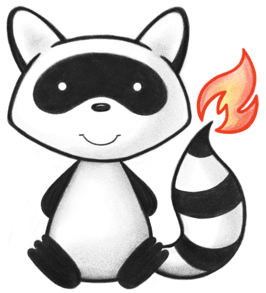
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 038import org.hl7.fhir.instance.model.api.ICompositeType; 039import org.hl7.fhir.r4.model.Enumerations.BindingStrength; 040import org.hl7.fhir.r4.model.Enumerations.BindingStrengthEnumFactory; 041import org.hl7.fhir.r4.utils.ToolingExtensions; 042// added from java-adornments.txt: 043import org.hl7.fhir.utilities.CommaSeparatedStringBuilder; 044import org.hl7.fhir.utilities.Utilities; 045 046import ca.uhn.fhir.model.api.annotation.Block; 047import ca.uhn.fhir.model.api.annotation.Child; 048import ca.uhn.fhir.model.api.annotation.DatatypeDef; 049import ca.uhn.fhir.model.api.annotation.Description; 050 051// end addition 052/** 053 * Captures constraints on each element within the resource, profile, or 054 * extension. 055 */ 056@DatatypeDef(name = "ElementDefinition") 057public class ElementDefinition extends BackboneType implements ICompositeType { 058 059 public enum PropertyRepresentation { 060 /** 061 * In XML, this property is represented as an attribute not an element. 062 */ 063 XMLATTR, 064 /** 065 * This element is represented using the XML text attribute (primitives only). 066 */ 067 XMLTEXT, 068 /** 069 * The type of this element is indicated using xsi:type. 070 */ 071 TYPEATTR, 072 /** 073 * Use CDA narrative instead of XHTML. 074 */ 075 CDATEXT, 076 /** 077 * The property is represented using XHTML. 078 */ 079 XHTML, 080 /** 081 * added to help the parsers with the generic types 082 */ 083 NULL; 084 085 public static PropertyRepresentation fromCode(String codeString) throws FHIRException { 086 if (codeString == null || "".equals(codeString)) 087 return null; 088 if ("xmlAttr".equals(codeString)) 089 return XMLATTR; 090 if ("xmlText".equals(codeString)) 091 return XMLTEXT; 092 if ("typeAttr".equals(codeString)) 093 return TYPEATTR; 094 if ("cdaText".equals(codeString)) 095 return CDATEXT; 096 if ("xhtml".equals(codeString)) 097 return XHTML; 098 if (Configuration.isAcceptInvalidEnums()) 099 return null; 100 else 101 throw new FHIRException("Unknown PropertyRepresentation code '" + codeString + "'"); 102 } 103 104 public String toCode() { 105 switch (this) { 106 case XMLATTR: 107 return "xmlAttr"; 108 case XMLTEXT: 109 return "xmlText"; 110 case TYPEATTR: 111 return "typeAttr"; 112 case CDATEXT: 113 return "cdaText"; 114 case XHTML: 115 return "xhtml"; 116 case NULL: 117 return null; 118 default: 119 return "?"; 120 } 121 } 122 123 public String getSystem() { 124 switch (this) { 125 case XMLATTR: 126 return "http://hl7.org/fhir/property-representation"; 127 case XMLTEXT: 128 return "http://hl7.org/fhir/property-representation"; 129 case TYPEATTR: 130 return "http://hl7.org/fhir/property-representation"; 131 case CDATEXT: 132 return "http://hl7.org/fhir/property-representation"; 133 case XHTML: 134 return "http://hl7.org/fhir/property-representation"; 135 case NULL: 136 return null; 137 default: 138 return "?"; 139 } 140 } 141 142 public String getDefinition() { 143 switch (this) { 144 case XMLATTR: 145 return "In XML, this property is represented as an attribute not an element."; 146 case XMLTEXT: 147 return "This element is represented using the XML text attribute (primitives only)."; 148 case TYPEATTR: 149 return "The type of this element is indicated using xsi:type."; 150 case CDATEXT: 151 return "Use CDA narrative instead of XHTML."; 152 case XHTML: 153 return "The property is represented using XHTML."; 154 case NULL: 155 return null; 156 default: 157 return "?"; 158 } 159 } 160 161 public String getDisplay() { 162 switch (this) { 163 case XMLATTR: 164 return "XML Attribute"; 165 case XMLTEXT: 166 return "XML Text"; 167 case TYPEATTR: 168 return "Type Attribute"; 169 case CDATEXT: 170 return "CDA Text Format"; 171 case XHTML: 172 return "XHTML"; 173 case NULL: 174 return null; 175 default: 176 return "?"; 177 } 178 } 179 } 180 181 public static class PropertyRepresentationEnumFactory implements EnumFactory<PropertyRepresentation> { 182 public PropertyRepresentation fromCode(String codeString) throws IllegalArgumentException { 183 if (codeString == null || "".equals(codeString)) 184 if (codeString == null || "".equals(codeString)) 185 return null; 186 if ("xmlAttr".equals(codeString)) 187 return PropertyRepresentation.XMLATTR; 188 if ("xmlText".equals(codeString)) 189 return PropertyRepresentation.XMLTEXT; 190 if ("typeAttr".equals(codeString)) 191 return PropertyRepresentation.TYPEATTR; 192 if ("cdaText".equals(codeString)) 193 return PropertyRepresentation.CDATEXT; 194 if ("xhtml".equals(codeString)) 195 return PropertyRepresentation.XHTML; 196 throw new IllegalArgumentException("Unknown PropertyRepresentation code '" + codeString + "'"); 197 } 198 199 public Enumeration<PropertyRepresentation> fromType(PrimitiveType<?> code) throws FHIRException { 200 if (code == null) 201 return null; 202 if (code.isEmpty()) 203 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.NULL, code); 204 String codeString = code.asStringValue(); 205 if (codeString == null || "".equals(codeString)) 206 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.NULL, code); 207 if ("xmlAttr".equals(codeString)) 208 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.XMLATTR, code); 209 if ("xmlText".equals(codeString)) 210 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.XMLTEXT, code); 211 if ("typeAttr".equals(codeString)) 212 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.TYPEATTR, code); 213 if ("cdaText".equals(codeString)) 214 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.CDATEXT, code); 215 if ("xhtml".equals(codeString)) 216 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.XHTML, code); 217 throw new FHIRException("Unknown PropertyRepresentation code '" + codeString + "'"); 218 } 219 220 public String toCode(PropertyRepresentation code) { 221 if (code == PropertyRepresentation.NULL) 222 return null; 223 if (code == PropertyRepresentation.XMLATTR) 224 return "xmlAttr"; 225 if (code == PropertyRepresentation.XMLTEXT) 226 return "xmlText"; 227 if (code == PropertyRepresentation.TYPEATTR) 228 return "typeAttr"; 229 if (code == PropertyRepresentation.CDATEXT) 230 return "cdaText"; 231 if (code == PropertyRepresentation.XHTML) 232 return "xhtml"; 233 return "?"; 234 } 235 236 public String toSystem(PropertyRepresentation code) { 237 return code.getSystem(); 238 } 239 } 240 241 public enum DiscriminatorType { 242 /** 243 * The slices have different values in the nominated element. 244 */ 245 VALUE, 246 /** 247 * The slices are differentiated by the presence or absence of the nominated 248 * element. 249 */ 250 EXISTS, 251 /** 252 * The slices have different values in the nominated element, as determined by 253 * testing them against the applicable ElementDefinition.pattern[x]. 254 */ 255 PATTERN, 256 /** 257 * The slices are differentiated by type of the nominated element. 258 */ 259 TYPE, 260 /** 261 * The slices are differentiated by conformance of the nominated element to a 262 * specified profile. Note that if the path specifies .resolve() then the 263 * profile is the target profile on the reference. In this case, validation by 264 * the possible profiles is required to differentiate the slices. 265 */ 266 PROFILE, 267 /** 268 * added to help the parsers with the generic types 269 */ 270 NULL; 271 272 public static DiscriminatorType fromCode(String codeString) throws FHIRException { 273 if (codeString == null || "".equals(codeString)) 274 return null; 275 if ("value".equals(codeString)) 276 return VALUE; 277 if ("exists".equals(codeString)) 278 return EXISTS; 279 if ("pattern".equals(codeString)) 280 return PATTERN; 281 if ("type".equals(codeString)) 282 return TYPE; 283 if ("profile".equals(codeString)) 284 return PROFILE; 285 if (Configuration.isAcceptInvalidEnums()) 286 return null; 287 else 288 throw new FHIRException("Unknown DiscriminatorType code '" + codeString + "'"); 289 } 290 291 public String toCode() { 292 switch (this) { 293 case VALUE: 294 return "value"; 295 case EXISTS: 296 return "exists"; 297 case PATTERN: 298 return "pattern"; 299 case TYPE: 300 return "type"; 301 case PROFILE: 302 return "profile"; 303 case NULL: 304 return null; 305 default: 306 return "?"; 307 } 308 } 309 310 public String getSystem() { 311 switch (this) { 312 case VALUE: 313 return "http://hl7.org/fhir/discriminator-type"; 314 case EXISTS: 315 return "http://hl7.org/fhir/discriminator-type"; 316 case PATTERN: 317 return "http://hl7.org/fhir/discriminator-type"; 318 case TYPE: 319 return "http://hl7.org/fhir/discriminator-type"; 320 case PROFILE: 321 return "http://hl7.org/fhir/discriminator-type"; 322 case NULL: 323 return null; 324 default: 325 return "?"; 326 } 327 } 328 329 public String getDefinition() { 330 switch (this) { 331 case VALUE: 332 return "The slices have different values in the nominated element."; 333 case EXISTS: 334 return "The slices are differentiated by the presence or absence of the nominated element."; 335 case PATTERN: 336 return "The slices have different values in the nominated element, as determined by testing them against the applicable ElementDefinition.pattern[x]."; 337 case TYPE: 338 return "The slices are differentiated by type of the nominated element."; 339 case PROFILE: 340 return "The slices are differentiated by conformance of the nominated element to a specified profile. Note that if the path specifies .resolve() then the profile is the target profile on the reference. In this case, validation by the possible profiles is required to differentiate the slices."; 341 case NULL: 342 return null; 343 default: 344 return "?"; 345 } 346 } 347 348 public String getDisplay() { 349 switch (this) { 350 case VALUE: 351 return "Value"; 352 case EXISTS: 353 return "Exists"; 354 case PATTERN: 355 return "Pattern"; 356 case TYPE: 357 return "Type"; 358 case PROFILE: 359 return "Profile"; 360 case NULL: 361 return null; 362 default: 363 return "?"; 364 } 365 } 366 } 367 368 public static class DiscriminatorTypeEnumFactory implements EnumFactory<DiscriminatorType> { 369 public DiscriminatorType fromCode(String codeString) throws IllegalArgumentException { 370 if (codeString == null || "".equals(codeString)) 371 if (codeString == null || "".equals(codeString)) 372 return null; 373 if ("value".equals(codeString)) 374 return DiscriminatorType.VALUE; 375 if ("exists".equals(codeString)) 376 return DiscriminatorType.EXISTS; 377 if ("pattern".equals(codeString)) 378 return DiscriminatorType.PATTERN; 379 if ("type".equals(codeString)) 380 return DiscriminatorType.TYPE; 381 if ("profile".equals(codeString)) 382 return DiscriminatorType.PROFILE; 383 throw new IllegalArgumentException("Unknown DiscriminatorType code '" + codeString + "'"); 384 } 385 386 public Enumeration<DiscriminatorType> fromType(PrimitiveType<?> code) throws FHIRException { 387 if (code == null) 388 return null; 389 if (code.isEmpty()) 390 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.NULL, code); 391 String codeString = code.asStringValue(); 392 if (codeString == null || "".equals(codeString)) 393 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.NULL, code); 394 if ("value".equals(codeString)) 395 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.VALUE, code); 396 if ("exists".equals(codeString)) 397 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.EXISTS, code); 398 if ("pattern".equals(codeString)) 399 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.PATTERN, code); 400 if ("type".equals(codeString)) 401 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.TYPE, code); 402 if ("profile".equals(codeString)) 403 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.PROFILE, code); 404 throw new FHIRException("Unknown DiscriminatorType code '" + codeString + "'"); 405 } 406 407 public String toCode(DiscriminatorType code) { 408 if (code == DiscriminatorType.NULL) 409 return null; 410 if (code == DiscriminatorType.VALUE) 411 return "value"; 412 if (code == DiscriminatorType.EXISTS) 413 return "exists"; 414 if (code == DiscriminatorType.PATTERN) 415 return "pattern"; 416 if (code == DiscriminatorType.TYPE) 417 return "type"; 418 if (code == DiscriminatorType.PROFILE) 419 return "profile"; 420 return "?"; 421 } 422 423 public String toSystem(DiscriminatorType code) { 424 return code.getSystem(); 425 } 426 } 427 428 public enum SlicingRules { 429 /** 430 * No additional content is allowed other than that described by the slices in 431 * this profile. 432 */ 433 CLOSED, 434 /** 435 * Additional content is allowed anywhere in the list. 436 */ 437 OPEN, 438 /** 439 * Additional content is allowed, but only at the end of the list. Note that 440 * using this requires that the slices be ordered, which makes it hard to share 441 * uses. This should only be done where absolutely required. 442 */ 443 OPENATEND, 444 /** 445 * added to help the parsers with the generic types 446 */ 447 NULL; 448 449 public static SlicingRules fromCode(String codeString) throws FHIRException { 450 if (codeString == null || "".equals(codeString)) 451 return null; 452 if ("closed".equals(codeString)) 453 return CLOSED; 454 if ("open".equals(codeString)) 455 return OPEN; 456 if ("openAtEnd".equals(codeString)) 457 return OPENATEND; 458 if (Configuration.isAcceptInvalidEnums()) 459 return null; 460 else 461 throw new FHIRException("Unknown SlicingRules code '" + codeString + "'"); 462 } 463 464 public String toCode() { 465 switch (this) { 466 case CLOSED: 467 return "closed"; 468 case OPEN: 469 return "open"; 470 case OPENATEND: 471 return "openAtEnd"; 472 case NULL: 473 return null; 474 default: 475 return "?"; 476 } 477 } 478 479 public String getSystem() { 480 switch (this) { 481 case CLOSED: 482 return "http://hl7.org/fhir/resource-slicing-rules"; 483 case OPEN: 484 return "http://hl7.org/fhir/resource-slicing-rules"; 485 case OPENATEND: 486 return "http://hl7.org/fhir/resource-slicing-rules"; 487 case NULL: 488 return null; 489 default: 490 return "?"; 491 } 492 } 493 494 public String getDefinition() { 495 switch (this) { 496 case CLOSED: 497 return "No additional content is allowed other than that described by the slices in this profile."; 498 case OPEN: 499 return "Additional content is allowed anywhere in the list."; 500 case OPENATEND: 501 return "Additional content is allowed, but only at the end of the list. Note that using this requires that the slices be ordered, which makes it hard to share uses. This should only be done where absolutely required."; 502 case NULL: 503 return null; 504 default: 505 return "?"; 506 } 507 } 508 509 public String getDisplay() { 510 switch (this) { 511 case CLOSED: 512 return "Closed"; 513 case OPEN: 514 return "Open"; 515 case OPENATEND: 516 return "Open at End"; 517 case NULL: 518 return null; 519 default: 520 return "?"; 521 } 522 } 523 } 524 525 public static class SlicingRulesEnumFactory implements EnumFactory<SlicingRules> { 526 public SlicingRules fromCode(String codeString) throws IllegalArgumentException { 527 if (codeString == null || "".equals(codeString)) 528 if (codeString == null || "".equals(codeString)) 529 return null; 530 if ("closed".equals(codeString)) 531 return SlicingRules.CLOSED; 532 if ("open".equals(codeString)) 533 return SlicingRules.OPEN; 534 if ("openAtEnd".equals(codeString)) 535 return SlicingRules.OPENATEND; 536 throw new IllegalArgumentException("Unknown SlicingRules code '" + codeString + "'"); 537 } 538 539 public Enumeration<SlicingRules> fromType(PrimitiveType<?> code) throws FHIRException { 540 if (code == null) 541 return null; 542 if (code.isEmpty()) 543 return new Enumeration<SlicingRules>(this, SlicingRules.NULL, code); 544 String codeString = code.asStringValue(); 545 if (codeString == null || "".equals(codeString)) 546 return new Enumeration<SlicingRules>(this, SlicingRules.NULL, code); 547 if ("closed".equals(codeString)) 548 return new Enumeration<SlicingRules>(this, SlicingRules.CLOSED, code); 549 if ("open".equals(codeString)) 550 return new Enumeration<SlicingRules>(this, SlicingRules.OPEN, code); 551 if ("openAtEnd".equals(codeString)) 552 return new Enumeration<SlicingRules>(this, SlicingRules.OPENATEND, code); 553 throw new FHIRException("Unknown SlicingRules code '" + codeString + "'"); 554 } 555 556 public String toCode(SlicingRules code) { 557 if (code == SlicingRules.NULL) 558 return null; 559 if (code == SlicingRules.CLOSED) 560 return "closed"; 561 if (code == SlicingRules.OPEN) 562 return "open"; 563 if (code == SlicingRules.OPENATEND) 564 return "openAtEnd"; 565 return "?"; 566 } 567 568 public String toSystem(SlicingRules code) { 569 return code.getSystem(); 570 } 571 } 572 573 public enum AggregationMode { 574 /** 575 * The reference is a local reference to a contained resource. 576 */ 577 CONTAINED, 578 /** 579 * The reference to a resource that has to be resolved externally to the 580 * resource that includes the reference. 581 */ 582 REFERENCED, 583 /** 584 * The resource the reference points to will be found in the same bundle as the 585 * resource that includes the reference. 586 */ 587 BUNDLED, 588 /** 589 * added to help the parsers with the generic types 590 */ 591 NULL; 592 593 public static AggregationMode fromCode(String codeString) throws FHIRException { 594 if (codeString == null || "".equals(codeString)) 595 return null; 596 if ("contained".equals(codeString)) 597 return CONTAINED; 598 if ("referenced".equals(codeString)) 599 return REFERENCED; 600 if ("bundled".equals(codeString)) 601 return BUNDLED; 602 if (Configuration.isAcceptInvalidEnums()) 603 return null; 604 else 605 throw new FHIRException("Unknown AggregationMode code '" + codeString + "'"); 606 } 607 608 public String toCode() { 609 switch (this) { 610 case CONTAINED: 611 return "contained"; 612 case REFERENCED: 613 return "referenced"; 614 case BUNDLED: 615 return "bundled"; 616 case NULL: 617 return null; 618 default: 619 return "?"; 620 } 621 } 622 623 public String getSystem() { 624 switch (this) { 625 case CONTAINED: 626 return "http://hl7.org/fhir/resource-aggregation-mode"; 627 case REFERENCED: 628 return "http://hl7.org/fhir/resource-aggregation-mode"; 629 case BUNDLED: 630 return "http://hl7.org/fhir/resource-aggregation-mode"; 631 case NULL: 632 return null; 633 default: 634 return "?"; 635 } 636 } 637 638 public String getDefinition() { 639 switch (this) { 640 case CONTAINED: 641 return "The reference is a local reference to a contained resource."; 642 case REFERENCED: 643 return "The reference to a resource that has to be resolved externally to the resource that includes the reference."; 644 case BUNDLED: 645 return "The resource the reference points to will be found in the same bundle as the resource that includes the reference."; 646 case NULL: 647 return null; 648 default: 649 return "?"; 650 } 651 } 652 653 public String getDisplay() { 654 switch (this) { 655 case CONTAINED: 656 return "Contained"; 657 case REFERENCED: 658 return "Referenced"; 659 case BUNDLED: 660 return "Bundled"; 661 case NULL: 662 return null; 663 default: 664 return "?"; 665 } 666 } 667 } 668 669 public static class AggregationModeEnumFactory implements EnumFactory<AggregationMode> { 670 public AggregationMode fromCode(String codeString) throws IllegalArgumentException { 671 if (codeString == null || "".equals(codeString)) 672 if (codeString == null || "".equals(codeString)) 673 return null; 674 if ("contained".equals(codeString)) 675 return AggregationMode.CONTAINED; 676 if ("referenced".equals(codeString)) 677 return AggregationMode.REFERENCED; 678 if ("bundled".equals(codeString)) 679 return AggregationMode.BUNDLED; 680 throw new IllegalArgumentException("Unknown AggregationMode code '" + codeString + "'"); 681 } 682 683 public Enumeration<AggregationMode> fromType(PrimitiveType<?> code) throws FHIRException { 684 if (code == null) 685 return null; 686 if (code.isEmpty()) 687 return new Enumeration<AggregationMode>(this, AggregationMode.NULL, code); 688 String codeString = code.asStringValue(); 689 if (codeString == null || "".equals(codeString)) 690 return new Enumeration<AggregationMode>(this, AggregationMode.NULL, code); 691 if ("contained".equals(codeString)) 692 return new Enumeration<AggregationMode>(this, AggregationMode.CONTAINED, code); 693 if ("referenced".equals(codeString)) 694 return new Enumeration<AggregationMode>(this, AggregationMode.REFERENCED, code); 695 if ("bundled".equals(codeString)) 696 return new Enumeration<AggregationMode>(this, AggregationMode.BUNDLED, code); 697 throw new FHIRException("Unknown AggregationMode code '" + codeString + "'"); 698 } 699 700 public String toCode(AggregationMode code) { 701 if (code == AggregationMode.NULL) 702 return null; 703 if (code == AggregationMode.CONTAINED) 704 return "contained"; 705 if (code == AggregationMode.REFERENCED) 706 return "referenced"; 707 if (code == AggregationMode.BUNDLED) 708 return "bundled"; 709 return "?"; 710 } 711 712 public String toSystem(AggregationMode code) { 713 return code.getSystem(); 714 } 715 } 716 717 public enum ReferenceVersionRules { 718 /** 719 * The reference may be either version independent or version specific. 720 */ 721 EITHER, 722 /** 723 * The reference must be version independent. 724 */ 725 INDEPENDENT, 726 /** 727 * The reference must be version specific. 728 */ 729 SPECIFIC, 730 /** 731 * added to help the parsers with the generic types 732 */ 733 NULL; 734 735 public static ReferenceVersionRules fromCode(String codeString) throws FHIRException { 736 if (codeString == null || "".equals(codeString)) 737 return null; 738 if ("either".equals(codeString)) 739 return EITHER; 740 if ("independent".equals(codeString)) 741 return INDEPENDENT; 742 if ("specific".equals(codeString)) 743 return SPECIFIC; 744 if (Configuration.isAcceptInvalidEnums()) 745 return null; 746 else 747 throw new FHIRException("Unknown ReferenceVersionRules code '" + codeString + "'"); 748 } 749 750 public String toCode() { 751 switch (this) { 752 case EITHER: 753 return "either"; 754 case INDEPENDENT: 755 return "independent"; 756 case SPECIFIC: 757 return "specific"; 758 case NULL: 759 return null; 760 default: 761 return "?"; 762 } 763 } 764 765 public String getSystem() { 766 switch (this) { 767 case EITHER: 768 return "http://hl7.org/fhir/reference-version-rules"; 769 case INDEPENDENT: 770 return "http://hl7.org/fhir/reference-version-rules"; 771 case SPECIFIC: 772 return "http://hl7.org/fhir/reference-version-rules"; 773 case NULL: 774 return null; 775 default: 776 return "?"; 777 } 778 } 779 780 public String getDefinition() { 781 switch (this) { 782 case EITHER: 783 return "The reference may be either version independent or version specific."; 784 case INDEPENDENT: 785 return "The reference must be version independent."; 786 case SPECIFIC: 787 return "The reference must be version specific."; 788 case NULL: 789 return null; 790 default: 791 return "?"; 792 } 793 } 794 795 public String getDisplay() { 796 switch (this) { 797 case EITHER: 798 return "Either Specific or independent"; 799 case INDEPENDENT: 800 return "Version independent"; 801 case SPECIFIC: 802 return "Version Specific"; 803 case NULL: 804 return null; 805 default: 806 return "?"; 807 } 808 } 809 } 810 811 public static class ReferenceVersionRulesEnumFactory implements EnumFactory<ReferenceVersionRules> { 812 public ReferenceVersionRules fromCode(String codeString) throws IllegalArgumentException { 813 if (codeString == null || "".equals(codeString)) 814 if (codeString == null || "".equals(codeString)) 815 return null; 816 if ("either".equals(codeString)) 817 return ReferenceVersionRules.EITHER; 818 if ("independent".equals(codeString)) 819 return ReferenceVersionRules.INDEPENDENT; 820 if ("specific".equals(codeString)) 821 return ReferenceVersionRules.SPECIFIC; 822 throw new IllegalArgumentException("Unknown ReferenceVersionRules code '" + codeString + "'"); 823 } 824 825 public Enumeration<ReferenceVersionRules> fromType(PrimitiveType<?> code) throws FHIRException { 826 if (code == null) 827 return null; 828 if (code.isEmpty()) 829 return new Enumeration<ReferenceVersionRules>(this, ReferenceVersionRules.NULL, code); 830 String codeString = code.asStringValue(); 831 if (codeString == null || "".equals(codeString)) 832 return new Enumeration<ReferenceVersionRules>(this, ReferenceVersionRules.NULL, code); 833 if ("either".equals(codeString)) 834 return new Enumeration<ReferenceVersionRules>(this, ReferenceVersionRules.EITHER, code); 835 if ("independent".equals(codeString)) 836 return new Enumeration<ReferenceVersionRules>(this, ReferenceVersionRules.INDEPENDENT, code); 837 if ("specific".equals(codeString)) 838 return new Enumeration<ReferenceVersionRules>(this, ReferenceVersionRules.SPECIFIC, code); 839 throw new FHIRException("Unknown ReferenceVersionRules code '" + codeString + "'"); 840 } 841 842 public String toCode(ReferenceVersionRules code) { 843 if (code == ReferenceVersionRules.NULL) 844 return null; 845 if (code == ReferenceVersionRules.EITHER) 846 return "either"; 847 if (code == ReferenceVersionRules.INDEPENDENT) 848 return "independent"; 849 if (code == ReferenceVersionRules.SPECIFIC) 850 return "specific"; 851 return "?"; 852 } 853 854 public String toSystem(ReferenceVersionRules code) { 855 return code.getSystem(); 856 } 857 } 858 859 public enum ConstraintSeverity { 860 /** 861 * If the constraint is violated, the resource is not conformant. 862 */ 863 ERROR, 864 /** 865 * If the constraint is violated, the resource is conformant, but it is not 866 * necessarily following best practice. 867 */ 868 WARNING, 869 /** 870 * added to help the parsers with the generic types 871 */ 872 NULL; 873 874 public static ConstraintSeverity fromCode(String codeString) throws FHIRException { 875 if (codeString == null || "".equals(codeString)) 876 return null; 877 if ("error".equals(codeString)) 878 return ERROR; 879 if ("warning".equals(codeString)) 880 return WARNING; 881 if (Configuration.isAcceptInvalidEnums()) 882 return null; 883 else 884 throw new FHIRException("Unknown ConstraintSeverity code '" + codeString + "'"); 885 } 886 887 public String toCode() { 888 switch (this) { 889 case ERROR: 890 return "error"; 891 case WARNING: 892 return "warning"; 893 case NULL: 894 return null; 895 default: 896 return "?"; 897 } 898 } 899 900 public String getSystem() { 901 switch (this) { 902 case ERROR: 903 return "http://hl7.org/fhir/constraint-severity"; 904 case WARNING: 905 return "http://hl7.org/fhir/constraint-severity"; 906 case NULL: 907 return null; 908 default: 909 return "?"; 910 } 911 } 912 913 public String getDefinition() { 914 switch (this) { 915 case ERROR: 916 return "If the constraint is violated, the resource is not conformant."; 917 case WARNING: 918 return "If the constraint is violated, the resource is conformant, but it is not necessarily following best practice."; 919 case NULL: 920 return null; 921 default: 922 return "?"; 923 } 924 } 925 926 public String getDisplay() { 927 switch (this) { 928 case ERROR: 929 return "Error"; 930 case WARNING: 931 return "Warning"; 932 case NULL: 933 return null; 934 default: 935 return "?"; 936 } 937 } 938 } 939 940 public static class ConstraintSeverityEnumFactory implements EnumFactory<ConstraintSeverity> { 941 public ConstraintSeverity fromCode(String codeString) throws IllegalArgumentException { 942 if (codeString == null || "".equals(codeString)) 943 if (codeString == null || "".equals(codeString)) 944 return null; 945 if ("error".equals(codeString)) 946 return ConstraintSeverity.ERROR; 947 if ("warning".equals(codeString)) 948 return ConstraintSeverity.WARNING; 949 throw new IllegalArgumentException("Unknown ConstraintSeverity code '" + codeString + "'"); 950 } 951 952 public Enumeration<ConstraintSeverity> fromType(PrimitiveType<?> code) throws FHIRException { 953 if (code == null) 954 return null; 955 if (code.isEmpty()) 956 return new Enumeration<ConstraintSeverity>(this, ConstraintSeverity.NULL, code); 957 String codeString = code.asStringValue(); 958 if (codeString == null || "".equals(codeString)) 959 return new Enumeration<ConstraintSeverity>(this, ConstraintSeverity.NULL, code); 960 if ("error".equals(codeString)) 961 return new Enumeration<ConstraintSeverity>(this, ConstraintSeverity.ERROR, code); 962 if ("warning".equals(codeString)) 963 return new Enumeration<ConstraintSeverity>(this, ConstraintSeverity.WARNING, code); 964 throw new FHIRException("Unknown ConstraintSeverity code '" + codeString + "'"); 965 } 966 967 public String toCode(ConstraintSeverity code) { 968 if (code == ConstraintSeverity.NULL) 969 return null; 970 if (code == ConstraintSeverity.ERROR) 971 return "error"; 972 if (code == ConstraintSeverity.WARNING) 973 return "warning"; 974 return "?"; 975 } 976 977 public String toSystem(ConstraintSeverity code) { 978 return code.getSystem(); 979 } 980 } 981 982 @Block() 983 public static class ElementDefinitionSlicingComponent extends Element implements IBaseDatatypeElement { 984 /** 985 * Designates which child elements are used to discriminate between the slices 986 * when processing an instance. If one or more discriminators are provided, the 987 * value of the child elements in the instance data SHALL completely distinguish 988 * which slice the element in the resource matches based on the allowed values 989 * for those elements in each of the slices. 990 */ 991 @Child(name = "discriminator", type = {}, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 992 @Description(shortDefinition = "Element values that are used to distinguish the slices", formalDefinition = "Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices.") 993 protected List<ElementDefinitionSlicingDiscriminatorComponent> discriminator; 994 995 /** 996 * A human-readable text description of how the slicing works. If there is no 997 * discriminator, this is required to be present to provide whatever information 998 * is possible about how the slices can be differentiated. 999 */ 1000 @Child(name = "description", type = { 1001 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1002 @Description(shortDefinition = "Text description of how slicing works (or not)", formalDefinition = "A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated.") 1003 protected StringType description; 1004 1005 /** 1006 * If the matching elements have to occur in the same order as defined in the 1007 * profile. 1008 */ 1009 @Child(name = "ordered", type = { 1010 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1011 @Description(shortDefinition = "If elements must be in same order as slices", formalDefinition = "If the matching elements have to occur in the same order as defined in the profile.") 1012 protected BooleanType ordered; 1013 1014 /** 1015 * Whether additional slices are allowed or not. When the slices are ordered, 1016 * profile authors can also say that additional slices are only allowed at the 1017 * end. 1018 */ 1019 @Child(name = "rules", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 1020 @Description(shortDefinition = "closed | open | openAtEnd", formalDefinition = "Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end.") 1021 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/resource-slicing-rules") 1022 protected Enumeration<SlicingRules> rules; 1023 1024 private static final long serialVersionUID = -311635839L; 1025 1026 /** 1027 * Constructor 1028 */ 1029 public ElementDefinitionSlicingComponent() { 1030 super(); 1031 } 1032 1033 /** 1034 * Constructor 1035 */ 1036 public ElementDefinitionSlicingComponent(Enumeration<SlicingRules> rules) { 1037 super(); 1038 this.rules = rules; 1039 } 1040 1041 /** 1042 * @return {@link #discriminator} (Designates which child elements are used to 1043 * discriminate between the slices when processing an instance. If one 1044 * or more discriminators are provided, the value of the child elements 1045 * in the instance data SHALL completely distinguish which slice the 1046 * element in the resource matches based on the allowed values for those 1047 * elements in each of the slices.) 1048 */ 1049 public List<ElementDefinitionSlicingDiscriminatorComponent> getDiscriminator() { 1050 if (this.discriminator == null) 1051 this.discriminator = new ArrayList<ElementDefinitionSlicingDiscriminatorComponent>(); 1052 return this.discriminator; 1053 } 1054 1055 /** 1056 * @return Returns a reference to <code>this</code> for easy method chaining 1057 */ 1058 public ElementDefinitionSlicingComponent setDiscriminator( 1059 List<ElementDefinitionSlicingDiscriminatorComponent> theDiscriminator) { 1060 this.discriminator = theDiscriminator; 1061 return this; 1062 } 1063 1064 public boolean hasDiscriminator() { 1065 if (this.discriminator == null) 1066 return false; 1067 for (ElementDefinitionSlicingDiscriminatorComponent item : this.discriminator) 1068 if (!item.isEmpty()) 1069 return true; 1070 return false; 1071 } 1072 1073 public ElementDefinitionSlicingDiscriminatorComponent addDiscriminator() { // 3 1074 ElementDefinitionSlicingDiscriminatorComponent t = new ElementDefinitionSlicingDiscriminatorComponent(); 1075 if (this.discriminator == null) 1076 this.discriminator = new ArrayList<ElementDefinitionSlicingDiscriminatorComponent>(); 1077 this.discriminator.add(t); 1078 return t; 1079 } 1080 1081 public ElementDefinitionSlicingComponent addDiscriminator(ElementDefinitionSlicingDiscriminatorComponent t) { // 3 1082 if (t == null) 1083 return this; 1084 if (this.discriminator == null) 1085 this.discriminator = new ArrayList<ElementDefinitionSlicingDiscriminatorComponent>(); 1086 this.discriminator.add(t); 1087 return this; 1088 } 1089 1090 /** 1091 * @return The first repetition of repeating field {@link #discriminator}, 1092 * creating it if it does not already exist 1093 */ 1094 public ElementDefinitionSlicingDiscriminatorComponent getDiscriminatorFirstRep() { 1095 if (getDiscriminator().isEmpty()) { 1096 addDiscriminator(); 1097 } 1098 return getDiscriminator().get(0); 1099 } 1100 1101 /** 1102 * @return {@link #description} (A human-readable text description of how the 1103 * slicing works. If there is no discriminator, this is required to be 1104 * present to provide whatever information is possible about how the 1105 * slices can be differentiated.). This is the underlying object with 1106 * id, value and extensions. The accessor "getDescription" gives direct 1107 * access to the value 1108 */ 1109 public StringType getDescriptionElement() { 1110 if (this.description == null) 1111 if (Configuration.errorOnAutoCreate()) 1112 throw new Error("Attempt to auto-create ElementDefinitionSlicingComponent.description"); 1113 else if (Configuration.doAutoCreate()) 1114 this.description = new StringType(); // bb 1115 return this.description; 1116 } 1117 1118 public boolean hasDescriptionElement() { 1119 return this.description != null && !this.description.isEmpty(); 1120 } 1121 1122 public boolean hasDescription() { 1123 return this.description != null && !this.description.isEmpty(); 1124 } 1125 1126 /** 1127 * @param value {@link #description} (A human-readable text description of how 1128 * the slicing works. If there is no discriminator, this is 1129 * required to be present to provide whatever information is 1130 * possible about how the slices can be differentiated.). This is 1131 * the underlying object with id, value and extensions. The 1132 * accessor "getDescription" gives direct access to the value 1133 */ 1134 public ElementDefinitionSlicingComponent setDescriptionElement(StringType value) { 1135 this.description = value; 1136 return this; 1137 } 1138 1139 /** 1140 * @return A human-readable text description of how the slicing works. If there 1141 * is no discriminator, this is required to be present to provide 1142 * whatever information is possible about how the slices can be 1143 * differentiated. 1144 */ 1145 public String getDescription() { 1146 return this.description == null ? null : this.description.getValue(); 1147 } 1148 1149 /** 1150 * @param value A human-readable text description of how the slicing works. If 1151 * there is no discriminator, this is required to be present to 1152 * provide whatever information is possible about how the slices 1153 * can be differentiated. 1154 */ 1155 public ElementDefinitionSlicingComponent setDescription(String value) { 1156 if (Utilities.noString(value)) 1157 this.description = null; 1158 else { 1159 if (this.description == null) 1160 this.description = new StringType(); 1161 this.description.setValue(value); 1162 } 1163 return this; 1164 } 1165 1166 /** 1167 * @return {@link #ordered} (If the matching elements have to occur in the same 1168 * order as defined in the profile.). This is the underlying object with 1169 * id, value and extensions. The accessor "getOrdered" gives direct 1170 * access to the value 1171 */ 1172 public BooleanType getOrderedElement() { 1173 if (this.ordered == null) 1174 if (Configuration.errorOnAutoCreate()) 1175 throw new Error("Attempt to auto-create ElementDefinitionSlicingComponent.ordered"); 1176 else if (Configuration.doAutoCreate()) 1177 this.ordered = new BooleanType(); // bb 1178 return this.ordered; 1179 } 1180 1181 public boolean hasOrderedElement() { 1182 return this.ordered != null && !this.ordered.isEmpty(); 1183 } 1184 1185 public boolean hasOrdered() { 1186 return this.ordered != null && !this.ordered.isEmpty(); 1187 } 1188 1189 /** 1190 * @param value {@link #ordered} (If the matching elements have to occur in the 1191 * same order as defined in the profile.). This is the underlying 1192 * object with id, value and extensions. The accessor "getOrdered" 1193 * gives direct access to the value 1194 */ 1195 public ElementDefinitionSlicingComponent setOrderedElement(BooleanType value) { 1196 this.ordered = value; 1197 return this; 1198 } 1199 1200 /** 1201 * @return If the matching elements have to occur in the same order as defined 1202 * in the profile. 1203 */ 1204 public boolean getOrdered() { 1205 return this.ordered == null || this.ordered.isEmpty() ? false : this.ordered.getValue(); 1206 } 1207 1208 /** 1209 * @param value If the matching elements have to occur in the same order as 1210 * defined in the profile. 1211 */ 1212 public ElementDefinitionSlicingComponent setOrdered(boolean value) { 1213 if (this.ordered == null) 1214 this.ordered = new BooleanType(); 1215 this.ordered.setValue(value); 1216 return this; 1217 } 1218 1219 /** 1220 * @return {@link #rules} (Whether additional slices are allowed or not. When 1221 * the slices are ordered, profile authors can also say that additional 1222 * slices are only allowed at the end.). This is the underlying object 1223 * with id, value and extensions. The accessor "getRules" gives direct 1224 * access to the value 1225 */ 1226 public Enumeration<SlicingRules> getRulesElement() { 1227 if (this.rules == null) 1228 if (Configuration.errorOnAutoCreate()) 1229 throw new Error("Attempt to auto-create ElementDefinitionSlicingComponent.rules"); 1230 else if (Configuration.doAutoCreate()) 1231 this.rules = new Enumeration<SlicingRules>(new SlicingRulesEnumFactory()); // bb 1232 return this.rules; 1233 } 1234 1235 public boolean hasRulesElement() { 1236 return this.rules != null && !this.rules.isEmpty(); 1237 } 1238 1239 public boolean hasRules() { 1240 return this.rules != null && !this.rules.isEmpty(); 1241 } 1242 1243 /** 1244 * @param value {@link #rules} (Whether additional slices are allowed or not. 1245 * When the slices are ordered, profile authors can also say that 1246 * additional slices are only allowed at the end.). This is the 1247 * underlying object with id, value and extensions. The accessor 1248 * "getRules" gives direct access to the value 1249 */ 1250 public ElementDefinitionSlicingComponent setRulesElement(Enumeration<SlicingRules> value) { 1251 this.rules = value; 1252 return this; 1253 } 1254 1255 /** 1256 * @return Whether additional slices are allowed or not. When the slices are 1257 * ordered, profile authors can also say that additional slices are only 1258 * allowed at the end. 1259 */ 1260 public SlicingRules getRules() { 1261 return this.rules == null ? null : this.rules.getValue(); 1262 } 1263 1264 /** 1265 * @param value Whether additional slices are allowed or not. When the slices 1266 * are ordered, profile authors can also say that additional slices 1267 * are only allowed at the end. 1268 */ 1269 public ElementDefinitionSlicingComponent setRules(SlicingRules value) { 1270 if (this.rules == null) 1271 this.rules = new Enumeration<SlicingRules>(new SlicingRulesEnumFactory()); 1272 this.rules.setValue(value); 1273 return this; 1274 } 1275 1276 protected void listChildren(List<Property> children) { 1277 super.listChildren(children); 1278 children.add(new Property("discriminator", "", 1279 "Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices.", 1280 0, java.lang.Integer.MAX_VALUE, discriminator)); 1281 children.add(new Property("description", "string", 1282 "A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated.", 1283 0, 1, description)); 1284 children.add(new Property("ordered", "boolean", 1285 "If the matching elements have to occur in the same order as defined in the profile.", 0, 1, ordered)); 1286 children.add(new Property("rules", "code", 1287 "Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end.", 1288 0, 1, rules)); 1289 } 1290 1291 @Override 1292 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1293 switch (_hash) { 1294 case -1888270692: 1295 /* discriminator */ return new Property("discriminator", "", 1296 "Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices.", 1297 0, java.lang.Integer.MAX_VALUE, discriminator); 1298 case -1724546052: 1299 /* description */ return new Property("description", "string", 1300 "A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated.", 1301 0, 1, description); 1302 case -1207109523: 1303 /* ordered */ return new Property("ordered", "boolean", 1304 "If the matching elements have to occur in the same order as defined in the profile.", 0, 1, ordered); 1305 case 108873975: 1306 /* rules */ return new Property("rules", "code", 1307 "Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end.", 1308 0, 1, rules); 1309 default: 1310 return super.getNamedProperty(_hash, _name, _checkValid); 1311 } 1312 1313 } 1314 1315 @Override 1316 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1317 switch (hash) { 1318 case -1888270692: 1319 /* discriminator */ return this.discriminator == null ? new Base[0] 1320 : this.discriminator.toArray(new Base[this.discriminator.size()]); // ElementDefinitionSlicingDiscriminatorComponent 1321 case -1724546052: 1322 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1323 case -1207109523: 1324 /* ordered */ return this.ordered == null ? new Base[0] : new Base[] { this.ordered }; // BooleanType 1325 case 108873975: 1326 /* rules */ return this.rules == null ? new Base[0] : new Base[] { this.rules }; // Enumeration<SlicingRules> 1327 default: 1328 return super.getProperty(hash, name, checkValid); 1329 } 1330 1331 } 1332 1333 @Override 1334 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1335 switch (hash) { 1336 case -1888270692: // discriminator 1337 this.getDiscriminator().add((ElementDefinitionSlicingDiscriminatorComponent) value); // ElementDefinitionSlicingDiscriminatorComponent 1338 return value; 1339 case -1724546052: // description 1340 this.description = castToString(value); // StringType 1341 return value; 1342 case -1207109523: // ordered 1343 this.ordered = castToBoolean(value); // BooleanType 1344 return value; 1345 case 108873975: // rules 1346 value = new SlicingRulesEnumFactory().fromType(castToCode(value)); 1347 this.rules = (Enumeration) value; // Enumeration<SlicingRules> 1348 return value; 1349 default: 1350 return super.setProperty(hash, name, value); 1351 } 1352 1353 } 1354 1355 @Override 1356 public Base setProperty(String name, Base value) throws FHIRException { 1357 if (name.equals("discriminator")) { 1358 this.getDiscriminator().add((ElementDefinitionSlicingDiscriminatorComponent) value); 1359 } else if (name.equals("description")) { 1360 this.description = castToString(value); // StringType 1361 } else if (name.equals("ordered")) { 1362 this.ordered = castToBoolean(value); // BooleanType 1363 } else if (name.equals("rules")) { 1364 value = new SlicingRulesEnumFactory().fromType(castToCode(value)); 1365 this.rules = (Enumeration) value; // Enumeration<SlicingRules> 1366 } else 1367 return super.setProperty(name, value); 1368 return value; 1369 } 1370 1371 @Override 1372 public void removeChild(String name, Base value) throws FHIRException { 1373 if (name.equals("discriminator")) { 1374 this.getDiscriminator().remove((ElementDefinitionSlicingDiscriminatorComponent) value); 1375 } else if (name.equals("description")) { 1376 this.description = null; 1377 } else if (name.equals("ordered")) { 1378 this.ordered = null; 1379 } else if (name.equals("rules")) { 1380 this.rules = null; 1381 } else 1382 super.removeChild(name, value); 1383 1384 } 1385 1386 @Override 1387 public Base makeProperty(int hash, String name) throws FHIRException { 1388 switch (hash) { 1389 case -1888270692: 1390 return addDiscriminator(); 1391 case -1724546052: 1392 return getDescriptionElement(); 1393 case -1207109523: 1394 return getOrderedElement(); 1395 case 108873975: 1396 return getRulesElement(); 1397 default: 1398 return super.makeProperty(hash, name); 1399 } 1400 1401 } 1402 1403 @Override 1404 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1405 switch (hash) { 1406 case -1888270692: 1407 /* discriminator */ return new String[] {}; 1408 case -1724546052: 1409 /* description */ return new String[] { "string" }; 1410 case -1207109523: 1411 /* ordered */ return new String[] { "boolean" }; 1412 case 108873975: 1413 /* rules */ return new String[] { "code" }; 1414 default: 1415 return super.getTypesForProperty(hash, name); 1416 } 1417 1418 } 1419 1420 @Override 1421 public Base addChild(String name) throws FHIRException { 1422 if (name.equals("discriminator")) { 1423 return addDiscriminator(); 1424 } else if (name.equals("description")) { 1425 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.description"); 1426 } else if (name.equals("ordered")) { 1427 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.ordered"); 1428 } else if (name.equals("rules")) { 1429 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.rules"); 1430 } else 1431 return super.addChild(name); 1432 } 1433 1434 public ElementDefinitionSlicingComponent copy() { 1435 ElementDefinitionSlicingComponent dst = new ElementDefinitionSlicingComponent(); 1436 copyValues(dst); 1437 return dst; 1438 } 1439 1440 public void copyValues(ElementDefinitionSlicingComponent dst) { 1441 super.copyValues(dst); 1442 if (discriminator != null) { 1443 dst.discriminator = new ArrayList<ElementDefinitionSlicingDiscriminatorComponent>(); 1444 for (ElementDefinitionSlicingDiscriminatorComponent i : discriminator) 1445 dst.discriminator.add(i.copy()); 1446 } 1447 ; 1448 dst.description = description == null ? null : description.copy(); 1449 dst.ordered = ordered == null ? null : ordered.copy(); 1450 dst.rules = rules == null ? null : rules.copy(); 1451 } 1452 1453 @Override 1454 public boolean equalsDeep(Base other_) { 1455 if (!super.equalsDeep(other_)) 1456 return false; 1457 if (!