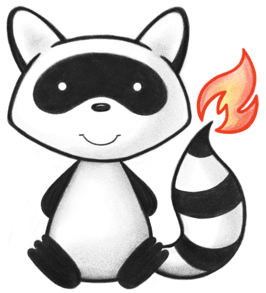
001package org.hl7.fhir.r4.model; 002 003import org.hl7.fhir.exceptions.FHIRException; 004import org.hl7.fhir.utilities.Utilities; 005 006public class Enumerations { 007 008// In here: 009// AbstractType: A list of the base types defined by this version of the FHIR specification - types that are defined, but for which only specializations actually are created. 010// AdministrativeGender: The gender of a person used for administrative purposes. 011// AgeUnits: A valueSet of UCUM codes for representing age value units. 012// BindingStrength: Indication of the degree of conformance expectations associated with a binding. 013// ConceptMapEquivalence: The degree of equivalence between concepts. 014// DataAbsentReason: Used to specify why the normally expected content of the data element is missing. 015// DataType: A version specific list of the data types defined by the FHIR specification for use as an element type (any of the FHIR defined data types). 016// DefinitionResourceType: A list of all the definition resource types defined in this version of the FHIR specification. 017// DocumentReferenceStatus: The status of the document reference. 018// EventResourceType: A list of all the event resource types defined in this version of the FHIR specification. 019// FHIRAllTypes: A list of all the concrete types defined in this version of the FHIR specification - Abstract Types, Data Types and Resource Types. 020// FHIRDefinedType: A list of all the concrete types defined in this version of the FHIR specification - Data Types and Resource Types. 021// FHIRVersion: All published FHIR Versions. 022// KnowledgeResourceType: A list of all the knowledge resource types defined in this version of the FHIR specification. 023// MessageEvent: One of the message events defined as part of this version of FHIR. 024// NoteType: The presentation types of notes. 025// PublicationStatus: The lifecycle status of an artifact. 026// RemittanceOutcome: The outcome of the processing. 027// RequestResourceType: A list of all the request resource types defined in this version of the FHIR specification. 028// ResourceType: One of the resource types defined as part of this version of FHIR. 029// SearchParamType: Data types allowed to be used for search parameters. 030// SpecialValues: A set of generally useful codes defined so they can be included in value sets. 031 032 public enum AbstractType { 033 /** 034 * A place holder that means any kind of data type 035 */ 036 TYPE, 037 /** 038 * A place holder that means any kind of resource 039 */ 040 ANY, 041 /** 042 * added to help the parsers 043 */ 044 NULL; 045 046 public static AbstractType fromCode(String codeString) throws FHIRException { 047 if (codeString == null || "".equals(codeString)) 048 return null; 049 if ("Type".equals(codeString)) 050 return TYPE; 051 if ("Any".equals(codeString)) 052 return ANY; 053 throw new FHIRException("Unknown AbstractType code '" + codeString + "'"); 054 } 055 056 public String toCode() { 057 switch (this) { 058 case TYPE: 059 return "Type"; 060 case ANY: 061 return "Any"; 062 case NULL: 063 return null; 064 default: 065 return "?"; 066 } 067 } 068 069 public String getSystem() { 070 switch (this) { 071 case TYPE: 072 return "http://hl7.org/fhir/abstract-types"; 073 case ANY: 074 return "http://hl7.org/fhir/abstract-types"; 075 case NULL: 076 return null; 077 default: 078 return "?"; 079 } 080 } 081 082 public String getDefinition() { 083 switch (this) { 084 case TYPE: 085 return "A place holder that means any kind of data type"; 086 case ANY: 087 return "A place holder that means any kind of resource"; 088 case NULL: 089 return null; 090 default: 091 return "?"; 092 } 093 } 094 095 public String getDisplay() { 096 switch (this) { 097 case TYPE: 098 return "Type"; 099 case ANY: 100 return "Any"; 101 case NULL: 102 return null; 103 default: 104 return "?"; 105 } 106 } 107 } 108 109 public static class AbstractTypeEnumFactory implements EnumFactory<AbstractType> { 110 public AbstractType fromCode(String codeString) throws IllegalArgumentException { 111 if (codeString == null || "".equals(codeString)) 112 if (codeString == null || "".equals(codeString)) 113 return null; 114 if ("Type".equals(codeString)) 115 return AbstractType.TYPE; 116 if ("Any".equals(codeString)) 117 return AbstractType.ANY; 118 throw new IllegalArgumentException("Unknown AbstractType code '" + codeString + "'"); 119 } 120 121 public Enumeration<AbstractType> fromType(PrimitiveType<?> code) throws FHIRException { 122 if (code == null) 123 return null; 124 if (code.isEmpty()) 125 return new Enumeration<AbstractType>(this, AbstractType.NULL, code); 126 String codeString = code.asStringValue(); 127 if (codeString == null || "".equals(codeString)) 128 return new Enumeration<AbstractType>(this, AbstractType.NULL, code); 129 if ("Type".equals(codeString)) 130 return new Enumeration<AbstractType>(this, AbstractType.TYPE, code); 131 if ("Any".equals(codeString)) 132 return new Enumeration<AbstractType>(this, AbstractType.ANY, code); 133 throw new FHIRException("Unknown AbstractType code '" + codeString + "'"); 134 } 135 136 public String toCode(AbstractType code) { 137 if (code == AbstractType.NULL) 138 return null; 139 if (code == AbstractType.TYPE) 140 return "Type"; 141 if (code == AbstractType.ANY) 142 return "Any"; 143 return "?"; 144 } 145 146 public String toSystem(AbstractType code) { 147 return code.getSystem(); 148 } 149 } 150 151 public enum AdministrativeGender { 152 /** 153 * Male. 154 */ 155 MALE, 156 /** 157 * Female. 158 */ 159 FEMALE, 160 /** 161 * Other. 162 */ 163 OTHER, 164 /** 165 * Unknown. 166 */ 167 UNKNOWN, 168 /** 169 * added to help the parsers 170 */ 171 NULL; 172 173 public static AdministrativeGender fromCode(String codeString) throws FHIRException { 174 if (codeString == null || "".equals(codeString)) 175 return null; 176 if ("male".equals(codeString)) 177 return MALE; 178 if ("female".equals(codeString)) 179 return FEMALE; 180 if ("other".equals(codeString)) 181 return OTHER; 182 if ("unknown".equals(codeString)) 183 return UNKNOWN; 184 throw new FHIRException("Unknown AdministrativeGender code '" + codeString + "'"); 185 } 186 187 public String toCode() { 188 switch (this) { 189 case MALE: 190 return "male"; 191 case FEMALE: 192 return "female"; 193 case OTHER: 194 return "other"; 195 case UNKNOWN: 196 return "unknown"; 197 case NULL: 198 return null; 199 default: 200 return "?"; 201 } 202 } 203 204 public String getSystem() { 205 switch (this) { 206 case MALE: 207 return "http://hl7.org/fhir/administrative-gender"; 208 case FEMALE: 209 return "http://hl7.org/fhir/administrative-gender"; 210 case OTHER: 211 return "http://hl7.org/fhir/administrative-gender"; 212 case UNKNOWN: 213 return "http://hl7.org/fhir/administrative-gender"; 214 case NULL: 215 return null; 216 default: 217 return "?"; 218 } 219 } 220 221 public String getDefinition() { 222 switch (this) { 223 case MALE: 224 return "Male."; 225 case FEMALE: 226 return "Female."; 227 case OTHER: 228 return "Other."; 229 case UNKNOWN: 230 return "Unknown."; 231 case NULL: 232 return null; 233 default: 234 return "?"; 235 } 236 } 237 238 public String getDisplay() { 239 switch (this) { 240 case MALE: 241 return "Male"; 242 case FEMALE: 243 return "Female"; 244 case OTHER: 245 return "Other"; 246 case UNKNOWN: 247 return "Unknown"; 248 case NULL: 249 return null; 250 default: 251 return "?"; 252 } 253 } 254 } 255 256 public static class AdministrativeGenderEnumFactory implements EnumFactory<AdministrativeGender> { 257 public AdministrativeGender fromCode(String codeString) throws IllegalArgumentException { 258 if (codeString == null || "".equals(codeString)) 259 if (codeString == null || "".equals(codeString)) 260 return null; 261 if ("male".equals(codeString)) 262 return AdministrativeGender.MALE; 263 if ("female".equals(codeString)) 264 return AdministrativeGender.FEMALE; 265 if ("other".equals(codeString)) 266 return AdministrativeGender.OTHER; 267 if ("unknown".equals(codeString)) 268 return AdministrativeGender.UNKNOWN; 269 throw new IllegalArgumentException("Unknown AdministrativeGender code '" + codeString + "'"); 270 } 271 272 public Enumeration<AdministrativeGender> fromType(PrimitiveType<?> code) throws FHIRException { 273 if (code == null) 274 return null; 275 if (code.isEmpty()) 276 return new Enumeration<AdministrativeGender>(this, AdministrativeGender.NULL, code); 277 String codeString = code.asStringValue(); 278 if (codeString == null || "".equals(codeString)) 279 return new Enumeration<AdministrativeGender>(this, AdministrativeGender.NULL, code); 280 if ("male".equals(codeString)) 281 return new Enumeration<AdministrativeGender>(this, AdministrativeGender.MALE, code); 282 if ("female".equals(codeString)) 283 return new Enumeration<AdministrativeGender>(this, AdministrativeGender.FEMALE, code); 284 if ("other".equals(codeString)) 285 return new Enumeration<AdministrativeGender>(this, AdministrativeGender.OTHER, code); 286 if ("unknown".equals(codeString)) 287 return new Enumeration<AdministrativeGender>(this, AdministrativeGender.UNKNOWN, code); 288 throw new FHIRException("Unknown AdministrativeGender code '" + codeString + "'"); 289 } 290 291 public String toCode(AdministrativeGender code) { 292 if (code == AdministrativeGender.NULL) 293 return null; 294 if (code == AdministrativeGender.MALE) 295 return "male"; 296 if (code == AdministrativeGender.FEMALE) 297 return "female"; 298 if (code == AdministrativeGender.OTHER) 299 return "other"; 300 if (code == AdministrativeGender.UNKNOWN) 301 return "unknown"; 302 return "?"; 303 } 304 305 public String toSystem(AdministrativeGender code) { 306 return code.getSystem(); 307 } 308 } 309 310 public enum AgeUnits { 311 /** 312 * null 313 */ 314 MIN, 315 /** 316 * null 317 */ 318 H, 319 /** 320 * null 321 */ 322 D, 323 /** 324 * null 325 */ 326 WK, 327 /** 328 * null 329 */ 330 MO, 331 /** 332 * null 333 */ 334 A, 335 /** 336 * added to help the parsers 337 */ 338 NULL; 339 340 public static AgeUnits fromCode(String codeString) throws FHIRException { 341 if (codeString == null || "".equals(codeString)) 342 return null; 343 if ("min".equals(codeString)) 344 return MIN; 345 if ("h".equals(codeString)) 346 return H; 347 if ("d".equals(codeString)) 348 return D; 349 if ("wk".equals(codeString)) 350 return WK; 351 if ("mo".equals(codeString)) 352 return MO; 353 if ("a".equals(codeString)) 354 return A; 355 throw new FHIRException("Unknown AgeUnits code '" + codeString + "'"); 356 } 357 358 public String toCode() { 359 switch (this) { 360 case MIN: 361 return "min"; 362 case H: 363 return "h"; 364 case D: 365 return "d"; 366 case WK: 367 return "wk"; 368 case MO: 369 return "mo"; 370 case A: 371 return "a"; 372 case NULL: 373 return null; 374 default: 375 return "?"; 376 } 377 } 378 379 public String getSystem() { 380 switch (this) { 381 case MIN: 382 return "http://unitsofmeasure.org"; 383 case H: 384 return "http://unitsofmeasure.org"; 385 case D: 386 return "http://unitsofmeasure.org"; 387 case WK: 388 return "http://unitsofmeasure.org"; 389 case MO: 390 return "http://unitsofmeasure.org"; 391 case A: 392 return "http://unitsofmeasure.org"; 393 case NULL: 394 return null; 395 default: 396 return "?"; 397 } 398 } 399 400 public String getDefinition() { 401 switch (this) { 402 case MIN: 403 return ""; 404 case H: 405 return ""; 406 case D: 407 return ""; 408 case WK: 409 return ""; 410 case MO: 411 return ""; 412 case A: 413 return ""; 414 case NULL: 415 return null; 416 default: 417 return "?"; 418 } 419 } 420 421 public String getDisplay() { 422 switch (this) { 423 case MIN: 424 return "Minute"; 425 case H: 426 return "Hour"; 427 case D: 428 return "Day"; 429 case WK: 430 return "Week"; 431 case MO: 432 return "Month"; 433 case A: 434 return "Year"; 435 case NULL: 436 return null; 437 default: 438 return "?"; 439 } 440 } 441 } 442 443 public static class AgeUnitsEnumFactory implements EnumFactory<AgeUnits> { 444 public AgeUnits fromCode(String codeString) throws IllegalArgumentException { 445 if (codeString == null || "".equals(codeString)) 446 if (codeString == null || "".equals(codeString)) 447 return null; 448 if ("min".equals(codeString)) 449 return AgeUnits.MIN; 450 if ("h".equals(codeString)) 451 return AgeUnits.H; 452 if ("d".equals(codeString)) 453 return AgeUnits.D; 454 if ("wk".equals(codeString)) 455 return AgeUnits.WK; 456 if ("mo".equals(codeString)) 457 return AgeUnits.MO; 458 if ("a".equals(codeString)) 459 return AgeUnits.A; 460 throw new IllegalArgumentException("Unknown AgeUnits code '" + codeString + "'"); 461 } 462 463 public Enumeration<AgeUnits> fromType(PrimitiveType<?> code) throws FHIRException { 464 if (code == null) 465 return null; 466 if (code.isEmpty()) 467 return new Enumeration<AgeUnits>(this, AgeUnits.NULL, code); 468 String codeString = code.asStringValue(); 469 if (codeString == null || "".equals(codeString)) 470 return new Enumeration<AgeUnits>(this, AgeUnits.NULL, code); 471 if ("min".equals(codeString)) 472 return new Enumeration<AgeUnits>(this, AgeUnits.MIN, code); 473 if ("h".equals(codeString)) 474 return new Enumeration<AgeUnits>(this, AgeUnits.H, code); 475 if ("d".equals(codeString)) 476 return new Enumeration<AgeUnits>(this, AgeUnits.D, code); 477 if ("wk".equals(codeString)) 478 return new Enumeration<AgeUnits>(this, AgeUnits.WK, code); 479 if ("mo".equals(codeString)) 480 return new Enumeration<AgeUnits>(this, AgeUnits.MO, code); 481 if ("a".equals(codeString)) 482 return new Enumeration<AgeUnits>(this, AgeUnits.A, code); 483 throw new FHIRException("Unknown AgeUnits code '" + codeString + "'"); 484 } 485 486 public String toCode(AgeUnits code) { 487 if (code == AgeUnits.NULL) 488 return null; 489 if (code == AgeUnits.MIN) 490 return "min"; 491 if (code == AgeUnits.H) 492 return "h"; 493 if (code == AgeUnits.D) 494 return "d"; 495 if (code == AgeUnits.WK) 496 return "wk"; 497 if (code == AgeUnits.MO) 498 return "mo"; 499 if (code == AgeUnits.A) 500 return "a"; 501 return "?"; 502 } 503 504 public String toSystem(AgeUnits code) { 505 return code.getSystem(); 506 } 507 } 508 509 public enum BindingStrength { 510 /** 511 * To be conformant, the concept in this element SHALL be from the specified 512 * value set. 513 */ 514 REQUIRED, 515 /** 516 * To be conformant, the concept in this element SHALL be from the specified 517 * value set if any of the codes within the value set can apply to the concept 518 * being communicated. If the value set does not cover the concept (based on 519 * human review), alternate codings (or, data type allowing, text) may be 520 * included instead. 521 */ 522 EXTENSIBLE, 523 /** 524 * Instances are encouraged to draw from the specified codes for 525 * interoperability purposes but are not required to do so to be considered 526 * conformant. 527 */ 528 PREFERRED, 529 /** 530 * Instances are not expected or even encouraged to draw from the specified 531 * value set. The value set merely provides examples of the types of concepts 532 * intended to be included. 533 */ 534 EXAMPLE, 535 /** 536 * added to help the parsers 537 */ 538 NULL; 539 540 public static BindingStrength fromCode(String codeString) throws FHIRException { 541 if (codeString == null || "".equals(codeString)) 542 return null; 543 if ("required".equals(codeString)) 544 return REQUIRED; 545 if ("extensible".equals(codeString)) 546 return EXTENSIBLE; 547 if ("preferred".equals(codeString)) 548 return PREFERRED; 549 if ("example".equals(codeString)) 550 return EXAMPLE; 551 throw new FHIRException("Unknown BindingStrength code '" + codeString + "'"); 552 } 553 554 public String toCode() { 555 switch (this) { 556 case REQUIRED: 557 return "required"; 558 case EXTENSIBLE: 559 return "extensible"; 560 case PREFERRED: 561 return "preferred"; 562 case EXAMPLE: 563 return "example"; 564 case NULL: 565 return null; 566 default: 567 return "?"; 568 } 569 } 570 571 public String getSystem() { 572 switch (this) { 573 case REQUIRED: 574 return "http://hl7.org/fhir/binding-strength"; 575 case EXTENSIBLE: 576 return "http://hl7.org/fhir/binding-strength"; 577 case PREFERRED: 578 return "http://hl7.org/fhir/binding-strength"; 579 case EXAMPLE: 580 return "http://hl7.org/fhir/binding-strength"; 581 case NULL: 582 return null; 583 default: 584 return "?"; 585 } 586 } 587 588 public String getDefinition() { 589 switch (this) { 590 case REQUIRED: 591 return "To be conformant, the concept in this element SHALL be from the specified value set."; 592 case EXTENSIBLE: 593 return "To be conformant, the concept in this element SHALL be from the specified value set if any of the codes within the value set can apply to the concept being communicated. If the value set does not cover the concept (based on human review), alternate codings (or, data type allowing, text) may be included instead."; 594 case PREFERRED: 595 return "Instances are encouraged to draw from the specified codes for interoperability purposes but are not required to do so to be considered conformant."; 596 case EXAMPLE: 597 return "Instances are not expected or even encouraged to draw from the specified value set. The value set merely provides examples of the types of concepts intended to be included."; 598 case NULL: 599 return null; 600 default: 601 return "?"; 602 } 603 } 604 605 public String getDisplay() { 606 switch (this) { 607 case REQUIRED: 608 return "Required"; 609 case EXTENSIBLE: 610 return "Extensible"; 611 case PREFERRED: 612 return "Preferred"; 613 case EXAMPLE: 614 return "Example"; 615 case NULL: 616 return null; 617 default: 618 return "?"; 619 } 620 } 621 } 622 623 public static class BindingStrengthEnumFactory implements EnumFactory<BindingStrength> { 624 public BindingStrength fromCode(String codeString) throws IllegalArgumentException { 625 if (codeString == null || "".equals(codeString)) 626 if (codeString == null || "".equals(codeString)) 627 return null; 628 if ("required".equals(codeString)) 629 return BindingStrength.REQUIRED; 630 if ("extensible".equals(codeString)) 631 return BindingStrength.EXTENSIBLE; 632 if ("preferred".equals(codeString)) 633 return BindingStrength.PREFERRED; 634 if ("example".equals(codeString)) 635 return BindingStrength.EXAMPLE; 636 throw new IllegalArgumentException("Unknown BindingStrength code '" + codeString + "'"); 637 } 638 639 public Enumeration<BindingStrength> fromType(PrimitiveType<?> code) throws FHIRException { 640 if (code == null) 641 return null; 642 if (code.isEmpty()) 643 return new Enumeration<BindingStrength>(this, BindingStrength.NULL, code); 644 String codeString = code.asStringValue(); 645 if (codeString == null || "".equals(codeString)) 646 return new Enumeration<BindingStrength>(this, BindingStrength.NULL, code); 647 if ("required".equals(codeString)) 648 return new Enumeration<BindingStrength>(this, BindingStrength.REQUIRED, code); 649 if ("extensible".equals(codeString)) 650 return new Enumeration<BindingStrength>(this, BindingStrength.EXTENSIBLE, code); 651 if ("preferred".equals(codeString)) 652 return new Enumeration<BindingStrength>(this, BindingStrength.PREFERRED, code); 653 if ("example".equals(codeString)) 654 return new Enumeration<BindingStrength>(this, BindingStrength.EXAMPLE, code); 655 throw new FHIRException("Unknown BindingStrength code '" + codeString + "'"); 656 } 657 658 public String toCode(BindingStrength code) { 659 if (code == BindingStrength.NULL) 660 return null; 661 if (code == BindingStrength.REQUIRED) 662 return "required"; 663 if (code == BindingStrength.EXTENSIBLE) 664 return "extensible"; 665 if (code == BindingStrength.PREFERRED) 666 return "preferred"; 667 if (code == BindingStrength.EXAMPLE) 668 return "example"; 669 return "?"; 670 } 671 672 public String toSystem(BindingStrength code) { 673 return code.getSystem(); 674 } 675 } 676 677 public enum ConceptMapEquivalence { 678 /** 679 * The concepts are related to each other, and have at least some overlap in 680 * meaning, but the exact relationship is not known. 681 */ 682 RELATEDTO, 683 /** 684 * The definitions of the concepts mean the same thing (including when 685 * structural implications of meaning are considered) (i.e. extensionally 686 * identical). 687 */ 688 EQUIVALENT, 689 /** 690 * The definitions of the concepts are exactly the same (i.e. only grammatical 691 * differences) and structural implications of meaning are identical or 692 * irrelevant (i.e. intentionally identical). 693 */ 694 EQUAL, 695 /** 696 * The target mapping is wider in meaning than the source concept. 697 */ 698 WIDER, 699 /** 700 * The target mapping subsumes the meaning of the source concept (e.g. the 701 * source is-a target). 702 */ 703 SUBSUMES, 704 /** 705 * The target mapping is narrower in meaning than the source concept. The sense 706 * in which the mapping is narrower SHALL be described in the comments in this 707 * case, and applications should be careful when attempting to use these 708 * mappings operationally. 709 */ 710 NARROWER, 711 /** 712 * The target mapping specializes the meaning of the source concept (e.g. the 713 * target is-a source). 714 */ 715 SPECIALIZES, 716 /** 717 * The target mapping overlaps with the source concept, but both source and 718 * target cover additional meaning, or the definitions are imprecise and it is 719 * uncertain whether they have the same boundaries to their meaning. The sense 720 * in which the mapping is inexact SHALL be described in the comments in this 721 * case, and applications should be careful when attempting to use these 722 * mappings operationally. 723 */ 724 INEXACT, 725 /** 726 * There is no match for this concept in the target code system. 727 */ 728 UNMATCHED, 729 /** 730 * This is an explicit assertion that there is no mapping between the source and 731 * target concept. 732 */ 733 DISJOINT, 734 /** 735 * added to help the parsers 736 */ 737 NULL; 738 739 public static ConceptMapEquivalence fromCode(String codeString) throws FHIRException { 740 if (codeString == null || "".equals(codeString)) 741 return null; 742 if ("relatedto".equals(codeString)) 743 return RELATEDTO; 744 if ("equivalent".equals(codeString)) 745 return EQUIVALENT; 746 if ("equal".equals(codeString)) 747 return EQUAL; 748 if ("wider".equals(codeString)) 749 return WIDER; 750 if ("subsumes".equals(codeString)) 751 return SUBSUMES; 752 if ("narrower".equals(codeString)) 753 return NARROWER; 754 if ("specializes".equals(codeString)) 755 return SPECIALIZES; 756 if ("inexact".equals(codeString)) 757 return INEXACT; 758 if ("unmatched".equals(codeString)) 759 return UNMATCHED; 760 if ("disjoint".equals(codeString)) 761 return DISJOINT; 762 throw new FHIRException("Unknown ConceptMapEquivalence code '" + codeString + "'"); 763 } 764 765 public String toCode() { 766 switch (this) { 767 case RELATEDTO: 768 return "relatedto"; 769 case EQUIVALENT: 770 return "equivalent"; 771 case EQUAL: 772 return "equal"; 773 case WIDER: 774 return "wider"; 775 case SUBSUMES: 776 return "subsumes"; 777 case NARROWER: 778 return "narrower"; 779 case SPECIALIZES: 780 return "specializes"; 781 case INEXACT: 782 return "inexact"; 783 case UNMATCHED: 784 return "unmatched"; 785 case DISJOINT: 786 return "disjoint"; 787 case NULL: 788 return null; 789 default: 790 return "?"; 791 } 792 } 793 794 public String getSystem() { 795 switch (this) { 796 case RELATEDTO: 797 return "http://hl7.org/fhir/concept-map-equivalence"; 798 case EQUIVALENT: 799 return "http://hl7.org/fhir/concept-map-equivalence"; 800 case EQUAL: 801 return "http://hl7.org/fhir/concept-map-equivalence"; 802 case WIDER: 803 return "http://hl7.org/fhir/concept-map-equivalence"; 804 case SUBSUMES: 805 return "http://hl7.org/fhir/concept-map-equivalence"; 806 case NARROWER: 807 return "http://hl7.org/fhir/concept-map-equivalence"; 808 case SPECIALIZES: 809 return "http://hl7.org/fhir/concept-map-equivalence"; 810 case INEXACT: 811 return "http://hl7.org/fhir/concept-map-equivalence"; 812 case UNMATCHED: 813 return "http://hl7.org/fhir/concept-map-equivalence"; 814 case DISJOINT: 815 return "http://hl7.org/fhir/concept-map-equivalence"; 816 case NULL: 817 return null; 818 default: 819 return "?"; 820 } 821 } 822 823 public String getDefinition() { 824 switch (this) { 825 case RELATEDTO: 826 return "The concepts are related to each other, and have at least some overlap in meaning, but the exact relationship is not known."; 827 case EQUIVALENT: 828 return "The definitions of the concepts mean the same thing (including when structural implications of meaning are considered) (i.e. extensionally identical)."; 829 case EQUAL: 830 return "The definitions of the concepts are exactly the same (i.e. only grammatical differences) and structural implications of meaning are identical or irrelevant (i.e. intentionally identical)."; 831 case WIDER: 832 return "The target mapping is wider in meaning than the source concept."; 833 case SUBSUMES: 834 return "The target mapping subsumes the meaning of the source concept (e.g. the source is-a target)."; 835 case NARROWER: 836 return "The target mapping is narrower in meaning than the source concept. The sense in which the mapping is narrower SHALL be described in the comments in this case, and applications should be careful when attempting to use these mappings operationally."; 837 case SPECIALIZES: 838 return "The target mapping specializes the meaning of the source concept (e.g. the target is-a source)."; 839 case INEXACT: 840 return "The target mapping overlaps with the source concept, but both source and target cover additional meaning, or the definitions are imprecise and it is uncertain whether they have the same boundaries to their meaning. The sense in which the mapping is inexact SHALL be described in the comments in this case, and applications should be careful when attempting to use these mappings operationally."; 841 case UNMATCHED: 842 return "There is no match for this concept in the target code system."; 843 case DISJOINT: 844 return "This is an explicit assertion that there is no mapping between the source and target concept."; 845 case NULL: 846 return null; 847 default: 848 return "?"; 849 } 850 } 851 852 public String getDisplay() { 853 switch (this) { 854 case RELATEDTO: 855 return "Related To"; 856 case EQUIVALENT: 857 return "Equivalent"; 858 case EQUAL: 859 return "Equal"; 860 case WIDER: 861 return "Wider"; 862 case SUBSUMES: 863 return "Subsumes"; 864 case NARROWER: 865 return "Narrower"; 866 case SPECIALIZES: 867 return "Specializes"; 868 case INEXACT: 869 return "Inexact"; 870 case UNMATCHED: 871 return "Unmatched"; 872 case DISJOINT: 873 return "Disjoint"; 874 case NULL: 875 return null; 876 default: 877 return "?"; 878 } 879 } 880 } 881 882 public static class ConceptMapEquivalenceEnumFactory implements EnumFactory<ConceptMapEquivalence> { 883 public ConceptMapEquivalence fromCode(String codeString) throws IllegalArgumentException { 884 if (codeString == null || "".equals(codeString)) 885 if (codeString == null || "".equals(codeString)) 886 return null; 887 if ("relatedto".equals(codeString)) 888 return ConceptMapEquivalence.RELATEDTO; 889 if ("equivalent".equals(codeString)) 890 return ConceptMapEquivalence.EQUIVALENT; 891 if ("equal".equals(codeString)) 892 return ConceptMapEquivalence.EQUAL; 893 if ("wider".equals(codeString)) 894 return ConceptMapEquivalence.WIDER; 895 if ("subsumes".equals(codeString)) 896 return ConceptMapEquivalence.SUBSUMES; 897 if ("narrower".equals(codeString)) 898 return ConceptMapEquivalence.NARROWER; 899 if ("specializes".equals(codeString)) 900 return ConceptMapEquivalence.SPECIALIZES; 901 if ("inexact".equals(codeString)) 902 return ConceptMapEquivalence.INEXACT; 903 if ("unmatched".equals(codeString)) 904 return ConceptMapEquivalence.UNMATCHED; 905 if ("disjoint".equals(codeString)) 906 return ConceptMapEquivalence.DISJOINT; 907 throw new IllegalArgumentException("Unknown ConceptMapEquivalence code '" + codeString + "'"); 908 } 909 910 public Enumeration<ConceptMapEquivalence> fromType(PrimitiveType<?> code) throws FHIRException { 911 if (code == null) 912 return null; 913 if (code.isEmpty()) 914 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.NULL, code); 915 String codeString = code.asStringValue(); 916 if (codeString == null || "".equals(codeString)) 917 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.NULL, code); 918 if ("relatedto".equals(codeString)) 919 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.RELATEDTO, code); 920 if ("equivalent".equals(codeString)) 921 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.EQUIVALENT, code); 922 if ("equal".equals(codeString)) 923 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.EQUAL, code); 924 if ("wider".equals(codeString)) 925 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.WIDER, code); 926 if ("subsumes".equals(codeString)) 927 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.SUBSUMES, code); 928 if ("narrower".equals(codeString)) 929 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.NARROWER, code); 930 if ("specializes".equals(codeString)) 931 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.SPECIALIZES, code); 932 if ("inexact".equals(codeString)) 933 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.INEXACT, code); 934 if ("unmatched".equals(codeString)) 935 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.UNMATCHED, code); 936 if ("disjoint".equals(codeString)) 937 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.DISJOINT, code); 938 throw new FHIRException("Unknown ConceptMapEquivalence code '" + codeString + "'"); 939 } 940 941 public String toCode(ConceptMapEquivalence code) { 942 if (code == ConceptMapEquivalence.NULL) 943 return null; 944 if (code == ConceptMapEquivalence.RELATEDTO) 945 return "relatedto"; 946 if (code == ConceptMapEquivalence.EQUIVALENT) 947 return "equivalent"; 948 if (code == ConceptMapEquivalence.EQUAL) 949 return "equal"; 950 if (code == ConceptMapEquivalence.WIDER) 951 return "wider"; 952 if (code == ConceptMapEquivalence.SUBSUMES) 953 return "subsumes"; 954 if (code == ConceptMapEquivalence.NARROWER) 955 return "narrower"; 956 if (code == ConceptMapEquivalence.SPECIALIZES) 957 return "specializes"; 958 if (code == ConceptMapEquivalence.INEXACT) 959 return "inexact"; 960 if (code == ConceptMapEquivalence.UNMATCHED) 961 return "unmatched"; 962 if (code == ConceptMapEquivalence.DISJOINT) 963 return "disjoint"; 964 return "?"; 965 } 966 967 public String toSystem(ConceptMapEquivalence code) { 968 return code.getSystem(); 969 } 970 } 971 972 public enum DataAbsentReason { 973 /** 974 * The value is expected to exist but is not known. 975 */ 976 UNKNOWN, 977 /** 978 * The source was asked but does not know the value. 979 */ 980 ASKEDUNKNOWN, 981 /** 982 * There is reason to expect (from the workflow) that the value may become 983 * known. 984 */ 985 TEMPUNKNOWN, 986 /** 987 * The workflow didn't lead to this value being known. 988 */ 989 NOTASKED, 990 /** 991 * The source was asked but declined to answer. 992 */ 993 ASKEDDECLINED, 994 /** 995 * The information is not available due to security, privacy or related reasons. 996 */ 997 MASKED, 998 /** 999 * There is no proper value for this element (e.g. last menstrual period for a 1000 * male). 1001 */ 1002 NOTAPPLICABLE, 1003 /** 1004 * The source system wasn't capable of supporting this element. 1005 */ 1006 UNSUPPORTED, 1007 /** 1008 * The content of the data is represented in the resource narrative. 1009 */ 1010 ASTEXT, 1011 /** 1012 * Some system or workflow process error means that the information is not 1013 * available. 1014 */ 1015 ERROR, 1016 /** 1017 * The numeric value is undefined or unrepresentable due to a floating point 1018 * processing error. 1019 */ 1020 NOTANUMBER, 1021 /** 1022 * The numeric value is excessively low and unrepresentable due to a floating 1023 * point processing error. 1024 */ 1025 NEGATIVEINFINITY, 1026 /** 1027 * The numeric value is excessively high and unrepresentable due to a floating 1028 * point processing error. 1029 */ 1030 POSITIVEINFINITY, 1031 /** 1032 * The value is not available because the observation procedure (test, etc.) was 1033 * not performed. 1034 */ 1035 NOTPERFORMED, 1036 /** 1037 * The value is not permitted in this context (e.g. due to profiles, or the base 1038 * data types). 1039 */ 1040 NOTPERMITTED, 1041 /** 1042 * added to help the parsers 1043 */ 1044 NULL; 1045 1046 public static DataAbsentReason fromCode(String codeString) throws FHIRException { 1047 if (codeString == null || "".equals(codeString)) 1048 return null; 1049 if ("unknown".equals(codeString)) 1050 return UNKNOWN; 1051 if ("asked-unknown".equals(codeString)) 1052 return ASKEDUNKNOWN; 1053 if ("temp-unknown".equals(codeString)) 1054 return TEMPUNKNOWN; 1055 if ("not-asked".equals(codeString)) 1056 return NOTASKED; 1057 if ("asked-declined".equals(codeString)) 1058 return ASKEDDECLINED; 1059 if ("masked".equals(codeString)) 1060 return MASKED; 1061 if ("not-applicable".equals(codeString)) 1062 return NOTAPPLICABLE; 1063 if ("unsupported".equals(codeString)) 1064 return UNSUPPORTED; 1065 if ("as-text".equals(codeString)) 1066 return ASTEXT; 1067 if ("error".equals(codeString)) 1068 return ERROR; 1069 if ("not-a-number".equals(codeString)) 1070 return NOTANUMBER; 1071 if ("negative-infinity".equals(codeString)) 1072 return NEGATIVEINFINITY; 1073 if ("positive-infinity".equals(codeString)) 1074 return POSITIVEINFINITY; 1075 if ("not-performed".equals(codeString)) 1076 return NOTPERFORMED; 1077 if ("not-permitted".equals(codeString)) 1078 return NOTPERMITTED; 1079 throw new FHIRException("Unknown DataAbsentReason code '" + codeString + "'"); 1080 } 1081 1082 public String toCode() { 1083 switch (this) { 1084 case UNKNOWN: 1085 return "unknown"; 1086 case ASKEDUNKNOWN: 1087 return "asked-unknown"; 1088 case TEMPUNKNOWN: 1089 return "temp-unknown"; 1090 case NOTASKED: 1091 return "not-asked"; 1092 case ASKEDDECLINED: 1093 return "asked-declined"; 1094 case MASKED: 1095 return "masked"; 1096 case NOTAPPLICABLE: 1097 return "not-applicable"; 1098 case UNSUPPORTED: 1099 return "unsupported"; 1100 case ASTEXT: 1101 return "as-text"; 1102 case ERROR: 1103 return "error"; 1104 case NOTANUMBER: 1105 return "not-a-number"; 1106 case NEGATIVEINFINITY: 1107 return "negative-infinity"; 1108 case POSITIVEINFINITY: 1109 return "positive-infinity"; 1110 case NOTPERFORMED: 1111 return "not-performed"; 1112 case NOTPERMITTED: 1113 return "not-permitted"; 1114 case NULL: 1115 return null; 1116 default: 1117 return "?"; 1118 } 1119 } 1120 1121 public String getSystem() { 1122 switch (this) { 1123 case UNKNOWN: 1124 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1125 case ASKEDUNKNOWN: 1126 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1127 case TEMPUNKNOWN: 1128 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1129 case NOTASKED: 1130 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1131 case ASKEDDECLINED: 1132 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1133 case MASKED: 1134 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1135 case NOTAPPLICABLE: 1136 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1137 case UNSUPPORTED: 1138 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1139 case ASTEXT: 1140 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1141 case ERROR: 1142 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1143 case NOTANUMBER: 1144 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1145 case NEGATIVEINFINITY: 1146 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1147 case POSITIVEINFINITY: 1148 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1149 case NOTPERFORMED: 1150 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1151 case NOTPERMITTED: 1152 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1153 case NULL: 1154 return null; 1155 default: 1156 return "?"; 1157 } 1158 } 1159 1160 public String getDefinition() { 1161 switch (this) { 1162 case UNKNOWN: 1163 return "The value is expected to exist but is not known."; 1164 case ASKEDUNKNOWN: 1165 return "The source was asked but does not know the value."; 1166 case TEMPUNKNOWN: 1167 return "There is reason to expect (from the workflow) that the value may become known."; 1168 case NOTASKED: 1169 return "The workflow didn't lead to this value being known."; 1170 case ASKEDDECLINED: 1171 return "The source was asked but declined to answer."; 1172 case MASKED: 1173 return "The information is not available due to security, privacy or related reasons."; 1174 case NOTAPPLICABLE: 1175 return "There is no proper value for this element (e.g. last menstrual period for a male)."; 1176 case UNSUPPORTED: 1177 return "The source system wasn't capable of supporting this element."; 1178 case ASTEXT: 1179 return "The content of the data is represented in the resource narrative."; 1180 case ERROR: 1181 return "Some system or workflow process error means that the information is not available."; 1182 case NOTANUMBER: 1183 return "The numeric value is undefined or unrepresentable due to a floating point processing error."; 1184 case NEGATIVEINFINITY: 1185 return "The numeric value is excessively low and unrepresentable due to a floating point processing error."; 1186 case POSITIVEINFINITY: 1187 return "The numeric value is excessively high and unrepresentable due to a floating point processing error."; 1188 case NOTPERFORMED: 1189 return "The value is not available because the observation procedure (test, etc.) was not performed."; 1190 case NOTPERMITTED: 1191 return "The value is not permitted in this context (e.g. due to profiles, or the base data types)."; 1192 case NULL: 1193 return null; 1194 default: 1195 return "?"; 1196 } 1197 } 1198 1199 public String getDisplay() { 1200 switch (this) { 1201 case UNKNOWN: 1202 return "Unknown"; 1203 case ASKEDUNKNOWN: 1204 return "Asked But Unknown"; 1205 case TEMPUNKNOWN: 1206 return "Temporarily Unknown"; 1207 case NOTASKED: 1208 return "Not Asked"; 1209 case ASKEDDECLINED: 1210 return "Asked But Declined"; 1211 case MASKED: 1212 return "Masked"; 1213 case NOTAPPLICABLE: 1214 return "Not Applicable"; 1215 case UNSUPPORTED: 1216 return "Unsupported"; 1217 case ASTEXT: 1218 return "As Text"; 1219 case ERROR: 1220 return "Error"; 1221 case NOTANUMBER: 1222 return "Not a Number (NaN)"; 1223 case NEGATIVEINFINITY: 1224 return "Negative Infinity (NINF)"; 1225 case POSITIVEINFINITY: 1226 return "Positive Infinity (PINF)"; 1227 case NOTPERFORMED: 1228 return "Not Performed"; 1229 case NOTPERMITTED: 1230 return "Not Permitted"; 1231 case NULL: 1232 return null; 1233 default: 1234 return "?"; 1235 } 1236 } 1237 } 1238 1239 public static class DataAbsentReasonEnumFactory implements EnumFactory<DataAbsentReason> { 1240 public DataAbsentReason fromCode(String codeString) throws IllegalArgumentException { 1241 if (codeString == null || "".equals(codeString)) 1242 if (codeString == null || "".equals(codeString)) 1243 return null; 1244 if ("unknown".equals(codeString)) 1245 return DataAbsentReason.UNKNOWN; 1246 if ("asked-unknown".equals(codeString)) 1247 return DataAbsentReason.ASKEDUNKNOWN; 1248 if ("temp-unknown".equals(codeString)) 1249 return DataAbsentReason.TEMPUNKNOWN; 1250 if ("not-asked".equals(codeString)) 1251 return DataAbsentReason.NOTASKED; 1252 if ("asked-declined".equals(codeString)) 1253 return DataAbsentReason.ASKEDDECLINED; 1254 if ("masked".equals(codeString)) 1255 return DataAbsentReason.MASKED; 1256 if ("not-applicable".equals(codeString)) 1257 return DataAbsentReason.NOTAPPLICABLE; 1258 if ("unsupported".equals(codeString)) 1259 return DataAbsentReason.UNSUPPORTED; 1260 if ("as-text".equals(codeString)) 1261 return DataAbsentReason.ASTEXT; 1262 if ("error".equals(codeString)) 1263 return DataAbsentReason.ERROR; 1264 if ("not-a-number".equals(codeString)) 1265 return DataAbsentReason.NOTANUMBER; 1266 if ("negative-infinity".equals(codeString)) 1267 return DataAbsentReason.NEGATIVEINFINITY; 1268 if ("positive-infinity".equals(codeString)) 1269 return DataAbsentReason.POSITIVEINFINITY; 1270 if ("not-performed".equals(codeString)) 1271 return DataAbsentReason.NOTPERFORMED; 1272 if ("not-permitted".equals(codeString)) 1273 return DataAbsentReason.NOTPERMITTED; 1274 throw new IllegalArgumentException("Unknown DataAbsentReason code '" + codeString + "'"); 1275 } 1276 1277 public Enumeration<DataAbsentReason> fromType(PrimitiveType<?> code) throws FHIRException { 1278 if (code == null) 1279 return null; 1280 if (code.isEmpty()) 1281 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.NULL, code); 1282 String codeString = code.asStringValue(); 1283 if (codeString == null || "".equals(codeString)) 1284 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.NULL, code); 1285 if ("unknown".equals(codeString)) 1286 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.UNKNOWN, code); 1287 if ("asked-unknown".equals(codeString)) 1288 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.ASKEDUNKNOWN, code); 1289 if ("temp-unknown".equals(codeString)) 1290 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.TEMPUNKNOWN, code); 1291 if ("not-asked".equals(codeString)) 1292 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.NOTASKED, code); 1293 if ("asked-declined".equals(codeString)) 1294 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.ASKEDDECLINED, code); 1295 if ("masked".equals(codeString)) 1296 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.MASKED, code); 1297 if ("not-applicable".equals(codeString)) 1298 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.NOTAPPLICABLE, code); 1299 if ("unsupported".equals(codeString)) 1300 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.UNSUPPORTED, code); 1301 if ("as-text".equals(codeString)) 1302 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.ASTEXT, code); 1303 if ("error".equals(codeString)) 1304 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.ERROR, code); 1305 if ("not-a-number".equals(codeString)) 1306 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.NOTANUMBER, code); 1307 if ("negative-infinity".equals(codeString)) 1308 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.NEGATIVEINFINITY, code); 1309 if ("positive-infinity".equals(codeString)) 1310 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.POSITIVEINFINITY, code); 1311 if ("not-performed".equals(codeString)) 1312 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.NOTPERFORMED, code); 1313 if ("not-permitted".equals(codeString)) 1314 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.NOTPERMITTED, code); 1315 throw new FHIRException("Unknown DataAbsentReason code '" + codeString + "'"); 1316 } 1317 1318 public String toCode(DataAbsentReason code) { 1319 if (code == DataAbsentReason.NULL) 1320 return null; 1321 if (code == DataAbsentReason.UNKNOWN) 1322 return "unknown"; 1323 if (code == DataAbsentReason.ASKEDUNKNOWN) 1324 return "asked-unknown"; 1325 if (code == DataAbsentReason.TEMPUNKNOWN) 1326 return "temp-unknown"; 1327 if (code == DataAbsentReason.NOTASKED) 1328 return "not-asked"; 1329 if (code == DataAbsentReason.ASKEDDECLINED) 1330 return "asked-declined"; 1331 if (code == DataAbsentReason.MASKED) 1332 return "masked"; 1333 if (code == DataAbsentReason.NOTAPPLICABLE) 1334 return "not-applicable"; 1335 if (code == DataAbsentReason.UNSUPPORTED) 1336 return "unsupported"; 1337 if (code == DataAbsentReason.ASTEXT) 1338 return "as-text"; 1339 if (code == DataAbsentReason.ERROR) 1340 return "error"; 1341 if (code == DataAbsentReason.NOTANUMBER) 1342 return "not-a-number"; 1343 if (code == DataAbsentReason.NEGATIVEINFINITY) 1344 return "negative-infinity"; 1345 if (code == DataAbsentReason.POSITIVEINFINITY) 1346 return "positive-infinity"; 1347 if (code == DataAbsentReason.NOTPERFORMED) 1348 return "not-performed"; 1349 if (code == DataAbsentReason.NOTPERMITTED) 1350 return "not-permitted"; 1351 return "?"; 1352 } 1353 1354 public String toSystem(DataAbsentReason code) { 1355 return code.getSystem(); 1356 } 1357 } 1358 1359 public enum DataType { 1360 /** 1361 * An address expressed using postal conventions (as opposed to GPS or other 1362 * location definition formats). This data type may be used to convey addresses 1363 * for use in delivering mail as well as for visiting locations which might not 1364 * be valid for mail delivery. There are a variety of postal address formats 1365 * defined around the world. 1366 */ 1367 ADDRESS, 1368 /** 1369 * A duration of time during which an organism (or a process) has existed. 1370 */ 1371 AGE, 1372 /** 1373 * A text note which also contains information about who made the statement and 1374 * when. 1375 */ 1376 ANNOTATION, 1377 /** 1378 * For referring to data content defined in other formats. 1379 */ 1380 ATTACHMENT, 1381 /** 1382 * Base definition for all elements that are defined inside a resource - but not 1383 * those in a data type. 1384 */ 1385 BACKBONEELEMENT, 1386 /** 1387 * A concept that may be defined by a formal reference to a terminology or 1388 * ontology or may be provided by text. 1389 */ 1390 CODEABLECONCEPT, 1391 /** 1392 * A reference to a code defined by a terminology system. 1393 */ 1394 CODING, 1395 /** 1396 * Specifies contact information for a person or organization. 1397 */ 1398 CONTACTDETAIL, 1399 /** 1400 * Details for all kinds of technology mediated contact points for a person or 1401 * organization, including telephone, email, etc. 1402 */ 1403 CONTACTPOINT, 1404 /** 1405 * A contributor to the content of a knowledge asset, including authors, 1406 * editors, reviewers, and endorsers. 1407 */ 1408 CONTRIBUTOR, 1409 /** 1410 * A measured amount (or an amount that can potentially be measured). Note that 1411 * measured amounts include amounts that are not precisely quantified, including 1412 * amounts involving arbitrary units and floating currencies. 1413 */ 1414 COUNT, 1415 /** 1416 * Describes a required data item for evaluation in terms of the type of data, 1417 * and optional code or date-based filters of the data. 1418 */ 1419 DATAREQUIREMENT, 1420 /** 1421 * A length - a value with a unit that is a physical distance. 1422 */ 1423 DISTANCE, 1424 /** 1425 * Indicates how the medication is/was taken or should be taken by the patient. 1426 */ 1427 DOSAGE, 1428 /** 1429 * A length of time. 1430 */ 1431 DURATION, 1432 /** 1433 * Base definition for all elements in a resource. 1434 */ 1435 ELEMENT, 1436 /** 1437 * Captures constraints on each element within the resource, profile, or 1438 * extension. 1439 */ 1440 ELEMENTDEFINITION, 1441 /** 1442 * A expression that is evaluated in a specified context and returns a value. 1443 * The context of use of the expression must specify the context in which the 1444 * expression is evaluated, and how the result of the expression is used. 1445 */ 1446 EXPRESSION, 1447 /** 1448 * Optional Extension Element - found in all resources. 1449 */ 1450 EXTENSION, 1451 /** 1452 * A human's name with the ability to identify parts and usage. 1453 */ 1454 HUMANNAME, 1455 /** 1456 * An identifier - identifies some entity uniquely and unambiguously. Typically 1457 * this is used for business identifiers. 1458 */ 1459 IDENTIFIER, 1460 /** 1461 * The marketing status describes the date when a medicinal product is actually 1462 * put on the market or the date as of which it is no longer available. 1463 */ 1464 MARKETINGSTATUS, 1465 /** 1466 * The metadata about a resource. This is content in the resource that is 1467 * maintained by the infrastructure. Changes to the content might not always be 1468 * associated with version changes to the resource. 1469 */ 1470 META, 1471 /** 1472 * An amount of economic utility in some recognized currency. 1473 */ 1474 MONEY, 1475 /** 1476 * null 1477 */ 1478 MONEYQUANTITY, 1479 /** 1480 * A human-readable summary of the resource conveying the essential clinical and 1481 * business information for the resource. 1482 */ 1483 NARRATIVE, 1484 /** 1485 * The parameters to the module. This collection specifies both the input and 1486 * output parameters. Input parameters are provided by the caller as part of the 1487 * $evaluate operation. Output parameters are included in the GuidanceResponse. 1488 */ 1489 PARAMETERDEFINITION, 1490 /** 1491 * A time period defined by a start and end date and optionally time. 1492 */ 1493 PERIOD, 1494 /** 1495 * A populatioof people with some set of grouping criteria. 1496 */ 1497 POPULATION, 1498 /** 1499 * The marketing status describes the date when a medicinal product is actually 1500 * put on the market or the date as of which it is no longer available. 1501 */ 1502 PRODCHARACTERISTIC, 1503 /** 1504 * The shelf-life and storage information for a medicinal product item or 1505 * container can be described using this class. 1506 */ 1507 PRODUCTSHELFLIFE, 1508 /** 1509 * A measured amount (or an amount that can potentially be measured). Note that 1510 * measured amounts include amounts that are not precisely quantified, including 1511 * amounts involving arbitrary units and floating currencies. 1512 */ 1513 QUANTITY, 1514 /** 1515 * A set of ordered Quantities defined by a low and high limit. 1516 */ 1517 RANGE, 1518 /** 1519 * A relationship of two Quantity values - expressed as a numerator and a 1520 * denominator. 1521 */ 1522 RATIO, 1523 /** 1524 * A reference from one resource to another. 1525 */ 1526 REFERENCE, 1527 /** 1528 * Related artifacts such as additional documentation, justification, or 1529 * bibliographic references. 1530 */ 1531 RELATEDARTIFACT, 1532 /** 1533 * A series of measurements taken by a device, with upper and lower limits. 1534 * There may be more than one dimension in the data. 1535 */ 1536 SAMPLEDDATA, 1537 /** 1538 * A signature along with supporting context. The signature may be a digital 1539 * signature that is cryptographic in nature, or some other signature acceptable 1540 * to the domain. This other signature may be as simple as a graphical image 1541 * representing a hand-written signature, or a signature ceremony Different 1542 * signature approaches have different utilities. 1543 */ 1544 SIGNATURE, 1545 /** 1546 * null 1547 */ 1548 SIMPLEQUANTITY, 1549 /** 1550 * Chemical substances are a single substance type whose primary defining 1551 * element is the molecular structure. Chemical substances shall be defined on 1552 * the basis of their complete covalent molecular structure; the presence of a 1553 * salt (counter-ion) and/or solvates (water, alcohols) is also captured. 1554 * Purity, grade, physical form or particle size are not taken into account in 1555 * the definition of a chemical substance or in the assignment of a Substance 1556 * ID. 1557 */ 1558 SUBSTANCEAMOUNT, 1559 /** 1560 * Specifies an event that may occur multiple times. Timing schedules are used 1561 * to record when things are planned, expected or requested to occur. The most 1562 * common usage is in dosage instructions for medications. They are also used 1563 * when planning care of various kinds, and may be used for reporting the 1564 * schedule to which past regular activities were carried out. 1565 */ 1566 TIMING, 1567 /** 1568 * A description of a triggering event. Triggering events can be named events, 1569 * data events, or periodic, as determined by the type element. 1570 */ 1571 TRIGGERDEFINITION, 1572 /** 1573 * Specifies clinical/business/etc. metadata that can be used to retrieve, index 1574 * and/or categorize an artifact. This metadata can either be specific to the 1575 * applicable population (e.g., age category, DRG) or the specific context of 1576 * care (e.g., venue, care setting, provider of care). 1577 */ 1578 USAGECONTEXT, 1579 /** 1580 * A stream of bytes 1581 */ 1582 BASE64BINARY, 1583 /** 1584 * Value of "true" or "false" 1585 */ 1586 BOOLEAN, 1587 /** 1588 * A URI that is a reference to a canonical URL on a FHIR resource 1589 */ 1590 CANONICAL, 1591 /** 1592 * A string which has at least one character and no leading or trailing 1593 * whitespace and where there is no whitespace other than single spaces in the 1594 * contents 1595 */ 1596 CODE, 1597 /** 1598 * A date or partial date (e.g. just year or year + month). There is no time 1599 * zone. The format is a union of the schema types gYear, gYearMonth and date. 1600 * Dates SHALL be valid dates. 1601 */ 1602 DATE, 1603 /** 1604 * A date, date-time or partial date (e.g. just year or year + month). If hours 1605 * and minutes are specified, a time zone SHALL be populated. The format is a 1606 * union of the schema types gYear, gYearMonth, date and dateTime. Seconds must 1607 * be provided due to schema type constraints but may be zero-filled and may be 1608 * ignored. Dates SHALL be valid dates. 1609 */ 1610 DATETIME, 1611 /** 1612 * A rational number with implicit precision 1613 */ 1614 DECIMAL, 1615 /** 1616 * Any combination of letters, numerals, "-" and ".", with a length limit of 64 1617 * characters. (This might be an integer, an unprefixed OID, UUID or any other 1618 * identifier pattern that meets these constraints.) Ids are case-insensitive. 1619 */ 1620 ID, 1621 /** 1622 * An instant in time - known at least to the second 1623 */ 1624 INSTANT, 1625 /** 1626 * A whole number 1627 */ 1628 INTEGER, 1629 /** 1630 * A string that may contain Github Flavored Markdown syntax for optional 1631 * processing by a mark down presentation engine 1632 */ 1633 MARKDOWN, 1634 /** 1635 * An OID represented as a URI 1636 */ 1637 OID, 1638 /** 1639 * An integer with a value that is positive (e.g. >0) 1640 */ 1641 POSITIVEINT, 1642 /** 1643 * A sequence of Unicode characters 1644 */ 1645 STRING, 1646 /** 1647 * A time during the day, with no date specified 1648 */ 1649 TIME, 1650 /** 1651 * An integer with a value that is not negative (e.g. >= 0) 1652 */ 1653 UNSIGNEDINT, 1654 /** 1655 * String of characters used to identify a name or a resource 1656 */ 1657 URI, 1658 /** 1659 * A URI that is a literal reference 1660 */ 1661 URL, 1662 /** 1663 * A UUID, represented as a URI 1664 */ 1665 UUID, 1666 /** 1667 * XHTML format, as defined by W3C, but restricted usage (mainly, no active 1668 * content) 1669 */ 1670 XHTML, 1671 /** 1672 * added to help the parsers 1673 */ 1674 NULL; 1675 1676 public static DataType fromCode(String codeString) throws FHIRException { 1677 if (codeString == null || "".equals(codeString)) 1678 return null; 1679 if ("Address".equals(codeString)) 1680 return ADDRESS; 1681 if ("Age".equals(codeString)) 1682 return AGE; 1683 if ("Annotation".equals(codeString)) 1684 return ANNOTATION; 1685 if ("Attachment".equals(codeString)) 1686 return ATTACHMENT; 1687 if ("BackboneElement".equals(codeString)) 1688 return BACKBONEELEMENT; 1689 if ("CodeableConcept".equals(codeString)) 1690 return CODEABLECONCEPT; 1691 if ("Coding".equals(codeString)) 1692 return CODING; 1693 if ("ContactDetail".equals(codeString)) 1694 return CONTACTDETAIL; 1695 if ("ContactPoint".equals(codeString)) 1696 return CONTACTPOINT; 1697 if ("Contributor".equals(codeString)) 1698 return CONTRIBUTOR; 1699 if ("Count".equals(codeString)) 1700 return COUNT; 1701 if ("DataRequirement".equals(codeString)) 1702 return DATAREQUIREMENT; 1703 if ("Distance".equals(codeString)) 1704 return DISTANCE; 1705 if ("Dosage".equals(codeString)) 1706 return DOSAGE; 1707 if ("Duration".equals(codeString)) 1708 return DURATION; 1709 if ("Element".equals(codeString)) 1710 return ELEMENT; 1711 if ("ElementDefinition".equals(codeString)) 1712 return ELEMENTDEFINITION; 1713 if ("Expression".equals(codeString)) 1714 return EXPRESSION; 1715 if ("Extension".equals(codeString)) 1716 return EXTENSION; 1717 if ("HumanName".equals(codeString)) 1718 return HUMANNAME; 1719 if ("Identifier".equals(codeString)) 1720 return IDENTIFIER; 1721 if ("MarketingStatus".equals(codeString)) 1722 return MARKETINGSTATUS; 1723 if ("Meta".equals(codeString)) 1724 return META; 1725 if ("Money".equals(codeString)) 1726 return MONEY; 1727 if ("MoneyQuantity".equals(codeString)) 1728 return MONEYQUANTITY; 1729 if ("Narrative".equals(codeString)) 1730 return NARRATIVE; 1731 if ("ParameterDefinition".equals(codeString)) 1732 return PARAMETERDEFINITION; 1733 if ("Period".equals(codeString)) 1734 return PERIOD; 1735 if ("Population".equals(codeString)) 1736 return POPULATION; 1737 if ("ProdCharacteristic".equals(codeString)) 1738 return PRODCHARACTERISTIC; 1739 if ("ProductShelfLife".equals(codeString)) 1740 return PRODUCTSHELFLIFE; 1741 if ("Quantity".equals(codeString)) 1742 return QUANTITY; 1743 if ("Range".equals(codeString)) 1744 return RANGE; 1745 if ("Ratio".equals(codeString)) 1746 return RATIO; 1747 if ("Reference".equals(codeString)) 1748 return REFERENCE; 1749 if ("RelatedArtifact".equals(codeString)) 1750 return RELATEDARTIFACT; 1751 if ("SampledData".equals(codeString)) 1752 return SAMPLEDDATA; 1753 if ("Signature".equals(codeString)) 1754 return SIGNATURE; 1755 if ("SimpleQuantity".equals(codeString)) 1756 return SIMPLEQUANTITY; 1757 if ("SubstanceAmount".equals(codeString)) 1758 return SUBSTANCEAMOUNT; 1759 if ("Timing".equals(codeString)) 1760 return TIMING; 1761 if ("TriggerDefinition".equals(codeString)) 1762 return TRIGGERDEFINITION; 1763 if ("UsageContext".equals(codeString)) 1764 return USAGECONTEXT; 1765 if ("base64Binary".equals(codeString)) 1766 return BASE64BINARY; 1767 if ("boolean".equals(codeString)) 1768 return BOOLEAN; 1769 if ("canonical".equals(codeString)) 1770 return CANONICAL; 1771 if ("code".equals(codeString)) 1772 return CODE; 1773 if ("date".equals(codeString)) 1774 return DATE; 1775 if ("dateTime".equals(codeString)) 1776 return DATETIME; 1777 if ("decimal".equals(codeString)) 1778 return DECIMAL; 1779 if ("id".equals(codeString)) 1780 return ID; 1781 if ("instant".equals(codeString)) 1782 return INSTANT; 1783 if ("integer".equals(codeString)) 1784 return INTEGER; 1785 if ("markdown".equals(codeString)) 1786 return MARKDOWN; 1787 if ("oid".equals(codeString)) 1788 return OID; 1789 if ("positiveInt".equals(codeString)) 1790 return POSITIVEINT; 1791 if ("string".equals(codeString)) 1792 return STRING; 1793 if ("time".equals(codeString)) 1794 return TIME; 1795 if ("unsignedInt".equals(codeString)) 1796 return UNSIGNEDINT; 1797 if ("uri".equals(codeString)) 1798 return URI; 1799 if ("url".equals(codeString)) 1800 return URL; 1801 if ("uuid".equals(codeString)) 1802 return UUID; 1803 if ("xhtml".equals(codeString)) 1804 return XHTML; 1805 throw new FHIRException("Unknown DataType code '" + codeString + "'"); 1806 } 1807 1808 public String toCode() { 1809 switch (this) { 1810 case ADDRESS: 1811 return "Address"; 1812 case AGE: 1813 return "Age"; 1814 case ANNOTATION: 1815 return "Annotation"; 1816 case ATTACHMENT: 1817 return "Attachment"; 1818 case BACKBONEELEMENT: 1819 return "BackboneElement"; 1820 case CODEABLECONCEPT: 1821 return "CodeableConcept"; 1822 case CODING: 1823 return "Coding"; 1824 case CONTACTDETAIL: 1825 return "ContactDetail"; 1826 case CONTACTPOINT: 1827 return "ContactPoint"; 1828 case CONTRIBUTOR: 1829 return "Contributor"; 1830 case COUNT: 1831 return "Count"; 1832 case DATAREQUIREMENT: 1833 return "DataRequirement"; 1834 case DISTANCE: 1835 return "Distance"; 1836 case DOSAGE: 1837 return "Dosage"; 1838 case DURATION: 1839 return "Duration"; 1840 case ELEMENT: 1841 return "Element"; 1842 case ELEMENTDEFINITION: 1843 return "ElementDefinition"; 1844 case EXPRESSION: 1845 return "Expression"; 1846 case EXTENSION: 1847 return "Extension"; 1848 case HUMANNAME: 1849 return "HumanName"; 1850 case IDENTIFIER: 1851 return "Identifier"; 1852 case MARKETINGSTATUS: 1853 return "MarketingStatus"; 1854 case META: 1855 return "Meta"; 1856 case MONEY: 1857 return "Money"; 1858 case MONEYQUANTITY: 1859 return "MoneyQuantity"; 1860 case NARRATIVE: 1861 return "Narrative"; 1862 case PARAMETERDEFINITION: 1863 return "ParameterDefinition"; 1864 case PERIOD: 1865 return "Period"; 1866 case POPULATION: 1867 return "Population"; 1868 case PRODCHARACTERISTIC: 1869 return "ProdCharacteristic"; 1870 case PRODUCTSHELFLIFE: 1871 return "ProductShelfLife"; 1872 case QUANTITY: 1873 return "Quantity"; 1874 case RANGE: 1875 return "Range"; 1876 case RATIO: 1877 return "Ratio"; 1878 case REFERENCE: 1879 return "Reference"; 1880 case RELATEDARTIFACT: 1881 return "RelatedArtifact"; 1882 case SAMPLEDDATA: 1883 return "SampledData"; 1884 case SIGNATURE: 1885 return "Signature"; 1886 case SIMPLEQUANTITY: 1887 return "SimpleQuantity"; 1888 case SUBSTANCEAMOUNT: 1889 return "SubstanceAmount"; 1890 case TIMING: 1891 return "Timing"; 1892 case TRIGGERDEFINITION: 1893 return "TriggerDefinition"; 1894 case USAGECONTEXT: 1895 return "UsageContext"; 1896 case BASE64BINARY: 1897 return "base64Binary"; 1898 case BOOLEAN: 1899 return "boolean"; 1900 case CANONICAL: 1901 return "canonical"; 1902 case CODE: 1903 return "code"; 1904 case DATE: 1905 return "date"; 1906 case DATETIME: 1907 return "dateTime"; 1908 case DECIMAL: 1909 return "decimal"; 1910 case ID: 1911 return "id"; 1912 case INSTANT: 1913 return "instant"; 1914 case INTEGER: 1915 return "integer"; 1916 case MARKDOWN: 1917 return "markdown"; 1918 case OID: 1919 return "oid"; 1920 case POSITIVEINT: 1921 return "positiveInt"; 1922 case STRING: 1923 return "string"; 1924 case TIME: 1925 return "time"; 1926 case UNSIGNEDINT: 1927 return "unsignedInt"; 1928 case URI: 1929 return "uri"; 1930 case URL: 1931 return "url"; 1932 case UUID: 1933 return "uuid"; 1934 case XHTML: 1935 return "xhtml"; 1936 case NULL: 1937 return null; 1938 default: 1939 return "?"; 1940 } 1941 } 1942 1943 public String getSystem() { 1944 switch (this) { 1945 case ADDRESS: 1946 return "http://hl7.org/fhir/data-types"; 1947 case AGE: 1948 return "http://hl7.org/fhir/data-types"; 1949 case ANNOTATION: 1950 return "http://hl7.org/fhir/data-types"; 1951 case ATTACHMENT: 1952 return "http://hl7.org/fhir/data-types"; 1953 case BACKBONEELEMENT: 1954 return "http://hl7.org/fhir/data-types"; 1955 case CODEABLECONCEPT: 1956 return "http://hl7.org/fhir/data-types"; 1957 case CODING: 1958 return "http://hl7.org/fhir/data-types"; 1959 case CONTACTDETAIL: 1960 return "http://hl7.org/fhir/data-types"; 1961 case CONTACTPOINT: 1962 return "http://hl7.org/fhir/data-types"; 1963 case CONTRIBUTOR: 1964 return "http://hl7.org/fhir/data-types"; 1965 case COUNT: 1966 return "http://hl7.org/fhir/data-types"; 1967 case DATAREQUIREMENT: 1968 return "http://hl7.org/fhir/data-types"; 1969 case DISTANCE: 1970 return "http://hl7.org/fhir/data-types"; 1971 case DOSAGE: 1972 return "http://hl7.org/fhir/data-types"; 1973 case DURATION: 1974 return "http://hl7.org/fhir/data-types"; 1975 case ELEMENT: 1976 return "http://hl7.org/fhir/data-types"; 1977 case ELEMENTDEFINITION: 1978 return "http://hl7.org/fhir/data-types"; 1979 case EXPRESSION: 1980 return "http://hl7.org/fhir/data-types"; 1981 case EXTENSION: 1982 return "http://hl7.org/fhir/data-types"; 1983 case HUMANNAME: 1984 return "http://hl7.org/fhir/data-types"; 1985 case IDENTIFIER: 1986 return "http://hl7.org/fhir/data-types"; 1987 case MARKETINGSTATUS: 1988 return "http://hl7.org/fhir/data-types"; 1989 case META: 1990 return "http://hl7.org/fhir/data-types"; 1991 case MONEY: 1992 return "http://hl7.org/fhir/data-types"; 1993 case MONEYQUANTITY: 1994 return "http://hl7.org/fhir/data-types"; 1995 case NARRATIVE: 1996 return "http://hl7.org/fhir/data-types"; 1997 case PARAMETERDEFINITION: 1998 return "http://hl7.org/fhir/data-types"; 1999 case PERIOD: 2000 return "http://hl7.org/fhir/data-types"; 2001 case POPULATION: 2002 return "http://hl7.org/fhir/data-types"; 2003 case PRODCHARACTERISTIC: 2004 return "http://hl7.org/fhir/data-types"; 2005 case PRODUCTSHELFLIFE: 2006 return "http://hl7.org/fhir/data-types"; 2007 case QUANTITY: 2008 return "http://hl7.org/fhir/data-types"; 2009 case RANGE: 2010 return "http://hl7.org/fhir/data-types"; 2011 case RATIO: 2012 return "http://hl7.org/fhir/data-types"; 2013 case REFERENCE: 2014 return "http://hl7.org/fhir/data-types"; 2015 case RELATEDARTIFACT: 2016 return "http://hl7.org/fhir/data-types"; 2017 case SAMPLEDDATA: 2018 return "http://hl7.org/fhir/data-types"; 2019 case SIGNATURE: 2020 return "http://hl7.org/fhir/data-types"; 2021 case SIMPLEQUANTITY: 2022 return "http://hl7.org/fhir/data-types"; 2023 case SUBSTANCEAMOUNT: 2024 return "http://hl7.org/fhir/data-types"; 2025 case TIMING: 2026 return "http://hl7.org/fhir/data-types"; 2027 case TRIGGERDEFINITION: 2028 return "http://hl7.org/fhir/data-types"; 2029 case USAGECONTEXT: 2030 return "http://hl7.org/fhir/data-types"; 2031 case BASE64BINARY: 2032 return "http://hl7.org/fhir/data-types"; 2033 case BOOLEAN: 2034 return "http://hl7.org/fhir/data-types"; 2035 case CANONICAL: 2036 return "http://hl7.org/fhir/data-types"; 2037 case CODE: 2038 return "http://hl7.org/fhir/data-types"; 2039 case DATE: 2040 return "http://hl7.org/fhir/data-types"; 2041 case DATETIME: 2042 return "http://hl7.org/fhir/data-types"; 2043 case DECIMAL: 2044 return "http://hl7.org/fhir/data-types"; 2045 case ID: 2046 return "http://hl7.org/fhir/data-types"; 2047 case INSTANT: 2048 return "http://hl7.org/fhir/data-types"; 2049 case INTEGER: 2050 return "http://hl7.org/fhir/data-types"; 2051 case MARKDOWN: 2052 return "http://hl7.org/fhir/data-types"; 2053 case OID: 2054 return "http://hl7.org/fhir/data-types"; 2055 case POSITIVEINT: 2056 return "http://hl7.org/fhir/data-types"; 2057 case STRING: 2058 return "http://hl7.org/fhir/data-types"; 2059 case TIME: 2060 return "http://hl7.org/fhir/data-types"; 2061 case UNSIGNEDINT: 2062 return "http://hl7.org/fhir/data-types"; 2063 case URI: 2064 return "http://hl7.org/fhir/data-types"; 2065 case URL: 2066 return "http://hl7.org/fhir/data-types"; 2067 case UUID: 2068 return "http://hl7.org/fhir/data-types"; 2069 case XHTML: 2070 return "http://hl7.org/fhir/data-types"; 2071 case NULL: 2072 return null; 2073 default: 2074 return "?"; 2075 } 2076 } 2077 2078 public String getDefinition() { 2079 switch (this) { 2080 case ADDRESS: 2081 return "An address expressed using postal conventions (as opposed to GPS or other location definition formats). This data type may be used to convey addresses for use in delivering mail as well as for visiting locations which might not be valid for mail delivery. There are a variety of postal address formats defined around the world."; 2082 case AGE: 2083 return "A duration of time during which an organism (or a process) has existed."; 2084 case ANNOTATION: 2085 return "A text note which also contains information about who made the statement and when."; 2086 case ATTACHMENT: 2087 return "For referring to data content defined in other formats."; 2088 case BACKBONEELEMENT: 2089 return "Base definition for all elements that are defined inside a resource - but not those in a data type."; 2090 case CODEABLECONCEPT: 2091 return "A concept that may be defined by a formal reference to a terminology or ontology or may be provided by text."; 2092 case CODING: 2093 return "A reference to a code defined by a terminology system."; 2094 case CONTACTDETAIL: 2095 return "Specifies contact information for a person or organization."; 2096 case CONTACTPOINT: 2097 return "Details for all kinds of technology mediated contact points for a person or organization, including telephone, email, etc."; 2098 case CONTRIBUTOR: 2099 return "A contributor to the content of a knowledge asset, including authors, editors, reviewers, and endorsers."; 2100 case COUNT: 2101 return "A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies."; 2102 case DATAREQUIREMENT: 2103 return "Describes a required data item for evaluation in terms of the type of data, and optional code or date-based filters of the data."; 2104 case DISTANCE: 2105 return "A length - a value with a unit that is a physical distance."; 2106 case DOSAGE: 2107 return "Indicates how the medication is/was taken or should be taken by the patient."; 2108 case DURATION: 2109 return "A length of time."; 2110 case ELEMENT: 2111 return "Base definition for all elements in a resource."; 2112 case ELEMENTDEFINITION: 2113 return "Captures constraints on each element within the resource, profile, or extension."; 2114 case EXPRESSION: 2115 return "A expression that is evaluated in a specified context and returns a value. The context of use of the expression must specify the context in which the expression is evaluated, and how the result of the expression is used."; 2116 case EXTENSION: 2117 return "Optional Extension Element - found in all resources."; 2118 case HUMANNAME: 2119 return "A human's name with the ability to identify parts and usage."; 2120 case IDENTIFIER: 2121 return "An identifier - identifies some entity uniquely and unambiguously. Typically this is used for business identifiers."; 2122 case MARKETINGSTATUS: 2123 return "The marketing status describes the date when a medicinal product is actually put on the market or the date as of which it is no longer available."; 2124 case META: 2125 return "The metadata about a resource. This is content in the resource that is maintained by the infrastructure. Changes to the content might not always be associated with version changes to the resource."; 2126 case MONEY: 2127 return "An amount of economic utility in some recognized currency."; 2128 case MONEYQUANTITY: 2129 return ""; 2130 case NARRATIVE: 2131 return "A human-readable summary of the resource conveying the essential clinical and business information for the resource."; 2132 case PARAMETERDEFINITION: 2133 return "The parameters to the module. This collection specifies both the input and output parameters. Input parameters are provided by the caller as part of the $evaluate operation. Output parameters are included in the GuidanceResponse."; 2134 case PERIOD: 2135 return "A time period defined by a start and end date and optionally time."; 2136 case POPULATION: 2137 return "A populatioof people with some set of grouping criteria."; 2138 case PRODCHARACTERISTIC: 2139 return "The marketing status describes the date when a medicinal product is actually put on the market or the date as of which it is no longer available."; 2140 case PRODUCTSHELFLIFE: 2141 return "The shelf-life and storage information for a medicinal product item or container can be described using this class."; 2142 case QUANTITY: 2143 return "A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies."; 2144 case RANGE: 2145 return "A set of ordered Quantities defined by a low and high limit."; 2146 case RATIO: 2147 return "A relationship of two Quantity values - expressed as a numerator and a denominator."; 2148 case REFERENCE: 2149 return "A reference from one resource to another."; 2150 case RELATEDARTIFACT: 2151 return "Related artifacts such as additional documentation, justification, or bibliographic references."; 2152 case SAMPLEDDATA: 2153 return "A series of measurements taken by a device, with upper and lower limits. There may be more than one dimension in the data."; 2154 case SIGNATURE: 2155 return "A signature along with supporting context. The signature may be a digital signature that is cryptographic in nature, or some other signature acceptable to the domain. This other signature may be as simple as a graphical image representing a hand-written signature, or a signature ceremony Different signature approaches have different utilities."; 2156 case SIMPLEQUANTITY: 2157 return ""; 2158 case SUBSTANCEAMOUNT: 2159 return "Chemical substances are a single substance type whose primary defining element is the molecular structure. Chemical substances shall be defined on the basis of their complete covalent molecular structure; the presence of a salt (counter-ion) and/or solvates (water, alcohols) is also captured. Purity, grade, physical form or particle size are not taken into account in the definition of a chemical substance or in the assignment of a Substance ID."; 2160 case TIMING: 2161 return "Specifies an event that may occur multiple times. Timing schedules are used to record when things are planned, expected or requested to occur. The most common usage is in dosage instructions for medications. They are also used when planning care of various kinds, and may be used for reporting the schedule to which past regular activities were carried out."; 2162 case TRIGGERDEFINITION: 2163 return "A description of a triggering event. Triggering events can be named events, data events, or periodic, as determined by the type element."; 2164 case USAGECONTEXT: 2165 return "Specifies clinical/business/etc. metadata that can be used to retrieve, index and/or categorize an artifact. This metadata can either be specific to the applicable population (e.g., age category, DRG) or the specific context of care (e.g., venue, care setting, provider of care)."; 2166 case BASE64BINARY: 2167 return "A stream of bytes"; 2168 case BOOLEAN: 2169 return "Value of \"true\" or \"false\""; 2170 case CANONICAL: 2171 return "A URI that is a reference to a canonical URL on a FHIR resource"; 2172 case CODE: 2173 return "A string which has at least one character and no leading or trailing whitespace and where there is no whitespace other than single spaces in the contents"; 2174 case DATE: 2175 return "A date or partial date (e.g. just year or year + month). There is no time zone. The format is a union of the schema types gYear, gYearMonth and date. Dates SHALL be valid dates."; 2176 case DATETIME: 2177 return "A date, date-time or partial date (e.g. just year or year + month). If hours and minutes are specified, a time zone SHALL be populated. The format is a union of the schema types gYear, gYearMonth, date and dateTime. Seconds must be provided due to schema type constraints but may be zero-filled and may be ignored. Dates SHALL be valid dates."; 2178 case DECIMAL: 2179 return "A rational number with implicit precision"; 2180 case ID: 2181 return "Any combination of letters, numerals, \"-\" and \".