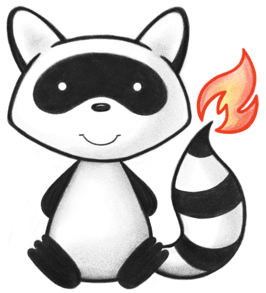
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 042import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.ResourceDef; 050import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 051 052/** 053 * The EffectEvidenceSynthesis resource describes the difference in an outcome 054 * between exposures states in a population where the effect estimate is derived 055 * from a combination of research studies. 056 */ 057@ResourceDef(name = "EffectEvidenceSynthesis", profile = "http://hl7.org/fhir/StructureDefinition/EffectEvidenceSynthesis") 058@ChildOrder(names = { "url", "identifier", "version", "name", "title", "status", "date", "publisher", "contact", 059 "description", "note", "useContext", "jurisdiction", "copyright", "approvalDate", "lastReviewDate", 060 "effectivePeriod", "topic", "author", "editor", "reviewer", "endorser", "relatedArtifact", "synthesisType", 061 "studyType", "population", "exposure", "exposureAlternative", "outcome", "sampleSize", "resultsByExposure", 062 "effectEstimate", "certainty" }) 063public class EffectEvidenceSynthesis extends MetadataResource { 064 065 public enum ExposureState { 066 /** 067 * used when the results by exposure is describing the results for the primary 068 * exposure of interest. 069 */ 070 EXPOSURE, 071 /** 072 * used when the results by exposure is describing the results for the 073 * alternative exposure state, control state or comparator state. 074 */ 075 EXPOSUREALTERNATIVE, 076 /** 077 * added to help the parsers with the generic types 078 */ 079 NULL; 080 081 public static ExposureState fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("exposure".equals(codeString)) 085 return EXPOSURE; 086 if ("exposure-alternative".equals(codeString)) 087 return EXPOSUREALTERNATIVE; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown ExposureState code '" + codeString + "'"); 092 } 093 094 public String toCode() { 095 switch (this) { 096 case EXPOSURE: 097 return "exposure"; 098 case EXPOSUREALTERNATIVE: 099 return "exposure-alternative"; 100 case NULL: 101 return null; 102 default: 103 return "?"; 104 } 105 } 106 107 public String getSystem() { 108 switch (this) { 109 case EXPOSURE: 110 return "http://hl7.org/fhir/exposure-state"; 111 case EXPOSUREALTERNATIVE: 112 return "http://hl7.org/fhir/exposure-state"; 113 case NULL: 114 return null; 115 default: 116 return "?"; 117 } 118 } 119 120 public String getDefinition() { 121 switch (this) { 122 case EXPOSURE: 123 return "used when the results by exposure is describing the results for the primary exposure of interest."; 124 case EXPOSUREALTERNATIVE: 125 return "used when the results by exposure is describing the results for the alternative exposure state, control state or comparator state."; 126 case NULL: 127 return null; 128 default: 129 return "?"; 130 } 131 } 132 133 public String getDisplay() { 134 switch (this) { 135 case EXPOSURE: 136 return "Exposure"; 137 case EXPOSUREALTERNATIVE: 138 return "Exposure Alternative"; 139 case NULL: 140 return null; 141 default: 142 return "?"; 143 } 144 } 145 } 146 147 public static class ExposureStateEnumFactory implements EnumFactory<ExposureState> { 148 public ExposureState fromCode(String codeString) throws IllegalArgumentException { 149 if (codeString == null || "".equals(codeString)) 150 if (codeString == null || "".equals(codeString)) 151 return null; 152 if ("exposure".equals(codeString)) 153 return ExposureState.EXPOSURE; 154 if ("exposure-alternative".equals(codeString)) 155 return ExposureState.EXPOSUREALTERNATIVE; 156 throw new IllegalArgumentException("Unknown ExposureState code '" + codeString + "'"); 157 } 158 159 public Enumeration<ExposureState> fromType(PrimitiveType<?> code) throws FHIRException { 160 if (code == null) 161 return null; 162 if (code.isEmpty()) 163 return new Enumeration<ExposureState>(this, ExposureState.NULL, code); 164 String codeString = code.asStringValue(); 165 if (codeString == null || "".equals(codeString)) 166 return new Enumeration<ExposureState>(this, ExposureState.NULL, code); 167 if ("exposure".equals(codeString)) 168 return new Enumeration<ExposureState>(this, ExposureState.EXPOSURE, code); 169 if ("exposure-alternative".equals(codeString)) 170 return new Enumeration<ExposureState>(this, ExposureState.EXPOSUREALTERNATIVE, code); 171 throw new FHIRException("Unknown ExposureState code '" + codeString + "'"); 172 } 173 174 public String toCode(ExposureState code) { 175 if (code == ExposureState.NULL) 176 return null; 177 if (code == ExposureState.EXPOSURE) 178 return "exposure"; 179 if (code == ExposureState.EXPOSUREALTERNATIVE) 180 return "exposure-alternative"; 181 return "?"; 182 } 183 184 public String toSystem(ExposureState code) { 185 return code.getSystem(); 186 } 187 } 188 189 @Block() 190 public static class EffectEvidenceSynthesisSampleSizeComponent extends BackboneElement 191 implements IBaseBackboneElement { 192 /** 193 * Human-readable summary of sample size. 194 */ 195 @Child(name = "description", type = { 196 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 197 @Description(shortDefinition = "Description of sample size", formalDefinition = "Human-readable summary of sample size.") 198 protected StringType description; 199 200 /** 201 * Number of studies included in this evidence synthesis. 202 */ 203 @Child(name = "numberOfStudies", type = { 204 IntegerType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 205 @Description(shortDefinition = "How many studies?", formalDefinition = "Number of studies included in this evidence synthesis.") 206 protected IntegerType numberOfStudies; 207 208 /** 209 * Number of participants included in this evidence synthesis. 210 */ 211 @Child(name = "numberOfParticipants", type = { 212 IntegerType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 213 @Description(shortDefinition = "How many participants?", formalDefinition = "Number of participants included in this evidence synthesis.") 214 protected IntegerType numberOfParticipants; 215 216 private static final long serialVersionUID = -1116074476L; 217 218 /** 219 * Constructor 220 */ 221 public EffectEvidenceSynthesisSampleSizeComponent() { 222 super(); 223 } 224 225 /** 226 * @return {@link #description} (Human-readable summary of sample size.). This 227 * is the underlying object with id, value and extensions. The accessor 228 * "getDescription" gives direct access to the value 229 */ 230 public StringType getDescriptionElement() { 231 if (this.description == null) 232 if (Configuration.errorOnAutoCreate()) 233 throw new Error("Attempt to auto-create EffectEvidenceSynthesisSampleSizeComponent.description"); 234 else if (Configuration.doAutoCreate()) 235 this.description = new StringType(); // bb 236 return this.description; 237 } 238 239 public boolean hasDescriptionElement() { 240 return this.description != null && !this.description.isEmpty(); 241 } 242 243 public boolean hasDescription() { 244 return this.description != null && !this.description.isEmpty(); 245 } 246 247 /** 248 * @param value {@link #description} (Human-readable summary of sample size.). 249 * This is the underlying object with id, value and extensions. The 250 * accessor "getDescription" gives direct access to the value 251 */ 252 public EffectEvidenceSynthesisSampleSizeComponent setDescriptionElement(StringType value) { 253 this.description = value; 254 return this; 255 } 256 257 /** 258 * @return Human-readable summary of sample size. 259 */ 260 public String getDescription() { 261 return this.description == null ? null : this.description.getValue(); 262 } 263 264 /** 265 * @param value Human-readable summary of sample size. 266 */ 267 public EffectEvidenceSynthesisSampleSizeComponent setDescription(String value) { 268 if (Utilities.noString(value)) 269 this.description = null; 270 else { 271 if (this.description == null) 272 this.description = new StringType(); 273 this.description.setValue(value); 274 } 275 return this; 276 } 277 278 /** 279 * @return {@link #numberOfStudies} (Number of studies included in this evidence 280 * synthesis.). This is the underlying object with id, value and 281 * extensions. The accessor "getNumberOfStudies" gives direct access to 282 * the value 283 */ 284 public IntegerType getNumberOfStudiesElement() { 285 if (this.numberOfStudies == null) 286 if (Configuration.errorOnAutoCreate()) 287 throw new Error("Attempt to auto-create EffectEvidenceSynthesisSampleSizeComponent.numberOfStudies"); 288 else if (Configuration.doAutoCreate()) 289 this.numberOfStudies = new IntegerType(); // bb 290 return this.numberOfStudies; 291 } 292 293 public boolean hasNumberOfStudiesElement() { 294 return this.numberOfStudies != null && !this.numberOfStudies.isEmpty(); 295 } 296 297 public boolean hasNumberOfStudies() { 298 return this.numberOfStudies != null && !this.numberOfStudies.isEmpty(); 299 } 300 301 /** 302 * @param value {@link #numberOfStudies} (Number of studies included in this 303 * evidence synthesis.). This is the underlying object with id, 304 * value and extensions. The accessor "getNumberOfStudies" gives 305 * direct access to the value 306 */ 307 public EffectEvidenceSynthesisSampleSizeComponent setNumberOfStudiesElement(IntegerType value) { 308 this.numberOfStudies = value; 309 return this; 310 } 311 312 /** 313 * @return Number of studies included in this evidence synthesis. 314 */ 315 public int getNumberOfStudies() { 316 return this.numberOfStudies == null || this.numberOfStudies.isEmpty() ? 0 : this.numberOfStudies.getValue(); 317 } 318 319 /** 320 * @param value Number of studies included in this evidence synthesis. 321 */ 322 public EffectEvidenceSynthesisSampleSizeComponent setNumberOfStudies(int value) { 323 if (this.numberOfStudies == null) 324 this.numberOfStudies = new IntegerType(); 325 this.numberOfStudies.setValue(value); 326 return this; 327 } 328 329 /** 330 * @return {@link #numberOfParticipants} (Number of participants included in 331 * this evidence synthesis.). This is the underlying object with id, 332 * value and extensions. The accessor "getNumberOfParticipants" gives 333 * direct access to the value 334 */ 335 public IntegerType getNumberOfParticipantsElement() { 336 if (this.numberOfParticipants == null) 337 if (Configuration.errorOnAutoCreate()) 338 throw new Error("Attempt to auto-create EffectEvidenceSynthesisSampleSizeComponent.numberOfParticipants"); 339 else if (Configuration.doAutoCreate()) 340 this.numberOfParticipants = new IntegerType(); // bb 341 return this.numberOfParticipants; 342 } 343 344 public boolean hasNumberOfParticipantsElement() { 345 return this.numberOfParticipants != null && !this.numberOfParticipants.isEmpty(); 346 } 347 348 public boolean hasNumberOfParticipants() { 349 return this.numberOfParticipants != null && !this.numberOfParticipants.isEmpty(); 350 } 351 352 /** 353 * @param value {@link #numberOfParticipants} (Number of participants included 354 * in this evidence synthesis.). This is the underlying object with 355 * id, value and extensions. The accessor "getNumberOfParticipants" 356 * gives direct access to the value 357 */ 358 public EffectEvidenceSynthesisSampleSizeComponent setNumberOfParticipantsElement(IntegerType value) { 359 this.numberOfParticipants = value; 360 return this; 361 } 362 363 /** 364 * @return Number of participants included in this evidence synthesis. 365 */ 366 public int getNumberOfParticipants() { 367 return this.numberOfParticipants == null || this.numberOfParticipants.isEmpty() ? 0 368 : this.numberOfParticipants.getValue(); 369 } 370 371 /** 372 * @param value Number of participants included in this evidence synthesis. 373 */ 374 public EffectEvidenceSynthesisSampleSizeComponent setNumberOfParticipants(int value) { 375 if (this.numberOfParticipants == null) 376 this.numberOfParticipants = new IntegerType(); 377 this.numberOfParticipants.setValue(value); 378 return this; 379 } 380 381 protected void listChildren(List<Property> children) { 382 super.listChildren(children); 383 children.add(new Property("description", "string", "Human-readable summary of sample size.", 0, 1, description)); 384 children.add(new Property("numberOfStudies", "integer", "Number of studies included in this evidence synthesis.", 385 0, 1, numberOfStudies)); 386 children.add(new Property("numberOfParticipants", "integer", 387 "Number of participants included in this evidence synthesis.", 0, 1, numberOfParticipants)); 388 } 389 390 @Override 391 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 392 switch (_hash) { 393 case -1724546052: 394 /* description */ return new Property("description", "string", "Human-readable summary of sample size.", 0, 1, 395 description); 396 case -177467129: 397 /* numberOfStudies */ return new Property("numberOfStudies", "integer", 398 "Number of studies included in this evidence synthesis.", 0, 1, numberOfStudies); 399 case 1799357120: 400 /* numberOfParticipants */ return new Property("numberOfParticipants", "integer", 401 "Number of participants included in this evidence synthesis.", 0, 1, numberOfParticipants); 402 default: 403 return super.getNamedProperty(_hash, _name, _checkValid); 404 } 405 406 } 407 408 @Override 409 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 410 switch (hash) { 411 case -1724546052: 412 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 413 case -177467129: 414 /* numberOfStudies */ return this.numberOfStudies == null ? new Base[0] : new Base[] { this.numberOfStudies }; // IntegerType 415 case 1799357120: 416 /* numberOfParticipants */ return this.numberOfParticipants == null ? new Base[0] 417 : new Base[] { this.numberOfParticipants }; // IntegerType 418 default: 419 return super.getProperty(hash, name, checkValid); 420 } 421 422 } 423 424 @Override 425 public Base setProperty(int hash, String name, Base value) throws FHIRException { 426 switch (hash) { 427 case -1724546052: // description 428 this.description = castToString(value); // StringType 429 return value; 430 case -177467129: // numberOfStudies 431 this.numberOfStudies = castToInteger(value); // IntegerType 432 return value; 433 case 1799357120: // numberOfParticipants 434 this.numberOfParticipants = castToInteger(value); // IntegerType 435 return value; 436 default: 437 return super.setProperty(hash, name, value); 438 } 439 440 } 441 442 @Override 443 public Base setProperty(String name, Base value) throws FHIRException { 444 if (name.equals("description")) { 445 this.description = castToString(value); // StringType 446 } else if (name.equals("numberOfStudies")) { 447 this.numberOfStudies = castToInteger(value); // IntegerType 448 } else if (name.equals("numberOfParticipants")) { 449 this.numberOfParticipants = castToInteger(value); // IntegerType 450 } else 451 return super.setProperty(name, value); 452 return value; 453 } 454 455 @Override 456 public void removeChild(String name, Base value) throws FHIRException { 457 if (name.equals("description")) { 458 this.description = null; 459 } else if (name.equals("numberOfStudies")) { 460 this.numberOfStudies = null; 461 } else if (name.equals("numberOfParticipants")) { 462 this.numberOfParticipants = null; 463 } else 464 super.removeChild(name, value); 465 466 } 467 468 @Override 469 public Base makeProperty(int hash, String name) throws FHIRException { 470 switch (hash) { 471 case -1724546052: 472 return getDescriptionElement(); 473 case -177467129: 474 return getNumberOfStudiesElement(); 475 case 1799357120: 476 return getNumberOfParticipantsElement(); 477 default: 478 return super.makeProperty(hash, name); 479 } 480 481 } 482 483 @Override 484 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 485 switch (hash) { 486 case -1724546052: 487 /* description */ return new String[] { "string" }; 488 case -177467129: 489 /* numberOfStudies */ return new String[] { "integer" }; 490 case 1799357120: 491 /* numberOfParticipants */ return new String[] { "integer" }; 492 default: 493 return super.getTypesForProperty(hash, name); 494 } 495 496 } 497 498 @Override 499 public Base addChild(String name) throws FHIRException { 500 if (name.equals("description")) { 501 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.description"); 502 } else if (name.equals("numberOfStudies")) { 503 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.numberOfStudies"); 504 } else if (name.equals("numberOfParticipants")) { 505 throw new FHIRException( 506 "Cannot call addChild on a singleton property EffectEvidenceSynthesis.numberOfParticipants"); 507 } else 508 return super.addChild(name); 509 } 510 511 public EffectEvidenceSynthesisSampleSizeComponent copy() { 512 EffectEvidenceSynthesisSampleSizeComponent dst = new EffectEvidenceSynthesisSampleSizeComponent(); 513 copyValues(dst); 514 return dst; 515 } 516 517 public void copyValues(EffectEvidenceSynthesisSampleSizeComponent dst) { 518 super.copyValues(dst); 519 dst.description = description == null ? null : description.copy(); 520 dst.numberOfStudies = numberOfStudies == null ? null : numberOfStudies.copy(); 521 dst.numberOfParticipants = numberOfParticipants == null ? null : numberOfParticipants.copy(); 522 } 523 524 @Override 525 public boolean equalsDeep(Base other_) { 526 if (!super.equalsDeep(other_)) 527 return false; 528 if (!(other_ instanceof EffectEvidenceSynthesisSampleSizeComponent)) 529 return false; 530 EffectEvidenceSynthesisSampleSizeComponent o = (EffectEvidenceSynthesisSampleSizeComponent) other_; 531 return compareDeep(description, o.description, true) && compareDeep(numberOfStudies, o.numberOfStudies, true) 532 && compareDeep(numberOfParticipants, o.numberOfParticipants, true); 533 } 534 535 @Override 536 public boolean equalsShallow(Base other_) { 537 if (!super.equalsShallow(other_)) 538 return false; 539 if (!(other_ instanceof EffectEvidenceSynthesisSampleSizeComponent)) 540 return false; 541 EffectEvidenceSynthesisSampleSizeComponent o = (EffectEvidenceSynthesisSampleSizeComponent) other_; 542 return compareValues(description, o.description, true) && compareValues(numberOfStudies, o.numberOfStudies, true) 543 && compareValues(numberOfParticipants, o.numberOfParticipants, true); 544 } 545 546 public boolean isEmpty() { 547 return super.isEmpty() 548 && ca.uhn.fhir.util.ElementUtil.isEmpty(description, numberOfStudies, numberOfParticipants); 549 } 550 551 public String fhirType() { 552 return "EffectEvidenceSynthesis.sampleSize"; 553 554 } 555 556 } 557 558 @Block() 559 public static class EffectEvidenceSynthesisResultsByExposureComponent extends BackboneElement 560 implements IBaseBackboneElement { 561 /** 562 * Human-readable summary of results by exposure state. 563 */ 564 @Child(name = "description", type = { 565 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 566 @Description(shortDefinition = "Description of results by exposure", formalDefinition = "Human-readable summary of results by exposure state.") 567 protected StringType description; 568 569 /** 570 * Whether these results are for the exposure state or alternative exposure 571 * state. 572 */ 573 @Child(name = "exposureState", type = { 574 CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 575 @Description(shortDefinition = "exposure | exposure-alternative", formalDefinition = "Whether these results are for the exposure state or alternative exposure state.") 576 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/exposure-state") 577 protected Enumeration<ExposureState> exposureState; 578 579 /** 580 * Used to define variant exposure states such as low-risk state. 581 */ 582 @Child(name = "variantState", type = { 583 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 584 @Description(shortDefinition = "Variant exposure states", formalDefinition = "Used to define variant exposure states such as low-risk state.") 585 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/evidence-variant-state") 586 protected CodeableConcept variantState; 587 588 /** 589 * Reference to a RiskEvidenceSynthesis resource. 590 */ 591 @Child(name = "riskEvidenceSynthesis", type = { 592 RiskEvidenceSynthesis.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 593 @Description(shortDefinition = "Risk evidence synthesis", formalDefinition = "Reference to a RiskEvidenceSynthesis resource.") 594 protected Reference riskEvidenceSynthesis; 595 596 /** 597 * The actual object that is the target of the reference (Reference to a 598 * RiskEvidenceSynthesis resource.) 599 */ 600 protected RiskEvidenceSynthesis riskEvidenceSynthesisTarget; 601 602 private static final long serialVersionUID = 144886133L; 603 604 /** 605 * Constructor 606 */ 607 public EffectEvidenceSynthesisResultsByExposureComponent() { 608 super(); 609 } 610 611 /** 612 * Constructor 613 */ 614 public EffectEvidenceSynthesisResultsByExposureComponent(Reference riskEvidenceSynthesis) { 615 super(); 616 this.riskEvidenceSynthesis = riskEvidenceSynthesis; 617 } 618 619 /** 620 * @return {@link #description} (Human-readable summary of results by exposure 621 * state.). This is the underlying object with id, value and extensions. 622 * The accessor "getDescription" gives direct access to the value 623 */ 624 public StringType getDescriptionElement() { 625 if (this.description == null) 626 if (Configuration.errorOnAutoCreate()) 627 throw new Error("Attempt to auto-create EffectEvidenceSynthesisResultsByExposureComponent.description"); 628 else if (Configuration.doAutoCreate()) 629 this.description = new StringType(); // bb 630 return this.description; 631 } 632 633 public boolean hasDescriptionElement() { 634 return this.description != null && !this.description.isEmpty(); 635 } 636 637 public boolean hasDescription() { 638 return this.description != null && !this.description.isEmpty(); 639 } 640 641 /** 642 * @param value {@link #description} (Human-readable summary of results by 643 * exposure state.). This is the underlying object with id, value 644 * and extensions. The accessor "getDescription" gives direct 645 * access to the value 646 */ 647 public EffectEvidenceSynthesisResultsByExposureComponent setDescriptionElement(StringType value) { 648 this.description = value; 649 return this; 650 } 651 652 /** 653 * @return Human-readable summary of results by exposure state. 654 */ 655 public String getDescription() { 656 return this.description == null ? null : this.description.getValue(); 657 } 658 659 /** 660 * @param value Human-readable summary of results by exposure state. 661 */ 662 public EffectEvidenceSynthesisResultsByExposureComponent setDescription(String value) { 663 if (Utilities.noString(value)) 664 this.description = null; 665 else { 666 if (this.description == null) 667 this.description = new StringType(); 668 this.description.setValue(value); 669 } 670 return this; 671 } 672 673 /** 674 * @return {@link #exposureState} (Whether these results are for the exposure 675 * state or alternative exposure state.). This is the underlying object 676 * with id, value and extensions. The accessor "getExposureState" gives 677 * direct access to the value 678 */ 679 public Enumeration<ExposureState> getExposureStateElement() { 680 if (this.exposureState == null) 681 if (Configuration.errorOnAutoCreate()) 682 throw new Error("Attempt to auto-create EffectEvidenceSynthesisResultsByExposureComponent.exposureState"); 683 else if (Configuration.doAutoCreate()) 684 this.exposureState = new Enumeration<ExposureState>(new ExposureStateEnumFactory()); // bb 685 return this.exposureState; 686 } 687 688 public boolean hasExposureStateElement() { 689 return this.exposureState != null && !this.exposureState.isEmpty(); 690 } 691 692 public boolean hasExposureState() { 693 return this.exposureState != null && !this.exposureState.isEmpty(); 694 } 695 696 /** 697 * @param value {@link #exposureState} (Whether these results are for the 698 * exposure state or alternative exposure state.). This is the 699 * underlying object with id, value and extensions. The accessor 700 * "getExposureState" gives direct access to the value 701 */ 702 public EffectEvidenceSynthesisResultsByExposureComponent setExposureStateElement(Enumeration<ExposureState> value) { 703 this.exposureState = value; 704 return this; 705 } 706 707 /** 708 * @return Whether these results are for the exposure state or alternative 709 * exposure state. 710 */ 711 public ExposureState getExposureState() { 712 return this.exposureState == null ? null : this.exposureState.getValue(); 713 } 714 715 /** 716 * @param value Whether these results are for the exposure state or alternative 717 * exposure state. 718 */ 719 public EffectEvidenceSynthesisResultsByExposureComponent setExposureState(ExposureState value) { 720 if (value == null) 721 this.exposureState = null; 722 else { 723 if (this.exposureState == null) 724 this.exposureState = new Enumeration<ExposureState>(new ExposureStateEnumFactory()); 725 this.exposureState.setValue(value); 726 } 727 return this; 728 } 729 730 /** 731 * @return {@link #variantState} (Used to define variant exposure states such as 732 * low-risk state.) 733 */ 734 public CodeableConcept getVariantState() { 735 if (this.variantState == null) 736 if (Configuration.errorOnAutoCreate()) 737 throw new Error("Attempt to auto-create EffectEvidenceSynthesisResultsByExposureComponent.variantState"); 738 else if (Configuration.doAutoCreate()) 739 this.variantState = new CodeableConcept(); // cc 740 return this.variantState; 741 } 742 743 public boolean hasVariantState() { 744 return this.variantState != null && !this.variantState.isEmpty(); 745 } 746 747 /** 748 * @param value {@link #variantState} (Used to define variant exposure states 749 * such as low-risk state.) 750 */ 751 public EffectEvidenceSynthesisResultsByExposureComponent setVariantState(CodeableConcept value) { 752 this.variantState = value; 753 return this; 754 } 755 756 /** 757 * @return {@link #riskEvidenceSynthesis} (Reference to a RiskEvidenceSynthesis 758 * resource.) 759 */ 760 public Reference getRiskEvidenceSynthesis() { 761 if (this.riskEvidenceSynthesis == null) 762 if (Configuration.errorOnAutoCreate()) 763 throw new Error( 764 "Attempt to auto-create EffectEvidenceSynthesisResultsByExposureComponent.riskEvidenceSynthesis"); 765 else if (Configuration.doAutoCreate()) 766 this.riskEvidenceSynthesis = new Reference(); // cc 767 return this.riskEvidenceSynthesis; 768 } 769 770 public boolean hasRiskEvidenceSynthesis() { 771 return this.riskEvidenceSynthesis != null && !this.riskEvidenceSynthesis.isEmpty(); 772 } 773 774 /** 775 * @param value {@link #riskEvidenceSynthesis} (Reference to a 776 * RiskEvidenceSynthesis resource.) 777 */ 778 public EffectEvidenceSynthesisResultsByExposureComponent setRiskEvidenceSynthesis(Reference value) { 779 this.riskEvidenceSynthesis = value; 780 return this; 781 } 782 783 /** 784 * @return {@link #riskEvidenceSynthesis} The actual object that is the target 785 * of the reference. The reference library doesn't populate this, but 786 * you can use it to hold the resource if you resolve it. (Reference to 787 * a RiskEvidenceSynthesis resource.) 788 */ 789 public RiskEvidenceSynthesis getRiskEvidenceSynthesisTarget() { 790 if (this.riskEvidenceSynthesisTarget == null) 791 if (Configuration.errorOnAutoCreate()) 792 throw new Error( 793 "Attempt to auto-create EffectEvidenceSynthesisResultsByExposureComponent.riskEvidenceSynthesis"); 794 else if (Configuration.doAutoCreate()) 795 this.riskEvidenceSynthesisTarget = new RiskEvidenceSynthesis(); // aa 796 return this.riskEvidenceSynthesisTarget; 797 } 798 799 /** 800 * @param value {@link #riskEvidenceSynthesis} The actual object that is the 801 * target of the reference. The reference library doesn't use 802 * these, but you can use it to hold the resource if you resolve 803 * it. (Reference to a RiskEvidenceSynthesis resource.) 