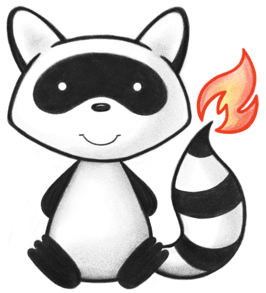
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 042import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.ResourceDef; 050import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 051 052/** 053 * The EffectEvidenceSynthesis resource describes the difference in an outcome 054 * between exposures states in a population where the effect estimate is derived 055 * from a combination of research studies. 056 */ 057@ResourceDef(name = "EffectEvidenceSynthesis", profile = "http://hl7.org/fhir/StructureDefinition/EffectEvidenceSynthesis") 058@ChildOrder(names = { "url", "identifier", "version", "name", "title", "status", "date", "publisher", "contact", 059 "description", "note", "useContext", "jurisdiction", "copyright", "approvalDate", "lastReviewDate", 060 "effectivePeriod", "topic", "author", "editor", "reviewer", "endorser", "relatedArtifact", "synthesisType", 061 "studyType", "population", "exposure", "exposureAlternative", "outcome", "sampleSize", "resultsByExposure", 062 "effectEstimate", "certainty" }) 063public class EffectEvidenceSynthesis extends MetadataResource { 064 065 public enum ExposureState { 066 /** 067 * used when the results by exposure is describing the results for the primary 068 * exposure of interest. 069 */ 070 EXPOSURE, 071 /** 072 * used when the results by exposure is describing the results for the 073 * alternative exposure state, control state or comparator state. 074 */ 075 EXPOSUREALTERNATIVE, 076 /** 077 * added to help the parsers with the generic types 078 */ 079 NULL; 080 081 public static ExposureState fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("exposure".equals(codeString)) 085 return EXPOSURE; 086 if ("exposure-alternative".equals(codeString)) 087 return EXPOSUREALTERNATIVE; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown ExposureState code '" + codeString + "'"); 092 } 093 094 public String toCode() { 095 switch (this) { 096 case EXPOSURE: 097 return "exposure"; 098 case EXPOSUREALTERNATIVE: 099 return "exposure-alternative"; 100 case NULL: 101 return null; 102 default: 103 return "?"; 104 } 105 } 106 107 public String getSystem() { 108 switch (this) { 109 case EXPOSURE: 110 return "http://hl7.org/fhir/exposure-state"; 111 case EXPOSUREALTERNATIVE: 112 return "http://hl7.org/fhir/exposure-state"; 113 case NULL: 114 return null; 115 default: 116 return "?"; 117 } 118 } 119 120 public String getDefinition() { 121 switch (this) { 122 case EXPOSURE: 123 return "used when the results by exposure is describing the results for the primary exposure of interest."; 124 case EXPOSUREALTERNATIVE: 125 return "used when the results by exposure is describing the results for the alternative exposure state, control state or comparator state."; 126 case NULL: 127 return null; 128 default: 129 return "?"; 130 } 131 } 132 133 public String getDisplay() { 134 switch (this) { 135 case EXPOSURE: 136 return "Exposure"; 137 case EXPOSUREALTERNATIVE: 138 return "Exposure Alternative"; 139 case NULL: 140 return null; 141 default: 142 return "?"; 143 } 144 } 145 } 146 147 public static class ExposureStateEnumFactory implements EnumFactory<ExposureState> { 148 public ExposureState fromCode(String codeString) throws IllegalArgumentException { 149 if (codeString == null || "".equals(codeString)) 150 if (codeString == null || "".equals(codeString)) 151 return null; 152 if ("exposure".equals(codeString)) 153 return ExposureState.EXPOSURE; 154 if ("exposure-alternative".equals(codeString)) 155 return ExposureState.EXPOSUREALTERNATIVE; 156 throw new IllegalArgumentException("Unknown ExposureState code '" + codeString + "'"); 157 } 158 159 public Enumeration<ExposureState> fromType(PrimitiveType<?> code) throws FHIRException { 160 if (code == null) 161 return null; 162 if (code.isEmpty()) 163 return new Enumeration<ExposureState>(this, ExposureState.NULL, code); 164 String codeString = code.asStringValue(); 165 if (codeString == null || "".equals(codeString)) 166 return new Enumeration<ExposureState>(this, ExposureState.NULL, code); 167 if ("exposure".equals(codeString)) 168 return new Enumeration<ExposureState>(this, ExposureState.EXPOSURE, code); 169 if ("exposure-alternative".equals(codeString)) 170 return new Enumeration<ExposureState>(this, ExposureState.EXPOSUREALTERNATIVE, code); 171 throw new FHIRException("Unknown ExposureState code '" + codeString + "'"); 172 } 173 174 public String toCode(ExposureState code) { 175 if (code == ExposureState.EXPOSURE) 176 return "exposure"; 177 if (code == ExposureState.EXPOSUREALTERNATIVE) 178 return "exposure-alternative"; 179 return "?"; 180 } 181 182 public String toSystem(ExposureState code) { 183 return code.getSystem(); 184 } 185 } 186 187 @Block() 188 public static class EffectEvidenceSynthesisSampleSizeComponent extends BackboneElement 189 implements IBaseBackboneElement { 190 /** 191 * Human-readable summary of sample size. 192 */ 193 @Child(name = "description", type = { 194 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 195 @Description(shortDefinition = "Description of sample size", formalDefinition = "Human-readable summary of sample size.") 196 protected StringType description; 197 198 /** 199 * Number of studies included in this evidence synthesis. 200 */ 201 @Child(name = "numberOfStudies", type = { 202 IntegerType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 203 @Description(shortDefinition = "How many studies?", formalDefinition = "Number of studies included in this evidence synthesis.") 204 protected IntegerType numberOfStudies; 205 206 /** 207 * Number of participants included in this evidence synthesis. 208 */ 209 @Child(name = "numberOfParticipants", type = { 210 IntegerType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 211 @Description(shortDefinition = "How many participants?", formalDefinition = "Number of participants included in this evidence synthesis.") 212 protected IntegerType numberOfParticipants; 213 214 private static final long serialVersionUID = -1116074476L; 215 216 /** 217 * Constructor 218 */ 219 public EffectEvidenceSynthesisSampleSizeComponent() { 220 super(); 221 } 222 223 /** 224 * @return {@link #description} (Human-readable summary of sample size.). This 225 * is the underlying object with id, value and extensions. The accessor 226 * "getDescription" gives direct access to the value 227 */ 228 public StringType getDescriptionElement() { 229 if (this.description == null) 230 if (Configuration.errorOnAutoCreate()) 231 throw new Error("Attempt to auto-create EffectEvidenceSynthesisSampleSizeComponent.description"); 232 else if (Configuration.doAutoCreate()) 233 this.description = new StringType(); // bb 234 return this.description; 235 } 236 237 public boolean hasDescriptionElement() { 238 return this.description != null && !this.description.isEmpty(); 239 } 240 241 public boolean hasDescription() { 242 return this.description != null && !this.description.isEmpty(); 243 } 244 245 /** 246 * @param value {@link #description} (Human-readable summary of sample size.). 247 * This is the underlying object with id, value and extensions. The 248 * accessor "getDescription" gives direct access to the value 249 */ 250 public EffectEvidenceSynthesisSampleSizeComponent setDescriptionElement(StringType value) { 251 this.description = value; 252 return this; 253 } 254 255 /** 256 * @return Human-readable summary of sample size. 257 */ 258 public String getDescription() { 259 return this.description == null ? null : this.description.getValue(); 260 } 261 262 /** 263 * @param value Human-readable summary of sample size. 264 */ 265 public EffectEvidenceSynthesisSampleSizeComponent setDescription(String value) { 266 if (Utilities.noString(value)) 267 this.description = null; 268 else { 269 if (this.description == null) 270 this.description = new StringType(); 271 this.description.setValue(value); 272 } 273 return this; 274 } 275 276 /** 277 * @return {@link #numberOfStudies} (Number of studies included in this evidence 278 * synthesis.). This is the underlying object with id, value and 279 * extensions. The accessor "getNumberOfStudies" gives direct access to 280 * the value 281 */ 282 public IntegerType getNumberOfStudiesElement() { 283 if (this.numberOfStudies == null) 284 if (Configuration.errorOnAutoCreate()) 285 throw new Error("Attempt to auto-create EffectEvidenceSynthesisSampleSizeComponent.numberOfStudies"); 286 else if (Configuration.doAutoCreate()) 287 this.numberOfStudies = new IntegerType(); // bb 288 return this.numberOfStudies; 289 } 290 291 public boolean hasNumberOfStudiesElement() { 292 return this.numberOfStudies != null && !this.numberOfStudies.isEmpty(); 293 } 294 295 public boolean hasNumberOfStudies() { 296 return this.numberOfStudies != null && !this.numberOfStudies.isEmpty(); 297 } 298 299 /** 300 * @param value {@link #numberOfStudies} (Number of studies included in this 301 * evidence synthesis.). This is the underlying object with id, 302 * value and extensions. The accessor "getNumberOfStudies" gives 303 * direct access to the value 304 */ 305 public EffectEvidenceSynthesisSampleSizeComponent setNumberOfStudiesElement(IntegerType value) { 306 this.numberOfStudies = value; 307 return this; 308 } 309 310 /** 311 * @return Number of studies included in this evidence synthesis. 312 */ 313 public int getNumberOfStudies() { 314 return this.numberOfStudies == null || this.numberOfStudies.isEmpty() ? 0 : this.numberOfStudies.getValue(); 315 } 316 317 /** 318 * @param value Number of studies included in this evidence synthesis. 319 */ 320 public EffectEvidenceSynthesisSampleSizeComponent setNumberOfStudies(int value) { 321 if (this.numberOfStudies == null) 322 this.numberOfStudies = new IntegerType(); 323 this.numberOfStudies.setValue(value); 324 return this; 325 } 326 327 /** 328 * @return {@link #numberOfParticipants} (Number of participants included in 329 * this evidence synthesis.). This is the underlying object with id, 330 * value and extensions. The accessor "getNumberOfParticipants" gives 331 * direct access to the value 332 */ 333 public IntegerType getNumberOfParticipantsElement() { 334 if (this.numberOfParticipants == null) 335 if (Configuration.errorOnAutoCreate()) 336 throw new Error("Attempt to auto-create EffectEvidenceSynthesisSampleSizeComponent.numberOfParticipants"); 337 else if (Configuration.doAutoCreate()) 338 this.numberOfParticipants = new IntegerType(); // bb 339 return this.numberOfParticipants; 340 } 341 342 public boolean hasNumberOfParticipantsElement() { 343 return this.numberOfParticipants != null && !this.numberOfParticipants.isEmpty(); 344 } 345 346 public boolean hasNumberOfParticipants() { 347 return this.numberOfParticipants != null && !this.numberOfParticipants.isEmpty(); 348 } 349 350 /** 351 * @param value {@link #numberOfParticipants} (Number of participants included 352 * in this evidence synthesis.). This is the underlying object with 353 * id, value and extensions. The accessor "getNumberOfParticipants" 354 * gives direct access to the value 355 */ 356 public EffectEvidenceSynthesisSampleSizeComponent setNumberOfParticipantsElement(IntegerType value) { 357 this.numberOfParticipants = value; 358 return this; 359 } 360 361 /** 362 * @return Number of participants included in this evidence synthesis. 363 */ 364 public int getNumberOfParticipants() { 365 return this.numberOfParticipants == null || this.numberOfParticipants.isEmpty() ? 0 366 : this.numberOfParticipants.getValue(); 367 } 368 369 /** 370 * @param value Number of participants included in this evidence synthesis. 371 */ 372 public EffectEvidenceSynthesisSampleSizeComponent setNumberOfParticipants(int value) { 373 if (this.numberOfParticipants == null) 374 this.numberOfParticipants = new IntegerType(); 375 this.numberOfParticipants.setValue(value); 376 return this; 377 } 378 379 protected void listChildren(List<Property> children) { 380 super.listChildren(children); 381 children.add(new Property("description", "string", "Human-readable summary of sample size.", 0, 1, description)); 382 children.add(new Property("numberOfStudies", "integer", "Number of studies included in this evidence synthesis.", 383 0, 1, numberOfStudies)); 384 children.add(new Property("numberOfParticipants", "integer", 385 "Number of participants included in this evidence synthesis.", 0, 1, numberOfParticipants)); 386 } 387 388 @Override 389 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 390 switch (_hash) { 391 case -1724546052: 392 /* description */ return new Property("description", "string", "Human-readable summary of sample size.", 0, 1, 393 description); 394 case -177467129: 395 /* numberOfStudies */ return new Property("numberOfStudies", "integer", 396 "Number of studies included in this evidence synthesis.", 0, 1, numberOfStudies); 397 case 1799357120: 398 /* numberOfParticipants */ return new Property("numberOfParticipants", "integer", 399 "Number of participants included in this evidence synthesis.", 0, 1, numberOfParticipants); 400 default: 401 return super.getNamedProperty(_hash, _name, _checkValid); 402 } 403 404 } 405 406 @Override 407 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 408 switch (hash) { 409 case -1724546052: 410 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 411 case -177467129: 412 /* numberOfStudies */ return this.numberOfStudies == null ? new Base[0] : new Base[] { this.numberOfStudies }; // IntegerType 413 case 1799357120: 414 /* numberOfParticipants */ return this.numberOfParticipants == null ? new Base[0] 415 : new Base[] { this.numberOfParticipants }; // IntegerType 416 default: 417 return super.getProperty(hash, name, checkValid); 418 } 419 420 } 421 422 @Override 423 public Base setProperty(int hash, String name, Base value) throws FHIRException { 424 switch (hash) { 425 case -1724546052: // description 426 this.description = castToString(value); // StringType 427 return value; 428 case -177467129: // numberOfStudies 429 this.numberOfStudies = castToInteger(value); // IntegerType 430 return value; 431 case 1799357120: // numberOfParticipants 432 this.numberOfParticipants = castToInteger(value); // IntegerType 433 return value; 434 default: 435 return super.setProperty(hash, name, value); 436 } 437 438 } 439 440 @Override 441 public Base setProperty(String name, Base value) throws FHIRException { 442 if (name.equals("description")) { 443 this.description = castToString(value); // StringType 444 } else if (name.equals("numberOfStudies")) { 445 this.numberOfStudies = castToInteger(value); // IntegerType 446 } else if (name.equals("numberOfParticipants")) { 447 this.numberOfParticipants = castToInteger(value); // IntegerType 448 } else 449 return super.setProperty(name, value); 450 return value; 451 } 452 453 @Override 454 public Base makeProperty(int hash, String name) throws FHIRException { 455 switch (hash) { 456 case -1724546052: 457 return getDescriptionElement(); 458 case -177467129: 459 return getNumberOfStudiesElement(); 460 case 1799357120: 461 return getNumberOfParticipantsElement(); 462 default: 463 return super.makeProperty(hash, name); 464 } 465 466 } 467 468 @Override 469 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 470 switch (hash) { 471 case -1724546052: 472 /* description */ return new String[] { "string" }; 473 case -177467129: 474 /* numberOfStudies */ return new String[] { "integer" }; 475 case 1799357120: 476 /* numberOfParticipants */ return new String[] { "integer" }; 477 default: 478 return super.getTypesForProperty(hash, name); 479 } 480 481 } 482 483 @Override 484 public Base addChild(String name) throws FHIRException { 485 if (name.equals("description")) { 486 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.description"); 487 } else if (name.equals("numberOfStudies")) { 488 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.numberOfStudies"); 489 } else if (name.equals("numberOfParticipants")) { 490 throw new FHIRException( 491 "Cannot call addChild on a singleton property EffectEvidenceSynthesis.numberOfParticipants"); 492 } else 493 return super.addChild(name); 494 } 495 496 public EffectEvidenceSynthesisSampleSizeComponent copy() { 497 EffectEvidenceSynthesisSampleSizeComponent dst = new EffectEvidenceSynthesisSampleSizeComponent(); 498 copyValues(dst); 499 return dst; 500 } 501 502 public void copyValues(EffectEvidenceSynthesisSampleSizeComponent dst) { 503 super.copyValues(dst); 504 dst.description = description == null ? null : description.copy(); 505 dst.numberOfStudies = numberOfStudies == null ? null : numberOfStudies.copy(); 506 dst.numberOfParticipants = numberOfParticipants == null ? null : numberOfParticipants.copy(); 507 } 508 509 @Override 510 public boolean equalsDeep(Base other_) { 511 if (!super.equalsDeep(other_)) 512 return false; 513 if (!(other_ instanceof EffectEvidenceSynthesisSampleSizeComponent)) 514 return false; 515 EffectEvidenceSynthesisSampleSizeComponent o = (EffectEvidenceSynthesisSampleSizeComponent) other_; 516 return compareDeep(description, o.description, true) && compareDeep(numberOfStudies, o.numberOfStudies, true) 517 && compareDeep(numberOfParticipants, o.numberOfParticipants, true); 518 } 519 520 @Override 521 public boolean equalsShallow(Base other_) { 522 if (!super.equalsShallow(other_)) 523 return false; 524 if (!(other_ instanceof EffectEvidenceSynthesisSampleSizeComponent)) 525 return false; 526 EffectEvidenceSynthesisSampleSizeComponent o = (EffectEvidenceSynthesisSampleSizeComponent) other_; 527 return compareValues(description, o.description, true) && compareValues(numberOfStudies, o.numberOfStudies, true) 528 && compareValues(numberOfParticipants, o.numberOfParticipants, true); 529 } 530 531 public boolean isEmpty() { 532 return super.isEmpty() 533 && ca.uhn.fhir.util.ElementUtil.isEmpty(description, numberOfStudies, numberOfParticipants); 534 } 535 536 public String fhirType() { 537 return "EffectEvidenceSynthesis.sampleSize"; 538 539 } 540 541 } 542 543 @Block() 544 public static class EffectEvidenceSynthesisResultsByExposureComponent extends BackboneElement 545 implements IBaseBackboneElement { 546 /** 547 * Human-readable summary of results by exposure state. 548 */ 549 @Child(name = "description", type = { 550 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 551 @Description(shortDefinition = "Description of results by exposure", formalDefinition = "Human-readable summary of results by exposure state.") 552 protected StringType description; 553 554 /** 555 * Whether these results are for the exposure state or alternative exposure 556 * state. 557 */ 558 @Child(name = "exposureState", type = { 559 CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 560 @Description(shortDefinition = "exposure | exposure-alternative", formalDefinition = "Whether these results are for the exposure state or alternative exposure state.") 561 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/exposure-state") 562 protected Enumeration<ExposureState> exposureState; 563 564 /** 565 * Used to define variant exposure states such as low-risk state. 566 */ 567 @Child(name = "variantState", type = { 568 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 569 @Description(shortDefinition = "Variant exposure states", formalDefinition = "Used to define variant exposure states such as low-risk state.") 570 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/evidence-variant-state") 571 protected CodeableConcept variantState; 572 573 /** 574 * Reference to a RiskEvidenceSynthesis resource. 575 */ 576 @Child(name = "riskEvidenceSynthesis", type = { 577 RiskEvidenceSynthesis.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 578 @Description(shortDefinition = "Risk evidence synthesis", formalDefinition = "Reference to a RiskEvidenceSynthesis resource.") 579 protected Reference riskEvidenceSynthesis; 580 581 /** 582 * The actual object that is the target of the reference (Reference to a 583 * RiskEvidenceSynthesis resource.) 584 */ 585 protected RiskEvidenceSynthesis riskEvidenceSynthesisTarget; 586 587 private static final long serialVersionUID = 144886133L; 588 589 /** 590 * Constructor 591 */ 592 public EffectEvidenceSynthesisResultsByExposureComponent() { 593 super(); 594 } 595 596 /** 597 * Constructor 598 */ 599 public EffectEvidenceSynthesisResultsByExposureComponent(Reference riskEvidenceSynthesis) { 600 super(); 601 this.riskEvidenceSynthesis = riskEvidenceSynthesis; 602 } 603 604 /** 605 * @return {@link #description} (Human-readable summary of results by exposure 606 * state.). This is the underlying object with id, value and extensions. 607 * The accessor "getDescription" gives direct access to the value 608 */ 609 public StringType getDescriptionElement() { 610 if (this.description == null) 611 if (Configuration.errorOnAutoCreate()) 612 throw new Error("Attempt to auto-create EffectEvidenceSynthesisResultsByExposureComponent.description"); 613 else if (Configuration.doAutoCreate()) 614 this.description = new StringType(); // bb 615 return this.description; 616 } 617 618 public boolean hasDescriptionElement() { 619 return this.description != null && !this.description.isEmpty(); 620 } 621 622 public boolean hasDescription() { 623 return this.description != null && !this.description.isEmpty(); 624 } 625 626 /** 627 * @param value {@link #description} (Human-readable summary of results by 628 * exposure state.). This is the underlying object with id, value 629 * and extensions. The accessor "getDescription" gives direct 630 * access to the value 631 */ 632 public EffectEvidenceSynthesisResultsByExposureComponent setDescriptionElement(StringType value) { 633 this.description = value; 634 return this; 635 } 636 637 /** 638 * @return Human-readable summary of results by exposure state. 639 */ 640 public String getDescription() { 641 return this.description == null ? null : this.description.getValue(); 642 } 643 644 /** 645 * @param value Human-readable summary of results by exposure state. 646 */ 647 public EffectEvidenceSynthesisResultsByExposureComponent setDescription(String value) { 648 if (Utilities.noString(value)) 649 this.description = null; 650 else { 651 if (this.description == null) 652 this.description = new StringType(); 653 this.description.setValue(value); 654 } 655 return this; 656 } 657 658 /** 659 * @return {@link #exposureState} (Whether these results are for the exposure 660 * state or alternative exposure state.). This is the underlying object 661 * with id, value and extensions. The accessor "getExposureState" gives 662 * direct access to the value 663 */ 664 public Enumeration<ExposureState> getExposureStateElement() { 665 if (this.exposureState == null) 666 if (Configuration.errorOnAutoCreate()) 667 throw new Error("Attempt to auto-create EffectEvidenceSynthesisResultsByExposureComponent.exposureState"); 668 else if (Configuration.doAutoCreate()) 669 this.exposureState = new Enumeration<ExposureState>(new ExposureStateEnumFactory()); // bb 670 return this.exposureState; 671 } 672 673 public boolean hasExposureStateElement() { 674 return this.exposureState != null && !this.exposureState.isEmpty(); 675 } 676 677 public boolean hasExposureState() { 678 return this.exposureState != null && !this.exposureState.isEmpty(); 679 } 680 681 /** 682 * @param value {@link #exposureState} (Whether these results are for the 683 * exposure state or alternative exposure state.). This is the 684 * underlying object with id, value and extensions. The accessor 685 * "getExposureState" gives direct access to the value 686 */ 687 public EffectEvidenceSynthesisResultsByExposureComponent setExposureStateElement(Enumeration<ExposureState> value) { 688 this.exposureState = value; 689 return this; 690 } 691 692 /** 693 * @return Whether these results are for the exposure state or alternative 694 * exposure state. 695 */ 696 public ExposureState getExposureState() { 697 return this.exposureState == null ? null : this.exposureState.getValue(); 698 } 699 700 /** 701 * @param value Whether these results are for the exposure state or alternative 702 * exposure state. 703 */ 704 public EffectEvidenceSynthesisResultsByExposureComponent setExposureState(ExposureState value) { 705 if (value == null) 706 this.exposureState = null; 707 else { 708 if (this.exposureState == null) 709 this.exposureState = new Enumeration<ExposureState>(new ExposureStateEnumFactory()); 710 this.exposureState.setValue(value); 711 } 712 return this; 713 } 714 715 /** 716 * @return {@link #variantState} (Used to define variant exposure states such as 717 * low-risk state.) 718 */ 719 public CodeableConcept getVariantState() { 720 if (this.variantState == null) 721 if (Configuration.errorOnAutoCreate()) 722 throw new Error("Attempt to auto-create EffectEvidenceSynthesisResultsByExposureComponent.variantState"); 723 else if (Configuration.doAutoCreate()) 724 this.variantState = new CodeableConcept(); // cc 725 return this.variantState; 726 } 727 728 public boolean hasVariantState() { 729 return this.variantState != null && !this.variantState.isEmpty(); 730 } 731 732 /** 733 * @param value {@link #variantState} (Used to define variant exposure states 734 * such as low-risk state.) 735 */ 736 public EffectEvidenceSynthesisResultsByExposureComponent setVariantState(CodeableConcept value) { 737 this.variantState = value; 738 return this; 739 } 740 741 /** 742 * @return {@link #riskEvidenceSynthesis} (Reference to a RiskEvidenceSynthesis 743 * resource.) 744 */ 745 public Reference getRiskEvidenceSynthesis() { 746 if (this.riskEvidenceSynthesis == null) 747 if (Configuration.errorOnAutoCreate()) 748 throw new Error( 749 "Attempt to auto-create EffectEvidenceSynthesisResultsByExposureComponent.riskEvidenceSynthesis"); 750 else if (Configuration.doAutoCreate()) 751 this.riskEvidenceSynthesis = new Reference(); // cc 752 return this.riskEvidenceSynthesis; 753 } 754 755 public boolean hasRiskEvidenceSynthesis() { 756 return this.riskEvidenceSynthesis != null && !this.riskEvidenceSynthesis.isEmpty(); 757 } 758 759 /** 760 * @param value {@link #riskEvidenceSynthesis} (Reference to a 761 * RiskEvidenceSynthesis resource.) 762 */ 763 public EffectEvidenceSynthesisResultsByExposureComponent setRiskEvidenceSynthesis(Reference value) { 764 this.riskEvidenceSynthesis = value; 765 return this; 766 } 767 768 /** 769 * @return {@link #riskEvidenceSynthesis} The actual object that is the target 770 * of the reference. The reference library doesn't populate this, but 771 * you can use it to hold the resource if you resolve it. (Reference to 772 * a RiskEvidenceSynthesis resource.) 773 */ 774 public RiskEvidenceSynthesis getRiskEvidenceSynthesisTarget() { 775 if (this.riskEvidenceSynthesisTarget == null) 776 if (Configuration.errorOnAutoCreate()) 777 throw new Error( 778 "Attempt to auto-create EffectEvidenceSynthesisResultsByExposureComponent.riskEvidenceSynthesis"); 779 else if (Configuration.doAutoCreate()) 780 this.riskEvidenceSynthesisTarget = new RiskEvidenceSynthesis(); // aa 781 return this.riskEvidenceSynthesisTarget; 782 } 783 784 /** 785 * @param value {@link #riskEvidenceSynthesis} The actual object that is the 786 * target of the reference. The reference library doesn't use 787 * these, but you can use it to hold the resource if you resolve 788 * it. (Reference to a RiskEvidenceSynthesis resource.) 