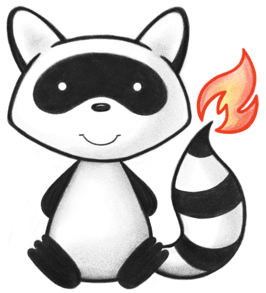
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.DocumentReferenceStatus; 040import org.hl7.fhir.r4.model.Enumerations.DocumentReferenceStatusEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048 049/** 050 * A reference to a document of any kind for any purpose. Provides metadata 051 * about the document so that the document can be discovered and managed. The 052 * scope of a document is any seralized object with a mime-type, so includes 053 * formal patient centric documents (CDA), cliical notes, scanned paper, and 054 * non-patient specific documents like policy text. 055 */ 056@ResourceDef(name = "DocumentReference", profile = "http://hl7.org/fhir/StructureDefinition/DocumentReference") 057public class DocumentReference extends DomainResource { 058 059 public enum ReferredDocumentStatus { 060 /** 061 * This is a preliminary composition or document (also known as initial or 062 * interim). The content may be incomplete or unverified. 063 */ 064 PRELIMINARY, 065 /** 066 * This version of the composition is complete and verified by an appropriate 067 * person and no further work is planned. Any subsequent updates would be on a 068 * new version of the composition. 069 */ 070 FINAL, 071 /** 072 * The composition content or the referenced resources have been modified 073 * (edited or added to) subsequent to being released as "final" and the 074 * composition is complete and verified by an authorized person. 075 */ 076 AMENDED, 077 /** 078 * The composition or document was originally created/issued in error, and this 079 * is an amendment that marks that the entire series should not be considered as 080 * valid. 081 */ 082 ENTEREDINERROR, 083 /** 084 * added to help the parsers with the generic types 085 */ 086 NULL; 087 088 public static ReferredDocumentStatus fromCode(String codeString) throws FHIRException { 089 if (codeString == null || "".equals(codeString)) 090 return null; 091 if ("preliminary".equals(codeString)) 092 return PRELIMINARY; 093 if ("final".equals(codeString)) 094 return FINAL; 095 if ("amended".equals(codeString)) 096 return AMENDED; 097 if ("entered-in-error".equals(codeString)) 098 return ENTEREDINERROR; 099 if (Configuration.isAcceptInvalidEnums()) 100 return null; 101 else 102 throw new FHIRException("Unknown ReferredDocumentStatus code '" + codeString + "'"); 103 } 104 105 public String toCode() { 106 switch (this) { 107 case PRELIMINARY: 108 return "preliminary"; 109 case FINAL: 110 return "final"; 111 case AMENDED: 112 return "amended"; 113 case ENTEREDINERROR: 114 return "entered-in-error"; 115 case NULL: 116 return null; 117 default: 118 return "?"; 119 } 120 } 121 122 public String getSystem() { 123 switch (this) { 124 case PRELIMINARY: 125 return "http://hl7.org/fhir/composition-status"; 126 case FINAL: 127 return "http://hl7.org/fhir/composition-status"; 128 case AMENDED: 129 return "http://hl7.org/fhir/composition-status"; 130 case ENTEREDINERROR: 131 return "http://hl7.org/fhir/composition-status"; 132 case NULL: 133 return null; 134 default: 135 return "?"; 136 } 137 } 138 139 public String getDefinition() { 140 switch (this) { 141 case PRELIMINARY: 142 return "This is a preliminary composition or document (also known as initial or interim). The content may be incomplete or unverified."; 143 case FINAL: 144 return "This version of the composition is complete and verified by an appropriate person and no further work is planned. Any subsequent updates would be on a new version of the composition."; 145 case AMENDED: 146 return "The composition content or the referenced resources have been modified (edited or added to) subsequent to being released as \"final\" and the composition is complete and verified by an authorized person."; 147 case ENTEREDINERROR: 148 return "The composition or document was originally created/issued in error, and this is an amendment that marks that the entire series should not be considered as valid."; 149 case NULL: 150 return null; 151 default: 152 return "?"; 153 } 154 } 155 156 public String getDisplay() { 157 switch (this) { 158 case PRELIMINARY: 159 return "Preliminary"; 160 case FINAL: 161 return "Final"; 162 case AMENDED: 163 return "Amended"; 164 case ENTEREDINERROR: 165 return "Entered in Error"; 166 case NULL: 167 return null; 168 default: 169 return "?"; 170 } 171 } 172 } 173 174 public static class ReferredDocumentStatusEnumFactory implements EnumFactory<ReferredDocumentStatus> { 175 public ReferredDocumentStatus fromCode(String codeString) throws IllegalArgumentException { 176 if (codeString == null || "".equals(codeString)) 177 if (codeString == null || "".equals(codeString)) 178 return null; 179 if ("preliminary".equals(codeString)) 180 return ReferredDocumentStatus.PRELIMINARY; 181 if ("final".equals(codeString)) 182 return ReferredDocumentStatus.FINAL; 183 if ("amended".equals(codeString)) 184 return ReferredDocumentStatus.AMENDED; 185 if ("entered-in-error".equals(codeString)) 186 return ReferredDocumentStatus.ENTEREDINERROR; 187 throw new IllegalArgumentException("Unknown ReferredDocumentStatus code '" + codeString + "'"); 188 } 189 190 public Enumeration<ReferredDocumentStatus> fromType(PrimitiveType<?> code) throws FHIRException { 191 if (code == null) 192 return null; 193 if (code.isEmpty()) 194 return new Enumeration<ReferredDocumentStatus>(this, ReferredDocumentStatus.NULL, code); 195 String codeString = code.asStringValue(); 196 if (codeString == null || "".equals(codeString)) 197 return new Enumeration<ReferredDocumentStatus>(this, ReferredDocumentStatus.NULL, code); 198 if ("preliminary".equals(codeString)) 199 return new Enumeration<ReferredDocumentStatus>(this, ReferredDocumentStatus.PRELIMINARY, code); 200 if ("final".equals(codeString)) 201 return new Enumeration<ReferredDocumentStatus>(this, ReferredDocumentStatus.FINAL, code); 202 if ("amended".equals(codeString)) 203 return new Enumeration<ReferredDocumentStatus>(this, ReferredDocumentStatus.AMENDED, code); 204 if ("entered-in-error".equals(codeString)) 205 return new Enumeration<ReferredDocumentStatus>(this, ReferredDocumentStatus.ENTEREDINERROR, code); 206 throw new FHIRException("Unknown ReferredDocumentStatus code '" + codeString + "'"); 207 } 208 209 public String toCode(ReferredDocumentStatus code) { 210 if (code == ReferredDocumentStatus.PRELIMINARY) 211 return "preliminary"; 212 if (code == ReferredDocumentStatus.FINAL) 213 return "final"; 214 if (code == ReferredDocumentStatus.AMENDED) 215 return "amended"; 216 if (code == ReferredDocumentStatus.ENTEREDINERROR) 217 return "entered-in-error"; 218 return "?"; 219 } 220 221 public String toSystem(ReferredDocumentStatus code) { 222 return code.getSystem(); 223 } 224 } 225 226 public enum DocumentRelationshipType { 227 /** 228 * This document logically replaces or supersedes the target document. 229 */ 230 REPLACES, 231 /** 232 * This document was generated by transforming the target document (e.g. format 233 * or language conversion). 234 */ 235 TRANSFORMS, 236 /** 237 * This document is a signature of the target document. 238 */ 239 SIGNS, 240 /** 241 * This document adds additional information to the target document. 242 */ 243 APPENDS, 244 /** 245 * added to help the parsers with the generic types 246 */ 247 NULL; 248 249 public static DocumentRelationshipType fromCode(String codeString) throws FHIRException { 250 if (codeString == null || "".equals(codeString)) 251 return null; 252 if ("replaces".equals(codeString)) 253 return REPLACES; 254 if ("transforms".equals(codeString)) 255 return TRANSFORMS; 256 if ("signs".equals(codeString)) 257 return SIGNS; 258 if ("appends".equals(codeString)) 259 return APPENDS; 260 if (Configuration.isAcceptInvalidEnums()) 261 return null; 262 else 263 throw new FHIRException("Unknown DocumentRelationshipType code '" + codeString + "'"); 264 } 265 266 public String toCode() { 267 switch (this) { 268 case REPLACES: 269 return "replaces"; 270 case TRANSFORMS: 271 return "transforms"; 272 case SIGNS: 273 return "signs"; 274 case APPENDS: 275 return "appends"; 276 case NULL: 277 return null; 278 default: 279 return "?"; 280 } 281 } 282 283 public String getSystem() { 284 switch (this) { 285 case REPLACES: 286 return "http://hl7.org/fhir/document-relationship-type"; 287 case TRANSFORMS: 288 return "http://hl7.org/fhir/document-relationship-type"; 289 case SIGNS: 290 return "http://hl7.org/fhir/document-relationship-type"; 291 case APPENDS: 292 return "http://hl7.org/fhir/document-relationship-type"; 293 case NULL: 294 return null; 295 default: 296 return "?"; 297 } 298 } 299 300 public String getDefinition() { 301 switch (this) { 302 case REPLACES: 303 return "This document logically replaces or supersedes the target document."; 304 case TRANSFORMS: 305 return "This document was generated by transforming the target document (e.g. format or language conversion)."; 306 case SIGNS: 307 return "This document is a signature of the target document."; 308 case APPENDS: 309 return "This document adds additional information to the target document."; 310 case NULL: 311 return null; 312 default: 313 return "?"; 314 } 315 } 316 317 public String getDisplay() { 318 switch (this) { 319 case REPLACES: 320 return "Replaces"; 321 case TRANSFORMS: 322 return "Transforms"; 323 case SIGNS: 324 return "Signs"; 325 case APPENDS: 326 return "Appends"; 327 case NULL: 328 return null; 329 default: 330 return "?"; 331 } 332 } 333 } 334 335 public static class DocumentRelationshipTypeEnumFactory implements EnumFactory<DocumentRelationshipType> { 336 public DocumentRelationshipType fromCode(String codeString) throws IllegalArgumentException { 337 if (codeString == null || "".equals(codeString)) 338 if (codeString == null || "".equals(codeString)) 339 return null; 340 if ("replaces".equals(codeString)) 341 return DocumentRelationshipType.REPLACES; 342 if ("transforms".equals(codeString)) 343 return DocumentRelationshipType.TRANSFORMS; 344 if ("signs".equals(codeString)) 345 return DocumentRelationshipType.SIGNS; 346 if ("appends".equals(codeString)) 347 return DocumentRelationshipType.APPENDS; 348 throw new IllegalArgumentException("Unknown DocumentRelationshipType code '" + codeString + "'"); 349 } 350 351 public Enumeration<DocumentRelationshipType> fromType(PrimitiveType<?> code) throws FHIRException { 352 if (code == null) 353 return null; 354 if (code.isEmpty()) 355 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.NULL, code); 356 String codeString = code.asStringValue(); 357 if (codeString == null || "".equals(codeString)) 358 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.NULL, code); 359 if ("replaces".equals(codeString)) 360 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.REPLACES, code); 361 if ("transforms".equals(codeString)) 362 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.TRANSFORMS, code); 363 if ("signs".equals(codeString)) 364 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.SIGNS, code); 365 if ("appends".equals(codeString)) 366 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.APPENDS, code); 367 throw new FHIRException("Unknown DocumentRelationshipType code '" + codeString + "'"); 368 } 369 370 public String toCode(DocumentRelationshipType code) { 371 if (code == DocumentRelationshipType.REPLACES) 372 return "replaces"; 373 if (code == DocumentRelationshipType.TRANSFORMS) 374 return "transforms"; 375 if (code == DocumentRelationshipType.SIGNS) 376 return "signs"; 377 if (code == DocumentRelationshipType.APPENDS) 378 return "appends"; 379 return "?"; 380 } 381 382 public String toSystem(DocumentRelationshipType code) { 383 return code.getSystem(); 384 } 385 } 386 387 @Block() 388 public static class DocumentReferenceRelatesToComponent extends BackboneElement implements IBaseBackboneElement { 389 /** 390 * The type of relationship that this document has with anther document. 391 */ 392 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 393 @Description(shortDefinition = "replaces | transforms | signs | appends", formalDefinition = "The type of relationship that this document has with anther document.") 394 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/document-relationship-type") 395 protected Enumeration<DocumentRelationshipType> code; 396 397 /** 398 * The target document of this relationship. 399 */ 400 @Child(name = "target", type = { 401 DocumentReference.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 402 @Description(shortDefinition = "Target of the relationship", formalDefinition = "The target document of this relationship.") 403 protected Reference target; 404 405 /** 406 * The actual object that is the target of the reference (The target document of 407 * this relationship.) 408 */ 409 protected DocumentReference targetTarget; 410 411 private static final long serialVersionUID = -347257495L; 412 413 /** 414 * Constructor 415 */ 416 public DocumentReferenceRelatesToComponent() { 417 super(); 418 } 419 420 /** 421 * Constructor 422 */ 423 public DocumentReferenceRelatesToComponent(Enumeration<DocumentRelationshipType> code, Reference target) { 424 super(); 425 this.code = code; 426 this.target = target; 427 } 428 429 /** 430 * @return {@link #code} (The type of relationship that this document has with 431 * anther document.). This is the underlying object with id, value and 432 * extensions. The accessor "getCode" gives direct access to the value 433 */ 434 public Enumeration<DocumentRelationshipType> getCodeElement() { 435 if (this.code == null) 436 if (Configuration.errorOnAutoCreate()) 437 throw new Error("Attempt to auto-create DocumentReferenceRelatesToComponent.code"); 438 else if (Configuration.doAutoCreate()) 439 this.code = new Enumeration<DocumentRelationshipType>(new DocumentRelationshipTypeEnumFactory()); // bb 440 return this.code; 441 } 442 443 public boolean hasCodeElement() { 444 return this.code != null && !this.code.isEmpty(); 445 } 446 447 public boolean hasCode() { 448 return this.code != null && !this.code.isEmpty(); 449 } 450 451 /** 452 * @param value {@link #code} (The type of relationship that this document has 453 * with anther document.). This is the underlying object with id, 454 * value and extensions. The accessor "getCode" gives direct access 455 * to the value 456 */ 457 public DocumentReferenceRelatesToComponent setCodeElement(Enumeration<DocumentRelationshipType> value) { 458 this.code = value; 459 return this; 460 } 461 462 /** 463 * @return The type of relationship that this document has with anther document. 464 */ 465 public DocumentRelationshipType getCode() { 466 return this.code == null ? null : this.code.getValue(); 467 } 468 469 /** 470 * @param value The type of relationship that this document has with anther 471 * document. 472 */ 473 public DocumentReferenceRelatesToComponent setCode(DocumentRelationshipType value) { 474 if (this.code == null) 475 this.code = new Enumeration<DocumentRelationshipType>(new DocumentRelationshipTypeEnumFactory()); 476 this.code.setValue(value); 477 return this; 478 } 479 480 /** 481 * @return {@link #target} (The target document of this relationship.) 482 */ 483 public Reference getTarget() { 484 if (this.target == null) 485 if (Configuration.errorOnAutoCreate()) 486 throw new Error("Attempt to auto-create DocumentReferenceRelatesToComponent.target"); 487 else if (Configuration.doAutoCreate()) 488 this.target = new Reference(); // cc 489 return this.target; 490 } 491 492 public boolean hasTarget() { 493 return this.target != null && !this.target.isEmpty(); 494 } 495 496 /** 497 * @param value {@link #target} (The target document of this relationship.) 498 */ 499 public DocumentReferenceRelatesToComponent setTarget(Reference value) { 500 this.target = value; 501 return this; 502 } 503 504 /** 505 * @return {@link #target} The actual object that is the target of the 506 * reference. The reference library doesn't populate this, but you can 507 * use it to hold the resource if you resolve it. (The target document 508 * of this relationship.) 509 */ 510 public DocumentReference getTargetTarget() { 511 if (this.targetTarget == null) 512 if (Configuration.errorOnAutoCreate()) 513 throw new Error("Attempt to auto-create DocumentReferenceRelatesToComponent.target"); 514 else if (Configuration.doAutoCreate()) 515 this.targetTarget = new DocumentReference(); // aa 516 return this.targetTarget; 517 } 518 519 /** 520 * @param value {@link #target} The actual object that is the target of the 521 * reference. The reference library doesn't use these, but you can 522 * use it to hold the resource if you resolve it. (The target 523 * document of this relationship.) 