804 */ 805 public EffectEvidenceSynthesisResultsByExposureComponent setRiskEvidenceSynthesisTarget( 806 RiskEvidenceSynthesis value) { 807 this.riskEvidenceSynthesisTarget = value; 808 return this; 809 } 810 811 protected void listChildren(List<Property> children) { 812 super.listChildren(children); 813 children.add(new Property("description", "string", "Human-readable summary of results by exposure state.", 0, 1, 814 description)); 815 children.add(new Property("exposureState", "code", 816 "Whether these results are for the exposure state or alternative exposure state.", 0, 1, exposureState)); 817 children.add(new Property("variantState", "CodeableConcept", 818 "Used to define variant exposure states such as low-risk state.", 0, 1, variantState)); 819 children.add(new Property("riskEvidenceSynthesis", "Reference(RiskEvidenceSynthesis)", 820 "Reference to a RiskEvidenceSynthesis resource.", 0, 1, riskEvidenceSynthesis)); 821 } 822 823 @Override 824 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 825 switch (_hash) { 826 case -1724546052: 827 /* description */ return new Property("description", "string", 828 "Human-readable summary of results by exposure state.", 0, 1, description); 829 case 422339530: 830 /* exposureState */ return new Property("exposureState", "code", 831 "Whether these results are for the exposure state or alternative exposure state.", 0, 1, exposureState); 832 case 1900629772: 833 /* variantState */ return new Property("variantState", "CodeableConcept", 834 "Used to define variant exposure states such as low-risk state.", 0, 1, variantState); 835 case 109085678: 836 /* riskEvidenceSynthesis */ return new Property("riskEvidenceSynthesis", "Reference(RiskEvidenceSynthesis)", 837 "Reference to a RiskEvidenceSynthesis resource.", 0, 1, riskEvidenceSynthesis); 838 default: 839 return super.getNamedProperty(_hash, _name, _checkValid); 840 } 841 842 } 843 844 @Override 845 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 846 switch (hash) { 847 case -1724546052: 848 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 849 case 422339530: 850 /* exposureState */ return this.exposureState == null ? new Base[0] : new Base[] { this.exposureState }; // Enumeration<ExposureState> 851 case 1900629772: 852 /* variantState */ return this.variantState == null ? new Base[0] : new Base[] { this.variantState }; // CodeableConcept 853 case 109085678: 854 /* riskEvidenceSynthesis */ return this.riskEvidenceSynthesis == null ? new Base[0] 855 : new Base[] { this.riskEvidenceSynthesis }; // Reference 856 default: 857 return super.getProperty(hash, name, checkValid); 858 } 859 860 } 861 862 @Override 863 public Base setProperty(int hash, String name, Base value) throws FHIRException { 864 switch (hash) { 865 case -1724546052: // description 866 this.description = castToString(value); // StringType 867 return value; 868 case 422339530: // exposureState 869 value = new ExposureStateEnumFactory().fromType(castToCode(value)); 870 this.exposureState = (Enumeration) value; // Enumeration<ExposureState> 871 return value; 872 case 1900629772: // variantState 873 this.variantState = castToCodeableConcept(value); // CodeableConcept 874 return value; 875 case 109085678: // riskEvidenceSynthesis 876 this.riskEvidenceSynthesis = castToReference(value); // Reference 877 return value; 878 default: 879 return super.setProperty(hash, name, value); 880 } 881 882 } 883 884 @Override 885 public Base setProperty(String name, Base value) throws FHIRException { 886 if (name.equals("description")) { 887 this.description = castToString(value); // StringType 888 } else if (name.equals("exposureState")) { 889 value = new ExposureStateEnumFactory().fromType(castToCode(value)); 890 this.exposureState = (Enumeration) value; // Enumeration<ExposureState> 891 } else if (name.equals("variantState")) { 892 this.variantState = castToCodeableConcept(value); // CodeableConcept 893 } else if (name.equals("riskEvidenceSynthesis")) { 894 this.riskEvidenceSynthesis = castToReference(value); // Reference 895 } else 896 return super.setProperty(name, value); 897 return value; 898 } 899 900 @Override 901 public void removeChild(String name, Base value) throws FHIRException { 902 if (name.equals("description")) { 903 this.description = null; 904 } else if (name.equals("exposureState")) { 905 this.exposureState = null; 906 } else if (name.equals("variantState")) { 907 this.variantState = null; 908 } else if (name.equals("riskEvidenceSynthesis")) { 909 this.riskEvidenceSynthesis = null; 910 } else 911 super.removeChild(name, value); 912 913 } 914 915 @Override 916 public Base makeProperty(int hash, String name) throws FHIRException { 917 switch (hash) { 918 case -1724546052: 919 return getDescriptionElement(); 920 case 422339530: 921 return getExposureStateElement(); 922 case 1900629772: 923 return getVariantState(); 924 case 109085678: 925 return getRiskEvidenceSynthesis(); 926 default: 927 return super.makeProperty(hash, name); 928 } 929 930 } 931 932 @Override 933 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 934 switch (hash) { 935 case -1724546052: 936 /* description */ return new String[] { "string" }; 937 case 422339530: 938 /* exposureState */ return new String[] { "code" }; 939 case 1900629772: 940 /* variantState */ return new String[] { "CodeableConcept" }; 941 case 109085678: 942 /* riskEvidenceSynthesis */ return new String[] { "Reference" }; 943 default: 944 return super.getTypesForProperty(hash, name); 945 } 946 947 } 948 949 @Override 950 public Base addChild(String name) throws FHIRException { 951 if (name.equals("description")) { 952 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.description"); 953 } else if (name.equals("exposureState")) { 954 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.exposureState"); 955 } else if (name.equals("variantState")) { 956 this.variantState = new CodeableConcept(); 957 return this.variantState; 958 } else if (name.equals("riskEvidenceSynthesis")) { 959 this.riskEvidenceSynthesis = new Reference(); 960 return this.riskEvidenceSynthesis; 961 } else 962 return super.addChild(name); 963 } 964 965 public EffectEvidenceSynthesisResultsByExposureComponent copy() { 966 EffectEvidenceSynthesisResultsByExposureComponent dst = new EffectEvidenceSynthesisResultsByExposureComponent(); 967 copyValues(dst); 968 return dst; 969 } 970 971 public void copyValues(EffectEvidenceSynthesisResultsByExposureComponent dst) { 972 super.copyValues(dst); 973 dst.description = description == null ? null : description.copy(); 974 dst.exposureState = exposureState == null ? null : exposureState.copy(); 975 dst.variantState = variantState == null ? null : variantState.copy(); 976 dst.riskEvidenceSynthesis = riskEvidenceSynthesis == null ? null : riskEvidenceSynthesis.copy(); 977 } 978 979 @Override 980 public boolean equalsDeep(Base other_) { 981 if (!super.equalsDeep(other_)) 982 return false; 983 if (!(other_ instanceof EffectEvidenceSynthesisResultsByExposureComponent)) 984 return false; 985 EffectEvidenceSynthesisResultsByExposureComponent o = (EffectEvidenceSynthesisResultsByExposureComponent) other_; 986 return compareDeep(description, o.description, true) && compareDeep(exposureState, o.exposureState, true) 987 && compareDeep(variantState, o.variantState, true) 988 && compareDeep(riskEvidenceSynthesis, o.riskEvidenceSynthesis, true); 989 } 990 991 @Override 992 public boolean equalsShallow(Base other_) { 993 if (!super.equalsShallow(other_)) 994 return false; 995 if (!(other_ instanceof EffectEvidenceSynthesisResultsByExposureComponent)) 996 return false; 997 EffectEvidenceSynthesisResultsByExposureComponent o = (EffectEvidenceSynthesisResultsByExposureComponent) other_; 998 return compareValues(description, o.description, true) && compareValues(exposureState, o.exposureState, true); 999 } 1000 1001 public boolean isEmpty() { 1002 return super.isEmpty() 1003 && ca.uhn.fhir.util.ElementUtil.isEmpty(description, exposureState, variantState, riskEvidenceSynthesis); 1004 } 1005 1006 public String fhirType() { 1007 return "EffectEvidenceSynthesis.resultsByExposure"; 1008 1009 } 1010 1011 } 1012 1013 @Block() 1014 public static class EffectEvidenceSynthesisEffectEstimateComponent extends BackboneElement 1015 implements IBaseBackboneElement { 1016 /** 1017 * Human-readable summary of effect estimate. 1018 */ 1019 @Child(name = "description", type = { 1020 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1021 @Description(shortDefinition = "Description of effect estimate", formalDefinition = "Human-readable summary of effect estimate.") 1022 protected StringType description; 1023 1024 /** 1025 * Examples include relative risk and mean difference. 1026 */ 1027 @Child(name = "type", type = { 1028 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1029 @Description(shortDefinition = "Type of efffect estimate", formalDefinition = "Examples include relative risk and mean difference.") 1030 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/effect-estimate-type") 1031 protected CodeableConcept type; 1032 1033 /** 1034 * Used to define variant exposure states such as low-risk state. 1035 */ 1036 @Child(name = "variantState", type = { 1037 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1038 @Description(shortDefinition = "Variant exposure states", formalDefinition = "Used to define variant exposure states such as low-risk state.") 1039 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/evidence-variant-state") 1040 protected CodeableConcept variantState; 1041 1042 /** 1043 * The point estimate of the effect estimate. 1044 */ 1045 @Child(name = "value", type = { DecimalType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1046 @Description(shortDefinition = "Point estimate", formalDefinition = "The point estimate of the effect estimate.") 1047 protected DecimalType value; 1048 1049 /** 1050 * Specifies the UCUM unit for the outcome. 1051 */ 1052 @Child(name = "unitOfMeasure", type = { 1053 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1054 @Description(shortDefinition = "What unit is the outcome described in?", formalDefinition = "Specifies the UCUM unit for the outcome.") 1055 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ucum-units") 1056 protected CodeableConcept unitOfMeasure; 1057 1058 /** 1059 * A description of the precision of the estimate for the effect. 1060 */ 1061 @Child(name = "precisionEstimate", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1062 @Description(shortDefinition = "How precise the estimate is", formalDefinition = "A description of the precision of the estimate for the effect.") 1063 protected List<EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent> precisionEstimate; 1064 1065 private static final long serialVersionUID = -1075065083L; 1066 1067 /** 1068 * Constructor 1069 */ 1070 public EffectEvidenceSynthesisEffectEstimateComponent() { 1071 super(); 1072 } 1073 1074 /** 1075 * @return {@link #description} (Human-readable summary of effect estimate.). 1076 * This is the underlying object with id, value and extensions. The 1077 * accessor "getDescription" gives direct access to the value 1078 */ 1079 public StringType getDescriptionElement() { 1080 if (this.description == null) 1081 if (Configuration.errorOnAutoCreate()) 1082 throw new Error("Attempt to auto-create EffectEvidenceSynthesisEffectEstimateComponent.description"); 1083 else if (Configuration.doAutoCreate()) 1084 this.description = new StringType(); // bb 1085 return this.description; 1086 } 1087 1088 public boolean hasDescriptionElement() { 1089 return this.description != null && !this.description.isEmpty(); 1090 } 1091 1092 public boolean hasDescription() { 1093 return this.description != null && !this.description.isEmpty(); 1094 } 1095 1096 /** 1097 * @param value {@link #description} (Human-readable summary of effect 1098 * estimate.). This is the underlying object with id, value and 1099 * extensions. The accessor "getDescription" gives direct access to 1100 * the value 1101 */ 1102 public EffectEvidenceSynthesisEffectEstimateComponent setDescriptionElement(StringType value) { 1103 this.description = value; 1104 return this; 1105 } 1106 1107 /** 1108 * @return Human-readable summary of effect estimate. 1109 */ 1110 public String getDescription() { 1111 return this.description == null ? null : this.description.getValue(); 1112 } 1113 1114 /** 1115 * @param value Human-readable summary of effect estimate. 1116 */ 1117 public EffectEvidenceSynthesisEffectEstimateComponent setDescription(String value) { 1118 if (Utilities.noString(value)) 1119 this.description = null; 1120 else { 1121 if (this.description == null) 1122 this.description = new StringType(); 1123 this.description.setValue(value); 1124 } 1125 return this; 1126 } 1127 1128 /** 1129 * @return {@link #type} (Examples include relative risk and mean difference.) 1130 */ 1131 public CodeableConcept getType() { 1132 if (this.type == null) 1133 if (Configuration.errorOnAutoCreate()) 1134 throw new Error("Attempt to auto-create EffectEvidenceSynthesisEffectEstimateComponent.type"); 1135 else if (Configuration.doAutoCreate()) 1136 this.type = new CodeableConcept(); // cc 1137 return this.type; 1138 } 1139 1140 public boolean hasType() { 1141 return this.type != null && !this.type.isEmpty(); 1142 } 1143 1144 /** 1145 * @param value {@link #type} (Examples include relative risk and mean 1146 * difference.) 1147 */ 1148 public EffectEvidenceSynthesisEffectEstimateComponent setType(CodeableConcept value) { 1149 this.type = value; 1150 return this; 1151 } 1152 1153 /** 1154 * @return {@link #variantState} (Used to define variant exposure states such as 1155 * low-risk state.) 1156 */ 1157 public CodeableConcept getVariantState() { 1158 if (this.variantState == null) 1159 if (Configuration.errorOnAutoCreate()) 1160 throw new Error("Attempt to auto-create EffectEvidenceSynthesisEffectEstimateComponent.variantState"); 1161 else if (Configuration.doAutoCreate()) 1162 this.variantState = new CodeableConcept(); // cc 1163 return this.variantState; 1164 } 1165 1166 public boolean hasVariantState() { 1167 return this.variantState != null && !this.variantState.isEmpty(); 1168 } 1169 1170 /** 1171 * @param value {@link #variantState} (Used to define variant exposure states 1172 * such as low-risk state.) 1173 */ 1174 public EffectEvidenceSynthesisEffectEstimateComponent setVariantState(CodeableConcept value) { 1175 this.variantState = value; 1176 return this; 1177 } 1178 1179 /** 1180 * @return {@link #value} (The point estimate of the effect estimate.). This is 1181 * the underlying object with id, value and extensions. The accessor 1182 * "getValue" gives direct access to the value 1183 */ 1184 public DecimalType getValueElement() { 1185 if (this.value == null) 1186 if (Configuration.errorOnAutoCreate()) 1187 throw new Error("Attempt to auto-create EffectEvidenceSynthesisEffectEstimateComponent.value"); 1188 else if (Configuration.doAutoCreate()) 1189 this.value = new DecimalType(); // bb 1190 return this.value; 1191 } 1192 1193 public boolean hasValueElement() { 1194 return this.value != null && !this.value.isEmpty(); 1195 } 1196 1197 public boolean hasValue() { 1198 return this.value != null && !this.value.isEmpty(); 1199 } 1200 1201 /** 1202 * @param value {@link #value} (The point estimate of the effect estimate.). 1203 * This is the underlying object with id, value and extensions. The 1204 * accessor "getValue" gives direct access to the value 1205 */ 1206 public EffectEvidenceSynthesisEffectEstimateComponent setValueElement(DecimalType value) { 1207 this.value = value; 1208 return this; 1209 } 1210 1211 /** 1212 * @return The point estimate of the effect estimate. 1213 */ 1214 public BigDecimal getValue() { 1215 return this.value == null ? null : this.value.getValue(); 1216 } 1217 1218 /** 1219 * @param value The point estimate of the effect estimate. 1220 */ 1221 public EffectEvidenceSynthesisEffectEstimateComponent setValue(BigDecimal value) { 1222 if (value == null) 1223 this.value = null; 1224 else { 1225 if (this.value == null) 1226 this.value = new DecimalType(); 1227 this.value.setValue(value); 1228 } 1229 return this; 1230 } 1231 1232 /** 1233 * @param value The point estimate of the effect estimate. 1234 */ 1235 public EffectEvidenceSynthesisEffectEstimateComponent setValue(long value) { 1236 this.value = new DecimalType(); 1237 this.value.setValue(value); 1238 return this; 1239 } 1240 1241 /** 1242 * @param value The point estimate of the effect estimate. 1243 */ 1244 public EffectEvidenceSynthesisEffectEstimateComponent setValue(double value) { 1245 this.value = new DecimalType(); 1246 this.value.setValue(value); 1247 return this; 1248 } 1249 1250 /** 1251 * @return {@link #unitOfMeasure} (Specifies the UCUM unit for the outcome.) 1252 */ 1253 public CodeableConcept getUnitOfMeasure() { 1254 if (this.unitOfMeasure == null) 1255 if (Configuration.errorOnAutoCreate()) 1256 throw new Error("Attempt to auto-create EffectEvidenceSynthesisEffectEstimateComponent.unitOfMeasure"); 1257 else if (Configuration.doAutoCreate()) 1258 this.unitOfMeasure = new CodeableConcept(); // cc 1259 return this.unitOfMeasure; 1260 } 1261 1262 public boolean hasUnitOfMeasure() { 1263 return this.unitOfMeasure != null && !this.unitOfMeasure.isEmpty(); 1264 } 1265 1266 /** 1267 * @param value {@link #unitOfMeasure} (Specifies the UCUM unit for the 1268 * outcome.) 1269 */ 1270 public EffectEvidenceSynthesisEffectEstimateComponent setUnitOfMeasure(CodeableConcept value) { 1271 this.unitOfMeasure = value; 1272 return this; 1273 } 1274 1275 /** 1276 * @return {@link #precisionEstimate} (A description of the precision of the 1277 * estimate for the effect.) 1278 */ 1279 public List<EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent> getPrecisionEstimate() { 1280 if (this.precisionEstimate == null) 1281 this.precisionEstimate = new ArrayList<EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent>(); 1282 return this.precisionEstimate; 1283 } 1284 1285 /** 1286 * @return Returns a reference to <code>this</code> for easy method chaining 1287 */ 1288 public EffectEvidenceSynthesisEffectEstimateComponent setPrecisionEstimate( 1289 List<EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent> thePrecisionEstimate) { 1290 this.precisionEstimate = thePrecisionEstimate; 1291 return this; 1292 } 1293 1294 public boolean hasPrecisionEstimate() { 1295 if (this.precisionEstimate == null) 1296 return false; 1297 for (EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent item : this.precisionEstimate) 1298 if (!item.isEmpty()) 1299 return true; 1300 return false; 1301 } 1302 1303 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent addPrecisionEstimate() { // 3 1304 EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent t = new EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent(); 1305 if (this.precisionEstimate == null) 1306 this.precisionEstimate = new ArrayList<EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent>(); 1307 this.precisionEstimate.add(t); 1308 return t; 1309 } 1310 1311 public EffectEvidenceSynthesisEffectEstimateComponent addPrecisionEstimate( 1312 EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent t) { // 3 1313 if (t == null) 1314 return this; 1315 if (this.precisionEstimate == null) 1316 this.precisionEstimate = new ArrayList<EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent>(); 1317 this.precisionEstimate.add(t); 1318 return this; 1319 } 1320 1321 /** 1322 * @return The first repetition of repeating field {@link #precisionEstimate}, 1323 * creating it if it does not already exist 1324 */ 1325 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent getPrecisionEstimateFirstRep() { 1326 if (getPrecisionEstimate().isEmpty()) { 1327 addPrecisionEstimate(); 1328 } 1329 return getPrecisionEstimate().get(0); 1330 } 1331 1332 protected void listChildren(List<Property> children) { 1333 super.listChildren(children); 1334 children 1335 .add(new Property("description", "string", "Human-readable summary of effect estimate.", 0, 1, description)); 1336 children.add( 1337 new Property("type", "CodeableConcept", "Examples include relative risk and mean difference.", 0, 1, type)); 1338 children.add(new Property("variantState", "CodeableConcept", 1339 "Used to define variant exposure states such as low-risk state.", 0, 1, variantState)); 1340 children.add(new Property("value", "decimal", "The point estimate of the effect estimate.", 0, 1, value)); 1341 children.add(new Property("unitOfMeasure", "CodeableConcept", "Specifies the UCUM unit for the outcome.", 0, 1, 1342 unitOfMeasure)); 1343 children 1344 .add(new Property("precisionEstimate", "", "A description of the precision of the estimate for the effect.", 1345 0, java.lang.Integer.MAX_VALUE, precisionEstimate)); 1346 } 1347 1348 @Override 1349 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1350 switch (_hash) { 1351 case -1724546052: 1352 /* description */ return new Property("description", "string", "Human-readable summary of effect estimate.", 0, 1353 1, description); 1354 case 3575610: 1355 /* type */ return new Property("type", "CodeableConcept", "Examples include relative risk and mean difference.", 1356 0, 1, type); 1357 case 1900629772: 1358 /* variantState */ return new Property("variantState", "CodeableConcept", 1359 "Used to define variant exposure states such as low-risk state.", 0, 1, variantState); 1360 case 111972721: 1361 /* value */ return new Property("value", "decimal", "The point estimate of the effect estimate.", 0, 1, value); 1362 case -750257565: 1363 /* unitOfMeasure */ return new Property("unitOfMeasure", "CodeableConcept", 1364 "Specifies the UCUM unit for the outcome.", 0, 1, unitOfMeasure); 1365 case 339632070: 1366 /* precisionEstimate */ return new Property("precisionEstimate", "", 1367 "A description of the precision of the estimate for the effect.", 0, java.lang.Integer.MAX_VALUE, 1368 precisionEstimate); 1369 default: 1370 return super.getNamedProperty(_hash, _name, _checkValid); 1371 } 1372 1373 } 1374 1375 @Override 1376 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1377 switch (hash) { 1378 case -1724546052: 1379 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1380 case 3575610: 1381 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1382 case 1900629772: 1383 /* variantState */ return this.variantState == null ? new Base[0] : new Base[] { this.variantState }; // CodeableConcept 1384 case 111972721: 1385 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // DecimalType 1386 case -750257565: 1387 /* unitOfMeasure */ return this.unitOfMeasure == null ? new Base[0] : new Base[] { this.unitOfMeasure }; // CodeableConcept 1388 case 339632070: 1389 /* precisionEstimate */ return this.precisionEstimate == null ? new Base[0] 1390 : this.precisionEstimate.toArray(new Base[this.precisionEstimate.size()]); // EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent 1391 default: 1392 return super.getProperty(hash, name, checkValid); 1393 } 1394 1395 } 1396 1397 @Override 1398 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1399 switch (hash) { 1400 case -1724546052: // description 1401 this.description = castToString(value); // StringType 1402 return value; 1403 case 3575610: // type 1404 this.type = castToCodeableConcept(value); // CodeableConcept 1405 return value; 1406 case 1900629772: // variantState 1407 this.variantState = castToCodeableConcept(value); // CodeableConcept 1408 return value; 1409 case 111972721: // value 1410 this.value = castToDecimal(value); // DecimalType 1411 return value; 1412 case -750257565: // unitOfMeasure 1413 this.unitOfMeasure = castToCodeableConcept(value); // CodeableConcept 1414 return value; 1415 case 339632070: // precisionEstimate 1416 this.getPrecisionEstimate().add((EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent) value); // EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent 1417 return value; 1418 default: 1419 return super.setProperty(hash, name, value); 1420 } 1421 1422 } 1423 1424 @Override 1425 public Base setProperty(String name, Base value) throws FHIRException { 1426 if (name.equals("description")) { 1427 this.description = castToString(value); // StringType 1428 } else if (name.equals("type")) { 1429 this.type = castToCodeableConcept(value); // CodeableConcept 1430 } else if (name.equals("variantState")) { 1431 this.variantState = castToCodeableConcept(value); // CodeableConcept 1432 } else if (name.equals("value")) { 1433 this.value = castToDecimal(value); // DecimalType 1434 } else if (name.equals("unitOfMeasure")) { 1435 this.unitOfMeasure = castToCodeableConcept(value); // CodeableConcept 1436 } else if (name.equals("precisionEstimate")) { 1437 this.getPrecisionEstimate().add((EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent) value); 1438 } else 1439 return super.setProperty(name, value); 1440 return value; 1441 } 1442 1443 @Override 1444 public void removeChild(String name, Base value) throws FHIRException { 1445 if (name.equals("description")) { 1446 this.description = null; 1447 } else if (name.equals("type")) { 1448 this.type = null; 1449 } else if (name.equals("variantState")) { 1450 this.variantState = null; 1451 } else if (name.equals("value")) { 1452 this.value = null; 1453 } else if (name.equals("unitOfMeasure")) { 1454 this.unitOfMeasure = null; 1455 } else if (name.equals("precisionEstimate")) { 1456 this.getPrecisionEstimate().remove((EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent) value); 1457 } else 1458 super.removeChild(name, value); 1459 1460 } 1461 1462 @Override 1463 public Base makeProperty(int hash, String name) throws FHIRException { 1464 switch (hash) { 1465 case -1724546052: 1466 return getDescriptionElement(); 1467 case 3575610: 1468 return getType(); 1469 case 1900629772: 1470 return getVariantState(); 1471 case 111972721: 1472 return getValueElement(); 1473 case -750257565: 1474 return getUnitOfMeasure(); 1475 case 339632070: 1476 return addPrecisionEstimate(); 1477 default: 1478 return super.makeProperty(hash, name); 1479 } 1480 1481 } 1482 1483 @Override 1484 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1485 switch (hash) { 1486 case -1724546052: 1487 /* description */ return new String[] { "string" }; 1488 case 3575610: 1489 /* type */ return new String[] { "CodeableConcept" }; 1490 case 1900629772: 1491 /* variantState */ return new String[] { "CodeableConcept" }; 1492 case 111972721: 1493 /* value */ return new String[] { "decimal" }; 1494 case -750257565: 1495 /* unitOfMeasure */ return new String[] { "CodeableConcept" }; 1496 case 339632070: 1497 /* precisionEstimate */ return new String[] {}; 1498 default: 1499 return super.getTypesForProperty(hash, name); 1500 } 1501 1502 } 1503 1504 @Override 1505 public Base addChild(String name) throws FHIRException { 1506 if (name.equals("description")) { 1507 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.description"); 1508 } else if (name.equals("type")) { 1509 this.type = new CodeableConcept(); 1510 return this.type; 1511 } else if (name.equals("variantState")) { 1512 this.variantState = new CodeableConcept(); 1513 return this.variantState; 1514 } else if (name.equals("value")) { 1515 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.value"); 1516 } else if (name.equals("unitOfMeasure")) { 1517 this.unitOfMeasure = new CodeableConcept(); 1518 return this.unitOfMeasure; 1519 } else if (name.equals("precisionEstimate")) { 1520 return addPrecisionEstimate(); 1521 } else 1522 return super.addChild(name); 1523 } 1524 1525 public EffectEvidenceSynthesisEffectEstimateComponent copy() { 1526 EffectEvidenceSynthesisEffectEstimateComponent dst = new EffectEvidenceSynthesisEffectEstimateComponent(); 1527 copyValues(dst); 1528 return dst; 1529 } 1530 1531 public void copyValues(EffectEvidenceSynthesisEffectEstimateComponent dst) { 1532 super.copyValues(dst); 1533 dst.description = description == null ? null : description.copy(); 1534 dst.type = type == null ? null : type.copy(); 1535 dst.variantState = variantState == null ? null : variantState.copy(); 1536 dst.value = value == null ? null : value.copy(); 1537 dst.unitOfMeasure = unitOfMeasure == null ? null : unitOfMeasure.copy(); 1538 if (precisionEstimate != null) { 1539 dst.precisionEstimate = new ArrayList<EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent>(); 1540 for (EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent i : precisionEstimate) 1541 dst.precisionEstimate.add(i.copy()); 1542 } 1543 ; 1544 } 1545 1546 @Override 1547 public boolean equalsDeep(Base other_) { 1548 if (!super.equalsDeep(other_)) 1549 return false; 1550 if (!(other_ instanceof EffectEvidenceSynthesisEffectEstimateComponent)) 1551 return false; 1552 EffectEvidenceSynthesisEffectEstimateComponent o = (EffectEvidenceSynthesisEffectEstimateComponent) other_; 1553 return compareDeep(description, o.description, true) && compareDeep(type, o.type, true) 1554 && compareDeep(variantState, o.variantState, true) && compareDeep(value, o.value, true) 1555 && compareDeep(unitOfMeasure, o.unitOfMeasure, true) 1556 && compareDeep(precisionEstimate, o.precisionEstimate, true); 1557 } 1558 1559 @Override 1560 public boolean equalsShallow(Base other_) { 1561 if (!super.equalsShallow(other_)) 1562 return false; 1563 if (!(other_ instanceof EffectEvidenceSynthesisEffectEstimateComponent)) 1564 return false; 1565 EffectEvidenceSynthesisEffectEstimateComponent o = (EffectEvidenceSynthesisEffectEstimateComponent) other_; 1566 return compareValues(description, o.description, true) && compareValues(value, o.value, true); 1567 } 1568 1569 public boolean isEmpty() { 1570 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, type, variantState, value, 1571 unitOfMeasure, precisionEstimate); 1572 } 1573 1574 public String fhirType() { 1575 return "EffectEvidenceSynthesis.effectEstimate"; 1576 1577 } 1578 1579 } 1580 1581 @Block() 1582 public static class EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent extends BackboneElement 1583 implements IBaseBackboneElement { 1584 /** 1585 * Examples include confidence interval and interquartile range. 