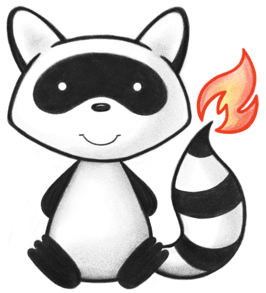
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044 045/** 046 * An interaction between a patient and healthcare provider(s) for the purpose 047 * of providing healthcare service(s) or assessing the health status of a 048 * patient. 049 */ 050@ResourceDef(name = "Encounter", profile = "http://hl7.org/fhir/StructureDefinition/Encounter") 051public class Encounter extends DomainResource { 052 053 public enum EncounterStatus { 054 /** 055 * The Encounter has not yet started. 056 */ 057 PLANNED, 058 /** 059 * The Patient is present for the encounter, however is not currently meeting 060 * with a practitioner. 061 */ 062 ARRIVED, 063 /** 064 * The patient has been assessed for the priority of their treatment based on 065 * the severity of their condition. 066 */ 067 TRIAGED, 068 /** 069 * The Encounter has begun and the patient is present / the practitioner and the 070 * patient are meeting. 071 */ 072 INPROGRESS, 073 /** 074 * The Encounter has begun, but the patient is temporarily on leave. 075 */ 076 ONLEAVE, 077 /** 078 * The Encounter has ended. 079 */ 080 FINISHED, 081 /** 082 * The Encounter has ended before it has begun. 083 */ 084 CANCELLED, 085 /** 086 * This instance should not have been part of this patient's medical record. 087 */ 088 ENTEREDINERROR, 089 /** 090 * The encounter status is unknown. Note that "unknown" is a value of last 091 * resort and every attempt should be made to provide a meaningful value other 092 * than "unknown". 093 */ 094 UNKNOWN, 095 /** 096 * added to help the parsers with the generic types 097 */ 098 NULL; 099 100 public static EncounterStatus fromCode(String codeString) throws FHIRException { 101 if (codeString == null || "".equals(codeString)) 102 return null; 103 if ("planned".equals(codeString)) 104 return PLANNED; 105 if ("arrived".equals(codeString)) 106 return ARRIVED; 107 if ("triaged".equals(codeString)) 108 return TRIAGED; 109 if ("in-progress".equals(codeString)) 110 return INPROGRESS; 111 if ("onleave".equals(codeString)) 112 return ONLEAVE; 113 if ("finished".equals(codeString)) 114 return FINISHED; 115 if ("cancelled".equals(codeString)) 116 return CANCELLED; 117 if ("entered-in-error".equals(codeString)) 118 return ENTEREDINERROR; 119 if ("unknown".equals(codeString)) 120 return UNKNOWN; 121 if (Configuration.isAcceptInvalidEnums()) 122 return null; 123 else 124 throw new FHIRException("Unknown EncounterStatus code '" + codeString + "'"); 125 } 126 127 public String toCode() { 128 switch (this) { 129 case PLANNED: 130 return "planned"; 131 case ARRIVED: 132 return "arrived"; 133 case TRIAGED: 134 return "triaged"; 135 case INPROGRESS: 136 return "in-progress"; 137 case ONLEAVE: 138 return "onleave"; 139 case FINISHED: 140 return "finished"; 141 case CANCELLED: 142 return "cancelled"; 143 case ENTEREDINERROR: 144 return "entered-in-error"; 145 case UNKNOWN: 146 return "unknown"; 147 case NULL: 148 return null; 149 default: 150 return "?"; 151 } 152 } 153 154 public String getSystem() { 155 switch (this) { 156 case PLANNED: 157 return "http://hl7.org/fhir/encounter-status"; 158 case ARRIVED: 159 return "http://hl7.org/fhir/encounter-status"; 160 case TRIAGED: 161 return "http://hl7.org/fhir/encounter-status"; 162 case INPROGRESS: 163 return "http://hl7.org/fhir/encounter-status"; 164 case ONLEAVE: 165 return "http://hl7.org/fhir/encounter-status"; 166 case FINISHED: 167 return "http://hl7.org/fhir/encounter-status"; 168 case CANCELLED: 169 return "http://hl7.org/fhir/encounter-status"; 170 case ENTEREDINERROR: 171 return "http://hl7.org/fhir/encounter-status"; 172 case UNKNOWN: 173 return "http://hl7.org/fhir/encounter-status"; 174 case NULL: 175 return null; 176 default: 177 return "?"; 178 } 179 } 180 181 public String getDefinition() { 182 switch (this) { 183 case PLANNED: 184 return "The Encounter has not yet started."; 185 case ARRIVED: 186 return "The Patient is present for the encounter, however is not currently meeting with a practitioner."; 187 case TRIAGED: 188 return "The patient has been assessed for the priority of their treatment based on the severity of their condition."; 189 case INPROGRESS: 190 return "The Encounter has begun and the patient is present / the practitioner and the patient are meeting."; 191 case ONLEAVE: 192 return "The Encounter has begun, but the patient is temporarily on leave."; 193 case FINISHED: 194 return "The Encounter has ended."; 195 case CANCELLED: 196 return "The Encounter has ended before it has begun."; 197 case ENTEREDINERROR: 198 return "This instance should not have been part of this patient's medical record."; 199 case UNKNOWN: 200 return "The encounter status is unknown. Note that \"unknown\" is a value of last resort and every attempt should be made to provide a meaningful value other than \"unknown\"."; 201 case NULL: 202 return null; 203 default: 204 return "?"; 205 } 206 } 207 208 public String getDisplay() { 209 switch (this) { 210 case PLANNED: 211 return "Planned"; 212 case ARRIVED: 213 return "Arrived"; 214 case TRIAGED: 215 return "Triaged"; 216 case INPROGRESS: 217 return "In Progress"; 218 case ONLEAVE: 219 return "On Leave"; 220 case FINISHED: 221 return "Finished"; 222 case CANCELLED: 223 return "Cancelled"; 224 case ENTEREDINERROR: 225 return "Entered in Error"; 226 case UNKNOWN: 227 return "Unknown"; 228 case NULL: 229 return null; 230 default: 231 return "?"; 232 } 233 } 234 } 235 236 public static class EncounterStatusEnumFactory implements EnumFactory<EncounterStatus> { 237 public EncounterStatus fromCode(String codeString) throws IllegalArgumentException { 238 if (codeString == null || "".equals(codeString)) 239 if (codeString == null || "".equals(codeString)) 240 return null; 241 if ("planned".equals(codeString)) 242 return EncounterStatus.PLANNED; 243 if ("arrived".equals(codeString)) 244 return EncounterStatus.ARRIVED; 245 if ("triaged".equals(codeString)) 246 return EncounterStatus.TRIAGED; 247 if ("in-progress".equals(codeString)) 248 return EncounterStatus.INPROGRESS; 249 if ("onleave".equals(codeString)) 250 return EncounterStatus.ONLEAVE; 251 if ("finished".equals(codeString)) 252 return EncounterStatus.FINISHED; 253 if ("cancelled".equals(codeString)) 254 return EncounterStatus.CANCELLED; 255 if ("entered-in-error".equals(codeString)) 256 return EncounterStatus.ENTEREDINERROR; 257 if ("unknown".equals(codeString)) 258 return EncounterStatus.UNKNOWN; 259 throw new IllegalArgumentException("Unknown EncounterStatus code '" + codeString + "'"); 260 } 261 262 public Enumeration<EncounterStatus> fromType(PrimitiveType<?> code) throws FHIRException { 263 if (code == null) 264 return null; 265 if (code.isEmpty()) 266 return new Enumeration<EncounterStatus>(this, EncounterStatus.NULL, code); 267 String codeString = code.asStringValue(); 268 if (codeString == null || "".equals(codeString)) 269 return new Enumeration<EncounterStatus>(this, EncounterStatus.NULL, code); 270 if ("planned".equals(codeString)) 271 return new Enumeration<EncounterStatus>(this, EncounterStatus.PLANNED, code); 272 if ("arrived".equals(codeString)) 273 return new Enumeration<EncounterStatus>(this, EncounterStatus.ARRIVED, code); 274 if ("triaged".equals(codeString)) 275 return new Enumeration<EncounterStatus>(this, EncounterStatus.TRIAGED, code); 276 if ("in-progress".equals(codeString)) 277 return new Enumeration<EncounterStatus>(this, EncounterStatus.INPROGRESS, code); 278 if ("onleave".equals(codeString)) 279 return new Enumeration<EncounterStatus>(this, EncounterStatus.ONLEAVE, code); 280 if ("finished".equals(codeString)) 281 return new Enumeration<EncounterStatus>(this, EncounterStatus.FINISHED, code); 282 if ("cancelled".equals(codeString)) 283 return new Enumeration<EncounterStatus>(this, EncounterStatus.CANCELLED, code); 284 if ("entered-in-error".equals(codeString)) 285 return new Enumeration<EncounterStatus>(this, EncounterStatus.ENTEREDINERROR, code); 286 if ("unknown".equals(codeString)) 287 return new Enumeration<EncounterStatus>(this, EncounterStatus.UNKNOWN, code); 288 throw new FHIRException("Unknown EncounterStatus code '" + codeString + "'"); 289 } 290 291 public String toCode(EncounterStatus code) { 292 if (code == EncounterStatus.NULL) 293 return null; 294 if (code == EncounterStatus.PLANNED) 295 return "planned"; 296 if (code == EncounterStatus.ARRIVED) 297 return "arrived"; 298 if (code == EncounterStatus.TRIAGED) 299 return "triaged"; 300 if (code == EncounterStatus.INPROGRESS) 301 return "in-progress"; 302 if (code == EncounterStatus.ONLEAVE) 303 return "onleave"; 304 if (code == EncounterStatus.FINISHED) 305 return "finished"; 306 if (code == EncounterStatus.CANCELLED) 307 return "cancelled"; 308 if (code == EncounterStatus.ENTEREDINERROR) 309 return "entered-in-error"; 310 if (code == EncounterStatus.UNKNOWN) 311 return "unknown"; 312 return "?"; 313 } 314 315 public String toSystem(EncounterStatus code) { 316 return code.getSystem(); 317 } 318 } 319 320 public enum EncounterLocationStatus { 321 /** 322 * The patient is planned to be moved to this location at some point in the 323 * future. 324 */ 325 PLANNED, 326 /** 327 * The patient is currently at this location, or was between the period 328 * specified. 329 * 330 * A system may update these records when the patient leaves the location to 331 * either reserved, or completed. 332 */ 333 ACTIVE, 334 /** 335 * This location is held empty for this patient. 336 */ 337 RESERVED, 338 /** 339 * The patient was at this location during the period specified. 340 * 341 * Not to be used when the patient is currently at the location. 342 */ 343 COMPLETED, 344 /** 345 * added to help the parsers with the generic types 346 */ 347 NULL; 348 349 public static EncounterLocationStatus fromCode(String codeString) throws FHIRException { 350 if (codeString == null || "".equals(codeString)) 351 return null; 352 if ("planned".equals(codeString)) 353 return PLANNED; 354 if ("active".equals(codeString)) 355 return ACTIVE; 356 if ("reserved".equals(codeString)) 357 return RESERVED; 358 if ("completed".equals(codeString)) 359 return COMPLETED; 360 if (Configuration.isAcceptInvalidEnums()) 361 return null; 362 else 363 throw new FHIRException("Unknown EncounterLocationStatus code '" + codeString + "'"); 364 } 365 366 public String toCode() { 367 switch (this) { 368 case PLANNED: 369 return "planned"; 370 case ACTIVE: 371 return "active"; 372 case RESERVED: 373 return "reserved"; 374 case COMPLETED: 375 return "completed"; 376 case NULL: 377 return null; 378 default: 379 return "?"; 380 } 381 } 382 383 public String getSystem() { 384 switch (this) { 385 case PLANNED: 386 return "http://hl7.org/fhir/encounter-location-status"; 387 case ACTIVE: 388 return "http://hl7.org/fhir/encounter-location-status"; 389 case RESERVED: 390 return "http://hl7.org/fhir/encounter-location-status"; 391 case COMPLETED: 392 return "http://hl7.org/fhir/encounter-location-status"; 393 case NULL: 394 return null; 395 default: 396 return "?"; 397 } 398 } 399 400 public String getDefinition() { 401 switch (this) { 402 case PLANNED: 403 return "The patient is planned to be moved to this location at some point in the future."; 404 case ACTIVE: 405 return "The patient is currently at this location, or was between the period specified.\r\rA system may update these records when the patient leaves the location to either reserved, or completed."; 406 case RESERVED: 407 return "This location is held empty for this patient."; 408 case COMPLETED: 409 return "The patient was at this location during the period specified.\r\rNot to be used when the patient is currently at the location."; 410 case NULL: 411 return null; 412 default: 413 return "?"; 414 } 415 } 416 417 public String getDisplay() { 418 switch (this) { 419 case PLANNED: 420 return "Planned"; 421 case ACTIVE: 422 return "Active"; 423 case RESERVED: 424 return "Reserved"; 425 case COMPLETED: 426 return "Completed"; 427 case NULL: 428 return null; 429 default: 430 return "?"; 431 } 432 } 433 } 434 435 public static class EncounterLocationStatusEnumFactory implements EnumFactory<EncounterLocationStatus> { 436 public EncounterLocationStatus fromCode(String codeString) throws IllegalArgumentException { 437 if (codeString == null || "".equals(codeString)) 438 if (codeString == null || "".equals(codeString)) 439 return null; 440 if ("planned".equals(codeString)) 441 return EncounterLocationStatus.PLANNED; 442 if ("active".equals(codeString)) 443 return EncounterLocationStatus.ACTIVE; 444 if ("reserved".equals(codeString)) 445 return EncounterLocationStatus.RESERVED; 446 if ("completed".equals(codeString)) 447 return EncounterLocationStatus.COMPLETED; 448 throw new IllegalArgumentException("Unknown EncounterLocationStatus code '" + codeString + "'"); 449 } 450 451 public Enumeration<EncounterLocationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 452 if (code == null) 453 return null; 454 if (code.isEmpty()) 455 return new Enumeration<EncounterLocationStatus>(this, EncounterLocationStatus.NULL, code); 456 String codeString = code.asStringValue(); 457 if (codeString == null || "".equals(codeString)) 458 return new Enumeration<EncounterLocationStatus>(this, EncounterLocationStatus.NULL, code); 459 if ("planned".equals(codeString)) 460 return new Enumeration<EncounterLocationStatus>(this, EncounterLocationStatus.PLANNED, code); 461 if ("active".equals(codeString)) 462 return new Enumeration<EncounterLocationStatus>(this, EncounterLocationStatus.ACTIVE, code); 463 if ("reserved".equals(codeString)) 464 return new Enumeration<EncounterLocationStatus>(this, EncounterLocationStatus.RESERVED, code); 465 if ("completed".equals(codeString)) 466 return new Enumeration<EncounterLocationStatus>(this, EncounterLocationStatus.COMPLETED, code); 467 throw new FHIRException("Unknown EncounterLocationStatus code '" + codeString + "'"); 468 } 469 470 public String toCode(EncounterLocationStatus code) { 471 if (code == EncounterLocationStatus.NULL) 472 return null; 473 if (code == EncounterLocationStatus.PLANNED) 474 return "planned"; 475 if (code == EncounterLocationStatus.ACTIVE) 476 return "active"; 477 if (code == EncounterLocationStatus.RESERVED) 478 return "reserved"; 479 if (code == EncounterLocationStatus.COMPLETED) 480 return "completed"; 481 return "?"; 482 } 483 484 public String toSystem(EncounterLocationStatus code) { 485 return code.getSystem(); 486 } 487 } 488 489 @Block() 490 public static class StatusHistoryComponent extends BackboneElement implements IBaseBackboneElement { 491 /** 492 * planned | arrived | triaged | in-progress | onleave | finished | cancelled +. 493 */ 494 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 495 @Description(shortDefinition = "planned | arrived | triaged | in-progress | onleave | finished | cancelled +", formalDefinition = "planned | arrived | triaged | in-progress | onleave | finished | cancelled +.") 496 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-status") 497 protected Enumeration<EncounterStatus> status; 498 499 /** 500 * The time that the episode was in the specified status. 501 */ 502 @Child(name = "period", type = { Period.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 503 @Description(shortDefinition = "The time that the episode was in the specified status", formalDefinition = "The time that the episode was in the specified status.") 504 protected Period period; 505 506 private static final long serialVersionUID = -1893906736L; 507 508 /** 509 * Constructor 510 */ 511 public StatusHistoryComponent() { 512 super(); 513 } 514 515 /** 516 * Constructor 517 */ 518 public StatusHistoryComponent(Enumeration<EncounterStatus> status, Period period) { 519 super(); 520 this.status = status; 521 this.period = period; 522 } 523 524 /** 525 * @return {@link #status} (planned | arrived | triaged | in-progress | onleave 526 * | finished | cancelled +.). This is the underlying object with id, 527 * value and extensions. The accessor "getStatus" gives direct access to 528 * the value 529 */ 530 public Enumeration<EncounterStatus> getStatusElement() { 531 if (this.status == null) 532 if (Configuration.errorOnAutoCreate()) 533 throw new Error("Attempt to auto-create StatusHistoryComponent.status"); 534 else if (Configuration.doAutoCreate()) 535 this.status = new Enumeration<EncounterStatus>(new EncounterStatusEnumFactory()); // bb 536 return this.status; 537 } 538 539 public boolean hasStatusElement() { 540 return this.status != null && !this.status.isEmpty(); 541 } 542 543 public boolean hasStatus() { 544 return this.status != null && !this.status.isEmpty(); 545 } 546 547 /** 548 * @param value {@link #status} (planned | arrived | triaged | in-progress | 549 * onleave | finished | cancelled +.). This is the underlying 550 * object with id, value and extensions. The accessor "getStatus" 551 * gives direct access to the value 552 */ 553 public StatusHistoryComponent setStatusElement(Enumeration<EncounterStatus> value) { 554 this.status = value; 555 return this; 556 } 557 558 /** 559 * @return planned | arrived | triaged | in-progress | onleave | finished | 560 * cancelled +. 561 */ 562 public EncounterStatus getStatus() { 563 return this.status == null ? null : this.status.getValue(); 564 } 565 566 /** 567 * @param value planned | arrived | triaged | in-progress | onleave | finished | 568 * cancelled +. 569 */ 570 public StatusHistoryComponent setStatus(EncounterStatus value) { 571 if (this.status == null) 572 this.status = new Enumeration<EncounterStatus>(new EncounterStatusEnumFactory()); 573 this.status.setValue(value); 574 return this; 575 } 576 577 /** 578 * @return {@link #period} (The time that the episode was in the specified 579 * status.) 580 */ 581 public Period getPeriod() { 582 if (this.period == null) 583 if (Configuration.errorOnAutoCreate()) 584 throw new Error("Attempt to auto-create StatusHistoryComponent.period"); 585 else if (Configuration.doAutoCreate()) 586 this.period = new Period(); // cc 587 return this.period; 588 } 589 590 public boolean hasPeriod() { 591 return this.period != null && !this.period.isEmpty(); 592 } 593 594 /** 595 * @param value {@link #period} (The time that the episode was in the specified 596 * status.) 597 */ 598 public StatusHistoryComponent setPeriod(Period value) { 599 this.period = value; 600 return this; 601 } 602 603 protected void listChildren(List<Property> children) { 604 super.listChildren(children); 605 children.add(new Property("status", "code", 606 "planned | arrived | triaged | in-progress | onleave | finished | cancelled +.", 0, 1, status)); 607 children.add( 608 new Property("period", "Period", "The time that the episode was in the specified status.", 0, 1, period)); 609 } 610 611 @Override 612 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 613 switch (_hash) { 614 case -892481550: 615 /* status */ return new Property("status", "code", 616 "planned | arrived | triaged | in-progress | onleave | finished | cancelled +.", 0, 1, status); 617 case -991726143: 618 /* period */ return new Property("period", "Period", "The time that the episode was in the specified status.", 619 0, 1, period); 620 default: 621 return super.getNamedProperty(_hash, _name, _checkValid); 622 } 623 624 } 625 626 @Override 627 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 628 switch (hash) { 629 case -892481550: 630 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<EncounterStatus> 631 case -991726143: 632 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 633 default: 634 return super.getProperty(hash, name, checkValid); 635 } 636 637 } 638 639 @Override 640 public Base setProperty(int hash, String name, Base value) throws FHIRException { 641 switch (hash) { 642 case -892481550: // status 643 value = new EncounterStatusEnumFactory().fromType(castToCode(value)); 644 this.status = (Enumeration) value; // Enumeration<EncounterStatus> 645 return value; 646 case -991726143: // period 647 this.period = castToPeriod(value); // Period 648 return value; 649 default: 650 return super.setProperty(hash, name, value); 651 } 652 653 } 654 655 @Override 656 public Base setProperty(String name, Base value) throws FHIRException { 657 if (name.equals("status")) { 658 value = new EncounterStatusEnumFactory().fromType(castToCode(value)); 659 this.status = (Enumeration) value; // Enumeration<EncounterStatus> 660 } else if (name.equals("period")) { 661 this.period = castToPeriod(value); // Period 662 } else 663 return super.setProperty(name, value); 664 return value; 665 } 666 667 @Override 668 public void removeChild(String name, Base value) throws FHIRException { 669 if (name.equals("status")) { 670 this.status = null; 671 } else if (name.equals("period")) { 672 this.period = null; 673 } else 674 super.removeChild(name, value); 675 676 } 677 678 @Override 679 public Base makeProperty(int hash, String name) throws FHIRException { 680 switch (hash) { 681 case -892481550: 682 return getStatusElement(); 683 case -991726143: 684 return getPeriod(); 685 default: 686 return super.makeProperty(hash, name); 687 } 688 689 } 690 691 @Override 692 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 693 switch (hash) { 694 case -892481550: 695 /* status */ return new String[] { "code" }; 696 case -991726143: 697 /* period */ return new String[] { "Period" }; 698 default: 699 return super.getTypesForProperty(hash, name); 700 } 701 702 } 703 704 @Override 705 public Base addChild(String name) throws FHIRException { 706 if (name.equals("status")) { 707 throw new FHIRException("Cannot call addChild on a singleton property Encounter.status"); 708 } else if (name.equals("period")) { 709 this.period = new Period(); 710 return this.period; 711 } else 712 return super.addChild(name); 713 } 714 715 public StatusHistoryComponent copy() { 716 StatusHistoryComponent dst = new StatusHistoryComponent(); 717 copyValues(dst); 718 return dst; 719 } 720 721 public void copyValues(StatusHistoryComponent dst) { 722 super.copyValues(dst); 723 dst.status = status == null ? null : status.copy(); 724 dst.period = period == null ? null : period.copy(); 725 } 726 727 @Override 728 public boolean equalsDeep(Base other_) { 729 if (!super.equalsDeep(other_)) 730 return false; 731 if (!(other_ instanceof StatusHistoryComponent)) 732 return false; 733 StatusHistoryComponent o = (StatusHistoryComponent) other_; 734 return compareDeep(status, o.status, true) && compareDeep(period, o.period, true); 735 } 736 737 @Override 738 public boolean equalsShallow(Base other_) { 739 if (!super.equalsShallow(other_)) 740 return false; 741 if (!(other_ instanceof StatusHistoryComponent)) 742 return false; 743 StatusHistoryComponent o = (StatusHistoryComponent) other_; 744 return compareValues(status, o.status, true); 745 } 746 747 public boolean isEmpty() { 748 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(status, period); 749 } 750 751 public String fhirType() { 752 return "Encounter.statusHistory"; 753 754 } 755 756 } 757 758 @Block() 759 public static class ClassHistoryComponent extends BackboneElement implements IBaseBackboneElement { 760 /** 761 * inpatient | outpatient | ambulatory | emergency +. 762 */ 763 @Child(name = "class", type = { Coding.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 764 @Description(shortDefinition = "inpatient | outpatient | ambulatory | emergency +", formalDefinition = "inpatient | outpatient | ambulatory | emergency +.") 765 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ActEncounterCode") 766 protected Coding class_; 767 768 /** 769 * The time that the episode was in the specified class. 770 */ 771 @Child(name = "period", type = { Period.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 772 @Description(shortDefinition = "The time that the episode was in the specified class", formalDefinition = "The time that the episode was in the specified class.") 773 protected Period period; 774 775 private static final long serialVersionUID = 1331020311L; 776 777 /** 778 * Constructor 779 */ 780 public ClassHistoryComponent() { 781 super(); 782 } 783 784 /** 785 * Constructor 786 */ 787 public ClassHistoryComponent(Coding class_, Period period) { 788 super(); 789 this.class_ = class_; 790 this.period = period; 791 } 792 793 /** 794 * @return {@link #class_} (inpatient | outpatient | ambulatory | emergency +.) 795 */ 796 public Coding getClass_() { 797 if (this.class_ == null) 798 if (Configuration.errorOnAutoCreate()) 799 throw new Error("Attempt to auto-create ClassHistoryComponent.class_"); 800 else if (Configuration.doAutoCreate()) 801 this.class_ = new Coding(); // cc 802 return this.class_; 803 } 804 805 public boolean hasClass_() { 806 return this.class_ != null && !this.class_.isEmpty(); 807 } 808 809 /** 810 * @param value {@link #class_} (inpatient | outpatient | ambulatory | emergency 811 * +.) 812 */ 813 public ClassHistoryComponent setClass_(Coding value) { 814 this.class_ = value; 815 return this; 816 } 817 818 /** 819 * @return {@link #period} (The time that the episode was in the specified 820 * class.) 821 */ 822 public Period getPeriod() { 823 if (this.period == null) 824 if (Configuration.errorOnAutoCreate()) 825 throw new Error("Attempt to auto-create ClassHistoryComponent.period"); 826 else if (Configuration.doAutoCreate()) 827 this.period = new Period(); // cc 828 return this.period; 829 } 830 831 public boolean hasPeriod() { 832 return this.period != null && !this.period.isEmpty(); 833 } 834 835 /** 836 * @param value {@link #period} (The time that the episode was in the specified 837 * class.) 