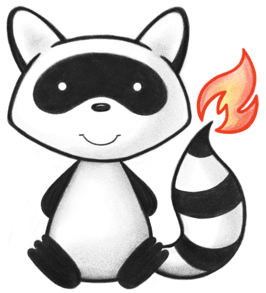
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.utilities.Utilities; 038 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.Description; 041import ca.uhn.fhir.model.api.annotation.ResourceDef; 042import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 043 044/** 045 * The technical details of an endpoint that can be used for electronic 046 * services, such as for web services providing XDS.b or a REST endpoint for 047 * another FHIR server. This may include any security context information. 048 */ 049@ResourceDef(name = "Endpoint", profile = "http://hl7.org/fhir/StructureDefinition/Endpoint") 050public class Endpoint extends DomainResource { 051 052 public enum EndpointStatus { 053 /** 054 * This endpoint is expected to be active and can be used. 055 */ 056 ACTIVE, 057 /** 058 * This endpoint is temporarily unavailable. 059 */ 060 SUSPENDED, 061 /** 062 * This endpoint has exceeded connectivity thresholds and is considered in an 063 * error state and should no longer be attempted to connect to until corrective 064 * action is taken. 065 */ 066 ERROR, 067 /** 068 * This endpoint is no longer to be used. 069 */ 070 OFF, 071 /** 072 * This instance should not have been part of this patient's medical record. 073 */ 074 ENTEREDINERROR, 075 /** 076 * This endpoint is not intended for production usage. 077 */ 078 TEST, 079 /** 080 * added to help the parsers with the generic types 081 */ 082 NULL; 083 084 public static EndpointStatus fromCode(String codeString) throws FHIRException { 085 if (codeString == null || "".equals(codeString)) 086 return null; 087 if ("active".equals(codeString)) 088 return ACTIVE; 089 if ("suspended".equals(codeString)) 090 return SUSPENDED; 091 if ("error".equals(codeString)) 092 return ERROR; 093 if ("off".equals(codeString)) 094 return OFF; 095 if ("entered-in-error".equals(codeString)) 096 return ENTEREDINERROR; 097 if ("test".equals(codeString)) 098 return TEST; 099 if (Configuration.isAcceptInvalidEnums()) 100 return null; 101 else 102 throw new FHIRException("Unknown EndpointStatus code '" + codeString + "'"); 103 } 104 105 public String toCode() { 106 switch (this) { 107 case ACTIVE: 108 return "active"; 109 case SUSPENDED: 110 return "suspended"; 111 case ERROR: 112 return "error"; 113 case OFF: 114 return "off"; 115 case ENTEREDINERROR: 116 return "entered-in-error"; 117 case TEST: 118 return "test"; 119 case NULL: 120 return null; 121 default: 122 return "?"; 123 } 124 } 125 126 public String getSystem() { 127 switch (this) { 128 case ACTIVE: 129 return "http://hl7.org/fhir/endpoint-status"; 130 case SUSPENDED: 131 return "http://hl7.org/fhir/endpoint-status"; 132 case ERROR: 133 return "http://hl7.org/fhir/endpoint-status"; 134 case OFF: 135 return "http://hl7.org/fhir/endpoint-status"; 136 case ENTEREDINERROR: 137 return "http://hl7.org/fhir/endpoint-status"; 138 case TEST: 139 return "http://hl7.org/fhir/endpoint-status"; 140 case NULL: 141 return null; 142 default: 143 return "?"; 144 } 145 } 146 147 public String getDefinition() { 148 switch (this) { 149 case ACTIVE: 150 return "This endpoint is expected to be active and can be used."; 151 case SUSPENDED: 152 return "This endpoint is temporarily unavailable."; 153 case ERROR: 154 return "This endpoint has exceeded connectivity thresholds and is considered in an error state and should no longer be attempted to connect to until corrective action is taken."; 155 case OFF: 156 return "This endpoint is no longer to be used."; 157 case ENTEREDINERROR: 158 return "This instance should not have been part of this patient's medical record."; 159 case TEST: 160 return "This endpoint is not intended for production usage."; 161 case NULL: 162 return null; 163 default: 164 return "?"; 165 } 166 } 167 168 public String getDisplay() { 169 switch (this) { 170 case ACTIVE: 171 return "Active"; 172 case SUSPENDED: 173 return "Suspended"; 174 case ERROR: 175 return "Error"; 176 case OFF: 177 return "Off"; 178 case ENTEREDINERROR: 179 return "Entered in error"; 180 case TEST: 181 return "Test"; 182 case NULL: 183 return null; 184 default: 185 return "?"; 186 } 187 } 188 } 189 190 public static class EndpointStatusEnumFactory implements EnumFactory<EndpointStatus> { 191 public EndpointStatus fromCode(String codeString) throws IllegalArgumentException { 192 if (codeString == null || "".equals(codeString)) 193 if (codeString == null || "".equals(codeString)) 194 return null; 195 if ("active".equals(codeString)) 196 return EndpointStatus.ACTIVE; 197 if ("suspended".equals(codeString)) 198 return EndpointStatus.SUSPENDED; 199 if ("error".equals(codeString)) 200 return EndpointStatus.ERROR; 201 if ("off".equals(codeString)) 202 return EndpointStatus.OFF; 203 if ("entered-in-error".equals(codeString)) 204 return EndpointStatus.ENTEREDINERROR; 205 if ("test".equals(codeString)) 206 return EndpointStatus.TEST; 207 throw new IllegalArgumentException("Unknown EndpointStatus code '" + codeString + "'"); 208 } 209 210 public Enumeration<EndpointStatus> fromType(PrimitiveType<?> code) throws FHIRException { 211 if (code == null) 212 return null; 213 if (code.isEmpty()) 214 return new Enumeration<EndpointStatus>(this, EndpointStatus.NULL, code); 215 String codeString = code.asStringValue(); 216 if (codeString == null || "".equals(codeString)) 217 return new Enumeration<EndpointStatus>(this, EndpointStatus.NULL, code); 218 if ("active".equals(codeString)) 219 return new Enumeration<EndpointStatus>(this, EndpointStatus.ACTIVE, code); 220 if ("suspended".equals(codeString)) 221 return new Enumeration<EndpointStatus>(this, EndpointStatus.SUSPENDED, code); 222 if ("error".equals(codeString)) 223 return new Enumeration<EndpointStatus>(this, EndpointStatus.ERROR, code); 224 if ("off".equals(codeString)) 225 return new Enumeration<EndpointStatus>(this, EndpointStatus.OFF, code); 226 if ("entered-in-error".equals(codeString)) 227 return new Enumeration<EndpointStatus>(this, EndpointStatus.ENTEREDINERROR, code); 228 if ("test".equals(codeString)) 229 return new Enumeration<EndpointStatus>(this, EndpointStatus.TEST, code); 230 throw new FHIRException("Unknown EndpointStatus code '" + codeString + "'"); 231 } 232 233 public String toCode(EndpointStatus code) { 234 if (code == EndpointStatus.NULL) 235 return null; 236 if (code == EndpointStatus.ACTIVE) 237 return "active"; 238 if (code == EndpointStatus.SUSPENDED) 239 return "suspended"; 240 if (code == EndpointStatus.ERROR) 241 return "error"; 242 if (code == EndpointStatus.OFF) 243 return "off"; 244 if (code == EndpointStatus.ENTEREDINERROR) 245 return "entered-in-error"; 246 if (code == EndpointStatus.TEST) 247 return "test"; 248 return "?"; 249 } 250 251 public String toSystem(EndpointStatus code) { 252 return code.getSystem(); 253 } 254 } 255 256 /** 257 * Identifier for the organization that is used to identify the endpoint across 258 * multiple disparate systems. 