", 2852 "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be required for delivering the requested service.", 2853 0, java.lang.Integer.MAX_VALUE, insurance); 2854 case 1922406657: 2855 /* supportingInfo */ return new Property("supportingInfo", "Reference(Any)", 2856 "Additional clinical information about the patient that may influence the request fulfilment. For example, this may include where on the subject's body the device will be used (i.e. the target site).", 2857 0, java.lang.Integer.MAX_VALUE, supportingInfo); 2858 case 3387378: 2859 /* note */ return new Property("note", "Annotation", 2860 "Details about this request that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.", 2861 0, java.lang.Integer.MAX_VALUE, note); 2862 case 1538891575: 2863 /* relevantHistory */ return new Property("relevantHistory", "Reference(Provenance)", 2864 "Key events in the history of the request.", 0, java.lang.Integer.MAX_VALUE, relevantHistory); 2865 default: 2866 return super.getNamedProperty(_hash, _name, _checkValid); 2867 } 2868 2869 } 2870 2871 @Override 2872 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2873 switch (hash) { 2874 case -1618432855: 2875 /* identifier */ return this.identifier == null ? new Base[0] 2876 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2877 case 8911915: 2878 /* instantiatesCanonical */ return this.instantiatesCanonical == null ? new Base[0] 2879 : this.instantiatesCanonical.toArray(new Base[this.instantiatesCanonical.size()]); // CanonicalType 2880 case -1926393373: 2881 /* instantiatesUri */ return this.instantiatesUri == null ? new Base[0] 2882 : this.instantiatesUri.toArray(new Base[this.instantiatesUri.size()]); // UriType 2883 case -332612366: 2884 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2885 case 237568101: 2886 /* priorRequest */ return this.priorRequest == null ? new Base[0] 2887 : this.priorRequest.toArray(new Base[this.priorRequest.size()]); // Reference 2888 case -445338488: 2889 /* groupIdentifier */ return this.groupIdentifier == null ? new Base[0] : new Base[] { this.groupIdentifier }; // Identifier 2890 case -892481550: 2891 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<DeviceRequestStatus> 2892 case -1183762788: 2893 /* intent */ return this.intent == null ? new Base[0] : new Base[] { this.intent }; // Enumeration<RequestIntent> 2894 case -1165461084: 2895 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // Enumeration<RequestPriority> 2896 case 3059181: 2897 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // Type 2898 case 1954460585: 2899 /* parameter */ return this.parameter == null ? new Base[0] 2900 : this.parameter.toArray(new Base[this.parameter.size()]); // DeviceRequestParameterComponent 2901 case -1867885268: 2902 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2903 case 1524132147: 2904 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 2905 case 1687874001: 2906 /* occurrence */ return this.occurrence == null ? new Base[0] : new Base[] { this.occurrence }; // Type 2907 case -1500852503: 2908 /* authoredOn */ return this.authoredOn == null ? new Base[0] : new Base[] { this.authoredOn }; // DateTimeType 2909 case 693933948: 2910 /* requester */ return this.requester == null ? new Base[0] : new Base[] { this.requester }; // Reference 2911 case -901444568: 2912 /* performerType */ return this.performerType == null ? new Base[0] : new Base[] { this.performerType }; // CodeableConcept 2913 case 481140686: 2914 /* performer */ return this.performer == null ? new Base[0] : new Base[] { this.performer }; // Reference 2915 case 722137681: 2916 /* reasonCode */ return this.reasonCode == null ? new Base[0] 2917 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 2918 case -1146218137: 2919 /* reasonReference */ return this.reasonReference == null ? new Base[0] 2920 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 2921 case 73049818: 2922 /* insurance */ return this.insurance == null ? new Base[0] 2923 : this.insurance.toArray(new Base[this.insurance.size()]); // Reference 2924 case 1922406657: 2925 /* supportingInfo */ return this.supportingInfo == null ? new Base[0] 2926 : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // Reference 2927 case 3387378: 2928 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2929 case 1538891575: 2930 /* relevantHistory */ return this.relevantHistory == null ? new Base[0] 2931 : this.relevantHistory.toArray(new Base[this.relevantHistory.size()]); // Reference 2932 default: 2933 return super.getProperty(hash, name, checkValid); 2934 } 2935 2936 } 2937 2938 @Override 2939 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2940 switch (hash) { 2941 case -1618432855: // identifier 2942 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2943 return value; 2944 case 8911915: // instantiatesCanonical 2945 this.getInstantiatesCanonical().add(castToCanonical(value)); // CanonicalType 2946 return value; 2947 case -1926393373: // instantiatesUri 2948 this.getInstantiatesUri().add(castToUri(value)); // UriType 2949 return value; 2950 case -332612366: // basedOn 2951 this.getBasedOn().add(castToReference(value)); // Reference 2952 return value; 2953 case 237568101: // priorRequest 2954 this.getPriorRequest().add(castToReference(value)); // Reference 2955 return value; 2956 case -445338488: // groupIdentifier 2957 this.groupIdentifier = castToIdentifier(value); // Identifier 2958 return value; 2959 case -892481550: // status 2960 value = new DeviceRequestStatusEnumFactory().fromType(castToCode(value)); 2961 this.status = (Enumeration) value; // Enumeration<DeviceRequestStatus> 