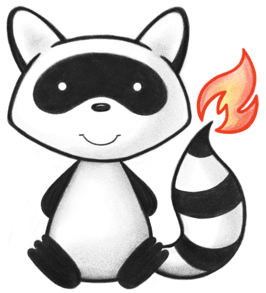
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.r4.model.Enumerations.RemittanceOutcome; 039import org.hl7.fhir.r4.model.Enumerations.RemittanceOutcomeEnumFactory; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * This resource provides enrollment and plan details from the processing of an 049 * EnrollmentRequest resource. 050 */ 051@ResourceDef(name = "EnrollmentResponse", profile = "http://hl7.org/fhir/StructureDefinition/EnrollmentResponse") 052public class EnrollmentResponse extends DomainResource { 053 054 public enum EnrollmentResponseStatus { 055 /** 056 * The instance is currently in-force. 057 */ 058 ACTIVE, 059 /** 060 * The instance is withdrawn, rescinded or reversed. 061 */ 062 CANCELLED, 063 /** 064 * A new instance the contents of which is not complete. 065 */ 066 DRAFT, 067 /** 068 * The instance was entered in error. 069 */ 070 ENTEREDINERROR, 071 /** 072 * added to help the parsers with the generic types 073 */ 074 NULL; 075 076 public static EnrollmentResponseStatus fromCode(String codeString) throws FHIRException { 077 if (codeString == null || "".equals(codeString)) 078 return null; 079 if ("active".equals(codeString)) 080 return ACTIVE; 081 if ("cancelled".equals(codeString)) 082 return CANCELLED; 083 if ("draft".equals(codeString)) 084 return DRAFT; 085 if ("entered-in-error".equals(codeString)) 086 return ENTEREDINERROR; 087 if (Configuration.isAcceptInvalidEnums()) 088 return null; 089 else 090 throw new FHIRException("Unknown EnrollmentResponseStatus code '" + codeString + "'"); 091 } 092 093 public String toCode() { 094 switch (this) { 095 case ACTIVE: 096 return "active"; 097 case CANCELLED: 098 return "cancelled"; 099 case DRAFT: 100 return "draft"; 101 case ENTEREDINERROR: 102 return "entered-in-error"; 103 case NULL: 104 return null; 105 default: 106 return "?"; 107 } 108 } 109 110 public String getSystem() { 111 switch (this) { 112 case ACTIVE: 113 return "http://hl7.org/fhir/fm-status"; 114 case CANCELLED: 115 return "http://hl7.org/fhir/fm-status"; 116 case DRAFT: 117 return "http://hl7.org/fhir/fm-status"; 118 case ENTEREDINERROR: 119 return "http://hl7.org/fhir/fm-status"; 120 case NULL: 121 return null; 122 default: 123 return "?"; 124 } 125 } 126 127 public String getDefinition() { 128 switch (this) { 129 case ACTIVE: 130 return "The instance is currently in-force."; 131 case CANCELLED: 132 return "The instance is withdrawn, rescinded or reversed."; 133 case DRAFT: 134 return "A new instance the contents of which is not complete."; 135 case ENTEREDINERROR: 136 return "The instance was entered in error."; 137 case NULL: 138 return null; 139 default: 140 return "?"; 141 } 142 } 143 144 public String getDisplay() { 145 switch (this) { 146 case ACTIVE: 147 return "Active"; 148 case CANCELLED: 149 return "Cancelled"; 150 case DRAFT: 151 return "Draft"; 152 case ENTEREDINERROR: 153 return "Entered in Error"; 154 case NULL: 155 return null; 156 default: 157 return "?"; 158 } 159 } 160 } 161 162 public static class EnrollmentResponseStatusEnumFactory implements EnumFactory<EnrollmentResponseStatus> { 163 public EnrollmentResponseStatus fromCode(String codeString) throws IllegalArgumentException { 164 if (codeString == null || "".equals(codeString)) 165 if (codeString == null || "".equals(codeString)) 166 return null; 167 if ("active".equals(codeString)) 168 return EnrollmentResponseStatus.ACTIVE; 169 if ("cancelled".equals(codeString)) 170 return EnrollmentResponseStatus.CANCELLED; 171 if ("draft".equals(codeString)) 172 return EnrollmentResponseStatus.DRAFT; 173 if ("entered-in-error".equals(codeString)) 174 return EnrollmentResponseStatus.ENTEREDINERROR; 175 throw new IllegalArgumentException("Unknown EnrollmentResponseStatus code '" + codeString + "'"); 176 } 177 178 public Enumeration<EnrollmentResponseStatus> fromType(PrimitiveType<?> code) throws FHIRException { 179 if (code == null) 180 return null; 181 if (code.isEmpty()) 182 return new Enumeration<EnrollmentResponseStatus>(this, EnrollmentResponseStatus.NULL, code); 183 String codeString = code.asStringValue(); 184 if (codeString == null || "".equals(codeString)) 185 return new Enumeration<EnrollmentResponseStatus>(this, EnrollmentResponseStatus.NULL, code); 186 if ("active".equals(codeString)) 187 return new Enumeration<EnrollmentResponseStatus>(this, EnrollmentResponseStatus.ACTIVE, code); 188 if ("cancelled".equals(codeString)) 189 return new Enumeration<EnrollmentResponseStatus>(this, EnrollmentResponseStatus.CANCELLED, code); 190 if ("draft".equals(codeString)) 191 return new Enumeration<EnrollmentResponseStatus>(this, EnrollmentResponseStatus.DRAFT, code); 192 if ("entered-in-error".equals(codeString)) 