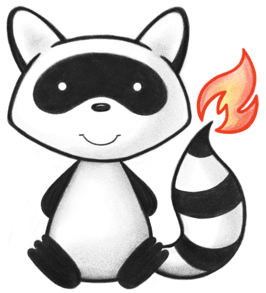
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * The findings and interpretation of diagnostic tests performed on patients, 049 * groups of patients, devices, and locations, and/or specimens derived from 050 * these. The report includes clinical context such as requesting and provider 051 * information, and some mix of atomic results, images, textual and coded 052 * interpretations, and formatted representation of diagnostic reports. 053 */ 054@ResourceDef(name = "DiagnosticReport", profile = "http://hl7.org/fhir/StructureDefinition/DiagnosticReport") 055public class DiagnosticReport extends DomainResource { 056 057 public enum DiagnosticReportStatus { 058 /** 059 * The existence of the report is registered, but there is nothing yet 060 * available. 061 */ 062 REGISTERED, 063 /** 064 * This is a partial (e.g. initial, interim or preliminary) report: data in the 065 * report may be incomplete or unverified. 066 */ 067 PARTIAL, 068 /** 069 * Verified early results are available, but not all results are final. 070 */ 071 PRELIMINARY, 072 /** 073 * The report is complete and verified by an authorized person. 074 */ 075 FINAL, 076 /** 077 * Subsequent to being final, the report has been modified. This includes any 078 * change in the results, diagnosis, narrative text, or other content of a 079 * report that has been issued. 080 */ 081 AMENDED, 082 /** 083 * Subsequent to being final, the report has been modified to correct an error 084 * in the report or referenced results. 085 */ 086 CORRECTED, 087 /** 088 * Subsequent to being final, the report has been modified by adding new 089 * content. The existing content is unchanged. 090 */ 091 APPENDED, 092 /** 093 * The report is unavailable because the measurement was not started or not 094 * completed (also sometimes called "aborted"). 095 */ 096 CANCELLED, 097 /** 098 * The report has been withdrawn following a previous final release. This 099 * electronic record should never have existed, though it is possible that 100 * real-world decisions were based on it. (If real-world activity has occurred, 101 * the status should be "cancelled" rather than "entered-in-error".). 102 */ 103 ENTEREDINERROR, 104 /** 105 * The authoring/source system does not know which of the status values 106 * currently applies for this observation. Note: This concept is not to be used 107 * for "other" - one of the listed statuses is presumed to apply, but the 108 * authoring/source system does not know which. 109 */ 110 UNKNOWN, 111 /** 112 * added to help the parsers with the generic types 113 */ 114 NULL; 115 116 public static DiagnosticReportStatus fromCode(String codeString) throws FHIRException { 117 if (codeString == null || "".equals(codeString)) 118 return null; 119 if ("registered".equals(codeString)) 120 return REGISTERED; 121 if ("partial".equals(codeString)) 122 return PARTIAL; 123 if ("preliminary".equals(codeString)) 124 return PRELIMINARY; 125 if ("final".equals(codeString)) 126 return FINAL; 127 if ("amended".equals(codeString)) 128 return AMENDED; 129 if ("corrected".equals(codeString)) 130 return CORRECTED; 131 if ("appended".equals(codeString)) 132 return APPENDED; 133 if ("cancelled".equals(codeString)) 134 return CANCELLED; 135 if ("entered-in-error".equals(codeString)) 136 return ENTEREDINERROR; 137 if ("unknown".equals(codeString)) 138 return UNKNOWN; 139 if (Configuration.isAcceptInvalidEnums()) 140 return null; 141 else 142 throw new FHIRException("Unknown DiagnosticReportStatus code '" + codeString + "'"); 143 } 144 145 public String toCode() { 146 switch (this) { 147 case REGISTERED: 148 return "registered"; 149 case PARTIAL: 150 return "partial"; 151 case PRELIMINARY: 152 return "preliminary"; 153 case FINAL: 154 return "final"; 155 case AMENDED: 156 return "amended"; 157 case CORRECTED: 158 return "corrected"; 159 case APPENDED: 160 return "appended"; 161 case CANCELLED: 162 return "cancelled"; 163 case ENTEREDINERROR: 164 return "entered-in-error"; 165 case UNKNOWN: 166 return "unknown"; 167 case NULL: 168 return null; 169 default: 170 return "?"; 171 } 172 } 173 174 public String getSystem() { 175 switch (this) { 176 case REGISTERED: 177 return "http://hl7.org/fhir/diagnostic-report-status"; 178 case PARTIAL: 179 return "http://hl7.org/fhir/diagnostic-report-status"; 180 case PRELIMINARY: 181 return "http://hl7.org/fhir/diagnostic-report-status"; 182 case FINAL: 183 return "http://hl7.org/fhir/diagnostic-report-status"; 184 case AMENDED: 185 return "http://hl7.org/fhir/diagnostic-report-status"; 186 case CORRECTED: 187 return "http://hl7.org/fhir/diagnostic-report-status"; 188 case APPENDED: 189 return "http://hl7.org/fhir/diagnostic-report-status"; 190 case CANCELLED: 191 return "http://hl7.org/fhir/diagnostic-report-status"; 192 case ENTEREDINERROR: 193 return "http://hl7.org/fhir/diagnostic-report-status"; 194 case UNKNOWN: 195 return "http://hl7.org/fhir/diagnostic-report-status"; 196 case NULL: 197 return null; 198 default: 199 return "?"; 200 } 201 } 202 203 public String getDefinition() { 204 switch (this) { 205 case REGISTERED: 206 return "The existence of the report is registered, but there is nothing yet available."; 207 case PARTIAL: 208 return "This is a partial (e.g. initial, interim or preliminary) report: data in the report may be incomplete or unverified."; 209 case PRELIMINARY: 210 return "Verified early results are available, but not all results are final."; 211 case FINAL: 212 return "The report is complete and verified by an authorized person."; 213 case AMENDED: 214 return "Subsequent to being final, the report has been modified. This includes any change in the results, diagnosis, narrative text, or other content of a report that has been issued."; 215 case CORRECTED: 216 return "Subsequent to being final, the report has been modified to correct an error in the report or referenced results."; 217 case APPENDED: 218 return "Subsequent to being final, the report has been modified by adding new content. The existing content is unchanged."; 219 case CANCELLED: 220 return "The report is unavailable because the measurement was not started or not completed (also sometimes called \"aborted\")."; 221 case ENTEREDINERROR: 222 return "The report has been withdrawn following a previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 223 case UNKNOWN: 224 return "The authoring/source system does not know which of the status values currently applies for this observation. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 225 case NULL: 226 return null; 227 default: 228 return "?"; 229 } 230 } 231 232 public String getDisplay() { 233 switch (this) { 234 case REGISTERED: 235 return "Registered"; 236 case PARTIAL: 237 return "Partial"; 238 case PRELIMINARY: 239 return "Preliminary"; 240 case FINAL: 241 return "Final"; 242 case AMENDED: 243 return "Amended"; 244 case CORRECTED: 245 return "Corrected"; 246 case APPENDED: 247 return "Appended"; 248 case CANCELLED: 249 return "Cancelled"; 250 case ENTEREDINERROR: 251 return "Entered in Error"; 252 case UNKNOWN: 253 return "Unknown"; 254 case NULL: 255 return null; 256 default: 257 return "?"; 258 } 259 } 260 } 261 262 public static class DiagnosticReportStatusEnumFactory implements EnumFactory<DiagnosticReportStatus> { 263 public DiagnosticReportStatus fromCode(String codeString) throws IllegalArgumentException { 264 if (codeString == null || "".equals(codeString)) 265 if (codeString == null || "".equals(codeString)) 266 return null; 267 if ("registered".equals(codeString)) 268 return DiagnosticReportStatus.REGISTERED; 269 if ("partial".equals(codeString)) 270 return DiagnosticReportStatus.PARTIAL; 271 if ("preliminary".equals(codeString)) 272 return DiagnosticReportStatus.PRELIMINARY; 273 if ("final".equals(codeString)) 274 return DiagnosticReportStatus.FINAL; 275 if ("amended".equals(codeString)) 276 return DiagnosticReportStatus.AMENDED; 277 if ("corrected".equals(codeString)) 278 return DiagnosticReportStatus.CORRECTED; 279 if ("appended".equals(codeString)) 280 return DiagnosticReportStatus.APPENDED; 281 if ("cancelled".equals(codeString)) 282 return DiagnosticReportStatus.CANCELLED; 283 if ("entered-in-error".equals(codeString)) 284 return DiagnosticReportStatus.ENTEREDINERROR; 285 if ("unknown".equals(codeString)) 286 return DiagnosticReportStatus.UNKNOWN; 287 throw new IllegalArgumentException("Unknown DiagnosticReportStatus code '" + codeString + "'"); 288 } 289 290 public Enumeration<DiagnosticReportStatus> fromType(PrimitiveType<?> code) throws FHIRException { 291 if (code == null) 292 return null; 293 if (code.isEmpty()) 294 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.NULL, code); 295 String codeString = code.asStringValue(); 296 if (codeString == null || "".equals(codeString)) 297 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.NULL, code); 298 if ("registered".equals(codeString)) 299 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.REGISTERED, code); 300 if ("partial".equals(codeString)) 301 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.PARTIAL, code); 302 if ("preliminary".equals(codeString)) 303 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.PRELIMINARY, code); 304 if ("final".equals(codeString)) 305 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.FINAL, code); 306 if ("amended".equals(codeString)) 307 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.AMENDED, code); 308 if ("corrected".equals(codeString)) 309 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.CORRECTED, code); 310 if ("appended".equals(codeString)) 311 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.APPENDED, code); 312 if ("cancelled".equals(codeString)) 313 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.CANCELLED, code); 314 if ("entered-in-error".equals(codeString)) 315 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.ENTEREDINERROR, code); 316 if ("unknown".equals(codeString)) 317 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.UNKNOWN, code); 318 throw new FHIRException("Unknown DiagnosticReportStatus code '" + codeString + "'"); 319 } 320 321 public String toCode(DiagnosticReportStatus code) { 322 if (code == DiagnosticReportStatus.NULL) 323 return null; 324 if (code == DiagnosticReportStatus.REGISTERED) 325 return "registered"; 326 if (code == DiagnosticReportStatus.PARTIAL) 327 return "partial"; 328 if (code == DiagnosticReportStatus.PRELIMINARY) 329 return "preliminary"; 330 if (code == DiagnosticReportStatus.FINAL) 331 return "final"; 332 if (code == DiagnosticReportStatus.AMENDED) 333 return "amended"; 334 if (code == DiagnosticReportStatus.CORRECTED) 335 return "corrected"; 336 if (code == DiagnosticReportStatus.APPENDED) 337 return "appended"; 338 if (code == DiagnosticReportStatus.CANCELLED) 339 return "cancelled"; 340 if (code == DiagnosticReportStatus.ENTEREDINERROR) 341 return "entered-in-error"; 342 if (code == DiagnosticReportStatus.UNKNOWN) 343 return "unknown"; 344 return "?"; 345 } 346 347 public String toSystem(DiagnosticReportStatus code) { 348 return code.getSystem(); 349 } 350 } 351 352 @Block() 353 public static class DiagnosticReportMediaComponent extends BackboneElement implements IBaseBackboneElement { 354 /** 355 * A comment about the image. Typically, this is used to provide an explanation 356 * for why the image is included, or to draw the viewer's attention to important 357 * features. 358 */ 359 @Child(name = "comment", type = { 360 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 361 @Description(shortDefinition = "Comment about the image (e.g. explanation)", formalDefinition = "A comment about the image. Typically, this is used to provide an explanation for why the image is included, or to draw the viewer's attention to important features.") 362 protected StringType comment; 363 364 /** 365 * Reference to the image source. 366 */ 367 @Child(name = "link", type = { Media.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 368 @Description(shortDefinition = "Reference to the image source", formalDefinition = "Reference to the image source.") 369 protected Reference link; 370 371 /** 372 * The actual object that is the target of the reference (Reference to the image 373 * source.) 374 */ 375 protected Media linkTarget; 376 377 private static final long serialVersionUID = 935791940L; 378 379 /** 380 * Constructor 381 */ 382 public DiagnosticReportMediaComponent() { 383 super(); 384 } 385 386 /** 387 * Constructor 388 */ 389 public DiagnosticReportMediaComponent(Reference link) { 390 super(); 391 this.link = link; 392 } 393 394 /** 395 * @return {@link #comment} (A comment about the image. Typically, this is used 396 * to provide an explanation for why the image is included, or to draw 397 * the viewer's attention to important features.). This is the 398 * underlying object with id, value and extensions. The accessor 399 * "getComment" gives direct access to the value 400 */ 401 public StringType getCommentElement() { 402 if (this.comment == null) 403 if (Configuration.errorOnAutoCreate()) 404 throw new Error("Attempt to auto-create DiagnosticReportMediaComponent.comment"); 405 else if (Configuration.doAutoCreate()) 406 this.comment = new StringType(); // bb 407 return this.comment; 408 } 409 410 public boolean hasCommentElement() { 411 return this.comment != null && !this.comment.isEmpty(); 412 } 413 414 public boolean hasComment() { 415 return this.comment != null && !this.comment.isEmpty(); 416 } 417 418 /** 419 * @param value {@link #comment} (A comment about the image. Typically, this is 420 * used to provide an explanation for why the image is included, or 421 * to draw the viewer's attention to important features.). This is 422 * the underlying object with id, value and extensions. The 423 * accessor "getComment" gives direct access to the value 424 */ 425 public DiagnosticReportMediaComponent setCommentElement(StringType value) { 426 this.comment = value; 427 return this; 428 } 429 430 /** 431 * @return A comment about the image. Typically, this is used to provide an 432 * explanation for why the image is included, or to draw the viewer's 433 * attention to important features. 