524 */ 525 public DocumentReferenceRelatesToComponent setTargetTarget(DocumentReference value) { 526 this.targetTarget = value; 527 return this; 528 } 529 530 protected void listChildren(List<Property> children) { 531 super.listChildren(children); 532 children.add(new Property("code", "code", "The type of relationship that this document has with anther document.", 533 0, 1, code)); 534 children.add(new Property("target", "Reference(DocumentReference)", "The target document of this relationship.", 535 0, 1, target)); 536 } 537 538 @Override 539 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 540 switch (_hash) { 541 case 3059181: 542 /* code */ return new Property("code", "code", 543 "The type of relationship that this document has with anther document.", 0, 1, code); 544 case -880905839: 545 /* target */ return new Property("target", "Reference(DocumentReference)", 546 "The target document of this relationship.", 0, 1, target); 547 default: 548 return super.getNamedProperty(_hash, _name, _checkValid); 549 } 550 551 } 552 553 @Override 554 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 555 switch (hash) { 556 case 3059181: 557 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // Enumeration<DocumentRelationshipType> 558 case -880905839: 559 /* target */ return this.target == null ? new Base[0] : new Base[] { this.target }; // Reference 560 default: 561 return super.getProperty(hash, name, checkValid); 562 } 563 564 } 565 566 @Override 567 public Base setProperty(int hash, String name, Base value) throws FHIRException { 568 switch (hash) { 569 case 3059181: // code 570 value = new DocumentRelationshipTypeEnumFactory().fromType(castToCode(value)); 571 this.code = (Enumeration) value; // Enumeration<DocumentRelationshipType> 572 return value; 573 case -880905839: // target 574 this.target = castToReference(value); // Reference 575 return value; 576 default: 577 return super.setProperty(hash, name, value); 578 } 579 580 } 581 582 @Override 583 public Base setProperty(String name, Base value) throws FHIRException { 584 if (name.equals("code")) { 585 value = new DocumentRelationshipTypeEnumFactory().fromType(castToCode(value)); 586 this.code = (Enumeration) value; // Enumeration<DocumentRelationshipType> 587 } else if (name.equals("target")) { 588 this.target = castToReference(value); // Reference 589 } else 590 return super.setProperty(name, value); 591 return value; 592 } 593 594 @Override 595 public Base makeProperty(int hash, String name) throws FHIRException { 596 switch (hash) { 597 case 3059181: 598 return getCodeElement(); 599 case -880905839: 600 return getTarget(); 601 default: 602 return super.makeProperty(hash, name); 603 } 604 605 } 606 607 @Override 608 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 609 switch (hash) { 610 case 3059181: 611 /* code */ return new String[] { "code" }; 612 case -880905839: 613 /* target */ return new String[] { "Reference" }; 614 default: 615 return super.getTypesForProperty(hash, name); 616 } 617 618 } 619 620 @Override 621 public Base addChild(String name) throws FHIRException { 622 if (name.equals("code")) { 623 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.code"); 624 } else if (name.equals("target")) { 625 this.target = new Reference(); 626 return this.target; 627 } else 628 return super.addChild(name); 629 } 630 631 public DocumentReferenceRelatesToComponent copy() { 632 DocumentReferenceRelatesToComponent dst = new DocumentReferenceRelatesToComponent(); 633 copyValues(dst); 634 return dst; 635 } 636 637 public void copyValues(DocumentReferenceRelatesToComponent dst) { 638 super.copyValues(dst); 639 dst.code = code == null ? null : code.copy(); 640 dst.target = target == null ? null : target.copy(); 641 } 642 643 @Override 644 public boolean equalsDeep(Base other_) { 645 if (!super.equalsDeep(other_)) 646 return false; 647 if (!(other_ instanceof DocumentReferenceRelatesToComponent)) 648 return false; 649 DocumentReferenceRelatesToComponent o = (DocumentReferenceRelatesToComponent) other_; 650 return compareDeep(code, o.code, true) && compareDeep(target, o.target, true); 651 } 652 653 @Override 654 public boolean equalsShallow(Base other_) { 655 if (!super.equalsShallow(other_)) 656 return false; 657 if (!(other_ instanceof DocumentReferenceRelatesToComponent)) 658 return false; 659 DocumentReferenceRelatesToComponent o = (DocumentReferenceRelatesToComponent) other_; 660 return compareValues(code, o.code, true); 661 } 662 663 public boolean isEmpty() { 664 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, target); 665 } 666 667 public String fhirType() { 668 return "DocumentReference.relatesTo"; 669 670 } 671 672 } 673 674 @Block() 675 public static class DocumentReferenceContentComponent extends BackboneElement implements IBaseBackboneElement { 676 /** 677 * The document or URL of the document along with critical metadata to prove 678 * content has integrity. 679 */ 680 @Child(name = "attachment", type = { 681 Attachment.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 682 @Description(shortDefinition = "Where to access the document", formalDefinition = "The document or URL of the document along with critical metadata to prove content has integrity.") 683 protected Attachment attachment; 684 685 /** 686 * An identifier of the document encoding, structure, and template that the 687 * document conforms to beyond the base format indicated in the mimeType. 688 */ 689 @Child(name = "format", type = { Coding.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 690 @Description(shortDefinition = "Format/content rules for the document", formalDefinition = "An identifier of the document encoding, structure, and template that the document conforms to beyond the base format indicated in the mimeType.") 691 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/formatcodes") 692 protected Coding format; 693 694 private static final long serialVersionUID = -1313860217L; 695 696 /** 697 * Constructor 698 */ 699 public DocumentReferenceContentComponent() { 700 super(); 701 } 702 703 /** 704 * Constructor 705 */ 706 public DocumentReferenceContentComponent(Attachment attachment) { 707 super(); 708 this.attachment = attachment; 709 } 710 711 /** 712 * @return {@link #attachment} (The document or URL of the document along with 713 * critical metadata to prove content has integrity.) 714 */ 715 public Attachment getAttachment() { 716 if (this.attachment == null) 717 if (Configuration.errorOnAutoCreate()) 718 throw new Error("Attempt to auto-create DocumentReferenceContentComponent.attachment"); 719 else if (Configuration.doAutoCreate()) 720 this.attachment = new Attachment(); // cc 721 return this.attachment; 722 } 723 724 public boolean hasAttachment() { 725 return this.attachment != null && !this.attachment.isEmpty(); 726 } 727 728 /** 729 * @param value {@link #attachment} (The document or URL of the document along 730 * with critical metadata to prove content has integrity.) 731 */ 732 public DocumentReferenceContentComponent setAttachment(Attachment value) { 733 this.attachment = value; 734 return this; 735 } 736 737 /** 738 * @return {@link #format} (An identifier of the document encoding, structure, 739 * and template that the document conforms to beyond the base format 740 * indicated in the mimeType.) 741 */ 742 public Coding getFormat() { 743 if (this.format == null) 744 if (Configuration.errorOnAutoCreate()) 745 throw new Error("Attempt to auto-create DocumentReferenceContentComponent.format"); 746 else if (Configuration.doAutoCreate()) 747 this.format = new Coding(); // cc 748 return this.format; 749 } 750 751 public boolean hasFormat() { 752 return this.format != null && !this.format.isEmpty(); 753 } 754 755 /** 756 * @param value {@link #format} (An identifier of the document encoding, 757 * structure, and template that the document conforms to beyond the 758 * base format indicated in the mimeType.) 759 */ 760 public DocumentReferenceContentComponent setFormat(Coding value) { 761 this.format = value; 762 return this; 763 } 764 765 protected void listChildren(List<Property> children) { 766 super.listChildren(children); 767 children.add(new Property("attachment", "Attachment", 768 "The document or URL of the document along with critical metadata to prove content has integrity.", 0, 1, 769 attachment)); 770 children.add(new Property("format", "Coding", 771 "An identifier of the document encoding, structure, and template that the document conforms to beyond the base format indicated in the mimeType.", 772 0, 1, format)); 773 } 774 775 @Override 776 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 777 switch (_hash) { 778 case -1963501277: 779 /* attachment */ return new Property("attachment", "Attachment", 780 "The document or URL of the document along with critical metadata to prove content has integrity.", 0, 1, 781 attachment); 782 case -1268779017: 783 /* format */ return new Property("format", "Coding", 784 "An identifier of the document encoding, structure, and template that the document conforms to beyond the base format indicated in the mimeType.", 785 0, 1, format); 786 default: 787 return super.getNamedProperty(_hash, _name, _checkValid); 788 } 789 790 } 791 792 @Override 793 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 794 switch (hash) { 795 case -1963501277: 796 /* attachment */ return this.attachment == null ? new Base[0] : new Base[] { this.attachment }; // Attachment 797 case -1268779017: 798 /* format */ return this.format == null ? new Base[0] : new Base[] { this.format }; // Coding 799 default: 800 return super.getProperty(hash, name, checkValid); 801 } 802 803 } 804 805 @Override 806 public Base setProperty(int hash, String name, Base value) throws FHIRException { 807 switch (hash) { 808 case -1963501277: // attachment 809 this.attachment = castToAttachment(value); // Attachment 810 return value; 811 case -1268779017: // format 812 this.format = castToCoding(value); // Coding 813 return value; 814 default: 815 return super.setProperty(hash, name, value); 816 } 817 818 } 819 820 @Override 821 public Base setProperty(String name, Base value) throws FHIRException { 822 if (name.equals("attachment")) { 823 this.attachment = castToAttachment(value); // Attachment 824 } else if (name.equals("format")) { 825 this.format = castToCoding(value); // Coding 826 } else 827 return super.setProperty(name, value); 828 return value; 829 } 830 831 @Override 832 public Base makeProperty(int hash, String name) throws FHIRException { 833 switch (hash) { 834 case -1963501277: 835 return getAttachment(); 836 case -1268779017: 837 return getFormat(); 838 default: 839 return super.makeProperty(hash, name); 840 } 841 842 } 843 844 @Override 845 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 846 switch (hash) { 847 case -1963501277: 848 /* attachment */ return new String[] { "Attachment" }; 849 case -1268779017: 850 /* format */ return new String[] { "Coding" }; 851 default: 852 return super.getTypesForProperty(hash, name); 853 } 854 855 } 856 857 @Override 858 public Base addChild(String name) throws FHIRException { 859 if (name.equals("attachment")) { 860 this.attachment = new Attachment(); 861 return this.attachment; 862 } else if (name.equals("format")) { 863 this.format = new Coding(); 864 return this.format; 865 } else 866 return super.addChild(name); 867 } 868 869 public DocumentReferenceContentComponent copy() { 870 DocumentReferenceContentComponent dst = new DocumentReferenceContentComponent(); 871 copyValues(dst); 872 return dst; 873 } 874 875 public void copyValues(DocumentReferenceContentComponent dst) { 876 super.copyValues(dst); 877 dst.attachment = attachment == null ? null : attachment.copy(); 878 dst.format = format == null ? null : format.copy(); 879 } 880 881 @Override 882 public boolean equalsDeep(Base other_) { 883 if (!super.equalsDeep(other_)) 884 return false; 885 if (!(other_ instanceof DocumentReferenceContentComponent)) 886 return false; 887 DocumentReferenceContentComponent o = (DocumentReferenceContentComponent) other_; 888 return compareDeep(attachment, o.attachment, true) && compareDeep(format, o.format, true); 889 } 890 891 @Override 892 public boolean equalsShallow(Base other_) { 893 if (!super.equalsShallow(other_)) 894 return false; 895 if (!(other_ instanceof DocumentReferenceContentComponent)) 896 return false; 897 DocumentReferenceContentComponent o = (DocumentReferenceContentComponent) other_; 898 return true; 899 } 900 901 public boolean isEmpty() { 902 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(attachment, format); 903 } 904 905 public String fhirType() { 906 return "DocumentReference.content"; 907 908 } 909 910 } 911 912 @Block() 913 public static class DocumentReferenceContextComponent extends BackboneElement implements IBaseBackboneElement { 914 /** 915 * Describes the clinical encounter or type of care that the document content is 916 * associated with. 917 */ 918 @Child(name = "encounter", type = { Encounter.class, 919 EpisodeOfCare.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 920 @Description(shortDefinition = "Context of the document content", formalDefinition = "Describes the clinical encounter or type of care that the document content is associated with.") 921 protected List<Reference> encounter; 922 /** 923 * The actual objects that are the target of the reference (Describes the 924 * clinical encounter or type of care that the document content is associated 925 * with.) 926 */ 927 protected List<Resource> encounterTarget; 928 929 /** 930 * This list of codes represents the main clinical acts, such as a colonoscopy 931 * or an appendectomy, being documented. In some cases, the event is inherent in 932 * the type Code, such as a "History and Physical Report" in which the procedure 933 * being documented is necessarily a "History and Physical" act. 934 */ 935 @Child(name = "event", type = { 936 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 937 @Description(shortDefinition = "Main clinical acts documented", formalDefinition = "This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the type Code, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act.") 938 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ActCode") 939 protected List<CodeableConcept> event; 940 941 /** 942 * The time period over which the service that is described by the document was 943 * provided. 944 */ 945 @Child(name = "period", type = { Period.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 946 @Description(shortDefinition = "Time of service that is being documented", formalDefinition = "The time period over which the service that is described by the document was provided.") 947 protected Period period; 948 949 /** 950 * The kind of facility where the patient was seen. 951 */ 952 @Child(name = "facilityType", type = { 953 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 954 @Description(shortDefinition = "Kind of facility where patient was seen", formalDefinition = "The kind of facility where the patient was seen.") 955 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/c80-facilitycodes") 956 protected CodeableConcept facilityType; 957 958 /** 959 * This property may convey specifics about the practice setting where the 960 * content was created, often reflecting the clinical specialty. 961 */ 962 @Child(name = "practiceSetting", type = { 963 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 964 @Description(shortDefinition = "Additional details about where the content was created (e.g. clinical specialty)", formalDefinition = "This property may convey specifics about the practice setting where the content was created, often reflecting the clinical specialty.") 965 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/c80-practice-codes") 966 protected CodeableConcept practiceSetting; 967 968 /** 969 * The Patient Information as known when the document was published. May be a 970 * reference to a version specific, or contained. 971 */ 972 @Child(name = "sourcePatientInfo", type = { 973 Patient.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 974 @Description(shortDefinition = "Patient demographics from source", formalDefinition = "The Patient Information as known when the document was published. May be a reference to a version specific, or contained.") 975 protected Reference sourcePatientInfo; 976 977 /** 978 * The actual object that is the target of the reference (The Patient 979 * Information as known when the document was published. May be a reference to a 980 * version specific, or contained.) 981 */ 982 protected Patient sourcePatientInfoTarget; 983 984 /** 985 * Related identifiers or resources associated with the DocumentReference. 986 */ 987 @Child(name = "related", type = { 988 Reference.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 989 @Description(shortDefinition = "Related identifiers or resources", formalDefinition = "Related identifiers or resources associated with the DocumentReference.") 990 protected List<Reference> related; 991 /** 992 * The actual objects that are the target of the reference (Related identifiers 993 * or resources associated with the DocumentReference.) 