193 return new Enumeration<EnrollmentResponseStatus>(this, EnrollmentResponseStatus.ENTEREDINERROR, code); 194 throw new FHIRException("Unknown EnrollmentResponseStatus code '" + codeString + "'"); 195 } 196 197 public String toCode(EnrollmentResponseStatus code) { 198 if (code == EnrollmentResponseStatus.NULL) 199 return null; 200 if (code == EnrollmentResponseStatus.ACTIVE) 201 return "active"; 202 if (code == EnrollmentResponseStatus.CANCELLED) 203 return "cancelled"; 204 if (code == EnrollmentResponseStatus.DRAFT) 205 return "draft"; 206 if (code == EnrollmentResponseStatus.ENTEREDINERROR) 207 return "entered-in-error"; 208 return "?"; 209 } 210 211 public String toSystem(EnrollmentResponseStatus code) { 212 return code.getSystem(); 213 } 214 } 215 216 /** 217 * The Response business identifier. 218 */ 219 @Child(name = "identifier", type = { 220 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 221 @Description(shortDefinition = "Business Identifier", formalDefinition = "The Response business identifier.") 222 protected List<Identifier> identifier; 223 224 /** 225 * The status of the resource instance. 226 */ 227 @Child(name = "status", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 228 @Description(shortDefinition = "active | cancelled | draft | entered-in-error", formalDefinition = "The status of the resource instance.") 229 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/fm-status") 230 protected Enumeration<EnrollmentResponseStatus> status; 231 232 /** 233 * Original request resource reference. 234 */ 235 @Child(name = "request", type = { 236 EnrollmentRequest.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 237 @Description(shortDefinition = "Claim reference", formalDefinition = "Original request resource reference.") 238 protected Reference request; 239 240 /** 241 * The actual object that is the target of the reference (Original request 242 * resource reference.) 243 */ 244 protected EnrollmentRequest requestTarget; 245 246 /** 247 * Processing status: error, complete. 248 */ 249 @Child(name = "outcome", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 250 @Description(shortDefinition = "queued | complete | error | partial", formalDefinition = "Processing status: error, complete.") 251 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/remittance-outcome") 252 protected Enumeration<RemittanceOutcome> outcome; 253 254 /** 255 * A description of the status of the adjudication. 256 */ 257 @Child(name = "disposition", type = { 258 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 259 @Description(shortDefinition = "Disposition Message", formalDefinition = "A description of the status of the adjudication.") 260 protected StringType disposition; 261 262 /** 263 * The date when the enclosed suite of services were performed or completed. 264 */ 265 @Child(name = "created", type = { 266 DateTimeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 267 @Description(shortDefinition = "Creation date", formalDefinition = "The date when the enclosed suite of services were performed or completed.") 268 protected DateTimeType created; 269 270 /** 271 * The Insurer who produced this adjudicated response. 272 */ 273 @Child(name = "organization", type = { 274 Organization.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 275 @Description(shortDefinition = "Insurer", formalDefinition = "The Insurer who produced this adjudicated response.") 276 protected Reference organization; 277 278 /** 279 * The actual object that is the target of the reference (The Insurer who 280 * produced this adjudicated response.) 281 */ 282 protected Organization organizationTarget; 283 284 /** 285 * The practitioner who is responsible for the services rendered to the patient. 286 */ 287 @Child(name = "requestProvider", type = { Practitioner.class, PractitionerRole.class, 288 Organization.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 289 @Description(shortDefinition = "Responsible practitioner", formalDefinition = "The practitioner who is responsible for the services rendered to the patient.") 290 protected Reference requestProvider; 291 292 /** 293 * The actual object that is the target of the reference (The practitioner who 294 * is responsible for the services rendered to the patient.) 295 */ 296 protected Resource requestProviderTarget; 297 298 private static final long serialVersionUID = -1759921458L; 299 300 /** 301 * Constructor 302 */ 303 public EnrollmentResponse() { 304 super(); 305 } 306 307 /** 308 * @return {@link #identifier} (The Response business identifier.) 