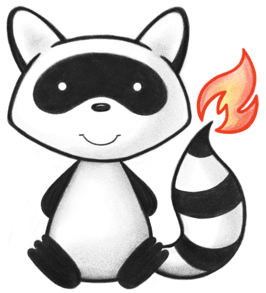
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.DocumentReferenceStatus; 040import org.hl7.fhir.r4.model.Enumerations.DocumentReferenceStatusEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048 049/** 050 * A reference to a document of any kind for any purpose. Provides metadata 051 * about the document so that the document can be discovered and managed. The 052 * scope of a document is any seralized object with a mime-type, so includes 053 * formal patient centric documents (CDA), cliical notes, scanned paper, and 054 * non-patient specific documents like policy text. 055 */ 056@ResourceDef(name = "DocumentReference", profile = "http://hl7.org/fhir/StructureDefinition/DocumentReference") 057public class DocumentReference extends DomainResource { 058 059 public enum ReferredDocumentStatus { 060 /** 061 * This is a preliminary composition or document (also known as initial or 062 * interim). The content may be incomplete or unverified. 063 */ 064 PRELIMINARY, 065 /** 066 * This version of the composition is complete and verified by an appropriate 067 * person and no further work is planned. Any subsequent updates would be on a 068 * new version of the composition. 069 */ 070 FINAL, 071 /** 072 * The composition content or the referenced resources have been modified 073 * (edited or added to) subsequent to being released as "final" and the 074 * composition is complete and verified by an authorized person. 075 */ 076 AMENDED, 077 /** 078 * The composition or document was originally created/issued in error, and this 079 * is an amendment that marks that the entire series should not be considered as 080 * valid. 081 */ 082 ENTEREDINERROR, 083 /** 084 * added to help the parsers with the generic types 085 */ 086 NULL; 087 088 public static ReferredDocumentStatus fromCode(String codeString) throws FHIRException { 089 if (codeString == null || "".equals(codeString)) 090 return null; 091 if ("preliminary".equals(codeString)) 092 return PRELIMINARY; 093 if ("final".equals(codeString)) 094 return FINAL; 095 if ("amended".equals(codeString)) 096 return AMENDED; 097 if ("entered-in-error".equals(codeString)) 098 return ENTEREDINERROR; 099 if (Configuration.isAcceptInvalidEnums()) 100 return null; 101 else 102 throw new FHIRException("Unknown ReferredDocumentStatus code '" + codeString + "'"); 103 } 104 105 public String toCode() { 106 switch (this) { 107 case PRELIMINARY: 108 return "preliminary"; 109 case FINAL: 110 return "final"; 111 case AMENDED: 112 return "amended"; 113 case ENTEREDINERROR: 114 return "entered-in-error"; 115 case NULL: 116 return null; 117 default: 118 return "?"; 119 } 120 } 121 122 public String getSystem() { 123 switch (this) { 124 case PRELIMINARY: 125 return "http://hl7.org/fhir/composition-status"; 126 case FINAL: 127 return "http://hl7.org/fhir/composition-status"; 128 case AMENDED: 129 return "http://hl7.org/fhir/composition-status"; 130 case ENTEREDINERROR: 131 return "http://hl7.org/fhir/composition-status"; 132 case NULL: 133 return null; 134 default: 135 return "?"; 136 } 137 } 138 139 public String getDefinition() { 140 switch (this) { 141 case PRELIMINARY: 142 return "This is a preliminary composition or document (also known as initial or interim). The content may be incomplete or unverified."; 143 case FINAL: 144 return "This version of the composition is complete and verified by an appropriate person and no further work is planned. Any subsequent updates would be on a new version of the composition."; 145 case AMENDED: 146 return "The composition content or the referenced resources have been modified (edited or added to) subsequent to being released as \"final\" and the composition is complete and verified by an authorized person."; 147 case ENTEREDINERROR: 148 return "The composition or document was originally created/issued in error, and this is an amendment that marks that the entire series should not be considered as valid."; 149 case NULL: 150 return null; 151 default: 152 return "?"; 153 } 154 } 155 156 public String getDisplay() { 157 switch (this) { 158 case PRELIMINARY: 159 return "Preliminary"; 160 case FINAL: 161 return "Final"; 162 case AMENDED: 163 return "Amended"; 164 case ENTEREDINERROR: 165 return "Entered in Error"; 166 case NULL: 167 return null; 168 default: 169 return "?"; 170 } 171 } 172 } 173 174 public static class ReferredDocumentStatusEnumFactory implements EnumFactory<ReferredDocumentStatus> { 175 public ReferredDocumentStatus fromCode(String codeString) throws IllegalArgumentException { 176 if (codeString == null || "".equals(codeString)) 177 return null; 178 if ("preliminary".equals(codeString)) 179 return ReferredDocumentStatus.PRELIMINARY; 180 if ("final".equals(codeString)) 181 return ReferredDocumentStatus.FINAL; 182 if ("amended".equals(codeString)) 183 return ReferredDocumentStatus.AMENDED; 184 if ("entered-in-error".equals(codeString)) 185 return ReferredDocumentStatus.ENTEREDINERROR; 186 throw new IllegalArgumentException("Unknown ReferredDocumentStatus code '" + codeString + "'"); 187 } 188 189 public Enumeration<ReferredDocumentStatus> fromType(PrimitiveType<?> code) throws FHIRException { 190 if (code == null) 191 return null; 192 if (code.isEmpty()) 193 return new Enumeration<ReferredDocumentStatus>(this, ReferredDocumentStatus.NULL, code); 194 String codeString = code.asStringValue(); 195 if (codeString == null || "".equals(codeString)) 196 return new Enumeration<ReferredDocumentStatus>(this, ReferredDocumentStatus.NULL, code); 197 if ("preliminary".equals(codeString)) 198 return new Enumeration<ReferredDocumentStatus>(this, ReferredDocumentStatus.PRELIMINARY, code); 199 if ("final".equals(codeString)) 200 return new Enumeration<ReferredDocumentStatus>(this, ReferredDocumentStatus.FINAL, code); 201 if ("amended".equals(codeString)) 202 return new Enumeration<ReferredDocumentStatus>(this, ReferredDocumentStatus.AMENDED, code); 203 if ("entered-in-error".equals(codeString)) 204 return new Enumeration<ReferredDocumentStatus>(this, ReferredDocumentStatus.ENTEREDINERROR, code); 205 throw new FHIRException("Unknown ReferredDocumentStatus code '" + codeString + "'"); 206 } 207 208 public String toCode(ReferredDocumentStatus code) { 209 if (code == ReferredDocumentStatus.NULL) 210 return null; 211 if (code == ReferredDocumentStatus.PRELIMINARY) 212 return "preliminary"; 213 if (code == ReferredDocumentStatus.FINAL) 214 return "final"; 215 if (code == ReferredDocumentStatus.AMENDED) 216 return "amended"; 217 if (code == ReferredDocumentStatus.ENTEREDINERROR) 218 return "entered-in-error"; 219 return "?"; 220 } 221 222 public String toSystem(ReferredDocumentStatus code) { 223 return code.getSystem(); 224 } 225 } 226 227 public enum DocumentRelationshipType { 228 /** 229 * This document logically replaces or supersedes the target document. 230 */ 231 REPLACES, 232 /** 233 * This document was generated by transforming the target document (e.g. format 234 * or language conversion). 235 */ 236 TRANSFORMS, 237 /** 238 * This document is a signature of the target document. 239 */ 240 SIGNS, 241 /** 242 * This document adds additional information to the target document. 243 */ 244 APPENDS, 245 /** 246 * added to help the parsers with the generic types 247 */ 248 NULL; 249 250 public static DocumentRelationshipType fromCode(String codeString) throws FHIRException { 251 if (codeString == null || "".equals(codeString)) 252 return null; 253 if ("replaces".equals(codeString)) 254 return REPLACES; 255 if ("transforms".equals(codeString)) 256 return TRANSFORMS; 257 if ("signs".equals(codeString)) 258 return SIGNS; 259 if ("appends".equals(codeString)) 260 return APPENDS; 261 if (Configuration.isAcceptInvalidEnums()) 262 return null; 263 else 264 throw new FHIRException("Unknown DocumentRelationshipType code '" + codeString + "'"); 265 } 266 267 public String toCode() { 268 switch (this) { 269 case REPLACES: 270 return "replaces"; 271 case TRANSFORMS: 272 return "transforms"; 273 case SIGNS: 274 return "signs"; 275 case APPENDS: 276 return "appends"; 277 case NULL: 278 return null; 279 default: 280 return "?"; 281 } 282 } 283 284 public String getSystem() { 285 switch (this) { 286 case REPLACES: 287 return "http://hl7.org/fhir/document-relationship-type"; 288 case TRANSFORMS: 289 return "http://hl7.org/fhir/document-relationship-type"; 290 case SIGNS: 291 return "http://hl7.org/fhir/document-relationship-type"; 292 case APPENDS: 293 return "http://hl7.org/fhir/document-relationship-type"; 294 case NULL: 295 return null; 296 default: 297 return "?"; 298 } 299 } 300 301 public String getDefinition() { 302 switch (this) { 303 case REPLACES: 304 return "This document logically replaces or supersedes the target document."; 305 case TRANSFORMS: 306 return "This document was generated by transforming the target document (e.g. format or language conversion)."; 307 case SIGNS: 308 return "This document is a signature of the target document."; 309 case APPENDS: 310 return "This document adds additional information to the target document."; 311 case NULL: 312 return null; 313 default: 314 return "?"; 315 } 316 } 317 318 public String getDisplay() { 319 switch (this) { 320 case REPLACES: 321 return "Replaces"; 322 case TRANSFORMS: 323 return "Transforms"; 324 case SIGNS: 325 return "Signs"; 326 case APPENDS: 327 return "Appends"; 328 case NULL: 329 return null; 330 default: 331 return "?"; 332 } 333 } 334 } 335 336 public static class DocumentRelationshipTypeEnumFactory implements EnumFactory<DocumentRelationshipType> { 337 public DocumentRelationshipType fromCode(String codeString) throws IllegalArgumentException { 338 if (codeString == null || "".equals(codeString)) 339 if (codeString == null || "".equals(codeString)) 340 return null; 341 if ("replaces".equals(codeString)) 342 return DocumentRelationshipType.REPLACES; 343 if ("transforms".equals(codeString)) 344 return DocumentRelationshipType.TRANSFORMS; 345 if ("signs".equals(codeString)) 346 return DocumentRelationshipType.SIGNS; 347 if ("appends".equals(codeString)) 348 return DocumentRelationshipType.APPENDS; 349 throw new IllegalArgumentException("Unknown DocumentRelationshipType code '" + codeString + "'"); 350 } 351 352 public Enumeration<DocumentRelationshipType> fromType(PrimitiveType<?> code) throws FHIRException { 353 if (code == null) 354 return null; 355 if (code.isEmpty()) 356 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.NULL, code); 357 String codeString = code.asStringValue(); 358 if (codeString == null || "".equals(codeString)) 359 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.NULL, code); 360 if ("replaces".equals(codeString)) 361 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.REPLACES, code); 362 if ("transforms".equals(codeString)) 363 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.TRANSFORMS, code); 364 if ("signs".equals(codeString)) 365 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.SIGNS, code); 366 if ("appends".equals(codeString)) 367 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.APPENDS, code); 368 throw new FHIRException("Unknown DocumentRelationshipType code '" + codeString + "'"); 369 } 370 371 public String toCode(DocumentRelationshipType code) { 372 if (code == DocumentRelationshipType.NULL) 373 return null; 374 if (code == DocumentRelationshipType.REPLACES) 375 return "replaces"; 376 if (code == DocumentRelationshipType.TRANSFORMS) 377 return "transforms"; 378 if (code == DocumentRelationshipType.SIGNS) 379 return "signs"; 380 if (code == DocumentRelationshipType.APPENDS) 381 return "appends"; 382 return "?"; 383 } 384 385 public String toSystem(DocumentRelationshipType code) { 386 return code.getSystem(); 387 } 388 } 389 390 @Block() 391 public static class DocumentReferenceRelatesToComponent extends BackboneElement implements IBaseBackboneElement { 392 /** 393 * The type of relationship that this document has with anther document. 394 */ 395 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 396 @Description(shortDefinition = "replaces | transforms | signs | appends", formalDefinition = "The type of relationship that this document has with anther document.") 397 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/document-relationship-type") 398 protected Enumeration<DocumentRelationshipType> code; 399 400 /** 401 * The target document of this relationship. 402 */ 403 @Child(name = "target", type = { 404 DocumentReference.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 405 @Description(shortDefinition = "Target of the relationship", formalDefinition = "The target document of this relationship.") 406 protected Reference target; 407 408 /** 409 * The actual object that is the target of the reference (The target document of 410 * this relationship.) 411 */ 412 protected DocumentReference targetTarget; 413 414 private static final long serialVersionUID = -347257495L; 415 416 /** 417 * Constructor 418 */ 419 public DocumentReferenceRelatesToComponent() { 420 super(); 421 } 422 423 /** 424 * Constructor 425 */ 426 public DocumentReferenceRelatesToComponent(Enumeration<DocumentRelationshipType> code, Reference target) { 427 super(); 428 this.code = code; 429 this.target = target; 430 } 431 432 /** 433 * @return {@link #code} (The type of relationship that this document has with 434 * anther document.). This is the underlying object with id, value and 435 * extensions. The accessor "getCode" gives direct access to the value 436 */ 437 public Enumeration<DocumentRelationshipType> getCodeElement() { 438 if (this.code == null) 439 if (Configuration.errorOnAutoCreate()) 440 throw new Error("Attempt to auto-create DocumentReferenceRelatesToComponent.code"); 441 else if (Configuration.doAutoCreate()) 442 this.code = new Enumeration<DocumentRelationshipType>(new DocumentRelationshipTypeEnumFactory()); // bb 443 return this.code; 444 } 445 446 public boolean hasCodeElement() { 447 return this.code != null && !this.code.isEmpty(); 448 } 449 450 public boolean hasCode() { 451 return this.code != null && !this.code.isEmpty(); 452 } 453 454 /** 455 * @param value {@link #code} (The type of relationship that this document has 456 * with anther document.). This is the underlying object with id, 457 * value and extensions. The accessor "getCode" gives direct access 458 * to the value 459 */ 460 public DocumentReferenceRelatesToComponent setCodeElement(Enumeration<DocumentRelationshipType> value) { 461 this.code = value; 462 return this; 463 } 464 465 /** 466 * @return The type of relationship that this document has with anther document. 467 */ 468 public DocumentRelationshipType getCode() { 469 return this.code == null ? null : this.code.getValue(); 470 } 471 472 /** 473 * @param value The type of relationship that this document has with anther 474 * document. 475 */ 476 public DocumentReferenceRelatesToComponent setCode(DocumentRelationshipType value) { 477 if (this.code == null) 478 this.code = new Enumeration<DocumentRelationshipType>(new DocumentRelationshipTypeEnumFactory()); 479 this.code.setValue(value); 480 return this; 481 } 482 483 /** 484 * @return {@link #target} (The target document of this relationship.) 485 */ 486 public Reference getTarget() { 487 if (this.target == null) 488 if (Configuration.errorOnAutoCreate()) 489 throw new Error("Attempt to auto-create DocumentReferenceRelatesToComponent.target"); 490 else if (Configuration.doAutoCreate()) 491 this.target = new Reference(); // cc 492 return this.target; 493 } 494 495 public boolean hasTarget() { 496 return this.target != null && !this.target.isEmpty(); 497 } 498 499 /** 500 * @param value {@link #target} (The target document of this relationship.) 501 */ 502 public DocumentReferenceRelatesToComponent setTarget(Reference value) { 503 this.target = value; 504 return this; 505 } 506 507 /** 508 * @return {@link #target} The actual object that is the target of the 509 * reference. The reference library doesn't populate this, but you can 510 * use it to hold the resource if you resolve it. (The target document 511 * of this relationship.) 512 */ 513 public DocumentReference getTargetTarget() { 514 if (this.targetTarget == null) 515 if (Configuration.errorOnAutoCreate()) 516 throw new Error("Attempt to auto-create DocumentReferenceRelatesToComponent.target"); 517 else if (Configuration.doAutoCreate()) 518 this.targetTarget = new DocumentReference(); // aa 519 return this.targetTarget; 520 } 521 522 /** 523 * @param value {@link #target} The actual object that is the target of the 524 * reference. The reference library doesn't use these, but you can 525 * use it to hold the resource if you resolve it. (The target 526 * document of this relationship.) 