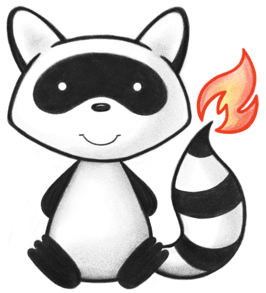
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.Description; 041import ca.uhn.fhir.model.api.annotation.ResourceDef; 042import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 043 044/** 045 * A record of a device being used by a patient where the record is the result 046 * of a report from the patient or another clinician. 047 */ 048@ResourceDef(name = "DeviceUseStatement", profile = "http://hl7.org/fhir/StructureDefinition/DeviceUseStatement") 049public class DeviceUseStatement extends DomainResource { 050 051 public enum DeviceUseStatementStatus { 052 /** 053 * The device is still being used. 054 */ 055 ACTIVE, 056 /** 057 * The device is no longer being used. 058 */ 059 COMPLETED, 060 /** 061 * The statement was recorded incorrectly. 062 */ 063 ENTEREDINERROR, 064 /** 065 * The device may be used at some time in the future. 066 */ 067 INTENDED, 068 /** 069 * Actions implied by the statement have been permanently halted, before all of 070 * them occurred. 071 */ 072 STOPPED, 073 /** 074 * Actions implied by the statement have been temporarily halted, but are 075 * expected to continue later. May also be called "suspended". 076 */ 077 ONHOLD, 078 /** 079 * added to help the parsers with the generic types 080 */ 081 NULL; 082 083 public static DeviceUseStatementStatus fromCode(String codeString) throws FHIRException { 084 if (codeString == null || "".equals(codeString)) 085 return null; 086 if ("active".equals(codeString)) 087 return ACTIVE; 088 if ("completed".equals(codeString)) 089 return COMPLETED; 090 if ("entered-in-error".equals(codeString)) 091 return ENTEREDINERROR; 092 if ("intended".equals(codeString)) 093 return INTENDED; 094 if ("stopped".equals(codeString)) 095 return STOPPED; 096 if ("on-hold".equals(codeString)) 097 return ONHOLD; 098 if (Configuration.isAcceptInvalidEnums()) 099 return null; 100 else 101 throw new FHIRException("Unknown DeviceUseStatementStatus code '" + codeString + "'"); 102 } 103 104 public String toCode() { 105 switch (this) { 106 case ACTIVE: 107 return "active"; 108 case COMPLETED: 109 return "completed"; 110 case ENTEREDINERROR: 111 return "entered-in-error"; 112 case INTENDED: 113 return "intended"; 114 case STOPPED: 115 return "stopped"; 116 case ONHOLD: 117 return "on-hold"; 118 case NULL: 119 return null; 120 default: 121 return "?"; 122 } 123 } 124 125 public String getSystem() { 126 switch (this) { 127 case ACTIVE: 128 return "http://hl7.org/fhir/device-statement-status"; 129 case COMPLETED: 130 return "http://hl7.org/fhir/device-statement-status"; 131 case ENTEREDINERROR: 132 return "http://hl7.org/fhir/device-statement-status"; 133 case INTENDED: 134 return "http://hl7.org/fhir/device-statement-status"; 135 case STOPPED: 136 return "http://hl7.org/fhir/device-statement-status"; 137 case ONHOLD: 138 return "http://hl7.org/fhir/device-statement-status"; 139 case NULL: 140 return null; 141 default: 142 return "?"; 143 } 144 } 145 146 public String getDefinition() { 147 switch (this) { 148 case ACTIVE: 149 return "The device is still being used."; 150 case COMPLETED: 151 return "The device is no longer being used."; 152 case ENTEREDINERROR: 153 return "The statement was recorded incorrectly."; 154 case INTENDED: 155 return "The device may be used at some time in the future."; 156 case STOPPED: 157 return "Actions implied by the statement have been permanently halted, before all of them occurred."; 158 case ONHOLD: 159 return "Actions implied by the statement have been temporarily halted, but are expected to continue later. May also be called \"suspended\"."; 160 case NULL: 161 return null; 162 default: 163 return "?"; 164 } 165 } 166 167 public String getDisplay() { 168 switch (this) { 169 case ACTIVE: 170 return "Active"; 171 case COMPLETED: 172 return "Completed"; 173 case ENTEREDINERROR: 174 return "Entered in Error"; 175 case INTENDED: 176 return "Intended"; 177 case STOPPED: 178 return "Stopped"; 179 case ONHOLD: 180 return "On Hold"; 181 case NULL: 182 return null; 183 default: 184 return "?"; 185 } 186 } 187 } 188 189 public static class DeviceUseStatementStatusEnumFactory implements EnumFactory<DeviceUseStatementStatus> { 190 public DeviceUseStatementStatus fromCode(String codeString) throws IllegalArgumentException { 191 if (codeString == null || "".equals(codeString)) 192 if (codeString == null || "".equals(codeString)) 193 return null; 194 if ("active".equals(codeString)) 195 return DeviceUseStatementStatus.ACTIVE; 196 if ("completed".equals(codeString)) 197 return DeviceUseStatementStatus.COMPLETED; 198 if ("entered-in-error".equals(codeString)) 199 return DeviceUseStatementStatus.ENTEREDINERROR; 200 if ("intended".equals(codeString)) 201 return DeviceUseStatementStatus.INTENDED; 202 if ("stopped".equals(codeString)) 203 return DeviceUseStatementStatus.STOPPED; 204 if ("on-hold".equals(codeString)) 205 return DeviceUseStatementStatus.ONHOLD; 206 throw new IllegalArgumentException("Unknown DeviceUseStatementStatus code '" + codeString + "'"); 207 } 208 209 public Enumeration<DeviceUseStatementStatus> fromType(PrimitiveType<?> code) throws FHIRException { 210 if (code == null) 211 return null; 212 if (code.isEmpty()) 213 return new Enumeration<DeviceUseStatementStatus>(this, DeviceUseStatementStatus.NULL, code); 214 String codeString = code.asStringValue(); 215 if (codeString == null || "".equals(codeString)) 216 return new Enumeration<DeviceUseStatementStatus>(this, DeviceUseStatementStatus.NULL, code); 217 if ("active".equals(codeString)) 218 return new Enumeration<DeviceUseStatementStatus>(this, DeviceUseStatementStatus.ACTIVE, code); 219 if ("completed".equals(codeString)) 220 return new Enumeration<DeviceUseStatementStatus>(this, DeviceUseStatementStatus.COMPLETED, code); 221 if ("entered-in-error".equals(codeString)) 222 return new Enumeration<DeviceUseStatementStatus>(this, DeviceUseStatementStatus.ENTEREDINERROR, code); 223 if ("intended".equals(codeString)) 224 return new Enumeration<DeviceUseStatementStatus>(this, DeviceUseStatementStatus.INTENDED, code); 225 if ("stopped".equals(codeString)) 226 return new Enumeration<DeviceUseStatementStatus>(this, DeviceUseStatementStatus.STOPPED, code); 227 if ("on-hold".equals(codeString)) 228 return new Enumeration<DeviceUseStatementStatus>(this, DeviceUseStatementStatus.ONHOLD, code); 229 throw new FHIRException("Unknown DeviceUseStatementStatus code '" + codeString + "'"); 230 } 231 232 public String toCode(DeviceUseStatementStatus code) { 233 if (code == DeviceUseStatementStatus.ACTIVE) 234 return "active"; 235 if (code == DeviceUseStatementStatus.COMPLETED) 236 return "completed"; 237 if (code == DeviceUseStatementStatus.ENTEREDINERROR) 238 return "entered-in-error"; 239 if (code == DeviceUseStatementStatus.INTENDED) 240 return "intended"; 241 if (code == DeviceUseStatementStatus.STOPPED) 242 return "stopped"; 243 if (code == DeviceUseStatementStatus.ONHOLD) 244 return "on-hold"; 245 return "?"; 246 } 247 248 public String toSystem(DeviceUseStatementStatus code) { 249 return code.getSystem(); 250 } 251 } 252 253 /** 254 * An external identifier for this statement such as an IRI. 255 */ 256 @Child(name = "identifier", type = { 257 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 258 @Description(shortDefinition = "External identifier for this record", formalDefinition = "An external identifier for this statement such as an IRI.") 259 protected List<Identifier> identifier; 260 261 /** 262 * A plan, proposal or order that is fulfilled in whole or in part by this 263 * DeviceUseStatement. 