\", with a length limit of 64 characters. (This might be an integer, an unprefixed OID, UUID or any other identifier pattern that meets these constraints.) Ids are case-insensitive."; 2182 case INSTANT: 2183 return "An instant in time - known at least to the second"; 2184 case INTEGER: 2185 return "A whole number"; 2186 case MARKDOWN: 2187 return "A string that may contain Github Flavored Markdown syntax for optional processing by a mark down presentation engine"; 2188 case OID: 2189 return "An OID represented as a URI"; 2190 case POSITIVEINT: 2191 return "An integer with a value that is positive (e.g. >0)"; 2192 case STRING: 2193 return "A sequence of Unicode characters"; 2194 case TIME: 2195 return "A time during the day, with no date specified"; 2196 case UNSIGNEDINT: 2197 return "An integer with a value that is not negative (e.g. >= 0)"; 2198 case URI: 2199 return "String of characters used to identify a name or a resource"; 2200 case URL: 2201 return "A URI that is a literal reference"; 2202 case UUID: 2203 return "A UUID, represented as a URI"; 2204 case XHTML: 2205 return "XHTML format, as defined by W3C, but restricted usage (mainly, no active content)"; 2206 case NULL: 2207 return null; 2208 default: 2209 return "?"; 2210 } 2211 } 2212 2213 public String getDisplay() { 2214 switch (this) { 2215 case ADDRESS: 2216 return "Address"; 2217 case AGE: 2218 return "Age"; 2219 case ANNOTATION: 2220 return "Annotation"; 2221 case ATTACHMENT: 2222 return "Attachment"; 2223 case BACKBONEELEMENT: 2224 return "BackboneElement"; 2225 case CODEABLECONCEPT: 2226 return "CodeableConcept"; 2227 case CODING: 2228 return "Coding"; 2229 case CONTACTDETAIL: 2230 return "ContactDetail"; 2231 case CONTACTPOINT: 2232 return "ContactPoint"; 2233 case CONTRIBUTOR: 2234 return "Contributor"; 2235 case COUNT: 2236 return "Count"; 2237 case DATAREQUIREMENT: 2238 return "DataRequirement"; 2239 case DISTANCE: 2240 return "Distance"; 2241 case DOSAGE: 2242 return "Dosage"; 2243 case DURATION: 2244 return "Duration"; 2245 case ELEMENT: 2246 return "Element"; 2247 case ELEMENTDEFINITION: 2248 return "ElementDefinition"; 2249 case EXPRESSION: 2250 return "Expression"; 2251 case EXTENSION: 2252 return "Extension"; 2253 case HUMANNAME: 2254 return "HumanName"; 2255 case IDENTIFIER: 2256 return "Identifier"; 2257 case MARKETINGSTATUS: 2258 return "MarketingStatus"; 2259 case META: 2260 return "Meta"; 2261 case MONEY: 2262 return "Money"; 2263 case MONEYQUANTITY: 2264 return "MoneyQuantity"; 2265 case NARRATIVE: 2266 return "Narrative"; 2267 case PARAMETERDEFINITION: 2268 return "ParameterDefinition"; 2269 case PERIOD: 2270 return "Period"; 2271 case POPULATION: 2272 return "Population"; 2273 case PRODCHARACTERISTIC: 2274 return "ProdCharacteristic"; 2275 case PRODUCTSHELFLIFE: 2276 return "ProductShelfLife"; 2277 case QUANTITY: 2278 return "Quantity"; 2279 case RANGE: 2280 return "Range"; 2281 case RATIO: 2282 return "Ratio"; 2283 case REFERENCE: 2284 return "Reference"; 2285 case RELATEDARTIFACT: 2286 return "RelatedArtifact"; 2287 case SAMPLEDDATA: 2288 return "SampledData"; 2289 case SIGNATURE: 2290 return "Signature"; 2291 case SIMPLEQUANTITY: 2292 return "SimpleQuantity"; 2293 case SUBSTANCEAMOUNT: 2294 return "SubstanceAmount"; 2295 case TIMING: 2296 return "Timing"; 2297 case TRIGGERDEFINITION: 2298 return "TriggerDefinition"; 2299 case USAGECONTEXT: 2300 return "UsageContext"; 2301 case BASE64BINARY: 2302 return "base64Binary"; 2303 case BOOLEAN: 2304 return "boolean"; 2305 case CANONICAL: 2306 return "canonical"; 2307 case CODE: 2308 return "code"; 2309 case DATE: 2310 return "date"; 2311 case DATETIME: 2312 return "dateTime"; 2313 case DECIMAL: 2314 return "decimal"; 2315 case ID: 2316 return "id"; 2317 case INSTANT: 2318 return "instant"; 2319 case INTEGER: 2320 return "integer"; 2321 case MARKDOWN: 2322 return "markdown"; 2323 case OID: 2324 return "oid"; 2325 case POSITIVEINT: 2326 return "positiveInt"; 2327 case STRING: 2328 return "string"; 2329 case TIME: 2330 return "time"; 2331 case UNSIGNEDINT: 2332 return "unsignedInt"; 2333 case URI: 2334 return "uri"; 2335 case URL: 2336 return "url"; 2337 case UUID: 2338 return "uuid"; 2339 case XHTML: 2340 return "XHTML"; 2341 case NULL: 2342 return null; 2343 default: 2344 return "?"; 2345 } 2346 } 2347 } 2348 2349 public static class DataTypeEnumFactory implements EnumFactory<DataType> { 2350 public DataType fromCode(String codeString) throws IllegalArgumentException { 2351 if (codeString == null || "".equals(codeString)) 2352 if (codeString == null || "".equals(codeString)) 2353 return null; 2354 if ("Address".equals(codeString)) 2355 return DataType.ADDRESS; 2356 if ("Age".equals(codeString)) 2357 return DataType.AGE; 2358 if ("Annotation".equals(codeString)) 2359 return DataType.ANNOTATION; 2360 if ("Attachment".equals(codeString)) 2361 return DataType.ATTACHMENT; 2362 if ("BackboneElement".equals(codeString)) 2363 return DataType.BACKBONEELEMENT; 2364 if ("CodeableConcept".equals(codeString)) 2365 return DataType.CODEABLECONCEPT; 2366 if ("Coding".equals(codeString)) 2367 return DataType.CODING; 2368 if ("ContactDetail".equals(codeString)) 2369 return DataType.CONTACTDETAIL; 2370 if ("ContactPoint".equals(codeString)) 2371 return DataType.CONTACTPOINT; 2372 if ("Contributor".equals(codeString)) 2373 return DataType.CONTRIBUTOR; 2374 if ("Count".equals(codeString)) 2375 return DataType.COUNT; 2376 if ("DataRequirement".equals(codeString)) 2377 return DataType.DATAREQUIREMENT; 2378 if ("Distance".equals(codeString)) 2379 return DataType.DISTANCE; 2380 if ("Dosage".equals(codeString)) 2381 return DataType.DOSAGE; 2382 if ("Duration".equals(codeString)) 2383 return DataType.DURATION; 2384 if ("Element".equals(codeString)) 2385 return DataType.ELEMENT; 2386 if ("ElementDefinition".equals(codeString)) 2387 return DataType.ELEMENTDEFINITION; 2388 if ("Expression".equals(codeString)) 2389 return DataType.EXPRESSION; 2390 if ("Extension".equals(codeString)) 2391 return DataType.EXTENSION; 2392 if ("HumanName".equals(codeString)) 2393 return DataType.HUMANNAME; 2394 if ("Identifier".equals(codeString)) 2395 return DataType.IDENTIFIER; 2396 if ("MarketingStatus".equals(codeString)) 2397 return DataType.MARKETINGSTATUS; 2398 if ("Meta".equals(codeString)) 2399 return DataType.META; 2400 if ("Money".equals(codeString)) 2401 return DataType.MONEY; 2402 if ("MoneyQuantity".equals(codeString)) 2403 return DataType.MONEYQUANTITY; 2404 if ("Narrative".equals(codeString)) 2405 return DataType.NARRATIVE; 2406 if ("ParameterDefinition".equals(codeString)) 2407 return DataType.PARAMETERDEFINITION; 2408 if ("Period".equals(codeString)) 2409 return DataType.PERIOD; 2410 if ("Population".equals(codeString)) 2411 return DataType.POPULATION; 2412 if ("ProdCharacteristic".equals(codeString)) 2413 return DataType.PRODCHARACTERISTIC; 2414 if ("ProductShelfLife".equals(codeString)) 2415 return DataType.PRODUCTSHELFLIFE; 2416 if ("Quantity".equals(codeString)) 2417 return DataType.QUANTITY; 2418 if ("Range".equals(codeString)) 2419 return DataType.RANGE; 2420 if ("Ratio".equals(codeString)) 2421 return DataType.RATIO; 2422 if ("Reference".equals(codeString)) 2423 return DataType.REFERENCE; 2424 if ("RelatedArtifact".equals(codeString)) 2425 return DataType.RELATEDARTIFACT; 2426 if ("SampledData".equals(codeString)) 2427 return DataType.SAMPLEDDATA; 2428 if ("Signature".equals(codeString)) 2429 return DataType.SIGNATURE; 2430 if ("SimpleQuantity".equals(codeString)) 2431 return DataType.SIMPLEQUANTITY; 2432 if ("SubstanceAmount".equals(codeString)) 2433 return DataType.SUBSTANCEAMOUNT; 2434 if ("Timing".equals(codeString)) 2435 return DataType.TIMING; 2436 if ("TriggerDefinition".equals(codeString)) 2437 return DataType.TRIGGERDEFINITION; 2438 if ("UsageContext".equals(codeString)) 2439 return DataType.USAGECONTEXT; 2440 if ("base64Binary".equals(codeString)) 2441 return DataType.BASE64BINARY; 2442 if ("boolean".equals(codeString)) 2443 return DataType.BOOLEAN; 2444 if ("canonical".equals(codeString)) 2445 return DataType.CANONICAL; 2446 if ("code".equals(codeString)) 2447 return DataType.CODE; 2448 if ("date".equals(codeString)) 2449 return DataType.DATE; 2450 if ("dateTime".equals(codeString)) 2451 return DataType.DATETIME; 2452 if ("decimal".equals(codeString)) 2453 return DataType.DECIMAL; 2454 if ("id".equals(codeString)) 2455 return DataType.ID; 2456 if ("instant".equals(codeString)) 2457 return DataType.INSTANT; 2458 if ("integer".equals(codeString)) 2459 return DataType.INTEGER; 2460 if ("markdown".equals(codeString)) 2461 return DataType.MARKDOWN; 2462 if ("oid".equals(codeString)) 2463 return DataType.OID; 2464 if ("positiveInt".equals(codeString)) 2465 return DataType.POSITIVEINT; 2466 if ("string".equals(codeString)) 2467 return DataType.STRING; 2468 if ("time".equals(codeString)) 2469 return DataType.TIME; 2470 if ("unsignedInt".equals(codeString)) 2471 return DataType.UNSIGNEDINT; 2472 if ("uri".equals(codeString)) 2473 return DataType.URI; 2474 if ("url".equals(codeString)) 2475 return DataType.URL; 2476 if ("uuid".equals(codeString)) 2477 return DataType.UUID; 2478 if ("xhtml".equals(codeString)) 2479 return DataType.XHTML; 2480 throw new IllegalArgumentException("Unknown DataType code '" + codeString + "'"); 2481 } 2482 2483 public Enumeration<DataType> fromType(PrimitiveType<?> code) throws FHIRException { 2484 if (code == null) 2485 return null; 2486 if (code.isEmpty()) 2487 return new Enumeration<DataType>(this, DataType.NULL, code); 2488 String codeString = code.asStringValue(); 2489 if (codeString == null || "".equals(codeString)) 2490 return new Enumeration<DataType>(this, DataType.NULL, code); 2491 if ("Address".equals(codeString)) 2492 return new Enumeration<DataType>(this, DataType.ADDRESS, code); 2493 if ("Age".equals(codeString)) 2494 return new Enumeration<DataType>(this, DataType.AGE, code); 2495 if ("Annotation".equals(codeString)) 2496 return new Enumeration<DataType>(this, DataType.ANNOTATION, code); 2497 if ("Attachment".equals(codeString)) 2498 return new Enumeration<DataType>(this, DataType.ATTACHMENT, code); 2499 if ("BackboneElement".equals(codeString)) 2500 return new Enumeration<DataType>(this, DataType.BACKBONEELEMENT, code); 2501 if ("CodeableConcept".equals(codeString)) 2502 return new Enumeration<DataType>(this, DataType.CODEABLECONCEPT, code); 2503 if ("Coding".equals(codeString)) 2504 return new Enumeration<DataType>(this, DataType.CODING, code); 2505 if ("ContactDetail".equals(codeString)) 2506 return new Enumeration<DataType>(this, DataType.CONTACTDETAIL, code); 2507 if ("ContactPoint".equals(codeString)) 2508 return new Enumeration<DataType>(this, DataType.CONTACTPOINT, code); 2509 if ("Contributor".equals(codeString)) 2510 return new Enumeration<DataType>(this, DataType.CONTRIBUTOR, code); 2511 if ("Count".equals(codeString)) 2512 return new Enumeration<DataType>(this, DataType.COUNT, code); 2513 if ("DataRequirement".equals(codeString)) 2514 return new Enumeration<DataType>(this, DataType.DATAREQUIREMENT, code); 2515 if ("Distance".equals(codeString)) 2516 return new Enumeration<DataType>(this, DataType.DISTANCE, code); 2517 if ("Dosage".equals(codeString)) 2518 return new Enumeration<DataType>(this, DataType.DOSAGE, code); 2519 if ("Duration".equals(codeString)) 2520 return new Enumeration<DataType>(this, DataType.DURATION, code); 2521 if ("Element".equals(codeString)) 2522 return new Enumeration<DataType>(this, DataType.ELEMENT, code); 2523 if ("ElementDefinition".equals(codeString)) 2524 return new Enumeration<DataType>(this, DataType.ELEMENTDEFINITION, code); 2525 if ("Expression".equals(codeString)) 2526 return new Enumeration<DataType>(this, DataType.EXPRESSION, code); 2527 if ("Extension".equals(codeString)) 2528 return new Enumeration<DataType>(this, DataType.EXTENSION, code); 2529 if ("HumanName".equals(codeString)) 2530 return new Enumeration<DataType>(this, DataType.HUMANNAME, code); 2531 if ("Identifier".equals(codeString)) 2532 return new Enumeration<DataType>(this, DataType.IDENTIFIER, code); 2533 if ("MarketingStatus".equals(codeString)) 2534 return new Enumeration<DataType>(this, DataType.MARKETINGSTATUS, code); 2535 if ("Meta".equals(codeString)) 2536 return new Enumeration<DataType>(this, DataType.META, code); 2537 if ("Money".equals(codeString)) 2538 return new Enumeration<DataType>(this, DataType.MONEY, code); 2539 if ("MoneyQuantity".equals(codeString)) 2540 return new Enumeration<DataType>(this, DataType.MONEYQUANTITY, code); 2541 if ("Narrative".equals(codeString)) 2542 return new Enumeration<DataType>(this, DataType.NARRATIVE, code); 2543 if ("ParameterDefinition".equals(codeString)) 2544 return new Enumeration<DataType>(this, DataType.PARAMETERDEFINITION, code); 2545 if ("Period".equals(codeString)) 2546 return new Enumeration<DataType>(this, DataType.PERIOD, code); 2547 if ("Population".equals(codeString)) 2548 return new Enumeration<DataType>(this, DataType.POPULATION, code); 2549 if ("ProdCharacteristic".equals(codeString)) 2550 return new Enumeration<DataType>(this, DataType.PRODCHARACTERISTIC, code); 2551 if ("ProductShelfLife".equals(codeString)) 2552 return new Enumeration<DataType>(this, DataType.PRODUCTSHELFLIFE, code); 2553 if ("Quantity".equals(codeString)) 2554 return new Enumeration<DataType>(this, DataType.QUANTITY, code); 2555 if ("Range".equals(codeString)) 2556 return new Enumeration<DataType>(this, DataType.RANGE, code); 2557 if ("Ratio".equals(codeString)) 2558 return new Enumeration<DataType>(this, DataType.RATIO, code); 2559 if ("Reference".equals(codeString)) 2560 return new Enumeration<DataType>(this, DataType.REFERENCE, code); 2561 if ("RelatedArtifact".equals(codeString)) 2562 return new Enumeration<DataType>(this, DataType.RELATEDARTIFACT, code); 2563 if ("SampledData".equals(codeString)) 2564 return new Enumeration<DataType>(this, DataType.SAMPLEDDATA, code); 2565 if ("Signature".equals(codeString)) 2566 return new Enumeration<DataType>(this, DataType.SIGNATURE, code); 2567 if ("SimpleQuantity".equals(codeString)) 2568 return new Enumeration<DataType>(this, DataType.SIMPLEQUANTITY, code); 2569 if ("SubstanceAmount".equals(codeString)) 2570 return new Enumeration<DataType>(this, DataType.SUBSTANCEAMOUNT, code); 2571 if ("Timing".equals(codeString)) 2572 return new Enumeration<DataType>(this, DataType.TIMING, code); 2573 if ("TriggerDefinition".equals(codeString)) 2574 return new Enumeration<DataType>(this, DataType.TRIGGERDEFINITION, code); 2575 if ("UsageContext".equals(codeString)) 2576 return new Enumeration<DataType>(this, DataType.USAGECONTEXT, code); 2577 if ("base64Binary".equals(codeString)) 2578 return new Enumeration<DataType>(this, DataType.BASE64BINARY, code); 2579 if ("boolean".equals(codeString)) 2580 return new Enumeration<DataType>(this, DataType.BOOLEAN, code); 2581 if ("canonical".equals(codeString)) 2582 return new Enumeration<DataType>(this, DataType.CANONICAL, code); 2583 if ("code".equals(codeString)) 2584 return new Enumeration<DataType>(this, DataType.CODE, code); 2585 if ("date".equals(codeString)) 2586 return new Enumeration<DataType>(this, DataType.DATE, code); 2587 if ("dateTime".equals(codeString)) 2588 return new Enumeration<DataType>(this, DataType.DATETIME, code); 2589 if ("decimal".equals(codeString)) 2590 return new Enumeration<DataType>(this, DataType.DECIMAL, code); 2591 if ("id".equals(codeString)) 2592 return new Enumeration<DataType>(this, DataType.ID, code); 2593 if ("instant".equals(codeString)) 2594 return new Enumeration<DataType>(this, DataType.INSTANT, code); 2595 if ("integer".equals(codeString)) 2596 return new Enumeration<DataType>(this, DataType.INTEGER, code); 2597 if ("markdown".equals(codeString)) 2598 return new Enumeration<DataType>(this, DataType.MARKDOWN, code); 2599 if ("oid".equals(codeString)) 2600 return new Enumeration<DataType>(this, DataType.OID, code); 2601 if ("positiveInt".equals(codeString)) 2602 return new Enumeration<DataType>(this, DataType.POSITIVEINT, code); 2603 if ("string".equals(codeString)) 2604 return new Enumeration<DataType>(this, DataType.STRING, code); 2605 if ("time".equals(codeString)) 2606 return new Enumeration<DataType>(this, DataType.TIME, code); 2607 if ("unsignedInt".equals(codeString)) 2608 return new Enumeration<DataType>(this, DataType.UNSIGNEDINT, code); 2609 if ("uri".equals(codeString)) 2610 return new Enumeration<DataType>(this, DataType.URI, code); 2611 if ("url".equals(codeString)) 2612 return new Enumeration<DataType>(this, DataType.URL, code); 2613 if ("uuid".equals(codeString)) 2614 return new Enumeration<DataType>(this, DataType.UUID, code); 2615 if ("xhtml".equals(codeString)) 2616 return new Enumeration<DataType>(this, DataType.XHTML, code); 2617 throw new FHIRException("Unknown DataType code '" + codeString + "'"); 2618 } 2619 2620 public String toCode(DataType code) { 2621 if (code == DataType.NULL) 2622 return null; 2623 if (code == DataType.ADDRESS) 2624 return "Address"; 2625 if (code == DataType.AGE) 2626 return "Age"; 2627 if (code == DataType.ANNOTATION) 2628 return "Annotation"; 2629 if (code == DataType.ATTACHMENT) 2630 return "Attachment"; 2631 if (code == DataType.BACKBONEELEMENT) 2632 return "BackboneElement"; 2633 if (code == DataType.CODEABLECONCEPT) 2634 return "CodeableConcept"; 2635 if (code == DataType.CODING) 2636 return "Coding"; 2637 if (code == DataType.CONTACTDETAIL) 2638 return "ContactDetail"; 2639 if (code == DataType.CONTACTPOINT) 2640 return "ContactPoint"; 2641 if (code == DataType.CONTRIBUTOR) 2642 return "Contributor"; 2643 if (code == DataType.COUNT) 2644 return "Count"; 2645 if (code == DataType.DATAREQUIREMENT) 2646 return "DataRequirement"; 2647 if (code == DataType.DISTANCE) 2648 return "Distance"; 2649 if (code == DataType.DOSAGE) 2650 return "Dosage"; 2651 if (code == DataType.DURATION) 2652 return "Duration"; 2653 if (code == DataType.ELEMENT) 2654 return "Element"; 2655 if (code == DataType.ELEMENTDEFINITION) 2656 return "ElementDefinition"; 2657 if (code == DataType.EXPRESSION) 2658 return "Expression"; 2659 if (code == DataType.EXTENSION) 2660 return "Extension"; 2661 if (code == DataType.HUMANNAME) 2662 return "HumanName"; 2663 if (code == DataType.IDENTIFIER) 2664 return "Identifier"; 2665 if (code == DataType.MARKETINGSTATUS) 2666 return "MarketingStatus"; 2667 if (code == DataType.META) 2668 return "Meta"; 2669 if (code == DataType.MONEY) 2670 return "Money"; 2671 if (code == DataType.MONEYQUANTITY) 2672 return "MoneyQuantity"; 2673 if (code == DataType.NARRATIVE) 2674 return "Narrative"; 2675 if (code == DataType.PARAMETERDEFINITION) 2676 return "ParameterDefinition"; 2677 if (code == DataType.PERIOD) 2678 return "Period"; 2679 if (code == DataType.POPULATION) 2680 return "Population"; 2681 if (code == DataType.PRODCHARACTERISTIC) 2682 return "ProdCharacteristic"; 2683 if (code == DataType.PRODUCTSHELFLIFE) 2684 return "ProductShelfLife"; 2685 if (code == DataType.QUANTITY) 2686 return "Quantity"; 2687 if (code == DataType.RANGE) 2688 return "Range"; 2689 if (code == DataType.RATIO) 2690 return "Ratio"; 2691 if (code == DataType.REFERENCE) 2692 return "Reference"; 2693 if (code == DataType.RELATEDARTIFACT) 2694 return "RelatedArtifact"; 2695 if (code == DataType.SAMPLEDDATA) 2696 return "SampledData"; 2697 if (code == DataType.SIGNATURE) 2698 return "Signature"; 2699 if (code == DataType.SIMPLEQUANTITY) 2700 return "SimpleQuantity"; 2701 if (code == DataType.SUBSTANCEAMOUNT) 2702 return "SubstanceAmount"; 2703 if (code == DataType.TIMING) 2704 return "Timing"; 2705 if (code == DataType.TRIGGERDEFINITION) 2706 return "TriggerDefinition"; 2707 if (code == DataType.USAGECONTEXT) 2708 return "UsageContext"; 2709 if (code == DataType.BASE64BINARY) 2710 return "base64Binary"; 2711 if (code == DataType.BOOLEAN) 2712 return "boolean"; 2713 if (code == DataType.CANONICAL) 2714 return "canonical"; 2715 if (code == DataType.CODE) 2716 return "code"; 2717 if (code == DataType.DATE) 2718 return "date"; 2719 if (code == DataType.DATETIME) 2720 return "dateTime"; 2721 if (code == DataType.DECIMAL) 2722 return "decimal"; 2723 if (code == DataType.ID) 2724 return "id"; 2725 if (code == DataType.INSTANT) 2726 return "instant"; 2727 if (code == DataType.INTEGER) 2728 return "integer"; 2729 if (code == DataType.MARKDOWN) 2730 return "markdown"; 2731 if (code == DataType.OID) 2732 return "oid"; 2733 if (code == DataType.POSITIVEINT) 2734 return "positiveInt"; 2735 if (code == DataType.STRING) 2736 return "string"; 2737 if (code == DataType.TIME) 2738 return "time"; 2739 if (code == DataType.UNSIGNEDINT) 2740 return "unsignedInt"; 2741 if (code == DataType.URI) 2742 return "uri"; 2743 if (code == DataType.URL) 2744 return "url"; 2745 if (code == DataType.UUID) 2746 return "uuid"; 2747 if (code == DataType.XHTML) 2748 return "xhtml"; 2749 return "?"; 2750 } 2751 2752 public String toSystem(DataType code) { 2753 return code.getSystem(); 2754 } 2755 } 2756 2757 public enum DefinitionResourceType { 2758 /** 2759 * This resource allows for the definition of some activity to be performed, 2760 * independent of a particular patient, practitioner, or other performance 2761 * context. 2762 */ 2763 ACTIVITYDEFINITION, 2764 /** 2765 * The EventDefinition resource provides a reusable description of when a 2766 * particular event can occur. 2767 */ 2768 EVENTDEFINITION, 2769 /** 2770 * The Measure resource provides the definition of a quality measure. 2771 */ 2772 MEASURE, 2773 /** 2774 * A formal computable definition of an operation (on the RESTful interface) or 2775 * a named query (using the search interaction). 2776 */ 2777 OPERATIONDEFINITION, 2778 /** 2779 * This resource allows for the definition of various types of plans as a 2780 * sharable, consumable, and executable artifact. The resource is general enough 2781 * to support the description of a broad range of clinical artifacts such as 2782 * clinical decision support rules, order sets and protocols. 2783 */ 2784 PLANDEFINITION, 2785 /** 2786 * A structured set of questions intended to guide the collection of answers 2787 * from end-users. Questionnaires provide detailed control over order, 2788 * presentation, phraseology and grouping to allow coherent, consistent data 2789 * collection. 2790 */ 2791 QUESTIONNAIRE, 2792 /** 2793 * added to help the parsers 2794 */ 2795 NULL; 2796 2797 public static DefinitionResourceType fromCode(String codeString) throws FHIRException { 2798 if (codeString == null || "".equals(codeString)) 2799 return null; 2800 if ("ActivityDefinition".equals(codeString)) 2801 return ACTIVITYDEFINITION; 2802 if ("EventDefinition".equals(codeString)) 2803 return EVENTDEFINITION; 2804 if ("Measure".equals(codeString)) 2805 return MEASURE; 2806 if ("OperationDefinition".equals(codeString)) 2807 return OPERATIONDEFINITION; 2808 if ("PlanDefinition".equals(codeString)) 2809 return PLANDEFINITION; 2810 if ("Questionnaire".equals(codeString)) 2811 return QUESTIONNAIRE; 2812 throw new FHIRException("Unknown DefinitionResourceType code '" + codeString + "'"); 2813 } 2814 2815 public String toCode() { 2816 switch (this) { 2817 case ACTIVITYDEFINITION: 2818 return "ActivityDefinition"; 2819 case EVENTDEFINITION: 2820 return "EventDefinition"; 2821 case MEASURE: 2822 return "Measure"; 2823 case OPERATIONDEFINITION: 2824 return "OperationDefinition"; 2825 case PLANDEFINITION: 2826 return "PlanDefinition"; 2827 case QUESTIONNAIRE: 2828 return "Questionnaire"; 2829 case NULL: 2830 return null; 2831 default: 2832 return "?"; 2833 } 2834 } 2835 2836 public String getSystem() { 2837 switch (this) { 2838 case ACTIVITYDEFINITION: 2839 return "http://hl7.org/fhir/definition-resource-types"; 2840 case EVENTDEFINITION: 2841 return "http://hl7.org/fhir/definition-resource-types"; 2842 case MEASURE: 2843 return "http://hl7.org/fhir/definition-resource-types"; 2844 case OPERATIONDEFINITION: 2845 return "http://hl7.org/fhir/definition-resource-types"; 2846 case PLANDEFINITION: 2847 return "http://hl7.org/fhir/definition-resource-types"; 2848 case QUESTIONNAIRE: 2849 return "http://hl7.org/fhir/definition-resource-types"; 2850 case NULL: 2851 return null; 2852 default: 2853 return "?"; 2854 } 2855 } 2856 2857 public String getDefinition() { 2858 switch (this) { 2859 case ACTIVITYDEFINITION: 2860 return "This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context."; 2861 case EVENTDEFINITION: 2862 return "The EventDefinition resource provides a reusable description of when a particular event can occur."; 2863 case MEASURE: 2864 return "The Measure resource provides the definition of a quality measure."; 2865 case OPERATIONDEFINITION: 2866 return "A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction)."; 2867 case PLANDEFINITION: 2868 return "This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical artifacts such as clinical decision support rules, order sets and protocols."; 2869 case QUESTIONNAIRE: 2870 return "A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection."; 2871 case NULL: 2872 return null; 2873 default: 2874 return "?"; 2875 } 2876 } 2877 2878 public String getDisplay() { 2879 switch (this) { 2880 case ACTIVITYDEFINITION: 2881 return "ActivityDefinition"; 2882 case EVENTDEFINITION: 2883 return "EventDefinition"; 2884 case MEASURE: 2885 return "Measure"; 2886 case OPERATIONDEFINITION: 2887 return "OperationDefinition"; 2888 case PLANDEFINITION: 2889 return "PlanDefinition"; 2890 case QUESTIONNAIRE: 2891 return "Questionnaire"; 2892 case NULL: 2893 return null; 2894 default: 2895 return "?"; 2896 } 2897 } 2898 } 2899 2900 public static class DefinitionResourceTypeEnumFactory implements EnumFactory<DefinitionResourceType> { 2901 public DefinitionResourceType fromCode(String codeString) throws IllegalArgumentException { 2902 if (codeString == null || "".equals(codeString)) 2903 if (codeString == null || "".equals(codeString)) 2904 return null; 2905 if ("ActivityDefinition".equals(codeString)) 2906 return DefinitionResourceType.ACTIVITYDEFINITION; 2907 if ("EventDefinition".equals(codeString)) 2908 return DefinitionResourceType.EVENTDEFINITION; 2909 if ("Measure".equals(codeString)) 2910 return DefinitionResourceType.MEASURE; 2911 if ("OperationDefinition".equals(codeString)) 2912 return DefinitionResourceType.OPERATIONDEFINITION; 2913 if ("PlanDefinition".equals(codeString)) 2914 return DefinitionResourceType.PLANDEFINITION; 2915 if ("Questionnaire".equals(codeString)) 2916 return DefinitionResourceType.QUESTIONNAIRE; 2917 throw new IllegalArgumentException("Unknown DefinitionResourceType code '" + codeString + "'"); 2918 } 2919 2920 public Enumeration<DefinitionResourceType> fromType(PrimitiveType<?> code) throws FHIRException { 2921 if (code == null) 2922 return null; 2923 if (code.isEmpty()) 2924 return new Enumeration<DefinitionResourceType>(this, DefinitionResourceType.NULL, code); 2925 String codeString = code.asStringValue(); 2926 if (codeString == null || "".equals(codeString)) 2927 return new Enumeration<DefinitionResourceType>(this, DefinitionResourceType.NULL, code); 2928 if ("ActivityDefinition".equals(codeString)) 2929 return new Enumeration<DefinitionResourceType>(this, DefinitionResourceType.ACTIVITYDEFINITION, code); 2930 if ("EventDefinition".equals(codeString)) 2931 return new Enumeration<DefinitionResourceType>(this, DefinitionResourceType.EVENTDEFINITION, code); 2932 if ("Measure".equals(codeString)) 2933 return new Enumeration<DefinitionResourceType>(this, DefinitionResourceType.MEASURE, code); 2934 if ("OperationDefinition".equals(codeString)) 2935 return new Enumeration<DefinitionResourceType>(this, DefinitionResourceType.OPERATIONDEFINITION, code); 2936 if ("PlanDefinition".equals(codeString)) 2937 return new Enumeration<DefinitionResourceType>(this, DefinitionResourceType.PLANDEFINITION, code); 2938 if ("Questionnaire".equals(codeString)) 2939 return new Enumeration<DefinitionResourceType>(this, DefinitionResourceType.QUESTIONNAIRE, code); 2940 throw new FHIRException("Unknown DefinitionResourceType code '" + codeString + "'"); 2941 } 2942 2943 public String toCode(DefinitionResourceType code) { 2944 if (code == DefinitionResourceType.NULL) 2945 return null; 2946 if (code == DefinitionResourceType.ACTIVITYDEFINITION) 2947 return "ActivityDefinition"; 2948 if (code == DefinitionResourceType.EVENTDEFINITION) 2949 return "EventDefinition"; 2950 if (code == DefinitionResourceType.MEASURE) 2951 return "Measure"; 2952 if (code == DefinitionResourceType.OPERATIONDEFINITION) 2953 return "OperationDefinition"; 2954 if (code == DefinitionResourceType.PLANDEFINITION) 2955 return "PlanDefinition"; 2956 if (code == DefinitionResourceType.QUESTIONNAIRE) 2957 return "Questionnaire"; 2958 return "?"; 2959 } 2960 2961 public String toSystem(DefinitionResourceType code) { 2962 return code.getSystem(); 2963 } 2964 } 2965 2966 public enum DocumentReferenceStatus { 2967 /** 2968 * This is the current reference for this document. 2969 */ 2970 CURRENT, 2971 /** 2972 * This reference has been superseded by another reference. 2973 */ 2974 SUPERSEDED, 2975 /** 2976 * This reference was created in error. 2977 */ 2978 ENTEREDINERROR, 2979 /** 2980 * added to help the parsers 2981 */ 2982 NULL; 2983 2984 public static DocumentReferenceStatus fromCode(String codeString) throws FHIRException { 2985 if (codeString == null || "".equals(codeString)) 2986 return null; 2987 if ("current".equals(codeString)) 2988 return CURRENT; 2989 if ("superseded".equals(codeString)) 2990 return SUPERSEDED; 2991 if ("entered-in-error".equals(codeString)) 2992 return ENTEREDINERROR; 2993 throw new FHIRException("Unknown DocumentReferenceStatus code '" + codeString + "'"); 2994 } 2995 2996 public String toCode() { 2997 switch (this) { 2998 case CURRENT: 2999 return "current"; 3000 case SUPERSEDED: 3001 return "superseded"; 3002 case ENTEREDINERROR: 3003 return "entered-in-error"; 3004 case NULL: 3005 return null; 3006 default: 3007 return "?"; 3008 } 3009 } 3010 3011 public String getSystem() { 3012 switch (this) { 3013 case CURRENT: 3014 return "http://hl7.org/fhir/document-reference-status"; 3015 case SUPERSEDED: 3016 return "http://hl7.org/fhir/document-reference-status"; 3017 case ENTEREDINERROR: 3018 return "http://hl7.org/fhir/document-reference-status"; 3019 case NULL: 3020 return null; 3021 default: 3022 return "?"; 3023 } 3024 } 3025 3026 public String getDefinition() { 3027 switch (this) { 3028 case CURRENT: 3029 return "This is the current reference for this document."; 3030 case SUPERSEDED: 3031 return "This reference has been superseded by another reference."; 3032 case ENTEREDINERROR: 3033 return "This reference was created in error."; 3034 case NULL: 3035 return null; 3036 default: 3037 return "?"; 3038 } 3039 } 3040 3041 public String getDisplay() { 3042 switch (this) { 3043 case CURRENT: 3044 return "Current"; 3045 case SUPERSEDED: 3046 return "Superseded"; 3047 case ENTEREDINERROR: 3048 return "Entered in Error"; 3049 case NULL: 3050 return null; 3051 default: 3052 return "?"; 3053 } 3054 } 3055 } 3056 3057 public static class DocumentReferenceStatusEnumFactory implements EnumFactory<DocumentReferenceStatus> { 3058 public DocumentReferenceStatus fromCode(String codeString) throws IllegalArgumentException { 3059 if (codeString == null || "".equals(codeString)) 3060 if (codeString == null || "".equals(codeString)) 3061 return null; 3062 if ("current".equals(codeString)) 3063 return DocumentReferenceStatus.CURRENT; 3064 if ("superseded".equals(codeString)) 3065 return DocumentReferenceStatus.SUPERSEDED; 3066 if ("entered-in-error".equals(codeString)) 3067 return DocumentReferenceStatus.ENTEREDINERROR; 3068 throw new IllegalArgumentException("Unknown DocumentReferenceStatus code '" + codeString + "'"); 3069 } 3070 3071 public Enumeration<DocumentReferenceStatus> fromType(PrimitiveType<?> code) throws FHIRException { 3072 if (code == null) 3073 return null; 3074 if (code.isEmpty()) 3075 return new Enumeration<DocumentReferenceStatus>(this, DocumentReferenceStatus.NULL, code); 3076 String codeString = code.asStringValue(); 3077 if (codeString == null || "".equals(codeString)) 3078 return new Enumeration<DocumentReferenceStatus>(this, DocumentReferenceStatus.NULL, code); 3079 if ("current".equals(codeString)) 3080 return new Enumeration<DocumentReferenceStatus>(this, DocumentReferenceStatus.CURRENT, code); 3081 if ("superseded".equals(codeString)) 3082 return new Enumeration<DocumentReferenceStatus>(this, DocumentReferenceStatus.SUPERSEDED, code); 3083 if ("entered-in-error".equals(codeString)) 3084 return new Enumeration<DocumentReferenceStatus>(this, DocumentReferenceStatus.ENTEREDINERROR, code); 3085 throw new FHIRException("Unknown DocumentReferenceStatus code '" + codeString + "'"); 3086 } 3087 3088 public String toCode(DocumentReferenceStatus code) { 3089 if (code == DocumentReferenceStatus.NULL) 3090 return null; 3091 if (code == DocumentReferenceStatus.CURRENT) 3092 return "current"; 3093 if (code == DocumentReferenceStatus.SUPERSEDED) 3094 return "superseded"; 3095 if (code == DocumentReferenceStatus.ENTEREDINERROR) 3096 return "entered-in-error"; 3097 return "?"; 3098 } 3099 3100 public String toSystem(DocumentReferenceStatus code) { 3101 return code.getSystem(); 3102 } 3103 } 3104 3105 public enum EventResourceType { 3106 /** 3107 * Item containing charge code(s) associated with the provision of healthcare 3108 * provider products. 3109 */ 3110 CHARGEITEM, 3111 /** 3112 * Remittance resource. 3113 */ 3114 CLAIMRESPONSE, 3115 /** 3116 * A clinical assessment performed when planning treatments and management 3117 * strategies for a patient. 3118 */ 3119 CLINICALIMPRESSION, 3120 /** 3121 * A record of information transmitted from a sender to a receiver. 3122 */ 3123 COMMUNICATION, 3124 /** 3125 * A set of resources composed into a single coherent clinical statement with 3126 * clinical attestation. 3127 */ 3128 COMPOSITION, 3129 /** 3130 * Detailed information about conditions, problems or diagnoses. 3131 */ 3132 CONDITION, 3133 /** 3134 * A healthcare consumer's policy choices to permits or denies recipients or 3135 * roles to perform actions for specific purposes and periods of time. 3136 */ 3137 CONSENT, 3138 /** 3139 * Insurance or medical plan or a payment agreement. 3140 */ 3141 COVERAGE, 3142 /** 3143 * Record of use of a device. 3144 */ 3145 DEVICEUSESTATEMENT, 3146 /** 3147 * A Diagnostic report - a combination of request information, atomic results, 3148 * images, interpretation, as well as formatted reports. 3149 */ 3150 DIAGNOSTICREPORT, 3151 /** 3152 * A list that defines a set of documents. 3153 */ 3154 DOCUMENTMANIFEST, 3155 /** 3156 * A reference to a document. 3157 */ 3158 DOCUMENTREFERENCE, 3159 /** 3160 * An interaction during which services are provided to the patient. 3161 */ 3162 ENCOUNTER, 3163 /** 3164 * EnrollmentResponse resource. 3165 */ 3166 ENROLLMENTRESPONSE, 3167 /** 3168 * An association of a Patient with an Organization and Healthcare Provider(s) 3169 * for a period of time that the Organization assumes some level of 3170 * responsibility. 3171 */ 3172 EPISODEOFCARE, 3173 /** 3174 * Explanation of Benefit resource. 3175 */ 3176 EXPLANATIONOFBENEFIT, 3177 /** 3178 * Information about patient's relatives, relevant for patient. 3179 */ 3180 FAMILYMEMBERHISTORY, 3181 /** 3182 * The formal response to a guidance request. 3183 */ 3184 GUIDANCERESPONSE, 3185 /** 3186 * A set of images produced in single study (one or more series of references 3187 * images). 3188 */ 3189 IMAGINGSTUDY, 3190 /** 3191 * Immunization event information. 3192 */ 3193 IMMUNIZATION, 3194 /** 3195 * Results of a measure evaluation. 3196 */ 3197 MEASUREREPORT, 3198 /** 3199 * A photo, video, or audio recording acquired or used in healthcare. The actual 3200 * content may be inline or provided by direct reference. 3201 */ 3202 MEDIA, 3203 /** 3204 * Administration of medication to a patient. 3205 */ 3206 MEDICATIONADMINISTRATION, 3207 /** 3208 * Dispensing a medication to a named patient. 3209 */ 3210 MEDICATIONDISPENSE, 3211 /** 3212 * Record of medication being taken by a patient. 3213 */ 3214 MEDICATIONSTATEMENT, 3215 /** 3216 * Measurements and simple assertions. 3217 */ 3218 OBSERVATION, 3219 /** 3220 * PaymentNotice request. 3221 */ 3222 PAYMENTNOTICE, 3223 /** 3224 * PaymentReconciliation resource. 3225 */ 3226 PAYMENTRECONCILIATION, 3227 /** 3228 * An action that is being or was performed on a patient. 3229 */ 3230 PROCEDURE, 3231 /** 3232 * ProcessResponse resource. 3233 */ 3234 PROCESSRESPONSE, 3235 /** 3236 * A structured set of questions and their answers. 3237 */ 3238 QUESTIONNAIRERESPONSE, 3239 /** 3240 * Potential outcomes for a subject with likelihood. 3241 */ 3242 RISKASSESSMENT, 3243 /** 3244 * Delivery of bulk Supplies. 3245 */ 3246 SUPPLYDELIVERY, 3247 /** 3248 * A task to be performed. 3249 */ 3250 TASK, 3251 /** 3252 * added to help the parsers 3253 */ 3254 NULL; 3255 3256 public static EventResourceType fromCode(String codeString) throws FHIRException { 3257 if (codeString == null || "".equals(codeString)) 3258 return null; 3259 if ("ChargeItem".equals(codeString)) 3260 return CHARGEITEM; 3261 if ("ClaimResponse".equals(codeString)) 3262 return CLAIMRESPONSE; 3263 if ("ClinicalImpression".equals(codeString)) 3264 return CLINICALIMPRESSION; 3265 if ("Communication".equals(codeString)) 3266 return COMMUNICATION; 3267 if ("Composition".equals(codeString)) 3268 return COMPOSITION; 3269 if ("Condition".equals(codeString)) 3270 return CONDITION; 3271 if ("Consent".equals(codeString)) 3272 return CONSENT; 3273 if ("Coverage".equals(codeString)) 3274 return COVERAGE; 3275 if ("DeviceUseStatement".equals(codeString)) 3276 return DEVICEUSESTATEMENT; 3277 if ("DiagnosticReport".equals(codeString)) 3278 return DIAGNOSTICREPORT; 3279 if ("DocumentManifest".equals(codeString)) 3280 return DOCUMENTMANIFEST; 3281 if ("DocumentReference".equals(codeString)) 3282 return DOCUMENTREFERENCE; 3283 if ("Encounter".equals(codeString)) 3284 return ENCOUNTER; 3285 if ("EnrollmentResponse".equals(codeString)) 3286 return ENROLLMENTRESPONSE; 3287 if ("EpisodeOfCare".equals(codeString)) 3288 return EPISODEOFCARE; 3289 if ("ExplanationOfBenefit".equals(codeString)) 3290 return EXPLANATIONOFBENEFIT; 3291 if ("FamilyMemberHistory".equals(codeString)) 3292 return FAMILYMEMBERHISTORY; 3293 if ("GuidanceResponse".equals(codeString)) 3294 return GUIDANCERESPONSE; 3295 if ("ImagingStudy".equals(codeString)) 3296 return IMAGINGSTUDY; 3297 if ("Immunization".equals(codeString)) 3298 return IMMUNIZATION; 3299 if ("MeasureReport".equals(codeString)) 3300 return MEASUREREPORT; 3301 if ("Media".equals(codeString)) 3302 return MEDIA; 3303 if ("MedicationAdministration".equals(codeString)) 3304 return MEDICATIONADMINISTRATION; 3305 if ("MedicationDispense".equals(codeString)) 3306 return MEDICATIONDISPENSE; 3307 if ("MedicationStatement".equals(codeString)) 3308 return MEDICATIONSTATEMENT; 3309 if ("Observation".equals(codeString)) 3310 return OBSERVATION; 3311 if ("PaymentNotice".equals(codeString)) 3312 return PAYMENTNOTICE; 3313 if ("PaymentReconciliation".equals(codeString)) 3314 return PAYMENTRECONCILIATION; 3315 if ("Procedure".equals(codeString)) 3316 return PROCEDURE; 3317 if ("ProcessResponse".equals(codeString)) 3318 return PROCESSRESPONSE; 3319 if ("QuestionnaireResponse".equals(codeString)) 3320 return QUESTIONNAIRERESPONSE; 3321 if ("RiskAssessment".equals(codeString)) 3322 return RISKASSESSMENT; 3323 if ("SupplyDelivery".equals(codeString)) 3324 return SUPPLYDELIVERY; 3325 if ("Task".equals(codeString)) 3326 return TASK; 3327 throw new FHIRException("Unknown EventResourceType code '" + codeString + "'"); 3328 } 3329 3330 public String toCode() { 3331 switch (this) { 3332 case CHARGEITEM: 3333 return "ChargeItem"; 3334 case CLAIMRESPONSE: 3335 return "ClaimResponse"; 3336 case CLINICALIMPRESSION: 3337 return "ClinicalImpression"; 3338 case COMMUNICATION: 3339 return "Communication"; 3340 case COMPOSITION: 3341 return "Composition"; 3342 case CONDITION: 3343 return "Condition"; 3344 case CONSENT: 3345 return "Consent"; 3346 case COVERAGE: 3347 return "Coverage"; 3348 case DEVICEUSESTATEMENT: 3349 return "DeviceUseStatement"; 3350 case DIAGNOSTICREPORT: 3351 return "DiagnosticReport"; 3352 case DOCUMENTMANIFEST: 3353 return "DocumentManifest"; 3354 case DOCUMENTREFERENCE: 3355 return "DocumentReference"; 3356 case ENCOUNTER: 3357 return "Encounter"; 3358 case ENROLLMENTRESPONSE: 3359 return "EnrollmentResponse"; 3360 case EPISODEOFCARE: 3361 return "EpisodeOfCare"; 3362 case EXPLANATIONOFBENEFIT: 3363 return "ExplanationOfBenefit"; 3364 case FAMILYMEMBERHISTORY: 3365 return "FamilyMemberHistory"; 3366 case GUIDANCERESPONSE: 3367 return "GuidanceResponse"; 3368 case IMAGINGSTUDY: 3369 return "ImagingStudy"; 3370 case IMMUNIZATION: 3371 return "Immunization"; 3372 case MEASUREREPORT: 3373 return "MeasureReport"; 3374 case MEDIA: 3375 return "Media"; 3376 case MEDICATIONADMINISTRATION: 3377 return "MedicationAdministration"; 3378 case MEDICATIONDISPENSE: 3379 return "MedicationDispense"; 3380 case MEDICATIONSTATEMENT: 3381 return "MedicationStatement"; 3382 case OBSERVATION: 3383 return "Observation"; 3384 case PAYMENTNOTICE: 3385 return "PaymentNotice"; 3386 case PAYMENTRECONCILIATION: 3387 return "PaymentReconciliation"; 3388 case PROCEDURE: 3389 return "Procedure"; 3390 case PROCESSRESPONSE: 3391 return "ProcessResponse"; 3392 case QUESTIONNAIRERESPONSE: 3393 return "QuestionnaireResponse"; 3394 case RISKASSESSMENT: 3395 return "RiskAssessment"; 3396 case SUPPLYDELIVERY: 3397 return "SupplyDelivery"; 3398 case TASK: 3399 return "Task"; 3400 case NULL: 3401 return null; 3402 default: 3403 return "?"; 3404 } 3405 } 3406 3407 public String getSystem() { 3408 switch (this) { 3409 case CHARGEITEM: 3410 return "http://hl7.org/fhir/event-resource-types"; 3411 case CLAIMRESPONSE: 3412 return "http://hl7.org/fhir/event-resource-types"; 3413 case CLINICALIMPRESSION: 3414 return "http://hl7.org/fhir/event-resource-types"; 3415 case COMMUNICATION: 3416 return "http://hl7.org/fhir/event-resource-types"; 3417 case COMPOSITION: 3418 return "http://hl7.org/fhir/event-resource-types"; 3419 case CONDITION: 3420 return "http://hl7.org/fhir/event-resource-types"; 3421 case CONSENT: 3422 return "http://hl7.org/fhir/event-resource-types"; 3423 case COVERAGE: 3424 return "http://hl7.org/fhir/event-resource-types"; 3425 case DEVICEUSESTATEMENT: 3426 return "http://hl7.org/fhir/event-resource-types"; 3427 case DIAGNOSTICREPORT: 3428 return "http://hl7.org/fhir/event-resource-types"; 3429 case DOCUMENTMANIFEST: 3430 return "http://hl7.org/fhir/event-resource-types"; 3431 case DOCUMENTREFERENCE: 3432 return "http://hl7.org/fhir/event-resource-types"; 3433 case ENCOUNTER: 3434 return "http://hl7.org/fhir/event-resource-types"; 3435 case ENROLLMENTRESPONSE: 3436 return "http://hl7.org/fhir/event-resource-types"; 3437 case EPISODEOFCARE: 3438 return "http://hl7.org/fhir/event-resource-types"; 3439 case EXPLANATIONOFBENEFIT: 3440 return "http://hl7.org/fhir/event-resource-types"; 3441 case FAMILYMEMBERHISTORY: 3442 return "http://hl7.org/fhir/event-resource-types"; 3443 case GUIDANCERESPONSE: 3444 return "http://hl7.org/fhir/event-resource-types"; 3445 case IMAGINGSTUDY: 3446 return "http://hl7.org/fhir/event-resource-types"; 3447 case IMMUNIZATION: 3448 return "http://hl7.org/fhir/event-resource-types"; 3449 case MEASUREREPORT: 3450 return "http://hl7.org/fhir/event-resource-types"; 3451 case MEDIA: 3452 return "http://hl7.org/fhir/event-resource-types"; 3453 case MEDICATIONADMINISTRATION: 3454 return "http://hl7.org/fhir/event-resource-types"; 3455 case MEDICATIONDISPENSE: 3456 return "http://hl7.org/fhir/event-resource-types"; 3457 case MEDICATIONSTATEMENT: 3458 return "http://hl7.org/fhir/event-resource-types"; 3459 case OBSERVATION: 3460 return "http://hl7.org/fhir/event-resource-types"; 3461 case PAYMENTNOTICE: 3462 return "http://hl7.org/fhir/event-resource-types"; 3463 case PAYMENTRECONCILIATION: 3464 return "http://hl7.org/fhir/event-resource-types"; 3465 case PROCEDURE: 3466 return "http://hl7.org/fhir/event-resource-types"; 3467 case PROCESSRESPONSE: 3468 return "http://hl7.org/fhir/event-resource-types"; 3469 case QUESTIONNAIRERESPONSE: 3470 return "http://hl7.org/fhir/event-resource-types"; 3471 case RISKASSESSMENT: 3472 return "http://hl7.org/fhir/event-resource-types"; 3473 case SUPPLYDELIVERY: 3474 return "http://hl7.org/fhir/event-resource-types"; 3475 case TASK: 3476 return "http://hl7.org/fhir/event-resource-types"; 3477 case NULL: 3478 return null; 3479 default: 3480 return "?"; 3481 } 3482 } 3483 3484 public String getDefinition() { 3485 switch (this) { 3486 case CHARGEITEM: 3487 return "Item containing charge code(s) associated with the provision of healthcare provider products."; 3488 case CLAIMRESPONSE: 3489 return "Remittance resource."; 3490 case CLINICALIMPRESSION: 3491 return "A clinical assessment performed when planning treatments and management strategies for a patient."; 3492 case COMMUNICATION: 3493 return "A record of information transmitted from a sender to a receiver."; 3494 case COMPOSITION: 3495 return "A set of resources composed into a single coherent clinical statement with clinical attestation."; 3496 case CONDITION: 3497 return "Detailed information about conditions, problems or diagnoses."; 3498 case CONSENT: 3499 return "A healthcare consumer's policy choices to permits or denies recipients or roles to perform actions for specific purposes and periods of time."; 3500 case COVERAGE: 3501 return "Insurance or medical plan or a payment agreement."; 3502 case DEVICEUSESTATEMENT: 3503 return "Record of use of a device."; 3504 case DIAGNOSTICREPORT: 3505 return "A Diagnostic report - a combination of request information, atomic results, images, interpretation, as well as formatted reports."; 3506 case DOCUMENTMANIFEST: 3507 return "A list that defines a set of documents."; 3508 case DOCUMENTREFERENCE: 3509 return "A reference to a document."; 3510 case ENCOUNTER: 3511 return "An interaction during which services are provided to the patient."; 3512 case ENROLLMENTRESPONSE: 3513 return "EnrollmentResponse resource."; 3514 case EPISODEOFCARE: 3515 return "An association of a Patient with an Organization and Healthcare Provider(s) for a period of time that the Organization assumes some level of responsibility."; 3516 case EXPLANATIONOFBENEFIT: 3517 return "Explanation of Benefit resource."; 3518 case FAMILYMEMBERHISTORY: 3519 return "Information about patient's relatives, relevant for patient."; 3520 case GUIDANCERESPONSE: 3521 return "The formal response to a guidance request."; 3522 case IMAGINGSTUDY: 3523 return "A set of images produced in single study (one or more series of references images)."; 3524 case IMMUNIZATION: 3525 return "Immunization event information."; 3526 case MEASUREREPORT: 3527 return "Results of a measure evaluation."; 3528 case MEDIA: 3529 return "A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference."; 3530 case MEDICATIONADMINISTRATION: 3531 return "Administration of medication to a patient."; 3532 case MEDICATIONDISPENSE: 3533 return "Dispensing a medication to a named patient."; 3534 case MEDICATIONSTATEMENT: 3535 return "Record of medication being taken by a patient."; 3536 case OBSERVATION: 3537 return "Measurements and simple assertions."; 3538 case PAYMENTNOTICE: 3539 return "PaymentNotice request."; 3540 case PAYMENTRECONCILIATION: 3541 return "PaymentReconciliation resource."; 3542 case PROCEDURE: 3543 return "An action that is being or was performed on a patient."; 3544 case PROCESSRESPONSE: 3545 return "ProcessResponse resource."; 3546 case QUESTIONNAIRERESPONSE: 3547 return "A structured set of questions and their answers."; 3548 case RISKASSESSMENT: 3549 return "Potential outcomes for a subject with likelihood."; 3550 case SUPPLYDELIVERY: 3551 return "Delivery of bulk Supplies."; 3552 case TASK: 3553 return "A task to be performed."; 3554 case NULL: 3555 return null; 3556 default: 3557 return "?"; 3558 } 3559 } 3560 3561 public String getDisplay() { 3562 switch (this) { 3563 case CHARGEITEM: 3564 return "ChargeItem"; 3565 case CLAIMRESPONSE: 3566 return "ClaimResponse"; 3567 case CLINICALIMPRESSION: 3568 return "ClinicalImpression"; 3569 case COMMUNICATION: 3570 return "Communication"; 3571 case COMPOSITION: 3572 return "Composition"; 3573 case CONDITION: 3574 return "Condition"; 3575 case CONSENT: 3576 return "Consent"; 3577 case COVERAGE: 3578 return "Coverage"; 3579 case DEVICEUSESTATEMENT: 3580 return "DeviceUseStatement"; 3581 case DIAGNOSTICREPORT: 3582 return "DiagnosticReport"; 3583 case DOCUMENTMANIFEST: 3584 return "DocumentManifest"; 3585 case DOCUMENTREFERENCE: 3586 return "DocumentReference"; 3587 case ENCOUNTER: 3588 return "Encounter"; 3589 case ENROLLMENTRESPONSE: 3590 return "EnrollmentResponse"; 3591 case EPISODEOFCARE: 3592 return "EpisodeOfCare"; 3593 case EXPLANATIONOFBENEFIT: 3594 return "ExplanationOfBenefit"; 3595 case FAMILYMEMBERHISTORY: 3596 return "FamilyMemberHistory"; 3597 case GUIDANCERESPONSE: 3598 return "GuidanceResponse"; 3599 case IMAGINGSTUDY: 3600 return "ImagingStudy"; 3601 case IMMUNIZATION: 3602 return "Immunization"; 3603 case MEASUREREPORT: 3604 return "MeasureReport"; 3605 case MEDIA: 3606 return "Media"; 3607 case MEDICATIONADMINISTRATION: 3608 return "MedicationAdministration"; 3609 case MEDICATIONDISPENSE: 3610 return "MedicationDispense"; 3611 case MEDICATIONSTATEMENT: 3612 return "MedicationStatement"; 3613 case OBSERVATION: 3614 return "Observation"; 3615 case PAYMENTNOTICE: 3616 return "PaymentNotice"; 3617 case PAYMENTRECONCILIATION: 3618 return "PaymentReconciliation"; 3619 case PROCEDURE: 3620 return "Procedure"; 3621 case PROCESSRESPONSE: 3622 return "ProcessResponse"; 3623 case QUESTIONNAIRERESPONSE: 3624 return "QuestionnaireResponse"; 3625 case RISKASSESSMENT: 3626 return "RiskAssessment"; 3627 case SUPPLYDELIVERY: 3628 return "SupplyDelivery"; 3629 case TASK: 3630 return "Task"; 3631 case NULL: 3632 return null; 3633 default: 3634 return "?"; 3635 } 3636 } 3637 } 3638 3639 public static class EventResourceTypeEnumFactory implements EnumFactory<EventResourceType> { 3640 public EventResourceType fromCode(String codeString) throws IllegalArgumentException { 3641 if (codeString == null || "".equals(codeString)) 3642 if (codeString == null || "".equals(codeString)) 3643 return null; 3644 if ("ChargeItem".equals(codeString)) 3645 return EventResourceType.CHARGEITEM; 3646 if ("ClaimResponse".equals(codeString)) 3647 return EventResourceType.CLAIMRESPONSE; 3648 if ("ClinicalImpression".equals(codeString)) 3649 return EventResourceType.CLINICALIMPRESSION; 3650 if ("Communication".equals(codeString)) 3651 return EventResourceType.COMMUNICATION; 3652 if ("Composition".equals(codeString)) 3653 return EventResourceType.COMPOSITION; 3654 if ("Condition".equals(codeString)) 3655 return EventResourceType.CONDITION; 3656 if ("Consent".equals(codeString)) 3657 return EventResourceType.CONSENT; 3658 if ("Coverage".equals(codeString)) 3659 return EventResourceType.COVERAGE; 3660 if ("DeviceUseStatement".equals(codeString)) 3661 return EventResourceType.DEVICEUSESTATEMENT; 3662 if ("DiagnosticReport".equals(codeString)) 3663 return EventResourceType.DIAGNOSTICREPORT; 3664 if ("DocumentManifest".equals(codeString)) 3665 return EventResourceType.DOCUMENTMANIFEST; 3666 if ("DocumentReference".equals(codeString)) 3667 return EventResourceType.DOCUMENTREFERENCE; 3668 if ("Encounter".equals(codeString)) 3669 return EventResourceType.ENCOUNTER; 3670 if ("EnrollmentResponse".equals(codeString)) 3671 return EventResourceType.ENROLLMENTRESPONSE; 3672 if ("EpisodeOfCare".equals(codeString)) 3673 return EventResourceType.EPISODEOFCARE; 3674 if ("ExplanationOfBenefit".equals(codeString)) 3675 return EventResourceType.EXPLANATIONOFBENEFIT; 3676 if ("FamilyMemberHistory".equals(codeString)) 3677 return EventResourceType.FAMILYMEMBERHISTORY; 3678 if ("GuidanceResponse".equals(codeString)) 3679 return EventResourceType.GUIDANCERESPONSE; 3680 if ("ImagingStudy".equals(codeString)) 3681 return EventResourceType.IMAGINGSTUDY; 3682 if ("Immunization".equals(codeString)) 3683 return EventResourceType.IMMUNIZATION; 3684 if ("MeasureReport".equals(codeString)) 3685 return EventResourceType.MEASUREREPORT; 3686 if ("Media".equals(codeString)) 3687 return EventResourceType.MEDIA; 3688 if ("MedicationAdministration".equals(codeString)) 3689 return EventResourceType.MEDICATIONADMINISTRATION; 3690 if ("MedicationDispense".equals(codeString)) 3691 return EventResourceType.MEDICATIONDISPENSE; 3692 if ("MedicationStatement".equals(codeString)) 3693 return EventResourceType.MEDICATIONSTATEMENT; 3694 if ("Observation".equals(codeString)) 3695 return EventResourceType.OBSERVATION; 3696 if ("PaymentNotice".equals(codeString)) 3697 return EventResourceType.PAYMENTNOTICE; 3698 if ("PaymentReconciliation".equals(codeString)) 3699 return EventResourceType.PAYMENTRECONCILIATION; 3700 if ("Procedure".equals(codeString)) 3701 return EventResourceType.PROCEDURE; 3702 if ("ProcessResponse".equals(codeString)) 3703 return EventResourceType.PROCESSRESPONSE; 3704 if ("QuestionnaireResponse".equals(codeString)) 3705 return EventResourceType.QUESTIONNAIRERESPONSE; 3706 if ("RiskAssessment".equals(codeString)) 3707 return EventResourceType.RISKASSESSMENT; 3708 if ("SupplyDelivery".equals(codeString)) 3709 return EventResourceType.SUPPLYDELIVERY; 3710 if ("Task".equals(codeString)) 3711 return EventResourceType.TASK; 3712 throw new IllegalArgumentException("Unknown EventResourceType code '" + codeString + "'"); 3713 } 3714 3715 public Enumeration<EventResourceType> fromType(PrimitiveType<?> code) throws FHIRException { 3716 if (code == null) 3717 return null; 3718 if (code.isEmpty()) 3719 return new Enumeration<EventResourceType>(this, EventResourceType.NULL, code); 3720 String codeString = code.asStringValue(); 3721 if (codeString == null || "".equals(codeString)) 3722 return new Enumeration<EventResourceType>(this, EventResourceType.NULL, code); 3723 if ("ChargeItem".equals(codeString)) 3724 return new Enumeration<EventResourceType>(this, EventResourceType.CHARGEITEM, code); 3725 if ("ClaimResponse".equals(codeString)) 3726 return new Enumeration<EventResourceType>(this, EventResourceType.CLAIMRESPONSE, code); 3727 if ("ClinicalImpression".equals(codeString)) 3728 return new Enumeration<EventResourceType>(this, EventResourceType.CLINICALIMPRESSION, code); 3729 if ("Communication".equals(codeString)) 3730 return new Enumeration<EventResourceType>(this, EventResourceType.COMMUNICATION, code); 3731 if ("Composition".equals(codeString)) 3732 return new Enumeration<EventResourceType>(this, EventResourceType.COMPOSITION, code); 3733 if ("Condition".equals(codeString)) 3734 return new Enumeration<EventResourceType>(this, EventResourceType.CONDITION, code); 3735 if ("Consent".equals(codeString)) 3736 return new Enumeration<EventResourceType>(this, EventResourceType.CONSENT, code); 3737 if ("Coverage".equals(codeString)) 3738 return new Enumeration<EventResourceType>(this, EventResourceType.COVERAGE, code); 3739 if ("DeviceUseStatement".equals(codeString)) 3740 return new Enumeration<EventResourceType>(this, EventResourceType.DEVICEUSESTATEMENT, code); 3741 if ("DiagnosticReport".equals(codeString)) 3742 return new Enumeration<EventResourceType>(this, EventResourceType.DIAGNOSTICREPORT, code); 3743 if ("DocumentManifest".equals(codeString)) 3744 return new Enumeration<EventResourceType>(this, EventResourceType.DOCUMENTMANIFEST, code); 3745 if ("DocumentReference".equals(codeString)) 3746 return new Enumeration<EventResourceType>(this, EventResourceType.DOCUMENTREFERENCE, code); 3747 if ("Encounter".equals(codeString)) 3748 return new Enumeration<EventResourceType>(this, EventResourceType.ENCOUNTER, code); 3749 if ("EnrollmentResponse".equals(codeString)) 3750 return new Enumeration<EventResourceType>(this, EventResourceType.ENROLLMENTRESPONSE, code); 3751 if ("EpisodeOfCare".equals(codeString)) 3752 return new Enumeration<EventResourceType>(this, EventResourceType.EPISODEOFCARE, code); 3753 if ("ExplanationOfBenefit".equals(codeString)) 3754 return new Enumeration<EventResourceType>(this, EventResourceType.EXPLANATIONOFBENEFIT, code); 3755 if ("FamilyMemberHistory".equals(codeString)) 3756 return new Enumeration<EventResourceType>(this, EventResourceType.FAMILYMEMBERHISTORY, code); 3757 if ("GuidanceResponse".equals(codeString)) 3758 return new Enumeration<EventResourceType>(this, EventResourceType.GUIDANCERESPONSE, code); 3759 if ("ImagingStudy".equals(codeString)) 3760 return new Enumeration<EventResourceType>(this, EventResourceType.IMAGINGSTUDY, code); 3761 if ("Immunization".equals(codeString)) 3762 return new Enumeration<EventResourceType>(this, EventResourceType.IMMUNIZATION, code); 3763 if ("MeasureReport".equals(codeString)) 3764 return new Enumeration<EventResourceType>(this, EventResourceType.MEASUREREPORT, code); 3765 if ("Media".equals(codeString)) 3766 return new Enumeration<EventResourceType>(this, EventResourceType.MEDIA, code); 3767 if ("MedicationAdministration".equals(codeString)) 3768 return new Enumeration<EventResourceType>(this, EventResourceType.MEDICATIONADMINISTRATION, code); 3769 if ("MedicationDispense".equals(codeString)) 3770 return new Enumeration<EventResourceType>(this, EventResourceType.MEDICATIONDISPENSE, code); 3771 if ("MedicationStatement".equals(codeString)) 3772 return new Enumeration<EventResourceType>(this, EventResourceType.MEDICATIONSTATEMENT, code); 3773 if ("Observation".equals(codeString)) 3774 return new Enumeration<EventResourceType>(this, EventResourceType.OBSERVATION, code); 3775 if ("PaymentNotice".equals(codeString)) 3776 return new Enumeration<EventResourceType>(this, EventResourceType.PAYMENTNOTICE, code); 3777 if ("PaymentReconciliation".equals(codeString)) 3778 return new Enumeration<EventResourceType>(this, EventResourceType.PAYMENTRECONCILIATION, code); 3779 if ("Procedure".equals(codeString)) 3780 return new Enumeration<EventResourceType>(this, EventResourceType.PROCEDURE, code); 3781 if ("ProcessResponse".equals(codeString)) 3782 return new Enumeration<EventResourceType>(this, EventResourceType.PROCESSRESPONSE, code); 3783 if ("QuestionnaireResponse".equals(codeString)) 3784 return new Enumeration<EventResourceType>(this, EventResourceType.QUESTIONNAIRERESPONSE, code); 3785 if ("RiskAssessment".equals(codeString)) 3786 return new Enumeration<EventResourceType>(this, EventResourceType.RISKASSESSMENT, code); 3787 if ("SupplyDelivery".equals(codeString)) 3788 return new Enumeration<EventResourceType>(this, EventResourceType.SUPPLYDELIVERY, code); 3789 if ("Task".equals(codeString)) 3790 return new Enumeration<EventResourceType>(this, EventResourceType.TASK, code); 3791 throw new FHIRException("Unknown EventResourceType code '" + codeString + "'"); 3792 } 3793 3794 public String toCode(EventResourceType code) { 3795 if (code == EventResourceType.NULL) 3796 return null; 3797 if (code == EventResourceType.CHARGEITEM) 3798 return "ChargeItem"; 3799 if (code == EventResourceType.CLAIMRESPONSE) 3800 return "ClaimResponse"; 3801 if (code == EventResourceType.CLINICALIMPRESSION) 3802 return "ClinicalImpression"; 3803 if (code == EventResourceType.COMMUNICATION) 3804 return "Communication"; 3805 if (code == EventResourceType.COMPOSITION) 3806 return "Composition"; 3807 if (code == EventResourceType.CONDITION) 3808 return "Condition"; 3809 if (code == EventResourceType.CONSENT) 3810 return "Consent"; 3811 if (code == EventResourceType.COVERAGE) 3812 return "Coverage"; 3813 if (code == EventResourceType.DEVICEUSESTATEMENT) 3814 return "DeviceUseStatement"; 3815 if (code == EventResourceType.DIAGNOSTICREPORT) 3816 return "DiagnosticReport"; 3817 if (code == EventResourceType.DOCUMENTMANIFEST) 3818 return "DocumentManifest"; 3819 if (code == EventResourceType.DOCUMENTREFERENCE) 3820 return "DocumentReference"; 3821 if (code == EventResourceType.ENCOUNTER) 3822 return "Encounter"; 3823 if (code == EventResourceType.ENROLLMENTRESPONSE) 3824 return "EnrollmentResponse"; 3825 if (code == EventResourceType.EPISODEOFCARE) 3826 return "EpisodeOfCare"; 3827 if (code == EventResourceType.EXPLANATIONOFBENEFIT) 3828 return "ExplanationOfBenefit"; 3829 if (code == EventResourceType.FAMILYMEMBERHISTORY) 3830 return "FamilyMemberHistory"; 3831 if (code == EventResourceType.GUIDANCERESPONSE) 3832 return "GuidanceResponse"; 3833 if (code == EventResourceType.IMAGINGSTUDY) 3834 return "ImagingStudy"; 3835 if (code == EventResourceType.IMMUNIZATION) 3836 return "Immunization"; 3837 if (code == EventResourceType.MEASUREREPORT) 3838 return "MeasureReport"; 3839 if (code == EventResourceType.MEDIA) 3840 return "Media"; 3841 if (code == EventResourceType.MEDICATIONADMINISTRATION) 3842 return "MedicationAdministration"; 3843 if (code == EventResourceType.MEDICATIONDISPENSE) 3844 return "MedicationDispense"; 3845 if (code == EventResourceType.MEDICATIONSTATEMENT) 3846 return "MedicationStatement"; 3847 if (code == EventResourceType.OBSERVATION) 3848 return "Observation"; 3849 if (code == EventResourceType.PAYMENTNOTICE) 3850 return "PaymentNotice"; 3851 if (code == EventResourceType.PAYMENTRECONCILIATION) 3852 return "PaymentReconciliation"; 3853 if (code == EventResourceType.PROCEDURE) 3854 return "Procedure"; 3855 if (code == EventResourceType.PROCESSRESPONSE) 3856 return "ProcessResponse"; 3857 if (code == EventResourceType.QUESTIONNAIRERESPONSE) 3858 return "QuestionnaireResponse"; 3859 if (code == EventResourceType.RISKASSESSMENT) 3860 return "RiskAssessment"; 3861 if (code == EventResourceType.SUPPLYDELIVERY) 3862 return "SupplyDelivery"; 3863 if (code == EventResourceType.TASK) 3864 return "Task"; 3865 return "?"; 3866 } 3867 3868 public String toSystem(EventResourceType code) { 3869 return code.getSystem(); 3870 } 3871 } 3872 3873 public enum FHIRAllTypes { 3874 /** 3875 * An address expressed using postal conventions (as opposed to GPS or other 3876 * location definition formats). This data type may be used to convey addresses 3877 * for use in delivering mail as well as for visiting locations which might not 3878 * be valid for mail delivery. There are a variety of postal address formats 3879 * defined around the world. 3880 */ 3881 ADDRESS, 3882 /** 3883 * A duration of time during which an organism (or a process) has existed. 3884 */ 3885 AGE, 3886 /** 3887 * A text note which also contains information about who made the statement and 3888 * when. 3889 */ 3890 ANNOTATION, 3891 /** 3892 * For referring to data content defined in other formats. 3893 */ 3894 ATTACHMENT, 3895 /** 3896 * Base definition for all elements that are defined inside a resource - but not 3897 * those in a data type. 3898 */ 3899 BACKBONEELEMENT, 3900 /** 3901 * A concept that may be defined by a formal reference to a terminology or 3902 * ontology or may be provided by text. 3903 */ 3904 CODEABLECONCEPT, 3905 /** 3906 * A reference to a code defined by a terminology system. 3907 */ 3908 CODING, 3909 /** 3910 * Specifies contact information for a person or organization. 3911 */ 3912 CONTACTDETAIL, 3913 /** 3914 * Details for all kinds of technology mediated contact points for a person or 3915 * organization, including telephone, email, etc. 3916 */ 3917 CONTACTPOINT, 3918 /** 3919 * A contributor to the content of a knowledge asset, including authors, 3920 * editors, reviewers, and endorsers. 3921 */ 3922 CONTRIBUTOR, 3923 /** 3924 * A measured amount (or an amount that can potentially be measured). Note that 3925 * measured amounts include amounts that are not precisely quantified, including 3926 * amounts involving arbitrary units and floating currencies. 3927 */ 3928 COUNT, 3929 /** 3930 * Describes a required data item for evaluation in terms of the type of data, 3931 * and optional code or date-based filters of the data. 3932 */ 3933 DATAREQUIREMENT, 3934 /** 3935 * A length - a value with a unit that is a physical distance. 3936 */ 3937 DISTANCE, 3938 /** 3939 * Indicates how the medication is/was taken or should be taken by the patient. 3940 */ 3941 DOSAGE, 3942 /** 3943 * A length of time. 3944 */ 3945 DURATION, 3946 /** 3947 * Base definition for all elements in a resource. 3948 */ 3949 ELEMENT, 3950 /** 3951 * Captures constraints on each element within the resource, profile, or 3952 * extension. 3953 */ 3954 ELEMENTDEFINITION, 3955 /** 3956 * A expression that is evaluated in a specified context and returns a value. 3957 * The context of use of the expression must specify the context in which the 3958 * expression is evaluated, and how the result of the expression is used. 3959 */ 3960 EXPRESSION, 3961 /** 3962 * Optional Extension Element - found in all resources. 3963 */ 3964 EXTENSION, 3965 /** 3966 * A human's name with the ability to identify parts and usage. 3967 */ 3968 HUMANNAME, 3969 /** 3970 * An identifier - identifies some entity uniquely and unambiguously. Typically 3971 * this is used for business identifiers. 3972 */ 3973 IDENTIFIER, 3974 /** 3975 * The marketing status describes the date when a medicinal product is actually 3976 * put on the market or the date as of which it is no longer available. 3977 */ 3978 MARKETINGSTATUS, 3979 /** 3980 * The metadata about a resource. This is content in the resource that is 3981 * maintained by the infrastructure. Changes to the content might not always be 3982 * associated with version changes to the resource. 3983 */ 3984 META, 3985 /** 3986 * An amount of economic utility in some recognized currency. 3987 */ 3988 MONEY, 3989 /** 3990 * null 3991 */ 3992 MONEYQUANTITY, 3993 /** 3994 * A human-readable summary of the resource conveying the essential clinical and 3995 * business information for the resource. 3996 */ 3997 NARRATIVE, 3998 /** 3999 * The parameters to the module. This collection specifies both the input and 4000 * output parameters. Input parameters are provided by the caller as part of the 4001 * $evaluate operation. Output parameters are included in the GuidanceResponse. 4002 */ 4003 PARAMETERDEFINITION, 4004 /** 4005 * A time period defined by a start and end date and optionally time. 4006 */ 4007 PERIOD, 4008 /** 4009 * A populatioof people with some set of grouping criteria. 4010 */ 4011 POPULATION, 4012 /** 4013 * The marketing status describes the date when a medicinal product is actually 4014 * put on the market or the date as of which it is no longer available. 4015 */ 4016 PRODCHARACTERISTIC, 4017 /** 4018 * The shelf-life and storage information for a medicinal product item or 4019 * container can be described using this class. 4020 */ 4021 PRODUCTSHELFLIFE, 4022 /** 4023 * A measured amount (or an amount that can potentially be measured). Note that 4024 * measured amounts include amounts that are not precisely quantified, including 4025 * amounts involving arbitrary units and floating currencies. 4026 */ 4027 QUANTITY, 4028 /** 4029 * A set of ordered Quantities defined by a low and high limit. 4030 */ 4031 RANGE, 4032 /** 4033 * A relationship of two Quantity values - expressed as a numerator and a 4034 * denominator. 4035 */ 4036 RATIO, 4037 /** 4038 * A reference from one resource to another. 4039 */ 4040 REFERENCE, 4041 /** 4042 * Related artifacts such as additional documentation, justification, or 4043 * bibliographic references. 4044 */ 4045 RELATEDARTIFACT, 4046 /** 4047 * A series of measurements taken by a device, with upper and lower limits. 4048 * There may be more than one dimension in the data. 4049 */ 4050 SAMPLEDDATA, 4051 /** 4052 * A signature along with supporting context. The signature may be a digital 4053 * signature that is cryptographic in nature, or some other signature acceptable 4054 * to the domain. This other signature may be as simple as a graphical image 4055 * representing a hand-written signature, or a signature ceremony Different 4056 * signature approaches have different utilities. 4057 */ 4058 SIGNATURE, 4059 /** 4060 * null 4061 */ 4062 SIMPLEQUANTITY, 4063 /** 4064 * Chemical substances are a single substance type whose primary defining 4065 * element is the molecular structure. Chemical substances shall be defined on 4066 * the basis of their complete covalent molecular structure; the presence of a 4067 * salt (counter-ion) and/or solvates (water, alcohols) is also captured. 4068 * Purity, grade, physical form or particle size are not taken into account in 4069 * the definition of a chemical substance or in the assignment of a Substance 4070 * ID. 4071 */ 4072 SUBSTANCEAMOUNT, 4073 /** 4074 * Specifies an event that may occur multiple times. Timing schedules are used 4075 * to record when things are planned, expected or requested to occur. The most 4076 * common usage is in dosage instructions for medications. They are also used 4077 * when planning care of various kinds, and may be used for reporting the 4078 * schedule to which past regular activities were carried out. 4079 */ 4080 TIMING, 4081 /** 4082 * A description of a triggering event. Triggering events can be named events, 4083 * data events, or periodic, as determined by the type element. 4084 */ 4085 TRIGGERDEFINITION, 4086 /** 4087 * Specifies clinical/business/etc. metadata that can be used to retrieve, index 4088 * and/or categorize an artifact. This metadata can either be specific to the 4089 * applicable population (e.g., age category, DRG) or the specific context of 4090 * care (e.g., venue, care setting, provider of care). 4091 */ 4092 USAGECONTEXT, 4093 /** 4094 * A stream of bytes 4095 */ 4096 BASE64BINARY, 4097 /** 4098 * Value of "true" or "false" 4099 */ 4100 BOOLEAN, 4101 /** 4102 * A URI that is a reference to a canonical URL on a FHIR resource 4103 */ 4104 CANONICAL, 4105 /** 4106 * A string which has at least one character and no leading or trailing 4107 * whitespace and where there is no whitespace other than single spaces in the 4108 * contents 4109 */ 4110 CODE, 4111 /** 4112 * A date or partial date (e.g. just year or year + month). There is no time 4113 * zone. The format is a union of the schema types gYear, gYearMonth and date. 4114 * Dates SHALL be valid dates. 4115 */ 4116 DATE, 4117 /** 4118 * A date, date-time or partial date (e.g. just year or year + month). If hours 4119 * and minutes are specified, a time zone SHALL be populated. The format is a 4120 * union of the schema types gYear, gYearMonth, date and dateTime. Seconds must 4121 * be provided due to schema type constraints but may be zero-filled and may be 4122 * ignored. Dates SHALL be valid dates. 4123 */ 4124 DATETIME, 4125 /** 4126 * A rational number with implicit precision 4127 */ 4128 DECIMAL, 4129 /** 4130 * Any combination of letters, numerals, "-" and ".", with a length limit of 64 4131 * characters. (This might be an integer, an unprefixed OID, UUID or any other 4132 * identifier pattern that meets these constraints.) Ids are case-insensitive. 4133 */ 4134 ID, 4135 /** 4136 * An instant in time - known at least to the second 4137 */ 4138 INSTANT, 4139 /** 4140 * A whole number 4141 */ 4142 INTEGER, 4143 /** 4144 * A string that may contain Github Flavored Markdown syntax for optional 4145 * processing by a mark down presentation engine 4146 */ 4147 MARKDOWN, 4148 /** 4149 * An OID represented as a URI 4150 */ 4151 OID, 4152 /** 4153 * An integer with a value that is positive (e.g. >0) 4154 */ 4155 POSITIVEINT, 4156 /** 4157 * A sequence of Unicode characters 4158 */ 4159 STRING, 4160 /** 4161 * A time during the day, with no date specified 4162 */ 4163 TIME, 4164 /** 4165 * An integer with a value that is not negative (e.g. >= 0) 4166 */ 4167 UNSIGNEDINT, 4168 /** 4169 * String of characters used to identify a name or a resource 4170 */ 4171 URI, 4172 /** 4173 * A URI that is a literal reference 4174 */ 4175 URL, 4176 /** 4177 * A UUID, represented as a URI 4178 */ 4179 UUID, 4180 /** 4181 * XHTML format, as defined by W3C, but restricted usage (mainly, no active 4182 * content) 4183 */ 4184 XHTML, 4185 /** 4186 * A financial tool for tracking value accrued for a particular purpose. In the 4187 * healthcare field, used to track charges for a patient, cost centers, etc. 4188 */ 4189 ACCOUNT, 4190 /** 4191 * This resource allows for the definition of some activity to be performed, 4192 * independent of a particular patient, practitioner, or other performance 4193 * context. 