838 */ 839 public ClassHistoryComponent setPeriod(Period value) { 840 this.period = value; 841 return this; 842 } 843 844 protected void listChildren(List<Property> children) { 845 super.listChildren(children); 846 children.add(new Property("class", "Coding", "inpatient | outpatient | ambulatory | emergency +.", 0, 1, class_)); 847 children 848 .add(new Property("period", "Period", "The time that the episode was in the specified class.", 0, 1, period)); 849 } 850 851 @Override 852 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 853 switch (_hash) { 854 case 94742904: 855 /* class */ return new Property("class", "Coding", "inpatient | outpatient | ambulatory | emergency +.", 0, 1, 856 class_); 857 case -991726143: 858 /* period */ return new Property("period", "Period", "The time that the episode was in the specified class.", 0, 859 1, period); 860 default: 861 return super.getNamedProperty(_hash, _name, _checkValid); 862 } 863 864 } 865 866 @Override 867 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 868 switch (hash) { 869 case 94742904: 870 /* class */ return this.class_ == null ? new Base[0] : new Base[] { this.class_ }; // Coding 871 case -991726143: 872 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 873 default: 874 return super.getProperty(hash, name, checkValid); 875 } 876 877 } 878 879 @Override 880 public Base setProperty(int hash, String name, Base value) throws FHIRException { 881 switch (hash) { 882 case 94742904: // class 883 this.class_ = castToCoding(value); // Coding 884 return value; 885 case -991726143: // period 886 this.period = castToPeriod(value); // Period 887 return value; 888 default: 889 return super.setProperty(hash, name, value); 890 } 891 892 } 893 894 @Override 895 public Base setProperty(String name, Base value) throws FHIRException { 896 if (name.equals("class")) { 897 this.class_ = castToCoding(value); // Coding 898 } else if (name.equals("period")) { 899 this.period = castToPeriod(value); // Period 900 } else 901 return super.setProperty(name, value); 902 return value; 903 } 904 905 @Override 906 public void removeChild(String name, Base value) throws FHIRException { 907 if (name.equals("class")) { 908 this.class_ = null; 909 } else if (name.equals("period")) { 910 this.period = null; 911 } else 912 super.removeChild(name, value); 913 914 } 915 916 @Override 917 public Base makeProperty(int hash, String name) throws FHIRException { 918 switch (hash) { 919 case 94742904: 920 return getClass_(); 921 case -991726143: 922 return getPeriod(); 923 default: 924 return super.makeProperty(hash, name); 925 } 926 927 } 928 929 @Override 930 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 931 switch (hash) { 932 case 94742904: 933 /* class */ return new String[] { "Coding" }; 934 case -991726143: 935 /* period */ return new String[] { "Period" }; 936 default: 937 return super.getTypesForProperty(hash, name); 938 } 939 940 } 941 942 @Override 943 public Base addChild(String name) throws FHIRException { 944 if (name.equals("class")) { 945 this.class_ = new Coding(); 946 return this.class_; 947 } else if (name.equals("period")) { 948 this.period = new Period(); 949 return this.period; 950 } else 951 return super.addChild(name); 952 } 953 954 public ClassHistoryComponent copy() { 955 ClassHistoryComponent dst = new ClassHistoryComponent(); 956 copyValues(dst); 957 return dst; 958 } 959 960 public void copyValues(ClassHistoryComponent dst) { 961 super.copyValues(dst); 962 dst.class_ = class_ == null ? null : class_.copy(); 963 dst.period = period == null ? null : period.copy(); 964 } 965 966 @Override 967 public boolean equalsDeep(Base other_) { 968 if (!super.equalsDeep(other_)) 969 return false; 970 if (!(other_ instanceof ClassHistoryComponent)) 971 return false; 972 ClassHistoryComponent o = (ClassHistoryComponent) other_; 973 return compareDeep(class_, o.class_, true) && compareDeep(period, o.period, true); 974 } 975 976 @Override 977 public boolean equalsShallow(Base other_) { 978 if (!super.equalsShallow(other_)) 979 return false; 980 if (!(other_ instanceof ClassHistoryComponent)) 981 return false; 982 ClassHistoryComponent o = (ClassHistoryComponent) other_; 983 return true; 984 } 985 986 public boolean isEmpty() { 987 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(class_, period); 988 } 989 990 public String fhirType() { 991 return "Encounter.classHistory"; 992 993 } 994 995 } 996 997 @Block() 998 public static class EncounterParticipantComponent extends BackboneElement implements IBaseBackboneElement { 999 /** 1000 * Role of participant in encounter. 1001 */ 1002 @Child(name = "type", type = { 1003 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1004 @Description(shortDefinition = "Role of participant in encounter", formalDefinition = "Role of participant in encounter.") 1005 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-participant-type") 1006 protected List<CodeableConcept> type; 1007 1008 /** 1009 * The period of time that the specified participant participated in the 1010 * encounter. These can overlap or be sub-sets of the overall encounter's 1011 * period. 1012 */ 1013 @Child(name = "period", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1014 @Description(shortDefinition = "Period of time during the encounter that the participant participated", formalDefinition = "The period of time that the specified participant participated in the encounter. These can overlap or be sub-sets of the overall encounter's period.") 1015 protected Period period; 1016 1017 /** 1018 * Persons involved in the encounter other than the patient. 1019 */ 1020 @Child(name = "individual", type = { Practitioner.class, PractitionerRole.class, 1021 RelatedPerson.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1022 @Description(shortDefinition = "Persons involved in the encounter other than the patient", formalDefinition = "Persons involved in the encounter other than the patient.") 1023 protected Reference individual; 1024 1025 /** 1026 * The actual object that is the target of the reference (Persons involved in 1027 * the encounter other than the patient.) 1028 */ 1029 protected Resource individualTarget; 1030 1031 private static final long serialVersionUID = 317095765L; 1032 1033 /** 1034 * Constructor 1035 */ 1036 public EncounterParticipantComponent() { 1037 super(); 1038 } 1039 1040 /** 1041 * @return {@link #type} (Role of participant in encounter.) 1042 */ 1043 public List<CodeableConcept> getType() { 1044 if (this.type == null) 1045 this.type = new ArrayList<CodeableConcept>(); 1046 return this.type; 1047 } 1048 1049 /** 1050 * @return Returns a reference to <code>this</code> for easy method chaining 1051 */ 1052 public EncounterParticipantComponent setType(List<CodeableConcept> theType) { 1053 this.type = theType; 1054 return this; 1055 } 1056 1057 public boolean hasType() { 1058 if (this.type == null) 1059 return false; 1060 for (CodeableConcept item : this.type) 1061 if (!item.isEmpty()) 1062 return true; 1063 return false; 1064 } 1065 1066 public CodeableConcept addType() { // 3 1067 CodeableConcept t = new CodeableConcept(); 1068 if (this.type == null) 1069 this.type = new ArrayList<CodeableConcept>(); 1070 this.type.add(t); 1071 return t; 1072 } 1073 1074 public EncounterParticipantComponent addType(CodeableConcept t) { // 3 1075 if (t == null) 1076 return this; 1077 if (this.type == null) 1078 this.type = new ArrayList<CodeableConcept>(); 1079 this.type.add(t); 1080 return this; 1081 } 1082 1083 /** 1084 * @return The first repetition of repeating field {@link #type}, creating it if 1085 * it does not already exist 1086 */ 1087 public CodeableConcept getTypeFirstRep() { 1088 if (getType().isEmpty()) { 1089 addType(); 1090 } 1091 return getType().get(0); 1092 } 1093 1094 /** 1095 * @return {@link #period} (The period of time that the specified participant 1096 * participated in the encounter. These can overlap or be sub-sets of 1097 * the overall encounter's period.) 1098 */ 1099 public Period getPeriod() { 1100 if (this.period == null) 1101 if (Configuration.errorOnAutoCreate()) 1102 throw new Error("Attempt to auto-create EncounterParticipantComponent.period"); 1103 else if (Configuration.doAutoCreate()) 1104 this.period = new Period(); // cc 1105 return this.period; 1106 } 1107 1108 public boolean hasPeriod() { 1109 return this.period != null && !this.period.isEmpty(); 1110 } 1111 1112 /** 1113 * @param value {@link #period} (The period of time that the specified 1114 * participant participated in the encounter. These can overlap or 1115 * be sub-sets of the overall encounter's period.) 1116 */ 1117 public EncounterParticipantComponent setPeriod(Period value) { 1118 this.period = value; 1119 return this; 1120 } 1121 1122 /** 1123 * @return {@link #individual} (Persons involved in the encounter other than the 1124 * patient.) 1125 */ 1126 public Reference getIndividual() { 1127 if (this.individual == null) 1128 if (Configuration.errorOnAutoCreate()) 1129 throw new Error("Attempt to auto-create EncounterParticipantComponent.individual"); 1130 else if (Configuration.doAutoCreate()) 1131 this.individual = new Reference(); // cc 1132 return this.individual; 1133 } 1134 1135 public boolean hasIndividual() { 1136 return this.individual != null && !this.individual.isEmpty(); 1137 } 1138 1139 /** 1140 * @param value {@link #individual} (Persons involved in the encounter other 1141 * than the patient.) 1142 */ 1143 public EncounterParticipantComponent setIndividual(Reference value) { 1144 this.individual = value; 1145 return this; 1146 } 1147 1148 /** 1149 * @return {@link #individual} The actual object that is the target of the 1150 * reference. The reference library doesn't populate this, but you can 1151 * use it to hold the resource if you resolve it. (Persons involved in 1152 * the encounter other than the patient.) 1153 */ 1154 public Resource getIndividualTarget() { 1155 return this.individualTarget; 1156 } 1157 1158 /** 1159 * @param value {@link #individual} The actual object that is the target of the 1160 * reference. The reference library doesn't use these, but you can 1161 * use it to hold the resource if you resolve it. (Persons involved 1162 * in the encounter other than the patient.) 1163 */ 1164 public EncounterParticipantComponent setIndividualTarget(Resource value) { 1165 this.individualTarget = value; 1166 return this; 1167 } 1168 1169 protected void listChildren(List<Property> children) { 1170 super.listChildren(children); 1171 children.add(new Property("type", "CodeableConcept", "Role of participant in encounter.", 0, 1172 java.lang.Integer.MAX_VALUE, type)); 1173 children.add(new Property("period", "Period", 1174 "The period of time that the specified participant participated in the encounter. These can overlap or be sub-sets of the overall encounter's period.", 1175 0, 1, period)); 1176 children.add(new Property("individual", "Reference(Practitioner|PractitionerRole|RelatedPerson)", 1177 "Persons involved in the encounter other than the patient.", 0, 1, individual)); 1178 } 1179 1180 @Override 1181 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1182 switch (_hash) { 1183 case 3575610: 1184 /* type */ return new Property("type", "CodeableConcept", "Role of participant in encounter.", 0, 1185 java.lang.Integer.MAX_VALUE, type); 1186 case -991726143: 1187 /* period */ return new Property("period", "Period", 1188 "The period of time that the specified participant participated in the encounter. These can overlap or be sub-sets of the overall encounter's period.", 1189 0, 1, period); 1190 case -46292327: 1191 /* individual */ return new Property("individual", "Reference(Practitioner|PractitionerRole|RelatedPerson)", 1192 "Persons involved in the encounter other than the patient.", 0, 1, individual); 1193 default: 1194 return super.getNamedProperty(_hash, _name, _checkValid); 1195 } 1196 1197 } 1198 1199 @Override 1200 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1201 switch (hash) { 1202 case 3575610: 1203 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 1204 case -991726143: 1205 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 1206 case -46292327: 1207 /* individual */ return this.individual == null ? new Base[0] : new Base[] { this.individual }; // Reference 1208 default: 1209 return super.getProperty(hash, name, checkValid); 1210 } 1211 1212 } 1213 1214 @Override 1215 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1216 switch (hash) { 1217 case 3575610: // type 1218 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 1219 return value; 1220 case -991726143: // period 1221 this.period = castToPeriod(value); // Period 1222 return value; 1223 case -46292327: // individual 1224 this.individual = castToReference(value); // Reference 1225 return value; 1226 default: 1227 return super.setProperty(hash, name, value); 1228 } 1229 1230 } 1231 1232 @Override 1233 public Base setProperty(String name, Base value) throws FHIRException { 1234 if (name.equals("type")) { 1235 this.getType().add(castToCodeableConcept(value)); 1236 } else if (name.equals("period")) { 1237 this.period = castToPeriod(value); // Period 1238 } else if (name.equals("individual")) { 1239 this.individual = castToReference(value); // Reference 1240 } else 1241 return super.setProperty(name, value); 1242 return value; 1243 } 1244 1245 @Override 1246 public void removeChild(String name, Base value) throws FHIRException { 1247 if (name.equals("type")) { 1248 this.getType().remove(castToCodeableConcept(value)); 1249 } else if (name.equals("period")) { 1250 this.period = null; 1251 } else if (name.equals("individual")) { 1252 this.individual = null; 1253 } else 1254 super.removeChild(name, value); 1255 1256 } 1257 1258 @Override 1259 public Base makeProperty(int hash, String name) throws FHIRException { 1260 switch (hash) { 1261 case 3575610: 1262 return addType(); 1263 case -991726143: 1264 return getPeriod(); 1265 case -46292327: 1266 return getIndividual(); 1267 default: 1268 return super.makeProperty(hash, name); 1269 } 1270 1271 } 1272 1273 @Override 1274 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1275 switch (hash) { 1276 case 3575610: 1277 /* type */ return new String[] { "CodeableConcept" }; 1278 case -991726143: 1279 /* period */ return new String[] { "Period" }; 1280 case -46292327: 1281 /* individual */ return new String[] { "Reference" }; 1282 default: 1283 return super.getTypesForProperty(hash, name); 1284 } 1285 1286 } 1287 1288 @Override 1289 public Base addChild(String name) throws FHIRException { 1290 if (name.equals("type")) { 1291 return addType(); 1292 } else if (name.equals("period")) { 1293 this.period = new Period(); 1294 return this.period; 1295 } else if (name.equals("individual")) { 1296 this.individual = new Reference(); 1297 return this.individual; 1298 } else 1299 return super.addChild(name); 1300 } 1301 1302 public EncounterParticipantComponent copy() { 1303 EncounterParticipantComponent dst = new EncounterParticipantComponent(); 1304 copyValues(dst); 1305 return dst; 1306 } 1307 1308 public void copyValues(EncounterParticipantComponent dst) { 1309 super.copyValues(dst); 1310 if (type != null) { 1311 dst.type = new ArrayList<CodeableConcept>(); 1312 for (CodeableConcept i : type) 1313 dst.type.add(i.copy()); 1314 } 1315 ; 1316 dst.period = period == null ? null : period.copy(); 1317 dst.individual = individual == null ? null : individual.copy(); 1318 } 1319 1320 @Override 1321 public boolean equalsDeep(Base other_) { 1322 if (!super.equalsDeep(other_)) 1323 return false; 1324 if (!(other_ instanceof EncounterParticipantComponent)) 1325 return false; 1326 EncounterParticipantComponent o = (EncounterParticipantComponent) other_; 1327 return compareDeep(type, o.type, true) && compareDeep(period, o.period, true) 1328 && compareDeep(individual, o.individual, true); 1329 } 1330 1331 @Override 1332 public boolean equalsShallow(Base other_) { 1333 if (!super.equalsShallow(other_)) 1334 return false; 1335 if (!(other_ instanceof EncounterParticipantComponent)) 1336 return false; 1337 EncounterParticipantComponent o = (EncounterParticipantComponent) other_; 1338 return true; 1339 } 1340 1341 public boolean isEmpty() { 1342 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, period, individual); 1343 } 1344 1345 public String fhirType() { 1346 return "Encounter.participant"; 1347 1348 } 1349 1350 } 1351 1352 @Block() 1353 public static class DiagnosisComponent extends BackboneElement implements IBaseBackboneElement { 1354 /** 1355 * Reason the encounter takes place, as specified using information from another 1356 * resource. For admissions, this is the admission diagnosis. The indication 1357 * will typically be a Condition (with other resources referenced in the 1358 * evidence.detail), or a Procedure. 1359 */ 1360 @Child(name = "condition", type = { Condition.class, 1361 Procedure.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1362 @Description(shortDefinition = "The diagnosis or procedure relevant to the encounter", formalDefinition = "Reason the encounter takes place, as specified using information from another resource. For admissions, this is the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.") 1363 protected Reference condition; 1364 1365 /** 1366 * The actual object that is the target of the reference (Reason the encounter 1367 * takes place, as specified using information from another resource. For 1368 * admissions, this is the admission diagnosis. The indication will typically be 1369 * a Condition (with other resources referenced in the evidence.detail), or a 1370 * Procedure.) 1371 */ 1372 protected Resource conditionTarget; 1373 1374 /** 1375 * Role that this diagnosis has within the encounter (e.g. admission, billing, 1376 * discharge ?). 1377 */ 1378 @Child(name = "use", type = { 1379 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1380 @Description(shortDefinition = "Role that this diagnosis has within the encounter (e.g. admission, billing, discharge ?)", formalDefinition = "Role that this diagnosis has within the encounter (e.g. admission, billing, discharge ?).") 1381 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/diagnosis-role") 1382 protected CodeableConcept use; 1383 1384 /** 1385 * Ranking of the diagnosis (for each role type). 1386 */ 1387 @Child(name = "rank", type = { 1388 PositiveIntType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1389 @Description(shortDefinition = "Ranking of the diagnosis (for each role type)", formalDefinition = "Ranking of the diagnosis (for each role type).") 1390 protected PositiveIntType rank; 1391 1392 private static final long serialVersionUID = 128213376L; 1393 1394 /** 1395 * Constructor 1396 */ 1397 public DiagnosisComponent() { 1398 super(); 1399 } 1400 1401 /** 1402 * Constructor 1403 */ 1404 public DiagnosisComponent(Reference condition) { 1405 super(); 1406 this.condition = condition; 1407 } 1408 1409 /** 1410 * @return {@link #condition} (Reason the encounter takes place, as specified 1411 * using information from another resource. For admissions, this is the 1412 * admission diagnosis. The indication will typically be a Condition 1413 * (with other resources referenced in the evidence.detail), or a 1414 * Procedure.) 1415 */ 1416 public Reference getCondition() { 1417 if (this.condition == null) 1418 if (Configuration.errorOnAutoCreate()) 1419 throw new Error("Attempt to auto-create DiagnosisComponent.condition"); 1420 else if (Configuration.doAutoCreate()) 1421 this.condition = new Reference(); // cc 1422 return this.condition; 1423 } 1424 1425 public boolean hasCondition() { 1426 return this.condition != null && !this.condition.isEmpty(); 1427 } 1428 1429 /** 1430 * @param value {@link #condition} (Reason the encounter takes place, as 1431 * specified using information from another resource. For 1432 * admissions, this is the admission diagnosis. The indication will 1433 * typically be a Condition (with other resources referenced in the 1434 * evidence.detail), or a Procedure.) 1435 */ 1436 public DiagnosisComponent setCondition(Reference value) { 1437 this.condition = value; 1438 return this; 1439 } 1440 1441 /** 1442 * @return {@link #condition} The actual object that is the target of the 1443 * reference. The reference library doesn't populate this, but you can 1444 * use it to hold the resource if you resolve it. (Reason the encounter 1445 * takes place, as specified using information from another resource. 1446 * For admissions, this is the admission diagnosis. The indication will 1447 * typically be a Condition (with other resources referenced in the 1448 * evidence.detail), or a Procedure.) 1449 */ 1450 public Resource getConditionTarget() { 1451 return this.conditionTarget; 1452 } 1453 1454 /** 1455 * @param value {@link #condition} The actual object that is the target of the 1456 * reference. The reference library doesn't use these, but you can 1457 * use it to hold the resource if you resolve it. (Reason the 1458 * encounter takes place, as specified using information from 1459 * another resource. For admissions, this is the admission 1460 * diagnosis. The indication will typically be a Condition (with 1461 * other resources referenced in the evidence.detail), or a 1462 * Procedure.) 1463 */ 1464 public DiagnosisComponent setConditionTarget(Resource value) { 1465 this.conditionTarget = value; 1466 return this; 1467 } 1468 1469 /** 1470 * @return {@link #use} (Role that this diagnosis has within the encounter (e.g. 1471 * admission, billing, discharge ?).) 1472 */ 1473 public CodeableConcept getUse() { 1474 if (this.use == null) 1475 if (Configuration.errorOnAutoCreate()) 1476 throw new Error("Attempt to auto-create DiagnosisComponent.use"); 1477 else if (Configuration.doAutoCreate()) 1478 this.use = new CodeableConcept(); // cc 1479 return this.use; 1480 } 1481 1482 public boolean hasUse() { 1483 return this.use != null && !this.use.isEmpty(); 1484 } 1485 1486 /** 1487 * @param value {@link #use} (Role that this diagnosis has within the encounter 1488 * (e.g. admission, billing, discharge ?).) 1489 */ 1490 public DiagnosisComponent setUse(CodeableConcept value) { 1491 this.use = value; 1492 return this; 1493 } 1494 1495 /** 1496 * @return {@link #rank} (Ranking of the diagnosis (for each role type).). This 1497 * is the underlying object with id, value and extensions. The accessor 1498 * "getRank" gives direct access to the value 1499 */ 1500 public PositiveIntType getRankElement() { 1501 if (this.rank == null) 1502 if (Configuration.errorOnAutoCreate()) 1503 throw new Error("Attempt to auto-create DiagnosisComponent.rank"); 1504 else if (Configuration.doAutoCreate()) 1505 this.rank = new PositiveIntType(); // bb 1506 return this.rank; 1507 } 1508 1509 public boolean hasRankElement() { 1510 return this.rank != null && !this.rank.isEmpty(); 1511 } 1512 1513 public boolean hasRank() { 1514 return this.rank != null && !this.rank.isEmpty(); 1515 } 1516 1517 /** 1518 * @param value {@link #rank} (Ranking of the diagnosis (for each role type).). 1519 * This is the underlying object with id, value and extensions. The 1520 * accessor "getRank" gives direct access to the value 1521 */ 1522 public DiagnosisComponent setRankElement(PositiveIntType value) { 1523 this.rank = value; 1524 return this; 1525 } 1526 1527 /** 1528 * @return Ranking of the diagnosis (for each role type). 1529 */ 1530 public int getRank() { 1531 return this.rank == null || this.rank.isEmpty() ? 0 : this.rank.getValue(); 1532 } 1533 1534 /** 1535 * @param value Ranking of the diagnosis (for each role type). 1536 */ 1537 public DiagnosisComponent setRank(int value) { 1538 if (this.rank == null) 1539 this.rank = new PositiveIntType(); 1540 this.rank.setValue(value); 1541 return this; 1542 } 1543 1544 protected void listChildren(List<Property> children) { 1545 super.listChildren(children); 1546 children.add(new Property("condition", "Reference(Condition|Procedure)", 1547 "Reason the encounter takes place, as specified using information from another resource. For admissions, this is the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.", 1548 0, 1, condition)); 1549 children.add(new Property("use", "CodeableConcept", 1550 "Role that this diagnosis has within the encounter (e.g. admission, billing, discharge ?).", 0, 1, use)); 1551 children.add(new Property("rank", "positiveInt", "Ranking of the diagnosis (for each role type).", 0, 1, rank)); 1552 } 1553 1554 @Override 1555 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1556 switch (_hash) { 1557 case -861311717: 1558 /* condition */ return new Property("condition", "Reference(Condition|Procedure)", 1559 "Reason the encounter takes place, as specified using information from another resource. For admissions, this is the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.", 1560 0, 1, condition); 1561 case 116103: 1562 /* use */ return new Property("use", "CodeableConcept", 1563 "Role that this diagnosis has within the encounter (e.g. admission, billing, discharge ?).", 0, 1, use); 1564 case 3492908: 1565 /* rank */ return new Property("rank", "positiveInt", "Ranking of the diagnosis (for each role type).", 0, 1, 1566 rank); 1567 default: 1568 return super.getNamedProperty(_hash, _name, _checkValid); 1569 } 1570 1571 } 1572 1573 @Override 1574 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1575 switch (hash) { 1576 case -861311717: 1577 /* condition */ return this.condition == null ? new Base[0] : new Base[] { this.condition }; // Reference 1578 case 116103: 1579 /* use */ return this.use == null ? new Base[0] : new Base[] { this.use }; // CodeableConcept 1580 case 3492908: 1581 /* rank */ return this.rank == null ? new Base[0] : new Base[] { this.rank }; // PositiveIntType 1582 default: 1583 return super.getProperty(hash, name, checkValid); 1584 } 1585 1586 } 1587 1588 @Override 1589 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1590 switch (hash) { 1591 case -861311717: // condition 1592 this.condition = castToReference(value); // Reference 1593 return value; 1594 case 116103: // use 1595 this.use = castToCodeableConcept(value); // CodeableConcept 1596 return value; 1597 case 3492908: // rank 1598 this.rank = castToPositiveInt(value); // PositiveIntType 1599 return value; 1600 default: 1601 return super.setProperty(hash, name, value); 1602 } 1603 1604 } 1605 1606 @Override 1607 public Base setProperty(String name, Base value) throws FHIRException { 1608 if (name.equals("condition")) { 1609 this.condition = castToReference(value); // Reference 1610 } else if (name.equals("use")) { 1611 this.use = castToCodeableConcept(value); // CodeableConcept 1612 } else if (name.equals("rank")) { 1613 this.rank = castToPositiveInt(value); // PositiveIntType 1614 } else 1615 return super.setProperty(name, value); 1616 return value; 1617 } 1618 1619 @Override 1620 public void removeChild(String name, Base value) throws FHIRException { 1621 if (name.equals("condition")) { 1622 this.condition = null; 1623 } else if (name.equals("use")) { 1624 this.use = null; 1625 } else if (name.equals("rank")) { 1626 this.rank = null; 1627 } else 1628 super.removeChild(name, value); 1629 1630 } 1631 1632 @Override 1633 public Base makeProperty(int hash, String name) throws FHIRException { 1634 switch (hash) { 1635 case -861311717: 1636 return getCondition(); 1637 case 116103: 1638 return getUse(); 1639 case 3492908: 1640 return getRankElement(); 1641 default: 1642 return super.makeProperty(hash, name); 1643 } 1644 1645 } 1646 1647 @Override 1648 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1649 switch (hash) { 1650 case -861311717: 1651 /* condition */ return new String[] { "Reference" }; 1652 case 116103: 1653 /* use */ return new String[] { "CodeableConcept" }; 1654 case 3492908: 1655 /* rank */ return new String[] { "positiveInt" }; 1656 default: 1657 return super.getTypesForProperty(hash, name); 1658 } 1659 1660 } 1661 1662 @Override 1663 public Base addChild(String name) throws FHIRException { 1664 if (name.equals("condition")) { 1665 this.condition = new Reference(); 1666 return this.condition; 1667 } else if (name.equals("use")) { 1668 this.use = new CodeableConcept(); 1669 return this.use; 1670 } else if (name.equals("rank")) { 1671 throw new FHIRException("Cannot call addChild on a singleton property Encounter.rank"); 1672 } else 1673 return super.addChild(name); 1674 } 1675 1676 public DiagnosisComponent copy() { 1677 DiagnosisComponent dst = new DiagnosisComponent(); 1678 copyValues(dst); 1679 return dst; 1680 } 1681 1682 public void copyValues(DiagnosisComponent dst) { 1683 super.copyValues(dst); 1684 dst.condition = condition == null ? null : condition.copy(); 1685 dst.use = use == null ? null : use.copy(); 1686 dst.rank = rank == null ? null : rank.copy(); 1687 } 1688 1689 @Override 1690 public boolean equalsDeep(Base other_) { 1691 if (!super.equalsDeep(other_)) 1692 return false; 1693 if (!