994 */ 995 protected List<Resource> relatedTarget; 996 997 private static final long serialVersionUID = 140463218L; 998 999 /** 1000 * Constructor 1001 */ 1002 public DocumentReferenceContextComponent() { 1003 super(); 1004 } 1005 1006 /** 1007 * @return {@link #encounter} (Describes the clinical encounter or type of care 1008 * that the document content is associated with.) 1009 */ 1010 public List<Reference> getEncounter() { 1011 if (this.encounter == null) 1012 this.encounter = new ArrayList<Reference>(); 1013 return this.encounter; 1014 } 1015 1016 /** 1017 * @return Returns a reference to <code>this</code> for easy method chaining 1018 */ 1019 public DocumentReferenceContextComponent setEncounter(List<Reference> theEncounter) { 1020 this.encounter = theEncounter; 1021 return this; 1022 } 1023 1024 public boolean hasEncounter() { 1025 if (this.encounter == null) 1026 return false; 1027 for (Reference item : this.encounter) 1028 if (!item.isEmpty()) 1029 return true; 1030 return false; 1031 } 1032 1033 public Reference addEncounter() { // 3 1034 Reference t = new Reference(); 1035 if (this.encounter == null) 1036 this.encounter = new ArrayList<Reference>(); 1037 this.encounter.add(t); 1038 return t; 1039 } 1040 1041 public DocumentReferenceContextComponent addEncounter(Reference t) { // 3 1042 if (t == null) 1043 return this; 1044 if (this.encounter == null) 1045 this.encounter = new ArrayList<Reference>(); 1046 this.encounter.add(t); 1047 return this; 1048 } 1049 1050 /** 1051 * @return The first repetition of repeating field {@link #encounter}, creating 1052 * it if it does not already exist 1053 */ 1054 public Reference getEncounterFirstRep() { 1055 if (getEncounter().isEmpty()) { 1056 addEncounter(); 1057 } 1058 return getEncounter().get(0); 1059 } 1060 1061 /** 1062 * @deprecated Use Reference#setResource(IBaseResource) instead 1063 */ 1064 @Deprecated 1065 public List<Resource> getEncounterTarget() { 1066 if (this.encounterTarget == null) 1067 this.encounterTarget = new ArrayList<Resource>(); 1068 return this.encounterTarget; 1069 } 1070 1071 /** 1072 * @return {@link #event} (This list of codes represents the main clinical acts, 1073 * such as a colonoscopy or an appendectomy, being documented. In some 1074 * cases, the event is inherent in the type Code, such as a "History and 1075 * Physical Report" in which the procedure being documented is 1076 * necessarily a "History and Physical" act.) 1077 */ 1078 public List<CodeableConcept> getEvent() { 1079 if (this.event == null) 1080 this.event = new ArrayList<CodeableConcept>(); 1081 return this.event; 1082 } 1083 1084 /** 1085 * @return Returns a reference to <code>this</code> for easy method chaining 1086 */ 1087 public DocumentReferenceContextComponent setEvent(List<CodeableConcept> theEvent) { 1088 this.event = theEvent; 1089 return this; 1090 } 1091 1092 public boolean hasEvent() { 1093 if (this.event == null) 1094 return false; 1095 for (CodeableConcept item : this.event) 1096 if (!item.isEmpty()) 1097 return true; 1098 return false; 1099 } 1100 1101 public CodeableConcept addEvent() { // 3 1102 CodeableConcept t = new CodeableConcept(); 1103 if (this.event == null) 1104 this.event = new ArrayList<CodeableConcept>(); 1105 this.event.add(t); 1106 return t; 1107 } 1108 1109 public DocumentReferenceContextComponent addEvent(CodeableConcept t) { // 3 1110 if (t == null) 1111 return this; 1112 if (this.event == null) 1113 this.event = new ArrayList<CodeableConcept>(); 1114 this.event.add(t); 1115 return this; 1116 } 1117 1118 /** 1119 * @return The first repetition of repeating field {@link #event}, creating it 1120 * if it does not already exist 1121 */ 1122 public CodeableConcept getEventFirstRep() { 1123 if (getEvent().isEmpty()) { 1124 addEvent(); 1125 } 1126 return getEvent().get(0); 1127 } 1128 1129 /** 1130 * @return {@link #period} (The time period over which the service that is 1131 * described by the document was provided.) 1132 */ 1133 public Period getPeriod() { 1134 if (this.period == null) 1135 if (Configuration.errorOnAutoCreate()) 1136 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.period"); 1137 else if (Configuration.doAutoCreate()) 1138 this.period = new Period(); // cc 1139 return this.period; 1140 } 1141 1142 public boolean hasPeriod() { 1143 return this.period != null && !this.period.isEmpty(); 1144 } 1145 1146 /** 1147 * @param value {@link #period} (The time period over which the service that is 1148 * described by the document was provided.) 1149 */ 1150 public DocumentReferenceContextComponent setPeriod(Period value) { 1151 this.period = value; 1152 return this; 1153 } 1154 1155 /** 1156 * @return {@link #facilityType} (The kind of facility where the patient was 1157 * seen.) 1158 */ 1159 public CodeableConcept getFacilityType() { 1160 if (this.facilityType == null) 1161 if (Configuration.errorOnAutoCreate()) 1162 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.facilityType"); 1163 else if (Configuration.doAutoCreate()) 1164 this.facilityType = new CodeableConcept(); // cc 1165 return this.facilityType; 1166 } 1167 1168 public boolean hasFacilityType() { 1169 return this.facilityType != null && !this.facilityType.isEmpty(); 1170 } 1171 1172 /** 1173 * @param value {@link #facilityType} (The kind of facility where the patient 1174 * was seen.) 1175 */ 1176 public DocumentReferenceContextComponent setFacilityType(CodeableConcept value) { 1177 this.facilityType = value; 1178 return this; 1179 } 1180 1181 /** 1182 * @return {@link #practiceSetting} (This property may convey specifics about 1183 * the practice setting where the content was created, often reflecting 1184 * the clinical specialty.) 1185 */ 1186 public CodeableConcept getPracticeSetting() { 1187 if (this.practiceSetting == null) 1188 if (Configuration.errorOnAutoCreate()) 1189 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.practiceSetting"); 1190 else if (Configuration.doAutoCreate()) 1191 this.practiceSetting = new CodeableConcept(); // cc 1192 return this.practiceSetting; 1193 } 1194 1195 public boolean hasPracticeSetting() { 1196 return this.practiceSetting != null && !this.practiceSetting.isEmpty(); 1197 } 1198 1199 /** 1200 * @param value {@link #practiceSetting} (This property may convey specifics 1201 * about the practice setting where the content was created, often 1202 * reflecting the clinical specialty.) 1203 */ 1204 public DocumentReferenceContextComponent setPracticeSetting(CodeableConcept value) { 1205 this.practiceSetting = value; 1206 return this; 1207 } 1208 1209 /** 1210 * @return {@link #sourcePatientInfo} (The Patient Information as known when the 1211 * document was published. May be a reference to a version specific, or 1212 * contained.) 1213 */ 1214 public Reference getSourcePatientInfo() { 1215 if (this.sourcePatientInfo == null) 1216 if (Configuration.errorOnAutoCreate()) 1217 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.sourcePatientInfo"); 1218 else if (Configuration.doAutoCreate()) 1219 this.sourcePatientInfo = new Reference(); // cc 1220 return this.sourcePatientInfo; 1221 } 1222 1223 public boolean hasSourcePatientInfo() { 1224 return this.sourcePatientInfo != null && !this.sourcePatientInfo.isEmpty(); 1225 } 1226 1227 /** 1228 * @param value {@link #sourcePatientInfo} (The Patient Information as known 1229 * when the document was published. May be a reference to a version 1230 * specific, or contained.) 1231 */ 1232 public DocumentReferenceContextComponent setSourcePatientInfo(Reference value) { 1233 this.sourcePatientInfo = value; 1234 return this; 1235 } 1236 1237 /** 1238 * @return {@link #sourcePatientInfo} The actual object that is the target of 1239 * the reference. The reference library doesn't populate this, but you 1240 * can use it to hold the resource if you resolve it. (The Patient 1241 * Information as known when the document was published. May be a 1242 * reference to a version specific, or contained.) 1243 */ 1244 public Patient getSourcePatientInfoTarget() { 1245 if (this.sourcePatientInfoTarget == null) 1246 if (Configuration.errorOnAutoCreate()) 1247 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.sourcePatientInfo"); 1248 else if (Configuration.doAutoCreate()) 1249 this.sourcePatientInfoTarget = new Patient(); // aa 1250 return this.sourcePatientInfoTarget; 1251 } 1252 1253 /** 1254 * @param value {@link #sourcePatientInfo} The actual object that is the target 1255 * of the reference. The reference library doesn't use these, but 1256 * you can use it to hold the resource if you resolve it. (The 1257 * Patient Information as known when the document was published. 1258 * May be a reference to a version specific, or contained.) 1259 */ 1260 public DocumentReferenceContextComponent setSourcePatientInfoTarget(Patient value) { 1261 this.sourcePatientInfoTarget = value; 1262 return this; 1263 } 1264 1265 /** 1266 * @return {@link #related} (Related identifiers or resources associated with 1267 * the DocumentReference.) 1268 */ 1269 public List<Reference> getRelated() { 1270 if (this.related == null) 1271 this.related = new ArrayList<Reference>(); 1272 return this.related; 1273 } 1274 1275 /** 1276 * @return Returns a reference to <code>this</code> for easy method chaining 1277 */ 1278 public DocumentReferenceContextComponent setRelated(List<Reference> theRelated) { 1279 this.related = theRelated; 1280 return this; 1281 } 1282 1283 public boolean hasRelated() { 1284 if (this.related == null) 1285 return false; 1286 for (Reference item : this.related) 1287 if (!item.isEmpty()) 1288 return true; 1289 return false; 1290 } 1291 1292 public Reference addRelated() { // 3 1293 Reference t = new Reference(); 1294 if (this.related == null) 1295 this.related = new ArrayList<Reference>(); 1296 this.related.add(t); 1297 return t; 1298 } 1299 1300 public DocumentReferenceContextComponent addRelated(Reference t) { // 3 1301 if (t == null) 1302 return this; 1303 if (this.related == null) 1304 this.related = new ArrayList<Reference>(); 1305 this.related.add(t); 1306 return this; 1307 } 1308 1309 /** 1310 * @return The first repetition of repeating field {@link #related}, creating it 1311 * if it does not already exist 1312 */ 1313 public Reference getRelatedFirstRep() { 1314 if (getRelated().isEmpty()) { 1315 addRelated(); 1316 } 1317 return getRelated().get(0); 1318 } 1319 1320 /** 1321 * @deprecated Use Reference#setResource(IBaseResource) instead 1322 */ 1323 @Deprecated 1324 public List<Resource> getRelatedTarget() { 1325 if (this.relatedTarget == null) 1326 this.relatedTarget = new ArrayList<Resource>(); 1327 return this.relatedTarget; 1328 } 1329 1330 protected void listChildren(List<Property> children) { 1331 super.listChildren(children); 1332 children.add(new Property("encounter", "Reference(Encounter|EpisodeOfCare)", 1333 "Describes the clinical encounter or type of care that the document content is associated with.", 0, 1334 java.lang.Integer.MAX_VALUE, encounter)); 1335 children.add(new Property("event", "CodeableConcept", 1336 "This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the type Code, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act.", 1337 0, java.lang.Integer.MAX_VALUE, event)); 1338 children.add(new Property("period", "Period", 1339 "The time period over which the service that is described by the document was provided.", 0, 1, period)); 1340 children.add(new Property("facilityType", "CodeableConcept", "The kind of facility where the patient was seen.", 1341 0, 1, facilityType)); 1342 children.add(new Property("practiceSetting", "CodeableConcept", 1343 "This property may convey specifics about the practice setting where the content was created, often reflecting the clinical specialty.", 1344 0, 1, practiceSetting)); 1345 children.add(new Property("sourcePatientInfo", "Reference(Patient)", 1346 "The Patient Information as known when the document was published. May be a reference to a version specific, or contained.", 1347 0, 1, sourcePatientInfo)); 1348 children.add(new Property("related", "Reference(Any)", 1349 "Related identifiers or resources associated with the DocumentReference.", 0, java.lang.Integer.MAX_VALUE, 1350 related)); 1351 } 1352 1353 @Override 1354 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1355 switch (_hash) { 1356 case 1524132147: 1357 /* encounter */ return new Property("encounter", "Reference(Encounter|EpisodeOfCare)", 1358 "Describes the clinical encounter or type of care that the document content is associated with.", 0, 1359 java.lang.Integer.MAX_VALUE, encounter); 1360 case 96891546: 1361 /* event */ return new Property("event", "CodeableConcept", 1362 "This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the type Code, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act.", 1363 0, java.lang.Integer.MAX_VALUE, event); 1364 case -991726143: 1365 /* period */ return new Property("period", "Period", 1366 "The time period over which the service that is described by the document was provided.", 0, 1, period); 1367 case 370698365: 1368 /* facilityType */ return new Property("facilityType", "CodeableConcept", 1369 "The kind of facility where the patient was seen.", 0, 1, facilityType); 1370 case 331373717: 1371 /* practiceSetting */ return new Property("practiceSetting", "CodeableConcept", 1372 "This property may convey specifics about the practice setting where the content was created, often reflecting the clinical specialty.", 1373 0, 1, practiceSetting); 1374 case 2031381048: 1375 /* sourcePatientInfo */ return new Property("sourcePatientInfo", "Reference(Patient)", 1376 "The Patient Information as known when the document was published. May be a reference to a version specific, or contained.", 1377 0, 1, sourcePatientInfo); 1378 case 1090493483: 1379 /* related */ return new Property("related", "Reference(Any)", 1380 "Related identifiers or resources associated with the DocumentReference.", 0, java.lang.Integer.MAX_VALUE, 1381 related); 1382 default: 1383 return super.getNamedProperty(_hash, _name, _checkValid); 1384 } 1385 1386 } 1387 1388 @Override 1389 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1390 switch (hash) { 1391 case 1524132147: 1392 /* encounter */ return this.encounter == null ? new Base[0] 1393 : this.encounter.toArray(new Base[this.encounter.size()]); // Reference 1394 case 96891546: 1395 /* event */ return this.event == null ? new Base[0] : this.event.toArray(new Base[this.event.size()]); // CodeableConcept 1396 case -991726143: 1397 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 1398 case 370698365: 1399 /* facilityType */ return this.facilityType == null ? new Base[0] : new Base[] { this.facilityType }; // CodeableConcept 1400 case 331373717: 1401 /* practiceSetting */ return this.practiceSetting == null ? new Base[0] : new Base[] { this.practiceSetting }; // CodeableConcept 1402 case 2031381048: 1403 /* sourcePatientInfo */ return this.sourcePatientInfo == null ? new Base[0] 1404 : new Base[] { this.sourcePatientInfo }; // Reference 1405 case 1090493483: 1406 /* related */ return this.related == null ? new Base[0] : this.related.toArray(new Base[this.related.size()]); // Reference 1407 default: 1408 return super.getProperty(hash, name, checkValid); 1409 } 1410 1411 } 1412 1413 @Override 1414 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1415 switch (hash) { 1416 case 1524132147: // encounter 1417 this.getEncounter().add(castToReference(value)); // Reference 1418 return value; 1419 case 96891546: // event 1420 this.getEvent().add(castToCodeableConcept(value)); // CodeableConcept 1421 return value; 1422 case -991726143: // period 1423 this.period = castToPeriod(value); // Period 1424 return value; 1425 case 370698365: // facilityType 1426 this.facilityType = castToCodeableConcept(value); // CodeableConcept 1427 return value; 1428 case 331373717: // practiceSetting 1429 this.practiceSetting = castToCodeableConcept(value); // CodeableConcept 1430 return value; 1431 case 2031381048: // sourcePatientInfo 1432 this.sourcePatientInfo = castToReference(value); // Reference 1433 return value; 1434 case 1090493483: // related 1435 this.getRelated().add(castToReference(value)); // Reference 1436 return value; 1437 default: 1438 return super.setProperty(hash, name, value); 1439 } 1440 1441 } 1442 1443 @Override 1444 public Base setProperty(String name, Base value) throws FHIRException { 1445 if (name.equals("encounter")) { 1446 this.getEncounter().add(castToReference(value)); 1447 } else if (name.equals("event")) { 1448 this.getEvent().add(castToCodeableConcept(value)); 1449 } else if (name.equals("period")) { 1450 this.period = castToPeriod(value); // Period 1451 } else if (name.equals("facilityType")) { 1452 this.facilityType = castToCodeableConcept(value); // CodeableConcept 1453 } else if (name.equals("practiceSetting")) { 1454 this.practiceSetting = castToCodeableConcept(value); // CodeableConcept 1455 } else if (name.equals("sourcePatientInfo")) { 1456 this.sourcePatientInfo = castToReference(value); // Reference 1457 } else if (name.equals("related")) { 1458 this.getRelated().add(castToReference(value)); 1459 } else 1460 return super.setProperty(name, value); 1461 return value; 1462 } 1463 1464 @Override 1465 public Base makeProperty(int hash, String name) throws FHIRException { 1466 switch (hash) { 1467 case 1524132147: 1468 return addEncounter(); 1469 case 96891546: 1470 return addEvent(); 1471 case -991726143: 1472 return getPeriod(); 1473 case 370698365: 1474 return getFacilityType(); 1475 case 331373717: 1476 return getPracticeSetting(); 1477 case 2031381048: 1478 return getSourcePatientInfo(); 1479 case 1090493483: 1480 return addRelated(); 1481 default: 1482 return super.makeProperty(hash, name); 1483 } 1484 1485 } 1486 1487 @Override 1488 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1489 switch (hash) { 1490 case 1524132147: 1491 /* encounter */ return new String[] { "Reference" }; 1492 case 96891546: 1493 /* event */ return new String[] { "CodeableConcept" }; 1494 case -991726143: 1495 /* period */ return new String[] { "Period" }; 1496 case 370698365: 1497 /* facilityType */ return new String[] { "CodeableConcept" }; 1498 case 331373717: 1499 /* practiceSetting */ return new String[] { "CodeableConcept" }; 1500 case 2031381048: 1501 /* sourcePatientInfo */ return new String[] { "Reference" }; 1502 case 1090493483: 1503 /* related */ return new String[] { "Reference" }; 1504 default: 1505 return super.getTypesForProperty(hash, name); 1506 } 1507 1508 } 1509 1510 @Override 1511 public Base addChild(String name) throws FHIRException { 1512 if (name.equals("encounter")) { 1513 return addEncounter(); 1514 } else if (name.equals("event")) { 1515 return addEvent(); 1516 } else if (name.equals("period")) { 1517 this.period = new Period(); 1518 return this.period; 1519 } else if (name.equals("facilityType")) { 1520 this.facilityType = new CodeableConcept(); 1521 return this.facilityType; 1522 } else if (name.equals("practiceSetting")) { 1523 this.practiceSetting = new CodeableConcept(); 1524 return this.practiceSetting; 1525 } else if (name.equals("sourcePatientInfo")) { 1526 this.sourcePatientInfo = new Reference(); 1527 return this.sourcePatientInfo; 1528 } else if (name.equals("related")) { 1529 return addRelated(); 1530 } else 1531 return super.addChild(name); 1532 } 1533 1534 public DocumentReferenceContextComponent copy() { 1535 DocumentReferenceContextComponent dst = new DocumentReferenceContextComponent(); 1536 copyValues(dst); 1537 return dst; 1538 } 1539 1540 public void copyValues(DocumentReferenceContextComponent dst) { 1541 super.copyValues(dst); 1542 if (encounter != null) { 1543 dst.encounter = new ArrayList<Reference>(); 1544 for (Reference i : encounter) 1545 dst.encounter.add(i.copy()); 1546 } 1547 ; 1548 if (event != null) { 1549 dst.event = new ArrayList<CodeableConcept>(); 1550 for (CodeableConcept i : event) 1551 dst.event.add(i.copy()); 1552 } 1553 ; 1554 dst.period = period == null ? null : period.copy(); 1555 dst.facilityType = facilityType == null ? null : facilityType.copy(); 1556 dst.practiceSetting = practiceSetting == null ? null : practiceSetting.copy(); 1557 dst.sourcePatientInfo = sourcePatientInfo == null ? null : sourcePatientInfo.copy(); 1558 if (related != null) { 1559 dst.related = new ArrayList<Reference>(); 1560 for (Reference i : related) 1561 dst.related.add(i.copy()); 1562 } 1563 ; 1564 } 1565 1566 @Override 1567 public boolean equalsDeep(Base other_) { 1568 if (!super.equalsDeep(other_)) 1569 return false; 1570 if (!