309 */ 310 public List<Identifier> getIdentifier() { 311 if (this.identifier == null) 312 this.identifier = new ArrayList<Identifier>(); 313 return this.identifier; 314 } 315 316 /** 317 * @return Returns a reference to <code>this</code> for easy method chaining 318 */ 319 public EnrollmentResponse setIdentifier(List<Identifier> theIdentifier) { 320 this.identifier = theIdentifier; 321 return this; 322 } 323 324 public boolean hasIdentifier() { 325 if (this.identifier == null) 326 return false; 327 for (Identifier item : this.identifier) 328 if (!item.isEmpty()) 329 return true; 330 return false; 331 } 332 333 public Identifier addIdentifier() { // 3 334 Identifier t = new Identifier(); 335 if (this.identifier == null) 336 this.identifier = new ArrayList<Identifier>(); 337 this.identifier.add(t); 338 return t; 339 } 340 341 public EnrollmentResponse addIdentifier(Identifier t) { // 3 342 if (t == null) 343 return this; 344 if (this.identifier == null) 345 this.identifier = new ArrayList<Identifier>(); 346 this.identifier.add(t); 347 return this; 348 } 349 350 /** 351 * @return The first repetition of repeating field {@link #identifier}, creating 352 * it if it does not already exist 353 */ 354 public Identifier getIdentifierFirstRep() { 355 if (getIdentifier().isEmpty()) { 356 addIdentifier(); 357 } 358 return getIdentifier().get(0); 359 } 360 361 /** 362 * @return {@link #status} (The status of the resource instance.). This is the 363 * underlying object with id, value and extensions. The accessor 364 * "getStatus" gives direct access to the value 365 */ 366 public Enumeration<EnrollmentResponseStatus> getStatusElement() { 367 if (this.status == null) 368 if (Configuration.errorOnAutoCreate()) 369 throw new Error("Attempt to auto-create EnrollmentResponse.status"); 370 else if (Configuration.doAutoCreate()) 371 this.status = new Enumeration<EnrollmentResponseStatus>(new EnrollmentResponseStatusEnumFactory()); // bb 372 return this.status; 373 } 374 375 public boolean hasStatusElement() { 376 return this.status != null && !this.status.isEmpty(); 377 } 378 379 public boolean hasStatus() { 380 return this.status != null && !this.status.isEmpty(); 381 } 382 383 /** 384 * @param value {@link #status} (The status of the resource instance.). This is 385 * the underlying object with id, value and extensions. The 386 * accessor "getStatus" gives direct access to the value 387 */ 388 public EnrollmentResponse setStatusElement(Enumeration<EnrollmentResponseStatus> value) { 389 this.status = value; 390 return this; 391 } 392 393 /** 394 * @return The status of the resource instance. 395 */ 396 public EnrollmentResponseStatus getStatus() { 397 return this.status == null ? null : this.status.getValue(); 398 } 399 400 /** 401 * @param value The status of the resource instance. 402 */ 403 public EnrollmentResponse setStatus(EnrollmentResponseStatus value) { 404 if (value == null) 405 this.status = null; 406 else { 407 if (this.status == null) 408 this.status = new Enumeration<EnrollmentResponseStatus>(new EnrollmentResponseStatusEnumFactory()); 409 this.status.setValue(value); 410 } 411 return this; 412 } 413 414 /** 415 * @return {@link #request} (Original request resource reference.) 416 */ 417 public Reference getRequest() { 418 if (this.request == null) 419 if (Configuration.errorOnAutoCreate()) 420 throw new Error("Attempt to auto-create EnrollmentResponse.request"); 421 else if (Configuration.doAutoCreate()) 422 this.request = new Reference(); // cc 423 return this.request; 424 } 425 426 public boolean hasRequest() { 427 return this.request != null && !this.request.isEmpty(); 428 } 429 430 /** 431 * @param value {@link #request} (Original request resource reference.) 432 */ 433 public EnrollmentResponse setRequest(Reference value) { 434 this.request = value; 435 return this; 436 } 437 438 /** 439 * @return {@link #request} The actual object that is the target of the 440 * reference. The reference library doesn't populate this, but you can 441 * use it to hold the resource if you resolve it. (Original request 442 * resource reference.) 443 */ 444 public EnrollmentRequest getRequestTarget() { 445 if (this.requestTarget == null) 446 if (Configuration.errorOnAutoCreate()) 447 throw new Error("Attempt to auto-create EnrollmentResponse.request"); 448 else if (Configuration.doAutoCreate()) 449 this.requestTarget = new EnrollmentRequest(); // aa 450 return this.requestTarget; 451 } 452 453 /** 454 * @param value {@link #request} The actual object that is the target of the 455 * reference. The reference library doesn't use these, but you can 456 * use it to hold the resource if you resolve it. (Original request 457 * resource reference.) 