(other_ instanceof DiagnosisComponent)) 1694 return false; 1695 DiagnosisComponent o = (DiagnosisComponent) other_; 1696 return compareDeep(condition, o.condition, true) && compareDeep(use, o.use, true) 1697 && compareDeep(rank, o.rank, true); 1698 } 1699 1700 @Override 1701 public boolean equalsShallow(Base other_) { 1702 if (!super.equalsShallow(other_)) 1703 return false; 1704 if (!(other_ instanceof DiagnosisComponent)) 1705 return false; 1706 DiagnosisComponent o = (DiagnosisComponent) other_; 1707 return compareValues(rank, o.rank, true); 1708 } 1709 1710 public boolean isEmpty() { 1711 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(condition, use, rank); 1712 } 1713 1714 public String fhirType() { 1715 return "Encounter.diagnosis"; 1716 1717 } 1718 1719 } 1720 1721 @Block() 1722 public static class EncounterHospitalizationComponent extends BackboneElement implements IBaseBackboneElement { 1723 /** 1724 * Pre-admission identifier. 1725 */ 1726 @Child(name = "preAdmissionIdentifier", type = { 1727 Identifier.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1728 @Description(shortDefinition = "Pre-admission identifier", formalDefinition = "Pre-admission identifier.") 1729 protected Identifier preAdmissionIdentifier; 1730 1731 /** 1732 * The location/organization from which the patient came before admission. 1733 */ 1734 @Child(name = "origin", type = { Location.class, 1735 Organization.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1736 @Description(shortDefinition = "The location/organization from which the patient came before admission", formalDefinition = "The location/organization from which the patient came before admission.") 1737 protected Reference origin; 1738 1739 /** 1740 * The actual object that is the target of the reference (The 1741 * location/organization from which the patient came before admission.) 1742 */ 1743 protected Resource originTarget; 1744 1745 /** 1746 * From where patient was admitted (physician referral, transfer). 1747 */ 1748 @Child(name = "admitSource", type = { 1749 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1750 @Description(shortDefinition = "From where patient was admitted (physician referral, transfer)", formalDefinition = "From where patient was admitted (physician referral, transfer).") 1751 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-admit-source") 1752 protected CodeableConcept admitSource; 1753 1754 /** 1755 * Whether this hospitalization is a readmission and why if known. 1756 */ 1757 @Child(name = "reAdmission", type = { 1758 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1759 @Description(shortDefinition = "The type of hospital re-admission that has occurred (if any). If the value is absent, then this is not identified as a readmission", formalDefinition = "Whether this hospitalization is a readmission and why if known.") 1760 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v2-0092") 1761 protected CodeableConcept reAdmission; 1762 1763 /** 1764 * Diet preferences reported by the patient. 1765 */ 1766 @Child(name = "dietPreference", type = { 1767 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1768 @Description(shortDefinition = "Diet preferences reported by the patient", formalDefinition = "Diet preferences reported by the patient.") 1769 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-diet") 1770 protected List<CodeableConcept> dietPreference; 1771 1772 /** 1773 * Special courtesies (VIP, board member). 1774 */ 1775 @Child(name = "specialCourtesy", type = { 1776 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1777 @Description(shortDefinition = "Special courtesies (VIP, board member)", formalDefinition = "Special courtesies (VIP, board member).") 1778 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-special-courtesy") 1779 protected List<CodeableConcept> specialCourtesy; 1780 1781 /** 1782 * Any special requests that have been made for this hospitalization encounter, 1783 * such as the provision of specific equipment or other things. 1784 */ 1785 @Child(name = "specialArrangement", type = { 1786 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1787 @Description(shortDefinition = "Wheelchair, translator, stretcher, etc.", formalDefinition = "Any special requests that have been made for this hospitalization encounter, such as the provision of specific equipment or other things.") 1788 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-special-arrangements") 1789 protected List<CodeableConcept> specialArrangement; 1790 1791 /** 1792 * Location/organization to which the patient is discharged. 1793 */ 1794 @Child(name = "destination", type = { Location.class, 1795 Organization.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1796 @Description(shortDefinition = "Location/organization to which the patient is discharged", formalDefinition = "Location/organization to which the patient is discharged.") 1797 protected Reference destination; 1798 1799 /** 1800 * The actual object that is the target of the reference (Location/organization 1801 * to which the patient is discharged.) 1802 */ 1803 protected Resource destinationTarget; 1804 1805 /** 1806 * Category or kind of location after discharge. 1807 */ 1808 @Child(name = "dischargeDisposition", type = { 1809 CodeableConcept.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1810 @Description(shortDefinition = "Category or kind of location after discharge", formalDefinition = "Category or kind of location after discharge.") 1811 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-discharge-disposition") 1812 protected CodeableConcept dischargeDisposition; 1813 1814 private static final long serialVersionUID = 1350555270L; 1815 1816 /** 1817 * Constructor 1818 */ 1819 public EncounterHospitalizationComponent() { 1820 super(); 1821 } 1822 1823 /** 1824 * @return {@link #preAdmissionIdentifier} (Pre-admission identifier.) 1825 */ 1826 public Identifier getPreAdmissionIdentifier() { 1827 if (this.preAdmissionIdentifier == null) 1828 if (Configuration.errorOnAutoCreate()) 1829 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.preAdmissionIdentifier"); 1830 else if (Configuration.doAutoCreate()) 1831 this.preAdmissionIdentifier = new Identifier(); // cc 1832 return this.preAdmissionIdentifier; 1833 } 1834 1835 public boolean hasPreAdmissionIdentifier() { 1836 return this.preAdmissionIdentifier != null && !this.preAdmissionIdentifier.isEmpty(); 1837 } 1838 1839 /** 1840 * @param value {@link #preAdmissionIdentifier} (Pre-admission identifier.) 1841 */ 1842 public EncounterHospitalizationComponent setPreAdmissionIdentifier(Identifier value) { 1843 this.preAdmissionIdentifier = value; 1844 return this; 1845 } 1846 1847 /** 1848 * @return {@link #origin} (The location/organization from which the patient 1849 * came before admission.) 1850 */ 1851 public Reference getOrigin() { 1852 if (this.origin == null) 1853 if (Configuration.errorOnAutoCreate()) 1854 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.origin"); 1855 else if (Configuration.doAutoCreate()) 1856 this.origin = new Reference(); // cc 1857 return this.origin; 1858 } 1859 1860 public boolean hasOrigin() { 1861 return this.origin != null && !this.origin.isEmpty(); 1862 } 1863 1864 /** 1865 * @param value {@link #origin} (The location/organization from which the 1866 * patient came before admission.) 1867 */ 1868 public EncounterHospitalizationComponent setOrigin(Reference value) { 1869 this.origin = value; 1870 return this; 1871 } 1872 1873 /** 1874 * @return {@link #origin} The actual object that is the target of the 1875 * reference. The reference library doesn't populate this, but you can 1876 * use it to hold the resource if you resolve it. (The 1877 * location/organization from which the patient came before admission.) 1878 */ 1879 public Resource getOriginTarget() { 1880 return this.originTarget; 1881 } 1882 1883 /** 1884 * @param value {@link #origin} The actual object that is the target of the 1885 * reference. The reference library doesn't use these, but you can 1886 * use it to hold the resource if you resolve it. (The 1887 * location/organization from which the patient came before 1888 * admission.) 1889 */ 1890 public EncounterHospitalizationComponent setOriginTarget(Resource value) { 1891 this.originTarget = value; 1892 return this; 1893 } 1894 1895 /** 1896 * @return {@link #admitSource} (From where patient was admitted (physician 1897 * referral, transfer).) 1898 */ 1899 public CodeableConcept getAdmitSource() { 1900 if (this.admitSource == null) 1901 if (Configuration.errorOnAutoCreate()) 1902 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.admitSource"); 1903 else if (Configuration.doAutoCreate()) 1904 this.admitSource = new CodeableConcept(); // cc 1905 return this.admitSource; 1906 } 1907 1908 public boolean hasAdmitSource() { 1909 return this.admitSource != null && !this.admitSource.isEmpty(); 1910 } 1911 1912 /** 1913 * @param value {@link #admitSource} (From where patient was admitted (physician 1914 * referral, transfer).) 1915 */ 1916 public EncounterHospitalizationComponent setAdmitSource(CodeableConcept value) { 1917 this.admitSource = value; 1918 return this; 1919 } 1920 1921 /** 1922 * @return {@link #reAdmission} (Whether this hospitalization is a readmission 1923 * and why if known.) 1924 */ 1925 public CodeableConcept getReAdmission() { 1926 if (this.reAdmission == null) 1927 if (Configuration.errorOnAutoCreate()) 1928 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.reAdmission"); 1929 else if (Configuration.doAutoCreate()) 1930 this.reAdmission = new CodeableConcept(); // cc 1931 return this.reAdmission; 1932 } 1933 1934 public boolean hasReAdmission() { 1935 return this.reAdmission != null && !this.reAdmission.isEmpty(); 1936 } 1937 1938 /** 1939 * @param value {@link #reAdmission} (Whether this hospitalization is a 1940 * readmission and why if known.) 1941 */ 1942 public EncounterHospitalizationComponent setReAdmission(CodeableConcept value) { 1943 this.reAdmission = value; 1944 return this; 1945 } 1946 1947 /** 1948 * @return {@link #dietPreference} (Diet preferences reported by the patient.) 1949 */ 1950 public List<CodeableConcept> getDietPreference() { 1951 if (this.dietPreference == null) 1952 this.dietPreference = new ArrayList<CodeableConcept>(); 1953 return this.dietPreference; 1954 } 1955 1956 /** 1957 * @return Returns a reference to <code>this</code> for easy method chaining 1958 */ 1959 public EncounterHospitalizationComponent setDietPreference(List<CodeableConcept> theDietPreference) { 1960 this.dietPreference = theDietPreference; 1961 return this; 1962 } 1963 1964 public boolean hasDietPreference() { 1965 if (this.dietPreference == null) 1966 return false; 1967 for (CodeableConcept item : this.dietPreference) 1968 if (!item.isEmpty()) 1969 return true; 1970 return false; 1971 } 1972 1973 public CodeableConcept addDietPreference() { // 3 1974 CodeableConcept t = new CodeableConcept(); 1975 if (this.dietPreference == null) 1976 this.dietPreference = new ArrayList<CodeableConcept>(); 1977 this.dietPreference.add(t); 1978 return t; 1979 } 1980 1981 public EncounterHospitalizationComponent addDietPreference(CodeableConcept t) { // 3 1982 if (t == null) 1983 return this; 1984 if (this.dietPreference == null) 1985 this.dietPreference = new ArrayList<CodeableConcept>(); 1986 this.dietPreference.add(t); 1987 return this; 1988 } 1989 1990 /** 1991 * @return The first repetition of repeating field {@link #dietPreference}, 1992 * creating it if it does not already exist 1993 */ 1994 public CodeableConcept getDietPreferenceFirstRep() { 1995 if (getDietPreference().isEmpty()) { 1996 addDietPreference(); 1997 } 1998 return getDietPreference().get(0); 1999 } 2000 2001 /** 2002 * @return {@link #specialCourtesy} (Special courtesies (VIP, board member).) 2003 */ 2004 public List<CodeableConcept> getSpecialCourtesy() { 2005 if (this.specialCourtesy == null) 2006 this.specialCourtesy = new ArrayList<CodeableConcept>(); 2007 return this.specialCourtesy; 2008 } 2009 2010 /** 2011 * @return Returns a reference to <code>this</code> for easy method chaining 2012 */ 2013 public EncounterHospitalizationComponent setSpecialCourtesy(List<CodeableConcept> theSpecialCourtesy) { 2014 this.specialCourtesy = theSpecialCourtesy; 2015 return this; 2016 } 2017 2018 public boolean hasSpecialCourtesy() { 2019 if (this.specialCourtesy == null) 2020 return false; 2021 for (CodeableConcept item : this.specialCourtesy) 2022 if (!item.isEmpty()) 2023 return true; 2024 return false; 2025 } 2026 2027 public CodeableConcept addSpecialCourtesy() { // 3 2028 CodeableConcept t = new CodeableConcept(); 2029 if (this.specialCourtesy == null) 2030 this.specialCourtesy = new ArrayList<CodeableConcept>(); 2031 this.specialCourtesy.add(t); 2032 return t; 2033 } 2034 2035 public EncounterHospitalizationComponent addSpecialCourtesy(CodeableConcept t) { // 3 2036 if (t == null) 2037 return this; 2038 if (this.specialCourtesy == null) 2039 this.specialCourtesy = new ArrayList<CodeableConcept>(); 2040 this.specialCourtesy.add(t); 2041 return this; 2042 } 2043 2044 /** 2045 * @return The first repetition of repeating field {@link #specialCourtesy}, 2046 * creating it if it does not already exist 2047 */ 2048 public CodeableConcept getSpecialCourtesyFirstRep() { 2049 if (getSpecialCourtesy().isEmpty()) { 2050 addSpecialCourtesy(); 2051 } 2052 return getSpecialCourtesy().get(0); 2053 } 2054 2055 /** 2056 * @return {@link #specialArrangement} (Any special requests that have been made 2057 * for this hospitalization encounter, such as the provision of specific 2058 * equipment or other things.) 2059 */ 2060 public List<CodeableConcept> getSpecialArrangement() { 2061 if (this.specialArrangement == null) 2062 this.specialArrangement = new ArrayList<CodeableConcept>(); 2063 return this.specialArrangement; 2064 } 2065 2066 /** 2067 * @return Returns a reference to <code>this</code> for easy method chaining 2068 */ 2069 public EncounterHospitalizationComponent setSpecialArrangement(List<CodeableConcept> theSpecialArrangement) { 2070 this.specialArrangement = theSpecialArrangement; 2071 return this; 2072 } 2073 2074 public boolean hasSpecialArrangement() { 2075 if (this.specialArrangement == null) 2076 return false; 2077 for (CodeableConcept item : this.specialArrangement) 2078 if (!item.isEmpty()) 2079 return true; 2080 return false; 2081 } 2082 2083 public CodeableConcept addSpecialArrangement() { // 3 2084 CodeableConcept t = new CodeableConcept(); 2085 if (this.specialArrangement == null) 2086 this.specialArrangement = new ArrayList<CodeableConcept>(); 2087 this.specialArrangement.add(t); 2088 return t; 2089 } 2090 2091 public EncounterHospitalizationComponent addSpecialArrangement(CodeableConcept t) { // 3 2092 if (t == null) 2093 return this; 2094 if (this.specialArrangement == null) 2095 this.specialArrangement = new ArrayList<CodeableConcept>(); 2096 this.specialArrangement.add(t); 2097 return this; 2098 } 2099 2100 /** 2101 * @return The first repetition of repeating field {@link #specialArrangement}, 2102 * creating it if it does not already exist 2103 */ 2104 public CodeableConcept getSpecialArrangementFirstRep() { 2105 if (getSpecialArrangement().isEmpty()) { 2106 addSpecialArrangement(); 2107 } 2108 return getSpecialArrangement().get(0); 2109 } 2110 2111 /** 2112 * @return {@link #destination} (Location/organization to which the patient is 2113 * discharged.) 2114 */ 2115 public Reference getDestination() { 2116 if (this.destination == null) 2117 if (Configuration.errorOnAutoCreate()) 2118 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.destination"); 2119 else if (Configuration.doAutoCreate()) 2120 this.destination = new Reference(); // cc 2121 return this.destination; 2122 } 2123 2124 public boolean hasDestination() { 2125 return this.destination != null && !this.destination.isEmpty(); 2126 } 2127 2128 /** 2129 * @param value {@link #destination} (Location/organization to which the patient 2130 * is discharged.) 2131 */ 2132 public EncounterHospitalizationComponent setDestination(Reference value) { 2133 this.destination = value; 2134 return this; 2135 } 2136 2137 /** 2138 * @return {@link #destination} The actual object that is the target of the 2139 * reference. The reference library doesn't populate this, but you can 2140 * use it to hold the resource if you resolve it. (Location/organization 2141 * to which the patient is discharged.) 2142 */ 2143 public Resource getDestinationTarget() { 2144 return this.destinationTarget; 2145 } 2146 2147 /** 2148 * @param value {@link #destination} The actual object that is the target of the 2149 * reference. The reference library doesn't use these, but you can 2150 * use it to hold the resource if you resolve it. 2151 * (Location/organization to which the patient is discharged.) 2152 */ 2153 public EncounterHospitalizationComponent setDestinationTarget(Resource value) { 2154 this.destinationTarget = value; 2155 return this; 2156 } 2157 2158 /** 2159 * @return {@link #dischargeDisposition} (Category or kind of location after 2160 * discharge.) 2161 */ 2162 public CodeableConcept getDischargeDisposition() { 2163 if (this.dischargeDisposition == null) 2164 if (Configuration.errorOnAutoCreate()) 2165 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.dischargeDisposition"); 2166 else if (Configuration.doAutoCreate()) 2167 this.dischargeDisposition = new CodeableConcept(); // cc 2168 return this.dischargeDisposition; 2169 } 2170 2171 public boolean hasDischargeDisposition() { 2172 return this.dischargeDisposition != null && !this.dischargeDisposition.isEmpty(); 2173 } 2174 2175 /** 2176 * @param value {@link #dischargeDisposition} (Category or kind of location 2177 * after discharge.) 2178 */ 2179 public EncounterHospitalizationComponent setDischargeDisposition(CodeableConcept value) { 2180 this.dischargeDisposition = value; 2181 return this; 2182 } 2183 2184 protected void listChildren(List<Property> children) { 2185 super.listChildren(children); 2186 children.add(new Property("preAdmissionIdentifier", "Identifier", "Pre-admission identifier.", 0, 1, 2187 preAdmissionIdentifier)); 2188 children.add(new Property("origin", "Reference(Location|Organization)", 2189 "The location/organization from which the patient came before admission.", 0, 1, origin)); 2190 children.add(new Property("admitSource", "CodeableConcept", 2191 "From where patient was admitted (physician referral, transfer).", 0, 1, admitSource)); 2192 children.add(new Property("reAdmission", "CodeableConcept", 2193 "Whether this hospitalization is a readmission and why if known.", 0, 1, reAdmission)); 2194 children.add(new Property("dietPreference", "CodeableConcept", "Diet preferences reported by the patient.", 0, 2195 java.lang.Integer.MAX_VALUE, dietPreference)); 2196 children.add(new Property("specialCourtesy", "CodeableConcept", "Special courtesies (VIP, board member).", 0, 2197 java.lang.Integer.MAX_VALUE, specialCourtesy)); 2198 children.add(new Property("specialArrangement", "CodeableConcept", 2199 "Any special requests that have been made for this hospitalization encounter, such as the provision of specific equipment or other things.", 2200 0, java.lang.Integer.MAX_VALUE, specialArrangement)); 2201 children.add(new Property("destination", "Reference(Location|Organization)", 2202 "Location/organization to which the patient is discharged.", 0, 1, destination)); 2203 children.add(new Property("dischargeDisposition", "CodeableConcept", 2204 "Category or kind of location after discharge.", 0, 1, dischargeDisposition)); 2205 } 2206 2207 @Override 2208 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2209 switch (_hash) { 2210 case -965394961: 2211 /* preAdmissionIdentifier */ return new Property("preAdmissionIdentifier", "Identifier", 2212 "Pre-admission identifier.", 0, 1, preAdmissionIdentifier); 2213 case -1008619738: 2214 /* origin */ return new Property("origin", "Reference(Location|Organization)", 2215 "The location/organization from which the patient came before admission.", 0, 1, origin); 2216 case 538887120: 2217 /* admitSource */ return new Property("admitSource", "CodeableConcept", 2218 "From where patient was admitted (physician referral, transfer).", 0, 1, admitSource); 2219 case 669348630: 2220 /* reAdmission */ return new Property("reAdmission", "CodeableConcept", 2221 "Whether this hospitalization is a readmission and why if known.", 0, 1, reAdmission); 2222 case -1360641041: 2223 /* dietPreference */ return new Property("dietPreference", "CodeableConcept", 2224 "Diet preferences reported by the patient.", 0, java.lang.Integer.MAX_VALUE, dietPreference); 2225 case 1583588345: 2226 /* specialCourtesy */ return new Property("specialCourtesy", "CodeableConcept", 2227 "Special courtesies (VIP, board member).", 0, java.lang.Integer.MAX_VALUE, specialCourtesy); 2228 case 47410321: 2229 /* specialArrangement */ return new Property("specialArrangement", "CodeableConcept", 2230 "Any special requests that have been made for this hospitalization encounter, such as the provision of specific equipment or other things.", 2231 0, java.lang.Integer.MAX_VALUE, specialArrangement); 2232 case -1429847026: 2233 /* destination */ return new Property("destination", "Reference(Location|Organization)", 2234 "Location/organization to which the patient is discharged.", 0, 1, destination); 2235 case 528065941: 2236 /* dischargeDisposition */ return new Property("dischargeDisposition", "CodeableConcept", 2237 "Category or kind of location after discharge.", 0, 1, dischargeDisposition); 2238 default: 2239 return super.getNamedProperty(_hash, _name, _checkValid); 2240 } 2241 2242 } 2243 2244 @Override 2245 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2246 switch (hash) { 2247 case -965394961: 2248 /* preAdmissionIdentifier */ return this.preAdmissionIdentifier == null ? new Base[0] 2249 : new Base[] { this.preAdmissionIdentifier }; // Identifier 2250 case -1008619738: 2251 /* origin */ return this.origin == null ? new Base[0] : new Base[] { this.origin }; // Reference 2252 case 538887120: 2253 /* admitSource */ return this.admitSource == null ? new Base[0] : new Base[] { this.admitSource }; // CodeableConcept 2254 case 669348630: 2255 /* reAdmission */ return this.reAdmission == null ? new Base[0] : new Base[] { this.reAdmission }; // CodeableConcept 2256 case -1360641041: 2257 /* dietPreference */ return this.dietPreference == null ? new Base[0] 2258 : this.dietPreference.toArray(new Base[this.dietPreference.size()]); // CodeableConcept 2259 case 1583588345: 2260 /* specialCourtesy */ return this.specialCourtesy == null ? new Base[0] 2261 : this.specialCourtesy.toArray(new Base[this.specialCourtesy.size()]); // CodeableConcept 2262 case 47410321: 2263 /* specialArrangement */ return this.specialArrangement == null ? new Base[0] 2264 : this.specialArrangement.toArray(new Base[this.specialArrangement.size()]); // CodeableConcept 2265 case -1429847026: 2266 /* destination */ return this.destination == null ? new Base[0] : new Base[] { this.destination }; // Reference 2267 case 528065941: 2268 /* dischargeDisposition */ return this.dischargeDisposition == null ? new Base[0] 2269 : new Base[] { this.dischargeDisposition }; // CodeableConcept 2270 default: 2271 return super.getProperty(hash, name, checkValid); 2272 } 2273 2274 } 2275 2276 @Override 2277 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2278 switch (hash) { 2279 case -965394961: // preAdmissionIdentifier 2280 this.preAdmissionIdentifier = castToIdentifier(value); // Identifier 2281 return value; 2282 case -1008619738: // origin 2283 this.origin = castToReference(value); // Reference 2284 return value; 2285 case 538887120: // admitSource 2286 this.admitSource = castToCodeableConcept(value); // CodeableConcept 2287 return value; 2288 case 669348630: // reAdmission 2289 this.reAdmission = castToCodeableConcept(value); // CodeableConcept 2290 return value; 2291 case -1360641041: // dietPreference 2292 this.getDietPreference().add(castToCodeableConcept(value)); // CodeableConcept 2293 return value; 2294 case 1583588345: // specialCourtesy 2295 this.getSpecialCourtesy().add(castToCodeableConcept(value)); // CodeableConcept 2296 return value; 2297 case 47410321: // specialArrangement 2298 this.getSpecialArrangement().add(castToCodeableConcept(value)); // CodeableConcept 2299 return value; 2300 case -1429847026: // destination 2301 this.destination = castToReference(value); // Reference 2302 return value; 2303 case 528065941: // dischargeDisposition 2304 this.dischargeDisposition = castToCodeableConcept(value); // CodeableConcept 2305 return value; 2306 default: 2307 return super.setProperty(hash, name, value); 2308 } 2309 2310 } 2311 2312 @Override 2313 public Base setProperty(String name, Base value) throws FHIRException { 2314 if (name.equals("preAdmissionIdentifier")) { 2315 this.preAdmissionIdentifier = castToIdentifier(value); // Identifier 2316 } else if (name.equals("origin")) { 2317 this.origin = castToReference(value); // Reference 2318 } else if (name.equals("admitSource")) { 2319 this.admitSource = castToCodeableConcept(value); // CodeableConcept 2320 } else if (name.equals("reAdmission")) { 2321 this.reAdmission = castToCodeableConcept(value); // CodeableConcept 2322 } else if (name.equals("dietPreference")) { 2323 this.getDietPreference().add(castToCodeableConcept(value)); 2324 } else if (name.equals("specialCourtesy")) { 2325 this.getSpecialCourtesy().add(castToCodeableConcept(value)); 2326 } else if (name.equals("specialArrangement")) { 2327 this.getSpecialArrangement().add(castToCodeableConcept(value)); 2328 } else if (name.equals("destination")) { 2329 this.destination = castToReference(value); // Reference 2330 } else if (name.equals("dischargeDisposition")) { 2331 this.dischargeDisposition = castToCodeableConcept(value); // CodeableConcept 2332 } else 2333 return super.setProperty(name, value); 2334 return value; 2335 } 2336 2337 @Override 2338 public void removeChild(String name, Base value) throws FHIRException { 2339 if (name.equals("preAdmissionIdentifier")) { 2340 this.preAdmissionIdentifier = null; 2341 } else if (name.equals("origin")) { 2342 this.origin = null; 2343 } else if (name.equals("admitSource")) { 2344 this.admitSource = null; 2345 } else if (name.equals("reAdmission")) { 2346 this.reAdmission = null; 2347 } else if (name.equals("dietPreference")) { 2348 this.getDietPreference().remove(castToCodeableConcept(value)); 2349 } else if (name.equals("specialCourtesy")) { 2350 this.getSpecialCourtesy().remove(castToCodeableConcept(value)); 2351 } else if (name.equals("specialArrangement")) { 2352 this.getSpecialArrangement().remove(castToCodeableConcept(value)); 2353 } else if (name.equals("destination")) { 2354 this.destination = null; 2355 } else if (name.equals("dischargeDisposition")) { 2356 this.dischargeDisposition = null; 2357 } else 2358 super.removeChild(name, value); 2359 2360 } 2361 2362 @Override 2363 public Base