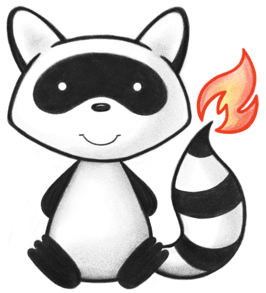
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * The findings and interpretation of diagnostic tests performed on patients, 049 * groups of patients, devices, and locations, and/or specimens derived from 050 * these. The report includes clinical context such as requesting and provider 051 * information, and some mix of atomic results, images, textual and coded 052 * interpretations, and formatted representation of diagnostic reports. 053 */ 054@ResourceDef(name = "DiagnosticReport", profile = "http://hl7.org/fhir/StructureDefinition/DiagnosticReport") 055public class DiagnosticReport extends DomainResource { 056 057 public enum DiagnosticReportStatus { 058 /** 059 * The existence of the report is registered, but there is nothing yet 060 * available. 061 */ 062 REGISTERED, 063 /** 064 * This is a partial (e.g. initial, interim or preliminary) report: data in the 065 * report may be incomplete or unverified. 066 */ 067 PARTIAL, 068 /** 069 * Verified early results are available, but not all results are final. 070 */ 071 PRELIMINARY, 072 /** 073 * The report is complete and verified by an authorized person. 074 */ 075 FINAL, 076 /** 077 * Subsequent to being final, the report has been modified. This includes any 078 * change in the results, diagnosis, narrative text, or other content of a 079 * report that has been issued. 080 */ 081 AMENDED, 082 /** 083 * Subsequent to being final, the report has been modified to correct an error 084 * in the report or referenced results. 085 */ 086 CORRECTED, 087 /** 088 * Subsequent to being final, the report has been modified by adding new 089 * content. The existing content is unchanged. 090 */ 091 APPENDED, 092 /** 093 * The report is unavailable because the measurement was not started or not 094 * completed (also sometimes called "aborted"). 095 */ 096 CANCELLED, 097 /** 098 * The report has been withdrawn following a previous final release. This 099 * electronic record should never have existed, though it is possible that 100 * real-world decisions were based on it. (If real-world activity has occurred, 101 * the status should be "cancelled" rather than "entered-in-error".). 102 */ 103 ENTEREDINERROR, 104 /** 105 * The authoring/source system does not know which of the status values 106 * currently applies for this observation. Note: This concept is not to be used 107 * for "other" - one of the listed statuses is presumed to apply, but the 108 * authoring/source system does not know which. 109 */ 110 UNKNOWN, 111 /** 112 * added to help the parsers with the generic types 113 */ 114 NULL; 115 116 public static DiagnosticReportStatus fromCode(String codeString) throws FHIRException { 117 if (codeString == null || "".equals(codeString)) 118 return null; 119 if ("registered".equals(codeString)) 120 return REGISTERED; 121 if ("partial".equals(codeString)) 122 return PARTIAL; 123 if ("preliminary".equals(codeString)) 124 return PRELIMINARY; 125 if ("final".equals(codeString)) 126 return FINAL; 127 if ("amended".equals(codeString)) 128 return AMENDED; 129 if ("corrected".equals(codeString)) 130 return CORRECTED; 131 if ("appended".equals(codeString)) 132 return APPENDED; 133 if ("cancelled".equals(codeString)) 134 return CANCELLED; 135 if ("entered-in-error".equals(codeString)) 136 return ENTEREDINERROR; 137 if ("unknown".equals(codeString)) 138 return UNKNOWN; 139 if (Configuration.isAcceptInvalidEnums()) 140 return null; 141 else 142 throw new FHIRException("Unknown DiagnosticReportStatus code '" + codeString + "'"); 143 } 144 145 public String toCode() { 146 switch (this) { 147 case REGISTERED: 148 return "registered"; 149 case PARTIAL: 150 return "partial"; 151 case PRELIMINARY: 152 return "preliminary"; 153 case FINAL: 154 return "final"; 155 case AMENDED: 156 return "amended"; 157 case CORRECTED: 158 return "corrected"; 159 case APPENDED: 160 return "appended"; 161 case CANCELLED: 162 return "cancelled"; 163 case ENTEREDINERROR: 164 return "entered-in-error"; 165 case UNKNOWN: 166 return "unknown"; 167 case NULL: 168 return null; 169 default: 170 return "?"; 171 } 172 } 173 174 public String getSystem() { 175 switch (this) { 176 case REGISTERED: 177 return "http://hl7.org/fhir/diagnostic-report-status"; 178 case PARTIAL: 179 return "http://hl7.org/fhir/diagnostic-report-status"; 180 case PRELIMINARY: 181 return "http://hl7.org/fhir/diagnostic-report-status"; 182 case FINAL: 183 return "http://hl7.org/fhir/diagnostic-report-status"; 184 case AMENDED: 185 return "http://hl7.org/fhir/diagnostic-report-status"; 186 case CORRECTED: 187 return "http://hl7.org/fhir/diagnostic-report-status"; 188 case APPENDED: 189 return "http://hl7.org/fhir/diagnostic-report-status"; 190 case CANCELLED: 191 return "http://hl7.org/fhir/diagnostic-report-status"; 192 case ENTEREDINERROR: 193 return "http://hl7.org/fhir/diagnostic-report-status"; 194 case UNKNOWN: 195 return "http://hl7.org/fhir/diagnostic-report-status"; 196 case NULL: 197 return null; 198 default: 199 return "?"; 200 } 201 } 202 203 public String getDefinition() { 204 switch (this) { 205 case REGISTERED: 206 return "The existence of the report is registered, but there is nothing yet available."; 207 case PARTIAL: 208 return "This is a partial (e.g. initial, interim or preliminary) report: data in the report may be incomplete or unverified."; 209 case PRELIMINARY: 210 return "Verified early results are available, but not all results are final."; 211 case FINAL: 212 return "The report is complete and verified by an authorized person."; 213 case AMENDED: 214 return "Subsequent to being final, the report has been modified. This includes any change in the results, diagnosis, narrative text, or other content of a report that has been issued."; 215 case CORRECTED: 216 return "Subsequent to being final, the report has been modified to correct an error in the report or referenced results."; 217 case APPENDED: 218 return "Subsequent to being final, the report has been modified by adding new content. The existing content is unchanged."; 219 case CANCELLED: 220 return "The report is unavailable because the measurement was not started or not completed (also sometimes called \"aborted\")."; 221 case ENTEREDINERROR: 222 return "The report has been withdrawn following a previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 223 case UNKNOWN: 224 return "The authoring/source system does not know which of the status values currently applies for this observation. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 225 case NULL: 226 return null; 227 default: 228 return "?"; 229 } 230 } 231 232 public String getDisplay() { 233 switch (this) { 234 case REGISTERED: 235 return "Registered"; 236 case PARTIAL: 237 return "Partial"; 238 case PRELIMINARY: 239 return "Preliminary"; 240 case FINAL: 241 return "Final"; 242 case AMENDED: 243 return "Amended"; 244 case CORRECTED: 245 return "Corrected"; 246 case APPENDED: 247 return "Appended"; 248 case CANCELLED: 249 return "Cancelled"; 250 case ENTEREDINERROR: 251 return "Entered in Error"; 252 case UNKNOWN: 253 return "Unknown"; 254 case NULL: 255 return null; 256 default: 257 return "?"; 258 } 259 } 260 } 261 262 public static class DiagnosticReportStatusEnumFactory implements EnumFactory<DiagnosticReportStatus> { 263 public DiagnosticReportStatus fromCode(String codeString) throws IllegalArgumentException { 264 if (codeString == null || "".equals(codeString)) 265 if (codeString == null || "".equals(codeString)) 266 return null; 267 if ("registered".equals(codeString)) 268 return DiagnosticReportStatus.REGISTERED; 269 if ("partial".equals(codeString)) 270 return DiagnosticReportStatus.PARTIAL; 271 if ("preliminary".equals(codeString)) 272 return DiagnosticReportStatus.PRELIMINARY; 273 if ("final".equals(codeString)) 274 return DiagnosticReportStatus.FINAL; 275 if ("amended".equals(codeString)) 276 return DiagnosticReportStatus.AMENDED; 277 if ("corrected".equals(codeString)) 278 return DiagnosticReportStatus.CORRECTED; 279 if ("appended".equals(codeString)) 280 return DiagnosticReportStatus.APPENDED; 281 if ("cancelled".equals(codeString)) 282 return DiagnosticReportStatus.CANCELLED; 283 if ("entered-in-error".equals(codeString)) 284 return DiagnosticReportStatus.ENTEREDINERROR; 285 if ("unknown".equals(codeString)) 286 return DiagnosticReportStatus.UNKNOWN; 287 throw new IllegalArgumentException("Unknown DiagnosticReportStatus code '" + codeString + "'"); 288 } 289 290 public Enumeration<DiagnosticReportStatus> fromType(PrimitiveType<?> code) throws FHIRException { 291 if (code == null) 292 return null; 293 if (code.isEmpty()) 294 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.NULL, code); 295 String codeString = code.asStringValue(); 296 if (codeString == null || "".equals(codeString)) 297 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.NULL, code); 298 if ("registered".equals(codeString)) 299 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.REGISTERED, code); 300 if ("partial".equals(codeString)) 301 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.PARTIAL, code); 302 if ("preliminary".equals(codeString)) 303 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.PRELIMINARY, code); 304 if ("final".equals(codeString)) 305 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.FINAL, code); 306 if ("amended".equals(codeString)) 307 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.AMENDED, code); 308 if ("corrected".equals(codeString)) 309 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.CORRECTED, code); 310 if ("appended".equals(codeString)) 311 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.APPENDED, code); 312 if ("cancelled".equals(codeString)) 313 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.CANCELLED, code); 314 if ("entered-in-error".equals(codeString)) 315 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.ENTEREDINERROR, code); 316 if ("unknown".equals(codeString)) 317 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.UNKNOWN, code); 318 throw new FHIRException("Unknown DiagnosticReportStatus code '" + codeString + "'"); 319 } 320 321 public String toCode(DiagnosticReportStatus code) { 322 if (code == DiagnosticReportStatus.NULL) 323 return null; 324 if (code == DiagnosticReportStatus.REGISTERED) 325 return "registered"; 326 if (code == DiagnosticReportStatus.PARTIAL) 327 return "partial"; 328 if (code == DiagnosticReportStatus.PRELIMINARY) 329 return "preliminary"; 330 if (code == DiagnosticReportStatus.FINAL) 331 return "final"; 332 if (code == DiagnosticReportStatus.AMENDED) 333 return "amended"; 334 if (code == DiagnosticReportStatus.CORRECTED) 335 return "corrected"; 336 if (code == DiagnosticReportStatus.APPENDED) 337 return "appended"; 338 if (code == DiagnosticReportStatus.CANCELLED) 339 return "cancelled"; 340 if (code == DiagnosticReportStatus.ENTEREDINERROR) 341 return "entered-in-error"; 342 if (code == DiagnosticReportStatus.UNKNOWN) 343 return "unknown"; 344 return "?"; 345 } 346 347 public String toSystem(DiagnosticReportStatus code) { 348 return code.getSystem(); 349 } 350 } 351 352 @Block() 353 public static class DiagnosticReportMediaComponent extends BackboneElement implements IBaseBackboneElement { 354 /** 355 * A comment about the image. Typically, this is used to provide an explanation 356 * for why the image is included, or to draw the viewer's attention to important 357 * features. 358 */ 359 @Child(name = "comment", type = { 360 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 361 @Description(shortDefinition = "Comment about the image (e.g. explanation)", formalDefinition = "A comment about the image. Typically, this is used to provide an explanation for why the image is included, or to draw the viewer's attention to important features.") 362 protected StringType comment; 363 364 /** 365 * Reference to the image source. 366 */ 367 @Child(name = "link", type = { Media.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 368 @Description(shortDefinition = "Reference to the image source", formalDefinition = "Reference to the image source.") 369 protected Reference link; 370 371 /** 372 * The actual object that is the target of the reference (Reference to the image 373 * source.) 374 */ 375 protected Media linkTarget; 376 377 private static final long serialVersionUID = 935791940L; 378 379 /** 380 * Constructor 381 */ 382 public DiagnosticReportMediaComponent() { 383 super(); 384 } 385 386 /** 387 * Constructor 388 */ 389 public DiagnosticReportMediaComponent(Reference link) { 390 super(); 391 this.link = link; 392 } 393 394 /** 395 * @return {@link #comment} (A comment about the image. Typically, this is used 396 * to provide an explanation for why the image is included, or to draw 397 * the viewer's attention to important features.). This is the 398 * underlying object with id, value and extensions. The accessor 399 * "getComment" gives direct access to the value 400 */ 401 public StringType getCommentElement() { 402 if (this.comment == null) 403 if (Configuration.errorOnAutoCreate()) 404 throw new Error("Attempt to auto-create DiagnosticReportMediaComponent.comment"); 405 else if (Configuration.doAutoCreate()) 406 this.comment = new StringType(); // bb 407 return this.comment; 408 } 409 410 public boolean hasCommentElement() { 411 return this.comment != null && !this.comment.isEmpty(); 412 } 413 414 public boolean hasComment() { 415 return this.comment != null && !this.comment.isEmpty(); 416 } 417 418 /** 419 * @param value {@link #comment} (A comment about the image. Typically, this is 420 * used to provide an explanation for why the image is included, or 421 * to draw the viewer's attention to important features.). This is 422 * the underlying object with id, value and extensions. The 423 * accessor "getComment" gives direct access to the value 424 */ 425 public DiagnosticReportMediaComponent setCommentElement(StringType value) { 426 this.comment = value; 427 return this; 428 } 429 430 /** 431 * @return A comment about the image. Typically, this is used to provide an 432 * explanation for why the image is included, or to draw the viewer's 433 * attention to important features. 