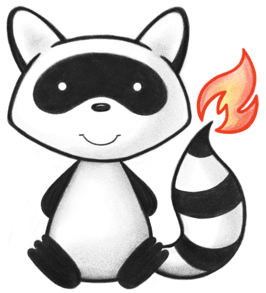
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 038import org.hl7.fhir.instance.model.api.ICompositeType; 039import org.hl7.fhir.r4.model.Enumerations.BindingStrength; 040import org.hl7.fhir.r4.model.Enumerations.BindingStrengthEnumFactory; 041import org.hl7.fhir.r4.utils.ToolingExtensions; 042// added from java-adornments.txt: 043import org.hl7.fhir.utilities.CommaSeparatedStringBuilder; 044import org.hl7.fhir.utilities.Utilities; 045 046import ca.uhn.fhir.model.api.annotation.Block; 047import ca.uhn.fhir.model.api.annotation.Child; 048import ca.uhn.fhir.model.api.annotation.DatatypeDef; 049import ca.uhn.fhir.model.api.annotation.Description; 050 051// end addition 052/** 053 * Captures constraints on each element within the resource, profile, or 054 * extension. 055 */ 056@DatatypeDef(name = "ElementDefinition") 057public class ElementDefinition extends BackboneType implements ICompositeType { 058 059 public enum PropertyRepresentation { 060 /** 061 * In XML, this property is represented as an attribute not an element. 062 */ 063 XMLATTR, 064 /** 065 * This element is represented using the XML text attribute (primitives only). 066 */ 067 XMLTEXT, 068 /** 069 * The type of this element is indicated using xsi:type. 070 */ 071 TYPEATTR, 072 /** 073 * Use CDA narrative instead of XHTML. 074 */ 075 CDATEXT, 076 /** 077 * The property is represented using XHTML. 078 */ 079 XHTML, 080 /** 081 * added to help the parsers with the generic types 082 */ 083 NULL; 084 085 public static PropertyRepresentation fromCode(String codeString) throws FHIRException { 086 if (codeString == null || "".equals(codeString)) 087 return null; 088 if ("xmlAttr".equals(codeString)) 089 return XMLATTR; 090 if ("xmlText".equals(codeString)) 091 return XMLTEXT; 092 if ("typeAttr".equals(codeString)) 093 return TYPEATTR; 094 if ("cdaText".equals(codeString)) 095 return CDATEXT; 096 if ("xhtml".equals(codeString)) 097 return XHTML; 098 if (Configuration.isAcceptInvalidEnums()) 099 return null; 100 else 101 throw new FHIRException("Unknown PropertyRepresentation code '" + codeString + "'"); 102 } 103 104 public String toCode() { 105 switch (this) { 106 case XMLATTR: 107 return "xmlAttr"; 108 case XMLTEXT: 109 return "xmlText"; 110 case TYPEATTR: 111 return "typeAttr"; 112 case CDATEXT: 113 return "cdaText"; 114 case XHTML: 115 return "xhtml"; 116 case NULL: 117 return null; 118 default: 119 return "?"; 120 } 121 } 122 123 public String getSystem() { 124 switch (this) { 125 case XMLATTR: 126 return "http://hl7.org/fhir/property-representation"; 127 case XMLTEXT: 128 return "http://hl7.org/fhir/property-representation"; 129 case TYPEATTR: 130 return "http://hl7.org/fhir/property-representation"; 131 case CDATEXT: 132 return "http://hl7.org/fhir/property-representation"; 133 case XHTML: 134 return "http://hl7.org/fhir/property-representation"; 135 case NULL: 136 return null; 137 default: 138 return "?"; 139 } 140 } 141 142 public String getDefinition() { 143 switch (this) { 144 case XMLATTR: 145 return "In XML, this property is represented as an attribute not an element."; 146 case XMLTEXT: 147 return "This element is represented using the XML text attribute (primitives only)."; 148 case TYPEATTR: 149 return "The type of this element is indicated using xsi:type."; 150 case CDATEXT: 151 return "Use CDA narrative instead of XHTML."; 152 case XHTML: 153 return "The property is represented using XHTML."; 154 case NULL: 155 return null; 156 default: 157 return "?"; 158 } 159 } 160 161 public String getDisplay() { 162 switch (this) { 163 case XMLATTR: 164 return "XML Attribute"; 165 case XMLTEXT: 166 return "XML Text"; 167 case TYPEATTR: 168 return "Type Attribute"; 169 case CDATEXT: 170 return "CDA Text Format"; 171 case XHTML: 172 return "XHTML"; 173 case NULL: 174 return null; 175 default: 176 return "?"; 177 } 178 } 179 } 180 181 public static class PropertyRepresentationEnumFactory implements EnumFactory<PropertyRepresentation> { 182 public PropertyRepresentation fromCode(String codeString) throws IllegalArgumentException { 183 if (codeString == null || "".equals(codeString)) 184 if (codeString == null || "".equals(codeString)) 185 return null; 186 if ("xmlAttr".equals(codeString)) 187 return PropertyRepresentation.XMLATTR; 188 if ("xmlText".equals(codeString)) 189 return PropertyRepresentation.XMLTEXT; 190 if ("typeAttr".equals(codeString)) 191 return PropertyRepresentation.TYPEATTR; 192 if ("cdaText".equals(codeString)) 193 return PropertyRepresentation.CDATEXT; 194 if ("xhtml".equals(codeString)) 195 return PropertyRepresentation.XHTML; 196 throw new IllegalArgumentException("Unknown PropertyRepresentation code '" + codeString + "'"); 197 } 198 199 public Enumeration<PropertyRepresentation> fromType(PrimitiveType<?> code) throws FHIRException { 200 if (code == null) 201 return null; 202 if (code.isEmpty()) 203 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.NULL, code); 204 String codeString = code.asStringValue(); 205 if (codeString == null || "".equals(codeString)) 206 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.NULL, code); 207 if ("xmlAttr".equals(codeString)) 208 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.XMLATTR, code); 209 if ("xmlText".equals(codeString)) 210 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.XMLTEXT, code); 211 if ("typeAttr".equals(codeString)) 212 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.TYPEATTR, code); 213 if ("cdaText".equals(codeString)) 214 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.CDATEXT, code); 215 if ("xhtml".equals(codeString)) 216 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.XHTML, code); 217 throw new FHIRException("Unknown PropertyRepresentation code '" + codeString + "'"); 218 } 219 220 public String toCode(PropertyRepresentation code) { 221 if (code == PropertyRepresentation.XMLATTR) 222 return "xmlAttr"; 223 if (code == PropertyRepresentation.XMLTEXT) 224 return "xmlText"; 225 if (code == PropertyRepresentation.TYPEATTR) 226 return "typeAttr"; 227 if (code == PropertyRepresentation.CDATEXT) 228 return "cdaText"; 229 if (code == PropertyRepresentation.XHTML) 230 return "xhtml"; 231 return "?"; 232 } 233 234 public String toSystem(PropertyRepresentation code) { 235 return code.getSystem(); 236 } 237 } 238 239 public enum DiscriminatorType { 240 /** 241 * The slices have different values in the nominated element. 242 */ 243 VALUE, 244 /** 245 * The slices are differentiated by the presence or absence of the nominated 246 * element. 247 */ 248 EXISTS, 249 /** 250 * The slices have different values in the nominated element, as determined by 251 * testing them against the applicable ElementDefinition.pattern[x]. 252 */ 253 PATTERN, 254 /** 255 * The slices are differentiated by type of the nominated element. 256 */ 257 TYPE, 258 /** 259 * The slices are differentiated by conformance of the nominated element to a 260 * specified profile. Note that if the path specifies .resolve() then the 261 * profile is the target profile on the reference. In this case, validation by 262 * the possible profiles is required to differentiate the slices. 263 */ 264 PROFILE, 265 /** 266 * added to help the parsers with the generic types 267 */ 268 NULL; 269 270 public static DiscriminatorType fromCode(String codeString) throws FHIRException { 271 if (codeString == null || "".equals(codeString)) 272 return null; 273 if ("value".equals(codeString)) 274 return VALUE; 275 if ("exists".equals(codeString)) 276 return EXISTS; 277 if ("pattern".equals(codeString)) 278 return PATTERN; 279 if ("type".equals(codeString)) 280 return TYPE; 281 if ("profile".equals(codeString)) 282 return PROFILE; 283 if (Configuration.isAcceptInvalidEnums()) 284 return null; 285 else 286 throw new FHIRException("Unknown DiscriminatorType code '" + codeString + "'"); 287 } 288 289 public String toCode() { 290 switch (this) { 291 case VALUE: 292 return "value"; 293 case EXISTS: 294 return "exists"; 295 case PATTERN: 296 return "pattern"; 297 case TYPE: 298 return "type"; 299 case PROFILE: 300 return "profile"; 301 case NULL: 302 return null; 303 default: 304 return "?"; 305 } 306 } 307 308 public String getSystem() { 309 switch (this) { 310 case VALUE: 311 return "http://hl7.org/fhir/discriminator-type"; 312 case EXISTS: 313 return "http://hl7.org/fhir/discriminator-type"; 314 case PATTERN: 315 return "http://hl7.org/fhir/discriminator-type"; 316 case TYPE: 317 return "http://hl7.org/fhir/discriminator-type"; 318 case PROFILE: 319 return "http://hl7.org/fhir/discriminator-type"; 320 case NULL: 321 return null; 322 default: 323 return "?"; 324 } 325 } 326 327 public String getDefinition() { 328 switch (this) { 329 case VALUE: 330 return "The slices have different values in the nominated element."; 331 case EXISTS: 332 return "The slices are differentiated by the presence or absence of the nominated element."; 333 case PATTERN: 334 return "The slices have different values in the nominated element, as determined by testing them against the applicable ElementDefinition.pattern[x]."; 335 case TYPE: 336 return "The slices are differentiated by type of the nominated element."; 337 case PROFILE: 338 return "The slices are differentiated by conformance of the nominated element to a specified profile. Note that if the path specifies .resolve() then the profile is the target profile on the reference. In this case, validation by the possible profiles is required to differentiate the slices."; 339 case NULL: 340 return null; 341 default: 342 return "?"; 343 } 344 } 345 346 public String getDisplay() { 347 switch (this) { 348 case VALUE: 349 return "Value"; 350 case EXISTS: 351 return "Exists"; 352 case PATTERN: 353 return "Pattern"; 354 case TYPE: 355 return "Type"; 356 case PROFILE: 357 return "Profile"; 358 case NULL: 359 return null; 360 default: 361 return "?"; 362 } 363 } 364 } 365 366 public static class DiscriminatorTypeEnumFactory implements EnumFactory<DiscriminatorType> { 367 public DiscriminatorType fromCode(String codeString) throws IllegalArgumentException { 368 if (codeString == null || "".equals(codeString)) 369 if (codeString == null || "".equals(codeString)) 370 return null; 371 if ("value".equals(codeString)) 372 return DiscriminatorType.VALUE; 373 if ("exists".equals(codeString)) 374 return DiscriminatorType.EXISTS; 375 if ("pattern".equals(codeString)) 376 return DiscriminatorType.PATTERN; 377 if ("type".equals(codeString)) 378 return DiscriminatorType.TYPE; 379 if ("profile".equals(codeString)) 380 return DiscriminatorType.PROFILE; 381 throw new IllegalArgumentException("Unknown DiscriminatorType code '" + codeString + "'"); 382 } 383 384 public Enumeration<DiscriminatorType> fromType(PrimitiveType<?> code) throws FHIRException { 385 if (code == null) 386 return null; 387 if (code.isEmpty()) 388 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.NULL, code); 389 String codeString = code.asStringValue(); 390 if (codeString == null || "".equals(codeString)) 391 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.NULL, code); 392 if ("value".equals(codeString)) 393 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.VALUE, code); 394 if ("exists".equals(codeString)) 395 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.EXISTS, code); 396 if ("pattern".equals(codeString)) 397 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.PATTERN, code); 398 if ("type".equals(codeString)) 399 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.TYPE, code); 400 if ("profile".equals(codeString)) 401 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.PROFILE, code); 402 throw new FHIRException("Unknown DiscriminatorType code '" + codeString + "'"); 403 } 404 405 public String toCode(DiscriminatorType code) { 406 if (code == DiscriminatorType.VALUE) 407 return "value"; 408 if (code == DiscriminatorType.EXISTS) 409 return "exists"; 410 if (code == DiscriminatorType.PATTERN) 411 return "pattern"; 412 if (code == DiscriminatorType.TYPE) 413 return "type"; 414 if (code == DiscriminatorType.PROFILE) 415 return "profile"; 416 return "?"; 417 } 418 419 public String toSystem(DiscriminatorType code) { 420 return code.getSystem(); 421 } 422 } 423 424 public enum SlicingRules { 425 /** 426 * No additional content is allowed other than that described by the slices in 427 * this profile. 428 */ 429 CLOSED, 430 /** 431 * Additional content is allowed anywhere in the list. 432 */ 433 OPEN, 434 /** 435 * Additional content is allowed, but only at the end of the list. Note that 436 * using this requires that the slices be ordered, which makes it hard to share 437 * uses. This should only be done where absolutely required. 438 */ 439 OPENATEND, 440 /** 441 * added to help the parsers with the generic types 442 */ 443 NULL; 444 445 public static SlicingRules fromCode(String codeString) throws FHIRException { 446 if (codeString == null || "".equals(codeString)) 447 return null; 448 if ("closed".equals(codeString)) 449 return CLOSED; 450 if ("open".equals(codeString)) 451 return OPEN; 452 if ("openAtEnd".equals(codeString)) 453 return OPENATEND; 454 if (Configuration.isAcceptInvalidEnums()) 455 return null; 456 else 457 throw new FHIRException("Unknown SlicingRules code '" + codeString + "'"); 458 } 459 460 public String toCode() { 461 switch (this) { 462 case CLOSED: 463 return "closed"; 464 case OPEN: 465 return "open"; 466 case OPENATEND: 467 return "openAtEnd"; 468 case NULL: 469 return null; 470 default: 471 return "?"; 472 } 473 } 474 475 public String getSystem() { 476 switch (this) { 477 case CLOSED: 478 return "http://hl7.org/fhir/resource-slicing-rules"; 479 case OPEN: 480 return "http://hl7.org/fhir/resource-slicing-rules"; 481 case OPENATEND: 482 return "http://hl7.org/fhir/resource-slicing-rules"; 483 case NULL: 484 return null; 485 default: 486 return "?"; 487 } 488 } 489 490 public String getDefinition() { 491 switch (this) { 492 case CLOSED: 493 return "No additional content is allowed other than that described by the slices in this profile."; 494 case OPEN: 495 return "Additional content is allowed anywhere in the list."; 496 case OPENATEND: 497 return "Additional content is allowed, but only at the end of the list. Note that using this requires that the slices be ordered, which makes it hard to share uses. This should only be done where absolutely required."; 498 case NULL: 499 return null; 500 default: 501 return "?"; 502 } 503 } 504 505 public String getDisplay() { 506 switch (this) { 507 case CLOSED: 508 return "Closed"; 509 case OPEN: 510 return "Open"; 511 case OPENATEND: 512 return "Open at End"; 513 case NULL: 514 return null; 515 default: 516 return "?"; 517 } 518 } 519 } 520 521 public static class SlicingRulesEnumFactory implements EnumFactory<SlicingRules> { 522 public SlicingRules fromCode(String codeString) throws IllegalArgumentException { 523 if (codeString == null || "".equals(codeString)) 524 if (codeString == null || "".equals(codeString)) 525 return null; 526 if ("closed".equals(codeString)) 527 return SlicingRules.CLOSED; 528 if ("open".equals(codeString)) 529 return SlicingRules.OPEN; 530 if ("openAtEnd".equals(codeString)) 531 return SlicingRules.OPENATEND; 532 throw new IllegalArgumentException("Unknown SlicingRules code '" + codeString + "'"); 533 } 534 535 public Enumeration<SlicingRules> fromType(PrimitiveType<?> code) throws FHIRException { 536 if (code == null) 537 return null; 538 if (code.isEmpty()) 539 return new Enumeration<SlicingRules>(this, SlicingRules.NULL, code); 540 String codeString = code.asStringValue(); 541 if (codeString == null || "".equals(codeString)) 542 return new Enumeration<SlicingRules>(this, SlicingRules.NULL, code); 543 if ("closed".equals(codeString)) 544 return new Enumeration<SlicingRules>(this, SlicingRules.CLOSED, code); 545 if ("open".equals(codeString)) 546 return new Enumeration<SlicingRules>(this, SlicingRules.OPEN, code); 547 if ("openAtEnd".equals(codeString)) 548 return new Enumeration<SlicingRules>(this, SlicingRules.OPENATEND, code); 549 throw new FHIRException("Unknown SlicingRules code '" + codeString + "'"); 550 } 551 552 public String toCode(SlicingRules code) { 553 if (code == SlicingRules.CLOSED) 554 return "closed"; 555 if (code == SlicingRules.OPEN) 556 return "open"; 557 if (code == SlicingRules.OPENATEND) 558 return "openAtEnd"; 559 return "?"; 560 } 561 562 public String toSystem(SlicingRules code) { 563 return code.getSystem(); 564 } 565 } 566 567 public enum AggregationMode { 568 /** 569 * The reference is a local reference to a contained resource. 570 */ 571 CONTAINED, 572 /** 573 * The reference to a resource that has to be resolved externally to the 574 * resource that includes the reference. 575 */ 576 REFERENCED, 577 /** 578 * The resource the reference points to will be found in the same bundle as the 579 * resource that includes the reference. 580 */ 581 BUNDLED, 582 /** 583 * added to help the parsers with the generic types 584 */ 585 NULL; 586 587 public static AggregationMode fromCode(String codeString) throws FHIRException { 588 if (codeString == null || "".equals(codeString)) 589 return null; 590 if ("contained".equals(codeString)) 591 return CONTAINED; 592 if ("referenced".equals(codeString)) 593 return REFERENCED; 594 if ("bundled".equals(codeString)) 595 return BUNDLED; 596 if (Configuration.isAcceptInvalidEnums()) 597 return null; 598 else 599 throw new FHIRException("Unknown AggregationMode code '" + codeString + "'"); 600 } 601 602 public String toCode() { 603 switch (this) { 604 case CONTAINED: 605 return "contained"; 606 case REFERENCED: 607 return "referenced"; 608 case BUNDLED: 609 return "bundled"; 610 case NULL: 611 return null; 612 default: 613 return "?"; 614 } 615 } 616 617 public String getSystem() { 618 switch (this) { 619 case CONTAINED: 620 return "http://hl7.org/fhir/resource-aggregation-mode"; 621 case REFERENCED: 622 return "http://hl7.org/fhir/resource-aggregation-mode"; 623 case BUNDLED: 624 return "http://hl7.org/fhir/resource-aggregation-mode"; 625 case NULL: 626 return null; 627 default: 628 return "?"; 629 } 630 } 631 632 public String getDefinition() { 633 switch (this) { 634 case CONTAINED: 635 return "The reference is a local reference to a contained resource."; 636 case REFERENCED: 637 return "The reference to a resource that has to be resolved externally to the resource that includes the reference."; 638 case BUNDLED: 639 return "The resource the reference points to will be found in the same bundle as the resource that includes the reference."; 640 case NULL: 641 return null; 642 default: 643 return "?"; 644 } 645 } 646 647 public String getDisplay() { 648 switch (this) { 649 case CONTAINED: 650 return "Contained"; 651 case REFERENCED: 652 return "Referenced"; 653 case BUNDLED: 654 return "Bundled"; 655 case NULL: 656 return null; 657 default: 658 return "?"; 659 } 660 } 661 } 662 663 public static class AggregationModeEnumFactory implements EnumFactory<AggregationMode> { 664 public AggregationMode fromCode(String codeString) throws IllegalArgumentException { 665 if (codeString == null || "".equals(codeString)) 666 if (codeString == null || "".equals(codeString)) 667 return null; 668 if ("contained".equals(codeString)) 669 return AggregationMode.CONTAINED; 670 if ("referenced".equals(codeString)) 671 return AggregationMode.REFERENCED; 672 if ("bundled".equals(codeString)) 673 return AggregationMode.BUNDLED; 674 throw new IllegalArgumentException("Unknown AggregationMode code '" + codeString + "'"); 675 } 676 677 public Enumeration<AggregationMode> fromType(PrimitiveType<?> code) throws FHIRException { 678 if (code == null) 679 return null; 680 if (code.isEmpty()) 681 return new Enumeration<AggregationMode>(this, AggregationMode.NULL, code); 682 String codeString = code.asStringValue(); 683 if (codeString == null || "".equals(codeString)) 684 return new Enumeration<AggregationMode>(this, AggregationMode.NULL, code); 685 if ("contained".equals(codeString)) 686 return new Enumeration<AggregationMode>(this, AggregationMode.CONTAINED, code); 687 if ("referenced".equals(codeString)) 688 return new Enumeration<AggregationMode>(this, AggregationMode.REFERENCED, code); 689 if ("bundled".equals(codeString)) 690 return new Enumeration<AggregationMode>(this, AggregationMode.BUNDLED, code); 691 throw new FHIRException("Unknown AggregationMode code '" + codeString + "'"); 692 } 693 694 public String toCode(AggregationMode code) { 695 if (code == AggregationMode.CONTAINED) 696 return "contained"; 697 if (code == AggregationMode.REFERENCED) 698 return "referenced"; 699 if (code == AggregationMode.BUNDLED) 700 return "bundled"; 701 return "?"; 702 } 703 704 public String toSystem(AggregationMode code) { 705 return code.getSystem(); 706 } 707 } 708 709 public enum ReferenceVersionRules { 710 /** 711 * The reference may be either version independent or version specific. 712 */ 713 EITHER, 714 /** 715 * The reference must be version independent. 716 */ 717 INDEPENDENT, 718 /** 719 * The reference must be version specific. 720 */ 721 SPECIFIC, 722 /** 723 * added to help the parsers with the generic types 724 */ 725 NULL; 726 727 public static ReferenceVersionRules fromCode(String codeString) throws FHIRException { 728 if (codeString == null || "".equals(codeString)) 729 return null; 730 if ("either".equals(codeString)) 731 return EITHER; 732 if ("independent".equals(codeString)) 733 return INDEPENDENT; 734 if ("specific".equals(codeString)) 735 return SPECIFIC; 736 if (Configuration.isAcceptInvalidEnums()) 737 return null; 738 else 739 throw new FHIRException("Unknown ReferenceVersionRules code '" + codeString + "'"); 740 } 741 742 public String toCode() { 743 switch (this) { 744 case EITHER: 745 return "either"; 746 case INDEPENDENT: 747 return "independent"; 748 case SPECIFIC: 749 return "specific"; 750 case NULL: 751 return null; 752 default: 753 return "?"; 754 } 755 } 756 757 public String getSystem() { 758 switch (this) { 759 case EITHER: 760 return "http://hl7.org/fhir/reference-version-rules"; 761 case INDEPENDENT: 762 return "http://hl7.org/fhir/reference-version-rules"; 763 case SPECIFIC: 764 return "http://hl7.org/fhir/reference-version-rules"; 765 case NULL: 766 return null; 767 default: 768 return "?"; 769 } 770 } 771 772 public String getDefinition() { 773 switch (this) { 774 case EITHER: 775 return "The reference may be either version independent or version specific."; 776 case INDEPENDENT: 777 return "The reference must be version independent."; 778 case SPECIFIC: 779 return "The reference must be version specific."; 780 case NULL: 781 return null; 782 default: 783 return "?"; 784 } 785 } 786 787 public String getDisplay() { 788 switch (this) { 789 case EITHER: 790 return "Either Specific or independent"; 791 case INDEPENDENT: 792 return "Version independent"; 793 case SPECIFIC: 794 return "Version Specific"; 795 case NULL: 796 return null; 797 default: 798 return "?"; 799 } 800 } 801 } 802 803 public static class ReferenceVersionRulesEnumFactory implements EnumFactory<ReferenceVersionRules> { 804 public ReferenceVersionRules fromCode(String codeString) throws IllegalArgumentException { 805 if (codeString == null || "".equals(codeString)) 806 if (codeString == null || "".equals(codeString)) 807 return null; 808 if ("either".equals(codeString)) 809 return ReferenceVersionRules.EITHER; 810 if ("independent".equals(codeString)) 811 return ReferenceVersionRules.INDEPENDENT; 812 if ("specific".equals(codeString)) 813 return ReferenceVersionRules.SPECIFIC; 814 throw new IllegalArgumentException("Unknown ReferenceVersionRules code '" + codeString + "'"); 815 } 816 817 public Enumeration<ReferenceVersionRules> fromType(PrimitiveType<?> code) throws FHIRException { 818 if (code == null) 819 return null; 820 if (code.isEmpty()) 821 return new Enumeration<ReferenceVersionRules>(this, ReferenceVersionRules.NULL, code); 822 String codeString = code.asStringValue(); 823 if (codeString == null || "".equals(codeString)) 824 return new Enumeration<ReferenceVersionRules>(this, ReferenceVersionRules.NULL, code); 825 if ("either".equals(codeString)) 826 return new Enumeration<ReferenceVersionRules>(this, ReferenceVersionRules.EITHER, code); 827 if ("independent".equals(codeString)) 828 return new Enumeration<ReferenceVersionRules>(this, ReferenceVersionRules.INDEPENDENT, code); 829 if ("specific".equals(codeString)) 830 return new Enumeration<ReferenceVersionRules>(this, ReferenceVersionRules.SPECIFIC, code); 831 throw new FHIRException("Unknown ReferenceVersionRules code '" + codeString + "'"); 832 } 833 834 public String toCode(ReferenceVersionRules code) { 835 if (code == ReferenceVersionRules.EITHER) 836 return "either"; 837 if (code == ReferenceVersionRules.INDEPENDENT) 838 return "independent"; 839 if (code == ReferenceVersionRules.SPECIFIC) 840 return "specific"; 841 return "?"; 842 } 843 844 public String toSystem(ReferenceVersionRules code) { 845 return code.getSystem(); 846 } 847 } 848 849 public enum ConstraintSeverity { 850 /** 851 * If the constraint is violated, the resource is not conformant. 852 */ 853 ERROR, 854 /** 855 * If the constraint is violated, the resource is conformant, but it is not 856 * necessarily following best practice. 857 */ 858 WARNING, 859 /** 860 * added to help the parsers with the generic types 861 */ 862 NULL; 863 864 public static ConstraintSeverity fromCode(String codeString) throws FHIRException { 865 if (codeString == null || "".equals(codeString)) 866 return null; 867 if ("error".equals(codeString)) 868 return ERROR; 869 if ("warning".equals(codeString)) 870 return WARNING; 871 if (Configuration.isAcceptInvalidEnums()) 872 return null; 873 else 874 throw new FHIRException("Unknown ConstraintSeverity code '" + codeString + "'"); 875 } 876 877 public String toCode() { 878 switch (this) { 879 case ERROR: 880 return "error"; 881 case WARNING: 882 return "warning"; 883 case NULL: 884 return null; 885 default: 886 return "?"; 887 } 888 } 889 890 public String getSystem() { 891 switch (this) { 892 case ERROR: 893 return "http://hl7.org/fhir/constraint-severity"; 894 case WARNING: 895 return "http://hl7.org/fhir/constraint-severity"; 896 case NULL: 897 return null; 898 default: 899 return "?"; 900 } 901 } 902 903 public String getDefinition() { 904 switch (this) { 905 case ERROR: 906 return "If the constraint is violated, the resource is not conformant."; 907 case WARNING: 908 return "If the constraint is violated, the resource is conformant, but it is not necessarily following best practice."; 909 case NULL: 910 return null; 911 default: 912 return "?"; 913 } 914 } 915 916 public String getDisplay() { 917 switch (this) { 918 case ERROR: 919 return "Error"; 920 case WARNING: 921 return "Warning"; 922 case NULL: 923 return null; 924 default: 925 return "?"; 926 } 927 } 928 } 929 930 public static class ConstraintSeverityEnumFactory implements EnumFactory<ConstraintSeverity> { 931 public ConstraintSeverity fromCode(String codeString) throws IllegalArgumentException { 932 if (codeString == null || "".equals(codeString)) 933 if (codeString == null || "".equals(codeString)) 934 return null; 935 if ("error".equals(codeString)) 936 return ConstraintSeverity.ERROR; 937 if ("warning".equals(codeString)) 938 return ConstraintSeverity.WARNING; 939 throw new IllegalArgumentException("Unknown ConstraintSeverity code '" + codeString + "'"); 940 } 941 942 public Enumeration<ConstraintSeverity> fromType(PrimitiveType<?> code) throws FHIRException { 943 if (code == null) 944 return null; 945 if (code.isEmpty()) 946 return new Enumeration<ConstraintSeverity>(this, ConstraintSeverity.NULL, code); 947 String codeString = code.asStringValue(); 948 if (codeString == null || "".equals(codeString)) 949 return new Enumeration<ConstraintSeverity>(this, ConstraintSeverity.NULL, code); 950 if ("error".equals(codeString)) 951 return new Enumeration<ConstraintSeverity>(this, ConstraintSeverity.ERROR, code); 952 if ("warning".equals(codeString)) 953 return new Enumeration<ConstraintSeverity>(this, ConstraintSeverity.WARNING, code); 954 throw new FHIRException("Unknown ConstraintSeverity code '" + codeString + "'"); 955 } 956 957 public String toCode(ConstraintSeverity code) { 958 if (code == ConstraintSeverity.ERROR) 959 return "error"; 960 if (code == ConstraintSeverity.WARNING) 961 return "warning"; 962 return "?"; 963 } 964 965 public String toSystem(ConstraintSeverity code) { 966 return code.getSystem(); 967 } 968 } 969 970 @Block() 971 public static class ElementDefinitionSlicingComponent extends Element implements IBaseDatatypeElement { 972 /** 973 * Designates which child elements are used to discriminate between the slices 974 * when processing an instance. If one or more discriminators are provided, the 975 * value of the child elements in the instance data SHALL completely distinguish 976 * which slice the element in the resource matches based on the allowed values 977 * for those elements in each of the slices. 978 */ 979 @Child(name = "discriminator", type = {}, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 980 @Description(shortDefinition = "Element values that are used to distinguish the slices", formalDefinition = "Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices.") 981 protected List<ElementDefinitionSlicingDiscriminatorComponent> discriminator; 982 983 /** 984 * A human-readable text description of how the slicing works. If there is no 985 * discriminator, this is required to be present to provide whatever information 986 * is possible about how the slices can be differentiated. 987 */ 988 @Child(name = "description", type = { 989 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 990 @Description(shortDefinition = "Text description of how slicing works (or not)", formalDefinition = "A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated.") 991 protected StringType description; 992 993 /** 994 * If the matching elements have to occur in the same order as defined in the 995 * profile. 996 */ 997 @Child(name = "ordered", type = { 998 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 999 @Description(shortDefinition = "If elements must be in same order as slices", formalDefinition = "If the matching elements have to occur in the same order as defined in the profile.") 1000 protected BooleanType ordered; 1001 1002 /** 1003 * Whether additional slices are allowed or not. When the slices are ordered, 1004 * profile authors can also say that additional slices are only allowed at the 1005 * end. 1006 */ 1007 @Child(name = "rules", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 1008 @Description(shortDefinition = "closed | open | openAtEnd", formalDefinition = "Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end.") 1009 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/resource-slicing-rules") 1010 protected Enumeration<SlicingRules> rules; 1011 1012 private static final long serialVersionUID = -311635839L; 1013 1014 /** 1015 * Constructor 1016 */ 1017 public ElementDefinitionSlicingComponent() { 1018 super(); 1019 } 1020 1021 /** 1022 * Constructor 1023 */ 1024 public ElementDefinitionSlicingComponent(Enumeration<SlicingRules> rules) { 1025 super(); 1026 this.rules = rules; 1027 } 1028 1029 /** 1030 * @return {@link #discriminator} (Designates which child elements are used to 1031 * discriminate between the slices when processing an instance. If one 1032 * or more discriminators are provided, the value of the child elements 1033 * in the instance data SHALL completely distinguish which slice the 1034 * element in the resource matches based on the allowed values for those 1035 * elements in each of the slices.) 1036 */ 1037 public List<ElementDefinitionSlicingDiscriminatorComponent> getDiscriminator() { 1038 if (this.discriminator == null) 1039 this.discriminator = new ArrayList<ElementDefinitionSlicingDiscriminatorComponent>(); 1040 return this.discriminator; 1041 } 1042 1043 /** 1044 * @return Returns a reference to <code>this</code> for easy method chaining 1045 */ 1046 public ElementDefinitionSlicingComponent setDiscriminator( 1047 List<ElementDefinitionSlicingDiscriminatorComponent> theDiscriminator) { 1048 this.discriminator = theDiscriminator; 1049 return this; 1050 } 1051 1052 public boolean hasDiscriminator() { 1053 if (this.discriminator == null) 1054 return false; 1055 for (ElementDefinitionSlicingDiscriminatorComponent item : this.discriminator) 1056 if (!item.isEmpty()) 1057 return true; 1058 return false; 1059 } 1060 1061 public ElementDefinitionSlicingDiscriminatorComponent addDiscriminator() { // 3 1062 ElementDefinitionSlicingDiscriminatorComponent t = new ElementDefinitionSlicingDiscriminatorComponent(); 1063 if (this.discriminator == null) 1064 this.discriminator = new ArrayList<ElementDefinitionSlicingDiscriminatorComponent>(); 1065 this.discriminator.add(t); 1066 return t; 1067 } 1068 1069 public ElementDefinitionSlicingComponent addDiscriminator(ElementDefinitionSlicingDiscriminatorComponent t) { // 3 1070 if (t == null) 1071 return this; 1072 if (this.discriminator == null) 1073 this.discriminator = new ArrayList<ElementDefinitionSlicingDiscriminatorComponent>(); 1074 this.discriminator.add(t); 1075 return this; 1076 } 1077 1078 /** 1079 * @return The first repetition of repeating field {@link #discriminator}, 1080 * creating it if it does not already exist 1081 */ 1082 public ElementDefinitionSlicingDiscriminatorComponent getDiscriminatorFirstRep() { 1083 if (getDiscriminator().isEmpty()) { 1084 addDiscriminator(); 1085 } 1086 return getDiscriminator().get(0); 1087 } 1088 1089 /** 1090 * @return {@link #description} (A human-readable text description of how the 1091 * slicing works. If there is no discriminator, this is required to be 1092 * present to provide whatever information is possible about how the 1093 * slices can be differentiated.). This is the underlying object with 1094 * id, value and extensions. The accessor "getDescription" gives direct 1095 * access to the value 1096 */ 1097 public StringType getDescriptionElement() { 1098 if (this.description == null) 1099 if (Configuration.errorOnAutoCreate()) 1100 throw new Error("Attempt to auto-create ElementDefinitionSlicingComponent.description"); 1101 else if (Configuration.doAutoCreate()) 1102 this.description = new StringType(); // bb 1103 return this.description; 1104 } 1105 1106 public boolean hasDescriptionElement() { 1107 return this.description != null && !this.description.isEmpty(); 1108 } 1109 1110 public boolean hasDescription() { 1111 return this.description != null && !this.description.isEmpty(); 1112 } 1113 1114 /** 1115 * @param value {@link #description} (A human-readable text description of how 1116 * the slicing works. If there is no discriminator, this is 1117 * required to be present to provide whatever information is 1118 * possible about how the slices can be differentiated.). This is 1119 * the underlying object with id, value and extensions. The 1120 * accessor "getDescription" gives direct access to the value 1121 */ 1122 public ElementDefinitionSlicingComponent setDescriptionElement(StringType value) { 1123 this.description = value; 1124 return this; 1125 } 1126 1127 /** 1128 * @return A human-readable text description of how the slicing works. If there 1129 * is no discriminator, this is required to be present to provide 1130 * whatever information is possible about how the slices can be 1131 * differentiated. 1132 */ 1133 public String getDescription() { 1134 return this.description == null ? null : this.description.getValue(); 1135 } 1136 1137 /** 1138 * @param value A human-readable text description of how the slicing works. If 1139 * there is no discriminator, this is required to be present to 1140 * provide whatever information is possible about how the slices 1141 * can be differentiated. 1142 */ 1143 public ElementDefinitionSlicingComponent setDescription(String value) { 1144 if (Utilities.noString(value)) 1145 this.description = null; 1146 else { 1147 if (this.description == null) 1148 this.description = new StringType(); 1149 this.description.setValue(value); 1150 } 1151 return this; 1152 } 1153 1154 /** 1155 * @return {@link #ordered} (If the matching elements have to occur in the same 1156 * order as defined in the profile.). This is the underlying object with 1157 * id, value and extensions. The accessor "getOrdered" gives direct 1158 * access to the value 1159 */ 1160 public BooleanType getOrderedElement() { 1161 if (this.ordered == null) 1162 if (Configuration.errorOnAutoCreate()) 1163 throw new Error("Attempt to auto-create ElementDefinitionSlicingComponent.ordered"); 1164 else if (Configuration.doAutoCreate()) 1165 this.ordered = new BooleanType(); // bb 1166 return this.ordered; 1167 } 1168 1169 public boolean hasOrderedElement() { 1170 return this.ordered != null && !this.ordered.isEmpty(); 1171 } 1172 1173 public boolean hasOrdered() { 1174 return this.ordered != null && !this.ordered.isEmpty(); 1175 } 1176 1177 /** 1178 * @param value {@link #ordered} (If the matching elements have to occur in the 1179 * same order as defined in the profile.). This is the underlying 1180 * object with id, value and extensions. The accessor "getOrdered" 1181 * gives direct access to the value 1182 */ 1183 public ElementDefinitionSlicingComponent setOrderedElement(BooleanType value) { 1184 this.ordered = value; 1185 return this; 1186 } 1187 1188 /** 1189 * @return If the matching elements have to occur in the same order as defined 1190 * in the profile. 1191 */ 1192 public boolean getOrdered() { 1193 return this.ordered == null || this.ordered.isEmpty() ? false : this.ordered.getValue(); 1194 } 1195 1196 /** 1197 * @param value If the matching elements have to occur in the same order as 1198 * defined in the profile. 1199 */ 1200 public ElementDefinitionSlicingComponent setOrdered(boolean value) { 1201 if (this.ordered == null) 1202 this.ordered = new BooleanType(); 1203 this.ordered.setValue(value); 1204 return this; 1205 } 1206 1207 /** 1208 * @return {@link #rules} (Whether additional slices are allowed or not. When 1209 * the slices are ordered, profile authors can also say that additional 1210 * slices are only allowed at the end.). This is the underlying object 1211 * with id, value and extensions. The accessor "getRules" gives direct 1212 * access to the value 1213 */ 1214 public Enumeration<SlicingRules> getRulesElement() { 1215 if (this.rules == null) 1216 if (Configuration.errorOnAutoCreate()) 1217 throw new Error("Attempt to auto-create ElementDefinitionSlicingComponent.rules"); 1218 else if (Configuration.doAutoCreate()) 1219 this.rules = new Enumeration<SlicingRules>(new SlicingRulesEnumFactory()); // bb 1220 return this.rules; 1221 } 1222 1223 public boolean hasRulesElement() { 1224 return this.rules != null && !this.rules.isEmpty(); 1225 } 1226 1227 public boolean hasRules() { 1228 return this.rules != null && !this.rules.isEmpty(); 1229 } 1230 1231 /** 1232 * @param value {@link #rules} (Whether additional slices are allowed or not. 1233 * When the slices are ordered, profile authors can also say that 1234 * additional slices are only allowed at the end.). This is the 1235 * underlying object with id, value and extensions. The accessor 1236 * "getRules" gives direct access to the value 1237 */ 1238 public ElementDefinitionSlicingComponent setRulesElement(Enumeration<SlicingRules> value) { 1239 this.rules = value; 1240 return this; 1241 } 1242 1243 /** 1244 * @return Whether additional slices are allowed or not. When the slices are 1245 * ordered, profile authors can also say that additional slices are only 1246 * allowed at the end. 1247 */ 1248 public SlicingRules getRules() { 1249 return this.rules == null ? null : this.rules.getValue(); 1250 } 1251 1252 /** 1253 * @param value Whether additional slices are allowed or not. When the slices 1254 * are ordered, profile authors can also say that additional slices 1255 * are only allowed at the end. 1256 */ 1257 public ElementDefinitionSlicingComponent setRules(SlicingRules value) { 1258 if (this.rules == null) 1259 this.rules = new Enumeration<SlicingRules>(new SlicingRulesEnumFactory()); 1260 this.rules.setValue(value); 1261 return this; 1262 } 1263 1264 protected void listChildren(List<Property> children) { 1265 super.listChildren(children); 1266 children.add(new Property("discriminator", "", 1267 "Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices.", 1268 0, java.lang.Integer.MAX_VALUE, discriminator)); 1269 children.add(new Property("description", "string", 1270 "A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated.", 1271 0, 1, description)); 1272 children.add(new Property("ordered", "boolean", 1273 "If the matching elements have to occur in the same order as defined in the profile.", 0, 1, ordered)); 1274 children.add(new Property("rules", "code", 1275 "Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end.", 1276 0, 1, rules)); 1277 } 1278 1279 @Override 1280 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1281 switch (_hash) { 1282 case -1888270692: 1283 /* discriminator */ return new Property("discriminator", "", 1284 "Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices.", 1285 0, java.lang.Integer.MAX_VALUE, discriminator); 1286 case -1724546052: 1287 /* description */ return new Property("description", "string", 1288 "A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated.", 1289 0, 1, description); 1290 case -1207109523: 1291 /* ordered */ return new Property("ordered", "boolean", 1292 "If the matching elements have to occur in the same order as defined in the profile.", 0, 1, ordered); 1293 case 108873975: 1294 /* rules */ return new Property("rules", "code", 1295 "Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end.", 1296 0, 1, rules); 1297 default: 1298 return super.getNamedProperty(_hash, _name, _checkValid); 1299 } 1300 1301 } 1302 1303 @Override 1304 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1305 switch (hash) { 1306 case -1888270692: 1307 /* discriminator */ return this.discriminator == null ? new Base[0] 1308 : this.discriminator.toArray(new Base[this.discriminator.size()]); // ElementDefinitionSlicingDiscriminatorComponent 1309 case -1724546052: 1310 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1311 case -1207109523: 1312 /* ordered */ return this.ordered == null ? new Base[0] : new Base[] { this.ordered }; // BooleanType 1313 case 108873975: 1314 /* rules */ return this.rules == null ? new Base[0] : new Base[] { this.rules }; // Enumeration<SlicingRules> 1315 default: 1316 return super.getProperty(hash, name, checkValid); 1317 } 1318 1319 } 1320 1321 @Override 1322 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1323 switch (hash) { 1324 case -1888270692: // discriminator 1325 this.getDiscriminator().add((ElementDefinitionSlicingDiscriminatorComponent) value); // ElementDefinitionSlicingDiscriminatorComponent 1326 return value; 1327 case -1724546052: // description 1328 this.description = castToString(value); // StringType 1329 return value; 1330 case -1207109523: // ordered 1331 this.ordered = castToBoolean(value); // BooleanType 1332 return value; 1333 case 108873975: // rules 1334 value = new SlicingRulesEnumFactory().fromType(castToCode(value)); 1335 this.rules = (Enumeration) value; // Enumeration<SlicingRules> 1336 return value; 1337 default: 1338 return super.setProperty(hash, name, value); 1339 } 1340 1341 } 1342 1343 @Override 1344 public Base setProperty(String name, Base value) throws FHIRException { 1345 if (name.equals("discriminator")) { 1346 this.getDiscriminator().add((ElementDefinitionSlicingDiscriminatorComponent) value); 1347 } else if (name.equals("description")) { 1348 this.description = castToString(value); // StringType 1349 } else if (name.equals("ordered")) { 1350 this.ordered = castToBoolean(value); // BooleanType 1351 } else if (name.equals("rules")) { 1352 value = new SlicingRulesEnumFactory().fromType(castToCode(value)); 1353 this.rules = (Enumeration) value; // Enumeration<SlicingRules> 1354 } else 1355 return super.setProperty(name, value); 1356 return value; 1357 } 1358 1359 @Override 1360 public Base makeProperty(int hash, String name) throws FHIRException { 1361 switch (hash) { 1362 case -1888270692: 1363 return addDiscriminator(); 1364 case -1724546052: 1365 return getDescriptionElement(); 1366 case -1207109523: 1367 return getOrderedElement(); 1368 case 108873975: 1369 return getRulesElement(); 1370 default: 1371 return super.makeProperty(hash, name); 1372 } 1373 1374 } 1375 1376 @Override 1377 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1378 switch (hash) { 1379 case -1888270692: 1380 /* discriminator */ return new String[] {}; 1381 case -1724546052: 1382 /* description */ return new String[] { "string" }; 1383 case -1207109523: 1384 /* ordered */ return new String[] { "boolean" }; 1385 case 108873975: 1386 /* rules */ return new String[] { "code" }; 1387 default: 1388 return super.getTypesForProperty(hash, name); 1389 } 1390 1391 } 1392 1393 @Override 1394 public Base addChild(String name) throws FHIRException { 1395 if (name.equals("discriminator")) { 1396 return addDiscriminator(); 1397 } else if (name.equals("description")) { 1398 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.description"); 1399 } else if (name.equals("ordered")) { 1400 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.ordered"); 1401 } else if (name.equals("rules")) { 1402 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.rules"); 1403 } else 1404 return super.addChild(name); 1405 } 1406 1407 public ElementDefinitionSlicingComponent copy() { 1408 ElementDefinitionSlicingComponent dst = new ElementDefinitionSlicingComponent(); 1409 copyValues(dst); 1410 return dst; 1411 } 1412 1413 public void copyValues(ElementDefinitionSlicingComponent dst) { 1414 super.copyValues(dst); 1415 if (discriminator != null) { 1416 dst.discriminator = new ArrayList<ElementDefinitionSlicingDiscriminatorComponent>(); 1417 for (ElementDefinitionSlicingDiscriminatorComponent i : discriminator) 1418 dst.discriminator.add(i.copy()); 1419 } 1420 ; 1421 dst.description = description == null ? null : description.copy(); 1422 dst.ordered = ordered == null ? null : ordered.copy(); 1423 dst.rules = rules == null ? null : rules.copy(); 1424 } 1425 1426 @Override 1427 public boolean equalsDeep(Base other_) { 1428 if (!super.equalsDeep(other_)) 1429 return false; 1430 if (!