264 */ 265 @Child(name = "basedOn", type = { 266 ServiceRequest.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 267 @Description(shortDefinition = "Fulfills plan, proposal or order", formalDefinition = "A plan, proposal or order that is fulfilled in whole or in part by this DeviceUseStatement.") 268 protected List<Reference> basedOn; 269 /** 270 * The actual objects that are the target of the reference (A plan, proposal or 271 * order that is fulfilled in whole or in part by this DeviceUseStatement.) 272 */ 273 protected List<ServiceRequest> basedOnTarget; 274 275 /** 276 * A code representing the patient or other source's judgment about the state of 277 * the device used that this statement is about. Generally this will be active 278 * or completed. 279 */ 280 @Child(name = "status", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = true, summary = true) 281 @Description(shortDefinition = "active | completed | entered-in-error +", formalDefinition = "A code representing the patient or other source's judgment about the state of the device used that this statement is about. Generally this will be active or completed.") 282 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/device-statement-status") 283 protected Enumeration<DeviceUseStatementStatus> status; 284 285 /** 286 * The patient who used the device. 287 */ 288 @Child(name = "subject", type = { Patient.class, 289 Group.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 290 @Description(shortDefinition = "Patient using device", formalDefinition = "The patient who used the device.") 291 protected Reference subject; 292 293 /** 294 * The actual object that is the target of the reference (The patient who used 295 * the device.) 296 */ 297 protected Resource subjectTarget; 298 299 /** 300 * Allows linking the DeviceUseStatement to the underlying Request, or to other 301 * information that supports or is used to derive the DeviceUseStatement. 302 */ 303 @Child(name = "derivedFrom", type = { ServiceRequest.class, Procedure.class, Claim.class, Observation.class, 304 QuestionnaireResponse.class, 305 DocumentReference.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 306 @Description(shortDefinition = "Supporting information", formalDefinition = "Allows linking the DeviceUseStatement to the underlying Request, or to other information that supports or is used to derive the DeviceUseStatement.") 307 protected List<Reference> derivedFrom; 308 /** 309 * The actual objects that are the target of the reference (Allows linking the 310 * DeviceUseStatement to the underlying Request, or to other information that 311 * supports or is used to derive the DeviceUseStatement.) 312 */ 313 protected List<Resource> derivedFromTarget; 314 315 /** 316 * How often the device was used. 317 */ 318 @Child(name = "timing", type = { Timing.class, Period.class, 319 DateTimeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 320 @Description(shortDefinition = "How often the device was used", formalDefinition = "How often the device was used.") 321 protected Type timing; 322 323 /** 324 * The time at which the statement was made/recorded. 325 */ 326 @Child(name = "recordedOn", type = { 327 DateTimeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 328 @Description(shortDefinition = "When statement was recorded", formalDefinition = "The time at which the statement was made/recorded.") 329 protected DateTimeType recordedOn; 330 331 /** 332 * Who reported the device was being used by the patient. 333 */ 334 @Child(name = "source", type = { Patient.class, Practitioner.class, PractitionerRole.class, 335 RelatedPerson.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 336 @Description(shortDefinition = "Who made the statement", formalDefinition = "Who reported the device was being used by the patient.") 337 protected Reference source; 338 339 /** 340 * The actual object that is the target of the reference (Who reported the 341 * device was being used by the patient.) 342 */ 343 protected Resource sourceTarget; 344 345 /** 346 * The details of the device used. 347 */ 348 @Child(name = "device", type = { Device.class }, order = 8, min = 1, max = 1, modifier = false, summary = true) 349 @Description(shortDefinition = "Reference to device used", formalDefinition = "The details of the device used.") 350 protected Reference device; 351 352 /** 353 * The actual object that is the target of the reference (The details of the 354 * device used.) 355 */ 356 protected Device deviceTarget; 357 358 /** 359 * Reason or justification for the use of the device. 360 */ 361 @Child(name = "reasonCode", type = { 362 CodeableConcept.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 363 @Description(shortDefinition = "Why device was used", formalDefinition = "Reason or justification for the use of the device.") 364 protected List<CodeableConcept> reasonCode; 365 366 /** 367 * Indicates another resource whose existence justifies this DeviceUseStatement. 368 */ 369 @Child(name = "reasonReference", type = { Condition.class, Observation.class, DiagnosticReport.class, 370 DocumentReference.class, 371 Media.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 372 @Description(shortDefinition = "Why was DeviceUseStatement performed?", formalDefinition = "Indicates another resource whose existence justifies this DeviceUseStatement.") 373 protected List<Reference> reasonReference; 374 /** 375 * The actual objects that are the target of the reference (Indicates another 376 * resource whose existence justifies this DeviceUseStatement.) 377 */ 378 protected List<Resource> reasonReferenceTarget; 379 380 /** 381 * Indicates the anotomic location on the subject's body where the device was 382 * used ( i.e. the target). 383 */ 384 @Child(name = "bodySite", type = { 385 CodeableConcept.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 386 @Description(shortDefinition = "Target body site", formalDefinition = "Indicates the anotomic location on the subject's body where the device was used ( i.e. the target).") 387 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/body-site") 388 protected CodeableConcept bodySite; 389 390 /** 391 * Details about the device statement that were not represented at all or 392 * sufficiently in one of the attributes provided in a class. These may include 393 * for example a comment, an instruction, or a note associated with the 394 * statement. 395 */ 396 @Child(name = "note", type = { 397 Annotation.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 398 @Description(shortDefinition = "Addition details (comments, instructions)", formalDefinition = "Details about the device statement that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.") 