(other_ instanceof ElementDefinitionSlicingComponent)) 1458 return false; 1459 ElementDefinitionSlicingComponent o = (ElementDefinitionSlicingComponent) other_; 1460 return compareDeep(discriminator, o.discriminator, true) && compareDeep(description, o.description, true) 1461 && compareDeep(ordered, o.ordered, true) && compareDeep(rules, o.rules, true); 1462 } 1463 1464 @Override 1465 public boolean equalsShallow(Base other_) { 1466 if (!super.equalsShallow(other_)) 1467 return false; 1468 if (!(other_ instanceof ElementDefinitionSlicingComponent)) 1469 return false; 1470 ElementDefinitionSlicingComponent o = (ElementDefinitionSlicingComponent) other_; 1471 return compareValues(description, o.description, true) && compareValues(ordered, o.ordered, true) 1472 && compareValues(rules, o.rules, true); 1473 } 1474 1475 public boolean isEmpty() { 1476 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(discriminator, description, ordered, rules); 1477 } 1478 1479 public String fhirType() { 1480 return "ElementDefinition.slicing"; 1481 1482 } 1483 1484 } 1485 1486 @Block() 1487 public static class ElementDefinitionSlicingDiscriminatorComponent extends Element implements IBaseDatatypeElement { 1488 /** 1489 * How the element value is interpreted when discrimination is evaluated. 1490 */ 1491 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1492 @Description(shortDefinition = "value | exists | pattern | type | profile", formalDefinition = "How the element value is interpreted when discrimination is evaluated.") 1493 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/discriminator-type") 1494 protected Enumeration<DiscriminatorType> type; 1495 1496 /** 1497 * A FHIRPath expression, using [the simple subset of 1498 * FHIRPath](fhirpath.html#simple), that is used to identify the element on 1499 * which discrimination is based. 1500 */ 1501 @Child(name = "path", type = { StringType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1502 @Description(shortDefinition = "Path to element value", formalDefinition = "A FHIRPath expression, using [the simple subset of FHIRPath](fhirpath.html#simple), that is used to identify the element on which discrimination is based.") 1503 protected StringType path; 1504 1505 private static final long serialVersionUID = 1151159293L; 1506 1507 /** 1508 * Constructor 1509 */ 1510 public ElementDefinitionSlicingDiscriminatorComponent() { 1511 super(); 1512 } 1513 1514 /** 1515 * Constructor 1516 */ 1517 public ElementDefinitionSlicingDiscriminatorComponent(Enumeration<DiscriminatorType> type, StringType path) { 1518 super(); 1519 this.type = type; 1520 this.path = path; 1521 } 1522 1523 /** 1524 * @return {@link #type} (How the element value is interpreted when 1525 * discrimination is evaluated.). This is the underlying object with id, 1526 * value and extensions. The accessor "getType" gives direct access to 1527 * the value 1528 */ 1529 public Enumeration<DiscriminatorType> getTypeElement() { 1530 if (this.type == null) 1531 if (Configuration.errorOnAutoCreate()) 1532 throw new Error("Attempt to auto-create ElementDefinitionSlicingDiscriminatorComponent.type"); 1533 else if (Configuration.doAutoCreate()) 1534 this.type = new Enumeration<DiscriminatorType>(new DiscriminatorTypeEnumFactory()); // bb 1535 return this.type; 1536 } 1537 1538 public boolean hasTypeElement() { 1539 return this.type != null && !this.type.isEmpty(); 1540 } 1541 1542 public boolean hasType() { 1543 return this.type != null && !this.type.isEmpty(); 1544 } 1545 1546 /** 1547 * @param value {@link #type} (How the element value is interpreted when 1548 * discrimination is evaluated.). This is the underlying object 1549 * with id, value and extensions. The accessor "getType" gives 1550 * direct access to the value 1551 */ 1552 public ElementDefinitionSlicingDiscriminatorComponent setTypeElement(Enumeration<DiscriminatorType> value) { 1553 this.type = value; 1554 return this; 1555 } 1556 1557 /** 1558 * @return How the element value is interpreted when discrimination is 1559 * evaluated. 1560 */ 1561 public DiscriminatorType getType() { 1562 return this.type == null ? null : this.type.getValue(); 1563 } 1564 1565 /** 1566 * @param value How the element value is interpreted when discrimination is 1567 * evaluated. 1568 */ 1569 public ElementDefinitionSlicingDiscriminatorComponent setType(DiscriminatorType value) { 1570 if (this.type == null) 1571 this.type = new Enumeration<DiscriminatorType>(new DiscriminatorTypeEnumFactory()); 1572 this.type.setValue(value); 1573 return this; 1574 } 1575 1576 /** 1577 * @return {@link #path} (A FHIRPath expression, using [the simple subset of 1578 * FHIRPath](fhirpath.html#simple), that is used to identify the element 1579 * on which discrimination is based.). This is the underlying object 1580 * with id, value and extensions. The accessor "getPath" gives direct 1581 * access to the value 1582 */ 1583 public StringType getPathElement() { 1584 if (this.path == null) 1585 if (Configuration.errorOnAutoCreate()) 1586 throw new Error("Attempt to auto-create ElementDefinitionSlicingDiscriminatorComponent.path"); 1587 else if (Configuration.doAutoCreate()) 1588 this.path = new StringType(); // bb 1589 return this.path; 1590 } 1591 1592 public boolean hasPathElement() { 1593 return this.path != null && !this.path.isEmpty(); 1594 } 1595 1596 public boolean hasPath() { 1597 return this.path != null && !this.path.isEmpty(); 1598 } 1599 1600 /** 1601 * @param value {@link #path} (A FHIRPath expression, using [the simple subset 1602 * of FHIRPath](fhirpath.html#simple), that is used to identify the 1603 * element on which discrimination is based.). This is the 1604 * underlying object with id, value and extensions. The accessor 1605 * "getPath" gives direct access to the value 1606 */ 1607 public ElementDefinitionSlicingDiscriminatorComponent setPathElement(StringType value) { 1608 this.path = value; 1609 return this; 1610 } 1611 1612 /** 1613 * @return A FHIRPath expression, using [the simple subset of 1614 * FHIRPath](fhirpath.html#simple), that is used to identify the element 1615 * on which discrimination is based. 1616 */ 1617 public String getPath() { 1618 return this.path == null ? null : this.path.getValue(); 1619 } 1620 1621 /** 1622 * @param value A FHIRPath expression, using [the simple subset of 1623 * FHIRPath](fhirpath.html#simple), that is used to identify the 1624 * element on which discrimination is based. 1625 */ 1626 public ElementDefinitionSlicingDiscriminatorComponent setPath(String value) { 1627 if (this.path == null) 1628 this.path = new StringType(); 1629 this.path.setValue(value); 1630 return this; 1631 } 1632 1633 protected void listChildren(List<Property> children) { 1634 super.listChildren(children); 1635 children.add(new Property("type", "code", 1636 "How the element value is interpreted when discrimination is evaluated.", 0, 1, type)); 1637 children.add(new Property("path", "string", 1638 "A FHIRPath expression, using [the simple subset of FHIRPath](fhirpath.html#simple), that is used to identify the element on which discrimination is based.", 1639 0, 1, path)); 1640 } 1641 1642 @Override 1643 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1644 switch (_hash) { 1645 case 3575610: 1646 /* type */ return new Property("type", "code", 1647 "How the element value is interpreted when discrimination is evaluated.", 0, 1, type); 1648 case 3433509: 1649 /* path */ return new Property("path", "string", 1650 "A FHIRPath expression, using [the simple subset of FHIRPath](fhirpath.html#simple), that is used to identify the element on which discrimination is based.", 1651 0, 1, path); 1652 default: 1653 return super.getNamedProperty(_hash, _name, _checkValid); 1654 } 1655 1656 } 1657 1658 @Override 1659 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1660 switch (hash) { 1661 case 3575610: 1662 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<DiscriminatorType> 1663 case 3433509: 1664 /* path */ return this.path == null ? new Base[0] : new Base[] { this.path }; // StringType 1665 default: 1666 return super.getProperty(hash, name, checkValid); 1667 } 1668 1669 } 1670 1671 @Override 1672 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1673 switch (hash) { 1674 case 3575610: // type 1675 value = new DiscriminatorTypeEnumFactory().fromType(castToCode(value)); 1676 this.type = (Enumeration) value; // Enumeration<DiscriminatorType> 1677 return value; 1678 case 3433509: // path 1679 this.path = castToString(value); // StringType 1680 return value; 1681 default: 1682 return super.setProperty(hash, name, value); 1683 } 1684 1685 } 1686 1687 @Override 1688 public Base setProperty(String name, Base value) throws FHIRException { 1689 if (name.equals("type")) { 1690 value = new DiscriminatorTypeEnumFactory().fromType(castToCode(value)); 1691 this.type = (Enumeration) value; // Enumeration<DiscriminatorType> 1692 } else if (name.equals("path")) { 1693 this.path = castToString(value); // StringType 1694 } else 1695 return super.setProperty(name, value); 1696 return value; 1697 } 1698 1699 @Override 1700 public void removeChild(String name, Base value) throws FHIRException { 1701 if (name.equals("type")) { 1702 this.type = null; 1703 } else if (name.equals("path")) { 1704 this.path = null; 1705 } else 1706 super.removeChild(name, value); 1707 1708 } 1709 1710 @Override 1711 public Base makeProperty(int hash, String name) throws FHIRException { 1712 switch (hash) { 1713 case 3575610: 1714 return getTypeElement(); 1715 case 3433509: 1716 return getPathElement(); 1717 default: 1718 return super.makeProperty(hash, name); 1719 } 1720 1721 } 1722 1723 @Override 1724 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1725 switch (hash) { 1726 case 3575610: 1727 /* type */ return new String[] { "code" }; 1728 case 3433509: 1729 /* path */ return new String[] { "string" }; 1730 default: 1731 return super.getTypesForProperty(hash, name); 1732 } 1733 1734 } 1735 1736 @Override 1737 public Base addChild(String name) throws FHIRException { 1738 if (name.equals("type")) { 1739 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.type"); 1740 } else if (name.equals("path")) { 1741 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.path"); 1742 } else 1743 return super.addChild(name); 1744 } 1745 1746 public ElementDefinitionSlicingDiscriminatorComponent copy() { 1747 ElementDefinitionSlicingDiscriminatorComponent dst = new ElementDefinitionSlicingDiscriminatorComponent(); 1748 copyValues(dst); 1749 return dst; 1750 } 1751 1752 public void copyValues(ElementDefinitionSlicingDiscriminatorComponent dst) { 1753 super.copyValues(dst); 1754 dst.type = type == null ? null : type.copy(); 1755 dst.path = path == null ? null : path.copy(); 1756 } 1757 1758 @Override 1759 public boolean equalsDeep(Base other_) { 1760 if (!super.equalsDeep(other_)) 1761 return false; 1762 if (!(other_ instanceof ElementDefinitionSlicingDiscriminatorComponent)) 1763 return false; 1764 ElementDefinitionSlicingDiscriminatorComponent o = (ElementDefinitionSlicingDiscriminatorComponent) other_; 1765 return compareDeep(type, o.type, true) && compareDeep(path, o.path, true); 1766 } 1767 1768 @Override 1769 public boolean equalsShallow(Base other_) { 1770 if (!super.equalsShallow(other_)) 1771 return false; 1772 if (!(other_ instanceof ElementDefinitionSlicingDiscriminatorComponent)) 1773 return false; 1774 ElementDefinitionSlicingDiscriminatorComponent o = (ElementDefinitionSlicingDiscriminatorComponent) other_; 1775 return compareValues(type, o.type, true) && compareValues(path, o.path, true); 1776 } 1777 1778 public boolean isEmpty() { 1779 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, path); 1780 } 1781 1782 public String fhirType() { 1783 return "ElementDefinition.slicing.discriminator"; 1784 1785 } 1786 1787 } 1788 1789 @Block() 1790 public static class ElementDefinitionBaseComponent extends Element implements IBaseDatatypeElement { 1791 /** 1792 * The Path that identifies the base element - this matches the 1793 * ElementDefinition.path for that element. Across FHIR, there is only one base 1794 * definition of any element - that is, an element definition on a 1795 * [[[StructureDefinition]]] without a StructureDefinition.base. 1796 */ 1797 @Child(name = "path", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1798 @Description(shortDefinition = "Path that identifies the base element", formalDefinition = "The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [[[StructureDefinition]]] without a StructureDefinition.base.") 1799 protected StringType path; 1800 1801 /** 1802 * Minimum cardinality of the base element identified by the path. 1803 */ 1804 @Child(name = "min", type = { 1805 UnsignedIntType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1806 @Description(shortDefinition = "Min cardinality of the base element", formalDefinition = "Minimum cardinality of the base element identified by the path.") 1807 protected UnsignedIntType min; 1808 1809 /** 1810 * Maximum cardinality of the base element identified by the path. 1811 */ 1812 @Child(name = "max", type = { StringType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 1813 @Description(shortDefinition = "Max cardinality of the base element", formalDefinition = "Maximum cardinality of the base element identified by the path.") 1814 protected StringType max; 1815 1816 private static final long serialVersionUID = -1412704221L; 1817 1818 /** 1819 * Constructor 1820 */ 1821 public ElementDefinitionBaseComponent() { 1822 super(); 1823 } 1824 1825 /** 1826 * Constructor 1827 */ 1828 public ElementDefinitionBaseComponent(StringType path, UnsignedIntType min, StringType max) { 1829 super(); 1830 this.path = path; 1831 this.min = min; 1832 this.max = max; 1833 } 1834 1835 /** 1836 * @return {@link #path} (The Path that identifies the base element - this 1837 * matches the ElementDefinition.path for that element. Across FHIR, 1838 * there is only one base definition of any element - that is, an 1839 * element definition on a [[[StructureDefinition]]] without a 1840 * StructureDefinition.base.). This is the underlying object with id, 1841 * value and extensions. The accessor "getPath" gives direct access to 1842 * the value 1843 */ 1844 public StringType getPathElement() { 1845 if (this.path == null) 1846 if (Configuration.errorOnAutoCreate()) 1847 throw new Error("Attempt to auto-create ElementDefinitionBaseComponent.path"); 1848 else if (Configuration.doAutoCreate()) 1849 this.path = new StringType(); // bb 1850 return this.path; 1851 } 1852 1853 public boolean hasPathElement() { 1854 return this.path != null && !this.path.isEmpty(); 1855 } 1856 1857 public boolean hasPath() { 1858 return this.path != null && !this.path.isEmpty(); 1859 } 1860 1861 /** 1862 * @param value {@link #path} (The Path that identifies the base element - this 1863 * matches the ElementDefinition.path for that element. Across 1864 * FHIR, there is only one base definition of any element - that 1865 * is, an element definition on a [[[StructureDefinition]]] without 1866 * a StructureDefinition.base.). This is the underlying object with 1867 * id, value and extensions. The accessor "getPath" gives direct 1868 * access to the value 1869 */ 1870 public ElementDefinitionBaseComponent setPathElement(StringType value) { 1871 this.path = value; 1872 return this; 1873 } 1874 1875 /** 1876 * @return The Path that identifies the base element - this matches the 1877 * ElementDefinition.path for that element. Across FHIR, there is only 1878 * one base definition of any element - that is, an element definition 1879 * on a [[[StructureDefinition]]] without a StructureDefinition.base. 1880 */ 1881 public String getPath() { 1882 return this.path == null ? null : this.path.getValue(); 1883 } 1884 1885 /** 1886 * @param value The Path that identifies the base element - this matches the 1887 * ElementDefinition.path for that element. Across FHIR, there is 1888 * only one base definition of any element - that is, an element 1889 * definition on a [[[StructureDefinition]]] without a 1890 * StructureDefinition.base. 1891 */ 1892 public ElementDefinitionBaseComponent setPath(String value) { 1893 if (this.path == null) 1894 this.path = new StringType(); 1895 this.path.setValue(value); 1896 return this; 1897 } 1898 1899 /** 1900 * @return {@link #min} (Minimum cardinality of the base element identified by 1901 * the path.). This is the underlying object with id, value and 1902 * extensions. The accessor "getMin" gives direct access to the value 1903 */ 1904 public UnsignedIntType getMinElement() { 1905 if (this.min == null) 1906 if (Configuration.errorOnAutoCreate()) 1907 throw new Error("Attempt to auto-create ElementDefinitionBaseComponent.min"); 1908 else if (Configuration.doAutoCreate()) 1909 this.min = new UnsignedIntType(); // bb 1910 return this.min; 1911 } 1912 1913 public boolean hasMinElement() { 1914 return this.min != null && !this.min.isEmpty(); 1915 } 1916 1917 public boolean hasMin() { 1918 return this.min != null && !this.min.isEmpty(); 1919 } 1920 1921 /** 1922 * @param value {@link #min} (Minimum cardinality of the base element identified 1923 * by the path.). This is the underlying object with id, value and 1924 * extensions. The accessor "getMin" gives direct access to the 1925 * value 1926 */ 1927 public ElementDefinitionBaseComponent setMinElement(UnsignedIntType value) { 1928 this.min = value; 1929 return this; 1930 } 1931 1932 /** 1933 * @return Minimum cardinality of the base element identified by the path. 1934 */ 1935 public int getMin() { 1936 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 1937 } 1938 1939 /** 1940 * @param value Minimum cardinality of the base element identified by the path. 1941 */ 1942 public ElementDefinitionBaseComponent setMin(int value) { 1943 if (this.min == null) 1944 this.min = new UnsignedIntType(); 1945 this.min.setValue(value); 1946 return this; 1947 } 1948 1949 /** 1950 * @return {@link #max} (Maximum cardinality of the base element identified by 1951 * the path.). This is the underlying object with id, value and 1952 * extensions. The accessor "getMax" gives direct access to the value 1953 */ 1954 public StringType getMaxElement() { 1955 if (this.max == null) 1956 if (Configuration.errorOnAutoCreate()) 1957 throw new Error("Attempt to auto-create ElementDefinitionBaseComponent.max"); 1958 else if (Configuration.doAutoCreate()) 1959 this.max = new StringType(); // bb 1960 return this.max; 1961 } 1962 1963 public boolean hasMaxElement() { 1964 return this.max != null && !this.max.isEmpty(); 1965 } 1966 1967 public boolean hasMax() { 1968 return this.max != null && !this.max.isEmpty(); 1969 } 1970 1971 /** 1972 * @param value {@link #max} (Maximum cardinality of the base element identified 1973 * by the path.). This is the underlying object with id, value and 1974 * extensions. The accessor "getMax" gives direct access to the 1975 * value 1976 */ 1977 public ElementDefinitionBaseComponent setMaxElement(StringType value) { 1978 this.max = value; 1979 return this; 1980 } 1981 1982 /** 1983 * @return Maximum cardinality of the base element identified by the path. 1984 */ 1985 public String getMax() { 1986 return this.max == null ? null : this.max.getValue(); 1987 } 1988 1989 /** 1990 * @param value Maximum cardinality of the base element identified by the path. 1991 */ 1992 public ElementDefinitionBaseComponent setMax(String value) { 1993 if (this.max == null) 1994 this.max = new StringType(); 1995 this.max.setValue(value); 1996 return this; 1997 } 1998 1999 protected void listChildren(List<Property> children) { 2000 super.listChildren(children); 2001 children.add(new Property("path", "string", 2002 "The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [[[StructureDefinition]]] without a StructureDefinition.base.", 2003 0, 1, path)); 2004 children.add(new Property("min", "unsignedInt", "Minimum cardinality of the base element identified by the path.", 2005 0, 1, min)); 2006 children.add( 2007 new Property("max", "string", "Maximum cardinality of the base element identified by the path.", 0, 1, max)); 2008 } 2009 2010 @Override 2011 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2012 switch (_hash) { 2013 case 3433509: 2014 /* path */ return new Property("path", "string", 2015 "The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [[[StructureDefinition]]] without a StructureDefinition.base.", 2016 0, 1, path); 2017 case 108114: 2018 /* min */ return new Property("min", "unsignedInt", 2019 "Minimum cardinality of the base element identified by the path.", 0, 1, min); 2020 case 107876: 2021 /* max */ return new Property("max", "string", 2022 "Maximum cardinality of the base element identified by the path.", 0, 1, max); 2023 default: 2024 return super.getNamedProperty(_hash, _name, _checkValid); 2025 } 2026 2027 } 2028 2029 @Override 2030 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2031 switch (hash) { 2032 case 3433509: 2033 /* path */ return this.path == null ? new Base[0] : new Base[] { this.path }; // StringType 2034 case 108114: 2035 /* min */ return this.min == null ? new Base[0] : new Base[] { this.min }; // UnsignedIntType 2036 case 107876: 2037 /* max */ return this.max == null ? new Base[0] : new Base[] { this.max }; // StringType 2038 default: 2039 return super.getProperty(hash, name, checkValid); 2040 } 2041 2042 } 2043 2044 @Override 2045 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2046 switch (hash) { 2047 case 3433509: // path 2048 this.path = castToString(value); // StringType 2049 return value; 2050 case 108114: // min 2051 this.min = castToUnsignedInt(value); // UnsignedIntType 2052 return value; 2053 case 107876: // max 2054 this.max = castToString(value); // StringType 2055 return value; 2056 default: 2057 return super.setProperty(hash, name, value); 2058 } 2059 2060 } 2061 2062 @Override 2063 public Base setProperty(String name, Base value) throws FHIRException { 2064 if (name.equals("path")) { 2065 this.path = castToString(value); // StringType 2066 } else if (name.equals("min")) { 2067 this.min = castToUnsignedInt(value); // UnsignedIntType 2068 } else if (name.equals("max")) { 2069 this.max = castToString(value); // StringType 2070 } else 2071 return super.setProperty(name, value); 2072 return value; 2073 } 2074 2075 @Override 2076 public void removeChild(String name, Base value) throws FHIRException { 2077 if (name.equals("path")) { 2078 this.path = null; 2079 } else if (name.equals("min")) { 2080 this.min = null; 2081 } else if (name.equals("max")) { 2082 this.max = null; 2083 } else 2084 super.removeChild(name, value); 2085 2086 } 2087 2088 @Override 2089 public Base makeProperty(int hash, String name) throws FHIRException { 2090 switch (hash) { 2091 case 3433509: 2092 return getPathElement(); 2093 case 108114: 2094 return getMinElement(); 2095 case 107876: 2096 return getMaxElement(); 2097 default: 2098 return super.makeProperty(hash, name); 2099 } 2100 2101 } 2102 2103 @Override 2104 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2105 switch (hash) { 2106 case 3433509: 2107 /* path */ return new String[] { "string" }; 2108 case 108114: 2109 /* min */ return new String[] { "unsignedInt" }; 2110 case 107876: 2111 /* max */ return new String[] { "string" }; 2112 default: 2113 return super.getTypesForProperty(hash, name); 2114 } 2115 2116 } 2117 2118 @Override 2119 public Base addChild(String name) throws FHIRException { 2120 if (name.equals("path")) { 2121 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.path"); 2122 } else if (name.equals("min")) { 2123 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.min"); 2124 } else if (name.equals("max")) { 2125 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.max"); 2126 } else 2127 return super.addChild(name); 2128 } 2129 2130 public ElementDefinitionBaseComponent copy() { 2131 ElementDefinitionBaseComponent dst = new ElementDefinitionBaseComponent(); 2132 copyValues(dst); 2133 return dst; 2134 } 2135 2136 public void copyValues(ElementDefinitionBaseComponent dst) { 2137 super.copyValues(dst); 2138 dst.path = path == null ? null : path.copy(); 2139 dst.min = min == null ? null : min.copy(); 2140 dst.max = max == null ? null : max.copy(); 2141 } 2142 2143 @Override 2144 public boolean equalsDeep(Base other_) { 2145 if (!super.equalsDeep(other_)) 2146 return false; 2147 if (!(other_ instanceof ElementDefinitionBaseComponent)) 2148 return false; 2149 ElementDefinitionBaseComponent o = (ElementDefinitionBaseComponent) other_; 2150 return compareDeep(path, o.path, true) && compareDeep(min, o.min, true) && compareDeep(max, o.max, true); 2151 } 2152 2153 @Override 2154 public boolean equalsShallow(Base other_) { 2155 if (!super.equalsShallow(other_)) 2156 return false; 2157 if (!(other_ instanceof ElementDefinitionBaseComponent)) 2158 return false; 2159 ElementDefinitionBaseComponent o = (ElementDefinitionBaseComponent) other_; 2160 return compareValues(path, o.path, true) && compareValues(min, o.min, true) && compareValues(max, o.max, true); 2161 } 2162 2163 public boolean isEmpty() { 2164 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, min, max); 2165 } 2166 2167 public String fhirType() { 2168 return "ElementDefinition.base"; 2169 2170 } 2171 2172 } 2173 2174 @Block() 2175 public static class TypeRefComponent extends Element implements IBaseDatatypeElement { 2176 /** 2177 * URL of Data type or Resource that is a(or the) type used for this element. 2178 * References are URLs that are relative to 2179 * http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to 2180 * http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only 2181 * allowed in logical models. 2182 */ 2183 @Child(name = "code", type = { UriType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2184 @Description(shortDefinition = "Data type or Resource (reference to definition)", formalDefinition = "URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. \"string\" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models.") 2185 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/defined-types") 2186 protected UriType code; 2187 2188 /** 2189 * Identifies a profile structure or implementation Guide that applies to the 2190 * datatype this element refers to. If any profiles are specified, then the 2191 * content must conform to at least one of them. The URL can be a local 2192 * reference - to a contained StructureDefinition, or a reference to another 2193 * StructureDefinition or Implementation Guide by a canonical URL. When an 2194 * implementation guide is specified, the type SHALL conform to at least one 2195 * profile defined in the implementation guide. 2196 */ 2197 @Child(name = "profile", type = { 2198 CanonicalType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2199 @Description(shortDefinition = "Profiles (StructureDefinition or IG) - one must apply", formalDefinition = "Identifies a profile structure or implementation Guide that applies to the datatype this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the type SHALL conform to at least one profile defined in the implementation guide.") 2200 protected List<CanonicalType> profile; 2201 2202 /** 2203 * Used when the type is "Reference" or "canonical", and identifies a profile 2204 * structure or implementation Guide that applies to the target of the reference 2205 * this element refers to. If any profiles are specified, then the content must 2206 * conform to at least one of them. The URL can be a local reference - to a 2207 * contained StructureDefinition, or a reference to another StructureDefinition 2208 * or Implementation Guide by a canonical URL. When an implementation guide is 2209 * specified, the target resource SHALL conform to at least one profile defined 2210 * in the implementation guide. 2211 */ 2212 @Child(name = "targetProfile", type = { 2213 CanonicalType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2214 @Description(shortDefinition = "Profile (StructureDefinition or IG) on the Reference/canonical target - one must apply", formalDefinition = "Used when the type is \"Reference\" or \"canonical\", and identifies a profile structure or implementation Guide that applies to the target of the reference this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.") 2215 protected List<CanonicalType> targetProfile; 2216 2217 /** 2218 * If the type is a reference to another resource, how the resource is or can be 2219 * aggregated - is it a contained resource, or a reference, and if the context 2220 * is a bundle, is it included in the bundle. 2221 */ 2222 @Child(name = "aggregation", type = { 2223 CodeType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2224 @Description(shortDefinition = "contained | referenced | bundled - how aggregated", formalDefinition = "If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle.") 2225 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/resource-aggregation-mode") 2226 protected List<Enumeration<AggregationMode>> aggregation; 2227 2228 /** 2229 * Whether this reference needs to be version specific or version independent, 2230 * or whether either can be used. 2231 */ 2232 @Child(name = "versioning", type = { 2233 CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 2234 @Description(shortDefinition = "either | independent | specific", formalDefinition = "Whether this reference needs to be version specific or version independent, or whether either can be used.") 2235 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/reference-version-rules") 2236 protected Enumeration<ReferenceVersionRules> versioning; 2237 2238 private static final long serialVersionUID = 957891653L; 2239 2240 /** 2241 * Constructor 2242 */ 2243 public TypeRefComponent() { 2244 super(); 2245 } 2246 2247 /** 2248 * Constructor 2249 */ 2250 public TypeRefComponent(UriType code) { 2251 super(); 2252 this.code = code; 2253 } 2254 2255 /** 2256 * @return {@link #code} (URL of Data type or Resource that is a(or the) type 2257 * used for this element. References are URLs that are relative to 2258 * http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference 2259 * to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are 2260 * only allowed in logical models.). This is the underlying object with 2261 * id, value and extensions. The accessor "getCode" gives direct access 2262 * to the value 2263 */ 2264 public UriType getCodeElement() { 2265 if (this.code == null) 2266 if (Configuration.errorOnAutoCreate()) 2267 throw new Error("Attempt to auto-create TypeRefComponent.code"); 2268 else if (Configuration.doAutoCreate()) 2269 this.code = new UriType(); // bb 2270 return this.code; 2271 } 2272 2273 public boolean hasCodeElement() { 2274 return this.code != null && !this.code.isEmpty(); 2275 } 2276 2277 public boolean hasCode() { 2278 return this.code != null && !this.code.isEmpty(); 2279 } 2280 2281 /** 2282 * @param value {@link #code} (URL of Data type or Resource that is a(or the) 2283 * type used for this element. References are URLs that are 2284 * relative to http://hl7.org/fhir/StructureDefinition e.g. 2285 * "string" is a reference to 2286 * http://hl7.org/fhir/StructureDefinition/string. Absolute URLs 2287 * are only allowed in logical models.). This is the underlying 2288 * object with id, value and extensions. The accessor "getCode" 2289 * gives direct access to the value 2290 */ 2291 public TypeRefComponent setCodeElement(UriType value) { 2292 this.code = value; 2293 return this; 2294 } 2295 2296 /** 2297 * @return URL of Data type or Resource that is a(or the) type used for this 2298 * element. References are URLs that are relative to 2299 * http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference 2300 * to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are 2301 * only allowed in logical models. 2302 */ 2303 public String getCode() { 2304 return this.code == null ? null : this.code.getValue(); 2305 } 2306 2307 /** 2308 * @param value URL of Data type or Resource that is a(or the) type used for 2309 * this element. References are URLs that are relative to 2310 * http://hl7.org/fhir/StructureDefinition e.g. "string" is a 2311 * reference to http://hl7.org/fhir/StructureDefinition/string. 2312 * Absolute URLs are only allowed in logical models. 2313 */ 2314 public TypeRefComponent setCode(String value) { 2315 if (this.code == null) 2316 this.code = new UriType(); 2317 this.code.setValue(value); 2318 return this; 2319 } 2320 2321 /** 2322 * @return {@link #profile} (Identifies a profile structure or implementation 2323 * Guide that applies to the datatype this element refers to. If any 2324 * profiles are specified, then the content must conform to at least one 2325 * of them. The URL can be a local reference - to a contained 2326 * StructureDefinition, or a reference to another StructureDefinition or 2327 * Implementation Guide by a canonical URL. When an implementation guide 2328 * is specified, the type SHALL conform to at least one profile defined 2329 * in the implementation guide.) 2330 */ 2331 public List<CanonicalType> getProfile() { 2332 if (this.profile == null) 2333 this.profile = new ArrayList<CanonicalType>(); 2334 return this.profile; 2335 } 2336 2337 /** 2338 * @return Returns a reference to <code>this</code> for easy method chaining 2339 */ 2340 public TypeRefComponent setProfile(List<CanonicalType> theProfile) { 2341 this.profile = theProfile; 2342 return this; 2343 } 2344 2345 public boolean hasProfile() { 2346 if (this.profile == null) 2347 return false; 2348 for (CanonicalType item : this.profile) 2349 if (!item.isEmpty()) 2350 return true; 2351 return false; 2352 } 2353 2354 /** 2355 * @return {@link #profile} (Identifies a profile structure or implementation 2356 * Guide that applies to the datatype this element refers to. If any 2357 * profiles are specified, then the content must conform to at least one 2358 * of them. The URL can be a local reference - to a contained 2359 * StructureDefinition, or a reference to another StructureDefinition or 2360 * Implementation Guide by a canonical URL. When an implementation guide 2361 * is specified, the type SHALL conform to at least one profile defined 2362 * in the implementation guide.) 2363 */ 2364 public CanonicalType addProfileElement() {// 2 2365 CanonicalType t = new CanonicalType(); 2366 if (this.profile == null) 2367 this.profile = new ArrayList<CanonicalType>(); 2368 this.profile.add(t); 2369 return t; 2370 } 2371 2372 /** 2373 * @param value {@link #profile} (Identifies a profile structure or 2374 * implementation Guide that applies to the datatype this element 2375 * refers to. If any profiles are specified, then the content must 2376 * conform to at least one of them. The URL can be a local 2377 * reference - to a contained StructureDefinition, or a reference 2378 * to another StructureDefinition or Implementation Guide by a 2379 * canonical URL. When an implementation guide is specified, the 2380 * type SHALL conform to at least one profile defined in the 2381 * implementation guide.) 2382 */ 2383 public TypeRefComponent addProfile(String value) { // 1 2384 CanonicalType t = new CanonicalType(); 2385 t.setValue(value); 2386 if (this.profile == null) 2387 this.profile = new ArrayList<CanonicalType>(); 2388 this.profile.add(t); 2389 return this; 2390 } 2391 2392 /** 2393 * @param value {@link #profile} (Identifies a profile structure or 2394 * implementation Guide that applies to the datatype this element 2395 * refers to. If any profiles are specified, then the content must 2396 * conform to at least one of them. The URL can be a local 2397 * reference - to a contained StructureDefinition, or a reference 2398 * to another StructureDefinition or Implementation Guide by a 2399 * canonical URL. When an implementation guide is specified, the 2400 * type SHALL conform to at least one profile defined in the 2401 * implementation guide.) 2402 */ 2403 public boolean hasProfile(String value) { 2404 if (this.profile == null) 2405 return false; 2406 for (CanonicalType v : this.profile) 2407 if (v.getValue().equals(value)) // canonical(StructureDefinition|ImplementationGuide) 2408 return true; 2409 return false; 2410 } 2411 2412 /** 2413 * @return {@link #targetProfile} (Used when the type is "Reference" or 2414 * "canonical", and identifies a profile structure or implementation 2415 * Guide that applies to the target of the reference this element refers 2416 * to. If any profiles are specified, then the content must conform to 2417 * at least one of them. The URL can be a local reference - to a 2418 * contained StructureDefinition, or a reference to another 2419 * StructureDefinition or Implementation Guide by a canonical URL. When 2420 * an implementation guide is specified, the target resource SHALL 2421 * conform to at least one profile defined in the implementation guide.) 2422 */ 2423 public List<CanonicalType> getTargetProfile() { 2424 if (this.targetProfile == null) 2425 this.targetProfile = new ArrayList<CanonicalType>(); 2426 return this.targetProfile; 2427 } 2428 2429 /** 2430 * @return Returns a reference to <code>this</code> for easy method chaining 2431 */ 2432 public TypeRefComponent setTargetProfile(List<CanonicalType> theTargetProfile) { 2433 this.targetProfile = theTargetProfile; 2434 return this; 2435 } 2436 2437 public boolean hasTargetProfile() { 2438 if (this.targetProfile == null) 2439 return false; 2440 for (CanonicalType item : this.targetProfile) 2441 if (!item.isEmpty()) 2442 return true; 2443 return false; 2444 } 2445 2446 /** 2447 * @return {@link #targetProfile} (Used when the type is "Reference" or 2448 * "canonical", and identifies a profile structure or implementation 2449 * Guide that applies to the target of the reference this element refers 2450 * to. If any profiles are specified, then the content must conform to 2451 * at least one of them. The URL can be a local reference - to a 2452 * contained StructureDefinition, or a reference to another 2453 * StructureDefinition or Implementation Guide by a canonical URL. When 2454 * an implementation guide is specified, the target resource SHALL 2455 * conform to at least one profile defined in the implementation guide.) 2456 */ 2457 public CanonicalType addTargetProfileElement() {// 2 2458 CanonicalType t = new CanonicalType(); 2459 if (this.targetProfile == null) 2460 this.targetProfile = new ArrayList<CanonicalType>(); 2461 this.targetProfile.add(t); 2462 return t; 2463 } 2464 2465 /** 2466 * @param value {@link #targetProfile} (Used when the type is "Reference" or 2467 * "canonical", and identifies a profile structure or 2468 * implementation Guide that applies to the target of the reference 2469 * this element refers to. If any profiles are specified, then the 2470 * content must conform to at least one of them. The URL can be a 2471 * local reference - to a contained StructureDefinition, or a 2472 * reference to another StructureDefinition or Implementation Guide 2473 * by a canonical URL. When an implementation guide is specified, 2474 * the target resource SHALL conform to at least one profile 2475 * defined in the implementation guide.) 2476 */ 2477 public TypeRefComponent addTargetProfile(String value) { // 1 2478 CanonicalType t = new CanonicalType(); 2479 t.setValue(value); 2480 if (this.targetProfile == null) 2481 this.targetProfile = new ArrayList<CanonicalType>(); 2482 this.targetProfile.add(t); 2483 return this; 2484 } 2485 2486 /** 2487 * @param value {@link #targetProfile} (Used when the type is "Reference" or 2488 * "canonical", and identifies a profile structure or 2489 * implementation Guide that applies to the target of the reference 2490 * this element refers to. If any profiles are specified, then the 2491 * content must conform to at least one of them. The URL can be a 2492 * local reference - to a contained StructureDefinition, or a 2493 * reference to another StructureDefinition or Implementation Guide 2494 * by a canonical URL. When an implementation guide is specified, 2495 * the target resource SHALL conform to at least one profile 2496 * defined in the implementation guide.) 2497 */ 2498 public boolean hasTargetProfile(String value) { 2499 if (this.targetProfile == null) 2500 return false; 2501 for (CanonicalType v : this.targetProfile) 2502 if (v.getValue().equals(value)) // canonical(StructureDefinition|ImplementationGuide) 2503 return true; 2504 return false; 2505 } 2506 2507 /** 2508 * @return {@link #aggregation} (If the type is a reference to another resource, 2509 * how the resource is or can be aggregated - is it a contained 2510 * resource, or a reference, and if the context is a bundle, is it 2511 * included in the bundle.) 2512 */ 2513 public List<Enumeration<AggregationMode>> getAggregation() { 2514 if (this.aggregation == null) 2515 this.aggregation = new ArrayList<Enumeration<AggregationMode>>(); 2516 return this.aggregation; 2517 } 2518 2519 /** 2520 * @return Returns a reference to <code>this</code> for easy method chaining 2521 */ 2522 public TypeRefComponent setAggregation(List<Enumeration<AggregationMode>> theAggregation) { 2523 this.aggregation = theAggregation; 2524 return this; 2525 } 2526 2527 public boolean hasAggregation() { 2528 if (this.aggregation == null) 2529 return false; 2530 for (Enumeration<AggregationMode> item : this.aggregation) 2531 if (!item.isEmpty()) 2532 return true; 2533 return false; 2534 } 2535 2536 /** 2537 * @return {@link #aggregation} (If the type is a reference to another resource, 2538 * how the resource is or can be aggregated - is it a contained 2539 * resource, or a reference, and if the context is a bundle, is it 2540 * included in the bundle.) 2541 */ 2542 public Enumeration<AggregationMode> addAggregationElement() {// 2 2543 Enumeration<AggregationMode> t = new Enumeration<AggregationMode>(new AggregationModeEnumFactory()); 2544 if (this.aggregation == null) 2545 this.aggregation = new ArrayList<Enumeration<AggregationMode>>(); 2546 this.aggregation.add(t); 2547 return t; 2548 } 2549 2550 /** 2551 * @param value {@link #aggregation} (If the type is a reference to another 2552 * resource, how the resource is or can be aggregated - is it a 2553 * contained resource, or a reference, and if the context is a 2554 * bundle, is it included in the bundle.) 2555 */ 2556 public TypeRefComponent addAggregation(AggregationMode value) { // 1 2557 Enumeration<AggregationMode> t = new Enumeration<AggregationMode>(new AggregationModeEnumFactory()); 2558 t.setValue(value); 2559 if (this.aggregation == null) 2560 this.aggregation = new ArrayList<Enumeration<AggregationMode>>(); 2561 this.aggregation.add(t); 2562 return this; 2563 } 2564 2565 /** 2566 * @param value {@link #aggregation} (If the type is a reference to another 2567 * resource, how the resource is or can be aggregated - is it a 2568 * contained resource, or a reference, and if the context is a 2569 * bundle, is it included in the bundle.) 2570 */ 2571 public boolean hasAggregation(AggregationMode value) { 2572 if (this.aggregation == null) 2573 return false; 2574 for (Enumeration<AggregationMode> v : this.aggregation) 2575 if (v.getValue().equals(value)) // code 2576 return true; 2577 return false; 2578 } 2579 2580 /** 2581 * @return {@link #versioning} (Whether this reference needs to be version 2582 * specific or version independent, or whether either can be used.). 