4194 */ 4195 ACTIVITYDEFINITION, 4196 /** 4197 * Actual or potential/avoided event causing unintended physical injury 4198 * resulting from or contributed to by medical care, a research study or other 4199 * healthcare setting factors that requires additional monitoring, treatment, or 4200 * hospitalization, or that results in death. 4201 */ 4202 ADVERSEEVENT, 4203 /** 4204 * Risk of harmful or undesirable, physiological response which is unique to an 4205 * individual and associated with exposure to a substance. 4206 */ 4207 ALLERGYINTOLERANCE, 4208 /** 4209 * A booking of a healthcare event among patient(s), practitioner(s), related 4210 * person(s) and/or device(s) for a specific date/time. This may result in one 4211 * or more Encounter(s). 4212 */ 4213 APPOINTMENT, 4214 /** 4215 * A reply to an appointment request for a patient and/or practitioner(s), such 4216 * as a confirmation or rejection. 4217 */ 4218 APPOINTMENTRESPONSE, 4219 /** 4220 * A record of an event made for purposes of maintaining a security log. Typical 4221 * uses include detection of intrusion attempts and monitoring for inappropriate 4222 * usage. 4223 */ 4224 AUDITEVENT, 4225 /** 4226 * Basic is used for handling concepts not yet defined in FHIR, narrative-only 4227 * resources that don't map to an existing resource, and custom resources not 4228 * appropriate for inclusion in the FHIR specification. 4229 */ 4230 BASIC, 4231 /** 4232 * A resource that represents the data of a single raw artifact as digital 4233 * content accessible in its native format. A Binary resource can contain any 4234 * content, whether text, image, pdf, zip archive, etc. 4235 */ 4236 BINARY, 4237 /** 4238 * A material substance originating from a biological entity intended to be 4239 * transplanted or infused into another (possibly the same) biological entity. 4240 */ 4241 BIOLOGICALLYDERIVEDPRODUCT, 4242 /** 4243 * Record details about an anatomical structure. This resource may be used when 4244 * a coded concept does not provide the necessary detail needed for the use 4245 * case. 4246 */ 4247 BODYSTRUCTURE, 4248 /** 4249 * A container for a collection of resources. 4250 */ 4251 BUNDLE, 4252 /** 4253 * A Capability Statement documents a set of capabilities (behaviors) of a FHIR 4254 * Server for a particular version of FHIR that may be used as a statement of 4255 * actual server functionality or a statement of required or desired server 4256 * implementation. 4257 */ 4258 CAPABILITYSTATEMENT, 4259 /** 4260 * Describes the intention of how one or more practitioners intend to deliver 4261 * care for a particular patient, group or community for a period of time, 4262 * possibly limited to care for a specific condition or set of conditions. 4263 */ 4264 CAREPLAN, 4265 /** 4266 * The Care Team includes all the people and organizations who plan to 4267 * participate in the coordination and delivery of care for a patient. 4268 */ 4269 CARETEAM, 4270 /** 4271 * Catalog entries are wrappers that contextualize items included in a catalog. 4272 */ 4273 CATALOGENTRY, 4274 /** 4275 * The resource ChargeItem describes the provision of healthcare provider 4276 * products for a certain patient, therefore referring not only to the product, 4277 * but containing in addition details of the provision, like date, time, amounts 4278 * and participating organizations and persons. Main Usage of the ChargeItem is 4279 * to enable the billing process and internal cost allocation. 4280 */ 4281 CHARGEITEM, 4282 /** 4283 * The ChargeItemDefinition resource provides the properties that apply to the 4284 * (billing) codes necessary to calculate costs and prices. The properties may 4285 * differ largely depending on type and realm, therefore this resource gives 4286 * only a rough structure and requires profiling for each type of billing code 4287 * system. 4288 */ 4289 CHARGEITEMDEFINITION, 4290 /** 4291 * A provider issued list of professional services and products which have been 4292 * provided, or are to be provided, to a patient which is sent to an insurer for 4293 * reimbursement. 4294 */ 4295 CLAIM, 4296 /** 4297 * This resource provides the adjudication details from the processing of a 4298 * Claim resource. 4299 */ 4300 CLAIMRESPONSE, 4301 /** 4302 * A record of a clinical assessment performed to determine what problem(s) may 4303 * affect the patient and before planning the treatments or management 4304 * strategies that are best to manage a patient's condition. Assessments are 4305 * often 1:1 with a clinical consultation / encounter, but this varies greatly 4306 * depending on the clinical workflow. This resource is called 4307 * "ClinicalImpression" rather than "ClinicalAssessment" to avoid confusion with 4308 * the recording of assessment tools such as Apgar score. 4309 */ 4310 CLINICALIMPRESSION, 4311 /** 4312 * The CodeSystem resource is used to declare the existence of and describe a 4313 * code system or code system supplement and its key properties, and optionally 4314 * define a part or all of its content. 4315 */ 4316 CODESYSTEM, 4317 /** 4318 * An occurrence of information being transmitted; e.g. an alert that was sent 4319 * to a responsible provider, a public health agency that was notified about a 4320 * reportable condition. 4321 */ 4322 COMMUNICATION, 4323 /** 4324 * A request to convey information; e.g. the CDS system proposes that an alert 4325 * be sent to a responsible provider, the CDS system proposes that the public 4326 * health agency be notified about a reportable condition. 4327 */ 4328 COMMUNICATIONREQUEST, 4329 /** 4330 * A compartment definition that defines how resources are accessed on a server. 4331 */ 4332 COMPARTMENTDEFINITION, 4333 /** 4334 * A set of healthcare-related information that is assembled together into a 4335 * single logical package that provides a single coherent statement of meaning, 4336 * establishes its own context and that has clinical attestation with regard to 4337 * who is making the statement. A Composition defines the structure and 4338 * narrative content necessary for a document. However, a Composition alone does 4339 * not constitute a document. Rather, the Composition must be the first entry in 4340 * a Bundle where Bundle.type=document, and any other resources referenced from 4341 * Composition must be included as subsequent entries in the Bundle (for example 4342 * Patient, Practitioner, Encounter, etc.). 4343 */ 4344 COMPOSITION, 4345 /** 4346 * A statement of relationships from one set of concepts to one or more other 4347 * concepts - either concepts in code systems, or data element/data element 4348 * concepts, or classes in class models. 4349 */ 4350 CONCEPTMAP, 4351 /** 4352 * A clinical condition, problem, diagnosis, or other event, situation, issue, 4353 * or clinical concept that has risen to a level of concern. 4354 */ 4355 CONDITION, 4356 /** 4357 * A record of a healthcare consumer?s choices, which permits or denies 4358 * identified recipient(s) or recipient role(s) to perform one or more actions 4359 * within a given policy context, for specific purposes and periods of time. 4360 */ 4361 CONSENT, 4362 /** 4363 * Legally enforceable, formally recorded unilateral or bilateral directive 4364 * i.e., a policy or agreement. 4365 */ 4366 CONTRACT, 4367 /** 4368 * Financial instrument which may be used to reimburse or pay for health care 4369 * products and services. Includes both insurance and self-payment. 4370 */ 4371 COVERAGE, 4372 /** 4373 * The CoverageEligibilityRequest provides patient and insurance coverage 4374 * information to an insurer for them to respond, in the form of an 4375 * CoverageEligibilityResponse, with information regarding whether the stated 4376 * coverage is valid and in-force and optionally to provide the insurance 4377 * details of the policy. 4378 */ 4379 COVERAGEELIGIBILITYREQUEST, 4380 /** 4381 * This resource provides eligibility and plan details from the processing of an 4382 * CoverageEligibilityRequest resource. 4383 */ 4384 COVERAGEELIGIBILITYRESPONSE, 4385 /** 4386 * Indicates an actual or potential clinical issue with or between one or more 4387 * active or proposed clinical actions for a patient; e.g. Drug-drug 4388 * interaction, Ineffective treatment frequency, Procedure-condition conflict, 4389 * etc. 4390 */ 4391 DETECTEDISSUE, 4392 /** 4393 * A type of a manufactured item that is used in the provision of healthcare 4394 * without being substantially changed through that activity. The device may be 4395 * a medical or non-medical device. 4396 */ 4397 DEVICE, 4398 /** 4399 * The characteristics, operational status and capabilities of a medical-related 4400 * component of a medical device. 4401 */ 4402 DEVICEDEFINITION, 4403 /** 4404 * Describes a measurement, calculation or setting capability of a medical 4405 * device. 4406 */ 4407 DEVICEMETRIC, 4408 /** 4409 * Represents a request for a patient to employ a medical device. The device may 4410 * be an implantable device, or an external assistive device, such as a walker. 4411 */ 4412 DEVICEREQUEST, 4413 /** 4414 * A record of a device being used by a patient where the record is the result 4415 * of a report from the patient or another clinician. 4416 */ 4417 DEVICEUSESTATEMENT, 4418 /** 4419 * The findings and interpretation of diagnostic tests performed on patients, 4420 * groups of patients, devices, and locations, and/or specimens derived from 4421 * these. The report includes clinical context such as requesting and provider 4422 * information, and some mix of atomic results, images, textual and coded 4423 * interpretations, and formatted representation of diagnostic reports. 4424 */ 4425 DIAGNOSTICREPORT, 4426 /** 4427 * A collection of documents compiled for a purpose together with metadata that 4428 * applies to the collection. 4429 */ 4430 DOCUMENTMANIFEST, 4431 /** 4432 * A reference to a document of any kind for any purpose. Provides metadata 4433 * about the document so that the document can be discovered and managed. The 4434 * scope of a document is any seralized object with a mime-type, so includes 4435 * formal patient centric documents (CDA), cliical notes, scanned paper, and 4436 * non-patient specific documents like policy text. 4437 */ 4438 DOCUMENTREFERENCE, 4439 /** 4440 * A resource that includes narrative, extensions, and contained resources. 4441 */ 4442 DOMAINRESOURCE, 4443 /** 4444 * The EffectEvidenceSynthesis resource describes the difference in an outcome 4445 * between exposures states in a population where the effect estimate is derived 4446 * from a combination of research studies. 4447 */ 4448 EFFECTEVIDENCESYNTHESIS, 4449 /** 4450 * An interaction between a patient and healthcare provider(s) for the purpose 4451 * of providing healthcare service(s) or assessing the health status of a 4452 * patient. 4453 */ 4454 ENCOUNTER, 4455 /** 4456 * The technical details of an endpoint that can be used for electronic 4457 * services, such as for web services providing XDS.b or a REST endpoint for 4458 * another FHIR server. This may include any security context information. 4459 */ 4460 ENDPOINT, 4461 /** 4462 * This resource provides the insurance enrollment details to the insurer 4463 * regarding a specified coverage. 4464 */ 4465 ENROLLMENTREQUEST, 4466 /** 4467 * This resource provides enrollment and plan details from the processing of an 4468 * EnrollmentRequest resource. 4469 */ 4470 ENROLLMENTRESPONSE, 4471 /** 4472 * An association between a patient and an organization / healthcare provider(s) 4473 * during which time encounters may occur. The managing organization assumes a 4474 * level of responsibility for the patient during this time. 4475 */ 4476 EPISODEOFCARE, 4477 /** 4478 * The EventDefinition resource provides a reusable description of when a 4479 * particular event can occur. 4480 */ 4481 EVENTDEFINITION, 4482 /** 4483 * The Evidence resource describes the conditional state (population and any 4484 * exposures being compared within the population) and outcome (if specified) 4485 * that the knowledge (evidence, assertion, recommendation) is about. 4486 */ 4487 EVIDENCE, 4488 /** 4489 * The EvidenceVariable resource describes a "PICO" element that knowledge 4490 * (evidence, assertion, recommendation) is about. 4491 */ 4492 EVIDENCEVARIABLE, 4493 /** 4494 * Example of workflow instance. 4495 */ 4496 EXAMPLESCENARIO, 4497 /** 4498 * This resource provides: the claim details; adjudication details from the 4499 * processing of a Claim; and optionally account balance information, for 4500 * informing the subscriber of the benefits provided. 4501 */ 4502 EXPLANATIONOFBENEFIT, 4503 /** 4504 * Significant health conditions for a person related to the patient relevant in 4505 * the context of care for the patient. 4506 */ 4507 FAMILYMEMBERHISTORY, 4508 /** 4509 * Prospective warnings of potential issues when providing care to the patient. 4510 */ 4511 FLAG, 4512 /** 4513 * Describes the intended objective(s) for a patient, group or organization 4514 * care, for example, weight loss, restoring an activity of daily living, 4515 * obtaining herd immunity via immunization, meeting a process improvement 4516 * objective, etc. 4517 */ 4518 GOAL, 4519 /** 4520 * A formal computable definition of a graph of resources - that is, a coherent 4521 * set of resources that form a graph by following references. The Graph 4522 * Definition resource defines a set and makes rules about the set. 4523 */ 4524 GRAPHDEFINITION, 4525 /** 4526 * Represents a defined collection of entities that may be discussed or acted 4527 * upon collectively but which are not expected to act collectively, and are not 4528 * formally or legally recognized; i.e. a collection of entities that isn't an 4529 * Organization. 4530 */ 4531 GROUP, 4532 /** 4533 * A guidance response is the formal response to a guidance request, including 4534 * any output parameters returned by the evaluation, as well as the description 4535 * of any proposed actions to be taken. 4536 */ 4537 GUIDANCERESPONSE, 4538 /** 4539 * The details of a healthcare service available at a location. 4540 */ 4541 HEALTHCARESERVICE, 4542 /** 4543 * Representation of the content produced in a DICOM imaging study. A study 4544 * comprises a set of series, each of which includes a set of Service-Object 4545 * Pair Instances (SOP Instances - images or other data) acquired or produced in 4546 * a common context. A series is of only one modality (e.g. X-ray, CT, MR, 4547 * ultrasound), but a study may have multiple series of different modalities. 4548 */ 4549 IMAGINGSTUDY, 4550 /** 4551 * Describes the event of a patient being administered a vaccine or a record of 4552 * an immunization as reported by a patient, a clinician or another party. 4553 */ 4554 IMMUNIZATION, 4555 /** 4556 * Describes a comparison of an immunization event against published 4557 * recommendations to determine if the administration is "valid" in relation to 4558 * those recommendations. 4559 */ 4560 IMMUNIZATIONEVALUATION, 4561 /** 4562 * A patient's point-in-time set of recommendations (i.e. forecasting) according 4563 * to a published schedule with optional supporting justification. 4564 */ 4565 IMMUNIZATIONRECOMMENDATION, 4566 /** 4567 * A set of rules of how a particular interoperability or standards problem is 4568 * solved - typically through the use of FHIR resources. This resource is used 4569 * to gather all the parts of an implementation guide into a logical whole and 4570 * to publish a computable definition of all the parts. 4571 */ 4572 IMPLEMENTATIONGUIDE, 4573 /** 4574 * Details of a Health Insurance product/plan provided by an organization. 4575 */ 4576 INSURANCEPLAN, 4577 /** 4578 * Invoice containing collected ChargeItems from an Account with calculated 4579 * individual and total price for Billing purpose. 4580 */ 4581 INVOICE, 4582 /** 4583 * The Library resource is a general-purpose container for knowledge asset 4584 * definitions. It can be used to describe and expose existing knowledge assets 4585 * such as logic libraries and information model descriptions, as well as to 4586 * describe a collection of knowledge assets. 4587 */ 4588 LIBRARY, 4589 /** 4590 * Identifies two or more records (resource instances) that refer to the same 4591 * real-world "occurrence". 4592 */ 4593 LINKAGE, 4594 /** 4595 * A list is a curated collection of resources. 4596 */ 4597 LIST, 4598 /** 4599 * Details and position information for a physical place where services are 4600 * provided and resources and participants may be stored, found, contained, or 4601 * accommodated. 4602 */ 4603 LOCATION, 4604 /** 4605 * The Measure resource provides the definition of a quality measure. 4606 */ 4607 MEASURE, 4608 /** 4609 * The MeasureReport resource contains the results of the calculation of a 4610 * measure; and optionally a reference to the resources involved in that 4611 * calculation. 4612 */ 4613 MEASUREREPORT, 4614 /** 4615 * A photo, video, or audio recording acquired or used in healthcare. The actual 4616 * content may be inline or provided by direct reference. 4617 */ 4618 MEDIA, 4619 /** 4620 * This resource is primarily used for the identification and definition of a 4621 * medication for the purposes of prescribing, dispensing, and administering a 4622 * medication as well as for making statements about medication use. 4623 */ 4624 MEDICATION, 4625 /** 4626 * Describes the event of a patient consuming or otherwise being administered a 4627 * medication. This may be as simple as swallowing a tablet or it may be a long 4628 * running infusion. Related resources tie this event to the authorizing 4629 * prescription, and the specific encounter between patient and health care 4630 * practitioner. 4631 */ 4632 MEDICATIONADMINISTRATION, 4633 /** 4634 * Indicates that a medication product is to be or has been dispensed for a 4635 * named person/patient. This includes a description of the medication product 4636 * (supply) provided and the instructions for administering the medication. The 4637 * medication dispense is the result of a pharmacy system responding to a 4638 * medication order. 4639 */ 4640 MEDICATIONDISPENSE, 4641 /** 4642 * Information about a medication that is used to support knowledge. 4643 */ 4644 MEDICATIONKNOWLEDGE, 4645 /** 4646 * An order or request for both supply of the medication and the instructions 4647 * for administration of the medication to a patient. The resource is called 4648 * "MedicationRequest" rather than "MedicationPrescription" or "MedicationOrder" 4649 * to generalize the use across inpatient and outpatient settings, including 4650 * care plans, etc., and to harmonize with workflow patterns. 4651 */ 4652 MEDICATIONREQUEST, 4653 /** 4654 * A record of a medication that is being consumed by a patient. A 4655 * MedicationStatement may indicate that the patient may be taking the 4656 * medication now or has taken the medication in the past or will be taking the 4657 * medication in the future. The source of this information can be the patient, 4658 * significant other (such as a family member or spouse), or a clinician. A 4659 * common scenario where this information is captured is during the history 4660 * taking process during a patient visit or stay. The medication information may 4661 * come from sources such as the patient's memory, from a prescription bottle, 4662 * or from a list of medications the patient, clinician or other party 4663 * maintains. 4664 * 4665 * The primary difference between a medication statement and a medication 4666 * administration is that the medication administration has complete 4667 * administration information and is based on actual administration information 4668 * from the person who administered the medication. A medication statement is 4669 * often, if not always, less specific. There is no required date/time when the 4670 * medication was administered, in fact we only know that a source has reported 4671 * the patient is taking this medication, where details such as time, quantity, 4672 * or rate or even medication product may be incomplete or missing or less 4673 * precise. As stated earlier, the medication statement information may come 4674 * from the patient's memory, from a prescription bottle or from a list of 4675 * medications the patient, clinician or other party maintains. Medication 4676 * administration is more formal and is not missing detailed information. 4677 */ 4678 MEDICATIONSTATEMENT, 4679 /** 4680 * Detailed definition of a medicinal product, typically for uses other than 4681 * direct patient care (e.g. regulatory use). 4682 */ 4683 MEDICINALPRODUCT, 4684 /** 4685 * The regulatory authorization of a medicinal product. 4686 */ 4687 MEDICINALPRODUCTAUTHORIZATION, 4688 /** 4689 * The clinical particulars - indications, contraindications etc. of a medicinal 4690 * product, including for regulatory purposes. 4691 */ 4692 MEDICINALPRODUCTCONTRAINDICATION, 4693 /** 4694 * Indication for the Medicinal Product. 4695 */ 4696 MEDICINALPRODUCTINDICATION, 4697 /** 4698 * An ingredient of a manufactured item or pharmaceutical product. 4699 */ 4700 MEDICINALPRODUCTINGREDIENT, 4701 /** 4702 * The interactions of the medicinal product with other medicinal products, or 4703 * other forms of interactions. 4704 */ 4705 MEDICINALPRODUCTINTERACTION, 4706 /** 4707 * The manufactured item as contained in the packaged medicinal product. 4708 */ 4709 MEDICINALPRODUCTMANUFACTURED, 4710 /** 4711 * A medicinal product in a container or package. 4712 */ 4713 MEDICINALPRODUCTPACKAGED, 4714 /** 4715 * A pharmaceutical product described in terms of its composition and dose form. 4716 */ 4717 MEDICINALPRODUCTPHARMACEUTICAL, 4718 /** 4719 * Describe the undesirable effects of the medicinal product. 4720 */ 4721 MEDICINALPRODUCTUNDESIRABLEEFFECT, 4722 /** 4723 * Defines the characteristics of a message that can be shared between systems, 4724 * including the type of event that initiates the message, the content to be 4725 * transmitted and what response(s), if any, are permitted. 4726 */ 4727 MESSAGEDEFINITION, 4728 /** 4729 * The header for a message exchange that is either requesting or responding to 4730 * an action. The reference(s) that are the subject of the action as well as 4731 * other information related to the action are typically transmitted in a bundle 4732 * in which the MessageHeader resource instance is the first resource in the 4733 * bundle. 4734 */ 4735 MESSAGEHEADER, 4736 /** 4737 * Raw data describing a biological sequence. 4738 */ 4739 MOLECULARSEQUENCE, 4740 /** 4741 * A curated namespace that issues unique symbols within that namespace for the 4742 * identification of concepts, people, devices, etc. Represents a "System" used 4743 * within the Identifier and Coding data types. 4744 */ 4745 NAMINGSYSTEM, 4746 /** 4747 * A request to supply a diet, formula feeding (enteral) or oral nutritional 4748 * supplement to a patient/resident. 4749 */ 4750 NUTRITIONORDER, 4751 /** 4752 * Measurements and simple assertions made about a patient, device or other 4753 * subject. 4754 */ 4755 OBSERVATION, 4756 /** 4757 * Set of definitional characteristics for a kind of observation or measurement 4758 * produced or consumed by an orderable health care service. 4759 */ 4760 OBSERVATIONDEFINITION, 4761 /** 4762 * A formal computable definition of an operation (on the RESTful interface) or 4763 * a named query (using the search interaction). 4764 */ 4765 OPERATIONDEFINITION, 4766 /** 4767 * A collection of error, warning, or information messages that result from a 4768 * system action. 4769 */ 4770 OPERATIONOUTCOME, 4771 /** 4772 * A formally or informally recognized grouping of people or organizations 4773 * formed for the purpose of achieving some form of collective action. Includes 4774 * companies, institutions, corporations, departments, community groups, 4775 * healthcare practice groups, payer/insurer, etc. 4776 */ 4777 ORGANIZATION, 4778 /** 4779 * Defines an affiliation/assotiation/relationship between 2 distinct 4780 * oganizations, that is not a part-of relationship/sub-division relationship. 4781 */ 4782 ORGANIZATIONAFFILIATION, 4783 /** 4784 * This resource is a non-persisted resource used to pass information into and 4785 * back from an [operation](operations.html). It has no other use, and there is 4786 * no RESTful endpoint associated with it. 4787 */ 4788 PARAMETERS, 4789 /** 4790 * Demographics and other administrative information about an individual or 4791 * animal receiving care or other health-related services. 4792 */ 4793 PATIENT, 4794 /** 4795 * This resource provides the status of the payment for goods and services 4796 * rendered, and the request and response resource references. 4797 */ 4798 PAYMENTNOTICE, 4799 /** 4800 * This resource provides the details including amount of a payment and 4801 * allocates the payment items being paid. 4802 */ 4803 PAYMENTRECONCILIATION, 4804 /** 4805 * Demographics and administrative information about a person independent of a 4806 * specific health-related context. 4807 */ 4808 PERSON, 4809 /** 4810 * This resource allows for the definition of various types of plans as a 4811 * sharable, consumable, and executable artifact. The resource is general enough 4812 * to support the description of a broad range of clinical artifacts such as 4813 * clinical decision support rules, order sets and protocols. 4814 */ 4815 PLANDEFINITION, 4816 /** 4817 * A person who is directly or indirectly involved in the provisioning of 4818 * healthcare. 4819 */ 4820 PRACTITIONER, 4821 /** 4822 * A specific set of Roles/Locations/specialties/services that a practitioner 4823 * may perform at an organization for a period of time. 4824 */ 4825 PRACTITIONERROLE, 4826 /** 4827 * An action that is or was performed on or for a patient. This can be a 4828 * physical intervention like an operation, or less invasive like long term 4829 * services, counseling, or hypnotherapy. 4830 */ 4831 PROCEDURE, 4832 /** 4833 * Provenance of a resource is a record that describes entities and processes 4834 * involved in producing and delivering or otherwise influencing that resource. 4835 * Provenance provides a critical foundation for assessing authenticity, 4836 * enabling trust, and allowing reproducibility. Provenance assertions are a 4837 * form of contextual metadata and can themselves become important records with 4838 * their own provenance. Provenance statement indicates clinical significance in 4839 * terms of confidence in authenticity, reliability, and trustworthiness, 4840 * integrity, and stage in lifecycle (e.g. Document Completion - has the 4841 * artifact been legally authenticated), all of which may impact security, 4842 * privacy, and trust policies. 4843 */ 4844 PROVENANCE, 4845 /** 4846 * A structured set of questions intended to guide the collection of answers 4847 * from end-users. Questionnaires provide detailed control over order, 4848 * presentation, phraseology and grouping to allow coherent, consistent data 4849 * collection. 4850 */ 4851 QUESTIONNAIRE, 4852 /** 4853 * A structured set of questions and their answers. The questions are ordered 4854 * and grouped into coherent subsets, corresponding to the structure of the 4855 * grouping of the questionnaire being responded to. 4856 */ 4857 QUESTIONNAIRERESPONSE, 4858 /** 4859 * Information about a person that is involved in the care for a patient, but 4860 * who is not the target of healthcare, nor has a formal responsibility in the 4861 * care process. 4862 */ 4863 RELATEDPERSON, 4864 /** 4865 * A group of related requests that can be used to capture intended activities 4866 * that have inter-dependencies such as "give this medication after that one". 4867 */ 4868 REQUESTGROUP, 4869 /** 4870 * The ResearchDefinition resource describes the conditional state (population 4871 * and any exposures being compared within the population) and outcome (if 4872 * specified) that the knowledge (evidence, assertion, recommendation) is about. 4873 */ 4874 RESEARCHDEFINITION, 4875 /** 4876 * The ResearchElementDefinition resource describes a "PICO" element that 4877 * knowledge (evidence, assertion, recommendation) is about. 4878 */ 4879 RESEARCHELEMENTDEFINITION, 4880 /** 4881 * A process where a researcher or organization plans and then executes a series 4882 * of steps intended to increase the field of healthcare-related knowledge. This 4883 * includes studies of safety, efficacy, comparative effectiveness and other 4884 * information about medications, devices, therapies and other interventional 4885 * and investigative techniques. A ResearchStudy involves the gathering of 4886 * information about human or animal subjects. 4887 */ 4888 RESEARCHSTUDY, 4889 /** 4890 * A physical entity which is the primary unit of operational and/or 4891 * administrative interest in a study. 4892 */ 4893 RESEARCHSUBJECT, 4894 /** 4895 * This is the base resource type for everything. 4896 */ 4897 RESOURCE, 4898 /** 4899 * An assessment of the likely outcome(s) for a patient or other subject as well 4900 * as the likelihood of each outcome. 4901 */ 4902 RISKASSESSMENT, 4903 /** 4904 * The RiskEvidenceSynthesis resource describes the likelihood of an outcome in 4905 * a population plus exposure state where the risk estimate is derived from a 4906 * combination of research studies. 4907 */ 4908 RISKEVIDENCESYNTHESIS, 4909 /** 4910 * A container for slots of time that may be available for booking appointments. 4911 */ 4912 SCHEDULE, 4913 /** 4914 * A search parameter that defines a named search item that can be used to 4915 * search/filter on a resource. 4916 */ 4917 SEARCHPARAMETER, 4918 /** 4919 * A record of a request for service such as diagnostic investigations, 4920 * treatments, or operations to be performed. 4921 */ 4922 SERVICEREQUEST, 4923 /** 4924 * A slot of time on a schedule that may be available for booking appointments. 4925 */ 4926 SLOT, 4927 /** 4928 * A sample to be used for analysis. 4929 */ 4930 SPECIMEN, 4931 /** 4932 * A kind of specimen with associated set of requirements. 4933 */ 4934 SPECIMENDEFINITION, 4935 /** 4936 * A definition of a FHIR structure. This resource is used to describe the 4937 * underlying resources, data types defined in FHIR, and also for describing 4938 * extensions and constraints on resources and data types. 4939 */ 4940 STRUCTUREDEFINITION, 4941 /** 4942 * A Map of relationships between 2 structures that can be used to transform 4943 * data. 4944 */ 4945 STRUCTUREMAP, 4946 /** 4947 * The subscription resource is used to define a push-based subscription from a 4948 * server to another system. Once a subscription is registered with the server, 4949 * the server checks every resource that is created or updated, and if the 4950 * resource matches the given criteria, it sends a message on the defined 4951 * "channel" so that another system can take an appropriate action. 4952 */ 4953 SUBSCRIPTION, 4954 /** 4955 * A homogeneous material with a definite composition. 4956 */ 4957 SUBSTANCE, 4958 /** 4959 * Nucleic acids are defined by three distinct elements: the base, sugar and 4960 * linkage. Individual substance/moiety IDs will be created for each of these 4961 * elements. The nucleotide sequence will be always entered in the 5?-3? 4962 * direction. 4963 */ 4964 SUBSTANCENUCLEICACID, 4965 /** 4966 * Todo. 4967 */ 4968 SUBSTANCEPOLYMER, 4969 /** 4970 * A SubstanceProtein is defined as a single unit of a linear amino acid 4971 * sequence, or a combination of subunits that are either covalently linked or 4972 * have a defined invariant stoichiometric relationship. This includes all 4973 * synthetic, recombinant and purified SubstanceProteins of defined sequence, 4974 * whether the use is therapeutic or prophylactic. This set of elements will be 4975 * used to describe albumins, coagulation factors, cytokines, growth factors, 4976 * peptide/SubstanceProtein hormones, enzymes, toxins, toxoids, recombinant 4977 * vaccines, and immunomodulators. 4978 */ 4979 SUBSTANCEPROTEIN, 4980 /** 4981 * Todo. 4982 */ 4983 SUBSTANCEREFERENCEINFORMATION, 4984 /** 4985 * Source material shall capture information on the taxonomic and anatomical 4986 * origins as well as the fraction of a material that can result in or can be 4987 * modified to form a substance. This set of data elements shall be used to 4988 * define polymer substances isolated from biological matrices. Taxonomic and 4989 * anatomical origins shall be described using a controlled vocabulary as 4990 * required. This information is captured for naturally derived polymers ( . 4991 * starch) and structurally diverse substances. For Organisms belonging to the 4992 * Kingdom Plantae the Substance level defines the fresh material of a single 4993 * species or infraspecies, the Herbal Drug and the Herbal preparation. For 4994 * Herbal preparations, the fraction information will be captured at the 4995 * Substance information level and additional information for herbal extracts 4996 * will be captured at the Specified Substance Group 1 information level. See 4997 * for further explanation the Substance Class: Structurally Diverse and the 4998 * herbal annex. 4999 */ 5000 SUBSTANCESOURCEMATERIAL, 5001 /** 5002 * The detailed description of a substance, typically at a level beyond what is 5003 * used for prescribing. 5004 */ 5005 SUBSTANCESPECIFICATION, 5006 /** 5007 * Record of delivery of what is supplied. 5008 */ 5009 SUPPLYDELIVERY, 5010 /** 5011 * A record of a request for a medication, substance or device used in the 5012 * healthcare setting. 5013 */ 5014 SUPPLYREQUEST, 5015 /** 5016 * A task to be performed. 5017 */ 5018 TASK, 5019 /** 5020 * A Terminology Capabilities documents a set of capabilities (behaviors) of a 5021 * FHIR Server that may be used as a statement of actual server functionality or 5022 * a statement of required or desired server implementation. 5023 */ 5024 TERMINOLOGYCAPABILITIES, 5025 /** 5026 * A summary of information based on the results of executing a TestScript. 5027 */ 5028 TESTREPORT, 5029 /** 5030 * A structured set of tests against a FHIR server or client implementation to 5031 * determine compliance against the FHIR specification. 5032 */ 5033 TESTSCRIPT, 5034 /** 5035 * A ValueSet resource instance specifies a set of codes drawn from one or more 5036 * code systems, intended for use in a particular context. Value sets link 5037 * between [[[CodeSystem]]] definitions and their use in [coded 5038 * elements](terminologies.html). 5039 */ 5040 VALUESET, 5041 /** 5042 * Describes validation requirements, source(s), status and dates for one or 5043 * more elements. 5044 */ 5045 VERIFICATIONRESULT, 5046 /** 5047 * An authorization for the provision of glasses and/or contact lenses to a 5048 * patient. 5049 */ 5050 VISIONPRESCRIPTION, 5051 /** 5052 * A place holder that means any kind of data type 5053 */ 5054 TYPE, 5055 /** 5056 * A place holder that means any kind of resource 5057 */ 5058 ANY, 5059 /** 5060 * added to help the parsers 5061 */ 5062 NULL; 5063 5064 public static FHIRAllTypes fromCode(String codeString) throws FHIRException { 5065 if (codeString == null || "".equals(codeString)) 5066 return null; 5067 if ("Address".equals(codeString)) 5068 return ADDRESS; 5069 if ("Age".equals(codeString)) 5070 return AGE; 5071 if ("Annotation".equals(codeString)) 5072 return ANNOTATION; 5073 if ("Attachment".equals(codeString)) 5074 return ATTACHMENT; 5075 if ("BackboneElement".equals(codeString)) 5076 return BACKBONEELEMENT; 5077 if ("CodeableConcept".equals(codeString)) 5078 return CODEABLECONCEPT; 5079 if ("Coding".equals(codeString)) 5080 return CODING; 5081 if ("ContactDetail".equals(codeString)) 5082 return CONTACTDETAIL; 5083 if ("ContactPoint".equals(codeString)) 5084 return CONTACTPOINT; 5085 if ("Contributor".equals(codeString)) 5086 return CONTRIBUTOR; 5087 if ("Count".equals(codeString)) 5088 return COUNT; 5089 if ("DataRequirement".equals(codeString)) 5090 return DATAREQUIREMENT; 5091 if ("Distance".equals(codeString)) 5092 return DISTANCE; 5093 if ("Dosage".equals(codeString)) 5094 return DOSAGE; 5095 if ("Duration".equals(codeString)) 5096 return DURATION; 5097 if ("Element".equals(codeString)) 5098 return ELEMENT; 5099 if ("ElementDefinition".equals(codeString)) 5100 return ELEMENTDEFINITION; 5101 if ("Expression".equals(codeString)) 5102 return EXPRESSION; 5103 if ("Extension".equals(codeString)) 5104 return EXTENSION; 5105 if ("HumanName".equals(codeString)) 5106 return HUMANNAME; 5107 if ("Identifier".equals(codeString)) 5108 return IDENTIFIER; 5109 if ("MarketingStatus".equals(codeString)) 5110 return MARKETINGSTATUS; 5111 if ("Meta".equals(codeString)) 5112 return META; 5113 if ("Money".equals(codeString)) 5114 return MONEY; 5115 if ("MoneyQuantity".equals(codeString)) 5116 return MONEYQUANTITY; 5117 if ("Narrative".equals(codeString)) 5118 return NARRATIVE; 5119 if ("ParameterDefinition".equals(codeString)) 5120 return PARAMETERDEFINITION; 5121 if ("Period".equals(codeString)) 5122 return PERIOD; 5123 if ("Population".equals(codeString)) 5124 return POPULATION; 5125 if ("ProdCharacteristic".equals(codeString)) 5126 return PRODCHARACTERISTIC; 5127 if ("ProductShelfLife".equals(codeString)) 5128 return PRODUCTSHELFLIFE; 5129 if ("Quantity".equals(codeString)) 5130 return QUANTITY; 5131 if ("Range".equals(codeString)) 5132 return RANGE; 5133 if ("Ratio".equals(codeString)) 5134 return RATIO; 5135 if ("Reference".equals(codeString)) 5136 return REFERENCE; 5137 if ("RelatedArtifact".equals(codeString)) 5138 return RELATEDARTIFACT; 5139 if ("SampledData".equals(codeString)) 5140 return SAMPLEDDATA; 5141 if ("Signature".equals(codeString)) 5142 return SIGNATURE; 5143 if ("SimpleQuantity".equals(codeString)) 5144 return SIMPLEQUANTITY; 5145 if ("SubstanceAmount".equals(codeString)) 5146 return SUBSTANCEAMOUNT; 5147 if ("Timing".equals(codeString)) 5148 return TIMING; 5149 if ("TriggerDefinition".equals(codeString)) 5150 return TRIGGERDEFINITION; 5151 if ("UsageContext".equals(codeString)) 5152 return USAGECONTEXT; 5153 if ("base64Binary".equals(codeString)) 5154 return BASE64BINARY; 5155 if ("boolean".equals(codeString)) 5156 return BOOLEAN; 5157 if ("canonical".equals(codeString)) 5158 return CANONICAL; 5159 if ("code".equals(codeString)) 5160 return CODE; 5161 if ("date".equals(codeString)) 5162 return DATE; 5163 if ("dateTime".equals(codeString)) 5164 return DATETIME; 5165 if ("decimal".equals(codeString)) 5166 return DECIMAL; 5167 if ("id".equals(codeString)) 5168 return ID; 5169 if ("instant".equals(codeString)) 5170 return INSTANT; 5171 if ("integer".equals(codeString)) 5172 return INTEGER; 5173 if ("markdown".equals(codeString)) 5174 return MARKDOWN; 5175 if ("oid".equals(codeString)) 5176 return OID; 5177 if ("positiveInt".equals(codeString)) 5178 return POSITIVEINT; 5179 if ("string".equals(codeString)) 5180 return STRING; 5181 if ("time".equals(codeString)) 5182 return TIME; 5183 if ("unsignedInt".equals(codeString)) 5184 return UNSIGNEDINT; 5185 if ("uri".equals(codeString)) 5186 return URI; 5187 if ("url".equals(codeString)) 5188 return URL; 5189 if ("uuid".equals(codeString)) 5190 return UUID; 5191 if ("xhtml".equals(codeString)) 5192 return XHTML; 5193 if ("Account".equals(codeString)) 5194 return ACCOUNT; 5195 if ("ActivityDefinition".equals(codeString)) 5196 return ACTIVITYDEFINITION; 5197 if ("AdverseEvent".equals(codeString)) 5198 return ADVERSEEVENT; 5199 if ("AllergyIntolerance".equals(codeString)) 5200 return ALLERGYINTOLERANCE; 5201 if ("Appointment".equals(codeString)) 5202 return APPOINTMENT; 5203 if ("AppointmentResponse".equals(codeString)) 5204 return APPOINTMENTRESPONSE; 5205 if ("AuditEvent".equals(codeString)) 5206 return AUDITEVENT; 5207 if ("Basic".equals(codeString)) 5208 return BASIC; 5209 if ("Binary".equals(codeString)) 5210 return BINARY; 5211 if ("BiologicallyDerivedProduct".equals(codeString)) 5212 return BIOLOGICALLYDERIVEDPRODUCT; 5213 if ("BodyStructure".equals(codeString)) 5214 return BODYSTRUCTURE; 5215 if ("Bundle".equals(codeString)) 5216 return BUNDLE; 5217 if ("CapabilityStatement".equals(codeString)) 5218 return CAPABILITYSTATEMENT; 5219 if ("CarePlan".equals(codeString)) 5220 return CAREPLAN; 5221 if ("CareTeam".equals(codeString)) 5222 return CARETEAM; 5223 if ("CatalogEntry".equals(codeString)) 5224 return CATALOGENTRY; 5225 if ("ChargeItem".equals(codeString)) 5226 return CHARGEITEM; 5227 if ("ChargeItemDefinition".equals(codeString)) 5228 return CHARGEITEMDEFINITION; 5229 if ("Claim".equals(codeString)) 5230 return CLAIM; 5231 if ("ClaimResponse".equals(codeString)) 5232 return CLAIMRESPONSE; 5233 if ("ClinicalImpression".equals(codeString)) 5234 return CLINICALIMPRESSION; 5235 if ("CodeSystem".equals(codeString)) 5236 return CODESYSTEM; 5237 if ("Communication".equals(codeString)) 5238 return COMMUNICATION; 5239 if ("CommunicationRequest".equals(codeString)) 5240 return COMMUNICATIONREQUEST; 5241 if ("CompartmentDefinition".equals(codeString)) 5242 return COMPARTMENTDEFINITION; 5243 if ("Composition".equals(codeString)) 5244 return COMPOSITION; 5245 if ("ConceptMap".equals(codeString)) 5246 return CONCEPTMAP; 5247 if ("Condition".equals(codeString)) 5248 return CONDITION; 5249 if ("Consent".equals(codeString)) 5250 return CONSENT; 5251 if ("Contract".equals(codeString)) 5252 return CONTRACT; 5253 if ("Coverage".equals(codeString)) 5254 return COVERAGE; 5255 if ("CoverageEligibilityRequest".equals(codeString)) 5256 return COVERAGEELIGIBILITYREQUEST; 5257 if ("CoverageEligibilityResponse".equals(codeString)) 5258 return COVERAGEELIGIBILITYRESPONSE; 5259 if ("DetectedIssue".equals(codeString)) 5260 return DETECTEDISSUE; 5261 if ("Device".equals(codeString)) 5262 return DEVICE; 5263 if ("DeviceDefinition".equals(codeString)) 5264 return DEVICEDEFINITION; 5265 if ("DeviceMetric".equals(codeString)) 5266 return DEVICEMETRIC; 5267 if ("DeviceRequest".equals(codeString)) 5268 return DEVICEREQUEST; 5269 if ("DeviceUseStatement".equals(codeString)) 5270 return DEVICEUSESTATEMENT; 5271 if ("DiagnosticReport".equals(codeString)) 5272 return DIAGNOSTICREPORT; 5273 if ("DocumentManifest".equals(codeString)) 5274 return DOCUMENTMANIFEST; 5275 if ("DocumentReference".equals(codeString)) 5276 return DOCUMENTREFERENCE; 5277 if ("DomainResource".equals(codeString)) 5278 return DOMAINRESOURCE; 5279 if ("EffectEvidenceSynthesis".equals(codeString)) 5280 return EFFECTEVIDENCESYNTHESIS; 5281 if ("Encounter".equals(codeString)) 5282 return ENCOUNTER; 5283 if ("Endpoint".equals(codeString)) 5284 return ENDPOINT; 5285 if ("EnrollmentRequest".equals(codeString)) 5286 return ENROLLMENTREQUEST; 5287 if ("EnrollmentResponse".equals(codeString)) 5288 return ENROLLMENTRESPONSE; 5289 if ("EpisodeOfCare".equals(codeString)) 5290 return EPISODEOFCARE; 5291 if ("EventDefinition".equals(codeString)) 5292 return EVENTDEFINITION; 5293 if ("Evidence".equals(codeString)) 5294 return EVIDENCE; 5295 if ("EvidenceVariable".equals(codeString)) 5296 return EVIDENCEVARIABLE; 5297 if ("ExampleScenario".equals(codeString)) 5298 return EXAMPLESCENARIO; 5299 if ("ExplanationOfBenefit".equals(codeString)) 5300 return EXPLANATIONOFBENEFIT; 5301 if ("FamilyMemberHistory".equals(codeString)) 5302 return FAMILYMEMBERHISTORY; 5303 if ("Flag".equals(codeString)) 5304 return FLAG; 5305 if ("Goal".equals(codeString)) 5306 return GOAL; 5307 if ("GraphDefinition".equals(codeString)) 5308 return GRAPHDEFINITION; 5309 if ("Group".equals(codeString)) 5310 return GROUP; 5311 if ("GuidanceResponse".equals(codeString)) 5312 return GUIDANCERESPONSE; 5313 if ("HealthcareService".equals(codeString)) 5314 return HEALTHCARESERVICE; 5315 if ("ImagingStudy".equals(codeString)) 5316 return IMAGINGSTUDY; 5317 if ("Immunization".equals(codeString)) 5318 return IMMUNIZATION; 5319 if ("ImmunizationEvaluation".equals(codeString)) 5320 return IMMUNIZATIONEVALUATION; 5321 if ("ImmunizationRecommendation".equals(codeString)) 5322 return IMMUNIZATIONRECOMMENDATION; 5323 if ("ImplementationGuide".equals(codeString)) 5324 return IMPLEMENTATIONGUIDE; 5325 if ("InsurancePlan".equals(codeString)) 5326 return INSURANCEPLAN; 5327 if ("Invoice".equals(codeString)) 5328 return INVOICE; 5329 if ("Library".equals(codeString)) 5330 return LIBRARY; 5331 if ("Linkage".equals(codeString)) 5332 return LINKAGE; 5333 if ("List".equals(codeString)) 5334 return LIST; 5335 if ("Location".equals(codeString)) 5336 return LOCATION; 5337 if ("Measure".equals(codeString)) 5338 return MEASURE; 5339 if ("MeasureReport".equals(codeString)) 5340 return MEASUREREPORT; 5341 if ("Media".equals(codeString)) 5342 return MEDIA; 5343 if ("Medication".equals(codeString)) 5344 return MEDICATION; 5345 if ("MedicationAdministration".equals(codeString)) 5346 return MEDICATIONADMINISTRATION; 5347 if ("MedicationDispense".equals(codeString)) 5348 return MEDICATIONDISPENSE; 5349 if ("MedicationKnowledge".equals(codeString)) 5350 return MEDICATIONKNOWLEDGE; 5351 if ("MedicationRequest".equals(codeString)) 5352 return MEDICATIONREQUEST; 5353 if ("MedicationStatement".equals(codeString)) 5354 return MEDICATIONSTATEMENT; 5355 if ("MedicinalProduct".equals(codeString)) 5356 return MEDICINALPRODUCT; 5357 if ("MedicinalProductAuthorization".equals(codeString)) 5358 return MEDICINALPRODUCTAUTHORIZATION; 5359 if ("MedicinalProductContraindication".equals(codeString)) 5360 return MEDICINALPRODUCTCONTRAINDICATION; 5361 if ("MedicinalProductIndication".equals(codeString)) 5362 return MEDICINALPRODUCTINDICATION; 5363 if ("MedicinalProductIngredient".equals(codeString)) 5364 return MEDICINALPRODUCTINGREDIENT; 5365 if ("MedicinalProductInteraction".equals(codeString)) 5366 return MEDICINALPRODUCTINTERACTION; 5367 if ("MedicinalProductManufactured".equals(codeString)) 5368 return MEDICINALPRODUCTMANUFACTURED; 5369 if ("MedicinalProductPackaged".equals(codeString)) 5370 return MEDICINALPRODUCTPACKAGED; 5371 if ("MedicinalProductPharmaceutical".equals(codeString)) 5372 return MEDICINALPRODUCTPHARMACEUTICAL; 5373 if ("MedicinalProductUndesirableEffect".equals(codeString)) 5374 return MEDICINALPRODUCTUNDESIRABLEEFFECT; 5375 if ("MessageDefinition".equals(codeString)) 5376 return MESSAGEDEFINITION; 5377 if ("MessageHeader".equals(codeString)) 5378 return MESSAGEHEADER; 5379 if ("MolecularSequence".equals(codeString)) 5380 return MOLECULARSEQUENCE; 5381 if ("NamingSystem".equals(codeString)) 5382 return NAMINGSYSTEM; 5383 if ("NutritionOrder".equals(codeString)) 5384 return NUTRITIONORDER; 5385 if ("Observation".equals(codeString)) 5386 return OBSERVATION; 5387 if ("ObservationDefinition".equals(codeString)) 5388 return OBSERVATIONDEFINITION; 5389 if ("OperationDefinition".equals(codeString)) 5390 return OPERATIONDEFINITION; 5391 if ("OperationOutcome".equals(codeString)) 5392 return OPERATIONOUTCOME; 5393 if ("Organization".equals(codeString)) 5394 return ORGANIZATION; 5395 if ("OrganizationAffiliation".equals(codeString)) 5396 return ORGANIZATIONAFFILIATION; 5397 if ("Parameters".equals(codeString)) 5398 return PARAMETERS; 5399 if ("Patient".equals(codeString)) 5400 return PATIENT; 5401 if ("PaymentNotice".equals(codeString)) 5402 return PAYMENTNOTICE; 5403 if ("PaymentReconciliation".equals(codeString)) 5404 return PAYMENTRECONCILIATION; 5405 if ("Person".equals(codeString)) 5406 return PERSON; 5407 if ("PlanDefinition".equals(codeString)) 5408 return PLANDEFINITION; 5409 if ("Practitioner".equals(codeString)) 5410 return PRACTITIONER; 5411 if ("PractitionerRole".equals(codeString)) 5412 return PRACTITIONERROLE; 5413 if ("Procedure".equals(codeString)) 5414 return PROCEDURE; 5415 if ("Provenance".equals(codeString)) 5416 return PROVENANCE; 5417 if ("Questionnaire".equals(codeString)) 5418 return QUESTIONNAIRE; 5419 if ("QuestionnaireResponse".equals(codeString)) 5420 return QUESTIONNAIRERESPONSE; 5421 if ("RelatedPerson".equals(codeString)) 5422 return RELATEDPERSON; 5423 if ("RequestGroup".equals(codeString)) 5424 return REQUESTGROUP; 5425 if ("ResearchDefinition".equals(codeString)) 5426 return RESEARCHDEFINITION; 5427 if ("ResearchElementDefinition".equals(codeString)) 5428 return RESEARCHELEMENTDEFINITION; 5429 if ("ResearchStudy".equals(codeString)) 5430 return RESEARCHSTUDY; 5431 if ("ResearchSubject".equals(codeString)) 5432 return RESEARCHSUBJECT; 5433 if ("Resource".equals(codeString)) 5434 return RESOURCE; 5435 if ("RiskAssessment".equals(codeString)) 5436 return RISKASSESSMENT; 5437 if ("RiskEvidenceSynthesis".equals(codeString)) 5438 return RISKEVIDENCESYNTHESIS; 5439 if ("Schedule".equals(codeString)) 5440 return SCHEDULE; 5441 if ("SearchParameter".equals(codeString)) 5442 return SEARCHPARAMETER; 5443 if ("ServiceRequest".equals(codeString)) 5444 return SERVICEREQUEST; 5445 if ("Slot".equals(codeString)) 5446 return SLOT; 5447 if ("Specimen".equals(codeString)) 5448 return SPECIMEN; 5449 if ("SpecimenDefinition".equals(codeString)) 5450 return SPECIMENDEFINITION; 5451 if ("StructureDefinition".equals(codeString)) 5452 return STRUCTUREDEFINITION; 5453 if ("StructureMap".equals(codeString)) 5454 return STRUCTUREMAP; 5455 if ("Subscription".equals(codeString)) 5456 return SUBSCRIPTION; 5457 if ("Substance".equals(codeString)) 5458 return SUBSTANCE; 5459 if ("SubstanceNucleicAcid".equals(codeString)) 5460 return SUBSTANCENUCLEICACID; 5461 if ("SubstancePolymer".equals(codeString)) 5462 return SUBSTANCEPOLYMER; 5463 if ("SubstanceProtein".equals(codeString)) 5464 return SUBSTANCEPROTEIN; 5465 if ("SubstanceReferenceInformation".equals(codeString)) 5466 return SUBSTANCEREFERENCEINFORMATION; 5467 if ("SubstanceSourceMaterial".equals(codeString)) 5468 return SUBSTANCESOURCEMATERIAL; 5469 if ("SubstanceSpecification".equals(codeString)) 5470 return SUBSTANCESPECIFICATION; 5471 if ("SupplyDelivery".equals(codeString)) 5472 return SUPPLYDELIVERY; 5473 if ("SupplyRequest".equals(codeString)) 5474 return SUPPLYREQUEST; 5475 if ("Task".equals(codeString)) 5476 return TASK; 5477 if ("TerminologyCapabilities".equals(codeString)) 5478 return TERMINOLOGYCAPABILITIES; 5479 if ("TestReport".equals(codeString)) 5480 return TESTREPORT; 5481 if ("TestScript".equals(codeString)) 5482 return TESTSCRIPT; 5483 if ("ValueSet".equals(codeString)) 5484 return VALUESET; 5485 if ("VerificationResult".equals(codeString)) 5486 return VERIFICATIONRESULT; 5487 if ("VisionPrescription".equals(codeString)) 5488 return VISIONPRESCRIPTION; 5489 if ("Type".equals(codeString)) 5490 return TYPE; 5491 if ("Any".equals(codeString)) 5492 return ANY; 5493 throw new FHIRException("Unknown FHIRAllTypes code '" + codeString + "'"); 5494 } 5495 5496 public String toCode() { 5497 switch (this) { 5498 case ADDRESS: 5499 return "Address"; 5500 case AGE: 5501 return "Age"; 5502 case ANNOTATION: 5503 return "Annotation"; 5504 case ATTACHMENT: 5505 return "Attachment"; 5506 case BACKBONEELEMENT: 5507 return "BackboneElement"; 5508 case CODEABLECONCEPT: 5509 return "CodeableConcept"; 5510 case CODING: 5511 return "Coding"; 5512 case CONTACTDETAIL: 5513 return "ContactDetail"; 5514 case CONTACTPOINT: 5515 return "ContactPoint"; 5516 case CONTRIBUTOR: 5517 return "Contributor"; 5518 case COUNT: 5519 return "Count"; 5520 case DATAREQUIREMENT: 5521 return "DataRequirement"; 5522 case DISTANCE: 5523 return "Distance"; 5524 case DOSAGE: 5525 return "Dosage"; 5526 case DURATION: 5527 return "Duration"; 5528 case ELEMENT: 5529 return "Element"; 5530 case ELEMENTDEFINITION: 5531 return "ElementDefinition"; 5532 case EXPRESSION: 5533 return "Expression"; 5534 case EXTENSION: 5535 return "Extension"; 5536 case HUMANNAME: 5537 return "HumanName"; 5538 case IDENTIFIER: 5539 return "Identifier"; 5540 case MARKETINGSTATUS: 5541 return "MarketingStatus"; 5542 case META: 5543 return "Meta"; 5544 case MONEY: 5545 return "Money"; 5546 case MONEYQUANTITY: 5547 return "MoneyQuantity"; 5548 case NARRATIVE: 5549 return "Narrative"; 5550 case PARAMETERDEFINITION: 5551 return "ParameterDefinition"; 5552 case PERIOD: 5553 return "Period"; 5554 case POPULATION: 5555 return "Population"; 5556 case PRODCHARACTERISTIC: 5557 return "ProdCharacteristic"; 5558 case PRODUCTSHELFLIFE: 5559 return "ProductShelfLife"; 5560 case QUANTITY: 5561 return "Quantity"; 5562 case RANGE: 5563 return "Range"; 5564 case RATIO: 5565 return "Ratio"; 5566 case REFERENCE: 5567 return "Reference"; 5568 case RELATEDARTIFACT: 5569 return "RelatedArtifact"; 5570 case SAMPLEDDATA: 5571 return "SampledData"; 5572 case SIGNATURE: 5573 return "Signature"; 5574 case SIMPLEQUANTITY: 5575 return "SimpleQuantity"; 5576 case SUBSTANCEAMOUNT: 5577 return "SubstanceAmount"; 5578 case TIMING: 5579 return "Timing"; 5580 case TRIGGERDEFINITION: 5581 return "TriggerDefinition"; 5582 case USAGECONTEXT: 5583 return "UsageContext"; 5584 case BASE64BINARY: 5585 return "base64Binary"; 5586 case BOOLEAN: 5587 return "boolean"; 5588 case CANONICAL: 5589 return "canonical"; 5590 case CODE: 5591 return "code"; 5592 case DATE: 5593 return "date"; 5594 case DATETIME: 5595 return "dateTime"; 5596 case DECIMAL: 5597 return "decimal"; 5598 case ID: 5599 return "id"; 5600 case INSTANT: 5601 return "instant"; 5602 case INTEGER: 5603 return "integer"; 5604 case MARKDOWN: 5605 return "markdown"; 5606 case OID: 5607 return "oid"; 5608 case POSITIVEINT: 5609 return "positiveInt"; 5610 case STRING: 5611 return "string"; 5612 case TIME: 5613 return "time"; 5614 case UNSIGNEDINT: 5615 return "unsignedInt"; 5616 case URI: 5617 return "uri"; 5618 case URL: 5619 return "url"; 5620 case UUID: 5621 return "uuid"; 5622 case XHTML: 5623 return "xhtml"; 5624 case ACCOUNT: 5625 return "Account"; 5626 case ACTIVITYDEFINITION: 5627 return "ActivityDefinition"; 5628 case ADVERSEEVENT: 5629 return "AdverseEvent"; 5630 case ALLERGYINTOLERANCE: 5631 return "AllergyIntolerance"; 5632 case APPOINTMENT: 5633 return "Appointment"; 5634 case APPOINTMENTRESPONSE: 5635 return "AppointmentResponse"; 5636 case AUDITEVENT: 5637 return "AuditEvent"; 5638 case BASIC: 5639 return "Basic"; 5640 case BINARY: 5641 return "Binary"; 5642 case BIOLOGICALLYDERIVEDPRODUCT: 5643 return "BiologicallyDerivedProduct"; 5644 case BODYSTRUCTURE: 5645 return "BodyStructure"; 5646 case BUNDLE: 5647 return "Bundle"; 5648 case CAPABILITYSTATEMENT: 5649 return "CapabilityStatement"; 5650 case CAREPLAN: 5651 return "CarePlan"; 5652 case CARETEAM: 5653 return "CareTeam"; 5654 case CATALOGENTRY: 5655 return "CatalogEntry"; 5656 case CHARGEITEM: 5657 return "ChargeItem"; 5658 case CHARGEITEMDEFINITION: 5659 return "ChargeItemDefinition"; 5660 case CLAIM: 5661 return "Claim"; 5662 case CLAIMRESPONSE: 5663 return "ClaimResponse"; 5664 case CLINICALIMPRESSION: 5665 return "ClinicalImpression"; 5666 case CODESYSTEM: 5667 return "CodeSystem"; 5668 case COMMUNICATION: 5669 return "Communication"; 5670 case COMMUNICATIONREQUEST: 5671 return "CommunicationRequest"; 5672 case COMPARTMENTDEFINITION: 5673 return "CompartmentDefinition"; 5674 case COMPOSITION: 5675 return "Composition"; 5676 case CONCEPTMAP: 5677 return "ConceptMap"; 5678 case CONDITION: 5679 return "Condition"; 5680 case CONSENT: 5681 return "Consent"; 5682 case CONTRACT: 5683 return "Contract"; 5684 case COVERAGE: 5685 return "Coverage"; 5686 case COVERAGEELIGIBILITYREQUEST: 5687 return "CoverageEligibilityRequest"; 5688 case COVERAGEELIGIBILITYRESPONSE: 5689 return "CoverageEligibilityResponse"; 5690 case DETECTEDISSUE: 5691 return "DetectedIssue"; 5692 case DEVICE: 5693 return "Device"; 5694 case DEVICEDEFINITION: 5695 return "DeviceDefinition"; 5696 case DEVICEMETRIC: 5697 return "DeviceMetric"; 5698 case DEVICEREQUEST: 5699 return "DeviceRequest"; 5700 case DEVICEUSESTATEMENT: 5701 return "DeviceUseStatement"; 5702 case DIAGNOSTICREPORT: 5703 return "DiagnosticReport"; 5704 case DOCUMENTMANIFEST: 5705 return "DocumentManifest"; 5706 case DOCUMENTREFERENCE: 5707 return "DocumentReference"; 5708 case DOMAINRESOURCE: 5709 return "DomainResource"; 5710 case EFFECTEVIDENCESYNTHESIS: 5711 return "EffectEvidenceSynthesis"; 5712 case ENCOUNTER: 5713 return "Encounter"; 5714 case ENDPOINT: 5715 return "Endpoint"; 5716 case ENROLLMENTREQUEST: 5717 return "EnrollmentRequest"; 5718 case ENROLLMENTRESPONSE: 5719 return "EnrollmentResponse"; 5720 case EPISODEOFCARE: 5721 return "EpisodeOfCare"; 5722 case EVENTDEFINITION: 5723 return "EventDefinition"; 5724 case EVIDENCE: 5725 return "Evidence"; 5726 case EVIDENCEVARIABLE: 5727 return "EvidenceVariable"; 5728 case EXAMPLESCENARIO: 5729 return "ExampleScenario"; 5730 case EXPLANATIONOFBENEFIT: 5731 return "ExplanationOfBenefit"; 5732 case FAMILYMEMBERHISTORY: 5733 return "FamilyMemberHistory"; 5734 case FLAG: 5735 return "Flag"; 5736 case GOAL: 5737 return "Goal"; 5738 case GRAPHDEFINITION: 5739 return "GraphDefinition"; 5740 case GROUP: 5741 return "Group"; 5742 case GUIDANCERESPONSE: 5743 return "GuidanceResponse"; 5744 case HEALTHCARESERVICE: 5745 return "HealthcareService"; 5746 case IMAGINGSTUDY: 5747 return "ImagingStudy"; 5748 case IMMUNIZATION: 5749 return "Immunization"; 5750 case IMMUNIZATIONEVALUATION: 5751 return "ImmunizationEvaluation"; 5752 case IMMUNIZATIONRECOMMENDATION: 5753 return "ImmunizationRecommendation"; 5754 case IMPLEMENTATIONGUIDE: 5755 return "ImplementationGuide"; 5756 case INSURANCEPLAN: 5757 return "InsurancePlan"; 5758 case INVOICE: 5759 return "Invoice"; 5760 case LIBRARY: 5761 return "Library"; 5762 case LINKAGE: 5763 return "Linkage"; 5764 case LIST: 5765 return "List"; 5766 case LOCATION: 5767 return "Location"; 5768 case MEASURE: 5769 return "Measure"; 5770 case MEASUREREPORT: 5771 return "MeasureReport"; 5772 case MEDIA: 5773 return "Media"; 5774 case MEDICATION: 5775 return "Medication"; 5776 case MEDICATIONADMINISTRATION: 5777 return "MedicationAdministration"; 5778 case MEDICATIONDISPENSE: 5779 return "MedicationDispense"; 5780 case MEDICATIONKNOWLEDGE: 5781 return "MedicationKnowledge"; 5782 case MEDICATIONREQUEST: 5783 return "MedicationRequest"; 5784 case MEDICATIONSTATEMENT: 5785 return "MedicationStatement"; 5786 case MEDICINALPRODUCT: 5787 return "MedicinalProduct"; 5788 case MEDICINALPRODUCTAUTHORIZATION: 5789 return "MedicinalProductAuthorization"; 5790 case MEDICINALPRODUCTCONTRAINDICATION: 5791 return "MedicinalProductContraindication"; 5792 case MEDICINALPRODUCTINDICATION: 5793 return "MedicinalProductIndication"; 5794 case MEDICINALPRODUCTINGREDIENT: 5795 return "MedicinalProductIngredient"; 5796 case MEDICINALPRODUCTINTERACTION: 5797 return "MedicinalProductInteraction"; 5798 case MEDICINALPRODUCTMANUFACTURED: 5799 return "MedicinalProductManufactured"; 5800 case MEDICINALPRODUCTPACKAGED: 5801 return "MedicinalProductPackaged"; 5802 case MEDICINALPRODUCTPHARMACEUTICAL: 5803 return "MedicinalProductPharmaceutical"; 5804 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 5805 return "MedicinalProductUndesirableEffect"; 5806 case MESSAGEDEFINITION: 5807 return "MessageDefinition"; 5808 case MESSAGEHEADER: 5809 return "MessageHeader"; 5810 case MOLECULARSEQUENCE: 5811 return "MolecularSequence"; 5812 case NAMINGSYSTEM: 5813 return "NamingSystem"; 5814 case NUTRITIONORDER: 5815 return "NutritionOrder"; 5816 case OBSERVATION: 5817 return "Observation"; 5818 case OBSERVATIONDEFINITION: 5819 return "ObservationDefinition"; 5820 case OPERATIONDEFINITION: 5821 return "OperationDefinition"; 5822 case OPERATIONOUTCOME: 5823 return "OperationOutcome"; 5824 case ORGANIZATION: 5825 return "Organization"; 5826 case ORGANIZATIONAFFILIATION: 5827 return "OrganizationAffiliation"; 5828 case PARAMETERS: 5829 return "Parameters"; 5830 case PATIENT: 5831 return "Patient"; 5832 case PAYMENTNOTICE: 5833 return "PaymentNotice"; 5834 case PAYMENTRECONCILIATION: 5835 return "PaymentReconciliation"; 5836 case PERSON: 5837 return "Person"; 5838 case PLANDEFINITION: 5839 return "PlanDefinition"; 5840 case PRACTITIONER: 5841 return "Practitioner"; 5842 case PRACTITIONERROLE: 5843 return "PractitionerRole"; 5844 case PROCEDURE: 5845 return "Procedure"; 5846 case PROVENANCE: 5847 return "Provenance"; 5848 case QUESTIONNAIRE: 5849 return "Questionnaire"; 5850 case QUESTIONNAIRERESPONSE: 5851 return "QuestionnaireResponse"; 5852 case RELATEDPERSON: 5853 return "RelatedPerson"; 5854 case REQUESTGROUP: 5855 return "RequestGroup"; 5856 case RESEARCHDEFINITION: 5857 return "ResearchDefinition"; 5858 case RESEARCHELEMENTDEFINITION: 5859 return "ResearchElementDefinition"; 5860 case RESEARCHSTUDY: 5861 return "ResearchStudy"; 5862 case RESEARCHSUBJECT: 5863 return "ResearchSubject"; 5864 case RESOURCE: 5865 return "Resource"; 5866 case RISKASSESSMENT: 5867 return "RiskAssessment"; 5868 case RISKEVIDENCESYNTHESIS: 5869 return "RiskEvidenceSynthesis"; 5870 case SCHEDULE: 5871 return "Schedule"; 5872 case SEARCHPARAMETER: 5873 return "SearchParameter"; 5874 case SERVICEREQUEST: 5875 return "ServiceRequest"; 5876 case SLOT: 5877 return "Slot"; 5878 case SPECIMEN: 5879 return "Specimen"; 5880 case SPECIMENDEFINITION: 5881 return "SpecimenDefinition"; 5882 case STRUCTUREDEFINITION: 5883 return "StructureDefinition"; 5884 case STRUCTUREMAP: 5885 return "StructureMap"; 5886 case SUBSCRIPTION: 5887 return "Subscription"; 5888 case SUBSTANCE: 5889 return "Substance"; 5890 case SUBSTANCENUCLEICACID: 5891 return "SubstanceNucleicAcid"; 5892 case SUBSTANCEPOLYMER: 5893 return "SubstancePolymer"; 5894 case SUBSTANCEPROTEIN: 5895 return "SubstanceProtein"; 5896 case SUBSTANCEREFERENCEINFORMATION: 5897 return "SubstanceReferenceInformation"; 5898 case SUBSTANCESOURCEMATERIAL: 5899 return "SubstanceSourceMaterial"; 5900 case SUBSTANCESPECIFICATION: 5901 return "SubstanceSpecification"; 5902 case SUPPLYDELIVERY: 5903 return "SupplyDelivery"; 5904 case SUPPLYREQUEST: 5905 return "SupplyRequest"; 5906 case TASK: 5907 return "Task"; 5908 case TERMINOLOGYCAPABILITIES: 5909 return "TerminologyCapabilities"; 5910 case TESTREPORT: 5911 return "TestReport"; 5912 case TESTSCRIPT: 5913 return "TestScript"; 5914 case VALUESET: 5915 return "ValueSet"; 5916 case VERIFICATIONRESULT: 5917 return "VerificationResult"; 5918 case VISIONPRESCRIPTION: 5919 return "VisionPrescription"; 5920 case TYPE: 5921 return "Type"; 5922 case ANY: 5923 return "Any"; 5924 case NULL: 5925 return null; 5926 default: 5927 return "?"; 5928 } 5929 } 5930 5931 public String getSystem() { 5932 switch (this) { 5933 case ADDRESS: 5934 return "http://hl7.org/fhir/data-types"; 5935 case AGE: 5936 return "http://hl7.org/fhir/data-types"; 5937 case ANNOTATION: 5938 return "http://hl7.org/fhir/data-types"; 5939 case ATTACHMENT: 5940 return "http://hl7.org/fhir/data-types"; 5941 case BACKBONEELEMENT: 5942 return "http://hl7.org/fhir/data-types"; 5943 case CODEABLECONCEPT: 5944 return "http://hl7.org/fhir/data-types"; 5945 case CODING: 5946 return "http://hl7.org/fhir/data-types"; 5947 case CONTACTDETAIL: 5948 return "http://hl7.org/fhir/data-types"; 5949 case CONTACTPOINT: 5950 return "http://hl7.org/fhir/data-types"; 5951 case CONTRIBUTOR: 5952 return "http://hl7.org/fhir/data-types"; 5953 case COUNT: 5954 return "http://hl7.org/fhir/data-types"; 5955 case DATAREQUIREMENT: 5956 return "http://hl7.org/fhir/data-types"; 5957 case DISTANCE: 5958 return "http://hl7.org/fhir/data-types"; 5959 case DOSAGE: 5960 return "http://hl7.org/fhir/data-types"; 5961 case DURATION: 5962 return "http://hl7.org/fhir/data-types"; 5963 case ELEMENT: 5964 return "http://hl7.org/fhir/data-types"; 5965 case ELEMENTDEFINITION: 5966 return "http://hl7.org/fhir/data-types"; 5967 case EXPRESSION: 5968 return "http://hl7.org/fhir/data-types"; 5969 case EXTENSION: 5970 return "http://hl7.org/fhir/data-types"; 5971 case HUMANNAME: 5972 return "http://hl7.org/fhir/data-types"; 5973 case IDENTIFIER: 5974 return "http://hl7.org/fhir/data-types"; 5975 case MARKETINGSTATUS: 5976 return "http://hl7.org/fhir/data-types"; 5977 case META: 5978 return "http://hl7.org/fhir/data-types"; 5979 case MONEY: 5980 return "http://hl7.org/fhir/data-types"; 5981 case MONEYQUANTITY: 5982 return "http://hl7.org/fhir/data-types"; 5983 case NARRATIVE: 5984 return "http://hl7.org/fhir/data-types"; 5985 case PARAMETERDEFINITION: 5986 return "http://hl7.org/fhir/data-types"; 5987 case PERIOD: 5988 return "http://hl7.org/fhir/data-types"; 5989 case POPULATION: 5990 return "http://hl7.org/fhir/data-types"; 5991 case PRODCHARACTERISTIC: 5992 return "http://hl7.org/fhir/data-types"; 5993 case PRODUCTSHELFLIFE: 5994 return "http://hl7.org/fhir/data-types"; 5995 case QUANTITY: 5996 return "http://hl7.org/fhir/data-types"; 5997 case RANGE: 5998 return "http://hl7.org/fhir/data-types"; 5999 case RATIO: 6000 return "http://hl7.org/fhir/data-types"; 6001 case REFERENCE: 6002 return "http://hl7.org/fhir/data-types"; 6003 case RELATEDARTIFACT: 6004 return "http://hl7.org/fhir/data-types"; 6005 case SAMPLEDDATA: 6006 return "http://hl7.org/fhir/data-types"; 6007 case SIGNATURE: 6008 return "http://hl7.org/fhir/data-types"; 6009 case SIMPLEQUANTITY: 6010 return "http://hl7.org/fhir/data-types"; 6011 case SUBSTANCEAMOUNT: 6012 return "http://hl7.org/fhir/data-types"; 6013 case TIMING: 6014 return "http://hl7.org/fhir/data-types"; 6015 case TRIGGERDEFINITION: 6016 return "http://hl7.org/fhir/data-types"; 6017 case USAGECONTEXT: 6018 return "http://hl7.org/fhir/data-types"; 6019 case BASE64BINARY: 6020 return "http://hl7.org/fhir/data-types"; 6021 case BOOLEAN: 6022 return "http://hl7.org/fhir/data-types"; 6023 case CANONICAL: 6024 return "http://hl7.org/fhir/data-types"; 6025 case CODE: 6026 return "http://hl7.org/fhir/data-types"; 6027 case DATE: 6028 return "http://hl7.org/fhir/data-types"; 6029 case DATETIME: 6030 return "http://hl7.org/fhir/data-types"; 6031 case DECIMAL: 6032 return "http://hl7.org/fhir/data-types"; 6033 case ID: 6034 return "http://hl7.org/fhir/data-types"; 6035 case INSTANT: 6036 return "http://hl7.org/fhir/data-types"; 6037 case INTEGER: 6038 return "http://hl7.org/fhir/data-types"; 6039 case MARKDOWN: 6040 return "http://hl7.org/fhir/data-types"; 6041 case OID: 6042 return "http://hl7.org/fhir/data-types"; 6043 case POSITIVEINT: 6044 return "http://hl7.org/fhir/data-types"; 6045 case STRING: 6046 return "http://hl7.org/fhir/data-types"; 6047 case TIME: 6048 return "http://hl7.org/fhir/data-types"; 6049 case UNSIGNEDINT: 6050 return "http://hl7.org/fhir/data-types"; 6051 case URI: 6052 return "http://hl7.org/fhir/data-types"; 6053 case URL: 6054 return "http://hl7.org/fhir/data-types"; 6055 case UUID: 6056 return "http://hl7.org/fhir/data-types"; 6057 case XHTML: 6058 return "http://hl7.org/fhir/data-types"; 6059 case ACCOUNT: 6060 return "http://hl7.org/fhir/resource-types"; 6061 case ACTIVITYDEFINITION: 6062 return "http://hl7.org/fhir/resource-types"; 6063 case ADVERSEEVENT: 6064 return "http://hl7.org/fhir/resource-types"; 6065 case ALLERGYINTOLERANCE: 6066 return "http://hl7.org/fhir/resource-types"; 6067 case APPOINTMENT: 6068 return "http://hl7.org/fhir/resource-types"; 6069 case APPOINTMENTRESPONSE: 6070 return "http://hl7.org/fhir/resource-types"; 6071 case AUDITEVENT: 6072 return "http://hl7.org/fhir/resource-types"; 6073 case BASIC: 6074 return "http://hl7.org/fhir/resource-types"; 6075 case BINARY: 6076 return "http://hl7.org/fhir/resource-types"; 6077 case BIOLOGICALLYDERIVEDPRODUCT: 6078 return "http://hl7.org/fhir/resource-types"; 6079 case BODYSTRUCTURE: 6080 return "http://hl7.org/fhir/resource-types"; 6081 case BUNDLE: 6082 return "http://hl7.org/fhir/resource-types"; 6083 case CAPABILITYSTATEMENT: 6084 return "http://hl7.org/fhir/resource-types"; 6085 case CAREPLAN: 6086 return "http://hl7.org/fhir/resource-types"; 6087 case CARETEAM: 6088 return "http://hl7.org/fhir/resource-types"; 6089 case CATALOGENTRY: 6090 return "http://hl7.org/fhir/resource-types"; 6091 case CHARGEITEM: 6092 return "http://hl7.org/fhir/resource-types"; 6093 case CHARGEITEMDEFINITION: 6094 return "http://hl7.org/fhir/resource-types"; 6095 case CLAIM: 6096 return "http://hl7.org/fhir/resource-types"; 6097 case CLAIMRESPONSE: 6098 return "http://hl7.org/fhir/resource-types"; 6099 case CLINICALIMPRESSION: 6100 return "http://hl7.org/fhir/resource-types"; 6101 case CODESYSTEM: 6102 return "http://hl7.org/fhir/resource-types"; 6103 case COMMUNICATION: 6104 return "http://hl7.org/fhir/resource-types"; 6105 case COMMUNICATIONREQUEST: 6106 return "http://hl7.org/fhir/resource-types"; 6107 case COMPARTMENTDEFINITION: 6108 return "http://hl7.org/fhir/resource-types"; 6109 case COMPOSITION: 6110 return "http://hl7.org/fhir/resource-types"; 6111 case CONCEPTMAP: 6112 return "http://hl7.org/fhir/resource-types"; 6113 case CONDITION: 6114 return "http://hl7.org/fhir/resource-types"; 6115 case CONSENT: 6116 return "http://hl7.org/fhir/resource-types"; 6117 case CONTRACT: 6118 return "http://hl7.org/fhir/resource-types"; 6119 case COVERAGE: 6120 return "http://hl7.org/fhir/resource-types"; 6121 case COVERAGEELIGIBILITYREQUEST: 6122 return "http://hl7.org/fhir/resource-types"; 6123 case COVERAGEELIGIBILITYRESPONSE: 6124 return "http://hl7.org/fhir/resource-types"; 6125 case DETECTEDISSUE: 6126 return "http://hl7.org/fhir/resource-types"; 6127 case DEVICE: 6128 return "http://hl7.org/fhir/resource-types"; 6129 case DEVICEDEFINITION: 6130 return "http://hl7.org/fhir/resource-types"; 6131 case DEVICEMETRIC: 6132 return "http://hl7.org/fhir/resource-types"; 6133 case DEVICEREQUEST: 6134 return "http://hl7.org/fhir/resource-types"; 6135 case DEVICEUSESTATEMENT: 6136 return "http://hl7.org/fhir/resource-types"; 6137 case DIAGNOSTICREPORT: 6138 return "http://hl7.org/fhir/resource-types"; 6139 case DOCUMENTMANIFEST: 6140 return "http://hl7.org/fhir/resource-types"; 6141 case DOCUMENTREFERENCE: 6142 return "http://hl7.org/fhir/resource-types"; 6143 case DOMAINRESOURCE: 6144 return "http://hl7.org/fhir/resource-types"; 6145 case EFFECTEVIDENCESYNTHESIS: 6146 return "http://hl7.org/fhir/resource-types"; 6147 case ENCOUNTER: 6148 return "http://hl7.org/fhir/resource-types"; 6149 case ENDPOINT: 6150 return "http://hl7.org/fhir/resource-types"; 6151 case ENROLLMENTREQUEST: 6152 return "http://hl7.org/fhir/resource-types"; 6153 case ENROLLMENTRESPONSE: 6154 return "http://hl7.org/fhir/resource-types"; 6155 case EPISODEOFCARE: 6156 return "http://hl7.org/fhir/resource-types"; 6157 case EVENTDEFINITION: 6158 return "http://hl7.org/fhir/resource-types"; 6159 case EVIDENCE: 6160 return "http://hl7.org/fhir/resource-types"; 6161 case EVIDENCEVARIABLE: 6162 return "http://hl7.org/fhir/resource-types"; 6163 case EXAMPLESCENARIO: 6164 return "http://hl7.org/fhir/resource-types"; 6165 case EXPLANATIONOFBENEFIT: 6166 return "http://hl7.org/fhir/resource-types"; 6167 case FAMILYMEMBERHISTORY: 6168 return "http://hl7.org/fhir/resource-types"; 6169 case FLAG: 6170 return "http://hl7.org/fhir/resource-types"; 6171 case GOAL: 6172 return "http://hl7.org/fhir/resource-types"; 6173 case GRAPHDEFINITION: 6174 return "http://hl7.org/fhir/resource-types"; 6175 case GROUP: 6176 return "http://hl7.org/fhir/resource-types"; 6177 case GUIDANCERESPONSE: 6178 return "http://hl7.org/fhir/resource-types"; 6179 case HEALTHCARESERVICE: 6180 return "http://hl7.org/fhir/resource-types"; 6181 case IMAGINGSTUDY: 6182 return "http://hl7.org/fhir/resource-types"; 6183 case IMMUNIZATION: 6184 return "http://hl7.org/fhir/resource-types"; 6185 case IMMUNIZATIONEVALUATION: 6186 return "http://hl7.org/fhir/resource-types"; 6187 case IMMUNIZATIONRECOMMENDATION: 6188 return "http://hl7.org/fhir/resource-types"; 6189 case IMPLEMENTATIONGUIDE: 6190 return "http://hl7.org/fhir/resource-types"; 6191 case INSURANCEPLAN: 6192 return "http://hl7.org/fhir/resource-types"; 6193 case INVOICE: 6194 return "http://hl7.org/fhir/resource-types"; 6195 case LIBRARY: 6196 return "http://hl7.org/fhir/resource-types"; 6197 case LINKAGE: 6198 return "http://hl7.org/fhir/resource-types"; 6199 case LIST: 6200 return "http://hl7.org/fhir/resource-types"; 6201 case LOCATION: 6202 return "http://hl7.org/fhir/resource-types"; 6203 case MEASURE: 6204 return "http://hl7.org/fhir/resource-types"; 6205 case MEASUREREPORT: 6206 return "http://hl7.org/fhir/resource-types"; 6207 case MEDIA: 6208 return "http://hl7.org/fhir/resource-types"; 6209 case MEDICATION: 6210 return "http://hl7.org/fhir/resource-types"; 6211 case MEDICATIONADMINISTRATION: 6212 return "http://hl7.org/fhir/resource-types"; 6213 case MEDICATIONDISPENSE: 6214 return "http://hl7.org/fhir/resource-types"; 6215 case MEDICATIONKNOWLEDGE: 6216 return "http://hl7.org/fhir/resource-types"; 6217 case MEDICATIONREQUEST: 6218 return "http://hl7.org/fhir/resource-types"; 6219 case MEDICATIONSTATEMENT: 6220 return "http://hl7.org/fhir/resource-types"; 6221 case MEDICINALPRODUCT: 6222 return "http://hl7.org/fhir/resource-types"; 6223 case MEDICINALPRODUCTAUTHORIZATION: 6224 return "http://hl7.org/fhir/resource-types"; 6225 case MEDICINALPRODUCTCONTRAINDICATION: 6226 return "http://hl7.org/fhir/resource-types"; 6227 case MEDICINALPRODUCTINDICATION: 6228 return "http://hl7.org/fhir/resource-types"; 6229 case MEDICINALPRODUCTINGREDIENT: 6230 return "http://hl7.org/fhir/resource-types"; 6231 case MEDICINALPRODUCTINTERACTION: 6232 return "http://hl7.org/fhir/resource-types"; 6233 case MEDICINALPRODUCTMANUFACTURED: 6234 return "http://hl7.org/fhir/resource-types"; 