399 protected List<Annotation> note; 400 401 private static final long serialVersionUID = -968330048L; 402 403 /** 404 * Constructor 405 */ 406 public DeviceUseStatement() { 407 super(); 408 } 409 410 /** 411 * Constructor 412 */ 413 public DeviceUseStatement(Enumeration<DeviceUseStatementStatus> status, Reference subject, Reference device) { 414 super(); 415 this.status = status; 416 this.subject = subject; 417 this.device = device; 418 } 419 420 /** 421 * @return {@link #identifier} (An external identifier for this statement such 422 * as an IRI.) 423 */ 424 public List<Identifier> getIdentifier() { 425 if (this.identifier == null) 426 this.identifier = new ArrayList<Identifier>(); 427 return this.identifier; 428 } 429 430 /** 431 * @return Returns a reference to <code>this</code> for easy method chaining 432 */ 433 public DeviceUseStatement setIdentifier(List<Identifier> theIdentifier) { 434 this.identifier = theIdentifier; 435 return this; 436 } 437 438 public boolean hasIdentifier() { 439 if (this.identifier == null) 440 return false; 441 for (Identifier item : this.identifier) 442 if (!item.isEmpty()) 443 return true; 444 return false; 445 } 446 447 public Identifier addIdentifier() { // 3 448 Identifier t = new Identifier(); 449 if (this.identifier == null) 450 this.identifier = new ArrayList<Identifier>(); 451 this.identifier.add(t); 452 return t; 453 } 454 455 public DeviceUseStatement addIdentifier(Identifier t) { // 3 456 if (t == null) 457 return this; 458 if (this.identifier == null) 459 this.identifier = new ArrayList<Identifier>(); 460 this.identifier.add(t); 461 return this; 462 } 463 464 /** 465 * @return The first repetition of repeating field {@link #identifier}, creating 466 * it if it does not already exist 467 */ 468 public Identifier getIdentifierFirstRep() { 469 if (getIdentifier().isEmpty()) { 470 addIdentifier(); 471 } 472 return getIdentifier().get(0); 473 } 474 475 /** 476 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in 477 * whole or in part by this DeviceUseStatement.) 478 */ 479 public List<Reference> getBasedOn() { 480 if (this.basedOn == null) 481 this.basedOn = new ArrayList<Reference>(); 482 return this.basedOn; 483 } 484 485 /** 486 * @return Returns a reference to <code>this</code> for easy method chaining 487 */ 488 public DeviceUseStatement setBasedOn(List<Reference> theBasedOn) { 489 this.basedOn = theBasedOn; 490 return this; 491 } 492 493 public boolean hasBasedOn() { 494 if (this.basedOn == null) 495 return false; 496 for (Reference item : this.basedOn) 497 if (!item.isEmpty()) 498 return true; 499 return false; 500 } 501 502 public Reference addBasedOn() { // 3 503 Reference t = new Reference(); 504 if (this.basedOn == null) 505 this.basedOn = new ArrayList<Reference>(); 506 this.basedOn.add(t); 507 return t; 508 } 509 510 public DeviceUseStatement addBasedOn(Reference t) { // 3 511 if (t == null) 512 return this; 513 if (this.basedOn == null) 514 this.basedOn = new ArrayList<Reference>(); 515 this.basedOn.add(t); 516 return this; 517 } 518 519 /** 520 * @return The first repetition of repeating field {@link #basedOn}, creating it 521 * if it does not already exist 522 */ 523 public Reference getBasedOnFirstRep() { 524 if (getBasedOn().isEmpty()) { 525 addBasedOn(); 526 } 527 return getBasedOn().get(0); 528 } 529 530 /** 531 * @deprecated Use Reference#setResource(IBaseResource) instead 532 */ 533 @Deprecated 534 public List<ServiceRequest> getBasedOnTarget() { 535 if (this.basedOnTarget == null) 536 this.basedOnTarget = new ArrayList<ServiceRequest>(); 537 return this.basedOnTarget; 538 } 539 540 /** 541 * @deprecated Use Reference#setResource(IBaseResource) instead 542 */ 543 @Deprecated 544 public ServiceRequest addBasedOnTarget() { 545 ServiceRequest r = new ServiceRequest(); 546 if (this.basedOnTarget == null) 547 this.basedOnTarget = new ArrayList<ServiceRequest>(); 548 this.basedOnTarget.add(r); 549 return r; 550 } 551 552 /** 553 * @return {@link #status} (A code representing the patient or other source's 554 * judgment about the state of the device used that this statement is 555 * about. Generally this will be active or completed.). This is the 556 * underlying object with id, value and extensions. The accessor 557 * "getStatus" gives direct access to the value 558 */ 559 public Enumeration<DeviceUseStatementStatus> getStatusElement() { 560 if (this.status == null) 561 if (Configuration.errorOnAutoCreate()) 562 throw new Error("Attempt to auto-create DeviceUseStatement.status"); 563 else if (Configuration.doAutoCreate()) 564 this.status = new Enumeration<DeviceUseStatementStatus>(new DeviceUseStatementStatusEnumFactory()); // bb 565 return this.status; 566 } 567 568 public boolean hasStatusElement() { 569 return this.status != null && !this.status.isEmpty(); 570 } 571 572 public boolean hasStatus() { 573 return this.status != null && !this.status.isEmpty(); 574 } 575 576 /** 577 * @param value {@link #status} (A code representing the patient or other 578 * source's judgment about the state of the device used that this 579 * statement is about. Generally this will be active or 580 * completed.). This is the underlying object with id, value and 581 * extensions. The accessor "getStatus" gives direct access to the 582 * value 583 */ 584 public DeviceUseStatement setStatusElement(Enumeration<DeviceUseStatementStatus> value) { 585 this.status = value; 586 return this; 587 } 588 589 /** 590 * @return A code representing the patient or other source's judgment about the 591 * state of the device used that this statement is about. Generally this 592 * will be active or completed. 593 */ 594 public DeviceUseStatementStatus getStatus() { 595 return this.status == null ? null : this.status.getValue(); 596 } 597 598 /** 599 * @param value A code representing the patient or other source's judgment about 600 * the state of the device used that this statement is about. 601 * Generally this will be active or completed. 602 */ 603 public DeviceUseStatement setStatus(DeviceUseStatementStatus value) { 604 if (this.status == null) 605 this.status = new Enumeration<DeviceUseStatementStatus>(new DeviceUseStatementStatusEnumFactory()); 606 this.status.setValue(value); 607 return this; 608 } 609 610 /** 611 * @return {@link #subject} (The patient who used the device.) 612 */ 613 public Reference getSubject() { 614 if (this.subject == null) 615 if (Configuration.errorOnAutoCreate()) 616 throw new Error("Attempt to auto-create DeviceUseStatement.subject"); 617 else if (Configuration.doAutoCreate()) 618 this.subject = new Reference(); // cc 619 return this.subject; 620 } 621 622 public boolean hasSubject() { 623 return this.subject != null && !this.subject.isEmpty(); 624 } 625 626 /** 627 * @param value {@link #subject} (The patient who used the device.) 628 */ 629 public DeviceUseStatement setSubject(Reference value) { 630 this.subject = value; 631 return this; 632 } 633 634 /** 635 * @return {@link #subject} The actual object that is the target of the 636 * reference. The reference library doesn't populate this, but you can 637 * use it to hold the resource if you resolve it. (The patient who used 638 * the device.) 639 */ 640 public Resource getSubjectTarget() { 641 return this.subjectTarget; 642 } 643 644 /** 645 * @param value {@link #subject} The actual object that is the target of the 646 * reference. The reference library doesn't use these, but you can 647 * use it to hold the resource if you resolve it. (The patient who 648 * used the device.) 649 */ 650 public DeviceUseStatement setSubjectTarget(Resource value) { 651 this.subjectTarget = value; 652 return this; 653 } 654 655 /** 656 * @return {@link #derivedFrom} (Allows linking the DeviceUseStatement to the 657 * underlying Request, or to other information that supports or is used 658 * to derive the DeviceUseStatement.) 