makeProperty(int hash, String name) throws FHIRException { 2364 switch (hash) { 2365 case -965394961: 2366 return getPreAdmissionIdentifier(); 2367 case -1008619738: 2368 return getOrigin(); 2369 case 538887120: 2370 return getAdmitSource(); 2371 case 669348630: 2372 return getReAdmission(); 2373 case -1360641041: 2374 return addDietPreference(); 2375 case 1583588345: 2376 return addSpecialCourtesy(); 2377 case 47410321: 2378 return addSpecialArrangement(); 2379 case -1429847026: 2380 return getDestination(); 2381 case 528065941: 2382 return getDischargeDisposition(); 2383 default: 2384 return super.makeProperty(hash, name); 2385 } 2386 2387 } 2388 2389 @Override 2390 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2391 switch (hash) { 2392 case -965394961: 2393 /* preAdmissionIdentifier */ return new String[] { "Identifier" }; 2394 case -1008619738: 2395 /* origin */ return new String[] { "Reference" }; 2396 case 538887120: 2397 /* admitSource */ return new String[] { "CodeableConcept" }; 2398 case 669348630: 2399 /* reAdmission */ return new String[] { "CodeableConcept" }; 2400 case -1360641041: 2401 /* dietPreference */ return new String[] { "CodeableConcept" }; 2402 case 1583588345: 2403 /* specialCourtesy */ return new String[] { "CodeableConcept" }; 2404 case 47410321: 2405 /* specialArrangement */ return new String[] { "CodeableConcept" }; 2406 case -1429847026: 2407 /* destination */ return new String[] { "Reference" }; 2408 case 528065941: 2409 /* dischargeDisposition */ return new String[] { "CodeableConcept" }; 2410 default: 2411 return super.getTypesForProperty(hash, name); 2412 } 2413 2414 } 2415 2416 @Override 2417 public Base addChild(String name) throws FHIRException { 2418 if (name.equals("preAdmissionIdentifier")) { 2419 this.preAdmissionIdentifier = new Identifier(); 2420 return this.preAdmissionIdentifier; 2421 } else if (name.equals("origin")) { 2422 this.origin = new Reference(); 2423 return this.origin; 2424 } else if (name.equals("admitSource")) { 2425 this.admitSource = new CodeableConcept(); 2426 return this.admitSource; 2427 } else if (name.equals("reAdmission")) { 2428 this.reAdmission = new CodeableConcept(); 2429 return this.reAdmission; 2430 } else if (name.equals("dietPreference")) { 2431 return addDietPreference(); 2432 } else if (name.equals("specialCourtesy")) { 2433 return addSpecialCourtesy(); 2434 } else if (name.equals("specialArrangement")) { 2435 return addSpecialArrangement(); 2436 } else if (name.equals("destination")) { 2437 this.destination = new Reference(); 2438 return this.destination; 2439 } else if (name.equals("dischargeDisposition")) { 2440 this.dischargeDisposition = new CodeableConcept(); 2441 return this.dischargeDisposition; 2442 } else 2443 return super.addChild(name); 2444 } 2445 2446 public EncounterHospitalizationComponent copy() { 2447 EncounterHospitalizationComponent dst = new EncounterHospitalizationComponent(); 2448 copyValues(dst); 2449 return dst; 2450 } 2451 2452 public void copyValues(EncounterHospitalizationComponent dst) { 2453 super.copyValues(dst); 2454 dst.preAdmissionIdentifier = preAdmissionIdentifier == null ? null : preAdmissionIdentifier.copy(); 2455 dst.origin = origin == null ? null : origin.copy(); 2456 dst.admitSource = admitSource == null ? null : admitSource.copy(); 2457 dst.reAdmission = reAdmission == null ? null : reAdmission.copy(); 2458 if (dietPreference != null) { 2459 dst.dietPreference = new ArrayList<CodeableConcept>(); 2460 for (CodeableConcept i : dietPreference) 2461 dst.dietPreference.add(i.copy()); 2462 } 2463 ; 2464 if (specialCourtesy != null) { 2465 dst.specialCourtesy = new ArrayList<CodeableConcept>(); 2466 for (CodeableConcept i : specialCourtesy) 2467 dst.specialCourtesy.add(i.copy()); 2468 } 2469 ; 2470 if (specialArrangement != null) { 2471 dst.specialArrangement = new ArrayList<CodeableConcept>(); 2472 for (CodeableConcept i : specialArrangement) 2473 dst.specialArrangement.add(i.copy()); 2474 } 2475 ; 2476 dst.destination = destination == null ? null : destination.copy(); 2477 dst.dischargeDisposition = dischargeDisposition == null ? null : dischargeDisposition.copy(); 2478 } 2479 2480 @Override 2481 public boolean equalsDeep(Base other_) { 2482 if (!super.equalsDeep(other_)) 2483 return false; 2484 if (!(other_ instanceof EncounterHospitalizationComponent)) 2485 return false; 2486 EncounterHospitalizationComponent o = (EncounterHospitalizationComponent) other_; 2487 return compareDeep(preAdmissionIdentifier, o.preAdmissionIdentifier, true) && compareDeep(origin, o.origin, true) 2488 && compareDeep(admitSource, o.admitSource, true) && compareDeep(reAdmission, o.reAdmission, true) 2489 && compareDeep(dietPreference, o.dietPreference, true) 2490 && compareDeep(specialCourtesy, o.specialCourtesy, true) 2491 && compareDeep(specialArrangement, o.specialArrangement, true) 2492 && compareDeep(destination, o.destination, true) 2493 && compareDeep(dischargeDisposition, o.dischargeDisposition, true); 2494 } 2495 2496 @Override 2497 public boolean equalsShallow(Base other_) { 2498 if (!super.equalsShallow(other_)) 2499 return false; 2500 if (!(other_ instanceof EncounterHospitalizationComponent)) 2501 return false; 2502 EncounterHospitalizationComponent o = (EncounterHospitalizationComponent) other_; 2503 return true; 2504 } 2505 2506 public boolean isEmpty() { 2507 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(preAdmissionIdentifier, origin, admitSource, 2508 reAdmission, dietPreference, specialCourtesy, specialArrangement, destination, dischargeDisposition); 2509 } 2510 2511 public String fhirType() { 2512 return "Encounter.hospitalization"; 2513 2514 } 2515 2516 } 2517 2518 @Block() 2519 public static class EncounterLocationComponent extends BackboneElement implements IBaseBackboneElement { 2520 /** 2521 * The location where the encounter takes place. 2522 */ 2523 @Child(name = "location", type = { Location.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2524 @Description(shortDefinition = "Location the encounter takes place", formalDefinition = "The location where the encounter takes place.") 2525 protected Reference location; 2526 2527 /** 2528 * The actual object that is the target of the reference (The location where the 2529 * encounter takes place.) 2530 */ 2531 protected Location locationTarget; 2532 2533 /** 2534 * The status of the participants' presence at the specified location during the 2535 * period specified. If the participant is no longer at the location, then the 2536 * period will have an end date/time. 2537 */ 2538 @Child(name = "status", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2539 @Description(shortDefinition = "planned | active | reserved | completed", formalDefinition = "The status of the participants' presence at the specified location during the period specified. If the participant is no longer at the location, then the period will have an end date/time.") 2540 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-location-status") 2541 protected Enumeration<EncounterLocationStatus> status; 2542 2543 /** 2544 * This will be used to specify the required levels (bed/ward/room/etc.) desired 2545 * to be recorded to simplify either messaging or query. 2546 */ 2547 @Child(name = "physicalType", type = { 2548 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2549 @Description(shortDefinition = "The physical type of the location (usually the level in the location hierachy - bed room ward etc.)", formalDefinition = "This will be used to specify the required levels (bed/ward/room/etc.) desired to be recorded to simplify either messaging or query.") 2550 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/location-physical-type") 2551 protected CodeableConcept physicalType; 2552 2553 /** 2554 * Time period during which the patient was present at the location. 2555 */ 2556 @Child(name = "period", type = { Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2557 @Description(shortDefinition = "Time period during which the patient was present at the location", formalDefinition = "Time period during which the patient was present at the location.") 2558 protected Period period; 2559 2560 private static final long serialVersionUID = -755081862L; 2561 2562 /** 2563 * Constructor 2564 */ 2565 public EncounterLocationComponent() { 2566 super(); 2567 } 2568 2569 /** 2570 * Constructor 2571 */ 2572 public EncounterLocationComponent(Reference location) { 2573 super(); 2574 this.location = location; 2575 } 2576 2577 /** 2578 * @return {@link #location} (The location where the encounter takes place.) 2579 */ 2580 public Reference getLocation() { 2581 if (this.location == null) 2582 if (Configuration.errorOnAutoCreate()) 2583 throw new Error("Attempt to auto-create EncounterLocationComponent.location"); 2584 else if (Configuration.doAutoCreate()) 2585 this.location = new Reference(); // cc 2586 return this.location; 2587 } 2588 2589 public boolean hasLocation() { 2590 return this.location != null && !this.location.isEmpty(); 2591 } 2592 2593 /** 2594 * @param value {@link #location} (The location where the encounter takes 2595 * place.) 2596 */ 2597 public EncounterLocationComponent setLocation(Reference value) { 2598 this.location = value; 2599 return this; 2600 } 2601 2602 /** 2603 * @return {@link #location} The actual object that is the target of the 2604 * reference. The reference library doesn't populate this, but you can 2605 * use it to hold the resource if you resolve it. (The location where 2606 * the encounter takes place.) 2607 */ 2608 public Location getLocationTarget() { 2609 if (this.locationTarget == null) 2610 if (Configuration.errorOnAutoCreate()) 2611 throw new Error("Attempt to auto-create EncounterLocationComponent.location"); 2612 else if (Configuration.doAutoCreate()) 2613 this.locationTarget = new Location(); // aa 2614 return this.locationTarget; 2615 } 2616 2617 /** 2618 * @param value {@link #location} The actual object that is the target of the 2619 * reference. The reference library doesn't use these, but you can 2620 * use it to hold the resource if you resolve it. (The location 2621 * where the encounter takes place.) 2622 */ 2623 public EncounterLocationComponent setLocationTarget(Location value) { 2624 this.locationTarget = value; 2625 return this; 2626 } 2627 2628 /** 2629 * @return {@link #status} (The status of the participants' presence at the 2630 * specified location during the period specified. If the participant is 2631 * no longer at the location, then the period will have an end 2632 * date/time.). This is the underlying object with id, value and 2633 * extensions. The accessor "getStatus" gives direct access to the value 2634 */ 2635 public Enumeration<EncounterLocationStatus> getStatusElement() { 2636 if (this.status == null) 2637 if (Configuration.errorOnAutoCreate()) 2638 throw new Error("Attempt to auto-create EncounterLocationComponent.status"); 2639 else if (Configuration.doAutoCreate()) 2640 this.status = new Enumeration<EncounterLocationStatus>(new EncounterLocationStatusEnumFactory()); // bb 2641 return this.status; 2642 } 2643 2644 public boolean hasStatusElement() { 2645 return this.status != null && !this.status.isEmpty(); 2646 } 2647 2648 public boolean hasStatus() { 2649 return this.status != null && !this.status.isEmpty(); 2650 } 2651 2652 /** 2653 * @param value {@link #status} (The status of the participants' presence at the 2654 * specified location during the period specified. If the 2655 * participant is no longer at the location, then the period will 2656 * have an end date/time.). This is the underlying object with id, 2657 * value and extensions. The accessor "getStatus" gives direct 2658 * access to the value 2659 */ 2660 public EncounterLocationComponent setStatusElement(Enumeration<EncounterLocationStatus> value) { 2661 this.status = value; 2662 return this; 2663 } 2664 2665 /** 2666 * @return The status of the participants' presence at the specified location 2667 * during the period specified. If the participant is no longer at the 2668 * location, then the period will have an end date/time. 2669 */ 2670 public EncounterLocationStatus getStatus() { 2671 return this.status == null ? null : this.status.getValue(); 2672 } 2673 2674 /** 2675 * @param value The status of the participants' presence at the specified 2676 * location during the period specified. If the participant is no 2677 * longer at the location, then the period will have an end 2678 * date/time. 2679 */ 2680 public EncounterLocationComponent setStatus(EncounterLocationStatus value) { 2681 if (value == null) 2682 this.status = null; 2683 else { 2684 if (this.status == null) 2685 this.status = new Enumeration<EncounterLocationStatus>(new EncounterLocationStatusEnumFactory()); 2686 this.status.setValue(value); 2687 } 2688 return this; 2689 } 2690 2691 /** 2692 * @return {@link #physicalType} (This will be used to specify the required 2693 * levels (bed/ward/room/etc.) desired to be recorded to simplify either 2694 * messaging or query.) 2695 */ 2696 public CodeableConcept getPhysicalType() { 2697 if (this.physicalType == null) 2698 if (Configuration.errorOnAutoCreate()) 2699 throw new Error("Attempt to auto-create EncounterLocationComponent.physicalType"); 2700 else if (Configuration.doAutoCreate()) 2701 this.physicalType = new CodeableConcept(); // cc 2702 return this.physicalType; 2703 } 2704 2705 public boolean hasPhysicalType() { 2706 return this.physicalType != null && !this.physicalType.isEmpty(); 2707 } 2708 2709 /** 2710 * @param value {@link #physicalType} (This will be used to specify the required 2711 * levels (bed/ward/room/etc.) desired to be recorded to simplify 2712 * either messaging or query.) 2713 */ 2714 public EncounterLocationComponent setPhysicalType(CodeableConcept value) { 2715 this.physicalType = value; 2716 return this; 2717 } 2718 2719 /** 2720 * @return {@link #period} (Time period during which the patient was present at 2721 * the location.) 2722 */ 2723 public Period getPeriod() { 2724 if (this.period == null) 2725 if (Configuration.errorOnAutoCreate()) 2726 throw new Error("Attempt to auto-create EncounterLocationComponent.period"); 2727 else if (Configuration.doAutoCreate()) 2728 this.period = new Period(); // cc 2729 return this.period; 2730 } 2731 2732 public boolean hasPeriod() { 2733 return this.period != null && !this.period.isEmpty(); 2734 } 2735 2736 /** 2737 * @param value {@link #period} (Time period during which the patient was 2738 * present at the location.) 2739 */ 2740 public EncounterLocationComponent setPeriod(Period value) { 2741 this.period = value; 2742 return this; 2743 } 2744 2745 protected void listChildren(List<Property> children) { 2746 super.listChildren(children); 2747 children.add(new Property("location", "Reference(Location)", "The location where the encounter takes place.", 0, 2748 1, location)); 2749 children.add(new Property("status", "code", 2750 "The status of the participants' presence at the specified location during the period specified. If the participant is no longer at the location, then the period will have an end date/time.", 2751 0, 1, status)); 2752 children.add(new Property("physicalType", "CodeableConcept", 2753 "This will be used to specify the required levels (bed/ward/room/etc.) desired to be recorded to simplify either messaging or query.", 2754 0, 1, physicalType)); 2755 children.add(new Property("period", "Period", "Time period during which the patient was present at the location.", 2756 0, 1, period)); 2757 } 2758 2759 @Override 2760 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2761 switch (_hash) { 2762 case 1901043637: 2763 /* location */ return new Property("location", "Reference(Location)", 2764 "The location where the encounter takes place.", 0, 1, location); 2765 case -892481550: 2766 /* status */ return new Property("status", "code", 2767 "The status of the participants' presence at the specified location during the period specified. If the participant is no longer at the location, then the period will have an end date/time.", 2768 0, 1, status); 2769 case -1474715471: 2770 /* physicalType */ return new Property("physicalType", "CodeableConcept", 2771 "This will be used to specify the required levels (bed/ward/room/etc.) desired to be recorded to simplify either messaging or query.", 2772 0, 1, physicalType); 2773 case -991726143: 2774 /* period */ return new Property("period", "Period", 2775 "Time period during which the patient was present at the location.", 0, 1, period); 2776 default: 2777 return super.getNamedProperty(_hash, _name, _checkValid); 2778 } 2779 2780 } 2781 2782 @Override 2783 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2784 switch (hash) { 2785 case 1901043637: 2786 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Reference 2787 case -892481550: 2788 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<EncounterLocationStatus> 2789 case -1474715471: 2790 /* physicalType */ return this.physicalType == null ? new Base[0] : new Base[] { this.physicalType }; // CodeableConcept 2791 case -991726143: 2792 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 2793 default: 2794 return super.getProperty(hash, name, checkValid); 2795 } 2796 2797 } 2798 2799 @Override 2800 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2801 switch (hash) { 2802 case 1901043637: // location 2803 this.location = castToReference(value); // Reference 2804 return value; 2805 case -892481550: // status 2806 value = new EncounterLocationStatusEnumFactory().fromType(castToCode(value)); 2807 this.status = (Enumeration) value; // Enumeration<EncounterLocationStatus> 2808 return value; 2809 case -1474715471: // physicalType 2810 this.physicalType = castToCodeableConcept(value); // CodeableConcept 2811 return value; 2812 case -991726143: // period 2813 this.period = castToPeriod(value); // Period 2814 return value; 2815 default: 2816 return super.setProperty(hash, name, value); 2817 } 2818 2819 } 2820 2821 @Override 2822 public Base setProperty(String name, Base value) throws FHIRException { 2823 if (name.equals("location")) { 2824 this.location = castToReference(value); // Reference 2825 } else if (name.equals("status")) { 2826 value = new EncounterLocationStatusEnumFactory().fromType(castToCode(value)); 2827 this.status = (Enumeration) value; // Enumeration<EncounterLocationStatus> 2828 } else if (name.equals("physicalType")) { 2829 this.physicalType = castToCodeableConcept(value); // CodeableConcept 2830 } else if (name.equals("period")) { 2831 this.period = castToPeriod(value); // Period 2832 } else 2833 return super.setProperty(name, value); 2834 return value; 2835 } 2836 2837 @Override 2838 public void removeChild(String name, Base value) throws FHIRException { 2839 if (name.equals("location")) { 2840 this.location = null; 2841 } else if (name.equals("status")) { 2842 this.status = null; 2843 } else if (name.equals("physicalType")) { 2844 this.physicalType = null; 2845 } else if (name.equals("period")) { 2846 this.period = null; 2847 } else 2848 super.removeChild(name, value); 2849 2850 } 2851 2852 @Override 2853 public Base makeProperty(int hash, String name) throws FHIRException { 2854 switch (hash) { 2855 case 1901043637: 2856 return getLocation(); 2857 case -892481550: 2858 return getStatusElement(); 2859 case -1474715471: 2860 return getPhysicalType(); 2861 case -991726143: 2862 return getPeriod(); 2863 default: 2864 return super.makeProperty(hash, name); 2865 } 2866 2867 } 2868 2869 @Override 2870 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2871 switch (hash) { 2872 case 1901043637: 2873 /* location */ return new String[] { "Reference" }; 2874 case -892481550: 2875 /* status */ return new String[] { "code" }; 2876 case -1474715471: 2877 /* physicalType */ return new String[] { "CodeableConcept" }; 2878 case -991726143: 2879 /* period */ return new String[] { "Period" }; 2880 default: 2881 return super.getTypesForProperty(hash, name); 2882 } 2883 2884 } 2885 2886 @Override 2887 public Base addChild(String name) throws FHIRException { 2888 if (name.equals("location")) { 2889 this.location = new Reference(); 2890 return this.location; 2891 } else if (name.equals("status")) { 2892 throw new FHIRException("Cannot call addChild on a singleton property Encounter.status"); 2893 } else if (name.equals("physicalType")) { 2894 this.physicalType = new CodeableConcept(); 2895 return this.physicalType; 2896 } else if (name.equals("period")) { 2897 this.period = new Period(); 2898 return this.period; 2899 } else 2900 return super.addChild(name); 2901 } 2902 2903 public EncounterLocationComponent copy() { 2904 EncounterLocationComponent dst = new EncounterLocationComponent(); 2905 copyValues(dst); 2906 return dst; 2907 } 2908 2909 public void copyValues(EncounterLocationComponent dst) { 2910 super.copyValues(dst); 2911 dst.location = location == null ? null : location.copy(); 2912 dst.status = status == null ? null : status.copy(); 2913 dst.physicalType = physicalType == null ? null : physicalType.copy(); 2914 dst.period = period == null ? null : period.copy(); 2915 } 2916 2917 @Override 2918 public boolean equalsDeep(Base other_) { 2919 if (!super.equalsDeep(other_)) 2920 return false; 2921 if (!(other_ instanceof EncounterLocationComponent)) 2922 return false; 2923 EncounterLocationComponent o = (EncounterLocationComponent) other_; 2924 return compareDeep(location, o.location, true) && compareDeep(status, o.status, true) 2925 && compareDeep(physicalType, o.physicalType, true) && compareDeep(period, o.period, true); 2926 } 2927 2928 @Override 2929 public boolean equalsShallow(Base other_) { 2930 if (!super.equalsShallow(other_)) 2931 return false; 2932 if (!(other_ instanceof EncounterLocationComponent)) 2933 return false; 2934 EncounterLocationComponent o = (EncounterLocationComponent) other_; 2935 return compareValues(status, o.status, true); 2936 } 2937 2938 public boolean isEmpty() { 2939 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(location, status, physicalType, period); 2940 } 2941 2942 public String fhirType() { 2943 return "Encounter.location"; 2944 2945 } 2946 2947 } 2948 2949 /** 2950 * Identifier(s) by which this encounter is known. 2951 */ 2952 @Child(name = "identifier", type = { 2953 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2954 @Description(shortDefinition = "Identifier(s) by which this encounter is known", formalDefinition = "Identifier(s) by which this encounter is known.") 2955 protected List<Identifier> identifier; 2956 2957 /** 2958 * planned | arrived | triaged | in-progress | onleave | finished | cancelled +. 2959 */ 2960 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 2961 @Description(shortDefinition = "planned | arrived | triaged | in-progress | onleave | finished | cancelled +", formalDefinition = "planned | arrived | triaged | in-progress | onleave | finished | cancelled +.") 2962 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-status") 2963 protected Enumeration<EncounterStatus> status; 2964 2965 /** 2966 * The status history permits the encounter resource to contain the status 2967 * history without needing to read through the historical versions of the 2968 * resource, or even have the server store them. 2969 */ 2970 @Child(name = "statusHistory", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2971 @Description(shortDefinition = "List of past encounter statuses", formalDefinition = "The status history permits the encounter resource to contain the status history without needing to read through the historical versions of the resource, or even have the server store them.") 2972 protected List<StatusHistoryComponent> statusHistory; 2973 2974 /** 2975 * Concepts representing classification of patient encounter such as ambulatory 2976 * (outpatient), inpatient, emergency, home health or others due to local 2977 * variations. 2978 */ 2979 @Child(name = "class", type = { Coding.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 2980 @Description(shortDefinition = "Classification of patient encounter", formalDefinition = "Concepts representing classification of patient encounter such as ambulatory (outpatient), inpatient, emergency, home health or others due to local variations.") 2981 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ActEncounterCode") 2982 protected Coding class_; 2983 2984 /** 2985 * The class history permits the tracking of the encounters transitions without 2986 * needing to go through the resource history. This would be used for a case 2987 * where an admission starts of as an emergency encounter, then transitions into 2988 * an inpatient scenario. Doing this and not restarting a new encounter ensures 2989 * that any lab/diagnostic results can more easily follow the patient and not 2990 * require re-processing and not get lost or cancelled during a kind of 2991 * discharge from emergency to inpatient. 2992 */ 2993 @Child(name = "classHistory", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2994 @Description(shortDefinition = "List of past encounter classes", formalDefinition = "The class history permits the tracking of the encounters transitions without needing to go through the resource history. This would be used for a case where an admission starts of as an emergency encounter, then transitions into an inpatient scenario. Doing this and not restarting a new encounter ensures that any lab/diagnostic results can more easily follow the patient and not require re-processing and not get lost or cancelled during a kind of discharge from emergency to inpatient.") 2995 protected List<ClassHistoryComponent> classHistory; 2996 2997 /** 2998 * Specific type of encounter (e.g. e-mail consultation, surgical day-care, 2999 * skilled nursing, rehabilitation). 3000 */ 3001 @Child(name = "type", type = { 3002 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3003 @Description(shortDefinition = "Specific type of encounter", formalDefinition = "Specific type of encounter (e.g. e-mail consultation, surgical day-care, skilled nursing, rehabilitation).") 3004 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-type") 3005 protected List<CodeableConcept> type; 3006 3007 /** 3008 * Broad categorization of the service that is to be provided (e.g. cardiology). 3009 */ 3010 @Child(name = "serviceType", type = { 3011 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 3012 @Description(shortDefinition = "Specific type of service", formalDefinition = "Broad categorization of the service that is to be provided (e.g. cardiology).") 3013 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-type") 3014 protected CodeableConcept serviceType; 3015 3016 /** 3017 * Indicates the urgency of the encounter. 3018 */ 3019 @Child(name = "priority", type = { 3020 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 3021 @Description(shortDefinition = "Indicates the urgency of the encounter", formalDefinition = "Indicates the urgency of the encounter.") 3022 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ActPriority") 3023 protected CodeableConcept priority; 3024 3025 /** 3026 * The patient or group present at the encounter. 