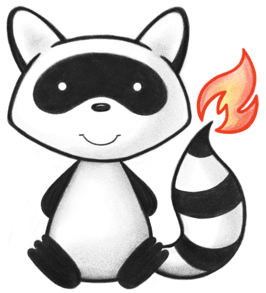
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 038import org.hl7.fhir.instance.model.api.ICompositeType; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.DatatypeDef; 044import ca.uhn.fhir.model.api.annotation.Description; 045 046/** 047 * Indicates how the medication is/was taken or should be taken by the patient. 048 */ 049@DatatypeDef(name = "Dosage") 050public class Dosage extends BackboneType implements ICompositeType { 051 052 @Block() 053 public static class DosageDoseAndRateComponent extends Element implements IBaseDatatypeElement { 054 /** 055 * The kind of dose or rate specified, for example, ordered or calculated. 056 */ 057 @Child(name = "type", type = { 058 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 059 @Description(shortDefinition = "The kind of dose or rate specified", formalDefinition = "The kind of dose or rate specified, for example, ordered or calculated.") 060 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/dose-rate-type") 061 protected CodeableConcept type; 062 063 /** 064 * Amount of medication per dose. 065 */ 066 @Child(name = "dose", type = { Range.class, 067 Quantity.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 068 @Description(shortDefinition = "Amount of medication per dose", formalDefinition = "Amount of medication per dose.") 069 protected Type dose; 070 071 /** 072 * Amount of medication per unit of time. 073 */ 074 @Child(name = "rate", type = { Ratio.class, Range.class, 075 Quantity.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 076 @Description(shortDefinition = "Amount of medication per unit of time", formalDefinition = "Amount of medication per unit of time.") 077 protected Type rate; 078 079 private static final long serialVersionUID = -2133698888L; 080 081 /** 082 * Constructor 083 */ 084 public DosageDoseAndRateComponent() { 085 super(); 086 } 087 088 /** 089 * @return {@link #type} (The kind of dose or rate specified, for example, 090 * ordered or calculated.) 091 */ 092 public CodeableConcept getType() { 093 if (this.type == null) 094 if (Configuration.errorOnAutoCreate()) 095 throw new Error("Attempt to auto-create DosageDoseAndRateComponent.type"); 096 else if (Configuration.doAutoCreate()) 097 this.type = new CodeableConcept(); // cc 098 return this.type; 099 } 100 101 public boolean hasType() { 102 return this.type != null && !this.type.isEmpty(); 103 } 104 105 /** 106 * @param value {@link #type} (The kind of dose or rate specified, for example, 107 * ordered or calculated.) 108 */ 109 public DosageDoseAndRateComponent setType(CodeableConcept value) { 110 this.type = value; 111 return this; 112 } 113 114 /** 115 * @return {@link #dose} (Amount of medication per dose.) 116 */ 117 public Type getDose() { 118 return this.dose; 119 } 120 121 /** 122 * @return {@link #dose} (Amount of medication per dose.) 123 */ 124 public Range getDoseRange() throws FHIRException { 125 if (this.dose == null) 126 this.dose = new Range(); 127 if (!(this.dose instanceof Range)) 128 throw new FHIRException( 129 "Type mismatch: the type Range was expected, but " + this.dose.getClass().getName() + " was encountered"); 130 return (Range) this.dose; 131 } 132 133 public boolean hasDoseRange() { 134 return this != null && this.dose instanceof Range; 135 } 136 137 /** 138 * @return {@link #dose} (Amount of medication per dose.) 139 */ 140 public Quantity getDoseQuantity() throws FHIRException { 141 if (this.dose == null) 142 this.dose = new Quantity(); 143 if (!(this.dose instanceof Quantity)) 144 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.dose.getClass().getName() 145 + " was encountered"); 146 return (Quantity) this.dose; 147 } 148 149 public boolean hasDoseQuantity() { 150 return this != null && this.dose instanceof Quantity; 151 } 152 153 public boolean hasDose() { 154 return this.dose != null && !this.dose.isEmpty(); 155 } 156 157 /** 158 * @param value {@link #dose} (Amount of medication per dose.) 159 */ 160 public DosageDoseAndRateComponent setDose(Type value) { 161 if (value != null && !(value instanceof Range || value instanceof Quantity)) 162 throw new Error("Not the right type for Dosage.doseAndRate.dose[x]: " + value.fhirType()); 163 this.dose = value; 164 return this; 165 } 166 167 /** 168 * @return {@link #rate} (Amount of medication per unit of time.) 169 */ 170 public Type getRate() { 171 return this.rate; 172 } 173 174 /** 175 * @return {@link #rate} (Amount of medication per unit of time.) 176 */ 177 public Ratio getRateRatio() throws FHIRException { 178 if (this.rate == null) 179 this.rate = new Ratio(); 180 if (!(this.rate instanceof Ratio)) 181 throw new FHIRException( 182 "Type mismatch: the type Ratio was expected, but " + this.rate.getClass().getName() + " was encountered"); 183 return (Ratio) this.rate; 184 } 185 186 public boolean hasRateRatio() { 187 return this != null && this.rate instanceof Ratio; 188 } 189 190 /** 191 * @return {@link #rate} (Amount of medication per unit of time.) 192 */ 193 public Range getRateRange() throws FHIRException { 194 if (this.rate == null) 195 this.rate = new Range(); 196 if (!(this.rate instanceof Range)) 197 throw new FHIRException( 198 "Type mismatch: the type Range was expected, but " + this.rate.getClass().getName() + " was encountered"); 199 return (Range) this.rate; 200 } 201 202 public boolean hasRateRange() { 203 return this != null && this.rate instanceof Range; 204 } 205 206 /** 207 * @return {@link #rate} (Amount of medication per unit of time.) 208 */ 209 public Quantity getRateQuantity() throws FHIRException { 210 if (this.rate == null) 211 this.rate = new Quantity(); 212 if (!(this.rate instanceof Quantity)) 213 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.rate.getClass().getName() 214 + " was encountered"); 215 return (Quantity) this.rate; 216 } 217 218 public boolean hasRateQuantity() { 219 return this != null && this.rate instanceof Quantity; 220 } 221 222 public boolean hasRate() { 223 return this.rate != null && !this.rate.isEmpty(); 224 } 225 226 /** 227 * @param value {@link #rate} (Amount of medication per unit of time.) 228 */ 229 public DosageDoseAndRateComponent setRate(Type value) { 230 if (value != null && !