659 */ 660 public List<Reference> getDerivedFrom() { 661 if (this.derivedFrom == null) 662 this.derivedFrom = new ArrayList<Reference>(); 663 return this.derivedFrom; 664 } 665 666 /** 667 * @return Returns a reference to <code>this</code> for easy method chaining 668 */ 669 public DeviceUseStatement setDerivedFrom(List<Reference> theDerivedFrom) { 670 this.derivedFrom = theDerivedFrom; 671 return this; 672 } 673 674 public boolean hasDerivedFrom() { 675 if (this.derivedFrom == null) 676 return false; 677 for (Reference item : this.derivedFrom) 678 if (!item.isEmpty()) 679 return true; 680 return false; 681 } 682 683 public Reference addDerivedFrom() { // 3 684 Reference t = new Reference(); 685 if (this.derivedFrom == null) 686 this.derivedFrom = new ArrayList<Reference>(); 687 this.derivedFrom.add(t); 688 return t; 689 } 690 691 public DeviceUseStatement addDerivedFrom(Reference t) { // 3 692 if (t == null) 693 return this; 694 if (this.derivedFrom == null) 695 this.derivedFrom = new ArrayList<Reference>(); 696 this.derivedFrom.add(t); 697 return this; 698 } 699 700 /** 701 * @return The first repetition of repeating field {@link #derivedFrom}, 702 * creating it if it does not already exist 703 */ 704 public Reference getDerivedFromFirstRep() { 705 if (getDerivedFrom().isEmpty()) { 706 addDerivedFrom(); 707 } 708 return getDerivedFrom().get(0); 709 } 710 711 /** 712 * @deprecated Use Reference#setResource(IBaseResource) instead 713 */ 714 @Deprecated 715 public List<Resource> getDerivedFromTarget() { 716 if (this.derivedFromTarget == null) 717 this.derivedFromTarget = new ArrayList<Resource>(); 718 return this.derivedFromTarget; 719 } 720 721 /** 722 * @return {@link #timing} (How often the device was used.) 723 */ 724 public Type getTiming() { 725 return this.timing; 726 } 727 728 /** 729 * @return {@link #timing} (How often the device was used.) 730 */ 731 public Timing getTimingTiming() throws FHIRException { 732 if (this.timing == null) 733 this.timing = new Timing(); 734 if (!(this.timing instanceof Timing)) 735 throw new FHIRException( 736 "Type mismatch: the type Timing was expected, but " + this.timing.getClass().getName() + " was encountered"); 737 return (Timing) this.timing; 738 } 739 740 public boolean hasTimingTiming() { 741 return this != null && this.timing instanceof Timing; 742 } 743 744 /** 745 * @return {@link #timing} (How often the device was used.) 746 */ 747 public Period getTimingPeriod() throws FHIRException { 748 if (this.timing == null) 749 this.timing = new Period(); 750 if (!(this.timing instanceof Period)) 751 throw new FHIRException( 752 "Type mismatch: the type Period was expected, but " + this.timing.getClass().getName() + " was encountered"); 753 return (Period) this.timing; 754 } 755 756 public boolean hasTimingPeriod() { 757 return this != null && this.timing instanceof Period; 758 } 759 760 /** 761 * @return {@link #timing} (How often the device was used.) 762 */ 763 public DateTimeType getTimingDateTimeType() throws FHIRException { 764 if (this.timing == null) 765 this.timing = new DateTimeType(); 766 if (!(this.timing instanceof DateTimeType)) 767 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 768 + this.timing.getClass().getName() + " was encountered"); 769 return (DateTimeType) this.timing; 770 } 771 772 public boolean hasTimingDateTimeType() { 773 return this != null && this.timing instanceof DateTimeType; 774 } 775 776 public boolean hasTiming() { 777 return this.timing != null && !this.timing.isEmpty(); 778 } 779 780 /** 781 * @param value {@link #timing} (How often the device was used.) 782 */ 783 public DeviceUseStatement setTiming(Type value) { 784 if (value != null && !(value instanceof Timing || value instanceof Period || value instanceof DateTimeType)) 785 throw new Error("Not the right type for DeviceUseStatement.timing[x]: " + value.fhirType()); 786 this.timing = value; 787 return this; 788 } 789 790 /** 791 * @return {@link #recordedOn} (The time at which the statement was 792 * made/recorded.). This is the underlying object with id, value and 793 * extensions. The accessor "getRecordedOn" gives direct access to the 794 * value 795 */ 796 public DateTimeType getRecordedOnElement() { 797 if (this.recordedOn == null) 798 if (Configuration.errorOnAutoCreate()) 799 throw new Error("Attempt to auto-create DeviceUseStatement.recordedOn"); 800 else if (Configuration.doAutoCreate()) 801 this.recordedOn = new DateTimeType(); // bb 802 return this.recordedOn; 803 } 804 805 public boolean hasRecordedOnElement() { 806 return this.recordedOn != null && !this.recordedOn.isEmpty(); 807 } 808 809 public boolean hasRecordedOn() { 810 return this.recordedOn != null && !this.recordedOn.isEmpty(); 811 } 812 813 /** 814 * @param value {@link #recordedOn} (The time at which the statement was 815 * made/recorded.). This is the underlying object with id, value 816 * and extensions. The accessor "getRecordedOn" gives direct access 817 * to the value 818 */ 819 public DeviceUseStatement setRecordedOnElement(DateTimeType value) { 820 this.recordedOn = value; 821 return this; 822 } 823 824 /** 825 * @return The time at which the statement was made/recorded. 826 */ 827 public Date getRecordedOn() { 828 return this.recordedOn == null ? null : this.recordedOn.getValue(); 829 } 830 831 /** 832 * @param value The time at which the statement was made/recorded. 833 */ 834 public DeviceUseStatement setRecordedOn(Date value) { 835 if (value == null) 836 this.recordedOn = null; 837 else { 838 if (this.recordedOn == null) 839 this.recordedOn = new DateTimeType(); 840 this.recordedOn.setValue(value); 841 } 842 return this; 843 } 844 845 /** 846 * @return {@link #source} (Who reported the device was being used by the 847 * patient.) 848 */ 849 public Reference getSource() { 850 if (this.source == null) 851 if (Configuration.errorOnAutoCreate()) 852 throw new Error("Attempt to auto-create DeviceUseStatement.source"); 853 else if (Configuration.doAutoCreate()) 854 this.source = new Reference(); // cc 855 return this.source; 856 } 857 858 public boolean hasSource() { 859 return this.source != null && !this.source.isEmpty(); 860 } 861 862 /** 863 * @param value {@link #source} (Who reported the device was being used by the 864 * patient.) 865 */ 866 public DeviceUseStatement setSource(Reference value) { 867 this.source = value; 868 return this; 869 } 870 871 /** 872 * @return {@link #source} The actual object that is the target of the 873 * reference. The reference library doesn't populate this, but you can 874 * use it to hold the resource if you resolve it. (Who reported the 875 * device was being used by the patient.) 876 */ 877 public Resource getSourceTarget() { 878 return this.sourceTarget; 879 } 880 881 /** 882 * @param value {@link #source} The actual object that is the target of the 883 * reference. The reference library doesn't use these, but you can 884 * use it to hold the resource if you resolve it. (Who reported the 885 * device was being used by the patient.) 