527 */ 528 public DocumentReferenceRelatesToComponent setTargetTarget(DocumentReference value) { 529 this.targetTarget = value; 530 return this; 531 } 532 533 protected void listChildren(List<Property> children) { 534 super.listChildren(children); 535 children.add(new Property("code", "code", "The type of relationship that this document has with anther document.", 536 0, 1, code)); 537 children.add(new Property("target", "Reference(DocumentReference)", "The target document of this relationship.", 538 0, 1, target)); 539 } 540 541 @Override 542 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 543 switch (_hash) { 544 case 3059181: 545 /* code */ return new Property("code", "code", 546 "The type of relationship that this document has with anther document.", 0, 1, code); 547 case -880905839: 548 /* target */ return new Property("target", "Reference(DocumentReference)", 549 "The target document of this relationship.", 0, 1, target); 550 default: 551 return super.getNamedProperty(_hash, _name, _checkValid); 552 } 553 554 } 555 556 @Override 557 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 558 switch (hash) { 559 case 3059181: 560 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // Enumeration<DocumentRelationshipType> 561 case -880905839: 562 /* target */ return this.target == null ? new Base[0] : new Base[] { this.target }; // Reference 563 default: 564 return super.getProperty(hash, name, checkValid); 565 } 566 567 } 568 569 @Override 570 public Base setProperty(int hash, String name, Base value) throws FHIRException { 571 switch (hash) { 572 case 3059181: // code 573 value = new DocumentRelationshipTypeEnumFactory().fromType(castToCode(value)); 574 this.code = (Enumeration) value; // Enumeration<DocumentRelationshipType> 575 return value; 576 case -880905839: // target 577 this.target = castToReference(value); // Reference 578 return value; 579 default: 580 return super.setProperty(hash, name, value); 581 } 582 583 } 584 585 @Override 586 public Base setProperty(String name, Base value) throws FHIRException { 587 if (name.equals("code")) { 588 value = new DocumentRelationshipTypeEnumFactory().fromType(castToCode(value)); 589 this.code = (Enumeration) value; // Enumeration<DocumentRelationshipType> 590 } else if (name.equals("target")) { 591 this.target = castToReference(value); // Reference 592 } else 593 return super.setProperty(name, value); 594 return value; 595 } 596 597 @Override 598 public void removeChild(String name, Base value) throws FHIRException { 599 if (name.equals("code")) { 600 this.code = null; 601 } else if (name.equals("target")) { 602 this.target = null; 603 } else 604 super.removeChild(name, value); 605 606 } 607 608 @Override 609 public Base makeProperty(int hash, String name) throws FHIRException { 610 switch (hash) { 611 case 3059181: 612 return getCodeElement(); 613 case -880905839: 614 return getTarget(); 615 default: 616 return super.makeProperty(hash, name); 617 } 618 619 } 620 621 @Override 622 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 623 switch (hash) { 624 case 3059181: 625 /* code */ return new String[] { "code" }; 626 case -880905839: 627 /* target */ return new String[] { "Reference" }; 628 default: 629 return super.getTypesForProperty(hash, name); 630 } 631 632 } 633 634 @Override 635 public Base addChild(String name) throws FHIRException { 636 if (name.equals("code")) { 637 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.code"); 638 } else if (name.equals("target")) { 639 this.target = new Reference(); 640 return this.target; 641 } else 642 return super.addChild(name); 643 } 644 645 public DocumentReferenceRelatesToComponent copy() { 646 DocumentReferenceRelatesToComponent dst = new DocumentReferenceRelatesToComponent(); 647 copyValues(dst); 648 return dst; 649 } 650 651 public void copyValues(DocumentReferenceRelatesToComponent dst) { 652 super.copyValues(dst); 653 dst.code = code == null ? null : code.copy(); 654 dst.target = target == null ? null : target.copy(); 655 } 656 657 @Override 658 public boolean equalsDeep(Base other_) { 659 if (!super.equalsDeep(other_)) 660 return false; 661 if (!(other_ instanceof DocumentReferenceRelatesToComponent)) 662 return false; 663 DocumentReferenceRelatesToComponent o = (DocumentReferenceRelatesToComponent) other_; 664 return compareDeep(code, o.code, true) && compareDeep(target, o.target, true); 665 } 666 667 @Override 668 public boolean equalsShallow(Base other_) { 669 if (!super.equalsShallow(other_)) 670 return false; 671 if (!(other_ instanceof DocumentReferenceRelatesToComponent)) 672 return false; 673 DocumentReferenceRelatesToComponent o = (DocumentReferenceRelatesToComponent) other_; 674 return compareValues(code, o.code, true); 675 } 676 677 public boolean isEmpty() { 678 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, target); 679 } 680 681 public String fhirType() { 682 return "DocumentReference.relatesTo"; 683 684 } 685 686 } 687 688 @Block() 689 public static class DocumentReferenceContentComponent extends BackboneElement implements IBaseBackboneElement { 690 /** 691 * The document or URL of the document along with critical metadata to prove 692 * content has integrity. 693 */ 694 @Child(name = "attachment", type = { 695 Attachment.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 696 @Description(shortDefinition = "Where to access the document", formalDefinition = "The document or URL of the document along with critical metadata to prove content has integrity.") 697 protected Attachment attachment; 698 699 /** 700 * An identifier of the document encoding, structure, and template that the 701 * document conforms to beyond the base format indicated in the mimeType. 702 */ 703 @Child(name = "format", type = { Coding.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 704 @Description(shortDefinition = "Format/content rules for the document", formalDefinition = "An identifier of the document encoding, structure, and template that the document conforms to beyond the base format indicated in the mimeType.") 705 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/formatcodes") 706 protected Coding format; 707 708 private static final long serialVersionUID = -1313860217L; 709 710 /** 711 * Constructor 712 */ 713 public DocumentReferenceContentComponent() { 714 super(); 715 } 716 717 /** 718 * Constructor 719 */ 720 public DocumentReferenceContentComponent(Attachment attachment) { 721 super(); 722 this.attachment = attachment; 723 } 724 725 /** 726 * @return {@link #attachment} (The document or URL of the document along with 727 * critical metadata to prove content has integrity.) 728 */ 729 public Attachment getAttachment() { 730 if (this.attachment == null) 731 if (Configuration.errorOnAutoCreate()) 732 throw new Error("Attempt to auto-create DocumentReferenceContentComponent.attachment"); 733 else if (Configuration.doAutoCreate()) 734 this.attachment = new Attachment(); // cc 735 return this.attachment; 736 } 737 738 public boolean hasAttachment() { 739 return this.attachment != null && !this.attachment.isEmpty(); 740 } 741 742 /** 743 * @param value {@link #attachment} (The document or URL of the document along 744 * with critical metadata to prove content has integrity.) 745 */ 746 public DocumentReferenceContentComponent setAttachment(Attachment value) { 747 this.attachment = value; 748 return this; 749 } 750 751 /** 752 * @return {@link #format} (An identifier of the document encoding, structure, 753 * and template that the document conforms to beyond the base format 754 * indicated in the mimeType.) 755 */ 756 public Coding getFormat() { 757 if (this.format == null) 758 if (Configuration.errorOnAutoCreate()) 759 throw new Error("Attempt to auto-create DocumentReferenceContentComponent.format"); 760 else if (Configuration.doAutoCreate()) 761 this.format = new Coding(); // cc 762 return this.format; 763 } 764 765 public boolean hasFormat() { 766 return this.format != null && !this.format.isEmpty(); 767 } 768 769 /** 770 * @param value {@link #format} (An identifier of the document encoding, 771 * structure, and template that the document conforms to beyond the 772 * base format indicated in the mimeType.) 773 */ 774 public DocumentReferenceContentComponent setFormat(Coding value) { 775 this.format = value; 776 return this; 777 } 778 779 protected void listChildren(List<Property> children) { 780 super.listChildren(children); 781 children.add(new Property("attachment", "Attachment", 782 "The document or URL of the document along with critical metadata to prove content has integrity.", 0, 1, 783 attachment)); 784 children.add(new Property("format", "Coding", 785 "An identifier of the document encoding, structure, and template that the document conforms to beyond the base format indicated in the mimeType.", 786 0, 1, format)); 787 } 788 789 @Override 790 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 791 switch (_hash) { 792 case -1963501277: 793 /* attachment */ return new Property("attachment", "Attachment", 794 "The document or URL of the document along with critical metadata to prove content has integrity.", 0, 1, 795 attachment); 796 case -1268779017: 797 /* format */ return new Property("format", "Coding", 798 "An identifier of the document encoding, structure, and template that the document conforms to beyond the base format indicated in the mimeType.", 799 0, 1, format); 800 default: 801 return super.getNamedProperty(_hash, _name, _checkValid); 802 } 803 804 } 805 806 @Override 807 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 808 switch (hash) { 809 case -1963501277: 810 /* attachment */ return this.attachment == null ? new Base[0] : new Base[] { this.attachment }; // Attachment 811 case -1268779017: 812 /* format */ return this.format == null ? new Base[0] : new Base[] { this.format }; // Coding 813 default: 814 return super.getProperty(hash, name, checkValid); 815 } 816 817 } 818 819 @Override 820 public Base setProperty(int hash, String name, Base value) throws FHIRException { 821 switch (hash) { 822 case -1963501277: // attachment 823 this.attachment = castToAttachment(value); // Attachment 824 return value; 825 case -1268779017: // format 826 this.format = castToCoding(value); // Coding 827 return value; 828 default: 829 return super.setProperty(hash, name, value); 830 } 831 832 } 833 834 @Override 835 public Base setProperty(String name, Base value) throws FHIRException { 836 if (name.equals("attachment")) { 837 this.attachment = castToAttachment(value); // Attachment 838 } else if (name.equals("format")) { 839 this.format = castToCoding(value); // Coding 840 } else 841 return super.setProperty(name, value); 842 return value; 843 } 844 845 @Override 846 public void removeChild(String name, Base value) throws FHIRException { 847 if (name.equals("attachment")) { 848 this.attachment = null; 849 } else if (name.equals("format")) { 850 this.format = null; 851 } else 852 super.removeChild(name, value); 853 854 } 855 856 @Override 857 public Base makeProperty(int hash, String name) throws FHIRException { 858 switch (hash) { 859 case -1963501277: 860 return getAttachment(); 861 case -1268779017: 862 return getFormat(); 863 default: 864 return super.makeProperty(hash, name); 865 } 866 867 } 868 869 @Override 870 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 871 switch (hash) { 872 case -1963501277: 873 /* attachment */ return new String[] { "Attachment" }; 874 case -1268779017: 875 /* format */ return new String[] { "Coding" }; 876 default: 877 return super.getTypesForProperty(hash, name); 878 } 879 880 } 881 882 @Override 883 public Base addChild(String name) throws FHIRException { 884 if (name.equals("attachment")) { 885 this.attachment = new Attachment(); 886 return this.attachment; 887 } else if (name.equals("format")) { 888 this.format = new Coding(); 889 return this.format; 890 } else 891 return super.addChild(name); 892 } 893 894 public DocumentReferenceContentComponent copy() { 895 DocumentReferenceContentComponent dst = new DocumentReferenceContentComponent(); 896 copyValues(dst); 897 return dst; 898 } 899 900 public void copyValues(DocumentReferenceContentComponent dst) { 901 super.copyValues(dst); 902 dst.attachment = attachment == null ? null : attachment.copy(); 903 dst.format = format == null ? null : format.copy(); 904 } 905 906 @Override 907 public boolean equalsDeep(Base other_) { 908 if (!super.equalsDeep(other_)) 909 return false; 910 if (!(other_ instanceof DocumentReferenceContentComponent)) 911 return false; 912 DocumentReferenceContentComponent o = (DocumentReferenceContentComponent) other_; 913 return compareDeep(attachment, o.attachment, true) && compareDeep(format, o.format, true); 914 } 915 916 @Override 917 public boolean equalsShallow(Base other_) { 918 if (!super.equalsShallow(other_)) 919 return false; 920 if (!(other_ instanceof DocumentReferenceContentComponent)) 921 return false; 922 DocumentReferenceContentComponent o = (DocumentReferenceContentComponent) other_; 923 return true; 924 } 925 926 public boolean isEmpty() { 927 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(attachment, format); 928 } 929 930 public String fhirType() { 931 return "DocumentReference.content"; 932 933 } 934 935 } 936 937 @Block() 938 public static class DocumentReferenceContextComponent extends BackboneElement implements IBaseBackboneElement { 939 /** 940 * Describes the clinical encounter or type of care that the document content is 941 * associated with. 942 */ 943 @Child(name = "encounter", type = { Encounter.class, 944 EpisodeOfCare.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 945 @Description(shortDefinition = "Context of the document content", formalDefinition = "Describes the clinical encounter or type of care that the document content is associated with.") 946 protected List<Reference> encounter; 947 /** 948 * The actual objects that are the target of the reference (Describes the 949 * clinical encounter or type of care that the document content is associated 950 * with.) 951 */ 952 protected List<Resource> encounterTarget; 953 954 /** 955 * This list of codes represents the main clinical acts, such as a colonoscopy 956 * or an appendectomy, being documented. In some cases, the event is inherent in 957 * the type Code, such as a "History and Physical Report" in which the procedure 958 * being documented is necessarily a "History and Physical" act. 959 */ 960 @Child(name = "event", type = { 961 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 962 @Description(shortDefinition = "Main clinical acts documented", formalDefinition = "This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the type Code, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act.") 963 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ActCode") 964 protected List<CodeableConcept> event; 965 966 /** 967 * The time period over which the service that is described by the document was 968 * provided. 969 */ 970 @Child(name = "period", type = { Period.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 971 @Description(shortDefinition = "Time of service that is being documented", formalDefinition = "The time period over which the service that is described by the document was provided.") 972 protected Period period; 973 974 /** 975 * The kind of facility where the patient was seen. 976 */ 977 @Child(name = "facilityType", type = { 978 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 979 @Description(shortDefinition = "Kind of facility where patient was seen", formalDefinition = "The kind of facility where the patient was seen.") 980 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/c80-facilitycodes") 981 protected CodeableConcept facilityType; 982 983 /** 984 * This property may convey specifics about the practice setting where the 985 * content was created, often reflecting the clinical specialty. 986 */ 987 @Child(name = "practiceSetting", type = { 988 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 989 @Description(shortDefinition = "Additional details about where the content was created (e.g. clinical specialty)", formalDefinition = "This property may convey specifics about the practice setting where the content was created, often reflecting the clinical specialty.") 990 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/c80-practice-codes") 991 protected CodeableConcept practiceSetting; 992 993 /** 994 * The Patient Information as known when the document was published. May be a 995 * reference to a version specific, or contained. 996 */ 997 @Child(name = "sourcePatientInfo", type = { 998 Patient.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 999 @Description(shortDefinition = "Patient demographics from source", formalDefinition = "The Patient Information as known when the document was published. May be a reference to a version specific, or contained.") 1000 protected Reference sourcePatientInfo; 1001 1002 /** 1003 * The actual object that is the target of the reference (The Patient 1004 * Information as known when the document was published. May be a reference to a 1005 * version specific, or contained.) 1006 */ 1007 protected Patient sourcePatientInfoTarget; 1008 1009 /** 1010 * Related identifiers or resources associated with the DocumentReference. 1011 */ 1012 @Child(name = "related", type = { 1013 Reference.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1014 @Description(shortDefinition = "Related identifiers or resources", formalDefinition = "Related identifiers or resources associated with the DocumentReference.") 1015 protected List<Reference> related; 1016 /** 1017 * The actual objects that are the target of the reference (Related identifiers 1018 * or resources associated with the DocumentReference.) 