(other_ instanceof DocumentReferenceContextComponent)) 1571 return false; 1572 DocumentReferenceContextComponent o = (DocumentReferenceContextComponent) other_; 1573 return compareDeep(encounter, o.encounter, true) && compareDeep(event, o.event, true) 1574 && compareDeep(period, o.period, true) && compareDeep(facilityType, o.facilityType, true) 1575 && compareDeep(practiceSetting, o.practiceSetting, true) 1576 && compareDeep(sourcePatientInfo, o.sourcePatientInfo, true) && compareDeep(related, o.related, true); 1577 } 1578 1579 @Override 1580 public boolean equalsShallow(Base other_) { 1581 if (!super.equalsShallow(other_)) 1582 return false; 1583 if (!(other_ instanceof DocumentReferenceContextComponent)) 1584 return false; 1585 DocumentReferenceContextComponent o = (DocumentReferenceContextComponent) other_; 1586 return true; 1587 } 1588 1589 public boolean isEmpty() { 1590 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(encounter, event, period, facilityType, 1591 practiceSetting, sourcePatientInfo, related); 1592 } 1593 1594 public String fhirType() { 1595 return "DocumentReference.context"; 1596 1597 } 1598 1599 } 1600 1601 /** 1602 * Document identifier as assigned by the source of the document. This 1603 * identifier is specific to this version of the document. This unique 1604 * identifier may be used elsewhere to identify this version of the document. 1605 */ 1606 @Child(name = "masterIdentifier", type = { 1607 Identifier.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 1608 @Description(shortDefinition = "Master Version Specific Identifier", formalDefinition = "Document identifier as assigned by the source of the document. This identifier is specific to this version of the document. This unique identifier may be used elsewhere to identify this version of the document.") 1609 protected Identifier masterIdentifier; 1610 1611 /** 1612 * Other identifiers associated with the document, including version independent 1613 * identifiers. 1614 */ 1615 @Child(name = "identifier", type = { 1616 Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1617 @Description(shortDefinition = "Other identifiers for the document", formalDefinition = "Other identifiers associated with the document, including version independent identifiers.") 1618 protected List<Identifier> identifier; 1619 1620 /** 1621 * The status of this document reference. 1622 */ 1623 @Child(name = "status", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = true, summary = true) 1624 @Description(shortDefinition = "current | superseded | entered-in-error", formalDefinition = "The status of this document reference.") 1625 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/document-reference-status") 1626 protected Enumeration<DocumentReferenceStatus> status; 1627 1628 /** 1629 * The status of the underlying document. 1630 */ 1631 @Child(name = "docStatus", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1632 @Description(shortDefinition = "preliminary | final | amended | entered-in-error", formalDefinition = "The status of the underlying document.") 1633 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/composition-status") 1634 protected Enumeration<ReferredDocumentStatus> docStatus; 1635 1636 /** 1637 * Specifies the particular kind of document referenced (e.g. History and 1638 * Physical, Discharge Summary, Progress Note). This usually equates to the 1639 * purpose of making the document referenced. 1640 */ 1641 @Child(name = "type", type = { CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1642 @Description(shortDefinition = "Kind of document (LOINC if possible)", formalDefinition = "Specifies the particular kind of document referenced (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the document referenced.") 1643 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/c80-doc-typecodes") 1644 protected CodeableConcept type; 1645 1646 /** 1647 * A categorization for the type of document referenced - helps for indexing and 1648 * searching. This may be implied by or derived from the code specified in the 1649 * DocumentReference.type. 1650 */ 1651 @Child(name = "category", type = { 1652 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1653 @Description(shortDefinition = "Categorization of document", formalDefinition = "A categorization for the type of document referenced - helps for indexing and searching. This may be implied by or derived from the code specified in the DocumentReference.type.") 1654 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/document-classcodes") 1655 protected List<CodeableConcept> category; 1656 1657 /** 1658 * Who or what the document is about. The document can be about a person, 1659 * (patient or healthcare practitioner), a device (e.g. a machine) or even a 1660 * group of subjects (such as a document about a herd of farm animals, or a set 1661 * of patients that share a common exposure). 1662 */ 1663 @Child(name = "subject", type = { Patient.class, Practitioner.class, Group.class, 1664 Device.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1665 @Description(shortDefinition = "Who/what is the subject of the document", formalDefinition = "Who or what the document is about. The document can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure).") 1666 protected Reference subject; 1667 1668 /** 1669 * The actual object that is the target of the reference (Who or what the 1670 * document is about. The document can be about a person, (patient or healthcare 1671 * practitioner), a device (e.g. a machine) or even a group of subjects (such as 1672 * a document about a herd of farm animals, or a set of patients that share a 1673 * common exposure).) 1674 */ 1675 protected Resource subjectTarget; 1676 1677 /** 1678 * When the document reference was created. 1679 */ 1680 @Child(name = "date", type = { InstantType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1681 @Description(shortDefinition = "When this document reference was created", formalDefinition = "When the document reference was created.") 1682 protected InstantType date; 1683 1684 /** 1685 * Identifies who is responsible for adding the information to the document. 1686 */ 1687 @Child(name = "author", type = { Practitioner.class, PractitionerRole.class, Organization.class, Device.class, 1688 Patient.class, 1689 RelatedPerson.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1690 @Description(shortDefinition = "Who and/or what authored the document", formalDefinition = "Identifies who is responsible for adding the information to the document.") 1691 protected List<Reference> author; 1692 /** 1693 * The actual objects that are the target of the reference (Identifies who is 1694 * responsible for adding the information to the document.) 1695 */ 1696 protected List<Resource> authorTarget; 1697 1698 /** 1699 * Which person or organization authenticates that this document is valid. 1700 */ 1701 @Child(name = "authenticator", type = { Practitioner.class, PractitionerRole.class, 1702 Organization.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1703 @Description(shortDefinition = "Who/what authenticated the document", formalDefinition = "Which person or organization authenticates that this document is valid.") 1704 protected Reference authenticator; 1705 1706 /** 1707 * The actual object that is the target of the reference (Which person or 1708 * organization authenticates that this document is valid.) 1709 */ 1710 protected Resource authenticatorTarget; 1711 1712 /** 1713 * Identifies the organization or group who is responsible for ongoing 1714 * maintenance of and access to the document. 1715 */ 1716 @Child(name = "custodian", type = { 1717 Organization.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 1718 @Description(shortDefinition = "Organization which maintains the document", formalDefinition = "Identifies the organization or group who is responsible for ongoing maintenance of and access to the document.") 1719 protected Reference custodian; 1720 1721 /** 1722 * The actual object that is the target of the reference (Identifies the 1723 * organization or group who is responsible for ongoing maintenance of and 1724 * access to the document.) 1725 */ 1726 protected Organization custodianTarget; 1727 1728 /** 1729 * Relationships that this document has with other document references that 1730 * already exist. 1731 */ 1732 @Child(name = "relatesTo", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1733 @Description(shortDefinition = "Relationships to other documents", formalDefinition = "Relationships that this document has with other document references that already exist.") 1734 protected List<DocumentReferenceRelatesToComponent> relatesTo; 1735 1736 /** 1737 * Human-readable description of the source document. 1738 */ 1739 @Child(name = "description", type = { 1740 StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 1741 @Description(shortDefinition = "Human-readable description", formalDefinition = "Human-readable description of the source document.") 1742 protected StringType description; 1743 1744 /** 1745 * A set of Security-Tag codes specifying the level of privacy/security of the 1746 * Document. Note that DocumentReference.meta.security contains the security 1747 * labels of the "reference" to the document, while 1748 * DocumentReference.securityLabel contains a snapshot of the security labels on 1749 * the document the reference refers to. 1750 */ 1751 @Child(name = "securityLabel", type = { 1752 CodeableConcept.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1753 @Description(shortDefinition = "Document security-tags", formalDefinition = "A set of Security-Tag codes specifying the level of privacy/security of the Document. Note that DocumentReference.meta.security contains the security labels of the \"reference\" to the document, while DocumentReference.securityLabel contains a snapshot of the security labels on the document the reference refers to.") 1754 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/security-labels") 1755 protected List<CodeableConcept> securityLabel; 1756 1757 /** 1758 * The document and format referenced. There may be multiple content element 1759 * repetitions, each with a different format. 1760 */ 1761 @Child(name = "content", type = {}, order = 14, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1762 @Description(shortDefinition = "Document referenced", formalDefinition = "The document and format referenced. There may be multiple content element repetitions, each with a different format.") 1763 protected List<DocumentReferenceContentComponent> content; 1764 1765 /** 1766 * The clinical context in which the document was prepared. 1767 */ 1768 @Child(name = "context", type = {}, order = 15, min = 0, max = 1, modifier = false, summary = true) 1769 @Description(shortDefinition = "Clinical context of document", formalDefinition = "The clinical context in which the document was prepared.") 1770 protected DocumentReferenceContextComponent context; 1771 1772 private static final long serialVersionUID = 307086535L; 1773 1774 /** 1775 * Constructor 1776 */ 1777 public DocumentReference() { 1778 super(); 1779 } 1780 1781 /** 1782 * Constructor 1783 */ 1784 public DocumentReference(Enumeration<DocumentReferenceStatus> status) { 1785 super(); 1786 this.status = status; 1787 } 1788 1789 /** 1790 * @return {@link #masterIdentifier} (Document identifier as assigned by the 1791 * source of the document. This identifier is specific to this version 1792 * of the document. This unique identifier may be used elsewhere to 1793 * identify this version of the document.) 1794 */ 1795 public Identifier getMasterIdentifier() { 1796 if (this.masterIdentifier == null) 1797 if (Configuration.errorOnAutoCreate()) 1798 throw new Error("Attempt to auto-create DocumentReference.masterIdentifier"); 1799 else if (Configuration.doAutoCreate()) 1800 this.masterIdentifier = new Identifier(); // cc 1801 return this.masterIdentifier; 1802 } 1803 1804 public boolean hasMasterIdentifier() { 1805 return this.masterIdentifier != null && !this.masterIdentifier.isEmpty(); 1806 } 1807 1808 /** 1809 * @param value {@link #masterIdentifier} (Document identifier as assigned by 1810 * the source of the document. This identifier is specific to this 1811 * version of the document. This unique identifier may be used 1812 * elsewhere to identify this version of the document.) 1813 */ 1814 public DocumentReference setMasterIdentifier(Identifier value) { 1815 this.masterIdentifier = value; 1816 return this; 1817 } 1818 1819 /** 1820 * @return {@link #identifier} (Other identifiers associated with the document, 1821 * including version independent identifiers.) 1822 */ 1823 public List<Identifier> getIdentifier() { 1824 if (this.identifier == null) 1825 this.identifier = new ArrayList<Identifier>(); 1826 return this.identifier; 1827 } 1828 1829 /** 1830 * @return Returns a reference to <code>this</code> for easy method chaining 1831 */ 1832 public DocumentReference setIdentifier(List<Identifier> theIdentifier) { 1833 this.identifier = theIdentifier; 1834 return this; 1835 } 1836 1837 public boolean hasIdentifier() { 1838 if (this.identifier == null) 1839 return false; 1840 for (Identifier item : this.identifier) 1841 if (!item.isEmpty()) 1842 return true; 1843 return false; 1844 } 1845 1846 public Identifier addIdentifier() { // 3 1847 Identifier t = new Identifier(); 1848 if (this.identifier == null) 1849 this.identifier = new ArrayList<Identifier>(); 1850 this.identifier.add(t); 1851 return t; 1852 } 1853 1854 public DocumentReference addIdentifier(Identifier t) { // 3 1855 if (t == null) 1856 return this; 1857 if (this.identifier == null) 1858 this.identifier = new ArrayList<Identifier>(); 1859 this.identifier.add(t); 1860 return this; 1861 } 1862 1863 /** 1864 * @return The first repetition of repeating field {@link #identifier}, creating 1865 * it if it does not already exist 1866 */ 1867 public Identifier getIdentifierFirstRep() { 1868 if (getIdentifier().isEmpty()) { 1869 addIdentifier(); 1870 } 1871 return getIdentifier().get(0); 1872 } 1873 1874 /** 1875 * @return {@link #status} (The status of this document reference.). This is the 1876 * underlying object with id, value and extensions. The accessor 1877 * "getStatus" gives direct access to the value 1878 */ 1879 public Enumeration<DocumentReferenceStatus> getStatusElement() { 1880 if (this.status == null) 1881 if (Configuration.errorOnAutoCreate()) 1882 throw new Error("Attempt to auto-create DocumentReference.status"); 1883 else if (Configuration.doAutoCreate()) 1884 this.status = new Enumeration<DocumentReferenceStatus>(new DocumentReferenceStatusEnumFactory()); // bb 1885 return this.status; 1886 } 1887 1888 public boolean hasStatusElement() { 1889 return this.status != null && !this.status.isEmpty(); 1890 } 1891 1892 public boolean hasStatus() { 1893 return this.status != null && !this.status.isEmpty(); 1894 } 1895 1896 /** 1897 * @param value {@link #status} (The status of this document reference.). This 1898 * is the underlying object with id, value and extensions. The 1899 * accessor "getStatus" gives direct access to the value 1900 */ 1901 public DocumentReference setStatusElement(Enumeration<DocumentReferenceStatus> value) { 1902 this.status = value; 1903 return this; 1904 } 1905 1906 /** 1907 * @return The status of this document reference. 