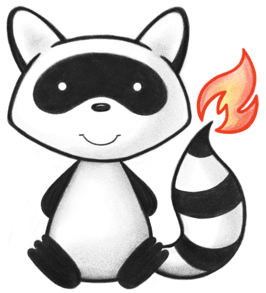
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.DocumentReferenceStatus; 040import org.hl7.fhir.r4.model.Enumerations.DocumentReferenceStatusEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048 049/** 050 * A reference to a document of any kind for any purpose. Provides metadata 051 * about the document so that the document can be discovered and managed. The 052 * scope of a document is any seralized object with a mime-type, so includes 053 * formal patient centric documents (CDA), cliical notes, scanned paper, and 054 * non-patient specific documents like policy text. 055 */ 056@ResourceDef(name = "DocumentReference", profile = "http://hl7.org/fhir/StructureDefinition/DocumentReference") 057public class DocumentReference extends DomainResource { 058 059 public enum ReferredDocumentStatus { 060 /** 061 * This is a preliminary composition or document (also known as initial or 062 * interim). The content may be incomplete or unverified. 063 */ 064 PRELIMINARY, 065 /** 066 * This version of the composition is complete and verified by an appropriate 067 * person and no further work is planned. Any subsequent updates would be on a 068 * new version of the composition. 069 */ 070 FINAL, 071 /** 072 * The composition content or the referenced resources have been modified 073 * (edited or added to) subsequent to being released as "final" and the 074 * composition is complete and verified by an authorized person. 075 */ 076 AMENDED, 077 /** 078 * The composition or document was originally created/issued in error, and this 079 * is an amendment that marks that the entire series should not be considered as 080 * valid. 081 */ 082 ENTEREDINERROR, 083 /** 084 * added to help the parsers with the generic types 085 */ 086 NULL; 087 088 public static ReferredDocumentStatus fromCode(String codeString) throws FHIRException { 089 if (codeString == null || "".equals(codeString)) 090 return null; 091 if ("preliminary".equals(codeString)) 092 return PRELIMINARY; 093 if ("final".equals(codeString)) 094 return FINAL; 095 if ("amended".equals(codeString)) 096 return AMENDED; 097 if ("entered-in-error".equals(codeString)) 098 return ENTEREDINERROR; 099 if (Configuration.isAcceptInvalidEnums()) 100 return null; 101 else 102 throw new FHIRException("Unknown ReferredDocumentStatus code '" + codeString + "'"); 103 } 104 105 public String toCode() { 106 switch (this) { 107 case PRELIMINARY: 108 return "preliminary"; 109 case FINAL: 110 return "final"; 111 case AMENDED: 112 return "amended"; 113 case ENTEREDINERROR: 114 return "entered-in-error"; 115 case NULL: 116 return null; 117 default: 118 return "?"; 119 } 120 } 121 122 public String getSystem() { 123 switch (this) { 124 case PRELIMINARY: 125 return "http://hl7.org/fhir/composition-status"; 126 case FINAL: 127 return "http://hl7.org/fhir/composition-status"; 128 case AMENDED: 129 return "http://hl7.org/fhir/composition-status"; 130 case ENTEREDINERROR: 131 return "http://hl7.org/fhir/composition-status"; 132 case NULL: 133 return null; 134 default: 135 return "?"; 136 } 137 } 138 139 public String getDefinition() { 140 switch (this) { 141 case PRELIMINARY: 142 return "This is a preliminary composition or document (also known as initial or interim). The content may be incomplete or unverified."; 143 case FINAL: 144 return "This version of the composition is complete and verified by an appropriate person and no further work is planned. Any subsequent updates would be on a new version of the composition."; 145 case AMENDED: 146 return "The composition content or the referenced resources have been modified (edited or added to) subsequent to being released as \"final\" and the composition is complete and verified by an authorized person."; 147 case ENTEREDINERROR: 148 return "The composition or document was originally created/issued in error, and this is an amendment that marks that the entire series should not be considered as valid."; 149 case NULL: 150 return null; 151 default: 152 return "?"; 153 } 154 } 155 156 public String getDisplay() { 157 switch (this) { 158 case PRELIMINARY: 159 return "Preliminary"; 160 case FINAL: 161 return "Final"; 162 case AMENDED: 163 return "Amended"; 164 case ENTEREDINERROR: 165 return "Entered in Error"; 166 case NULL: 167 return null; 168 default: 169 return "?"; 170 } 171 } 172 } 173 174 public static class ReferredDocumentStatusEnumFactory implements EnumFactory<ReferredDocumentStatus> { 175 public ReferredDocumentStatus fromCode(String codeString) throws IllegalArgumentException { 176 if (codeString == null || "".equals(codeString)) 177 return null; 178 if ("preliminary".equals(codeString)) 179 return ReferredDocumentStatus.PRELIMINARY; 180 if ("final".equals(codeString)) 181 return ReferredDocumentStatus.FINAL; 182 if ("amended".equals(codeString)) 183 return ReferredDocumentStatus.AMENDED; 184 if ("entered-in-error".equals(codeString)) 185 return ReferredDocumentStatus.ENTEREDINERROR; 186 throw new IllegalArgumentException("Unknown ReferredDocumentStatus code '" + codeString + "'"); 187 } 188 189 public Enumeration<ReferredDocumentStatus> fromType(PrimitiveType<?> code) throws FHIRException { 190 if (code == null) 191 return null; 192 if (code.isEmpty()) 193 return new Enumeration<ReferredDocumentStatus>(this, ReferredDocumentStatus.NULL, code); 194 String codeString = code.asStringValue(); 195 if (codeString == null || "".equals(codeString)) 196 return new Enumeration<ReferredDocumentStatus>(this, ReferredDocumentStatus.NULL, code); 197 if ("preliminary".equals(codeString)) 198 return new Enumeration<ReferredDocumentStatus>(this, ReferredDocumentStatus.PRELIMINARY, code); 199 if ("final".equals(codeString)) 200 return new Enumeration<ReferredDocumentStatus>(this, ReferredDocumentStatus.FINAL, code); 201 if ("amended".equals(codeString)) 202 return new Enumeration<ReferredDocumentStatus>(this, ReferredDocumentStatus.AMENDED, code); 203 if ("entered-in-error".equals(codeString)) 204 return new Enumeration<ReferredDocumentStatus>(this, ReferredDocumentStatus.ENTEREDINERROR, code); 205 throw new FHIRException("Unknown ReferredDocumentStatus code '" + codeString + "'"); 206 } 207 208 public String toCode(ReferredDocumentStatus code) { 209 if (code == ReferredDocumentStatus.NULL) 210 return null; 211 if (code == ReferredDocumentStatus.PRELIMINARY) 212 return "preliminary"; 213 if (code == ReferredDocumentStatus.FINAL) 214 return "final"; 215 if (code == ReferredDocumentStatus.AMENDED) 216 return "amended"; 217 if (code == ReferredDocumentStatus.ENTEREDINERROR) 218 return "entered-in-error"; 219 return "?"; 220 } 221 222 public String toSystem(ReferredDocumentStatus code) { 223 return code.getSystem(); 224 } 225 } 226 227 public enum DocumentRelationshipType { 228 /** 229 * This document logically replaces or supersedes the target document. 230 */ 231 REPLACES, 232 /** 233 * This document was generated by transforming the target document (e.g. format 234 * or language conversion). 235 */ 236 TRANSFORMS, 237 /** 238 * This document is a signature of the target document. 239 */ 240 SIGNS, 241 /** 242 * This document adds additional information to the target document. 243 */ 244 APPENDS, 245 /** 246 * added to help the parsers with the generic types 247 */ 248 NULL; 249 250 public static DocumentRelationshipType fromCode(String codeString) throws FHIRException { 251 if (codeString == null || "".equals(codeString)) 252 return null; 253 if ("replaces".equals(codeString)) 254 return REPLACES; 255 if ("transforms".equals(codeString)) 256 return TRANSFORMS; 257 if ("signs".equals(codeString)) 258 return SIGNS; 259 if ("appends".equals(codeString)) 260 return APPENDS; 261 if (Configuration.isAcceptInvalidEnums()) 262 return null; 263 else 264 throw new FHIRException("Unknown DocumentRelationshipType code '" + codeString + "'"); 265 } 266 267 public String toCode() { 268 switch (this) { 269 case REPLACES: 270 return "replaces"; 271 case TRANSFORMS: 272 return "transforms"; 273 case SIGNS: 274 return "signs"; 275 case APPENDS: 276 return "appends"; 277 case NULL: 278 return null; 279 default: 280 return "?"; 281 } 282 } 283 284 public String getSystem() { 285 switch (this) { 286 case REPLACES: 287 return "http://hl7.org/fhir/document-relationship-type"; 288 case TRANSFORMS: 289 return "http://hl7.org/fhir/document-relationship-type"; 290 case SIGNS: 291 return "http://hl7.org/fhir/document-relationship-type"; 292 case APPENDS: 293 return "http://hl7.org/fhir/document-relationship-type"; 294 case NULL: 295 return null; 296 default: 297 return "?"; 298 } 299 } 300 301 public String getDefinition() { 302 switch (this) { 303 case REPLACES: 304 return "This document logically replaces or supersedes the target document."; 305 case TRANSFORMS: 306 return "This document was generated by transforming the target document (e.g. format or language conversion)."; 307 case SIGNS: 308 return "This document is a signature of the target document."; 309 case APPENDS: 310 return "This document adds additional information to the target document."; 311 case NULL: 312 return null; 313 default: 314 return "?"; 315 } 316 } 317 318 public String getDisplay() { 319 switch (this) { 320 case REPLACES: 321 return "Replaces"; 322 case TRANSFORMS: 323 return "Transforms"; 324 case SIGNS: 325 return "Signs"; 326 case APPENDS: 327 return "Appends"; 328 case NULL: 329 return null; 330 default: 331 return "?"; 332 } 333 } 334 } 335 336 public static class DocumentRelationshipTypeEnumFactory implements EnumFactory<DocumentRelationshipType> { 337 public DocumentRelationshipType fromCode(String codeString) throws IllegalArgumentException { 338 if (codeString == null || "".equals(codeString)) 339 if (codeString == null || "".equals(codeString)) 340 return null; 341 if ("replaces".equals(codeString)) 342 return DocumentRelationshipType.REPLACES; 343 if ("transforms".equals(codeString)) 344 return DocumentRelationshipType.TRANSFORMS; 345 if ("signs".equals(codeString)) 346 return DocumentRelationshipType.SIGNS; 347 if ("appends".equals(codeString)) 348 return DocumentRelationshipType.APPENDS; 349 throw new IllegalArgumentException("Unknown DocumentRelationshipType code '" + codeString + "'"); 350 } 351 352 public Enumeration<DocumentRelationshipType> fromType(PrimitiveType<?> code) throws FHIRException { 353 if (code == null) 354 return null; 355 if (code.isEmpty()) 356 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.NULL, code); 357 String codeString = code.asStringValue(); 358 if (codeString == null || "".equals(codeString)) 359 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.NULL, code); 360 if ("replaces".equals(codeString)) 361 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.REPLACES, code); 362 if ("transforms".equals(codeString)) 363 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.TRANSFORMS, code); 364 if ("signs".equals(codeString)) 365 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.SIGNS, code); 366 if ("appends".equals(codeString)) 367 return new Enumeration<DocumentRelationshipType>(this, DocumentRelationshipType.APPENDS, code); 368 throw new FHIRException("Unknown DocumentRelationshipType code '" + codeString + "'"); 369 } 370 371 public String toCode(DocumentRelationshipType code) { 372 if (code == DocumentRelationshipType.NULL) 373 return null; 374 if (code == DocumentRelationshipType.REPLACES) 375 return "replaces"; 376 if (code == DocumentRelationshipType.TRANSFORMS) 377 return "transforms"; 378 if (code == DocumentRelationshipType.SIGNS) 379 return "signs"; 380 if (code == DocumentRelationshipType.APPENDS) 381 return "appends"; 382 return "?"; 383 } 384 385 public String toSystem(DocumentRelationshipType code) { 386 return code.getSystem(); 387 } 388 } 389 390 @Block() 391 public static class DocumentReferenceRelatesToComponent extends BackboneElement implements IBaseBackboneElement { 392 /** 393 * The type of relationship that this document has with anther document. 394 */ 395 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 396 @Description(shortDefinition = "replaces | transforms | signs | appends", formalDefinition = "The type of relationship that this document has with anther document.") 397 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/document-relationship-type") 398 protected Enumeration<DocumentRelationshipType> code; 399 400 /** 401 * The target document of this relationship. 402 */ 403 @Child(name = "target", type = { 404 DocumentReference.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 405 @Description(shortDefinition = "Target of the relationship", formalDefinition = "The target document of this relationship.") 406 protected Reference target; 407 408 /** 409 * The actual object that is the target of the reference (The target document of 410 * this relationship.) 411 */ 412 protected DocumentReference targetTarget; 413 414 private static final long serialVersionUID = -347257495L; 415 416 /** 417 * Constructor 418 */ 419 public DocumentReferenceRelatesToComponent() { 420 super(); 421 } 422 423 /** 424 * Constructor 425 */ 426 public DocumentReferenceRelatesToComponent(Enumeration<DocumentRelationshipType> code, Reference target) { 427 super(); 428 this.code = code; 429 this.target = target; 430 } 431 432 /** 433 * @return {@link #code} (The type of relationship that this document has with 434 * anther document.). This is the underlying object with id, value and 435 * extensions. The accessor "getCode" gives direct access to the value 436 */ 437 public Enumeration<DocumentRelationshipType> getCodeElement() { 438 if (this.code == null) 439 if (Configuration.errorOnAutoCreate()) 440 throw new Error("Attempt to auto-create DocumentReferenceRelatesToComponent.code"); 441 else if (Configuration.doAutoCreate()) 442 this.code = new Enumeration<DocumentRelationshipType>(new DocumentRelationshipTypeEnumFactory()); // bb 443 return this.code; 444 } 445 446 public boolean hasCodeElement() { 447 return this.code != null && !this.code.isEmpty(); 448 } 449 450 public boolean hasCode() { 451 return this.code != null && !this.code.isEmpty(); 452 } 453 454 /** 455 * @param value {@link #code} (The type of relationship that this document has 456 * with anther document.). This is the underlying object with id, 457 * value and extensions. The accessor "getCode" gives direct access 458 * to the value 459 */ 460 public DocumentReferenceRelatesToComponent setCodeElement(Enumeration<DocumentRelationshipType> value) { 461 this.code = value; 462 return this; 463 } 464 465 /** 466 * @return The type of relationship that this document has with anther document. 467 */ 468 public DocumentRelationshipType getCode() { 469 return this.code == null ? null : this.code.getValue(); 470 } 471 472 /** 473 * @param value The type of relationship that this document has with anther 474 * document. 475 */ 476 public DocumentReferenceRelatesToComponent setCode(DocumentRelationshipType value) { 477 if (this.code == null) 478 this.code = new Enumeration<DocumentRelationshipType>(new DocumentRelationshipTypeEnumFactory()); 479 this.code.setValue(value); 480 return this; 481 } 482 483 /** 484 * @return {@link #target} (The target document of this relationship.) 485 */ 486 public Reference getTarget() { 487 if (this.target == null) 488 if (Configuration.errorOnAutoCreate()) 489 throw new Error("Attempt to auto-create DocumentReferenceRelatesToComponent.target"); 490 else if (Configuration.doAutoCreate()) 491 this.target = new Reference(); // cc 492 return this.target; 493 } 494 495 public boolean hasTarget() { 496 return this.target != null && !this.target.isEmpty(); 497 } 498 499 /** 500 * @param value {@link #target} (The target document of this relationship.) 501 */ 502 public DocumentReferenceRelatesToComponent setTarget(Reference value) { 503 this.target = value; 504 return this; 505 } 506 507 /** 508 * @return {@link #target} The actual object that is the target of the 509 * reference. The reference library doesn't populate this, but you can 510 * use it to hold the resource if you resolve it. (The target document 511 * of this relationship.) 512 */ 513 public DocumentReference getTargetTarget() { 514 if (this.targetTarget == null) 515 if (Configuration.errorOnAutoCreate()) 516 throw new Error("Attempt to auto-create DocumentReferenceRelatesToComponent.target"); 517 else if (Configuration.doAutoCreate()) 518 this.targetTarget = new DocumentReference(); // aa 519 return this.targetTarget; 520 } 521 522 /** 523 * @param value {@link #target} The actual object that is the target of the 524 * reference. The reference library doesn't use these, but you can 525 * use it to hold the resource if you resolve it. (The target 526 * document of this relationship.) 