1019 */ 1020 protected List<Resource> relatedTarget; 1021 1022 private static final long serialVersionUID = 140463218L; 1023 1024 /** 1025 * Constructor 1026 */ 1027 public DocumentReferenceContextComponent() { 1028 super(); 1029 } 1030 1031 /** 1032 * @return {@link #encounter} (Describes the clinical encounter or type of care 1033 * that the document content is associated with.) 1034 */ 1035 public List<Reference> getEncounter() { 1036 if (this.encounter == null) 1037 this.encounter = new ArrayList<Reference>(); 1038 return this.encounter; 1039 } 1040 1041 /** 1042 * @return Returns a reference to <code>this</code> for easy method chaining 1043 */ 1044 public DocumentReferenceContextComponent setEncounter(List<Reference> theEncounter) { 1045 this.encounter = theEncounter; 1046 return this; 1047 } 1048 1049 public boolean hasEncounter() { 1050 if (this.encounter == null) 1051 return false; 1052 for (Reference item : this.encounter) 1053 if (!item.isEmpty()) 1054 return true; 1055 return false; 1056 } 1057 1058 public Reference addEncounter() { // 3 1059 Reference t = new Reference(); 1060 if (this.encounter == null) 1061 this.encounter = new ArrayList<Reference>(); 1062 this.encounter.add(t); 1063 return t; 1064 } 1065 1066 public DocumentReferenceContextComponent addEncounter(Reference t) { // 3 1067 if (t == null) 1068 return this; 1069 if (this.encounter == null) 1070 this.encounter = new ArrayList<Reference>(); 1071 this.encounter.add(t); 1072 return this; 1073 } 1074 1075 /** 1076 * @return The first repetition of repeating field {@link #encounter}, creating 1077 * it if it does not already exist 1078 */ 1079 public Reference getEncounterFirstRep() { 1080 if (getEncounter().isEmpty()) { 1081 addEncounter(); 1082 } 1083 return getEncounter().get(0); 1084 } 1085 1086 /** 1087 * @return {@link #event} (This list of codes represents the main clinical acts, 1088 * such as a colonoscopy or an appendectomy, being documented. In some 1089 * cases, the event is inherent in the type Code, such as a "History and 1090 * Physical Report" in which the procedure being documented is 1091 * necessarily a "History and Physical" act.) 1092 */ 1093 public List<CodeableConcept> getEvent() { 1094 if (this.event == null) 1095 this.event = new ArrayList<CodeableConcept>(); 1096 return this.event; 1097 } 1098 1099 /** 1100 * @return Returns a reference to <code>this</code> for easy method chaining 1101 */ 1102 public DocumentReferenceContextComponent setEvent(List<CodeableConcept> theEvent) { 1103 this.event = theEvent; 1104 return this; 1105 } 1106 1107 public boolean hasEvent() { 1108 if (this.event == null) 1109 return false; 1110 for (CodeableConcept item : this.event) 1111 if (!item.isEmpty()) 1112 return true; 1113 return false; 1114 } 1115 1116 public CodeableConcept addEvent() { // 3 1117 CodeableConcept t = new CodeableConcept(); 1118 if (this.event == null) 1119 this.event = new ArrayList<CodeableConcept>(); 1120 this.event.add(t); 1121 return t; 1122 } 1123 1124 public DocumentReferenceContextComponent addEvent(CodeableConcept t) { // 3 1125 if (t == null) 1126 return this; 1127 if (this.event == null) 1128 this.event = new ArrayList<CodeableConcept>(); 1129 this.event.add(t); 1130 return this; 1131 } 1132 1133 /** 1134 * @return The first repetition of repeating field {@link #event}, creating it 1135 * if it does not already exist 1136 */ 1137 public CodeableConcept getEventFirstRep() { 1138 if (getEvent().isEmpty()) { 1139 addEvent(); 1140 } 1141 return getEvent().get(0); 1142 } 1143 1144 /** 1145 * @return {@link #period} (The time period over which the service that is 1146 * described by the document was provided.) 1147 */ 1148 public Period getPeriod() { 1149 if (this.period == null) 1150 if (Configuration.errorOnAutoCreate()) 1151 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.period"); 1152 else if (Configuration.doAutoCreate()) 1153 this.period = new Period(); // cc 1154 return this.period; 1155 } 1156 1157 public boolean hasPeriod() { 1158 return this.period != null && !this.period.isEmpty(); 1159 } 1160 1161 /** 1162 * @param value {@link #period} (The time period over which the service that is 1163 * described by the document was provided.) 1164 */ 1165 public DocumentReferenceContextComponent setPeriod(Period value) { 1166 this.period = value; 1167 return this; 1168 } 1169 1170 /** 1171 * @return {@link #facilityType} (The kind of facility where the patient was 1172 * seen.) 1173 */ 1174 public CodeableConcept getFacilityType() { 1175 if (this.facilityType == null) 1176 if (Configuration.errorOnAutoCreate()) 1177 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.facilityType"); 1178 else if (Configuration.doAutoCreate()) 1179 this.facilityType = new CodeableConcept(); // cc 1180 return this.facilityType; 1181 } 1182 1183 public boolean hasFacilityType() { 1184 return this.facilityType != null && !this.facilityType.isEmpty(); 1185 } 1186 1187 /** 1188 * @param value {@link #facilityType} (The kind of facility where the patient 1189 * was seen.) 1190 */ 1191 public DocumentReferenceContextComponent setFacilityType(CodeableConcept value) { 1192 this.facilityType = value; 1193 return this; 1194 } 1195 1196 /** 1197 * @return {@link #practiceSetting} (This property may convey specifics about 1198 * the practice setting where the content was created, often reflecting 1199 * the clinical specialty.) 1200 */ 1201 public CodeableConcept getPracticeSetting() { 1202 if (this.practiceSetting == null) 1203 if (Configuration.errorOnAutoCreate()) 1204 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.practiceSetting"); 1205 else if (Configuration.doAutoCreate()) 1206 this.practiceSetting = new CodeableConcept(); // cc 1207 return this.practiceSetting; 1208 } 1209 1210 public boolean hasPracticeSetting() { 1211 return this.practiceSetting != null && !this.practiceSetting.isEmpty(); 1212 } 1213 1214 /** 1215 * @param value {@link #practiceSetting} (This property may convey specifics 1216 * about the practice setting where the content was created, often 1217 * reflecting the clinical specialty.) 1218 */ 1219 public DocumentReferenceContextComponent setPracticeSetting(CodeableConcept value) { 1220 this.practiceSetting = value; 1221 return this; 1222 } 1223 1224 /** 1225 * @return {@link #sourcePatientInfo} (The Patient Information as known when the 1226 * document was published. May be a reference to a version specific, or 1227 * contained.) 1228 */ 1229 public Reference getSourcePatientInfo() { 1230 if (this.sourcePatientInfo == null) 1231 if (Configuration.errorOnAutoCreate()) 1232 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.sourcePatientInfo"); 1233 else if (Configuration.doAutoCreate()) 1234 this.sourcePatientInfo = new Reference(); // cc 1235 return this.sourcePatientInfo; 1236 } 1237 1238 public boolean hasSourcePatientInfo() { 1239 return this.sourcePatientInfo != null && !this.sourcePatientInfo.isEmpty(); 1240 } 1241 1242 /** 1243 * @param value {@link #sourcePatientInfo} (The Patient Information as known 1244 * when the document was published. May be a reference to a version 1245 * specific, or contained.) 1246 */ 1247 public DocumentReferenceContextComponent setSourcePatientInfo(Reference value) { 1248 this.sourcePatientInfo = value; 1249 return this; 1250 } 1251 1252 /** 1253 * @return {@link #sourcePatientInfo} The actual object that is the target of 1254 * the reference. The reference library doesn't populate this, but you 1255 * can use it to hold the resource if you resolve it. (The Patient 1256 * Information as known when the document was published. May be a 1257 * reference to a version specific, or contained.) 1258 */ 1259 public Patient getSourcePatientInfoTarget() { 1260 if (this.sourcePatientInfoTarget == null) 1261 if (Configuration.errorOnAutoCreate()) 1262 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.sourcePatientInfo"); 1263 else if (Configuration.doAutoCreate()) 1264 this.sourcePatientInfoTarget = new Patient(); // aa 1265 return this.sourcePatientInfoTarget; 1266 } 1267 1268 /** 1269 * @param value {@link #sourcePatientInfo} The actual object that is the target 1270 * of the reference. The reference library doesn't use these, but 1271 * you can use it to hold the resource if you resolve it. (The 1272 * Patient Information as known when the document was published. 1273 * May be a reference to a version specific, or contained.) 1274 */ 1275 public DocumentReferenceContextComponent setSourcePatientInfoTarget(Patient value) { 1276 this.sourcePatientInfoTarget = value; 1277 return this; 1278 } 1279 1280 /** 1281 * @return {@link #related} (Related identifiers or resources associated with 1282 * the DocumentReference.) 1283 */ 1284 public List<Reference> getRelated() { 1285 if (this.related == null) 1286 this.related = new ArrayList<Reference>(); 1287 return this.related; 1288 } 1289 1290 /** 1291 * @return Returns a reference to <code>this</code> for easy method chaining 1292 */ 1293 public DocumentReferenceContextComponent setRelated(List<Reference> theRelated) { 1294 this.related = theRelated; 1295 return this; 1296 } 1297 1298 public boolean hasRelated() { 1299 if (this.related == null) 1300 return false; 1301 for (Reference item : this.related) 1302 if (!item.isEmpty()) 1303 return true; 1304 return false; 1305 } 1306 1307 public Reference addRelated() { // 3 1308 Reference t = new Reference(); 1309 if (this.related == null) 1310 this.related = new ArrayList<Reference>(); 1311 this.related.add(t); 1312 return t; 1313 } 1314 1315 public DocumentReferenceContextComponent addRelated(Reference t) { // 3 1316 if (t == null) 1317 return this; 1318 if (this.related == null) 1319 this.related = new ArrayList<Reference>(); 1320 this.related.add(t); 1321 return this; 1322 } 1323 1324 /** 1325 * @return The first repetition of repeating field {@link #related}, creating it 1326 * if it does not already exist 1327 */ 1328 public Reference getRelatedFirstRep() { 1329 if (getRelated().isEmpty()) { 1330 addRelated(); 1331 } 1332 return getRelated().get(0); 1333 } 1334 1335 protected void listChildren(List<Property> children) { 1336 super.listChildren(children); 1337 children.add(new Property("encounter", "Reference(Encounter|EpisodeOfCare)", 1338 "Describes the clinical encounter or type of care that the document content is associated with.", 0, 1339 java.lang.Integer.MAX_VALUE, encounter)); 1340 children.add(new Property("event", "CodeableConcept", 1341 "This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the type Code, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act.", 1342 0, java.lang.Integer.MAX_VALUE, event)); 1343 children.add(new Property("period", "Period", 1344 "The time period over which the service that is described by the document was provided.", 0, 1, period)); 1345 children.add(new Property("facilityType", "CodeableConcept", "The kind of facility where the patient was seen.", 1346 0, 1, facilityType)); 1347 children.add(new Property("practiceSetting", "CodeableConcept", 1348 "This property may convey specifics about the practice setting where the content was created, often reflecting the clinical specialty.", 1349 0, 1, practiceSetting)); 1350 children.add(new Property("sourcePatientInfo", "Reference(Patient)", 1351 "The Patient Information as known when the document was published. May be a reference to a version specific, or contained.", 1352 0, 1, sourcePatientInfo)); 1353 children.add(new Property("related", "Reference(Any)", 1354 "Related identifiers or resources associated with the DocumentReference.", 0, java.lang.Integer.MAX_VALUE, 1355 related)); 1356 } 1357 1358 @Override 1359 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1360 switch (_hash) { 1361 case 1524132147: 1362 /* encounter */ return new Property("encounter", "Reference(Encounter|EpisodeOfCare)", 1363 "Describes the clinical encounter or type of care that the document content is associated with.", 0, 1364 java.lang.Integer.MAX_VALUE, encounter); 1365 case 96891546: 1366 /* event */ return new Property("event", "CodeableConcept", 1367 "This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the type Code, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act.", 1368 0, java.lang.Integer.MAX_VALUE, event); 1369 case -991726143: 1370 /* period */ return new Property("period", "Period", 1371 "The time period over which the service that is described by the document was provided.", 0, 1, period); 1372 case 370698365: 1373 /* facilityType */ return new Property("facilityType", "CodeableConcept", 1374 "The kind of facility where the patient was seen.", 0, 1, facilityType); 1375 case 331373717: 1376 /* practiceSetting */ return new Property("practiceSetting", "CodeableConcept", 1377 "This property may convey specifics about the practice setting where the content was created, often reflecting the clinical specialty.", 1378 0, 1, practiceSetting); 1379 case 2031381048: 1380 /* sourcePatientInfo */ return new Property("sourcePatientInfo", "Reference(Patient)", 1381 "The Patient Information as known when the document was published. May be a reference to a version specific, or contained.", 1382 0, 1, sourcePatientInfo); 1383 case 1090493483: 1384 /* related */ return new Property("related", "Reference(Any)", 1385 "Related identifiers or resources associated with the DocumentReference.", 0, java.lang.Integer.MAX_VALUE, 1386 related); 1387 default: 1388 return super.getNamedProperty(_hash, _name, _checkValid); 1389 } 1390 1391 } 1392 1393 @Override 1394 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1395 switch (hash) { 1396 case 1524132147: 1397 /* encounter */ return this.encounter == null ? new Base[0] 1398 : this.encounter.toArray(new Base[this.encounter.size()]); // Reference 1399 case 96891546: 1400 /* event */ return this.event == null ? new Base[0] : this.event.toArray(new Base[this.event.size()]); // CodeableConcept 1401 case -991726143: 1402 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 1403 case 370698365: 1404 /* facilityType */ return this.facilityType == null ? new Base[0] : new Base[] { this.facilityType }; // CodeableConcept 1405 case 331373717: 1406 /* practiceSetting */ return this.practiceSetting == null ? new Base[0] : new Base[] { this.practiceSetting }; // CodeableConcept 1407 case 2031381048: 1408 /* sourcePatientInfo */ return this.sourcePatientInfo == null ? new Base[0] 1409 : new Base[] { this.sourcePatientInfo }; // Reference 1410 case 1090493483: 1411 /* related */ return this.related == null ? new Base[0] : this.related.toArray(new Base[this.related.size()]); // Reference 1412 default: 1413 return super.getProperty(hash, name, checkValid); 1414 } 1415 1416 } 1417 1418 @Override 1419 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1420 switch (hash) { 1421 case 1524132147: // encounter 1422 this.getEncounter().add(castToReference(value)); // Reference 1423 return value; 1424 case 96891546: // event 1425 this.getEvent().add(castToCodeableConcept(value)); // CodeableConcept 1426 return value; 1427 case -991726143: // period 1428 this.period = castToPeriod(value); // Period 1429 return value; 1430 case 370698365: // facilityType 1431 this.facilityType = castToCodeableConcept(value); // CodeableConcept 1432 return value; 1433 case 331373717: // practiceSetting 1434 this.practiceSetting = castToCodeableConcept(value); // CodeableConcept 1435 return value; 1436 case 2031381048: // sourcePatientInfo 1437 this.sourcePatientInfo = castToReference(value); // Reference 1438 return value; 1439 case 1090493483: // related 1440 this.getRelated().add(castToReference(value)); // Reference 1441 return value; 1442 default: 1443 return super.setProperty(hash, name, value); 1444 } 1445 1446 } 1447 1448 @Override 1449 public Base setProperty(String name, Base value) throws FHIRException { 1450 if (name.equals("encounter")) { 1451 this.getEncounter().add(castToReference(value)); 1452 } else if (name.equals("event")) { 1453 this.getEvent().add(castToCodeableConcept(value)); 1454 } else if (name.equals("period")) { 1455 this.period = castToPeriod(value); // Period 1456 } else if (name.equals("facilityType")) { 1457 this.facilityType = castToCodeableConcept(value); // CodeableConcept 1458 } else if (name.equals("practiceSetting")) { 1459 this.practiceSetting = castToCodeableConcept(value); // CodeableConcept 1460 } else if (name.equals("sourcePatientInfo")) { 1461 this.sourcePatientInfo = castToReference(value); // Reference 1462 } else if (name.equals("related")) { 1463 this.getRelated().add(castToReference(value)); 1464 } else 1465 return super.setProperty(name, value); 1466 return value; 1467 } 1468 1469 @Override 1470 public void removeChild(String name, Base value) throws FHIRException { 1471 if (name.equals("encounter")) { 1472 this.getEncounter().remove(castToReference(value)); 1473 } else if (name.equals("event")) { 1474 this.getEvent().remove(castToCodeableConcept(value)); 1475 } else if (name.equals("period")) { 1476 this.period = null; 1477 } else if (name.equals("facilityType")) { 1478 this.facilityType = null; 1479 } else if (name.equals("practiceSetting")) { 1480 this.practiceSetting = null; 1481 } else if (name.equals("sourcePatientInfo")) { 1482 this.sourcePatientInfo = null; 1483 } else if (name.equals("related")) { 1484 this.getRelated().remove(castToReference(value)); 1485 } else 1486 super.removeChild(name, value); 1487 1488 } 1489 1490 @Override 1491 public Base makeProperty(int hash, String name) throws FHIRException { 1492 switch (hash) { 1493 case 1524132147: 1494 return addEncounter(); 1495 case 96891546: 1496 return addEvent(); 1497 case -991726143: 1498 return getPeriod(); 1499 case 370698365: 1500 return getFacilityType(); 1501 case 331373717: 1502 return getPracticeSetting(); 1503 case 2031381048: 1504 return getSourcePatientInfo(); 1505 case 1090493483: 1506 return addRelated(); 1507 default: 1508 return super.makeProperty(hash, name); 1509 } 1510 1511 } 1512 1513 @Override 1514 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1515 switch (hash) { 1516 case 1524132147: 1517 /* encounter */ return new String[] { "Reference" }; 1518 case 96891546: 1519 /* event */ return new String[] { "CodeableConcept" }; 1520 case -991726143: 1521 /* period */ return new String[] { "Period" }; 1522 case 370698365: 1523 /* facilityType */ return new String[] { "CodeableConcept" }; 1524 case 331373717: 1525 /* practiceSetting */ return new String[] { "CodeableConcept" }; 1526 case 2031381048: 1527 /* sourcePatientInfo */ return new String[] { "Reference" }; 1528 case 1090493483: 1529 /* related */ return new String[] { "Reference" }; 1530 default: 1531 return super.getTypesForProperty(hash, name); 1532 } 1533 1534 } 1535 1536 @Override 1537 public Base addChild(String name) throws FHIRException { 1538 if (name.equals("encounter")) { 1539 return addEncounter(); 1540 } else if (name.equals("event")) { 1541 return addEvent(); 1542 } else if (name.equals("period")) { 1543 this.period = new Period(); 1544 return this.period; 1545 } else if (name.equals("facilityType")) { 1546 this.facilityType = new CodeableConcept(); 1547 return this.facilityType; 1548 } else if (name.equals("practiceSetting")) { 1549 this.practiceSetting = new CodeableConcept(); 1550 return this.practiceSetting; 1551 } else if (name.equals("sourcePatientInfo")) { 1552 this.sourcePatientInfo = new Reference(); 1553 return this.sourcePatientInfo; 1554 } else if (name.equals("related")) { 1555 return addRelated(); 1556 } else 1557 return super.addChild(name); 1558 } 1559 1560 public DocumentReferenceContextComponent copy() { 1561 DocumentReferenceContextComponent dst = new DocumentReferenceContextComponent(); 1562 copyValues(dst); 1563 return dst; 1564 } 1565 1566 public void copyValues(DocumentReferenceContextComponent dst) { 1567 super.copyValues(dst); 1568 if (encounter != null) { 1569 dst.encounter = new ArrayList<Reference>(); 1570 for (Reference i : encounter) 1571 dst.encounter.add(i.copy()); 1572 } 1573 ; 1574 if (event != null) { 1575 dst.event = new ArrayList<CodeableConcept>(); 1576 for (CodeableConcept i : event) 1577 dst.event.add(i.copy()); 1578 } 1579 ; 1580 dst.period = period == null ? null : period.copy(); 1581 dst.facilityType = facilityType == null ? null : facilityType.copy(); 1582 dst.practiceSetting = practiceSetting == null ? null : practiceSetting.copy(); 1583 dst.sourcePatientInfo = sourcePatientInfo == null ? null : sourcePatientInfo.copy(); 1584 if (related != null) { 1585 dst.related = new ArrayList<Reference>(); 1586 for (Reference i : related) 1587 dst.related.add(i.copy()); 1588 } 1589 ; 1590 } 1591 1592 @Override 1593 public boolean equalsDeep(Base other_) { 1594 if (!super.equalsDeep(other_)) 1595 return false; 1596 if (!