434 */ 435 public String getComment() { 436 return this.comment == null ? null : this.comment.getValue(); 437 } 438 439 /** 440 * @param value A comment about the image. Typically, this is used to provide an 441 * explanation for why the image is included, or to draw the 442 * viewer's attention to important features. 443 */ 444 public DiagnosticReportMediaComponent setComment(String value) { 445 if (Utilities.noString(value)) 446 this.comment = null; 447 else { 448 if (this.comment == null) 449 this.comment = new StringType(); 450 this.comment.setValue(value); 451 } 452 return this; 453 } 454 455 /** 456 * @return {@link #link} (Reference to the image source.) 457 */ 458 public Reference getLink() { 459 if (this.link == null) 460 if (Configuration.errorOnAutoCreate()) 461 throw new Error("Attempt to auto-create DiagnosticReportMediaComponent.link"); 462 else if (Configuration.doAutoCreate()) 463 this.link = new Reference(); // cc 464 return this.link; 465 } 466 467 public boolean hasLink() { 468 return this.link != null && !this.link.isEmpty(); 469 } 470 471 /** 472 * @param value {@link #link} (Reference to the image source.) 473 */ 474 public DiagnosticReportMediaComponent setLink(Reference value) { 475 this.link = value; 476 return this; 477 } 478 479 /** 480 * @return {@link #link} The actual object that is the target of the reference. 481 * The reference library doesn't populate this, but you can use it to 482 * hold the resource if you resolve it. (Reference to the image source.) 483 */ 484 public Media getLinkTarget() { 485 if (this.linkTarget == null) 486 if (Configuration.errorOnAutoCreate()) 487 throw new Error("Attempt to auto-create DiagnosticReportMediaComponent.link"); 488 else if (Configuration.doAutoCreate()) 489 this.linkTarget = new Media(); // aa 490 return this.linkTarget; 491 } 492 493 /** 494 * @param value {@link #link} The actual object that is the target of the 495 * reference. The reference library doesn't use these, but you can 496 * use it to hold the resource if you resolve it. (Reference to the 497 * image source.) 498 */ 499 public DiagnosticReportMediaComponent setLinkTarget(Media value) { 500 this.linkTarget = value; 501 return this; 502 } 503 504 protected void listChildren(List<Property> children) { 505 super.listChildren(children); 506 children.add(new Property("comment", "string", 507 "A comment about the image. Typically, this is used to provide an explanation for why the image is included, or to draw the viewer's attention to important features.", 508 0, 1, comment)); 509 children.add(new Property("link", "Reference(Media)", "Reference to the image source.", 0, 1, link)); 510 } 511 512 @Override 513 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 514 switch (_hash) { 515 case 950398559: 516 /* comment */ return new Property("comment", "string", 517 "A comment about the image. Typically, this is used to provide an explanation for why the image is included, or to draw the viewer's attention to important features.", 518 0, 1, comment); 519 case 3321850: 520 /* link */ return new Property("link", "Reference(Media)", "Reference to the image source.", 0, 1, link); 521 default: 522 return super.getNamedProperty(_hash, _name, _checkValid); 523 } 524 525 } 526 527 @Override 528 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 529 switch (hash) { 530 case 950398559: 531 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // StringType 532 case 3321850: 533 /* link */ return this.link == null ? new Base[0] : new Base[] { this.link }; // Reference 534 default: 535 return super.getProperty(hash, name, checkValid); 536 } 537 538 } 539 540 @Override 541 public Base setProperty(int hash, String name, Base value) throws FHIRException { 542 switch (hash) { 543 case 950398559: // comment 544 this.comment = castToString(value); // StringType 545 return value; 546 case 3321850: // link 547 this.link = castToReference(value); // Reference 548 return value; 549 default: 550 return super.setProperty(hash, name, value); 551 } 552 553 } 554 555 @Override 556 public Base setProperty(String name, Base value) throws FHIRException { 557 if (name.equals("comment")) { 558 this.comment = castToString(value); // StringType 559 } else if (name.equals("link")) { 560 this.link = castToReference(value); // Reference 561 } else 562 return super.setProperty(name, value); 563 return value; 564 } 565 566 @Override 567 public void removeChild(String name, Base value) throws FHIRException { 568 if (name.equals("comment")) { 569 this.comment = null; 570 } else if (name.equals("link")) { 571 this.link = null; 572 } else 573 super.removeChild(name, value); 574 575 } 576 577 @Override 578 public Base makeProperty(int hash, String name) throws FHIRException { 579 switch (hash) { 580 case 950398559: 581 return getCommentElement(); 582 case 3321850: 583 return getLink(); 584 default: 585 return super.makeProperty(hash, name); 586 } 587 588 } 589 590 @Override 591 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 592 switch (hash) { 593 case 950398559: 594 /* comment */ return new String[] { "string" }; 595 case 3321850: 596 /* link */ return new String[] { "Reference" }; 597 default: 598 return super.getTypesForProperty(hash, name); 599 } 600 601 } 602 603 @Override 604 public Base addChild(String name) throws FHIRException { 605 if (name.equals("comment")) { 606 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticReport.comment"); 607 } else if (name.equals("link")) { 608 this.link = new Reference(); 609 return this.link; 610 } else 611 return super.addChild(name); 612 } 613 614 public DiagnosticReportMediaComponent copy() { 615 DiagnosticReportMediaComponent dst = new DiagnosticReportMediaComponent(); 616 copyValues(dst); 617 return dst; 618 } 619 620 public void copyValues(DiagnosticReportMediaComponent dst) { 621 super.copyValues(dst); 622 dst.comment = comment == null ? null : comment.copy(); 623 dst.link = link == null ? null : link.copy(); 624 } 625 626 @Override 627 public boolean equalsDeep(Base other_) { 628 if (!super.equalsDeep(other_)) 629 return false; 630 if (!(other_ instanceof DiagnosticReportMediaComponent)) 631 return false; 632 DiagnosticReportMediaComponent o = (DiagnosticReportMediaComponent) other_; 633 return compareDeep(comment, o.comment, true) && compareDeep(link, o.link, true); 634 } 635 636 @Override 637 public boolean equalsShallow(Base other_) { 638 if (!super.equalsShallow(other_)) 639 return false; 640 if (!(other_ instanceof DiagnosticReportMediaComponent)) 641 return false; 642 DiagnosticReportMediaComponent o = (DiagnosticReportMediaComponent) other_; 643 return compareValues(comment, o.comment, true); 644 } 645 646 public boolean isEmpty() { 647 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(comment, link); 648 } 649 650 public String fhirType() { 651 return "DiagnosticReport.media"; 652 653 } 654 655 } 656 657 /** 658 * Identifiers assigned to this report by the performer or other systems. 659 */ 660 @Child(name = "identifier", type = { 661 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 662 @Description(shortDefinition = "Business identifier for report", formalDefinition = "Identifiers assigned to this report by the performer or other systems.") 663 protected List<Identifier> identifier; 664 665 /** 666 * Details concerning a service requested. 667 */ 668 @Child(name = "basedOn", type = { CarePlan.class, ImmunizationRecommendation.class, MedicationRequest.class, 669 NutritionOrder.class, 670 ServiceRequest.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 671 @Description(shortDefinition = "What was requested", formalDefinition = "Details concerning a service requested.") 672 protected List<Reference> basedOn; 673 /** 674 * The actual objects that are the target of the reference (Details concerning a 675 * service requested.) 676 */ 677 protected List<Resource> basedOnTarget; 678 679 /** 680 * The status of the diagnostic report. 681 */ 682 @Child(name = "status", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = true, summary = true) 683 @Description(shortDefinition = "registered | partial | preliminary | final +", formalDefinition = "The status of the diagnostic report.") 684 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/diagnostic-report-status") 685 protected Enumeration<DiagnosticReportStatus> status; 686 687 /** 688 * A code that classifies the clinical discipline, department or diagnostic 689 * service that created the report (e.g. cardiology, biochemistry, hematology, 690 * MRI). This is used for searching, sorting and display purposes. 691 */ 692 @Child(name = "category", type = { 693 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 694 @Description(shortDefinition = "Service category", formalDefinition = "A code that classifies the clinical discipline, department or diagnostic service that created the report (e.g. cardiology, biochemistry, hematology, MRI). This is used for searching, sorting and display purposes.") 695 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/diagnostic-service-sections") 696 protected List<CodeableConcept> category; 697 698 /** 699 * A code or name that describes this diagnostic report. 700 */ 701 @Child(name = "code", type = { CodeableConcept.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 702 @Description(shortDefinition = "Name/Code for this diagnostic report", formalDefinition = "A code or name that describes this diagnostic report.") 703 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/report-codes") 704 protected CodeableConcept code; 705 706 /** 707 * The subject of the report. Usually, but not always, this is a patient. 708 * However, diagnostic services also perform analyses on specimens collected 709 * from a variety of other sources. 710 */ 711 @Child(name = "subject", type = { Patient.class, Group.class, Device.class, 712 Location.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 713 @Description(shortDefinition = "The subject of the report - usually, but not always, the patient", formalDefinition = "The subject of the report. Usually, but not always, this is a patient. However, diagnostic services also perform analyses on specimens collected from a variety of other sources.") 714 protected Reference subject; 715 716 /** 717 * The actual object that is the target of the reference (The subject of the 718 * report. Usually, but not always, this is a patient. However, diagnostic 719 * services also perform analyses on specimens collected from a variety of other 720 * sources.) 721 */ 722 protected Resource subjectTarget; 723 724 /** 725 * The healthcare event (e.g. a patient and healthcare provider interaction) 726 * which this DiagnosticReport is about. 727 */ 728 @Child(name = "encounter", type = { Encounter.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 729 @Description(shortDefinition = "Health care event when test ordered", formalDefinition = "The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport is about.") 730 protected Reference encounter; 731 732 /** 733 * The actual object that is the target of the reference (The healthcare event 734 * (e.g. a patient and healthcare provider interaction) which this 735 * DiagnosticReport is about.) 736 */ 737 protected Encounter encounterTarget; 738 739 /** 740 * The time or time-period the observed values are related to. When the subject 741 * of the report is a patient, this is usually either the time of the procedure 742 * or of specimen collection(s), but very often the source of the date/time is 743 * not known, only the date/time itself. 744 */ 745 @Child(name = "effective", type = { DateTimeType.class, 746 Period.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 747 @Description(shortDefinition = "Clinically relevant time/time-period for report", formalDefinition = "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.") 748 protected Type effective; 749 750 /** 751 * The date and time that this version of the report was made available to 752 * providers, typically after the report was reviewed and verified. 753 */ 754 @Child(name = "issued", type = { InstantType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 755 @Description(shortDefinition = "DateTime this version was made", formalDefinition = "The date and time that this version of the report was made available to providers, typically after the report was reviewed and verified.") 756 protected InstantType issued; 757 758 /** 759 * The diagnostic service that is responsible for issuing the report. 760 */ 761 @Child(name = "performer", type = { Practitioner.class, PractitionerRole.class, Organization.class, 762 CareTeam.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 763 @Description(shortDefinition = "Responsible Diagnostic Service", formalDefinition = "The diagnostic service that is responsible for issuing the report.") 764 protected List<Reference> performer; 765 /** 766 * The actual objects that are the target of the reference (The diagnostic 767 * service that is responsible for issuing the report.) 768 */ 769 protected List<Resource> performerTarget; 770 771 /** 772 * The practitioner or organization that is responsible for the report's 773 * conclusions and interpretations. 