458 */ 459 public EnrollmentResponse setRequestTarget(EnrollmentRequest value) { 460 this.requestTarget = value; 461 return this; 462 } 463 464 /** 465 * @return {@link #outcome} (Processing status: error, complete.). This is the 466 * underlying object with id, value and extensions. The accessor 467 * "getOutcome" gives direct access to the value 468 */ 469 public Enumeration<RemittanceOutcome> getOutcomeElement() { 470 if (this.outcome == null) 471 if (Configuration.errorOnAutoCreate()) 472 throw new Error("Attempt to auto-create EnrollmentResponse.outcome"); 473 else if (Configuration.doAutoCreate()) 474 this.outcome = new Enumeration<RemittanceOutcome>(new RemittanceOutcomeEnumFactory()); // bb 475 return this.outcome; 476 } 477 478 public boolean hasOutcomeElement() { 479 return this.outcome != null && !this.outcome.isEmpty(); 480 } 481 482 public boolean hasOutcome() { 483 return this.outcome != null && !this.outcome.isEmpty(); 484 } 485 486 /** 487 * @param value {@link #outcome} (Processing status: error, complete.). This is 488 * the underlying object with id, value and extensions. The 489 * accessor "getOutcome" gives direct access to the value 490 */ 491 public EnrollmentResponse setOutcomeElement(Enumeration<RemittanceOutcome> value) { 492 this.outcome = value; 493 return this; 494 } 495 496 /** 497 * @return Processing status: error, complete. 498 */ 499 public RemittanceOutcome getOutcome() { 500 return this.outcome == null ? null : this.outcome.getValue(); 501 } 502 503 /** 504 * @param value Processing status: error, complete. 505 */ 506 public EnrollmentResponse setOutcome(RemittanceOutcome value) { 507 if (value == null) 508 this.outcome = null; 509 else { 510 if (this.outcome == null) 511 this.outcome = new Enumeration<RemittanceOutcome>(new RemittanceOutcomeEnumFactory()); 512 this.outcome.setValue(value); 513 } 514 return this; 515 } 516 517 /** 518 * @return {@link #disposition} (A description of the status of the 519 * adjudication.). This is the underlying object with id, value and 520 * extensions. The accessor "getDisposition" gives direct access to the 521 * value 522 */ 523 public StringType getDispositionElement() { 524 if (this.disposition == null) 525 if (Configuration.errorOnAutoCreate()) 526 throw new Error("Attempt to auto-create EnrollmentResponse.disposition"); 527 else if (Configuration.doAutoCreate()) 528 this.disposition = new StringType(); // bb 529 return this.disposition; 530 } 531 532 public boolean hasDispositionElement() { 533 return this.disposition != null && !this.disposition.isEmpty(); 534 } 535 536 public boolean hasDisposition() { 537 return this.disposition != null && !this.disposition.isEmpty(); 538 } 539 540 /** 541 * @param value {@link #disposition} (A description of the status of the 542 * adjudication.). This is the underlying object with id, value and 543 * extensions. The accessor "getDisposition" gives direct access to 544 * the value 545 */ 546 public EnrollmentResponse setDispositionElement(StringType value) { 547 this.disposition = value; 548 return this; 549 } 550 551 /** 552 * @return A description of the status of the adjudication. 553 */ 554 public String getDisposition() { 555 return this.disposition == null ? null : this.disposition.getValue(); 556 } 557 558 /** 559 * @param value A description of the status of the adjudication. 560 */ 561 public EnrollmentResponse setDisposition(String value) { 562 if (Utilities.noString(value)) 563 this.disposition = null; 564 else { 565 if (this.disposition == null) 566 this.disposition = new StringType(); 567 this.disposition.setValue(value); 568 } 569 return this; 570 } 571 572 /** 573 * @return {@link #created} (The date when the enclosed suite of services were 574 * performed or completed.). This is the underlying object with id, 575 * value and extensions. The accessor "getCreated" gives direct access 576 * to the value 577 */ 578 public DateTimeType getCreatedElement() { 579 if (this.created == null) 580 if (Configuration.errorOnAutoCreate()) 581 throw new Error("Attempt to auto-create EnrollmentResponse.created"); 582 else if (Configuration.doAutoCreate()) 583 this.created = new DateTimeType(); // bb 584 return this.created; 585 } 586 587 public boolean hasCreatedElement() { 588 return this.created != null && !this.created.isEmpty(); 589 } 590 591 public boolean hasCreated() { 592 return this.created != null && !this.created.isEmpty(); 593 } 594 595 /** 596 * @param value {@link #created} (The date when the enclosed suite of services 597 * were performed or completed.). This is the underlying object 598 * with id, value and extensions. The accessor "getCreated" gives 599 * direct access to the value 600 */ 601 public EnrollmentResponse setCreatedElement(DateTimeType value) { 602 this.created = value; 603 return this; 604 } 605 606 /** 607 * @return The date when the enclosed suite of services were performed or 608 * completed. 