(other_ instanceof DocumentReferenceContextComponent)) 1597 return false; 1598 DocumentReferenceContextComponent o = (DocumentReferenceContextComponent) other_; 1599 return compareDeep(encounter, o.encounter, true) && compareDeep(event, o.event, true) 1600 && compareDeep(period, o.period, true) && compareDeep(facilityType, o.facilityType, true) 1601 && compareDeep(practiceSetting, o.practiceSetting, true) 1602 && compareDeep(sourcePatientInfo, o.sourcePatientInfo, true) && compareDeep(related, o.related, true); 1603 } 1604 1605 @Override 1606 public boolean equalsShallow(Base other_) { 1607 if (!super.equalsShallow(other_)) 1608 return false; 1609 if (!(other_ instanceof DocumentReferenceContextComponent)) 1610 return false; 1611 DocumentReferenceContextComponent o = (DocumentReferenceContextComponent) other_; 1612 return true; 1613 } 1614 1615 public boolean isEmpty() { 1616 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(encounter, event, period, facilityType, 1617 practiceSetting, sourcePatientInfo, related); 1618 } 1619 1620 public String fhirType() { 1621 return "DocumentReference.context"; 1622 1623 } 1624 1625 } 1626 1627 /** 1628 * Document identifier as assigned by the source of the document. This 1629 * identifier is specific to this version of the document. This unique 1630 * identifier may be used elsewhere to identify this version of the document. 1631 */ 1632 @Child(name = "masterIdentifier", type = { 1633 Identifier.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 1634 @Description(shortDefinition = "Master Version Specific Identifier", formalDefinition = "Document identifier as assigned by the source of the document. This identifier is specific to this version of the document. This unique identifier may be used elsewhere to identify this version of the document.") 1635 protected Identifier masterIdentifier; 1636 1637 /** 1638 * Other identifiers associated with the document, including version independent 1639 * identifiers. 1640 */ 1641 @Child(name = "identifier", type = { 1642 Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1643 @Description(shortDefinition = "Other identifiers for the document", formalDefinition = "Other identifiers associated with the document, including version independent identifiers.") 1644 protected List<Identifier> identifier; 1645 1646 /** 1647 * The status of this document reference. 1648 */ 1649 @Child(name = "status", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = true, summary = true) 1650 @Description(shortDefinition = "current | superseded | entered-in-error", formalDefinition = "The status of this document reference.") 1651 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/document-reference-status") 1652 protected Enumeration<DocumentReferenceStatus> status; 1653 1654 /** 1655 * The status of the underlying document. 1656 */ 1657 @Child(name = "docStatus", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1658 @Description(shortDefinition = "preliminary | final | amended | entered-in-error", formalDefinition = "The status of the underlying document.") 1659 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/composition-status") 1660 protected Enumeration<ReferredDocumentStatus> docStatus; 1661 1662 /** 1663 * Specifies the particular kind of document referenced (e.g. History and 1664 * Physical, Discharge Summary, Progress Note). This usually equates to the 1665 * purpose of making the document referenced. 1666 */ 1667 @Child(name = "type", type = { CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1668 @Description(shortDefinition = "Kind of document (LOINC if possible)", formalDefinition = "Specifies the particular kind of document referenced (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the document referenced.") 1669 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/c80-doc-typecodes") 1670 protected CodeableConcept type; 1671 1672 /** 1673 * A categorization for the type of document referenced - helps for indexing and 1674 * searching. This may be implied by or derived from the code specified in the 1675 * DocumentReference.type. 1676 */ 1677 @Child(name = "category", type = { 1678 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1679 @Description(shortDefinition = "Categorization of document", formalDefinition = "A categorization for the type of document referenced - helps for indexing and searching. This may be implied by or derived from the code specified in the DocumentReference.type.") 1680 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/document-classcodes") 1681 protected List<CodeableConcept> category; 1682 1683 /** 1684 * Who or what the document is about. The document can be about a person, 1685 * (patient or healthcare practitioner), a device (e.g. a machine) or even a 1686 * group of subjects (such as a document about a herd of farm animals, or a set 1687 * of patients that share a common exposure). 1688 */ 1689 @Child(name = "subject", type = { Patient.class, Practitioner.class, Group.class, 1690 Device.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1691 @Description(shortDefinition = "Who/what is the subject of the document", formalDefinition = "Who or what the document is about. The document can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure).") 1692 protected Reference subject; 1693 1694 /** 1695 * The actual object that is the target of the reference (Who or what the 1696 * document is about. The document can be about a person, (patient or healthcare 1697 * practitioner), a device (e.g. a machine) or even a group of subjects (such as 1698 * a document about a herd of farm animals, or a set of patients that share a 1699 * common exposure).) 1700 */ 1701 protected Resource subjectTarget; 1702 1703 /** 1704 * When the document reference was created. 1705 */ 1706 @Child(name = "date", type = { InstantType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1707 @Description(shortDefinition = "When this document reference was created", formalDefinition = "When the document reference was created.") 1708 protected InstantType date; 1709 1710 /** 1711 * Identifies who is responsible for adding the information to the document. 1712 */ 1713 @Child(name = "author", type = { Practitioner.class, PractitionerRole.class, Organization.class, Device.class, 1714 Patient.class, 1715 RelatedPerson.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1716 @Description(shortDefinition = "Who and/or what authored the document", formalDefinition = "Identifies who is responsible for adding the information to the document.") 1717 protected List<Reference> author; 1718 /** 1719 * The actual objects that are the target of the reference (Identifies who is 1720 * responsible for adding the information to the document.) 1721 */ 1722 protected List<Resource> authorTarget; 1723 1724 /** 1725 * Which person or organization authenticates that this document is valid. 1726 */ 1727 @Child(name = "authenticator", type = { Practitioner.class, PractitionerRole.class, 1728 Organization.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1729 @Description(shortDefinition = "Who/what authenticated the document", formalDefinition = "Which person or organization authenticates that this document is valid.") 1730 protected Reference authenticator; 1731 1732 /** 1733 * The actual object that is the target of the reference (Which person or 1734 * organization authenticates that this document is valid.) 1735 */ 1736 protected Resource authenticatorTarget; 1737 1738 /** 1739 * Identifies the organization or group who is responsible for ongoing 1740 * maintenance of and access to the document. 1741 */ 1742 @Child(name = "custodian", type = { 1743 Organization.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 1744 @Description(shortDefinition = "Organization which maintains the document", formalDefinition = "Identifies the organization or group who is responsible for ongoing maintenance of and access to the document.") 1745 protected Reference custodian; 1746 1747 /** 1748 * The actual object that is the target of the reference (Identifies the 1749 * organization or group who is responsible for ongoing maintenance of and 1750 * access to the document.) 1751 */ 1752 protected Organization custodianTarget; 1753 1754 /** 1755 * Relationships that this document has with other document references that 1756 * already exist. 1757 */ 1758 @Child(name = "relatesTo", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1759 @Description(shortDefinition = "Relationships to other documents", formalDefinition = "Relationships that this document has with other document references that already exist.") 1760 protected List<DocumentReferenceRelatesToComponent> relatesTo; 1761 1762 /** 1763 * Human-readable description of the source document. 1764 */ 1765 @Child(name = "description", type = { 1766 StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 1767 @Description(shortDefinition = "Human-readable description", formalDefinition = "Human-readable description of the source document.") 1768 protected StringType description; 1769 1770 /** 1771 * A set of Security-Tag codes specifying the level of privacy/security of the 1772 * Document. Note that DocumentReference.meta.security contains the security 1773 * labels of the "reference" to the document, while 1774 * DocumentReference.securityLabel contains a snapshot of the security labels on 1775 * the document the reference refers to. 1776 */ 1777 @Child(name = "securityLabel", type = { 1778 CodeableConcept.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1779 @Description(shortDefinition = "Document security-tags", formalDefinition = "A set of Security-Tag codes specifying the level of privacy/security of the Document. Note that DocumentReference.meta.security contains the security labels of the \"reference\" to the document, while DocumentReference.securityLabel contains a snapshot of the security labels on the document the reference refers to.") 1780 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/security-labels") 1781 protected List<CodeableConcept> securityLabel; 1782 1783 /** 1784 * The document and format referenced. There may be multiple content element 1785 * repetitions, each with a different format. 1786 */ 1787 @Child(name = "content", type = {}, order = 14, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1788 @Description(shortDefinition = "Document referenced", formalDefinition = "The document and format referenced. There may be multiple content element repetitions, each with a different format.") 1789 protected List<DocumentReferenceContentComponent> content; 1790 1791 /** 1792 * The clinical context in which the document was prepared. 1793 */ 1794 @Child(name = "context", type = {}, order = 15, min = 0, max = 1, modifier = false, summary = true) 1795 @Description(shortDefinition = "Clinical context of document", formalDefinition = "The clinical context in which the document was prepared.") 1796 protected DocumentReferenceContextComponent context; 1797 1798 private static final long serialVersionUID = 307086535L; 1799 1800 /** 1801 * Constructor 1802 */ 1803 public DocumentReference() { 1804 super(); 1805 } 1806 1807 /** 1808 * Constructor 1809 */ 1810 public DocumentReference(Enumeration<DocumentReferenceStatus> status) { 1811 super(); 1812 this.status = status; 1813 } 1814 1815 /** 1816 * @return {@link #masterIdentifier} (Document identifier as assigned by the 1817 * source of the document. This identifier is specific to this version 1818 * of the document. This unique identifier may be used elsewhere to 1819 * identify this version of the document.) 1820 */ 1821 public Identifier getMasterIdentifier() { 1822 if (this.masterIdentifier == null) 1823 if (Configuration.errorOnAutoCreate()) 1824 throw new Error("Attempt to auto-create DocumentReference.masterIdentifier"); 1825 else if (Configuration.doAutoCreate()) 1826 this.masterIdentifier = new Identifier(); // cc 1827 return this.masterIdentifier; 1828 } 1829 1830 public boolean hasMasterIdentifier() { 1831 return this.masterIdentifier != null && !this.masterIdentifier.isEmpty(); 1832 } 1833 1834 /** 1835 * @param value {@link #masterIdentifier} (Document identifier as assigned by 1836 * the source of the document. This identifier is specific to this 1837 * version of the document. This unique identifier may be used 1838 * elsewhere to identify this version of the document.) 1839 */ 1840 public DocumentReference setMasterIdentifier(Identifier value) { 1841 this.masterIdentifier = value; 1842 return this; 1843 } 1844 1845 /** 1846 * @return {@link #identifier} (Other identifiers associated with the document, 1847 * including version independent identifiers.) 1848 */ 1849 public List<Identifier> getIdentifier() { 1850 if (this.identifier == null) 1851 this.identifier = new ArrayList<Identifier>(); 1852 return this.identifier; 1853 } 1854 1855 /** 1856 * @return Returns a reference to <code>this</code> for easy method chaining 1857 */ 1858 public DocumentReference setIdentifier(List<Identifier> theIdentifier) { 1859 this.identifier = theIdentifier; 1860 return this; 1861 } 1862 1863 public boolean hasIdentifier() { 1864 if (this.identifier == null) 1865 return false; 1866 for (Identifier item : this.identifier) 1867 if (!item.isEmpty()) 1868 return true; 1869 return false; 1870 } 1871 1872 public Identifier addIdentifier() { // 3 1873 Identifier t = new Identifier(); 1874 if (this.identifier == null) 1875 this.identifier = new ArrayList<Identifier>(); 1876 this.identifier.add(t); 1877 return t; 1878 } 1879 1880 public DocumentReference addIdentifier(Identifier t) { // 3 1881 if (t == null) 1882 return this; 1883 if (this.identifier == null) 1884 this.identifier = new ArrayList<Identifier>(); 1885 this.identifier.add(t); 1886 return this; 1887 } 1888 1889 /** 1890 * @return The first repetition of repeating field {@link #identifier}, creating 1891 * it if it does not already exist 1892 */ 1893 public Identifier getIdentifierFirstRep() { 1894 if (getIdentifier().isEmpty()) { 1895 addIdentifier(); 1896 } 1897 return getIdentifier().get(0); 1898 } 1899 1900 /** 1901 * @return {@link #status} (The status of this document reference.). This is the 1902 * underlying object with id, value and extensions. The accessor 1903 * "getStatus" gives direct access to the value 1904 */ 1905 public Enumeration<DocumentReferenceStatus> getStatusElement() { 1906 if (this.status == null) 1907 if (Configuration.errorOnAutoCreate()) 1908 throw new Error("Attempt to auto-create DocumentReference.status"); 1909 else if (Configuration.doAutoCreate()) 1910 this.status = new Enumeration<DocumentReferenceStatus>(new DocumentReferenceStatusEnumFactory()); // bb 1911 return this.status; 1912 } 1913 1914 public boolean hasStatusElement() { 1915 return this.status != null && !this.status.isEmpty(); 1916 } 1917 1918 public boolean hasStatus() { 1919 return this.status != null && !this.status.isEmpty(); 1920 } 1921 1922 /** 1923 * @param value {@link #status} (The status of this document reference.). This 1924 * is the underlying object with id, value and extensions. The 1925 * accessor "getStatus" gives direct access to the value 1926 */ 1927 public DocumentReference setStatusElement(Enumeration<DocumentReferenceStatus> value) { 1928 this.status = value; 1929 return this; 1930 } 1931 1932 /** 1933 * @return The status of this document reference. 1934 */ 1935 public DocumentReferenceStatus getStatus() { 1936 return this.status == null ? null : this.status.getValue(); 1937 } 1938 1939 /** 1940 * @param value The status of this document reference. 