(value instanceof Ratio || value instanceof Range || value instanceof Quantity)) 231 throw new Error("Not the right type for Dosage.doseAndRate.rate[x]: " + value.fhirType()); 232 this.rate = value; 233 return this; 234 } 235 236 protected void listChildren(List<Property> children) { 237 super.listChildren(children); 238 children.add(new Property("type", "CodeableConcept", 239 "The kind of dose or rate specified, for example, ordered or calculated.", 0, 1, type)); 240 children.add(new Property("dose[x]", "Range|SimpleQuantity", "Amount of medication per dose.", 0, 1, dose)); 241 children.add( 242 new Property("rate[x]", "Ratio|Range|SimpleQuantity", "Amount of medication per unit of time.", 0, 1, rate)); 243 } 244 245 @Override 246 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 247 switch (_hash) { 248 case 3575610: 249 /* type */ return new Property("type", "CodeableConcept", 250 "The kind of dose or rate specified, for example, ordered or calculated.", 0, 1, type); 251 case 1843195715: 252 /* dose[x] */ return new Property("dose[x]", "Range|SimpleQuantity", "Amount of medication per dose.", 0, 1, 253 dose); 254 case 3089437: 255 /* dose */ return new Property("dose[x]", "Range|SimpleQuantity", "Amount of medication per dose.", 0, 1, dose); 256 case 1775578912: 257 /* doseRange */ return new Property("dose[x]", "Range|SimpleQuantity", "Amount of medication per dose.", 0, 1, 258 dose); 259 case -2083618872: 260 /* doseQuantity */ return new Property("dose[x]", "Range|SimpleQuantity", "Amount of medication per dose.", 0, 261 1, dose); 262 case 983460768: 263 /* rate[x] */ return new Property("rate[x]", "Ratio|Range|SimpleQuantity", 264 "Amount of medication per unit of time.", 0, 1, rate); 265 case 3493088: 266 /* rate */ return new Property("rate[x]", "Ratio|Range|SimpleQuantity", 267 "Amount of medication per unit of time.", 0, 1, rate); 268 case 204021515: 269 /* rateRatio */ return new Property("rate[x]", "Ratio|Range|SimpleQuantity", 270 "Amount of medication per unit of time.", 0, 1, rate); 271 case 204015677: 272 /* rateRange */ return new Property("rate[x]", "Ratio|Range|SimpleQuantity", 273 "Amount of medication per unit of time.", 0, 1, rate); 274 case -1085459061: 275 /* rateQuantity */ return new Property("rate[x]", "Ratio|Range|SimpleQuantity", 276 "Amount of medication per unit of time.", 0, 1, rate); 277 default: 278 return super.getNamedProperty(_hash, _name, _checkValid); 279 } 280 281 } 282 283 @Override 284 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 285 switch (hash) { 286 case 3575610: 287 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 288 case 3089437: 289 /* dose */ return this.dose == null ? new Base[0] : new Base[] { this.dose }; // Type 290 case 3493088: 291 /* rate */ return this.rate == null ? new Base[0] : new Base[] { this.rate }; // Type 292 default: 293 return super.getProperty(hash, name, checkValid); 294 } 295 296 } 297 298 @Override 299 public Base setProperty(int hash, String name, Base value) throws FHIRException { 300 switch (hash) { 301 case 3575610: // type 302 this.type = castToCodeableConcept(value); // CodeableConcept 303 return value; 304 case 3089437: // dose 305 this.dose = castToType(value); // Type 306 return value; 307 case 3493088: // rate 308 this.rate = castToType(value); // Type 309 return value; 310 default: 311 return super.setProperty(hash, name, value); 312 } 313 314 } 315 316 @Override 317 public Base setProperty(String name, Base value) throws FHIRException { 318 if (name.equals("type")) { 319 this.type = castToCodeableConcept(value); // CodeableConcept 320 } else if (name.equals("dose[x]")) { 321 this.dose = castToType(value); // Type 322 } else if (name.equals("rate[x]")) { 323 this.rate = castToType(value); // Type 324 } else 325 return super.setProperty(name, value); 326 return value; 327 } 328 329 @Override 330 public void removeChild(String name, Base value) throws FHIRException { 331 if (name.equals("type")) { 332 this.type = null; 333 } else if (name.equals("dose[x]")) { 334 this.dose = null; 335 } else if (name.equals("rate[x]")) { 336 this.rate = null; 337 } else 338 super.removeChild(name, value); 339 340 } 341 342 @Override 343 public Base makeProperty(int hash, String name) throws FHIRException { 344 switch (hash) { 345 case 3575610: 346 return getType(); 347 case 1843195715: 348 return getDose(); 349 case 3089437: 350 return getDose(); 351 case 983460768: 352 return getRate(); 353 case 3493088: 354 return getRate(); 355 default: 356 return super.makeProperty(hash, name); 357 } 358 359 } 360 361 @Override 362 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 363 switch (hash) { 364 case 3575610: 365 /* type */ return new String[] { "CodeableConcept" }; 366 case 3089437: 367 /* dose */ return new String[] { "Range", "SimpleQuantity" }; 368 case 3493088: 369 /* rate */ return new String[] { "Ratio", "Range", "SimpleQuantity" }; 370 default: 371 return super.getTypesForProperty(hash, name); 372 } 373 374 } 375 376 @Override 377 public Base addChild(String name) throws FHIRException { 378 if (name.equals("type")) { 379 this.type = new CodeableConcept(); 380 return this.type; 381 } else if (name.equals("doseRange")) { 382 this.dose = new Range(); 383 return this.dose; 384 } else if (name.equals("doseQuantity")) { 385 this.dose = new Quantity(); 386 return this.dose; 387 } else if (name.equals("rateRatio")) { 388 this.rate = new Ratio(); 389 return this.rate; 390 } else if (name.equals("rateRange")) { 391 this.rate = new Range(); 392 return this.rate; 393 } else if (name.equals("rateQuantity")) { 394 this.rate = new Quantity(); 395 return this.rate; 396 } else 397 return super.addChild(name); 398 } 399 400 public DosageDoseAndRateComponent copy() { 401 DosageDoseAndRateComponent dst = new DosageDoseAndRateComponent(); 402 copyValues(dst); 403 return dst; 404 } 405 406 public void copyValues(DosageDoseAndRateComponent dst) { 407 super.copyValues(dst); 408 dst.type = type == null ? null : type.copy(); 409 dst.dose = dose == null ? null : dose.copy(); 410 dst.rate = rate == null ? null : rate.copy(); 411 } 412 413 @Override 414 public boolean equalsDeep(Base other_) { 415 if (!super.equalsDeep(other_)) 416 return false; 417 if (!(other_ instanceof DosageDoseAndRateComponent)) 418 return false; 419 DosageDoseAndRateComponent o = (DosageDoseAndRateComponent) other_; 420 return compareDeep(type, o.type, true) && compareDeep(dose, o.dose, true) && compareDeep(rate, o.rate, true); 421 } 422 423 @Override 424 public boolean equalsShallow(Base other_) { 425 if (!super.equalsShallow(other_)) 426 return false; 427 if (!(other_ instanceof DosageDoseAndRateComponent)) 428 return false; 429 DosageDoseAndRateComponent o = (DosageDoseAndRateComponent) other_; 430 return true; 431 } 432 433 public boolean isEmpty() { 434 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, dose, rate); 435 } 436 437 public String fhirType() { 438 return "Dosage.doseAndRate"; 439 440 } 441 442 } 443 444 /** 445 * Indicates the order in which the dosage instructions should be applied or 446 * interpreted. 447 */ 448 @Child(name = "sequence", type = { IntegerType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 449 @Description(shortDefinition = "The order of the dosage instructions", formalDefinition = "Indicates the order in which the dosage instructions should be applied or interpreted.") 