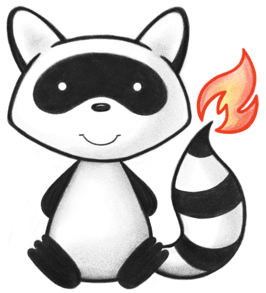
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Collections; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseHasExtensions; 039import org.hl7.fhir.instance.model.api.IBaseHasModifierExtensions; 040import org.hl7.fhir.instance.model.api.IDomainResource; 041 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044 045/** 046 * A resource that includes narrative, extensions, and contained resources. 047 */ 048public abstract class DomainResource extends Resource 049 implements IBaseHasExtensions, IBaseHasModifierExtensions, IDomainResource { 050 051 /** 052 * A human-readable narrative that contains a summary of the resource and can be 053 * used to represent the content of the resource to a human. The narrative need 054 * not encode all the structured data, but is required to contain sufficient 055 * detail to make it "clinically safe" for a human to just read the narrative. 056 * Resource definitions may define what content should be represented in the 057 * narrative to ensure clinical safety. 058 */ 059 @Child(name = "text", type = { Narrative.class }, order = 0, min = 0, max = 1, modifier = false, summary = false) 060 @Description(shortDefinition = "Text summary of the resource, for human interpretation", formalDefinition = "A human-readable narrative that contains a summary of the resource and can be used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative. Resource definitions may define what content should be represented in the narrative to ensure clinical safety.") 061 protected Narrative text; 062 063 /** 064 * These resources do not have an independent existence apart from the resource 065 * that contains them - they cannot be identified independently, and nor can 066 * they have their own independent transaction scope. 067 */ 068 @Child(name = "contained", type = { 069 Resource.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 070 @Description(shortDefinition = "Contained, inline Resources", formalDefinition = "These resources do not have an independent existence apart from the resource that contains them - they cannot be identified independently, and nor can they have their own independent transaction scope.") 071 protected List<Resource> contained; 072 073 /** 074 * May be used to represent additional information that is not part of the basic 075 * definition of the resource. To make the use of extensions safe and 076 * manageable, there is a strict set of governance applied to the definition and 077 * use of extensions. Though any implementer can define an extension, there is a 078 * set of requirements that SHALL be met as part of the definition of the 079 * extension. 080 */ 081 @Child(name = "extension", type = { 082 Extension.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 083 @Description(shortDefinition = "Additional content defined by implementations", formalDefinition = "May be used to represent additional information that is not part of the basic definition of the resource. To make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.") 084 protected List<Extension> extension; 085 086 /** 087 * May be used to represent additional information that is not part of the basic 088 * definition of the resource and that modifies the understanding of the element 089 * that contains it and/or the understanding of the containing element's 090 * descendants. Usually modifier elements provide negation or qualification. To 091 * make the use of extensions safe and manageable, there is a strict set of 092 * governance applied to the definition and use of extensions. Though any 093 * implementer is allowed to define an extension, there is a set of requirements 094 * that SHALL be met as part of the definition of the extension. Applications 095 * processing a resource are required to check for modifier extensions. 096 * 097 * Modifier extensions SHALL NOT change the meaning of any elements on Resource 098 * or DomainResource (including cannot change the meaning of modifierExtension 099 * itself). 100 */ 101 @Child(name = "modifierExtension", type = { 102 Extension.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = true, summary = false) 103 @Description(shortDefinition = "Extensions that cannot be ignored", formalDefinition = "May be used to represent additional information that is not part of the basic definition of the resource and that modifies the understanding of the element that contains it and/or the understanding of the containing element's descendants. Usually modifier elements provide negation or qualification. To make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.