434 */ 435 public String getComment() { 436 return this.comment == null ? null : this.comment.getValue(); 437 } 438 439 /** 440 * @param value A comment about the image. Typically, this is used to provide an 441 * explanation for why the image is included, or to draw the 442 * viewer's attention to important features. 443 */ 444 public DiagnosticReportMediaComponent setComment(String value) { 445 if (Utilities.noString(value)) 446 this.comment = null; 447 else { 448 if (this.comment == null) 449 this.comment = new StringType(); 450 this.comment.setValue(value); 451 } 452 return this; 453 } 454 455 /** 456 * @return {@link #link} (Reference to the image source.) 457 */ 458 public Reference getLink() { 459 if (this.link == null) 460 if (Configuration.errorOnAutoCreate()) 461 throw new Error("Attempt to auto-create DiagnosticReportMediaComponent.link"); 462 else if (Configuration.doAutoCreate()) 463 this.link = new Reference(); // cc 464 return this.link; 465 } 466 467 public boolean hasLink() { 468 return this.link != null && !this.link.isEmpty(); 469 } 470 471 /** 472 * @param value {@link #link} (Reference to the image source.) 473 */ 474 public DiagnosticReportMediaComponent setLink(Reference value) { 475 this.link = value; 476 return this; 477 } 478 479 /** 480 * @return {@link #link} The actual object that is the target of the reference. 481 * The reference library doesn't populate this, but you can use it to 482 * hold the resource if you resolve it. (Reference to the image source.) 483 */ 484 public Media getLinkTarget() { 485 if (this.linkTarget == null) 486 if (Configuration.errorOnAutoCreate()) 487 throw new Error("Attempt to auto-create DiagnosticReportMediaComponent.link"); 488 else if (Configuration.doAutoCreate()) 489 this.linkTarget = new Media(); // aa 490 return this.linkTarget; 491 } 492 493 /** 494 * @param value {@link #link} The actual object that is the target of the 495 * reference. The reference library doesn't use these, but you can 496 * use it to hold the resource if you resolve it. (Reference to the 497 * image source.) 498 */ 499 public DiagnosticReportMediaComponent setLinkTarget(Media value) { 500 this.linkTarget = value; 501 return this; 502 } 503 504 protected void listChildren(List<Property> children) { 505 super.listChildren(children); 506 children.add(new Property("comment", "string", 507 "A comment about the image. Typically, this is used to provide an explanation for why the image is included, or to draw the viewer's attention to important features.", 508 0, 1, comment)); 509 children.add(new Property("link", "Reference(Media)", "Reference to the image source.", 0, 1, link)); 510 } 511 512 @Override 513 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 514 switch (_hash) { 515 case 950398559: 516 /* comment */ return new Property("comment", "string", 517 "A comment about the image. Typically, this is used to provide an explanation for why the image is included, or to draw the viewer's attention to important features.", 518 0, 1, comment); 519 case 3321850: 520 /* link */ return new Property("link", "Reference(Media)", "Reference to the image source.", 0, 1, link); 521 default: 522 return super.getNamedProperty(_hash, _name, _checkValid); 523 } 524 525 } 526 527 @Override 528 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 529 switch (hash) { 530 case 950398559: 531 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // StringType 532 case 3321850: 533 /* link */ return this.link == null ? new Base[0] : new Base[] { this.link }; // Reference 534 default: 535 return super.getProperty(hash, name, checkValid); 536 } 537 538 } 539 540 @Override 541 public Base setProperty(int hash, String name, Base value) throws FHIRException { 542 switch (hash) { 543 case 950398559: // comment 544 this.comment = castToString(value); // StringType 545 return value; 546 case 3321850: // link 547 this.link = castToReference(value); // Reference 548 return value; 549 default: 550 return super.setProperty(hash, name, value); 551 } 552 553 } 554 555 @Override 556 public Base setProperty(String name, Base value) throws FHIRException { 557 if (name.equals("comment")) { 558 this.comment = castToString(value); // StringType 559 } else if (name.equals("link")) { 560 this.link = castToReference(value); // Reference 561 } else 562 return super.setProperty(name, value); 563 return value; 564 } 565 566 @Override 567 public void removeChild(String name, Base value) throws FHIRException { 568 if (name.equals("comment")) { 569 this.comment = null; 570 } else if (name.equals("link")) { 571 this.link = null; 572 } else 573 super.removeChild(name, value); 574 575 } 576 577 @Override 578 public Base makeProperty(int hash, String name) throws FHIRException { 579 switch (hash) { 580 case 950398559: 581 return getCommentElement(); 582 case 3321850: 583 return getLink(); 584 default: 585 return super.makeProperty(hash, name); 586 } 587 588 } 589 590 @Override 591 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 592 switch (hash) { 593 case 950398559: 594 /* comment */ return new String[] { "string" }; 595 case 3321850: 596 /* link */ return new String[] { "Reference" }; 597 default: 598 return super.getTypesForProperty(hash, name); 599 } 600 601 } 602 603 @Override 604 public Base addChild(String name) throws FHIRException { 605 if (name.equals("comment")) { 606 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticReport.comment"); 607 } else if (name.equals("link")) { 608 this.link = new Reference(); 609 return this.link; 610 } else 611 return super.addChild(name); 612 } 613 614 public DiagnosticReportMediaComponent copy() { 615 DiagnosticReportMediaComponent dst = new DiagnosticReportMediaComponent(); 616 copyValues(dst); 617 return dst; 618 } 619 620 public void copyValues(DiagnosticReportMediaComponent dst) { 621 super.copyValues(dst); 622 dst.comment = comment == null ? null : comment.copy(); 623 dst.link = link == null ? null : link.copy(); 624 } 625 626 @Override 627 public boolean equalsDeep(Base other_) { 628 if (!super.equalsDeep(other_)) 629 return false; 630 if (!(other_ instanceof DiagnosticReportMediaComponent)) 631 return false; 632 DiagnosticReportMediaComponent o = (DiagnosticReportMediaComponent) other_; 633 return compareDeep(comment, o.comment, true) && compareDeep(link, o.link, true); 634 } 635 636 @Override 637 public boolean equalsShallow(Base other_) { 638 if (!super.equalsShallow(other_)) 639 return false; 640 if (!(other_ instanceof DiagnosticReportMediaComponent)) 641 return false; 642 DiagnosticReportMediaComponent o = (DiagnosticReportMediaComponent) other_; 643 return compareValues(comment, o.comment, true); 644 } 645 646 public boolean isEmpty() { 647 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(comment, link); 648 } 649 650 public String fhirType() { 651 return "DiagnosticReport.media"; 652 653 } 654 655 } 656 657 /** 658 * Identifiers assigned to this report by the performer or other systems. 659 */ 660 @Child(name = "identifier", type = { 661 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 662 @Description(shortDefinition = "Business identifier for report", formalDefinition = "Identifiers assigned to this report by the performer or other systems.") 663 protected List<Identifier> identifier; 664 665 /** 666 * Details concerning a service requested. 667 */ 668 @Child(name = "basedOn", type = { CarePlan.class, ImmunizationRecommendation.class, MedicationRequest.class, 669 NutritionOrder.class, 670 ServiceRequest.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 671 @Description(shortDefinition = "What was requested", formalDefinition = "Details concerning a service requested.") 672 protected List<Reference> basedOn; 673 /** 674 * The actual objects that are the target of the reference (Details concerning a 675 * service requested.) 676 */ 677 protected List<Resource> basedOnTarget; 678 679 /** 680 * The status of the diagnostic report. 681 */ 682 @Child(name = "status", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = true, summary = true) 683 @Description(shortDefinition = "registered | partial | preliminary | final +", formalDefinition = "The status of the diagnostic report.") 684 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/diagnostic-report-status") 685 protected Enumeration<DiagnosticReportStatus> status; 686 687 /** 688 * A code that classifies the clinical discipline, department or diagnostic 689 * service that created the report (e.g. cardiology, biochemistry, hematology, 690 * MRI). This is used for searching, sorting and display purposes. 691 */ 692 @Child(name = "category", type = { 693 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 694 @Description(shortDefinition = "Service category", formalDefinition = "A code that classifies the clinical discipline, department or diagnostic service that created the report (e.g. cardiology, biochemistry, hematology, MRI). This is used for searching, sorting and display purposes.") 695 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/diagnostic-service-sections") 696 protected List<CodeableConcept> category; 697 698 /** 699 * A code or name that describes this diagnostic report. 700 */ 701 @Child(name = "code", type = { CodeableConcept.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 702 @Description(shortDefinition = "Name/Code for this diagnostic report", formalDefinition = "A code or name that describes this diagnostic report.") 703 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/report-codes") 704 protected CodeableConcept code; 705 706 /** 707 * The subject of the report. Usually, but not always, this is a patient. 708 * However, diagnostic services also perform analyses on specimens collected 709 * from a variety of other sources. 710 */ 711 @Child(name = "subject", type = { Patient.class, Group.class, Device.class, 712 Location.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 713 @Description(shortDefinition = "The subject of the report - usually, but not always, the patient", formalDefinition = "The subject of the report. Usually, but not always, this is a patient. However, diagnostic services also perform analyses on specimens collected from a variety of other sources.") 714 protected Reference subject; 715 716 /** 717 * The actual object that is the target of the reference (The subject of the 718 * report. Usually, but not always, this is a patient. However, diagnostic 719 * services also perform analyses on specimens collected from a variety of other 720 * sources.) 721 */ 722 protected Resource subjectTarget; 723 724 /** 725 * The healthcare event (e.g. a patient and healthcare provider interaction) 726 * which this DiagnosticReport is about. 727 */ 728 @Child(name = "encounter", type = { Encounter.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 729 @Description(shortDefinition = "Health care event when test ordered", formalDefinition = "The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport is about.") 730 protected Reference encounter; 731 732 /** 733 * The actual object that is the target of the reference (The healthcare event 734 * (e.g. a patient and healthcare provider interaction) which this 735 * DiagnosticReport is about.) 736 */ 737 protected Encounter encounterTarget; 738 739 /** 740 * The time or time-period the observed values are related to. When the subject 741 * of the report is a patient, this is usually either the time of the procedure 742 * or of specimen collection(s), but very often the source of the date/time is 743 * not known, only the date/time itself. 744 */ 745 @Child(name = "effective", type = { DateTimeType.class, 746 Period.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 747 @Description(shortDefinition = "Clinically relevant time/time-period for report", formalDefinition = "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.") 748 protected Type effective; 749 750 /** 751 * The date and time that this version of the report was made available to 752 * providers, typically after the report was reviewed and verified. 753 */ 754 @Child(name = "issued", type = { InstantType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 755 @Description(shortDefinition = "DateTime this version was made", formalDefinition = "The date and time that this version of the report was made available to providers, typically after the report was reviewed and verified.") 756 protected InstantType issued; 757 758 /** 759 * The diagnostic service that is responsible for issuing the report. 760 */ 761 @Child(name = "performer", type = { Practitioner.class, PractitionerRole.class, Organization.class, 762 CareTeam.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 763 @Description(shortDefinition = "Responsible Diagnostic Service", formalDefinition = "The diagnostic service that is responsible for issuing the report.") 764 protected List<Reference> performer; 765 /** 766 * The actual objects that are the target of the reference (The diagnostic 767 * service that is responsible for issuing the report.) 768 */ 769 protected List<Resource> performerTarget; 770 771 /** 772 * The practitioner or organization that is responsible for the report's 773 * conclusions and interpretations. 774 */ 775 @Child(name = "resultsInterpreter", type = { Practitioner.class, PractitionerRole.class, Organization.class, 776 CareTeam.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 777 @Description(shortDefinition = "Primary result interpreter", formalDefinition = "The practitioner or organization that is responsible for the report's conclusions and interpretations.") 778 protected List<Reference> resultsInterpreter; 779 /** 780 * The actual objects that are the target of the reference (The practitioner or 781 * organization that is responsible for the report's conclusions and 782 * interpretations.) 