6235 case MEDICINALPRODUCTPACKAGED: 6236 return "http://hl7.org/fhir/resource-types"; 6237 case MEDICINALPRODUCTPHARMACEUTICAL: 6238 return "http://hl7.org/fhir/resource-types"; 6239 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 6240 return "http://hl7.org/fhir/resource-types"; 6241 case MESSAGEDEFINITION: 6242 return "http://hl7.org/fhir/resource-types"; 6243 case MESSAGEHEADER: 6244 return "http://hl7.org/fhir/resource-types"; 6245 case MOLECULARSEQUENCE: 6246 return "http://hl7.org/fhir/resource-types"; 6247 case NAMINGSYSTEM: 6248 return "http://hl7.org/fhir/resource-types"; 6249 case NUTRITIONORDER: 6250 return "http://hl7.org/fhir/resource-types"; 6251 case OBSERVATION: 6252 return "http://hl7.org/fhir/resource-types"; 6253 case OBSERVATIONDEFINITION: 6254 return "http://hl7.org/fhir/resource-types"; 6255 case OPERATIONDEFINITION: 6256 return "http://hl7.org/fhir/resource-types"; 6257 case OPERATIONOUTCOME: 6258 return "http://hl7.org/fhir/resource-types"; 6259 case ORGANIZATION: 6260 return "http://hl7.org/fhir/resource-types"; 6261 case ORGANIZATIONAFFILIATION: 6262 return "http://hl7.org/fhir/resource-types"; 6263 case PARAMETERS: 6264 return "http://hl7.org/fhir/resource-types"; 6265 case PATIENT: 6266 return "http://hl7.org/fhir/resource-types"; 6267 case PAYMENTNOTICE: 6268 return "http://hl7.org/fhir/resource-types"; 6269 case PAYMENTRECONCILIATION: 6270 return "http://hl7.org/fhir/resource-types"; 6271 case PERSON: 6272 return "http://hl7.org/fhir/resource-types"; 6273 case PLANDEFINITION: 6274 return "http://hl7.org/fhir/resource-types"; 6275 case PRACTITIONER: 6276 return "http://hl7.org/fhir/resource-types"; 6277 case PRACTITIONERROLE: 6278 return "http://hl7.org/fhir/resource-types"; 6279 case PROCEDURE: 6280 return "http://hl7.org/fhir/resource-types"; 6281 case PROVENANCE: 6282 return "http://hl7.org/fhir/resource-types"; 6283 case QUESTIONNAIRE: 6284 return "http://hl7.org/fhir/resource-types"; 6285 case QUESTIONNAIRERESPONSE: 6286 return "http://hl7.org/fhir/resource-types"; 6287 case RELATEDPERSON: 6288 return "http://hl7.org/fhir/resource-types"; 6289 case REQUESTGROUP: 6290 return "http://hl7.org/fhir/resource-types"; 6291 case RESEARCHDEFINITION: 6292 return "http://hl7.org/fhir/resource-types"; 6293 case RESEARCHELEMENTDEFINITION: 6294 return "http://hl7.org/fhir/resource-types"; 6295 case RESEARCHSTUDY: 6296 return "http://hl7.org/fhir/resource-types"; 6297 case RESEARCHSUBJECT: 6298 return "http://hl7.org/fhir/resource-types"; 6299 case RESOURCE: 6300 return "http://hl7.org/fhir/resource-types"; 6301 case RISKASSESSMENT: 6302 return "http://hl7.org/fhir/resource-types"; 6303 case RISKEVIDENCESYNTHESIS: 6304 return "http://hl7.org/fhir/resource-types"; 6305 case SCHEDULE: 6306 return "http://hl7.org/fhir/resource-types"; 6307 case SEARCHPARAMETER: 6308 return "http://hl7.org/fhir/resource-types"; 6309 case SERVICEREQUEST: 6310 return "http://hl7.org/fhir/resource-types"; 6311 case SLOT: 6312 return "http://hl7.org/fhir/resource-types"; 6313 case SPECIMEN: 6314 return "http://hl7.org/fhir/resource-types"; 6315 case SPECIMENDEFINITION: 6316 return "http://hl7.org/fhir/resource-types"; 6317 case STRUCTUREDEFINITION: 6318 return "http://hl7.org/fhir/resource-types"; 6319 case STRUCTUREMAP: 6320 return "http://hl7.org/fhir/resource-types"; 6321 case SUBSCRIPTION: 6322 return "http://hl7.org/fhir/resource-types"; 6323 case SUBSTANCE: 6324 return "http://hl7.org/fhir/resource-types"; 6325 case SUBSTANCENUCLEICACID: 6326 return "http://hl7.org/fhir/resource-types"; 6327 case SUBSTANCEPOLYMER: 6328 return "http://hl7.org/fhir/resource-types"; 6329 case SUBSTANCEPROTEIN: 6330 return "http://hl7.org/fhir/resource-types"; 6331 case SUBSTANCEREFERENCEINFORMATION: 6332 return "http://hl7.org/fhir/resource-types"; 6333 case SUBSTANCESOURCEMATERIAL: 6334 return "http://hl7.org/fhir/resource-types"; 6335 case SUBSTANCESPECIFICATION: 6336 return "http://hl7.org/fhir/resource-types"; 6337 case SUPPLYDELIVERY: 6338 return "http://hl7.org/fhir/resource-types"; 6339 case SUPPLYREQUEST: 6340 return "http://hl7.org/fhir/resource-types"; 6341 case TASK: 6342 return "http://hl7.org/fhir/resource-types"; 6343 case TERMINOLOGYCAPABILITIES: 6344 return "http://hl7.org/fhir/resource-types"; 6345 case TESTREPORT: 6346 return "http://hl7.org/fhir/resource-types"; 6347 case TESTSCRIPT: 6348 return "http://hl7.org/fhir/resource-types"; 6349 case VALUESET: 6350 return "http://hl7.org/fhir/resource-types"; 6351 case VERIFICATIONRESULT: 6352 return "http://hl7.org/fhir/resource-types"; 6353 case VISIONPRESCRIPTION: 6354 return "http://hl7.org/fhir/resource-types"; 6355 case TYPE: 6356 return "http://hl7.org/fhir/abstract-types"; 6357 case ANY: 6358 return "http://hl7.org/fhir/abstract-types"; 6359 case NULL: 6360 return null; 6361 default: 6362 return "?"; 6363 } 6364 } 6365 6366 public String getDefinition() { 6367 switch (this) { 6368 case ADDRESS: 6369 return "An address expressed using postal conventions (as opposed to GPS or other location definition formats). This data type may be used to convey addresses for use in delivering mail as well as for visiting locations which might not be valid for mail delivery. There are a variety of postal address formats defined around the world."; 6370 case AGE: 6371 return "A duration of time during which an organism (or a process) has existed."; 6372 case ANNOTATION: 6373 return "A text note which also contains information about who made the statement and when."; 6374 case ATTACHMENT: 6375 return "For referring to data content defined in other formats."; 6376 case BACKBONEELEMENT: 6377 return "Base definition for all elements that are defined inside a resource - but not those in a data type."; 6378 case CODEABLECONCEPT: 6379 return "A concept that may be defined by a formal reference to a terminology or ontology or may be provided by text."; 6380 case CODING: 6381 return "A reference to a code defined by a terminology system."; 6382 case CONTACTDETAIL: 6383 return "Specifies contact information for a person or organization."; 6384 case CONTACTPOINT: 6385 return "Details for all kinds of technology mediated contact points for a person or organization, including telephone, email, etc."; 6386 case CONTRIBUTOR: 6387 return "A contributor to the content of a knowledge asset, including authors, editors, reviewers, and endorsers."; 6388 case COUNT: 6389 return "A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies."; 6390 case DATAREQUIREMENT: 6391 return "Describes a required data item for evaluation in terms of the type of data, and optional code or date-based filters of the data."; 6392 case DISTANCE: 6393 return "A length - a value with a unit that is a physical distance."; 6394 case DOSAGE: 6395 return "Indicates how the medication is/was taken or should be taken by the patient."; 6396 case DURATION: 6397 return "A length of time."; 6398 case ELEMENT: 6399 return "Base definition for all elements in a resource."; 6400 case ELEMENTDEFINITION: 6401 return "Captures constraints on each element within the resource, profile, or extension."; 6402 case EXPRESSION: 6403 return "A expression that is evaluated in a specified context and returns a value. The context of use of the expression must specify the context in which the expression is evaluated, and how the result of the expression is used."; 6404 case EXTENSION: 6405 return "Optional Extension Element - found in all resources."; 6406 case HUMANNAME: 6407 return "A human's name with the ability to identify parts and usage."; 6408 case IDENTIFIER: 6409 return "An identifier - identifies some entity uniquely and unambiguously. Typically this is used for business identifiers."; 6410 case MARKETINGSTATUS: 6411 return "The marketing status describes the date when a medicinal product is actually put on the market or the date as of which it is no longer available."; 6412 case META: 6413 return "The metadata about a resource. This is content in the resource that is maintained by the infrastructure. Changes to the content might not always be associated with version changes to the resource."; 6414 case MONEY: 6415 return "An amount of economic utility in some recognized currency."; 6416 case MONEYQUANTITY: 6417 return ""; 6418 case NARRATIVE: 6419 return "A human-readable summary of the resource conveying the essential clinical and business information for the resource."; 6420 case PARAMETERDEFINITION: 6421 return "The parameters to the module. This collection specifies both the input and output parameters. Input parameters are provided by the caller as part of the $evaluate operation. Output parameters are included in the GuidanceResponse."; 6422 case PERIOD: 6423 return "A time period defined by a start and end date and optionally time."; 6424 case POPULATION: 6425 return "A populatioof people with some set of grouping criteria."; 6426 case PRODCHARACTERISTIC: 6427 return "The marketing status describes the date when a medicinal product is actually put on the market or the date as of which it is no longer available."; 6428 case PRODUCTSHELFLIFE: 6429 return "The shelf-life and storage information for a medicinal product item or container can be described using this class."; 6430 case QUANTITY: 6431 return "A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies."; 6432 case RANGE: 6433 return "A set of ordered Quantities defined by a low and high limit."; 6434 case RATIO: 6435 return "A relationship of two Quantity values - expressed as a numerator and a denominator."; 6436 case REFERENCE: 6437 return "A reference from one resource to another."; 6438 case RELATEDARTIFACT: 6439 return "Related artifacts such as additional documentation, justification, or bibliographic references."; 6440 case SAMPLEDDATA: 6441 return "A series of measurements taken by a device, with upper and lower limits. There may be more than one dimension in the data."; 6442 case SIGNATURE: 6443 return "A signature along with supporting context. The signature may be a digital signature that is cryptographic in nature, or some other signature acceptable to the domain. This other signature may be as simple as a graphical image representing a hand-written signature, or a signature ceremony Different signature approaches have different utilities."; 6444 case SIMPLEQUANTITY: 6445 return ""; 6446 case SUBSTANCEAMOUNT: 6447 return "Chemical substances are a single substance type whose primary defining element is the molecular structure. Chemical substances shall be defined on the basis of their complete covalent molecular structure; the presence of a salt (counter-ion) and/or solvates (water, alcohols) is also captured. Purity, grade, physical form or particle size are not taken into account in the definition of a chemical substance or in the assignment of a Substance ID."; 6448 case TIMING: 6449 return "Specifies an event that may occur multiple times. Timing schedules are used to record when things are planned, expected or requested to occur. The most common usage is in dosage instructions for medications. They are also used when planning care of various kinds, and may be used for reporting the schedule to which past regular activities were carried out."; 6450 case TRIGGERDEFINITION: 6451 return "A description of a triggering event. Triggering events can be named events, data events, or periodic, as determined by the type element."; 6452 case USAGECONTEXT: 6453 return "Specifies clinical/business/etc. metadata that can be used to retrieve, index and/or categorize an artifact. This metadata can either be specific to the applicable population (e.g., age category, DRG) or the specific context of care (e.g., venue, care setting, provider of care)."; 6454 case BASE64BINARY: 6455 return "A stream of bytes"; 6456 case BOOLEAN: 6457 return "Value of \"true\" or \"false\""; 6458 case CANONICAL: 6459 return "A URI that is a reference to a canonical URL on a FHIR resource"; 6460 case CODE: 6461 return "A string which has at least one character and no leading or trailing whitespace and where there is no whitespace other than single spaces in the contents"; 6462 case DATE: 6463 return "A date or partial date (e.g. just year or year + month). There is no time zone. The format is a union of the schema types gYear, gYearMonth and date. Dates SHALL be valid dates."; 6464 case DATETIME: 6465 return "A date, date-time or partial date (e.g. just year or year + month). If hours and minutes are specified, a time zone SHALL be populated. The format is a union of the schema types gYear, gYearMonth, date and dateTime. Seconds must be provided due to schema type constraints but may be zero-filled and may be ignored. Dates SHALL be valid dates."; 6466 case DECIMAL: 6467 return "A rational number with implicit precision"; 6468 case ID: 6469 return "Any combination of letters, numerals, \"-\" and \".\", with a length limit of 64 characters. (This might be an integer, an unprefixed OID, UUID or any other identifier pattern that meets these constraints.) Ids are case-insensitive."; 6470 case INSTANT: 6471 return "An instant in time - known at least to the second"; 6472 case INTEGER: 6473 return "A whole number"; 6474 case MARKDOWN: 6475 return "A string that may contain Github Flavored Markdown syntax for optional processing by a mark down presentation engine"; 6476 case OID: 6477 return "An OID represented as a URI"; 6478 case POSITIVEINT: 6479 return "An integer with a value that is positive (e.g. >0)"; 6480 case STRING: 6481 return "A sequence of Unicode characters"; 6482 case TIME: 6483 return "A time during the day, with no date specified"; 6484 case UNSIGNEDINT: 6485 return "An integer with a value that is not negative (e.g. >= 0)"; 6486 case URI: 6487 return "String of characters used to identify a name or a resource"; 6488 case URL: 6489 return "A URI that is a literal reference"; 6490 case UUID: 6491 return "A UUID, represented as a URI"; 6492 case XHTML: 6493 return "XHTML format, as defined by W3C, but restricted usage (mainly, no active content)"; 6494 case ACCOUNT: 6495 return "A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centers, etc."; 6496 case ACTIVITYDEFINITION: 6497 return "This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context."; 6498 case ADVERSEEVENT: 6499 return "Actual or potential/avoided event causing unintended physical injury resulting from or contributed to by medical care, a research study or other healthcare setting factors that requires additional monitoring, treatment, or hospitalization, or that results in death."; 6500 case ALLERGYINTOLERANCE: 6501 return "Risk of harmful or undesirable, physiological response which is unique to an individual and associated with exposure to a substance."; 6502 case APPOINTMENT: 6503 return "A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s)."; 6504 case APPOINTMENTRESPONSE: 6505 return "A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection."; 6506 case AUDITEVENT: 6507 return "A record of an event made for purposes of maintaining a security log. Typical uses include detection of intrusion attempts and monitoring for inappropriate usage."; 6508 case BASIC: 6509 return "Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification."; 6510 case BINARY: 6511 return "A resource that represents the data of a single raw artifact as digital content accessible in its native format. A Binary resource can contain any content, whether text, image, pdf, zip archive, etc."; 6512 case BIOLOGICALLYDERIVEDPRODUCT: 6513 return "A material substance originating from a biological entity intended to be transplanted or infused\ninto another (possibly the same) biological entity."; 6514 case BODYSTRUCTURE: 6515 return "Record details about an anatomical structure. This resource may be used when a coded concept does not provide the necessary detail needed for the use case."; 6516 case BUNDLE: 6517 return "A container for a collection of resources."; 6518 case CAPABILITYSTATEMENT: 6519 return "A Capability Statement documents a set of capabilities (behaviors) of a FHIR Server for a particular version of FHIR that may be used as a statement of actual server functionality or a statement of required or desired server implementation."; 6520 case CAREPLAN: 6521 return "Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions."; 6522 case CARETEAM: 6523 return "The Care Team includes all the people and organizations who plan to participate in the coordination and delivery of care for a patient."; 6524 case CATALOGENTRY: 6525 return "Catalog entries are wrappers that contextualize items included in a catalog."; 6526 case CHARGEITEM: 6527 return "The resource ChargeItem describes the provision of healthcare provider products for a certain patient, therefore referring not only to the product, but containing in addition details of the provision, like date, time, amounts and participating organizations and persons. Main Usage of the ChargeItem is to enable the billing process and internal cost allocation."; 6528 case CHARGEITEMDEFINITION: 6529 return "The ChargeItemDefinition resource provides the properties that apply to the (billing) codes necessary to calculate costs and prices. The properties may differ largely depending on type and realm, therefore this resource gives only a rough structure and requires profiling for each type of billing code system."; 6530 case CLAIM: 6531 return "A provider issued list of professional services and products which have been provided, or are to be provided, to a patient which is sent to an insurer for reimbursement."; 6532 case CLAIMRESPONSE: 6533 return "This resource provides the adjudication details from the processing of a Claim resource."; 6534 case CLINICALIMPRESSION: 6535 return "A record of a clinical assessment performed to determine what problem(s) may affect the patient and before planning the treatments or management strategies that are best to manage a patient's condition. Assessments are often 1:1 with a clinical consultation / encounter, but this varies greatly depending on the clinical workflow. This resource is called \"ClinicalImpression\" rather than \"ClinicalAssessment\" to avoid confusion with the recording of assessment tools such as Apgar score."; 6536 case CODESYSTEM: 6537 return "The CodeSystem resource is used to declare the existence of and describe a code system or code system supplement and its key properties, and optionally define a part or all of its content."; 6538 case COMMUNICATION: 6539 return "An occurrence of information being transmitted; e.g. an alert that was sent to a responsible provider, a public health agency that was notified about a reportable condition."; 6540 case COMMUNICATIONREQUEST: 6541 return "A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition."; 6542 case COMPARTMENTDEFINITION: 6543 return "A compartment definition that defines how resources are accessed on a server."; 6544 case COMPOSITION: 6545 return "A set of healthcare-related information that is assembled together into a single logical package that provides a single coherent statement of meaning, establishes its own context and that has clinical attestation with regard to who is making the statement. A Composition defines the structure and narrative content necessary for a document. However, a Composition alone does not constitute a document. Rather, the Composition must be the first entry in a Bundle where Bundle.type=document, and any other resources referenced from Composition must be included as subsequent entries in the Bundle (for example Patient, Practitioner, Encounter, etc.)."; 6546 case CONCEPTMAP: 6547 return "A statement of relationships from one set of concepts to one or more other concepts - either concepts in code systems, or data element/data element concepts, or classes in class models."; 6548 case CONDITION: 6549 return "A clinical condition, problem, diagnosis, or other event, situation, issue, or clinical concept that has risen to a level of concern."; 6550 case CONSENT: 6551 return "A record of a healthcare consumer?s choices, which permits or denies identified recipient(s) or recipient role(s) to perform one or more actions within a given policy context, for specific purposes and periods of time."; 6552 case CONTRACT: 6553 return "Legally enforceable, formally recorded unilateral or bilateral directive i.e., a policy or agreement."; 6554 case COVERAGE: 6555 return "Financial instrument which may be used to reimburse or pay for health care products and services. Includes both insurance and self-payment."; 6556 case COVERAGEELIGIBILITYREQUEST: 6557 return "The CoverageEligibilityRequest provides patient and insurance coverage information to an insurer for them to respond, in the form of an CoverageEligibilityResponse, with information regarding whether the stated coverage is valid and in-force and optionally to provide the insurance details of the policy."; 6558 case COVERAGEELIGIBILITYRESPONSE: 6559 return "This resource provides eligibility and plan details from the processing of an CoverageEligibilityRequest resource."; 6560 case DETECTEDISSUE: 6561 return "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, etc."; 6562 case DEVICE: 6563 return "A type of a manufactured item that is used in the provision of healthcare without being substantially changed through that activity. The device may be a medical or non-medical device."; 6564 case DEVICEDEFINITION: 6565 return "The characteristics, operational status and capabilities of a medical-related component of a medical device."; 6566 case DEVICEMETRIC: 6567 return "Describes a measurement, calculation or setting capability of a medical device."; 6568 case DEVICEREQUEST: 6569 return "Represents a request for a patient to employ a medical device. The device may be an implantable device, or an external assistive device, such as a walker."; 6570 case DEVICEUSESTATEMENT: 6571 return "A record of a device being used by a patient where the record is the result of a report from the patient or another clinician."; 6572 case DIAGNOSTICREPORT: 6573 return "The findings and interpretation of diagnostic tests performed on patients, groups of patients, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting and provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports."; 6574 case DOCUMENTMANIFEST: 6575 return "A collection of documents compiled for a purpose together with metadata that applies to the collection."; 6576 case DOCUMENTREFERENCE: 6577 return "A reference to a document of any kind for any purpose. Provides metadata about the document so that the document can be discovered and managed. The scope of a document is any seralized object with a mime-type, so includes formal patient centric documents (CDA), cliical notes, scanned paper, and non-patient specific documents like policy text."; 6578 case DOMAINRESOURCE: 6579 return "A resource that includes narrative, extensions, and contained resources."; 6580 case EFFECTEVIDENCESYNTHESIS: 6581 return "The EffectEvidenceSynthesis resource describes the difference in an outcome between exposures states in a population where the effect estimate is derived from a combination of research studies."; 6582 case ENCOUNTER: 6583 return "An interaction between a patient and healthcare provider(s) for the purpose of providing healthcare service(s) or assessing the health status of a patient."; 6584 case ENDPOINT: 6585 return "The technical details of an endpoint that can be used for electronic services, such as for web services providing XDS.b or a REST endpoint for another FHIR server. This may include any security context information."; 6586 case ENROLLMENTREQUEST: 6587 return "This resource provides the insurance enrollment details to the insurer regarding a specified coverage."; 6588 case ENROLLMENTRESPONSE: 6589 return "This resource provides enrollment and plan details from the processing of an EnrollmentRequest resource."; 6590 case EPISODEOFCARE: 6591 return "An association between a patient and an organization / healthcare provider(s) during which time encounters may occur. The managing organization assumes a level of responsibility for the patient during this time."; 6592 case EVENTDEFINITION: 6593 return "The EventDefinition resource provides a reusable description of when a particular event can occur."; 6594 case EVIDENCE: 6595 return "The Evidence resource describes the conditional state (population and any exposures being compared within the population) and outcome (if specified) that the knowledge (evidence, assertion, recommendation) is about."; 6596 case EVIDENCEVARIABLE: 6597 return "The EvidenceVariable resource describes a \"PICO\" element that knowledge (evidence, assertion, recommendation) is about."; 6598 case EXAMPLESCENARIO: 6599 return "Example of workflow instance."; 6600 case EXPLANATIONOFBENEFIT: 6601 return "This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided."; 6602 case FAMILYMEMBERHISTORY: 6603 return "Significant health conditions for a person related to the patient relevant in the context of care for the patient."; 6604 case FLAG: 6605 return "Prospective warnings of potential issues when providing care to the patient."; 6606 case GOAL: 6607 return "Describes the intended objective(s) for a patient, group or organization care, for example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc."; 6608 case GRAPHDEFINITION: 6609 return "A formal computable definition of a graph of resources - that is, a coherent set of resources that form a graph by following references. The Graph Definition resource defines a set and makes rules about the set."; 6610 case GROUP: 6611 return "Represents a defined collection of entities that may be discussed or acted upon collectively but which are not expected to act collectively, and are not formally or legally recognized; i.e. a collection of entities that isn't an Organization."; 6612 case GUIDANCERESPONSE: 6613 return "A guidance response is the formal response to a guidance request, including any output parameters returned by the evaluation, as well as the description of any proposed actions to be taken."; 6614 case HEALTHCARESERVICE: 6615 return "The details of a healthcare service available at a location."; 6616 case IMAGINGSTUDY: 6617 return "Representation of the content produced in a DICOM imaging study. A study comprises a set of series, each of which includes a set of Service-Object Pair Instances (SOP Instances - images or other data) acquired or produced in a common context. A series is of only one modality (e.g. X-ray, CT, MR, ultrasound), but a study may have multiple series of different modalities."; 6618 case IMMUNIZATION: 6619 return "Describes the event of a patient being administered a vaccine or a record of an immunization as reported by a patient, a clinician or another party."; 6620 case IMMUNIZATIONEVALUATION: 6621 return "Describes a comparison of an immunization event against published recommendations to determine if the administration is \"valid\" in relation to those recommendations."; 6622 case IMMUNIZATIONRECOMMENDATION: 6623 return "A patient's point-in-time set of recommendations (i.e. forecasting) according to a published schedule with optional supporting justification."; 6624 case IMPLEMENTATIONGUIDE: 6625 return "A set of rules of how a particular interoperability or standards problem is solved - typically through the use of FHIR resources. This resource is used to gather all the parts of an implementation guide into a logical whole and to publish a computable definition of all the parts."; 6626 case INSURANCEPLAN: 6627 return "Details of a Health Insurance product/plan provided by an organization."; 6628 case INVOICE: 6629 return "Invoice containing collected ChargeItems from an Account with calculated individual and total price for Billing purpose."; 6630 case LIBRARY: 6631 return "The Library resource is a general-purpose container for knowledge asset definitions. It can be used to describe and expose existing knowledge assets such as logic libraries and information model descriptions, as well as to describe a collection of knowledge assets."; 6632 case LINKAGE: 6633 return "Identifies two or more records (resource instances) that refer to the same real-world \"occurrence\"."; 6634 case LIST: 6635 return "A list is a curated collection of resources."; 6636 case LOCATION: 6637 return "Details and position information for a physical place where services are provided and resources and participants may be stored, found, contained, or accommodated."; 6638 case MEASURE: 6639 return "The Measure resource provides the definition of a quality measure."; 6640 case MEASUREREPORT: 6641 return "The MeasureReport resource contains the results of the calculation of a measure; and optionally a reference to the resources involved in that calculation."; 6642 case MEDIA: 6643 return "A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference."; 6644 case MEDICATION: 6645 return "This resource is primarily used for the identification and definition of a medication for the purposes of prescribing, dispensing, and administering a medication as well as for making statements about medication use."; 6646 case MEDICATIONADMINISTRATION: 6647 return "Describes the event of a patient consuming or otherwise being administered a medication. This may be as simple as swallowing a tablet or it may be a long running infusion. Related resources tie this event to the authorizing prescription, and the specific encounter between patient and health care practitioner."; 6648 case MEDICATIONDISPENSE: 6649 return "Indicates that a medication product is to be or has been dispensed for a named person/patient. This includes a description of the medication product (supply) provided and the instructions for administering the medication. The medication dispense is the result of a pharmacy system responding to a medication order."; 6650 case MEDICATIONKNOWLEDGE: 6651 return "Information about a medication that is used to support knowledge."; 6652 case MEDICATIONREQUEST: 6653 return "An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called \"MedicationRequest\" rather than \"MedicationPrescription\" or \"MedicationOrder\" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns."; 6654 case MEDICATIONSTATEMENT: 6655 return "A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from sources such as the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains. \n\nThe primary difference between a medication statement and a medication administration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medication statement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the medication statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information."; 6656 case MEDICINALPRODUCT: 6657 return "Detailed definition of a medicinal product, typically for uses other than direct patient care (e.g. regulatory use)."; 6658 case MEDICINALPRODUCTAUTHORIZATION: 6659 return "The regulatory authorization of a medicinal product."; 6660 case MEDICINALPRODUCTCONTRAINDICATION: 6661 return "The clinical particulars - indications, contraindications etc. of a medicinal product, including for regulatory purposes."; 6662 case MEDICINALPRODUCTINDICATION: 6663 return "Indication for the Medicinal Product."; 6664 case MEDICINALPRODUCTINGREDIENT: 6665 return "An ingredient of a manufactured item or pharmaceutical product."; 6666 case MEDICINALPRODUCTINTERACTION: 6667 return "The interactions of the medicinal product with other medicinal products, or other forms of interactions."; 6668 case MEDICINALPRODUCTMANUFACTURED: 6669 return "The manufactured item as contained in the packaged medicinal product."; 6670 case MEDICINALPRODUCTPACKAGED: 6671 return "A medicinal product in a container or package."; 6672 case MEDICINALPRODUCTPHARMACEUTICAL: 6673 return "A pharmaceutical product described in terms of its composition and dose form."; 6674 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 6675 return "Describe the undesirable effects of the medicinal product."; 6676 case MESSAGEDEFINITION: 6677 return "Defines the characteristics of a message that can be shared between systems, including the type of event that initiates the message, the content to be transmitted and what response(s), if any, are permitted."; 6678 case MESSAGEHEADER: 6679 return "The header for a message exchange that is either requesting or responding to an action. The reference(s) that are the subject of the action as well as other information related to the action are typically transmitted in a bundle in which the MessageHeader resource instance is the first resource in the bundle."; 6680 case MOLECULARSEQUENCE: 6681 return "Raw data describing a biological sequence."; 6682 case NAMINGSYSTEM: 6683 return "A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a \"System\" used within the Identifier and Coding data types."; 6684 case NUTRITIONORDER: 6685 return "A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident."; 6686 case OBSERVATION: 6687 return "Measurements and simple assertions made about a patient, device or other subject."; 6688 case OBSERVATIONDEFINITION: 6689 return "Set of definitional characteristics for a kind of observation or measurement produced or consumed by an orderable health care service."; 6690 case OPERATIONDEFINITION: 6691 return "A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction)."; 6692 case OPERATIONOUTCOME: 6693 return "A collection of error, warning, or information messages that result from a system action."; 6694 case ORGANIZATION: 6695 return "A formally or informally recognized grouping of people or organizations formed for the purpose of achieving some form of collective action. Includes companies, institutions, corporations, departments, community groups, healthcare practice groups, payer/insurer, etc."; 6696 case ORGANIZATIONAFFILIATION: 6697 return "Defines an affiliation/assotiation/relationship between 2 distinct oganizations, that is not a part-of relationship/sub-division relationship."; 6698 case PARAMETERS: 6699 return "This resource is a non-persisted resource used to pass information into and back from an [operation](operations.html). It has no other use, and there is no RESTful endpoint associated with it."; 6700 case PATIENT: 6701 return "Demographics and other administrative information about an individual or animal receiving care or other health-related services."; 6702 case PAYMENTNOTICE: 6703 return "This resource provides the status of the payment for goods and services rendered, and the request and response resource references."; 6704 case PAYMENTRECONCILIATION: 6705 return "This resource provides the details including amount of a payment and allocates the payment items being paid."; 6706 case PERSON: 6707 return "Demographics and administrative information about a person independent of a specific health-related context."; 6708 case PLANDEFINITION: 6709 return "This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical artifacts such as clinical decision support rules, order sets and protocols."; 6710 case PRACTITIONER: 6711 return "A person who is directly or indirectly involved in the provisioning of healthcare."; 6712 case PRACTITIONERROLE: 6713 return "A specific set of Roles/Locations/specialties/services that a practitioner may perform at an organization for a period of time."; 6714 case PROCEDURE: 6715 return "An action that is or was performed on or for a patient. This can be a physical intervention like an operation, or less invasive like long term services, counseling, or hypnotherapy."; 6716 case PROVENANCE: 6717 return "Provenance of a resource is a record that describes entities and processes involved in producing and delivering or otherwise influencing that resource. Provenance provides a critical foundation for assessing authenticity, enabling trust, and allowing reproducibility. Provenance assertions are a form of contextual metadata and can themselves become important records with their own provenance. Provenance statement indicates clinical significance in terms of confidence in authenticity, reliability, and trustworthiness, integrity, and stage in lifecycle (e.g. Document Completion - has the artifact been legally authenticated), all of which may impact security, privacy, and trust policies."; 6718 case QUESTIONNAIRE: 6719 return "A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection."; 6720 case QUESTIONNAIRERESPONSE: 6721 return "A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the questionnaire being responded to."; 6722 case RELATEDPERSON: 6723 return "Information about a person that is involved in the care for a patient, but who is not the target of healthcare, nor has a formal responsibility in the care process."; 6724 case REQUESTGROUP: 6725 return "A group of related requests that can be used to capture intended activities that have inter-dependencies such as \"give this medication after that one\"."; 6726 case RESEARCHDEFINITION: 6727 return "The ResearchDefinition resource describes the conditional state (population and any exposures being compared within the population) and outcome (if specified) that the knowledge (evidence, assertion, recommendation) is about."; 6728 case RESEARCHELEMENTDEFINITION: 6729 return "The ResearchElementDefinition resource describes a \"PICO\" element that knowledge (evidence, assertion, recommendation) is about."; 6730 case RESEARCHSTUDY: 6731 return "A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects."; 6732 case RESEARCHSUBJECT: 6733 return "A physical entity which is the primary unit of operational and/or administrative interest in a study."; 6734 case RESOURCE: 6735 return "This is the base resource type for everything."; 6736 case RISKASSESSMENT: 6737 return "An assessment of the likely outcome(s) for a patient or other subject as well as the likelihood of each outcome."; 6738 case RISKEVIDENCESYNTHESIS: 6739 return "The RiskEvidenceSynthesis resource describes the likelihood of an outcome in a population plus exposure state where the risk estimate is derived from a combination of research studies."; 6740 case SCHEDULE: 6741 return "A container for slots of time that may be available for booking appointments."; 6742 case SEARCHPARAMETER: 6743 return "A search parameter that defines a named search item that can be used to search/filter on a resource."; 6744 case SERVICEREQUEST: 6745 return "A record of a request for service such as diagnostic investigations, treatments, or operations to be performed."; 6746 case SLOT: 6747 return "A slot of time on a schedule that may be available for booking appointments."; 6748 case SPECIMEN: 6749 return "A sample to be used for analysis."; 6750 case SPECIMENDEFINITION: 6751 return "A kind of specimen with associated set of requirements."; 6752 case STRUCTUREDEFINITION: 6753 return "A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions and constraints on resources and data types."; 6754 case STRUCTUREMAP: 6755 return "A Map of relationships between 2 structures that can be used to transform data."; 6756 case SUBSCRIPTION: 6757 return "The subscription resource is used to define a push-based subscription from a server to another system. Once a subscription is registered with the server, the server checks every resource that is created or updated, and if the resource matches the given criteria, it sends a message on the defined \"channel\" so that another system can take an appropriate action."; 6758 case SUBSTANCE: 6759 return "A homogeneous material with a definite composition."; 6760 case SUBSTANCENUCLEICACID: 6761 return "Nucleic acids are defined by three distinct elements: the base, sugar and linkage. Individual substance/moiety IDs will be created for each of these elements. The nucleotide sequence will be always entered in the 5?-3? direction."; 6762 case SUBSTANCEPOLYMER: 6763 return "Todo."; 6764 case SUBSTANCEPROTEIN: 6765 return "A SubstanceProtein is defined as a single unit of a linear amino acid sequence, or a combination of subunits that are either covalently linked or have a defined invariant stoichiometric relationship. This includes all synthetic, recombinant and purified SubstanceProteins of defined sequence, whether the use is therapeutic or prophylactic. This set of elements will be used to describe albumins, coagulation factors, cytokines, growth factors, peptide/SubstanceProtein hormones, enzymes, toxins, toxoids, recombinant vaccines, and immunomodulators."; 6766 case SUBSTANCEREFERENCEINFORMATION: 6767 return "Todo."; 6768 case SUBSTANCESOURCEMATERIAL: 6769 return "Source material shall capture information on the taxonomic and anatomical origins as well as the fraction of a material that can result in or can be modified to form a substance. This set of data elements shall be used to define polymer substances isolated from biological matrices. Taxonomic and anatomical origins shall be described using a controlled vocabulary as required. This information is captured for naturally derived polymers ( . starch) and structurally diverse substances. For Organisms belonging to the Kingdom Plantae the Substance level defines the fresh material of a single species or infraspecies, the Herbal Drug and the Herbal preparation. For Herbal preparations, the fraction information will be captured at the Substance information level and additional information for herbal extracts will be captured at the Specified Substance Group 1 information level. See for further explanation the Substance Class: Structurally Diverse and the herbal annex."; 6770 case SUBSTANCESPECIFICATION: 6771 return "The detailed description of a substance, typically at a level beyond what is used for prescribing."; 6772 case SUPPLYDELIVERY: 6773 return "Record of delivery of what is supplied."; 6774 case SUPPLYREQUEST: 6775 return "A record of a request for a medication, substance or device used in the healthcare setting."; 6776 case TASK: 6777 return "A task to be performed."; 6778 case TERMINOLOGYCAPABILITIES: 6779 return "A Terminology Capabilities documents a set of capabilities (behaviors) of a FHIR Server that may be used as a statement of actual server functionality or a statement of required or desired server implementation."; 6780 case TESTREPORT: 6781 return "A summary of information based on the results of executing a TestScript."; 6782 case TESTSCRIPT: 6783 return "A structured set of tests against a FHIR server or client implementation to determine compliance against the FHIR specification."; 6784 case VALUESET: 6785 return "A ValueSet resource instance specifies a set of codes drawn from one or more code systems, intended for use in a particular context. Value sets link between [[[CodeSystem]]] definitions and their use in [coded elements](terminologies.html)."; 6786 case VERIFICATIONRESULT: 6787 return "Describes validation requirements, source(s), status and dates for one or more elements."; 6788 case VISIONPRESCRIPTION: 6789 return "An authorization for the provision of glasses and/or contact lenses to a patient."; 6790 case TYPE: 6791 return "A place holder that means any kind of data type"; 6792 case ANY: 6793 return "A place holder that means any kind of resource"; 6794 case NULL: 6795 return null; 6796 default: 6797 return "?"; 6798 } 6799 } 6800 6801 public String getDisplay() { 6802 switch (this) { 6803 case ADDRESS: 6804 return "Address"; 6805 case AGE: 6806 return "Age"; 6807 case ANNOTATION: 6808 return "Annotation"; 6809 case ATTACHMENT: 6810 return "Attachment"; 6811 case BACKBONEELEMENT: 6812 return "BackboneElement"; 6813 case CODEABLECONCEPT: 6814 return "CodeableConcept"; 6815 case CODING: 6816 return "Coding"; 6817 case CONTACTDETAIL: 6818 return "ContactDetail"; 6819 case CONTACTPOINT: 6820 return "ContactPoint"; 6821 case CONTRIBUTOR: 6822 return "Contributor"; 6823 case COUNT: 6824 return "Count"; 6825 case DATAREQUIREMENT: 6826 return "DataRequirement"; 6827 case DISTANCE: 6828 return "Distance"; 6829 case DOSAGE: 6830 return "Dosage"; 6831 case DURATION: 6832 return "Duration"; 6833 case ELEMENT: 6834 return "Element"; 6835 case ELEMENTDEFINITION: 6836 return "ElementDefinition"; 6837 case EXPRESSION: 6838 return "Expression"; 6839 case EXTENSION: 6840 return "Extension"; 6841 case HUMANNAME: 6842 return "HumanName"; 6843 case IDENTIFIER: 6844 return "Identifier"; 6845 case MARKETINGSTATUS: 6846 return "MarketingStatus"; 6847 case META: 6848 return "Meta"; 6849 case MONEY: 6850 return "Money"; 6851 case MONEYQUANTITY: 6852 return "MoneyQuantity"; 6853 case NARRATIVE: 6854 return "Narrative"; 6855 case PARAMETERDEFINITION: 6856 return "ParameterDefinition"; 6857 case PERIOD: 6858 return "Period"; 6859 case POPULATION: 6860 return "Population"; 6861 case PRODCHARACTERISTIC: 6862 return "ProdCharacteristic"; 6863 case PRODUCTSHELFLIFE: 6864 return "ProductShelfLife"; 6865 case QUANTITY: 6866 return "Quantity"; 6867 case RANGE: 6868 return "Range"; 6869 case RATIO: 6870 return "Ratio"; 6871 case REFERENCE: 6872 return "Reference"; 6873 case RELATEDARTIFACT: 6874 return "RelatedArtifact"; 6875 case SAMPLEDDATA: 6876 return "SampledData"; 6877 case SIGNATURE: 6878 return "Signature"; 6879 case SIMPLEQUANTITY: 6880 return "SimpleQuantity"; 6881 case SUBSTANCEAMOUNT: 6882 return "SubstanceAmount"; 6883 case TIMING: 6884 return "Timing"; 6885 case TRIGGERDEFINITION: 6886 return "TriggerDefinition"; 6887 case USAGECONTEXT: 6888 return "UsageContext"; 6889 case BASE64BINARY: 6890 return "base64Binary"; 6891 case BOOLEAN: 6892 return "boolean"; 6893 case CANONICAL: 6894 return "canonical"; 6895 case CODE: 6896 return "code"; 6897 case DATE: 6898 return "date"; 6899 case DATETIME: 6900 return "dateTime"; 6901 case DECIMAL: 6902 return "decimal"; 6903 case ID: 6904 return "id"; 6905 case INSTANT: 6906 return "instant"; 6907 case INTEGER: 6908 return "integer"; 6909 case MARKDOWN: 6910 return "markdown"; 6911 case OID: 6912 return "oid"; 6913 case POSITIVEINT: 6914 return "positiveInt"; 6915 case STRING: 6916 return "string"; 6917 case TIME: 6918 return "time"; 6919 case UNSIGNEDINT: 6920 return "unsignedInt"; 6921 case URI: 6922 return "uri"; 6923 case URL: 6924 return "url"; 6925 case UUID: 6926 return "uuid"; 6927 case XHTML: 6928 return "XHTML"; 6929 case ACCOUNT: 6930 return "Account"; 6931 case ACTIVITYDEFINITION: 6932 return "ActivityDefinition"; 6933 case ADVERSEEVENT: 6934 return "AdverseEvent"; 6935 case ALLERGYINTOLERANCE: 6936 return "AllergyIntolerance"; 6937 case APPOINTMENT: 6938 return "Appointment"; 6939 case APPOINTMENTRESPONSE: 6940 return "AppointmentResponse"; 6941 case AUDITEVENT: 6942 return "AuditEvent"; 6943 case BASIC: 6944 return "Basic"; 6945 case BINARY: 6946 return "Binary"; 6947 case BIOLOGICALLYDERIVEDPRODUCT: 6948 return "BiologicallyDerivedProduct"; 6949 case BODYSTRUCTURE: 6950 return "BodyStructure"; 6951 case BUNDLE: 6952 return "Bundle"; 6953 case CAPABILITYSTATEMENT: 6954 return "CapabilityStatement"; 6955 case CAREPLAN: 6956 return "CarePlan"; 6957 case CARETEAM: 6958 return "CareTeam"; 6959 case CATALOGENTRY: 6960 return "CatalogEntry"; 6961 case CHARGEITEM: 6962 return "ChargeItem"; 6963 case CHARGEITEMDEFINITION: 6964 return "ChargeItemDefinition"; 6965 case CLAIM: 6966 return "Claim"; 6967 case CLAIMRESPONSE: 6968 return "ClaimResponse"; 6969 case CLINICALIMPRESSION: 6970 return "ClinicalImpression"; 6971 case CODESYSTEM: 6972 return "CodeSystem"; 6973 case COMMUNICATION: 6974 return "Communication"; 6975 case COMMUNICATIONREQUEST: 6976 return "CommunicationRequest"; 6977 case COMPARTMENTDEFINITION: 6978 return "CompartmentDefinition"; 6979 case COMPOSITION: 6980 return "Composition"; 6981 case CONCEPTMAP: 6982 return "ConceptMap"; 6983 case CONDITION: 6984 return "Condition"; 6985 case CONSENT: 6986 return "Consent"; 6987 case CONTRACT: 6988 return "Contract"; 6989 case COVERAGE: 6990 return "Coverage"; 6991 case COVERAGEELIGIBILITYREQUEST: 6992 return "CoverageEligibilityRequest"; 6993 case COVERAGEELIGIBILITYRESPONSE: 6994 return "CoverageEligibilityResponse"; 6995 case DETECTEDISSUE: 6996 return "DetectedIssue"; 6997 case DEVICE: 6998 return "Device"; 6999 case DEVICEDEFINITION: 7000 return "DeviceDefinition"; 7001 case DEVICEMETRIC: 7002 return "DeviceMetric"; 7003 case DEVICEREQUEST: 7004 return "DeviceRequest"; 7005 case DEVICEUSESTATEMENT: 7006 return "DeviceUseStatement"; 7007 case DIAGNOSTICREPORT: 7008 return "DiagnosticReport"; 7009 case DOCUMENTMANIFEST: 7010 return "DocumentManifest"; 7011 case DOCUMENTREFERENCE: 7012 return "DocumentReference"; 7013 case DOMAINRESOURCE: 7014 return "DomainResource"; 7015 case EFFECTEVIDENCESYNTHESIS: 7016 return "EffectEvidenceSynthesis"; 7017 case ENCOUNTER: 7018 return "Encounter"; 7019 case ENDPOINT: 7020 return "Endpoint"; 7021 case ENROLLMENTREQUEST: 7022 return "EnrollmentRequest"; 7023 case ENROLLMENTRESPONSE: 7024 return "EnrollmentResponse"; 7025 case EPISODEOFCARE: 7026 return "EpisodeOfCare"; 7027 case EVENTDEFINITION: 7028 return "EventDefinition"; 7029 case EVIDENCE: 7030 return "Evidence"; 7031 case EVIDENCEVARIABLE: 7032 return "EvidenceVariable"; 7033 case EXAMPLESCENARIO: 7034 return "ExampleScenario"; 7035 case EXPLANATIONOFBENEFIT: 7036 return "ExplanationOfBenefit"; 7037 case FAMILYMEMBERHISTORY: 7038 return "FamilyMemberHistory"; 7039 case FLAG: 7040 return "Flag"; 7041 case GOAL: 7042 return "Goal"; 7043 case GRAPHDEFINITION: 7044 return "GraphDefinition"; 7045 case GROUP: 7046 return "Group"; 7047 case GUIDANCERESPONSE: 7048 return "GuidanceResponse"; 7049 case HEALTHCARESERVICE: 7050 return "HealthcareService"; 7051 case IMAGINGSTUDY: 7052 return "ImagingStudy"; 7053 case IMMUNIZATION: 7054 return "Immunization"; 7055 case IMMUNIZATIONEVALUATION: 7056 return "ImmunizationEvaluation"; 7057 case IMMUNIZATIONRECOMMENDATION: 7058 return "ImmunizationRecommendation"; 7059 case IMPLEMENTATIONGUIDE: 7060 return "ImplementationGuide"; 7061 case INSURANCEPLAN: 7062 return "InsurancePlan"; 7063 case INVOICE: 7064 return "Invoice"; 7065 case LIBRARY: 7066 return "Library"; 7067 case LINKAGE: 7068 return "Linkage"; 7069 case LIST: 7070 return "List"; 7071 case LOCATION: 7072 return "Location"; 7073 case MEASURE: 7074 return "Measure"; 7075 case MEASUREREPORT: 7076 return "MeasureReport"; 7077 case MEDIA: 7078 return "Media"; 7079 case MEDICATION: 7080 return "Medication"; 7081 case MEDICATIONADMINISTRATION: 7082 return "MedicationAdministration"; 7083 case MEDICATIONDISPENSE: 7084 return "MedicationDispense"; 7085 case MEDICATIONKNOWLEDGE: 7086 return "MedicationKnowledge"; 7087 case MEDICATIONREQUEST: 7088 return "MedicationRequest"; 7089 case MEDICATIONSTATEMENT: 7090 return "MedicationStatement"; 7091 case MEDICINALPRODUCT: 7092 return "MedicinalProduct"; 7093 case MEDICINALPRODUCTAUTHORIZATION: 7094 return "MedicinalProductAuthorization"; 7095 case MEDICINALPRODUCTCONTRAINDICATION: 7096 return "MedicinalProductContraindication"; 7097 case MEDICINALPRODUCTINDICATION: 7098 return "MedicinalProductIndication"; 7099 case MEDICINALPRODUCTINGREDIENT: 7100 return "MedicinalProductIngredient"; 7101 case MEDICINALPRODUCTINTERACTION: 7102 return "MedicinalProductInteraction"; 7103 case MEDICINALPRODUCTMANUFACTURED: 7104 return "MedicinalProductManufactured"; 7105 case MEDICINALPRODUCTPACKAGED: 7106 return "MedicinalProductPackaged"; 7107 case MEDICINALPRODUCTPHARMACEUTICAL: 7108 return "MedicinalProductPharmaceutical"; 7109 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 7110 return "MedicinalProductUndesirableEffect"; 7111 case MESSAGEDEFINITION: 7112 return "MessageDefinition"; 7113 case MESSAGEHEADER: 7114 return "MessageHeader"; 7115 case MOLECULARSEQUENCE: 7116 return "MolecularSequence"; 7117 case NAMINGSYSTEM: 7118 return "NamingSystem"; 7119 case NUTRITIONORDER: 7120 return "NutritionOrder"; 7121 case OBSERVATION: 7122 return "Observation"; 7123 case OBSERVATIONDEFINITION: 7124 return "ObservationDefinition"; 7125 case OPERATIONDEFINITION: 7126 return "OperationDefinition"; 7127 case OPERATIONOUTCOME: 7128 return "OperationOutcome"; 7129 case ORGANIZATION: 7130 return "Organization"; 7131 case ORGANIZATIONAFFILIATION: 7132 return "OrganizationAffiliation"; 7133 case PARAMETERS: 7134 return "Parameters"; 7135 case PATIENT: 7136 return "Patient"; 7137 case PAYMENTNOTICE: 7138 return "PaymentNotice"; 7139 case PAYMENTRECONCILIATION: 7140 return "PaymentReconciliation"; 7141 case PERSON: 7142 return "Person"; 7143 case PLANDEFINITION: 7144 return "PlanDefinition"; 7145 case PRACTITIONER: 7146 return "Practitioner"; 7147 case PRACTITIONERROLE: 7148 return "PractitionerRole"; 7149 case PROCEDURE: 7150 return "Procedure"; 7151 case PROVENANCE: 7152 return "Provenance"; 7153 case QUESTIONNAIRE: 7154 return "Questionnaire"; 7155 case QUESTIONNAIRERESPONSE: 7156 return "QuestionnaireResponse"; 7157 case RELATEDPERSON: 7158 return "RelatedPerson"; 7159 case REQUESTGROUP: 7160 return "RequestGroup"; 7161 case RESEARCHDEFINITION: 7162 return "ResearchDefinition"; 7163 case RESEARCHELEMENTDEFINITION: 7164 return "ResearchElementDefinition"; 7165 case RESEARCHSTUDY: 7166 return "ResearchStudy"; 7167 case RESEARCHSUBJECT: 7168 return "ResearchSubject"; 7169 case RESOURCE: 7170 return "Resource"; 7171 case RISKASSESSMENT: 7172 return "RiskAssessment"; 7173 case RISKEVIDENCESYNTHESIS: 7174 return "RiskEvidenceSynthesis"; 7175 case SCHEDULE: 7176 return "Schedule"; 7177 case SEARCHPARAMETER: 7178 return "SearchParameter"; 7179 case SERVICEREQUEST: 7180 return "ServiceRequest"; 7181 case SLOT: 7182 return "Slot"; 7183 case SPECIMEN: 7184 return "Specimen"; 7185 case SPECIMENDEFINITION: 7186 return "SpecimenDefinition"; 7187 case STRUCTUREDEFINITION: 7188 return "StructureDefinition"; 7189 case STRUCTUREMAP: 7190 return "StructureMap"; 7191 case SUBSCRIPTION: 7192 return "Subscription"; 7193 case SUBSTANCE: 7194 return "Substance"; 7195 case SUBSTANCENUCLEICACID: 7196 return "SubstanceNucleicAcid"; 7197 case SUBSTANCEPOLYMER: 7198 return "SubstancePolymer"; 7199 case SUBSTANCEPROTEIN: 7200 return "SubstanceProtein"; 7201 case SUBSTANCEREFERENCEINFORMATION: 7202 return "SubstanceReferenceInformation"; 7203 case SUBSTANCESOURCEMATERIAL: 7204 return "SubstanceSourceMaterial"; 7205 case SUBSTANCESPECIFICATION: 7206 return "SubstanceSpecification"; 7207 case SUPPLYDELIVERY: 7208 return "SupplyDelivery"; 7209 case SUPPLYREQUEST: 7210 return "SupplyRequest"; 7211 case TASK: 7212 return "Task"; 7213 case TERMINOLOGYCAPABILITIES: 7214 return "TerminologyCapabilities"; 7215 case TESTREPORT: 7216 return "TestReport"; 7217 case TESTSCRIPT: 7218 return "TestScript"; 7219 case VALUESET: 7220 return "ValueSet"; 7221 case VERIFICATIONRESULT: 7222 return "VerificationResult"; 7223 case VISIONPRESCRIPTION: 7224 return "VisionPrescription"; 7225 case TYPE: 7226 return "Type"; 7227 case ANY: 7228 return "Any"; 7229 case NULL: 7230 return null; 7231 default: 7232 return "?"; 7233 } 7234 } 7235 } 7236 7237 public static class FHIRAllTypesEnumFactory implements EnumFactory<FHIRAllTypes> { 7238 public FHIRAllTypes fromCode(String codeString) throws IllegalArgumentException { 7239 if (codeString == null || "".equals(codeString)) 7240 if (codeString == null || "".equals(codeString)) 7241 return null; 7242 if ("Address".equals(codeString)) 7243 return FHIRAllTypes.ADDRESS; 7244 if ("Age".equals(codeString)) 7245 return FHIRAllTypes.AGE; 7246 if ("Annotation".equals(codeString)) 7247 return FHIRAllTypes.ANNOTATION; 7248 if ("Attachment".equals(codeString)) 7249 return FHIRAllTypes.ATTACHMENT; 7250 if ("BackboneElement".equals(codeString)) 7251 return FHIRAllTypes.BACKBONEELEMENT; 7252 if ("CodeableConcept".equals(codeString)) 7253 return FHIRAllTypes.CODEABLECONCEPT; 7254 if ("Coding".equals(codeString)) 7255 return FHIRAllTypes.CODING; 7256 if ("ContactDetail".equals(codeString)) 7257 return FHIRAllTypes.CONTACTDETAIL; 7258 if ("ContactPoint".equals(codeString)) 7259 return FHIRAllTypes.CONTACTPOINT; 7260 if ("Contributor".equals(codeString)) 7261 return FHIRAllTypes.CONTRIBUTOR; 7262 if ("Count".equals(codeString)) 7263 return FHIRAllTypes.COUNT; 7264 if ("DataRequirement".equals(codeString)) 7265 return FHIRAllTypes.DATAREQUIREMENT; 7266 if ("Distance".equals(codeString)) 7267 return FHIRAllTypes.DISTANCE; 7268 if ("Dosage".equals(codeString)) 7269 return FHIRAllTypes.DOSAGE; 7270 if ("Duration".equals(codeString)) 7271 return FHIRAllTypes.DURATION; 7272 if ("Element".equals(codeString)) 7273 return FHIRAllTypes.ELEMENT; 7274 if ("ElementDefinition".equals(codeString)) 7275 return FHIRAllTypes.ELEMENTDEFINITION; 7276 if ("Expression".equals(codeString)) 7277 return FHIRAllTypes.EXPRESSION; 7278 if ("Extension".equals(codeString)) 7279 return FHIRAllTypes.EXTENSION; 7280 if ("HumanName".equals(codeString)) 7281 return FHIRAllTypes.HUMANNAME; 7282 if ("Identifier".equals(codeString)) 7283 return FHIRAllTypes.IDENTIFIER; 7284 if ("MarketingStatus".equals(codeString)) 7285 return FHIRAllTypes.MARKETINGSTATUS; 7286 if ("Meta".equals(codeString)) 7287 return FHIRAllTypes.META; 7288 if ("Money".equals(codeString)) 7289 return FHIRAllTypes.MONEY; 7290 if ("MoneyQuantity".equals(codeString)) 7291 return FHIRAllTypes.MONEYQUANTITY; 7292 if ("Narrative".equals(codeString)) 7293 return FHIRAllTypes.NARRATIVE; 7294 if ("ParameterDefinition".equals(codeString)) 7295 return FHIRAllTypes.PARAMETERDEFINITION; 7296 if ("Period".equals(codeString)) 7297 return FHIRAllTypes.PERIOD; 7298 if ("Population".equals(codeString)) 7299 return FHIRAllTypes.POPULATION; 7300 if ("ProdCharacteristic".equals(codeString)) 7301 return FHIRAllTypes.PRODCHARACTERISTIC; 7302 if ("ProductShelfLife".equals(codeString)) 7303 return FHIRAllTypes.PRODUCTSHELFLIFE; 7304 if ("Quantity".equals(codeString)) 7305 return FHIRAllTypes.QUANTITY; 7306 if ("Range".equals(codeString)) 7307 return FHIRAllTypes.RANGE; 7308 if ("Ratio".equals(codeString)) 7309 return FHIRAllTypes.RATIO; 7310 if ("Reference".equals(codeString)) 7311 return FHIRAllTypes.REFERENCE; 7312 if ("RelatedArtifact".equals(codeString)) 7313 return FHIRAllTypes.RELATEDARTIFACT; 7314 if ("SampledData".equals(codeString)) 7315 return FHIRAllTypes.SAMPLEDDATA; 7316 if ("Signature".equals(codeString)) 7317 return FHIRAllTypes.SIGNATURE; 7318 if ("SimpleQuantity".equals(codeString)) 7319 return FHIRAllTypes.SIMPLEQUANTITY; 7320 if ("SubstanceAmount".equals(codeString)) 7321 return FHIRAllTypes.SUBSTANCEAMOUNT; 7322 if ("Timing".equals(codeString)) 7323 return FHIRAllTypes.TIMING; 7324 if ("TriggerDefinition".equals(codeString)) 7325 return FHIRAllTypes.TRIGGERDEFINITION; 7326 if ("UsageContext".equals(codeString)) 7327 return FHIRAllTypes.USAGECONTEXT; 7328 if ("base64Binary".equals(codeString)) 7329 return FHIRAllTypes.BASE64BINARY; 7330 if ("boolean".equals(codeString)) 7331 return FHIRAllTypes.BOOLEAN; 7332 if ("canonical".equals(codeString)) 7333 return FHIRAllTypes.CANONICAL; 7334 if ("code".equals(codeString)) 7335 return FHIRAllTypes.CODE; 7336 if ("date".equals(codeString)) 7337 return FHIRAllTypes.DATE; 7338 if ("dateTime".equals(codeString)) 7339 return FHIRAllTypes.DATETIME; 7340 if ("decimal".equals(codeString)) 7341 return FHIRAllTypes.DECIMAL; 7342 if ("id".equals(codeString)) 7343 return FHIRAllTypes.ID; 7344 if ("instant".equals(codeString)) 7345 return FHIRAllTypes.INSTANT; 7346 if ("integer".equals(codeString)) 7347 return FHIRAllTypes.INTEGER; 7348 if ("markdown".equals(codeString)) 7349 return FHIRAllTypes.MARKDOWN; 7350 if ("oid".equals(codeString)) 7351 return FHIRAllTypes.OID; 7352 if ("positiveInt".equals(codeString)) 7353 return FHIRAllTypes.POSITIVEINT; 7354 if ("string".equals(codeString)) 7355 return FHIRAllTypes.STRING; 7356 if ("time".equals(codeString)) 7357 return FHIRAllTypes.TIME; 7358 if ("unsignedInt".equals(codeString)) 7359 return FHIRAllTypes.UNSIGNEDINT; 7360 if ("uri".equals(codeString)) 7361 return FHIRAllTypes.URI; 7362 if ("url".equals(codeString)) 7363 return FHIRAllTypes.URL; 7364 if ("uuid".equals(codeString)) 7365 return FHIRAllTypes.UUID; 7366 if ("xhtml".equals(codeString)) 7367 return FHIRAllTypes.XHTML; 7368 if ("Account".equals(codeString)) 7369 return FHIRAllTypes.ACCOUNT; 7370 if ("ActivityDefinition".equals(codeString)) 7371 return FHIRAllTypes.ACTIVITYDEFINITION; 7372 if ("AdverseEvent".equals(codeString)) 7373 return FHIRAllTypes.ADVERSEEVENT; 7374 if ("AllergyIntolerance".equals(codeString)) 7375 return FHIRAllTypes.ALLERGYINTOLERANCE; 7376 if ("Appointment".equals(codeString)) 7377 return FHIRAllTypes.APPOINTMENT; 7378 if ("AppointmentResponse".equals(codeString)) 7379 return FHIRAllTypes.APPOINTMENTRESPONSE; 7380 if ("AuditEvent".equals(codeString)) 7381 return FHIRAllTypes.AUDITEVENT; 7382 if ("Basic".equals(codeString)) 7383 return FHIRAllTypes.BASIC; 7384 if ("Binary".equals(codeString)) 7385 return FHIRAllTypes.BINARY; 7386 if ("BiologicallyDerivedProduct".equals(codeString)) 7387 return FHIRAllTypes.BIOLOGICALLYDERIVEDPRODUCT; 7388 if ("BodyStructure".equals(codeString)) 7389 return FHIRAllTypes.BODYSTRUCTURE; 7390 if ("Bundle".equals(codeString)) 7391 return FHIRAllTypes.BUNDLE; 7392 if ("CapabilityStatement".equals(codeString)) 7393 return FHIRAllTypes.CAPABILITYSTATEMENT; 7394 if ("CarePlan".equals(codeString)) 7395 return FHIRAllTypes.CAREPLAN; 7396 if ("CareTeam".equals(codeString)) 7397 return FHIRAllTypes.CARETEAM; 7398 if ("CatalogEntry".equals(codeString)) 7399 return FHIRAllTypes.CATALOGENTRY; 7400 if ("ChargeItem".equals(codeString)) 7401 return FHIRAllTypes.CHARGEITEM; 7402 if ("ChargeItemDefinition".equals(codeString)) 7403 return FHIRAllTypes.CHARGEITEMDEFINITION; 7404 if ("Claim".equals(codeString)) 7405 return FHIRAllTypes.CLAIM; 7406 if ("ClaimResponse".equals(codeString)) 7407 return FHIRAllTypes.CLAIMRESPONSE; 7408 if ("ClinicalImpression".equals(codeString)) 7409 return FHIRAllTypes.CLINICALIMPRESSION; 7410 if ("CodeSystem".equals(codeString)) 7411 return FHIRAllTypes.CODESYSTEM; 7412 if ("Communication".equals(codeString)) 7413 return FHIRAllTypes.COMMUNICATION; 7414 if ("CommunicationRequest".equals(codeString)) 7415 return FHIRAllTypes.COMMUNICATIONREQUEST; 7416 if ("CompartmentDefinition".equals(codeString)) 7417 return FHIRAllTypes.COMPARTMENTDEFINITION; 7418 if ("Composition".equals(codeString)) 7419 return FHIRAllTypes.COMPOSITION; 7420 if ("ConceptMap".equals(codeString)) 7421 return FHIRAllTypes.CONCEPTMAP; 7422 if ("Condition".equals(codeString)) 7423 return FHIRAllTypes.CONDITION; 7424 if ("Consent".equals(codeString)) 7425 return FHIRAllTypes.CONSENT; 7426 if ("Contract".equals(codeString)) 7427 return FHIRAllTypes.CONTRACT; 7428 if ("Coverage".equals(codeString)) 7429 return FHIRAllTypes.COVERAGE; 7430 if ("CoverageEligibilityRequest".equals(codeString)) 7431 return FHIRAllTypes.COVERAGEELIGIBILITYREQUEST; 7432 if ("CoverageEligibilityResponse".equals(codeString)) 7433 return FHIRAllTypes.COVERAGEELIGIBILITYRESPONSE; 7434 if ("DetectedIssue".equals(codeString)) 7435 return FHIRAllTypes.DETECTEDISSUE; 7436 if ("Device".equals(codeString)) 7437 return FHIRAllTypes.DEVICE; 7438 if ("DeviceDefinition".equals(codeString)) 7439 return FHIRAllTypes.DEVICEDEFINITION; 7440 if ("DeviceMetric".equals(codeString)) 7441 return FHIRAllTypes.DEVICEMETRIC; 7442 if ("DeviceRequest".equals(codeString)) 7443 return FHIRAllTypes.DEVICEREQUEST; 7444 if ("DeviceUseStatement".equals(codeString)) 7445 return FHIRAllTypes.DEVICEUSESTATEMENT; 7446 if ("DiagnosticReport".equals(codeString)) 7447 return FHIRAllTypes.DIAGNOSTICREPORT; 7448 if ("DocumentManifest".equals(codeString)) 7449 return FHIRAllTypes.DOCUMENTMANIFEST; 7450 if ("DocumentReference".equals(codeString)) 7451 return FHIRAllTypes.DOCUMENTREFERENCE; 7452 if ("DomainResource".equals(codeString)) 7453 return FHIRAllTypes.DOMAINRESOURCE; 7454 if ("EffectEvidenceSynthesis".equals(codeString)) 7455 return FHIRAllTypes.EFFECTEVIDENCESYNTHESIS; 7456 if ("Encounter".equals(codeString)) 7457 return FHIRAllTypes.ENCOUNTER; 7458 if ("Endpoint".equals(codeString)) 7459 return FHIRAllTypes.ENDPOINT; 7460 if ("EnrollmentRequest".equals(codeString)) 7461 return FHIRAllTypes.ENROLLMENTREQUEST; 7462 if ("EnrollmentResponse".equals(codeString)) 7463 return FHIRAllTypes.ENROLLMENTRESPONSE; 7464 if ("EpisodeOfCare".equals(codeString)) 7465 return FHIRAllTypes.EPISODEOFCARE; 7466 if ("EventDefinition".equals(codeString)) 7467 return FHIRAllTypes.EVENTDEFINITION; 7468 if ("Evidence".equals(codeString)) 7469 return FHIRAllTypes.EVIDENCE; 7470 if ("EvidenceVariable".equals(codeString)) 7471 return FHIRAllTypes.EVIDENCEVARIABLE; 7472 if ("ExampleScenario".equals(codeString)) 7473 return FHIRAllTypes.EXAMPLESCENARIO; 7474 if ("ExplanationOfBenefit".equals(codeString)) 7475 return FHIRAllTypes.EXPLANATIONOFBENEFIT; 7476 if ("FamilyMemberHistory".equals(codeString)) 7477 return FHIRAllTypes.FAMILYMEMBERHISTORY; 7478 if ("Flag".equals(codeString)) 7479 return FHIRAllTypes.FLAG; 7480 if ("Goal".equals(codeString)) 7481 return FHIRAllTypes.GOAL; 7482 if ("GraphDefinition".equals(codeString)) 7483 return FHIRAllTypes.GRAPHDEFINITION; 7484 if ("Group".equals(codeString)) 7485 return FHIRAllTypes.GROUP; 7486 if ("GuidanceResponse".equals(codeString)) 7487 return FHIRAllTypes.GUIDANCERESPONSE; 7488 if ("HealthcareService".equals(codeString)) 7489 return FHIRAllTypes.HEALTHCARESERVICE; 7490 if ("ImagingStudy".equals(codeString)) 7491 return FHIRAllTypes.IMAGINGSTUDY; 7492 if ("Immunization".equals(codeString)) 7493 return FHIRAllTypes.IMMUNIZATION; 7494 if ("ImmunizationEvaluation".equals(codeString)) 7495 return FHIRAllTypes.IMMUNIZATIONEVALUATION; 7496 if ("ImmunizationRecommendation".equals(codeString)) 7497 return FHIRAllTypes.IMMUNIZATIONRECOMMENDATION; 7498 if ("ImplementationGuide".equals(codeString)) 7499 return FHIRAllTypes.IMPLEMENTATIONGUIDE; 7500 if ("InsurancePlan".equals(codeString)) 7501 return FHIRAllTypes.INSURANCEPLAN; 7502 if ("Invoice".equals(codeString)) 7503 return FHIRAllTypes.INVOICE; 7504 if ("Library".equals(codeString)) 7505 return FHIRAllTypes.LIBRARY; 7506 if ("Linkage".equals(codeString)) 7507 return FHIRAllTypes.LINKAGE; 7508 if ("List".equals(codeString)) 7509 return FHIRAllTypes.LIST; 7510 if ("Location".equals(codeString)) 7511 return FHIRAllTypes.LOCATION; 7512 if ("Measure".equals(codeString)) 7513 return FHIRAllTypes.MEASURE; 7514 if ("MeasureReport".equals(codeString)) 7515 return FHIRAllTypes.MEASUREREPORT; 7516 if ("Media".equals(codeString)) 7517 return FHIRAllTypes.MEDIA; 7518 if ("Medication".equals(codeString)) 7519 return FHIRAllTypes.MEDICATION; 7520 if ("MedicationAdministration".equals(codeString)) 7521 return FHIRAllTypes.MEDICATIONADMINISTRATION; 7522 if ("MedicationDispense".equals(codeString)) 7523 return FHIRAllTypes.MEDICATIONDISPENSE; 7524 if ("MedicationKnowledge".equals(codeString)) 7525 return FHIRAllTypes.MEDICATIONKNOWLEDGE; 7526 if ("MedicationRequest".equals(codeString)) 7527 return FHIRAllTypes.MEDICATIONREQUEST; 7528 if ("MedicationStatement".equals(codeString)) 7529 return FHIRAllTypes.MEDICATIONSTATEMENT; 7530 if ("MedicinalProduct".equals(codeString)) 7531 return FHIRAllTypes.MEDICINALPRODUCT; 7532 if ("MedicinalProductAuthorization".equals(codeString)) 7533 return FHIRAllTypes.MEDICINALPRODUCTAUTHORIZATION; 7534 if ("MedicinalProductContraindication".equals(codeString)) 7535 return FHIRAllTypes.MEDICINALPRODUCTCONTRAINDICATION; 7536 if ("MedicinalProductIndication".equals(codeString)) 7537 return FHIRAllTypes.MEDICINALPRODUCTINDICATION; 7538 if ("MedicinalProductIngredient".equals(codeString)) 7539 return FHIRAllTypes.MEDICINALPRODUCTINGREDIENT; 7540 if ("MedicinalProductInteraction".equals(codeString)) 7541 return FHIRAllTypes.MEDICINALPRODUCTINTERACTION; 7542 if ("MedicinalProductManufactured".equals(codeString)) 7543 return FHIRAllTypes.MEDICINALPRODUCTMANUFACTURED; 7544 if ("MedicinalProductPackaged".equals(codeString)) 7545 return FHIRAllTypes.MEDICINALPRODUCTPACKAGED; 7546 if ("MedicinalProductPharmaceutical".equals(codeString)) 7547 return FHIRAllTypes.MEDICINALPRODUCTPHARMACEUTICAL; 7548 if ("MedicinalProductUndesirableEffect".equals(codeString)) 7549 return FHIRAllTypes.MEDICINALPRODUCTUNDESIRABLEEFFECT; 7550 if ("MessageDefinition".equals(codeString)) 7551 return FHIRAllTypes.MESSAGEDEFINITION; 7552 if ("MessageHeader".equals(codeString)) 7553 return FHIRAllTypes.MESSAGEHEADER; 7554 if ("MolecularSequence".equals(codeString)) 7555 return FHIRAllTypes.MOLECULARSEQUENCE; 7556 if ("NamingSystem".equals(codeString)) 7557 return FHIRAllTypes.NAMINGSYSTEM; 7558 if ("NutritionOrder".equals(codeString)) 7559 return FHIRAllTypes.NUTRITIONORDER; 7560 if ("Observation".equals(codeString)) 7561 return FHIRAllTypes.OBSERVATION; 7562 if ("ObservationDefinition".equals(codeString)) 7563 return FHIRAllTypes.OBSERVATIONDEFINITION; 7564 if ("OperationDefinition".equals(codeString)) 7565 return FHIRAllTypes.OPERATIONDEFINITION; 7566 if ("OperationOutcome".equals(codeString)) 7567 return FHIRAllTypes.OPERATIONOUTCOME; 7568 if ("Organization".equals(codeString)) 7569 return FHIRAllTypes.ORGANIZATION; 7570 if ("OrganizationAffiliation".equals(codeString)) 7571 return FHIRAllTypes.ORGANIZATIONAFFILIATION; 7572 if ("Parameters".equals(codeString)) 7573 return FHIRAllTypes.PARAMETERS; 7574 if ("Patient".equals(codeString)) 7575 return FHIRAllTypes.PATIENT; 7576 if ("PaymentNotice".equals(codeString)) 7577 return FHIRAllTypes.PAYMENTNOTICE; 7578 if ("PaymentReconciliation".equals(codeString)) 7579 return FHIRAllTypes.PAYMENTRECONCILIATION; 7580 if ("Person".equals(codeString)) 7581 return FHIRAllTypes.PERSON; 7582 if ("PlanDefinition".equals(codeString)) 7583 return FHIRAllTypes.PLANDEFINITION; 7584 if ("Practitioner".equals(codeString)) 7585 return FHIRAllTypes.PRACTITIONER; 7586 if ("PractitionerRole".equals(codeString)) 7587 return FHIRAllTypes.PRACTITIONERROLE; 7588 if ("Procedure".equals(codeString)) 7589 return FHIRAllTypes.PROCEDURE; 7590 if ("Provenance".equals(codeString)) 7591 return FHIRAllTypes.PROVENANCE; 7592 if ("Questionnaire".equals(codeString)) 7593 return FHIRAllTypes.QUESTIONNAIRE; 7594 if ("QuestionnaireResponse".equals(codeString)) 7595 return FHIRAllTypes.QUESTIONNAIRERESPONSE; 7596 if ("RelatedPerson".equals(codeString)) 7597 return FHIRAllTypes.RELATEDPERSON; 7598 if ("RequestGroup".equals(codeString)) 7599 return FHIRAllTypes.REQUESTGROUP; 7600 if ("ResearchDefinition".equals(codeString)) 7601 return FHIRAllTypes.RESEARCHDEFINITION; 7602 if ("ResearchElementDefinition".equals(codeString)) 7603 return FHIRAllTypes.RESEARCHELEMENTDEFINITION; 7604 if ("ResearchStudy".equals(codeString)) 7605 return FHIRAllTypes.RESEARCHSTUDY; 7606 if ("ResearchSubject".equals(codeString)) 7607 return FHIRAllTypes.RESEARCHSUBJECT; 7608 if ("Resource".equals(codeString)) 7609 return FHIRAllTypes.RESOURCE; 7610 if ("RiskAssessment".equals(codeString)) 7611 return FHIRAllTypes.RISKASSESSMENT; 7612 if ("RiskEvidenceSynthesis".equals(codeString)) 7613 return FHIRAllTypes.RISKEVIDENCESYNTHESIS; 7614 if ("Schedule".equals(codeString)) 7615 return FHIRAllTypes.SCHEDULE; 7616 if ("SearchParameter".equals(codeString)) 7617 return FHIRAllTypes.SEARCHPARAMETER; 7618 if ("ServiceRequest".equals(codeString)) 7619 return FHIRAllTypes.SERVICEREQUEST; 7620 if ("Slot".equals(codeString)) 7621 return FHIRAllTypes.SLOT; 7622 if ("Specimen".equals(codeString)) 7623 return FHIRAllTypes.SPECIMEN; 7624 if ("SpecimenDefinition".equals(codeString)) 7625 return FHIRAllTypes.SPECIMENDEFINITION; 7626 if ("StructureDefinition".equals(codeString)) 7627 return FHIRAllTypes.STRUCTUREDEFINITION; 7628 if ("StructureMap".equals(codeString)) 7629 return FHIRAllTypes.STRUCTUREMAP; 7630 if ("Subscription".equals(codeString)) 7631 return FHIRAllTypes.SUBSCRIPTION; 7632 if ("Substance".equals(codeString)) 7633 return FHIRAllTypes.SUBSTANCE; 7634 if ("SubstanceNucleicAcid".equals(codeString)) 7635 return FHIRAllTypes.SUBSTANCENUCLEICACID; 7636 if ("SubstancePolymer".equals(codeString)) 7637 return FHIRAllTypes.SUBSTANCEPOLYMER; 7638 if ("SubstanceProtein".equals(codeString)) 7639 return FHIRAllTypes.SUBSTANCEPROTEIN; 7640 if ("SubstanceReferenceInformation".equals(codeString)) 7641 return FHIRAllTypes.SUBSTANCEREFERENCEINFORMATION; 7642 if ("SubstanceSourceMaterial".equals(codeString)) 7643 return FHIRAllTypes.SUBSTANCESOURCEMATERIAL; 7644 if ("SubstanceSpecification".equals(codeString)) 7645 return FHIRAllTypes.SUBSTANCESPECIFICATION; 7646 if ("SupplyDelivery".equals(codeString)) 7647 return FHIRAllTypes.SUPPLYDELIVERY; 7648 if ("SupplyRequest".equals(codeString)) 7649 return FHIRAllTypes.SUPPLYREQUEST; 7650 if ("Task".equals(codeString)) 7651 return FHIRAllTypes.TASK; 7652 if ("TerminologyCapabilities".equals(codeString)) 7653 return FHIRAllTypes.TERMINOLOGYCAPABILITIES; 7654 if ("TestReport".equals(codeString)) 7655 return FHIRAllTypes.TESTREPORT; 7656 if ("TestScript".equals(codeString)) 7657 return FHIRAllTypes.TESTSCRIPT; 7658 if ("ValueSet".equals(codeString)) 7659 return FHIRAllTypes.VALUESET; 7660 if ("VerificationResult".equals(codeString)) 7661 return FHIRAllTypes.VERIFICATIONRESULT; 7662 if ("VisionPrescription".equals(codeString)) 7663 return FHIRAllTypes.VISIONPRESCRIPTION; 7664 if ("Type".equals(codeString)) 7665 return FHIRAllTypes.TYPE; 7666 if ("Any".equals(codeString)) 7667 return FHIRAllTypes.ANY; 7668 throw new IllegalArgumentException("Unknown FHIRAllTypes code '" + codeString + "'"); 7669 } 7670 7671 public Enumeration<FHIRAllTypes> fromType(PrimitiveType<?