259 */ 260 @Child(name = "identifier", type = { 261 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 262 @Description(shortDefinition = "Identifies this endpoint across multiple systems", formalDefinition = "Identifier for the organization that is used to identify the endpoint across multiple disparate systems.") 263 protected List<Identifier> identifier; 264 265 /** 266 * active | suspended | error | off | test. 267 */ 268 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 269 @Description(shortDefinition = "active | suspended | error | off | entered-in-error | test", formalDefinition = "active | suspended | error | off | test.") 270 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/endpoint-status") 271 protected Enumeration<EndpointStatus> status; 272 273 /** 274 * A coded value that represents the technical details of the usage of this 275 * endpoint, such as what WSDLs should be used in what way. (e.g. 276 * XDS.b/DICOM/cds-hook). 277 */ 278 @Child(name = "connectionType", type = { 279 Coding.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 280 @Description(shortDefinition = "Protocol/Profile/Standard to be used with this endpoint connection", formalDefinition = "A coded value that represents the technical details of the usage of this endpoint, such as what WSDLs should be used in what way. (e.g. XDS.b/DICOM/cds-hook).") 281 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/endpoint-connection-type") 282 protected Coding connectionType; 283 284 /** 285 * A friendly name that this endpoint can be referred to with. 286 */ 287 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 288 @Description(shortDefinition = "A name that this endpoint can be identified by", formalDefinition = "A friendly name that this endpoint can be referred to with.") 289 protected StringType name; 290 291 /** 292 * The organization that manages this endpoint (even if technically another 293 * organization is hosting this in the cloud, it is the organization associated 294 * with the data). 295 */ 296 @Child(name = "managingOrganization", type = { 297 Organization.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 298 @Description(shortDefinition = "Organization that manages this endpoint (might not be the organization that exposes the endpoint)", formalDefinition = "The organization that manages this endpoint (even if technically another organization is hosting this in the cloud, it is the organization associated with the data).") 299 protected Reference managingOrganization; 300 301 /** 302 * The actual object that is the target of the reference (The organization that 303 * manages this endpoint (even if technically another organization is hosting 304 * this in the cloud, it is the organization associated with the data).) 305 */ 306 protected Organization managingOrganizationTarget; 307 308 /** 309 * Contact details for a human to contact about the subscription. The primary 310 * use of this for system administrator troubleshooting. 311 */ 312 @Child(name = "contact", type = { 313 ContactPoint.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 314 @Description(shortDefinition = "Contact details for source (e.g. troubleshooting)", formalDefinition = "Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting.") 315 protected List<ContactPoint> contact; 316 317 /** 318 * The interval during which the endpoint is expected to be operational. 319 */ 320 @Child(name = "period", type = { Period.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 321 @Description(shortDefinition = "Interval the endpoint is expected to be operational", formalDefinition = "The interval during which the endpoint is expected to be operational.") 322 protected Period period; 323 324 /** 325 * The payload type describes the acceptable content that can be communicated on 326 * the endpoint. 327 */ 328 @Child(name = "payloadType", type = { 329 CodeableConcept.class }, order = 7, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 330 @Description(shortDefinition = "The type of content that may be used at this endpoint (e.g. XDS Discharge summaries)", formalDefinition = "The payload type describes the acceptable content that can be communicated on the endpoint.") 331 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/endpoint-payload-type") 332 protected List<CodeableConcept> payloadType; 333 334 /** 335 * The mime type to send the payload in - e.g. application/fhir+xml, 336 * application/fhir+json. If the mime type is not specified, then the sender 337 * could send any content (including no content depending on the 338 * connectionType). 339 */ 340 @Child(name = "payloadMimeType", type = { 341 CodeType.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 342 @Description(shortDefinition = "Mimetype to send. If not specified, the content could be anything (including no payload, if the connectionType defined this)", formalDefinition = "The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType).") 343 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/mimetypes") 344 protected List<CodeType> payloadMimeType; 345 346 /** 347 * The uri that describes the actual end-point to connect to. 348 */ 349 @Child(name = "address", type = { UrlType.class }, order = 9, min = 1, max = 1, modifier = false, summary = true) 350 @Description(shortDefinition = "The technical base address for connecting to this endpoint", formalDefinition = "The uri that describes the actual end-point to connect to.") 351 protected UrlType address; 352 353 /** 354 * Additional headers / information to send as part of the notification. 355 */ 356 @Child(name = "header", type = { 357 StringType.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 358 @Description(shortDefinition = "Usage depends on the channel type", formalDefinition = "Additional headers / information to send as part of the notification.") 359 protected List<StringType> header; 360 361 private static final long serialVersionUID = 755181080L; 362 363 /** 364 * Constructor 365 */ 366 public Endpoint() { 367 super(); 368 } 369 370 /** 371 * Constructor 372 */ 373 public Endpoint(Enumeration<EndpointStatus> status, Coding connectionType, UrlType address) { 374 super(); 375 this.status = status; 376 this.connectionType = connectionType; 377 this.address = address; 378 } 379 380 /** 381 * @return {@link #identifier} (Identifier for the organization that is used to 382 * identify the endpoint across multiple disparate systems.) 