2583 * This is the underlying object with id, value and extensions. The 2584 * accessor "getVersioning" gives direct access to the value 2585 */ 2586 public Enumeration<ReferenceVersionRules> getVersioningElement() { 2587 if (this.versioning == null) 2588 if (Configuration.errorOnAutoCreate()) 2589 throw new Error("Attempt to auto-create TypeRefComponent.versioning"); 2590 else if (Configuration.doAutoCreate()) 2591 this.versioning = new Enumeration<ReferenceVersionRules>(new ReferenceVersionRulesEnumFactory()); // bb 2592 return this.versioning; 2593 } 2594 2595 public boolean hasVersioningElement() { 2596 return this.versioning != null && !this.versioning.isEmpty(); 2597 } 2598 2599 public boolean hasVersioning() { 2600 return this.versioning != null && !this.versioning.isEmpty(); 2601 } 2602 2603 /** 2604 * @param value {@link #versioning} (Whether this reference needs to be version 2605 * specific or version independent, or whether either can be 2606 * used.). This is the underlying object with id, value and 2607 * extensions. The accessor "getVersioning" gives direct access to 2608 * the value 2609 */ 2610 public TypeRefComponent setVersioningElement(Enumeration<ReferenceVersionRules> value) { 2611 this.versioning = value; 2612 return this; 2613 } 2614 2615 /** 2616 * @return Whether this reference needs to be version specific or version 2617 * independent, or whether either can be used. 2618 */ 2619 public ReferenceVersionRules getVersioning() { 2620 return this.versioning == null ? null : this.versioning.getValue(); 2621 } 2622 2623 /** 2624 * @param value Whether this reference needs to be version specific or version 2625 * independent, or whether either can be used. 2626 */ 2627 public TypeRefComponent setVersioning(ReferenceVersionRules value) { 2628 if (value == null) 2629 this.versioning = null; 2630 else { 2631 if (this.versioning == null) 2632 this.versioning = new Enumeration<ReferenceVersionRules>(new ReferenceVersionRulesEnumFactory()); 2633 this.versioning.setValue(value); 2634 } 2635 return this; 2636 } 2637 2638 protected void listChildren(List<Property> children) { 2639 super.listChildren(children); 2640 children.add(new Property("code", "uri", 2641 "URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. \"string\" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models.", 2642 0, 1, code)); 2643 children.add(new Property("profile", "canonical(StructureDefinition|ImplementationGuide)", 2644 "Identifies a profile structure or implementation Guide that applies to the datatype this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the type SHALL conform to at least one profile defined in the implementation guide.", 2645 0, java.lang.Integer.MAX_VALUE, profile)); 2646 children.add(new Property("targetProfile", "canonical(StructureDefinition|ImplementationGuide)", 2647 "Used when the type is \"Reference\" or \"canonical\", and identifies a profile structure or implementation Guide that applies to the target of the reference this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.", 2648 0, java.lang.Integer.MAX_VALUE, targetProfile)); 2649 children.add(new Property("aggregation", "code", 2650 "If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle.", 2651 0, java.lang.Integer.MAX_VALUE, aggregation)); 2652 children.add(new Property("versioning", "code", 2653 "Whether this reference needs to be version specific or version independent, or whether either can be used.", 2654 0, 1, versioning)); 2655 } 2656 2657 @Override 2658 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2659 switch (_hash) { 2660 case 3059181: 2661 /* code */ return new Property("code", "uri", 2662 "URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. \"string\" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models.", 2663 0, 1, code); 2664 case -309425751: 2665 /* profile */ return new Property("profile", "canonical(StructureDefinition|ImplementationGuide)", 2666 "Identifies a profile structure or implementation Guide that applies to the datatype this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the type SHALL conform to at least one profile defined in the implementation guide.", 2667 0, java.lang.Integer.MAX_VALUE, profile); 2668 case 1994521304: 2669 /* targetProfile */ return new Property("targetProfile", "canonical(StructureDefinition|ImplementationGuide)", 2670 "Used when the type is \"Reference\" or \"canonical\", and identifies a profile structure or implementation Guide that applies to the target of the reference this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.", 2671 0, java.lang.Integer.MAX_VALUE, targetProfile); 2672 case 841524962: 2673 /* aggregation */ return new Property("aggregation", "code", 2674 "If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle.", 2675 0, java.lang.Integer.MAX_VALUE, aggregation); 2676 case -670487542: 2677 /* versioning */ return new Property("versioning", "code", 2678 "Whether this reference needs to be version specific or version independent, or whether either can be used.", 2679 0, 1, versioning); 2680 default: 2681 return super.getNamedProperty(_hash, _name, _checkValid); 2682 } 2683 2684 } 2685 2686 @Override 2687 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2688 switch (hash) { 2689 case 3059181: 2690 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // UriType 2691 case -309425751: 2692 /* profile */ return this.profile == null ? new Base[0] : this.profile.toArray(new Base[this.profile.size()]); // CanonicalType 2693 case 1994521304: 2694 /* targetProfile */ return this.targetProfile == null ? new Base[0] 2695 : this.targetProfile.toArray(new Base[this.targetProfile.size()]); // CanonicalType 2696 case 841524962: 2697 /* aggregation */ return this.aggregation == null ? new Base[0] 2698 : this.aggregation.toArray(new Base[this.aggregation.size()]); // Enumeration<AggregationMode> 2699 case -670487542: 2700 /* versioning */ return this.versioning == null ? new Base[0] : new Base[] { this.versioning }; // Enumeration<ReferenceVersionRules> 2701 default: 2702 return super.getProperty(hash, name, checkValid); 2703 } 2704 2705 } 2706 2707 @Override 2708 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2709 switch (hash) { 2710 case 3059181: // code 2711 this.code = castToUri(value); // UriType 2712 return value; 2713 case -309425751: // profile 2714 this.getProfile().add(castToCanonical(value)); // CanonicalType 2715 return value; 2716 case 1994521304: // targetProfile 2717 this.getTargetProfile().add(castToCanonical(value)); // CanonicalType 2718 return value; 2719 case 841524962: // aggregation 2720 value = new AggregationModeEnumFactory().fromType(castToCode(value)); 2721 this.getAggregation().add((Enumeration) value); // Enumeration<AggregationMode> 2722 return value; 2723 case -670487542: // versioning 2724 value = new ReferenceVersionRulesEnumFactory().fromType(castToCode(value)); 2725 this.versioning = (Enumeration) value; // Enumeration<ReferenceVersionRules> 2726 return value; 2727 default: 2728 return super.setProperty(hash, name, value); 2729 } 2730 2731 } 2732 2733 @Override 2734 public Base setProperty(String name, Base value) throws FHIRException { 2735 if (name.equals("code")) { 2736 this.code = castToUri(value); // UriType 2737 } else if (name.equals("profile")) { 2738 this.getProfile().add(castToCanonical(value)); 2739 } else if (name.equals("targetProfile")) { 2740 this.getTargetProfile().add(castToCanonical(value)); 2741 } else if (name.equals("aggregation")) { 2742 value = new AggregationModeEnumFactory().fromType(castToCode(value)); 2743 this.getAggregation().add((Enumeration) value); 2744 } else if (name.equals("versioning")) { 2745 value = new ReferenceVersionRulesEnumFactory().fromType(castToCode(value)); 2746 this.versioning = (Enumeration) value; // Enumeration<ReferenceVersionRules> 2747 } else 2748 return super.setProperty(name, value); 2749 return value; 2750 } 2751 2752 @Override 2753 public void removeChild(String name, Base value) throws FHIRException { 2754 if (name.equals("code")) { 2755 this.code = null; 2756 } else if (name.equals("profile")) { 2757 this.getProfile().remove(castToCanonical(value)); 2758 } else if (name.equals("targetProfile")) { 2759 this.getTargetProfile().remove(castToCanonical(value)); 2760 } else if (name.equals("aggregation")) { 2761 this.getAggregation().remove((Enumeration) value); 2762 } else if (name.equals("versioning")) { 2763 value = null; 2764 this.versioning = (Enumeration) value; // Enumeration<ReferenceVersionRules> 2765 } else 2766 super.removeChild(name, value); 2767 2768 } 2769 2770 @Override 2771 public Base makeProperty(int hash, String name) throws FHIRException { 2772 switch (hash) { 2773 case 3059181: 2774 return getCodeElement(); 2775 case -309425751: 2776 return addProfileElement(); 2777 case 1994521304: 2778 return addTargetProfileElement(); 2779 case 841524962: 2780 return addAggregationElement(); 2781 case -670487542: 2782 return getVersioningElement(); 2783 default: 2784 return super.makeProperty(hash, name); 2785 } 2786 2787 } 2788 2789 @Override 2790 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2791 switch (hash) { 2792 case 3059181: 2793 /* code */ return new String[] { "uri" }; 2794 case -309425751: 2795 /* profile */ return new String[] { "canonical" }; 2796 case 1994521304: 2797 /* targetProfile */ return new String[] { "canonical" }; 2798 case 841524962: 2799 /* aggregation */ return new String[] { "code" }; 2800 case -670487542: 2801 /* versioning */ return new String[] { "code" }; 2802 default: 2803 return super.getTypesForProperty(hash, name); 2804 } 2805 2806 } 2807 2808 @Override 2809 public Base addChild(String name) throws FHIRException { 2810 if (name.equals("code")) { 2811 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.code"); 2812 } else if (name.equals("profile")) { 2813 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.profile"); 2814 } else if (name.equals("targetProfile")) { 2815 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.targetProfile"); 2816 } else if (name.equals("aggregation")) { 2817 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.aggregation"); 2818 } else if (name.equals("versioning")) { 2819 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.versioning"); 2820 } else 2821 return super.addChild(name); 2822 } 2823 2824 public TypeRefComponent copy() { 2825 TypeRefComponent dst = new TypeRefComponent(); 2826 copyValues(dst); 2827 return dst; 2828 } 2829 2830 public void copyValues(TypeRefComponent dst) { 2831 super.copyValues(dst); 2832 dst.code = code == null ? null : code.copy(); 2833 if (profile != null) { 2834 dst.profile = new ArrayList<CanonicalType>(); 2835 for (CanonicalType i : profile) 2836 dst.profile.add(i.copy()); 2837 } 2838 ; 2839 if (targetProfile != null) { 2840 dst.targetProfile = new ArrayList<CanonicalType>(); 2841 for (CanonicalType i : targetProfile) 2842 dst.targetProfile.add(i.copy()); 2843 } 2844 ; 2845 if (aggregation != null) { 2846 dst.aggregation = new ArrayList<Enumeration<AggregationMode>>(); 2847 for (Enumeration<AggregationMode> i : aggregation) 2848 dst.aggregation.add(i.copy()); 2849 } 2850 ; 2851 dst.versioning = versioning == null ? null : versioning.copy(); 2852 } 2853 2854 @Override 2855 public boolean equalsDeep(Base other_) { 2856 if (!super.equalsDeep(other_)) 2857 return false; 2858 if (!(other_ instanceof TypeRefComponent)) 2859 return false; 2860 TypeRefComponent o = (TypeRefComponent) other_; 2861 return compareDeep(code, o.code, true) && compareDeep(profile, o.profile, true) 2862 && compareDeep(targetProfile, o.targetProfile, true) && compareDeep(aggregation, o.aggregation, true) 2863 && compareDeep(versioning, o.versioning, true); 2864 } 2865 2866 @Override 2867 public boolean equalsShallow(Base other_) { 2868 if (!super.equalsShallow(other_)) 2869 return false; 2870 if (!(other_ instanceof TypeRefComponent)) 2871 return false; 2872 TypeRefComponent o = (TypeRefComponent) other_; 2873 return compareValues(code, o.code, true) && compareValues(aggregation, o.aggregation, true) 2874 && compareValues(versioning, o.versioning, true); 2875 } 2876 2877 public boolean isEmpty() { 2878 return super.isEmpty() 2879 && ca.uhn.fhir.util.ElementUtil.isEmpty(code, profile, targetProfile, aggregation, versioning); 2880 } 2881 2882 public String fhirType() { 2883 return "ElementDefinition.type"; 2884 2885 } 2886 2887// added from java-adornments.txt: 2888 2889 public boolean hasTarget() { 2890 return Utilities.existsInList(getCode(), "Reference", "canonical"); 2891 } 2892 2893 /** 2894 * This code checks for the system prefix and returns the FHIR type 2895 * 2896 * @return 2897 */ 2898 public String getWorkingCode() { 2899 if (hasExtension(ToolingExtensions.EXT_FHIR_TYPE)) 2900 return getExtensionString(ToolingExtensions.EXT_FHIR_TYPE); 2901 if (!hasCodeElement()) 2902 return null; 2903 if (getCodeElement().hasExtension(ToolingExtensions.EXT_XML_TYPE)) { 2904 String s = getCodeElement().getExtensionString(ToolingExtensions.EXT_XML_TYPE); 2905 if ("xsd:gYear OR xsd:gYearMonth OR xsd:date OR xsd:dateTime".equals(s)) 2906 return "dateTime"; 2907 if ("xsd:gYear OR xsd:gYearMonth OR xsd:date".equals(s)) 2908 return "date"; 2909 if ("xsd:dateTime".equals(s)) 2910 return "instant"; 2911 if ("xsd:token".equals(s)) 2912 return "code"; 2913 if ("xsd:boolean".equals(s)) 2914 return "boolean"; 2915 if ("xsd:string".equals(s)) 2916 return "string"; 2917 if ("xsd:time".equals(s)) 2918 return "time"; 2919 if ("xsd:int".equals(s)) 2920 return "integer"; 2921 if ("xsd:decimal OR xsd:double".equals(s)) 2922 return "decimal"; 2923 if ("xsd:base64Binary".equals(s)) 2924 return "base64Binary"; 2925 if ("xsd:positiveInteger".equals(s)) 2926 return "positiveInt"; 2927 if ("xsd:nonNegativeInteger".equals(s)) 2928 return "unsignedInt"; 2929 if ("xsd:anyURI".equals(s)) 2930 return "uri"; 2931 2932 throw new Error("Unknown xml type '" + s + "'"); 2933 } 2934 return getCode(); 2935 } 2936 2937// end addition 2938 } 2939 2940 @Block() 2941 public static class ElementDefinitionExampleComponent extends Element implements IBaseDatatypeElement { 2942 /** 2943 * Describes the purpose of this example amoung the set of examples. 2944 */ 2945 @Child(name = "label", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2946 @Description(shortDefinition = "Describes the purpose of this example", formalDefinition = "Describes the purpose of this example amoung the set of examples.") 2947 protected StringType label; 2948 2949 /** 2950 * The actual value for the element, which must be one of the types allowed for 2951 * this element. 2952 */ 2953 @Child(name = "value", type = {}, order = 2, min = 1, max = 1, modifier = false, summary = true) 2954 @Description(shortDefinition = "Value of Example (one of allowed types)", formalDefinition = "The actual value for the element, which must be one of the types allowed for this element.") 2955 protected org.hl7.fhir.r4.model.Type value; 2956 2957 private static final long serialVersionUID = 457572481L; 2958 2959 /** 2960 * Constructor 2961 */ 2962 public ElementDefinitionExampleComponent() { 2963 super(); 2964 } 2965 2966 /** 2967 * Constructor 2968 */ 2969 public ElementDefinitionExampleComponent(StringType label, org.hl7.fhir.r4.model.Type value) { 2970 super(); 2971 this.label = label; 2972 this.value = value; 2973 } 2974 2975 /** 2976 * @return {@link #label} (Describes the purpose of this example amoung the set 2977 * of examples.). This is the underlying object with id, value and 2978 * extensions. The accessor "getLabel" gives direct access to the value 2979 */ 2980 public StringType getLabelElement() { 2981 if (this.label == null) 2982 if (Configuration.errorOnAutoCreate()) 2983 throw new Error("Attempt to auto-create ElementDefinitionExampleComponent.label"); 2984 else if (Configuration.doAutoCreate()) 2985 this.label = new StringType(); // bb 2986 return this.label; 2987 } 2988 2989 public boolean hasLabelElement() { 2990 return this.label != null && !this.label.isEmpty(); 2991 } 2992 2993 public boolean hasLabel() { 2994 return this.label != null && !this.label.isEmpty(); 2995 } 2996 2997 /** 2998 * @param value {@link #label} (Describes the purpose of this example amoung the 2999 * set of examples.). This is the underlying object with id, value 3000 * and extensions. The accessor "getLabel" gives direct access to 3001 * the value 3002 */ 3003 public ElementDefinitionExampleComponent setLabelElement(StringType value) { 3004 this.label = value; 3005 return this; 3006 } 3007 3008 /** 3009 * @return Describes the purpose of this example amoung the set of examples. 3010 */ 3011 public String getLabel() { 3012 return this.label == null ? null : this.label.getValue(); 3013 } 3014 3015 /** 3016 * @param value Describes the purpose of this example amoung the set of 3017 * examples. 3018 */ 3019 public ElementDefinitionExampleComponent setLabel(String value) { 3020 if (this.label == null) 3021 this.label = new StringType(); 3022 this.label.setValue(value); 3023 return this; 3024 } 3025 3026 /** 3027 * @return {@link #value} (The actual value for the element, which must be one 3028 * of the types allowed for this element.) 3029 */ 3030 public org.hl7.fhir.r4.model.Type getValue() { 3031 return this.value; 3032 } 3033 3034 public boolean hasValue() { 3035 return this.value != null && !this.value.isEmpty(); 3036 } 3037 3038 /** 3039 * @param value {@link #value} (The actual value for the element, which must be 3040 * one of the types allowed for this element.) 3041 */ 3042 public ElementDefinitionExampleComponent setValue(org.hl7.fhir.r4.model.Type value) { 3043 this.value = value; 3044 return this; 3045 } 3046 3047 protected void listChildren(List<Property> children) { 3048 super.listChildren(children); 3049 children.add(new Property("label", "string", "Describes the purpose of this example amoung the set of examples.", 3050 0, 1, label)); 3051 children.add(new Property("value[x]", "*", 3052 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value)); 3053 } 3054 3055 @Override 3056 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3057 switch (_hash) { 3058 case 102727412: 3059 /* label */ return new Property("label", "string", 3060 "Describes the purpose of this example amoung the set of examples.", 0, 1, label); 3061 case -1410166417: 3062 /* value[x] */ return new Property("value[x]", "*", 3063 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3064 case 111972721: 3065 /* value */ return new Property("value[x]", "*", 3066 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3067 case -1535024575: 3068 /* valueBase64Binary */ return new Property("value[x]", "*", 3069 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3070 case 733421943: 3071 /* valueBoolean */ return new Property("value[x]", "*", 3072 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3073 case -786218365: 3074 /* valueCanonical */ return new Property("value[x]", "*", 3075 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3076 case -766209282: 3077 /* valueCode */ return new Property("value[x]", "*", 3078 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3079 case -766192449: 3080 /* valueDate */ return new Property("value[x]", "*", 3081 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3082 case 1047929900: 3083 /* valueDateTime */ return new Property("value[x]", "*", 3084 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3085 case -2083993440: 3086 /* valueDecimal */ return new Property("value[x]", "*", 3087 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3088 case 231604844: 3089 /* valueId */ return new Property("value[x]", "*", 3090 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3091 case -1668687056: 3092 /* valueInstant */ return new Property("value[x]", "*", 3093 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3094 case -1668204915: 3095 /* valueInteger */ return new Property("value[x]", "*", 3096 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3097 case -497880704: 3098 /* valueMarkdown */ return new Property("value[x]", "*", 3099 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3100 case -1410178407: 3101 /* valueOid */ return new Property("value[x]", "*", 3102 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3103 case -1249932027: 3104 /* valuePositiveInt */ return new Property("value[x]", "*", 3105 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3106 case -1424603934: 3107 /* valueString */ return new Property("value[x]", "*", 3108 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3109 case -765708322: 3110 /* valueTime */ return new Property("value[x]", "*", 3111 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3112 case 26529417: 3113 /* valueUnsignedInt */ return new Property("value[x]", "*", 3114 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3115 case -1410172357: 3116 /* valueUri */ return new Property("value[x]", "*", 3117 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3118 case -1410172354: 3119 /* valueUrl */ return new Property("value[x]", "*", 3120 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3121 case -765667124: 3122 /* valueUuid */ return new Property("value[x]", "*", 3123 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3124 case -478981821: 3125 /* valueAddress */ return new Property("value[x]", "*", 3126 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3127 case -67108992: 3128 /* valueAnnotation */ return new Property("value[x]", "*", 3129 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3130 case -475566732: 3131 /* valueAttachment */ return new Property("value[x]", "*", 3132 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3133 case 924902896: 3134 /* valueCodeableConcept */ return new Property("value[x]", "*", 3135 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3136 case -1887705029: 3137 /* valueCoding */ return new Property("value[x]", "*", 3138 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3139 case 944904545: 3140 /* valueContactPoint */ return new Property("value[x]", "*", 3141 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3142 case -2026205465: 3143 /* valueHumanName */ return new Property("value[x]", "*", 3144 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3145 case -130498310: 3146 /* valueIdentifier */ return new Property("value[x]", "*", 3147 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3148 case -1524344174: 3149 /* valuePeriod */ return new Property("value[x]", "*", 3150 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3151 case -2029823716: 3152 /* valueQuantity */ return new Property("value[x]", "*", 3153 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3154 case 2030761548: 3155 /* valueRange */ return new Property("value[x]", "*", 3156 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3157 case 2030767386: 3158 /* valueRatio */ return new Property("value[x]", "*", 3159 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3160 case 1755241690: 3161 /* valueReference */ return new Property("value[x]", "*", 3162 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3163 case -962229101: 3164 /* valueSampledData */ return new Property("value[x]", "*", 3165 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3166 case -540985785: 3167 /* valueSignature */ return new Property("value[x]", "*", 3168 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3169 case -1406282469: 3170 /* valueTiming */ return new Property("value[x]", "*", 3171 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3172 case -1858636920: 3173 /* valueDosage */ return new Property("value[x]", "*", 3174 "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3175 default: 3176 return super.getNamedProperty(_hash, _name, _checkValid); 3177 } 3178 3179 } 3180 3181 @Override 3182 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3183 switch (hash) { 3184 case 102727412: 3185 /* label */ return this.label == null ? new Base[0] : new Base[] { this.label }; // StringType 3186 case 111972721: 3187 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // org.hl7.fhir.r4.model.Type 3188 default: 3189 return super.getProperty(hash, name, checkValid); 3190 } 3191 3192 } 3193 3194 @Override 3195 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3196 switch (hash) { 3197 case 102727412: // label 3198 this.label = castToString(value); // StringType 3199 return value; 3200 case 111972721: // value 3201 this.value = castToType(value); // org.hl7.fhir.r4.model.Type 3202 return value; 3203 default: 3204 return super.setProperty(hash, name, value); 3205 } 3206 3207 } 3208 3209 @Override 3210 public Base setProperty(String name, Base value) throws FHIRException { 3211 if (name.equals("label")) { 3212 this.label = castToString(value); // StringType 3213 } else if (name.equals("value[x]")) { 3214 this.value = castToType(value); // org.hl7.fhir.r4.model.Type 3215 } else 3216 return super.setProperty(name, value); 3217 return value; 3218 } 3219 3220 @Override 3221 public void removeChild(String name, Base value) throws FHIRException { 3222 if (name.equals("label")) { 3223 this.label = null; 3224 } else if (name.equals("value[x]")) { 3225 this.value = null; 3226 } else 3227 super.removeChild(name, value); 3228 3229 } 3230 3231 @Override 3232 public Base makeProperty(int hash, String name) throws FHIRException { 3233 switch (hash) { 3234 case 102727412: 3235 return getLabelElement(); 3236 case -1410166417: 3237 return getValue(); 3238 case 111972721: 3239 return getValue(); 3240 default: 3241 return super.makeProperty(hash, name); 3242 } 3243 3244 } 3245 3246 @Override 3247 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3248 switch (hash) { 3249 case 102727412: 3250 /* label */ return new String[] { "string" }; 3251 case 111972721: 3252 /* value */ return new String[] { "*" }; 3253 default: 3254 return super.getTypesForProperty(hash, name); 3255 } 3256 3257 } 3258 3259 @Override 3260 public Base addChild(String name) throws FHIRException { 3261 if (name.equals("label")) { 3262 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.label"); 3263 } else if (name.equals("valueBase64Binary")) { 3264 this.value = new Base64BinaryType(); 3265 return this.value; 3266 } else if (name.equals("valueBoolean")) { 3267 this.value = new BooleanType(); 3268 return this.value; 3269 } else if (name.equals("valueCanonical")) { 3270 this.value = new CanonicalType(); 3271 return this.value; 3272 } else if (name.equals("valueCode")) { 3273 this.value = new CodeType(); 3274 return this.value; 3275 } else if (name.equals("valueDate")) { 3276 this.value = new DateType(); 3277 return this.value; 3278 } else if (name.equals("valueDateTime")) { 3279 this.value = new DateTimeType(); 3280 return this.value; 3281 } else if (name.equals("valueDecimal")) { 3282 this.value = new DecimalType(); 3283 return this.value; 3284 } else if (name.equals("valueId")) { 3285 this.value = new IdType(); 3286 return this.value; 3287 } else if (name.equals("valueInstant")) { 3288 this.value = new InstantType(); 3289 return this.value; 3290 } else if (name.equals("valueInteger")) { 3291 this.value = new IntegerType(); 3292 return this.value; 3293 } else if (name.equals("valueMarkdown")) { 3294 this.value = new MarkdownType(); 3295 return this.value; 3296 } else if (name.equals("valueOid")) { 3297 this.value = new OidType(); 3298 return this.value; 3299 } else if (name.equals("valuePositiveInt")) { 3300 this.value = new PositiveIntType(); 3301 return this.value; 3302 } else if (name.equals("valueString")) { 3303 this.value = new StringType(); 3304 return this.value; 3305 } else if (name.equals("valueTime")) { 3306 this.value = new TimeType(); 3307 return this.value; 3308 } else if (name.equals("valueUnsignedInt")) { 3309 this.value = new UnsignedIntType(); 3310 return this.value; 3311 } else if (name.equals("valueUri")) { 3312 this.value = new UriType(); 3313 return this.value; 3314 } else if (name.equals("valueUrl")) { 3315 this.value = new UrlType(); 3316 return this.value; 3317 } else if (name.equals("valueUuid")) { 3318 this.value = new UuidType(); 3319 return this.value; 3320 } else if (name.equals("valueAddress")) { 3321 this.value = new Address(); 3322 return this.value; 3323 } else if (name.equals("valueAge")) { 3324 this.value = new Age(); 3325 return this.value; 3326 } else if (name.equals("valueAnnotation")) { 3327 this.value = new Annotation(); 3328 return this.value; 3329 } else if (name.equals("valueAttachment")) { 3330 this.value = new Attachment(); 3331 return this.value; 3332 } else if (name.equals("valueCodeableConcept")) { 3333 this.value = new CodeableConcept(); 3334 return this.value; 3335 } else if (name.equals("valueCoding")) { 3336 this.value = new Coding(); 3337 return this.value; 3338 } else if (name.equals("valueContactPoint")) { 3339 this.value = new ContactPoint(); 3340 return this.value; 3341 } else if (name.equals("valueCount")) { 3342 this.value = new Count(); 3343 return this.value; 3344 } else if (name.equals("valueDistance")) { 3345 this.value = new Distance(); 3346 return this.value; 3347 } else if (name.equals("valueDuration")) { 3348 this.value = new Duration(); 3349 return this.value; 3350 } else if (name.equals("valueHumanName")) { 3351 this.value = new HumanName(); 3352 return this.value; 3353 } else if (name.equals("valueIdentifier")) { 3354 this.value = new Identifier(); 3355 return this.value; 3356 } else if (name.equals("valueMoney")) { 3357 this.value = new Money(); 3358 return this.value; 3359 } else if (name.equals("valuePeriod")) { 3360 this.value = new Period(); 3361 return this.value; 3362 } else if (name.equals("valueQuantity")) { 3363 this.value = new Quantity(); 3364 return this.value; 3365 } else if (name.equals("valueRange")) { 3366 this.value = new Range(); 3367 return this.value; 3368 } else if (name.equals("valueRatio")) { 3369 this.value = new Ratio(); 3370 return this.value; 3371 } else if (name.equals("valueReference")) { 3372 this.value = new Reference(); 3373 return this.value; 3374 } else if (name.equals("valueSampledData")) { 3375 this.value = new SampledData(); 3376 return this.value; 3377 } else if (name.equals("valueSignature")) { 3378 this.value = new Signature(); 3379 return this.value; 3380 } else if (name.equals("valueTiming")) { 3381 this.value = new Timing(); 3382 return this.value; 3383 } else if (name.equals("valueContactDetail")) { 3384 this.value = new ContactDetail(); 3385 return this.value; 3386 } else if (name.equals("valueContributor")) { 3387 this.value = new Contributor(); 3388 return this.value; 3389 } else if (name.equals("valueDataRequirement")) { 3390 this.value = new DataRequirement(); 3391 return this.value; 3392 } else if (name.equals("valueExpression")) { 3393 this.value = new Expression(); 3394 return this.value; 3395 } else if (name.equals("valueParameterDefinition")) { 3396 this.value = new ParameterDefinition(); 3397 return this.value; 3398 } else if (name.equals("valueRelatedArtifact")) { 3399 this.value = new RelatedArtifact(); 3400 return this.value; 3401 } else if (name.equals("valueTriggerDefinition")) { 3402 this.value = new TriggerDefinition(); 3403 return this.value; 3404 } else if (name.equals("valueUsageContext")) { 3405 this.value = new UsageContext(); 3406 return this.value; 3407 } else if (name.equals("valueDosage")) { 3408 this.value = new Dosage(); 3409 return this.value; 3410 } else if (name.equals("valueMeta")) { 3411 this.value = new Meta(); 3412 return this.value; 3413 } else 3414 return super.addChild(name); 3415 } 3416 3417 public ElementDefinitionExampleComponent copy() { 3418 ElementDefinitionExampleComponent dst = new ElementDefinitionExampleComponent(); 3419 copyValues(dst); 3420 return dst; 3421 } 3422 3423 public void copyValues(ElementDefinitionExampleComponent dst) { 3424 super.copyValues(dst); 3425 dst.label = label == null ? null : label.copy(); 3426 dst.value = value == null ? null : value.copy(); 3427 } 3428 3429 @Override 3430 public boolean equalsDeep(Base other_) { 3431 if (!super.equalsDeep(other_)) 3432 return false; 3433 if (!(other_ instanceof ElementDefinitionExampleComponent)) 3434 return false; 3435 ElementDefinitionExampleComponent o = (ElementDefinitionExampleComponent) other_; 3436 return compareDeep(label, o.label, true) && compareDeep(value, o.value, true); 3437 } 3438 3439 @Override 3440 public boolean equalsShallow(Base other_) { 3441 if (!super.equalsShallow(other_)) 3442 return false; 3443 if (!(other_ instanceof ElementDefinitionExampleComponent)) 3444 return false; 3445 ElementDefinitionExampleComponent o = (ElementDefinitionExampleComponent) other_; 3446 return compareValues(label, o.label, true); 3447 } 3448 3449 public boolean isEmpty() { 3450 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(label, value); 3451 } 3452 3453 public String fhirType() { 3454 return "ElementDefinition.example"; 3455 3456 } 3457 3458 } 3459 3460 @Block() 3461 public static class ElementDefinitionConstraintComponent extends Element implements IBaseDatatypeElement { 3462 /** 3463 * Allows identification of which elements have their cardinalities impacted by 3464 * the constraint. Will not be referenced for constraints that do not affect 3465 * cardinality. 3466 */ 3467 @Child(name = "key", type = { IdType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 3468 @Description(shortDefinition = "Target of 'condition' reference above", formalDefinition = "Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality.") 3469 protected IdType key; 3470 3471 /** 3472 * Description of why this constraint is necessary or appropriate. 3473 */ 3474 @Child(name = "requirements", type = { 3475 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 3476 @Description(shortDefinition = "Why this constraint is necessary or appropriate", formalDefinition = "Description of why this constraint is necessary or appropriate.") 3477 protected StringType requirements; 3478 3479 /** 3480 * Identifies the impact constraint violation has on the conformance of the 3481 * instance. 3482 */ 3483 @Child(name = "severity", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 3484 @Description(shortDefinition = "error | warning", formalDefinition = "Identifies the impact constraint violation has on the conformance of the instance.") 3485 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/constraint-severity") 3486 protected Enumeration<ConstraintSeverity> severity; 3487 3488 /** 3489 * Text that can be used to describe the constraint in messages identifying that 3490 * the constraint has been violated. 3491 */ 3492 @Child(name = "human", type = { StringType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 3493 @Description(shortDefinition = "Human description of constraint", formalDefinition = "Text that can be used to describe the constraint in messages identifying that the constraint has been violated.") 3494 protected StringType human; 3495 3496 /** 3497 * A [FHIRPath](fhirpath.html) expression of constraint that can be executed to 3498 * see if this constraint is met. 3499 */ 3500 @Child(name = "expression", type = { 3501 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 3502 @Description(shortDefinition = "FHIRPath expression of constraint", formalDefinition = "A [FHIRPath](fhirpath.html) expression of constraint that can be executed to see if this constraint is met.") 3503 protected StringType expression; 3504 3505 /** 3506 * An XPath expression of constraint that can be executed to see if this 3507 * constraint is met. 3508 */ 3509 @Child(name = "xpath", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 3510 @Description(shortDefinition = "XPath expression of constraint", formalDefinition = "An XPath expression of constraint that can be executed to see if this constraint is met.") 3511 protected StringType xpath; 3512 3513 /** 3514 * A reference to the original source of the constraint, for traceability 3515 * purposes. 3516 */ 3517 @Child(name = "source", type = { 3518 CanonicalType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 3519 @Description(shortDefinition = "Reference to original source of constraint", formalDefinition = "A reference to the original source of the constraint, for traceability purposes.") 3520 protected CanonicalType source; 3521 3522 private static final long serialVersionUID = 1048354565L; 3523 3524 /** 3525 * Constructor 3526 */ 3527 public ElementDefinitionConstraintComponent() { 3528 super(); 3529 } 3530 3531 /** 3532 * Constructor 3533 */ 3534 public ElementDefinitionConstraintComponent(IdType key, Enumeration<ConstraintSeverity> severity, 3535 StringType human) { 3536 super(); 3537 this.key = key; 3538 this.severity = severity; 3539 this.human = human; 3540 } 3541 3542 /** 3543 * @return {@link #key} (Allows identification of which elements have their 3544 * cardinalities impacted by the constraint. Will not be referenced for 3545 * constraints that do not affect cardinality.). This is the underlying 3546 * object with id, value and extensions. The accessor "getKey" gives 3547 * direct access to the value 3548 */ 3549 public IdType getKeyElement() { 3550 if (this.key == null) 3551 if (Configuration.errorOnAutoCreate()) 3552 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.key"); 3553 else if (Configuration.doAutoCreate()) 3554 this.key = new IdType(); // bb 3555 return this.key; 3556 } 3557 3558 public boolean hasKeyElement() { 3559 return this.key != null && !this.key.isEmpty(); 3560 } 3561 3562 public boolean hasKey() { 3563 return this.key != null && !this.key.isEmpty(); 3564 } 3565 3566 /** 3567 * @param value {@link #key} (Allows identification of which elements have their 3568 * cardinalities impacted by the constraint. Will not be referenced 3569 * for constraints that do not affect cardinality.). This is the 3570 * underlying object with id, value and extensions. The accessor 3571 * "getKey" gives direct access to the value 3572 */ 3573 public ElementDefinitionConstraintComponent setKeyElement(IdType value) { 3574 this.key = value; 3575 return this; 3576 } 3577 3578 /** 3579 * @return Allows identification of which elements have their cardinalities 3580 * impacted by the constraint. Will not be referenced for constraints 3581 * that do not affect cardinality. 3582 */ 3583 public String getKey() { 3584 return this.key == null ? null : this.key.getValue(); 3585 } 3586 3587 /** 3588 * @param value Allows identification of which elements have their cardinalities 3589 * impacted by the constraint. Will not be referenced for 3590 * constraints that do not affect cardinality. 3591 */ 3592 public ElementDefinitionConstraintComponent setKey(String value) { 3593 if (this.key == null) 3594 this.key = new IdType(); 3595 this.key.setValue(value); 3596 return this; 3597 } 3598 3599 /** 3600 * @return {@link #requirements} (Description of why this constraint is 3601 * necessary or appropriate.). This is the underlying object with id, 3602 * value and extensions. The accessor "getRequirements" gives direct 3603 * access to the value 3604 */ 3605 public StringType getRequirementsElement() { 3606 if (this.requirements == null) 3607 if (Configuration.errorOnAutoCreate()) 3608 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.requirements"); 3609 else if (Configuration.doAutoCreate()) 3610 this.requirements = new StringType(); // bb 3611 return this.requirements; 3612 } 3613 3614 public boolean hasRequirementsElement() { 3615 return this.requirements != null && !this.requirements.isEmpty(); 3616 } 3617 3618 public boolean hasRequirements() { 3619 return this.requirements != null && !this.requirements.isEmpty(); 3620 } 3621 3622 /** 3623 * @param value {@link #requirements} (Description of why this constraint is 3624 * necessary or appropriate.). This is the underlying object with 3625 * id, value and extensions. The accessor "getRequirements" gives 3626 * direct access to the value 3627 */ 3628 public ElementDefinitionConstraintComponent setRequirementsElement(StringType value) { 3629 this.requirements = value; 3630 return this; 3631 } 3632 3633 /** 3634 * @return Description of why this constraint is necessary or appropriate. 3635 */ 3636 public String getRequirements() { 3637 return this.requirements == null ? null : this.requirements.getValue(); 3638 } 3639 3640 /** 3641 * @param value Description of why this constraint is necessary or appropriate. 3642 */ 3643 public ElementDefinitionConstraintComponent setRequirements(String value) { 3644 if (Utilities.noString(value)) 3645 this.requirements = null; 3646 else { 3647 if (this.requirements == null) 3648 this.requirements = new StringType(); 3649 this.requirements.setValue(value); 3650 } 3651 return this; 3652 } 3653 3654 /** 3655 * @return {@link #severity} (Identifies the impact constraint violation has on 3656 * the conformance of the instance.). This is the underlying object with 3657 * id, value and extensions. The accessor "getSeverity" gives direct 3658 * access to the value 3659 */ 3660 public Enumeration<ConstraintSeverity> getSeverityElement() { 3661 if (this.severity == null) 3662 if (Configuration.errorOnAutoCreate()) 3663 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.severity"); 3664 else if (Configuration.doAutoCreate()) 3665 this.severity = new Enumeration<ConstraintSeverity>(new ConstraintSeverityEnumFactory()); // bb 3666 return this.severity; 3667 } 3668 3669 public boolean hasSeverityElement() { 3670 return this.severity != null && !this.severity.isEmpty(); 3671 } 3672 3673 public boolean hasSeverity() { 3674 return this.severity != null && !this.severity.isEmpty(); 3675 } 3676 3677 /** 3678 * @param value {@link #severity} (Identifies the impact constraint violation 3679 * has on the conformance of the instance.). This is the underlying 3680 * object with id, value and extensions. The accessor "getSeverity" 3681 * gives direct access to the value 3682 */ 3683 public ElementDefinitionConstraintComponent setSeverityElement(Enumeration<ConstraintSeverity> value) { 3684 this.severity = value; 3685 return this; 3686 } 3687 3688 /** 3689 * @return Identifies the impact constraint violation has on the conformance of 3690 * the instance. 3691 */ 3692 public ConstraintSeverity getSeverity() { 3693 return this.severity == null ? null : this.severity.getValue(); 3694 } 3695 3696 /** 3697 * @param value Identifies the impact constraint violation has on the 3698 * conformance of the instance. 3699 */ 3700 public ElementDefinitionConstraintComponent setSeverity(ConstraintSeverity value) { 3701 if (this.severity == null) 3702 this.severity = new Enumeration<ConstraintSeverity>(new ConstraintSeverityEnumFactory()); 3703 this.severity.setValue(value); 3704 return this; 3705 } 3706 3707 /** 3708 * @return {@link #human} (Text that can be used to describe the constraint in 3709 * messages identifying that the constraint has been violated.). This is 3710 * the underlying object with id, value and extensions. The accessor 3711 * "getHuman" gives direct access to the value 3712 */ 3713 public StringType getHumanElement() { 3714 if (this.human == null) 3715 if (Configuration.errorOnAutoCreate()) 3716 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.human"); 3717 else if (Configuration.doAutoCreate()) 3718 this.human = new StringType(); // bb 3719 return this.human; 3720 } 3721 3722 public boolean hasHumanElement() { 3723 return this.human != null && !this.human.isEmpty(); 3724 } 3725 3726 public boolean hasHuman() { 3727 return this.human != null && !this.human.isEmpty(); 3728 } 3729 3730 /** 3731 * @param value {@link #human} (Text that can be used to describe the constraint 3732 * in messages identifying that the constraint has been violated.). 3733 * This is the underlying object with id, value and extensions. The 3734 * accessor "getHuman" gives direct access to the value 3735 */ 3736 public ElementDefinitionConstraintComponent setHumanElement(StringType value) { 3737 this.human = value; 3738 return this; 3739 } 3740 3741 /** 3742 * @return Text that can be used to describe the constraint in messages 3743 * identifying that the constraint has been violated. 3744 */ 3745 public String getHuman() { 3746 return this.human == null ? null : this.human.getValue(); 3747 } 3748 3749 /** 3750 * @param value Text that can be used to describe the constraint in messages 3751 * identifying that the constraint has been violated. 3752 */ 3753 public ElementDefinitionConstraintComponent setHuman(String value) { 3754 if (this.human == null) 3755 this.human = new StringType(); 3756 this.human.setValue(value); 3757 return this; 3758 } 3759 3760 /** 3761 * @return {@link #expression} (A [FHIRPath](fhirpath.html) expression of 3762 * constraint that can be executed to see if this constraint is met.). 3763 * This is the underlying object with id, value and extensions. The 3764 * accessor "getExpression" gives direct access to the value 3765 */ 3766 public StringType getExpressionElement() { 3767 if (this.expression == null) 3768 if (Configuration.errorOnAutoCreate()) 3769 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.expression"); 3770 else if (Configuration.doAutoCreate()) 3771 this.expression = new StringType(); // bb 3772 return this.expression; 3773 } 3774 3775 public boolean hasExpressionElement() { 3776 return this.expression != null && !this.expression.isEmpty(); 3777 } 3778 3779 public boolean hasExpression() { 3780 return this.expression != null && !this.expression.isEmpty(); 3781 } 3782 3783 /** 3784 * @param value {@link #expression} (A [FHIRPath](fhirpath.html) expression of 3785 * constraint that can be executed to see if this constraint is 3786 * met.). This is the underlying object with id, value and 3787 * extensions. The accessor "getExpression" gives direct access to 3788 * the value 3789 */ 3790 public ElementDefinitionConstraintComponent setExpressionElement(StringType value) { 3791 this.expression = value; 3792 return this; 3793 } 3794 3795 /** 3796 * @return A [FHIRPath](fhirpath.html) expression of constraint that can be 3797 * executed to see if this constraint is met. 3798 */ 3799 public String getExpression() { 3800 return this.expression == null ? null : this.expression.getValue(); 3801 } 3802 3803 /** 3804 * @param value A [FHIRPath](fhirpath.html) expression of constraint that can be 3805 * executed to see if this constraint is met. 3806 */ 3807 public ElementDefinitionConstraintComponent setExpression(String value) { 3808 if (Utilities.noString(value)) 3809 this.expression = null; 3810 else { 3811 if (this.expression == null) 3812 this.expression = new StringType(); 3813 this.expression.setValue(value); 3814 } 3815 return this; 3816 } 3817 3818 /** 3819 * @return {@link #xpath} (An XPath expression of constraint that can be 3820 * executed to see if this constraint is met.). This is the underlying 3821 * object with id, value and extensions. The accessor "getXpath" gives 3822 * direct access to the value 3823 */ 3824 public StringType getXpathElement() { 3825 if (this.xpath == null) 3826 if (Configuration.errorOnAutoCreate()) 3827 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.xpath"); 3828 else if (Configuration.doAutoCreate()) 3829 this.xpath = new StringType(); // bb 3830 return this.xpath; 3831 } 3832 3833 public boolean hasXpathElement() { 3834 return this.xpath != null && !this.xpath.isEmpty(); 3835 } 3836 3837 public boolean hasXpath() { 3838 return this.xpath != null && !this.xpath.isEmpty(); 3839 } 3840 3841 /** 3842 * @param value {@link #xpath} (An XPath expression of constraint that can be 3843 * executed to see if this constraint is met.). This is the 3844 * underlying object with id, value and extensions. The accessor 3845 * "getXpath" gives direct access to the value 3846 */ 3847 public ElementDefinitionConstraintComponent setXpathElement(StringType value) { 3848 this.xpath = value; 3849 return this; 3850 } 3851 3852 /** 3853 * @return An XPath expression of constraint that can be executed to see if this 3854 * constraint is met. 3855 */ 3856 public String getXpath() { 3857 return this.xpath == null ? null : this.xpath.getValue(); 3858 } 3859 3860 /** 3861 * @param value An XPath expression of constraint that can be executed to see if 3862 * this constraint is met. 3863 */ 3864 public ElementDefinitionConstraintComponent setXpath(String value) { 3865 if (Utilities.noString(value)) 3866 this.xpath = null; 3867 else { 3868 if (this.xpath == null) 3869 this.xpath = new StringType(); 3870 this.xpath.setValue(value); 3871 } 3872 return this; 3873 } 3874 3875 /** 3876 * @return {@link #source} (A reference to the original source of the 3877 * constraint, for traceability purposes.). This is the underlying 3878 * object with id, value and extensions. The accessor "getSource" gives 3879 * direct access to the value 3880 */ 3881 public CanonicalType getSourceElement() { 3882 if (this.source == null) 3883 if (Configuration.errorOnAutoCreate()) 3884 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.source"); 3885 else if (Configuration.doAutoCreate()) 3886 this.source = new CanonicalType(); // bb 3887 return this.source; 3888 } 3889 3890 public boolean hasSourceElement() { 3891 return this.source != null && !this.source.isEmpty(); 3892 } 3893 3894 public boolean hasSource() { 3895 return this.source != null && !this.source.isEmpty(); 3896 } 3897 3898 /** 3899 * @param value {@link #source} (A reference to the original source of the 3900 * constraint, for traceability purposes.). This is the underlying 3901 * object with id, value and extensions. The accessor "getSource" 3902 * gives direct access to the value 3903 */ 3904 public ElementDefinitionConstraintComponent setSourceElement(CanonicalType value) { 3905 this.source = value; 3906 return this; 3907 } 3908 3909 /** 3910 * @return A reference to the original source of the constraint, for 3911 * traceability purposes. 3912 */ 3913 public String getSource() { 3914 return this.source == null ? null : this.source.getValue(); 3915 } 3916 3917 /** 3918 * @param value A reference to the original source of the constraint, for 3919 * traceability purposes. 