3027 */ 3028 @Child(name = "subject", type = { Patient.class, 3029 Group.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 3030 @Description(shortDefinition = "The patient or group present at the encounter", formalDefinition = "The patient or group present at the encounter.") 3031 protected Reference subject; 3032 3033 /** 3034 * The actual object that is the target of the reference (The patient or group 3035 * present at the encounter.) 3036 */ 3037 protected Resource subjectTarget; 3038 3039 /** 3040 * Where a specific encounter should be classified as a part of a specific 3041 * episode(s) of care this field should be used. This association can facilitate 3042 * grouping of related encounters together for a specific purpose, such as 3043 * government reporting, issue tracking, association via a common problem. The 3044 * association is recorded on the encounter as these are typically created after 3045 * the episode of care and grouped on entry rather than editing the episode of 3046 * care to append another encounter to it (the episode of care could span 3047 * years). 3048 */ 3049 @Child(name = "episodeOfCare", type = { 3050 EpisodeOfCare.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3051 @Description(shortDefinition = "Episode(s) of care that this encounter should be recorded against", formalDefinition = "Where a specific encounter should be classified as a part of a specific episode(s) of care this field should be used. This association can facilitate grouping of related encounters together for a specific purpose, such as government reporting, issue tracking, association via a common problem. The association is recorded on the encounter as these are typically created after the episode of care and grouped on entry rather than editing the episode of care to append another encounter to it (the episode of care could span years).") 3052 protected List<Reference> episodeOfCare; 3053 /** 3054 * The actual objects that are the target of the reference (Where a specific 3055 * encounter should be classified as a part of a specific episode(s) of care 3056 * this field should be used. This association can facilitate grouping of 3057 * related encounters together for a specific purpose, such as government 3058 * reporting, issue tracking, association via a common problem. The association 3059 * is recorded on the encounter as these are typically created after the episode 3060 * of care and grouped on entry rather than editing the episode of care to 3061 * append another encounter to it (the episode of care could span years).) 3062 */ 3063 protected List<EpisodeOfCare> episodeOfCareTarget; 3064 3065 /** 3066 * The request this encounter satisfies (e.g. incoming referral or procedure 3067 * request). 3068 */ 3069 @Child(name = "basedOn", type = { 3070 ServiceRequest.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3071 @Description(shortDefinition = "The ServiceRequest that initiated this encounter", formalDefinition = "The request this encounter satisfies (e.g. incoming referral or procedure request).") 3072 protected List<Reference> basedOn; 3073 /** 3074 * The actual objects that are the target of the reference (The request this 3075 * encounter satisfies (e.g. incoming referral or procedure request).) 3076 */ 3077 protected List<ServiceRequest> basedOnTarget; 3078 3079 /** 3080 * The list of people responsible for providing the service. 3081 */ 3082 @Child(name = "participant", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3083 @Description(shortDefinition = "List of participants involved in the encounter", formalDefinition = "The list of people responsible for providing the service.") 3084 protected List<EncounterParticipantComponent> participant; 3085 3086 /** 3087 * The appointment that scheduled this encounter. 3088 */ 3089 @Child(name = "appointment", type = { 3090 Appointment.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3091 @Description(shortDefinition = "The appointment that scheduled this encounter", formalDefinition = "The appointment that scheduled this encounter.") 3092 protected List<Reference> appointment; 3093 /** 3094 * The actual objects that are the target of the reference (The appointment that 3095 * scheduled this encounter.) 3096 */ 3097 protected List<Appointment> appointmentTarget; 3098 3099 /** 3100 * The start and end time of the encounter. 3101 */ 3102 @Child(name = "period", type = { Period.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 3103 @Description(shortDefinition = "The start and end time of the encounter", formalDefinition = "The start and end time of the encounter.") 3104 protected Period period; 3105 3106 /** 3107 * Quantity of time the encounter lasted. This excludes the time during leaves 3108 * of absence. 3109 */ 3110 @Child(name = "length", type = { Duration.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 3111 @Description(shortDefinition = "Quantity of time the encounter lasted (less time absent)", formalDefinition = "Quantity of time the encounter lasted. This excludes the time during leaves of absence.") 3112 protected Duration length; 3113 3114 /** 3115 * Reason the encounter takes place, expressed as a code. For admissions, this 3116 * can be used for a coded admission diagnosis. 3117 */ 3118 @Child(name = "reasonCode", type = { 3119 CodeableConcept.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3120 @Description(shortDefinition = "Coded reason the encounter takes place", formalDefinition = "Reason the encounter takes place, expressed as a code. For admissions, this can be used for a coded admission diagnosis.") 3121 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-reason") 3122 protected List<CodeableConcept> reasonCode; 3123 3124 /** 3125 * Reason the encounter takes place, expressed as a code. For admissions, this 3126 * can be used for a coded admission diagnosis. 3127 */ 3128 @Child(name = "reasonReference", type = { Condition.class, Procedure.class, Observation.class, 3129 ImmunizationRecommendation.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3130 @Description(shortDefinition = "Reason the encounter takes place (reference)", formalDefinition = "Reason the encounter takes place, expressed as a code. For admissions, this can be used for a coded admission diagnosis.") 3131 protected List<Reference> reasonReference; 3132 /** 3133 * The actual objects that are the target of the reference (Reason the encounter 3134 * takes place, expressed as a code. For admissions, this can be used for a 3135 * coded admission diagnosis.) 3136 */ 3137 protected List<Resource> reasonReferenceTarget; 3138 3139 /** 3140 * The list of diagnosis relevant to this encounter. 3141 */ 3142 @Child(name = "diagnosis", type = {}, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3143 @Description(shortDefinition = "The list of diagnosis relevant to this encounter", formalDefinition = "The list of diagnosis relevant to this encounter.") 3144 protected List<DiagnosisComponent> diagnosis; 3145 3146 /** 3147 * The set of accounts that may be used for billing for this Encounter. 3148 */ 3149 @Child(name = "account", type = { 3150 Account.class }, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3151 @Description(shortDefinition = "The set of accounts that may be used for billing for this Encounter", formalDefinition = "The set of accounts that may be used for billing for this Encounter.") 3152 protected List<Reference> account; 3153 /** 3154 * The actual objects that are the target of the reference (The set of accounts 3155 * that may be used for billing for this Encounter.) 3156 */ 3157 protected List<Account> accountTarget; 3158 3159 /** 3160 * Details about the admission to a healthcare service. 3161 */ 3162 @Child(name = "hospitalization", type = {}, order = 19, min = 0, max = 1, modifier = false, summary = false) 3163 @Description(shortDefinition = "Details about the admission to a healthcare service", formalDefinition = "Details about the admission to a healthcare service.") 3164 protected EncounterHospitalizationComponent hospitalization; 3165 3166 /** 3167 * List of locations where the patient has been during this encounter. 3168 */ 3169 @Child(name = "location", type = {}, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3170 @Description(shortDefinition = "List of locations where the patient has been", formalDefinition = "List of locations where the patient has been during this encounter.") 3171 protected List<EncounterLocationComponent> location; 3172 3173 /** 3174 * The organization that is primarily responsible for this Encounter's services. 3175 * This MAY be the same as the organization on the Patient record, however it 3176 * could be different, such as if the actor performing the services was from an 3177 * external organization (which may be billed seperately) for an external 3178 * consultation. Refer to the example bundle showing an abbreviated set of 3179 * Encounters for a colonoscopy. 3180 */ 3181 @Child(name = "serviceProvider", type = { 3182 Organization.class }, order = 21, min = 0, max = 1, modifier = false, summary = false) 3183 @Description(shortDefinition = "The organization (facility) responsible for this encounter", formalDefinition = "The organization that is primarily responsible for this Encounter's services. This MAY be the same as the organization on the Patient record, however it could be different, such as if the actor performing the services was from an external organization (which may be billed seperately) for an external consultation. Refer to the example bundle showing an abbreviated set of Encounters for a colonoscopy.") 3184 protected Reference serviceProvider; 3185 3186 /** 3187 * The actual object that is the target of the reference (The organization that 3188 * is primarily responsible for this Encounter's services. This MAY be the same 3189 * as the organization on the Patient record, however it could be different, 3190 * such as if the actor performing the services was from an external 3191 * organization (which may be billed seperately) for an external consultation. 3192 * Refer to the example bundle showing an abbreviated set of Encounters for a 3193 * colonoscopy.) 3194 */ 3195 protected Organization serviceProviderTarget; 3196 3197 /** 3198 * Another Encounter of which this encounter is a part of (administratively or 3199 * in time). 3200 */ 3201 @Child(name = "partOf", type = { Encounter.class }, order = 22, min = 0, max = 1, modifier = false, summary = false) 3202 @Description(shortDefinition = "Another Encounter this encounter is part of", formalDefinition = "Another Encounter of which this encounter is a part of (administratively or in time).") 3203 protected Reference partOf; 3204 3205 /** 3206 * The actual object that is the target of the reference (Another Encounter of 3207 * which this encounter is a part of (administratively or in time).) 3208 */ 3209 protected Encounter partOfTarget; 3210 3211 private static final long serialVersionUID = 1358318037L; 3212 3213 /** 3214 * Constructor 3215 */ 3216 public Encounter() { 3217 super(); 3218 } 3219 3220 /** 3221 * Constructor 3222 */ 3223 public Encounter(Enumeration<EncounterStatus> status, Coding class_) { 3224 super(); 3225 this.status = status; 3226 this.class_ = class_; 3227 } 3228 3229 /** 3230 * @return {@link #identifier} (Identifier(s) by which this encounter is known.) 3231 */ 3232 public List<Identifier> getIdentifier() { 3233 if (this.identifier == null) 3234 this.identifier = new ArrayList<Identifier>(); 3235 return this.identifier; 3236 } 3237 3238 /** 3239 * @return Returns a reference to <code>this</code> for easy method chaining 3240 */ 3241 public Encounter setIdentifier(List<Identifier> theIdentifier) { 3242 this.identifier = theIdentifier; 3243 return this; 3244 } 3245 3246 public boolean hasIdentifier() { 3247 if (this.identifier == null) 3248 return false; 3249 for (Identifier item : this.identifier) 3250 if (!item.isEmpty()) 3251 return true; 3252 return false; 3253 } 3254 3255 public Identifier addIdentifier() { // 3 3256 Identifier t = new Identifier(); 3257 if (this.identifier == null) 3258 this.identifier = new ArrayList<Identifier>(); 3259 this.identifier.add(t); 3260 return t; 3261 } 3262 3263 public Encounter addIdentifier(Identifier t) { // 3 3264 if (t == null) 3265 return this; 3266 if (this.identifier == null) 3267 this.identifier = new ArrayList<Identifier>(); 3268 this.identifier.add(t); 3269 return this; 3270 } 3271 3272 /** 3273 * @return The first repetition of repeating field {@link #identifier}, creating 3274 * it if it does not already exist 3275 */ 3276 public Identifier getIdentifierFirstRep() { 3277 if (getIdentifier().isEmpty()) { 3278 addIdentifier(); 3279 } 3280 return getIdentifier().get(0); 3281 } 3282 3283 /** 3284 * @return {@link #status} (planned | arrived | triaged | in-progress | onleave 3285 * | finished | cancelled +.). This is the underlying object with id, 3286 * value and extensions. The accessor "getStatus" gives direct access to 3287 * the value 3288 */ 3289 public Enumeration<EncounterStatus> getStatusElement() { 3290 if (this.status == null) 3291 if (Configuration.errorOnAutoCreate()) 3292 throw new Error("Attempt to auto-create Encounter.status"); 3293 else if (Configuration.doAutoCreate()) 3294 this.status = new Enumeration<EncounterStatus>(new EncounterStatusEnumFactory()); // bb 3295 return this.status; 3296 } 3297 3298 public boolean hasStatusElement() { 3299 return this.status != null && !this.status.isEmpty(); 3300 } 3301 3302 public boolean hasStatus() { 3303 return this.status != null && !this.status.isEmpty(); 3304 } 3305 3306 /** 3307 * @param value {@link #status} (planned | arrived | triaged | in-progress | 3308 * onleave | finished | cancelled +.). This is the underlying 3309 * object with id, value and extensions. The accessor "getStatus" 3310 * gives direct access to the value 3311 */ 3312 public Encounter setStatusElement(Enumeration<EncounterStatus> value) { 3313 this.status = value; 3314 return this; 3315 } 3316 3317 /** 3318 * @return planned | arrived | triaged | in-progress | onleave | finished | 3319 * cancelled +. 3320 */ 3321 public EncounterStatus getStatus() { 3322 return this.status == null ? null : this.status.getValue(); 3323 } 3324 3325 /** 3326 * @param value planned | arrived | triaged | in-progress | onleave | finished | 3327 * cancelled +. 3328 */ 3329 public Encounter setStatus(EncounterStatus value) { 3330 if (this.status == null) 3331 this.status = new Enumeration<EncounterStatus>(new EncounterStatusEnumFactory()); 3332 this.status.setValue(value); 3333 return this; 3334 } 3335 3336 /** 3337 * @return {@link #statusHistory} (The status history permits the encounter 3338 * resource to contain the status history without needing to read 3339 * through the historical versions of the resource, or even have the 3340 * server store them.) 3341 */ 3342 public List<StatusHistoryComponent> getStatusHistory() { 3343 if (this.statusHistory == null) 3344 this.statusHistory = new ArrayList<StatusHistoryComponent>(); 3345 return this.statusHistory; 3346 } 3347 3348 /** 3349 * @return Returns a reference to <code>this</code> for easy method chaining 3350 */ 3351 public Encounter setStatusHistory(List<StatusHistoryComponent> theStatusHistory) { 3352 this.statusHistory = theStatusHistory; 3353 return this; 3354 } 3355 3356 public boolean hasStatusHistory() { 3357 if (this.statusHistory == null) 3358 return false; 3359 for (StatusHistoryComponent item : this.statusHistory) 3360 if (!item.isEmpty()) 3361 return true; 3362 return false; 3363 } 3364 3365 public StatusHistoryComponent addStatusHistory() { // 3 3366 StatusHistoryComponent t = new StatusHistoryComponent(); 3367 if (this.statusHistory == null) 3368 this.statusHistory = new ArrayList<StatusHistoryComponent>(); 3369 this.statusHistory.add(t); 3370 return t; 3371 } 3372 3373 public Encounter addStatusHistory(StatusHistoryComponent t) { // 3 3374 if (t == null) 3375 return this; 3376 if (this.statusHistory == null) 3377 this.statusHistory = new ArrayList<StatusHistoryComponent>(); 3378 this.statusHistory.add(t); 3379 return this; 3380 } 3381 3382 /** 3383 * @return The first repetition of repeating field {@link #statusHistory}, 3384 * creating it if it does not already exist 3385 */ 3386 public StatusHistoryComponent getStatusHistoryFirstRep() { 3387 if (getStatusHistory().isEmpty()) { 3388 addStatusHistory(); 3389 } 3390 return getStatusHistory().get(0); 3391 } 3392 3393 /** 3394 * @return {@link #class_} (Concepts representing classification of patient 3395 * encounter such as ambulatory (outpatient), inpatient, emergency, home 3396 * health or others due to local variations.) 3397 */ 3398 public Coding getClass_() { 3399 if (this.class_ == null) 3400 if (Configuration.errorOnAutoCreate()) 3401 throw new Error("Attempt to auto-create Encounter.class_"); 3402 else if (Configuration.doAutoCreate()) 3403 this.class_ = new Coding(); // cc 3404 return this.class_; 3405 } 3406 3407 public boolean hasClass_() { 3408 return this.class_ != null && !this.class_.isEmpty(); 3409 } 3410 3411 /** 3412 * @param value {@link #class_} (Concepts representing classification of patient 3413 * encounter such as ambulatory (outpatient), inpatient, emergency, 3414 * home health or others due to local variations.) 3415 */ 3416 public Encounter setClass_(Coding value) { 3417 this.class_ = value; 3418 return this; 3419 } 3420 3421 /** 3422 * @return {@link #classHistory} (The class history permits the tracking of the 3423 * encounters transitions without needing to go through the resource 3424 * history. This would be used for a case where an admission starts of 3425 * as an emergency encounter, then transitions into an inpatient 3426 * scenario. Doing this and not restarting a new encounter ensures that 3427 * any lab/diagnostic results can more easily follow the patient and not 3428 * require re-processing and not get lost or cancelled during a kind of 3429 * discharge from emergency to inpatient.) 3430 */ 3431 public List<ClassHistoryComponent> getClassHistory() { 3432 if (this.classHistory == null) 3433 this.classHistory = new ArrayList<ClassHistoryComponent>(); 3434 return this.classHistory; 3435 } 3436 3437 /** 3438 * @return Returns a reference to <code>this</code> for easy method chaining 3439 */ 3440 public Encounter setClassHistory(List<ClassHistoryComponent> theClassHistory) { 3441 this.classHistory = theClassHistory; 3442 return this; 3443 } 3444 3445 public boolean hasClassHistory() { 3446 if (this.classHistory == null) 3447 return false; 3448 for (ClassHistoryComponent item : this.classHistory) 3449 if (!item.isEmpty()) 3450 return true; 3451 return false; 3452 } 3453 3454 public ClassHistoryComponent addClassHistory() { // 3 3455 ClassHistoryComponent t = new ClassHistoryComponent(); 3456 if (this.classHistory == null) 3457 this.classHistory = new ArrayList<ClassHistoryComponent>(); 3458 this.classHistory.add(t); 3459 return t; 3460 } 3461 3462 public Encounter addClassHistory(ClassHistoryComponent t) { // 3 3463 if (t == null) 3464 return this; 3465 if (this.classHistory == null) 3466 this.classHistory = new ArrayList<ClassHistoryComponent>(); 3467 this.classHistory.add(t); 3468 return this; 3469 } 3470 3471 /** 3472 * @return The first repetition of repeating field {@link #classHistory}, 3473 * creating it if it does not already exist 3474 */ 3475 public ClassHistoryComponent getClassHistoryFirstRep() { 3476 if (getClassHistory().isEmpty()) { 3477 addClassHistory(); 3478 } 3479 return getClassHistory().get(0); 3480 } 3481 3482 /** 3483 * @return {@link #type} (Specific type of encounter (e.g. e-mail consultation, 3484 * surgical day-care, skilled nursing, rehabilitation).) 3485 */ 3486 public List<CodeableConcept> getType() { 3487 if (this.type == null) 3488 this.type = new ArrayList<CodeableConcept>(); 3489 return this.type; 3490 } 3491 3492 /** 3493 * @return Returns a reference to <code>this</code> for easy method chaining 3494 */ 3495 public Encounter setType(List<CodeableConcept> theType) { 3496 this.type = theType; 3497 return this; 3498 } 3499 3500 public boolean hasType() { 3501 if (this.type == null) 3502 return false; 3503 for (CodeableConcept item : this.type) 3504 if (!item.isEmpty()) 3505 return true; 3506 return false; 3507 } 3508 3509 public CodeableConcept addType() { // 3 3510 CodeableConcept t = new CodeableConcept(); 3511 if (this.type == null) 3512 this.type = new ArrayList<CodeableConcept>(); 3513 this.type.add(t); 3514 return t; 3515 } 3516 3517 public Encounter addType(CodeableConcept t) { // 3 3518 if (t == null) 3519 return this; 3520 if (this.type == null) 3521 this.type = new ArrayList<CodeableConcept>(); 3522 this.type.add(t); 3523 return this; 3524 } 3525 3526 /** 3527 * @return The first repetition of repeating field {@link #type}, creating it if 3528 * it does not already exist 3529 */ 3530 public CodeableConcept getTypeFirstRep() { 3531 if (getType().isEmpty()) { 3532 addType(); 3533 } 3534 return getType().get(0); 3535 } 3536 3537 /** 3538 * @return {@link #serviceType} (Broad categorization of the service that is to 3539 * be provided (e.g. cardiology).) 3540 */ 3541 public CodeableConcept getServiceType() { 3542 if (this.serviceType == null) 3543 if (Configuration.errorOnAutoCreate()) 3544 throw new Error("Attempt to auto-create Encounter.serviceType"); 3545 else if (Configuration.doAutoCreate()) 3546 this.serviceType = new CodeableConcept(); // cc 3547 return this.serviceType; 3548 } 3549 3550 public boolean hasServiceType() { 3551 return this.serviceType != null && !this.serviceType.isEmpty(); 3552 } 3553 3554 /** 3555 * @param value {@link #serviceType} (Broad categorization of the service that 3556 * is to be provided (e.g. cardiology).) 3557 */ 3558 public Encounter setServiceType(CodeableConcept value) { 3559 this.serviceType = value; 3560 return this; 3561 } 3562 3563 /** 3564 * @return {@link #priority} (Indicates the urgency of the encounter.) 3565 */ 3566 public CodeableConcept getPriority() { 3567 if (this.priority == null) 3568 if (Configuration.errorOnAutoCreate()) 3569 throw new Error("Attempt to auto-create Encounter.priority"); 3570 else if (Configuration.doAutoCreate()) 3571 this.priority = new CodeableConcept(); // cc 3572 return this.priority; 3573 } 3574 3575 public boolean hasPriority() { 3576 return this.priority != null && !this.priority.isEmpty(); 3577 } 3578 3579 /** 3580 * @param value {@link #priority} (Indicates the urgency of the encounter.) 3581 */ 3582 public Encounter setPriority(CodeableConcept value) { 3583 this.priority = value; 3584 return this; 3585 } 3586 3587 /** 3588 * @return {@link #subject} (The patient or group present at the encounter.) 3589 */ 3590 public Reference getSubject() { 3591 if (this.subject == null) 3592 if (Configuration.errorOnAutoCreate()) 3593 throw new Error("Attempt to auto-create Encounter.subject"); 3594 else if (Configuration.doAutoCreate()) 3595 this.subject = new Reference(); // cc 3596 return this.subject; 3597 } 3598 3599 public boolean hasSubject() { 3600 return this.subject != null && !this.subject.isEmpty(); 3601 } 3602 3603 /** 3604 * @param value {@link #subject} (The patient or group present at the 3605 * encounter.) 3606 */ 3607 public Encounter setSubject(Reference value) { 3608 this.subject = value; 3609 return this; 3610 } 3611 3612 /** 3613 * @return {@link #subject} The actual object that is the target of the 3614 * reference. The reference library doesn't populate this, but you can 3615 * use it to hold the resource if you resolve it. (The patient or group 3616 * present at the encounter.) 3617 */ 3618 public Resource getSubjectTarget() { 3619 return this.subjectTarget; 3620 } 3621 3622 /** 3623 * @param value {@link #subject} The actual object that is the target of the 3624 * reference. The reference library doesn't use these, but you can 3625 * use it to hold the resource if you resolve it. (The patient or 3626 * group present at the encounter.) 3627 */ 3628 public Encounter setSubjectTarget(Resource value) { 3629 this.subjectTarget = value; 3630 return this; 3631 } 3632 3633 /** 3634 * @return {@link #episodeOfCare} (Where a specific encounter should be 3635 * classified as a part of a specific episode(s) of care this field 3636 * should be used. This association can facilitate grouping of related 3637 * encounters together for a specific purpose, such as government 3638 * reporting, issue tracking, association via a common problem. The 3639 * association is recorded on the encounter as these are typically 3640 * created after the episode of care and grouped on entry rather than 3641 * editing the episode of care to append another encounter to it (the 3642 * episode of care could span years).) 3643 */ 3644 public List<Reference> getEpisodeOfCare() { 3645 if (this.episodeOfCare == null) 3646 this.episodeOfCare = new ArrayList<Reference>(); 3647 return this.episodeOfCare; 3648 } 3649 3650 /** 3651 * @return Returns a reference to <code>this</code> for easy method chaining 3652 */ 3653 public Encounter setEpisodeOfCare(List<Reference> theEpisodeOfCare) { 3654 this.episodeOfCare = theEpisodeOfCare; 3655 return this; 3656 } 3657 3658 public boolean hasEpisodeOfCare() { 3659 if (this.episodeOfCare == null) 3660 return false; 3661 for (Reference item : this.episodeOfCare) 3662 if (!item.isEmpty()) 3663 return true; 3664 return false; 3665 } 3666 3667 public Reference addEpisodeOfCare() { // 3 3668 Reference t = new Reference(); 3669 if (this.episodeOfCare == null) 3670 this.episodeOfCare = new ArrayList<Reference>(); 3671 this.episodeOfCare.add(t); 3672 return t; 3673 } 3674 3675 public Encounter addEpisodeOfCare(Reference t) { // 3 3676 if (t == null) 3677 return this; 3678 if (this.episodeOfCare == null) 3679 this.episodeOfCare = new ArrayList<Reference>(); 3680 this.episodeOfCare.add(t); 3681 return this; 3682 } 3683 3684 /** 3685 * @return The first repetition of repeating field {@link #episodeOfCare}, 3686 * creating it if it does not already exist 3687 */ 3688 public Reference getEpisodeOfCareFirstRep() { 3689 if (getEpisodeOfCare().isEmpty()) { 3690 addEpisodeOfCare(); 3691 } 3692 return getEpisodeOfCare().get(0); 3693 } 3694 3695 /** 3696 * @deprecated Use Reference#setResource(IBaseResource) instead 3697 */ 3698 @Deprecated 3699 public List<EpisodeOfCare> getEpisodeOfCareTarget() { 3700 if (this.episodeOfCareTarget == null) 3701 this.episodeOfCareTarget = new ArrayList<EpisodeOfCare>(); 3702 return this.episodeOfCareTarget; 3703 } 3704 3705 /** 3706 * @deprecated Use Reference#setResource(IBaseResource) instead 3707 */ 3708 @Deprecated 3709 public EpisodeOfCare addEpisodeOfCareTarget() { 3710 EpisodeOfCare r = new EpisodeOfCare(); 3711 if (this.episodeOfCareTarget == null) 3712 this.episodeOfCareTarget = new ArrayList<EpisodeOfCare>(); 3713 this.episodeOfCareTarget.add(r); 3714 return r; 3715 } 3716 3717 /** 3718 * @return {@link #basedOn} (The request this encounter satisfies (e.g. incoming 3719 * referral or procedure request).) 