383 */ 384 public List<Identifier> getIdentifier() { 385 if (this.identifier == null) 386 this.identifier = new ArrayList<Identifier>(); 387 return this.identifier; 388 } 389 390 /** 391 * @return Returns a reference to <code>this</code> for easy method chaining 392 */ 393 public Endpoint setIdentifier(List<Identifier> theIdentifier) { 394 this.identifier = theIdentifier; 395 return this; 396 } 397 398 public boolean hasIdentifier() { 399 if (this.identifier == null) 400 return false; 401 for (Identifier item : this.identifier) 402 if (!item.isEmpty()) 403 return true; 404 return false; 405 } 406 407 public Identifier addIdentifier() { // 3 408 Identifier t = new Identifier(); 409 if (this.identifier == null) 410 this.identifier = new ArrayList<Identifier>(); 411 this.identifier.add(t); 412 return t; 413 } 414 415 public Endpoint addIdentifier(Identifier t) { // 3 416 if (t == null) 417 return this; 418 if (this.identifier == null) 419 this.identifier = new ArrayList<Identifier>(); 420 this.identifier.add(t); 421 return this; 422 } 423 424 /** 425 * @return The first repetition of repeating field {@link #identifier}, creating 426 * it if it does not already exist 427 */ 428 public Identifier getIdentifierFirstRep() { 429 if (getIdentifier().isEmpty()) { 430 addIdentifier(); 431 } 432 return getIdentifier().get(0); 433 } 434 435 /** 436 * @return {@link #status} (active | suspended | error | off | test.). This is 437 * the underlying object with id, value and extensions. The accessor 438 * "getStatus" gives direct access to the value 439 */ 440 public Enumeration<EndpointStatus> getStatusElement() { 441 if (this.status == null) 442 if (Configuration.errorOnAutoCreate()) 443 throw new Error("Attempt to auto-create Endpoint.status"); 444 else if (Configuration.doAutoCreate()) 445 this.status = new Enumeration<EndpointStatus>(new EndpointStatusEnumFactory()); // bb 446 return this.status; 447 } 448 449 public boolean hasStatusElement() { 450 return this.status != null && !this.status.isEmpty(); 451 } 452 453 public boolean hasStatus() { 454 return this.status != null && !this.status.isEmpty(); 455 } 456 457 /** 458 * @param value {@link #status} (active | suspended | error | off | test.). This 459 * is the underlying object with id, value and extensions. The 460 * accessor "getStatus" gives direct access to the value 461 */ 462 public Endpoint setStatusElement(Enumeration<EndpointStatus> value) { 463 this.status = value; 464 return this; 465 } 466 467 /** 468 * @return active | suspended | error | off | test. 469 */ 470 public EndpointStatus getStatus() { 471 return this.status == null ? null : this.status.getValue(); 472 } 473 474 /** 475 * @param value active | suspended | error | off | test. 476 */ 477 public Endpoint setStatus(EndpointStatus value) { 478 if (this.status == null) 479 this.status = new Enumeration<EndpointStatus>(new EndpointStatusEnumFactory()); 480 this.status.setValue(value); 481 return this; 482 } 483 484 /** 485 * @return {@link #connectionType} (A coded value that represents the technical 486 * details of the usage of this endpoint, such as what WSDLs should be 487 * used in what way. (e.g. XDS.b/DICOM/cds-hook).) 488 */ 489 public Coding getConnectionType() { 490 if (this.connectionType == null) 491 if (Configuration.errorOnAutoCreate()) 492 throw new Error("Attempt to auto-create Endpoint.connectionType"); 493 else if (Configuration.doAutoCreate()) 494 this.connectionType = new Coding(); // cc 495 return this.connectionType; 496 } 497 498 public boolean hasConnectionType() { 499 return this.connectionType != null && !this.connectionType.isEmpty(); 500 } 501 502 /** 503 * @param value {@link #connectionType} (A coded value that represents the 504 * technical details of the usage of this endpoint, such as what 505 * WSDLs should be used in what way. (e.g. XDS.b/DICOM/cds-hook).) 506 */ 507 public Endpoint setConnectionType(Coding value) { 508 this.connectionType = value; 509 return this; 510 } 511 512 /** 513 * @return {@link #name} (A friendly name that this endpoint can be referred to 514 * with.). This is the underlying object with id, value and extensions. 515 * The accessor "getName" gives direct access to the value 516 */ 517 public StringType getNameElement() { 518 if (this.name == null) 519 if (Configuration.errorOnAutoCreate()) 520 throw new Error("Attempt to auto-create Endpoint.name"); 521 else if (Configuration.doAutoCreate()) 522 this.name = new StringType(); // bb 523 return this.name; 524 } 525 526 public boolean hasNameElement() { 527 return this.name != null && !this.name.isEmpty(); 528 } 529 530 public boolean hasName() { 531 return this.name != null && !this.name.isEmpty(); 532 } 533 534 /** 535 * @param value {@link #name} (A friendly name that this endpoint can be 536 * referred to with.). This is the underlying object with id, value 537 * and extensions. The accessor "getName" gives direct access to 538 * the value 539 */ 540 public Endpoint setNameElement(StringType value) { 541 this.name = value; 542 return this; 543 } 544 545 /** 546 * @return A friendly name that this endpoint can be referred to with. 547 */ 548 public String getName() { 549 return this.name == null ? null : this.name.getValue(); 550 } 551 552 /** 553 * @param value A friendly name that this endpoint can be referred to with. 554 */ 555 public Endpoint setName(String value) { 556 if (Utilities.noString(value)) 557 this.name = null; 558 else { 559 if (this.name == null) 560 this.name = new StringType(); 561 this.name.setValue(value); 562 } 563 return this; 564 } 565 566 /** 567 * @return {@link #managingOrganization} (The organization that manages this 568 * endpoint (even if technically another organization is hosting this in 569 * the cloud, it is the organization associated with the data).) 570 */ 571 public Reference getManagingOrganization() { 572 if (this.managingOrganization == null) 573 if (Configuration.errorOnAutoCreate()) 574 throw new Error("Attempt to auto-create Endpoint.managingOrganization"); 575 else if (Configuration.doAutoCreate()) 576 this.managingOrganization = new Reference(); // cc 577 return this.managingOrganization; 578 } 579 580 public boolean hasManagingOrganization() { 581 return this.managingOrganization != null && !this.managingOrganization.isEmpty(); 582 } 583 584 /** 585 * @param value {@link #managingOrganization} (The organization that manages 586 * this endpoint (even if technically another organization is 587 * hosting this in the cloud, it is the organization associated 588 * with the data).) 