3920 */ 3921 public ElementDefinitionConstraintComponent setSource(String value) { 3922 if (Utilities.noString(value)) 3923 this.source = null; 3924 else { 3925 if (this.source == null) 3926 this.source = new CanonicalType(); 3927 this.source.setValue(value); 3928 } 3929 return this; 3930 } 3931 3932 protected void listChildren(List<Property> children) { 3933 super.listChildren(children); 3934 children.add(new Property("key", "id", 3935 "Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality.", 3936 0, 1, key)); 3937 children.add(new Property("requirements", "string", 3938 "Description of why this constraint is necessary or appropriate.", 0, 1, requirements)); 3939 children.add(new Property("severity", "code", 3940 "Identifies the impact constraint violation has on the conformance of the instance.", 0, 1, severity)); 3941 children.add(new Property("human", "string", 3942 "Text that can be used to describe the constraint in messages identifying that the constraint has been violated.", 3943 0, 1, human)); 3944 children.add(new Property("expression", "string", 3945 "A [FHIRPath](fhirpath.html) expression of constraint that can be executed to see if this constraint is met.", 3946 0, 1, expression)); 3947 children.add(new Property("xpath", "string", 3948 "An XPath expression of constraint that can be executed to see if this constraint is met.", 0, 1, xpath)); 3949 children.add(new Property("source", "canonical(StructureDefinition)", 3950 "A reference to the original source of the constraint, for traceability purposes.", 0, 1, source)); 3951 } 3952 3953 @Override 3954 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3955 switch (_hash) { 3956 case 106079: 3957 /* key */ return new Property("key", "id", 3958 "Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality.", 3959 0, 1, key); 3960 case -1619874672: 3961 /* requirements */ return new Property("requirements", "string", 3962 "Description of why this constraint is necessary or appropriate.", 0, 1, requirements); 3963 case 1478300413: 3964 /* severity */ return new Property("severity", "code", 3965 "Identifies the impact constraint violation has on the conformance of the instance.", 0, 1, severity); 3966 case 99639597: 3967 /* human */ return new Property("human", "string", 3968 "Text that can be used to describe the constraint in messages identifying that the constraint has been violated.", 3969 0, 1, human); 3970 case -1795452264: 3971 /* expression */ return new Property("expression", "string", 3972 "A [FHIRPath](fhirpath.html) expression of constraint that can be executed to see if this constraint is met.", 3973 0, 1, expression); 3974 case 114256029: 3975 /* xpath */ return new Property("xpath", "string", 3976 "An XPath expression of constraint that can be executed to see if this constraint is met.", 0, 1, xpath); 3977 case -896505829: 3978 /* source */ return new Property("source", "canonical(StructureDefinition)", 3979 "A reference to the original source of the constraint, for traceability purposes.", 0, 1, source); 3980 default: 3981 return super.getNamedProperty(_hash, _name, _checkValid); 3982 } 3983 3984 } 3985 3986 @Override 3987 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3988 switch (hash) { 3989 case 106079: 3990 /* key */ return this.key == null ? new Base[0] : new Base[] { this.key }; // IdType 3991 case -1619874672: 3992 /* requirements */ return this.requirements == null ? new Base[0] : new Base[] { this.requirements }; // StringType 3993 case 1478300413: 3994 /* severity */ return this.severity == null ? new Base[0] : new Base[] { this.severity }; // Enumeration<ConstraintSeverity> 3995 case 99639597: 3996 /* human */ return this.human == null ? new Base[0] : new Base[] { this.human }; // StringType 3997 case -1795452264: 3998 /* expression */ return this.expression == null ? new Base[0] : new Base[] { this.expression }; // StringType 3999 case 114256029: 4000 /* xpath */ return this.xpath == null ? new Base[0] : new Base[] { this.xpath }; // StringType 4001 case -896505829: 4002 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // CanonicalType 4003 default: 4004 return super.getProperty(hash, name, checkValid); 4005 } 4006 4007 } 4008 4009 @Override 4010 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4011 switch (hash) { 4012 case 106079: // key 4013 this.key = castToId(value); // IdType 4014 return value; 4015 case -1619874672: // requirements 4016 this.requirements = castToString(value); // StringType 4017 return value; 4018 case 1478300413: // severity 4019 value = new ConstraintSeverityEnumFactory().fromType(castToCode(value)); 4020 this.severity = (Enumeration) value; // Enumeration<ConstraintSeverity> 4021 return value; 4022 case 99639597: // human 4023 this.human = castToString(value); // StringType 4024 return value; 4025 case -1795452264: // expression 4026 this.expression = castToString(value); // StringType 4027 return value; 4028 case 114256029: // xpath 4029 this.xpath = castToString(value); // StringType 4030 return value; 4031 case -896505829: // source 4032 this.source = castToCanonical(value); // CanonicalType 4033 return value; 4034 default: 4035 return super.setProperty(hash, name, value); 4036 } 4037 4038 } 4039 4040 @Override 4041 public Base setProperty(String name, Base value) throws FHIRException { 4042 if (name.equals("key")) { 4043 this.key = castToId(value); // IdType 4044 } else if (name.equals("requirements")) { 4045 this.requirements = castToString(value); // StringType 4046 } else if (name.equals("severity")) { 4047 value = new ConstraintSeverityEnumFactory().fromType(castToCode(value)); 4048 this.severity = (Enumeration) value; // Enumeration<ConstraintSeverity> 4049 } else if (name.equals("human")) { 4050 this.human = castToString(value); // StringType 4051 } else if (name.equals("expression")) { 4052 this.expression = castToString(value); // StringType 4053 } else if (name.equals("xpath")) { 4054 this.xpath = castToString(value); // StringType 4055 } else if (name.equals("source")) { 4056 this.source = castToCanonical(value); // CanonicalType 4057 } else 4058 return super.setProperty(name, value); 4059 return value; 4060 } 4061 4062 @Override 4063 public void removeChild(String name, Base value) throws FHIRException { 4064 if (name.equals("key")) { 4065 this.key = null; 4066 } else if (name.equals("requirements")) { 4067 this.requirements = null; 4068 } else if (name.equals("severity")) { 4069 this.severity = null; 4070 } else if (name.equals("human")) { 4071 this.human = null; 4072 } else if (name.equals("expression")) { 4073 this.expression = null; 4074 } else if (name.equals("xpath")) { 4075 this.xpath = null; 4076 } else if (name.equals("source")) { 4077 this.source = null; 4078 } else 4079 super.removeChild(name, value); 4080 4081 } 4082 4083 @Override 4084 public Base makeProperty(int hash, String name) throws FHIRException { 4085 switch (hash) { 4086 case 106079: 4087 return getKeyElement(); 4088 case -1619874672: 4089 return getRequirementsElement(); 4090 case 1478300413: 4091 return getSeverityElement(); 4092 case 99639597: 4093 return getHumanElement(); 4094 case -1795452264: 4095 return getExpressionElement(); 4096 case 114256029: 4097 return getXpathElement(); 4098 case -896505829: 4099 return getSourceElement(); 4100 default: 4101 return super.makeProperty(hash, name); 4102 } 4103 4104 } 4105 4106 @Override 4107 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4108 switch (hash) { 4109 case 106079: 4110 /* key */ return new String[] { "id" }; 4111 case -1619874672: 4112 /* requirements */ return new String[] { "string" }; 4113 case 1478300413: 4114 /* severity */ return new String[] { "code" }; 4115 case 99639597: 4116 /* human */ return new String[] { "string" }; 4117 case -1795452264: 4118 /* expression */ return new String[] { "string" }; 4119 case 114256029: 4120 /* xpath */ return new String[] { "string" }; 4121 case -896505829: 4122 /* source */ return new String[] { "canonical" }; 4123 default: 4124 return super.getTypesForProperty(hash, name); 4125 } 4126 4127 } 4128 4129 @Override 4130 public Base addChild(String name) throws FHIRException { 4131 if (name.equals("key")) { 4132 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.key"); 4133 } else if (name.equals("requirements")) { 4134 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.requirements"); 4135 } else if (name.equals("severity")) { 4136 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.severity"); 4137 } else if (name.equals("human")) { 4138 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.human"); 4139 } else if (name.equals("expression")) { 4140 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.expression"); 4141 } else if (name.equals("xpath")) { 4142 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.xpath"); 4143 } else if (name.equals("source")) { 4144 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.source"); 4145 } else 4146 return super.addChild(name); 4147 } 4148 4149 public ElementDefinitionConstraintComponent copy() { 4150 ElementDefinitionConstraintComponent dst = new ElementDefinitionConstraintComponent(); 4151 copyValues(dst); 4152 return dst; 4153 } 4154 4155 public void copyValues(ElementDefinitionConstraintComponent dst) { 4156 super.copyValues(dst); 4157 dst.key = key == null ? null : key.copy(); 4158 dst.requirements = requirements == null ? null : requirements.copy(); 4159 dst.severity = severity == null ? null : severity.copy(); 4160 dst.human = human == null ? null : human.copy(); 4161 dst.expression = expression == null ? null : expression.copy(); 4162 dst.xpath = xpath == null ? null : xpath.copy(); 4163 dst.source = source == null ? null : source.copy(); 4164 } 4165 4166 @Override 4167 public boolean equalsDeep(Base other_) { 4168 if (!super.equalsDeep(other_)) 4169 return false; 4170 if (!(other_ instanceof ElementDefinitionConstraintComponent)) 4171 return false; 4172 ElementDefinitionConstraintComponent o = (ElementDefinitionConstraintComponent) other_; 4173 return compareDeep(key, o.key, true) && compareDeep(requirements, o.requirements, true) 4174 && compareDeep(severity, o.severity, true) && compareDeep(human, o.human, true) 4175 && compareDeep(expression, o.expression, true) && compareDeep(xpath, o.xpath, true) 4176 && compareDeep(source, o.source, true); 4177 } 4178 4179 @Override 4180 public boolean equalsShallow(Base other_) { 4181 if (!super.equalsShallow(other_)) 4182 return false; 4183 if (!(other_ instanceof ElementDefinitionConstraintComponent)) 4184 return false; 4185 ElementDefinitionConstraintComponent o = (ElementDefinitionConstraintComponent) other_; 4186 return compareValues(key, o.key, true) && compareValues(requirements, o.requirements, true) 4187 && compareValues(severity, o.severity, true) && compareValues(human, o.human, true) 4188 && compareValues(expression, o.expression, true) && compareValues(xpath, o.xpath, true); 4189 } 4190 4191 public boolean isEmpty() { 4192 return super.isEmpty() 4193 && ca.uhn.fhir.util.ElementUtil.isEmpty(key, requirements, severity, human, expression, xpath, source); 4194 } 4195 4196 public String fhirType() { 4197 return "ElementDefinition.constraint"; 4198 4199 } 4200 4201 } 4202 4203 @Block() 4204 public static class ElementDefinitionBindingComponent extends Element implements IBaseDatatypeElement { 4205 /** 4206 * Indicates the degree of conformance expectations associated with this binding 4207 * - that is, the degree to which the provided value set must be adhered to in 4208 * the instances. 4209 */ 4210 @Child(name = "strength", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 4211 @Description(shortDefinition = "required | extensible | preferred | example", formalDefinition = "Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.") 4212 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/binding-strength") 4213 protected Enumeration<BindingStrength> strength; 4214 4215 /** 4216 * Describes the intended use of this particular set of codes. 4217 */ 4218 @Child(name = "description", type = { 4219 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 4220 @Description(shortDefinition = "Human explanation of the value set", formalDefinition = "Describes the intended use of this particular set of codes.") 4221 protected StringType description; 4222 4223 /** 4224 * Refers to the value set that identifies the set of codes the binding refers 4225 * to. 4226 */ 4227 @Child(name = "valueSet", type = { 4228 CanonicalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 4229 @Description(shortDefinition = "Source of value set", formalDefinition = "Refers to the value set that identifies the set of codes the binding refers to.") 4230 protected CanonicalType valueSet; 4231 4232 private static final long serialVersionUID = -514477030L; 4233 4234 /** 4235 * Constructor 4236 */ 4237 public ElementDefinitionBindingComponent() { 4238 super(); 4239 } 4240 4241 /** 4242 * Constructor 4243 */ 4244 public ElementDefinitionBindingComponent(Enumeration<BindingStrength> strength) { 4245 super(); 4246 this.strength = strength; 4247 } 4248 4249 /** 4250 * @return {@link #strength} (Indicates the degree of conformance expectations 4251 * associated with this binding - that is, the degree to which the 4252 * provided value set must be adhered to in the instances.). This is the 4253 * underlying object with id, value and extensions. The accessor 4254 * "getStrength" gives direct access to the value 4255 */ 4256 public Enumeration<BindingStrength> getStrengthElement() { 4257 if (this.strength == null) 4258 if (Configuration.errorOnAutoCreate()) 4259 throw new Error("Attempt to auto-create ElementDefinitionBindingComponent.strength"); 4260 else if (Configuration.doAutoCreate()) 4261 this.strength = new Enumeration<BindingStrength>(new BindingStrengthEnumFactory()); // bb 4262 return this.strength; 4263 } 4264 4265 public boolean hasStrengthElement() { 4266 return this.strength != null && !this.strength.isEmpty(); 4267 } 4268 4269 public boolean hasStrength() { 4270 return this.strength != null && !this.strength.isEmpty(); 4271 } 4272 4273 /** 4274 * @param value {@link #strength} (Indicates the degree of conformance 4275 * expectations associated with this binding - that is, the degree 4276 * to which the provided value set must be adhered to in the 4277 * instances.). This is the underlying object with id, value and 4278 * extensions. The accessor "getStrength" gives direct access to 4279 * the value 4280 */ 4281 public ElementDefinitionBindingComponent setStrengthElement(Enumeration<BindingStrength> value) { 4282 this.strength = value; 4283 return this; 4284 } 4285 4286 /** 4287 * @return Indicates the degree of conformance expectations associated with this 4288 * binding - that is, the degree to which the provided value set must be 4289 * adhered to in the instances. 4290 */ 4291 public BindingStrength getStrength() { 4292 return this.strength == null ? null : this.strength.getValue(); 4293 } 4294 4295 /** 4296 * @param value Indicates the degree of conformance expectations associated with 4297 * this binding - that is, the degree to which the provided value 4298 * set must be adhered to in the instances. 4299 */ 4300 public ElementDefinitionBindingComponent setStrength(BindingStrength value) { 4301 if (this.strength == null) 4302 this.strength = new Enumeration<BindingStrength>(new BindingStrengthEnumFactory()); 4303 this.strength.setValue(value); 4304 return this; 4305 } 4306 4307 /** 4308 * @return {@link #description} (Describes the intended use of this particular 4309 * set of codes.). This is the underlying object with id, value and 4310 * extensions. The accessor "getDescription" gives direct access to the 4311 * value 4312 */ 4313 public StringType getDescriptionElement() { 4314 if (this.description == null) 4315 if (Configuration.errorOnAutoCreate()) 4316 throw new Error("Attempt to auto-create ElementDefinitionBindingComponent.description"); 4317 else if (Configuration.doAutoCreate()) 4318 this.description = new StringType(); // bb 4319 return this.description; 4320 } 4321 4322 public boolean hasDescriptionElement() { 4323 return this.description != null && !this.description.isEmpty(); 4324 } 4325 4326 public boolean hasDescription() { 4327 return this.description != null && !this.description.isEmpty(); 4328 } 4329 4330 /** 4331 * @param value {@link #description} (Describes the intended use of this 4332 * particular set of codes.). This is the underlying object with 4333 * id, value and extensions. The accessor "getDescription" gives 4334 * direct access to the value 4335 */ 4336 public ElementDefinitionBindingComponent setDescriptionElement(StringType value) { 4337 this.description = value; 4338 return this; 4339 } 4340 4341 /** 4342 * @return Describes the intended use of this particular set of codes. 4343 */ 4344 public String getDescription() { 4345 return this.description == null ? null : this.description.getValue(); 4346 } 4347 4348 /** 4349 * @param value Describes the intended use of this particular set of codes. 4350 */ 4351 public ElementDefinitionBindingComponent setDescription(String value) { 4352 if (Utilities.noString(value)) 4353 this.description = null; 4354 else { 4355 if (this.description == null) 4356 this.description = new StringType(); 4357 this.description.setValue(value); 4358 } 4359 return this; 4360 } 4361 4362 /** 4363 * @return {@link #valueSet} (Refers to the value set that identifies the set of 4364 * codes the binding refers to.). This is the underlying object with id, 4365 * value and extensions. The accessor "getValueSet" gives direct access 4366 * to the value 4367 */ 4368 public CanonicalType getValueSetElement() { 4369 if (this.valueSet == null) 4370 if (Configuration.errorOnAutoCreate()) 4371 throw new Error("Attempt to auto-create ElementDefinitionBindingComponent.valueSet"); 4372 else if (Configuration.doAutoCreate()) 4373 this.valueSet = new CanonicalType(); // bb 4374 return this.valueSet; 4375 } 4376 4377 public boolean hasValueSetElement() { 4378 return this.valueSet != null && !this.valueSet.isEmpty(); 4379 } 4380 4381 public boolean hasValueSet() { 4382 return this.valueSet != null && !this.valueSet.isEmpty(); 4383 } 4384 4385 /** 4386 * @param value {@link #valueSet} (Refers to the value set that identifies the 4387 * set of codes the binding refers to.). This is the underlying 4388 * object with id, value and extensions. The accessor "getValueSet" 4389 * gives direct access to the value 4390 */ 4391 public ElementDefinitionBindingComponent setValueSetElement(CanonicalType value) { 4392 this.valueSet = value; 4393 return this; 4394 } 4395 4396 /** 4397 * @return Refers to the value set that identifies the set of codes the binding 4398 * refers to. 4399 */ 4400 public String getValueSet() { 4401 return this.valueSet == null ? null : this.valueSet.getValue(); 4402 } 4403 4404 /** 4405 * @param value Refers to the value set that identifies the set of codes the 4406 * binding refers to. 4407 */ 4408 public ElementDefinitionBindingComponent setValueSet(String value) { 4409 if (Utilities.noString(value)) 4410 this.valueSet = null; 4411 else { 4412 if (this.valueSet == null) 4413 this.valueSet = new CanonicalType(); 4414 this.valueSet.setValue(value); 4415 } 4416 return this; 4417 } 4418 4419 protected void listChildren(List<Property> children) { 4420 super.listChildren(children); 4421 children.add(new Property("strength", "code", 4422 "Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.", 4423 0, 1, strength)); 4424 children.add(new Property("description", "string", "Describes the intended use of this particular set of codes.", 4425 0, 1, description)); 4426 children.add(new Property("valueSet", "canonical(ValueSet)", 4427 "Refers to the value set that identifies the set of codes the binding refers to.", 0, 1, valueSet)); 4428 } 4429 4430 @Override 4431 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4432 switch (_hash) { 4433 case 1791316033: 4434 /* strength */ return new Property("strength", "code", 4435 "Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.", 4436 0, 1, strength); 4437 case -1724546052: 4438 /* description */ return new Property("description", "string", 4439 "Describes the intended use of this particular set of codes.", 0, 1, description); 4440 case -1410174671: 4441 /* valueSet */ return new Property("valueSet", "canonical(ValueSet)", 4442 "Refers to the value set that identifies the set of codes the binding refers to.", 0, 1, valueSet); 4443 default: 4444 return super.getNamedProperty(_hash, _name, _checkValid); 4445 } 4446 4447 } 4448 4449 @Override 4450 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4451 switch (hash) { 4452 case 1791316033: 4453 /* strength */ return this.strength == null ? new Base[0] : new Base[] { this.strength }; // Enumeration<BindingStrength> 4454 case -1724546052: 4455 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 4456 case -1410174671: 4457 /* valueSet */ return this.valueSet == null ? new Base[0] : new Base[] { this.valueSet }; // CanonicalType 4458 default: 4459 return super.getProperty(hash, name, checkValid); 4460 } 4461 4462 } 4463 4464 @Override 4465 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4466 switch (hash) { 4467 case 1791316033: // strength 4468 value = new BindingStrengthEnumFactory().fromType(castToCode(value)); 4469 this.strength = (Enumeration) value; // Enumeration<BindingStrength> 4470 return value; 4471 case -1724546052: // description 4472 this.description = castToString(value); // StringType 4473 return value; 4474 case -1410174671: // valueSet 4475 this.valueSet = castToCanonical(value); // CanonicalType 4476 return value; 4477 default: 4478 return super.setProperty(hash, name, value); 4479 } 4480 4481 } 4482 4483 @Override 4484 public Base setProperty(String name, Base value) throws FHIRException { 4485 if (name.equals("strength")) { 4486 value = new BindingStrengthEnumFactory().fromType(castToCode(value)); 4487 this.strength = (Enumeration) value; // Enumeration<BindingStrength> 4488 } else if (name.equals("description")) { 4489 this.description = castToString(value); // StringType 4490 } else if (name.equals("valueSet")) { 4491 this.valueSet = castToCanonical(value); // CanonicalType 4492 } else 4493 return super.setProperty(name, value); 4494 return value; 4495 } 4496 4497 @Override 4498 public void removeChild(String name, Base value) throws FHIRException { 4499 if (name.equals("strength")) { 4500 this.strength = null; 4501 } else if (name.equals("description")) { 4502 this.description = null; 4503 } else if (name.equals("valueSet")) { 4504 this.valueSet = null; 4505 } else 4506 super.removeChild(name, value); 4507 4508 } 4509 4510 @Override 4511 public Base makeProperty(int hash, String name) throws FHIRException { 4512 switch (hash) { 4513 case 1791316033: 4514 return getStrengthElement(); 4515 case -1724546052: 4516 return getDescriptionElement(); 4517 case -1410174671: 4518 return getValueSetElement(); 4519 default: 4520 return super.makeProperty(hash, name); 4521 } 4522 4523 } 4524 4525 @Override 4526 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4527 switch (hash) { 4528 case 1791316033: 4529 /* strength */ return new String[] { "code" }; 4530 case -1724546052: 4531 /* description */ return new String[] { "string" }; 4532 case -1410174671: 4533 /* valueSet */ return new String[] { "canonical" }; 4534 default: 4535 return super.getTypesForProperty(hash, name); 4536 } 4537 4538 } 4539 4540 @Override 4541 public Base addChild(String name) throws FHIRException { 4542 if (name.equals("strength")) { 4543 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.strength"); 4544 } else if (name.equals("description")) { 4545 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.description"); 4546 } else if (name.equals("valueSet")) { 4547 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.valueSet"); 4548 } else 4549 return super.addChild(name); 4550 } 4551 4552 public ElementDefinitionBindingComponent copy() { 4553 ElementDefinitionBindingComponent dst = new ElementDefinitionBindingComponent(); 4554 copyValues(dst); 4555 return dst; 4556 } 4557 4558 public void copyValues(ElementDefinitionBindingComponent dst) { 4559 super.copyValues(dst); 4560 dst.strength = strength == null ? null : strength.copy(); 4561 dst.description = description == null ? null : description.copy(); 4562 dst.valueSet = valueSet == null ? null : valueSet.copy(); 4563 } 4564 4565 @Override 4566 public boolean equalsDeep(Base other_) { 4567 if (!super.equalsDeep(other_)) 4568 return false; 4569 if (!(other_ instanceof ElementDefinitionBindingComponent)) 4570 return false; 4571 ElementDefinitionBindingComponent o = (ElementDefinitionBindingComponent) other_; 4572 return compareDeep(strength, o.strength, true) && compareDeep(description, o.description, true) 4573 && compareDeep(valueSet, o.valueSet, true); 4574 } 4575 4576 @Override 4577 public boolean equalsShallow(Base other_) { 4578 if (!super.equalsShallow(other_)) 4579 return false; 4580 if (!(other_ instanceof ElementDefinitionBindingComponent)) 4581 return false; 4582 ElementDefinitionBindingComponent o = (ElementDefinitionBindingComponent) other_; 4583 return compareValues(strength, o.strength, true) && compareValues(description, o.description, true); 4584 } 4585 4586 public boolean isEmpty() { 4587 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(strength, description, valueSet); 4588 } 4589 4590 public String fhirType() { 4591 return "ElementDefinition.binding"; 4592 4593 } 4594 4595 } 4596 4597 @Block() 4598 public static class ElementDefinitionMappingComponent extends Element implements IBaseDatatypeElement { 4599 /** 4600 * An internal reference to the definition of a mapping. 4601 */ 4602 @Child(name = "identity", type = { IdType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 4603 @Description(shortDefinition = "Reference to mapping declaration", formalDefinition = "An internal reference to the definition of a mapping.") 4604 protected IdType identity; 4605 4606 /** 4607 * Identifies the computable language in which mapping.map is expressed. 4608 */ 4609 @Child(name = "language", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 4610 @Description(shortDefinition = "Computable language of mapping", formalDefinition = "Identifies the computable language in which mapping.map is expressed.") 4611 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/mimetypes") 4612 protected CodeType language; 4613 4614 /** 4615 * Expresses what part of the target specification corresponds to this element. 4616 */ 4617 @Child(name = "map", type = { StringType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 4618 @Description(shortDefinition = "Details of the mapping", formalDefinition = "Expresses what part of the target specification corresponds to this element.") 4619 protected StringType map; 4620 4621 /** 4622 * Comments that provide information about the mapping or its use. 4623 */ 4624 @Child(name = "comment", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 4625 @Description(shortDefinition = "Comments about the mapping or its use", formalDefinition = "Comments that provide information about the mapping or its use.") 4626 protected StringType comment; 4627 4628 private static final long serialVersionUID = 1386816887L; 4629 4630 /** 4631 * Constructor 4632 */ 4633 public ElementDefinitionMappingComponent() { 4634 super(); 4635 } 4636 4637 /** 4638 * Constructor 4639 */ 4640 public ElementDefinitionMappingComponent(IdType identity, StringType map) { 4641 super(); 4642 this.identity = identity; 4643 this.map = map; 4644 } 4645 4646 /** 4647 * @return {@link #identity} (An internal reference to the definition of a 4648 * mapping.). This is the underlying object with id, value and 4649 * extensions. The accessor "getIdentity" gives direct access to the 4650 * value 4651 */ 4652 public IdType getIdentityElement() { 4653 if (this.identity == null) 4654 if (Configuration.errorOnAutoCreate()) 4655 throw new Error("Attempt to auto-create ElementDefinitionMappingComponent.identity"); 4656 else if (Configuration.doAutoCreate()) 4657 this.identity = new IdType(); // bb 4658 return this.identity; 4659 } 4660 4661 public boolean hasIdentityElement() { 4662 return this.identity != null && !this.identity.isEmpty(); 4663 } 4664 4665 public boolean hasIdentity() { 4666 return this.identity != null && !this.identity.isEmpty(); 4667 } 4668 4669 /** 4670 * @param value {@link #identity} (An internal reference to the definition of a 4671 * mapping.). This is the underlying object with id, value and 4672 * extensions. The accessor "getIdentity" gives direct access to 4673 * the value 4674 */ 4675 public ElementDefinitionMappingComponent setIdentityElement(IdType value) { 4676 this.identity = value; 4677 return this; 4678 } 4679 4680 /** 4681 * @return An internal reference to the definition of a mapping. 4682 */ 4683 public String getIdentity() { 4684 return this.identity == null ? null : this.identity.getValue(); 4685 } 4686 4687 /** 4688 * @param value An internal reference to the definition of a mapping. 4689 */ 4690 public ElementDefinitionMappingComponent setIdentity(String value) { 4691 if (this.identity == null) 4692 this.identity = new IdType(); 4693 this.identity.setValue(value); 4694 return this; 4695 } 4696 4697 /** 4698 * @return {@link #language} (Identifies the computable language in which 4699 * mapping.map is expressed.). This is the underlying object with id, 4700 * value and extensions. The accessor "getLanguage" gives direct access 4701 * to the value 4702 */ 4703 public CodeType getLanguageElement() { 4704 if (this.language == null) 4705 if (Configuration.errorOnAutoCreate()) 4706 throw new Error("Attempt to auto-create ElementDefinitionMappingComponent.language"); 4707 else if (Configuration.doAutoCreate()) 4708 this.language = new CodeType(); // bb 4709 return this.language; 4710 } 4711 4712 public boolean hasLanguageElement() { 4713 return this.language != null && !this.language.isEmpty(); 4714 } 4715 4716 public boolean hasLanguage() { 4717 return this.language != null && !this.language.isEmpty(); 4718 } 4719 4720 /** 4721 * @param value {@link #language} (Identifies the computable language in which 4722 * mapping.map is expressed.). This is the underlying object with 4723 * id, value and extensions. The accessor "getLanguage" gives 4724 * direct access to the value 4725 */ 4726 public ElementDefinitionMappingComponent setLanguageElement(CodeType value) { 4727 this.language = value; 4728 return this; 4729 } 4730 4731 /** 4732 * @return Identifies the computable language in which mapping.map is expressed. 4733 */ 4734 public String getLanguage() { 4735 return this.language == null ? null : this.language.getValue(); 4736 } 4737 4738 /** 4739 * @param value Identifies the computable language in which mapping.map is 4740 * expressed. 4741 */ 4742 public ElementDefinitionMappingComponent setLanguage(String value) { 4743 if (Utilities.noString(value)) 4744 this.language = null; 4745 else { 4746 if (this.language == null) 4747 this.language = new CodeType(); 4748 this.language.setValue(value); 4749 } 4750 return this; 4751 } 4752 4753 /** 4754 * @return {@link #map} (Expresses what part of the target specification 4755 * corresponds to this element.). This is the underlying object with id, 4756 * value and extensions. The accessor "getMap" gives direct access to 4757 * the value 4758 */ 4759 public StringType getMapElement() { 4760 if (this.map == null) 4761 if (Configuration.errorOnAutoCreate()) 4762 throw new Error("Attempt to auto-create ElementDefinitionMappingComponent.map"); 4763 else if (Configuration.doAutoCreate()) 4764 this.map = new StringType(); // bb 4765 return this.map; 4766 } 4767 4768 public boolean hasMapElement() { 4769 return this.map != null && !this.map.isEmpty(); 4770 } 4771 4772 public boolean hasMap() { 4773 return this.map != null && !this.map.isEmpty(); 4774 } 4775 4776 /** 4777 * @param value {@link #map} (Expresses what part of the target specification 4778 * corresponds to this element.). This is the underlying object 4779 * with id, value and extensions. The accessor "getMap" gives 4780 * direct access to the value 4781 */ 4782 public ElementDefinitionMappingComponent setMapElement(StringType value) { 4783 this.map = value; 4784 return this; 4785 } 4786 4787 /** 4788 * @return Expresses what part of the target specification corresponds to this 4789 * element. 4790 */ 4791 public String getMap() { 4792 return this.map == null ? null : this.map.getValue(); 4793 } 4794 4795 /** 4796 * @param value Expresses what part of the target specification corresponds to 4797 * this element. 4798 */ 4799 public ElementDefinitionMappingComponent setMap(String value) { 4800 if (this.map == null) 4801 this.map = new StringType(); 4802 this.map.setValue(value); 4803 return this; 4804 } 4805 4806 /** 4807 * @return {@link #comment} (Comments that provide information about the mapping 4808 * or its use.). This is the underlying object with id, value and 4809 * extensions. The accessor "getComment" gives direct access to the 4810 * value 4811 */ 4812 public StringType getCommentElement() { 4813 if (this.comment == null) 4814 if (Configuration.errorOnAutoCreate()) 4815 throw new Error("Attempt to auto-create ElementDefinitionMappingComponent.comment"); 4816 else if (Configuration.doAutoCreate()) 4817 this.comment = new StringType(); // bb 4818 return this.comment; 4819 } 4820 4821 public boolean hasCommentElement() { 4822 return this.comment != null && !this.comment.isEmpty(); 4823 } 4824 4825 public boolean hasComment() { 4826 return this.comment != null && !this.comment.isEmpty(); 4827 } 4828 4829 /** 4830 * @param value {@link #comment} (Comments that provide information about the 4831 * mapping or its use.). This is the underlying object with id, 4832 * value and extensions. The accessor "getComment" gives direct 4833 * access to the value 4834 */ 4835 public ElementDefinitionMappingComponent setCommentElement(StringType value) { 4836 this.comment = value; 4837 return this; 4838 } 4839 4840 /** 4841 * @return Comments that provide information about the mapping or its use. 4842 */ 4843 public String getComment() { 4844 return this.comment == null ? null : this.comment.getValue(); 4845 } 4846 4847 /** 4848 * @param value Comments that provide information about the mapping or its use. 4849 */ 4850 public ElementDefinitionMappingComponent setComment(String value) { 4851 if (Utilities.noString(value)) 4852 this.comment = null; 4853 else { 4854 if (this.comment == null) 4855 this.comment = new StringType(); 4856 this.comment.setValue(value); 4857 } 4858 return this; 4859 } 4860 4861 protected void listChildren(List<Property> children) { 4862 super.listChildren(children); 4863 children 4864 .add(new Property("identity", "id", "An internal reference to the definition of a mapping.", 0, 1, identity)); 4865 children.add(new Property("language", "code", 4866 "Identifies the computable language in which mapping.map is expressed.", 0, 1, language)); 4867 children.add(new Property("map", "string", 4868 "Expresses what part of the target specification corresponds to this element.", 0, 1, map)); 4869 children.add(new Property("comment", "string", "Comments that provide information about the mapping or its use.", 4870 0, 1, comment)); 4871 } 4872 4873 @Override 4874 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4875 switch (_hash) { 4876 case -135761730: 4877 /* identity */ return new Property("identity", "id", "An internal reference to the definition of a mapping.", 0, 4878 1, identity); 4879 case -1613589672: 4880 /* language */ return new Property("language", "code", 4881 "Identifies the computable language in which mapping.map is expressed.", 0, 1, language); 4882 case 107868: 4883 /* map */ return new Property("map", "string", 4884 "Expresses what part of the target specification corresponds to this element.", 0, 1, map); 4885 case 950398559: 4886 /* comment */ return new Property("comment", "string", 4887 "Comments that provide information about the mapping or its use.", 0, 1, comment); 4888 default: 4889 return super.getNamedProperty(_hash, _name, _checkValid); 4890 } 4891 4892 } 4893 4894 @Override 4895 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4896 switch (hash) { 4897 case -135761730: 4898 /* identity */ return this.identity == null ? new Base[0] : new Base[] { this.identity }; // IdType 4899 case -1613589672: 4900 /* language */ return this.language == null ? new Base[0] : new Base[] { this.language }; // CodeType 4901 case 107868: 4902 /* map */ return this.map == null ? new Base[0] : new Base[] { this.map }; // StringType 4903 case 950398559: 4904 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // StringType 4905 default: 4906 return super.getProperty(hash, name, checkValid); 4907 } 4908 4909 } 4910 4911 @Override 4912 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4913 switch (hash) { 4914 case -135761730: // identity 4915 this.identity = castToId(value); // IdType 4916 return value; 4917 case -1613589672: // language 4918 this.language = castToCode(value); // CodeType 4919 return value; 4920 case 107868: // map 4921 this.map = castToString(value); // StringType 4922 return value; 4923 case 950398559: // comment 4924 this.comment = castToString(value); // StringType 4925 return value; 4926 default: 4927 return super.setProperty(hash, name, value); 4928 } 4929 4930 } 4931 4932 @Override 4933 public Base setProperty(String name, Base value) throws FHIRException { 4934 if (name.equals("identity")) { 4935 this.identity = castToId(value); // IdType 4936 } else if (name.equals("language")) { 4937 this.language = castToCode(value); // CodeType 4938 } else if (name.equals("map")) { 4939 this.map = castToString(value); // StringType 4940 } else if (name.equals("comment")) { 4941 this.comment = castToString(value); // StringType 4942 } else 4943 return super.setProperty(name, value); 4944 return value; 4945 } 4946 4947 @Override 4948 public void removeChild(String name, Base value) throws FHIRException { 4949 if (name.equals("identity")) { 4950 this.identity = null; 4951 } else if (name.equals("language")) { 4952 this.language = null; 4953 } else if (name.equals("map")) { 4954 this.map = null; 4955 } else if (name.equals("comment")) { 4956 this.comment = null; 4957 } else 4958 super.removeChild(name, value); 4959 4960 } 4961 4962 @Override 4963 public Base makeProperty(int hash, String name) throws FHIRException { 4964 switch (hash) { 4965 case -135761730: 4966 return getIdentityElement(); 4967 case -1613589672: 4968 return getLanguageElement(); 4969 case 107868: 4970 return getMapElement(); 4971 case 950398559: 4972 return getCommentElement(); 4973 default: 4974 return super.makeProperty(hash, name); 4975 } 4976 4977 } 4978 4979 @Override 4980 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4981 switch (hash) { 4982 case -135761730: 4983 /* identity */ return new String[] { "id" }; 4984 case -1613589672: 4985 /* language */ return new String[] { "code" }; 4986 case 107868: 4987 /* map */ return new String[] { "string" }; 4988 case 950398559: 4989 /* comment */ return new String[] { "string" }; 4990 default: 4991 return super.getTypesForProperty(hash, name); 4992 } 4993 4994 } 4995 4996 @Override 4997 public Base addChild(String name) throws FHIRException { 4998 if (name.equals("identity")) { 4999 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.identity"); 5000 } else if (name.equals("language")) { 5001 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.language"); 5002 } else if (name.equals("map")) { 5003 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.map"); 5004 } else if (name.equals("comment")) { 5005 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.comment"); 5006 } else 5007 return super.addChild(name); 5008 } 5009 5010 public ElementDefinitionMappingComponent copy() { 5011 ElementDefinitionMappingComponent dst = new ElementDefinitionMappingComponent(); 5012 copyValues(dst); 5013 return dst; 5014 } 5015 5016 public void copyValues(ElementDefinitionMappingComponent dst) { 5017 super.copyValues(dst); 5018 dst.identity = identity == null ? null : identity.copy(); 5019 dst.language = language == null ? null : language.copy(); 5020 dst.map = map == null ? null : map.copy(); 5021 dst.comment = comment == null ? null : comment.copy(); 5022 } 5023 5024 @Override 5025 public boolean equalsDeep(Base other_) { 5026 if (!super.equalsDeep(other_)) 5027 return false; 5028 if (!(other_ instanceof ElementDefinitionMappingComponent)) 5029 return false; 5030 ElementDefinitionMappingComponent o = (ElementDefinitionMappingComponent) other_; 5031 return compareDeep(identity, o.identity, true) && compareDeep(language, o.language, true) 5032 && compareDeep(map, o.map, true) && compareDeep(comment, o.comment, true); 5033 } 5034 5035 @Override 5036 public boolean equalsShallow(Base other_) { 5037 if (!super.equalsShallow(other_)) 5038 return false; 5039 if (!(other_ instanceof ElementDefinitionMappingComponent)) 5040 return false; 5041 ElementDefinitionMappingComponent o = (ElementDefinitionMappingComponent) other_; 5042 return compareValues(identity, o.identity, true) && compareValues(language, o.language, true) 5043 && compareValues(map, o.map, true) && compareValues(comment, o.comment, true); 5044 } 5045 5046 public boolean isEmpty() { 5047 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identity, language, map, comment); 5048 } 5049 5050 public String fhirType() { 5051 return "ElementDefinition.mapping"; 5052 5053 } 5054 5055 } 5056 5057 /** 5058 * The path identifies the element and is expressed as a "."-separated list of 5059 * ancestor elements, beginning with the name of the resource or extension. 5060 */ 5061 @Child(name = "path", type = { StringType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 5062 @Description(shortDefinition = "Path of the element in the hierarchy of elements", formalDefinition = "The path identifies the element and is expressed as a \".\"-separated list of ancestor elements, beginning with the name of the resource or extension.") 5063 protected StringType path; 5064 5065 /** 5066 * Codes that define how this element is represented in instances, when the 5067 * deviation varies from the normal case. 5068 */ 5069 @Child(name = "representation", type = { 5070 CodeType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 5071 @Description(shortDefinition = "xmlAttr | xmlText | typeAttr | cdaText | xhtml", formalDefinition = "Codes that define how this element is represented in instances, when the deviation varies from the normal case.") 5072 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/property-representation") 5073 protected List<Enumeration<PropertyRepresentation>> representation; 5074 5075 /** 5076 * The name of this element definition slice, when slicing is working. The name 5077 * must be a token with no dots or spaces. This is a unique name referring to a 5078 * specific set of constraints applied to this element, used to provide a name 5079 * to different slices of the same element. 5080 */ 5081 @Child(name = "sliceName", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 5082 @Description(shortDefinition = "Name for this particular element (in a set of slices)", formalDefinition = "The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element.") 5083 protected StringType sliceName; 5084 5085 /** 5086 * If true, indicates that this slice definition is constraining a slice 5087 * definition with the same name in an inherited profile. If false, the slice is 5088 * not overriding any slice in an inherited profile. If missing, the slice might 5089 * or might not be overriding a slice in an inherited profile, depending on the 5090 * sliceName. 5091 */ 5092 @Child(name = "sliceIsConstraining", type = { 5093 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 5094 @Description(shortDefinition = "If this slice definition constrains an inherited slice definition (or not)", formalDefinition = "If true, indicates that this slice definition is constraining a slice definition with the same name in an inherited profile. If false, the slice is not overriding any slice in an inherited profile. If missing, the slice might or might not be overriding a slice in an inherited profile, depending on the sliceName.") 5095 protected BooleanType sliceIsConstraining; 5096 5097 /** 5098 * A single preferred label which is the text to display beside the element 5099 * indicating its meaning or to use to prompt for the element in a user display 5100 * or form. 5101 */ 5102 @Child(name = "label", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 5103 @Description(shortDefinition = "Name for element to display with or prompt for element", formalDefinition = "A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form.") 5104 protected StringType label; 5105 5106 /** 5107 * A code that has the same meaning as the element in a particular terminology. 5108 */ 5109 @Child(name = "code", type = { 5110 Coding.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 5111 @Description(shortDefinition = "Corresponding codes in terminologies", formalDefinition = "A code that has the same meaning as the element in a particular terminology.") 5112 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-codes") 5113 protected List<Coding> code; 5114 5115 /** 5116 * Indicates that the element is sliced into a set of alternative definitions 5117 * (i.e. in a structure definition, there are multiple different constraints on 5118 * a single element in the base resource). Slicing can be used in any resource 5119 * that has cardinality ..* on the base resource, or any resource with a choice 5120 * of types. The set of slices is any elements that come after this in the 5121 * element sequence that have the same path, until a shorter path occurs (the 5122 * shorter path terminates the set). 5123 */ 5124 @Child(name = "slicing", type = {}, order = 6, min = 0, max = 1, modifier = false, summary = true) 5125 @Description(shortDefinition = "This element is sliced - slices follow", formalDefinition = "Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set).") 5126 protected ElementDefinitionSlicingComponent slicing; 5127 5128 /** 5129 * A concise description of what this element means (e.g. for use in 5130 * autogenerated summaries). 5131 */ 5132 @Child(name = "short", type = { StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 5133 @Description(shortDefinition = "Concise definition for space-constrained presentation", formalDefinition = "A concise description of what this element means (e.g. for use in autogenerated summaries).") 5134 protected StringType short_; 5135 5136 /** 5137 * Provides a complete explanation of the meaning of the data element for human 5138 * readability. For the case of elements derived from existing elements (e.g. 5139 * constraints), the definition SHALL be consistent with the base definition, 5140 * but convey the meaning of the element in the particular context of use of the 5141 * resource. (Note: The text you are reading is specified in 5142 * ElementDefinition.definition). 5143 */ 5144 @Child(name = "definition", type = { 5145 MarkdownType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 5146 @Description(shortDefinition = "Full formal definition as narrative text", formalDefinition = "Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource. (Note: The text you are reading is specified in ElementDefinition.definition).") 5147 protected MarkdownType definition; 5148 5149 /** 5150 * Explanatory notes and implementation guidance about the data element, 5151 * including notes about how to use the data properly, exceptions to proper use, 5152 * etc. (Note: The text you are reading is specified in 5153 * ElementDefinition.comment). 5154 */ 5155 @Child(name = "comment", type = { MarkdownType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 5156 @Description(shortDefinition = "Comments about the use of this element", formalDefinition = "Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. (Note: The text you are reading is specified in ElementDefinition.comment).") 5157 protected MarkdownType comment; 5158 5159 /** 5160 * This element is for traceability of why the element was created and why the 5161 * constraints exist as they do. This may be used to point to source materials 5162 * or specifications that drove the structure of this element. 5163 */ 5164 @Child(name = "requirements", type = { 5165 MarkdownType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 5166 @Description(shortDefinition = "Why this resource has been created", formalDefinition = "This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element.") 5167 protected MarkdownType requirements; 5168 5169 /** 5170 * Identifies additional names by which this element might also be known. 5171 */ 5172 @Child(name = "alias", type = { 5173 StringType.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 5174 @Description(shortDefinition = "Other names", formalDefinition = "Identifies additional names by which this element might also be known.") 5175 protected List<StringType> alias; 5176 5177 /** 5178 * The minimum number of times this element SHALL appear in the instance. 5179 */ 5180 @Child(name = "min", type = { UnsignedIntType.