> code) throws FHIRException { 7672 if (code == null) 7673 return null; 7674 if (code.isEmpty()) 7675 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.NULL, code); 7676 String codeString = code.asStringValue(); 7677 if (codeString == null || "".equals(codeString)) 7678 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.NULL, code); 7679 if ("Address".equals(codeString)) 7680 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ADDRESS, code); 7681 if ("Age".equals(codeString)) 7682 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.AGE, code); 7683 if ("Annotation".equals(codeString)) 7684 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ANNOTATION, code); 7685 if ("Attachment".equals(codeString)) 7686 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ATTACHMENT, code); 7687 if ("BackboneElement".equals(codeString)) 7688 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BACKBONEELEMENT, code); 7689 if ("CodeableConcept".equals(codeString)) 7690 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CODEABLECONCEPT, code); 7691 if ("Coding".equals(codeString)) 7692 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CODING, code); 7693 if ("ContactDetail".equals(codeString)) 7694 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONTACTDETAIL, code); 7695 if ("ContactPoint".equals(codeString)) 7696 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONTACTPOINT, code); 7697 if ("Contributor".equals(codeString)) 7698 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONTRIBUTOR, code); 7699 if ("Count".equals(codeString)) 7700 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COUNT, code); 7701 if ("DataRequirement".equals(codeString)) 7702 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DATAREQUIREMENT, code); 7703 if ("Distance".equals(codeString)) 7704 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DISTANCE, code); 7705 if ("Dosage".equals(codeString)) 7706 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DOSAGE, code); 7707 if ("Duration".equals(codeString)) 7708 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DURATION, code); 7709 if ("Element".equals(codeString)) 7710 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ELEMENT, code); 7711 if ("ElementDefinition".equals(codeString)) 7712 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ELEMENTDEFINITION, code); 7713 if ("Expression".equals(codeString)) 7714 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.EXPRESSION, code); 7715 if ("Extension".equals(codeString)) 7716 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.EXTENSION, code); 7717 if ("HumanName".equals(codeString)) 7718 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.HUMANNAME, code); 7719 if ("Identifier".equals(codeString)) 7720 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.IDENTIFIER, code); 7721 if ("MarketingStatus".equals(codeString)) 7722 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MARKETINGSTATUS, code); 7723 if ("Meta".equals(codeString)) 7724 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.META, code); 7725 if ("Money".equals(codeString)) 7726 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MONEY, code); 7727 if ("MoneyQuantity".equals(codeString)) 7728 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MONEYQUANTITY, code); 7729 if ("Narrative".equals(codeString)) 7730 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.NARRATIVE, code); 7731 if ("ParameterDefinition".equals(codeString)) 7732 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PARAMETERDEFINITION, code); 7733 if ("Period".equals(codeString)) 7734 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PERIOD, code); 7735 if ("Population".equals(codeString)) 7736 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.POPULATION, code); 7737 if ("ProdCharacteristic".equals(codeString)) 7738 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PRODCHARACTERISTIC, code); 7739 if ("ProductShelfLife".equals(codeString)) 7740 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PRODUCTSHELFLIFE, code); 7741 if ("Quantity".equals(codeString)) 7742 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.QUANTITY, code); 7743 if ("Range".equals(codeString)) 7744 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RANGE, code); 7745 if ("Ratio".equals(codeString)) 7746 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RATIO, code); 7747 if ("Reference".equals(codeString)) 7748 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.REFERENCE, code); 7749 if ("RelatedArtifact".equals(codeString)) 7750 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RELATEDARTIFACT, code); 7751 if ("SampledData".equals(codeString)) 7752 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SAMPLEDDATA, code); 7753 if ("Signature".equals(codeString)) 7754 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SIGNATURE, code); 7755 if ("SimpleQuantity".equals(codeString)) 7756 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SIMPLEQUANTITY, code); 7757 if ("SubstanceAmount".equals(codeString)) 7758 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUBSTANCEAMOUNT, code); 7759 if ("Timing".equals(codeString)) 7760 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TIMING, code); 7761 if ("TriggerDefinition".equals(codeString)) 7762 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TRIGGERDEFINITION, code); 7763 if ("UsageContext".equals(codeString)) 7764 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.USAGECONTEXT, code); 7765 if ("base64Binary".equals(codeString)) 7766 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BASE64BINARY, code); 7767 if ("boolean".equals(codeString)) 7768 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BOOLEAN, code); 7769 if ("canonical".equals(codeString)) 7770 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CANONICAL, code); 7771 if ("code".equals(codeString)) 7772 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CODE, code); 7773 if ("date".equals(codeString)) 7774 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DATE, code); 7775 if ("dateTime".equals(codeString)) 7776 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DATETIME, code); 7777 if ("decimal".equals(codeString)) 7778 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DECIMAL, code); 7779 if ("id".equals(codeString)) 7780 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ID, code); 7781 if ("instant".equals(codeString)) 7782 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.INSTANT, code); 7783 if ("integer".equals(codeString)) 7784 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.INTEGER, code); 7785 if ("markdown".equals(codeString)) 7786 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MARKDOWN, code); 7787 if ("oid".equals(codeString)) 7788 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.OID, code); 7789 if ("positiveInt".equals(codeString)) 7790 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.POSITIVEINT, code); 7791 if ("string".equals(codeString)) 7792 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.STRING, code); 7793 if ("time".equals(codeString)) 7794 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TIME, code); 7795 if ("unsignedInt".equals(codeString)) 7796 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.UNSIGNEDINT, code); 7797 if ("uri".equals(codeString)) 7798 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.URI, code); 7799 if ("url".equals(codeString)) 7800 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.URL, code); 7801 if ("uuid".equals(codeString)) 7802 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.UUID, code); 7803 if ("xhtml".equals(codeString)) 7804 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.XHTML, code); 7805 if ("Account".equals(codeString)) 7806 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ACCOUNT, code); 7807 if ("ActivityDefinition".equals(codeString)) 7808 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ACTIVITYDEFINITION, code); 7809 if ("AdverseEvent".equals(codeString)) 7810 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ADVERSEEVENT, code); 7811 if ("AllergyIntolerance".equals(codeString)) 7812 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ALLERGYINTOLERANCE, code); 7813 if ("Appointment".equals(codeString)) 7814 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.APPOINTMENT, code); 7815 if ("AppointmentResponse".equals(codeString)) 7816 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.APPOINTMENTRESPONSE, code); 7817 if ("AuditEvent".equals(codeString)) 7818 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.AUDITEVENT, code); 7819 if ("Basic".equals(codeString)) 7820 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BASIC, code); 7821 if ("Binary".equals(codeString)) 7822 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BINARY, code); 7823 if ("BiologicallyDerivedProduct".equals(codeString)) 7824 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BIOLOGICALLYDERIVEDPRODUCT, code); 7825 if ("BodyStructure".equals(codeString)) 7826 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BODYSTRUCTURE, code); 7827 if ("Bundle".equals(codeString)) 7828 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BUNDLE, code); 7829 if ("CapabilityStatement".equals(codeString)) 7830 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CAPABILITYSTATEMENT, code); 7831 if ("CarePlan".equals(codeString)) 7832 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CAREPLAN, code); 7833 if ("CareTeam".equals(codeString)) 7834 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CARETEAM, code); 7835 if ("CatalogEntry".equals(codeString)) 7836 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CATALOGENTRY, code); 7837 if ("ChargeItem".equals(codeString)) 7838 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CHARGEITEM, code); 7839 if ("ChargeItemDefinition".equals(codeString)) 7840 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CHARGEITEMDEFINITION, code); 7841 if ("Claim".equals(codeString)) 7842 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CLAIM, code); 7843 if ("ClaimResponse".equals(codeString)) 7844 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CLAIMRESPONSE, code); 7845 if ("ClinicalImpression".equals(codeString)) 7846 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CLINICALIMPRESSION, code); 7847 if ("CodeSystem".equals(codeString)) 7848 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CODESYSTEM, code); 7849 if ("Communication".equals(codeString)) 7850 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COMMUNICATION, code); 7851 if ("CommunicationRequest".equals(codeString)) 7852 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COMMUNICATIONREQUEST, code); 7853 if ("CompartmentDefinition".equals(codeString)) 7854 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COMPARTMENTDEFINITION, code); 7855 if ("Composition".equals(codeString)) 7856 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COMPOSITION, code); 7857 if ("ConceptMap".equals(codeString)) 7858 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONCEPTMAP, code); 7859 if ("Condition".equals(codeString)) 7860 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONDITION, code); 7861 if ("Consent".equals(codeString)) 7862 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONSENT, code); 7863 if ("Contract".equals(codeString)) 7864 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONTRACT, code); 7865 if ("Coverage".equals(codeString)) 7866 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COVERAGE, code); 7867 if ("CoverageEligibilityRequest".equals(codeString)) 7868 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COVERAGEELIGIBILITYREQUEST, code); 7869 if ("CoverageEligibilityResponse".equals(codeString)) 7870 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COVERAGEELIGIBILITYRESPONSE, code); 7871 if ("DetectedIssue".equals(codeString)) 7872 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DETECTEDISSUE, code); 7873 if ("Device".equals(codeString)) 7874 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DEVICE, code); 7875 if ("DeviceDefinition".equals(codeString)) 7876 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DEVICEDEFINITION, code); 7877 if ("DeviceMetric".equals(codeString)) 7878 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DEVICEMETRIC, code); 7879 if ("DeviceRequest".equals(codeString)) 7880 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DEVICEREQUEST, code); 7881 if ("DeviceUseStatement".equals(codeString)) 7882 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DEVICEUSESTATEMENT, code); 7883 if ("DiagnosticReport".equals(codeString)) 7884 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DIAGNOSTICREPORT, code); 7885 if ("DocumentManifest".equals(codeString)) 7886 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DOCUMENTMANIFEST, code); 7887 if ("DocumentReference".equals(codeString)) 7888 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DOCUMENTREFERENCE, code); 7889 if ("DomainResource".equals(codeString)) 7890 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DOMAINRESOURCE, code); 7891 if ("EffectEvidenceSynthesis".equals(codeString)) 7892 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.EFFECTEVIDENCESYNTHESIS, code); 7893 if ("Encounter".equals(codeString)) 7894 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ENCOUNTER, code); 7895 if ("Endpoint".equals(codeString)) 7896 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ENDPOINT, code); 7897 if ("EnrollmentRequest".equals(codeString)) 7898 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ENROLLMENTREQUEST, code); 7899 if ("EnrollmentResponse".equals(codeString)) 7900 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ENROLLMENTRESPONSE, code); 7901 if ("EpisodeOfCare".equals(codeString)) 7902 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.EPISODEOFCARE, code); 7903 if ("EventDefinition".equals(codeString)) 7904 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.EVENTDEFINITION, code); 7905 if ("Evidence".equals(codeString)) 7906 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.EVIDENCE, code); 7907 if ("EvidenceVariable".equals(codeString)) 7908 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.EVIDENCEVARIABLE, code); 7909 if ("ExampleScenario".equals(codeString)) 7910 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.EXAMPLESCENARIO, code); 7911 if ("ExplanationOfBenefit".equals(codeString)) 7912 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.EXPLANATIONOFBENEFIT, code); 7913 if ("FamilyMemberHistory".equals(codeString)) 7914 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.FAMILYMEMBERHISTORY, code); 7915 if ("Flag".equals(codeString)) 7916 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.FLAG, code); 7917 if ("Goal".equals(codeString)) 7918 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.GOAL, code); 7919 if ("GraphDefinition".equals(codeString)) 7920 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.GRAPHDEFINITION, code); 7921 if ("Group".equals(codeString)) 7922 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.GROUP, code); 7923 if ("GuidanceResponse".equals(codeString)) 7924 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.GUIDANCERESPONSE, code); 7925 if ("HealthcareService".equals(codeString)) 7926 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.HEALTHCARESERVICE, code); 7927 if ("ImagingStudy".equals(codeString)) 7928 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.IMAGINGSTUDY, code); 7929 if ("Immunization".equals(codeString)) 7930 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.IMMUNIZATION, code); 7931 if ("ImmunizationEvaluation".equals(codeString)) 7932 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.IMMUNIZATIONEVALUATION, code); 7933 if ("ImmunizationRecommendation".equals(codeString)) 7934 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.IMMUNIZATIONRECOMMENDATION, code); 7935 if ("ImplementationGuide".equals(codeString)) 7936 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.IMPLEMENTATIONGUIDE, code); 7937 if ("InsurancePlan".equals(codeString)) 7938 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.INSURANCEPLAN, code); 7939 if ("Invoice".equals(codeString)) 7940 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.INVOICE, code); 7941 if ("Library".equals(codeString)) 7942 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.LIBRARY, code); 7943 if ("Linkage".equals(codeString)) 7944 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.LINKAGE, code); 7945 if ("List".equals(codeString)) 7946 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.LIST, code); 7947 if ("Location".equals(codeString)) 7948 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.LOCATION, code); 7949 if ("Measure".equals(codeString)) 7950 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEASURE, code); 7951 if ("MeasureReport".equals(codeString)) 7952 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEASUREREPORT, code); 7953 if ("Media".equals(codeString)) 7954 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDIA, code); 7955 if ("Medication".equals(codeString)) 7956 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICATION, code); 7957 if ("MedicationAdministration".equals(codeString)) 7958 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICATIONADMINISTRATION, code); 7959 if ("MedicationDispense".equals(codeString)) 7960 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICATIONDISPENSE, code); 7961 if ("MedicationKnowledge".equals(codeString)) 7962 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICATIONKNOWLEDGE, code); 7963 if ("MedicationRequest".equals(codeString)) 7964 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICATIONREQUEST, code); 7965 if ("MedicationStatement".equals(codeString)) 7966 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICATIONSTATEMENT, code); 7967 if ("MedicinalProduct".equals(codeString)) 7968 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICINALPRODUCT, code); 7969 if ("MedicinalProductAuthorization".equals(codeString)) 7970 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICINALPRODUCTAUTHORIZATION, code); 7971 if ("MedicinalProductContraindication".equals(codeString)) 7972 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICINALPRODUCTCONTRAINDICATION, code); 7973 if ("MedicinalProductIndication".equals(codeString)) 7974 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICINALPRODUCTINDICATION, code); 7975 if ("MedicinalProductIngredient".equals(codeString)) 7976 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICINALPRODUCTINGREDIENT, code); 7977 if ("MedicinalProductInteraction".equals(codeString)) 7978 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICINALPRODUCTINTERACTION, code); 7979 if ("MedicinalProductManufactured".equals(codeString)) 7980 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICINALPRODUCTMANUFACTURED, code); 7981 if ("MedicinalProductPackaged".equals(codeString)) 7982 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICINALPRODUCTPACKAGED, code); 7983 if ("MedicinalProductPharmaceutical".equals(codeString)) 7984 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICINALPRODUCTPHARMACEUTICAL, code); 7985 if ("MedicinalProductUndesirableEffect".equals(codeString)) 7986 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICINALPRODUCTUNDESIRABLEEFFECT, code); 7987 if ("MessageDefinition".equals(codeString)) 7988 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MESSAGEDEFINITION, code); 7989 if ("MessageHeader".equals(codeString)) 7990 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MESSAGEHEADER, code); 7991 if ("MolecularSequence".equals(codeString)) 7992 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MOLECULARSEQUENCE, code); 7993 if ("NamingSystem".equals(codeString)) 7994 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.NAMINGSYSTEM, code); 7995 if ("NutritionOrder".equals(codeString)) 7996 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.NUTRITIONORDER, code); 7997 if ("Observation".equals(codeString)) 7998 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.OBSERVATION, code); 7999 if ("ObservationDefinition".equals(codeString)) 8000 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.OBSERVATIONDEFINITION, code); 8001 if ("OperationDefinition".equals(codeString)) 8002 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.OPERATIONDEFINITION, code); 8003 if ("OperationOutcome".equals(codeString)) 8004 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.OPERATIONOUTCOME, code); 8005 if ("Organization".equals(codeString)) 8006 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ORGANIZATION, code); 8007 if ("OrganizationAffiliation".equals(codeString)) 8008 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ORGANIZATIONAFFILIATION, code); 8009 if ("Parameters".equals(codeString)) 8010 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PARAMETERS, code); 8011 if ("Patient".equals(codeString)) 8012 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PATIENT, code); 8013 if ("PaymentNotice".equals(codeString)) 8014 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PAYMENTNOTICE, code); 8015 if ("PaymentReconciliation".equals(codeString)) 8016 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PAYMENTRECONCILIATION, code); 8017 if ("Person".equals(codeString)) 8018 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PERSON, code); 8019 if ("PlanDefinition".equals(codeString)) 8020 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PLANDEFINITION, code); 8021 if ("Practitioner".equals(codeString)) 8022 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PRACTITIONER, code); 8023 if ("PractitionerRole".equals(codeString)) 8024 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PRACTITIONERROLE, code); 8025 if ("Procedure".equals(codeString)) 8026 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PROCEDURE, code); 8027 if ("Provenance".equals(codeString)) 8028 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PROVENANCE, code); 8029 if ("Questionnaire".equals(codeString)) 8030 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.QUESTIONNAIRE, code); 8031 if ("QuestionnaireResponse".equals(codeString)) 8032 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.QUESTIONNAIRERESPONSE, code); 8033 if ("RelatedPerson".equals(codeString)) 8034 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RELATEDPERSON, code); 8035 if ("RequestGroup".equals(codeString)) 8036 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.REQUESTGROUP, code); 8037 if ("ResearchDefinition".equals(codeString)) 8038 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RESEARCHDEFINITION, code); 8039 if ("ResearchElementDefinition".equals(codeString)) 8040 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RESEARCHELEMENTDEFINITION, code); 8041 if ("ResearchStudy".equals(codeString)) 8042 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RESEARCHSTUDY, code); 8043 if ("ResearchSubject".equals(codeString)) 8044 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RESEARCHSUBJECT, code); 8045 if ("Resource".equals(codeString)) 8046 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RESOURCE, code); 8047 if ("RiskAssessment".equals(codeString)) 8048 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RISKASSESSMENT, code); 8049 if ("RiskEvidenceSynthesis".equals(codeString)) 8050 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RISKEVIDENCESYNTHESIS, code); 8051 if ("Schedule".equals(codeString)) 8052 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SCHEDULE, code); 8053 if ("SearchParameter".equals(codeString)) 8054 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SEARCHPARAMETER, code); 8055 if ("ServiceRequest".equals(codeString)) 8056 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SERVICEREQUEST, code); 8057 if ("Slot".equals(codeString)) 8058 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SLOT, code); 8059 if ("Specimen".equals(codeString)) 8060 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SPECIMEN, code); 8061 if ("SpecimenDefinition".equals(codeString)) 8062 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SPECIMENDEFINITION, code); 8063 if ("StructureDefinition".equals(codeString)) 8064 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.STRUCTUREDEFINITION, code); 8065 if ("StructureMap".equals(codeString)) 8066 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.STRUCTUREMAP, code); 8067 if ("Subscription".equals(codeString)) 8068 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUBSCRIPTION, code); 8069 if ("Substance".equals(codeString)) 8070 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUBSTANCE, code); 8071 if ("SubstanceNucleicAcid".equals(codeString)) 8072 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUBSTANCENUCLEICACID, code); 8073 if ("SubstancePolymer".equals(codeString)) 8074 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUBSTANCEPOLYMER, code); 8075 if ("SubstanceProtein".equals(codeString)) 8076 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUBSTANCEPROTEIN, code); 8077 if ("SubstanceReferenceInformation".equals(codeString)) 8078 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUBSTANCEREFERENCEINFORMATION, code); 8079 if ("SubstanceSourceMaterial".equals(codeString)) 8080 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUBSTANCESOURCEMATERIAL, code); 8081 if ("SubstanceSpecification".equals(codeString)) 8082 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUBSTANCESPECIFICATION, code); 8083 if ("SupplyDelivery".equals(codeString)) 8084 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUPPLYDELIVERY, code); 8085 if ("SupplyRequest".equals(codeString)) 8086 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUPPLYREQUEST, code); 8087 if ("Task".equals(codeString)) 8088 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TASK, code); 8089 if ("TerminologyCapabilities".equals(codeString)) 8090 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TERMINOLOGYCAPABILITIES, code); 8091 if ("TestReport".equals(codeString)) 8092 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TESTREPORT, code); 8093 if ("TestScript".equals(codeString)) 8094 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TESTSCRIPT, code); 8095 if ("ValueSet".equals(codeString)) 8096 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.VALUESET, code); 8097 if ("VerificationResult".equals(codeString)) 8098 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.VERIFICATIONRESULT, code); 8099 if ("VisionPrescription".equals(codeString)) 8100 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.VISIONPRESCRIPTION, code); 8101 if ("Type".equals(codeString)) 8102 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TYPE, code); 8103 if ("Any".equals(codeString)) 8104 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ANY, code); 8105 throw new FHIRException("Unknown FHIRAllTypes code '" + codeString + "'"); 8106 } 8107 8108 public String toCode(FHIRAllTypes code) { 8109 if (code == FHIRAllTypes.NULL) 8110 return null; 8111 if (code == FHIRAllTypes.ADDRESS) 8112 return "Address"; 8113 if (code == FHIRAllTypes.AGE) 8114 return "Age"; 8115 if (code == FHIRAllTypes.ANNOTATION) 8116 return "Annotation"; 8117 if (code == FHIRAllTypes.ATTACHMENT) 8118 return "Attachment"; 8119 if (code == FHIRAllTypes.BACKBONEELEMENT) 8120 return "BackboneElement"; 8121 if (code == FHIRAllTypes.CODEABLECONCEPT) 8122 return "CodeableConcept"; 8123 if (code == FHIRAllTypes.CODING) 8124 return "Coding"; 8125 if (code == FHIRAllTypes.CONTACTDETAIL) 8126 return "ContactDetail"; 8127 if (code == FHIRAllTypes.CONTACTPOINT) 8128 return "ContactPoint"; 8129 if (code == FHIRAllTypes.CONTRIBUTOR) 8130 return "Contributor"; 8131 if (code == FHIRAllTypes.COUNT) 8132 return "Count"; 8133 if (code == FHIRAllTypes.DATAREQUIREMENT) 8134 return "DataRequirement"; 8135 if (code == FHIRAllTypes.DISTANCE) 8136 return "Distance"; 8137 if (code == FHIRAllTypes.DOSAGE) 8138 return "Dosage"; 8139 if (code == FHIRAllTypes.DURATION) 8140 return "Duration"; 8141 if (code == FHIRAllTypes.ELEMENT) 8142 return "Element"; 8143 if (code == FHIRAllTypes.ELEMENTDEFINITION) 8144 return "ElementDefinition"; 8145 if (code == FHIRAllTypes.EXPRESSION) 8146 return "Expression"; 8147 if (code == FHIRAllTypes.EXTENSION) 8148 return "Extension"; 8149 if (code == FHIRAllTypes.HUMANNAME) 8150 return "HumanName"; 8151 if (code == FHIRAllTypes.IDENTIFIER) 8152 return "Identifier"; 8153 if (code == FHIRAllTypes.MARKETINGSTATUS) 8154 return "MarketingStatus"; 8155 if (code == FHIRAllTypes.META) 8156 return "Meta"; 8157 if (code == FHIRAllTypes.MONEY) 8158 return "Money"; 8159 if (code == FHIRAllTypes.MONEYQUANTITY) 8160 return "MoneyQuantity"; 8161 if (code == FHIRAllTypes.NARRATIVE) 8162 return "Narrative"; 8163 if (code == FHIRAllTypes.PARAMETERDEFINITION) 8164 return "ParameterDefinition"; 8165 if (code == FHIRAllTypes.PERIOD) 8166 return "Period"; 8167 if (code == FHIRAllTypes.POPULATION) 8168 return "Population"; 8169 if (code == FHIRAllTypes.PRODCHARACTERISTIC) 8170 return "ProdCharacteristic"; 8171 if (code == FHIRAllTypes.PRODUCTSHELFLIFE) 8172 return "ProductShelfLife"; 8173 if (code == FHIRAllTypes.QUANTITY) 8174 return "Quantity"; 8175 if (code == FHIRAllTypes.RANGE) 8176 return "Range"; 8177 if (code == FHIRAllTypes.RATIO) 8178 return "Ratio"; 8179 if (code == FHIRAllTypes.REFERENCE) 8180 return "Reference"; 8181 if (code == FHIRAllTypes.RELATEDARTIFACT) 8182 return "RelatedArtifact"; 8183 if (code == FHIRAllTypes.SAMPLEDDATA) 8184 return "SampledData"; 8185 if (code == FHIRAllTypes.SIGNATURE) 8186 return "Signature"; 8187 if (code == FHIRAllTypes.SIMPLEQUANTITY) 8188 return "SimpleQuantity"; 8189 if (code == FHIRAllTypes.SUBSTANCEAMOUNT) 8190 return "SubstanceAmount"; 8191 if (code == FHIRAllTypes.TIMING) 8192 return "Timing"; 8193 if (code == FHIRAllTypes.TRIGGERDEFINITION) 8194 return "TriggerDefinition"; 8195 if (code == FHIRAllTypes.USAGECONTEXT) 8196 return "UsageContext"; 8197 if (code == FHIRAllTypes.BASE64BINARY) 8198 return "base64Binary"; 8199 if (code == FHIRAllTypes.BOOLEAN) 8200 return "boolean"; 8201 if (code == FHIRAllTypes.CANONICAL) 8202 return "canonical"; 8203 if (code == FHIRAllTypes.CODE) 8204 return "code"; 8205 if (code == FHIRAllTypes.DATE) 8206 return "date"; 8207 if (code == FHIRAllTypes.DATETIME) 8208 return "dateTime"; 8209 if (code == FHIRAllTypes.DECIMAL) 8210 return "decimal"; 8211 if (code == FHIRAllTypes.ID) 8212 return "id"; 8213 if (code == FHIRAllTypes.INSTANT) 8214 return "instant"; 8215 if (code == FHIRAllTypes.INTEGER) 8216 return "integer"; 8217 if (code == FHIRAllTypes.MARKDOWN) 8218 return "markdown"; 8219 if (code == FHIRAllTypes.OID) 8220 return "oid"; 8221 if (code == FHIRAllTypes.POSITIVEINT) 8222 return "positiveInt"; 8223 if (code == FHIRAllTypes.STRING) 8224 return "string"; 8225 if (code == FHIRAllTypes.TIME) 8226 return "time"; 8227 if (code == FHIRAllTypes.UNSIGNEDINT) 8228 return "unsignedInt"; 8229 if (code == FHIRAllTypes.URI) 8230 return "uri"; 8231 if (code == FHIRAllTypes.URL) 8232 return "url"; 8233 if (code == FHIRAllTypes.UUID) 8234 return "uuid"; 8235 if (code == FHIRAllTypes.XHTML) 8236 return "xhtml"; 8237 if (code == FHIRAllTypes.ACCOUNT) 8238 return "Account"; 8239 if (code == FHIRAllTypes.ACTIVITYDEFINITION) 8240 return "ActivityDefinition"; 8241 if (code == FHIRAllTypes.ADVERSEEVENT) 8242 return "AdverseEvent"; 8243 if (code == FHIRAllTypes.ALLERGYINTOLERANCE) 8244 return "AllergyIntolerance"; 8245 if (code == FHIRAllTypes.APPOINTMENT) 8246 return "Appointment"; 8247 if (code == FHIRAllTypes.APPOINTMENTRESPONSE) 8248 return "AppointmentResponse"; 8249 if (code == FHIRAllTypes.AUDITEVENT) 8250 return "AuditEvent"; 8251 if (code == FHIRAllTypes.BASIC) 8252 return "Basic"; 8253 if (code == FHIRAllTypes.BINARY) 8254 return "Binary"; 8255 if (code == FHIRAllTypes.BIOLOGICALLYDERIVEDPRODUCT) 8256 return "BiologicallyDerivedProduct"; 8257 if (code == FHIRAllTypes.BODYSTRUCTURE) 8258 return "BodyStructure"; 8259 if (code == FHIRAllTypes.BUNDLE) 8260 return "Bundle"; 8261 if (code == FHIRAllTypes.CAPABILITYSTATEMENT) 8262 return "CapabilityStatement"; 8263 if (code == FHIRAllTypes.CAREPLAN) 8264 return "CarePlan"; 8265 if (code == FHIRAllTypes.CARETEAM) 8266 return "CareTeam"; 8267 if (code == FHIRAllTypes.CATALOGENTRY) 8268 return "CatalogEntry"; 8269 if (code == FHIRAllTypes.CHARGEITEM) 8270 return "ChargeItem"; 8271 if (code == FHIRAllTypes.CHARGEITEMDEFINITION) 8272 return "ChargeItemDefinition"; 8273 if (code == FHIRAllTypes.CLAIM) 8274 return "Claim"; 8275 if (code == FHIRAllTypes.CLAIMRESPONSE) 8276 return "ClaimResponse"; 8277 if (code == FHIRAllTypes.CLINICALIMPRESSION) 8278 return "ClinicalImpression"; 8279 if (code == FHIRAllTypes.CODESYSTEM) 8280 return "CodeSystem"; 8281 if (code == FHIRAllTypes.COMMUNICATION) 8282 return "Communication"; 8283 if (code == FHIRAllTypes.COMMUNICATIONREQUEST) 8284 return "CommunicationRequest"; 8285 if (code == FHIRAllTypes.COMPARTMENTDEFINITION) 8286 return "CompartmentDefinition"; 8287 if (code == FHIRAllTypes.COMPOSITION) 8288 return "Composition"; 8289 if (code == FHIRAllTypes.CONCEPTMAP) 8290 return "ConceptMap"; 8291 if (code == FHIRAllTypes.CONDITION) 8292 return "Condition"; 8293 if (code == FHIRAllTypes.CONSENT) 8294 return "Consent"; 8295 if (code == FHIRAllTypes.CONTRACT) 8296 return "Contract"; 8297 if (code == FHIRAllTypes.COVERAGE) 8298 return "Coverage"; 8299 if (code == FHIRAllTypes.COVERAGEELIGIBILITYREQUEST) 8300 return "CoverageEligibilityRequest"; 8301 if (code == FHIRAllTypes.COVERAGEELIGIBILITYRESPONSE) 8302 return "CoverageEligibilityResponse"; 8303 if (code == FHIRAllTypes.DETECTEDISSUE) 8304 return "DetectedIssue"; 8305 if (code == FHIRAllTypes.DEVICE) 8306 return "Device"; 8307 if (code == FHIRAllTypes.DEVICEDEFINITION) 8308 return "DeviceDefinition"; 8309 if (code == FHIRAllTypes.DEVICEMETRIC) 8310 return "DeviceMetric"; 8311 if (code == FHIRAllTypes.DEVICEREQUEST) 8312 return "DeviceRequest"; 8313 if (code == FHIRAllTypes.DEVICEUSESTATEMENT) 8314 return "DeviceUseStatement"; 8315 if (code == FHIRAllTypes.DIAGNOSTICREPORT) 8316 return "DiagnosticReport"; 8317 if (code == FHIRAllTypes.DOCUMENTMANIFEST) 8318 return "DocumentManifest"; 8319 if (code == FHIRAllTypes.DOCUMENTREFERENCE) 8320 return "DocumentReference"; 8321 if (code == FHIRAllTypes.DOMAINRESOURCE) 8322 return "DomainResource"; 8323 if (code == FHIRAllTypes.EFFECTEVIDENCESYNTHESIS) 8324 return "EffectEvidenceSynthesis"; 8325 if (code == FHIRAllTypes.ENCOUNTER) 8326 return "Encounter"; 8327 if (code == FHIRAllTypes.ENDPOINT) 8328 return "Endpoint"; 8329 if (code == FHIRAllTypes.ENROLLMENTREQUEST) 8330 return "EnrollmentRequest"; 8331 if (code == FHIRAllTypes.ENROLLMENTRESPONSE) 8332 return "EnrollmentResponse"; 8333 if (code == FHIRAllTypes.EPISODEOFCARE) 8334 return "EpisodeOfCare"; 8335 if (code == FHIRAllTypes.EVENTDEFINITION) 8336 return "EventDefinition"; 8337 if (code == FHIRAllTypes.EVIDENCE) 8338 return "Evidence"; 8339 if (code == FHIRAllTypes.EVIDENCEVARIABLE) 8340 return "EvidenceVariable"; 8341 if (code == FHIRAllTypes.EXAMPLESCENARIO) 8342 return "ExampleScenario"; 8343 if (code == FHIRAllTypes.EXPLANATIONOFBENEFIT) 8344 return "ExplanationOfBenefit"; 8345 if (code == FHIRAllTypes.FAMILYMEMBERHISTORY) 8346 return "FamilyMemberHistory"; 8347 if (code == FHIRAllTypes.FLAG) 8348 return "Flag"; 8349 if (code == FHIRAllTypes.GOAL) 8350 return "Goal"; 8351 if (code == FHIRAllTypes.GRAPHDEFINITION) 8352 return "GraphDefinition"; 8353 if (code == FHIRAllTypes.GROUP) 8354 return "Group"; 8355 if (code == FHIRAllTypes.GUIDANCERESPONSE) 8356 return "GuidanceResponse"; 8357 if (code == FHIRAllTypes.HEALTHCARESERVICE) 8358 return "HealthcareService"; 8359 if (code == FHIRAllTypes.IMAGINGSTUDY) 8360 return "ImagingStudy"; 8361 if (code == FHIRAllTypes.IMMUNIZATION) 8362 return "Immunization"; 8363 if (code == FHIRAllTypes.IMMUNIZATIONEVALUATION) 8364 return "ImmunizationEvaluation"; 8365 if (code == FHIRAllTypes.IMMUNIZATIONRECOMMENDATION) 8366 return "ImmunizationRecommendation"; 8367 if (code == FHIRAllTypes.IMPLEMENTATIONGUIDE) 8368 return "ImplementationGuide"; 8369 if (code == FHIRAllTypes.INSURANCEPLAN) 8370 return "InsurancePlan"; 8371 if (code == FHIRAllTypes.INVOICE) 8372 return "Invoice"; 8373 if (code == FHIRAllTypes.LIBRARY) 8374 return "Library"; 8375 if (code == FHIRAllTypes.LINKAGE) 8376 return "Linkage"; 8377 if (code == FHIRAllTypes.LIST) 8378 return "List"; 8379 if (code == FHIRAllTypes.LOCATION) 8380 return "Location"; 8381 if (code == FHIRAllTypes.MEASURE) 8382 return "Measure"; 8383 if (code == FHIRAllTypes.MEASUREREPORT) 8384 return "MeasureReport"; 8385 if (code == FHIRAllTypes.MEDIA) 8386 return "Media"; 8387 if (code == FHIRAllTypes.MEDICATION) 8388 return "Medication"; 8389 if (code == FHIRAllTypes.MEDICATIONADMINISTRATION) 8390 return "MedicationAdministration"; 8391 if (code == FHIRAllTypes.MEDICATIONDISPENSE) 8392 return "MedicationDispense"; 8393 if (code == FHIRAllTypes.MEDICATIONKNOWLEDGE) 8394 return "MedicationKnowledge"; 8395 if (code == FHIRAllTypes.MEDICATIONREQUEST) 8396 return "MedicationRequest"; 8397 if (code == FHIRAllTypes.MEDICATIONSTATEMENT) 8398 return "MedicationStatement"; 8399 if (code == FHIRAllTypes.MEDICINALPRODUCT) 8400 return "MedicinalProduct"; 8401 if (code == FHIRAllTypes.MEDICINALPRODUCTAUTHORIZATION) 8402 return "MedicinalProductAuthorization"; 8403 if (code == FHIRAllTypes.MEDICINALPRODUCTCONTRAINDICATION) 8404 return "MedicinalProductContraindication"; 8405 if (code == FHIRAllTypes.MEDICINALPRODUCTINDICATION) 8406 return "MedicinalProductIndication"; 8407 if (code == FHIRAllTypes.MEDICINALPRODUCTINGREDIENT) 8408 return "MedicinalProductIngredient"; 8409 if (code == FHIRAllTypes.MEDICINALPRODUCTINTERACTION) 8410 return "MedicinalProductInteraction"; 8411 if (code == FHIRAllTypes.MEDICINALPRODUCTMANUFACTURED) 8412 return "MedicinalProductManufactured"; 8413 if (code == FHIRAllTypes.MEDICINALPRODUCTPACKAGED) 8414 return "MedicinalProductPackaged"; 8415 if (code == FHIRAllTypes.MEDICINALPRODUCTPHARMACEUTICAL) 8416 return "MedicinalProductPharmaceutical"; 8417 if (code == FHIRAllTypes.MEDICINALPRODUCTUNDESIRABLEEFFECT) 8418 return "MedicinalProductUndesirableEffect"; 8419 if (code == FHIRAllTypes.MESSAGEDEFINITION) 8420 return "MessageDefinition"; 8421 if (code == FHIRAllTypes.MESSAGEHEADER) 8422 return "MessageHeader"; 8423 if (code == FHIRAllTypes.MOLECULARSEQUENCE) 8424 return "MolecularSequence"; 8425 if (code == FHIRAllTypes.NAMINGSYSTEM) 8426 return "NamingSystem"; 8427 if (code == FHIRAllTypes.NUTRITIONORDER) 8428 return "NutritionOrder"; 8429 if (code == FHIRAllTypes.OBSERVATION) 8430 return "Observation"; 8431 if (code == FHIRAllTypes.OBSERVATIONDEFINITION) 8432 return "ObservationDefinition"; 8433 if (code == FHIRAllTypes.OPERATIONDEFINITION) 8434 return "OperationDefinition"; 8435 if (code == FHIRAllTypes.OPERATIONOUTCOME) 8436 return "OperationOutcome"; 8437 if (code == FHIRAllTypes.ORGANIZATION) 8438 return "Organization"; 8439 if (code == FHIRAllTypes.ORGANIZATIONAFFILIATION) 8440 return "OrganizationAffiliation"; 8441 if (code == FHIRAllTypes.PARAMETERS) 8442 return "Parameters"; 8443 if (code == FHIRAllTypes.PATIENT) 8444 return "Patient"; 8445 if (code == FHIRAllTypes.PAYMENTNOTICE) 8446 return "PaymentNotice"; 8447 if (code == FHIRAllTypes.PAYMENTRECONCILIATION) 8448 return "PaymentReconciliation"; 8449 if (code == FHIRAllTypes.PERSON) 8450 return "Person"; 8451 if (code == FHIRAllTypes.PLANDEFINITION) 8452 return "PlanDefinition"; 8453 if (code == FHIRAllTypes.PRACTITIONER) 8454 return "Practitioner"; 8455 if (code == FHIRAllTypes.PRACTITIONERROLE) 8456 return "PractitionerRole"; 8457 if (code == FHIRAllTypes.PROCEDURE) 8458 return "Procedure"; 8459 if (code == FHIRAllTypes.PROVENANCE) 8460 return "Provenance"; 8461 if (code == FHIRAllTypes.QUESTIONNAIRE) 8462 return "Questionnaire"; 8463 if (code == FHIRAllTypes.QUESTIONNAIRERESPONSE) 8464 return "QuestionnaireResponse"; 8465 if (code == FHIRAllTypes.RELATEDPERSON) 8466 return "RelatedPerson"; 8467 if (code == FHIRAllTypes.REQUESTGROUP) 8468 return "RequestGroup"; 8469 if (code == FHIRAllTypes.RESEARCHDEFINITION) 8470 return "ResearchDefinition"; 8471 if (code == FHIRAllTypes.RESEARCHELEMENTDEFINITION) 8472 return "ResearchElementDefinition"; 8473 if (code == FHIRAllTypes.RESEARCHSTUDY) 8474 return "ResearchStudy"; 8475 if (code == FHIRAllTypes.RESEARCHSUBJECT) 8476 return "ResearchSubject"; 8477 if (code == FHIRAllTypes.RESOURCE) 8478 return "Resource"; 8479 if (code == FHIRAllTypes.RISKASSESSMENT) 8480 return "RiskAssessment"; 8481 if (code == FHIRAllTypes.RISKEVIDENCESYNTHESIS) 8482 return "RiskEvidenceSynthesis"; 8483 if (code == FHIRAllTypes.SCHEDULE) 8484 return "Schedule"; 8485 if (code == FHIRAllTypes.SEARCHPARAMETER) 8486 return "SearchParameter"; 8487 if (code == FHIRAllTypes.SERVICEREQUEST) 8488 return "ServiceRequest"; 8489 if (code == FHIRAllTypes.SLOT) 8490 return "Slot"; 8491 if (code == FHIRAllTypes.SPECIMEN) 8492 return "Specimen"; 8493 if (code == FHIRAllTypes.SPECIMENDEFINITION) 8494 return "SpecimenDefinition"; 8495 if (code == FHIRAllTypes.STRUCTUREDEFINITION) 8496 return "StructureDefinition"; 8497 if (code == FHIRAllTypes.STRUCTUREMAP) 8498 return "StructureMap"; 8499 if (code == FHIRAllTypes.SUBSCRIPTION) 8500 return "Subscription"; 8501 if (code == FHIRAllTypes.SUBSTANCE) 8502 return "Substance"; 8503 if (code == FHIRAllTypes.SUBSTANCENUCLEICACID) 8504 return "SubstanceNucleicAcid"; 8505 if (code == FHIRAllTypes.SUBSTANCEPOLYMER) 8506 return "SubstancePolymer"; 8507 if (code == FHIRAllTypes.SUBSTANCEPROTEIN) 8508 return "SubstanceProtein"; 8509 if (code == FHIRAllTypes.SUBSTANCEREFERENCEINFORMATION) 8510 return "SubstanceReferenceInformation"; 8511 if (code == FHIRAllTypes.SUBSTANCESOURCEMATERIAL) 8512 return "SubstanceSourceMaterial"; 8513 if (code == FHIRAllTypes.SUBSTANCESPECIFICATION) 8514 return "SubstanceSpecification"; 8515 if (code == FHIRAllTypes.SUPPLYDELIVERY) 8516 return "SupplyDelivery"; 8517 if (code == FHIRAllTypes.SUPPLYREQUEST) 8518 return "SupplyRequest"; 8519 if (code == FHIRAllTypes.TASK) 8520 return "Task"; 8521 if (code == FHIRAllTypes.TERMINOLOGYCAPABILITIES) 8522 return "TerminologyCapabilities"; 8523 if (code == FHIRAllTypes.TESTREPORT) 8524 return "TestReport"; 8525 if (code == FHIRAllTypes.TESTSCRIPT) 8526 return "TestScript"; 8527 if (code == FHIRAllTypes.VALUESET) 8528 return "ValueSet"; 8529 if (code == FHIRAllTypes.VERIFICATIONRESULT) 8530 return "VerificationResult"; 8531 if (code == FHIRAllTypes.VISIONPRESCRIPTION) 8532 return "VisionPrescription"; 8533 if (code == FHIRAllTypes.TYPE) 8534 return "Type"; 8535 if (code == FHIRAllTypes.ANY) 8536 return "Any"; 8537 return "?"; 8538 } 8539 8540 public String toSystem(FHIRAllTypes code) { 8541 return code.getSystem(); 8542 } 8543 } 8544 8545 public enum FHIRDefinedType { 8546 /** 8547 * An address expressed using postal conventions (as opposed to GPS or other 8548 * location definition formats). This data type may be used to convey addresses 8549 * for use in delivering mail as well as for visiting locations which might not 8550 * be valid for mail delivery. There are a variety of postal address formats 8551 * defined around the world. 8552 */ 8553 ADDRESS, 8554 /** 8555 * A duration of time during which an organism (or a process) has existed. 8556 */ 8557 AGE, 8558 /** 8559 * A text note which also contains information about who made the statement and 8560 * when. 8561 */ 8562 ANNOTATION, 8563 /** 8564 * For referring to data content defined in other formats. 8565 */ 8566 ATTACHMENT, 8567 /** 8568 * Base definition for all elements that are defined inside a resource - but not 8569 * those in a data type. 8570 */ 8571 BACKBONEELEMENT, 8572 /** 8573 * A concept that may be defined by a formal reference to a terminology or 8574 * ontology or may be provided by text. 8575 */ 8576 CODEABLECONCEPT, 8577 /** 8578 * A reference to a code defined by a terminology system. 8579 */ 8580 CODING, 8581 /** 8582 * Specifies contact information for a person or organization. 8583 */ 8584 CONTACTDETAIL, 8585 /** 8586 * Details for all kinds of technology mediated contact points for a person or 8587 * organization, including telephone, email, etc. 8588 */ 8589 CONTACTPOINT, 8590 /** 8591 * A contributor to the content of a knowledge asset, including authors, 8592 * editors, reviewers, and endorsers. 8593 */ 8594 CONTRIBUTOR, 8595 /** 8596 * A measured amount (or an amount that can potentially be measured). Note that 8597 * measured amounts include amounts that are not precisely quantified, including 8598 * amounts involving arbitrary units and floating currencies. 8599 */ 8600 COUNT, 8601 /** 8602 * Describes a required data item for evaluation in terms of the type of data, 8603 * and optional code or date-based filters of the data. 8604 */ 8605 DATAREQUIREMENT, 8606 /** 8607 * A length - a value with a unit that is a physical distance. 8608 */ 8609 DISTANCE, 8610 /** 8611 * Indicates how the medication is/was taken or should be taken by the patient. 8612 */ 8613 DOSAGE, 8614 /** 8615 * A length of time. 8616 */ 8617 DURATION, 8618 /** 8619 * Base definition for all elements in a resource. 8620 */ 8621 ELEMENT, 8622 /** 8623 * Captures constraints on each element within the resource, profile, or 8624 * extension. 8625 */ 8626 ELEMENTDEFINITION, 8627 /** 8628 * A expression that is evaluated in a specified context and returns a value. 8629 * The context of use of the expression must specify the context in which the 8630 * expression is evaluated, and how the result of the expression is used. 8631 */ 8632 EXPRESSION, 8633 /** 8634 * Optional Extension Element - found in all resources. 8635 */ 8636 EXTENSION, 8637 /** 8638 * A human's name with the ability to identify parts and usage. 8639 */ 8640 HUMANNAME, 8641 /** 8642 * An identifier - identifies some entity uniquely and unambiguously. Typically 8643 * this is used for business identifiers. 8644 */ 8645 IDENTIFIER, 8646 /** 8647 * The marketing status describes the date when a medicinal product is actually 8648 * put on the market or the date as of which it is no longer available. 8649 */ 8650 MARKETINGSTATUS, 8651 /** 8652 * The metadata about a resource. This is content in the resource that is 8653 * maintained by the infrastructure. Changes to the content might not always be 8654 * associated with version changes to the resource. 8655 */ 8656 META, 8657 /** 8658 * An amount of economic utility in some recognized currency. 8659 */ 8660 MONEY, 8661 /** 8662 * null 8663 */ 8664 MONEYQUANTITY, 8665 /** 8666 * A human-readable summary of the resource conveying the essential clinical and 8667 * business information for the resource. 8668 */ 8669 NARRATIVE, 8670 /** 8671 * The parameters to the module. This collection specifies both the input and 8672 * output parameters. Input parameters are provided by the caller as part of the 8673 * $evaluate operation. Output parameters are included in the GuidanceResponse. 8674 */ 8675 PARAMETERDEFINITION, 8676 /** 8677 * A time period defined by a start and end date and optionally time. 8678 */ 8679 PERIOD, 8680 /** 8681 * A populatioof people with some set of grouping criteria. 8682 */ 8683 POPULATION, 8684 /** 8685 * The marketing status describes the date when a medicinal product is actually 8686 * put on the market or the date as of which it is no longer available. 8687 */ 8688 PRODCHARACTERISTIC, 8689 /** 8690 * The shelf-life and storage information for a medicinal product item or 8691 * container can be described using this class. 8692 */ 8693 PRODUCTSHELFLIFE, 8694 /** 8695 * A measured amount (or an amount that can potentially be measured). Note that 8696 * measured amounts include amounts that are not precisely quantified, including 8697 * amounts involving arbitrary units and floating currencies. 8698 */ 8699 QUANTITY, 8700 /** 8701 * A set of ordered Quantities defined by a low and high limit. 8702 */ 8703 RANGE, 8704 /** 8705 * A relationship of two Quantity values - expressed as a numerator and a 8706 * denominator. 8707 */ 8708 RATIO, 8709 /** 8710 * A reference from one resource to another. 8711 */ 8712 REFERENCE, 8713 /** 8714 * Related artifacts such as additional documentation, justification, or 8715 * bibliographic references. 8716 */ 8717 RELATEDARTIFACT, 8718 /** 8719 * A series of measurements taken by a device, with upper and lower limits. 8720 * There may be more than one dimension in the data. 8721 */ 8722 SAMPLEDDATA, 8723 /** 8724 * A signature along with supporting context. The signature may be a digital 8725 * signature that is cryptographic in nature, or some other signature acceptable 8726 * to the domain. This other signature may be as simple as a graphical image 8727 * representing a hand-written signature, or a signature ceremony Different 8728 * signature approaches have different utilities. 8729 */ 8730 SIGNATURE, 8731 /** 8732 * null 8733 */ 8734 SIMPLEQUANTITY, 8735 /** 8736 * Chemical substances are a single substance type whose primary defining 8737 * element is the molecular structure. Chemical substances shall be defined on 8738 * the basis of their complete covalent molecular structure; the presence of a 8739 * salt (counter-ion) and/or solvates (water, alcohols) is also captured. 8740 * Purity, grade, physical form or particle size are not taken into account in 8741 * the definition of a chemical substance or in the assignment of a Substance 8742 * ID. 8743 */ 8744 SUBSTANCEAMOUNT, 8745 /** 8746 * Specifies an event that may occur multiple times. Timing schedules are used 8747 * to record when things are planned, expected or requested to occur. The most 8748 * common usage is in dosage instructions for medications. They are also used 8749 * when planning care of various kinds, and may be used for reporting the 8750 * schedule to which past regular activities were carried out. 8751 */ 8752 TIMING, 8753 /** 8754 * A description of a triggering event. Triggering events can be named events, 8755 * data events, or periodic, as determined by the type element. 8756 */ 8757 TRIGGERDEFINITION, 8758 /** 8759 * Specifies clinical/business/etc. metadata that can be used to retrieve, index 8760 * and/or categorize an artifact. This metadata can either be specific to the 8761 * applicable population (e.g., age category, DRG) or the specific context of 8762 * care (e.g., venue, care setting, provider of care). 8763 */ 8764 USAGECONTEXT, 8765 /** 8766 * A stream of bytes 8767 */ 8768 BASE64BINARY, 8769 /** 8770 * Value of "true" or "false" 8771 */ 8772 BOOLEAN, 8773 /** 8774 * A URI that is a reference to a canonical URL on a FHIR resource 8775 */ 8776 CANONICAL, 8777 /** 8778 * A string which has at least one character and no leading or trailing 8779 * whitespace and where there is no whitespace other than single spaces in the 8780 * contents 8781 */ 8782 CODE, 8783 /** 8784 * A date or partial date (e.g. just year or year + month). There is no time 8785 * zone. The format is a union of the schema types gYear, gYearMonth and date. 8786 * Dates SHALL be valid dates. 8787 */ 8788 DATE, 8789 /** 8790 * A date, date-time or partial date (e.g. just year or year + month). If hours 8791 * and minutes are specified, a time zone SHALL be populated. The format is a 8792 * union of the schema types gYear, gYearMonth, date and dateTime. Seconds must 8793 * be provided due to schema type constraints but may be zero-filled and may be 8794 * ignored. Dates SHALL be valid dates. 8795 */ 8796 DATETIME, 8797 /** 8798 * A rational number with implicit precision 8799 */ 8800 DECIMAL, 8801 /** 8802 * Any combination of letters, numerals, "-" and ".", with a length limit of 64 8803 * characters. (This might be an integer, an unprefixed OID, UUID or any other 8804 * identifier pattern that meets these constraints.) Ids are case-insensitive. 8805 */ 8806 ID, 8807 /** 8808 * An instant in time - known at least to the second 8809 */ 8810 INSTANT, 8811 /** 8812 * A whole number 8813 */ 8814 INTEGER, 8815 /** 8816 * A string that may contain Github Flavored Markdown syntax for optional 8817 * processing by a mark down presentation engine 8818 */ 8819 MARKDOWN, 8820 /** 8821 * An OID represented as a URI 8822 */ 8823 OID, 8824 /** 8825 * An integer with a value that is positive (e.g. >0) 8826 */ 8827 POSITIVEINT, 8828 /** 8829 * A sequence of Unicode characters 8830 */ 8831 STRING, 8832 /** 8833 * A time during the day, with no date specified 8834 */ 8835 TIME, 8836 /** 8837 * An integer with a value that is not negative (e.g. >= 0) 8838 */ 8839 UNSIGNEDINT, 8840 /** 8841 * String of characters used to identify a name or a resource 8842 */ 8843 URI, 8844 /** 8845 * A URI that is a literal reference 8846 */ 8847 URL, 8848 /** 8849 * A UUID, represented as a URI 8850 */ 8851 UUID, 8852 /** 8853 * XHTML format, as defined by W3C, but restricted usage (mainly, no active 8854 * content) 8855 */ 8856 XHTML, 8857 /** 8858 * A financial tool for tracking value accrued for a particular purpose. In the 8859 * healthcare field, used to track charges for a patient, cost centers, etc. 8860 */ 8861 ACCOUNT, 8862 /** 8863 * This resource allows for the definition of some activity to be performed, 8864 * independent of a particular patient, practitioner, or other performance 8865 * context. 8866 */ 8867 ACTIVITYDEFINITION, 8868 /** 8869 * Actual or potential/avoided event causing unintended physical injury 8870 * resulting from or contributed to by medical care, a research study or other 8871 * healthcare setting factors that requires additional monitoring, treatment, or 8872 * hospitalization, or that results in death. 8873 */ 8874 ADVERSEEVENT, 8875 /** 8876 * Risk of harmful or undesirable, physiological response which is unique to an 8877 * individual and associated with exposure to a substance. 8878 */ 8879 ALLERGYINTOLERANCE, 8880 /** 8881 * A booking of a healthcare event among patient(s), practitioner(s), related 8882 * person(s) and/or device(s) for a specific date/time. This may result in one 8883 * or more Encounter(s). 8884 */ 8885 APPOINTMENT, 8886 /** 8887 * A reply to an appointment request for a patient and/or practitioner(s), such 8888 * as a confirmation or rejection. 8889 */ 8890 APPOINTMENTRESPONSE, 8891 /** 8892 * A record of an event made for purposes of maintaining a security log. Typical 8893 * uses include detection of intrusion attempts and monitoring for inappropriate 8894 * usage. 8895 */ 8896 AUDITEVENT, 8897 /** 8898 * Basic is used for handling concepts not yet defined in FHIR, narrative-only 8899 * resources that don't map to an existing resource, and custom resources not 8900 * appropriate for inclusion in the FHIR specification. 8901 */ 8902 BASIC, 8903 /** 8904 * A resource that represents the data of a single raw artifact as digital 8905 * content accessible in its native format. A Binary resource can contain any 8906 * content, whether text, image, pdf, zip archive, etc. 8907 */ 8908 BINARY, 8909 /** 8910 * A material substance originating from a biological entity intended to be 8911 * transplanted or infused into another (possibly the same) biological entity. 8912 */ 8913 BIOLOGICALLYDERIVEDPRODUCT, 8914 /** 8915 * Record details about an anatomical structure. This resource may be used when 8916 * a coded concept does not provide the necessary detail needed for the use 8917 * case. 8918 */ 8919 BODYSTRUCTURE, 8920 /** 8921 * A container for a collection of resources. 8922 */ 8923 BUNDLE, 8924 /** 8925 * A Capability Statement documents a set of capabilities (behaviors) of a FHIR 8926 * Server for a particular version of FHIR that may be used as a statement of 8927 * actual server functionality or a statement of required or desired server 8928 * implementation. 8929 */ 8930 CAPABILITYSTATEMENT, 8931 /** 8932 * Describes the intention of how one or more practitioners intend to deliver 8933 * care for a particular patient, group or community for a period of time, 8934 * possibly limited to care for a specific condition or set of conditions. 8935 */ 8936 CAREPLAN, 8937 /** 8938 * The Care Team includes all the people and organizations who plan to 8939 * participate in the coordination and delivery of care for a patient. 8940 */ 8941 CARETEAM, 8942 /** 8943 * Catalog entries are wrappers that contextualize items included in a catalog. 8944 */ 8945 CATALOGENTRY, 8946 /** 8947 * The resource ChargeItem describes the provision of healthcare provider 8948 * products for a certain patient, therefore referring not only to the product, 8949 * but containing in addition details of the provision, like date, time, amounts 8950 * and participating organizations and persons. Main Usage of the ChargeItem is 8951 * to enable the billing process and internal cost allocation. 8952 */ 8953 CHARGEITEM, 8954 /** 8955 * The ChargeItemDefinition resource provides the properties that apply to the 8956 * (billing) codes necessary to calculate costs and prices. The properties may 8957 * differ largely depending on type and realm, therefore this resource gives 8958 * only a rough structure and requires profiling for each type of billing code 8959 * system. 8960 */ 8961 CHARGEITEMDEFINITION, 8962 /** 8963 * A provider issued list of professional services and products which have been 8964 * provided, or are to be provided, to a patient which is sent to an insurer for 8965 * reimbursement. 8966 */ 8967 CLAIM, 8968 /** 8969 * This resource provides the adjudication details from the processing of a 8970 * Claim resource. 8971 */ 8972 CLAIMRESPONSE, 8973 /** 8974 * A record of a clinical assessment performed to determine what problem(s) may 8975 * affect the patient and before planning the treatments or management 8976 * strategies that are best to manage a patient's condition. Assessments are 8977 * often 1:1 with a clinical consultation / encounter, but this varies greatly 8978 * depending on the clinical workflow. This resource is called 8979 * "ClinicalImpression" rather than "ClinicalAssessment" to avoid confusion with 8980 * the recording of assessment tools such as Apgar score. 8981 */ 8982 CLINICALIMPRESSION, 8983 /** 8984 * The CodeSystem resource is used to declare the existence of and describe a 8985 * code system or code system supplement and its key properties, and optionally 8986 * define a part or all of its content. 8987 */ 8988 CODESYSTEM, 8989 /** 8990 * An occurrence of information being transmitted; e.g. an alert that was sent 8991 * to a responsible provider, a public health agency that was notified about a 8992 * reportable condition. 8993 */ 8994 COMMUNICATION, 8995 /** 8996 * A request to convey information; e.g. the CDS system proposes that an alert 8997 * be sent to a responsible provider, the CDS system proposes that the public 8998 * health agency be notified about a reportable condition. 8999 */ 9000 COMMUNICATIONREQUEST, 9001 /** 9002 * A compartment definition that defines how resources are accessed on a server. 9003 */ 9004 COMPARTMENTDEFINITION, 9005 /** 9006 * A set of healthcare-related information that is assembled together into a 9007 * single logical package that provides a single coherent statement of meaning, 9008 * establishes its own context and that has clinical attestation with regard to 9009 * who is making the statement. A Composition defines the structure and 9010 * narrative content necessary for a document. However, a Composition alone does 9011 * not constitute a document. Rather, the Composition must be the first entry in 9012 * a Bundle where Bundle.type=document, and any other resources referenced from 9013 * Composition must be included as subsequent entries in the Bundle (for example 9014 * Patient, Practitioner, Encounter, etc.). 9015 */ 9016 COMPOSITION, 9017 /** 9018 * A statement of relationships from one set of concepts to one or more other 9019 * concepts - either concepts in code systems, or data element/data element 9020 * concepts, or classes in class models. 9021 */ 9022 CONCEPTMAP, 9023 /** 9024 * A clinical condition, problem, diagnosis, or other event, situation, issue, 9025 * or clinical concept that has risen to a level of concern. 9026 */ 9027 CONDITION, 9028 /** 9029 * A record of a healthcare consumer?s choices, which permits or denies 9030 * identified recipient(s) or recipient role(s) to perform one or more actions 9031 * within a given policy context, for specific purposes and periods of time. 9032 */ 9033 CONSENT, 9034 /** 9035 * Legally enforceable, formally recorded unilateral or bilateral directive 9036 * i.e., a policy or agreement. 9037 */ 9038 CONTRACT, 9039 /** 9040 * Financial instrument which may be used to reimburse or pay for health care 9041 * products and services. Includes both insurance and self-payment. 9042 */ 9043 COVERAGE, 9044 /** 9045 * The CoverageEligibilityRequest provides patient and insurance coverage 9046 * information to an insurer for them to respond, in the form of an 9047 * CoverageEligibilityResponse, with information regarding whether the stated 9048 * coverage is valid and in-force and optionally to provide the insurance 9049 * details of the policy. 9050 */ 9051 COVERAGEELIGIBILITYREQUEST, 9052 /** 9053 * This resource provides eligibility and plan details from the processing of an 9054 * CoverageEligibilityRequest resource. 9055 */ 9056 COVERAGEELIGIBILITYRESPONSE, 9057 /** 9058 * Indicates an actual or potential clinical issue with or between one or more 9059 * active or proposed clinical actions for a patient; e.g. Drug-drug 9060 * interaction, Ineffective treatment frequency, Procedure-condition conflict, 9061 * etc. 9062 */ 9063 DETECTEDISSUE, 9064 /** 9065 * A type of a manufactured item that is used in the provision of healthcare 9066 * without being substantially changed through that activity. The device may be 9067 * a medical or non-medical device. 9068 */ 9069 DEVICE, 9070 /** 9071 * The characteristics, operational status and capabilities of a medical-related 9072 * component of a medical device. 9073 */ 9074 DEVICEDEFINITION, 9075 /** 9076 * Describes a measurement, calculation or setting capability of a medical 9077 * device. 9078 */ 9079 DEVICEMETRIC, 9080 /** 9081 * Represents a request for a patient to employ a medical device. The device may 9082 * be an implantable device, or an external assistive device, such as a walker. 9083 */ 9084 DEVICEREQUEST, 9085 /** 9086 * A record of a device being used by a patient where the record is the result 9087 * of a report from the patient or another clinician. 9088 */ 9089 DEVICEUSESTATEMENT, 9090 /** 9091 * The findings and interpretation of diagnostic tests performed on patients, 9092 * groups of patients, devices, and locations, and/or specimens derived from 9093 * these. The report includes clinical context such as requesting and provider 9094 * information, and some mix of atomic results, images, textual and coded 9095 * interpretations, and formatted representation of diagnostic reports. 9096 */ 9097 DIAGNOSTICREPORT, 9098 /** 9099 * A collection of documents compiled for a purpose together with metadata that 9100 * applies to the collection. 9101 */ 9102 DOCUMENTMANIFEST, 9103 /** 9104 * A reference to a document of any kind for any purpose. Provides metadata 9105 * about the document so that the document can be discovered and managed. The 9106 * scope of a document is any seralized object with a mime-type, so includes 9107 * formal patient centric documents (CDA), cliical notes, scanned paper, and 9108 * non-patient specific documents like policy text. 9109 */ 9110 DOCUMENTREFERENCE, 9111 /** 9112 * A resource that includes narrative, extensions, and contained resources. 9113 */ 9114 DOMAINRESOURCE, 9115 /** 9116 * The EffectEvidenceSynthesis resource describes the difference in an outcome 9117 * between exposures states in a population where the effect estimate is derived 9118 * from a combination of research studies. 9119 */ 9120 EFFECTEVIDENCESYNTHESIS, 9121 /** 9122 * An interaction between a patient and healthcare provider(s) for the purpose 9123 * of providing healthcare service(s) or assessing the health status of a 9124 * patient. 9125 */ 9126 ENCOUNTER, 9127 /** 9128 * The technical details of an endpoint that can be used for electronic 9129 * services, such as for web services providing XDS.b or a REST endpoint for 9130 * another FHIR server. This may include any security context information. 9131 */ 9132 ENDPOINT, 9133 /** 9134 * This resource provides the insurance enrollment details to the insurer 9135 * regarding a specified coverage. 9136 */ 9137 ENROLLMENTREQUEST, 9138 /** 9139 * This resource provides enrollment and plan details from the processing of an 9140 * EnrollmentRequest resource. 9141 */ 9142 ENROLLMENTRESPONSE, 9143 /** 9144 * An association between a patient and an organization / healthcare provider(s) 9145 * during which time encounters may occur. The managing organization assumes a 9146 * level of responsibility for the patient during this time. 9147 */ 9148 EPISODEOFCARE, 9149 /** 9150 * The EventDefinition resource provides a reusable description of when a 9151 * particular event can occur. 9152 */ 9153 EVENTDEFINITION, 9154 /** 9155 * The Evidence resource describes the conditional state (population and any 9156 * exposures being compared within the population) and outcome (if specified) 9157 * that the knowledge (evidence, assertion, recommendation) is about. 9158 */ 9159 EVIDENCE, 9160 /** 9161 * The EvidenceVariable resource describes a "PICO" element that knowledge 9162 * (evidence, assertion, recommendation) is about. 9163 */ 9164 EVIDENCEVARIABLE, 9165 /** 9166 * Example of workflow instance. 9167 */ 9168 EXAMPLESCENARIO, 9169 /** 9170 * This resource provides: the claim details; adjudication details from the 9171 * processing of a Claim; and optionally account balance information, for 9172 * informing the subscriber of the benefits provided. 9173 */ 9174 EXPLANATIONOFBENEFIT, 9175 /** 9176 * Significant health conditions for a person related to the patient relevant in 9177 * the context of care for the patient. 9178 */ 9179 FAMILYMEMBERHISTORY, 9180 /** 9181 * Prospective warnings of potential issues when providing care to the patient. 9182 */ 9183 FLAG, 9184 /** 9185 * Describes the intended objective(s) for a patient, group or organization 9186 * care, for example, weight loss, restoring an activity of daily living, 9187 * obtaining herd immunity via immunization, meeting a process improvement 9188 * objective, etc. 9189 */ 9190 GOAL, 9191 /** 9192 * A formal computable definition of a graph of resources - that is, a coherent 9193 * set of resources that form a graph by following references. The Graph 9194 * Definition resource defines a set and makes rules about the set. 9195 */ 9196 GRAPHDEFINITION, 9197 /** 9198 * Represents a defined collection of entities that may be discussed or acted 9199 * upon collectively but which are not expected to act collectively, and are not 9200 * formally or legally recognized; i.e. a collection of entities that isn't an 9201 * Organization. 9202 */ 9203 GROUP, 9204 /** 9205 * A guidance response is the formal response to a guidance request, including 9206 * any output parameters returned by the evaluation, as well as the description 9207 * of any proposed actions to be taken. 9208 */ 9209 GUIDANCERESPONSE, 9210 /** 9211 * The details of a healthcare service available at a location. 9212 */ 9213 HEALTHCARESERVICE, 9214 /** 9215 * Representation of the content produced in a DICOM imaging study. A study 9216 * comprises a set of series, each of which includes a set of Service-Object 9217 * Pair Instances (SOP Instances - images or other data) acquired or produced in 9218 * a common context. A series is of only one modality (e.g. X-ray, CT, MR, 9219 * ultrasound), but a study may have multiple series of different modalities. 9220 */ 9221 IMAGINGSTUDY, 9222 /** 9223 * Describes the event of a patient being administered a vaccine or a record of 9224 * an immunization as reported by a patient, a clinician or another party. 9225 */ 9226 IMMUNIZATION, 9227 /** 9228 * Describes a comparison of an immunization event against published 9229 * recommendations to determine if the administration is "valid" in relation to 9230 * those recommendations. 9231 */ 9232 IMMUNIZATIONEVALUATION, 9233 /** 9234 * A patient's point-in-time set of recommendations (i.e. forecasting) according 9235 * to a published schedule with optional supporting justification. 9236 */ 9237 IMMUNIZATIONRECOMMENDATION, 9238 /** 9239 * A set of rules of how a particular interoperability or standards problem is 9240 * solved - typically through the use of FHIR resources. This resource is used 9241 * to gather all the parts of an implementation guide into a logical whole and 9242 * to publish a computable definition of all the parts. 9243 */ 9244 IMPLEMENTATIONGUIDE, 9245 /** 9246 * Details of a Health Insurance product/plan provided by an organization. 9247 */ 9248 INSURANCEPLAN, 9249 /** 9250 * Invoice containing collected ChargeItems from an Account with calculated 9251 * individual and total price for Billing purpose. 9252 */ 9253 INVOICE, 9254 /** 9255 * The Library resource is a general-purpose container for knowledge asset 9256 * definitions. It can be used to describe and expose existing knowledge assets 9257 * such as logic libraries and information model descriptions, as well as to 9258 * describe a collection of knowledge assets. 9259 */ 9260 LIBRARY, 9261 /** 9262 * Identifies two or more records (resource instances) that refer to the same 9263 * real-world "occurrence". 9264 */ 9265 LINKAGE, 9266 /** 9267 * A list is a curated collection of resources. 9268 */ 9269 LIST, 9270 /** 9271 * Details and position information for a physical place where services are 9272 * provided and resources and participants may be stored, found, contained, or 9273 * accommodated. 9274 */ 9275 LOCATION, 9276 /** 9277 * The Measure resource provides the definition of a quality measure. 9278 */ 9279 MEASURE, 9280 /** 9281 * The MeasureReport resource contains the results of the calculation of a 9282 * measure; and optionally a reference to the resources involved in that 9283 * calculation. 9284 */ 9285 MEASUREREPORT, 9286 /** 9287 * A photo, video, or audio recording acquired or used in healthcare. The actual 9288 * content may be inline or provided by direct reference. 9289 */ 9290 MEDIA, 9291 /** 9292 * This resource is primarily used for the identification and definition of a 9293 * medication for the purposes of prescribing, dispensing, and administering a 9294 * medication as well as for making statements about medication use. 9295 */ 9296 MEDICATION, 9297 /** 9298 * Describes the event of a patient consuming or otherwise being administered a 9299 * medication. This may be as simple as swallowing a tablet or it may be a long 9300 * running infusion. Related resources tie this event to the authorizing 9301 * prescription, and the specific encounter between patient and health care 9302 * practitioner. 9303 */ 9304 MEDICATIONADMINISTRATION, 9305 /** 9306 * Indicates that a medication product is to be or has been dispensed for a 9307 * named person/patient. This includes a description of the medication product 9308 * (supply) provided and the instructions for administering the medication. The 9309 * medication dispense is the result of a pharmacy system responding to a 9310 * medication order. 9311 */ 9312 MEDICATIONDISPENSE, 9313 /** 9314 * Information about a medication that is used to support knowledge. 9315 */ 9316 MEDICATIONKNOWLEDGE, 9317 /** 9318 * An order or request for both supply of the medication and the instructions 9319 * for administration of the medication to a patient. The resource is called 9320 * "MedicationRequest" rather than "MedicationPrescription" or "MedicationOrder" 9321 * to generalize the use across inpatient and outpatient settings, including 9322 * care plans, etc., and to harmonize with workflow patterns. 9323 */ 9324 MEDICATIONREQUEST, 9325 /** 9326 * A record of a medication that is being consumed by a patient. A 9327 * MedicationStatement may indicate that the patient may be taking the 9328 * medication now or has taken the medication in the past or will be taking the 9329 * medication in the future. The source of this information can be the patient, 9330 * significant other (such as a family member or spouse), or a clinician. A 9331 * common scenario where this information is captured is during the history 9332 * taking process during a patient visit or stay. The medication information may 9333 * come from sources such as the patient's memory, from a prescription bottle, 9334 * or from a list of medications the patient, clinician or other party 9335 * maintains. 