774 */ 775 @Child(name = "resultsInterpreter", type = { Practitioner.class, PractitionerRole.class, Organization.class, 776 CareTeam.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 777 @Description(shortDefinition = "Primary result interpreter", formalDefinition = "The practitioner or organization that is responsible for the report's conclusions and interpretations.") 778 protected List<Reference> resultsInterpreter; 779 /** 780 * The actual objects that are the target of the reference (The practitioner or 781 * organization that is responsible for the report's conclusions and 782 * interpretations.) 783 */ 784 protected List<Resource> resultsInterpreterTarget; 785 786 /** 787 * Details about the specimens on which this diagnostic report is based. 788 */ 789 @Child(name = "specimen", type = { 790 Specimen.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 791 @Description(shortDefinition = "Specimens this report is based on", formalDefinition = "Details about the specimens on which this diagnostic report is based.") 792 protected List<Reference> specimen; 793 /** 794 * The actual objects that are the target of the reference (Details about the 795 * specimens on which this diagnostic report is based.) 796 */ 797 protected List<Specimen> specimenTarget; 798 799 /** 800 * [Observations](observation.html) that are part of this diagnostic report. 801 */ 802 @Child(name = "result", type = { 803 Observation.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 804 @Description(shortDefinition = "Observations", formalDefinition = "[Observations](observation.html) that are part of this diagnostic report.") 805 protected List<Reference> result; 806 /** 807 * The actual objects that are the target of the reference 808 * ([Observations](observation.html) that are part of this diagnostic report.) 809 */ 810 protected List<Observation> resultTarget; 811 812 /** 813 * One or more links to full details of any imaging performed during the 814 * diagnostic investigation. Typically, this is imaging performed by DICOM 815 * enabled modalities, but this is not required. A fully enabled PACS viewer can 816 * use this information to provide views of the source images. 817 */ 818 @Child(name = "imagingStudy", type = { 819 ImagingStudy.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 820 @Description(shortDefinition = "Reference to full details of imaging associated with the diagnostic report", formalDefinition = "One or more links to full details of any imaging performed during the diagnostic investigation. Typically, this is imaging performed by DICOM enabled modalities, but this is not required. A fully enabled PACS viewer can use this information to provide views of the source images.") 821 protected List<Reference> imagingStudy; 822 /** 823 * The actual objects that are the target of the reference (One or more links to 824 * full details of any imaging performed during the diagnostic investigation. 825 * Typically, this is imaging performed by DICOM enabled modalities, but this is 826 * not required. A fully enabled PACS viewer can use this information to provide 827 * views of the source images.) 828 */ 829 protected List<ImagingStudy> imagingStudyTarget; 830 831 /** 832 * A list of key images associated with this report. The images are generally 833 * created during the diagnostic process, and may be directly of the patient, or 834 * of treated specimens (i.e. slides of interest). 835 */ 836 @Child(name = "media", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 837 @Description(shortDefinition = "Key images associated with this report", formalDefinition = "A list of key images associated with this report. The images are generally created during the diagnostic process, and may be directly of the patient, or of treated specimens (i.e. slides of interest).") 838 protected List<DiagnosticReportMediaComponent> media; 839 840 /** 841 * Concise and clinically contextualized summary conclusion 842 * (interpretation/impression) of the diagnostic report. 843 */ 844 @Child(name = "conclusion", type = { 845 StringType.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 846 @Description(shortDefinition = "Clinical conclusion (interpretation) of test results", formalDefinition = "Concise and clinically contextualized summary conclusion (interpretation/impression) of the diagnostic report.") 847 protected StringType conclusion; 848 849 /** 850 * One or more codes that represent the summary conclusion 851 * (interpretation/impression) of the diagnostic report. 852 */ 853 @Child(name = "conclusionCode", type = { 854 CodeableConcept.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 855 @Description(shortDefinition = "Codes for the clinical conclusion of test results", formalDefinition = "One or more codes that represent the summary conclusion (interpretation/impression) of the diagnostic report.") 856 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/clinical-findings") 857 protected List<CodeableConcept> conclusionCode; 858 859 /** 860 * Rich text representation of the entire result as issued by the diagnostic 861 * service. Multiple formats are allowed but they SHALL be semantically 862 * equivalent. 863 */ 864 @Child(name = "presentedForm", type = { 865 Attachment.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 866 @Description(shortDefinition = "Entire report as issued", formalDefinition = "Rich text representation of the entire result as issued by the diagnostic service. Multiple formats are allowed but they SHALL be semantically equivalent.") 867 protected List<Attachment> presentedForm; 868 869 private static final long serialVersionUID = 589102296L; 870 871 /** 872 * Constructor 873 */ 874 public DiagnosticReport() { 875 super(); 876 } 877 878 /** 879 * Constructor 880 */ 881 public DiagnosticReport(Enumeration<DiagnosticReportStatus> status, CodeableConcept code) { 882 super(); 883 this.status = status; 884 this.code = code; 885 } 886 887 /** 888 * @return {@link #identifier} (Identifiers assigned to this report by the 889 * performer or other systems.) 890 */ 891 public List<Identifier> getIdentifier() { 892 if (this.identifier == null) 893 this.identifier = new ArrayList<Identifier>(); 894 return this.identifier; 895 } 896 897 /** 898 * @return Returns a reference to <code>this</code> for easy method chaining 899 */ 900 public DiagnosticReport setIdentifier(List<Identifier> theIdentifier) { 901 this.identifier = theIdentifier; 902 return this; 903 } 904 905 public boolean hasIdentifier() { 906 if (this.identifier == null) 907 return false; 908 for (Identifier item : this.identifier) 909 if (!item.isEmpty()) 910 return true; 911 return false; 912 } 913 914 public Identifier addIdentifier() { // 3 915 Identifier t = new Identifier(); 916 if (this.identifier == null) 917 this.identifier = new ArrayList<Identifier>(); 918 this.identifier.add(t); 919 return t; 920 } 921 922 public DiagnosticReport addIdentifier(Identifier t) { // 3 923 if (t == null) 924 return this; 925 if (this.identifier == null) 926 this.identifier = new ArrayList<Identifier>(); 927 this.identifier.add(t); 928 return this; 929 } 930 931 /** 932 * @return The first repetition of repeating field {@link #identifier}, creating 933 * it if it does not already exist 934 */ 935 public Identifier getIdentifierFirstRep() { 936 if (getIdentifier().isEmpty()) { 937 addIdentifier(); 938 } 939 return getIdentifier().get(0); 940 } 941 942 /** 943 * @return {@link #basedOn} (Details concerning a service requested.) 944 */ 945 public List<Reference> getBasedOn() { 946 if (this.basedOn == null) 947 this.basedOn = new ArrayList<Reference>(); 948 return this.basedOn; 949 } 950 951 /** 952 * @return Returns a reference to <code>this</code> for easy method chaining 953 */ 954 public DiagnosticReport setBasedOn(List<Reference> theBasedOn) { 955 this.basedOn = theBasedOn; 956 return this; 957 } 958 959 public boolean hasBasedOn() { 960 if (this.basedOn == null) 961 return false; 962 for (Reference item : this.basedOn) 963 if (!item.isEmpty()) 964 return true; 965 return false; 966 } 967 968 public Reference addBasedOn() { // 3 969 Reference t = new Reference(); 970 if (this.basedOn == null) 971 this.basedOn = new ArrayList<Reference>(); 972 this.basedOn.add(t); 973 return t; 974 } 975 976 public DiagnosticReport addBasedOn(Reference t) { // 3 977 if (t == null) 978 return this; 979 if (this.basedOn == null) 980 this.basedOn = new ArrayList<Reference>(); 981 this.basedOn.add(t); 982 return this; 983 } 984 985 /** 986 * @return The first repetition of repeating field {@link #basedOn}, creating it 987 * if it does not already exist 988 */ 989 public Reference getBasedOnFirstRep() { 990 if (getBasedOn().isEmpty()) { 991 addBasedOn(); 992 } 993 return getBasedOn().get(0); 994 } 995 996 /** 997 * @return {@link #status} (The status of the diagnostic report.). This is the 998 * underlying object with id, value and extensions. The accessor 999 * "getStatus" gives direct access to the value 1000 */ 1001 public Enumeration<DiagnosticReportStatus> getStatusElement() { 1002 if (this.status == null) 1003 if (Configuration.errorOnAutoCreate()) 1004 throw new Error("Attempt to auto-create DiagnosticReport.status"); 1005 else if (Configuration.doAutoCreate()) 1006 this.status = new Enumeration<DiagnosticReportStatus>(new DiagnosticReportStatusEnumFactory()); // bb 1007 return this.status; 1008 } 1009 1010 public boolean hasStatusElement() { 1011 return this.status != null && !this.status.isEmpty(); 1012 } 1013 1014 public boolean hasStatus() { 1015 return this.status != null && !this.status.isEmpty(); 1016 } 1017 1018 /** 1019 * @param value {@link #status} (The status of the diagnostic report.). This is 1020 * the underlying object with id, value and extensions. The 1021 * accessor "getStatus" gives direct access to the value 1022 */ 1023 public DiagnosticReport setStatusElement(Enumeration<DiagnosticReportStatus> value) { 1024 this.status = value; 1025 return this; 1026 } 1027 1028 /** 1029 * @return The status of the diagnostic report. 1030 */ 1031 public DiagnosticReportStatus getStatus() { 1032 return this.status == null ? null : this.status.getValue(); 1033 } 1034 1035 /** 1036 * @param value The status of the diagnostic report. 1037 */ 1038 public DiagnosticReport setStatus(DiagnosticReportStatus value) { 1039 if (this.status == null) 1040 this.status = new Enumeration<DiagnosticReportStatus>(new DiagnosticReportStatusEnumFactory()); 1041 this.status.setValue(value); 1042 return this; 1043 } 1044 1045 /** 1046 * @return {@link #category} (A code that classifies the clinical discipline, 1047 * department or diagnostic service that created the report (e.g. 1048 * cardiology, biochemistry, hematology, MRI). This is used for 1049 * searching, sorting and display purposes.) 1050 */ 1051 public List<CodeableConcept> getCategory() { 1052 if (this.category == null) 1053 this.category = new ArrayList<CodeableConcept>(); 1054 return this.category; 1055 } 1056 1057 /** 1058 * @return Returns a reference to <code>this</code> for easy method chaining 1059 */ 1060 public DiagnosticReport setCategory(List<CodeableConcept> theCategory) { 1061 this.category = theCategory; 1062 return this; 1063 } 1064 1065 public boolean hasCategory() { 1066 if (this.category == null) 1067 return false; 1068 for (CodeableConcept item : this.category) 1069 if (!item.isEmpty()) 1070 return true; 1071 return false; 1072 } 1073 1074 public CodeableConcept addCategory() { // 3 1075 CodeableConcept t = new CodeableConcept(); 1076 if (this.category == null) 1077 this.category = new ArrayList<CodeableConcept>(); 1078 this.category.add(t); 1079 return t; 1080 } 1081 1082 public DiagnosticReport addCategory(CodeableConcept t) { // 3 1083 if (t == null) 1084 return this; 1085 if (this.category == null) 1086 this.category = new ArrayList<CodeableConcept>(); 1087 this.category.add(t); 1088 return this; 1089 } 1090 1091 /** 1092 * @return The first repetition of repeating field {@link #category}, creating 1093 * it if it does not already exist 1094 */ 1095 public CodeableConcept getCategoryFirstRep() { 1096 if (getCategory().isEmpty()) { 1097 addCategory(); 1098 } 1099 return getCategory().get(0); 1100 } 1101 1102 /** 1103 * @return {@link #code} (A code or name that describes this diagnostic report.) 1104 */ 1105 public CodeableConcept getCode() { 1106 if (this.code == null) 1107 if (Configuration.errorOnAutoCreate()) 1108 throw new Error("Attempt to auto-create DiagnosticReport.code"); 1109 else if (Configuration.doAutoCreate()) 1110 this.code = new CodeableConcept(); // cc 1111 return this.code; 1112 } 1113 1114 public boolean hasCode() { 1115 return this.code != null && !this.code.isEmpty(); 1116 } 1117 1118 /** 1119 * @param value {@link #code} (A code or name that describes this diagnostic 1120 * report.) 1121 */ 1122 public DiagnosticReport setCode(CodeableConcept value) { 1123 this.code = value; 1124 return this; 1125 } 1126 1127 /** 1128 * @return {@link #subject} (The subject of the report. Usually, but not always, 1129 * this is a patient. However, diagnostic services also perform analyses 1130 * on specimens collected from a variety of other sources.) 1131 */ 1132 public Reference getSubject() { 1133 if (this.subject == null) 1134 if (Configuration.errorOnAutoCreate()) 1135 throw new Error("Attempt to auto-create DiagnosticReport.subject"); 1136 else if (Configuration.doAutoCreate()) 1137 this.subject = new Reference(); // cc 1138 return this.subject; 1139 } 1140 1141 public boolean hasSubject() { 1142 return this.subject != null && !this.subject.isEmpty(); 1143 } 1144 1145 /** 1146 * @param value {@link #subject} (The subject of the report. Usually, but not 1147 * always, this is a patient. However, diagnostic services also 1148 * perform analyses on specimens collected from a variety of other 1149 * sources.) 