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 5181 @Description(shortDefinition = "Minimum Cardinality", formalDefinition = "The minimum number of times this element SHALL appear in the instance.") 5182 protected UnsignedIntType min; 5183 5184 /** 5185 * The maximum number of times this element is permitted to appear in the 5186 * instance. 5187 */ 5188 @Child(name = "max", type = { StringType.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 5189 @Description(shortDefinition = "Maximum Cardinality (a number or *)", formalDefinition = "The maximum number of times this element is permitted to appear in the instance.") 5190 protected StringType max; 5191 5192 /** 5193 * Information about the base definition of the element, provided to make it 5194 * unnecessary for tools to trace the deviation of the element through the 5195 * derived and related profiles. When the element definition is not the original 5196 * definition of an element - i.g. either in a constraint on another type, or 5197 * for elements from a super type in a snap shot - then the information in 5198 * provided in the element definition may be different to the base definition. 5199 * On the original definition of the element, it will be same. 5200 */ 5201 @Child(name = "base", type = {}, order = 14, min = 0, max = 1, modifier = false, summary = true) 5202 @Description(shortDefinition = "Base definition information for tools", formalDefinition = "Information about the base definition of the element, provided to make it unnecessary for tools to trace the deviation of the element through the derived and related profiles. When the element definition is not the original definition of an element - i.g. either in a constraint on another type, or for elements from a super type in a snap shot - then the information in provided in the element definition may be different to the base definition. On the original definition of the element, it will be same.") 5203 protected ElementDefinitionBaseComponent base; 5204 5205 /** 5206 * Identifies an element defined elsewhere in the definition whose content rules 5207 * should be applied to the current element. ContentReferences bring across all 5208 * the rules that are in the ElementDefinition for the element, including 5209 * definitions, cardinality constraints, bindings, invariants etc. 5210 */ 5211 @Child(name = "contentReference", type = { 5212 UriType.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 5213 @Description(shortDefinition = "Reference to definition of content for the element", formalDefinition = "Identifies an element defined elsewhere in the definition whose content rules should be applied to the current element. ContentReferences bring across all the rules that are in the ElementDefinition for the element, including definitions, cardinality constraints, bindings, invariants etc.") 5214 protected UriType contentReference; 5215 5216 /** 5217 * The data type or resource that the value of this element is permitted to be. 5218 */ 5219 @Child(name = "type", type = {}, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 5220 @Description(shortDefinition = "Data type and Profile for this element", formalDefinition = "The data type or resource that the value of this element is permitted to be.") 5221 protected List<TypeRefComponent> type; 5222 5223 /** 5224 * The value that should be used if there is no value stated in the instance 5225 * (e.g. 'if not otherwise specified, the abstract is false'). 5226 */ 5227 @Child(name = "defaultValue", type = {}, order = 17, min = 0, max = 1, modifier = false, summary = true) 5228 @Description(shortDefinition = "Specified value if missing from instance", formalDefinition = "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').") 5229 protected org.hl7.fhir.r4.model.Type defaultValue; 5230 5231 /** 5232 * The Implicit meaning that is to be understood when this element is missing 5233 * (e.g. 'when this element is missing, the period is ongoing'). 5234 */ 5235 @Child(name = "meaningWhenMissing", type = { 5236 MarkdownType.class }, order = 18, min = 0, max = 1, modifier = false, summary = true) 5237 @Description(shortDefinition = "Implicit meaning when this element is missing", formalDefinition = "The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing').") 5238 protected MarkdownType meaningWhenMissing; 5239 5240 /** 5241 * If present, indicates that the order of the repeating element has meaning and 5242 * describes what that meaning is. If absent, it means that the order of the 5243 * element has no meaning. 5244 */ 5245 @Child(name = "orderMeaning", type = { 5246 StringType.class }, order = 19, min = 0, max = 1, modifier = false, summary = true) 5247 @Description(shortDefinition = "What the order of the elements means", formalDefinition = "If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning.") 5248 protected StringType orderMeaning; 5249 5250 /** 5251 * Specifies a value that SHALL be exactly the value for this element in the 5252 * instance. For purposes of comparison, non-significant whitespace is ignored, 5253 * and all values must be an exact match (case and accent sensitive). Missing 5254 * elements/attributes must also be missing. 5255 */ 5256 @Child(name = "fixed", type = {}, order = 20, min = 0, max = 1, modifier = false, summary = true) 5257 @Description(shortDefinition = "Value must be exactly this", formalDefinition = "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.") 5258 protected org.hl7.fhir.r4.model.Type fixed; 5259 5260 /** 5261 * Specifies a value that the value in the instance SHALL follow - that is, any 5262 * value in the pattern must be found in the instance. Other additional values 5263 * may be found too. This is effectively constraint by example. 5264 * 5265 * When pattern[x] is used to constrain a primitive, it means that the value 5266 * provided in the pattern[x] must match the instance value exactly. 5267 * 5268 * When pattern[x] is used to constrain an array, it means that each element 5269 * provided in the pattern[x] array must (recursively) match at least one 5270 * element from the instance array. 5271 * 5272 * When pattern[x] is used to constrain a complex object, it means that each 5273 * property in the pattern must be present in the complex object, and its value 5274 * must recursively match -- i.e., 5275 * 5276 * 1. If primitive: it must match exactly the pattern value 2. If a complex 5277 * object: it must match (recursively) the pattern value 3. If an array: it must 5278 * match (recursively) the pattern value. 5279 */ 5280 @Child(name = "pattern", type = {}, order = 21, min = 0, max = 1, modifier = false, summary = true) 5281 @Description(shortDefinition = "Value must have at least these property values", formalDefinition = "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.") 5282 protected org.hl7.fhir.r4.model.Type pattern; 5283 5284 /** 5285 * A sample value for this element demonstrating the type of information that 5286 * would typically be found in the element. 5287 */ 5288 @Child(name = "example", type = {}, order = 22, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 5289 @Description(shortDefinition = "Example value (as defined for type)", formalDefinition = "A sample value for this element demonstrating the type of information that would typically be found in the element.") 5290 protected List<ElementDefinitionExampleComponent> example; 5291 5292 /** 5293 * The minimum allowed value for the element. The value is inclusive. This is 5294 * allowed for the types date, dateTime, instant, time, decimal, integer, and 5295 * Quantity. 5296 */ 5297 @Child(name = "minValue", type = { DateType.class, DateTimeType.class, InstantType.class, TimeType.class, 5298 DecimalType.class, IntegerType.class, PositiveIntType.class, UnsignedIntType.class, 5299 Quantity.class }, order = 23, min = 0, max = 1, modifier = false, summary = true) 5300 @Description(shortDefinition = "Minimum Allowed Value (for some types)", formalDefinition = "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.") 5301 protected Type minValue; 5302 5303 /** 5304 * The maximum allowed value for the element. The value is inclusive. This is 5305 * allowed for the types date, dateTime, instant, time, decimal, integer, and 5306 * Quantity. 5307 */ 5308 @Child(name = "maxValue", type = { DateType.class, DateTimeType.class, InstantType.class, TimeType.class, 5309 DecimalType.class, IntegerType.class, PositiveIntType.class, UnsignedIntType.class, 5310 Quantity.class }, order = 24, min = 0, max = 1, modifier = false, summary = true) 5311 @Description(shortDefinition = "Maximum Allowed Value (for some types)", formalDefinition = "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.") 5312 protected Type maxValue; 5313 5314 /** 5315 * Indicates the maximum length in characters that is permitted to be present in 5316 * conformant instances and which is expected to be supported by conformant 5317 * consumers that support the element. 5318 */ 5319 @Child(name = "maxLength", type = { 5320 IntegerType.class }, order = 25, min = 0, max = 1, modifier = false, summary = true) 5321 @Description(shortDefinition = "Max length for strings", formalDefinition = "Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element.") 5322 protected IntegerType maxLength; 5323 5324 /** 5325 * A reference to an invariant that may make additional statements about the 5326 * cardinality or value in the instance. 5327 */ 5328 @Child(name = "condition", type = { 5329 IdType.class }, order = 26, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 5330 @Description(shortDefinition = "Reference to invariant about presence", formalDefinition = "A reference to an invariant that may make additional statements about the cardinality or value in the instance.") 5331 protected List<IdType> condition; 5332 5333 /** 5334 * Formal constraints such as co-occurrence and other constraints that can be 5335 * computationally evaluated within the context of the instance. 5336 */ 5337 @Child(name = "constraint", type = {}, order = 27, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 5338 @Description(shortDefinition = "Condition that must evaluate to true", formalDefinition = "Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance.") 5339 protected List<ElementDefinitionConstraintComponent> constraint; 5340 5341 /** 5342 * If true, implementations that produce or consume resources SHALL provide 5343 * "support" for the element in some meaningful way. If false, the element may 5344 * be ignored and not supported. If false, whether to populate or use the data 5345 * element in any way is at the discretion of the implementation. 5346 */ 5347 @Child(name = "mustSupport", type = { 5348 BooleanType.class }, order = 28, min = 0, max = 1, modifier = false, summary = true) 5349 @Description(shortDefinition = "If the element must be supported", formalDefinition = "If true, implementations that produce or consume resources SHALL provide \"support\" for the element in some meaningful way. If false, the element may be ignored and not supported. If false, whether to populate or use the data element in any way is at the discretion of the implementation.") 5350 protected BooleanType mustSupport; 5351 5352 /** 5353 * If true, the value of this element affects the interpretation of the element 5354 * or resource that contains it, and the value of the element cannot be ignored. 5355 * Typically, this is used for status, negation and qualification codes. The 5356 * effect of this is that the element cannot be ignored by systems: they SHALL 5357 * either recognize the element and process it, and/or a pre-determination has 5358 * been made that it is not relevant to their particular system. 5359 */ 5360 @Child(name = "isModifier", type = { 5361 BooleanType.class }, order = 29, min = 0, max = 1, modifier = false, summary = true) 5362 @Description(shortDefinition = "If this modifies the meaning of other elements", formalDefinition = "If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system.") 5363 protected BooleanType isModifier; 5364 5365 /** 5366 * Explains how that element affects the interpretation of the resource or 5367 * element that contains it. 5368 */ 5369 @Child(name = "isModifierReason", type = { 5370 StringType.class }, order = 30, min = 0, max = 1, modifier = false, summary = true) 5371 @Description(shortDefinition = "Reason that this element is marked as a modifier", formalDefinition = "Explains how that element affects the interpretation of the resource or element that contains it.") 5372 protected StringType isModifierReason; 5373 5374 /** 5375 * Whether the element should be included if a client requests a search with the 5376 * parameter _summary=true. 5377 */ 5378 @Child(name = "isSummary", type = { 5379 BooleanType.class }, order = 31, min = 0, max = 1, modifier = false, summary = true) 5380 @Description(shortDefinition = "Include when _summary = true?", formalDefinition = "Whether the element should be included if a client requests a search with the parameter _summary=true.") 5381 protected BooleanType isSummary; 5382 5383 /** 5384 * Binds to a value set if this element is coded (code, Coding, CodeableConcept, 5385 * Quantity), or the data types (string, uri). 5386 */ 5387 @Child(name = "binding", type = {}, order = 32, min = 0, max = 1, modifier = false, summary = true) 5388 @Description(shortDefinition = "ValueSet details if this is coded", formalDefinition = "Binds to a value set if this element is coded (code, Coding, CodeableConcept, Quantity), or the data types (string, uri).") 5389 protected ElementDefinitionBindingComponent binding; 5390 5391 /** 5392 * Identifies a concept from an external specification that roughly corresponds 5393 * to this element. 5394 */ 5395 @Child(name = "mapping", type = {}, order = 33, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 5396 @Description(shortDefinition = "Map element to another set of definitions", formalDefinition = "Identifies a concept from an external specification that roughly corresponds to this element.") 5397 protected List<ElementDefinitionMappingComponent> mapping; 5398 5399 private static final long serialVersionUID = 1482114790L; 5400 5401 /** 5402 * Constructor 5403 */ 5404 public ElementDefinition() { 5405 super(); 5406 } 5407 5408 /** 5409 * Constructor 5410 */ 5411 public ElementDefinition(StringType path) { 5412 super(); 5413 this.path = path; 5414 } 5415 5416 /** 5417 * @return {@link #path} (The path identifies the element and is expressed as a 5418 * "."-separated list of ancestor elements, beginning with the name of 5419 * the resource or extension.). This is the underlying object with id, 5420 * value and extensions. The accessor "getPath" gives direct access to 5421 * the value 5422 */ 5423 public StringType getPathElement() { 5424 if (this.path == null) 5425 if (Configuration.errorOnAutoCreate()) 5426 throw new Error("Attempt to auto-create ElementDefinition.path"); 5427 else if (Configuration.doAutoCreate()) 5428 this.path = new StringType(); // bb 5429 return this.path; 5430 } 5431 5432 public boolean hasPathElement() { 5433 return this.path != null && !this.path.isEmpty(); 5434 } 5435 5436 public boolean hasPath() { 5437 return this.path != null && !this.path.isEmpty(); 5438 } 5439 5440 /** 5441 * @param value {@link #path} (The path identifies the element and is expressed 5442 * as a "."-separated list of ancestor elements, beginning with the 5443 * name of the resource or extension.). This is the underlying 5444 * object with id, value and extensions. The accessor "getPath" 5445 * gives direct access to the value 5446 */ 5447 public ElementDefinition setPathElement(StringType value) { 5448 this.path = value; 5449 return this; 5450 } 5451 5452 /** 5453 * @return The path identifies the element and is expressed as a "."-separated 5454 * list of ancestor elements, beginning with the name of the resource or 5455 * extension. 5456 */ 5457 public String getPath() { 5458 return this.path == null ? null : this.path.getValue(); 5459 } 5460 5461 /** 5462 * @param value The path identifies the element and is expressed as a 5463 * "."-separated list of ancestor elements, beginning with the name 5464 * of the resource or extension. 5465 */ 5466 public ElementDefinition setPath(String value) { 5467 if (this.path == null) 5468 this.path = new StringType(); 5469 this.path.setValue(value); 5470 return this; 5471 } 5472 5473 /** 5474 * @return {@link #representation} (Codes that define how this element is 5475 * represented in instances, when the deviation varies from the normal 5476 * case.) 5477 */ 5478 public List<Enumeration<PropertyRepresentation>> getRepresentation() { 5479 if (this.representation == null) 5480 this.representation = new ArrayList<Enumeration<PropertyRepresentation>>(); 5481 return this.representation; 5482 } 5483 5484 /** 5485 * @return Returns a reference to <code>this</code> for easy method chaining 5486 */ 5487 public ElementDefinition setRepresentation(List<Enumeration<PropertyRepresentation>> theRepresentation) { 5488 this.representation = theRepresentation; 5489 return this; 5490 } 5491 5492 public boolean hasRepresentation() { 5493 if (this.representation == null) 5494 return false; 5495 for (Enumeration<PropertyRepresentation> item : this.representation) 5496 if (!item.isEmpty()) 5497 return true; 5498 return false; 5499 } 5500 5501 /** 5502 * @return {@link #representation} (Codes that define how this element is 5503 * represented in instances, when the deviation varies from the normal 5504 * case.) 5505 */ 5506 public Enumeration<PropertyRepresentation> addRepresentationElement() {// 2 5507 Enumeration<PropertyRepresentation> t = new Enumeration<PropertyRepresentation>( 5508 new PropertyRepresentationEnumFactory()); 5509 if (this.representation == null) 5510 this.representation = new ArrayList<Enumeration<PropertyRepresentation>>(); 5511 this.representation.add(t); 5512 return t; 5513 } 5514 5515 /** 5516 * @param value {@link #representation} (Codes that define how this element is 5517 * represented in instances, when the deviation varies from the 5518 * normal case.) 5519 */ 5520 public ElementDefinition addRepresentation(PropertyRepresentation value) { // 1 5521 Enumeration<PropertyRepresentation> t = new Enumeration<PropertyRepresentation>( 5522 new PropertyRepresentationEnumFactory()); 5523 t.setValue(value); 5524 if (this.representation == null) 5525 this.representation = new ArrayList<Enumeration<PropertyRepresentation>>(); 5526 this.representation.add(t); 5527 return this; 5528 } 5529 5530 /** 5531 * @param value {@link #representation} (Codes that define how this element is 5532 * represented in instances, when the deviation varies from the 5533 * normal case.) 5534 */ 5535 public boolean hasRepresentation(PropertyRepresentation value) { 5536 if (this.representation == null) 5537 return false; 5538 for (Enumeration<PropertyRepresentation> v : this.representation) 5539 if (v.getValue().equals(value)) // code 5540 return true; 5541 return false; 5542 } 5543 5544 /** 5545 * @return {@link #sliceName} (The name of this element definition slice, when 5546 * slicing is working. The name must be a token with no dots or spaces. 5547 * This is a unique name referring to a specific set of constraints 5548 * applied to this element, used to provide a name to different slices 5549 * of the same element.). This is the underlying object with id, value 5550 * and extensions. The accessor "getSliceName" gives direct access to 5551 * the value 5552 */ 5553 public StringType getSliceNameElement() { 5554 if (this.sliceName == null) 5555 if (Configuration.errorOnAutoCreate()) 5556 throw new Error("Attempt to auto-create ElementDefinition.sliceName"); 5557 else if (Configuration.doAutoCreate()) 5558 this.sliceName = new StringType(); // bb 5559 return this.sliceName; 5560 } 5561 5562 public boolean hasSliceNameElement() { 5563 return this.sliceName != null && !this.sliceName.isEmpty(); 5564 } 5565 5566 public boolean hasSliceName() { 5567 return this.sliceName != null && !this.sliceName.isEmpty(); 5568 } 5569 5570 /** 5571 * @param value {@link #sliceName} (The name of this element definition slice, 5572 * when slicing is working. The name must be a token with no dots 5573 * or spaces. This is a unique name referring to a specific set of 5574 * constraints applied to this element, used to provide a name to 5575 * different slices of the same element.). This is the underlying 5576 * object with id, value and extensions. The accessor 5577 * "getSliceName" gives direct access to the value 5578 */ 5579 public ElementDefinition setSliceNameElement(StringType value) { 5580 this.sliceName = value; 5581 return this; 5582 } 5583 5584 /** 5585 * @return The name of this element definition slice, when slicing is working. 5586 * The name must be a token with no dots or spaces. This is a unique 5587 * name referring to a specific set of constraints applied to this 5588 * element, used to provide a name to different slices of the same 5589 * element. 5590 */ 5591 public String getSliceName() { 5592 return this.sliceName == null ? null : this.sliceName.getValue(); 5593 } 5594 5595 /** 5596 * @param value The name of this element definition slice, when slicing is 5597 * working. The name must be a token with no dots or spaces. This 5598 * is a unique name referring to a specific set of constraints 5599 * applied to this element, used to provide a name to different 5600 * slices of the same element. 5601 */ 5602 public ElementDefinition setSliceName(String value) { 5603 if (Utilities.noString(value)) 5604 this.sliceName = null; 5605 else { 5606 if (this.sliceName == null) 5607 this.sliceName = new StringType(); 5608 this.sliceName.setValue(value); 5609 } 5610 return this; 5611 } 5612 5613 /** 5614 * @return {@link #sliceIsConstraining} (If true, indicates that this slice 5615 * definition is constraining a slice definition with the same name in 5616 * an inherited profile. If false, the slice is not overriding any slice 5617 * in an inherited profile. If missing, the slice might or might not be 5618 * overriding a slice in an inherited profile, depending on the 5619 * sliceName.). This is the underlying object with id, value and 5620 * extensions. The accessor "getSliceIsConstraining" gives direct access 5621 * to the value 5622 */ 5623 public BooleanType getSliceIsConstrainingElement() { 5624 if (this.sliceIsConstraining == null) 5625 if (Configuration.errorOnAutoCreate()) 5626 throw new Error("Attempt to auto-create ElementDefinition.sliceIsConstraining"); 5627 else if (Configuration.doAutoCreate()) 5628 this.sliceIsConstraining = new BooleanType(); // bb 5629 return this.sliceIsConstraining; 5630 } 5631 5632 public boolean hasSliceIsConstrainingElement() { 5633 return this.sliceIsConstraining != null && !this.sliceIsConstraining.isEmpty(); 5634 } 5635 5636 public boolean hasSliceIsConstraining() { 5637 return this.sliceIsConstraining != null && !this.sliceIsConstraining.isEmpty(); 5638 } 5639 5640 /** 5641 * @param value {@link #sliceIsConstraining} (If true, indicates that this slice 5642 * definition is constraining a slice definition with the same name 5643 * in an inherited profile. If false, the slice is not overriding 5644 * any slice in an inherited profile. If missing, the slice might 5645 * or might not be overriding a slice in an inherited profile, 5646 * depending on the sliceName.). This is the underlying object with 5647 * id, value and extensions. The accessor "getSliceIsConstraining" 5648 * gives direct access to the value 5649 */ 5650 public ElementDefinition setSliceIsConstrainingElement(BooleanType value) { 5651 this.sliceIsConstraining = value; 5652 return this; 5653 } 5654 5655 /** 5656 * @return If true, indicates that this slice definition is constraining a slice 5657 * definition with the same name in an inherited profile. If false, the 5658 * slice is not overriding any slice in an inherited profile. If 5659 * missing, the slice might or might not be overriding a slice in an 5660 * inherited profile, depending on the sliceName. 5661 */ 5662 public boolean getSliceIsConstraining() { 5663 return this.sliceIsConstraining == null || this.sliceIsConstraining.isEmpty() ? false 5664 : this.sliceIsConstraining.getValue(); 5665 } 5666 5667 /** 5668 * @param value If true, indicates that this slice definition is constraining a 5669 * slice definition with the same name in an inherited profile. If 5670 * false, the slice is not overriding any slice in an inherited 5671 * profile. If missing, the slice might or might not be overriding 5672 * a slice in an inherited profile, depending on the sliceName. 5673 */ 5674 public ElementDefinition setSliceIsConstraining(boolean value) { 5675 if (this.sliceIsConstraining == null) 5676 this.sliceIsConstraining = new BooleanType(); 5677 this.sliceIsConstraining.setValue(value); 5678 return this; 5679 } 5680 5681 /** 5682 * @return {@link #label} (A single preferred label which is the text to display 5683 * beside the element indicating its meaning or to use to prompt for the 5684 * element in a user display or form.). This is the underlying object 5685 * with id, value and extensions. The accessor "getLabel" gives direct 5686 * access to the value 5687 */ 5688 public StringType getLabelElement() { 5689 if (this.label == null) 5690 if (Configuration.errorOnAutoCreate()) 5691 throw new Error("Attempt to auto-create ElementDefinition.label"); 5692 else if (Configuration.doAutoCreate()) 5693 this.label = new StringType(); // bb 5694 return this.label; 5695 } 5696 5697 public boolean hasLabelElement() { 5698 return this.label != null && !this.label.isEmpty(); 5699 } 5700 5701 public boolean hasLabel() { 5702 return this.label != null && !this.label.isEmpty(); 5703 } 5704 5705 /** 5706 * @param value {@link #label} (A single preferred label which is the text to 5707 * display beside the element indicating its meaning or to use to 5708 * prompt for the element in a user display or form.). This is the 5709 * underlying object with id, value and extensions. The accessor 5710 * "getLabel" gives direct access to the value 5711 */ 5712 public ElementDefinition setLabelElement(StringType value) { 5713 this.label = value; 5714 return this; 5715 } 5716 5717 /** 5718 * @return A single preferred label which is the text to display beside the 5719 * element indicating its meaning or to use to prompt for the element in 5720 * a user display or form. 5721 */ 5722 public String getLabel() { 5723 return this.label == null ? null : this.label.getValue(); 5724 } 5725 5726 /** 5727 * @param value A single preferred label which is the text to display beside the 5728 * element indicating its meaning or to use to prompt for the 5729 * element in a user display or form. 5730 */ 5731 public ElementDefinition setLabel(String value) { 5732 if (Utilities.noString(value)) 5733 this.label = null; 5734 else { 5735 if (this.label == null) 5736 this.label = new StringType(); 5737 this.label.setValue(value); 5738 } 5739 return this; 5740 } 5741 5742 /** 5743 * @return {@link #code} (A code that has the same meaning as the element in a 5744 * particular terminology.) 5745 */ 5746 public List<Coding> getCode() { 5747 if (this.code == null) 5748 this.code = new ArrayList<Coding>(); 5749 return this.code; 5750 } 5751 5752 /** 5753 * @return Returns a reference to <code>this</code> for easy method chaining 5754 */ 5755 public ElementDefinition setCode(List<Coding> theCode) { 5756 this.code = theCode; 5757 return this; 5758 } 5759 5760 public boolean hasCode() { 5761 if (this.code == null) 5762 return false; 5763 for (Coding item : this.code) 5764 if (!item.isEmpty()) 5765 return true; 5766 return false; 5767 } 5768 5769 public Coding addCode() { // 3 5770 Coding t = new Coding(); 5771 if (this.code == null) 5772 this.code = new ArrayList<Coding>(); 5773 this.code.add(t); 5774 return t; 5775 } 5776 5777 public ElementDefinition addCode(Coding t) { // 3 5778 if (t == null) 5779 return this; 5780 if (this.code == null) 5781 this.code = new ArrayList<Coding>(); 5782 this.code.add(t); 5783 return this; 5784 } 5785 5786 /** 5787 * @return The first repetition of repeating field {@link #code}, creating it if 5788 * it does not already exist 5789 */ 5790 public Coding getCodeFirstRep() { 5791 if (getCode().isEmpty()) { 5792 addCode(); 5793 } 5794 return getCode().get(0); 5795 } 5796 5797 /** 5798 * @return {@link #slicing} (Indicates that the element is sliced into a set of 5799 * alternative definitions (i.e. in a structure definition, there are 5800 * multiple different constraints on a single element in the base 5801 * resource). Slicing can be used in any resource that has cardinality 5802 * ..* on the base resource, or any resource with a choice of types. The 5803 * set of slices is any elements that come after this in the element 5804 * sequence that have the same path, until a shorter path occurs (the 5805 * shorter path terminates the set).) 5806 */ 5807 public ElementDefinitionSlicingComponent getSlicing() { 5808 if (this.slicing == null) 5809 if (Configuration.errorOnAutoCreate()) 5810 throw new Error("Attempt to auto-create ElementDefinition.slicing"); 5811 else if (Configuration.doAutoCreate()) 5812 this.slicing = new ElementDefinitionSlicingComponent(); // cc 5813 return this.slicing; 5814 } 5815 5816 public boolean hasSlicing() { 5817 return this.slicing != null && !this.slicing.isEmpty(); 5818 } 5819 5820 /** 5821 * @param value {@link #slicing} (Indicates that the element is sliced into a 5822 * set of alternative definitions (i.e. in a structure definition, 5823 * there are multiple different constraints on a single element in 5824 * the base resource). Slicing can be used in any resource that has 5825 * cardinality ..* on the base resource, or any resource with a 5826 * choice of types. The set of slices is any elements that come 5827 * after this in the element sequence that have the same path, 5828 * until a shorter path occurs (the shorter path terminates the 5829 * set).) 5830 */ 5831 public ElementDefinition setSlicing(ElementDefinitionSlicingComponent value) { 5832 this.slicing = value; 5833 return this; 5834 } 5835 5836 /** 5837 * @return {@link #short_} (A concise description of what this element means 5838 * (e.g. for use in autogenerated summaries).). This is the underlying 5839 * object with id, value and extensions. The accessor "getShort" gives 5840 * direct access to the value 5841 */ 5842 public StringType getShortElement() { 5843 if (this.short_ == null) 5844 if (Configuration.errorOnAutoCreate()) 5845 throw new Error("Attempt to auto-create ElementDefinition.short_"); 5846 else if (Configuration.doAutoCreate()) 5847 this.short_ = new StringType(); // bb 5848 return this.short_; 5849 } 5850 5851 public boolean hasShortElement() { 5852 return this.short_ != null && !this.short_.isEmpty(); 5853 } 5854 5855 public boolean hasShort() { 5856 return this.short_ != null && !this.short_.isEmpty(); 5857 } 5858 5859 /** 5860 * @param value {@link #short_} (A concise description of what this element 5861 * means (e.g. for use in autogenerated summaries).). This is the 5862 * underlying object with id, value and extensions. The accessor 5863 * "getShort" gives direct access to the value 5864 */ 5865 public ElementDefinition setShortElement(StringType value) { 5866 this.short_ = value; 5867 return this; 5868 } 5869 5870 /** 5871 * @return A concise description of what this element means (e.g. for use in 5872 * autogenerated summaries). 5873 */ 5874 public String getShort() { 5875 return this.short_ == null ? null : this.short_.getValue(); 5876 } 5877 5878 /** 5879 * @param value A concise description of what this element means (e.g. for use 5880 * in autogenerated summaries). 5881 */ 5882 public ElementDefinition setShort(String value) { 5883 if (Utilities.noString(value)) 5884 this.short_ = null; 5885 else { 5886 if (this.short_ == null) 5887 this.short_ = new StringType(); 5888 this.short_.setValue(value); 5889 } 5890 return this; 5891 } 5892 5893 /** 5894 * @return {@link #definition} (Provides a complete explanation of the meaning 5895 * of the data element for human readability. For the case of elements 5896 * derived from existing elements (e.g. constraints), the definition 5897 * SHALL be consistent with the base definition, but convey the meaning 5898 * of the element in the particular context of use of the resource. 5899 * (Note: The text you are reading is specified in 5900 * ElementDefinition.definition).). This is the underlying object with 5901 * id, value and extensions. The accessor "getDefinition" gives direct 5902 * access to the value 5903 */ 5904 public MarkdownType getDefinitionElement() { 5905 if (this.definition == null) 5906 if (Configuration.errorOnAutoCreate()) 5907 throw new Error("Attempt to auto-create ElementDefinition.definition"); 5908 else if (Configuration.doAutoCreate()) 5909 this.definition = new MarkdownType(); // bb 5910 return this.definition; 5911 } 5912 5913 public boolean hasDefinitionElement() { 5914 return this.definition != null && !this.definition.isEmpty(); 5915 } 5916 5917 public boolean hasDefinition() { 5918 return this.definition != null && !this.definition.isEmpty(); 5919 } 5920 5921 /** 5922 * @param value {@link #definition} (Provides a complete explanation of the 5923 * meaning of the data element for human readability. For the case 5924 * of elements derived from existing elements (e.g. constraints), 5925 * the definition SHALL be consistent with the base definition, but 5926 * convey the meaning of the element in the particular context of 5927 * use of the resource. (Note: The text you are reading is 5928 * specified in ElementDefinition.definition).). This is the 5929 * underlying object with id, value and extensions. The accessor 5930 * "getDefinition" gives direct access to the value 5931 */ 5932 public ElementDefinition setDefinitionElement(MarkdownType value) { 5933 this.definition = value; 5934 return this; 5935 } 5936 5937 /** 5938 * @return Provides a complete explanation of the meaning of the data element 5939 * for human readability. For the case of elements derived from existing 5940 * elements (e.g. constraints), the definition SHALL be consistent with 5941 * the base definition, but convey the meaning of the element in the 5942 * particular context of use of the resource. (Note: The text you are 5943 * reading is specified in ElementDefinition.definition). 5944 */ 5945 public String getDefinition() { 5946 return this.definition == null ? null : this.definition.getValue(); 5947 } 5948 5949 /** 5950 * @param value Provides a complete explanation of the meaning of the data 5951 * element for human readability. For the case of elements derived 5952 * from existing elements (e.g. constraints), the definition SHALL 5953 * be consistent with the base definition, but convey the meaning 5954 * of the element in the particular context of use of the resource. 5955 * (Note: The text you are reading is specified in 5956 * ElementDefinition.definition). 5957 */ 5958 public ElementDefinition setDefinition(String value) { 5959 if (value == null) 5960 this.definition = null; 5961 else { 5962 if (this.definition == null) 5963 this.definition = new MarkdownType(); 5964 this.definition.setValue(value); 5965 } 5966 return this; 5967 } 5968 5969 /** 5970 * @return {@link #comment} (Explanatory notes and implementation guidance about 5971 * the data element, including notes about how to use the data properly, 5972 * exceptions to proper use, etc. (Note: The text you are reading is 5973 * specified in ElementDefinition.comment).). This is the underlying 5974 * object with id, value and extensions. The accessor "getComment" gives 5975 * direct access to the value 5976 */ 5977 public MarkdownType getCommentElement() { 5978 if (this.comment == null) 5979 if (Configuration.errorOnAutoCreate()) 5980 throw new Error("Attempt to auto-create ElementDefinition.comment"); 5981 else if (Configuration.doAutoCreate()) 5982 this.comment = new MarkdownType(); // bb 5983 return this.comment; 5984 } 5985 5986 public boolean hasCommentElement() { 5987 return this.comment != null && !this.comment.isEmpty(); 5988 } 5989 5990 public boolean hasComment() { 5991 return this.comment != null && !this.comment.isEmpty(); 5992 } 5993 5994 /** 5995 * @param value {@link #comment} (Explanatory notes and implementation guidance 5996 * about the data element, including notes about how to use the 5997 * data properly, exceptions to proper use, etc. (Note: The text 5998 * you are reading is specified in ElementDefinition.comment).). 5999 * This is the underlying object with id, value and extensions. The 6000 * accessor "getComment" gives direct access to the value 6001 */ 6002 public ElementDefinition setCommentElement(MarkdownType value) { 6003 this.comment = value; 6004 return this; 6005 } 6006 6007 /** 6008 * @return Explanatory notes and implementation guidance about the data element, 6009 * including notes about how to use the data properly, exceptions to 6010 * proper use, etc. (Note: The text you are reading is specified in 6011 * ElementDefinition.comment). 6012 */ 6013 public String getComment() { 6014 return this.comment == null ? null : this.comment.getValue(); 6015 } 6016 6017 /** 6018 * @param value Explanatory notes and implementation guidance about the data 6019 * element, including notes about how to use the data properly, 6020 * exceptions to proper use, etc. (Note: The text you are reading 6021 * is specified in ElementDefinition.comment). 6022 */ 6023 public ElementDefinition setComment(String value) { 6024 if (value == null) 6025 this.comment = null; 6026 else { 6027 if (this.comment == null) 6028 this.comment = new MarkdownType(); 6029 this.comment.setValue(value); 6030 } 6031 return this; 6032 } 6033 6034 /** 6035 * @return {@link #requirements} (This element is for traceability of why the 6036 * element was created and why the constraints exist as they do. This 6037 * may be used to point to source materials or specifications that drove 6038 * the structure of this element.). This is the underlying object with 6039 * id, value and extensions. The accessor "getRequirements" gives direct 6040 * access to the value 6041 */ 6042 public MarkdownType getRequirementsElement() { 6043 if (this.requirements == null) 6044 if (Configuration.errorOnAutoCreate()) 6045 throw new Error("Attempt to auto-create ElementDefinition.requirements"); 6046 else if (Configuration.doAutoCreate()) 6047 this.requirements = new MarkdownType(); // bb 6048 return this.requirements; 6049 } 6050 6051 public boolean hasRequirementsElement() { 6052 return this.requirements != null && !this.requirements.isEmpty(); 6053 } 6054 6055 public boolean hasRequirements() { 6056 return this.requirements != null && !this.requirements.isEmpty(); 6057 } 6058 6059 /** 6060 * @param value {@link #requirements} (This element is for traceability of why 6061 * the element was created and why the constraints exist as they 6062 * do. This may be used to point to source materials or 6063 * specifications that drove the structure of this element.). This 6064 * is the underlying object with id, value and extensions. The 6065 * accessor "getRequirements" gives direct access to the value 6066 */ 6067 public ElementDefinition setRequirementsElement(MarkdownType value) { 6068 this.requirements = value; 6069 return this; 6070 } 6071 6072 /** 6073 * @return This element is for traceability of why the element was created and 6074 * why the constraints exist as they do. This may be used to point to 6075 * source materials or specifications that drove the structure of this 6076 * element. 6077 */ 6078 public String getRequirements() { 6079 return this.requirements == null ? null : this.requirements.getValue(); 6080 } 6081 6082 /** 6083 * @param value This element is for traceability of why the element was created 6084 * and why the constraints exist as they do. This may be used to 6085 * point to source materials or specifications that drove the 6086 * structure of this element. 6087 */ 6088 public ElementDefinition setRequirements(String value) { 6089 if (value == null) 6090 this.requirements = null; 6091 else { 6092 if (this.requirements == null) 6093 this.requirements = new MarkdownType(); 6094 this.requirements.setValue(value); 6095 } 6096 return this; 6097 } 6098 6099 /** 6100 * @return {@link #alias} (Identifies additional names by which this element 6101 * might also be known.) 6102 */ 6103 public List<StringType> getAlias() { 6104 if (this.alias == null) 6105 this.alias = new ArrayList<StringType>(); 6106 return this.alias; 6107 } 6108 6109 /** 6110 * @return Returns a reference to <code>this</code> for easy method chaining 6111 */ 6112 public ElementDefinition setAlias(List<StringType> theAlias) { 6113 this.alias = theAlias; 6114 return this; 6115 } 6116 6117 public boolean hasAlias() { 6118 if (this.alias == null) 6119 return false; 6120 for (StringType item : this.alias) 6121 if (!item.isEmpty()) 6122 return true; 6123 return false; 6124 } 6125 6126 /** 6127 * @return {@link #alias} (Identifies additional names by which this element 6128 * might also be known.) 6129 */ 6130 public StringType addAliasElement() {// 2 6131 StringType t = new StringType(); 6132 if (this.alias == null) 6133 this.alias = new ArrayList<StringType>(); 6134 this.alias.add(t); 6135 return t; 6136 } 6137 6138 /** 6139 * @param value {@link #alias} (Identifies additional names by which this 6140 * element might also be known.) 6141 */ 6142 public ElementDefinition addAlias(String value) { // 1 6143 StringType t = new StringType(); 6144 t.setValue(value); 6145 if (this.alias == null) 6146 this.alias = new ArrayList<StringType>(); 6147 this.alias.add(t); 6148 return this; 6149 } 6150 6151 /** 6152 * @param value {@link #alias} (Identifies additional names by which this 6153 * element might also be known.) 6154 */ 6155 public boolean hasAlias(String value) { 6156 if (this.alias == null) 6157 return false; 6158 for (StringType v : this.alias) 6159 if (v.getValue().equals(value)) // string 6160 return true; 6161 return false; 6162 } 6163 6164 /** 6165 * @return {@link #min} (The minimum number of times this element SHALL appear 6166 * in the instance.). This is the underlying object with id, value and 6167 * extensions. The accessor "getMin" gives direct access to the value 6168 */ 6169 public UnsignedIntType getMinElement() { 6170 if (this.min == null) 6171 if (Configuration.errorOnAutoCreate()) 6172 throw new Error("Attempt to auto-create ElementDefinition.min"); 6173 else if (Configuration.doAutoCreate()) 6174 this.min = new UnsignedIntType(); // bb 6175 return this.min; 6176 } 6177 6178 public boolean hasMinElement() { 6179 return this.min != null && !this.min.isEmpty(); 6180 } 6181 6182 public boolean hasMin() { 6183 return this.min != null && !this.min.isEmpty(); 6184 } 6185 6186 /** 6187 * @param value {@link #min} (The minimum number of times this element SHALL 6188 * appear in the instance.). This is the underlying object with id, 6189 * value and extensions. The accessor "getMin" gives direct access 6190 * to the value 6191 */ 6192 public ElementDefinition setMinElement(UnsignedIntType value) { 6193 this.min = value; 6194 return this; 6195 } 6196 6197 /** 6198 * @return The minimum number of times this element SHALL appear in the 6199 * instance. 6200 */ 6201 public int getMin() { 6202 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 6203 } 6204 6205 /** 6206 * @param value The minimum number of times this element SHALL appear in the 6207 * instance. 6208 */ 6209 public ElementDefinition setMin(int value) { 6210 if (this.min == null) 6211 this.min = new UnsignedIntType(); 6212 this.min.setValue(value); 6213 return this; 6214 } 6215 6216 /** 6217 * @return {@link #max} (The maximum number of times this element is permitted 6218 * to appear in the instance.). This is the underlying object with id, 6219 * value and extensions. The accessor "getMax" gives direct access to 6220 * the value 6221 */ 6222 public StringType getMaxElement() { 6223 if (this.max == null) 6224 if (Configuration.errorOnAutoCreate()) 6225 throw new Error("Attempt to auto-create ElementDefinition.max"); 6226 else if (Configuration.doAutoCreate()) 6227 this.max = new StringType(); // bb 6228 return this.max; 6229 } 6230 6231 public boolean hasMaxElement() { 6232 return this.max != null && !this.max.isEmpty(); 6233 } 6234 6235 public boolean hasMax() { 6236 return this.max != null && !this.max.isEmpty(); 6237 } 6238 6239 /** 6240 * @param value {@link #max} (The maximum number of times this element is 6241 * permitted to appear in the instance.). This is the underlying 6242 * object with id, value and extensions. The accessor "getMax" 6243 * gives direct access to the value 6244 */ 6245 public ElementDefinition setMaxElement(StringType value) { 6246 this.max = value; 6247 return this; 6248 } 6249 6250 /** 6251 * @return The maximum number of times this element is permitted to appear in 6252 * the instance. 6253 */ 6254 public String getMax() { 6255 return this.max == null ? null : this.max.getValue(); 6256 } 6257 6258 /** 6259 * @param value The maximum number of times this element is permitted to appear 6260 * in the instance. 6261 */ 6262 public ElementDefinition setMax(String value) { 6263 if (Utilities.noString(value)) 6264 this.max = null; 6265 else { 6266 if (this.max == null) 6267 this.max = new StringType(); 6268 this.max.setValue(value); 6269 } 6270 return this; 6271 } 6272 6273 /** 6274 * @return {@link #base} (Information about the base definition of the element, 6275 * provided to make it unnecessary for tools to trace the deviation of 6276 * the element through the derived and related profiles. When the 6277 * element definition is not the original definition of an element - 6278 * i.g. either in a constraint on another type, or for elements from a 6279 * super type in a snap shot - then the information in provided in the 6280 * element definition may be different to the base definition. On the 6281 * original definition of the element, it will be same.) 6282 */ 6283 public ElementDefinitionBaseComponent getBase() { 6284 if (this.base == null) 6285 if (Configuration.errorOnAutoCreate()) 6286 throw new Error("Attempt to auto-create ElementDefinition.base"); 6287 else if (Configuration.doAutoCreate()) 6288 this.base = new ElementDefinitionBaseComponent(); // cc 6289 return this.base; 6290 } 6291 6292 public boolean hasBase() { 6293 return this.base != null && !this.base.isEmpty(); 6294 } 6295 6296 /** 6297 * @param value {@link #base} (Information about the base definition of the 6298 * element, provided to make it unnecessary for tools to trace the 6299 * deviation of the element through the derived and related 6300 * profiles. When the element definition is not the original 6301 * definition of an element - i.g. either in a constraint on 6302 * another type, or for elements from a super type in a snap shot - 6303 * then the information in provided in the element definition may 6304 * be different to the base definition. On the original definition 6305 * of the element, it will be same.) 6306 */ 6307 public ElementDefinition setBase(ElementDefinitionBaseComponent value) { 6308 this.base = value; 6309 return this; 6310 } 6311 6312 /** 6313 * @return {@link #contentReference} (Identifies an element defined elsewhere in 6314 * the definition whose content rules should be applied to the current 6315 * element. ContentReferences bring across all the rules that are in the 6316 * ElementDefinition for the element, including definitions, cardinality 6317 * constraints, bindings, invariants etc.). This is the underlying 6318 * object with id, value and extensions. The accessor 6319 * "getContentReference" gives direct access to the value 6320 */ 6321 public UriType getContentReferenceElement() { 6322 if (this.contentReference == null) 6323 if (Configuration.errorOnAutoCreate()) 6324 throw new Error("Attempt to auto-create ElementDefinition.contentReference"); 6325 else if (Configuration.doAutoCreate()) 6326 this.contentReference = new UriType(); // bb 6327 return this.contentReference; 6328 } 6329 6330 public boolean hasContentReferenceElement() { 6331 return this.contentReference != null && !this.contentReference.isEmpty(); 6332 } 6333 6334 public boolean hasContentReference() { 6335 return this.contentReference != null && !this.contentReference.isEmpty(); 6336 } 6337 6338 /** 6339 * @param value {@link #contentReference} (Identifies an element defined 6340 * elsewhere in the definition whose content rules should be 6341 * applied to the current element. ContentReferences bring across 6342 * all the rules that are in the ElementDefinition for the element, 6343 * including definitions, cardinality constraints, bindings, 6344 * invariants etc.). This is the underlying object with id, value 6345 * and extensions. The accessor "getContentReference" gives direct 6346 * access to the value 6347 */ 6348 public ElementDefinition setContentReferenceElement(UriType value) { 6349 this.contentReference = value; 6350 return this; 6351 } 6352 6353 /** 6354 * @return Identifies an element defined elsewhere in the definition whose 6355 * content rules should be applied to the current element. 