9336 * 9337 * The primary difference between a medication statement and a medication 9338 * administration is that the medication administration has complete 9339 * administration information and is based on actual administration information 9340 * from the person who administered the medication. A medication statement is 9341 * often, if not always, less specific. There is no required date/time when the 9342 * medication was administered, in fact we only know that a source has reported 9343 * the patient is taking this medication, where details such as time, quantity, 9344 * or rate or even medication product may be incomplete or missing or less 9345 * precise. As stated earlier, the medication statement information may come 9346 * from the patient's memory, from a prescription bottle or from a list of 9347 * medications the patient, clinician or other party maintains. Medication 9348 * administration is more formal and is not missing detailed information. 9349 */ 9350 MEDICATIONSTATEMENT, 9351 /** 9352 * Detailed definition of a medicinal product, typically for uses other than 9353 * direct patient care (e.g. regulatory use). 9354 */ 9355 MEDICINALPRODUCT, 9356 /** 9357 * The regulatory authorization of a medicinal product. 9358 */ 9359 MEDICINALPRODUCTAUTHORIZATION, 9360 /** 9361 * The clinical particulars - indications, contraindications etc. of a medicinal 9362 * product, including for regulatory purposes. 9363 */ 9364 MEDICINALPRODUCTCONTRAINDICATION, 9365 /** 9366 * Indication for the Medicinal Product. 9367 */ 9368 MEDICINALPRODUCTINDICATION, 9369 /** 9370 * An ingredient of a manufactured item or pharmaceutical product. 9371 */ 9372 MEDICINALPRODUCTINGREDIENT, 9373 /** 9374 * The interactions of the medicinal product with other medicinal products, or 9375 * other forms of interactions. 9376 */ 9377 MEDICINALPRODUCTINTERACTION, 9378 /** 9379 * The manufactured item as contained in the packaged medicinal product. 9380 */ 9381 MEDICINALPRODUCTMANUFACTURED, 9382 /** 9383 * A medicinal product in a container or package. 9384 */ 9385 MEDICINALPRODUCTPACKAGED, 9386 /** 9387 * A pharmaceutical product described in terms of its composition and dose form. 9388 */ 9389 MEDICINALPRODUCTPHARMACEUTICAL, 9390 /** 9391 * Describe the undesirable effects of the medicinal product. 9392 */ 9393 MEDICINALPRODUCTUNDESIRABLEEFFECT, 9394 /** 9395 * Defines the characteristics of a message that can be shared between systems, 9396 * including the type of event that initiates the message, the content to be 9397 * transmitted and what response(s), if any, are permitted. 9398 */ 9399 MESSAGEDEFINITION, 9400 /** 9401 * The header for a message exchange that is either requesting or responding to 9402 * an action. The reference(s) that are the subject of the action as well as 9403 * other information related to the action are typically transmitted in a bundle 9404 * in which the MessageHeader resource instance is the first resource in the 9405 * bundle. 9406 */ 9407 MESSAGEHEADER, 9408 /** 9409 * Raw data describing a biological sequence. 9410 */ 9411 MOLECULARSEQUENCE, 9412 /** 9413 * A curated namespace that issues unique symbols within that namespace for the 9414 * identification of concepts, people, devices, etc. Represents a "System" used 9415 * within the Identifier and Coding data types. 9416 */ 9417 NAMINGSYSTEM, 9418 /** 9419 * A request to supply a diet, formula feeding (enteral) or oral nutritional 9420 * supplement to a patient/resident. 9421 */ 9422 NUTRITIONORDER, 9423 /** 9424 * Measurements and simple assertions made about a patient, device or other 9425 * subject. 9426 */ 9427 OBSERVATION, 9428 /** 9429 * Set of definitional characteristics for a kind of observation or measurement 9430 * produced or consumed by an orderable health care service. 9431 */ 9432 OBSERVATIONDEFINITION, 9433 /** 9434 * A formal computable definition of an operation (on the RESTful interface) or 9435 * a named query (using the search interaction). 9436 */ 9437 OPERATIONDEFINITION, 9438 /** 9439 * A collection of error, warning, or information messages that result from a 9440 * system action. 9441 */ 9442 OPERATIONOUTCOME, 9443 /** 9444 * A formally or informally recognized grouping of people or organizations 9445 * formed for the purpose of achieving some form of collective action. Includes 9446 * companies, institutions, corporations, departments, community groups, 9447 * healthcare practice groups, payer/insurer, etc. 9448 */ 9449 ORGANIZATION, 9450 /** 9451 * Defines an affiliation/assotiation/relationship between 2 distinct 9452 * oganizations, that is not a part-of relationship/sub-division relationship. 9453 */ 9454 ORGANIZATIONAFFILIATION, 9455 /** 9456 * This resource is a non-persisted resource used to pass information into and 9457 * back from an [operation](operations.html). It has no other use, and there is 9458 * no RESTful endpoint associated with it. 9459 */ 9460 PARAMETERS, 9461 /** 9462 * Demographics and other administrative information about an individual or 9463 * animal receiving care or other health-related services. 9464 */ 9465 PATIENT, 9466 /** 9467 * This resource provides the status of the payment for goods and services 9468 * rendered, and the request and response resource references. 9469 */ 9470 PAYMENTNOTICE, 9471 /** 9472 * This resource provides the details including amount of a payment and 9473 * allocates the payment items being paid. 9474 */ 9475 PAYMENTRECONCILIATION, 9476 /** 9477 * Demographics and administrative information about a person independent of a 9478 * specific health-related context. 9479 */ 9480 PERSON, 9481 /** 9482 * This resource allows for the definition of various types of plans as a 9483 * sharable, consumable, and executable artifact. The resource is general enough 9484 * to support the description of a broad range of clinical artifacts such as 9485 * clinical decision support rules, order sets and protocols. 9486 */ 9487 PLANDEFINITION, 9488 /** 9489 * A person who is directly or indirectly involved in the provisioning of 9490 * healthcare. 9491 */ 9492 PRACTITIONER, 9493 /** 9494 * A specific set of Roles/Locations/specialties/services that a practitioner 9495 * may perform at an organization for a period of time. 9496 */ 9497 PRACTITIONERROLE, 9498 /** 9499 * An action that is or was performed on or for a patient. This can be a 9500 * physical intervention like an operation, or less invasive like long term 9501 * services, counseling, or hypnotherapy. 9502 */ 9503 PROCEDURE, 9504 /** 9505 * Provenance of a resource is a record that describes entities and processes 9506 * involved in producing and delivering or otherwise influencing that resource. 9507 * Provenance provides a critical foundation for assessing authenticity, 9508 * enabling trust, and allowing reproducibility. Provenance assertions are a 9509 * form of contextual metadata and can themselves become important records with 9510 * their own provenance. Provenance statement indicates clinical significance in 9511 * terms of confidence in authenticity, reliability, and trustworthiness, 9512 * integrity, and stage in lifecycle (e.g. Document Completion - has the 9513 * artifact been legally authenticated), all of which may impact security, 9514 * privacy, and trust policies. 9515 */ 9516 PROVENANCE, 9517 /** 9518 * A structured set of questions intended to guide the collection of answers 9519 * from end-users. Questionnaires provide detailed control over order, 9520 * presentation, phraseology and grouping to allow coherent, consistent data 9521 * collection. 9522 */ 9523 QUESTIONNAIRE, 9524 /** 9525 * A structured set of questions and their answers. The questions are ordered 9526 * and grouped into coherent subsets, corresponding to the structure of the 9527 * grouping of the questionnaire being responded to. 9528 */ 9529 QUESTIONNAIRERESPONSE, 9530 /** 9531 * Information about a person that is involved in the care for a patient, but 9532 * who is not the target of healthcare, nor has a formal responsibility in the 9533 * care process. 9534 */ 9535 RELATEDPERSON, 9536 /** 9537 * A group of related requests that can be used to capture intended activities 9538 * that have inter-dependencies such as "give this medication after that one". 9539 */ 9540 REQUESTGROUP, 9541 /** 9542 * The ResearchDefinition resource describes the conditional state (population 9543 * and any exposures being compared within the population) and outcome (if 9544 * specified) that the knowledge (evidence, assertion, recommendation) is about. 9545 */ 9546 RESEARCHDEFINITION, 9547 /** 9548 * The ResearchElementDefinition resource describes a "PICO" element that 9549 * knowledge (evidence, assertion, recommendation) is about. 9550 */ 9551 RESEARCHELEMENTDEFINITION, 9552 /** 9553 * A process where a researcher or organization plans and then executes a series 9554 * of steps intended to increase the field of healthcare-related knowledge. This 9555 * includes studies of safety, efficacy, comparative effectiveness and other 9556 * information about medications, devices, therapies and other interventional 9557 * and investigative techniques. A ResearchStudy involves the gathering of 9558 * information about human or animal subjects. 9559 */ 9560 RESEARCHSTUDY, 9561 /** 9562 * A physical entity which is the primary unit of operational and/or 9563 * administrative interest in a study. 9564 */ 9565 RESEARCHSUBJECT, 9566 /** 9567 * This is the base resource type for everything. 9568 */ 9569 RESOURCE, 9570 /** 9571 * An assessment of the likely outcome(s) for a patient or other subject as well 9572 * as the likelihood of each outcome. 9573 */ 9574 RISKASSESSMENT, 9575 /** 9576 * The RiskEvidenceSynthesis resource describes the likelihood of an outcome in 9577 * a population plus exposure state where the risk estimate is derived from a 9578 * combination of research studies. 9579 */ 9580 RISKEVIDENCESYNTHESIS, 9581 /** 9582 * A container for slots of time that may be available for booking appointments. 9583 */ 9584 SCHEDULE, 9585 /** 9586 * A search parameter that defines a named search item that can be used to 9587 * search/filter on a resource. 9588 */ 9589 SEARCHPARAMETER, 9590 /** 9591 * A record of a request for service such as diagnostic investigations, 9592 * treatments, or operations to be performed. 9593 */ 9594 SERVICEREQUEST, 9595 /** 9596 * A slot of time on a schedule that may be available for booking appointments. 9597 */ 9598 SLOT, 9599 /** 9600 * A sample to be used for analysis. 9601 */ 9602 SPECIMEN, 9603 /** 9604 * A kind of specimen with associated set of requirements. 9605 */ 9606 SPECIMENDEFINITION, 9607 /** 9608 * A definition of a FHIR structure. This resource is used to describe the 9609 * underlying resources, data types defined in FHIR, and also for describing 9610 * extensions and constraints on resources and data types. 9611 */ 9612 STRUCTUREDEFINITION, 9613 /** 9614 * A Map of relationships between 2 structures that can be used to transform 9615 * data. 9616 */ 9617 STRUCTUREMAP, 9618 /** 9619 * The subscription resource is used to define a push-based subscription from a 9620 * server to another system. Once a subscription is registered with the server, 9621 * the server checks every resource that is created or updated, and if the 9622 * resource matches the given criteria, it sends a message on the defined 9623 * "channel" so that another system can take an appropriate action. 9624 */ 9625 SUBSCRIPTION, 9626 /** 9627 * A homogeneous material with a definite composition. 9628 */ 9629 SUBSTANCE, 9630 /** 9631 * Nucleic acids are defined by three distinct elements: the base, sugar and 9632 * linkage. Individual substance/moiety IDs will be created for each of these 9633 * elements. The nucleotide sequence will be always entered in the 5?-3? 9634 * direction. 9635 */ 9636 SUBSTANCENUCLEICACID, 9637 /** 9638 * Todo. 9639 */ 9640 SUBSTANCEPOLYMER, 9641 /** 9642 * A SubstanceProtein is defined as a single unit of a linear amino acid 9643 * sequence, or a combination of subunits that are either covalently linked or 9644 * have a defined invariant stoichiometric relationship. This includes all 9645 * synthetic, recombinant and purified SubstanceProteins of defined sequence, 9646 * whether the use is therapeutic or prophylactic. This set of elements will be 9647 * used to describe albumins, coagulation factors, cytokines, growth factors, 9648 * peptide/SubstanceProtein hormones, enzymes, toxins, toxoids, recombinant 9649 * vaccines, and immunomodulators. 9650 */ 9651 SUBSTANCEPROTEIN, 9652 /** 9653 * Todo. 9654 */ 9655 SUBSTANCEREFERENCEINFORMATION, 9656 /** 9657 * Source material shall capture information on the taxonomic and anatomical 9658 * origins as well as the fraction of a material that can result in or can be 9659 * modified to form a substance. This set of data elements shall be used to 9660 * define polymer substances isolated from biological matrices. Taxonomic and 9661 * anatomical origins shall be described using a controlled vocabulary as 9662 * required. This information is captured for naturally derived polymers ( . 9663 * starch) and structurally diverse substances. For Organisms belonging to the 9664 * Kingdom Plantae the Substance level defines the fresh material of a single 9665 * species or infraspecies, the Herbal Drug and the Herbal preparation. For 9666 * Herbal preparations, the fraction information will be captured at the 9667 * Substance information level and additional information for herbal extracts 9668 * will be captured at the Specified Substance Group 1 information level. See 9669 * for further explanation the Substance Class: Structurally Diverse and the 9670 * herbal annex. 9671 */ 9672 SUBSTANCESOURCEMATERIAL, 9673 /** 9674 * The detailed description of a substance, typically at a level beyond what is 9675 * used for prescribing. 9676 */ 9677 SUBSTANCESPECIFICATION, 9678 /** 9679 * Record of delivery of what is supplied. 9680 */ 9681 SUPPLYDELIVERY, 9682 /** 9683 * A record of a request for a medication, substance or device used in the 9684 * healthcare setting. 9685 */ 9686 SUPPLYREQUEST, 9687 /** 9688 * A task to be performed. 9689 */ 9690 TASK, 9691 /** 9692 * A Terminology Capabilities documents a set of capabilities (behaviors) of a 9693 * FHIR Server that may be used as a statement of actual server functionality or 9694 * a statement of required or desired server implementation. 9695 */ 9696 TERMINOLOGYCAPABILITIES, 9697 /** 9698 * A summary of information based on the results of executing a TestScript. 9699 */ 9700 TESTREPORT, 9701 /** 9702 * A structured set of tests against a FHIR server or client implementation to 9703 * determine compliance against the FHIR specification. 9704 */ 9705 TESTSCRIPT, 9706 /** 9707 * A ValueSet resource instance specifies a set of codes drawn from one or more 9708 * code systems, intended for use in a particular context. Value sets link 9709 * between [[[CodeSystem]]] definitions and their use in [coded 9710 * elements](terminologies.html). 9711 */ 9712 VALUESET, 9713 /** 9714 * Describes validation requirements, source(s), status and dates for one or 9715 * more elements. 9716 */ 9717 VERIFICATIONRESULT, 9718 /** 9719 * An authorization for the provision of glasses and/or contact lenses to a 9720 * patient. 9721 */ 9722 VISIONPRESCRIPTION, 9723 /** 9724 * added to help the parsers 9725 */ 9726 NULL; 9727 9728 public static FHIRDefinedType fromCode(String codeString) throws FHIRException { 9729 if (codeString == null || "".equals(codeString)) 9730 return null; 9731 if ("Address".equals(codeString)) 9732 return ADDRESS; 9733 if ("Age".equals(codeString)) 9734 return AGE; 9735 if ("Annotation".equals(codeString)) 9736 return ANNOTATION; 9737 if ("Attachment".equals(codeString)) 9738 return ATTACHMENT; 9739 if ("BackboneElement".equals(codeString)) 9740 return BACKBONEELEMENT; 9741 if ("CodeableConcept".equals(codeString)) 9742 return CODEABLECONCEPT; 9743 if ("Coding".equals(codeString)) 9744 return CODING; 9745 if ("ContactDetail".equals(codeString)) 9746 return CONTACTDETAIL; 9747 if ("ContactPoint".equals(codeString)) 9748 return CONTACTPOINT; 9749 if ("Contributor".equals(codeString)) 9750 return CONTRIBUTOR; 9751 if ("Count".equals(codeString)) 9752 return COUNT; 9753 if ("DataRequirement".equals(codeString)) 9754 return DATAREQUIREMENT; 9755 if ("Distance".equals(codeString)) 9756 return DISTANCE; 9757 if ("Dosage".equals(codeString)) 9758 return DOSAGE; 9759 if ("Duration".equals(codeString)) 9760 return DURATION; 9761 if ("Element".equals(codeString)) 9762 return ELEMENT; 9763 if ("ElementDefinition".equals(codeString)) 9764 return ELEMENTDEFINITION; 9765 if ("Expression".equals(codeString)) 9766 return EXPRESSION; 9767 if ("Extension".equals(codeString)) 9768 return EXTENSION; 9769 if ("HumanName".equals(codeString)) 9770 return HUMANNAME; 9771 if ("Identifier".equals(codeString)) 9772 return IDENTIFIER; 9773 if ("MarketingStatus".equals(codeString)) 9774 return MARKETINGSTATUS; 9775 if ("Meta".equals(codeString)) 9776 return META; 9777 if ("Money".equals(codeString)) 9778 return MONEY; 9779 if ("MoneyQuantity".equals(codeString)) 9780 return MONEYQUANTITY; 9781 if ("Narrative".equals(codeString)) 9782 return NARRATIVE; 9783 if ("ParameterDefinition".equals(codeString)) 9784 return PARAMETERDEFINITION; 9785 if ("Period".equals(codeString)) 9786 return PERIOD; 9787 if ("Population".equals(codeString)) 9788 return POPULATION; 9789 if ("ProdCharacteristic".equals(codeString)) 9790 return PRODCHARACTERISTIC; 9791 if ("ProductShelfLife".equals(codeString)) 9792 return PRODUCTSHELFLIFE; 9793 if ("Quantity".equals(codeString)) 9794 return QUANTITY; 9795 if ("Range".equals(codeString)) 9796 return RANGE; 9797 if ("Ratio".equals(codeString)) 9798 return RATIO; 9799 if ("Reference".equals(codeString)) 9800 return REFERENCE; 9801 if ("RelatedArtifact".equals(codeString)) 9802 return RELATEDARTIFACT; 9803 if ("SampledData".equals(codeString)) 9804 return SAMPLEDDATA; 9805 if ("Signature".equals(codeString)) 9806 return SIGNATURE; 9807 if ("SimpleQuantity".equals(codeString)) 9808 return SIMPLEQUANTITY; 9809 if ("SubstanceAmount".equals(codeString)) 9810 return SUBSTANCEAMOUNT; 9811 if ("Timing".equals(codeString)) 9812 return TIMING; 9813 if ("TriggerDefinition".equals(codeString)) 9814 return TRIGGERDEFINITION; 9815 if ("UsageContext".equals(codeString)) 9816 return USAGECONTEXT; 9817 if ("base64Binary".equals(codeString)) 9818 return BASE64BINARY; 9819 if ("boolean".equals(codeString)) 9820 return BOOLEAN; 9821 if ("canonical".equals(codeString)) 9822 return CANONICAL; 9823 if ("code".equals(codeString)) 9824 return CODE; 9825 if ("date".equals(codeString)) 9826 return DATE; 9827 if ("dateTime".equals(codeString)) 9828 return DATETIME; 9829 if ("decimal".equals(codeString)) 9830 return DECIMAL; 9831 if ("id".equals(codeString)) 9832 return ID; 9833 if ("instant".equals(codeString)) 9834 return INSTANT; 9835 if ("integer".equals(codeString)) 9836 return INTEGER; 9837 if ("markdown".equals(codeString)) 9838 return MARKDOWN; 9839 if ("oid".equals(codeString)) 9840 return OID; 9841 if ("positiveInt".equals(codeString)) 9842 return POSITIVEINT; 9843 if ("string".equals(codeString)) 9844 return STRING; 9845 if ("time".equals(codeString)) 9846 return TIME; 9847 if ("unsignedInt".equals(codeString)) 9848 return UNSIGNEDINT; 9849 if ("uri".equals(codeString)) 9850 return URI; 9851 if ("url".equals(codeString)) 9852 return URL; 9853 if ("uuid".equals(codeString)) 9854 return UUID; 9855 if ("xhtml".equals(codeString)) 9856 return XHTML; 9857 if ("Account".equals(codeString)) 9858 return ACCOUNT; 9859 if ("ActivityDefinition".equals(codeString)) 9860 return ACTIVITYDEFINITION; 9861 if ("AdverseEvent".equals(codeString)) 9862 return ADVERSEEVENT; 9863 if ("AllergyIntolerance".equals(codeString)) 9864 return ALLERGYINTOLERANCE; 9865 if ("Appointment".equals(codeString)) 9866 return APPOINTMENT; 9867 if ("AppointmentResponse".equals(codeString)) 9868 return APPOINTMENTRESPONSE; 9869 if ("AuditEvent".equals(codeString)) 9870 return AUDITEVENT; 9871 if ("Basic".equals(codeString)) 9872 return BASIC; 9873 if ("Binary".equals(codeString)) 9874 return BINARY; 9875 if ("BiologicallyDerivedProduct".equals(codeString)) 9876 return BIOLOGICALLYDERIVEDPRODUCT; 9877 if ("BodyStructure".equals(codeString)) 9878 return BODYSTRUCTURE; 9879 if ("Bundle".equals(codeString)) 9880 return BUNDLE; 9881 if ("CapabilityStatement".equals(codeString)) 9882 return CAPABILITYSTATEMENT; 9883 if ("CarePlan".equals(codeString)) 9884 return CAREPLAN; 9885 if ("CareTeam".equals(codeString)) 9886 return CARETEAM; 9887 if ("CatalogEntry".equals(codeString)) 9888 return CATALOGENTRY; 9889 if ("ChargeItem".equals(codeString)) 9890 return CHARGEITEM; 9891 if ("ChargeItemDefinition".equals(codeString)) 9892 return CHARGEITEMDEFINITION; 9893 if ("Claim".equals(codeString)) 9894 return CLAIM; 9895 if ("ClaimResponse".equals(codeString)) 9896 return CLAIMRESPONSE; 9897 if ("ClinicalImpression".equals(codeString)) 9898 return CLINICALIMPRESSION; 9899 if ("CodeSystem".equals(codeString)) 9900 return CODESYSTEM; 9901 if ("Communication".equals(codeString)) 9902 return COMMUNICATION; 9903 if ("CommunicationRequest".equals(codeString)) 9904 return COMMUNICATIONREQUEST; 9905 if ("CompartmentDefinition".equals(codeString)) 9906 return COMPARTMENTDEFINITION; 9907 if ("Composition".equals(codeString)) 9908 return COMPOSITION; 9909 if ("ConceptMap".equals(codeString)) 9910 return CONCEPTMAP; 9911 if ("Condition".equals(codeString)) 9912 return CONDITION; 9913 if ("Consent".equals(codeString)) 9914 return CONSENT; 9915 if ("Contract".equals(codeString)) 9916 return CONTRACT; 9917 if ("Coverage".equals(codeString)) 9918 return COVERAGE; 9919 if ("CoverageEligibilityRequest".equals(codeString)) 9920 return COVERAGEELIGIBILITYREQUEST; 9921 if ("CoverageEligibilityResponse".equals(codeString)) 9922 return COVERAGEELIGIBILITYRESPONSE; 9923 if ("DetectedIssue".equals(codeString)) 9924 return DETECTEDISSUE; 9925 if ("Device".equals(codeString)) 9926 return DEVICE; 9927 if ("DeviceDefinition".equals(codeString)) 9928 return DEVICEDEFINITION; 9929 if ("DeviceMetric".equals(codeString)) 9930 return DEVICEMETRIC; 9931 if ("DeviceRequest".equals(codeString)) 9932 return DEVICEREQUEST; 9933 if ("DeviceUseStatement".equals(codeString)) 9934 return DEVICEUSESTATEMENT; 9935 if ("DiagnosticReport".equals(codeString)) 9936 return DIAGNOSTICREPORT; 9937 if ("DocumentManifest".equals(codeString)) 9938 return DOCUMENTMANIFEST; 9939 if ("DocumentReference".equals(codeString)) 9940 return DOCUMENTREFERENCE; 9941 if ("DomainResource".equals(codeString)) 9942 return DOMAINRESOURCE; 9943 if ("EffectEvidenceSynthesis".equals(codeString)) 9944 return EFFECTEVIDENCESYNTHESIS; 9945 if ("Encounter".equals(codeString)) 9946 return ENCOUNTER; 9947 if ("Endpoint".equals(codeString)) 9948 return ENDPOINT; 9949 if ("EnrollmentRequest".equals(codeString)) 9950 return ENROLLMENTREQUEST; 9951 if ("EnrollmentResponse".equals(codeString)) 9952 return ENROLLMENTRESPONSE; 9953 if ("EpisodeOfCare".equals(codeString)) 9954 return EPISODEOFCARE; 9955 if ("EventDefinition".equals(codeString)) 9956 return EVENTDEFINITION; 9957 if ("Evidence".equals(codeString)) 9958 return EVIDENCE; 9959 if ("EvidenceVariable".equals(codeString)) 9960 return EVIDENCEVARIABLE; 9961 if ("ExampleScenario".equals(codeString)) 9962 return EXAMPLESCENARIO; 9963 if ("ExplanationOfBenefit".equals(codeString)) 9964 return EXPLANATIONOFBENEFIT; 9965 if ("FamilyMemberHistory".equals(codeString)) 9966 return FAMILYMEMBERHISTORY; 9967 if ("Flag".equals(codeString)) 9968 return FLAG; 9969 if ("Goal".equals(codeString)) 9970 return GOAL; 9971 if ("GraphDefinition".equals(codeString)) 9972 return GRAPHDEFINITION; 9973 if ("Group".equals(codeString)) 9974 return GROUP; 9975 if ("GuidanceResponse".equals(codeString)) 9976 return GUIDANCERESPONSE; 9977 if ("HealthcareService".equals(codeString)) 9978 return HEALTHCARESERVICE; 9979 if ("ImagingStudy".equals(codeString)) 9980 return IMAGINGSTUDY; 9981 if ("Immunization".equals(codeString)) 9982 return IMMUNIZATION; 9983 if ("ImmunizationEvaluation".equals(codeString)) 9984 return IMMUNIZATIONEVALUATION; 9985 if ("ImmunizationRecommendation".equals(codeString)) 9986 return IMMUNIZATIONRECOMMENDATION; 9987 if ("ImplementationGuide".equals(codeString)) 9988 return IMPLEMENTATIONGUIDE; 9989 if ("InsurancePlan".equals(codeString)) 9990 return INSURANCEPLAN; 9991 if ("Invoice".equals(codeString)) 9992 return INVOICE; 9993 if ("Library".equals(codeString)) 9994 return LIBRARY; 9995 if ("Linkage".equals(codeString)) 9996 return LINKAGE; 9997 if ("List".equals(codeString)) 9998 return LIST; 9999 if ("Location".equals(codeString)) 10000 return LOCATION; 10001 if ("Measure".equals(codeString)) 10002 return MEASURE; 10003 if ("MeasureReport".equals(codeString)) 10004 return MEASUREREPORT; 10005 if ("Media".equals(codeString)) 10006 return MEDIA; 10007 if ("Medication".equals(codeString)) 10008 return MEDICATION; 10009 if ("MedicationAdministration".equals(codeString)) 10010 return MEDICATIONADMINISTRATION; 10011 if ("MedicationDispense".equals(codeString)) 10012 return MEDICATIONDISPENSE; 10013 if ("MedicationKnowledge".equals(codeString)) 10014 return MEDICATIONKNOWLEDGE; 10015 if ("MedicationRequest".equals(codeString)) 10016 return MEDICATIONREQUEST; 10017 if ("MedicationStatement".equals(codeString)) 10018 return MEDICATIONSTATEMENT; 10019 if ("MedicinalProduct".equals(codeString)) 10020 return MEDICINALPRODUCT; 10021 if ("MedicinalProductAuthorization".equals(codeString)) 10022 return MEDICINALPRODUCTAUTHORIZATION; 10023 if ("MedicinalProductContraindication".equals(codeString)) 10024 return MEDICINALPRODUCTCONTRAINDICATION; 10025 if ("MedicinalProductIndication".equals(codeString)) 10026 return MEDICINALPRODUCTINDICATION; 10027 if ("MedicinalProductIngredient".equals(codeString)) 10028 return MEDICINALPRODUCTINGREDIENT; 10029 if ("MedicinalProductInteraction".equals(codeString)) 10030 return MEDICINALPRODUCTINTERACTION; 10031 if ("MedicinalProductManufactured".equals(codeString)) 10032 return MEDICINALPRODUCTMANUFACTURED; 10033 if ("MedicinalProductPackaged".equals(codeString)) 10034 return MEDICINALPRODUCTPACKAGED; 10035 if ("MedicinalProductPharmaceutical".equals(codeString)) 10036 return MEDICINALPRODUCTPHARMACEUTICAL; 10037 if ("MedicinalProductUndesirableEffect".equals(codeString)) 10038 return MEDICINALPRODUCTUNDESIRABLEEFFECT; 10039 if ("MessageDefinition".equals(codeString)) 10040 return MESSAGEDEFINITION; 10041 if ("MessageHeader".equals(codeString)) 10042 return MESSAGEHEADER; 10043 if ("MolecularSequence".equals(codeString)) 10044 return MOLECULARSEQUENCE; 10045 if ("NamingSystem".equals(codeString)) 10046 return NAMINGSYSTEM; 10047 if ("NutritionOrder".equals(codeString)) 10048 return NUTRITIONORDER; 10049 if ("Observation".equals(codeString)) 10050 return OBSERVATION; 10051 if ("ObservationDefinition".equals(codeString)) 10052 return OBSERVATIONDEFINITION; 10053 if ("OperationDefinition".equals(codeString)) 10054 return OPERATIONDEFINITION; 10055 if ("OperationOutcome".equals(codeString)) 10056 return OPERATIONOUTCOME; 10057 if ("Organization".equals(codeString)) 10058 return ORGANIZATION; 10059 if ("OrganizationAffiliation".equals(codeString)) 10060 return ORGANIZATIONAFFILIATION; 10061 if ("Parameters".equals(codeString)) 10062 return PARAMETERS; 10063 if ("Patient".equals(codeString)) 10064 return PATIENT; 10065 if ("PaymentNotice".equals(codeString)) 10066 return PAYMENTNOTICE; 10067 if ("PaymentReconciliation".equals(codeString)) 10068 return PAYMENTRECONCILIATION; 10069 if ("Person".equals(codeString)) 10070 return PERSON; 10071 if ("PlanDefinition".equals(codeString)) 10072 return PLANDEFINITION; 10073 if ("Practitioner".equals(codeString)) 10074 return PRACTITIONER; 10075 if ("PractitionerRole".equals(codeString)) 10076 return PRACTITIONERROLE; 10077 if ("Procedure".equals(codeString)) 10078 return PROCEDURE; 10079 if ("Provenance".equals(codeString)) 10080 return PROVENANCE; 10081 if ("Questionnaire".equals(codeString)) 10082 return QUESTIONNAIRE; 10083 if ("QuestionnaireResponse".equals(codeString)) 10084 return QUESTIONNAIRERESPONSE; 10085 if ("RelatedPerson".equals(codeString)) 10086 return RELATEDPERSON; 10087 if ("RequestGroup".equals(codeString)) 10088 return REQUESTGROUP; 10089 if ("ResearchDefinition".equals(codeString)) 10090 return RESEARCHDEFINITION; 10091 if ("ResearchElementDefinition".equals(codeString)) 10092 return RESEARCHELEMENTDEFINITION; 10093 if ("ResearchStudy".equals(codeString)) 10094 return RESEARCHSTUDY; 10095 if ("ResearchSubject".equals(codeString)) 10096 return RESEARCHSUBJECT; 10097 if ("Resource".equals(codeString)) 10098 return RESOURCE; 10099 if ("RiskAssessment".equals(codeString)) 10100 return RISKASSESSMENT; 10101 if ("RiskEvidenceSynthesis".equals(codeString)) 10102 return RISKEVIDENCESYNTHESIS; 10103 if ("Schedule".equals(codeString)) 10104 return SCHEDULE; 10105 if ("SearchParameter".equals(codeString)) 10106 return SEARCHPARAMETER; 10107 if ("ServiceRequest".equals(codeString)) 10108 return SERVICEREQUEST; 10109 if ("Slot".equals(codeString)) 10110 return SLOT; 10111 if ("Specimen".equals(codeString)) 10112 return SPECIMEN; 10113 if ("SpecimenDefinition".equals(codeString)) 10114 return SPECIMENDEFINITION; 10115 if ("StructureDefinition".equals(codeString)) 10116 return STRUCTUREDEFINITION; 10117 if ("StructureMap".equals(codeString)) 10118 return STRUCTUREMAP; 10119 if ("Subscription".equals(codeString)) 10120 return SUBSCRIPTION; 10121 if ("Substance".equals(codeString)) 10122 return SUBSTANCE; 10123 if ("SubstanceNucleicAcid".equals(codeString)) 10124 return SUBSTANCENUCLEICACID; 10125 if ("SubstancePolymer".equals(codeString)) 10126 return SUBSTANCEPOLYMER; 10127 if ("SubstanceProtein".equals(codeString)) 10128 return SUBSTANCEPROTEIN; 10129 if ("SubstanceReferenceInformation".equals(codeString)) 10130 return SUBSTANCEREFERENCEINFORMATION; 10131 if ("SubstanceSourceMaterial".equals(codeString)) 10132 return SUBSTANCESOURCEMATERIAL; 10133 if ("SubstanceSpecification".equals(codeString)) 10134 return SUBSTANCESPECIFICATION; 10135 if ("SupplyDelivery".equals(codeString)) 10136 return SUPPLYDELIVERY; 10137 if ("SupplyRequest".equals(codeString)) 10138 return SUPPLYREQUEST; 10139 if ("Task".equals(codeString)) 10140 return TASK; 10141 if ("TerminologyCapabilities".equals(codeString)) 10142 return TERMINOLOGYCAPABILITIES; 10143 if ("TestReport".equals(codeString)) 10144 return TESTREPORT; 10145 if ("TestScript".equals(codeString)) 10146 return TESTSCRIPT; 10147 if ("ValueSet".equals(codeString)) 10148 return VALUESET; 10149 if ("VerificationResult".equals(codeString)) 10150 return VERIFICATIONRESULT; 10151 if ("VisionPrescription".equals(codeString)) 10152 return VISIONPRESCRIPTION; 10153 throw new FHIRException("Unknown FHIRDefinedType code '" + codeString + "'"); 10154 } 10155 10156 public String toCode() { 10157 switch (this) { 10158 case ADDRESS: 10159 return "Address"; 10160 case AGE: 10161 return "Age"; 10162 case ANNOTATION: 10163 return "Annotation"; 10164 case ATTACHMENT: 10165 return "Attachment"; 10166 case BACKBONEELEMENT: 10167 return "BackboneElement"; 10168 case CODEABLECONCEPT: 10169 return "CodeableConcept"; 10170 case CODING: 10171 return "Coding"; 10172 case CONTACTDETAIL: 10173 return "ContactDetail"; 10174 case CONTACTPOINT: 10175 return "ContactPoint"; 10176 case CONTRIBUTOR: 10177 return "Contributor"; 10178 case COUNT: 10179 return "Count"; 10180 case DATAREQUIREMENT: 10181 return "DataRequirement"; 10182 case DISTANCE: 10183 return "Distance"; 10184 case DOSAGE: 10185 return "Dosage"; 10186 case DURATION: 10187 return "Duration"; 10188 case ELEMENT: 10189 return "Element"; 10190 case ELEMENTDEFINITION: 10191 return "ElementDefinition"; 10192 case EXPRESSION: 10193 return "Expression"; 10194 case EXTENSION: 10195 return "Extension"; 10196 case HUMANNAME: 10197 return "HumanName"; 10198 case IDENTIFIER: 10199 return "Identifier"; 10200 case MARKETINGSTATUS: 10201 return "MarketingStatus"; 10202 case META: 10203 return "Meta"; 10204 case MONEY: 10205 return "Money"; 10206 case MONEYQUANTITY: 10207 return "MoneyQuantity"; 10208 case NARRATIVE: 10209 return "Narrative"; 10210 case PARAMETERDEFINITION: 10211 return "ParameterDefinition"; 10212 case PERIOD: 10213 return "Period"; 10214 case POPULATION: 10215 return "Population"; 10216 case PRODCHARACTERISTIC: 10217 return "ProdCharacteristic"; 10218 case PRODUCTSHELFLIFE: 10219 return "ProductShelfLife"; 10220 case QUANTITY: 10221 return "Quantity"; 10222 case RANGE: 10223 return "Range"; 10224 case RATIO: 10225 return "Ratio"; 10226 case REFERENCE: 10227 return "Reference"; 10228 case RELATEDARTIFACT: 10229 return "RelatedArtifact"; 10230 case SAMPLEDDATA: 10231 return "SampledData"; 10232 case SIGNATURE: 10233 return "Signature"; 10234 case SIMPLEQUANTITY: 10235 return "SimpleQuantity"; 10236 case SUBSTANCEAMOUNT: 10237 return "SubstanceAmount"; 10238 case TIMING: 10239 return "Timing"; 10240 case TRIGGERDEFINITION: 10241 return "TriggerDefinition"; 10242 case USAGECONTEXT: 10243 return "UsageContext"; 10244 case BASE64BINARY: 10245 return "base64Binary"; 10246 case BOOLEAN: 10247 return "boolean"; 10248 case CANONICAL: 10249 return "canonical"; 10250 case CODE: 10251 return "code"; 10252 case DATE: 10253 return "date"; 10254 case DATETIME: 10255 return "dateTime"; 10256 case DECIMAL: 10257 return "decimal"; 10258 case ID: 10259 return "id"; 10260 case INSTANT: 10261 return "instant"; 10262 case INTEGER: 10263 return "integer"; 10264 case MARKDOWN: 10265 return "markdown"; 10266 case OID: 10267 return "oid"; 10268 case POSITIVEINT: 10269 return "positiveInt"; 10270 case STRING: 10271 return "string"; 10272 case TIME: 10273 return "time"; 10274 case UNSIGNEDINT: 10275 return "unsignedInt"; 10276 case URI: 10277 return "uri"; 10278 case URL: 10279 return "url"; 10280 case UUID: 10281 return "uuid"; 10282 case XHTML: 10283 return "xhtml"; 10284 case ACCOUNT: 10285 return "Account"; 10286 case ACTIVITYDEFINITION: 10287 return "ActivityDefinition"; 10288 case ADVERSEEVENT: 10289 return "AdverseEvent"; 10290 case ALLERGYINTOLERANCE: 10291 return "AllergyIntolerance"; 10292 case APPOINTMENT: 10293 return "Appointment"; 10294 case APPOINTMENTRESPONSE: 10295 return "AppointmentResponse"; 10296 case AUDITEVENT: 10297 return "AuditEvent"; 10298 case BASIC: 10299 return "Basic"; 10300 case BINARY: 10301 return "Binary"; 10302 case BIOLOGICALLYDERIVEDPRODUCT: 10303 return "BiologicallyDerivedProduct"; 10304 case BODYSTRUCTURE: 10305 return "BodyStructure"; 10306 case BUNDLE: 10307 return "Bundle"; 10308 case CAPABILITYSTATEMENT: 10309 return "CapabilityStatement"; 10310 case CAREPLAN: 10311 return "CarePlan"; 10312 case CARETEAM: 10313 return "CareTeam"; 10314 case CATALOGENTRY: 10315 return "CatalogEntry"; 10316 case CHARGEITEM: 10317 return "ChargeItem"; 10318 case CHARGEITEMDEFINITION: 10319 return "ChargeItemDefinition"; 10320 case CLAIM: 10321 return "Claim"; 10322 case CLAIMRESPONSE: 10323 return "ClaimResponse"; 10324 case CLINICALIMPRESSION: 10325 return "ClinicalImpression"; 10326 case CODESYSTEM: 10327 return "CodeSystem"; 10328 case COMMUNICATION: 10329 return "Communication"; 10330 case COMMUNICATIONREQUEST: 10331 return "CommunicationRequest"; 10332 case COMPARTMENTDEFINITION: 10333 return "CompartmentDefinition"; 10334 case COMPOSITION: 10335 return "Composition"; 10336 case CONCEPTMAP: 10337 return "ConceptMap"; 10338 case CONDITION: 10339 return "Condition"; 10340 case CONSENT: 10341 return "Consent"; 10342 case CONTRACT: 10343 return "Contract"; 10344 case COVERAGE: 10345 return "Coverage"; 10346 case COVERAGEELIGIBILITYREQUEST: 10347 return "CoverageEligibilityRequest"; 10348 case COVERAGEELIGIBILITYRESPONSE: 10349 return "CoverageEligibilityResponse"; 10350 case DETECTEDISSUE: 10351 return "DetectedIssue"; 10352 case DEVICE: 10353 return "Device"; 10354 case DEVICEDEFINITION: 10355 return "DeviceDefinition"; 10356 case DEVICEMETRIC: 10357 return "DeviceMetric"; 10358 case DEVICEREQUEST: 10359 return "DeviceRequest"; 10360 case DEVICEUSESTATEMENT: 10361 return "DeviceUseStatement"; 10362 case DIAGNOSTICREPORT: 10363 return "DiagnosticReport"; 10364 case DOCUMENTMANIFEST: 10365 return "DocumentManifest"; 10366 case DOCUMENTREFERENCE: 10367 return "DocumentReference"; 10368 case DOMAINRESOURCE: 10369 return "DomainResource"; 10370 case EFFECTEVIDENCESYNTHESIS: 10371 return "EffectEvidenceSynthesis"; 10372 case ENCOUNTER: 10373 return "Encounter"; 10374 case ENDPOINT: 10375 return "Endpoint"; 10376 case ENROLLMENTREQUEST: 10377 return "EnrollmentRequest"; 10378 case ENROLLMENTRESPONSE: 10379 return "EnrollmentResponse"; 10380 case EPISODEOFCARE: 10381 return "EpisodeOfCare"; 10382 case EVENTDEFINITION: 10383 return "EventDefinition"; 10384 case EVIDENCE: 10385 return "Evidence"; 10386 case EVIDENCEVARIABLE: 10387 return "EvidenceVariable"; 10388 case EXAMPLESCENARIO: 10389 return "ExampleScenario"; 10390 case EXPLANATIONOFBENEFIT: 10391 return "ExplanationOfBenefit"; 10392 case FAMILYMEMBERHISTORY: 10393 return "FamilyMemberHistory"; 10394 case FLAG: 10395 return "Flag"; 10396 case GOAL: 10397 return "Goal"; 10398 case GRAPHDEFINITION: 10399 return "GraphDefinition"; 10400 case GROUP: 10401 return "Group"; 10402 case GUIDANCERESPONSE: 10403 return "GuidanceResponse"; 10404 case HEALTHCARESERVICE: 10405 return "HealthcareService"; 10406 case IMAGINGSTUDY: 10407 return "ImagingStudy"; 10408 case IMMUNIZATION: 10409 return "Immunization"; 10410 case IMMUNIZATIONEVALUATION: 10411 return "ImmunizationEvaluation"; 10412 case IMMUNIZATIONRECOMMENDATION: 10413 return "ImmunizationRecommendation"; 10414 case IMPLEMENTATIONGUIDE: 10415 return "ImplementationGuide"; 10416 case INSURANCEPLAN: 10417 return "InsurancePlan"; 10418 case INVOICE: 10419 return "Invoice"; 10420 case LIBRARY: 10421 return "Library"; 10422 case LINKAGE: 10423 return "Linkage"; 10424 case LIST: 10425 return "List"; 10426 case LOCATION: 10427 return "Location"; 10428 case MEASURE: 10429 return "Measure"; 10430 case MEASUREREPORT: 10431 return "MeasureReport"; 10432 case MEDIA: 10433 return "Media"; 10434 case MEDICATION: 10435 return "Medication"; 10436 case MEDICATIONADMINISTRATION: 10437 return "MedicationAdministration"; 10438 case MEDICATIONDISPENSE: 10439 return "MedicationDispense"; 10440 case MEDICATIONKNOWLEDGE: 10441 return "MedicationKnowledge"; 10442 case MEDICATIONREQUEST: 10443 return "MedicationRequest"; 10444 case MEDICATIONSTATEMENT: 10445 return "MedicationStatement"; 10446 case MEDICINALPRODUCT: 10447 return "MedicinalProduct"; 10448 case MEDICINALPRODUCTAUTHORIZATION: 10449 return "MedicinalProductAuthorization"; 10450 case MEDICINALPRODUCTCONTRAINDICATION: 10451 return "MedicinalProductContraindication"; 10452 case MEDICINALPRODUCTINDICATION: 10453 return "MedicinalProductIndication"; 10454 case MEDICINALPRODUCTINGREDIENT: 10455 return "MedicinalProductIngredient"; 10456 case MEDICINALPRODUCTINTERACTION: 10457 return "MedicinalProductInteraction"; 10458 case MEDICINALPRODUCTMANUFACTURED: 10459 return "MedicinalProductManufactured"; 10460 case MEDICINALPRODUCTPACKAGED: 10461 return "MedicinalProductPackaged"; 10462 case MEDICINALPRODUCTPHARMACEUTICAL: 10463 return "MedicinalProductPharmaceutical"; 10464 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 10465 return "MedicinalProductUndesirableEffect"; 10466 case MESSAGEDEFINITION: 10467 return "MessageDefinition"; 10468 case MESSAGEHEADER: 10469 return "MessageHeader"; 10470 case MOLECULARSEQUENCE: 10471 return "MolecularSequence"; 10472 case NAMINGSYSTEM: 10473 return "NamingSystem"; 10474 case NUTRITIONORDER: 10475 return "NutritionOrder"; 10476 case OBSERVATION: 10477 return "Observation"; 10478 case OBSERVATIONDEFINITION: 10479 return "ObservationDefinition"; 10480 case OPERATIONDEFINITION: 10481 return "OperationDefinition"; 10482 case OPERATIONOUTCOME: 10483 return "OperationOutcome"; 10484 case ORGANIZATION: 10485 return "Organization"; 10486 case ORGANIZATIONAFFILIATION: 10487 return "OrganizationAffiliation"; 10488 case PARAMETERS: 10489 return "Parameters"; 10490 case PATIENT: 10491 return "Patient"; 10492 case PAYMENTNOTICE: 10493 return "PaymentNotice"; 10494 case PAYMENTRECONCILIATION: 10495 return "PaymentReconciliation"; 10496 case PERSON: 10497 return "Person"; 10498 case PLANDEFINITION: 10499 return "PlanDefinition"; 10500 case PRACTITIONER: 10501 return "Practitioner"; 10502 case PRACTITIONERROLE: 10503 return "PractitionerRole"; 10504 case PROCEDURE: 10505 return "Procedure"; 10506 case PROVENANCE: 10507 return "Provenance"; 10508 case QUESTIONNAIRE: 10509 return "Questionnaire"; 10510 case QUESTIONNAIRERESPONSE: 10511 return "QuestionnaireResponse"; 10512 case RELATEDPERSON: 10513 return "RelatedPerson"; 10514 case REQUESTGROUP: 10515 return "RequestGroup"; 10516 case RESEARCHDEFINITION: 10517 return "ResearchDefinition"; 10518 case RESEARCHELEMENTDEFINITION: 10519 return "ResearchElementDefinition"; 10520 case RESEARCHSTUDY: 10521 return "ResearchStudy"; 10522 case RESEARCHSUBJECT: 10523 return "ResearchSubject"; 10524 case RESOURCE: 10525 return "Resource"; 10526 case RISKASSESSMENT: 10527 return "RiskAssessment"; 10528 case RISKEVIDENCESYNTHESIS: 10529 return "RiskEvidenceSynthesis"; 10530 case SCHEDULE: 10531 return "Schedule"; 10532 case SEARCHPARAMETER: 10533 return "SearchParameter"; 10534 case SERVICEREQUEST: 10535 return "ServiceRequest"; 10536 case SLOT: 10537 return "Slot"; 10538 case SPECIMEN: 10539 return "Specimen"; 10540 case SPECIMENDEFINITION: 10541 return "SpecimenDefinition"; 10542 case STRUCTUREDEFINITION: 10543 return "StructureDefinition"; 10544 case STRUCTUREMAP: 10545 return "StructureMap"; 10546 case SUBSCRIPTION: 10547 return "Subscription"; 10548 case SUBSTANCE: 10549 return "Substance"; 10550 case SUBSTANCENUCLEICACID: 10551 return "SubstanceNucleicAcid"; 10552 case SUBSTANCEPOLYMER: 10553 return "SubstancePolymer"; 10554 case SUBSTANCEPROTEIN: 10555 return "SubstanceProtein"; 10556 case SUBSTANCEREFERENCEINFORMATION: 10557 return "SubstanceReferenceInformation"; 10558 case SUBSTANCESOURCEMATERIAL: 10559 return "SubstanceSourceMaterial"; 10560 case SUBSTANCESPECIFICATION: 10561 return "SubstanceSpecification"; 10562 case SUPPLYDELIVERY: 10563 return "SupplyDelivery"; 10564 case SUPPLYREQUEST: 10565 return "SupplyRequest"; 10566 case TASK: 10567 return "Task"; 10568 case TERMINOLOGYCAPABILITIES: 10569 return "TerminologyCapabilities"; 10570 case TESTREPORT: 10571 return "TestReport"; 10572 case TESTSCRIPT: 10573 return "TestScript"; 10574 case VALUESET: 10575 return "ValueSet"; 10576 case VERIFICATIONRESULT: 10577 return "VerificationResult"; 10578 case VISIONPRESCRIPTION: 10579 return "VisionPrescription"; 10580 case NULL: 10581 return null; 10582 default: 10583 return "?"; 10584 } 10585 } 10586 10587 public String getSystem() { 10588 switch (this) { 10589 case ADDRESS: 10590 return "http://hl7.org/fhir/data-types"; 10591 case AGE: 10592 return "http://hl7.org/fhir/data-types"; 10593 case ANNOTATION: 10594 return "http://hl7.org/fhir/data-types"; 10595 case ATTACHMENT: 10596 return "http://hl7.org/fhir/data-types"; 10597 case BACKBONEELEMENT: 10598 return "http://hl7.org/fhir/data-types"; 10599 case CODEABLECONCEPT: 10600 return "http://hl7.org/fhir/data-types"; 10601 case CODING: 10602 return "http://hl7.org/fhir/data-types"; 10603 case CONTACTDETAIL: 10604 return "http://hl7.org/fhir/data-types"; 10605 case CONTACTPOINT: 10606 return "http://hl7.org/fhir/data-types"; 10607 case CONTRIBUTOR: 10608 return "http://hl7.org/fhir/data-types"; 10609 case COUNT: 10610 return "http://hl7.org/fhir/data-types"; 10611 case DATAREQUIREMENT: 10612 return "http://hl7.org/fhir/data-types"; 10613 case DISTANCE: 10614 return "http://hl7.org/fhir/data-types"; 10615 case DOSAGE: 10616 return "http://hl7.org/fhir/data-types"; 10617 case DURATION: 10618 return "http://hl7.org/fhir/data-types"; 10619 case ELEMENT: 10620 return "http://hl7.org/fhir/data-types"; 10621 case ELEMENTDEFINITION: 10622 return "http://hl7.org/fhir/data-types"; 10623 case EXPRESSION: 10624 return "http://hl7.org/fhir/data-types"; 10625 case EXTENSION: 10626 return "http://hl7.org/fhir/data-types"; 10627 case HUMANNAME: 10628 return "http://hl7.org/fhir/data-types"; 10629 case IDENTIFIER: 10630 return "http://hl7.org/fhir/data-types"; 10631 case MARKETINGSTATUS: 10632 return "http://hl7.org/fhir/data-types"; 10633 case META: 10634 return "http://hl7.org/fhir/data-types"; 10635 case MONEY: 10636 return "http://hl7.org/fhir/data-types"; 10637 case MONEYQUANTITY: 10638 return "http://hl7.org/fhir/data-types"; 10639 case NARRATIVE: 10640 return "http://hl7.org/fhir/data-types"; 10641 case PARAMETERDEFINITION: 10642 return "http://hl7.org/fhir/data-types"; 10643 case PERIOD: 10644 return "http://hl7.org/fhir/data-types"; 10645 case POPULATION: 10646 return "http://hl7.org/fhir/data-types"; 10647 case PRODCHARACTERISTIC: 10648 return "http://hl7.org/fhir/data-types"; 10649 case PRODUCTSHELFLIFE: 10650 return "http://hl7.org/fhir/data-types"; 10651 case QUANTITY: 10652 return "http://hl7.org/fhir/data-types"; 10653 case RANGE: 10654 return "http://hl7.org/fhir/data-types"; 10655 case RATIO: 10656 return "http://hl7.org/fhir/data-types"; 10657 case REFERENCE: 10658 return "http://hl7.org/fhir/data-types"; 10659 case RELATEDARTIFACT: 10660 return "http://hl7.org/fhir/data-types"; 10661 case SAMPLEDDATA: 10662 return "http://hl7.org/fhir/data-types"; 10663 case SIGNATURE: 10664 return "http://hl7.org/fhir/data-types"; 10665 case SIMPLEQUANTITY: 10666 return "http://hl7.org/fhir/data-types"; 10667 case SUBSTANCEAMOUNT: 10668 return "http://hl7.org/fhir/data-types"; 10669 case TIMING: 10670 return "http://hl7.org/fhir/data-types"; 10671 case TRIGGERDEFINITION: 10672 return "http://hl7.org/fhir/data-types"; 10673 case USAGECONTEXT: 10674 return "http://hl7.org/fhir/data-types"; 10675 case BASE64BINARY: 10676 return "http://hl7.org/fhir/data-types"; 10677 case BOOLEAN: 10678 return "http://hl7.org/fhir/data-types"; 10679 case CANONICAL: 10680 return "http://hl7.org/fhir/data-types"; 10681 case CODE: 10682 return "http://hl7.org/fhir/data-types"; 10683 case DATE: 10684 return "http://hl7.org/fhir/data-types"; 10685 case DATETIME: 10686 return "http://hl7.org/fhir/data-types"; 10687 case DECIMAL: 10688 return "http://hl7.org/fhir/data-types"; 10689 case ID: 10690 return "http://hl7.org/fhir/data-types"; 10691 case INSTANT: 10692 return "http://hl7.org/fhir/data-types"; 10693 case INTEGER: 10694 return "http://hl7.org/fhir/data-types"; 10695 case MARKDOWN: 10696 return "http://hl7.org/fhir/data-types"; 10697 case OID: 10698 return "http://hl7.org/fhir/data-types"; 10699 case POSITIVEINT: 10700 return "http://hl7.org/fhir/data-types"; 10701 case STRING: 10702 return "http://hl7.org/fhir/data-types"; 10703 case TIME: 10704 return "http://hl7.org/fhir/data-types"; 10705 case UNSIGNEDINT: 10706 return "http://hl7.org/fhir/data-types"; 10707 case URI: 10708 return "http://hl7.org/fhir/data-types"; 10709 case URL: 10710 return "http://hl7.org/fhir/data-types"; 10711 case UUID: 10712 return "http://hl7.org/fhir/data-types"; 10713 case XHTML: 10714 return "http://hl7.org/fhir/data-types"; 10715 case ACCOUNT: 10716 return "http://hl7.org/fhir/resource-types"; 10717 case ACTIVITYDEFINITION: 10718 return "http://hl7.org/fhir/resource-types"; 10719 case ADVERSEEVENT: 10720 return "http://hl7.org/fhir/resource-types"; 10721 case ALLERGYINTOLERANCE: 10722 return "http://hl7.org/fhir/resource-types"; 10723 case APPOINTMENT: 10724 return "http://hl7.org/fhir/resource-types"; 10725 case APPOINTMENTRESPONSE: 10726 return "http://hl7.org/fhir/resource-types"; 10727 case AUDITEVENT: 10728 return "http://hl7.org/fhir/resource-types"; 10729 case BASIC: 10730 return "http://hl7.org/fhir/resource-types"; 10731 case BINARY: 10732 return "http://hl7.org/fhir/resource-types"; 10733 case BIOLOGICALLYDERIVEDPRODUCT: 10734 return "http://hl7.org/fhir/resource-types"; 10735 case BODYSTRUCTURE: 10736 return "http://hl7.org/fhir/resource-types"; 10737 case BUNDLE: 10738 return "http://hl7.org/fhir/resource-types"; 10739 case CAPABILITYSTATEMENT: 10740 return "http://hl7.org/fhir/resource-types"; 10741 case CAREPLAN: 10742 return "http://hl7.org/fhir/resource-types"; 10743 case CARETEAM: 10744 return "http://hl7.org/fhir/resource-types"; 10745 case CATALOGENTRY: 10746 return "http://hl7.org/fhir/resource-types"; 10747 case CHARGEITEM: 10748 return "http://hl7.org/fhir/resource-types"; 10749 case CHARGEITEMDEFINITION: 10750 return "http://hl7.org/fhir/resource-types"; 10751 case CLAIM: 10752 return "http://hl7.org/fhir/resource-types"; 10753 case CLAIMRESPONSE: 10754 return "http://hl7.org/fhir/resource-types"; 10755 case CLINICALIMPRESSION: 10756 return "http://hl7.org/fhir/resource-types"; 10757 case CODESYSTEM: 10758 return "http://hl7.org/fhir/resource-types"; 10759 case COMMUNICATION: 10760 return "http://hl7.org/fhir/resource-types"; 10761 case COMMUNICATIONREQUEST: 10762 return "http://hl7.org/fhir/resource-types"; 10763 case COMPARTMENTDEFINITION: 10764 return "http://hl7.org/fhir/resource-types"; 10765 case COMPOSITION: 10766 return "http://hl7.org/fhir/resource-types"; 10767 case CONCEPTMAP: 10768 return "http://hl7.org/fhir/resource-types"; 10769 case CONDITION: 10770 return "http://hl7.org/fhir/resource-types"; 10771 case CONSENT: 10772 return "http://hl7.org/fhir/resource-types"; 10773 case CONTRACT: 10774 return "http://hl7.org/fhir/resource-types"; 10775 case COVERAGE: 10776 return "http://hl7.org/fhir/resource-types"; 10777 case COVERAGEELIGIBILITYREQUEST: 10778 return "http://hl7.org/fhir/resource-types"; 10779 case COVERAGEELIGIBILITYRESPONSE: 10780 return "http://hl7.org/fhir/resource-types"; 10781 case DETECTEDISSUE: 10782 return "http://hl7.org/fhir/resource-types"; 10783 case DEVICE: 10784 return "http://hl7.org/fhir/resource-types"; 10785 case DEVICEDEFINITION: 10786 return "http://hl7.org/fhir/resource-types"; 10787 case DEVICEMETRIC: 10788 return "http://hl7.org/fhir/resource-types"; 10789 case DEVICEREQUEST: 10790 return "http://hl7.org/fhir/resource-types"; 10791 case DEVICEUSESTATEMENT: 10792 return "http://hl7.org/fhir/resource-types"; 10793 case DIAGNOSTICREPORT: 10794 return "http://hl7.org/fhir/resource-types"; 10795 case DOCUMENTMANIFEST: 10796 return "http://hl7.org/fhir/resource-types"; 10797 case DOCUMENTREFERENCE: 10798 return "http://hl7.org/fhir/resource-types"; 10799 case DOMAINRESOURCE: 10800 return "http://hl7.org/fhir/resource-types"; 10801 case EFFECTEVIDENCESYNTHESIS: 10802 return "http://hl7.org/fhir/resource-types"; 10803 case ENCOUNTER: 10804 return "http://hl7.org/fhir/resource-types"; 10805 case ENDPOINT: 10806 return "http://hl7.org/fhir/resource-types"; 10807 case ENROLLMENTREQUEST: 10808 return "http://hl7.org/fhir/resource-types"; 10809 case ENROLLMENTRESPONSE: 10810 return "http://hl7.org/fhir/resource-types"; 10811 case EPISODEOFCARE: 10812 return "http://hl7.org/fhir/resource-types"; 10813 case EVENTDEFINITION: 10814 return "http://hl7.org/fhir/resource-types"; 10815 case EVIDENCE: 10816 return "http://hl7.org/fhir/resource-types"; 10817 case EVIDENCEVARIABLE: 10818 return "http://hl7.org/fhir/resource-types"; 10819 case EXAMPLESCENARIO: 10820 return "http://hl7.org/fhir/resource-types"; 10821 case EXPLANATIONOFBENEFIT: 10822 return "http://hl7.org/fhir/resource-types"; 10823 case FAMILYMEMBERHISTORY: 10824 return "http://hl7.org/fhir/resource-types"; 10825 case FLAG: 10826 return "http://hl7.org/fhir/resource-types"; 10827 case GOAL: 10828 return "http://hl7.org/fhir/resource-types"; 10829 case GRAPHDEFINITION: 10830 return "http://hl7.org/fhir/resource-types"; 10831 case GROUP: 10832 return "http://hl7.org/fhir/resource-types"; 10833 case GUIDANCERESPONSE: 10834 return "http://hl7.org/fhir/resource-types"; 10835 case HEALTHCARESERVICE: 10836 return "http://hl7.org/fhir/resource-types"; 10837 case IMAGINGSTUDY: 10838 return "http://hl7.org/fhir/resource-types"; 10839 case IMMUNIZATION: 10840 return "http://hl7.org/fhir/resource-types"; 10841 case IMMUNIZATIONEVALUATION: 10842 return "http://hl7.org/fhir/resource-types"; 10843 case IMMUNIZATIONRECOMMENDATION: 10844 return "http://hl7.org/fhir/resource-types"; 10845 case IMPLEMENTATIONGUIDE: 10846 return "http://hl7.org/fhir/resource-types"; 10847 case INSURANCEPLAN: 10848 return "http://hl7.org/fhir/resource-types"; 10849 case INVOICE: 10850 return "http://hl7.org/fhir/resource-types"; 10851 case LIBRARY: 10852 return "http://hl7.org/fhir/resource-types"; 10853 case LINKAGE: 10854 return "http://hl7.org/fhir/resource-types"; 10855 case LIST: 10856 return "http://hl7.org/fhir/resource-types"; 10857 case LOCATION: 10858 return "http://hl7.org/fhir/resource-types"; 10859 case MEASURE: 10860 return "http://hl7.org/fhir/resource-types"; 10861 case MEASUREREPORT: 10862 return "http://hl7.org/fhir/resource-types"; 10863 case MEDIA: 10864 return "http://hl7.org/fhir/resource-types"; 10865 case MEDICATION: 10866 return "http://hl7.org/fhir/resource-types"; 10867 case MEDICATIONADMINISTRATION: 10868 return "http://hl7.org/fhir/resource-types"; 10869 case MEDICATIONDISPENSE: 10870 return "http://hl7.org/fhir/resource-types"; 10871 case MEDICATIONKNOWLEDGE: 10872 return "http://hl7.org/fhir/resource-types"; 10873 case MEDICATIONREQUEST: 10874 return "http://hl7.org/fhir/resource-types"; 10875 case MEDICATIONSTATEMENT: 10876 return "http://hl7.org/fhir/resource-types"; 10877 case MEDICINALPRODUCT: 10878 return "http://hl7.org/fhir/resource-types"; 10879 case MEDICINALPRODUCTAUTHORIZATION: 10880 return "http://hl7.org/fhir/resource-types"; 10881 case MEDICINALPRODUCTCONTRAINDICATION: 10882 return "http://hl7.org/fhir/resource-types"; 10883 case MEDICINALPRODUCTINDICATION: 10884 return "http://hl7.org/fhir/resource-types"; 10885 case MEDICINALPRODUCTINGREDIENT: 10886 return "http://hl7.org/fhir/resource-types"; 10887 case MEDICINALPRODUCTINTERACTION: 10888 return "http://hl7.org/fhir/resource-types"; 10889 case MEDICINALPRODUCTMANUFACTURED: 10890 return "http://hl7.org/fhir/resource-types"; 10891 case MEDICINALPRODUCTPACKAGED: 10892 return "http://hl7.org/fhir/resource-types"; 10893 case MEDICINALPRODUCTPHARMACEUTICAL: 10894 return "http://hl7.org/fhir/resource-types"; 10895 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 10896 return "http://hl7.org/fhir/resource-types"; 10897 case MESSAGEDEFINITION: 10898 return "http://hl7.org/fhir/resource-types"; 10899 case MESSAGEHEADER: 10900 return "http://hl7.org/fhir/resource-types"; 10901 case MOLECULARSEQUENCE: 10902 return "http://hl7.org/fhir/resource-types"; 10903 case NAMINGSYSTEM: 10904 return "http://hl7.org/fhir/resource-types"; 10905 case NUTRITIONORDER: 10906 return "http://hl7.org/fhir/resource-types"; 10907 case OBSERVATION: 10908 return "http://hl7.org/fhir/resource-types"; 10909 case OBSERVATIONDEFINITION: 10910 return "http://hl7.org/fhir/resource-types"; 10911 case OPERATIONDEFINITION: 10912 return "http://hl7.org/fhir/resource-types"; 10913 case OPERATIONOUTCOME: 10914 return "http://hl7.org/fhir/resource-types"; 10915 case ORGANIZATION: 10916 return "http://hl7.org/fhir/resource-types"; 10917 case ORGANIZATIONAFFILIATION: 10918 return "http://hl7.org/fhir/resource-types"; 10919 case PARAMETERS: 10920 return "http://hl7.org/fhir/resource-types"; 10921 case PATIENT: 10922 return "http://hl7.org/fhir/resource-types"; 10923 case PAYMENTNOTICE: 10924 return "http://hl7.org/fhir/resource-types"; 10925 case PAYMENTRECONCILIATION: 10926 return "http://hl7.org/fhir/resource-types"; 10927 case PERSON: 10928 return "http://hl7.org/fhir/resource-types"; 10929 case PLANDEFINITION: 10930 return "http://hl7.org/fhir/resource-types"; 10931 case PRACTITIONER: 10932 return "http://hl7.org/fhir/resource-types"; 10933 case PRACTITIONERROLE: 10934 return "http://hl7.org/fhir/resource-types"; 10935 case PROCEDURE: 10936 return "http://hl7.org/fhir/resource-types"; 10937 case PROVENANCE: 10938 return "http://hl7.org/fhir/resource-types"; 10939 case QUESTIONNAIRE: 10940 return "http://hl7.org/fhir/resource-types"; 10941 case QUESTIONNAIRERESPONSE: 10942 return "http://hl7.org/fhir/resource-types"; 10943 case RELATEDPERSON: 10944 return "http://hl7.org/fhir/resource-types"; 10945 case REQUESTGROUP: 10946 return "http://hl7.org/fhir/resource-types"; 10947 case RESEARCHDEFINITION: 10948 return "http://hl7.org/fhir/resource-types"; 10949 case RESEARCHELEMENTDEFINITION: 10950 return "http://hl7.org/fhir/resource-types"; 10951 case RESEARCHSTUDY: 10952 return "http://hl7.org/fhir/resource-types"; 10953 case RESEARCHSUBJECT: 10954 return "http://hl7.org/fhir/resource-types"; 10955 case RESOURCE: 10956 return "http://hl7.org/fhir/resource-types"; 10957 case RISKASSESSMENT: 10958 return "http://hl7.org/fhir/resource-types"; 10959 case RISKEVIDENCESYNTHESIS: 10960 return "http://hl7.org/fhir/resource-types"; 10961 case SCHEDULE: 10962 return "http://hl7.org/fhir/resource-types"; 10963 case SEARCHPARAMETER: 10964 return "http://hl7.org/fhir/resource-types"; 10965 case SERVICEREQUEST: 10966 return "http://hl7.org/fhir/resource-types"; 10967 case SLOT: 10968 return "http://hl7.org/fhir/resource-types"; 10969 case SPECIMEN: 10970 return "http://hl7.org/fhir/resource-types"; 10971 case SPECIMENDEFINITION: 10972 return "http://hl7.org/fhir/resource-types"; 10973 case STRUCTUREDEFINITION: 10974 return "http://hl7.org/fhir/resource-types"; 10975 case STRUCTUREMAP: 10976 return "http://hl7.org/fhir/resource-types"; 10977 case SUBSCRIPTION: 10978 return "http://hl7.org/fhir/resource-types"; 10979 case SUBSTANCE: 10980 return "http://hl7.org/fhir/resource-types"; 10981 case SUBSTANCENUCLEICACID: 10982 return "http://hl7.org/fhir/resource-types"; 10983 case SUBSTANCEPOLYMER: 10984 return "http://hl7.org/fhir/resource-types"; 10985 case SUBSTANCEPROTEIN: 10986 return "http://hl7.org/fhir/resource-types"; 10987 case SUBSTANCEREFERENCEINFORMATION: 10988 return "http://hl7.org/fhir/resource-types"; 10989 case SUBSTANCESOURCEMATERIAL: 10990 return "http://hl7.org/fhir/resource-types"; 10991 case SUBSTANCESPECIFICATION: 10992 return "http://hl7.org/fhir/resource-types"; 10993 case SUPPLYDELIVERY: 10994 return "http://hl7.org/fhir/resource-types"; 10995 case SUPPLYREQUEST: 10996 return "http://hl7.org/fhir/resource-types"; 10997 case TASK: 10998 return "http://hl7.org/fhir/resource-types"; 10999 case TERMINOLOGYCAPABILITIES: 11000 return "http://hl7.org/fhir/resource-types"; 11001 case TESTREPORT: 11002 return "http://hl7.org/fhir/resource-types"; 11003 case TESTSCRIPT: 11004 return "http://hl7.org/fhir/resource-types"; 11005 case VALUESET: 11006 return "http://hl7.org/fhir/resource-types"; 11007 case VERIFICATIONRESULT: 11008 return "http://hl7.org/fhir/resource-types"; 11009 case VISIONPRESCRIPTION: 11010 return "http://hl7.org/fhir/resource-types"; 11011 case NULL: 11012 return null; 11013 default: 11014 return "?"; 11015 } 11016 } 11017 11018 public String getDefinition() { 11019 switch (this) { 11020 case ADDRESS: 11021 return "An address expressed using postal conventions (as opposed to GPS or other location definition formats). This data type may be used to convey addresses for use in delivering mail as well as for visiting locations which might not be valid for mail delivery. There are a variety of postal address formats defined around the world."; 11022 case AGE: 11023 return "A duration of time during which an organism (or a process) has existed."; 11024 case ANNOTATION: 11025 return "A text note which also contains information about who made the statement and when."; 11026 case ATTACHMENT: 11027 return "For referring to data content defined in other formats."; 11028 case BACKBONEELEMENT: 11029 return "Base definition for all elements that are defined inside a resource - but not those in a data type."; 11030 case CODEABLECONCEPT: 11031 return "A concept that may be defined by a formal reference to a terminology or ontology or may be provided by text."; 11032 case CODING: 11033 return "A reference to a code defined by a terminology system."; 11034 case CONTACTDETAIL: 11035 return "Specifies contact information for a person or organization."; 11036 case CONTACTPOINT: 11037 return "Details for all kinds of technology mediated contact points for a person or organization, including telephone, email, etc."; 11038 case CONTRIBUTOR: 11039 return "A contributor to the content of a knowledge asset, including authors, editors, reviewers, and endorsers."; 11040 case COUNT: 11041 return "A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies."; 11042 case DATAREQUIREMENT: 11043 return "Describes a required data item for evaluation in terms of the type of data, and optional code or date-based filters of the data."; 11044 case DISTANCE: 11045 return "A length - a value with a unit that is a physical distance."; 11046 case DOSAGE: 11047 return "Indicates how the medication is/was taken or should be taken by the patient."; 11048 case DURATION: 11049 return "A length of time."; 11050 case ELEMENT: 11051 return "Base definition for all elements in a resource."; 11052 case ELEMENTDEFINITION: 11053 return "Captures constraints on each element within the resource, profile, or extension."; 11054 case EXPRESSION: 11055 return "A expression that is evaluated in a specified context and returns a value. The context of use of the expression must specify the context in which the expression is evaluated, and how the result of the expression is used."; 11056 case EXTENSION: 11057 return "Optional Extension Element - found in all resources."; 11058 case HUMANNAME: 11059 return "A human's name with the ability to identify parts and usage."; 11060 case IDENTIFIER: 11061 return "An identifier - identifies some entity uniquely and unambiguously. Typically this is used for business identifiers."; 11062 case MARKETINGSTATUS: 11063 return "The marketing status describes the date when a medicinal product is actually put on the market or the date as of which it is no longer available."; 11064 case META: 11065 return "The metadata about a resource. This is content in the resource that is maintained by the infrastructure. Changes to the content might not always be associated with version changes to the resource."; 11066 case MONEY: 11067 return "An amount of economic utility in some recognized currency."; 11068 case MONEYQUANTITY: 11069 return ""; 11070 case NARRATIVE: 11071 return "A human-readable summary of the resource conveying the essential clinical and business information for the resource."; 11072 case PARAMETERDEFINITION: 11073 return "The parameters to the module. This collection specifies both the input and output parameters. Input parameters are provided by the caller as part of the $evaluate operation. Output parameters are included in the GuidanceResponse."; 11074 case PERIOD: 11075 return "A time period defined by a start and end date and optionally time."; 11076 case POPULATION: 11077 return "A populatioof people with some set of grouping criteria."; 11078 case PRODCHARACTERISTIC: 11079 return "The marketing status describes the date when a medicinal product is actually put on the market or the date as of which it is no longer available."; 11080 case PRODUCTSHELFLIFE: 11081 return "The shelf-life and storage information for a medicinal product item or container can be described using this class."; 11082 case QUANTITY: 11083 return "A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies."; 11084 case RANGE: 11085 return "A set of ordered Quantities defined by a low and high limit."; 11086 case RATIO: 11087 return "A relationship of two Quantity values - expressed as a numerator and a denominator."; 11088 case REFERENCE: 11089 return "A reference from one resource to another."; 11090 case RELATEDARTIFACT: 11091 return "Related artifacts such as additional documentation, justification, or bibliographic references."; 11092 case SAMPLEDDATA: 11093 return "A series of measurements taken by a device, with upper and lower limits. There may be more than one dimension in the data."; 11094 case SIGNATURE: 11095 return "A signature along with supporting context. The signature may be a digital signature that is cryptographic in nature, or some other signature acceptable to the domain. This other signature may be as simple as a graphical image representing a hand-written signature, or a signature ceremony Different signature approaches have different utilities."; 11096 case SIMPLEQUANTITY: 11097 return ""; 11098 case SUBSTANCEAMOUNT: 11099 return "Chemical substances are a single substance type whose primary defining element is the molecular structure. Chemical substances shall be defined on the basis of their complete covalent molecular structure; the presence of a salt (counter-ion) and/or solvates (water, alcohols) is also captured. Purity, grade, physical form or particle size are not taken into account in the definition of a chemical substance or in the assignment of a Substance ID."; 11100 case TIMING: 11101 return "Specifies an event that may occur multiple times. Timing schedules are used to record when things are planned, expected or requested to occur. The most common usage is in dosage instructions for medications. They are also used when planning care of various kinds, and may be used for reporting the schedule to which past regular activities were carried out."; 11102 case TRIGGERDEFINITION: 11103 return "A description of a triggering event. Triggering events can be named events, data events, or periodic, as determined by the type element."; 11104 case USAGECONTEXT: 11105 return "Specifies clinical/business/etc. metadata that can be used to retrieve, index and/or categorize an artifact. This metadata can either be specific to the applicable population (e.g., age category, DRG) or the specific context of care (e.g., venue, care setting, provider of care)."; 11106 case BASE64BINARY: 11107 return "A stream of bytes"; 11108 case BOOLEAN: 11109 return "Value of \"true\" or \"false\""; 11110 case CANONICAL: 11111 return "A URI that is a reference to a canonical URL on a FHIR resource"; 11112 case CODE: 11113 return "A string which has at least one character and no leading or trailing whitespace and where there is no whitespace other than single spaces in the contents"; 11114 case DATE: 11115 return "A date or partial date (e.g. just year or year + month). There is no time zone. The format is a union of the schema types gYear, gYearMonth and date. Dates SHALL be valid dates."; 11116 case DATETIME: 11117 return "A date, date-time or partial date (e.g. just year or year + month). If hours and minutes are specified, a time zone SHALL be populated. The format is a union of the schema types gYear, gYearMonth, date and dateTime. Seconds must be provided due to schema type constraints but may be zero-filled and may be ignored. Dates SHALL be valid dates."; 11118 case DECIMAL: 11119 return "A rational number with implicit precision"; 11120 case ID: 11121 return "Any combination of letters, numerals, \"-\" and \".\", with a length limit of 64 characters. (This might be an integer, an unprefixed OID, UUID or any other identifier pattern that meets these constraints.) Ids are case-insensitive."; 11122 case INSTANT: 11123 return "An instant in time - known at least to the second"; 11124 case INTEGER: 11125 return "A whole number"; 11126 case MARKDOWN: 11127 return "A string that may contain Github Flavored Markdown syntax for optional processing by a mark down presentation engine"; 11128 case OID: 11129 return "An OID represented as a URI"; 11130 case POSITIVEINT: 11131 return "An integer with a value that is positive (e.g. >0)"; 11132 case STRING: 11133 return "A sequence of Unicode characters"; 11134 case TIME: 11135 return "A time during the day, with no date specified"; 11136 case UNSIGNEDINT: 11137 return "An integer with a value that is not negative (e.g. >= 0)"; 11138 case URI: 11139 return "String of characters used to identify a name or a resource"; 11140 case URL: 11141 return "A URI that is a literal reference"; 11142 case UUID: 11143 return "A UUID, represented as a URI"; 11144 case XHTML: 11145 return "XHTML format, as defined by W3C, but restricted usage (mainly, no active content)"; 11146 case ACCOUNT: 11147 return "A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centers, etc."; 11148 case ACTIVITYDEFINITION: 11149 return "This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context."; 11150 case ADVERSEEVENT: 11151 return "Actual or potential/avoided event causing unintended physical injury resulting from or contributed to by medical care, a research study or other healthcare setting factors that requires additional monitoring, treatment, or hospitalization, or that results in death."; 11152 case ALLERGYINTOLERANCE: 11153 return "Risk of harmful or undesirable, physiological response which is unique to an individual and associated with exposure to a substance."; 11154 case APPOINTMENT: 11155 return "A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s)."; 11156 case APPOINTMENTRESPONSE: 11157 return "A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection."; 11158 case AUDITEVENT: 11159 return "A record of an event made for purposes of maintaining a security log. Typical uses include detection of intrusion attempts and monitoring for inappropriate usage."; 11160 case BASIC: 11161 return "Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification."; 11162 case BINARY: 11163 return "A resource that represents the data of a single raw artifact as digital content accessible in its native format. A Binary resource can contain any content, whether text, image, pdf, zip archive, etc."; 11164 case BIOLOGICALLYDERIVEDPRODUCT: 11165 return "A material substance originating from a biological entity intended to be transplanted or infused\ninto another (possibly the same) biological entity."; 11166 case BODYSTRUCTURE: 11167 return "Record details about an anatomical structure. This resource may be used when a coded concept does not provide the necessary detail needed for the use case."; 11168 case BUNDLE: 11169 return "A container for a collection of resources."; 11170 case CAPABILITYSTATEMENT: 11171 return "A Capability Statement documents a set of capabilities (behaviors) of a FHIR Server for a particular version of FHIR that may be used as a statement of actual server functionality or a statement of required or desired server implementation."; 11172 case CAREPLAN: 11173 return "Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions."; 11174 case CARETEAM: 11175 return "The Care Team includes all the people and organizations who plan to participate in the coordination and delivery of care for a patient."; 11176 case CATALOGENTRY: 11177 return "Catalog entries are wrappers that contextualize items included in a catalog."; 11178 case CHARGEITEM: 11179 return "The resource ChargeItem describes the provision of healthcare provider products for a certain patient, therefore referring not only to the product, but containing in addition details of the provision, like date, time, amounts and participating organizations and persons. Main Usage of the ChargeItem is to enable the billing process and internal cost allocation."; 11180 case CHARGEITEMDEFINITION: 11181 return "The ChargeItemDefinition resource provides the properties that apply to the (billing) codes necessary to calculate costs and prices. The properties may differ largely depending on type and realm, therefore this resource gives only a rough structure and requires profiling for each type of billing code system."; 11182 case CLAIM: 11183 return "A provider issued list of professional services and products which have been provided, or are to be provided, to a patient which is sent to an insurer for reimbursement."; 11184 case CLAIMRESPONSE: 11185 return "This resource provides the adjudication details from the processing of a Claim resource."; 11186 case CLINICALIMPRESSION: 11187 return "A record of a clinical assessment performed to determine what problem(s) may affect the patient and before planning the treatments or management strategies that are best to manage a patient's condition. Assessments are often 1:1 with a clinical consultation / encounter, but this varies greatly depending on the clinical workflow. This resource is called \"ClinicalImpression\" rather than \"ClinicalAssessment\" to avoid confusion with the recording of assessment tools such as Apgar score."; 11188 case CODESYSTEM: 11189 return "The CodeSystem resource is used to declare the existence of and describe a code system or code system supplement and its key properties, and optionally define a part or all of its content."; 11190 case COMMUNICATION: 11191 return "An occurrence of information being transmitted; e.g. an alert that was sent to a responsible provider, a public health agency that was notified about a reportable condition."; 11192 case COMMUNICATIONREQUEST: 11193 return "A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition."; 11194 case COMPARTMENTDEFINITION: 11195 return "A compartment definition that defines how resources are accessed on a server."; 11196 case COMPOSITION: 11197 return "A set of healthcare-related information that is assembled together into a single logical package that provides a single coherent statement of meaning, establishes its own context and that has clinical attestation with regard to who is making the statement. A Composition defines the structure and narrative content necessary for a document. However, a Composition alone does not constitute a document. Rather, the Composition must be the first entry in a Bundle where Bundle.type=document, and any other resources referenced from Composition must be included as subsequent entries in the Bundle (for example Patient, Practitioner, Encounter, etc.)