609 */ 610 public Date getCreated() { 611 return this.created == null ? null : this.created.getValue(); 612 } 613 614 /** 615 * @param value The date when the enclosed suite of services were performed or 616 * completed. 617 */ 618 public EnrollmentResponse setCreated(Date value) { 619 if (value == null) 620 this.created = null; 621 else { 622 if (this.created == null) 623 this.created = new DateTimeType(); 624 this.created.setValue(value); 625 } 626 return this; 627 } 628 629 /** 630 * @return {@link #organization} (The Insurer who produced this adjudicated 631 * response.) 632 */ 633 public Reference getOrganization() { 634 if (this.organization == null) 635 if (Configuration.errorOnAutoCreate()) 636 throw new Error("Attempt to auto-create EnrollmentResponse.organization"); 637 else if (Configuration.doAutoCreate()) 638 this.organization = new Reference(); // cc 639 return this.organization; 640 } 641 642 public boolean hasOrganization() { 643 return this.organization != null && !this.organization.isEmpty(); 644 } 645 646 /** 647 * @param value {@link #organization} (The Insurer who produced this adjudicated 648 * response.) 649 */ 650 public EnrollmentResponse setOrganization(Reference value) { 651 this.organization = value; 652 return this; 653 } 654 655 /** 656 * @return {@link #organization} The actual object that is the target of the 657 * reference. The reference library doesn't populate this, but you can 658 * use it to hold the resource if you resolve it. (The Insurer who 659 * produced this adjudicated response.) 660 */ 661 public Organization getOrganizationTarget() { 662 if (this.organizationTarget == null) 663 if (Configuration.errorOnAutoCreate()) 664 throw new Error("Attempt to auto-create EnrollmentResponse.organization"); 665 else if (Configuration.doAutoCreate()) 666 this.organizationTarget = new Organization(); // aa 667 return this.organizationTarget; 668 } 669 670 /** 671 * @param value {@link #organization} The actual object that is the target of 672 * the reference. The reference library doesn't use these, but you 673 * can use it to hold the resource if you resolve it. (The Insurer 674 * who produced this adjudicated response.) 675 */ 676 public EnrollmentResponse setOrganizationTarget(Organization value) { 677 this.organizationTarget = value; 678 return this; 679 } 680 681 /** 682 * @return {@link #requestProvider} (The practitioner who is responsible for the 683 * services rendered to the patient.) 684 */ 685 public Reference getRequestProvider() { 686 if (this.requestProvider == null) 687 if (Configuration.errorOnAutoCreate()) 688 throw new Error("Attempt to auto-create EnrollmentResponse.requestProvider"); 689 else if (Configuration.doAutoCreate()) 690 this.requestProvider = new Reference(); // cc 691 return this.requestProvider; 692 } 693 694 public boolean hasRequestProvider() { 695 return this.requestProvider != null && !this.requestProvider.isEmpty(); 696 } 697 698 /** 699 * @param value {@link #requestProvider} (The practitioner who is responsible 700 * for the services rendered to the patient.) 701 */ 702 public EnrollmentResponse setRequestProvider(Reference value) { 703 this.requestProvider = value; 704 return this; 705 } 706 707 /** 708 * @return {@link #requestProvider} The actual object that is the target of the 709 * reference. The reference library doesn't populate this, but you can 710 * use it to hold the resource if you resolve it. (The practitioner who 711 * is responsible for the services rendered to the patient.) 712 */ 713 public Resource getRequestProviderTarget() { 714 return this.requestProviderTarget; 715 } 716 717 /** 718 * @param value {@link #requestProvider} The actual object that is the target of 719 * the reference. The reference library doesn't use these, but you 720 * can use it to hold the resource if you resolve it. (The 721 * practitioner who is responsible for the services rendered to the 722 * patient.) 723 */ 724 public EnrollmentResponse setRequestProviderTarget(Resource value) { 725 this.requestProviderTarget = value; 726 return this; 727 } 728 729 protected void listChildren(List<Property> children) { 730 super.listChildren(children); 731 children.add(new Property("identifier", "Identifier", "The Response business identifier.", 0, 732 java.lang.Integer.MAX_VALUE, identifier)); 733 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 734 children.add( 735 new Property("request", "Reference(EnrollmentRequest)", "Original request resource reference.", 0, 1, request)); 736 children.add(new Property("outcome", "code", "Processing status: error, complete.", 0, 1, outcome)); 737 children.add( 738 new Property("disposition", "string", "A description of