2962 return value; 2963 case -1183762788: // intent 2964 value = new RequestIntentEnumFactory().fromType(castToCode(value)); 2965 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 2966 return value; 2967 case -1165461084: // priority 2968 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 2969 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 2970 return value; 2971 case 3059181: // code 2972 this.code = castToType(value); // Type 2973 return value; 2974 case 1954460585: // parameter 2975 this.getParameter().add((DeviceRequestParameterComponent) value); // DeviceRequestParameterComponent 2976 return value; 2977 case -1867885268: // subject 2978 this.subject = castToReference(value); // Reference 2979 return value; 2980 case 1524132147: // encounter 2981 this.encounter = castToReference(value); // Reference 2982 return value; 2983 case 1687874001: // occurrence 2984 this.occurrence = castToType(value); // Type 2985 return value; 2986 case -1500852503: // authoredOn 2987 this.authoredOn = castToDateTime(value); // DateTimeType 2988 return value; 2989 case 693933948: // requester 2990 this.requester = castToReference(value); // Reference 2991 return value; 2992 case -901444568: // performerType 2993 this.performerType = castToCodeableConcept(value); // CodeableConcept 2994 return value; 2995 case 481140686: // performer 2996 this.performer = castToReference(value); // Reference 2997 return value; 2998 case 722137681: // reasonCode 2999 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 3000 return value; 3001 case -1146218137: // reasonReference 3002 this.getReasonReference().add(castToReference(value)); // Reference 3003 return value; 3004 case 73049818: // insurance 3005 this.getInsurance().add(castToReference(value)); // Reference 3006 return value; 3007 case 1922406657: // supportingInfo 3008 this.getSupportingInfo().add(castToReference(value)); // Reference 3009 return value; 3010 case 3387378: // note 3011 this.getNote().add(castToAnnotation(value)); // Annotation 3012 return value; 3013 case 1538891575: // relevantHistory 3014 this.getRelevantHistory().add(castToReference(value)); // Reference 3015 return value; 3016 default: 3017 return super.setProperty(hash, name, value); 3018 } 3019 3020 } 3021 3022 @Override 3023 public Base setProperty(String name, Base value) throws FHIRException { 3024 if (name.equals("identifier")) { 3025 this.getIdentifier().add(castToIdentifier(value)); 3026 } else if (name.equals("instantiatesCanonical")) { 3027 this.getInstantiatesCanonical().add(castToCanonical(value)); 3028 } else if (name.equals("instantiatesUri")) { 3029 this.getInstantiatesUri().add(castToUri(value)); 3030 } else if (name.equals("basedOn")) { 3031 this.getBasedOn().add(castToReference(value)); 3032 } else if (name.equals("priorRequest")) { 3033 this.getPriorRequest().add(castToReference(value)); 3034 } else if (name.equals("groupIdentifier")) { 3035 this.groupIdentifier = castToIdentifier(value); // Identifier 3036 } else if (name.equals("status")) { 3037 value = new DeviceRequestStatusEnumFactory().fromType(castToCode(value)); 3038 this.status = (Enumeration) value; // Enumeration<DeviceRequestStatus> 3039 } else if (name.equals("intent")) { 3040 value = new RequestIntentEnumFactory().fromType(castToCode(value)); 3041 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 3042 } else if (name.equals("priority")) { 3043 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 3044 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 3045 } else if (name.equals("code[x]")) { 3046 this.code = castToType(value); // Type 3047 } else if (name.equals("parameter")) { 3048 this.getParameter().add((DeviceRequestParameterComponent) value); 3049 } else if (name.equals("subject")) { 3050 this.subject = castToReference(value); // Reference 3051 } else if (name.equals("encounter")) { 3052 this.encounter = castToReference(value); // Reference 3053 } else if (name.equals("occurrence[x]")) { 3054 this.occurrence = castToType(value); // Type 3055 } else if (name.equals("authoredOn")) { 3056 this.authoredOn = castToDateTime(value); // DateTimeType 3057 } else if (name.equals("requester")) { 3058 this.requester = castToReference(value); // Reference 3059 } else if (name.equals("performerType")) { 3060 this.performerType = castToCodeableConcept(value); // CodeableConcept 3061 } else if (name.equals("performer")) { 3062 this.performer = castToReference(value); // Reference 3063 } else if (name.equals("reasonCode")) { 3064 this.getReasonCode().add(castToCodeableConcept(value)); 3065 } else if (name.equals("reasonReference")) { 3066 this.getReasonReference().add(castToReference(value)); 3067 } else if (name.equals("insurance")) { 3068 this.getInsurance().add(castToReference(value)); 3069 } else if (name.equals("supportingInfo")) { 3070 this.getSupportingInfo().add(castToReference(value)); 3071 } else if (name.equals("note")) { 3072 this.getNote().add(castToAnnotation(value)); 3073 } else if (name.equals("relevantHistory")) { 3074 this.getRelevantHistory().add(castToReference(value)); 3075 } else 3076 return super.setProperty(name, value); 3077 return value; 3078 } 3079 3080 @Override 3081 public void removeChild(String name, Base value) throws FHIRException { 3082 if (name.equals("identifier")) { 3083 this.getIdentifier().remove(castToIdentifier(value)); 3084 } else if (name.equals("instantiatesCanonical")) { 3085 this.getInstantiatesCanonical().remove(castToCanonical(value)); 3086 } else if (name.equals("instantiatesUri")) { 3087 this.getInstantiatesUri().remove(castToUri(value)); 3088 } else if (name.equals("basedOn")) { 3089 this.getBasedOn().remove(castToReference(value)); 3090 } else if (name.equals("priorRequest")) { 3091 this.getPriorRequest().remove(castToReference(value)); 3092 } else if (name.equals("groupIdentifier")) { 3093 this.groupIdentifier = null; 3094 } else if (name.equals("status")) { 3095 this.status = null; 3096 } else if (name.equals("intent")) { 3097 this.intent = null; 3098 } else if (name.equals("priority")) { 3099 this.priority = null; 3100 } else if (name.equals("code[x]")) { 3101 this.code = null; 3102 } else if (name.equals("parameter")) { 3103 this.getParameter().remove((DeviceRequestParameterComponent) value); 3104 } else if (name.equals("subject")) { 3105 this.subject = null; 3106 } else if (name.equals("encounter")) { 3107 this.encounter = null; 3108 } else if (name.equals("occurrence[x]")) { 3109 this.occurrence = null; 3110 } else if (name.equals("authoredOn")) { 3111 this.authoredOn = null; 3112 } else if (name.equals("requester")) { 3113 this.requester = null; 3114 } else if (name.equals("performerType")) { 3115 this.performerType = null; 3116 } else if (name.equals("performer")) { 3117 this.performer = null; 3118 } else if (name.equals("reasonCode")) { 3119 this.getReasonCode().remove(castToCodeableConcept(value)); 3120 } else if (name.equals("reasonReference")) { 3121 this.getReasonReference().remove(castToReference(value)); 3122 } else if (name.equals("insurance")) { 3123 this.getInsurance().remove(castToReference(value)); 3124 } else if (name.equals("supportingInfo")) { 3125 this.getSupportingInfo().remove(castToReference(value)); 3126 } else if (name.equals("note")) { 3127 this.getNote().remove(castToAnnotation(value)); 3128 } else if (name.equals("relevantHistory")) { 3129 this.getRelevantHistory().remove(castToReference(value)); 3130 } else 3131 super.removeChild(name, value); 3132 3133 } 3134 3135 @Override 3136 public Base makeProperty(int hash, String name) throws FHIRException { 3137 switch (hash) { 3138 case -1618432855: 3139 return addIdentifier(); 3140 case 8911915: 3141 return addInstantiatesCanonicalElement(); 3142 case -1926393373: 3143 return addInstantiatesUriElement(); 3144 case -332612366: 3145 return addBasedOn(); 3146 case 237568101: 3147 return addPriorRequest(); 3148 case -445338488: 3149 return getGroupIdentifier(); 3150 case -892481550: 3151 return getStatusElement(); 3152 case -1183762788: 3153 return getIntentElement(); 3154 case -1165461084: 3155 return getPriorityElement(); 3156 case 941839219: 3157 return getCode(); 3158 case 3059181: 3159 return getCode(); 3160 case 1954460585: 3161 return addParameter(); 3162 case -1867885268: 3163 return getSubject(); 3164 case 1524132147: 3165 return getEncounter(); 3166 case -2022646513: 3167 return getOccurrence(); 3168 case 1687874001: 3169 return getOccurrence(); 3170 case -1500852503: 3171 return getAuthoredOnElement(); 3172 case 693933948: 3173 return getRequester(); 3174 case -901444568: 3175 return getPerformerType(); 3176 case 481140686: 3177 return getPerformer(); 3178 case 722137681: 3179 return addReasonCode(); 3180 case -1146218137: 3181 return addReasonReference(); 3182 case 73049818: 3183 return addInsurance(); 3184 case 1922406657: 3185 return addSupportingInfo(); 3186 case 3387378: 3187 return addNote(); 3188 case 1538891575: 3189 return addRelevantHistory(); 3190 default: 3191 return super.makeProperty(hash, name); 3192 } 3193 3194 } 3195 3196 @Override 3197 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3198 switch (hash) { 3199 case -1618432855: 3200 /* identifier */ return new String[] { "Identifier" }; 3201 case 8911915: 3202 /* instantiatesCanonical */ return new String[] { "canonical" }; 3203 case -1926393373: 3204 /* instantiatesUri */ return new String[] { "uri" }; 3205 case -332612366: 3206 /* basedOn */ return new String[] { "Reference" }; 3207 case 237568101: 3208 /* priorRequest */ return new String[] { "Reference" }; 3209 case -445338488: 3210 /* groupIdentifier */ return new String[] { "Identifier" }; 3211 case -892481550: 3212 /* status */ return new String[] { "code" }; 3213 case -1183762788: 3214 /* intent */ return new String[] { "code" }; 3215 case -1165461084: 3216 /* priority */ return new String[] { "code" }; 3217 case 3059181: 3218 /* code */ return new String[] { "Reference", "CodeableConcept" }; 3219 case 1954460585: 3220 /* parameter */ return new String[] {}; 3221 case -1867885268: 3222 /* subject */ return new String[] { "Reference" }; 3223 case 1524132147: 3224 /* encounter */ return new String[] { "Reference" }; 3225 case 1687874001: 3226 /* occurrence */ return new String[] { "dateTime", "Period", "Timing" }; 3227 case -1500852503: 3228 /* authoredOn */ return new String[] { "dateTime" }; 3229 case 693933948: 3230 /* requester */ return new String[] { "Reference" }; 3231 case -901444568: 3232 /* performerType */ return new String[] { "CodeableConcept" }; 3233 case 481140686: 3234 /* performer */ return new String[] { "Reference" }; 3235 case 722137681: 3236 /* reasonCode */ return new String[] { "CodeableConcept" }; 3237 case -1146218137: 3238 /* reasonReference */ return new String[] { "Reference" }; 3239 case 73049818: 3240 /* insurance */ return new String[] { "Reference" }; 3241 case 1922406657: 3242 /* supportingInfo */ return new String[] { "Reference" }; 3243 case 3387378: 3244 /* note */ return new String[] { "Annotation" }; 3245 case 1538891575: 3246 /* relevantHistory */ return new String[] { "Reference" }; 3247 default: 3248 return super.getTypesForProperty(hash, name); 3249 } 3250 3251 } 3252 3253 @Override 3254 public