783 */ 784 protected List<Resource> resultsInterpreterTarget; 785 786 /** 787 * Details about the specimens on which this diagnostic report is based. 788 */ 789 @Child(name = "specimen", type = { 790 Specimen.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 791 @Description(shortDefinition = "Specimens this report is based on", formalDefinition = "Details about the specimens on which this diagnostic report is based.") 792 protected List<Reference> specimen; 793 /** 794 * The actual objects that are the target of the reference (Details about the 795 * specimens on which this diagnostic report is based.) 796 */ 797 protected List<Specimen> specimenTarget; 798 799 /** 800 * [Observations](observation.html) that are part of this diagnostic report. 801 */ 802 @Child(name = "result", type = { 803 Observation.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 804 @Description(shortDefinition = "Observations", formalDefinition = "[Observations](observation.html) that are part of this diagnostic report.") 805 protected List<Reference> result; 806 /** 807 * The actual objects that are the target of the reference 808 * ([Observations](observation.html) that are part of this diagnostic report.) 809 */ 810 protected List<Observation> resultTarget; 811 812 /** 813 * One or more links to full details of any imaging performed during the 814 * diagnostic investigation. Typically, this is imaging performed by DICOM 815 * enabled modalities, but this is not required. A fully enabled PACS viewer can 816 * use this information to provide views of the source images. 817 */ 818 @Child(name = "imagingStudy", type = { 819 ImagingStudy.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 820 @Description(shortDefinition = "Reference to full details of imaging associated with the diagnostic report", formalDefinition = "One or more links to full details of any imaging performed during the diagnostic investigation. Typically, this is imaging performed by DICOM enabled modalities, but this is not required. A fully enabled PACS viewer can use this information to provide views of the source images.") 821 protected List<Reference> imagingStudy; 822 /** 823 * The actual objects that are the target of the reference (One or more links to 824 * full details of any imaging performed during the diagnostic investigation. 825 * Typically, this is imaging performed by DICOM enabled modalities, but this is 826 * not required. A fully enabled PACS viewer can use this information to provide 827 * views of the source images.) 828 */ 829 protected List<ImagingStudy> imagingStudyTarget; 830 831 /** 832 * A list of key images associated with this report. The images are generally 833 * created during the diagnostic process, and may be directly of the patient, or 834 * of treated specimens (i.e. slides of interest). 835 */ 836 @Child(name = "media", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 837 @Description(shortDefinition = "Key images associated with this report", formalDefinition = "A list of key images associated with this report. The images are generally created during the diagnostic process, and may be directly of the patient, or of treated specimens (i.e. slides of interest).") 838 protected List<DiagnosticReportMediaComponent> media; 839 840 /** 841 * Concise and clinically contextualized summary conclusion 842 * (interpretation/impression) of the diagnostic report. 843 */ 844 @Child(name = "conclusion", type = { 845 StringType.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 846 @Description(shortDefinition = "Clinical conclusion (interpretation) of test results", formalDefinition = "Concise and clinically contextualized summary conclusion (interpretation/impression) of the diagnostic report.") 847 protected StringType conclusion; 848 849 /** 850 * One or more codes that represent the summary conclusion 851 * (interpretation/impression) of the diagnostic report. 852 */ 853 @Child(name = "conclusionCode", type = { 854 CodeableConcept.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 855 @Description(shortDefinition = "Codes for the clinical conclusion of test results", formalDefinition = "One or more codes that represent the summary conclusion (interpretation/impression) of the diagnostic report.") 856 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/clinical-findings") 857 protected List<CodeableConcept> conclusionCode; 858 859 /** 860 * Rich text representation of the entire result as issued by the diagnostic 861 * service. Multiple formats are allowed but they SHALL be semantically 862 * equivalent. 863 */ 864 @Child(name = "presentedForm", type = { 865 Attachment.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 866 @Description(shortDefinition = "Entire report as issued", formalDefinition = "Rich text representation of the entire result as issued by the diagnostic service. Multiple formats are allowed but they SHALL be semantically equivalent.") 867 protected List<Attachment> presentedForm; 868 869 private static final long serialVersionUID = 589102296L; 870 871 /** 872 * Constructor 873 */ 874 public DiagnosticReport() { 875 super(); 876 } 877 878 /** 879 * Constructor 880 */ 881 public DiagnosticReport(Enumeration<DiagnosticReportStatus> status, CodeableConcept code) { 882 super(); 883 this.status = status; 884 this.code = code; 885 } 886 887 /** 888 * @return {@link #identifier} (Identifiers assigned to this report by the 889 * performer or other systems.) 890 */ 891 public List<Identifier> getIdentifier() { 892 if (this.identifier == null) 893 this.identifier = new ArrayList<Identifier>(); 894 return this.identifier; 895 } 896 897 /** 898 * @return Returns a reference to <code>this</code> for easy method chaining 899 */ 900 public DiagnosticReport setIdentifier(List<Identifier> theIdentifier) { 901 this.identifier = theIdentifier; 902 return this; 903 } 904 905 public boolean hasIdentifier() { 906 if (this.identifier == null) 907 return false; 908 for (Identifier item : this.identifier) 909 if (!item.isEmpty()) 910 return true; 911 return false; 912 } 913 914 public Identifier addIdentifier() { // 3 915 Identifier t = new Identifier(); 916 if (this.identifier == null) 917 this.identifier = new ArrayList<Identifier>(); 918 this.identifier.add(t); 919 return t; 920 } 921 922 public DiagnosticReport addIdentifier(Identifier t) { // 3 923 if (t == null) 924 return this; 925 if (this.identifier == null) 926 this.identifier = new ArrayList<Identifier>(); 927 this.identifier.add(t); 928 return this; 929 } 930 931 /** 932 * @return The first repetition of repeating field {@link #identifier}, creating 933 * it if it does not already exist 934 */ 935 public Identifier getIdentifierFirstRep() { 936 if (getIdentifier().isEmpty()) { 937 addIdentifier(); 938 } 939 return getIdentifier().get(0); 940 } 941 942 /** 943 * @return {@link #basedOn} (Details concerning a service requested.) 944 */ 945 public List<Reference> getBasedOn() { 946 if (this.basedOn == null) 947 this.basedOn = new ArrayList<Reference>(); 948 return this.basedOn; 949 } 950 951 /** 952 * @return Returns a reference to <code>this</code> for easy method chaining 953 */ 954 public DiagnosticReport setBasedOn(List<Reference> theBasedOn) { 955 this.basedOn = theBasedOn; 956 return this; 957 } 958 959 public boolean hasBasedOn() { 960 if (this.basedOn == null) 961 return false; 962 for (Reference item : this.basedOn) 963 if (!item.isEmpty()) 964 return true; 965 return false; 966 } 967 968 public Reference addBasedOn() { // 3 969 Reference t = new Reference(); 970 if (this.basedOn == null) 971 this.basedOn = new ArrayList<Reference>(); 972 this.basedOn.add(t); 973 return t; 974 } 975 976 public DiagnosticReport addBasedOn(Reference t) { // 3 977 if (t == null) 978 return this; 979 if (this.basedOn == null) 980 this.basedOn = new ArrayList<Reference>(); 981 this.basedOn.add(t); 982 return this; 983 } 984 985 /** 986 * @return The first repetition of repeating field {@link #basedOn}, creating it 987 * if it does not already exist 988 */ 989 public Reference getBasedOnFirstRep() { 990 if (getBasedOn().isEmpty()) { 991 addBasedOn(); 992 } 993 return getBasedOn().get(0); 994 } 995 996 /** 997 * @deprecated Use Reference#setResource(IBaseResource) instead 998 */ 999 @Deprecated 1000 public List<Resource> getBasedOnTarget() { 1001 if (this.basedOnTarget == null) 1002 this.basedOnTarget = new ArrayList<Resource>(); 1003 return this.basedOnTarget; 1004 } 1005 1006 /** 1007 * @return {@link #status} (The status of the diagnostic report.). This is the 1008 * underlying object with id, value and extensions. The accessor 1009 * "getStatus" gives direct access to the value 1010 */ 1011 public Enumeration<DiagnosticReportStatus> getStatusElement() { 1012 if (this.status == null) 1013 if (Configuration.errorOnAutoCreate()) 1014 throw new Error("Attempt to auto-create DiagnosticReport.status"); 1015 else if (Configuration.doAutoCreate()) 1016 this.status = new Enumeration<DiagnosticReportStatus>(new DiagnosticReportStatusEnumFactory()); // bb 1017 return this.status; 1018 } 1019 1020 public boolean hasStatusElement() { 1021 return this.status != null && !this.status.isEmpty(); 1022 } 1023 1024 public boolean hasStatus() { 1025 return this.status != null && !this.status.isEmpty(); 1026 } 1027 1028 /** 1029 * @param value {@link #status} (The status of the diagnostic report.). This is 1030 * the underlying object with id, value and extensions. The 1031 * accessor "getStatus" gives direct access to the value 1032 */ 1033 public DiagnosticReport setStatusElement(Enumeration<DiagnosticReportStatus> value) { 1034 this.status = value; 1035 return this; 1036 } 1037 1038 /** 1039 * @return The status of the diagnostic report. 1040 */ 1041 public DiagnosticReportStatus getStatus() { 1042 return this.status == null ? null : this.status.getValue(); 1043 } 1044 1045 /** 1046 * @param value The status of the diagnostic report. 1047 */ 1048 public DiagnosticReport setStatus(DiagnosticReportStatus value) { 1049 if (this.status == null) 1050 this.status = new Enumeration<DiagnosticReportStatus>(new DiagnosticReportStatusEnumFactory()); 1051 this.status.setValue(value); 1052 return this; 1053 } 1054 1055 /** 1056 * @return {@link #category} (A code that classifies the clinical discipline, 1057 * department or diagnostic service that created the report (e.g. 1058 * cardiology, biochemistry, hematology, MRI). This is used for 1059 * searching, sorting and display purposes.) 1060 */ 1061 public List<CodeableConcept> getCategory() { 1062 if (this.category == null) 1063 this.category = new ArrayList<CodeableConcept>(); 1064 return this.category; 1065 } 1066 1067 /** 1068 * @return Returns a reference to <code>this</code> for easy method chaining 1069 */ 1070 public DiagnosticReport setCategory(List<CodeableConcept> theCategory) { 1071 this.category = theCategory; 1072 return this; 1073 } 1074 1075 public boolean hasCategory() { 1076 if (this.category == null) 1077 return false; 1078 for (CodeableConcept item : this.category) 1079 if (!item.isEmpty()) 1080 return true; 1081 return false; 1082 } 1083 1084 public CodeableConcept addCategory() { // 3 1085 CodeableConcept t = new CodeableConcept(); 1086 if (this.category == null) 1087 this.category = new ArrayList<CodeableConcept>(); 1088 this.category.add(t); 1089 return t; 1090 } 1091 1092 public DiagnosticReport addCategory(CodeableConcept t) { // 3 1093 if (t == null) 1094 return this; 1095 if (this.category == null) 1096 this.category = new ArrayList<CodeableConcept>(); 1097 this.category.add(t); 1098 return this; 1099 } 1100 1101 /** 1102 * @return The first repetition of repeating field {@link #category}, creating 1103 * it if it does not already exist 1104 */ 1105 public CodeableConcept getCategoryFirstRep() { 1106 if (getCategory().isEmpty()) { 1107 addCategory(); 1108 } 1109 return getCategory().get(0); 1110 } 1111 1112 /** 1113 * @return {@link #code} (A code or name that describes this diagnostic report.) 1114 */ 1115 public CodeableConcept getCode() { 1116 if (this.code == null) 1117 if (Configuration.errorOnAutoCreate()) 1118 throw new Error("Attempt to auto-create DiagnosticReport.code"); 1119 else if (Configuration.doAutoCreate()) 1120 this.code = new CodeableConcept(); // cc 1121 return this.code; 1122 } 1123 1124 public boolean hasCode() { 1125 return this.code != null && !this.code.isEmpty(); 1126 } 1127 1128 /** 1129 * @param value {@link #code} (A code or name that describes this diagnostic 1130 * report.) 1131 */ 1132 public DiagnosticReport setCode(CodeableConcept value) { 1133 this.code = value; 1134 return this; 1135 } 1136 1137 /** 1138 * @return {@link #subject} (The subject of the report. Usually, but not always, 1139 * this is a patient. However, diagnostic services also perform analyses 1140 * on specimens collected from a variety of other sources.) 1141 */ 1142 public Reference getSubject() { 1143 if (this.subject == null) 1144 if (Configuration.errorOnAutoCreate()) 1145 throw new Error("Attempt to auto-create DiagnosticReport.subject"); 1146 else if (Configuration.doAutoCreate()) 1147 this.subject = new Reference(); // cc 1148 return this.subject; 1149 } 1150 1151 public boolean hasSubject() { 1152 return this.subject != null && !this.subject.isEmpty(); 1153 } 1154 1155 /** 1156 * @param value {@link #subject} (The subject of the report. Usually, but not 1157 * always, this is a patient. However, diagnostic services also 1158 * perform analyses on specimens collected from a variety of other 1159 * sources.) 1160 */ 1161 public DiagnosticReport setSubject(Reference value) { 1162 this.subject = value; 1163 return this; 1164 } 1165 1166 /** 1167 * @return {@link #subject} The actual object that is the target of the 1168 * reference. The reference library doesn't populate this, but you can 1169 * use it to hold the resource if you resolve it. (The subject of the 1170 * report. Usually, but not always, this is a patient. However, 1171 * diagnostic services also perform analyses on specimens collected from 1172 * a variety of other sources.) 