789 */ 790 public EffectEvidenceSynthesisResultsByExposureComponent setRiskEvidenceSynthesisTarget( 791 RiskEvidenceSynthesis value) { 792 this.riskEvidenceSynthesisTarget = value; 793 return this; 794 } 795 796 protected void listChildren(List<Property> children) { 797 super.listChildren(children); 798 children.add(new Property("description", "string", "Human-readable summary of results by exposure state.", 0, 1, 799 description)); 800 children.add(new Property("exposureState", "code", 801 "Whether these results are for the exposure state or alternative exposure state.", 0, 1, exposureState)); 802 children.add(new Property("variantState", "CodeableConcept", 803 "Used to define variant exposure states such as low-risk state.", 0, 1, variantState)); 804 children.add(new Property("riskEvidenceSynthesis", "Reference(RiskEvidenceSynthesis)", 805 "Reference to a RiskEvidenceSynthesis resource.", 0, 1, riskEvidenceSynthesis)); 806 } 807 808 @Override 809 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 810 switch (_hash) { 811 case -1724546052: 812 /* description */ return new Property("description", "string", 813 "Human-readable summary of results by exposure state.", 0, 1, description); 814 case 422339530: 815 /* exposureState */ return new Property("exposureState", "code", 816 "Whether these results are for the exposure state or alternative exposure state.", 0, 1, exposureState); 817 case 1900629772: 818 /* variantState */ return new Property("variantState", "CodeableConcept", 819 "Used to define variant exposure states such as low-risk state.", 0, 1, variantState); 820 case 109085678: 821 /* riskEvidenceSynthesis */ return new Property("riskEvidenceSynthesis", "Reference(RiskEvidenceSynthesis)", 822 "Reference to a RiskEvidenceSynthesis resource.", 0, 1, riskEvidenceSynthesis); 823 default: 824 return super.getNamedProperty(_hash, _name, _checkValid); 825 } 826 827 } 828 829 @Override 830 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 831 switch (hash) { 832 case -1724546052: 833 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 834 case 422339530: 835 /* exposureState */ return this.exposureState == null ? new Base[0] : new Base[] { this.exposureState }; // Enumeration<ExposureState> 836 case 1900629772: 837 /* variantState */ return this.variantState == null ? new Base[0] : new Base[] { this.variantState }; // CodeableConcept 838 case 109085678: 839 /* riskEvidenceSynthesis */ return this.riskEvidenceSynthesis == null ? new Base[0] 840 : new Base[] { this.riskEvidenceSynthesis }; // Reference 841 default: 842 return super.getProperty(hash, name, checkValid); 843 } 844 845 } 846 847 @Override 848 public Base setProperty(int hash, String name, Base value) throws FHIRException { 849 switch (hash) { 850 case -1724546052: // description 851 this.description = castToString(value); // StringType 852 return value; 853 case 422339530: // exposureState 854 value = new ExposureStateEnumFactory().fromType(castToCode(value)); 855 this.exposureState = (Enumeration) value; // Enumeration<ExposureState> 856 return value; 857 case 1900629772: // variantState 858 this.variantState = castToCodeableConcept(value); // CodeableConcept 859 return value; 860 case 109085678: // riskEvidenceSynthesis 861 this.riskEvidenceSynthesis = castToReference(value); // Reference 862 return value; 863 default: 864 return super.setProperty(hash, name, value); 865 } 866 867 } 868 869 @Override 870 public Base setProperty(String name, Base value) throws FHIRException { 871 if (name.equals("description")) { 872 this.description = castToString(value); // StringType 873 } else if (name.equals("exposureState")) { 874 value = new ExposureStateEnumFactory().fromType(castToCode(value)); 875 this.exposureState = (Enumeration) value; // Enumeration<ExposureState> 876 } else if (name.equals("variantState")) { 877 this.variantState = castToCodeableConcept(value); // CodeableConcept 878 } else if (name.equals("riskEvidenceSynthesis")) { 879 this.riskEvidenceSynthesis = castToReference(value); // Reference 880 } else 881 return super.setProperty(name, value); 882 return value; 883 } 884 885 @Override 886 public Base makeProperty(int hash, String name) throws FHIRException { 887 switch (hash) { 888 case -1724546052: 889 return getDescriptionElement(); 890 case 422339530: 891 return getExposureStateElement(); 892 case 1900629772: 893 return getVariantState(); 894 case 109085678: 895 return getRiskEvidenceSynthesis(); 896 default: 897 return super.makeProperty(hash, name); 898 } 899 900 } 901 902 @Override 903 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 904 switch (hash) { 905 case -1724546052: 906 /* description */ return new String[] { "string" }; 907 case 422339530: 908 /* exposureState */ return new String[] { "code" }; 909 case 1900629772: 910 /* variantState */ return new String[] { "CodeableConcept" }; 911 case 109085678: 912 /* riskEvidenceSynthesis */ return new String[] { "Reference" }; 913 default: 914 return super.getTypesForProperty(hash, name); 915 } 916 917 } 918 919 @Override 920 public Base addChild(String name) throws FHIRException { 921 if (name.equals("description")) { 922 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.description"); 923 } else if (name.equals("exposureState")) { 924 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.exposureState"); 925 } else if (name.equals("variantState")) { 926 this.variantState = new CodeableConcept(); 927 return this.variantState; 928 } else if (name.equals("riskEvidenceSynthesis")) { 929 this.riskEvidenceSynthesis = new Reference(); 930 return this.riskEvidenceSynthesis; 931 } else 932 return super.addChild(name); 933 } 934 935 public EffectEvidenceSynthesisResultsByExposureComponent copy() { 936 EffectEvidenceSynthesisResultsByExposureComponent dst = new EffectEvidenceSynthesisResultsByExposureComponent(); 937 copyValues(dst); 938 return dst; 939 } 940 941 public void copyValues(EffectEvidenceSynthesisResultsByExposureComponent dst) { 942 super.copyValues(dst); 943 dst.description = description == null ? null : description.copy(); 944 dst.exposureState = exposureState == null ? null : exposureState.copy(); 945 dst.variantState = variantState == null ? null : variantState.copy(); 946 dst.riskEvidenceSynthesis = riskEvidenceSynthesis == null ? null : riskEvidenceSynthesis.copy(); 947 } 948 949 @Override 950 public boolean equalsDeep(Base other_) { 951 if (!super.equalsDeep(other_)) 952 return false; 953 if (!(other_ instanceof EffectEvidenceSynthesisResultsByExposureComponent)) 954 return false; 955 EffectEvidenceSynthesisResultsByExposureComponent o = (EffectEvidenceSynthesisResultsByExposureComponent) other_; 956 return compareDeep(description, o.description, true) && compareDeep(exposureState, o.exposureState, true) 957 && compareDeep(variantState, o.variantState, true) 958 && compareDeep(riskEvidenceSynthesis, o.riskEvidenceSynthesis, true); 959 } 960 961 @Override 962 public boolean equalsShallow(Base other_) { 963 if (!super.equalsShallow(other_)) 964 return false; 965 if (!(other_ instanceof EffectEvidenceSynthesisResultsByExposureComponent)) 966 return false; 967 EffectEvidenceSynthesisResultsByExposureComponent o = (EffectEvidenceSynthesisResultsByExposureComponent) other_; 968 return compareValues(description, o.description, true) && compareValues(exposureState, o.exposureState, true); 969 } 970 971 public boolean isEmpty() { 972 return super.isEmpty() 973 && ca.uhn.fhir.util.ElementUtil.isEmpty(description, exposureState, variantState, riskEvidenceSynthesis); 974 } 975 976 public String fhirType() { 977 return "EffectEvidenceSynthesis.resultsByExposure"; 978 979 } 980 981 } 982 983 @Block() 984 public static class EffectEvidenceSynthesisEffectEstimateComponent extends BackboneElement 985 implements IBaseBackboneElement { 986 /** 987 * Human-readable summary of effect estimate. 988 */ 989 @Child(name = "description", type = { 990 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 991 @Description(shortDefinition = "Description of effect estimate", formalDefinition = "Human-readable summary of effect estimate.") 992 protected StringType description; 993 994 /** 995 * Examples include relative risk and mean difference. 996 */ 997 @Child(name = "type", type = { 998 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 999 @Description(shortDefinition = "Type of efffect estimate", formalDefinition = "Examples include relative risk and mean difference.") 1000 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/effect-estimate-type") 1001 protected CodeableConcept type; 1002 1003 /** 1004 * Used to define variant exposure states such as low-risk state. 1005 */ 1006 @Child(name = "variantState", type = { 1007 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1008 @Description(shortDefinition = "Variant exposure states", formalDefinition = "Used to define variant exposure states such as low-risk state.") 1009 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/evidence-variant-state") 1010 protected CodeableConcept variantState; 1011 1012 /** 1013 * The point estimate of the effect estimate. 1014 */ 1015 @Child(name = "value", type = { DecimalType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1016 @Description(shortDefinition = "Point estimate", formalDefinition = "The point estimate of the effect estimate.") 1017 protected DecimalType value; 1018 1019 /** 1020 * Specifies the UCUM unit for the outcome. 1021 */ 1022 @Child(name = "unitOfMeasure", type = { 1023 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1024 @Description(shortDefinition = "What unit is the outcome described in?", formalDefinition = "Specifies the UCUM unit for the outcome.") 1025 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ucum-units") 1026 protected CodeableConcept unitOfMeasure; 1027 1028 /** 1029 * A description of the precision of the estimate for the effect. 1030 */ 1031 @Child(name = "precisionEstimate", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1032 @Description(shortDefinition = "How precise the estimate is", formalDefinition = "A description of the precision of the estimate for the effect.") 1033 protected List<EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent> precisionEstimate; 1034 1035 private static final long serialVersionUID = -1075065083L; 1036 1037 /** 1038 * Constructor 1039 */ 1040 public EffectEvidenceSynthesisEffectEstimateComponent() { 1041 super(); 1042 } 1043 1044 /** 1045 * @return {@link #description} (Human-readable summary of effect estimate.). 1046 * This is the underlying object with id, value and extensions. The 1047 * accessor "getDescription" gives direct access to the value 1048 */ 1049 public StringType getDescriptionElement() { 1050 if (this.description == null) 1051 if (Configuration.errorOnAutoCreate()) 1052 throw new Error("Attempt to auto-create EffectEvidenceSynthesisEffectEstimateComponent.description"); 1053 else if (Configuration.doAutoCreate()) 1054 this.description = new StringType(); // bb 1055 return this.description; 1056 } 1057 1058 public boolean hasDescriptionElement() { 1059 return this.description != null && !this.description.isEmpty(); 1060 } 1061 1062 public boolean hasDescription() { 1063 return this.description != null && !this.description.isEmpty(); 1064 } 1065 1066 /** 1067 * @param value {@link #description} (Human-readable summary of effect 1068 * estimate.). This is the underlying object with id, value and 1069 * extensions. The accessor "getDescription" gives direct access to 1070 * the value 1071 */ 1072 public EffectEvidenceSynthesisEffectEstimateComponent setDescriptionElement(StringType value) { 1073 this.description = value; 1074 return this; 1075 } 1076 1077 /** 1078 * @return Human-readable summary of effect estimate. 1079 */ 1080 public String getDescription() { 1081 return this.description == null ? null : this.description.getValue(); 1082 } 1083 1084 /** 1085 * @param value Human-readable summary of effect estimate. 1086 */ 1087 public EffectEvidenceSynthesisEffectEstimateComponent setDescription(String value) { 1088 if (Utilities.noString(value)) 1089 this.description = null; 1090 else { 1091 if (this.description == null) 1092 this.description = new StringType(); 1093 this.description.setValue(value); 1094 } 1095 return this; 1096 } 1097 1098 /** 1099 * @return {@link #type} (Examples include relative risk and mean difference.) 1100 */ 1101 public CodeableConcept getType() { 1102 if (this.type == null) 1103 if (Configuration.errorOnAutoCreate()) 1104 throw new Error("Attempt to auto-create EffectEvidenceSynthesisEffectEstimateComponent.type"); 1105 else if (Configuration.doAutoCreate()) 1106 this.type = new CodeableConcept(); // cc 1107 return this.type; 1108 } 1109 1110 public boolean hasType() { 1111 return this.type != null && !this.type.isEmpty(); 1112 } 1113 1114 /** 1115 * @param value {@link #type} (Examples include relative risk and mean 1116 * difference.) 1117 */ 1118 public EffectEvidenceSynthesisEffectEstimateComponent setType(CodeableConcept value) { 1119 this.type = value; 1120 return this; 1121 } 1122 1123 /** 1124 * @return {@link #variantState} (Used to define variant exposure states such as 1125 * low-risk state.) 1126 */ 1127 public CodeableConcept getVariantState() { 1128 if (this.variantState == null) 1129 if (Configuration.errorOnAutoCreate()) 1130 throw new Error("Attempt to auto-create EffectEvidenceSynthesisEffectEstimateComponent.variantState"); 1131 else if (Configuration.doAutoCreate()) 1132 this.variantState = new CodeableConcept(); // cc 1133 return this.variantState; 1134 } 1135 1136 public boolean hasVariantState() { 1137 return this.variantState != null && !this.variantState.isEmpty(); 1138 } 1139 1140 /** 1141 * @param value {@link #variantState} (Used to define variant exposure states 1142 * such as low-risk state.) 1143 */ 1144 public EffectEvidenceSynthesisEffectEstimateComponent setVariantState(CodeableConcept value) { 1145 this.variantState = value; 1146 return this; 1147 } 1148 1149 /** 1150 * @return {@link #value} (The point estimate of the effect estimate.). This is 1151 * the underlying object with id, value and extensions. The accessor 1152 * "getValue" gives direct access to the value 1153 */ 1154 public DecimalType getValueElement() { 1155 if (this.value == null) 1156 if (Configuration.errorOnAutoCreate()) 1157 throw new Error("Attempt to auto-create EffectEvidenceSynthesisEffectEstimateComponent.value"); 1158 else if (Configuration.doAutoCreate()) 1159 this.value = new DecimalType(); // bb 1160 return this.value; 1161 } 1162 1163 public boolean hasValueElement() { 1164 return this.value != null && !this.value.isEmpty(); 1165 } 1166 1167 public boolean hasValue() { 1168 return this.value != null && !this.value.isEmpty(); 1169 } 1170 1171 /** 1172 * @param value {@link #value} (The point estimate of the effect estimate.). 1173 * This is the underlying object with id, value and extensions. The 1174 * accessor "getValue" gives direct access to the value 1175 */ 1176 public EffectEvidenceSynthesisEffectEstimateComponent setValueElement(DecimalType value) { 1177 this.value = value; 1178 return this; 1179 } 1180 1181 /** 1182 * @return The point estimate of the effect estimate. 1183 */ 1184 public BigDecimal getValue() { 1185 return this.value == null ? null : this.value.getValue(); 1186 } 1187 1188 /** 1189 * @param value The point estimate of the effect estimate. 1190 */ 1191 public EffectEvidenceSynthesisEffectEstimateComponent setValue(BigDecimal value) { 1192 if (value == null) 1193 this.value = null; 1194 else { 1195 if (this.value == null) 1196 this.value = new DecimalType(); 1197 this.value.setValue(value); 1198 } 1199 return this; 1200 } 1201 1202 /** 1203 * @param value The point estimate of the effect estimate. 1204 */ 1205 public EffectEvidenceSynthesisEffectEstimateComponent setValue(long value) { 1206 this.value = new DecimalType(); 1207 this.value.setValue(value); 1208 return this; 1209 } 1210 1211 /** 1212 * @param value The point estimate of the effect estimate. 1213 */ 1214 public EffectEvidenceSynthesisEffectEstimateComponent setValue(double value) { 1215 this.value = new DecimalType(); 1216 this.value.setValue(value); 1217 return this; 1218 } 1219 1220 /** 1221 * @return {@link #unitOfMeasure} (Specifies the UCUM unit for the outcome.) 1222 */ 1223 public CodeableConcept getUnitOfMeasure() { 1224 if (this.unitOfMeasure == null) 1225 if (Configuration.errorOnAutoCreate()) 1226 throw new Error("Attempt to auto-create EffectEvidenceSynthesisEffectEstimateComponent.unitOfMeasure"); 1227 else if (Configuration.doAutoCreate()) 1228 this.unitOfMeasure = new CodeableConcept(); // cc 1229 return this.unitOfMeasure; 1230 } 1231 1232 public boolean hasUnitOfMeasure() { 1233 return this.unitOfMeasure != null && !this.unitOfMeasure.isEmpty(); 1234 } 1235 1236 /** 1237 * @param value {@link #unitOfMeasure} (Specifies the UCUM unit for the 1238 * outcome.) 1239 */ 1240 public EffectEvidenceSynthesisEffectEstimateComponent setUnitOfMeasure(CodeableConcept value) { 1241 this.unitOfMeasure = value; 1242 return this; 1243 } 1244 1245 /** 1246 * @return {@link #precisionEstimate} (A description of the precision of the 1247 * estimate for the effect.) 1248 */ 1249 public List<EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent> getPrecisionEstimate() { 1250 if (this.precisionEstimate == null) 1251 this.precisionEstimate = new ArrayList<EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent>(); 1252 return this.precisionEstimate; 1253 } 1254 1255 /** 1256 * @return Returns a reference to <code>this</code> for easy method chaining 1257 */ 1258 public EffectEvidenceSynthesisEffectEstimateComponent setPrecisionEstimate( 1259 List<EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent> thePrecisionEstimate) { 1260 this.precisionEstimate = thePrecisionEstimate; 1261 return this; 1262 } 1263 1264 public boolean hasPrecisionEstimate() { 1265 if (this.precisionEstimate == null) 1266 return false; 1267 for (EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent item : this.precisionEstimate) 1268 if (!item.isEmpty()) 1269 return true; 1270 return false; 1271 } 1272 1273 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent addPrecisionEstimate() { // 3 1274 EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent t = new EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent(); 1275 if (this.precisionEstimate == null) 1276 this.precisionEstimate = new ArrayList<EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent>(); 1277 this.precisionEstimate.add(t); 1278 return t; 1279 } 1280 1281 public EffectEvidenceSynthesisEffectEstimateComponent addPrecisionEstimate( 1282 EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent t) { // 3 1283 if (t == null) 1284 return this; 1285 if (this.precisionEstimate == null) 1286 this.precisionEstimate = new ArrayList<EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent>(); 1287 this.precisionEstimate.add(t); 1288 return this; 1289 } 1290 1291 /** 1292 * @return The first repetition of repeating field {@link #precisionEstimate}, 1293 * creating it if it does not already exist 1294 */ 1295 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent getPrecisionEstimateFirstRep() { 1296 if (getPrecisionEstimate().isEmpty()) { 1297 addPrecisionEstimate(); 1298 } 1299 return getPrecisionEstimate().get(0); 1300 } 1301 1302 protected void listChildren(List<Property> children) { 1303 super.listChildren(children); 1304 children 1305 .add(new Property("description", "string", "Human-readable summary of effect estimate.", 0, 1, description)); 1306 children.add( 1307 new Property("type", "CodeableConcept", "Examples include relative risk and mean difference.", 0, 1, type)); 1308 children.add(new Property("variantState", "CodeableConcept", 1309 "Used to define variant exposure states such as low-risk state.", 0, 1, variantState)); 1310 children.add(new Property("value", "decimal", "The point estimate of the effect estimate.", 0, 1, value)); 1311 children.add(new Property("unitOfMeasure", "CodeableConcept", "Specifies the UCUM unit for the outcome.", 0, 1, 1312 unitOfMeasure)); 1313 children 1314 .add(new Property("precisionEstimate", "", "A description of the precision of the estimate for the effect.", 1315 0, java.lang.Integer.MAX_VALUE, precisionEstimate)); 1316 } 1317 1318 @Override 1319 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1320 switch (_hash) { 1321 case -1724546052: 1322 /* description */ return new Property("description", "string", "Human-readable summary of effect estimate.", 0, 1323 1, description); 1324 case 3575610: 1325 /* type */ return new Property("type", "CodeableConcept", "Examples include relative risk and mean difference.", 1326 0, 1, type); 1327 case 1900629772: 1328 /* variantState */ return new Property("variantState", "CodeableConcept", 1329 "Used to define variant exposure states such as low-risk state.", 0, 1, variantState); 1330 case 111972721: 1331 /* value */ return new Property("value", "decimal", "The point estimate of the effect estimate.", 0, 1, value); 1332 case -750257565: 1333 /* unitOfMeasure */ return new Property("unitOfMeasure", "CodeableConcept", 1334 "Specifies the UCUM unit for the outcome.", 0, 1, unitOfMeasure); 1335 case 339632070: 1336 /* precisionEstimate */ return new Property("precisionEstimate", "", 1337 "A description of the precision of the estimate for the effect.", 0, java.lang.Integer.MAX_VALUE, 1338 precisionEstimate); 1339 default: 1340 return super.getNamedProperty(_hash, _name, _checkValid); 1341 } 1342 1343 } 1344 1345 @Override 1346 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1347 switch (hash) { 1348 case -1724546052: 1349 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1350 case 3575610: 1351 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1352 case 1900629772: 1353 /* variantState */ return this.variantState == null ? new Base[0] : new Base[] { this.variantState }; // CodeableConcept 1354 case 111972721: 1355 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // DecimalType 1356 case -750257565: 1357 /* unitOfMeasure */ return this.unitOfMeasure == null ? new Base[0] : new Base[] { this.unitOfMeasure }; // CodeableConcept 1358 case 339632070: 1359 /* precisionEstimate */ return this.precisionEstimate == null ? new Base[0] 1360 : this.precisionEstimate.toArray(new Base[this.precisionEstimate.size()]); // EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent 1361 default: 1362 return super.getProperty(hash, name, checkValid); 1363 } 1364 1365 } 1366 1367 @Override 1368 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1369 switch (hash) { 1370 case -1724546052: // description 1371 this.description = castToString(value); // StringType 1372 return value; 1373 case 3575610: // type 1374 this.type = castToCodeableConcept(value); // CodeableConcept 1375 return value; 1376 case 1900629772: // variantState 1377 this.variantState = castToCodeableConcept(value); // CodeableConcept 1378 return value; 1379 case 111972721: // value 1380 this.value = castToDecimal(value); // DecimalType 1381 return value; 1382 case -750257565: // unitOfMeasure 1383 this.unitOfMeasure = castToCodeableConcept(value); // CodeableConcept 1384 return value; 1385 case 339632070: // precisionEstimate 1386 this.getPrecisionEstimate().add((EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent) value); // EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent 1387 return value; 1388 default: 1389 return super.setProperty(hash, name, value); 1390 } 1391 1392 } 1393 1394 @Override 1395 public Base setProperty(String name, Base value) throws FHIRException { 1396 if (name.equals("description")) { 1397 this.description = castToString(value); // StringType 1398 } else if (name.equals("type")) { 1399 this.type = castToCodeableConcept(value); // CodeableConcept 1400 } else if (name.equals("variantState")) { 1401 this.variantState = castToCodeableConcept(value); // CodeableConcept 1402 } else if (name.equals("value")) { 1403 this.value = castToDecimal(value); // DecimalType 1404 } else if (name.equals("unitOfMeasure")) { 1405 this.unitOfMeasure = castToCodeableConcept(value); // CodeableConcept 1406 } else if (name.equals("precisionEstimate")) { 1407 this.getPrecisionEstimate().add((EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent) value); 1408 } else 1409 return super.setProperty(name, value); 1410 return value; 1411 } 1412 1413 @Override 1414 public Base makeProperty(int hash, String name) throws FHIRException { 1415 switch (hash) { 1416 case -1724546052: 1417 return getDescriptionElement(); 1418 case 3575610: 1419 return getType(); 1420 case 1900629772: 1421 return getVariantState(); 1422 case 111972721: 1423 return getValueElement(); 1424 case -750257565: 1425 return getUnitOfMeasure(); 1426 case 339632070: 1427 return addPrecisionEstimate(); 1428 default: 1429 return super.makeProperty(hash, name); 1430 } 1431 1432 } 1433 1434 @Override 1435 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1436 switch (hash) { 1437 case -1724546052: 1438 /* description */ return new String[] { "string" }; 1439 case 3575610: 1440 /* type */ return new String[] { "CodeableConcept" }; 1441 case 1900629772: 1442 /* variantState */ return new String[] { "CodeableConcept" }; 1443 case 111972721: 1444 /* value */ return new String[] { "decimal" }; 1445 case -750257565: 1446 /* unitOfMeasure */ return new String[] { "CodeableConcept" }; 1447 case 339632070: 1448 /* precisionEstimate */ return new String[] {}; 1449 default: 1450 return super.getTypesForProperty(hash, name); 1451 } 1452 1453 } 1454 1455 @Override 1456 public Base addChild(String name) throws FHIRException { 1457 if (name.equals("description")) { 1458 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.description"); 1459 } else if (name.equals("type")) { 1460 this.type = new CodeableConcept(); 1461 return this.type; 1462 } else if (name.equals("variantState")) { 1463 this.variantState = new CodeableConcept(); 1464 return this.variantState; 1465 } else if (name.equals("value")) { 1466 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.value"); 1467 } else if (name.equals("unitOfMeasure")) { 1468 this.unitOfMeasure = new CodeableConcept(); 1469 return this.unitOfMeasure; 1470 } else if (name.equals("precisionEstimate")) { 1471 return addPrecisionEstimate(); 1472 } else 1473 return super.addChild(name); 1474 } 1475 1476 public EffectEvidenceSynthesisEffectEstimateComponent copy() { 1477 EffectEvidenceSynthesisEffectEstimateComponent dst = new EffectEvidenceSynthesisEffectEstimateComponent(); 1478 copyValues(dst); 1479 return dst; 1480 } 1481 1482 public void copyValues(EffectEvidenceSynthesisEffectEstimateComponent dst) { 1483 super.copyValues(dst); 1484 dst.description = description == null ? null : description.copy(); 1485 dst.type = type == null ? null : type.copy(); 1486 dst.variantState = variantState == null ? null : variantState.copy(); 1487 dst.value = value == null ? null : value.copy(); 1488 dst.unitOfMeasure = unitOfMeasure == null ? null : unitOfMeasure.copy(); 1489 if (precisionEstimate != null) { 1490 dst.precisionEstimate = new ArrayList<EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent>(); 1491 for (EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent i : precisionEstimate) 1492 dst.precisionEstimate.add(i.copy()); 1493 } 1494 ; 1495 } 1496 1497 @Override 1498 public boolean equalsDeep(Base other_) { 1499 if (!super.equalsDeep(other_)) 1500 return false; 1501 if (!(other_ instanceof EffectEvidenceSynthesisEffectEstimateComponent)) 1502 return false; 1503 EffectEvidenceSynthesisEffectEstimateComponent o = (EffectEvidenceSynthesisEffectEstimateComponent) other_; 1504 return compareDeep(description, o.description, true) && compareDeep(type, o.type, true) 1505 && compareDeep(variantState, o.variantState, true) && compareDeep(value, o.value, true) 1506 && compareDeep(unitOfMeasure, o.unitOfMeasure, true) 1507 && compareDeep(precisionEstimate, o.precisionEstimate, true); 1508 } 1509 1510 @Override 1511 public boolean equalsShallow(Base other_) { 1512 if (!super.equalsShallow(other_)) 1513 return false; 1514 if (!(other_ instanceof EffectEvidenceSynthesisEffectEstimateComponent)) 1515 return false; 1516 EffectEvidenceSynthesisEffectEstimateComponent o = (EffectEvidenceSynthesisEffectEstimateComponent) other_; 1517 return compareValues(description, o.description, true) && compareValues(value, o.value, true); 1518 } 1519 1520 public boolean isEmpty() { 1521 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, type, variantState, value, 1522 unitOfMeasure, precisionEstimate); 1523 } 1524 1525 public String fhirType() { 1526 return "EffectEvidenceSynthesis.effectEstimate"; 1527 1528 } 1529 1530 } 1531 1532 @Block() 1533 public static class EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent extends BackboneElement 1534 implements IBaseBackboneElement { 1535 /** 1536 * Examples include confidence interval and interquartile range. 1537 */ 1538 @Child(name = "type", type = { 1539 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1540 @Description(shortDefinition = "Type of precision estimate", formalDefinition = "Examples include confidence interval and interquartile range.") 