the status of the adjudication.", 0, 1, disposition)); 739 children.add(new Property("created", "dateTime", 740 "The date when the enclosed suite of services were performed or completed.", 0, 1, created)); 741 children.add(new Property("organization", "Reference(Organization)", 742 "The Insurer who produced this adjudicated response.", 0, 1, organization)); 743 children.add(new Property("requestProvider", "Reference(Practitioner|PractitionerRole|Organization)", 744 "The practitioner who is responsible for the services rendered to the patient.", 0, 1, requestProvider)); 745 } 746 747 @Override 748 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 749 switch (_hash) { 750 case -1618432855: 751 /* identifier */ return new Property("identifier", "Identifier", "The Response business identifier.", 0, 752 java.lang.Integer.MAX_VALUE, identifier); 753 case -892481550: 754 /* status */ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 755 case 1095692943: 756 /* request */ return new Property("request", "Reference(EnrollmentRequest)", 757 "Original request resource reference.", 0, 1, request); 758 case -1106507950: 759 /* outcome */ return new Property("outcome", "code", "Processing status: error, complete.", 0, 1, outcome); 760 case 583380919: 761 /* disposition */ return new Property("disposition", "string", "A description of the status of the adjudication.", 762 0, 1, disposition); 763 case 1028554472: 764 /* created */ return new Property("created", "dateTime", 765 "The date when the enclosed suite of services were performed or completed.", 0, 1, created); 766 case 1178922291: 767 /* organization */ return new Property("organization", "Reference(Organization)", 768 "The Insurer who produced this adjudicated response.", 0, 1, organization); 769 case 1601527200: 770 /* requestProvider */ return new Property("requestProvider", 771 "Reference(Practitioner|PractitionerRole|Organization)", 772 "The practitioner who is responsible for the services rendered to the patient.", 0, 1, requestProvider); 773 default: 774 return super.getNamedProperty(_hash, _name, _checkValid); 775 } 776 777 } 778 779 @Override 780 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 781 switch (hash) { 782 case -1618432855: 783 /* identifier */ return this.identifier == null ? new Base[0] 784 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 785 case -892481550: 786 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<EnrollmentResponseStatus> 787 case 1095692943: 788 /* request */ return this.request == null ? new Base[0] : new Base[] { this.request }; // Reference 789 case -1106507950: 790 /* outcome */ return this.outcome == null ? new Base[0] : new Base[] { this.outcome }; // Enumeration<RemittanceOutcome> 791 case 583380919: 792 /* disposition */ return this.disposition == null ? new Base[0] : new Base[] { this.disposition }; // StringType 793 case 1028554472: 794 /* created */ return this.created == null ? new Base[0] : new Base[] { this.created }; // DateTimeType 795 case 1178922291: 796 /* organization */ return this.organization == null ? new Base[0] : new Base[] { this.organization }; // Reference 797 case 1601527200: 798 /* requestProvider */ return this.requestProvider == null ? new Base[0] : new Base[] { this.requestProvider }; // Reference 799 default: 800 return super.getProperty(hash, name, checkValid); 801 } 802 803 } 804 805 @Override 806 public Base setProperty(int hash, String name, Base value) throws FHIRException { 807 switch (hash) { 808 case -1618432855: // identifier 809 this.getIdentifier().add(castToIdentifier(value)); // Identifier 810 return value; 811 case -892481550: // status 812 value = new EnrollmentResponseStatusEnumFactory().fromType(castToCode(value)); 813 this.status = (Enumeration) value; // Enumeration<EnrollmentResponseStatus> 814 return value; 815 case 1095692943: // request 816 this.request = castToReference(value); // Reference 817 return value; 818 case -1106507950: // outcome 819 value = new RemittanceOutcomeEnumFactory().fromType(castToCode(value)); 820 this.outcome = (Enumeration) value; // Enumeration<RemittanceOutcome> 821 return value; 822 case 583380919: // disposition 823 this.disposition = castToString(value); // StringType 824 return value; 825 case 1028554472: // created 826 this.created = castToDateTime(value); // DateTimeType 827 return value; 828 case 1178922291: // organization 829 this.organization = castToReference(value); // Reference 830 return value; 831 case 1601527200: // requestProvider 832 this.requestProvider = castToReference(value); // Reference 833 return value; 834 default: 835 return super.setProperty(hash, name, value); 836 } 837 838 } 839 840 @Override 841 public Base setProperty(String