1173 */ 1174 public Resource getSubjectTarget() { 1175 return this.subjectTarget; 1176 } 1177 1178 /** 1179 * @param value {@link #subject} The actual object that is the target of the 1180 * reference. The reference library doesn't use these, but you can 1181 * use it to hold the resource if you resolve it. (The subject of 1182 * the report. Usually, but not always, this is a patient. However, 1183 * diagnostic services also perform analyses on specimens collected 1184 * from a variety of other sources.) 1185 */ 1186 public DiagnosticReport setSubjectTarget(Resource value) { 1187 this.subjectTarget = value; 1188 return this; 1189 } 1190 1191 /** 1192 * @return {@link #encounter} (The healthcare event (e.g. a patient and 1193 * healthcare provider interaction) which this DiagnosticReport is 1194 * about.) 1195 */ 1196 public Reference getEncounter() { 1197 if (this.encounter == null) 1198 if (Configuration.errorOnAutoCreate()) 1199 throw new Error("Attempt to auto-create DiagnosticReport.encounter"); 1200 else if (Configuration.doAutoCreate()) 1201 this.encounter = new Reference(); // cc 1202 return this.encounter; 1203 } 1204 1205 public boolean hasEncounter() { 1206 return this.encounter != null && !this.encounter.isEmpty(); 1207 } 1208 1209 /** 1210 * @param value {@link #encounter} (The healthcare event (e.g. a patient and 1211 * healthcare provider interaction) which this DiagnosticReport is 1212 * about.) 1213 */ 1214 public DiagnosticReport setEncounter(Reference value) { 1215 this.encounter = value; 1216 return this; 1217 } 1218 1219 /** 1220 * @return {@link #encounter} The actual object that is the target of the 1221 * reference. The reference library doesn't populate this, but you can 1222 * use it to hold the resource if you resolve it. (The healthcare event 1223 * (e.g. a patient and healthcare provider interaction) which this 1224 * DiagnosticReport is about.) 1225 */ 1226 public Encounter getEncounterTarget() { 1227 if (this.encounterTarget == null) 1228 if (Configuration.errorOnAutoCreate()) 1229 throw new Error("Attempt to auto-create DiagnosticReport.encounter"); 1230 else if (Configuration.doAutoCreate()) 1231 this.encounterTarget = new Encounter(); // aa 1232 return this.encounterTarget; 1233 } 1234 1235 /** 1236 * @param value {@link #encounter} The actual object that is the target of the 1237 * reference. The reference library doesn't use these, but you can 1238 * use it to hold the resource if you resolve it. (The healthcare 1239 * event (e.g. a patient and healthcare provider interaction) which 1240 * this DiagnosticReport is about.) 1241 */ 1242 public DiagnosticReport setEncounterTarget(Encounter value) { 1243 this.encounterTarget = value; 1244 return this; 1245 } 1246 1247 /** 1248 * @return {@link #effective} (The time or time-period the observed values are 1249 * related to. When the subject of the report is a patient, this is 1250 * usually either the time of the procedure or of specimen 1251 * collection(s), but very often the source of the date/time is not 1252 * known, only the date/time itself.) 1253 */ 1254 public Type getEffective() { 1255 return this.effective; 1256 } 1257 1258 /** 1259 * @return {@link #effective} (The time or time-period the observed values are 1260 * related to. When the subject of the report is a patient, this is 1261 * usually either the time of the procedure or of specimen 1262 * collection(s), but very often the source of the date/time is not 1263 * known, only the date/time itself.) 1264 */ 1265 public DateTimeType getEffectiveDateTimeType() throws FHIRException { 1266 if (this.effective == null) 1267 this.effective = new DateTimeType(); 1268 if (!(this.effective instanceof DateTimeType)) 1269 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1270 + this.effective.getClass().getName() + " was encountered"); 1271 return (DateTimeType) this.effective; 1272 } 1273 1274 public boolean hasEffectiveDateTimeType() { 1275 return this != null && this.effective instanceof DateTimeType; 1276 } 1277 1278 /** 1279 * @return {@link #effective} (The time or time-period the observed values are 1280 * related to. When the subject of the report is a patient, this is 1281 * usually either the time of the procedure or of specimen 1282 * collection(s), but very often the source of the date/time is not 1283 * known, only the date/time itself.) 1284 */ 1285 public Period getEffectivePeriod() throws FHIRException { 1286 if (this.effective == null) 1287 this.effective = new Period(); 1288 if (!(this.effective instanceof Period)) 1289 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.effective.getClass().getName() 1290 + " was encountered"); 1291 return (Period) this.effective; 1292 } 1293 1294 public boolean hasEffectivePeriod() { 1295 return this != null && this.effective instanceof Period; 1296 } 1297 1298 public boolean hasEffective() { 1299 return this.effective != null && !this.effective.isEmpty(); 1300 } 1301 1302 /** 1303 * @param value {@link #effective} (The time or time-period the observed values 1304 * are related to. When the subject of the report is a patient, 1305 * this is usually either the time of the procedure or of specimen 1306 * collection(s), but very often the source of the date/time is not 1307 * known, only the date/time itself.) 1308 */ 1309 public DiagnosticReport setEffective(Type value) { 1310 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1311 throw new Error("Not the right type for DiagnosticReport.effective[x]: " + value.fhirType()); 1312 this.effective = value; 1313 return this; 1314 } 1315 1316 /** 1317 * @return {@link #issued} (The date and time that this version of the report 1318 * was made available to providers, typically after the report was 1319 * reviewed and verified.). This is the underlying object with id, value 1320 * and extensions. The accessor "getIssued" gives direct access to the 1321 * value 1322 */ 1323 public InstantType getIssuedElement() { 1324 if (this.issued == null) 1325 if (Configuration.errorOnAutoCreate()) 1326 throw new Error("Attempt to auto-create DiagnosticReport.issued"); 1327 else if (Configuration.doAutoCreate()) 1328 this.issued = new InstantType(); // bb 1329 return this.issued; 1330 } 1331 1332 public boolean hasIssuedElement() { 1333 return this.issued != null && !this.issued.isEmpty(); 1334 } 1335 1336 public boolean hasIssued() { 1337 return this.issued != null && !this.issued.isEmpty(); 1338 } 1339 1340 /** 1341 * @param value {@link #issued} (The date and time that this version of the 1342 * report was made available to providers, typically after the 1343 * report was reviewed and verified.). This is the underlying 1344 * object with id, value and extensions. The accessor "getIssued" 1345 * gives direct access to the value 1346 */ 1347 public DiagnosticReport setIssuedElement(InstantType value) { 1348 this.issued = value; 1349 return this; 1350 } 1351 1352 /** 1353 * @return The date and time that this version of the report was made available 1354 * to providers, typically after the report was reviewed and verified. 1355 */ 1356 public Date getIssued() { 1357 return this.issued == null ? null : this.issued.getValue(); 1358 } 1359 1360 /** 1361 * @param value The date and time that this version of the report was made 1362 * available to providers, typically after the report was reviewed 1363 * and verified. 1364 */ 1365 public DiagnosticReport setIssued(Date value) { 1366 if (value == null) 1367 this.issued = null; 1368 else { 1369 if (this.issued == null) 1370 this.issued = new InstantType(); 1371 this.issued.setValue(value); 1372 } 1373 return this; 1374 } 1375 1376 /** 1377 * @return {@link #performer} (The diagnostic service that is responsible for 1378 * issuing the report.) 1379 */ 1380 public List<Reference> getPerformer() { 1381 if (this.performer == null) 1382 this.performer = new ArrayList<Reference>(); 1383 return this.performer; 1384 } 1385 1386 /** 1387 * @return Returns a reference to <code>this</code> for easy method chaining 1388 */ 1389 public DiagnosticReport setPerformer(List<Reference> thePerformer) { 1390 this.performer = thePerformer; 1391 return this; 1392 } 1393 1394 public boolean hasPerformer() { 1395 if (this.performer == null) 1396 return false; 1397 for (Reference item : this.performer) 1398 if (!item.isEmpty()) 1399 return true; 1400 return false; 1401 } 1402 1403 public Reference addPerformer() { // 3 1404 Reference t = new Reference(); 1405 if (this.performer == null) 1406 this.performer = new ArrayList<Reference>(); 1407 this.performer.add(t); 1408 return t; 1409 } 1410 1411 public DiagnosticReport addPerformer(Reference t) { // 3 1412 if (t == null) 1413 return this; 1414 if (this.performer == null) 1415 this.performer = new ArrayList<Reference>(); 1416 this.performer.add(t); 1417 return this; 1418 } 1419 1420 /** 1421 * @return The first repetition of repeating field {@link #performer}, creating 1422 * it if it does not already exist 1423 */ 1424 public Reference getPerformerFirstRep() { 1425 if (getPerformer().isEmpty()) { 1426 addPerformer(); 1427 } 1428 return getPerformer().get(0); 1429 } 1430 1431 /** 1432 * @deprecated Use Reference#setResource(IBaseResource) instead 1433 */ 1434 @Deprecated 1435 public List<Resource> getPerformerTarget() { 1436 if (this.performerTarget == null) 1437 this.performerTarget = new ArrayList<Resource>(); 1438 return this.performerTarget; 1439 } 1440 1441 /** 1442 * @return {@link #resultsInterpreter} (The practitioner or organization that is 1443 * responsible for the report's conclusions and interpretations.) 1444 */ 1445 public List<Reference> getResultsInterpreter() { 1446 if (this.resultsInterpreter == null) 1447 this.resultsInterpreter = new ArrayList<Reference>(); 1448 return this.resultsInterpreter; 1449 } 1450 1451 /** 1452 * @return Returns a reference to <code>this</code> for easy method chaining 1453 */ 1454 public DiagnosticReport setResultsInterpreter(List<Reference> theResultsInterpreter) { 1455 this.resultsInterpreter = theResultsInterpreter; 1456 return this; 1457 } 1458 1459 public boolean hasResultsInterpreter() { 1460 if (this.resultsInterpreter == null) 1461 return false; 1462 for (Reference item : this.resultsInterpreter) 1463 if (!item.isEmpty()) 1464 return true; 1465 return false; 1466 } 1467 1468 public Reference addResultsInterpreter() { // 3 1469 Reference t = new Reference(); 1470 if (this.resultsInterpreter == null) 1471 this.resultsInterpreter = new ArrayList<Reference>(); 1472 this.resultsInterpreter.add(t); 1473 return t; 1474 } 1475 1476 public DiagnosticReport addResultsInterpreter(Reference t) { // 3 1477 if (t == null) 1478 return this; 1479 if (this.resultsInterpreter == null) 1480 this.resultsInterpreter = new ArrayList<Reference>(); 1481 this.resultsInterpreter.add(t); 1482 return this; 1483 } 1484 1485 /** 1486 * @return The first repetition of repeating field {@link #resultsInterpreter}, 1487 * creating it if it does not already exist 1488 */ 1489 public Reference getResultsInterpreterFirstRep() { 1490 if (getResultsInterpreter().isEmpty()) { 1491 addResultsInterpreter(); 1492 } 1493 return getResultsInterpreter().get(0); 1494 } 1495 1496 /** 1497 * @deprecated Use Reference#setResource(IBaseResource) instead 1498 */ 1499 @Deprecated 1500 public List<Resource> getResultsInterpreterTarget() { 1501 if (this.resultsInterpreterTarget == null) 1502 this.resultsInterpreterTarget = new ArrayList<Resource>(); 1503 return this.resultsInterpreterTarget; 1504 } 1505 1506 /** 1507 * @return {@link #specimen} (Details about the specimens on which this 1508 * diagnostic report is based.) 1509 */ 1510 public List<Reference> getSpecimen() { 1511 if (this.specimen == null) 1512 this.specimen = new ArrayList<Reference>(); 1513 return this.specimen; 1514 } 1515 1516 /** 1517 * @return Returns a reference to <code>this</code> for easy method chaining 1518 */ 1519 public DiagnosticReport setSpecimen(List<Reference> theSpecimen) { 1520 this.specimen = theSpecimen; 1521 return this; 1522 } 1523 1524 public boolean hasSpecimen() { 1525 if (this.specimen == null) 1526 return false; 1527 for (Reference item : this.specimen) 1528 if (!item.isEmpty()) 1529 return true; 1530 return false; 1531 } 1532 1533 public Reference addSpecimen() { // 3 1534 Reference t = new Reference(); 1535 if (this.specimen == null) 1536 this.specimen = new ArrayList<Reference>(); 1537 this.specimen.add(t); 1538 return t; 1539 } 1540 1541 public DiagnosticReport addSpecimen(Reference t) { // 3 1542 if (t == null) 1543 return this; 1544 if (this.specimen == null) 1545 this.specimen = new ArrayList<Reference>(); 1546 this.specimen.add(t); 1547 return this; 1548 } 1549 1550 /** 1551 * @return The first repetition of repeating field {@link #specimen}, creating 1552 * it if it does not already exist 1553 */ 1554 public Reference getSpecimenFirstRep() { 1555 if (getSpecimen().isEmpty()) { 1556 addSpecimen(); 1557 } 1558 return getSpecimen().get(0); 1559 } 1560 1561 /** 1562 * @deprecated Use Reference#setResource(IBaseResource) instead 1563 */ 1564 @Deprecated 1565 public List<Specimen> getSpecimenTarget() { 1566 if (this.specimenTarget == null) 1567 this.specimenTarget = new ArrayList<Specimen>(); 1568 return this.specimenTarget; 1569 } 1570 1571 /** 1572 * @deprecated Use Reference#setResource(IBaseResource) instead 1573 */ 1574 @Deprecated 1575 public Specimen addSpecimenTarget() { 1576 Specimen r = new Specimen(); 1577 if (this.specimenTarget == null) 1578 this.specimenTarget = new ArrayList<Specimen>(); 1579 this.specimenTarget.add(r); 1580 return r; 1581 } 1582 1583 /** 1584 * @return {@link #result} ([Observations](observation.html) that are part of 1585 * this diagnostic report.) 