527 */ 528 public DocumentReferenceRelatesToComponent setTargetTarget(DocumentReference value) { 529 this.targetTarget = value; 530 return this; 531 } 532 533 protected void listChildren(List<Property> children) { 534 super.listChildren(children); 535 children.add(new Property("code", "code", "The type of relationship that this document has with anther document.", 536 0, 1, code)); 537 children.add(new Property("target", "Reference(DocumentReference)", "The target document of this relationship.", 538 0, 1, target)); 539 } 540 541 @Override 542 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 543 switch (_hash) { 544 case 3059181: 545 /* code */ return new Property("code", "code", 546 "The type of relationship that this document has with anther document.", 0, 1, code); 547 case -880905839: 548 /* target */ return new Property("target", "Reference(DocumentReference)", 549 "The target document of this relationship.", 0, 1, target); 550 default: 551 return super.getNamedProperty(_hash, _name, _checkValid); 552 } 553 554 } 555 556 @Override 557 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 558 switch (hash) { 559 case 3059181: 560 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // Enumeration<DocumentRelationshipType> 561 case -880905839: 562 /* target */ return this.target == null ? new Base[0] : new Base[] { this.target }; // Reference 563 default: 564 return super.getProperty(hash, name, checkValid); 565 } 566 567 } 568 569 @Override 570 public Base setProperty(int hash, String name, Base value) throws FHIRException { 571 switch (hash) { 572 case 3059181: // code 573 value = new DocumentRelationshipTypeEnumFactory().fromType(castToCode(value)); 574 this.code = (Enumeration) value; // Enumeration<DocumentRelationshipType> 575 return value; 576 case -880905839: // target 577 this.target = castToReference(value); // Reference 578 return value; 579 default: 580 return super.setProperty(hash, name, value); 581 } 582 583 } 584 585 @Override 586 public Base setProperty(String name, Base value) throws FHIRException { 587 if (name.equals("code")) { 588 value = new DocumentRelationshipTypeEnumFactory().fromType(castToCode(value)); 589 this.code = (Enumeration) value; // Enumeration<DocumentRelationshipType> 590 } else if (name.equals("target")) { 591 this.target = castToReference(value); // Reference 592 } else 593 return super.setProperty(name, value); 594 return value; 595 } 596 597 @Override 598 public void removeChild(String name, Base value) throws FHIRException { 599 if (name.equals("code")) { 600 this.code = null; 601 } else if (name.equals("target")) { 602 this.target = null; 603 } else 604 super.removeChild(name, value); 605 606 } 607 608 @Override 609 public Base makeProperty(int hash, String name) throws FHIRException { 610 switch (hash) { 611 case 3059181: 612 return getCodeElement(); 613 case -880905839: 614 return getTarget(); 615 default: 616 return super.makeProperty(hash, name); 617 } 618 619 } 620 621 @Override 622 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 623 switch (hash) { 624 case 3059181: 625 /* code */ return new String[] { "code" }; 626 case -880905839: 627 /* target */ return new String[] { "Reference" }; 628 default: 629 return super.getTypesForProperty(hash, name); 630 } 631 632 } 633 634 @Override 635 public Base addChild(String name) throws FHIRException { 636 if (name.equals("code")) { 637 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.code"); 638 } else if (name.equals("target")) { 639 this.target = new Reference(); 640 return this.target; 641 } else 642 return super.addChild(name); 643 } 644 645 public DocumentReferenceRelatesToComponent copy() { 646 DocumentReferenceRelatesToComponent dst = new DocumentReferenceRelatesToComponent(); 647 copyValues(dst); 648 return dst; 649 } 650 651 public void copyValues(DocumentReferenceRelatesToComponent dst) { 652 super.copyValues(dst); 653 dst.code = code == null ? null : code.copy(); 654 dst.target = target == null ? null : target.copy(); 655 } 656 657 @Override 658 public boolean equalsDeep(Base other_) { 659 if (!super.equalsDeep(other_)) 660 return false; 661 if (!(other_ instanceof DocumentReferenceRelatesToComponent)) 662 return false; 663 DocumentReferenceRelatesToComponent o = (DocumentReferenceRelatesToComponent) other_; 664 return compareDeep(code, o.code, true) && compareDeep(target, o.target, true); 665 } 666 667 @Override 668 public boolean equalsShallow(Base other_) { 669 if (!super.equalsShallow(other_)) 670 return false; 671 if (!(other_ instanceof DocumentReferenceRelatesToComponent)) 672 return false; 673 DocumentReferenceRelatesToComponent o = (DocumentReferenceRelatesToComponent) other_; 674 return compareValues(code, o.code, true); 675 } 676 677 public boolean isEmpty() { 678 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, target); 679 } 680 681 public String fhirType() { 682 return "DocumentReference.relatesTo"; 683 684 } 685 686 } 687 688 @Block() 689 public static class DocumentReferenceContentComponent extends BackboneElement implements IBaseBackboneElement { 690 /** 691 * The document or URL of the document along with critical metadata to prove 692 * content has integrity. 693 */ 694 @Child(name = "attachment", type = { 695 Attachment.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 696 @Description(shortDefinition = "Where to access the document", formalDefinition = "The document or URL of the document along with critical metadata to prove content has integrity.") 697 protected Attachment attachment; 698 699 /** 700 * An identifier of the document encoding, structure, and template that the 701 * document conforms to beyond the base format indicated in the mimeType. 702 */ 703 @Child(name = "format", type = { Coding.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 704 @Description(shortDefinition = "Format/content rules for the document", formalDefinition = "An identifier of the document encoding, structure, and template that the document conforms to beyond the base format indicated in the mimeType.") 705 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/formatcodes") 706 protected Coding format; 707 708 private static final long serialVersionUID = -1313860217L; 709 710 /** 711 * Constructor 712 */ 713 public DocumentReferenceContentComponent() { 714 super(); 715 } 716 717 /** 718 * Constructor 719 */ 720 public DocumentReferenceContentComponent(Attachment attachment) { 721 super(); 722 this.attachment = attachment; 723 } 724 725 /** 726 * @return {@link #attachment} (The document or URL of the document along with 727 * critical metadata to prove content has integrity.) 728 */ 729 public Attachment getAttachment() { 730 if (this.attachment == null) 731 if (Configuration.errorOnAutoCreate()) 732 throw new Error("Attempt to auto-create DocumentReferenceContentComponent.attachment"); 733 else if (Configuration.doAutoCreate()) 734 this.attachment = new Attachment(); // cc 735 return this.attachment; 736 } 737 738 public boolean hasAttachment() { 739 return this.attachment != null && !this.attachment.isEmpty(); 740 } 741 742 /** 743 * @param value {@link #attachment} (The document or URL of the document along 744 * with critical metadata to prove content has integrity.) 745 */ 746 public DocumentReferenceContentComponent setAttachment(Attachment value) { 747 this.attachment = value; 748 return this; 749 } 750 751 /** 752 * @return {@link #format} (An identifier of the document encoding, structure, 753 * and template that the document conforms to beyond the base format 754 * indicated in the mimeType.) 755 */ 756 public Coding getFormat() { 757 if (this.format == null) 758 if (Configuration.errorOnAutoCreate()) 759 throw new Error("Attempt to auto-create DocumentReferenceContentComponent.format"); 760 else if (Configuration.doAutoCreate()) 761 this.format = new Coding(); // cc 762 return this.format; 763 } 764 765 public boolean hasFormat() { 766 return this.format != null && !this.format.isEmpty(); 767 } 768 769 /** 770 * @param value {@link #format} (An identifier of the document encoding, 771 * structure, and template that the document conforms to beyond the 772 * base format indicated in the mimeType.) 773 */ 774 public DocumentReferenceContentComponent setFormat(Coding value) { 775 this.format = value; 776 return this; 777 } 778 779 protected void listChildren(List<Property> children) { 780 super.listChildren(children); 781 children.add(new Property("attachment", "Attachment", 782 "The document or URL of the document along with critical metadata to prove content has integrity.", 0, 1, 783 attachment)); 784 children.add(new Property("format", "Coding", 785 "An identifier of the document encoding, structure, and template that the document conforms to beyond the base format indicated in the mimeType.", 786 0, 1, format)); 787 } 788 789 @Override 790 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 791 switch (_hash) { 792 case -1963501277: 793 /* attachment */ return new Property("attachment", "Attachment", 794 "The document or URL of the document along with critical metadata to prove content has integrity.", 0, 1, 795 attachment); 796 case -1268779017: 797 /* format */ return new Property("format", "Coding", 798 "An identifier of the document encoding, structure, and template that the document conforms to beyond the base format indicated in the mimeType.", 799 0, 1, format); 800 default: 801 return super.getNamedProperty(_hash, _name, _checkValid); 802 } 803 804 } 805 806 @Override 807 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 808 switch (hash) { 809 case -1963501277: 810 /* attachment */ return this.attachment == null ? new Base[0] : new Base[] { this.attachment }; // Attachment 811 case -1268779017: 812 /* format */ return this.format == null ? new Base[0] : new Base[] { this.format }; // Coding 813 default: 814 return super.getProperty(hash, name, checkValid); 815 } 816 817 } 818 819 @Override 820 public Base setProperty(int hash, String name, Base value) throws FHIRException { 821 switch (hash) { 822 case -1963501277: // attachment 823 this.attachment = castToAttachment(value); // Attachment 824 return value; 825 case -1268779017: // format 826 this.format = castToCoding(value); // Coding 827 return value; 828 default: 829 return super.setProperty(hash, name, value); 830 } 831 832 } 833 834 @Override 835 public Base setProperty(String name, Base value) throws FHIRException { 836 if (name.equals("attachment")) { 837 this.attachment = castToAttachment(value); // Attachment 838 } else if (name.equals("format")) { 839 this.format = castToCoding(value); // Coding 840 } else 841 return super.setProperty(name, value); 842 return value; 843 } 844 845 @Override 846 public void removeChild(String name, Base value) throws FHIRException { 847 if (name.equals("attachment")) { 848 this.attachment = null; 849 } else if (name.equals("format")) { 850 this.format = null; 851 } else 852 super.removeChild(name, value); 853 854 } 855 856 @Override 857 public Base makeProperty(int hash, String name) throws FHIRException { 858 switch (hash) { 859 case -1963501277: 860 return getAttachment(); 861 case -1268779017: 862 return getFormat(); 863 default: 864 return super.makeProperty(hash, name); 865 } 866 867 } 868 869 @Override 870 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 871 switch (hash) { 872 case -1963501277: 873 /* attachment */ return new String[] { "Attachment" }; 874 case -1268779017: 875 /* format */ return new String[] { "Coding" }; 876 default: 877 return super.getTypesForProperty(hash, name); 878 } 879 880 } 881 882 @Override 883 public Base addChild(String name) throws FHIRException { 884 if (name.equals("attachment")) { 885 this.attachment = new Attachment(); 886 return this.attachment; 887 } else if (name.equals("format")) { 888 this.format = new Coding(); 889 return this.format; 890 } else 891 return super.addChild(name); 892 } 893 894 public DocumentReferenceContentComponent copy() { 895 DocumentReferenceContentComponent dst = new DocumentReferenceContentComponent(); 896 copyValues(dst); 897 return dst; 898 } 899 900 public void copyValues(DocumentReferenceContentComponent dst) { 901 super.copyValues(dst); 902 dst.attachment = attachment == null ? null : attachment.copy(); 903 dst.format = format == null ? null : format.copy(); 904 } 905 906 @Override 907 public boolean equalsDeep(Base other_) { 908 if (!super.equalsDeep(other_)) 909 return false; 910 if (!(other_ instanceof DocumentReferenceContentComponent)) 911 return false; 912 DocumentReferenceContentComponent o = (DocumentReferenceContentComponent) other_; 913 return compareDeep(attachment, o.attachment, true) && compareDeep(format, o.format, true); 914 } 915 916 @Override 917 public boolean equalsShallow(Base other_) { 918 if (!super.equalsShallow(other_)) 919 return false; 920 if (!(other_ instanceof DocumentReferenceContentComponent)) 921 return false; 922 DocumentReferenceContentComponent o = (DocumentReferenceContentComponent) other_; 923 return true; 924 } 925 926 public boolean isEmpty() { 927 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(attachment, format); 928 } 929 930 public String fhirType() { 931 return "DocumentReference.content"; 932 933 } 934 935 } 936 937 @Block() 938 public static class DocumentReferenceContextComponent extends BackboneElement implements IBaseBackboneElement { 939 /** 940 * Describes the clinical encounter or type of care that the document content is 941 * associated with. 942 */ 943 @Child(name = "encounter", type = { Encounter.class, 944 EpisodeOfCare.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 945 @Description(shortDefinition = "Context of the document content", formalDefinition = "Describes the clinical encounter or type of care that the document content is associated with.") 946 protected List<Reference> encounter; 947 /** 948 * The actual objects that are the target of the reference (Describes the 949 * clinical encounter or type of care that the document content is associated 950 * with.) 951 */ 952 protected List<Resource> encounterTarget; 953 954 /** 955 * This list of codes represents the main clinical acts, such as a colonoscopy 956 * or an appendectomy, being documented. In some cases, the event is inherent in 957 * the type Code, such as a "History and Physical Report" in which the procedure 958 * being documented is necessarily a "History and Physical" act. 959 */ 960 @Child(name = "event", type = { 961 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 962 @Description(shortDefinition = "Main clinical acts documented", formalDefinition = "This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the type Code, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act.") 963 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ActCode") 964 protected List<CodeableConcept> event; 965 966 /** 967 * The time period over which the service that is described by the document was 968 * provided. 969 */ 970 @Child(name = "period", type = { Period.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 971 @Description(shortDefinition = "Time of service that is being documented", formalDefinition = "The time period over which the service that is described by the document was provided.") 972 protected Period period; 973 974 /** 975 * The kind of facility where the patient was seen. 976 */ 977 @Child(name = "facilityType", type = { 978 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 979 @Description(shortDefinition = "Kind of facility where patient was seen", formalDefinition = "The kind of facility where the patient was seen.") 980 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/c80-facilitycodes") 981 protected CodeableConcept facilityType; 982 983 /** 984 * This property may convey specifics about the practice setting where the 985 * content was created, often reflecting the clinical specialty. 986 */ 987 @Child(name = "practiceSetting", type = { 988 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 989 @Description(shortDefinition = "Additional details about where the content was created (e.g. clinical specialty)", formalDefinition = "This property may convey specifics about the practice setting where the content was created, often reflecting the clinical specialty.") 990 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/c80-practice-codes") 991 protected CodeableConcept practiceSetting; 992 993 /** 994 * The Patient Information as known when the document was published. May be a 995 * reference to a version specific, or contained. 996 */ 997 @Child(name = "sourcePatientInfo", type = { 998 Patient.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 999 @Description(shortDefinition = "Patient demographics from source", formalDefinition = "The Patient Information as known when the document was published. May be a reference to a version specific, or contained.") 1000 protected Reference sourcePatientInfo; 1001 1002 /** 1003 * The actual object that is the target of the reference (The Patient 1004 * Information as known when the document was published. May be a reference to a 1005 * version specific, or contained.) 1006 */ 1007 protected Patient sourcePatientInfoTarget; 1008 1009 /** 1010 * Related identifiers or resources associated with the DocumentReference. 1011 */ 1012 @Child(name = "related", type = { 1013 Reference.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1014 @Description(shortDefinition = "Related identifiers or resources", formalDefinition = "Related identifiers or resources associated with the DocumentReference.") 1015 protected List<Reference> related; 1016 /** 1017 * The actual objects that are the target of the reference (Related identifiers 1018 * or resources associated with the DocumentReference.) 