450 protected IntegerType sequence; 451 452 /** 453 * Free text dosage instructions e.g. SIG. 454 */ 455 @Child(name = "text", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 456 @Description(shortDefinition = "Free text dosage instructions e.g. SIG", formalDefinition = "Free text dosage instructions e.g. SIG.") 457 protected StringType text; 458 459 /** 460 * Supplemental instructions to the patient on how to take the medication (e.g. 461 * "with meals" or"take half to one hour before food") or warnings for the 462 * patient about the medication (e.g. "may cause drowsiness" or "avoid exposure 463 * of skin to direct sunlight or sunlamps"). 464 */ 465 @Child(name = "additionalInstruction", type = { 466 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 467 @Description(shortDefinition = "Supplemental instruction or warnings to the patient - e.g. \"with meals\", \"may cause drowsiness\"", formalDefinition = "Supplemental instructions to the patient on how to take the medication (e.g. \"with meals\" or\"take half to one hour before food\") or warnings for the patient about the medication (e.g. \"may cause drowsiness\" or \"avoid exposure of skin to direct sunlight or sunlamps\").") 468 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/additional-instruction-codes") 469 protected List<CodeableConcept> additionalInstruction; 470 471 /** 472 * Instructions in terms that are understood by the patient or consumer. 473 */ 474 @Child(name = "patientInstruction", type = { 475 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 476 @Description(shortDefinition = "Patient or consumer oriented instructions", formalDefinition = "Instructions in terms that are understood by the patient or consumer.") 477 protected StringType patientInstruction; 478 479 /** 480 * When medication should be administered. 481 */ 482 @Child(name = "timing", type = { Timing.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 483 @Description(shortDefinition = "When medication should be administered", formalDefinition = "When medication should be administered.") 484 protected Timing timing; 485 486 /** 487 * Indicates whether the Medication is only taken when needed within a specific 488 * dosing schedule (Boolean option), or it indicates the precondition for taking 489 * the Medication (CodeableConcept). 490 */ 491 @Child(name = "asNeeded", type = { BooleanType.class, 492 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 493 @Description(shortDefinition = "Take \"as needed\" (for x)", formalDefinition = "Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option), or it indicates the precondition for taking the Medication (CodeableConcept).") 494 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medication-as-needed-reason") 495 protected Type asNeeded; 496 497 /** 498 * Body site to administer to. 499 */ 500 @Child(name = "site", type = { CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 501 @Description(shortDefinition = "Body site to administer to", formalDefinition = "Body site to administer to.") 502 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/approach-site-codes") 503 protected CodeableConcept site; 504 505 /** 506 * How drug should enter body. 507 */ 508 @Child(name = "route", type = { 509 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 510 @Description(shortDefinition = "How drug should enter body", formalDefinition = "How drug should enter body.") 511 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/route-codes") 512 protected CodeableConcept route; 513 514 /** 515 * Technique for administering medication. 516 */ 517 @Child(name = "method", type = { 518 CodeableConcept.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 519 @Description(shortDefinition = "Technique for administering medication", formalDefinition = "Technique for administering medication.") 520 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/administration-method-codes") 521 protected CodeableConcept method; 522 523 /** 524 * The amount of medication administered. 525 */ 526 @Child(name = "doseAndRate", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 527 @Description(shortDefinition = "Amount of medication administered", formalDefinition = "The amount of medication administered.") 528 protected List<DosageDoseAndRateComponent> doseAndRate; 529 530 /** 531 * Upper limit on medication per unit of time. 532 */ 533 @Child(name = "maxDosePerPeriod", type = { 534 Ratio.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 535 @Description(shortDefinition = "Upper limit on medication per unit of time", formalDefinition = "Upper limit on medication per unit of time.") 536 protected Ratio maxDosePerPeriod; 537 538 /** 539 * Upper limit on medication per administration. 540 */ 541 @Child(name = "maxDosePerAdministration", type = { 542 Quantity.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 543 @Description(shortDefinition = "Upper limit on medication per administration", formalDefinition = "Upper limit on medication per administration.") 544 protected Quantity maxDosePerAdministration; 545 546 /** 547 * Upper limit on medication per lifetime of the patient. 548 */ 549 @Child(name = "maxDosePerLifetime", type = { 550 Quantity.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 551 @Description(shortDefinition = "Upper limit on medication per lifetime of the patient", formalDefinition = "Upper limit on medication per lifetime of the patient.") 552 protected Quantity maxDosePerLifetime; 553 554 private static final long serialVersionUID = -1095063329L; 555 556 /** 557 * Constructor 558 */ 559 public Dosage() { 560 super(); 561 } 562 563 /** 564 * @return {@link #sequence} (Indicates the order in which the dosage 565 * instructions should be applied or interpreted.). This is the 566 * underlying object with id, value and extensions. The accessor 567 * "getSequence" gives direct access to the value 568 */ 569 public IntegerType getSequenceElement() { 570 if (this.sequence == null) 571 if (Configuration.errorOnAutoCreate()) 572 throw new Error("Attempt to auto-create Dosage.sequence"); 573 else if (Configuration.doAutoCreate()) 574 this.sequence = new IntegerType(); // bb 575 return this.sequence; 576 } 577 578 public boolean hasSequenceElement() { 579 return this.sequence != null && !this.sequence.isEmpty(); 580 } 581 582 public boolean hasSequence() { 583 return this.sequence != null && !this.sequence.isEmpty(); 584 } 585 586 /** 587 * @param value {@link #sequence} (Indicates the order in which the dosage 588 * instructions should be applied or interpreted.). This is the 589 * underlying object with id, value and extensions. The accessor 590 * "getSequence" gives direct access to the value 591 */ 592 public Dosage setSequenceElement(IntegerType value) { 593 this.sequence = value; 594 return this; 595 } 596 597 /** 598 * @return Indicates the order in which the dosage instructions should be 599 * applied or interpreted. 600 */ 601 public int getSequence() { 602 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 603 } 604 605 /** 606 * @param value Indicates the order in which the dosage instructions should be 607 * applied or interpreted. 