1586 */ 1587 public List<Reference> getResult() { 1588 if (this.result == null) 1589 this.result = new ArrayList<Reference>(); 1590 return this.result; 1591 } 1592 1593 /** 1594 * @return Returns a reference to <code>this</code> for easy method chaining 1595 */ 1596 public DiagnosticReport setResult(List<Reference> theResult) { 1597 this.result = theResult; 1598 return this; 1599 } 1600 1601 public boolean hasResult() { 1602 if (this.result == null) 1603 return false; 1604 for (Reference item : this.result) 1605 if (!item.isEmpty()) 1606 return true; 1607 return false; 1608 } 1609 1610 public Reference addResult() { // 3 1611 Reference t = new Reference(); 1612 if (this.result == null) 1613 this.result = new ArrayList<Reference>(); 1614 this.result.add(t); 1615 return t; 1616 } 1617 1618 public DiagnosticReport addResult(Reference t) { // 3 1619 if (t == null) 1620 return this; 1621 if (this.result == null) 1622 this.result = new ArrayList<Reference>(); 1623 this.result.add(t); 1624 return this; 1625 } 1626 1627 /** 1628 * @return The first repetition of repeating field {@link #result}, creating it 1629 * if it does not already exist 1630 */ 1631 public Reference getResultFirstRep() { 1632 if (getResult().isEmpty()) { 1633 addResult(); 1634 } 1635 return getResult().get(0); 1636 } 1637 1638 /** 1639 * @deprecated Use Reference#setResource(IBaseResource) instead 1640 */ 1641 @Deprecated 1642 public List<Observation> getResultTarget() { 1643 if (this.resultTarget == null) 1644 this.resultTarget = new ArrayList<Observation>(); 1645 return this.resultTarget; 1646 } 1647 1648 /** 1649 * @deprecated Use Reference#setResource(IBaseResource) instead 1650 */ 1651 @Deprecated 1652 public Observation addResultTarget() { 1653 Observation r = new Observation(); 1654 if (this.resultTarget == null) 1655 this.resultTarget = new ArrayList<Observation>(); 1656 this.resultTarget.add(r); 1657 return r; 1658 } 1659 1660 /** 1661 * @return {@link #imagingStudy} (One or more links to full details of any 1662 * imaging performed during the diagnostic investigation. Typically, 1663 * this is imaging performed by DICOM enabled modalities, but this is 1664 * not required. A fully enabled PACS viewer can use this information to 1665 * provide views of the source images.) 1666 */ 1667 public List<Reference> getImagingStudy() { 1668 if (this.imagingStudy == null) 1669 this.imagingStudy = new ArrayList<Reference>(); 1670 return this.imagingStudy; 1671 } 1672 1673 /** 1674 * @return Returns a reference to <code>this</code> for easy method chaining 1675 */ 1676 public DiagnosticReport setImagingStudy(List<Reference> theImagingStudy) { 1677 this.imagingStudy = theImagingStudy; 1678 return this; 1679 } 1680 1681 public boolean hasImagingStudy() { 1682 if (this.imagingStudy == null) 1683 return false; 1684 for (Reference item : this.imagingStudy) 1685 if (!item.isEmpty()) 1686 return true; 1687 return false; 1688 } 1689 1690 public Reference addImagingStudy() { // 3 1691 Reference t = new Reference(); 1692 if (this.imagingStudy == null) 1693 this.imagingStudy = new ArrayList<Reference>(); 1694 this.imagingStudy.add(t); 1695 return t; 1696 } 1697 1698 public DiagnosticReport addImagingStudy(Reference t) { // 3 1699 if (t == null) 1700 return this; 1701 if (this.imagingStudy == null) 1702 this.imagingStudy = new ArrayList<Reference>(); 1703 this.imagingStudy.add(t); 1704 return this; 1705 } 1706 1707 /** 1708 * @return The first repetition of repeating field {@link #imagingStudy}, 1709 * creating it if it does not already exist 1710 */ 1711 public Reference getImagingStudyFirstRep() { 1712 if (getImagingStudy().isEmpty()) { 1713 addImagingStudy(); 1714 } 1715 return getImagingStudy().get(0); 1716 } 1717 1718 /** 1719 * @deprecated Use Reference#setResource(IBaseResource) instead 1720 */ 1721 @Deprecated 1722 public List<ImagingStudy> getImagingStudyTarget() { 1723 if (this.imagingStudyTarget == null) 1724 this.imagingStudyTarget = new ArrayList<ImagingStudy>(); 1725 return this.imagingStudyTarget; 1726 } 1727 1728 /** 1729 * @deprecated Use Reference#setResource(IBaseResource) instead 1730 */ 1731 @Deprecated 1732 public ImagingStudy addImagingStudyTarget() { 1733 ImagingStudy r = new ImagingStudy(); 1734 if (this.imagingStudyTarget == null) 1735 this.imagingStudyTarget = new ArrayList<ImagingStudy>(); 1736 this.imagingStudyTarget.add(r); 1737 return r; 1738 } 1739 1740 /** 1741 * @return {@link #media} (A list of key images associated with this report. The 1742 * images are generally created during the diagnostic process, and may 1743 * be directly of the patient, or of treated specimens (i.e. slides of 1744 * interest).) 1745 */ 1746 public List<DiagnosticReportMediaComponent> getMedia() { 1747 if (this.media == null) 1748 this.media = new ArrayList<DiagnosticReportMediaComponent>(); 1749 return this.media; 1750 } 1751 1752 /** 1753 * @return Returns a reference to <code>this</code> for easy method chaining 1754 */ 1755 public DiagnosticReport setMedia(List<DiagnosticReportMediaComponent> theMedia) { 1756 this.media = theMedia; 1757 return this; 1758 } 1759 1760 public boolean hasMedia() { 1761 if (this.media == null) 1762 return false; 1763 for (DiagnosticReportMediaComponent item : this.media) 1764 if (!item.isEmpty()) 1765 return true; 1766 return false; 1767 } 1768 1769 public DiagnosticReportMediaComponent addMedia() { // 3 1770 DiagnosticReportMediaComponent t = new DiagnosticReportMediaComponent(); 1771 if (this.media == null) 1772 this.media = new ArrayList<DiagnosticReportMediaComponent>(); 1773 this.media.add(t); 1774 return t; 1775 } 1776 1777 public DiagnosticReport addMedia(DiagnosticReportMediaComponent t) { // 3 1778 if (t == null) 1779 return this; 1780 if (this.media == null) 1781 this.media = new ArrayList<DiagnosticReportMediaComponent>(); 1782 this.media.add(t); 1783 return this; 1784 } 1785 1786 /** 1787 * @return The first repetition of repeating field {@link #media}, creating it 1788 * if it does not already exist 1789 */ 1790 public DiagnosticReportMediaComponent getMediaFirstRep() { 1791 if (getMedia().isEmpty()) { 1792 addMedia(); 1793 } 1794 return getMedia().get(0); 1795 } 1796 1797 /** 1798 * @return {@link #conclusion} (Concise and clinically contextualized summary 1799 * conclusion (interpretation/impression) of the diagnostic report.). 1800 * This is the underlying object with id, value and extensions. The 1801 * accessor "getConclusion" gives direct access to the value 1802 */ 1803 public StringType getConclusionElement() { 1804 if (this.conclusion == null) 1805 if (Configuration.errorOnAutoCreate()) 1806 throw new Error("Attempt to auto-create DiagnosticReport.conclusion"); 1807 else if (Configuration.doAutoCreate()) 1808 this.conclusion = new StringType(); // bb 1809 return this.conclusion; 1810 } 1811 1812 public boolean hasConclusionElement() { 1813 return this.conclusion != null && !this.conclusion.isEmpty(); 1814 } 1815 1816 public boolean hasConclusion() { 1817 return this.conclusion != null && !this.conclusion.isEmpty(); 1818 } 1819 1820 /** 1821 * @param value {@link #conclusion} (Concise and clinically contextualized 1822 * summary conclusion (interpretation/impression) of the diagnostic 1823 * report.). This is the underlying object with id, value and 1824 * extensions. The accessor "getConclusion" gives direct access to 1825 * the value 1826 */ 1827 public DiagnosticReport setConclusionElement(StringType value) { 1828 this.conclusion = value; 1829 return this; 1830 } 1831 1832 /** 1833 * @return Concise and clinically contextualized summary conclusion 1834 * (interpretation/impression) of the diagnostic report. 1835 */ 1836 public String getConclusion() { 1837 return this.conclusion == null ? null : this.conclusion.getValue(); 1838 } 1839 1840 /** 1841 * @param value Concise and clinically contextualized summary conclusion 1842 * (interpretation/impression) of the diagnostic report. 1843 */ 1844 public DiagnosticReport setConclusion(String value) { 1845 if (Utilities.noString(value)) 1846 this.conclusion = null; 1847 else { 1848 if (this.conclusion == null) 1849 this.conclusion = new StringType(); 1850 this.conclusion.setValue(value); 1851 } 1852 return this; 1853 } 1854 1855 /** 1856 * @return {@link #conclusionCode} (One or more codes that represent the summary 1857 * conclusion (interpretation/impression) of the diagnostic report.) 1858 */ 1859 public List<CodeableConcept> getConclusionCode() { 1860 if (this.conclusionCode == null) 1861 this.conclusionCode = new ArrayList<CodeableConcept>(); 1862 return this.conclusionCode; 1863 } 1864 1865 /** 1866 * @return Returns a reference to <code>this</code> for easy method chaining 1867 */ 1868 public DiagnosticReport setConclusionCode(List<CodeableConcept> theConclusionCode) { 1869 this.conclusionCode = theConclusionCode; 1870 return this; 1871 } 1872 1873 public boolean hasConclusionCode() { 1874 if (this.conclusionCode == null) 1875 return false; 1876 for (CodeableConcept item : this.conclusionCode) 1877 if (!item.isEmpty()) 1878 return true; 1879 return false; 1880 } 1881 1882 public CodeableConcept addConclusionCode() { // 3 1883 CodeableConcept t = new CodeableConcept(); 1884 if (this.conclusionCode == null) 1885 this.conclusionCode = new ArrayList<CodeableConcept>(); 1886 this.conclusionCode.add(t); 1887 return t; 1888 } 1889 1890 public DiagnosticReport addConclusionCode(CodeableConcept t) { // 3 1891 if (t == null) 1892 return this; 1893 if (this.conclusionCode == null) 1894 this.conclusionCode = new ArrayList<CodeableConcept>(); 1895 this.conclusionCode.add(t); 1896 return this; 1897 } 1898 1899 /** 1900 * @return The first repetition of repeating field {@link #conclusionCode}, 1901 * creating it if it does not already exist 1902 */ 1903 public CodeableConcept getConclusionCodeFirstRep() { 1904 if (getConclusionCode().isEmpty()) { 1905 addConclusionCode(); 1906 } 1907 return getConclusionCode().get(0); 1908 } 1909 1910 /** 1911 * @return {@link #presentedForm} (Rich text representation of the entire result 1912 * as issued by the diagnostic service. Multiple formats are allowed but 1913 * they SHALL be semantically equivalent.) 1914 */ 1915 public List<Attachment> getPresentedForm() { 1916 if (this.presentedForm == null) 1917 this.presentedForm = new ArrayList<Attachment>(); 1918 return this.presentedForm; 1919 } 1920 1921 /** 1922 * @return Returns a reference to <code>this</code> for easy method chaining 1923 */ 1924 public DiagnosticReport setPresentedForm(List<Attachment> thePresentedForm) { 1925 this.presentedForm = thePresentedForm; 1926 return this; 1927 } 1928 1929 public boolean hasPresentedForm() { 1930 if (this.presentedForm == null) 1931 return false; 1932 for (Attachment item : this.presentedForm) 1933 if (!item.isEmpty()) 1934 return true; 1935 return false; 1936 } 1937 1938 public Attachment addPresentedForm() { // 3 1939 Attachment t = new Attachment(); 1940 if (this.presentedForm == null) 1941 this.presentedForm = new ArrayList<Attachment>(); 1942 this.presentedForm.add(t); 1943 return t; 1944 } 1945 1946 public DiagnosticReport addPresentedForm(Attachment t) { // 3 1947 if (t == null) 1948 return this; 1949 if (this.presentedForm == null) 1950 this.presentedForm = new ArrayList<Attachment>(); 1951 this.presentedForm.add(t); 1952 return this; 1953 } 1954 1955 /** 1956 * @return The first repetition of repeating field {@link #presentedForm}, 1957 * creating it if it does not already exist 1958 */ 1959 public Attachment getPresentedFormFirstRep() { 1960 if (getPresentedForm().isEmpty()) { 1961 addPresentedForm(); 1962 } 1963 return getPresentedForm().get(0); 1964 } 1965 1966 protected void listChildren(List<Property> children) { 1967 super.listChildren(children); 1968 children.add(new Property("identifier", "Identifier", 1969 "Identifiers assigned to this report by the performer or other systems.", 0, java.lang.Integer.MAX_VALUE, 1970 identifier)); 1971 children.add(new Property("basedOn", 1972 "Reference(CarePlan|ImmunizationRecommendation|MedicationRequest|NutritionOrder|ServiceRequest)", 1973 "Details concerning a service requested.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1974 children.add(new Property("status", "code", "The status of the diagnostic report.", 0, 1, status)); 1975 children.add(new Property("category", "CodeableConcept", 1976 "A code that classifies the clinical discipline, department or diagnostic service that created the report (e.g. cardiology, biochemistry, hematology, MRI). This is used for searching, sorting and display purposes.", 1977 0, java.lang.Integer.MAX_VALUE, category)); 1978 children.add( 1979 new Property("code", "CodeableConcept", "A code or name that describes this diagnostic report.", 0, 1, code)); 1980 children.add(new Property("subject", "Reference(Patient|Group|Device|Location)", 1981 "The subject of the report. Usually, but not always, this is a patient. However, diagnostic services also perform analyses on specimens collected from a variety of other sources.", 1982 0, 1, subject)); 1983 children.add(new Property("encounter", "Reference(Encounter)", 1984 "The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport is about.", 1985 0, 1, encounter)); 1986 children.add(new Property("effective[x]", "dateTime|Period", 1987 "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.", 1988 0, 1, effective)); 1989 children.add(new Property("issued", "instant", 1990 "The date and time that this version of the report was made available to providers, typically after the report was reviewed and verified.", 1991 0, 1, issued)); 1992 children.add(new Property("performer", "Reference(Practitioner|PractitionerRole|Organization|CareTeam)", 1993 "The diagnostic service that is responsible for issuing the report.", 0, java.lang.Integer.MAX_VALUE, 1994 performer)); 1995 children.add(new Property("resultsInterpreter", "Reference(Practitioner|PractitionerRole|Organization|CareTeam)", 1996 "The practitioner or organization that is responsible for the report's conclusions and interpretations.", 0, 1997 java.lang.Integer.MAX_VALUE, resultsInterpreter)); 1998 children.add(new Property("specimen", "Reference(Specimen)", 1999 "Details about the specimens on which this diagnostic report