589 */ 590 public Endpoint setManagingOrganization(Reference value) { 591 this.managingOrganization = value; 592 return this; 593 } 594 595 /** 596 * @return {@link #managingOrganization} The actual object that is the target of 597 * the reference. The reference library doesn't populate this, but you 598 * can use it to hold the resource if you resolve it. (The organization 599 * that manages this endpoint (even if technically another organization 600 * is hosting this in the cloud, it is the organization associated with 601 * the data).) 602 */ 603 public Organization getManagingOrganizationTarget() { 604 if (this.managingOrganizationTarget == null) 605 if (Configuration.errorOnAutoCreate()) 606 throw new Error("Attempt to auto-create Endpoint.managingOrganization"); 607 else if (Configuration.doAutoCreate()) 608 this.managingOrganizationTarget = new Organization(); // aa 609 return this.managingOrganizationTarget; 610 } 611 612 /** 613 * @param value {@link #managingOrganization} The actual object that is the 614 * target of the reference. The reference library doesn't use 615 * these, but you can use it to hold the resource if you resolve 616 * it. (The organization that manages this endpoint (even if 617 * technically another organization is hosting this in the cloud, 618 * it is the organization associated with the data).) 619 */ 620 public Endpoint setManagingOrganizationTarget(Organization value) { 621 this.managingOrganizationTarget = value; 622 return this; 623 } 624 625 /** 626 * @return {@link #contact} (Contact details for a human to contact about the 627 * subscription. The primary use of this for system administrator 628 * troubleshooting.) 629 */ 630 public List<ContactPoint> getContact() { 631 if (this.contact == null) 632 this.contact = new ArrayList<ContactPoint>(); 633 return this.contact; 634 } 635 636 /** 637 * @return Returns a reference to <code>this</code> for easy method chaining 638 */ 639 public Endpoint setContact(List<ContactPoint> theContact) { 640 this.contact = theContact; 641 return this; 642 } 643 644 public boolean hasContact() { 645 if (this.contact == null) 646 return false; 647 for (ContactPoint item : this.contact) 648 if (!item.isEmpty()) 649 return true; 650 return false; 651 } 652 653 public ContactPoint addContact() { // 3 654 ContactPoint t = new ContactPoint(); 655 if (this.contact == null) 656 this.contact = new ArrayList<ContactPoint>(); 657 this.contact.add(t); 658 return t; 659 } 660 661 public Endpoint addContact(ContactPoint t) { // 3 662 if (t == null) 663 return this; 664 if (this.contact == null) 665 this.contact = new ArrayList<ContactPoint>(); 666 this.contact.add(t); 667 return this; 668 } 669 670 /** 671 * @return The first repetition of repeating field {@link #contact}, creating it 672 * if it does not already exist 673 */ 674 public ContactPoint getContactFirstRep() { 675 if (getContact().isEmpty()) { 676 addContact(); 677 } 678 return getContact().get(0); 679 } 680 681 /** 682 * @return {@link #period} (The interval during which the endpoint is expected 683 * to be operational.) 684 */ 685 public Period getPeriod() { 686 if (this.period == null) 687 if (Configuration.errorOnAutoCreate()) 688 throw new Error("Attempt to auto-create Endpoint.period"); 689 else if (Configuration.doAutoCreate()) 690 this.period = new Period(); // cc 691 return this.period; 692 } 693 694 public boolean hasPeriod() { 695 return this.period != null && !this.period.isEmpty(); 696 } 697 698 /** 699 * @param value {@link #period} (The interval during which the endpoint is 700 * expected to be operational.) 701 */ 702 public Endpoint setPeriod(Period value) { 703 this.period = value; 704 return this; 705 } 706 707 /** 708 * @return {@link #payloadType} (The payload type describes the acceptable 709 * content that can be communicated on the endpoint.) 710 */ 711 public List<CodeableConcept> getPayloadType() { 712 if (this.payloadType == null) 713 this.payloadType = new ArrayList<CodeableConcept>(); 714 return this.payloadType; 715 } 716 717 /** 718 * @return Returns a reference to <code>this</code> for easy method chaining 719 */ 720 public Endpoint setPayloadType(List<CodeableConcept> thePayloadType) { 721 this.payloadType = thePayloadType; 722 return this; 723 } 724 725 public boolean hasPayloadType() { 726 if (this.payloadType == null) 727 return false; 728 for (CodeableConcept item : this.payloadType) 729 if (!item.isEmpty()) 730 return true; 731 return false; 732 } 733 734 public CodeableConcept addPayloadType() { // 3 735 CodeableConcept t = new CodeableConcept(); 736 if (this.payloadType == null) 737 this.payloadType = new ArrayList<CodeableConcept>(); 738 this.payloadType.add(t); 739 return t; 740 } 741 742 public Endpoint addPayloadType(CodeableConcept t) { // 3 743 if (t == null) 744 return this; 745 if (this.payloadType == null) 746 this.payloadType = new ArrayList<CodeableConcept>(); 747 this.payloadType.add(t); 748 return this; 749 } 750 751 /** 752 * @return The first repetition of repeating field {@link #payloadType}, 753 * creating it if it does not already exist 754 */ 755 public CodeableConcept getPayloadTypeFirstRep() { 756 if (getPayloadType().isEmpty()) { 757 addPayloadType(); 758 } 759 return getPayloadType().get(0); 760 } 761 762 /** 763 * @return {@link #payloadMimeType} (The mime type to send the payload in - e.g. 764 * application/fhir+xml, application/fhir+json. If the mime type is not 765 * specified, then the sender could send any content (including no 766 * content depending on the connectionType).) 767 */ 768 public List<CodeType> getPayloadMimeType() { 769 if (this.payloadMimeType == null) 770 this.payloadMimeType = new ArrayList<CodeType>(); 771 return this.payloadMimeType; 772 } 773 774 /** 775 * @return Returns a reference to <code>this</code> for easy method chaining 776 */ 777 public Endpoint setPayloadMimeType(List<CodeType> thePayloadMimeType) { 778 this.payloadMimeType = thePayloadMimeType; 779 return this; 780 } 781 782 public boolean hasPayloadMimeType() { 783 if (this.payloadMimeType == null) 784 return false; 785 for (CodeType item : this.payloadMimeType) 786 if (!item.isEmpty()) 787 return true; 788 return false; 789 } 790 791 /** 792 * @return {@link #payloadMimeType} (The mime type to send the payload in - e.g. 793 * application/fhir+xml, application/fhir+json. If the mime type is not 794 * specified, then the sender could send any content (including no 795 * content depending on the connectionType).) 