(other_ instanceof ElementDefinitionSlicingComponent)) 1431 return false; 1432 ElementDefinitionSlicingComponent o = (ElementDefinitionSlicingComponent) other_; 1433 return compareDeep(discriminator, o.discriminator, true) && compareDeep(description, o.description, true) 1434 && compareDeep(ordered, o.ordered, true) && compareDeep(rules, o.rules, true); 1435 } 1436 1437 @Override 1438 public boolean equalsShallow(Base other_) { 1439 if (!super.equalsShallow(other_)) 1440 return false; 1441 if (!(other_ instanceof ElementDefinitionSlicingComponent)) 1442 return false; 1443 ElementDefinitionSlicingComponent o = (ElementDefinitionSlicingComponent) other_; 1444 return compareValues(description, o.description, true) && compareValues(ordered, o.ordered, true) 1445 && compareValues(rules, o.rules, true); 1446 } 1447 1448 public boolean isEmpty() { 1449 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(discriminator, description, ordered, rules); 1450 } 1451 1452 public String fhirType() { 1453 return "ElementDefinition.slicing"; 1454 1455 } 1456 1457 } 1458 1459 @Block() 1460 public static class ElementDefinitionSlicingDiscriminatorComponent extends Element implements IBaseDatatypeElement { 1461 /** 1462 * How the element value is interpreted when discrimination is evaluated. 1463 */ 1464 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1465 @Description(shortDefinition = "value | exists | pattern | type | profile", formalDefinition = "How the element value is interpreted when discrimination is evaluated.") 1466 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/discriminator-type") 1467 protected Enumeration<DiscriminatorType> type; 1468 1469 /** 1470 * A FHIRPath expression, using [the simple subset of 1471 * FHIRPath](fhirpath.html#simple), that is used to identify the element on 1472 * which discrimination is based. 1473 */ 1474 @Child(name = "path", type = { StringType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1475 @Description(shortDefinition = "Path to element value", formalDefinition = "A FHIRPath expression, using [the simple subset of FHIRPath](fhirpath.html#simple), that is used to identify the element on which discrimination is based.") 1476 protected StringType path; 1477 1478 private static final long serialVersionUID = 1151159293L; 1479 1480 /** 1481 * Constructor 1482 */ 1483 public ElementDefinitionSlicingDiscriminatorComponent() { 1484 super(); 1485 } 1486 1487 /** 1488 * Constructor 1489 */ 1490 public ElementDefinitionSlicingDiscriminatorComponent(Enumeration<DiscriminatorType> type, StringType path) { 1491 super(); 1492 this.type = type; 1493 this.path = path; 1494 } 1495 1496 /** 1497 * @return {@link #type} (How the element value is interpreted when 1498 * discrimination is evaluated.). This is the underlying object with id, 1499 * value and extensions. The accessor "getType" gives direct access to 1500 * the value 1501 */ 1502 public Enumeration<DiscriminatorType> getTypeElement() { 1503 if (this.type == null) 1504 if (Configuration.errorOnAutoCreate()) 1505 throw new Error("Attempt to auto-create ElementDefinitionSlicingDiscriminatorComponent.type"); 1506 else if (Configuration.doAutoCreate()) 1507 this.type = new Enumeration<DiscriminatorType>(new DiscriminatorTypeEnumFactory()); // bb 1508 return this.type; 1509 } 1510 1511 public boolean hasTypeElement() { 1512 return this.type != null && !this.type.isEmpty(); 1513 } 1514 1515 public boolean hasType() { 1516 return this.type != null && !this.type.isEmpty(); 1517 } 1518 1519 /** 1520 * @param value {@link #type} (How the element value is interpreted when 1521 * discrimination is evaluated.). This is the underlying object 1522 * with id, value and extensions. The accessor "getType" gives 1523 * direct access to the value 1524 */ 1525 public ElementDefinitionSlicingDiscriminatorComponent setTypeElement(Enumeration<DiscriminatorType> value) { 1526 this.type = value; 1527 return this; 1528 } 1529 1530 /** 1531 * @return How the element value is interpreted when discrimination is 1532 * evaluated. 1533 */ 1534 public DiscriminatorType getType() { 1535 return this.type == null ? null : this.type.getValue(); 1536 } 1537 1538 /** 1539 * @param value How the element value is interpreted when discrimination is 1540 * evaluated. 1541 */ 1542 public ElementDefinitionSlicingDiscriminatorComponent setType(DiscriminatorType value) { 1543 if (this.type == null) 1544 this.type = new Enumeration<DiscriminatorType>(new DiscriminatorTypeEnumFactory()); 1545 this.type.setValue(value); 1546 return this; 1547 } 1548 1549 /** 1550 * @return {@link #path} (A FHIRPath expression, using [the simple subset of 1551 * FHIRPath](fhirpath.html#simple), that is used to identify the element 1552 * on which discrimination is based.). This is the underlying object 1553 * with id, value and extensions. The accessor "getPath" gives direct 1554 * access to the value 1555 */ 1556 public StringType getPathElement() { 1557 if (this.path == null) 1558 if (Configuration.errorOnAutoCreate()) 1559 throw new Error("Attempt to auto-create ElementDefinitionSlicingDiscriminatorComponent.path"); 1560 else if (Configuration.doAutoCreate()) 1561 this.path = new StringType(); // bb 1562 return this.path; 1563 } 1564 1565 public boolean hasPathElement() { 1566 return this.path != null && !this.path.isEmpty(); 1567 } 1568 1569 public boolean hasPath() { 1570 return this.path != null && !this.path.isEmpty(); 1571 } 1572 1573 /** 1574 * @param value {@link #path} (A FHIRPath expression, using [the simple subset 1575 * of FHIRPath](fhirpath.html#simple), that is used to identify the 1576 * element on which discrimination is based.). This is the 1577 * underlying object with id, value and extensions. The accessor 1578 * "getPath" gives direct access to the value 1579 */ 1580 public ElementDefinitionSlicingDiscriminatorComponent setPathElement(StringType value) { 1581 this.path = value; 1582 return this; 1583 } 1584 1585 /** 1586 * @return A FHIRPath expression, using [the simple subset of 1587 * FHIRPath](fhirpath.html#simple), that is used to identify the element 1588 * on which discrimination is based. 1589 */ 1590 public String getPath() { 1591 return this.path == null ? null : this.path.getValue(); 1592 } 1593 1594 /** 1595 * @param value A FHIRPath expression, using [the simple subset of 1596 * FHIRPath](fhirpath.html#simple), that is used to identify the 1597 * element on which discrimination is based. 1598 */ 1599 public ElementDefinitionSlicingDiscriminatorComponent setPath(String value) { 1600 if (this.path == null) 1601 this.path = new StringType(); 1602 this.path.setValue(value); 1603 return this; 1604 } 1605 1606 protected void listChildren(List<Property> children) { 1607 super.listChildren(children); 1608 children.add(new Property("type", "code", 1609 "How the element value is interpreted when discrimination is evaluated.", 0, 1, type)); 1610 children.add(new Property("path", "string", 1611 "A FHIRPath expression, using [the simple subset of FHIRPath](fhirpath.html#simple), that is used to identify the element on which discrimination is based.", 1612 0, 1, path)); 1613 } 1614 1615 @Override 1616 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1617 switch (_hash) { 1618 case 3575610: 1619 /* type */ return new Property("type", "code", 1620 "How the element value is interpreted when discrimination is evaluated.", 0, 1, type); 1621 case 3433509: 1622 /* path */ return new Property("path", "string", 1623 "A FHIRPath expression, using [the simple subset of FHIRPath](fhirpath.html#simple), that is used to identify the element on which discrimination is based.", 1624 0, 1, path); 1625 default: 1626 return super.getNamedProperty(_hash, _name, _checkValid); 1627 } 1628 1629 } 1630 1631 @Override 1632 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1633 switch (hash) { 1634 case 3575610: 1635 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<DiscriminatorType> 1636 case 3433509: 1637 /* path */ return this.path == null ? new Base[0] : new Base[] { this.path }; // StringType 1638 default: 1639 return super.getProperty(hash, name, checkValid); 1640 } 1641 1642 } 1643 1644 @Override 1645 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1646 switch (hash) { 1647 case 3575610: // type 1648 value = new DiscriminatorTypeEnumFactory().fromType(castToCode(value)); 1649 this.type = (Enumeration) value; // Enumeration<DiscriminatorType> 1650 return value; 1651 case 3433509: // path 1652 this.path = castToString(value); // StringType 1653 return value; 1654 default: 1655 return super.setProperty(hash, name, value); 1656 } 1657 1658 } 1659 1660 @Override 1661 public Base setProperty(String name, Base value) throws FHIRException { 1662 if (name.equals("type")) { 1663 value = new DiscriminatorTypeEnumFactory().fromType(castToCode(value)); 1664 this.type = (Enumeration) value; // Enumeration<DiscriminatorType> 1665 } else if (name.equals("path")) { 1666 this.path = castToString(value); // StringType 1667 } else 1668 return super.setProperty(name, value); 1669 return value; 1670 } 1671 1672 @Override 1673 public Base makeProperty(int hash, String name) throws FHIRException { 1674 switch (hash) { 1675 case 3575610: 1676 return getTypeElement(); 1677 case 3433509: 1678 return getPathElement(); 1679 default: 1680 return super.makeProperty(hash, name); 1681 } 1682 1683 } 1684 1685 @Override 1686 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1687 switch (hash) { 1688 case 3575610: 1689 /* type */ return new String[] { "code" }; 1690 case 3433509: 1691 /* path */ return new String[] { "string" }; 1692 default: 1693 return super.getTypesForProperty(hash, name); 1694 } 1695 1696 } 1697 1698 @Override 1699 public Base addChild(String name) throws FHIRException { 1700 if (name.equals("type")) { 1701 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.type"); 1702 } else if (name.equals("path")) { 1703 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.path"); 1704 } else 1705 return super.addChild(name); 1706 } 1707 1708 public ElementDefinitionSlicingDiscriminatorComponent copy() { 1709 ElementDefinitionSlicingDiscriminatorComponent dst = new ElementDefinitionSlicingDiscriminatorComponent(); 1710 copyValues(dst); 1711 return dst; 1712 } 1713 1714 public void copyValues(ElementDefinitionSlicingDiscriminatorComponent dst) { 1715 super.copyValues(dst); 1716 dst.type = type == null ? null : type.copy(); 1717 dst.path = path == null ? null : path.copy(); 1718 } 1719 1720 @Override 1721 public boolean equalsDeep(Base other_) { 1722 if (!super.equalsDeep(other_)) 1723 return false; 1724 if (!(other_ instanceof ElementDefinitionSlicingDiscriminatorComponent)) 1725 return false; 1726 ElementDefinitionSlicingDiscriminatorComponent o = (ElementDefinitionSlicingDiscriminatorComponent) other_; 1727 return compareDeep(type, o.type, true) && compareDeep(path, o.path, true); 1728 } 1729 1730 @Override 1731 public boolean equalsShallow(Base other_) { 1732 if (!super.equalsShallow(other_)) 1733 return false; 1734 if (!(other_ instanceof ElementDefinitionSlicingDiscriminatorComponent)) 1735 return false; 1736 ElementDefinitionSlicingDiscriminatorComponent o = (ElementDefinitionSlicingDiscriminatorComponent) other_; 1737 return compareValues(type, o.type, true) && compareValues(path, o.path, true); 1738 } 1739 1740 public boolean isEmpty() { 1741 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, path); 1742 } 1743 1744 public String fhirType() { 1745 return "ElementDefinition.slicing.discriminator"; 1746 1747 } 1748 1749 } 1750 1751 @Block() 1752 public static class ElementDefinitionBaseComponent extends Element implements IBaseDatatypeElement { 1753 /** 1754 * The Path that identifies the base element - this matches the 1755 * ElementDefinition.path for that element. Across FHIR, there is only one base 1756 * definition of any element - that is, an element definition on a 1757 * [[[StructureDefinition]]] without a StructureDefinition.base. 1758 */ 1759 @Child(name = "path", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1760 @Description(shortDefinition = "Path that identifies the base element", formalDefinition = "The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [[[StructureDefinition]]] without a StructureDefinition.base.") 1761 protected StringType path; 1762 1763 /** 1764 * Minimum cardinality of the base element identified by the path. 1765 */ 1766 @Child(name = "min", type = { 1767 UnsignedIntType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1768 @Description(shortDefinition = "Min cardinality of the base element", formalDefinition = "Minimum cardinality of the base element identified by the path.") 1769 protected UnsignedIntType min; 1770 1771 /** 1772 * Maximum cardinality of the base element identified by the path. 1773 */ 1774 @Child(name = "max", type = { StringType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 1775 @Description(shortDefinition = "Max cardinality of the base element", formalDefinition = "Maximum cardinality of the base element identified by the path.") 1776 protected StringType max; 1777 1778 private static final long serialVersionUID = -1412704221L; 1779 1780 /** 1781 * Constructor 1782 */ 1783 public ElementDefinitionBaseComponent() { 1784 super(); 1785 } 1786 1787 /** 1788 * Constructor 1789 */ 1790 public ElementDefinitionBaseComponent(StringType path, UnsignedIntType min, StringType max) { 1791 super(); 1792 this.path = path; 1793 this.min = min; 1794 this.max = max; 1795 } 1796 1797 /** 1798 * @return {@link #path} (The Path that identifies the base element - this 1799 * matches the ElementDefinition.path for that element. Across FHIR, 1800 * there is only one base definition of any element - that is, an 1801 * element definition on a [[[StructureDefinition]]] without a 1802 * StructureDefinition.base.). This is the underlying object with id, 1803 * value and extensions. The accessor "getPath" gives direct access to 1804 * the value 1805 */ 1806 public StringType getPathElement() { 1807 if (this.path == null) 1808 if (Configuration.errorOnAutoCreate()) 1809 throw new Error("Attempt to auto-create ElementDefinitionBaseComponent.path"); 1810 else if (Configuration.doAutoCreate()) 1811 this.path = new StringType(); // bb 1812 return this.path; 1813 } 1814 1815 public boolean hasPathElement() { 1816 return this.path != null && !this.path.isEmpty(); 1817 } 1818 1819 public boolean hasPath() { 1820 return this.path != null && !this.path.isEmpty(); 1821 } 1822 1823 /** 1824 * @param value {@link #path} (The Path that identifies the base element - this 1825 * matches the ElementDefinition.path for that element. Across 1826 * FHIR, there is only one base definition of any element - that 1827 * is, an element definition on a [[[StructureDefinition]]] without 1828 * a StructureDefinition.base.). This is the underlying object with 1829 * id, value and extensions. The accessor "getPath" gives direct 1830 * access to the value 1831 */ 1832 public ElementDefinitionBaseComponent setPathElement(StringType value) { 1833 this.path = value; 1834 return this; 1835 } 1836 1837 /** 1838 * @return The Path that identifies the base element - this matches the 1839 * ElementDefinition.path for that element. Across FHIR, there is only 1840 * one base definition of any element - that is, an element definition 1841 * on a [[[StructureDefinition]]] without a StructureDefinition.base. 1842 */ 1843 public String getPath() { 1844 return this.path == null ? null : this.path.getValue(); 1845 } 1846 1847 /** 1848 * @param value The Path that identifies the base element - this matches the 1849 * ElementDefinition.path for that element. Across FHIR, there is 1850 * only one base definition of any element - that is, an element 1851 * definition on a [[[StructureDefinition]]] without a 1852 * StructureDefinition.base. 1853 */ 1854 public ElementDefinitionBaseComponent setPath(String value) { 1855 if (this.path == null) 1856 this.path = new StringType(); 1857 this.path.setValue(value); 1858 return this; 1859 } 1860 1861 /** 1862 * @return {@link #min} (Minimum cardinality of the base element identified by 1863 * the path.). This is the underlying object with id, value and 1864 * extensions. The accessor "getMin" gives direct access to the value 1865 */ 1866 public UnsignedIntType getMinElement() { 1867 if (this.min == null) 1868 if (Configuration.errorOnAutoCreate()) 1869 throw new Error("Attempt to auto-create ElementDefinitionBaseComponent.min"); 1870 else if (Configuration.doAutoCreate()) 1871 this.min = new UnsignedIntType(); // bb 1872 return this.min; 1873 } 1874 1875 public boolean hasMinElement() { 1876 return this.min != null && !this.min.isEmpty(); 1877 } 1878 1879 public boolean hasMin() { 1880 return this.min != null && !this.min.isEmpty(); 1881 } 1882 1883 /** 1884 * @param value {@link #min} (Minimum cardinality of the base element identified 1885 * by the path.). This is the underlying object with id, value and 1886 * extensions. The accessor "getMin" gives direct access to the 1887 * value 1888 */ 1889 public ElementDefinitionBaseComponent setMinElement(UnsignedIntType value) { 1890 this.min = value; 1891 return this; 1892 } 1893 1894 /** 1895 * @return Minimum cardinality of the base element identified by the path. 1896 */ 1897 public int getMin() { 1898 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 1899 } 1900 1901 /** 1902 * @param value Minimum cardinality of the base element identified by the path. 1903 */ 1904 public ElementDefinitionBaseComponent setMin(int value) { 1905 if (this.min == null) 1906 this.min = new UnsignedIntType(); 1907 this.min.setValue(value); 1908 return this; 1909 } 1910 1911 /** 1912 * @return {@link #max} (Maximum cardinality of the base element identified by 1913 * the path.). This is the underlying object with id, value and 1914 * extensions. The accessor "getMax" gives direct access to the value 1915 */ 1916 public StringType getMaxElement() { 1917 if (this.max == null) 1918 if (Configuration.errorOnAutoCreate()) 1919 throw new Error("Attempt to auto-create ElementDefinitionBaseComponent.max"); 1920 else if (Configuration.doAutoCreate()) 1921 this.max = new StringType(); // bb 1922 return this.max; 1923 } 1924 1925 public boolean hasMaxElement() { 1926 return this.max != null && !this.max.isEmpty(); 1927 } 1928 1929 public boolean hasMax() { 1930 return this.max != null && !this.max.isEmpty(); 1931 } 1932 1933 /** 1934 * @param value {@link #max} (Maximum cardinality of the base element identified 1935 * by the path.). This is the underlying object with id, value and 1936 * extensions. The accessor "getMax" gives direct access to the 1937 * value 1938 */ 1939 public ElementDefinitionBaseComponent setMaxElement(StringType value) { 1940 this.max = value; 1941 return this; 1942 } 1943 1944 /** 1945 * @return Maximum cardinality of the base element identified by the path. 1946 */ 1947 public String getMax() { 1948 return this.max == null ? null : this.max.getValue(); 1949 } 1950 1951 /** 1952 * @param value Maximum cardinality of the base element identified by the path. 1953 */ 1954 public ElementDefinitionBaseComponent setMax(String value) { 1955 if (this.max == null) 1956 this.max = new StringType(); 1957 this.max.setValue(value); 1958 return this; 1959 } 1960 1961 protected void listChildren(List<Property> children) { 1962 super.listChildren(children); 1963 children.add(new Property("path", "string", 1964 "The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [[[StructureDefinition]]] without a StructureDefinition.base.", 1965 0, 1, path)); 1966 children.add(new Property("min", "unsignedInt", "Minimum cardinality of the base element identified by the path.", 1967 0, 1, min)); 1968 children.add( 1969 new Property("max", "string", "Maximum cardinality of the base element identified by the path.", 0, 1, max)); 1970 } 1971 1972 @Override 1973 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1974 switch (_hash) { 1975 case 3433509: 1976 /* path */ return new Property("path", "string", 1977 "The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [[[StructureDefinition]]] without a StructureDefinition.base.", 1978 0, 1, path); 1979 case 108114: 1980 /* min */ return new Property("min", "unsignedInt", 1981 "Minimum cardinality of the base element identified by the path.", 0, 1, min); 1982 case 107876: 1983 /* max */ return new Property("max", "string", 1984 "Maximum cardinality of the base element identified by the path.", 0, 1, max); 1985 default: 1986 return super.getNamedProperty(_hash, _name, _checkValid); 1987 } 1988 1989 } 1990 1991 @Override 1992 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1993 switch (hash) { 1994 case 3433509: 1995 /* path */ return this.path == null ? new Base[0] : new Base[] { this.path }; // StringType 1996 case 108114: 1997 /* min */ return this.min == null ? new Base[0] : new Base[] { this.min }; // UnsignedIntType 1998 case 107876: 1999 /* max */ return this.max == null ? new Base[0] : new Base[] { this.max }; // StringType 2000 default: 2001 return super.getProperty(hash, name, checkValid); 2002 } 2003 2004 } 2005 2006 @Override 2007 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2008 switch (hash) { 2009 case 3433509: // path 2010 this.path = castToString(value); // StringType 2011 return value; 2012 case 108114: // min 2013 this.min = castToUnsignedInt(value); // UnsignedIntType 2014 return value; 2015 case 107876: // max 2016 this.max = castToString(value); // StringType 2017 return value; 2018 default: 2019 return super.setProperty(hash, name, value); 2020 } 2021 2022 } 2023 2024 @Override 2025 public Base setProperty(String name, Base value) throws FHIRException { 2026 if (name.equals("path")) { 2027 this.path = castToString(value); // StringType 2028 } else if (name.equals("min")) { 2029 this.min = castToUnsignedInt(value); // UnsignedIntType 2030 } else if (name.equals("max")) { 2031 this.max = castToString(value); // StringType 2032 } else 2033 return super.setProperty(name, value); 2034 return value; 2035 } 2036 2037 @Override 2038 public Base makeProperty(int hash, String name) throws FHIRException { 2039 switch (hash) { 2040 case 3433509: 2041 return getPathElement(); 2042 case 108114: 2043 return getMinElement(); 2044 case 107876: 2045 return getMaxElement(); 2046 default: 2047 return super.makeProperty(hash, name); 2048 } 2049 2050 } 2051 2052 @Override 2053 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2054 switch (hash) { 2055 case 3433509: 2056 /* path */ return new String[] { "string" }; 2057 case 108114: 2058 /* min */ return new String[] { "unsignedInt" }; 2059 case 107876: 2060 /* max */ return new String[] { "string" }; 2061 default: 2062 return super.getTypesForProperty(hash, name); 2063 } 2064 2065 } 2066 2067 @Override 2068 public Base addChild(String name) throws FHIRException { 2069 if (name.equals("path")) { 2070 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.path"); 2071 } else if (name.equals("min")) { 2072 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.min"); 2073 } else if (name.equals("max")) { 2074 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.max"); 2075 } else 2076 return super.addChild(name); 2077 } 2078 2079 public ElementDefinitionBaseComponent copy() { 2080 ElementDefinitionBaseComponent dst = new ElementDefinitionBaseComponent(); 2081 copyValues(dst); 2082 return dst; 2083 } 2084 2085 public void copyValues(ElementDefinitionBaseComponent dst) { 2086 super.copyValues(dst); 2087 dst.path = path == null ? null : path.copy(); 2088 dst.min = min == null ? null : min.copy(); 2089 dst.max = max == null ? null : max.copy(); 2090 } 2091 2092 @Override 2093 public boolean equalsDeep(Base other_) { 2094 if (!super.equalsDeep(other_)) 2095 return false; 2096 if (!(other_ instanceof ElementDefinitionBaseComponent)) 2097 return false; 2098 ElementDefinitionBaseComponent o = (ElementDefinitionBaseComponent) other_; 2099 return compareDeep(path, o.path, true) && compareDeep(min, o.min, true) && compareDeep(max, o.max, true); 2100 } 2101 2102 @Override 2103 public boolean equalsShallow(Base other_) { 2104 if (!super.equalsShallow(other_)) 2105 return false; 2106 if (!(other_ instanceof ElementDefinitionBaseComponent)) 2107 return false; 2108 ElementDefinitionBaseComponent o = (ElementDefinitionBaseComponent) other_; 2109 return compareValues(path, o.path, true) && compareValues(min, o.min, true) && compareValues(max, o.max, true); 2110 } 2111 2112 public boolean isEmpty() { 2113 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, min, max); 2114 } 2115 2116 public String fhirType() { 2117 return "ElementDefinition.base"; 2118 2119 } 2120 2121 } 2122 2123 @Block() 2124 public static class TypeRefComponent extends Element implements IBaseDatatypeElement { 2125 /** 2126 * URL of Data type or Resource that is a(or the) type used for this element. 2127 * References are URLs that are relative to 2128 * http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to 2129 * http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only 2130 * allowed in logical models. 2131 */ 2132 @Child(name = "code", type = { UriType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2133 @Description(shortDefinition = "Data type or Resource (reference to definition)", formalDefinition = "URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. \"string\" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models.") 2134 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/defined-types") 2135 protected UriType code; 2136 2137 /** 2138 * Identifies a profile structure or implementation Guide that applies to the 2139 * datatype this element refers to. If any profiles are specified, then the 2140 * content must conform to at least one of them. The URL can be a local 2141 * reference - to a contained StructureDefinition, or a reference to another 2142 * StructureDefinition or Implementation Guide by a canonical URL. When an 2143 * implementation guide is specified, the type SHALL conform to at least one 2144 * profile defined in the implementation guide. 2145 */ 2146 @Child(name = "profile", type = { 2147 CanonicalType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2148 @Description(shortDefinition = "Profiles (StructureDefinition or IG) - one must apply", formalDefinition = "Identifies a profile structure or implementation Guide that applies to the datatype this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the type SHALL conform to at least one profile defined in the implementation guide.") 2149 protected List<CanonicalType> profile; 2150 2151 /** 2152 * Used when the type is "Reference" or "canonical", and identifies a profile 2153 * structure or implementation Guide that applies to the target of the reference 2154 * this element refers to. If any profiles are specified, then the content must 2155 * conform to at least one of them. The URL can be a local reference - to a 2156 * contained StructureDefinition, or a reference to another StructureDefinition 2157 * or Implementation Guide by a canonical URL. When an implementation guide is 2158 * specified, the target resource SHALL conform to at least one profile defined 2159 * in the implementation guide. 2160 */ 2161 @Child(name = "targetProfile", type = { 2162 CanonicalType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2163 @Description(shortDefinition = "Profile (StructureDefinition or IG) on the Reference/canonical target - one must apply", formalDefinition = "Used when the type is \"Reference\" or \"canonical\", and identifies a profile structure or implementation Guide that applies to the target of the reference this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.") 2164 protected List<CanonicalType> targetProfile; 2165 2166 /** 2167 * If the type is a reference to another resource, how the resource is or can be 2168 * aggregated - is it a contained resource, or a reference, and if the context 2169 * is a bundle, is it included in the bundle. 2170 */ 2171 @Child(name = "aggregation", type = { 2172 CodeType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2173 @Description(shortDefinition = "contained | referenced | bundled - how aggregated", formalDefinition = "If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle.") 2174 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/resource-aggregation-mode") 2175 protected List<Enumeration<AggregationMode>> aggregation; 2176 2177 /** 2178 * Whether this reference needs to be version specific or version independent, 2179 * or whether either can be used. 2180 */ 2181 @Child(name = "versioning", type = { 2182 CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 2183 @Description(shortDefinition = "either | independent | specific", formalDefinition = "Whether this reference needs to be version specific or version independent, or whether either can be used.") 2184 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/reference-version-rules") 2185 protected Enumeration<ReferenceVersionRules> versioning; 2186 2187 private static final long serialVersionUID = 957891653L; 2188 2189 /** 2190 * Constructor 2191 */ 2192 public TypeRefComponent() { 2193 super(); 2194 } 2195 2196 /** 2197 * Constructor 2198 */ 2199 public TypeRefComponent(UriType code) { 2200 super(); 2201 this.code = code; 2202 } 2203 2204 /** 2205 * @return {@link #code} (URL of Data type or Resource that is a(or the) type 2206 * used for this element. References are URLs that are relative to 2207 * http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference 2208 * to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are 2209 * only allowed in logical models.). This is the underlying object with 2210 * id, value and extensions. The accessor "getCode" gives direct access 2211 * to the value 2212 */ 2213 public UriType getCodeElement() { 2214 if (this.code == null) 2215 if (Configuration.errorOnAutoCreate()) 2216 throw new Error("Attempt to auto-create TypeRefComponent.code"); 2217 else if (Configuration.doAutoCreate()) 2218 this.code = new UriType(); // bb 2219 return this.code; 2220 } 2221 2222 public boolean hasCodeElement() { 2223 return this.code != null && !this.code.isEmpty(); 2224 } 2225 2226 public boolean hasCode() { 2227 return this.code != null && !this.code.isEmpty(); 2228 } 2229 2230 /** 2231 * @param value {@link #code} (URL of Data type or Resource that is a(or the) 2232 * type used for this element. References are URLs that are 2233 * relative to http://hl7.org/fhir/StructureDefinition e.g. 2234 * "string" is a reference to 2235 * http://hl7.org/fhir/StructureDefinition/string. Absolute URLs 2236 * are only allowed in logical models.). This is the underlying 2237 * object with id, value and extensions. The accessor "getCode" 2238 * gives direct access to the value 2239 */ 2240 public TypeRefComponent setCodeElement(UriType value) { 2241 this.code = value; 2242 return this; 2243 } 2244 2245 /** 2246 * @return URL of Data type or Resource that is a(or the) type used for this 2247 * element. References are URLs that are relative to 2248 * http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference 2249 * to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are 2250 * only allowed in logical models. 2251 */ 2252 public String getCode() { 2253 return this.code == null ? null : this.code.getValue(); 2254 } 2255 2256 /** 2257 * @param value URL of Data type or Resource that is a(or the) type used for 2258 * this element. References are URLs that are relative to 2259 * http://hl7.org/fhir/StructureDefinition e.g. "string" is a 2260 * reference to http://hl7.org/fhir/StructureDefinition/string. 2261 * Absolute URLs are only allowed in logical models. 2262 */ 2263 public TypeRefComponent setCode(String value) { 2264 if (this.code == null) 2265 this.code = new UriType(); 2266 this.code.setValue(value); 2267 return this; 2268 } 2269 2270 /** 2271 * @return {@link #profile} (Identifies a profile structure or implementation 2272 * Guide that applies to the datatype this element refers to. If any 2273 * profiles are specified, then the content must conform to at least one 2274 * of them. The URL can be a local reference - to a contained 2275 * StructureDefinition, or a reference to another StructureDefinition or 2276 * Implementation Guide by a canonical URL. When an implementation guide 2277 * is specified, the type SHALL conform to at least one profile defined 2278 * in the implementation guide.) 2279 */ 2280 public List<CanonicalType> getProfile() { 2281 if (this.profile == null) 2282 this.profile = new ArrayList<CanonicalType>(); 2283 return this.profile; 2284 } 2285 2286 /** 2287 * @return Returns a reference to <code>this</code> for easy method chaining 2288 */ 2289 public TypeRefComponent setProfile(List<CanonicalType> theProfile) { 2290 this.profile = theProfile; 2291 return this; 2292 } 2293 2294 public boolean hasProfile() { 2295 if (this.profile == null) 2296 return false; 2297 for (CanonicalType item : this.profile) 2298 if (!item.isEmpty()) 2299 return true; 2300 return false; 2301 } 2302 2303 /** 2304 * @return {@link #profile} (Identifies a profile structure or implementation 2305 * Guide that applies to the datatype this element refers to. If any 2306 * profiles are specified, then the content must conform to at least one 2307 * of them. The URL can be a local reference - to a contained 2308 * StructureDefinition, or a reference to another StructureDefinition or 2309 * Implementation Guide by a canonical URL. When an implementation guide 2310 * is specified, the type SHALL conform to at least one profile defined 2311 * in the implementation guide.) 2312 */ 2313 public CanonicalType addProfileElement() {// 2 2314 CanonicalType t = new CanonicalType(); 2315 if (this.profile == null) 2316 this.profile = new ArrayList<CanonicalType>(); 2317 this.profile.add(t); 2318 return t; 2319 } 2320 2321 /** 2322 * @param value {@link #profile} (Identifies a profile structure or 2323 * implementation Guide that applies to the datatype this element 2324 * refers to. If any profiles are specified, then the content must 2325 * conform to at least one of them. The URL can be a local 2326 * reference - to a contained StructureDefinition, or a reference 2327 * to another StructureDefinition or Implementation Guide by a 2328 * canonical URL. When an implementation guide is specified, the 2329 * type SHALL conform to at least one profile defined in the 2330 * implementation guide.) 2331 */ 2332 public TypeRefComponent addProfile(String value) { // 1 2333 CanonicalType t = new CanonicalType(); 2334 t.setValue(value); 2335 if (this.profile == null) 2336 this.profile = new ArrayList<CanonicalType>(); 2337 this.profile.add(t); 2338 return this; 2339 } 2340 2341 /** 2342 * @param value {@link #profile} (Identifies a profile structure or 2343 * implementation Guide that applies to the datatype this element 2344 * refers to. If any profiles are specified, then the content must 2345 * conform to at least one of them. The URL can be a local 2346 * reference - to a contained StructureDefinition, or a reference 2347 * to another StructureDefinition or Implementation Guide by a 2348 * canonical URL. When an implementation guide is specified, the 2349 * type SHALL conform to at least one profile defined in the 2350 * implementation guide.) 2351 */ 2352 public boolean hasProfile(String value) { 2353 if (this.profile == null) 2354 return false; 2355 for (CanonicalType v : this.profile) 2356 if (v.getValue().equals(value)) // canonical(StructureDefinition|ImplementationGuide) 2357 return true; 2358 return false; 2359 } 2360 2361 /** 2362 * @return {@link #targetProfile} (Used when the type is "Reference" or 2363 * "canonical", and identifies a profile structure or implementation 2364 * Guide that applies to the target of the reference this element refers 2365 * to. If any profiles are specified, then the content must conform to 2366 * at least one of them. The URL can be a local reference - to a 2367 * contained StructureDefinition, or a reference to another 2368 * StructureDefinition or Implementation Guide by a canonical URL. When 2369 * an implementation guide is specified, the target resource SHALL 2370 * conform to at least one profile defined in the implementation guide.) 2371 */ 2372 public List<CanonicalType> getTargetProfile() { 2373 if (this.targetProfile == null) 2374 this.targetProfile = new ArrayList<CanonicalType>(); 2375 return this.targetProfile; 2376 } 2377 2378 /** 2379 * @return Returns a reference to <code>this</code> for easy method chaining 2380 */ 2381 public TypeRefComponent setTargetProfile(List<CanonicalType> theTargetProfile) { 2382 this.targetProfile = theTargetProfile; 2383 return this; 2384 } 2385 2386 public boolean hasTargetProfile() { 2387 if (this.targetProfile == null) 2388 return false; 2389 for (CanonicalType item : this.targetProfile) 2390 if (!item.isEmpty()) 2391 return true; 2392 return false; 2393 } 2394 2395 /** 2396 * @return {@link #targetProfile} (Used when the type is "Reference" or 2397 * "canonical", and identifies a profile structure or implementation 2398 * Guide that applies to the target of the reference this element refers 2399 * to. If any profiles are specified, then the content must conform to 2400 * at least one of them. The URL can be a local reference - to a 2401 * contained StructureDefinition, or a reference to another 2402 * StructureDefinition or Implementation Guide by a canonical URL. When 2403 * an implementation guide is specified, the target resource SHALL 2404 * conform to at least one profile defined in the implementation guide.) 2405 */ 2406 public CanonicalType addTargetProfileElement() {// 2 2407 CanonicalType t = new CanonicalType(); 2408 if (this.targetProfile == null) 2409 this.targetProfile = new ArrayList<CanonicalType>(); 2410 this.targetProfile.add(t); 2411 return t; 2412 } 2413 2414 /** 2415 * @param value {@link #targetProfile} (Used when the type is "Reference" or 2416 * "canonical", and identifies a profile structure or 2417 * implementation Guide that applies to the target of the reference 2418 * this element refers to. If any profiles are specified, then the 2419 * content must conform to at least one of them. The URL can be a 2420 * local reference - to a contained StructureDefinition, or a 2421 * reference to another StructureDefinition or Implementation Guide 2422 * by a canonical URL. When an implementation guide is specified, 2423 * the target resource SHALL conform to at least one profile 2424 * defined in the implementation guide.) 2425 */ 2426 public TypeRefComponent addTargetProfile(String value) { // 1 2427 CanonicalType t = new CanonicalType(); 2428 t.setValue(value); 2429 if (this.targetProfile == null) 2430 this.targetProfile = new ArrayList<CanonicalType>(); 2431 this.targetProfile.add(t); 2432 return this; 2433 } 2434 2435 /** 2436 * @param value {@link #targetProfile} (Used when the type is "Reference" or 2437 * "canonical", and identifies a profile structure or 2438 * implementation Guide that applies to the target of the reference 2439 * this element refers to. If any profiles are specified, then the 2440 * content must conform to at least one of them. The URL can be a 2441 * local reference - to a contained StructureDefinition, or a 2442 * reference to another StructureDefinition or Implementation Guide 2443 * by a canonical URL. When an implementation guide is specified, 2444 * the target resource SHALL conform to at least one profile 2445 * defined in the implementation guide.) 2446 */ 2447 public boolean hasTargetProfile(String value) { 2448 if (this.targetProfile == null) 2449 return false; 2450 for (CanonicalType v : this.targetProfile) 2451 if (v.getValue().equals(value)) // canonical(StructureDefinition|ImplementationGuide) 2452 return true; 2453 return false; 2454 } 2455 2456 /** 2457 * @return {@link #aggregation} (If the type is a reference to another resource, 2458 * how the resource is or can be aggregated - is it a contained 2459 * resource, or a reference, and if the context is a bundle, is it 2460 * included in the bundle.) 2461 */ 2462 public List<Enumeration<AggregationMode>> getAggregation() { 2463 if (this.aggregation == null) 2464 this.aggregation = new ArrayList<Enumeration<AggregationMode>>(); 2465 return this.aggregation; 2466 } 2467 2468 /** 2469 * @return Returns a reference to <code>this</code> for easy method chaining 2470 */ 2471 public TypeRefComponent setAggregation(List<Enumeration<AggregationMode>> theAggregation) { 2472 this.aggregation = theAggregation; 2473 return this; 2474 } 2475 2476 public boolean hasAggregation() { 2477 if (this.aggregation == null) 2478 return false; 2479 for (Enumeration<AggregationMode> item : this.aggregation) 2480 if (!item.isEmpty()) 2481 return true; 2482 return false; 2483 } 2484 2485 /** 2486 * @return {@link #aggregation} (If the type is a reference to another resource, 2487 * how the resource is or can be aggregated - is it a contained 2488 * resource, or a reference, and if the context is a bundle, is it 2489 * included in the bundle.) 2490 */ 2491 public Enumeration<AggregationMode> addAggregationElement() {// 2 2492 Enumeration<AggregationMode> t = new Enumeration<AggregationMode>(new AggregationModeEnumFactory()); 2493 if (this.aggregation == null) 2494 this.aggregation = new ArrayList<Enumeration<AggregationMode>>(); 2495 this.aggregation.add(t); 2496 return t; 2497 } 2498 2499 /** 2500 * @param value {@link #aggregation} (If the type is a reference to another 2501 * resource, how the resource is or can be aggregated - is it a 2502 * contained resource, or a reference, and if the context is a 2503 * bundle, is it included in the bundle.) 2504 */ 2505 public TypeRefComponent addAggregation(AggregationMode value) { // 1 2506 Enumeration<AggregationMode> t = new Enumeration<AggregationMode>(new AggregationModeEnumFactory()); 2507 t.setValue(value); 2508 if (this.aggregation == null) 2509 this.aggregation = new ArrayList<Enumeration<AggregationMode>>(); 2510 this.aggregation.add(t); 2511 return this; 2512 } 2513 2514 /** 2515 * @param value {@link #aggregation} (If the type is a reference to another 2516 * resource, how the resource is or can be aggregated - is it a 2517 * contained resource, or a reference, and if the context is a 2518 * bundle, is it included in the bundle.) 2519 */ 2520 public boolean hasAggregation(AggregationMode value) { 2521 if (this.aggregation == null) 2522 return false; 2523 for (Enumeration<AggregationMode> v : this.aggregation) 2524 if (v.getValue().equals(value)) // code 2525 return true; 2526 return false; 2527 } 2528 2529 /** 2530 * @return {@link #versioning} (Whether this reference needs to be version 2531 * specific or version independent, or whether either can be used.). 