Base addChild(String name) throws FHIRException { 3255 if (name.equals("identifier")) { 3256 return addIdentifier(); 3257 } else if (name.equals("instantiatesCanonical")) { 3258 throw new FHIRException("Cannot call addChild on a singleton property DeviceRequest.instantiatesCanonical"); 3259 } else if (name.equals("instantiatesUri")) { 3260 throw new FHIRException("Cannot call addChild on a singleton property DeviceRequest.instantiatesUri"); 3261 } else if (name.equals("basedOn")) { 3262 return addBasedOn(); 3263 } else if (name.equals("priorRequest")) { 3264 return addPriorRequest(); 3265 } else if (name.equals("groupIdentifier")) { 3266 this.groupIdentifier = new Identifier(); 3267 return this.groupIdentifier; 3268 } else if (name.equals("status")) { 3269 throw new FHIRException("Cannot call addChild on a singleton property DeviceRequest.status"); 3270 } else if (name.equals("intent")) { 3271 throw new FHIRException("Cannot call addChild on a singleton property DeviceRequest.intent"); 3272 } else if (name.equals("priority")) { 3273 throw new FHIRException("Cannot call addChild on a singleton property DeviceRequest.priority"); 3274 } else if (name.equals("codeReference")) { 3275 this.code = new Reference(); 3276 return this.code; 3277 } else if (name.equals("codeCodeableConcept")) { 3278 this.code = new CodeableConcept(); 3279 return this.code; 3280 } else if (name.equals("parameter")) { 3281 return addParameter(); 3282 } else if (name.equals("subject")) { 3283 this.subject = new Reference(); 3284 return this.subject; 3285 } else if (name.equals("encounter")) { 3286 this.encounter = new Reference(); 3287 return this.encounter; 3288 } else if (name.equals("occurrenceDateTime")) { 3289 this.occurrence = new DateTimeType(); 3290 return this.occurrence; 3291 } else if (name.equals("occurrencePeriod")) { 3292 this.occurrence = new Period(); 3293 return this.occurrence; 3294 } else if (name.equals("occurrenceTiming")) { 3295 this.occurrence = new Timing(); 3296 return this.occurrence; 3297 } else if (name.equals("authoredOn")) { 3298 throw new FHIRException("Cannot call addChild on a singleton property DeviceRequest.authoredOn"); 3299 } else if (name.equals("requester")) { 3300 this.requester = new Reference(); 3301 return this.requester; 3302 } else if (name.equals("performerType")) { 3303 this.performerType = new CodeableConcept(); 3304 return this.performerType; 3305 } else if (name.equals("performer")) { 3306 this.performer = new Reference(); 3307 return this.performer; 3308 } else if (name.equals("reasonCode")) { 3309 return addReasonCode(); 3310 } else if (name.equals("reasonReference")) { 3311 return addReasonReference(); 3312 } else if (name.equals("insurance")) { 3313 return addInsurance(); 3314 } else if (name.equals("supportingInfo")) { 3315 return addSupportingInfo(); 3316 } else if (name.equals("note")) { 3317 return addNote(); 3318 } else if (name.equals("relevantHistory")) { 3319 return addRelevantHistory(); 3320 } else 3321 return super.addChild(name); 3322 } 3323 3324 public String fhirType() { 3325 return "DeviceRequest"; 3326 3327 } 3328 3329 public DeviceRequest copy() { 3330 DeviceRequest dst = new DeviceRequest(); 3331 copyValues(dst); 3332 return dst; 3333 } 3334 3335 public void copyValues(DeviceRequest dst) { 3336 super.copyValues(dst); 3337 if (identifier != null) { 3338 dst.identifier = new ArrayList<Identifier>(); 3339 for (Identifier i : identifier) 3340 dst.identifier.add(i.copy()); 3341 } 3342 ; 3343 if (instantiatesCanonical != null) { 3344 dst.instantiatesCanonical = new ArrayList<CanonicalType>(); 3345 for (CanonicalType i : instantiatesCanonical) 3346 dst.instantiatesCanonical.add(i.copy()); 3347 } 3348 ; 3349 if (instantiatesUri != null) { 3350 dst.instantiatesUri = new ArrayList<UriType>(); 3351 for (UriType i : instantiatesUri) 3352 dst.instantiatesUri.add(i.copy()); 3353 } 3354 ; 3355 if (basedOn != null) { 3356 dst.basedOn = new ArrayList<Reference>(); 3357 for (Reference i : basedOn) 3358 dst.basedOn.add(i.copy()); 3359 } 3360 ; 3361 if (priorRequest != null) { 3362 dst.priorRequest = new ArrayList<Reference>(); 3363 for (Reference i : priorRequest) 3364 dst.priorRequest.add(i.copy()); 3365 } 3366 ; 3367 dst.groupIdentifier = groupIdentifier == null ? null : groupIdentifier.copy(); 3368 dst.status = status == null ? null : status.copy(); 3369 dst.intent = intent == null ? null : intent.copy(); 3370 dst.priority = priority == null ? null : priority.copy(); 3371 dst.code = code == null ? null : code.copy(); 3372 if (parameter != null) { 3373 dst.parameter = new ArrayList<DeviceRequestParameterComponent>(); 3374 for (DeviceRequestParameterComponent i : parameter) 3375 dst.parameter.add(i.copy()); 3376 } 3377 ; 3378 dst.subject = subject == null ? null : subject.copy(); 3379 dst.encounter = encounter == null ? null : encounter.copy(); 3380 dst.occurrence = occurrence == null ? null : occurrence.copy(); 3381 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 3382 dst.requester = requester == null ? null : requester.copy(); 3383 dst.performerType = performerType == null ? null : performerType.copy(); 3384 dst.performer = performer == null ? null : performer.copy(); 3385 if (reasonCode != null) { 3386 dst.reasonCode = new ArrayList<CodeableConcept>(); 3387 for (CodeableConcept i : reasonCode) 3388 dst.reasonCode.add(i.copy()); 3389 } 3390 ; 3391 if (reasonReference != null) { 3392 dst.reasonReference = new ArrayList<Reference>(); 3393 for (Reference i : reasonReference) 3394 dst.reasonReference.add(i.copy()); 3395 } 3396 ; 3397 if (insurance != null) { 3398 dst.insurance = new ArrayList<Reference>(); 3399 for (Reference i : insurance) 3400 dst.insurance.add(i.copy()); 3401 } 3402 ; 3403 if (supportingInfo != null) { 3404 dst.supportingInfo = new ArrayList<Reference>(); 3405 for (Reference i : supportingInfo) 3406 dst.supportingInfo.add(i.copy()); 3407 } 3408 ; 3409 if (note != null) { 3410 dst.note = new ArrayList<Annotation>(); 3411 for (Annotation i : note) 3412 dst.note.add(i.copy()); 3413 } 3414 ; 3415 if (relevantHistory != null) { 3416 dst.relevantHistory = new ArrayList<Reference>(); 3417 for (Reference i : relevantHistory) 3418 dst.relevantHistory.add(i.copy()); 3419 } 3420 ; 3421 } 3422 3423 protected DeviceRequest typedCopy() { 3424 return copy(); 3425 } 3426 3427 @Override 3428 public boolean equalsDeep(Base other_) { 3429 if (!super.equalsDeep(other_)) 3430 return false; 3431 if (!