1541 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/precision-estimate-type") 1542 protected CodeableConcept type; 1543 1544 /** 1545 * Use 95 for a 95% confidence interval. 1546 */ 1547 @Child(name = "level", type = { DecimalType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1548 @Description(shortDefinition = "Level of confidence interval", formalDefinition = "Use 95 for a 95% confidence interval.") 1549 protected DecimalType level; 1550 1551 /** 1552 * Lower bound of confidence interval. 1553 */ 1554 @Child(name = "from", type = { DecimalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1555 @Description(shortDefinition = "Lower bound", formalDefinition = "Lower bound of confidence interval.") 1556 protected DecimalType from; 1557 1558 /** 1559 * Upper bound of confidence interval. 1560 */ 1561 @Child(name = "to", type = { DecimalType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1562 @Description(shortDefinition = "Upper bound", formalDefinition = "Upper bound of confidence interval.") 1563 protected DecimalType to; 1564 1565 private static final long serialVersionUID = -110178057L; 1566 1567 /** 1568 * Constructor 1569 */ 1570 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent() { 1571 super(); 1572 } 1573 1574 /** 1575 * @return {@link #type} (Examples include confidence interval and interquartile 1576 * range.) 1577 */ 1578 public CodeableConcept getType() { 1579 if (this.type == null) 1580 if (Configuration.errorOnAutoCreate()) 1581 throw new Error( 1582 "Attempt to auto-create EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent.type"); 1583 else if (Configuration.doAutoCreate()) 1584 this.type = new CodeableConcept(); // cc 1585 return this.type; 1586 } 1587 1588 public boolean hasType() { 1589 return this.type != null && !this.type.isEmpty(); 1590 } 1591 1592 /** 1593 * @param value {@link #type} (Examples include confidence interval and 1594 * interquartile range.) 1595 */ 1596 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent setType(CodeableConcept value) { 1597 this.type = value; 1598 return this; 1599 } 1600 1601 /** 1602 * @return {@link #level} (Use 95 for a 95% confidence interval.). This is the 1603 * underlying object with id, value and extensions. The accessor 1604 * "getLevel" gives direct access to the value 1605 */ 1606 public DecimalType getLevelElement() { 1607 if (this.level == null) 1608 if (Configuration.errorOnAutoCreate()) 1609 throw new Error( 1610 "Attempt to auto-create EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent.level"); 1611 else if (Configuration.doAutoCreate()) 1612 this.level = new DecimalType(); // bb 1613 return this.level; 1614 } 1615 1616 public boolean hasLevelElement() { 1617 return this.level != null && !this.level.isEmpty(); 1618 } 1619 1620 public boolean hasLevel() { 1621 return this.level != null && !this.level.isEmpty(); 1622 } 1623 1624 /** 1625 * @param value {@link #level} (Use 95 for a 95% confidence interval.). This is 1626 * the underlying object with id, value and extensions. The 1627 * accessor "getLevel" gives direct access to the value 1628 */ 1629 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent setLevelElement(DecimalType value) { 1630 this.level = value; 1631 return this; 1632 } 1633 1634 /** 1635 * @return Use 95 for a 95% confidence interval. 1636 */ 1637 public BigDecimal getLevel() { 1638 return this.level == null ? null : this.level.getValue(); 1639 } 1640 1641 /** 1642 * @param value Use 95 for a 95% confidence interval. 1643 */ 1644 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent setLevel(BigDecimal value) { 1645 if (value == null) 1646 this.level = null; 1647 else { 1648 if (this.level == null) 1649 this.level = new DecimalType(); 1650 this.level.setValue(value); 1651 } 1652 return this; 1653 } 1654 1655 /** 1656 * @param value Use 95 for a 95% confidence interval. 1657 */ 1658 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent setLevel(long value) { 1659 this.level = new DecimalType(); 1660 this.level.setValue(value); 1661 return this; 1662 } 1663 1664 /** 1665 * @param value Use 95 for a 95% confidence interval. 1666 */ 1667 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent setLevel(double value) { 1668 this.level = new DecimalType(); 1669 this.level.setValue(value); 1670 return this; 1671 } 1672 1673 /** 1674 * @return {@link #from} (Lower bound of confidence interval.). This is the 1675 * underlying object with id, value and extensions. The accessor 1676 * "getFrom" gives direct access to the value 1677 */ 1678 public DecimalType getFromElement() { 1679 if (this.from == null) 1680 if (Configuration.errorOnAutoCreate()) 1681 throw new Error( 1682 "Attempt to auto-create EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent.from"); 1683 else if (Configuration.doAutoCreate()) 1684 this.from = new DecimalType(); // bb 1685 return this.from; 1686 } 1687 1688 public boolean hasFromElement() { 1689 return this.from != null && !this.from.isEmpty(); 1690 } 1691 1692 public boolean hasFrom() { 1693 return this.from != null && !this.from.isEmpty(); 1694 } 1695 1696 /** 1697 * @param value {@link #from} (Lower bound of confidence interval.). This is the 1698 * underlying object with id, value and extensions. The accessor 1699 * "getFrom" gives direct access to the value 1700 */ 1701 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent setFromElement(DecimalType value) { 1702 this.from = value; 1703 return this; 1704 } 1705 1706 /** 1707 * @return Lower bound of confidence interval. 1708 */ 1709 public BigDecimal getFrom() { 1710 return this.from == null ? null : this.from.getValue(); 1711 } 1712 1713 /** 1714 * @param value Lower bound of confidence interval. 1715 */ 1716 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent setFrom(BigDecimal value) { 1717 if (value == null) 1718 this.from = null; 1719 else { 1720 if (this.from == null) 1721 this.from = new DecimalType(); 1722 this.from.setValue(value); 1723 } 1724 return this; 1725 } 1726 1727 /** 1728 * @param value Lower bound of confidence interval. 1729 */ 1730 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent setFrom(long value) { 1731 this.from = new DecimalType(); 1732 this.from.setValue(value); 1733 return this; 1734 } 1735 1736 /** 1737 * @param value Lower bound of confidence interval. 1738 */ 1739 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent setFrom(double value) { 1740 this.from = new DecimalType(); 1741 this.from.setValue(value); 1742 return this; 1743 } 1744 1745 /** 1746 * @return {@link #to} (Upper bound of confidence interval.). This is the 1747 * underlying object with id, value and extensions. The accessor "getTo" 1748 * gives direct access to the value 1749 */ 1750 public DecimalType getToElement() { 1751 if (this.to == null) 1752 if (Configuration.errorOnAutoCreate()) 1753 throw new Error("Attempt to auto-create EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent.to"); 1754 else if (Configuration.doAutoCreate()) 1755 this.to = new DecimalType(); // bb 1756 return this.to; 1757 } 1758 1759 public boolean hasToElement() { 1760 return this.to != null && !this.to.isEmpty(); 1761 } 1762 1763 public boolean hasTo() { 1764 return this.to != null && !this.to.isEmpty(); 1765 } 1766 1767 /** 1768 * @param value {@link #to} (Upper bound of confidence interval.). This is the 1769 * underlying object with id, value and extensions. The accessor 1770 * "getTo" gives direct access to the value 1771 */ 1772 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent setToElement(DecimalType value) { 1773 this.to = value; 1774 return this; 1775 } 1776 1777 /** 1778 * @return Upper bound of confidence interval. 1779 */ 1780 public BigDecimal getTo() { 1781 return this.to == null ? null : this.to.getValue(); 1782 } 1783 1784 /** 1785 * @param value Upper bound of confidence interval. 1786 */ 1787 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent setTo(BigDecimal value) { 1788 if (value == null) 1789 this.to = null; 1790 else { 1791 if (this.to == null) 1792 this.to = new DecimalType(); 1793 this.to.setValue(value); 1794 } 1795 return this; 1796 } 1797 1798 /** 1799 * @param value Upper bound of confidence interval. 1800 */ 1801 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent setTo(long value) { 1802 this.to = new DecimalType(); 1803 this.to.setValue(value); 1804 return this; 1805 } 1806 1807 /** 1808 * @param value Upper bound of confidence interval. 1809 */ 1810 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent setTo(double value) { 1811 this.to = new DecimalType(); 1812 this.to.setValue(value); 1813 return this; 1814 } 1815 1816 protected void listChildren(List<Property> children) { 1817 super.listChildren(children); 1818 children.add(new Property("type", "CodeableConcept", 1819 "Examples include confidence interval and interquartile range.", 0, 1, type)); 1820 children.add(new Property("level", "decimal", "Use 95 for a 95% confidence interval.", 0, 1, level)); 1821 children.add(new Property("from", "decimal", "Lower bound of confidence interval.", 0, 1, from)); 1822 children.add(new Property("to", "decimal", "Upper bound of confidence interval.", 0, 1, to)); 1823 } 1824 1825 @Override 1826 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1827 switch (_hash) { 1828 case 3575610: 1829 /* type */ return new Property("type", "CodeableConcept", 1830 "Examples include confidence interval and interquartile range.", 0, 1, type); 1831 case 102865796: 1832 /* level */ return new Property("level", "decimal", "Use 95 for a 95% confidence interval.", 0, 1, level); 1833 case 3151786: 1834 /* from */ return new Property("from", "decimal", "Lower bound of confidence interval.", 0, 1, from); 1835 case 3707: 1836 /* to */ return new Property("to", "decimal", "Upper bound of confidence interval.", 0, 1, to); 1837 default: 1838 return super.getNamedProperty(_hash, _name, _checkValid); 1839 } 1840 1841 } 1842 1843 @Override 1844 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1845 switch (hash) { 1846 case 3575610: 1847 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1848 case 102865796: 1849 /* level */ return this.level == null ? new Base[0] : new Base[] { this.level }; // DecimalType 1850 case 3151786: 1851 /* from */ return this.from == null ? new Base[0] : new Base[] { this.from }; // DecimalType 1852 case 3707: 1853 /* to */ return this.to == null ? new Base[0] : new Base[] { this.to }; // DecimalType 1854 default: 1855 return super.getProperty(hash, name, checkValid); 1856 } 1857 1858 } 1859 1860 @Override 1861 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1862 switch (hash) { 1863 case 3575610: // type 1864 this.type = castToCodeableConcept(value); // CodeableConcept 1865 return value; 1866 case 102865796: // level 1867 this.level = castToDecimal(value); // DecimalType 1868 return value; 1869 case 3151786: // from 1870 this.from = castToDecimal(value); // DecimalType 1871 return value; 1872 case 3707: // to 1873 this.to = castToDecimal(value); // DecimalType 1874 return value; 1875 default: 1876 return super.setProperty(hash, name, value); 1877 } 1878 1879 } 1880 1881 @Override 1882 public Base setProperty(String name, Base value) throws FHIRException { 1883 if (name.equals("type")) { 1884 this.type = castToCodeableConcept(value); // CodeableConcept 1885 } else if (name.equals("level")) { 1886 this.level = castToDecimal(value); // DecimalType 1887 } else if (name.equals("from")) { 1888 this.from = castToDecimal(value); // DecimalType 1889 } else if (name.equals("to")) { 1890 this.to = castToDecimal(value); // DecimalType 1891 } else 1892 return super.setProperty(name, value); 1893 return value; 1894 } 1895 1896 @Override 1897 public Base makeProperty(int hash, String name) throws FHIRException { 1898 switch (hash) { 1899 case 3575610: 1900 return getType(); 1901 case 102865796: 1902 return getLevelElement(); 1903 case 3151786: 1904 return getFromElement(); 1905 case 3707: 1906 return getToElement(); 1907 default: 1908 return super.makeProperty(hash, name); 1909 } 1910 1911 } 1912 1913 @Override 1914 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1915 switch (hash) { 1916 case 3575610: 1917 /* type */ return new String[] { "CodeableConcept" }; 1918 case 102865796: 1919 /* level */ return new String[] { "decimal" }; 1920 case 3151786: 1921 /* from */ return new String[] { "decimal" }; 1922 case 3707: 1923 /* to */ return new String[] { "decimal" }; 1924 default: 1925 return super.getTypesForProperty(hash, name); 1926 } 1927 1928 } 1929 1930 @Override 1931 public Base addChild(String name) throws FHIRException { 1932 if (name.equals("type")) { 1933 this.type = new CodeableConcept(); 1934 return this.type; 1935 } else if (name.equals("level")) { 1936 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.level"); 1937 } else if (name.equals("from")) { 1938 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.from"); 1939 } else if (name.equals("to")) { 1940 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.to"); 1941 } else 1942 return super.addChild(name); 1943 } 1944 1945 public EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent copy() { 1946 EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent dst = new EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent(); 1947 copyValues(dst); 1948 return dst; 1949 } 1950 1951 public void copyValues(EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent dst) { 1952 super.copyValues(dst); 1953 dst.type = type == null ? null : type.copy(); 1954 dst.level = level == null ? null : level.copy(); 1955 dst.from = from == null ? null : from.copy(); 1956 dst.to = to == null ? null : to.copy(); 1957 } 1958 1959 @Override 1960 public boolean equalsDeep(Base other_) { 1961 if (!super.equalsDeep(other_)) 1962 return false; 1963 if (!(other_ instanceof EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent)) 1964 return false; 1965 EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent o = (EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent) other_; 1966 return compareDeep(type, o.type, true) && compareDeep(level, o.level, true) && compareDeep(from, o.from, true) 1967 && compareDeep(to, o.to, true); 1968 } 1969 1970 @Override 1971 public boolean equalsShallow(Base other_) { 1972 if (!super.equalsShallow(other_)) 1973 return false; 1974 if (!(other_ instanceof EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent)) 1975 return false; 1976 EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent o = (EffectEvidenceSynthesisEffectEstimatePrecisionEstimateComponent) other_; 1977 return compareValues(level, o.level, true) && compareValues(from, o.from, true) && compareValues(to, o.to, true); 1978 } 1979 1980 public boolean isEmpty() { 1981 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, level, from, to); 1982 } 1983 1984 public String fhirType() { 1985 return "EffectEvidenceSynthesis.effectEstimate.precisionEstimate"; 1986 1987 } 1988 1989 } 1990 1991 @Block() 1992 public static class EffectEvidenceSynthesisCertaintyComponent extends BackboneElement 1993 implements IBaseBackboneElement { 1994 /** 1995 * A rating of the certainty of the effect estimate. 1996 */ 1997 @Child(name = "rating", type = { 1998 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1999 @Description(shortDefinition = "Certainty rating", formalDefinition = "A rating of the certainty of the effect estimate.") 2000 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/evidence-quality") 2001 protected List<CodeableConcept> rating; 2002 2003 /** 2004 * A human-readable string to clarify or explain concepts about the resource. 2005 */ 2006 @Child(name = "note", type = { 2007 Annotation.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2008 @Description(shortDefinition = "Used for footnotes or explanatory notes", formalDefinition = "A human-readable string to clarify or explain concepts about the resource.") 2009 protected List<Annotation> note; 2010 2011 /** 2012 * A description of a component of the overall certainty. 2013 */ 2014 @Child(name = "certaintySubcomponent", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2015 @Description(shortDefinition = "A component that contributes to the overall certainty", formalDefinition = "A description of a component of the overall certainty.") 2016 protected List<EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent> certaintySubcomponent; 2017 2018 private static final long serialVersionUID = 647101417L; 2019 2020 /** 2021 * Constructor 2022 */ 2023 public EffectEvidenceSynthesisCertaintyComponent() { 2024 super(); 2025 } 2026 2027 /** 2028 * @return {@link #rating} (A rating of the certainty of the effect estimate.) 2029 */ 2030 public List<CodeableConcept> getRating() { 2031 if (this.rating == null) 2032 this.rating = new ArrayList<CodeableConcept>(); 2033 return this.rating; 2034 } 2035 2036 /** 2037 * @return Returns a reference to <code>this</code> for easy method chaining 2038 */ 2039 public EffectEvidenceSynthesisCertaintyComponent setRating(List<CodeableConcept> theRating) { 2040 this.rating = theRating; 2041 return this; 2042 } 2043 2044 public boolean hasRating() { 2045 if (this.rating == null) 2046 return false; 2047 for (CodeableConcept item : this.rating) 2048 if (!item.isEmpty()) 2049 return true; 2050 return false; 2051 } 2052 2053 public CodeableConcept addRating() { // 3 2054 CodeableConcept t = new CodeableConcept(); 2055 if (this.rating == null) 2056 this.rating = new ArrayList<CodeableConcept>(); 2057 this.rating.add(t); 2058 return t; 2059 } 2060 2061 public EffectEvidenceSynthesisCertaintyComponent addRating(CodeableConcept t) { // 3 2062 if (t == null) 2063 return this; 2064 if (this.rating == null) 2065 this.rating = new ArrayList<CodeableConcept>(); 2066 this.rating.add(t); 2067 return this; 2068 } 2069 2070 /** 2071 * @return The first repetition of repeating field {@link #rating}, creating it 2072 * if it does not already exist 2073 */ 2074 public CodeableConcept getRatingFirstRep() { 2075 if (getRating().isEmpty()) { 2076 addRating(); 2077 } 2078 return getRating().get(0); 2079 } 2080 2081 /** 2082 * @return {@link #note} (A human-readable string to clarify or explain concepts 2083 * about the resource.) 2084 */ 2085 public List<Annotation> getNote() { 2086 if (this.note == null) 2087 this.note = new ArrayList<Annotation>(); 2088 return this.note; 2089 } 2090 2091 /** 2092 * @return Returns a reference to <code>this</code> for easy method chaining 2093 */ 2094 public EffectEvidenceSynthesisCertaintyComponent setNote(List<Annotation> theNote) { 2095 this.note = theNote; 2096 return this; 2097 } 2098 2099 public boolean hasNote() { 2100 if (this.note == null) 2101 return false; 2102 for (Annotation item : this.note) 2103 if (!item.isEmpty()) 2104 return true; 2105 return false; 2106 } 2107 2108 public Annotation addNote() { // 3 2109 Annotation t = new Annotation(); 2110 if (this.note == null) 2111 this.note = new ArrayList<Annotation>(); 2112 this.note.add(t); 2113 return t; 2114 } 2115 2116 public EffectEvidenceSynthesisCertaintyComponent addNote(Annotation t) { // 3 2117 if (t == null) 2118 return this; 2119 if (this.note == null) 2120 this.note = new ArrayList<Annotation>(); 2121 this.note.add(t); 2122 return this; 2123 } 2124 2125 /** 2126 * @return The first repetition of repeating field {@link #note}, creating it if 2127 * it does not already exist 2128 */ 2129 public Annotation getNoteFirstRep() { 2130 if (getNote().isEmpty()) { 2131 addNote(); 2132 } 2133 return getNote().get(0); 2134 } 2135 2136 /** 2137 * @return {@link #certaintySubcomponent} (A description of a component of the 2138 * overall certainty.) 2139 */ 2140 public List<EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent> getCertaintySubcomponent() { 2141 if (this.certaintySubcomponent == null) 2142 this.certaintySubcomponent = new ArrayList<EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent>(); 2143 return this.certaintySubcomponent; 2144 } 2145 2146 /** 2147 * @return Returns a reference to <code>this</code> for easy method chaining 2148 */ 2149 public EffectEvidenceSynthesisCertaintyComponent setCertaintySubcomponent( 2150 List<EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent> theCertaintySubcomponent) { 2151 this.certaintySubcomponent = theCertaintySubcomponent; 2152 return this; 2153 } 2154 2155 public boolean hasCertaintySubcomponent() { 2156 if (this.certaintySubcomponent == null) 2157 return false; 2158 for (EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent item : this.certaintySubcomponent) 2159 if (!item.isEmpty()) 2160 return true; 2161 return false; 2162 } 2163 2164 public EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent addCertaintySubcomponent() { // 3 2165 EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent t = new EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent(); 2166 if (this.certaintySubcomponent == null) 2167 this.certaintySubcomponent = new ArrayList<EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent>(); 2168 this.certaintySubcomponent.add(t); 2169 return t; 2170 } 2171 2172 public EffectEvidenceSynthesisCertaintyComponent addCertaintySubcomponent( 2173 EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent t) { // 3 2174 if (t == null) 2175 return this; 2176 if (this.certaintySubcomponent == null) 2177 this.certaintySubcomponent = new ArrayList<EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent>(); 2178 this.certaintySubcomponent.add(t); 2179 return this; 2180 } 2181 2182 /** 2183 * @return The first repetition of repeating field 2184 * {@link #certaintySubcomponent}, creating it if it does not already 2185 * exist 2186 */ 2187 public EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent getCertaintySubcomponentFirstRep() { 2188 if (getCertaintySubcomponent().isEmpty()) { 2189 addCertaintySubcomponent(); 2190 } 2191 return getCertaintySubcomponent().get(0); 2192 } 2193 2194 protected void listChildren(List<Property> children) { 2195 super.listChildren(children); 2196 children.add(new Property("rating", "CodeableConcept", "A rating of the certainty of the effect estimate.", 0, 2197 java.lang.Integer.MAX_VALUE, rating)); 2198 children.add(new Property("note", "Annotation", 2199 "A human-readable string to clarify or explain concepts about the resource.", 0, java.lang.Integer.MAX_VALUE, 2200 note)); 2201 children.add(new Property("certaintySubcomponent", "", "A description of a component of the overall certainty.", 2202 0, java.lang.Integer.MAX_VALUE, certaintySubcomponent)); 2203 } 2204 2205 @Override 2206 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2207 switch (_hash) { 2208 case -938102371: 2209 /* rating */ return new Property("rating", "CodeableConcept", 2210 "A rating of the certainty of the effect estimate.", 0, java.lang.Integer.MAX_VALUE, rating); 2211 case 3387378: 2212 /* note */ return new Property("note", "Annotation", 2213 "A human-readable string to clarify or explain concepts about the resource.", 0, 2214 java.lang.Integer.MAX_VALUE, note); 2215 case 1806398212: 2216 /* certaintySubcomponent */ return new Property("certaintySubcomponent", "", 2217 "A description of a component of the overall certainty.", 0, java.lang.Integer.MAX_VALUE, 2218 certaintySubcomponent); 2219 default: 2220 return super.getNamedProperty(_hash, _name, _checkValid); 2221 } 2222 2223 } 2224 2225 @Override 2226 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2227 switch (hash) { 2228 case -938102371: 2229 /* rating */ return this.rating == null ? new Base[0] : this.rating.toArray(new Base[this.rating.size()]); // CodeableConcept 2230 case 3387378: 2231 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2232 case 1806398212: 2233 /* certaintySubcomponent */ return this.certaintySubcomponent == null ? new Base[0] 2234 : this.certaintySubcomponent.toArray(new Base[this.certaintySubcomponent.size()]); // EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent 2235 default: 2236 return super.getProperty(hash, name, checkValid); 2237 } 2238 2239 } 2240 2241 @Override 2242 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2243 switch (hash) { 2244 case -938102371: // rating 2245 this.getRating().add(castToCodeableConcept(value)); // CodeableConcept 2246 return value; 2247 case 3387378: // note 2248 this.getNote().add(castToAnnotation(value)); // Annotation 2249 return value; 2250 case 1806398212: // certaintySubcomponent 2251 this.getCertaintySubcomponent().add((EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent) value); // EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent 2252 return value; 2253 default: 2254 return super.setProperty(hash, name, value); 2255 } 2256 2257 } 2258 2259 @Override 2260 public Base setProperty(String name, Base value) throws FHIRException { 2261 if (name.equals("rating")) { 2262 this.getRating().add(castToCodeableConcept(value)); 2263 } else if (name.equals("note")) { 2264 this.getNote().add(castToAnnotation(value)); 2265 } else if (name.equals("certaintySubcomponent")) { 2266 this.getCertaintySubcomponent().add((EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent) value); 2267 } else 2268 return super.setProperty(name, value); 2269 return value; 2270 } 2271 2272 @Override 2273 public Base makeProperty(int hash, String name) throws FHIRException { 2274 switch (hash) { 2275 case -938102371: 2276 return addRating(); 2277 case 3387378: 2278 return addNote(); 2279 case 1806398212: 2280 return addCertaintySubcomponent(); 2281 default: 2282 return super.makeProperty(hash, name); 2283 } 2284 2285 } 2286 2287 @Override 2288 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2289 switch (hash) { 2290 case -938102371: 2291 /* rating */ return new String[] { "CodeableConcept" }; 2292 case 3387378: 2293 /* note */ return new String[] { "Annotation" }; 2294 case 1806398212: 2295 /* certaintySubcomponent */ return new String[] {}; 2296 default: 2297 return super.getTypesForProperty(hash, name); 2298 } 2299 2300 } 2301 2302 @Override 2303 public Base addChild(String name) throws FHIRException { 2304 if (name.equals("rating")) { 2305 return addRating(); 2306 } else if (name.equals("note")) { 2307 return addNote(); 2308 } else if (name.equals("certaintySubcomponent")) { 2309 return addCertaintySubcomponent(); 2310 } else 2311 return super.addChild(name); 2312 } 2313 2314 public EffectEvidenceSynthesisCertaintyComponent copy() { 2315 EffectEvidenceSynthesisCertaintyComponent dst = new EffectEvidenceSynthesisCertaintyComponent(); 2316 copyValues(dst); 2317 return dst; 2318 } 2319 2320 public void copyValues(EffectEvidenceSynthesisCertaintyComponent dst) { 2321 super.copyValues(dst); 2322 if (rating != null) { 2323 dst.rating = new ArrayList<CodeableConcept>(); 2324 for (CodeableConcept i : rating) 2325 dst.rating.add(i.copy()); 2326 } 2327 ; 2328 if (note != null) { 2329 dst.note = new ArrayList<Annotation>(); 2330 for (Annotation i : note) 2331 dst.note.add(i.copy()); 2332 } 2333 ; 2334 if (certaintySubcomponent != null) { 2335 dst.certaintySubcomponent = new ArrayList<EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent>(); 2336 for (EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent i : certaintySubcomponent) 2337 dst.certaintySubcomponent.add(i.copy()); 2338 } 2339 ; 2340 } 2341 2342 @Override 2343 public boolean equalsDeep(Base other_) { 2344 if (!super.equalsDeep(other_)) 2345 return false; 2346 if (!(other_ instanceof EffectEvidenceSynthesisCertaintyComponent)) 2347 return false; 2348 EffectEvidenceSynthesisCertaintyComponent o = (EffectEvidenceSynthesisCertaintyComponent) other_; 2349 return compareDeep(rating, o.rating, true) && compareDeep(note, o.note, true) 2350 && compareDeep(certaintySubcomponent, o.certaintySubcomponent, true); 2351 } 2352 2353 @Override 2354 public boolean equalsShallow(Base other_) { 2355 if (!super.equalsShallow(other_)) 2356 return false; 2357 if (!(other_ instanceof EffectEvidenceSynthesisCertaintyComponent)) 2358 return false; 2359 EffectEvidenceSynthesisCertaintyComponent o = (EffectEvidenceSynthesisCertaintyComponent) other_; 2360 return true; 2361 } 2362 2363 public boolean isEmpty() { 2364 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(rating, note, certaintySubcomponent); 2365 } 2366 2367 public String fhirType() { 2368 return "EffectEvidenceSynthesis.certainty"; 2369 2370 } 2371 2372 } 2373 2374 @Block() 2375 public static class EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent extends BackboneElement 2376 implements IBaseBackboneElement { 2377 /** 2378 * Type of subcomponent of certainty rating. 