1586 */ 1587 @Child(name = "type", type = { 1588 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1589 @Description(shortDefinition = "Type of precision estimate", formalDefinition = "Examples include confidence interval and interquartile range.") 1590 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/precision-estimate-type") 1591 protected CodeableConcept type; 1592 1593 /** 1594 * Use 95 for a 95% confidence interval. 1595 */ 1596 @Child(name = "level", type = { DecimalType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1597 @Description(shortDefinition = "Level of confidence interval", formalDefinition = "Use 95 for a 95% confidence interval.") 1598 protected DecimalType level; 1599 1600 /** 1601 * Lower bound of confidence interval. 1602 */ 1603 @Child(name = "from", type = { DecimalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1604 @Description(shortDefinition = "Lower bound", formalDefinition = "Lower bound of confidence interval.") 1605 protected DecimalType from; 1606 1607 /** 1608 * Upper bound of confidence interval. 1609 */ 1610 @Child(name = "to", type = { DecimalType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1611 @Description(shortDefinition = "Upper bound", formalDefinition = "Upper bound of confidence interval.") 1612 protected DecimalType to; 1613 1614 private static final long serialVersionUID = -110178057L; 1615 1616 /** 1617 * Constructor 1618 */ 1619 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent() { 1620 super(); 1621 } 1622 1623 /** 1624 * @return {@link #type} (Examples include confidence interval and interquartile 1625 * range.) 1626 */ 1627 public CodeableConcept getType() { 1628 if (this.type == null) 1629 if (Configuration.errorOnAutoCreate()) 1630 throw new Error( 1631 "Attempt to auto-create EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent.type"); 1632 else if (Configuration.doAutoCreate()) 1633 this.type = new CodeableConcept(); // cc 1634 return this.type; 1635 } 1636 1637 public boolean hasType() { 1638 return this.type != null && !this.type.isEmpty(); 1639 } 1640 1641 /** 1642 * @param value {@link #type} (Examples include confidence interval and 1643 * interquartile range.) 1644 */ 1645 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent setType(CodeableConcept value) { 1646 this.type = value; 1647 return this; 1648 } 1649 1650 /** 1651 * @return {@link #level} (Use 95 for a 95% confidence interval.). This is the 1652 * underlying object with id, value and extensions. The accessor 1653 * "getLevel" gives direct access to the value 1654 */ 1655 public DecimalType getLevelElement() { 1656 if (this.level == null) 1657 if (Configuration.errorOnAutoCreate()) 1658 throw new Error( 1659 "Attempt to auto-create EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent.level"); 1660 else if (Configuration.doAutoCreate()) 1661 this.level = new DecimalType(); // bb 1662 return this.level; 1663 } 1664 1665 public boolean hasLevelElement() { 1666 return this.level != null && !this.level.isEmpty(); 1667 } 1668 1669 public boolean hasLevel() { 1670 return this.level != null && !this.level.isEmpty(); 1671 } 1672 1673 /** 1674 * @param value {@link #level} (Use 95 for a 95% confidence interval.). This is 1675 * the underlying object with id, value and extensions. The 1676 * accessor "getLevel" gives direct access to the value 1677 */ 1678 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent setLevelElement(DecimalType value) { 1679 this.level = value; 1680 return this; 1681 } 1682 1683 /** 1684 * @return Use 95 for a 95% confidence interval. 1685 */ 1686 public BigDecimal getLevel() { 1687 return this.level == null ? null : this.level.getValue(); 1688 } 1689 1690 /** 1691 * @param value Use 95 for a 95% confidence interval. 1692 */ 1693 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent setLevel(BigDecimal value) { 1694 if (value == null) 1695 this.level = null; 1696 else { 1697 if (this.level == null) 1698 this.level = new DecimalType(); 1699 this.level.setValue(value); 1700 } 1701 return this; 1702 } 1703 1704 /** 1705 * @param value Use 95 for a 95% confidence interval. 1706 */ 1707 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent setLevel(long value) { 1708 this.level = new DecimalType(); 1709 this.level.setValue(value); 1710 return this; 1711 } 1712 1713 /** 1714 * @param value Use 95 for a 95% confidence interval. 1715 */ 1716 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent setLevel(double value) { 1717 this.level = new DecimalType(); 1718 this.level.setValue(value); 1719 return this; 1720 } 1721 1722 /** 1723 * @return {@link #from} (Lower bound of confidence interval.). This is the 1724 * underlying object with id, value and extensions. The accessor 1725 * "getFrom" gives direct access to the value 1726 */ 1727 public DecimalType getFromElement() { 1728 if (this.from == null) 1729 if (Configuration.errorOnAutoCreate()) 1730 throw new Error( 1731 "Attempt to auto-create EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent.from"); 1732 else if (Configuration.doAutoCreate()) 1733 this.from = new DecimalType(); // bb 1734 return this.from; 1735 } 1736 1737 public boolean hasFromElement() { 1738 return this.from != null && !this.from.isEmpty(); 1739 } 1740 1741 public boolean hasFrom() { 1742 return this.from != null && !this.from.isEmpty(); 1743 } 1744 1745 /** 1746 * @param value {@link #from} (Lower bound of confidence interval.). This is the 1747 * underlying object with id, value and extensions. The accessor 1748 * "getFrom" gives direct access to the value 1749 */ 1750 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent setFromElement(DecimalType value) { 1751 this.from = value; 1752 return this; 1753 } 1754 1755 /** 1756 * @return Lower bound of confidence interval. 1757 */ 1758 public BigDecimal getFrom() { 1759 return this.from == null ? null : this.from.getValue(); 1760 } 1761 1762 /** 1763 * @param value Lower bound of confidence interval. 1764 */ 1765 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent setFrom(BigDecimal value) { 1766 if (value == null) 1767 this.from = null; 1768 else { 1769 if (this.from == null) 1770 this.from = new DecimalType(); 1771 this.from.setValue(value); 1772 } 1773 return this; 1774 } 1775 1776 /** 1777 * @param value Lower bound of confidence interval. 1778 */ 1779 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent setFrom(long value) { 1780 this.from = new DecimalType(); 1781 this.from.setValue(value); 1782 return this; 1783 } 1784 1785 /** 1786 * @param value Lower bound of confidence interval. 1787 */ 1788 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent setFrom(double value) { 1789 this.from = new DecimalType(); 1790 this.from.setValue(value); 1791 return this; 1792 } 1793 1794 /** 1795 * @return {@link #to} (Upper bound of confidence interval.). This is the 1796 * underlying object with id, value and extensions. The accessor "getTo" 1797 * gives direct access to the value 1798 */ 1799 public DecimalType getToElement() { 1800 if (this.to == null) 1801 if (Configuration.errorOnAutoCreate()) 1802 throw new Error("Attempt to auto-create EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent.to"); 1803 else if (Configuration.doAutoCreate()) 1804 this.to = new DecimalType(); // bb 1805 return this.to; 1806 } 1807 1808 public boolean hasToElement() { 1809 return this.to != null && !this.to.isEmpty(); 1810 } 1811 1812 public boolean hasTo() { 1813 return this.to != null && !this.to.isEmpty(); 1814 } 1815 1816 /** 1817 * @param value {@link #to} (Upper bound of confidence interval.). This is the 1818 * underlying object with id, value and extensions. The accessor 1819 * "getTo" gives direct access to the value 1820 */ 1821 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent setToElement(DecimalType value) { 1822 this.to = value; 1823 return this; 1824 } 1825 1826 /** 1827 * @return Upper bound of confidence interval. 1828 */ 1829 public BigDecimal getTo() { 1830 return this.to == null ? null : this.to.getValue(); 1831 } 1832 1833 /** 1834 * @param value Upper bound of confidence interval. 1835 */ 1836 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent setTo(BigDecimal value) { 1837 if (value == null) 1838 this.to = null; 1839 else { 1840 if (this.to == null) 1841 this.to = new DecimalType(); 1842 this.to.setValue(value); 1843 } 1844 return this; 1845 } 1846 1847 /** 1848 * @param value Upper bound of confidence interval. 1849 */ 1850 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent setTo(long value) { 1851 this.to = new DecimalType(); 1852 this.to.setValue(value); 1853 return this; 1854 } 1855 1856 /** 1857 * @param value Upper bound of confidence interval. 1858 */ 1859 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent setTo(double value) { 1860 this.to = new DecimalType(); 1861 this.to.setValue(value); 1862 return this; 1863 } 1864 1865 protected void listChildren(List<Property> children) { 1866 super.listChildren(children); 1867 children.add(new Property("type", "CodeableConcept", 1868 "Examples include confidence interval and interquartile range.", 0, 1, type)); 1869 children.add(new Property("level", "decimal", "Use 95 for a 95% confidence interval.", 0, 1, level)); 1870 children.add(new Property("from", "decimal", "Lower bound of confidence interval.", 0, 1, from)); 1871 children.add(new Property("to", "decimal", "Upper bound of confidence interval.", 0, 1, to)); 1872 } 1873 1874 @Override 1875 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1876 switch (_hash) { 1877 case 3575610: 1878 /* type */ return new Property("type", "CodeableConcept", 1879 "Examples include confidence interval and interquartile range.", 0, 1, type); 1880 case 102865796: 1881 /* level */ return new Property("level", "decimal", "Use 95 for a 95% confidence interval.", 0, 1, level); 1882 case 3151786: 1883 /* from */ return new Property("from", "decimal", "Lower bound of confidence interval.", 0, 1, from); 1884 case 3707: 1885 /* to */ return new Property("to", "decimal", "Upper bound of confidence interval.", 0, 1, to); 1886 default: 1887 return super.getNamedProperty(_hash, _name, _checkValid); 1888 } 1889 1890 } 1891 1892 @Override 1893 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1894 switch (hash) { 1895 case 3575610: 1896 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1897 case 102865796: 1898 /* level */ return this.level == null ? new Base[0] : new Base[] { this.level }; // DecimalType 1899 case 3151786: 1900 /* from */ return this.from == null ? new Base[0] : new Base[] { this.from }; // DecimalType 1901 case 3707: 1902 /* to */ return this.to == null ? new Base[0] : new Base[] { this.to }; // DecimalType 1903 default: 1904 return super.getProperty(hash, name, checkValid); 1905 } 1906 1907 } 1908 1909 @Override 1910 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1911 switch (hash) { 1912 case 3575610: // type 1913 this.type = castToCodeableConcept(value); // CodeableConcept 1914 return value; 1915 case 102865796: // level 1916 this.level = castToDecimal(value); // DecimalType 1917 return value; 1918 case 3151786: // from 1919 this.from = castToDecimal(value); // DecimalType 1920 return value; 1921 case 3707: // to 1922 this.to = castToDecimal(value); // DecimalType 1923 return value; 1924 default: 1925 return super.setProperty(hash, name, value); 1926 } 1927 1928 } 1929 1930 @Override 1931 public Base setProperty(String name, Base value) throws FHIRException { 1932 if (name.equals("type")) { 1933 this.type = castToCodeableConcept(value); // CodeableConcept 1934 } else if (name.equals("level")) { 1935 this.level = castToDecimal(value); // DecimalType 1936 } else if (name.equals("from")) { 1937 this.from = castToDecimal(value); // DecimalType 1938 } else if (name.equals("to")) { 1939 this.to = castToDecimal(value); // DecimalType 1940 } else 1941 return super.setProperty(name, value); 1942 return value; 1943 } 1944 1945 @Override 1946 public void removeChild(String name, Base value) throws FHIRException { 1947 if (name.equals("type")) { 1948 this.type = null; 1949 } else if (name.equals("level")) { 1950 this.level = null; 1951 } else if (name.equals("from")) { 1952 this.from = null; 1953 } else if (name.equals("to")) { 1954 this.to = null; 1955 } else 1956 super.removeChild(name, value); 1957 1958 } 1959 1960 @Override 1961 public Base makeProperty(int hash, String name) throws FHIRException { 1962 switch (hash) { 1963 case 3575610: 1964 return getType(); 1965 case 102865796: 1966 return getLevelElement(); 1967 case 3151786: 1968 return getFromElement(); 1969 case 3707: 1970 return getToElement(); 1971 default: 1972 return super.makeProperty(hash, name); 1973 } 1974 1975 } 1976 1977 @Override 1978 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1979 switch (hash) { 1980 case 3575610: 1981 /* type */ return new String[] { "CodeableConcept" }; 1982 case 102865796: 1983 /* level */ return new String[] { "decimal" }; 1984 case 3151786: 1985 /* from */ return new String[] { "decimal" }; 1986 case 3707: 1987 /* to */ return new String[] { "decimal" }; 1988 default: 1989 return super.getTypesForProperty(hash, name); 1990 } 1991 1992 } 1993 1994 @Override 1995 public Base addChild(String name) throws FHIRException { 1996 if (name.equals("type")) { 1997 this.type = new CodeableConcept(); 1998 return this.type; 1999 } else if (name.equals("level")) { 2000 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.level"); 2001 } else if (name.equals("from")) { 2002 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.from"); 2003 } else if (name.equals("to")) { 2004 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.to"); 2005 } else 2006 return super.addChild(name); 2007 } 2008 2009 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent copy() { 2010 EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent dst = new EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent(); 2011 copyValues(dst); 2012 return dst; 2013 } 2014 2015 public void copyValues(EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent dst) { 2016 super.copyValues(dst); 2017 dst.type = type == null ? null : type.copy(); 2018 dst.level = level == null ? null : level.copy(); 2019 dst.from = from == null ? null : from.copy(); 2020 dst.to = to == null ? null : to.copy(); 2021 } 2022 2023 @Override 2024 public boolean equalsDeep(Base other_) { 2025 if (!super.equalsDeep(other_)) 2026 return false; 2027 if (!(other_ instanceof EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent)) 2028 return false; 2029 EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent o = (EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent) other_; 2030 return compareDeep(type, o.type, true) && compareDeep(level, o.level, true) && compareDeep(from, o.from, true) 2031 && compareDeep(to, o.to, true); 2032 } 2033 2034 @Override 2035 public boolean equalsShallow(Base other_) { 2036 if (!super.equalsShallow(other_)) 2037 return false; 2038 if (!(other_ instanceof EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent)) 2039 return false; 2040 EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent o = (EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent) other_; 2041 return compareValues(level, o.level, true) && compareValues(from, o.from, true) && compareValues(to, o.to, true); 2042 } 2043 2044 public boolean isEmpty() { 2045 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, level, from, to); 2046 } 2047 2048 public String fhirType() { 2049 return "EffectEvidenceSynthesis.effectEstimate.precisionEstimate"; 2050 2051 } 2052 2053 } 2054 2055 @Block() 2056 public static class EffectEvidenceSynthesisCertaintyComponent extends BackboneElement 2057 implements IBaseBackboneElement { 2058 /** 2059 * A rating of the certainty of the effect estimate. 2060 */ 2061 @Child(name = "rating", type = { 2062 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2063 @Description(shortDefinition = "Certainty rating", formalDefinition = "A rating of the certainty of the effect estimate.") 2064 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/evidence-quality") 2065 protected List<CodeableConcept> rating; 2066 2067 /** 2068 * A human-readable string to clarify or explain concepts about the resource. 2069 */ 2070 @Child(name = "note", type = { 2071 Annotation.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2072 @Description(shortDefinition = "Used for footnotes or explanatory notes", formalDefinition = "A human-readable string to clarify or explain concepts about the resource.") 2073 protected List<Annotation> note; 2074 2075 /** 2076 * A description of a component of the overall certainty. 2077 */ 2078 @Child(name = "certaintySubcomponent", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2079 @Description(shortDefinition = "A component that contributes to the overall certainty", formalDefinition = "A description of a component of the overall certainty.") 2080 protected List<EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent> certaintySubcomponent; 2081 2082 private static final long serialVersionUID = 647101417L; 2083 2084 /** 2085 * Constructor 2086 */ 2087 public EffectEvidenceSynthesisCertaintyComponent() { 2088 super(); 2089 } 2090 2091 /** 2092 * @return {@link #rating} (A rating of the certainty of the effect estimate.) 2093 */ 2094 public List<CodeableConcept> getRating() { 2095 if (this.rating == null) 2096 this.rating = new ArrayList<CodeableConcept>(); 2097 return this.rating; 2098 } 2099 2100 /** 2101 * @return Returns a reference to <code>this</code> for easy method chaining 2102 */ 2103 public EffectEvidenceSynthesisCertaintyComponent setRating(List<CodeableConcept> theRating) { 2104 this.rating = theRating; 2105 return this; 2106 } 2107 2108 public boolean hasRating() { 2109 if (this.rating == null) 2110 return false; 2111 for (CodeableConcept item : this.rating) 2112 if (!item.isEmpty()) 2113 return true; 2114 return false; 2115 } 2116 2117 public CodeableConcept addRating() { // 3 2118 CodeableConcept t = new CodeableConcept(); 2119 if (this.rating == null) 2120 this.rating = new ArrayList<CodeableConcept>(); 2121 this.rating.add(t); 2122 return t; 2123 } 2124 2125 public EffectEvidenceSynthesisCertaintyComponent addRating(CodeableConcept t) { // 3 2126 if (t == null) 2127 return this; 2128 if (this.rating == null) 2129 this.rating = new ArrayList<CodeableConcept>(); 2130 this.rating.add(t); 2131 return this; 2132 } 2133 2134 /** 2135 * @return The first repetition of repeating field {@link #rating}, creating it 2136 * if it does not already exist 2137 */ 2138 public CodeableConcept getRatingFirstRep() { 2139 if (getRating().isEmpty()) { 2140 addRating(); 2141 } 2142 return getRating().get(0); 2143 } 2144 2145 /** 2146 * @return {@link #note} (A human-readable string to clarify or explain concepts 2147 * about the resource.) 2148 */ 2149 public List<Annotation> getNote() { 2150 if (this.note == null) 2151 this.note = new ArrayList<Annotation>(); 2152 return this.note; 2153 } 2154 2155 /** 2156 * @return Returns a reference to <code>this</code> for easy method chaining 2157 */ 2158 public EffectEvidenceSynthesisCertaintyComponent setNote(List<Annotation> theNote) { 2159 this.note = theNote; 2160 return this; 2161 } 2162 2163 public boolean hasNote() { 2164 if (this.note == null) 2165 return false; 2166 for (Annotation item : this.note) 2167 if (!item.isEmpty()) 2168 return true; 2169 return false; 2170 } 2171 2172 public Annotation addNote() { // 3 2173 Annotation t = new Annotation(); 2174 if (this.note == null) 2175 this.note = new ArrayList<Annotation>(); 2176 this.note.add(t); 2177 return t; 2178 } 2179 2180 public EffectEvidenceSynthesisCertaintyComponent addNote(Annotation t) { // 3 2181 if (t == null) 2182 return this; 2183 if (this.note == null) 2184 this.note = new ArrayList<Annotation>(); 2185 this.note.add(t); 2186 return this; 2187 } 2188 2189 /** 2190 * @return The first repetition of repeating field {@link #note}, creating it if 2191 * it does not already exist 2192 */ 2193 public Annotation getNoteFirstRep() { 2194 if (getNote().isEmpty()) { 2195 addNote(); 2196 } 2197 return getNote().get(0); 2198 } 2199 2200 /** 2201 * @return {@link #certaintySubcomponent} (A description of a component of the 2202 * overall certainty.) 2203 */ 2204 public List<EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent> getCertaintySubcomponent() { 2205 if (this.certaintySubcomponent == null) 2206 this.certaintySubcomponent = new ArrayList<EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent>(); 2207 return this.certaintySubcomponent; 2208 } 2209 2210 /** 2211 * @return Returns a reference to <code>this</code> for easy method chaining 2212 */ 2213 public EffectEvidenceSynthesisCertaintyComponent setCertaintySubcomponent( 2214 List<EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent> theCertaintySubcomponent) { 2215 this.certaintySubcomponent = theCertaintySubcomponent; 2216 return this; 2217 } 2218 2219 public boolean hasCertaintySubcomponent() { 2220 if (this.certaintySubcomponent == null) 2221 return false; 2222 for (EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent item : this.certaintySubcomponent) 2223 if (!item.isEmpty()) 2224 return true; 2225 return false; 2226 } 2227 2228 public EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent addCertaintySubcomponent() { // 3 2229 EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent t = new EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent(); 2230 if (this.certaintySubcomponent == null) 2231 this.certaintySubcomponent = new ArrayList<EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent>(); 2232 this.certaintySubcomponent.add(t); 2233 return t; 2234 } 2235 2236 public EffectEvidenceSynthesisCertaintyComponent addCertaintySubcomponent( 2237 EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent t) { // 3 2238 if (t == null) 2239 return this; 2240 if (this.certaintySubcomponent == null) 2241 this.certaintySubcomponent = new ArrayList<EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent>(); 2242 this.certaintySubcomponent.add(t); 2243 return this; 2244 } 2245 2246 /** 2247 * @return The first repetition of repeating field 2248 * {@link #certaintySubcomponent}, creating it if it does not already 2249 * exist 2250 */ 2251 public EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent getCertaintySubcomponentFirstRep() { 2252 if (getCertaintySubcomponent().isEmpty()) { 2253 addCertaintySubcomponent(); 2254 } 2255 return getCertaintySubcomponent().get(0); 2256 } 2257 2258 protected void listChildren(List<Property> children) { 2259 super.listChildren(children); 2260 children.add(new Property("rating", "CodeableConcept", "A rating of the certainty of the effect estimate.", 0, 2261 java.lang.Integer.MAX_VALUE, rating)); 2262 children.add(new Property("note", "Annotation", 2263 "A human-readable string to clarify or explain concepts about the resource.", 0, java.lang.Integer.MAX_VALUE, 2264 note)); 2265 children.add(new Property("certaintySubcomponent", "", "A description of a component of the overall certainty.", 2266 0, java.lang.Integer.MAX_VALUE, certaintySubcomponent)); 2267 } 2268 2269 @Override 2270 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2271 switch (_hash) { 2272 case -938102371: 2273 /* rating */ return new Property("rating", "CodeableConcept", 2274 "A rating of the certainty of the effect estimate.", 0, java.lang.Integer.MAX_VALUE, rating); 2275 case 3387378: 2276 /* note */ return new Property("note", "Annotation", 2277 "A human-readable string to clarify or explain concepts about the resource.", 0, 2278 java.lang.Integer.MAX_VALUE, note); 2279 case 1806398212: 2280 /* certaintySubcomponent */ return new Property("certaintySubcomponent", "", 2281 "A description of a component of the overall certainty.", 0, java.lang.Integer.MAX_VALUE, 2282 certaintySubcomponent); 2283 default: 2284 return super.getNamedProperty(_hash, _name, _checkValid); 2285 } 2286 2287 } 2288 2289 @Override 2290 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2291 switch (hash) { 2292 case -938102371: 2293 /* rating */ return this.rating == null ? new Base[0] : this.rating.toArray(new Base[this.rating.size()]); // CodeableConcept 2294 case 3387378: 2295 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2296 case 1806398212: 2297 /* certaintySubcomponent */ return this.certaintySubcomponent == null ? new Base[0] 2298 : this.certaintySubcomponent.toArray(new Base[this.certaintySubcomponent.size()]); // EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent 2299 default: 2300 return super.getProperty(hash, name, checkValid); 2301 } 2302 2303 } 2304 2305 @Override 2306 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2307 switch (hash) { 2308 case -938102371: // rating 2309 this.getRating().add(castToCodeableConcept(value)); // CodeableConcept 2310 return value; 2311 case 3387378: // note 2312 this.getNote().add(castToAnnotation(value)); // Annotation 2313 return value; 2314 case 1806398212: // certaintySubcomponent 2315 this.getCertaintySubcomponent().add((EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent) value); // EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent 2316 return value; 2317 default: 2318 return super.setProperty(hash, name, value); 2319 } 2320 2321 } 2322 2323 @Override 2324 public Base setProperty(String name, Base value) throws FHIRException { 2325 if (name.equals("rating")) { 2326 this.getRating().add(castToCodeableConcept(value)); 2327 } else if (name.equals("note")) { 2328 this.getNote().add(castToAnnotation(value)); 2329 } else if (name.equals("certaintySubcomponent")) { 2330 this.getCertaintySubcomponent().add((EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent) value); 2331 } else 2332 return super.setProperty(name, value); 2333 return value; 2334 } 2335 2336 @Override 2337 public void removeChild(String name, Base value) throws FHIRException { 2338 if (name.equals("rating")) { 2339 this.getRating().remove(castToCodeableConcept(value)); 2340 } else if (name.equals("note")) { 2341 this.getNote().remove(castToAnnotation(value)); 2342 } else if (name.equals("certaintySubcomponent")) { 2343 this.getCertaintySubcomponent().remove((EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent) value); 2344 } else 2345 super.removeChild(name, value); 2346 2347 } 2348 2349 @Override 2350 public Base makeProperty(int hash, String name) throws FHIRException { 2351 switch (hash) { 2352 case -938102371: 2353 return addRating(); 2354 case 3387378: 2355 return addNote(); 2356 case 1806398212: 2357 return addCertaintySubcomponent(); 2358 default: 2359 return super.makeProperty(hash, name); 2360 } 2361 2362 } 2363 2364 @Override 2365 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2366 switch (hash) { 2367 case -938102371: 2368 /* rating */ return new String[] { "CodeableConcept" }; 2369 case 3387378: 2370 /* note */ return new String[] { "Annotation" }; 2371 case 1806398212: 2372 /* certaintySubcomponent */ return new String[] {}; 2373 default: 2374 return super.getTypesForProperty(hash, name); 2375 } 2376 2377 } 2378 2379 @Override 2380 public Base addChild(String name) throws FHIRException { 2381 if (name.equals("rating")) { 2382 return addRating(); 2383 } else if (name.equals("note")) { 2384 return addNote(); 2385 } else if (name.equals("certaintySubcomponent")) { 2386 return addCertaintySubcomponent(); 2387 } else 2388 return super.addChild(name); 2389 } 2390 2391 public EffectEvidenceSynthesisCertaintyComponent copy() { 2392 EffectEvidenceSynthesisCertaintyComponent dst = new EffectEvidenceSynthesisCertaintyComponent(); 2393 copyValues(dst); 2394 return dst; 2395 } 2396 2397 public void copyValues(EffectEvidenceSynthesisCertaintyComponent dst) { 2398 super.copyValues(dst); 2399 if (rating != null) { 2400 dst.rating = new ArrayList<CodeableConcept>(); 2401 for (CodeableConcept i : rating) 2402 dst.rating.add(i.copy()); 2403 } 2404 ; 2405 if (note != null) { 2406 dst.note = new ArrayList<Annotation>(); 2407 for (Annotation i : note) 2408 dst.note.add(i.copy()); 2409 } 2410 ; 2411 if (certaintySubcomponent != null) { 2412 dst.certaintySubcomponent = new ArrayList<EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent>(); 2413 for (EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent i : certaintySubcomponent) 2414 dst.certaintySubcomponent.add(i.copy()); 2415 } 2416 ; 2417 } 2418 2419 @Override 2420 public boolean equalsDeep(Base other_) { 2421 if (!super.equalsDeep(other_)) 2422 return false; 2423 if (!(other_ instanceof EffectEvidenceSynthesisCertaintyComponent)) 2424 return false; 2425 EffectEvidenceSynthesisCertaintyComponent o = (EffectEvidenceSynthesisCertaintyComponent) other_; 2426 return compareDeep(rating, o.rating, true) && compareDeep(note, o.note, true) 2427 && compareDeep(certaintySubcomponent, o.certaintySubcomponent, true); 2428 } 2429 2430 @Override 2431 public boolean equalsShallow(Base other_) { 2432 if (!super.equalsShallow(other_)) 2433 return false; 2434 if (!