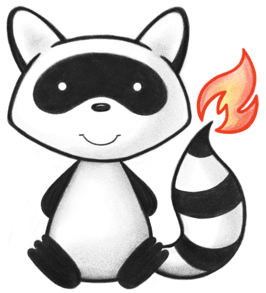
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import java.io.IOException; 033import java.io.ObjectInput; 034import java.io.ObjectOutput; 035import java.util.ArrayList; 036 037import org.hl7.fhir.instance.model.api.IBaseEnumeration; 038 039import ca.uhn.fhir.model.api.annotation.DatatypeDef; 040 041/* 042Copyright (c) 2011+, HL7, Inc 043All rights reserved. 044 045Redistribution and use in source and binary forms, with or without modification, 046are permitted provided that the following conditions are met: 047 048 * Redistributions of source code must retain the above copyright notice, this 049 list of conditions and the following disclaimer. 050 * Redistributions in binary form must reproduce the above copyright notice, 051 this list of conditions and the following disclaimer in the documentation 052 and/or other materials provided with the distribution. 053 * Neither the name of HL7 nor the names of its contributors may be used to 054 endorse or promote products derived from this software without specific 055 prior written permission. 056 057THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 058ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 059WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 060IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 061INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 062NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 063PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 064WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 065ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 066POSSIBILITY OF SUCH DAMAGE. 067 068*/ 069 070/** 071 * Primitive type "code" in FHIR, where the code is tied to an enumerated list 072 * of possible values 073 * 074 */ 075@DatatypeDef(name = "code", isSpecialization = true) 076public class Enumeration<T extends Enum<?>> extends PrimitiveType<T> implements IBaseEnumeration<T>, ICoding { 077 078 private static final long serialVersionUID = 1L; 079 private EnumFactory<T> myEnumFactory; 080 081 /** 082 * Constructor 083 * 084 * @deprecated This no-arg constructor is provided for serialization only - Do 085 * not use 086 */ 087 @Deprecated 088 public Enumeration() { 089 // nothing 090 } 091 092 /** 093 * Constructor 094 */ 095 public Enumeration(EnumFactory<T> theEnumFactory) { 096 if (theEnumFactory == null) 097 throw new IllegalArgumentException("An enumeration factory must be provided"); 098 myEnumFactory = theEnumFactory; 099 } 100 101 /** 102 * Constructor 103 */ 104 public Enumeration(EnumFactory<T> theEnumFactory, String theValue) { 105 if (theEnumFactory == null) 106 throw new IllegalArgumentException("An enumeration factory must be provided"); 107 myEnumFactory = theEnumFactory; 108 setValueAsString(theValue); 109 } 110 111 /** 112 * Constructor 113 */ 114 public Enumeration(EnumFactory<T> theEnumFactory, T theValue) { 115 if (theEnumFactory == null) 116 throw new IllegalArgumentException("An enumeration factory must be provided"); 117 myEnumFactory = theEnumFactory; 118 setValue(theValue); 119 } 120 121 /** 122 * Constructor 123 */ 124 public Enumeration(EnumFactory<T> theEnumFactory, T theValue, Element source) { 125 if (theEnumFactory == null) 126 throw new IllegalArgumentException("An enumeration factory must be provided"); 127 myEnumFactory = theEnumFactory; 128 setValue(theValue); 129 setId(source.getId()); 130 getExtension().addAll(source.getExtension()); 131 } 132 133 @Override 134 public Enumeration<T> copy() { 135 Enumeration dst = new Enumeration(this.myEnumFactory, (Enum) this.getValue()); 136 // Copy the Extension 137 if (extension != null) { 138 dst.extension = new ArrayList(); 139 for (Extension i : extension) 140 dst.extension.add(i.copy()); 141 } 142 ; 143 return dst; 144 } 145 146 @Override 147 protected String encode(T theValue) { 148 return myEnumFactory.toCode(theValue); 149 } 150 151 public String fhirType() { 152 return "code"; 153 } 154 155 /** 156 * Provides the enum factory which binds this enumeration to a specific ValueSet 157 */ 158 public EnumFactory<T> getEnumFactory() { 159 return myEnumFactory; 160 } 161 162 @Override 163 protected T parse(String theValue) { 164 if (myEnumFactory != null) { 165 return myEnumFactory.fromCode(theValue); 166 } 167 return null; 168 } 169 170 @SuppressWarnings("unchecked") 171 @Override 172 public void readExternal(ObjectInput theIn) throws IOException, ClassNotFoundException { 173 myEnumFactory = (EnumFactory<T>) theIn.readObject(); 174 super.readExternal(theIn); 175 } 176 177 public String toSystem() { 178 return getEnumFactory().toSystem(getValue()); 179 } 180 181 @Override 182 public void writeExternal(ObjectOutput theOut) throws IOException { 183 theOut.writeObject(myEnumFactory); 184 super.writeExternal(theOut); 185 } 186 187 @Override 188 public String getSystem() { 189 return myEnumFactory.toSystem(myEnumFactory.fromCode(asStringValue())); 190 } 191 192 @Override 193 public boolean hasSystem() { 194 return myEnumFactory.toSystem(myEnumFactory.fromCode(asStringValue())) != null; 195 } 196 197 @Override 198 public String getVersion() { 199 return null; 200 } 201 202 @Override 203 public boolean hasVersion() { 204 return false; 205 } 206 207 @Override 208 public boolean supportsVersion() { 209 return false; 210 } 211 212 @Override 213 public String getCode() { 214 return asStringValue(); 215 } 216 217 @Override 218 public boolean hasCode() { 219 return asStringValue() != null; 220 } 221 222 @Override 223 public String getDisplay() { 224 return null; 225 } 226 227 @Override 228 public boolean hasDisplay() { 229 return false; 230 } 231 232 @Override 233 public boolean supportsDisplay() { 234 return false; 235 } 236}