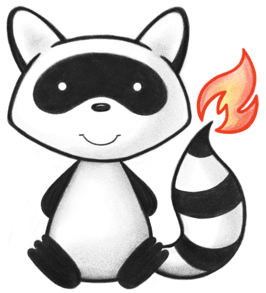
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseElement; 038import org.hl7.fhir.instance.model.api.IBaseHasExtensions; 039import org.hl7.fhir.utilities.StandardsStatus; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044 045/** 046 * Base definition for all elements in a resource. 047 */ 048public abstract class Element extends Base implements IBaseHasExtensions, IBaseElement { 049 050 /** 051 * Unique id for the element within a resource (for internal references). This 052 * may be any string value that does not contain spaces. 053 */ 054 @Child(name = "id", type = { StringType.class }, order = 0, min = 0, max = 1, modifier = false, summary = false) 055 @Description(shortDefinition = "Unique id for inter-element referencing", formalDefinition = "Unique id for the element within a resource (for internal references). This may be any string value that does not contain spaces.") 056 protected StringType id; 057 058 /** 059 * May be used to represent additional information that is not part of the basic 060 * definition of the element. To make the use of extensions safe and manageable, 061 * there is a strict set of governance applied to the definition and use of 062 * extensions. Though any implementer can define an extension, there is a set of 063 * requirements that SHALL be met as part of the definition of the extension. 064 */ 065 @Child(name = "extension", type = { 066 Extension.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 067 @Description(shortDefinition = "Additional content defined by implementations", formalDefinition = "May be used to represent additional information that is not part of the basic definition of the element. To make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.") 068 protected List<Extension> extension; 069 070 private static final long serialVersionUID = -1452745816L; 071 072 /** 073 * Constructor 074 */ 075 public Element() { 076 super(); 077 } 078 079 /** 080 * @return {@link #id} (Unique id for the element within a resource (for 081 * internal references). This may be any string value that does not 082 * contain spaces.). This is the underlying object with id, value and 083 * extensions. The accessor "getId" gives direct access to the value 084 */ 085 public StringType getIdElement() { 086 if (this.id == null) 087 if (Configuration.errorOnAutoCreate()) 088 throw new Error("Attempt to auto-create Element.id"); 089 else if (Configuration.doAutoCreate()) 090 this.id = new StringType(); // bb 091 return this.id; 092 } 093 094 public boolean hasIdElement() { 095 return this.id != null && !this.id.isEmpty(); 096 } 097 098 public boolean hasId() { 099 return this.id != null && !this.id.isEmpty(); 100 } 101 102 /** 103 * @param value {@link #id} (Unique id for the element within a resource (for 104 * internal references). This may be any string value that does not 105 * contain spaces.). This is the underlying object with id, value 106 * and extensions. The accessor "getId" gives direct access to the 107 * value 108 */ 109 public Element setIdElement(StringType value) { 110 this.id = value; 111 return this; 112 } 113 114 /** 115 * @return Unique id for the element within a resource (for internal 116 * references). This may be any string value that does not contain 117 * spaces. 118 */ 119 public String getId() { 120 return this.id == null ? null : this.id.getValue(); 121 } 122 123 /** 124 * @param value Unique id for the element within a resource (for internal 125 * references). This may be any string value that does not contain 126 * spaces. 127 */ 128 public Element setId(String value) { 129 if (Utilities.noString(value)) 130 this.id = null; 131 else { 132 if (this.id == null) 133 this.id = new StringType(); 134 this.id.setValue(value); 135 } 136 return this; 137 } 138 139 /** 140 * @return {@link #extension} (May be used to represent additional information 141 * that is not part of the basic definition of the element. To make the 142 * use of extensions safe and manageable, there is a strict set of 143 * governance applied to the definition and use of extensions. Though 144 * any implementer can define an extension, there is a set of 145 * requirements that SHALL be met as part of the definition of the 146 * extension.) 