3720 */ 3721 public List<Reference> getBasedOn() { 3722 if (this.basedOn == null) 3723 this.basedOn = new ArrayList<Reference>(); 3724 return this.basedOn; 3725 } 3726 3727 /** 3728 * @return Returns a reference to <code>this</code> for easy method chaining 3729 */ 3730 public Encounter setBasedOn(List<Reference> theBasedOn) { 3731 this.basedOn = theBasedOn; 3732 return this; 3733 } 3734 3735 public boolean hasBasedOn() { 3736 if (this.basedOn == null) 3737 return false; 3738 for (Reference item : this.basedOn) 3739 if (!item.isEmpty()) 3740 return true; 3741 return false; 3742 } 3743 3744 public Reference addBasedOn() { // 3 3745 Reference t = new Reference(); 3746 if (this.basedOn == null) 3747 this.basedOn = new ArrayList<Reference>(); 3748 this.basedOn.add(t); 3749 return t; 3750 } 3751 3752 public Encounter addBasedOn(Reference t) { // 3 3753 if (t == null) 3754 return this; 3755 if (this.basedOn == null) 3756 this.basedOn = new ArrayList<Reference>(); 3757 this.basedOn.add(t); 3758 return this; 3759 } 3760 3761 /** 3762 * @return The first repetition of repeating field {@link #basedOn}, creating it 3763 * if it does not already exist 3764 */ 3765 public Reference getBasedOnFirstRep() { 3766 if (getBasedOn().isEmpty()) { 3767 addBasedOn(); 3768 } 3769 return getBasedOn().get(0); 3770 } 3771 3772 /** 3773 * @deprecated Use Reference#setResource(IBaseResource) instead 3774 */ 3775 @Deprecated 3776 public List<ServiceRequest> getBasedOnTarget() { 3777 if (this.basedOnTarget == null) 3778 this.basedOnTarget = new ArrayList<ServiceRequest>(); 3779 return this.basedOnTarget; 3780 } 3781 3782 /** 3783 * @deprecated Use Reference#setResource(IBaseResource) instead 3784 */ 3785 @Deprecated 3786 public ServiceRequest addBasedOnTarget() { 3787 ServiceRequest r = new ServiceRequest(); 3788 if (this.basedOnTarget == null) 3789 this.basedOnTarget = new ArrayList<ServiceRequest>(); 3790 this.basedOnTarget.add(r); 3791 return r; 3792 } 3793 3794 /** 3795 * @return {@link #participant} (The list of people responsible for providing 3796 * the service.) 3797 */ 3798 public List<EncounterParticipantComponent> getParticipant() { 3799 if (this.participant == null) 3800 this.participant = new ArrayList<EncounterParticipantComponent>(); 3801 return this.participant; 3802 } 3803 3804 /** 3805 * @return Returns a reference to <code>this</code> for easy method chaining 3806 */ 3807 public Encounter setParticipant(List<EncounterParticipantComponent> theParticipant) { 3808 this.participant = theParticipant; 3809 return this; 3810 } 3811 3812 public boolean hasParticipant() { 3813 if (this.participant == null) 3814 return false; 3815 for (EncounterParticipantComponent item : this.participant) 3816 if (!item.isEmpty()) 3817 return true; 3818 return false; 3819 } 3820 3821 public EncounterParticipantComponent addParticipant() { // 3 3822 EncounterParticipantComponent t = new EncounterParticipantComponent(); 3823 if (this.participant == null) 3824 this.participant = new ArrayList<EncounterParticipantComponent>(); 3825 this.participant.add(t); 3826 return t; 3827 } 3828 3829 public Encounter addParticipant(EncounterParticipantComponent t) { // 3 3830 if (t == null) 3831 return this; 3832 if (this.participant == null) 3833 this.participant = new ArrayList<EncounterParticipantComponent>(); 3834 this.participant.add(t); 3835 return this; 3836 } 3837 3838 /** 3839 * @return The first repetition of repeating field {@link #participant}, 3840 * creating it if it does not already exist 3841 */ 3842 public EncounterParticipantComponent getParticipantFirstRep() { 3843 if (getParticipant().isEmpty()) { 3844 addParticipant(); 3845 } 3846 return getParticipant().get(0); 3847 } 3848 3849 /** 3850 * @return {@link #appointment} (The appointment that scheduled this encounter.) 3851 */ 3852 public List<Reference> getAppointment() { 3853 if (this.appointment == null) 3854 this.appointment = new ArrayList<Reference>(); 3855 return this.appointment; 3856 } 3857 3858 /** 3859 * @return Returns a reference to <code>this</code> for easy method chaining 3860 */ 3861 public Encounter setAppointment(List<Reference> theAppointment) { 3862 this.appointment = theAppointment; 3863 return this; 3864 } 3865 3866 public boolean hasAppointment() { 3867 if (this.appointment == null) 3868 return false; 3869 for (Reference item : this.appointment) 3870 if (!item.isEmpty()) 3871 return true; 3872 return false; 3873 } 3874 3875 public Reference addAppointment() { // 3 3876 Reference t = new Reference(); 3877 if (this.appointment == null) 3878 this.appointment = new ArrayList<Reference>(); 3879 this.appointment.add(t); 3880 return t; 3881 } 3882 3883 public Encounter addAppointment(Reference t) { // 3 3884 if (t == null) 3885 return this; 3886 if (this.appointment == null) 3887 this.appointment = new ArrayList<Reference>(); 3888 this.appointment.add(t); 3889 return this; 3890 } 3891 3892 /** 3893 * @return The first repetition of repeating field {@link #appointment}, 3894 * creating it if it does not already exist 3895 */ 3896 public Reference getAppointmentFirstRep() { 3897 if (getAppointment().isEmpty()) { 3898 addAppointment(); 3899 } 3900 return getAppointment().get(0); 3901 } 3902 3903 /** 3904 * @deprecated Use Reference#setResource(IBaseResource) instead 3905 */ 3906 @Deprecated 3907 public List<Appointment> getAppointmentTarget() { 3908 if (this.appointmentTarget == null) 3909 this.appointmentTarget = new ArrayList<Appointment>(); 3910 return this.appointmentTarget; 3911 } 3912 3913 /** 3914 * @deprecated Use Reference#setResource(IBaseResource) instead 3915 */ 3916 @Deprecated 3917 public Appointment addAppointmentTarget() { 3918 Appointment r = new Appointment(); 3919 if (this.appointmentTarget == null) 3920 this.appointmentTarget = new ArrayList<Appointment>(); 3921 this.appointmentTarget.add(r); 3922 return r; 3923 } 3924 3925 /** 3926 * @return {@link #period} (The start and end time of the encounter.) 3927 */ 3928 public Period getPeriod() { 3929 if (this.period == null) 3930 if (Configuration.errorOnAutoCreate()) 3931 throw new Error("Attempt to auto-create Encounter.period"); 3932 else if (Configuration.doAutoCreate()) 3933 this.period = new Period(); // cc 3934 return this.period; 3935 } 3936 3937 public boolean hasPeriod() { 3938 return this.period != null && !this.period.isEmpty(); 3939 } 3940 3941 /** 3942 * @param value {@link #period} (The start and end time of the encounter.) 3943 */ 3944 public Encounter setPeriod(Period value) { 3945 this.period = value; 3946 return this; 3947 } 3948 3949 /** 3950 * @return {@link #length} (Quantity of time the encounter lasted. This excludes 3951 * the time during leaves of absence.) 3952 */ 3953 public Duration getLength() { 3954 if (this.length == null) 3955 if (Configuration.errorOnAutoCreate()) 3956 throw new Error("Attempt to auto-create Encounter.length"); 3957 else if (Configuration.doAutoCreate()) 3958 this.length = new Duration(); // cc 3959 return this.length; 3960 } 3961 3962 public boolean hasLength() { 3963 return this.length != null && !this.length.isEmpty(); 3964 } 3965 3966 /** 3967 * @param value {@link #length} (Quantity of time the encounter lasted. This 3968 * excludes the time during leaves of absence.) 3969 */ 3970 public Encounter setLength(Duration value) { 3971 this.length = value; 3972 return this; 3973 } 3974 3975 /** 3976 * @return {@link #reasonCode} (Reason the encounter takes place, expressed as a 3977 * code. For admissions, this can be used for a coded admission 3978 * diagnosis.) 3979 */ 3980 public List<CodeableConcept> getReasonCode() { 3981 if (this.reasonCode == null) 3982 this.reasonCode = new ArrayList<CodeableConcept>(); 3983 return this.reasonCode; 3984 } 3985 3986 /** 3987 * @return Returns a reference to <code>this</code> for easy method chaining 3988 */ 3989 public Encounter setReasonCode(List<CodeableConcept> theReasonCode) { 3990 this.reasonCode = theReasonCode; 3991 return this; 3992 } 3993 3994 public boolean hasReasonCode() { 3995 if (this.reasonCode == null) 3996 return false; 3997 for (CodeableConcept item : this.reasonCode) 3998 if (!item.isEmpty()) 3999 return true; 4000 return false; 4001 } 4002 4003 public CodeableConcept addReasonCode() { // 3 4004 CodeableConcept t = new CodeableConcept(); 4005 if (this.reasonCode == null) 4006 this.reasonCode = new ArrayList<CodeableConcept>(); 4007 this.reasonCode.add(t); 4008 return t; 4009 } 4010 4011 public Encounter addReasonCode(CodeableConcept t) { // 3 4012 if (t == null) 4013 return this; 4014 if (this.reasonCode == null) 4015 this.reasonCode = new ArrayList<CodeableConcept>(); 4016 this.reasonCode.add(t); 4017 return this; 4018 } 4019 4020 /** 4021 * @return The first repetition of repeating field {@link #reasonCode}, creating 4022 * it if it does not already exist 4023 */ 4024 public CodeableConcept getReasonCodeFirstRep() { 4025 if (getReasonCode().isEmpty()) { 4026 addReasonCode(); 4027 } 4028 return getReasonCode().get(0); 4029 } 4030 4031 /** 4032 * @return {@link #reasonReference} (Reason the encounter takes place, expressed 4033 * as a code. For admissions, this can be used for a coded admission 4034 * diagnosis.) 4035 */ 4036 public List<Reference> getReasonReference() { 4037 if (this.reasonReference == null) 4038 this.reasonReference = new ArrayList<Reference>(); 4039 return this.reasonReference; 4040 } 4041 4042 /** 4043 * @return Returns a reference to <code>this</code> for easy method chaining 4044 */ 4045 public Encounter setReasonReference(List<Reference> theReasonReference) { 4046 this.reasonReference = theReasonReference; 4047 return this; 4048 } 4049 4050 public boolean hasReasonReference() { 4051 if (this.reasonReference == null) 4052 return false; 4053 for (Reference item : this.reasonReference) 4054 if (!item.isEmpty()) 4055 return true; 4056 return false; 4057 } 4058 4059 public Reference addReasonReference() { // 3 4060 Reference t = new Reference(); 4061 if (this.reasonReference == null) 4062 this.reasonReference = new ArrayList<Reference>(); 4063 this.reasonReference.add(t); 4064 return t; 4065 } 4066 4067 public Encounter addReasonReference(Reference t) { // 3 4068 if (t == null) 4069 return this; 4070 if (this.reasonReference == null) 4071 this.reasonReference = new ArrayList<Reference>(); 4072 this.reasonReference.add(t); 4073 return this; 4074 } 4075 4076 /** 4077 * @return The first repetition of repeating field {@link #reasonReference}, 4078 * creating it if it does not already exist 4079 */ 4080 public Reference getReasonReferenceFirstRep() { 4081 if (getReasonReference().isEmpty()) { 4082 addReasonReference(); 4083 } 4084 return getReasonReference().get(0); 4085 } 4086 4087 /** 4088 * @deprecated Use Reference#setResource(IBaseResource) instead 4089 */ 4090 @Deprecated 4091 public List<Resource> getReasonReferenceTarget() { 4092 if (this.reasonReferenceTarget == null) 4093 this.reasonReferenceTarget = new ArrayList<Resource>(); 4094 return this.reasonReferenceTarget; 4095 } 4096 4097 /** 4098 * @return {@link #diagnosis} (The list of diagnosis relevant to this 4099 * encounter.) 4100 */ 4101 public List<DiagnosisComponent> getDiagnosis() { 4102 if (this.diagnosis == null) 4103 this.diagnosis = new ArrayList<DiagnosisComponent>(); 4104 return this.diagnosis; 4105 } 4106 4107 /** 4108 * @return Returns a reference to <code>this</code> for easy method chaining 4109 */ 4110 public Encounter setDiagnosis(List<DiagnosisComponent> theDiagnosis) { 4111 this.diagnosis = theDiagnosis; 4112 return this; 4113 } 4114 4115 public boolean hasDiagnosis() { 4116 if (this.diagnosis == null) 4117 return false; 4118 for (DiagnosisComponent item : this.diagnosis) 4119 if (!item.isEmpty()) 4120 return true; 4121 return false; 4122 } 4123 4124 public DiagnosisComponent addDiagnosis() { // 3 4125 DiagnosisComponent t = new DiagnosisComponent(); 4126 if (this.diagnosis == null) 4127 this.diagnosis = new ArrayList<DiagnosisComponent>(); 4128 this.diagnosis.add(t); 4129 return t; 4130 } 4131 4132 public Encounter addDiagnosis(DiagnosisComponent t) { // 3 4133 if (t == null) 4134 return this; 4135 if (this.diagnosis == null) 4136 this.diagnosis = new ArrayList<DiagnosisComponent>(); 4137 this.diagnosis.add(t); 4138 return this; 4139 } 4140 4141 /** 4142 * @return The first repetition of repeating field {@link #diagnosis}, creating 4143 * it if it does not already exist 4144 */ 4145 public DiagnosisComponent getDiagnosisFirstRep() { 4146 if (getDiagnosis().isEmpty()) { 4147 addDiagnosis(); 4148 } 4149 return getDiagnosis().get(0); 4150 } 4151 4152 /** 4153 * @return {@link #account} (The set of accounts that may be used for billing 4154 * for this Encounter.) 4155 */ 4156 public List<Reference> getAccount() { 4157 if (this.account == null) 4158 this.account = new ArrayList<Reference>(); 4159 return this.account; 4160 } 4161 4162 /** 4163 * @return Returns a reference to <code>this</code> for easy method chaining 4164 */ 4165 public Encounter setAccount(List<Reference> theAccount) { 4166 this.account = theAccount; 4167 return this; 4168 } 4169 4170 public boolean hasAccount() { 4171 if (this.account == null) 4172 return false; 4173 for (Reference item : this.account) 4174 if (!item.isEmpty()) 4175 return true; 4176 return false; 4177 } 4178 4179 public Reference addAccount() { // 3 4180 Reference t = new Reference(); 4181 if (this.account == null) 4182 this.account = new ArrayList<Reference>(); 4183 this.account.add(t); 4184 return t; 4185 } 4186 4187 public Encounter addAccount(Reference t) { // 3 4188 if (t == null) 4189 return this; 4190 if (this.account == null) 4191 this.account = new ArrayList<Reference>(); 4192 this.account.add(t); 4193 return this; 4194 } 4195 4196 /** 4197 * @return The first repetition of repeating field {@link #account}, creating it 4198 * if it does not already exist 4199 */ 4200 public Reference getAccountFirstRep() { 4201 if (getAccount().isEmpty()) { 4202 addAccount(); 4203 } 4204 return getAccount().get(0); 4205 } 4206 4207 /** 4208 * @deprecated Use Reference#setResource(IBaseResource) instead 4209 */ 4210 @Deprecated 4211 public List<Account> getAccountTarget() { 4212 if (this.accountTarget == null) 4213 this.accountTarget = new ArrayList<Account>(); 4214 return this.accountTarget; 4215 } 4216 4217 /** 4218 * @deprecated Use Reference#setResource(IBaseResource) instead 4219 */ 4220 @Deprecated 4221 public Account addAccountTarget() { 4222 Account r = new Account(); 4223 if (this.accountTarget == null) 4224 this.accountTarget = new ArrayList<Account>(); 4225 this.accountTarget.add(r); 4226 return r; 4227 } 4228 4229 /** 4230 * @return {@link #hospitalization} (Details about the admission to a healthcare 4231 * service.) 4232 */ 4233 public EncounterHospitalizationComponent getHospitalization() { 4234 if (this.hospitalization == null) 4235 if (Configuration.errorOnAutoCreate()) 4236 throw new Error("Attempt to auto-create Encounter.hospitalization"); 4237 else if (Configuration.doAutoCreate()) 4238 this.hospitalization = new EncounterHospitalizationComponent(); // cc 4239 return this.hospitalization; 4240 } 4241 4242 public boolean hasHospitalization() { 4243 return this.hospitalization != null && !this.hospitalization.isEmpty(); 4244 } 4245 4246 /** 4247 * @param value {@link #hospitalization} (Details about the admission to a 4248 * healthcare service.) 4249 */ 4250 public Encounter setHospitalization(EncounterHospitalizationComponent value) { 4251 this.hospitalization = value; 4252 return this; 4253 } 4254 4255 /** 4256 * @return {@link #location} (List of locations where the patient has been 4257 * during this encounter.) 4258 */ 4259 public List<EncounterLocationComponent> getLocation() { 4260 if (this.location == null) 4261 this.location = new ArrayList<EncounterLocationComponent>(); 4262 return this.location; 4263 } 4264 4265 /** 4266 * @return Returns a reference to <code>this</code> for easy method chaining 4267 */ 4268 public Encounter setLocation(List<EncounterLocationComponent> theLocation) { 4269 this.location = theLocation; 4270 return this; 4271 } 4272 4273 public boolean hasLocation() { 4274 if (this.location == null) 4275 return false; 4276 for (EncounterLocationComponent item : this.location) 4277 if (!item.isEmpty()) 4278 return true; 4279 return false; 4280 } 4281 4282 public EncounterLocationComponent addLocation() { // 3 4283 EncounterLocationComponent t = new EncounterLocationComponent(); 4284 if (this.location == null) 4285 this.location = new ArrayList<EncounterLocationComponent>(); 4286 this.location.add(t); 4287 return t; 4288 } 4289 4290 public Encounter addLocation(EncounterLocationComponent t) { // 3 4291 if (t == null) 4292 return this; 4293 if (this.location == null) 4294 this.location = new ArrayList<EncounterLocationComponent>(); 4295 this.location.add(t); 4296 return this; 4297 } 4298 4299 /** 4300 * @return The first repetition of repeating field {@link #location}, creating 4301 * it if it does not already exist 4302 */ 4303 public EncounterLocationComponent getLocationFirstRep() { 4304 if (getLocation().isEmpty()) { 4305 addLocation(); 4306 } 4307 return getLocation().get(0); 4308 } 4309 4310 /** 4311 * @return {@link #serviceProvider} (The organization that is primarily 4312 * responsible for this Encounter's services. This MAY be the same as 4313 * the organization on the Patient record, however it could be 4314 * different, such as if the actor performing the services was from an 4315 * external organization (which may be billed seperately) for an 4316 * external consultation. Refer to the example bundle showing an 4317 * abbreviated set of Encounters for a colonoscopy.) 4318 */ 4319 public Reference getServiceProvider() { 4320 if (this.serviceProvider == null) 4321 if (Configuration.errorOnAutoCreate()) 4322 throw new Error("Attempt to auto-create Encounter.serviceProvider"); 4323 else if (Configuration.doAutoCreate()) 4324 this.serviceProvider = new Reference(); // cc 4325 return this.serviceProvider; 4326 } 4327 4328 public boolean hasServiceProvider() { 4329 return this.serviceProvider != null && !this.serviceProvider.isEmpty(); 4330 } 4331 4332 /** 4333 * @param value {@link #serviceProvider} (The organization that is primarily 4334 * responsible for this Encounter's services. This MAY be the same 4335 * as the organization on the Patient record, however it could be 4336 * different, such as if the actor performing the services was from 4337 * an external organization (which may be billed seperately) for an 4338 * external consultation. Refer to the example bundle showing an 4339 * abbreviated set of Encounters for a colonoscopy.) 4340 */ 4341 public Encounter setServiceProvider(Reference value) { 4342 this.serviceProvider = value; 4343 return this; 4344 } 4345 4346 /** 4347 * @return {@link #serviceProvider} The actual object that is the target of the 4348 * reference. The reference library doesn't populate this, but you can 4349 * use it to hold the resource if you resolve it. (The organization that 4350 * is primarily responsible for this Encounter's services. This MAY be 4351 * the same as the organization on the Patient record, however it could 4352 * be different, such as if the actor performing the services was from 4353 * an external organization (which may be billed seperately) for an 4354 * external consultation. Refer to the example bundle showing an 4355 * abbreviated set of Encounters for a colonoscopy.) 4356 */ 4357 public Organization getServiceProviderTarget() { 4358 if (this.serviceProviderTarget == null) 4359 if (Configuration.errorOnAutoCreate()) 4360 throw new Error("Attempt to auto-create Encounter.serviceProvider"); 4361 else if (Configuration.doAutoCreate()) 4362 this.serviceProviderTarget = new Organization(); // aa 4363 return this.serviceProviderTarget; 4364 } 4365 4366 /** 4367 * @param value {@link #serviceProvider} The actual object that is the target of 4368 * the reference. The reference library doesn't use these, but you 4369 * can use it to hold the resource if you resolve it. (The 4370 * organization that is primarily responsible for this Encounter's 4371 * services. This MAY be the same as the organization on the 4372 * Patient record, however it could be different, such as if the 4373 * actor performing the services was from an external organization 4374 * (which may be billed seperately) for an external consultation. 4375 * Refer to the example bundle showing an abbreviated set of 4376 * Encounters for a colonoscopy.) 4377 */ 4378 public Encounter setServiceProviderTarget(Organization value) { 4379 this.serviceProviderTarget = value; 4380 return this; 4381 } 4382 4383 /** 4384 * @return {@link #partOf} (Another Encounter of which this encounter is a part 4385 * of (administratively or in time).) 4386 */ 4387 public Reference getPartOf() { 4388 if (this.partOf == null) 4389 if (Configuration.errorOnAutoCreate()) 4390 throw new Error("Attempt to auto-create Encounter.partOf"); 4391 else if (Configuration.doAutoCreate()) 4392 this.partOf = new Reference(); // cc 4393 return this.partOf; 4394 } 4395 4396 public boolean hasPartOf() { 4397 return this.partOf != null && !this.partOf.isEmpty(); 4398 } 4399 4400 /** 4401 * @param value {@link #partOf} (Another Encounter of which this encounter is a 4402 * part of (administratively or in time).) 4403 */ 4404 public Encounter setPartOf(Reference value) { 4405 this.partOf = value; 4406 return this; 4407 } 4408 4409 /** 4410 * @return {@link #partOf} The actual object that is the target of the 4411 * reference. The reference library doesn't populate this, but you can 4412 * use it to hold the resource if you resolve it. (Another Encounter of 4413 * which this encounter is a part of (administratively or in time).) 4414 */ 4415 public Encounter getPartOfTarget() { 4416 if (this.partOfTarget == null) 4417 if (Configuration.errorOnAutoCreate()) 4418 throw new Error("Attempt to auto-create Encounter.partOf"); 4419 else if (Configuration.doAutoCreate()) 4420 this.partOfTarget = new Encounter(); // aa 4421 return this.partOfTarget; 4422 } 4423 4424 /** 4425 * @param value {@link #partOf} The actual object that is the target of the 4426 * reference. The reference library doesn't use these, but you can 4427 * use it to hold the resource if you resolve it. (Another 4428 * Encounter of which this encounter is a part of (administratively 4429 * or in time).) 4430 */ 4431 public Encounter setPartOfTarget(Encounter value) { 4432 this.partOfTarget = value; 4433 return this; 4434 } 4435 4436 protected void listChildren(List<Property> children) { 4437 super.listChildren(children); 4438 children.add(new Property("identifier", "Identifier", "Identifier(s) by which this encounter is known.", 0, 4439 java.lang.Integer.MAX_VALUE, identifier)); 4440 children.add(new Property("status", "code", 4441 "planned | arrived | triaged | in-progress | onleave | finished | cancelled +.", 0, 1, status)); 4442 children.add(new Property("statusHistory", "", 4443 "The status history permits the encounter resource to contain the status history without needing to read through the historical versions of the resource, or even have the server store them.", 4444 0, java.lang.Integer.MAX_VALUE, statusHistory)); 4445 children.add(new Property("class", "Coding", 4446 "Concepts representing classification of patient encounter such as ambulatory (outpatient), inpatient, emergency, home health or others due to local variations.", 4447 0, 1, class_)); 4448 children.add(new Property("classHistory", "", 4449 "The class history permits the tracking of the encounters transitions without needing to go through the resource history. This would be used for a case where an admission starts of as an emergency encounter, then transitions into an inpatient scenario. Doing this and not restarting a new encounter ensures that any lab/diagnostic results can more easily follow the patient and not require re-processing and not get lost or cancelled during a kind of discharge from emergency to inpatient.", 4450 0, java.lang.Integer.MAX_VALUE, classHistory)); 4451 children.add(new Property("type", "CodeableConcept", 4452 "Specific type of encounter (e.g. e-mail consultation, surgical day-care, skilled nursing, rehabilitation).", 0, 4453 java.lang.Integer.MAX_VALUE, type)); 4454 children.add(new Property("serviceType", "CodeableConcept", 4455 "Broad categorization of the service that is to be provided (e.g. cardiology).", 0, 1, serviceType)); 4456 children 4457 .add(new Property("priority", "CodeableConcept", "Indicates the urgency of the encounter.", 0, 1, priority)); 4458 children.add(new Property("subject", "Reference(Patient|Group)", "The patient or group present at the encounter.", 4459 0, 1, subject)); 4460 children.add(new Property("episodeOfCare", "Reference(EpisodeOfCare)", 4461 "Where a specific encounter should be classified as a part of a specific episode(s) of care this field should be used. This association can facilitate grouping of related encounters together for a specific purpose, such as government reporting, issue tracking, association via a common problem. The association is recorded on the encounter as these are typically created after the episode of care and grouped on entry rather than editing the episode of care to append another encounter to it (the episode of care could span years).", 4462 0, java.lang.Integer.MAX_VALUE, episodeOfCare)); 4463 children.add(new Property("basedOn", "Reference(ServiceRequest)", 4464 "The request this encounter satisfies (e.g. incoming referral or procedure request).", 0, 4465 java.lang.Integer.MAX_VALUE, basedOn)); 4466 children.add(new Property("participant", "", "The list of people responsible for providing the service.", 0, 4467 java.lang.Integer.MAX_VALUE, participant)); 4468 children.add(new Property("appointment", "Reference(Appointment)", "The appointment that scheduled this encounter.", 4469 0, java.lang.Integer.MAX_VALUE, appointment)); 4470 children.add(new Property("period", "Period", "The start and end time of the encounter.", 0, 1, period)); 4471 children.add(new Property("length", "Duration", 4472 "Quantity of time the encounter lasted. This excludes the time during leaves of absence.", 0, 1, length)); 4473 children.add(new Property("reasonCode", "CodeableConcept", 4474 "Reason the encounter takes place, expressed as a code. For admissions, this can be used for a coded admission diagnosis.", 4475 0, java.lang.Integer.MAX_VALUE, reasonCode)); 4476 children.add(new Property("reasonReference", 4477 "Reference(Condition|Procedure|Observation|ImmunizationRecommendation)", 4478 "Reason the encounter takes place, expressed as a code. For admissions, this can be used for a coded admission diagnosis.", 4479 0, java.lang.Integer.MAX_VALUE, reasonReference)); 4480 children.add(new Property("diagnosis", "", "The list of diagnosis relevant to this encounter.", 0, 4481 java.lang.Integer.MAX_VALUE, diagnosis)); 4482 children.add(new Property("account", "Reference(Account)", 4483 "The set of accounts that may be used for billing for this Encounter.", 0, java.lang.Integer.MAX_VALUE, 4484 account)); 4485 children.add(new Property("hospitalization", "", "Details about the admission to a healthcare service.", 0, 1, 4486 hospitalization)); 4487 children.add(new Property("location", "", "List of locations where the