1908 */ 1909 public DocumentReferenceStatus getStatus() { 1910 return this.status == null ? null : this.status.getValue(); 1911 } 1912 1913 /** 1914 * @param value The status of this document reference. 1915 */ 1916 public DocumentReference setStatus(DocumentReferenceStatus value) { 1917 if (this.status == null) 1918 this.status = new Enumeration<DocumentReferenceStatus>(new DocumentReferenceStatusEnumFactory()); 1919 this.status.setValue(value); 1920 return this; 1921 } 1922 1923 /** 1924 * @return {@link #docStatus} (The status of the underlying document.). This is 1925 * the underlying object with id, value and extensions. The accessor 1926 * "getDocStatus" gives direct access to the value 1927 */ 1928 public Enumeration<ReferredDocumentStatus> getDocStatusElement() { 1929 if (this.docStatus == null) 1930 if (Configuration.errorOnAutoCreate()) 1931 throw new Error("Attempt to auto-create DocumentReference.docStatus"); 1932 else if (Configuration.doAutoCreate()) 1933 this.docStatus = new Enumeration<ReferredDocumentStatus>(new ReferredDocumentStatusEnumFactory()); // bb 1934 return this.docStatus; 1935 } 1936 1937 public boolean hasDocStatusElement() { 1938 return this.docStatus != null && !this.docStatus.isEmpty(); 1939 } 1940 1941 public boolean hasDocStatus() { 1942 return this.docStatus != null && !this.docStatus.isEmpty(); 1943 } 1944 1945 /** 1946 * @param value {@link #docStatus} (The status of the underlying document.). 1947 * This is the underlying object with id, value and extensions. The 1948 * accessor "getDocStatus" gives direct access to the value 1949 */ 1950 public DocumentReference setDocStatusElement(Enumeration<ReferredDocumentStatus> value) { 1951 this.docStatus = value; 1952 return this; 1953 } 1954 1955 /** 1956 * @return The status of the underlying document. 1957 */ 1958 public ReferredDocumentStatus getDocStatus() { 1959 return this.docStatus == null ? null : this.docStatus.getValue(); 1960 } 1961 1962 /** 1963 * @param value The status of the underlying document. 1964 */ 1965 public DocumentReference setDocStatus(ReferredDocumentStatus value) { 1966 if (value == null) 1967 this.docStatus = null; 1968 else { 1969 if (this.docStatus == null) 1970 this.docStatus = new Enumeration<ReferredDocumentStatus>(new ReferredDocumentStatusEnumFactory()); 1971 this.docStatus.setValue(value); 1972 } 1973 return this; 1974 } 1975 1976 /** 1977 * @return {@link #type} (Specifies the particular kind of document referenced 1978 * (e.g. History and Physical, Discharge Summary, Progress Note). This 1979 * usually equates to the purpose of making the document referenced.) 1980 */ 1981 public CodeableConcept getType() { 1982 if (this.type == null) 1983 if (Configuration.errorOnAutoCreate()) 1984 throw new Error("Attempt to auto-create DocumentReference.type"); 1985 else if (Configuration.doAutoCreate()) 1986 this.type = new CodeableConcept(); // cc 1987 return this.type; 1988 } 1989 1990 public boolean hasType() { 1991 return this.type != null && !this.type.isEmpty(); 1992 } 1993 1994 /** 1995 * @param value {@link #type} (Specifies the particular kind of document 1996 * referenced (e.g. History and Physical, Discharge Summary, 1997 * Progress Note). This usually equates to the purpose of making 1998 * the document referenced.) 1999 */ 2000 public DocumentReference setType(CodeableConcept value) { 2001 this.type = value; 2002 return this; 2003 } 2004 2005 /** 2006 * @return {@link #category} (A categorization for the type of document 2007 * referenced - helps for indexing and searching. This may be implied by 2008 * or derived from the code specified in the DocumentReference.type.) 2009 */ 2010 public List<CodeableConcept> getCategory() { 2011 if (this.category == null) 2012 this.category = new ArrayList<CodeableConcept>(); 2013 return this.category; 2014 } 2015 2016 /** 2017 * @return Returns a reference to <code>this</code> for easy method chaining 2018 */ 2019 public DocumentReference setCategory(List<CodeableConcept> theCategory) { 2020 this.category = theCategory; 2021 return this; 2022 } 2023 2024 public boolean hasCategory() { 2025 if (this.category == null) 2026 return false; 2027 for (CodeableConcept item : this.category) 2028 if (!item.isEmpty()) 2029 return true; 2030 return false; 2031 } 2032 2033 public CodeableConcept addCategory() { // 3 2034 CodeableConcept t = new CodeableConcept(); 2035 if (this.category == null) 2036 this.category = new ArrayList<CodeableConcept>(); 2037 this.category.add(t); 2038 return t; 2039 } 2040 2041 public DocumentReference addCategory(CodeableConcept t) { // 3 2042 if (t == null) 2043 return this; 2044 if (this.category == null) 2045 this.category = new ArrayList<CodeableConcept>(); 2046 this.category.add(t); 2047 return this; 2048 } 2049 2050 /** 2051 * @return The first repetition of repeating field {@link #category}, creating 2052 * it if it does not already exist 2053 */ 2054 public CodeableConcept getCategoryFirstRep() { 2055 if (getCategory().isEmpty()) { 2056 addCategory(); 2057 } 2058 return getCategory().get(0); 2059 } 2060 2061 /** 2062 * @return {@link #subject} (Who or what the document is about. The document can 2063 * be about a person, (patient or healthcare practitioner), a device 2064 * (e.g. a machine) or even a group of subjects (such as a document 2065 * about a herd of farm animals, or a set of patients that share a 2066 * common exposure).) 2067 */ 2068 public Reference getSubject() { 2069 if (this.subject == null) 2070 if (Configuration.errorOnAutoCreate()) 2071 throw new Error("Attempt to auto-create DocumentReference.subject"); 2072 else if (Configuration.doAutoCreate()) 2073 this.subject = new Reference(); // cc 2074 return this.subject; 2075 } 2076 2077 public boolean hasSubject() { 2078 return this.subject != null && !this.subject.isEmpty(); 2079 } 2080 2081 /** 2082 * @param value {@link #subject} (Who or what the document is about. The 2083 * document can be about a person, (patient or healthcare 2084 * practitioner), a device (e.g. a machine) or even a group of 2085 * subjects (such as a document about a herd of farm animals, or a 2086 * set of patients that share a common exposure).) 2087 */ 2088 public DocumentReference setSubject(Reference value) { 2089 this.subject = value; 2090 return this; 2091 } 2092 2093 /** 2094 * @return {@link #subject} The actual object that is the target of the 2095 * reference. The reference library doesn't populate this, but you can 2096 * use it to hold the resource if you resolve it. (Who or what the 2097 * document is about. The document can be about a person, (patient or 2098 * healthcare practitioner), a device (e.g. a machine) or even a group 2099 * of subjects (such as a document about a herd of farm animals, or a 2100 * set of patients that share a common exposure).) 2101 */ 2102 public Resource getSubjectTarget() { 2103 return this.subjectTarget; 2104 } 2105 2106 /** 2107 * @param value {@link #subject} The actual object that is the target of the 2108 * reference. The reference library doesn't use these, but you can 2109 * use it to hold the resource if you resolve it. (Who or what the 2110 * document is about. The document can be about a person, (patient 2111 * or healthcare practitioner), a device (e.g. a machine) or even a 2112 * group of subjects (such as a document about a herd of farm 2113 * animals, or a set of patients that share a common exposure).) 2114 */ 2115 public DocumentReference setSubjectTarget(Resource value) { 2116 this.subjectTarget = value; 2117 return this; 2118 } 2119 2120 /** 2121 * @return {@link #date} (When the document reference was created.). This is the 2122 * underlying object with id, value and extensions. The accessor 2123 * "getDate" gives direct access to the value 2124 */ 2125 public InstantType getDateElement() { 2126 if (this.date == null) 2127 if (Configuration.errorOnAutoCreate()) 2128 throw new Error("Attempt to auto-create DocumentReference.date"); 2129 else if (Configuration.doAutoCreate()) 2130 this.date = new InstantType(); // bb 2131 return this.date; 2132 } 2133 2134 public boolean hasDateElement() { 2135 return this.date != null && !this.date.isEmpty(); 2136 } 2137 2138 public boolean hasDate() { 2139 return this.date != null && !this.date.isEmpty(); 2140 } 2141 2142 /** 2143 * @param value {@link #date} (When the document reference was created.). This 2144 * is the underlying object with id, value and extensions. The 2145 * accessor "getDate" gives direct access to the value 2146 */ 2147 public DocumentReference setDateElement(InstantType value) { 2148 this.date = value; 2149 return this; 2150 } 2151 2152 /** 2153 * @return When the document reference was created. 2154 */ 2155 public Date getDate() { 2156 return this.date == null ? null : this.date.getValue(); 2157 } 2158 2159 /** 2160 * @param value When the document reference was created. 2161 */ 2162 public DocumentReference setDate(Date value) { 2163 if (value == null) 2164 this.date = null; 2165 else { 2166 if (this.date == null) 2167 this.date = new InstantType(); 2168 this.date.setValue(value); 2169 } 2170 return this; 2171 } 2172 2173 /** 2174 * @return {@link #author} (Identifies who is responsible for adding the 2175 * information to the document.) 2176 */ 2177 public List<Reference> getAuthor() { 2178 if (this.author == null) 2179 this.author = new ArrayList<Reference>(); 2180 return this.author; 2181 } 2182 2183 /** 2184 * @return Returns a reference to <code>this</code> for easy method chaining 2185 */ 2186 public DocumentReference setAuthor(List<Reference> theAuthor) { 2187 this.author = theAuthor; 2188 return this; 2189 } 2190 2191 public boolean hasAuthor() { 2192 if (this.author == null) 2193 return false; 2194 for (Reference item : this.author) 2195 if (!item.isEmpty()) 2196 return true; 2197 return false; 2198 } 2199 2200 public Reference addAuthor() { // 3 2201 Reference t = new Reference(); 2202 if (this.author == null) 2203 this.author = new ArrayList<Reference>(); 2204 this.author.add(t); 2205 return t; 2206 } 2207 2208 public DocumentReference addAuthor(Reference t) { // 3 2209 if (t == null) 2210 return this; 2211 if (this.author == null) 2212 this.author = new ArrayList<Reference>(); 2213 this.author.add(t); 2214 return this; 2215 } 2216 2217 /** 2218 * @return The first repetition of repeating field {@link #author}, creating it 2219 * if it does not already exist 2220 */ 2221 public Reference getAuthorFirstRep() { 2222 if (getAuthor().isEmpty()) { 2223 addAuthor(); 2224 } 2225 return getAuthor().get(0); 2226 } 2227 2228 /** 2229 * @deprecated Use Reference#setResource(IBaseResource) instead 2230 */ 2231 @Deprecated 2232 public List<Resource> getAuthorTarget() { 2233 if (this.authorTarget == null) 2234 this.authorTarget = new ArrayList<Resource>(); 2235 return this.authorTarget; 2236 } 2237 2238 /** 2239 * @return {@link #authenticator} (Which person or organization authenticates 2240 * that this document is valid.) 2241 */ 2242 public Reference getAuthenticator() { 2243 if (this.authenticator == null) 2244 if (Configuration.errorOnAutoCreate()) 2245 throw new Error("Attempt to auto-create DocumentReference.authenticator"); 2246 else if (Configuration.doAutoCreate()) 2247 this.authenticator = new Reference(); // cc 2248 return this.authenticator; 2249 } 2250 2251 public boolean hasAuthenticator() { 2252 return this.authenticator != null && !this.authenticator.isEmpty(); 2253 } 2254 2255 /** 2256 * @param value {@link #authenticator} (Which person or organization 2257 * authenticates that this document is valid.) 2258 */ 2259 public DocumentReference setAuthenticator(Reference value) { 2260 this.authenticator = value; 2261 return this; 2262 } 2263 2264 /** 2265 * @return {@link #authenticator} The actual object that is the target of the 2266 * reference. The reference library doesn't populate this, but you can 2267 * use it to hold the resource if you resolve it. (Which person or 2268 * organization authenticates that this document is valid.) 2269 */ 2270 public Resource getAuthenticatorTarget() { 2271 return this.authenticatorTarget; 2272 } 2273 2274 /** 2275 * @param value {@link #authenticator} The actual object that is the target of 2276 * the reference. The reference library doesn't use these, but you 2277 * can use it to hold the resource if you resolve it. (Which person 2278 * or organization authenticates that this document is valid.) 2279 */ 2280 public DocumentReference setAuthenticatorTarget(Resource value) { 2281 this.authenticatorTarget = value; 2282 return this; 2283 } 2284 2285 /** 2286 * @return {@link #custodian} (Identifies the organization or group who is 2287 * responsible for ongoing maintenance of and access to the document.) 2288 */ 2289 public Reference getCustodian() { 2290 if (this.custodian == null) 2291 if (Configuration.errorOnAutoCreate()) 2292 throw new Error("Attempt to auto-create DocumentReference.custodian"); 2293 else if (Configuration.doAutoCreate()) 2294 this.custodian = new Reference(); // cc 2295 return this.custodian; 2296 } 2297 2298 public boolean hasCustodian() { 2299 return this.custodian != null && !this.custodian.isEmpty(); 2300 } 2301 2302 /** 2303 * @param value {@link #custodian} (Identifies the organization or group who is 2304 * responsible for ongoing maintenance of and access to the 2305 * document.) 2306 */ 2307 public DocumentReference setCustodian(Reference value) { 2308 this.custodian = value; 2309 return this; 2310 } 2311 2312 /** 2313 * @return {@link #custodian} The actual object that is the target of the 2314 * reference. The reference library doesn't populate this, but you can 2315 * use it to hold the resource if you resolve it. (Identifies the 2316 * organization or group who is responsible for ongoing maintenance of 2317 * and access to the document.) 2318 */ 2319 public Organization getCustodianTarget() { 2320 if (this.custodianTarget == null) 2321 if (Configuration.errorOnAutoCreate()) 2322 throw new Error("Attempt to auto-create DocumentReference.custodian"); 2323 else if (Configuration.doAutoCreate()) 2324 this.custodianTarget = new Organization(); // aa 2325 return this.custodianTarget; 2326 } 2327 2328 /** 2329 * @param value {@link #custodian} The actual object that is the target of the 2330 * reference. The reference library doesn't use these, but you can 2331 * use it to hold the resource if you resolve it. (Identifies the 2332 * organization or group who is responsible for ongoing maintenance 2333 * of and access to the document.) 2334 */ 2335 public DocumentReference setCustodianTarget(Organization value) { 2336 this.custodianTarget = value; 2337 return this; 2338 } 2339 2340 /** 2341 * @return {@link #relatesTo} (Relationships that this document has with other 2342 * document references that already exist.) 2343 */ 2344 public List<DocumentReferenceRelatesToComponent> getRelatesTo() { 2345 if (this.relatesTo == null) 2346 this.relatesTo = new ArrayList<DocumentReferenceRelatesToComponent>(); 2347 return this.relatesTo; 2348 } 2349 2350 /** 2351 * @return Returns a reference to <code>this</code> for easy method chaining 2352 */ 2353 public DocumentReference setRelatesTo(List<DocumentReferenceRelatesToComponent> theRelatesTo) { 2354 this.relatesTo = theRelatesTo; 2355 return this; 2356 } 2357 2358 public boolean hasRelatesTo() { 2359 if (this.relatesTo == null) 2360 return false; 2361 for (DocumentReferenceRelatesToComponent item : this.relatesTo) 2362 if (!item.isEmpty()) 2363 return true; 2364 return false; 2365 } 2366 2367 public DocumentReferenceRelatesToComponent addRelatesTo() { // 3 2368 DocumentReferenceRelatesToComponent t = new DocumentReferenceRelatesToComponent(); 2369 if (this.relatesTo == null) 2370 this.relatesTo = new ArrayList<DocumentReferenceRelatesToComponent>(); 2371 this.relatesTo.add(t); 2372 return t; 2373 } 2374 2375 public DocumentReference addRelatesTo(DocumentReferenceRelatesToComponent t) { // 3 2376 if (t == null) 2377 return this; 2378 if (this.relatesTo == null) 2379 this.relatesTo = new ArrayList<DocumentReferenceRelatesToComponent>(); 2380 this.relatesTo.add(t); 2381 return this; 2382 } 2383 2384 /** 2385 * @return The first repetition of repeating field {@link #relatesTo}, creating 2386 * it if it does not already exist 2387 */ 2388 public DocumentReferenceRelatesToComponent getRelatesToFirstRep() { 2389 if (getRelatesTo().isEmpty()) { 2390 addRelatesTo(); 2391 } 2392 return getRelatesTo().get(0); 2393 } 2394 2395 /** 2396 * @return {@link #description} (Human-readable description of the source 2397 * document.). This is the underlying object with id, value and 2398 * extensions. The accessor "getDescription" gives direct access to the 2399 * value 2400 */ 2401 public StringType getDescriptionElement() { 2402 if (this.description == null) 2403 if (Configuration.errorOnAutoCreate()) 2404 throw new Error("Attempt to auto-create DocumentReference.description"); 2405 else if (Configuration.doAutoCreate()) 2406 this.description = new StringType(); // bb 2407 return this.description; 2408 } 2409 2410 public boolean hasDescriptionElement() { 2411 return this.description != null && !this.description.isEmpty(); 2412 } 2413 2414 public boolean hasDescription() { 2415 return this.description != null && !this.description.isEmpty(); 2416 } 2417 2418 /** 2419 * @param value {@link #description} (Human-readable description of the source 2420 * document.). This is the underlying object with id, value and 2421 * extensions. The accessor "getDescription" gives direct access to 2422 * the value 2423 */ 2424 public DocumentReference setDescriptionElement(StringType value) { 2425 this.description = value; 2426 return this; 2427 } 2428 2429 /** 2430 * @return Human-readable description of the source document. 