\n\nModifier extensions SHALL NOT change the meaning of any elements on Resource or DomainResource (including cannot change the meaning of modifierExtension itself).") 104 protected List<Extension> modifierExtension; 105 106 private static final long serialVersionUID = -970285559L; 107 108 /** 109 * Constructor 110 */ 111 public DomainResource() { 112 super(); 113 } 114 115 /** 116 * @return {@link #text} (A human-readable narrative that contains a summary of 117 * the resource and can be used to represent the content of the resource 118 * to a human. The narrative need not encode all the structured data, 119 * but is required to contain sufficient detail to make it "clinically 120 * safe" for a human to just read the narrative. Resource definitions 121 * may define what content should be represented in the narrative to 122 * ensure clinical safety.) 123 */ 124 public Narrative getText() { 125 if (this.text == null) 126 if (Configuration.errorOnAutoCreate()) 127 throw new Error("Attempt to auto-create DomainResource.text"); 128 else if (Configuration.doAutoCreate()) 129 this.text = new Narrative(); // cc 130 return this.text; 131 } 132 133 public boolean hasText() { 134 return this.text != null && !this.text.isEmpty(); 135 } 136 137 /** 138 * @param value {@link #text} (A human-readable narrative that contains a 139 * summary of the resource and can be used to represent the content 140 * of the resource to a human. The narrative need not encode all 141 * the structured data, but is required to contain sufficient 142 * detail to make it "clinically safe" for a human to just read the 143 * narrative. Resource definitions may define what content should 144 * be represented in the narrative to ensure clinical safety.) 145 */ 146 public DomainResource setText(Narrative value) { 147 this.text = value; 148 return this; 149 } 150 151 /** 152 * @return {@link #contained} (These resources do not have an independent 153 * existence apart from the resource that contains them - they cannot be 154 * identified independently, and nor can they have their own independent 155 * transaction scope.) 156 */ 157 public List<Resource> getContained() { 158 if (this.contained == null) 159 this.contained = new ArrayList<Resource>(); 160 return this.contained; 161 } 162 163 /** 164 * @return Returns a reference to <code>this</code> for easy method chaining 165 */ 166 public DomainResource setContained(List<Resource> theContained) { 167 this.contained = theContained; 168 return this; 169 } 170 171 public boolean hasContained() { 172 if (this.contained == null) 173 return false; 174 for (Resource item : this.contained) 175 if (!item.isEmpty()) 176 return true; 177 return false; 178 } 179 180 public DomainResource addContained(Resource t) { // 3 181 if (t == null) 182 return this; 183 if (this.contained == null) 184 this.contained = new ArrayList<Resource>(); 185 this.contained.add(t); 186 return this; 187 } 188 189 /** 190 * @return {@link #extension} (May be used to represent additional information 191 * that is not part of the basic definition of the resource. To make the 192 * use of extensions safe and manageable, there is a strict set of 193 * governance applied to the definition and use of extensions. Though 194 * any implementer can define an extension, there is a set of 195 * requirements that SHALL be met as part of the definition of the 196 * extension.) 197 */ 198 public List<Extension> getExtension() { 199 if (this.extension == null) 200 this.extension = new ArrayList<Extension>(); 201 return this.extension; 202 } 203 204 /** 205 * @return Returns a reference to <code>this</code> for easy method chaining 206 */ 207 public DomainResource setExtension(List<Extension> theExtension) { 208 this.extension = theExtension; 209 return this; 210 } 211 212 public boolean hasExtension() { 213 if (this.extension == null) 214 return false; 215 for (Extension item : this.extension) 216 if (!item.isEmpty()) 217 return true; 218 return false; 219 } 220 221 public Extension addExtension() { // 3 222 Extension t = new Extension(); 223 if (this.extension == null) 224 this.extension = new ArrayList<Extension>(); 225 this.extension.add(t); 226 return t; 227 } 228 229 public DomainResource addExtension(Extension t) { // 3 230 if (t == null) 231 return this; 232 if (this.extension == null) 233 this.extension = new ArrayList<Extension>(); 234 this.extension.add(t); 235 return this; 236 } 237 238 /** 239 * @return {@link #modifierExtension} (May be used to represent additional 240 * information that is not part of the basic definition of the resource 241 * and that modifies the understanding of the element that contains it 242 * and/or the understanding of the containing element's descendants. 