."; 11198 case CONCEPTMAP: 11199 return "A statement of relationships from one set of concepts to one or more other concepts - either concepts in code systems, or data element/data element concepts, or classes in class models."; 11200 case CONDITION: 11201 return "A clinical condition, problem, diagnosis, or other event, situation, issue, or clinical concept that has risen to a level of concern."; 11202 case CONSENT: 11203 return "A record of a healthcare consumer?s choices, which permits or denies identified recipient(s) or recipient role(s) to perform one or more actions within a given policy context, for specific purposes and periods of time."; 11204 case CONTRACT: 11205 return "Legally enforceable, formally recorded unilateral or bilateral directive i.e., a policy or agreement."; 11206 case COVERAGE: 11207 return "Financial instrument which may be used to reimburse or pay for health care products and services. Includes both insurance and self-payment."; 11208 case COVERAGEELIGIBILITYREQUEST: 11209 return "The CoverageEligibilityRequest provides patient and insurance coverage information to an insurer for them to respond, in the form of an CoverageEligibilityResponse, with information regarding whether the stated coverage is valid and in-force and optionally to provide the insurance details of the policy."; 11210 case COVERAGEELIGIBILITYRESPONSE: 11211 return "This resource provides eligibility and plan details from the processing of an CoverageEligibilityRequest resource."; 11212 case DETECTEDISSUE: 11213 return "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, etc."; 11214 case DEVICE: 11215 return "A type of a manufactured item that is used in the provision of healthcare without being substantially changed through that activity. The device may be a medical or non-medical device."; 11216 case DEVICEDEFINITION: 11217 return "The characteristics, operational status and capabilities of a medical-related component of a medical device."; 11218 case DEVICEMETRIC: 11219 return "Describes a measurement, calculation or setting capability of a medical device."; 11220 case DEVICEREQUEST: 11221 return "Represents a request for a patient to employ a medical device. The device may be an implantable device, or an external assistive device, such as a walker."; 11222 case DEVICEUSESTATEMENT: 11223 return "A record of a device being used by a patient where the record is the result of a report from the patient or another clinician."; 11224 case DIAGNOSTICREPORT: 11225 return "The findings and interpretation of diagnostic tests performed on patients, groups of patients, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting and provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports."; 11226 case DOCUMENTMANIFEST: 11227 return "A collection of documents compiled for a purpose together with metadata that applies to the collection."; 11228 case DOCUMENTREFERENCE: 11229 return "A reference to a document of any kind for any purpose. Provides metadata about the document so that the document can be discovered and managed. The scope of a document is any seralized object with a mime-type, so includes formal patient centric documents (CDA), cliical notes, scanned paper, and non-patient specific documents like policy text."; 11230 case DOMAINRESOURCE: 11231 return "A resource that includes narrative, extensions, and contained resources."; 11232 case EFFECTEVIDENCESYNTHESIS: 11233 return "The EffectEvidenceSynthesis resource describes the difference in an outcome between exposures states in a population where the effect estimate is derived from a combination of research studies."; 11234 case ENCOUNTER: 11235 return "An interaction between a patient and healthcare provider(s) for the purpose of providing healthcare service(s) or assessing the health status of a patient."; 11236 case ENDPOINT: 11237 return "The technical details of an endpoint that can be used for electronic services, such as for web services providing XDS.b or a REST endpoint for another FHIR server. This may include any security context information."; 11238 case ENROLLMENTREQUEST: 11239 return "This resource provides the insurance enrollment details to the insurer regarding a specified coverage."; 11240 case ENROLLMENTRESPONSE: 11241 return "This resource provides enrollment and plan details from the processing of an EnrollmentRequest resource."; 11242 case EPISODEOFCARE: 11243 return "An association between a patient and an organization / healthcare provider(s) during which time encounters may occur. The managing organization assumes a level of responsibility for the patient during this time."; 11244 case EVENTDEFINITION: 11245 return "The EventDefinition resource provides a reusable description of when a particular event can occur."; 11246 case EVIDENCE: 11247 return "The Evidence resource describes the conditional state (population and any exposures being compared within the population) and outcome (if specified) that the knowledge (evidence, assertion, recommendation) is about."; 11248 case EVIDENCEVARIABLE: 11249 return "The EvidenceVariable resource describes a \"PICO\" element that knowledge (evidence, assertion, recommendation) is about."; 11250 case EXAMPLESCENARIO: 11251 return "Example of workflow instance."; 11252 case EXPLANATIONOFBENEFIT: 11253 return "This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided."; 11254 case FAMILYMEMBERHISTORY: 11255 return "Significant health conditions for a person related to the patient relevant in the context of care for the patient."; 11256 case FLAG: 11257 return "Prospective warnings of potential issues when providing care to the patient."; 11258 case GOAL: 11259 return "Describes the intended objective(s) for a patient, group or organization care, for example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc."; 11260 case GRAPHDEFINITION: 11261 return "A formal computable definition of a graph of resources - that is, a coherent set of resources that form a graph by following references. The Graph Definition resource defines a set and makes rules about the set."; 11262 case GROUP: 11263 return "Represents a defined collection of entities that may be discussed or acted upon collectively but which are not expected to act collectively, and are not formally or legally recognized; i.e. a collection of entities that isn't an Organization."; 11264 case GUIDANCERESPONSE: 11265 return "A guidance response is the formal response to a guidance request, including any output parameters returned by the evaluation, as well as the description of any proposed actions to be taken."; 11266 case HEALTHCARESERVICE: 11267 return "The details of a healthcare service available at a location."; 11268 case IMAGINGSTUDY: 11269 return "Representation of the content produced in a DICOM imaging study. A study comprises a set of series, each of which includes a set of Service-Object Pair Instances (SOP Instances - images or other data) acquired or produced in a common context. A series is of only one modality (e.g. X-ray, CT, MR, ultrasound), but a study may have multiple series of different modalities."; 11270 case IMMUNIZATION: 11271 return "Describes the event of a patient being administered a vaccine or a record of an immunization as reported by a patient, a clinician or another party."; 11272 case IMMUNIZATIONEVALUATION: 11273 return "Describes a comparison of an immunization event against published recommendations to determine if the administration is \"valid\" in relation to those recommendations."; 11274 case IMMUNIZATIONRECOMMENDATION: 11275 return "A patient's point-in-time set of recommendations (i.e. forecasting) according to a published schedule with optional supporting justification."; 11276 case IMPLEMENTATIONGUIDE: 11277 return "A set of rules of how a particular interoperability or standards problem is solved - typically through the use of FHIR resources. This resource is used to gather all the parts of an implementation guide into a logical whole and to publish a computable definition of all the parts."; 11278 case INSURANCEPLAN: 11279 return "Details of a Health Insurance product/plan provided by an organization."; 11280 case INVOICE: 11281 return "Invoice containing collected ChargeItems from an Account with calculated individual and total price for Billing purpose."; 11282 case LIBRARY: 11283 return "The Library resource is a general-purpose container for knowledge asset definitions. It can be used to describe and expose existing knowledge assets such as logic libraries and information model descriptions, as well as to describe a collection of knowledge assets."; 11284 case LINKAGE: 11285 return "Identifies two or more records (resource instances) that refer to the same real-world \"occurrence\"."; 11286 case LIST: 11287 return "A list is a curated collection of resources."; 11288 case LOCATION: 11289 return "Details and position information for a physical place where services are provided and resources and participants may be stored, found, contained, or accommodated."; 11290 case MEASURE: 11291 return "The Measure resource provides the definition of a quality measure."; 11292 case MEASUREREPORT: 11293 return "The MeasureReport resource contains the results of the calculation of a measure; and optionally a reference to the resources involved in that calculation."; 11294 case MEDIA: 11295 return "A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference."; 11296 case MEDICATION: 11297 return "This resource is primarily used for the identification and definition of a medication for the purposes of prescribing, dispensing, and administering a medication as well as for making statements about medication use."; 11298 case MEDICATIONADMINISTRATION: 11299 return "Describes the event of a patient consuming or otherwise being administered a medication. This may be as simple as swallowing a tablet or it may be a long running infusion. Related resources tie this event to the authorizing prescription, and the specific encounter between patient and health care practitioner."; 11300 case MEDICATIONDISPENSE: 11301 return "Indicates that a medication product is to be or has been dispensed for a named person/patient. This includes a description of the medication product (supply) provided and the instructions for administering the medication. The medication dispense is the result of a pharmacy system responding to a medication order."; 11302 case MEDICATIONKNOWLEDGE: 11303 return "Information about a medication that is used to support knowledge."; 11304 case MEDICATIONREQUEST: 11305 return "An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called \"MedicationRequest\" rather than \"MedicationPrescription\" or \"MedicationOrder\" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns."; 11306 case MEDICATIONSTATEMENT: 11307 return "A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from sources such as the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains. \n\nThe primary difference between a medication statement and a medication administration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medication statement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the medication statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information."; 11308 case MEDICINALPRODUCT: 11309 return "Detailed definition of a medicinal product, typically for uses other than direct patient care (e.g. regulatory use)."; 11310 case MEDICINALPRODUCTAUTHORIZATION: 11311 return "The regulatory authorization of a medicinal product."; 11312 case MEDICINALPRODUCTCONTRAINDICATION: 11313 return "The clinical particulars - indications, contraindications etc. of a medicinal product, including for regulatory purposes."; 11314 case MEDICINALPRODUCTINDICATION: 11315 return "Indication for the Medicinal Product."; 11316 case MEDICINALPRODUCTINGREDIENT: 11317 return "An ingredient of a manufactured item or pharmaceutical product."; 11318 case MEDICINALPRODUCTINTERACTION: 11319 return "The interactions of the medicinal product with other medicinal products, or other forms of interactions."; 11320 case MEDICINALPRODUCTMANUFACTURED: 11321 return "The manufactured item as contained in the packaged medicinal product."; 11322 case MEDICINALPRODUCTPACKAGED: 11323 return "A medicinal product in a container or package."; 11324 case MEDICINALPRODUCTPHARMACEUTICAL: 11325 return "A pharmaceutical product described in terms of its composition and dose form."; 11326 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 11327 return "Describe the undesirable effects of the medicinal product."; 11328 case MESSAGEDEFINITION: 11329 return "Defines the characteristics of a message that can be shared between systems, including the type of event that initiates the message, the content to be transmitted and what response(s), if any, are permitted."; 11330 case MESSAGEHEADER: 11331 return "The header for a message exchange that is either requesting or responding to an action. The reference(s) that are the subject of the action as well as other information related to the action are typically transmitted in a bundle in which the MessageHeader resource instance is the first resource in the bundle."; 11332 case MOLECULARSEQUENCE: 11333 return "Raw data describing a biological sequence."; 11334 case NAMINGSYSTEM: 11335 return "A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a \"System\" used within the Identifier and Coding data types."; 11336 case NUTRITIONORDER: 11337 return "A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident."; 11338 case OBSERVATION: 11339 return "Measurements and simple assertions made about a patient, device or other subject."; 11340 case OBSERVATIONDEFINITION: 11341 return "Set of definitional characteristics for a kind of observation or measurement produced or consumed by an orderable health care service."; 11342 case OPERATIONDEFINITION: 11343 return "A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction)."; 11344 case OPERATIONOUTCOME: 11345 return "A collection of error, warning, or information messages that result from a system action."; 11346 case ORGANIZATION: 11347 return "A formally or informally recognized grouping of people or organizations formed for the purpose of achieving some form of collective action. Includes companies, institutions, corporations, departments, community groups, healthcare practice groups, payer/insurer, etc."; 11348 case ORGANIZATIONAFFILIATION: 11349 return "Defines an affiliation/assotiation/relationship between 2 distinct oganizations, that is not a part-of relationship/sub-division relationship."; 11350 case PARAMETERS: 11351 return "This resource is a non-persisted resource used to pass information into and back from an [operation](operations.html). It has no other use, and there is no RESTful endpoint associated with it."; 11352 case PATIENT: 11353 return "Demographics and other administrative information about an individual or animal receiving care or other health-related services."; 11354 case PAYMENTNOTICE: 11355 return "This resource provides the status of the payment for goods and services rendered, and the request and response resource references."; 11356 case PAYMENTRECONCILIATION: 11357 return "This resource provides the details including amount of a payment and allocates the payment items being paid."; 11358 case PERSON: 11359 return "Demographics and administrative information about a person independent of a specific health-related context."; 11360 case PLANDEFINITION: 11361 return "This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical artifacts such as clinical decision support rules, order sets and protocols."; 11362 case PRACTITIONER: 11363 return "A person who is directly or indirectly involved in the provisioning of healthcare."; 11364 case PRACTITIONERROLE: 11365 return "A specific set of Roles/Locations/specialties/services that a practitioner may perform at an organization for a period of time."; 11366 case PROCEDURE: 11367 return "An action that is or was performed on or for a patient. This can be a physical intervention like an operation, or less invasive like long term services, counseling, or hypnotherapy."; 11368 case PROVENANCE: 11369 return "Provenance of a resource is a record that describes entities and processes involved in producing and delivering or otherwise influencing that resource. Provenance provides a critical foundation for assessing authenticity, enabling trust, and allowing reproducibility. Provenance assertions are a form of contextual metadata and can themselves become important records with their own provenance. Provenance statement indicates clinical significance in terms of confidence in authenticity, reliability, and trustworthiness, integrity, and stage in lifecycle (e.g. Document Completion - has the artifact been legally authenticated), all of which may impact security, privacy, and trust policies."; 11370 case QUESTIONNAIRE: 11371 return "A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection."; 11372 case QUESTIONNAIRERESPONSE: 11373 return "A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the questionnaire being responded to."; 11374 case RELATEDPERSON: 11375 return "Information about a person that is involved in the care for a patient, but who is not the target of healthcare, nor has a formal responsibility in the care process."; 11376 case REQUESTGROUP: 11377 return "A group of related requests that can be used to capture intended activities that have inter-dependencies such as \"give this medication after that one\"."; 11378 case RESEARCHDEFINITION: 11379 return "The ResearchDefinition resource describes the conditional state (population and any exposures being compared within the population) and outcome (if specified) that the knowledge (evidence, assertion, recommendation) is about."; 11380 case RESEARCHELEMENTDEFINITION: 11381 return "The ResearchElementDefinition resource describes a \"PICO\" element that knowledge (evidence, assertion, recommendation) is about."; 11382 case RESEARCHSTUDY: 11383 return "A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects."; 11384 case RESEARCHSUBJECT: 11385 return "A physical entity which is the primary unit of operational and/or administrative interest in a study."; 11386 case RESOURCE: 11387 return "This is the base resource type for everything."; 11388 case RISKASSESSMENT: 11389 return "An assessment of the likely outcome(s) for a patient or other subject as well as the likelihood of each outcome."; 11390 case RISKEVIDENCESYNTHESIS: 11391 return "The RiskEvidenceSynthesis resource describes the likelihood of an outcome in a population plus exposure state where the risk estimate is derived from a combination of research studies."; 11392 case SCHEDULE: 11393 return "A container for slots of time that may be available for booking appointments."; 11394 case SEARCHPARAMETER: 11395 return "A search parameter that defines a named search item that can be used to search/filter on a resource."; 11396 case SERVICEREQUEST: 11397 return "A record of a request for service such as diagnostic investigations, treatments, or operations to be performed."; 11398 case SLOT: 11399 return "A slot of time on a schedule that may be available for booking appointments."; 11400 case SPECIMEN: 11401 return "A sample to be used for analysis."; 11402 case SPECIMENDEFINITION: 11403 return "A kind of specimen with associated set of requirements."; 11404 case STRUCTUREDEFINITION: 11405 return "A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions and constraints on resources and data types."; 11406 case STRUCTUREMAP: 11407 return "A Map of relationships between 2 structures that can be used to transform data."; 11408 case SUBSCRIPTION: 11409 return "The subscription resource is used to define a push-based subscription from a server to another system. Once a subscription is registered with the server, the server checks every resource that is created or updated, and if the resource matches the given criteria, it sends a message on the defined \"channel\" so that another system can take an appropriate action."; 11410 case SUBSTANCE: 11411 return "A homogeneous material with a definite composition."; 11412 case SUBSTANCENUCLEICACID: 11413 return "Nucleic acids are defined by three distinct elements: the base, sugar and linkage. Individual substance/moiety IDs will be created for each of these elements. The nucleotide sequence will be always entered in the 5?-3? direction."; 11414 case SUBSTANCEPOLYMER: 11415 return "Todo."; 11416 case SUBSTANCEPROTEIN: 11417 return "A SubstanceProtein is defined as a single unit of a linear amino acid sequence, or a combination of subunits that are either covalently linked or have a defined invariant stoichiometric relationship. This includes all synthetic, recombinant and purified SubstanceProteins of defined sequence, whether the use is therapeutic or prophylactic. This set of elements will be used to describe albumins, coagulation factors, cytokines, growth factors, peptide/SubstanceProtein hormones, enzymes, toxins, toxoids, recombinant vaccines, and immunomodulators."; 11418 case SUBSTANCEREFERENCEINFORMATION: 11419 return "Todo."; 11420 case SUBSTANCESOURCEMATERIAL: 11421 return "Source material shall capture information on the taxonomic and anatomical origins as well as the fraction of a material that can result in or can be modified to form a substance. This set of data elements shall be used to define polymer substances isolated from biological matrices. Taxonomic and anatomical origins shall be described using a controlled vocabulary as required. This information is captured for naturally derived polymers ( . starch) and structurally diverse substances. For Organisms belonging to the Kingdom Plantae the Substance level defines the fresh material of a single species or infraspecies, the Herbal Drug and the Herbal preparation. For Herbal preparations, the fraction information will be captured at the Substance information level and additional information for herbal extracts will be captured at the Specified Substance Group 1 information level. See for further explanation the Substance Class: Structurally Diverse and the herbal annex."; 11422 case SUBSTANCESPECIFICATION: 11423 return "The detailed description of a substance, typically at a level beyond what is used for prescribing."; 11424 case SUPPLYDELIVERY: 11425 return "Record of delivery of what is supplied."; 11426 case SUPPLYREQUEST: 11427 return "A record of a request for a medication, substance or device used in the healthcare setting."; 11428 case TASK: 11429 return "A task to be performed."; 11430 case TERMINOLOGYCAPABILITIES: 11431 return "A Terminology Capabilities documents a set of capabilities (behaviors) of a FHIR Server that may be used as a statement of actual server functionality or a statement of required or desired server implementation."; 11432 case TESTREPORT: 11433 return "A summary of information based on the results of executing a TestScript."; 11434 case TESTSCRIPT: 11435 return "A structured set of tests against a FHIR server or client implementation to determine compliance against the FHIR specification."; 11436 case VALUESET: 11437 return "A ValueSet resource instance specifies a set of codes drawn from one or more code systems, intended for use in a particular context. Value sets link between [[[CodeSystem]]] definitions and their use in [coded elements](terminologies.html)."; 11438 case VERIFICATIONRESULT: 11439 return "Describes validation requirements, source(s), status and dates for one or more elements."; 11440 case VISIONPRESCRIPTION: 11441 return "An authorization for the provision of glasses and/or contact lenses to a patient."; 11442 case NULL: 11443 return null; 11444 default: 11445 return "?"; 11446 } 11447 } 11448 11449 public String getDisplay() { 11450 switch (this) { 11451 case ADDRESS: 11452 return "Address"; 11453 case AGE: 11454 return "Age"; 11455 case ANNOTATION: 11456 return "Annotation"; 11457 case ATTACHMENT: 11458 return "Attachment"; 11459 case BACKBONEELEMENT: 11460 return "BackboneElement"; 11461 case CODEABLECONCEPT: 11462 return "CodeableConcept"; 11463 case CODING: 11464 return "Coding"; 11465 case CONTACTDETAIL: 11466 return "ContactDetail"; 11467 case CONTACTPOINT: 11468 return "ContactPoint"; 11469 case CONTRIBUTOR: 11470 return "Contributor"; 11471 case COUNT: 11472 return "Count"; 11473 case DATAREQUIREMENT: 11474 return "DataRequirement"; 11475 case DISTANCE: 11476 return "Distance"; 11477 case DOSAGE: 11478 return "Dosage"; 11479 case DURATION: 11480 return "Duration"; 11481 case ELEMENT: 11482 return "Element"; 11483 case ELEMENTDEFINITION: 11484 return "ElementDefinition"; 11485 case EXPRESSION: 11486 return "Expression"; 11487 case EXTENSION: 11488 return "Extension"; 11489 case HUMANNAME: 11490 return "HumanName"; 11491 case IDENTIFIER: 11492 return "Identifier"; 11493 case MARKETINGSTATUS: 11494 return "MarketingStatus"; 11495 case META: 11496 return "Meta"; 11497 case MONEY: 11498 return "Money"; 11499 case MONEYQUANTITY: 11500 return "MoneyQuantity"; 11501 case NARRATIVE: 11502 return "Narrative"; 11503 case PARAMETERDEFINITION: 11504 return "ParameterDefinition"; 11505 case PERIOD: 11506 return "Period"; 11507 case POPULATION: 11508 return "Population"; 11509 case PRODCHARACTERISTIC: 11510 return "ProdCharacteristic"; 11511 case PRODUCTSHELFLIFE: 11512 return "ProductShelfLife"; 11513 case QUANTITY: 11514 return "Quantity"; 11515 case RANGE: 11516 return "Range"; 11517 case RATIO: 11518 return "Ratio"; 11519 case REFERENCE: 11520 return "Reference"; 11521 case RELATEDARTIFACT: 11522 return "RelatedArtifact"; 11523 case SAMPLEDDATA: 11524 return "SampledData"; 11525 case SIGNATURE: 11526 return "Signature"; 11527 case SIMPLEQUANTITY: 11528 return "SimpleQuantity"; 11529 case SUBSTANCEAMOUNT: 11530 return "SubstanceAmount"; 11531 case TIMING: 11532 return "Timing"; 11533 case TRIGGERDEFINITION: 11534 return "TriggerDefinition"; 11535 case USAGECONTEXT: 11536 return "UsageContext"; 11537 case BASE64BINARY: 11538 return "base64Binary"; 11539 case BOOLEAN: 11540 return "boolean"; 11541 case CANONICAL: 11542 return "canonical"; 11543 case CODE: 11544 return "code"; 11545 case DATE: 11546 return "date"; 11547 case DATETIME: 11548 return "dateTime"; 11549 case DECIMAL: 11550 return "decimal"; 11551 case ID: 11552 return "id"; 11553 case INSTANT: 11554 return "instant"; 11555 case INTEGER: 11556 return "integer"; 11557 case MARKDOWN: 11558 return "markdown"; 11559 case OID: 11560 return "oid"; 11561 case POSITIVEINT: 11562 return "positiveInt"; 11563 case STRING: 11564 return "string"; 11565 case TIME: 11566 return "time"; 11567 case UNSIGNEDINT: 11568 return "unsignedInt"; 11569 case URI: 11570 return "uri"; 11571 case URL: 11572 return "url"; 11573 case UUID: 11574 return "uuid"; 11575 case XHTML: 11576 return "XHTML"; 11577 case ACCOUNT: 11578 return "Account"; 11579 case ACTIVITYDEFINITION: 11580 return "ActivityDefinition"; 11581 case ADVERSEEVENT: 11582 return "AdverseEvent"; 11583 case ALLERGYINTOLERANCE: 11584 return "AllergyIntolerance"; 11585 case APPOINTMENT: 11586 return "Appointment"; 11587 case APPOINTMENTRESPONSE: 11588 return "AppointmentResponse"; 11589 case AUDITEVENT: 11590 return "AuditEvent"; 11591 case BASIC: 11592 return "Basic"; 11593 case BINARY: 11594 return "Binary"; 11595 case BIOLOGICALLYDERIVEDPRODUCT: 11596 return "BiologicallyDerivedProduct"; 11597 case BODYSTRUCTURE: 11598 return "BodyStructure"; 11599 case BUNDLE: 11600 return "Bundle"; 11601 case CAPABILITYSTATEMENT: 11602 return "CapabilityStatement"; 11603 case CAREPLAN: 11604 return "CarePlan"; 11605 case CARETEAM: 11606 return "CareTeam"; 11607 case CATALOGENTRY: 11608 return "CatalogEntry"; 11609 case CHARGEITEM: 11610 return "ChargeItem"; 11611 case CHARGEITEMDEFINITION: 11612 return "ChargeItemDefinition"; 11613 case CLAIM: 11614 return "Claim"; 11615 case CLAIMRESPONSE: 11616 return "ClaimResponse"; 11617 case CLINICALIMPRESSION: 11618 return "ClinicalImpression"; 11619 case CODESYSTEM: 11620 return "CodeSystem"; 11621 case COMMUNICATION: 11622 return "Communication"; 11623 case COMMUNICATIONREQUEST: 11624 return "CommunicationRequest"; 11625 case COMPARTMENTDEFINITION: 11626 return "CompartmentDefinition"; 11627 case COMPOSITION: 11628 return "Composition"; 11629 case CONCEPTMAP: 11630 return "ConceptMap"; 11631 case CONDITION: 11632 return "Condition"; 11633 case CONSENT: 11634 return "Consent"; 11635 case CONTRACT: 11636 return "Contract"; 11637 case COVERAGE: 11638 return "Coverage"; 11639 case COVERAGEELIGIBILITYREQUEST: 11640 return "CoverageEligibilityRequest"; 11641 case COVERAGEELIGIBILITYRESPONSE: 11642 return "CoverageEligibilityResponse"; 11643 case DETECTEDISSUE: 11644 return "DetectedIssue"; 11645 case DEVICE: 11646 return "Device"; 11647 case DEVICEDEFINITION: 11648 return "DeviceDefinition"; 11649 case DEVICEMETRIC: 11650 return "DeviceMetric"; 11651 case DEVICEREQUEST: 11652 return "DeviceRequest"; 11653 case DEVICEUSESTATEMENT: 11654 return "DeviceUseStatement"; 11655 case DIAGNOSTICREPORT: 11656 return "DiagnosticReport"; 11657 case DOCUMENTMANIFEST: 11658 return "DocumentManifest"; 11659 case DOCUMENTREFERENCE: 11660 return "DocumentReference"; 11661 case DOMAINRESOURCE: 11662 return "DomainResource"; 11663 case EFFECTEVIDENCESYNTHESIS: 11664 return "EffectEvidenceSynthesis"; 11665 case ENCOUNTER: 11666 return "Encounter"; 11667 case ENDPOINT: 11668 return "Endpoint"; 11669 case ENROLLMENTREQUEST: 11670 return "EnrollmentRequest"; 11671 case ENROLLMENTRESPONSE: 11672 return "EnrollmentResponse"; 11673 case EPISODEOFCARE: 11674 return "EpisodeOfCare"; 11675 case EVENTDEFINITION: 11676 return "EventDefinition"; 11677 case EVIDENCE: 11678 return "Evidence"; 11679 case EVIDENCEVARIABLE: 11680 return "EvidenceVariable"; 11681 case EXAMPLESCENARIO: 11682 return "ExampleScenario"; 11683 case EXPLANATIONOFBENEFIT: 11684 return "ExplanationOfBenefit"; 11685 case FAMILYMEMBERHISTORY: 11686 return "FamilyMemberHistory"; 11687 case FLAG: 11688 return "Flag"; 11689 case GOAL: 11690 return "Goal"; 11691 case GRAPHDEFINITION: 11692 return "GraphDefinition"; 11693 case GROUP: 11694 return "Group"; 11695 case GUIDANCERESPONSE: 11696 return "GuidanceResponse"; 11697 case HEALTHCARESERVICE: 11698 return "HealthcareService"; 11699 case IMAGINGSTUDY: 11700 return "ImagingStudy"; 11701 case IMMUNIZATION: 11702 return "Immunization"; 11703 case IMMUNIZATIONEVALUATION: 11704 return "ImmunizationEvaluation"; 11705 case IMMUNIZATIONRECOMMENDATION: 11706 return "ImmunizationRecommendation"; 11707 case IMPLEMENTATIONGUIDE: 11708 return "ImplementationGuide"; 11709 case INSURANCEPLAN: 11710 return "InsurancePlan"; 11711 case INVOICE: 11712 return "Invoice"; 11713 case LIBRARY: 11714 return "Library"; 11715 case LINKAGE: 11716 return "Linkage"; 11717 case LIST: 11718 return "List"; 11719 case LOCATION: 11720 return "Location"; 11721 case MEASURE: 11722 return "Measure"; 11723 case MEASUREREPORT: 11724 return "MeasureReport"; 11725 case MEDIA: 11726 return "Media"; 11727 case MEDICATION: 11728 return "Medication"; 11729 case MEDICATIONADMINISTRATION: 11730 return "MedicationAdministration"; 11731 case MEDICATIONDISPENSE: 11732 return "MedicationDispense"; 11733 case MEDICATIONKNOWLEDGE: 11734 return "MedicationKnowledge"; 11735 case MEDICATIONREQUEST: 11736 return "MedicationRequest"; 11737 case MEDICATIONSTATEMENT: 11738 return "MedicationStatement"; 11739 case MEDICINALPRODUCT: 11740 return "MedicinalProduct"; 11741 case MEDICINALPRODUCTAUTHORIZATION: 11742 return "MedicinalProductAuthorization"; 11743 case MEDICINALPRODUCTCONTRAINDICATION: 11744 return "MedicinalProductContraindication"; 11745 case MEDICINALPRODUCTINDICATION: 11746 return "MedicinalProductIndication"; 11747 case MEDICINALPRODUCTINGREDIENT: 11748 return "MedicinalProductIngredient"; 11749 case MEDICINALPRODUCTINTERACTION: 11750 return "MedicinalProductInteraction"; 11751 case MEDICINALPRODUCTMANUFACTURED: 11752 return "MedicinalProductManufactured"; 11753 case MEDICINALPRODUCTPACKAGED: 11754 return "MedicinalProductPackaged"; 11755 case MEDICINALPRODUCTPHARMACEUTICAL: 11756 return "MedicinalProductPharmaceutical"; 11757 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 11758 return "MedicinalProductUndesirableEffect"; 11759 case MESSAGEDEFINITION: 11760 return "MessageDefinition"; 11761 case MESSAGEHEADER: 11762 return "MessageHeader"; 11763 case MOLECULARSEQUENCE: 11764 return "MolecularSequence"; 11765 case NAMINGSYSTEM: 11766 return "NamingSystem"; 11767 case NUTRITIONORDER: 11768 return "NutritionOrder"; 11769 case OBSERVATION: 11770 return "Observation"; 11771 case OBSERVATIONDEFINITION: 11772 return "ObservationDefinition"; 11773 case OPERATIONDEFINITION: 11774 return "OperationDefinition"; 11775 case OPERATIONOUTCOME: 11776 return "OperationOutcome"; 11777 case ORGANIZATION: 11778 return "Organization"; 11779 case ORGANIZATIONAFFILIATION: 11780 return "OrganizationAffiliation"; 11781 case PARAMETERS: 11782 return "Parameters"; 11783 case PATIENT: 11784 return "Patient"; 11785 case PAYMENTNOTICE: 11786 return "PaymentNotice"; 11787 case PAYMENTRECONCILIATION: 11788 return "PaymentReconciliation"; 11789 case PERSON: 11790 return "Person"; 11791 case PLANDEFINITION: 11792 return "PlanDefinition"; 11793 case PRACTITIONER: 11794 return "Practitioner"; 11795 case PRACTITIONERROLE: 11796 return "PractitionerRole"; 11797 case PROCEDURE: 11798 return "Procedure"; 11799 case PROVENANCE: 11800 return "Provenance"; 11801 case QUESTIONNAIRE: 11802 return "Questionnaire"; 11803 case QUESTIONNAIRERESPONSE: 11804 return "QuestionnaireResponse"; 11805 case RELATEDPERSON: 11806 return "RelatedPerson"; 11807 case REQUESTGROUP: 11808 return "RequestGroup"; 11809 case RESEARCHDEFINITION: 11810 return "ResearchDefinition"; 11811 case RESEARCHELEMENTDEFINITION: 11812 return "ResearchElementDefinition"; 11813 case RESEARCHSTUDY: 11814 return "ResearchStudy"; 11815 case RESEARCHSUBJECT: 11816 return "ResearchSubject"; 11817 case RESOURCE: 11818 return "Resource"; 11819 case RISKASSESSMENT: 11820 return "RiskAssessment"; 11821 case RISKEVIDENCESYNTHESIS: 11822 return "RiskEvidenceSynthesis"; 11823 case SCHEDULE: 11824 return "Schedule"; 11825 case SEARCHPARAMETER: 11826 return "SearchParameter"; 11827 case SERVICEREQUEST: 11828 return "ServiceRequest"; 11829 case SLOT: 11830 return "Slot"; 11831 case SPECIMEN: 11832 return "Specimen"; 11833 case SPECIMENDEFINITION: 11834 return "SpecimenDefinition"; 11835 case STRUCTUREDEFINITION: 11836 return "StructureDefinition"; 11837 case STRUCTUREMAP: 11838 return "StructureMap"; 11839 case SUBSCRIPTION: 11840 return "Subscription"; 11841 case SUBSTANCE: 11842 return "Substance"; 11843 case SUBSTANCENUCLEICACID: 11844 return "SubstanceNucleicAcid"; 11845 case SUBSTANCEPOLYMER: 11846 return "SubstancePolymer"; 11847 case SUBSTANCEPROTEIN: 11848 return "SubstanceProtein"; 11849 case SUBSTANCEREFERENCEINFORMATION: 11850 return "SubstanceReferenceInformation"; 11851 case SUBSTANCESOURCEMATERIAL: 11852 return "SubstanceSourceMaterial"; 11853 case SUBSTANCESPECIFICATION: 11854 return "SubstanceSpecification"; 11855 case SUPPLYDELIVERY: 11856 return "SupplyDelivery"; 11857 case SUPPLYREQUEST: 11858 return "SupplyRequest"; 11859 case TASK: 11860 return "Task"; 11861 case TERMINOLOGYCAPABILITIES: 11862 return "TerminologyCapabilities"; 11863 case TESTREPORT: 11864 return "TestReport"; 11865 case TESTSCRIPT: 11866 return "TestScript"; 11867 case VALUESET: 11868 return "ValueSet"; 11869 case VERIFICATIONRESULT: 11870 return "VerificationResult"; 11871 case VISIONPRESCRIPTION: 11872 return "VisionPrescription"; 11873 case NULL: 11874 return null; 11875 default: 11876 return "?"; 11877 } 11878 } 11879 } 11880 11881 public static class FHIRDefinedTypeEnumFactory implements EnumFactory<FHIRDefinedType> { 11882 public FHIRDefinedType fromCode(String codeString) throws IllegalArgumentException { 11883 if (codeString == null || "".equals(codeString)) 11884 if (codeString == null || "".equals(codeString)) 11885 return null; 11886 if ("Address".equals(codeString)) 11887 return FHIRDefinedType.ADDRESS; 11888 if ("Age".equals(codeString)) 11889 return FHIRDefinedType.AGE; 11890 if ("Annotation".equals(codeString)) 11891 return FHIRDefinedType.ANNOTATION; 11892 if ("Attachment".equals(codeString)) 11893 return FHIRDefinedType.ATTACHMENT; 11894 if ("BackboneElement".equals(codeString)) 11895 return FHIRDefinedType.BACKBONEELEMENT; 11896 if ("CodeableConcept".equals(codeString)) 11897 return FHIRDefinedType.CODEABLECONCEPT; 11898 if ("Coding".equals(codeString)) 11899 return FHIRDefinedType.CODING; 11900 if ("ContactDetail".equals(codeString)) 11901 return FHIRDefinedType.CONTACTDETAIL; 11902 if ("ContactPoint".equals(codeString)) 11903 return FHIRDefinedType.CONTACTPOINT; 11904 if ("Contributor".equals(codeString)) 11905 return FHIRDefinedType.CONTRIBUTOR; 11906 if ("Count".equals(codeString)) 11907 return FHIRDefinedType.COUNT; 11908 if ("DataRequirement".equals(codeString)) 11909 return FHIRDefinedType.DATAREQUIREMENT; 11910 if ("Distance".equals(codeString)) 11911 return FHIRDefinedType.DISTANCE; 11912 if ("Dosage".equals(codeString)) 11913 return FHIRDefinedType.DOSAGE; 11914 if ("Duration".equals(codeString)) 11915 return FHIRDefinedType.DURATION; 11916 if ("Element".equals(codeString)) 11917 return FHIRDefinedType.ELEMENT; 11918 if ("ElementDefinition".equals(codeString)) 11919 return FHIRDefinedType.ELEMENTDEFINITION; 11920 if ("Expression".equals(codeString)) 11921 return FHIRDefinedType.EXPRESSION; 11922 if ("Extension".equals(codeString)) 11923 return FHIRDefinedType.EXTENSION; 11924 if ("HumanName".equals(codeString)) 11925 return FHIRDefinedType.HUMANNAME; 11926 if ("Identifier".equals(codeString)) 11927 return FHIRDefinedType.IDENTIFIER; 11928 if ("MarketingStatus".equals(codeString)) 11929 return FHIRDefinedType.MARKETINGSTATUS; 11930 if ("Meta".equals(codeString)) 11931 return FHIRDefinedType.META; 11932 if ("Money".equals(codeString)) 11933 return FHIRDefinedType.MONEY; 11934 if ("MoneyQuantity".equals(codeString)) 11935 return FHIRDefinedType.MONEYQUANTITY; 11936 if ("Narrative".equals(codeString)) 11937 return FHIRDefinedType.NARRATIVE; 11938 if ("ParameterDefinition".equals(codeString)) 11939 return FHIRDefinedType.PARAMETERDEFINITION; 11940 if ("Period".equals(codeString)) 11941 return FHIRDefinedType.PERIOD; 11942 if ("Population".equals(codeString)) 11943 return FHIRDefinedType.POPULATION; 11944 if ("ProdCharacteristic".equals(codeString)) 11945 return FHIRDefinedType.PRODCHARACTERISTIC; 11946 if ("ProductShelfLife".equals(codeString)) 11947 return FHIRDefinedType.PRODUCTSHELFLIFE; 11948 if ("Quantity".equals(codeString)) 11949 return FHIRDefinedType.QUANTITY; 11950 if ("Range".equals(codeString)) 11951 return FHIRDefinedType.RANGE; 11952 if ("Ratio".equals(codeString)) 11953 return FHIRDefinedType.RATIO; 11954 if ("Reference".equals(codeString)) 11955 return FHIRDefinedType.REFERENCE; 11956 if ("RelatedArtifact".equals(codeString)) 11957 return FHIRDefinedType.RELATEDARTIFACT; 11958 if ("SampledData".equals(codeString)) 11959 return FHIRDefinedType.SAMPLEDDATA; 11960 if ("Signature".equals(codeString)) 11961 return FHIRDefinedType.SIGNATURE; 11962 if ("SimpleQuantity".equals(codeString)) 11963 return FHIRDefinedType.SIMPLEQUANTITY; 11964 if ("SubstanceAmount".equals(codeString)) 11965 return FHIRDefinedType.SUBSTANCEAMOUNT; 11966 if ("Timing".equals(codeString)) 11967 return FHIRDefinedType.TIMING; 11968 if ("TriggerDefinition".equals(codeString)) 11969 return FHIRDefinedType.TRIGGERDEFINITION; 11970 if ("UsageContext".equals(codeString)) 11971 return FHIRDefinedType.USAGECONTEXT; 11972 if ("base64Binary".equals(codeString)) 11973 return FHIRDefinedType.BASE64BINARY; 11974 if ("boolean".equals(codeString)) 11975 return FHIRDefinedType.BOOLEAN; 11976 if ("canonical".equals(codeString)) 11977 return FHIRDefinedType.CANONICAL; 11978 if ("code".equals(codeString)) 11979 return FHIRDefinedType.CODE; 11980 if ("date".equals(codeString)) 11981 return FHIRDefinedType.DATE; 11982 if ("dateTime".equals(codeString)) 11983 return FHIRDefinedType.DATETIME; 11984 if ("decimal".equals(codeString)) 11985 return FHIRDefinedType.DECIMAL; 11986 if ("id".equals(codeString)) 11987 return FHIRDefinedType.ID; 11988 if ("instant".equals(codeString)) 11989 return FHIRDefinedType.INSTANT; 11990 if ("integer".equals(codeString)) 11991 return FHIRDefinedType.INTEGER; 11992 if ("markdown".equals(codeString)) 11993 return FHIRDefinedType.MARKDOWN; 11994 if ("oid".equals(codeString)) 11995 return FHIRDefinedType.OID; 11996 if ("positiveInt".equals(codeString)) 11997 return FHIRDefinedType.POSITIVEINT; 11998 if ("string".equals(codeString)) 11999 return FHIRDefinedType.STRING; 12000 if ("time".equals(codeString)) 12001 return FHIRDefinedType.TIME; 12002 if ("unsignedInt".equals(codeString)) 12003 return FHIRDefinedType.UNSIGNEDINT; 12004 if ("uri".equals(codeString)) 12005 return FHIRDefinedType.URI; 12006 if ("url".equals(codeString)) 12007 return FHIRDefinedType.URL; 12008 if ("uuid".equals(codeString)) 12009 return FHIRDefinedType.UUID; 12010 if ("xhtml".equals(codeString)) 12011 return FHIRDefinedType.XHTML; 12012 if ("Account".equals(codeString)) 12013 return FHIRDefinedType.ACCOUNT; 12014 if ("ActivityDefinition".equals(codeString)) 12015 return FHIRDefinedType.ACTIVITYDEFINITION; 12016 if ("AdverseEvent".equals(codeString)) 12017 return FHIRDefinedType.ADVERSEEVENT; 12018 if ("AllergyIntolerance".equals(codeString)) 12019 return FHIRDefinedType.ALLERGYINTOLERANCE; 12020 if ("Appointment".equals(codeString)) 12021 return FHIRDefinedType.APPOINTMENT; 12022 if ("AppointmentResponse".equals(codeString)) 12023 return FHIRDefinedType.APPOINTMENTRESPONSE; 12024 if ("AuditEvent".equals(codeString)) 12025 return FHIRDefinedType.AUDITEVENT; 12026 if ("Basic".equals(codeString)) 12027 return FHIRDefinedType.BASIC; 12028 if ("Binary".equals(codeString)) 12029 return FHIRDefinedType.BINARY; 12030 if ("BiologicallyDerivedProduct".equals(codeString)) 12031 return FHIRDefinedType.BIOLOGICALLYDERIVEDPRODUCT; 12032 if ("BodyStructure".equals(codeString)) 12033 return FHIRDefinedType.BODYSTRUCTURE; 12034 if ("Bundle".equals(codeString)) 12035 return FHIRDefinedType.BUNDLE; 12036 if ("CapabilityStatement".equals(codeString)) 12037 return FHIRDefinedType.CAPABILITYSTATEMENT; 12038 if ("CarePlan".equals(codeString)) 12039 return FHIRDefinedType.CAREPLAN; 12040 if ("CareTeam".equals(codeString)) 12041 return FHIRDefinedType.CARETEAM; 12042 if ("CatalogEntry".equals(codeString)) 12043 return FHIRDefinedType.CATALOGENTRY; 12044 if ("ChargeItem".equals(codeString)) 12045 return FHIRDefinedType.CHARGEITEM; 12046 if ("ChargeItemDefinition".equals(codeString)) 12047 return FHIRDefinedType.CHARGEITEMDEFINITION; 12048 if ("Claim".equals(codeString)) 12049 return FHIRDefinedType.CLAIM; 12050 if ("ClaimResponse".equals(codeString)) 12051 return FHIRDefinedType.CLAIMRESPONSE; 12052 if ("ClinicalImpression".equals(codeString)) 12053 return FHIRDefinedType.CLINICALIMPRESSION; 12054 if ("CodeSystem".equals(codeString)) 12055 return FHIRDefinedType.CODESYSTEM; 12056 if ("Communication".equals(codeString)) 12057 return FHIRDefinedType.COMMUNICATION; 12058 if ("CommunicationRequest".equals(codeString)) 12059 return FHIRDefinedType.COMMUNICATIONREQUEST; 12060 if ("CompartmentDefinition".equals(codeString)) 12061 return FHIRDefinedType.COMPARTMENTDEFINITION; 12062 if ("Composition".equals(codeString)) 12063 return FHIRDefinedType.COMPOSITION; 12064 if ("ConceptMap".equals(codeString)) 12065 return FHIRDefinedType.CONCEPTMAP; 12066 if ("Condition".equals(codeString)) 12067 return FHIRDefinedType.CONDITION; 12068 if ("Consent".equals(codeString)) 12069 return FHIRDefinedType.CONSENT; 12070 if ("Contract".equals(codeString)) 12071 return FHIRDefinedType.CONTRACT; 12072 if ("Coverage".equals(codeString)) 12073 return FHIRDefinedType.COVERAGE; 12074 if ("CoverageEligibilityRequest".equals(codeString)) 12075 return FHIRDefinedType.COVERAGEELIGIBILITYREQUEST; 12076 if ("CoverageEligibilityResponse".equals(codeString)) 12077 return FHIRDefinedType.COVERAGEELIGIBILITYRESPONSE; 12078 if ("DetectedIssue".equals(codeString)) 12079 return FHIRDefinedType.DETECTEDISSUE; 12080 if ("Device".equals(codeString)) 12081 return FHIRDefinedType.DEVICE; 12082 if ("DeviceDefinition".equals(codeString)) 12083 return FHIRDefinedType.DEVICEDEFINITION; 12084 if ("DeviceMetric".equals(codeString)) 12085 return FHIRDefinedType.DEVICEMETRIC; 12086 if ("DeviceRequest".equals(codeString)) 12087 return FHIRDefinedType.DEVICEREQUEST; 12088 if ("DeviceUseStatement".equals(codeString)) 12089 return FHIRDefinedType.DEVICEUSESTATEMENT; 12090 if ("DiagnosticReport".equals(codeString)) 12091 return FHIRDefinedType.DIAGNOSTICREPORT; 12092 if ("DocumentManifest".equals(codeString)) 12093 return FHIRDefinedType.DOCUMENTMANIFEST; 12094 if ("DocumentReference".equals(codeString)) 12095 return FHIRDefinedType.DOCUMENTREFERENCE; 12096 if ("DomainResource".equals(codeString)) 12097 return FHIRDefinedType.DOMAINRESOURCE; 12098 if ("EffectEvidenceSynthesis".equals(codeString)) 12099 return FHIRDefinedType.EFFECTEVIDENCESYNTHESIS; 12100 if ("Encounter".equals(codeString)) 12101 return FHIRDefinedType.ENCOUNTER; 12102 if ("Endpoint".equals(codeString)) 12103 return FHIRDefinedType.ENDPOINT; 12104 if ("EnrollmentRequest".equals(codeString)) 12105 return FHIRDefinedType.ENROLLMENTREQUEST; 12106 if ("EnrollmentResponse".equals(codeString)) 12107 return FHIRDefinedType.ENROLLMENTRESPONSE; 12108 if ("EpisodeOfCare".equals(codeString)) 12109 return FHIRDefinedType.EPISODEOFCARE; 12110 if ("EventDefinition".equals(codeString)) 12111 return FHIRDefinedType.EVENTDEFINITION; 12112 if ("Evidence".equals(codeString)) 12113 return FHIRDefinedType.EVIDENCE; 12114 if ("EvidenceVariable".equals(codeString)) 12115 return FHIRDefinedType.EVIDENCEVARIABLE; 12116 if ("ExampleScenario".equals(codeString)) 12117 return FHIRDefinedType.EXAMPLESCENARIO; 12118 if ("ExplanationOfBenefit".equals(codeString)) 12119 return FHIRDefinedType.EXPLANATIONOFBENEFIT; 12120 if ("FamilyMemberHistory".equals(codeString)) 12121 return FHIRDefinedType.FAMILYMEMBERHISTORY; 12122 if ("Flag".equals(codeString)) 12123 return FHIRDefinedType.FLAG; 12124 if ("Goal".equals(codeString)) 12125 return FHIRDefinedType.GOAL; 12126 if ("GraphDefinition".equals(codeString)) 12127 return FHIRDefinedType.GRAPHDEFINITION; 12128 if ("Group".equals(codeString)) 12129 return FHIRDefinedType.GROUP; 12130 if ("GuidanceResponse".equals(codeString)) 12131 return FHIRDefinedType.GUIDANCERESPONSE; 12132 if ("HealthcareService".equals(codeString)) 12133 return FHIRDefinedType.HEALTHCARESERVICE; 12134 if ("ImagingStudy".equals(codeString)) 12135 return FHIRDefinedType.IMAGINGSTUDY; 12136 if ("Immunization".equals(codeString)) 12137 return FHIRDefinedType.IMMUNIZATION; 12138 if ("ImmunizationEvaluation".equals(codeString)) 12139 return FHIRDefinedType.IMMUNIZATIONEVALUATION; 12140 if ("ImmunizationRecommendation".equals(codeString)) 12141 return FHIRDefinedType.IMMUNIZATIONRECOMMENDATION; 12142 if ("ImplementationGuide".equals(codeString)) 12143 return FHIRDefinedType.IMPLEMENTATIONGUIDE; 12144 if ("InsurancePlan".equals(codeString)) 12145 return FHIRDefinedType.INSURANCEPLAN; 12146 if ("Invoice".equals(codeString)) 12147 return FHIRDefinedType.INVOICE; 12148 if ("Library".equals(codeString)) 12149 return FHIRDefinedType.LIBRARY; 12150 if ("Linkage".equals(codeString)) 12151 return FHIRDefinedType.LINKAGE; 12152 if ("List".equals(codeString)) 12153 return FHIRDefinedType.LIST; 12154 if ("Location".equals(codeString)) 12155 return FHIRDefinedType.LOCATION; 12156 if ("Measure".equals(codeString)) 12157 return FHIRDefinedType.MEASURE; 12158 if ("MeasureReport".equals(codeString)) 12159 return FHIRDefinedType.MEASUREREPORT; 12160 if ("Media".equals(codeString)) 12161 return FHIRDefinedType.MEDIA; 12162 if ("Medication".equals(codeString)) 12163 return FHIRDefinedType.MEDICATION; 12164 if ("MedicationAdministration".equals(codeString)) 12165 return FHIRDefinedType.MEDICATIONADMINISTRATION; 12166 if ("MedicationDispense".equals(codeString)) 12167 return FHIRDefinedType.MEDICATIONDISPENSE; 12168 if ("MedicationKnowledge".equals(codeString)) 12169 return FHIRDefinedType.MEDICATIONKNOWLEDGE; 12170 if ("MedicationRequest".equals(codeString)) 12171 return FHIRDefinedType.MEDICATIONREQUEST; 12172 if ("MedicationStatement".equals(codeString)) 12173 return FHIRDefinedType.MEDICATIONSTATEMENT; 12174 if ("MedicinalProduct".equals(codeString)) 12175 return FHIRDefinedType.MEDICINALPRODUCT; 12176 if ("MedicinalProductAuthorization".equals(codeString)) 12177 return FHIRDefinedType.MEDICINALPRODUCTAUTHORIZATION; 12178 if ("MedicinalProductContraindication".equals(codeString)) 12179 return FHIRDefinedType.MEDICINALPRODUCTCONTRAINDICATION; 12180 if ("MedicinalProductIndication".equals(codeString)) 12181 return FHIRDefinedType.MEDICINALPRODUCTINDICATION; 12182 if ("MedicinalProductIngredient".equals(codeString)) 12183 return FHIRDefinedType.MEDICINALPRODUCTINGREDIENT; 12184 if ("MedicinalProductInteraction".equals(codeString)) 12185 return FHIRDefinedType.MEDICINALPRODUCTINTERACTION; 12186 if ("MedicinalProductManufactured".equals(codeString)) 12187 return FHIRDefinedType.MEDICINALPRODUCTMANUFACTURED; 12188 if ("MedicinalProductPackaged".equals(codeString)) 12189 return FHIRDefinedType.MEDICINALPRODUCTPACKAGED; 12190 if ("MedicinalProductPharmaceutical".equals(codeString)) 12191 return FHIRDefinedType.MEDICINALPRODUCTPHARMACEUTICAL; 12192 if ("MedicinalProductUndesirableEffect".equals(codeString)) 12193 return FHIRDefinedType.MEDICINALPRODUCTUNDESIRABLEEFFECT; 12194 if ("MessageDefinition".equals(codeString)) 12195 return FHIRDefinedType.MESSAGEDEFINITION; 12196 if ("MessageHeader".equals(codeString)) 12197 return FHIRDefinedType.MESSAGEHEADER; 12198 if ("MolecularSequence".equals(codeString)) 12199 return FHIRDefinedType.MOLECULARSEQUENCE; 12200 if ("NamingSystem".equals(codeString)) 12201 return FHIRDefinedType.NAMINGSYSTEM; 12202 if ("NutritionOrder".equals(codeString)) 12203 return FHIRDefinedType.NUTRITIONORDER; 12204 if ("Observation".equals(codeString)) 12205 return FHIRDefinedType.OBSERVATION; 12206 if ("ObservationDefinition".equals(codeString)) 12207 return FHIRDefinedType.OBSERVATIONDEFINITION; 12208 if ("OperationDefinition".equals(codeString)) 12209 return FHIRDefinedType.OPERATIONDEFINITION; 12210 if ("OperationOutcome".equals(codeString)) 12211 return FHIRDefinedType.OPERATIONOUTCOME; 12212 if ("Organization".equals(codeString)) 12213 return FHIRDefinedType.ORGANIZATION; 12214 if ("OrganizationAffiliation".equals(codeString)) 12215 return FHIRDefinedType.ORGANIZATIONAFFILIATION; 12216 if ("Parameters".equals(codeString)) 12217 return FHIRDefinedType.PARAMETERS; 12218 if ("Patient".equals(codeString)) 12219 return FHIRDefinedType.PATIENT; 12220 if ("PaymentNotice".equals(codeString)) 12221 return FHIRDefinedType.PAYMENTNOTICE; 12222 if ("PaymentReconciliation".equals(codeString)) 12223 return FHIRDefinedType.PAYMENTRECONCILIATION; 12224 if ("Person".equals(codeString)) 12225 return FHIRDefinedType.PERSON; 12226 if ("PlanDefinition".equals(codeString)) 12227 return FHIRDefinedType.PLANDEFINITION; 12228 if ("Practitioner".equals(codeString)) 12229 return FHIRDefinedType.PRACTITIONER; 12230 if ("PractitionerRole".equals(codeString)) 12231 return FHIRDefinedType.PRACTITIONERROLE; 12232 if ("Procedure".equals(codeString)) 12233 return FHIRDefinedType.PROCEDURE; 12234 if ("Provenance".equals(codeString)) 12235 return FHIRDefinedType.PROVENANCE; 12236 if ("Questionnaire".equals(codeString)) 12237 return FHIRDefinedType.QUESTIONNAIRE; 12238 if ("QuestionnaireResponse".equals(codeString)) 12239 return FHIRDefinedType.QUESTIONNAIRERESPONSE; 12240 if ("RelatedPerson".equals(codeString)) 12241 return FHIRDefinedType.RELATEDPERSON; 12242 if ("RequestGroup".equals(codeString)) 12243 return FHIRDefinedType.REQUESTGROUP; 12244 if ("ResearchDefinition".equals(codeString)) 12245 return FHIRDefinedType.RESEARCHDEFINITION; 12246 if ("ResearchElementDefinition".equals(codeString)) 12247 return FHIRDefinedType.RESEARCHELEMENTDEFINITION; 12248 if ("ResearchStudy".equals(codeString)) 12249 return FHIRDefinedType.RESEARCHSTUDY; 12250 if ("ResearchSubject".equals(codeString)) 12251 return FHIRDefinedType.RESEARCHSUBJECT; 12252 if ("Resource".equals(codeString)) 12253 return FHIRDefinedType.RESOURCE; 12254 if ("RiskAssessment".equals(codeString)) 12255 return FHIRDefinedType.RISKASSESSMENT; 12256 if ("RiskEvidenceSynthesis".equals(codeString)) 12257 return FHIRDefinedType.RISKEVIDENCESYNTHESIS; 12258 if ("Schedule".equals(codeString)) 12259 return FHIRDefinedType.SCHEDULE; 12260 if ("SearchParameter".equals(codeString)) 12261 return FHIRDefinedType.SEARCHPARAMETER; 12262 if ("ServiceRequest".equals(codeString)) 12263 return FHIRDefinedType.SERVICEREQUEST; 12264 if ("Slot".equals(codeString)) 12265 return FHIRDefinedType.SLOT; 12266 if ("Specimen".equals(codeString)) 12267 return FHIRDefinedType.SPECIMEN; 12268 if ("SpecimenDefinition".equals(codeString)) 12269 return FHIRDefinedType.SPECIMENDEFINITION; 12270 if ("StructureDefinition".equals(codeString)) 12271 return FHIRDefinedType.STRUCTUREDEFINITION; 12272 if ("StructureMap".equals(codeString)) 12273 return FHIRDefinedType.STRUCTUREMAP; 12274 if ("Subscription".equals(codeString)) 12275 return FHIRDefinedType.SUBSCRIPTION; 12276 if ("Substance".equals(codeString)) 12277 return FHIRDefinedType.SUBSTANCE; 12278 if ("SubstanceNucleicAcid".equals(codeString)) 12279 return FHIRDefinedType.SUBSTANCENUCLEICACID; 12280 if ("SubstancePolymer".equals(codeString)) 12281 return FHIRDefinedType.SUBSTANCEPOLYMER; 12282 if ("SubstanceProtein".equals(codeString)) 12283 return FHIRDefinedType.SUBSTANCEPROTEIN; 12284 if ("SubstanceReferenceInformation".equals(codeString)) 12285 return FHIRDefinedType.SUBSTANCEREFERENCEINFORMATION; 12286 if ("SubstanceSourceMaterial".equals(codeString)) 12287 return FHIRDefinedType.SUBSTANCESOURCEMATERIAL; 12288 if ("SubstanceSpecification".equals(codeString)) 12289 return FHIRDefinedType.SUBSTANCESPECIFICATION; 12290 if ("SupplyDelivery".equals(codeString)) 12291 return FHIRDefinedType.SUPPLYDELIVERY; 12292 if ("SupplyRequest".equals(codeString)) 12293 return FHIRDefinedType.SUPPLYREQUEST; 12294 if ("Task".equals(codeString)) 12295 return FHIRDefinedType.TASK; 12296 if ("TerminologyCapabilities".equals(codeString)) 12297 return FHIRDefinedType.TERMINOLOGYCAPABILITIES; 12298 if ("TestReport".equals(codeString)) 12299 return FHIRDefinedType.TESTREPORT; 12300 if ("TestScript".equals(codeString)) 12301 return FHIRDefinedType.TESTSCRIPT; 12302 if ("ValueSet".equals(codeString)) 12303 return FHIRDefinedType.VALUESET; 12304 if ("VerificationResult".equals(codeString)) 12305 return FHIRDefinedType.VERIFICATIONRESULT; 12306 if ("VisionPrescription".equals(codeString)) 12307 return FHIRDefinedType.VISIONPRESCRIPTION; 12308 throw new IllegalArgumentException("Unknown FHIRDefinedType code '" + codeString + "'"); 12309 } 12310 12311 public Enumeration<FHIRDefinedType> fromType(PrimitiveType<?> code) throws FHIRException { 12312 if (code == null) 12313 return null; 12314 if (code.isEmpty()) 12315 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.NULL, code); 12316 String codeString = code.asStringValue(); 12317 if (codeString == null || "".equals(codeString)) 12318 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.NULL, code); 12319 if ("Address".equals(codeString)) 12320 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ADDRESS, code); 12321 if ("Age".equals(codeString)) 12322 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.AGE, code); 12323 if ("Annotation".equals(codeString)) 12324 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ANNOTATION, code); 12325 if ("Attachment".equals(codeString)) 12326 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ATTACHMENT, code); 12327 if ("BackboneElement".equals(codeString)) 12328 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BACKBONEELEMENT, code); 12329 if ("CodeableConcept".equals(codeString)) 12330 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CODEABLECONCEPT, code); 12331 if ("Coding".equals(codeString)) 12332 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CODING, code); 12333 if ("ContactDetail".equals(codeString)) 12334 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONTACTDETAIL, code); 12335 if ("ContactPoint".equals(codeString)) 12336 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONTACTPOINT, code); 12337 if ("Contributor".equals(codeString)) 12338 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONTRIBUTOR, code); 12339 if ("Count".equals(codeString)) 12340 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COUNT, code); 12341 if ("DataRequirement".equals(codeString)) 12342 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DATAREQUIREMENT, code); 12343 if ("Distance".equals(codeString)) 12344 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DISTANCE, code); 12345 if ("Dosage".equals(codeString)) 12346 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DOSAGE, code); 12347 if ("Duration".equals(codeString)) 12348 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DURATION, code); 12349 if ("Element".equals(codeString)) 12350 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ELEMENT, code); 12351 if ("ElementDefinition".equals(codeString)) 12352 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ELEMENTDEFINITION, code); 12353 if ("Expression".equals(codeString)) 12354 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EXPRESSION, code); 12355 if ("Extension".equals(codeString)) 12356 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EXTENSION, code); 12357 if ("HumanName".equals(codeString)) 12358 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.HUMANNAME, code); 12359 if ("Identifier".equals(codeString)) 12360 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IDENTIFIER, code); 12361 if ("MarketingStatus".equals(codeString)) 12362 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MARKETINGSTATUS, code); 12363 if ("Meta".equals(codeString)) 12364 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.META, code); 12365 if ("Money".equals(codeString)) 12366 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MONEY, code); 12367 if ("MoneyQuantity".equals(codeString)) 12368 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MONEYQUANTITY, code); 12369 if ("Narrative".equals(codeString)) 12370 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.NARRATIVE, code); 12371 if ("ParameterDefinition".equals(codeString)) 12372 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PARAMETERDEFINITION, code); 12373 if ("Period".equals(codeString)) 12374 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PERIOD, code); 12375 if ("Population".equals(codeString)) 12376 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.POPULATION, code); 12377 if ("ProdCharacteristic".equals(codeString)) 12378 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PRODCHARACTERISTIC, code); 12379 if ("ProductShelfLife".equals(codeString)) 12380 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PRODUCTSHELFLIFE, code); 12381 if ("Quantity".equals(codeString)) 12382 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.QUANTITY, code); 12383 if ("Range".equals(codeString)) 12384 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RANGE, code); 12385 if ("Ratio".equals(codeString)) 12386 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RATIO, code); 12387 if ("Reference".equals(codeString)) 12388 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.REFERENCE, code); 12389 if ("RelatedArtifact".equals(codeString)) 12390 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RELATEDARTIFACT, code); 12391 if ("SampledData".equals(codeString)) 12392 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SAMPLEDDATA, code); 12393 if ("Signature".equals(codeString)) 12394 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SIGNATURE, code); 12395 if ("SimpleQuantity".equals(codeString)) 12396 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SIMPLEQUANTITY, code); 12397 if ("SubstanceAmount".equals(codeString)) 12398 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUBSTANCEAMOUNT, code); 12399 if ("Timing".equals(codeString)) 12400 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TIMING, code); 12401 if ("TriggerDefinition".equals(codeString)) 12402 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TRIGGERDEFINITION, code); 12403 if ("UsageContext".equals(codeString)) 12404 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.USAGECONTEXT, code); 12405 if ("base64Binary".equals(codeString)) 12406 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BASE64BINARY, code); 12407 if ("boolean".equals(codeString)) 12408 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BOOLEAN, code); 12409 if ("canonical".equals(codeString)) 12410 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CANONICAL, code); 12411 if ("code".equals(codeString)) 12412 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CODE, code); 12413 if ("date".equals(codeString)) 12414 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DATE, code); 12415 if ("dateTime".equals(codeString)) 12416 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DATETIME, code); 12417 if ("decimal".equals(codeString)) 12418 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DECIMAL, code); 12419 if ("id".equals(codeString)) 12420 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ID, code); 12421 if ("instant".equals(codeString)) 12422 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.INSTANT, code); 12423 if ("integer".equals(codeString)) 12424 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.INTEGER, code); 12425 if ("markdown".equals(codeString)) 12426 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MARKDOWN, code); 12427 if ("oid".equals(codeString)) 12428 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.OID, code); 12429 if ("positiveInt".equals(codeString)) 12430 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.POSITIVEINT, code); 12431 if ("string".equals(codeString)) 12432 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.STRING, code); 12433 if ("time".equals(codeString)) 12434 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TIME, code); 12435 if ("unsignedInt".equals(codeString)) 12436 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.UNSIGNEDINT, code); 12437 if ("uri".equals(codeString)) 12438 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.URI, code); 12439 if ("url".equals(codeString)) 12440 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.URL, code); 12441 if ("uuid".equals(codeString)) 12442 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.UUID, code); 12443 if ("xhtml".equals(codeString)) 12444 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.XHTML, code); 12445 if ("Account".equals(codeString)) 12446 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ACCOUNT, code); 12447 if ("ActivityDefinition".equals(codeString)) 12448 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ACTIVITYDEFINITION, code); 12449 if ("AdverseEvent".equals(codeString)) 12450 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ADVERSEEVENT, code); 12451 if ("AllergyIntolerance".equals(codeString)) 12452 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ALLERGYINTOLERANCE, code); 12453 if ("Appointment".equals(codeString)) 12454 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.APPOINTMENT, code); 12455 if ("AppointmentResponse".equals(codeString)) 12456 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.APPOINTMENTRESPONSE, code); 12457 if ("AuditEvent".equals(codeString)) 12458 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.AUDITEVENT, code); 12459 if ("Basic".equals(codeString)) 12460 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BASIC, code); 12461 if ("Binary".equals(codeString)) 12462 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BINARY, code); 12463 if ("BiologicallyDerivedProduct".equals(codeString)) 12464 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BIOLOGICALLYDERIVEDPRODUCT, code); 12465 if ("BodyStructure".equals(codeString)) 12466 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BODYSTRUCTURE, code); 12467 if ("Bundle".equals(codeString)) 12468 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BUNDLE, code); 12469 if ("CapabilityStatement".equals(codeString)) 12470 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CAPABILITYSTATEMENT, code); 12471 if ("CarePlan".equals(codeString)) 12472 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CAREPLAN, code); 12473 if ("CareTeam".equals(codeString)) 12474 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CARETEAM, code); 12475 if ("CatalogEntry".equals(codeString)) 12476 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CATALOGENTRY, code); 12477 if ("ChargeItem".equals(codeString)) 12478 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CHARGEITEM, code); 12479 if ("ChargeItemDefinition".equals(codeString)) 12480 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CHARGEITEMDEFINITION, code); 12481 if ("Claim".equals(codeString)) 12482 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CLAIM, code); 12483 if ("ClaimResponse".equals(codeString)) 12484 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CLAIMRESPONSE, code); 12485 if ("ClinicalImpression".equals(codeString)) 12486 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CLINICALIMPRESSION, code); 12487 if ("CodeSystem".equals(codeString)) 12488 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CODESYSTEM, code); 12489 if ("Communication".equals(codeString)) 12490 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COMMUNICATION, code); 12491 if ("CommunicationRequest".equals(codeString)) 12492 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COMMUNICATIONREQUEST, code); 12493 if ("CompartmentDefinition".equals(codeString)) 12494 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COMPARTMENTDEFINITION, code); 12495 if ("Composition".equals(codeString)) 12496 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COMPOSITION, code); 12497 if ("ConceptMap".equals(codeString)) 12498 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONCEPTMAP, code); 12499 if ("Condition".equals(codeString)) 12500 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONDITION, code); 12501 if ("Consent".equals(codeString)) 12502 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONSENT, code); 12503 if ("Contract".equals(codeString)) 12504 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONTRACT, code); 12505 if ("Coverage".equals(codeString)) 12506 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COVERAGE, code); 12507 if ("CoverageEligibilityRequest".equals(codeString)) 12508 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COVERAGEELIGIBILITYREQUEST, code); 12509 if ("CoverageEligibilityResponse".equals(codeString)) 12510 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COVERAGEELIGIBILITYRESPONSE, code); 12511 if ("DetectedIssue".equals(codeString)) 12512 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DETECTEDISSUE, code); 12513 if ("Device".equals(codeString)) 12514 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DEVICE, code); 12515 if ("DeviceDefinition".equals(codeString)) 12516 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DEVICEDEFINITION, code); 12517 if ("DeviceMetric".equals(codeString)) 12518 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DEVICEMETRIC, code); 12519 if ("DeviceRequest".equals(codeString)) 12520 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DEVICEREQUEST, code); 12521 if ("DeviceUseStatement".equals(codeString)) 12522 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DEVICEUSESTATEMENT, code); 12523 if ("DiagnosticReport".equals(codeString)) 12524 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DIAGNOSTICREPORT, code); 12525 if ("DocumentManifest".equals(codeString)) 12526 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DOCUMENTMANIFEST, code); 12527 if ("DocumentReference".equals(codeString)) 12528 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DOCUMENTREFERENCE, code); 12529 if ("DomainResource".equals(codeString)) 12530 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DOMAINRESOURCE, code); 12531 if ("EffectEvidenceSynthesis".equals(codeString)) 12532 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EFFECTEVIDENCESYNTHESIS, code); 12533 if ("Encounter".equals(codeString)) 12534 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ENCOUNTER, code); 12535 if ("Endpoint".equals(codeString)) 12536 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ENDPOINT, code); 12537 if ("EnrollmentRequest".equals(codeString)) 12538 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ENROLLMENTREQUEST, code); 12539 if ("EnrollmentResponse".equals(codeString)) 12540 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ENROLLMENTRESPONSE, code); 12541 if ("EpisodeOfCare".equals(codeString)) 12542 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EPISODEOFCARE, code); 12543 if ("EventDefinition".equals(codeString)) 12544 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EVENTDEFINITION, code); 12545 if ("Evidence".equals(codeString)) 12546 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EVIDENCE, code); 12547 if ("EvidenceVariable".equals(codeString)) 12548 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EVIDENCEVARIABLE, code); 12549 if ("ExampleScenario".equals(codeString)) 12550 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EXAMPLESCENARIO, code); 12551 if ("ExplanationOfBenefit".equals(codeString)) 12552 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EXPLANATIONOFBENEFIT, code); 12553 if ("FamilyMemberHistory".equals(codeString)) 12554 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.FAMILYMEMBERHISTORY, code); 12555 if ("Flag".equals(codeString)) 12556 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.FLAG, code); 12557 if ("Goal".equals(codeString)) 12558 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.GOAL, code); 12559 if ("GraphDefinition".equals(codeString)) 12560 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.GRAPHDEFINITION, code); 12561 if ("Group".equals(codeString)) 12562 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.GROUP, code); 12563 if ("GuidanceResponse".equals(codeString)) 12564 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.GUIDANCERESPONSE, code); 12565 if ("HealthcareService".equals(codeString)) 12566 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.HEALTHCARESERVICE, code); 12567 if ("ImagingStudy".equals(codeString)) 12568 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IMAGINGSTUDY, code); 12569 if ("Immunization".equals(codeString)) 12570 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IMMUNIZATION, code); 12571 if ("ImmunizationEvaluation".equals(codeString)) 12572 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IMMUNIZATIONEVALUATION, code); 12573 if ("ImmunizationRecommendation".equals(codeString)) 12574 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IMMUNIZATIONRECOMMENDATION, code); 12575 if ("ImplementationGuide".equals(codeString)) 12576 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IMPLEMENTATIONGUIDE, code); 12577 if ("InsurancePlan".equals(codeString)) 12578 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.INSURANCEPLAN, code); 12579 if ("Invoice".equals(codeString)) 12580 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.INVOICE, code); 12581 if ("Library".equals(codeString)) 12582 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.LIBRARY, code); 12583 if ("Linkage".equals(codeString)) 12584 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.LINKAGE, code); 12585 if ("List".equals(codeString)) 12586 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.LIST, code); 12587 if ("Location".equals(codeString)) 12588 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.LOCATION, code); 12589 if ("Measure".equals(codeString)) 