(other_ instanceof DeviceRequest)) 3432 return false; 3433 DeviceRequest o = (DeviceRequest) other_; 3434 return compareDeep(identifier, o.identifier, true) 3435 && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 3436 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(basedOn, o.basedOn, true) 3437 && compareDeep(priorRequest, o.priorRequest, true) && compareDeep(groupIdentifier, o.groupIdentifier, true) 3438 && compareDeep(status, o.status, true) && compareDeep(intent, o.intent, true) 3439 && compareDeep(priority, o.priority, true) && compareDeep(code, o.code, true) 3440 && compareDeep(parameter, o.parameter, true) && compareDeep(subject, o.subject, true) 3441 && compareDeep(encounter, o.encounter, true) && compareDeep(occurrence, o.occurrence, true) 3442 && compareDeep(authoredOn, o.authoredOn, true) && compareDeep(requester, o.requester, true) 3443 && compareDeep(performerType, o.performerType, true) && compareDeep(performer, o.performer, true) 3444 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 3445 && compareDeep(insurance, o.insurance, true) && compareDeep(supportingInfo, o.supportingInfo, true) 3446 && compareDeep(note, o.note, true) && compareDeep(relevantHistory, o.relevantHistory, true); 3447 } 3448 3449 @Override 3450 public boolean equalsShallow(Base other_) { 3451 if (!super.equalsShallow(other_)) 3452 return false; 3453 if (!(other_ instanceof DeviceRequest)) 3454 return false; 3455 DeviceRequest o = (DeviceRequest) other_; 3456 return compareValues(instantiatesUri, o.instantiatesUri, true) && compareValues(status, o.status, true) 3457 && compareValues(intent, o.intent, true) && compareValues(priority, o.priority, true) 3458 && compareValues(authoredOn, o.authoredOn, true); 3459 } 3460 3461 public boolean isEmpty() { 3462 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiatesCanonical, instantiatesUri, 3463 basedOn, priorRequest, groupIdentifier, status, intent, priority, code, parameter, subject, encounter, 3464 occurrence, authoredOn, requester, performerType, performer, reasonCode, reasonReference, insurance, 3465 supportingInfo, note, relevantHistory); 3466 } 3467 3468 @Override 3469 public ResourceType getResourceType() { 3470 return ResourceType.DeviceRequest; 3471 } 3472 3473 /** 3474 * Search parameter: <b>requester</b> 3475 * <p> 3476 * Description: <b>Who/what is requesting service</b><br> 3477 * Type: <b>reference</b><br> 3478 * Path: <b>DeviceRequest.requester</b><br> 3479 * </p> 3480 */ 3481 @SearchParamDefinition(name = "requester", path = "DeviceRequest.requester", description = "Who/what is requesting service", type = "reference", providesMembershipIn = { 3482 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 3483 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Device.class, 3484 Organization.class, Practitioner.class, PractitionerRole.class }) 3485 public static final String SP_REQUESTER = "requester"; 3486 /** 3487 * <b>Fluent Client</b> search parameter constant for <b>requester</b> 3488 * <p> 3489 * Description: <b>Who/what is requesting service</b><br> 3490 * Type: <b>reference</b><br> 3491 * Path: <b>DeviceRequest.requester</b><br> 3492 * </p> 3493 */ 3494 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3495 SP_REQUESTER); 3496 3497 /** 3498 * Constant for fluent queries to be used to add include statements. Specifies 3499 * the path value of "<b>DeviceRequest:requester</b>". 3500 */ 3501 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTER = new ca.uhn.fhir.model.api.Include( 3502 "DeviceRequest:requester").toLocked(); 3503 3504 /** 3505 * Search parameter: <b>insurance</b> 3506 * <p> 3507 * Description: <b>Associated insurance coverage</b><br> 3508 * Type: <b>reference</b><br> 3509 * Path: <b>DeviceRequest.insurance</b><br> 3510 * </p> 3511 */ 3512 @SearchParamDefinition(name = "insurance", path = "DeviceRequest.insurance", description = "Associated insurance coverage", type = "reference", target = { 3513 ClaimResponse.class, Coverage.class }) 3514 public static final String SP_INSURANCE = "insurance"; 3515 /** 3516 * <b>Fluent Client</b> search parameter constant for <b>insurance</b> 3517 * <p> 3518 * Description: <b>Associated insurance coverage</b><br> 3519 * Type: <b>reference</b><br> 3520 * Path: <b>DeviceRequest.insurance</b><br> 3521 * </p> 3522 */ 3523 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSURANCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3524 SP_INSURANCE); 3525 3526 /** 3527 * Constant for fluent queries to be used to add include statements. Specifies 3528 * the path value of "<b>DeviceRequest:insurance</b>". 