2532 * This is the underlying object with id, value and extensions. The 2533 * accessor "getVersioning" gives direct access to the value 2534 */ 2535 public Enumeration<ReferenceVersionRules> getVersioningElement() { 2536 if (this.versioning == null) 2537 if (Configuration.errorOnAutoCreate()) 2538 throw new Error("Attempt to auto-create TypeRefComponent.versioning"); 2539 else if (Configuration.doAutoCreate()) 2540 this.versioning = new Enumeration<ReferenceVersionRules>(new ReferenceVersionRulesEnumFactory()); // bb 2541 return this.versioning; 2542 } 2543 2544 public boolean hasVersioningElement() { 2545 return this.versioning != null && !this.versioning.isEmpty(); 2546 } 2547 2548 public boolean hasVersioning() { 2549 return this.versioning != null && !this.versioning.isEmpty(); 2550 } 2551 2552 /** 2553 * @param value {@link #versioning} (Whether this reference needs to be version 2554 * specific or version independent, or whether either can be 2555 * used.). This is the underlying object with id, value and 2556 * extensions. The accessor "getVersioning" gives direct access to 2557 * the value 2558 */ 2559 public TypeRefComponent setVersioningElement(Enumeration<ReferenceVersionRules> value) { 2560 this.versioning = value; 2561 return this; 2562 } 2563 2564 /** 2565 * @return Whether this reference needs to be version specific or version 2566 * independent, or whether either can be used. 2567 */ 2568 public ReferenceVersionRules getVersioning() { 2569 return this.versioning == null ? null : this.versioning.getValue(); 2570 } 2571 2572 /** 2573 * @param value Whether this reference needs to be version specific or version 2574 * independent, or whether either can be used. 2575 */ 2576 public TypeRefComponent setVersioning(ReferenceVersionRules value) { 2577 if (value == null) 2578 this.versioning = null; 2579 else { 2580 if (this.versioning == null) 2581 this.versioning = new Enumeration<ReferenceVersionRules>(new ReferenceVersionRulesEnumFactory()); 2582 this.versioning.setValue(value); 2583 } 2584 return this; 2585 } 2586 2587 protected void listChildren(List<Property> children) { 2588 super.listChildren(children); 2589 children.add(new Property("code", "uri", 2590 "URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. \"string\" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models.", 2591 0, 1, code)); 2592 children.add(new Property("profile", "canonical(StructureDefinition|ImplementationGuide)", 2593 "Identifies a profile structure or implementation Guide that applies to the datatype this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the type SHALL conform to at least one profile defined in the implementation guide.", 2594 0, java.lang.Integer.MAX_VALUE, profile)); 2595 children.add(new Property("targetProfile", "canonical(StructureDefinition|ImplementationGuide)", 2596 "Used when the type is \"Reference\" or \"canonical\", and identifies a profile structure or implementation Guide that applies to the target of the reference this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.", 2597 0, java.lang.Integer.MAX_VALUE, targetProfile)); 2598 children.add(new Property("aggregation", "code", 2599 "If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle.", 2600 0, java.lang.Integer.MAX_VALUE, aggregation)); 2601 children.add(new Property("versioning", "code", 2602 "Whether this reference needs to be version specific or version independent, or whether either can be used.", 2603 0, 1, versioning)); 2604 } 2605 2606 @Override 2607 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2608 switch (_hash) { 2609 case 3059181: 2610 /* code */ return new Property("code", "uri", 2611 "URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. \"string\" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models.", 2612 0, 1, code); 2613 case -309425751: 2614 /* profile */ return new Property("profile", "canonical(StructureDefinition|ImplementationGuide)", 2615 "Identifies a profile structure or implementation Guide that applies to the datatype this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the type SHALL conform to at least one profile defined in the implementation guide.", 2616 0, java.lang.Integer.MAX_VALUE, profile); 2617 case 1994521304: 2618 /* targetProfile */ return new Property("targetProfile", "canonical(StructureDefinition|ImplementationGuide)", 2619 "Used when the type is \"Reference\" or \"canonical\", and identifies a profile structure or implementation Guide that applies to the target of the reference this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.", 2620 0, java.lang.Integer.MAX_VALUE, targetProfile); 2621 case 841524962: 2622 /* aggregation */ return new Property("aggregation", "code", 2623 "If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle.", 2624 0, java.lang.Integer.MAX_VALUE, aggregation); 2625 case -670487542: 2626 /* versioning */ return new Property("versioning", "code", 2627 "Whether this reference needs to be version specific or version independent, or whether either can be used.", 2628 0, 1, versioning); 2629 default: 2630 return super.getNamedProperty(_hash, _name, _checkValid); 2631 } 2632 2633 } 2634 2635 @Override 2636 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2637 switch (hash) { 2638 case 3059181: 2639 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // UriType 2640 case -309425751: 2641 /* profile */ return this.profile == null ? new Base[0] : this.profile.toArray(new Base[this.profile.size()]); // CanonicalType 2642 case 1994521304: 2643 /* targetProfile */ return this.targetProfile == null ? new Base[0] 2644 : this.targetProfile.toArray(new Base[this.targetProfile.size()]); // CanonicalType 2645 case 841524962: 2646 /* aggregation */ return this.aggregation == null ? new Base[0] 2647 : this.aggregation.toArray(new Base[this.aggregation.size()]); // Enumeration<AggregationMode> 2648 case -670487542: 2649 /* versioning */ return this.versioning == null ? new Base[0] : new Base[] { this.versioning }; // Enumeration<ReferenceVersionRules> 2650 default: 2651 return super.getProperty(hash, name, checkValid); 2652 } 2653 2654 } 2655 2656 @Override 2657 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2658 switch (hash) { 2659 case 3059181: // code 2660 this.code = castToUri(value); // UriType 2661 return value; 2662 case -309425751: // profile 2663 this.getProfile().add(castToCanonical(value)); // CanonicalType 2664 return value; 2665 case 1994521304: // targetProfile 2666 this.getTargetProfile().add(castToCanonical(value)); // CanonicalType 2667 return value; 2668 case 841524962: // aggregation 2669 value = new AggregationModeEnumFactory().fromType(castToCode(value)); 2670 this.getAggregation().add((Enumeration) value); // Enumeration<AggregationMode> 2671 return value; 2672 case -670487542: // versioning 2673 value = new ReferenceVersionRulesEnumFactory().fromType(castToCode(value)); 2674 this.versioning = (Enumeration) value; // Enumeration<ReferenceVersionRules> 2675 return value; 2676 default: 2677 return super.setProperty(hash, name, value); 2678 } 2679 2680 } 2681 2682 @Override 2683 public Base setProperty(String name, Base value) throws FHIRException { 2684 if (name.equals("code")) { 2685 this.code = castToUri(value); // UriType 2686 } else if (name.equals("profile")) { 2687 this.getProfile().add(castToCanonical(value)); 2688 } else if (name.equals("targetProfile")) { 2689 this.getTargetProfile().add(castToCanonical(value)); 2690 } else if (name.equals("aggregation")) { 2691 value = new AggregationModeEnumFactory().fromType(castToCode(value)); 2692 this.getAggregation().add((Enumeration) value); 2693 } else if (name.equals("versioning")) { 2694 value = new ReferenceVersionRulesEnumFactory().fromType(castToCode(value)); 2695 this.versioning = (Enumeration) value; // Enumeration<ReferenceVersionRules> 2696 } else 2697 return super.setProperty(name, value); 2698 return value; 2699 } 2700 2701 @Override 2702 public Base makeProperty(int hash, String name) throws FHIRException { 2703 switch (hash) { 2704 case 3059181: 2705 return getCodeElement(); 2706 case -309425751: 2707 return addProfileElement(); 2708 case 1994521304: 2709 return addTargetProfileElement(); 2710 case 841524962: 2711 return addAggregationElement(); 2712 case -670487542: 2713 return getVersioningElement(); 2714 default: 2715 return super.makeProperty(hash, name); 2716 } 2717 2718 } 2719 2720 @Override 2721 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2722 switch (hash) { 2723 case 3059181: 2724 /* code */ return new String[] { "uri" }; 2725 case -309425751: 2726 /* profile */ return new String[] { "canonical" }; 2727 case 1994521304: 2728 /* targetProfile */ return new String[] { "canonical" }; 2729 case 841524962: 2730 /* aggregation */ return new String[] { "code" }; 2731 case -670487542: 2732 /* versioning */ return new String[] { "code" }; 2733 default: 2734 return super.getTypesForProperty(hash, name); 2735 } 2736 2737 } 2738 2739 @Override 2740 public Base addChild(String name) throws FHIRException { 2741 if (name.equals("code")) { 2742 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.code"); 2743 } else if (name.equals("profile")) { 2744 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.profile"); 2745 } else if (name.equals("targetProfile")) { 2746 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.targetProfile"); 2747 } else if (name.equals("aggregation")) { 2748 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.aggregation"); 2749 } else if (name.equals("versioning")) { 2750 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.versioning"); 2751 } else 2752 return super.addChild(name); 2753 } 2754 2755 public TypeRefComponent copy() { 2756 TypeRefComponent dst = new TypeRefComponent(); 2757 copyValues(dst); 2758 return dst; 2759 } 2760 2761 public void copyValues(TypeRefComponent dst) { 2762 super.copyValues(dst); 2763 dst.code = code == null ? null : code.copy(); 2764 if (profile != null) { 2765 dst.profile = new ArrayList<CanonicalType>(); 2766 for (CanonicalType i : profile) 2767 dst.profile.add(i.copy()); 2768 } 2769 ; 2770 if (targetProfile != null) { 2771 dst.targetProfile = new ArrayList<CanonicalType>(); 2772 for (CanonicalType i : targetProfile) 2773 dst.targetProfile.add(i.copy()); 2774 } 2775 ; 2776 if (aggregation != null) { 2777 dst.aggregation = new ArrayList<Enumeration<AggregationMode>>(); 2778 for (Enumeration<AggregationMode> i : aggregation) 2779 dst.aggregation.add(i.copy()); 2780 } 2781 ; 2782 dst.versioning = versioning == null ? null : versioning.copy(); 2783 } 2784 2785 @Override 2786 public boolean equalsDeep(Base other_) { 2787 if (!super.equalsDeep(other_)) 2788 return false; 2789 if (!(other_ instanceof TypeRefComponent)) 2790 return false; 2791 TypeRefComponent o = (TypeRefComponent) other_; 2792 return compareDeep(code, o.code, true) && compareDeep(profile, o.profile, true) 2793 && compareDeep(targetProfile, o.targetProfile, true) && compareDeep(aggregation, o.aggregation, true) 2794 && compareDeep(versioning, o.versioning, true); 2795 } 2796 2797 @Override 2798 public boolean equalsShallow(Base other_) { 2799 if (!super.equalsShallow(other_)) 2800 return false; 2801 if (!(other_ instanceof TypeRefComponent)) 2802 return false; 2803 TypeRefComponent o = (TypeRefComponent) other_; 2804 return compareValues(code, o.code, true) && compareValues(aggregation, o.aggregation, true) 2805 && compareValues(versioning, o.versioning, true); 2806 } 2807 2808 public boolean isEmpty() { 2809 return super.isEmpty() 2810 && ca.uhn.fhir.util.ElementUtil.isEmpty(code, profile, targetProfile, aggregation, versioning); 2811 } 2812 2813 public String fhirType() { 2814 return "ElementDefinition.type"; 2815 2816 } 2817 2818// added from java-adornments.txt: 2819 2820 public boolean hasTarget() { 2821 return Utilities.existsInList(getCode(), "Reference", "canonical"); 2822 } 2823 2824 /** 2825 * This code checks for the system prefix and returns the FHIR type 2826 * 2827 * @return 2828 */ 2829 public String getWorkingCode() { 2830 if (hasExtension(ToolingExtensions.EXT_FHIR_TYPE)) 2831 return getExtensionString(ToolingExtensions.EXT_FHIR_TYPE); 2832 if (!hasCodeElement()) 2833 return null; 2834 if (getCodeElement().hasExtension(ToolingExtensions.EXT_XML_TYPE)) { 2835 String s = getCodeElement().getExtensionString(ToolingExtensions.EXT_XML_TYPE); 2836 if ("xsd:gYear OR xsd:gYearMonth OR xsd:date OR xsd:dateTime".equals(s)) 2837 return "dateTime"; 2838 if ("xsd:gYear OR xsd:gYearMonth OR xsd:date".equals(s)) 2839 return "date"; 2840 if ("xsd:dateTime".equals(s)) 2841 return "instant"; 2842 if ("xsd:token".equals(s)) 2843 return "code"; 2844 if ("xsd:boolean".equals(s)) 2845 return "boolean"; 2846 if ("xsd:string".equals(s)) 2847 return "string"; 2848 if ("xsd:time".equals(s)) 2849 return "time"; 2850 if ("xsd:int".equals(s)) 2851 return "integer"; 2852 if ("xsd:decimal OR xsd:double".equals(s)) 2853 return "decimal"; 2854 if ("xsd:base64Binary".equals(s)) 2855 return "base64Binary"; 2856 if ("xsd:positiveInteger".equals(s)) 2857 return "positiveInt"; 2858 if ("xsd:nonNegativeInteger".equals(s)) 2859 return "unsignedInt"; 2860 if ("xsd:anyURI".equals(s)) 2861 return "uri"; 2862 2863 throw new Error("Unknown xml type '" + s + "'"); 2864 } 2865 return getCode(); 2866 } 2867 2868// end addition 2869 } 2870 2871 @Block() 2872 public static class ElementDefinitionExampleComponent extends Element implements IBaseDatatypeElement { 2873 /** 2874 * Describes the purpose of this example amoung the set of examples. 2875 */ 2876 @Child(name = "label", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2877 @Description(shortDefinition = "Describes the purpose of this example", formalDefinition = "Describes the purpose of this example amoung the set of examples.") 2878 protected StringType label; 2879 2880 /** 2881 * The actual value for the element, which must be one of the types allowed for 2882 * this element. 2883 */ 2884 @Child(name = "value", type = {}, order = 2, min = 1, max = 1, modifier = false, summary = true) 2885 @Description(shortDefinition = "Value of Example (one of allowed types)", formalDefinition = "The actual value for the element, which must be one of the types allowed for this element.") 2886 protected org.hl7.fhir.r4.model.Type value; 2887 2888 private static final long serialVersionUID = 457572481L; 2889 2890 /** 2891 * Constructor 2892 */ 2893 public ElementDefinitionExampleComponent() { 2894 super(); 2895 } 2896 2897 /** 2898 * Constructor 2899 */ 2900 public ElementDefinitionExampleComponent(StringType label, org.hl7.fhir.r4.model.Type value) { 2901 super(); 2902 this.label = label; 2903 this.value = value; 2904 } 2905 2906 /** 2907 * @return {@link #label} (Describes the purpose of this example amoung the set 2908 * of examples.). This is the underlying object with id, value and 2909 * extensions. The accessor "getLabel" gives direct access to the value 2910 */ 2911 public StringType getLabelElement() { 2912 if (this.label == null) 2913 if (Configuration.errorOnAutoCreate()) 2914 throw new Error("Attempt to auto-create ElementDefinitionExampleComponent.label"); 2915 else if (Configuration.doAutoCreate()) 2916 this.label = new StringType(); // bb 2917 return this.label; 2918 } 2919 2920 public boolean hasLabelElement() { 2921 return this.label != null && !this.label.isEmpty(); 2922 } 2923 2924 public boolean hasLabel() { 2925 return this.label != null && !this.label.isEmpty(); 2926 } 2927 2928 /** 2929 * @param value {@link #label} (Describes the purpose of this example amoung the 2930 * set of examples.). This is the underlying object with id, value 2931 * and extensions. The accessor "getLabel" gives direct access to 2932 * the value 2933 */ 2934 public ElementDefinitionExampleComponent setLabelElement(StringType value) { 2935 this.label = value; 2936 return this; 2937 } 2938 2939 /** 2940 * @return Describes the purpose of this example amoung the set of examples. 2941 */ 2942 public String getLabel() { 2943 return this.label == null ? null : this.label.getValue(); 2944 } 2945 2946 /** 2947 * @param value Describes the purpose of this example amoung the set of 2948 * examples. 2949 */ 2950 public ElementDefinitionExampleComponent setLabel(String value) { 2951 if (this.label == null) 2952 this.label = new StringType(); 2953 this.label.setValue(value); 2954 return this; 2955 } 2956 2957 /** 2958 * @return {@link #value} (The actual value for the element, which must be one 2959 * of the types allowed for this element.) 2960 */ 2961 public org.hl7.fhir.r4.model.Type getValue() { 2962 return this.value; 2963 } 2964 2965 public boolean hasValue() { 2966 return this.value != null && !this.value.isEmpty(); 2967 } 2968 2969 /** 2970 * @param value {@link #value} (The actual value for the element, which must be 2971 * one of the types allowed for this element.) 2972 */ 2973 public ElementDefinitionExampleComponent setValue(org.hl7.fhir.r4.model.Type value) { 2974 this.value = value; 2975 return this; 2976 } 2977 2978 protected void listChildren(List<Property> children) { 2979 super.listChildren(children); 2980 children.add(new Property("label", "string", "Describes the purpose of this example amoung the set of examples.", 2981 0, 1, label)); 2982 children.add(new Property("value[x]", "*", 2983 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value)); 2984 } 2985 2986 @Override 2987 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2988 switch (_hash) { 2989 case 102727412: 2990 /* label */ return new Property("label", "string", 2991 "Describes the purpose of this example amoung the set of examples.", 0, 1, label); 2992 case -1410166417: 2993 /* value[x] */ return new Property("value[x]", "*", 2994 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2995 case 111972721: 2996 /* value */ return new Property("value[x]", "*", 2997 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2998 case -1535024575: 2999 /* valueBase64Binary */ return new Property("value[x]", "*", 3000 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3001 case 733421943: 3002 /* valueBoolean */ return new Property("value[x]", "*", 3003 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3004 case -786218365: 3005 /* valueCanonical */ return new Property("value[x]", "*", 3006 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3007 case -766209282: 3008 /* valueCode */ return new Property("value[x]", "*", 3009 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3010 case -766192449: 3011 /* valueDate */ return new Property("value[x]", "*", 3012 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3013 case 1047929900: 3014 /* valueDateTime */ return new Property("value[x]", "*", 3015 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3016 case -2083993440: 3017 /* valueDecimal */ return new Property("value[x]", "*", 3018 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3019 case 231604844: 3020 /* valueId */ return new Property("value[x]", "*", 3021 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3022 case -1668687056: 3023 /* valueInstant */ return new Property("value[x]", "*", 3024 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3025 case -1668204915: 3026 /* valueInteger */ return new Property("value[x]", "*", 3027 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3028 case -497880704: 3029 /* valueMarkdown */ return new Property("value[x]", "*", 3030 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3031 case -1410178407: 3032 /* valueOid */ return new Property("value[x]", "*", 3033 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3034 case -1249932027: 3035 /* valuePositiveInt */ return new Property("value[x]", "*", 3036 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3037 case -1424603934: 3038 /* valueString */ return new Property("value[x]", "*", 3039 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3040 case -765708322: 3041 /* valueTime */ return new Property("value[x]", "*", 3042 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3043 case 26529417: 3044 /* valueUnsignedInt */ return new Property("value[x]", "*", 3045 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3046 case -1410172357: 3047 /* valueUri */ return new Property("value[x]", "*", 3048 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3049 case -1410172354: 3050 /* valueUrl */ return new Property("value[x]", "*", 3051 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3052 case -765667124: 3053 /* valueUuid */ return new Property("value[x]", "*", 3054 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3055 case -478981821: 3056 /* valueAddress */ return new Property("value[x]", "*", 3057 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3058 case -67108992: 3059 /* valueAnnotation */ return new Property("value[x]", "*", 3060 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3061 case -475566732: 3062 /* valueAttachment */ return new Property("value[x]", "*", 3063 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3064 case 924902896: 3065 /* valueCodeableConcept */ return new Property("value[x]", "*", 3066 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3067 case -1887705029: 3068 /* valueCoding */ return new Property("value[x]", "*", 3069 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3070 case 944904545: 3071 /* valueContactPoint */ return new Property("value[x]", "*", 3072 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3073 case -2026205465: 3074 /* valueHumanName */ return new Property("value[x]", "*", 3075 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3076 case -130498310: 3077 /* valueIdentifier */ return new Property("value[x]", "*", 3078 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3079 case -1524344174: 3080 /* valuePeriod */ return new Property("value[x]", "*", 3081 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3082 case -2029823716: 3083 /* valueQuantity */ return new Property("value[x]", "*", 3084 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3085 case 2030761548: 3086 /* valueRange */ return new Property("value[x]", "*", 3087 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3088 case 2030767386: 3089 /* valueRatio */ return new Property("value[x]", "*", 3090 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3091 case 1755241690: 3092 /* valueReference */ return new Property("value[x]", "*", 3093 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3094 case -962229101: 3095 /* valueSampledData */ return new Property("value[x]", "*", 3096 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3097 case -540985785: 3098 /* valueSignature */ return new Property("value[x]", "*", 3099 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3100 case -1406282469: 3101 /* valueTiming */ return new Property("value[x]", "*", 3102 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3103 case -1858636920: 3104 /* valueDosage */ return new Property("value[x]", "*", 3105 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3106 default: 3107 return super.getNamedProperty(_hash, _name, _checkValid); 3108 } 3109 3110 } 3111 3112 @Override 3113 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3114 switch (hash) { 3115 case 102727412: 3116 /* label */ return this.label == null ? new Base[0] : new Base[] { this.label }; // StringType 3117 case 111972721: 3118 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // org.hl7.fhir.r4.model.Type 3119 default: 3120 return super.getProperty(hash, name, checkValid); 3121 } 3122 3123 } 3124 3125 @Override 3126 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3127 switch (hash) { 3128 case 102727412: // label 3129 this.label = castToString(value); // StringType 3130 return value; 3131 case 111972721: // value 3132 this.value = castToType(value); // org.hl7.fhir.r4.model.Type 3133 return value; 3134 default: 3135 return super.setProperty(hash, name, value); 3136 } 3137 3138 } 3139 3140 @Override 3141 public Base setProperty(String name, Base value) throws FHIRException { 3142 if (name.equals("label")) { 3143 this.label = castToString(value); // StringType 3144 } else if (name.equals("value[x]")) { 3145 this.value = castToType(value); // org.hl7.fhir.r4.model.Type 3146 } else 3147 return super.setProperty(name, value); 3148 return value; 3149 } 3150 3151 @Override 3152 public Base makeProperty(int hash, String name) throws FHIRException { 3153 switch (hash) { 3154 case 102727412: 3155 return getLabelElement(); 3156 case -1410166417: 3157 return getValue(); 3158 case 111972721: 3159 return getValue(); 3160 default: 3161 return super.makeProperty(hash, name); 3162 } 3163 3164 } 3165 3166 @Override 3167 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3168 switch (hash) { 3169 case 102727412: 3170 /* label */ return new String[] { "string" }; 3171 case 111972721: 3172 /* value */ return new String[] { "*" }; 3173 default: 3174 return super.getTypesForProperty(hash, name); 3175 } 3176 3177 } 3178 3179 @Override 3180 public Base addChild(String name) throws FHIRException { 3181 if (name.equals("label")) { 3182 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.label"); 3183 } else if (name.equals("valueBase64Binary")) { 3184 this.value = new Base64BinaryType(); 3185 return this.value; 3186 } else if (name.equals("valueBoolean")) { 3187 this.value = new BooleanType(); 3188 return this.value; 3189 } else if (name.equals("valueCanonical")) { 3190 this.value = new CanonicalType(); 3191 return this.value; 3192 } else if (name.equals("valueCode")) { 3193 this.value = new CodeType(); 3194 return this.value; 3195 } else if (name.equals("valueDate")) { 3196 this.value = new DateType(); 3197 return this.value; 3198 } else if (name.equals("valueDateTime")) { 3199 this.value = new DateTimeType(); 3200 return this.value; 3201 } else if (name.equals("valueDecimal")) { 3202 this.value = new DecimalType(); 3203 return this.value; 3204 } else if (name.equals("valueId")) { 3205 this.value = new IdType(); 3206 return this.value; 3207 } else if (name.equals("valueInstant")) { 3208 this.value = new InstantType(); 3209 return this.value; 3210 } else if (name.equals("valueInteger")) { 3211 this.value = new IntegerType(); 3212 return this.value; 3213 } else if (name.equals("valueMarkdown")) { 3214 this.value = new MarkdownType(); 3215 return this.value; 3216 } else if (name.equals("valueOid")) { 3217 this.value = new OidType(); 3218 return this.value; 3219 } else if (name.equals("valuePositiveInt")) { 3220 this.value = new PositiveIntType(); 3221 return this.value; 3222 } else if (name.equals("valueString")) { 3223 this.value = new StringType(); 3224 return this.value; 3225 } else if (name.equals("valueTime")) { 3226 this.value = new TimeType(); 3227 return this.value; 3228 } else if (name.equals("valueUnsignedInt")) { 3229 this.value = new UnsignedIntType(); 3230 return this.value; 3231 } else if (name.equals("valueUri")) { 3232 this.value = new UriType(); 3233 return this.value; 3234 } else if (name.equals("valueUrl")) { 3235 this.value = new UrlType(); 3236 return this.value; 3237 } else if (name.equals("valueUuid")) { 3238 this.value = new UuidType(); 3239 return this.value; 3240 } else if (name.equals("valueAddress")) { 3241 this.value = new Address(); 3242 return this.value; 3243 } else if (name.equals("valueAge")) { 3244 this.value = new Age(); 3245 return this.value; 3246 } else if (name.equals("valueAnnotation")) { 3247 this.value = new Annotation(); 3248 return this.value; 3249 } else if (name.equals("valueAttachment")) { 3250 this.value = new Attachment(); 3251 return this.value; 3252 } else if (name.equals("valueCodeableConcept")) { 3253 this.value = new CodeableConcept(); 3254 return this.value; 3255 } else if (name.equals("valueCoding")) { 3256 this.value = new Coding(); 3257 return this.value; 3258 } else if (name.equals("valueContactPoint")) { 3259 this.value = new ContactPoint(); 3260 return this.value; 3261 } else if (name.equals("valueCount")) { 3262 this.value = new Count(); 3263 return this.value; 3264 } else if (name.equals("valueDistance")) { 3265 this.value = new Distance(); 3266 return this.value; 3267 } else if (name.equals("valueDuration")) { 3268 this.value = new Duration(); 3269 return this.value; 3270 } else if (name.equals("valueHumanName")) { 3271 this.value = new HumanName(); 3272 return this.value; 3273 } else if (name.equals("valueIdentifier")) { 3274 this.value = new Identifier(); 3275 return this.value; 3276 } else if (name.equals("valueMoney")) { 3277 this.value = new Money(); 3278 return this.value; 3279 } else if (name.equals("valuePeriod")) { 3280 this.value = new Period(); 3281 return this.value; 3282 } else if (name.equals("valueQuantity")) { 3283 this.value = new Quantity(); 3284 return this.value; 3285 } else if (name.equals("valueRange")) { 3286 this.value = new Range(); 3287 return this.value; 3288 } else if (name.equals("valueRatio")) { 3289 this.value = new Ratio(); 3290 return this.value; 3291 } else if (name.equals("valueReference")) { 3292 this.value = new Reference(); 3293 return this.value; 3294 } else if (name.equals("valueSampledData")) { 3295 this.value = new SampledData(); 3296 return this.value; 3297 } else if (name.equals("valueSignature")) { 3298 this.value = new Signature(); 3299 return this.value; 3300 } else if (name.equals("valueTiming")) { 3301 this.value = new Timing(); 3302 return this.value; 3303 } else if (name.equals("valueContactDetail")) { 3304 this.value = new ContactDetail(); 3305 return this.value; 3306 } else if (name.equals("valueContributor")) { 3307 this.value = new Contributor(); 3308 return this.value; 3309 } else if (name.equals("valueDataRequirement")) { 3310 this.value = new DataRequirement(); 3311 return this.value; 3312 } else if (name.equals("valueExpression")) { 3313 this.value = new Expression(); 3314 return this.value; 3315 } else if (name.equals("valueParameterDefinition")) { 3316 this.value = new ParameterDefinition(); 3317 return this.value; 3318 } else if (name.equals("valueRelatedArtifact")) { 3319 this.value = new RelatedArtifact(); 3320 return this.value; 3321 } else if (name.equals("valueTriggerDefinition")) { 3322 this.value = new TriggerDefinition(); 3323 return this.value; 3324 } else if (name.equals("valueUsageContext")) { 3325 this.value = new UsageContext(); 3326 return this.value; 3327 } else if (name.equals("valueDosage")) { 3328 this.value = new Dosage(); 3329 return this.value; 3330 } else if (name.equals("valueMeta")) { 3331 this.value = new Meta(); 3332 return this.value; 3333 } else 3334 return super.addChild(name); 3335 } 3336 3337 public ElementDefinitionExampleComponent copy() { 3338 ElementDefinitionExampleComponent dst = new ElementDefinitionExampleComponent(); 3339 copyValues(dst); 3340 return dst; 3341 } 3342 3343 public void copyValues(ElementDefinitionExampleComponent dst) { 3344 super.copyValues(dst); 3345 dst.label = label == null ? null : label.copy(); 3346 dst.value = value == null ? null : value.copy(); 3347 } 3348 3349 @Override 3350 public boolean equalsDeep(Base other_) { 3351 if (!super.equalsDeep(other_)) 3352 return false; 3353 if (!(other_ instanceof ElementDefinitionExampleComponent)) 3354 return false; 3355 ElementDefinitionExampleComponent o = (ElementDefinitionExampleComponent) other_; 3356 return compareDeep(label, o.label, true) && compareDeep(value, o.value, true); 3357 } 3358 3359 @Override 3360 public boolean equalsShallow(Base other_) { 3361 if (!super.equalsShallow(other_)) 3362 return false; 3363 if (!(other_ instanceof ElementDefinitionExampleComponent)) 3364 return false; 3365 ElementDefinitionExampleComponent o = (ElementDefinitionExampleComponent) other_; 3366 return compareValues(label, o.label, true); 3367 } 3368 3369 public boolean isEmpty() { 3370 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(label, value); 3371 } 3372 3373 public String fhirType() { 3374 return "ElementDefinition.example"; 3375 3376 } 3377 3378 } 3379 3380 @Block() 3381 public static class ElementDefinitionConstraintComponent extends Element implements IBaseDatatypeElement { 3382 /** 3383 * Allows identification of which elements have their cardinalities impacted by 3384 * the constraint. Will not be referenced for constraints that do not affect 3385 * cardinality. 3386 */ 3387 @Child(name = "key", type = { IdType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 3388 @Description(shortDefinition = "Target of 'condition' reference above", formalDefinition = "Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality.") 3389 protected IdType key; 3390 3391 /** 3392 * Description of why this constraint is necessary or appropriate. 3393 */ 3394 @Child(name = "requirements", type = { 3395 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 3396 @Description(shortDefinition = "Why this constraint is necessary or appropriate", formalDefinition = "Description of why this constraint is necessary or appropriate.") 3397 protected StringType requirements; 3398 3399 /** 3400 * Identifies the impact constraint violation has on the conformance of the 3401 * instance. 3402 */ 3403 @Child(name = "severity", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 3404 @Description(shortDefinition = "error | warning", formalDefinition = "Identifies the impact constraint violation has on the conformance of the instance.") 3405 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/constraint-severity") 3406 protected Enumeration<ConstraintSeverity> severity; 3407 3408 /** 3409 * Text that can be used to describe the constraint in messages identifying that 3410 * the constraint has been violated. 3411 */ 3412 @Child(name = "human", type = { StringType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 3413 @Description(shortDefinition = "Human description of constraint", formalDefinition = "Text that can be used to describe the constraint in messages identifying that the constraint has been violated.") 3414 protected StringType human; 3415 3416 /** 3417 * A [FHIRPath](fhirpath.html) expression of constraint that can be executed to 3418 * see if this constraint is met. 3419 */ 3420 @Child(name = "expression", type = { 3421 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 3422 @Description(shortDefinition = "FHIRPath expression of constraint", formalDefinition = "A [FHIRPath](fhirpath.html) expression of constraint that can be executed to see if this constraint is met.") 3423 protected StringType expression; 3424 3425 /** 3426 * An XPath expression of constraint that can be executed to see if this 3427 * constraint is met. 3428 */ 3429 @Child(name = "xpath", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 3430 @Description(shortDefinition = "XPath expression of constraint", formalDefinition = "An XPath expression of constraint that can be executed to see if this constraint is met.") 3431 protected StringType xpath; 3432 3433 /** 3434 * A reference to the original source of the constraint, for traceability 3435 * purposes. 3436 */ 3437 @Child(name = "source", type = { 3438 CanonicalType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 3439 @Description(shortDefinition = "Reference to original source of constraint", formalDefinition = "A reference to the original source of the constraint, for traceability purposes.") 3440 protected CanonicalType source; 3441 3442 private static final long serialVersionUID = 1048354565L; 3443 3444 /** 3445 * Constructor 3446 */ 3447 public ElementDefinitionConstraintComponent() { 3448 super(); 3449 } 3450 3451 /** 3452 * Constructor 3453 */ 3454 public ElementDefinitionConstraintComponent(IdType key, Enumeration<ConstraintSeverity> severity, 3455 StringType human) { 3456 super(); 3457 this.key = key; 3458 this.severity = severity; 3459 this.human = human; 3460 } 3461 3462 /** 3463 * @return {@link #key} (Allows identification of which elements have their 3464 * cardinalities impacted by the constraint. Will not be referenced for 3465 * constraints that do not affect cardinality.). This is the underlying 3466 * object with id, value and extensions. The accessor "getKey" gives 3467 * direct access to the value 3468 */ 3469 public IdType getKeyElement() { 3470 if (this.key == null) 3471 if (Configuration.errorOnAutoCreate()) 3472 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.key"); 3473 else if (Configuration.doAutoCreate()) 3474 this.key = new IdType(); // bb 3475 return this.key; 3476 } 3477 3478 public boolean hasKeyElement() { 3479 return this.key != null && !this.key.isEmpty(); 3480 } 3481 3482 public boolean hasKey() { 3483 return this.key != null && !this.key.isEmpty(); 3484 } 3485 3486 /** 3487 * @param value {@link #key} (Allows identification of which elements have their 3488 * cardinalities impacted by the constraint. Will not be referenced 3489 * for constraints that do not affect cardinality.). This is the 3490 * underlying object with id, value and extensions. The accessor 3491 * "getKey" gives direct access to the value 3492 */ 3493 public ElementDefinitionConstraintComponent setKeyElement(IdType value) { 3494 this.key = value; 3495 return this; 3496 } 3497 3498 /** 3499 * @return Allows identification of which elements have their cardinalities 3500 * impacted by the constraint. Will not be referenced for constraints 3501 * that do not affect cardinality. 3502 */ 3503 public String getKey() { 3504 return this.key == null ? null : this.key.getValue(); 3505 } 3506 3507 /** 3508 * @param value Allows identification of which elements have their cardinalities 3509 * impacted by the constraint. Will not be referenced for 3510 * constraints that do not affect cardinality. 3511 */ 3512 public ElementDefinitionConstraintComponent setKey(String value) { 3513 if (this.key == null) 3514 this.key = new IdType(); 3515 this.key.setValue(value); 3516 return this; 3517 } 3518 3519 /** 3520 * @return {@link #requirements} (Description of why this constraint is 3521 * necessary or appropriate.). This is the underlying object with id, 3522 * value and extensions. The accessor "getRequirements" gives direct 3523 * access to the value 3524 */ 3525 public StringType getRequirementsElement() { 3526 if (this.requirements == null) 3527 if (Configuration.errorOnAutoCreate()) 3528 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.requirements"); 3529 else if (Configuration.doAutoCreate()) 3530 this.requirements = new StringType(); // bb 3531 return this.requirements; 3532 } 3533 3534 public boolean hasRequirementsElement() { 3535 return this.requirements != null && !this.requirements.isEmpty(); 3536 } 3537 3538 public boolean hasRequirements() { 3539 return this.requirements != null && !this.requirements.isEmpty(); 3540 } 3541 3542 /** 3543 * @param value {@link #requirements} (Description of why this constraint is 3544 * necessary or appropriate.). This is the underlying object with 3545 * id, value and extensions. The accessor "getRequirements" gives 3546 * direct access to the value 3547 */ 3548 public ElementDefinitionConstraintComponent setRequirementsElement(StringType value) { 3549 this.requirements = value; 3550 return this; 3551 } 3552 3553 /** 3554 * @return Description of why this constraint is necessary or appropriate. 3555 */ 3556 public String getRequirements() { 3557 return this.requirements == null ? null : this.requirements.getValue(); 3558 } 3559 3560 /** 3561 * @param value Description of why this constraint is necessary or appropriate. 3562 */ 3563 public ElementDefinitionConstraintComponent setRequirements(String value) { 3564 if (Utilities.noString(value)) 3565 this.requirements = null; 3566 else { 3567 if (this.requirements == null) 3568 this.requirements = new StringType(); 3569 this.requirements.setValue(value); 3570 } 3571 return this; 3572 } 3573 3574 /** 3575 * @return {@link #severity} (Identifies the impact constraint violation has on 3576 * the conformance of the instance.). This is the underlying object with 3577 * id, value and extensions. The accessor "getSeverity" gives direct 3578 * access to the value 3579 */ 3580 public Enumeration<ConstraintSeverity> getSeverityElement() { 3581 if (this.severity == null) 3582 if (Configuration.errorOnAutoCreate()) 3583 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.severity"); 3584 else if (Configuration.doAutoCreate()) 3585 this.severity = new Enumeration<ConstraintSeverity>(new ConstraintSeverityEnumFactory()); // bb 3586 return this.severity; 3587 } 3588 3589 public boolean hasSeverityElement() { 3590 return this.severity != null && !this.severity.isEmpty(); 3591 } 3592 3593 public boolean hasSeverity() { 3594 return this.severity != null && !this.severity.isEmpty(); 3595 } 3596 3597 /** 3598 * @param value {@link #severity} (Identifies the impact constraint violation 3599 * has on the conformance of the instance.). This is the underlying 3600 * object with id, value and extensions. The accessor "getSeverity" 3601 * gives direct access to the value 3602 */ 3603 public ElementDefinitionConstraintComponent setSeverityElement(Enumeration<ConstraintSeverity> value) { 3604 this.severity = value; 3605 return this; 3606 } 3607 3608 /** 3609 * @return Identifies the impact constraint violation has on the conformance of 3610 * the instance. 3611 */ 3612 public ConstraintSeverity getSeverity() { 3613 return this.severity == null ? null : this.severity.getValue(); 3614 } 3615 3616 /** 3617 * @param value Identifies the impact constraint violation has on the 3618 * conformance of the instance. 3619 */ 3620 public ElementDefinitionConstraintComponent setSeverity(ConstraintSeverity value) { 3621 if (this.severity == null) 3622 this.severity = new Enumeration<ConstraintSeverity>(new ConstraintSeverityEnumFactory()); 3623 this.severity.setValue(value); 3624 return this; 3625 } 3626 3627 /** 3628 * @return {@link #human} (Text that can be used to describe the constraint in 3629 * messages identifying that the constraint has been violated.). This is 3630 * the underlying object with id, value and extensions. The accessor 3631 * "getHuman" gives direct access to the value 3632 */ 3633 public StringType getHumanElement() { 3634 if (this.human == null) 3635 if (Configuration.errorOnAutoCreate()) 3636 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.human"); 3637 else if (Configuration.doAutoCreate()) 3638 this.human = new StringType(); // bb 3639 return this.human; 3640 } 3641 3642 public boolean hasHumanElement() { 3643 return this.human != null && !this.human.isEmpty(); 3644 } 3645 3646 public boolean hasHuman() { 3647 return this.human != null && !this.human.isEmpty(); 3648 } 3649 3650 /** 3651 * @param value {@link #human} (Text that can be used to describe the constraint 3652 * in messages identifying that the constraint has been violated.). 3653 * This is the underlying object with id, value and extensions. The 3654 * accessor "getHuman" gives direct access to the value 3655 */ 3656 public ElementDefinitionConstraintComponent setHumanElement(StringType value) { 3657 this.human = value; 3658 return this; 3659 } 3660 3661 /** 3662 * @return Text that can be used to describe the constraint in messages 3663 * identifying that the constraint has been violated. 3664 */ 3665 public String getHuman() { 3666 return this.human == null ? null : this.human.getValue(); 3667 } 3668 3669 /** 3670 * @param value Text that can be used to describe the constraint in messages 3671 * identifying that the constraint has been violated. 3672 */ 3673 public ElementDefinitionConstraintComponent setHuman(String value) { 3674 if (this.human == null) 3675 this.human = new StringType(); 3676 this.human.setValue(value); 3677 return this; 3678 } 3679 3680 /** 3681 * @return {@link #expression} (A [FHIRPath](fhirpath.html) expression of 3682 * constraint that can be executed to see if this constraint is met.). 3683 * This is the underlying object with id, value and extensions. The 3684 * accessor "getExpression" gives direct access to the value 3685 */ 3686 public StringType getExpressionElement() { 3687 if (this.expression == null) 3688 if (Configuration.errorOnAutoCreate()) 3689 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.expression"); 3690 else if (Configuration.doAutoCreate()) 3691 this.expression = new StringType(); // bb 3692 return this.expression; 3693 } 3694 3695 public boolean hasExpressionElement() { 3696 return this.expression != null && !this.expression.isEmpty(); 3697 } 3698 3699 public boolean hasExpression() { 3700 return this.expression != null && !this.expression.isEmpty(); 3701 } 3702 3703 /** 3704 * @param value {@link #expression} (A [FHIRPath](fhirpath.html) expression of 3705 * constraint that can be executed to see if this constraint is 3706 * met.). This is the underlying object with id, value and 3707 * extensions. The accessor "getExpression" gives direct access to 3708 * the value 3709 */ 3710 public ElementDefinitionConstraintComponent setExpressionElement(StringType value) { 3711 this.expression = value; 3712 return this; 3713 } 3714 3715 /** 3716 * @return A [FHIRPath](fhirpath.html) expression of constraint that can be 3717 * executed to see if this constraint is met. 3718 */ 3719 public String getExpression() { 3720 return this.expression == null ? null : this.expression.getValue(); 3721 } 3722 3723 /** 3724 * @param value A [FHIRPath](fhirpath.html) expression of constraint that can be 3725 * executed to see if this constraint is met. 3726 */ 3727 public ElementDefinitionConstraintComponent setExpression(String value) { 3728 if (Utilities.noString(value)) 3729 this.expression = null; 3730 else { 3731 if (this.expression == null) 3732 this.expression = new StringType(); 3733 this.expression.setValue(value); 3734 } 3735 return this; 3736 } 3737 3738 /** 3739 * @return {@link #xpath} (An XPath expression of constraint that can be 3740 * executed to see if this constraint is met.). This is the underlying 3741 * object with id, value and extensions. The accessor "getXpath" gives 3742 * direct access to the value 3743 */ 3744 public StringType getXpathElement() { 3745 if (this.xpath == null) 3746 if (Configuration.errorOnAutoCreate()) 3747 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.xpath"); 3748 else if (Configuration.doAutoCreate()) 3749 this.xpath = new StringType(); // bb 3750 return this.xpath; 3751 } 3752 3753 public boolean hasXpathElement() { 3754 return this.xpath != null && !this.xpath.isEmpty(); 3755 } 3756 3757 public boolean hasXpath() { 3758 return this.xpath != null && !this.xpath.isEmpty(); 3759 } 3760 3761 /** 3762 * @param value {@link #xpath} (An XPath expression of constraint that can be 3763 * executed to see if this constraint is met.). This is the 3764 * underlying object with id, value and extensions. The accessor 3765 * "getXpath" gives direct access to the value 3766 */ 3767 public ElementDefinitionConstraintComponent setXpathElement(StringType value) { 3768 this.xpath = value; 3769 return this; 3770 } 3771 3772 /** 3773 * @return An XPath expression of constraint that can be executed to see if this 3774 * constraint is met. 3775 */ 3776 public String getXpath() { 3777 return this.xpath == null ? null : this.xpath.getValue(); 3778 } 3779 3780 /** 3781 * @param value An XPath expression of constraint that can be executed to see if 3782 * this constraint is met. 3783 */ 3784 public ElementDefinitionConstraintComponent setXpath(String value) { 3785 if (Utilities.noString(value)) 3786 this.xpath = null; 3787 else { 3788 if (this.xpath == null) 3789 this.xpath = new StringType(); 3790 this.xpath.setValue(value); 3791 } 3792 return this; 3793 } 3794 3795 /** 3796 * @return {@link #source} (A reference to the original source of the 3797 * constraint, for traceability purposes.). This is the underlying 3798 * object with id, value and extensions. The accessor "getSource" gives 3799 * direct access to the value 3800 */ 3801 public CanonicalType getSourceElement() { 3802 if (this.source == null) 3803 if (Configuration.errorOnAutoCreate()) 3804 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.source"); 3805 else if (Configuration.doAutoCreate()) 3806 this.source = new CanonicalType(); // bb 3807 return this.source; 3808 } 3809 3810 public boolean hasSourceElement() { 3811 return this.source != null && !this.source.isEmpty(); 3812 } 3813 3814 public boolean hasSource() { 3815 return this.source != null && !this.source.isEmpty(); 3816 } 3817 3818 /** 3819 * @param value {@link #source} (A reference to the original source of the 3820 * constraint, for traceability purposes.). This is the underlying 3821 * object with id, value and extensions. The accessor "getSource" 3822 * gives direct access to the value 3823 */ 3824 public ElementDefinitionConstraintComponent setSourceElement(CanonicalType value) { 3825 this.source = value; 3826 return this; 3827 } 3828 3829 /** 3830 * @return A reference to the original source of the constraint, for 3831 * traceability purposes. 3832 */ 3833 public String getSource() { 3834 return this.source == null ? null : this.source.getValue(); 3835 } 3836 3837 /** 3838 * @param value A reference to the original source of the constraint, for 3839 * traceability purposes. 