608 */ 609 public Dosage setSequence(int value) { 610 if (this.sequence == null) 611 this.sequence = new IntegerType(); 612 this.sequence.setValue(value); 613 return this; 614 } 615 616 /** 617 * @return {@link #text} (Free text dosage instructions e.g. SIG.). This is the 618 * underlying object with id, value and extensions. The accessor 619 * "getText" gives direct access to the value 620 */ 621 public StringType getTextElement() { 622 if (this.text == null) 623 if (Configuration.errorOnAutoCreate()) 624 throw new Error("Attempt to auto-create Dosage.text"); 625 else if (Configuration.doAutoCreate()) 626 this.text = new StringType(); // bb 627 return this.text; 628 } 629 630 public boolean hasTextElement() { 631 return this.text != null && !this.text.isEmpty(); 632 } 633 634 public boolean hasText() { 635 return this.text != null && !this.text.isEmpty(); 636 } 637 638 /** 639 * @param value {@link #text} (Free text dosage instructions e.g. SIG.). This is 640 * the underlying object with id, value and extensions. The 641 * accessor "getText" gives direct access to the value 642 */ 643 public Dosage setTextElement(StringType value) { 644 this.text = value; 645 return this; 646 } 647 648 /** 649 * @return Free text dosage instructions e.g. SIG. 650 */ 651 public String getText() { 652 return this.text == null ? null : this.text.getValue(); 653 } 654 655 /** 656 * @param value Free text dosage instructions e.g. SIG. 657 */ 658 public Dosage setText(String value) { 659 if (Utilities.noString(value)) 660 this.text = null; 661 else { 662 if (this.text == null) 663 this.text = new StringType(); 664 this.text.setValue(value); 665 } 666 return this; 667 } 668 669 /** 670 * @return {@link #additionalInstruction} (Supplemental instructions to the 671 * patient on how to take the medication (e.g. "with meals" or"take half 672 * to one hour before food") or warnings for the patient about the 673 * medication (e.g. "may cause drowsiness" or "avoid exposure of skin to 674 * direct sunlight or sunlamps").) 675 */ 676 public List<CodeableConcept> getAdditionalInstruction() { 677 if (this.additionalInstruction == null) 678 this.additionalInstruction = new ArrayList<CodeableConcept>(); 679 return this.additionalInstruction; 680 } 681 682 /** 683 * @return Returns a reference to <code>this</code> for easy method chaining 684 */ 685 public Dosage setAdditionalInstruction(List<CodeableConcept> theAdditionalInstruction) { 686 this.additionalInstruction = theAdditionalInstruction; 687 return this; 688 } 689 690 public boolean hasAdditionalInstruction() { 691 if (this.additionalInstruction == null) 692 return false; 693 for (CodeableConcept item : this.additionalInstruction) 694 if (!item.isEmpty()) 695 return true; 696 return false; 697 } 698 699 public CodeableConcept addAdditionalInstruction() { // 3 700 CodeableConcept t = new CodeableConcept(); 701 if (this.additionalInstruction == null) 702 this.additionalInstruction = new ArrayList<CodeableConcept>(); 703 this.additionalInstruction.add(t); 704 return t; 705 } 706 707 public Dosage addAdditionalInstruction(CodeableConcept t) { // 3 708 if (t == null) 709 return this; 710 if (this.additionalInstruction == null) 711 this.additionalInstruction = new ArrayList<CodeableConcept>(); 712 this.additionalInstruction.add(t); 713 return this; 714 } 715 716 /** 717 * @return The first repetition of repeating field 718 * {@link #additionalInstruction}, creating it if it does not already 719 * exist 720 */ 721 public CodeableConcept getAdditionalInstructionFirstRep() { 722 if (getAdditionalInstruction().isEmpty()) { 723 addAdditionalInstruction(); 724 } 725 return getAdditionalInstruction().get(0); 726 } 727 728 /** 729 * @return {@link #patientInstruction} (Instructions in terms that are 730 * understood by the patient or consumer.). This is the underlying 731 * object with id, value and extensions. The accessor 732 * "getPatientInstruction" gives direct access to the value 733 */ 734 public StringType getPatientInstructionElement() { 735 if (this.patientInstruction == null) 736 if (Configuration.errorOnAutoCreate()) 737 throw new Error("Attempt to auto-create Dosage.patientInstruction"); 738 else if (Configuration.doAutoCreate()) 739 this.patientInstruction = new StringType(); // bb 740 return this.patientInstruction; 741 } 742 743 public boolean hasPatientInstructionElement() { 744 return this.patientInstruction != null && !this.patientInstruction.isEmpty(); 745 } 746 747 public boolean hasPatientInstruction() { 748 return this.patientInstruction != null && !this.patientInstruction.isEmpty(); 749 } 750 751 /** 752 * @param value {@link #patientInstruction} (Instructions in terms that are 753 * understood by the patient or consumer.). This is the underlying 754 * object with id, value and extensions. The accessor 755 * "getPatientInstruction" gives direct access to the value 756 */ 757 public Dosage setPatientInstructionElement(StringType value) { 758 this.patientInstruction = value; 759 return this; 760 } 761 762 /** 763 * @return Instructions in terms that are understood by the patient or consumer. 764 */ 765 public String getPatientInstruction() { 766 return this.patientInstruction == null ? null : this.patientInstruction.getValue(); 767 } 768 769 /** 770 * @param value Instructions in terms that are understood by the patient or 771 * consumer. 772 */ 773 public Dosage setPatientInstruction(String value) { 774 if (Utilities.noString(value)) 775 this.patientInstruction = null; 776 else { 777 if (this.patientInstruction == null) 778 this.patientInstruction = new StringType(); 779 this.patientInstruction.setValue(value); 780 } 781 return this; 782 } 783 784 /** 785 * @return {@link #timing} (When medication should be administered.) 786 */ 787 public Timing getTiming() { 788 if (this.timing == null) 789 if (Configuration.errorOnAutoCreate()) 790 throw new Error("Attempt to auto-create Dosage.timing"); 791 else if (Configuration.doAutoCreate()) 792 this.timing = new Timing(); // cc 793 return this.timing; 794 } 795 796 public boolean hasTiming() { 797 return this.timing != null && !this.timing.isEmpty(); 798 } 799 800 /** 801 * @param value {@link #timing} (When medication should be administered.) 802 */ 803 public Dosage setTiming(Timing value) { 804 this.timing = value; 805 return this; 806 } 807 808 /** 809 * @return {@link #asNeeded} (Indicates whether the Medication is only taken 810 * when needed within a specific dosing schedule (Boolean option), or it 811 * indicates the precondition for taking the Medication 812 * (CodeableConcept).) 813 */ 814 public Type getAsNeeded() { 815 return this.asNeeded; 816 } 817 818 /** 819 * @return {@link #asNeeded} (Indicates whether the Medication is only taken 820 * when needed within a specific dosing schedule (Boolean option), or it 821 * indicates the precondition for taking the Medication 822 * (CodeableConcept).) 