1019 */ 1020 protected List<Resource> relatedTarget; 1021 1022 private static final long serialVersionUID = 140463218L; 1023 1024 /** 1025 * Constructor 1026 */ 1027 public DocumentReferenceContextComponent() { 1028 super(); 1029 } 1030 1031 /** 1032 * @return {@link #encounter} (Describes the clinical encounter or type of care 1033 * that the document content is associated with.) 1034 */ 1035 public List<Reference> getEncounter() { 1036 if (this.encounter == null) 1037 this.encounter = new ArrayList<Reference>(); 1038 return this.encounter; 1039 } 1040 1041 /** 1042 * @return Returns a reference to <code>this</code> for easy method chaining 1043 */ 1044 public DocumentReferenceContextComponent setEncounter(List<Reference> theEncounter) { 1045 this.encounter = theEncounter; 1046 return this; 1047 } 1048 1049 public boolean hasEncounter() { 1050 if (this.encounter == null) 1051 return false; 1052 for (Reference item : this.encounter) 1053 if (!item.isEmpty()) 1054 return true; 1055 return false; 1056 } 1057 1058 public Reference addEncounter() { // 3 1059 Reference t = new Reference(); 1060 if (this.encounter == null) 1061 this.encounter = new ArrayList<Reference>(); 1062 this.encounter.add(t); 1063 return t; 1064 } 1065 1066 public DocumentReferenceContextComponent addEncounter(Reference t) { // 3 1067 if (t == null) 1068 return this; 1069 if (this.encounter == null) 1070 this.encounter = new ArrayList<Reference>(); 1071 this.encounter.add(t); 1072 return this; 1073 } 1074 1075 /** 1076 * @return The first repetition of repeating field {@link #encounter}, creating 1077 * it if it does not already exist 1078 */ 1079 public Reference getEncounterFirstRep() { 1080 if (getEncounter().isEmpty()) { 1081 addEncounter(); 1082 } 1083 return getEncounter().get(0); 1084 } 1085 1086 /** 1087 * @deprecated Use Reference#setResource(IBaseResource) instead 1088 */ 1089 @Deprecated 1090 public List<Resource> getEncounterTarget() { 1091 if (this.encounterTarget == null) 1092 this.encounterTarget = new ArrayList<Resource>(); 1093 return this.encounterTarget; 1094 } 1095 1096 /** 1097 * @return {@link #event} (This list of codes represents the main clinical acts, 1098 * such as a colonoscopy or an appendectomy, being documented. In some 1099 * cases, the event is inherent in the type Code, such as a "History and 1100 * Physical Report" in which the procedure being documented is 1101 * necessarily a "History and Physical" act.) 1102 */ 1103 public List<CodeableConcept> getEvent() { 1104 if (this.event == null) 1105 this.event = new ArrayList<CodeableConcept>(); 1106 return this.event; 1107 } 1108 1109 /** 1110 * @return Returns a reference to <code>this</code> for easy method chaining 1111 */ 1112 public DocumentReferenceContextComponent setEvent(List<CodeableConcept> theEvent) { 1113 this.event = theEvent; 1114 return this; 1115 } 1116 1117 public boolean hasEvent() { 1118 if (this.event == null) 1119 return false; 1120 for (CodeableConcept item : this.event) 1121 if (!item.isEmpty()) 1122 return true; 1123 return false; 1124 } 1125 1126 public CodeableConcept addEvent() { // 3 1127 CodeableConcept t = new CodeableConcept(); 1128 if (this.event == null) 1129 this.event = new ArrayList<CodeableConcept>(); 1130 this.event.add(t); 1131 return t; 1132 } 1133 1134 public DocumentReferenceContextComponent addEvent(CodeableConcept t) { // 3 1135 if (t == null) 1136 return this; 1137 if (this.event == null) 1138 this.event = new ArrayList<CodeableConcept>(); 1139 this.event.add(t); 1140 return this; 1141 } 1142 1143 /** 1144 * @return The first repetition of repeating field {@link #event}, creating it 1145 * if it does not already exist 1146 */ 1147 public CodeableConcept getEventFirstRep() { 1148 if (getEvent().isEmpty()) { 1149 addEvent(); 1150 } 1151 return getEvent().get(0); 1152 } 1153 1154 /** 1155 * @return {@link #period} (The time period over which the service that is 1156 * described by the document was provided.) 1157 */ 1158 public Period getPeriod() { 1159 if (this.period == null) 1160 if (Configuration.errorOnAutoCreate()) 1161 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.period"); 1162 else if (Configuration.doAutoCreate()) 1163 this.period = new Period(); // cc 1164 return this.period; 1165 } 1166 1167 public boolean hasPeriod() { 1168 return this.period != null && !this.period.isEmpty(); 1169 } 1170 1171 /** 1172 * @param value {@link #period} (The time period over which the service that is 1173 * described by the document was provided.) 1174 */ 1175 public DocumentReferenceContextComponent setPeriod(Period value) { 1176 this.period = value; 1177 return this; 1178 } 1179 1180 /** 1181 * @return {@link #facilityType} (The kind of facility where the patient was 1182 * seen.) 1183 */ 1184 public CodeableConcept getFacilityType() { 1185 if (this.facilityType == null) 1186 if (Configuration.errorOnAutoCreate()) 1187 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.facilityType"); 1188 else if (Configuration.doAutoCreate()) 1189 this.facilityType = new CodeableConcept(); // cc 1190 return this.facilityType; 1191 } 1192 1193 public boolean hasFacilityType() { 1194 return this.facilityType != null && !this.facilityType.isEmpty(); 1195 } 1196 1197 /** 1198 * @param value {@link #facilityType} (The kind of facility where the patient 1199 * was seen.) 1200 */ 1201 public DocumentReferenceContextComponent setFacilityType(CodeableConcept value) { 1202 this.facilityType = value; 1203 return this; 1204 } 1205 1206 /** 1207 * @return {@link #practiceSetting} (This property may convey specifics about 1208 * the practice setting where the content was created, often reflecting 1209 * the clinical specialty.) 1210 */ 1211 public CodeableConcept getPracticeSetting() { 1212 if (this.practiceSetting == null) 1213 if (Configuration.errorOnAutoCreate()) 1214 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.practiceSetting"); 1215 else if (Configuration.doAutoCreate()) 1216 this.practiceSetting = new CodeableConcept(); // cc 1217 return this.practiceSetting; 1218 } 1219 1220 public boolean hasPracticeSetting() { 1221 return this.practiceSetting != null && !this.practiceSetting.isEmpty(); 1222 } 1223 1224 /** 1225 * @param value {@link #practiceSetting} (This property may convey specifics 1226 * about the practice setting where the content was created, often 1227 * reflecting the clinical specialty.) 1228 */ 1229 public DocumentReferenceContextComponent setPracticeSetting(CodeableConcept value) { 1230 this.practiceSetting = value; 1231 return this; 1232 } 1233 1234 /** 1235 * @return {@link #sourcePatientInfo} (The Patient Information as known when the 1236 * document was published. May be a reference to a version specific, or 1237 * contained.) 1238 */ 1239 public Reference getSourcePatientInfo() { 1240 if (this.sourcePatientInfo == null) 1241 if (Configuration.errorOnAutoCreate()) 1242 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.sourcePatientInfo"); 1243 else if (Configuration.doAutoCreate()) 1244 this.sourcePatientInfo = new Reference(); // cc 1245 return this.sourcePatientInfo; 1246 } 1247 1248 public boolean hasSourcePatientInfo() { 1249 return this.sourcePatientInfo != null && !this.sourcePatientInfo.isEmpty(); 1250 } 1251 1252 /** 1253 * @param value {@link #sourcePatientInfo} (The Patient Information as known 1254 * when the document was published. May be a reference to a version 1255 * specific, or contained.) 1256 */ 1257 public DocumentReferenceContextComponent setSourcePatientInfo(Reference value) { 1258 this.sourcePatientInfo = value; 1259 return this; 1260 } 1261 1262 /** 1263 * @return {@link #sourcePatientInfo} The actual object that is the target of 1264 * the reference. The reference library doesn't populate this, but you 1265 * can use it to hold the resource if you resolve it. (The Patient 1266 * Information as known when the document was published. May be a 1267 * reference to a version specific, or contained.) 1268 */ 1269 public Patient getSourcePatientInfoTarget() { 1270 if (this.sourcePatientInfoTarget == null) 1271 if (Configuration.errorOnAutoCreate()) 1272 throw new Error("Attempt to auto-create DocumentReferenceContextComponent.sourcePatientInfo"); 1273 else if (Configuration.doAutoCreate()) 1274 this.sourcePatientInfoTarget = new Patient(); // aa 1275 return this.sourcePatientInfoTarget; 1276 } 1277 1278 /** 1279 * @param value {@link #sourcePatientInfo} The actual object that is the target 1280 * of the reference. The reference library doesn't use these, but 1281 * you can use it to hold the resource if you resolve it. (The 1282 * Patient Information as known when the document was published. 1283 * May be a reference to a version specific, or contained.) 1284 */ 1285 public DocumentReferenceContextComponent setSourcePatientInfoTarget(Patient value) { 1286 this.sourcePatientInfoTarget = value; 1287 return this; 1288 } 1289 1290 /** 1291 * @return {@link #related} (Related identifiers or resources associated with 1292 * the DocumentReference.) 1293 */ 1294 public List<Reference> getRelated() { 1295 if (this.related == null) 1296 this.related = new ArrayList<Reference>(); 1297 return this.related; 1298 } 1299 1300 /** 1301 * @return Returns a reference to <code>this</code> for easy method chaining 1302 */ 1303 public DocumentReferenceContextComponent setRelated(List<Reference> theRelated) { 1304 this.related = theRelated; 1305 return this; 1306 } 1307 1308 public boolean hasRelated() { 1309 if (this.related == null) 1310 return false; 1311 for (Reference item : this.related) 1312 if (!item.isEmpty()) 1313 return true; 1314 return false; 1315 } 1316 1317 public Reference addRelated() { // 3 1318 Reference t = new Reference(); 1319 if (this.related == null) 1320 this.related = new ArrayList<Reference>(); 1321 this.related.add(t); 1322 return t; 1323 } 1324 1325 public DocumentReferenceContextComponent addRelated(Reference t) { // 3 1326 if (t == null) 1327 return this; 1328 if (this.related == null) 1329 this.related = new ArrayList<Reference>(); 1330 this.related.add(t); 1331 return this; 1332 } 1333 1334 /** 1335 * @return The first repetition of repeating field {@link #related}, creating it 1336 * if it does not already exist 1337 */ 1338 public Reference getRelatedFirstRep() { 1339 if (getRelated().isEmpty()) { 1340 addRelated(); 1341 } 1342 return getRelated().get(0); 1343 } 1344 1345 /** 1346 * @deprecated Use Reference#setResource(IBaseResource) instead 1347 */ 1348 @Deprecated 1349 public List<Resource> getRelatedTarget() { 1350 if (this.relatedTarget == null) 1351 this.relatedTarget = new ArrayList<Resource>(); 1352 return this.relatedTarget; 1353 } 1354 1355 protected void listChildren(List<Property> children) { 1356 super.listChildren(children); 1357 children.add(new Property("encounter", "Reference(Encounter|EpisodeOfCare)", 1358 "Describes the clinical encounter or type of care that the document content is associated with.", 0, 1359 java.lang.Integer.MAX_VALUE, encounter)); 1360 children.add(new Property("event", "CodeableConcept", 1361 "This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the type Code, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act.", 1362 0, java.lang.Integer.MAX_VALUE, event)); 1363 children.add(new Property("period", "Period", 1364 "The time period over which the service that is described by the document was provided.", 0, 1, period)); 1365 children.add(new Property("facilityType", "CodeableConcept", "The kind of facility where the patient was seen.", 1366 0, 1, facilityType)); 1367 children.add(new Property("practiceSetting", "CodeableConcept", 1368 "This property may convey specifics about the practice setting where the content was created, often reflecting the clinical specialty.", 1369 0, 1, practiceSetting)); 1370 children.add(new Property("sourcePatientInfo", "Reference(Patient)", 1371 "The Patient Information as known when the document was published. May be a reference to a version specific, or contained.", 1372 0, 1, sourcePatientInfo)); 1373 children.add(new Property("related", "Reference(Any)", 1374 "Related identifiers or resources associated with the DocumentReference.", 0, java.lang.Integer.MAX_VALUE, 1375 related)); 1376 } 1377 1378 @Override 1379 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1380 switch (_hash) { 1381 case 1524132147: 1382 /* encounter */ return new Property("encounter", "Reference(Encounter|EpisodeOfCare)", 1383 "Describes the clinical encounter or type of care that the document content is associated with.", 0, 1384 java.lang.Integer.MAX_VALUE, encounter); 1385 case 96891546: 1386 /* event */ return new Property("event", "CodeableConcept", 1387 "This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the type Code, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act.", 1388 0, java.lang.Integer.MAX_VALUE, event); 1389 case -991726143: 1390 /* period */ return new Property("period", "Period", 1391 "The time period over which the service that is described by the document was provided.", 0, 1, period); 1392 case 370698365: 1393 /* facilityType */ return new Property("facilityType", "CodeableConcept", 1394 "The kind of facility where the patient was seen.", 0, 1, facilityType); 1395 case 331373717: 1396 /* practiceSetting */ return new Property("practiceSetting", "CodeableConcept", 1397 "This property may convey specifics about the practice setting where the content was created, often reflecting the clinical specialty.", 1398 0, 1, practiceSetting); 1399 case 2031381048: 1400 /* sourcePatientInfo */ return new Property("sourcePatientInfo", "Reference(Patient)", 1401 "The Patient Information as known when the document was published. May be a reference to a version specific, or contained.", 1402 0, 1, sourcePatientInfo); 1403 case 1090493483: 1404 /* related */ return new Property("related", "Reference(Any)", 1405 "Related identifiers or resources associated with the DocumentReference.", 0, java.lang.Integer.MAX_VALUE, 1406 related); 1407 default: 1408 return super.getNamedProperty(_hash, _name, _checkValid); 1409 } 1410 1411 } 1412 1413 @Override 1414 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1415 switch (hash) { 1416 case 1524132147: 1417 /* encounter */ return this.encounter == null ? new Base[0] 1418 : this.encounter.toArray(new Base[this.encounter.size()]); // Reference 1419 case 96891546: 1420 /* event */ return this.event == null ? new Base[0] : this.event.toArray(new Base[this.event.size()]); // CodeableConcept 1421 case -991726143: 1422 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 1423 case 370698365: 1424 /* facilityType */ return this.facilityType == null ? new Base[0] : new Base[] { this.facilityType }; // CodeableConcept 1425 case 331373717: 1426 /* practiceSetting */ return this.practiceSetting == null ? new Base[0] : new Base[] { this.practiceSetting }; // CodeableConcept 1427 case 2031381048: 1428 /* sourcePatientInfo */ return this.sourcePatientInfo == null ? new Base[0] 1429 : new Base[] { this.sourcePatientInfo }; // Reference 1430 case 1090493483: 1431 /* related */ return this.related == null ? new Base[0] : this.related.toArray(new Base[this.related.size()]); // Reference 1432 default: 1433 return super.getProperty(hash, name, checkValid); 1434 } 1435 1436 } 1437 1438 @Override 1439 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1440 switch (hash) { 1441 case 1524132147: // encounter 1442 this.getEncounter().add(castToReference(value)); // Reference 1443 return value; 1444 case 96891546: // event 1445 this.getEvent().add(castToCodeableConcept(value)); // CodeableConcept 1446 return value; 1447 case -991726143: // period 1448 this.period = castToPeriod(value); // Period 1449 return value; 1450 case 370698365: // facilityType 1451 this.facilityType = castToCodeableConcept(value); // CodeableConcept 1452 return value; 1453 case 331373717: // practiceSetting 1454 this.practiceSetting = castToCodeableConcept(value); // CodeableConcept 1455 return value; 1456 case 2031381048: // sourcePatientInfo 1457 this.sourcePatientInfo = castToReference(value); // Reference 1458 return value; 1459 case 1090493483: // related 1460 this.getRelated().add(castToReference(value)); // Reference 1461 return value; 1462 default: 1463 return super.setProperty(hash, name, value); 1464 } 1465 1466 } 1467 1468 @Override 1469 public Base setProperty(String name, Base value) throws FHIRException { 1470 if (name.equals("encounter")) { 1471 this.getEncounter().add(castToReference(value)); 1472 } else if (name.equals("event")) { 1473 this.getEvent().add(castToCodeableConcept(value)); 1474 } else if (name.equals("period")) { 1475 this.period = castToPeriod(value); // Period 1476 } else if (name.equals("facilityType")) { 1477 this.facilityType = castToCodeableConcept(value); // CodeableConcept 1478 } else if (name.equals("practiceSetting")) { 1479 this.practiceSetting = castToCodeableConcept(value); // CodeableConcept 1480 } else if (name.equals("sourcePatientInfo")) { 1481 this.sourcePatientInfo = castToReference(value); // Reference 1482 } else if (name.equals("related")) { 1483 this.getRelated().add(castToReference(value)); 1484 } else 1485 return super.setProperty(name, value); 1486 return value; 1487 } 1488 1489 @Override 1490 public void removeChild(String name, Base value) throws FHIRException { 1491 if (name.equals("encounter")) { 1492 this.getEncounter().remove(castToReference(value)); 1493 } else if (name.equals("event")) { 1494 this.getEvent().remove(castToCodeableConcept(value)); 1495 } else if (name.equals("period")) { 1496 this.period = null; 1497 } else if (name.equals("facilityType")) { 1498 this.facilityType = null; 1499 } else if (name.equals("practiceSetting")) { 1500 this.practiceSetting = null; 1501 } else if (name.equals("sourcePatientInfo")) { 1502 this.sourcePatientInfo = null; 1503 } else if (name.equals("related")) { 1504 this.getRelated().remove(castToReference(value)); 1505 } else 1506 super.removeChild(name, value); 1507 1508 } 1509 1510 @Override 1511 public Base makeProperty(int hash, String name) throws FHIRException { 1512 switch (hash) { 1513 case 1524132147: 1514 return addEncounter(); 1515 case 96891546: 1516 return addEvent(); 1517 case -991726143: 1518 return getPeriod(); 1519 case 370698365: 1520 return getFacilityType(); 1521 case 331373717: 1522 return getPracticeSetting(); 1523 case 2031381048: 1524 return getSourcePatientInfo(); 1525 case 1090493483: 1526 return addRelated(); 1527 default: 1528 return super.makeProperty(hash, name); 1529 } 1530 1531 } 1532 1533 @Override 1534 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1535 switch (hash) { 1536 case 1524132147: 1537 /* encounter */ return new String[] { "Reference" }; 1538 case 96891546: 1539 /* event */ return new String[] { "CodeableConcept" }; 1540 case -991726143: 1541 /* period */ return new String[] { "Period" }; 1542 case 370698365: 1543 /* facilityType */ return new String[] { "CodeableConcept" }; 1544 case 331373717: 1545 /* practiceSetting */ return new String[] { "CodeableConcept" }; 1546 case 2031381048: 1547 /* sourcePatientInfo */ return new String[] { "Reference" }; 1548 case 1090493483: 1549 /* related */ return new String[] { "Reference" }; 1550 default: 1551 return super.getTypesForProperty(hash, name); 1552 } 1553 1554 } 1555 1556 @Override 1557 public Base addChild(String name) throws FHIRException { 1558 if (name.equals("encounter")) { 1559 return addEncounter(); 1560 } else if (name.equals("event")) { 1561 return addEvent(); 1562 } else if (name.equals("period")) { 1563 this.period = new Period(); 1564 return this.period; 1565 } else if (name.equals("facilityType")) { 1566 this.facilityType = new CodeableConcept(); 1567 return this.facilityType; 1568 } else if (name.equals("practiceSetting")) { 1569 this.practiceSetting = new CodeableConcept(); 1570 return this.practiceSetting; 1571 } else if (name.equals("sourcePatientInfo")) { 1572 this.sourcePatientInfo = new Reference(); 1573 return this.sourcePatientInfo; 1574 } else if (name.equals("related")) { 1575 return addRelated(); 1576 } else 1577 return super.addChild(name); 1578 } 1579 1580 public DocumentReferenceContextComponent copy() { 1581 DocumentReferenceContextComponent dst = new DocumentReferenceContextComponent(); 1582 copyValues(dst); 1583 return dst; 1584 } 1585 1586 public void copyValues(DocumentReferenceContextComponent dst) { 1587 super.copyValues(dst); 1588 if (encounter != null) { 1589 dst.encounter = new ArrayList<Reference>(); 1590 for (Reference i : encounter) 1591 dst.encounter.add(i.copy()); 1592 } 1593 ; 1594 if (event != null) { 1595 dst.event = new ArrayList<CodeableConcept>(); 1596 for (CodeableConcept i : event) 1597 dst.event.add(i.copy()); 1598 } 1599 ; 1600 dst.period = period == null ? null : period.copy(); 1601 dst.facilityType = facilityType == null ? null : facilityType.copy(); 1602 dst.practiceSetting = practiceSetting == null ? null : practiceSetting.copy(); 1603 dst.sourcePatientInfo = sourcePatientInfo == null ? null : sourcePatientInfo.copy(); 1604 if (related != null) { 1605 dst.related = new ArrayList<Reference>(); 1606 for (Reference i : related) 1607 dst.related.add(i.copy()); 1608 } 1609 ; 1610 } 1611 1612 @Override 1613 public boolean equalsDeep(Base other_) { 1614 if (!super.equalsDeep(other_)) 1615 return false; 1616 if (!