3840 */ 3841 public ElementDefinitionConstraintComponent setSource(String value) { 3842 if (Utilities.noString(value)) 3843 this.source = null; 3844 else { 3845 if (this.source == null) 3846 this.source = new CanonicalType(); 3847 this.source.setValue(value); 3848 } 3849 return this; 3850 } 3851 3852 protected void listChildren(List<Property> children) { 3853 super.listChildren(children); 3854 children.add(new Property("key", "id", 3855 "Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality.", 3856 0, 1, key)); 3857 children.add(new Property("requirements", "string", 3858 "Description of why this constraint is necessary or appropriate.", 0, 1, requirements)); 3859 children.add(new Property("severity", "code", 3860 "Identifies the impact constraint violation has on the conformance of the instance.", 0, 1, severity)); 3861 children.add(new Property("human", "string", 3862 "Text that can be used to describe the constraint in messages identifying that the constraint has been violated.", 3863 0, 1, human)); 3864 children.add(new Property("expression", "string", 3865 "A [FHIRPath](fhirpath.html) expression of constraint that can be executed to see if this constraint is met.", 3866 0, 1, expression)); 3867 children.add(new Property("xpath", "string", 3868 "An XPath expression of constraint that can be executed to see if this constraint is met.", 0, 1, xpath)); 3869 children.add(new Property("source", "canonical(StructureDefinition)", 3870 "A reference to the original source of the constraint, for traceability purposes.", 0, 1, source)); 3871 } 3872 3873 @Override 3874 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3875 switch (_hash) { 3876 case 106079: 3877 /* key */ return new Property("key", "id", 3878 "Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality.", 3879 0, 1, key); 3880 case -1619874672: 3881 /* requirements */ return new Property("requirements", "string", 3882 "Description of why this constraint is necessary or appropriate.", 0, 1, requirements); 3883 case 1478300413: 3884 /* severity */ return new Property("severity", "code", 3885 "Identifies the impact constraint violation has on the conformance of the instance.", 0, 1, severity); 3886 case 99639597: 3887 /* human */ return new Property("human", "string", 3888 "Text that can be used to describe the constraint in messages identifying that the constraint has been violated.", 3889 0, 1, human); 3890 case -1795452264: 3891 /* expression */ return new Property("expression", "string", 3892 "A [FHIRPath](fhirpath.html) expression of constraint that can be executed to see if this constraint is met.", 3893 0, 1, expression); 3894 case 114256029: 3895 /* xpath */ return new Property("xpath", "string", 3896 "An XPath expression of constraint that can be executed to see if this constraint is met.", 0, 1, xpath); 3897 case -896505829: 3898 /* source */ return new Property("source", "canonical(StructureDefinition)", 3899 "A reference to the original source of the constraint, for traceability purposes.", 0, 1, source); 3900 default: 3901 return super.getNamedProperty(_hash, _name, _checkValid); 3902 } 3903 3904 } 3905 3906 @Override 3907 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3908 switch (hash) { 3909 case 106079: 3910 /* key */ return this.key == null ? new Base[0] : new Base[] { this.key }; // IdType 3911 case -1619874672: 3912 /* requirements */ return this.requirements == null ? new Base[0] : new Base[] { this.requirements }; // StringType 3913 case 1478300413: 3914 /* severity */ return this.severity == null ? new Base[0] : new Base[] { this.severity }; // Enumeration<ConstraintSeverity> 3915 case 99639597: 3916 /* human */ return this.human == null ? new Base[0] : new Base[] { this.human }; // StringType 3917 case -1795452264: 3918 /* expression */ return this.expression == null ? new Base[0] : new Base[] { this.expression }; // StringType 3919 case 114256029: 3920 /* xpath */ return this.xpath == null ? new Base[0] : new Base[] { this.xpath }; // StringType 3921 case -896505829: 3922 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // CanonicalType 3923 default: 3924 return super.getProperty(hash, name, checkValid); 3925 } 3926 3927 } 3928 3929 @Override 3930 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3931 switch (hash) { 3932 case 106079: // key 3933 this.key = castToId(value); // IdType 3934 return value; 3935 case -1619874672: // requirements 3936 this.requirements = castToString(value); // StringType 3937 return value; 3938 case 1478300413: // severity 3939 value = new ConstraintSeverityEnumFactory().fromType(castToCode(value)); 3940 this.severity = (Enumeration) value; // Enumeration<ConstraintSeverity> 3941 return value; 3942 case 99639597: // human 3943 this.human = castToString(value); // StringType 3944 return value; 3945 case -1795452264: // expression 3946 this.expression = castToString(value); // StringType 3947 return value; 3948 case 114256029: // xpath 3949 this.xpath = castToString(value); // StringType 3950 return value; 3951 case -896505829: // source 3952 this.source = castToCanonical(value); // CanonicalType 3953 return value; 3954 default: 3955 return super.setProperty(hash, name, value); 3956 } 3957 3958 } 3959 3960 @Override 3961 public Base setProperty(String name, Base value) throws FHIRException { 3962 if (name.equals("key")) { 3963 this.key = castToId(value); // IdType 3964 } else if (name.equals("requirements")) { 3965 this.requirements = castToString(value); // StringType 3966 } else if (name.equals("severity")) { 3967 value = new ConstraintSeverityEnumFactory().fromType(castToCode(value)); 3968 this.severity = (Enumeration) value; // Enumeration<ConstraintSeverity> 3969 } else if (name.equals("human")) { 3970 this.human = castToString(value); // StringType 3971 } else if (name.equals("expression")) { 3972 this.expression = castToString(value); // StringType 3973 } else if (name.equals("xpath")) { 3974 this.xpath = castToString(value); // StringType 3975 } else if (name.equals("source")) { 3976 this.source = castToCanonical(value); // CanonicalType 3977 } else 3978 return super.setProperty(name, value); 3979 return value; 3980 } 3981 3982 @Override 3983 public Base makeProperty(int hash, String name) throws FHIRException { 3984 switch (hash) { 3985 case 106079: 3986 return getKeyElement(); 3987 case -1619874672: 3988 return getRequirementsElement(); 3989 case 1478300413: 3990 return getSeverityElement(); 3991 case 99639597: 3992 return getHumanElement(); 3993 case -1795452264: 3994 return getExpressionElement(); 3995 case 114256029: 3996 return getXpathElement(); 3997 case -896505829: 3998 return getSourceElement(); 3999 default: 4000 return super.makeProperty(hash, name); 4001 } 4002 4003 } 4004 4005 @Override 4006 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4007 switch (hash) { 4008 case 106079: 4009 /* key */ return new String[] { "id" }; 4010 case -1619874672: 4011 /* requirements */ return new String[] { "string" }; 4012 case 1478300413: 4013 /* severity */ return new String[] { "code" }; 4014 case 99639597: 4015 /* human */ return new String[] { "string" }; 4016 case -1795452264: 4017 /* expression */ return new String[] { "string" }; 4018 case 114256029: 4019 /* xpath */ return new String[] { "string" }; 4020 case -896505829: 4021 /* source */ return new String[] { "canonical" }; 4022 default: 4023 return super.getTypesForProperty(hash, name); 4024 } 4025 4026 } 4027 4028 @Override 4029 public Base addChild(String name) throws FHIRException { 4030 if (name.equals("key")) { 4031 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.key"); 4032 } else if (name.equals("requirements")) { 4033 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.requirements"); 4034 } else if (name.equals("severity")) { 4035 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.severity"); 4036 } else if (name.equals("human")) { 4037 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.human"); 4038 } else if (name.equals("expression")) { 4039 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.expression"); 4040 } else if (name.equals("xpath")) { 4041 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.xpath"); 4042 } else if (name.equals("source")) { 4043 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.source"); 4044 } else 4045 return super.addChild(name); 4046 } 4047 4048 public ElementDefinitionConstraintComponent copy() { 4049 ElementDefinitionConstraintComponent dst = new ElementDefinitionConstraintComponent(); 4050 copyValues(dst); 4051 return dst; 4052 } 4053 4054 public void copyValues(ElementDefinitionConstraintComponent dst) { 4055 super.copyValues(dst); 4056 dst.key = key == null ? null : key.copy(); 4057 dst.requirements = requirements == null ? null : requirements.copy(); 4058 dst.severity = severity == null ? null : severity.copy(); 4059 dst.human = human == null ? null : human.copy(); 4060 dst.expression = expression == null ? null : expression.copy(); 4061 dst.xpath = xpath == null ? null : xpath.copy(); 4062 dst.source = source == null ? null : source.copy(); 4063 } 4064 4065 @Override 4066 public boolean equalsDeep(Base other_) { 4067 if (!super.equalsDeep(other_)) 4068 return false; 4069 if (!(other_ instanceof ElementDefinitionConstraintComponent)) 4070 return false; 4071 ElementDefinitionConstraintComponent o = (ElementDefinitionConstraintComponent) other_; 4072 return compareDeep(key, o.key, true) && compareDeep(requirements, o.requirements, true) 4073 && compareDeep(severity, o.severity, true) && compareDeep(human, o.human, true) 4074 && compareDeep(expression, o.expression, true) && compareDeep(xpath, o.xpath, true) 4075 && compareDeep(source, o.source, true); 4076 } 4077 4078 @Override 4079 public boolean equalsShallow(Base other_) { 4080 if (!super.equalsShallow(other_)) 4081 return false; 4082 if (!(other_ instanceof ElementDefinitionConstraintComponent)) 4083 return false; 4084 ElementDefinitionConstraintComponent o = (ElementDefinitionConstraintComponent) other_; 4085 return compareValues(key, o.key, true) && compareValues(requirements, o.requirements, true) 4086 && compareValues(severity, o.severity, true) && compareValues(human, o.human, true) 4087 && compareValues(expression, o.expression, true) && compareValues(xpath, o.xpath, true); 4088 } 4089 4090 public boolean isEmpty() { 4091 return super.isEmpty() 4092 && ca.uhn.fhir.util.ElementUtil.isEmpty(key, requirements, severity, human, expression, xpath, source); 4093 } 4094 4095 public String fhirType() { 4096 return "ElementDefinition.constraint"; 4097 4098 } 4099 4100 } 4101 4102 @Block() 4103 public static class ElementDefinitionBindingComponent extends Element implements IBaseDatatypeElement { 4104 /** 4105 * Indicates the degree of conformance expectations associated with this binding 4106 * - that is, the degree to which the provided value set must be adhered to in 4107 * the instances. 4108 */ 4109 @Child(name = "strength", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 4110 @Description(shortDefinition = "required | extensible | preferred | example", formalDefinition = "Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.") 4111 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/binding-strength") 4112 protected Enumeration<BindingStrength> strength; 4113 4114 /** 4115 * Describes the intended use of this particular set of codes. 4116 */ 4117 @Child(name = "description", type = { 4118 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 4119 @Description(shortDefinition = "Human explanation of the value set", formalDefinition = "Describes the intended use of this particular set of codes.") 4120 protected StringType description; 4121 4122 /** 4123 * Refers to the value set that identifies the set of codes the binding refers 4124 * to. 4125 */ 4126 @Child(name = "valueSet", type = { 4127 CanonicalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 4128 @Description(shortDefinition = "Source of value set", formalDefinition = "Refers to the value set that identifies the set of codes the binding refers to.") 4129 protected CanonicalType valueSet; 4130 4131 private static final long serialVersionUID = -514477030L; 4132 4133 /** 4134 * Constructor 4135 */ 4136 public ElementDefinitionBindingComponent() { 4137 super(); 4138 } 4139 4140 /** 4141 * Constructor 4142 */ 4143 public ElementDefinitionBindingComponent(Enumeration<BindingStrength> strength) { 4144 super(); 4145 this.strength = strength; 4146 } 4147 4148 /** 4149 * @return {@link #strength} (Indicates the degree of conformance expectations 4150 * associated with this binding - that is, the degree to which the 4151 * provided value set must be adhered to in the instances.). This is the 4152 * underlying object with id, value and extensions. The accessor 4153 * "getStrength" gives direct access to the value 4154 */ 4155 public Enumeration<BindingStrength> getStrengthElement() { 4156 if (this.strength == null) 4157 if (Configuration.errorOnAutoCreate()) 4158 throw new Error("Attempt to auto-create ElementDefinitionBindingComponent.strength"); 4159 else if (Configuration.doAutoCreate()) 4160 this.strength = new Enumeration<BindingStrength>(new BindingStrengthEnumFactory()); // bb 4161 return this.strength; 4162 } 4163 4164 public boolean hasStrengthElement() { 4165 return this.strength != null && !this.strength.isEmpty(); 4166 } 4167 4168 public boolean hasStrength() { 4169 return this.strength != null && !this.strength.isEmpty(); 4170 } 4171 4172 /** 4173 * @param value {@link #strength} (Indicates the degree of conformance 4174 * expectations associated with this binding - that is, the degree 4175 * to which the provided value set must be adhered to in the 4176 * instances.). This is the underlying object with id, value and 4177 * extensions. The accessor "getStrength" gives direct access to 4178 * the value 4179 */ 4180 public ElementDefinitionBindingComponent setStrengthElement(Enumeration<BindingStrength> value) { 4181 this.strength = value; 4182 return this; 4183 } 4184 4185 /** 4186 * @return Indicates the degree of conformance expectations associated with this 4187 * binding - that is, the degree to which the provided value set must be 4188 * adhered to in the instances. 4189 */ 4190 public BindingStrength getStrength() { 4191 return this.strength == null ? null : this.strength.getValue(); 4192 } 4193 4194 /** 4195 * @param value Indicates the degree of conformance expectations associated with 4196 * this binding - that is, the degree to which the provided value 4197 * set must be adhered to in the instances. 4198 */ 4199 public ElementDefinitionBindingComponent setStrength(BindingStrength value) { 4200 if (this.strength == null) 4201 this.strength = new Enumeration<BindingStrength>(new BindingStrengthEnumFactory()); 4202 this.strength.setValue(value); 4203 return this; 4204 } 4205 4206 /** 4207 * @return {@link #description} (Describes the intended use of this particular 4208 * set of codes.). This is the underlying object with id, value and 4209 * extensions. The accessor "getDescription" gives direct access to the 4210 * value 4211 */ 4212 public StringType getDescriptionElement() { 4213 if (this.description == null) 4214 if (Configuration.errorOnAutoCreate()) 4215 throw new Error("Attempt to auto-create ElementDefinitionBindingComponent.description"); 4216 else if (Configuration.doAutoCreate()) 4217 this.description = new StringType(); // bb 4218 return this.description; 4219 } 4220 4221 public boolean hasDescriptionElement() { 4222 return this.description != null && !this.description.isEmpty(); 4223 } 4224 4225 public boolean hasDescription() { 4226 return this.description != null && !this.description.isEmpty(); 4227 } 4228 4229 /** 4230 * @param value {@link #description} (Describes the intended use of this 4231 * particular set of codes.). This is the underlying object with 4232 * id, value and extensions. The accessor "getDescription" gives 4233 * direct access to the value 4234 */ 4235 public ElementDefinitionBindingComponent setDescriptionElement(StringType value) { 4236 this.description = value; 4237 return this; 4238 } 4239 4240 /** 4241 * @return Describes the intended use of this particular set of codes. 4242 */ 4243 public String getDescription() { 4244 return this.description == null ? null : this.description.getValue(); 4245 } 4246 4247 /** 4248 * @param value Describes the intended use of this particular set of codes. 4249 */ 4250 public ElementDefinitionBindingComponent setDescription(String value) { 4251 if (Utilities.noString(value)) 4252 this.description = null; 4253 else { 4254 if (this.description == null) 4255 this.description = new StringType(); 4256 this.description.setValue(value); 4257 } 4258 return this; 4259 } 4260 4261 /** 4262 * @return {@link #valueSet} (Refers to the value set that identifies the set of 4263 * codes the binding refers to.). This is the underlying object with id, 4264 * value and extensions. The accessor "getValueSet" gives direct access 4265 * to the value 4266 */ 4267 public CanonicalType getValueSetElement() { 4268 if (this.valueSet == null) 4269 if (Configuration.errorOnAutoCreate()) 4270 throw new Error("Attempt to auto-create ElementDefinitionBindingComponent.valueSet"); 4271 else if (Configuration.doAutoCreate()) 4272 this.valueSet = new CanonicalType(); // bb 4273 return this.valueSet; 4274 } 4275 4276 public boolean hasValueSetElement() { 4277 return this.valueSet != null && !this.valueSet.isEmpty(); 4278 } 4279 4280 public boolean hasValueSet() { 4281 return this.valueSet != null && !this.valueSet.isEmpty(); 4282 } 4283 4284 /** 4285 * @param value {@link #valueSet} (Refers to the value set that identifies the 4286 * set of codes the binding refers to.). This is the underlying 4287 * object with id, value and extensions. The accessor "getValueSet" 4288 * gives direct access to the value 4289 */ 4290 public ElementDefinitionBindingComponent setValueSetElement(CanonicalType value) { 4291 this.valueSet = value; 4292 return this; 4293 } 4294 4295 /** 4296 * @return Refers to the value set that identifies the set of codes the binding 4297 * refers to. 4298 */ 4299 public String getValueSet() { 4300 return this.valueSet == null ? null : this.valueSet.getValue(); 4301 } 4302 4303 /** 4304 * @param value Refers to the value set that identifies the set of codes the 4305 * binding refers to. 4306 */ 4307 public ElementDefinitionBindingComponent setValueSet(String value) { 4308 if (Utilities.noString(value)) 4309 this.valueSet = null; 4310 else { 4311 if (this.valueSet == null) 4312 this.valueSet = new CanonicalType(); 4313 this.valueSet.setValue(value); 4314 } 4315 return this; 4316 } 4317 4318 protected void listChildren(List<Property> children) { 4319 super.listChildren(children); 4320 children.add(new Property("strength", "code", 4321 "Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.", 4322 0, 1, strength)); 4323 children.add(new Property("description", "string", "Describes the intended use of this particular set of codes.", 4324 0, 1, description)); 4325 children.add(new Property("valueSet", "canonical(ValueSet)", 4326 "Refers to the value set that identifies the set of codes the binding refers to.", 0, 1, valueSet)); 4327 } 4328 4329 @Override 4330 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4331 switch (_hash) { 4332 case 1791316033: 4333 /* strength */ return new Property("strength", "code", 4334 "Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.", 4335 0, 1, strength); 4336 case -1724546052: 4337 /* description */ return new Property("description", "string", 4338 "Describes the intended use of this particular set of codes.", 0, 1, description); 4339 case -1410174671: 4340 /* valueSet */ return new Property("valueSet", "canonical(ValueSet)", 4341 "Refers to the value set that identifies the set of codes the binding refers to.", 0, 1, valueSet); 4342 default: 4343 return super.getNamedProperty(_hash, _name, _checkValid); 4344 } 4345 4346 } 4347 4348 @Override 4349 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4350 switch (hash) { 4351 case 1791316033: 4352 /* strength */ return this.strength == null ? new Base[0] : new Base[] { this.strength }; // Enumeration<BindingStrength> 4353 case -1724546052: 4354 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 4355 case -1410174671: 4356 /* valueSet */ return this.valueSet == null ? new Base[0] : new Base[] { this.valueSet }; // CanonicalType 4357 default: 4358 return super.getProperty(hash, name, checkValid); 4359 } 4360 4361 } 4362 4363 @Override 4364 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4365 switch (hash) { 4366 case 1791316033: // strength 4367 value = new BindingStrengthEnumFactory().fromType(castToCode(value)); 4368 this.strength = (Enumeration) value; // Enumeration<BindingStrength> 4369 return value; 4370 case -1724546052: // description 4371 this.description = castToString(value); // StringType 4372 return value; 4373 case -1410174671: // valueSet 4374 this.valueSet = castToCanonical(value); // CanonicalType 4375 return value; 4376 default: 4377 return super.setProperty(hash, name, value); 4378 } 4379 4380 } 4381 4382 @Override 4383 public Base setProperty(String name, Base value) throws FHIRException { 4384 if (name.equals("strength")) { 4385 value = new BindingStrengthEnumFactory().fromType(castToCode(value)); 4386 this.strength = (Enumeration) value; // Enumeration<BindingStrength> 4387 } else if (name.equals("description")) { 4388 this.description = castToString(value); // StringType 4389 } else if (name.equals("valueSet")) { 4390 this.valueSet = castToCanonical(value); // CanonicalType 4391 } else 4392 return super.setProperty(name, value); 4393 return value; 4394 } 4395 4396 @Override 4397 public Base makeProperty(int hash, String name) throws FHIRException { 4398 switch (hash) { 4399 case 1791316033: 4400 return getStrengthElement(); 4401 case -1724546052: 4402 return getDescriptionElement(); 4403 case -1410174671: 4404 return getValueSetElement(); 4405 default: 4406 return super.makeProperty(hash, name); 4407 } 4408 4409 } 4410 4411 @Override 4412 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4413 switch (hash) { 4414 case 1791316033: 4415 /* strength */ return new String[] { "code" }; 4416 case -1724546052: 4417 /* description */ return new String[] { "string" }; 4418 case -1410174671: 4419 /* valueSet */ return new String[] { "canonical" }; 4420 default: 4421 return super.getTypesForProperty(hash, name); 4422 } 4423 4424 } 4425 4426 @Override 4427 public Base addChild(String name) throws FHIRException { 4428 if (name.equals("strength")) { 4429 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.strength"); 4430 } else if (name.equals("description")) { 4431 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.description"); 4432 } else if (name.equals("valueSet")) { 4433 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.valueSet"); 4434 } else 4435 return super.addChild(name); 4436 } 4437 4438 public ElementDefinitionBindingComponent copy() { 4439 ElementDefinitionBindingComponent dst = new ElementDefinitionBindingComponent(); 4440 copyValues(dst); 4441 return dst; 4442 } 4443 4444 public void copyValues(ElementDefinitionBindingComponent dst) { 4445 super.copyValues(dst); 4446 dst.strength = strength == null ? null : strength.copy(); 4447 dst.description = description == null ? null : description.copy(); 4448 dst.valueSet = valueSet == null ? null : valueSet.copy(); 4449 } 4450 4451 @Override 4452 public boolean equalsDeep(Base other_) { 4453 if (!super.equalsDeep(other_)) 4454 return false; 4455 if (!(other_ instanceof ElementDefinitionBindingComponent)) 4456 return false; 4457 ElementDefinitionBindingComponent o = (ElementDefinitionBindingComponent) other_; 4458 return compareDeep(strength, o.strength, true) && compareDeep(description, o.description, true) 4459 && compareDeep(valueSet, o.valueSet, true); 4460 } 4461 4462 @Override 4463 public boolean equalsShallow(Base other_) { 4464 if (!super.equalsShallow(other_)) 4465 return false; 4466 if (!(other_ instanceof ElementDefinitionBindingComponent)) 4467 return false; 4468 ElementDefinitionBindingComponent o = (ElementDefinitionBindingComponent) other_; 4469 return compareValues(strength, o.strength, true) && compareValues(description, o.description, true); 4470 } 4471 4472 public boolean isEmpty() { 4473 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(strength, description, valueSet); 4474 } 4475 4476 public String fhirType() { 4477 return "ElementDefinition.binding"; 4478 4479 } 4480 4481 } 4482 4483 @Block() 4484 public static class ElementDefinitionMappingComponent extends Element implements IBaseDatatypeElement { 4485 /** 4486 * An internal reference to the definition of a mapping. 4487 */ 4488 @Child(name = "identity", type = { IdType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 4489 @Description(shortDefinition = "Reference to mapping declaration", formalDefinition = "An internal reference to the definition of a mapping.") 4490 protected IdType identity; 4491 4492 /** 4493 * Identifies the computable language in which mapping.map is expressed. 4494 */ 4495 @Child(name = "language", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 4496 @Description(shortDefinition = "Computable language of mapping", formalDefinition = "Identifies the computable language in which mapping.map is expressed.") 4497 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/mimetypes") 4498 protected CodeType language; 4499 4500 /** 4501 * Expresses what part of the target specification corresponds to this element. 4502 */ 4503 @Child(name = "map", type = { StringType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 4504 @Description(shortDefinition = "Details of the mapping", formalDefinition = "Expresses what part of the target specification corresponds to this element.") 4505 protected StringType map; 4506 4507 /** 4508 * Comments that provide information about the mapping or its use. 4509 */ 4510 @Child(name = "comment", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 4511 @Description(shortDefinition = "Comments about the mapping or its use", formalDefinition = "Comments that provide information about the mapping or its use.") 4512 protected StringType comment; 4513 4514 private static final long serialVersionUID = 1386816887L; 4515 4516 /** 4517 * Constructor 4518 */ 4519 public ElementDefinitionMappingComponent() { 4520 super(); 4521 } 4522 4523 /** 4524 * Constructor 4525 */ 4526 public ElementDefinitionMappingComponent(IdType identity, StringType map) { 4527 super(); 4528 this.identity = identity; 4529 this.map = map; 4530 } 4531 4532 /** 4533 * @return {@link #identity} (An internal reference to the definition of a 4534 * mapping.). This is the underlying object with id, value and 4535 * extensions. The accessor "getIdentity" gives direct access to the 4536 * value 4537 */ 4538 public IdType getIdentityElement() { 4539 if (this.identity == null) 4540 if (Configuration.errorOnAutoCreate()) 4541 throw new Error("Attempt to auto-create ElementDefinitionMappingComponent.identity"); 4542 else if (Configuration.doAutoCreate()) 4543 this.identity = new IdType(); // bb 4544 return this.identity; 4545 } 4546 4547 public boolean hasIdentityElement() { 4548 return this.identity != null && !this.identity.isEmpty(); 4549 } 4550 4551 public boolean hasIdentity() { 4552 return this.identity != null && !this.identity.isEmpty(); 4553 } 4554 4555 /** 4556 * @param value {@link #identity} (An internal reference to the definition of a 4557 * mapping.). This is the underlying object with id, value and 4558 * extensions. The accessor "getIdentity" gives direct access to 4559 * the value 4560 */ 4561 public ElementDefinitionMappingComponent setIdentityElement(IdType value) { 4562 this.identity = value; 4563 return this; 4564 } 4565 4566 /** 4567 * @return An internal reference to the definition of a mapping. 4568 */ 4569 public String getIdentity() { 4570 return this.identity == null ? null : this.identity.getValue(); 4571 } 4572 4573 /** 4574 * @param value An internal reference to the definition of a mapping. 4575 */ 4576 public ElementDefinitionMappingComponent setIdentity(String value) { 4577 if (this.identity == null) 4578 this.identity = new IdType(); 4579 this.identity.setValue(value); 4580 return this; 4581 } 4582 4583 /** 4584 * @return {@link #language} (Identifies the computable language in which 4585 * mapping.map is expressed.). This is the underlying object with id, 4586 * value and extensions. The accessor "getLanguage" gives direct access 4587 * to the value 4588 */ 4589 public CodeType getLanguageElement() { 4590 if (this.language == null) 4591 if (Configuration.errorOnAutoCreate()) 4592 throw new Error("Attempt to auto-create ElementDefinitionMappingComponent.language"); 4593 else if (Configuration.doAutoCreate()) 4594 this.language = new CodeType(); // bb 4595 return this.language; 4596 } 4597 4598 public boolean hasLanguageElement() { 4599 return this.language != null && !this.language.isEmpty(); 4600 } 4601 4602 public boolean hasLanguage() { 4603 return this.language != null && !this.language.isEmpty(); 4604 } 4605 4606 /** 4607 * @param value {@link #language} (Identifies the computable language in which 4608 * mapping.map is expressed.). This is the underlying object with 4609 * id, value and extensions. The accessor "getLanguage" gives 4610 * direct access to the value 4611 */ 4612 public ElementDefinitionMappingComponent setLanguageElement(CodeType value) { 4613 this.language = value; 4614 return this; 4615 } 4616 4617 /** 4618 * @return Identifies the computable language in which mapping.map is expressed. 4619 */ 4620 public String getLanguage() { 4621 return this.language == null ? null : this.language.getValue(); 4622 } 4623 4624 /** 4625 * @param value Identifies the computable language in which mapping.map is 4626 * expressed. 4627 */ 4628 public ElementDefinitionMappingComponent setLanguage(String value) { 4629 if (Utilities.noString(value)) 4630 this.language = null; 4631 else { 4632 if (this.language == null) 4633 this.language = new CodeType(); 4634 this.language.setValue(value); 4635 } 4636 return this; 4637 } 4638 4639 /** 4640 * @return {@link #map} (Expresses what part of the target specification 4641 * corresponds to this element.). This is the underlying object with id, 4642 * value and extensions. The accessor "getMap" gives direct access to 4643 * the value 4644 */ 4645 public StringType getMapElement() { 4646 if (this.map == null) 4647 if (Configuration.errorOnAutoCreate()) 4648 throw new Error("Attempt to auto-create ElementDefinitionMappingComponent.map"); 4649 else if (Configuration.doAutoCreate()) 4650 this.map = new StringType(); // bb 4651 return this.map; 4652 } 4653 4654 public boolean hasMapElement() { 4655 return this.map != null && !this.map.isEmpty(); 4656 } 4657 4658 public boolean hasMap() { 4659 return this.map != null && !this.map.isEmpty(); 4660 } 4661 4662 /** 4663 * @param value {@link #map} (Expresses what part of the target specification 4664 * corresponds to this element.). This is the underlying object 4665 * with id, value and extensions. The accessor "getMap" gives 4666 * direct access to the value 4667 */ 4668 public ElementDefinitionMappingComponent setMapElement(StringType value) { 4669 this.map = value; 4670 return this; 4671 } 4672 4673 /** 4674 * @return Expresses what part of the target specification corresponds to this 4675 * element. 4676 */ 4677 public String getMap() { 4678 return this.map == null ? null : this.map.getValue(); 4679 } 4680 4681 /** 4682 * @param value Expresses what part of the target specification corresponds to 4683 * this element. 4684 */ 4685 public ElementDefinitionMappingComponent setMap(String value) { 4686 if (this.map == null) 4687 this.map = new StringType(); 4688 this.map.setValue(value); 4689 return this; 4690 } 4691 4692 /** 4693 * @return {@link #comment} (Comments that provide information about the mapping 4694 * or its use.). This is the underlying object with id, value and 4695 * extensions. The accessor "getComment" gives direct access to the 4696 * value 4697 */ 4698 public StringType getCommentElement() { 4699 if (this.comment == null) 4700 if (Configuration.errorOnAutoCreate()) 4701 throw new Error("Attempt to auto-create ElementDefinitionMappingComponent.comment"); 4702 else if (Configuration.doAutoCreate()) 4703 this.comment = new StringType(); // bb 4704 return this.comment; 4705 } 4706 4707 public boolean hasCommentElement() { 4708 return this.comment != null && !this.comment.isEmpty(); 4709 } 4710 4711 public boolean hasComment() { 4712 return this.comment != null && !this.comment.isEmpty(); 4713 } 4714 4715 /** 4716 * @param value {@link #comment} (Comments that provide information about the 4717 * mapping or its use.). This is the underlying object with id, 4718 * value and extensions. The accessor "getComment" gives direct 4719 * access to the value 4720 */ 4721 public ElementDefinitionMappingComponent setCommentElement(StringType value) { 4722 this.comment = value; 4723 return this; 4724 } 4725 4726 /** 4727 * @return Comments that provide information about the mapping or its use. 4728 */ 4729 public String getComment() { 4730 return this.comment == null ? null : this.comment.getValue(); 4731 } 4732 4733 /** 4734 * @param value Comments that provide information about the mapping or its use. 4735 */ 4736 public ElementDefinitionMappingComponent setComment(String value) { 4737 if (Utilities.noString(value)) 4738 this.comment = null; 4739 else { 4740 if (this.comment == null) 4741 this.comment = new StringType(); 4742 this.comment.setValue(value); 4743 } 4744 return this; 4745 } 4746 4747 protected void listChildren(List<Property> children) { 4748 super.listChildren(children); 4749 children 4750 .add(new Property("identity", "id", "An internal reference to the definition of a mapping.", 0, 1, identity)); 4751 children.add(new Property("language", "code", 4752 "Identifies the computable language in which mapping.map is expressed.", 0, 1, language)); 4753 children.add(new Property("map", "string", 4754 "Expresses what part of the target specification corresponds to this element.", 0, 1, map)); 4755 children.add(new Property("comment", "string", "Comments that provide information about the mapping or its use.", 4756 0, 1, comment)); 4757 } 4758 4759 @Override 4760 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4761 switch (_hash) { 4762 case -135761730: 4763 /* identity */ return new Property("identity", "id", "An internal reference to the definition of a mapping.", 0, 4764 1, identity); 4765 case -1613589672: 4766 /* language */ return new Property("language", "code", 4767 "Identifies the computable language in which mapping.map is expressed.", 0, 1, language); 4768 case 107868: 4769 /* map */ return new Property("map", "string", 4770 "Expresses what part of the target specification corresponds to this element.", 0, 1, map); 4771 case 950398559: 4772 /* comment */ return new Property("comment", "string", 4773 "Comments that provide information about the mapping or its use.", 0, 1, comment); 4774 default: 4775 return super.getNamedProperty(_hash, _name, _checkValid); 4776 } 4777 4778 } 4779 4780 @Override 4781 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4782 switch (hash) { 4783 case -135761730: 4784 /* identity */ return this.identity == null ? new Base[0] : new Base[] { this.identity }; // IdType 4785 case -1613589672: 4786 /* language */ return this.language == null ? new Base[0] : new Base[] { this.language }; // CodeType 4787 case 107868: 4788 /* map */ return this.map == null ? new Base[0] : new Base[] { this.map }; // StringType 4789 case 950398559: 4790 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // StringType 4791 default: 4792 return super.getProperty(hash, name, checkValid); 4793 } 4794 4795 } 4796 4797 @Override 4798 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4799 switch (hash) { 4800 case -135761730: // identity 4801 this.identity = castToId(value); // IdType 4802 return value; 4803 case -1613589672: // language 4804 this.language = castToCode(value); // CodeType 4805 return value; 4806 case 107868: // map 4807 this.map = castToString(value); // StringType 4808 return value; 4809 case 950398559: // comment 4810 this.comment = castToString(value); // StringType 4811 return value; 4812 default: 4813 return super.setProperty(hash, name, value); 4814 } 4815 4816 } 4817 4818 @Override 4819 public Base setProperty(String name, Base value) throws FHIRException { 4820 if (name.equals("identity")) { 4821 this.identity = castToId(value); // IdType 4822 } else if (name.equals("language")) { 4823 this.language = castToCode(value); // CodeType 4824 } else if (name.equals("map")) { 4825 this.map = castToString(value); // StringType 4826 } else if (name.equals("comment")) { 4827 this.comment = castToString(value); // StringType 4828 } else 4829 return super.setProperty(name, value); 4830 return value; 4831 } 4832 4833 @Override 4834 public Base makeProperty(int hash, String name) throws FHIRException { 4835 switch (hash) { 4836 case -135761730: 4837 return getIdentityElement(); 4838 case -1613589672: 4839 return getLanguageElement(); 4840 case 107868: 4841 return getMapElement(); 4842 case 950398559: 4843 return getCommentElement(); 4844 default: 4845 return super.makeProperty(hash, name); 4846 } 4847 4848 } 4849 4850 @Override 4851 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4852 switch (hash) { 4853 case -135761730: 4854 /* identity */ return new String[] { "id" }; 4855 case -1613589672: 4856 /* language */ return new String[] { "code" }; 4857 case 107868: 4858 /* map */ return new String[] { "string" }; 4859 case 950398559: 4860 /* comment */ return new String[] { "string" }; 4861 default: 4862 return super.getTypesForProperty(hash, name); 4863 } 4864 4865 } 4866 4867 @Override 4868 public Base addChild(String name) throws FHIRException { 4869 if (name.equals("identity")) { 4870 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.identity"); 4871 } else if (name.equals("language")) { 4872 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.language"); 4873 } else if (name.equals("map")) { 4874 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.map"); 4875 } else if (name.equals("comment")) { 4876 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.comment"); 4877 } else 4878 return super.addChild(name); 4879 } 4880 4881 public ElementDefinitionMappingComponent copy() { 4882 ElementDefinitionMappingComponent dst = new ElementDefinitionMappingComponent(); 4883 copyValues(dst); 4884 return dst; 4885 } 4886 4887 public void copyValues(ElementDefinitionMappingComponent dst) { 4888 super.copyValues(dst); 4889 dst.identity = identity == null ? null : identity.copy(); 4890 dst.language = language == null ? null : language.copy(); 4891 dst.map = map == null ? null : map.copy(); 4892 dst.comment = comment == null ? null : comment.copy(); 4893 } 4894 4895 @Override 4896 public boolean equalsDeep(Base other_) { 4897 if (!super.equalsDeep(other_)) 4898 return false; 4899 if (!(other_ instanceof ElementDefinitionMappingComponent)) 4900 return false; 4901 ElementDefinitionMappingComponent o = (ElementDefinitionMappingComponent) other_; 4902 return compareDeep(identity, o.identity, true) && compareDeep(language, o.language, true) 4903 && compareDeep(map, o.map, true) && compareDeep(comment, o.comment, true); 4904 } 4905 4906 @Override 4907 public boolean equalsShallow(Base other_) { 4908 if (!super.equalsShallow(other_)) 4909 return false; 4910 if (!(other_ instanceof ElementDefinitionMappingComponent)) 4911 return false; 4912 ElementDefinitionMappingComponent o = (ElementDefinitionMappingComponent) other_; 4913 return compareValues(identity, o.identity, true) && compareValues(language, o.language, true) 4914 && compareValues(map, o.map, true) && compareValues(comment, o.comment, true); 4915 } 4916 4917 public boolean isEmpty() { 4918 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identity, language, map, comment); 4919 } 4920 4921 public String fhirType() { 4922 return "ElementDefinition.mapping"; 4923 4924 } 4925 4926 } 4927 4928 /** 4929 * The path identifies the element and is expressed as a "."-separated list of 4930 * ancestor elements, beginning with the name of the resource or extension. 4931 */ 4932 @Child(name = "path", type = { StringType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 4933 @Description(shortDefinition = "Path of the element in the hierarchy of elements", formalDefinition = "The path identifies the element and is expressed as a \".\"-separated list of ancestor elements, beginning with the name of the resource or extension.") 4934 protected StringType path; 4935 4936 /** 4937 * Codes that define how this element is represented in instances, when the 4938 * deviation varies from the normal case. 4939 */ 4940 @Child(name = "representation", type = { 4941 CodeType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4942 @Description(shortDefinition = "xmlAttr | xmlText | typeAttr | cdaText | xhtml", formalDefinition = "Codes that define how this element is represented in instances, when the deviation varies from the normal case.") 4943 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/property-representation") 4944 protected List<Enumeration<PropertyRepresentation>> representation; 4945 4946 /** 4947 * The name of this element definition slice, when slicing is working. The name 4948 * must be a token with no dots or spaces. This is a unique name referring to a 4949 * specific set of constraints applied to this element, used to provide a name 4950 * to different slices of the same element. 4951 */ 4952 @Child(name = "sliceName", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 4953 @Description(shortDefinition = "Name for this particular element (in a set of slices)", formalDefinition = "The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element.") 4954 protected StringType sliceName; 4955 4956 /** 4957 * If true, indicates that this slice definition is constraining a slice 4958 * definition with the same name in an inherited profile. If false, the slice is 4959 * not overriding any slice in an inherited profile. If missing, the slice might 4960 * or might not be overriding a slice in an inherited profile, depending on the 4961 * sliceName. 4962 */ 4963 @Child(name = "sliceIsConstraining", type = { 4964 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 4965 @Description(shortDefinition = "If this slice definition constrains an inherited slice definition (or not)", formalDefinition = "If true, indicates that this slice definition is constraining a slice definition with the same name in an inherited profile. If false, the slice is not overriding any slice in an inherited profile. If missing, the slice might or might not be overriding a slice in an inherited profile, depending on the sliceName.") 4966 protected BooleanType sliceIsConstraining; 4967 4968 /** 4969 * A single preferred label which is the text to display beside the element 4970 * indicating its meaning or to use to prompt for the element in a user display 4971 * or form. 4972 */ 4973 @Child(name = "label", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 4974 @Description(shortDefinition = "Name for element to display with or prompt for element", formalDefinition = "A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form.") 4975 protected StringType label; 4976 4977 /** 4978 * A code that has the same meaning as the element in a particular terminology. 4979 */ 4980 @Child(name = "code", type = { 4981 Coding.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4982 @Description(shortDefinition = "Corresponding codes in terminologies", formalDefinition = "A code that has the same meaning as the element in a particular terminology.") 4983 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-codes") 4984 protected List<Coding> code; 4985 4986 /** 4987 * Indicates that the element is sliced into a set of alternative definitions 4988 * (i.e. in a structure definition, there are multiple different constraints on 4989 * a single element in the base resource). Slicing can be used in any resource 4990 * that has cardinality ..* on the base resource, or any resource with a choice 4991 * of types. The set of slices is any elements that come after this in the 4992 * element sequence that have the same path, until a shorter path occurs (the 4993 * shorter path terminates the set). 4994 */ 4995 @Child(name = "slicing", type = {}, order = 6, min = 0, max = 1, modifier = false, summary = true) 4996 @Description(shortDefinition = "This element is sliced - slices follow", formalDefinition = "Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set).") 4997 protected ElementDefinitionSlicingComponent slicing; 4998 4999 /** 5000 * A concise description of what this element means (e.g. for use in 5001 * autogenerated summaries). 5002 */ 5003 @Child(name = "short", type = { StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 5004 @Description(shortDefinition = "Concise definition for space-constrained presentation", formalDefinition = "A concise description of what this element means (e.g. for use in autogenerated summaries).") 5005 protected StringType short_; 5006 5007 /** 5008 * Provides a complete explanation of the meaning of the data element for human 5009 * readability. For the case of elements derived from existing elements (e.g. 5010 * constraints), the definition SHALL be consistent with the base definition, 5011 * but convey the meaning of the element in the particular context of use of the 5012 * resource. (Note: The text you are reading is specified in 5013 * ElementDefinition.definition). 5014 */ 5015 @Child(name = "definition", type = { 5016 MarkdownType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 5017 @Description(shortDefinition = "Full formal definition as narrative text", formalDefinition = "Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource. (Note: The text you are reading is specified in ElementDefinition.definition).") 5018 protected MarkdownType definition; 5019 5020 /** 5021 * Explanatory notes and implementation guidance about the data element, 5022 * including notes about how to use the data properly, exceptions to proper use, 5023 * etc. (Note: The text you are reading is specified in 5024 * ElementDefinition.comment). 5025 */ 5026 @Child(name = "comment", type = { MarkdownType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 5027 @Description(shortDefinition = "Comments about the use of this element", formalDefinition = "Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. (Note: The text you are reading is specified in ElementDefinition.comment).") 5028 protected MarkdownType comment; 5029 5030 /** 5031 * This element is for traceability of why the element was created and why the 5032 * constraints exist as they do. This may be used to point to source materials 5033 * or specifications that drove the structure of this element. 5034 */ 5035 @Child(name = "requirements", type = { 5036 MarkdownType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 5037 @Description(shortDefinition = "Why this resource has been created", formalDefinition = "This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element.") 5038 protected MarkdownType requirements; 5039 5040 /** 5041 * Identifies additional names by which this element might also be known. 5042 */ 5043 @Child(name = "alias", type = { 5044 StringType.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 5045 @Description(shortDefinition = "Other names", formalDefinition = "Identifies additional names by which this element might also be known.") 5046 protected List<StringType> alias; 5047 5048 /** 5049 * The minimum number of times this element SHALL appear in the instance. 5050 */ 5051 @Child(name = "min", type = { UnsignedIntType.