2379 */ 2380 @Child(name = "type", type = { 2381 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2382 @Description(shortDefinition = "Type of subcomponent of certainty rating", formalDefinition = "Type of subcomponent of certainty rating.") 2383 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/certainty-subcomponent-type") 2384 protected CodeableConcept type; 2385 2386 /** 2387 * A rating of a subcomponent of rating certainty. 2388 */ 2389 @Child(name = "rating", type = { 2390 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2391 @Description(shortDefinition = "Subcomponent certainty rating", formalDefinition = "A rating of a subcomponent of rating certainty.") 2392 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/certainty-subcomponent-rating") 2393 protected List<CodeableConcept> rating; 2394 2395 /** 2396 * A human-readable string to clarify or explain concepts about the resource. 2397 */ 2398 @Child(name = "note", type = { 2399 Annotation.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2400 @Description(shortDefinition = "Used for footnotes or explanatory notes", formalDefinition = "A human-readable string to clarify or explain concepts about the resource.") 2401 protected List<Annotation> note; 2402 2403 private static final long serialVersionUID = -411994816L; 2404 2405 /** 2406 * Constructor 2407 */ 2408 public EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent() { 2409 super(); 2410 } 2411 2412 /** 2413 * @return {@link #type} (Type of subcomponent of certainty rating.) 2414 */ 2415 public CodeableConcept getType() { 2416 if (this.type == null) 2417 if (Configuration.errorOnAutoCreate()) 2418 throw new Error("Attempt to auto-create EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent.type"); 2419 else if (Configuration.doAutoCreate()) 2420 this.type = new CodeableConcept(); // cc 2421 return this.type; 2422 } 2423 2424 public boolean hasType() { 2425 return this.type != null && !this.type.isEmpty(); 2426 } 2427 2428 /** 2429 * @param value {@link #type} (Type of subcomponent of certainty rating.) 2430 */ 2431 public EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent setType(CodeableConcept value) { 2432 this.type = value; 2433 return this; 2434 } 2435 2436 /** 2437 * @return {@link #rating} (A rating of a subcomponent of rating certainty.) 2438 */ 2439 public List<CodeableConcept> getRating() { 2440 if (this.rating == null) 2441 this.rating = new ArrayList<CodeableConcept>(); 2442 return this.rating; 2443 } 2444 2445 /** 2446 * @return Returns a reference to <code>this</code> for easy method chaining 2447 */ 2448 public EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent setRating(List<CodeableConcept> theRating) { 2449 this.rating = theRating; 2450 return this; 2451 } 2452 2453 public boolean hasRating() { 2454 if (this.rating == null) 2455 return false; 2456 for (CodeableConcept item : this.rating) 2457 if (!item.isEmpty()) 2458 return true; 2459 return false; 2460 } 2461 2462 public CodeableConcept addRating() { // 3 2463 CodeableConcept t = new CodeableConcept(); 2464 if (this.rating == null) 2465 this.rating = new ArrayList<CodeableConcept>(); 2466 this.rating.add(t); 2467 return t; 2468 } 2469 2470 public EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent addRating(CodeableConcept t) { // 3 2471 if (t == null) 2472 return this; 2473 if (this.rating == null) 2474 this.rating = new ArrayList<CodeableConcept>(); 2475 this.rating.add(t); 2476 return this; 2477 } 2478 2479 /** 2480 * @return The first repetition of repeating field {@link #rating}, creating it 2481 * if it does not already exist 2482 */ 2483 public CodeableConcept getRatingFirstRep() { 2484 if (getRating().isEmpty()) { 2485 addRating(); 2486 } 2487 return getRating().get(0); 2488 } 2489 2490 /** 2491 * @return {@link #note} (A human-readable string to clarify or explain concepts 2492 * about the resource.) 2493 */ 2494 public List<Annotation> getNote() { 2495 if (this.note == null) 2496 this.note = new ArrayList<Annotation>(); 2497 return this.note; 2498 } 2499 2500 /** 2501 * @return Returns a reference to <code>this</code> for easy method chaining 2502 */ 2503 public EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent setNote(List<Annotation> theNote) { 2504 this.note = theNote; 2505 return this; 2506 } 2507 2508 public boolean hasNote() { 2509 if (this.note == null) 2510 return false; 2511 for (Annotation item : this.note) 2512 if (!item.isEmpty()) 2513 return true; 2514 return false; 2515 } 2516 2517 public Annotation addNote() { // 3 2518 Annotation t = new Annotation(); 2519 if (this.note == null) 2520 this.note = new ArrayList<Annotation>(); 2521 this.note.add(t); 2522 return t; 2523 } 2524 2525 public EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent addNote(Annotation t) { // 3 2526 if (t == null) 2527 return this; 2528 if (this.note == null) 2529 this.note = new ArrayList<Annotation>(); 2530 this.note.add(t); 2531 return this; 2532 } 2533 2534 /** 2535 * @return The first repetition of repeating field {@link #note}, creating it if 2536 * it does not already exist 2537 */ 2538 public Annotation getNoteFirstRep() { 2539 if (getNote().isEmpty()) { 2540 addNote(); 2541 } 2542 return getNote().get(0); 2543 } 2544 2545 protected void listChildren(List<Property> children) { 2546 super.listChildren(children); 2547 children.add(new Property("type", "CodeableConcept", "Type of subcomponent of certainty rating.", 0, 1, type)); 2548 children.add(new Property("rating", "CodeableConcept", "A rating of a subcomponent of rating certainty.", 0, 2549 java.lang.Integer.MAX_VALUE, rating)); 2550 children.add(new Property("note", "Annotation", 2551 "A human-readable string to clarify or explain concepts about the resource.", 0, java.lang.Integer.MAX_VALUE, 2552 note)); 2553 } 2554 2555 @Override 2556 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2557 switch (_hash) { 2558 case 3575610: 2559 /* type */ return new Property("type", "CodeableConcept", "Type of subcomponent of certainty rating.", 0, 1, 2560 type); 2561 case -938102371: 2562 /* rating */ return new Property("rating", "CodeableConcept", "A rating of a subcomponent of rating certainty.", 2563 0, java.lang.Integer.MAX_VALUE, rating); 2564 case 3387378: 2565 /* note */ return new Property("note", "Annotation", 2566 "A human-readable string to clarify or explain concepts about the resource.", 0, 2567 java.lang.Integer.MAX_VALUE, note); 2568 default: 2569 return super.getNamedProperty(_hash, _name, _checkValid); 2570 } 2571 2572 } 2573 2574 @Override 2575 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2576 switch (hash) { 2577 case 3575610: 2578 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2579 case -938102371: 2580 /* rating */ return this.rating == null ? new Base[0] : this.rating.toArray(new Base[this.rating.size()]); // CodeableConcept 2581 case 3387378: 2582 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2583 default: 2584 return super.getProperty(hash, name, checkValid); 2585 } 2586 2587 } 2588 2589 @Override 2590 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2591 switch (hash) { 2592 case 3575610: // type 2593 this.type = castToCodeableConcept(value); // CodeableConcept 2594 return value; 2595 case -938102371: // rating 2596 this.getRating().add(castToCodeableConcept(value)); // CodeableConcept 2597 return value; 2598 case 3387378: // note 2599 this.getNote().add(castToAnnotation(value)); // Annotation 2600 return value; 2601 default: 2602 return super.setProperty(hash, name, value); 2603 } 2604 2605 } 2606 2607 @Override 2608 public Base setProperty(String name, Base value) throws FHIRException { 2609 if (name.equals("type")) { 2610 this.type = castToCodeableConcept(value); // CodeableConcept 2611 } else if (name.equals("rating")) { 2612 this.getRating().add(castToCodeableConcept(value)); 2613 } else if (name.equals("note")) { 2614 this.getNote().add(castToAnnotation(value)); 2615 } else 2616 return super.setProperty(name, value); 2617 return value; 2618 } 2619 2620 @Override 2621 public Base makeProperty(int hash, String name) throws FHIRException { 2622 switch (hash) { 2623 case 3575610: 2624 return getType(); 2625 case -938102371: 2626 return addRating(); 2627 case 3387378: 2628 return addNote(); 2629 default: 2630 return super.makeProperty(hash, name); 2631 } 2632 2633 } 2634 2635 @Override 2636 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2637 switch (hash) { 2638 case 3575610: 2639 /* type */ return new String[] { "CodeableConcept" }; 2640 case -938102371: 2641 /* rating */ return new String[] { "CodeableConcept" }; 2642 case 3387378: 2643 /* note */ return new String[] { "Annotation" }; 2644 default: 2645 return super.getTypesForProperty(hash, name); 2646 } 2647 2648 } 2649 2650 @Override 2651 public Base addChild(String name) throws FHIRException { 2652 if (name.equals("type")) { 2653 this.type = new CodeableConcept(); 2654 return this.type; 2655 } else if (name.equals("rating")) { 2656 return addRating(); 2657 } else if (name.equals("note")) { 2658 return addNote(); 2659 } else 2660 return super.addChild(name); 2661 } 2662 2663 public EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent copy() { 2664 EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent dst = new EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent(); 2665 copyValues(dst); 2666 return dst; 2667 } 2668 2669 public void copyValues(EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent dst) { 2670 super.copyValues(dst); 2671 dst.type = type == null ? null : type.copy(); 2672 if (rating != null) { 2673 dst.rating = new ArrayList<CodeableConcept>(); 2674 for (CodeableConcept i : rating) 2675 dst.rating.add(i.copy()); 2676 } 2677 ; 2678 if (note != null) { 2679 dst.note = new ArrayList<Annotation>(); 2680 for (Annotation i : note) 2681 dst.note.add(i.copy()); 2682 } 2683 ; 2684 } 2685 2686 @Override 2687 public boolean equalsDeep(Base other_) { 2688 if (!super.equalsDeep(other_)) 2689 return false; 2690 if (!(other_ instanceof EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent)) 2691 return false; 2692 EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent o = (EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent) other_; 2693 return compareDeep(type, o.type, true) && compareDeep(rating, o.rating, true) && compareDeep(note, o.note, true); 2694 } 2695 2696 @Override 2697 public boolean equalsShallow(Base other_) { 2698 if (!super.equalsShallow(other_)) 2699 return false; 2700 if (!(other_ instanceof EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent)) 2701 return false; 2702 EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent o = (EffectEvidenceSynthesisCertaintyCertaintySubcomponentComponent) other_; 2703 return true; 2704 } 2705 2706 public boolean isEmpty() { 2707 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, rating, note); 2708 } 2709 2710 public String fhirType() { 2711 return "EffectEvidenceSynthesis.certainty.certaintySubcomponent"; 2712 2713 } 2714 2715 } 2716 2717 /** 2718 * A formal identifier that is used to identify this effect evidence synthesis 2719 * when it is represented in other formats, or referenced in a specification, 2720 * model, design or an instance. 2721 */ 2722 @Child(name = "identifier", type = { 2723 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2724 @Description(shortDefinition = "Additional identifier for the effect evidence synthesis", formalDefinition = "A formal identifier that is used to identify this effect evidence synthesis when it is represented in other formats, or referenced in a specification, model, design or an instance.") 2725 protected List<Identifier> identifier; 2726 2727 /** 2728 * A human-readable string to clarify or explain concepts about the resource. 2729 */ 2730 @Child(name = "note", type = { 2731 Annotation.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2732 @Description(shortDefinition = "Used for footnotes or explanatory notes", formalDefinition = "A human-readable string to clarify or explain concepts about the resource.") 2733 protected List<Annotation> note; 2734 2735 /** 2736 * A copyright statement relating to the effect evidence synthesis and/or its 2737 * contents. Copyright statements are generally legal restrictions on the use 2738 * and publishing of the effect evidence synthesis. 2739 */ 2740 @Child(name = "copyright", type = { 2741 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2742 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the effect evidence synthesis and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the effect evidence synthesis.") 2743 protected MarkdownType copyright; 2744 2745 /** 2746 * The date on which the resource content was approved by the publisher. 2747 * Approval happens once when the content is officially approved for usage. 2748 */ 2749 @Child(name = "approvalDate", type = { 2750 DateType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2751 @Description(shortDefinition = "When the effect evidence synthesis was approved by publisher", formalDefinition = "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.") 2752 protected DateType approvalDate; 2753 2754 /** 2755 * The date on which the resource content was last reviewed. Review happens 2756 * periodically after approval but does not change the original approval date. 2757 */ 2758 @Child(name = "lastReviewDate", type = { 2759 DateType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2760 @Description(shortDefinition = "When the effect evidence synthesis was last reviewed", formalDefinition = "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.") 2761 protected DateType lastReviewDate; 2762 2763 /** 2764 * The period during which the effect evidence synthesis content was or is 2765 * planned to be in active use. 2766 */ 2767 @Child(name = "effectivePeriod", type = { 2768 Period.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 2769 @Description(shortDefinition = "When the effect evidence synthesis is expected to be used", formalDefinition = "The period during which the effect evidence synthesis content was or is planned to be in active use.") 2770 protected Period effectivePeriod; 2771 2772 /** 2773 * Descriptive topics related to the content of the EffectEvidenceSynthesis. 2774 * Topics provide a high-level categorization grouping types of 2775 * EffectEvidenceSynthesiss that can be useful for filtering and searching. 2776 */ 2777 @Child(name = "topic", type = { 2778 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2779 @Description(shortDefinition = "The category of the EffectEvidenceSynthesis, such as Education, Treatment, Assessment, etc.", formalDefinition = "Descriptive topics related to the content of the EffectEvidenceSynthesis. Topics provide a high-level categorization grouping types of EffectEvidenceSynthesiss that can be useful for filtering and searching.") 2780 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/definition-topic") 2781 protected List<CodeableConcept> topic; 2782 2783 /** 2784 * An individiual or organization primarily involved in the creation and 2785 * maintenance of the content. 2786 */ 2787 @Child(name = "author", type = { 2788 ContactDetail.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2789 @Description(shortDefinition = "Who authored the content", formalDefinition = "An individiual or organization primarily involved in the creation and maintenance of the content.") 2790 protected List<ContactDetail> author; 2791 2792 /** 2793 * An individual or organization primarily responsible for internal coherence of 2794 * the content. 2795 */ 2796 @Child(name = "editor", type = { 2797 ContactDetail.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2798 @Description(shortDefinition = "Who edited the content", formalDefinition = "An individual or organization primarily responsible for internal coherence of the content.") 2799 protected List<ContactDetail> editor; 2800 2801 /** 2802 * An individual or organization primarily responsible for review of some aspect 2803 * of the content. 2804 */ 2805 @Child(name = "reviewer", type = { 2806 ContactDetail.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2807 @Description(shortDefinition = "Who reviewed the content", formalDefinition = "An individual or organization primarily responsible for review of some aspect of the content.") 2808 protected List<ContactDetail> reviewer; 2809 2810 /** 2811 * An individual or organization responsible for officially endorsing the 2812 * content for use in some setting. 2813 */ 2814 @Child(name = "endorser", type = { 2815 ContactDetail.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2816 @Description(shortDefinition = "Who endorsed the content", formalDefinition = "An individual or organization responsible for officially endorsing the content for use in some setting.") 2817 protected List<ContactDetail> endorser; 2818 2819 /** 2820 * Related artifacts such as additional documentation, justification, or 2821 * bibliographic references. 2822 */ 2823 @Child(name = "relatedArtifact", type = { 2824 RelatedArtifact.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2825 @Description(shortDefinition = "Additional documentation, citations, etc.", formalDefinition = "Related artifacts such as additional documentation, justification, or bibliographic references.") 2826 protected List<RelatedArtifact> relatedArtifact; 2827 2828 /** 2829 * Type of synthesis eg meta-analysis. 2830 */ 2831 @Child(name = "synthesisType", type = { 2832 CodeableConcept.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 2833 @Description(shortDefinition = "Type of synthesis", formalDefinition = "Type of synthesis eg meta-analysis.") 2834 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/synthesis-type") 2835 protected CodeableConcept synthesisType; 2836 2837 /** 2838 * Type of study eg randomized trial. 2839 */ 2840 @Child(name = "studyType", type = { 2841 CodeableConcept.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 2842 @Description(shortDefinition = "Type of study", formalDefinition = "Type of study eg randomized trial.") 2843 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/study-type") 2844 protected CodeableConcept studyType; 2845 2846 /** 2847 * A reference to a EvidenceVariable resource that defines the population for 2848 * the research. 2849 */ 2850 @Child(name = "population", type = { 2851 EvidenceVariable.class }, order = 14, min = 1, max = 1, modifier = false, summary = true) 2852 @Description(shortDefinition = "What population?", formalDefinition = "A reference to a EvidenceVariable resource that defines the population for the research.") 2853 protected Reference population; 2854 2855 /** 2856 * The actual object that is the target of the reference (A reference to a 2857 * EvidenceVariable resource that defines the population for the research.) 2858 */ 2859 protected EvidenceVariable populationTarget; 2860 2861 /** 2862 * A reference to a EvidenceVariable resource that defines the exposure for the 2863 * research. 2864 */ 2865 @Child(name = "exposure", type = { 2866 EvidenceVariable.class }, order = 15, min = 1, max = 1, modifier = false, summary = true) 2867 @Description(shortDefinition = "What exposure?", formalDefinition = "A reference to a EvidenceVariable resource that defines the exposure for the research.") 2868 protected Reference exposure; 2869 2870 /** 2871 * The actual object that is the target of the reference (A reference to a 2872 * EvidenceVariable resource that defines the exposure for the research.) 2873 */ 2874 protected EvidenceVariable exposureTarget; 2875 2876 /** 2877 * A reference to a EvidenceVariable resource that defines the comparison 2878 * exposure for the research. 2879 */ 2880 @Child(name = "exposureAlternative", type = { 2881 EvidenceVariable.class }, order = 16, min = 1, max = 1, modifier = false, summary = true) 2882 @Description(shortDefinition = "What comparison exposure?", formalDefinition = "A reference to a EvidenceVariable resource that defines the comparison exposure for the research.") 2883 protected Reference exposureAlternative; 2884 2885 /** 2886 * The actual object that is the target of the reference (A reference to a 2887 * EvidenceVariable resource that defines the comparison exposure for the 2888 * research.) 2889 */ 2890 protected EvidenceVariable exposureAlternativeTarget; 2891 2892 /** 2893 * A reference to a EvidenceVariable resomece that defines the outcome for the 2894 * research. 2895 */ 2896 @Child(name = "outcome", type = { 2897 EvidenceVariable.class }, order = 17, min = 1, max = 1, modifier = false, summary = true) 2898 @Description(shortDefinition = "What outcome?", formalDefinition = "A reference to a EvidenceVariable resomece that defines the outcome for the research.") 2899 protected Reference outcome; 2900 2901 /** 2902 * The actual object that is the target of the reference (A reference to a 2903 * EvidenceVariable resomece that defines the outcome for the research.) 2904 */ 2905 protected EvidenceVariable outcomeTarget; 2906 2907 /** 2908 * A description of the size of the sample involved in the synthesis. 2909 */ 2910 @Child(name = "sampleSize", type = {}, order = 18, min = 0, max = 1, modifier = false, summary = false) 2911 @Description(shortDefinition = "What sample size was involved?", formalDefinition = "A description of the size of the sample involved in the synthesis.") 2912 protected EffectEvidenceSynthesisSampleSizeComponent sampleSize; 2913 2914 /** 2915 * A description of the results for each exposure considered in the effect 2916 * estimate. 2917 */ 2918 @Child(name = "resultsByExposure", type = {}, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2919 @Description(shortDefinition = "What was the result per exposure?", formalDefinition = "A description of the results for each exposure considered in the effect estimate.") 2920 protected List<EffectEvidenceSynthesisResultsByExposureComponent> resultsByExposure; 2921 2922 /** 2923 * The estimated effect of the exposure variant. 2924 */ 2925 @Child(name = "effectEstimate", type = {}, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2926 @Description(shortDefinition = "What was the estimated effect", formalDefinition = "The estimated effect of the exposure variant.") 2927 protected List<EffectEvidenceSynthesisEffectEstimateComponent> effectEstimate; 2928 2929 /** 2930 * A description of the certainty of the effect estimate. 2931 */ 2932 @Child(name = "certainty", type = {}, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2933 @Description(shortDefinition = "How certain is the effect", formalDefinition = "A description of the certainty of the effect estimate.") 2934 protected List<EffectEvidenceSynthesisCertaintyComponent> certainty; 2935 2936 private static final long serialVersionUID = 23150467L; 2937 2938 /** 2939 * Constructor 2940 */ 2941 public EffectEvidenceSynthesis() { 2942 super(); 2943 } 2944 2945 /** 2946 * Constructor 2947 */ 2948 public EffectEvidenceSynthesis(Enumeration<PublicationStatus> status, Reference population, Reference exposure, 2949 Reference exposureAlternative, Reference outcome) { 2950 super(); 2951 this.status = status; 2952 this.population = population; 2953 this.exposure = exposure; 2954 this.exposureAlternative = exposureAlternative; 2955 this.outcome = outcome; 2956 } 2957 2958 /** 2959 * @return {@link #url} (An absolute URI that is used to identify this effect 2960 * evidence synthesis when it is referenced in a specification, model, 2961 * design or an instance; also called its canonical identifier. This 2962 * SHOULD be globally unique and SHOULD be a literal address at which at 2963 * which an authoritative instance of this effect evidence synthesis is 2964 * (or will be) published. This URL can be the target of a canonical 2965 * reference. It SHALL remain the same when the effect evidence 2966 * synthesis is stored on different servers.). This is the underlying 2967 * object with id, value and extensions. The accessor "getUrl" gives 2968 * direct access to the value 2969 */ 2970 public UriType getUrlElement() { 2971 if (this.url == null) 2972 if (Configuration.errorOnAutoCreate()) 2973 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.url"); 2974 else if (Configuration.doAutoCreate()) 2975 this.url = new UriType(); // bb 2976 return this.url; 2977 } 2978 2979 public boolean hasUrlElement() { 2980 return this.url != null && !this.url.isEmpty(); 2981 } 2982 2983 public boolean hasUrl() { 2984 return this.url != null && !this.url.isEmpty(); 2985 } 2986 2987 /** 2988 * @param value {@link #url} (An absolute URI that is used to identify this 2989 * effect evidence synthesis when it is referenced in a 2990 * specification, model, design or an instance; also called its 2991 * canonical identifier. This SHOULD be globally unique and SHOULD 2992 * be a literal address at which at which an authoritative instance 2993 * of this effect evidence synthesis is (or will be) published. 2994 * This URL can be the target of a canonical reference. It SHALL 2995 * remain the same when the effect evidence synthesis is stored on 2996 * different servers.). This is the underlying object with id, 2997 * value and extensions. The accessor "getUrl" gives direct access 2998 * to the value 2999 */ 3000 public EffectEvidenceSynthesis setUrlElement(UriType value) { 3001 this.url = value; 3002 return this; 3003 } 3004 3005 /** 3006 * @return An absolute URI that is used to identify this effect evidence 3007 * synthesis when it is referenced in a specification, model, design or 3008 * an instance; also called its canonical identifier. This SHOULD be 3009 * globally unique and SHOULD be a literal address at which at which an 3010 * authoritative instance of this effect evidence synthesis is (or will 3011 * be) published. This URL can be the target of a canonical reference. 3012 * It SHALL remain the same when the effect evidence synthesis is stored 3013 * on different servers. 3014 */ 3015 public String getUrl() { 3016 return this.url == null ? null : this.url.getValue(); 3017 } 3018 3019 /** 3020 * @param value An absolute URI that is used to identify this effect evidence 3021 * synthesis when it is referenced in a specification, model, 3022 * design or an instance; also called its canonical identifier. 3023 * This SHOULD be globally unique and SHOULD be a literal address 3024 * at which at which an authoritative instance of this effect 3025 * evidence synthesis is (or will be) published. This URL can be 3026 * the target of a canonical reference. It SHALL remain the same 3027 * when the effect evidence synthesis is stored on different 3028 * servers. 3029 */ 3030 public EffectEvidenceSynthesis setUrl(String value) { 3031 if (Utilities.noString(value)) 3032 this.url = null; 3033 else { 3034 if (this.url == null) 3035 this.url = new UriType(); 3036 this.url.setValue(value); 3037 } 3038 return this; 3039 } 3040 3041 /** 3042 * @return {@link #identifier} (A formal identifier that is used to identify 3043 * this effect evidence synthesis when it is represented in other 3044 * formats, or referenced in a specification, model, design or an 3045 * instance.) 