796 */ 797 public CodeType addPayloadMimeTypeElement() {// 2 798 CodeType t = new CodeType(); 799 if (this.payloadMimeType == null) 800 this.payloadMimeType = new ArrayList<CodeType>(); 801 this.payloadMimeType.add(t); 802 return t; 803 } 804 805 /** 806 * @param value {@link #payloadMimeType} (The mime type to send the payload in - 807 * e.g. application/fhir+xml, application/fhir+json. If the mime 808 * type is not specified, then the sender could send any content 809 * (including no content depending on the connectionType).) 810 */ 811 public Endpoint addPayloadMimeType(String value) { // 1 812 CodeType t = new CodeType(); 813 t.setValue(value); 814 if (this.payloadMimeType == null) 815 this.payloadMimeType = new ArrayList<CodeType>(); 816 this.payloadMimeType.add(t); 817 return this; 818 } 819 820 /** 821 * @param value {@link #payloadMimeType} (The mime type to send the payload in - 822 * e.g. application/fhir+xml, application/fhir+json. If the mime 823 * type is not specified, then the sender could send any content 824 * (including no content depending on the connectionType).) 825 */ 826 public boolean hasPayloadMimeType(String value) { 827 if (this.payloadMimeType == null) 828 return false; 829 for (CodeType v : this.payloadMimeType) 830 if (v.getValue().equals(value)) // code 831 return true; 832 return false; 833 } 834 835 /** 836 * @return {@link #address} (The uri that describes the actual end-point to 837 * connect to.). This is the underlying object with id, value and 838 * extensions. The accessor "getAddress" gives direct access to the 839 * value 840 */ 841 public UrlType getAddressElement() { 842 if (this.address == null) 843 if (Configuration.errorOnAutoCreate()) 844 throw new Error("Attempt to auto-create Endpoint.address"); 845 else if (Configuration.doAutoCreate()) 846 this.address = new UrlType(); // bb 847 return this.address; 848 } 849 850 public boolean hasAddressElement() { 851 return this.address != null && !this.address.isEmpty(); 852 } 853 854 public boolean hasAddress() { 855 return this.address != null && !this.address.isEmpty(); 856 } 857 858 /** 859 * @param value {@link #address} (The uri that describes the actual end-point to 860 * connect to.). This is the underlying object with id, value and 861 * extensions. The accessor "getAddress" gives direct access to the 862 * value 863 */ 864 public Endpoint setAddressElement(UrlType value) { 865 this.address = value; 866 return this; 867 } 868 869 /** 870 * @return The uri that describes the actual end-point to connect to. 871 */ 872 public String getAddress() { 873 return this.address == null ? null : this.address.getValue(); 874 } 875 876 /** 877 * @param value The uri that describes the actual end-point to connect to. 878 */ 879 public Endpoint setAddress(String value) { 880 if (this.address == null) 881 this.address = new UrlType(); 882 this.address.setValue(value); 883 return this; 884 } 885 886 /** 887 * @return {@link #header} (Additional headers / information to send as part of 888 * the notification.) 889 */ 890 public List<StringType> getHeader() { 891 if (this.header == null) 892 this.header = new ArrayList<StringType>(); 893 return this.header; 894 } 895 896 /** 897 * @return Returns a reference to <code>this</code> for easy method chaining 898 */ 899 public Endpoint setHeader(List<StringType> theHeader) { 900 this.header = theHeader; 901 return this; 902 } 903 904 public boolean hasHeader() { 905 if (this.header == null) 906 return false; 907 for (StringType item : this.header) 908 if (!item.isEmpty()) 909 return true; 910 return false; 911 } 912 913 /** 914 * @return {@link #header} (Additional headers / information to send as part of 915 * the notification.) 916 */ 917 public StringType addHeaderElement() {// 2 918 StringType t = new StringType(); 919 if (this.header == null) 920 this.header = new ArrayList<StringType>(); 921 this.header.add(t); 922 return t; 923 } 924 925 /** 926 * @param value {@link #header} (Additional headers / information to send as 927 * part of the notification.) 928 */ 929 public Endpoint addHeader(String value) { // 1 930 StringType t = new StringType(); 931 t.setValue(value); 932 if (this.header == null) 933 this.header = new ArrayList<StringType>(); 934 this.header.add(t); 935 return this; 936 } 937 938 /** 939 * @param value {@link #header} (Additional headers / information to send as 940 * part of the notification.) 941 */ 942 public boolean hasHeader(String value) { 943 if (this.header == null) 944 return false; 945 for (StringType v : this.header) 946 if (v.getValue().equals(value)) // string 947 return true; 948 return false; 949 } 950 951 protected void listChildren(List<Property> children) { 952 super.listChildren(children); 953 children.add(new Property("identifier", "Identifier", 954 "Identifier for the organization that is used to identify the endpoint across multiple disparate systems.", 0, 955 java.lang.Integer.MAX_VALUE, identifier)); 956 children.add(new Property("status", "code", "active | suspended | error | off | test.", 0, 1, status)); 957 children.add(new Property("connectionType", "Coding", 958 "A coded value that represents the technical details of the usage of this endpoint, such as what WSDLs should be used in what way. (e.g. XDS.b/DICOM/cds-hook).", 959 0, 1, connectionType)); 960 children 961 .add(new Property("name", "string", "A friendly name that this endpoint can be referred to with.", 0, 1, name)); 962 children.add(new Property("managingOrganization", "Reference(Organization)", 963 "The organization that manages this endpoint (even if technically another organization is hosting this in the cloud, it is the organization associated with the data).", 964 0, 1, managingOrganization)); 965 children.add(new Property("contact", "ContactPoint", 966 "Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting.", 967 0, java.lang.Integer.MAX_VALUE, contact)); 968 children.add(new Property("period", "Period", 969 "The interval during which the endpoint is expected to be operational.", 0, 1, period)); 970 children.add(new Property("payloadType", "CodeableConcept", 971 "The payload type describes the acceptable content that can be communicated on the endpoint.", 0, 972 java.lang.Integer.MAX_VALUE, payloadType)); 973 children.add(new Property("payloadMimeType", "code", 974 "The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType).", 975 0, java.lang.Integer.MAX_VALUE, payloadMimeType)); 976 children.add( 977 new Property("address", "url", "The uri that describes the actual end-point to connect to.", 0, 1, address)); 978 children 979 .add(new Property("header", "string", "Additional headers / information to send as part of the notification.", 980 0, java.lang.Integer.MAX_VALUE, header)); 981 } 982 983 @Override 984 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 985 switch (_hash) { 986 case -1618432855: 