3529 */ 3530 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSURANCE = new ca.uhn.fhir.model.api.Include( 3531 "DeviceRequest:insurance").toLocked(); 3532 3533 /** 3534 * Search parameter: <b>identifier</b> 3535 * <p> 3536 * Description: <b>Business identifier for request/order</b><br> 3537 * Type: <b>token</b><br> 3538 * Path: <b>DeviceRequest.identifier</b><br> 3539 * </p> 3540 */ 3541 @SearchParamDefinition(name = "identifier", path = "DeviceRequest.identifier", description = "Business identifier for request/order", type = "token") 3542 public static final String SP_IDENTIFIER = "identifier"; 3543 /** 3544 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3545 * <p> 3546 * Description: <b>Business identifier for request/order</b><br> 3547 * Type: <b>token</b><br> 3548 * Path: <b>DeviceRequest.identifier</b><br> 3549 * </p> 3550 */ 3551 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3552 SP_IDENTIFIER); 3553 3554 /** 3555 * Search parameter: <b>code</b> 3556 * <p> 3557 * Description: <b>Code for what is being requested/ordered</b><br> 3558 * Type: <b>token</b><br> 3559 * Path: <b>DeviceRequest.codeCodeableConcept</b><br> 3560 * </p> 3561 */ 3562 @SearchParamDefinition(name = "code", path = "(DeviceRequest.code as CodeableConcept)", description = "Code for what is being requested/ordered", type = "token") 3563 public static final String SP_CODE = "code"; 3564 /** 3565 * <b>Fluent Client</b> search parameter constant for <b>code</b> 3566 * <p> 3567 * Description: <b>Code for what is being requested/ordered</b><br> 3568 * Type: <b>token</b><br> 3569 * Path: <b>DeviceRequest.codeCodeableConcept</b><br> 3570 * </p> 3571 */ 3572 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3573 SP_CODE); 3574 3575 /** 3576 * Search parameter: <b>performer</b> 3577 * <p> 3578 * Description: <b>Desired performer for service</b><br> 3579 * Type: <b>reference</b><br> 3580 * Path: <b>DeviceRequest.performer</b><br> 3581 * </p> 3582 */ 3583 @SearchParamDefinition(name = "performer", path = "DeviceRequest.performer", description = "Desired performer for service", type = "reference", providesMembershipIn = { 3584 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 3585 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3586 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { CareTeam.class, Device.class, 3587 HealthcareService.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, 3588 RelatedPerson.class }) 3589 public static final String SP_PERFORMER = "performer"; 3590 /** 3591 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 3592 * <p> 3593 * Description: <b>Desired performer for service</b><br> 3594 * Type: <b>reference</b><br> 3595 * Path: <b>DeviceRequest.performer</b><br> 3596 * </p> 3597 */ 3598 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3599 SP_PERFORMER); 3600 3601 /** 3602 * Constant for fluent queries to be used to add include statements. Specifies 3603 * the path value of "<b>DeviceRequest:performer</b>". 3604 */ 3605 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include( 3606 "DeviceRequest:performer").toLocked(); 3607 3608 /** 3609 * Search parameter: <b>event-date</b> 3610 * <p> 3611 * Description: <b>When service should occur</b><br> 3612 * Type: <b>date</b><br> 3613 * Path: <b>DeviceRequest.occurrenceDateTime, 3614 * DeviceRequest.occurrencePeriod</b><br> 3615 * </p> 3616 */ 3617 @SearchParamDefinition(name = "event-date", path = "(DeviceRequest.occurrence as dateTime) | (DeviceRequest.occurrence as Period)", description = "When service should occur", type = "date") 3618 public static final String SP_EVENT_DATE = "event-date"; 3619 /** 3620 * <b>Fluent Client</b> search parameter constant for <b>event-date</b> 3621 * <p> 3622 * Description: <b>When service should occur</b><br> 3623 * Type: <b>date</b><br> 3624 * Path: <b>DeviceRequest.occurrenceDateTime, 3625 * DeviceRequest.occurrencePeriod</b><br> 3626 * </p> 3627 */ 3628 public static final ca.uhn.fhir.rest.gclient.DateClientParam EVENT_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3629 SP_EVENT_DATE); 3630 3631 /** 3632 * Search parameter: <b>subject</b> 3633 * <p> 3634 * Description: <b>Individual the service is ordered for</b><br> 3635 * Type: <b>reference</b><br> 3636 * Path: <b>DeviceRequest.subject</b><br> 3637 * </p> 3638 */ 3639 @SearchParamDefinition(name = "subject", path = "DeviceRequest.subject", description = "Individual the service is ordered for", type = "reference", providesMembershipIn = { 3640 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 3641 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Device.class, Group.class, 3642 Location.class, Patient.class }) 3643 public static final String SP_SUBJECT = "subject"; 3644 /** 3645 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3646 * <p> 3647 * Description: <b>Individual the service is ordered for</b><br> 3648 * Type: <b>reference</b><br> 3649 * Path: <b>DeviceRequest.subject</b><br> 3650 * </p> 3651 */ 3652 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3653 SP_SUBJECT); 3654 3655 /** 3656 * Constant for fluent queries to be used to add include statements. Specifies 3657 * the path value of "<b>DeviceRequest:subject</b>". 