147 */ 148 public List<Extension> getExtension() { 149 if (this.extension == null) 150 this.extension = new ArrayList<Extension>(); 151 return this.extension; 152 } 153 154 /** 155 * @return Returns a reference to <code>this</code> for easy method chaining 156 */ 157 public Element setExtension(List<Extension> theExtension) { 158 this.extension = theExtension; 159 return this; 160 } 161 162 public boolean hasExtension() { 163 if (this.extension == null) 164 return false; 165 for (Extension item : this.extension) 166 if (!item.isEmpty()) 167 return true; 168 return false; 169 } 170 171 public Extension addExtension() { // 3 172 Extension t = new Extension(); 173 if (this.extension == null) 174 this.extension = new ArrayList<Extension>(); 175 this.extension.add(t); 176 return t; 177 } 178 179 public Element addExtension(Extension t) { // 3 180 if (t == null) 181 return this; 182 if (this.extension == null) 183 this.extension = new ArrayList<Extension>(); 184 this.extension.add(t); 185 return this; 186 } 187 188 /** 189 * @return The first repetition of repeating field {@link #extension}, creating 190 * it if it does not already exist 191 */ 192 public Extension getExtensionFirstRep() { 193 if (getExtension().isEmpty()) { 194 addExtension(); 195 } 196 return getExtension().get(0); 197 } 198 199 /** 200 * Returns an unmodifiable list containing all extensions on this element which 201 * match the given URL. 202 * 203 * @param theUrl The URL. Must not be blank or null. 204 * @return an unmodifiable list containing all extensions on this element which 205 * match the given URL 206 */ 207 public List<Extension> getExtensionsByUrl(String theUrl) { 208 org.apache.commons.lang3.Validate.notBlank(theUrl, "theUrl must not be blank or null"); 209 ArrayList<Extension> retVal = new ArrayList<Extension>(); 210 for (Extension next : getExtension()) { 211 if (theUrl.equals(next.getUrl())) { 212 retVal.add(next); 213 } 214 } 215 return java.util.Collections.unmodifiableList(retVal); 216 } 217 218 public boolean hasExtension(String theUrl) { 219 return !getExtensionsByUrl(theUrl).isEmpty(); 220 } 221 222 public String getExtensionString(String theUrl) throws FHIRException { 223 List<Extension> ext = getExtensionsByUrl(theUrl); 224 if (ext.isEmpty()) 225 return null; 226 if (ext.size() > 1) 227 throw new FHIRException("Multiple matching extensions found"); 228 if (!ext.get(0).getValue().isPrimitive()) 229 throw new FHIRException("Extension could not be converted to a string"); 230 return ext.get(0).getValue().primitiveValue(); 231 } 232 233 protected void listChildren(List<Property> children) { 234 children.add(new Property("id", "string", 235 "Unique id for the element within a resource (for internal references). This may be any string value that does not contain spaces.", 236 0, 1, id)); 237 children.add(new Property("extension", "Extension", 238 "May be used to represent additional information that is not part of the basic definition of the element. To make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.", 239 0, java.lang.Integer.MAX_VALUE, extension)); 240 } 241 242 @Override 243 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 244 switch (_hash) { 245 case 3355: 246 /* id */ return new Property("id", "string", 247 "Unique id for the element within a resource (for internal references). This may be any string value that does not contain spaces.", 248 0, 1, id); 249 case -612557761: 250 /* extension */ return new Property("extension", "Extension", 251 "May be used to represent additional information that is not part of the basic definition of the element. To make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.", 252 0, java.lang.Integer.MAX_VALUE, extension); 253 default: 254 return super.getNamedProperty(_hash, _name, _checkValid); 255 } 256 257 } 258 259 @Override 260 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 261 switch (hash) { 262 case 3355: 263 /* id */ return this.id == null ? new Base[0] : new Base[] { this.id }; // StringType 264 case -612557761: 265 /* extension */ return this.extension == null ? new Base[0] 266 : this.extension.toArray(new Base[this.extension.size()]); // Extension 267 default: 268 return super.getProperty(hash, name, checkValid); 269 } 270 271 } 272 273 @Override 274 public Base setProperty(int hash, String name, Base value) throws FHIRException { 275 switch (hash) { 276 case 3355: // id 277 this.id = castToString(value); // StringType 278 return value; 279 case -612557761: // extension 280 this.getExtension().add(castToExtension(value)); // Extension 281 return value; 282 default: 283 return super.setProperty(hash, name, value); 284 } 285 286 } 287 288 @Override 289 public Base setProperty(String name, Base value) throws FHIRException { 290 if (name.equals("id")) { 291 this.id = castToString(value); // StringType 292 } else if (name.equals("extension")) { 293 this.getExtension().add(castToExtension(value)); 294 } else 295 return super.setProperty(name, value); 296 return value; 297 } 298 299 @Override 300 public void removeChild(String name, Base value) throws FHIRException { 301 if (name.equals("id")) { 302 this.id = null; 303 } else if (name.equals("extension")) { 304 this.getExtension().remove(castToExtension(value)); 305 } else 306 super.removeChild(name, value); 307 308 } 309 310 @Override 311 public Base makeProperty(int hash, String name) throws FHIRException { 312 switch (hash) { 313 case 3355: 314 return