987 /* identifier */ return new Property("identifier", "Identifier", 988 "Identifier for the organization that is used to identify the endpoint across multiple disparate systems.", 0, 989 java.lang.Integer.MAX_VALUE, identifier); 990 case -892481550: 991 /* status */ return new Property("status", "code", "active | suspended | error | off | test.", 0, 1, status); 992 case 1270211384: 993 /* connectionType */ return new Property("connectionType", "Coding", 994 "A coded value that represents the technical details of the usage of this endpoint, such as what WSDLs should be used in what way. (e.g. XDS.b/DICOM/cds-hook).", 995 0, 1, connectionType); 996 case 3373707: 997 /* name */ return new Property("name", "string", "A friendly name that this endpoint can be referred to with.", 0, 998 1, name); 999 case -2058947787: 1000 /* managingOrganization */ return new Property("managingOrganization", "Reference(Organization)", 1001 "The organization that manages this endpoint (even if technically another organization is hosting this in the cloud, it is the organization associated with the data).", 1002 0, 1, managingOrganization); 1003 case 951526432: 1004 /* contact */ return new Property("contact", "ContactPoint", 1005 "Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting.", 1006 0, java.lang.Integer.MAX_VALUE, contact); 1007 case -991726143: 1008 /* period */ return new Property("period", "Period", 1009 "The interval during which the endpoint is expected to be operational.", 0, 1, period); 1010 case 909929960: 1011 /* payloadType */ return new Property("payloadType", "CodeableConcept", 1012 "The payload type describes the acceptable content that can be communicated on the endpoint.", 0, 1013 java.lang.Integer.MAX_VALUE, payloadType); 1014 case -1702836932: 1015 /* payloadMimeType */ return new Property("payloadMimeType", "code", 1016 "The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType).", 1017 0, java.lang.Integer.MAX_VALUE, payloadMimeType); 1018 case -1147692044: 1019 /* address */ return new Property("address", "url", "The uri that describes the actual end-point to connect to.", 1020 0, 1, address); 1021 case -1221270899: 1022 /* header */ return new Property("header", "string", 1023 "Additional headers / information to send as part of the notification.", 0, java.lang.Integer.MAX_VALUE, 1024 header); 1025 default: 1026 return super.getNamedProperty(_hash, _name, _checkValid); 1027 } 1028 1029 } 1030 1031 @Override 1032 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1033 switch (hash) { 1034 case -1618432855: 1035 /* identifier */ return this.identifier == null ? new Base[0] 1036 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1037 case -892481550: 1038 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<EndpointStatus> 1039 case 1270211384: 1040 /* connectionType */ return this.connectionType == null ? new Base[0] : new Base[] { this.connectionType }; // Coding 1041 case 3373707: 1042 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 1043 case -2058947787: 1044 /* managingOrganization */ return this.managingOrganization == null ? new Base[0] 1045 : new Base[] { this.managingOrganization }; // Reference 1046 case 951526432: 1047 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactPoint 1048 case -991726143: 1049 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 1050 case 909929960: 1051 /* payloadType */ return this.payloadType == null ? new Base[0] 1052 : this.payloadType.toArray(new Base[this.payloadType.size()]); // CodeableConcept 1053 case -1702836932: 1054 /* payloadMimeType */ return this.payloadMimeType == null ? new Base[0] 1055 : this.payloadMimeType.toArray(new Base[this.payloadMimeType.size()]); // CodeType 1056 case -1147692044: 1057 /* address */ return this.address == null ? new Base[0] : new Base[] { this.address }; // UrlType 1058 case -1221270899: 1059 /* header */ return this.header == null ? new Base[0] : this.header.toArray(new Base[this.header.size()]); // StringType 1060 default: 1061 return super.getProperty(hash, name, checkValid); 1062 } 1063 1064 } 1065 1066 @Override 1067 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1068 switch (hash) { 1069 case -1618432855: // identifier 1070 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1071 return value; 1072 case -892481550: // status 1073 value = new EndpointStatusEnumFactory().fromType(castToCode(value)); 1074 this.status = (Enumeration) value; // Enumeration<EndpointStatus> 1075 return value; 1076 case 1270211384: // connectionType 1077 this.connectionType = castToCoding(value); // Coding 1078 return value; 1079 case 3373707: // name 1080 this.name = castToString(value); // StringType 1081 return value; 1082 case -2058947787: // managingOrganization 1083 this.managingOrganization = castToReference(value); // Reference 1084 return value; 1085 case 951526432: // contact 1086 this.getContact().add(castToContactPoint(value)); // ContactPoint 1087 return value; 1088 case -991726143: // period 1089 this.period = castToPeriod(value); // Period 1090 return value; 1091 case 909929960: // payloadType 1092 this.getPayloadType().add(castToCodeableConcept(value)); // CodeableConcept 1093 return value; 1094 case -1702836932: // payloadMimeType 1095 this.getPayloadMimeType().add(castToCode(value)); // CodeType 1096 return value; 1097 case -1147692044: // address 1098 this.address = castToUrl(value); // UrlType 1099 return value; 1100 case -1221270899: // header 1101 this.getHeader().add(castToString(value)); // StringType 1102 return value; 1103 default: 1104 return super.setProperty(hash, name, value); 1105 } 1106 1107 } 1108 1109 @Override 1110 public Base setProperty(String name, Base value) throws FHIRException { 1111 if (name.equals("identifier")) { 1112 this.getIdentifier().add(castToIdentifier(value)); 1113 } else if (name.equals("status")) { 1114 value = new EndpointStatusEnumFactory().fromType(castToCode(value)); 1115 this.status = (Enumeration) value; // Enumeration<EndpointStatus> 1116 } else if (name.equals("connectionType")) { 1117 this.connectionType = castToCoding(value); // Coding 1118 } else if (name.equals("name")) { 1119 this.name = castToString(value); // StringType 1120 } else if (name.equals("managingOrganization")) { 1121 this.managingOrganization = castToReference(value); // Reference 1122 } else if (name.equals("contact")) { 1123 this.getContact().add(castToContactPoint(value)); 1124 } else if (name.equals("period")) { 1125 this.period = castToPeriod(value); // Period 1126 } else if (name.equals("payloadType")) { 1127 this.getPayloadType().add(castToCodeableConcept(value)); 1128 } else if (name.equals("payloadMimeType")) { 