(other_ instanceof DocumentReferenceContextComponent)) 1617 return false; 1618 DocumentReferenceContextComponent o = (DocumentReferenceContextComponent) other_; 1619 return compareDeep(encounter, o.encounter, true) && compareDeep(event, o.event, true) 1620 && compareDeep(period, o.period, true) && compareDeep(facilityType, o.facilityType, true) 1621 && compareDeep(practiceSetting, o.practiceSetting, true) 1622 && compareDeep(sourcePatientInfo, o.sourcePatientInfo, true) && compareDeep(related, o.related, true); 1623 } 1624 1625 @Override 1626 public boolean equalsShallow(Base other_) { 1627 if (!super.equalsShallow(other_)) 1628 return false; 1629 if (!(other_ instanceof DocumentReferenceContextComponent)) 1630 return false; 1631 DocumentReferenceContextComponent o = (DocumentReferenceContextComponent) other_; 1632 return true; 1633 } 1634 1635 public boolean isEmpty() { 1636 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(encounter, event, period, facilityType, 1637 practiceSetting, sourcePatientInfo, related); 1638 } 1639 1640 public String fhirType() { 1641 return "DocumentReference.context"; 1642 1643 } 1644 1645 } 1646 1647 /** 1648 * Document identifier as assigned by the source of the document. This 1649 * identifier is specific to this version of the document. This unique 1650 * identifier may be used elsewhere to identify this version of the document. 1651 */ 1652 @Child(name = "masterIdentifier", type = { 1653 Identifier.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 1654 @Description(shortDefinition = "Master Version Specific Identifier", formalDefinition = "Document identifier as assigned by the source of the document. This identifier is specific to this version of the document. This unique identifier may be used elsewhere to identify this version of the document.") 1655 protected Identifier masterIdentifier; 1656 1657 /** 1658 * Other identifiers associated with the document, including version independent 1659 * identifiers. 1660 */ 1661 @Child(name = "identifier", type = { 1662 Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1663 @Description(shortDefinition = "Other identifiers for the document", formalDefinition = "Other identifiers associated with the document, including version independent identifiers.") 1664 protected List<Identifier> identifier; 1665 1666 /** 1667 * The status of this document reference. 1668 */ 1669 @Child(name = "status", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = true, summary = true) 1670 @Description(shortDefinition = "current | superseded | entered-in-error", formalDefinition = "The status of this document reference.") 1671 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/document-reference-status") 1672 protected Enumeration<DocumentReferenceStatus> status; 1673 1674 /** 1675 * The status of the underlying document. 1676 */ 1677 @Child(name = "docStatus", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1678 @Description(shortDefinition = "preliminary | final | amended | entered-in-error", formalDefinition = "The status of the underlying document.") 1679 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/composition-status") 1680 protected Enumeration<ReferredDocumentStatus> docStatus; 1681 1682 /** 1683 * Specifies the particular kind of document referenced (e.g. History and 1684 * Physical, Discharge Summary, Progress Note). This usually equates to the 1685 * purpose of making the document referenced. 1686 */ 1687 @Child(name = "type", type = { CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1688 @Description(shortDefinition = "Kind of document (LOINC if possible)", formalDefinition = "Specifies the particular kind of document referenced (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the document referenced.") 1689 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/c80-doc-typecodes") 1690 protected CodeableConcept type; 1691 1692 /** 1693 * A categorization for the type of document referenced - helps for indexing and 1694 * searching. This may be implied by or derived from the code specified in the 1695 * DocumentReference.type. 1696 */ 1697 @Child(name = "category", type = { 1698 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1699 @Description(shortDefinition = "Categorization of document", formalDefinition = "A categorization for the type of document referenced - helps for indexing and searching. This may be implied by or derived from the code specified in the DocumentReference.type.") 1700 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/document-classcodes") 1701 protected List<CodeableConcept> category; 1702 1703 /** 1704 * Who or what the document is about. The document can be about a person, 1705 * (patient or healthcare practitioner), a device (e.g. a machine) or even a 1706 * group of subjects (such as a document about a herd of farm animals, or a set 1707 * of patients that share a common exposure). 1708 */ 1709 @Child(name = "subject", type = { Patient.class, Practitioner.class, Group.class, 1710 Device.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1711 @Description(shortDefinition = "Who/what is the subject of the document", formalDefinition = "Who or what the document is about. The document can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure).") 1712 protected Reference subject; 1713 1714 /** 1715 * The actual object that is the target of the reference (Who or what the 1716 * document is about. The document can be about a person, (patient or healthcare 1717 * practitioner), a device (e.g. a machine) or even a group of subjects (such as 1718 * a document about a herd of farm animals, or a set of patients that share a 1719 * common exposure).) 1720 */ 1721 protected Resource subjectTarget; 1722 1723 /** 1724 * When the document reference was created. 1725 */ 1726 @Child(name = "date", type = { InstantType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1727 @Description(shortDefinition = "When this document reference was created", formalDefinition = "When the document reference was created.") 1728 protected InstantType date; 1729 1730 /** 1731 * Identifies who is responsible for adding the information to the document. 1732 */ 1733 @Child(name = "author", type = { Practitioner.class, PractitionerRole.class, Organization.class, Device.class, 1734 Patient.class, 1735 RelatedPerson.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1736 @Description(shortDefinition = "Who and/or what authored the document", formalDefinition = "Identifies who is responsible for adding the information to the document.") 1737 protected List<Reference> author; 1738 /** 1739 * The actual objects that are the target of the reference (Identifies who is 1740 * responsible for adding the information to the document.) 1741 */ 1742 protected List<Resource> authorTarget; 1743 1744 /** 1745 * Which person or organization authenticates that this document is valid. 1746 */ 1747 @Child(name = "authenticator", type = { Practitioner.class, PractitionerRole.class, 1748 Organization.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1749 @Description(shortDefinition = "Who/what authenticated the document", formalDefinition = "Which person or organization authenticates that this document is valid.") 1750 protected Reference authenticator; 1751 1752 /** 1753 * The actual object that is the target of the reference (Which person or 1754 * organization authenticates that this document is valid.) 1755 */ 1756 protected Resource authenticatorTarget; 1757 1758 /** 1759 * Identifies the organization or group who is responsible for ongoing 1760 * maintenance of and access to the document. 1761 */ 1762 @Child(name = "custodian", type = { 1763 Organization.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 1764 @Description(shortDefinition = "Organization which maintains the document", formalDefinition = "Identifies the organization or group who is responsible for ongoing maintenance of and access to the document.") 1765 protected Reference custodian; 1766 1767 /** 1768 * The actual object that is the target of the reference (Identifies the 1769 * organization or group who is responsible for ongoing maintenance of and 1770 * access to the document.) 1771 */ 1772 protected Organization custodianTarget; 1773 1774 /** 1775 * Relationships that this document has with other document references that 1776 * already exist. 1777 */ 1778 @Child(name = "relatesTo", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1779 @Description(shortDefinition = "Relationships to other documents", formalDefinition = "Relationships that this document has with other document references that already exist.") 1780 protected List<DocumentReferenceRelatesToComponent> relatesTo; 1781 1782 /** 1783 * Human-readable description of the source document. 1784 */ 1785 @Child(name = "description", type = { 1786 StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 1787 @Description(shortDefinition = "Human-readable description", formalDefinition = "Human-readable description of the source document.") 1788 protected StringType description; 1789 1790 /** 1791 * A set of Security-Tag codes specifying the level of privacy/security of the 1792 * Document. Note that DocumentReference.meta.security contains the security 1793 * labels of the "reference" to the document, while 1794 * DocumentReference.securityLabel contains a snapshot of the security labels on 1795 * the document the reference refers to. 1796 */ 1797 @Child(name = "securityLabel", type = { 1798 CodeableConcept.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1799 @Description(shortDefinition = "Document security-tags", formalDefinition = "A set of Security-Tag codes specifying the level of privacy/security of the Document. Note that DocumentReference.meta.security contains the security labels of the \"reference\" to the document, while DocumentReference.securityLabel contains a snapshot of the security labels on the document the reference refers to.") 1800 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/security-labels") 1801 protected List<CodeableConcept> securityLabel; 1802 1803 /** 1804 * The document and format referenced. There may be multiple content element 1805 * repetitions, each with a different format. 1806 */ 1807 @Child(name = "content", type = {}, order = 14, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1808 @Description(shortDefinition = "Document referenced", formalDefinition = "The document and format referenced. There may be multiple content element repetitions, each with a different format.") 1809 protected List<DocumentReferenceContentComponent> content; 1810 1811 /** 1812 * The clinical context in which the document was prepared. 1813 */ 1814 @Child(name = "context", type = {}, order = 15, min = 0, max = 1, modifier = false, summary = true) 1815 @Description(shortDefinition = "Clinical context of document", formalDefinition = "The clinical context in which the document was prepared.") 1816 protected DocumentReferenceContextComponent context; 1817 1818 private static final long serialVersionUID = 307086535L; 1819 1820 /** 1821 * Constructor 1822 */ 1823 public DocumentReference() { 1824 super(); 1825 } 1826 1827 /** 1828 * Constructor 1829 */ 1830 public DocumentReference(Enumeration<DocumentReferenceStatus> status) { 1831 super(); 1832 this.status = status; 1833 } 1834 1835 /** 1836 * @return {@link #masterIdentifier} (Document identifier as assigned by the 1837 * source of the document. This identifier is specific to this version 1838 * of the document. This unique identifier may be used elsewhere to 1839 * identify this version of the document.) 1840 */ 1841 public Identifier getMasterIdentifier() { 1842 if (this.masterIdentifier == null) 1843 if (Configuration.errorOnAutoCreate()) 1844 throw new Error("Attempt to auto-create DocumentReference.masterIdentifier"); 1845 else if (Configuration.doAutoCreate()) 1846 this.masterIdentifier = new Identifier(); // cc 1847 return this.masterIdentifier; 1848 } 1849 1850 public boolean hasMasterIdentifier() { 1851 return this.masterIdentifier != null && !this.masterIdentifier.isEmpty(); 1852 } 1853 1854 /** 1855 * @param value {@link #masterIdentifier} (Document identifier as assigned by 1856 * the source of the document. This identifier is specific to this 1857 * version of the document. This unique identifier may be used 1858 * elsewhere to identify this version of the document.) 1859 */ 1860 public DocumentReference setMasterIdentifier(Identifier value) { 1861 this.masterIdentifier = value; 1862 return this; 1863 } 1864 1865 /** 1866 * @return {@link #identifier} (Other identifiers associated with the document, 1867 * including version independent identifiers.) 1868 */ 1869 public List<Identifier> getIdentifier() { 1870 if (this.identifier == null) 1871 this.identifier = new ArrayList<Identifier>(); 1872 return this.identifier; 1873 } 1874 1875 /** 1876 * @return Returns a reference to <code>this</code> for easy method chaining 1877 */ 1878 public DocumentReference setIdentifier(List<Identifier> theIdentifier) { 1879 this.identifier = theIdentifier; 1880 return this; 1881 } 1882 1883 public boolean hasIdentifier() { 1884 if (this.identifier == null) 1885 return false; 1886 for (Identifier item : this.identifier) 1887 if (!item.isEmpty()) 1888 return true; 1889 return false; 1890 } 1891 1892 public Identifier addIdentifier() { // 3 1893 Identifier t = new Identifier(); 1894 if (this.identifier == null) 1895 this.identifier = new ArrayList<Identifier>(); 1896 this.identifier.add(t); 1897 return t; 1898 } 1899 1900 public DocumentReference addIdentifier(Identifier t) { // 3 1901 if (t == null) 1902 return this; 1903 if (this.identifier == null) 1904 this.identifier = new ArrayList<Identifier>(); 1905 this.identifier.add(t); 1906 return this; 1907 } 1908 1909 /** 1910 * @return The first repetition of repeating field {@link #identifier}, creating 1911 * it if it does not already exist 1912 */ 1913 public Identifier getIdentifierFirstRep() { 1914 if (getIdentifier().isEmpty()) { 1915 addIdentifier(); 1916 } 1917 return getIdentifier().get(0); 1918 } 1919 1920 /** 1921 * @return {@link #status} (The status of this document reference.). This is the 1922 * underlying object with id, value and extensions. The accessor 1923 * "getStatus" gives direct access to the value 1924 */ 1925 public Enumeration<DocumentReferenceStatus> getStatusElement() { 1926 if (this.status == null) 1927 if (Configuration.errorOnAutoCreate()) 1928 throw new Error("Attempt to auto-create DocumentReference.status"); 1929 else if (Configuration.doAutoCreate()) 1930 this.status = new Enumeration<DocumentReferenceStatus>(new DocumentReferenceStatusEnumFactory()); // bb 1931 return this.status; 1932 } 1933 1934 public boolean hasStatusElement() { 1935 return this.status != null && !this.status.isEmpty(); 1936 } 1937 1938 public boolean hasStatus() { 1939 return this.status != null && !this.status.isEmpty(); 1940 } 1941 1942 /** 1943 * @param value {@link #status} (The status of this document reference.). This 1944 * is the underlying object with id, value and extensions. The 1945 * accessor "getStatus" gives direct access to the value 1946 */ 1947 public DocumentReference setStatusElement(Enumeration<DocumentReferenceStatus> value) { 1948 this.status = value; 1949 return this; 1950 } 1951 1952 /** 1953 * @return The status of this document reference. 1954 */ 1955 public DocumentReferenceStatus getStatus() { 1956 return this.status == null ? null : this.status.getValue(); 1957 } 1958 1959 /** 1960 * @param value The status of this document reference. 