1941 */ 1942 public DocumentReference setStatus(DocumentReferenceStatus value) { 1943 if (this.status == null) 1944 this.status = new Enumeration<DocumentReferenceStatus>(new DocumentReferenceStatusEnumFactory()); 1945 this.status.setValue(value); 1946 return this; 1947 } 1948 1949 /** 1950 * @return {@link #docStatus} (The status of the underlying document.). This is 1951 * the underlying object with id, value and extensions. The accessor 1952 * "getDocStatus" gives direct access to the value 1953 */ 1954 public Enumeration<ReferredDocumentStatus> getDocStatusElement() { 1955 if (this.docStatus == null) 1956 if (Configuration.errorOnAutoCreate()) 1957 throw new Error("Attempt to auto-create DocumentReference.docStatus"); 1958 else if (Configuration.doAutoCreate()) 1959 this.docStatus = new Enumeration<ReferredDocumentStatus>(new ReferredDocumentStatusEnumFactory()); // bb 1960 return this.docStatus; 1961 } 1962 1963 public boolean hasDocStatusElement() { 1964 return this.docStatus != null && !this.docStatus.isEmpty(); 1965 } 1966 1967 public boolean hasDocStatus() { 1968 return this.docStatus != null && !this.docStatus.isEmpty(); 1969 } 1970 1971 /** 1972 * @param value {@link #docStatus} (The status of the underlying document.). 1973 * This is the underlying object with id, value and extensions. The 1974 * accessor "getDocStatus" gives direct access to the value 1975 */ 1976 public DocumentReference setDocStatusElement(Enumeration<ReferredDocumentStatus> value) { 1977 this.docStatus = value; 1978 return this; 1979 } 1980 1981 /** 1982 * @return The status of the underlying document. 1983 */ 1984 public ReferredDocumentStatus getDocStatus() { 1985 return this.docStatus == null ? null : this.docStatus.getValue(); 1986 } 1987 1988 /** 1989 * @param value The status of the underlying document. 1990 */ 1991 public DocumentReference setDocStatus(ReferredDocumentStatus value) { 1992 if (value == null) 1993 this.docStatus = null; 1994 else { 1995 if (this.docStatus == null) 1996 this.docStatus = new Enumeration<ReferredDocumentStatus>(new ReferredDocumentStatusEnumFactory()); 1997 this.docStatus.setValue(value); 1998 } 1999 return this; 2000 } 2001 2002 /** 2003 * @return {@link #type} (Specifies the particular kind of document referenced 2004 * (e.g. History and Physical, Discharge Summary, Progress Note). This 2005 * usually equates to the purpose of making the document referenced.) 2006 */ 2007 public CodeableConcept getType() { 2008 if (this.type == null) 2009 if (Configuration.errorOnAutoCreate()) 2010 throw new Error("Attempt to auto-create DocumentReference.type"); 2011 else if (Configuration.doAutoCreate()) 2012 this.type = new CodeableConcept(); // cc 2013 return this.type; 2014 } 2015 2016 public boolean hasType() { 2017 return this.type != null && !this.type.isEmpty(); 2018 } 2019 2020 /** 2021 * @param value {@link #type} (Specifies the particular kind of document 2022 * referenced (e.g. History and Physical, Discharge Summary, 2023 * Progress Note). This usually equates to the purpose of making 2024 * the document referenced.) 2025 */ 2026 public DocumentReference setType(CodeableConcept value) { 2027 this.type = value; 2028 return this; 2029 } 2030 2031 /** 2032 * @return {@link #category} (A categorization for the type of document 2033 * referenced - helps for indexing and searching. This may be implied by 2034 * or derived from the code specified in the DocumentReference.type.) 2035 */ 2036 public List<CodeableConcept> getCategory() { 2037 if (this.category == null) 2038 this.category = new ArrayList<CodeableConcept>(); 2039 return this.category; 2040 } 2041 2042 /** 2043 * @return Returns a reference to <code>this</code> for easy method chaining 2044 */ 2045 public DocumentReference setCategory(List<CodeableConcept> theCategory) { 2046 this.category = theCategory; 2047 return this; 2048 } 2049 2050 public boolean hasCategory() { 2051 if (this.category == null) 2052 return false; 2053 for (CodeableConcept item : this.category) 2054 if (!item.isEmpty()) 2055 return true; 2056 return false; 2057 } 2058 2059 public CodeableConcept addCategory() { // 3 2060 CodeableConcept t = new CodeableConcept(); 2061 if (this.category == null) 2062 this.category = new ArrayList<CodeableConcept>(); 2063 this.category.add(t); 2064 return t; 2065 } 2066 2067 public DocumentReference addCategory(CodeableConcept t) { // 3 2068 if (t == null) 2069 return this; 2070 if (this.category == null) 2071 this.category = new ArrayList<CodeableConcept>(); 2072 this.category.add(t); 2073 return this; 2074 } 2075 2076 /** 2077 * @return The first repetition of repeating field {@link #category}, creating 2078 * it if it does not already exist 2079 */ 2080 public CodeableConcept getCategoryFirstRep() { 2081 if (getCategory().isEmpty()) { 2082 addCategory(); 2083 } 2084 return getCategory().get(0); 2085 } 2086 2087 /** 2088 * @return {@link #subject} (Who or what the document is about. The document can 2089 * be about a person, (patient or healthcare practitioner), a device 2090 * (e.g. a machine) or even a group of subjects (such as a document 2091 * about a herd of farm animals, or a set of patients that share a 2092 * common exposure).) 2093 */ 2094 public Reference getSubject() { 2095 if (this.subject == null) 2096 if (Configuration.errorOnAutoCreate()) 2097 throw new Error("Attempt to auto-create DocumentReference.subject"); 2098 else if (Configuration.doAutoCreate()) 2099 this.subject = new Reference(); // cc 2100 return this.subject; 2101 } 2102 2103 public boolean hasSubject() { 2104 return this.subject != null && !this.subject.isEmpty(); 2105 } 2106 2107 /** 2108 * @param value {@link #subject} (Who or what the document is about. The 2109 * document can be about a person, (patient or healthcare 2110 * practitioner), a device (e.g. a machine) or even a group of 2111 * subjects (such as a document about a herd of farm animals, or a 2112 * set of patients that share a common exposure).) 2113 */ 2114 public DocumentReference setSubject(Reference value) { 2115 this.subject = value; 2116 return this; 2117 } 2118 2119 /** 2120 * @return {@link #subject} The actual object that is the target of the 2121 * reference. The reference library doesn't populate this, but you can 2122 * use it to hold the resource if you resolve it. (Who or what the 2123 * document is about. The document can be about a person, (patient or 2124 * healthcare practitioner), a device (e.g. a machine) or even a group 2125 * of subjects (such as a document about a herd of farm animals, or a 2126 * set of patients that share a common exposure).) 2127 */ 2128 public Resource getSubjectTarget() { 2129 return this.subjectTarget; 2130 } 2131 2132 /** 2133 * @param value {@link #subject} The actual object that is the target of the 2134 * reference. The reference library doesn't use these, but you can 2135 * use it to hold the resource if you resolve it. (Who or what the 2136 * document is about. The document can be about a person, (patient 2137 * or healthcare practitioner), a device (e.g. a machine) or even a 2138 * group of subjects (such as a document about a herd of farm 2139 * animals, or a set of patients that share a common exposure).) 2140 */ 2141 public DocumentReference setSubjectTarget(Resource value) { 2142 this.subjectTarget = value; 2143 return this; 2144 } 2145 2146 /** 2147 * @return {@link #date} (When the document reference was created.). This is the 2148 * underlying object with id, value and extensions. The accessor 2149 * "getDate" gives direct access to the value 2150 */ 2151 public InstantType getDateElement() { 2152 if (this.date == null) 2153 if (Configuration.errorOnAutoCreate()) 2154 throw new Error("Attempt to auto-create DocumentReference.date"); 2155 else if (Configuration.doAutoCreate()) 2156 this.date = new InstantType(); // bb 2157 return this.date; 2158 } 2159 2160 public boolean hasDateElement() { 2161 return this.date != null && !this.date.isEmpty(); 2162 } 2163 2164 public boolean hasDate() { 2165 return this.date != null && !this.date.isEmpty(); 2166 } 2167 2168 /** 2169 * @param value {@link #date} (When the document reference was created.). This 2170 * is the underlying object with id, value and extensions. The 2171 * accessor "getDate" gives direct access to the value 2172 */ 2173 public DocumentReference setDateElement(InstantType value) { 2174 this.date = value; 2175 return this; 2176 } 2177 2178 /** 2179 * @return When the document reference was created. 2180 */ 2181 public Date getDate() { 2182 return this.date == null ? null : this.date.getValue(); 2183 } 2184 2185 /** 2186 * @param value When the document reference was created. 2187 */ 2188 public DocumentReference setDate(Date value) { 2189 if (value == null) 2190 this.date = null; 2191 else { 2192 if (this.date == null) 2193 this.date = new InstantType(); 2194 this.date.setValue(value); 2195 } 2196 return this; 2197 } 2198 2199 /** 2200 * @return {@link #author} (Identifies who is responsible for adding the 2201 * information to the document.) 2202 */ 2203 public List<Reference> getAuthor() { 2204 if (this.author == null) 2205 this.author = new ArrayList<Reference>(); 2206 return this.author; 2207 } 2208 2209 /** 2210 * @return Returns a reference to <code>this</code> for easy method chaining 2211 */ 2212 public DocumentReference setAuthor(List<Reference> theAuthor) { 2213 this.author = theAuthor; 2214 return this; 2215 } 2216 2217 public boolean hasAuthor() { 2218 if (this.author == null) 2219 return false; 2220 for (Reference item : this.author) 2221 if (!item.isEmpty()) 2222 return true; 2223 return false; 2224 } 2225 2226 public Reference addAuthor() { // 3 2227 Reference t = new Reference(); 2228 if (this.author == null) 2229 this.author = new ArrayList<Reference>(); 2230 this.author.add(t); 2231 return t; 2232 } 2233 2234 public DocumentReference addAuthor(Reference t) { // 3 2235 if (t == null) 2236 return this; 2237 if (this.author == null) 2238 this.author = new ArrayList<Reference>(); 2239 this.author.add(t); 2240 return this; 2241 } 2242 2243 /** 2244 * @return The first repetition of repeating field {@link #author}, creating it 2245 * if it does not already exist 2246 */ 2247 public Reference getAuthorFirstRep() { 2248 if (getAuthor().isEmpty()) { 2249 addAuthor(); 2250 } 2251 return getAuthor().get(0); 2252 } 2253 2254 /** 2255 * @return {@link #authenticator} (Which person or organization authenticates 2256 * that this document is valid.) 2257 */ 2258 public Reference getAuthenticator() { 2259 if (this.authenticator == null) 2260 if (Configuration.errorOnAutoCreate()) 2261 throw new Error("Attempt to auto-create DocumentReference.authenticator"); 2262 else if (Configuration.doAutoCreate()) 2263 this.authenticator = new Reference(); // cc 2264 return this.authenticator; 2265 } 2266 2267 public boolean hasAuthenticator() { 2268 return this.authenticator != null && !this.authenticator.isEmpty(); 2269 } 2270 2271 /** 2272 * @param value {@link #authenticator} (Which person or organization 2273 * authenticates that this document is valid.) 2274 */ 2275 public DocumentReference setAuthenticator(Reference value) { 2276 this.authenticator = value; 2277 return this; 2278 } 2279 2280 /** 2281 * @return {@link #authenticator} The actual object that is the target of the 2282 * reference. The reference library doesn't populate this, but you can 2283 * use it to hold the resource if you resolve it. (Which person or 2284 * organization authenticates that this document is valid.) 2285 */ 2286 public Resource getAuthenticatorTarget() { 2287 return this.authenticatorTarget; 2288 } 2289 2290 /** 2291 * @param value {@link #authenticator} The actual object that is the target of 2292 * the reference. The reference library doesn't use these, but you 2293 * can use it to hold the resource if you resolve it. (Which person 2294 * or organization authenticates that this document is valid.) 2295 */ 2296 public DocumentReference setAuthenticatorTarget(Resource value) { 2297 this.authenticatorTarget = value; 2298 return this; 2299 } 2300 2301 /** 2302 * @return {@link #custodian} (Identifies the organization or group who is 2303 * responsible for ongoing maintenance of and access to the document.) 2304 */ 2305 public Reference getCustodian() { 2306 if (this.custodian == null) 2307 if (Configuration.errorOnAutoCreate()) 2308 throw new Error("Attempt to auto-create DocumentReference.custodian"); 2309 else if (Configuration.doAutoCreate()) 2310 this.custodian = new Reference(); // cc 2311 return this.custodian; 2312 } 2313 2314 public boolean hasCustodian() { 2315 return this.custodian != null && !this.custodian.isEmpty(); 2316 } 2317 2318 /** 2319 * @param value {@link #custodian} (Identifies the organization or group who is 2320 * responsible for ongoing maintenance of and access to the 2321 * document.) 2322 */ 2323 public DocumentReference setCustodian(Reference value) { 2324 this.custodian = value; 2325 return this; 2326 } 2327 2328 /** 2329 * @return {@link #custodian} The actual object that is the target of the 2330 * reference. The reference library doesn't populate this, but you can 2331 * use it to hold the resource if you resolve it. (Identifies the 2332 * organization or group who is responsible for ongoing maintenance of 2333 * and access to the document.) 2334 */ 2335 public Organization getCustodianTarget() { 2336 if (this.custodianTarget == null) 2337 if (Configuration.errorOnAutoCreate()) 2338 throw new Error("Attempt to auto-create DocumentReference.custodian"); 2339 else if (Configuration.doAutoCreate()) 2340 this.custodianTarget = new Organization(); // aa 2341 return this.custodianTarget; 2342 } 2343 2344 /** 2345 * @param value {@link #custodian} The actual object that is the target of the 2346 * reference. The reference library doesn't use these, but you can 2347 * use it to hold the resource if you resolve it. (Identifies the 2348 * organization or group who is responsible for ongoing maintenance 2349 * of and access to the document.) 2350 */ 2351 public DocumentReference setCustodianTarget(Organization value) { 2352 this.custodianTarget = value; 2353 return this; 2354 } 2355 2356 /** 2357 * @return {@link #relatesTo} (Relationships that this document has with other 2358 * document references that already exist.) 2359 */ 2360 public List<DocumentReferenceRelatesToComponent> getRelatesTo() { 2361 if (this.relatesTo == null) 2362 this.relatesTo = new ArrayList<DocumentReferenceRelatesToComponent>(); 2363 return this.relatesTo; 2364 } 2365 2366 /** 2367 * @return Returns a reference to <code>this</code> for easy method chaining 2368 */ 2369 public DocumentReference setRelatesTo(List<DocumentReferenceRelatesToComponent> theRelatesTo) { 2370 this.relatesTo = theRelatesTo; 2371 return this; 2372 } 2373 2374 public boolean hasRelatesTo() { 2375 if (this.relatesTo == null) 2376 return false; 2377 for (DocumentReferenceRelatesToComponent item : this.relatesTo) 2378 if (!item.isEmpty()) 2379 return true; 2380 return false; 2381 } 2382 2383 public DocumentReferenceRelatesToComponent addRelatesTo() { // 3 2384 DocumentReferenceRelatesToComponent t = new DocumentReferenceRelatesToComponent(); 2385 if (this.relatesTo == null) 2386 this.relatesTo = new ArrayList<DocumentReferenceRelatesToComponent>(); 2387 this.relatesTo.add(t); 2388 return t; 2389 } 2390 2391 public DocumentReference addRelatesTo(DocumentReferenceRelatesToComponent t) { // 3 2392 if (t == null) 2393 return this; 2394 if (this.relatesTo == null) 2395 this.relatesTo = new ArrayList<DocumentReferenceRelatesToComponent>(); 2396 this.relatesTo.add(t); 2397 return this; 2398 } 2399 2400 /** 2401 * @return The first repetition of repeating field {@link #relatesTo}, creating 2402 * it if it does not already exist 2403 */ 2404 public DocumentReferenceRelatesToComponent getRelatesToFirstRep() { 2405 if (getRelatesTo().isEmpty()) { 2406 addRelatesTo(); 2407 } 2408 return getRelatesTo().get(0); 2409 } 2410 2411 /** 2412 * @return {@link #description} (Human-readable description of the source 2413 * document.). This is the underlying object with id, value and 2414 * extensions. The accessor "getDescription" gives direct access to the 2415 * value 2416 */ 2417 public StringType getDescriptionElement() { 2418 if (this.description == null) 2419 if (Configuration.errorOnAutoCreate()) 2420 throw new Error("Attempt to auto-create DocumentReference.description"); 2421 else if (Configuration.doAutoCreate()) 2422 this.description = new StringType(); // bb 2423 return this.description; 2424 } 2425 2426 public boolean hasDescriptionElement() { 2427 return this.description != null && !this.description.isEmpty(); 2428 } 2429 2430 public boolean hasDescription() { 2431 return this.description != null && !this.description.isEmpty(); 2432 } 2433 2434 /** 2435 * @param value {@link #description} (Human-readable description of the source 2436 * document.). This is the underlying object with id, value and 2437 * extensions. The accessor "getDescription" gives direct access to 2438 * the value 2439 */ 2440 public DocumentReference setDescriptionElement(StringType value) { 2441 this.description = value; 2442 return this; 2443 } 2444 2445 /** 2446 * @return Human-readable description of the source document. 2447 */ 2448 public String getDescription() { 2449 return this.description == null ? null : this.description.getValue(); 2450 } 2451 2452 /** 2453 * @param value Human-readable description of the source document. 