(other_ instanceof EffectEvidenceSynthesisCertaintyComponent)) 2435 return false; 2436 EffectEvidenceSynthesisCertaintyComponent o = (EffectEvidenceSynthesisCertaintyComponent) other_; 2437 return true; 2438 } 2439 2440 public boolean isEmpty() { 2441 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(rating, note, certaintySubcomponent); 2442 } 2443 2444 public String fhirType() { 2445 return "EffectEvidenceSynthesis.certainty"; 2446 2447 } 2448 2449 } 2450 2451 @Block() 2452 public static class EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent extends BackboneElement 2453 implements IBaseBackboneElement { 2454 /** 2455 * Type of subcomponent of certainty rating. 2456 */ 2457 @Child(name = "type", type = { 2458 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2459 @Description(shortDefinition = "Type of subcomponent of certainty rating", formalDefinition = "Type of subcomponent of certainty rating.") 2460 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/certainty-subcomponent-type") 2461 protected CodeableConcept type; 2462 2463 /** 2464 * A rating of a subcomponent of rating certainty. 2465 */ 2466 @Child(name = "rating", type = { 2467 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2468 @Description(shortDefinition = "Subcomponent certainty rating", formalDefinition = "A rating of a subcomponent of rating certainty.") 2469 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/certainty-subcomponent-rating") 2470 protected List<CodeableConcept> rating; 2471 2472 /** 2473 * A human-readable string to clarify or explain concepts about the resource. 2474 */ 2475 @Child(name = "note", type = { 2476 Annotation.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2477 @Description(shortDefinition = "Used for footnotes or explanatory notes", formalDefinition = "A human-readable string to clarify or explain concepts about the resource.") 2478 protected List<Annotation> note; 2479 2480 private static final long serialVersionUID = -411994816L; 2481 2482 /** 2483 * Constructor 2484 */ 2485 public EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent() { 2486 super(); 2487 } 2488 2489 /** 2490 * @return {@link #type} (Type of subcomponent of certainty rating.) 2491 */ 2492 public CodeableConcept getType() { 2493 if (this.type == null) 2494 if (Configuration.errorOnAutoCreate()) 2495 throw new Error("Attempt to auto-create EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent.type"); 2496 else if (Configuration.doAutoCreate()) 2497 this.type = new CodeableConcept(); // cc 2498 return this.type; 2499 } 2500 2501 public boolean hasType() { 2502 return this.type != null && !this.type.isEmpty(); 2503 } 2504 2505 /** 2506 * @param value {@link #type} (Type of subcomponent of certainty rating.) 2507 */ 2508 public EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent setType(CodeableConcept value) { 2509 this.type = value; 2510 return this; 2511 } 2512 2513 /** 2514 * @return {@link #rating} (A rating of a subcomponent of rating certainty.) 2515 */ 2516 public List<CodeableConcept> getRating() { 2517 if (this.rating == null) 2518 this.rating = new ArrayList<CodeableConcept>(); 2519 return this.rating; 2520 } 2521 2522 /** 2523 * @return Returns a reference to <code>this</code> for easy method chaining 2524 */ 2525 public EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent setRating(List<CodeableConcept> theRating) { 2526 this.rating = theRating; 2527 return this; 2528 } 2529 2530 public boolean hasRating() { 2531 if (this.rating == null) 2532 return false; 2533 for (CodeableConcept item : this.rating) 2534 if (!item.isEmpty()) 2535 return true; 2536 return false; 2537 } 2538 2539 public CodeableConcept addRating() { // 3 2540 CodeableConcept t = new CodeableConcept(); 2541 if (this.rating == null) 2542 this.rating = new ArrayList<CodeableConcept>(); 2543 this.rating.add(t); 2544 return t; 2545 } 2546 2547 public EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent addRating(CodeableConcept t) { // 3 2548 if (t == null) 2549 return this; 2550 if (this.rating == null) 2551 this.rating = new ArrayList<CodeableConcept>(); 2552 this.rating.add(t); 2553 return this; 2554 } 2555 2556 /** 2557 * @return The first repetition of repeating field {@link #rating}, creating it 2558 * if it does not already exist 2559 */ 2560 public CodeableConcept getRatingFirstRep() { 2561 if (getRating().isEmpty()) { 2562 addRating(); 2563 } 2564 return getRating().get(0); 2565 } 2566 2567 /** 2568 * @return {@link #note} (A human-readable string to clarify or explain concepts 2569 * about the resource.) 2570 */ 2571 public List<Annotation> getNote() { 2572 if (this.note == null) 2573 this.note = new ArrayList<Annotation>(); 2574 return this.note; 2575 } 2576 2577 /** 2578 * @return Returns a reference to <code>this</code> for easy method chaining 2579 */ 2580 public EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent setNote(List<Annotation> theNote) { 2581 this.note = theNote; 2582 return this; 2583 } 2584 2585 public boolean hasNote() { 2586 if (this.note == null) 2587 return false; 2588 for (Annotation item : this.note) 2589 if (!item.isEmpty()) 2590 return true; 2591 return false; 2592 } 2593 2594 public Annotation addNote() { // 3 2595 Annotation t = new Annotation(); 2596 if (this.note == null) 2597 this.note = new ArrayList<Annotation>(); 2598 this.note.add(t); 2599 return t; 2600 } 2601 2602 public EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent addNote(Annotation t) { // 3 2603 if (t == null) 2604 return this; 2605 if (this.note == null) 2606 this.note = new ArrayList<Annotation>(); 2607 this.note.add(t); 2608 return this; 2609 } 2610 2611 /** 2612 * @return The first repetition of repeating field {@link #note}, creating it if 2613 * it does not already exist 2614 */ 2615 public Annotation getNoteFirstRep() { 2616 if (getNote().isEmpty()) { 2617 addNote(); 2618 } 2619 return getNote().get(0); 2620 } 2621 2622 protected void listChildren(List<Property> children) { 2623 super.listChildren(children); 2624 children.add(new Property("type", "CodeableConcept", "Type of subcomponent of certainty rating.", 0, 1, type)); 2625 children.add(new Property("rating", "CodeableConcept", "A rating of a subcomponent of rating certainty.", 0, 2626 java.lang.Integer.MAX_VALUE, rating)); 2627 children.add(new Property("note", "Annotation", 2628 "A human-readable string to clarify or explain concepts about the resource.", 0, java.lang.Integer.MAX_VALUE, 2629 note)); 2630 } 2631 2632 @Override 2633 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2634 switch (_hash) { 2635 case 3575610: 2636 /* type */ return new Property("type", "CodeableConcept", "Type of subcomponent of certainty rating.", 0, 1, 2637 type); 2638 case -938102371: 2639 /* rating */ return new Property("rating", "CodeableConcept", "A rating of a subcomponent of rating certainty.", 2640 0, java.lang.Integer.MAX_VALUE, rating); 2641 case 3387378: 2642 /* note */ return new Property("note", "Annotation", 2643 "A human-readable string to clarify or explain concepts about the resource.", 0, 2644 java.lang.Integer.MAX_VALUE, note); 2645 default: 2646 return super.getNamedProperty(_hash, _name, _checkValid); 2647 } 2648 2649 } 2650 2651 @Override 2652 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2653 switch (hash) { 2654 case 3575610: 2655 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2656 case -938102371: 2657 /* rating */ return this.rating == null ? new Base[0] : this.rating.toArray(new Base[this.rating.size()]); // CodeableConcept 2658 case 3387378: 2659 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2660 default: 2661 return super.getProperty(hash, name, checkValid); 2662 } 2663 2664 } 2665 2666 @Override 2667 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2668 switch (hash) { 2669 case 3575610: // type 2670 this.type = castToCodeableConcept(value); // CodeableConcept 2671 return value; 2672 case -938102371: // rating 2673 this.getRating().add(castToCodeableConcept(value)); // CodeableConcept 2674 return value; 2675 case 3387378: // note 2676 this.getNote().add(castToAnnotation(value)); // Annotation 2677 return value; 2678 default: 2679 return super.setProperty(hash, name, value); 2680 } 2681 2682 } 2683 2684 @Override 2685 public Base setProperty(String name, Base value) throws FHIRException { 2686 if (name.equals("type")) { 2687 this.type = castToCodeableConcept(value); // CodeableConcept 2688 } else if (name.equals("rating")) { 2689 this.getRating().add(castToCodeableConcept(value)); 2690 } else if (name.equals("note")) { 2691 this.getNote().add(castToAnnotation(value)); 2692 } else 2693 return super.setProperty(name, value); 2694 return value; 2695 } 2696 2697 @Override 2698 public void removeChild(String name, Base value) throws FHIRException { 2699 if (name.equals("type")) { 2700 this.type = null; 2701 } else if (name.equals("rating")) { 2702 this.getRating().remove(castToCodeableConcept(value)); 2703 } else if (name.equals("note")) { 2704 this.getNote().remove(castToAnnotation(value)); 2705 } else 2706 super.removeChild(name, value); 2707 2708 } 2709 2710 @Override 2711 public Base makeProperty(int hash, String name) throws FHIRException { 2712 switch (hash) { 2713 case 3575610: 2714 return getType(); 2715 case -938102371: 2716 return addRating(); 2717 case 3387378: 2718 return addNote(); 2719 default: 2720 return super.makeProperty(hash, name); 2721 } 2722 2723 } 2724 2725 @Override 2726 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2727 switch (hash) { 2728 case 3575610: 2729 /* type */ return new String[] { "CodeableConcept" }; 2730 case -938102371: 2731 /* rating */ return new String[] { "CodeableConcept" }; 2732 case 3387378: 2733 /* note */ return new String[] { "Annotation" }; 2734 default: 2735 return super.getTypesForProperty(hash, name); 2736 } 2737 2738 } 2739 2740 @Override 2741 public Base addChild(String name) throws FHIRException { 2742 if (name.equals("type")) { 2743 this.type = new CodeableConcept(); 2744 return this.type; 2745 } else if (name.equals("rating")) { 2746 return addRating(); 2747 } else if (name.equals("note")) { 2748 return addNote(); 2749 } else 2750 return super.addChild(name); 2751 } 2752 2753 public EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent copy() { 2754 EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent dst = new EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent(); 2755 copyValues(dst); 2756 return dst; 2757 } 2758 2759 public void copyValues(EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent dst) { 2760 super.copyValues(dst); 2761 dst.type = type == null ? null : type.copy(); 2762 if (rating != null) { 2763 dst.rating = new ArrayList<CodeableConcept>(); 2764 for (CodeableConcept i : rating) 2765 dst.rating.add(i.copy()); 2766 } 2767 ; 2768 if (note != null) { 2769 dst.note = new ArrayList<Annotation>(); 2770 for (Annotation i : note) 2771 dst.note.add(i.copy()); 2772 } 2773 ; 2774 } 2775 2776 @Override 2777 public boolean equalsDeep(Base other_) { 2778 if (!super.equalsDeep(other_)) 2779 return false; 2780 if (!(other_ instanceof EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent)) 2781 return false; 2782 EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent o = (EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent) other_; 2783 return compareDeep(type, o.type, true) && compareDeep(rating, o.rating, true) && compareDeep(note, o.note, true); 2784 } 2785 2786 @Override 2787 public boolean equalsShallow(Base other_) { 2788 if (!super.equalsShallow(other_)) 2789 return false; 2790 if (!(other_ instanceof EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent)) 2791 return false; 2792 EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent o = (EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent) other_; 2793 return true; 2794 } 2795 2796 public boolean isEmpty() { 2797 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, rating, note); 2798 } 2799 2800 public String fhirType() { 2801 return "EffectEvidenceSynthesis.certainty.certaintySubcomponent"; 2802 2803 } 2804 2805 } 2806 2807 /** 2808 * A formal identifier that is used to identify this effect evidence synthesis 2809 * when it is represented in other formats, or referenced in a specification, 2810 * model, design or an instance. 2811 */ 2812 @Child(name = "identifier", type = { 2813 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2814 @Description(shortDefinition = "Additional identifier for the effect evidence synthesis", formalDefinition = "A formal identifier that is used to identify this effect evidence synthesis when it is represented in other formats, or referenced in a specification, model, design or an instance.") 2815 protected List<Identifier> identifier; 2816 2817 /** 2818 * A human-readable string to clarify or explain concepts about the resource. 2819 */ 2820 @Child(name = "note", type = { 2821 Annotation.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2822 @Description(shortDefinition = "Used for footnotes or explanatory notes", formalDefinition = "A human-readable string to clarify or explain concepts about the resource.") 2823 protected List<Annotation> note; 2824 2825 /** 2826 * A copyright statement relating to the effect evidence synthesis and/or its 2827 * contents. Copyright statements are generally legal restrictions on the use 2828 * and publishing of the effect evidence synthesis. 2829 */ 2830 @Child(name = "copyright", type = { 2831 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2832 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the effect evidence synthesis and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the effect evidence synthesis.") 2833 protected MarkdownType copyright; 2834 2835 /** 2836 * The date on which the resource content was approved by the publisher. 2837 * Approval happens once when the content is officially approved for usage. 2838 */ 2839 @Child(name = "approvalDate", type = { 2840 DateType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2841 @Description(shortDefinition = "When the effect evidence synthesis was approved by publisher", formalDefinition = "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.") 2842 protected DateType approvalDate; 2843 2844 /** 2845 * The date on which the resource content was last reviewed. Review happens 2846 * periodically after approval but does not change the original approval date. 2847 */ 2848 @Child(name = "lastReviewDate", type = { 2849 DateType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2850 @Description(shortDefinition = "When the effect evidence synthesis was last reviewed", formalDefinition = "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.") 2851 protected DateType lastReviewDate; 2852 2853 /** 2854 * The period during which the effect evidence synthesis content was or is 2855 * planned to be in active use. 2856 */ 2857 @Child(name = "effectivePeriod", type = { 2858 Period.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 2859 @Description(shortDefinition = "When the effect evidence synthesis is expected to be used", formalDefinition = "The period during which the effect evidence synthesis content was or is planned to be in active use.") 2860 protected Period effectivePeriod; 2861 2862 /** 2863 * Descriptive topics related to the content of the EffectEvidenceSynthesis. 2864 * Topics provide a high-level categorization grouping types of 2865 * EffectEvidenceSynthesiss that can be useful for filtering and searching. 2866 */ 2867 @Child(name = "topic", type = { 2868 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2869 @Description(shortDefinition = "The category of the EffectEvidenceSynthesis, such as Education, Treatment, Assessment, etc.", formalDefinition = "Descriptive topics related to the content of the EffectEvidenceSynthesis. Topics provide a high-level categorization grouping types of EffectEvidenceSynthesiss that can be useful for filtering and searching.") 2870 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/definition-topic") 2871 protected List<CodeableConcept> topic; 2872 2873 /** 2874 * An individiual or organization primarily involved in the creation and 2875 * maintenance of the content. 2876 */ 2877 @Child(name = "author", type = { 2878 ContactDetail.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2879 @Description(shortDefinition = "Who authored the content", formalDefinition = "An individiual or organization primarily involved in the creation and maintenance of the content.") 2880 protected List<ContactDetail> author; 2881 2882 /** 2883 * An individual or organization primarily responsible for internal coherence of 2884 * the content. 2885 */ 2886 @Child(name = "editor", type = { 2887 ContactDetail.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2888 @Description(shortDefinition = "Who edited the content", formalDefinition = "An individual or organization primarily responsible for internal coherence of the content.") 2889 protected List<ContactDetail> editor; 2890 2891 /** 2892 * An individual or organization primarily responsible for review of some aspect 2893 * of the content. 2894 */ 2895 @Child(name = "reviewer", type = { 2896 ContactDetail.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2897 @Description(shortDefinition = "Who reviewed the content", formalDefinition = "An individual or organization primarily responsible for review of some aspect of the content.") 2898 protected List<ContactDetail> reviewer; 2899 2900 /** 2901 * An individual or organization responsible for officially endorsing the 2902 * content for use in some setting. 2903 */ 2904 @Child(name = "endorser", type = { 2905 ContactDetail.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2906 @Description(shortDefinition = "Who endorsed the content", formalDefinition = "An individual or organization responsible for officially endorsing the content for use in some setting.") 2907 protected List<ContactDetail> endorser; 2908 2909 /** 2910 * Related artifacts such as additional documentation, justification, or 2911 * bibliographic references. 2912 */ 2913 @Child(name = "relatedArtifact", type = { 2914 RelatedArtifact.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2915 @Description(shortDefinition = "Additional documentation, citations, etc.", formalDefinition = "Related artifacts such as additional documentation, justification, or bibliographic references.") 2916 protected List<RelatedArtifact> relatedArtifact; 2917 2918 /** 2919 * Type of synthesis eg meta-analysis. 2920 */ 2921 @Child(name = "synthesisType", type = { 2922 CodeableConcept.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 2923 @Description(shortDefinition = "Type of synthesis", formalDefinition = "Type of synthesis eg meta-analysis.") 2924 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/synthesis-type") 2925 protected CodeableConcept synthesisType; 2926 2927 /** 2928 * Type of study eg randomized trial. 2929 */ 2930 @Child(name = "studyType", type = { 2931 CodeableConcept.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 2932 @Description(shortDefinition = "Type of study", formalDefinition = "Type of study eg randomized trial.") 2933 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/study-type") 2934 protected CodeableConcept studyType; 2935 2936 /** 2937 * A reference to a EvidenceVariable resource that defines the population for 2938 * the research. 2939 */ 2940 @Child(name = "population", type = { 2941 EvidenceVariable.class }, order = 14, min = 1, max = 1, modifier = false, summary = true) 2942 @Description(shortDefinition = "What population?", formalDefinition = "A reference to a EvidenceVariable resource that defines the population for the research.") 2943 protected Reference population; 2944 2945 /** 2946 * The actual object that is the target of the reference (A reference to a 2947 * EvidenceVariable resource that defines the population for the research.) 2948 */ 2949 protected EvidenceVariable populationTarget; 2950 2951 /** 2952 * A reference to a EvidenceVariable resource that defines the exposure for the 2953 * research. 2954 */ 2955 @Child(name = "exposure", type = { 2956 EvidenceVariable.class }, order = 15, min = 1, max = 1, modifier = false, summary = true) 2957 @Description(shortDefinition = "What exposure?", formalDefinition = "A reference to a EvidenceVariable resource that defines the exposure for the research.") 2958 protected Reference exposure; 2959 2960 /** 2961 * The actual object that is the target of the reference (A reference to a 2962 * EvidenceVariable resource that defines the exposure for the research.) 2963 */ 2964 protected EvidenceVariable exposureTarget; 2965 2966 /** 2967 * A reference to a EvidenceVariable resource that defines the comparison 2968 * exposure for the research. 2969 */ 2970 @Child(name = "exposureAlternative", type = { 2971 EvidenceVariable.class }, order = 16, min = 1, max = 1, modifier = false, summary = true) 2972 @Description(shortDefinition = "What comparison exposure?", formalDefinition = "A reference to a EvidenceVariable resource that defines the comparison exposure for the research.") 2973 protected Reference exposureAlternative; 2974 2975 /** 2976 * The actual object that is the target of the reference (A reference to a 2977 * EvidenceVariable resource that defines the comparison exposure for the 2978 * research.) 2979 */ 2980 protected EvidenceVariable exposureAlternativeTarget; 2981 2982 /** 2983 * A reference to a EvidenceVariable resomece that defines the outcome for the 2984 * research. 2985 */ 2986 @Child(name = "outcome", type = { 2987 EvidenceVariable.class }, order = 17, min = 1, max = 1, modifier = false, summary = true) 2988 @Description(shortDefinition = "What outcome?", formalDefinition = "A reference to a EvidenceVariable resomece that defines the outcome for the research.") 2989 protected Reference outcome; 2990 2991 /** 2992 * The actual object that is the target of the reference (A reference to a 2993 * EvidenceVariable resomece that defines the outcome for the research.) 2994 */ 2995 protected EvidenceVariable outcomeTarget; 2996 2997 /** 2998 * A description of the size of the sample involved in the synthesis. 2999 */ 3000 @Child(name = "sampleSize", type = {}, order = 18, min = 0, max = 1, modifier = false, summary = false) 3001 @Description(shortDefinition = "What sample size was involved?", formalDefinition = "A description of the size of the sample involved in the synthesis.") 3002 protected EffectEvidenceSynthesisSampleSizeComponent sampleSize; 3003 3004 /** 3005 * A description of the results for each exposure considered in the effect 3006 * estimate. 3007 */ 3008 @Child(name = "resultsByExposure", type = {}, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3009 @Description(shortDefinition = "What was the result per exposure?", formalDefinition = "A description of the results for each exposure considered in the effect estimate.") 3010 protected List<EffectEvidenceSynthesisResultsByExposureComponent> resultsByExposure; 3011 3012 /** 3013 * The estimated effect of the exposure variant. 3014 */ 3015 @Child(name = "effectEstimate", type = {}, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3016 @Description(shortDefinition = "What was the estimated effect", formalDefinition = "The estimated effect of the exposure variant.") 3017 protected List<EffectEvidenceSynthesisEffectEstimateComponent> effectEstimate; 3018 3019 /** 3020 * A description of the certainty of the effect estimate. 3021 */ 3022 @Child(name = "certainty", type = {}, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3023 @Description(shortDefinition = "How certain is the effect", formalDefinition = "A description of the certainty of the effect estimate.") 3024 protected List<EffectEvidenceSynthesisCertaintyComponent> certainty; 3025 3026 private static final long serialVersionUID = 23150467L; 3027 3028 /** 3029 * Constructor 3030 */ 3031 public EffectEvidenceSynthesis() { 3032 super(); 3033 } 3034 3035 /** 3036 * Constructor 3037 */ 3038 public EffectEvidenceSynthesis(Enumeration<PublicationStatus> status, Reference population, Reference exposure, 3039 Reference exposureAlternative, Reference outcome) { 3040 super(); 3041 this.status = status; 3042 this.population = population; 3043 this.exposure = exposure; 3044 this.exposureAlternative = exposureAlternative; 3045 this.outcome = outcome; 3046 } 3047 3048 /** 3049 * @return {@link #url} (An absolute URI that is used to identify this effect 3050 * evidence synthesis when it is referenced in a specification, model, 3051 * design or an instance; also called its canonical identifier. This 3052 * SHOULD be globally unique and SHOULD be a literal address at which at 3053 * which an authoritative instance of this effect evidence synthesis is 3054 * (or will be) published. This URL can be the target of a canonical 3055 * reference. It SHALL remain the same when the effect evidence 3056 * synthesis is stored on different servers.). This is the underlying 3057 * object with id, value and extensions. The accessor "getUrl" gives 3058 * direct access to the value 3059 */ 3060 public UriType getUrlElement() { 3061 if (this.url == null) 3062 if (Configuration.errorOnAutoCreate()) 3063 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.url"); 3064 else if (Configuration.doAutoCreate()) 3065 this.url = new UriType(); // bb 3066 return this.url; 3067 } 3068 3069 public boolean hasUrlElement() { 3070 return this.url != null && !this.url.isEmpty(); 3071 } 3072 3073 public boolean hasUrl() { 3074 return this.url != null && !this.url.isEmpty(); 3075 } 3076 3077 /** 3078 * @param value {@link #url} (An absolute URI that is used to identify this 3079 * effect evidence synthesis when it is referenced in a 3080 * specification, model, design or an instance; also called its 3081 * canonical identifier. This SHOULD be globally unique and SHOULD 3082 * be a literal address at which at which an authoritative instance 3083 * of this effect evidence synthesis is (or will be) published. 3084 * This URL can be the target of a canonical reference. It SHALL 3085 * remain the same when the effect evidence synthesis is stored on 3086 * different servers.). This is the underlying object with id, 3087 * value and extensions. The accessor "getUrl" gives direct access 3088 * to the value 3089 */ 3090 public EffectEvidenceSynthesis setUrlElement(UriType value) { 3091 this.url = value; 3092 return this; 3093 } 3094 3095 /** 3096 * @return An absolute URI that is used to identify this effect evidence 3097 * synthesis when it is referenced in a specification, model, design or 3098 * an instance; also called its canonical identifier. This SHOULD be 3099 * globally unique and SHOULD be a literal address at which at which an 3100 * authoritative instance of this effect evidence synthesis is (or will 3101 * be) published. This URL can be the target of a canonical reference. 3102 * It SHALL remain the same when the effect evidence synthesis is stored 3103 * on different servers. 3104 */ 3105 public String getUrl() { 3106 return this.url == null ? null : this.url.getValue(); 3107 } 3108 3109 /** 3110 * @param value An absolute URI that is used to identify this effect evidence 3111 * synthesis when it is referenced in a specification, model, 3112 * design or an instance; also called its canonical identifier. 3113 * This SHOULD be globally unique and SHOULD be a literal address 3114 * at which at which an authoritative instance of this effect 3115 * evidence synthesis is (or will be) published. This URL can be 3116 * the target of a canonical reference. It SHALL remain the same 3117 * when the effect evidence synthesis is stored on different 3118 * servers. 3119 */ 3120 public EffectEvidenceSynthesis setUrl(String value) { 3121 if (Utilities.noString(value)) 3122 this.url = null; 3123 else { 3124 if (this.url == null) 3125 this.url = new UriType(); 3126 this.url.setValue(value); 3127 } 3128 return this; 3129 } 3130 3131 /** 3132 * @return {@link #identifier} (A formal identifier that is used to identify 3133 * this effect evidence synthesis when it is represented in other 3134 * formats, or referenced in a specification, model, design or an 3135 * instance.) 