1961 */ 1962 public DocumentReference setStatus(DocumentReferenceStatus value) { 1963 if (this.status == null) 1964 this.status = new Enumeration<DocumentReferenceStatus>(new DocumentReferenceStatusEnumFactory()); 1965 this.status.setValue(value); 1966 return this; 1967 } 1968 1969 /** 1970 * @return {@link #docStatus} (The status of the underlying document.). This is 1971 * the underlying object with id, value and extensions. The accessor 1972 * "getDocStatus" gives direct access to the value 1973 */ 1974 public Enumeration<ReferredDocumentStatus> getDocStatusElement() { 1975 if (this.docStatus == null) 1976 if (Configuration.errorOnAutoCreate()) 1977 throw new Error("Attempt to auto-create DocumentReference.docStatus"); 1978 else if (Configuration.doAutoCreate()) 1979 this.docStatus = new Enumeration<ReferredDocumentStatus>(new ReferredDocumentStatusEnumFactory()); // bb 1980 return this.docStatus; 1981 } 1982 1983 public boolean hasDocStatusElement() { 1984 return this.docStatus != null && !this.docStatus.isEmpty(); 1985 } 1986 1987 public boolean hasDocStatus() { 1988 return this.docStatus != null && !this.docStatus.isEmpty(); 1989 } 1990 1991 /** 1992 * @param value {@link #docStatus} (The status of the underlying document.). 1993 * This is the underlying object with id, value and extensions. The 1994 * accessor "getDocStatus" gives direct access to the value 1995 */ 1996 public DocumentReference setDocStatusElement(Enumeration<ReferredDocumentStatus> value) { 1997 this.docStatus = value; 1998 return this; 1999 } 2000 2001 /** 2002 * @return The status of the underlying document. 2003 */ 2004 public ReferredDocumentStatus getDocStatus() { 2005 return this.docStatus == null ? null : this.docStatus.getValue(); 2006 } 2007 2008 /** 2009 * @param value The status of the underlying document. 2010 */ 2011 public DocumentReference setDocStatus(ReferredDocumentStatus value) { 2012 if (value == null) 2013 this.docStatus = null; 2014 else { 2015 if (this.docStatus == null) 2016 this.docStatus = new Enumeration<ReferredDocumentStatus>(new ReferredDocumentStatusEnumFactory()); 2017 this.docStatus.setValue(value); 2018 } 2019 return this; 2020 } 2021 2022 /** 2023 * @return {@link #type} (Specifies the particular kind of document referenced 2024 * (e.g. History and Physical, Discharge Summary, Progress Note). This 2025 * usually equates to the purpose of making the document referenced.) 2026 */ 2027 public CodeableConcept getType() { 2028 if (this.type == null) 2029 if (Configuration.errorOnAutoCreate()) 2030 throw new Error("Attempt to auto-create DocumentReference.type"); 2031 else if (Configuration.doAutoCreate()) 2032 this.type = new CodeableConcept(); // cc 2033 return this.type; 2034 } 2035 2036 public boolean hasType() { 2037 return this.type != null && !this.type.isEmpty(); 2038 } 2039 2040 /** 2041 * @param value {@link #type} (Specifies the particular kind of document 2042 * referenced (e.g. History and Physical, Discharge Summary, 2043 * Progress Note). This usually equates to the purpose of making 2044 * the document referenced.) 2045 */ 2046 public DocumentReference setType(CodeableConcept value) { 2047 this.type = value; 2048 return this; 2049 } 2050 2051 /** 2052 * @return {@link #category} (A categorization for the type of document 2053 * referenced - helps for indexing and searching. This may be implied by 2054 * or derived from the code specified in the DocumentReference.type.) 2055 */ 2056 public List<CodeableConcept> getCategory() { 2057 if (this.category == null) 2058 this.category = new ArrayList<CodeableConcept>(); 2059 return this.category; 2060 } 2061 2062 /** 2063 * @return Returns a reference to <code>this</code> for easy method chaining 2064 */ 2065 public DocumentReference setCategory(List<CodeableConcept> theCategory) { 2066 this.category = theCategory; 2067 return this; 2068 } 2069 2070 public boolean hasCategory() { 2071 if (this.category == null) 2072 return false; 2073 for (CodeableConcept item : this.category) 2074 if (!item.isEmpty()) 2075 return true; 2076 return false; 2077 } 2078 2079 public CodeableConcept addCategory() { // 3 2080 CodeableConcept t = new CodeableConcept(); 2081 if (this.category == null) 2082 this.category = new ArrayList<CodeableConcept>(); 2083 this.category.add(t); 2084 return t; 2085 } 2086 2087 public DocumentReference addCategory(CodeableConcept t) { // 3 2088 if (t == null) 2089 return this; 2090 if (this.category == null) 2091 this.category = new ArrayList<CodeableConcept>(); 2092 this.category.add(t); 2093 return this; 2094 } 2095 2096 /** 2097 * @return The first repetition of repeating field {@link #category}, creating 2098 * it if it does not already exist 2099 */ 2100 public CodeableConcept getCategoryFirstRep() { 2101 if (getCategory().isEmpty()) { 2102 addCategory(); 2103 } 2104 return getCategory().get(0); 2105 } 2106 2107 /** 2108 * @return {@link #subject} (Who or what the document is about. The document can 2109 * be about a person, (patient or healthcare practitioner), a device 2110 * (e.g. a machine) or even a group of subjects (such as a document 2111 * about a herd of farm animals, or a set of patients that share a 2112 * common exposure).) 2113 */ 2114 public Reference getSubject() { 2115 if (this.subject == null) 2116 if (Configuration.errorOnAutoCreate()) 2117 throw new Error("Attempt to auto-create DocumentReference.subject"); 2118 else if (Configuration.doAutoCreate()) 2119 this.subject = new Reference(); // cc 2120 return this.subject; 2121 } 2122 2123 public boolean hasSubject() { 2124 return this.subject != null && !this.subject.isEmpty(); 2125 } 2126 2127 /** 2128 * @param value {@link #subject} (Who or what the document is about. The 2129 * document can be about a person, (patient or healthcare 2130 * practitioner), a device (e.g. a machine) or even a group of 2131 * subjects (such as a document about a herd of farm animals, or a 2132 * set of patients that share a common exposure).) 2133 */ 2134 public DocumentReference setSubject(Reference value) { 2135 this.subject = value; 2136 return this; 2137 } 2138 2139 /** 2140 * @return {@link #subject} The actual object that is the target of the 2141 * reference. The reference library doesn't populate this, but you can 2142 * use it to hold the resource if you resolve it. (Who or what the 2143 * document is about. The document can be about a person, (patient or 2144 * healthcare practitioner), a device (e.g. a machine) or even a group 2145 * of subjects (such as a document about a herd of farm animals, or a 2146 * set of patients that share a common exposure).) 2147 */ 2148 public Resource getSubjectTarget() { 2149 return this.subjectTarget; 2150 } 2151 2152 /** 2153 * @param value {@link #subject} The actual object that is the target of the 2154 * reference. The reference library doesn't use these, but you can 2155 * use it to hold the resource if you resolve it. (Who or what the 2156 * document is about. The document can be about a person, (patient 2157 * or healthcare practitioner), a device (e.g. a machine) or even a 2158 * group of subjects (such as a document about a herd of farm 2159 * animals, or a set of patients that share a common exposure).) 2160 */ 2161 public DocumentReference setSubjectTarget(Resource value) { 2162 this.subjectTarget = value; 2163 return this; 2164 } 2165 2166 /** 2167 * @return {@link #date} (When the document reference was created.). This is the 2168 * underlying object with id, value and extensions. The accessor 2169 * "getDate" gives direct access to the value 2170 */ 2171 public InstantType getDateElement() { 2172 if (this.date == null) 2173 if (Configuration.errorOnAutoCreate()) 2174 throw new Error("Attempt to auto-create DocumentReference.date"); 2175 else if (Configuration.doAutoCreate()) 2176 this.date = new InstantType(); // bb 2177 return this.date; 2178 } 2179 2180 public boolean hasDateElement() { 2181 return this.date != null && !this.date.isEmpty(); 2182 } 2183 2184 public boolean hasDate() { 2185 return this.date != null && !this.date.isEmpty(); 2186 } 2187 2188 /** 2189 * @param value {@link #date} (When the document reference was created.). This 2190 * is the underlying object with id, value and extensions. The 2191 * accessor "getDate" gives direct access to the value 2192 */ 2193 public DocumentReference setDateElement(InstantType value) { 2194 this.date = value; 2195 return this; 2196 } 2197 2198 /** 2199 * @return When the document reference was created. 2200 */ 2201 public Date getDate() { 2202 return this.date == null ? null : this.date.getValue(); 2203 } 2204 2205 /** 2206 * @param value When the document reference was created. 2207 */ 2208 public DocumentReference setDate(Date value) { 2209 if (value == null) 2210 this.date = null; 2211 else { 2212 if (this.date == null) 2213 this.date = new InstantType(); 2214 this.date.setValue(value); 2215 } 2216 return this; 2217 } 2218 2219 /** 2220 * @return {@link #author} (Identifies who is responsible for adding the 2221 * information to the document.) 2222 */ 2223 public List<Reference> getAuthor() { 2224 if (this.author == null) 2225 this.author = new ArrayList<Reference>(); 2226 return this.author; 2227 } 2228 2229 /** 2230 * @return Returns a reference to <code>this</code> for easy method chaining 2231 */ 2232 public DocumentReference setAuthor(List<Reference> theAuthor) { 2233 this.author = theAuthor; 2234 return this; 2235 } 2236 2237 public boolean hasAuthor() { 2238 if (this.author == null) 2239 return false; 2240 for (Reference item : this.author) 2241 if (!item.isEmpty()) 2242 return true; 2243 return false; 2244 } 2245 2246 public Reference addAuthor() { // 3 2247 Reference t = new Reference(); 2248 if (this.author == null) 2249 this.author = new ArrayList<Reference>(); 2250 this.author.add(t); 2251 return t; 2252 } 2253 2254 public DocumentReference addAuthor(Reference t) { // 3 2255 if (t == null) 2256 return this; 2257 if (this.author == null) 2258 this.author = new ArrayList<Reference>(); 2259 this.author.add(t); 2260 return this; 2261 } 2262 2263 /** 2264 * @return The first repetition of repeating field {@link #author}, creating it 2265 * if it does not already exist 2266 */ 2267 public Reference getAuthorFirstRep() { 2268 if (getAuthor().isEmpty()) { 2269 addAuthor(); 2270 } 2271 return getAuthor().get(0); 2272 } 2273 2274 /** 2275 * @deprecated Use Reference#setResource(IBaseResource) instead 2276 */ 2277 @Deprecated 2278 public List<Resource> getAuthorTarget() { 2279 if (this.authorTarget == null) 2280 this.authorTarget = new ArrayList<Resource>(); 2281 return this.authorTarget; 2282 } 2283 2284 /** 2285 * @return {@link #authenticator} (Which person or organization authenticates 2286 * that this document is valid.) 2287 */ 2288 public Reference getAuthenticator() { 2289 if (this.authenticator == null) 2290 if (Configuration.errorOnAutoCreate()) 2291 throw new Error("Attempt to auto-create DocumentReference.authenticator"); 2292 else if (Configuration.doAutoCreate()) 2293 this.authenticator = new Reference(); // cc 2294 return this.authenticator; 2295 } 2296 2297 public boolean hasAuthenticator() { 2298 return this.authenticator != null && !this.authenticator.isEmpty(); 2299 } 2300 2301 /** 2302 * @param value {@link #authenticator} (Which person or organization 2303 * authenticates that this document is valid.) 2304 */ 2305 public DocumentReference setAuthenticator(Reference value) { 2306 this.authenticator = value; 2307 return this; 2308 } 2309 2310 /** 2311 * @return {@link #authenticator} The actual object that is the target of the 2312 * reference. The reference library doesn't populate this, but you can 2313 * use it to hold the resource if you resolve it. (Which person or 2314 * organization authenticates that this document is valid.) 2315 */ 2316 public Resource getAuthenticatorTarget() { 2317 return this.authenticatorTarget; 2318 } 2319 2320 /** 2321 * @param value {@link #authenticator} The actual object that is the target of 2322 * the reference. The reference library doesn't use these, but you 2323 * can use it to hold the resource if you resolve it. (Which person 2324 * or organization authenticates that this document is valid.) 2325 */ 2326 public DocumentReference setAuthenticatorTarget(Resource value) { 2327 this.authenticatorTarget = value; 2328 return this; 2329 } 2330 2331 /** 2332 * @return {@link #custodian} (Identifies the organization or group who is 2333 * responsible for ongoing maintenance of and access to the document.) 2334 */ 2335 public Reference getCustodian() { 2336 if (this.custodian == null) 2337 if (Configuration.errorOnAutoCreate()) 2338 throw new Error("Attempt to auto-create DocumentReference.custodian"); 2339 else if (Configuration.doAutoCreate()) 2340 this.custodian = new Reference(); // cc 2341 return this.custodian; 2342 } 2343 2344 public boolean hasCustodian() { 2345 return this.custodian != null && !this.custodian.isEmpty(); 2346 } 2347 2348 /** 2349 * @param value {@link #custodian} (Identifies the organization or group who is 2350 * responsible for ongoing maintenance of and access to the 2351 * document.) 2352 */ 2353 public DocumentReference setCustodian(Reference value) { 2354 this.custodian = value; 2355 return this; 2356 } 2357 2358 /** 2359 * @return {@link #custodian} The actual object that is the target of the 2360 * reference. The reference library doesn't populate this, but you can 2361 * use it to hold the resource if you resolve it. (Identifies the 2362 * organization or group who is responsible for ongoing maintenance of 2363 * and access to the document.) 2364 */ 2365 public Organization getCustodianTarget() { 2366 if (this.custodianTarget == null) 2367 if (Configuration.errorOnAutoCreate()) 2368 throw new Error("Attempt to auto-create DocumentReference.custodian"); 2369 else if (Configuration.doAutoCreate()) 2370 this.custodianTarget = new Organization(); // aa 2371 return this.custodianTarget; 2372 } 2373 2374 /** 2375 * @param value {@link #custodian} The actual object that is the target of the 2376 * reference. The reference library doesn't use these, but you can 2377 * use it to hold the resource if you resolve it. (Identifies the 2378 * organization or group who is responsible for ongoing maintenance 2379 * of and access to the document.) 2380 */ 2381 public DocumentReference setCustodianTarget(Organization value) { 2382 this.custodianTarget = value; 2383 return this; 2384 } 2385 2386 /** 2387 * @return {@link #relatesTo} (Relationships that this document has with other 2388 * document references that already exist.) 2389 */ 2390 public List<DocumentReferenceRelatesToComponent> getRelatesTo() { 2391 if (this.relatesTo == null) 2392 this.relatesTo = new ArrayList<DocumentReferenceRelatesToComponent>(); 2393 return this.relatesTo; 2394 } 2395 2396 /** 2397 * @return Returns a reference to <code>this</code> for easy method chaining 2398 */ 2399 public DocumentReference setRelatesTo(List<DocumentReferenceRelatesToComponent> theRelatesTo) { 2400 this.relatesTo = theRelatesTo; 2401 return this; 2402 } 2403 2404 public boolean hasRelatesTo() { 2405 if (this.relatesTo == null) 2406 return false; 2407 for (DocumentReferenceRelatesToComponent item : this.relatesTo) 2408 if (!item.isEmpty()) 2409 return true; 2410 return false; 2411 } 2412 2413 public DocumentReferenceRelatesToComponent addRelatesTo() { // 3 2414 DocumentReferenceRelatesToComponent t = new DocumentReferenceRelatesToComponent(); 2415 if (this.relatesTo == null) 2416 this.relatesTo = new ArrayList<DocumentReferenceRelatesToComponent>(); 2417 this.relatesTo.add(t); 2418 return t; 2419 } 2420 2421 public DocumentReference addRelatesTo(DocumentReferenceRelatesToComponent t) { // 3 2422 if (t == null) 2423 return this; 2424 if (this.relatesTo == null) 2425 this.relatesTo = new ArrayList<DocumentReferenceRelatesToComponent>(); 2426 this.relatesTo.add(t); 2427 return this; 2428 } 2429 2430 /** 2431 * @return The first repetition of repeating field {@link #relatesTo}, creating 2432 * it if it does not already exist 2433 */ 2434 public DocumentReferenceRelatesToComponent getRelatesToFirstRep() { 2435 if (getRelatesTo().isEmpty()) { 2436 addRelatesTo(); 2437 } 2438 return getRelatesTo().get(0); 2439 } 2440 2441 /** 2442 * @return {@link #description} (Human-readable description of the source 2443 * document.). This is the underlying object with id, value and 2444 * extensions. The accessor "getDescription" gives direct access to the 2445 * value 2446 */ 2447 public StringType getDescriptionElement() { 2448 if (this.description == null) 2449 if (Configuration.errorOnAutoCreate()) 2450 throw new Error("Attempt to auto-create DocumentReference.description"); 2451 else if (Configuration.doAutoCreate()) 2452 this.description = new StringType(); // bb 2453 return this.description; 2454 } 2455 2456 public boolean hasDescriptionElement() { 2457 return this.description != null && !this.description.isEmpty(); 2458 } 2459 2460 public boolean hasDescription() { 2461 return this.description != null && !this.description.isEmpty(); 2462 } 2463 2464 /** 2465 * @param value {@link #description} (Human-readable description of the source 2466 * document.). This is the underlying object with id, value and 2467 * extensions. The accessor "getDescription" gives direct access to 2468 * the value 2469 */ 2470 public DocumentReference setDescriptionElement(StringType value) { 2471 this.description = value; 2472 return this; 2473 } 2474 2475 /** 2476 * @return Human-readable description of the source document. 2477 */ 2478 public String getDescription() { 2479 return this.description == null ? null : this.description.getValue(); 2480 } 2481 2482 /** 2483 * @param value Human-readable description of the source document. 