823 */ 824 public BooleanType getAsNeededBooleanType() throws FHIRException { 825 if (this.asNeeded == null) 826 this.asNeeded = new BooleanType(); 827 if (!(this.asNeeded instanceof BooleanType)) 828 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 829 + this.asNeeded.getClass().getName() + " was encountered"); 830 return (BooleanType) this.asNeeded; 831 } 832 833 public boolean hasAsNeededBooleanType() { 834 return this != null && this.asNeeded instanceof BooleanType; 835 } 836 837 /** 838 * @return {@link #asNeeded} (Indicates whether the Medication is only taken 839 * when needed within a specific dosing schedule (Boolean option), or it 840 * indicates the precondition for taking the Medication 841 * (CodeableConcept).) 842 */ 843 public CodeableConcept getAsNeededCodeableConcept() throws FHIRException { 844 if (this.asNeeded == null) 845 this.asNeeded = new CodeableConcept(); 846 if (!(this.asNeeded instanceof CodeableConcept)) 847 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 848 + this.asNeeded.getClass().getName() + " was encountered"); 849 return (CodeableConcept) this.asNeeded; 850 } 851 852 public boolean hasAsNeededCodeableConcept() { 853 return this != null && this.asNeeded instanceof CodeableConcept; 854 } 855 856 public boolean hasAsNeeded() { 857 return this.asNeeded != null && !this.asNeeded.isEmpty(); 858 } 859 860 /** 861 * @param value {@link #asNeeded} (Indicates whether the Medication is only 862 * taken when needed within a specific dosing schedule (Boolean 863 * option), or it indicates the precondition for taking the 864 * Medication (CodeableConcept).) 865 */ 866 public Dosage setAsNeeded(Type value) { 867 if (value != null && !(value instanceof BooleanType || value instanceof CodeableConcept)) 868 throw new Error("Not the right type for Dosage.asNeeded[x]: " + value.fhirType()); 869 this.asNeeded = value; 870 return this; 871 } 872 873 /** 874 * @return {@link #site} (Body site to administer to.) 875 */ 876 public CodeableConcept getSite() { 877 if (this.site == null) 878 if (Configuration.errorOnAutoCreate()) 879 throw new Error("Attempt to auto-create Dosage.site"); 880 else if (Configuration.doAutoCreate()) 881 this.site = new CodeableConcept(); // cc 882 return this.site; 883 } 884 885 public boolean hasSite() { 886 return this.site != null && !this.site.isEmpty(); 887 } 888 889 /** 890 * @param value {@link #site} (Body site to administer to.) 891 */ 892 public Dosage setSite(CodeableConcept value) { 893 this.site = value; 894 return this; 895 } 896 897 /** 898 * @return {@link #route} (How drug should enter body.) 899 */ 900 public CodeableConcept getRoute() { 901 if (this.route == null) 902 if (Configuration.errorOnAutoCreate()) 903 throw new Error("Attempt to auto-create Dosage.route"); 904 else if (Configuration.doAutoCreate()) 905 this.route = new CodeableConcept(); // cc 906 return this.route; 907 } 908 909 public boolean hasRoute() { 910 return this.route != null && !this.route.isEmpty(); 911 } 912 913 /** 914 * @param value {@link #route} (How drug should enter body.) 915 */ 916 public Dosage setRoute(CodeableConcept value) { 917 this.route = value; 918 return this; 919 } 920 921 /** 922 * @return {@link #method} (Technique for administering medication.) 923 */ 924 public CodeableConcept getMethod() { 925 if (this.method == null) 926 if (Configuration.errorOnAutoCreate()) 927 throw new Error("Attempt to auto-create Dosage.method"); 928 else if (Configuration.doAutoCreate()) 929 this.method = new CodeableConcept(); // cc 930 return this.method; 931 } 932 933 public boolean hasMethod() { 934 return this.method != null && !this.method.isEmpty(); 935 } 936 937 /** 938 * @param value {@link #method} (Technique for administering medication.) 939 */ 940 public Dosage setMethod(CodeableConcept value) { 941 this.method = value; 942 return this; 943 } 944 945 /** 946 * @return {@link #doseAndRate} (The amount of medication administered.) 947 */ 948 public List<DosageDoseAndRateComponent> getDoseAndRate() { 949 if (this.doseAndRate == null) 950 this.doseAndRate = new ArrayList<DosageDoseAndRateComponent>(); 951 return this.doseAndRate; 952 } 953 954 /** 955 * @return Returns a reference to <code>this</code> for easy method chaining 956 */ 957 public Dosage setDoseAndRate(List<DosageDoseAndRateComponent> theDoseAndRate) { 958 this.doseAndRate = theDoseAndRate; 959 return this; 960 } 961 962 public boolean hasDoseAndRate() { 963 if (this.doseAndRate == null) 964 return false; 965 for (DosageDoseAndRateComponent item : this.doseAndRate) 966 if (!item.isEmpty()) 967 return true; 968 return false; 969 } 970 971 public DosageDoseAndRateComponent addDoseAndRate() { // 3 972 DosageDoseAndRateComponent t = new DosageDoseAndRateComponent(); 973 if (this.doseAndRate == null) 974 this.doseAndRate = new ArrayList<DosageDoseAndRateComponent>(); 975 this.doseAndRate.add(t); 976 return t; 977 } 978 979 public Dosage addDoseAndRate(DosageDoseAndRateComponent t) { // 3 980 if (t == null) 981 return this; 982 if (this.doseAndRate == null) 983 this.doseAndRate = new ArrayList<DosageDoseAndRateComponent>(); 984 this.doseAndRate.add(t); 985 return this; 986 } 987 988 /** 989 * @return The first repetition of repeating field {@link #doseAndRate}, 990 * creating it if it does not already exist 991 */ 992 public DosageDoseAndRateComponent getDoseAndRateFirstRep() { 993 if (getDoseAndRate().isEmpty()) { 994 addDoseAndRate(); 995 } 996 return getDoseAndRate().get(0); 997 } 998 999 /** 1000 * @return {@link #maxDosePerPeriod} (Upper limit on medication per unit of 1001 * time.) 1002 */ 1003 public Ratio getMaxDosePerPeriod() { 1004 if (this.maxDosePerPeriod == null) 1005 if (Configuration.errorOnAutoCreate()) 1006 throw new Error("Attempt to auto-create Dosage.maxDosePerPeriod"); 1007 else if (Configuration.doAutoCreate()) 1008 this.maxDosePerPeriod = new Ratio(); // cc 1009 return this.maxDosePerPeriod; 1010 } 1011 1012 public boolean hasMaxDosePerPeriod() { 1013 return this.maxDosePerPeriod != null && !this.maxDosePerPeriod.isEmpty(); 1014 } 1015 1016 /** 1017 * @param value {@link #maxDosePerPeriod} (Upper limit on medication per unit of 1018 * time.) 1019 */ 1020 public Dosage setMaxDosePerPeriod(Ratio value) { 1021 this.maxDosePerPeriod = value; 1022 return this; 1023 } 1024 1025 /** 1026 * @return {@link #maxDosePerAdministration} (Upper limit on medication per 1027 * administration.) 1028 */ 1029 public Quantity getMaxDosePerAdministration() { 1030 if (this.maxDosePerAdministration == null) 1031 if (Configuration.errorOnAutoCreate()) 1032 throw new Error("Attempt to auto-create Dosage.maxDosePerAdministration"); 1033 else if (Configuration.doAutoCreate()) 1034 this.maxDosePerAdministration = new Quantity(); // cc 1035 return this.maxDosePerAdministration; 1036 } 1037 1038 public boolean hasMaxDosePerAdministration() { 1039 return this.maxDosePerAdministration != null && !this.maxDosePerAdministration.isEmpty(); 1040 } 1041 1042 /** 1043 * @param value {@link #maxDosePerAdministration} (Upper limit on medication per 1044 * administration.) 