3136 */ 3137 public List<Identifier> getIdentifier() { 3138 if (this.identifier == null) 3139 this.identifier = new ArrayList<Identifier>(); 3140 return this.identifier; 3141 } 3142 3143 /** 3144 * @return Returns a reference to <code>this</code> for easy method chaining 3145 */ 3146 public EffectEvidenceSynthesis setIdentifier(List<Identifier> theIdentifier) { 3147 this.identifier = theIdentifier; 3148 return this; 3149 } 3150 3151 public boolean hasIdentifier() { 3152 if (this.identifier == null) 3153 return false; 3154 for (Identifier item : this.identifier) 3155 if (!item.isEmpty()) 3156 return true; 3157 return false; 3158 } 3159 3160 public Identifier addIdentifier() { // 3 3161 Identifier t = new Identifier(); 3162 if (this.identifier == null) 3163 this.identifier = new ArrayList<Identifier>(); 3164 this.identifier.add(t); 3165 return t; 3166 } 3167 3168 public EffectEvidenceSynthesis addIdentifier(Identifier t) { // 3 3169 if (t == null) 3170 return this; 3171 if (this.identifier == null) 3172 this.identifier = new ArrayList<Identifier>(); 3173 this.identifier.add(t); 3174 return this; 3175 } 3176 3177 /** 3178 * @return The first repetition of repeating field {@link #identifier}, creating 3179 * it if it does not already exist 3180 */ 3181 public Identifier getIdentifierFirstRep() { 3182 if (getIdentifier().isEmpty()) { 3183 addIdentifier(); 3184 } 3185 return getIdentifier().get(0); 3186 } 3187 3188 /** 3189 * @return {@link #version} (The identifier that is used to identify this 3190 * version of the effect evidence synthesis when it is referenced in a 3191 * specification, model, design or instance. This is an arbitrary value 3192 * managed by the effect evidence synthesis author and is not expected 3193 * to be globally unique. For example, it might be a timestamp (e.g. 3194 * yyyymmdd) if a managed version is not available. There is also no 3195 * expectation that versions can be placed in a lexicographical 3196 * sequence.). This is the underlying object with id, value and 3197 * extensions. The accessor "getVersion" gives direct access to the 3198 * value 3199 */ 3200 public StringType getVersionElement() { 3201 if (this.version == null) 3202 if (Configuration.errorOnAutoCreate()) 3203 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.version"); 3204 else if (Configuration.doAutoCreate()) 3205 this.version = new StringType(); // bb 3206 return this.version; 3207 } 3208 3209 public boolean hasVersionElement() { 3210 return this.version != null && !this.version.isEmpty(); 3211 } 3212 3213 public boolean hasVersion() { 3214 return this.version != null && !this.version.isEmpty(); 3215 } 3216 3217 /** 3218 * @param value {@link #version} (The identifier that is used to identify this 3219 * version of the effect evidence synthesis when it is referenced 3220 * in a specification, model, design or instance. This is an 3221 * arbitrary value managed by the effect evidence synthesis author 3222 * and is not expected to be globally unique. For example, it might 3223 * be a timestamp (e.g. yyyymmdd) if a managed version is not 3224 * available. There is also no expectation that versions can be 3225 * placed in a lexicographical sequence.). This is the underlying 3226 * object with id, value and extensions. The accessor "getVersion" 3227 * gives direct access to the value 3228 */ 3229 public EffectEvidenceSynthesis setVersionElement(StringType value) { 3230 this.version = value; 3231 return this; 3232 } 3233 3234 /** 3235 * @return The identifier that is used to identify this version of the effect 3236 * evidence synthesis when it is referenced in a specification, model, 3237 * design or instance. This is an arbitrary value managed by the effect 3238 * evidence synthesis author and is not expected to be globally unique. 3239 * For example, it might be a timestamp (e.g. yyyymmdd) if a managed 3240 * version is not available. There is also no expectation that versions 3241 * can be placed in a lexicographical sequence. 3242 */ 3243 public String getVersion() { 3244 return this.version == null ? null : this.version.getValue(); 3245 } 3246 3247 /** 3248 * @param value The identifier that is used to identify this version of the 3249 * effect evidence synthesis when it is referenced in a 3250 * specification, model, design or instance. This is an arbitrary 3251 * value managed by the effect evidence synthesis author and is not 3252 * expected to be globally unique. For example, it might be a 3253 * timestamp (e.g. yyyymmdd) if a managed version is not available. 3254 * There is also no expectation that versions can be placed in a 3255 * lexicographical sequence. 3256 */ 3257 public EffectEvidenceSynthesis setVersion(String value) { 3258 if (Utilities.noString(value)) 3259 this.version = null; 3260 else { 3261 if (this.version == null) 3262 this.version = new StringType(); 3263 this.version.setValue(value); 3264 } 3265 return this; 3266 } 3267 3268 /** 3269 * @return {@link #name} (A natural language name identifying the effect 3270 * evidence synthesis. This name should be usable as an identifier for 3271 * the module by machine processing applications such as code 3272 * generation.). This is the underlying object with id, value and 3273 * extensions. The accessor "getName" gives direct access to the value 3274 */ 3275 public StringType getNameElement() { 3276 if (this.name == null) 3277 if (Configuration.errorOnAutoCreate()) 3278 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.name"); 3279 else if (Configuration.doAutoCreate()) 3280 this.name = new StringType(); // bb 3281 return this.name; 3282 } 3283 3284 public boolean hasNameElement() { 3285 return this.name != null && !this.name.isEmpty(); 3286 } 3287 3288 public boolean hasName() { 3289 return this.name != null && !this.name.isEmpty(); 3290 } 3291 3292 /** 3293 * @param value {@link #name} (A natural language name identifying the effect 3294 * evidence synthesis. This name should be usable as an identifier 3295 * for the module by machine processing applications such as code 3296 * generation.). This is the underlying object with id, value and 3297 * extensions. The accessor "getName" gives direct access to the 3298 * value 3299 */ 3300 public EffectEvidenceSynthesis setNameElement(StringType value) { 3301 this.name = value; 3302 return this; 3303 } 3304 3305 /** 3306 * @return A natural language name identifying the effect evidence synthesis. 3307 * This name should be usable as an identifier for the module by machine 3308 * processing applications such as code generation. 3309 */ 3310 public String getName() { 3311 return this.name == null ? null : this.name.getValue(); 3312 } 3313 3314 /** 3315 * @param value A natural language name identifying the effect evidence 3316 * synthesis. This name should be usable as an identifier for the 3317 * module by machine processing applications such as code 3318 * generation. 3319 */ 3320 public EffectEvidenceSynthesis setName(String value) { 3321 if (Utilities.noString(value)) 3322 this.name = null; 3323 else { 3324 if (this.name == null) 3325 this.name = new StringType(); 3326 this.name.setValue(value); 3327 } 3328 return this; 3329 } 3330 3331 /** 3332 * @return {@link #title} (A short, descriptive, user-friendly title for the 3333 * effect evidence synthesis.). This is the underlying object with id, 3334 * value and extensions. The accessor "getTitle" gives direct access to 3335 * the value 3336 */ 3337 public StringType getTitleElement() { 3338 if (this.title == null) 3339 if (Configuration.errorOnAutoCreate()) 3340 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.title"); 3341 else if (Configuration.doAutoCreate()) 3342 this.title = new StringType(); // bb 3343 return this.title; 3344 } 3345 3346 public boolean hasTitleElement() { 3347 return this.title != null && !this.title.isEmpty(); 3348 } 3349 3350 public boolean hasTitle() { 3351 return this.title != null && !this.title.isEmpty(); 3352 } 3353 3354 /** 3355 * @param value {@link #title} (A short, descriptive, user-friendly title for 3356 * the effect evidence synthesis.). This is the underlying object 3357 * with id, value and extensions. The accessor "getTitle" gives 3358 * direct access to the value 3359 */ 3360 public EffectEvidenceSynthesis setTitleElement(StringType value) { 3361 this.title = value; 3362 return this; 3363 } 3364 3365 /** 3366 * @return A short, descriptive, user-friendly title for the effect evidence 3367 * synthesis. 3368 */ 3369 public String getTitle() { 3370 return this.title == null ? null : this.title.getValue(); 3371 } 3372 3373 /** 3374 * @param value A short, descriptive, user-friendly title for the effect 3375 * evidence synthesis. 3376 */ 3377 public EffectEvidenceSynthesis setTitle(String value) { 3378 if (Utilities.noString(value)) 3379 this.title = null; 3380 else { 3381 if (this.title == null) 3382 this.title = new StringType(); 3383 this.title.setValue(value); 3384 } 3385 return this; 3386 } 3387 3388 /** 3389 * @return {@link #status} (The status of this effect evidence synthesis. 3390 * Enables tracking the life-cycle of the content.). This is the 3391 * underlying object with id, value and extensions. The accessor 3392 * "getStatus" gives direct access to the value 3393 */ 3394 public Enumeration<PublicationStatus> getStatusElement() { 3395 if (this.status == null) 3396 if (Configuration.errorOnAutoCreate()) 3397 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.status"); 3398 else if (Configuration.doAutoCreate()) 3399 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 3400 return this.status; 3401 } 3402 3403 public boolean hasStatusElement() { 3404 return this.status != null && !this.status.isEmpty(); 3405 } 3406 3407 public boolean hasStatus() { 3408 return this.status != null && !this.status.isEmpty(); 3409 } 3410 3411 /** 3412 * @param value {@link #status} (The status of this effect evidence synthesis. 3413 * Enables tracking the life-cycle of the content.). This is the 3414 * underlying object with id, value and extensions. The accessor 3415 * "getStatus" gives direct access to the value 3416 */ 3417 public EffectEvidenceSynthesis setStatusElement(Enumeration<PublicationStatus> value) { 3418 this.status = value; 3419 return this; 3420 } 3421 3422 /** 3423 * @return The status of this effect evidence synthesis. Enables tracking the 3424 * life-cycle of the content. 3425 */ 3426 public PublicationStatus getStatus() { 3427 return this.status == null ? null : this.status.getValue(); 3428 } 3429 3430 /** 3431 * @param value The status of this effect evidence synthesis. Enables tracking 3432 * the life-cycle of the content. 3433 */ 3434 public EffectEvidenceSynthesis setStatus(PublicationStatus value) { 3435 if (this.status == null) 3436 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 3437 this.status.setValue(value); 3438 return this; 3439 } 3440 3441 /** 3442 * @return {@link #date} (The date (and optionally time) when the effect 3443 * evidence synthesis was published. The date must change when the 3444 * business version changes and it must change if the status code 3445 * changes. In addition, it should change when the substantive content 3446 * of the effect evidence synthesis changes.). This is the underlying 3447 * object with id, value and extensions. The accessor "getDate" gives 3448 * direct access to the value 3449 */ 3450 public DateTimeType getDateElement() { 3451 if (this.date == null) 3452 if (Configuration.errorOnAutoCreate()) 3453 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.date"); 3454 else if (Configuration.doAutoCreate()) 3455 this.date = new DateTimeType(); // bb 3456 return this.date; 3457 } 3458 3459 public boolean hasDateElement() { 3460 return this.date != null && !this.date.isEmpty(); 3461 } 3462 3463 public boolean hasDate() { 3464 return this.date != null && !this.date.isEmpty(); 3465 } 3466 3467 /** 3468 * @param value {@link #date} (The date (and optionally time) when the effect 3469 * evidence synthesis was published. The date must change when the 3470 * business version changes and it must change if the status code 3471 * changes. In addition, it should change when the substantive 3472 * content of the effect evidence synthesis changes.). This is the 3473 * underlying object with id, value and extensions. The accessor 3474 * "getDate" gives direct access to the value 3475 */ 3476 public EffectEvidenceSynthesis setDateElement(DateTimeType value) { 3477 this.date = value; 3478 return this; 3479 } 3480 3481 /** 3482 * @return The date (and optionally time) when the effect evidence synthesis was 3483 * published. The date must change when the business version changes and 3484 * it must change if the status code changes. In addition, it should 3485 * change when the substantive content of the effect evidence synthesis 3486 * changes. 3487 */ 3488 public Date getDate() { 3489 return this.date == null ? null : this.date.getValue(); 3490 } 3491 3492 /** 3493 * @param value The date (and optionally time) when the effect evidence 3494 * synthesis was published. The date must change when the business 3495 * version changes and it must change if the status code changes. 3496 * In addition, it should change when the substantive content of 3497 * the effect evidence synthesis changes. 3498 */ 3499 public EffectEvidenceSynthesis setDate(Date value) { 3500 if (value == null) 3501 this.date = null; 3502 else { 3503 if (this.date == null) 3504 this.date = new DateTimeType(); 3505 this.date.setValue(value); 3506 } 3507 return this; 3508 } 3509 3510 /** 3511 * @return {@link #publisher} (The name of the organization or individual that 3512 * published the effect evidence synthesis.). This is the underlying 3513 * object with id, value and extensions. The accessor "getPublisher" 3514 * gives direct access to the value 3515 */ 3516 public StringType getPublisherElement() { 3517 if (this.publisher == null) 3518 if (Configuration.errorOnAutoCreate()) 3519 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.publisher"); 3520 else if (Configuration.doAutoCreate()) 3521 this.publisher = new StringType(); // bb 3522 return this.publisher; 3523 } 3524 3525 public boolean hasPublisherElement() { 3526 return this.publisher != null && !this.publisher.isEmpty(); 3527 } 3528 3529 public boolean hasPublisher() { 3530 return this.publisher != null && !this.publisher.isEmpty(); 3531 } 3532 3533 /** 3534 * @param value {@link #publisher} (The name of the organization or individual 3535 * that published the effect evidence synthesis.). This is the 3536 * underlying object with id, value and extensions. The accessor 3537 * "getPublisher" gives direct access to the value 3538 */ 3539 public EffectEvidenceSynthesis setPublisherElement(StringType value) { 3540 this.publisher = value; 3541 return this; 3542 } 3543 3544 /** 3545 * @return The name of the organization or individual that published the effect 3546 * evidence synthesis. 3547 */ 3548 public String getPublisher() { 3549 return this.publisher == null ? null : this.publisher.getValue(); 3550 } 3551 3552 /** 3553 * @param value The name of the organization or individual that published the 3554 * effect evidence synthesis. 3555 */ 3556 public EffectEvidenceSynthesis setPublisher(String value) { 3557 if (Utilities.noString(value)) 3558 this.publisher = null; 3559 else { 3560 if (this.publisher == null) 3561 this.publisher = new StringType(); 3562 this.publisher.setValue(value); 3563 } 3564 return this; 3565 } 3566 3567 /** 3568 * @return {@link #contact} (Contact details to assist a user in finding and 3569 * communicating with the publisher.) 3570 */ 3571 public List<ContactDetail> getContact() { 3572 if (this.contact == null) 3573 this.contact = new ArrayList<ContactDetail>(); 3574 return this.contact; 3575 } 3576 3577 /** 3578 * @return Returns a reference to <code>this</code> for easy method chaining 3579 */ 3580 public EffectEvidenceSynthesis setContact(List<ContactDetail> theContact) { 3581 this.contact = theContact; 3582 return this; 3583 } 3584 3585 public boolean hasContact() { 3586 if (this.contact == null) 3587 return false; 3588 for (ContactDetail item : this.contact) 3589 if (!item.isEmpty()) 3590 return true; 3591 return false; 3592 } 3593 3594 public ContactDetail addContact() { // 3 3595 ContactDetail t = new ContactDetail(); 3596 if (this.contact == null) 3597 this.contact = new ArrayList<ContactDetail>(); 3598 this.contact.add(t); 3599 return t; 3600 } 3601 3602 public EffectEvidenceSynthesis addContact(ContactDetail t) { // 3 3603 if (t == null) 3604 return this; 3605 if (this.contact == null) 3606 this.contact = new ArrayList<ContactDetail>(); 3607 this.contact.add(t); 3608 return this; 3609 } 3610 3611 /** 3612 * @return The first repetition of repeating field {@link #contact}, creating it 3613 * if it does not already exist 3614 */ 3615 public ContactDetail getContactFirstRep() { 3616 if (getContact().isEmpty()) { 3617 addContact(); 3618 } 3619 return getContact().get(0); 3620 } 3621 3622 /** 3623 * @return {@link #description} (A free text natural language description of the 3624 * effect evidence synthesis from a consumer's perspective.). This is 3625 * the underlying object with id, value and extensions. The accessor 3626 * "getDescription" gives direct access to the value 3627 */ 3628 public MarkdownType getDescriptionElement() { 3629 if (this.description == null) 3630 if (Configuration.errorOnAutoCreate()) 3631 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.description"); 3632 else if (Configuration.doAutoCreate()) 3633 this.description = new MarkdownType(); // bb 3634 return this.description; 3635 } 3636 3637 public boolean hasDescriptionElement() { 3638 return this.description != null && !this.description.isEmpty(); 3639 } 3640 3641 public boolean hasDescription() { 3642 return this.description != null && !this.description.isEmpty(); 3643 } 3644 3645 /** 3646 * @param value {@link #description} (A free text natural language description 3647 * of the effect evidence synthesis from a consumer's 3648 * perspective.). This is the underlying object with id, value and 3649 * extensions. The accessor "getDescription" gives direct access to 3650 * the value 3651 */ 3652 public EffectEvidenceSynthesis setDescriptionElement(MarkdownType value) { 3653 this.description = value; 3654 return this; 3655 } 3656 3657 /** 3658 * @return A free text natural language description of the effect evidence 3659 * synthesis from a consumer's perspective. 3660 */ 3661 public String getDescription() { 3662 return this.description == null ? null : this.description.getValue(); 3663 } 3664 3665 /** 3666 * @param value A free text natural language description of the effect evidence 3667 * synthesis from a consumer's perspective. 3668 */ 3669 public EffectEvidenceSynthesis setDescription(String value) { 3670 if (value == null) 3671 this.description = null; 3672 else { 3673 if (this.description == null) 3674 this.description = new MarkdownType(); 3675 this.description.setValue(value); 3676 } 3677 return this; 3678 } 3679 3680 /** 3681 * @return {@link #note} (A human-readable string to clarify or explain concepts 3682 * about the resource.) 3683 */ 3684 public List<Annotation> getNote() { 3685 if (this.note == null) 3686 this.note = new ArrayList<Annotation>(); 3687 return this.note; 3688 } 3689 3690 /** 3691 * @return Returns a reference to <code>this</code> for easy method chaining 3692 */ 3693 public EffectEvidenceSynthesis setNote(List<Annotation> theNote) { 3694 this.note = theNote; 3695 return this; 3696 } 3697 3698 public boolean hasNote() { 3699 if (this.note == null) 3700 return false; 3701 for (Annotation item : this.note) 3702 if (!item.isEmpty()) 3703 return true; 3704 return false; 3705 } 3706 3707 public Annotation addNote() { // 3 3708 Annotation t = new Annotation(); 3709 if (this.note == null) 3710 this.note = new ArrayList<Annotation>(); 3711 this.note.add(t); 3712 return t; 3713 } 3714 3715 public EffectEvidenceSynthesis addNote(Annotation t) { // 3 3716 if (t == null) 3717 return this; 3718 if (this.note == null) 3719 this.note = new ArrayList<Annotation>(); 3720 this.note.add(t); 3721 return this; 3722 } 3723 3724 /** 3725 * @return The first repetition of repeating field {@link #note}, creating it if 3726 * it does not already exist 3727 */ 3728 public Annotation getNoteFirstRep() { 3729 if (getNote().isEmpty()) { 3730 addNote(); 3731 } 3732 return getNote().get(0); 3733 } 3734 3735 /** 3736 * @return {@link #useContext} (The content was developed with a focus and 3737 * intent of supporting the contexts that are listed. These contexts may 3738 * be general categories (gender, age, ...) or may be references to 3739 * specific programs (insurance plans, studies, ...) and may be used to 3740 * assist with indexing and searching for appropriate effect evidence 3741 * synthesis instances.) 3742 */ 3743 public List<UsageContext> getUseContext() { 3744 if (this.useContext == null) 3745 this.useContext = new ArrayList<UsageContext>(); 3746 return this.useContext; 3747 } 3748 3749 /** 3750 * @return Returns a reference to <code>this</code> for easy method chaining 3751 */ 3752 public EffectEvidenceSynthesis setUseContext(List<UsageContext> theUseContext) { 3753 this.useContext = theUseContext; 3754 return this; 3755 } 3756 3757 public boolean hasUseContext() { 3758 if (this.useContext == null) 3759 return false; 3760 for (UsageContext item : this.useContext) 3761 if (!item.isEmpty()) 3762 return true; 3763 return false; 3764 } 3765 3766 public UsageContext addUseContext() { // 3 3767 UsageContext t = new UsageContext(); 3768 if (this.useContext == null) 3769 this.useContext = new ArrayList<UsageContext>(); 3770 this.useContext.add(t); 3771 return t; 3772 } 3773 3774 public EffectEvidenceSynthesis addUseContext(UsageContext t) { // 3 3775 if (t == null) 3776 return this; 3777 if (this.useContext == null) 3778 this.useContext = new ArrayList<UsageContext>(); 3779 this.useContext.add(t); 3780 return this; 3781 } 3782 3783 /** 3784 * @return The first repetition of repeating field {@link #useContext}, creating 3785 * it if it does not already exist 3786 */ 3787 public UsageContext getUseContextFirstRep() { 3788 if (getUseContext().isEmpty()) { 3789 addUseContext(); 3790 } 3791 return getUseContext().get(0); 3792 } 3793 3794 /** 3795 * @return {@link #jurisdiction} (A legal or geographic region in which the 3796 * effect evidence synthesis is intended to be used.) 3797 */ 3798 public List<CodeableConcept> getJurisdiction() { 3799 if (this.jurisdiction == null) 3800 this.jurisdiction = new ArrayList<CodeableConcept>(); 3801 return this.jurisdiction; 3802 } 3803 3804 /** 3805 * @return Returns a reference to <code>this</code> for easy method chaining 3806 */ 3807 public EffectEvidenceSynthesis setJurisdiction(List<CodeableConcept> theJurisdiction) { 3808 this.jurisdiction = theJurisdiction; 3809 return this; 3810 } 3811 3812 public boolean hasJurisdiction() { 3813 if (this.jurisdiction == null) 3814 return false; 3815 for (CodeableConcept item : this.jurisdiction) 3816 if (!item.isEmpty()) 3817 return true; 3818 return false; 3819 } 3820 3821 public CodeableConcept addJurisdiction() { // 3 3822 CodeableConcept t = new CodeableConcept(); 3823 if (this.jurisdiction == null) 3824 this.jurisdiction = new ArrayList<CodeableConcept>(); 3825 this.jurisdiction.add(t); 3826 return t; 3827 } 3828 3829 public EffectEvidenceSynthesis addJurisdiction(CodeableConcept t) { // 3 3830 if (t == null) 3831 return this; 3832 if (this.jurisdiction == null) 3833 this.jurisdiction = new ArrayList<CodeableConcept>(); 3834 this.jurisdiction.add(t); 3835 return this; 3836 } 3837 3838 /** 3839 * @return The first repetition of repeating field {@link #jurisdiction}, 3840 * creating it if it does not already exist 3841 */ 3842 public CodeableConcept getJurisdictionFirstRep() { 3843 if (getJurisdiction().isEmpty()) { 3844 addJurisdiction(); 3845 } 3846 return getJurisdiction().get(0); 3847 } 3848 3849 /** 3850 * @return {@link #copyright} (A copyright statement relating to the effect 3851 * evidence synthesis and/or its contents. Copyright statements are 3852 * generally legal restrictions on the use and publishing of the effect 3853 * evidence synthesis.). This is the underlying object with id, value 3854 * and extensions. The accessor "getCopyright" gives direct access to 3855 * the value 3856 */ 3857 public MarkdownType getCopyrightElement() { 3858 if (this.copyright == null) 3859 if (Configuration.errorOnAutoCreate()) 3860 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.copyright"); 3861 else if (Configuration.doAutoCreate()) 3862 this.copyright = new MarkdownType(); // bb 3863 return this.copyright; 3864 } 3865 3866 public boolean hasCopyrightElement() { 3867 return this.copyright != null && !this.copyright.isEmpty(); 3868 } 3869 3870 public boolean hasCopyright() { 3871 return this.copyright != null && !this.copyright.isEmpty(); 3872 } 3873 3874 /** 3875 * @param value {@link #copyright} (A copyright statement relating to the effect 3876 * evidence synthesis and/or its contents. Copyright statements are 3877 * generally legal restrictions on the use and publishing of the 3878 * effect evidence synthesis.). This is the underlying object with 3879 * id, value and extensions. The accessor "getCopyright" gives 3880 * direct access to the value 3881 */ 3882 public EffectEvidenceSynthesis setCopyrightElement(MarkdownType value) { 3883 this.copyright = value; 3884 return this; 3885 } 3886 3887 /** 3888 * @return A copyright statement relating to the effect evidence synthesis 3889 * and/or its contents. Copyright statements are generally legal 3890 * restrictions on the use and publishing of the effect evidence 3891 * synthesis. 3892 */ 3893 public String getCopyright() { 3894 return this.copyright == null ? null : this.copyright.getValue(); 3895 } 3896 3897 /** 3898 * @param value A copyright statement relating to the effect evidence synthesis 3899 * and/or its contents. Copyright statements are generally legal 3900 * restrictions on the use and publishing of the effect evidence 3901 * synthesis. 3902 */ 3903 public EffectEvidenceSynthesis setCopyright(String value) { 3904 if (value == null) 3905 this.copyright = null; 3906 else { 3907 if (this.copyright == null) 3908 this.copyright = new MarkdownType(); 3909 this.copyright.setValue(value); 3910 } 3911 return this; 3912 } 3913 3914 /** 3915 * @return {@link #approvalDate} (The date on which the resource content was 3916 * approved by the publisher. Approval happens once when the content is 3917 * officially approved for usage.). This is the underlying object with 3918 * id, value and extensions. The accessor "getApprovalDate" gives direct 3919 * access to the value 3920 */ 3921 public DateType getApprovalDateElement() { 3922 if (this.approvalDate == null) 3923 if (Configuration.errorOnAutoCreate()) 3924 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.approvalDate"); 3925 else if (Configuration.doAutoCreate()) 3926 this.approvalDate = new DateType(); // bb 3927 return this.approvalDate; 3928 } 3929 3930 public boolean hasApprovalDateElement() { 3931 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3932 } 3933 3934 public boolean hasApprovalDate() { 3935 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3936 } 3937 3938 /** 3939 * @param value {@link #approvalDate} (The date on which the resource content 3940 * was approved by the publisher. Approval happens once when the 3941 * content is officially approved for usage.). This is the 3942 * underlying object with id, value and extensions. The accessor 3943 * "getApprovalDate" gives direct access to the value 3944 */ 3945 public EffectEvidenceSynthesis setApprovalDateElement(DateType value) { 3946 this.approvalDate = value; 3947 return this; 3948 } 3949 3950 /** 3951 * @return The date on which the resource content was approved by the publisher. 3952 * Approval happens once when the content is officially approved for 3953 * usage. 3954 */ 3955 public Date getApprovalDate() { 3956 return this.approvalDate == null ? null : this.approvalDate.getValue(); 3957 } 3958 3959 /** 3960 * @param value The date on which the resource content was approved by the 3961 * publisher. Approval happens once when the content is officially 3962 * approved for usage. 