is based.", 0, java.lang.Integer.MAX_VALUE, 2000 specimen)); 2001 children.add(new Property("result", "Reference(Observation)", 2002 "[Observations](observation.html) that are part of this diagnostic report.", 0, java.lang.Integer.MAX_VALUE, 2003 result)); 2004 children.add(new Property("imagingStudy", "Reference(ImagingStudy)", 2005 "One or more links to full details of any imaging performed during the diagnostic investigation. Typically, this is imaging performed by DICOM enabled modalities, but this is not required. A fully enabled PACS viewer can use this information to provide views of the source images.", 2006 0, java.lang.Integer.MAX_VALUE, imagingStudy)); 2007 children.add(new Property("media", "", 2008 "A list of key images associated with this report. The images are generally created during the diagnostic process, and may be directly of the patient, or of treated specimens (i.e. slides of interest).", 2009 0, java.lang.Integer.MAX_VALUE, media)); 2010 children.add(new Property("conclusion", "string", 2011 "Concise and clinically contextualized summary conclusion (interpretation/impression) of the diagnostic report.", 2012 0, 1, conclusion)); 2013 children.add(new Property("conclusionCode", "CodeableConcept", 2014 "One or more codes that represent the summary conclusion (interpretation/impression) of the diagnostic report.", 2015 0, java.lang.Integer.MAX_VALUE, conclusionCode)); 2016 children.add(new Property("presentedForm", "Attachment", 2017 "Rich text representation of the entire result as issued by the diagnostic service. Multiple formats are allowed but they SHALL be semantically equivalent.", 2018 0, java.lang.Integer.MAX_VALUE, presentedForm)); 2019 } 2020 2021 @Override 2022 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2023 switch (_hash) { 2024 case -1618432855: 2025 /* identifier */ return new Property("identifier", "Identifier", 2026 "Identifiers assigned to this report by the performer or other systems.", 0, java.lang.Integer.MAX_VALUE, 2027 identifier); 2028 case -332612366: 2029 /* basedOn */ return new Property("basedOn", 2030 "Reference(CarePlan|ImmunizationRecommendation|MedicationRequest|NutritionOrder|ServiceRequest)", 2031 "Details concerning a service requested.", 0, java.lang.Integer.MAX_VALUE, basedOn); 2032 case -892481550: 2033 /* status */ return new Property("status", "code", "The status of the diagnostic report.", 0, 1, status); 2034 case 50511102: 2035 /* category */ return new Property("category", "CodeableConcept", 2036 "A code that classifies the clinical discipline, department or diagnostic service that created the report (e.g. cardiology, biochemistry, hematology, MRI). This is used for searching, sorting and display purposes.", 2037 0, java.lang.Integer.MAX_VALUE, category); 2038 case 3059181: 2039 /* code */ return new Property("code", "CodeableConcept", "A code or name that describes this diagnostic report.", 2040 0, 1, code); 2041 case -1867885268: 2042 /* subject */ return new Property("subject", "Reference(Patient|Group|Device|Location)", 2043 "The subject of the report. Usually, but not always, this is a patient. However, diagnostic services also perform analyses on specimens collected from a variety of other sources.", 2044 0, 1, subject); 2045 case 1524132147: 2046 /* encounter */ return new Property("encounter", "Reference(Encounter)", 2047 "The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport is about.", 2048 0, 1, encounter); 2049 case 247104889: 2050 /* effective[x] */ return new Property("effective[x]", "dateTime|Period", 2051 "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.", 2052 0, 1, effective); 2053 case -1468651097: 2054 /* effective */ return new Property("effective[x]", "dateTime|Period", 2055 "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.", 2056 0, 1, effective); 2057 case -275306910: 2058 /* effectiveDateTime */ return new Property("effective[x]", "dateTime|Period", 2059 "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.", 2060 0, 1, effective); 2061 case -403934648: 2062 /* effectivePeriod */ return new Property("effective[x]", "dateTime|Period", 2063 "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.", 2064 0, 1, effective); 2065 case -1179159893: 2066 /* issued */ return new Property("issued", "instant", 2067 "The date and time that this version of the report was made available to providers, typically after the report was reviewed and verified.", 2068 0, 1, issued); 2069 case 481140686: 2070 /* performer */ return new Property("performer", "Reference(Practitioner|PractitionerRole|Organization|CareTeam)", 2071 "The diagnostic service that is responsible for issuing the report.", 0, java.lang.Integer.MAX_VALUE, 2072 performer); 2073 case 2134944932: 2074 /* resultsInterpreter */ return new Property("resultsInterpreter", 2075 "Reference(Practitioner|PractitionerRole|Organization|CareTeam)", 2076 "The practitioner or organization that is responsible for the report's conclusions and interpretations.", 0, 2077 java.lang.Integer.MAX_VALUE, resultsInterpreter); 2078 case -2132868344: 2079 /* specimen */ return new Property("specimen", "Reference(Specimen)", 2080 "Details about the specimens on which this diagnostic report is based.", 0, java.lang.Integer.MAX_VALUE, 2081 specimen); 2082 case -934426595: 2083 /* result */ return new Property("result", "Reference(Observation)", 2084 "[Observations](observation.html) that are part of this diagnostic report.", 0, java.lang.Integer.MAX_VALUE, 2085 result); 2086 case -814900911: 2087 /* imagingStudy */ return new Property("imagingStudy", "Reference(ImagingStudy)", 2088 "One or more links to full details of any imaging performed during the diagnostic investigation. Typically, this is imaging performed by DICOM enabled modalities, but this is not required. A fully enabled PACS viewer can use this information to provide views of the source images.", 2089 0, java.lang.Integer.MAX_VALUE, imagingStudy); 2090 case 103772132: 2091 /* media */ return new Property("media", "", 2092 "A list of key images associated with this report. The images are generally created during the diagnostic process, and may be directly of the patient, or of treated specimens (i.e. slides of interest).", 2093 0, java.lang.Integer.MAX_VALUE, media); 2094 case -1731259873: 2095 /* conclusion */ return new Property("conclusion", "string", 2096 "Concise and clinically contextualized summary conclusion (interpretation/impression) of the diagnostic report.", 2097 0, 1, conclusion); 2098 case -1731523412: 2099 /* conclusionCode */ return new Property("conclusionCode", "CodeableConcept", 2100 "One or more codes that represent the summary conclusion (interpretation/impression) of the diagnostic report.", 2101 0, java.lang.Integer.MAX_VALUE, conclusionCode); 2102 case 230090366: 2103 /* presentedForm */ return new Property("presentedForm", "Attachment", 2104 "Rich text representation of the entire result as issued by the diagnostic service. Multiple formats are allowed but they SHALL be semantically equivalent.", 2105 0, java.lang.Integer.MAX_VALUE, presentedForm); 2106 default: 2107 return super.getNamedProperty(_hash, _name, _checkValid); 2108 } 2109 2110 } 2111 2112 @Override 2113 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2114 switch (hash) { 2115 case -1618432855: 2116 /* identifier */ return this.identifier == null ? new Base[0] 2117 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2118 case -332612366: 2119 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2120 case -892481550: 2121 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<DiagnosticReportStatus> 2122 case 50511102: 2123 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2124 case 3059181: 2125 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 2126 case -1867885268: 2127 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2128 case 1524132147: 2129 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 2130 case -1468651097: 2131 /* effective */ return this.effective == null ? new Base[0] : new Base[] { this.effective }; // Type 2132 case -1179159893: 2133 /* issued */ return this.issued == null ? new Base[0] : new Base[] { this.issued }; // InstantType 2134 case 481140686: 2135 /* performer */ return this.performer == null ? new Base[0] 2136 : this.performer.toArray(new Base[this.performer.size()]); // Reference 2137 case 2134944932: 2138 /* resultsInterpreter */ return this.resultsInterpreter == null ? new Base[0] 2139 : this.resultsInterpreter.toArray(new Base[this.resultsInterpreter.size()]); // Reference 2140 case -2132868344: 2141 /* specimen */ return this.specimen == null ? new Base[0] : this.specimen.toArray(new Base[this.specimen.size()]); // Reference 2142 case -934426595: 2143 /* result */ return this.result == null ? new Base[0] : this.result.toArray(new Base[this.result.size()]); // Reference 2144 case -814900911: 2145 /* imagingStudy */ return this.imagingStudy == null ? new Base[0] 2146 : this.imagingStudy.toArray(new Base[this.imagingStudy.size()]); // Reference 2147 case 103772132: 2148 /* media */ return this.media == null ? new Base[0] : this.media.toArray(new Base[this.media.size()]); // DiagnosticReportMediaComponent 2149 case -1731259873: 2150 /* conclusion */ return this.conclusion == null ? new Base[0] : new Base[] { this.conclusion }; // StringType 2151 case -1731523412: 2152 /* conclusionCode */ return this.conclusionCode == null ? new Base[0] 2153 : this.conclusionCode.toArray(new Base[this.conclusionCode.size()]); // CodeableConcept 2154 case 230090366: 2155 /* presentedForm */ return this.presentedForm == null ? new Base[0] 2156 : this.presentedForm.toArray(new Base[this.presentedForm.size()]); // Attachment 2157 default: 2158 return super.getProperty(hash, name, checkValid); 2159 } 2160 2161 } 2162 2163 @Override 2164 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2165 switch (hash) { 2166 case -1618432855: // identifier 2167 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2168 return value; 2169 case -332612366: // basedOn 2170 this.getBasedOn().add(castToReference(value)); // Reference 2171 return value; 2172 case -892481550: // status 2173 value = new DiagnosticReportStatusEnumFactory().fromType(castToCode(value)); 2174 this.status = (Enumeration) value; // Enumeration<DiagnosticReportStatus> 2175 return value; 2176 case 50511102: // category 2177 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 2178 return value; 2179 case 3059181: // code 2180 this.code = castToCodeableConcept(value); // CodeableConcept 2181 return value; 2182 case -1867885268: // subject 2183 this.subject = castToReference(value); // Reference 2184 return value; 2185 case 1524132147: // encounter 2186 this.encounter = castToReference(value); // Reference 2187 return value; 2188 case -1468651097: // effective 2189 this.effective = castToType(value); // Type 2190 return value; 2191 case -1179159893: // issued 2192 this.issued = castToInstant(value); // InstantType 2193 return value; 2194 case 481140686: // performer 2195 this.getPerformer().add(castToReference(value)); // Reference 2196 return value; 2197 case 2134944932: // resultsInterpreter 2198 this.getResultsInterpreter().add(castToReference(value)); // Reference 2199 return value; 2200 case -2132868344: // specimen 2201 this.getSpecimen().add(castToReference(value)); // Reference 2202 return value; 2203 case -934426595: // result 2204 this.getResult().add(castToReference(value)); // Reference 2205 return value; 2206 case -814900911: // imagingStudy 2207 this.getImagingStudy().add(castToReference(value)); // Reference 2208 return value; 2209 case 103772132: // media 2210 this.getMedia().add((DiagnosticReportMediaComponent) value); // DiagnosticReportMediaComponent 2211 return value; 2212 case -1731259873: // conclusion 2213 this.conclusion = castToString(value); // StringType 2214 return value; 2215 case -1731523412: // conclusionCode 2216 this.getConclusionCode().add(castToCodeableConcept(value)); // CodeableConcept 2217 return value; 2218 case 230090366: // presentedForm 2219 this.getPresentedForm().add(castToAttachment(value)); // Attachment 2220 return value; 2221 default: 2222 return super.setProperty(hash, name, value); 2223 } 2224 2225 } 2226 2227 @Override 2228 public Base setProperty(String name, Base value) throws FHIRException { 2229 if (name.equals("identifier")) { 2230 this.getIdentifier().add(castToIdentifier(value)); 2231 } else if (name.equals("basedOn")) { 2232 this.getBasedOn().add(castToReference(value)); 2233 } else if (name.equals("status")) { 2234 value = new DiagnosticReportStatusEnumFactory().fromType(castToCode(value)); 2235 this.status = (Enumeration) value; // Enumeration<DiagnosticReportStatus> 2236 } else if (name.equals("category")) { 2237 this.getCategory().add(castToCodeableConcept(value)); 2238 } else if (name.equals("code")) { 2239 this.code = castToCodeableConcept(value); // CodeableConcept 2240 } else if (name.equals("subject")) { 2241 this.subject = castToReference(value); // Reference 2242 } else if (name.equals("encounter")) { 2243 this.encounter = castToReference(value); // Reference 2244 } else if (name.equals("effective[x]")) { 2245 this.effective = castToType(value); // Type 2246 } else if (name.equals("issued")) { 2247 this.issued = castToInstant(value); // InstantType 2248 } else if (name.equals("performer")) { 2249 this.getPerformer().add(castToReference(value)); 2250 } else if (name.equals("resultsInterpreter")) { 2251 this.getResultsInterpreter().add(castToReference(value)); 2252 } else if (name.equals("specimen")) { 2253 this.getSpecimen().add(castToReference(value)); 2254 } else if (name.equals("result")) { 2255 this.getResult().add(castToReference(value)); 2256 } else if (name.equals("imagingStudy")) { 2257 this.getImagingStudy().add(castToReference(value)); 2258 } else if (name.equals("media")) { 2259 this.getMedia().add((DiagnosticReportMediaComponent) value); 2260 } else if (name.equals("conclusion")) { 2261 this.conclusion = castToString(value); // StringType 2262 } else if (name.equals("conclusionCode")) { 2263 this.getConclusionCode().add(castToCodeableConcept(value)); 2264 } else if (name.equals("presentedForm")) { 2265 this.getPresentedForm().add(castToAttachment(value)); 2266 } else 2267 return super.setProperty(name, value); 2268 return value; 2269 } 2270 2271 @Override 2272 public void removeChild(String name, Base value) throws FHIRException { 2273 if (name.equals("identifier")) { 2274 this.getIdentifier().remove(castToIdentifier(value)); 2275 } else if (name.equals("basedOn")) { 2276 this.getBasedOn().remove(castToReference(value)); 2277 } else if (name.equals("status")) { 2278 this.status = null; 2279 } else if (name.equals("category")) { 2280 this.getCategory().remove(castToCodeableConcept(value)); 2281 } else if (name.equals("code")) { 2282 this.code = null; 2283 } else if (name.equals("subject")) { 2284 this.subject = null; 2285 } else if (name.equals("encounter")) { 2286 this.encounter = null; 2287 } else if (name.equals("effective[x]")) { 2288 this.effective = null; 2289 } else if (name.equals("issued")) { 2290 this.issued = null; 2291 } else if (name.equals("performer")) { 2292 this.getPerformer().remove(castToReference(value)); 2293 } else if (name.equals("resultsInterpreter")) { 2294 this.getResultsInterpreter().remove(castToReference(value)); 2295 } else if (name.equals("specimen")) { 2296 this.getSpecimen().remove(castToReference(value)); 2297 } else if (name.equals("result")) { 2298 this.getResult().remove(castToReference(value)); 2299 } else if (name.equals("imagingStudy")) { 2300 this.getImagingStudy().remove(castToReference(value)); 2301 } else if (name.equals("media")) { 2302 this.getMedia().remove((DiagnosticReportMediaComponent) value); 2303 } else if (name.equals("conclusion")) { 2304 this.conclusion = null; 2305 } else if (name.equals("conclusionCode")) { 2306 this.getConclusionCode().remove(castToCodeableConcept(value)); 2307 } else if (name.equals("presentedForm")) { 2308 this.getPresentedForm().remove(castToAttachment(value)); 2309 } else 2310 super.removeChild(name, value); 2311 2312 } 2313 2314 @Override 2315 public Base makeProperty(int hash, String name) throws FHIRException { 2316 switch (hash) { 2317 case -1618432855: 2318 return addIdentifier(); 2319 case -332612366: 2320 return addBasedOn(); 2321 case -892481550: 2322 return getStatusElement(); 2323 case 50511102: 2324 return addCategory(); 2325 case 3059181: 2326 return getCode(); 2327 case -1867885268: 2328 return getSubject(); 2329 case 1524132147: 2330 return getEncounter(); 2331 case 247104889: 2332 return getEffective(); 2333 case -1468651097: 2334 return getEffective(); 2335 case -1179159893: 2336 return getIssuedElement(); 2337 case 481140686: 2338 return addPerformer(); 2339 case 2134944932: 2340 return addResultsInterpreter(); 2341 case -2132868344: 2342 return addSpecimen(); 2343 case -934426595: 2344 return addResult(); 2345 case -814900911: 2346 return addImagingStudy(); 2347 case 103772132: 2348 return addMedia(); 2349 case -1731259873: 2350 return getConclusionElement(); 2351 case -1731523412: 2352 return addConclusionCode(); 2353 case 230090366: 2354 return addPresentedForm(); 2355 default: 2356 return super.makeProperty(hash, name); 2357 } 2358 2359 } 2360 2361 @Override 2362 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2363 switch (hash) { 2364 case -1618432855: 2365 /* identifier */ return new String[] { "Identifier" }; 2366 case -332612366: 2367 /* basedOn */ return new String[] { "Reference" }; 2368 case -892481550: 2369 /* status */ return new String[] { "code" }; 2370 case 50511102: 2371 /* category */ return new String[] { "CodeableConcept" }; 2372 case 3059181: 2373 /* code */ return new String[] { "CodeableConcept" }; 2374 case -1867885268: 2375 /* subject */ return new String[] { "Reference" }; 2376 case 1524132147: 2377 /* encounter */ return new String[] { "Reference" }; 2378 case -1468651097: 2379 /* effective */ return new String[] { "dateTime", "Period" }; 2380 case -1179159893: 2381 /* issued */ return new String[] { "instant" }; 2382 case 481140686: 2383 /* performer */ return new String[] { "Reference" }; 2384 case 2134944932: 2385 /* resultsInterpreter */ return new String[] { "Reference" }; 2386 case -2132868344: 2387 /* specimen */ return new String[] { "Reference" }; 2388 case -934426595: 2389 /* result */ return new String[] { "Reference" }; 2390 case -814900911: 2391 /* imagingStudy */ return new String[] { "Reference" }; 2392 case 103772132: 2393 /* media */ return new String[] {}; 2394 case -1731259873: 2395 /* conclusion */ return new String[] { "string" }; 2396 case -1731523412: 2397 /* conclusionCode */ return new String[] { "CodeableConcept" }; 2398 case 230090366: 2399 /* presentedForm */ return new String[] { "Attachment" }; 2400 default: 2401 return super.getTypesForProperty(hash, name); 2402 } 2403 2404 } 2405 2406 @Override 2407 public Base addChild(String name) throws FHIRException { 2408 if (name.equals("identifier")) { 2409 return addIdentifier(); 2410 } else if (name.equals("basedOn")) { 2411 return addBasedOn(); 2412 } else if (name.equals("status")) { 2413 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticReport.status"); 2414 } else if (name.equals("category")) { 2415 return addCategory(); 2416 } else if (name.equals("code")) { 2417 this.code = new CodeableConcept(); 2418 return this.code; 2419 } else if (name.equals("subject")) { 2420 this.subject = new Reference(); 2421 return this.subject; 2422 } else if (name.equals("encounter")) { 2423 this.encounter = new Reference(); 2424 return this.encounter; 2425 } else if (name.equals("effectiveDateTime")) { 2426 this.effective = new DateTimeType(); 2427 return this.effective; 2428 } else if (name.equals("effectivePeriod")) { 2429 this.effective = new Period(); 2430 return this.effective; 2431 } else if (name.equals("issued")) { 2432 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticReport.issued"); 2433 } else if (name.equals("performer")) { 2434 return addPerformer(); 2435 } else if (name.equals("resultsInterpreter")) { 2436 return addResultsInterpreter(); 2437 } else if (name.equals("specimen")) { 2438 return addSpecimen(); 2439 } else if (name.equals("result")) { 2440 return addResult(); 2441 } else if (name.equals("imagingStudy")) { 2442 return addImagingStudy(); 2443 } else if (name.equals("media")) { 2444 return addMedia(); 2445 } else if (name.equals("conclusion")) { 2446 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticReport.conclusion"); 2447 } else if (name.equals("conclusionCode")) { 2448 return addConclusionCode(); 2449 } else if (name.equals("presentedForm")) { 2450 return addPresentedForm(); 2451 } else 2452 return super.addChild(name); 2453 } 2454 2455 public String fhirType() { 2456 return "DiagnosticReport"; 2457 2458 } 2459 2460 public DiagnosticReport copy() { 2461 DiagnosticReport dst = new DiagnosticReport(); 2462 copyValues(dst); 2463 return dst; 2464 } 2465 2466 public void copyValues(DiagnosticReport dst) { 2467 super.copyValues(dst); 2468 if (identifier != null) { 2469 dst.identifier = new ArrayList<Identifier>(); 2470 for (Identifier i : identifier) 2471 dst.identifier.add(i.copy()); 2472 } 2473 ; 2474 if (basedOn != null) { 2475 dst.basedOn = new ArrayList<Reference>(); 2476 for (Reference i : basedOn) 2477 dst.basedOn.add(i.copy()); 2478 } 2479 ; 2480 dst.status = status == null ? null : status.copy(); 2481 if (category != null) { 2482 dst.category = new ArrayList<CodeableConcept>(); 2483 for (CodeableConcept i : category) 2484 dst.category.add(i.copy()); 2485 } 2486 ; 2487 dst.code = code == null ? null : code.copy(); 2488 dst.subject = subject == null ? null : subject.copy(); 2489 dst.encounter = encounter == null ? null : encounter.copy(); 2490 dst.effective = effective == null ? null : effective.copy(); 2491 dst.issued = issued == null ? null : issued.copy(); 2492 if (performer != null) { 2493 dst.performer = new ArrayList<Reference>(); 2494 for (Reference i : performer) 2495 dst.performer.add(i.copy()); 2496 } 2497 ; 2498 if (resultsInterpreter != null) { 2499 dst.resultsInterpreter = new ArrayList<Reference>(); 2500 for (Reference i : resultsInterpreter) 2501 dst.resultsInterpreter.add(i.copy()); 2502 } 2503 ; 2504 if (specimen != null) { 2505 dst.specimen = new ArrayList<Reference>(); 2506 for (Reference i : specimen) 2507 dst.specimen.add(i.copy()); 2508 } 2509 ; 2510 if (result != null) { 2511 dst.result = new ArrayList<Reference>(); 2512 for (Reference i : result) 2513 dst.result.add(i.copy()); 2514 } 2515 ; 2516 if (imagingStudy != null) { 2517 dst.imagingStudy = new ArrayList<Reference>(); 2518 for (Reference i : imagingStudy) 2519 dst.imagingStudy.add(i.copy()); 2520 } 2521 ; 2522 if (media != null) { 2523 dst.media = new ArrayList<DiagnosticReportMediaComponent>(); 2524 for (DiagnosticReportMediaComponent i : media) 2525 dst.media.add(i.copy()); 2526 } 2527 ; 2528 dst.conclusion = conclusion == null ? null : conclusion.copy(); 2529 if (conclusionCode != null) { 2530 dst.conclusionCode = new ArrayList<CodeableConcept>(); 2531 for (CodeableConcept i : conclusionCode) 2532 dst.conclusionCode.add(i.copy()); 2533 } 2534 ; 2535 if (presentedForm != null) { 2536 dst.presentedForm = new ArrayList<Attachment>(); 2537 for (Attachment i : presentedForm) 2538 dst.presentedForm.add(i.copy()); 2539 } 2540 ; 2541 } 2542 2543 protected DiagnosticReport typedCopy() { 2544 return copy(); 2545 } 2546 2547 @Override 2548 public boolean equalsDeep(Base other_) { 2549 if (!super.equalsDeep(other_)) 2550 return false; 2551 if (!(other_ instanceof DiagnosticReport)) 2552 return false; 2553 DiagnosticReport o = (DiagnosticReport) other_; 2554 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) 2555 && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) 2556 && compareDeep(code, o.code, true) && compareDeep(subject, o.subject, true) 2557 && compareDeep(encounter, o.encounter, true) && compareDeep(effective, o.effective, true) 2558 && compareDeep(issued, o.issued, true) && compareDeep(performer, o.performer, true) 2559 && compareDeep(resultsInterpreter, o.resultsInterpreter, true) && compareDeep(specimen, o.specimen, true) 2560 && compareDeep(result, o.result, true) && compareDeep(imagingStudy, o.imagingStudy, true) 2561 && compareDeep(media, o.media, true) && compareDeep(conclusion, o.conclusion, true) 2562 && compareDeep(conclusionCode, o.conclusionCode, true) && compareDeep(presentedForm, o.presentedForm, true); 2563 } 2564 2565 @Override 2566 public boolean equalsShallow(Base other_) { 2567 if (!super.equalsShallow(other_)) 2568 return false; 2569 if (!(other_ instanceof DiagnosticReport)) 2570 return false; 2571 DiagnosticReport o = (DiagnosticReport) other_; 2572 return compareValues(status, o.status, true) && compareValues(issued, o.issued, true) 2573 && compareValues(conclusion, o.conclusion, true); 2574 } 2575 2576 public boolean isEmpty() { 2577 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, status, category, code, subject, 2578 encounter, effective, issued, performer, resultsInterpreter, specimen, result, imagingStudy, media, conclusion, 2579 conclusionCode, presentedForm); 2580 } 2581 2582 @Override 2583 public ResourceType getResourceType() { 2584 return ResourceType.DiagnosticReport; 2585 } 2586 2587 /** 2588 * Search parameter: <b>date</b> 2589 * <p> 2590 * Description: <b>The clinically relevant time of the report</b><br> 2591 * Type: <b>date</b><br> 2592 * Path: <b>DiagnosticReport.effective[x]</b><br> 2593 * </p> 2594 */ 2595 @SearchParamDefinition(name = "date", path = "DiagnosticReport.effective", description = "The clinically relevant time of the report", type = "date") 2596 public static final String SP_DATE = "date"; 2597 /** 2598 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2599 * <p> 2600 * Description: <b>The clinically relevant time of the report</b><br> 2601 * Type: <b>date</b><br> 2602 * Path: <b>DiagnosticReport.effective[x]</b><br> 2603 * </p> 2604 */ 2605 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2606 SP_DATE); 2607 2608 /** 2609 * Search parameter: <b>identifier</b> 2610 * <p> 2611 * Description: <b>An identifier for the report</b><br> 2612 * Type: <b>token</b><br> 2613 * Path: <b>DiagnosticReport.identifier</b><br> 2614 * </p> 2615 */ 2616 @SearchParamDefinition(name = "identifier", path = "DiagnosticReport.identifier", description = "An identifier for the report", type = "token") 2617 public static final String SP_IDENTIFIER = "identifier"; 2618 /** 2619 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2620 * <p> 2621 * Description: <b>An identifier for the report</b><br> 2622 * Type: <b>token</b><br> 2623 * Path: <b>DiagnosticReport.identifier</b><br> 2624 * </p> 2625 */ 2626 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2627 SP_IDENTIFIER); 2628 2629 /** 2630 * Search parameter: <b>performer</b> 2631 * <p> 2632 * Description: <b>Who is responsible for the report</b><br> 2633 * Type: <b>reference</b><br> 2634 * Path: <b>DiagnosticReport.performer</b><br> 2635 * </p> 2636 */ 2637 @SearchParamDefinition(name = "performer", path = "DiagnosticReport.performer", description = "Who is responsible for the report", type = "reference", providesMembershipIn = { 2638 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { CareTeam.class, 2639 Organization.class, Practitioner.class, PractitionerRole.class }) 2640 public static final String SP_PERFORMER = "performer"; 2641 /** 2642 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 2643 * <p> 2644 * Description: <b>Who is responsible for the report</b><br> 2645 * Type: <b>reference</b><br> 2646 * Path: <b>DiagnosticReport.performer</b><br> 2647 * </p> 2648 */ 2649 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2650 SP_PERFORMER); 2651 2652 /** 2653 * Constant for fluent queries to be used to add include statements. Specifies 2654 * the path value of "<b>DiagnosticReport:performer</b>". 