2454 */ 2455 public DocumentReference setDescription(String value) { 2456 if (Utilities.noString(value)) 2457 this.description = null; 2458 else { 2459 if (this.description == null) 2460 this.description = new StringType(); 2461 this.description.setValue(value); 2462 } 2463 return this; 2464 } 2465 2466 /** 2467 * @return {@link #securityLabel} (A set of Security-Tag codes specifying the 2468 * level of privacy/security of the Document. Note that 2469 * DocumentReference.meta.security contains the security labels of the 2470 * "reference" to the document, while DocumentReference.securityLabel 2471 * contains a snapshot of the security labels on the document the 2472 * reference refers to.) 2473 */ 2474 public List<CodeableConcept> getSecurityLabel() { 2475 if (this.securityLabel == null) 2476 this.securityLabel = new ArrayList<CodeableConcept>(); 2477 return this.securityLabel; 2478 } 2479 2480 /** 2481 * @return Returns a reference to <code>this</code> for easy method chaining 2482 */ 2483 public DocumentReference setSecurityLabel(List<CodeableConcept> theSecurityLabel) { 2484 this.securityLabel = theSecurityLabel; 2485 return this; 2486 } 2487 2488 public boolean hasSecurityLabel() { 2489 if (this.securityLabel == null) 2490 return false; 2491 for (CodeableConcept item : this.securityLabel) 2492 if (!item.isEmpty()) 2493 return true; 2494 return false; 2495 } 2496 2497 public CodeableConcept addSecurityLabel() { // 3 2498 CodeableConcept t = new CodeableConcept(); 2499 if (this.securityLabel == null) 2500 this.securityLabel = new ArrayList<CodeableConcept>(); 2501 this.securityLabel.add(t); 2502 return t; 2503 } 2504 2505 public DocumentReference addSecurityLabel(CodeableConcept t) { // 3 2506 if (t == null) 2507 return this; 2508 if (this.securityLabel == null) 2509 this.securityLabel = new ArrayList<CodeableConcept>(); 2510 this.securityLabel.add(t); 2511 return this; 2512 } 2513 2514 /** 2515 * @return The first repetition of repeating field {@link #securityLabel}, 2516 * creating it if it does not already exist 2517 */ 2518 public CodeableConcept getSecurityLabelFirstRep() { 2519 if (getSecurityLabel().isEmpty()) { 2520 addSecurityLabel(); 2521 } 2522 return getSecurityLabel().get(0); 2523 } 2524 2525 /** 2526 * @return {@link #content} (The document and format referenced. There may be 2527 * multiple content element repetitions, each with a different format.) 2528 */ 2529 public List<DocumentReferenceContentComponent> getContent() { 2530 if (this.content == null) 2531 this.content = new ArrayList<DocumentReferenceContentComponent>(); 2532 return this.content; 2533 } 2534 2535 /** 2536 * @return Returns a reference to <code>this</code> for easy method chaining 2537 */ 2538 public DocumentReference setContent(List<DocumentReferenceContentComponent> theContent) { 2539 this.content = theContent; 2540 return this; 2541 } 2542 2543 public boolean hasContent() { 2544 if (this.content == null) 2545 return false; 2546 for (DocumentReferenceContentComponent item : this.content) 2547 if (!item.isEmpty()) 2548 return true; 2549 return false; 2550 } 2551 2552 public DocumentReferenceContentComponent addContent() { // 3 2553 DocumentReferenceContentComponent t = new DocumentReferenceContentComponent(); 2554 if (this.content == null) 2555 this.content = new ArrayList<DocumentReferenceContentComponent>(); 2556 this.content.add(t); 2557 return t; 2558 } 2559 2560 public DocumentReference addContent(DocumentReferenceContentComponent t) { // 3 2561 if (t == null) 2562 return this; 2563 if (this.content == null) 2564 this.content = new ArrayList<DocumentReferenceContentComponent>(); 2565 this.content.add(t); 2566 return this; 2567 } 2568 2569 /** 2570 * @return The first repetition of repeating field {@link #content}, creating it 2571 * if it does not already exist 2572 */ 2573 public DocumentReferenceContentComponent getContentFirstRep() { 2574 if (getContent().isEmpty()) { 2575 addContent(); 2576 } 2577 return getContent().get(0); 2578 } 2579 2580 /** 2581 * @return {@link #context} (The clinical context in which the document was 2582 * prepared.) 2583 */ 2584 public DocumentReferenceContextComponent getContext() { 2585 if (this.context == null) 2586 if (Configuration.errorOnAutoCreate()) 2587 throw new Error("Attempt to auto-create DocumentReference.context"); 2588 else if (Configuration.doAutoCreate()) 2589 this.context = new DocumentReferenceContextComponent(); // cc 2590 return this.context; 2591 } 2592 2593 public boolean hasContext() { 2594 return this.context != null && !this.context.isEmpty(); 2595 } 2596 2597 /** 2598 * @param value {@link #context} (The clinical context in which the document was 2599 * prepared.) 2600 */ 2601 public DocumentReference setContext(DocumentReferenceContextComponent value) { 2602 this.context = value; 2603 return this; 2604 } 2605 2606 protected void listChildren(List<Property> children) { 2607 super.listChildren(children); 2608 children.add(new Property("masterIdentifier", "Identifier", 2609 "Document identifier as assigned by the source of the document. This identifier is specific to this version of the document. This unique identifier may be used elsewhere to identify this version of the document.", 2610 0, 1, masterIdentifier)); 2611 children.add(new Property("identifier", "Identifier", 2612 "Other identifiers associated with the document, including version independent identifiers.", 0, 2613 java.lang.Integer.MAX_VALUE, identifier)); 2614 children.add(new Property("status", "code", "The status of this document reference.", 0, 1, status)); 2615 children.add(new Property("docStatus", "code", "The status of the underlying document.", 0, 1, docStatus)); 2616 children.add(new Property("type", "CodeableConcept", 2617 "Specifies the particular kind of document referenced (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the document referenced.", 2618 0, 1, type)); 2619 children.add(new Property("category", "CodeableConcept", 2620 "A categorization for the type of document referenced - helps for indexing and searching. This may be implied by or derived from the code specified in the DocumentReference.type.", 2621 0, java.lang.Integer.MAX_VALUE, category)); 2622 children.add(new Property("subject", "Reference(Patient|Practitioner|Group|Device)", 2623 "Who or what the document is about. The document can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure).", 2624 0, 1, subject)); 2625 children.add(new Property("date", "instant", "When the document reference was created.", 0, 1, date)); 2626 children.add( 2627 new Property("author", "Reference(Practitioner|PractitionerRole|Organization|Device|Patient|RelatedPerson)", 2628 "Identifies who is responsible for adding the information to the document.", 0, java.lang.Integer.MAX_VALUE, 2629 author)); 2630 children.add(new Property("authenticator", "Reference(Practitioner|PractitionerRole|Organization)", 2631 "Which person or organization authenticates that this document is valid.", 0, 1, authenticator)); 2632 children.add(new Property("custodian", "Reference(Organization)", 2633 "Identifies the organization or group who is responsible for ongoing maintenance of and access to the document.", 2634 0, 1, custodian)); 2635 children.add(new Property("relatesTo", "", 2636 "Relationships that this document has with other document references that already exist.", 0, 2637 java.lang.Integer.MAX_VALUE, relatesTo)); 2638 children.add( 2639 new Property("description", "string", "Human-readable description of the source document.", 0, 1, description)); 2640 children.add(new Property("securityLabel", "CodeableConcept", 2641 "A set of Security-Tag codes specifying the level of privacy/security of the Document. Note that DocumentReference.meta.security contains the security labels of the \"reference\" to the document, while DocumentReference.securityLabel contains a snapshot of the security labels on the document the reference refers to.", 2642 0, java.lang.Integer.MAX_VALUE, securityLabel)); 2643 children.add(new Property("content", "", 2644 "The document and format referenced. There may be multiple content element repetitions, each with a different format.", 2645 0, java.lang.Integer.MAX_VALUE, content)); 2646 children 2647 .add(new Property("context", "", "The clinical context in which the document was prepared.", 0, 1, context)); 2648 } 2649 2650 @Override 2651 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2652 switch (_hash) { 2653 case 243769515: 2654 /* masterIdentifier */ return new Property("masterIdentifier", "Identifier", 2655 "Document identifier as assigned by the source of the document. This identifier is specific to this version of the document. This unique identifier may be used elsewhere to identify this version of the document.", 2656 0, 1, masterIdentifier); 2657 case -1618432855: 2658 /* identifier */ return new Property("identifier", "Identifier", 2659 "Other identifiers associated with the document, including version independent identifiers.", 0, 2660 java.lang.Integer.MAX_VALUE, identifier); 2661 case -892481550: 2662 /* status */ return new Property("status", "code", "The status of this document reference.", 0, 1, status); 2663 case -23496886: 2664 /* docStatus */ return new Property("docStatus", "code", "The status of the underlying document.", 0, 1, 2665 docStatus); 2666 case 3575610: 2667 /* type */ return new Property("type", "CodeableConcept", 2668 "Specifies the particular kind of document referenced (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the document referenced.", 2669 0, 1, type); 2670 case 50511102: 2671 /* category */ return new Property("category", "CodeableConcept", 2672 "A categorization for the type of document referenced - helps for indexing and searching. This may be implied by or derived from the code specified in the DocumentReference.type.", 2673 0, java.lang.Integer.MAX_VALUE, category); 2674 case -1867885268: 2675 /* subject */ return new Property("subject", "Reference(Patient|Practitioner|Group|Device)", 2676 "Who or what the document is about. The document can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure).", 2677 0, 1, subject); 2678 case 3076014: 2679 /* date */ return new Property("date", "instant", "When the document reference was created.", 0, 1, date); 2680 case -1406328437: 2681 /* author */ return new Property("author", 2682 "Reference(Practitioner|PractitionerRole|Organization|Device|Patient|RelatedPerson)", 2683 "Identifies who is responsible for adding the information to the document.", 0, java.lang.Integer.MAX_VALUE, 2684 author); 2685 case 1815000435: 2686 /* authenticator */ return new Property("authenticator", "Reference(Practitioner|PractitionerRole|Organization)", 2687 "Which person or organization authenticates that this document is valid.", 0, 1, authenticator); 2688 case 1611297262: 2689 /* custodian */ return new Property("custodian", "Reference(Organization)", 2690 "Identifies the organization or group who is responsible for ongoing maintenance of and access to the document.", 2691 0, 1, custodian); 2692 case -7765931: 2693 /* relatesTo */ return new Property("relatesTo", "", 2694 "Relationships that this document has with other document references that already exist.", 0, 2695 java.lang.Integer.MAX_VALUE, relatesTo); 2696 case -1724546052: 2697 /* description */ return new Property("description", "string", 2698 "Human-readable description of the source document.", 0, 1, description); 2699 case -722296940: 2700 /* securityLabel */ return new Property("securityLabel", "CodeableConcept", 2701 "A set of Security-Tag codes specifying the level of privacy/security of the Document. Note that DocumentReference.meta.security contains the security labels of the \"reference\" to the document, while DocumentReference.securityLabel contains a snapshot of the security labels on the document the reference refers to.", 2702 0, java.lang.Integer.MAX_VALUE, securityLabel); 2703 case 951530617: 2704 /* content */ return new Property("content", "", 2705 "The document and format referenced. There may be multiple content element repetitions, each with a different format.", 2706 0, java.lang.Integer.MAX_VALUE, content); 2707 case 951530927: 2708 /* context */ return new Property("context", "", "The clinical context in which the document was prepared.", 0, 1, 2709 context); 2710 default: 2711 return super.getNamedProperty(_hash, _name, _checkValid); 2712 } 2713 2714 } 2715 2716 @Override 2717 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2718 switch (hash) { 2719 case 243769515: 2720 /* masterIdentifier */ return this.masterIdentifier == null ? new Base[0] : new Base[] { this.masterIdentifier }; // Identifier 2721 case -1618432855: 2722 /* identifier */ return this.identifier == null ? new Base[0] 2723 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2724 case -892481550: 2725 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<DocumentReferenceStatus> 2726 case -23496886: 2727 /* docStatus */ return this.docStatus == null ? new Base[0] : new Base[] { this.docStatus }; // Enumeration<ReferredDocumentStatus> 2728 case 3575610: 2729 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2730 case 50511102: 2731 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2732 case -1867885268: 2733 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2734 case 3076014: 2735 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // InstantType 2736 case -1406328437: 2737 /* author */ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // Reference 2738 case 1815000435: 2739 /* authenticator */ return this.authenticator == null ? new Base[0] : new Base[] { this.authenticator }; // Reference 2740 case 1611297262: 2741 /* custodian */ return this.custodian == null ? new Base[0] : new Base[] { this.custodian }; // Reference 2742 case -7765931: 2743 /* relatesTo */ return this.relatesTo == null ? new Base[0] 2744 : this.relatesTo.toArray(new Base[this.relatesTo.size()]); // DocumentReferenceRelatesToComponent 2745 case -1724546052: 2746 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 2747 case -722296940: 2748 /* securityLabel */ return this.securityLabel == null ? new Base[0] 2749 : this.securityLabel.toArray(new Base[this.securityLabel.size()]); // CodeableConcept 2750 case 951530617: 2751 /* content */ return this.content == null ? new Base[0] : this.content.toArray(new Base[this.content.size()]); // DocumentReferenceContentComponent 2752 case 951530927: 2753 /* context */ return this.context == null ? new Base[0] : new Base[] { this.context }; // DocumentReferenceContextComponent 2754 default: 2755 return super.getProperty(hash, name, checkValid); 2756 } 2757 2758 } 2759 2760 @Override 2761 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2762 switch (hash) { 2763 case 243769515: // masterIdentifier 2764 this.masterIdentifier = castToIdentifier(value); // Identifier 2765 return value; 2766 case -1618432855: // identifier 2767 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2768 return value; 2769 case -892481550: // status 2770 value = new DocumentReferenceStatusEnumFactory().fromType(castToCode(value)); 2771 this.status = (Enumeration) value; // Enumeration<DocumentReferenceStatus> 2772 return value; 2773 case -23496886: // docStatus 2774 value = new ReferredDocumentStatusEnumFactory().fromType(castToCode(value)); 2775 this.docStatus = (Enumeration) value; // Enumeration<ReferredDocumentStatus> 2776 return value; 2777 case 3575610: // type 2778 this.type = castToCodeableConcept(value); // CodeableConcept 2779 return value; 2780 case 50511102: // category 2781 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 2782 return value; 2783 case -1867885268: // subject 2784 this.subject = castToReference(value); // Reference 2785 return value; 2786 case 3076014: // date 2787 this.date = castToInstant(value); // InstantType 2788 return value; 2789 case -1406328437: // author 2790 this.getAuthor().add(castToReference(value)); // Reference 2791 return value; 2792 case 1815000435: // authenticator 2793 this.authenticator = castToReference(value); // Reference 2794 return value; 2795 case 1611297262: // custodian 2796 this.custodian = castToReference(value); // Reference 2797 return value; 2798 case -7765931: // relatesTo 2799 this.getRelatesTo().add((DocumentReferenceRelatesToComponent) value); // DocumentReferenceRelatesToComponent 2800 return value; 2801 case -1724546052: // description 2802 this.description = castToString(value); // StringType 2803 return value; 2804 case -722296940: // securityLabel 2805 this.getSecurityLabel().add(castToCodeableConcept(value)); // CodeableConcept 2806 return value; 2807 case 951530617: // content 2808 this.getContent().add((DocumentReferenceContentComponent) value); // DocumentReferenceContentComponent 2809 return value; 2810 case 951530927: // context 2811 this.context = (DocumentReferenceContextComponent) value; // DocumentReferenceContextComponent 2812 return value; 2813 default: 2814 return super.setProperty(hash, name, value); 2815 } 2816 2817 } 2818 2819 @Override 2820 public Base setProperty(String name, Base value) throws FHIRException { 2821 if (name.equals("masterIdentifier")) { 2822 this.masterIdentifier = castToIdentifier(value); // Identifier 2823 } else if (name.equals("identifier")) { 2824 this.getIdentifier().add(castToIdentifier(value)); 2825 } else if (name.equals("status")) { 2826 value = new DocumentReferenceStatusEnumFactory().fromType(castToCode(value)); 2827 this.status = (Enumeration) value; // Enumeration<DocumentReferenceStatus> 2828 } else if (name.equals("docStatus")) { 2829 value = new ReferredDocumentStatusEnumFactory().fromType(castToCode(value)); 2830 this.docStatus = (Enumeration) value; // Enumeration<ReferredDocumentStatus> 2831 } else if (name.equals("type")) { 2832 this.type = castToCodeableConcept(value); // CodeableConcept 2833 } else if (name.equals("category")) { 2834 this.getCategory().add(castToCodeableConcept(value)); 2835 } else if (name.equals("subject")) { 2836 this.subject = castToReference(value); // Reference 2837 } else if (name.equals("date")) { 2838 this.date = castToInstant(value); // InstantType 2839 } else if (name.equals("author")) { 2840 this.getAuthor().add(castToReference(value)); 2841 } else if (name.equals("authenticator")) { 2842 this.authenticator = castToReference(value); // Reference 2843 } else if (name.equals("custodian")) { 2844 this.custodian = castToReference(value); // Reference 2845 } else if (name.equals("relatesTo")) { 2846 this.getRelatesTo().add((DocumentReferenceRelatesToComponent) value); 2847 } else if (name.equals("description")) { 2848 this.description = castToString(value); // StringType 2849 } else if (name.equals("securityLabel")) { 2850 this.getSecurityLabel().add(castToCodeableConcept(value)); 2851 } else if (name.equals("content")) { 2852 this.getContent().add((DocumentReferenceContentComponent) value); 2853 } else if (name.equals("context")) { 2854 this.context = (DocumentReferenceContextComponent) value; // DocumentReferenceContextComponent 2855 } else 2856 return super.setProperty(name, value); 2857 return value; 2858 } 2859 2860 @Override 2861 public void removeChild(String name, Base value) throws FHIRException { 2862 if (name.equals("masterIdentifier")) { 2863 this.masterIdentifier = null; 2864 } else if (name.equals("identifier")) { 2865 this.getIdentifier().remove(castToIdentifier(value)); 2866 } else if (name.equals("status")) { 2867 this.status = null; 2868 } else if (name.equals("docStatus")) { 2869 this.docStatus = null; 2870 } else if (name.equals("type")) { 2871 this.type = null; 2872 } else if (name.equals("category")) { 2873 this.getCategory().remove(castToCodeableConcept(value)); 2874 } else if (name.equals("subject")) { 2875 this.subject = null; 2876 } else if (name.equals("date")) { 2877 this.date = null; 2878 } else if (name.equals("author")) { 2879 this.getAuthor().remove(castToReference(value)); 2880 } else if (name.equals("authenticator")) { 2881 this.authenticator = null; 2882 } else if (name.equals("custodian")) { 2883 this.custodian = null; 2884 } else if (name.equals("relatesTo")) { 2885 this.getRelatesTo().remove((DocumentReferenceRelatesToComponent) value); 2886 } else if (name.equals("description")) { 2887 this.description = null; 2888 } else if (name.equals("securityLabel")) { 2889 this.getSecurityLabel().remove(castToCodeableConcept(value)); 2890 } else if (name.equals("content")) { 2891 this.getContent().remove((DocumentReferenceContentComponent) value); 2892 } else if (name.equals("context")) { 2893 this.context = (DocumentReferenceContextComponent) value; // DocumentReferenceContextComponent 2894 } else 2895 super.removeChild(name, value); 2896 2897 } 2898 2899 @Override 2900 public Base makeProperty(int hash, String name) throws FHIRException { 2901 switch (hash) { 2902 case 243769515: 2903 return getMasterIdentifier(); 2904 case -1618432855: 2905 return addIdentifier(); 2906 case -892481550: 2907 return getStatusElement(); 2908 case -23496886: 2909 return getDocStatusElement(); 2910 case 3575610: 2911 return getType(); 2912 case 50511102: 2913 return addCategory(); 2914 case -1867885268: 2915 return getSubject(); 2916 case 3076014: 2917 return getDateElement(); 2918 case -1406328437: 2919 return addAuthor(); 2920 case 1815000435: 2921 return getAuthenticator(); 2922 case 1611297262: 2923 return getCustodian(); 2924 case -7765931: 2925 return addRelatesTo(); 2926 case -1724546052: 2927 return getDescriptionElement(); 2928 case -722296940: 2929 return addSecurityLabel(); 2930 case 951530617: 2931 return addContent(); 2932 case 951530927: 2933 return getContext(); 2934 default: 2935 return super.makeProperty(hash, name); 2936 } 2937 2938 } 2939 2940 @Override 2941 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2942 switch (hash) { 2943 case 243769515: 2944 /* masterIdentifier */ return new String[] { "Identifier" }; 2945 case -1618432855: 2946 /* identifier */ return new String[] { "Identifier" }; 2947 case -892481550: 2948 /* status */ return new String[] { "code" }; 2949 case -23496886: 2950 /* docStatus */ return new String[] { "code" }; 2951 case 3575610: 2952 /* type */ return new String[] { "CodeableConcept" }; 2953 case 50511102: 2954 /* category */ return new String[] { "CodeableConcept" }; 2955 case -1867885268: 2956 /* subject */ return new String[] { "Reference" }; 2957 case 3076014: 2958 /* date */ return new String[] { "instant" }; 2959 case -1406328437: 2960 /* author */ return new String[] { "Reference" }; 2961 case 1815000435: 2962 /* authenticator */ return new String[] { "Reference" }; 2963 case 1611297262: 2964 /* custodian */ return new String[] { "Reference" }; 2965 case -7765931: 2966 /* relatesTo */ return new String[] {}; 2967 case -1724546052: 2968 /* description */ return new String[] { "string" }; 2969 case -722296940: 2970 /* securityLabel */ return new String[] { "CodeableConcept" }; 2971 case 951530617: 2972 /* content */ return new String[] {}; 2973 case 951530927: 2974 /* context */ return new String[] {}; 2975 default: 2976 return super.getTypesForProperty(hash, name); 2977 } 2978 2979 } 2980 2981 @Override 2982 public Base addChild(String name) throws FHIRException { 2983 if (name.equals("masterIdentifier")) { 2984 this.masterIdentifier = new Identifier(); 2985 return this.masterIdentifier; 2986 } else if (name.equals("identifier")) { 2987 return addIdentifier(); 2988 } else if (name.equals("status")) { 2989 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.status"); 2990 } else if (name.equals("docStatus")) { 2991 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.docStatus"); 2992 } else if (name.equals("type")) { 2993 this.type = new CodeableConcept(); 2994 return this.type; 2995 } else if (name.equals("category")) { 2996 return addCategory(); 2997 } else if (name.equals("subject")) { 2998 this.subject = new Reference(); 2999 return this.subject; 3000 } else if (name.equals("date")) { 3001 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.date"); 3002 } else if (name.equals("author")) { 3003 return addAuthor(); 3004 } else if (name.equals("authenticator")) { 3005 this.authenticator = new Reference(); 3006 return this.authenticator; 3007 } else if (name.equals("custodian")) { 3008 this.custodian = new Reference(); 3009 return this.custodian; 3010 } else if (name.equals("relatesTo")) { 3011 return addRelatesTo(); 3012 } else if (name.equals("description")) { 3013 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.description"); 3014 } else if (name.equals("securityLabel")) { 3015 return addSecurityLabel(); 3016 } else if (name.equals("content")) { 3017 return addContent(); 3018 } else if (name.equals("context")) { 3019 this.context = new DocumentReferenceContextComponent(); 3020 return this.context; 3021 } else 3022 return super.addChild(name); 3023 } 3024 3025 public String fhirType() { 3026 return "DocumentReference"; 3027 3028 } 3029 3030 public DocumentReference copy() { 3031 DocumentReference dst = new DocumentReference(); 3032 copyValues(dst); 3033 return dst; 3034 } 3035 3036 public void copyValues(DocumentReference dst) { 3037 super.copyValues(dst); 3038 dst.masterIdentifier = masterIdentifier == null ? null : masterIdentifier.copy(); 3039 if (identifier != null) { 3040 dst.identifier = new ArrayList<Identifier>(); 3041 for (Identifier i : identifier) 3042 dst.identifier.add(i.copy()); 3043 } 3044 ; 3045 dst.status = status == null ? null : status.copy(); 3046 dst.docStatus = docStatus == null ? null : docStatus.copy(); 3047 dst.type = type == null ? null : type.copy(); 3048 if (category != null) { 3049 dst.category = new ArrayList<CodeableConcept>(); 3050 for (CodeableConcept i : category) 3051 dst.category.add(i.copy()); 3052 } 3053 ; 3054 dst.subject = subject == null ? null : subject.copy(); 3055 dst.date = date == null ? null : date.copy(); 3056 if (author != null) { 3057 dst.author = new ArrayList<Reference>(); 3058 for (Reference i : author) 3059 dst.author.add(i.copy()); 3060 } 3061 ; 3062 dst.authenticator = authenticator == null ? null : authenticator.copy(); 3063 dst.custodian = custodian == null ? null : custodian.copy(); 3064 if (relatesTo != null) { 3065 dst.relatesTo = new ArrayList<DocumentReferenceRelatesToComponent>(); 3066 for (DocumentReferenceRelatesToComponent i : relatesTo) 3067 dst.relatesTo.add(i.copy()); 3068 } 3069 ; 3070 dst.description = description == null ? null : description.copy(); 3071 if (securityLabel != null) { 3072 dst.securityLabel = new ArrayList<CodeableConcept>(); 3073 for (CodeableConcept i : securityLabel) 3074 dst.securityLabel.add(i.copy()); 3075 } 3076 ; 3077 if (content != null) { 3078 dst.content = new ArrayList<DocumentReferenceContentComponent>(); 3079 for (DocumentReferenceContentComponent i : content) 3080 dst.content.add(i.copy()); 3081 } 3082 ; 3083 dst.context = context == null ? null : context.copy(); 3084 } 3085 3086 protected DocumentReference typedCopy() { 3087 return copy(); 3088 } 3089 3090 @Override 3091 public boolean equalsDeep(Base other_) { 3092 if (!super.equalsDeep(other_)) 3093 return false; 3094 if (!(other_ instanceof DocumentReference)) 3095 return false; 3096 DocumentReference o = (DocumentReference) other_; 3097 return compareDeep(masterIdentifier, o.masterIdentifier, true) && compareDeep(identifier, o.identifier, true) 3098 && compareDeep(status, o.status, true) && compareDeep(docStatus, o.docStatus, true) 3099 && compareDeep(type, o.type, true) && compareDeep(category, o.category, true) 3100 && compareDeep(subject, o.subject, true) && compareDeep(date, o.date, true) 3101 && compareDeep(author, o.author, true) && compareDeep(authenticator, o.authenticator, true) 3102 && compareDeep(custodian, o.custodian, true) && compareDeep(relatesTo, o.relatesTo, true) 3103 && compareDeep(description, o.description, true) && compareDeep(securityLabel, o.securityLabel, true) 3104 && compareDeep(content, o.content, true) && compareDeep(context, o.context, true); 3105 } 3106 3107 @Override 3108 public boolean equalsShallow(Base other_) { 3109 if (!super.equalsShallow(other_)) 3110 return false; 3111 if (!(other_ instanceof DocumentReference)) 3112 return false; 3113 DocumentReference o = (DocumentReference) other_; 3114 return compareValues(status, o.status, true) && compareValues(docStatus, o.docStatus, true) 3115 && compareValues(date, o.date, true) && compareValues(description, o.description, true); 3116 } 3117 3118 public boolean isEmpty() { 3119 return super.isEmpty() 3120 && ca.uhn.fhir.util.ElementUtil.isEmpty(masterIdentifier, identifier, status, docStatus, type, category, 3121 subject, date, author, authenticator, custodian, relatesTo, description, securityLabel, content, context); 3122 } 3123 3124 @Override 3125 public ResourceType getResourceType() { 3126 return ResourceType.DocumentReference; 3127 } 3128 3129 /** 3130 * Search parameter: <b>date</b> 3131 * <p> 3132 * Description: <b>When this document reference was created</b><br> 3133 * Type: <b>date</b><br> 3134 * Path: <b>DocumentReference.date</b><br> 3135 * </p> 3136 */ 3137 @SearchParamDefinition(name = "date", path = "DocumentReference.date", description = "When this document reference was created", type = "date") 3138 public static final String SP_DATE = "date"; 3139 /** 3140 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3141 * <p> 3142 * Description: <b>When this document reference was created</b><br> 3143 * Type: <b>date</b><br> 3144 * Path: <b>DocumentReference.date</b><br> 3145 * </p> 3146 */ 3147 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3148 SP_DATE); 3149 3150 /** 3151 * Search parameter: <b>subject</b> 3152 * <p> 3153 * Description: <b>Who/what is the subject of the document</b><br> 3154 * Type: <b>reference</b><br> 3155 * Path: <b>DocumentReference.subject</b><br> 3156 * </p> 3157 */ 3158 @SearchParamDefinition(name = "subject", path = "DocumentReference.subject", description = "Who/what is the subject of the document", type = "reference", providesMembershipIn = { 3159 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 3160 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3161 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Device.class, Group.class, 3162 Patient.class, Practitioner.class }) 3163 public static final String SP_SUBJECT = "subject"; 3164 /** 3165 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3166 * <p> 3167 * Description: <b>Who/what is the subject of the document</b><br> 3168 * Type: <b>reference</b><br> 3169 * Path: <b>DocumentReference.subject</b><br> 3170 * </p> 3171 */ 3172 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3173 SP_SUBJECT); 3174 3175 /** 3176 * Constant for fluent queries to be used to add include statements. Specifies 3177 * the path value of "<b>DocumentReference:subject</b>". 3178 */ 3179 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 3180 "DocumentReference:subject").toLocked(); 3181 3182 /** 3183 * Search parameter: <b>description</b> 3184 * <p> 3185 * Description: <b>Human-readable description</b><br> 3186 * Type: <b>string</b><br> 3187 * Path: <b>DocumentReference.description</b><br> 3188 * </p> 3189 */ 3190 @SearchParamDefinition(name = "description", path = "DocumentReference.description", description = "Human-readable description", type = "string") 3191 public static final String SP_DESCRIPTION = "description"; 3192 /** 3193 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3194 * <p> 3195 * Description: <b>Human-readable description</b><br> 3196 * Type: <b>string</b><br> 3197 * Path: <b>DocumentReference.description</b><br> 3198 * </p> 3199 */ 3200 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 3201 SP_DESCRIPTION); 3202 3203 /** 3204 * Search parameter: <b>language</b> 3205 * <p> 3206 * Description: <b>Human language of the content (BCP-47)</b><br> 3207 * Type: <b>token</b><br> 3208 * Path: <b>DocumentReference.content.attachment.language</b><br> 3209 * </p> 3210 */ 3211 @SearchParamDefinition(name = "language", path = "DocumentReference.content.attachment.language", description = "Human language of the content (BCP-47)", type = "token") 3212 public static final String SP_LANGUAGE = "language"; 3213 /** 3214 * <b>Fluent Client</b> search parameter constant for <b>language</b> 3215 * <p> 3216 * Description: <b>Human language of the content (BCP-47)</b><br> 3217 * Type: <b>token</b><br> 3218 * Path: <b>DocumentReference.content.attachment.language</b><br> 3219 * </p> 3220 */ 3221 public static final ca.uhn.fhir.rest.gclient.TokenClientParam LANGUAGE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3222 SP_LANGUAGE); 3223 3224 /** 3225 * Search parameter: <b>type</b> 3226 * <p> 3227 * Description: <b>Kind of document (LOINC if possible)</b><br> 3228 * Type: <b>token</b><br> 3229 * Path: <b>DocumentReference.type</b><br> 3230 * </p> 3231 */ 3232 @SearchParamDefinition(name = "type", path = "DocumentReference.type", description = "Kind of document (LOINC if possible)", type = "token") 3233 public static final String SP_TYPE = "type"; 3234 /** 3235 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3236 * <p> 3237 * Description: <b>Kind of document (LOINC if possible)</b><br> 3238 * Type: <b>token</b><br> 3239 * Path: <b>DocumentReference.type</b><br> 3240 * </p> 3241 */ 3242 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3243 SP_TYPE); 3244 3245 /** 3246 * Search parameter: <b>relation</b> 3247 * <p> 3248 * Description: <b>replaces | transforms | signs | appends</b><br> 3249 * Type: <b>token</b><br> 3250 * Path: <b>DocumentReference.relatesTo.code</b><br> 3251 * </p> 3252 */ 3253 @SearchParamDefinition(name = "relation", path = "DocumentReference.relatesTo.code", description = "replaces | transforms | signs | appends", type = "token") 3254 public static final String SP_RELATION = "relation"; 3255 /** 3256 * <b>Fluent Client</b> search parameter constant for <b>relation</b> 3257 * <p> 3258 * Description: <b>replaces | transforms | signs | appends</b><br> 3259 * Type: <b>token</b><br> 3260 * Path: <b>DocumentReference.relatesTo.code</b><br> 3261 * </p> 3262 */ 3263 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RELATION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3264 SP_RELATION); 3265 3266 /** 3267 * Search parameter: <b>setting</b> 3268 * <p> 3269 * Description: <b>Additional details about where the content was created (e.g. 3270 * clinical specialty)</b><br> 3271 * Type: <b>token</b><br> 3272 * Path: <b>DocumentReference.context.practiceSetting</b><br> 3273 * </p> 3274 */ 3275 @SearchParamDefinition(name = "setting", path = "DocumentReference.context.practiceSetting", description = "Additional details about where the content was created (e.g. clinical specialty)", type = "token") 3276 public static final String SP_SETTING = "setting"; 3277 /** 3278 * <b>Fluent Client</b> search parameter constant for <b>setting</b> 3279 * <p> 3280 * Description: <b>Additional details about where the content was created (e.g. 3281 * clinical specialty)</b><br> 3282 * Type: <b>token</b><br> 3283 * Path: <b>DocumentReference.context.practiceSetting</b><br> 3284 * </p> 3285 */ 3286 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SETTING = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3287 SP_SETTING); 3288 3289 /** 3290 * Search parameter: <b>related</b> 3291 * <p> 3292 * Description: <b>Related identifiers or resources</b><br> 3293 * Type: <b>reference</b><br> 3294 * Path: <b>DocumentReference.context.related</b><br> 3295 * </p> 3296 */ 3297 @SearchParamDefinition(name = "related", path = "DocumentReference.context.related", description = "Related identifiers or resources", type = "reference") 3298 public static final String SP_RELATED = "related"; 3299 /** 3300 * <b>Fluent Client</b> search parameter constant for <b>related</b> 3301 * <p> 3302 * Description: <b>Related identifiers or resources</b><br> 3303 * Type: <b>reference</b><br> 3304 * Path: <b>DocumentReference.context.related</b><br> 3305 * </p> 3306 */ 3307 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RELATED = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3308 SP_RELATED); 3309 3310 /** 3311 * Constant for fluent queries to be used to add include statements. Specifies 3312 * the path value of "<b>DocumentReference:related</b>". 