886 */ 887 public DeviceUseStatement setSourceTarget(Resource value) { 888 this.sourceTarget = value; 889 return this; 890 } 891 892 /** 893 * @return {@link #device} (The details of the device used.) 894 */ 895 public Reference getDevice() { 896 if (this.device == null) 897 if (Configuration.errorOnAutoCreate()) 898 throw new Error("Attempt to auto-create DeviceUseStatement.device"); 899 else if (Configuration.doAutoCreate()) 900 this.device = new Reference(); // cc 901 return this.device; 902 } 903 904 public boolean hasDevice() { 905 return this.device != null && !this.device.isEmpty(); 906 } 907 908 /** 909 * @param value {@link #device} (The details of the device used.) 910 */ 911 public DeviceUseStatement setDevice(Reference value) { 912 this.device = value; 913 return this; 914 } 915 916 /** 917 * @return {@link #device} The actual object that is the target of the 918 * reference. The reference library doesn't populate this, but you can 919 * use it to hold the resource if you resolve it. (The details of the 920 * device used.) 921 */ 922 public Device getDeviceTarget() { 923 if (this.deviceTarget == null) 924 if (Configuration.errorOnAutoCreate()) 925 throw new Error("Attempt to auto-create DeviceUseStatement.device"); 926 else if (Configuration.doAutoCreate()) 927 this.deviceTarget = new Device(); // aa 928 return this.deviceTarget; 929 } 930 931 /** 932 * @param value {@link #device} The actual object that is the target of the 933 * reference. The reference library doesn't use these, but you can 934 * use it to hold the resource if you resolve it. (The details of 935 * the device used.) 936 */ 937 public DeviceUseStatement setDeviceTarget(Device value) { 938 this.deviceTarget = value; 939 return this; 940 } 941 942 /** 943 * @return {@link #reasonCode} (Reason or justification for the use of the 944 * device.) 945 */ 946 public List<CodeableConcept> getReasonCode() { 947 if (this.reasonCode == null) 948 this.reasonCode = new ArrayList<CodeableConcept>(); 949 return this.reasonCode; 950 } 951 952 /** 953 * @return Returns a reference to <code>this</code> for easy method chaining 954 */ 955 public DeviceUseStatement setReasonCode(List<CodeableConcept> theReasonCode) { 956 this.reasonCode = theReasonCode; 957 return this; 958 } 959 960 public boolean hasReasonCode() { 961 if (this.reasonCode == null) 962 return false; 963 for (CodeableConcept item : this.reasonCode) 964 if (!item.isEmpty()) 965 return true; 966 return false; 967 } 968 969 public CodeableConcept addReasonCode() { // 3 970 CodeableConcept t = new CodeableConcept(); 971 if (this.reasonCode == null) 972 this.reasonCode = new ArrayList<CodeableConcept>(); 973 this.reasonCode.add(t); 974 return t; 975 } 976 977 public DeviceUseStatement addReasonCode(CodeableConcept t) { // 3 978 if (t == null) 979 return this; 980 if (this.reasonCode == null) 981 this.reasonCode = new ArrayList<CodeableConcept>(); 982 this.reasonCode.add(t); 983 return this; 984 } 985 986 /** 987 * @return The first repetition of repeating field {@link #reasonCode}, creating 988 * it if it does not already exist 989 */ 990 public CodeableConcept getReasonCodeFirstRep() { 991 if (getReasonCode().isEmpty()) { 992 addReasonCode(); 993 } 994 return getReasonCode().get(0); 995 } 996 997 /** 998 * @return {@link #reasonReference} (Indicates another resource whose existence 999 * justifies this DeviceUseStatement.) 1000 */ 1001 public List<Reference> getReasonReference() { 1002 if (this.reasonReference == null) 1003 this.reasonReference = new ArrayList<Reference>(); 1004 return this.reasonReference; 1005 } 1006 1007 /** 1008 * @return Returns a reference to <code>this</code> for easy method chaining 1009 */ 1010 public DeviceUseStatement setReasonReference(List<Reference> theReasonReference) { 1011 this.reasonReference = theReasonReference; 1012 return this; 1013 } 1014 1015 public boolean hasReasonReference() { 1016 if (this.reasonReference == null) 1017 return false; 1018 for (Reference item : this.reasonReference) 1019 if (!item.isEmpty()) 1020 return true; 1021 return false; 1022 } 1023 1024 public Reference addReasonReference() { // 3 1025 Reference t = new Reference(); 1026 if (this.reasonReference == null) 1027 this.reasonReference = new ArrayList<Reference>(); 1028 this.reasonReference.add(t); 1029 return t; 1030 } 1031 1032 public DeviceUseStatement addReasonReference(Reference t) { // 3 1033 if (t == null) 1034 return this; 1035 if (this.reasonReference == null) 1036 this.reasonReference = new ArrayList<Reference>(); 1037 this.reasonReference.add(t); 1038 return this; 1039 } 1040 1041 /** 1042 * @return The first repetition of repeating field {@link #reasonReference}, 1043 * creating it if it does not already exist 1044 */ 1045 public Reference getReasonReferenceFirstRep() { 1046 if (getReasonReference().isEmpty()) { 1047 addReasonReference(); 1048 } 1049 return getReasonReference().get(0); 1050 } 1051 1052 /** 1053 * @deprecated Use Reference#setResource(IBaseResource) instead 1054 */ 1055 @Deprecated 1056 public List<Resource> getReasonReferenceTarget() { 1057 if (this.reasonReferenceTarget == null) 1058 this.reasonReferenceTarget = new ArrayList<Resource>(); 1059 return this.reasonReferenceTarget; 1060 } 1061 1062 /** 1063 * @return {@link #bodySite} (Indicates the anotomic location on the subject's 1064 * body where the device was used ( i.e. the target).) 1065 */ 1066 public CodeableConcept getBodySite() { 1067 if (this.bodySite == null) 1068 if (Configuration.errorOnAutoCreate()) 1069 throw new Error("Attempt to auto-create DeviceUseStatement.bodySite"); 1070 else if (Configuration.doAutoCreate()) 1071 this.bodySite = new CodeableConcept(); // cc 1072 return this.bodySite; 1073 } 1074 1075 public boolean hasBodySite() { 1076 return this.bodySite != null && !this.bodySite.isEmpty(); 1077 } 1078 1079 /** 1080 * @param value {@link #bodySite} (Indicates the anotomic location on the 1081 * subject's body where the device was used ( i.e. the target).) 1082 */ 1083 public DeviceUseStatement setBodySite(CodeableConcept value) { 1084 this.bodySite = value; 1085 return this; 1086 } 1087 1088 /** 1089 * @return {@link #note} (Details about the device statement that were not 1090 * represented at all or sufficiently in one of the attributes provided 1091 * in a class. These may include for example a comment, an instruction, 1092 * or a note associated with the statement.) 1093 */ 1094 public List<Annotation> getNote() { 1095 if (this.note == null) 1096 this.note = new ArrayList<Annotation>(); 1097 return this.note; 1098 } 1099 1100 /** 1101 * @return Returns a reference to <code>this</code> for easy method chaining 1102 */ 1103 public DeviceUseStatement setNote(List<Annotation> theNote) { 1104 this.note = theNote; 1105 return this; 1106 } 1107 1108 public boolean hasNote() { 1109 if (this.note == null) 1110 return false; 1111 for (Annotation item : this.note) 1112 if (!item.isEmpty()) 1113 return true; 1114 return false; 1115 } 1116 1117 public Annotation addNote() { // 3 1118 Annotation t = new Annotation(); 1119 if (this.note == null) 1120 this.note = new ArrayList<Annotation>(); 1121 this.note.add(t); 1122 return t; 1123 } 1124 1125 public DeviceUseStatement addNote(Annotation t) { // 3 1126 if (t == null) 1127 return this; 1128 if (this.note == null) 1129 this.note = new ArrayList<Annotation>(); 1130 this.note.add(t); 1131 return this; 1132 } 1133 1134 /** 1135 * @return The first repetition of repeating field {@link #note}, creating it if 1136 * it does not already exist 1137 */ 1138 public Annotation getNoteFirstRep() { 1139 if (getNote().isEmpty()) { 1140 addNote(); 1141 } 1142 return getNote().get(0); 1143 } 1144 1145 protected void listChildren(List<Property> children) { 1146 super.listChildren(children); 1147 children.add(new Property("identifier", "Identifier", "An external identifier for this statement such as an IRI.", 1148 0, java.lang.Integer.MAX_VALUE, identifier)); 1149 children.add(new