2484 */ 2485 public DocumentReference setDescription(String value) { 2486 if (Utilities.noString(value)) 2487 this.description = null; 2488 else { 2489 if (this.description == null) 2490 this.description = new StringType(); 2491 this.description.setValue(value); 2492 } 2493 return this; 2494 } 2495 2496 /** 2497 * @return {@link #securityLabel} (A set of Security-Tag codes specifying the 2498 * level of privacy/security of the Document. Note that 2499 * DocumentReference.meta.security contains the security labels of the 2500 * "reference" to the document, while DocumentReference.securityLabel 2501 * contains a snapshot of the security labels on the document the 2502 * reference refers to.) 2503 */ 2504 public List<CodeableConcept> getSecurityLabel() { 2505 if (this.securityLabel == null) 2506 this.securityLabel = new ArrayList<CodeableConcept>(); 2507 return this.securityLabel; 2508 } 2509 2510 /** 2511 * @return Returns a reference to <code>this</code> for easy method chaining 2512 */ 2513 public DocumentReference setSecurityLabel(List<CodeableConcept> theSecurityLabel) { 2514 this.securityLabel = theSecurityLabel; 2515 return this; 2516 } 2517 2518 public boolean hasSecurityLabel() { 2519 if (this.securityLabel == null) 2520 return false; 2521 for (CodeableConcept item : this.securityLabel) 2522 if (!item.isEmpty()) 2523 return true; 2524 return false; 2525 } 2526 2527 public CodeableConcept addSecurityLabel() { // 3 2528 CodeableConcept t = new CodeableConcept(); 2529 if (this.securityLabel == null) 2530 this.securityLabel = new ArrayList<CodeableConcept>(); 2531 this.securityLabel.add(t); 2532 return t; 2533 } 2534 2535 public DocumentReference addSecurityLabel(CodeableConcept t) { // 3 2536 if (t == null) 2537 return this; 2538 if (this.securityLabel == null) 2539 this.securityLabel = new ArrayList<CodeableConcept>(); 2540 this.securityLabel.add(t); 2541 return this; 2542 } 2543 2544 /** 2545 * @return The first repetition of repeating field {@link #securityLabel}, 2546 * creating it if it does not already exist 2547 */ 2548 public CodeableConcept getSecurityLabelFirstRep() { 2549 if (getSecurityLabel().isEmpty()) { 2550 addSecurityLabel(); 2551 } 2552 return getSecurityLabel().get(0); 2553 } 2554 2555 /** 2556 * @return {@link #content} (The document and format referenced. There may be 2557 * multiple content element repetitions, each with a different format.) 2558 */ 2559 public List<DocumentReferenceContentComponent> getContent() { 2560 if (this.content == null) 2561 this.content = new ArrayList<DocumentReferenceContentComponent>(); 2562 return this.content; 2563 } 2564 2565 /** 2566 * @return Returns a reference to <code>this</code> for easy method chaining 2567 */ 2568 public DocumentReference setContent(List<DocumentReferenceContentComponent> theContent) { 2569 this.content = theContent; 2570 return this; 2571 } 2572 2573 public boolean hasContent() { 2574 if (this.content == null) 2575 return false; 2576 for (DocumentReferenceContentComponent item : this.content) 2577 if (!item.isEmpty()) 2578 return true; 2579 return false; 2580 } 2581 2582 public DocumentReferenceContentComponent addContent() { // 3 2583 DocumentReferenceContentComponent t = new DocumentReferenceContentComponent(); 2584 if (this.content == null) 2585 this.content = new ArrayList<DocumentReferenceContentComponent>(); 2586 this.content.add(t); 2587 return t; 2588 } 2589 2590 public DocumentReference addContent(DocumentReferenceContentComponent t) { // 3 2591 if (t == null) 2592 return this; 2593 if (this.content == null) 2594 this.content = new ArrayList<DocumentReferenceContentComponent>(); 2595 this.content.add(t); 2596 return this; 2597 } 2598 2599 /** 2600 * @return The first repetition of repeating field {@link #content}, creating it 2601 * if it does not already exist 2602 */ 2603 public DocumentReferenceContentComponent getContentFirstRep() { 2604 if (getContent().isEmpty()) { 2605 addContent(); 2606 } 2607 return getContent().get(0); 2608 } 2609 2610 /** 2611 * @return {@link #context} (The clinical context in which the document was 2612 * prepared.) 2613 */ 2614 public DocumentReferenceContextComponent getContext() { 2615 if (this.context == null) 2616 if (Configuration.errorOnAutoCreate()) 2617 throw new Error("Attempt to auto-create DocumentReference.context"); 2618 else if (Configuration.doAutoCreate()) 2619 this.context = new DocumentReferenceContextComponent(); // cc 2620 return this.context; 2621 } 2622 2623 public boolean hasContext() { 2624 return this.context != null && !this.context.isEmpty(); 2625 } 2626 2627 /** 2628 * @param value {@link #context} (The clinical context in which the document was 2629 * prepared.) 2630 */ 2631 public DocumentReference setContext(DocumentReferenceContextComponent value) { 2632 this.context = value; 2633 return this; 2634 } 2635 2636 protected void listChildren(List<Property> children) { 2637 super.listChildren(children); 2638 children.add(new Property("masterIdentifier", "Identifier", 2639 "Document identifier as assigned by the source of the document. This identifier is specific to this version of the document. This unique identifier may be used elsewhere to identify this version of the document.", 2640 0, 1, masterIdentifier)); 2641 children.add(new Property("identifier", "Identifier", 2642 "Other identifiers associated with the document, including version independent identifiers.", 0, 2643 java.lang.Integer.MAX_VALUE, identifier)); 2644 children.add(new Property("status", "code", "The status of this document reference.", 0, 1, status)); 2645 children.add(new Property("docStatus", "code", "The status of the underlying document.", 0, 1, docStatus)); 2646 children.add(new Property("type", "CodeableConcept", 2647 "Specifies the particular kind of document referenced (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the document referenced.", 2648 0, 1, type)); 2649 children.add(new Property("category", "CodeableConcept", 2650 "A categorization for the type of document referenced - helps for indexing and searching. This may be implied by or derived from the code specified in the DocumentReference.type.", 2651 0, java.lang.Integer.MAX_VALUE, category)); 2652 children.add(new Property("subject", "Reference(Patient|Practitioner|Group|Device)", 2653 "Who or what the document is about. The document can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure).", 2654 0, 1, subject)); 2655 children.add(new Property("date", "instant", "When the document reference was created.", 0, 1, date)); 2656 children.add( 2657 new Property("author", "Reference(Practitioner|PractitionerRole|Organization|Device|Patient|RelatedPerson)", 2658 "Identifies who is responsible for adding the information to the document.", 0, java.lang.Integer.MAX_VALUE, 2659 author)); 2660 children.add(new Property("authenticator", "Reference(Practitioner|PractitionerRole|Organization)", 2661 "Which person or organization authenticates that this document is valid.", 0, 1, authenticator)); 2662 children.add(new Property("custodian", "Reference(Organization)", 2663 "Identifies the organization or group who is responsible for ongoing maintenance of and access to the document.", 2664 0, 1, custodian)); 2665 children.add(new Property("relatesTo", "", 2666 "Relationships that this document has with other document references that already exist.", 0, 2667 java.lang.Integer.MAX_VALUE, relatesTo)); 2668 children.add( 2669 new Property("description", "string", "Human-readable description of the source document.", 0, 1, description)); 2670 children.add(new Property("securityLabel", "CodeableConcept", 2671 "A set of Security-Tag codes specifying the level of privacy/security of the Document. Note that DocumentReference.meta.security contains the security labels of the \"reference\" to the document, while DocumentReference.securityLabel contains a snapshot of the security labels on the document the reference refers to.", 2672 0, java.lang.Integer.MAX_VALUE, securityLabel)); 2673 children.add(new Property("content", "", 2674 "The document and format referenced. There may be multiple content element repetitions, each with a different format.", 2675 0, java.lang.Integer.MAX_VALUE, content)); 2676 children 2677 .add(new Property("context", "", "The clinical context in which the document was prepared.", 0, 1, context)); 2678 } 2679 2680 @Override 2681 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2682 switch (_hash) { 2683 case 243769515: 2684 /* masterIdentifier */ return new Property("masterIdentifier", "Identifier", 2685 "Document identifier as assigned by the source of the document. This identifier is specific to this version of the document. This unique identifier may be used elsewhere to identify this version of the document.", 2686 0, 1, masterIdentifier); 2687 case -1618432855: 2688 /* identifier */ return new Property("identifier", "Identifier", 2689 "Other identifiers associated with the document, including version independent identifiers.", 0, 2690 java.lang.Integer.MAX_VALUE, identifier); 2691 case -892481550: 2692 /* status */ return new Property("status", "code", "The status of this document reference.", 0, 1, status); 2693 case -23496886: 2694 /* docStatus */ return new Property("docStatus", "code", "The status of the underlying document.", 0, 1, 2695 docStatus); 2696 case 3575610: 2697 /* type */ return new Property("type", "CodeableConcept", 2698 "Specifies the particular kind of document referenced (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the document referenced.", 2699 0, 1, type); 2700 case 50511102: 2701 /* category */ return new Property("category", "CodeableConcept", 2702 "A categorization for the type of document referenced - helps for indexing and searching. This may be implied by or derived from the code specified in the DocumentReference.type.", 2703 0, java.lang.Integer.MAX_VALUE, category); 2704 case -1867885268: 2705 /* subject */ return new Property("subject", "Reference(Patient|Practitioner|Group|Device)", 2706 "Who or what the document is about. The document can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure).", 2707 0, 1, subject); 2708 case 3076014: 2709 /* date */ return new Property("date", "instant", "When the document reference was created.", 0, 1, date); 2710 case -1406328437: 2711 /* author */ return new Property("author", 2712 "Reference(Practitioner|PractitionerRole|Organization|Device|Patient|RelatedPerson)", 2713 "Identifies who is responsible for adding the information to the document.", 0, java.lang.Integer.MAX_VALUE, 2714 author); 2715 case 1815000435: 2716 /* authenticator */ return new Property("authenticator", "Reference(Practitioner|PractitionerRole|Organization)", 2717 "Which person or organization authenticates that this document is valid.", 0, 1, authenticator); 2718 case 1611297262: 2719 /* custodian */ return new Property("custodian", "Reference(Organization)", 2720 "Identifies the organization or group who is responsible for ongoing maintenance of and access to the document.", 2721 0, 1, custodian); 2722 case -7765931: 2723 /* relatesTo */ return new Property("relatesTo", "", 2724 "Relationships that this document has with other document references that already exist.", 0, 2725 java.lang.Integer.MAX_VALUE, relatesTo); 2726 case -1724546052: 2727 /* description */ return new Property("description", "string", 2728 "Human-readable description of the source document.", 0, 1, description); 2729 case -722296940: 2730 /* securityLabel */ return new Property("securityLabel", "CodeableConcept", 2731 "A set of Security-Tag codes specifying the level of privacy/security of the Document. Note that DocumentReference.meta.security contains the security labels of the \"reference\" to the document, while DocumentReference.securityLabel contains a snapshot of the security labels on the document the reference refers to.", 2732 0, java.lang.Integer.MAX_VALUE, securityLabel); 2733 case 951530617: 2734 /* content */ return new Property("content", "", 2735 "The document and format referenced. There may be multiple content element repetitions, each with a different format.", 2736 0, java.lang.Integer.MAX_VALUE, content); 2737 case 951530927: 2738 /* context */ return new Property("context", "", "The clinical context in which the document was prepared.", 0, 1, 2739 context); 2740 default: 2741 return super.getNamedProperty(_hash, _name, _checkValid); 2742 } 2743 2744 } 2745 2746 @Override 2747 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2748 switch (hash) { 2749 case 243769515: 2750 /* masterIdentifier */ return this.masterIdentifier == null ? new Base[0] : new Base[] { this.masterIdentifier }; // Identifier 2751 case -1618432855: 2752 /* identifier */ return this.identifier == null ? new Base[0] 2753 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2754 case -892481550: 2755 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<DocumentReferenceStatus> 2756 case -23496886: 2757 /* docStatus */ return this.docStatus == null ? new Base[0] : new Base[] { this.docStatus }; // Enumeration<ReferredDocumentStatus> 2758 case 3575610: 2759 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2760 case 50511102: 2761 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2762 case -1867885268: 2763 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2764 case 3076014: 2765 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // InstantType 2766 case -1406328437: 2767 /* author */ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // Reference 2768 case 1815000435: 2769 /* authenticator */ return this.authenticator == null ? new Base[0] : new Base[] { this.authenticator }; // Reference 2770 case 1611297262: 2771 /* custodian */ return this.custodian == null ? new Base[0] : new Base[] { this.custodian }; // Reference 2772 case -7765931: 2773 /* relatesTo */ return this.relatesTo == null ? new Base[0] 2774 : this.relatesTo.toArray(new Base[this.relatesTo.size()]); // DocumentReferenceRelatesToComponent 2775 case -1724546052: 2776 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 2777 case -722296940: 2778 /* securityLabel */ return this.securityLabel == null ? new Base[0] 2779 : this.securityLabel.toArray(new Base[this.securityLabel.size()]); // CodeableConcept 2780 case 951530617: 2781 /* content */ return this.content == null ? new Base[0] : this.content.toArray(new Base[this.content.size()]); // DocumentReferenceContentComponent 2782 case 951530927: 2783 /* context */ return this.context == null ? new Base[0] : new Base[] { this.context }; // DocumentReferenceContextComponent 2784 default: 2785 return super.getProperty(hash, name, checkValid); 2786 } 2787 2788 } 2789 2790 @Override 2791 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2792 switch (hash) { 2793 case 243769515: // masterIdentifier 2794 this.masterIdentifier = castToIdentifier(value); // Identifier 2795 return value; 2796 case -1618432855: // identifier 2797 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2798 return value; 2799 case -892481550: // status 2800 value = new DocumentReferenceStatusEnumFactory().fromType(castToCode(value)); 2801 this.status = (Enumeration) value; // Enumeration<DocumentReferenceStatus> 2802 return value; 2803 case -23496886: // docStatus 2804 value = new ReferredDocumentStatusEnumFactory().fromType(castToCode(value)); 2805 this.docStatus = (Enumeration) value; // Enumeration<ReferredDocumentStatus> 2806 return value; 2807 case 3575610: // type 2808 this.type = castToCodeableConcept(value); // CodeableConcept 2809 return value; 2810 case 50511102: // category 2811 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 2812 return value; 2813 case -1867885268: // subject 2814 this.subject = castToReference(value); // Reference 2815 return value; 2816 case 3076014: // date 2817 this.date = castToInstant(value); // InstantType 2818 return value; 2819 case -1406328437: // author 2820 this.getAuthor().add(castToReference(value)); // Reference 2821 return value; 2822 case 1815000435: // authenticator 2823 this.authenticator = castToReference(value); // Reference 2824 return value; 2825 case 1611297262: // custodian 2826 this.custodian = castToReference(value); // Reference 2827 return value; 2828 case -7765931: // relatesTo 2829 this.getRelatesTo().add((DocumentReferenceRelatesToComponent) value); // DocumentReferenceRelatesToComponent 2830 return value; 2831 case -1724546052: // description 2832 this.description = castToString(value); // StringType 2833 return value; 2834 case -722296940: // securityLabel 2835 this.getSecurityLabel().add(castToCodeableConcept(value)); // CodeableConcept 2836 return value; 2837 case 951530617: // content 2838 this.getContent().add((DocumentReferenceContentComponent) value); // DocumentReferenceContentComponent 2839 return value; 2840 case 951530927: // context 2841 this.context = (DocumentReferenceContextComponent) value; // DocumentReferenceContextComponent 2842 return value; 2843 default: 2844 return super.setProperty(hash, name, value); 2845 } 2846 2847 } 2848 2849 @Override 2850 public Base setProperty(String name, Base value) throws FHIRException { 2851 if (name.equals("masterIdentifier")) { 2852 this.masterIdentifier = castToIdentifier(value); // Identifier 2853 } else if (name.equals("identifier")) { 2854 this.getIdentifier().add(castToIdentifier(value)); 2855 } else if (name.equals("status")) { 2856 value = new DocumentReferenceStatusEnumFactory().fromType(castToCode(value)); 2857 this.status = (Enumeration) value; // Enumeration<DocumentReferenceStatus> 2858 } else if (name.equals("docStatus")) { 2859 value = new ReferredDocumentStatusEnumFactory().fromType(castToCode(value)); 2860 this.docStatus = (Enumeration) value; // Enumeration<ReferredDocumentStatus> 2861 } else if (name.equals("type")) { 2862 this.type = castToCodeableConcept(value); // CodeableConcept 2863 } else if (name.equals("category")) { 2864 this.getCategory().add(castToCodeableConcept(value)); 2865 } else if (name.equals("subject")) { 2866 this.subject = castToReference(value); // Reference 2867 } else if (name.equals("date")) { 2868 this.date = castToInstant(value); // InstantType 2869 } else if (name.equals("author")) { 2870 this.getAuthor().add(castToReference(value)); 2871 } else if (name.equals("authenticator")) { 2872 this.authenticator = castToReference(value); // Reference 2873 } else if (name.equals("custodian")) { 2874 this.custodian = castToReference(value); // Reference 2875 } else if (name.equals("relatesTo")) { 2876 this.getRelatesTo().add((DocumentReferenceRelatesToComponent) value); 2877 } else if (name.equals("description")) { 2878 this.description = castToString(value); // StringType 2879 } else if (name.equals("securityLabel")) { 2880 this.getSecurityLabel().add(castToCodeableConcept(value)); 2881 } else if (name.equals("content")) { 2882 this.getContent().add((DocumentReferenceContentComponent) value); 2883 } else if (name.equals("context")) { 2884 this.context = (DocumentReferenceContextComponent) value; // DocumentReferenceContextComponent 2885 } else 2886 return super.setProperty(name, value); 2887 return value; 2888 } 2889 2890 @Override 2891 public void removeChild(String name, Base value) throws FHIRException { 2892 if (name.equals("masterIdentifier")) { 2893 this.masterIdentifier = null; 2894 } else if (name.equals("identifier")) { 2895 this.getIdentifier().remove(castToIdentifier(value)); 2896 } else if (name.equals("status")) { 2897 this.status = null; 2898 } else if (name.equals("docStatus")) { 2899 this.docStatus = null; 2900 } else if (name.equals("type")) { 2901 this.type = null; 2902 } else if (name.equals("category")) { 2903 this.getCategory().remove(castToCodeableConcept(value)); 2904 } else if (name.equals("subject")) { 2905 this.subject = null; 2906 } else if (name.equals("date")) { 2907 this.date = null; 2908 } else if (name.equals("author")) { 2909 this.getAuthor().remove(castToReference(value)); 2910 } else if (name.equals("authenticator")) { 2911 this.authenticator = null; 2912 } else if (name.equals("custodian")) { 2913 this.custodian = null; 2914 } else if (name.equals("relatesTo")) { 2915 this.getRelatesTo().remove((DocumentReferenceRelatesToComponent) value); 2916 } else if (name.equals("description")) { 2917 this.description = null; 2918 } else if (name.equals("securityLabel")) { 2919 this.getSecurityLabel().remove(castToCodeableConcept(value)); 2920 } else if (name.equals("content")) { 2921 this.getContent().remove((DocumentReferenceContentComponent) value); 2922 } else if (name.equals("context")) { 2923 this.context = (DocumentReferenceContextComponent) value; // DocumentReferenceContextComponent 2924 } else 2925 super.removeChild(name, value); 2926 2927 } 2928 2929 @Override 2930 public Base makeProperty(int hash, String name) throws FHIRException { 2931 switch (hash) { 2932 case 243769515: 2933 return getMasterIdentifier(); 2934 case -1618432855: 2935 return addIdentifier(); 2936 case -892481550: 2937 return getStatusElement(); 2938 case -23496886: 2939 return getDocStatusElement(); 2940 case 3575610: 2941 return getType(); 2942 case 50511102: 2943 return addCategory(); 2944 case -1867885268: 2945 return getSubject(); 2946 case 3076014: 2947 return getDateElement(); 2948 case -1406328437: 2949 return addAuthor(); 2950 case 1815000435: 2951 return getAuthenticator(); 2952 case 1611297262: 2953 return getCustodian(); 2954 case -7765931: 2955 return addRelatesTo(); 2956 case -1724546052: 2957 return getDescriptionElement(); 2958 case -722296940: 2959 return addSecurityLabel(); 2960 case 951530617: 2961 return addContent(); 2962 case 951530927: 2963 return getContext(); 2964 default: 2965 return super.makeProperty(hash, name); 2966 } 2967 2968 } 2969 2970 @Override 2971 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2972 switch (hash) { 2973 case 243769515: 2974 /* masterIdentifier */ return new String[] { "Identifier" }; 2975 case -1618432855: 2976 /* identifier */ return new String[] { "Identifier" }; 2977 case -892481550: 2978 /* status */ return new String[] { "code" }; 2979 case -23496886: 2980 /* docStatus */ return new String[] { "code" }; 2981 case 3575610: 2982 /* type */ return new String[] { "CodeableConcept" }; 2983 case 50511102: 2984 /* category */ return new String[] { "CodeableConcept" }; 2985 case -1867885268: 2986 /* subject */ return new String[] { "Reference" }; 2987 case 3076014: 2988 /* date */ return new String[] { "instant" }; 2989 case -1406328437: 2990 /* author */ return new String[] { "Reference" }; 2991 case 1815000435: 2992 /* authenticator */ return new String[] { "Reference" }; 2993 case 1611297262: 2994 /* custodian */ return new String[] { "Reference" }; 2995 case -7765931: 2996 /* relatesTo */ return new String[] {}; 2997 case -1724546052: 2998 /* description */ return new String[] { "string" }; 2999 case -722296940: 3000 /* securityLabel */ return new String[] { "CodeableConcept" }; 3001 case 951530617: 3002 /* content */ return new String[] {}; 3003 case 951530927: 3004 /* context */ return new String[] {}; 3005 default: 3006 return super.getTypesForProperty(hash, name); 3007 } 3008 3009 } 3010 3011 @Override 3012 public Base addChild(String name) throws FHIRException { 3013 if (name.equals("masterIdentifier")) { 3014 this.masterIdentifier = new Identifier(); 3015 return this.masterIdentifier; 3016 } else if (name.equals("identifier")) { 3017 return addIdentifier(); 3018 } else if (name.equals("status")) { 3019 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.status"); 3020 } else if (name.equals("docStatus")) { 3021 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.docStatus"); 3022 } else if (name.equals("type")) { 3023 this.type = new CodeableConcept(); 3024 return this.type; 3025 } else if (name.equals("category")) { 3026 return addCategory(); 3027 } else if (name.equals("subject")) { 3028 this.subject = new Reference(); 3029 return this.subject; 3030 } else if (name.equals("date")) { 3031 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.date"); 3032 } else if (name.equals("author")) { 3033 return addAuthor(); 3034 } else if (name.equals("authenticator")) { 3035 this.authenticator = new Reference(); 3036 return this.authenticator; 3037 } else if (name.equals("custodian")) { 3038 this.custodian = new Reference(); 3039 return this.custodian; 3040 } else if (name.equals("relatesTo")) { 3041 return addRelatesTo(); 3042 } else if (name.equals("description")) { 3043 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.description"); 3044 } else if (name.equals("securityLabel")) { 3045 return addSecurityLabel(); 3046 } else if (name.equals("content")) { 3047 return addContent(); 3048 } else if (name.equals("context")) { 3049 this.context = new DocumentReferenceContextComponent(); 3050 return this.context; 3051 } else 3052 return super.addChild(name); 3053 } 3054 3055 public String fhirType() { 3056 return "DocumentReference"; 3057 3058 } 3059 3060 public DocumentReference copy() { 3061 DocumentReference dst = new DocumentReference(); 3062 copyValues(dst); 3063 return dst; 3064 } 3065 3066 public void copyValues(DocumentReference dst) { 3067 super.copyValues(dst); 3068 dst.masterIdentifier = masterIdentifier == null ? null : masterIdentifier.copy(); 3069 if (identifier != null) { 3070 dst.identifier = new ArrayList<Identifier>(); 3071 for (Identifier i : identifier) 3072 dst.identifier.add(i.copy()); 3073 } 3074 ; 3075 dst.status = status == null ? null : status.copy(); 3076 dst.docStatus = docStatus == null ? null : docStatus.copy(); 3077 dst.type = type == null ? null : type.copy(); 3078 if (category != null) { 3079 dst.category = new ArrayList<CodeableConcept>(); 3080 for (CodeableConcept i : category) 3081 dst.category.add(i.copy()); 3082 } 3083 ; 3084 dst.subject = subject == null ? null : subject.copy(); 3085 dst.date = date == null ? null : date.copy(); 3086 if (author != null) { 3087 dst.author = new ArrayList<Reference>(); 3088 for (Reference i : author) 3089 dst.author.add(i.copy()); 3090 } 3091 ; 3092 dst.authenticator = authenticator == null ? null : authenticator.copy(); 3093 dst.custodian = custodian == null ? null : custodian.copy(); 3094 if (relatesTo != null) { 3095 dst.relatesTo = new ArrayList<DocumentReferenceRelatesToComponent>(); 3096 for (DocumentReferenceRelatesToComponent i : relatesTo) 3097 dst.relatesTo.add(i.copy()); 3098 } 3099 ; 3100 dst.description = description == null ? null : description.copy(); 3101 if (securityLabel != null) { 3102 dst.securityLabel = new ArrayList<CodeableConcept>(); 3103 for (CodeableConcept i : securityLabel) 3104 dst.securityLabel.add(i.copy()); 3105 } 3106 ; 3107 if (content != null) { 3108 dst.content = new ArrayList<DocumentReferenceContentComponent>(); 3109 for (DocumentReferenceContentComponent i : content) 3110 dst.content.add(i.copy()); 3111 } 3112 ; 3113 dst.context = context == null ? null : context.copy(); 3114 } 3115 3116 protected DocumentReference typedCopy() { 3117 return copy(); 3118 } 3119 3120 @Override 3121 public boolean equalsDeep(Base other_) { 3122 if (!super.equalsDeep(other_)) 3123 return false; 3124 if (!(other_ instanceof DocumentReference)) 3125 return false; 3126 DocumentReference o = (DocumentReference) other_; 3127 return compareDeep(masterIdentifier, o.masterIdentifier, true) && compareDeep(identifier, o.identifier, true) 3128 && compareDeep(status, o.status, true) && compareDeep(docStatus, o.docStatus, true) 3129 && compareDeep(type, o.type, true) && compareDeep(category, o.category, true) 3130 && compareDeep(subject, o.subject, true) && compareDeep(date, o.date, true) 3131 && compareDeep(author, o.author, true) && compareDeep(authenticator, o.authenticator, true) 3132 && compareDeep(custodian, o.custodian, true) && compareDeep(relatesTo, o.relatesTo, true) 3133 && compareDeep(description, o.description, true) && compareDeep(securityLabel, o.securityLabel, true) 3134 && compareDeep(content, o.content, true) && compareDeep(context, o.context, true); 3135 } 3136 3137 @Override 3138 public boolean equalsShallow(Base other_) { 3139 if (!super.equalsShallow(other_)) 3140 return false; 3141 if (!(other_ instanceof DocumentReference)) 3142 return false; 3143 DocumentReference o = (DocumentReference) other_; 3144 return compareValues(status, o.status, true) && compareValues(docStatus, o.docStatus, true) 3145 && compareValues(date, o.date, true) && compareValues(description, o.description, true); 3146 } 3147 3148 public boolean isEmpty() { 3149 return super.isEmpty() 3150 && ca.uhn.fhir.util.ElementUtil.isEmpty(masterIdentifier, identifier, status, docStatus, type, category, 3151 subject, date, author, authenticator, custodian, relatesTo, description, securityLabel, content, context); 3152 } 3153 3154 @Override 3155 public ResourceType getResourceType() { 3156 return ResourceType.DocumentReference; 3157 } 3158 3159 /** 3160 * Search parameter: <b>date</b> 3161 * <p> 3162 * Description: <b>When this document reference was created</b><br> 3163 * Type: <b>date</b><br> 3164 * Path: <b>DocumentReference.date</b><br> 3165 * </p> 3166 */ 3167 @SearchParamDefinition(name = "date", path = "DocumentReference.date", description = "When this document reference was created", type = "date") 3168 public static final String SP_DATE = "date"; 3169 /** 3170 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3171 * <p> 3172 * Description: <b>When this document reference was created</b><br> 3173 * Type: <b>date</b><br> 3174 * Path: <b>DocumentReference.date</b><br> 3175 * </p> 3176 */ 3177 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3178 SP_DATE); 3179 3180 /** 3181 * Search parameter: <b>subject</b> 3182 * <p> 3183 * Description: <b>Who/what is the subject of the document</b><br> 3184 * Type: <b>reference</b><br> 3185 * Path: <b>DocumentReference.subject</b><br> 3186 * </p> 3187 */ 3188 @SearchParamDefinition(name = "subject", path = "DocumentReference.subject", description = "Who/what is the subject of the document", type = "reference", providesMembershipIn = { 3189 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 3190 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3191 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Device.class, Group.class, 3192 Patient.class, Practitioner.class }) 3193 public static final String SP_SUBJECT = "subject"; 3194 /** 3195 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3196 * <p> 3197 * Description: <b>Who/what is the subject of the document</b><br> 3198 * Type: <b>reference</b><br> 3199 * Path: <b>DocumentReference.subject</b><br> 3200 * </p> 3201 */ 3202 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3203 SP_SUBJECT); 3204 3205 /** 3206 * Constant for fluent queries to be used to add include statements. Specifies 3207 * the path value of "<b>DocumentReference:subject</b>". 3208 */ 3209 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 3210 "DocumentReference:subject").toLocked(); 3211 3212 /** 3213 * Search parameter: <b>description</b> 3214 * <p> 3215 * Description: <b>Human-readable description</b><br> 3216 * Type: <b>string</b><br> 3217 * Path: <b>DocumentReference.description</b><br> 3218 * </p> 3219 */ 3220 @SearchParamDefinition(name = "description", path = "DocumentReference.description", description = "Human-readable description", type = "string") 3221 public static final String SP_DESCRIPTION = "description"; 3222 /** 3223 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3224 * <p> 3225 * Description: <b>Human-readable description</b><br> 3226 * Type: <b>string</b><br> 3227 * Path: <b>DocumentReference.description</b><br> 3228 * </p> 3229 */ 3230 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 3231 SP_DESCRIPTION); 3232 3233 /** 3234 * Search parameter: <b>language</b> 3235 * <p> 3236 * Description: <b>Human language of the content (BCP-47)</b><br> 3237 * Type: <b>token</b><br> 3238 * Path: <b>DocumentReference.content.attachment.language</b><br> 3239 * </p> 3240 */ 3241 @SearchParamDefinition(name = "language", path = "DocumentReference.content.attachment.language", description = "Human language of the content (BCP-47)", type = "token") 3242 public static final String SP_LANGUAGE = "language"; 3243 /** 3244 * <b>Fluent Client</b> search parameter constant for <b>language</b> 3245 * <p> 3246 * Description: <b>Human language of the content (BCP-47)</b><br> 3247 * Type: <b>token</b><br> 3248 * Path: <b>DocumentReference.content.attachment.language</b><br> 3249 * </p> 3250 */ 3251 public static final ca.uhn.fhir.rest.gclient.TokenClientParam LANGUAGE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3252 SP_LANGUAGE); 3253 3254 /** 3255 * Search parameter: <b>type</b> 3256 * <p> 3257 * Description: <b>Kind of document (LOINC if possible)</b><br> 3258 * Type: <b>token</b><br> 3259 * Path: <b>DocumentReference.type</b><br> 3260 * </p> 3261 */ 3262 @SearchParamDefinition(name = "type", path = "DocumentReference.type", description = "Kind of document (LOINC if possible)", type = "token") 3263 public static final String SP_TYPE = "type"; 3264 /** 3265 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3266 * <p> 3267 * Description: <b>Kind of document (LOINC if possible)</b><br> 3268 * Type: <b>token</b><br> 3269 * Path: <b>DocumentReference.type</b><br> 3270 * </p> 3271 */ 3272 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3273 SP_TYPE); 3274 3275 /** 3276 * Search parameter: <b>relation</b> 3277 * <p> 3278 * Description: <b>replaces | transforms | signs | appends</b><br> 3279 * Type: <b>token</b><br> 3280 * Path: <b>DocumentReference.relatesTo.code</b><br> 3281 * </p> 3282 */ 3283 @SearchParamDefinition(name = "relation", path = "DocumentReference.relatesTo.code", description = "replaces | transforms | signs | appends", type = "token") 3284 public static final String SP_RELATION = "relation"; 3285 /** 3286 * <b>Fluent Client</b> search parameter constant for <b>relation</b> 3287 * <p> 3288 * Description: <b>replaces | transforms | signs | appends</b><br> 3289 * Type: <b>token</b><br> 3290 * Path: <b>DocumentReference.relatesTo.code</b><br> 3291 * </p> 3292 */ 3293 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RELATION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3294 SP_RELATION); 3295 3296 /** 3297 * Search parameter: <b>setting</b> 3298 * <p> 3299 * Description: <b>Additional details about where the content was created (e.g. 3300 * clinical specialty)</b><br> 3301 * Type: <b>token</b><br> 3302 * Path: <b>DocumentReference.context.practiceSetting</b><br> 3303 * </p> 3304 */ 3305 @SearchParamDefinition(name = "setting", path = "DocumentReference.context.practiceSetting", description = "Additional details about where the content was created (e.g. clinical specialty)", type = "token") 3306 public static final String SP_SETTING = "setting"; 3307 /** 3308 * <b>Fluent Client</b> search parameter constant for <b>setting</b> 3309 * <p> 3310 * Description: <b>Additional details about where the content was created (e.g. 3311 * clinical specialty)</b><br> 3312 * Type: <b>token</b><br> 3313 * Path: <b>DocumentReference.context.practiceSetting</b><br> 3314 * </p> 3315 */ 3316 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SETTING = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3317 SP_SETTING); 3318 3319 /** 3320 * Search parameter: <b>related</b> 3321 * <p> 3322 * Description: <b>Related identifiers or resources</b><br> 3323 * Type: <b>reference</b><br> 3324 * Path: <b>DocumentReference.context.related</b><br> 3325 * </p> 3326 */ 3327 @SearchParamDefinition(name = "related", path = "DocumentReference.context.related", description = "Related identifiers or resources", type = "reference") 3328 public static final String SP_RELATED = "related"; 3329 /** 3330 * <b>Fluent Client</b> search parameter constant for <b>related</b> 3331 * <p> 3332 * Description: <b>Related identifiers or resources</b><br> 3333 * Type: <b>reference</b><br> 3334 * Path: <b>DocumentReference.context.related</b><br> 3335 * </p> 3336 */ 3337 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RELATED = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3338 SP_RELATED); 3339 3340 /** 3341 * Constant for fluent queries to be used to add include statements. Specifies 3342 * the path value of "<b>DocumentReference:related</b>". 