1129 this.getPayloadMimeType().add(castToCode(value)); 1130 } else if (name.equals("address")) { 1131 this.address = castToUrl(value); // UrlType 1132 } else if (name.equals("header")) { 1133 this.getHeader().add(castToString(value)); 1134 } else 1135 return super.setProperty(name, value); 1136 return value; 1137 } 1138 1139 @Override 1140 public void removeChild(String name, Base value) throws FHIRException { 1141 if (name.equals("identifier")) { 1142 this.getIdentifier().remove(castToIdentifier(value)); 1143 } else if (name.equals("status")) { 1144 this.status = null; 1145 } else if (name.equals("connectionType")) { 1146 this.connectionType = null; 1147 } else if (name.equals("name")) { 1148 this.name = null; 1149 } else if (name.equals("managingOrganization")) { 1150 this.managingOrganization = null; 1151 } else if (name.equals("contact")) { 1152 this.getContact().remove(castToContactPoint(value)); 1153 } else if (name.equals("period")) { 1154 this.period = null; 1155 } else if (name.equals("payloadType")) { 1156 this.getPayloadType().remove(castToCodeableConcept(value)); 1157 } else if (name.equals("payloadMimeType")) { 1158 this.getPayloadMimeType().remove(castToCode(value)); 1159 } else if (name.equals("address")) { 1160 this.address = null; 1161 } else if (name.equals("header")) { 1162 this.getHeader().remove(castToString(value)); 1163 } else 1164 super.removeChild(name, value); 1165 1166 } 1167 1168 @Override 1169 public Base makeProperty(int hash, String name) throws FHIRException { 1170 switch (hash) { 1171 case -1618432855: 1172 return addIdentifier(); 1173 case -892481550: 1174 return getStatusElement(); 1175 case 1270211384: 1176 return getConnectionType(); 1177 case 3373707: 1178 return getNameElement(); 1179 case -2058947787: 1180 return getManagingOrganization(); 1181 case 951526432: 1182 return addContact(); 1183 case -991726143: 1184 return getPeriod(); 1185 case 909929960: 1186 return addPayloadType(); 1187 case -1702836932: 1188 return addPayloadMimeTypeElement(); 1189 case -1147692044: 1190 return getAddressElement(); 1191 case -1221270899: 1192 return addHeaderElement(); 1193 default: 1194 return super.makeProperty(hash, name); 1195 } 1196 1197 } 1198 1199 @Override 1200 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1201 switch (hash) { 1202 case -1618432855: 1203 /* identifier */ return new String[] { "Identifier" }; 1204 case -892481550: 1205 /* status */ return new String[] { "code" }; 1206 case 1270211384: 1207 /* connectionType */ return new String[] { "Coding" }; 1208 case 3373707: 1209 /* name */ return new String[] { "string" }; 1210 case -2058947787: 1211 /* managingOrganization */ return new String[] { "Reference" }; 1212 case 951526432: 1213 /* contact */ return new String[] { "ContactPoint" }; 1214 case -991726143: 1215 /* period */ return new String[] { "Period" }; 1216 case 909929960: 1217 /* payloadType */ return new String[] { "CodeableConcept" }; 1218 case -1702836932: 1219 /* payloadMimeType */ return new String[] { "code" }; 1220 case -1147692044: 1221 /* address */ return new String[] { "url" }; 1222 case -1221270899: 1223 /* header */ return new String[] { "string" }; 1224 default: 1225 return super.getTypesForProperty(hash, name); 1226 } 1227 1228 } 1229 1230 @Override 1231 public Base addChild(String name) throws FHIRException { 1232 if (name.equals("identifier")) { 1233 return addIdentifier(); 1234 } else if (name.equals("status")) { 1235 throw new FHIRException("Cannot call addChild on a singleton property Endpoint.status"); 1236 } else if (name.equals("connectionType")) { 1237 this.connectionType = new Coding(); 1238 return this.connectionType; 1239 } else if (name.equals("name")) { 1240 throw new FHIRException("Cannot call addChild on a singleton property Endpoint.name"); 1241 } else if (name.equals("managingOrganization")) { 1242 this.managingOrganization = new Reference(); 1243 return this.managingOrganization; 1244 } else if (name.equals("contact")) { 1245 return addContact(); 1246 } else if (name.equals("period")) { 1247 this.period = new Period(); 1248 return this.period; 1249 } else if (name.equals("payloadType")) { 1250 return addPayloadType(); 1251 } else if (name.equals("payloadMimeType")) { 1252 throw new FHIRException("Cannot call addChild on a singleton property Endpoint.payloadMimeType"); 1253 } else if (name.equals("address")) { 1254 throw new FHIRException("Cannot call addChild on a singleton property Endpoint.address"); 1255 } else if (name.equals("header")) { 1256 throw new FHIRException("Cannot call addChild on a singleton property Endpoint.header"); 1257 } else 1258 return super.addChild(name); 1259 } 1260 1261 public String fhirType() { 1262 return "Endpoint"; 1263 1264 } 1265 1266 public Endpoint copy() { 1267 Endpoint dst = new Endpoint(); 1268 copyValues(dst); 1269 return dst; 1270 } 1271 1272 public void copyValues(Endpoint dst) { 1273 super.copyValues(dst); 1274 if (identifier != null) { 1275 dst.identifier = new ArrayList<Identifier>(); 1276 for (Identifier i : identifier) 1277 dst.identifier.add(i.copy()); 1278 } 1279 ; 1280 dst.status = status == null ? null : status.copy(); 1281 dst.connectionType = connectionType == null ? null : connectionType.copy(); 1282 dst.name = name == null ? null : name.copy(); 1283 dst.managingOrganization = managingOrganization == null ? null : managingOrganization.copy(); 1284 if (contact != null) { 1285 dst.contact = new ArrayList<ContactPoint>(); 1286 for (ContactPoint i : contact) 1287 dst.contact.add(i.copy()); 1288 } 1289 ; 1290 dst.period = period == null ? null : period.copy(); 1291 if (payloadType != null) { 1292 dst.payloadType = new ArrayList<CodeableConcept>(); 1293 for (CodeableConcept i : payloadType) 1294 dst.payloadType.add(i.copy()); 1295 } 1296 ; 1297 if (payloadMimeType != null) { 1298 dst.payloadMimeType = new ArrayList<CodeType>(); 1299 for (CodeType i : payloadMimeType) 1300 dst.payloadMimeType.add(i.copy()); 1301 } 1302 ; 1303 dst.address = address == null ? null : address.copy(); 1304 if (header != null) { 1305 dst.header = new ArrayList<StringType>(); 1306 for (StringType i : header) 1307 dst.header.add(i.copy()); 1308 } 1309 ; 1310 } 1311 1312 protected Endpoint typedCopy() { 1313 return copy(); 1314 } 1315 1316 @Override 1317 public boolean equalsDeep(Base other_) { 1318 if (!super.equalsDeep(other_)) 1319 return false; 1320 if (!