Property("basedOn", "Reference(ServiceRequest)", 1150 "A plan, proposal or order that is fulfilled in whole or in part by this DeviceUseStatement.", 0, 1151 java.lang.Integer.MAX_VALUE, basedOn)); 1152 children.add(new Property("status", "code", 1153 "A code representing the patient or other source's judgment about the state of the device used that this statement is about. Generally this will be active or completed.", 1154 0, 1, status)); 1155 children 1156 .add(new Property("subject", "Reference(Patient|Group)", "The patient who used the device.", 0, 1, subject)); 1157 children.add(new Property("derivedFrom", 1158 "Reference(ServiceRequest|Procedure|Claim|Observation|QuestionnaireResponse|DocumentReference)", 1159 "Allows linking the DeviceUseStatement to the underlying Request, or to other information that supports or is used to derive the DeviceUseStatement.", 1160 0, java.lang.Integer.MAX_VALUE, derivedFrom)); 1161 children.add(new Property("timing[x]", "Timing|Period|dateTime", "How often the device was used.", 0, 1, timing)); 1162 children.add( 1163 new Property("recordedOn", "dateTime", "The time at which the statement was made/recorded.", 0, 1, recordedOn)); 1164 children.add(new Property("source", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson)", 1165 "Who reported the device was being used by the patient.", 0, 1, source)); 1166 children.add(new Property("device", "Reference(Device)", "The details of the device used.", 0, 1, device)); 1167 children.add(new Property("reasonCode", "CodeableConcept", "Reason or justification for the use of the device.", 0, 1168 java.lang.Integer.MAX_VALUE, reasonCode)); 1169 children.add( 1170 new Property("reasonReference", "Reference(Condition|Observation|DiagnosticReport|DocumentReference|Media)", 1171 "Indicates another resource whose existence justifies this DeviceUseStatement.", 0, 1172 java.lang.Integer.MAX_VALUE, reasonReference)); 1173 children.add(new Property("bodySite", "CodeableConcept", 1174 "Indicates the anotomic location on the subject's body where the device was used ( i.e. the target).", 0, 1, 1175 bodySite)); 1176 children.add(new Property("note", "Annotation", 1177 "Details about the device statement that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.", 1178 0, java.lang.Integer.MAX_VALUE, note)); 1179 } 1180 1181 @Override 1182 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1183 switch (_hash) { 1184 case -1618432855: 1185 /* identifier */ return new Property("identifier", "Identifier", 1186 "An external identifier for this statement such as an IRI.", 0, java.lang.Integer.MAX_VALUE, identifier); 1187 case -332612366: 1188 /* basedOn */ return new Property("basedOn", "Reference(ServiceRequest)", 1189 "A plan, proposal or order that is fulfilled in whole or in part by this DeviceUseStatement.", 0, 1190 java.lang.Integer.MAX_VALUE, basedOn); 1191 case -892481550: 1192 /* status */ return new Property("status", "code", 1193 "A code representing the patient or other source's judgment about the state of the device used that this statement is about. Generally this will be active or completed.", 1194 0, 1, status); 1195 case -1867885268: 1196 /* subject */ return new Property("subject", "Reference(Patient|Group)", "The patient who used the device.", 0, 1, 1197 subject); 1198 case 1077922663: 1199 /* derivedFrom */ return new Property("derivedFrom", 1200 "Reference(ServiceRequest|Procedure|Claim|Observation|QuestionnaireResponse|DocumentReference)", 1201 "Allows linking the DeviceUseStatement to the underlying Request, or to other information that supports or is used to derive the DeviceUseStatement.", 1202 0, java.lang.Integer.MAX_VALUE, derivedFrom); 1203 case 164632566: 1204 /* timing[x] */ return new Property("timing[x]", "Timing|Period|dateTime", "How often the device was used.", 0, 1, 1205 timing); 1206 case -873664438: 1207 /* timing */ return new Property("timing[x]", "Timing|Period|dateTime", "How often the device was used.", 0, 1, 1208 timing); 1209 case -497554124: 1210 /* timingTiming */ return new Property("timing[x]", "Timing|Period|dateTime", "How often the device was used.", 0, 1211 1, timing); 1212 case -615615829: 1213 /* timingPeriod */ return new Property("timing[x]", "Timing|Period|dateTime", "How often the device was used.", 0, 1214 1, timing); 1215 case -1837458939: 1216 /* timingDateTime */ return new Property("timing[x]", "Timing|Period|dateTime", "How often the device was used.", 1217 0, 1, timing); 1218 case 735397551: 1219 /* recordedOn */ return new Property("recordedOn", "dateTime", 1220 "The time at which the statement was made/recorded.", 0, 1, recordedOn); 1221 case -896505829: 1222 /* source */ return new Property("source", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson)", 1223 "Who reported the device was being used by the patient.", 0, 1, source); 1224 case -1335157162: 1225 /* device */ return new Property("device", "Reference(Device)", "The details of the device used.", 0, 1, device); 1226 case 722137681: 1227 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 1228 "Reason or justification for the use of the device.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 1229 case -1146218137: 1230 /* reasonReference */ return new Property("reasonReference", 1231 "Reference(Condition|Observation|DiagnosticReport|DocumentReference|Media)", 1232 "Indicates another resource whose existence justifies this DeviceUseStatement.", 0, 1233 java.lang.Integer.MAX_VALUE, reasonReference); 1234 case 1702620169: 1235 /* bodySite */ return new Property("bodySite", "CodeableConcept", 1236 "Indicates the anotomic location on the subject's body where the device was used ( i.e. the target).", 0, 1, 1237 bodySite); 1238 case 3387378: 1239 /* note */ return new Property("note", "Annotation", 1240 "Details about the device statement that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.", 1241 0, java.lang.Integer.MAX_VALUE, note); 1242 default: 1243 return super.getNamedProperty(_hash, _name, _checkValid); 1244 } 1245 1246 } 1247 1248 @Override 1249 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1250 switch (hash) { 1251 case -1618432855: 1252 /* identifier */ return this.identifier == null ? new Base[0] 1253 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1254 case -332612366: 1255 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1256 case -892481550: 1257 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<DeviceUseStatementStatus> 1258 case -1867885268: 1259 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 1260 case 1077922663: 1261 /* derivedFrom */ return this.derivedFrom == null ? new Base[0] 1262 : this.derivedFrom.toArray(new Base[this.derivedFrom.size()]); // Reference 1263 case -873664438: 1264 /* timing */ return this.timing == null ? new Base[0] : new Base[] { this.timing }; // Type 1265 case 735397551: 1266 /* recordedOn */ return this.recordedOn == null ? new Base[0] : new Base[] { this.recordedOn }; // DateTimeType 1267 case -896505829: 1268 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // Reference 1269 case -1335157162: 1270 /* device */ return this.device == null ? new Base[0] : new Base[] { this.device }; // Reference 1271 case 722137681: 1272 /* reasonCode */ return this.reasonCode == null ? new Base[0] 1273 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 1274 case -1146218137: 1275 /* reasonReference */ return this.reasonReference == null ? new Base[0] 1276 