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 5052 @Description(shortDefinition = "Minimum Cardinality", formalDefinition = "The minimum number of times this element SHALL appear in the instance.") 5053 protected UnsignedIntType min; 5054 5055 /** 5056 * The maximum number of times this element is permitted to appear in the 5057 * instance. 5058 */ 5059 @Child(name = "max", type = { StringType.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 5060 @Description(shortDefinition = "Maximum Cardinality (a number or *)", formalDefinition = "The maximum number of times this element is permitted to appear in the instance.") 5061 protected StringType max; 5062 5063 /** 5064 * Information about the base definition of the element, provided to make it 5065 * unnecessary for tools to trace the deviation of the element through the 5066 * derived and related profiles. When the element definition is not the original 5067 * definition of an element - i.g. either in a constraint on another type, or 5068 * for elements from a super type in a snap shot - then the information in 5069 * provided in the element definition may be different to the base definition. 5070 * On the original definition of the element, it will be same. 5071 */ 5072 @Child(name = "base", type = {}, order = 14, min = 0, max = 1, modifier = false, summary = true) 5073 @Description(shortDefinition = "Base definition information for tools", formalDefinition = "Information about the base definition of the element, provided to make it unnecessary for tools to trace the deviation of the element through the derived and related profiles. When the element definition is not the original definition of an element - i.g. either in a constraint on another type, or for elements from a super type in a snap shot - then the information in provided in the element definition may be different to the base definition. On the original definition of the element, it will be same.") 5074 protected ElementDefinitionBaseComponent base; 5075 5076 /** 5077 * Identifies an element defined elsewhere in the definition whose content rules 5078 * should be applied to the current element. ContentReferences bring across all 5079 * the rules that are in the ElementDefinition for the element, including 5080 * definitions, cardinality constraints, bindings, invariants etc. 5081 */ 5082 @Child(name = "contentReference", type = { 5083 UriType.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 5084 @Description(shortDefinition = "Reference to definition of content for the element", formalDefinition = "Identifies an element defined elsewhere in the definition whose content rules should be applied to the current element. ContentReferences bring across all the rules that are in the ElementDefinition for the element, including definitions, cardinality constraints, bindings, invariants etc.") 5085 protected UriType contentReference; 5086 5087 /** 5088 * The data type or resource that the value of this element is permitted to be. 5089 */ 5090 @Child(name = "type", type = {}, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 5091 @Description(shortDefinition = "Data type and Profile for this element", formalDefinition = "The data type or resource that the value of this element is permitted to be.") 5092 protected List<TypeRefComponent> type; 5093 5094 /** 5095 * The value that should be used if there is no value stated in the instance 5096 * (e.g. 'if not otherwise specified, the abstract is false'). 5097 */ 5098 @Child(name = "defaultValue", type = {}, order = 17, min = 0, max = 1, modifier = false, summary = true) 5099 @Description(shortDefinition = "Specified value if missing from instance", formalDefinition = "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').") 5100 protected org.hl7.fhir.r4.model.Type defaultValue; 5101 5102 /** 5103 * The Implicit meaning that is to be understood when this element is missing 5104 * (e.g. 'when this element is missing, the period is ongoing'). 5105 */ 5106 @Child(name = "meaningWhenMissing", type = { 5107 MarkdownType.class }, order = 18, min = 0, max = 1, modifier = false, summary = true) 5108 @Description(shortDefinition = "Implicit meaning when this element is missing", formalDefinition = "The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing').") 5109 protected MarkdownType meaningWhenMissing; 5110 5111 /** 5112 * If present, indicates that the order of the repeating element has meaning and 5113 * describes what that meaning is. If absent, it means that the order of the 5114 * element has no meaning. 5115 */ 5116 @Child(name = "orderMeaning", type = { 5117 StringType.class }, order = 19, min = 0, max = 1, modifier = false, summary = true) 5118 @Description(shortDefinition = "What the order of the elements means", formalDefinition = "If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning.") 5119 protected StringType orderMeaning; 5120 5121 /** 5122 * Specifies a value that SHALL be exactly the value for this element in the 5123 * instance. For purposes of comparison, non-significant whitespace is ignored, 5124 * and all values must be an exact match (case and accent sensitive). Missing 5125 * elements/attributes must also be missing. 5126 */ 5127 @Child(name = "fixed", type = {}, order = 20, min = 0, max = 1, modifier = false, summary = true) 5128 @Description(shortDefinition = "Value must be exactly this", formalDefinition = "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.") 5129 protected org.hl7.fhir.r4.model.Type fixed; 5130 5131 /** 5132 * Specifies a value that the value in the instance SHALL follow - that is, any 5133 * value in the pattern must be found in the instance. Other additional values 5134 * may be found too. This is effectively constraint by example. 5135 * 5136 * When pattern[x] is used to constrain a primitive, it means that the value 5137 * provided in the pattern[x] must match the instance value exactly. 5138 * 5139 * When pattern[x] is used to constrain an array, it means that each element 5140 * provided in the pattern[x] array must (recursively) match at least one 5141 * element from the instance array. 5142 * 5143 * When pattern[x] is used to constrain a complex object, it means that each 5144 * property in the pattern must be present in the complex object, and its value 5145 * must recursively match -- i.e., 5146 * 5147 * 1. If primitive: it must match exactly the pattern value 2. If a complex 5148 * object: it must match (recursively) the pattern value 3. If an array: it must 5149 * match (recursively) the pattern value. 5150 */ 5151 @Child(name = "pattern", type = {}, order = 21, min = 0, max = 1, modifier = false, summary = true) 5152 @Description(shortDefinition = "Value must have at least these property values", formalDefinition = "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.") 5153 protected org.hl7.fhir.r4.model.Type pattern; 5154 5155 /** 5156 * A sample value for this element demonstrating the type of information that 5157 * would typically be found in the element. 5158 */ 5159 @Child(name = "example", type = {}, order = 22, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 5160 @Description(shortDefinition = "Example value (as defined for type)", formalDefinition = "A sample value for this element demonstrating the type of information that would typically be found in the element.") 5161 protected List<ElementDefinitionExampleComponent> example; 5162 5163 /** 5164 * The minimum allowed value for the element. The value is inclusive. This is 5165 * allowed for the types date, dateTime, instant, time, decimal, integer, and 5166 * Quantity. 5167 */ 5168 @Child(name = "minValue", type = { DateType.class, DateTimeType.class, InstantType.class, TimeType.class, 5169 DecimalType.class, IntegerType.class, PositiveIntType.class, UnsignedIntType.class, 5170 Quantity.class }, order = 23, min = 0, max = 1, modifier = false, summary = true) 5171 @Description(shortDefinition = "Minimum Allowed Value (for some types)", formalDefinition = "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.") 5172 protected Type minValue; 5173 5174 /** 5175 * The maximum allowed value for the element. The value is inclusive. This is 5176 * allowed for the types date, dateTime, instant, time, decimal, integer, and 5177 * Quantity. 5178 */ 5179 @Child(name = "maxValue", type = { DateType.class, DateTimeType.class, InstantType.class, TimeType.class, 5180 DecimalType.class, IntegerType.class, PositiveIntType.class, UnsignedIntType.class, 5181 Quantity.class }, order = 24, min = 0, max = 1, modifier = false, summary = true) 5182 @Description(shortDefinition = "Maximum Allowed Value (for some types)", formalDefinition = "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.") 5183 protected Type maxValue; 5184 5185 /** 5186 * Indicates the maximum length in characters that is permitted to be present in 5187 * conformant instances and which is expected to be supported by conformant 5188 * consumers that support the element. 5189 */ 5190 @Child(name = "maxLength", type = { 5191 IntegerType.class }, order = 25, min = 0, max = 1, modifier = false, summary = true) 5192 @Description(shortDefinition = "Max length for strings", formalDefinition = "Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element.") 5193 protected IntegerType maxLength; 5194 5195 /** 5196 * A reference to an invariant that may make additional statements about the 5197 * cardinality or value in the instance. 5198 */ 5199 @Child(name = "condition", type = { 5200 IdType.class }, order = 26, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 5201 @Description(shortDefinition = "Reference to invariant about presence", formalDefinition = "A reference to an invariant that may make additional statements about the cardinality or value in the instance.") 5202 protected List<IdType> condition; 5203 5204 /** 5205 * Formal constraints such as co-occurrence and other constraints that can be 5206 * computationally evaluated within the context of the instance. 5207 */ 5208 @Child(name = "constraint", type = {}, order = 27, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 5209 @Description(shortDefinition = "Condition that must evaluate to true", formalDefinition = "Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance.") 5210 protected List<ElementDefinitionConstraintComponent> constraint; 5211 5212 /** 5213 * If true, implementations that produce or consume resources SHALL provide 5214 * "support" for the element in some meaningful way. If false, the element may 5215 * be ignored and not supported. If false, whether to populate or use the data 5216 * element in any way is at the discretion of the implementation. 5217 */ 5218 @Child(name = "mustSupport", type = { 5219 BooleanType.class }, order = 28, min = 0, max = 1, modifier = false, summary = true) 5220 @Description(shortDefinition = "If the element must be supported", formalDefinition = "If true, implementations that produce or consume resources SHALL provide \"support\" for the element in some meaningful way. If false, the element may be ignored and not supported. If false, whether to populate or use the data element in any way is at the discretion of the implementation.") 5221 protected BooleanType mustSupport; 5222 5223 /** 5224 * If true, the value of this element affects the interpretation of the element 5225 * or resource that contains it, and the value of the element cannot be ignored. 5226 * Typically, this is used for status, negation and qualification codes. The 5227 * effect of this is that the element cannot be ignored by systems: they SHALL 5228 * either recognize the element and process it, and/or a pre-determination has 5229 * been made that it is not relevant to their particular system. 5230 */ 5231 @Child(name = "isModifier", type = { 5232 BooleanType.class }, order = 29, min = 0, max = 1, modifier = false, summary = true) 5233 @Description(shortDefinition = "If this modifies the meaning of other elements", formalDefinition = "If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system.") 5234 protected BooleanType isModifier; 5235 5236 /** 5237 * Explains how that element affects the interpretation of the resource or 5238 * element that contains it. 5239 */ 5240 @Child(name = "isModifierReason", type = { 5241 StringType.class }, order = 30, min = 0, max = 1, modifier = false, summary = true) 5242 @Description(shortDefinition = "Reason that this element is marked as a modifier", formalDefinition = "Explains how that element affects the interpretation of the resource or element that contains it.") 5243 protected StringType isModifierReason; 5244 5245 /** 5246 * Whether the element should be included if a client requests a search with the 5247 * parameter _summary=true. 5248 */ 5249 @Child(name = "isSummary", type = { 5250 BooleanType.class }, order = 31, min = 0, max = 1, modifier = false, summary = true) 5251 @Description(shortDefinition = "Include when _summary = true?", formalDefinition = "Whether the element should be included if a client requests a search with the parameter _summary=true.") 5252 protected BooleanType isSummary; 5253 5254 /** 5255 * Binds to a value set if this element is coded (code, Coding, CodeableConcept, 5256 * Quantity), or the data types (string, uri). 5257 */ 5258 @Child(name = "binding", type = {}, order = 32, min = 0, max = 1, modifier = false, summary = true) 5259 @Description(shortDefinition = "ValueSet details if this is coded", formalDefinition = "Binds to a value set if this element is coded (code, Coding, CodeableConcept, Quantity), or the data types (string, uri).") 5260 protected ElementDefinitionBindingComponent binding; 5261 5262 /** 5263 * Identifies a concept from an external specification that roughly corresponds 5264 * to this element. 5265 */ 5266 @Child(name = "mapping", type = {}, order = 33, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 5267 @Description(shortDefinition = "Map element to another set of definitions", formalDefinition = "Identifies a concept from an external specification that roughly corresponds to this element.") 5268 protected List<ElementDefinitionMappingComponent> mapping; 5269 5270 private static final long serialVersionUID = 1482114790L; 5271 5272 /** 5273 * Constructor 5274 */ 5275 public ElementDefinition() { 5276 super(); 5277 } 5278 5279 /** 5280 * Constructor 5281 */ 5282 public ElementDefinition(StringType path) { 5283 super(); 5284 this.path = path; 5285 } 5286 5287 /** 5288 * @return {@link #path} (The path identifies the element and is expressed as a 5289 * "."-separated list of ancestor elements, beginning with the name of 5290 * the resource or extension.). This is the underlying object with id, 5291 * value and extensions. The accessor "getPath" gives direct access to 5292 * the value 5293 */ 5294 public StringType getPathElement() { 5295 if (this.path == null) 5296 if (Configuration.errorOnAutoCreate()) 5297 throw new Error("Attempt to auto-create ElementDefinition.path"); 5298 else if (Configuration.doAutoCreate()) 5299 this.path = new StringType(); // bb 5300 return this.path; 5301 } 5302 5303 public boolean hasPathElement() { 5304 return this.path != null && !this.path.isEmpty(); 5305 } 5306 5307 public boolean hasPath() { 5308 return this.path != null && !this.path.isEmpty(); 5309 } 5310 5311 /** 5312 * @param value {@link #path} (The path identifies the element and is expressed 5313 * as a "."-separated list of ancestor elements, beginning with the 5314 * name of the resource or extension.). This is the underlying 5315 * object with id, value and extensions. The accessor "getPath" 5316 * gives direct access to the value 5317 */ 5318 public ElementDefinition setPathElement(StringType value) { 5319 this.path = value; 5320 return this; 5321 } 5322 5323 /** 5324 * @return The path identifies the element and is expressed as a "."-separated 5325 * list of ancestor elements, beginning with the name of the resource or 5326 * extension. 5327 */ 5328 public String getPath() { 5329 return this.path == null ? null : this.path.getValue(); 5330 } 5331 5332 /** 5333 * @param value The path identifies the element and is expressed as a 5334 * "."-separated list of ancestor elements, beginning with the name 5335 * of the resource or extension. 5336 */ 5337 public ElementDefinition setPath(String value) { 5338 if (this.path == null) 5339 this.path = new StringType(); 5340 this.path.setValue(value); 5341 return this; 5342 } 5343 5344 /** 5345 * @return {@link #representation} (Codes that define how this element is 5346 * represented in instances, when the deviation varies from the normal 5347 * case.) 5348 */ 5349 public List<Enumeration<PropertyRepresentation>> getRepresentation() { 5350 if (this.representation == null) 5351 this.representation = new ArrayList<Enumeration<PropertyRepresentation>>(); 5352 return this.representation; 5353 } 5354 5355 /** 5356 * @return Returns a reference to <code>this</code> for easy method chaining 5357 */ 5358 public ElementDefinition setRepresentation(List<Enumeration<PropertyRepresentation>> theRepresentation) { 5359 this.representation = theRepresentation; 5360 return this; 5361 } 5362 5363 public boolean hasRepresentation() { 5364 if (this.representation == null) 5365 return false; 5366 for (Enumeration<PropertyRepresentation> item : this.representation) 5367 if (!item.isEmpty()) 5368 return true; 5369 return false; 5370 } 5371 5372 /** 5373 * @return {@link #representation} (Codes that define how this element is 5374 * represented in instances, when the deviation varies from the normal 5375 * case.) 5376 */ 5377 public Enumeration<PropertyRepresentation> addRepresentationElement() {// 2 5378 Enumeration<PropertyRepresentation> t = new Enumeration<PropertyRepresentation>( 5379 new PropertyRepresentationEnumFactory()); 5380 if (this.representation == null) 5381 this.representation = new ArrayList<Enumeration<PropertyRepresentation>>(); 5382 this.representation.add(t); 5383 return t; 5384 } 5385 5386 /** 5387 * @param value {@link #representation} (Codes that define how this element is 5388 * represented in instances, when the deviation varies from the 5389 * normal case.) 5390 */ 5391 public ElementDefinition addRepresentation(PropertyRepresentation value) { // 1 5392 Enumeration<PropertyRepresentation> t = new Enumeration<PropertyRepresentation>( 5393 new PropertyRepresentationEnumFactory()); 5394 t.setValue(value); 5395 if (this.representation == null) 5396 this.representation = new ArrayList<Enumeration<PropertyRepresentation>>(); 5397 this.representation.add(t); 5398 return this; 5399 } 5400 5401 /** 5402 * @param value {@link #representation} (Codes that define how this element is 5403 * represented in instances, when the deviation varies from the 5404 * normal case.) 5405 */ 5406 public boolean hasRepresentation(PropertyRepresentation value) { 5407 if (this.representation == null) 5408 return false; 5409 for (Enumeration<PropertyRepresentation> v : this.representation) 5410 if (v.getValue().equals(value)) // code 5411 return true; 5412 return false; 5413 } 5414 5415 /** 5416 * @return {@link #sliceName} (The name of this element definition slice, when 5417 * slicing is working. The name must be a token with no dots or spaces. 5418 * This is a unique name referring to a specific set of constraints 5419 * applied to this element, used to provide a name to different slices 5420 * of the same element.). This is the underlying object with id, value 5421 * and extensions. The accessor "getSliceName" gives direct access to 5422 * the value 5423 */ 5424 public StringType getSliceNameElement() { 5425 if (this.sliceName == null) 5426 if (Configuration.errorOnAutoCreate()) 5427 throw new Error("Attempt to auto-create ElementDefinition.sliceName"); 5428 else if (Configuration.doAutoCreate()) 5429 this.sliceName = new StringType(); // bb 5430 return this.sliceName; 5431 } 5432 5433 public boolean hasSliceNameElement() { 5434 return this.sliceName != null && !this.sliceName.isEmpty(); 5435 } 5436 5437 public boolean hasSliceName() { 5438 return this.sliceName != null && !this.sliceName.isEmpty(); 5439 } 5440 5441 /** 5442 * @param value {@link #sliceName} (The name of this element definition slice, 5443 * when slicing is working. The name must be a token with no dots 5444 * or spaces. This is a unique name referring to a specific set of 5445 * constraints applied to this element, used to provide a name to 5446 * different slices of the same element.). This is the underlying 5447 * object with id, value and extensions. The accessor 5448 * "getSliceName" gives direct access to the value 5449 */ 5450 public ElementDefinition setSliceNameElement(StringType value) { 5451 this.sliceName = value; 5452 return this; 5453 } 5454 5455 /** 5456 * @return The name of this element definition slice, when slicing is working. 5457 * The name must be a token with no dots or spaces. This is a unique 5458 * name referring to a specific set of constraints applied to this 5459 * element, used to provide a name to different slices of the same 5460 * element. 5461 */ 5462 public String getSliceName() { 5463 return this.sliceName == null ? null : this.sliceName.getValue(); 5464 } 5465 5466 /** 5467 * @param value The name of this element definition slice, when slicing is 5468 * working. The name must be a token with no dots or spaces. This 5469 * is a unique name referring to a specific set of constraints 5470 * applied to this element, used to provide a name to different 5471 * slices of the same element. 5472 */ 5473 public ElementDefinition setSliceName(String value) { 5474 if (Utilities.noString(value)) 5475 this.sliceName = null; 5476 else { 5477 if (this.sliceName == null) 5478 this.sliceName = new StringType(); 5479 this.sliceName.setValue(value); 5480 } 5481 return this; 5482 } 5483 5484 /** 5485 * @return {@link #sliceIsConstraining} (If true, indicates that this slice 5486 * definition is constraining a slice definition with the same name in 5487 * an inherited profile. If false, the slice is not overriding any slice 5488 * in an inherited profile. If missing, the slice might or might not be 5489 * overriding a slice in an inherited profile, depending on the 5490 * sliceName.). This is the underlying object with id, value and 5491 * extensions. The accessor "getSliceIsConstraining" gives direct access 5492 * to the value 5493 */ 5494 public BooleanType getSliceIsConstrainingElement() { 5495 if (this.sliceIsConstraining == null) 5496 if (Configuration.errorOnAutoCreate()) 5497 throw new Error("Attempt to auto-create ElementDefinition.sliceIsConstraining"); 5498 else if (Configuration.doAutoCreate()) 5499 this.sliceIsConstraining = new BooleanType(); // bb 5500 return this.sliceIsConstraining; 5501 } 5502 5503 public boolean hasSliceIsConstrainingElement() { 5504 return this.sliceIsConstraining != null && !this.sliceIsConstraining.isEmpty(); 5505 } 5506 5507 public boolean hasSliceIsConstraining() { 5508 return this.sliceIsConstraining != null && !this.sliceIsConstraining.isEmpty(); 5509 } 5510 5511 /** 5512 * @param value {@link #sliceIsConstraining} (If true, indicates that this slice 5513 * definition is constraining a slice definition with the same name 5514 * in an inherited profile. If false, the slice is not overriding 5515 * any slice in an inherited profile. If missing, the slice might 5516 * or might not be overriding a slice in an inherited profile, 5517 * depending on the sliceName.). This is the underlying object with 5518 * id, value and extensions. The accessor "getSliceIsConstraining" 5519 * gives direct access to the value 5520 */ 5521 public ElementDefinition setSliceIsConstrainingElement(BooleanType value) { 5522 this.sliceIsConstraining = value; 5523 return this; 5524 } 5525 5526 /** 5527 * @return If true, indicates that this slice definition is constraining a slice 5528 * definition with the same name in an inherited profile. If false, the 5529 * slice is not overriding any slice in an inherited profile. If 5530 * missing, the slice might or might not be overriding a slice in an 5531 * inherited profile, depending on the sliceName. 5532 */ 5533 public boolean getSliceIsConstraining() { 5534 return this.sliceIsConstraining == null || this.sliceIsConstraining.isEmpty() ? false 5535 : this.sliceIsConstraining.getValue(); 5536 } 5537 5538 /** 5539 * @param value If true, indicates that this slice definition is constraining a 5540 * slice definition with the same name in an inherited profile. If 5541 * false, the slice is not overriding any slice in an inherited 5542 * profile. If missing, the slice might or might not be overriding 5543 * a slice in an inherited profile, depending on the sliceName. 5544 */ 5545 public ElementDefinition setSliceIsConstraining(boolean value) { 5546 if (this.sliceIsConstraining == null) 5547 this.sliceIsConstraining = new BooleanType(); 5548 this.sliceIsConstraining.setValue(value); 5549 return this; 5550 } 5551 5552 /** 5553 * @return {@link #label} (A single preferred label which is the text to display 5554 * beside the element indicating its meaning or to use to prompt for the 5555 * element in a user display or form.). This is the underlying object 5556 * with id, value and extensions. The accessor "getLabel" gives direct 5557 * access to the value 5558 */ 5559 public StringType getLabelElement() { 5560 if (this.label == null) 5561 if (Configuration.errorOnAutoCreate()) 5562 throw new Error("Attempt to auto-create ElementDefinition.label"); 5563 else if (Configuration.doAutoCreate()) 5564 this.label = new StringType(); // bb 5565 return this.label; 5566 } 5567 5568 public boolean hasLabelElement() { 5569 return this.label != null && !this.label.isEmpty(); 5570 } 5571 5572 public boolean hasLabel() { 5573 return this.label != null && !this.label.isEmpty(); 5574 } 5575 5576 /** 5577 * @param value {@link #label} (A single preferred label which is the text to 5578 * display beside the element indicating its meaning or to use to 5579 * prompt for the element in a user display or form.). This is the 5580 * underlying object with id, value and extensions. The accessor 5581 * "getLabel" gives direct access to the value 5582 */ 5583 public ElementDefinition setLabelElement(StringType value) { 5584 this.label = value; 5585 return this; 5586 } 5587 5588 /** 5589 * @return A single preferred label which is the text to display beside the 5590 * element indicating its meaning or to use to prompt for the element in 5591 * a user display or form. 5592 */ 5593 public String getLabel() { 5594 return this.label == null ? null : this.label.getValue(); 5595 } 5596 5597 /** 5598 * @param value A single preferred label which is the text to display beside the 5599 * element indicating its meaning or to use to prompt for the 5600 * element in a user display or form. 5601 */ 5602 public ElementDefinition setLabel(String value) { 5603 if (Utilities.noString(value)) 5604 this.label = null; 5605 else { 5606 if (this.label == null) 5607 this.label = new StringType(); 5608 this.label.setValue(value); 5609 } 5610 return this; 5611 } 5612 5613 /** 5614 * @return {@link #code} (A code that has the same meaning as the element in a 5615 * particular terminology.) 5616 */ 5617 public List<Coding> getCode() { 5618 if (this.code == null) 5619 this.code = new ArrayList<Coding>(); 5620 return this.code; 5621 } 5622 5623 /** 5624 * @return Returns a reference to <code>this</code> for easy method chaining 5625 */ 5626 public ElementDefinition setCode(List<Coding> theCode) { 5627 this.code = theCode; 5628 return this; 5629 } 5630 5631 public boolean hasCode() { 5632 if (this.code == null) 5633 return false; 5634 for (Coding item : this.code) 5635 if (!item.isEmpty()) 5636 return true; 5637 return false; 5638 } 5639 5640 public Coding addCode() { // 3 5641 Coding t = new Coding(); 5642 if (this.code == null) 5643 this.code = new ArrayList<Coding>(); 5644 this.code.add(t); 5645 return t; 5646 } 5647 5648 public ElementDefinition addCode(Coding t) { // 3 5649 if (t == null) 5650 return this; 5651 if (this.code == null) 5652 this.code = new ArrayList<Coding>(); 5653 this.code.add(t); 5654 return this; 5655 } 5656 5657 /** 5658 * @return The first repetition of repeating field {@link #code}, creating it if 5659 * it does not already exist 5660 */ 5661 public Coding getCodeFirstRep() { 5662 if (getCode().isEmpty()) { 5663 addCode(); 5664 } 5665 return getCode().get(0); 5666 } 5667 5668 /** 5669 * @return {@link #slicing} (Indicates that the element is sliced into a set of 5670 * alternative definitions (i.e. in a structure definition, there are 5671 * multiple different constraints on a single element in the base 5672 * resource). Slicing can be used in any resource that has cardinality 5673 * ..* on the base resource, or any resource with a choice of types. The 5674 * set of slices is any elements that come after this in the element 5675 * sequence that have the same path, until a shorter path occurs (the 5676 * shorter path terminates the set).) 5677 */ 5678 public ElementDefinitionSlicingComponent getSlicing() { 5679 if (this.slicing == null) 5680 if (Configuration.errorOnAutoCreate()) 5681 throw new Error("Attempt to auto-create ElementDefinition.slicing"); 5682 else if (Configuration.doAutoCreate()) 5683 this.slicing = new ElementDefinitionSlicingComponent(); // cc 5684 return this.slicing; 5685 } 5686 5687 public boolean hasSlicing() { 5688 return this.slicing != null && !this.slicing.isEmpty(); 5689 } 5690 5691 /** 5692 * @param value {@link #slicing} (Indicates that the element is sliced into a 5693 * set of alternative definitions (i.e. in a structure definition, 5694 * there are multiple different constraints on a single element in 5695 * the base resource). Slicing can be used in any resource that has 5696 * cardinality ..* on the base resource, or any resource with a 5697 * choice of types. The set of slices is any elements that come 5698 * after this in the element sequence that have the same path, 5699 * until a shorter path occurs (the shorter path terminates the 5700 * set).) 5701 */ 5702 public ElementDefinition setSlicing(ElementDefinitionSlicingComponent value) { 5703 this.slicing = value; 5704 return this; 5705 } 5706 5707 /** 5708 * @return {@link #short_} (A concise description of what this element means 5709 * (e.g. for use in autogenerated summaries).). This is the underlying 5710 * object with id, value and extensions. The accessor "getShort" gives 5711 * direct access to the value 5712 */ 5713 public StringType getShortElement() { 5714 if (this.short_ == null) 5715 if (Configuration.errorOnAutoCreate()) 5716 throw new Error("Attempt to auto-create ElementDefinition.short_"); 5717 else if (Configuration.doAutoCreate()) 5718 this.short_ = new StringType(); // bb 5719 return this.short_; 5720 } 5721 5722 public boolean hasShortElement() { 5723 return this.short_ != null && !this.short_.isEmpty(); 5724 } 5725 5726 public boolean hasShort() { 5727 return this.short_ != null && !this.short_.isEmpty(); 5728 } 5729 5730 /** 5731 * @param value {@link #short_} (A concise description of what this element 5732 * means (e.g. for use in autogenerated summaries).). This is the 5733 * underlying object with id, value and extensions. The accessor 5734 * "getShort" gives direct access to the value 5735 */ 5736 public ElementDefinition setShortElement(StringType value) { 5737 this.short_ = value; 5738 return this; 5739 } 5740 5741 /** 5742 * @return A concise description of what this element means (e.g. for use in 5743 * autogenerated summaries). 5744 */ 5745 public String getShort() { 5746 return this.short_ == null ? null : this.short_.getValue(); 5747 } 5748 5749 /** 5750 * @param value A concise description of what this element means (e.g. for use 5751 * in autogenerated summaries). 5752 */ 5753 public ElementDefinition setShort(String value) { 5754 if (Utilities.noString(value)) 5755 this.short_ = null; 5756 else { 5757 if (this.short_ == null) 5758 this.short_ = new StringType(); 5759 this.short_.setValue(value); 5760 } 5761 return this; 5762 } 5763 5764 /** 5765 * @return {@link #definition} (Provides a complete explanation of the meaning 5766 * of the data element for human readability. For the case of elements 5767 * derived from existing elements (e.g. constraints), the definition 5768 * SHALL be consistent with the base definition, but convey the meaning 5769 * of the element in the particular context of use of the resource. 5770 * (Note: The text you are reading is specified in 5771 * ElementDefinition.definition).). This is the underlying object with 5772 * id, value and extensions. The accessor "getDefinition" gives direct 5773 * access to the value 5774 */ 5775 public MarkdownType getDefinitionElement() { 5776 if (this.definition == null) 5777 if (Configuration.errorOnAutoCreate()) 5778 throw new Error("Attempt to auto-create ElementDefinition.definition"); 5779 else if (Configuration.doAutoCreate()) 5780 this.definition = new MarkdownType(); // bb 5781 return this.definition; 5782 } 5783 5784 public boolean hasDefinitionElement() { 5785 return this.definition != null && !this.definition.isEmpty(); 5786 } 5787 5788 public boolean hasDefinition() { 5789 return this.definition != null && !this.definition.isEmpty(); 5790 } 5791 5792 /** 5793 * @param value {@link #definition} (Provides a complete explanation of the 5794 * meaning of the data element for human readability. For the case 5795 * of elements derived from existing elements (e.g. constraints), 5796 * the definition SHALL be consistent with the base definition, but 5797 * convey the meaning of the element in the particular context of 5798 * use of the resource. (Note: The text you are reading is 5799 * specified in ElementDefinition.definition).). This is the 5800 * underlying object with id, value and extensions. The accessor 5801 * "getDefinition" gives direct access to the value 5802 */ 5803 public ElementDefinition setDefinitionElement(MarkdownType value) { 5804 this.definition = value; 5805 return this; 5806 } 5807 5808 /** 5809 * @return Provides a complete explanation of the meaning of the data element 5810 * for human readability. For the case of elements derived from existing 5811 * elements (e.g. constraints), the definition SHALL be consistent with 5812 * the base definition, but convey the meaning of the element in the 5813 * particular context of use of the resource. (Note: The text you are 5814 * reading is specified in ElementDefinition.definition). 5815 */ 5816 public String getDefinition() { 5817 return this.definition == null ? null : this.definition.getValue(); 5818 } 5819 5820 /** 5821 * @param value Provides a complete explanation of the meaning of the data 5822 * element for human readability. For the case of elements derived 5823 * from existing elements (e.g. constraints), the definition SHALL 5824 * be consistent with the base definition, but convey the meaning 5825 * of the element in the particular context of use of the resource. 5826 * (Note: The text you are reading is specified in 5827 * ElementDefinition.definition). 5828 */ 5829 public ElementDefinition setDefinition(String value) { 5830 if (value == null) 5831 this.definition = null; 5832 else { 5833 if (this.definition == null) 5834 this.definition = new MarkdownType(); 5835 this.definition.setValue(value); 5836 } 5837 return this; 5838 } 5839 5840 /** 5841 * @return {@link #comment} (Explanatory notes and implementation guidance about 5842 * the data element, including notes about how to use the data properly, 5843 * exceptions to proper use, etc. (Note: The text you are reading is 5844 * specified in ElementDefinition.comment).). This is the underlying 5845 * object with id, value and extensions. The accessor "getComment" gives 5846 * direct access to the value 5847 */ 5848 public MarkdownType getCommentElement() { 5849 if (this.comment == null) 5850 if (Configuration.errorOnAutoCreate()) 5851 throw new Error("Attempt to auto-create ElementDefinition.comment"); 5852 else if (Configuration.doAutoCreate()) 5853 this.comment = new MarkdownType(); // bb 5854 return this.comment; 5855 } 5856 5857 public boolean hasCommentElement() { 5858 return this.comment != null && !this.comment.isEmpty(); 5859 } 5860 5861 public boolean hasComment() { 5862 return this.comment != null && !this.comment.isEmpty(); 5863 } 5864 5865 /** 5866 * @param value {@link #comment} (Explanatory notes and implementation guidance 5867 * about the data element, including notes about how to use the 5868 * data properly, exceptions to proper use, etc. (Note: The text 5869 * you are reading is specified in ElementDefinition.comment).). 5870 * This is the underlying object with id, value and extensions. The 5871 * accessor "getComment" gives direct access to the value 5872 */ 5873 public ElementDefinition setCommentElement(MarkdownType value) { 5874 this.comment = value; 5875 return this; 5876 } 5877 5878 /** 5879 * @return Explanatory notes and implementation guidance about the data element, 5880 * including notes about how to use the data properly, exceptions to 5881 * proper use, etc. (Note: The text you are reading is specified in 5882 * ElementDefinition.comment). 5883 */ 5884 public String getComment() { 5885 return this.comment == null ? null : this.comment.getValue(); 5886 } 5887 5888 /** 5889 * @param value Explanatory notes and implementation guidance about the data 5890 * element, including notes about how to use the data properly, 5891 * exceptions to proper use, etc. (Note: The text you are reading 5892 * is specified in ElementDefinition.comment). 5893 */ 5894 public ElementDefinition setComment(String value) { 5895 if (value == null) 5896 this.comment = null; 5897 else { 5898 if (this.comment == null) 5899 this.comment = new MarkdownType(); 5900 this.comment.setValue(value); 5901 } 5902 return this; 5903 } 5904 5905 /** 5906 * @return {@link #requirements} (This element is for traceability of why the 5907 * element was created and why the constraints exist as they do. This 5908 * may be used to point to source materials or specifications that drove 5909 * the structure of this element.). This is the underlying object with 5910 * id, value and extensions. The accessor "getRequirements" gives direct 5911 * access to the value 5912 */ 5913 public MarkdownType getRequirementsElement() { 5914 if (this.requirements == null) 5915 if (Configuration.errorOnAutoCreate()) 5916 throw new Error("Attempt to auto-create ElementDefinition.requirements"); 5917 else if (Configuration.doAutoCreate()) 5918 this.requirements = new MarkdownType(); // bb 5919 return this.requirements; 5920 } 5921 5922 public boolean hasRequirementsElement() { 5923 return this.requirements != null && !this.requirements.isEmpty(); 5924 } 5925 5926 public boolean hasRequirements() { 5927 return this.requirements != null && !this.requirements.isEmpty(); 5928 } 5929 5930 /** 5931 * @param value {@link #requirements} (This element is for traceability of why 5932 * the element was created and why the constraints exist as they 5933 * do. This may be used to point to source materials or 5934 * specifications that drove the structure of this element.). This 5935 * is the underlying object with id, value and extensions. The 5936 * accessor "getRequirements" gives direct access to the value 5937 */ 5938 public ElementDefinition setRequirementsElement(MarkdownType value) { 5939 this.requirements = value; 5940 return this; 5941 } 5942 5943 /** 5944 * @return This element is for traceability of why the element was created and 5945 * why the constraints exist as they do. This may be used to point to 5946 * source materials or specifications that drove the structure of this 5947 * element. 5948 */ 5949 public String getRequirements() { 5950 return this.requirements == null ? null : this.requirements.getValue(); 5951 } 5952 5953 /** 5954 * @param value This element is for traceability of why the element was created 5955 * and why the constraints exist as they do. This may be used to 5956 * point to source materials or specifications that drove the 5957 * structure of this element. 5958 */ 5959 public ElementDefinition setRequirements(String value) { 5960 if (value == null) 5961 this.requirements = null; 5962 else { 5963 if (this.requirements == null) 5964 this.requirements = new MarkdownType(); 5965 this.requirements.setValue(value); 5966 } 5967 return this; 5968 } 5969 5970 /** 5971 * @return {@link #alias} (Identifies additional names by which this element 5972 * might also be known.) 5973 */ 5974 public List<StringType> getAlias() { 5975 if (this.alias == null) 5976 this.alias = new ArrayList<StringType>(); 5977 return this.alias; 5978 } 5979 5980 /** 5981 * @return Returns a reference to <code>this</code> for easy method chaining 5982 */ 5983 public ElementDefinition setAlias(List<StringType> theAlias) { 5984 this.alias = theAlias; 5985 return this; 5986 } 5987 5988 public boolean hasAlias() { 5989 if (this.alias == null) 5990 return false; 5991 for (StringType item : this.alias) 5992 if (!item.isEmpty()) 5993 return true; 5994 return false; 5995 } 5996 5997 /** 5998 * @return {@link #alias} (Identifies additional names by which this element 5999 * might also be known.) 6000 */ 6001 public StringType addAliasElement() {// 2 6002 StringType t = new StringType(); 6003 if (this.alias == null) 6004 this.alias = new ArrayList<StringType>(); 6005 this.alias.add(t); 6006 return t; 6007 } 6008 6009 /** 6010 * @param value {@link #alias} (Identifies additional names by which this 6011 * element might also be known.) 6012 */ 6013 public ElementDefinition addAlias(String value) { // 1 6014 StringType t = new StringType(); 6015 t.setValue(value); 6016 if (this.alias == null) 6017 this.alias = new ArrayList<StringType>(); 6018 this.alias.add(t); 6019 return this; 6020 } 6021 6022 /** 6023 * @param value {@link #alias} (Identifies additional names by which this 6024 * element might also be known.) 6025 */ 6026 public boolean hasAlias(String value) { 6027 if (this.alias == null) 6028 return false; 6029 for (StringType v : this.alias) 6030 if (v.getValue().equals(value)) // string 6031 return true; 6032 return false; 6033 } 6034 6035 /** 6036 * @return {@link #min} (The minimum number of times this element SHALL appear 6037 * in the instance.). This is the underlying object with id, value and 6038 * extensions. The accessor "getMin" gives direct access to the value 6039 */ 6040 public UnsignedIntType getMinElement() { 6041 if (this.min == null) 6042 if (Configuration.errorOnAutoCreate()) 6043 throw new Error("Attempt to auto-create ElementDefinition.min"); 6044 else if (Configuration.doAutoCreate()) 6045 this.min = new UnsignedIntType(); // bb 6046 return this.min; 6047 } 6048 6049 public boolean hasMinElement() { 6050 return this.min != null && !this.min.isEmpty(); 6051 } 6052 6053 public boolean hasMin() { 6054 return this.min != null && !this.min.isEmpty(); 6055 } 6056 6057 /** 6058 * @param value {@link #min} (The minimum number of times this element SHALL 6059 * appear in the instance.). This is the underlying object with id, 6060 * value and extensions. The accessor "getMin" gives direct access 6061 * to the value 6062 */ 6063 public ElementDefinition setMinElement(UnsignedIntType value) { 6064 this.min = value; 6065 return this; 6066 } 6067 6068 /** 6069 * @return The minimum number of times this element SHALL appear in the 6070 * instance. 6071 */ 6072 public int getMin() { 6073 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 6074 } 6075 6076 /** 6077 * @param value The minimum number of times this element SHALL appear in the 6078 * instance. 6079 */ 6080 public ElementDefinition setMin(int value) { 6081 if (this.min == null) 6082 this.min = new UnsignedIntType(); 6083 this.min.setValue(value); 6084 return this; 6085 } 6086 6087 /** 6088 * @return {@link #max} (The maximum number of times this element is permitted 6089 * to appear in the instance.). This is the underlying object with id, 6090 * value and extensions. The accessor "getMax" gives direct access to 6091 * the value 6092 */ 6093 public StringType getMaxElement() { 6094 if (this.max == null) 6095 if (Configuration.errorOnAutoCreate()) 6096 throw new Error("Attempt to auto-create ElementDefinition.max"); 6097 else if (Configuration.doAutoCreate()) 6098 this.max = new StringType(); // bb 6099 return this.max; 6100 } 6101 6102 public boolean hasMaxElement() { 6103 return this.max != null && !this.max.isEmpty(); 6104 } 6105 6106 public boolean hasMax() { 6107 return this.max != null && !this.max.isEmpty(); 6108 } 6109 6110 /** 6111 * @param value {@link #max} (The maximum number of times this element is 6112 * permitted to appear in the instance.). This is the underlying 6113 * object with id, value and extensions. The accessor "getMax" 6114 * gives direct access to the value 6115 */ 6116 public ElementDefinition setMaxElement(StringType value) { 6117 this.max = value; 6118 return this; 6119 } 6120 6121 /** 6122 * @return The maximum number of times this element is permitted to appear in 6123 * the instance. 6124 */ 6125 public String getMax() { 6126 return this.max == null ? null : this.max.getValue(); 6127 } 6128 6129 /** 6130 * @param value The maximum number of times this element is permitted to appear 6131 * in the instance. 