243 * Usually modifier elements provide negation or qualification. To make 244 * the use of extensions safe and manageable, there is a strict set of 245 * governance applied to the definition and use of extensions. Though 246 * any implementer is allowed to define an extension, there is a set of 247 * requirements that SHALL be met as part of the definition of the 248 * extension. Applications processing a resource are required to check 249 * for modifier extensions. 250 * 251 * Modifier extensions SHALL NOT change the meaning of any elements on 252 * Resource or DomainResource (including cannot change the meaning of 253 * modifierExtension itself).) 254 */ 255 public List<Extension> getModifierExtension() { 256 if (this.modifierExtension == null) 257 this.modifierExtension = new ArrayList<Extension>(); 258 return this.modifierExtension; 259 } 260 261 /** 262 * @return Returns a reference to <code>this</code> for easy method chaining 263 */ 264 public DomainResource setModifierExtension(List<Extension> theModifierExtension) { 265 this.modifierExtension = theModifierExtension; 266 return this; 267 } 268 269 public boolean hasModifierExtension() { 270 if (this.modifierExtension == null) 271 return false; 272 for (Extension item : this.modifierExtension) 273 if (!item.isEmpty()) 274 return true; 275 return false; 276 } 277 278 public Extension addModifierExtension() { // 3 279 Extension t = new Extension(); 280 if (this.modifierExtension == null) 281 this.modifierExtension = new ArrayList<Extension>(); 282 this.modifierExtension.add(t); 283 return t; 284 } 285 286 public DomainResource addModifierExtension(Extension t) { // 3 287 if (t == null) 288 return this; 289 if (this.modifierExtension == null) 290 this.modifierExtension = new ArrayList<Extension>(); 291 this.modifierExtension.add(t); 292 return this; 293 } 294 295 /** 296 * Returns a list of extensions from this element which have the given URL. Note 297 * that this list may not be modified (you can not add or remove elements from 298 * it) 299 */ 300 public List<Extension> getExtensionsByUrl(String theUrl) { 301 org.apache.commons.lang3.Validate.notBlank(theUrl, "theUrl must be provided with a value"); 302 ArrayList<Extension> retVal = new ArrayList<Extension>(); 303 for (Extension next : getExtension()) { 304 if (theUrl.equals(next.getUrl())) { 305 retVal.add(next); 306 } 307 } 308 return Collections.unmodifiableList(retVal); 309 } 310 311 /** 312 * Returns a list of modifier extensions from this element which have the given 313 * URL. Note that this list may not be modified (you can not add or remove 314 * elements from it) 315 */ 316 public List<Extension> getModifierExtensionsByUrl(String theUrl) { 317 org.apache.commons.lang3.Validate.notBlank(theUrl, "theUrl must be provided with a value"); 318 ArrayList<Extension> retVal = new ArrayList<Extension>(); 319 for (Extension next : getModifierExtension()) { 320 if (theUrl.equals(next.getUrl())) { 321 retVal.add(next); 322 } 323 } 324 return Collections.unmodifiableList(retVal); 325 } 326 327 protected void listChildren(List<Property> children) { 328 super.listChildren(children); 329 children.add(new Property("text", "Narrative", 330 "A human-readable narrative that contains a summary of the resource and can be used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative. Resource definitions may define what content should be represented in the narrative to ensure clinical safety.", 331 0, 1, text)); 332 children.add(new Property("contained", "Resource", 333 "These resources do not have an independent existence apart from the resource that contains them - they cannot be identified independently, and nor can they have their own independent transaction scope.", 334 0, java.lang.Integer.MAX_VALUE, contained)); 335 children.add(new Property("extension", "Extension", 336 "May be used to represent additional information that is not part of the basic definition of the resource. To make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.", 337 0, java.lang.Integer.MAX_VALUE, extension)); 338 children.add(new Property("modifierExtension", "Extension", 339 "May be used to represent additional information that is not part of the basic definition of the resource and that modifies the understanding of the element that contains it and/or the understanding of the containing element's descendants. Usually modifier elements provide negation or qualification. To make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.