(other_ instanceof Endpoint)) 1321 return false; 1322 Endpoint o = (Endpoint) other_; 1323 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 1324 && compareDeep(connectionType, o.connectionType, true) && compareDeep(name, o.name, true) 1325 && compareDeep(managingOrganization, o.managingOrganization, true) && compareDeep(contact, o.contact, true) 1326 && compareDeep(period, o.period, true) && compareDeep(payloadType, o.payloadType, true) 1327 && compareDeep(payloadMimeType, o.payloadMimeType, true) && compareDeep(address, o.address, true) 1328 && compareDeep(header, o.header, true); 1329 } 1330 1331 @Override 1332 public boolean equalsShallow(Base other_) { 1333 if (!super.equalsShallow(other_)) 1334 return false; 1335 if (!(other_ instanceof Endpoint)) 1336 return false; 1337 Endpoint o = (Endpoint) other_; 1338 return compareValues(status, o.status, true) && compareValues(name, o.name, true) 1339 && compareValues(payloadMimeType, o.payloadMimeType, true) && compareValues(address, o.address, true) 1340 && compareValues(header, o.header, true); 1341 } 1342 1343 public boolean isEmpty() { 1344 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, connectionType, name, 1345 managingOrganization, contact, period, payloadType, payloadMimeType, address, header); 1346 } 1347 1348 @Override 1349 public ResourceType getResourceType() { 1350 return ResourceType.Endpoint; 1351 } 1352 1353 /** 1354 * Search parameter: <b>payload-type</b> 1355 * <p> 1356 * Description: <b>The type of content that may be used at this endpoint (e.g. 1357 * XDS Discharge summaries)</b><br> 1358 * Type: <b>token</b><br> 1359 * Path: <b>Endpoint.payloadType</b><br> 1360 * </p> 1361 */ 1362 @SearchParamDefinition(name = "payload-type", path = "Endpoint.payloadType", description = "The type of content that may be used at this endpoint (e.g. XDS Discharge summaries)", type = "token") 1363 public static final String SP_PAYLOAD_TYPE = "payload-type"; 1364 /** 1365 * <b>Fluent Client</b> search parameter constant for <b>payload-type</b> 1366 * <p> 1367 * Description: <b>The type of content that may be used at this endpoint (e.g. 1368 * XDS Discharge summaries)</b><br> 1369 * Type: <b>token</b><br> 1370 * Path: <b>Endpoint.payloadType</b><br> 1371 * </p> 1372 */ 1373 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PAYLOAD_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1374 SP_PAYLOAD_TYPE); 1375 1376 /** 1377 * Search parameter: <b>identifier</b> 1378 * <p> 1379 * Description: <b>Identifies this endpoint across multiple systems</b><br> 1380 * Type: <b>token</b><br> 1381 * Path: <b>Endpoint.identifier</b><br> 1382 * </p> 1383 */ 1384 @SearchParamDefinition(name = "identifier", path = "Endpoint.identifier", description = "Identifies this endpoint across multiple systems", type = "token") 1385 public static final String SP_IDENTIFIER = "identifier"; 1386 /** 1387 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1388 * <p> 1389 * Description: <b>Identifies this endpoint across multiple systems</b><br> 1390 * Type: <b>token</b><br> 1391 * Path: <b>Endpoint.identifier</b><br> 1392 * </p> 1393 */ 1394 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1395 SP_IDENTIFIER); 1396 1397 /** 1398 * Search parameter: <b>organization</b> 1399 * <p> 1400 * Description: <b>The organization that is managing the endpoint</b><br> 1401 * Type: <b>reference</b><br> 1402 * Path: <b>Endpoint.managingOrganization</b><br> 1403 * </p> 1404 */ 1405 @SearchParamDefinition(name = "organization", path = "Endpoint.managingOrganization", description = "The organization that is managing the endpoint", type = "reference", target = { 1406 Organization.class }) 1407 public static final String SP_ORGANIZATION = "organization"; 1408 /** 1409 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 1410 * <p> 1411 * Description: <b>The organization that is managing the endpoint</b><br> 1412 * Type: <b>reference</b><br> 1413 * Path: <b>Endpoint.managingOrganization</b><br> 1414 * </p> 1415 */ 1416 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1417 SP_ORGANIZATION); 1418 1419 /** 1420 * Constant for fluent queries to be used to add include statements. Specifies 1421 * the path value of "<b>Endpoint:organization</b>". 1422 */ 1423 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include( 1424 "Endpoint:organization").toLocked(); 1425 1426 /** 1427 * Search parameter: <b>connection-type</b> 1428 * <p> 1429 * Description: <b>Protocol/Profile/Standard to be used with this endpoint 1430 * connection</b><br> 1431 * Type: <b>token</b><br> 1432 * Path: <b>Endpoint.connectionType</b><br> 1433 * </p> 1434 */ 1435 @SearchParamDefinition(name = "connection-type", path = "Endpoint.connectionType", description = "Protocol/Profile/Standard to be used with this endpoint connection", type = "token") 1436 public static final String SP_CONNECTION_TYPE = "connection-type"; 1437 /** 1438 * <b>Fluent Client</b> search parameter constant for <b>connection-type</b> 1439 * <p> 1440 * Description: <b>Protocol/Profile/Standard to be used with this endpoint 1441 * connection</b><br> 1442 * Type: <b>token</b><br> 1443 * Path: <b>Endpoint.connectionType</b><br> 1444 * </p> 1445 */ 1446 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONNECTION_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1447 SP_CONNECTION_TYPE); 1448 1449 /** 1450 * Search parameter: <b>name</b> 1451 * <p> 1452 * Description: <b>A name that this endpoint can be identified by</b><br> 1453 * Type: <b>string</b><br> 1454 * Path: <b>Endpoint.name</b><br> 1455 * </p> 1456 */ 1457 @SearchParamDefinition(name = "name", path = "Endpoint.name", description = "A name that this endpoint can be identified by", type = "string") 1458 public static final String SP_NAME = "name"; 1459 /** 1460 * <b>Fluent Client</b> search parameter constant for <b>name</b> 1461 * <p> 1462 * Description: <b>A name that this endpoint can be identified by</b><br> 1463 * Type: <b>string</b><br> 1464 * Path: <b>Endpoint.name</b><br> 1465 * </p> 1466 */ 1467 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 1468 SP_NAME); 1469 1470 /** 1471 * Search parameter: <b>status</b> 1472 * <p> 1473 * Description: <b>The current status of the Endpoint (usually expected to be 1474 * active)</b><br> 1475 * Type: <b>token</b><br> 1476 * Path: <b>Endpoint.status</b><br> 1477 * </p> 1478 */ 1479 @SearchParamDefinition(name = "status", path = "Endpoint.status", description = "The current status of the Endpoint (usually expected to be active)", type = "token") 1480 public static final String SP_STATUS = "status"; 1481 /** 1482 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1483 * <p> 1484 * Description: <b>The current status of the Endpoint (usually expected to be 1485 * active)</b><br> 1486 * Type: <b>token</b><br> 1487 * Path: <b>Endpoint.status</b><br> 1488 * </p> 1489 */ 1490 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1491 SP_STATUS); 1492 1493}