6356 * ContentReferences bring across all the rules that are in the 6357 * ElementDefinition for the element, including definitions, cardinality 6358 * constraints, bindings, invariants etc. 6359 */ 6360 public String getContentReference() { 6361 return this.contentReference == null ? null : this.contentReference.getValue(); 6362 } 6363 6364 /** 6365 * @param value Identifies an element defined elsewhere in the definition whose 6366 * content rules should be applied to the current element. 6367 * ContentReferences bring across all the rules that are in the 6368 * ElementDefinition for the element, including definitions, 6369 * cardinality constraints, bindings, invariants etc. 6370 */ 6371 public ElementDefinition setContentReference(String value) { 6372 if (Utilities.noString(value)) 6373 this.contentReference = null; 6374 else { 6375 if (this.contentReference == null) 6376 this.contentReference = new UriType(); 6377 this.contentReference.setValue(value); 6378 } 6379 return this; 6380 } 6381 6382 /** 6383 * @return {@link #type} (The data type or resource that the value of this 6384 * element is permitted to be.) 6385 */ 6386 public List<TypeRefComponent> getType() { 6387 if (this.type == null) 6388 this.type = new ArrayList<TypeRefComponent>(); 6389 return this.type; 6390 } 6391 6392 /** 6393 * @return Returns a reference to <code>this</code> for easy method chaining 6394 */ 6395 public ElementDefinition setType(List<TypeRefComponent> theType) { 6396 this.type = theType; 6397 return this; 6398 } 6399 6400 public boolean hasType() { 6401 if (this.type == null) 6402 return false; 6403 for (TypeRefComponent item : this.type) 6404 if (!item.isEmpty()) 6405 return true; 6406 return false; 6407 } 6408 6409 public TypeRefComponent addType() { // 3 6410 TypeRefComponent t = new TypeRefComponent(); 6411 if (this.type == null) 6412 this.type = new ArrayList<TypeRefComponent>(); 6413 this.type.add(t); 6414 return t; 6415 } 6416 6417 public ElementDefinition addType(TypeRefComponent t) { // 3 6418 if (t == null) 6419 return this; 6420 if (this.type == null) 6421 this.type = new ArrayList<TypeRefComponent>(); 6422 this.type.add(t); 6423 return this; 6424 } 6425 6426 /** 6427 * @return The first repetition of repeating field {@link #type}, creating it if 6428 * it does not already exist 6429 */ 6430 public TypeRefComponent getTypeFirstRep() { 6431 if (getType().isEmpty()) { 6432 addType(); 6433 } 6434 return getType().get(0); 6435 } 6436 6437 /** 6438 * @return {@link #defaultValue} (The value that should be used if there is no 6439 * value stated in the instance (e.g. 'if not otherwise specified, the 6440 * abstract is false').) 6441 */ 6442 public org.hl7.fhir.r4.model.Type getDefaultValue() { 6443 return this.defaultValue; 6444 } 6445 6446 public boolean hasDefaultValue() { 6447 return this.defaultValue != null && !this.defaultValue.isEmpty(); 6448 } 6449 6450 /** 6451 * @param value {@link #defaultValue} (The value that should be used if there is 6452 * no value stated in the instance (e.g. 'if not otherwise 6453 * specified, the abstract is false').) 6454 */ 6455 public ElementDefinition setDefaultValue(org.hl7.fhir.r4.model.Type value) { 6456 this.defaultValue = value; 6457 return this; 6458 } 6459 6460 /** 6461 * @return {@link #meaningWhenMissing} (The Implicit meaning that is to be 6462 * understood when this element is missing (e.g. 'when this element is 6463 * missing, the period is ongoing').). This is the underlying object 6464 * with id, value and extensions. The accessor "getMeaningWhenMissing" 6465 * gives direct access to the value 6466 */ 6467 public MarkdownType getMeaningWhenMissingElement() { 6468 if (this.meaningWhenMissing == null) 6469 if (Configuration.errorOnAutoCreate()) 6470 throw new Error("Attempt to auto-create ElementDefinition.meaningWhenMissing"); 6471 else if (Configuration.doAutoCreate()) 6472 this.meaningWhenMissing = new MarkdownType(); // bb 6473 return this.meaningWhenMissing; 6474 } 6475 6476 public boolean hasMeaningWhenMissingElement() { 6477 return this.meaningWhenMissing != null && !this.meaningWhenMissing.isEmpty(); 6478 } 6479 6480 public boolean hasMeaningWhenMissing() { 6481 return this.meaningWhenMissing != null && !this.meaningWhenMissing.isEmpty(); 6482 } 6483 6484 /** 6485 * @param value {@link #meaningWhenMissing} (The Implicit meaning that is to be 6486 * understood when this element is missing (e.g. 'when this element 6487 * is missing, the period is ongoing').). This is the underlying 6488 * object with id, value and extensions. The accessor 6489 * "getMeaningWhenMissing" gives direct access to the value 6490 */ 6491 public ElementDefinition setMeaningWhenMissingElement(MarkdownType value) { 6492 this.meaningWhenMissing = value; 6493 return this; 6494 } 6495 6496 /** 6497 * @return The Implicit meaning that is to be understood when this element is 6498 * missing (e.g. 'when this element is missing, the period is ongoing'). 6499 */ 6500 public String getMeaningWhenMissing() { 6501 return this.meaningWhenMissing == null ? null : this.meaningWhenMissing.getValue(); 6502 } 6503 6504 /** 6505 * @param value The Implicit meaning that is to be understood when this element 6506 * is missing (e.g. 'when this element is missing, the period is 6507 * ongoing'). 6508 */ 6509 public ElementDefinition setMeaningWhenMissing(String value) { 6510 if (value == null) 6511 this.meaningWhenMissing = null; 6512 else { 6513 if (this.meaningWhenMissing == null) 6514 this.meaningWhenMissing = new MarkdownType(); 6515 this.meaningWhenMissing.setValue(value); 6516 } 6517 return this; 6518 } 6519 6520 /** 6521 * @return {@link #orderMeaning} (If present, indicates that the order of the 6522 * repeating element has meaning and describes what that meaning is. If 6523 * absent, it means that the order of the element has no meaning.). This 6524 * is the underlying object with id, value and extensions. The accessor 6525 * "getOrderMeaning" gives direct access to the value 6526 */ 6527 public StringType getOrderMeaningElement() { 6528 if (this.orderMeaning == null) 6529 if (Configuration.errorOnAutoCreate()) 6530 throw new Error("Attempt to auto-create ElementDefinition.orderMeaning"); 6531 else if (Configuration.doAutoCreate()) 6532 this.orderMeaning = new StringType(); // bb 6533 return this.orderMeaning; 6534 } 6535 6536 public boolean hasOrderMeaningElement() { 6537 return this.orderMeaning != null && !this.orderMeaning.isEmpty(); 6538 } 6539 6540 public boolean hasOrderMeaning() { 6541 return this.orderMeaning != null && !this.orderMeaning.isEmpty(); 6542 } 6543 6544 /** 6545 * @param value {@link #orderMeaning} (If present, indicates that the order of 6546 * the repeating element has meaning and describes what that 6547 * meaning is. If absent, it means that the order of the element 6548 * has no meaning.). This is the underlying object with id, value 6549 * and extensions. The accessor "getOrderMeaning" gives direct 6550 * access to the value 6551 */ 6552 public ElementDefinition setOrderMeaningElement(StringType value) { 6553 this.orderMeaning = value; 6554 return this; 6555 } 6556 6557 /** 6558 * @return If present, indicates that the order of the repeating element has 6559 * meaning and describes what that meaning is. If absent, it means that 6560 * the order of the element has no meaning. 6561 */ 6562 public String getOrderMeaning() { 6563 return this.orderMeaning == null ? null : this.orderMeaning.getValue(); 6564 } 6565 6566 /** 6567 * @param value If present, indicates that the order of the repeating element 6568 * has meaning and describes what that meaning is. If absent, it 6569 * means that the order of the element has no meaning. 6570 */ 6571 public ElementDefinition setOrderMeaning(String value) { 6572 if (Utilities.noString(value)) 6573 this.orderMeaning = null; 6574 else { 6575 if (this.orderMeaning == null) 6576 this.orderMeaning = new StringType(); 6577 this.orderMeaning.setValue(value); 6578 } 6579 return this; 6580 } 6581 6582 /** 6583 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for 6584 * this element in the instance. For purposes of comparison, 6585 * non-significant whitespace is ignored, and all values must be an 6586 * exact match (case and accent sensitive). Missing elements/attributes 6587 * must also be missing.) 6588 */ 6589 public org.hl7.fhir.r4.model.Type getFixed() { 6590 return this.fixed; 6591 } 6592 6593 public boolean hasFixed() { 6594 return this.fixed != null && !this.fixed.isEmpty(); 6595 } 6596 6597 /** 6598 * @param value {@link #fixed} (Specifies a value that SHALL be exactly the 6599 * value for this element in the instance. For purposes of 6600 * comparison, non-significant whitespace is ignored, and all 6601 * values must be an exact match (case and accent sensitive). 6602 * Missing elements/attributes must also be missing.) 6603 */ 6604 public ElementDefinition setFixed(org.hl7.fhir.r4.model.Type value) { 6605 this.fixed = value; 6606 return this; 6607 } 6608 6609 /** 6610 * @return {@link #pattern} (Specifies a value that the value in the instance 6611 * SHALL follow - that is, any value in the pattern must be found in the 6612 * instance. Other additional values may be found too. This is 6613 * effectively constraint by example. 6614 * 6615 * When pattern[x] is used to constrain a primitive, it means that the 6616 * value provided in the pattern[x] must match the instance value 6617 * exactly. 6618 * 6619 * When pattern[x] is used to constrain an array, it means that each 6620 * element provided in the pattern[x] array must (recursively) match at 6621 * least one element from the instance array. 6622 * 6623 * When pattern[x] is used to constrain a complex object, it means that 6624 * each property in the pattern must be present in the complex object, 6625 * and its value must recursively match -- i.e., 6626 * 6627 * 1. If primitive: it must match exactly the pattern value 2. If a 6628 * complex object: it must match (recursively) the pattern value 3. If 6629 * an array: it must match (recursively) the pattern value.) 6630 */ 6631 public org.hl7.fhir.r4.model.Type getPattern() { 6632 return this.pattern; 6633 } 6634 6635 public boolean hasPattern() { 6636 return this.pattern != null && !this.pattern.isEmpty(); 6637 } 6638 6639 /** 6640 * @param value {@link #pattern} (Specifies a value that the value in the 6641 * instance SHALL follow - that is, any value in the pattern must 6642 * be found in the instance. Other additional values may be found 6643 * too. This is effectively constraint by example. 6644 * 6645 * When pattern[x] is used to constrain a primitive, it means that 6646 * the value provided in the pattern[x] must match the instance 6647 * value exactly. 6648 * 6649 * When pattern[x] is used to constrain an array, it means that 6650 * each element provided in the pattern[x] array must (recursively) 6651 * match at least one element from the instance array. 6652 * 6653 * When pattern[x] is used to constrain a complex object, it means 6654 * that each property in the pattern must be present in the complex 6655 * object, and its value must recursively match -- i.e., 6656 * 6657 * 1. If primitive: it must match exactly the pattern value 2. If a 6658 * complex object: it must match (recursively) the pattern value 3. 6659 * If an array: it must match (recursively) the pattern value.) 6660 */ 6661 public ElementDefinition setPattern(org.hl7.fhir.r4.model.Type value) { 6662 this.pattern = value; 6663 return this; 6664 } 6665 6666 /** 6667 * @return {@link #example} (A sample value for this element demonstrating the 6668 * type of information that would typically be found in the element.) 6669 */ 6670 public List<ElementDefinitionExampleComponent> getExample() { 6671 if (this.example == null) 6672 this.example = new ArrayList<ElementDefinitionExampleComponent>(); 6673 return this.example; 6674 } 6675 6676 /** 6677 * @return Returns a reference to <code>this</code> for easy method chaining 6678 */ 6679 public ElementDefinition setExample(List<ElementDefinitionExampleComponent> theExample) { 6680 this.example = theExample; 6681 return this; 6682 } 6683 6684 public boolean hasExample() { 6685 if (this.example == null) 6686 return false; 6687 for (ElementDefinitionExampleComponent item : this.example) 6688 if (!item.isEmpty()) 6689 return true; 6690 return false; 6691 } 6692 6693 public ElementDefinitionExampleComponent addExample() { // 3 6694 ElementDefinitionExampleComponent t = new ElementDefinitionExampleComponent(); 6695 if (this.example == null) 6696 this.example = new ArrayList<ElementDefinitionExampleComponent>(); 6697 this.example.add(t); 6698 return t; 6699 } 6700 6701 public ElementDefinition addExample(ElementDefinitionExampleComponent t) { // 3 6702 if (t == null) 6703 return this; 6704 if (this.example == null) 6705 this.example = new ArrayList<ElementDefinitionExampleComponent>(); 6706 this.example.add(t); 6707 return this; 6708 } 6709 6710 /** 6711 * @return The first repetition of repeating field {@link #example}, creating it 6712 * if it does not already exist 6713 */ 6714 public ElementDefinitionExampleComponent getExampleFirstRep() { 6715 if (getExample().isEmpty()) { 6716 addExample(); 6717 } 6718 return getExample().get(0); 6719 } 6720 6721 /** 6722 * @return {@link #minValue} (The minimum allowed value for the element. The 6723 * value is inclusive. This is allowed for the types date, dateTime, 6724 * instant, time, decimal, integer, and Quantity.) 6725 */ 6726 public Type getMinValue() { 6727 return this.minValue; 6728 } 6729 6730 /** 6731 * @return {@link #minValue} (The minimum allowed value for the element. The 6732 * value is inclusive. This is allowed for the types date, dateTime, 6733 * instant, time, decimal, integer, and Quantity.) 6734 */ 6735 public DateType getMinValueDateType() throws FHIRException { 6736 if (this.minValue == null) 6737 this.minValue = new DateType(); 6738 if (!(this.minValue instanceof DateType)) 6739 throw new FHIRException("Type mismatch: the type DateType was expected, but " + this.minValue.getClass().getName() 6740 + " was encountered"); 6741 return (DateType) this.minValue; 6742 } 6743 6744 public boolean hasMinValueDateType() { 6745 return this.minValue instanceof DateType; 6746 } 6747 6748 /** 6749 * @return {@link #minValue} (The minimum allowed value for the element. The 6750 * value is inclusive. This is allowed for the types date, dateTime, 6751 * instant, time, decimal, integer, and Quantity.) 6752 */ 6753 public DateTimeType getMinValueDateTimeType() throws FHIRException { 6754 if (this.minValue == null) 6755 this.minValue = new DateTimeType(); 6756 if (!(this.minValue instanceof DateTimeType)) 6757 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 6758 + this.minValue.getClass().getName() + " was encountered"); 6759 return (DateTimeType) this.minValue; 6760 } 6761 6762 public boolean hasMinValueDateTimeType() { 6763 return this.minValue instanceof DateTimeType; 6764 } 6765 6766 /** 6767 * @return {@link #minValue} (The minimum allowed value for the element. The 6768 * value is inclusive. This is allowed for the types date, dateTime, 6769 * instant, time, decimal, integer, and Quantity.) 6770 */ 6771 public InstantType getMinValueInstantType() throws FHIRException { 6772 if (this.minValue == null) 6773 this.minValue = new InstantType(); 6774 if (!(this.minValue instanceof InstantType)) 6775 throw new FHIRException("Type mismatch: the type InstantType was expected, but " 6776 + this.minValue.getClass().getName() + " was encountered"); 6777 return (InstantType) this.minValue; 6778 } 6779 6780 public boolean hasMinValueInstantType() { 6781 return this.minValue instanceof InstantType; 6782 } 6783 6784 /** 6785 * @return {@link #minValue} (The minimum allowed value for the element. The 6786 * value is inclusive. This is allowed for the types date, dateTime, 6787 * instant, time, decimal, integer, and Quantity.) 6788 */ 6789 public TimeType getMinValueTimeType() throws FHIRException { 6790 if (this.minValue == null) 6791 this.minValue = new TimeType(); 6792 if (!(this.minValue instanceof TimeType)) 6793 throw new FHIRException("Type mismatch: the type TimeType was expected, but " + this.minValue.getClass().getName() 6794 + " was encountered"); 6795 return (TimeType) this.minValue; 6796 } 6797 6798 public boolean hasMinValueTimeType() { 6799 return this.minValue instanceof TimeType; 6800 } 6801 6802 /** 6803 * @return {@link #minValue} (The minimum allowed value for the element. The 6804 * value is inclusive. This is allowed for the types date, dateTime, 6805 * instant, time, decimal, integer, and Quantity.) 6806 */ 6807 public DecimalType getMinValueDecimalType() throws FHIRException { 6808 if (this.minValue == null) 6809 this.minValue = new DecimalType(); 6810 if (!(this.minValue instanceof DecimalType)) 6811 throw new FHIRException("Type mismatch: the type DecimalType was expected, but " 6812 + this.minValue.getClass().getName() + " was encountered"); 6813 return (DecimalType) this.minValue; 6814 } 6815 6816 public boolean hasMinValueDecimalType() { 6817 return this.minValue instanceof DecimalType; 6818 } 6819 6820 /** 6821 * @return {@link #minValue} (The minimum allowed value for the element. The 6822 * value is inclusive. This is allowed for the types date, dateTime, 6823 * instant, time, decimal, integer, and Quantity.) 6824 */ 6825 public IntegerType getMinValueIntegerType() throws FHIRException { 6826 if (this.minValue == null) 6827 this.minValue = new IntegerType(); 6828 if (!(this.minValue instanceof IntegerType)) 6829 throw new FHIRException("Type mismatch: the type IntegerType was expected, but " 6830 + this.minValue.getClass().getName() + " was encountered"); 6831 return (IntegerType) this.minValue; 6832 } 6833 6834 public boolean hasMinValueIntegerType() { 6835 return this.minValue instanceof IntegerType; 6836 } 6837 6838 /** 6839 * @return {@link #minValue} (The minimum allowed value for the element. The 6840 * value is inclusive. This is allowed for the types date, dateTime, 6841 * instant, time, decimal, integer, and Quantity.) 6842 */ 6843 public PositiveIntType getMinValuePositiveIntType() throws FHIRException { 6844 if (this.minValue == null) 6845 this.minValue = new PositiveIntType(); 6846 if (!(this.minValue instanceof PositiveIntType)) 6847 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but " 6848 + this.minValue.getClass().getName() + " was encountered"); 6849 return (PositiveIntType) this.minValue; 6850 } 6851 6852 public boolean hasMinValuePositiveIntType() { 6853 return this.minValue instanceof PositiveIntType; 6854 } 6855 6856 /** 6857 * @return {@link #minValue} (The minimum allowed value for the element. The 6858 * value is inclusive. This is allowed for the types date, dateTime, 6859 * instant, time, decimal, integer, and Quantity.) 6860 */ 6861 public UnsignedIntType getMinValueUnsignedIntType() throws FHIRException { 6862 if (this.minValue == null) 6863 this.minValue = new UnsignedIntType(); 6864 if (!(this.minValue instanceof UnsignedIntType)) 6865 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but " 6866 + this.minValue.getClass().getName() + " was encountered"); 6867 return (UnsignedIntType) this.minValue; 6868 } 6869 6870 public boolean hasMinValueUnsignedIntType() { 6871 return this.minValue instanceof UnsignedIntType; 6872 } 6873 6874 /** 6875 * @return {@link #minValue} (The minimum allowed value for the element. The 6876 * value is inclusive. This is allowed for the types date, dateTime, 6877 * instant, time, decimal, integer, and Quantity.) 6878 */ 6879 public Quantity getMinValueQuantity() throws FHIRException { 6880 if (this.minValue == null) 6881 this.minValue = new Quantity(); 6882 if (!(this.minValue instanceof Quantity)) 6883 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.minValue.getClass().getName() 6884 + " was encountered"); 6885 return (Quantity) this.minValue; 6886 } 6887 6888 public boolean hasMinValueQuantity() { 6889 return this.minValue instanceof Quantity; 6890 } 6891 6892 public boolean hasMinValue() { 6893 return this.minValue != null && !this.minValue.isEmpty(); 6894 } 6895 6896 /** 6897 * @param value {@link #minValue} (The minimum allowed value for the element. 6898 * The value is inclusive. This is allowed for the types date, 6899 * dateTime, instant, time, decimal, integer, and Quantity.) 6900 */ 6901 public ElementDefinition setMinValue(Type value) { 6902 if (value != null && !(value instanceof DateType || value instanceof DateTimeType || value instanceof InstantType 6903 || value instanceof TimeType || value instanceof DecimalType || value instanceof IntegerType 6904 || value instanceof PositiveIntType || value instanceof UnsignedIntType || value instanceof Quantity)) 6905 throw new Error("Not the right type for ElementDefinition.minValue[x]: " + value.fhirType()); 6906 this.minValue = value; 6907 return this; 6908 } 6909 6910 /** 6911 * @return {@link #maxValue} (The maximum allowed value for the element. The 6912 * value is inclusive. This is allowed for the types date, dateTime, 6913 * instant, time, decimal, integer, and Quantity.) 6914 */ 6915 public Type getMaxValue() { 6916 return this.maxValue; 6917 } 6918 6919 /** 6920 * @return {@link #maxValue} (The maximum allowed value for the element. The 6921 * value is inclusive. This is allowed for the types date, dateTime, 6922 * instant, time, decimal, integer, and Quantity.) 6923 */ 6924 public DateType getMaxValueDateType() throws FHIRException { 6925 if (this.maxValue == null) 6926 this.maxValue = new DateType(); 6927 if (!(this.maxValue instanceof DateType)) 6928 throw new FHIRException("Type mismatch: the type DateType was expected, but " + this.maxValue.getClass().getName() 6929 + " was encountered"); 6930 return (DateType) this.maxValue; 6931 } 6932 6933 public boolean hasMaxValueDateType() { 6934 return this.maxValue instanceof DateType; 6935 } 6936 6937 /** 6938 * @return {@link #maxValue} (The maximum allowed value for the element. The 6939 * value is inclusive. This is allowed for the types date, dateTime, 6940 * instant, time, decimal, integer, and Quantity.) 6941 */ 6942 public DateTimeType getMaxValueDateTimeType() throws FHIRException { 6943 if (this.maxValue == null) 6944 this.maxValue = new DateTimeType(); 6945 if (!(this.maxValue instanceof DateTimeType)) 6946 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 6947 + this.maxValue.getClass().getName() + " was encountered"); 6948 return (DateTimeType) this.maxValue; 6949 } 6950 6951 public boolean hasMaxValueDateTimeType() { 6952 return this.maxValue instanceof DateTimeType; 6953 } 6954 6955 /** 6956 * @return {@link #maxValue} (The maximum allowed value for the element. The 6957 * value is inclusive. This is allowed for the types date, dateTime, 6958 * instant, time, decimal, integer, and Quantity.) 6959 */ 6960 public InstantType getMaxValueInstantType() throws FHIRException { 6961 if (this.maxValue == null) 6962 this.maxValue = new InstantType(); 6963 if (!(this.maxValue instanceof InstantType)) 6964 throw new FHIRException("Type mismatch: the type InstantType was expected, but " 6965 + this.maxValue.getClass().getName() + " was encountered"); 6966 return (InstantType) this.maxValue; 6967 } 6968 6969 public boolean hasMaxValueInstantType() { 6970 return this.maxValue instanceof InstantType; 6971 } 6972 6973 /** 6974 * @return {@link #maxValue} (The maximum allowed value for the element. The 6975 * value is inclusive. This is allowed for the types date, dateTime, 6976 * instant, time, decimal, integer, and Quantity.) 6977 */ 6978 public TimeType getMaxValueTimeType() throws FHIRException { 6979 if (this.maxValue == null) 6980 this.maxValue = new TimeType(); 6981 if (!(this.maxValue instanceof TimeType)) 6982 throw new FHIRException("Type mismatch: the type TimeType was expected, but " + this.maxValue.getClass().getName() 6983 + " was encountered"); 6984 return (TimeType) this.maxValue; 6985 } 6986 6987 public boolean hasMaxValueTimeType() { 6988 return this.maxValue instanceof TimeType; 6989 } 6990 6991 /** 6992 * @return {@link #maxValue} (The maximum allowed value for the element. The 6993 * value is inclusive. This is allowed for the types date, dateTime, 6994 * instant, time, decimal, integer, and Quantity.) 6995 */ 6996 public DecimalType getMaxValueDecimalType() throws FHIRException { 6997 if (this.maxValue == null) 6998 this.maxValue = new DecimalType(); 6999 if (!(this.maxValue instanceof DecimalType)) 7000 throw new FHIRException("Type mismatch: the type DecimalType was expected, but " 7001 + this.maxValue.getClass().getName() + " was encountered"); 7002 return (DecimalType) this.maxValue; 7003 } 7004 7005 public boolean hasMaxValueDecimalType() { 7006 return this.maxValue instanceof DecimalType; 7007 } 7008 7009 /** 7010 * @return {@link #maxValue} (The maximum allowed value for the element. The 7011 * value is inclusive. This is allowed for the types date, dateTime, 7012 * instant, time, decimal, integer, and Quantity.) 7013 */ 7014 public IntegerType getMaxValueIntegerType() throws FHIRException { 7015 if (this.maxValue == null) 7016 this.maxValue = new IntegerType(); 7017 if (!(this.maxValue instanceof IntegerType)) 7018 throw new FHIRException("Type mismatch: the type IntegerType was expected, but " 7019 + this.maxValue.getClass().getName() + " was encountered"); 7020 return (IntegerType) this.maxValue; 7021 } 7022 7023 public boolean hasMaxValueIntegerType() { 7024 return this.maxValue instanceof IntegerType; 7025 } 7026 7027 /** 7028 * @return {@link #maxValue} (The maximum allowed value for the element. The 7029 * value is inclusive. This is allowed for the types date, dateTime, 7030 * instant, time, decimal, integer, and Quantity.) 7031 */ 7032 public PositiveIntType getMaxValuePositiveIntType() throws FHIRException { 7033 if (this.maxValue == null) 7034 this.maxValue = new PositiveIntType(); 7035 if (!(this.maxValue instanceof PositiveIntType)) 7036 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but " 7037 + this.maxValue.getClass().getName() + " was encountered"); 7038 return (PositiveIntType) this.maxValue; 7039 } 7040 7041 public boolean hasMaxValuePositiveIntType() { 7042 return this.maxValue instanceof PositiveIntType; 7043 } 7044 7045 /** 7046 * @return {@link #maxValue} (The maximum allowed value for the element. The 7047 * value is inclusive. This is allowed for the types date, dateTime, 7048 * instant, time, decimal, integer, and Quantity.) 7049 */ 7050 public UnsignedIntType getMaxValueUnsignedIntType() throws FHIRException { 7051 if (this.maxValue == null) 7052 this.maxValue = new UnsignedIntType(); 7053 if (!(this.maxValue instanceof UnsignedIntType)) 7054 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but " 7055 + this.maxValue.getClass().getName() + " was encountered"); 7056 return (UnsignedIntType) this.maxValue; 7057 } 7058 7059 public boolean hasMaxValueUnsignedIntType() { 7060 return this.maxValue instanceof UnsignedIntType; 7061 } 7062 7063 /** 7064 * @return {@link #maxValue} (The maximum allowed value for the element. The 7065 * value is inclusive. This is allowed for the types date, dateTime, 7066 * instant, time, decimal, integer, and Quantity.) 7067 */ 7068 public Quantity getMaxValueQuantity() throws FHIRException { 7069 if (this.maxValue == null) 7070 this.maxValue = new Quantity(); 7071 if (!(this.maxValue instanceof Quantity)) 7072 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.maxValue.getClass().getName() 7073 + " was encountered"); 7074 return (Quantity) this.maxValue; 7075 } 7076 7077 public boolean hasMaxValueQuantity() { 7078 return this.maxValue instanceof Quantity; 7079 } 7080 7081 public boolean hasMaxValue() { 7082 return this.maxValue != null && !this.maxValue.isEmpty(); 7083 } 7084 7085 /** 7086 * @param value {@link #maxValue} (The maximum allowed value for the element. 7087 * The value is inclusive. This is allowed for the types date, 7088 * dateTime, instant, time, decimal, integer, and Quantity.) 7089 */ 7090 public ElementDefinition setMaxValue(Type value) { 7091 if (value != null && !(value instanceof DateType || value instanceof DateTimeType || value instanceof InstantType 7092 || value instanceof TimeType || value instanceof DecimalType || value instanceof IntegerType 7093 || value instanceof PositiveIntType || value instanceof UnsignedIntType || value instanceof Quantity)) 7094 throw new Error("Not the right type for ElementDefinition.maxValue[x]: " + value.fhirType()); 7095 this.maxValue = value; 7096 return this; 7097 } 7098 7099 /** 7100 * @return {@link #maxLength} (Indicates the maximum length in characters that 7101 * is permitted to be present in conformant instances and which is 7102 * expected to be supported by conformant consumers that support the 7103 * element.). This is the underlying object with id, value and 7104 * extensions. The accessor "getMaxLength" gives direct access to the 7105 * value 7106 */ 7107 public IntegerType getMaxLengthElement() { 7108 if (this.maxLength == null) 7109 if (Configuration.errorOnAutoCreate()) 7110 throw new Error("Attempt to auto-create ElementDefinition.maxLength"); 7111 else if (Configuration.doAutoCreate()) 7112 this.maxLength = new IntegerType(); // bb 7113 return this.maxLength; 7114 } 7115 7116 public boolean hasMaxLengthElement() { 7117 return this.maxLength != null && !this.maxLength.isEmpty(); 7118 } 7119 7120 public boolean hasMaxLength() { 7121 return this.maxLength != null && !this.maxLength.isEmpty(); 7122 } 7123 7124 /** 7125 * @param value {@link #maxLength} (Indicates the maximum length in characters 7126 * that is permitted to be present in conformant instances and 7127 * which is expected to be supported by conformant consumers that 7128 * support the element.). This is the underlying object with id, 7129 * value and extensions. The accessor "getMaxLength" gives direct 7130 * access to the value 7131 */ 7132 public ElementDefinition setMaxLengthElement(IntegerType value) { 7133 this.maxLength = value; 7134 return this; 7135 } 7136 7137 /** 7138 * @return Indicates the maximum length in characters that is permitted to be 7139 * present in conformant instances and which is expected to be supported 7140 * by conformant consumers that support the element. 7141 */ 7142 public int getMaxLength() { 7143 return this.maxLength == null || this.maxLength.isEmpty() ? 0 : this.maxLength.getValue(); 7144 } 7145 7146 /** 7147 * @param value Indicates the maximum length in characters that is permitted to 7148 * be present in conformant instances and which is expected to be 7149 * supported by conformant consumers that support the element. 7150 */ 7151 public ElementDefinition setMaxLength(int value) { 7152 if (this.maxLength == null) 7153 this.maxLength = new IntegerType(); 7154 this.maxLength.setValue(value); 7155 return this; 7156 } 7157 7158 /** 7159 * @return {@link #condition} (A reference to an invariant that may make 7160 * additional statements about the cardinality or value in the 7161 * instance.) 7162 */ 7163 public List<IdType> getCondition() { 7164 if (this.condition == null) 7165 this.condition = new ArrayList<IdType>(); 7166 return this.condition; 7167 } 7168 7169 /** 7170 * @return Returns a reference to <code>this</code> for easy method chaining 7171 */ 7172 public ElementDefinition setCondition(List<IdType> theCondition) { 7173 this.condition = theCondition; 7174 return this; 7175 } 7176 7177 public boolean hasCondition() { 7178 if (this.condition == null) 7179 return false; 7180 for (IdType item : this.condition) 7181 if (!item.isEmpty()) 7182 return true; 7183 return false; 7184 } 7185 7186 /** 7187 * @return {@link #condition} (A reference to an invariant that may make 7188 * additional statements about the cardinality or value in the 7189 * instance.) 7190 */ 7191 public IdType addConditionElement() {// 2 7192 IdType t = new IdType(); 7193 if (this.condition == null) 7194 this.condition = new ArrayList<IdType>(); 7195 this.condition.add(t); 7196 return t; 7197 } 7198 7199 /** 7200 * @param value {@link #condition} (A reference to an invariant that may make 7201 * additional statements about the cardinality or value in the 7202 * instance.) 7203 */ 7204 public ElementDefinition addCondition(String value) { // 1 7205 IdType t = new IdType(); 7206 t.setValue(value); 7207 if (this.condition == null) 7208 this.condition = new ArrayList<IdType>(); 7209 this.condition.add(t); 7210 return this; 7211 } 7212 7213 /** 7214 * @param value {@link #condition} (A reference to an invariant that may make 7215 * additional statements about the cardinality or value in the 7216 * instance.) 7217 */ 7218 public boolean hasCondition(String value) { 7219 if (this.condition == null) 7220 return false; 7221 for (IdType v : this.condition) 7222 if (v.getValue().equals(value)) // id 7223 return true; 7224 return false; 7225 } 7226 7227 /** 7228 * @return {@link #constraint} (Formal constraints such as co-occurrence and 7229 * other constraints that can be computationally evaluated within the 7230 * context of the instance.) 7231 */ 7232 public List<ElementDefinitionConstraintComponent> getConstraint() { 7233 if (this.constraint == null) 7234 this.constraint = new ArrayList<ElementDefinitionConstraintComponent>(); 7235 return this.constraint; 7236 } 7237 7238 /** 7239 * @return Returns a reference to <code>this</code> for easy method chaining 7240 */ 7241 public ElementDefinition setConstraint(List<ElementDefinitionConstraintComponent> theConstraint) { 7242 this.constraint = theConstraint; 7243 return this; 7244 } 7245 7246 public boolean hasConstraint() { 7247 if (this.constraint == null) 7248 return false; 7249 for (ElementDefinitionConstraintComponent item : this.constraint) 7250 if (!item.isEmpty()) 7251 return true; 7252 return false; 7253 } 7254 7255 public ElementDefinitionConstraintComponent addConstraint() { // 3 7256 ElementDefinitionConstraintComponent t = new ElementDefinitionConstraintComponent(); 7257 if (this.constraint == null) 7258 this.constraint = new ArrayList<ElementDefinitionConstraintComponent>(); 7259 this.constraint.add(t); 7260 return t; 7261 } 7262 7263 public ElementDefinition addConstraint(ElementDefinitionConstraintComponent t) { // 3 7264 if (t == null) 7265 return this; 7266 if (this.constraint == null) 7267 this.constraint = new ArrayList<ElementDefinitionConstraintComponent>(); 7268 this.constraint.add(t); 7269 return this; 7270 } 7271 7272 /** 7273 * @return The first repetition of repeating field {@link #constraint}, creating 7274 * it if it does not already exist 7275 */ 7276 public ElementDefinitionConstraintComponent getConstraintFirstRep() { 7277 if (getConstraint().isEmpty()) { 7278 addConstraint(); 7279 } 7280 return getConstraint().get(0); 7281 } 7282 7283 /** 7284 * @return {@link #mustSupport} (If true, implementations that produce or 7285 * consume resources SHALL provide "support" for the element in some 7286 * meaningful way. If false, the element may be ignored and not 7287 * supported. If false, whether to populate or use the data element in 7288 * any way is at the discretion of the implementation.). This is the 7289 * underlying object with id, value and extensions. The accessor 7290 * "getMustSupport" gives direct access to the value 7291 */ 7292 public BooleanType getMustSupportElement() { 7293 if (this.mustSupport == null) 7294 if (Configuration.errorOnAutoCreate()) 7295 throw new Error("Attempt to auto-create ElementDefinition.mustSupport"); 7296 else if (Configuration.doAutoCreate()) 7297 this.mustSupport = new BooleanType(); // bb 7298 return this.mustSupport; 7299 } 7300 7301 public boolean hasMustSupportElement() { 7302 return this.mustSupport != null && !this.mustSupport.isEmpty(); 7303 } 7304 7305 public boolean hasMustSupport() { 7306 return this.mustSupport != null && !this.mustSupport.isEmpty(); 7307 } 7308 7309 /** 7310 * @param value {@link #mustSupport} (If true, implementations that produce or 7311 * consume resources SHALL provide "support" for the element in 7312 * some meaningful way. If false, the element may be ignored and 7313 * not supported. If false, whether to populate or use the data 7314 * element in any way is at the discretion of the implementation.). 7315 * This is the underlying object with id, value and extensions. The 7316 * accessor "getMustSupport" gives direct access to the value 7317 */ 7318 public ElementDefinition setMustSupportElement(BooleanType value) { 7319 this.mustSupport = value; 7320 return this; 7321 } 7322 7323 /** 7324 * @return If true, implementations that produce or consume resources SHALL 7325 * provide "support" for the element in some meaningful way. If false, 7326 * the element may be ignored and not supported. If false, whether to 7327 * populate or use the data element in any way is at the discretion of 7328 * the implementation. 7329 */ 7330 public boolean getMustSupport() { 7331 return this.mustSupport == null || this.mustSupport.isEmpty() ? false : this.mustSupport.getValue(); 7332 } 7333 7334 /** 7335 * @param value If true, implementations that produce or consume resources SHALL 7336 * provide "support" for the element in some meaningful way. If 7337 * false, the element may be ignored and not supported. If false, 7338 * whether to populate or use the data element in any way is at the 7339 * discretion of the implementation. 7340 */ 7341 public ElementDefinition setMustSupport(boolean value) { 7342 if (this.mustSupport == null) 7343 this.mustSupport = new BooleanType(); 7344 this.mustSupport.setValue(value); 7345 return this; 7346 } 7347 7348 /** 7349 * @return {@link #isModifier} (If true, the value of this element affects the 7350 * interpretation of the element or resource that contains it, and the 7351 * value of the element cannot be ignored. Typically, this is used for 7352 * status, negation and qualification codes. The effect of this is that 7353 * the element cannot be ignored by systems: they SHALL either recognize 7354 * the element and process it, and/or a pre-determination has been made 7355 * that it is not relevant to their particular system.). This is the 7356 * underlying object with id, value and extensions. The accessor 7357 * "getIsModifier" gives direct access to the value 7358 */ 7359 public BooleanType getIsModifierElement() { 7360 if (this.isModifier == null) 7361 if (Configuration.errorOnAutoCreate()) 7362 throw new Error("Attempt to auto-create ElementDefinition.isModifier"); 7363 else if (Configuration.doAutoCreate()) 7364 this.isModifier = new BooleanType(); // bb 7365 return this.isModifier; 7366 } 7367 7368 public boolean hasIsModifierElement() { 7369 return this.isModifier != null && !this.isModifier.isEmpty(); 7370 } 7371 7372 public boolean hasIsModifier() { 7373 return this.isModifier != null && !this.isModifier.isEmpty(); 7374 } 7375 7376 /** 7377 * @param value {@link #isModifier} (If true, the value of this element affects 7378 * the interpretation of the element or resource that contains it, 7379 * and the value of the element cannot be ignored. Typically, this 7380 * is used for status, negation and qualification codes. The effect 7381 * of this is that the element cannot be ignored by systems: they 7382 * SHALL either recognize the element and process it, and/or a 7383 * pre-determination has been made that it is not relevant to their 7384 * particular system.). This is the underlying object with id, 7385 * value and extensions. The accessor "getIsModifier" gives direct 7386 * access to the value 7387 */ 7388 public ElementDefinition setIsModifierElement(BooleanType value) { 7389 this.isModifier = value; 7390 return this; 7391 } 7392 7393 /** 7394 * @return If true, the value of this element affects the interpretation of the 7395 * element or resource that contains it, and the value of the element 7396 * cannot be ignored. Typically, this is used for status, negation and 7397 * qualification codes. The effect of this is that the element cannot be 7398 * ignored by systems: they SHALL either recognize the element and 7399 * process it, and/or a pre-determination has been made that it is not 7400 * relevant to their particular system. 7401 */ 7402 public boolean getIsModifier() { 7403 return this.isModifier == null || this.isModifier.isEmpty() ? false : this.isModifier.getValue(); 7404 } 7405 7406 /** 7407 * @param value If true, the value of this element affects the interpretation of 7408 * the element or resource that contains it, and the value of the 7409 * element cannot be ignored. Typically, this is used for status, 7410 * negation and qualification codes. The effect of this is that the 7411 * element cannot be ignored by systems: they SHALL either 7412 * recognize the element and process it, and/or a pre-determination 7413 * has been made that it is not relevant to their particular 7414 * system. 7415 */ 7416 public ElementDefinition setIsModifier(boolean value) { 7417 if (this.isModifier == null) 7418 this.isModifier = new BooleanType(); 7419 this.isModifier.setValue(value); 7420 return this; 7421 } 7422 7423 /** 7424 * @return {@link #isModifierReason} (Explains how that element affects the 7425 * interpretation of the resource or element that contains it.). This is 7426 * the underlying object with id, value and extensions. The accessor 7427 * "getIsModifierReason" gives direct access to the value 7428 */ 7429 public StringType getIsModifierReasonElement() { 7430 if (this.isModifierReason == null) 7431 if (Configuration.errorOnAutoCreate()) 7432 throw new Error("Attempt to auto-create ElementDefinition.isModifierReason"); 7433 else if (Configuration.doAutoCreate()) 7434 this.isModifierReason = new StringType(); // bb 7435 return this.isModifierReason; 7436 } 7437 7438 public boolean hasIsModifierReasonElement() { 7439 return this.isModifierReason != null && !this.isModifierReason.isEmpty(); 7440 } 7441 7442 public boolean hasIsModifierReason() { 7443 return this.isModifierReason != null && !this.isModifierReason.isEmpty(); 7444 } 7445 7446 /** 7447 * @param value {@link #isModifierReason} (Explains how that element affects the 7448 * interpretation of the resource or element that contains it.). 7449 * This is the underlying object with id, value and extensions. The 7450 * accessor "getIsModifierReason" gives direct access to the value 7451 */ 7452 public ElementDefinition setIsModifierReasonElement(StringType value) { 7453 this.isModifierReason = value; 7454 return this; 7455 } 7456 7457 /** 7458 * @return Explains how that element affects the interpretation of the resource 7459 * or element that contains it. 7460 */ 7461 public String getIsModifierReason() { 7462 return this.isModifierReason == null ? null : this.isModifierReason.getValue(); 7463 } 7464 7465 /** 7466 * @param value Explains how that element affects the interpretation of the 7467 * resource or element that contains it. 7468 */ 7469 public ElementDefinition setIsModifierReason(String value) { 7470 if (Utilities.noString(value)) 7471 this.isModifierReason = null; 7472 else { 7473 if (this.isModifierReason == null) 7474 this.isModifierReason = new StringType(); 7475 this.isModifierReason.setValue(value); 7476 } 7477 return this; 7478 } 7479 7480 /** 7481 * @return {@link #isSummary} (Whether the element should be included if a 7482 * client requests a search with the parameter _summary=true.). This is 7483 * the underlying object with id, value and extensions. The accessor 7484 * "getIsSummary" gives direct access to the value 7485 */ 7486 public BooleanType getIsSummaryElement() { 7487 if (this.isSummary == null) 7488 if (Configuration.errorOnAutoCreate()) 7489 throw new Error("Attempt to auto-create ElementDefinition.isSummary"); 7490 else if (Configuration.doAutoCreate()) 7491 this.isSummary = new BooleanType(); // bb 7492 return this.isSummary; 7493 } 7494 7495 public boolean hasIsSummaryElement() { 7496 return this.isSummary != null && !this.isSummary.isEmpty(); 7497 } 7498 7499 public boolean hasIsSummary() { 7500 return this.isSummary != null && !this.isSummary.isEmpty(); 7501 } 7502 7503 /** 7504 * @param value {@link #isSummary} (Whether the element should be included if a 7505 * client requests a search with the parameter _summary=true.). 7506 * This is the underlying object with id, value and extensions. The 7507 * accessor "getIsSummary" gives direct access to the value 7508 */ 7509 public ElementDefinition setIsSummaryElement(BooleanType value) { 7510 this.isSummary = value; 7511 return this; 7512 } 7513 7514 /** 7515 * @return Whether the element should be included if a client requests a search 7516 * with the parameter _summary=true. 7517 */ 7518 public boolean getIsSummary() { 7519 return this.isSummary == null || this.isSummary.isEmpty() ? false : this.isSummary.getValue(); 7520 } 7521 7522 /** 7523 * @param value Whether the element should be included if a client requests a 7524 * search with the parameter _summary=true. 7525 */ 7526 public ElementDefinition setIsSummary(boolean value) { 7527 if (this.isSummary == null) 7528 this.isSummary = new BooleanType(); 7529 this.isSummary.setValue(value); 7530 return this; 7531 } 7532 7533 /** 7534 * @return {@link #binding} (Binds to a value set if this element is coded 7535 * (code, Coding, CodeableConcept, Quantity), or the data types (string, 7536 * uri).) 7537 */ 7538 public ElementDefinitionBindingComponent getBinding() { 7539 if (this.binding == null) 7540 if (Configuration.errorOnAutoCreate()) 7541 throw new Error("Attempt to auto-create ElementDefinition.binding"); 7542 else if (Configuration.doAutoCreate()) 7543 this.binding = new ElementDefinitionBindingComponent(); // cc 7544 return this.binding; 7545 } 7546 7547 public boolean hasBinding() { 7548 return this.binding != null && !this.binding.isEmpty(); 7549 } 7550 7551 /** 7552 * @param value {@link #binding} (Binds to a value set if this element is coded 7553 * (code, Coding, CodeableConcept, Quantity), or the data types 7554 * (string, uri).) 