1150 */ 1151 public DiagnosticReport setSubject(Reference value) { 1152 this.subject = value; 1153 return this; 1154 } 1155 1156 /** 1157 * @return {@link #subject} The actual object that is the target of the 1158 * reference. The reference library doesn't populate this, but you can 1159 * use it to hold the resource if you resolve it. (The subject of the 1160 * report. Usually, but not always, this is a patient. However, 1161 * diagnostic services also perform analyses on specimens collected from 1162 * a variety of other sources.) 1163 */ 1164 public Resource getSubjectTarget() { 1165 return this.subjectTarget; 1166 } 1167 1168 /** 1169 * @param value {@link #subject} The actual object that is the target of the 1170 * reference. The reference library doesn't use these, but you can 1171 * use it to hold the resource if you resolve it. (The subject of 1172 * the report. Usually, but not always, this is a patient. However, 1173 * diagnostic services also perform analyses on specimens collected 1174 * from a variety of other sources.) 1175 */ 1176 public DiagnosticReport setSubjectTarget(Resource value) { 1177 this.subjectTarget = value; 1178 return this; 1179 } 1180 1181 /** 1182 * @return {@link #encounter} (The healthcare event (e.g. a patient and 1183 * healthcare provider interaction) which this DiagnosticReport is 1184 * about.) 1185 */ 1186 public Reference getEncounter() { 1187 if (this.encounter == null) 1188 if (Configuration.errorOnAutoCreate()) 1189 throw new Error("Attempt to auto-create DiagnosticReport.encounter"); 1190 else if (Configuration.doAutoCreate()) 1191 this.encounter = new Reference(); // cc 1192 return this.encounter; 1193 } 1194 1195 public boolean hasEncounter() { 1196 return this.encounter != null && !this.encounter.isEmpty(); 1197 } 1198 1199 /** 1200 * @param value {@link #encounter} (The healthcare event (e.g. a patient and 1201 * healthcare provider interaction) which this DiagnosticReport is 1202 * about.) 1203 */ 1204 public DiagnosticReport setEncounter(Reference value) { 1205 this.encounter = value; 1206 return this; 1207 } 1208 1209 /** 1210 * @return {@link #encounter} The actual object that is the target of the 1211 * reference. The reference library doesn't populate this, but you can 1212 * use it to hold the resource if you resolve it. (The healthcare event 1213 * (e.g. a patient and healthcare provider interaction) which this 1214 * DiagnosticReport is about.) 1215 */ 1216 public Encounter getEncounterTarget() { 1217 if (this.encounterTarget == null) 1218 if (Configuration.errorOnAutoCreate()) 1219 throw new Error("Attempt to auto-create DiagnosticReport.encounter"); 1220 else if (Configuration.doAutoCreate()) 1221 this.encounterTarget = new Encounter(); // aa 1222 return this.encounterTarget; 1223 } 1224 1225 /** 1226 * @param value {@link #encounter} The actual object that is the target of the 1227 * reference. The reference library doesn't use these, but you can 1228 * use it to hold the resource if you resolve it. (The healthcare 1229 * event (e.g. a patient and healthcare provider interaction) which 1230 * this DiagnosticReport is about.) 1231 */ 1232 public DiagnosticReport setEncounterTarget(Encounter value) { 1233 this.encounterTarget = value; 1234 return this; 1235 } 1236 1237 /** 1238 * @return {@link #effective} (The time or time-period the observed values are 1239 * related to. When the subject of the report is a patient, this is 1240 * usually either the time of the procedure or of specimen 1241 * collection(s), but very often the source of the date/time is not 1242 * known, only the date/time itself.) 1243 */ 1244 public Type getEffective() { 1245 return this.effective; 1246 } 1247 1248 /** 1249 * @return {@link #effective} (The time or time-period the observed values are 1250 * related to. When the subject of the report is a patient, this is 1251 * usually either the time of the procedure or of specimen 1252 * collection(s), but very often the source of the date/time is not 1253 * known, only the date/time itself.) 1254 */ 1255 public DateTimeType getEffectiveDateTimeType() throws FHIRException { 1256 if (this.effective == null) 1257 this.effective = new DateTimeType(); 1258 if (!(this.effective instanceof DateTimeType)) 1259 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1260 + this.effective.getClass().getName() + " was encountered"); 1261 return (DateTimeType) this.effective; 1262 } 1263 1264 public boolean hasEffectiveDateTimeType() { 1265 return this.effective instanceof DateTimeType; 1266 } 1267 1268 /** 1269 * @return {@link #effective} (The time or time-period the observed values are 1270 * related to. When the subject of the report is a patient, this is 1271 * usually either the time of the procedure or of specimen 1272 * collection(s), but very often the source of the date/time is not 1273 * known, only the date/time itself.) 1274 */ 1275 public Period getEffectivePeriod() throws FHIRException { 1276 if (this.effective == null) 1277 this.effective = new Period(); 1278 if (!(this.effective instanceof Period)) 1279 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.effective.getClass().getName() 1280 + " was encountered"); 1281 return (Period) this.effective; 1282 } 1283 1284 public boolean hasEffectivePeriod() { 1285 return this.effective instanceof Period; 1286 } 1287 1288 public boolean hasEffective() { 1289 return this.effective != null && !this.effective.isEmpty(); 1290 } 1291 1292 /** 1293 * @param value {@link #effective} (The time or time-period the observed values 1294 * are related to. When the subject of the report is a patient, 1295 * this is usually either the time of the procedure or of specimen 1296 * collection(s), but very often the source of the date/time is not 1297 * known, only the date/time itself.) 1298 */ 1299 public DiagnosticReport setEffective(Type value) { 1300 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1301 throw new Error("Not the right type for DiagnosticReport.effective[x]: " + value.fhirType()); 1302 this.effective = value; 1303 return this; 1304 } 1305 1306 /** 1307 * @return {@link #issued} (The date and time that this version of the report 1308 * was made available to providers, typically after the report was 1309 * reviewed and verified.). This is the underlying object with id, value 1310 * and extensions. The accessor "getIssued" gives direct access to the 1311 * value 1312 */ 1313 public InstantType getIssuedElement() { 1314 if (this.issued == null) 1315 if (Configuration.errorOnAutoCreate()) 1316 throw new Error("Attempt to auto-create DiagnosticReport.issued"); 1317 else if (Configuration.doAutoCreate()) 1318 this.issued = new InstantType(); // bb 1319 return this.issued; 1320 } 1321 1322 public boolean hasIssuedElement() { 1323 return this.issued != null && !this.issued.isEmpty(); 1324 } 1325 1326 public boolean hasIssued() { 1327 return this.issued != null && !this.issued.isEmpty(); 1328 } 1329 1330 /** 1331 * @param value {@link #issued} (The date and time that this version of the 1332 * report was made available to providers, typically after the 1333 * report was reviewed and verified.). This is the underlying 1334 * object with id, value and extensions. The accessor "getIssued" 1335 * gives direct access to the value 1336 */ 1337 public DiagnosticReport setIssuedElement(InstantType value) { 1338 this.issued = value; 1339 return this; 1340 } 1341 1342 /** 1343 * @return The date and time that this version of the report was made available 1344 * to providers, typically after the report was reviewed and verified. 1345 */ 1346 public Date getIssued() { 1347 return this.issued == null ? null : this.issued.getValue(); 1348 } 1349 1350 /** 1351 * @param value The date and time that this version of the report was made 1352 * available to providers, typically after the report was reviewed 1353 * and verified. 1354 */ 1355 public DiagnosticReport setIssued(Date value) { 1356 if (value == null) 1357 this.issued = null; 1358 else { 1359 if (this.issued == null) 1360 this.issued = new InstantType(); 1361 this.issued.setValue(value); 1362 } 1363 return this; 1364 } 1365 1366 /** 1367 * @return {@link #performer} (The diagnostic service that is responsible for 1368 * issuing the report.) 1369 */ 1370 public List<Reference> getPerformer() { 1371 if (this.performer == null) 1372 this.performer = new ArrayList<Reference>(); 1373 return this.performer; 1374 } 1375 1376 /** 1377 * @return Returns a reference to <code>this</code> for easy method chaining 1378 */ 1379 public DiagnosticReport setPerformer(List<Reference> thePerformer) { 1380 this.performer = thePerformer; 1381 return this; 1382 } 1383 1384 public boolean hasPerformer() { 1385 if (this.performer == null) 1386 return false; 1387 for (Reference item : this.performer) 1388 if (!item.isEmpty()) 1389 return true; 1390 return false; 1391 } 1392 1393 public Reference addPerformer() { // 3 1394 Reference t = new Reference(); 1395 if (this.performer == null) 1396 this.performer = new ArrayList<Reference>(); 1397 this.performer.add(t); 1398 return t; 1399 } 1400 1401 public DiagnosticReport addPerformer(Reference t) { // 3 1402 if (t == null) 1403 return this; 1404 if (this.performer == null) 1405 this.performer = new ArrayList<Reference>(); 1406 this.performer.add(t); 1407 return this; 1408 } 1409 1410 /** 1411 * @return The first repetition of repeating field {@link #performer}, creating 1412 * it if it does not already exist 1413 */ 1414 public Reference getPerformerFirstRep() { 1415 if (getPerformer().isEmpty()) { 1416 addPerformer(); 1417 } 1418 return getPerformer().get(0); 1419 } 1420 1421 /** 1422 * @return {@link #resultsInterpreter} (The practitioner or organization that is 1423 * responsible for the report's conclusions and interpretations.) 1424 */ 1425 public List<Reference> getResultsInterpreter() { 1426 if (this.resultsInterpreter == null) 1427 this.resultsInterpreter = new ArrayList<Reference>(); 1428 return this.resultsInterpreter; 1429 } 1430 1431 /** 1432 * @return Returns a reference to <code>this</code> for easy method chaining 1433 */ 1434 public DiagnosticReport setResultsInterpreter(List<Reference> theResultsInterpreter) { 1435 this.resultsInterpreter = theResultsInterpreter; 1436 return this; 1437 } 1438 1439 public boolean hasResultsInterpreter() { 1440 if (this.resultsInterpreter == null) 1441 return false; 1442 for (Reference item : this.resultsInterpreter) 1443 if (!item.isEmpty()) 1444 return true; 1445 return false; 1446 } 1447 1448 public Reference addResultsInterpreter() { // 3 1449 Reference t = new Reference(); 1450 if (this.resultsInterpreter == null) 1451 this.resultsInterpreter = new ArrayList<Reference>(); 1452 this.resultsInterpreter.add(t); 1453 return t; 1454 } 1455 1456 public DiagnosticReport addResultsInterpreter(Reference t) { // 3 1457 if (t == null) 1458 return this; 1459 if (this.resultsInterpreter == null) 1460 this.resultsInterpreter = new ArrayList<Reference>(); 1461 this.resultsInterpreter.add(t); 1462 return this; 1463 } 1464 1465 /** 1466 * @return The first repetition of repeating field {@link #resultsInterpreter}, 1467 * creating it if it does not already exist 1468 */ 1469 public Reference getResultsInterpreterFirstRep() { 1470 if (getResultsInterpreter().isEmpty()) { 1471 addResultsInterpreter(); 1472 } 1473 return getResultsInterpreter().get(0); 1474 } 1475 1476 /** 1477 * @return {@link #specimen} (Details about the specimens on which this 1478 * diagnostic report is based.) 1479 */ 1480 public List<Reference> getSpecimen() { 1481 if (this.specimen == null) 1482 this.specimen = new ArrayList<Reference>(); 1483 return this.specimen; 1484 } 1485 1486 /** 1487 * @return Returns a reference to <code>this</code> for easy method chaining 1488 */ 1489 public DiagnosticReport setSpecimen(List<Reference> theSpecimen) { 1490 this.specimen = theSpecimen; 1491 return this; 1492 } 1493 1494 public boolean hasSpecimen() { 1495 if (this.specimen == null) 1496 return false; 1497 for (Reference item : this.specimen) 1498 if (!item.isEmpty()) 1499 return true; 1500 return false; 1501 } 1502 1503 public Reference addSpecimen() { // 3 1504 Reference t = new Reference(); 1505 if (this.specimen == null) 1506 this.specimen = new ArrayList<Reference>(); 1507 this.specimen.add(t); 1508 return t; 1509 } 1510 1511 public DiagnosticReport addSpecimen(Reference t) { // 3 1512 if (t == null) 1513 return this; 1514 if (this.specimen == null) 1515 this.specimen = new ArrayList<Reference>(); 1516 this.specimen.add(t); 1517 return this; 1518 } 1519 1520 /** 1521 * @return The first repetition of repeating field {@link #specimen}, creating 1522 * it if it does not already exist 1523 */ 1524 public Reference getSpecimenFirstRep() { 1525 if (getSpecimen().isEmpty()) { 1526 addSpecimen(); 1527 } 1528 return getSpecimen().get(0); 1529 } 1530 1531 /** 1532 * @return {@link #result} ([Observations](observation.html) that are part of 1533 * this diagnostic report.) 