2431 */ 2432 public String getDescription() { 2433 return this.description == null ? null : this.description.getValue(); 2434 } 2435 2436 /** 2437 * @param value Human-readable description of the source document. 2438 */ 2439 public DocumentReference setDescription(String value) { 2440 if (Utilities.noString(value)) 2441 this.description = null; 2442 else { 2443 if (this.description == null) 2444 this.description = new StringType(); 2445 this.description.setValue(value); 2446 } 2447 return this; 2448 } 2449 2450 /** 2451 * @return {@link #securityLabel} (A set of Security-Tag codes specifying the 2452 * level of privacy/security of the Document. Note that 2453 * DocumentReference.meta.security contains the security labels of the 2454 * "reference" to the document, while DocumentReference.securityLabel 2455 * contains a snapshot of the security labels on the document the 2456 * reference refers to.) 2457 */ 2458 public List<CodeableConcept> getSecurityLabel() { 2459 if (this.securityLabel == null) 2460 this.securityLabel = new ArrayList<CodeableConcept>(); 2461 return this.securityLabel; 2462 } 2463 2464 /** 2465 * @return Returns a reference to <code>this</code> for easy method chaining 2466 */ 2467 public DocumentReference setSecurityLabel(List<CodeableConcept> theSecurityLabel) { 2468 this.securityLabel = theSecurityLabel; 2469 return this; 2470 } 2471 2472 public boolean hasSecurityLabel() { 2473 if (this.securityLabel == null) 2474 return false; 2475 for (CodeableConcept item : this.securityLabel) 2476 if (!item.isEmpty()) 2477 return true; 2478 return false; 2479 } 2480 2481 public CodeableConcept addSecurityLabel() { // 3 2482 CodeableConcept t = new CodeableConcept(); 2483 if (this.securityLabel == null) 2484 this.securityLabel = new ArrayList<CodeableConcept>(); 2485 this.securityLabel.add(t); 2486 return t; 2487 } 2488 2489 public DocumentReference addSecurityLabel(CodeableConcept t) { // 3 2490 if (t == null) 2491 return this; 2492 if (this.securityLabel == null) 2493 this.securityLabel = new ArrayList<CodeableConcept>(); 2494 this.securityLabel.add(t); 2495 return this; 2496 } 2497 2498 /** 2499 * @return The first repetition of repeating field {@link #securityLabel}, 2500 * creating it if it does not already exist 2501 */ 2502 public CodeableConcept getSecurityLabelFirstRep() { 2503 if (getSecurityLabel().isEmpty()) { 2504 addSecurityLabel(); 2505 } 2506 return getSecurityLabel().get(0); 2507 } 2508 2509 /** 2510 * @return {@link #content} (The document and format referenced. There may be 2511 * multiple content element repetitions, each with a different format.) 2512 */ 2513 public List<DocumentReferenceContentComponent> getContent() { 2514 if (this.content == null) 2515 this.content = new ArrayList<DocumentReferenceContentComponent>(); 2516 return this.content; 2517 } 2518 2519 /** 2520 * @return Returns a reference to <code>this</code> for easy method chaining 2521 */ 2522 public DocumentReference setContent(List<DocumentReferenceContentComponent> theContent) { 2523 this.content = theContent; 2524 return this; 2525 } 2526 2527 public boolean hasContent() { 2528 if (this.content == null) 2529 return false; 2530 for (DocumentReferenceContentComponent item : this.content) 2531 if (!item.isEmpty()) 2532 return true; 2533 return false; 2534 } 2535 2536 public DocumentReferenceContentComponent addContent() { // 3 2537 DocumentReferenceContentComponent t = new DocumentReferenceContentComponent(); 2538 if (this.content == null) 2539 this.content = new ArrayList<DocumentReferenceContentComponent>(); 2540 this.content.add(t); 2541 return t; 2542 } 2543 2544 public DocumentReference addContent(DocumentReferenceContentComponent t) { // 3 2545 if (t == null) 2546 return this; 2547 if (this.content == null) 2548 this.content = new ArrayList<DocumentReferenceContentComponent>(); 2549 this.content.add(t); 2550 return this; 2551 } 2552 2553 /** 2554 * @return The first repetition of repeating field {@link #content}, creating it 2555 * if it does not already exist 2556 */ 2557 public DocumentReferenceContentComponent getContentFirstRep() { 2558 if (getContent().isEmpty()) { 2559 addContent(); 2560 } 2561 return getContent().get(0); 2562 } 2563 2564 /** 2565 * @return {@link #context} (The clinical context in which the document was 2566 * prepared.) 2567 */ 2568 public DocumentReferenceContextComponent getContext() { 2569 if (this.context == null) 2570 if (Configuration.errorOnAutoCreate()) 2571 throw new Error("Attempt to auto-create DocumentReference.context"); 2572 else if (Configuration.doAutoCreate()) 2573 this.context = new DocumentReferenceContextComponent(); // cc 2574 return this.context; 2575 } 2576 2577 public boolean hasContext() { 2578 return this.context != null && !this.context.isEmpty(); 2579 } 2580 2581 /** 2582 * @param value {@link #context} (The clinical context in which the document was 2583 * prepared.) 2584 */ 2585 public DocumentReference setContext(DocumentReferenceContextComponent value) { 2586 this.context = value; 2587 return this; 2588 } 2589 2590 protected void listChildren(List<Property> children) { 2591 super.listChildren(children); 2592 children.add(new Property("masterIdentifier", "Identifier", 2593 "Document identifier as assigned by the source of the document. This identifier is specific to this version of the document. This unique identifier may be used elsewhere to identify this version of the document.", 2594 0, 1, masterIdentifier)); 2595 children.add(new Property("identifier", "Identifier", 2596 "Other identifiers associated with the document, including version independent identifiers.", 0, 2597 java.lang.Integer.MAX_VALUE, identifier)); 2598 children.add(new Property("status", "code", "The status of this document reference.", 0, 1, status)); 2599 children.add(new Property("docStatus", "code", "The status of the underlying document.", 0, 1, docStatus)); 2600 children.add(new Property("type", "CodeableConcept", 2601 "Specifies the particular kind of document referenced (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the document referenced.", 2602 0, 1, type)); 2603 children.add(new Property("category", "CodeableConcept", 2604 "A categorization for the type of document referenced - helps for indexing and searching. This may be implied by or derived from the code specified in the DocumentReference.type.", 2605 0, java.lang.Integer.MAX_VALUE, category)); 2606 children.add(new Property("subject", "Reference(Patient|Practitioner|Group|Device)", 2607 "Who or what the document is about. The document can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure).", 2608 0, 1, subject)); 2609 children.add(new Property("date", "instant", "When the document reference was created.", 0, 1, date)); 2610 children.add( 2611 new Property("author", "Reference(Practitioner|PractitionerRole|Organization|Device|Patient|RelatedPerson)", 2612 "Identifies who is responsible for adding the information to the document.", 0, java.lang.Integer.MAX_VALUE, 2613 author)); 2614 children.add(new Property("authenticator", "Reference(Practitioner|PractitionerRole|Organization)", 2615 "Which person or organization authenticates that this document is valid.", 0, 1, authenticator)); 2616 children.add(new Property("custodian", "Reference(Organization)", 2617 "Identifies the organization or group who is responsible for ongoing maintenance of and access to the document.", 2618 0, 1, custodian)); 2619 children.add(new Property("relatesTo", "", 2620 "Relationships that this document has with other document references that already exist.", 0, 2621 java.lang.Integer.MAX_VALUE, relatesTo)); 2622 children.add( 2623 new Property("description", "string", "Human-readable description of the source document.", 0, 1, description)); 2624 children.add(new Property("securityLabel", "CodeableConcept", 2625 "A set of Security-Tag codes specifying the level of privacy/security of the Document. Note that DocumentReference.meta.security contains the security labels of the \"reference\" to the document, while DocumentReference.securityLabel contains a snapshot of the security labels on the document the reference refers to.", 2626 0, java.lang.Integer.MAX_VALUE, securityLabel)); 2627 children.add(new Property("content", "", 2628 "The document and format referenced. There may be multiple content element repetitions, each with a different format.", 2629 0, java.lang.Integer.MAX_VALUE, content)); 2630 children 2631 .add(new Property("context", "", "The clinical context in which the document was prepared.", 0, 1, context)); 2632 } 2633 2634 @Override 2635 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2636 switch (_hash) { 2637 case 243769515: 2638 /* masterIdentifier */ return new Property("masterIdentifier", "Identifier", 2639 "Document identifier as assigned by the source of the document. This identifier is specific to this version of the document. This unique identifier may be used elsewhere to identify this version of the document.", 2640 0, 1, masterIdentifier); 2641 case -1618432855: 2642 /* identifier */ return new Property("identifier", "Identifier", 2643 "Other identifiers associated with the document, including version independent identifiers.", 0, 2644 java.lang.Integer.MAX_VALUE, identifier); 2645 case -892481550: 2646 /* status */ return new Property("status", "code", "The status of this document reference.", 0, 1, status); 2647 case -23496886: 2648 /* docStatus */ return new Property("docStatus", "code", "The status of the underlying document.", 0, 1, 2649 docStatus); 2650 case 3575610: 2651 /* type */ return new Property("type", "CodeableConcept", 2652 "Specifies the particular kind of document referenced (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the document referenced.", 2653 0, 1, type); 2654 case 50511102: 2655 /* category */ return new Property("category", "CodeableConcept", 2656 "A categorization for the type of document referenced - helps for indexing and searching. This may be implied by or derived from the code specified in the DocumentReference.type.", 2657 0, java.lang.Integer.MAX_VALUE, category); 2658 case -1867885268: 2659 /* subject */ return new Property("subject", "Reference(Patient|Practitioner|Group|Device)", 2660 "Who or what the document is about. The document can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure).", 2661 0, 1, subject); 2662 case 3076014: 2663 /* date */ return new Property("date", "instant", "When the document reference was created.", 0, 1, date); 2664 case -1406328437: 2665 /* author */ return new Property("author", 2666 "Reference(Practitioner|PractitionerRole|Organization|Device|Patient|RelatedPerson)", 2667 "Identifies who is responsible for adding the information to the document.", 0, java.lang.Integer.MAX_VALUE, 2668 author); 2669 case 1815000435: 2670 /* authenticator */ return new Property("authenticator", "Reference(Practitioner|PractitionerRole|Organization)", 2671 "Which person or organization authenticates that this document is valid.", 0, 1, authenticator); 2672 case 1611297262: 2673 /* custodian */ return new Property("custodian", "Reference(Organization)", 2674 "Identifies the organization or group who is responsible for ongoing maintenance of and access to the document.", 2675 0, 1, custodian); 2676 case -7765931: 2677 /* relatesTo */ return new Property("relatesTo", "", 2678 "Relationships that this document has with other document references that already exist.", 0, 2679 java.lang.Integer.MAX_VALUE, relatesTo); 2680 case -1724546052: 2681 /* description */ return new Property("description", "string", 2682 "Human-readable description of the source document.", 0, 1, description); 2683 case -722296940: 2684 /* securityLabel */ return new Property("securityLabel", "CodeableConcept", 2685 "A set of Security-Tag codes specifying the level of privacy/security of the Document. Note that DocumentReference.meta.security contains the security labels of the \"reference\" to the document, while DocumentReference.securityLabel contains a snapshot of the security labels on the document the reference refers to.", 2686 0, java.lang.Integer.MAX_VALUE, securityLabel); 2687 case 951530617: 2688 /* content */ return new Property("content", "", 2689 "The document and format referenced. There may be multiple content element repetitions, each with a different format.", 2690 0, java.lang.Integer.MAX_VALUE, content); 2691 case 951530927: 2692 /* context */ return new Property("context", "", "The clinical context in which the document was prepared.", 0, 1, 2693 context); 2694 default: 2695 return super.getNamedProperty(_hash, _name, _checkValid); 2696 } 2697 2698 } 2699 2700 @Override 2701 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2702 switch (hash) { 2703 case 243769515: 2704 /* masterIdentifier */ return this.masterIdentifier == null ? new Base[0] : new Base[] { this.masterIdentifier }; // Identifier 2705 case -1618432855: 2706 /* identifier */ return this.identifier == null ? new Base[0] 2707 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2708 case -892481550: 2709 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<DocumentReferenceStatus> 2710 case -23496886: 2711 /* docStatus */ return this.docStatus == null ? new Base[0] : new Base[] { this.docStatus }; // Enumeration<ReferredDocumentStatus> 2712 case 3575610: 2713 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2714 case 50511102: 2715 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2716 case -1867885268: 2717 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2718 case 3076014: 2719 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // InstantType 2720 case -1406328437: 2721 /* author */ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // Reference 2722 case 1815000435: 2723 /* authenticator */ return this.authenticator == null ? new Base[0] : new Base[] { this.authenticator }; // Reference 2724 case 1611297262: 2725 /* custodian */ return this.custodian == null ? new Base[0] : new Base[] { this.custodian }; // Reference 2726 case -7765931: 2727 /* relatesTo */ return this.relatesTo == null ? new Base[0] 2728 : this.relatesTo.toArray(new Base[this.relatesTo.size()]); // DocumentReferenceRelatesToComponent 2729 case -1724546052: 2730 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 2731 case -722296940: 2732 /* securityLabel */ return this.securityLabel == null ? new Base[0] 2733 : this.securityLabel.toArray(new Base[this.securityLabel.size()]); // CodeableConcept 2734 case 951530617: 2735 /* content */ return this.content == null ? new Base[0] : this.content.toArray(new Base[this.content.size()]); // DocumentReferenceContentComponent 2736 case 951530927: 2737 /* context */ return this.context == null ? new Base[0] : new Base[] { this.context }; // DocumentReferenceContextComponent 2738 default: 2739 return super.getProperty(hash, name, checkValid); 2740 } 2741 2742 } 2743 2744 @Override 2745 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2746 switch (hash) { 2747 case 243769515: // masterIdentifier 2748 this.masterIdentifier = castToIdentifier(value); // Identifier 2749 return value; 2750 case -1618432855: // identifier 2751 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2752 return value; 2753 case -892481550: // status 2754 value = new DocumentReferenceStatusEnumFactory().fromType(castToCode(value)); 2755 this.status = (Enumeration) value; // Enumeration<DocumentReferenceStatus> 2756 return value; 2757 case -23496886: // docStatus 2758 value = new ReferredDocumentStatusEnumFactory().fromType(castToCode(value)); 2759 this.docStatus = (Enumeration) value; // Enumeration<ReferredDocumentStatus> 2760 return value; 2761 case 3575610: // type 2762 this.type = castToCodeableConcept(value); // CodeableConcept 2763 return value; 2764 case 50511102: // category 2765 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 2766 return value; 2767 case -1867885268: // subject 2768 this.subject = castToReference(value); // Reference 2769 return value; 2770 case 3076014: // date 2771 this.date = castToInstant(value); // InstantType 2772 return value; 2773 case -1406328437: // author 2774 this.getAuthor().add(castToReference(value)); // Reference 2775 return value; 2776 case 1815000435: // authenticator 2777 this.authenticator = castToReference(value); // Reference 2778 return value; 2779 case 1611297262: // custodian 2780 this.custodian = castToReference(value); // Reference 2781 return value; 2782 case -7765931: // relatesTo 2783 this.getRelatesTo().add((DocumentReferenceRelatesToComponent) value); // DocumentReferenceRelatesToComponent 2784 return value; 2785 case -1724546052: // description 2786 this.description = castToString(value); // StringType 2787 return value; 2788 case -722296940: // securityLabel 2789 this.getSecurityLabel().add(castToCodeableConcept(value)); // CodeableConcept 2790 return value; 2791 case 951530617: // content 2792 this.getContent().add((DocumentReferenceContentComponent) value); // DocumentReferenceContentComponent 2793 return value; 2794 case 951530927: // context 2795 this.context = (DocumentReferenceContextComponent) value; // DocumentReferenceContextComponent 2796 return value; 2797 default: 2798 return