patient has been during this encounter.", 0, 4488 java.lang.Integer.MAX_VALUE, location)); 4489 children.add(new Property("serviceProvider", "Reference(Organization)", 4490 "The organization that is primarily responsible for this Encounter's services. This MAY be the same as the organization on the Patient record, however it could be different, such as if the actor performing the services was from an external organization (which may be billed seperately) for an external consultation. Refer to the example bundle showing an abbreviated set of Encounters for a colonoscopy.", 4491 0, 1, serviceProvider)); 4492 children.add(new Property("partOf", "Reference(Encounter)", 4493 "Another Encounter of which this encounter is a part of (administratively or in time).", 0, 1, partOf)); 4494 } 4495 4496 @Override 4497 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4498 switch (_hash) { 4499 case -1618432855: 4500 /* identifier */ return new Property("identifier", "Identifier", 4501 "Identifier(s) by which this encounter is known.", 0, java.lang.Integer.MAX_VALUE, identifier); 4502 case -892481550: 4503 /* status */ return new Property("status", "code", 4504 "planned | arrived | triaged | in-progress | onleave | finished | cancelled +.", 0, 1, status); 4505 case -986695614: 4506 /* statusHistory */ return new Property("statusHistory", "", 4507 "The status history permits the encounter resource to contain the status history without needing to read through the historical versions of the resource, or even have the server store them.", 4508 0, java.lang.Integer.MAX_VALUE, statusHistory); 4509 case 94742904: 4510 /* class */ return new Property("class", "Coding", 4511 "Concepts representing classification of patient encounter such as ambulatory (outpatient), inpatient, emergency, home health or others due to local variations.", 4512 0, 1, class_); 4513 case 962575356: 4514 /* classHistory */ return new Property("classHistory", "", 4515 "The class history permits the tracking of the encounters transitions without needing to go through the resource history. This would be used for a case where an admission starts of as an emergency encounter, then transitions into an inpatient scenario. Doing this and not restarting a new encounter ensures that any lab/diagnostic results can more easily follow the patient and not require re-processing and not get lost or cancelled during a kind of discharge from emergency to inpatient.", 4516 0, java.lang.Integer.MAX_VALUE, classHistory); 4517 case 3575610: 4518 /* type */ return new Property("type", "CodeableConcept", 4519 "Specific type of encounter (e.g. e-mail consultation, surgical day-care, skilled nursing, rehabilitation).", 4520 0, java.lang.Integer.MAX_VALUE, type); 4521 case -1928370289: 4522 /* serviceType */ return new Property("serviceType", "CodeableConcept", 4523 "Broad categorization of the service that is to be provided (e.g. cardiology).", 0, 1, serviceType); 4524 case -1165461084: 4525 /* priority */ return new Property("priority", "CodeableConcept", "Indicates the urgency of the encounter.", 0, 1, 4526 priority); 4527 case -1867885268: 4528 /* subject */ return new Property("subject", "Reference(Patient|Group)", 4529 "The patient or group present at the encounter.", 0, 1, subject); 4530 case -1892140189: 4531 /* episodeOfCare */ return new Property("episodeOfCare", "Reference(EpisodeOfCare)", 4532 "Where a specific encounter should be classified as a part of a specific episode(s) of care this field should be used. This association can facilitate grouping of related encounters together for a specific purpose, such as government reporting, issue tracking, association via a common problem. The association is recorded on the encounter as these are typically created after the episode of care and grouped on entry rather than editing the episode of care to append another encounter to it (the episode of care could span years).", 4533 0, java.lang.Integer.MAX_VALUE, episodeOfCare); 4534 case -332612366: 4535 /* basedOn */ return new Property("basedOn", "Reference(ServiceRequest)", 4536 "The request this encounter satisfies (e.g. incoming referral or procedure request).", 0, 4537 java.lang.Integer.MAX_VALUE, basedOn); 4538 case 767422259: 4539 /* participant */ return new Property("participant", "", 4540 "The list of people responsible for providing the service.", 0, java.lang.Integer.MAX_VALUE, participant); 4541 case -1474995297: 4542 /* appointment */ return new Property("appointment", "Reference(Appointment)", 4543 "The appointment that scheduled this encounter.", 0, java.lang.Integer.MAX_VALUE, appointment); 4544 case -991726143: 4545 /* period */ return new Property("period", "Period", "The start and end time of the encounter.", 0, 1, period); 4546 case -1106363674: 4547 /* length */ return new Property("length", "Duration", 4548 "Quantity of time the encounter lasted. This excludes the time during leaves of absence.", 0, 1, length); 4549 case 722137681: 4550 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 4551 "Reason the encounter takes place, expressed as a code. For admissions, this can be used for a coded admission diagnosis.", 4552 0, java.lang.Integer.MAX_VALUE, reasonCode); 4553 case -1146218137: 4554 /* reasonReference */ return new Property("reasonReference", 4555 "Reference(Condition|Procedure|Observation|ImmunizationRecommendation)", 4556 "Reason the encounter takes place, expressed as a code. For admissions, this can be used for a coded admission diagnosis.", 4557 0, java.lang.Integer.MAX_VALUE, reasonReference); 4558 case 1196993265: 4559 /* diagnosis */ return new Property("diagnosis", "", "The list of diagnosis relevant to this encounter.", 0, 4560 java.lang.Integer.MAX_VALUE, diagnosis); 4561 case -1177318867: 4562 /* account */ return new Property("account", "Reference(Account)", 4563 "The set of accounts that may be used for billing for this Encounter.", 0, java.lang.Integer.MAX_VALUE, 4564 account); 4565 case 1057894634: 4566 /* hospitalization */ return new Property("hospitalization", "", 4567 "Details about the admission to a healthcare service.", 0, 1, hospitalization); 4568 case 1901043637: 4569 /* location */ return new Property("location", "", 4570 "List of locations where the patient has been during this encounter.", 0, java.lang.Integer.MAX_VALUE, 4571 location); 4572 case 243182534: 4573 /* serviceProvider */ return new Property("serviceProvider", "Reference(Organization)", 4574 "The organization that is primarily responsible for this Encounter's services. This MAY be the same as the organization on the Patient record, however it could be different, such as if the actor performing the services was from an external organization (which may be billed seperately) for an external consultation. Refer to the example bundle showing an abbreviated set of Encounters for a colonoscopy.", 4575 0, 1, serviceProvider); 4576 case -995410646: 4577 /* partOf */ return new Property("partOf", "Reference(Encounter)", 4578 "Another Encounter of which this encounter is a part of (administratively or in time).", 0, 1, partOf); 4579 default: 4580 return super.getNamedProperty(_hash, _name, _checkValid); 4581 } 4582 4583 } 4584 4585 @Override 4586 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4587 switch (hash) { 4588 case -1618432855: 4589 /* identifier */ return this.identifier == null ? new Base[0] 4590 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4591 case -892481550: 4592 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<EncounterStatus> 4593 case -986695614: 4594 /* statusHistory */ return this.statusHistory == null ? new Base[0] 4595 : this.statusHistory.toArray(new Base[this.statusHistory.size()]); // StatusHistoryComponent 4596 case 94742904: 4597 /* class */ return this.class_ == null ? new Base[0] : new Base[] { this.class_ }; // Coding 4598 case 962575356: 4599 /* classHistory */ return this.classHistory == null ? new Base[0] 4600 : this.classHistory.toArray(new Base[this.classHistory.size()]); // ClassHistoryComponent 4601 case 3575610: 4602 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 4603 case -1928370289: 4604 /* serviceType */ return this.serviceType == null ? new Base[0] : new Base[] { this.serviceType }; // CodeableConcept 4605 case -1165461084: 4606 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // CodeableConcept 4607 case -1867885268: 4608 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 4609 case -1892140189: 4610 /* episodeOfCare */ return this.episodeOfCare == null ? new Base[0] 4611 : this.episodeOfCare.toArray(new Base[this.episodeOfCare.size()]); // Reference 4612 case -332612366: 4613 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 4614 case 767422259: 4615 /* participant */ return this.participant == null ? new Base[0] 4616 : this.participant.toArray(new Base[this.participant.size()]); // EncounterParticipantComponent 4617 case -1474995297: 4618 /* appointment */ return this.appointment == null ? new Base[0] 4619 : this.appointment.toArray(new Base[this.appointment.size()]); // Reference 4620 case -991726143: 4621 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 4622 case -1106363674: 4623 /* length */ return this.length == null ? new Base[0] : new Base[] { this.length }; // Duration 4624 case 722137681: 4625 /* reasonCode */ return this.reasonCode == null ? new Base[0] 4626 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 4627 case -1146218137: 4628 /* reasonReference */ return this.reasonReference == null ? new Base[0] 4629 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 4630 case 1196993265: 4631 /* diagnosis */ return this.diagnosis == null ? new Base[0] 4632 : this.diagnosis.toArray(new Base[this.diagnosis.size()]); // DiagnosisComponent 4633 case -1177318867: 4634 /* account */ return this.account == null ? new Base[0] : this.account.toArray(new Base[this.account.size()]); // Reference 4635 case 1057894634: 4636 /* hospitalization */ return this.hospitalization == null ? new Base[0] : new Base[] { this.hospitalization }; // EncounterHospitalizationComponent 4637 case 1901043637: 4638 /* location */ return this.location == null ? new Base[0] : this.location.toArray(new Base[this.location.size()]); // EncounterLocationComponent 4639 case 243182534: 4640 /* serviceProvider */ return this.serviceProvider == null ? new Base[0] : new Base[] { this.serviceProvider }; // Reference 4641 case -995410646: 4642 /* partOf */ return this.partOf == null ? new Base[0] : new Base[] { this.partOf }; // Reference 4643 default: 4644 return super.getProperty(hash, name, checkValid); 4645 } 4646 4647 } 4648 4649 @Override 4650 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4651 switch (hash) { 4652 case -1618432855: // identifier 4653 this.getIdentifier().add(castToIdentifier(value)); // Identifier 4654 return value; 4655 case -892481550: // status 4656 value = new EncounterStatusEnumFactory().fromType(castToCode(value)); 4657 this.status = (Enumeration) value; // Enumeration<EncounterStatus> 4658 return value; 4659 case -986695614: // statusHistory 4660 this.getStatusHistory().add((StatusHistoryComponent) value); // StatusHistoryComponent 4661 return value; 4662 case 94742904: // class 4663 this.class_ = castToCoding(value); // Coding 4664 return value; 4665 case 962575356: // classHistory 4666 this.getClassHistory().add((ClassHistoryComponent) value); // ClassHistoryComponent 4667 return value; 4668 case 3575610: // type 4669 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 4670 return value; 4671 case -1928370289: // serviceType 4672 this.serviceType = castToCodeableConcept(value); // CodeableConcept 4673 return value; 4674 case -1165461084: // priority 4675 this.priority = castToCodeableConcept(value); // CodeableConcept 4676 return value; 4677 case -1867885268: // subject 4678 this.subject = castToReference(value); // Reference 4679 return value; 4680 case -1892140189: // episodeOfCare 4681 this.getEpisodeOfCare().add(castToReference(value)); // Reference 4682 return value; 4683 case -332612366: // basedOn 4684 this.getBasedOn().add(castToReference(value)); // Reference 4685 return value; 4686 case 767422259: // participant 4687 this.getParticipant().add((EncounterParticipantComponent) value); // EncounterParticipantComponent 4688 return value; 4689 case -1474995297: // appointment 4690 this.getAppointment().add(castToReference(value)); // Reference 4691 return value; 4692 case -991726143: // period 4693 this.period = castToPeriod(value); // Period 4694 return value; 4695 case -1106363674: // length 4696 this.length = castToDuration(value); // Duration 4697 return value; 4698 case 722137681: // reasonCode 4699 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 4700 return value; 4701 case -1146218137: // reasonReference 4702 this.getReasonReference().add(castToReference(value)); // Reference 4703 return value; 4704 case 1196993265: // diagnosis 4705 this.getDiagnosis().add((DiagnosisComponent) value); // DiagnosisComponent 4706 return value; 4707 case -1177318867: // account 4708 this.getAccount().add(castToReference(value)); // Reference 4709 return value; 4710 case 1057894634: // hospitalization 4711 this.hospitalization = (EncounterHospitalizationComponent) value; // EncounterHospitalizationComponent 4712 return value; 4713 case 1901043637: // location 4714 this.getLocation().add((EncounterLocationComponent) value); // EncounterLocationComponent 4715 return value; 4716 case 243182534: // serviceProvider 4717 this.serviceProvider = castToReference(value); // Reference 4718 return value; 4719 case -995410646: // partOf 4720 this.partOf = castToReference(value); // Reference 4721 return value; 4722 default: 4723 return super.setProperty(hash, name, value); 4724 } 4725 4726 } 4727 4728 @Override 4729 public Base setProperty(String name, Base value) throws FHIRException { 4730 if (name.equals("identifier")) { 4731 this.getIdentifier().add(castToIdentifier(value)); 4732 } else if (name.equals("status")) { 4733 value = new EncounterStatusEnumFactory().fromType(castToCode(value)); 4734 this.status = (Enumeration) value; // Enumeration<EncounterStatus> 4735 } else if (name.equals("statusHistory")) { 4736 this.getStatusHistory().add((StatusHistoryComponent) value); 4737 } else if (name.equals("class")) { 4738 this.class_ = castToCoding(value); // Coding 4739 } else if (name.equals("classHistory")) { 4740 this.getClassHistory().add((ClassHistoryComponent) value); 4741 } else if (name.equals("type")) { 4742 this.getType().add(castToCodeableConcept(value)); 4743 } else if (name.equals("serviceType")) { 4744 this.serviceType = castToCodeableConcept(value); // CodeableConcept 4745 } else if (name.equals("priority")) { 4746 this.priority = castToCodeableConcept(value); // CodeableConcept 4747 } else if (name.equals("subject")) { 4748 this.subject = castToReference(value); // Reference 4749 } else if (name.equals("episodeOfCare")) { 4750 this.getEpisodeOfCare().add(castToReference(value)); 4751 } else if (name.equals("basedOn")) { 4752 this.getBasedOn().add(castToReference(value)); 4753 } else if (name.equals("participant")) { 4754 this.getParticipant().add((EncounterParticipantComponent) value); 4755 } else if (name.equals("appointment")) { 4756 this.getAppointment().add(castToReference(value)); 4757 } else if (name.equals("period")) { 4758 this.period = castToPeriod(value); // Period 4759 } else if (name.equals("length")) { 4760 this.length = castToDuration(value); // Duration 4761 } else if (name.equals("reasonCode")) { 4762 this.getReasonCode().add(castToCodeableConcept(value)); 4763 } else if (name.equals("reasonReference")) { 4764 this.getReasonReference().add(castToReference(value)); 4765 } else if (name.equals("diagnosis")) { 4766 this.getDiagnosis().add((DiagnosisComponent) value); 4767 } else if (name.equals("account")) { 4768 this.getAccount().add(castToReference(value)); 4769 } else if (name.equals("hospitalization")) { 4770 this.hospitalization = (EncounterHospitalizationComponent) value; // EncounterHospitalizationComponent 4771 } else if (name.equals("location")) { 4772 this.getLocation().add((EncounterLocationComponent) value); 4773 } else if (name.equals("serviceProvider")) { 4774 this.serviceProvider = castToReference(value); // Reference 4775 } else if (name.equals("partOf")) { 4776 this.partOf = castToReference(value); // Reference 4777 } else 4778 return super.setProperty(name, value); 4779 return value; 4780 } 4781 4782 @Override 4783 public void removeChild(String name, Base value) throws FHIRException { 4784 if (name.equals("identifier")) { 4785 this.getIdentifier().remove(castToIdentifier(value)); 4786 } else if (name.equals("status")) { 4787 this.status = null; 4788 } else if (name.equals("statusHistory")) { 4789 this.getStatusHistory().remove((StatusHistoryComponent) value); 4790 } else if (name.equals("class")) { 4791 this.class_ = null; 4792 } else if (name.equals("classHistory")) { 4793 this.getClassHistory().remove((ClassHistoryComponent) value); 4794 } else if (name.equals("type")) { 4795 this.getType().remove(castToCodeableConcept(value)); 4796 } else if (name.equals("serviceType")) { 4797 this.serviceType = null; 4798 } else if (name.equals("priority")) { 4799 this.priority = null; 4800 } else if (name.equals("subject")) { 4801 this.subject = null; 4802 } else if (name.equals("episodeOfCare")) { 4803 this.getEpisodeOfCare().remove(castToReference(value)); 4804 } else if (name.equals("basedOn")) { 4805 this.getBasedOn().remove(castToReference(value)); 4806 } else if (name.equals("participant")) { 4807 this.getParticipant().remove((EncounterParticipantComponent) value); 4808 } else if (name.equals("appointment")) { 4809 this.getAppointment().remove(castToReference(value)); 4810 } else if (name.equals("period")) { 4811 this.period = null; 4812 } else if (name.equals("length")) { 4813 this.length = null; 4814 } else if (name.equals("reasonCode")) { 4815 this.getReasonCode().remove(castToCodeableConcept(value)); 4816 } else if (name.equals("reasonReference")) { 4817 this.getReasonReference().remove(castToReference(value)); 4818 } else if (name.equals("diagnosis")) { 4819 this.getDiagnosis().remove((DiagnosisComponent) value); 4820 } else if (name.equals("account")) { 4821 this.getAccount().remove(castToReference(value)); 4822 } else if (name.equals("hospitalization")) { 4823 this.hospitalization = (EncounterHospitalizationComponent) value; // EncounterHospitalizationComponent 4824 } else if (name.equals("location")) { 4825 this.getLocation().remove((EncounterLocationComponent) value); 4826 } else if (name.equals("serviceProvider")) { 4827 this.serviceProvider = null; 4828 } else if (name.equals("partOf")) { 4829 this.partOf = null; 4830 } else 4831 super.removeChild(name, value); 4832 4833 } 4834 4835 @Override 4836 public Base makeProperty(int hash, String name) throws FHIRException { 4837 switch (hash) { 4838 case -1618432855: 4839 return addIdentifier(); 4840 case -892481550: 4841 return getStatusElement(); 4842 case -986695614: 4843 return addStatusHistory(); 4844 case 94742904: 4845 return getClass_(); 4846 case 962575356: 4847 return addClassHistory(); 4848 case 3575610: 4849 return addType(); 4850 case -1928370289: 4851 return getServiceType(); 4852 case -1165461084: 4853 return getPriority(); 4854 case -1867885268: 4855 return getSubject(); 4856 case -1892140189: 4857 return addEpisodeOfCare(); 4858 case -332612366: 4859 return addBasedOn(); 4860 case 767422259: 4861 return addParticipant(); 4862 case -1474995297: 4863 return addAppointment(); 4864 case -991726143: 4865 return getPeriod(); 4866 case -1106363674: 4867 return getLength(); 4868 case 722137681: 4869 return addReasonCode(); 4870 case -1146218137: 4871 return addReasonReference(); 4872 case 1196993265: 4873 return addDiagnosis(); 4874 case -1177318867: 4875 return addAccount(); 4876 case 1057894634: 4877 return getHospitalization(); 4878 case 1901043637: 4879 return addLocation(); 4880 case 243182534: 4881 return getServiceProvider(); 4882 case -995410646: 4883 return getPartOf(); 4884 default: 4885 return super.makeProperty(hash, name); 4886 } 4887 4888 } 4889 4890 @Override 4891 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4892 switch (hash) { 4893 case -1618432855: 4894 /* identifier */ return new String[] { "Identifier" }; 4895 case -892481550: 4896 /* status */ return new String[] { "code" }; 4897 case -986695614: 4898 /* statusHistory */ return new String[] {}; 4899 case 94742904: 4900 /* class */ return new String[] { "Coding" }; 4901 case 962575356: 4902 /* classHistory */ return new String[] {}; 4903 case 3575610: 4904 /* type */ return new String[] { "CodeableConcept" }; 4905 case -1928370289: 4906 /* serviceType */ return new String[] { "CodeableConcept" }; 4907 case -1165461084: 4908 /* priority */ return new String[] { "CodeableConcept" }; 4909 case -1867885268: 4910 /* subject */ return new String[] { "Reference" }; 4911 case -1892140189: 4912 /* episodeOfCare */ return new String[] { "Reference" }; 4913 case -332612366: 4914 /* basedOn */ return new String[] { "Reference" }; 4915 case 767422259: 4916 /* participant */ return new String[] {}; 4917 case -1474995297: 4918 /* appointment */ return new String[] { "Reference" }; 4919 case -991726143: 4920 /* period */ return new String[] { "Period" }; 4921 case -1106363674: 4922 /* length */ return new String[] { "Duration" }; 4923 case 722137681: 4924 /* reasonCode */ return new String[] { "CodeableConcept" }; 4925 case -1146218137: 4926 /* reasonReference */ return new String[] { "Reference" }; 4927 case 1196993265: 4928 /* diagnosis */ return new String[] {}; 4929 case -1177318867: 4930 /* account */ return new String[] { "Reference" }; 4931 case 1057894634: 4932 /* hospitalization */ return new String[] {}; 4933 case 1901043637: 4934 /* location */ return new String[] {}; 4935 case 243182534: 4936 /* serviceProvider */ return new String[] { "Reference" }; 4937 case -995410646: 4938 /* partOf */ return new String[] { "Reference" }; 4939 default: 4940 return super.getTypesForProperty(hash, name); 4941 } 4942 4943 } 4944 4945 @Override 4946 public Base addChild(String name) throws FHIRException { 4947 if (name.equals("identifier")) { 4948 return addIdentifier(); 4949 } else if (name.equals("status")) { 4950 throw new FHIRException("Cannot call addChild on a singleton property Encounter.status"); 4951 } else if (name.equals("statusHistory")) { 4952 return addStatusHistory(); 4953 } else if (name.equals("class")) { 4954 this.class_ = new Coding(); 4955 return this.class_; 4956 } else if (name.equals("classHistory")) { 4957 return addClassHistory(); 4958 } else if (name.equals("type")) { 4959 return addType(); 4960 } else if (name.equals("serviceType")) { 4961 this.serviceType = new CodeableConcept(); 4962 return this.serviceType; 4963 } else if (name.equals("priority")) { 4964 this.priority = new CodeableConcept(); 4965 return this.priority; 4966 } else if (name.equals("subject")) { 4967 this.subject = new Reference(); 4968 return this.subject; 4969 } else if (name.equals("episodeOfCare")) { 4970 return addEpisodeOfCare(); 4971 } else if (name.equals("basedOn")) { 4972 return addBasedOn(); 4973 } else if (name.equals("participant")) { 4974 return addParticipant(); 4975 } else if (name.equals("appointment")) { 4976 return addAppointment(); 4977 } else if (name.equals("period")) { 4978 this.period = new Period(); 4979 return this.period; 4980 } else if (name.equals("length")) { 4981 this.length = new Duration(); 4982 return this.length; 4983 } else if (name.equals("reasonCode")) { 4984 return addReasonCode(); 4985 } else if (name.equals("reasonReference")) { 4986 return addReasonReference(); 4987 } else if (name.equals("diagnosis")) { 4988 return addDiagnosis(); 4989 } else if (name.equals("account")) { 4990 return addAccount(); 4991 } else if (name.equals("hospitalization")) { 4992 this.hospitalization = new EncounterHospitalizationComponent(); 4993 return this.hospitalization; 4994 } else if (name.equals("location")) { 4995 return addLocation(); 4996 } else if (name.equals("serviceProvider")) { 4997 this.serviceProvider = new Reference(); 4998 return this.serviceProvider; 4999 } else if (name.equals("partOf")) { 5000 this.partOf = new Reference(); 5001 return this.partOf; 5002 } else 5003 return super.addChild(name); 5004 } 5005 5006 public String fhirType() { 5007 return "Encounter"; 5008 5009 } 5010 5011 public Encounter copy() { 5012 Encounter dst = new Encounter(); 5013 copyValues(dst); 5014 return dst; 5015 } 5016 5017 public void copyValues(Encounter dst) { 5018 super.copyValues(dst); 5019 if (identifier != null) { 5020 dst.identifier = new ArrayList<Identifier>(); 5021 for (Identifier i : identifier) 5022 dst.identifier.add(i.copy()); 5023 } 5024 ; 5025 dst.status = status == null ? null : status.copy(); 5026 if (statusHistory != null) { 5027 dst.statusHistory = new ArrayList<StatusHistoryComponent>(); 5028 for (StatusHistoryComponent i : statusHistory) 5029 dst.statusHistory.add(i.copy()); 5030 } 5031 ; 5032 dst.class_ = class_ == null ? null : class_.copy(); 5033 if (classHistory != null) { 5034 dst.classHistory = new ArrayList<ClassHistoryComponent>(); 5035 for (ClassHistoryComponent i : classHistory) 5036 dst.classHistory.add(i.copy()); 5037 } 5038 ; 5039 if (type != null) { 5040 dst.type = new ArrayList<CodeableConcept>(); 5041 for (CodeableConcept i : type) 5042 dst.type.add(i.copy()); 5043 } 5044 ; 5045 dst.serviceType = serviceType == null ? null : serviceType.copy(); 5046 dst.priority = priority == null ? null : priority.copy(); 5047 dst.subject = subject == null ? null : subject.copy(); 5048 if (episodeOfCare != null) { 5049 dst.episodeOfCare = new ArrayList<Reference>(); 5050 for (Reference i : episodeOfCare) 5051 dst.episodeOfCare.add(i.copy()); 5052 } 5053 ; 5054 if (basedOn != null) { 5055 dst.basedOn = new ArrayList<Reference>(); 5056 for (Reference i : basedOn) 5057 dst.basedOn.add(i.copy()); 5058 } 5059 ; 5060 if (participant != null) { 5061 dst.participant = new ArrayList<EncounterParticipantComponent>(); 5062 for (EncounterParticipantComponent i : participant) 5063 dst.participant.add(i.copy()); 5064 } 5065 ; 5066 if (appointment != null) { 5067 dst.appointment = new ArrayList<Reference>(); 5068 for (Reference i : appointment) 5069 dst.appointment.add(i.copy()); 5070 } 5071 ; 5072 dst.period = period == null ? null : period.copy(); 5073 dst.length = length == null ? null : length.copy(); 5074 if (reasonCode != null) { 5075 dst.reasonCode = new ArrayList<CodeableConcept>(); 5076 for (CodeableConcept i : reasonCode) 5077 dst.reasonCode.add(i.copy()); 5078 } 5079 ; 5080 if (reasonReference != null) { 5081 dst.reasonReference = new ArrayList<Reference>(); 5082 for (Reference i : reasonReference) 5083 dst.reasonReference.add(i.copy()); 5084 } 5085 ; 5086 if (diagnosis != null) { 5087 dst.diagnosis = new ArrayList<DiagnosisComponent>(); 5088 for (DiagnosisComponent i : diagnosis) 5089 dst.diagnosis.add(i.copy()); 5090 } 5091 ; 5092 if (account != null) { 5093 dst.account = new ArrayList<Reference>(); 5094 for (Reference i : account) 5095 dst.account.add(i.copy()); 5096 } 5097 ; 5098 dst.hospitalization = hospitalization == null ? null : hospitalization.copy(); 5099 if (location != null) { 5100 dst.location = new ArrayList<EncounterLocationComponent>(); 5101 for (EncounterLocationComponent i : location) 5102 dst.location.add(i.copy()); 5103 } 5104 ; 5105 dst.serviceProvider = serviceProvider == null ? null : serviceProvider.copy(); 5106 dst.partOf = partOf == null ? null : partOf.copy(); 5107 } 5108 5109 protected Encounter typedCopy() { 5110 return copy(); 5111 } 5112 5113 @Override 5114 public boolean equalsDeep(Base other_) { 5115 if (!super.equalsDeep(other_)) 5116 return false; 5117 if (!