2655 */ 2656 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include( 2657 "DiagnosticReport:performer").toLocked(); 2658 2659 /** 2660 * Search parameter: <b>code</b> 2661 * <p> 2662 * Description: <b>The code for the report, as opposed to codes for the atomic 2663 * results, which are the names on the observation resource referred to from the 2664 * result</b><br> 2665 * Type: <b>token</b><br> 2666 * Path: <b>DiagnosticReport.code</b><br> 2667 * </p> 2668 */ 2669 @SearchParamDefinition(name = "code", path = "DiagnosticReport.code", description = "The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result", type = "token") 2670 public static final String SP_CODE = "code"; 2671 /** 2672 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2673 * <p> 2674 * Description: <b>The code for the report, as opposed to codes for the atomic 2675 * results, which are the names on the observation resource referred to from the 2676 * result</b><br> 2677 * Type: <b>token</b><br> 2678 * Path: <b>DiagnosticReport.code</b><br> 2679 * </p> 2680 */ 2681 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2682 SP_CODE); 2683 2684 /** 2685 * Search parameter: <b>subject</b> 2686 * <p> 2687 * Description: <b>The subject of the report</b><br> 2688 * Type: <b>reference</b><br> 2689 * Path: <b>DiagnosticReport.subject</b><br> 2690 * </p> 2691 */ 2692 @SearchParamDefinition(name = "subject", path = "DiagnosticReport.subject", description = "The subject of the report", type = "reference", providesMembershipIn = { 2693 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 2694 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Device.class, Group.class, 2695 Location.class, Patient.class }) 2696 public static final String SP_SUBJECT = "subject"; 2697 /** 2698 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2699 * <p> 2700 * Description: <b>The subject of the report</b><br> 2701 * Type: <b>reference</b><br> 2702 * Path: <b>DiagnosticReport.subject</b><br> 2703 * </p> 2704 */ 2705 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2706 SP_SUBJECT); 2707 2708 /** 2709 * Constant for fluent queries to be used to add include statements. Specifies 2710 * the path value of "<b>DiagnosticReport:subject</b>". 2711 */ 2712 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 2713 "DiagnosticReport:subject").toLocked(); 2714 2715 /** 2716 * Search parameter: <b>media</b> 2717 * <p> 2718 * Description: <b>A reference to the image source.</b><br> 2719 * Type: <b>reference</b><br> 2720 * Path: <b>DiagnosticReport.media.link</b><br> 2721 * </p> 2722 */ 2723 @SearchParamDefinition(name = "media", path = "DiagnosticReport.media.link", description = "A reference to the image source.", type = "reference", target = { 2724 Media.class }) 2725 public static final String SP_MEDIA = "media"; 2726 /** 2727 * <b>Fluent Client</b> search parameter constant for <b>media</b> 2728 * <p> 2729 * Description: <b>A reference to the image source.</b><br> 2730 * Type: <b>reference</b><br> 2731 * Path: <b>DiagnosticReport.media.link</b><br> 2732 * </p> 2733 */ 2734 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEDIA = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2735 SP_MEDIA); 2736 2737 /** 2738 * Constant for fluent queries to be used to add include statements. Specifies 2739 * the path value of "<b>DiagnosticReport:media</b>". 2740 */ 2741 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEDIA = new ca.uhn.fhir.model.api.Include( 2742 "DiagnosticReport:media").toLocked(); 2743 2744 /** 2745 * Search parameter: <b>encounter</b> 2746 * <p> 2747 * Description: <b>The Encounter when the order was made</b><br> 2748 * Type: <b>reference</b><br> 2749 * Path: <b>DiagnosticReport.encounter</b><br> 2750 * </p> 2751 */ 2752 @SearchParamDefinition(name = "encounter", path = "DiagnosticReport.encounter", description = "The Encounter when the order was made", type = "reference", providesMembershipIn = { 2753 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 2754 public static final String SP_ENCOUNTER = "encounter"; 2755 /** 2756 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2757 * <p> 2758 * Description: <b>The Encounter when the order was made</b><br> 2759 * Type: <b>reference</b><br> 2760 * Path: <b>DiagnosticReport.encounter</b><br> 2761 * </p> 2762 */ 2763 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2764 SP_ENCOUNTER); 2765 2766 /** 2767 * Constant for fluent queries to be used to add include statements. Specifies 2768 * the path value of "<b>DiagnosticReport:encounter</b>". 2769 */ 2770 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 2771 "DiagnosticReport:encounter").toLocked(); 2772 2773 /** 2774 * Search parameter: <b>result</b> 2775 * <p> 2776 * Description: <b>Link to an atomic result (observation resource)</b><br> 2777 * Type: <b>reference</b><br> 2778 * Path: <b>DiagnosticReport.result</b><br> 2779 * </p> 2780 */ 2781 @SearchParamDefinition(name = "result", path = "DiagnosticReport.result", description = "Link to an atomic result (observation resource)", type = "reference", target = { 2782 Observation.class }) 2783 public static final String SP_RESULT = "result"; 2784 /** 2785 * <b>Fluent Client</b> search parameter constant for <b>result</b> 2786 * <p> 2787 * Description: <b>Link to an atomic result (observation resource)</b><br> 2788 * Type: <b>reference</b><br> 2789 * Path: <b>DiagnosticReport.result</b><br> 2790 * </p> 2791 */ 2792 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESULT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2793 SP_RESULT); 2794 2795 /** 2796 * Constant for fluent queries to be used to add include statements. Specifies 2797 * the path value of "<b>DiagnosticReport:result</b>". 2798 */ 2799 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESULT = new ca.uhn.fhir.model.api.Include( 2800 "DiagnosticReport:result").toLocked(); 2801 2802 /** 2803 * Search parameter: <b>conclusion</b> 2804 * <p> 2805 * Description: <b>A coded conclusion (interpretation/impression) on the 2806 * report</b><br> 2807 * Type: <b>token</b><br> 2808 * Path: <b>DiagnosticReport.conclusionCode</b><br> 2809 * </p> 2810 */ 2811 @SearchParamDefinition(name = "conclusion", path = "DiagnosticReport.conclusionCode", description = "A coded conclusion (interpretation/impression) on the report", type = "token") 2812 public static final String SP_CONCLUSION = "conclusion"; 2813 /** 2814 * <b>Fluent Client</b> search parameter constant for <b>conclusion</b> 2815 * <p> 2816 * Description: <b>A coded conclusion (interpretation/impression) on the 2817 * report</b><br> 2818 * Type: <b>token</b><br> 2819 * Path: <b>DiagnosticReport.conclusionCode</b><br> 2820 * </p> 2821 */ 2822 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONCLUSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2823 SP_CONCLUSION); 2824 2825 /** 2826 * Search parameter: <b>based-on</b> 2827 * <p> 2828 * Description: <b>Reference to the service request.</b><br> 2829 * Type: <b>reference</b><br> 2830 * Path: <b>DiagnosticReport.basedOn</b><br> 2831 * </p> 2832 */ 2833 @SearchParamDefinition(name = "based-on", path = "DiagnosticReport.basedOn", description = "Reference to the service request.", type = "reference", target = { 2834 CarePlan.class, ImmunizationRecommendation.class, MedicationRequest.class, NutritionOrder.class, 2835 ServiceRequest.class }) 2836 public static final String SP_BASED_ON = "based-on"; 2837 /** 2838 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 2839 * <p> 2840 * Description: <b>Reference to the service request.</b><br> 2841 * Type: <b>reference</b><br> 2842 * Path: <b>DiagnosticReport.basedOn</b><br> 2843 * </p> 2844 */ 2845 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2846 SP_BASED_ON); 2847 2848 /** 2849 * Constant for fluent queries to be used to add include statements. Specifies 2850 * the path value of "<b>DiagnosticReport:based-on</b>". 2851 */ 2852 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include( 2853 "DiagnosticReport:based-on").toLocked(); 2854 2855 /** 2856 * Search parameter: <b>patient</b> 2857 * <p> 2858 * Description: <b>The subject of the report if a patient</b><br> 2859 * Type: <b>reference</b><br> 2860 * Path: <b>DiagnosticReport.subject</b><br> 2861 * </p> 2862 */ 2863 @SearchParamDefinition(name = "patient", path = "DiagnosticReport.subject.where(resolve() is Patient)", description = "The subject of the report if a patient", type = "reference", target = { 2864 Patient.class }) 2865 public static final String SP_PATIENT = "patient"; 2866 /** 2867 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2868 * <p> 2869 * Description: <b>The subject of the report if a patient</b><br> 2870 * Type: <b>reference</b><br> 2871 * Path: <b>DiagnosticReport.subject</b><br> 2872 * </p> 2873 */ 2874 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2875 SP_PATIENT); 2876 2877 /** 2878 * Constant for fluent queries to be used to add include statements. Specifies 2879 * the path value of "<b>DiagnosticReport:patient</b>". 2880 */ 2881 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 2882 "DiagnosticReport:patient").toLocked(); 2883 2884 /** 2885 * Search parameter: <b>specimen</b> 2886 * <p> 2887 * Description: <b>The specimen details</b><br> 2888 * Type: <b>reference</b><br> 2889 * Path: <b>DiagnosticReport.specimen</b><br> 2890 * </p> 2891 */ 2892 @SearchParamDefinition(name = "specimen", path = "DiagnosticReport.specimen", description = "The specimen details", type = "reference", target = { 2893 Specimen.class }) 2894 public static final String SP_SPECIMEN = "specimen"; 2895 /** 2896 * <b>Fluent Client</b> search parameter constant for <b>specimen</b> 2897 * <p> 2898 * Description: <b>The specimen details</b><br> 2899 * Type: <b>reference</b><br> 2900 * Path: <b>DiagnosticReport.specimen</b><br> 2901 * </p> 2902 */ 2903 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SPECIMEN = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2904 SP_SPECIMEN); 2905 2906 /** 2907 * Constant for fluent queries to be used to add include statements. Specifies 2908 * the path value of "<b>DiagnosticReport:specimen</b>". 2909 */ 2910 public static final ca.uhn.fhir.model.api.Include INCLUDE_SPECIMEN = new ca.uhn.fhir.model.api.Include( 2911 "DiagnosticReport:specimen").toLocked(); 2912 2913 /** 2914 * Search parameter: <b>issued</b> 2915 * <p> 2916 * Description: <b>When the report was issued</b><br> 2917 * Type: <b>date</b><br> 2918 * Path: <b>DiagnosticReport.issued</b><br> 2919 * </p> 2920 */ 2921 @SearchParamDefinition(name = "issued", path = "DiagnosticReport.issued", description = "When the report was issued", type = "date") 2922 public static final String SP_ISSUED = "issued"; 2923 /** 2924 * <b>Fluent Client</b> search parameter constant for <b>issued</b> 2925 * <p> 2926 * Description: <b>When the report was issued</b><br> 2927 * Type: <b>date</b><br> 2928 * Path: <b>DiagnosticReport.issued</b><br> 2929 * </p> 2930 */ 2931 public static final ca.uhn.fhir.rest.gclient.DateClientParam ISSUED = new ca.uhn.fhir.rest.gclient.DateClientParam( 2932 SP_ISSUED); 2933 2934 /** 2935 * Search parameter: <b>category</b> 2936 * <p> 2937 * Description: <b>Which diagnostic discipline/department created the 2938 * report</b><br> 2939 * Type: <b>token</b><br> 2940 * Path: <b>DiagnosticReport.category</b><br> 2941 * </p> 2942 */ 2943 @SearchParamDefinition(name = "category", path = "DiagnosticReport.category", description = "Which diagnostic discipline/department created the report", type = "token") 2944 public static final String SP_CATEGORY = "category"; 2945 /** 2946 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2947 * <p> 2948 * Description: <b>Which diagnostic discipline/department created the 2949 * report</b><br> 2950 * Type: <b>token</b><br> 2951 * Path: <b>DiagnosticReport.category</b><br> 2952 * </p> 2953 */ 2954 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2955 SP_CATEGORY); 2956 2957 /** 2958 * Search parameter: <b>results-interpreter</b> 2959 * <p> 2960 * Description: <b>Who was the source of the report</b><br> 2961 * Type: <b>reference</b><br> 2962 * Path: <b>DiagnosticReport.resultsInterpreter</b><br> 2963 * </p> 2964 */ 2965 @SearchParamDefinition(name = "results-interpreter", path = "DiagnosticReport.resultsInterpreter", description = "Who was the source of the report", type = "reference", target = { 2966 CareTeam.class, Organization.class, Practitioner.class, PractitionerRole.class }) 2967 public static final String SP_RESULTS_INTERPRETER = "results-interpreter"; 2968 /** 2969 * <b>Fluent Client</b> search parameter constant for <b>results-interpreter</b> 2970 * <p> 2971 * Description: <b>Who was the source of the report</b><br> 2972 * Type: <b>reference</b><br> 2973 * Path: <b>DiagnosticReport.resultsInterpreter</b><br> 2974 * </p> 2975 */ 2976 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESULTS_INTERPRETER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2977 SP_RESULTS_INTERPRETER); 2978 2979 /** 2980 * Constant for fluent queries to be used to add include statements. Specifies 2981 * the path value of "<b>DiagnosticReport:results-interpreter</b>". 2982 */ 2983 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESULTS_INTERPRETER = new ca.uhn.fhir.model.api.Include( 2984 "DiagnosticReport:results-interpreter").toLocked(); 2985 2986 /** 2987 * Search parameter: <b>status</b> 2988 * <p> 2989 * Description: <b>The status of the report</b><br> 2990 * Type: <b>token</b><br> 2991 * Path: <b>DiagnosticReport.status</b><br> 2992 * </p> 2993 */ 2994 @SearchParamDefinition(name = "status", path = "DiagnosticReport.status", description = "The status of the report", type = "token") 2995 public static final String SP_STATUS = "status"; 2996 /** 2997 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2998 * <p> 2999 * Description: <b>The status of the report</b><br> 3000 * Type: <b>token</b><br> 3001 * Path: <b>DiagnosticReport.status</b><br> 3002 * </p> 3003 */ 3004 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3005 SP_STATUS); 3006 3007}