super.setProperty(hash, name, value); 2799 } 2800 2801 } 2802 2803 @Override 2804 public Base setProperty(String name, Base value) throws FHIRException { 2805 if (name.equals("masterIdentifier")) { 2806 this.masterIdentifier = castToIdentifier(value); // Identifier 2807 } else if (name.equals("identifier")) { 2808 this.getIdentifier().add(castToIdentifier(value)); 2809 } else if (name.equals("status")) { 2810 value = new DocumentReferenceStatusEnumFactory().fromType(castToCode(value)); 2811 this.status = (Enumeration) value; // Enumeration<DocumentReferenceStatus> 2812 } else if (name.equals("docStatus")) { 2813 value = new ReferredDocumentStatusEnumFactory().fromType(castToCode(value)); 2814 this.docStatus = (Enumeration) value; // Enumeration<ReferredDocumentStatus> 2815 } else if (name.equals("type")) { 2816 this.type = castToCodeableConcept(value); // CodeableConcept 2817 } else if (name.equals("category")) { 2818 this.getCategory().add(castToCodeableConcept(value)); 2819 } else if (name.equals("subject")) { 2820 this.subject = castToReference(value); // Reference 2821 } else if (name.equals("date")) { 2822 this.date = castToInstant(value); // InstantType 2823 } else if (name.equals("author")) { 2824 this.getAuthor().add(castToReference(value)); 2825 } else if (name.equals("authenticator")) { 2826 this.authenticator = castToReference(value); // Reference 2827 } else if (name.equals("custodian")) { 2828 this.custodian = castToReference(value); // Reference 2829 } else if (name.equals("relatesTo")) { 2830 this.getRelatesTo().add((DocumentReferenceRelatesToComponent) value); 2831 } else if (name.equals("description")) { 2832 this.description = castToString(value); // StringType 2833 } else if (name.equals("securityLabel")) { 2834 this.getSecurityLabel().add(castToCodeableConcept(value)); 2835 } else if (name.equals("content")) { 2836 this.getContent().add((DocumentReferenceContentComponent) value); 2837 } else if (name.equals("context")) { 2838 this.context = (DocumentReferenceContextComponent) value; // DocumentReferenceContextComponent 2839 } else 2840 return super.setProperty(name, value); 2841 return value; 2842 } 2843 2844 @Override 2845 public Base makeProperty(int hash, String name) throws FHIRException { 2846 switch (hash) { 2847 case 243769515: 2848 return getMasterIdentifier(); 2849 case -1618432855: 2850 return addIdentifier(); 2851 case -892481550: 2852 return getStatusElement(); 2853 case -23496886: 2854 return getDocStatusElement(); 2855 case 3575610: 2856 return getType(); 2857 case 50511102: 2858 return addCategory(); 2859 case -1867885268: 2860 return getSubject(); 2861 case 3076014: 2862 return getDateElement(); 2863 case -1406328437: 2864 return addAuthor(); 2865 case 1815000435: 2866 return getAuthenticator(); 2867 case 1611297262: 2868 return getCustodian(); 2869 case -7765931: 2870 return addRelatesTo(); 2871 case -1724546052: 2872 return getDescriptionElement(); 2873 case -722296940: 2874 return addSecurityLabel(); 2875 case 951530617: 2876 return addContent(); 2877 case 951530927: 2878 return getContext(); 2879 default: 2880 return super.makeProperty(hash, name); 2881 } 2882 2883 } 2884 2885 @Override 2886 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2887 switch (hash) { 2888 case 243769515: 2889 /* masterIdentifier */ return new String[] { "Identifier" }; 2890 case -1618432855: 2891 /* identifier */ return new String[] { "Identifier" }; 2892 case -892481550: 2893 /* status */ return new String[] { "code" }; 2894 case -23496886: 2895 /* docStatus */ return new String[] { "code" }; 2896 case 3575610: 2897 /* type */ return new String[] { "CodeableConcept" }; 2898 case 50511102: 2899 /* category */ return new String[] { "CodeableConcept" }; 2900 case -1867885268: 2901 /* subject */ return new String[] { "Reference" }; 2902 case 3076014: 2903 /* date */ return new String[] { "instant" }; 2904 case -1406328437: 2905 /* author */ return new String[] { "Reference" }; 2906 case 1815000435: 2907 /* authenticator */ return new String[] { "Reference" }; 2908 case 1611297262: 2909 /* custodian */ return new String[] { "Reference" }; 2910 case -7765931: 2911 /* relatesTo */ return new String[] {}; 2912 case -1724546052: 2913 /* description */ return new String[] { "string" }; 2914 case -722296940: 2915 /* securityLabel */ return new String[] { "CodeableConcept" }; 2916 case 951530617: 2917 /* content */ return new String[] {}; 2918 case 951530927: 2919 /* context */ return new String[] {}; 2920 default: 2921 return super.getTypesForProperty(hash, name); 2922 } 2923 2924 } 2925 2926 @Override 2927 public Base addChild(String name) throws FHIRException { 2928 if (name.equals("masterIdentifier")) { 2929 this.masterIdentifier = new Identifier(); 2930 return this.masterIdentifier; 2931 } else if (name.equals("identifier")) { 2932 return addIdentifier(); 2933 } else if (name.equals("status")) { 2934 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.status"); 2935 } else if (name.equals("docStatus")) { 2936 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.docStatus"); 2937 } else if (name.equals("type")) { 2938 this.type = new CodeableConcept(); 2939 return this.type; 2940 } else if (name.equals("category")) { 2941 return addCategory(); 2942 } else if (name.equals("subject")) { 2943 this.subject = new Reference(); 2944 return this.subject; 2945 } else if (name.equals("date")) { 2946 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.date"); 2947 } else if (name.equals("author")) { 2948 return addAuthor(); 2949 } else if (name.equals("authenticator")) { 2950 this.authenticator = new Reference(); 2951 return this.authenticator; 2952 } else if (name.equals("custodian")) { 2953 this.custodian = new Reference(); 2954 return this.custodian; 2955 } else if (name.equals("relatesTo")) { 2956 return addRelatesTo(); 2957 } else if (name.equals("description")) { 2958 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.description"); 2959 } else if (name.equals("securityLabel")) { 2960 return addSecurityLabel(); 2961 } else if (name.equals("content")) { 2962 return addContent(); 2963 } else if (name.equals("context")) { 2964 this.context = new DocumentReferenceContextComponent(); 2965 return this.context; 2966 } else 2967 return super.addChild(name); 2968 } 2969 2970 public String fhirType() { 2971 return "DocumentReference"; 2972 2973 } 2974 2975 public DocumentReference copy() { 2976 DocumentReference dst = new DocumentReference(); 2977 copyValues(dst); 2978 return dst; 2979 } 2980 2981 public void copyValues(DocumentReference dst) { 2982 super.copyValues(dst); 2983 dst.masterIdentifier = masterIdentifier == null ? null : masterIdentifier.copy(); 2984 if (identifier != null) { 2985 dst.identifier = new ArrayList<Identifier>(); 2986 for (Identifier i : identifier) 2987 dst.identifier.add(i.copy()); 2988 } 2989 ; 2990 dst.status = status == null ? null : status.copy(); 2991 dst.docStatus = docStatus == null ? null : docStatus.copy(); 2992 dst.type = type == null ? null : type.copy(); 2993 if (category != null) { 2994 dst.category = new ArrayList<CodeableConcept>(); 2995 for (CodeableConcept i : category) 2996 dst.category.add(i.copy()); 2997 } 2998 ; 2999 dst.subject = subject == null ? null : subject.copy(); 3000 dst.date = date == null ? null : date.copy(); 3001 if (author != null) { 3002 dst.author = new ArrayList<Reference>(); 3003 for (Reference i : author) 3004 dst.author.add(i.copy()); 3005 } 3006 ; 3007 dst.authenticator = authenticator == null ? null : authenticator.copy(); 3008 dst.custodian = custodian == null ? null : custodian.copy(); 3009 if (relatesTo != null) { 3010 dst.relatesTo = new ArrayList<DocumentReferenceRelatesToComponent>(); 3011 for (DocumentReferenceRelatesToComponent i : relatesTo) 3012 dst.relatesTo.add(i.copy()); 3013 } 3014 ; 3015 dst.description = description == null ? null : description.copy(); 3016 if (securityLabel != null) { 3017 dst.securityLabel = new ArrayList<CodeableConcept>(); 3018 for (CodeableConcept i : securityLabel) 3019 dst.securityLabel.add(i.copy()); 3020 } 3021 ; 3022 if (content != null) { 3023 dst.content = new ArrayList<DocumentReferenceContentComponent>(); 3024 for (DocumentReferenceContentComponent i : content) 3025 dst.content.add(i.copy()); 3026 } 3027 ; 3028 dst.context = context == null ? null : context.copy(); 3029 } 3030 3031 protected DocumentReference typedCopy() { 3032 return copy(); 3033 } 3034 3035 @Override 3036 public boolean equalsDeep(Base other_) { 3037 if (!super.equalsDeep(other_)) 3038 return false; 3039 if (!(other_ instanceof DocumentReference)) 3040 return false; 3041 DocumentReference o = (DocumentReference) other_; 3042 return compareDeep(masterIdentifier, o.masterIdentifier, true) && compareDeep(identifier, o.identifier, true) 3043 && compareDeep(status, o.status, true) && compareDeep(docStatus, o.docStatus, true) 3044 && compareDeep(type, o.type, true) && compareDeep(category, o.category, true) 3045 && compareDeep(subject, o.subject, true) && compareDeep(date, o.date, true) 3046 && compareDeep(author, o.author, true) && compareDeep(authenticator, o.authenticator, true) 3047 && compareDeep(custodian, o.custodian, true) && compareDeep(relatesTo, o.relatesTo, true) 3048 && compareDeep(description, o.description, true) && compareDeep(securityLabel, o.securityLabel, true) 3049 && compareDeep(content, o.content, true) && compareDeep(context, o.context, true); 3050 } 3051 3052 @Override 3053 public boolean equalsShallow(Base other_) { 3054 if (!super.equalsShallow(other_)) 3055 return false; 3056 if (!(other_ instanceof DocumentReference)) 3057 return false; 3058 DocumentReference o = (DocumentReference) other_; 3059 return compareValues(status, o.status, true) && compareValues(docStatus, o.docStatus, true) 3060 && compareValues(date, o.date, true) && compareValues(description, o.description, true); 3061 } 3062 3063 public boolean isEmpty() { 3064 return super.isEmpty() 3065 && ca.uhn.fhir.util.ElementUtil.isEmpty(masterIdentifier, identifier, status, docStatus, type, category, 3066 subject, date, author, authenticator, custodian, relatesTo, description, securityLabel, content, context); 3067 } 3068 3069 @Override 3070 public ResourceType getResourceType() { 3071 return ResourceType.DocumentReference; 3072 } 3073 3074 /** 3075 * Search parameter: <b>date</b> 3076 * <p> 3077 * Description: <b>When this document reference was created</b><br> 3078 * Type: <b>date</b><br> 3079 * Path: <b>DocumentReference.date</b><br> 3080 * </p> 3081 */ 3082 @SearchParamDefinition(name = "date", path = "DocumentReference.date", description = "When this document reference was created", type = "date") 3083 public static final String SP_DATE = "date"; 3084 /** 3085 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3086 * <p> 3087 * Description: <b>When this document reference was created</b><br> 3088 * Type: <b>date</b><br> 3089 * Path: <b>DocumentReference.date</b><br> 3090 * </p> 3091 */ 3092 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3093 SP_DATE); 3094 3095 /** 3096 * Search parameter: <b>subject</b> 3097 * <p> 3098 * Description: <b>Who/what is the subject of the document</b><br> 3099 * Type: <b>reference</b><br> 3100 * Path: <b>DocumentReference.subject</b><br> 3101 * </p> 3102 */ 3103 @SearchParamDefinition(name = "subject", path = "DocumentReference.subject", description = "Who/what is the subject of the document", type = "reference", providesMembershipIn = { 3104 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 3105 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3106 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Device.class, Group.class, 3107 Patient.class, Practitioner.class }) 3108 public static final String SP_SUBJECT = "subject"; 3109 /** 3110 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3111 * <p> 3112 * Description: <b>Who/what is the subject of the document</b><br> 3113 * Type: <b>reference</b><br> 3114 * Path: <b>DocumentReference.subject</b><br> 3115 * </p> 3116 */ 3117 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3118 SP_SUBJECT); 3119 3120 /** 3121 * Constant for fluent queries to be used to add include statements. Specifies 3122 * the path value of "<b>DocumentReference:subject</b>". 3123 */ 3124 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 3125 "DocumentReference:subject").toLocked(); 3126 3127 /** 3128 * Search parameter: <b>description</b> 3129 * <p> 3130 * Description: <b>Human-readable description</b><br> 3131 * Type: <b>string</b><br> 3132 * Path: <b>DocumentReference.description</b><br> 3133 * </p> 3134 */ 3135 @SearchParamDefinition(name = "description", path = "DocumentReference.description", description = "Human-readable description", type = "string") 3136 public static final String SP_DESCRIPTION = "description"; 3137 /** 3138 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3139 * <p> 3140 * Description: <b>Human-readable description</b><br> 3141 * Type: <b>string</b><br> 3142 * Path: <b>DocumentReference.description</b><br> 3143 * </p> 3144 */ 3145 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 3146 SP_DESCRIPTION); 3147 3148 /** 3149 * Search parameter: <b>language</b> 3150 * <p> 3151 * Description: <b>Human language of the content (BCP-47)</b><br> 3152 * Type: <b>token</b><br> 3153 * Path: <b>DocumentReference.content.attachment.language</b><br> 3154 * </p> 3155 */ 3156 @SearchParamDefinition(name = "language", path = "DocumentReference.content.attachment.language", description = "Human language of the content (BCP-47)", type = "token") 3157 public static final String SP_LANGUAGE = "language"; 3158 /** 3159 * <b>Fluent Client</b> search parameter constant for <b>language</b> 3160 * <p> 3161 * Description: <b>Human language of the content (BCP-47)</b><br> 3162 * Type: <b>token</b><br> 3163 * Path: <b>DocumentReference.content.attachment.language</b><br> 3164 * </p> 3165 */ 3166 public static final ca.uhn.fhir.rest.gclient.TokenClientParam LANGUAGE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3167 SP_LANGUAGE); 3168 3169 /** 3170 * Search parameter: <b>type</b> 3171 * <p> 3172 * Description: <b>Kind of document (LOINC if possible)</b><br> 3173 * Type: <b>token</b><br> 3174 * Path: <b>DocumentReference.type</b><br> 3175 * </p> 3176 */ 3177 @SearchParamDefinition(name = "type", path = "DocumentReference.type", description = "Kind of document (LOINC if possible)", type = "token") 3178 public static final String SP_TYPE = "type"; 3179 /** 3180 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3181 * <p> 3182 * Description: <b>Kind of document (LOINC if possible)</b><br> 3183 * Type: <b>token</b><br> 3184 * Path: <b>DocumentReference.type</b><br> 3185 * </p> 3186 */ 3187 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3188 SP_TYPE); 3189 3190 /** 3191 * Search parameter: <b>relation</b> 3192 * <p> 3193 * Description: <b>replaces | transforms | signs | appends</b><br> 3194 * Type: <b>token</b><br> 3195 * Path: <b>DocumentReference.relatesTo.code</b><br> 3196 * </p> 3197 */ 3198 @SearchParamDefinition(name = "relation", path = "DocumentReference.relatesTo.code", description = "replaces | transforms | signs | appends", type = "token") 3199 public static final String SP_RELATION = "relation"; 3200 /** 3201 * <b>Fluent Client</b> search parameter constant for <b>relation</b> 3202 * <p> 3203 * Description: <b>replaces | transforms | signs | appends</b><br> 3204 * Type: <b>token</b><br> 3205 * Path: <b>DocumentReference.relatesTo.code</b><br> 3206 * </p> 3207 */ 3208 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RELATION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3209 SP_RELATION); 3210 3211 /** 3212 * Search parameter: <b>setting</b> 3213 * <p> 3214 * Description: <b>Additional details about where the content was created (e.g. 3215 * clinical specialty)</b><br> 3216 * Type: <b>token</b><br> 3217 * Path: <b>DocumentReference.context.practiceSetting</b><br> 3218 * </p> 3219 */ 3220 @SearchParamDefinition(name = "setting", path = "DocumentReference.context.practiceSetting", description = "Additional details about where the content was created (e.g. clinical specialty)", type = "token") 3221 public static final String SP_SETTING = "setting"; 3222 /** 3223 * <b>Fluent Client</b> search parameter constant for <b>setting</b> 3224 * <p> 3225 * Description: <b>Additional details about where the content was created (e.g. 3226 * clinical specialty)</b><br> 3227 * Type: <b>token</b><br> 3228 * Path: <b>DocumentReference.context.practiceSetting</b><br> 3229 * </p> 3230 */ 3231 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SETTING = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3232 SP_SETTING); 3233 3234 /** 3235 * Search parameter: <b>related</b> 3236 * <p> 3237 * Description: <b>Related identifiers or resources</b><br> 3238 * Type: <b>reference</b><br> 3239 * Path: <b>DocumentReference.context.related</b><br> 3240 * </p> 3241 */ 3242 @SearchParamDefinition(name = "related", path = "DocumentReference.context.related", description = "Related identifiers or resources", type = "reference") 3243 public static final String SP_RELATED = "related"; 3244 /** 3245 * <b>Fluent Client</b> search parameter constant for <b>related</b> 3246 * <p> 3247 * Description: <b>Related identifiers or resources</b><br> 3248 * Type: <b>reference</b><br> 3249 * Path: <b>DocumentReference.context.related</b><br> 3250 * </p> 3251 */ 3252 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RELATED = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3253 SP_RELATED); 3254 3255 /** 3256 * Constant for fluent queries to be used to add include statements. Specifies 3257 * the path value of "<b>DocumentReference:related</b>". 