1534 */ 1535 public List<Reference> getResult() { 1536 if (this.result == null) 1537 this.result = new ArrayList<Reference>(); 1538 return this.result; 1539 } 1540 1541 /** 1542 * @return Returns a reference to <code>this</code> for easy method chaining 1543 */ 1544 public DiagnosticReport setResult(List<Reference> theResult) { 1545 this.result = theResult; 1546 return this; 1547 } 1548 1549 public boolean hasResult() { 1550 if (this.result == null) 1551 return false; 1552 for (Reference item : this.result) 1553 if (!item.isEmpty()) 1554 return true; 1555 return false; 1556 } 1557 1558 public Reference addResult() { // 3 1559 Reference t = new Reference(); 1560 if (this.result == null) 1561 this.result = new ArrayList<Reference>(); 1562 this.result.add(t); 1563 return t; 1564 } 1565 1566 public DiagnosticReport addResult(Reference t) { // 3 1567 if (t == null) 1568 return this; 1569 if (this.result == null) 1570 this.result = new ArrayList<Reference>(); 1571 this.result.add(t); 1572 return this; 1573 } 1574 1575 /** 1576 * @return The first repetition of repeating field {@link #result}, creating it 1577 * if it does not already exist 1578 */ 1579 public Reference getResultFirstRep() { 1580 if (getResult().isEmpty()) { 1581 addResult(); 1582 } 1583 return getResult().get(0); 1584 } 1585 1586 /** 1587 * @return {@link #imagingStudy} (One or more links to full details of any 1588 * imaging performed during the diagnostic investigation. Typically, 1589 * this is imaging performed by DICOM enabled modalities, but this is 1590 * not required. A fully enabled PACS viewer can use this information to 1591 * provide views of the source images.) 1592 */ 1593 public List<Reference> getImagingStudy() { 1594 if (this.imagingStudy == null) 1595 this.imagingStudy = new ArrayList<Reference>(); 1596 return this.imagingStudy; 1597 } 1598 1599 /** 1600 * @return Returns a reference to <code>this</code> for easy method chaining 1601 */ 1602 public DiagnosticReport setImagingStudy(List<Reference> theImagingStudy) { 1603 this.imagingStudy = theImagingStudy; 1604 return this; 1605 } 1606 1607 public boolean hasImagingStudy() { 1608 if (this.imagingStudy == null) 1609 return false; 1610 for (Reference item : this.imagingStudy) 1611 if (!item.isEmpty()) 1612 return true; 1613 return false; 1614 } 1615 1616 public Reference addImagingStudy() { // 3 1617 Reference t = new Reference(); 1618 if (this.imagingStudy == null) 1619 this.imagingStudy = new ArrayList<Reference>(); 1620 this.imagingStudy.add(t); 1621 return t; 1622 } 1623 1624 public DiagnosticReport addImagingStudy(Reference t) { // 3 1625 if (t == null) 1626 return this; 1627 if (this.imagingStudy == null) 1628 this.imagingStudy = new ArrayList<Reference>(); 1629 this.imagingStudy.add(t); 1630 return this; 1631 } 1632 1633 /** 1634 * @return The first repetition of repeating field {@link #imagingStudy}, 1635 * creating it if it does not already exist 1636 */ 1637 public Reference getImagingStudyFirstRep() { 1638 if (getImagingStudy().isEmpty()) { 1639 addImagingStudy(); 1640 } 1641 return getImagingStudy().get(0); 1642 } 1643 1644 /** 1645 * @return {@link #media} (A list of key images associated with this report. The 1646 * images are generally created during the diagnostic process, and may 1647 * be directly of the patient, or of treated specimens (i.e. slides of 1648 * interest).) 1649 */ 1650 public List<DiagnosticReportMediaComponent> getMedia() { 1651 if (this.media == null) 1652 this.media = new ArrayList<DiagnosticReportMediaComponent>(); 1653 return this.media; 1654 } 1655 1656 /** 1657 * @return Returns a reference to <code>this</code> for easy method chaining 1658 */ 1659 public DiagnosticReport setMedia(List<DiagnosticReportMediaComponent> theMedia) { 1660 this.media = theMedia; 1661 return this; 1662 } 1663 1664 public boolean hasMedia() { 1665 if (this.media == null) 1666 return false; 1667 for (DiagnosticReportMediaComponent item : this.media) 1668 if (!item.isEmpty()) 1669 return true; 1670 return false; 1671 } 1672 1673 public DiagnosticReportMediaComponent addMedia() { // 3 1674 DiagnosticReportMediaComponent t = new DiagnosticReportMediaComponent(); 1675 if (this.media == null) 1676 this.media = new ArrayList<DiagnosticReportMediaComponent>(); 1677 this.media.add(t); 1678 return t; 1679 } 1680 1681 public DiagnosticReport addMedia(DiagnosticReportMediaComponent t) { // 3 1682 if (t == null) 1683 return this; 1684 if (this.media == null) 1685 this.media = new ArrayList<DiagnosticReportMediaComponent>(); 1686 this.media.add(t); 1687 return this; 1688 } 1689 1690 /** 1691 * @return The first repetition of repeating field {@link #media}, creating it 1692 * if it does not already exist 1693 */ 1694 public DiagnosticReportMediaComponent getMediaFirstRep() { 1695 if (getMedia().isEmpty()) { 1696 addMedia(); 1697 } 1698 return getMedia().get(0); 1699 } 1700 1701 /** 1702 * @return {@link #conclusion} (Concise and clinically contextualized summary 1703 * conclusion (interpretation/impression) of the diagnostic report.). 1704 * This is the underlying object with id, value and extensions. The 1705 * accessor "getConclusion" gives direct access to the value 1706 */ 1707 public StringType getConclusionElement() { 1708 if (this.conclusion == null) 1709 if (Configuration.errorOnAutoCreate()) 1710 throw new Error("Attempt to auto-create DiagnosticReport.conclusion"); 1711 else if (Configuration.doAutoCreate()) 1712 this.conclusion = new StringType(); // bb 1713 return this.conclusion; 1714 } 1715 1716 public boolean hasConclusionElement() { 1717 return this.conclusion != null && !this.conclusion.isEmpty(); 1718 } 1719 1720 public boolean hasConclusion() { 1721 return this.conclusion != null && !this.conclusion.isEmpty(); 1722 } 1723 1724 /** 1725 * @param value {@link #conclusion} (Concise and clinically contextualized 1726 * summary conclusion (interpretation/impression) of the diagnostic 1727 * report.). This is the underlying object with id, value and 1728 * extensions. The accessor "getConclusion" gives direct access to 1729 * the value 1730 */ 1731 public DiagnosticReport setConclusionElement(StringType value) { 1732 this.conclusion = value; 1733 return this; 1734 } 1735 1736 /** 1737 * @return Concise and clinically contextualized summary conclusion 1738 * (interpretation/impression) of the diagnostic report. 1739 */ 1740 public String getConclusion() { 1741 return this.conclusion == null ? null : this.conclusion.getValue(); 1742 } 1743 1744 /** 1745 * @param value Concise and clinically contextualized summary conclusion 1746 * (interpretation/impression) of the diagnostic report. 1747 */ 1748 public DiagnosticReport setConclusion(String value) { 1749 if (Utilities.noString(value)) 1750 this.conclusion = null; 1751 else { 1752 if (this.conclusion == null) 1753 this.conclusion = new StringType(); 1754 this.conclusion.setValue(value); 1755 } 1756 return this; 1757 } 1758 1759 /** 1760 * @return {@link #conclusionCode} (One or more codes that represent the summary 1761 * conclusion (interpretation/impression) of the diagnostic report.) 1762 */ 1763 public List<CodeableConcept> getConclusionCode() { 1764 if (this.conclusionCode == null) 1765 this.conclusionCode = new ArrayList<CodeableConcept>(); 1766 return this.conclusionCode; 1767 } 1768 1769 /** 1770 * @return Returns a reference to <code>this</code> for easy method chaining 1771 */ 1772 public DiagnosticReport setConclusionCode(List<CodeableConcept> theConclusionCode) { 1773 this.conclusionCode = theConclusionCode; 1774 return this; 1775 } 1776 1777 public boolean hasConclusionCode() { 1778 if (this.conclusionCode == null) 1779 return false; 1780 for (CodeableConcept item : this.conclusionCode) 1781 if (!item.isEmpty()) 1782 return true; 1783 return false; 1784 } 1785 1786 public CodeableConcept addConclusionCode() { // 3 1787 CodeableConcept t = new CodeableConcept(); 1788 if (this.conclusionCode == null) 1789 this.conclusionCode = new ArrayList<CodeableConcept>(); 1790 this.conclusionCode.add(t); 1791 return t; 1792 } 1793 1794 public DiagnosticReport addConclusionCode(CodeableConcept t) { // 3 1795 if (t == null) 1796 return this; 1797 if (this.conclusionCode == null) 1798 this.conclusionCode = new ArrayList<CodeableConcept>(); 1799 this.conclusionCode.add(t); 1800 return this; 1801 } 1802 1803 /** 1804 * @return The first repetition of repeating field {@link #conclusionCode}, 1805 * creating it if it does not already exist 1806 */ 1807 public CodeableConcept getConclusionCodeFirstRep() { 1808 if (getConclusionCode().isEmpty()) { 1809 addConclusionCode(); 1810 } 1811 return getConclusionCode().get(0); 1812 } 1813 1814 /** 1815 * @return {@link #presentedForm} (Rich text representation of the entire result 1816 * as issued by the diagnostic service. Multiple formats are allowed but 1817 * they SHALL be semantically equivalent.) 1818 */ 1819 public List<Attachment> getPresentedForm() { 1820 if (this.presentedForm == null) 1821 this.presentedForm = new ArrayList<Attachment>(); 1822 return this.presentedForm; 1823 } 1824 1825 /** 1826 * @return Returns a reference to <code>this</code> for easy method chaining 1827 */ 1828 public DiagnosticReport setPresentedForm(List<Attachment> thePresentedForm) { 1829 this.presentedForm = thePresentedForm; 1830 return this; 1831 } 1832 1833 public boolean hasPresentedForm() { 1834 if (this.presentedForm == null) 1835 return false; 1836 for (Attachment item : this.presentedForm) 1837 if (!item.isEmpty()) 1838 return true; 1839 return false; 1840 } 1841 1842 public Attachment addPresentedForm() { // 3 1843 Attachment t = new Attachment(); 1844 if (this.presentedForm == null) 1845 this.presentedForm = new ArrayList<Attachment>(); 1846 this.presentedForm.add(t); 1847 return t; 1848 } 1849 1850 public DiagnosticReport addPresentedForm(Attachment t) { // 3 1851 if (t == null) 1852 return this; 1853 if (this.presentedForm == null) 1854 this.presentedForm = new ArrayList<Attachment>(); 1855 this.presentedForm.add(t); 1856 return this; 1857 } 1858 1859 /** 1860 * @return The first repetition of repeating field {@link #presentedForm}, 1861 * creating it if it does not already exist 1862 */ 1863 public Attachment getPresentedFormFirstRep() { 1864 if (getPresentedForm().isEmpty()) { 1865 addPresentedForm(); 1866 } 1867 return getPresentedForm().get(0); 1868 } 1869 1870 protected void listChildren(List<Property> children) { 1871 super.listChildren(children); 1872 children.add(new Property("identifier", "Identifier", 1873 "Identifiers assigned to this report by the performer or other systems.", 0, java.lang.Integer.MAX_VALUE, 1874 identifier)); 1875 children.add(new Property("basedOn", 1876 "Reference(CarePlan|ImmunizationRecommendation|MedicationRequest|NutritionOrder|ServiceRequest)", 1877 "Details concerning a service requested.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1878 children.add(new Property("status", "code", "The status of the diagnostic report.", 0, 1, status)); 1879 children.add(new Property("category", "CodeableConcept", 1880 "A code that classifies the clinical discipline, department or diagnostic service that created the report (e.g. cardiology, biochemistry, hematology, MRI). This is used for searching, sorting and display purposes.", 1881 0, java.lang.Integer.MAX_VALUE, category)); 1882 children.add( 1883 new Property("code", "CodeableConcept", "A code or name that describes this diagnostic report.", 0, 1, code)); 1884 children.add(new Property("subject", "Reference(Patient|Group|Device|Location)", 1885 "The subject of the report. Usually, but not always, this is a patient. However, diagnostic services also perform analyses on specimens collected from a variety of other sources.", 1886 0, 1, subject)); 1887 children.add(new Property("encounter", "Reference(Encounter)", 1888 "The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport is about.", 1889 0, 1, encounter)); 1890 children.add(new Property("effective[x]", "dateTime|Period", 1891 "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.", 1892 0, 1, effective)); 1893 children.add(new Property("issued", "instant", 1894 "The date and time that this version of the report was made available to providers, typically after the report was reviewed and verified.", 1895 0, 1, issued)); 1896 children.add(new Property("performer", "Reference(Practitioner|PractitionerRole|Organization|CareTeam)", 1897 "The diagnostic service that is responsible for issuing the report.", 0, java.lang.Integer.MAX_VALUE, 1898 performer)); 1899 children.add(new Property("resultsInterpreter", "Reference(Practitioner|PractitionerRole|Organization|CareTeam)", 1900 "The practitioner or organization that is responsible for the report's conclusions and interpretations.", 0, 1901 java.lang.Integer.MAX_VALUE, resultsInterpreter)); 1902 children.add(new Property("specimen", "Reference(Specimen)", 1903 "Details about the specimens on which this diagnostic report is based.", 0, java.lang.Integer.MAX_VALUE, 1904 specimen)); 1905 children.add(new Property("result", "Reference(Observation)", 1906 "[Observations](observation.html) that are part of this diagnostic report.", 0, java.lang.Integer.MAX_VALUE, 1907 result)); 1908 children.add(new Property("imagingStudy", "Reference(ImagingStudy)", 1909 "One or more links to full details of any imaging performed during the diagnostic investigation. Typically, this is imaging performed by DICOM enabled modalities, but this is not required. A fully enabled PACS viewer can use this information to provide views of the source images.", 1910 0, java.lang.Integer.MAX_VALUE, imagingStudy)); 1911 children.add(new Property("media", "", 1912 "A list of key images associated with this report. The images are generally created during the diagnostic process, and may be directly of the patient, or of treated specimens (i.e. slides of interest).", 1913 0, java.lang.Integer.MAX_VALUE, media)); 1914 children.add(new Property("conclusion", "string", 1915 "Concise and clinically contextualized