3046 */ 3047 public List<Identifier> getIdentifier() { 3048 if (this.identifier == null) 3049 this.identifier = new ArrayList<Identifier>(); 3050 return this.identifier; 3051 } 3052 3053 /** 3054 * @return Returns a reference to <code>this</code> for easy method chaining 3055 */ 3056 public EffectEvidenceSynthesis setIdentifier(List<Identifier> theIdentifier) { 3057 this.identifier = theIdentifier; 3058 return this; 3059 } 3060 3061 public boolean hasIdentifier() { 3062 if (this.identifier == null) 3063 return false; 3064 for (Identifier item : this.identifier) 3065 if (!item.isEmpty()) 3066 return true; 3067 return false; 3068 } 3069 3070 public Identifier addIdentifier() { // 3 3071 Identifier t = new Identifier(); 3072 if (this.identifier == null) 3073 this.identifier = new ArrayList<Identifier>(); 3074 this.identifier.add(t); 3075 return t; 3076 } 3077 3078 public EffectEvidenceSynthesis addIdentifier(Identifier t) { // 3 3079 if (t == null) 3080 return this; 3081 if (this.identifier == null) 3082 this.identifier = new ArrayList<Identifier>(); 3083 this.identifier.add(t); 3084 return this; 3085 } 3086 3087 /** 3088 * @return The first repetition of repeating field {@link #identifier}, creating 3089 * it if it does not already exist 3090 */ 3091 public Identifier getIdentifierFirstRep() { 3092 if (getIdentifier().isEmpty()) { 3093 addIdentifier(); 3094 } 3095 return getIdentifier().get(0); 3096 } 3097 3098 /** 3099 * @return {@link #version} (The identifier that is used to identify this 3100 * version of the effect evidence synthesis when it is referenced in a 3101 * specification, model, design or instance. This is an arbitrary value 3102 * managed by the effect evidence synthesis author and is not expected 3103 * to be globally unique. For example, it might be a timestamp (e.g. 3104 * yyyymmdd) if a managed version is not available. There is also no 3105 * expectation that versions can be placed in a lexicographical 3106 * sequence.). This is the underlying object with id, value and 3107 * extensions. The accessor "getVersion" gives direct access to the 3108 * value 3109 */ 3110 public StringType getVersionElement() { 3111 if (this.version == null) 3112 if (Configuration.errorOnAutoCreate()) 3113 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.version"); 3114 else if (Configuration.doAutoCreate()) 3115 this.version = new StringType(); // bb 3116 return this.version; 3117 } 3118 3119 public boolean hasVersionElement() { 3120 return this.version != null && !this.version.isEmpty(); 3121 } 3122 3123 public boolean hasVersion() { 3124 return this.version != null && !this.version.isEmpty(); 3125 } 3126 3127 /** 3128 * @param value {@link #version} (The identifier that is used to identify this 3129 * version of the effect evidence synthesis when it is referenced 3130 * in a specification, model, design or instance. This is an 3131 * arbitrary value managed by the effect evidence synthesis author 3132 * and is not expected to be globally unique. For example, it might 3133 * be a timestamp (e.g. yyyymmdd) if a managed version is not 3134 * available. There is also no expectation that versions can be 3135 * placed in a lexicographical sequence.). This is the underlying 3136 * object with id, value and extensions. The accessor "getVersion" 3137 * gives direct access to the value 3138 */ 3139 public EffectEvidenceSynthesis setVersionElement(StringType value) { 3140 this.version = value; 3141 return this; 3142 } 3143 3144 /** 3145 * @return The identifier that is used to identify this version of the effect 3146 * evidence synthesis when it is referenced in a specification, model, 3147 * design or instance. This is an arbitrary value managed by the effect 3148 * evidence synthesis author and is not expected to be globally unique. 3149 * For example, it might be a timestamp (e.g. yyyymmdd) if a managed 3150 * version is not available. There is also no expectation that versions 3151 * can be placed in a lexicographical sequence. 3152 */ 3153 public String getVersion() { 3154 return this.version == null ? null : this.version.getValue(); 3155 } 3156 3157 /** 3158 * @param value The identifier that is used to identify this version of the 3159 * effect evidence synthesis when it is referenced in a 3160 * specification, model, design or instance. This is an arbitrary 3161 * value managed by the effect evidence synthesis author and is not 3162 * expected to be globally unique. For example, it might be a 3163 * timestamp (e.g. yyyymmdd) if a managed version is not available. 3164 * There is also no expectation that versions can be placed in a 3165 * lexicographical sequence. 3166 */ 3167 public EffectEvidenceSynthesis setVersion(String value) { 3168 if (Utilities.noString(value)) 3169 this.version = null; 3170 else { 3171 if (this.version == null) 3172 this.version = new StringType(); 3173 this.version.setValue(value); 3174 } 3175 return this; 3176 } 3177 3178 /** 3179 * @return {@link #name} (A natural language name identifying the effect 3180 * evidence synthesis. This name should be usable as an identifier for 3181 * the module by machine processing applications such as code 3182 * generation.). This is the underlying object with id, value and 3183 * extensions. The accessor "getName" gives direct access to the value 3184 */ 3185 public StringType getNameElement() { 3186 if (this.name == null) 3187 if (Configuration.errorOnAutoCreate()) 3188 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.name"); 3189 else if (Configuration.doAutoCreate()) 3190 this.name = new StringType(); // bb 3191 return this.name; 3192 } 3193 3194 public boolean hasNameElement() { 3195 return this.name != null && !this.name.isEmpty(); 3196 } 3197 3198 public boolean hasName() { 3199 return this.name != null && !this.name.isEmpty(); 3200 } 3201 3202 /** 3203 * @param value {@link #name} (A natural language name identifying the effect 3204 * evidence synthesis. This name should be usable as an identifier 3205 * for the module by machine processing applications such as code 3206 * generation.). This is the underlying object with id, value and 3207 * extensions. The accessor "getName" gives direct access to the 3208 * value 3209 */ 3210 public EffectEvidenceSynthesis setNameElement(StringType value) { 3211 this.name = value; 3212 return this; 3213 } 3214 3215 /** 3216 * @return A natural language name identifying the effect evidence synthesis. 3217 * This name should be usable as an identifier for the module by machine 3218 * processing applications such as code generation. 3219 */ 3220 public String getName() { 3221 return this.name == null ? null : this.name.getValue(); 3222 } 3223 3224 /** 3225 * @param value A natural language name identifying the effect evidence 3226 * synthesis. This name should be usable as an identifier for the 3227 * module by machine processing applications such as code 3228 * generation. 3229 */ 3230 public EffectEvidenceSynthesis setName(String value) { 3231 if (Utilities.noString(value)) 3232 this.name = null; 3233 else { 3234 if (this.name == null) 3235 this.name = new StringType(); 3236 this.name.setValue(value); 3237 } 3238 return this; 3239 } 3240 3241 /** 3242 * @return {@link #title} (A short, descriptive, user-friendly title for the 3243 * effect evidence synthesis.). This is the underlying object with id, 3244 * value and extensions. The accessor "getTitle" gives direct access to 3245 * the value 3246 */ 3247 public StringType getTitleElement() { 3248 if (this.title == null) 3249 if (Configuration.errorOnAutoCreate()) 3250 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.title"); 3251 else if (Configuration.doAutoCreate()) 3252 this.title = new StringType(); // bb 3253 return this.title; 3254 } 3255 3256 public boolean hasTitleElement() { 3257 return this.title != null && !this.title.isEmpty(); 3258 } 3259 3260 public boolean hasTitle() { 3261 return this.title != null && !this.title.isEmpty(); 3262 } 3263 3264 /** 3265 * @param value {@link #title} (A short, descriptive, user-friendly title for 3266 * the effect evidence synthesis.). This is the underlying object 3267 * with id, value and extensions. The accessor "getTitle" gives 3268 * direct access to the value 3269 */ 3270 public EffectEvidenceSynthesis setTitleElement(StringType value) { 3271 this.title = value; 3272 return this; 3273 } 3274 3275 /** 3276 * @return A short, descriptive, user-friendly title for the effect evidence 3277 * synthesis. 3278 */ 3279 public String getTitle() { 3280 return this.title == null ? null : this.title.getValue(); 3281 } 3282 3283 /** 3284 * @param value A short, descriptive, user-friendly title for the effect 3285 * evidence synthesis. 3286 */ 3287 public EffectEvidenceSynthesis setTitle(String value) { 3288 if (Utilities.noString(value)) 3289 this.title = null; 3290 else { 3291 if (this.title == null) 3292 this.title = new StringType(); 3293 this.title.setValue(value); 3294 } 3295 return this; 3296 } 3297 3298 /** 3299 * @return {@link #status} (The status of this effect evidence synthesis. 3300 * Enables tracking the life-cycle of the content.). This is the 3301 * underlying object with id, value and extensions. The accessor 3302 * "getStatus" gives direct access to the value 3303 */ 3304 public Enumeration<PublicationStatus> getStatusElement() { 3305 if (this.status == null) 3306 if (Configuration.errorOnAutoCreate()) 3307 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.status"); 3308 else if (Configuration.doAutoCreate()) 3309 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 3310 return this.status; 3311 } 3312 3313 public boolean hasStatusElement() { 3314 return this.status != null && !this.status.isEmpty(); 3315 } 3316 3317 public boolean hasStatus() { 3318 return this.status != null && !this.status.isEmpty(); 3319 } 3320 3321 /** 3322 * @param value {@link #status} (The status of this effect evidence synthesis. 3323 * Enables tracking the life-cycle of the content.). This is the 3324 * underlying object with id, value and extensions. The accessor 3325 * "getStatus" gives direct access to the value 3326 */ 3327 public EffectEvidenceSynthesis setStatusElement(Enumeration<PublicationStatus> value) { 3328 this.status = value; 3329 return this; 3330 } 3331 3332 /** 3333 * @return The status of this effect evidence synthesis. Enables tracking the 3334 * life-cycle of the content. 3335 */ 3336 public PublicationStatus getStatus() { 3337 return this.status == null ? null : this.status.getValue(); 3338 } 3339 3340 /** 3341 * @param value The status of this effect evidence synthesis. Enables tracking 3342 * the life-cycle of the content. 3343 */ 3344 public EffectEvidenceSynthesis setStatus(PublicationStatus value) { 3345 if (this.status == null) 3346 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 3347 this.status.setValue(value); 3348 return this; 3349 } 3350 3351 /** 3352 * @return {@link #date} (The date (and optionally time) when the effect 3353 * evidence synthesis was published. The date must change when the 3354 * business version changes and it must change if the status code 3355 * changes. In addition, it should change when the substantive content 3356 * of the effect evidence synthesis changes.). This is the underlying 3357 * object with id, value and extensions. The accessor "getDate" gives 3358 * direct access to the value 3359 */ 3360 public DateTimeType getDateElement() { 3361 if (this.date == null) 3362 if (Configuration.errorOnAutoCreate()) 3363 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.date"); 3364 else if (Configuration.doAutoCreate()) 3365 this.date = new DateTimeType(); // bb 3366 return this.date; 3367 } 3368 3369 public boolean hasDateElement() { 3370 return this.date != null && !this.date.isEmpty(); 3371 } 3372 3373 public boolean hasDate() { 3374 return this.date != null && !this.date.isEmpty(); 3375 } 3376 3377 /** 3378 * @param value {@link #date} (The date (and optionally time) when the effect 3379 * evidence synthesis was published. The date must change when the 3380 * business version changes and it must change if the status code 3381 * changes. In addition, it should change when the substantive 3382 * content of the effect evidence synthesis changes.). This is the 3383 * underlying object with id, value and extensions. The accessor 3384 * "getDate" gives direct access to the value 3385 */ 3386 public EffectEvidenceSynthesis setDateElement(DateTimeType value) { 3387 this.date = value; 3388 return this; 3389 } 3390 3391 /** 3392 * @return The date (and optionally time) when the effect evidence synthesis was 3393 * published. The date must change when the business version changes and 3394 * it must change if the status code changes. In addition, it should 3395 * change when the substantive content of the effect evidence synthesis 3396 * changes. 3397 */ 3398 public Date getDate() { 3399 return this.date == null ? null : this.date.getValue(); 3400 } 3401 3402 /** 3403 * @param value The date (and optionally time) when the effect evidence 3404 * synthesis was published. The date must change when the business 3405 * version changes and it must change if the status code changes. 3406 * In addition, it should change when the substantive content of 3407 * the effect evidence synthesis changes. 3408 */ 3409 public EffectEvidenceSynthesis setDate(Date value) { 3410 if (value == null) 3411 this.date = null; 3412 else { 3413 if (this.date == null) 3414 this.date = new DateTimeType(); 3415 this.date.setValue(value); 3416 } 3417 return this; 3418 } 3419 3420 /** 3421 * @return {@link #publisher} (The name of the organization or individual that 3422 * published the effect evidence synthesis.). This is the underlying 3423 * object with id, value and extensions. The accessor "getPublisher" 3424 * gives direct access to the value 3425 */ 3426 public StringType getPublisherElement() { 3427 if (this.publisher == null) 3428 if (Configuration.errorOnAutoCreate()) 3429 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.publisher"); 3430 else if (Configuration.doAutoCreate()) 3431 this.publisher = new StringType(); // bb 3432 return this.publisher; 3433 } 3434 3435 public boolean hasPublisherElement() { 3436 return this.publisher != null && !this.publisher.isEmpty(); 3437 } 3438 3439 public boolean hasPublisher() { 3440 return this.publisher != null && !this.publisher.isEmpty(); 3441 } 3442 3443 /** 3444 * @param value {@link #publisher} (The name of the organization or individual 3445 * that published the effect evidence synthesis.). This is the 3446 * underlying object with id, value and extensions. The accessor 3447 * "getPublisher" gives direct access to the value 3448 */ 3449 public EffectEvidenceSynthesis setPublisherElement(StringType value) { 3450 this.publisher = value; 3451 return this; 3452 } 3453 3454 /** 3455 * @return The name of the organization or individual that published the effect 3456 * evidence synthesis. 3457 */ 3458 public String getPublisher() { 3459 return this.publisher == null ? null : this.publisher.getValue(); 3460 } 3461 3462 /** 3463 * @param value The name of the organization or individual that published the 3464 * effect evidence synthesis. 3465 */ 3466 public EffectEvidenceSynthesis setPublisher(String value) { 3467 if (Utilities.noString(value)) 3468 this.publisher = null; 3469 else { 3470 if (this.publisher == null) 3471 this.publisher = new StringType(); 3472 this.publisher.setValue(value); 3473 } 3474 return this; 3475 } 3476 3477 /** 3478 * @return {@link #contact} (Contact details to assist a user in finding and 3479 * communicating with the publisher.) 3480 */ 3481 public List<ContactDetail> getContact() { 3482 if (this.contact == null) 3483 this.contact = new ArrayList<ContactDetail>(); 3484 return this.contact; 3485 } 3486 3487 /** 3488 * @return Returns a reference to <code>this</code> for easy method chaining 3489 */ 3490 public EffectEvidenceSynthesis setContact(List<ContactDetail> theContact) { 3491 this.contact = theContact; 3492 return this; 3493 } 3494 3495 public boolean hasContact() { 3496 if (this.contact == null) 3497 return false; 3498 for (ContactDetail item : this.contact) 3499 if (!item.isEmpty()) 3500 return true; 3501 return false; 3502 } 3503 3504 public ContactDetail addContact() { // 3 3505 ContactDetail t = new ContactDetail(); 3506 if (this.contact == null) 3507 this.contact = new ArrayList<ContactDetail>(); 3508 this.contact.add(t); 3509 return t; 3510 } 3511 3512 public EffectEvidenceSynthesis addContact(ContactDetail t) { // 3 3513 if (t == null) 3514 return this; 3515 if (this.contact == null) 3516 this.contact = new ArrayList<ContactDetail>(); 3517 this.contact.add(t); 3518 return this; 3519 } 3520 3521 /** 3522 * @return The first repetition of repeating field {@link #contact}, creating it 3523 * if it does not already exist 3524 */ 3525 public ContactDetail getContactFirstRep() { 3526 if (getContact().isEmpty()) { 3527 addContact(); 3528 } 3529 return getContact().get(0); 3530 } 3531 3532 /** 3533 * @return {@link #description} (A free text natural language description of the 3534 * effect evidence synthesis from a consumer's perspective.). This is 3535 * the underlying object with id, value and extensions. The accessor 3536 * "getDescription" gives direct access to the value 3537 */ 3538 public MarkdownType getDescriptionElement() { 3539 if (this.description == null) 3540 if (Configuration.errorOnAutoCreate()) 3541 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.description"); 3542 else if (Configuration.doAutoCreate()) 3543 this.description = new MarkdownType(); // bb 3544 return this.description; 3545 } 3546 3547 public boolean hasDescriptionElement() { 3548 return this.description != null && !this.description.isEmpty(); 3549 } 3550 3551 public boolean hasDescription() { 3552 return this.description != null && !this.description.isEmpty(); 3553 } 3554 3555 /** 3556 * @param value {@link #description} (A free text natural language description 3557 * of the effect evidence synthesis from a consumer's 3558 * perspective.). This is the underlying object with id, value and 3559 * extensions. The accessor "getDescription" gives direct access to 3560 * the value 3561 */ 3562 public EffectEvidenceSynthesis setDescriptionElement(MarkdownType value) { 3563 this.description = value; 3564 return this; 3565 } 3566 3567 /** 3568 * @return A free text natural language description of the effect evidence 3569 * synthesis from a consumer's perspective. 3570 */ 3571 public String getDescription() { 3572 return this.description == null ? null : this.description.getValue(); 3573 } 3574 3575 /** 3576 * @param value A free text natural language description of the effect evidence 3577 * synthesis from a consumer's perspective. 3578 */ 3579 public EffectEvidenceSynthesis setDescription(String value) { 3580 if (value == null) 3581 this.description = null; 3582 else { 3583 if (this.description == null) 3584 this.description = new MarkdownType(); 3585 this.description.setValue(value); 3586 } 3587 return this; 3588 } 3589 3590 /** 3591 * @return {@link #note} (A human-readable string to clarify or explain concepts 3592 * about the resource.) 3593 */ 3594 public List<Annotation> getNote() { 3595 if (this.note == null) 3596 this.note = new ArrayList<Annotation>(); 3597 return this.note; 3598 } 3599 3600 /** 3601 * @return Returns a reference to <code>this</code> for easy method chaining 3602 */ 3603 public EffectEvidenceSynthesis setNote(List<Annotation> theNote) { 3604 this.note = theNote; 3605 return this; 3606 } 3607 3608 public boolean hasNote() { 3609 if (this.note == null) 3610 return false; 3611 for (Annotation item : this.note) 3612 if (!item.isEmpty()) 3613 return true; 3614 return false; 3615 } 3616 3617 public Annotation addNote() { // 3 3618 Annotation t = new Annotation(); 3619 if (this.note == null) 3620 this.note = new ArrayList<Annotation>(); 3621 this.note.add(t); 3622 return t; 3623 } 3624 3625 public EffectEvidenceSynthesis addNote(Annotation t) { // 3 3626 if (t == null) 3627 return this; 3628 if (this.note == null) 3629 this.note = new ArrayList<Annotation>(); 3630 this.note.add(t); 3631 return this; 3632 } 3633 3634 /** 3635 * @return The first repetition of repeating field {@link #note}, creating it if 3636 * it does not already exist 3637 */ 3638 public Annotation getNoteFirstRep() { 3639 if (getNote().isEmpty()) { 3640 addNote(); 3641 } 3642 return getNote().get(0); 3643 } 3644 3645 /** 3646 * @return {@link #useContext} (The content was developed with a focus and 3647 * intent of supporting the contexts that are listed. These contexts may 3648 * be general categories (gender, age, ...) or may be references to 3649 * specific programs (insurance plans, studies, ...) and may be used to 3650 * assist with indexing and searching for appropriate effect evidence 3651 * synthesis instances.) 3652 */ 3653 public List<UsageContext> getUseContext() { 3654 if (this.useContext == null) 3655 this.useContext = new ArrayList<UsageContext>(); 3656 return this.useContext; 3657 } 3658 3659 /** 3660 * @return Returns a reference to <code>this</code> for easy method chaining 3661 */ 3662 public EffectEvidenceSynthesis setUseContext(List<UsageContext> theUseContext) { 3663 this.useContext = theUseContext; 3664 return this; 3665 } 3666 3667 public boolean hasUseContext() { 3668 if (this.useContext == null) 3669 return false; 3670 for (UsageContext item : this.useContext) 3671 if (!item.isEmpty()) 3672 return true; 3673 return false; 3674 } 3675 3676 public UsageContext addUseContext() { // 3 3677 UsageContext t = new UsageContext(); 3678 if (this.useContext == null) 3679 this.useContext = new ArrayList<UsageContext>(); 3680 this.useContext.add(t); 3681 return t; 3682 } 3683 3684 public EffectEvidenceSynthesis addUseContext(UsageContext t) { // 3 3685 if (t == null) 3686 return this; 3687 if (this.useContext == null) 3688 this.useContext = new ArrayList<UsageContext>(); 3689 this.useContext.add(t); 3690 return this; 3691 } 3692 3693 /** 3694 * @return The first repetition of repeating field {@link #useContext}, creating 3695 * it if it does not already exist 3696 */ 3697 public UsageContext getUseContextFirstRep() { 3698 if (getUseContext().isEmpty()) { 3699 addUseContext(); 3700 } 3701 return getUseContext().get(0); 3702 } 3703 3704 /** 3705 * @return {@link #jurisdiction} (A legal or geographic region in which the 3706 * effect evidence synthesis is intended to be used.) 3707 */ 3708 public List<CodeableConcept> getJurisdiction() { 3709 if (this.jurisdiction == null) 3710 this.jurisdiction = new ArrayList<CodeableConcept>(); 3711 return this.jurisdiction; 3712 } 3713 3714 /** 3715 * @return Returns a reference to <code>this</code> for easy method chaining 3716 */ 3717 public EffectEvidenceSynthesis setJurisdiction(List<CodeableConcept> theJurisdiction) { 3718 this.jurisdiction = theJurisdiction; 3719 return this; 3720 } 3721 3722 public boolean hasJurisdiction() { 3723 if (this.jurisdiction == null) 3724 return false; 3725 for (CodeableConcept item : this.jurisdiction) 3726 if (!item.isEmpty()) 3727 return true; 3728 return false; 3729 } 3730 3731 public CodeableConcept addJurisdiction() { // 3 3732 CodeableConcept t = new CodeableConcept(); 3733 if (this.jurisdiction == null) 3734 this.jurisdiction = new ArrayList<CodeableConcept>(); 3735 this.jurisdiction.add(t); 3736 return t; 3737 } 3738 3739 public EffectEvidenceSynthesis addJurisdiction(CodeableConcept t) { // 3 3740 if (t == null) 3741 return this; 3742 if (this.jurisdiction == null) 3743 this.jurisdiction = new ArrayList<CodeableConcept>(); 3744 this.jurisdiction.add(t); 3745 return this; 3746 } 3747 3748 /** 3749 * @return The first repetition of repeating field {@link #jurisdiction}, 3750 * creating it if it does not already exist 3751 */ 3752 public CodeableConcept getJurisdictionFirstRep() { 3753 if (getJurisdiction().isEmpty()) { 3754 addJurisdiction(); 3755 } 3756 return getJurisdiction().get(0); 3757 } 3758 3759 /** 3760 * @return {@link #copyright} (A copyright statement relating to the effect 3761 * evidence synthesis and/or its contents. Copyright statements are 3762 * generally legal restrictions on the use and publishing of the effect 3763 * evidence synthesis.). This is the underlying object with id, value 3764 * and extensions. The accessor "getCopyright" gives direct access to 3765 * the value 3766 */ 3767 public MarkdownType getCopyrightElement() { 3768 if (this.copyright == null) 3769 if (Configuration.errorOnAutoCreate()) 3770 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.copyright"); 3771 else if (Configuration.doAutoCreate()) 3772 this.copyright = new MarkdownType(); // bb 3773 return this.copyright; 3774 } 3775 3776 public boolean hasCopyrightElement() { 3777 return this.copyright != null && !this.copyright.isEmpty(); 3778 } 3779 3780 public boolean hasCopyright() { 3781 return this.copyright != null && !this.copyright.isEmpty(); 3782 } 3783 3784 /** 3785 * @param value {@link #copyright} (A copyright statement relating to the effect 3786 * evidence synthesis and/or its contents. Copyright statements are 3787 * generally legal restrictions on the use and publishing of the 3788 * effect evidence synthesis.). This is the underlying object with 3789 * id, value and extensions. The accessor "getCopyright" gives 3790 * direct access to the value 3791 */ 3792 public EffectEvidenceSynthesis setCopyrightElement(MarkdownType value) { 3793 this.copyright = value; 3794 return this; 3795 } 3796 3797 /** 3798 * @return A copyright statement relating to the effect evidence synthesis 3799 * and/or its contents. Copyright statements are generally legal 3800 * restrictions on the use and publishing of the effect evidence 3801 * synthesis. 3802 */ 3803 public String getCopyright() { 3804 return this.copyright == null ? null : this.copyright.getValue(); 3805 } 3806 3807 /** 3808 * @param value A copyright statement relating to the effect evidence synthesis 3809 * and/or its contents. Copyright statements are generally legal 3810 * restrictions on the use and publishing of the effect evidence 3811 * synthesis. 3812 */ 3813 public EffectEvidenceSynthesis setCopyright(String value) { 3814 if (value == null) 3815 this.copyright = null; 3816 else { 3817 if (this.copyright == null) 3818 this.copyright = new MarkdownType(); 3819 this.copyright.setValue(value); 3820 } 3821 return this; 3822 } 3823 3824 /** 3825 * @return {@link #approvalDate} (The date on which the resource content was 3826 * approved by the publisher. Approval happens once when the content is 3827 * officially approved for usage.). This is the underlying object with 3828 * id, value and extensions. The accessor "getApprovalDate" gives direct 3829 * access to the value 3830 */ 3831 public DateType getApprovalDateElement() { 3832 if (this.approvalDate == null) 3833 if (Configuration.errorOnAutoCreate()) 3834 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.approvalDate"); 3835 else if (Configuration.doAutoCreate()) 3836 this.approvalDate = new DateType(); // bb 3837 return this.approvalDate; 3838 } 3839 3840 public boolean hasApprovalDateElement() { 3841 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3842 } 3843 3844 public boolean hasApprovalDate() { 3845 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3846 } 3847 3848 /** 3849 * @param value {@link #approvalDate} (The date on which the resource content 3850 * was approved by the publisher. Approval happens once when the 3851 * content is officially approved for usage.). This is the 3852 * underlying object with id, value and extensions. The accessor 3853 * "getApprovalDate" gives direct access to the value 3854 */ 3855 public EffectEvidenceSynthesis setApprovalDateElement(DateType value) { 3856 this.approvalDate = value; 3857 return this; 3858 } 3859 3860 /** 3861 * @return The date on which the resource content was approved by the publisher. 3862 * Approval happens once when the content is officially approved for 3863 * usage. 3864 */ 3865 public Date getApprovalDate() { 3866 return this.approvalDate == null ? null : this.approvalDate.getValue(); 3867 } 3868 3869 /** 3870 * @param value The date on which the resource content was approved by the 3871 * publisher. Approval happens once when the content is officially 3872 * approved for usage. 