7555 */ 7556 public ElementDefinition setBinding(ElementDefinitionBindingComponent value) { 7557 this.binding = value; 7558 return this; 7559 } 7560 7561 /** 7562 * @return {@link #mapping} (Identifies a concept from an external specification 7563 * that roughly corresponds to this element.) 7564 */ 7565 public List<ElementDefinitionMappingComponent> getMapping() { 7566 if (this.mapping == null) 7567 this.mapping = new ArrayList<ElementDefinitionMappingComponent>(); 7568 return this.mapping; 7569 } 7570 7571 /** 7572 * @return Returns a reference to <code>this</code> for easy method chaining 7573 */ 7574 public ElementDefinition setMapping(List<ElementDefinitionMappingComponent> theMapping) { 7575 this.mapping = theMapping; 7576 return this; 7577 } 7578 7579 public boolean hasMapping() { 7580 if (this.mapping == null) 7581 return false; 7582 for (ElementDefinitionMappingComponent item : this.mapping) 7583 if (!item.isEmpty()) 7584 return true; 7585 return false; 7586 } 7587 7588 public ElementDefinitionMappingComponent addMapping() { // 3 7589 ElementDefinitionMappingComponent t = new ElementDefinitionMappingComponent(); 7590 if (this.mapping == null) 7591 this.mapping = new ArrayList<ElementDefinitionMappingComponent>(); 7592 this.mapping.add(t); 7593 return t; 7594 } 7595 7596 public ElementDefinition addMapping(ElementDefinitionMappingComponent t) { // 3 7597 if (t == null) 7598 return this; 7599 if (this.mapping == null) 7600 this.mapping = new ArrayList<ElementDefinitionMappingComponent>(); 7601 this.mapping.add(t); 7602 return this; 7603 } 7604 7605 /** 7606 * @return The first repetition of repeating field {@link #mapping}, creating it 7607 * if it does not already exist 7608 */ 7609 public ElementDefinitionMappingComponent getMappingFirstRep() { 7610 if (getMapping().isEmpty()) { 7611 addMapping(); 7612 } 7613 return getMapping().get(0); 7614 } 7615 7616 protected void listChildren(List<Property> children) { 7617 super.listChildren(children); 7618 children.add(new Property("path", "string", 7619 "The path identifies the element and is expressed as a \".\"-separated list of ancestor elements, beginning with the name of the resource or extension.", 7620 0, 1, path)); 7621 children.add(new Property("representation", "code", 7622 "Codes that define how this element is represented in instances, when the deviation varies from the normal case.", 7623 0, java.lang.Integer.MAX_VALUE, representation)); 7624 children.add(new Property("sliceName", "string", 7625 "The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element.", 7626 0, 1, sliceName)); 7627 children.add(new Property("sliceIsConstraining", "boolean", 7628 "If true, indicates that this slice definition is constraining a slice definition with the same name in an inherited profile. If false, the slice is not overriding any slice in an inherited profile. If missing, the slice might or might not be overriding a slice in an inherited profile, depending on the sliceName.", 7629 0, 1, sliceIsConstraining)); 7630 children.add(new Property("label", "string", 7631 "A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form.", 7632 0, 1, label)); 7633 children.add( 7634 new Property("code", "Coding", "A code that has the same meaning as the element in a particular terminology.", 7635 0, java.lang.Integer.MAX_VALUE, code)); 7636 children.add(new Property("slicing", "", 7637 "Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set).", 7638 0, 1, slicing)); 7639 children.add(new Property("short", "string", 7640 "A concise description of what this element means (e.g. for use in autogenerated summaries).", 0, 1, short_)); 7641 children.add(new Property("definition", "markdown", 7642 "Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource. (Note: The text you are reading is specified in ElementDefinition.definition).", 7643 0, 1, definition)); 7644 children.add(new Property("comment", "markdown", 7645 "Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. (Note: The text you are reading is specified in ElementDefinition.comment).", 7646 0, 1, comment)); 7647 children.add(new Property("requirements", "markdown", 7648 "This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element.", 7649 0, 1, requirements)); 7650 children 7651 .add(new Property("alias", "string", "Identifies additional names by which this element might also be known.", 7652 0, java.lang.Integer.MAX_VALUE, alias)); 7653 children.add(new Property("min", "unsignedInt", 7654 "The minimum number of times this element SHALL appear in the instance.", 0, 1, min)); 7655 children.add(new Property("max", "string", 7656 "The maximum number of times this element is permitted to appear in the instance.", 0, 1, max)); 7657 children.add(new Property("base", "", 7658 "Information about the base definition of the element, provided to make it unnecessary for tools to trace the deviation of the element through the derived and related profiles. When the element definition is not the original definition of an element - i.g. either in a constraint on another type, or for elements from a super type in a snap shot - then the information in provided in the element definition may be different to the base definition. On the original definition of the element, it will be same.", 7659 0, 1, base)); 7660 children.add(new Property("contentReference", "uri", 7661 "Identifies an element defined elsewhere in the definition whose content rules should be applied to the current element. ContentReferences bring across all the rules that are in the ElementDefinition for the element, including definitions, cardinality constraints, bindings, invariants etc.", 7662 0, 1, contentReference)); 7663 children 7664 .add(new Property("type", "", "The data type or resource that the value of this element is permitted to be.", 0, 7665 java.lang.Integer.MAX_VALUE, type)); 7666 children.add(new Property("defaultValue[x]", "*", 7667 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7668 0, 1, defaultValue)); 7669 children.add(new Property("meaningWhenMissing", "markdown", 7670 "The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing').", 7671 0, 1, meaningWhenMissing)); 7672 children.add(new Property("orderMeaning", "string", 7673 "If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning.", 7674 0, 1, orderMeaning)); 7675 children.add(new Property("fixed[x]", "*", 7676 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7677 0, 1, fixed)); 7678 children.add(new Property("pattern[x]", "*", 7679 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 7680 0, 1, pattern)); 7681 children.add(new Property("example", "", 7682 "A sample value for this element demonstrating the type of information that would typically be found in the element.", 7683 0, java.lang.Integer.MAX_VALUE, example)); 7684 children.add(new Property("minValue[x]", 7685 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 7686 "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 7687 0, 1, minValue)); 7688 children.add(new Property("maxValue[x]", 7689 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 7690 "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 7691 0, 1, maxValue)); 7692 children.add(new Property("maxLength", "integer", 7693 "Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element.", 7694 0, 1, maxLength)); 7695 children.add(new Property("condition", "id", 7696 "A reference to an invariant that may make additional statements about the cardinality or value in the instance.", 7697 0, java.lang.Integer.MAX_VALUE, condition)); 7698 children.add(new Property("constraint", "", 7699 "Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance.", 7700 0, java.lang.Integer.MAX_VALUE, constraint)); 7701 children.add(new Property("mustSupport", "boolean", 7702 "If true, implementations that produce or consume resources SHALL provide \"support\" for the element in some meaningful way. If false, the element may be ignored and not supported. If false, whether to populate or use the data element in any way is at the discretion of the implementation.", 7703 0, 1, mustSupport)); 7704 children.add(new Property("isModifier", "boolean", 7705 "If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system.", 7706 0, 1, isModifier)); 7707 children.add(new Property("isModifierReason", "string", 7708 "Explains how that element affects the interpretation of the resource or element that contains it.", 0, 1, 7709 isModifierReason)); 7710 children.add(new Property("isSummary", "boolean", 7711 "Whether the element should be included if a client requests a search with the parameter _summary=true.", 0, 1, 7712 isSummary)); 7713 children.add(new Property("binding", "", 7714 "Binds to a value set if this element is coded (code, Coding, CodeableConcept, Quantity), or the data types (string, uri).", 7715 0, 1, binding)); 7716 children.add(new Property("mapping", "", 7717 "Identifies a concept from an external specification that roughly corresponds to this element.", 0, 7718 java.lang.Integer.MAX_VALUE, mapping)); 7719 } 7720 7721 @Override 7722 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7723 switch (_hash) { 7724 case 3433509: 7725 /* path */ return new Property("path", "string", 7726 "The path identifies the element and is expressed as a \".\"-separated list of ancestor elements, beginning with the name of the resource or extension.", 7727 0, 1, path); 7728 case -671065907: 7729 /* representation */ return new Property("representation", "code", 7730 "Codes that define how this element is represented in instances, when the deviation varies from the normal case.", 7731 0, java.lang.Integer.MAX_VALUE, representation); 7732 case -825289923: 7733 /* sliceName */ return new Property("sliceName", "string", 7734 "The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element.", 7735 0, 1, sliceName); 7736 case 333040519: 7737 /* sliceIsConstraining */ return new Property("sliceIsConstraining", "boolean", 7738 "If true, indicates that this slice definition is constraining a slice definition with the same name in an inherited profile. If false, the slice is not overriding any slice in an inherited profile. If missing, the slice might or might not be overriding a slice in an inherited profile, depending on the sliceName.", 7739 0, 1, sliceIsConstraining); 7740 case 102727412: 7741 /* label */ return new Property("label", "string", 7742 "A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form.", 7743 0, 1, label); 7744 case 3059181: 7745 /* code */ return new Property("code", "Coding", 7746 "A code that has the same meaning as the element in a particular terminology.", 0, 7747 java.lang.Integer.MAX_VALUE, code); 7748 case -2119287345: 7749 /* slicing */ return new Property("slicing", "", 7750 "Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set).", 7751 0, 1, slicing); 7752 case 109413500: 7753 /* short */ return new Property("short", "string", 7754 "A concise description of what this element means (e.g. for use in autogenerated summaries).", 0, 1, short_); 7755 case -1014418093: 7756 /* definition */ return new Property("definition", "markdown", 7757 "Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource. (Note: The text you are reading is specified in ElementDefinition.definition).", 7758 0, 1, definition); 7759 case 950398559: 7760 /* comment */ return new Property("comment", "markdown", 7761 "Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. (Note: The text you are reading is specified in ElementDefinition.comment).", 7762 0, 1, comment); 7763 case -1619874672: 7764 /* requirements */ return new Property("requirements", "markdown", 7765 "This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element.", 7766 0, 1, requirements); 7767 case 92902992: 7768 /* alias */ return new Property("alias", "string", 7769 "Identifies additional names by which this element might also be known.", 0, java.lang.Integer.MAX_VALUE, 7770 alias); 7771 case 108114: 7772 /* min */ return new Property("min", "unsignedInt", 7773 "The minimum number of times this element SHALL appear in the instance.", 0, 1, min); 7774 case 107876: 7775 /* max */ return new Property("max", "string", 7776 "The maximum number of times this element is permitted to appear in the instance.", 0, 1, max); 7777 case 3016401: 7778 /* base */ return new Property("base", "", 7779 "Information about the base definition of the element, provided to make it unnecessary for tools to trace the deviation of the element through the derived and related profiles. When the element definition is not the original definition of an element - i.g. either in a constraint on another type, or for elements from a super type in a snap shot - then the information in provided in the element definition may be different to the base definition. On the original definition of the element, it will be same.", 7780 0, 1, base); 7781 case 1193747154: 7782 /* contentReference */ return new Property("contentReference", "uri", 7783 "Identifies an element defined elsewhere in the definition whose content rules should be applied to the current element. ContentReferences bring across all the rules that are in the ElementDefinition for the element, including definitions, cardinality constraints, bindings, invariants etc.", 7784 0, 1, contentReference); 7785 case 3575610: 7786 /* type */ return new Property("type", "", 7787 "The data type or resource that the value of this element is permitted to be.", 0, 7788 java.lang.Integer.MAX_VALUE, type); 7789 case 587922128: 7790 /* defaultValue[x] */ return new Property("defaultValue[x]", "*", 7791 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7792 0, 1, defaultValue); 7793 case -659125328: 7794 /* defaultValue */ return new Property("defaultValue[x]", "*", 7795 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7796 0, 1, defaultValue); 7797 case 1470297600: 7798 /* defaultValueBase64Binary */ return new Property("defaultValue[x]", "*", 7799 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7800 0, 1, defaultValue); 7801 case 600437336: 7802 /* defaultValueBoolean */ return new Property("defaultValue[x]", "*", 7803 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7804 0, 1, defaultValue); 7805 case 264593188: 7806 /* defaultValueCanonical */ return new Property("defaultValue[x]", "*", 7807 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7808 0, 1, defaultValue); 7809 case 1044993469: 7810 /* defaultValueCode */ return new Property("defaultValue[x]", "*", 7811 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7812 0, 1, defaultValue); 7813 case 1045010302: 7814 /* defaultValueDate */ return new Property("defaultValue[x]", "*", 7815 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7816 0, 1, defaultValue); 7817 case 1220374379: 7818 /* defaultValueDateTime */ return new Property("defaultValue[x]", "*", 7819 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7820 0, 1, defaultValue); 7821 case 2077989249: 7822 /* defaultValueDecimal */ return new Property("defaultValue[x]", "*", 7823 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7824 0, 1, defaultValue); 7825 case -2059245333: 7826 /* defaultValueId */ return new Property("defaultValue[x]", "*", 7827 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7828 0, 1, defaultValue); 7829 case -1801671663: 7830 /* defaultValueInstant */ return new Property("defaultValue[x]", "*", 7831 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7832 0, 1, defaultValue); 7833 case -1801189522: 7834 /* defaultValueInteger */ return new Property("defaultValue[x]", "*", 7835 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7836 0, 1, defaultValue); 7837 case -325436225: 7838 /* defaultValueMarkdown */ return new Property("defaultValue[x]", "*", 7839 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7840 0, 1, defaultValue); 7841 case 587910138: 7842 /* defaultValueOid */ return new Property("defaultValue[x]", "*", 7843 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7844 0, 1, defaultValue); 7845 case -737344154: 7846 /* defaultValuePositiveInt */ return new Property("defaultValue[x]", "*", 7847 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7848 0, 1, defaultValue); 7849 case -320515103: 7850 /* defaultValueString */ return new Property("defaultValue[x]", "*", 7851 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7852 0, 1, defaultValue); 7853 case 1045494429: 7854 /* defaultValueTime */ return new Property("defaultValue[x]", "*", 7855 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7856 0, 1, defaultValue); 7857 case 539117290: 7858 /* defaultValueUnsignedInt */ return new Property("defaultValue[x]", "*", 7859 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7860 0, 1, defaultValue); 7861 case 587916188: 7862 /* defaultValueUri */ return new Property("defaultValue[x]", "*", 7863 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7864 0, 1, defaultValue); 7865 case 587916191: 7866 /* defaultValueUrl */ return new Property("defaultValue[x]", "*", 7867 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7868 0, 1, defaultValue); 7869 case 1045535627: 7870 /* defaultValueUuid */ return new Property("defaultValue[x]", "*", 7871 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7872 0, 1, defaultValue); 7873 case -611966428: 7874 /* defaultValueAddress */ return new Property("defaultValue[x]", "*", 7875 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7876 0, 1, defaultValue); 7877 case -1851689217: 7878 /* defaultValueAnnotation */ return new Property("defaultValue[x]", "*", 7879 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7880 0, 1, defaultValue); 7881 case 2034820339: 7882 /* defaultValueAttachment */ return new Property("defaultValue[x]", "*", 7883 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7884 0, 1, defaultValue); 7885 case -410434095: 7886 /* defaultValueCodeableConcept */ return new Property("defaultValue[x]", "*", 7887 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7888 0, 1, defaultValue); 7889 case -783616198: 7890 /* defaultValueCoding */ return new Property("defaultValue[x]", "*", 7891 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7892 0, 1, defaultValue); 7893 case -344740576: 7894 /* defaultValueContactPoint */ return new Property("defaultValue[x]", "*", 7895 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7896 0, 1, defaultValue); 7897 case -975393912: 7898 /* defaultValueHumanName */ return new Property("defaultValue[x]", "*", 7899 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7900 0, 1, defaultValue); 7901 case -1915078535: 7902 /* defaultValueIdentifier */ return new Property("defaultValue[x]", "*", 7903 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7904 0, 1, defaultValue); 7905 case -420255343: 7906 /* defaultValuePeriod */ return new Property("defaultValue[x]", "*", 7907 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7908 0, 1, defaultValue); 7909 case -1857379237: 7910 /* defaultValueQuantity */ return new Property("defaultValue[x]", "*", 7911 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7912 0, 1, defaultValue); 7913 case -1951495315: 7914 /* defaultValueRange */ return new Property("defaultValue[x]", "*", 7915 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7916 0, 1, defaultValue); 7917 case -1951489477: 7918 /* defaultValueRatio */ return new Property("defaultValue[x]", "*", 7919 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7920 0, 1, defaultValue); 7921 case -1488914053: 7922 /* defaultValueReference */ return new Property("defaultValue[x]", "*", 7923 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7924 0, 1, defaultValue); 7925 case -449641228: 7926 /* defaultValueSampledData */ return new Property("defaultValue[x]", "*", 7927 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7928 0, 1, defaultValue); 7929 case 509825768: 7930 /* defaultValueSignature */ return new Property("defaultValue[x]", "*", 7931 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7932 0, 1, defaultValue); 7933 case -302193638: 7934 /* defaultValueTiming */ return new Property("defaultValue[x]", "*", 7935 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7936 0, 1, defaultValue); 7937 case -754548089: 7938 /* defaultValueDosage */ return new Property("defaultValue[x]", "*", 7939 "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 7940 0, 1, defaultValue); 7941 case 1857257103: 7942 /* meaningWhenMissing */ return new Property("meaningWhenMissing", "markdown", 7943 "The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing').", 7944 0, 1, meaningWhenMissing); 7945 case 1828196047: 7946 /* orderMeaning */ return new Property("orderMeaning", "string", 7947 "If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning.", 7948 0, 1, orderMeaning); 7949 case -391522164: 7950 /* fixed[x] */ return new Property("fixed[x]", "*", 7951 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7952 0, 1, fixed); 7953 case 97445748: 7954 /* fixed */ return new Property("fixed[x]", "*", 7955 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7956 0, 1, fixed); 7957 case -799290428: 7958 /* fixedBase64Binary */ return new Property("fixed[x]", "*", 7959 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7960 0, 1, fixed); 7961 case 520851988: 7962 /* fixedBoolean */ return new Property("fixed[x]", "*", 7963 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7964 0, 1, fixed); 7965 case 1092485088: 7966 /* fixedCanonical */ return new Property("fixed[x]", "*", 7967 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7968 0, 1, fixed); 7969 case 746991489: 7970 /* fixedCode */ return new Property("fixed[x]", "*", 7971 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7972 0, 1, fixed); 7973 case 747008322: 7974 /* fixedDate */ return new Property("fixed[x]", "*", 7975 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7976 0, 1, fixed); 7977 case -1246771409: 7978 /* fixedDateTime */ return new Property("fixed[x]", "*", 7979 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7980 0, 1, fixed); 7981 case 1998403901: 7982 /* fixedDecimal */ return new Property("fixed[x]", "*", 7983 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7984 0, 1, fixed); 7985 case -843914321: 7986 /* fixedId */ return new Property("fixed[x]", "*", 7987 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7988 0, 1, fixed); 7989 case -1881257011: 7990 /* fixedInstant */ return new Property("fixed[x]", "*", 7991 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7992 0, 1, fixed); 7993 case -1880774870: 7994 /* fixedInteger */ return new Property("fixed[x]", "*", 7995 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 7996 0, 1, fixed); 7997 case 1502385283: 7998 /* fixedMarkdown */ return new Property("fixed[x]", "*", 7999 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 8000 0, 1, fixed); 8001 case -391534154: 8002 /* fixedOid */ return new Property("fixed[x]", "*", 8003 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 8004 0, 1, fixed); 8005 case 297821986: 8006 /* fixedPositiveInt */ return new Property("fixed[x]", "*", 8007 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 8008 0, 1, fixed); 8009 case 1062390949: 8010 /* fixedString */ return new Property("fixed[x]", "*", 8011 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 8012 0, 1, fixed); 8013 case 747492449: 8014 /* fixedTime */ return new Property("fixed[x]", "*", 8015 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 8016 0, 1, fixed); 8017 case 1574283430: 8018 /* fixedUnsignedInt */ return new Property("fixed[x]", "*", 8019 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 8020 0, 1, fixed); 8021 case -391528104: 8022 /* fixedUri */ return new Property("fixed[x]", "*", 8023 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 8024 0, 1, fixed); 8025 case -391528101: 8026 /* fixedUrl */ return new Property("fixed[x]", "*", 8027 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 8028 0, 1, fixed); 8029 case 747533647: 8030 /* fixedUuid */ return new Property("fixed[x]", "*", 8031 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 8032 0, 1, fixed); 8033 case -691551776: 8034 /* fixedAddress */ return new Property("fixed[x]", "*", 8035 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 8036 0, 1, fixed); 8037 case -1956844093: 8038 /* fixedAnnotation */ return new Property("fixed[x]", "*", 8039 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 8040 0, 1, fixed); 8041 case 1929665463: 8042 /* fixedAttachment */ return new Property("fixed[x]", "*", 8043 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 8044 0, 1, fixed); 8045 case 1962764685: 8046 /* fixedCodeableConcept */ return new Property("fixed[x]", "*", 8047 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 8048 0, 1, fixed); 8049 case 599289854: 8050 /* fixedCoding */ return new Property("fixed[x]", "*", 8051 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 8052 0, 1, fixed); 8053 case 1680638692: 8054 /* fixedContactPoint */ return new Property("fixed[x]", "*", 8055 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 8056 0, 1, fixed); 8057 case -147502012: 8058 /* fixedHumanName */ return new Property("fixed[x]", "*", 8059 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 8060 0, 1, fixed); 8061 case -2020233411: 8062 /* fixedIdentifier */ return new Property("fixed[x]", "*", 8063 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 8064 0, 1, fixed); 8065 case 962650709: 8066 /* fixedPeriod */ return new Property("fixed[x]", "*", 8067 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 8068 0, 1, fixed); 8069 case -29557729: 8070 /* fixedQuantity */ return new Property("fixed[x]", "*", 8071 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 8072 0, 1, fixed); 8073 case 1695345193: 8074 /* fixedRange */ return new Property("fixed[x]", "*", 8075 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 8076 0, 1, fixed); 8077 case 1695351031: 8078 /* fixedRatio */ return new Property("fixed[x]", "*", 8079 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 8080 0, 1, fixed); 8081 case -661022153: 8082 /* fixedReference */ return new Property("fixed[x]", "*", 8083 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 8084 0, 1, fixed); 8085 case 585524912: 8086 /* fixedSampledData */ return new Property("fixed[x]", "*", 8087 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 8088 0, 1, fixed); 8089 case 1337717668: 8090 /* fixedSignature */ return new Property("fixed[x]", "*", 8091 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 8092 0, 1, fixed); 8093 case 1080712414: 8094 /* fixedTiming */ return new Property("fixed[x]", "*", 8095 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 8096 0, 1, fixed); 8097 case 628357963: 8098 /* fixedDosage */ return new Property("fixed[x]", "*", 8099 "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 8100 0, 1, fixed); 8101 case -885125392: 8102 /* pattern[x] */ return new Property("pattern[x]", "*", 8103 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8104 0, 1, pattern); 8105 case -791090288: 8106 /* pattern */ return new Property("pattern[x]", "*", 8107 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8108 0, 1, pattern); 8109 case 2127857120: 8110 /* patternBase64Binary */ return new Property("pattern[x]", "*", 8111 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8112 0, 1, pattern); 8113 case -1776945544: 8114 /* patternBoolean */ return new Property("pattern[x]", "*", 8115 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8116 0, 1, pattern); 8117 case 522246980: 8118 /* patternCanonical */ return new Property("pattern[x]", "*", 8119 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8120 0, 1, pattern); 8121 case -1669806691: 8122 /* patternCode */ return new Property("pattern[x]", "*", 8123 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8124 0, 1, pattern); 8125 case -1669789858: 8126 /* patternDate */ return new Property("pattern[x]", "*", 8127 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8128 0, 1, pattern); 8129 case 535949131: 8130 /* patternDateTime */ return new Property("pattern[x]", "*", 8131 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8132 0, 1, pattern); 8133 case -299393631: 8134 /* patternDecimal */ return new Property("pattern[x]", "*", 8135 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8136 0, 1, pattern); 8137 case -28553013: 8138 /* patternId */ return new Property("pattern[x]", "*", 8139 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8140 0, 1, pattern); 8141 case 115912753: 8142 /* patternInstant */ return new Property("pattern[x]", "*", 8143 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8144 0, 1, pattern); 8145 case 116394894: 8146 /* patternInteger */ return new Property("pattern[x]", "*", 8147 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8148 0, 1, pattern); 8149 case -1009861473: 8150 /* patternMarkdown */ return new Property("pattern[x]", "*", 8151 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8152 0, 1, pattern); 8153 case -885137382: 8154 /* patternOid */ return new Property("pattern[x]", "*", 8155 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8156 0, 1, pattern); 8157 case 2054814086: 8158 /* patternPositiveInt */ return new Property("pattern[x]", "*", 8159 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8160 0, 1, pattern); 8161 case 2096647105: 8162 /* patternString */ return new Property("pattern[x]", "*", 8163 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8164 0, 1, pattern); 8165 case -1669305731: 8166 /* patternTime */ return new Property("pattern[x]", "*", 8167 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8168 0, 1, pattern); 8169 case -963691766: 8170 /* patternUnsignedInt */ return new Property("pattern[x]", "*", 8171 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8172 0, 1, pattern); 8173 case -885131332: 8174 /* patternUri */ return new Property("pattern[x]", "*", 8175 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8176 0, 1, pattern); 8177 case -885131329: 8178 /* patternUrl */ return new Property("pattern[x]", "*", 8179 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8180 0, 1, pattern); 8181 case -1669264533: 8182 /* patternUuid */ return new Property("pattern[x]", "*", 8183 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8184 0, 1, pattern); 8185 case 1305617988: 8186 /* patternAddress */ return new Property("pattern[x]", "*", 8187 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8188 0, 1, pattern); 8189 case 1840611039: 8190 /* patternAnnotation */ return new Property("pattern[x]", "*", 8191 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8192 0, 1, pattern); 8193 case 1432153299: 8194 /* patternAttachment */ return new Property("pattern[x]", "*", 8195 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8196 0, 1, pattern); 8197 case -400610831: 8198 /* patternCodeableConcept */ return new Property("pattern[x]", "*", 8199 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8200 0, 1, pattern); 8201 case 1633546010: 8202 /* patternCoding */ return new Property("pattern[x]", "*", 8203 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8204 0, 1, pattern); 8205 case 312818944: 8206 /* patternContactPoint */ return new Property("pattern[x]", "*", 8207 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8208 0, 1, pattern); 8209 case -717740120: 8210 /* patternHumanName */ return new Property("pattern[x]", "*", 8211 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8212 0, 1, pattern); 8213 case 1777221721: 8214 /* patternIdentifier */ return new Property("pattern[x]", "*", 8215 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8216 0, 1, pattern); 8217 case 1996906865: 8218 /* patternPeriod */ return new Property("pattern[x]", "*", 8219 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8220 0, 1, pattern); 8221 case 1753162811: 8222 /* patternQuantity */ return new Property("pattern[x]", "*", 8223 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8224 0, 1, pattern); 8225 case -210954355: 8226 /* patternRange */ return new Property("pattern[x]", "*", 8227 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8228 0, 1, pattern); 8229 case -210948517: 8230 /* patternRatio */ return new Property("pattern[x]", "*", 8231 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8232 0, 1, pattern); 8233 case -1231260261: 8234 /* patternReference */ return new Property("pattern[x]", "*", 8235 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8236 0, 1, pattern); 8237 case -1952450284: 8238 /* patternSampledData */ return new Property("pattern[x]", "*", 8239 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8240 0, 1, pattern); 8241 case 767479560: 8242 /* patternSignature */ return new Property("pattern[x]", "*", 8243 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8244 0, 1, pattern); 8245 case 2114968570: 8246 /* patternTiming */ return new Property("pattern[x]", "*", 8247 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8248 0, 1, pattern); 8249 case 1662614119: 8250 /* patternDosage */ return new Property("pattern[x]", "*", 8251 "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] array must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value.", 8252 0, 1, pattern); 8253 case -1322970774: 8254 /* example */ return new Property("example", "", 8255 "A sample value for this element demonstrating the type of information that would typically be found in the element.", 8256 0, java.lang.Integer.MAX_VALUE, example); 8257 case -55301663: 8258 /* minValue[x] */ return new Property("minValue[x]", 8259 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8260 "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8261 0, 1, minValue); 8262 case -1376969153: 8263 /* minValue */ return new Property("minValue[x]", 8264 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8265 "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8266 0, 1, minValue); 8267 case -1715058035: 8268 /* minValueDate */ return new Property("minValue[x]", 8269 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8270 "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8271 0, 1, minValue); 8272 case 1635517178: 8273 /* minValueDateTime */ return new Property("minValue[x]", 8274 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8275 "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8276 0, 1, minValue); 8277 case 151382690: 8278 /* minValueInstant */ return new Property("minValue[x]", 8279 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8280 "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8281 0, 1, minValue); 8282 case -1714573908: 8283 /* minValueTime */ return new Property("minValue[x]", 8284 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8285 "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8286 0, 1, minValue); 8287 case -263923694: 8288 /* minValueDecimal */ return new Property("minValue[x]", 8289 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8290 "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8291 0, 1, minValue); 8292 case 151864831: 8293 /* minValueInteger */ return new Property("minValue[x]", 8294 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8295 "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8296 0, 1, minValue); 8297 case 1570935671: 8298 /* minValuePositiveInt */ return new Property("minValue[x]", 8299 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8300 "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8301 0, 1, minValue); 8302 case -1447570181: 8303 /* minValueUnsignedInt */ return new Property("minValue[x]", 8304 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8305 "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8306 0, 1, minValue); 8307 case -1442236438: 8308 /* minValueQuantity */ return new Property("minValue[x]", 8309 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8310 "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8311 0, 1, minValue); 8312 case 622130931: 8313 /* maxValue[x] */ return new Property("maxValue[x]", 8314 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8315 "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8316 0, 1, maxValue); 8317 case 399227501: 8318 /* maxValue */ return new Property("maxValue[x]", 8319 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8320 "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8321 0, 1, maxValue); 8322 case 2105483195: 8323 /* maxValueDate */ return new Property("maxValue[x]", 8324 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8325 "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8326 0, 1, maxValue); 8327 case 1699385640: 8328 /* maxValueDateTime */ return new Property("maxValue[x]", 8329 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8330 "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8331 0, 1, maxValue); 8332 case 1261821620: 8333 /* maxValueInstant */ return new Property("maxValue[x]", 8334 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8335 "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8336 0, 1, maxValue); 8337 case 2105967322: 8338 /* maxValueTime */ return new Property("maxValue[x]", 8339 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8340 "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8341 0, 1, maxValue); 8342 case 846515236: 8343 /* maxValueDecimal */ return new Property("maxValue[x]", 8344 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8345 "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8346 0, 1, maxValue); 8347 case 1262303761: 8348 /* maxValueInteger */ return new Property("maxValue[x]", 8349 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8350 "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8351 0, 1, maxValue); 8352 case 1605774985: 8353 /* maxValuePositiveInt */ return new Property("maxValue[x]", 8354 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8355 "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8356 0, 1, maxValue); 8357 case -1412730867: 8358 /* maxValueUnsignedInt */ return new Property("maxValue[x]", 8359 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8360 "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8361 0, 1, maxValue); 8362 case -1378367976: 8363 /* maxValueQuantity */ return new Property("maxValue[x]", 8364 "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", 8365 "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 8366 0, 1, maxValue); 8367 case -791400086: 8368 /* maxLength */ return new Property("maxLength", "integer", 8369 "Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element.", 8370 0, 1, maxLength); 8371 case -861311717: 8372 /* condition */ return new Property("condition", "id", 8373 "A reference to an invariant that may make additional statements about the cardinality or value in the instance.", 8374 0, java.lang.Integer.MAX_VALUE, condition); 8375 case -190376483: 8376 /* constraint */ return new Property("constraint", "", 8377 "Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance.", 8378 0, java.lang.Integer.MAX_VALUE, constraint); 8379 case -1402857082: 8380 /* mustSupport */ return new Property("mustSupport", "boolean", 8381 "If true, implementations that produce or consume resources SHALL provide \"support\" for the element in some meaningful way. If false, the element may be ignored and not supported. If false, whether to populate or use the data element in any way is at the discretion of the implementation.", 8382 0, 1, mustSupport); 8383 case -1408783839: 8384 /* isModifier */ return new Property("isModifier", "boolean", 8385 "If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system.", 8386 0, 1, isModifier); 8387 case -1854387259: 8388 /* isModifierReason */ return new Property("isModifierReason", "string", 8389 "Explains how that element affects the interpretation of the resource or element that contains it.", 0, 1, 8390 isModifierReason); 8391 case 1857548060: 8392 /* isSummary */ return new Property("isSummary", "boolean", 8393 "Whether the element should be included if a client requests a search with the parameter _summary=true.", 0, 8394 1, isSummary); 8395 case -108220795: 8396 /* binding */ return new Property("binding", "", 8397 "Binds to a value set if this element is coded (code, Coding, CodeableConcept, Quantity), or the data types (string, uri).", 8398 0, 1, binding); 8399 case 837556430: 8400 /* mapping */ return new Property("mapping", "", 8401 "Identifies a concept from an external specification that roughly corresponds to this element.", 0, 8402 java.lang.Integer.MAX_VALUE, mapping); 8403 default: 8404 return super.getNamedProperty(_hash, _name, _checkValid); 8405 } 8406 8407 } 8408 8409 @Override 8410 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8411 switch (hash) { 8412 case 3433509: 8413 /* path */ return this.path == null ? new Base[0] : new Base[] { this.path }; // StringType 8414 case -671065907: 8415 /* representation */ return this.representation == null ? new Base[0] 8416 : this.representation.toArray(new Base[this.representation.size()]); // Enumeration<PropertyRepresentation> 8417 case -825289923: 8418 /* sliceName */ return this.sliceName == null ? new Base[0] : new Base[] { this.sliceName }; // StringType 8419 case 333040519: 8420 /* sliceIsConstraining */ return this.sliceIsConstraining == null ? new Base[0] 8421 : new Base[] { this.sliceIsConstraining }; // BooleanType 8422 case 102727412: 8423 /* label */ return this.label == null ? new Base[0] : new Base[] { this.label }; // StringType 8424 case 3059181: 8425 /* code */ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // Coding 8426 case -2119287345: 8427 /* slicing */ return this.slicing == null ? new Base[0] : new Base[] { this.slicing }; // ElementDefinitionSlicingComponent 8428 case 109413500: 8429 /* short */ return this.short_ == null ? new Base[0] : new Base[] { this.short_ }; // StringType 8430 case -1014418093: 8431 /* definition */ return this.definition == null ? new Base[0] : new Base[] { this.definition }; // MarkdownType 8432 case 950398559: 8433 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // MarkdownType 8434 case -1619874672: 8435 /* requirements */ return this.requirements == null ? new Base[0] : new Base[] { this.requirements }; // MarkdownType 8436 case 92902992: 8437 /* alias */ return this.alias == null ? new Base[0] : this.alias.toArray(new Base[this.alias.size()]); // StringType 8438 case 108114: 8439 /* min */ return this.min == null ? new Base[0] : new Base[] { this.min }; // UnsignedIntType 8440 case 107876: 8441 /* max */ return this.max == null ? new Base[0] : new Base[] { this.max }; // StringType 8442 case 3016401: 8443 /* base */ return this.base == null ? new Base[0] : new Base[] { this.base }; // ElementDefinitionBaseComponent 8444 case 1193747154: 8445 /* contentReference */ return this.contentReference == null ? new Base[0] : new Base[] { this.contentReference }; // UriType 8446 case 3575610: 8447 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // TypeRefComponent 8448 case -659125328: 8449 /* defaultValue */ return this.defaultValue == null ? new Base[0] : new Base[] { this.defaultValue }; // org.hl7.fhir.r4.model.Type 8450 case 1857257103: 8451 /* meaningWhenMissing */ return this.meaningWhenMissing == null ? new Base[0] 8452 : new Base[] { this.meaningWhenMissing }; // MarkdownType 8453 case 1828196047: 8454 /* orderMeaning */ return this.orderMeaning == null ? new Base[0] : new Base[] { this.orderMeaning }; // StringType 8455 case 97445748: 8456 /* fixed */ return this.fixed == null ? new Base[0] : new Base[] { this.fixed }; // org.hl7.fhir.r4.model.Type 8457 case -791090288: 8458 /* pattern */ return this.pattern == null ? new Base[0] : new Base[] { this.pattern }; // org.hl7.fhir.r4.model.Type 8459 case -1322970774: 8460 /* example */ return this.example == null ? new Base[0] : this.example.toArray(new Base[this.example.size()]); // ElementDefinitionExampleComponent 8461 case -1376969153: 8462 /* minValue */ return this.minValue == null ? new Base[0] : new Base[] { this.minValue }; // Type 8463 case 399227501: 8464 /* maxValue */ return this.maxValue == null ? new Base[0] : new Base[] { this.maxValue }; // Type 8465 case -791400086: 8466 /* maxLength */ return this.maxLength == null ? new Base[0] : new Base[] { this.maxLength }; // IntegerType 8467 case -861311717: 8468 /* condition */ return this.condition == null ? new Base[0] 8469 : this.condition.toArray(new Base[this.condition.size()]); // IdType 8470 case -190376483: 8471 /* constraint */ return this.constraint == null ? new Base[0] 8472 : this.constraint.toArray(new Base[this.constraint.size()]); // ElementDefinitionConstraintComponent 8473 case -1402857082: 8474 /* mustSupport */ return this.mustSupport == null ? new Base[0] : new Base[] { this.mustSupport }; // BooleanType 8475 case -1408783839: 8476 /* isModifier */ return this.isModifier == null ? new Base[0] : new Base[] { this.isModifier }; // BooleanType 8477 case -1854387259: 8478 /* isModifierReason */ return this.isModifierReason == null ? new Base[0] : new Base[] { this.isModifierReason }; // StringType 8479 case 1857548060: 8480 /* isSummary */ return this.isSummary == null ? new Base[0] : new Base[] { this.isSummary }; // BooleanType 8481 case -108220795: 8482 /* binding */ return this.binding == null ? new Base[0] : new Base[] { this.binding }; // ElementDefinitionBindingComponent 8483 case 837556430: 8484 /* mapping */ return this.mapping == null ? new Base[0] : this.mapping.toArray(new Base[this.mapping.size()]); // ElementDefinitionMappingComponent 8485 default: 8486 return super.getProperty(hash, name, checkValid); 8487 } 8488 8489 } 8490 8491 @Override 8492 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8493 switch (hash) { 8494 case 3433509: // path 8495 this.path = castToString(value); // StringType 8496 return value; 8497 case -671065907: // representation 8498 value = new PropertyRepresentationEnumFactory().fromType(castToCode(value)); 8499 this.getRepresentation().add((Enumeration) value); // Enumeration<PropertyRepresentation> 8500 return value; 8501 case -825289923: // sliceName 8502 this.sliceName = castToString(value); // StringType 8503 return value; 8504 case 333040519: // sliceIsConstraining 8505 this.sliceIsConstraining = castToBoolean(value); // BooleanType 8506 return value; 8507 case 102727412: // label 8508 this.label = castToString(value); // StringType 8509 return value; 8510 case 3059181: // code 8511 this.getCode().add(castToCoding(value)); // Coding 8512 return value; 8513 case -2119287345: // slicing 8514 this.slicing = (ElementDefinitionSlicingComponent) value; // ElementDefinitionSlicingComponent 8515 return value; 8516 case 109413500: // short 8517 this.short_ = castToString(value); // StringType 8518 return value; 8519 case -1014418093: // definition 8520 this.definition = castToMarkdown(value); // MarkdownType 8521 return value; 8522 case 950398559: // comment 8523 this.comment = castToMarkdown(value); // MarkdownType 8524 return value; 8525 case -1619874672: // requirements 8526 this.requirements = castToMarkdown(value); // MarkdownType 8527 return value; 8528 case 92902992: // alias 8529 this.getAlias().add(castToString(value)); // StringType 8530 return value; 8531 case 108114: // min 8532 this.min = castToUnsignedInt(value); // UnsignedIntType 8533 return value; 8534 case 107876: // max 8535 this.max = castToString(value); // StringType 8536 return value; 8537 case 3016401: // base 8538 