(other_ instanceof Encounter)) 5118 return false; 5119 Encounter o = (Encounter) other_; 5120 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 5121 && compareDeep(statusHistory, o.statusHistory, true) && compareDeep(class_, o.class_, true) 5122 && compareDeep(classHistory, o.classHistory, true) && compareDeep(type, o.type, true) 5123 && compareDeep(serviceType, o.serviceType, true) && compareDeep(priority, o.priority, true) 5124 && compareDeep(subject, o.subject, true) && compareDeep(episodeOfCare, o.episodeOfCare, true) 5125 && compareDeep(basedOn, o.basedOn, true) && compareDeep(participant, o.participant, true) 5126 && compareDeep(appointment, o.appointment, true) && compareDeep(period, o.period, true) 5127 && compareDeep(length, o.length, true) && compareDeep(reasonCode, o.reasonCode, true) 5128 && compareDeep(reasonReference, o.reasonReference, true) && compareDeep(diagnosis, o.diagnosis, true) 5129 && compareDeep(account, o.account, true) && compareDeep(hospitalization, o.hospitalization, true) 5130 && compareDeep(location, o.location, true) && compareDeep(serviceProvider, o.serviceProvider, true) 5131 && compareDeep(partOf, o.partOf, true); 5132 } 5133 5134 @Override 5135 public boolean equalsShallow(Base other_) { 5136 if (!super.equalsShallow(other_)) 5137 return false; 5138 if (!(other_ instanceof Encounter)) 5139 return false; 5140 Encounter o = (Encounter) other_; 5141 return compareValues(status, o.status, true); 5142 } 5143 5144 public boolean isEmpty() { 5145 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, statusHistory, class_, 5146 classHistory, type, serviceType, priority, subject, episodeOfCare, basedOn, participant, appointment, period, 5147 length, reasonCode, reasonReference, diagnosis, account, hospitalization, location, serviceProvider, partOf); 5148 } 5149 5150 @Override 5151 public ResourceType getResourceType() { 5152 return ResourceType.Encounter; 5153 } 5154 5155 /** 5156 * Search parameter: <b>date</b> 5157 * <p> 5158 * Description: <b>A date within the period the Encounter lasted</b><br> 5159 * Type: <b>date</b><br> 5160 * Path: <b>Encounter.period</b><br> 5161 * </p> 5162 */ 5163 @SearchParamDefinition(name = "date", path = "Encounter.period", description = "A date within the period the Encounter lasted", type = "date") 5164 public static final String SP_DATE = "date"; 5165 /** 5166 * <b>Fluent Client</b> search parameter constant for <b>date</b> 5167 * <p> 5168 * Description: <b>A date within the period the Encounter lasted</b><br> 5169 * Type: <b>date</b><br> 5170 * Path: <b>Encounter.period</b><br> 5171 * </p> 5172 */ 5173 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 5174 SP_DATE); 5175 5176 /** 5177 * Search parameter: <b>identifier</b> 5178 * <p> 5179 * Description: <b>Identifier(s) by which this encounter is known</b><br> 5180 * Type: <b>token</b><br> 5181 * Path: <b>Encounter.identifier</b><br> 5182 * </p> 5183 */ 5184 @SearchParamDefinition(name = "identifier", path = "Encounter.identifier", description = "Identifier(s) by which this encounter is known", type = "token") 5185 public static final String SP_IDENTIFIER = "identifier"; 5186 /** 5187 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5188 * <p> 5189 * Description: <b>Identifier(s) by which this encounter is known</b><br> 5190 * Type: <b>token</b><br> 5191 * Path: <b>Encounter.identifier</b><br> 5192 * </p> 5193 */ 5194 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5195 SP_IDENTIFIER); 5196 5197 /** 5198 * Search parameter: <b>participant-type</b> 5199 * <p> 5200 * Description: <b>Role of participant in encounter</b><br> 5201 * Type: <b>token</b><br> 5202 * Path: <b>Encounter.participant.type</b><br> 5203 * </p> 5204 */ 5205 @SearchParamDefinition(name = "participant-type", path = "Encounter.participant.type", description = "Role of participant in encounter", type = "token") 5206 public static final String SP_PARTICIPANT_TYPE = "participant-type"; 5207 /** 5208 * <b>Fluent Client</b> search parameter constant for <b>participant-type</b> 5209 * <p> 5210 * Description: <b>Role of participant in encounter</b><br> 5211 * Type: <b>token</b><br> 5212 * Path: <b>Encounter.participant.type</b><br> 5213 * </p> 5214 */ 5215 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PARTICIPANT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5216 SP_PARTICIPANT_TYPE); 5217 5218 /** 5219 * Search parameter: <b>practitioner</b> 5220 * <p> 5221 * Description: <b>Persons involved in the encounter other than the 5222 * patient</b><br> 5223 * Type: <b>reference</b><br> 5224 * Path: <b>Encounter.participant.individual</b><br> 5225 * </p> 5226 */ 5227 @SearchParamDefinition(name = "practitioner", path = "Encounter.participant.individual.where(resolve() is Practitioner)", description = "Persons involved in the encounter other than the patient", type = "reference", providesMembershipIn = { 5228 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Practitioner.class }) 5229 public static final String SP_PRACTITIONER = "practitioner"; 5230 /** 5231 * <b>Fluent Client</b> search parameter constant for <b>practitioner</b> 5232 * <p> 5233 * Description: <b>Persons involved in the encounter other than the 5234 * patient</b><br> 5235 * Type: <b>reference</b><br> 5236 * Path: <b>Encounter.participant.individual</b><br> 5237 * </p> 5238 */ 5239 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRACTITIONER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5240 SP_PRACTITIONER); 5241 5242 /** 5243 * Constant for fluent queries to be used to add include statements. Specifies 5244 * the path value of "<b>Encounter:practitioner</b>". 5245 */ 5246 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRACTITIONER = new ca.uhn.fhir.model.api.Include( 5247 "Encounter:practitioner").toLocked(); 5248 5249 /** 5250 * Search parameter: <b>subject</b> 5251 * <p> 5252 * Description: <b>The patient or group present at the encounter</b><br> 5253 * Type: <b>reference</b><br> 5254 * Path: <b>Encounter.subject</b><br> 5255 * </p> 5256 */ 5257 @SearchParamDefinition(name = "subject", path = "Encounter.subject", description = "The patient or group present at the encounter", type = "reference", target = { 5258 Group.class, Patient.class }) 5259 public static final String SP_SUBJECT = "subject"; 5260 /** 5261 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 5262 * <p> 5263 * Description: <b>The patient or group present at the encounter</b><br> 5264 * Type: <b>reference</b><br> 5265 * Path: <b>Encounter.subject</b><br> 5266 * </p> 5267 */ 5268 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5269 SP_SUBJECT); 5270 5271 /** 5272 * Constant for fluent queries to be used to add include statements. Specifies 5273 * the path value of "<b>Encounter:subject</b>". 5274 */ 5275 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 5276 "Encounter:subject").toLocked(); 5277 5278 /** 5279 * Search parameter: <b>length</b> 5280 * <p> 5281 * Description: <b>Length of encounter in days</b><br> 5282 * Type: <b>quantity</b><br> 5283 * Path: <b>Encounter.length</b><br> 5284 * </p> 5285 */ 5286 @SearchParamDefinition(name = "length", path = "Encounter.length", description = "Length of encounter in days", type = "quantity") 5287 public static final String SP_LENGTH = "length"; 5288 /** 5289 * <b>Fluent Client</b> search parameter constant for <b>length</b> 5290 * <p> 5291 * Description: <b>Length of encounter in days</b><br> 5292 * Type: <b>quantity</b><br> 5293 * Path: <b>Encounter.length</b><br> 5294 * </p> 5295 */ 5296 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam LENGTH = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 5297 SP_LENGTH); 5298 5299 /** 5300 * Search parameter: <b>episode-of-care</b> 5301 * <p> 5302 * Description: <b>Episode(s) of care that this encounter should be recorded 5303 * against</b><br> 5304 * Type: <b>reference</b><br> 5305 * Path: <b>Encounter.episodeOfCare</b><br> 5306 * </p> 5307 */ 5308 @SearchParamDefinition(name = "episode-of-care", path = "Encounter.episodeOfCare", description = "Episode(s) of care that this encounter should be recorded against", type = "reference", target = { 5309 EpisodeOfCare.class }) 5310 public static final String SP_EPISODE_OF_CARE = "episode-of-care"; 5311 /** 5312 * <b>Fluent Client</b> search parameter constant for <b>episode-of-care</b> 5313 * <p> 5314 * Description: <b>Episode(s) of care that this encounter should be recorded 5315 * against</b><br> 5316 * Type: <b>reference</b><br> 5317 * Path: <b>Encounter.episodeOfCare</b><br> 5318 * </p> 5319 */ 5320 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam EPISODE_OF_CARE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5321 SP_EPISODE_OF_CARE); 5322 5323 /** 5324 * Constant for fluent queries to be used to add include statements. Specifies 5325 * the path value of "<b>Encounter:episode-of-care</b>". 5326 */ 5327 public static final ca.uhn.fhir.model.api.Include INCLUDE_EPISODE_OF_CARE = new ca.uhn.fhir.model.api.Include( 5328 "Encounter:episode-of-care").toLocked(); 5329 5330 /** 5331 * Search parameter: <b>diagnosis</b> 5332 * <p> 5333 * Description: <b>The diagnosis or procedure relevant to the encounter</b><br> 5334 * Type: <b>reference</b><br> 5335 * Path: <b>Encounter.diagnosis.condition</b><br> 5336 * </p> 5337 */ 5338 @SearchParamDefinition(name = "diagnosis", path = "Encounter.diagnosis.condition", description = "The diagnosis or procedure relevant to the encounter", type = "reference", target = { 5339 Condition.class, Procedure.class }) 5340 public static final String SP_DIAGNOSIS = "diagnosis"; 5341 /** 5342 * <b>Fluent Client</b> search parameter constant for <b>diagnosis</b> 5343 * <p> 5344 * Description: <b>The diagnosis or procedure relevant to the encounter</b><br> 5345 * Type: <b>reference</b><br> 5346 * Path: <b>Encounter.diagnosis.condition</b><br> 5347 * </p> 5348 */ 5349 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DIAGNOSIS = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5350 SP_DIAGNOSIS); 5351 5352 /** 5353 * Constant for fluent queries to be used to add include statements. Specifies 5354 * the path value of "<b>Encounter:diagnosis</b>". 5355 */ 5356 public static final ca.uhn.fhir.model.api.Include INCLUDE_DIAGNOSIS = new ca.uhn.fhir.model.api.Include( 5357 "Encounter:diagnosis").toLocked(); 5358 5359 /** 5360 * Search parameter: <b>appointment</b> 5361 * <p> 5362 * Description: <b>The appointment that scheduled this encounter</b><br> 5363 * Type: <b>reference</b><br> 5364 * Path: <b>Encounter.appointment</b><br> 5365 * </p> 5366 */ 5367 @SearchParamDefinition(name = "appointment", path = "Encounter.appointment", description = "The appointment that scheduled this encounter", type = "reference", target = { 5368 Appointment.class }) 5369 public static final String SP_APPOINTMENT = "appointment"; 5370 /** 5371 * <b>Fluent Client</b> search parameter constant for <b>appointment</b> 5372 * <p> 5373 * Description: <b>The appointment that scheduled this encounter</b><br> 5374 * Type: <b>reference</b><br> 5375 * Path: <b>Encounter.appointment</b><br> 5376 * </p> 5377 */ 5378 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam APPOINTMENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5379 SP_APPOINTMENT); 5380 5381 /** 5382 * Constant for fluent queries to be used to add include statements. Specifies 5383 * the path value of "<b>Encounter:appointment</b>". 5384 */ 5385 public static final ca.uhn.fhir.model.api.Include INCLUDE_APPOINTMENT = new ca.uhn.fhir.model.api.Include( 5386 "Encounter:appointment").toLocked(); 5387 5388 /** 5389 * Search parameter: <b>part-of</b> 5390 * <p> 5391 * Description: <b>Another Encounter this encounter is part of</b><br> 5392 * Type: <b>reference</b><br> 5393 * Path: <b>Encounter.partOf</b><br> 5394 * </p> 5395 */ 5396 @SearchParamDefinition(name = "part-of", path = "Encounter.partOf", description = "Another Encounter this encounter is part of", type = "reference", target = { 5397 Encounter.class }) 5398 public static final String SP_PART_OF = "part-of"; 5399 /** 5400 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 5401 * <p> 5402 * Description: <b>Another Encounter this encounter is part of</b><br> 5403 * Type: <b>reference</b><br> 5404 * Path: <b>Encounter.partOf</b><br> 5405 * </p> 5406 */ 5407 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5408 SP_PART_OF); 5409 5410 /** 5411 * Constant for fluent queries to be used to add include statements. Specifies 5412 * the path value of "<b>Encounter:part-of</b>". 5413 */ 5414 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include( 5415 "Encounter:part-of").toLocked(); 5416 5417 /** 5418 * Search parameter: <b>type</b> 5419 * <p> 5420 * Description: <b>Specific type of encounter</b><br> 5421 * Type: <b>token</b><br> 5422 * Path: <b>Encounter.type</b><br> 5423 * </p> 5424 */ 5425 @SearchParamDefinition(name = "type", path = "Encounter.type", description = "Specific type of encounter", type = "token") 5426 public static final String SP_TYPE = "type"; 5427 /** 5428 * <b>Fluent Client</b> search parameter constant for <b>type</b> 5429 * <p> 5430 * Description: <b>Specific type of encounter</b><br> 5431 * Type: <b>token</b><br> 5432 * Path: <b>Encounter.type</b><br> 5433 * </p> 5434 */ 5435 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5436 SP_TYPE); 5437 5438 /** 5439 * Search parameter: <b>reason-code</b> 5440 * <p> 5441 * Description: <b>Coded reason the encounter takes place</b><br> 5442 * Type: <b>token</b><br> 5443 * Path: <b>Encounter.reasonCode</b><br> 5444 * </p> 5445 */ 5446 @SearchParamDefinition(name = "reason-code", path = "Encounter.reasonCode", description = "Coded reason the encounter takes place", type = "token") 5447 public static final String SP_REASON_CODE = "reason-code"; 5448 /** 5449 * <b>Fluent Client</b> search parameter constant for <b>reason-code</b> 5450 * <p> 5451 * Description: <b>Coded reason the encounter takes place</b><br> 5452 * Type: <b>token</b><br> 5453 * Path: <b>Encounter.reasonCode</b><br> 5454 * </p> 5455 */ 5456 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5457 SP_REASON_CODE); 5458 5459 /** 5460 * Search parameter: <b>participant</b> 5461 * <p> 5462 * Description: <b>Persons involved in the encounter other than the 5463 * patient</b><br> 5464 * Type: <b>reference</b><br> 5465 * Path: <b>Encounter.participant.individual</b><br> 5466 * </p> 5467 */ 5468 @SearchParamDefinition(name = "participant", path = "Encounter.participant.individual", description = "Persons involved in the encounter other than the patient", type = "reference", providesMembershipIn = { 5469 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 5470 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Practitioner.class, 5471 PractitionerRole.class, RelatedPerson.class }) 5472 public static final String SP_PARTICIPANT = "participant"; 5473 /** 5474 * <b>Fluent Client</b> search parameter constant for <b>participant</b> 5475 * <p> 5476 * Description: <b>Persons involved in the encounter other than the 5477 * patient</b><br> 5478 * Type: <b>reference</b><br> 5479 * Path: <b>Encounter.participant.individual</b><br> 5480 * </p> 5481 */ 5482 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTICIPANT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5483 SP_PARTICIPANT); 5484 5485 /** 5486 * Constant for fluent queries to be used to add include statements. Specifies 5487 * the path value of "<b>Encounter:participant</b>". 5488 */ 5489 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTICIPANT = new ca.uhn.fhir.model.api.Include( 5490 "Encounter:participant").toLocked(); 5491 5492 /** 5493 * Search parameter: <b>based-on</b> 5494 * <p> 5495 * Description: <b>The ServiceRequest that initiated this encounter</b><br> 5496 * Type: <b>reference</b><br> 5497 * Path: <b>Encounter.basedOn</b><br> 5498 * </p> 5499 */ 5500 @SearchParamDefinition(name = "based-on", path = "Encounter.basedOn", description = "The ServiceRequest that initiated this encounter", type = "reference", target = { 5501 ServiceRequest.class }) 5502 public static final String SP_BASED_ON = "based-on"; 5503 /** 5504 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 5505 * <p> 5506 * Description: <b>The ServiceRequest that initiated this encounter</b><br> 5507 * Type: <b>reference</b><br> 5508 * Path: <b>Encounter.basedOn</b><br> 5509 * </p> 5510 */ 5511 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5512 SP_BASED_ON); 5513 5514 /** 5515 * Constant for fluent queries to be used to add include statements. Specifies 5516 * the path value of "<b>Encounter:based-on</b>". 5517 */ 5518 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include( 5519 "Encounter:based-on").toLocked(); 5520 5521 /** 5522 * Search parameter: <b>patient</b> 5523 * <p> 5524 * Description: <b>The patient or group present at the encounter</b><br> 5525 * Type: <b>reference</b><br> 5526 * Path: <b>Encounter.subject</b><br> 5527 * </p> 5528 */ 5529 @SearchParamDefinition(name = "patient", path = "Encounter.subject.where(resolve() is Patient)", description = "The patient or group present at the encounter", type = "reference", providesMembershipIn = { 5530 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 5531 public static final String SP_PATIENT = "patient"; 5532 /** 5533 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 5534 * <p> 5535 * Description: <b>The patient or group present at the encounter</b><br> 5536 * Type: <b>reference</b><br> 5537 * Path: <b>Encounter.subject</b><br> 5538 * </p> 5539 */ 5540 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5541 SP_PATIENT); 5542 5543 /** 5544 * Constant for fluent queries to be used to add include statements. Specifies 5545 * the path value of "<b>Encounter:patient</b>". 5546 */ 5547 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 5548 "Encounter:patient").toLocked(); 5549 5550 /** 5551 * Search parameter: <b>reason-reference</b> 5552 * <p> 5553 * Description: <b>Reason the encounter takes place (reference)</b><br> 5554 * Type: <b>reference</b><br> 5555 * Path: <b>Encounter.reasonReference</b><br> 5556 * </p> 5557 */ 5558 @SearchParamDefinition(name = "reason-reference", path = "Encounter.reasonReference", description = "Reason the encounter takes place (reference)", type = "reference", target = { 5559 Condition.class, ImmunizationRecommendation.class, Observation.class, Procedure.class }) 5560 public static final String SP_REASON_REFERENCE = "reason-reference"; 5561 /** 5562 * <b>Fluent Client</b> search parameter constant for <b>reason-reference</b> 5563 * <p> 5564 * Description: <b>Reason the encounter takes place (reference)</b><br> 5565 * Type: <b>reference</b><br> 5566 * Path: <b>Encounter.reasonReference</b><br> 5567 * </p> 5568 */ 5569 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REASON_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5570 SP_REASON_REFERENCE); 5571 5572 /** 5573 * Constant for fluent queries to be used to add include statements. Specifies 5574 * the path value of "<b>Encounter:reason-reference</b>". 5575 */ 5576 public static final ca.uhn.fhir.model.api.Include INCLUDE_REASON_REFERENCE = new ca.uhn.fhir.model.api.Include( 5577 "Encounter:reason-reference").toLocked(); 5578 5579 /** 5580 * Search parameter: <b>location-period</b> 5581 * <p> 5582 * Description: <b>Time period during which the patient was present at the 5583 * location</b><br> 5584 * Type: <b>date</b><br> 5585 * Path: <b>Encounter.location.period</b><br> 5586 * </p> 5587 */ 5588 @SearchParamDefinition(name = "location-period", path = "Encounter.location.period", description = "Time period during which the patient was present at the location", type = "date") 5589 public static final String SP_LOCATION_PERIOD = "location-period"; 5590 /** 5591 * <b>Fluent Client</b> search parameter constant for <b>location-period</b> 5592 * <p> 5593 * Description: <b>Time period during which the patient was present at the 5594 * location</b><br> 5595 * Type: <b>date</b><br> 5596 * Path: <b>Encounter.location.period</b><br> 5597 * </p> 5598 */ 5599 public static final ca.uhn.fhir.rest.gclient.DateClientParam LOCATION_PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam( 5600 SP_LOCATION_PERIOD); 5601 5602 /** 5603 * Search parameter: <b>location</b> 5604 * <p> 5605 * Description: <b>Location the encounter takes place</b><br> 5606 * Type: <b>reference</b><br> 5607 * Path: <b>Encounter.location.location</b><br> 5608 * </p> 5609 */ 5610 @SearchParamDefinition(name = "location", path = "Encounter.location.location", description = "Location the encounter takes place", type = "reference", target = { 5611 Location.class }) 5612 public static final String SP_LOCATION = "location"; 5613 /** 5614 * <b>Fluent Client</b> search parameter constant for <b>location</b> 5615 * <p> 5616 * Description: <b>Location the encounter takes place</b><br> 5617 * Type: <b>reference</b><br> 5618 * Path: <b>Encounter.location.location</b><br> 5619 * </p> 5620 */ 5621 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5622 SP_LOCATION); 5623 5624 /** 5625 * Constant for fluent queries to be used to add include statements. Specifies 5626 * the path value of "<b>Encounter:location</b>". 5627 */ 5628 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include( 5629 "Encounter:location").toLocked(); 5630 5631 /** 5632 * Search parameter: <b>service-provider</b> 5633 * <p> 5634 * Description: <b>The organization (facility) responsible for this 5635 * encounter</b><br> 5636 * Type: <b>reference</b><br> 5637 * Path: <b>Encounter.serviceProvider</b><br> 5638 * </p> 5639 */ 5640 @SearchParamDefinition(name = "service-provider", path = "Encounter.serviceProvider", description = "The organization (facility) responsible for this encounter", type = "reference", target = { 5641 Organization.class }) 5642 public static final String SP_SERVICE_PROVIDER = "service-provider"; 5643 /** 5644 * <b>Fluent Client</b> search parameter constant for <b>service-provider</b> 5645 * <p> 5646 * Description: <b>The organization (facility) responsible for this 5647 * encounter</b><br> 5648 * Type: <b>reference</b><br> 5649 * Path: <b>Encounter.serviceProvider</b><br> 5650 * </p> 5651 */ 5652 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SERVICE_PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5653 SP_SERVICE_PROVIDER); 5654 5655 /** 5656 * Constant for fluent queries to be used to add include statements. Specifies 5657 * the path value of "<b>Encounter:service-provider</b>". 5658 */ 5659 public static final ca.uhn.fhir.model.api.Include INCLUDE_SERVICE_PROVIDER = new ca.uhn.fhir.model.api.Include( 5660 "Encounter:service-provider").toLocked(); 5661 5662 /** 5663 * Search parameter: <b>special-arrangement</b> 5664 * <p> 5665 * Description: <b>Wheelchair, translator, stretcher, etc.</b><br> 5666 * Type: <b>token</b><br> 5667 * Path: <b>Encounter.hospitalization.specialArrangement</b><br> 5668 * </p> 5669 */ 5670 @SearchParamDefinition(name = "special-arrangement", path = "Encounter.hospitalization.specialArrangement", description = "Wheelchair, translator, stretcher, etc.", type = "token") 5671 public static final String SP_SPECIAL_ARRANGEMENT = "special-arrangement"; 5672 /** 5673 * <b>Fluent Client</b> search parameter constant for <b>special-arrangement</b> 5674 * <p> 5675 * Description: <b>Wheelchair, translator, stretcher, etc.</b><br> 5676 * Type: <b>token</b><br> 5677 * Path: <b>Encounter.hospitalization.specialArrangement</b><br> 5678 * </p> 5679 */ 5680 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SPECIAL_ARRANGEMENT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5681 SP_SPECIAL_ARRANGEMENT); 5682 5683 /** 5684 * Search parameter: <b>class</b> 5685 * <p> 5686 * Description: <b>Classification of patient encounter</b><br> 5687 * Type: <b>token</b><br> 5688 * Path: <b>Encounter.class</b><br> 5689 * </p> 5690 */ 5691 @SearchParamDefinition(name = "class", path = "Encounter.class", description = "Classification of patient encounter", type = "token") 5692 public static final String SP_CLASS = "class"; 5693 /** 5694 * <b>Fluent Client</b> search parameter constant for <b>class</b> 5695 * <p> 5696 * Description: <b>Classification of patient encounter</b><br> 5697 * Type: <b>token</b><br> 5698 * Path: <b>Encounter.class</b><br> 5699 * </p> 5700 */ 5701 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLASS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5702 SP_CLASS); 5703 5704 /** 5705 * Search parameter: <b>account</b> 5706 * <p> 5707 * Description: <b>The set of accounts that may be used for billing for this 5708 * Encounter</b><br> 5709 * Type: <b>reference</b><br> 5710 * Path: <b>Encounter.account</b><br> 5711 * </p> 5712 */ 5713 @SearchParamDefinition(name = "account", path = "Encounter.account", description = "The set of accounts that may be used for billing for this Encounter", type = "reference", target = { 5714 Account.class }) 5715 public static final String SP_ACCOUNT = "account"; 5716 /** 5717 * <b>Fluent Client</b> search parameter constant for <b>account</b> 5718 * <p> 5719 * Description: <b>The set of accounts that may be used for billing for this 5720 * Encounter</b><br> 5721 * Type: <b>reference</b><br> 5722 * Path: <b>Encounter.account</b><br> 5723 * </p> 5724 */ 5725 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACCOUNT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5726 SP_ACCOUNT); 5727 5728 /** 5729 * Constant for fluent queries to be used to add include statements. Specifies 5730 * the path value of "<b>Encounter:account</b>". 5731 */ 5732 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACCOUNT = new ca.uhn.fhir.model.api.Include( 5733 "Encounter:account").toLocked(); 5734 5735 /** 5736 * Search parameter: <b>status</b> 5737 * <p> 5738 * Description: <b>planned | arrived | triaged | in-progress | onleave | 5739 * finished | cancelled +</b><br> 5740 * Type: <b>token</b><br> 5741 * Path: <b>Encounter.status</b><br> 5742 * </p> 5743 */ 5744 @SearchParamDefinition(name = "status", path = "Encounter.status", description = "planned | arrived | triaged | in-progress | onleave | finished | cancelled +", type = "token") 5745 public static final String SP_STATUS = "status"; 5746 /** 5747 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5748 * <p> 5749 * Description: <b>planned | arrived | triaged | in-progress | onleave | 5750 * finished | cancelled +</b><br> 5751 * Type: <b>token</b><br> 5752 * Path: <b>Encounter.status</b><br> 5753 * </p> 5754 */ 5755 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5756 SP_STATUS); 5757 5758}