3873 */ 3874 public EffectEvidenceSynthesis setApprovalDate(Date value) { 3875 if (value == null) 3876 this.approvalDate = null; 3877 else { 3878 if (this.approvalDate == null) 3879 this.approvalDate = new DateType(); 3880 this.approvalDate.setValue(value); 3881 } 3882 return this; 3883 } 3884 3885 /** 3886 * @return {@link #lastReviewDate} (The date on which the resource content was 3887 * last reviewed. Review happens periodically after approval but does 3888 * not change the original approval date.). This is the underlying 3889 * object with id, value and extensions. The accessor 3890 * "getLastReviewDate" gives direct access to the value 3891 */ 3892 public DateType getLastReviewDateElement() { 3893 if (this.lastReviewDate == null) 3894 if (Configuration.errorOnAutoCreate()) 3895 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.lastReviewDate"); 3896 else if (Configuration.doAutoCreate()) 3897 this.lastReviewDate = new DateType(); // bb 3898 return this.lastReviewDate; 3899 } 3900 3901 public boolean hasLastReviewDateElement() { 3902 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3903 } 3904 3905 public boolean hasLastReviewDate() { 3906 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3907 } 3908 3909 /** 3910 * @param value {@link #lastReviewDate} (The date on which the resource content 3911 * was last reviewed. Review happens periodically after approval 3912 * but does not change the original approval date.). This is the 3913 * underlying object with id, value and extensions. The accessor 3914 * "getLastReviewDate" gives direct access to the value 3915 */ 3916 public EffectEvidenceSynthesis setLastReviewDateElement(DateType value) { 3917 this.lastReviewDate = value; 3918 return this; 3919 } 3920 3921 /** 3922 * @return The date on which the resource content was last reviewed. Review 3923 * happens periodically after approval but does not change the original 3924 * approval date. 3925 */ 3926 public Date getLastReviewDate() { 3927 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 3928 } 3929 3930 /** 3931 * @param value The date on which the resource content was last reviewed. Review 3932 * happens periodically after approval but does not change the 3933 * original approval date. 3934 */ 3935 public EffectEvidenceSynthesis setLastReviewDate(Date value) { 3936 if (value == null) 3937 this.lastReviewDate = null; 3938 else { 3939 if (this.lastReviewDate == null) 3940 this.lastReviewDate = new DateType(); 3941 this.lastReviewDate.setValue(value); 3942 } 3943 return this; 3944 } 3945 3946 /** 3947 * @return {@link #effectivePeriod} (The period during which the effect evidence 3948 * synthesis content was or is planned to be in active use.) 3949 */ 3950 public Period getEffectivePeriod() { 3951 if (this.effectivePeriod == null) 3952 if (Configuration.errorOnAutoCreate()) 3953 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.effectivePeriod"); 3954 else if (Configuration.doAutoCreate()) 3955 this.effectivePeriod = new Period(); // cc 3956 return this.effectivePeriod; 3957 } 3958 3959 public boolean hasEffectivePeriod() { 3960 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 3961 } 3962 3963 /** 3964 * @param value {@link #effectivePeriod} (The period during which the effect 3965 * evidence synthesis content was or is planned to be in active 3966 * use.) 3967 */ 3968 public EffectEvidenceSynthesis setEffectivePeriod(Period value) { 3969 this.effectivePeriod = value; 3970 return this; 3971 } 3972 3973 /** 3974 * @return {@link #topic} (Descriptive topics related to the content of the 3975 * EffectEvidenceSynthesis. Topics provide a high-level categorization 3976 * grouping types of EffectEvidenceSynthesiss that can be useful for 3977 * filtering and searching.) 3978 */ 3979 public List<CodeableConcept> getTopic() { 3980 if (this.topic == null) 3981 this.topic = new ArrayList<CodeableConcept>(); 3982 return this.topic; 3983 } 3984 3985 /** 3986 * @return Returns a reference to <code>this</code> for easy method chaining 3987 */ 3988 public EffectEvidenceSynthesis setTopic(List<CodeableConcept> theTopic) { 3989 this.topic = theTopic; 3990 return this; 3991 } 3992 3993 public boolean hasTopic() { 3994 if (this.topic == null) 3995 return false; 3996 for (CodeableConcept item : this.topic) 3997 if (!item.isEmpty()) 3998 return true; 3999 return false; 4000 } 4001 4002 public CodeableConcept addTopic() { // 3 4003 CodeableConcept t = new CodeableConcept(); 4004 if (this.topic == null) 4005 this.topic = new ArrayList<CodeableConcept>(); 4006 this.topic.add(t); 4007 return t; 4008 } 4009 4010 public EffectEvidenceSynthesis addTopic(CodeableConcept t) { // 3 4011 if (t == null) 4012 return this; 4013 if (this.topic == null) 4014 this.topic = new ArrayList<CodeableConcept>(); 4015 this.topic.add(t); 4016 return this; 4017 } 4018 4019 /** 4020 * @return The first repetition of repeating field {@link #topic}, creating it 4021 * if it does not already exist 4022 */ 4023 public CodeableConcept getTopicFirstRep() { 4024 if (getTopic().isEmpty()) { 4025 addTopic(); 4026 } 4027 return getTopic().get(0); 4028 } 4029 4030 /** 4031 * @return {@link #author} (An individiual or organization primarily involved in 4032 * the creation and maintenance of the content.) 4033 */ 4034 public List<ContactDetail> getAuthor() { 4035 if (this.author == null) 4036 this.author = new ArrayList<ContactDetail>(); 4037 return this.author; 4038 } 4039 4040 /** 4041 * @return Returns a reference to <code>this</code> for easy method chaining 4042 */ 4043 public EffectEvidenceSynthesis setAuthor(List<ContactDetail> theAuthor) { 4044 this.author = theAuthor; 4045 return this; 4046 } 4047 4048 public boolean hasAuthor() { 4049 if (this.author == null) 4050 return false; 4051 for (ContactDetail item : this.author) 4052 if (!item.isEmpty()) 4053 return true; 4054 return false; 4055 } 4056 4057 public ContactDetail addAuthor() { // 3 4058 ContactDetail t = new ContactDetail(); 4059 if (this.author == null) 4060 this.author = new ArrayList<ContactDetail>(); 4061 this.author.add(t); 4062 return t; 4063 } 4064 4065 public EffectEvidenceSynthesis addAuthor(ContactDetail t) { // 3 4066 if (t == null) 4067 return this; 4068 if (this.author == null) 4069 this.author = new ArrayList<ContactDetail>(); 4070 this.author.add(t); 4071 return this; 4072 } 4073 4074 /** 4075 * @return The first repetition of repeating field {@link #author}, creating it 4076 * if it does not already exist 4077 */ 4078 public ContactDetail getAuthorFirstRep() { 4079 if (getAuthor().isEmpty()) { 4080 addAuthor(); 4081 } 4082 return getAuthor().get(0); 4083 } 4084 4085 /** 4086 * @return {@link #editor} (An individual or organization primarily responsible 4087 * for internal coherence of the content.) 4088 */ 4089 public List<ContactDetail> getEditor() { 4090 if (this.editor == null) 4091 this.editor = new ArrayList<ContactDetail>(); 4092 return this.editor; 4093 } 4094 4095 /** 4096 * @return Returns a reference to <code>this</code> for easy method chaining 4097 */ 4098 public EffectEvidenceSynthesis setEditor(List<ContactDetail> theEditor) { 4099 this.editor = theEditor; 4100 return this; 4101 } 4102 4103 public boolean hasEditor() { 4104 if (this.editor == null) 4105 return false; 4106 for (ContactDetail item : this.editor) 4107 if (!item.isEmpty()) 4108 return true; 4109 return false; 4110 } 4111 4112 public ContactDetail addEditor() { // 3 4113 ContactDetail t = new ContactDetail(); 4114 if (this.editor == null) 4115 this.editor = new ArrayList<ContactDetail>(); 4116 this.editor.add(t); 4117 return t; 4118 } 4119 4120 public EffectEvidenceSynthesis addEditor(ContactDetail t) { // 3 4121 if (t == null) 4122 return this; 4123 if (this.editor == null) 4124 this.editor = new ArrayList<ContactDetail>(); 4125 this.editor.add(t); 4126 return this; 4127 } 4128 4129 /** 4130 * @return The first repetition of repeating field {@link #editor}, creating it 4131 * if it does not already exist 4132 */ 4133 public ContactDetail getEditorFirstRep() { 4134 if (getEditor().isEmpty()) { 4135 addEditor(); 4136 } 4137 return getEditor().get(0); 4138 } 4139 4140 /** 4141 * @return {@link #reviewer} (An individual or organization primarily 4142 * responsible for review of some aspect of the content.) 4143 */ 4144 public List<ContactDetail> getReviewer() { 4145 if (this.reviewer == null) 4146 this.reviewer = new ArrayList<ContactDetail>(); 4147 return this.reviewer; 4148 } 4149 4150 /** 4151 * @return Returns a reference to <code>this</code> for easy method chaining 4152 */ 4153 public EffectEvidenceSynthesis setReviewer(List<ContactDetail> theReviewer) { 4154 this.reviewer = theReviewer; 4155 return this; 4156 } 4157 4158 public boolean hasReviewer() { 4159 if (this.reviewer == null) 4160 return false; 4161 for (ContactDetail item : this.reviewer) 4162 if (!item.isEmpty()) 4163 return true; 4164 return false; 4165 } 4166 4167 public ContactDetail addReviewer() { // 3 4168 ContactDetail t = new ContactDetail(); 4169 if (this.reviewer == null) 4170 this.reviewer = new ArrayList<ContactDetail>(); 4171 this.reviewer.add(t); 4172 return t; 4173 } 4174 4175 public EffectEvidenceSynthesis addReviewer(ContactDetail t) { // 3 4176 if (t == null) 4177 return this; 4178 if (this.reviewer == null) 4179 this.reviewer = new ArrayList<ContactDetail>(); 4180 this.reviewer.add(t); 4181 return this; 4182 } 4183 4184 /** 4185 * @return The first repetition of repeating field {@link #reviewer}, creating 4186 * it if it does not already exist 4187 */ 4188 public ContactDetail getReviewerFirstRep() { 4189 if (getReviewer().isEmpty()) { 4190 addReviewer(); 4191 } 4192 return getReviewer().get(0); 4193 } 4194 4195 /** 4196 * @return {@link #endorser} (An individual or organization responsible for 4197 * officially endorsing the content for use in some setting.) 4198 */ 4199 public List<ContactDetail> getEndorser() { 4200 if (this.endorser == null) 4201 this.endorser = new ArrayList<ContactDetail>(); 4202 return this.endorser; 4203 } 4204 4205 /** 4206 * @return Returns a reference to <code>this</code> for easy method chaining 4207 */ 4208 public EffectEvidenceSynthesis setEndorser(List<ContactDetail> theEndorser) { 4209 this.endorser = theEndorser; 4210 return this; 4211 } 4212 4213 public boolean hasEndorser() { 4214 if (this.endorser == null) 4215 return false; 4216 for (ContactDetail item : this.endorser) 4217 if (!item.isEmpty()) 4218 return true; 4219 return false; 4220 } 4221 4222 public ContactDetail addEndorser() { // 3 4223 ContactDetail t = new ContactDetail(); 4224 if (this.endorser == null) 4225 this.endorser = new ArrayList<ContactDetail>(); 4226 this.endorser.add(t); 4227 return t; 4228 } 4229 4230 public EffectEvidenceSynthesis addEndorser(ContactDetail t) { // 3 4231 if (t == null) 4232 return this; 4233 if (this.endorser == null) 4234 this.endorser = new ArrayList<ContactDetail>(); 4235 this.endorser.add(t); 4236 return this; 4237 } 4238 4239 /** 4240 * @return The first repetition of repeating field {@link #endorser}, creating 4241 * it if it does not already exist 4242 */ 4243 public ContactDetail getEndorserFirstRep() { 4244 if (getEndorser().isEmpty()) { 4245 addEndorser(); 4246 } 4247 return getEndorser().get(0); 4248 } 4249 4250 /** 4251 * @return {@link #relatedArtifact} (Related artifacts such as additional 4252 * documentation, justification, or bibliographic references.) 4253 */ 4254 public List<RelatedArtifact> getRelatedArtifact() { 4255 if (this.relatedArtifact == null) 4256 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 4257 return this.relatedArtifact; 4258 } 4259 4260 /** 4261 * @return Returns a reference to <code>this</code> for easy method chaining 4262 */ 4263 public EffectEvidenceSynthesis setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 4264 this.relatedArtifact = theRelatedArtifact; 4265 return this; 4266 } 4267 4268 public boolean hasRelatedArtifact() { 4269 if (this.relatedArtifact == null) 4270 return false; 4271 for (RelatedArtifact item : this.relatedArtifact) 4272 if (!item.isEmpty()) 4273 return true; 4274 return false; 4275 } 4276 4277 public RelatedArtifact addRelatedArtifact() { // 3 4278 RelatedArtifact t = new RelatedArtifact(); 4279 if (this.relatedArtifact == null) 4280 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 4281 this.relatedArtifact.add(t); 4282 return t; 4283 } 4284 4285 public EffectEvidenceSynthesis addRelatedArtifact(RelatedArtifact t) { // 3 4286 if (t == null) 4287 return this; 4288 if (this.relatedArtifact == null) 4289 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 4290 this.relatedArtifact.add(t); 4291 return this; 4292 } 4293 4294 /** 4295 * @return The first repetition of repeating field {@link #relatedArtifact}, 4296 * creating it if it does not already exist 4297 */ 4298 public RelatedArtifact getRelatedArtifactFirstRep() { 4299 if (getRelatedArtifact().isEmpty()) { 4300 addRelatedArtifact(); 4301 } 4302 return getRelatedArtifact().get(0); 4303 } 4304 4305 /** 4306 * @return {@link #synthesisType} (Type of synthesis eg meta-analysis.) 4307 */ 4308 public CodeableConcept getSynthesisType() { 4309 if (this.synthesisType == null) 4310 if (Configuration.errorOnAutoCreate()) 4311 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.synthesisType"); 4312 else if (Configuration.doAutoCreate()) 4313 this.synthesisType = new CodeableConcept(); // cc 4314 return this.synthesisType; 4315 } 4316 4317 public boolean hasSynthesisType() { 4318 return this.synthesisType != null && !this.synthesisType.isEmpty(); 4319 } 4320 4321 /** 4322 * @param value {@link #synthesisType} (Type of synthesis eg meta-analysis.) 4323 */ 4324 public EffectEvidenceSynthesis setSynthesisType(CodeableConcept value) { 4325 this.synthesisType = value; 4326 return this; 4327 } 4328 4329 /** 4330 * @return {@link #studyType} (Type of study eg randomized trial.) 4331 */ 4332 public CodeableConcept getStudyType() { 4333 if (this.studyType == null) 4334 if (Configuration.errorOnAutoCreate()) 4335 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.studyType"); 4336 else if (Configuration.doAutoCreate()) 4337 this.studyType = new CodeableConcept(); // cc 4338 return this.studyType; 4339 } 4340 4341 public boolean hasStudyType() { 4342 return this.studyType != null && !this.studyType.isEmpty(); 4343 } 4344 4345 /** 4346 * @param value {@link #studyType} (Type of study eg randomized trial.) 4347 */ 4348 public EffectEvidenceSynthesis setStudyType(CodeableConcept value) { 4349 this.studyType = value; 4350 return this; 4351 } 4352 4353 /** 4354 * @return {@link #population} (A reference to a EvidenceVariable resource that 4355 * defines the population for the research.) 4356 */ 4357 public Reference getPopulation() { 4358 if (this.population == null) 4359 if (Configuration.errorOnAutoCreate()) 4360 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.population"); 4361 else if (Configuration.doAutoCreate()) 4362 this.population = new Reference(); // cc 4363 return this.population; 4364 } 4365 4366 public boolean hasPopulation() { 4367 return this.population != null && !this.population.isEmpty(); 4368 } 4369 4370 /** 4371 * @param value {@link #population} (A reference to a EvidenceVariable resource 4372 * that defines the population for the research.) 4373 */ 4374 public EffectEvidenceSynthesis setPopulation(Reference value) { 4375 this.population = value; 4376 return this; 4377 } 4378 4379 /** 4380 * @return {@link #population} The actual object that is the target of the 4381 * reference. The reference library doesn't populate this, but you can 4382 * use it to hold the resource if you resolve it. (A reference to a 4383 * EvidenceVariable resource that defines the population for the 4384 * research.) 4385 */ 4386 public EvidenceVariable getPopulationTarget() { 4387 if (this.populationTarget == null) 4388 if (Configuration.errorOnAutoCreate()) 4389 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.population"); 4390 else if (Configuration.doAutoCreate()) 4391 this.populationTarget = new EvidenceVariable(); // aa 4392 return this.populationTarget; 4393 } 4394 4395 /** 4396 * @param value {@link #population} The actual object that is the target of the 4397 * reference. The reference library doesn't use these, but you can 4398 * use it to hold the resource if you resolve it. (A reference to a 4399 * EvidenceVariable resource that defines the population for the 4400 * research.) 4401 */ 4402 public EffectEvidenceSynthesis setPopulationTarget(EvidenceVariable value) { 4403 this.populationTarget = value; 4404 return this; 4405 } 4406 4407 /** 4408 * @return {@link #exposure} (A reference to a EvidenceVariable resource that 4409 * defines the exposure for the research.) 4410 */ 4411 public Reference getExposure() { 4412 if (this.exposure == null) 4413 if (Configuration.errorOnAutoCreate()) 4414 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.exposure"); 4415 else if (Configuration.doAutoCreate()) 4416 this.exposure = new Reference(); // cc 4417 return this.exposure; 4418 } 4419 4420 public boolean hasExposure() { 4421 return this.exposure != null && !this.exposure.isEmpty(); 4422 } 4423 4424 /** 4425 * @param value {@link #exposure} (A reference to a EvidenceVariable resource 4426 * that defines the exposure for the research.) 4427 */ 4428 public EffectEvidenceSynthesis setExposure(Reference value) { 4429 this.exposure = value; 4430 return this; 4431 } 4432 4433 /** 4434 * @return {@link #exposure} The actual object that is the target of the 4435 * reference. The reference library doesn't populate this, but you can 4436 * use it to hold the resource if you resolve it. (A reference to a 4437 * EvidenceVariable resource that defines the exposure for the 4438 * research.) 4439 */ 4440 public EvidenceVariable getExposureTarget() { 4441 if (this.exposureTarget == null) 4442 if (Configuration.errorOnAutoCreate()) 4443 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.exposure"); 4444 else if (Configuration.doAutoCreate()) 4445 this.exposureTarget = new EvidenceVariable(); // aa 4446 return this.exposureTarget; 4447 } 4448 4449 /** 4450 * @param value {@link #exposure} The actual object that is the target of the 4451 * reference. The reference library doesn't use these, but you can 4452 * use it to hold the resource if you resolve it. (A reference to a 4453 * EvidenceVariable resource that defines the exposure for the 4454 * research.) 4455 */ 4456 public EffectEvidenceSynthesis setExposureTarget(EvidenceVariable value) { 4457 this.exposureTarget = value; 4458 return this; 4459 } 4460 4461 /** 4462 * @return {@link #exposureAlternative} (A reference to a EvidenceVariable 4463 * resource that defines the comparison exposure for the research.) 4464 */ 4465 public Reference getExposureAlternative() { 4466 if (this.exposureAlternative == null) 4467 if (Configuration.errorOnAutoCreate()) 4468 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.exposureAlternative"); 4469 else if (Configuration.doAutoCreate()) 4470 this.exposureAlternative = new Reference(); // cc 4471 return this.exposureAlternative; 4472 } 4473 4474 public boolean hasExposureAlternative() { 4475 return this.exposureAlternative != null && !this.exposureAlternative.isEmpty(); 4476 } 4477 4478 /** 4479 * @param value {@link #exposureAlternative} (A reference to a EvidenceVariable 4480 * resource that defines the comparison exposure for the research.) 4481 */ 4482 public EffectEvidenceSynthesis setExposureAlternative(Reference value) { 4483 this.exposureAlternative = value; 4484 return this; 4485 } 4486 4487 /** 4488 * @return {@link #exposureAlternative} The actual object that is the target of 4489 * the reference. The reference library doesn't populate this, but you 4490 * can use it to hold the resource if you resolve it. (A reference to a 4491 * EvidenceVariable resource that defines the comparison exposure for 4492 * the research.) 4493 */ 4494 public EvidenceVariable getExposureAlternativeTarget() { 4495 if (this.exposureAlternativeTarget == null) 4496 if (Configuration.errorOnAutoCreate()) 4497 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.exposureAlternative"); 4498 else if (Configuration.doAutoCreate()) 4499 this.exposureAlternativeTarget = new EvidenceVariable(); // aa 4500 return this.exposureAlternativeTarget; 4501 } 4502 4503 /** 4504 * @param value {@link #exposureAlternative} The actual object that is the 4505 * target of the reference. The reference library doesn't use 4506 * these, but you can use it to hold the resource if you resolve 4507 * it. (A reference to a EvidenceVariable resource that defines the 4508 * comparison exposure for the research.) 4509 */ 4510 public EffectEvidenceSynthesis setExposureAlternativeTarget(EvidenceVariable value) { 4511 this.exposureAlternativeTarget = value; 4512 return this; 4513 } 4514 4515 /** 4516 * @return {@link #outcome} (A reference to a EvidenceVariable resomece that 4517 * defines the outcome for the research.) 4518 */ 4519 public Reference getOutcome() { 4520 if (this.outcome == null) 4521 if (Configuration.errorOnAutoCreate()) 4522 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.outcome"); 4523 else if (Configuration.doAutoCreate()) 4524 this.outcome = new Reference(); // cc 4525 return this.outcome; 4526 } 4527 4528 public boolean hasOutcome() { 4529 return this.outcome != null && !this.outcome.isEmpty(); 4530 } 4531 4532 /** 4533 * @param value {@link #outcome} (A reference to a EvidenceVariable resomece 4534 * that defines the outcome for the research.) 4535 */ 4536 public EffectEvidenceSynthesis setOutcome(Reference value) { 4537 this.outcome = value; 4538 return this; 4539 } 4540 4541 /** 4542 * @return {@link #outcome} The actual object that is the target of the 4543 * reference. The reference library doesn't populate this, but you can 4544 * use it to hold the resource if you resolve it. (A reference to a 4545 * EvidenceVariable resomece that defines the outcome for the research.) 4546 */ 4547 public EvidenceVariable getOutcomeTarget() { 4548 if (this.outcomeTarget == null) 4549 if (Configuration.errorOnAutoCreate()) 4550 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.outcome"); 4551 else if (Configuration.doAutoCreate()) 4552 this.outcomeTarget = new EvidenceVariable(); // aa 4553 return this.outcomeTarget; 4554 } 4555 4556 /** 4557 * @param value {@link #outcome} The actual object that is the target of the 4558 * reference. The reference library doesn't use these, but you can 4559 * use it to hold the resource if you resolve it. (A reference to a 4560 * EvidenceVariable resomece that defines the outcome for the 4561 * research.) 4562 */ 4563 public EffectEvidenceSynthesis setOutcomeTarget(EvidenceVariable value) { 4564 this.outcomeTarget = value; 4565 return this; 4566 } 4567 4568 /** 4569 * @return {@link #sampleSize} (A description of the size of the sample involved 4570 * in the synthesis.) 4571 */ 4572 public EffectEvidenceSynthesisSampleSizeComponent getSampleSize() { 4573 if (this.sampleSize == null) 4574 if (Configuration.errorOnAutoCreate()) 4575 throw new Error("Attempt to auto-create EffectEvidenceSynthesis.sampleSize"); 4576 else if (Configuration.doAutoCreate()) 4577 this.sampleSize = new EffectEvidenceSynthesisSampleSizeComponent(); // cc 4578 return this.sampleSize; 4579 } 4580 4581 public boolean hasSampleSize() { 4582 return this.sampleSize != null && !this.sampleSize.isEmpty(); 4583 } 4584 4585 /** 4586 * @param value {@link #sampleSize} (A description of the size of the sample 4587 * involved in the synthesis.) 4588 */ 4589 public EffectEvidenceSynthesis setSampleSize(EffectEvidenceSynthesisSampleSizeComponent value) { 4590 this.sampleSize = value; 4591 return this; 4592 } 4593 4594 /** 4595 * @return {@link #resultsByExposure} (A description of the results for each 4596 * exposure considered in the effect estimate.) 4597 */ 4598 public List<EffectEvidenceSynthesisResultsByExposureComponent> getResultsByExposure() { 4599 if (this.resultsByExposure == null) 4600 this.resultsByExposure = new ArrayList<EffectEvidenceSynthesisResultsByExposureComponent>(); 4601 return this.resultsByExposure; 4602 } 4603 4604 /** 4605 * @return Returns a reference to <code>this</code> for easy method chaining 4606 */ 4607 public EffectEvidenceSynthesis setResultsByExposure( 4608 List<EffectEvidenceSynthesisResultsByExposureComponent> theResultsByExposure) { 4609 this.resultsByExposure = theResultsByExposure; 4610 return this; 4611 } 4612 4613 public boolean hasResultsByExposure() { 4614 if (this.resultsByExposure == null) 4615 return false; 4616 for (EffectEvidenceSynthesisResultsByExposureComponent item : this.resultsByExposure) 4617 if (!item.isEmpty()) 4618 return true; 4619 return false; 4620 } 4621 4622 public EffectEvidenceSynthesisResultsByExposureComponent addResultsByExposure() { // 3 4623 EffectEvidenceSynthesisResultsByExposureComponent t = new EffectEvidenceSynthesisResultsByExposureComponent(); 4624 if (this.resultsByExposure == null) 4625 this.resultsByExposure = new ArrayList<EffectEvidenceSynthesisResultsByExposureComponent>(); 4626 this.resultsByExposure.add(t); 4627 return t; 4628 } 4629 4630 public EffectEvidenceSynthesis addResultsByExposure(EffectEvidenceSynthesisResultsByExposureComponent t) { // 3 4631 if (t == null) 4632 return this; 4633 if (this.resultsByExposure == null) 4634 this.resultsByExposure = new ArrayList<EffectEvidenceSynthesisResultsByExposureComponent>(); 4635 this.resultsByExposure.add(t); 4636 return this; 4637 } 4638 4639 /** 4640 * @return The first repetition of repeating field {@link #resultsByExposure}, 4641 * creating it if it does not already exist 4642 */ 4643 public EffectEvidenceSynthesisResultsByExposureComponent getResultsByExposureFirstRep() { 4644 if (getResultsByExposure().isEmpty()) { 4645 addResultsByExposure(); 4646 } 4647 return getResultsByExposure().get(0); 4648 } 4649 4650 /** 4651 * @return {@link #effectEstimate} (The estimated effect of the exposure 4652 * variant.) 4653 */ 4654 public List<EffectEvidenceSynthesisEffectEstimateComponent> getEffectEstimate() { 4655 if (this.effectEstimate == null) 4656 this.effectEstimate = new ArrayList<EffectEvidenceSynthesisEffectEstimateComponent>(); 4657 return this.effectEstimate; 4658 } 4659 4660 /** 4661 * @return Returns a reference to <code>this</code> for easy method chaining 4662 */ 4663 public EffectEvidenceSynthesis setEffectEstimate( 4664 List<EffectEvidenceSynthesisEffectEstimateComponent> theEffectEstimate) { 4665 this.effectEstimate = theEffectEstimate; 4666 return this; 4667 } 4668 4669 public boolean hasEffectEstimate() { 4670 if (this.effectEstimate == null) 4671 return false; 4672 for (EffectEvidenceSynthesisEffectEstimateComponent item : this.effectEstimate) 4673 if (!item.isEmpty()) 4674 return true; 4675 return false; 4676 } 4677 4678 public EffectEvidenceSynthesisEffectEstimateComponent addEffectEstimate() { // 3 4679 EffectEvidenceSynthesisEffectEstimateComponent t = new EffectEvidenceSynthesisEffectEstimateComponent(); 4680 if (this.effectEstimate == null) 4681 this.effectEstimate = new ArrayList<EffectEvidenceSynthesisEffectEstimateComponent>(); 4682 this.effectEstimate.add(t); 4683 return t; 4684 } 4685 4686 public EffectEvidenceSynthesis addEffectEstimate(EffectEvidenceSynthesisEffectEstimateComponent t) { // 3 4687 if (t == null) 4688 return this; 4689 if (this.effectEstimate == null) 4690 this.effectEstimate = new ArrayList<EffectEvidenceSynthesisEffectEstimateComponent>(); 4691 this.effectEstimate.add(t); 4692 return this; 4693 } 4694 4695 /** 4696 * @return The first repetition of repeating field {@link #effectEstimate}, 4697 * creating it if it does not already exist 4698 */ 4699 public EffectEvidenceSynthesisEffectEstimateComponent getEffectEstimateFirstRep() { 4700 if (getEffectEstimate().isEmpty()) { 4701 addEffectEstimate(); 4702 } 4703 return getEffectEstimate().get(0); 4704 } 4705 4706 /** 4707 * @return {@link #certainty} (A description of the certainty of the effect 4708 * estimate.) 