6132 */ 6133 public ElementDefinition setMax(String value) { 6134 if (Utilities.noString(value)) 6135 this.max = null; 6136 else { 6137 if (this.max == null) 6138 this.max = new StringType(); 6139 this.max.setValue(value); 6140 } 6141 return this; 6142 } 6143 6144 /** 6145 * @return {@link #base} (Information about the base definition of the element, 6146 * provided to make it unnecessary for tools to trace the deviation of 6147 * the element through the derived and related profiles. When the 6148 * element definition is not the original definition of an element - 6149 * i.g. either in a constraint on another type, or for elements from a 6150 * super type in a snap shot - then the information in provided in the 6151 * element definition may be different to the base definition. On the 6152 * original definition of the element, it will be same.) 6153 */ 6154 public ElementDefinitionBaseComponent getBase() { 6155 if (this.base == null) 6156 if (Configuration.errorOnAutoCreate()) 6157 throw new Error("Attempt to auto-create ElementDefinition.base"); 6158 else if (Configuration.doAutoCreate()) 6159 this.base = new ElementDefinitionBaseComponent(); // cc 6160 return this.base; 6161 } 6162 6163 public boolean hasBase() { 6164 return this.base != null && !this.base.isEmpty(); 6165 } 6166 6167 /** 6168 * @param value {@link #base} (Information about the base definition of the 6169 * element, provided to make it unnecessary for tools to trace the 6170 * deviation of the element through the derived and related 6171 * profiles. When the element definition is not the original 6172 * definition of an element - i.g. either in a constraint on 6173 * another type, or for elements from a super type in a snap shot - 6174 * then the information in provided in the element definition may 6175 * be different to the base definition. On the original definition 6176 * of the element, it will be same.) 6177 */ 6178 public ElementDefinition setBase(ElementDefinitionBaseComponent value) { 6179 this.base = value; 6180 return this; 6181 } 6182 6183 /** 6184 * @return {@link #contentReference} (Identifies an element defined elsewhere in 6185 * the definition whose content rules should be applied to the current 6186 * element. ContentReferences bring across all the rules that are in the 6187 * ElementDefinition for the element, including definitions, cardinality 6188 * constraints, bindings, invariants etc.). This is the underlying 6189 * object with id, value and extensions. The accessor 6190 * "getContentReference" gives direct access to the value 6191 */ 6192 public UriType getContentReferenceElement() { 6193 if (this.contentReference == null) 6194 if (Configuration.errorOnAutoCreate()) 6195 throw new Error("Attempt to auto-create ElementDefinition.contentReference"); 6196 else if (Configuration.doAutoCreate()) 6197 this.contentReference = new UriType(); // bb 6198 return this.contentReference; 6199 } 6200 6201 public boolean hasContentReferenceElement() { 6202 return this.contentReference != null && !this.contentReference.isEmpty(); 6203 } 6204 6205 public boolean hasContentReference() { 6206 return this.contentReference != null && !this.contentReference.isEmpty(); 6207 } 6208 6209 /** 6210 * @param value {@link #contentReference} (Identifies an element defined 6211 * elsewhere in the definition whose content rules should be 6212 * applied to the current element. ContentReferences bring across 6213 * all the rules that are in the ElementDefinition for the element, 6214 * including definitions, cardinality constraints, bindings, 6215 * invariants etc.). This is the underlying object with id, value 6216 * and extensions. The accessor "getContentReference" gives direct 6217 * access to the value 6218 */ 6219 public ElementDefinition setContentReferenceElement(UriType value) { 6220 this.contentReference = value; 6221 return this; 6222 } 6223 6224 /** 6225 * @return Identifies an element defined elsewhere in the definition whose 6226 * content rules should be applied to the current element. 6227 * ContentReferences bring across all the rules that are in the 6228 * ElementDefinition for the element, including definitions, cardinality 6229 * constraints, bindings, invariants etc. 6230 */ 6231 public String getContentReference() { 6232 return this.contentReference == null ? null : this.contentReference.getValue(); 6233 } 6234 6235 /** 6236 * @param value Identifies an element defined elsewhere in the definition whose 6237 * content rules should be applied to the current element. 6238 * ContentReferences bring across all the rules that are in the 6239 * ElementDefinition for the element, including definitions, 6240 * cardinality constraints, bindings, invariants etc. 6241 */ 6242 public ElementDefinition setContentReference(String value) { 6243 if (Utilities.noString(value)) 6244 this.contentReference = null; 6245 else { 6246 if (this.contentReference == null) 6247 this.contentReference = new UriType(); 6248 this.contentReference.setValue(value); 6249 } 6250 return this; 6251 } 6252 6253 /** 6254 * @return {@link #type} (The data type or resource that the value of this 6255 * element is permitted to be.) 6256 */ 6257 public List<TypeRefComponent> getType() { 6258 if (this.type == null) 6259 this.type = new ArrayList<TypeRefComponent>(); 6260 return this.type; 6261 } 6262 6263 /** 6264 * @return Returns a reference to <code>this</code> for easy method chaining 6265 */ 6266 public ElementDefinition setType(List<TypeRefComponent> theType) { 6267 this.type = theType; 6268 return this; 6269 } 6270 6271 public boolean hasType() { 6272 if (this.type == null) 6273 return false; 6274 for (TypeRefComponent item : this.type) 6275 if (!item.isEmpty()) 6276 return true; 6277 return false; 6278 } 6279 6280 public TypeRefComponent addType() { // 3 6281 TypeRefComponent t = new TypeRefComponent(); 6282 if (this.type == null) 6283 this.type = new ArrayList<TypeRefComponent>(); 6284 this.type.add(t); 6285 return t; 6286 } 6287 6288 public ElementDefinition addType(TypeRefComponent t) { // 3 6289 if (t == null) 6290 return this; 6291 if (this.type == null) 6292 this.type = new ArrayList<TypeRefComponent>(); 6293 this.type.add(t); 6294 return this; 6295 } 6296 6297 /** 6298 * @return The first repetition of repeating field {@link #type}, creating it if 6299 * it does not already exist 6300 */ 6301 public TypeRefComponent getTypeFirstRep() { 6302 if (getType().isEmpty()) { 6303 addType(); 6304 } 6305 return getType().get(0); 6306 } 6307 6308 /** 6309 * @return {@link #defaultValue} (The value that should be used if there is no 6310 * value stated in the instance (e.g. 'if not otherwise specified, the 6311 * abstract is false').) 6312 */ 6313 public org.hl7.fhir.r4.model.Type getDefaultValue() { 6314 return this.defaultValue; 6315 } 6316 6317 public boolean hasDefaultValue() { 6318 return this.defaultValue != null && !this.defaultValue.isEmpty(); 6319 } 6320 6321 /** 6322 * @param value {@link #defaultValue} (The value that should be used if there is 6323 * no value stated in the instance (e.g. 'if not otherwise 6324 * specified, the abstract is false').) 6325 */ 6326 public ElementDefinition setDefaultValue(org.hl7.fhir.r4.model.Type value) { 6327 this.defaultValue = value; 6328 return this; 6329 } 6330 6331 /** 6332 * @return {@link #meaningWhenMissing} (The Implicit meaning that is to be 6333 * understood when this element is missing (e.g. 'when this element is 6334 * missing, the period is ongoing').). This is the underlying object 6335 * with id, value and extensions. The accessor "getMeaningWhenMissing" 6336 * gives direct access to the value 6337 */ 6338 public MarkdownType getMeaningWhenMissingElement() { 6339 if (this.meaningWhenMissing == null) 6340 if (Configuration.errorOnAutoCreate()) 6341 throw new Error("Attempt to auto-create ElementDefinition.meaningWhenMissing"); 6342 else if (Configuration.doAutoCreate()) 6343 this.meaningWhenMissing = new MarkdownType(); // bb 6344 return this.meaningWhenMissing; 6345 } 6346 6347 public boolean hasMeaningWhenMissingElement() { 6348 return this.meaningWhenMissing != null && !this.meaningWhenMissing.isEmpty(); 6349 } 6350 6351 public boolean hasMeaningWhenMissing() { 6352 return this.meaningWhenMissing != null && !this.meaningWhenMissing.isEmpty(); 6353 } 6354 6355 /** 6356 * @param value {@link #meaningWhenMissing} (The Implicit meaning that is to be 6357 * understood when this element is missing (e.g. 'when this element 6358 * is missing, the period is ongoing').). This is the underlying 6359 * object with id, value and extensions. The accessor 6360 * "getMeaningWhenMissing" gives direct access to the value 6361 */ 6362 public ElementDefinition setMeaningWhenMissingElement(MarkdownType value) { 6363 this.meaningWhenMissing = value; 6364 return this; 6365 } 6366 6367 /** 6368 * @return The Implicit meaning that is to be understood when this element is 6369 * missing (e.g. 'when this element is missing, the period is ongoing'). 6370 */ 6371 public String getMeaningWhenMissing() { 6372 return this.meaningWhenMissing == null ? null : this.meaningWhenMissing.getValue(); 6373 } 6374 6375 /** 6376 * @param value The Implicit meaning that is to be understood when this element 6377 * is missing (e.g. 'when this element is missing, the period is 6378 * ongoing'). 6379 */ 6380 public ElementDefinition setMeaningWhenMissing(String value) { 6381 if (value == null) 6382 this.meaningWhenMissing = null; 6383 else { 6384 if (this.meaningWhenMissing == null) 6385 this.meaningWhenMissing = new MarkdownType(); 6386 this.meaningWhenMissing.setValue(value); 6387 } 6388 return this; 6389 } 6390 6391 /** 6392 * @return {@link #orderMeaning} (If present, indicates that the order of the 6393 * repeating element has meaning and describes what that meaning is. If 6394 * absent, it means that the order of the element has no meaning.). This 6395 * is the underlying object with id, value and extensions. The accessor 6396 * "getOrderMeaning" gives direct access to the value 6397 */ 6398 public StringType getOrderMeaningElement() { 6399 if (this.orderMeaning == null) 6400 if (Configuration.errorOnAutoCreate()) 6401 throw new Error("Attempt to auto-create ElementDefinition.orderMeaning"); 6402 else if (Configuration.doAutoCreate()) 6403 this.orderMeaning = new StringType(); // bb 6404 return this.orderMeaning; 6405 } 6406 6407 public boolean hasOrderMeaningElement() { 6408 return this.orderMeaning != null && !this.orderMeaning.isEmpty(); 6409 } 6410 6411 public boolean hasOrderMeaning() { 6412 return this.orderMeaning != null && !this.orderMeaning.isEmpty(); 6413 } 6414 6415 /** 6416 * @param value {@link #orderMeaning} (If present, indicates that the order of 6417 * the repeating element has meaning and describes what that 6418 * meaning is. If absent, it means that the order of the element 6419 * has no meaning.). This is the underlying object with id, value 6420 * and extensions. The accessor "getOrderMeaning" gives direct 6421 * access to the value 6422 */ 6423 public ElementDefinition setOrderMeaningElement(StringType value) { 6424 this.orderMeaning = value; 6425 return this; 6426 } 6427 6428 /** 6429 * @return If present, indicates that the order of the repeating element has 6430 * meaning and describes what that meaning is. If absent, it means that 6431 * the order of the element has no meaning. 6432 */ 6433 public String getOrderMeaning() { 6434 return this.orderMeaning == null ? null : this.orderMeaning.getValue(); 6435 } 6436 6437 /** 6438 * @param value If present, indicates that the order of the repeating element 6439 * has meaning and describes what that meaning is. If absent, it 6440 * means that the order of the element has no meaning. 6441 */ 6442 public ElementDefinition setOrderMeaning(String value) { 6443 if (Utilities.noString(value)) 6444 this.orderMeaning = null; 6445 else { 6446 if (this.orderMeaning == null) 6447 this.orderMeaning = new StringType(); 6448 this.orderMeaning.setValue(value); 6449 } 6450 return this; 6451 } 6452 6453 /** 6454 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for 6455 * this element in the instance. For purposes of comparison, 6456 * non-significant whitespace is ignored, and all values must be an 6457 * exact match (case and accent sensitive). Missing elements/attributes 6458 * must also be missing.) 6459 */ 6460 public org.hl7.fhir.r4.model.Type getFixed() { 6461 return this.fixed; 6462 } 6463 6464 public boolean hasFixed() { 6465 return this.fixed != null && !this.fixed.isEmpty(); 6466 } 6467 6468 /** 6469 * @param value {@link #fixed} (Specifies a value that SHALL be exactly the 6470 * value for this element in the instance. For purposes of 6471 * comparison, non-significant whitespace is ignored, and all 6472 * values must be an exact match (case and accent sensitive). 6473 * Missing elements/attributes must also be missing.) 6474 */ 6475 public ElementDefinition setFixed(org.hl7.fhir.r4.model.Type value) { 6476 this.fixed = value; 6477 return this; 6478 } 6479 6480 /** 6481 * @return {@link #pattern} (Specifies a value that the value in the instance 6482 * SHALL follow - that is, any value in the pattern must be found in the 6483 * instance. Other additional values may be found too. This is 6484 * effectively constraint by example. 6485 * 6486 * When pattern[x] is used to constrain a primitive, it means that the 6487 * value provided in the pattern[x] must match the instance value 6488 * exactly. 6489 * 6490 * When pattern[x] is used to constrain an array, it means that each 6491 * element provided in the pattern[x] array must (recursively) match at 6492 * least one element from the instance array. 6493 * 6494 * When pattern[x] is used to constrain a complex object, it means that 6495 * each property in the pattern must be present in the complex object, 6496 * and its value must recursively match -- i.e., 6497 * 6498 * 1. If primitive: it must match exactly the pattern value 2. If a 6499 * complex object: it must match (recursively) the pattern value 3. If 6500 * an array: it must match (recursively) the pattern value.) 6501 */ 6502 public org.hl7.fhir.r4.model.Type getPattern() { 6503 return this.pattern; 6504 } 6505 6506 public boolean hasPattern() { 6507 return this.pattern != null && !this.pattern.isEmpty(); 6508 } 6509 6510 /** 6511 * @param value {@link #pattern} (Specifies a value that the value in the 6512 * instance SHALL follow - that is, any value in the pattern must 6513 * be found in the instance. Other additional values may be found 6514 * too. This is effectively constraint by example. 6515 * 6516 * When pattern[x] is used to constrain a primitive, it means that 6517 * the value provided in the pattern[x] must match the instance 6518 * value exactly. 6519 * 6520 * When pattern[x] is used to constrain an array, it means that 6521 * each element provided in the pattern[x] array must (recursively) 6522 * match at least one element from the instance array. 6523 * 6524 * When pattern[x] is used to constrain a complex object, it means 6525 * that each property in the pattern must be present in the complex 6526 * object, and its value must recursively match -- i.e., 6527 * 6528 * 1. If primitive: it must match exactly the pattern value 2. If a 6529 * complex object: it must match (recursively) the pattern value 3. 6530 * If an array: it must match (recursively) the pattern value.) 6531 */ 6532 public ElementDefinition setPattern(org.hl7.fhir.r4.model.Type value) { 6533 this.pattern = value; 6534 return this; 6535 } 6536 6537 /** 6538 * @return {@link #example} (A sample value for this element demonstrating the 6539 * type of information that would typically be found in the element.) 6540 */ 6541 public List<ElementDefinitionExampleComponent> getExample() { 6542 if (this.example == null) 6543 this.example = new ArrayList<ElementDefinitionExampleComponent>(); 6544 return this.example; 6545 } 6546 6547 /** 6548 * @return Returns a reference to <code>this</code> for easy method chaining 6549 */ 6550 public ElementDefinition setExample(List<ElementDefinitionExampleComponent> theExample) { 6551 this.example = theExample; 6552 return this; 6553 } 6554 6555 public boolean hasExample() { 6556 if (this.example == null) 6557 return false; 6558 for (ElementDefinitionExampleComponent item : this.example) 6559 if (!item.isEmpty()) 6560 return true; 6561 return false; 6562 } 6563 6564 public ElementDefinitionExampleComponent addExample() { // 3 6565 ElementDefinitionExampleComponent t = new ElementDefinitionExampleComponent(); 6566 if (this.example == null) 6567 this.example = new ArrayList<ElementDefinitionExampleComponent>(); 6568 this.example.add(t); 6569 return t; 6570 } 6571 6572 public ElementDefinition addExample(ElementDefinitionExampleComponent t) { // 3 6573 if (t == null) 6574 return this; 6575 if (this.example == null) 6576 this.example = new ArrayList<ElementDefinitionExampleComponent>(); 6577 this.example.add(t); 6578 return this; 6579 } 6580 6581 /** 6582 * @return The first repetition of repeating field {@link #example}, creating it 6583 * if it does not already exist 6584 */ 6585 public ElementDefinitionExampleComponent getExampleFirstRep() { 6586 if (getExample().isEmpty()) { 6587 addExample(); 6588 } 6589 return getExample().get(0); 6590 } 6591 6592 /** 6593 * @return {@link #minValue} (The minimum allowed value for the element. The 6594 * value is inclusive. This is allowed for the types date, dateTime, 6595 * instant, time, decimal, integer, and Quantity.) 6596 */ 6597 public Type getMinValue() { 6598 return this.minValue; 6599 } 6600 6601 /** 6602 * @return {@link #minValue} (The minimum allowed value for the element. The 6603 * value is inclusive. This is allowed for the types date, dateTime, 6604 * instant, time, decimal, integer, and Quantity.) 6605 */ 6606 public DateType getMinValueDateType() throws FHIRException { 6607 if (this.minValue == null) 6608 this.minValue = new DateType(); 6609 if (!(this.minValue instanceof DateType)) 6610 throw new FHIRException("Type mismatch: the type DateType was expected, but " + this.minValue.getClass().getName() 6611 + " was encountered"); 6612 return (DateType) this.minValue; 6613 } 6614 6615 public boolean hasMinValueDateType() { 6616 return this != null && this.minValue instanceof DateType; 6617 } 6618 6619 /** 6620 * @return {@link #minValue} (The minimum allowed value for the element. The 6621 * value is inclusive. This is allowed for the types date, dateTime, 6622 * instant, time, decimal, integer, and Quantity.) 6623 */ 6624 public DateTimeType getMinValueDateTimeType() throws FHIRException { 6625 if (this.minValue == null) 6626 this.minValue = new DateTimeType(); 6627 if (!(this.minValue instanceof DateTimeType)) 6628 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 6629 + this.minValue.getClass().getName() + " was encountered"); 6630 return (DateTimeType) this.minValue; 6631 } 6632 6633 public boolean hasMinValueDateTimeType() { 6634 return this != null && this.minValue instanceof DateTimeType; 6635 } 6636 6637 /** 6638 * @return {@link #minValue} (The minimum allowed value for the element. The 6639 * value is inclusive. This is allowed for the types date, dateTime, 6640 * instant, time, decimal, integer, and Quantity.) 6641 */ 6642 public InstantType getMinValueInstantType() throws FHIRException { 6643 if (this.minValue == null) 6644 this.minValue = new InstantType(); 6645 if (!(this.minValue instanceof InstantType)) 6646 throw new FHIRException("Type mismatch: the type InstantType was expected, but " 6647 + this.minValue.getClass().getName() + " was encountered"); 6648 return (InstantType) this.minValue; 6649 } 6650 6651 public boolean hasMinValueInstantType() { 6652 return this != null && this.minValue instanceof InstantType; 6653 } 6654 6655 /** 6656 * @return {@link #minValue} (The minimum allowed value for the element. The 6657 * value is inclusive. This is allowed for the types date, dateTime, 6658 * instant, time, decimal, integer, and Quantity.) 6659 */ 6660 public TimeType getMinValueTimeType() throws FHIRException { 6661 if (this.minValue == null) 6662 this.minValue = new TimeType(); 6663 if (!(this.minValue instanceof TimeType)) 6664 throw new FHIRException("Type mismatch: the type TimeType was expected, but " + this.minValue.getClass().getName() 6665 + " was encountered"); 6666 return (TimeType) this.minValue; 6667 } 6668 6669 public boolean hasMinValueTimeType() { 6670 return this != null && this.minValue instanceof TimeType; 6671 } 6672 6673 /** 6674 * @return {@link #minValue} (The minimum allowed value for the element. The 6675 * value is inclusive. This is allowed for the types date, dateTime, 6676 * instant, time, decimal, integer, and Quantity.) 6677 */ 6678 public DecimalType getMinValueDecimalType() throws FHIRException { 6679 if (this.minValue == null) 6680 this.minValue = new DecimalType(); 6681 if (!(this.minValue instanceof DecimalType)) 6682 throw new FHIRException("Type mismatch: the type DecimalType was expected, but " 6683 + this.minValue.getClass().getName() + " was encountered"); 6684 return (DecimalType) this.minValue; 6685 } 6686 6687 public boolean hasMinValueDecimalType() { 6688 return this != null && this.minValue instanceof DecimalType; 6689 } 6690 6691 /** 6692 * @return {@link #minValue} (The minimum allowed value for the element. The 6693 * value is inclusive. This is allowed for the types date, dateTime, 6694 * instant, time, decimal, integer, and Quantity.) 6695 */ 6696 public IntegerType getMinValueIntegerType() throws FHIRException { 6697 if (this.minValue == null) 6698 this.minValue = new IntegerType(); 6699 if (!(this.minValue instanceof IntegerType)) 6700 throw new FHIRException("Type mismatch: the type IntegerType was expected, but " 6701 + this.minValue.getClass().getName() + " was encountered"); 6702 return (IntegerType) this.minValue; 6703 } 6704 6705 public boolean hasMinValueIntegerType() { 6706 return this != null && this.minValue instanceof IntegerType; 6707 } 6708 6709 /** 6710 * @return {@link #minValue} (The minimum allowed value for the element. The 6711 * value is inclusive. This is allowed for the types date, dateTime, 6712 * instant, time, decimal, integer, and Quantity.) 6713 */ 6714 public PositiveIntType getMinValuePositiveIntType() throws FHIRException { 6715 if (this.minValue == null) 6716 this.minValue = new PositiveIntType(); 6717 if (!(this.minValue instanceof PositiveIntType)) 6718 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but " 6719 + this.minValue.getClass().getName() + " was encountered"); 6720 return (PositiveIntType) this.minValue; 6721 } 6722 6723 public boolean hasMinValuePositiveIntType() { 6724 return this != null && this.minValue instanceof PositiveIntType; 6725 } 6726 6727 /** 6728 * @return {@link #minValue} (The minimum allowed value for the element. The 6729 * value is inclusive. This is allowed for the types date, dateTime, 6730 * instant, time, decimal, integer, and Quantity.) 6731 */ 6732 public UnsignedIntType getMinValueUnsignedIntType() throws FHIRException { 6733 if (this.minValue == null) 6734 this.minValue = new UnsignedIntType(); 6735 if (!(this.minValue instanceof UnsignedIntType)) 6736 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but " 6737 + this.minValue.getClass().getName() + " was encountered"); 6738 return (UnsignedIntType) this.minValue; 6739 } 6740 6741 public boolean hasMinValueUnsignedIntType() { 6742 return this != null && this.minValue instanceof UnsignedIntType; 6743 } 6744 6745 /** 6746 * @return {@link #minValue} (The minimum allowed value for the element. The 6747 * value is inclusive. This is allowed for the types date, dateTime, 6748 * instant, time, decimal, integer, and Quantity.) 6749 */ 6750 public Quantity getMinValueQuantity() throws FHIRException { 6751 if (this.minValue == null) 6752 this.minValue = new Quantity(); 6753 if (!(this.minValue instanceof Quantity)) 6754 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.minValue.getClass().getName() 6755 + " was encountered"); 6756 return (Quantity) this.minValue; 6757 } 6758 6759 public boolean hasMinValueQuantity() { 6760 return this != null && this.minValue instanceof Quantity; 6761 } 6762 6763 public boolean hasMinValue() { 6764 return this.minValue != null && !this.minValue.isEmpty(); 6765 } 6766 6767 /** 6768 * @param value {@link #minValue} (The minimum allowed value for the element. 6769 * The value is inclusive. This is allowed for the types date, 6770 * dateTime, instant, time, decimal, integer, and Quantity.) 6771 */ 6772 public ElementDefinition setMinValue(Type value) { 6773 if (value != null && !(value instanceof DateType || value instanceof DateTimeType || value instanceof InstantType 6774 || value instanceof TimeType || value instanceof DecimalType || value instanceof IntegerType 6775 || value instanceof PositiveIntType || value instanceof UnsignedIntType || value instanceof Quantity)) 6776 throw new Error("Not the right type for ElementDefinition.minValue[x]: " + value.fhirType()); 6777 this.minValue = value; 6778 return this; 6779 } 6780 6781 /** 6782 * @return {@link #maxValue} (The maximum allowed value for the element. The 6783 * value is inclusive. This is allowed for the types date, dateTime, 6784 * instant, time, decimal, integer, and Quantity.) 6785 */ 6786 public Type getMaxValue() { 6787 return this.maxValue; 6788 } 6789 6790 /** 6791 * @return {@link #maxValue} (The maximum allowed value for the element. The 6792 * value is inclusive. This is allowed for the types date, dateTime, 6793 * instant, time, decimal, integer, and Quantity.) 6794 */ 6795 public DateType getMaxValueDateType() throws FHIRException { 6796 if (this.maxValue == null) 6797 this.maxValue = new DateType(); 6798 if (!(this.maxValue instanceof DateType)) 6799 throw new FHIRException("Type mismatch: the type DateType was expected, but " + this.maxValue.getClass().getName() 6800 + " was encountered"); 6801 return (DateType) this.maxValue; 6802 } 6803 6804 public boolean hasMaxValueDateType() { 6805 return this != null && this.maxValue instanceof DateType; 6806 } 6807 6808 /** 6809 * @return {@link #maxValue} (The maximum allowed value for the element. The 6810 * value is inclusive. This is allowed for the types date, dateTime, 6811 * instant, time, decimal, integer, and Quantity.) 6812 */ 6813 public DateTimeType getMaxValueDateTimeType() throws FHIRException { 6814 if (this.maxValue == null) 6815 this.maxValue = new DateTimeType(); 6816 if (!(this.maxValue instanceof DateTimeType)) 6817 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 6818 + this.maxValue.getClass().getName() + " was encountered"); 6819 return (DateTimeType) this.maxValue; 6820 } 6821 6822 public boolean hasMaxValueDateTimeType() { 6823 return this != null && this.maxValue instanceof DateTimeType; 6824 } 6825 6826 /** 6827 * @return {@link #maxValue} (The maximum allowed value for the element. The 6828 * value is inclusive. This is allowed for the types date, dateTime, 6829 * instant, time, decimal, integer, and Quantity.) 6830 */ 6831 public InstantType getMaxValueInstantType() throws FHIRException { 6832 if (this.maxValue == null) 6833 this.maxValue = new InstantType(); 6834 if (!(this.maxValue instanceof InstantType)) 6835 throw new FHIRException("Type mismatch: the type InstantType was expected, but " 6836 + this.maxValue.getClass().getName() + " was encountered"); 6837 return (InstantType) this.maxValue; 6838 } 6839 6840 public boolean hasMaxValueInstantType() { 6841 return this != null && this.maxValue instanceof InstantType; 6842 } 6843 6844 /** 6845 * @return {@link #maxValue} (The maximum allowed value for the element. The 6846 * value is inclusive. This is allowed for the types date, dateTime, 6847 * instant, time, decimal, integer, and Quantity.) 6848 */ 6849 public TimeType getMaxValueTimeType() throws FHIRException { 6850 if (this.maxValue == null) 6851 this.maxValue = new TimeType(); 6852 if (!(this.maxValue instanceof TimeType)) 6853 throw new FHIRException("Type mismatch: the type TimeType was expected, but " + this.maxValue.getClass().getName() 6854 + " was encountered"); 6855 return (TimeType) this.maxValue; 6856 } 6857 6858 public boolean hasMaxValueTimeType() { 6859 return this != null && this.maxValue instanceof TimeType; 6860 } 6861 6862 /** 6863 * @return {@link #maxValue} (The maximum allowed value for the element. The 6864 * value is inclusive. This is allowed for the types date, dateTime, 6865 * instant, time, decimal, integer, and Quantity.) 6866 */ 6867 public DecimalType getMaxValueDecimalType() throws FHIRException { 6868 if (this.maxValue == null) 6869 this.maxValue = new DecimalType(); 6870 if (!(this.maxValue instanceof DecimalType)) 6871 throw new FHIRException("Type mismatch: the type DecimalType was expected, but " 6872 + this.maxValue.getClass().getName() + " was encountered"); 6873 return (DecimalType) this.maxValue; 6874 } 6875 6876 public boolean hasMaxValueDecimalType() { 6877 return this != null && this.maxValue instanceof DecimalType; 6878 } 6879 6880 /** 6881 * @return {@link #maxValue} (The maximum allowed value for the element. The 6882 * value is inclusive. This is allowed for the types date, dateTime, 6883 * instant, time, decimal, integer, and Quantity.) 6884 */ 6885 public IntegerType getMaxValueIntegerType() throws FHIRException { 6886 if (this.maxValue == null) 6887 this.maxValue = new IntegerType(); 6888 if (!(this.maxValue instanceof IntegerType)) 6889 throw new FHIRException("Type mismatch: the type IntegerType was expected, but " 6890 + this.maxValue.getClass().getName() + " was encountered"); 6891 return (IntegerType) this.maxValue; 6892 } 6893 6894 public boolean hasMaxValueIntegerType() { 6895 return this != null && this.maxValue instanceof IntegerType; 6896 } 6897 6898 /** 6899 * @return {@link #maxValue} (The maximum allowed value for the element. The 6900 * value is inclusive. This is allowed for the types date, dateTime, 6901 * instant, time, decimal, integer, and Quantity.) 6902 */ 6903 public PositiveIntType getMaxValuePositiveIntType() throws FHIRException { 6904 if (this.maxValue == null) 6905 this.maxValue = new PositiveIntType(); 6906 if (!(this.maxValue instanceof PositiveIntType)) 6907 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but " 6908 + this.maxValue.getClass().getName() + " was encountered"); 6909 return (PositiveIntType) this.maxValue; 6910 } 6911 6912 public boolean hasMaxValuePositiveIntType() { 6913 return this != null && this.maxValue instanceof PositiveIntType; 6914 } 6915 6916 /** 6917 * @return {@link #maxValue} (The maximum allowed value for the element. The 6918 * value is inclusive. This is allowed for the types date, dateTime, 6919 * instant, time, decimal, integer, and Quantity.) 6920 */ 6921 public UnsignedIntType getMaxValueUnsignedIntType() throws FHIRException { 6922 if (this.maxValue == null) 6923 this.maxValue = new UnsignedIntType(); 6924 if (!(this.maxValue instanceof UnsignedIntType)) 6925 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but " 6926 + this.maxValue.getClass().getName() + " was encountered"); 6927 return (UnsignedIntType) this.maxValue; 6928 } 6929 6930 public boolean hasMaxValueUnsignedIntType() { 6931 return this != null && this.maxValue instanceof UnsignedIntType; 6932 } 6933 6934 /** 6935 * @return {@link #maxValue} (The maximum allowed value for the element. The 6936 * value is inclusive. This is allowed for the types date, dateTime, 6937 * instant, time, decimal, integer, and Quantity.) 6938 */ 6939 public Quantity getMaxValueQuantity() throws FHIRException { 6940 if (this.maxValue == null) 6941 this.maxValue = new Quantity(); 6942 if (!(this.maxValue instanceof Quantity)) 6943 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.maxValue.getClass().getName() 6944 + " was encountered"); 6945 return (Quantity) this.maxValue; 6946 } 6947 6948 public boolean hasMaxValueQuantity() { 6949 return this != null && this.maxValue instanceof Quantity; 6950 } 6951 6952 public boolean hasMaxValue() { 6953 return this.maxValue != null && !this.maxValue.isEmpty(); 6954 } 6955 6956 /** 6957 * @param value {@link #maxValue} (The maximum allowed value for the element. 6958 * The value is inclusive. This is allowed for the types date, 6959 * dateTime, instant, time, decimal, integer, and Quantity.) 6960 */ 6961 public ElementDefinition setMaxValue(Type value) { 6962 if (value != null && !(value instanceof DateType || value instanceof DateTimeType || value instanceof InstantType 6963 || value instanceof TimeType || value instanceof DecimalType || value instanceof IntegerType 6964 || value instanceof PositiveIntType || value instanceof UnsignedIntType || value instanceof Quantity)) 6965 throw new Error("Not the right type for ElementDefinition.maxValue[x]: " + value.fhirType()); 6966 this.maxValue = value; 6967 return this; 6968 } 6969 6970 /** 6971 * @return {@link #maxLength} (Indicates the maximum length in characters that 6972 * is permitted to be present in conformant instances and which is 6973 * expected to be supported by conformant consumers that support the 6974 * element.). This is the underlying object with id, value and 6975 * extensions. The accessor "getMaxLength" gives direct access to the 6976 * value 6977 */ 6978 public IntegerType getMaxLengthElement() { 6979 if (this.maxLength == null) 6980 if (Configuration.errorOnAutoCreate()) 6981 throw new Error("Attempt to auto-create ElementDefinition.maxLength"); 6982 else if (Configuration.doAutoCreate()) 6983 this.maxLength = new IntegerType(); // bb 6984 return this.maxLength; 6985 } 6986 6987 public boolean hasMaxLengthElement() { 6988 return this.maxLength != null && !this.maxLength.isEmpty(); 6989 } 6990 6991 public boolean hasMaxLength() { 6992 return this.maxLength != null && !this.maxLength.isEmpty(); 6993 } 6994 6995 /** 6996 * @param value {@link #maxLength} (Indicates the maximum length in characters 6997 * that is permitted to be present in conformant instances and 6998 * which is expected to be supported by conformant consumers that 6999 * support the element.). This is the underlying object with id, 7000 * value and extensions. The accessor "getMaxLength" gives direct 7001 * access to the value 7002 */ 7003 public ElementDefinition setMaxLengthElement(IntegerType value) { 7004 this.maxLength = value; 7005 return this; 7006 } 7007 7008 /** 7009 * @return Indicates the maximum length in characters that is permitted to be 7010 * present in conformant instances and which is expected to be supported 7011 * by conformant consumers that support the element. 7012 */ 7013 public int getMaxLength() { 7014 return this.maxLength == null || this.maxLength.isEmpty() ? 0 : this.maxLength.getValue(); 7015 } 7016 7017 /** 7018 * @param value Indicates the maximum length in characters that is permitted to 7019 * be present in conformant instances and which is expected to be 7020 * supported by conformant consumers that support the element. 7021 */ 7022 public ElementDefinition setMaxLength(int value) { 7023 if (this.maxLength == null) 7024 this.maxLength = new IntegerType(); 7025 this.maxLength.setValue(value); 7026 return this; 7027 } 7028 7029 /** 7030 * @return {@link #condition} (A reference to an invariant that may make 7031 * additional statements about the cardinality or value in the 7032 * instance.) 7033 */ 7034 public List<IdType> getCondition() { 7035 if (this.condition == null) 7036 this.condition = new ArrayList<IdType>(); 7037 return this.condition; 7038 } 7039 7040 /** 7041 * @return Returns a reference to <code>this</code> for easy method chaining 7042 */ 7043 public ElementDefinition setCondition(List<IdType> theCondition) { 7044 this.condition = theCondition; 7045 return this; 7046 } 7047 7048 public boolean hasCondition() { 7049 if (this.condition == null) 7050 return false; 7051 for (IdType item : this.condition) 7052 if (!item.isEmpty()) 7053 return true; 7054 return false; 7055 } 7056 7057 /** 7058 * @return {@link #condition} (A reference to an invariant that may make 7059 * additional statements about the cardinality or value in the 7060 * instance.) 7061 */ 7062 public IdType addConditionElement() {// 2 7063 IdType t = new IdType(); 7064 if (this.condition == null) 7065 this.condition = new ArrayList<IdType>(); 7066 this.condition.add(t); 7067 return t; 7068 } 7069 7070 /** 7071 * @param value {@link #condition} (A reference to an invariant that may make 7072 * additional statements about the cardinality or value in the 7073 * instance.) 7074 */ 7075 public ElementDefinition addCondition(String value) { // 1 7076 IdType t = new IdType(); 7077 t.setValue(value); 7078 if (this.condition == null) 7079 this.condition = new ArrayList<IdType>(); 7080 this.condition.add(t); 7081 return this; 7082 } 7083 7084 /** 7085 * @param value {@link #condition} (A reference to an invariant that may make 7086 * additional statements about the cardinality or value in the 7087 * instance.) 7088 */ 7089 public boolean hasCondition(String value) { 7090 if (this.condition == null) 7091 return false; 7092 for (IdType v : this.condition) 7093 if (v.getValue().equals(value)) // id 7094 return true; 7095 return false; 7096 } 7097 7098 /** 7099 * @return {@link #constraint} (Formal constraints such as co-occurrence and 7100 * other constraints that can be computationally evaluated within the 7101 * context of the instance.) 7102 */ 7103 public List<ElementDefinitionConstraintComponent> getConstraint() { 7104 if (this.constraint == null) 7105 this.constraint = new ArrayList<ElementDefinitionConstraintComponent>(); 7106 return this.constraint; 7107 } 7108 7109 /** 7110 * @return Returns a reference to <code>this</code> for easy method chaining 7111 */ 7112 public ElementDefinition setConstraint(List<ElementDefinitionConstraintComponent> theConstraint) { 7113 this.constraint = theConstraint; 7114 return this; 7115 } 7116 7117 public boolean hasConstraint() { 7118 if (this.constraint == null) 7119 return false; 7120 for (ElementDefinitionConstraintComponent item : this.constraint) 7121 if (!item.isEmpty()) 7122 return true; 7123 return false; 7124 } 7125 7126 public ElementDefinitionConstraintComponent addConstraint() { // 3 7127 ElementDefinitionConstraintComponent t = new ElementDefinitionConstraintComponent(); 7128 if (this.constraint == null) 7129 this.constraint = new ArrayList<ElementDefinitionConstraintComponent>(); 7130 this.constraint.add(t); 7131 return t; 7132 } 7133 7134 public ElementDefinition addConstraint(ElementDefinitionConstraintComponent t) { // 3 7135 if (t == null) 7136 return this; 7137 if (this.constraint == null) 7138 this.constraint = new ArrayList<ElementDefinitionConstraintComponent>(); 7139 this.constraint.add(t); 7140 return this; 7141 } 7142 7143 /** 7144 * @return The first repetition of repeating field {@link #constraint}, creating 7145 * it if it does not already exist 7146 */ 7147 public ElementDefinitionConstraintComponent getConstraintFirstRep() { 7148 if (getConstraint().isEmpty()) { 7149 addConstraint(); 7150 } 7151 return getConstraint().get(0); 7152 } 7153 7154 /** 7155 * @return {@link #mustSupport} (If true, implementations that produce or 7156 * consume resources SHALL provide "support" for the element in some 7157 * meaningful way. If false, the element may be ignored and not 7158 * supported. If false, whether to populate or use the data element in 7159 * any way is at the discretion of the implementation.). This is the 7160 * underlying object with id, value and extensions. The accessor 7161 * "getMustSupport" gives direct access to the value 7162 */ 7163 public BooleanType getMustSupportElement() { 7164 if (this.mustSupport == null) 7165 if (Configuration.errorOnAutoCreate()) 7166 throw new Error("Attempt to auto-create ElementDefinition.mustSupport"); 7167 else if (Configuration.doAutoCreate()) 7168 this.mustSupport = new BooleanType(); // bb 7169 return this.mustSupport; 7170 } 7171 7172 public boolean hasMustSupportElement() { 7173 return this.mustSupport != null && !this.mustSupport.isEmpty(); 7174 } 7175 7176 public boolean hasMustSupport() { 7177 return this.mustSupport != null && !this.mustSupport.isEmpty(); 7178 } 7179 7180 /** 7181 * @param value {@link #mustSupport} (If true, implementations that produce or 7182 * consume resources SHALL provide "support" for the element in 7183 * some meaningful way. If false, the element may be ignored and 7184 * not supported. If false, whether to populate or use the data 7185 * element in any way is at the discretion of the implementation.). 7186 * This is the underlying object with id, value and extensions. The 7187 * accessor "getMustSupport" gives direct access to the value 7188 */ 7189 public ElementDefinition setMustSupportElement(BooleanType value) { 7190 this.mustSupport = value; 7191 return this; 7192 } 7193 7194 /** 7195 * @return If true, implementations that produce or consume resources SHALL 7196 * provide "support" for the element in some meaningful way. If false, 7197 * the element may be ignored and not supported. If false, whether to 7198 * populate or use the data element in any way is at the discretion of 7199 * the implementation. 7200 */ 7201 public boolean getMustSupport() { 7202 return this.mustSupport == null || this.mustSupport.isEmpty() ? false : this.mustSupport.getValue(); 7203 } 7204 7205 /** 7206 * @param value If true, implementations that produce or consume resources SHALL 7207 * provide "support" for the element in some meaningful way. If 7208 * false, the element may be ignored and not supported. If false, 7209 * whether to populate or use the data element in any way is at the 7210 * discretion of the implementation. 7211 */ 7212 public ElementDefinition setMustSupport(boolean value) { 7213 if (this.mustSupport == null) 7214 this.mustSupport = new BooleanType(); 7215 this.mustSupport.setValue(value); 7216 return this; 7217 } 7218 7219 /** 7220 * @return {@link #isModifier} (If true, the value of this element affects the 7221 * interpretation of the element or resource that contains it, and the 7222 * value of the element cannot be ignored. Typically, this is used for 7223 * status, negation and qualification codes. The effect of this is that 7224 * the element cannot be ignored by systems: they SHALL either recognize 7225 * the element and process it, and/or a pre-determination has been made 7226 * that it is not relevant to their particular system.). This is the 7227 * underlying object with id, value and extensions. The accessor 7228 * "getIsModifier" gives direct access to the value 7229 */ 7230 public BooleanType getIsModifierElement() { 7231 if (this.isModifier == null) 7232 if (Configuration.errorOnAutoCreate()) 7233 throw new Error("Attempt to auto-create ElementDefinition.isModifier"); 7234 else if (Configuration.doAutoCreate()) 7235 this.isModifier = new BooleanType(); // bb 7236 return this.isModifier; 7237 } 7238 7239 public boolean hasIsModifierElement() { 7240 return this.isModifier != null && !this.isModifier.isEmpty(); 7241 } 7242 7243 public boolean hasIsModifier() { 7244 return this.isModifier != null && !this.isModifier.isEmpty(); 7245 } 7246 7247 /** 7248 * @param value {@link #isModifier} (If true, the value of this element affects 7249 * the interpretation of the element or resource that contains it, 7250 * and the value of the element cannot be ignored. Typically, this 7251 * is used for status, negation and qualification codes. The effect 7252 * of this is that the element cannot be ignored by systems: they 7253 * SHALL either recognize the element and process it, and/or a 7254 * pre-determination has been made that it is not relevant to their 7255 * particular system.). This is the underlying object with id, 7256 * value and extensions. The accessor "getIsModifier" gives direct 7257 * access to the value 7258 */ 7259 public ElementDefinition setIsModifierElement(BooleanType value) { 7260 this.isModifier = value; 7261 return this; 7262 } 7263 7264 /** 7265 * @return If true, the value of this element affects the interpretation of the 7266 * element or resource that contains it, and the value of the element 7267 * cannot be ignored. Typically, this is used for status, negation and 7268 * qualification codes. The effect of this is that the element cannot be 7269 * ignored by systems: they SHALL either recognize the element and 7270 * process it, and/or a pre-determination has been made that it is not 7271 * relevant to their particular system. 7272 */ 7273 public boolean getIsModifier() { 7274 return this.isModifier == null || this.isModifier.isEmpty() ? false : this.isModifier.getValue(); 7275 } 7276 7277 /** 7278 * @param value If true, the value of this element affects the interpretation of 7279 * the element or resource that contains it, and the value of the 7280 * element cannot be ignored. Typically, this is used for status, 7281 * negation and qualification codes. The effect of this is that the 7282 * element cannot be ignored by systems: they SHALL either 7283 * recognize the element and process it, and/or a pre-determination 7284 * has been made that it is not relevant to their particular 7285 * system. 7286 */ 7287 public ElementDefinition setIsModifier(boolean value) { 7288 if (this.isModifier == null) 7289 this.isModifier = new BooleanType(); 7290 this.isModifier.setValue(value); 7291 return this; 7292 } 7293 7294 /** 7295 * @return {@link #isModifierReason} (Explains how that element affects the 7296 * interpretation of the resource or element that contains it.). This is 7297 * the underlying object with id, value and extensions. The accessor 7298 * "getIsModifierReason" gives direct access to the value 7299 */ 7300 public StringType getIsModifierReasonElement() { 7301 if (this.isModifierReason == null) 7302 if (Configuration.errorOnAutoCreate()) 7303 throw new Error("Attempt to auto-create ElementDefinition.isModifierReason"); 7304 else if (Configuration.doAutoCreate()) 7305 this.isModifierReason = new StringType(); // bb 7306 return this.isModifierReason; 7307 } 7308 7309 public boolean hasIsModifierReasonElement() { 7310 return this.isModifierReason != null && !this.isModifierReason.isEmpty(); 7311 } 7312 7313 public boolean hasIsModifierReason() { 7314 return this.isModifierReason != null && !this.isModifierReason.isEmpty(); 7315 } 7316 7317 /** 7318 * @param value {@link #isModifierReason} (Explains how that element affects the 7319 * interpretation of the resource or element that contains it.). 7320 * This is the underlying object with id, value and extensions. The 7321 * accessor "getIsModifierReason" gives direct access to the value 7322 */ 7323 public ElementDefinition setIsModifierReasonElement(StringType value) { 7324 this.isModifierReason = value; 7325 return this; 7326 } 7327 7328 /** 7329 * @return Explains how that element affects the interpretation of the resource 7330 * or element that contains it. 7331 */ 7332 public String getIsModifierReason() { 7333 return this.isModifierReason == null ? null : this.isModifierReason.getValue(); 7334 } 7335 7336 /** 7337 * @param value Explains how that element affects the interpretation of the 7338 * resource or element that contains it. 7339 */ 7340 public ElementDefinition setIsModifierReason(String value) { 7341 if (Utilities.noString(value)) 7342 this.isModifierReason = null; 7343 else { 7344 if (this.isModifierReason == null) 7345 this.isModifierReason = new StringType(); 7346 this.isModifierReason.setValue(value); 7347 } 7348 return this; 7349 } 7350 7351 /** 7352 * @return {@link #isSummary} (Whether the element should be included if a 7353 * client requests a search with the parameter _summary=true.). This is 7354 * the underlying object with id, value and extensions. The accessor 7355 * "getIsSummary" gives direct access to the value 7356 */ 7357 public BooleanType getIsSummaryElement() { 7358 if (this.isSummary == null) 7359 if (Configuration.errorOnAutoCreate()) 7360 throw new Error("Attempt to auto-create ElementDefinition.isSummary"); 7361 else if (Configuration.doAutoCreate()) 7362 this.isSummary = new BooleanType(); // bb 7363 return this.isSummary; 7364 } 7365 7366 public boolean hasIsSummaryElement() { 7367 return this.isSummary != null && !this.isSummary.isEmpty(); 7368 } 7369 7370 public boolean hasIsSummary() { 7371 return this.isSummary != null && !this.isSummary.isEmpty(); 7372 } 7373 7374 /** 7375 * @param value {@link #isSummary} (Whether the element should be included if a 7376 * client requests a search with the parameter _summary=true.). 