3963 */ 3964 public EffectEvidenceSynthesis setApprovalDate(Date value) { 3965 if (value == null) 3966 this.approvalDate = null; 3967 else { 3968 if (this.approvalDate == null) 3969 this.approvalDate = new DateType(); 3970 this.approvalDate.setValue(value); 3971 } 3972 return this; 3973 } 3974 3975 /** 3976 * @return {@link #lastReviewDate} (The date on which the resource content was 3977 * last reviewed. Review happens periodically after approval but does 3978 * not change the original approval date.). This is the underlying 3979 * object with id, value and extensions. The accessor 3980 * "getLastReviewDate" gives direct access to the value 3981 */ 3982 public DateType getLastReviewDateElement() { 3983 if (this.lastReviewDate == null) 3984 if (Configuration.errorOnAutoCreate()) 3985 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.lastReviewDate"); 3986 else if (Configuration.doAutoCreate()) 3987 this.lastReviewDate = new DateType(); // bb 3988 return this.lastReviewDate; 3989 } 3990 3991 public boolean hasLastReviewDateElement() { 3992 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3993 } 3994 3995 public boolean hasLastReviewDate() { 3996 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3997 } 3998 3999 /** 4000 * @param value {@link #lastReviewDate} (The date on which the resource content 4001 * was last reviewed. Review happens periodically after approval 4002 * but does not change the original approval date.). This is the 4003 * underlying object with id, value and extensions. The accessor 4004 * "getLastReviewDate" gives direct access to the value 4005 */ 4006 public EffectEvidenceSynthesis setLastReviewDateElement(DateType value) { 4007 this.lastReviewDate = value; 4008 return this; 4009 } 4010 4011 /** 4012 * @return The date on which the resource content was last reviewed. Review 4013 * happens periodically after approval but does not change the original 4014 * approval date. 4015 */ 4016 public Date getLastReviewDate() { 4017 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 4018 } 4019 4020 /** 4021 * @param value The date on which the resource content was last reviewed. Review 4022 * happens periodically after approval but does not change the 4023 * original approval date. 4024 */ 4025 public EffectEvidenceSynthesis setLastReviewDate(Date value) { 4026 if (value == null) 4027 this.lastReviewDate = null; 4028 else { 4029 if (this.lastReviewDate == null) 4030 this.lastReviewDate = new DateType(); 4031 this.lastReviewDate.setValue(value); 4032 } 4033 return this; 4034 } 4035 4036 /** 4037 * @return {@link #effectivePeriod} (The period during which the effect evidence 4038 * synthesis content was or is planned to be in active use.) 4039 */ 4040 public Period getEffectivePeriod() { 4041 if (this.effectivePeriod == null) 4042 if (Configuration.errorOnAutoCreate()) 4043 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.effectivePeriod"); 4044 else if (Configuration.doAutoCreate()) 4045 this.effectivePeriod = new Period(); // cc 4046 return this.effectivePeriod; 4047 } 4048 4049 public boolean hasEffectivePeriod() { 4050 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 4051 } 4052 4053 /** 4054 * @param value {@link #effectivePeriod} (The period during which the effect 4055 * evidence synthesis content was or is planned to be in active 4056 * use.) 4057 */ 4058 public EffectEvidenceSynthesis setEffectivePeriod(Period value) { 4059 this.effectivePeriod = value; 4060 return this; 4061 } 4062 4063 /** 4064 * @return {@link #topic} (Descriptive topics related to the content of the 4065 * EffectEvidenceSynthesis. Topics provide a high-level categorization 4066 * grouping types of EffectEvidenceSynthesiss that can be useful for 4067 * filtering and searching.) 4068 */ 4069 public List<CodeableConcept> getTopic() { 4070 if (this.topic == null) 4071 this.topic = new ArrayList<CodeableConcept>(); 4072 return this.topic; 4073 } 4074 4075 /** 4076 * @return Returns a reference to <code>this</code> for easy method chaining 4077 */ 4078 public EffectEvidenceSynthesis setTopic(List<CodeableConcept> theTopic) { 4079 this.topic = theTopic; 4080 return this; 4081 } 4082 4083 public boolean hasTopic() { 4084 if (this.topic == null) 4085 return false; 4086 for (CodeableConcept item : this.topic) 4087 if (!item.isEmpty()) 4088 return true; 4089 return false; 4090 } 4091 4092 public CodeableConcept addTopic() { // 3 4093 CodeableConcept t = new CodeableConcept(); 4094 if (this.topic == null) 4095 this.topic = new ArrayList<CodeableConcept>(); 4096 this.topic.add(t); 4097 return t; 4098 } 4099 4100 public EffectEvidenceSynthesis addTopic(CodeableConcept t) { // 3 4101 if (t == null) 4102 return this; 4103 if (this.topic == null) 4104 this.topic = new ArrayList<CodeableConcept>(); 4105 this.topic.add(t); 4106 return this; 4107 } 4108 4109 /** 4110 * @return The first repetition of repeating field {@link #topic}, creating it 4111 * if it does not already exist 4112 */ 4113 public CodeableConcept getTopicFirstRep() { 4114 if (getTopic().isEmpty()) { 4115 addTopic(); 4116 } 4117 return getTopic().get(0); 4118 } 4119 4120 /** 4121 * @return {@link #author} (An individiual or organization primarily involved in 4122 * the creation and maintenance of the content.) 4123 */ 4124 public List<ContactDetail> getAuthor() { 4125 if (this.author == null) 4126 this.author = new ArrayList<ContactDetail>(); 4127 return this.author; 4128 } 4129 4130 /** 4131 * @return Returns a reference to <code>this</code> for easy method chaining 4132 */ 4133 public EffectEvidenceSynthesis setAuthor(List<ContactDetail> theAuthor) { 4134 this.author = theAuthor; 4135 return this; 4136 } 4137 4138 public boolean hasAuthor() { 4139 if (this.author == null) 4140 return false; 4141 for (ContactDetail item : this.author) 4142 if (!item.isEmpty()) 4143 return true; 4144 return false; 4145 } 4146 4147 public ContactDetail addAuthor() { // 3 4148 ContactDetail t = new ContactDetail(); 4149 if (this.author == null) 4150 this.author = new ArrayList<ContactDetail>(); 4151 this.author.add(t); 4152 return t; 4153 } 4154 4155 public EffectEvidenceSynthesis addAuthor(ContactDetail t) { // 3 4156 if (t == null) 4157 return this; 4158 if (this.author == null) 4159 this.author = new ArrayList<ContactDetail>(); 4160 this.author.add(t); 4161 return this; 4162 } 4163 4164 /** 4165 * @return The first repetition of repeating field {@link #author}, creating it 4166 * if it does not already exist 4167 */ 4168 public ContactDetail getAuthorFirstRep() { 4169 if (getAuthor().isEmpty()) { 4170 addAuthor(); 4171 } 4172 return getAuthor().get(0); 4173 } 4174 4175 /** 4176 * @return {@link #editor} (An individual or organization primarily responsible 4177 * for internal coherence of the content.) 4178 */ 4179 public List<ContactDetail> getEditor() { 4180 if (this.editor == null) 4181 this.editor = new ArrayList<ContactDetail>(); 4182 return this.editor; 4183 } 4184 4185 /** 4186 * @return Returns a reference to <code>this</code> for easy method chaining 4187 */ 4188 public EffectEvidenceSynthesis setEditor(List<ContactDetail> theEditor) { 4189 this.editor = theEditor; 4190 return this; 4191 } 4192 4193 public boolean hasEditor() { 4194 if (this.editor == null) 4195 return false; 4196 for (ContactDetail item : this.editor) 4197 if (!item.isEmpty()) 4198 return true; 4199 return false; 4200 } 4201 4202 public ContactDetail addEditor() { // 3 4203 ContactDetail t = new ContactDetail(); 4204 if (this.editor == null) 4205 this.editor = new ArrayList<ContactDetail>(); 4206 this.editor.add(t); 4207 return t; 4208 } 4209 4210 public EffectEvidenceSynthesis addEditor(ContactDetail t) { // 3 4211 if (t == null) 4212 return this; 4213 if (this.editor == null) 4214 this.editor = new ArrayList<ContactDetail>(); 4215 this.editor.add(t); 4216 return this; 4217 } 4218 4219 /** 4220 * @return The first repetition of repeating field {@link #editor}, creating it 4221 * if it does not already exist 4222 */ 4223 public ContactDetail getEditorFirstRep() { 4224 if (getEditor().isEmpty()) { 4225 addEditor(); 4226 } 4227 return getEditor().get(0); 4228 } 4229 4230 /** 4231 * @return {@link #reviewer} (An individual or organization primarily 4232 * responsible for review of some aspect of the content.) 4233 */ 4234 public List<ContactDetail> getReviewer() { 4235 if (this.reviewer == null) 4236 this.reviewer = new ArrayList<ContactDetail>(); 4237 return this.reviewer; 4238 } 4239 4240 /** 4241 * @return Returns a reference to <code>this</code> for easy method chaining 4242 */ 4243 public EffectEvidenceSynthesis setReviewer(List<ContactDetail> theReviewer) { 4244 this.reviewer = theReviewer; 4245 return this; 4246 } 4247 4248 public boolean hasReviewer() { 4249 if (this.reviewer == null) 4250 return false; 4251 for (ContactDetail item : this.reviewer) 4252 if (!item.isEmpty()) 4253 return true; 4254 return false; 4255 } 4256 4257 public ContactDetail addReviewer() { // 3 4258 ContactDetail t = new ContactDetail(); 4259 if (this.reviewer == null) 4260 this.reviewer = new ArrayList<ContactDetail>(); 4261 this.reviewer.add(t); 4262 return t; 4263 } 4264 4265 public EffectEvidenceSynthesis addReviewer(ContactDetail t) { // 3 4266 if (t == null) 4267 return this; 4268 if (this.reviewer == null) 4269 this.reviewer = new ArrayList<ContactDetail>(); 4270 this.reviewer.add(t); 4271 return this; 4272 } 4273 4274 /** 4275 * @return The first repetition of repeating field {@link #reviewer}, creating 4276 * it if it does not already exist 4277 */ 4278 public ContactDetail getReviewerFirstRep() { 4279 if (getReviewer().isEmpty()) { 4280 addReviewer(); 4281 } 4282 return getReviewer().get(0); 4283 } 4284 4285 /** 4286 * @return {@link #endorser} (An individual or organization responsible for 4287 * officially endorsing the content for use in some setting.) 4288 */ 4289 public List<ContactDetail> getEndorser() { 4290 if (this.endorser == null) 4291 this.endorser = new ArrayList<ContactDetail>(); 4292 return this.endorser; 4293 } 4294 4295 /** 4296 * @return Returns a reference to <code>this</code> for easy method chaining 4297 */ 4298 public EffectEvidenceSynthesis setEndorser(List<ContactDetail> theEndorser) { 4299 this.endorser = theEndorser; 4300 return this; 4301 } 4302 4303 public boolean hasEndorser() { 4304 if (this.endorser == null) 4305 return false; 4306 for (ContactDetail item : this.endorser) 4307 if (!item.isEmpty()) 4308 return true; 4309 return false; 4310 } 4311 4312 public ContactDetail addEndorser() { // 3 4313 ContactDetail t = new ContactDetail(); 4314 if (this.endorser == null) 4315 this.endorser = new ArrayList<ContactDetail>(); 4316 this.endorser.add(t); 4317 return t; 4318 } 4319 4320 public EffectEvidenceSynthesis addEndorser(ContactDetail t) { // 3 4321 if (t == null) 4322 return this; 4323 if (this.endorser == null) 4324 this.endorser = new ArrayList<ContactDetail>(); 4325 this.endorser.add(t); 4326 return this; 4327 } 4328 4329 /** 4330 * @return The first repetition of repeating field {@link #endorser}, creating 4331 * it if it does not already exist 4332 */ 4333 public ContactDetail getEndorserFirstRep() { 4334 if (getEndorser().isEmpty()) { 4335 addEndorser(); 4336 } 4337 return getEndorser().get(0); 4338 } 4339 4340 /** 4341 * @return {@link #relatedArtifact} (Related artifacts such as additional 4342 * documentation, justification, or bibliographic references.) 4343 */ 4344 public List<RelatedArtifact> getRelatedArtifact() { 4345 if (this.relatedArtifact == null) 4346 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 4347 return this.relatedArtifact; 4348 } 4349 4350 /** 4351 * @return Returns a reference to <code>this</code> for easy method chaining 4352 */ 4353 public EffectEvidenceSynthesis setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 4354 this.relatedArtifact = theRelatedArtifact; 4355 return this; 4356 } 4357 4358 public boolean hasRelatedArtifact() { 4359 if (this.relatedArtifact == null) 4360 return false; 4361 for (RelatedArtifact item : this.relatedArtifact) 4362 if (!item.isEmpty()) 4363 return true; 4364 return false; 4365 } 4366 4367 public RelatedArtifact addRelatedArtifact() { // 3 4368 RelatedArtifact t = new RelatedArtifact(); 4369 if (this.relatedArtifact == null) 4370 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 4371 this.relatedArtifact.add(t); 4372 return t; 4373 } 4374 4375 public EffectEvidenceSynthesis addRelatedArtifact(RelatedArtifact t) { // 3 4376 if (t == null) 4377 return this; 4378 if (this.relatedArtifact == null) 4379 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 4380 this.relatedArtifact.add(t); 4381 return this; 4382 } 4383 4384 /** 4385 * @return The first repetition of repeating field {@link #relatedArtifact}, 4386 * creating it if it does not already exist 4387 */ 4388 public RelatedArtifact getRelatedArtifactFirstRep() { 4389 if (getRelatedArtifact().isEmpty()) { 4390 addRelatedArtifact(); 4391 } 4392 return getRelatedArtifact().get(0); 4393 } 4394 4395 /** 4396 * @return {@link #synthesisType} (Type of synthesis eg meta-analysis.) 4397 */ 4398 public CodeableConcept getSynthesisType() { 4399 if (this.synthesisType == null) 4400 if (Configuration.errorOnAutoCreate()) 4401 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.synthesisType"); 4402 else if (Configuration.doAutoCreate()) 4403 this.synthesisType = new CodeableConcept(); // cc 4404 return this.synthesisType; 4405 } 4406 4407 public boolean hasSynthesisType() { 4408 return this.synthesisType != null && !this.synthesisType.isEmpty(); 4409 } 4410 4411 /** 4412 * @param value {@link #synthesisType} (Type of synthesis eg meta-analysis.) 4413 */ 4414 public EffectEvidenceSynthesis setSynthesisType(CodeableConcept value) { 4415 this.synthesisType = value; 4416 return this; 4417 } 4418 4419 /** 4420 * @return {@link #studyType} (Type of study eg randomized trial.) 4421 */ 4422 public CodeableConcept getStudyType() { 4423 if (this.studyType == null) 4424 if (Configuration.errorOnAutoCreate()) 4425 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.studyType"); 4426 else if (Configuration.doAutoCreate()) 4427 this.studyType = new CodeableConcept(); // cc 4428 return this.studyType; 4429 } 4430 4431 public boolean hasStudyType() { 4432 return this.studyType != null && !this.studyType.isEmpty(); 4433 } 4434 4435 /** 4436 * @param value {@link #studyType} (Type of study eg randomized trial.) 4437 */ 4438 public EffectEvidenceSynthesis setStudyType(CodeableConcept value) { 4439 this.studyType = value; 4440 return this; 4441 } 4442 4443 /** 4444 * @return {@link #population} (A reference to a EvidenceVariable resource that 4445 * defines the population for the research.) 4446 */ 4447 public Reference getPopulation() { 4448 if (this.population == null) 4449 if (Configuration.errorOnAutoCreate()) 4450 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.population"); 4451 else if (Configuration.doAutoCreate()) 4452 this.population = new Reference(); // cc 4453 return this.population; 4454 } 4455 4456 public boolean hasPopulation() { 4457 return this.population != null && !this.population.isEmpty(); 4458 } 4459 4460 /** 4461 * @param value {@link #population} (A reference to a EvidenceVariable resource 4462 * that defines the population for the research.) 4463 */ 4464 public EffectEvidenceSynthesis setPopulation(Reference value) { 4465 this.population = value; 4466 return this; 4467 } 4468 4469 /** 4470 * @return {@link #population} The actual object that is the target of the 4471 * reference. The reference library doesn't populate this, but you can 4472 * use it to hold the resource if you resolve it. (A reference to a 4473 * EvidenceVariable resource that defines the population for the 4474 * research.) 4475 */ 4476 public EvidenceVariable getPopulationTarget() { 4477 if (this.populationTarget == null) 4478 if (Configuration.errorOnAutoCreate()) 4479 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.population"); 4480 else if (Configuration.doAutoCreate()) 4481 this.populationTarget = new EvidenceVariable(); // aa 4482 return this.populationTarget; 4483 } 4484 4485 /** 4486 * @param value {@link #population} The actual object that is the target of the 4487 * reference. The reference library doesn't use these, but you can 4488 * use it to hold the resource if you resolve it. (A reference to a 4489 * EvidenceVariable resource that defines the population for the 4490 * research.) 4491 */ 4492 public EffectEvidenceSynthesis setPopulationTarget(EvidenceVariable value) { 4493 this.populationTarget = value; 4494 return this; 4495 } 4496 4497 /** 4498 * @return {@link #exposure} (A reference to a EvidenceVariable resource that 4499 * defines the exposure for the research.) 4500 */ 4501 public Reference getExposure() { 4502 if (this.exposure == null) 4503 if (Configuration.errorOnAutoCreate()) 4504 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.exposure"); 4505 else if (Configuration.doAutoCreate()) 4506 this.exposure = new Reference(); // cc 4507 return this.exposure; 4508 } 4509 4510 public boolean hasExposure() { 4511 return this.exposure != null && !this.exposure.isEmpty(); 4512 } 4513 4514 /** 4515 * @param value {@link #exposure} (A reference to a EvidenceVariable resource 4516 * that defines the exposure for the research.) 4517 */ 4518 public EffectEvidenceSynthesis setExposure(Reference value) { 4519 this.exposure = value; 4520 return this; 4521 } 4522 4523 /** 4524 * @return {@link #exposure} The actual object that is the target of the 4525 * reference. The reference library doesn't populate this, but you can 4526 * use it to hold the resource if you resolve it. (A reference to a 4527 * EvidenceVariable resource that defines the exposure for the 4528 * research.) 4529 */ 4530 public EvidenceVariable getExposureTarget() { 4531 if (this.exposureTarget == null) 4532 if (Configuration.errorOnAutoCreate()) 4533 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.exposure"); 4534 else if (Configuration.doAutoCreate()) 4535 this.exposureTarget = new EvidenceVariable(); // aa 4536 return this.exposureTarget; 4537 } 4538 4539 /** 4540 * @param value {@link #exposure} The actual object that is the target of the 4541 * reference. The reference library doesn't use these, but you can 4542 * use it to hold the resource if you resolve it. (A reference to a 4543 * EvidenceVariable resource that defines the exposure for the 4544 * research.) 4545 */ 4546 public EffectEvidenceSynthesis setExposureTarget(EvidenceVariable value) { 4547 this.exposureTarget = value; 4548 return this; 4549 } 4550 4551 /** 4552 * @return {@link #exposureAlternative} (A reference to a EvidenceVariable 4553 * resource that defines the comparison exposure for the research.) 4554 */ 4555 public Reference getExposureAlternative() { 4556 if (this.exposureAlternative == null) 4557 if (Configuration.errorOnAutoCreate()) 4558 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.exposureAlternative"); 4559 else if (Configuration.doAutoCreate()) 4560 this.exposureAlternative = new Reference(); // cc 4561 return this.exposureAlternative; 4562 } 4563 4564 public boolean hasExposureAlternative() { 4565 return this.exposureAlternative != null && !this.exposureAlternative.isEmpty(); 4566 } 4567 4568 /** 4569 * @param value {@link #exposureAlternative} (A reference to a EvidenceVariable 4570 * resource that defines the comparison exposure for the research.) 4571 */ 4572 public EffectEvidenceSynthesis setExposureAlternative(Reference value) { 4573 this.exposureAlternative = value; 4574 return this; 4575 } 4576 4577 /** 4578 * @return {@link #exposureAlternative} The actual object that is the target of 4579 * the reference. The reference library doesn't populate this, but you 4580 * can use it to hold the resource if you resolve it. (A reference to a 4581 * EvidenceVariable resource that defines the comparison exposure for 4582 * the research.) 4583 */ 4584 public EvidenceVariable getExposureAlternativeTarget() { 4585 if (this.exposureAlternativeTarget == null) 4586 if (Configuration.errorOnAutoCreate()) 4587 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.exposureAlternative"); 4588 else if (Configuration.doAutoCreate()) 4589 this.exposureAlternativeTarget = new EvidenceVariable(); // aa 4590 return this.exposureAlternativeTarget; 4591 } 4592 4593 /** 4594 * @param value {@link #exposureAlternative} The actual object that is the 4595 * target of the reference. The reference library doesn't use 4596 * these, but you can use it to hold the resource if you resolve 4597 * it. (A reference to a EvidenceVariable resource that defines the 4598 * comparison exposure for the research.) 4599 */ 4600 public EffectEvidenceSynthesis setExposureAlternativeTarget(EvidenceVariable value) { 4601 this.exposureAlternativeTarget = value; 4602 return this; 4603 } 4604 4605 /** 4606 * @return {@link #outcome} (A reference to a EvidenceVariable resomece that 4607 * defines the outcome for the research.) 4608 */ 4609 public Reference getOutcome() { 4610 if (this.outcome == null) 4611 if (Configuration.errorOnAutoCreate()) 4612 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.outcome"); 4613 else if (Configuration.doAutoCreate()) 4614 this.outcome = new Reference(); // cc 4615 return this.outcome; 4616 } 4617 4618 public boolean hasOutcome() { 4619 return this.outcome != null && !this.outcome.isEmpty(); 4620 } 4621 4622 /** 4623 * @param value {@link #outcome} (A reference to a EvidenceVariable resomece 4624 * that defines the outcome for the research.) 4625 */ 4626 public EffectEvidenceSynthesis setOutcome(Reference value) { 4627 this.outcome = value; 4628 return this; 4629 } 4630 4631 /** 4632 * @return {@link #outcome} The actual object that is the target of the 4633 * reference. The reference library doesn't populate this, but you can 4634 * use it to hold the resource if you resolve it. (A reference to a 4635 * EvidenceVariable resomece that defines the outcome for the research.) 4636 */ 4637 public EvidenceVariable getOutcomeTarget() { 4638 if (this.outcomeTarget == null) 4639 if (Configuration.errorOnAutoCreate()) 4640 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.outcome"); 4641 else if (Configuration.doAutoCreate()) 4642 this.outcomeTarget = new EvidenceVariable(); // aa 4643 return this.outcomeTarget; 4644 } 4645 4646 /** 4647 * @param value {@link #outcome} The actual object that is the target of the 4648 * reference. The reference library doesn't use these, but you can 4649 * use it to hold the resource if you resolve it. (A reference to a 4650 * EvidenceVariable resomece that defines the outcome for the 4651 * research.) 4652 */ 4653 public EffectEvidenceSynthesis setOutcomeTarget(EvidenceVariable value) { 4654 this.outcomeTarget = value; 4655 return this; 4656 } 4657 4658 /** 4659 * @return {@link #sampleSize} (A description of the size of the sample involved 4660 * in the synthesis.) 4661 */ 4662 public EffectEvidenceSynthesisSampleSizeComponent getSampleSize() { 4663 if (this.sampleSize == null) 4664 if (Configuration.errorOnAutoCreate()) 4665 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.sampleSize"); 4666 else if (Configuration.doAutoCreate()) 4667 this.sampleSize = new EffectEvidenceSynthesisSampleSizeComponent(); // cc 4668 return this.sampleSize; 4669 } 4670 4671 public boolean hasSampleSize() { 4672 return this.sampleSize != null && !this.sampleSize.isEmpty(); 4673 } 4674 4675 /** 4676 * @param value {@link #sampleSize} (A description of the size of the sample 4677 * involved in the synthesis.) 4678 */ 4679 public EffectEvidenceSynthesis setSampleSize(EffectEvidenceSynthesisSampleSizeComponent value) { 4680 this.sampleSize = value; 4681 return this; 4682 } 4683 4684 /** 4685 * @return {@link #resultsByExposure} (A description of the results for each 4686 * exposure considered in the effect estimate.) 4687 */ 4688 public List<EffectEvidenceSynthesisResultsByExposureComponent> getResultsByExposure() { 4689 if (this.resultsByExposure == null) 4690 this.resultsByExposure = new ArrayList<EffectEvidenceSynthesisResultsByExposureComponent>(); 4691 return this.resultsByExposure; 4692 } 4693 4694 /** 4695 * @return Returns a reference to <code>this</code> for easy method chaining 4696 */ 4697 public EffectEvidenceSynthesis setResultsByExposure( 4698 List<EffectEvidenceSynthesisResultsByExposureComponent> theResultsByExposure) { 4699 this.resultsByExposure = theResultsByExposure; 4700 return this; 4701 } 4702 4703 public boolean hasResultsByExposure() { 4704 if (this.resultsByExposure == null) 4705 return false; 4706 for (EffectEvidenceSynthesisResultsByExposureComponent item : this.resultsByExposure) 4707 if (!item.isEmpty()) 4708 return true; 4709 return false; 4710 } 4711 4712 public EffectEvidenceSynthesisResultsByExposureComponent addResultsByExposure() { // 3 4713 EffectEvidenceSynthesisResultsByExposureComponent t = new EffectEvidenceSynthesisResultsByExposureComponent(); 4714 if (this.resultsByExposure == null) 4715 this.resultsByExposure = new ArrayList<EffectEvidenceSynthesisResultsByExposureComponent>(); 4716 this.resultsByExposure.add(t); 4717 return t; 4718 } 4719 4720 public EffectEvidenceSynthesis addResultsByExposure(EffectEvidenceSynthesisResultsByExposureComponent t) { // 3 4721 if (t == null) 4722 return this; 4723 if (this.resultsByExposure == null) 4724 this.resultsByExposure = new ArrayList<EffectEvidenceSynthesisResultsByExposureComponent>(); 4725 this.resultsByExposure.add(t); 4726 return this; 4727 } 4728 4729 /** 4730 * @return The first repetition of repeating field {@link #resultsByExposure}, 4731 * creating it if it does not already exist 4732 */ 4733 public EffectEvidenceSynthesisResultsByExposureComponent getResultsByExposureFirstRep() { 4734 if (getResultsByExposure().isEmpty()) { 4735 addResultsByExposure(); 4736 } 4737 return getResultsByExposure().get(0); 4738 } 4739 4740 /** 4741 * @return {@link #effectEstimate} (The estimated effect of the exposure 4742 * variant.) 4743 */ 4744 public List<EffectEvidenceSynthesisEffectEstimateComponent> getEffectEstimate() { 4745 if (this.effectEstimate == null) 4746 this.effectEstimate = new ArrayList<EffectEvidenceSynthesisEffectEstimateComponent>(); 4747 return this.effectEstimate; 4748 } 4749 4750 /** 4751 * @return Returns a reference to <code>this</code> for easy method chaining 4752 */ 4753 public EffectEvidenceSynthesis setEffectEstimate( 4754 List<EffectEvidenceSynthesisEffectEstimateComponent> theEffectEstimate) { 4755 this.effectEstimate = theEffectEstimate; 4756 return this; 4757 } 4758 4759 public boolean hasEffectEstimate() { 4760 if (this.effectEstimate == null) 4761 return false; 4762 for (EffectEvidenceSynthesisEffectEstimateComponent item : this.effectEstimate) 4763 if (!item.isEmpty()) 4764 return true; 4765 return false; 4766 } 4767 4768 public EffectEvidenceSynthesisEffectEstimateComponent addEffectEstimate() { // 3 4769 EffectEvidenceSynthesisEffectEstimateComponent t = new EffectEvidenceSynthesisEffectEstimateComponent(); 4770 if (this.effectEstimate == null) 4771 this.effectEstimate = new ArrayList<EffectEvidenceSynthesisEffectEstimateComponent>(); 4772 this.effectEstimate.add(t); 4773 return t; 4774 } 4775 4776 public EffectEvidenceSynthesis addEffectEstimate(EffectEvidenceSynthesisEffectEstimateComponent t) { // 3 4777 if (t == null) 4778 return this; 4779 if (this.effectEstimate == null) 4780 this.effectEstimate = new ArrayList<EffectEvidenceSynthesisEffectEstimateComponent>(); 4781 this.effectEstimate.add(t); 4782 return this; 4783 } 4784 4785 /** 4786 * @return The first repetition of repeating field {@link #effectEstimate}, 4787 * creating it if it does not already exist 4788 */ 4789 public EffectEvidenceSynthesisEffectEstimateComponent getEffectEstimateFirstRep() { 4790 if (getEffectEstimate().isEmpty()) { 4791 addEffectEstimate(); 4792 } 4793 return getEffectEstimate().get(0); 4794 } 4795 4796 /** 4797 * @return {@link #certainty} (A description of the certainty of the effect 4798 * estimate.) 4799 */ 4800 public List<EffectEvidenceSynthesisCertaintyComponent> getCertainty() { 4801 if (this.certainty == null) 4802 this.certainty = new ArrayList<EffectEvidenceSynthesisCertaintyComponent>(); 4803 return this.certainty; 4804 } 4805 4806 /** 4807 * @return Returns a reference to <code>this</code> for easy method chaining 4808 */ 4809 public EffectEvidenceSynthesis setCertainty(List<EffectEvidenceSynthesisCertaintyComponent> theCertainty) { 4810 this.certainty = theCertainty; 4811 return this; 4812 } 4813 4814 public boolean hasCertainty() { 4815 if (this.certainty == null) 4816 return false; 4817 for (EffectEvidenceSynthesisCertaintyComponent item : this.certainty) 4818 if (!item.isEmpty()) 4819 return true; 4820 return false; 4821 } 4822 4823 public EffectEvidenceSynthesisCertaintyComponent addCertainty() { // 3 4824 EffectEvidenceSynthesisCertaintyComponent t = new EffectEvidenceSynthesisCertaintyComponent(); 4825 if (this.certainty == null) 4826 this.certainty = new ArrayList<EffectEvidenceSynthesisCertaintyComponent>(); 4827 this.certainty.add(t); 4828 return t; 4829 } 4830 4831 public EffectEvidenceSynthesis addCertainty(EffectEvidenceSynthesisCertaintyComponent t) { // 3 4832 if (t == null) 4833 return this; 4834 if (this.certainty == null) 4835 this.certainty = new ArrayList<EffectEvidenceSynthesisCertaintyComponent>(); 4836 this.certainty.add(t); 4837 return this; 4838 } 4839 4840 /** 4841 * @return The first repetition of repeating field {@link #certainty}, creating 4842 * it if it does not already exist 4843 */ 4844 public EffectEvidenceSynthesisCertaintyComponent getCertaintyFirstRep() { 4845 if (getCertainty().isEmpty()) { 4846 addCertainty(); 4847 } 4848 return getCertainty().get(0); 4849 } 4850 4851 protected void listChildren(List<Property> children) { 4852 super.listChildren(children); 4853 children.add(new Property("url", "uri", 4854 "An absolute URI that is used to identify this effect evidence synthesis when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this effect evidence synthesis is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the effect evidence synthesis is stored on different servers.", 4855 0, 1, url)); 4856 children.add(new Property("identifier", "Identifier", 4857 "A formal identifier that is used to identify this effect evidence synthesis when it is represented in other formats, or referenced in a specification, model, design or an instance.", 4858 0, java.lang.Integer.MAX_VALUE, identifier)); 4859 children.add(new Property("version", "string", 4860 "The identifier that is used to identify this version of the effect evidence synthesis when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the effect evidence synthesis author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 4861 0, 1, version)); 4862 children.add(new Property("name", "string", 4863 "A natural language name identifying the effect evidence synthesis. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 4864 0, 1, name)); 4865 children.add(new Property("title", "string", 4866 "A short, descriptive, user-friendly title for the effect evidence synthesis.", 0, 1, title)); 4867 children.add(new Property("status", "code", 4868 "The status of this effect evidence synthesis. Enables tracking the life-cycle of the content.", 0, 1, status)); 4869 children.add(new Property("date", "dateTime", 4870 "The date (and optionally time) when the effect evidence synthesis was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the effect evidence synthesis changes.", 4871 0, 1, date)); 4872 children.add(new Property("publisher", "string", 4873 "The name of the organization or individual that published the effect evidence synthesis.", 0, 1, publisher)); 4874 children.add(new Property("contact", "ContactDetail", 4875 "Contact details to assist a user in finding and communicating with the publisher.", 0, 4876 java.lang.Integer.MAX_VALUE, contact)); 4877 children.add(new Property("description", "markdown", 4878 "A free text natural language description of the effect evidence synthesis from a consumer's perspective.", 0, 4879 1, description)); 4880 children.add( 4881 new Property("note", "Annotation", "A human-readable string to clarify or explain concepts about the resource.", 4882 0, java.lang.Integer.MAX_VALUE, note)); 4883 children.add(new Property("useContext", "UsageContext", 4884 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate effect evidence synthesis instances.", 4885 0, java.lang.Integer.MAX_VALUE, useContext)); 4886 children.add(new Property("jurisdiction", "CodeableConcept", 4887 "A legal or geographic region in which the effect evidence synthesis is intended to be used.", 0, 4888 java.lang.Integer.MAX_VALUE, jurisdiction)); 4889 children.add(new Property("copyright", "markdown", 4890 "A copyright statement relating to the effect evidence synthesis and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the effect evidence synthesis.", 4891 0, 1, copyright)); 4892 children.add(new Property("approvalDate", "date", 4893 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 4894 0, 1, approvalDate)); 4895 children.add(new Property("lastReviewDate", "date", 4896 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 4897 0, 1, lastReviewDate)); 4898 children.add(new Property("effectivePeriod", "Period", 4899 "The period during which the effect evidence synthesis content was or is planned to be in active use.", 0, 1, 4900 effectivePeriod)); 4901 children.add(new Property("topic", "CodeableConcept", 4902 "Descriptive topics related to the content of the EffectEvidenceSynthesis. Topics provide a high-level categorization grouping types of EffectEvidenceSynthesiss that can be useful for filtering and searching.", 4903 0, java.lang.Integer.MAX_VALUE, topic)); 4904 children.add(new Property("author", "ContactDetail", 4905 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 4906 java.lang.Integer.MAX_VALUE, author)); 4907 children.add(new Property("editor", "ContactDetail", 4908 "An individual or organization primarily responsible for internal coherence of the content.", 0, 4909 java.lang.Integer.MAX_VALUE, editor)); 4910 children.add(new Property("reviewer", "ContactDetail", 4911 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 4912 java.lang.Integer.MAX_VALUE, reviewer)); 4913 children.add(new Property("endorser", "ContactDetail", 4914 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 4915 java.lang.Integer.MAX_VALUE, endorser)); 4916 children.add(new Property("relatedArtifact", "RelatedArtifact", 4917 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 4918 java.lang.Integer.MAX_VALUE, relatedArtifact)); 4919 children.add( 4920 new Property("synthesisType", "CodeableConcept", "Type of synthesis eg meta-analysis.", 0, 1, synthesisType)); 4921 children.add(new Property("studyType", "CodeableConcept", "Type of study eg randomized trial.", 0, 1, studyType)); 4922 children.add(new Property("population", "Reference(EvidenceVariable)", 4923 "A reference to a EvidenceVariable resource that defines the population for the research.", 0, 1, population)); 4924 children.add(new Property("exposure", "Reference(EvidenceVariable)", 4925 "A reference to a EvidenceVariable resource that defines the exposure for the research.", 0, 1, exposure)); 4926 children.add(new Property("exposureAlternative", "Reference(EvidenceVariable)", 4927 "A reference to a EvidenceVariable resource that defines the comparison exposure for the research.", 0, 1, 4928 exposureAlternative)); 4929 children.add(new Property("outcome", "Reference(EvidenceVariable)", 4930 "A reference to a EvidenceVariable resomece that defines the outcome for the research.", 0, 1, outcome)); 4931 children.add(new Property("sampleSize", "", "A description of the size of the sample involved in the synthesis.", 0, 4932 1, sampleSize)); 4933 children.add(new Property("resultsByExposure", "", 4934 "A description of the results for each exposure considered in the effect estimate.", 0, 4935 java.lang.Integer.MAX_VALUE, resultsByExposure)); 4936 children.add(new Property("effectEstimate", "", "The estimated effect of the exposure variant.", 0, 4937 java.lang.Integer.MAX_VALUE, effectEstimate)); 4938 children.add(new Property("certainty", "", "A description of the certainty of the effect estimate.", 0, 4939 java.lang.Integer.MAX_VALUE, certainty)); 4940 } 4941 4942 @Override 4943 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4944 switch (_hash) { 4945 case 116079: 4946 /* url */ return new Property("url", "uri", 4947 "An absolute URI that is used to identify this effect evidence synthesis when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this effect evidence synthesis is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the effect evidence synthesis is stored on different servers.", 4948 0, 1, url); 4949 case -1618432855: 4950 /* identifier */ return new Property("identifier", "Identifier", 4951 "A formal identifier that is used to identify this effect evidence synthesis when it is represented in other formats, or referenced in a specification, model, design or an instance.", 4952 0, java.lang.Integer.MAX_VALUE, identifier); 4953 case 351608024: 4954 /* version */ return new Property("version", "string", 4955 "The identifier that is used to identify this version of the effect evidence synthesis when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the effect evidence synthesis author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 4956 0, 1, version); 4957 case 3373707: 4958 /* name */ return new Property("name", "string", 4959 "A natural language name identifying the effect evidence synthesis. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 4960 0, 1, name); 4961 case 110371416: 4962 /* title */ return new Property("title", "string", 4963 "A short, descriptive, user-friendly title for the effect evidence synthesis.", 0, 1, title); 4964 case -892481550: 4965 /* status */ return new Property("status", "code", 4966 "The status of this effect evidence synthesis. Enables tracking the life-cycle of the content.", 0, 1, 4967 status); 4968 case 3076014: 4969 /* date */ return new Property("date", "dateTime", 4970 "The date (and optionally time) when the effect evidence synthesis was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the effect evidence synthesis changes.", 4971 0, 1, date); 4972 case 1447404028: 4973 /* publisher */ return new Property("publisher", "string", 4974 "The name of the organization or individual that published the effect evidence synthesis.", 0, 1, publisher); 4975 case 951526432: 4976 /* contact */ return new Property("contact", "ContactDetail", 4977 "Contact details to assist a user in finding and communicating with the publisher.", 0, 4978 java.lang.Integer.MAX_VALUE, contact); 4979 case -1724546052: 4980 /* description */ return new Property("description", "markdown", 4981 "A free text natural language description of the effect evidence synthesis from a consumer's perspective.", 0, 4982 1, description); 4983 case 3387378: 4984 /* note */ return new Property("note", "Annotation", 4985 "A human-readable string to clarify or explain concepts about the resource.", 0, java.lang.Integer.MAX_VALUE, 4986 note); 4987 case -669707736: 4988 /* useContext */ return new Property("useContext", "UsageContext", 4989 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate effect evidence synthesis instances.", 4990 0, java.lang.Integer.MAX_VALUE, useContext); 4991 case -507075711: 4992 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 4993 "A legal or geographic region in which the effect evidence synthesis is intended to be used.", 0, 4994 java.lang.Integer.MAX_VALUE, jurisdiction); 4995 case 1522889671: 4996 /* copyright */ return new Property("copyright", "markdown", 4997 "A copyright statement relating to the effect evidence synthesis and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the effect evidence synthesis.", 4998 0, 1, copyright); 4999 case 223539345: 5000 /* approvalDate */ return new Property("approvalDate", "date", 5001 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 5002 0, 1, approvalDate); 5003 case -1687512484: 5004 /* lastReviewDate */ return new Property("lastReviewDate", "date", 5005 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 5006 0, 1, lastReviewDate); 5007 case -403934648: 5008 /* effectivePeriod */ return new Property("effectivePeriod", "Period", 5009 "The period during which the effect evidence synthesis content was or is planned to be in active use.", 0, 1, 5010 effectivePeriod); 5011 case 110546223: 5012 /* topic */ return new Property("topic", "CodeableConcept", 5013 "Descriptive topics related to the content of the EffectEvidenceSynthesis. Topics provide a high-level categorization grouping types of EffectEvidenceSynthesiss that can be useful for filtering and searching.", 5014 0, java.lang.Integer.MAX_VALUE, topic); 5015 case -1406328437: 5016 /* author */ return new Property("author", "ContactDetail", 5017 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 5018 java.lang.Integer.MAX_VALUE, author); 5019 case -1307827859: 5020 /* editor */ return new Property("editor", "ContactDetail", 5021 "An individual or organization primarily responsible for internal coherence of the content.", 0, 5022 java.lang.Integer.MAX_VALUE, editor); 5023 case -261190139: 5024 /* reviewer */ return new Property("reviewer", "ContactDetail", 5025 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 5026 java.lang.Integer.MAX_VALUE, reviewer); 5027 case 1740277666: 5028 /* endorser */ return new Property("endorser", "ContactDetail", 5029 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 5030 java.lang.Integer.MAX_VALUE, endorser); 5031 case 666807069: 5032 /* relatedArtifact */ return new Property("relatedArtifact", "RelatedArtifact", 5033 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 5034 java.lang.Integer.MAX_VALUE, relatedArtifact); 5035 case 672726254: 5036 /* synthesisType */ return new Property("synthesisType", "CodeableConcept", "Type of synthesis eg meta-analysis.", 5037 0, 1, synthesisType); 5038 case -1955265373: 5039 /* studyType */ return new Property("studyType", "CodeableConcept", "Type of study eg randomized trial.", 0, 1, 5040 studyType); 5041 case -2023558323: 5042 /* population */ return new Property("population", "Reference(EvidenceVariable)", 5043 "A reference to a EvidenceVariable resource that defines the population for the research.", 0, 1, population); 5044 case -1926005497: 5045 /* exposure */ return new Property("exposure", "Reference(EvidenceVariable)", 5046 "A reference to a EvidenceVariable resource that defines the exposure for the research.", 0, 1, exposure); 5047 case -1875462106: 5048 /* exposureAlternative */ return new Property("exposureAlternative", "Reference(EvidenceVariable)", 5049 "A reference to a EvidenceVariable resource that defines the comparison exposure for the research.", 0, 1, 5050 exposureAlternative); 5051 case -1106507950: 5052 /* outcome */ return new Property("outcome", "Reference(EvidenceVariable)", 5053 "A reference to a EvidenceVariable resomece that defines the outcome for the research.", 0, 1, outcome); 5054 case 143123659: 5055 /* sampleSize */ return new Property("sampleSize", "", 5056 "A description of the size of the sample involved in the synthesis.", 0, 1, sampleSize); 5057 case 553042708: 5058 /* resultsByExposure */ return new Property("resultsByExposure", "", 5059 "A description of the results for each exposure considered in the effect estimate.", 0, 5060 java.lang.Integer.MAX_VALUE, resultsByExposure); 5061 case 707469785: 5062 /* effectEstimate */ return new Property("effectEstimate", "", "The estimated effect of the exposure variant.", 0, 5063 java.lang.Integer.MAX_VALUE, effectEstimate); 5064 case -1404142937: 5065 /* certainty */ return new Property("certainty", "", "A description of the certainty of the effect estimate.", 0, 5066 java.lang.Integer.MAX_VALUE, certainty); 5067 default: 5068 return super.getNamedProperty(_hash, _name, _checkValid); 5069 } 5070 5071 } 5072 5073 @Override 5074 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5075 switch (hash) { 5076 case 116079: 5077 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 5078 case -1618432855: 5079 /* identifier */ return this.identifier == null ? new Base[0] 5080 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 5081 case 351608024: 5082 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 5083 case 3373707: 5084 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 5085 case 110371416: 5086 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 5087 case -892481550: 5088 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 5089 case 3076014: 5090 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 5091 case 1447404028: 5092 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 5093 case 951526432: 5094 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 5095 case -1724546052: 5096 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 5097 case 3387378: 5098 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 5099 case -669707736: 5100 /* useContext */ return this.useContext == null ? new Base[0] 5101 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 5102 case -507075711: 5103 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 5104 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 5105 case 1522889671: 5106 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 5107 case 223539345: 5108 /* approvalDate */ return this.approvalDate == null ? new Base[0] : new Base[] { this.approvalDate }; // DateType 5109 case -1687512484: 5110 /* lastReviewDate */ return this.lastReviewDate == null ? new Base[0] : new Base[] { this.lastReviewDate }; // DateType 5111 case -403934648: 5112 /* effectivePeriod */ return this.effectivePeriod == null ? new Base[0] : new Base[] { this.effectivePeriod }; // Period 5113 case 110546223: 5114 /* topic */ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 5115 case -1406328437: 5116 /* author */ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 5117 case -1307827859: 5118 /* editor */ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 5119 case -261190139: 5120 /* reviewer */ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 5121 case 1740277666: 5122 /* endorser */ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 5123 case 666807069: 5124 /* relatedArtifact */ return this.relatedArtifact == null ? new Base[0] 5125 : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 5126 case 672726254: 5127 /* synthesisType */ return this.synthesisType == null ? new Base[0] : new Base[] { this.synthesisType }; // CodeableConcept 5128 case -1955265373: 5129 /* studyType */ return this.studyType == null ? new Base[0] : new Base[] { this.studyType }; // CodeableConcept 5130 case -2023558323: 5131 /* population */ return this.population == null ? new Base[0] : new Base[] { this.population }; // Reference 5132 case -1926005497: 5133 /* exposure */ return this.exposure == null ? new Base[0] : new Base[] { this.exposure }; // Reference 5134 case -1875462106: 5135 /* exposureAlternative */ return this.exposureAlternative == null ? new Base[0] 5136 : new Base[] { this.exposureAlternative }; // Reference 5137 case -1106507950: 5138 /* outcome */ return this.outcome == null ? new Base[0] : new Base[] { this.outcome }; // Reference 5139 case 143123659: 5140 /* sampleSize */ return this.sampleSize == null ? new Base[0] : new Base[] { this.sampleSize }; // EffectEvidenceSynthesisSampleSizeComponent 5141 case 553042708: 5142 /* resultsByExposure */ return this.resultsByExposure == null ? new Base[0] 5143 : this.resultsByExposure.toArray(new Base[this.resultsByExposure.size()]); // EffectEvidenceSynthesisResultsByExposureComponent 5144 case 707469785: 5145 /* effectEstimate */ return this.effectEstimate == null ? new Base[0] 5146 : this.effectEstimate.toArray(new Base[this.effectEstimate.size()]); // EffectEvidenceSynthesisEffectEstimateComponent 5147 case -1404142937: 5148 /* certainty */ return this.certainty == null ? new Base[0] 5149 : this.certainty.toArray(new Base[this.certainty.size()]); // EffectEvidenceSynthesisCertaintyComponent 5150 default: 5151 return super.getProperty(hash, name, checkValid); 5152 } 5153 5154 } 5155 5156 @Override 5157 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5158 switch (hash) { 5159 case 116079: // url 5160 this.url = castToUri(value); // UriType 5161 return value; 5162 case -1618432855: // identifier 5163 this.getIdentifier().add(castToIdentifier(value)); // Identifier 5164 return value; 5165 case 351608024: // version 5166 this.version = castToString(value); // StringType 5167 return value; 5168 case 3373707: // name 5169 this.name = castToString(value); // StringType 5170 return value; 5171 case 110371416: // title 5172 this.title = castToString(value); // StringType 5173 return value; 5174 case -892481550: // status 5175 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 5176 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5177 return value; 5178 case 3076014: // date 5179 this.date = castToDateTime(value); // DateTimeType 5180 return value; 5181 case 1447404028: // publisher 5182 this.publisher = castToString(value); // StringType 5183 return value; 5184 case 951526432: // contact 5185 this.getContact().add(castToContactDetail(value)); // ContactDetail 5186 return value; 5187 case -1724546052: // description 5188 this.description = castToMarkdown(value); // MarkdownType 5189 return value; 5190 case 3387378: // note 5191 this.getNote().add(castToAnnotation(value)); // Annotation 5192 return value; 5193 case -669707736: // useContext 5194 this.getUseContext().add(castToUsageContext(value)); // UsageContext 5195 return value; 5196 case -507075711: // jurisdiction 5197 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 5198 return value; 5199 case 1522889671: // copyright 5200 this.copyright = castToMarkdown(value); // MarkdownType 5201 return value; 5202 case 223539345: // approvalDate 5203 this.approvalDate = castToDate(value); // DateType 5204 return value; 5205 case -1687512484: // lastReviewDate 5206 this.lastReviewDate = castToDate(value); // DateType 5207 return value; 5208 case -403934648: // effectivePeriod 5209 this.effectivePeriod = castToPeriod(value); // Period 5210 return value; 5211 case 110546223: // topic 5212 this.getTopic().add(castToCodeableConcept(value)); // CodeableConcept 5213 return value; 5214 case -1406328437: // author 5215 this.getAuthor().add(castToContactDetail(value)); // ContactDetail 5216 return value; 5217 case -1307827859: // editor 5218 this.getEditor().add(castToContactDetail(value)); // ContactDetail 5219 return value; 5220 case -261190139: // reviewer 5221 this.getReviewer().add(castToContactDetail(value)); // ContactDetail 5222 return value; 5223 case 1740277666: // endorser 5224 this.getEndorser().add(castToContactDetail(value)); // ContactDetail 5225 return value; 5226 case 666807069: // relatedArtifact 5227 this.getRelatedArtifact().add(castToRelatedArtifact(value)); // RelatedArtifact 5228 return value; 5229 case 672726254: // synthesisType 5230 this.synthesisType = castToCodeableConcept(value); // CodeableConcept 5231 return value; 5232 case -1955265373: // studyType 5233 this.studyType = castToCodeableConcept(value); // CodeableConcept 5234 return value; 5235 case -2023558323: // population 5236 this.population = castToReference(value); // Reference 5237 return value; 5238 case -1926005497: // exposure 5239 this.exposure = castToReference(value); // Reference 5240 return value; 5241 case -1875462106: // exposureAlternative 5242 this.exposureAlternative = castToReference(value); // Reference 5243 return value; 5244 case -1106507950: // outcome 5245 this.outcome = castToReference(value); // Reference 5246 return value; 5247 case 143123659: // sampleSize 5248 this.sampleSize = (EffectEvidenceSynthesisSampleSizeComponent) value; // EffectEvidenceSynthesisSampleSizeComponent 5249 return value; 5250 case 553042708: // resultsByExposure 5251 this.getResultsByExposure().add((EffectEvidenceSynthesisResultsByExposureComponent) value); // EffectEvidenceSynthesisResultsByExposureComponent 5252 return value; 5253 case 707469785: // effectEstimate 5254 this.getEffectEstimate().add((EffectEvidenceSynthesisEffectEstimateComponent) value); // EffectEvidenceSynthesisEffectEstimateComponent 5255 return value; 5256 case -1404142937: // certainty 5257 this.getCertainty().add((EffectEvidenceSynthesisCertaintyComponent) value); // EffectEvidenceSynthesisCertaintyComponent 5258 return value; 5259 default: 5260 return super.setProperty(hash, name, value); 5261 } 5262 5263 } 5264 5265 @Override 5266 public Base setProperty(String name, Base value) throws FHIRException { 5267 if (name.equals("url")) { 5268 this.url = castToUri(value); // UriType 5269 } else if (name.equals("identifier")) { 5270 this.getIdentifier().add(castToIdentifier(value)); 5271 } else if (name.equals("version")) { 5272 this.version = castToString(value); // StringType 5273 } else if (name.equals("name")) { 5274 this.name = castToString(value); // StringType 5275 } else if (name.equals("title")) { 5276 this.title = castToString(value); // StringType 5277 } else if (name.equals("status")) { 5278 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 5279 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5280 } else if (name.equals("date")) { 5281 this.date = castToDateTime(value); // DateTimeType 5282 } else if (name.equals("publisher")) { 5283 this.publisher = castToString(value); // StringType 5284 } else if (name.equals("contact")) { 5285 this.getContact().add(castToContactDetail(value)); 5286 } else if (name.equals("description")) { 5287 this.description = castToMarkdown(value); // MarkdownType 5288 } else if (name.equals("note")) { 5289 this.getNote().add(castToAnnotation(value)); 5290 } else if (name.equals("useContext")) { 5291 this.getUseContext().add(castToUsageContext(value)); 5292 } else if (name.equals("jurisdiction")) { 5293 this.getJurisdiction().add(castToCodeableConcept(value)); 5294 } else if (name.equals("copyright")) { 5295 this.copyright = castToMarkdown(value); // MarkdownType 5296 } else if (name.equals("approvalDate")) { 5297 this.approvalDate = castToDate(value); // DateType 5298 } else if (name.equals("lastReviewDate")) { 5299 this.lastReviewDate = castToDate(value); // DateType 5300 } else if (name.equals("effectivePeriod")) { 5301 this.effectivePeriod = castToPeriod(value); // Period 5302 } else if (name.equals("topic")) { 5303 this.getTopic().add(castToCodeableConcept(value)); 5304 } else if (name.equals("author")) { 5305 this.getAuthor().add(castToContactDetail(value)); 5306 } else if (name.equals("editor")) { 5307 this.getEditor().add(castToContactDetail(value)); 5308 } else if (name.equals("reviewer")) { 5309 this.getReviewer().add(castToContactDetail(value)); 5310 } else if (name.equals("endorser")) { 5311 this.getEndorser().add(castToContactDetail(value)); 5312 } else if (name.equals("relatedArtifact")) { 5313 this.getRelatedArtifact().add(castToRelatedArtifact(value)); 5314 } else if (name.equals("synthesisType")) { 5315 this.synthesisType = castToCodeableConcept(value); // CodeableConcept 5316 } else if (name.equals("studyType")) { 5317 this.studyType = castToCodeableConcept(value); // CodeableConcept 5318 } else if (name.equals("population")) { 5319 this.population = castToReference(value); // Reference 5320 } else if (name.equals("exposure")) { 5321 this.exposure = castToReference(value); // Reference 5322 } else if (name.equals("exposureAlternative")) { 5323 this.exposureAlternative = castToReference(value); // Reference 5324 } else if (name.equals("outcome")) { 5325 this.outcome = castToReference(value); // Reference 5326 } else if (name.equals("sampleSize")) { 5327 this.sampleSize = (EffectEvidenceSynthesisSampleSizeComponent) value; // EffectEvidenceSynthesisSampleSizeComponent 5328 } else if (name.equals("resultsByExposure")) { 5329 this.getResultsByExposure().add((EffectEvidenceSynthesisResultsByExposureComponent) value); 5330 } else if (name.equals("effectEstimate")) { 5331 this.getEffectEstimate().add((EffectEvidenceSynthesisEffectEstimateComponent) value); 5332 } else if (name.equals("certainty")) { 5333 this.getCertainty().add((EffectEvidenceSynthesisCertaintyComponent) value); 5334 } else 5335 return super.setProperty(name, value); 5336 return value; 5337 } 5338 5339 @Override 5340 public void removeChild(String name, Base value) throws FHIRException { 5341 if (name.equals("url")) { 5342 this.url = null; 5343 } else if (name.equals("identifier")) { 5344 this.getIdentifier().remove(castToIdentifier(value)); 5345 } else if (name.equals("version")) { 5346 this.version = null; 5347 } else if (name.equals("name")) { 5348 this.name = null; 5349 } else if (name.equals("title")) { 5350 this.title = null; 5351 } else if (name.equals("status")) { 5352 this.status = null; 5353 } else if (name.equals("date")) { 5354 this.date = null; 5355 } else if (name.equals("publisher")) { 5356 this.publisher = null; 5357 } else if (name.equals("contact")) { 5358 this.getContact().remove(castToContactDetail(value)); 5359 } else if (name.equals("description")) { 5360 this.description = null; 5361 } else if (name.equals("note")) { 5362 this.getNote().remove(castToAnnotation(value)); 5363 } else if (name.equals("useContext")) { 5364 this.getUseContext().remove(castToUsageContext(value)); 5365 } else if (name.equals("jurisdiction")) { 5366 this.getJurisdiction().remove(castToCodeableConcept(value)); 5367 } else if (name.equals("copyright")) { 5368 this.copyright = null; 5369 } else if (name.equals("approvalDate")) { 5370 this.approvalDate = null; 5371 } else if (name.equals("lastReviewDate")) { 5372 this.lastReviewDate = null; 5373 } else if (name.equals("effectivePeriod")) { 5374 this.effectivePeriod = null; 5375 } else if (name.equals("topic")) { 5376 this.getTopic().remove(castToCodeableConcept(value)); 5377 } else if (name.equals("author")) { 5378 this.getAuthor().remove(castToContactDetail(value)); 5379 } else if (name.equals("editor")) { 5380 this.getEditor().remove(castToContactDetail(value)); 5381 } else if (name.equals("reviewer")) { 5382 this.getReviewer().remove(castToContactDetail(value)); 5383 } else if (name.equals("endorser")) { 5384 this.getEndorser().remove(castToContactDetail(value)); 5385 } else if (name.equals("relatedArtifact")) { 5386 this.getRelatedArtifact().remove(castToRelatedArtifact(value)); 5387 } else if (name.equals("synthesisType")) { 5388 this.synthesisType = null; 5389 } else if (name.equals("studyType")) { 5390 this.studyType = null; 5391 } else if (name.equals("population")) { 5392 this.population = null; 5393 } else if (name.equals("exposure")) { 5394 this.exposure = null; 5395 } else if (name.equals("exposureAlternative")) { 5396 this.exposureAlternative = null; 5397 } else if (name.equals("outcome")) { 5398 this.outcome = null; 5399 } else if (name.equals("sampleSize")) { 5400 this.sampleSize = (EffectEvidenceSynthesisSampleSizeComponent) value; // EffectEvidenceSynthesisSampleSizeComponent 5401 } else if (name.equals("resultsByExposure")) { 5402 this.getResultsByExposure().remove((EffectEvidenceSynthesisResultsByExposureComponent) value); 5403 } else if (name.equals("effectEstimate")) { 5404 this.getEffectEstimate().remove((EffectEvidenceSynthesisEffectEstimateComponent) value); 5405 } else if (name.equals("certainty")) { 5406 this.getCertainty().remove((EffectEvidenceSynthesisCertaintyComponent) value); 5407 } else 5408 super.removeChild(name, value); 5409 5410 } 5411 5412 @Override 5413 