this.base = (ElementDefinitionBaseComponent) value; // ElementDefinitionBaseComponent 8539 return value; 8540 case 1193747154: // contentReference 8541 this.contentReference = castToUri(value); // UriType 8542 return value; 8543 case 3575610: // type 8544 this.getType().add((TypeRefComponent) value); // TypeRefComponent 8545 return value; 8546 case -659125328: // defaultValue 8547 this.defaultValue = castToType(value); // org.hl7.fhir.r4.model.Type 8548 return value; 8549 case 1857257103: // meaningWhenMissing 8550 this.meaningWhenMissing = castToMarkdown(value); // MarkdownType 8551 return value; 8552 case 1828196047: // orderMeaning 8553 this.orderMeaning = castToString(value); // StringType 8554 return value; 8555 case 97445748: // fixed 8556 this.fixed = castToType(value); // org.hl7.fhir.r4.model.Type 8557 return value; 8558 case -791090288: // pattern 8559 this.pattern = castToType(value); // org.hl7.fhir.r4.model.Type 8560 return value; 8561 case -1322970774: // example 8562 this.getExample().add((ElementDefinitionExampleComponent) value); // ElementDefinitionExampleComponent 8563 return value; 8564 case -1376969153: // minValue 8565 this.minValue = castToType(value); // Type 8566 return value; 8567 case 399227501: // maxValue 8568 this.maxValue = castToType(value); // Type 8569 return value; 8570 case -791400086: // maxLength 8571 this.maxLength = castToInteger(value); // IntegerType 8572 return value; 8573 case -861311717: // condition 8574 this.getCondition().add(castToId(value)); // IdType 8575 return value; 8576 case -190376483: // constraint 8577 this.getConstraint().add((ElementDefinitionConstraintComponent) value); // ElementDefinitionConstraintComponent 8578 return value; 8579 case -1402857082: // mustSupport 8580 this.mustSupport = castToBoolean(value); // BooleanType 8581 return value; 8582 case -1408783839: // isModifier 8583 this.isModifier = castToBoolean(value); // BooleanType 8584 return value; 8585 case -1854387259: // isModifierReason 8586 this.isModifierReason = castToString(value); // StringType 8587 return value; 8588 case 1857548060: // isSummary 8589 this.isSummary = castToBoolean(value); // BooleanType 8590 return value; 8591 case -108220795: // binding 8592 this.binding = (ElementDefinitionBindingComponent) value; // ElementDefinitionBindingComponent 8593 return value; 8594 case 837556430: // mapping 8595 this.getMapping().add((ElementDefinitionMappingComponent) value); // ElementDefinitionMappingComponent 8596 return value; 8597 default: 8598 return super.setProperty(hash, name, value); 8599 } 8600 8601 } 8602 8603 @Override 8604 public Base setProperty(String name, Base value) throws FHIRException { 8605 if (name.equals("path")) { 8606 this.path = castToString(value); // StringType 8607 } else if (name.equals("representation")) { 8608 value = new PropertyRepresentationEnumFactory().fromType(castToCode(value)); 8609 this.getRepresentation().add((Enumeration) value); 8610 } else if (name.equals("sliceName")) { 8611 this.sliceName = castToString(value); // StringType 8612 } else if (name.equals("sliceIsConstraining")) { 8613 this.sliceIsConstraining = castToBoolean(value); // BooleanType 8614 } else if (name.equals("label")) { 8615 this.label = castToString(value); // StringType 8616 } else if (name.equals("code")) { 8617 this.getCode().add(castToCoding(value)); 8618 } else if (name.equals("slicing")) { 8619 this.slicing = (ElementDefinitionSlicingComponent) value; // ElementDefinitionSlicingComponent 8620 } else if (name.equals("short")) { 8621 this.short_ = castToString(value); // StringType 8622 } else if (name.equals("definition")) { 8623 this.definition = castToMarkdown(value); // MarkdownType 8624 } else if (name.equals("comment")) { 8625 this.comment = castToMarkdown(value); // MarkdownType 8626 } else if (name.equals("requirements")) { 8627 this.requirements = castToMarkdown(value); // MarkdownType 8628 } else if (name.equals("alias")) { 8629 this.getAlias().add(castToString(value)); 8630 } else if (name.equals("min")) { 8631 this.min = castToUnsignedInt(value); // UnsignedIntType 8632 } else if (name.equals("max")) { 8633 this.max = castToString(value); // StringType 8634 } else if (name.equals("base")) { 8635 this.base = (ElementDefinitionBaseComponent) value; // ElementDefinitionBaseComponent 8636 } else if (name.equals("contentReference")) { 8637 this.contentReference = castToUri(value); // UriType 8638 } else if (name.equals("type")) { 8639 this.getType().add((TypeRefComponent) value); 8640 } else if (name.equals("defaultValue[x]")) { 8641 this.defaultValue = castToType(value); // org.hl7.fhir.r4.model.Type 8642 } else if (name.equals("meaningWhenMissing")) { 8643 this.meaningWhenMissing = castToMarkdown(value); // MarkdownType 8644 } else if (name.equals("orderMeaning")) { 8645 this.orderMeaning = castToString(value); // StringType 8646 } else if (name.equals("fixed[x]")) { 8647 this.fixed = castToType(value); // org.hl7.fhir.r4.model.Type 8648 } else if (name.equals("pattern[x]")) { 8649 this.pattern = castToType(value); // org.hl7.fhir.r4.model.Type 8650 } else if (name.equals("example")) { 8651 this.getExample().add((ElementDefinitionExampleComponent) value); 8652 } else if (name.equals("minValue[x]")) { 8653 this.minValue = castToType(value); // Type 8654 } else if (name.equals("maxValue[x]")) { 8655 this.maxValue = castToType(value); // Type 8656 } else if (name.equals("maxLength")) { 8657 this.maxLength = castToInteger(value); // IntegerType 8658 } else if (name.equals("condition")) { 8659 this.getCondition().add(castToId(value)); 8660 } else if (name.equals("constraint")) { 8661 this.getConstraint().add((ElementDefinitionConstraintComponent) value); 8662 } else if (name.equals("mustSupport")) { 8663 this.mustSupport = castToBoolean(value); // BooleanType 8664 } else if (name.equals("isModifier")) { 8665 this.isModifier = castToBoolean(value); // BooleanType 8666 } else if (name.equals("isModifierReason")) { 8667 this.isModifierReason = castToString(value); // StringType 8668 } else if (name.equals("isSummary")) { 8669 this.isSummary = castToBoolean(value); // BooleanType 8670 } else if (name.equals("binding")) { 8671 this.binding = (ElementDefinitionBindingComponent) value; // ElementDefinitionBindingComponent 8672 } else if (name.equals("mapping")) { 8673 this.getMapping().add((ElementDefinitionMappingComponent) value); 8674 } else 8675 return super.setProperty(name, value); 8676 return value; 8677 } 8678 8679 @Override 8680 public void removeChild(String name, Base value) throws FHIRException { 8681 if (name.equals("path")) { 8682 this.path = null; 8683 } else if (name.equals("representation")) { 8684 this.getRepresentation().remove((Enumeration) value); 8685 } else if (name.equals("sliceName")) { 8686 this.sliceName = null; 8687 } else if (name.equals("sliceIsConstraining")) { 8688 this.sliceIsConstraining = null; 8689 } else if (name.equals("label")) { 8690 this.label = null; 8691 } else if (name.equals("code")) { 8692 this.getCode().remove(castToCoding(value)); 8693 } else if (name.equals("slicing")) { 8694 this.slicing = (ElementDefinitionSlicingComponent) value; // ElementDefinitionSlicingComponent 8695 } else if (name.equals("short")) { 8696 this.short_ = null; 8697 } else if (name.equals("definition")) { 8698 this.definition = null; 8699 } else if (name.equals("comment")) { 8700 this.comment = null; 8701 } else if (name.equals("requirements")) { 8702 this.requirements = null; 8703 } else if (name.equals("alias")) { 8704 this.getAlias().remove(castToString(value)); 8705 } else if (name.equals("min")) { 8706 this.min = null; 8707 } else if (name.equals("max")) { 8708 this.max = null; 8709 } else if (name.equals("base")) { 8710 this.base = (ElementDefinitionBaseComponent) value; // ElementDefinitionBaseComponent 8711 } else if (name.equals("contentReference")) { 8712 this.contentReference = null; 8713 } else if (name.equals("type")) { 8714 this.getType().remove((TypeRefComponent) value); 8715 } else if (name.equals("defaultValue[x]")) { 8716 this.defaultValue = null; 8717 } else if (name.equals("meaningWhenMissing")) { 8718 this.meaningWhenMissing = null; 8719 } else if (name.equals("orderMeaning")) { 8720 this.orderMeaning = null; 8721 } else if (name.equals("fixed[x]")) { 8722 this.fixed = null; 8723 } else if (name.equals("pattern[x]")) { 8724 this.pattern = null; 8725 } else if (name.equals("example")) { 8726 this.getExample().remove((ElementDefinitionExampleComponent) value); 8727 } else if (name.equals("minValue[x]")) { 8728 this.minValue = null; 8729 } else if (name.equals("maxValue[x]")) { 8730 this.maxValue = null; 8731 } else if (name.equals("maxLength")) { 8732 this.maxLength = null; 8733 } else if (name.equals("condition")) { 8734 this.getCondition().remove(castToId(value)); 8735 } else if (name.equals("constraint")) { 8736 this.getConstraint().remove((ElementDefinitionConstraintComponent) value); 8737 } else if (name.equals("mustSupport")) { 8738 this.mustSupport = null; 8739 } else if (name.equals("isModifier")) { 8740 this.isModifier = null; 8741 } else if (name.equals("isModifierReason")) { 8742 this.isModifierReason = null; 8743 } else if (name.equals("isSummary")) { 8744 this.isSummary = null; 8745 } else if (name.equals("binding")) { 8746 this.binding = (ElementDefinitionBindingComponent) value; // ElementDefinitionBindingComponent 8747 } else if (name.equals("mapping")) { 8748 this.getMapping().remove((ElementDefinitionMappingComponent) value); 8749 } else 8750 super.removeChild(name, value); 8751 8752 } 8753 8754 @Override 8755 public Base makeProperty(int hash, String name) throws FHIRException { 8756 switch (hash) { 8757 case 3433509: 8758 return getPathElement(); 8759 case -671065907: 8760 return addRepresentationElement(); 8761 case -825289923: 8762 return getSliceNameElement(); 8763 case 333040519: 8764 return getSliceIsConstrainingElement(); 8765 case 102727412: 8766 return getLabelElement(); 8767 case 3059181: 8768 return addCode(); 8769 case -2119287345: 8770 return getSlicing(); 8771 case 109413500: 8772 return getShortElement(); 8773 case -1014418093: 8774 return getDefinitionElement(); 8775 case 950398559: 8776 return getCommentElement(); 8777 case -1619874672: 8778 return getRequirementsElement(); 8779 case 92902992: 8780 return addAliasElement(); 8781 case 108114: 8782 return getMinElement(); 8783 case 107876: 8784 return getMaxElement(); 8785 case 3016401: 8786 return getBase(); 8787 case 1193747154: 8788 return getContentReferenceElement(); 8789 case 3575610: 8790 return addType(); 8791 case 587922128: 8792 return getDefaultValue(); 8793 case -659125328: 8794 return getDefaultValue(); 8795 case 1857257103: 8796 return getMeaningWhenMissingElement(); 8797 case 1828196047: 8798 return getOrderMeaningElement(); 8799 case -391522164: 8800 return getFixed(); 8801 case 97445748: 8802 return getFixed(); 8803 case -885125392: 8804 return getPattern(); 8805 case -791090288: 8806 return getPattern(); 8807 case -1322970774: 8808 return addExample(); 8809 case -55301663: 8810 return getMinValue(); 8811 case -1376969153: 8812 return getMinValue(); 8813 case 622130931: 8814 return getMaxValue(); 8815 case 399227501: 8816 return getMaxValue(); 8817 case -791400086: 8818 return getMaxLengthElement(); 8819 case -861311717: 8820 return addConditionElement(); 8821 case -190376483: 8822 return addConstraint(); 8823 case -1402857082: 8824 return getMustSupportElement(); 8825 case -1408783839: 8826 return getIsModifierElement(); 8827 case -1854387259: 8828 return getIsModifierReasonElement(); 8829 case 1857548060: 8830 return getIsSummaryElement(); 8831 case -108220795: 8832 return getBinding(); 8833 case 837556430: 8834 return addMapping(); 8835 default: 8836 return super.makeProperty(hash, name); 8837 } 8838 8839 } 8840 8841 @Override 8842 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8843 switch (hash) { 8844 case 3433509: 8845 /* path */ return new String[] { "string" }; 8846 case -671065907: 8847 /* representation */ return new String[] { "code" }; 8848 case -825289923: 8849 /* sliceName */ return new String[] { "string" }; 8850 case 333040519: 8851 /* sliceIsConstraining */ return new String[] { "boolean" }; 8852 case 102727412: 8853 /* label */ return new String[] { "string" }; 8854 case 3059181: 8855 /* code */ return new String[] { "Coding" }; 8856 case -2119287345: 8857 /* slicing */ return new String[] {}; 8858 case 109413500: 8859 /* short */ return new String[] { "string" }; 8860 case -1014418093: 8861 /* definition */ return new String[] { "markdown" }; 8862 case 950398559: 8863 /* comment */ return new String[] { "markdown" }; 8864 case -1619874672: 8865 /* requirements */ return new String[] { "markdown" }; 8866 case 92902992: 8867 /* alias */ return new String[] { "string" }; 8868 case 108114: 8869 /* min */ return new String[] { "unsignedInt" }; 8870 case 107876: 8871 /* max */ return new String[] { "string" }; 8872 case 3016401: 8873 /* base */ return new String[] {}; 8874 case 1193747154: 8875 /* contentReference */ return new String[] { "uri" }; 8876 case 3575610: 8877 /* type */ return new String[] {}; 8878 case -659125328: 8879 /* defaultValue */ return new String[] { "*" }; 8880 case 1857257103: 8881 /* meaningWhenMissing */ return new String[] { "markdown" }; 8882 case 1828196047: 8883 /* orderMeaning */ return new String[] { "string" }; 8884 case 97445748: 8885 /* fixed */ return new String[] { "*" }; 8886 case -791090288: 8887 /* pattern */ return new String[] { "*" }; 8888 case -1322970774: 8889 /* example */ return new String[] {}; 8890 case -1376969153: 8891 /* minValue */ return new String[] { "date", "dateTime", "instant", "time", "decimal", "integer", "positiveInt", 8892 "unsignedInt", "Quantity" }; 8893 case 399227501: 8894 /* maxValue */ return new String[] { "date", "dateTime", "instant", "time", "decimal", "integer", "positiveInt", 8895 "unsignedInt", "Quantity" }; 8896 case -791400086: 8897 /* maxLength */ return new String[] { "integer" }; 8898 case -861311717: 8899 /* condition */ return new String[] { "id" }; 8900 case -190376483: 8901 /* constraint */ return new String[] {}; 8902 case -1402857082: 8903 /* mustSupport */ return new String[] { "boolean" }; 8904 case -1408783839: 8905 /* isModifier */ return new String[] { "boolean" }; 8906 case -1854387259: 8907 /* isModifierReason */ return new String[] { "string" }; 8908 case 1857548060: 8909 /* isSummary */ return new String[] { "boolean" }; 8910 case -108220795: 8911 /* binding */ return new String[] {}; 8912 case 837556430: 8913 /* mapping */ return new String[] {}; 8914 default: 8915 return super.getTypesForProperty(hash, name); 8916 } 8917 8918 } 8919 8920 @Override 8921 public Base addChild(String name) throws FHIRException { 8922 if (name.equals("path")) { 8923 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.path"); 8924 } else if (name.equals("representation")) { 8925 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.representation"); 8926 } else if (name.equals("sliceName")) { 8927 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.sliceName"); 8928 } else if (name.equals("sliceIsConstraining")) { 8929 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.sliceIsConstraining"); 8930 } else if (name.equals("label")) { 8931 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.label"); 8932 } else if (name.equals("code")) { 8933 return addCode(); 8934 } else if (name.equals("slicing")) { 8935 this.slicing = new ElementDefinitionSlicingComponent(); 8936 return this.slicing; 8937 } else if (name.equals("short")) { 8938 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.short"); 8939 } else if (name.equals("definition")) { 8940 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.definition"); 8941 } else if (name.equals("comment")) { 8942 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.comment"); 8943 } else if (name.equals("requirements")) { 8944 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.requirements"); 8945 } else if (name.equals("alias")) { 8946 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.alias"); 8947 } else if (name.equals("min")) { 8948 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.min"); 8949 } else if (name.equals("max")) { 8950 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.max"); 8951 } else if (name.equals("base")) { 8952 this.base = new ElementDefinitionBaseComponent(); 8953 return this.base; 8954 } else if (name.equals("contentReference")) { 8955 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.contentReference"); 8956 } else if (name.equals("type")) { 8957 return addType(); 8958 } else if (name.equals("defaultValueBase64Binary")) { 8959 this.defaultValue = new Base64BinaryType(); 8960 return this.defaultValue; 8961 } else if (name.equals("defaultValueBoolean")) { 8962 this.defaultValue = new BooleanType(); 8963 return this.defaultValue; 8964 } else if (name.equals("defaultValueCanonical")) { 8965 this.defaultValue = new CanonicalType(); 8966 return this.defaultValue; 8967 } else if (name.equals("defaultValueCode")) { 8968 this.defaultValue = new CodeType(); 8969 return this.defaultValue; 8970 } else if (name.equals("defaultValueDate")) { 8971 this.defaultValue = new DateType(); 8972 return this.defaultValue; 8973 } else if (name.equals("defaultValueDateTime")) { 8974 this.defaultValue = new DateTimeType(); 8975 return this.defaultValue; 8976 } else if (name.equals("defaultValueDecimal")) { 8977 this.defaultValue = new DecimalType(); 8978 return this.defaultValue; 8979 } else if (name.equals("defaultValueId")) { 8980 this.defaultValue = new IdType(); 8981 return this.defaultValue; 8982 } else if (name.equals("defaultValueInstant")) { 8983 this.defaultValue = new InstantType(); 8984 return this.defaultValue; 8985 } else if (name.equals("defaultValueInteger")) { 8986 this.defaultValue = new IntegerType(); 8987 return this.defaultValue; 8988 } else if (name.equals("defaultValueMarkdown")) { 8989 this.defaultValue = new MarkdownType(); 8990 return this.defaultValue; 8991 } else if (name.equals("defaultValueOid")) { 8992 this.defaultValue = new OidType(); 8993 return this.defaultValue; 8994 } else if (name.equals("defaultValuePositiveInt")) { 8995 this.defaultValue = new PositiveIntType(); 8996 return this.defaultValue; 8997 } else if (name.equals("defaultValueString")) { 8998 this.defaultValue = new StringType(); 8999 return this.defaultValue; 9000 } else if (name.equals("defaultValueTime")) { 9001 this.defaultValue = new TimeType(); 9002 return this.defaultValue; 9003 } else if (name.equals("defaultValueUnsignedInt")) { 9004 this.defaultValue = new UnsignedIntType(); 9005 return this.defaultValue; 9006 } else if (name.equals("defaultValueUri")) { 9007 this.defaultValue = new UriType(); 9008 return this.defaultValue; 9009 } else if (name.equals("defaultValueUrl")) { 9010 this.defaultValue = new UrlType(); 9011 return this.defaultValue; 9012 } else if (name.equals("defaultValueUuid")) { 9013 this.defaultValue = new UuidType(); 9014 return this.defaultValue; 9015 } else if (name.equals("defaultValueAddress")) { 9016 this.defaultValue = new Address(); 9017 return this.defaultValue; 9018 } else if (name.equals("defaultValueAge")) { 9019 this.defaultValue = new Age(); 9020 return this.defaultValue; 9021 } else if (name.equals("defaultValueAnnotation")) { 9022 this.defaultValue = new Annotation(); 9023 return this.defaultValue; 9024 } else if (name.equals("defaultValueAttachment")) { 9025 this.defaultValue = new Attachment(); 9026 return this.defaultValue; 9027 } else if (name.equals("defaultValueCodeableConcept")) { 9028 this.defaultValue = new CodeableConcept(); 9029 return this.defaultValue; 9030 } else if (name.equals("defaultValueCoding")) { 9031 this.defaultValue = new Coding(); 9032 return this.defaultValue; 9033 } else if (name.equals("defaultValueContactPoint")) { 9034 this.defaultValue = new ContactPoint(); 9035 return this.defaultValue; 9036 } else if (name.equals("defaultValueCount")) { 9037 this.defaultValue = new Count(); 9038 return this.defaultValue; 9039 } else if (name.equals("defaultValueDistance")) { 9040 this.defaultValue = new Distance(); 9041 return this.defaultValue; 9042 } else if (name.equals("defaultValueDuration")) { 9043 this.defaultValue = new Duration(); 9044 return this.defaultValue; 9045 } else if (name.equals("defaultValueHumanName")) { 9046 this.defaultValue = new HumanName(); 9047 return this.defaultValue; 9048 } else if (name.equals("defaultValueIdentifier")) { 9049 this.defaultValue = new Identifier(); 9050 return this.defaultValue; 9051 } else if (name.equals("defaultValueMoney")) { 9052 this.defaultValue = new Money(); 9053 return this.defaultValue; 9054 } else if (name.equals("defaultValuePeriod")) { 9055 this.defaultValue = new Period(); 9056 return this.defaultValue; 9057 } else if (name.equals("defaultValueQuantity")) { 9058 this.defaultValue = new Quantity(); 9059 return this.defaultValue; 9060 } else if (name.equals("defaultValueRange")) { 9061 this.defaultValue = new Range(); 9062 return this.defaultValue; 9063 } else if (name.equals("defaultValueRatio")) { 9064 this.defaultValue = new Ratio(); 9065 return this.defaultValue; 9066 } else if (name.equals("defaultValueReference")) { 9067 this.defaultValue = new Reference(); 9068 return this.defaultValue; 9069 } else if (name.equals("defaultValueSampledData")) { 9070 this.defaultValue = new SampledData(); 9071 return this.defaultValue; 9072 } else if (name.equals("defaultValueSignature")) { 9073 this.defaultValue = new Signature(); 9074 return this.defaultValue; 9075 } else if (name.equals("defaultValueTiming")) { 9076 this.defaultValue = new Timing(); 9077 return this.defaultValue; 9078 } else if (name.equals("defaultValueContactDetail")) { 9079 this.defaultValue = new ContactDetail(); 9080 return this.defaultValue; 9081 } else if (name.equals("defaultValueContributor")) { 9082 this.defaultValue = new Contributor(); 9083 return this.defaultValue; 9084 } else if (name.equals("defaultValueDataRequirement")) { 9085 this.defaultValue = new DataRequirement(); 9086 return this.defaultValue; 9087 } else if (name.equals("defaultValueExpression")) { 9088 this.defaultValue = new Expression(); 9089 return this.defaultValue; 9090 } else if (name.equals("defaultValueParameterDefinition")) { 9091 this.defaultValue = new ParameterDefinition(); 9092 return this.defaultValue; 9093 } else if (name.equals("defaultValueRelatedArtifact")) { 9094 this.defaultValue = new RelatedArtifact(); 9095 return this.defaultValue; 9096 } else if (name.equals("defaultValueTriggerDefinition")) { 9097 this.defaultValue = new TriggerDefinition(); 9098 return this.defaultValue; 9099 } else if (name.equals("defaultValueUsageContext")) { 9100 this.defaultValue = new UsageContext(); 9101 return this.defaultValue; 9102 } else if (name.equals("defaultValueDosage")) { 9103 this.defaultValue = new Dosage(); 9104 return this.defaultValue; 9105 } else if (name.equals("defaultValueMeta")) { 9106 this.defaultValue = new Meta(); 9107 return this.defaultValue; 9108 } else if (name.equals("meaningWhenMissing")) { 9109 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.meaningWhenMissing"); 9110 } else if (name.equals("orderMeaning")) { 9111 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.orderMeaning"); 9112 } else if (name.equals("fixedBase64Binary")) { 9113 this.fixed = new Base64BinaryType(); 9114 return this.fixed; 9115 } else if (name.equals("fixedBoolean")) { 9116 this.fixed = new BooleanType(); 9117 return this.fixed; 9118 } else if (name.equals("fixedCanonical")) { 9119 this.fixed = new CanonicalType(); 9120 return this.fixed; 9121 } else if (name.equals("fixedCode")) { 9122 this.fixed = new CodeType(); 9123 return this.fixed; 9124 } else if (name.equals("fixedDate")) { 9125 this.fixed = new DateType(); 9126 return this.fixed; 9127 } else if (name.equals("fixedDateTime")) { 9128 this.fixed = new DateTimeType(); 9129 return this.fixed; 9130 } else if (name.equals("fixedDecimal")) { 9131 this.fixed = new DecimalType(); 9132 return this.fixed; 9133 } else if (name.equals("fixedId")) { 9134 this.fixed = new IdType(); 9135 return this.fixed; 9136 } else if (name.equals("fixedInstant")) { 9137 this.fixed = new InstantType(); 9138 return this.fixed; 9139 } else if (name.equals("fixedInteger")) { 9140 this.fixed = new IntegerType(); 9141 return this.fixed; 9142 } else if (name.equals("fixedMarkdown")) { 9143 this.fixed = new MarkdownType(); 9144 return this.fixed; 9145 } else if (name.equals("fixedOid")) { 9146 this.fixed = new OidType(); 9147 return this.fixed; 9148 } else if (name.equals("fixedPositiveInt")) { 9149 this.fixed = new PositiveIntType(); 9150 return this.fixed; 9151 } else if (name.equals("fixedString")) { 9152 this.fixed = new StringType(); 9153 return this.fixed; 9154 } else if (name.equals("fixedTime")) { 9155 this.fixed = new TimeType(); 9156 return this.fixed; 9157 } else if (name.equals("fixedUnsignedInt")) { 9158 this.fixed = new UnsignedIntType(); 9159 return this.fixed; 9160 } else if (name.equals("fixedUri")) { 9161 this.fixed = new UriType(); 9162 return this.fixed; 9163 } else if (name.equals("fixedUrl")) { 9164 this.fixed = new UrlType(); 9165 return this.fixed; 9166 } else if (name.equals("fixedUuid")) { 9167 this.fixed = new UuidType(); 9168 return this.fixed; 9169 } else if (name.equals("fixedAddress")) { 9170 this.fixed = new Address(); 9171 return this.fixed; 9172 } else if (name.equals("fixedAge")) { 9173 this.fixed = new Age(); 9174 return this.fixed; 9175 } else if (name.equals("fixedAnnotation")) { 9176 this.fixed = new Annotation(); 9177 return this.fixed; 9178 } else if (name.equals("fixedAttachment")) { 9179 this.fixed = new Attachment(); 9180 return this.fixed; 9181 } else if (name.equals("fixedCodeableConcept")) { 9182 this.fixed = new CodeableConcept(); 9183 return this.fixed; 9184 } else if (name.equals("fixedCoding")) { 9185 this.fixed = new Coding(); 9186 return this.fixed; 9187 } else if (name.equals("fixedContactPoint")) { 9188 this.fixed = new ContactPoint(); 9189 return this.fixed; 9190 } else if (name.equals("fixedCount")) { 9191 this.fixed = new Count(); 9192 return this.fixed; 9193 } else if (name.equals("fixedDistance")) { 9194 this.fixed = new Distance(); 9195 return this.fixed; 9196 } else if (name.equals("fixedDuration")) { 9197 this.fixed = new Duration(); 9198 return this.fixed; 9199 } else if (name.equals("fixedHumanName")) { 9200 this.fixed = new HumanName(); 9201 return this.fixed; 9202 } else if (name.equals("fixedIdentifier")) { 9203 this.fixed = new Identifier(); 9204 return this.fixed; 9205 } else if (name.equals("fixedMoney")) { 9206 this.fixed = new Money(); 9207 return this.fixed; 9208 } else if (name.equals("fixedPeriod")) { 9209 this.fixed = new Period(); 9210 return this.fixed; 9211 } else if (name.equals("fixedQuantity")) { 9212 this.fixed = new Quantity(); 9213 return this.fixed; 9214 } else if (name.equals("fixedRange")) { 9215 this.fixed = new Range(); 9216 return this.fixed; 9217 } else if (name.equals("fixedRatio")) { 9218 this.fixed = new Ratio(); 9219 return this.fixed; 9220 } else if (name.equals("fixedReference")) { 9221 this.fixed = new Reference(); 9222 return this.fixed; 9223 } else if (name.equals("fixedSampledData")) { 9224 this.fixed = new SampledData(); 9225 return this.fixed; 9226 } else if (name.equals("fixedSignature")) { 9227 this.fixed = new Signature(); 9228 return this.fixed; 9229 } else if (name.equals("fixedTiming")) { 9230 this.fixed = new Timing(); 9231 return this.fixed; 9232 } else if (name.equals("fixedContactDetail")) { 9233 this.fixed = new ContactDetail(); 9234 return this.fixed; 9235 } else if (name.equals("fixedContributor")) { 9236 this.fixed = new Contributor(); 9237 return this.fixed; 9238 } else if (name.equals("fixedDataRequirement")) { 9239 this.fixed = new DataRequirement(); 9240 return this.fixed; 9241 } else if (name.equals("fixedExpression")) { 9242 this.fixed = new Expression(); 9243 return this.fixed; 9244 } else if (name.equals("fixedParameterDefinition")) { 9245 this.fixed = new ParameterDefinition(); 9246 return this.fixed; 9247 } else if (name.equals("fixedRelatedArtifact")) { 9248 this.fixed = new RelatedArtifact(); 9249 return this.fixed; 9250 } else if (name.equals("fixedTriggerDefinition")) { 9251 this.fixed = new TriggerDefinition(); 9252 return this.fixed; 9253 } else if (name.equals("fixedUsageContext")) { 9254 this.fixed = new UsageContext(); 9255 return this.fixed; 9256 } else if (name.equals("fixedDosage")) { 9257 this.fixed = new Dosage(); 9258 return this.fixed; 9259 } else if (name.equals("fixedMeta")) { 9260 this.fixed = new Meta(); 9261 return this.fixed; 9262 } else if (name.equals("patternBase64Binary")) { 9263 this.pattern = new Base64BinaryType(); 9264 return this.pattern; 9265 } else if (name.equals("patternBoolean")) { 9266 this.pattern = new BooleanType(); 9267 return this.pattern; 9268 } else if (name.equals("patternCanonical")) { 9269 this.pattern = new CanonicalType(); 9270 return this.pattern; 9271 } else if (name.equals("patternCode")) { 9272 this.pattern = new CodeType(); 9273 return this.pattern; 9274 } else if (name.equals("patternDate")) { 9275 this.pattern = new DateType(); 9276 return this.pattern; 9277 } else if (name.equals("patternDateTime")) { 9278 this.pattern = new DateTimeType(); 9279 return this.pattern; 9280 } else if (name.equals("patternDecimal")) { 9281 this.pattern = new DecimalType(); 9282 return this.pattern; 9283 } else if (name.equals("patternId")) { 9284 this.pattern = new IdType(); 9285 return this.pattern; 9286 } else if (name.equals("patternInstant")) { 9287 this.pattern = new InstantType(); 9288 return this.pattern; 9289 } else if (name.equals("patternInteger")) { 9290 this.pattern = new IntegerType(); 9291 return this.pattern; 9292 } else if (name.equals("patternMarkdown")) { 9293 this.pattern = new MarkdownType(); 9294 return this.pattern; 9295 } else if (name.equals("patternOid")) { 9296 this.pattern = new OidType(); 9297 return this.pattern; 9298 } else if (name.equals("patternPositiveInt")) { 9299 this.pattern = new PositiveIntType(); 9300 return this.pattern; 9301 } else if (name.equals("patternString")) { 9302 this.pattern = new StringType(); 9303 return this.pattern; 9304 } else if (name.equals("patternTime")) { 9305 this.pattern = new TimeType(); 9306 return this.pattern; 9307 } else if (name.equals("patternUnsignedInt")) { 9308 this.pattern = new UnsignedIntType(); 9309 return this.pattern; 9310 } else if (name.equals("patternUri")) { 9311 this.pattern = new UriType(); 9312 return this.pattern; 9313 } else if (name.equals("patternUrl")) { 9314 this.pattern = new UrlType(); 9315 return this.pattern; 9316 } else if (name.equals("patternUuid")) { 9317 this.pattern = new UuidType(); 9318 return this.pattern; 9319 } else if (name.equals("patternAddress")) { 9320 this.pattern = new Address(); 9321 return this.pattern; 9322 } else if (name.equals("patternAge")) { 9323 this.pattern = new Age(); 9324 return this.pattern; 9325 } else if (name.equals("patternAnnotation")) { 9326 this.pattern = new Annotation(); 9327 return this.pattern; 9328 } else if (name.equals("patternAttachment")) { 9329 this.pattern = new Attachment(); 9330 return this.pattern; 9331 } else if (name.equals("patternCodeableConcept")) { 9332 this.pattern = new CodeableConcept(); 9333 return this.pattern; 9334 } else if (name.equals("patternCoding")) { 9335 this.pattern = new Coding(); 9336 return this.pattern; 9337 } else if (name.equals("patternContactPoint")) { 9338 this.pattern = new ContactPoint(); 9339 return this.pattern; 9340 } else if (name.equals("patternCount")) { 9341 this.pattern = new Count(); 9342 return this.pattern; 9343 } else if (name.equals("patternDistance")) { 9344 this.pattern = new Distance(); 9345 return this.pattern; 9346 } else if (name.equals("patternDuration")) { 9347 this.pattern = new Duration(); 9348 return this.pattern; 9349 } else if (name.equals("patternHumanName")) { 9350 this.pattern = new HumanName(); 9351 return this.pattern; 9352 } else if (name.equals("patternIdentifier")) { 9353 this.pattern = new Identifier(); 9354 return this.pattern; 9355 } else if (name.equals("patternMoney")) { 9356 this.pattern = new Money(); 9357 return this.pattern; 9358 } else if (name.equals("patternPeriod")) { 9359 this.pattern = new Period(); 9360 return this.pattern; 9361 } else if (name.equals("patternQuantity")) { 9362 this.pattern = new Quantity(); 9363 return this.pattern; 9364 } else if (name.equals("patternRange")) { 9365 this.pattern = new Range(); 9366 return this.pattern; 9367 } else if (name.equals("patternRatio")) { 9368 this.pattern = new Ratio(); 9369 return this.pattern; 9370 } else if (name.equals("patternReference")) { 9371 this.pattern = new Reference(); 9372 return this.pattern; 9373 } else if (name.equals("patternSampledData")) { 9374 this.pattern = new SampledData(); 9375 return this.pattern; 9376 } else if (name.equals("patternSignature")) { 9377 this.pattern = new Signature(); 9378 return this.pattern; 9379 } else if (name.equals("patternTiming")) { 9380 this.pattern = new Timing(); 9381 return this.pattern; 9382 } else if (name.equals("patternContactDetail")) { 9383 this.pattern = new ContactDetail(); 9384 return this.pattern; 9385 } else if (name.equals("patternContributor")) { 9386 this.pattern = new Contributor(); 9387 return this.pattern; 9388 } else if (name.equals("patternDataRequirement")) { 9389 this.pattern = new DataRequirement(); 9390 return this.pattern; 9391 } else if (name.equals("patternExpression")) { 9392 this.pattern = new Expression(); 9393 return this.pattern; 9394 } else if (name.equals("patternParameterDefinition")) { 9395 this.pattern = new ParameterDefinition(); 9396 return this.pattern; 9397 } else if (name.equals("patternRelatedArtifact")) { 9398 this.pattern = new RelatedArtifact(); 9399 return this.pattern; 9400 } else if (name.equals("patternTriggerDefinition")) { 9401 this.pattern = new TriggerDefinition(); 9402 return this.pattern; 9403 } else if (name.equals("patternUsageContext")) { 9404 this.pattern = new UsageContext(); 9405 return this.pattern; 9406 } else if (name.equals("patternDosage")) { 9407 this.pattern = new Dosage(); 9408 return this.pattern; 9409 } else if (name.equals("patternMeta")) { 9410 this.pattern = new Meta(); 9411 return this.pattern; 9412 } else if (name.equals("example")) { 9413 return addExample(); 9414 } else if (name.equals("minValueDate")) { 9415 this.minValue = new DateType(); 9416 return this.minValue; 9417 } else if (name.equals("minValueDateTime")) { 9418 this.minValue = new DateTimeType(); 9419 return this.minValue; 9420 } else if (name.equals("minValueInstant")) { 9421 this.minValue = new InstantType(); 9422 return this.minValue; 9423 } else if (name.equals("minValueTime")) { 9424 this.minValue = new TimeType(); 9425 return this.minValue; 9426 } else if (name.equals("minValueDecimal")) { 9427 this.minValue = new DecimalType(); 9428 return this.minValue; 9429 } else if (name.equals("minValueInteger")) { 9430 this.minValue = new IntegerType(); 9431 return this.minValue; 9432 } else if (name.equals("minValuePositiveInt")) { 9433 this.minValue = new PositiveIntType(); 9434 return this.minValue; 9435 } else if (name.equals("minValueUnsignedInt")) { 9436 this.minValue = new UnsignedIntType(); 9437 return this.minValue; 9438 } else if (name.equals("minValueQuantity")) { 9439 this.minValue = new Quantity(); 9440 return this.minValue; 9441 } else if (name.equals("maxValueDate")) { 9442 this.maxValue = new DateType(); 9443 return this.maxValue; 9444 } else if (name.equals("maxValueDateTime")) { 9445 this.maxValue = new DateTimeType(); 9446 return this.maxValue; 9447 } else if (name.equals("maxValueInstant")) { 9448 this.maxValue = new InstantType(); 9449 return this.maxValue; 9450 } else if (name.equals("maxValueTime")) { 9451 this.maxValue = new TimeType(); 9452 return this.maxValue; 9453 } else if (name.equals("maxValueDecimal")) { 9454 this.maxValue = new DecimalType(); 9455 return this.maxValue; 9456 } else if (name.equals("maxValueInteger")) { 9457 this.maxValue = new IntegerType(); 9458 return this.maxValue; 9459 } else if (name.equals("maxValuePositiveInt")) { 9460 this.maxValue = new PositiveIntType(); 9461 return this.maxValue; 9462 } else if (name.equals("maxValueUnsignedInt")) { 9463 this.maxValue = new UnsignedIntType(); 9464 return this.maxValue; 9465 } else if (name.equals("maxValueQuantity")) { 9466 this.maxValue = new Quantity(); 9467 return this.maxValue; 9468 } else if (name.equals("maxLength")) { 9469 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.maxLength"); 9470 } else if (name.equals("condition")) { 9471 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.condition"); 9472 } else if (name.equals("constraint")) { 9473 return addConstraint(); 9474 } else if (name.equals("mustSupport")) { 9475 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.mustSupport"); 9476 } else if (name.equals("isModifier")) { 9477 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.isModifier"); 9478 } else if (name.equals("isModifierReason")) { 9479 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.isModifierReason"); 9480 } else if (name.equals("isSummary")) { 9481 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.isSummary"); 9482 } else if (name.equals("binding")) { 9483 this.binding = new ElementDefinitionBindingComponent(); 9484 return this.binding; 9485 } else if (name.equals("mapping")) { 9486 return addMapping(); 9487 } else 9488 return super.addChild(name); 9489 } 9490 9491 public String fhirType() { 9492 return "ElementDefinition"; 9493 9494 } 9495 9496 public ElementDefinition copy() { 9497 ElementDefinition dst = new ElementDefinition(); 9498 copyValues(dst); 9499 return dst; 9500 } 9501 9502 public void copyValues(ElementDefinition dst) { 9503 super.copyValues(dst); 9504 dst.path = path == null ? null : path.copy(); 9505 if (representation != null) { 9506 dst.representation = new ArrayList<Enumeration<PropertyRepresentation>>(); 9507 for (Enumeration<PropertyRepresentation> i : representation) 9508 dst.representation.add(i.copy()); 9509 } 9510 ; 9511 dst.sliceName = sliceName == null ? null : sliceName.copy(); 9512 dst.sliceIsConstraining = sliceIsConstraining == null ? null : sliceIsConstraining.copy(); 9513 dst.label = label == null ? null : label.copy(); 9514 if (code != null) { 9515 dst.code = new ArrayList<Coding>(); 9516 for (Coding i : code) 9517 dst.code.add(i.copy()); 9518 } 9519 ; 9520 dst.slicing = slicing == null ? null : slicing.copy(); 9521 dst.short_ = short_ == null ? null : short_.copy(); 9522 dst.definition = definition == null ? null : definition.copy(); 9523 dst.comment = comment == null ? null : comment.copy(); 9524 dst.requirements = requirements == null ? null : requirements.copy(); 9525 if (alias != null) { 9526 dst.alias = new ArrayList<StringType>(); 9527 for (StringType i : alias) 9528 dst.alias.add(i.copy()); 9529 } 9530 ; 9531 dst.min = min == null ? null : min.copy(); 9532 dst.max = max == null ? null : max.copy(); 9533 dst.base = base == null ? null : base.copy(); 9534 dst.contentReference = contentReference == null ? null : contentReference.copy(); 9535 if (type != null) { 9536 dst.type = new ArrayList<TypeRefComponent>(); 9537 for (TypeRefComponent i : type) 9538 dst.type.add(i.copy()); 9539 } 9540 ; 9541 dst.defaultValue = defaultValue == null ? null : defaultValue.copy(); 9542 dst.meaningWhenMissing = meaningWhenMissing == null ? null : meaningWhenMissing.copy(); 9543 dst.orderMeaning = orderMeaning == null ? null : orderMeaning.copy(); 9544 dst.fixed = fixed == null ? null : fixed.copy(); 9545 dst.pattern = pattern == null ? null : pattern.copy(); 9546 if (example != null) { 9547 dst.example = new ArrayList<ElementDefinitionExampleComponent>(); 9548 for (ElementDefinitionExampleComponent i : example) 9549 dst.example.add(i.copy()); 9550 } 9551 ; 9552 dst.minValue = minValue == null ? null : minValue.copy(); 9553 dst.maxValue = maxValue == null ? null : maxValue.copy(); 9554 dst.maxLength = maxLength == null ? null : maxLength.copy(); 9555 if (condition != null) { 9556 dst.condition = new ArrayList<IdType>(); 9557 for (IdType i : condition) 9558 dst.condition.add(i.copy()); 9559 } 9560 ; 9561 if (constraint != null) { 9562 dst.constraint = new ArrayList<ElementDefinitionConstraintComponent>(); 9563 for (ElementDefinitionConstraintComponent i : constraint) 9564 dst.constraint.add(i.copy()); 9565 } 9566 ; 9567 dst.mustSupport = mustSupport == null ? null : mustSupport.copy(); 9568 dst.isModifier = isModifier == null ? null : isModifier.copy(); 9569 dst.isModifierReason = isModifierReason == null ? null : isModifierReason.copy(); 9570 dst.isSummary = isSummary == null ? null : isSummary.copy(); 9571 dst.binding = binding == null ? null : binding.copy(); 9572 if (mapping != null) { 9573 dst.mapping = new ArrayList<ElementDefinitionMappingComponent>(); 9574 for (ElementDefinitionMappingComponent i : mapping) 9575 dst.mapping.add(i.copy()); 9576 } 9577 ; 9578 } 9579 9580 protected ElementDefinition typedCopy() { 9581 return copy(); 9582 } 9583 9584 @Override 9585 public boolean equalsDeep(Base other_) { 9586 if (!super.equalsDeep(other_)) 9587 return false; 9588 if (!(other_ instanceof ElementDefinition)) 9589 return false; 9590 ElementDefinition o = (ElementDefinition) other_; 9591 return compareDeep(path, o.path, true) && compareDeep(representation, o.representation, true) 9592 && compareDeep(sliceName, o.sliceName, true) && compareDeep(sliceIsConstraining, o.sliceIsConstraining, true) 9593 && compareDeep(label, o.label, true) && compareDeep(code, o.code, true) && compareDeep(slicing, o.slicing, true) 9594 && compareDeep(short_, o.short_, true) && compareDeep(definition, o.definition, true) 9595 && compareDeep(comment, o.comment, true) && compareDeep(requirements, o.requirements, true) 9596 && compareDeep(alias, o.alias, true) && compareDeep(min, o.min, true) && compareDeep(max, o.max, true) 9597 && compareDeep(base, o.base, true) && compareDeep(contentReference, o.contentReference, true) 9598 && compareDeep(type, o.type, true) && compareDeep(defaultValue, o.defaultValue, true) 9599 && compareDeep(meaningWhenMissing, o.meaningWhenMissing, true) 9600 && compareDeep(orderMeaning, o.orderMeaning, true) && compareDeep(fixed, o.fixed, true) 9601 && compareDeep(pattern, o.pattern, true) && compareDeep(example, o.example, true) 9602 && compareDeep(minValue, o.minValue, true) && compareDeep(maxValue, o.maxValue, true) 9603 && compareDeep(maxLength, o.maxLength, true) && compareDeep(condition, o.condition, true) 9604 && compareDeep(constraint, o.constraint, true) && compareDeep(mustSupport, o.mustSupport, true) 9605 && compareDeep(isModifier, o.isModifier, true) && compareDeep(isModifierReason, o.isModifierReason, true) 9606 && compareDeep(isSummary, o.isSummary, true) && compareDeep(binding, o.binding, true) 9607 && compareDeep(mapping, o.mapping, true); 9608 } 9609 9610 @Override 9611 public boolean equalsShallow(Base other_) { 9612 if (!super.equalsShallow(other_)) 9613 return false; 9614 if (!(other_ instanceof ElementDefinition)) 9615 return false; 9616 ElementDefinition o = (ElementDefinition) other_; 9617 return compareValues(path, o.path, true) && compareValues(representation, o.representation, true) 9618 && compareValues(sliceName, o.sliceName, true) 9619 && compareValues(sliceIsConstraining, o.sliceIsConstraining, true) && compareValues(label, o.label, true) 9620 && compareValues(short_, o.short_, true) && compareValues(definition, o.definition, true) 9621 && compareValues(comment, o.comment, true) && compareValues(requirements, o.requirements, true) 9622 && compareValues(alias, o.alias, true) && compareValues(min, o.min, true) && compareValues(max, o.max, true) 9623 && compareValues(contentReference, o.contentReference, true) 9624 && compareValues(meaningWhenMissing, o.meaningWhenMissing, true) 9625 && compareValues(orderMeaning, o.orderMeaning, true) && compareValues(maxLength, o.maxLength, true) 9626 && compareValues(condition, o.condition, true) && compareValues(mustSupport, o.mustSupport, true) 9627 && compareValues(isModifier, o.isModifier, true) && compareValues(isModifierReason, o.isModifierReason, true) 9628 && compareValues(isSummary, o.isSummary, true); 9629 } 9630 9631 public boolean isEmpty() { 9632 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, representation, sliceName, sliceIsConstraining, 9633 label, code, slicing, short_, definition, comment, requirements, alias, min, max, base, contentReference, type, 9634 defaultValue, meaningWhenMissing, orderMeaning, fixed, pattern, example, minValue, maxValue, maxLength, 9635 condition, constraint, mustSupport, isModifier, isModifierReason, isSummary, binding, mapping); 9636 } 9637 9638// added from java-adornments.txt: 9639 9640 public String toString() { 9641 if (hasId()) 9642 return getId(); 9643 if (hasSliceName()) 9644 return getPath() + ":" + getSliceName(); 9645 else 9646 return getPath(); 9647 } 9648 9649 public void makeBase(String path, int min, String max) { 9650 ElementDefinitionBaseComponent self = getBase(); 9651 self.setPath(path); 9652 self.setMin(min); 9653 self.setMax(max); 9654 } 9655 9656 public void makeBase() { 9657 ElementDefinitionBaseComponent self = getBase(); 9658 self.setPath(getPath()); 9659 self.setMin(getMin()); 9660 self.setMax(getMax()); 9661 } 9662 9663 public String typeSummary() { 9664 CommaSeparatedStringBuilder b = new CommaSeparatedStringBuilder(); 9665 for (TypeRefComponent tr : getType()) { 9666 if (tr.hasCode()) 9667 b.append(tr.getWorkingCode()); 9668 } 9669 return b.toString(); 9670 } 9671 9672 public TypeRefComponent getType(String code) { 9673 for (TypeRefComponent tr : getType()) 9674 if (tr.getCode().equals(code)) 9675 return tr; 9676 TypeRefComponent tr = new TypeRefComponent(); 9677 tr.setCode(code); 9678 type.add(tr); 9679 return tr; 9680 } 9681 9682 public static final boolean NOT_MODIFIER = false; 9683 public static final boolean NOT_IN_SUMMARY = false; 9684 public static final boolean IS_MODIFIER = true; 9685 public static final boolean IS_IN_SUMMARY = true; 9686 9687 public ElementDefinition(boolean defaults, boolean modifier, boolean inSummary) { 9688 super(); 9689 if (defaults) { 9690 setIsModifier(modifier); 9691 setIsSummary(inSummary); 9692 } 9693 } 9694 9695 public String present() { 9696 return hasId() ? getId() : getPath(); 9697 } 9698 9699 public boolean hasFixedOrPattern() { 9700 return hasFixed() || hasPattern(); 9701 } 9702 9703 public Type getFixedOrPattern() { 9704 return hasFixed() ? getFixed() : getPattern(); 9705 } 9706 9707 public String getName() { 9708 return hasPath() ? getPath().contains(".") ? getPath().substring(getPath().lastIndexOf(".")+1) : getPath() : null; 9709 } 9710 9711 public boolean getMustHaveValue() { 9712 return false; 9713 } 9714 public boolean isChoice() { 9715 return getPath().endsWith("[x]"); 9716 } 9717 9718 9719 public String getNameBase() { 9720 return getName().replace("[x]", ""); 9721 } 9722 9723 public boolean unbounded() { 9724 return getMax().equals("*") || Integer.parseInt(getMax()) > 1; 9725 } 9726 9727 public boolean isMandatory() { 9728 return getMin() > 0; 9729 } 9730 9731 9732 public boolean prohibited() { 9733 return "0".equals(getMax()); 9734 } 9735 9736 public boolean isProhibited() { 9737 return "0".equals(getMax()); 9738 } 9739 9740 public boolean isRequired() { 9741 return getMin() == 1; 9742 } 9743// end addition 9744 9745}