4709 */ 4710 public List<EffectEvidenceSynthesisCertaintyComponent> getCertainty() { 4711 if (this.certainty == null) 4712 this.certainty = new ArrayList<EffectEvidenceSynthesisCertaintyComponent>(); 4713 return this.certainty; 4714 } 4715 4716 /** 4717 * @return Returns a reference to <code>this</code> for easy method chaining 4718 */ 4719 public EffectEvidenceSynthesis setCertainty(List<EffectEvidenceSynthesisCertaintyComponent> theCertainty) { 4720 this.certainty = theCertainty; 4721 return this; 4722 } 4723 4724 public boolean hasCertainty() { 4725 if (this.certainty == null) 4726 return false; 4727 for (EffectEvidenceSynthesisCertaintyComponent item : this.certainty) 4728 if (!item.isEmpty()) 4729 return true; 4730 return false; 4731 } 4732 4733 public EffectEvidenceSynthesisCertaintyComponent addCertainty() { // 3 4734 EffectEvidenceSynthesisCertaintyComponent t = new EffectEvidenceSynthesisCertaintyComponent(); 4735 if (this.certainty == null) 4736 this.certainty = new ArrayList<EffectEvidenceSynthesisCertaintyComponent>(); 4737 this.certainty.add(t); 4738 return t; 4739 } 4740 4741 public EffectEvidenceSynthesis addCertainty(EffectEvidenceSynthesisCertaintyComponent t) { // 3 4742 if (t == null) 4743 return this; 4744 if (this.certainty == null) 4745 this.certainty = new ArrayList<EffectEvidenceSynthesisCertaintyComponent>(); 4746 this.certainty.add(t); 4747 return this; 4748 } 4749 4750 /** 4751 * @return The first repetition of repeating field {@link #certainty}, creating 4752 * it if it does not already exist 4753 */ 4754 public EffectEvidenceSynthesisCertaintyComponent getCertaintyFirstRep() { 4755 if (getCertainty().isEmpty()) { 4756 addCertainty(); 4757 } 4758 return getCertainty().get(0); 4759 } 4760 4761 protected void listChildren(List<Property> children) { 4762 super.listChildren(children); 4763 children.add(new Property("url", "uri", 4764 "An absolute URI that is used to identify this effect evidence synthesis when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this effect evidence synthesis is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the effect evidence synthesis is stored on different servers.", 4765 0, 1, url)); 4766 children.add(new Property("identifier", "Identifier", 4767 "A formal identifier that is used to identify this effect evidence synthesis when it is represented in other formats, or referenced in a specification, model, design or an instance.", 4768 0, java.lang.Integer.MAX_VALUE, identifier)); 4769 children.add(new Property("version", "string", 4770 "The identifier that is used to identify this version of the effect evidence synthesis when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the effect evidence synthesis author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 4771 0, 1, version)); 4772 children.add(new Property("name", "string", 4773 "A natural language name identifying the effect evidence synthesis. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 4774 0, 1, name)); 4775 children.add(new Property("title", "string", 4776 "A short, descriptive, user-friendly title for the effect evidence synthesis.", 0, 1, title)); 4777 children.add(new Property("status", "code", 4778 "The status of this effect evidence synthesis. Enables tracking the life-cycle of the content.", 0, 1, status)); 4779 children.add(new Property("date", "dateTime", 4780 "The date (and optionally time) when the effect evidence synthesis was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the effect evidence synthesis changes.", 4781 0, 1, date)); 4782 children.add(new Property("publisher", "string", 4783 "The name of the organization or individual that published the effect evidence synthesis.", 0, 1, publisher)); 4784 children.add(new Property("contact", "ContactDetail", 4785 "Contact details to assist a user in finding and communicating with the publisher.", 0, 4786 java.lang.Integer.MAX_VALUE, contact)); 4787 children.add(new Property("description", "markdown", 4788 "A free text natural language description of the effect evidence synthesis from a consumer's perspective.", 0, 4789 1, description)); 4790 children.add( 4791 new Property("note", "Annotation", "A human-readable string to clarify or explain concepts about the resource.", 4792 0, java.lang.Integer.MAX_VALUE, note)); 4793 children.add(new Property("useContext", "UsageContext", 4794 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate effect evidence synthesis instances.", 4795 0, java.lang.Integer.MAX_VALUE, useContext)); 4796 children.add(new Property("jurisdiction", "CodeableConcept", 4797 "A legal or geographic region in which the effect evidence synthesis is intended to be used.", 0, 4798 java.lang.Integer.MAX_VALUE, jurisdiction)); 4799 children.add(new Property("copyright", "markdown", 4800 "A copyright statement relating to the effect evidence synthesis and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the effect evidence synthesis.", 4801 0, 1, copyright)); 4802 children.add(new Property("approvalDate", "date", 4803 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 4804 0, 1, approvalDate)); 4805 children.add(new Property("lastReviewDate", "date", 4806 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 4807 0, 1, lastReviewDate)); 4808 children.add(new Property("effectivePeriod", "Period", 4809 "The period during which the effect evidence synthesis content was or is planned to be in active use.", 0, 1, 4810 effectivePeriod)); 4811 children.add(new Property("topic", "CodeableConcept", 4812 "Descriptive topics related to the content of the EffectEvidenceSynthesis. Topics provide a high-level categorization grouping types of EffectEvidenceSynthesiss that can be useful for filtering and searching.", 4813 0, java.lang.Integer.MAX_VALUE, topic)); 4814 children.add(new Property("author", "ContactDetail", 4815 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 4816 java.lang.Integer.MAX_VALUE, author)); 4817 children.add(new Property("editor", "ContactDetail", 4818 "An individual or organization primarily responsible for internal coherence of the content.", 0, 4819 java.lang.Integer.MAX_VALUE, editor)); 4820 children.add(new Property("reviewer", "ContactDetail", 4821 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 4822 java.lang.Integer.MAX_VALUE, reviewer)); 4823 children.add(new Property("endorser", "ContactDetail", 4824 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 4825 java.lang.Integer.MAX_VALUE, endorser)); 4826 children.add(new Property("relatedArtifact", "RelatedArtifact", 4827 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 4828 java.lang.Integer.MAX_VALUE, relatedArtifact)); 4829 children.add( 4830 new Property("synthesisType", "CodeableConcept", "Type of synthesis eg meta-analysis.", 0, 1, synthesisType)); 4831 children.add(new Property("studyType", "CodeableConcept", "Type of study eg randomized trial.", 0, 1, studyType)); 4832 children.add(new Property("population", "Reference(EvidenceVariable)", 4833 "A reference to a EvidenceVariable resource that defines the population for the research.", 0, 1, population)); 4834 children.add(new Property("exposure", "Reference(EvidenceVariable)", 4835 "A reference to a EvidenceVariable resource that defines the exposure for the research.", 0, 1, exposure)); 4836 children.add(new Property("exposureAlternative", "Reference(EvidenceVariable)", 4837 "A reference to a EvidenceVariable resource that defines the comparison exposure for the research.", 0, 1, 4838 exposureAlternative)); 4839 children.add(new Property("outcome", "Reference(EvidenceVariable)", 4840 "A reference to a EvidenceVariable resomece that defines the outcome for the research.", 0, 1, outcome)); 4841 children.add(new Property("sampleSize", "", "A description of the size of the sample involved in the synthesis.", 0, 4842 1, sampleSize)); 4843 children.add(new Property("resultsByExposure", "", 4844 "A description of the results for each exposure considered in the effect estimate.", 0, 4845 java.lang.Integer.MAX_VALUE, resultsByExposure)); 4846 children.add(new Property("effectEstimate", "", "The estimated effect of the exposure variant.", 0, 4847 java.lang.Integer.MAX_VALUE, effectEstimate)); 4848 children.add(new Property("certainty", "", "A description of the certainty of the effect estimate.", 0, 4849 java.lang.Integer.MAX_VALUE, certainty)); 4850 } 4851 4852 @Override 4853 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4854 switch (_hash) { 4855 case 116079: 4856 /* url */ return new Property("url", "uri", 4857 "An absolute URI that is used to identify this effect evidence synthesis when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this effect evidence synthesis is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the effect evidence synthesis is stored on different servers.", 4858 0, 1, url); 4859 case -1618432855: 4860 /* identifier */ return new Property("identifier", "Identifier", 4861 "A formal identifier that is used to identify this effect evidence synthesis when it is represented in other formats, or referenced in a specification, model, design or an instance.", 4862 0, java.lang.Integer.MAX_VALUE, identifier); 4863 case 351608024: 4864 /* version */ return new Property("version", "string", 4865 "The identifier that is used to identify this version of the effect evidence synthesis when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the effect evidence synthesis author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 4866 0, 1, version); 4867 case 3373707: 4868 /* name */ return new Property("name", "string", 4869 "A natural language name identifying the effect evidence synthesis. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 4870 0, 1, name); 4871 case 110371416: 4872 /* title */ return new Property("title", "string", 4873 "A short, descriptive, user-friendly title for the effect evidence synthesis.", 0, 1, title); 4874 case -892481550: 4875 /* status */ return new Property("status", "code", 4876 "The status of this effect evidence synthesis. Enables tracking the life-cycle of the content.", 0, 1, 4877 status); 4878 case 3076014: 4879 /* date */ return new Property("date", "dateTime", 4880 "The date (and optionally time) when the effect evidence synthesis was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the effect evidence synthesis changes.", 4881 0, 1, date); 4882 case 1447404028: 4883 /* publisher */ return new Property("publisher", "string", 4884 "The name of the organization or individual that published the effect evidence synthesis.", 0, 1, publisher); 4885 case 951526432: 4886 /* contact */ return new Property("contact", "ContactDetail", 4887 "Contact details to assist a user in finding and communicating with the publisher.", 0, 4888 java.lang.Integer.MAX_VALUE, contact); 4889 case -1724546052: 4890 /* description */ return new Property("description", "markdown", 4891 "A free text natural language description of the effect evidence synthesis from a consumer's perspective.", 0, 4892 1, description); 4893 case 3387378: 4894 /* note */ return new Property("note", "Annotation", 4895 "A human-readable string to clarify or explain concepts about the resource.", 0, java.lang.Integer.MAX_VALUE, 4896 note); 4897 case -669707736: 4898 /* useContext */ return new Property("useContext", "UsageContext", 4899 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate effect evidence synthesis instances.", 4900 0, java.lang.Integer.MAX_VALUE, useContext); 4901 case -507075711: 4902 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 4903 "A legal or geographic region in which the effect evidence synthesis is intended to be used.", 0, 4904 java.lang.Integer.MAX_VALUE, jurisdiction); 4905 case 1522889671: 4906 /* copyright */ return new Property("copyright", "markdown", 4907 "A copyright statement relating to the effect evidence synthesis and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the effect evidence synthesis.", 4908 0, 1, copyright); 4909 case 223539345: 4910 /* approvalDate */ return new Property("approvalDate", "date", 4911 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 4912 0, 1, approvalDate); 4913 case -1687512484: 4914 /* lastReviewDate */ return new Property("lastReviewDate", "date", 4915 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 4916 0, 1, lastReviewDate); 4917 case -403934648: 4918 /* effectivePeriod */ return new Property("effectivePeriod", "Period", 4919 "The period during which the effect evidence synthesis content was or is planned to be in active use.", 0, 1, 4920 effectivePeriod); 4921 case 110546223: 4922 /* topic */ return new Property("topic", "CodeableConcept", 4923 "Descriptive topics related to the content of the EffectEvidenceSynthesis. Topics provide a high-level categorization grouping types of EffectEvidenceSynthesiss that can be useful for filtering and searching.", 4924 0, java.lang.Integer.MAX_VALUE, topic); 4925 case -1406328437: 4926 /* author */ return new Property("author", "ContactDetail", 4927 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 4928 java.lang.Integer.MAX_VALUE, author); 4929 case -1307827859: 4930 /* editor */ return new Property("editor", "ContactDetail", 4931 "An individual or organization primarily responsible for internal coherence of the content.", 0, 4932 java.lang.Integer.MAX_VALUE, editor); 4933 case -261190139: 4934 /* reviewer */ return new Property("reviewer", "ContactDetail", 4935 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 4936 java.lang.Integer.MAX_VALUE, reviewer); 4937 case 1740277666: 4938 /* endorser */ return new Property("endorser", "ContactDetail", 4939 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 4940 java.lang.Integer.MAX_VALUE, endorser); 4941 case 666807069: 4942 /* relatedArtifact */ return new Property("relatedArtifact", "RelatedArtifact", 4943 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 4944 java.lang.Integer.MAX_VALUE, relatedArtifact); 4945 case 672726254: 4946 /* synthesisType */ return new Property("synthesisType", "CodeableConcept", "Type of synthesis eg meta-analysis.", 4947 0, 1, synthesisType); 4948 case -1955265373: 4949 /* studyType */ return new Property("studyType", "CodeableConcept", "Type of study eg randomized trial.", 0, 1, 4950 studyType); 4951 case -2023558323: 4952 /* population */ return new Property("population", "Reference(EvidenceVariable)", 4953 "A reference to a EvidenceVariable resource that defines the population for the research.", 0, 1, population); 4954 case -1926005497: 4955 /* exposure */ return new Property("exposure", "Reference(EvidenceVariable)", 4956 "A reference to a EvidenceVariable resource that defines the exposure for the research.", 0, 1, exposure); 4957 case -1875462106: 4958 /* exposureAlternative */ return new Property("exposureAlternative", "Reference(EvidenceVariable)", 4959 "A reference to a EvidenceVariable resource that defines the comparison exposure for the research.", 0, 1, 4960 exposureAlternative); 4961 case -1106507950: 4962 /* outcome */ return new Property("outcome", "Reference(EvidenceVariable)", 4963 "A reference to a EvidenceVariable resomece that defines the outcome for the research.", 0, 1, outcome); 4964 case 143123659: 4965 /* sampleSize */ return new Property("sampleSize", "", 4966 "A description of the size of the sample involved in the synthesis.", 0, 1, sampleSize); 4967 case 553042708: 4968 /* resultsByExposure */ return new Property("resultsByExposure", "", 4969 "A description of the results for each exposure considered in the effect estimate.", 0, 4970 java.lang.Integer.MAX_VALUE, resultsByExposure); 4971 case 707469785: 4972 /* effectEstimate */ return new Property("effectEstimate", "", "The estimated effect of the exposure variant.", 0, 4973 java.lang.Integer.MAX_VALUE, effectEstimate); 4974 case -1404142937: 4975 /* certainty */ return new Property("certainty", "", "A description of the certainty of the effect estimate.", 0, 4976 java.lang.Integer.MAX_VALUE, certainty); 4977 default: 4978 return super.getNamedProperty(_hash, _name, _checkValid); 4979 } 4980 4981 } 4982 4983 @Override 4984 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4985 switch (hash) { 4986 case 116079: 4987 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 4988 case -1618432855: 4989 /* identifier */ return this.identifier == null ? new Base[0] 4990 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4991 case 351608024: 4992 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 4993 case 3373707: 4994 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 4995 case 110371416: 4996 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 4997 case -892481550: 4998 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 4999 case 3076014: 5000 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 5001 case 1447404028: 5002 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 5003 case 951526432: 5004 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 5005 case -1724546052: 5006 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 5007 case 3387378: 5008 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 5009 case -669707736: 5010 /* useContext */ return this.useContext == null ? new Base[0] 5011 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 5012 case -507075711: 5013 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 5014 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 5015 case 1522889671: 5016 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 5017 case 223539345: 5018 /* approvalDate */ return this.approvalDate == null ? new Base[0] : new Base[] { this.approvalDate }; // DateType 5019 case -1687512484: 5020 /* lastReviewDate */ return this.lastReviewDate == null ? new Base[0] : new Base[] { this.lastReviewDate }; // DateType 5021 case -403934648: 5022 /* effectivePeriod */ return this.effectivePeriod == null ? new Base[0] : new Base[] { this.effectivePeriod }; // Period 5023 case 110546223: 5024 /* topic */ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 5025 case -1406328437: 5026 /* author */ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 5027 case -1307827859: 5028 /* editor */ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 5029 case -261190139: 5030 /* reviewer */ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 5031 case 1740277666: 5032 /* endorser */ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 5033 case 666807069: 5034 /* relatedArtifact */ return this.relatedArtifact == null ? new Base[0] 5035 : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 5036 case 672726254: 5037 /* synthesisType */ return this.synthesisType == null ? new Base[0] : new Base[] { this.synthesisType }; // CodeableConcept 5038 case -1955265373: 5039 /* studyType */ return this.studyType == null ? new Base[0] : new Base[] { this.studyType }; // CodeableConcept 5040 case -2023558323: 5041 /* population */ return this.population == null ? new Base[0] : new Base[] { this.population }; // Reference 5042 case -1926005497: 5043 /* exposure */ return this.exposure == null ? new Base[0] : new Base[] { this.exposure }; // Reference 5044 case -1875462106: 5045 /* exposureAlternative */ return this.exposureAlternative == null ? new Base[0] 5046 : new Base[] { this.exposureAlternative }; // Reference 5047 case -1106507950: 5048 /* outcome */ return this.outcome == null ? new Base[0] : new Base[] { this.outcome }; // Reference 5049 case 143123659: 5050 /* sampleSize */ return this.sampleSize == null ? new Base[0] : new Base[] { this.sampleSize }; // EffectEvidenceSynthesisSampleSizeComponent 5051 case 553042708: 5052 /* resultsByExposure */ return this.resultsByExposure == null ? new Base[0] 5053 : this.resultsByExposure.toArray(new Base[this.resultsByExposure.size()]); // EffectEvidenceSynthesisResultsByExposureComponent 5054 case 707469785: 5055 /* effectEstimate */ return this.effectEstimate == null ? new Base[0] 5056 : this.effectEstimate.toArray(new Base[this.effectEstimate.size()]); // EffectEvidenceSynthesisEffectEstimateComponent 5057 case -1404142937: 5058 /* certainty */ return this.certainty == null ? new Base[0] 5059 : this.certainty.toArray(new Base[this.certainty.size()]); // EffectEvidenceSynthesisCertaintyComponent 5060 default: 5061 return super.getProperty(hash, name, checkValid); 5062 } 5063 5064 } 5065 5066 @Override 5067 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5068 switch (hash) { 5069 case 116079: // url 5070 this.url = castToUri(value); // UriType 5071 return value; 5072 case -1618432855: // identifier 5073 this.getIdentifier().add(castToIdentifier(value)); // Identifier 5074 return value; 5075 case 351608024: // version 5076 this.version = castToString(value); // StringType 5077 return value; 5078 case 3373707: // name 5079 this.name = castToString(value); // StringType 5080 return value; 5081 case 110371416: // title 5082 this.title = castToString(value); // StringType 5083 return value; 5084 case -892481550: // status 5085 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 5086 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5087 return value; 5088 case 3076014: // date 5089 this.date = castToDateTime(value); // DateTimeType 5090 return value; 5091 case 1447404028: // publisher 5092 this.publisher = castToString(value); // StringType 5093 return value; 5094 case 951526432: // contact 5095 this.getContact().add(castToContactDetail(value)); // ContactDetail 5096 return value; 5097 case -1724546052: // description 5098 this.description = castToMarkdown(value); // MarkdownType 5099 return value; 5100 case 3387378: // note 5101 this.getNote().add(castToAnnotation(value)); // Annotation 5102 return value; 5103 case -669707736: // useContext 5104 this.getUseContext().add(castToUsageContext(value)); // UsageContext 5105 return value; 5106 case -507075711: // jurisdiction 5107 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 5108 return value; 5109 case 1522889671: // copyright 5110 this.copyright = castToMarkdown(value); // MarkdownType 5111 return value; 5112 case 223539345: // approvalDate 5113 this.approvalDate = castToDate(value); // DateType 5114 return value; 5115 case -1687512484: // lastReviewDate 5116 this.lastReviewDate = castToDate(value); // DateType 5117 return value; 5118 case -403934648: // effectivePeriod 5119 this.effectivePeriod = castToPeriod(value); // Period 5120 return value; 5121 case 110546223: // topic 5122 this.getTopic().add(castToCodeableConcept(value)); // CodeableConcept 5123 return value; 5124 case -1406328437: // author 5125 this.getAuthor().add(castToContactDetail(value)); // ContactDetail 5126 return value; 5127 case -1307827859: // editor 5128 this.getEditor().add(castToContactDetail(value)); // ContactDetail 5129 return value; 5130 case -261190139: // reviewer 5131 this.getReviewer().add(castToContactDetail(value)); // ContactDetail 5132 return value; 5133 case 1740277666: // endorser 5134 this.getEndorser().add(castToContactDetail(value)); // ContactDetail 5135 return value; 5136 case 666807069: // relatedArtifact 5137 this.getRelatedArtifact().add(castToRelatedArtifact(value)); // RelatedArtifact 5138 return value; 5139 case 672726254: // synthesisType 5140 this.synthesisType = castToCodeableConcept(value); // CodeableConcept 5141 return value; 5142 case -1955265373: // studyType 5143 this.studyType = castToCodeableConcept(value); // CodeableConcept 5144 return value; 5145 case -2023558323: // population 5146 this.population = castToReference(value); // Reference 5147 return value; 5148 case -1926005497: // exposure 5149 this.exposure = castToReference(value); // Reference 5150 return value; 5151 case -1875462106: // exposureAlternative 5152 this.exposureAlternative = castToReference(value); // Reference 5153 return value; 5154 case -1106507950: // outcome 5155 this.outcome = castToReference(value); // Reference 5156 return value; 5157 case 143123659: // sampleSize 5158 this.sampleSize = (EffectEvidenceSynthesisSampleSizeComponent) value; // EffectEvidenceSynthesisSampleSizeComponent 5159 return value; 5160 case 553042708: // resultsByExposure 5161 this.getResultsByExposure().add((EffectEvidenceSynthesisResultsByExposureComponent) value); // EffectEvidenceSynthesisResultsByExposureComponent 5162 return value; 5163 case 707469785: // effectEstimate 5164 this.getEffectEstimate().add((EffectEvidenceSynthesisEffectEstimateComponent) value); // EffectEvidenceSynthesisEffectEstimateComponent 5165 return value; 5166 case -1404142937: // certainty 5167 this.getCertainty().add((EffectEvidenceSynthesisCertaintyComponent) value); // EffectEvidenceSynthesisCertaintyComponent 5168 return value; 5169 default: 5170 return super.setProperty(hash, name, value); 5171 } 5172 5173 } 5174 5175 @Override 5176 public Base setProperty(String name, Base value) throws FHIRException { 5177 if (name.equals("url")) { 5178 this.url = castToUri(value); // UriType 5179 } else if (name.equals("identifier")) { 5180 this.getIdentifier().add(castToIdentifier(value)); 5181 } else if (name.equals("version")) { 5182 this.version = castToString(value); // StringType 5183 } else if (name.equals("name")) { 5184 this.name = castToString(value); // StringType 5185 } else if (name.equals("title")) { 5186 this.title = castToString(value); // StringType 5187 } else if (name.equals("status")) { 5188 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 5189 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5190 } else if (name.equals("date")) { 5191 this.date = castToDateTime(value); // DateTimeType 5192 } else if (name.equals("publisher")) { 5193 this.publisher = castToString(value); // StringType 5194 } else if (name.equals("contact")) { 5195 this.getContact().add(castToContactDetail(value)); 5196 } else if (name.equals("description")) { 5197 this.description = castToMarkdown(value); // MarkdownType 5198 } else if (name.equals("note")) { 5199 this.getNote().add(castToAnnotation(value)); 5200 } else if (name.equals("useContext")) { 5201 this.getUseContext().add(castToUsageContext(value)); 5202 } else if (name.equals("jurisdiction")) { 5203 this.getJurisdiction().add(castToCodeableConcept(value)); 5204 } else if (name.equals("copyright")) { 5205 this.copyright = castToMarkdown(value); // MarkdownType 5206 } else if (name.equals("approvalDate")) { 5207 this.approvalDate = castToDate(value); // DateType 5208 } else if (name.equals("lastReviewDate")) { 5209 this.lastReviewDate = castToDate(value); // DateType 5210 } else if (name.equals("effectivePeriod")) { 5211 this.effectivePeriod = castToPeriod(value); // Period 5212 } else if (name.equals("topic")) { 5213 this.getTopic().add(castToCodeableConcept(value)); 5214 } else if (name.equals("author")) { 5215 this.getAuthor().add(castToContactDetail(value)); 5216 } else if (name.equals("editor")) { 5217 this.getEditor().add(castToContactDetail(value)); 5218 } else if (name.equals("reviewer")) { 5219 this.getReviewer().add(castToContactDetail(value)); 5220 } else if (name.equals("endorser")) { 5221 this.getEndorser().add(castToContactDetail(value)); 5222 } else if (name.equals("relatedArtifact")) { 5223 this.getRelatedArtifact().add(castToRelatedArtifact(value)); 5224 } else if (name.equals("synthesisType")) { 5225 this.synthesisType = castToCodeableConcept(value); // CodeableConcept 5226 } else if (name.equals("studyType")) { 5227 this.studyType = castToCodeableConcept(value); // CodeableConcept 5228 } else if (name.equals("population")) { 5229 this.population = castToReference(value); // Reference 5230 } else if (name.equals("exposure")) { 5231 this.exposure = castToReference(value); // Reference 5232 } else if (name.equals("exposureAlternative")) { 5233 this.exposureAlternative = castToReference(value); // Reference 5234 } else if (name.equals("outcome")) { 5235 this.outcome = castToReference(value); // Reference 5236 } else if (name.equals("sampleSize")) { 5237 this.sampleSize = (EffectEvidenceSynthesisSampleSizeComponent) value; // EffectEvidenceSynthesisSampleSizeComponent 5238 } else if (name.equals("resultsByExposure")) { 5239 this.getResultsByExposure().add((EffectEvidenceSynthesisResultsByExposureComponent) value); 5240 } else if (name.equals("effectEstimate")) { 5241 this.getEffectEstimate().add((EffectEvidenceSynthesisEffectEstimateComponent) value); 5242 } else if (name.equals("certainty")) { 5243 this.getCertainty().add((EffectEvidenceSynthesisCertaintyComponent) value); 5244 } else 5245 return super.setProperty(name, value); 5246 return value; 5247 } 5248 5249 @Override 5250 public Base makeProperty(int hash, String name) throws FHIRException { 5251 switch (hash) { 5252 case 116079: 5253 return getUrlElement(); 5254 case -1618432855: 5255 return addIdentifier(); 5256 case 351608024: 5257 return getVersionElement(); 5258 case 3373707: 5259 return getNameElement(); 5260 case 110371416: 5261 return getTitleElement(); 5262 case -892481550: 5263 return getStatusElement(); 5264 case 3076014: 5265 return getDateElement(); 5266 case 1447404028: 5267 return getPublisherElement(); 5268 case 951526432: 5269 return addContact(); 5270 case -1724546052: 5271 return getDescriptionElement(); 5272 case 3387378: 5273 return addNote(); 5274 case -669707736: 5275 return addUseContext(); 5276 case -507075711: 5277 return addJurisdiction(); 5278 case 1522889671: 