summary conclusion (interpretation/impression) of the diagnostic report.", 1916 0, 1, conclusion)); 1917 children.add(new Property("conclusionCode", "CodeableConcept", 1918 "One or more codes that represent the summary conclusion (interpretation/impression) of the diagnostic report.", 1919 0, java.lang.Integer.MAX_VALUE, conclusionCode)); 1920 children.add(new Property("presentedForm", "Attachment", 1921 "Rich text representation of the entire result as issued by the diagnostic service. Multiple formats are allowed but they SHALL be semantically equivalent.", 1922 0, java.lang.Integer.MAX_VALUE, presentedForm)); 1923 } 1924 1925 @Override 1926 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1927 switch (_hash) { 1928 case -1618432855: 1929 /* identifier */ return new Property("identifier", "Identifier", 1930 "Identifiers assigned to this report by the performer or other systems.", 0, java.lang.Integer.MAX_VALUE, 1931 identifier); 1932 case -332612366: 1933 /* basedOn */ return new Property("basedOn", 1934 "Reference(CarePlan|ImmunizationRecommendation|MedicationRequest|NutritionOrder|ServiceRequest)", 1935 "Details concerning a service requested.", 0, java.lang.Integer.MAX_VALUE, basedOn); 1936 case -892481550: 1937 /* status */ return new Property("status", "code", "The status of the diagnostic report.", 0, 1, status); 1938 case 50511102: 1939 /* category */ return new Property("category", "CodeableConcept", 1940 "A code that classifies the clinical discipline, department or diagnostic service that created the report (e.g. cardiology, biochemistry, hematology, MRI). This is used for searching, sorting and display purposes.", 1941 0, java.lang.Integer.MAX_VALUE, category); 1942 case 3059181: 1943 /* code */ return new Property("code", "CodeableConcept", "A code or name that describes this diagnostic report.", 1944 0, 1, code); 1945 case -1867885268: 1946 /* subject */ return new Property("subject", "Reference(Patient|Group|Device|Location)", 1947 "The subject of the report. Usually, but not always, this is a patient. However, diagnostic services also perform analyses on specimens collected from a variety of other sources.", 1948 0, 1, subject); 1949 case 1524132147: 1950 /* encounter */ return new Property("encounter", "Reference(Encounter)", 1951 "The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport is about.", 1952 0, 1, encounter); 1953 case 247104889: 1954 /* effective[x] */ return new Property("effective[x]", "dateTime|Period", 1955 "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.", 1956 0, 1, effective); 1957 case -1468651097: 1958 /* effective */ return new Property("effective[x]", "dateTime|Period", 1959 "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.", 1960 0, 1, effective); 1961 case -275306910: 1962 /* effectiveDateTime */ return new Property("effective[x]", "dateTime|Period", 1963 "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.", 1964 0, 1, effective); 1965 case -403934648: 1966 /* effectivePeriod */ return new Property("effective[x]", "dateTime|Period", 1967 "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.", 1968 0, 1, effective); 1969 case -1179159893: 1970 /* issued */ return new Property("issued", "instant", 1971 "The date and time that this version of the report was made available to providers, typically after the report was reviewed and verified.", 1972 0, 1, issued); 1973 case 481140686: 1974 /* performer */ return new Property("performer", "Reference(Practitioner|PractitionerRole|Organization|CareTeam)", 1975 "The diagnostic service that is responsible for issuing the report.", 0, java.lang.Integer.MAX_VALUE, 1976 performer); 1977 case 2134944932: 1978 /* resultsInterpreter */ return new Property("resultsInterpreter", 1979 "Reference(Practitioner|PractitionerRole|Organization|CareTeam)", 1980 "The practitioner or organization that is responsible for the report's conclusions and interpretations.", 0, 1981 java.lang.Integer.MAX_VALUE, resultsInterpreter); 1982 case -2132868344: 1983 /* specimen */ return new Property("specimen", "Reference(Specimen)", 1984 "Details about the specimens on which this diagnostic report is based.", 0, java.lang.Integer.MAX_VALUE, 1985 specimen); 1986 case -934426595: 1987 /* result */ return new Property("result", "Reference(Observation)", 1988 "[Observations](observation.html) that are part of this diagnostic report.", 0, java.lang.Integer.MAX_VALUE, 1989 result); 1990 case -814900911: 1991 /* imagingStudy */ return new Property("imagingStudy", "Reference(ImagingStudy)", 1992 "One or more links to full details of any imaging performed during the diagnostic investigation. Typically, this is imaging performed by DICOM enabled modalities, but this is not required. A fully enabled PACS viewer can use this information to provide views of the source images.", 1993 0, java.lang.Integer.MAX_VALUE, imagingStudy); 1994 case 103772132: 1995 /* media */ return new Property("media", "", 1996 "A list of key images associated with this report. The images are generally created during the diagnostic process, and may be directly of the patient, or of treated specimens (i.e. slides of interest).", 1997 0, java.lang.Integer.MAX_VALUE, media); 1998 case -1731259873: 1999 /* conclusion */ return new Property("conclusion", "string", 2000 "Concise and clinically contextualized summary conclusion (interpretation/impression) of the diagnostic report.", 2001 0, 1, conclusion); 2002 case -1731523412: 2003 /* conclusionCode */ return new Property("conclusionCode", "CodeableConcept", 2004 "One or more codes that represent the summary conclusion (interpretation/impression) of the diagnostic report.", 2005 0, java.lang.Integer.MAX_VALUE, conclusionCode); 2006 case 230090366: 2007 /* presentedForm */ return new Property("presentedForm", "Attachment", 2008 "Rich text representation of the entire result as issued by the diagnostic service. Multiple formats are allowed but they SHALL be semantically equivalent.", 2009 0, java.lang.Integer.MAX_VALUE, presentedForm); 2010 default: 2011 return super.getNamedProperty(_hash, _name, _checkValid); 2012 } 2013 2014 } 2015 2016 @Override 2017 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2018 switch (hash) { 2019 case -1618432855: 2020 /* identifier */ return this.identifier == null ? new Base[0] 2021 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2022 case -332612366: 2023 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2024 case -892481550: 2025 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<DiagnosticReportStatus> 2026 case 50511102: 2027 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2028 case 3059181: 2029 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 2030 case -1867885268: 2031 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2032 case 1524132147: 2033 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 2034 case -1468651097: 2035 /* effective */ return this.effective == null ? new Base[0] : new Base[] { this.effective }; // Type 2036 case -1179159893: 2037 /* issued */ return this.issued == null ? new Base[0] : new Base[] { this.issued }; // InstantType 2038 case 481140686: 2039 /* performer */ return this.performer == null ? new Base[0] 2040 : this.performer.toArray(new Base[this.performer.size()]); // Reference 2041 case 2134944932: 2042 /* resultsInterpreter */ return this.resultsInterpreter == null ? new Base[0] 2043 : this.resultsInterpreter.toArray(new Base[this.resultsInterpreter.size()]); // Reference 2044 case -2132868344: 2045 /* specimen */ return this.specimen == null ? new Base[0] : this.specimen.toArray(new Base[this.specimen.size()]); // Reference 2046 case -934426595: 2047 /* result */ return this.result == null ? new Base[0] : this.result.toArray(new Base[this.result.size()]); // Reference 2048 case -814900911: 2049 /* imagingStudy */ return this.imagingStudy == null ? new Base[0] 2050 : this.imagingStudy.toArray(new Base[this.imagingStudy.size()]); // Reference 2051 case 103772132: 2052 /* media */ return this.media == null ? new Base[0] : this.media.toArray(new Base[this.media.size()]); // DiagnosticReportMediaComponent 2053 case -1731259873: 2054 /* conclusion */ return this.conclusion == null ? new Base[0] : new Base[] { this.conclusion }; // StringType 2055 case -1731523412: 2056 /* conclusionCode */ return this.conclusionCode == null ? new Base[0] 2057 : this.conclusionCode.toArray(new Base[this.conclusionCode.size()]); // CodeableConcept 2058 case 230090366: 2059 /* presentedForm */ return this.presentedForm == null ? new Base[0] 2060 : this.presentedForm.toArray(new Base[this.presentedForm.size()]); // Attachment 2061 default: 2062 return super.getProperty(hash, name, checkValid); 2063 } 2064 2065 } 2066 2067 @Override 2068 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2069 switch (hash) { 2070 case -1618432855: // identifier 2071 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2072 return value; 2073 case -332612366: // basedOn 2074 this.getBasedOn().add(castToReference(value)); // Reference 2075 return value; 2076 case -892481550: // status 2077 value = new DiagnosticReportStatusEnumFactory().fromType(castToCode(value)); 2078 this.status = (Enumeration) value; // Enumeration<DiagnosticReportStatus> 2079 return value; 2080 case 50511102: // category 2081 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 2082 return value; 2083 case 3059181: // code 2084 this.code = castToCodeableConcept(value); // CodeableConcept 2085 return value; 2086 case -1867885268: // subject 2087 this.subject = castToReference(value); // Reference 2088 return value; 2089 case 1524132147: // encounter 2090 this.encounter = castToReference(value); // Reference 2091 return value; 2092 case -1468651097: // effective 2093 this.effective = castToType(value); // Type 2094 return value; 2095 case -1179159893: // issued 2096 this.issued = castToInstant(value); // InstantType 2097 return value; 2098 case 481140686: // performer 2099 this.getPerformer().add(castToReference(value)); // Reference 2100 return value; 2101 case 2134944932: // resultsInterpreter 2102 this.getResultsInterpreter().add(castToReference(value)); // Reference 2103 return value; 2104 case -2132868344: // specimen 2105 this.getSpecimen().add(castToReference(value)); // Reference 2106 return value; 2107 case -934426595: // result 2108 this.getResult().add(castToReference(value)); // Reference 2109 return value; 2110 case -814900911: // imagingStudy 2111 this.getImagingStudy().add(castToReference(value)); // Reference 2112 return value; 2113 case 103772132: // media 2114 this.getMedia().add((DiagnosticReportMediaComponent) value); // DiagnosticReportMediaComponent 2115 return value; 2116 case -1731259873: // conclusion 2117 this.conclusion = castToString(value); // StringType 2118 return value; 2119 case -1731523412: // conclusionCode 2120 this.getConclusionCode().add(castToCodeableConcept(value)); // CodeableConcept 2121 return value; 2122 case 230090366: // presentedForm 2123 this.getPresentedForm().add(castToAttachment(value)); // Attachment 2124 return value; 2125 default: 2126 return super.setProperty(hash, name, value); 2127 } 2128 2129 } 2130 2131 @Override 2132 public Base setProperty(String name, Base value) throws FHIRException { 2133 if (name.equals("identifier")) { 2134 this.getIdentifier().add(castToIdentifier(value)); 2135 } else if (name.equals("basedOn")) { 2136 this.getBasedOn().add(castToReference(value)); 2137 } else if (name.equals("status")) { 2138 value = new DiagnosticReportStatusEnumFactory().fromType(castToCode(value)); 2139 this.status = (Enumeration) value; // Enumeration<DiagnosticReportStatus> 2140 } else if (name.equals("category")) { 2141 this.getCategory().add(castToCodeableConcept(value)); 2142 } else if (name.equals("code")) { 2143 this.code = castToCodeableConcept(value); // CodeableConcept 2144 } else if (name.equals("subject")) { 2145 this.subject = castToReference(value); // Reference 2146 } else if (name.equals("encounter")) { 2147 this.encounter = castToReference(value); // Reference 2148 } else if (name.equals("effective[x]")) { 2149 this.effective = castToType(value); // Type 2150 } else if (name.equals("issued")) { 2151 this.issued = castToInstant(value); // InstantType 2152 } else if (name.equals("performer")) { 2153 this.getPerformer().add(castToReference(value)); 2154 } else if (name.equals("resultsInterpreter")) { 2155 this.getResultsInterpreter().add(castToReference(value)); 2156 } else if (name.equals("specimen")) { 2157 this.getSpecimen().add(castToReference(value)); 2158 } else if (name.equals("result")) { 2159 this.getResult().add(castToReference(value)); 2160 } else if (name.equals("imagingStudy")) { 2161 this.getImagingStudy().add(castToReference(value)); 2162 } else if (name.equals("media")) { 2163 this.getMedia().add((DiagnosticReportMediaComponent) value); 2164 } else if (name.equals("conclusion")) { 2165 this.conclusion = castToString(value); // StringType 2166 } else if (name.equals("conclusionCode")) { 2167 this.getConclusionCode().add(castToCodeableConcept(value)); 2168 } else if (name.equals("presentedForm")) { 2169 this.getPresentedForm().add(castToAttachment(value)); 2170 } else 2171 return super.setProperty(name, value); 2172 return value; 2173 } 2174 2175 @Override 2176 public void removeChild(String name, Base value) throws FHIRException { 2177 if (name.equals("identifier")) { 2178 this.getIdentifier().remove(castToIdentifier(value)); 2179 } else if (name.equals("basedOn")) { 2180 this.getBasedOn().remove(castToReference(value)); 2181 } else if (name.equals("status")) { 2182 this.status = null; 2183 } else if (name.equals("category")) { 2184 this.getCategory().remove(castToCodeableConcept(value)); 2185 } else if (name.equals("code")) { 2186 this.code = null; 2187 } else if (name.equals("subject")) { 2188 this.subject = null; 2189 } else if (name.equals("encounter")) { 2190 this.encounter = null; 2191 } else if (name.equals("effective[x]")) { 2192 this.effective = null; 2193 } else if (name.equals("issued")) { 2194 this.issued = null; 2195 } else if (name.equals("performer")) { 2196 this.getPerformer().remove(castToReference(value)); 2197 } else if (name.equals("resultsInterpreter")) { 2198 this.getResultsInterpreter().remove(castToReference(value)); 2199 } else if (name.equals("specimen")) { 2200 this.getSpecimen().remove(castToReference(value)); 2201 } else if (name.equals("result")) { 2202 this.getResult().remove(castToReference(value)); 2203 } else if (name.equals("imagingStudy")) { 2204 this.getImagingStudy().remove(castToReference(value)); 2205 } else if (name.equals("media")) { 2206 this.getMedia().remove((DiagnosticReportMediaComponent) value); 2207 } else if (name.equals("conclusion")) { 2208 this.conclusion = null; 2209 } else if (name.equals("conclusionCode")) { 2210 this.getConclusionCode().remove(castToCodeableConcept(value)); 2211 } else if (name.equals("presentedForm")) { 2212 this.getPresentedForm().remove(castToAttachment(value)); 2213 } else 2214 super.removeChild(name, value); 2215 2216 } 2217 2218 @Override 2219 public Base makeProperty(int hash, String name) throws FHIRException { 2220 switch (hash) { 2221 case -1618432855: 2222 return addIdentifier(); 2223 case -332612366: 2224 return addBasedOn(); 2225 case -892481550: 2226 return getStatusElement(); 2227 case 50511102: 2228 return addCategory(); 2229 case 3059181: 2230 return getCode(); 2231 case -1867885268: 2232 return getSubject(); 2233 case 1524132147: 2234 return getEncounter(); 2235 case 247104889: 2236 return getEffective(); 2237 case -1468651097: 2238 return getEffective(); 2239 case -1179159893: 2240 return getIssuedElement(); 2241 case 481140686: 2242 return addPerformer(); 2243 case 2134944932: 2244 return addResultsInterpreter(); 2245 case -2132868344: 2246 return addSpecimen(); 2247 case -934426595: 2248 return addResult(); 2249 case -814900911: 2250 return addImagingStudy(); 2251 case 103772132: 2252 return addMedia(); 2253 case -1731259873: 2254 return getConclusionElement(); 2255 case -1731523412: 2256 return addConclusionCode(); 2257 case 230090366: 2258 return addPresentedForm(); 2259 default: 2260 return super.makeProperty(hash, name); 2261 } 2262 2263 } 2264 2265 @Override 2266 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2267 switch (hash) { 2268 case -1618432855: 2269 /* identifier */ return new String[] { "Identifier" }; 2270 case -332612366: 2271 /* basedOn */ return new String[] { "Reference" }; 2272 case -892481550: 2273 /* status */ return new String[] { "code" }; 2274 case 50511102: 2275 /* category */ return new String[] { "CodeableConcept" }; 2276 case 3059181: 2277 /* code */ return new String[] { "CodeableConcept" }; 2278 case -1867885268: 2279 /* subject */ return new String[] { "Reference" }; 2280 case 1524132147: 2281 /* encounter */ return new String[] { "Reference" }; 2282 case -1468651097: 2283 /* effective */ return new String[] { "dateTime", "Period" }; 2284 case -1179159893: 2285 /* issued */ return new String[] { "instant" }; 2286 case 481140686: 2287 /* performer */ return new String[] { "Reference" }; 2288 case 2134944932: 2289 /* resultsInterpreter */ return new String[] { "Reference" }; 2290 case -2132868344: 2291 /* specimen */ return new String[] { "Reference" }; 2292 case -934426595: 2293 /* result */ return new String[] { "Reference" }; 2294 case -814900911: 2295 /* imagingStudy */ return new String[] { "Reference" }; 2296 case 103772132: 2297 /* media */ return new String[] {}; 2298 case -1731259873: 2299 /* conclusion */ return new String[] { "string" }; 2300 case -1731523412: 2301 /* conclusionCode */ return new String[] { "CodeableConcept" }; 2302 case 230090366: 2303 /* presentedForm */ return new String[] { "Attachment" }; 2304 default: 2305 return super.getTypesForProperty(hash, name); 2306 } 2307 2308 } 2309 2310 @Override 2311 public Base addChild(String name) throws FHIRException { 2312 if (name.equals("identifier")) { 2313 return addIdentifier(); 2314 } else if (name.equals("basedOn")) { 2315 return addBasedOn(); 2316 } else if (name.equals("status")) { 2317 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticReport.status"); 2318 } else if (name.equals("category")) { 2319 return addCategory(); 2320 } else if (name.equals("code")) { 2321 this.code = new CodeableConcept(); 2322 return this.code; 2323 } else if (name.equals("subject")) { 2324 this.subject = new Reference(); 2325 return this.subject; 2326 } else if (name.equals("encounter")) { 2327 this.encounter = new Reference(); 2328 return this.encounter; 2329 } else if (name.equals("effectiveDateTime")) { 2330 this.effective = new DateTimeType(); 2331 return this.effective; 2332 } else if (name.equals("effectivePeriod")) { 2333 this.effective = new Period(); 2334 return this.effective; 2335 } else if (name.equals("issued")) { 2336 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticReport.issued"); 2337 } else if (name.equals("performer")) { 2338 return addPerformer(); 2339 } else if (name.equals("resultsInterpreter")) { 2340 return addResultsInterpreter(); 2341 } else if (name.equals("specimen")) { 2342 return addSpecimen(); 2343 } else if (name.equals("result")) { 2344 return addResult(); 2345 } else if (name.equals("imagingStudy")) { 2346 return addImagingStudy(); 2347 } else if (name.equals("media")) { 2348 return addMedia(); 2349 } else if (name.equals("conclusion")) { 2350 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticReport.conclusion"); 2351 } else if (name.equals("conclusionCode")) { 2352 return addConclusionCode(); 2353 } else if (name.equals("presentedForm")) { 2354 return addPresentedForm(); 2355 } else 2356 return super.addChild(name); 2357 } 2358 2359 public String fhirType() { 2360 return "DiagnosticReport"; 2361 2362 } 2363 2364 public DiagnosticReport copy() { 2365 DiagnosticReport dst = new DiagnosticReport(); 2366 copyValues(dst); 2367 return dst; 2368 } 2369 2370 public void copyValues(DiagnosticReport dst) { 2371 super.copyValues(dst); 2372 if (identifier != null) { 2373 dst.identifier = new ArrayList<Identifier>(); 2374 for (Identifier i : identifier) 2375 dst.identifier.add(i.copy()); 2376 } 2377 ; 2378 if (basedOn != null) { 2379 dst.basedOn = new ArrayList<Reference>(); 2380 for (Reference i : basedOn) 2381 dst.basedOn.add(i.copy()); 2382 } 2383 ; 2384 dst.status = status == null ? null : status.copy(); 2385 if (category != null) { 2386 dst.category = new ArrayList<CodeableConcept>(); 2387 for (CodeableConcept i : category) 2388 dst.category.add(i.copy()); 2389 } 2390 ; 2391 dst.code = code == null ? null : code.copy(); 2392 dst.subject = subject == null ? null : subject.copy(); 2393 dst.encounter = encounter == null ? null : encounter.copy(); 2394 dst.effective = effective == null ? null : effective.copy(); 2395 dst.issued = issued == null ? null : issued.copy(); 2396 if (performer != null) { 2397 dst.performer = new ArrayList<Reference>(); 2398 for (Reference i : performer) 2399 dst.performer.add(i.copy()); 2400 } 2401 ; 2402 if (resultsInterpreter != null) { 2403 dst.resultsInterpreter = new ArrayList<Reference>(); 2404 for (Reference i : resultsInterpreter) 2405 dst.resultsInterpreter.add(i.copy()); 2406 } 2407 ; 2408 if (specimen != null) { 2409 dst.specimen = new ArrayList<Reference>(); 2410 for (Reference i : specimen) 2411 dst.specimen.add(i.copy()); 2412 } 2413 ; 2414 if (result != null) { 2415 dst.result = new ArrayList<Reference>(); 2416 for (Reference i : result) 2417 dst.result.add(i.copy()); 2418 } 2419 ; 2420 if (imagingStudy != null) { 2421 dst.imagingStudy = new ArrayList<Reference>(); 2422 for (Reference i : imagingStudy) 2423 dst.imagingStudy.add(i.copy()); 2424 } 2425 ; 2426 if (media != null) { 2427 dst.media = new ArrayList<DiagnosticReportMediaComponent>(); 2428 for (DiagnosticReportMediaComponent i : media) 2429 dst.media.add(i.copy()); 2430 } 2431 ; 2432 dst.conclusion = conclusion == null ? null : conclusion.copy(); 2433 if (conclusionCode != null) { 2434 dst.conclusionCode = new ArrayList<CodeableConcept>(); 2435 for (CodeableConcept i : conclusionCode) 2436 dst.conclusionCode.add(i.copy()); 2437 } 2438 ; 2439 if (presentedForm != null) { 2440 dst.presentedForm = new ArrayList<Attachment>(); 2441 for (Attachment i : presentedForm) 2442 dst.presentedForm.add(i.copy()); 2443 } 2444 ; 2445 } 2446 2447 protected DiagnosticReport typedCopy() { 2448 return copy(); 2449 } 2450 2451 @Override 2452 public boolean equalsDeep(Base other_) { 2453 if (!super.equalsDeep(other_)) 2454 return false; 2455 if (!(other_ instanceof DiagnosticReport)) 2456 return false; 2457 DiagnosticReport o = (DiagnosticReport) other_; 2458 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) 2459 && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) 2460 && compareDeep(code, o.code, true) && compareDeep(subject, o.subject, true) 2461 && compareDeep(encounter, o.encounter, true) && compareDeep(effective, o.effective, true) 2462 && compareDeep(issued, o.issued, true) && compareDeep(performer, o.performer, true) 2463 && compareDeep(resultsInterpreter, o.resultsInterpreter, true) && compareDeep(specimen, o.specimen, true) 2464 && compareDeep(result, o.result, true) && compareDeep(imagingStudy, o.imagingStudy, true) 2465 && compareDeep(media, o.media, true) && compareDeep(conclusion, o.conclusion, true) 2466 && compareDeep(conclusionCode, o.conclusionCode, true) && compareDeep(presentedForm, o.presentedForm, true); 2467 } 2468 2469 @Override 2470 public boolean equalsShallow(Base other_) { 2471 if (!super.equalsShallow(other_)) 2472 return false; 2473 if (!(other_ instanceof DiagnosticReport)) 2474 return false; 2475 DiagnosticReport o = (DiagnosticReport) other_; 2476 return compareValues(status, o.status, true) && compareValues(issued, o.issued, true) 2477 && compareValues(conclusion, o.conclusion, true); 2478 } 2479 2480 public boolean isEmpty() { 2481 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, status, category, code, subject, 2482 encounter, effective, issued, performer, resultsInterpreter, specimen, result, imagingStudy, media, conclusion, 2483 conclusionCode, presentedForm); 2484 } 2485 2486 @Override 2487 public ResourceType getResourceType() { 2488 return ResourceType.DiagnosticReport; 2489 } 2490 2491 /** 2492 * Search parameter: <b>date</b> 2493 * <p> 2494 * Description: <b>The clinically relevant time of the report</b><br> 2495 * Type: <b>date</b><br> 2496 * Path: <b>DiagnosticReport.effective[x]</b><br> 2497 * </p> 2498 */ 2499 @SearchParamDefinition(name = "date", path = "DiagnosticReport.effective", description = "The clinically relevant time of the report", type = "date") 2500 public static final String SP_DATE = "date"; 2501 /** 2502 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2503 * <p> 2504 * Description: <b>The clinically relevant time of the report</b><br> 2505 * Type: <b>date</b><br> 2506 * Path: <b>DiagnosticReport.effective[x]</b><br> 2507 * </p> 2508 */ 2509 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2510 SP_DATE); 2511 2512 /** 2513 * Search parameter: <b>identifier</b> 2514 * <p> 2515 * Description: <b>An identifier for the report</b><br> 2516 * Type: <b>token</b><br> 2517 * Path: <b>DiagnosticReport.identifier</b><br> 2518 * </p> 2519 */ 2520 @SearchParamDefinition(name = "identifier", path = "DiagnosticReport.identifier", description = "An identifier for the report", type = "token") 2521 public static final String SP_IDENTIFIER = "identifier"; 2522 /** 2523 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2524 * <p> 2525 * Description: <b>An identifier for the report</b><br> 2526 * Type: <b>token</b><br> 2527 * Path: <b>DiagnosticReport.identifier</b><br> 2528 * </p> 2529 */ 2530 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2531 SP_IDENTIFIER); 2532 2533 /** 2534 * Search parameter: <b>performer</b> 2535 * <p> 2536 * Description: <b>Who is responsible for the report</b><br> 2537 * Type: <b>reference</b><br> 2538 * Path: <b>DiagnosticReport.performer</b><br> 2539 * </p> 2540 */ 2541 @SearchParamDefinition(name = "performer", path = "DiagnosticReport.performer", description = "Who is responsible for the report", type = "reference", providesMembershipIn = { 2542 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { CareTeam.class, 2543 Organization.class, Practitioner.class, PractitionerRole.class }) 2544 public static final String SP_PERFORMER = "performer"; 2545 /** 2546 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 2547 * <p> 2548 * Description: <b>Who is responsible for the report</b><br> 2549 * Type: <b>reference</b><br> 2550 * Path: <b>DiagnosticReport.performer</b><br> 2551 * </p> 2552 */ 2553 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2554 SP_PERFORMER); 2555 2556 /** 2557 * Constant for fluent queries to be used to add include statements. Specifies 2558 * the path value of "<b>DiagnosticReport:performer</b>". 