public Base makeProperty(int hash, String name) throws FHIRException { 5414 switch (hash) { 5415 case 116079: 5416 return getUrlElement(); 5417 case -1618432855: 5418 return addIdentifier(); 5419 case 351608024: 5420 return getVersionElement(); 5421 case 3373707: 5422 return getNameElement(); 5423 case 110371416: 5424 return getTitleElement(); 5425 case -892481550: 5426 return getStatusElement(); 5427 case 3076014: 5428 return getDateElement(); 5429 case 1447404028: 5430 return getPublisherElement(); 5431 case 951526432: 5432 return addContact(); 5433 case -1724546052: 5434 return getDescriptionElement(); 5435 case 3387378: 5436 return addNote(); 5437 case -669707736: 5438 return addUseContext(); 5439 case -507075711: 5440 return addJurisdiction(); 5441 case 1522889671: 5442 return getCopyrightElement(); 5443 case 223539345: 5444 return getApprovalDateElement(); 5445 case -1687512484: 5446 return getLastReviewDateElement(); 5447 case -403934648: 5448 return getEffectivePeriod(); 5449 case 110546223: 5450 return addTopic(); 5451 case -1406328437: 5452 return addAuthor(); 5453 case -1307827859: 5454 return addEditor(); 5455 case -261190139: 5456 return addReviewer(); 5457 case 1740277666: 5458 return addEndorser(); 5459 case 666807069: 5460 return addRelatedArtifact(); 5461 case 672726254: 5462 return getSynthesisType(); 5463 case -1955265373: 5464 return getStudyType(); 5465 case -2023558323: 5466 return getPopulation(); 5467 case -1926005497: 5468 return getExposure(); 5469 case -1875462106: 5470 return getExposureAlternative(); 5471 case -1106507950: 5472 return getOutcome(); 5473 case 143123659: 5474 return getSampleSize(); 5475 case 553042708: 5476 return addResultsByExposure(); 5477 case 707469785: 5478 return addEffectEstimate(); 5479 case -1404142937: 5480 return addCertainty(); 5481 default: 5482 return super.makeProperty(hash, name); 5483 } 5484 5485 } 5486 5487 @Override 5488 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5489 switch (hash) { 5490 case 116079: 5491 /* url */ return new String[] { "uri" }; 5492 case -1618432855: 5493 /* identifier */ return new String[] { "Identifier" }; 5494 case 351608024: 5495 /* version */ return new String[] { "string" }; 5496 case 3373707: 5497 /* name */ return new String[] { "string" }; 5498 case 110371416: 5499 /* title */ return new String[] { "string" }; 5500 case -892481550: 5501 /* status */ return new String[] { "code" }; 5502 case 3076014: 5503 /* date */ return new String[] { "dateTime" }; 5504 case 1447404028: 5505 /* publisher */ return new String[] { "string" }; 5506 case 951526432: 5507 /* contact */ return new String[] { "ContactDetail" }; 5508 case -1724546052: 5509 /* description */ return new String[] { "markdown" }; 5510 case 3387378: 5511 /* note */ return new String[] { "Annotation" }; 5512 case -669707736: 5513 /* useContext */ return new String[] { "UsageContext" }; 5514 case -507075711: 5515 /* jurisdiction */ return new String[] { "CodeableConcept" }; 5516 case 1522889671: 5517 /* copyright */ return new String[] { "markdown" }; 5518 case 223539345: 5519 /* approvalDate */ return new String[] { "date" }; 5520 case -1687512484: 5521 /* lastReviewDate */ return new String[] { "date" }; 5522 case -403934648: 5523 /* effectivePeriod */ return new String[] { "Period" }; 5524 case 110546223: 5525 /* topic */ return new String[] { "CodeableConcept" }; 5526 case -1406328437: 5527 /* author */ return new String[] { "ContactDetail" }; 5528 case -1307827859: 5529 /* editor */ return new String[] { "ContactDetail" }; 5530 case -261190139: 5531 /* reviewer */ return new String[] { "ContactDetail" }; 5532 case 1740277666: 5533 /* endorser */ return new String[] { "ContactDetail" }; 5534 case 666807069: 5535 /* relatedArtifact */ return new String[] { "RelatedArtifact" }; 5536 case 672726254: 5537 /* synthesisType */ return new String[] { "CodeableConcept" }; 5538 case -1955265373: 5539 /* studyType */ return new String[] { "CodeableConcept" }; 5540 case -2023558323: 5541 /* population */ return new String[] { "Reference" }; 5542 case -1926005497: 5543 /* exposure */ return new String[] { "Reference" }; 5544 case -1875462106: 5545 /* exposureAlternative */ return new String[] { "Reference" }; 5546 case -1106507950: 5547 /* outcome */ return new String[] { "Reference" }; 5548 case 143123659: 5549 /* sampleSize */ return new String[] {}; 5550 case 553042708: 5551 /* resultsByExposure */ return new String[] {}; 5552 case 707469785: 5553 /* effectEstimate */ return new String[] {}; 5554 case -1404142937: 5555 /* certainty */ return new String[] {}; 5556 default: 5557 return super.getTypesForProperty(hash, name); 5558 } 5559 5560 } 5561 5562 @Override 5563 public Base addChild(String name) throws FHIRException { 5564 if (name.equals("url")) { 5565 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.url"); 5566 } else if (name.equals("identifier")) { 5567 return addIdentifier(); 5568 } else if (name.equals("version")) { 5569 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.version"); 5570 } else if (name.equals("name")) { 5571 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.name"); 5572 } else if (name.equals("title")) { 5573 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.title"); 5574 } else if (name.equals("status")) { 5575 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.status"); 5576 } else if (name.equals("date")) { 5577 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.date"); 5578 } else if (name.equals("publisher")) { 5579 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.publisher"); 5580 } else if (name.equals("contact")) { 5581 return addContact(); 5582 } else if (name.equals("description")) { 5583 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.description"); 5584 } else if (name.equals("note")) { 5585 return addNote(); 5586 } else if (name.equals("useContext")) { 5587 return addUseContext(); 5588 } else if (name.equals("jurisdiction")) { 5589 return addJurisdiction(); 5590 } else if (name.equals("copyright")) { 5591 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.copyright"); 5592 } else if (name.equals("approvalDate")) { 5593 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.approvalDate"); 5594 } else if (name.equals("lastReviewDate")) { 5595 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.lastReviewDate"); 5596 } else if (name.equals("effectivePeriod")) { 5597 this.effectivePeriod = new Period(); 5598 return this.effectivePeriod; 5599 } else if (name.equals("topic")) { 5600 return addTopic(); 5601 } else if (name.equals("author")) { 5602 return addAuthor(); 5603 } else if (name.equals("editor")) { 5604 return addEditor(); 5605 } else if (name.equals("reviewer")) { 5606 return addReviewer(); 5607 } else if (name.equals("endorser")) { 5608 return addEndorser(); 5609 } else if (name.equals("relatedArtifact")) { 5610 return addRelatedArtifact(); 5611 } else if (name.equals("synthesisType")) { 5612 this.synthesisType = new CodeableConcept(); 5613 return this.synthesisType; 5614 } else if (name.equals("studyType")) { 5615 this.studyType = new CodeableConcept(); 5616 return this.studyType; 5617 } else if (name.equals("population")) { 5618 this.population = new Reference(); 5619 return this.population; 5620 } else if (name.equals("exposure")) { 5621 this.exposure = new Reference(); 5622 return this.exposure; 5623 } else if (name.equals("exposureAlternative")) { 5624 this.exposureAlternative = new Reference(); 5625 return this.exposureAlternative; 5626 } else if (name.equals("outcome")) { 5627 this.outcome = new Reference(); 5628 return this.outcome; 5629 } else if (name.equals("sampleSize")) { 5630 this.sampleSize = new EffectEvidenceSynthesisSampleSizeComponent(); 5631 return this.sampleSize; 5632 } else if (name.equals("resultsByExposure")) { 5633 return addResultsByExposure(); 5634 } else if (name.equals("effectEstimate")) { 5635 return addEffectEstimate(); 5636 } else if (name.equals("certainty")) { 5637 return addCertainty(); 5638 } else 5639 return super.addChild(name); 5640 } 5641 5642 public String fhirType() { 5643 return "EffectEvidenceSynthesis"; 5644 5645 } 5646 5647 public EffectEvidenceSynthesis copy() { 5648 EffectEvidenceSynthesis dst = new EffectEvidenceSynthesis(); 5649 copyValues(dst); 5650 return dst; 5651 } 5652 5653 public void copyValues(EffectEvidenceSynthesis dst) { 5654 super.copyValues(dst); 5655 dst.url = url == null ? null : url.copy(); 5656 if (identifier != null) { 5657 dst.identifier = new ArrayList<Identifier>(); 5658 for (Identifier i : identifier) 5659 dst.identifier.add(i.copy()); 5660 } 5661 ; 5662 dst.version = version == null ? null : version.copy(); 5663 dst.name = name == null ? null : name.copy(); 5664 dst.title = title == null ? null : title.copy(); 5665 dst.status = status == null ? null : status.copy(); 5666 dst.date = date == null ? null : date.copy(); 5667 dst.publisher = publisher == null ? null : publisher.copy(); 5668 if (contact != null) { 5669 dst.contact = new ArrayList<ContactDetail>(); 5670 for (ContactDetail i : contact) 5671 dst.contact.add(i.copy()); 5672 } 5673 ; 5674 dst.description = description == null ? null : description.copy(); 5675 if (note != null) { 5676 dst.note = new ArrayList<Annotation>(); 5677 for (Annotation i : note) 5678 dst.note.add(i.copy()); 5679 } 5680 ; 5681 if (useContext != null) { 5682 dst.useContext = new ArrayList<UsageContext>(); 5683 for (UsageContext i : useContext) 5684 dst.useContext.add(i.copy()); 5685 } 5686 ; 5687 if (jurisdiction != null) { 5688 dst.jurisdiction = new ArrayList<CodeableConcept>(); 5689 for (CodeableConcept i : jurisdiction) 5690 dst.jurisdiction.add(i.copy()); 5691 } 5692 ; 5693 dst.copyright = copyright == null ? null : copyright.copy(); 5694 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 5695 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 5696 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 5697 if (topic != null) { 5698 dst.topic = new ArrayList<CodeableConcept>(); 5699 for (CodeableConcept i : topic) 5700 dst.topic.add(i.copy()); 5701 } 5702 ; 5703 if (author != null) { 5704 dst.author = new ArrayList<ContactDetail>(); 5705 for (ContactDetail i : author) 5706 dst.author.add(i.copy()); 5707 } 5708 ; 5709 if (editor != null) { 5710 dst.editor = new ArrayList<ContactDetail>(); 5711 for (ContactDetail i : editor) 5712 dst.editor.add(i.copy()); 5713 } 5714 ; 5715 if (reviewer != null) { 5716 dst.reviewer = new ArrayList<ContactDetail>(); 5717 for (ContactDetail i : reviewer) 5718 dst.reviewer.add(i.copy()); 5719 } 5720 ; 5721 if (endorser != null) { 5722 dst.endorser = new ArrayList<ContactDetail>(); 5723 for (ContactDetail i : endorser) 5724 dst.endorser.add(i.copy()); 5725 } 5726 ; 5727 if (relatedArtifact != null) { 5728 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 5729 for (RelatedArtifact i : relatedArtifact) 5730 dst.relatedArtifact.add(i.copy()); 5731 } 5732 ; 5733 dst.synthesisType = synthesisType == null ? null : synthesisType.copy(); 5734 dst.studyType = studyType == null ? null : studyType.copy(); 5735 dst.population = population == null ? null : population.copy(); 5736 dst.exposure = exposure == null ? null : exposure.copy(); 5737 dst.exposureAlternative = exposureAlternative == null ? null : exposureAlternative.copy(); 5738 dst.outcome = outcome == null ? null : outcome.copy(); 5739 dst.sampleSize = sampleSize == null ? null : sampleSize.copy(); 5740 if (resultsByExposure != null) { 5741 dst.resultsByExposure = new ArrayList<EffectEvidenceSynthesisResultsByExposureComponent>(); 5742 for (EffectEvidenceSynthesisResultsByExposureComponent i : resultsByExposure) 5743 dst.resultsByExposure.add(i.copy()); 5744 } 5745 ; 5746 if (effectEstimate != null) { 5747 dst.effectEstimate = new ArrayList<EffectEvidenceSynthesisEffectEstimateComponent>(); 5748 for (EffectEvidenceSynthesisEffectEstimateComponent i : effectEstimate) 5749 dst.effectEstimate.add(i.copy()); 5750 } 5751 ; 5752 if (certainty != null) { 5753 dst.certainty = new ArrayList<EffectEvidenceSynthesisCertaintyComponent>(); 5754 for (EffectEvidenceSynthesisCertaintyComponent i : certainty) 5755 dst.certainty.add(i.copy()); 5756 } 5757 ; 5758 } 5759 5760 protected EffectEvidenceSynthesis typedCopy() { 5761 return copy(); 5762 } 5763 5764 @Override 5765 public boolean equalsDeep(Base other_) { 5766 if (!super.equalsDeep(other_)) 5767 return false; 5768 if (!(other_ instanceof EffectEvidenceSynthesis)) 5769 return false; 5770 EffectEvidenceSynthesis o = (EffectEvidenceSynthesis) other_; 5771 return compareDeep(identifier, o.identifier, true) && compareDeep(note, o.note, true) 5772 && compareDeep(copyright, o.copyright, true) && compareDeep(approvalDate, o.approvalDate, true) 5773 && compareDeep(lastReviewDate, o.lastReviewDate, true) && compareDeep(effectivePeriod, o.effectivePeriod, true) 5774 && compareDeep(topic, o.topic, true) && compareDeep(author, o.author, true) 5775 && compareDeep(editor, o.editor, true) && compareDeep(reviewer, o.reviewer, true) 5776 && compareDeep(endorser, o.endorser, true) && compareDeep(relatedArtifact, o.relatedArtifact, true) 5777 && compareDeep(synthesisType, o.synthesisType, true) && compareDeep(studyType, o.studyType, true) 5778 && compareDeep(population, o.population, true) && compareDeep(exposure, o.exposure, true) 5779 && compareDeep(exposureAlternative, o.exposureAlternative, true) && compareDeep(outcome, o.outcome, true) 5780 && compareDeep(sampleSize, o.sampleSize, true) && compareDeep(resultsByExposure, o.resultsByExposure, true) 5781 && compareDeep(effectEstimate, o.effectEstimate, true) && compareDeep(certainty, o.certainty, true); 5782 } 5783 5784 @Override 5785 public boolean equalsShallow(Base other_) { 5786 if (!super.equalsShallow(other_)) 5787 return false; 5788 if (!(other_ instanceof EffectEvidenceSynthesis)) 5789 return false; 5790 EffectEvidenceSynthesis o = (EffectEvidenceSynthesis) other_; 5791 return compareValues(copyright, o.copyright, true) && compareValues(approvalDate, o.approvalDate, true) 5792 && compareValues(lastReviewDate, o.lastReviewDate, true); 5793 } 5794 5795 public boolean isEmpty() { 5796 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, note, copyright, approvalDate, 5797 lastReviewDate, effectivePeriod, topic, author, editor, reviewer, endorser, relatedArtifact, synthesisType, 5798 studyType, population, exposure, exposureAlternative, outcome, sampleSize, resultsByExposure, effectEstimate, 5799 certainty); 5800 } 5801 5802 @Override 5803 public ResourceType getResourceType() { 5804 return ResourceType.EffectEvidenceSynthesis; 5805 } 5806 5807 /** 5808 * Search parameter: <b>date</b> 5809 * <p> 5810 * Description: <b>The effect evidence synthesis publication date</b><br> 5811 * Type: <b>date</b><br> 5812 * Path: <b>EffectEvidenceSynthesis.date</b><br> 5813 * </p> 5814 */ 5815 @SearchParamDefinition(name = "date", path = "EffectEvidenceSynthesis.date", description = "The effect evidence synthesis publication date", type = "date") 5816 public static final String SP_DATE = "date"; 5817 /** 5818 * <b>Fluent Client</b> search parameter constant for <b>date</b> 5819 * <p> 5820 * Description: <b>The effect evidence synthesis publication date</b><br> 5821 * Type: <b>date</b><br> 5822 * Path: <b>EffectEvidenceSynthesis.date</b><br> 5823 * </p> 5824 */ 5825 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 5826 SP_DATE); 5827 5828 /** 5829 * Search parameter: <b>identifier</b> 5830 * <p> 5831 * Description: <b>External identifier for the effect evidence synthesis</b><br> 5832 * Type: <b>token</b><br> 5833 * Path: <b>EffectEvidenceSynthesis.identifier</b><br> 5834 * </p> 5835 */ 5836 @SearchParamDefinition(name = "identifier", path = "EffectEvidenceSynthesis.identifier", description = "External identifier for the effect evidence synthesis", type = "token") 5837 public static final String SP_IDENTIFIER = "identifier"; 5838 /** 5839 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5840 * <p> 5841 * Description: <b>External identifier for the effect evidence synthesis</b><br> 5842 * Type: <b>token</b><br> 5843 * Path: <b>EffectEvidenceSynthesis.identifier</b><br> 5844 * </p> 5845 */ 5846 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5847 SP_IDENTIFIER); 5848 5849 /** 5850 * Search parameter: <b>context-type-value</b> 5851 * <p> 5852 * Description: <b>A use context type and value assigned to the effect evidence 5853 * synthesis</b><br> 5854 * Type: <b>composite</b><br> 5855 * Path: <b></b><br> 5856 * </p> 5857 */ 5858 @SearchParamDefinition(name = "context-type-value", path = "EffectEvidenceSynthesis.useContext", description = "A use context type and value assigned to the effect evidence synthesis", type = "composite", compositeOf = { 5859 "context-type", "context" }) 5860 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 5861 /** 5862 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 5863 * <p> 5864 * Description: <b>A use context type and value assigned to the effect evidence 5865 * synthesis</b><br> 5866 * Type: <b>composite</b><br> 5867 * Path: <b></b><br> 5868 * </p> 5869 */ 5870 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 5871 SP_CONTEXT_TYPE_VALUE); 5872 5873 /** 5874 * Search parameter: <b>jurisdiction</b> 5875 * <p> 5876 * Description: <b>Intended jurisdiction for the effect evidence 5877 * synthesis</b><br> 5878 * Type: <b>token</b><br> 5879 * Path: <b>EffectEvidenceSynthesis.jurisdiction</b><br> 5880 * </p> 5881 */ 5882 @SearchParamDefinition(name = "jurisdiction", path = "EffectEvidenceSynthesis.jurisdiction", description = "Intended jurisdiction for the effect evidence synthesis", type = "token") 5883 public static final String SP_JURISDICTION = "jurisdiction"; 5884 /** 5885 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 5886 * <p> 5887 * Description: <b>Intended jurisdiction for the effect evidence 5888 * synthesis</b><br> 5889 * Type: <b>token</b><br> 5890 * Path: <b>EffectEvidenceSynthesis.jurisdiction</b><br> 5891 * </p> 5892 */ 5893 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5894 SP_JURISDICTION); 5895 5896 /** 5897 * Search parameter: <b>description</b> 5898 * <p> 5899 * Description: <b>The description of the effect evidence synthesis</b><br> 5900 * Type: <b>string</b><br> 5901 * Path: <b>EffectEvidenceSynthesis.description</b><br> 5902 * </p> 5903 */ 5904 @SearchParamDefinition(name = "description", path = "EffectEvidenceSynthesis.description", description = "The description of the effect evidence synthesis", type = "string") 5905 public static final String SP_DESCRIPTION = "description"; 5906 /** 5907 * <b>Fluent Client</b> search parameter constant for <b>description</b> 5908 * <p> 5909 * Description: <b>The description of the effect evidence synthesis</b><br> 5910 * Type: <b>string</b><br> 5911 * Path: <b>EffectEvidenceSynthesis.description</b><br> 5912 * </p> 5913 */ 5914 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 5915 SP_DESCRIPTION); 5916 5917 /** 5918 * Search parameter: <b>context-type</b> 5919 * <p> 5920 * Description: <b>A type of use context assigned to the effect evidence 5921 * synthesis</b><br> 5922 * Type: <b>token</b><br> 5923 * Path: <b>EffectEvidenceSynthesis.useContext.code</b><br> 5924 * </p> 5925 */ 5926 @SearchParamDefinition(name = "context-type", path = "EffectEvidenceSynthesis.useContext.code", description = "A type of use context assigned to the effect evidence synthesis", type = "token") 5927 public static final String SP_CONTEXT_TYPE = "context-type"; 5928 /** 5929 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 5930 * <p> 5931 * Description: <b>A type of use context assigned to the effect evidence 5932 * synthesis</b><br> 5933 * Type: <b>token</b><br> 5934 * Path: <b>EffectEvidenceSynthesis.useContext.code</b><br> 5935 * </p> 5936 */ 5937 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5938 SP_CONTEXT_TYPE); 5939 5940 /** 5941 * Search parameter: <b>title</b> 5942 * <p> 5943 * Description: <b>The human-friendly name of the effect evidence 5944 * synthesis</b><br> 5945 * Type: <b>string</b><br> 5946 * Path: <b>EffectEvidenceSynthesis.title</b><br> 5947 * </p> 5948 */ 5949 @SearchParamDefinition(name = "title", path = "EffectEvidenceSynthesis.title", description = "The human-friendly name of the effect evidence synthesis", type = "string") 5950 public static final String SP_TITLE = "title"; 5951 /** 5952 * <b>Fluent Client</b> search parameter constant for <b>title</b> 5953 * <p> 5954 * Description: <b>The human-friendly name of the effect evidence 5955 * synthesis</b><br> 5956 * Type: <b>string</b><br> 5957 * Path: <b>EffectEvidenceSynthesis.title</b><br> 5958 * </p> 5959 */ 5960 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 5961 SP_TITLE); 5962 5963 /** 5964 * Search parameter: <b>version</b> 5965 * <p> 5966 * Description: <b>The business version of the effect evidence synthesis</b><br> 5967 * Type: <b>token</b><br> 5968 * Path: <b>EffectEvidenceSynthesis.version</b><br> 5969 * </p> 5970 */ 5971 @SearchParamDefinition(name = "version", path = "EffectEvidenceSynthesis.version", description = "The business version of the effect evidence synthesis", type = "token") 5972 public static final String SP_VERSION = "version"; 5973 /** 5974 * <b>Fluent Client</b> search parameter constant for <b>version</b> 5975 * <p> 5976 * Description: <b>The business version of the effect evidence synthesis</b><br> 5977 * Type: <b>token</b><br> 5978 * Path: <b>EffectEvidenceSynthesis.version</b><br> 5979 * </p> 5980 */ 5981 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5982 SP_VERSION); 5983 5984 /** 5985 * Search parameter: <b>url</b> 5986 * <p> 5987 * Description: <b>The uri that identifies the effect evidence synthesis</b><br> 5988 * Type: <b>uri</b><br> 5989 * Path: <b>EffectEvidenceSynthesis.url</b><br> 5990 * </p> 5991 */ 5992 @SearchParamDefinition(name = "url", path = "EffectEvidenceSynthesis.url", description = "The uri that identifies the effect evidence synthesis", type = "uri") 5993 public static final String SP_URL = "url"; 5994 /** 5995 * <b>Fluent Client</b> search parameter constant for <b>url</b> 5996 * <p> 5997 * Description: <b>The uri that identifies the effect evidence synthesis</b><br> 5998 * Type: <b>uri</b><br> 5999 * Path: <b>EffectEvidenceSynthesis.url</b><br> 6000 * </p> 6001 */ 6002 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 6003 6004 /** 6005 * Search parameter: <b>context-quantity</b> 6006 * <p> 6007 * Description: <b>A quantity- or range-valued use context assigned to the 6008 * effect evidence synthesis</b><br> 6009 * Type: <b>quantity</b><br> 6010 * Path: <b>EffectEvidenceSynthesis.useContext.valueQuantity, 6011 * EffectEvidenceSynthesis.useContext.valueRange</b><br> 6012 * </p> 6013 */ 6014 @SearchParamDefinition(name = "context-quantity", path = "(EffectEvidenceSynthesis.useContext.value as Quantity) | (EffectEvidenceSynthesis.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the effect evidence synthesis", type = "quantity") 6015 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 6016 /** 6017 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 6018 * <p> 6019 * Description: <b>A quantity- or range-valued use context assigned to the 6020 * effect evidence synthesis</b><br> 6021 * Type: <b>quantity</b><br> 6022 * Path: <b>EffectEvidenceSynthesis.useContext.valueQuantity, 6023 * EffectEvidenceSynthesis.useContext.valueRange</b><br> 6024 * </p> 6025 */ 6026 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 6027 SP_CONTEXT_QUANTITY); 6028 6029 /** 6030 * Search parameter: <b>effective</b> 6031 * <p> 6032 * Description: <b>The time during which the effect evidence synthesis is 6033 * intended to be in use</b><br> 6034 * Type: <b>date</b><br> 6035 * Path: <b>EffectEvidenceSynthesis.effectivePeriod</b><br> 6036 * </p> 6037 */ 6038 @SearchParamDefinition(name = "effective", path = "EffectEvidenceSynthesis.effectivePeriod", description = "The time during which the effect evidence synthesis is intended to be in use", type = "date") 6039 public static final String SP_EFFECTIVE = "effective"; 6040 /** 6041 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 6042 * <p> 6043 * Description: <b>The time during which the effect evidence synthesis is 6044 * intended to be in use</b><br> 6045 * Type: <b>date</b><br> 6046 * Path: <b>EffectEvidenceSynthesis.effectivePeriod</b><br> 6047 * </p> 6048 */ 6049 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam( 6050 SP_EFFECTIVE); 6051 6052 /** 6053 * Search parameter: <b>name</b> 6054 * <p> 6055 * Description: <b>Computationally friendly name of the effect evidence 6056 * synthesis</b><br> 6057 * Type: <b>string</b><br> 6058 * Path: <b>EffectEvidenceSynthesis.name</b><br> 6059 * </p> 6060 */ 6061 @SearchParamDefinition(name = "name", path = "EffectEvidenceSynthesis.name", description = "Computationally friendly name of the effect evidence synthesis", type = "string") 6062 public static final String SP_NAME = "name"; 6063 /** 6064 * <b>Fluent Client</b> search parameter constant for <b>name</b> 6065 * <p> 6066 * Description: <b>Computationally friendly name of the effect evidence 6067 * synthesis</b><br> 6068 * Type: <b>string</b><br> 6069 * Path: <b>EffectEvidenceSynthesis.name</b><br> 6070 * </p> 6071 */ 6072 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 6073 SP_NAME); 6074 6075 /** 6076 * Search parameter: <b>context</b> 6077 * <p> 6078 * Description: <b>A use context assigned to the effect evidence 6079 * synthesis</b><br> 6080 * Type: <b>token</b><br> 6081 * Path: <b>EffectEvidenceSynthesis.useContext.valueCodeableConcept</b><br> 6082 * </p> 6083 */ 6084 @SearchParamDefinition(name = "context", path = "(EffectEvidenceSynthesis.useContext.value as CodeableConcept)", description = "A use context assigned to the effect evidence synthesis", type = "token") 6085 public static final String SP_CONTEXT = "context"; 6086 /** 6087 * <b>Fluent Client</b> search parameter constant for <b>context</b> 6088 * <p> 6089 * Description: <b>A use context assigned to the effect evidence 6090 * synthesis</b><br> 6091 * Type: <b>token</b><br> 6092 * Path: <b>EffectEvidenceSynthesis.useContext.valueCodeableConcept</b><br> 6093 * </p> 6094 */ 6095 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6096 SP_CONTEXT); 6097 6098 /** 6099 * Search parameter: <b>publisher</b> 6100 * <p> 6101 * Description: <b>Name of the publisher of the effect evidence 6102 * synthesis</b><br> 6103 * Type: <b>string</b><br> 6104 * Path: <b>EffectEvidenceSynthesis.publisher</b><br> 6105 * </p> 6106 */ 6107 @SearchParamDefinition(name = "publisher", path = "EffectEvidenceSynthesis.publisher", description = "Name of the publisher of the effect evidence synthesis", type = "string") 6108 public static final String SP_PUBLISHER = "publisher"; 6109 /** 6110 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 6111 * <p> 6112 * Description: <b>Name of the publisher of the effect evidence 6113 * synthesis</b><br> 6114 * Type: <b>string</b><br> 6115 * Path: <b>EffectEvidenceSynthesis.publisher</b><br> 6116 * </p> 6117 */ 6118 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 6119 SP_PUBLISHER); 6120 6121 /** 6122 * Search parameter: <b>context-type-quantity</b> 6123 * <p> 6124 * Description: <b>A use context type and quantity- or range-based value 6125 * assigned to the effect evidence synthesis</b><br> 6126 * Type: <b>composite</b><br> 6127 * Path: <b></b><br> 6128 * </p> 6129 */ 6130 @SearchParamDefinition(name = "context-type-quantity", path = "EffectEvidenceSynthesis.useContext", description = "A use context type and quantity- or range-based value assigned to the effect evidence synthesis", type = "composite", compositeOf = { 6131 "context-type", "context-quantity" }) 6132 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 6133 /** 6134 * <b>Fluent Client</b> search parameter constant for 6135 * <b>context-type-quantity</b> 6136 * <p> 6137 * Description: <b>A use context type and quantity- or range-based value 6138 * assigned to the effect evidence synthesis</b><br> 6139 * Type: <b>composite</b><br> 6140 * Path: <b></b><br> 6141 * </p> 6142 */ 6143 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 6144 SP_CONTEXT_TYPE_QUANTITY); 6145 6146 /** 6147 * Search parameter: <b>status</b> 6148 * <p> 6149 * Description: <b>The current status of the effect evidence synthesis</b><br> 6150 * Type: <b>token</b><br> 6151 * Path: <b>EffectEvidenceSynthesis.status</b><br> 6152 * </p> 6153 */ 6154 @SearchParamDefinition(name = "status", path = "EffectEvidenceSynthesis.status", description = "The current status of the effect evidence synthesis", type = "token") 6155 public static final String SP_STATUS = "status"; 6156 /** 6157 * <b>Fluent Client</b> search parameter constant for <b>status</b> 6158 * <p> 6159 * Description: <b>The current status of the effect evidence synthesis</b><br> 6160 * Type: <b>token</b><br> 6161 * Path: <b>EffectEvidenceSynthesis.status</b><br> 6162 * </p> 6163 */ 6164 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6165 SP_STATUS); 6166 6167}