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 1277 case 1702620169: 1278 /* bodySite */ return this.bodySite == null ? new Base[0] : new Base[] { this.bodySite }; // CodeableConcept 1279 case 3387378: 1280 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1281 default: 1282 return super.getProperty(hash, name, checkValid); 1283 } 1284 1285 } 1286 1287 @Override 1288 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1289 switch (hash) { 1290 case -1618432855: // identifier 1291 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1292 return value; 1293 case -332612366: // basedOn 1294 this.getBasedOn().add(castToReference(value)); // Reference 1295 return value; 1296 case -892481550: // status 1297 value = new DeviceUseStatementStatusEnumFactory().fromType(castToCode(value)); 1298 this.status = (Enumeration) value; // Enumeration<DeviceUseStatementStatus> 1299 return value; 1300 case -1867885268: // subject 1301 this.subject = castToReference(value); // Reference 1302 return value; 1303 case 1077922663: // derivedFrom 1304 this.getDerivedFrom().add(castToReference(value)); // Reference 1305 return value; 1306 case -873664438: // timing 1307 this.timing = castToType(value); // Type 1308 return value; 1309 case 735397551: // recordedOn 1310 this.recordedOn = castToDateTime(value); // DateTimeType 1311 return value; 1312 case -896505829: // source 1313 this.source = castToReference(value); // Reference 1314 return value; 1315 case -1335157162: // device 1316 this.device = castToReference(value); // Reference 1317 return value; 1318 case 722137681: // reasonCode 1319 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 1320 return value; 1321 case -1146218137: // reasonReference 1322 this.getReasonReference().add(castToReference(value)); // Reference 1323 return value; 1324 case 1702620169: // bodySite 1325 this.bodySite = castToCodeableConcept(value); // CodeableConcept 1326 return value; 1327 case 3387378: // note 1328 this.getNote().add(castToAnnotation(value)); // Annotation 1329 return value; 1330 default: 1331 return super.setProperty(hash, name, value); 1332 } 1333 1334 } 1335 1336 @Override 1337 public Base setProperty(String name, Base value) throws FHIRException { 1338 if (name.equals("identifier")) { 1339 this.getIdentifier().add(castToIdentifier(value)); 1340 } else if (name.equals("basedOn")) { 1341 this.getBasedOn().add(castToReference(value)); 1342 } else if (name.equals("status")) { 1343 value = new DeviceUseStatementStatusEnumFactory().fromType(castToCode(value)); 1344 this.status = (Enumeration) value; // Enumeration<DeviceUseStatementStatus> 1345 } else if (name.equals("subject")) { 1346 this.subject = castToReference(value); // Reference 1347 } else if (name.equals("derivedFrom")) { 1348 this.getDerivedFrom().add(castToReference(value)); 1349 } else if (name.equals("timing[x]")) { 1350 this.timing = castToType(value); // Type 1351 } else if (name.equals("recordedOn")) { 1352 this.recordedOn = castToDateTime(value); // DateTimeType 1353 } else if (name.equals("source")) { 1354 this.source = castToReference(value); // Reference 1355 } else if (name.equals("device")) { 1356 this.device = castToReference(value); // Reference 1357 } else if (name.equals("reasonCode")) { 1358 this.getReasonCode().add(castToCodeableConcept(value)); 1359 } else if (name.equals("reasonReference")) { 1360 this.getReasonReference().add(castToReference(value)); 1361 } else if (name.equals("bodySite")) { 1362 this.bodySite = castToCodeableConcept(value); // CodeableConcept 1363 } else if (name.equals("note")) { 1364 this.getNote().add(castToAnnotation(value)); 1365 } else 1366 return super.setProperty(name, value); 1367 return value; 1368 } 1369 1370 @Override 1371 public Base makeProperty(int hash, String name) throws FHIRException { 1372 switch (hash) { 1373 case -1618432855: 1374 return addIdentifier(); 1375 case -332612366: 1376 return addBasedOn(); 1377 case -892481550: 1378 return getStatusElement(); 1379 case -1867885268: 1380 return getSubject(); 1381 case 1077922663: 1382 return addDerivedFrom(); 1383 case 164632566: 1384 return getTiming(); 1385 case -873664438: 1386 return getTiming(); 1387 case 735397551: 1388 return getRecordedOnElement(); 1389 case -896505829: 1390 return getSource(); 1391 case -1335157162: 1392 return getDevice(); 1393 case 722137681: 1394 return addReasonCode(); 1395 case -1146218137: 1396 return addReasonReference(); 1397 case 1702620169: 1398 return getBodySite(); 1399 case 3387378: 1400 return addNote(); 1401 default: 1402 return super.makeProperty(hash, name); 1403 } 1404 1405 } 1406 1407 @Override 1408 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1409 switch (hash) { 1410 case -1618432855: 1411 /* identifier */ return new String[] { "Identifier" }; 1412 case -332612366: 1413 /* basedOn */ return new String[] { "Reference" }; 1414 case -892481550: 1415 /* status */ return new String[] { "code" }; 1416 case -1867885268: 1417 /* subject */ return new String[] { "Reference" }; 1418 case 1077922663: 1419 /* derivedFrom */ return new String[] { "Reference" }; 1420 case -873664438: 1421 /* timing */ return new String[] { "Timing", "Period", "dateTime" }; 1422 case 735397551: 1423 /* recordedOn */ return new String[] { "dateTime" }; 1424 case -896505829: 1425 /* source */ return new String[] { "Reference" }; 1426 case -1335157162: 1427 /* device */ return new String[] { "Reference" }; 1428 case 722137681: 1429 /* reasonCode */ return new String[] { "CodeableConcept" }; 1430 case -1146218137: 1431 /* reasonReference */ return new String[] { "Reference" }; 1432 case 1702620169: 1433 /* bodySite */ return new String[] { "CodeableConcept" }; 1434 case 3387378: 1435 /* note */ return new String[] { "Annotation" }; 1436 default: 1437 return super.getTypesForProperty(hash, name); 1438 } 1439 1440 } 1441 1442 @Override 1443 public Base addChild(String name) throws FHIRException { 1444 if (name.equals("identifier")) { 1445 return addIdentifier(); 1446 } else if (name.equals("basedOn")) { 1447 return addBasedOn(); 1448 } else if (name.equals("status")) { 1449 throw new FHIRException("Cannot call addChild on a singleton property DeviceUseStatement.status"); 1450 } else if (name.equals("subject")) { 1451 this.subject = new Reference(); 1452 return this.subject; 1453 } else if (name.equals("derivedFrom")) { 1454 return addDerivedFrom(); 1455 } else if (name.equals("timingTiming")) { 1456 this.timing = new Timing(); 1457 return this.timing; 1458 } else if (name.equals("timingPeriod")) { 1459 this.timing = new Period(); 1460 return this.timing; 1461 } else if (name.equals("timingDateTime")) { 1462 this.timing = new DateTimeType(); 1463 return this.timing; 1464 } else if (name.equals("recordedOn")) { 1465 throw new FHIRException("Cannot call addChild on a singleton property DeviceUseStatement.recordedOn"); 1466 } else if (name.equals("source")) { 1467 this.source = new Reference(); 1468 return this.source; 1469 } else if (name.equals("device")) { 1470 this.device = new Reference(); 1471 return this.device; 1472 } else if (name.equals("reasonCode")) { 1473 return addReasonCode(); 1474 } else if (name.equals("reasonReference")) { 1475 return addReasonReference(); 1476 } else if (name.equals("bodySite")) { 1477 this.bodySite = new CodeableConcept(); 1478 return this.bodySite; 1479 } else if (name.equals("note")) { 1480 return addNote(); 1481 } else 1482 return super.addChild(name); 