name, Base value) throws FHIRException { 842 if (name.equals("identifier")) { 843 this.getIdentifier().add(castToIdentifier(value)); 844 } else if (name.equals("status")) { 845 value = new EnrollmentResponseStatusEnumFactory().fromType(castToCode(value)); 846 this.status = (Enumeration) value; // Enumeration<EnrollmentResponseStatus> 847 } else if (name.equals("request")) { 848 this.request = castToReference(value); // Reference 849 } else if (name.equals("outcome")) { 850 value = new RemittanceOutcomeEnumFactory().fromType(castToCode(value)); 851 this.outcome = (Enumeration) value; // Enumeration<RemittanceOutcome> 852 } else if (name.equals("disposition")) { 853 this.disposition = castToString(value); // StringType 854 } else if (name.equals("created")) { 855 this.created = castToDateTime(value); // DateTimeType 856 } else if (name.equals("organization")) { 857 this.organization = castToReference(value); // Reference 858 } else if (name.equals("requestProvider")) { 859 this.requestProvider = castToReference(value); // Reference 860 } else 861 return super.setProperty(name, value); 862 return value; 863 } 864 865 @Override 866 public void removeChild(String name, Base value) throws FHIRException { 867 if (name.equals("identifier")) { 868 this.getIdentifier().remove(castToIdentifier(value)); 869 } else if (name.equals("status")) { 870 this.status = null; 871 } else if (name.equals("request")) { 872 this.request = null; 873 } else if (name.equals("outcome")) { 874 this.outcome = null; 875 } else if (name.equals("disposition")) { 876 this.disposition = null; 877 } else if (name.equals("created")) { 878 this.created = null; 879 } else if (name.equals("organization")) { 880 this.organization = null; 881 } else if (name.equals("requestProvider")) { 882 this.requestProvider = null; 883 } else 884 super.removeChild(name, value); 885 886 } 887 888 @Override 889 public Base makeProperty(int hash, String name) throws FHIRException { 890 switch (hash) { 891 case -1618432855: 892 return addIdentifier(); 893 case -892481550: 894 return getStatusElement(); 895 case 1095692943: 896 return getRequest(); 897 case -1106507950: 898 return getOutcomeElement(); 899 case 583380919: 900 return getDispositionElement(); 901 case 1028554472: 902 return getCreatedElement(); 903 case 1178922291: 904 return getOrganization(); 905 case 1601527200: 906 return getRequestProvider(); 907 default: 908 return super.makeProperty(hash, name); 909 } 910 911 } 912 913 @Override 914 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 915 switch (hash) { 916 case -1618432855: 917 /* identifier */ return new String[] { "Identifier" }; 918 case -892481550: 919 /* status */ return new String[] { "code" }; 920 case 1095692943: 921 /* request */ return new String[] { "Reference" }; 922 case -1106507950: 923 /* outcome */ return new String[] { "code" }; 924 case 583380919: 925 /* disposition */ return new String[] { "string" }; 926 case 1028554472: 927 /* created */ return new String[] { "dateTime" }; 928 case 1178922291: 929 /* organization */ return new String[] { "Reference" }; 930 case 1601527200: 931 /* requestProvider */ return new String[] { "Reference" }; 932 default: 933 return super.getTypesForProperty(hash, name); 934 } 935 936 } 937 938 @Override 939 public Base addChild(String name) throws FHIRException { 940 if (name.equals("identifier")) { 941 return addIdentifier(); 942 } else if (name.equals("status")) { 943 throw new FHIRException("Cannot call addChild on a singleton property EnrollmentResponse.status"); 944 } else if (name.equals("request")) { 945 this.request = new Reference(); 946 return this.request; 947 } else if (name.equals("outcome")) { 948 throw new FHIRException("Cannot call addChild on a singleton property EnrollmentResponse.outcome"); 949 } else if (name.equals("disposition")) { 950 throw new FHIRException("Cannot call addChild on a singleton property EnrollmentResponse.disposition"); 951 } else if (name.equals("created")) { 952 throw new FHIRException("Cannot call addChild on a singleton property EnrollmentResponse.created"); 953 } else if (name.equals("organization")) { 954 this.organization = new Reference(); 955 return this.organization; 956 } else if (name.equals("requestProvider")) { 957 this.requestProvider = new Reference(); 958 return this.requestProvider; 959 } else 960 return super.addChild(name); 961 } 962 963 public String fhirType() { 964 return "EnrollmentResponse"; 965 966 } 967 968 public EnrollmentResponse copy() { 969 EnrollmentResponse dst = new EnrollmentResponse(); 970 copyValues(dst); 971 return dst; 972 } 973 974 public void copyValues(EnrollmentResponse dst) { 975 super.copyValues(dst); 976 if (identifier != null) { 977 dst.identifier = new ArrayList<Identifier>(); 978 for (Identifier i : identifier) 979 dst.identifier.add(i.copy()); 980 } 981 ; 982 dst.status = status == null ? null : status.copy(); 983 dst.request = request == null ? null : request.copy(); 984 dst.outcome = outcome == null ? null : outcome.copy(); 985 dst.disposition = disposition == null ? null : disposition.copy(); 986 dst.created = created == null ? null : created.copy(); 987 dst.organization = organization == null ? null : organization.copy(); 988 dst.requestProvider = requestProvider == null ? null : requestProvider.copy(); 989 } 990 991 protected EnrollmentResponse typedCopy() { 992 return copy(); 993 } 994 995 @Override 996 public boolean equalsDeep(Base other_) { 997 if (!super.equalsDeep(other_)) 998 return false; 999 if (!(other_ instanceof EnrollmentResponse)) 1000 return false; 1001 EnrollmentResponse o = (EnrollmentResponse) other_; 1002 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 1003 && compareDeep(request, o.request, true) && compareDeep(outcome, o.outcome, true) 1004 && compareDeep(disposition, o.disposition, true) && compareDeep(created, o.created, true) 1005 && compareDeep(organization, o.organization, true) && compareDeep(requestProvider, o.requestProvider, true); 1006 } 1007 1008 @Override 1009 public boolean equalsShallow(Base other_) { 1010 if (!super.equalsShallow(other_)) 1011 return false; 1012 if (!(other_ instanceof EnrollmentResponse)) 1013 return false; 1014 EnrollmentResponse o = (EnrollmentResponse) other_; 1015 return compareValues(status, o.status, true) && compareValues(outcome, o.outcome, true) 1016 && compareValues(disposition, o.disposition, true) && compareValues(created, o.created, true); 1017 } 1018 1019 public boolean isEmpty() { 1020 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, request, outcome, disposition, 1021 created, organization, requestProvider); 1022 } 1023 1024 @Override 1025 public ResourceType getResourceType() { 1026 return ResourceType.EnrollmentResponse; 1027 } 1028 1029 /** 1030 * Search parameter: <b>identifier</b> 1031 * <p> 1032 * Description: <b>The business identifier of the EnrollmentResponse</b><br> 1033 * Type: <b>token</b><br> 1034 * Path: <b>EnrollmentResponse.identifier</b><br> 1035 * </p> 1036 */ 1037 @SearchParamDefinition(name = "identifier", path = "EnrollmentResponse.identifier", description = "The business identifier of the EnrollmentResponse", type = "token") 1038 public static final String SP_IDENTIFIER = "identifier"; 1039 /** 1040 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1041 * <p> 1042 * Description: <b>The business identifier of the EnrollmentResponse</b><br> 1043 * Type: <b>token</b><br> 1044 * Path: <b>EnrollmentResponse.identifier</b><br> 1045 * </p> 1046 */ 1047 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1048 SP_IDENTIFIER); 1049 1050 /** 1051 * Search parameter: <b>request</b> 1052 * <p> 1053 * Description: <b>The reference to the claim</b><br> 1054 * Type: <b>reference</b><br> 1055 * Path: <b>EnrollmentResponse.request</b><br> 1056 * </p> 1057 */ 1058 @SearchParamDefinition(name = "request", path = "EnrollmentResponse.request", description = "The reference to the claim", type = "reference", target = { 1059 EnrollmentRequest.class }) 1060 public static final String SP_REQUEST = "request"; 1061 /** 1062 * <b>Fluent Client</b> search parameter constant for <b>request</b> 1063 * <p> 1064 * Description: <b>The reference to the claim</b><br> 1065 * Type: <b>reference</b><br> 1066 * Path: <b>EnrollmentResponse.request</b><br> 1067 * </p> 1068 */ 1069 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1070 SP_REQUEST); 1071 1072 /** 1073 * Constant for fluent queries to be used to add include statements. Specifies 1074 * the path value of "<b>EnrollmentResponse:request</b>". 1075 */ 1076 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST = new ca.uhn.fhir.model.api.Include( 1077 "EnrollmentResponse:request").toLocked(); 1078 1079 /** 1080 * Search parameter: <b>status</b> 1081 * <p> 1082 * Description: <b>The status of the enrollment response</b><br> 1083 * Type: <b>token</b><br> 1084 * Path: <b>EnrollmentResponse.status</b><br> 1085 * </p> 1086 */ 1087 @SearchParamDefinition(name = "status", path = "EnrollmentResponse.status", description = "The status of the enrollment response", type = "token") 1088 public static final String SP_STATUS = "status"; 1089 /** 1090 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1091 * <p> 1092 * Description: <b>The status of the enrollment response</b><br> 1093 * Type: <b>token</b><br> 1094 * Path: <b>EnrollmentResponse.status</b><br> 1095 * </p> 1096 */ 1097 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1098 SP_STATUS); 1099 1100}