1045 */ 1046 public Dosage setMaxDosePerAdministration(Quantity value) { 1047 this.maxDosePerAdministration = value; 1048 return this; 1049 } 1050 1051 /** 1052 * @return {@link #maxDosePerLifetime} (Upper limit on medication per lifetime 1053 * of the patient.) 1054 */ 1055 public Quantity getMaxDosePerLifetime() { 1056 if (this.maxDosePerLifetime == null) 1057 if (Configuration.errorOnAutoCreate()) 1058 throw new Error("Attempt to auto-create Dosage.maxDosePerLifetime"); 1059 else if (Configuration.doAutoCreate()) 1060 this.maxDosePerLifetime = new Quantity(); // cc 1061 return this.maxDosePerLifetime; 1062 } 1063 1064 public boolean hasMaxDosePerLifetime() { 1065 return this.maxDosePerLifetime != null && !this.maxDosePerLifetime.isEmpty(); 1066 } 1067 1068 /** 1069 * @param value {@link #maxDosePerLifetime} (Upper limit on medication per 1070 * lifetime of the patient.) 1071 */ 1072 public Dosage setMaxDosePerLifetime(Quantity value) { 1073 this.maxDosePerLifetime = value; 1074 return this; 1075 } 1076 1077 protected void listChildren(List<Property> children) { 1078 super.listChildren(children); 1079 children.add(new Property("sequence", "integer", 1080 "Indicates the order in which the dosage instructions should be applied or interpreted.", 0, 1, sequence)); 1081 children.add(new Property("text", "string", "Free text dosage instructions e.g. SIG.", 0, 1, text)); 1082 children.add(new Property("additionalInstruction", "CodeableConcept", 1083 "Supplemental instructions to the patient on how to take the medication (e.g. \"with meals\" or\"take half to one hour before food\") or warnings for the patient about the medication (e.g. \"may cause drowsiness\" or \"avoid exposure of skin to direct sunlight or sunlamps\").", 1084 0, java.lang.Integer.MAX_VALUE, additionalInstruction)); 1085 children.add(new Property("patientInstruction", "string", 1086 "Instructions in terms that are understood by the patient or consumer.", 0, 1, patientInstruction)); 1087 children.add(new Property("timing", "Timing", "When medication should be administered.", 0, 1, timing)); 1088 children.add(new Property("asNeeded[x]", "boolean|CodeableConcept", 1089 "Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option), or it indicates the precondition for taking the Medication (CodeableConcept).", 1090 0, 1, asNeeded)); 1091 children.add(new Property("site", "CodeableConcept", "Body site to administer to.", 0, 1, site)); 1092 children.add(new Property("route", "CodeableConcept", "How drug should enter body.", 0, 1, route)); 1093 children.add(new Property("method", "CodeableConcept", "Technique for administering medication.", 0, 1, method)); 1094 children.add(new Property("doseAndRate", "", "The amount of medication administered.", 0, 1095 java.lang.Integer.MAX_VALUE, doseAndRate)); 1096 children.add(new Property("maxDosePerPeriod", "Ratio", "Upper limit on medication per unit of time.", 0, 1, 1097 maxDosePerPeriod)); 1098 children.add(new Property("maxDosePerAdministration", "SimpleQuantity", 1099 "Upper limit on medication per administration.", 0, 1, maxDosePerAdministration)); 1100 children.add(new Property("maxDosePerLifetime", "SimpleQuantity", 1101 "Upper limit on medication per lifetime of the patient.", 0, 1, maxDosePerLifetime)); 1102 } 1103 1104 @Override 1105 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1106 switch (_hash) { 1107 case 1349547969: 1108 /* sequence */ return new Property("sequence", "integer", 1109 "Indicates the order in which the dosage instructions should be applied or interpreted.", 0, 1, sequence); 1110 case 3556653: 1111 /* text */ return new Property("text", "string", "Free text dosage instructions e.g. SIG.", 0, 1, text); 1112 case 1623641575: 1113 /* additionalInstruction */ return new Property("additionalInstruction", "CodeableConcept", 1114 "Supplemental instructions to the patient on how to take the medication (e.g. \"with meals\" or\"take half to one hour before food\") or warnings for the patient about the medication (e.g. \"may cause drowsiness\" or \"avoid exposure of skin to direct sunlight or sunlamps\").", 1115 0, java.lang.Integer.MAX_VALUE, additionalInstruction); 1116 case 737543241: 1117 /* patientInstruction */ return new Property("patientInstruction", "string", 1118 "Instructions in terms that are understood by the patient or consumer.", 0, 1, patientInstruction); 1119 case -873664438: 1120 /* timing */ return new Property("timing", "Timing", "When medication should be administered.", 0, 1, timing); 1121 case -544329575: 1122 /* asNeeded[x] */ return new Property("asNeeded[x]", "boolean|CodeableConcept", 1123 "Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option), or it indicates the precondition for taking the Medication (CodeableConcept).", 1124 0, 1, asNeeded); 1125 case -1432923513: 1126 /* asNeeded */ return new Property("asNeeded[x]", "boolean|CodeableConcept", 1127 "Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option), or it indicates the precondition for taking the Medication (CodeableConcept).", 1128 0, 1, asNeeded); 1129 case -591717471: 1130 /* asNeededBoolean */ return new Property("asNeeded[x]", "boolean|CodeableConcept", 1131 "Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option), or it indicates the precondition for taking the Medication (CodeableConcept).", 1132 0, 1, asNeeded); 1133 case 1556420122: 1134 /* asNeededCodeableConcept */ return new Property("asNeeded[x]", "boolean|CodeableConcept", 1135 "Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option), or it indicates the precondition for taking the Medication (CodeableConcept).", 1136 0, 1, asNeeded); 1137 case 3530567: 1138 /* site */ return new Property("site", "CodeableConcept", "Body site to administer to.", 0, 1, site); 1139 case 108704329: 1140 /* route */ return new Property("route", "CodeableConcept", "How drug should enter body.", 0, 1, route); 1141 case -1077554975: 1142 /* method */ return new Property("method", "CodeableConcept", "Technique for administering medication.", 0, 1, 1143 method); 1144 case -611024774: 1145 /* doseAndRate */ return new Property("doseAndRate", "", "The amount of medication administered.", 0, 1146 java.lang.Integer.MAX_VALUE, doseAndRate); 1147 case 1506263709: 1148 /* maxDosePerPeriod */ return new Property("maxDosePerPeriod", "Ratio", 1149 "Upper limit on medication per unit of time.", 0, 1, maxDosePerPeriod); 1150 case 2004889914: 1151 /* maxDosePerAdministration */ return new Property("maxDosePerAdministration", "SimpleQuantity", 1152 "Upper limit on medication per administration.", 0, 1, maxDosePerAdministration); 1153 case 642099621: 1154 /* maxDosePerLifetime */ return new Property("maxDosePerLifetime", "SimpleQuantity", 1155 "Upper limit on medication per lifetime of the patient.", 0, 1, maxDosePerLifetime); 1156 default: 1157 return