3258 */ 3259 public static final ca.uhn.fhir.model.api.Include INCLUDE_RELATED = new ca.uhn.fhir.model.api.Include( 3260 "DocumentReference:related").toLocked(); 3261 3262 /** 3263 * Search parameter: <b>patient</b> 3264 * <p> 3265 * Description: <b>Who/what is the subject of the document</b><br> 3266 * Type: <b>reference</b><br> 3267 * Path: <b>DocumentReference.subject</b><br> 3268 * </p> 3269 */ 3270 @SearchParamDefinition(name = "patient", path = "DocumentReference.subject.where(resolve() is Patient)", description = "Who/what is the subject of the document", type = "reference", target = { 3271 Patient.class }) 3272 public static final String SP_PATIENT = "patient"; 3273 /** 3274 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3275 * <p> 3276 * Description: <b>Who/what is the subject of the document</b><br> 3277 * Type: <b>reference</b><br> 3278 * Path: <b>DocumentReference.subject</b><br> 3279 * </p> 3280 */ 3281 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3282 SP_PATIENT); 3283 3284 /** 3285 * Constant for fluent queries to be used to add include statements. Specifies 3286 * the path value of "<b>DocumentReference:patient</b>". 3287 */ 3288 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 3289 "DocumentReference:patient").toLocked(); 3290 3291 /** 3292 * Search parameter: <b>relationship</b> 3293 * <p> 3294 * Description: <b>Combination of relation and relatesTo</b><br> 3295 * Type: <b>composite</b><br> 3296 * Path: <b></b><br> 3297 * </p> 3298 */ 3299 @SearchParamDefinition(name = "relationship", path = "DocumentReference.relatesTo", description = "Combination of relation and relatesTo", type = "composite", compositeOf = { 3300 "relatesto", "relation" }) 3301 public static final String SP_RELATIONSHIP = "relationship"; 3302 /** 3303 * <b>Fluent Client</b> search parameter constant for <b>relationship</b> 3304 * <p> 3305 * Description: <b>Combination of relation and relatesTo</b><br> 3306 * Type: <b>composite</b><br> 3307 * Path: <b></b><br> 3308 * </p> 3309 */ 3310 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.ReferenceClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> RELATIONSHIP = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.ReferenceClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 3311 SP_RELATIONSHIP); 3312 3313 /** 3314 * Search parameter: <b>event</b> 3315 * <p> 3316 * Description: <b>Main clinical acts documented</b><br> 3317 * Type: <b>token</b><br> 3318 * Path: <b>DocumentReference.context.event</b><br> 3319 * </p> 3320 */ 3321 @SearchParamDefinition(name = "event", path = "DocumentReference.context.event", description = "Main clinical acts documented", type = "token") 3322 public static final String SP_EVENT = "event"; 3323 /** 3324 * <b>Fluent Client</b> search parameter constant for <b>event</b> 3325 * <p> 3326 * Description: <b>Main clinical acts documented</b><br> 3327 * Type: <b>token</b><br> 3328 * Path: <b>DocumentReference.context.event</b><br> 3329 * </p> 3330 */ 3331 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EVENT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3332 SP_EVENT); 3333 3334 /** 3335 * Search parameter: <b>authenticator</b> 3336 * <p> 3337 * Description: <b>Who/what authenticated the document</b><br> 3338 * Type: <b>reference</b><br> 3339 * Path: <b>DocumentReference.authenticator</b><br> 3340 * </p> 3341 */ 3342 @SearchParamDefinition(name = "authenticator", path = "DocumentReference.authenticator", description = "Who/what authenticated the document", type = "reference", providesMembershipIn = { 3343 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 3344 Practitioner.class, PractitionerRole.class }) 3345 public static final String SP_AUTHENTICATOR = "authenticator"; 3346 /** 3347 * <b>Fluent Client</b> search parameter constant for <b>authenticator</b> 3348 * <p> 3349 * Description: <b>Who/what authenticated the document</b><br> 3350 * Type: <b>reference</b><br> 3351 * Path: <b>DocumentReference.authenticator</b><br> 3352 * </p> 3353 */ 3354 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHENTICATOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3355 SP_AUTHENTICATOR); 3356 3357 /** 3358 * Constant for fluent queries to be used to add include statements. Specifies 3359 * the path value of "<b>DocumentReference:authenticator</b>". 3360 */ 3361 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHENTICATOR = new ca.uhn.fhir.model.api.Include( 3362 "DocumentReference:authenticator").toLocked(); 3363 3364 /** 3365 * Search parameter: <b>identifier</b> 3366 * <p> 3367 * Description: <b>Master Version Specific Identifier</b><br> 3368 * Type: <b>token</b><br> 3369 * Path: <b>DocumentReference.masterIdentifier, 3370 * DocumentReference.identifier</b><br> 3371 * </p> 3372 */ 3373 @SearchParamDefinition(name = "identifier", path = "DocumentReference.masterIdentifier | DocumentReference.identifier", description = "Master Version Specific Identifier", type = "token") 3374 public static final String SP_IDENTIFIER = "identifier"; 3375 /** 3376 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3377 * <p> 3378 * Description: <b>Master Version Specific Identifier</b><br> 3379 * Type: <b>token</b><br> 3380 * Path: <b>DocumentReference.masterIdentifier, 3381 * DocumentReference.identifier</b><br> 3382 * </p> 3383 */ 3384 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3385 SP_IDENTIFIER); 3386 3387 /** 3388 * Search parameter: <b>period</b> 3389 * <p> 3390 * Description: <b>Time of service that is being documented</b><br> 3391 * Type: <b>date</b><br> 3392 * Path: <b>DocumentReference.context.period</b><br> 3393 * </p> 3394 */ 3395 @SearchParamDefinition(name = "period", path = "DocumentReference.context.period", description = "Time of service that is being documented", type = "date") 3396 public static final String SP_PERIOD = "period"; 3397 /** 3398 * <b>Fluent Client</b> search parameter constant for <b>period</b> 3399 * <p> 3400 * Description: <b>Time of service that is being documented</b><br> 3401 * Type: <b>date</b><br> 3402 * Path: <b>DocumentReference.context.period</b><br> 3403 * </p> 3404 */ 3405 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam( 3406 SP_PERIOD); 3407 3408 /** 3409 * Search parameter: <b>custodian</b> 3410 * <p> 3411 * Description: <b>Organization which maintains the document</b><br> 3412 * Type: <b>reference</b><br> 3413 * Path: <b>DocumentReference.custodian</b><br> 3414 * </p> 3415 */ 3416 @SearchParamDefinition(name = "custodian", path = "DocumentReference.custodian", description = "Organization which maintains the document", type = "reference", target = { 3417 Organization.class }) 3418 public static final String SP_CUSTODIAN = "custodian"; 3419 /** 3420 * <b>Fluent Client</b> search parameter constant for <b>custodian</b> 3421 * <p> 3422 * Description: <b>Organization which maintains the document</b><br> 3423 * Type: <b>reference</b><br> 3424 * Path: <b>DocumentReference.custodian</b><br> 3425 * </p> 3426 */ 3427 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CUSTODIAN = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3428 SP_CUSTODIAN); 3429 3430 /** 3431 * Constant for fluent queries to be used to add include statements. Specifies 3432 * the path value of "<b>DocumentReference:custodian</b>". 3433 */ 3434 public static final ca.uhn.fhir.model.api.Include INCLUDE_CUSTODIAN = new ca.uhn.fhir.model.api.Include( 3435 "DocumentReference:custodian").toLocked(); 3436 3437 /** 3438 * Search parameter: <b>author</b> 3439 * <p> 3440 * Description: <b>Who and/or what authored the document</b><br> 3441 * Type: <b>reference</b><br> 3442 * Path: <b>DocumentReference.author</b><br> 3443 * </p> 3444 */ 3445 @SearchParamDefinition(name = "author", path = "DocumentReference.author", description = "Who and/or what authored the document", type = "reference", providesMembershipIn = { 3446 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 3447 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3448 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 3449 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, 3450 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 3451 public static final String SP_AUTHOR = "author"; 3452 /** 3453 * <b>Fluent Client</b> search parameter constant for <b>author</b> 3454 * <p> 3455 * Description: <b>Who and/or what authored the document</b><br> 3456 * Type: <b>reference</b><br> 3457 * Path: <b>DocumentReference.author</b><br> 3458 * </p> 3459 */ 3460 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3461 SP_AUTHOR); 3462 3463 /** 3464 * Constant for fluent queries to be used to add include statements. Specifies 3465 * the path value of "<b>DocumentReference:author</b>". 3466 */ 3467 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include( 3468 "DocumentReference:author").toLocked(); 3469 3470 /** 3471 * Search parameter: <b>format</b> 3472 * <p> 3473 * Description: <b>Format/content rules for the document</b><br> 3474 * Type: <b>token</b><br> 3475 * Path: <b>DocumentReference.content.format</b><br> 3476 * </p> 3477 */ 3478 @SearchParamDefinition(name = "format", path = "DocumentReference.content.format", description = "Format/content rules for the document", type = "token") 3479 public static final String SP_FORMAT = "format"; 3480 /** 3481 * <b>Fluent Client</b> search parameter constant for <b>format</b> 3482 * <p> 3483 * Description: <b>Format/content rules for the document</b><br> 3484 * Type: <b>token</b><br> 3485 * Path: <b>DocumentReference.content.format</b><br> 3486 * </p> 3487 */ 3488 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FORMAT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3489 SP_FORMAT); 3490 3491 /** 3492 * Search parameter: <b>encounter</b> 3493 * <p> 3494 * Description: <b>Context of the document content</b><br> 3495 * Type: <b>reference</b><br> 3496 * Path: <b>DocumentReference.context.encounter</b><br> 3497 * </p> 3498 */ 3499 @SearchParamDefinition(name = "encounter", path = "DocumentReference.context.encounter", description = "Context of the document content", type = "reference", providesMembershipIn = { 3500 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class, 3501 EpisodeOfCare.class }) 3502 public static final String SP_ENCOUNTER = "encounter"; 3503 /** 3504 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 3505 * <p> 3506 * Description: <b>Context of the document content</b><br> 3507 * Type: <b>reference</b><br> 3508 * Path: <b>DocumentReference.context.encounter</b><br> 3509 * </p> 3510 */ 3511 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3512 SP_ENCOUNTER); 3513 3514 /** 3515 * Constant for fluent queries to be used to add include statements. Specifies 3516 * the path value of "<b>DocumentReference:encounter</b>". 3517 */ 3518 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 3519 "DocumentReference:encounter").toLocked(); 3520 3521 /** 3522 * Search parameter: <b>contenttype</b> 3523 * <p> 3524 * Description: <b>Mime type of the content, with charset etc.</b><br> 3525 * Type: <b>token</b><br> 3526 * Path: <b>DocumentReference.content.attachment.contentType</b><br> 3527 * </p> 3528 */ 3529 @SearchParamDefinition(name = "contenttype", path = "DocumentReference.content.attachment.contentType", description = "Mime type of the content, with charset etc.", type = "token") 3530 public static final String SP_CONTENTTYPE = "contenttype"; 3531 /** 3532 * <b>Fluent Client</b> search parameter constant for <b>contenttype</b> 3533 * <p> 3534 * Description: <b>Mime type of the content, with charset etc.</b><br> 3535 * Type: <b>token</b><br> 3536 * Path: <b>DocumentReference.content.attachment.contentType</b><br> 3537 * </p> 3538 */ 3539 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTENTTYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3540 SP_CONTENTTYPE); 3541 3542 /** 3543 * Search parameter: <b>security-label</b> 3544 * <p> 3545 * Description: <b>Document security-tags</b><br> 3546 * Type: <b>token</b><br> 3547 * Path: <b>DocumentReference.securityLabel</b><br> 3548 * </p> 3549 */ 3550 @SearchParamDefinition(name = "security-label", path = "DocumentReference.securityLabel", description = "Document security-tags", type = "token") 3551 public static final String SP_SECURITY_LABEL = "security-label"; 3552 /** 3553 * <b>Fluent Client</b> search parameter constant for <b>security-label</b> 3554 * <p> 3555 * Description: <b>Document security-tags</b><br> 3556 * Type: <b>token</b><br> 3557 * Path: <b>DocumentReference.securityLabel</b><br> 3558 * </p> 3559 */ 3560 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SECURITY_LABEL = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3561 SP_SECURITY_LABEL); 3562 3563 /** 3564 * Search parameter: <b>location</b> 3565 * <p> 3566 * Description: <b>Uri where the data can be found</b><br> 3567 * Type: <b>uri</b><br> 3568 * Path: <b>DocumentReference.content.attachment.url</b><br> 3569 * </p> 3570 */ 3571 @SearchParamDefinition(name = "location", path = "DocumentReference.content.attachment.url", description = "Uri where the data can be found", type = "uri") 3572 public static final String SP_LOCATION = "location"; 3573 /** 3574 * <b>Fluent Client</b> search parameter constant for <b>location</b> 3575 * <p> 3576 * Description: <b>Uri where the data can be found</b><br> 3577 * Type: <b>uri</b><br> 3578 * Path: <b>DocumentReference.content.attachment.url</b><br> 3579 * </p> 3580 */ 3581 public static final ca.uhn.fhir.rest.gclient.UriClientParam LOCATION = new ca.uhn.fhir.rest.gclient.UriClientParam( 3582 SP_LOCATION); 3583 3584 /** 3585 * Search parameter: <b>category</b> 3586 * <p> 3587 * Description: <b>Categorization of document</b><br> 3588 * Type: <b>token</b><br> 3589 * Path: <b>DocumentReference.category</b><br> 3590 * </p> 3591 */ 3592 @SearchParamDefinition(name = "category", path = "DocumentReference.category", description = "Categorization of document", type = "token") 3593 public static final String SP_CATEGORY = "category"; 3594 /** 3595 * <b>Fluent Client</b> search parameter constant for <b>category</b> 3596 * <p> 3597 * Description: <b>Categorization of document</b><br> 3598 * Type: <b>token</b><br> 3599 * Path: <b>DocumentReference.category</b><br> 3600 * </p> 3601 */ 3602 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3603 SP_CATEGORY); 3604 3605 /** 3606 * Search parameter: <b>relatesto</b> 3607 * <p> 3608 * Description: <b>Target of the relationship</b><br> 3609 * Type: <b>reference</b><br> 3610 * Path: <b>DocumentReference.relatesTo.target</b><br> 3611 * </p> 3612 */ 3613 @SearchParamDefinition(name = "relatesto", path = "DocumentReference.relatesTo.target", description = "Target of the relationship", type = "reference", target = { 3614 DocumentReference.class }) 3615 public static final String SP_RELATESTO = "relatesto"; 3616 /** 3617 * <b>Fluent Client</b> search parameter constant for <b>relatesto</b> 3618 * <p> 3619 * Description: <b>Target of the relationship</b><br> 3620 * Type: <b>reference</b><br> 3621 * Path: <b>DocumentReference.relatesTo.target</b><br> 3622 * </p> 3623 */ 3624 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RELATESTO = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3625 SP_RELATESTO); 3626 3627 /** 3628 * Constant for fluent queries to be used to add include statements. Specifies 3629 * the path value of "<b>DocumentReference:relatesto</b>". 3630 */ 3631 public static final ca.uhn.fhir.model.api.Include INCLUDE_RELATESTO = new ca.uhn.fhir.model.api.Include( 3632 "DocumentReference:relatesto").toLocked(); 3633 3634 /** 3635 * Search parameter: <b>facility</b> 3636 * <p> 3637 * Description: <b>Kind of facility where patient was seen</b><br> 3638 * Type: <b>token</b><br> 3639 * Path: <b>DocumentReference.context.facilityType</b><br> 3640 * </p> 3641 */ 3642 @SearchParamDefinition(name = "facility", path = "DocumentReference.context.facilityType", description = "Kind of facility where patient was seen", type = "token") 3643 public static final String SP_FACILITY = "facility"; 3644 /** 3645 * <b>Fluent Client</b> search parameter constant for <b>facility</b> 3646 * <p> 3647 * Description: <b>Kind of facility where patient was seen</b><br> 3648 * Type: <b>token</b><br> 3649 * Path: <b>DocumentReference.context.facilityType</b><br> 3650 * </p> 3651 */ 3652 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FACILITY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3653 SP_FACILITY); 3654 3655 /** 3656 * Search parameter: <b>status</b> 3657 * <p> 3658 * Description: <b>current | superseded | entered-in-error</b><br> 3659 * Type: <b>token</b><br> 3660 * Path: <b>DocumentReference.status</b><br> 3661 * </p> 3662 */ 3663 @SearchParamDefinition(name = "status", path = "DocumentReference.status", description = "current | superseded | entered-in-error", type = "token") 3664 public static final String SP_STATUS = "status"; 3665 /** 3666 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3667 * <p> 3668 * Description: <b>current | superseded | entered-in-error</b><br> 3669 * Type: <b>token</b><br> 3670 * Path: <b>DocumentReference.status</b><br> 3671 * </p> 3672 */ 3673 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3674 SP_STATUS); 3675 3676}