3313 */ 3314 public static final ca.uhn.fhir.model.api.Include INCLUDE_RELATED = new ca.uhn.fhir.model.api.Include( 3315 "DocumentReference:related").toLocked(); 3316 3317 /** 3318 * Search parameter: <b>patient</b> 3319 * <p> 3320 * Description: <b>Who/what is the subject of the document</b><br> 3321 * Type: <b>reference</b><br> 3322 * Path: <b>DocumentReference.subject</b><br> 3323 * </p> 3324 */ 3325 @SearchParamDefinition(name = "patient", path = "DocumentReference.subject.where(resolve() is Patient)", description = "Who/what is the subject of the document", type = "reference", target = { 3326 Patient.class }) 3327 public static final String SP_PATIENT = "patient"; 3328 /** 3329 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3330 * <p> 3331 * Description: <b>Who/what is the subject of the document</b><br> 3332 * Type: <b>reference</b><br> 3333 * Path: <b>DocumentReference.subject</b><br> 3334 * </p> 3335 */ 3336 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3337 SP_PATIENT); 3338 3339 /** 3340 * Constant for fluent queries to be used to add include statements. Specifies 3341 * the path value of "<b>DocumentReference:patient</b>". 3342 */ 3343 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 3344 "DocumentReference:patient").toLocked(); 3345 3346 /** 3347 * Search parameter: <b>relationship</b> 3348 * <p> 3349 * Description: <b>Combination of relation and relatesTo</b><br> 3350 * Type: <b>composite</b><br> 3351 * Path: <b></b><br> 3352 * </p> 3353 */ 3354 @SearchParamDefinition(name = "relationship", path = "DocumentReference.relatesTo", description = "Combination of relation and relatesTo", type = "composite", compositeOf = { 3355 "relatesto", "relation" }) 3356 public static final String SP_RELATIONSHIP = "relationship"; 3357 /** 3358 * <b>Fluent Client</b> search parameter constant for <b>relationship</b> 3359 * <p> 3360 * Description: <b>Combination of relation and relatesTo</b><br> 3361 * Type: <b>composite</b><br> 3362 * Path: <b></b><br> 3363 * </p> 3364 */ 3365 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.ReferenceClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> RELATIONSHIP = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.ReferenceClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 3366 SP_RELATIONSHIP); 3367 3368 /** 3369 * Search parameter: <b>event</b> 3370 * <p> 3371 * Description: <b>Main clinical acts documented</b><br> 3372 * Type: <b>token</b><br> 3373 * Path: <b>DocumentReference.context.event</b><br> 3374 * </p> 3375 */ 3376 @SearchParamDefinition(name = "event", path = "DocumentReference.context.event", description = "Main clinical acts documented", type = "token") 3377 public static final String SP_EVENT = "event"; 3378 /** 3379 * <b>Fluent Client</b> search parameter constant for <b>event</b> 3380 * <p> 3381 * Description: <b>Main clinical acts documented</b><br> 3382 * Type: <b>token</b><br> 3383 * Path: <b>DocumentReference.context.event</b><br> 3384 * </p> 3385 */ 3386 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EVENT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3387 SP_EVENT); 3388 3389 /** 3390 * Search parameter: <b>authenticator</b> 3391 * <p> 3392 * Description: <b>Who/what authenticated the document</b><br> 3393 * Type: <b>reference</b><br> 3394 * Path: <b>DocumentReference.authenticator</b><br> 3395 * </p> 3396 */ 3397 @SearchParamDefinition(name = "authenticator", path = "DocumentReference.authenticator", description = "Who/what authenticated the document", type = "reference", providesMembershipIn = { 3398 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 3399 Practitioner.class, PractitionerRole.class }) 3400 public static final String SP_AUTHENTICATOR = "authenticator"; 3401 /** 3402 * <b>Fluent Client</b> search parameter constant for <b>authenticator</b> 3403 * <p> 3404 * Description: <b>Who/what authenticated the document</b><br> 3405 * Type: <b>reference</b><br> 3406 * Path: <b>DocumentReference.authenticator</b><br> 3407 * </p> 3408 */ 3409 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHENTICATOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3410 SP_AUTHENTICATOR); 3411 3412 /** 3413 * Constant for fluent queries to be used to add include statements. Specifies 3414 * the path value of "<b>DocumentReference:authenticator</b>". 3415 */ 3416 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHENTICATOR = new ca.uhn.fhir.model.api.Include( 3417 "DocumentReference:authenticator").toLocked(); 3418 3419 /** 3420 * Search parameter: <b>identifier</b> 3421 * <p> 3422 * Description: <b>Master Version Specific Identifier</b><br> 3423 * Type: <b>token</b><br> 3424 * Path: <b>DocumentReference.masterIdentifier, 3425 * DocumentReference.identifier</b><br> 3426 * </p> 3427 */ 3428 @SearchParamDefinition(name = "identifier", path = "DocumentReference.masterIdentifier | DocumentReference.identifier", description = "Master Version Specific Identifier", type = "token") 3429 public static final String SP_IDENTIFIER = "identifier"; 3430 /** 3431 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3432 * <p> 3433 * Description: <b>Master Version Specific Identifier</b><br> 3434 * Type: <b>token</b><br> 3435 * Path: <b>DocumentReference.masterIdentifier, 3436 * DocumentReference.identifier</b><br> 3437 * </p> 3438 */ 3439 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3440 SP_IDENTIFIER); 3441 3442 /** 3443 * Search parameter: <b>period</b> 3444 * <p> 3445 * Description: <b>Time of service that is being documented</b><br> 3446 * Type: <b>date</b><br> 3447 * Path: <b>DocumentReference.context.period</b><br> 3448 * </p> 3449 */ 3450 @SearchParamDefinition(name = "period", path = "DocumentReference.context.period", description = "Time of service that is being documented", type = "date") 3451 public static final String SP_PERIOD = "period"; 3452 /** 3453 * <b>Fluent Client</b> search parameter constant for <b>period</b> 3454 * <p> 3455 * Description: <b>Time of service that is being documented</b><br> 3456 * Type: <b>date</b><br> 3457 * Path: <b>DocumentReference.context.period</b><br> 3458 * </p> 3459 */ 3460 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam( 3461 SP_PERIOD); 3462 3463 /** 3464 * Search parameter: <b>custodian</b> 3465 * <p> 3466 * Description: <b>Organization which maintains the document</b><br> 3467 * Type: <b>reference</b><br> 3468 * Path: <b>DocumentReference.custodian</b><br> 3469 * </p> 3470 */ 3471 @SearchParamDefinition(name = "custodian", path = "DocumentReference.custodian", description = "Organization which maintains the document", type = "reference", target = { 3472 Organization.class }) 3473 public static final String SP_CUSTODIAN = "custodian"; 3474 /** 3475 * <b>Fluent Client</b> search parameter constant for <b>custodian</b> 3476 * <p> 3477 * Description: <b>Organization which maintains the document</b><br> 3478 * Type: <b>reference</b><br> 3479 * Path: <b>DocumentReference.custodian</b><br> 3480 * </p> 3481 */ 3482 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CUSTODIAN = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3483 SP_CUSTODIAN); 3484 3485 /** 3486 * Constant for fluent queries to be used to add include statements. Specifies 3487 * the path value of "<b>DocumentReference:custodian</b>". 3488 */ 3489 public static final ca.uhn.fhir.model.api.Include INCLUDE_CUSTODIAN = new ca.uhn.fhir.model.api.Include( 3490 "DocumentReference:custodian").toLocked(); 3491 3492 /** 3493 * Search parameter: <b>author</b> 3494 * <p> 3495 * Description: <b>Who and/or what authored the document</b><br> 3496 * Type: <b>reference</b><br> 3497 * Path: <b>DocumentReference.author</b><br> 3498 * </p> 3499 */ 3500 @SearchParamDefinition(name = "author", path = "DocumentReference.author", description = "Who and/or what authored the document", type = "reference", providesMembershipIn = { 3501 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 3502 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3503 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 3504 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, 3505 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 3506 public static final String SP_AUTHOR = "author"; 3507 /** 3508 * <b>Fluent Client</b> search parameter constant for <b>author</b> 3509 * <p> 3510 * Description: <b>Who and/or what authored the document</b><br> 3511 * Type: <b>reference</b><br> 3512 * Path: <b>DocumentReference.author</b><br> 3513 * </p> 3514 */ 3515 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3516 SP_AUTHOR); 3517 3518 /** 3519 * Constant for fluent queries to be used to add include statements. Specifies 3520 * the path value of "<b>DocumentReference:author</b>". 3521 */ 3522 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include( 3523 "DocumentReference:author").toLocked(); 3524 3525 /** 3526 * Search parameter: <b>format</b> 3527 * <p> 3528 * Description: <b>Format/content rules for the document</b><br> 3529 * Type: <b>token</b><br> 3530 * Path: <b>DocumentReference.content.format</b><br> 3531 * </p> 3532 */ 3533 @SearchParamDefinition(name = "format", path = "DocumentReference.content.format", description = "Format/content rules for the document", type = "token") 3534 public static final String SP_FORMAT = "format"; 3535 /** 3536 * <b>Fluent Client</b> search parameter constant for <b>format</b> 3537 * <p> 3538 * Description: <b>Format/content rules for the document</b><br> 3539 * Type: <b>token</b><br> 3540 * Path: <b>DocumentReference.content.format</b><br> 3541 * </p> 3542 */ 3543 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FORMAT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3544 SP_FORMAT); 3545 3546 /** 3547 * Search parameter: <b>encounter</b> 3548 * <p> 3549 * Description: <b>Context of the document content</b><br> 3550 * Type: <b>reference</b><br> 3551 * Path: <b>DocumentReference.context.encounter</b><br> 3552 * </p> 3553 */ 3554 @SearchParamDefinition(name = "encounter", path = "DocumentReference.context.encounter", description = "Context of the document content", type = "reference", providesMembershipIn = { 3555 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class, 3556 EpisodeOfCare.class }) 3557 public static final String SP_ENCOUNTER = "encounter"; 3558 /** 3559 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 3560 * <p> 3561 * Description: <b>Context of the document content</b><br> 3562 * Type: <b>reference</b><br> 3563 * Path: <b>DocumentReference.context.encounter</b><br> 3564 * </p> 3565 */ 3566 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3567 SP_ENCOUNTER); 3568 3569 /** 3570 * Constant for fluent queries to be used to add include statements. Specifies 3571 * the path value of "<b>DocumentReference:encounter</b>". 3572 */ 3573 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 3574 "DocumentReference:encounter").toLocked(); 3575 3576 /** 3577 * Search parameter: <b>contenttype</b> 3578 * <p> 3579 * Description: <b>Mime type of the content, with charset etc.</b><br> 3580 * Type: <b>token</b><br> 3581 * Path: <b>DocumentReference.content.attachment.contentType</b><br> 3582 * </p> 3583 */ 3584 @SearchParamDefinition(name = "contenttype", path = "DocumentReference.content.attachment.contentType", description = "Mime type of the content, with charset etc.", type = "token") 3585 public static final String SP_CONTENTTYPE = "contenttype"; 3586 /** 3587 * <b>Fluent Client</b> search parameter constant for <b>contenttype</b> 3588 * <p> 3589 * Description: <b>Mime type of the content, with charset etc.</b><br> 3590 * Type: <b>token</b><br> 3591 * Path: <b>DocumentReference.content.attachment.contentType</b><br> 3592 * </p> 3593 */ 3594 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTENTTYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3595 SP_CONTENTTYPE); 3596 3597 /** 3598 * Search parameter: <b>security-label</b> 3599 * <p> 3600 * Description: <b>Document security-tags</b><br> 3601 * Type: <b>token</b><br> 3602 * Path: <b>DocumentReference.securityLabel</b><br> 3603 * </p> 3604 */ 3605 @SearchParamDefinition(name = "security-label", path = "DocumentReference.securityLabel", description = "Document security-tags", type = "token") 3606 public static final String SP_SECURITY_LABEL = "security-label"; 3607 /** 3608 * <b>Fluent Client</b> search parameter constant for <b>security-label</b> 3609 * <p> 3610 * Description: <b>Document security-tags</b><br> 3611 * Type: <b>token</b><br> 3612 * Path: <b>DocumentReference.securityLabel</b><br> 3613 * </p> 3614 */ 3615 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SECURITY_LABEL = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3616 SP_SECURITY_LABEL); 3617 3618 /** 3619 * Search parameter: <b>location</b> 3620 * <p> 3621 * Description: <b>Uri where the data can be found</b><br> 3622 * Type: <b>uri</b><br> 3623 * Path: <b>DocumentReference.content.attachment.url</b><br> 3624 * </p> 3625 */ 3626 @SearchParamDefinition(name = "location", path = "DocumentReference.content.attachment.url", description = "Uri where the data can be found", type = "uri") 3627 public static final String SP_LOCATION = "location"; 3628 /** 3629 * <b>Fluent Client</b> search parameter constant for <b>location</b> 3630 * <p> 3631 * Description: <b>Uri where the data can be found</b><br> 3632 * Type: <b>uri</b><br> 3633 * Path: <b>DocumentReference.content.attachment.url</b><br> 3634 * </p> 3635 */ 3636 public static final ca.uhn.fhir.rest.gclient.UriClientParam LOCATION = new ca.uhn.fhir.rest.gclient.UriClientParam( 3637 SP_LOCATION); 3638 3639 /** 3640 * Search parameter: <b>category</b> 3641 * <p> 3642 * Description: <b>Categorization of document</b><br> 3643 * Type: <b>token</b><br> 3644 * Path: <b>DocumentReference.category</b><br> 3645 * </p> 3646 */ 3647 @SearchParamDefinition(name = "category", path = "DocumentReference.category", description = "Categorization of document", type = "token") 3648 public static final String SP_CATEGORY = "category"; 3649 /** 3650 * <b>Fluent Client</b> search parameter constant for <b>category</b> 3651 * <p> 3652 * Description: <b>Categorization of document</b><br> 3653 * Type: <b>token</b><br> 3654 * Path: <b>DocumentReference.category</b><br> 3655 * </p> 3656 */ 3657 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3658 SP_CATEGORY); 3659 3660 /** 3661 * Search parameter: <b>relatesto</b> 3662 * <p> 3663 * Description: <b>Target of the relationship</b><br> 3664 * Type: <b>reference</b><br> 3665 * Path: <b>DocumentReference.relatesTo.target</b><br> 3666 * </p> 3667 */ 3668 @SearchParamDefinition(name = "relatesto", path = "DocumentReference.relatesTo.target", description = "Target of the relationship", type = "reference", target = { 3669 DocumentReference.class }) 3670 public static final String SP_RELATESTO = "relatesto"; 3671 /** 3672 * <b>Fluent Client</b> search parameter constant for <b>relatesto</b> 3673 * <p> 3674 * Description: <b>Target of the relationship</b><br> 3675 * Type: <b>reference</b><br> 3676 * Path: <b>DocumentReference.relatesTo.target</b><br> 3677 * </p> 3678 */ 3679 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RELATESTO = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3680 SP_RELATESTO); 3681 3682 /** 3683 * Constant for fluent queries to be used to add include statements. Specifies 3684 * the path value of "<b>DocumentReference:relatesto</b>". 3685 */ 3686 public static final ca.uhn.fhir.model.api.Include INCLUDE_RELATESTO = new ca.uhn.fhir.model.api.Include( 3687 "DocumentReference:relatesto").toLocked(); 3688 3689 /** 3690 * Search parameter: <b>facility</b> 3691 * <p> 3692 * Description: <b>Kind of facility where patient was seen</b><br> 3693 * Type: <b>token</b><br> 3694 * Path: <b>DocumentReference.context.facilityType</b><br> 3695 * </p> 3696 */ 3697 @SearchParamDefinition(name = "facility", path = "DocumentReference.context.facilityType", description = "Kind of facility where patient was seen", type = "token") 3698 public static final String SP_FACILITY = "facility"; 3699 /** 3700 * <b>Fluent Client</b> search parameter constant for <b>facility</b> 3701 * <p> 3702 * Description: <b>Kind of facility where patient was seen</b><br> 3703 * Type: <b>token</b><br> 3704 * Path: <b>DocumentReference.context.facilityType</b><br> 3705 * </p> 3706 */ 3707 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FACILITY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3708 SP_FACILITY); 3709 3710 /** 3711 * Search parameter: <b>status</b> 3712 * <p> 3713 * Description: <b>current | superseded | entered-in-error</b><br> 3714 * Type: <b>token</b><br> 3715 * Path: <b>DocumentReference.status</b><br> 3716 * </p> 3717 */ 3718 @SearchParamDefinition(name = "status", path = "DocumentReference.status", description = "current | superseded | entered-in-error", type = "token") 3719 public static final String SP_STATUS = "status"; 3720 /** 3721 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3722 * <p> 3723 * Description: <b>current | superseded | entered-in-error</b><br> 3724 * Type: <b>token</b><br> 3725 * Path: <b>DocumentReference.status</b><br> 3726 * </p> 3727 */ 3728 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3729 SP_STATUS); 3730 3731}