2559 */ 2560 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include( 2561 "DiagnosticReport:performer").toLocked(); 2562 2563 /** 2564 * Search parameter: <b>code</b> 2565 * <p> 2566 * Description: <b>The code for the report, as opposed to codes for the atomic 2567 * results, which are the names on the observation resource referred to from the 2568 * result</b><br> 2569 * Type: <b>token</b><br> 2570 * Path: <b>DiagnosticReport.code</b><br> 2571 * </p> 2572 */ 2573 @SearchParamDefinition(name = "code", path = "DiagnosticReport.code", description = "The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result", type = "token") 2574 public static final String SP_CODE = "code"; 2575 /** 2576 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2577 * <p> 2578 * Description: <b>The code for the report, as opposed to codes for the atomic 2579 * results, which are the names on the observation resource referred to from the 2580 * result</b><br> 2581 * Type: <b>token</b><br> 2582 * Path: <b>DiagnosticReport.code</b><br> 2583 * </p> 2584 */ 2585 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2586 SP_CODE); 2587 2588 /** 2589 * Search parameter: <b>subject</b> 2590 * <p> 2591 * Description: <b>The subject of the report</b><br> 2592 * Type: <b>reference</b><br> 2593 * Path: <b>DiagnosticReport.subject</b><br> 2594 * </p> 2595 */ 2596 @SearchParamDefinition(name = "subject", path = "DiagnosticReport.subject", description = "The subject of the report", type = "reference", providesMembershipIn = { 2597 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 2598 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Device.class, Group.class, 2599 Location.class, Patient.class }) 2600 public static final String SP_SUBJECT = "subject"; 2601 /** 2602 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2603 * <p> 2604 * Description: <b>The subject of the report</b><br> 2605 * Type: <b>reference</b><br> 2606 * Path: <b>DiagnosticReport.subject</b><br> 2607 * </p> 2608 */ 2609 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2610 SP_SUBJECT); 2611 2612 /** 2613 * Constant for fluent queries to be used to add include statements. Specifies 2614 * the path value of "<b>DiagnosticReport:subject</b>". 2615 */ 2616 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 2617 "DiagnosticReport:subject").toLocked(); 2618 2619 /** 2620 * Search parameter: <b>media</b> 2621 * <p> 2622 * Description: <b>A reference to the image source.</b><br> 2623 * Type: <b>reference</b><br> 2624 * Path: <b>DiagnosticReport.media.link</b><br> 2625 * </p> 2626 */ 2627 @SearchParamDefinition(name = "media", path = "DiagnosticReport.media.link", description = "A reference to the image source.", type = "reference", target = { 2628 Media.class }) 2629 public static final String SP_MEDIA = "media"; 2630 /** 2631 * <b>Fluent Client</b> search parameter constant for <b>media</b> 2632 * <p> 2633 * Description: <b>A reference to the image source.</b><br> 2634 * Type: <b>reference</b><br> 2635 * Path: <b>DiagnosticReport.media.link</b><br> 2636 * </p> 2637 */ 2638 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEDIA = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2639 SP_MEDIA); 2640 2641 /** 2642 * Constant for fluent queries to be used to add include statements. Specifies 2643 * the path value of "<b>DiagnosticReport:media</b>". 2644 */ 2645 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEDIA = new ca.uhn.fhir.model.api.Include( 2646 "DiagnosticReport:media").toLocked(); 2647 2648 /** 2649 * Search parameter: <b>encounter</b> 2650 * <p> 2651 * Description: <b>The Encounter when the order was made</b><br> 2652 * Type: <b>reference</b><br> 2653 * Path: <b>DiagnosticReport.encounter</b><br> 2654 * </p> 2655 */ 2656 @SearchParamDefinition(name = "encounter", path = "DiagnosticReport.encounter", description = "The Encounter when the order was made", type = "reference", providesMembershipIn = { 2657 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 2658 public static final String SP_ENCOUNTER = "encounter"; 2659 /** 2660 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2661 * <p> 2662 * Description: <b>The Encounter when the order was made</b><br> 2663 * Type: <b>reference</b><br> 2664 * Path: <b>DiagnosticReport.encounter</b><br> 2665 * </p> 2666 */ 2667 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2668 SP_ENCOUNTER); 2669 2670 /** 2671 * Constant for fluent queries to be used to add include statements. Specifies 2672 * the path value of "<b>DiagnosticReport:encounter</b>". 2673 */ 2674 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 2675 "DiagnosticReport:encounter").toLocked(); 2676 2677 /** 2678 * Search parameter: <b>result</b> 2679 * <p> 2680 * Description: <b>Link to an atomic result (observation resource)</b><br> 2681 * Type: <b>reference</b><br> 2682 * Path: <b>DiagnosticReport.result</b><br> 2683 * </p> 2684 */ 2685 @SearchParamDefinition(name = "result", path = "DiagnosticReport.result", description = "Link to an atomic result (observation resource)", type = "reference", target = { 2686 Observation.class }) 2687 public static final String SP_RESULT = "result"; 2688 /** 2689 * <b>Fluent Client</b> search parameter constant for <b>result</b> 2690 * <p> 2691 * Description: <b>Link to an atomic result (observation resource)</b><br> 2692 * Type: <b>reference</b><br> 2693 * Path: <b>DiagnosticReport.result</b><br> 2694 * </p> 2695 */ 2696 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESULT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2697 SP_RESULT); 2698 2699 /** 2700 * Constant for fluent queries to be used to add include statements. Specifies 2701 * the path value of "<b>DiagnosticReport:result</b>". 2702 */ 2703 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESULT = new ca.uhn.fhir.model.api.Include( 2704 "DiagnosticReport:result").toLocked(); 2705 2706 /** 2707 * Search parameter: <b>conclusion</b> 2708 * <p> 2709 * Description: <b>A coded conclusion (interpretation/impression) on the 2710 * report</b><br> 2711 * Type: <b>token</b><br> 2712 * Path: <b>DiagnosticReport.conclusionCode</b><br> 2713 * </p> 2714 */ 2715 @SearchParamDefinition(name = "conclusion", path = "DiagnosticReport.conclusionCode", description = "A coded conclusion (interpretation/impression) on the report", type = "token") 2716 public static final String SP_CONCLUSION = "conclusion"; 2717 /** 2718 * <b>Fluent Client</b> search parameter constant for <b>conclusion</b> 2719 * <p> 2720 * Description: <b>A coded conclusion (interpretation/impression) on the 2721 * report</b><br> 2722 * Type: <b>token</b><br> 2723 * Path: <b>DiagnosticReport.conclusionCode</b><br> 2724 * </p> 2725 */ 2726 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONCLUSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2727 SP_CONCLUSION); 2728 2729 /** 2730 * Search parameter: <b>based-on</b> 2731 * <p> 2732 * Description: <b>Reference to the service request.</b><br> 2733 * Type: <b>reference</b><br> 2734 * Path: <b>DiagnosticReport.basedOn</b><br> 2735 * </p> 2736 */ 2737 @SearchParamDefinition(name = "based-on", path = "DiagnosticReport.basedOn", description = "Reference to the service request.", type = "reference", target = { 2738 CarePlan.class, ImmunizationRecommendation.class, MedicationRequest.class, NutritionOrder.class, 2739 ServiceRequest.class }) 2740 public static final String SP_BASED_ON = "based-on"; 2741 /** 2742 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 2743 * <p> 2744 * Description: <b>Reference to the service request.</b><br> 2745 * Type: <b>reference</b><br> 2746 * Path: <b>DiagnosticReport.basedOn</b><br> 2747 * </p> 2748 */ 2749 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2750 SP_BASED_ON); 2751 2752 /** 2753 * Constant for fluent queries to be used to add include statements. Specifies 2754 * the path value of "<b>DiagnosticReport:based-on</b>". 2755 */ 2756 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include( 2757 "DiagnosticReport:based-on").toLocked(); 2758 2759 /** 2760 * Search parameter: <b>patient</b> 2761 * <p> 2762 * Description: <b>The subject of the report if a patient</b><br> 2763 * Type: <b>reference</b><br> 2764 * Path: <b>DiagnosticReport.subject</b><br> 2765 * </p> 2766 */ 2767 @SearchParamDefinition(name = "patient", path = "DiagnosticReport.subject.where(resolve() is Patient)", description = "The subject of the report if a patient", type = "reference", target = { 2768 Patient.class }) 2769 public static final String SP_PATIENT = "patient"; 2770 /** 2771 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2772 * <p> 2773 * Description: <b>The subject of the report if a patient</b><br> 2774 * Type: <b>reference</b><br> 2775 * Path: <b>DiagnosticReport.subject</b><br> 2776 * </p> 2777 */ 2778 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2779 SP_PATIENT); 2780 2781 /** 2782 * Constant for fluent queries to be used to add include statements. Specifies 2783 * the path value of "<b>DiagnosticReport:patient</b>". 2784 */ 2785 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 2786 "DiagnosticReport:patient").toLocked(); 2787 2788 /** 2789 * Search parameter: <b>specimen</b> 2790 * <p> 2791 * Description: <b>The specimen details</b><br> 2792 * Type: <b>reference</b><br> 2793 * Path: <b>DiagnosticReport.specimen</b><br> 2794 * </p> 2795 */ 2796 @SearchParamDefinition(name = "specimen", path = "DiagnosticReport.specimen", description = "The specimen details", type = "reference", target = { 2797 Specimen.class }) 2798 public static final String SP_SPECIMEN = "specimen"; 2799 /** 2800 * <b>Fluent Client</b> search parameter constant for <b>specimen</b> 2801 * <p> 2802 * Description: <b>The specimen details</b><br> 2803 * Type: <b>reference</b><br> 2804 * Path: <b>DiagnosticReport.specimen</b><br> 2805 * </p> 2806 */ 2807 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SPECIMEN = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2808 SP_SPECIMEN); 2809 2810 /** 2811 * Constant for fluent queries to be used to add include statements. Specifies 2812 * the path value of "<b>DiagnosticReport:specimen</b>". 2813 */ 2814 public static final ca.uhn.fhir.model.api.Include INCLUDE_SPECIMEN = new ca.uhn.fhir.model.api.Include( 2815 "DiagnosticReport:specimen").toLocked(); 2816 2817 /** 2818 * Search parameter: <b>issued</b> 2819 * <p> 2820 * Description: <b>When the report was issued</b><br> 2821 * Type: <b>date</b><br> 2822 * Path: <b>DiagnosticReport.issued</b><br> 2823 * </p> 2824 */ 2825 @SearchParamDefinition(name = "issued", path = "DiagnosticReport.issued", description = "When the report was issued", type = "date") 2826 public static final String SP_ISSUED = "issued"; 2827 /** 2828 * <b>Fluent Client</b> search parameter constant for <b>issued</b> 2829 * <p> 2830 * Description: <b>When the report was issued</b><br> 2831 * Type: <b>date</b><br> 2832 * Path: <b>DiagnosticReport.issued</b><br> 2833 * </p> 2834 */ 2835 public static final ca.uhn.fhir.rest.gclient.DateClientParam ISSUED = new ca.uhn.fhir.rest.gclient.DateClientParam( 2836 SP_ISSUED); 2837 2838 /** 2839 * Search parameter: <b>category</b> 2840 * <p> 2841 * Description: <b>Which diagnostic discipline/department created the 2842 * report</b><br> 2843 * Type: <b>token</b><br> 2844 * Path: <b>DiagnosticReport.category</b><br> 2845 * </p> 2846 */ 2847 @SearchParamDefinition(name = "category", path = "DiagnosticReport.category", description = "Which diagnostic discipline/department created the report", type = "token") 2848 public static final String SP_CATEGORY = "category"; 2849 /** 2850 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2851 * <p> 2852 * Description: <b>Which diagnostic discipline/department created the 2853 * report</b><br> 2854 * Type: <b>token</b><br> 2855 * Path: <b>DiagnosticReport.category</b><br> 2856 * </p> 2857 */ 2858 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2859 SP_CATEGORY); 2860 2861 /** 2862 * Search parameter: <b>results-interpreter</b> 2863 * <p> 2864 * Description: <b>Who was the source of the report</b><br> 2865 * Type: <b>reference</b><br> 2866 * Path: <b>DiagnosticReport.resultsInterpreter</b><br> 2867 * </p> 2868 */ 2869 @SearchParamDefinition(name = "results-interpreter", path = "DiagnosticReport.resultsInterpreter", description = "Who was the source of the report", type = "reference", target = { 2870 CareTeam.class, Organization.class, Practitioner.class, PractitionerRole.class }) 2871 public static final String SP_RESULTS_INTERPRETER = "results-interpreter"; 2872 /** 2873 * <b>Fluent Client</b> search parameter constant for <b>results-interpreter</b> 2874 * <p> 2875 * Description: <b>Who was the source of the report</b><br> 2876 * Type: <b>reference</b><br> 2877 * Path: <b>DiagnosticReport.resultsInterpreter</b><br> 2878 * </p> 2879 */ 2880 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESULTS_INTERPRETER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2881 SP_RESULTS_INTERPRETER); 2882 2883 /** 2884 * Constant for fluent queries to be used to add include statements. Specifies 2885 * the path value of "<b>DiagnosticReport:results-interpreter</b>". 2886 */ 2887 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESULTS_INTERPRETER = new ca.uhn.fhir.model.api.Include( 2888 "DiagnosticReport:results-interpreter").toLocked(); 2889 2890 /** 2891 * Search parameter: <b>status</b> 2892 * <p> 2893 * Description: <b>The status of the report</b><br> 2894 * Type: <b>token</b><br> 2895 * Path: <b>DiagnosticReport.status</b><br> 2896 * </p> 2897 */ 2898 @SearchParamDefinition(name = "status", path = "DiagnosticReport.status", description = "The status of the report", type = "token") 2899 public static final String SP_STATUS = "status"; 2900 /** 2901 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2902 * <p> 2903 * Description: <b>The status of the report</b><br> 2904 * Type: <b>token</b><br> 2905 * Path: <b>DiagnosticReport.status</b><br> 2906 * </p> 2907 */ 2908 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2909 SP_STATUS); 2910 2911}