3343 */ 3344 public static final ca.uhn.fhir.model.api.Include INCLUDE_RELATED = new ca.uhn.fhir.model.api.Include( 3345 "DocumentReference:related").toLocked(); 3346 3347 /** 3348 * Search parameter: <b>patient</b> 3349 * <p> 3350 * Description: <b>Who/what is the subject of the document</b><br> 3351 * Type: <b>reference</b><br> 3352 * Path: <b>DocumentReference.subject</b><br> 3353 * </p> 3354 */ 3355 @SearchParamDefinition(name = "patient", path = "DocumentReference.subject.where(resolve() is Patient)", description = "Who/what is the subject of the document", type = "reference", target = { 3356 Patient.class }) 3357 public static final String SP_PATIENT = "patient"; 3358 /** 3359 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3360 * <p> 3361 * Description: <b>Who/what is the subject of the document</b><br> 3362 * Type: <b>reference</b><br> 3363 * Path: <b>DocumentReference.subject</b><br> 3364 * </p> 3365 */ 3366 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3367 SP_PATIENT); 3368 3369 /** 3370 * Constant for fluent queries to be used to add include statements. Specifies 3371 * the path value of "<b>DocumentReference:patient</b>". 3372 */ 3373 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 3374 "DocumentReference:patient").toLocked(); 3375 3376 /** 3377 * Search parameter: <b>relationship</b> 3378 * <p> 3379 * Description: <b>Combination of relation and relatesTo</b><br> 3380 * Type: <b>composite</b><br> 3381 * Path: <b></b><br> 3382 * </p> 3383 */ 3384 @SearchParamDefinition(name = "relationship", path = "DocumentReference.relatesTo", description = "Combination of relation and relatesTo", type = "composite", compositeOf = { 3385 "relatesto", "relation" }) 3386 public static final String SP_RELATIONSHIP = "relationship"; 3387 /** 3388 * <b>Fluent Client</b> search parameter constant for <b>relationship</b> 3389 * <p> 3390 * Description: <b>Combination of relation and relatesTo</b><br> 3391 * Type: <b>composite</b><br> 3392 * Path: <b></b><br> 3393 * </p> 3394 */ 3395 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.ReferenceClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> RELATIONSHIP = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.ReferenceClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 3396 SP_RELATIONSHIP); 3397 3398 /** 3399 * Search parameter: <b>event</b> 3400 * <p> 3401 * Description: <b>Main clinical acts documented</b><br> 3402 * Type: <b>token</b><br> 3403 * Path: <b>DocumentReference.context.event</b><br> 3404 * </p> 3405 */ 3406 @SearchParamDefinition(name = "event", path = "DocumentReference.context.event", description = "Main clinical acts documented", type = "token") 3407 public static final String SP_EVENT = "event"; 3408 /** 3409 * <b>Fluent Client</b> search parameter constant for <b>event</b> 3410 * <p> 3411 * Description: <b>Main clinical acts documented</b><br> 3412 * Type: <b>token</b><br> 3413 * Path: <b>DocumentReference.context.event</b><br> 3414 * </p> 3415 */ 3416 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EVENT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3417 SP_EVENT); 3418 3419 /** 3420 * Search parameter: <b>authenticator</b> 3421 * <p> 3422 * Description: <b>Who/what authenticated the document</b><br> 3423 * Type: <b>reference</b><br> 3424 * Path: <b>DocumentReference.authenticator</b><br> 3425 * </p> 3426 */ 3427 @SearchParamDefinition(name = "authenticator", path = "DocumentReference.authenticator", description = "Who/what authenticated the document", type = "reference", providesMembershipIn = { 3428 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 3429 Practitioner.class, PractitionerRole.class }) 3430 public static final String SP_AUTHENTICATOR = "authenticator"; 3431 /** 3432 * <b>Fluent Client</b> search parameter constant for <b>authenticator</b> 3433 * <p> 3434 * Description: <b>Who/what authenticated the document</b><br> 3435 * Type: <b>reference</b><br> 3436 * Path: <b>DocumentReference.authenticator</b><br> 3437 * </p> 3438 */ 3439 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHENTICATOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3440 SP_AUTHENTICATOR); 3441 3442 /** 3443 * Constant for fluent queries to be used to add include statements. Specifies 3444 * the path value of "<b>DocumentReference:authenticator</b>". 3445 */ 3446 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHENTICATOR = new ca.uhn.fhir.model.api.Include( 3447 "DocumentReference:authenticator").toLocked(); 3448 3449 /** 3450 * Search parameter: <b>identifier</b> 3451 * <p> 3452 * Description: <b>Master Version Specific Identifier</b><br> 3453 * Type: <b>token</b><br> 3454 * Path: <b>DocumentReference.masterIdentifier, 3455 * DocumentReference.identifier</b><br> 3456 * </p> 3457 */ 3458 @SearchParamDefinition(name = "identifier", path = "DocumentReference.masterIdentifier | DocumentReference.identifier", description = "Master Version Specific Identifier", type = "token") 3459 public static final String SP_IDENTIFIER = "identifier"; 3460 /** 3461 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3462 * <p> 3463 * Description: <b>Master Version Specific Identifier</b><br> 3464 * Type: <b>token</b><br> 3465 * Path: <b>DocumentReference.masterIdentifier, 3466 * DocumentReference.identifier</b><br> 3467 * </p> 3468 */ 3469 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3470 SP_IDENTIFIER); 3471 3472 /** 3473 * Search parameter: <b>period</b> 3474 * <p> 3475 * Description: <b>Time of service that is being documented</b><br> 3476 * Type: <b>date</b><br> 3477 * Path: <b>DocumentReference.context.period</b><br> 3478 * </p> 3479 */ 3480 @SearchParamDefinition(name = "period", path = "DocumentReference.context.period", description = "Time of service that is being documented", type = "date") 3481 public static final String SP_PERIOD = "period"; 3482 /** 3483 * <b>Fluent Client</b> search parameter constant for <b>period</b> 3484 * <p> 3485 * Description: <b>Time of service that is being documented</b><br> 3486 * Type: <b>date</b><br> 3487 * Path: <b>DocumentReference.context.period</b><br> 3488 * </p> 3489 */ 3490 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam( 3491 SP_PERIOD); 3492 3493 /** 3494 * Search parameter: <b>custodian</b> 3495 * <p> 3496 * Description: <b>Organization which maintains the document</b><br> 3497 * Type: <b>reference</b><br> 3498 * Path: <b>DocumentReference.custodian</b><br> 3499 * </p> 3500 */ 3501 @SearchParamDefinition(name = "custodian", path = "DocumentReference.custodian", description = "Organization which maintains the document", type = "reference", target = { 3502 Organization.class }) 3503 public static final String SP_CUSTODIAN = "custodian"; 3504 /** 3505 * <b>Fluent Client</b> search parameter constant for <b>custodian</b> 3506 * <p> 3507 * Description: <b>Organization which maintains the document</b><br> 3508 * Type: <b>reference</b><br> 3509 * Path: <b>DocumentReference.custodian</b><br> 3510 * </p> 3511 */ 3512 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CUSTODIAN = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3513 SP_CUSTODIAN); 3514 3515 /** 3516 * Constant for fluent queries to be used to add include statements. Specifies 3517 * the path value of "<b>DocumentReference:custodian</b>". 3518 */ 3519 public static final ca.uhn.fhir.model.api.Include INCLUDE_CUSTODIAN = new ca.uhn.fhir.model.api.Include( 3520 "DocumentReference:custodian").toLocked(); 3521 3522 /** 3523 * Search parameter: <b>author</b> 3524 * <p> 3525 * Description: <b>Who and/or what authored the document</b><br> 3526 * Type: <b>reference</b><br> 3527 * Path: <b>DocumentReference.author</b><br> 3528 * </p> 3529 */ 3530 @SearchParamDefinition(name = "author", path = "DocumentReference.author", description = "Who and/or what authored the document", type = "reference", providesMembershipIn = { 3531 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 3532 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3533 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 3534 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, 3535 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 3536 public static final String SP_AUTHOR = "author"; 3537 /** 3538 * <b>Fluent Client</b> search parameter constant for <b>author</b> 3539 * <p> 3540 * Description: <b>Who and/or what authored the document</b><br> 3541 * Type: <b>reference</b><br> 3542 * Path: <b>DocumentReference.author</b><br> 3543 * </p> 3544 */ 3545 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3546 SP_AUTHOR); 3547 3548 /** 3549 * Constant for fluent queries to be used to add include statements. Specifies 3550 * the path value of "<b>DocumentReference:author</b>". 3551 */ 3552 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include( 3553 "DocumentReference:author").toLocked(); 3554 3555 /** 3556 * Search parameter: <b>format</b> 3557 * <p> 3558 * Description: <b>Format/content rules for the document</b><br> 3559 * Type: <b>token</b><br> 3560 * Path: <b>DocumentReference.content.format</b><br> 3561 * </p> 3562 */ 3563 @SearchParamDefinition(name = "format", path = "DocumentReference.content.format", description = "Format/content rules for the document", type = "token") 3564 public static final String SP_FORMAT = "format"; 3565 /** 3566 * <b>Fluent Client</b> search parameter constant for <b>format</b> 3567 * <p> 3568 * Description: <b>Format/content rules for the document</b><br> 3569 * Type: <b>token</b><br> 3570 * Path: <b>DocumentReference.content.format</b><br> 3571 * </p> 3572 */ 3573 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FORMAT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3574 SP_FORMAT); 3575 3576 /** 3577 * Search parameter: <b>encounter</b> 3578 * <p> 3579 * Description: <b>Context of the document content</b><br> 3580 * Type: <b>reference</b><br> 3581 * Path: <b>DocumentReference.context.encounter</b><br> 3582 * </p> 3583 */ 3584 @SearchParamDefinition(name = "encounter", path = "DocumentReference.context.encounter", description = "Context of the document content", type = "reference", providesMembershipIn = { 3585 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class, 3586 EpisodeOfCare.class }) 3587 public static final String SP_ENCOUNTER = "encounter"; 3588 /** 3589 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 3590 * <p> 3591 * Description: <b>Context of the document content</b><br> 3592 * Type: <b>reference</b><br> 3593 * Path: <b>DocumentReference.context.encounter</b><br> 3594 * </p> 3595 */ 3596 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3597 SP_ENCOUNTER); 3598 3599 /** 3600 * Constant for fluent queries to be used to add include statements. Specifies 3601 * the path value of "<b>DocumentReference:encounter</b>". 3602 */ 3603 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 3604 "DocumentReference:encounter").toLocked(); 3605 3606 /** 3607 * Search parameter: <b>contenttype</b> 3608 * <p> 3609 * Description: <b>Mime type of the content, with charset etc.</b><br> 3610 * Type: <b>token</b><br> 3611 * Path: <b>DocumentReference.content.attachment.contentType</b><br> 3612 * </p> 3613 */ 3614 @SearchParamDefinition(name = "contenttype", path = "DocumentReference.content.attachment.contentType", description = "Mime type of the content, with charset etc.", type = "token") 3615 public static final String SP_CONTENTTYPE = "contenttype"; 3616 /** 3617 * <b>Fluent Client</b> search parameter constant for <b>contenttype</b> 3618 * <p> 3619 * Description: <b>Mime type of the content, with charset etc.</b><br> 3620 * Type: <b>token</b><br> 3621 * Path: <b>DocumentReference.content.attachment.contentType</b><br> 3622 * </p> 3623 */ 3624 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTENTTYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3625 SP_CONTENTTYPE); 3626 3627 /** 3628 * Search parameter: <b>security-label</b> 3629 * <p> 3630 * Description: <b>Document security-tags</b><br> 3631 * Type: <b>token</b><br> 3632 * Path: <b>DocumentReference.securityLabel</b><br> 3633 * </p> 3634 */ 3635 @SearchParamDefinition(name = "security-label", path = "DocumentReference.securityLabel", description = "Document security-tags", type = "token") 3636 public static final String SP_SECURITY_LABEL = "security-label"; 3637 /** 3638 * <b>Fluent Client</b> search parameter constant for <b>security-label</b> 3639 * <p> 3640 * Description: <b>Document security-tags</b><br> 3641 * Type: <b>token</b><br> 3642 * Path: <b>DocumentReference.securityLabel</b><br> 3643 * </p> 3644 */ 3645 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SECURITY_LABEL = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3646 SP_SECURITY_LABEL); 3647 3648 /** 3649 * Search parameter: <b>location</b> 3650 * <p> 3651 * Description: <b>Uri where the data can be found</b><br> 3652 * Type: <b>uri</b><br> 3653 * Path: <b>DocumentReference.content.attachment.url</b><br> 3654 * </p> 3655 */ 3656 @SearchParamDefinition(name = "location", path = "DocumentReference.content.attachment.url", description = "Uri where the data can be found", type = "uri") 3657 public static final String SP_LOCATION = "location"; 3658 /** 3659 * <b>Fluent Client</b> search parameter constant for <b>location</b> 3660 * <p> 3661 * Description: <b>Uri where the data can be found</b><br> 3662 * Type: <b>uri</b><br> 3663 * Path: <b>DocumentReference.content.attachment.url</b><br> 3664 * </p> 3665 */ 3666 public static final ca.uhn.fhir.rest.gclient.UriClientParam LOCATION = new ca.uhn.fhir.rest.gclient.UriClientParam( 3667 SP_LOCATION); 3668 3669 /** 3670 * Search parameter: <b>category</b> 3671 * <p> 3672 * Description: <b>Categorization of document</b><br> 3673 * Type: <b>token</b><br> 3674 * Path: <b>DocumentReference.category</b><br> 3675 * </p> 3676 */ 3677 @SearchParamDefinition(name = "category", path = "DocumentReference.category", description = "Categorization of document", type = "token") 3678 public static final String SP_CATEGORY = "category"; 3679 /** 3680 * <b>Fluent Client</b> search parameter constant for <b>category</b> 3681 * <p> 3682 * Description: <b>Categorization of document</b><br> 3683 * Type: <b>token</b><br> 3684 * Path: <b>DocumentReference.category</b><br> 3685 * </p> 3686 */ 3687 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3688 SP_CATEGORY); 3689 3690 /** 3691 * Search parameter: <b>relatesto</b> 3692 * <p> 3693 * Description: <b>Target of the relationship</b><br> 3694 * Type: <b>reference</b><br> 3695 * Path: <b>DocumentReference.relatesTo.target</b><br> 3696 * </p> 3697 */ 3698 @SearchParamDefinition(name = "relatesto", path = "DocumentReference.relatesTo.target", description = "Target of the relationship", type = "reference", target = { 3699 DocumentReference.class }) 3700 public static final String SP_RELATESTO = "relatesto"; 3701 /** 3702 * <b>Fluent Client</b> search parameter constant for <b>relatesto</b> 3703 * <p> 3704 * Description: <b>Target of the relationship</b><br> 3705 * Type: <b>reference</b><br> 3706 * Path: <b>DocumentReference.relatesTo.target</b><br> 3707 * </p> 3708 */ 3709 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RELATESTO = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3710 SP_RELATESTO); 3711 3712 /** 3713 * Constant for fluent queries to be used to add include statements. Specifies 3714 * the path value of "<b>DocumentReference:relatesto</b>". 3715 */ 3716 public static final ca.uhn.fhir.model.api.Include INCLUDE_RELATESTO = new ca.uhn.fhir.model.api.Include( 3717 "DocumentReference:relatesto").toLocked(); 3718 3719 /** 3720 * Search parameter: <b>facility</b> 3721 * <p> 3722 * Description: <b>Kind of facility where patient was seen</b><br> 3723 * Type: <b>token</b><br> 3724 * Path: <b>DocumentReference.context.facilityType</b><br> 3725 * </p> 3726 */ 3727 @SearchParamDefinition(name = "facility", path = "DocumentReference.context.facilityType", description = "Kind of facility where patient was seen", type = "token") 3728 public static final String SP_FACILITY = "facility"; 3729 /** 3730 * <b>Fluent Client</b> search parameter constant for <b>facility</b> 3731 * <p> 3732 * Description: <b>Kind of facility where patient was seen</b><br> 3733 * Type: <b>token</b><br> 3734 * Path: <b>DocumentReference.context.facilityType</b><br> 3735 * </p> 3736 */ 3737 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FACILITY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3738 SP_FACILITY); 3739 3740 /** 3741 * Search parameter: <b>status</b> 3742 * <p> 3743 * Description: <b>current | superseded | entered-in-error</b><br> 3744 * Type: <b>token</b><br> 3745 * Path: <b>DocumentReference.status</b><br> 3746 * </p> 3747 */ 3748 @SearchParamDefinition(name = "status", path = "DocumentReference.status", description = "current | superseded | entered-in-error", type = "token") 3749 public static final String SP_STATUS = "status"; 3750 /** 3751 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3752 * <p> 3753 * Description: <b>current | superseded | entered-in-error</b><br> 3754 * Type: <b>token</b><br> 3755 * Path: <b>DocumentReference.status</b><br> 3756 * </p> 3757 */ 3758 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3759 SP_STATUS); 3760 3761}