1483 } 1484 1485 public String fhirType() { 1486 return "DeviceUseStatement"; 1487 1488 } 1489 1490 public DeviceUseStatement copy() { 1491 DeviceUseStatement dst = new DeviceUseStatement(); 1492 copyValues(dst); 1493 return dst; 1494 } 1495 1496 public void copyValues(DeviceUseStatement dst) { 1497 super.copyValues(dst); 1498 if (identifier != null) { 1499 dst.identifier = new ArrayList<Identifier>(); 1500 for (Identifier i : identifier) 1501 dst.identifier.add(i.copy()); 1502 } 1503 ; 1504 if (basedOn != null) { 1505 dst.basedOn = new ArrayList<Reference>(); 1506 for (Reference i : basedOn) 1507 dst.basedOn.add(i.copy()); 1508 } 1509 ; 1510 dst.status = status == null ? null : status.copy(); 1511 dst.subject = subject == null ? null : subject.copy(); 1512 if (derivedFrom != null) { 1513 dst.derivedFrom = new ArrayList<Reference>(); 1514 for (Reference i : derivedFrom) 1515 dst.derivedFrom.add(i.copy()); 1516 } 1517 ; 1518 dst.timing = timing == null ? null : timing.copy(); 1519 dst.recordedOn = recordedOn == null ? null : recordedOn.copy(); 1520 dst.source = source == null ? null : source.copy(); 1521 dst.device = device == null ? null : device.copy(); 1522 if (reasonCode != null) { 1523 dst.reasonCode = new ArrayList<CodeableConcept>(); 1524 for (CodeableConcept i : reasonCode) 1525 dst.reasonCode.add(i.copy()); 1526 } 1527 ; 1528 if (reasonReference != null) { 1529 dst.reasonReference = new ArrayList<Reference>(); 1530 for (Reference i : reasonReference) 1531 dst.reasonReference.add(i.copy()); 1532 } 1533 ; 1534 dst.bodySite = bodySite == null ? null : bodySite.copy(); 1535 if (note != null) { 1536 dst.note = new ArrayList<Annotation>(); 1537 for (Annotation i : note) 1538 dst.note.add(i.copy()); 1539 } 1540 ; 1541 } 1542 1543 protected DeviceUseStatement typedCopy() { 1544 return copy(); 1545 } 1546 1547 @Override 1548 public boolean equalsDeep(Base other_) { 1549 if (!super.equalsDeep(other_)) 1550 return false; 1551 if (!(other_ instanceof DeviceUseStatement)) 1552 return false; 1553 DeviceUseStatement o = (DeviceUseStatement) other_; 1554 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) 1555 && compareDeep(status, o.status, true) && compareDeep(subject, o.subject, true) 1556 && compareDeep(derivedFrom, o.derivedFrom, true) && compareDeep(timing, o.timing, true) 1557 && compareDeep(recordedOn, o.recordedOn, true) && compareDeep(source, o.source, true) 1558 && compareDeep(device, o.device, true) && compareDeep(reasonCode, o.reasonCode, true) 1559 && compareDeep(reasonReference, o.reasonReference, true) && compareDeep(bodySite, o.bodySite, true) 1560 && compareDeep(note, o.note, true); 1561 } 1562 1563 @Override 1564 public boolean equalsShallow(Base other_) { 1565 if (!super.equalsShallow(other_)) 1566 return false; 1567 if (!(other_ instanceof DeviceUseStatement)) 1568 return false; 1569 DeviceUseStatement o = (DeviceUseStatement) other_; 1570 return compareValues(status, o.status, true) && compareValues(recordedOn, o.recordedOn, true); 1571 } 1572 1573 public boolean isEmpty() { 1574 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, status, subject, derivedFrom, 1575 timing, recordedOn, source, device, reasonCode, reasonReference, bodySite, note); 1576 } 1577 1578 @Override 1579 public ResourceType getResourceType() { 1580 return ResourceType.DeviceUseStatement; 1581 } 1582 1583 /** 1584 * Search parameter: <b>identifier</b> 1585 * <p> 1586 * Description: <b>Search by identifier</b><br> 1587 * Type: <b>token</b><br> 1588 * Path: <b>DeviceUseStatement.identifier</b><br> 1589 * </p> 1590 */ 1591 @SearchParamDefinition(name = "identifier", path = "DeviceUseStatement.identifier", description = "Search by identifier", type = "token") 1592 public static final String SP_IDENTIFIER = "identifier"; 1593 /** 1594 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1595 * <p> 1596 * Description: <b>Search by identifier</b><br> 1597 * Type: <b>token</b><br> 1598 * Path: <b>DeviceUseStatement.identifier</b><br> 1599 * </p> 1600 */ 1601 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1602 SP_IDENTIFIER); 1603 1604 /** 1605 * Search parameter: <b>subject</b> 1606 * <p> 1607 * Description: <b>Search by subject</b><br> 1608 * Type: <b>reference</b><br> 1609 * Path: <b>DeviceUseStatement.subject</b><br> 1610 * </p> 1611 */ 1612 @SearchParamDefinition(name = "subject", path = "DeviceUseStatement.subject", description = "Search by subject", type = "reference", providesMembershipIn = { 1613 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Group.class, Patient.class }) 1614 public static final String SP_SUBJECT = "subject"; 1615 /** 1616 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1617 * <p> 1618 * Description: <b>Search by subject</b><br> 1619 * Type: <b>reference</b><br> 1620 * Path: <b>DeviceUseStatement.subject</b><br> 1621 * </p> 1622 */ 1623 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1624 SP_SUBJECT); 1625 1626 /** 1627 * Constant for fluent queries to be used to add include statements. Specifies 1628 * the path value of "<b>DeviceUseStatement:subject</b>". 1629 */ 1630 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 1631 "DeviceUseStatement:subject").toLocked(); 1632 1633 /** 1634 * Search parameter: <b>patient</b> 1635 * <p> 1636 * Description: <b>Search by subject - a patient</b><br> 1637 * Type: <b>reference</b><br> 1638 * Path: <b>DeviceUseStatement.subject</b><br> 1639 * </p> 1640 */ 1641 @SearchParamDefinition(name = "patient", path = "DeviceUseStatement.subject", description = "Search by subject - a patient", type = "reference", target = { 1642 Group.class, Patient.class }) 1643 public static final String SP_PATIENT = "patient"; 1644 /** 1645 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1646 * <p> 1647 * Description: <b>Search by subject - a patient</b><br> 1648 * Type: <b>reference</b><br> 1649 * Path: <b>DeviceUseStatement.subject</b><br> 1650 * </p> 1651 */ 1652 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1653 SP_PATIENT); 1654 1655 /** 1656 * Constant for fluent queries to be used to add include statements. Specifies 1657 * the path value of "<b>DeviceUseStatement:patient</b>". 1658 */ 1659 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 1660 "DeviceUseStatement:patient").toLocked(); 1661 1662 /** 1663 * Search parameter: <b>device</b> 1664 * <p> 1665 * Description: <b>Search by device</b><br> 1666 * Type: <b>reference</b><br> 1667 * Path: <b>DeviceUseStatement.device</b><br> 1668 * </p> 1669 */ 1670 @SearchParamDefinition(name = "device", path = "DeviceUseStatement.device", description = "Search by device", type = "reference", providesMembershipIn = { 1671 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device") }, target = { Device.class }) 1672 public static final String SP_DEVICE = "device"; 1673 /** 1674 * <b>Fluent Client</b> search parameter constant for <b>device</b> 1675 * <p> 1676 * Description: <b>Search by device</b><br> 1677 * Type: <b>reference</b><br> 1678 * Path: <b>DeviceUseStatement.device</b><br> 1679 * </p> 1680 */ 1681 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1682 SP_DEVICE); 1683 1684 /** 1685 * Constant for fluent queries to be used to add include statements. Specifies 1686 * the path value of "<b>DeviceUseStatement:device</b>". 1687 */ 1688 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEVICE = new ca.uhn.fhir.model.api.Include( 1689 "DeviceUseStatement:device").toLocked(); 1690 1691}