super.getNamedProperty(_hash, _name, _checkValid); 1158 } 1159 1160 } 1161 1162 @Override 1163 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1164 switch (hash) { 1165 case 1349547969: 1166 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // IntegerType 1167 case 3556653: 1168 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType 1169 case 1623641575: 1170 /* additionalInstruction */ return this.additionalInstruction == null ? new Base[0] 1171 : this.additionalInstruction.toArray(new Base[this.additionalInstruction.size()]); // CodeableConcept 1172 case 737543241: 1173 /* patientInstruction */ return this.patientInstruction == null ? new Base[0] 1174 : new Base[] { this.patientInstruction }; // StringType 1175 case -873664438: 1176 /* timing */ return this.timing == null ? new Base[0] : new Base[] { this.timing }; // Timing 1177 case -1432923513: 1178 /* asNeeded */ return this.asNeeded == null ? new Base[0] : new Base[] { this.asNeeded }; // Type 1179 case 3530567: 1180 /* site */ return this.site == null ? new Base[0] : new Base[] { this.site }; // CodeableConcept 1181 case 108704329: 1182 /* route */ return this.route == null ? new Base[0] : new Base[] { this.route }; // CodeableConcept 1183 case -1077554975: 1184 /* method */ return this.method == null ? new Base[0] : new Base[] { this.method }; // CodeableConcept 1185 case -611024774: 1186 /* doseAndRate */ return this.doseAndRate == null ? new Base[0] 1187 : this.doseAndRate.toArray(new Base[this.doseAndRate.size()]); // DosageDoseAndRateComponent 1188 case 1506263709: 1189 /* maxDosePerPeriod */ return this.maxDosePerPeriod == null ? new Base[0] : new Base[] { this.maxDosePerPeriod }; // Ratio 1190 case 2004889914: 1191 /* maxDosePerAdministration */ return this.maxDosePerAdministration == null ? new Base[0] 1192 : new Base[] { this.maxDosePerAdministration }; // Quantity 1193 case 642099621: 1194 /* maxDosePerLifetime */ return this.maxDosePerLifetime == null ? new Base[0] 1195 : new Base[] { this.maxDosePerLifetime }; // Quantity 1196 default: 1197 return super.getProperty(hash, name, checkValid); 1198 } 1199 1200 } 1201 1202 @Override 1203 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1204 switch (hash) { 1205 case 1349547969: // sequence 1206 this.sequence = castToInteger(value); // IntegerType 1207 return value; 1208 case 3556653: // text 1209 this.text = castToString(value); // StringType 1210 return value; 1211 case 1623641575: // additionalInstruction 1212 this.getAdditionalInstruction().add(castToCodeableConcept(value)); // CodeableConcept 1213 return value; 1214 case 737543241: // patientInstruction 1215 this.patientInstruction = castToString(value); // StringType 1216 return value; 1217 case -873664438: // timing 1218 this.timing = castToTiming(value); // Timing 1219 return value; 1220 case -1432923513: // asNeeded 1221 this.asNeeded = castToType(value); // Type 1222 return value; 1223 case 3530567: // site 1224 this.site = castToCodeableConcept(value); // CodeableConcept 1225 return value; 1226 case 108704329: // route 1227 this.route = castToCodeableConcept(value); // CodeableConcept 1228 return value; 1229 case -1077554975: // method 1230 this.method = castToCodeableConcept(value); // CodeableConcept 1231 return value; 1232 case -611024774: // doseAndRate 1233 this.getDoseAndRate().add((DosageDoseAndRateComponent) value); // DosageDoseAndRateComponent 1234 return value; 1235 case 1506263709: // maxDosePerPeriod 1236 this.maxDosePerPeriod = castToRatio(value); // Ratio 1237 return value; 1238 case 2004889914: // maxDosePerAdministration 1239 this.maxDosePerAdministration = castToQuantity(value); // Quantity 1240 return value; 1241 case 642099621: // maxDosePerLifetime 1242 this.maxDosePerLifetime = castToQuantity(value); // Quantity 1243 return value; 1244 default: 1245 return super.setProperty(hash, name, value); 1246 } 1247 1248 } 1249 1250 @Override 1251 public Base setProperty(String name, Base value) throws FHIRException { 1252 if (name.equals("sequence")) { 1253 this.sequence = castToInteger(value); // IntegerType 1254 } else if (name.equals("text")) { 1255 this.text = castToString(value); // StringType 1256 } else if (name.equals("additionalInstruction")) { 1257 this.getAdditionalInstruction().add(castToCodeableConcept(value)); 1258 } else if (name.equals("patientInstruction")) { 1259 this.patientInstruction = castToString(value); // StringType 1260 } else if (name.equals("timing")) { 1261 this.timing = castToTiming(value); // Timing 1262 } else if (name.equals("asNeeded[x]")) { 1263 this.asNeeded = castToType(value); // Type 1264 } else if (name.equals("site")) { 1265 this.site = castToCodeableConcept(value); // CodeableConcept 1266 } else if (name.equals("route")) { 1267 this.route = castToCodeableConcept(value); // CodeableConcept 1268 } else if (name.equals("method")) { 1269 this.method = castToCodeableConcept(value); // CodeableConcept 1270 } else if (name.equals("doseAndRate")) { 1271 this.getDoseAndRate().add((DosageDoseAndRateComponent) value); 1272 } else if (name.equals("maxDosePerPeriod")) { 1273 this.maxDosePerPeriod = castToRatio(value); // Ratio 1274 } else if (name.equals("maxDosePerAdministration")) { 1275 this.maxDosePerAdministration = castToQuantity(value); // Quantity 1276 } else if (name.equals("maxDosePerLifetime")) { 1277 this.maxDosePerLifetime = castToQuantity(value); // Quantity 1278 } else 1279 return super.setProperty(name, value); 1280 return value; 1281 } 1282 1283 @Override 1284 public void removeChild(String name, Base value) throws FHIRException { 1285 if (name.equals("sequence")) { 1286 this.sequence = null; 1287 } else if (name.equals("text")) { 1288 this.text = null; 1289 } else if (name.equals("additionalInstruction")) { 1290 this.getAdditionalInstruction().remove(castToCodeableConcept(value)); 1291 } else if (name.equals("patientInstruction")) { 1292 this.patientInstruction = null; 1293 } else if (name.equals("timing")) { 1294 this.timing = null; 1295 } else if (name.equals("asNeeded[x]")) { 1296 this.asNeeded = null; 1297 } else if (name.equals("site")) { 1298 this.site = null; 1299 } else if (name.equals("route")) { 1300 this.route = null; 1301 } else if (name.equals("method")) { 1302 this.method = null; 1303 } else if (name.equals("doseAndRate")) { 1304 this.getDoseAndRate().remove((DosageDoseAndRateComponent) value); 1305 } else if (name.equals("maxDosePerPeriod")) { 1306 this.maxDosePerPeriod = null; 1307 } else if (name.equals("maxDosePerAdministration")) { 1308 this.maxDosePerAdministration = null; 1309 } else if (name.equals("maxDosePerLifetime")) { 1310 this.maxDosePerLifetime = null; 1311 } else 1312 super.removeChild(name, value); 1313 1314 } 1315 1316 @Override 1317 public Base makeProperty(int hash, String name) throws FHIRException { 1318 switch (hash) { 1319 case 1349547969: 1320 return getSequenceElement(); 1321 case 3556653: 1322 return getTextElement(); 1323 