\n\nModifier extensions SHALL NOT change the meaning of any elements on Resource or DomainResource (including cannot change the meaning of modifierExtension itself).", 340 0, java.lang.Integer.MAX_VALUE, modifierExtension)); 341 } 342 343 @Override 344 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 345 switch (_hash) { 346 case 3556653: 347 /* text */ return new Property("text", "Narrative", 348 "A human-readable narrative that contains a summary of the resource and can be used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative. Resource definitions may define what content should be represented in the narrative to ensure clinical safety.", 349 0, 1, text); 350 case -410956685: 351 /* contained */ return new Property("contained", "Resource", 352 "These resources do not have an independent existence apart from the resource that contains them - they cannot be identified independently, and nor can they have their own independent transaction scope.", 353 0, java.lang.Integer.MAX_VALUE, contained); 354 case -612557761: 355 /* extension */ return new Property("extension", "Extension", 356 "May be used to represent additional information that is not part of the basic definition of the resource. To make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.", 357 0, java.lang.Integer.MAX_VALUE, extension); 358 case -298878168: 359 /* modifierExtension */ return new Property("modifierExtension", "Extension", 360 "May be used to represent additional information that is not part of the basic definition of the resource and that modifies the understanding of the element that contains it and/or the understanding of the containing element's descendants. Usually modifier elements provide negation or qualification. To make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.\n\nModifier extensions SHALL NOT change the meaning of any elements on Resource or DomainResource (including cannot change the meaning of modifierExtension itself).", 361 0, java.lang.Integer.MAX_VALUE, modifierExtension); 362 default: 363 return super.getNamedProperty(_hash, _name, _checkValid); 364 } 365 366 } 367 368 @Override 369 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 370 switch (hash) { 371 case 3556653: 372 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // Narrative 373 case -410956685: 374 /* contained */ return this.contained == null ? new Base[0] 375 : this.contained.toArray(new Base[this.contained.size()]); // Resource 376 case -612557761: 377 /* extension */ return this.extension == null ? new Base[0] 378 : this.extension.toArray(new Base[this.extension.size()]); // Extension 379 case -298878168: 380 /* modifierExtension */ return this.modifierExtension == null ? new Base[0] 381 : this.modifierExtension.toArray(new Base[this.modifierExtension.size()]); // Extension 382 default: 383 return super.getProperty(hash, name, checkValid); 384 } 385 386 } 387 388 @Override 389 public Base setProperty(int hash, String name, Base value) throws FHIRException { 390 switch (hash) { 391 case 3556653: // text 392 this.text = castToNarrative(value); // Narrative 393 return value; 394 case -410956685: // contained 395 this.getContained().add(castToResource(value)); // Resource 396 return value; 397 case -612557761: // extension 398 this.getExtension().add(castToExtension(value)); // Extension 399 return value; 400 case -298878168: // modifierExtension 401 this.getModifierExtension().add(castToExtension(value)); // Extension 402 return value; 403 default: 404 return super.setProperty(hash, name, value); 405 } 406 407 } 408 409 @Override 410 public Base setProperty(String name, Base value) throws FHIRException { 411 if (name.equals("text")) { 412 this.text = castToNarrative(value); // Narrative 413 } else if (name.equals("contained")) { 414 this.getContained().add(castToResource(value)); 415 } else if (name.equals("extension")) { 416 this.getExtension().add(castToExtension(value)); 417 } else if (name.equals("modifierExtension")) { 418 this.getModifierExtension().add(castToExtension(value)); 419 } else 420 return super.setProperty(name, value); 421 return value; 422 } 423 424 @Override 425 public void removeChild(String name, Base value) throws FHIRException { 426 if (name.equals("text")) { 427 this.text = null; 428 } else if (name.equals("contained")) { 429 this.getContained().remove(castToResource(value)); 430 } else if (name.equals("extension")) { 431 this.getExtension().remove(castToExtension(value)); 