3658 */ 3659 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 3660 "DeviceRequest:subject").toLocked(); 3661 3662 /** 3663 * Search parameter: <b>instantiates-canonical</b> 3664 * <p> 3665 * Description: <b>Instantiates FHIR protocol or definition</b><br> 3666 * Type: <b>reference</b><br> 3667 * Path: <b>DeviceRequest.instantiatesCanonical</b><br> 3668 * </p> 3669 */ 3670 @SearchParamDefinition(name = "instantiates-canonical", path = "DeviceRequest.instantiatesCanonical", description = "Instantiates FHIR protocol or definition", type = "reference", target = { 3671 ActivityDefinition.class, PlanDefinition.class }) 3672 public static final String SP_INSTANTIATES_CANONICAL = "instantiates-canonical"; 3673 /** 3674 * <b>Fluent Client</b> search parameter constant for 3675 * <b>instantiates-canonical</b> 3676 * <p> 3677 * Description: <b>Instantiates FHIR protocol or definition</b><br> 3678 * Type: <b>reference</b><br> 3679 * Path: <b>DeviceRequest.instantiatesCanonical</b><br> 3680 * </p> 3681 */ 3682 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSTANTIATES_CANONICAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3683 SP_INSTANTIATES_CANONICAL); 3684 3685 /** 3686 * Constant for fluent queries to be used to add include statements. Specifies 3687 * the path value of "<b>DeviceRequest:instantiates-canonical</b>". 3688 */ 3689 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSTANTIATES_CANONICAL = new ca.uhn.fhir.model.api.Include( 3690 "DeviceRequest:instantiates-canonical").toLocked(); 3691 3692 /** 3693 * Search parameter: <b>encounter</b> 3694 * <p> 3695 * Description: <b>Encounter during which request was created</b><br> 3696 * Type: <b>reference</b><br> 3697 * Path: <b>DeviceRequest.encounter</b><br> 3698 * </p> 3699 */ 3700 @SearchParamDefinition(name = "encounter", path = "DeviceRequest.encounter", description = "Encounter during which request was created", type = "reference", providesMembershipIn = { 3701 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 3702 public static final String SP_ENCOUNTER = "encounter"; 3703 /** 3704 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 3705 * <p> 3706 * Description: <b>Encounter during which request was created</b><br> 3707 * Type: <b>reference</b><br> 3708 * Path: <b>DeviceRequest.encounter</b><br> 3709 * </p> 3710 */ 3711 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3712 SP_ENCOUNTER); 3713 3714 /** 3715 * Constant for fluent queries to be used to add include statements. Specifies 3716 * the path value of "<b>DeviceRequest:encounter</b>". 3717 */ 3718 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 3719 "DeviceRequest:encounter").toLocked(); 3720 3721 /** 3722 * Search parameter: <b>authored-on</b> 3723 * <p> 3724 * Description: <b>When the request transitioned to being actionable</b><br> 3725 * Type: <b>date</b><br> 3726 * Path: <b>DeviceRequest.authoredOn</b><br> 3727 * </p> 3728 */ 3729 @SearchParamDefinition(name = "authored-on", path = "DeviceRequest.authoredOn", description = "When the request transitioned to being actionable", type = "date") 3730 public static final String SP_AUTHORED_ON = "authored-on"; 3731 /** 3732 * <b>Fluent Client</b> search parameter constant for <b>authored-on</b> 3733 * <p> 3734 * Description: <b>When the request transitioned to being actionable</b><br> 3735 * Type: <b>date</b><br> 3736 * Path: <b>DeviceRequest.authoredOn</b><br> 3737 * </p> 3738 */ 3739 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED_ON = new ca.uhn.fhir.rest.gclient.DateClientParam( 3740 SP_AUTHORED_ON); 3741 3742 /** 3743 * Search parameter: <b>intent</b> 3744 * <p> 3745 * Description: <b>proposal | plan | original-order |reflex-order</b><br> 3746 * Type: <b>token</b><br> 3747 * Path: <b>DeviceRequest.intent</b><br> 3748 * </p> 3749 */ 3750 @SearchParamDefinition(name = "intent", path = "DeviceRequest.intent", description = "proposal | plan | original-order |reflex-order", type = "token") 3751 public static final String SP_INTENT = "intent"; 3752 /** 3753 * <b>Fluent Client</b> search parameter constant for <b>intent</b> 3754 * <p> 3755 * Description: <b>proposal | plan | original-order |reflex-order</b><br> 3756 * Type: <b>token</b><br> 3757 * Path: <b>DeviceRequest.intent</b><br> 3758 * </p> 3759 */ 3760 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3761 SP_INTENT); 3762 3763 /** 3764 * Search parameter: <b>group-identifier</b> 3765 * <p> 3766 * Description: <b>Composite request this is part of</b><br> 3767 * Type: <b>token</b><br> 3768 * Path: <b>DeviceRequest.groupIdentifier</b><br> 3769 * </p> 3770 */ 3771 @SearchParamDefinition(name = "group-identifier", path = "DeviceRequest.groupIdentifier", description = "Composite request this is part of", type = "token") 3772 public static final String SP_GROUP_IDENTIFIER = "group-identifier"; 3773 /** 3774 * <b>Fluent Client</b> search parameter constant for <b>group-identifier</b> 3775 * <p> 3776 * Description: <b>Composite request this is part of</b><br> 3777 * Type: <b>token</b><br> 3778 * Path: <b>DeviceRequest.groupIdentifier</b><br> 3779 * </p> 3780 */ 3781 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GROUP_IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3782 SP_GROUP_IDENTIFIER); 3783 3784 /** 3785 * Search parameter: <b>based-on</b> 3786 * <p> 3787 * Description: <b>Plan/proposal/order fulfilled by this request</b><br> 3788 * Type: <b>reference</b><br> 3789 * Path: <b>DeviceRequest.basedOn</b><br> 3790 * </p> 3791 */ 3792 @SearchParamDefinition(name = "based-on", path = "DeviceRequest.basedOn", description = "Plan/proposal/order fulfilled by this request", type = "reference") 3793 public static final String SP_BASED_ON = "based-on"; 3794 /** 3795 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 3796 * <p> 3797 * Description: <b>Plan/proposal/order fulfilled by this request</b><br> 3798 * Type: <b>reference</b><br> 3799 * Path: <b>DeviceRequest.basedOn</b><br> 3800 * </p> 3801 */ 3802 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3803 SP_BASED_ON); 3804 3805 /** 3806 * Constant for fluent queries to be used to add include statements. Specifies 3807 * the path value of "<b>DeviceRequest:based-on</b>". 