case 1623641575: 1324 return addAdditionalInstruction(); 1325 case 737543241: 1326 return getPatientInstructionElement(); 1327 case -873664438: 1328 return getTiming(); 1329 case -544329575: 1330 return getAsNeeded(); 1331 case -1432923513: 1332 return getAsNeeded(); 1333 case 3530567: 1334 return getSite(); 1335 case 108704329: 1336 return getRoute(); 1337 case -1077554975: 1338 return getMethod(); 1339 case -611024774: 1340 return addDoseAndRate(); 1341 case 1506263709: 1342 return getMaxDosePerPeriod(); 1343 case 2004889914: 1344 return getMaxDosePerAdministration(); 1345 case 642099621: 1346 return getMaxDosePerLifetime(); 1347 default: 1348 return super.makeProperty(hash, name); 1349 } 1350 1351 } 1352 1353 @Override 1354 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1355 switch (hash) { 1356 case 1349547969: 1357 /* sequence */ return new String[] { "integer" }; 1358 case 3556653: 1359 /* text */ return new String[] { "string" }; 1360 case 1623641575: 1361 /* additionalInstruction */ return new String[] { "CodeableConcept" }; 1362 case 737543241: 1363 /* patientInstruction */ return new String[] { "string" }; 1364 case -873664438: 1365 /* timing */ return new String[] { "Timing" }; 1366 case -1432923513: 1367 /* asNeeded */ return new String[] { "boolean", "CodeableConcept" }; 1368 case 3530567: 1369 /* site */ return new String[] { "CodeableConcept" }; 1370 case 108704329: 1371 /* route */ return new String[] { "CodeableConcept" }; 1372 case -1077554975: 1373 /* method */ return new String[] { "CodeableConcept" }; 1374 case -611024774: 1375 /* doseAndRate */ return new String[] {}; 1376 case 1506263709: 1377 /* maxDosePerPeriod */ return new String[] { "Ratio" }; 1378 case 2004889914: 1379 /* maxDosePerAdministration */ return new String[] { "SimpleQuantity" }; 1380 case 642099621: 1381 /* maxDosePerLifetime */ return new String[] { "SimpleQuantity" }; 1382 default: 1383 return super.getTypesForProperty(hash, name); 1384 } 1385 1386 } 1387 1388 @Override 1389 public Base addChild(String name) throws FHIRException { 1390 if (name.equals("sequence")) { 1391 throw new FHIRException("Cannot call addChild on a singleton property Dosage.sequence"); 1392 } else if (name.equals("text")) { 1393 throw new FHIRException("Cannot call addChild on a singleton property Dosage.text"); 1394 } else if (name.equals("additionalInstruction")) { 1395 return addAdditionalInstruction(); 1396 } else if (name.equals("patientInstruction")) { 1397 throw new FHIRException("Cannot call addChild on a singleton property Dosage.patientInstruction"); 1398 } else if (name.equals("timing")) { 1399 this.timing = new Timing(); 1400 return this.timing; 1401 } else if (name.equals("asNeededBoolean")) { 1402 this.asNeeded = new BooleanType(); 1403 return this.asNeeded; 1404 } else if (name.equals("asNeededCodeableConcept")) { 1405 this.asNeeded = new CodeableConcept(); 1406 return this.asNeeded; 1407 } else if (name.equals("site")) { 1408 this.site = new CodeableConcept(); 1409 return this.site; 1410 } else if (name.equals("route")) { 1411 this.route = new CodeableConcept(); 1412 return this.route; 1413 } else if (name.equals("method")) { 1414 this.method = new CodeableConcept(); 1415 return this.method; 1416 } else if (name.equals("doseAndRate")) { 1417 return addDoseAndRate(); 1418 } else if (name.equals("maxDosePerPeriod")) { 1419 this.maxDosePerPeriod = new Ratio(); 1420 return this.maxDosePerPeriod; 1421 } else if (name.equals("maxDosePerAdministration")) { 1422 this.maxDosePerAdministration = new Quantity(); 1423 return this.maxDosePerAdministration; 1424 } else if (name.equals("maxDosePerLifetime")) { 1425 this.maxDosePerLifetime = new Quantity(); 1426 return this.maxDosePerLifetime; 1427 } else 1428 return super.addChild(name); 1429 } 1430 1431 public String fhirType() { 1432 return "Dosage"; 1433 1434 } 1435 1436 public Dosage copy() { 1437 Dosage dst = new Dosage(); 1438 copyValues(dst); 1439 return dst; 1440 } 1441 1442 public void copyValues(Dosage dst) { 1443 super.copyValues(dst); 1444 dst.sequence = sequence == null ? null : sequence.copy(); 1445 dst.text = text == null ? null : text.copy(); 1446 if (additionalInstruction != null) { 1447 dst.additionalInstruction = new ArrayList<CodeableConcept>(); 1448 for (CodeableConcept i : additionalInstruction) 1449 dst.additionalInstruction.add(i.copy()); 1450 } 1451 ; 1452 dst.patientInstruction = patientInstruction == null ? null : patientInstruction.copy(); 1453 dst.timing = timing == null ? null : timing.copy(); 1454 dst.asNeeded = asNeeded == null ? null : asNeeded.copy(); 1455 dst.site = site == null ? null : site.copy(); 1456 dst.route = route == null ? null : route.copy(); 1457 dst.method = method == null ? null : method.copy(); 1458 if (doseAndRate != null) { 1459 dst.doseAndRate = new ArrayList<DosageDoseAndRateComponent>(); 1460 for (DosageDoseAndRateComponent i : doseAndRate) 1461 dst.doseAndRate.add(i.copy()); 1462 } 1463 ; 1464 dst.maxDosePerPeriod = maxDosePerPeriod == null ? null : maxDosePerPeriod.copy(); 1465 dst.maxDosePerAdministration = maxDosePerAdministration == null ? null : maxDosePerAdministration.copy(); 1466 dst.maxDosePerLifetime = maxDosePerLifetime == null ? null : maxDosePerLifetime.copy(); 1467 } 1468 1469 protected Dosage typedCopy() { 1470 return copy(); 1471 } 1472 1473 @Override 1474 public boolean equalsDeep(Base other_) { 1475 if (!super.equalsDeep(other_)) 1476 return false; 1477 if (!(other_ instanceof Dosage)) 1478 return false; 1479 Dosage o = (Dosage) other_; 1480 return compareDeep(sequence, o.sequence, true) && compareDeep(text, o.text, true) 1481 && compareDeep(additionalInstruction, o.additionalInstruction, true) 1482 && compareDeep(patientInstruction, o.patientInstruction, true) && compareDeep(timing, o.timing, true) 1483 && compareDeep(asNeeded, o.asNeeded, true) && compareDeep(site, o.site, true) 1484 && compareDeep(route, o.route, true) && compareDeep(method, o.method, true) 1485 && compareDeep(doseAndRate, o.doseAndRate, true) && compareDeep(maxDosePerPeriod, o.maxDosePerPeriod, true) 1486 && compareDeep(maxDosePerAdministration, o.maxDosePerAdministration, true) 1487 && compareDeep(maxDosePerLifetime, o.maxDosePerLifetime, true); 1488 } 1489 1490 @Override 1491 public boolean equalsShallow(Base other_) { 1492 if (!super.equalsShallow(other_)) 1493 return false; 1494 if (!(other_ instanceof Dosage)) 1495 return false; 1496 Dosage o = (Dosage) other_; 1497 return compareValues(sequence, o.sequence, true) && compareValues(text, o.text, true) 1498 && compareValues(patientInstruction, o.patientInstruction, true); 1499 } 1500 1501 public boolean isEmpty() { 1502 return super.isEmpty() 1503 && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, text, additionalInstruction, patientInstruction, timing, 1504 asNeeded, site, route, method, doseAndRate, maxDosePerPeriod, maxDosePerAdministration, maxDosePerLifetime); 1505 } 1506 1507}