getIdElement(); 315 case -612557761: 316 return addExtension(); 317 default: 318 return super.makeProperty(hash, name); 319 } 320 321 } 322 323 @Override 324 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 325 switch (hash) { 326 case 3355: 327 /* id */ return new String[] { "string" }; 328 case -612557761: 329 /* extension */ return new String[] { "Extension" }; 330 default: 331 return super.getTypesForProperty(hash, name); 332 } 333 334 } 335 336 @Override 337 public Base addChild(String name) throws FHIRException { 338 if (name.equals("id")) { 339 throw new FHIRException("Cannot call addChild on a singleton property Element.id"); 340 } else if (name.equals("extension")) { 341 return addExtension(); 342 } else 343 return super.addChild(name); 344 } 345 346 public String fhirType() { 347 return "Element"; 348 349 } 350 351 public abstract Element copy(); 352 353 public void copyValues(Element dst) { 354 dst.id = id == null ? null : id.copy(); 355 if (extension != null) { 356 dst.extension = new ArrayList<Extension>(); 357 for (Extension i : extension) 358 dst.extension.add(i.copy()); 359 } 360 ; 361 } 362 363 @Override 364 public boolean equalsDeep(Base other_) { 365 if (!super.equalsDeep(other_)) 366 return false; 367 if (!(other_ instanceof Element)) 368 return false; 369 Element o = (Element) other_; 370 return compareDeep(id, o.id, true) && compareDeep(extension, o.extension, true); 371 } 372 373 @Override 374 public boolean equalsShallow(Base other_) { 375 if (!super.equalsShallow(other_)) 376 return false; 377 if (!(other_ instanceof Element)) 378 return false; 379 Element o = (Element) other_; 380 return compareValues(id, o.id, true); 381 } 382 383 public boolean isEmpty() { 384 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(id, extension); 385 } 386 387 @Override 388 public String getIdBase() { 389 return getId(); 390 } 391 392 @Override 393 public void setIdBase(String value) { 394 setId(value); 395 } 396 397 // added from java-adornments.txt: 398 public void addExtension(String url, Type value) { 399 if (disallowExtensions) 400 throw new Error("Extensions are not allowed in this context"); 401 Extension ex = new Extension(); 402 ex.setUrl(url); 403 ex.setValue(value); 404 getExtension().add(ex); 405 } 406 407 public Extension getExtensionByUrl(String theUrl) { 408 org.apache.commons.lang3.Validate.notBlank(theUrl, "theUrl must not be blank or null"); 409 ArrayList<Extension> retVal = new ArrayList<Extension>(); 410 for (Extension next : getExtension()) { 411 if (theUrl.equals(next.getUrl())) { 412 retVal.add(next); 413 } 414 } 415 if (retVal.size() == 0) 416 return null; 417 else { 418 org.apache.commons.lang3.Validate.isTrue(retVal.size() == 1, "Url " + theUrl + " must have only one match"); 419 return retVal.get(0); 420 } 421 } 422 423 public void removeExtension(String theUrl) { 424 for (int i = getExtension().size() - 1; i >= 0; i--) { 425 if (theUrl.equals(getExtension().get(i).getUrl())) 426 getExtension().remove(i); 427 } 428 } 429 430 /** 431 * This is used in the FHIRPath engine to record that no extensions are allowed 432 * for this item in the context in which it is used. todo: enforce this.... 433 */ 434 private boolean disallowExtensions; 435 436 public boolean isDisallowExtensions() { 437 return disallowExtensions; 438 } 439 440 public Element setDisallowExtensions(boolean disallowExtensions) { 441 this.disallowExtensions = disallowExtensions; 442 return this; 443 } 444 445 public Element noExtensions() { 446 this.disallowExtensions = true; 447 return this; 448 } 449 450 451 public List<Extension> getExtensionsByUrl(String... theUrls) { 452 453 ArrayList<Extension> retVal = new ArrayList<>(); 454 for (Extension next : getExtension()) { 455 if (Utilities.existsInList(next.getUrl(), theUrls)) { 456 retVal.add(next); 457 } 458 } 459 return java.util.Collections.unmodifiableList(retVal); 460 } 461 462 463 public boolean hasExtension(String... theUrls) { 464 for (Extension next : getExtension()) { 465 if (Utilities.existsInList(next.getUrl(), theUrls)) { 466 return true; 467 } 468 } 469 return false; 470 } 471 472 473 474 475 public boolean hasExtension(Extension ext) { 476 if (hasExtension()) { 477 for (Extension t : getExtension()) { 478 if (Base.compareDeep(t, ext, false)) { 479 return true; 480 } 481 } 482 } 483 return false; 484 } 485 486 public void copyExtensions(org.hl7.fhir.r4.model.Element src, String... urls) { 487 for (Extension e : src.getExtension()) { 488 if (Utilities.existsInList(e.getUrl(), urls)) { 489 addExtension(e.copy()); 490 } 491 } 492 } 493 494 public void copyNewExtensions(org.hl7.fhir.r4.model.Element src, String... urls) { 495 for (Extension e : src.getExtension()) { 496 if (Utilities.existsInList(e.getUrl(), urls) && !!hasExtension(e.getUrl())) { 497 addExtension(e.copy()); 498 } 499 } 500 } 501 502 // end addition 503 504}