3808 */ 3809 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include( 3810 "DeviceRequest:based-on").toLocked(); 3811 3812 /** 3813 * Search parameter: <b>patient</b> 3814 * <p> 3815 * Description: <b>Individual the service is ordered for</b><br> 3816 * Type: <b>reference</b><br> 3817 * Path: <b>DeviceRequest.subject</b><br> 3818 * </p> 3819 */ 3820 @SearchParamDefinition(name = "patient", path = "DeviceRequest.subject.where(resolve() is Patient)", description = "Individual the service is ordered for", type = "reference", target = { 3821 Patient.class }) 3822 public static final String SP_PATIENT = "patient"; 3823 /** 3824 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3825 * <p> 3826 * Description: <b>Individual the service is ordered for</b><br> 3827 * Type: <b>reference</b><br> 3828 * Path: <b>DeviceRequest.subject</b><br> 3829 * </p> 3830 */ 3831 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3832 SP_PATIENT); 3833 3834 /** 3835 * Constant for fluent queries to be used to add include statements. Specifies 3836 * the path value of "<b>DeviceRequest:patient</b>". 3837 */ 3838 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 3839 "DeviceRequest:patient").toLocked(); 3840 3841 /** 3842 * Search parameter: <b>instantiates-uri</b> 3843 * <p> 3844 * Description: <b>Instantiates external protocol or definition</b><br> 3845 * Type: <b>uri</b><br> 3846 * Path: <b>DeviceRequest.instantiatesUri</b><br> 3847 * </p> 3848 */ 3849 @SearchParamDefinition(name = "instantiates-uri", path = "DeviceRequest.instantiatesUri", description = "Instantiates external protocol or definition", type = "uri") 3850 public static final String SP_INSTANTIATES_URI = "instantiates-uri"; 3851 /** 3852 * <b>Fluent Client</b> search parameter constant for <b>instantiates-uri</b> 3853 * <p> 3854 * Description: <b>Instantiates external protocol or definition</b><br> 3855 * Type: <b>uri</b><br> 3856 * Path: <b>DeviceRequest.instantiatesUri</b><br> 3857 * </p> 3858 */ 3859 public static final ca.uhn.fhir.rest.gclient.UriClientParam INSTANTIATES_URI = new ca.uhn.fhir.rest.gclient.UriClientParam( 3860 SP_INSTANTIATES_URI); 3861 3862 /** 3863 * Search parameter: <b>prior-request</b> 3864 * <p> 3865 * Description: <b>Request takes the place of referenced completed or terminated 3866 * requests</b><br> 3867 * Type: <b>reference</b><br> 3868 * Path: <b>DeviceRequest.priorRequest</b><br> 3869 * </p> 3870 */ 3871 @SearchParamDefinition(name = "prior-request", path = "DeviceRequest.priorRequest", description = "Request takes the place of referenced completed or terminated requests", type = "reference") 3872 public static final String SP_PRIOR_REQUEST = "prior-request"; 3873 /** 3874 * <b>Fluent Client</b> search parameter constant for <b>prior-request</b> 3875 * <p> 3876 * Description: <b>Request takes the place of referenced completed or terminated 3877 * requests</b><br> 3878 * Type: <b>reference</b><br> 3879 * Path: <b>DeviceRequest.priorRequest</b><br> 3880 * </p> 3881 */ 3882 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRIOR_REQUEST = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3883 SP_PRIOR_REQUEST); 3884 3885 /** 3886 * Constant for fluent queries to be used to add include statements. Specifies 3887 * the path value of "<b>DeviceRequest:prior-request</b>". 3888 */ 3889 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRIOR_REQUEST = new ca.uhn.fhir.model.api.Include( 3890 "DeviceRequest:prior-request").toLocked(); 3891 3892 /** 3893 * Search parameter: <b>device</b> 3894 * <p> 3895 * Description: <b>Reference to resource that is being requested/ordered</b><br> 3896 * Type: <b>reference</b><br> 3897 * Path: <b>DeviceRequest.codeReference</b><br> 3898 * </p> 3899 */ 3900 @SearchParamDefinition(name = "device", path = "(DeviceRequest.code as Reference)", description = "Reference to resource that is being requested/ordered", type = "reference", providesMembershipIn = { 3901 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device") }, target = { Device.class }) 3902 public static final String SP_DEVICE = "device"; 3903 /** 3904 * <b>Fluent Client</b> search parameter constant for <b>device</b> 3905 * <p> 3906 * Description: <b>Reference to resource that is being requested/ordered</b><br> 3907 * Type: <b>reference</b><br> 3908 * Path: <b>DeviceRequest.codeReference</b><br> 3909 * </p> 3910 */ 3911 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3912 SP_DEVICE); 3913 3914 /** 3915 * Constant for fluent queries to be used to add include statements. Specifies 3916 * the path value of "<b>DeviceRequest:device</b>". 3917 */ 3918 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEVICE = new ca.uhn.fhir.model.api.Include( 3919 "DeviceRequest:device").toLocked(); 3920 3921 /** 3922 * Search parameter: <b>status</b> 3923 * <p> 3924 * Description: <b>entered-in-error | draft | active |suspended | 3925 * completed</b><br> 3926 * Type: <b>token</b><br> 3927 * Path: <b>DeviceRequest.status</b><br> 3928 * </p> 3929 */ 3930 @SearchParamDefinition(name = "status", path = "DeviceRequest.status", description = "entered-in-error | draft | active |suspended | completed", type = "token") 3931 public static final String SP_STATUS = "status"; 3932 /** 3933 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3934 * <p> 3935 * Description: <b>entered-in-error | draft | active |suspended | 3936 * completed</b><br> 3937 * Type: <b>token</b><br> 3938 * Path: <b>DeviceRequest.status</b><br> 3939 * </p> 3940 */ 3941 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3942 SP_STATUS); 3943 3944}