432 } else if (name.equals("modifierExtension")) { 433 this.getModifierExtension().remove(castToExtension(value)); 434 } else 435 super.removeChild(name, value); 436 437 } 438 439 @Override 440 public Base makeProperty(int hash, String name) throws FHIRException { 441 switch (hash) { 442 case 3556653: 443 return getText(); 444 case -410956685: 445 throw new FHIRException("Cannot make property contained as it is not a complex type"); // Resource 446 case -612557761: 447 return addExtension(); 448 case -298878168: 449 return addModifierExtension(); 450 default: 451 return super.makeProperty(hash, name); 452 } 453 454 } 455 456 @Override 457 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 458 switch (hash) { 459 case 3556653: 460 /* text */ return new String[] { "Narrative" }; 461 case -410956685: 462 /* contained */ return new String[] { "Resource" }; 463 case -612557761: 464 /* extension */ return new String[] { "Extension" }; 465 case -298878168: 466 /* modifierExtension */ return new String[] { "Extension" }; 467 default: 468 return super.getTypesForProperty(hash, name); 469 } 470 471 } 472 473 @Override 474 public Base addChild(String name) throws FHIRException { 475 if (name.equals("text")) { 476 this.text = new Narrative(); 477 return this.text; 478 } else if (name.equals("contained")) { 479 throw new FHIRException("Cannot call addChild on an abstract type DomainResource.contained"); 480 } else if (name.equals("extension")) { 481 return addExtension(); 482 } else if (name.equals("modifierExtension")) { 483 return addModifierExtension(); 484 } else 485 return super.addChild(name); 486 } 487 488 public String fhirType() { 489 return "DomainResource"; 490 491 } 492 493 public abstract DomainResource copy(); 494 495 public void copyValues(DomainResource dst) { 496 super.copyValues(dst); 497 dst.text = text == null ? null : text.copy(); 498 if (contained != null) { 499 dst.contained = new ArrayList<Resource>(); 500 for (Resource i : contained) 501 dst.contained.add(i.copy()); 502 } 503 ; 504 if (extension != null) { 505 dst.extension = new ArrayList<Extension>(); 506 for (Extension i : extension) 507 dst.extension.add(i.copy()); 508 } 509 ; 510 if (modifierExtension != null) { 511 dst.modifierExtension = new ArrayList<Extension>(); 512 for (Extension i : modifierExtension) 513 dst.modifierExtension.add(i.copy()); 514 } 515 ; 516 } 517 518 @Override 519 public boolean equalsDeep(Base other_) { 520 if (!super.equalsDeep(other_)) 521 return false; 522 if (!(other_ instanceof DomainResource)) 523 return false; 524 DomainResource o = (DomainResource) other_; 525 return compareDeep(text, o.text, true) && compareDeep(contained, o.contained, true) 526 && compareDeep(extension, o.extension, true) && compareDeep(modifierExtension, o.modifierExtension, true); 527 } 528 529 @Override 530 public boolean equalsShallow(Base other_) { 531 if (!super.equalsShallow(other_)) 532 return false; 533 if (!(other_ instanceof DomainResource)) 534 return false; 535 DomainResource o = (DomainResource) other_; 536 return true; 537 } 538 539 public boolean isEmpty() { 540 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(text, contained, extension, modifierExtension); 541 } 542 543// added from java-adornments.txt: 544 545 public void checkNoModifiers(String noun, String verb) throws FHIRException { 546 if (hasModifierExtension()) { 547 throw new FHIRException("Found unknown Modifier Exceptions on " + noun + " doing " + verb); 548 } 549 550 } 551 552 public void addExtension(String url, Type value) { 553 Extension ex = new Extension(); 554 ex.setUrl(url); 555 ex.setValue(value); 556 getExtension().add(ex); 557 } 558 559 public boolean hasExtension(String url) { 560 for (Extension e : getExtension()) 561 if (url.equals(e.getUrl())) 562 return true; 563 return false; 564 } 565 566 public Extension getExtensionByUrl(String theUrl) { 567 org.apache.commons.lang3.Validate.notBlank(theUrl, "theUrl must not be blank or null"); 568 ArrayList<Extension> retVal = new ArrayList<Extension>(); 569 for (Extension next : getExtension()) { 570 if (theUrl.equals(next.getUrl())) { 571 retVal.add(next); 572 } 573 } 574 if (retVal.size() == 0) 575 return null; 576 else { 577 org.apache.commons.lang3.Validate.isTrue(retVal.size() == 1, "Url " + theUrl + " must have only one match"); 578 return retVal.get(0); 579 } 580 } 581 582 public Resource getContained(String reference) { 583 for (Resource c : getContained()) { 584 if (reference.equals("#"+c.getId())) { 585 return c; 586 } 587 } 588 return null; 589 } 590 591// end addition 592 593}