5279 return getCopyrightElement(); 5280 case 223539345: 5281 return getApprovalDateElement(); 5282 case -1687512484: 5283 return getLastReviewDateElement(); 5284 case -403934648: 5285 return getEffectivePeriod(); 5286 case 110546223: 5287 return addTopic(); 5288 case -1406328437: 5289 return addAuthor(); 5290 case -1307827859: 5291 return addEditor(); 5292 case -261190139: 5293 return addReviewer(); 5294 case 1740277666: 5295 return addEndorser(); 5296 case 666807069: 5297 return addRelatedArtifact(); 5298 case 672726254: 5299 return getSynthesisType(); 5300 case -1955265373: 5301 return getStudyType(); 5302 case -2023558323: 5303 return getPopulation(); 5304 case -1926005497: 5305 return getExposure(); 5306 case -1875462106: 5307 return getExposureAlternative(); 5308 case -1106507950: 5309 return getOutcome(); 5310 case 143123659: 5311 return getSampleSize(); 5312 case 553042708: 5313 return addResultsByExposure(); 5314 case 707469785: 5315 return addEffectEstimate(); 5316 case -1404142937: 5317 return addCertainty(); 5318 default: 5319 return super.makeProperty(hash, name); 5320 } 5321 5322 } 5323 5324 @Override 5325 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5326 switch (hash) { 5327 case 116079: 5328 /* url */ return new String[] { "uri" }; 5329 case -1618432855: 5330 /* identifier */ return new String[] { "Identifier" }; 5331 case 351608024: 5332 /* version */ return new String[] { "string" }; 5333 case 3373707: 5334 /* name */ return new String[] { "string" }; 5335 case 110371416: 5336 /* title */ return new String[] { "string" }; 5337 case -892481550: 5338 /* status */ return new String[] { "code" }; 5339 case 3076014: 5340 /* date */ return new String[] { "dateTime" }; 5341 case 1447404028: 5342 /* publisher */ return new String[] { "string" }; 5343 case 951526432: 5344 /* contact */ return new String[] { "ContactDetail" }; 5345 case -1724546052: 5346 /* description */ return new String[] { "markdown" }; 5347 case 3387378: 5348 /* note */ return new String[] { "Annotation" }; 5349 case -669707736: 5350 /* useContext */ return new String[] { "UsageContext" }; 5351 case -507075711: 5352 /* jurisdiction */ return new String[] { "CodeableConcept" }; 5353 case 1522889671: 5354 /* copyright */ return new String[] { "markdown" }; 5355 case 223539345: 5356 /* approvalDate */ return new String[] { "date" }; 5357 case -1687512484: 5358 /* lastReviewDate */ return new String[] { "date" }; 5359 case -403934648: 5360 /* effectivePeriod */ return new String[] { "Period" }; 5361 case 110546223: 5362 /* topic */ return new String[] { "CodeableConcept" }; 5363 case -1406328437: 5364 /* author */ return new String[] { "ContactDetail" }; 5365 case -1307827859: 5366 /* editor */ return new String[] { "ContactDetail" }; 5367 case -261190139: 5368 /* reviewer */ return new String[] { "ContactDetail" }; 5369 case 1740277666: 5370 /* endorser */ return new String[] { "ContactDetail" }; 5371 case 666807069: 5372 /* relatedArtifact */ return new String[] { "RelatedArtifact" }; 5373 case 672726254: 5374 /* synthesisType */ return new String[] { "CodeableConcept" }; 5375 case -1955265373: 5376 /* studyType */ return new String[] { "CodeableConcept" }; 5377 case -2023558323: 5378 /* population */ return new String[] { "Reference" }; 5379 case -1926005497: 5380 /* exposure */ return new String[] { "Reference" }; 5381 case -1875462106: 5382 /* exposureAlternative */ return new String[] { "Reference" }; 5383 case -1106507950: 5384 /* outcome */ return new String[] { "Reference" }; 5385 case 143123659: 5386 /* sampleSize */ return new String[] {}; 5387 case 553042708: 5388 /* resultsByExposure */ return new String[] {}; 5389 case 707469785: 5390 /* effectEstimate */ return new String[] {}; 5391 case -1404142937: 5392 /* certainty */ return new String[] {}; 5393 default: 5394 return super.getTypesForProperty(hash, name); 5395 } 5396 5397 } 5398 5399 @Override 5400 public Base addChild(String name) throws FHIRException { 5401 if (name.equals("url")) { 5402 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.url"); 5403 } else if (name.equals("identifier")) { 5404 return addIdentifier(); 5405 } else if (name.equals("version")) { 5406 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.version"); 5407 } else if (name.equals("name")) { 5408 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.name"); 5409 } else if (name.equals("title")) { 5410 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.title"); 5411 } else if (name.equals("status")) { 5412 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.status"); 5413 } else if (name.equals("date")) { 5414 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.date"); 5415 } else if (name.equals("publisher")) { 5416 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.publisher"); 5417 } else if (name.equals("contact")) { 5418 return addContact(); 5419 } else if (name.equals("description")) { 5420 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.description"); 5421 } else if (name.equals("note")) { 5422 return addNote(); 5423 } else if (name.equals("useContext")) { 5424 return addUseContext(); 5425 } else if (name.equals("jurisdiction")) { 5426 return addJurisdiction(); 5427 } else if (name.equals("copyright")) { 5428 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.copyright"); 5429 } else if (name.equals("approvalDate")) { 5430 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.approvalDate"); 5431 } else if (name.equals("lastReviewDate")) { 5432 throw new FHIRException("Cannot call addChild on a singleton property EffectEvidenceSynthesis.lastReviewDate"); 5433 } else if (name.equals("effectivePeriod")) { 5434 this.effectivePeriod = new Period(); 5435 return this.effectivePeriod; 5436 } else if (name.equals("topic")) { 5437 return addTopic(); 5438 } else if (name.equals("author")) { 5439 return addAuthor(); 5440 } else if (name.equals("editor")) { 5441 return addEditor(); 5442 } else if (name.equals("reviewer")) { 5443 return addReviewer(); 5444 } else if (name.equals("endorser")) { 5445 return addEndorser(); 5446 } else if (name.equals("relatedArtifact")) { 5447 return addRelatedArtifact(); 5448 } else if (name.equals("synthesisType")) { 5449 this.synthesisType = new CodeableConcept(); 5450 return this.synthesisType; 5451 } else if (name.equals("studyType")) { 5452 this.studyType = new CodeableConcept(); 5453 return this.studyType; 5454 } else if (name.equals("population")) { 5455 this.population = new Reference(); 5456 return this.population; 5457 } else if (name.equals("exposure")) { 5458 this.exposure = new Reference(); 5459 return this.exposure; 5460 } else if (name.equals("exposureAlternative")) { 5461 this.exposureAlternative = new Reference(); 5462 return this.exposureAlternative; 5463 } else if (name.equals("outcome")) { 5464 this.outcome = new Reference(); 5465 return this.outcome; 5466 } else if (name.equals("sampleSize")) { 5467 this.sampleSize = new EffectEvidenceSynthesisSampleSizeComponent(); 5468 return this.sampleSize; 5469 } else if (name.equals("resultsByExposure")) { 5470 return addResultsByExposure(); 5471 } else if (name.equals("effectEstimate")) { 5472 return addEffectEstimate(); 5473 } else if (name.equals("certainty")) { 5474 return addCertainty(); 5475 } else 5476 return super.addChild(name); 5477 } 5478 5479 public String fhirType() { 5480 return "EffectEvidenceSynthesis"; 5481 5482 } 5483 5484 public EffectEvidenceSynthesis copy() { 5485 EffectEvidenceSynthesis dst = new EffectEvidenceSynthesis(); 5486 copyValues(dst); 5487 return dst; 5488 } 5489 5490 public void copyValues(EffectEvidenceSynthesis dst) { 5491 super.copyValues(dst); 5492 dst.url = url == null ? null : url.copy(); 5493 if (identifier != null) { 5494 dst.identifier = new ArrayList<Identifier>(); 5495 for (Identifier i : identifier) 5496 dst.identifier.add(i.copy()); 5497 } 5498 ; 5499 dst.version = version == null ? null : version.copy(); 5500 dst.name = name == null ? null : name.copy(); 5501 dst.title = title == null ? null : title.copy(); 5502 dst.status = status == null ? null : status.copy(); 5503 dst.date = date == null ? null : date.copy(); 5504 dst.publisher = publisher == null ? null : publisher.copy(); 5505 if (contact != null) { 5506 dst.contact = new ArrayList<ContactDetail>(); 5507 for (ContactDetail i : contact) 5508 dst.contact.add(i.copy()); 5509 } 5510 ; 5511 dst.description = description == null ? null : description.copy(); 5512 if (note != null) { 5513 dst.note = new ArrayList<Annotation>(); 5514 for (Annotation i : note) 5515 dst.note.add(i.copy()); 5516 } 5517 ; 5518 if (useContext != null) { 5519 dst.useContext = new ArrayList<UsageContext>(); 5520 for (UsageContext i : useContext) 5521 dst.useContext.add(i.copy()); 5522 } 5523 ; 5524 if (jurisdiction != null) { 5525 dst.jurisdiction = new ArrayList<CodeableConcept>(); 5526 for (CodeableConcept i : jurisdiction) 5527 dst.jurisdiction.add(i.copy()); 5528 } 5529 ; 5530 dst.copyright = copyright == null ? null : copyright.copy(); 5531 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 5532 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 5533 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 5534 if (topic != null) { 5535 dst.topic = new ArrayList<CodeableConcept>(); 5536 for (CodeableConcept i : topic) 5537 dst.topic.add(i.copy()); 5538 } 5539 ; 5540 if (author != null) { 5541 dst.author = new ArrayList<ContactDetail>(); 5542 for (ContactDetail i : author) 5543 dst.author.add(i.copy()); 5544 } 5545 ; 5546 if (editor != null) { 5547 dst.editor = new ArrayList<ContactDetail>(); 5548 for (ContactDetail i : editor) 5549 dst.editor.add(i.copy()); 5550 } 5551 ; 5552 if (reviewer != null) { 5553 dst.reviewer = new ArrayList<ContactDetail>(); 5554 for (ContactDetail i : reviewer) 5555 dst.reviewer.add(i.copy()); 5556 } 5557 ; 5558 if (endorser != null) { 5559 dst.endorser = new ArrayList<ContactDetail>(); 5560 for (ContactDetail i : endorser) 5561 dst.endorser.add(i.copy()); 5562 } 5563 ; 5564 if (relatedArtifact != null) { 5565 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 5566 for (RelatedArtifact i : relatedArtifact) 5567 dst.relatedArtifact.add(i.copy()); 5568 } 5569 ; 5570 dst.synthesisType = synthesisType == null ? null : synthesisType.copy(); 5571 dst.studyType = studyType == null ? null : studyType.copy(); 5572 dst.population = population == null ? null : population.copy(); 5573 dst.exposure = exposure == null ? null : exposure.copy(); 5574 dst.exposureAlternative = exposureAlternative == null ? null : exposureAlternative.copy(); 5575 dst.outcome = outcome == null ? null : outcome.copy(); 5576 dst.sampleSize = sampleSize == null ? null : sampleSize.copy(); 5577 if (resultsByExposure != null) { 5578 dst.resultsByExposure = new ArrayList<EffectEvidenceSynthesisResultsByExposureComponent>(); 5579 for (EffectEvidenceSynthesisResultsByExposureComponent i : resultsByExposure) 5580 dst.resultsByExposure.add(i.copy()); 5581 } 5582 ; 5583 if (effectEstimate != null) { 5584 dst.effectEstimate = new ArrayList<EffectEvidenceSynthesisEffectEstimateComponent>(); 5585 for (EffectEvidenceSynthesisEffectEstimateComponent i : effectEstimate) 5586 dst.effectEstimate.add(i.copy()); 5587 } 5588 ; 5589 if (certainty != null) { 5590 dst.certainty = new ArrayList<EffectEvidenceSynthesisCertaintyComponent>(); 5591 for (EffectEvidenceSynthesisCertaintyComponent i : certainty) 5592 dst.certainty.add(i.copy()); 5593 } 5594 ; 5595 } 5596 5597 protected EffectEvidenceSynthesis typedCopy() { 5598 return copy(); 5599 } 5600 5601 @Override 5602 public boolean equalsDeep(Base other_) { 5603 if (!super.equalsDeep(other_)) 5604 return false; 5605 if (!(other_ instanceof EffectEvidenceSynthesis)) 5606 return false; 5607 EffectEvidenceSynthesis o = (EffectEvidenceSynthesis) other_; 5608 return compareDeep(identifier, o.identifier, true) && compareDeep(note, o.note, true) 5609 && compareDeep(copyright, o.copyright, true) && compareDeep(approvalDate, o.approvalDate, true) 5610 && compareDeep(lastReviewDate, o.lastReviewDate, true) && compareDeep(effectivePeriod, o.effectivePeriod, true) 5611 && compareDeep(topic, o.topic, true) && compareDeep(author, o.author, true) 5612 && compareDeep(editor, o.editor, true) && compareDeep(reviewer, o.reviewer, true) 5613 && compareDeep(endorser, o.endorser, true) && compareDeep(relatedArtifact, o.relatedArtifact, true) 5614 && compareDeep(synthesisType, o.synthesisType, true) && compareDeep(studyType, o.studyType, true) 5615 && compareDeep(population, o.population, true) && compareDeep(exposure, o.exposure, true) 5616 && compareDeep(exposureAlternative, o.exposureAlternative, true) && compareDeep(outcome, o.outcome, true) 5617 && compareDeep(sampleSize, o.sampleSize, true) && compareDeep(resultsByExposure, o.resultsByExposure, true) 5618 && compareDeep(effectEstimate, o.effectEstimate, true) && compareDeep(certainty, o.certainty, true); 5619 } 5620 5621 @Override 5622 public boolean equalsShallow(Base other_) { 5623 if (!super.equalsShallow(other_)) 5624 return false; 5625 if (!(other_ instanceof EffectEvidenceSynthesis)) 5626 return false; 5627 EffectEvidenceSynthesis o = (EffectEvidenceSynthesis) other_; 5628 return compareValues(copyright, o.copyright, true) && compareValues(approvalDate, o.approvalDate, true) 5629 && compareValues(lastReviewDate, o.lastReviewDate, true); 5630 } 5631 5632 public boolean isEmpty() { 5633 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, note, copyright, approvalDate, 5634 lastReviewDate, effectivePeriod, topic, author, editor, reviewer, endorser, relatedArtifact, synthesisType, 5635 studyType, population, exposure, exposureAlternative, outcome, sampleSize, resultsByExposure, effectEstimate, 5636 certainty); 5637 } 5638 5639 @Override 5640 public ResourceType getResourceType() { 5641 return ResourceType.EffectEvidenceSynthesis; 5642 } 5643 5644 /** 5645 * Search parameter: <b>date</b> 5646 * <p> 5647 * Description: <b>The effect evidence synthesis publication date</b><br> 5648 * Type: <b>date</b><br> 5649 * Path: <b>EffectEvidenceSynthesis.date</b><br> 5650 * </p> 5651 */ 5652 @SearchParamDefinition(name = "date", path = "EffectEvidenceSynthesis.date", description = "The effect evidence synthesis publication date", type = "date") 5653 public static final String SP_DATE = "date"; 5654 /** 5655 * <b>Fluent Client</b> search parameter constant for <b>date</b> 5656 * <p> 5657 * Description: <b>The effect evidence synthesis publication date</b><br> 5658 * Type: <b>date</b><br> 5659 * Path: <b>EffectEvidenceSynthesis.date</b><br> 5660 * </p> 5661 */ 5662 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 5663 SP_DATE); 5664 5665 /** 5666 * Search parameter: <b>identifier</b> 5667 * <p> 5668 * Description: <b>External identifier for the effect evidence synthesis</b><br> 5669 * Type: <b>token</b><br> 5670 * Path: <b>EffectEvidenceSynthesis.identifier</b><br> 5671 * </p> 5672 */ 5673 @SearchParamDefinition(name = "identifier", path = "EffectEvidenceSynthesis.identifier", description = "External identifier for the effect evidence synthesis", type = "token") 5674 public static final String SP_IDENTIFIER = "identifier"; 5675 /** 5676 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5677 * <p> 5678 * Description: <b>External identifier for the effect evidence synthesis</b><br> 5679 * Type: <b>token</b><br> 5680 * Path: <b>EffectEvidenceSynthesis.identifier</b><br> 5681 * </p> 5682 */ 5683 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5684 SP_IDENTIFIER); 5685 5686 /** 5687 * Search parameter: <b>context-type-value</b> 5688 * <p> 5689 * Description: <b>A use context type and value assigned to the effect evidence 5690 * synthesis</b><br> 5691 * Type: <b>composite</b><br> 5692 * Path: <b></b><br> 5693 * </p> 5694 */ 5695 @SearchParamDefinition(name = "context-type-value", path = "EffectEvidenceSynthesis.useContext", description = "A use context type and value assigned to the effect evidence synthesis", type = "composite", compositeOf = { 5696 "context-type", "context" }) 5697 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 5698 /** 5699 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 5700 * <p> 5701 * Description: <b>A use context type and value assigned to the effect evidence 5702 * synthesis</b><br> 5703 * Type: <b>composite</b><br> 5704 * Path: <b></b><br> 5705 * </p> 5706 */ 5707 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 5708 SP_CONTEXT_TYPE_VALUE); 5709 5710 /** 5711 * Search parameter: <b>jurisdiction</b> 5712 * <p> 5713 * Description: <b>Intended jurisdiction for the effect evidence 5714 * synthesis</b><br> 5715 * Type: <b>token</b><br> 5716 * Path: <b>EffectEvidenceSynthesis.jurisdiction</b><br> 5717 * </p> 5718 */ 5719 @SearchParamDefinition(name = "jurisdiction", path = "EffectEvidenceSynthesis.jurisdiction", description = "Intended jurisdiction for the effect evidence synthesis", type = "token") 5720 public static final String SP_JURISDICTION = "jurisdiction"; 5721 /** 5722 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 5723 * <p> 5724 * Description: <b>Intended jurisdiction for the effect evidence 5725 * synthesis</b><br> 5726 * Type: <b>token</b><br> 5727 * Path: <b>EffectEvidenceSynthesis.jurisdiction</b><br> 5728 * </p> 5729 */ 5730 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5731 SP_JURISDICTION); 5732 5733 /** 5734 * Search parameter: <b>description</b> 5735 * <p> 5736 * Description: <b>The description of the effect evidence synthesis</b><br> 5737 * Type: <b>string</b><br> 5738 * Path: <b>EffectEvidenceSynthesis.description</b><br> 5739 * </p> 5740 */ 5741 @SearchParamDefinition(name = "description", path = "EffectEvidenceSynthesis.description", description = "The description of the effect evidence synthesis", type = "string") 5742 public static final String SP_DESCRIPTION = "description"; 5743 /** 5744 * <b>Fluent Client</b> search parameter constant for <b>description</b> 5745 * <p> 5746 * Description: <b>The description of the effect evidence synthesis</b><br> 5747 * Type: <b>string</b><br> 5748 * Path: <b>EffectEvidenceSynthesis.description</b><br> 5749 * </p> 5750 */ 5751 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 5752 SP_DESCRIPTION); 5753 5754 /** 5755 * Search parameter: <b>context-type</b> 5756 * <p> 5757 * Description: <b>A type of use context assigned to the effect evidence 5758 * synthesis</b><br> 5759 * Type: <b>token</b><br> 5760 * Path: <b>EffectEvidenceSynthesis.useContext.code</b><br> 5761 * </p> 5762 */ 5763 @SearchParamDefinition(name = "context-type", path = "EffectEvidenceSynthesis.useContext.code", description = "A type of use context assigned to the effect evidence synthesis", type = "token") 5764 public static final String SP_CONTEXT_TYPE = "context-type"; 5765 /** 5766 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 5767 * <p> 5768 * Description: <b>A type of use context assigned to the effect evidence 5769 * synthesis</b><br> 5770 * Type: <b>token</b><br> 5771 * Path: <b>EffectEvidenceSynthesis.useContext.code</b><br> 5772 * </p> 5773 */ 5774 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5775 SP_CONTEXT_TYPE); 5776 5777 /** 5778 * Search parameter: <b>title</b> 5779 * <p> 5780 * Description: <b>The human-friendly name of the effect evidence 5781 * synthesis</b><br> 5782 * Type: <b>string</b><br> 5783 * Path: <b>EffectEvidenceSynthesis.title</b><br> 5784 * </p> 5785 */ 5786 @SearchParamDefinition(name = "title", path = "EffectEvidenceSynthesis.title", description = "The human-friendly name of the effect evidence synthesis", type = "string") 5787 public static final String SP_TITLE = "title"; 5788 /** 5789 * <b>Fluent Client</b> search parameter constant for <b>title</b> 5790 * <p> 5791 * Description: <b>The human-friendly name of the effect evidence 5792 * synthesis</b><br> 5793 * Type: <b>string</b><br> 5794 * Path: <b>EffectEvidenceSynthesis.title</b><br> 5795 * </p> 5796 */ 5797 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 5798 SP_TITLE); 5799 5800 /** 5801 * Search parameter: <b>version</b> 5802 * <p> 5803 * Description: <b>The business version of the effect evidence synthesis</b><br> 5804 * Type: <b>token</b><br> 5805 * Path: <b>EffectEvidenceSynthesis.version</b><br> 5806 * </p> 5807 */ 5808 @SearchParamDefinition(name = "version", path = "EffectEvidenceSynthesis.version", description = "The business version of the effect evidence synthesis", type = "token") 5809 public static final String SP_VERSION = "version"; 5810 /** 5811 * <b>Fluent Client</b> search parameter constant for <b>version</b> 5812 * <p> 5813 * Description: <b>The business version of the effect evidence synthesis</b><br> 5814 * Type: <b>token</b><br> 5815 * Path: <b>EffectEvidenceSynthesis.version</b><br> 5816 * </p> 5817 */ 5818 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5819 SP_VERSION); 5820 5821 /** 5822 * Search parameter: <b>url</b> 5823 * <p> 5824 * Description: <b>The uri that identifies the effect evidence synthesis</b><br> 5825 * Type: <b>uri</b><br> 5826 * Path: <b>EffectEvidenceSynthesis.url</b><br> 5827 * </p> 5828 */ 5829 @SearchParamDefinition(name = "url", path = "EffectEvidenceSynthesis.url", description = "The uri that identifies the effect evidence synthesis", type = "uri") 5830 public static final String SP_URL = "url"; 5831 /** 5832 * <b>Fluent Client</b> search parameter constant for <b>url</b> 5833 * <p> 5834 * Description: <b>The uri that identifies the effect evidence synthesis</b><br> 5835 * Type: <b>uri</b><br> 5836 * Path: <b>EffectEvidenceSynthesis.url</b><br> 5837 * </p> 5838 */ 5839 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 5840 5841 /** 5842 * Search parameter: <b>context-quantity</b> 5843 * <p> 5844 * Description: <b>A quantity- or range-valued use context assigned to the 5845 * effect evidence synthesis</b><br> 5846 * Type: <b>quantity</b><br> 5847 * Path: <b>EffectEvidenceSynthesis.useContext.valueQuantity, 5848 * EffectEvidenceSynthesis.useContext.valueRange</b><br> 5849 * </p> 5850 */ 5851 @SearchParamDefinition(name = "context-quantity", path = "(EffectEvidenceSynthesis.useContext.value as Quantity) | (EffectEvidenceSynthesis.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the effect evidence synthesis", type = "quantity") 5852 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 5853 /** 5854 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 5855 * <p> 5856 * Description: <b>A quantity- or range-valued use context assigned to the 5857 * effect evidence synthesis</b><br> 5858 * Type: <b>quantity</b><br> 5859 * Path: <b>EffectEvidenceSynthesis.useContext.valueQuantity, 5860 * EffectEvidenceSynthesis.useContext.valueRange</b><br> 5861 * </p> 5862 */ 5863 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 5864 SP_CONTEXT_QUANTITY); 5865 5866 /** 5867 * Search parameter: <b>effective</b> 5868 * <p> 5869 * Description: <b>The time during which the effect evidence synthesis is 5870 * intended to be in use</b><br> 5871 * Type: <b>date</b><br> 5872 * Path: <b>EffectEvidenceSynthesis.effectivePeriod</b><br> 5873 * </p> 5874 */ 5875 @SearchParamDefinition(name = "effective", path = "EffectEvidenceSynthesis.effectivePeriod", description = "The time during which the effect evidence synthesis is intended to be in use", type = "date") 5876 public static final String SP_EFFECTIVE = "effective"; 5877 /** 5878 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 5879 * <p> 5880 * Description: <b>The time during which the effect evidence synthesis is 5881 * intended to be in use</b><br> 5882 * Type: <b>date</b><br> 5883 * Path: <b>EffectEvidenceSynthesis.effectivePeriod</b><br> 5884 * </p> 5885 */ 5886 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam( 5887 SP_EFFECTIVE); 5888 5889 /** 5890 * Search parameter: <b>name</b> 5891 * <p> 5892 * Description: <b>Computationally friendly name of the effect evidence 5893 * synthesis</b><br> 5894 * Type: <b>string</b><br> 5895 * Path: <b>EffectEvidenceSynthesis.name</b><br> 5896 * </p> 5897 */ 5898 @SearchParamDefinition(name = "name", path = "EffectEvidenceSynthesis.name", description = "Computationally friendly name of the effect evidence synthesis", type = "string") 5899 public static final String SP_NAME = "name"; 5900 /** 5901 * <b>Fluent Client</b> search parameter constant for <b>name</b> 5902 * <p> 5903 * Description: <b>Computationally friendly name of the effect evidence 5904 * synthesis</b><br> 5905 * Type: <b>string</b><br> 5906 * Path: <b>EffectEvidenceSynthesis.name</b><br> 5907 * </p> 5908 */ 5909 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 5910 SP_NAME); 5911 5912 /** 5913 * Search parameter: <b>context</b> 5914 * <p> 5915 * Description: <b>A use context assigned to the effect evidence 5916 * synthesis</b><br> 5917 * Type: <b>token</b><br> 5918 * Path: <b>EffectEvidenceSynthesis.useContext.valueCodeableConcept</b><br> 5919 * </p> 5920 */ 5921 @SearchParamDefinition(name = "context", path = "(EffectEvidenceSynthesis.useContext.value as CodeableConcept)", description = "A use context assigned to the effect evidence synthesis", type = "token") 5922 public static final String SP_CONTEXT = "context"; 5923 /** 5924 * <b>Fluent Client</b> search parameter constant for <b>context</b> 5925 * <p> 5926 * Description: <b>A use context assigned to the effect evidence 5927 * synthesis</b><br> 5928 * Type: <b>token</b><br> 5929 * Path: <b>EffectEvidenceSynthesis.useContext.valueCodeableConcept</b><br> 5930 * </p> 5931 */ 5932 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5933 SP_CONTEXT); 5934 5935 /** 5936 * Search parameter: <b>publisher</b> 5937 * <p> 5938 * Description: <b>Name of the publisher of the effect evidence 5939 * synthesis</b><br> 5940 * Type: <b>string</b><br> 5941 * Path: <b>EffectEvidenceSynthesis.publisher</b><br> 5942 * </p> 5943 */ 5944 @SearchParamDefinition(name = "publisher", path = "EffectEvidenceSynthesis.publisher", description = "Name of the publisher of the effect evidence synthesis", type = "string") 5945 public static final String SP_PUBLISHER = "publisher"; 5946 /** 5947 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 5948 * <p> 5949 * Description: <b>Name of the publisher of the effect evidence 5950 * synthesis</b><br> 5951 * Type: <b>string</b><br> 5952 * Path: <b>EffectEvidenceSynthesis.publisher</b><br> 5953 * </p> 5954 */ 5955 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 5956 SP_PUBLISHER); 5957 5958 /** 5959 * Search parameter: <b>context-type-quantity</b> 5960 * <p> 5961 * Description: <b>A use context type and quantity- or range-based value 5962 * assigned to the effect evidence synthesis</b><br> 5963 * Type: <b>composite</b><br> 5964 * Path: <b></b><br> 5965 * </p> 5966 */ 5967 @SearchParamDefinition(name = "context-type-quantity", path = "EffectEvidenceSynthesis.useContext", description = "A use context type and quantity- or range-based value assigned to the effect evidence synthesis", type = "composite", compositeOf = { 5968 "context-type", "context-quantity" }) 5969 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 5970 /** 5971 * <b>Fluent Client</b> search parameter constant for 5972 * <b>context-type-quantity</b> 5973 * <p> 5974 * Description: <b>A use context type and quantity- or range-based value 5975 * assigned to the effect evidence synthesis</b><br> 5976 * Type: <b>composite</b><br> 5977 * Path: <b></b><br> 5978 * </p> 5979 */ 5980 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 5981 SP_CONTEXT_TYPE_QUANTITY); 5982 5983 /** 5984 * Search parameter: <b>status</b> 5985 * <p> 5986 * Description: <b>The current status of the effect evidence synthesis</b><br> 5987 * Type: <b>token</b><br> 5988 * Path: <b>EffectEvidenceSynthesis.status</b><br> 5989 * </p> 5990 */ 5991 @SearchParamDefinition(name = "status", path = "EffectEvidenceSynthesis.status", description = "The current status of the effect evidence synthesis", type = "token") 5992 public static final String SP_STATUS = "status"; 5993 /** 5994 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5995 * <p> 5996 * Description: <b>The current status of the effect evidence synthesis</b><br> 5997 * Type: <b>token</b><br> 5998 * Path: <b>EffectEvidenceSynthesis.status</b><br> 5999 * </p> 6000 */ 6001 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6002 SP_STATUS); 6003 6004}