7377 * This is the underlying object with id, value and extensions. The 7378 * accessor "getIsSummary" gives direct access to the value 7379 */ 7380 public ElementDefinition setIsSummaryElement(BooleanType value) { 7381 this.isSummary = value; 7382 return this; 7383 } 7384 7385 /** 7386 * @return Whether the element should be included if a client requests a search 7387 * with the parameter _summary=true. 7388 */ 7389 public boolean getIsSummary() { 7390 return this.isSummary == null || this.isSummary.isEmpty() ? false : this.isSummary.getValue(); 7391 } 7392 7393 /** 7394 * @param value Whether the element should be included if a client requests a 7395 * search with the parameter _summary=true. 7396 */ 7397 public ElementDefinition setIsSummary(boolean value) { 7398 if (this.isSummary == null) 7399 this.isSummary = new BooleanType(); 7400 this.isSummary.setValue(value); 7401 return this; 7402 } 7403 7404 /** 7405 * @return {@link #binding} (Binds to a value set if this element is coded 7406 * (code, Coding, CodeableConcept, Quantity), or the data types (string, 7407 * uri).) 7408 */ 7409 public ElementDefinitionBindingComponent getBinding() { 7410 if (this.binding == null) 7411 if (Configuration.errorOnAutoCreate()) 7412 throw new Error("Attempt to auto-create ElementDefinition.binding"); 7413 else if (Configuration.doAutoCreate()) 7414 this.binding = new ElementDefinitionBindingComponent(); // cc 7415 return this.binding; 7416 } 7417 7418 public boolean hasBinding() { 7419 return this.binding != null && !this.binding.isEmpty(); 7420 } 7421 7422 /** 7423 * @param value {@link #binding} (Binds to a value set if this element is coded 7424 * (code, Coding, CodeableConcept, Quantity), or the data types 7425 * (string, uri).) 7426 */ 7427 public ElementDefinition setBinding(ElementDefinitionBindingComponent value) { 7428 this.binding = value; 7429 return this; 7430 } 7431 7432 /** 7433 * @return {@link #mapping} (Identifies a concept from an external specification 7434 * that roughly corresponds to this element.) 7435 */ 7436 public List<ElementDefinitionMappingComponent> getMapping() { 7437 if (this.mapping == null) 7438 this.mapping = new ArrayList<ElementDefinitionMappingComponent>(); 7439 return this.mapping; 7440 } 7441 7442 /** 7443 * @return Returns a reference to <code>this</code> for easy method chaining 7444 */ 7445 public ElementDefinition setMapping(List<ElementDefinitionMappingComponent> theMapping) { 7446 this.mapping = theMapping; 7447 return this; 7448 } 7449 7450 public boolean hasMapping() { 7451 if (this.mapping == null) 7452 return false; 7453 for (ElementDefinitionMappingComponent item : this.mapping) 7454 if (!item.isEmpty()) 7455 return true; 7456 return false; 7457 } 7458 7459 public ElementDefinitionMappingComponent addMapping() { // 3 7460 ElementDefinitionMappingComponent t = new ElementDefinitionMappingComponent(); 7461 if (this.mapping == null) 7462 this.mapping = new ArrayList<ElementDefinitionMappingComponent>(); 7463 this.mapping.add(t); 7464 return t; 7465 } 7466 7467 public ElementDefinition addMapping(ElementDefinitionMappingComponent t) { // 3 7468 if (t == null) 7469 return this; 7470 if (this.mapping == null) 7471 this.mapping = new ArrayList<ElementDefinitionMappingComponent>(); 7472 this.mapping.add(t); 7473 return this; 7474 } 7475 7476 /** 7477 * @return The first repetition of repeating field {@link #mapping}, creating it 7478 * if it does not already exist 7479 */ 7480 public ElementDefinitionMappingComponent getMappingFirstRep() { 7481 if (getMapping().isEmpty()) { 7482 addMapping(); 7483 } 7484 return getMapping().get(0); 7485 } 7486 7487 protected void listChildren(List<Property> children) { 7488 super.listChildren(children); 7489 children.add(new Property("path", "string", 7490 "The path identifies the element and is expressed as a \".\"-separated list of ancestor elements, beginning with the name of the resource or extension.", 7491 0, 1, path)); 7492 children.add(new Property("representation", "code", 7493 "Codes that define how this element is represented in instances, when the deviation varies from the normal case.", 7494 0, java.lang.Integer.MAX_VALUE, representation)); 7495 children.add(new Property("sliceName", "string", 7496 "The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element.", 7497 0, 1, sliceName)); 7498 children.add(new Property("sliceIsConstraining", "boolean", 7499 "If true, indicates that this slice definition is constraining a slice definition with the same name in an inherited profile. If false, the slice is not overriding any slice in an inherited profile. If missing, the slice might or might not be overriding a slice in an inherited profile, depending on the sliceName.", 7500 0, 1, sliceIsConstraining)); 7501 children.add(new Property("label", "string", 7502 "A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form.", 7503 0, 1, label)); 7504 children.add( 7505 new Property("code", "Coding", "A code that has the same meaning as the element in a particular terminology.", 7506 0, java.lang.Integer.MAX_VALUE, code)); 7507 children.add(new Property("slicing", "", 7508 "Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set).", 7509 0, 1, slicing)); 7510 children.add(new Property("short", "string", 7511 "A concise description of what this element means (e.g. for use in autogenerated summaries).", 0, 1, short_)); 7512 children.add(new Property("definition", "markdown", 7513 "Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource. (Note: The text you are reading is specified in ElementDefinition.definition).", 7514 0, 1, definition)); 7515 children.add(new Property("comment", "markdown", 7516 "Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. (Note: The text you are reading is specified in ElementDefinition.comment).", 7517 0, 1, comment)); 7518 children.add(new Property("requirements", "markdown", 7519 "This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element.", 7520 0, 1, requirements)); 7521 children 7522 .add(new Property("alias", "string", "Identifies additional names by which this element might also be known.", 7523 0, java.lang.Integer.MAX_VALUE, alias)); 7524 children.add(new Property("min", "unsignedInt", 7525 "The minimum number of times this element SHALL appear in the instance.", 0, 1, min)); 7526 children.add(new Property("max", "string", 7527 "The maximum number of times this element is permitted to appear in the instance.", 0, 1, max)); 7528 children.add(new Property("base", "", 7529 "Information about the base definition of the element, provided to make it unnecessary for tools to trace the deviation of the element through the derived and related profiles. When the element definition is not the original definition of an element - i.g. either in a constraint on another type, or for elements from a super type in a snap shot - then the information in provided in the element definition may be different to the base definition. On the original definition of the element, it will be same.", 7530 0, 1, base)); 7531 children.add(new Property("contentReference", "uri", 7532 "Identifies an element defined elsewhere in the definition whose content rules should be applied to the current element. ContentReferences bring across all the rules that are in the ElementDefinition for the element, including definitions, cardinality constraints, bindings, invariants etc.", 7533 0, 1, contentReference)); 7534 children 7535 .add(new Property("type", "", "The data type or resource that the value of this element is permitted to be.", 0, 7536 java.lang.Integer.MAX_VALUE, type)); 7537 children.add(new Property("defaultValue[x]", "*", 7538 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7539 0, 1, defaultValue)); 7540 children.add(new Property("meaningWhenMissing", "markdown", 7541 "The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing').", 7542 0, 1, meaningWhenMissing)); 7543 children.add(new Property("orderMeaning", "string", 7544 "If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning.", 7545 0, 1, orderMeaning)); 7546 children.add(new Property("fixed[x]", "*", 7547 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7548 0, 1, fixed)); 7549 children.add(new Property("pattern[x]", "*", 7550 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 7551 0, 1, pattern)); 7552 children.add(new Property("example", "", 7553 "A sample value for this element demonstrating the type of information that would typically be found in the element.", 7554 0, java.lang.Integer.MAX_VALUE, example)); 7555 children.add(new Property("minValue[x]", 7556 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 7557 "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 7558 0, 1, minValue)); 7559 children.add(new Property("maxValue[x]", 7560 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 7561 "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 7562 0, 1, maxValue)); 7563 children.add(new Property("maxLength", "integer", 7564 "Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element.", 7565 0, 1, maxLength)); 7566 children.add(new Property("condition", "id", 7567 "A reference to an invariant that may make additional statements about the cardinality or value in the instance.", 7568 0, java.lang.Integer.MAX_VALUE, condition)); 7569 children.add(new Property("constraint", "", 7570 "Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance.", 7571 0, java.lang.Integer.MAX_VALUE, constraint)); 7572 children.add(new Property("mustSupport", "boolean", 7573 "If true, implementations that produce or consume resources SHALL provide \"support\" for the element in some meaningful way. If false, the element may be ignored and not supported. If false, whether to populate or use the data element in any way is at the discretion of the implementation.", 7574 0, 1, mustSupport)); 7575 children.add(new Property("isModifier", "boolean", 7576 "If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system.", 7577 0, 1, isModifier)); 7578 children.add(new Property("isModifierReason", "string", 7579 "Explains how that element affects the interpretation of the resource or element that contains it.", 0, 1, 7580 isModifierReason)); 7581 children.add(new Property("isSummary", "boolean", 7582 "Whether the element should be included if a client requests a search with the parameter _summary=true.", 0, 1, 7583 isSummary)); 7584 children.add(new Property("binding", "", 7585 "Binds to a value set if this element is coded (code, Coding, CodeableConcept, Quantity), or the data types (string, uri).", 7586 0, 1, binding)); 7587 children.add(new Property("mapping", "", 7588 "Identifies a concept from an external specification that roughly corresponds to this element.", 0, 7589 java.lang.Integer.MAX_VALUE, mapping)); 7590 } 7591 7592 @Override 7593 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7594 switch (_hash) { 7595 case 3433509: 7596 /* path */ return new Property("path", "string", 7597 "The path identifies the element and is expressed as a \".\"-separated list of ancestor elements, beginning with the name of the resource or extension.", 7598 0, 1, path); 7599 case -671065907: 7600 /* representation */ return new Property("representation", "code", 7601 "Codes that define how this element is represented in instances, when the deviation varies from the normal case.", 7602 0, java.lang.Integer.MAX_VALUE, representation); 7603 case -825289923: 7604 /* sliceName */ return new Property("sliceName", "string", 7605 "The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element.", 7606 0, 1, sliceName); 7607 case 333040519: 7608 /* sliceIsConstraining */ return new Property("sliceIsConstraining", "boolean", 7609 "If true, indicates that this slice definition is constraining a slice definition with the same name in an inherited profile. If false, the slice is not overriding any slice in an inherited profile. If missing, the slice might or might not be overriding a slice in an inherited profile, depending on the sliceName.", 7610 0, 1, sliceIsConstraining); 7611 case 102727412: 7612 /* label */ return new Property("label", "string", 7613 "A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form.", 7614 0, 1, label); 7615 case 3059181: 7616 /* code */ return new Property("code", "Coding", 7617 "A code that has the same meaning as the element in a particular terminology.", 0, 7618 java.lang.Integer.MAX_VALUE, code); 7619 case -2119287345: 7620 /* slicing */ return new Property("slicing", "", 7621 "Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set).", 7622 0, 1, slicing); 7623 case 109413500: 7624 /* short */ return new Property("short", "string", 7625 "A concise description of what this element means (e.g. for use in autogenerated summaries).", 0, 1, short_); 7626 case -1014418093: 7627 /* definition */ return new Property("definition", "markdown", 7628 "Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource. (Note: The text you are reading is specified in ElementDefinition.definition).", 7629 0, 1, definition); 7630 case 950398559: 7631 /* comment */ return new Property("comment", "markdown", 7632 "Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. (Note: The text you are reading is specified in ElementDefinition.comment).", 7633 0, 1, comment); 7634 case -1619874672: 7635 /* requirements */ return new Property("requirements", "markdown", 7636 "This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element.", 7637 0, 1, requirements); 7638 case 92902992: 7639 /* alias */ return new Property("alias", "string", 7640 "Identifies additional names by which this element might also be known.", 0, java.lang.Integer.MAX_VALUE, 7641 alias); 7642 case 108114: 7643 /* min */ return new Property("min", "unsignedInt", 7644 "The minimum number of times this element SHALL appear in the instance.", 0, 1, min); 7645 case 107876: 7646 /* max */ return new Property("max", "string", 7647 "The maximum number of times this element is permitted to appear in the instance.", 0, 1, max); 7648 case 3016401: 7649 /* base */ return new Property("base", "", 7650 "Information about the base definition of the element, provided to make it unnecessary for tools to trace the deviation of the element through the derived and related profiles. When the element definition is not the original definition of an element - i.g. either in a constraint on another type, or for elements from a super type in a snap shot - then the information in provided in the element definition may be different to the base definition. On the original definition of the element, it will be same.", 7651 0, 1, base); 7652 case 1193747154: 7653 /* contentReference */ return new Property("contentReference", "uri", 7654 "Identifies an element defined elsewhere in the definition whose content rules should be applied to the current element. ContentReferences bring across all the rules that are in the ElementDefinition for the element, including definitions, cardinality constraints, bindings, invariants etc.", 7655 0, 1, contentReference); 7656 case 3575610: 7657 /* type */ return new Property("type", "", 7658 "The data type or resource that the value of this element is permitted to be.", 0, 7659 java.lang.Integer.MAX_VALUE, type); 7660 case 587922128: 7661 /* defaultValue[x] */ return new Property("defaultValue[x]", "*", 7662 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7663 0, 1, defaultValue); 7664 case -659125328: 7665 /* defaultValue */ return new Property("defaultValue[x]", "*", 7666 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7667 0, 1, defaultValue); 7668 case 1470297600: 7669 /* defaultValueBase64Binary */ return new Property("defaultValue[x]", "*", 7670 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7671 0, 1, defaultValue); 7672 case 600437336: 7673 /* defaultValueBoolean */ return new Property("defaultValue[x]", "*", 7674 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7675 0, 1, defaultValue); 7676 case 264593188: 7677 /* defaultValueCanonical */ return new Property("defaultValue[x]", "*", 7678 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7679 0, 1, defaultValue); 7680 case 1044993469: 7681 /* defaultValueCode */ return new Property("defaultValue[x]", "*", 7682 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7683 0, 1, defaultValue); 7684 case 1045010302: 7685 /* defaultValueDate */ return new Property("defaultValue[x]", "*", 7686 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7687 0, 1, defaultValue); 7688 case 1220374379: 7689 /* defaultValueDateTime */ return new Property("defaultValue[x]", "*", 7690 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7691 0, 1, defaultValue); 7692 case 2077989249: 7693 /* defaultValueDecimal */ return new Property("defaultValue[x]", "*", 7694 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7695 0, 1, defaultValue); 7696 case -2059245333: 7697 /* defaultValueId */ return new Property("defaultValue[x]", "*", 7698 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7699 0, 1, defaultValue); 7700 case -1801671663: 7701 /* defaultValueInstant */ return new Property("defaultValue[x]", "*", 7702 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7703 0, 1, defaultValue); 7704 case -1801189522: 7705 /* defaultValueInteger */ return new Property("defaultValue[x]", "*", 7706 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7707 0, 1, defaultValue); 7708 case -325436225: 7709 /* defaultValueMarkdown */ return new Property("defaultValue[x]", "*", 7710 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7711 0, 1, defaultValue); 7712 case 587910138: 7713 /* defaultValueOid */ return new Property("defaultValue[x]", "*", 7714 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7715 0, 1, defaultValue); 7716 case -737344154: 7717 /* defaultValuePositiveInt */ return new Property("defaultValue[x]", "*", 7718 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7719 0, 1, defaultValue); 7720 case -320515103: 7721 /* defaultValueString */ return new Property("defaultValue[x]", "*", 7722 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7723 0, 1, defaultValue); 7724 case 1045494429: 7725 /* defaultValueTime */ return new Property("defaultValue[x]", "*", 7726 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7727 0, 1, defaultValue); 7728 case 539117290: 7729 /* defaultValueUnsignedInt */ return new Property("defaultValue[x]", "*", 7730 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7731 0, 1, defaultValue); 7732 case 587916188: 7733 /* defaultValueUri */ return new Property("defaultValue[x]", "*", 7734 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7735 0, 1, defaultValue); 7736 case 587916191: 7737 /* defaultValueUrl */ return new Property("defaultValue[x]", "*", 7738 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7739 0, 1, defaultValue); 7740 case 1045535627: 7741 /* defaultValueUuid */ return new Property("defaultValue[x]", "*", 7742 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7743 0, 1, defaultValue); 7744 case -611966428: 7745 /* defaultValueAddress */ return new Property("defaultValue[x]", "*", 7746 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7747 0, 1, defaultValue); 7748 case -1851689217: 7749 /* defaultValueAnnotation */ return new Property("defaultValue[x]", "*", 7750 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7751 0, 1, defaultValue); 7752 case 2034820339: 7753 /* defaultValueAttachment */ return new Property("defaultValue[x]", "*", 7754 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7755 0, 1, defaultValue); 7756 case -410434095: 7757 /* defaultValueCodeableConcept */ return new Property("defaultValue[x]", "*", 7758 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7759 0, 1, defaultValue); 7760 case -783616198: 7761 /* defaultValueCoding */ return new Property("defaultValue[x]", "*", 7762 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7763 0, 1, defaultValue); 7764 case -344740576: 7765 /* defaultValueContactPoint */ return new Property("defaultValue[x]", "*", 7766 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7767 0, 1, defaultValue); 7768 case -975393912: 7769 /* defaultValueHumanName */ return new Property("defaultValue[x]", "*", 7770 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7771 0, 1, defaultValue); 7772 case -1915078535: 7773 /* defaultValueIdentifier */ return new Property("defaultValue[x]", "*", 7774 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7775 0, 1, defaultValue); 7776 case -420255343: 7777 /* defaultValuePeriod */ return new Property("defaultValue[x]", "*", 7778 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7779 0, 1, defaultValue); 7780 case -1857379237: 7781 /* defaultValueQuantity */ return new Property("defaultValue[x]", "*", 7782 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7783 0, 1, defaultValue); 7784 case -1951495315: 7785 /* defaultValueRange */ return new Property("defaultValue[x]", "*", 7786 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7787 0, 1, defaultValue); 7788 case -1951489477: 7789 /* defaultValueRatio */ return new Property("defaultValue[x]", "*", 7790 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7791 0, 1, defaultValue); 7792 case -1488914053: 7793 /* defaultValueReference */ return new Property("defaultValue[x]", "*", 7794 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7795 0, 1, defaultValue); 7796 case -449641228: 7797 /* defaultValueSampledData */ return new Property("defaultValue[x]", "*", 7798 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7799 0, 1, defaultValue); 7800 case 509825768: 7801 /* defaultValueSignature */ return new Property("defaultValue[x]", "*", 7802 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7803 0, 1, defaultValue); 7804 case -302193638: 7805 /* defaultValueTiming */ return new Property("defaultValue[x]", "*", 7806 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7807 0, 1, defaultValue); 7808 case -754548089: 7809 /* defaultValueDosage */ return new Property("defaultValue[x]", "*", 7810 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7811 0, 1, defaultValue); 7812 case 1857257103: 7813 /* meaningWhenMissing */ return new Property("meaningWhenMissing", "markdown", 7814 "The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing').", 7815 0, 1, meaningWhenMissing); 7816 case 1828196047: 7817 /* orderMeaning */ return new Property("orderMeaning", "string", 7818 "If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning.", 7819 0, 1, orderMeaning); 7820 case -391522164: 7821 /* fixed[x] */ return new Property("fixed[x]", "*", 7822 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7823 0, 1, fixed); 7824 case 97445748: 7825 /* fixed */ return new Property("fixed[x]", "*", 7826 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7827 0, 1, fixed); 7828 case -799290428: 7829 /* fixedBase64Binary */ return new Property("fixed[x]", "*", 7830 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7831 0, 1, fixed); 7832 case 520851988: 7833 /* fixedBoolean */ return new Property("fixed[x]", "*", 7834 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7835 0, 1, fixed); 7836 case 1092485088: 7837 /* fixedCanonical */ return new Property("fixed[x]", "*", 7838 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7839 0, 1, fixed); 7840 case 746991489: 7841 /* fixedCode */ return new Property("fixed[x]", "*", 7842 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7843 0, 1, fixed); 7844 case 747008322: 7845 /* fixedDate */ return new Property("fixed[x]", "*", 7846 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7847 0, 1, fixed); 7848 case -1246771409: 7849 /* fixedDateTime */ return new Property("fixed[x]", "*", 7850 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7851 0, 1, fixed); 7852 case 1998403901: 7853 /* fixedDecimal */ return new Property("fixed[x]", "*", 7854 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7855 0, 1, fixed); 7856 case -843914321: 7857 /* fixedId */ return new Property("fixed[x]", "*", 7858 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7859 0, 1, fixed); 7860 case -1881257011: 7861 /* fixedInstant */ return new Property("fixed[x]", "*", 7862 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7863 0, 1, fixed); 7864 case -1880774870: 7865 /* fixedInteger */ return new Property("fixed[x]", "*", 7866 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7867 0, 1, fixed); 7868 case 1502385283: 7869 /* fixedMarkdown */ return new Property("fixed[x]", "*", 7870 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7871 0, 1, fixed); 7872 case -391534154: 7873 /* fixedOid */ return new Property("fixed[x]", "*", 7874 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7875 0, 1, fixed); 7876 case 297821986: 7877 /* fixedPositiveInt */ return new Property("fixed[x]", "*", 7878 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7879 0, 1, fixed); 7880 case 1062390949: 7881 /* fixedString */ return new Property("fixed[x]", "*", 7882 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7883 0, 1, fixed); 7884 case 747492449: 7885 /* fixedTime */ return new Property("fixed[x]", "*", 7886 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7887 0, 1, fixed); 7888 case 1574283430: 7889 /* fixedUnsignedInt */ return new Property("fixed[x]", "*", 7890 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7891 0, 1, fixed); 7892 case -391528104: 7893 /* fixedUri */ return new Property("fixed[x]", "*", 7894 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7895 0, 1, fixed); 7896 case -391528101: 7897 /* fixedUrl */ return new Property("fixed[x]", "*", 7898 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7899 0, 1, fixed); 7900 case 747533647: 7901 /* fixedUuid */ return new Property("fixed[x]", "*", 7902 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7903 0, 1, fixed); 7904 case -691551776: 7905 /* fixedAddress */ return new Property("fixed[x]", "*", 7906 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7907 0, 1, fixed); 7908 case -1956844093: 7909 /* fixedAnnotation */ return new Property("fixed[x]", "*", 7910 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7911 0, 1, fixed); 7912 case 1929665463: 7913 /* fixedAttachment */ return new Property("fixed[x]", "*", 7914 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7915 0, 1, fixed); 7916 case 1962764685: 7917 /* fixedCodeableConcept */ return new Property("fixed[x]", "*", 7918 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7919 0, 1, fixed); 7920 case 599289854: 7921 /* fixedCoding */ return new Property("fixed[x]", "*", 7922 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7923 0, 1, fixed); 7924 case 1680638692: 7925 /* fixedContactPoint */ return new Property("fixed[x]", "*", 7926 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7927 0, 1, fixed); 7928 case -147502012: 7929 /* fixedHumanName */ return new Property("fixed[x]", "*", 7930 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7931 0, 1, fixed); 7932 case -2020233411: 7933 /* fixedIdentifier */ return new Property("fixed[x]", "*", 7934 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7935 0, 1, fixed); 7936 case 962650709: 7937 /* fixedPeriod */ return new Property("fixed[x]", "*", 7938 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7939 0, 1, fixed); 7940 case -29557729: 7941 /* fixedQuantity */ return new Property("fixed[x]", "*", 7942 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7943 0, 1, fixed); 7944 case 1695345193: 7945 /* fixedRange */ return new Property("fixed[x]", "*", 7946 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7947 0, 1, fixed); 7948 case 1695351031: 7949 /* fixedRatio */ return new Property("fixed[x]", "*", 7950 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7951 0, 1, fixed); 7952 case -661022153: 7953 /* fixedReference */ return new Property("fixed[x]", "*", 7954 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7955 0, 1, fixed); 7956 case 585524912: 7957 /* fixedSampledData */ return new Property("fixed[x]", "*", 7958 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7959 0, 1, fixed); 7960 case 1337717668: 7961 /* fixedSignature */ return new Property("fixed[x]", "*", 7962 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7963 0, 1, fixed); 7964 case 1080712414: 7965 /* fixedTiming */ return new Property("fixed[x]", "*", 7966 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7967 0, 1, fixed); 7968 case 628357963: 7969 /* fixedDosage */ return new Property("fixed[x]", "*", 7970 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7971 0, 1, fixed); 7972 case -885125392: 7973 /* pattern[x] */ return new Property("pattern[x]", "*", 7974 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 7975 0, 1, pattern); 7976 case -791090288: 7977 /* pattern */ return new Property("pattern[x]", "*", 7978 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 7979 0, 1, pattern); 7980 case 2127857120: 7981 /* patternBase64Binary */ return new Property("pattern[x]", "*", 7982 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 7983 0, 1, pattern); 7984 case -1776945544: 7985 /* patternBoolean */ return new Property("pattern[x]", "*", 7986 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 7987 0, 1, pattern); 7988 case 522246980: 7989 /* patternCanonical */ return new Property("pattern[x]", "*", 7990 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 7991 0, 1, pattern); 7992 case -1669806691: 7993 /* patternCode */ return new Property("pattern[x]", "*", 7994 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 7995 0, 1, pattern); 7996 case -1669789858: 7997 /* patternDate */ return new Property("pattern[x]", "*", 7998 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 7999 0, 1, pattern); 8000 case 535949131: 8001 /* patternDateTime */ return new Property("pattern[x]", "*", 8002 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8003 0, 1, pattern); 8004 case -299393631: 8005 /* patternDecimal */ return new Property("pattern[x]", "*", 8006 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8007 0, 1, pattern); 8008 case -28553013: 8009 /* patternId */ return new Property("pattern[x]", "*", 8010 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8011 0, 1, pattern); 8012 case 115912753: 8013 /* patternInstant */ return new Property("pattern[x]", "*", 8014 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8015 0, 1, pattern); 8016 case 116394894: 8017 /* patternInteger */ return new Property("pattern[x]", "*", 8018 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8019 0, 1, pattern); 8020 case -1009861473: 8021 /* patternMarkdown */ return new Property("pattern[x]", "*", 8022 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8023 0, 1, pattern); 8024 case -885137382: 8025 /* patternOid */ return new Property("pattern[x]", "*", 8026 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8027 0, 1, pattern); 8028 case 2054814086: 8029 /* patternPositiveInt */ return new Property("pattern[x]", "*", 8030 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8031 0, 1, pattern); 8032 case 2096647105: 8033 /* patternString */ return new Property("pattern[x]", "*", 8034 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8035 0, 1, pattern); 8036 case -1669305731: 8037 /* patternTime */ return new Property("pattern[x]", "*", 8038 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8039 0, 1, pattern); 8040 case -963691766: 8041 /* patternUnsignedInt */ return new Property("pattern[x]", "*", 8042 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8043 0, 1, pattern); 8044 case -885131332: 8045 /* patternUri */ return new Property("pattern[x]", "*", 8046 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8047 0, 1, pattern); 8048 case -885131329: 8049 /* patternUrl */ return new Property("pattern[x]", "*", 8050 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8051 0, 1, pattern); 8052 case -1669264533: 8053 /* patternUuid */ return new Property("pattern[x]", "*", 8054 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8055 0, 1, pattern); 8056 case 1305617988: 8057 /* patternAddress */ return new Property("pattern[x]", "*", 8058 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8059 0, 1, pattern); 8060 case 1840611039: 8061 /* patternAnnotation */ return new Property("pattern[x]", "*", 8062 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8063 0, 1, pattern); 8064 case 1432153299: 8065 /* patternAttachment */ return new Property("pattern[x]", "*", 8066 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8067 0, 1, pattern); 8068 case -400610831: 8069 /* patternCodeableConcept */ return new Property("pattern[x]", "*", 8070 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8071 0, 1, pattern); 8072 case 1633546010: 8073 /* patternCoding */ return new Property("pattern[x]", "*", 8074 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8075 0, 1, pattern); 8076 case 312818944: 8077 /* patternContactPoint */ return new Property("pattern[x]", "*", 8078 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8079 0, 1, pattern); 8080 case -717740120: 8081 /* patternHumanName */ return new Property("pattern[x]", "*", 8082 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8083 0, 1, pattern); 8084 case 1777221721: 8085 /* patternIdentifier */ return new Property("pattern[x]", "*", 8086 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8087 0, 1, pattern); 8088 case 1996906865: 8089 /* patternPeriod */ return new Property("pattern[x]", "*", 8090 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8091 0, 1, pattern); 8092 case 1753162811: 8093 /* patternQuantity */ return new Property("pattern[x]", "*", 8094 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8095 0, 1, pattern); 8096 case -210954355: 8097 /* patternRange */ return new Property("pattern[x]", "*", 8098 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8099 0, 1, pattern); 8100 case -210948517: 8101 /* patternRatio */ return new Property("pattern[x]", "*", 8102 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8103 0, 1, pattern); 8104 case -1231260261: 8105 /* patternReference */ return new Property("pattern[x]", "*", 8106 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8107 0, 1, pattern); 8108 case -1952450284: 8109 /* patternSampledData */ return new Property("pattern[x]", "*", 8110 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8111 0, 1, pattern); 8112 case 767479560: 8113 /* patternSignature */ return new Property("pattern[x]", "*", 8114 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8115 0, 1, pattern); 8116 case 2114968570: 8117 /* patternTiming */ return new Property("pattern[x]", "*", 8118 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8119 0, 1, pattern); 8120 case 1662614119: 8121 /* patternDosage */ return new Property("pattern[x]", "*", 8122 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8123 0, 1, pattern); 8124 case -1322970774: 8125 /* example */ return new Property("example", "", 8126 "A sample value for this element demonstrating the type of information that would typically be found in the element.", 8127 0, java.lang.Integer.MAX_VALUE, example); 8128 case -55301663: 8129 /* minValue[x] */ return new Property("minValue[x]", 8130 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8131 "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8132 0, 1, minValue); 8133 case -1376969153: 8134 /* minValue */ return new Property("minValue[x]", 8135 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8136 "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8137 0, 1, minValue); 8138 case -1715058035: 8139 /* minValueDate */ return new Property("minValue[x]", 8140 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8141 "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8142 0, 1, minValue); 8143 case 1635517178: 8144 /* minValueDateTime */ return new Property("minValue[x]", 8145 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8146 "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8147 0, 1, minValue); 8148 case 151382690: 8149 /* minValueInstant */ return new Property("minValue[x]", 8150 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8151 "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8152 0, 1, minValue); 8153 case -1714573908: 8154 /* minValueTime */ return new Property("minValue[x]", 8155 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8156 "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8157 0, 1, minValue); 8158 case -263923694: 8159 /* minValueDecimal */ return new Property("minValue[x]", 8160 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8161 "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8162 0, 1, minValue); 8163 case 151864831: 8164 /* minValueInteger */ return new Property("minValue[x]", 8165 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8166 "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8167 0, 1, minValue); 8168 case 1570935671: 8169 /* minValuePositiveInt */ return new Property("minValue[x]", 8170 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8171 "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8172 0, 1, minValue); 8173 case -1447570181: 8174 /* minValueUnsignedInt */ return new Property("minValue[x]", 8175 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8176 "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8177 0, 1, minValue); 8178 case -1442236438: 8179 /* minValueQuantity */ return new Property("minValue[x]", 8180 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8181 "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8182 0, 1, minValue); 8183 case 622130931: 8184 /* maxValue[x] */ return new Property("maxValue[x]", 8185 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8186 "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8187 0, 1, maxValue); 8188 case 399227501: 8189 /* maxValue */ return new Property("maxValue[x]", 8190 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8191 "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8192 0, 1, maxValue); 8193 case 2105483195: 8194 /* maxValueDate */ return new Property("maxValue[x]", 8195 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8196 "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8197 0, 1, maxValue); 8198 case 1699385640: 8199 /* maxValueDateTime */ return new Property("maxValue[x]", 8200 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8201 "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8202 0, 1, maxValue); 8203 case 1261821620: 8204 /* maxValueInstant */ return new Property("maxValue[x]", 8205 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8206 "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8207 0, 1, maxValue); 8208 case 2105967322: 8209 /* maxValueTime */ return new Property("maxValue[x]", 8210 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8211 "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8212 0, 1, maxValue); 8213 case 846515236: 8214 /* maxValueDecimal */ return new Property("maxValue[x]", 8215 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8216 "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8217 0, 1, maxValue); 8218 case 1262303761: 8219 /* maxValueInteger */ return new Property("maxValue[x]", 8220 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8221 "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8222 0, 1, maxValue); 8223 case 1605774985: 8224 /* maxValuePositiveInt */ return new Property("maxValue[x]", 8225 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8226 "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8227 0, 1, maxValue); 8228 case -1412730867: 8229 /* maxValueUnsignedInt */ return new Property("maxValue[x]", 8230 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8231 "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8232 0, 1, maxValue); 8233 case -1378367976: 8234 /* maxValueQuantity */ return new Property("maxValue[x]", 8235 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8236 "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8237 0, 1, maxValue); 8238 case -791400086: 8239 /* maxLength */ return new Property("maxLength", "integer", 8240 "Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element.", 8241 0, 1, maxLength); 8242 case -861311717: 8243 /* condition */ return new Property("condition", "id", 8244 "A reference to an invariant that may make additional statements about the cardinality or value in the instance.", 8245 0, java.lang.Integer.MAX_VALUE, condition); 8246 case -190376483: 8247 /* constraint */ return new Property("constraint", "", 8248 "Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance.", 8249 0, java.lang.Integer.MAX_VALUE, constraint); 8250 case -1402857082: 8251 /* mustSupport */ return new Property("mustSupport", "boolean", 8252 "If true, implementations that produce or consume resources SHALL provide \"support\" for the element in some meaningful way. If false, the element may be ignored and not supported. If false, whether to populate or use the data element in any way is at the discretion of the implementation.", 8253 0, 1, mustSupport); 8254 case -1408783839: 8255 /* isModifier */ return new Property("isModifier", "boolean", 8256 "If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system.", 8257 0, 1, isModifier); 8258 case -1854387259: 8259 /* isModifierReason */ return new Property("isModifierReason", "string", 8260 "Explains how that element affects the interpretation of the resource or element that contains it.", 0, 1, 8261 isModifierReason); 8262 case 1857548060: 8263 /* isSummary */ return new Property("isSummary", "boolean", 8264 "Whether the element should be included if a client requests a search with the parameter _summary=true.", 0, 8265 1, isSummary); 8266 case -108220795: 8267 /* binding */ return new Property("binding", "", 8268 "Binds to a value set if this element is coded (code, Coding, CodeableConcept, Quantity), or the data types (string, uri).", 8269 0, 1, binding); 8270 case 837556430: 8271 /* mapping */ return new Property("mapping", "", 8272 "Identifies a concept from an external specification that roughly corresponds to this element.", 0, 8273 java.lang.Integer.MAX_VALUE, mapping); 8274 default: 8275 return super.getNamedProperty(_hash, _name, _checkValid); 8276 } 8277 8278 } 8279 8280 @Override 8281 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8282 switch (hash) { 8283 case 3433509: 8284 /* path */ return this.path == null ? new Base[0] : new Base[] { this.path }; // StringType 8285 case -671065907: 8286 /* representation */ return this.representation == null ? new Base[0] 8287 : this.representation.toArray(new Base[this.representation.size()]); // Enumeration<PropertyRepresentation> 8288 case -825289923: 8289 /* sliceName */ return this.sliceName == null ? new Base[0] : new Base[] { this.sliceName }; // StringType 8290 case 333040519: 8291 /* sliceIsConstraining */ return this.sliceIsConstraining == null ? new Base[0] 8292 : new Base[] { this.sliceIsConstraining }; // BooleanType 8293 case 102727412: 8294 /* label */ return this.label == null ? new Base[0] : new Base[] { this.label }; // StringType 8295 case 3059181: 8296 /* code */ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // Coding 8297 case -2119287345: 8298 /* slicing */ return this.slicing == null ? new Base[0] : new Base[] { this.slicing }; // ElementDefinitionSlicingComponent 8299 case 109413500: 8300 /* short */ return this.short_ == null ? new Base[0] : new Base[] { this.short_ }; // StringType 8301 case -1014418093: 8302 /* definition */ return this.definition == null ? new Base[0] : new Base[] { this.definition }; // MarkdownType 8303 case 950398559: 8304 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // MarkdownType 8305 case -1619874672: 8306 /* requirements */ return this.requirements == null ? new Base[0] : new Base[] { this.requirements }; // MarkdownType 8307 case 92902992: 8308 /* alias */ return this.alias == null ? new Base[0] : this.alias.toArray(new Base[this.alias.size()]); // StringType 8309 case 108114: 8310 /* min */ return this.min == null ? new Base[0] : new Base[] { this.min }; // UnsignedIntType 8311 case 107876: 8312 /* max */ return this.max == null ? new Base[0] : new Base[] { this.max }; // StringType 8313 case 3016401: 8314 /* base */ return this.base == null ? new Base[0] : new Base[] { this.base }; // ElementDefinitionBaseComponent 8315 case 1193747154: 8316 /* contentReference */ return this.contentReference == null ? new Base[0] : new Base[] { this.contentReference }; // UriType 8317 case 3575610: 8318 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // TypeRefComponent 8319 case -659125328: 8320 /* defaultValue */ return this.defaultValue == null ? new Base[0] : new Base[] { this.defaultValue }; // org.hl7.fhir.r4.model.Type 8321 case 1857257103: 8322 /* meaningWhenMissing */ return this.meaningWhenMissing == null ? new Base[0] 8323 : new Base[] { this.meaningWhenMissing }; // MarkdownType 8324 case 1828196047: 8325 /* orderMeaning */ return this.orderMeaning == null ? new Base[0] : new Base[] { this.orderMeaning }; // StringType 8326 case 97445748: 8327 /* fixed */ return this.fixed == null ? new Base[0] : new Base[] { this.fixed }; // org.hl7.fhir.r4.model.Type 8328 case -791090288: 8329 /* pattern */ return this.pattern == null ? new Base[0] : new Base[] { this.pattern }; // org.hl7.fhir.r4.model.Type 8330 case -1322970774: 8331 /* example */ return this.example == null ? new Base[0] : this.example.toArray(new Base[this.example.size()]); // ElementDefinitionExampleComponent 8332 case -1376969153: 8333 /* minValue */ return this.minValue == null ? new Base[0] : new Base[] { this.minValue }; // Type 8334 case 399227501: 8335 /* maxValue */ return this.maxValue == null ? new Base[0] : new Base[] { this.maxValue }; // Type 8336 case -791400086: 8337 /* maxLength */ return this.maxLength == null ? new Base[0] : new Base[] { this.maxLength }; // IntegerType 8338 case -861311717: 8339 /* condition */ return this.condition == null ? new Base[0] 8340 : this.condition.toArray(new Base[this.condition.size()]); // IdType 8341 case -190376483: 8342 /* constraint */ return this.constraint == null ? new Base[0] 8343 : this.constraint.toArray(new Base[this.constraint.size()]); // ElementDefinitionConstraintComponent 8344 case -1402857082: 8345 /* mustSupport */ return this.mustSupport == null ? new Base[0] : new Base[] { this.mustSupport }; // BooleanType 8346 case -1408783839: 8347 /* isModifier */ return this.isModifier == null ? new Base[0] : new Base[] { this.isModifier }; // BooleanType 8348 case -1854387259: 8349 /* isModifierReason */ return this.isModifierReason == null ? new Base[0] : new Base[] { this.isModifierReason }; // StringType 8350 case 1857548060: 8351 /* isSummary */ return this.isSummary == null ? new Base[0] : new Base[] { this.isSummary }; // BooleanType 8352 case -108220795: 8353 /* binding */ return this.binding == null ? new Base[0] : new Base[] { this.binding }; // ElementDefinitionBindingComponent 8354 case 837556430: 8355 /* mapping */ return this.mapping == null ? new Base[0] : this.mapping.toArray(new Base[this.mapping.size()]); // ElementDefinitionMappingComponent 8356 default: 8357 return super.getProperty(hash, name, checkValid); 8358 } 8359 8360 } 8361 8362 @Override 8363 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8364 switch (hash) { 8365 case 3433509: // path 8366 this.path = castToString(value); // StringType 8367 return value; 8368 case -671065907: // representation 8369 value = new PropertyRepresentationEnumFactory().fromType(castToCode(value)); 8370 this.getRepresentation().add((Enumeration) value); // Enumeration<PropertyRepresentation> 8371 return value; 8372 case -825289923: // sliceName 8373 this.sliceName = castToString(value); // StringType 8374 return value; 8375 case 333040519: // sliceIsConstraining 8376 this.sliceIsConstraining = castToBoolean(value); // BooleanType 8377 return value; 8378 case 102727412: // label 8379 this.label = castToString(value); // StringType 8380 return value; 8381 case 3059181: // code 8382 this.getCode().add(castToCoding(value)); // Coding 8383 return value; 8384 case -2119287345: // slicing 8385 this.slicing = (ElementDefinitionSlicingComponent) value; // ElementDefinitionSlicingComponent 8386 return value; 8387 case 109413500: // short 8388 this.short_ = castToString(value); // StringType 8389 return value; 8390 case -1014418093: // definition 8391 this.definition = castToMarkdown(value); // MarkdownType 8392 return value; 8393 case 950398559: // comment 8394 this.comment = castToMarkdown(value); // MarkdownType 8395 return value; 8396 case -1619874672: // requirements 8397 this.requirements = castToMarkdown(value); // MarkdownType 8398 return value; 8399 case 92902992: // alias 8400 this.getAlias().add(castToString(value)); // StringType 8401 return value; 8402 case 108114: // min 8403 this.min = castToUnsignedInt(value); // UnsignedIntType 8404 return value; 8405 case 107876: // max 8406 this.max = castToString(value); // StringType 8407 return value; 8408 case 3016401: // base 8409 this.base = (ElementDefinitionBaseComponent) value; // ElementDefinitionBaseComponent 8410 return value; 8411 case 1193747154: // contentReference 8412 this.contentReference = castToUri(value); // UriType 8413 return value; 8414 case 3575610: // type 8415 this.getType().add((TypeRefComponent) value); // TypeRefComponent 8416 return value; 8417 case -659125328: // defaultValue 8418 this.defaultValue = castToType(value); // org.hl7.fhir.r4.model.Type 8419 return value; 8420 case 1857257103: // meaningWhenMissing 8421 this.meaningWhenMissing = castToMarkdown(value); // MarkdownType 8422 return value; 8423 case 1828196047: // orderMeaning 8424 this.orderMeaning = castToString(value); // StringType 8425 return value; 8426 case 97445748: // fixed 8427 this.fixed = castToType(value); // org.hl7.fhir.r4.model.Type 8428 return value; 8429 case -791090288: // pattern 8430 this.pattern = castToType(value); // org.hl7.fhir.r4.model.Type 8431 return value; 8432 case -1322970774: // example 8433 this.getExample().add((ElementDefinitionExampleComponent) value); // ElementDefinitionExampleComponent 8434 return value; 8435 case -1376969153: // minValue 8436 this.minValue = castToType(value); // Type 8437 return value; 8438 case 399227501: // maxValue 8439 this.maxValue = castToType(value); // Type 8440 return value; 8441 case -791400086: // maxLength 8442 this.maxLength = castToInteger(value); // IntegerType 8443 return value; 8444 case -861311717: // condition 8445 this.getCondition().add(castToId(value)); // IdType 8446 return value; 8447 case -190376483: // constraint 8448 this.getConstraint().add((ElementDefinitionConstraintComponent) value); // ElementDefinitionConstraintComponent 8449 return value; 8450 case -1402857082: // mustSupport 8451 this.mustSupport = castToBoolean(value); // BooleanType 8452 return value; 8453 case -1408783839: // isModifier 8454 this.isModifier = castToBoolean(value); // BooleanType 8455 return value; 8456 case -1854387259: // isModifierReason 8457 this.isModifierReason = castToString(value); // StringType 8458 return value; 8459 case 1857548060: // isSummary 8460 this.isSummary = castToBoolean(value); // BooleanType 8461 return value; 8462 case -108220795: // binding 8463 this.binding = (ElementDefinitionBindingComponent) value; // ElementDefinitionBindingComponent 8464 return value; 8465 case 837556430: // mapping 8466 this.getMapping().add((ElementDefinitionMappingComponent) value); // ElementDefinitionMappingComponent 8467 return value; 8468 default: 8469 return super.setProperty(hash, name, value); 8470 } 8471 8472 } 8473 8474 @Override 8475 public Base setProperty(String name, Base value) throws FHIRException { 8476 if (name.equals("path")) { 8477 this.path = castToString(value); // StringType 8478 } else if (name.equals("representation")) { 8479 value = new PropertyRepresentationEnumFactory().fromType(castToCode(value)); 8480 this.getRepresentation().add((Enumeration) value); 8481 } else if (name.equals("sliceName")) { 8482 this.sliceName = castToString(value); // StringType 8483 } else if (name.equals("sliceIsConstraining")) { 8484 this.sliceIsConstraining = castToBoolean(value); // BooleanType 8485 } else if (name.equals("label")) { 8486 this.label = castToString(value); // StringType 8487 } else if (name.equals("code")) { 8488 this.getCode().add(castToCoding(value)); 8489 } else if (name.equals("slicing")) { 8490 this.slicing = (ElementDefinitionSlicingComponent) value; // ElementDefinitionSlicingComponent 8491 } else if (name.equals("short")) { 8492 this.short_ = castToString(value); // StringType 8493 } else if (name.equals("definition")) { 8494 this.definition = castToMarkdown(value); // MarkdownType 8495 } else if (name.equals("comment")) { 8496 this.comment = castToMarkdown(value); // MarkdownType 8497 } else if (name.equals("requirements")) { 8498 this.requirements = castToMarkdown(value); // MarkdownType 8499 } else if (name.equals("alias")) { 8500 this.getAlias().add(castToString(value)); 8501 } else if (name.equals("min")) { 8502 this.min = castToUnsignedInt(value); // UnsignedIntType 8503 } else if (name.equals("max")) { 8504 this.max = castToString(value); // StringType 8505 } else if (name.equals("base")) { 8506 this.base = (ElementDefinitionBaseComponent) value; // ElementDefinitionBaseComponent 8507 } else if (name.equals("contentReference")) { 8508 this.contentReference = castToUri(value); // UriType 8509 } else if (name.equals("type")) { 8510 this.getType().add((TypeRefComponent) value); 8511 } else if (name.equals("defaultValue[x]")) { 8512 this.defaultValue = castToType(value); // org.hl7.fhir.r4.model.Type 8513 } else if (name.equals("meaningWhenMissing")) { 8514 this.meaningWhenMissing = castToMarkdown(value); // MarkdownType 8515 } else if (name.equals("orderMeaning")) { 8516 this.orderMeaning = castToString(value); // StringType 8517 } else if (name.equals("fixed[x]")) { 8518 this.fixed = castToType(value); // org.hl7.fhir.r4.model.Type 8519 } else if (name.equals("pattern[x]")) { 8520 this.pattern = castToType(value); // org.hl7.fhir.r4.model.Type 8521 } else if (name.equals("example")) { 8522 this.getExample().add((ElementDefinitionExampleComponent) value); 8523 } else if (name.equals("minValue[x]")) { 8524 this.minValue = castToType(value); // Type 8525 } else if (name.equals("maxValue[x]")) { 8526 this.maxValue = castToType(value); // Type 8527 } else if (name.equals("maxLength")) { 8528 this.maxLength = castToInteger(value); // IntegerType 8529 } else if (name.equals("condition")) { 8530 this.getCondition().add(castToId(value)); 8531 } else if (name.equals("constraint")) { 8532 this.getConstraint().add((ElementDefinitionConstraintComponent) value); 8533 } else if (name.equals("mustSupport")) { 8534 this.mustSupport = castToBoolean(value); // BooleanType 8535 } else if (name.equals("isModifier")) { 8536 this.isModifier = castToBoolean(value); // BooleanType 8537 } else if (name.equals("isModifierReason")) { 8538 this.isModifierReason = castToString(value); // StringType 8539 } else if (name.equals("isSummary")) { 8540 this.isSummary = castToBoolean(value); // BooleanType 8541 } else if (name.equals("binding")) { 8542 this.binding = (ElementDefinitionBindingComponent) value; // ElementDefinitionBindingComponent 8543 } else if (name.equals("mapping")) { 8544 this.getMapping().add((ElementDefinitionMappingComponent) value); 8545 } else 8546 return super.setProperty(name, value); 8547 return value; 8548 } 8549 8550 @Override 8551 public Base makeProperty(int hash, String name) throws FHIRException { 8552 switch (hash) { 8553 case 3433509: 8554 return getPathElement(); 8555 case -671065907: 8556 return addRepresentationElement(); 8557 case -825289923: 8558 return getSliceNameElement(); 8559 case 333040519: 8560 return getSliceIsConstrainingElement(); 8561 case 102727412: 8562 return getLabelElement(); 8563 case 3059181: 8564 return addCode(); 8565 case -2119287345: 8566 return getSlicing(); 8567 case 109413500: 8568 return getShortElement(); 8569 case -1014418093: 8570 return getDefinitionElement(); 8571 case 950398559: 8572 return getCommentElement(); 8573 case -1619874672: 8574 return getRequirementsElement(); 8575 case 92902992: 8576 return addAliasElement(); 8577 case 108114: 8578 return getMinElement(); 8579 case 107876: 8580 return getMaxElement(); 8581 case 3016401: 8582 return getBase(); 8583 case 1193747154: 8584 return getContentReferenceElement(); 8585 case 3575610: 8586 return addType(); 8587 case 587922128: 8588 return getDefaultValue(); 8589 case -659125328: 8590 return getDefaultValue(); 8591 case 1857257103: 8592 return getMeaningWhenMissingElement(); 8593 case 1828196047: 8594 return getOrderMeaningElement(); 8595 case -391522164: 8596 return getFixed(); 8597 case 97445748: 8598 return getFixed(); 8599 case -885125392: 8600 return getPattern(); 8601 case -791090288: 8602 return getPattern(); 8603 case -1322970774: 8604 return addExample(); 8605 case -55301663: 8606 return getMinValue(); 8607 case -1376969153: 8608 return getMinValue(); 8609 case 622130931: 8610 return getMaxValue(); 8611 case 399227501: 8612 return getMaxValue(); 8613 case -791400086: 8614 return getMaxLengthElement(); 8615 case -861311717: 8616 return addConditionElement(); 8617 case -190376483: 8618 return addConstraint(); 8619 case -1402857082: 8620 return getMustSupportElement(); 8621 case -1408783839: 8622 return getIsModifierElement(); 8623 case -1854387259: 8624 return getIsModifierReasonElement(); 8625 case 1857548060: 8626 return getIsSummaryElement(); 8627 case -108220795: 8628 return getBinding(); 8629 case 837556430: 8630 return addMapping(); 8631 default: 8632 return super.makeProperty(hash, name); 8633 } 8634 8635 } 8636 8637 @Override 8638 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8639 switch (hash) { 8640 case 3433509: 8641 /* path */ return new String[] { "string" }; 8642 case -671065907: 8643 /* representation */ return new String[] { "code" }; 8644 case -825289923: 8645 /* sliceName */ return new String[] { "string" }; 8646 case 333040519: 8647 /* sliceIsConstraining */ return new String[] { "boolean" }; 8648 case 102727412: 8649 /* label */ return new String[] { "string" }; 8650 case 3059181: 8651 /* code */ return new String[] { "Coding" }; 8652 case -2119287345: 8653 /* slicing */ return new String[] {}; 8654 case 109413500: 8655 /* short */ return new String[] { "string" }; 8656 case -1014418093: 8657 /* definition */ return new String[] { "markdown" }; 8658 case 950398559: 8659 /* comment */ return new String[] { "markdown" }; 8660 case -1619874672: 8661 /* requirements */ return new String[] { "markdown" }; 8662 case 92902992: 8663 /* alias */ return new String[] { "string" }; 8664 case 108114: 8665 /* min */ return new String[] { "unsignedInt" }; 8666 case 107876: 8667 /* max */ return new String[] { "string" }; 8668 case 3016401: 8669 /* base */ return new String[] {}; 8670 case 1193747154: 8671 /* contentReference */ return new String[] { "uri" }; 8672 case 3575610: 8673 /* type */ return new String[] {}; 8674 case -659125328: 8675 /* defaultValue */ return new String[] { "*" }; 8676 case 1857257103: 8677 /* meaningWhenMissing */ return new String[] { "markdown" }; 8678 case 1828196047: 8679 /* orderMeaning */ return new String[] { "string" }; 8680 case 97445748: 8681 /* fixed */ return new String[] { "*" }; 8682 case -791090288: 8683 /* pattern */ return new String[] { "*" }; 8684 case -1322970774: 8685 /* example */ return new String[] {}; 8686 case -1376969153: 8687 /* minValue */ return new String[] { "date", "dateTime", "instant", "time", "decimal", "integer", "positiveInt", 8688 "unsignedInt", "Quantity" }; 8689 case 399227501: 8690 /* maxValue */ return new String[] { "date", "dateTime", "instant", "time", "decimal", "integer", "positiveInt", 8691 "unsignedInt", "Quantity" }; 8692 case -791400086: 8693 /* maxLength */ return new String[] { "integer" }; 8694 case -861311717: 8695 /* condition */ return new String[] { "id" }; 8696 case -190376483: 8697 /* constraint */ return new String[] {}; 8698 case -1402857082: 8699 /* mustSupport */ return new String[] { "boolean" }; 8700 case -1408783839: 8701 /* isModifier */ return new String[] { "boolean" }; 8702 case -1854387259: 8703 /* isModifierReason */ return new String[] { "string" }; 8704 case 1857548060: 8705 /* isSummary */ return new String[] { "boolean" }; 8706 case -108220795: 8707 /* binding */ return new String[] {}; 8708 case 837556430: 8709 /* mapping */ return new String[] {}; 8710 default: 8711 return super.getTypesForProperty(hash, name); 8712 } 8713 8714 } 8715 8716 @Override 8717 public Base addChild(String name) throws FHIRException { 8718 if (name.equals("path")) { 8719 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.path"); 8720 } else if (name.equals("representation")) { 8721 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.representation"); 8722 } else if (name.equals("sliceName")) { 8723 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.sliceName"); 8724 } else if (name.equals("sliceIsConstraining")) { 8725 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.sliceIsConstraining"); 8726 } else if (name.equals("label")) { 8727 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.label"); 8728 } else if (name.equals("code")) { 8729 return addCode(); 8730 } else if (name.equals("slicing")) { 8731 this.slicing = new ElementDefinitionSlicingComponent(); 8732 return this.slicing; 8733 } else if (name.equals("short")) { 8734 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.short"); 8735 } else if (name.equals("definition")) { 8736 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.definition"); 8737 } else if (name.equals("comment")) { 8738 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.comment"); 8739 } else if (name.equals("requirements")) { 8740 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.requirements"); 8741 } else if (name.equals("alias")) { 8742 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.alias"); 8743 } else if (name.equals("min")) { 8744 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.min"); 8745 } else if (name.equals("max")) { 8746 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.max"); 8747 } else if (name.equals("base")) { 8748 this.base = new ElementDefinitionBaseComponent(); 8749 return this.base; 8750 } else if (name.equals("contentReference")) { 8751 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.contentReference"); 8752 } else if (name.equals("type")) { 8753 return addType(); 8754 } else if (name.equals("defaultValueBase64Binary")) { 8755 this.defaultValue = new Base64BinaryType(); 8756 return this.defaultValue; 8757 } else if (name.equals("defaultValueBoolean")) { 8758 this.defaultValue = new BooleanType(); 8759 return this.defaultValue; 8760 } else if (name.equals("defaultValueCanonical")) { 8761 this.defaultValue = new CanonicalType(); 8762 return this.defaultValue; 8763 } else if (name.equals("defaultValueCode")) { 8764 this.defaultValue = new CodeType(); 8765 return this.defaultValue; 8766 } else if (name.equals("defaultValueDate")) { 8767 this.defaultValue = new DateType(); 8768 return this.defaultValue; 8769 } else if (name.equals("defaultValueDateTime")) { 8770 this.defaultValue = new DateTimeType(); 8771 return this.defaultValue; 8772 } else if (name.equals("defaultValueDecimal")) { 8773 this.defaultValue = new DecimalType(); 8774 return this.defaultValue; 8775 } else if (name.equals("defaultValueId")) { 8776 this.defaultValue = new IdType(); 8777 return this.defaultValue; 8778 } else if (name.equals("defaultValueInstant")) { 8779 this.defaultValue = new InstantType(); 8780 return this.defaultValue; 8781 } else if (name.equals("defaultValueInteger")) { 8782 this.defaultValue = new IntegerType(); 8783 return this.defaultValue; 8784 } else if (name.equals("defaultValueMarkdown")) { 8785 this.defaultValue = new MarkdownType(); 8786 return this.defaultValue; 8787 } else if (name.equals("defaultValueOid")) { 8788 this.defaultValue = new OidType(); 8789 return this.defaultValue; 8790 } else if (name.equals("defaultValuePositiveInt")) { 8791 this.defaultValue = new PositiveIntType(); 8792 return this.defaultValue; 8793 } else if (name.equals("defaultValueString")) { 8794 this.defaultValue = new StringType(); 8795 return this.defaultValue; 8796 } else if (name.equals("defaultValueTime")) { 8797 this.defaultValue = new TimeType(); 8798 return this.defaultValue; 8799 } else if (name.equals("defaultValueUnsignedInt")) { 8800 this.defaultValue = new UnsignedIntType(); 8801 return this.defaultValue; 8802 } else if (name.equals("defaultValueUri")) { 8803 this.defaultValue = new UriType(); 8804 return this.defaultValue; 8805 } else if (name.equals("defaultValueUrl")) { 8806 this.defaultValue = new UrlType(); 8807 return this.defaultValue; 8808 } else if (name.equals("defaultValueUuid")) { 8809 this.defaultValue = new UuidType(); 8810 return this.defaultValue; 8811 } else if (name.equals("defaultValueAddress")) { 8812 this.defaultValue = new Address(); 8813 return this.defaultValue; 8814 } else if (name.equals("defaultValueAge")) { 8815 this.defaultValue = new Age(); 8816 return this.defaultValue; 8817 } else if (name.equals("defaultValueAnnotation")) { 8818 this.defaultValue = new Annotation(); 8819 return this.defaultValue; 8820 } else if (name.equals("defaultValueAttachment")) { 8821 this.defaultValue = new Attachment(); 8822 return this.defaultValue; 8823 } else if (name.equals("defaultValueCodeableConcept")) { 8824 this.defaultValue = new CodeableConcept(); 8825 return this.defaultValue; 8826 } else if (name.equals("defaultValueCoding")) { 8827 this.defaultValue = new Coding(); 8828 return this.defaultValue; 8829 } else if (name.equals("defaultValueContactPoint")) { 8830 this.defaultValue = new ContactPoint(); 8831 return this.defaultValue; 8832 } else if (name.equals("defaultValueCount")) { 8833 this.defaultValue = new Count(); 8834 return this.defaultValue; 8835 } else if (name.equals("defaultValueDistance")) { 8836 this.defaultValue = new Distance(); 8837 return this.defaultValue; 8838 } else if (name.equals("defaultValueDuration")) { 8839 this.defaultValue = new Duration(); 8840 return this.defaultValue; 8841 } else if (name.equals("defaultValueHumanName")) { 8842 this.defaultValue = new HumanName(); 8843 return this.defaultValue; 8844 } else if (name.equals("defaultValueIdentifier")) { 8845 this.defaultValue = new Identifier(); 8846 return this.defaultValue; 8847 } else if (name.equals("defaultValueMoney")) { 8848 this.defaultValue = new Money(); 8849 return this.defaultValue; 8850 } else if (name.equals("defaultValuePeriod")) { 8851 this.defaultValue = new Period(); 8852 return this.defaultValue; 8853 } else if (name.equals("defaultValueQuantity")) { 8854 this.defaultValue = new Quantity(); 8855 return this.defaultValue; 8856 } else if (name.equals("defaultValueRange")) { 8857 this.defaultValue = new Range(); 8858 return this.defaultValue; 8859 } else if (name.equals("defaultValueRatio")) { 8860 this.defaultValue = new Ratio(); 8861 return this.defaultValue; 8862 } else if (name.equals("defaultValueReference")) { 8863 this.defaultValue = new Reference(); 8864 return this.defaultValue; 8865 } else if (name.equals("defaultValueSampledData")) { 8866 this.defaultValue = new SampledData(); 8867 return this.defaultValue; 8868 } else if (name.equals("defaultValueSignature")) { 8869 this.defaultValue = new Signature(); 8870 return this.defaultValue; 8871 } else if (name.equals("defaultValueTiming")) { 8872 this.defaultValue = new Timing(); 8873 return this.defaultValue; 8874 } else if (name.equals("defaultValueContactDetail")) { 8875 this.defaultValue = new ContactDetail(); 8876 return this.defaultValue; 8877 } else if (name.equals("defaultValueContributor")) { 8878 this.defaultValue = new Contributor(); 8879 return this.defaultValue; 8880 } else if (name.equals("defaultValueDataRequirement")) { 8881 this.defaultValue = new DataRequirement(); 8882 return this.defaultValue; 8883 } else if (name.equals("defaultValueExpression")) { 8884 this.defaultValue = new Expression(); 8885 return this.defaultValue; 8886 } else if (name.equals("defaultValueParameterDefinition")) { 8887 this.defaultValue = new ParameterDefinition(); 8888 return this.defaultValue; 8889 } else if (name.equals("defaultValueRelatedArtifact")) { 8890 this.defaultValue = new RelatedArtifact(); 8891 return this.defaultValue; 8892 } else if (name.equals("defaultValueTriggerDefinition")) { 8893 this.defaultValue = new TriggerDefinition(); 8894 return this.defaultValue; 8895 } else if (name.equals("defaultValueUsageContext")) { 8896 this.defaultValue = new UsageContext(); 8897 return this.defaultValue; 8898 } else if (name.equals("defaultValueDosage")) { 8899 this.defaultValue = new Dosage(); 8900 return this.defaultValue; 8901 } else if (name.equals("defaultValueMeta")) { 8902 this.defaultValue = new Meta(); 8903 return this.defaultValue; 8904 } else if (name.equals("meaningWhenMissing")) { 8905 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.meaningWhenMissing"); 8906 } else if (name.equals("orderMeaning")) { 8907 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.orderMeaning"); 8908 } else if (name.equals("fixedBase64Binary")) { 8909 this.fixed = new Base64BinaryType(); 8910 return this.fixed; 8911 } else if (name.equals("fixedBoolean")) { 8912 this.fixed = new BooleanType(); 8913 return this.fixed; 8914 } else if (name.equals("fixedCanonical")) { 8915 this.fixed = new CanonicalType(); 8916 return this.fixed; 8917 } else if (name.equals("fixedCode")) { 8918 this.fixed = new CodeType(); 8919 return this.fixed; 8920 } else if (name.equals("fixedDate")) { 8921 this.fixed = new DateType(); 8922 return this.fixed; 8923 } else if (name.equals("fixedDateTime")) { 8924 this.fixed = new DateTimeType(); 8925 return this.fixed; 8926 } else if (name.equals("fixedDecimal")) { 8927 this.fixed = new DecimalType(); 8928 return this.fixed; 8929 } else if (name.equals("fixedId")) { 8930 this.fixed = new IdType(); 8931 return this.fixed; 8932 } else if (name.equals("fixedInstant")) { 8933 this.fixed = new InstantType(); 8934 return this.fixed; 8935 } else if (name.equals("fixedInteger")) { 8936 this.fixed = new IntegerType(); 8937 return this.fixed; 8938 } else if (name.equals("fixedMarkdown")) { 8939 this.fixed = new MarkdownType(); 8940 return this.fixed; 8941 } else if (name.equals("fixedOid")) { 8942 this.fixed = new OidType(); 8943 return this.fixed; 8944 } else if (name.equals("fixedPositiveInt")) { 8945 this.fixed = new PositiveIntType(); 8946 return this.fixed; 8947 } else if (name.equals("fixedString")) { 8948 this.fixed = new StringType(); 8949 return this.fixed; 8950 } else if (name.equals("fixedTime")) { 8951 this.fixed = new TimeType(); 8952 return this.fixed; 8953 } else if (name.equals("fixedUnsignedInt")) { 8954 this.fixed = new UnsignedIntType(); 8955 return this.fixed; 8956 } else if (name.equals("fixedUri")) { 8957 this.fixed = new UriType(); 8958 return this.fixed; 8959 } else if (name.equals("fixedUrl")) { 8960 this.fixed = new UrlType(); 8961 return this.fixed; 8962 } else if (name.equals("fixedUuid")) { 8963 this.fixed = new UuidType(); 8964 return this.fixed; 8965 } else if (name.equals("fixedAddress")) { 8966 this.fixed = new Address(); 8967 return this.fixed; 8968 } else if (name.equals("fixedAge")) { 8969 this.fixed = new Age(); 8970 return this.fixed; 8971 } else if (name.equals("fixedAnnotation")) { 8972 this.fixed = new Annotation(); 8973 return this.fixed; 8974 } else if (name.equals("fixedAttachment")) { 8975 this.fixed = new Attachment(); 8976 return this.fixed; 8977 } else if (name.equals("fixedCodeableConcept")) { 8978 this.fixed = new CodeableConcept(); 8979 return this.fixed; 8980 } else if (name.equals("fixedCoding")) { 8981 this.fixed = new Coding(); 8982 return this.fixed; 8983 } else if (name.equals("fixedContactPoint")) { 8984 this.fixed = new ContactPoint(); 8985 return this.fixed; 8986 } else if (name.equals("fixedCount")) { 8987 this.fixed = new Count(); 8988 return this.fixed; 8989 } else if (name.equals("fixedDistance")) { 8990 this.fixed = new Distance(); 8991 return this.fixed; 8992 } else if (name.equals("fixedDuration")) { 8993 this.fixed = new Duration(); 8994 return this.fixed; 8995 } else if (name.equals("fixedHumanName")) { 8996 this.fixed = new HumanName(); 8997 return this.fixed; 8998 } else if (name.equals("fixedIdentifier")) { 8999 this.fixed = new Identifier(); 9000 return this.fixed; 9001 } else if (name.equals("fixedMoney")) { 9002 this.fixed = new Money(); 9003 return this.fixed; 9004 } else if (name.equals("fixedPeriod")) { 9005 this.fixed = new Period(); 9006 return this.fixed; 9007 } else if (name.equals("fixedQuantity")) { 9008 this.fixed = new Quantity(); 9009 return this.fixed; 9010 } else if (name.equals("fixedRange")) { 9011 this.fixed = new Range(); 9012 return this.fixed; 9013 } else if (name.equals("fixedRatio")) { 9014 this.fixed = new Ratio(); 9015 return this.fixed; 9016 } else if (name.equals("fixedReference")) { 9017 this.fixed = new Reference(); 9018 return this.fixed; 9019 } else if (name.equals("fixedSampledData")) { 9020 this.fixed = new SampledData(); 9021 return this.fixed; 9022 } else if (name.equals("fixedSignature")) { 9023 this.fixed = new Signature(); 9024 return this.fixed; 9025 } else if (name.equals("fixedTiming")) { 9026 this.fixed = new Timing(); 9027 return this.fixed; 9028 } else if (name.equals("fixedContactDetail")) { 9029 this.fixed = new ContactDetail(); 9030 return this.fixed; 9031 } else if (name.equals("fixedContributor")) { 9032 this.fixed = new Contributor(); 9033 return this.fixed; 9034 } else if (name.equals("fixedDataRequirement")) { 9035 this.fixed = new DataRequirement(); 9036 return this.fixed; 9037 } else if (name.equals("fixedExpression")) { 9038 this.fixed = new Expression(); 9039 return this.fixed; 9040 } else if (name.equals("fixedParameterDefinition")) { 9041 this.fixed = new ParameterDefinition(); 9042 return this.fixed; 9043 } else if (name.equals("fixedRelatedArtifact")) { 9044 this.fixed = new RelatedArtifact(); 9045 return this.fixed; 9046 } else if (name.equals("fixedTriggerDefinition")) { 9047 this.fixed = new TriggerDefinition(); 9048 return this.fixed; 9049 } else if (name.equals("fixedUsageContext")) { 9050 this.fixed = new UsageContext(); 9051 return this.fixed; 9052 } else if (name.equals("fixedDosage")) { 9053 this.fixed = new Dosage(); 9054 return this.fixed; 9055 } else if (name.equals("fixedMeta")) { 9056 this.fixed = new Meta(); 9057 return this.fixed; 9058 } else if (name.equals("patternBase64Binary")) { 9059 this.pattern = new Base64BinaryType(); 9060 return this.pattern; 9061 } else if (name.equals("patternBoolean")) { 9062 this.pattern = new BooleanType(); 9063 return this.pattern; 9064 } else if (name.equals("patternCanonical")) { 9065 this.pattern = new CanonicalType(); 9066 return this.pattern; 9067 } else if (name.equals("patternCode")) { 9068 this.pattern = new CodeType(); 9069 return this.pattern; 9070 } else if (name.equals("patternDate")) { 9071 this.pattern = new DateType(); 9072 return this.pattern; 9073 } else if (name.equals("patternDateTime")) { 9074 this.pattern = new DateTimeType(); 9075 return this.pattern; 9076 } else if (name.equals("patternDecimal")) { 9077 this.pattern = new DecimalType(); 9078 return this.pattern; 9079 } else if (name.equals("patternId")) { 9080 this.pattern = new IdType(); 9081 return this.pattern; 9082 } else if (name.equals("patternInstant")) { 9083 this.pattern = new InstantType(); 9084 return this.pattern; 9085 } else if (name.equals("patternInteger")) { 9086 this.pattern = new IntegerType(); 9087 return this.pattern; 9088 } else if (name.equals("patternMarkdown")) { 9089 this.pattern = new MarkdownType(); 9090 return this.pattern; 9091 } else if (name.equals("patternOid")) { 9092 this.pattern = new OidType(); 9093 return this.pattern; 9094 } else if (name.equals("patternPositiveInt")) { 9095 this.pattern = new PositiveIntType(); 9096 return this.pattern; 9097 } else if (name.equals("patternString")) { 9098 this.pattern = new StringType(); 9099 return this.pattern; 9100 } else if (name.equals("patternTime")) { 9101 this.pattern = new TimeType(); 9102 return this.pattern; 9103 } else if (name.equals("patternUnsignedInt")) { 9104 this.pattern = new UnsignedIntType(); 9105 return this.pattern; 9106 } else if (name.equals("patternUri")) { 9107 this.pattern = new UriType(); 9108 return this.pattern; 9109 } else if (name.equals("patternUrl")) { 9110 this.pattern = new UrlType(); 9111 return this.pattern; 9112 } else if (name.equals("patternUuid")) { 9113 this.pattern = new UuidType(); 9114 return this.pattern; 9115 } else if (name.equals("patternAddress")) { 9116 this.pattern = new Address(); 9117 return this.pattern; 9118 } else if (name.equals("patternAge")) { 9119 this.pattern = new Age(); 9120 return this.pattern; 9121 } else if (name.equals("patternAnnotation")) { 9122 this.pattern = new Annotation(); 9123 return this.pattern; 9124 } else if (name.equals("patternAttachment")) { 9125 this.pattern = new Attachment(); 9126 return this.pattern; 9127 } else if (name.equals("patternCodeableConcept")) { 9128 this.pattern = new CodeableConcept(); 9129 return this.pattern; 9130 } else if (name.equals("patternCoding")) { 9131 this.pattern = new Coding(); 9132 return this.pattern; 9133 } else if (name.equals("patternContactPoint")) { 9134 this.pattern = new ContactPoint(); 9135 return this.pattern; 9136 } else if (name.equals("patternCount")) { 9137 this.pattern = new Count(); 9138 return this.pattern; 9139 } else if (name.equals("patternDistance")) { 9140 this.pattern = new Distance(); 9141 return this.pattern; 9142 } else if (name.equals("patternDuration")) { 9143 this.pattern = new Duration(); 9144 return this.pattern; 9145 } else if (name.equals("patternHumanName")) { 9146 this.pattern = new HumanName(); 9147 return this.pattern; 9148 } else if (name.equals("patternIdentifier")) { 9149 this.pattern = new Identifier(); 9150 return this.pattern; 9151 } else if (name.equals("patternMoney")) { 9152 this.pattern = new Money(); 9153 return this.pattern; 9154 } else if (name.equals("patternPeriod")) { 9155 this.pattern = new Period(); 9156 return this.pattern; 9157 } else if (name.equals("patternQuantity")) { 9158 this.pattern = new Quantity(); 9159 return this.pattern; 9160 } else if (name.equals("patternRange")) { 9161 this.pattern = new Range(); 9162 return this.pattern; 9163 } else if (name.equals("patternRatio")) { 9164 this.pattern = new Ratio(); 9165 return this.pattern; 9166 } else if (name.equals("patternReference")) { 9167 this.pattern = new Reference(); 9168 return this.pattern; 9169 } else if (name.equals("patternSampledData")) { 9170 this.pattern = new SampledData(); 9171 return this.pattern; 9172 } else if (name.equals("patternSignature")) { 9173 this.pattern = new Signature(); 9174 return this.pattern; 9175 } else if (name.equals("patternTiming")) { 9176 this.pattern = new Timing(); 9177 return this.pattern; 9178 } else if (name.equals("patternContactDetail")) { 9179 this.pattern = new ContactDetail(); 9180 return this.pattern; 9181 } else if (name.equals("patternContributor")) { 9182 this.pattern = new Contributor(); 9183 return this.pattern; 9184 } else if (name.equals("patternDataRequirement")) { 9185 this.pattern = new DataRequirement(); 9186 return this.pattern; 9187 } else if (name.equals("patternExpression")) { 9188 this.pattern = new Expression(); 9189 return this.pattern; 9190 } else if (name.equals("patternParameterDefinition")) { 9191 this.pattern = new ParameterDefinition(); 9192 return this.pattern; 9193 } else if (name.equals("patternRelatedArtifact")) { 9194 this.pattern = new RelatedArtifact(); 9195 return this.pattern; 9196 } else if (name.equals("patternTriggerDefinition")) { 9197 this.pattern = new TriggerDefinition(); 9198 return this.pattern; 9199 } else if (name.equals("patternUsageContext")) { 9200 this.pattern = new UsageContext(); 9201 return this.pattern; 9202 } else if (name.equals("patternDosage")) { 9203 this.pattern = new Dosage(); 9204 return this.pattern; 9205 } else if (name.equals("patternMeta")) { 9206 this.pattern = new Meta(); 9207 return this.pattern; 9208 } else if (name.equals("example")) { 9209 return addExample(); 9210 } else if (name.equals("minValueDate")) { 9211 this.minValue = new DateType(); 9212 return this.minValue; 9213 } else if (name.equals("minValueDateTime")) { 9214 this.minValue = new DateTimeType(); 9215 return this.minValue; 9216 } else if (name.equals("minValueInstant")) { 9217 this.minValue = new InstantType(); 9218 return this.minValue; 9219 } else if (name.equals("minValueTime")) { 9220 this.minValue = new TimeType(); 9221 return this.minValue; 9222 } else if (name.equals("minValueDecimal")) { 9223 this.minValue = new DecimalType(); 9224 return this.minValue; 9225 } else if (name.equals("minValueInteger")) { 9226 this.minValue = new IntegerType(); 9227 return this.minValue; 9228 } else if (name.equals("minValuePositiveInt")) { 9229 this.minValue = new PositiveIntType(); 9230 return this.minValue; 9231 } else if (name.equals("minValueUnsignedInt")) { 9232 this.minValue = new UnsignedIntType(); 9233 return this.minValue; 9234 } else if (name.equals("minValueQuantity")) { 9235 this.minValue = new Quantity(); 9236 return this.minValue; 9237 } else if (name.equals("maxValueDate")) { 9238 this.maxValue = new DateType(); 9239 return this.maxValue; 9240 } else if (name.equals("maxValueDateTime")) { 9241 this.maxValue = new DateTimeType(); 9242 return this.maxValue; 9243 } else if (name.equals("maxValueInstant")) { 9244 this.maxValue = new InstantType(); 9245 return this.maxValue; 9246 } else if (name.equals("maxValueTime")) { 9247 this.maxValue = new TimeType(); 9248 return this.maxValue; 9249 } else if (name.equals("maxValueDecimal")) { 9250 this.maxValue = new DecimalType(); 9251 return this.maxValue; 9252 } else if (name.equals("maxValueInteger")) { 9253 this.maxValue = new IntegerType(); 9254 return this.maxValue; 9255 } else if (name.equals("maxValuePositiveInt")) { 9256 this.maxValue = new PositiveIntType(); 9257 return this.maxValue; 9258 } else if (name.equals("maxValueUnsignedInt")) { 9259 this.maxValue = new UnsignedIntType(); 9260 return this.maxValue; 9261 } else if (name.equals("maxValueQuantity")) { 9262 this.maxValue = new Quantity(); 9263 return this.maxValue; 9264 } else if (name.equals("maxLength")) { 9265 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.maxLength"); 9266 } else if (name.equals("condition")) { 9267 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.condition"); 9268 } else if (name.equals("constraint")) { 9269 return addConstraint(); 9270 } else if (name.equals("mustSupport")) { 9271 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.mustSupport"); 9272 } else if (name.equals("isModifier")) { 9273 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.isModifier"); 9274 } else if (name.equals("isModifierReason")) { 9275 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.isModifierReason"); 9276 } else if (name.equals("isSummary")) { 9277 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.isSummary"); 9278 } else if (name.equals("binding")) { 9279 this.binding = new ElementDefinitionBindingComponent(); 9280 return this.binding; 9281 } else if (name.equals("mapping")) { 9282 return addMapping(); 9283 } else 9284 return super.addChild(name); 9285 } 9286 9287 public String fhirType() { 9288 return "ElementDefinition"; 9289 9290 } 9291 9292 public ElementDefinition copy() { 9293 ElementDefinition dst = new ElementDefinition(); 9294 copyValues(dst); 9295 return dst; 9296 } 9297 9298 public void copyValues(ElementDefinition dst) { 9299 super.copyValues(dst); 9300 dst.path = path == null ? null : path.copy(); 9301 if (representation != null) { 9302 dst.representation = new ArrayList<Enumeration<PropertyRepresentation>>(); 9303 for (Enumeration<PropertyRepresentation> i : representation) 9304 dst.representation.add(i.copy()); 9305 } 9306 ; 9307 dst.sliceName = sliceName == null ? null : sliceName.copy(); 9308 dst.sliceIsConstraining = sliceIsConstraining == null ? null : sliceIsConstraining.copy(); 9309 dst.label = label == null ? null : label.copy(); 9310 if (code != null) { 9311 dst.code = new ArrayList<Coding>(); 9312 for (Coding i : code) 9313 dst.code.add(i.copy()); 9314 } 9315 ; 9316 dst.slicing = slicing == null ? null : slicing.copy(); 9317 dst.short_ = short_ == null ? null : short_.copy(); 9318 dst.definition = definition == null ? null : definition.copy(); 9319 dst.comment = comment == null ? null : comment.copy(); 9320 dst.requirements = requirements == null ? null : requirements.copy(); 9321 if (alias != null) { 9322 dst.alias = new ArrayList<StringType>(); 9323 for (StringType i : alias) 9324 dst.alias.add(i.copy()); 9325 } 9326 ; 9327 dst.min = min == null ? null : min.copy(); 9328 dst.max = max == null ? null : max.copy(); 9329 dst.base = base == null ? null : base.copy(); 9330 dst.contentReference = contentReference == null ? null : contentReference.copy(); 9331 if (type != null) { 9332 dst.type = new ArrayList<TypeRefComponent>(); 9333 for (TypeRefComponent i : type) 9334 dst.type.add(i.copy()); 9335 } 9336 ; 9337 dst.defaultValue = defaultValue == null ? null : defaultValue.copy(); 9338 dst.meaningWhenMissing = meaningWhenMissing == null ? null : meaningWhenMissing.copy(); 9339 dst.orderMeaning = orderMeaning == null ? null : orderMeaning.copy(); 9340 dst.fixed = fixed == null ? null : fixed.copy(); 9341 dst.pattern = pattern == null ? null : pattern.copy(); 9342 if (example != null) { 9343 dst.example = new ArrayList<ElementDefinitionExampleComponent>(); 9344 for (ElementDefinitionExampleComponent i : example) 9345 dst.example.add(i.copy()); 9346 } 9347 ; 9348 dst.minValue = minValue == null ? null : minValue.copy(); 9349 dst.maxValue = maxValue == null ? null : maxValue.copy(); 9350 dst.maxLength = maxLength == null ? null : maxLength.copy(); 9351 if (condition != null) { 9352 dst.condition = new ArrayList<IdType>(); 9353 for (IdType i : condition) 9354 dst.condition.add(i.copy()); 9355 } 9356 ; 9357 if (constraint != null) { 9358 dst.constraint = new ArrayList<ElementDefinitionConstraintComponent>(); 9359 for (ElementDefinitionConstraintComponent i : constraint) 9360 dst.constraint.add(i.copy()); 9361 } 9362 ; 9363 dst.mustSupport = mustSupport == null ? null : mustSupport.copy(); 9364 dst.isModifier = isModifier == null ? null : isModifier.copy(); 9365 dst.isModifierReason = isModifierReason == null ? null : isModifierReason.copy(); 9366 dst.isSummary = isSummary == null ? null : isSummary.copy(); 9367 dst.binding = binding == null ? null : binding.copy(); 9368 if (mapping != null) { 9369 dst.mapping = new ArrayList<ElementDefinitionMappingComponent>(); 9370 for (ElementDefinitionMappingComponent i : mapping) 9371 dst.mapping.add(i.copy()); 9372 } 9373 ; 9374 } 9375 9376 protected ElementDefinition typedCopy() { 9377 return copy(); 9378 } 9379 9380 @Override 9381 public boolean equalsDeep(Base other_) { 9382 if (!super.equalsDeep(other_)) 9383 return false; 9384 if (!(other_ instanceof ElementDefinition)) 9385 return false; 9386 ElementDefinition o = (ElementDefinition) other_; 9387 return compareDeep(path, o.path, true) && compareDeep(representation, o.representation, true) 9388 && compareDeep(sliceName, o.sliceName, true) && compareDeep(sliceIsConstraining, o.sliceIsConstraining, true) 9389 && compareDeep(label, o.label, true) && compareDeep(code, o.code, true) && compareDeep(slicing, o.slicing, true) 9390 && compareDeep(short_, o.short_, true) && compareDeep(definition, o.definition, true) 9391 && compareDeep(comment, o.comment, true) && compareDeep(requirements, o.requirements, true) 9392 && compareDeep(alias, o.alias, true) && compareDeep(min, o.min, true) && compareDeep(max, o.max, true) 9393 && compareDeep(base, o.base, true) && compareDeep(contentReference, o.contentReference, true) 9394 && compareDeep(type, o.type, true) && compareDeep(defaultValue, o.defaultValue, true) 9395 && compareDeep(meaningWhenMissing, o.meaningWhenMissing, true) 9396 && compareDeep(orderMeaning, o.orderMeaning, true) && compareDeep(fixed, o.fixed, true) 9397 && compareDeep(pattern, o.pattern, true) && compareDeep(example, o.example, true) 9398 && compareDeep(minValue, o.minValue, true) && compareDeep(maxValue, o.maxValue, true) 9399 && compareDeep(maxLength, o.maxLength, true) && compareDeep(condition, o.condition, true) 9400 && compareDeep(constraint, o.constraint, true) && compareDeep(mustSupport, o.mustSupport, true) 9401 && compareDeep(isModifier, o.isModifier, true) && compareDeep(isModifierReason, o.isModifierReason, true) 9402 && compareDeep(isSummary, o.isSummary, true) && compareDeep(binding, o.binding, true) 9403 && compareDeep(mapping, o.mapping, true); 9404 } 9405 9406 @Override 9407 public boolean equalsShallow(Base other_) { 9408 if (!super.equalsShallow(other_)) 9409 return false; 9410 if (!(other_ instanceof ElementDefinition)) 9411 return false; 9412 ElementDefinition o = (ElementDefinition) other_; 9413 return compareValues(path, o.path, true) && compareValues(representation, o.representation, true) 9414 && compareValues(sliceName, o.sliceName, true) 9415 && compareValues(sliceIsConstraining, o.sliceIsConstraining, true) && compareValues(label, o.label, true) 9416 && compareValues(short_, o.short_, true) && compareValues(definition, o.definition, true) 9417 && compareValues(comment, o.comment, true) && compareValues(requirements, o.requirements, true) 9418 && compareValues(alias, o.alias, true) && compareValues(min, o.min, true) && compareValues(max, o.max, true) 9419 && compareValues(contentReference, o.contentReference, true) 9420 && compareValues(meaningWhenMissing, o.meaningWhenMissing, true) 9421 && compareValues(orderMeaning, o.orderMeaning, true) && compareValues(maxLength, o.maxLength, true) 9422 && compareValues(condition, o.condition, true) && compareValues(mustSupport, o.mustSupport, true) 9423 && compareValues(isModifier, o.isModifier, true) && compareValues(isModifierReason, o.isModifierReason, true) 9424 && compareValues(isSummary, o.isSummary, true); 9425 } 9426 9427 public boolean isEmpty() { 9428 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, representation, sliceName, sliceIsConstraining, 9429 label, code, slicing, short_, definition, comment, requirements, alias, min, max, base, contentReference, type, 9430 defaultValue, meaningWhenMissing, orderMeaning, fixed, pattern, example, minValue, maxValue, maxLength, 9431 condition, constraint, mustSupport, isModifier, isModifierReason, isSummary, binding, mapping); 9432 } 9433 9434// added from java-adornments.txt: 9435 9436 public String toString() { 9437 if (hasId()) 9438 return getId(); 9439 if (hasSliceName()) 9440 return getPath() + ":" + getSliceName(); 9441 else 9442 return getPath(); 9443 } 9444 9445 public void makeBase(String path, int min, String max) { 9446 ElementDefinitionBaseComponent self = getBase(); 9447 self.setPath(path); 9448 self.setMin(min); 9449 self.setMax(max); 9450 } 9451 9452 public void makeBase() { 9453 ElementDefinitionBaseComponent self = getBase(); 9454 self.setPath(getPath()); 9455 self.setMin(getMin()); 9456 self.setMax(getMax()); 9457 } 9458 9459 public String typeSummary() { 9460 CommaSeparatedStringBuilder b = new CommaSeparatedStringBuilder(); 9461 for (TypeRefComponent tr : getType()) { 9462 if (tr.hasCode()) 9463 b.append(tr.getWorkingCode()); 9464 } 9465 return b.toString(); 9466 } 9467 9468 public TypeRefComponent getType(String code) { 9469 for (TypeRefComponent tr : getType()) 9470 if (tr.getCode().equals(code)) 9471 return tr; 9472 TypeRefComponent tr = new TypeRefComponent(); 9473 tr.setCode(code); 9474 type.add(tr); 9475 return tr; 9476 } 9477 9478 public static final boolean NOT_MODIFIER = false; 9479 public static final boolean NOT_IN_SUMMARY = false; 9480 public static final boolean IS_MODIFIER = true; 9481 public static final boolean IS_IN_SUMMARY = true; 9482 9483 public ElementDefinition(boolean defaults, boolean modifier, boolean inSummary) { 9484 super(); 9485 if (defaults) { 9486 setIsModifier(modifier); 9487 setIsSummary(inSummary); 9488 } 9489 } 9490 9491 public String present() { 9492 return hasId() ? getId() : getPath(); 9493 } 9494 9495 public boolean hasFixedOrPattern() { 9496 return hasFixed() || hasPattern(); 9497 } 9498 9499 public Type getFixedOrPattern() { 9500 return hasFixed() ? getFixed() : getPattern(); 9501 } 9502 9503// end addition 9504 9505}