12590 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEASURE, code); 12591 if ("MeasureReport".equals(codeString)) 12592 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEASUREREPORT, code); 12593 if ("Media".equals(codeString)) 12594 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDIA, code); 12595 if ("Medication".equals(codeString)) 12596 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATION, code); 12597 if ("MedicationAdministration".equals(codeString)) 12598 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATIONADMINISTRATION, code); 12599 if ("MedicationDispense".equals(codeString)) 12600 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATIONDISPENSE, code); 12601 if ("MedicationKnowledge".equals(codeString)) 12602 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATIONKNOWLEDGE, code); 12603 if ("MedicationRequest".equals(codeString)) 12604 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATIONREQUEST, code); 12605 if ("MedicationStatement".equals(codeString)) 12606 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATIONSTATEMENT, code); 12607 if ("MedicinalProduct".equals(codeString)) 12608 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICINALPRODUCT, code); 12609 if ("MedicinalProductAuthorization".equals(codeString)) 12610 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICINALPRODUCTAUTHORIZATION, code); 12611 if ("MedicinalProductContraindication".equals(codeString)) 12612 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICINALPRODUCTCONTRAINDICATION, code); 12613 if ("MedicinalProductIndication".equals(codeString)) 12614 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICINALPRODUCTINDICATION, code); 12615 if ("MedicinalProductIngredient".equals(codeString)) 12616 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICINALPRODUCTINGREDIENT, code); 12617 if ("MedicinalProductInteraction".equals(codeString)) 12618 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICINALPRODUCTINTERACTION, code); 12619 if ("MedicinalProductManufactured".equals(codeString)) 12620 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICINALPRODUCTMANUFACTURED, code); 12621 if ("MedicinalProductPackaged".equals(codeString)) 12622 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICINALPRODUCTPACKAGED, code); 12623 if ("MedicinalProductPharmaceutical".equals(codeString)) 12624 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICINALPRODUCTPHARMACEUTICAL, code); 12625 if ("MedicinalProductUndesirableEffect".equals(codeString)) 12626 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICINALPRODUCTUNDESIRABLEEFFECT, code); 12627 if ("MessageDefinition".equals(codeString)) 12628 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MESSAGEDEFINITION, code); 12629 if ("MessageHeader".equals(codeString)) 12630 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MESSAGEHEADER, code); 12631 if ("MolecularSequence".equals(codeString)) 12632 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MOLECULARSEQUENCE, code); 12633 if ("NamingSystem".equals(codeString)) 12634 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.NAMINGSYSTEM, code); 12635 if ("NutritionOrder".equals(codeString)) 12636 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.NUTRITIONORDER, code); 12637 if ("Observation".equals(codeString)) 12638 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.OBSERVATION, code); 12639 if ("ObservationDefinition".equals(codeString)) 12640 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.OBSERVATIONDEFINITION, code); 12641 if ("OperationDefinition".equals(codeString)) 12642 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.OPERATIONDEFINITION, code); 12643 if ("OperationOutcome".equals(codeString)) 12644 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.OPERATIONOUTCOME, code); 12645 if ("Organization".equals(codeString)) 12646 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ORGANIZATION, code); 12647 if ("OrganizationAffiliation".equals(codeString)) 12648 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ORGANIZATIONAFFILIATION, code); 12649 if ("Parameters".equals(codeString)) 12650 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PARAMETERS, code); 12651 if ("Patient".equals(codeString)) 12652 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PATIENT, code); 12653 if ("PaymentNotice".equals(codeString)) 12654 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PAYMENTNOTICE, code); 12655 if ("PaymentReconciliation".equals(codeString)) 12656 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PAYMENTRECONCILIATION, code); 12657 if ("Person".equals(codeString)) 12658 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PERSON, code); 12659 if ("PlanDefinition".equals(codeString)) 12660 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PLANDEFINITION, code); 12661 if ("Practitioner".equals(codeString)) 12662 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PRACTITIONER, code); 12663 if ("PractitionerRole".equals(codeString)) 12664 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PRACTITIONERROLE, code); 12665 if ("Procedure".equals(codeString)) 12666 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PROCEDURE, code); 12667 if ("Provenance".equals(codeString)) 12668 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PROVENANCE, code); 12669 if ("Questionnaire".equals(codeString)) 12670 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.QUESTIONNAIRE, code); 12671 if ("QuestionnaireResponse".equals(codeString)) 12672 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.QUESTIONNAIRERESPONSE, code); 12673 if ("RelatedPerson".equals(codeString)) 12674 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RELATEDPERSON, code); 12675 if ("RequestGroup".equals(codeString)) 12676 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.REQUESTGROUP, code); 12677 if ("ResearchDefinition".equals(codeString)) 12678 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RESEARCHDEFINITION, code); 12679 if ("ResearchElementDefinition".equals(codeString)) 12680 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RESEARCHELEMENTDEFINITION, code); 12681 if ("ResearchStudy".equals(codeString)) 12682 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RESEARCHSTUDY, code); 12683 if ("ResearchSubject".equals(codeString)) 12684 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RESEARCHSUBJECT, code); 12685 if ("Resource".equals(codeString)) 12686 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RESOURCE, code); 12687 if ("RiskAssessment".equals(codeString)) 12688 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RISKASSESSMENT, code); 12689 if ("RiskEvidenceSynthesis".equals(codeString)) 12690 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RISKEVIDENCESYNTHESIS, code); 12691 if ("Schedule".equals(codeString)) 12692 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SCHEDULE, code); 12693 if ("SearchParameter".equals(codeString)) 12694 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SEARCHPARAMETER, code); 12695 if ("ServiceRequest".equals(codeString)) 12696 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SERVICEREQUEST, code); 12697 if ("Slot".equals(codeString)) 12698 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SLOT, code); 12699 if ("Specimen".equals(codeString)) 12700 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SPECIMEN, code); 12701 if ("SpecimenDefinition".equals(codeString)) 12702 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SPECIMENDEFINITION, code); 12703 if ("StructureDefinition".equals(codeString)) 12704 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.STRUCTUREDEFINITION, code); 12705 if ("StructureMap".equals(codeString)) 12706 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.STRUCTUREMAP, code); 12707 if ("Subscription".equals(codeString)) 12708 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUBSCRIPTION, code); 12709 if ("Substance".equals(codeString)) 12710 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUBSTANCE, code); 12711 if ("SubstanceNucleicAcid".equals(codeString)) 12712 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUBSTANCENUCLEICACID, code); 12713 if ("SubstancePolymer".equals(codeString)) 12714 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUBSTANCEPOLYMER, code); 12715 if ("SubstanceProtein".equals(codeString)) 12716 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUBSTANCEPROTEIN, code); 12717 if ("SubstanceReferenceInformation".equals(codeString)) 12718 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUBSTANCEREFERENCEINFORMATION, code); 12719 if ("SubstanceSourceMaterial".equals(codeString)) 12720 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUBSTANCESOURCEMATERIAL, code); 12721 if ("SubstanceSpecification".equals(codeString)) 12722 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUBSTANCESPECIFICATION, code); 12723 if ("SupplyDelivery".equals(codeString)) 12724 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUPPLYDELIVERY, code); 12725 if ("SupplyRequest".equals(codeString)) 12726 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUPPLYREQUEST, code); 12727 if ("Task".equals(codeString)) 12728 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TASK, code); 12729 if ("TerminologyCapabilities".equals(codeString)) 12730 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TERMINOLOGYCAPABILITIES, code); 12731 if ("TestReport".equals(codeString)) 12732 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TESTREPORT, code); 12733 if ("TestScript".equals(codeString)) 12734 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TESTSCRIPT, code); 12735 if ("ValueSet".equals(codeString)) 12736 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.VALUESET, code); 12737 if ("VerificationResult".equals(codeString)) 12738 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.VERIFICATIONRESULT, code); 12739 if ("VisionPrescription".equals(codeString)) 12740 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.VISIONPRESCRIPTION, code); 12741 throw new FHIRException("Unknown FHIRDefinedType code '" + codeString + "'"); 12742 } 12743 12744 public String toCode(FHIRDefinedType code) { 12745 if (code == FHIRDefinedType.NULL) 12746 return null; 12747 if (code == FHIRDefinedType.ADDRESS) 12748 return "Address"; 12749 if (code == FHIRDefinedType.AGE) 12750 return "Age"; 12751 if (code == FHIRDefinedType.ANNOTATION) 12752 return "Annotation"; 12753 if (code == FHIRDefinedType.ATTACHMENT) 12754 return "Attachment"; 12755 if (code == FHIRDefinedType.BACKBONEELEMENT) 12756 return "BackboneElement"; 12757 if (code == FHIRDefinedType.CODEABLECONCEPT) 12758 return "CodeableConcept"; 12759 if (code == FHIRDefinedType.CODING) 12760 return "Coding"; 12761 if (code == FHIRDefinedType.CONTACTDETAIL) 12762 return "ContactDetail"; 12763 if (code == FHIRDefinedType.CONTACTPOINT) 12764 return "ContactPoint"; 12765 if (code == FHIRDefinedType.CONTRIBUTOR) 12766 return "Contributor"; 12767 if (code == FHIRDefinedType.COUNT) 12768 return "Count"; 12769 if (code == FHIRDefinedType.DATAREQUIREMENT) 12770 return "DataRequirement"; 12771 if (code == FHIRDefinedType.DISTANCE) 12772 return "Distance"; 12773 if (code == FHIRDefinedType.DOSAGE) 12774 return "Dosage"; 12775 if (code == FHIRDefinedType.DURATION) 12776 return "Duration"; 12777 if (code == FHIRDefinedType.ELEMENT) 12778 return "Element"; 12779 if (code == FHIRDefinedType.ELEMENTDEFINITION) 12780 return "ElementDefinition"; 12781 if (code == FHIRDefinedType.EXPRESSION) 12782 return "Expression"; 12783 if (code == FHIRDefinedType.EXTENSION) 12784 return "Extension"; 12785 if (code == FHIRDefinedType.HUMANNAME) 12786 return "HumanName"; 12787 if (code == FHIRDefinedType.IDENTIFIER) 12788 return "Identifier"; 12789 if (code == FHIRDefinedType.MARKETINGSTATUS) 12790 return "MarketingStatus"; 12791 if (code == FHIRDefinedType.META) 12792 return "Meta"; 12793 if (code == FHIRDefinedType.MONEY) 12794 return "Money"; 12795 if (code == FHIRDefinedType.MONEYQUANTITY) 12796 return "MoneyQuantity"; 12797 if (code == FHIRDefinedType.NARRATIVE) 12798 return "Narrative"; 12799 if (code == FHIRDefinedType.PARAMETERDEFINITION) 12800 return "ParameterDefinition"; 12801 if (code == FHIRDefinedType.PERIOD) 12802 return "Period"; 12803 if (code == FHIRDefinedType.POPULATION) 12804 return "Population"; 12805 if (code == FHIRDefinedType.PRODCHARACTERISTIC) 12806 return "ProdCharacteristic"; 12807 if (code == FHIRDefinedType.PRODUCTSHELFLIFE) 12808 return "ProductShelfLife"; 12809 if (code == FHIRDefinedType.QUANTITY) 12810 return "Quantity"; 12811 if (code == FHIRDefinedType.RANGE) 12812 return "Range"; 12813 if (code == FHIRDefinedType.RATIO) 12814 return "Ratio"; 12815 if (code == FHIRDefinedType.REFERENCE) 12816 return "Reference"; 12817 if (code == FHIRDefinedType.RELATEDARTIFACT) 12818 return "RelatedArtifact"; 12819 if (code == FHIRDefinedType.SAMPLEDDATA) 12820 return "SampledData"; 12821 if (code == FHIRDefinedType.SIGNATURE) 12822 return "Signature"; 12823 if (code == FHIRDefinedType.SIMPLEQUANTITY) 12824 return "SimpleQuantity"; 12825 if (code == FHIRDefinedType.SUBSTANCEAMOUNT) 12826 return "SubstanceAmount"; 12827 if (code == FHIRDefinedType.TIMING) 12828 return "Timing"; 12829 if (code == FHIRDefinedType.TRIGGERDEFINITION) 12830 return "TriggerDefinition"; 12831 if (code == FHIRDefinedType.USAGECONTEXT) 12832 return "UsageContext"; 12833 if (code == FHIRDefinedType.BASE64BINARY) 12834 return "base64Binary"; 12835 if (code == FHIRDefinedType.BOOLEAN) 12836 return "boolean"; 12837 if (code == FHIRDefinedType.CANONICAL) 12838 return "canonical"; 12839 if (code == FHIRDefinedType.CODE) 12840 return "code"; 12841 if (code == FHIRDefinedType.DATE) 12842 return "date"; 12843 if (code == FHIRDefinedType.DATETIME) 12844 return "dateTime"; 12845 if (code == FHIRDefinedType.DECIMAL) 12846 return "decimal"; 12847 if (code == FHIRDefinedType.ID) 12848 return "id"; 12849 if (code == FHIRDefinedType.INSTANT) 12850 return "instant"; 12851 if (code == FHIRDefinedType.INTEGER) 12852 return "integer"; 12853 if (code == FHIRDefinedType.MARKDOWN) 12854 return "markdown"; 12855 if (code == FHIRDefinedType.OID) 12856 return "oid"; 12857 if (code == FHIRDefinedType.POSITIVEINT) 12858 return "positiveInt"; 12859 if (code == FHIRDefinedType.STRING) 12860 return "string"; 12861 if (code == FHIRDefinedType.TIME) 12862 return "time"; 12863 if (code == FHIRDefinedType.UNSIGNEDINT) 12864 return "unsignedInt"; 12865 if (code == FHIRDefinedType.URI) 12866 return "uri"; 12867 if (code == FHIRDefinedType.URL) 12868 return "url"; 12869 if (code == FHIRDefinedType.UUID) 12870 return "uuid"; 12871 if (code == FHIRDefinedType.XHTML) 12872 return "xhtml"; 12873 if (code == FHIRDefinedType.ACCOUNT) 12874 return "Account"; 12875 if (code == FHIRDefinedType.ACTIVITYDEFINITION) 12876 return "ActivityDefinition"; 12877 if (code == FHIRDefinedType.ADVERSEEVENT) 12878 return "AdverseEvent"; 12879 if (code == FHIRDefinedType.ALLERGYINTOLERANCE) 12880 return "AllergyIntolerance"; 12881 if (code == FHIRDefinedType.APPOINTMENT) 12882 return "Appointment"; 12883 if (code == FHIRDefinedType.APPOINTMENTRESPONSE) 12884 return "AppointmentResponse"; 12885 if (code == FHIRDefinedType.AUDITEVENT) 12886 return "AuditEvent"; 12887 if (code == FHIRDefinedType.BASIC) 12888 return "Basic"; 12889 if (code == FHIRDefinedType.BINARY) 12890 return "Binary"; 12891 if (code == FHIRDefinedType.BIOLOGICALLYDERIVEDPRODUCT) 12892 return "BiologicallyDerivedProduct"; 12893 if (code == FHIRDefinedType.BODYSTRUCTURE) 12894 return "BodyStructure"; 12895 if (code == FHIRDefinedType.BUNDLE) 12896 return "Bundle"; 12897 if (code == FHIRDefinedType.CAPABILITYSTATEMENT) 12898 return "CapabilityStatement"; 12899 if (code == FHIRDefinedType.CAREPLAN) 12900 return "CarePlan"; 12901 if (code == FHIRDefinedType.CARETEAM) 12902 return "CareTeam"; 12903 if (code == FHIRDefinedType.CATALOGENTRY) 12904 return "CatalogEntry"; 12905 if (code == FHIRDefinedType.CHARGEITEM) 12906 return "ChargeItem"; 12907 if (code == FHIRDefinedType.CHARGEITEMDEFINITION) 12908 return "ChargeItemDefinition"; 12909 if (code == FHIRDefinedType.CLAIM) 12910 return "Claim"; 12911 if (code == FHIRDefinedType.CLAIMRESPONSE) 12912 return "ClaimResponse"; 12913 if (code == FHIRDefinedType.CLINICALIMPRESSION) 12914 return "ClinicalImpression"; 12915 if (code == FHIRDefinedType.CODESYSTEM) 12916 return "CodeSystem"; 12917 if (code == FHIRDefinedType.COMMUNICATION) 12918 return "Communication"; 12919 if (code == FHIRDefinedType.COMMUNICATIONREQUEST) 12920 return "CommunicationRequest"; 12921 if (code == FHIRDefinedType.COMPARTMENTDEFINITION) 12922 return "CompartmentDefinition"; 12923 if (code == FHIRDefinedType.COMPOSITION) 12924 return "Composition"; 12925 if (code == FHIRDefinedType.CONCEPTMAP) 12926 return "ConceptMap"; 12927 if (code == FHIRDefinedType.CONDITION) 12928 return "Condition"; 12929 if (code == FHIRDefinedType.CONSENT) 12930 return "Consent"; 12931 if (code == FHIRDefinedType.CONTRACT) 12932 return "Contract"; 12933 if (code == FHIRDefinedType.COVERAGE) 12934 return "Coverage"; 12935 if (code == FHIRDefinedType.COVERAGEELIGIBILITYREQUEST) 12936 return "CoverageEligibilityRequest"; 12937 if (code == FHIRDefinedType.COVERAGEELIGIBILITYRESPONSE) 12938 return "CoverageEligibilityResponse"; 12939 if (code == FHIRDefinedType.DETECTEDISSUE) 12940 return "DetectedIssue"; 12941 if (code == FHIRDefinedType.DEVICE) 12942 return "Device"; 12943 if (code == FHIRDefinedType.DEVICEDEFINITION) 12944 return "DeviceDefinition"; 12945 if (code == FHIRDefinedType.DEVICEMETRIC) 12946 return "DeviceMetric"; 12947 if (code == FHIRDefinedType.DEVICEREQUEST) 12948 return "DeviceRequest"; 12949 if (code == FHIRDefinedType.DEVICEUSESTATEMENT) 12950 return "DeviceUseStatement"; 12951 if (code == FHIRDefinedType.DIAGNOSTICREPORT) 12952 return "DiagnosticReport"; 12953 if (code == FHIRDefinedType.DOCUMENTMANIFEST) 12954 return "DocumentManifest"; 12955 if (code == FHIRDefinedType.DOCUMENTREFERENCE) 12956 return "DocumentReference"; 12957 if (code == FHIRDefinedType.DOMAINRESOURCE) 12958 return "DomainResource"; 12959 if (code == FHIRDefinedType.EFFECTEVIDENCESYNTHESIS) 12960 return "EffectEvidenceSynthesis"; 12961 if (code == FHIRDefinedType.ENCOUNTER) 12962 return "Encounter"; 12963 if (code == FHIRDefinedType.ENDPOINT) 12964 return "Endpoint"; 12965 if (code == FHIRDefinedType.ENROLLMENTREQUEST) 12966 return "EnrollmentRequest"; 12967 if (code == FHIRDefinedType.ENROLLMENTRESPONSE) 12968 return "EnrollmentResponse"; 12969 if (code == FHIRDefinedType.EPISODEOFCARE) 12970 return "EpisodeOfCare"; 12971 if (code == FHIRDefinedType.EVENTDEFINITION) 12972 return "EventDefinition"; 12973 if (code == FHIRDefinedType.EVIDENCE) 12974 return "Evidence"; 12975 if (code == FHIRDefinedType.EVIDENCEVARIABLE) 12976 return "EvidenceVariable"; 12977 if (code == FHIRDefinedType.EXAMPLESCENARIO) 12978 return "ExampleScenario"; 12979 if (code == FHIRDefinedType.EXPLANATIONOFBENEFIT) 12980 return "ExplanationOfBenefit"; 12981 if (code == FHIRDefinedType.FAMILYMEMBERHISTORY) 12982 return "FamilyMemberHistory"; 12983 if (code == FHIRDefinedType.FLAG) 12984 return "Flag"; 12985 if (code == FHIRDefinedType.GOAL) 12986 return "Goal"; 12987 if (code == FHIRDefinedType.GRAPHDEFINITION) 12988 return "GraphDefinition"; 12989 if (code == FHIRDefinedType.GROUP) 12990 return "Group"; 12991 if (code == FHIRDefinedType.GUIDANCERESPONSE) 12992 return "GuidanceResponse"; 12993 if (code == FHIRDefinedType.HEALTHCARESERVICE) 12994 return "HealthcareService"; 12995 if (code == FHIRDefinedType.IMAGINGSTUDY) 12996 return "ImagingStudy"; 12997 if (code == FHIRDefinedType.IMMUNIZATION) 12998 return "Immunization"; 12999 if (code == FHIRDefinedType.IMMUNIZATIONEVALUATION) 13000 return "ImmunizationEvaluation"; 13001 if (code == FHIRDefinedType.IMMUNIZATIONRECOMMENDATION) 13002 return "ImmunizationRecommendation"; 13003 if (code == FHIRDefinedType.IMPLEMENTATIONGUIDE) 13004 return "ImplementationGuide"; 13005 if (code == FHIRDefinedType.INSURANCEPLAN) 13006 return "InsurancePlan"; 13007 if (code == FHIRDefinedType.INVOICE) 13008 return "Invoice"; 13009 if (code == FHIRDefinedType.LIBRARY) 13010 return "Library"; 13011 if (code == FHIRDefinedType.LINKAGE) 13012 return "Linkage"; 13013 if (code == FHIRDefinedType.LIST) 13014 return "List"; 13015 if (code == FHIRDefinedType.LOCATION) 13016 return "Location"; 13017 if (code == FHIRDefinedType.MEASURE) 13018 return "Measure"; 13019 if (code == FHIRDefinedType.MEASUREREPORT) 13020 return "MeasureReport"; 13021 if (code == FHIRDefinedType.MEDIA) 13022 return "Media"; 13023 if (code == FHIRDefinedType.MEDICATION) 13024 return "Medication"; 13025 if (code == FHIRDefinedType.MEDICATIONADMINISTRATION) 13026 return "MedicationAdministration"; 13027 if (code == FHIRDefinedType.MEDICATIONDISPENSE) 13028 return "MedicationDispense"; 13029 if (code == FHIRDefinedType.MEDICATIONKNOWLEDGE) 13030 return "MedicationKnowledge"; 13031 if (code == FHIRDefinedType.MEDICATIONREQUEST) 13032 return "MedicationRequest"; 13033 if (code == FHIRDefinedType.MEDICATIONSTATEMENT) 13034 return "MedicationStatement"; 13035 if (code == FHIRDefinedType.MEDICINALPRODUCT) 13036 return "MedicinalProduct"; 13037 if (code == FHIRDefinedType.MEDICINALPRODUCTAUTHORIZATION) 13038 return "MedicinalProductAuthorization"; 13039 if (code == FHIRDefinedType.MEDICINALPRODUCTCONTRAINDICATION) 13040 return "MedicinalProductContraindication"; 13041 if (code == FHIRDefinedType.MEDICINALPRODUCTINDICATION) 13042 return "MedicinalProductIndication"; 13043 if (code == FHIRDefinedType.MEDICINALPRODUCTINGREDIENT) 13044 return "MedicinalProductIngredient"; 13045 if (code == FHIRDefinedType.MEDICINALPRODUCTINTERACTION) 13046 return "MedicinalProductInteraction"; 13047 if (code == FHIRDefinedType.MEDICINALPRODUCTMANUFACTURED) 13048 return "MedicinalProductManufactured"; 13049 if (code == FHIRDefinedType.MEDICINALPRODUCTPACKAGED) 13050 return "MedicinalProductPackaged"; 13051 if (code == FHIRDefinedType.MEDICINALPRODUCTPHARMACEUTICAL) 13052 return "MedicinalProductPharmaceutical"; 13053 if (code == FHIRDefinedType.MEDICINALPRODUCTUNDESIRABLEEFFECT) 13054 return "MedicinalProductUndesirableEffect"; 13055 if (code == FHIRDefinedType.MESSAGEDEFINITION) 13056 return "MessageDefinition"; 13057 if (code == FHIRDefinedType.MESSAGEHEADER) 13058 return "MessageHeader"; 13059 if (code == FHIRDefinedType.MOLECULARSEQUENCE) 13060 return "MolecularSequence"; 13061 if (code == FHIRDefinedType.NAMINGSYSTEM) 13062 return "NamingSystem"; 13063 if (code == FHIRDefinedType.NUTRITIONORDER) 13064 return "NutritionOrder"; 13065 if (code == FHIRDefinedType.OBSERVATION) 13066 return "Observation"; 13067 if (code == FHIRDefinedType.OBSERVATIONDEFINITION) 13068 return "ObservationDefinition"; 13069 if (code == FHIRDefinedType.OPERATIONDEFINITION) 13070 return "OperationDefinition"; 13071 if (code == FHIRDefinedType.OPERATIONOUTCOME) 13072 return "OperationOutcome"; 13073 if (code == FHIRDefinedType.ORGANIZATION) 13074 return "Organization"; 13075 if (code == FHIRDefinedType.ORGANIZATIONAFFILIATION) 13076 return "OrganizationAffiliation"; 13077 if (code == FHIRDefinedType.PARAMETERS) 13078 return "Parameters"; 13079 if (code == FHIRDefinedType.PATIENT) 13080 return "Patient"; 13081 if (code == FHIRDefinedType.PAYMENTNOTICE) 13082 return "PaymentNotice"; 13083 if (code == FHIRDefinedType.PAYMENTRECONCILIATION) 13084 return "PaymentReconciliation"; 13085 if (code == FHIRDefinedType.PERSON) 13086 return "Person"; 13087 if (code == FHIRDefinedType.PLANDEFINITION) 13088 return "PlanDefinition"; 13089 if (code == FHIRDefinedType.PRACTITIONER) 13090 return "Practitioner"; 13091 if (code == FHIRDefinedType.PRACTITIONERROLE) 13092 return "PractitionerRole"; 13093 if (code == FHIRDefinedType.PROCEDURE) 13094 return "Procedure"; 13095 if (code == FHIRDefinedType.PROVENANCE) 13096 return "Provenance"; 13097 if (code == FHIRDefinedType.QUESTIONNAIRE) 13098 return "Questionnaire"; 13099 if (code == FHIRDefinedType.QUESTIONNAIRERESPONSE) 13100 return "QuestionnaireResponse"; 13101 if (code == FHIRDefinedType.RELATEDPERSON) 13102 return "RelatedPerson"; 13103 if (code == FHIRDefinedType.REQUESTGROUP) 13104 return "RequestGroup"; 13105 if (code == FHIRDefinedType.RESEARCHDEFINITION) 13106 return "ResearchDefinition"; 13107 if (code == FHIRDefinedType.RESEARCHELEMENTDEFINITION) 13108 return "ResearchElementDefinition"; 13109 if (code == FHIRDefinedType.RESEARCHSTUDY) 13110 return "ResearchStudy"; 13111 if (code == FHIRDefinedType.RESEARCHSUBJECT) 13112 return "ResearchSubject"; 13113 if (code == FHIRDefinedType.RESOURCE) 13114 return "Resource"; 13115 if (code == FHIRDefinedType.RISKASSESSMENT) 13116 return "RiskAssessment"; 13117 if (code == FHIRDefinedType.RISKEVIDENCESYNTHESIS) 13118 return "RiskEvidenceSynthesis"; 13119 if (code == FHIRDefinedType.SCHEDULE) 13120 return "Schedule"; 13121 if (code == FHIRDefinedType.SEARCHPARAMETER) 13122 return "SearchParameter"; 13123 if (code == FHIRDefinedType.SERVICEREQUEST) 13124 return "ServiceRequest"; 13125 if (code == FHIRDefinedType.SLOT) 13126 return "Slot"; 13127 if (code == FHIRDefinedType.SPECIMEN) 13128 return "Specimen"; 13129 if (code == FHIRDefinedType.SPECIMENDEFINITION) 13130 return "SpecimenDefinition"; 13131 if (code == FHIRDefinedType.STRUCTUREDEFINITION) 13132 return "StructureDefinition"; 13133 if (code == FHIRDefinedType.STRUCTUREMAP) 13134 return "StructureMap"; 13135 if (code == FHIRDefinedType.SUBSCRIPTION) 13136 return "Subscription"; 13137 if (code == FHIRDefinedType.SUBSTANCE) 13138 return "Substance"; 13139 if (code == FHIRDefinedType.SUBSTANCENUCLEICACID) 13140 return "SubstanceNucleicAcid"; 13141 if (code == FHIRDefinedType.SUBSTANCEPOLYMER) 13142 return "SubstancePolymer"; 13143 if (code == FHIRDefinedType.SUBSTANCEPROTEIN) 13144 return "SubstanceProtein"; 13145 if (code == FHIRDefinedType.SUBSTANCEREFERENCEINFORMATION) 13146 return "SubstanceReferenceInformation"; 13147 if (code == FHIRDefinedType.SUBSTANCESOURCEMATERIAL) 13148 return "SubstanceSourceMaterial"; 13149 if (code == FHIRDefinedType.SUBSTANCESPECIFICATION) 13150 return "SubstanceSpecification"; 13151 if (code == FHIRDefinedType.SUPPLYDELIVERY) 13152 return "SupplyDelivery"; 13153 if (code == FHIRDefinedType.SUPPLYREQUEST) 13154 return "SupplyRequest"; 13155 if (code == FHIRDefinedType.TASK) 13156 return "Task"; 13157 if (code == FHIRDefinedType.TERMINOLOGYCAPABILITIES) 13158 return "TerminologyCapabilities"; 13159 if (code == FHIRDefinedType.TESTREPORT) 13160 return "TestReport"; 13161 if (code == FHIRDefinedType.TESTSCRIPT) 13162 return "TestScript"; 13163 if (code == FHIRDefinedType.VALUESET) 13164 return "ValueSet"; 13165 if (code == FHIRDefinedType.VERIFICATIONRESULT) 13166 return "VerificationResult"; 13167 if (code == FHIRDefinedType.VISIONPRESCRIPTION) 13168 return "VisionPrescription"; 13169 return "?"; 13170 } 13171 13172 public String toSystem(FHIRDefinedType code) { 13173 return code.getSystem(); 13174 } 13175 } 13176 13177 public enum FHIRVersion { 13178 /** 13179 * Oldest archived version of FHIR. 13180 */ 13181 _0_01, 13182 /** 13183 * 1st Draft for Comment (Sept 2012 Ballot). 13184 */ 13185 _0_05, 13186 /** 13187 * 2nd Draft for Comment (January 2013 Ballot). 13188 */ 13189 _0_06, 13190 /** 13191 * DSTU 1 Ballot version. 13192 */ 13193 _0_11, 13194 /** 13195 * DSTU 1 version. 13196 */ 13197 _0_0, 13198 /** 13199 * DSTU 1 Official version. 13200 */ 13201 _0_0_80, 13202 /** 13203 * DSTU 1 Official version Technical Errata #1. 13204 */ 13205 _0_0_81, 13206 /** 13207 * DSTU 1 Official version Technical Errata #2. 13208 */ 13209 _0_0_82, 13210 /** 13211 * January 2015 Ballot. 13212 */ 13213 _0_4, 13214 /** 13215 * Draft For Comment (January 2015 Ballot). 13216 */ 13217 _0_4_0, 13218 /** 13219 * May 2015 Ballot. 13220 */ 13221 _0_5, 13222 /** 13223 * DSTU 2 Ballot version (May 2015 Ballot). 13224 */ 13225 _0_5_0, 13226 /** 13227 * DSTU 2 version. 13228 */ 13229 _1_0, 13230 /** 13231 * DSTU 2 QA Preview + CQIF Ballot (Sep 2015). 13232 */ 13233 _1_0_0, 13234 /** 13235 * DSTU 2 (Official version). 13236 */ 13237 _1_0_1, 13238 /** 13239 * DSTU 2 (Official version) with 1 technical errata. 13240 */ 13241 _1_0_2, 13242 /** 13243 * GAO Ballot version. 13244 */ 13245 _1_1, 13246 /** 13247 * GAO Ballot + draft changes to main FHIR standard. 13248 */ 13249 _1_1_0, 13250 /** 13251 * Connectathon 12 (Montreal) version. 13252 */ 13253 _1_4, 13254 /** 13255 * CQF on FHIR Ballot + Connectathon 12 (Montreal). 13256 */ 13257 _1_4_0, 13258 /** 13259 * Connectathon 13 (Baltimore) version. 13260 */ 13261 _1_6, 13262 /** 13263 * FHIR STU3 Ballot + Connectathon 13 (Baltimore). 13264 */ 13265 _1_6_0, 13266 /** 13267 * Connectathon 14 (San Antonio) version. 13268 */ 13269 _1_8, 13270 /** 13271 * FHIR STU3 Candidate + Connectathon 14 (San Antonio). 13272 */ 13273 _1_8_0, 13274 /** 13275 * STU3 version. 13276 */ 13277 _3_0, 13278 /** 13279 * FHIR Release 3 (STU). 13280 */ 13281 _3_0_0, 13282 /** 13283 * FHIR Release 3 (STU) with 1 technical errata. 13284 */ 13285 _3_0_1, 13286 /** 13287 * FHIR Release 3 (STU) with 2 technical errata. 13288 */ 13289 _3_0_2, 13290 /** 13291 * R4 Ballot #1 version. 13292 */ 13293 _3_3, 13294 /** 13295 * R4 Ballot #1 + Connectaton 18 (Cologne). 13296 */ 13297 _3_3_0, 13298 /** 13299 * R4 Ballot #2 version. 13300 */ 13301 _3_5, 13302 /** 13303 * R4 Ballot #2 + Connectathon 19 (Baltimore). 13304 */ 13305 _3_5_0, 13306 /** 13307 * R4 version. 13308 */ 13309 _4_0, 13310 /** 13311 * FHIR Release 4 (Normative + STU). 13312 */ 13313 _4_0_0, 13314 /** 13315 * FHIR Release 4 (Normative + STU) with 1 technical errata. 13316 */ 13317 _4_0_1, 13318 /** 13319 * R4B Ballot #1 version. 13320 */ 13321 _4_1, 13322 /** 13323 * R4B Ballot #1 + Connectathon 27 (Virtual). 13324 */ 13325 _4_1_0, 13326 /** 13327 * R5 Preview #1 version. 13328 */ 13329 _4_2, 13330 /** 13331 * R5 Preview #1 + Connectathon 23 (Sydney). 13332 */ 13333 _4_2_0, 13334 /** 13335 * R4B version. 13336 */ 13337 _4_3, 13338 /** 13339 * FHIR Release 4B (Normative + STU). 13340 */ 13341 _4_3_0, 13342 /** 13343 * FHIR Release 4B CI-Builld. 13344 */ 13345 _4_3_0CIBUILD, 13346 /** 13347 * FHIR Release 4B Snapshot #1. 13348 */ 13349 _4_3_0SNAPSHOT1, 13350 /** 13351 * R5 Preview #2 version. 13352 */ 13353 _4_4, 13354 /** 13355 * R5 Preview #2 + Connectathon 24 (Virtual). 13356 */ 13357 _4_4_0, 13358 /** 13359 * R5 Preview #3 version. 13360 */ 13361 _4_5, 13362 /** 13363 * R5 Preview #3 + Connectathon 25 (Virtual). 13364 */ 13365 _4_5_0, 13366 /** 13367 * R5 Draft Ballot version. 13368 */ 13369 _4_6, 13370 /** 13371 * R5 Draft Ballot + Connectathon 27 (Virtual). 13372 */ 13373 _4_6_0, 13374 /** 13375 * R5 Versions. 13376 */ 13377 _5_0, 13378 /** 13379 * R5 Final Version. 13380 */ 13381 _5_0_0, 13382 /** 13383 * R5 Rolling ci-build. 13384 */ 13385 _5_0_0CIBUILD, 13386 /** 13387 * R5 Preview #2. 13388 */ 13389 _5_0_0SNAPSHOT1, 13390 /** 13391 * R5 Interim tooling stage. 13392 */ 13393 _5_0_0SNAPSHOT2, 13394 /** 13395 * R5 Ballot. 13396 */ 13397 _5_0_0BALLOT, 13398 /** 13399 * R5 January 2023 Staging Release + Connectathon 32. 13400 */ 13401 _5_0_0SNAPSHOT3, 13402 /** 13403 * R5 Final QA. 13404 */ 13405 _5_0_0DRAFTFINAL, 13406 /** 13407 * R6 Rolling ci-build. 13408 */ 13409 _6_0_0CIBUILD, 13410 /** 13411 * R6 Formal version (does not exist yet) 13412 */ 13413 _6_0_0, 13414 _6_0_0_BALLOT1, 13415 _6_0_0_BALLOT2, 13416 /** 13417 * added to help the parsers 13418 */ 13419 NULL; 13420 public static FHIRVersion fromCode(String codeString) throws FHIRException { 13421 if (codeString == null || "".equals(codeString)) 13422 return null; 13423 if ("0.01".equals(codeString)) 13424 return _0_01; 13425 if ("0.05".equals(codeString)) 13426 return _0_05; 13427 if ("0.06".equals(codeString)) 13428 return _0_06; 13429 if ("0.11".equals(codeString)) 13430 return _0_11; 13431 if ("0.0".equals(codeString)) 13432 return _0_0; 13433 if ("0.0.80".equals(codeString)) 13434 return _0_0_80; 13435 if ("0.0.81".equals(codeString)) 13436 return _0_0_81; 13437 if ("0.0.82".equals(codeString)) 13438 return _0_0_82; 13439 if ("0.4".equals(codeString)) 13440 return _0_4; 13441 if ("0.4.0".equals(codeString)) 13442 return _0_4_0; 13443 if ("0.5".equals(codeString)) 13444 return _0_5; 13445 if ("0.5.0".equals(codeString)) 13446 return _0_5_0; 13447 if ("1.0".equals(codeString)) 13448 return _1_0; 13449 if ("1.0.0".equals(codeString)) 13450 return _1_0_0; 13451 if ("1.0.1".equals(codeString)) 13452 return _1_0_1; 13453 if ("1.0.2".equals(codeString)) 13454 return _1_0_2; 13455 if ("1.1".equals(codeString)) 13456 return _1_1; 13457 if ("1.1.0".equals(codeString)) 13458 return _1_1_0; 13459 if ("1.4".equals(codeString)) 13460 return _1_4; 13461 if ("1.4.0".equals(codeString)) 13462 return _1_4_0; 13463 if ("1.6".equals(codeString)) 13464 return _1_6; 13465 if ("1.6.0".equals(codeString)) 13466 return _1_6_0; 13467 if ("1.8".equals(codeString)) 13468 return _1_8; 13469 if ("1.8.0".equals(codeString)) 13470 return _1_8_0; 13471 if ("3.0".equals(codeString)) 13472 return _3_0; 13473 if ("3.0.0".equals(codeString)) 13474 return _3_0_0; 13475 if ("3.0.1".equals(codeString)) 13476 return _3_0_1; 13477 if ("3.0.2".equals(codeString)) 13478 return _3_0_2; 13479 if ("3.3".equals(codeString)) 13480 return _3_3; 13481 if ("3.3.0".equals(codeString)) 13482 return _3_3_0; 13483 if ("3.5".equals(codeString)) 13484 return _3_5; 13485 if ("3.5.0".equals(codeString)) 13486 return _3_5_0; 13487 if ("4.0".equals(codeString)) 13488 return _4_0; 13489 if ("4.0.0".equals(codeString)) 13490 return _4_0_0; 13491 if ("4.0.1".equals(codeString)) 13492 return _4_0_1; 13493 if ("4.1".equals(codeString)) 13494 return _4_1; 13495 if ("4.1.0".equals(codeString)) 13496 return _4_1_0; 13497 if ("4.2".equals(codeString)) 13498 return _4_2; 13499 if ("4.2.0".equals(codeString)) 13500 return _4_2_0; 13501 if ("4.3".equals(codeString)) 13502 return _4_3; 13503 if ("4.3.0".equals(codeString)) 13504 return _4_3_0; 13505 if ("4.3.0-cibuild".equals(codeString)) 13506 return _4_3_0CIBUILD; 13507 if ("4.3.0-snapshot1".equals(codeString)) 13508 return _4_3_0SNAPSHOT1; 13509 if ("4.4".equals(codeString)) 13510 return _4_4; 13511 if ("4.4.0".equals(codeString)) 13512 return _4_4_0; 13513 if ("4.5".equals(codeString)) 13514 return _4_5; 13515 if ("4.5.0".equals(codeString)) 13516 return _4_5_0; 13517 if ("4.6".equals(codeString)) 13518 return _4_6; 13519 if ("4.6.0".equals(codeString)) 13520 return _4_6_0; 13521 if ("5.0".equals(codeString)) 13522 return _5_0; 13523 if ("5.0.0".equals(codeString)) 13524 return _5_0_0; 13525 if ("5.0.0-cibuild".equals(codeString)) 13526 return _5_0_0CIBUILD; 13527 if ("5.0.0-snapshot1".equals(codeString)) 13528 return _5_0_0SNAPSHOT1; 13529 if ("5.0.0-snapshot2".equals(codeString)) 13530 return _5_0_0SNAPSHOT2; 13531 if ("5.0.0-ballot".equals(codeString)) 13532 return _5_0_0BALLOT; 13533 if ("5.0.0-snapshot3".equals(codeString)) 13534 return _5_0_0SNAPSHOT3; 13535 if ("5.0.0-draft-final".equals(codeString)) 13536 return _5_0_0DRAFTFINAL; 13537 if ("6.0.0-cibuild".equals(codeString)) 13538 return _6_0_0CIBUILD; 13539 if ("6.0.0".equals(codeString)) 13540 return _6_0_0; 13541 if ("6.0.0-ballot1".equals(codeString)) 13542 return _6_0_0_BALLOT1; 13543 if ("6.0.0-ballot2".equals(codeString)) 13544 return _6_0_0_BALLOT2; 13545 throw new FHIRException("Unknown FHIRVersion code '"+codeString+"'"); 13546 } 13547 public static boolean isValidCode(String codeString) { 13548 if (codeString == null || "".equals(codeString)) 13549 return false; 13550 return Utilities.existsInList(codeString, "0.01", "0.05", "0.06", "0.11", "0.0", "0.0.80", "0.0.81", "0.0.82", "0.4", "0.4.0", "0.5", "0.5.0", "1.0", "1.0.0", "1.0.1", "1.0.2", "1.1", "1.1.0", "1.4", "1.4.0", "1.6", "1.6.0", "1.8", "1.8.0", "3.0", "3.0.0", "3.0.1", "3.0.2", "3.3", "3.3.0", "3.5", "3.5.0", "4.0", "4.0.0", "4.0.1", "4.1", "4.1.0", "4.2", "4.2.0", "4.3", "4.3.0", "4.3.0-cibuild", "4.3.0-snapshot1", "4.4", "4.4.0", "4.5", "4.5.0", "4.6", "4.6.0", "5.0", "5.0.0", "5.0.0-cibuild", "5.0.0-snapshot1", "5.0.0-snapshot2", "5.0.0-ballot", "5.0.0-snapshot3", "5.0.0-draft-final"); 13551 } 13552 public String toCode() { 13553 switch (this) { 13554 case _0_01: return "0.01"; 13555 case _0_05: return "0.05"; 13556 case _0_06: return "0.06"; 13557 case _0_11: return "0.11"; 13558 case _0_0: return "0.0"; 13559 case _0_0_80: return "0.0.80"; 13560 case _0_0_81: return "0.0.81"; 13561 case _0_0_82: return "0.0.82"; 13562 case _0_4: return "0.4"; 13563 case _0_4_0: return "0.4.0"; 13564 case _0_5: return "0.5"; 13565 case _0_5_0: return "0.5.0"; 13566 case _1_0: return "1.0"; 13567 case _1_0_0: return "1.0.0"; 13568 case _1_0_1: return "1.0.1"; 13569 case _1_0_2: return "1.0.2"; 13570 case _1_1: return "1.1"; 13571 case _1_1_0: return "1.1.0"; 13572 case _1_4: return "1.4"; 13573 case _1_4_0: return "1.4.0"; 13574 case _1_6: return "1.6"; 13575 case _1_6_0: return "1.6.0"; 13576 case _1_8: return "1.8"; 13577 case _1_8_0: return "1.8.0"; 13578 case _3_0: return "3.0"; 13579 case _3_0_0: return "3.0.0"; 13580 case _3_0_1: return "3.0.1"; 13581 case _3_0_2: return "3.0.2"; 13582 case _3_3: return "3.3"; 13583 case _3_3_0: return "3.3.0"; 13584 case _3_5: return "3.5"; 13585 case _3_5_0: return "3.5.0"; 13586 case _4_0: return "4.0"; 13587 case _4_0_0: return "4.0.0"; 13588 case _4_0_1: return "4.0.1"; 13589 case _4_1: return "4.1"; 13590 case _4_1_0: return "4.1.0"; 13591 case _4_2: return "4.2"; 13592 case _4_2_0: return "4.2.0"; 13593 case _4_3: return "4.3"; 13594 case _4_3_0: return "4.3.0"; 13595 case _4_3_0CIBUILD: return "4.3.0-cibuild"; 13596 case _4_3_0SNAPSHOT1: return "4.3.0-snapshot1"; 13597 case _4_4: return "4.4"; 13598 case _4_4_0: return "4.4.0"; 13599 case _4_5: return "4.5"; 13600 case _4_5_0: return "4.5.0"; 13601 case _4_6: return "4.6"; 13602 case _4_6_0: return "4.6.0"; 13603 case _5_0: return "5.0"; 13604 case _5_0_0: return "5.0.0"; 13605 case _5_0_0CIBUILD: return "5.0.0-cibuild"; 13606 case _5_0_0SNAPSHOT1: return "5.0.0-snapshot1"; 13607 case _5_0_0SNAPSHOT2: return "5.0.0-snapshot2"; 13608 case _5_0_0BALLOT: return "5.0.0-ballot"; 13609 case _5_0_0SNAPSHOT3: return "5.0.0-snapshot3"; 13610 case _5_0_0DRAFTFINAL: return "5.0.0-draft-final"; 13611 case _6_0_0CIBUILD: return "6.0.0-cibuild"; 13612 case _6_0_0: return "6.0.0"; 13613 case _6_0_0_BALLOT1: return "6.0.0-ballot1"; 13614 case _6_0_0_BALLOT2: return "6.0.0-ballot2"; 13615 case NULL: return null; 13616 default: return "?"; 13617 } 13618 } 13619 public String getSystem() { 13620 switch (this) { 13621 case _0_01: return "http://hl7.org/fhir/FHIR-version"; 13622 case _0_05: return "http://hl7.org/fhir/FHIR-version"; 13623 case _0_06: return "http://hl7.org/fhir/FHIR-version"; 13624 case _0_11: return "http://hl7.org/fhir/FHIR-version"; 13625 case _0_0: return "http://hl7.org/fhir/FHIR-version"; 13626 case _0_0_80: return "http://hl7.org/fhir/FHIR-version"; 13627 case _0_0_81: return "http://hl7.org/fhir/FHIR-version"; 13628 case _0_0_82: return "http://hl7.org/fhir/FHIR-version"; 13629 case _0_4: return "http://hl7.org/fhir/FHIR-version"; 13630 case _0_4_0: return "http://hl7.org/fhir/FHIR-version"; 13631 case _0_5: return "http://hl7.org/fhir/FHIR-version"; 13632 case _0_5_0: return "http://hl7.org/fhir/FHIR-version"; 13633 case _1_0: return "http://hl7.org/fhir/FHIR-version"; 13634 case _1_0_0: return "http://hl7.org/fhir/FHIR-version"; 13635 case _1_0_1: return "http://hl7.org/fhir/FHIR-version"; 13636 case _1_0_2: return "http://hl7.org/fhir/FHIR-version"; 13637 case _1_1: return "http://hl7.org/fhir/FHIR-version"; 13638 case _1_1_0: return "http://hl7.org/fhir/FHIR-version"; 13639 case _1_4: return "http://hl7.org/fhir/FHIR-version"; 13640 case _1_4_0: return "http://hl7.org/fhir/FHIR-version"; 13641 case _1_6: return "http://hl7.org/fhir/FHIR-version"; 13642 case _1_6_0: return "http://hl7.org/fhir/FHIR-version"; 13643 case _1_8: return "http://hl7.org/fhir/FHIR-version"; 13644 case _1_8_0: return "http://hl7.org/fhir/FHIR-version"; 13645 case _3_0: return "http://hl7.org/fhir/FHIR-version"; 13646 case _3_0_0: return "http://hl7.org/fhir/FHIR-version"; 13647 case _3_0_1: return "http://hl7.org/fhir/FHIR-version"; 13648 case _3_0_2: return "http://hl7.org/fhir/FHIR-version"; 13649 case _3_3: return "http://hl7.org/fhir/FHIR-version"; 13650 case _3_3_0: return "http://hl7.org/fhir/FHIR-version"; 13651 case _3_5: return "http://hl7.org/fhir/FHIR-version"; 13652 case _3_5_0: return "http://hl7.org/fhir/FHIR-version"; 13653 case _4_0: return "http://hl7.org/fhir/FHIR-version"; 13654 case _4_0_0: return "http://hl7.org/fhir/FHIR-version"; 13655 case _4_0_1: return "http://hl7.org/fhir/FHIR-version"; 13656 case _4_1: return "http://hl7.org/fhir/FHIR-version"; 13657 case _4_1_0: return "http://hl7.org/fhir/FHIR-version"; 13658 case _4_2: return "http://hl7.org/fhir/FHIR-version"; 13659 case _4_2_0: return "http://hl7.org/fhir/FHIR-version"; 13660 case _4_3: return "http://hl7.org/fhir/FHIR-version"; 13661 case _4_3_0: return "http://hl7.org/fhir/FHIR-version"; 13662 case _4_3_0CIBUILD: return "http://hl7.org/fhir/FHIR-version"; 13663 case _4_3_0SNAPSHOT1: return "http://hl7.org/fhir/FHIR-version"; 13664 case _4_4: return "http://hl7.org/fhir/FHIR-version"; 13665 case _4_4_0: return "http://hl7.org/fhir/FHIR-version"; 13666 case _4_5: return "http://hl7.org/fhir/FHIR-version"; 13667 case _4_5_0: return "http://hl7.org/fhir/FHIR-version"; 13668 case _4_6: return "http://hl7.org/fhir/FHIR-version"; 13669 case _4_6_0: return "http://hl7.org/fhir/FHIR-version"; 13670 case _5_0: return "http://hl7.org/fhir/FHIR-version"; 13671 case _5_0_0: return "http://hl7.org/fhir/FHIR-version"; 13672 case _5_0_0CIBUILD: return "http://hl7.org/fhir/FHIR-version"; 13673 case _5_0_0SNAPSHOT1: return "http://hl7.org/fhir/FHIR-version"; 13674 case _5_0_0SNAPSHOT2: return "http://hl7.org/fhir/FHIR-version"; 13675 case _5_0_0BALLOT: return "http://hl7.org/fhir/FHIR-version"; 13676 case _5_0_0SNAPSHOT3: return "http://hl7.org/fhir/FHIR-version"; 13677 case _5_0_0DRAFTFINAL: return "http://hl7.org/fhir/FHIR-version"; 13678 case _6_0_0CIBUILD: return "http://hl7.org/fhir/FHIR-version"; 13679 case _6_0_0: return "http://hl7.org/fhir/FHIR-version"; 13680 case _6_0_0_BALLOT1: return "http://hl7.org/fhir/FHIR-version"; 13681 case NULL: return null; 13682 default: return "?"; 13683 } 13684 } 13685 public String getDefinition() { 13686 switch (this) { 13687 case _0_01: return "Oldest archived version of FHIR."; 13688 case _0_05: return "1st Draft for Comment (Sept 2012 Ballot)."; 13689 case _0_06: return "2nd Draft for Comment (January 2013 Ballot)."; 13690 case _0_11: return "DSTU 1 Ballot version."; 13691 case _0_0: return "DSTU 1 version."; 13692 case _0_0_80: return "DSTU 1 Official version."; 13693 case _0_0_81: return "DSTU 1 Official version Technical Errata #1."; 13694 case _0_0_82: return "DSTU 1 Official version Technical Errata #2."; 13695 case _0_4: return "January 2015 Ballot."; 13696 case _0_4_0: return "Draft For Comment (January 2015 Ballot)."; 13697 case _0_5: return "May 2015 Ballot."; 13698 case _0_5_0: return "DSTU 2 Ballot version (May 2015 Ballot)."; 13699 case _1_0: return "DSTU 2 version."; 13700 case _1_0_0: return "DSTU 2 QA Preview + CQIF Ballot (Sep 2015)."; 13701 case _1_0_1: return "DSTU 2 (Official version)."; 13702 case _1_0_2: return "DSTU 2 (Official version) with 1 technical errata."; 13703 case _1_1: return "GAO Ballot version."; 13704 case _1_1_0: return "GAO Ballot + draft changes to main FHIR standard."; 13705 case _1_4: return "Connectathon 12 (Montreal) version."; 13706 case _1_4_0: return "CQF on FHIR Ballot + Connectathon 12 (Montreal)."; 13707 case _1_6: return "Connectathon 13 (Baltimore) version."; 13708 case _1_6_0: return "FHIR STU3 Ballot + Connectathon 13 (Baltimore)."; 13709 case _1_8: return "Connectathon 14 (San Antonio) version."; 13710 case _1_8_0: return "FHIR STU3 Candidate + Connectathon 14 (San Antonio)."; 13711 case _3_0: return "STU3 version."; 13712 case _3_0_0: return "FHIR Release 3 (STU)."; 13713 case _3_0_1: return "FHIR Release 3 (STU) with 1 technical errata."; 13714 case _3_0_2: return "FHIR Release 3 (STU) with 2 technical errata."; 13715 case _3_3: return "R4 Ballot #1 version."; 13716 case _3_3_0: return "R4 Ballot #1 + Connectaton 18 (Cologne)."; 13717 case _3_5: return "R4 Ballot #2 version."; 13718 case _3_5_0: return "R4 Ballot #2 + Connectathon 19 (Baltimore)."; 13719 case _4_0: return "R4 version."; 13720 case _4_0_0: return "FHIR Release 4 (Normative + STU)."; 13721 case _4_0_1: return "FHIR Release 4 (Normative + STU) with 1 technical errata."; 13722 case _4_1: return "R4B Ballot #1 version."; 13723 case _4_1_0: return "R4B Ballot #1 + Connectathon 27 (Virtual)."; 13724 case _4_2: return "R5 Preview #1 version."; 13725 case _4_2_0: return "R5 Preview #1 + Connectathon 23 (Sydney)."; 13726 case _4_3: return "R4B version."; 13727 case _4_3_0: return "FHIR Release 4B (Normative + STU)."; 13728 case _4_3_0CIBUILD: return "FHIR Release 4B CI-Builld."; 13729 case _4_3_0SNAPSHOT1: return "FHIR Release 4B Snapshot #1."; 13730 case _4_4: return "R5 Preview #2 version."; 13731 case _4_4_0: return "R5 Preview #2 + Connectathon 24 (Virtual)."; 13732 case _4_5: return "R5 Preview #3 version."; 13733 case _4_5_0: return "R5 Preview #3 + Connectathon 25 (Virtual)."; 13734 case _4_6: return "R5 Draft Ballot version."; 13735 case _4_6_0: return "R5 Draft Ballot + Connectathon 27 (Virtual)."; 13736 case _5_0: return "R5 Versions."; 13737 case _5_0_0: return "R5 Final Version."; 13738 case _5_0_0CIBUILD: return "R5 Rolling ci-build."; 13739 case _5_0_0SNAPSHOT1: return "R5 Preview #2."; 13740 case _5_0_0SNAPSHOT2: return "R5 Interim tooling stage."; 13741 case _5_0_0BALLOT: return "R5 Ballot."; 13742 case _5_0_0SNAPSHOT3: return "R5 January 2023 Staging Release + Connectathon 32."; 13743 case _5_0_0DRAFTFINAL: return "R5 Final QA."; 13744 case _6_0_0CIBUILD: return "R6 Rolling ci-build."; 13745 case _6_0_0: return "R6 Final Version."; 13746 case _6_0_0_BALLOT1: return "R6 Ballot #1."; 13747 case NULL: return null; 13748 default: return "?"; 13749 } 13750 } 13751 public String getDisplay() { 13752 switch (this) { 13753 case _0_01: return "0.01"; 13754 case _0_05: return "0.05"; 13755 case _0_06: return "0.06"; 13756 case _0_11: return "0.11"; 13757 case _0_0: return "0.0"; 13758 case _0_0_80: return "0.0.80"; 13759 case _0_0_81: return "0.0.81"; 13760 case _0_0_82: return "0.0.82"; 13761 case _0_4: return "0.4"; 13762 case _0_4_0: return "0.4.0"; 13763 case _0_5: return "0.5"; 13764 case _0_5_0: return "0.5.0"; 13765 case _1_0: return "1.0"; 13766 case _1_0_0: return "1.0.0"; 13767 case _1_0_1: return "1.0.1"; 13768 case _1_0_2: return "1.0.2"; 13769 case _1_1: return "1.1"; 13770 case _1_1_0: return "1.1.0"; 13771 case _1_4: return "1.4"; 13772 case _1_4_0: return "1.4.0"; 13773 case _1_6: return "1.6"; 13774 case _1_6_0: return "1.6.0"; 13775 case _1_8: return "1.8"; 13776 case _1_8_0: return "1.8.0"; 13777 case _3_0: return "3.0"; 13778 case _3_0_0: return "3.0.0"; 13779 case _3_0_1: return "3.0.1"; 13780 case _3_0_2: return "3.0.2"; 13781 case _3_3: return "3.3"; 13782 case _3_3_0: return "3.3.0"; 13783 case _3_5: return "3.5"; 13784 case _3_5_0: return "3.5.0"; 13785 case _4_0: return "4.0"; 13786 case _4_0_0: return "4.0.0"; 13787 case _4_0_1: return "4.0.1"; 13788 case _4_1: return "4.1"; 13789 case _4_1_0: return "4.1.0"; 13790 case _4_2: return "4.2"; 13791 case _4_2_0: return "4.2.0"; 13792 case _4_3: return "4.3"; 13793 case _4_3_0: return "4.3.0"; 13794 case _4_3_0CIBUILD: return "4.3.0-cibuild"; 13795 case _4_3_0SNAPSHOT1: return "4.3.0-snapshot1"; 13796 case _4_4: return "4.4"; 13797 case _4_4_0: return "4.4.0"; 13798 case _4_5: return "4.5"; 13799 case _4_5_0: return "4.5.0"; 13800 case _4_6: return "4.6"; 13801 case _4_6_0: return "4.6.0"; 13802 case _5_0: return "5.0"; 13803 case _5_0_0: return "5.0.0"; 13804 case _5_0_0CIBUILD: return "5.0.0-cibuild"; 13805 case _5_0_0SNAPSHOT1: return "5.0.0-snapshot1"; 13806 case _5_0_0SNAPSHOT2: return "5.0.0-snapshot2"; 13807 case _5_0_0BALLOT: return "5.0.0-ballot"; 13808 case _5_0_0SNAPSHOT3: return "5.0.0-snapshot3"; 13809 case _5_0_0DRAFTFINAL: return "5.0.0-draft-final"; 13810 case _6_0_0CIBUILD: return "6.0.0-cibuild"; 13811 case _6_0_0: return "6.0.0"; 13812 case _6_0_0_BALLOT1: return "6.0.0-ballot1"; 13813 case _6_0_0_BALLOT2: return "6.0.0-ballot2"; 13814 case NULL: return null; 13815 default: return "?"; 13816 } 13817 } 13818// manual code from configuration.txt: 13819//public String toCode(int len) { 13820// return toCode().substring(0, len); 13821// } 13822// 13823// 13824// @Override 13825// public String toString() { 13826// return toCode(); 13827// } 13828// 13829// 13830// public boolean isR4B() { 13831// return toCode().startsWith("4.1"); 13832// } 13833// 13834// end addition 13835 } 13836 13837 public static class FHIRVersionEnumFactory implements EnumFactory<FHIRVersion> { 13838 public FHIRVersion fromCode(String codeString) throws IllegalArgumentException { 13839 if (codeString == null || "".equals(codeString)) 13840 if (codeString == null || "".equals(codeString)) 13841 return null; 13842 if ("0.01".equals(codeString)) 13843 return FHIRVersion._0_01; 13844 if ("0.05".equals(codeString)) 13845 return FHIRVersion._0_05; 13846 if ("0.06".equals(codeString)) 13847 return FHIRVersion._0_06; 13848 if ("0.11".equals(codeString)) 13849 return FHIRVersion._0_11; 13850 if ("0.0".equals(codeString)) 13851 return FHIRVersion._0_0; 13852 if ("0.0.80".equals(codeString)) 13853 return FHIRVersion._0_0_80; 13854 if ("0.0.81".equals(codeString)) 13855 return FHIRVersion._0_0_81; 13856 if ("0.0.82".equals(codeString)) 13857 return FHIRVersion._0_0_82; 13858 if ("0.4".equals(codeString)) 13859 return FHIRVersion._0_4; 13860 if ("0.4.0".equals(codeString)) 13861 return FHIRVersion._0_4_0; 13862 if ("0.5".equals(codeString)) 13863 return FHIRVersion._0_5; 13864 if ("0.5.0".equals(codeString)) 13865 return FHIRVersion._0_5_0; 13866 if ("1.0".equals(codeString)) 13867 return FHIRVersion._1_0; 13868 if ("1.0.0".equals(codeString)) 13869 return FHIRVersion._1_0_0; 13870 if ("1.0.1".equals(codeString)) 13871 return FHIRVersion._1_0_1; 13872 if ("1.0.2".equals(codeString)) 13873 return FHIRVersion._1_0_2; 13874 if ("1.1".equals(codeString)) 13875 return FHIRVersion._1_1; 13876 if ("1.1.0".equals(codeString)) 13877 return FHIRVersion._1_1_0; 13878 if ("1.4".equals(codeString)) 13879 return FHIRVersion._1_4; 13880 if ("1.4.0".equals(codeString)) 13881 return FHIRVersion._1_4_0; 13882 if ("1.6".equals(codeString)) 13883 return FHIRVersion._1_6; 13884 if ("1.6.0".equals(codeString)) 13885 return FHIRVersion._1_6_0; 13886 if ("1.8".equals(codeString)) 13887 return FHIRVersion._1_8; 13888 if ("1.8.0".equals(codeString)) 13889 return FHIRVersion._1_8_0; 13890 if ("3.0".equals(codeString)) 13891 return FHIRVersion._3_0; 13892 if ("3.0.0".equals(codeString)) 13893 return FHIRVersion._3_0_0; 13894 if ("3.0.1".equals(codeString)) 13895 return FHIRVersion._3_0_1; 13896 if ("3.0.2".equals(codeString)) 13897 return FHIRVersion._3_0_2; 13898 if ("3.3".equals(codeString)) 13899 return FHIRVersion._3_3; 13900 if ("3.3.0".equals(codeString)) 13901 return FHIRVersion._3_3_0; 13902 if ("3.5".equals(codeString)) 13903 return FHIRVersion._3_5; 13904 if ("3.5.0".equals(codeString)) 13905 return FHIRVersion._3_5_0; 13906 if ("4.0".equals(codeString)) 13907 return FHIRVersion._4_0; 13908 if ("4.0.0".equals(codeString)) 13909 return FHIRVersion._4_0_0; 13910 if ("4.0.1".equals(codeString)) 13911 return FHIRVersion._4_0_1; 13912 if ("4.1".equals(codeString)) 13913 return FHIRVersion._4_1; 13914 if ("4.1.0".equals(codeString)) 13915 return FHIRVersion._4_1_0; 13916 if ("4.2".equals(codeString)) 13917 return FHIRVersion._4_2; 13918 if ("4.2.0".equals(codeString)) 13919 return FHIRVersion._4_2_0; 13920 if ("4.3".equals(codeString)) 13921 return FHIRVersion._4_3; 13922 if ("4.3.0".equals(codeString)) 13923 return FHIRVersion._4_3_0; 13924 if ("4.3.0-cibuild".equals(codeString)) 13925 return FHIRVersion._4_3_0CIBUILD; 13926 if ("4.3.0-snapshot1".equals(codeString)) 13927 return FHIRVersion._4_3_0SNAPSHOT1; 13928 if ("4.4".equals(codeString)) 13929 return FHIRVersion._4_4; 13930 if ("4.4.0".equals(codeString)) 13931 return FHIRVersion._4_4_0; 13932 if ("4.5".equals(codeString)) 13933 return FHIRVersion._4_5; 13934 if ("4.5.0".equals(codeString)) 13935 return FHIRVersion._4_5_0; 13936 if ("4.6".equals(codeString)) 13937 return FHIRVersion._4_6; 13938 if ("4.6.0".equals(codeString)) 13939 return FHIRVersion._4_6_0; 13940 if ("5.0".equals(codeString)) 13941 return FHIRVersion._5_0; 13942 if ("5.0.0".equals(codeString)) 13943 return FHIRVersion._5_0_0; 13944 if ("5.0.0-cibuild".equals(codeString)) 13945 return FHIRVersion._5_0_0CIBUILD; 13946 if ("5.0.0-snapshot1".equals(codeString)) 13947 return FHIRVersion._5_0_0SNAPSHOT1; 13948 if ("5.0.0-snapshot2".equals(codeString)) 13949 return FHIRVersion._5_0_0SNAPSHOT2; 13950 if ("5.0.0-ballot".equals(codeString)) 13951 return FHIRVersion._5_0_0BALLOT; 13952 if ("5.0.0-snapshot3".equals(codeString)) 13953 return FHIRVersion._5_0_0SNAPSHOT3; 13954 if ("5.0.0-draft-final".equals(codeString)) 13955 return FHIRVersion._5_0_0DRAFTFINAL; 13956 if ("6.0.0-cibuild".equals(codeString)) 13957 return FHIRVersion._6_0_0CIBUILD; 13958 if ("6.0.0".equals(codeString)) 13959 return FHIRVersion._6_0_0; 13960 if ("6.0.0-ballot1".equals(codeString)) 13961 return FHIRVersion._6_0_0_BALLOT1; 13962 if ("6.0.0-ballot2".equals(codeString)) 13963 return FHIRVersion._6_0_0_BALLOT2; 13964 throw new IllegalArgumentException("Unknown FHIRVersion code '"+codeString+"'"); 13965 } 13966 13967 public Enumeration<FHIRVersion> fromType(PrimitiveType<?> code) throws FHIRException { 13968 if (code == null) 13969 return null; 13970 if (code.isEmpty()) 13971 return new Enumeration<FHIRVersion>(this, FHIRVersion.NULL, code); 13972 String codeString = ((PrimitiveType) code).asStringValue(); 13973 if (codeString == null || "".equals(codeString)) 13974 return new Enumeration<FHIRVersion>(this, FHIRVersion.NULL, code); 13975 if ("0.01".equals(codeString)) 13976 return new Enumeration<FHIRVersion>(this, FHIRVersion._0_01, code); 13977 if ("0.05".equals(codeString)) 13978 return new Enumeration<FHIRVersion>(this, FHIRVersion._0_05, code); 13979 if ("0.06".equals(codeString)) 13980 return new Enumeration<FHIRVersion>(this, FHIRVersion._0_06, code); 13981 if ("0.11".equals(codeString)) 13982 return new Enumeration<FHIRVersion>(this, FHIRVersion._0_11, code); 13983 if ("0.0".equals(codeString)) 13984 return new Enumeration<FHIRVersion>(this, FHIRVersion._0_0, code); 13985 if ("0.0.80".equals(codeString)) 13986 return new Enumeration<FHIRVersion>(this, FHIRVersion._0_0_80, code); 13987 if ("0.0.81".equals(codeString)) 13988 return new Enumeration<FHIRVersion>(this, FHIRVersion._0_0_81, code); 13989 if ("0.0.82".equals(codeString)) 13990 return new Enumeration<FHIRVersion>(this, FHIRVersion._0_0_82, code); 13991 if ("0.4".equals(codeString)) 13992 return new Enumeration<FHIRVersion>(this, FHIRVersion._0_4, code); 13993 if ("0.4.0".equals(codeString)) 13994 return new Enumeration<FHIRVersion>(this, FHIRVersion._0_4_0, code); 13995 if ("0.5".equals(codeString)) 13996 return new Enumeration<FHIRVersion>(this, FHIRVersion._0_5, code); 13997 if ("0.5.0".equals(codeString)) 13998 return new Enumeration<FHIRVersion>(this, FHIRVersion._0_5_0, code); 13999 if ("1.0".equals(codeString)) 14000 return new Enumeration<FHIRVersion>(this, FHIRVersion._1_0, code); 14001 if ("1.0.0".equals(codeString)) 14002 return new Enumeration<FHIRVersion>(this, FHIRVersion._1_0_0, code); 14003 if ("1.0.1".equals(codeString)) 14004 return new Enumeration<FHIRVersion>(this, FHIRVersion._1_0_1, code); 14005 if ("1.0.2".equals(codeString)) 14006 return new Enumeration<FHIRVersion>(this, FHIRVersion._1_0_2, code); 14007 if ("1.1".equals(codeString)) 14008 return new Enumeration<FHIRVersion>(this, FHIRVersion._1_1, code); 14009 if ("1.1.0".equals(codeString)) 14010 return new Enumeration<FHIRVersion>(this, FHIRVersion._1_1_0, code); 14011 if ("1.4".equals(codeString)) 14012 return new Enumeration<FHIRVersion>(this, FHIRVersion._1_4, code); 14013 if ("1.4.0".equals(codeString)) 14014 return new Enumeration<FHIRVersion>(this, FHIRVersion._1_4_0, code); 14015 if ("1.6".equals(codeString)) 14016 return new Enumeration<FHIRVersion>(this, FHIRVersion._1_6, code); 14017 if ("1.6.0".equals(codeString)) 14018 return new Enumeration<FHIRVersion>(this, FHIRVersion._1_6_0, code); 14019 if ("1.8".equals(codeString)) 14020 return new Enumeration<FHIRVersion>(this, FHIRVersion._1_8, code); 14021 if ("1.8.0".equals(codeString)) 14022 return new Enumeration<FHIRVersion>(this, FHIRVersion._1_8_0, code); 14023 if ("3.0".equals(codeString)) 14024 return new Enumeration<FHIRVersion>(this, FHIRVersion._3_0, code); 14025 if ("3.0.0".equals(codeString)) 14026 return new Enumeration<FHIRVersion>(this, FHIRVersion._3_0_0, code); 14027 if ("3.0.1".equals(codeString)) 14028 return new Enumeration<FHIRVersion>(this, FHIRVersion._3_0_1, code); 14029 if ("3.0.2".equals(codeString)) 14030 return new Enumeration<FHIRVersion>(this, FHIRVersion._3_0_2, code); 14031 if ("3.3".equals(codeString)) 14032 return new Enumeration<FHIRVersion>(this, FHIRVersion._3_3, code); 14033 if ("3.3.0".equals(codeString)) 14034 return new Enumeration<FHIRVersion>(this, FHIRVersion._3_3_0, code); 14035 if ("3.5".equals(codeString)) 14036 return new Enumeration<FHIRVersion>(this, FHIRVersion._3_5, code); 14037 if ("3.5.0".equals(codeString)) 14038 return new Enumeration<FHIRVersion>(this, FHIRVersion._3_5_0, code); 14039 if ("4.0".equals(codeString)) 14040 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_0, code); 14041 if ("4.0.0".equals(codeString)) 14042 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_0_0, code); 14043 if ("4.0.1".equals(codeString)) 14044 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_0_1, code); 14045 if ("4.1".equals(codeString)) 14046 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_1, code); 14047 if ("4.1.0".equals(codeString)) 14048 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_1_0, code); 14049 if ("4.2".equals(codeString)) 14050 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_2, code); 14051 if ("4.2.0".equals(codeString)) 14052 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_2_0, code); 14053 if ("4.3".equals(codeString)) 14054 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_3, code); 14055 if ("4.3.0".equals(codeString)) 14056 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_3_0, code); 14057 if ("4.3.0-cibuild".equals(codeString)) 14058 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_3_0CIBUILD, code); 14059 if ("4.3.0-snapshot1".equals(codeString)) 14060 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_3_0SNAPSHOT1, code); 14061 if ("4.4".equals(codeString)) 14062 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_4, code); 14063 if ("4.4.0".equals(codeString)) 14064 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_4_0, code); 14065 if ("4.5".equals(codeString)) 14066 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_5, code); 14067 if ("4.5.0".equals(codeString)) 14068 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_5_0, code); 14069 if ("4.6".equals(codeString)) 14070 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_6, code); 14071 if ("4.6.0".equals(codeString)) 14072 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_6_0, code); 14073 if ("5.0".equals(codeString)) 14074 return new Enumeration<FHIRVersion>(this, FHIRVersion._5_0, code); 14075 if ("5.0.0".equals(codeString)) 14076 return new Enumeration<FHIRVersion>(this, FHIRVersion._5_0_0, code); 14077 if ("5.0.0-cibuild".equals(codeString)) 14078 return new Enumeration<FHIRVersion>(this, FHIRVersion._5_0_0CIBUILD, code); 14079 if ("5.0.0-snapshot1".equals(codeString)) 14080 return new Enumeration<FHIRVersion>(this, FHIRVersion._5_0_0SNAPSHOT1, code); 14081 if ("5.0.0-snapshot2".equals(codeString)) 14082 return new Enumeration<FHIRVersion>(this, FHIRVersion._5_0_0SNAPSHOT2, code); 14083 if ("5.0.0-ballot".equals(codeString)) 14084 return new Enumeration<FHIRVersion>(this, FHIRVersion._5_0_0BALLOT, code); 14085 if ("5.0.0-snapshot3".equals(codeString)) 14086 return new Enumeration<FHIRVersion>(this, FHIRVersion._5_0_0SNAPSHOT3, code); 14087 if ("5.0.0-draft-final".equals(codeString)) 14088 return new Enumeration<FHIRVersion>(this, FHIRVersion._5_0_0DRAFTFINAL, code); 14089 if ("6.0.0-cibuild".equals(codeString)) 14090 return new Enumeration<FHIRVersion>(this, FHIRVersion._6_0_0CIBUILD, code); 14091 if ("6.0.0".equals(codeString)) 14092 return new Enumeration<FHIRVersion>(this, FHIRVersion._6_0_0, code); 14093 if ("6.0.0-ballot1".equals(codeString)) 14094 return new Enumeration<FHIRVersion>(this, FHIRVersion._6_0_0_BALLOT1, code); 14095 if ("6.0.0-ballot2".equals(codeString)) 14096 return new Enumeration<FHIRVersion>(this, FHIRVersion._6_0_0_BALLOT2, code); 14097 throw new FHIRException("Unknown FHIRVersion code '"+codeString+"'"); 14098 } 14099 public String toCode(FHIRVersion code) { 14100 if (code == FHIRVersion.NULL) 14101 return null; 14102 if (code == FHIRVersion._0_01) 14103 return "0.01"; 14104 if (code == FHIRVersion._0_05) 14105 return "0.05"; 14106 if (code == FHIRVersion._0_06) 14107 return "0.06"; 14108 if (code == FHIRVersion._0_11) 14109 return "0.11"; 14110 if (code == FHIRVersion._0_0) 14111 return "0.0"; 14112 if (code == FHIRVersion._0_0_80) 14113 return "0.0.80"; 14114 if (code == FHIRVersion._0_0_81) 14115 return "0.0.81"; 14116 if (code == FHIRVersion._0_0_82) 14117 return "0.0.82"; 14118 if (code == FHIRVersion._0_4) 14119 return "0.4"; 14120 if (code == FHIRVersion._0_4_0) 14121 return "0.4.0"; 14122 if (code == FHIRVersion._0_5) 14123 return "0.5"; 14124 if (code == FHIRVersion._0_5_0) 14125 return "0.5.0"; 14126 if (code == FHIRVersion._1_0) 14127 return "1.0"; 14128 if (code == FHIRVersion._1_0_0) 14129 return "1.0.0"; 14130 if (code == FHIRVersion._1_0_1) 14131 return "1.0.1"; 14132 if (code == FHIRVersion._1_0_2) 14133 return "1.0.2"; 14134 if (code == FHIRVersion._1_1) 14135 return "1.1"; 14136 if (code == FHIRVersion._1_1_0) 14137 return "1.1.0"; 14138 if (code == FHIRVersion._1_4) 14139 return "1.4"; 14140 if (code == FHIRVersion._1_4_0) 14141 return "1.4.0"; 14142 if (code == FHIRVersion._1_6) 14143 return "1.6"; 14144 if (code == FHIRVersion._1_6_0) 14145 return "1.6.0"; 14146 if (code == FHIRVersion._1_8) 14147 return "1.8"; 14148 if (code == FHIRVersion._1_8_0) 14149 return "1.8.0"; 14150 if (code == FHIRVersion._3_0) 14151 return "3.0"; 14152 if (code == FHIRVersion._3_0_0) 14153 return "3.0.0"; 14154 if (code == FHIRVersion._3_0_1) 14155 return "3.0.1"; 14156 if (code == FHIRVersion._3_0_2) 14157 return "3.0.2"; 14158 if (code == FHIRVersion._3_3) 14159 return "3.3"; 14160 if (code == FHIRVersion._3_3_0) 14161 return "3.3.0"; 14162 if (code == FHIRVersion._3_5) 14163 return "3.5"; 14164 if (code == FHIRVersion._3_5_0) 14165 return "3.5.0"; 14166 if (code == FHIRVersion._4_0) 14167 return "4.0"; 14168 if (code == FHIRVersion._4_0_0) 14169 return "4.0.0"; 14170 if (code == FHIRVersion._4_0_1) 14171 return "4.0.1"; 14172 if (code == FHIRVersion._4_1) 14173 return "4.1"; 14174 if (code == FHIRVersion._4_1_0) 14175 return "4.1.0"; 14176 if (code == FHIRVersion._4_2) 14177 return "4.2"; 14178 if (code == FHIRVersion._4_2_0) 14179 return "4.2.0"; 14180 if (code == FHIRVersion._4_3) 14181 return "4.3"; 14182 if (code == FHIRVersion._4_3_0) 14183 return "4.3.0"; 14184 if (code == FHIRVersion._4_3_0CIBUILD) 14185 return "4.3.0-cibuild"; 14186 if (code == FHIRVersion._4_3_0SNAPSHOT1) 14187 return "4.3.0-snapshot1"; 14188 if (code == FHIRVersion._4_4) 14189 return "4.4"; 14190 if (code == FHIRVersion._4_4_0) 14191 return "4.4.0"; 14192 if (code == FHIRVersion._4_5) 14193 return "4.5"; 14194 if (code == FHIRVersion._4_5_0) 14195 return "4.5.0"; 14196 if (code == FHIRVersion._4_6) 14197 return "4.6"; 14198 if (code == FHIRVersion._4_6_0) 14199 return "4.6.0"; 14200 if (code == FHIRVersion._5_0) 14201 return "5.0"; 14202 if (code == FHIRVersion._5_0_0) 14203 return "5.0.0"; 14204 if (code == FHIRVersion._5_0_0CIBUILD) 14205 return "5.0.0-cibuild"; 14206 if (code == FHIRVersion._5_0_0SNAPSHOT1) 14207 return "5.0.0-snapshot1"; 14208 if (code == FHIRVersion._5_0_0SNAPSHOT2) 14209 return "5.0.0-snapshot2"; 14210 if (code == FHIRVersion._5_0_0BALLOT) 14211 return "5.0.0-ballot"; 14212 if (code == FHIRVersion._5_0_0SNAPSHOT3) 14213 return "5.0.0-snapshot3"; 14214 if (code == FHIRVersion._5_0_0DRAFTFINAL) 14215 return "5.0.0-draft-final"; 14216 if (code == FHIRVersion._6_0_0CIBUILD) 14217 return "6.0.0-cibuild"; 14218 if (code == FHIRVersion._6_0_0) 14219 return "6.0.0"; 14220 if (code == FHIRVersion._6_0_0_BALLOT1) { 14221 return "6.0.0-ballot1"; 14222 } 14223 if (code == FHIRVersion._6_0_0_BALLOT2) { 14224 return "6.0.0-ballot2"; 14225 } 14226 return "?"; 14227 } 14228 14229 public String toSystem(FHIRVersion code) { 14230 return code.getSystem(); 14231 } 14232 } 14233 14234 public enum KnowledgeResourceType { 14235 /** 14236 * The definition of a specific activity to be taken, independent of any 14237 * particular patient or context. 14238 */ 14239 ACTIVITYDEFINITION, 14240 /** 14241 * A set of codes drawn from one or more code systems. 14242 */ 14243 CODESYSTEM, 14244 /** 14245 * A map from one set of concepts to one or more other concepts. 14246 */ 14247 CONCEPTMAP, 14248 /** 14249 * Represents a library of quality improvement components. 14250 */ 14251 LIBRARY, 14252 /** 14253 * A quality measure definition. 14254 */ 14255 MEASURE, 14256 /** 14257 * The definition of a plan for a series of actions, independent of any specific 14258 * patient or context. 14259 */ 14260 PLANDEFINITION, 14261 /** 14262 * Structural Definition. 14263 */ 14264 STRUCTUREDEFINITION, 14265 /** 14266 * A Map of relationships between 2 structures that can be used to transform 14267 * data. 14268 */ 14269 STRUCTUREMAP, 14270 /** 14271 * A set of codes drawn from one or more code systems. 14272 */ 14273 VALUESET, 14274 /** 14275 * added to help the parsers 14276 */ 14277 NULL; 14278 14279 public static KnowledgeResourceType fromCode(String codeString) throws FHIRException { 14280 if (codeString == null || "".equals(codeString)) 14281 return null; 14282 if ("ActivityDefinition".equals(codeString)) 14283 return ACTIVITYDEFINITION; 14284 if ("CodeSystem".equals(codeString)) 14285 return CODESYSTEM; 14286 if ("ConceptMap".equals(codeString)) 14287 return CONCEPTMAP; 14288 if ("Library".equals(codeString)) 14289 return LIBRARY; 14290 if ("Measure".equals(codeString)) 14291 return MEASURE; 14292 if ("PlanDefinition".equals(codeString)) 14293 return PLANDEFINITION; 14294 if ("StructureDefinition".equals(codeString)) 14295 return STRUCTUREDEFINITION; 14296 if ("StructureMap".equals(codeString)) 14297 return STRUCTUREMAP; 14298 if ("ValueSet".equals(codeString)) 14299 return VALUESET; 14300 throw new FHIRException("Unknown KnowledgeResourceType code '" + codeString + "'"); 14301 } 14302 14303 public String toCode() { 14304 switch (this) { 14305 case ACTIVITYDEFINITION: 14306 return "ActivityDefinition"; 14307 case CODESYSTEM: 14308 return "CodeSystem"; 14309 case CONCEPTMAP: 14310 return "ConceptMap"; 14311 case LIBRARY: 14312 return "Library"; 14313 case MEASURE: 14314 return "Measure"; 14315 case PLANDEFINITION: 14316 return "PlanDefinition"; 14317 case STRUCTUREDEFINITION: 14318 return "StructureDefinition"; 14319 case STRUCTUREMAP: 14320 return "StructureMap"; 14321 case VALUESET: 14322 return "ValueSet"; 14323 case NULL: 14324 return null; 14325 default: 14326 return "?"; 14327 } 14328 } 14329 14330 public String getSystem() { 14331 switch (this) { 14332 case ACTIVITYDEFINITION: 14333 return "http://hl7.org/fhir/knowledge-resource-types"; 14334 case CODESYSTEM: 14335 return "http://hl7.org/fhir/knowledge-resource-types"; 14336 case CONCEPTMAP: 14337 return "http://hl7.org/fhir/knowledge-resource-types"; 14338 case LIBRARY: 14339 return "http://hl7.org/fhir/knowledge-resource-types"; 14340 case MEASURE: 14341 return "http://hl7.org/fhir/knowledge-resource-types"; 14342 case PLANDEFINITION: 14343 return "http://hl7.org/fhir/knowledge-resource-types"; 14344 case STRUCTUREDEFINITION: 14345 return "http://hl7.org/fhir/knowledge-resource-types"; 14346 case STRUCTUREMAP: 14347 return "http://hl7.org/fhir/knowledge-resource-types"; 14348 case VALUESET: 14349 return "http://hl7.org/fhir/knowledge-resource-types"; 14350 case NULL: 14351 return null; 14352 default: 14353 return "?"; 14354 } 14355 } 14356 14357 public String getDefinition() { 14358 switch (this) { 14359 case ACTIVITYDEFINITION: 14360 return "The definition of a specific activity to be taken, independent of any particular patient or context."; 14361 case CODESYSTEM: 14362 return "A set of codes drawn from one or more code systems."; 14363 case CONCEPTMAP: 14364 return "A map from one set of concepts to one or more other concepts."; 14365 case LIBRARY: 14366 return "Represents a library of quality improvement components."; 14367 case MEASURE: 14368 return "A quality measure definition."; 14369 case PLANDEFINITION: 14370 return "The definition of a plan for a series of actions, independent of any specific patient or context."; 14371 case STRUCTUREDEFINITION: 14372 return "Structural Definition."; 14373 case STRUCTUREMAP: 14374 return "A Map of relationships between 2 structures that can be used to transform data."; 14375 case VALUESET: 14376 return "A set of codes drawn from one or more code systems."; 14377 case NULL: 14378 return null; 14379 default: 14380 return "?"; 14381 } 14382 } 14383 14384 public String getDisplay() { 14385 switch (this) { 14386 case ACTIVITYDEFINITION: 14387 return "ActivityDefinition"; 14388 case CODESYSTEM: 14389 return "CodeSystem"; 14390 case CONCEPTMAP: 14391 return "ConceptMap"; 14392 case LIBRARY: 14393 return "Library"; 14394 case MEASURE: 14395 return "Measure"; 14396 case PLANDEFINITION: 14397 return "PlanDefinition"; 14398 case STRUCTUREDEFINITION: 14399 return "StructureDefinition"; 14400 case STRUCTUREMAP: 14401 return "StructureMap"; 14402 case VALUESET: 14403 return "ValueSet"; 14404 case NULL: 14405 return null; 14406 default: 14407 return "?"; 14408 } 14409 } 14410 } 14411 14412 public static class KnowledgeResourceTypeEnumFactory implements EnumFactory<KnowledgeResourceType> { 14413 public KnowledgeResourceType fromCode(String codeString) throws IllegalArgumentException { 14414 if (codeString == null || "".equals(codeString)) 14415 if (codeString == null || "".equals(codeString)) 14416 return null; 14417 if ("ActivityDefinition".equals(codeString)) 14418 return KnowledgeResourceType.ACTIVITYDEFINITION; 14419 if ("CodeSystem".equals(codeString)) 14420 return KnowledgeResourceType.CODESYSTEM; 14421 if ("ConceptMap".equals(codeString)) 14422 return KnowledgeResourceType.CONCEPTMAP; 14423 if ("Library".equals(codeString)) 14424 return KnowledgeResourceType.LIBRARY; 14425 if ("Measure".equals(codeString)) 14426 return KnowledgeResourceType.MEASURE; 14427 if ("PlanDefinition".equals(codeString)) 14428 return KnowledgeResourceType.PLANDEFINITION; 14429 if ("StructureDefinition".equals(codeString)) 14430 return KnowledgeResourceType.STRUCTUREDEFINITION; 14431 if ("StructureMap".equals(codeString)) 14432 return KnowledgeResourceType.STRUCTUREMAP; 14433 if ("ValueSet".equals(codeString)) 14434 return KnowledgeResourceType.VALUESET; 14435 throw new IllegalArgumentException("Unknown KnowledgeResourceType code '" + codeString + "'"); 14436 } 14437 14438 public Enumeration<KnowledgeResourceType> fromType(PrimitiveType<?> code) throws FHIRException { 14439 if (code == null) 14440 return null; 14441 if (code.isEmpty()) 14442 return new Enumeration<KnowledgeResourceType>(this, KnowledgeResourceType.NULL, code); 14443 String codeString = code.asStringValue(); 14444 if (codeString == null || "".equals(codeString)) 14445 return new Enumeration<KnowledgeResourceType>(this, KnowledgeResourceType.NULL, code); 14446 if ("ActivityDefinition".equals(codeString)) 14447 return new Enumeration<KnowledgeResourceType>(this, KnowledgeResourceType.ACTIVITYDEFINITION, code); 14448 if ("CodeSystem".equals(codeString)) 14449 return new Enumeration<KnowledgeResourceType>(this, KnowledgeResourceType.CODESYSTEM, code); 14450 if ("ConceptMap".equals(codeString)) 14451 return new Enumeration<KnowledgeResourceType>(this, KnowledgeResourceType.CONCEPTMAP, code); 14452 if ("Library".equals(codeString)) 14453 return new Enumeration<KnowledgeResourceType>(this, KnowledgeResourceType.LIBRARY, code); 14454 if ("Measure".equals(codeString)) 14455 return new Enumeration<KnowledgeResourceType>(this, KnowledgeResourceType.MEASURE, code); 14456 if ("PlanDefinition".equals(codeString)) 14457 return new Enumeration<KnowledgeResourceType>(this, KnowledgeResourceType.PLANDEFINITION, code); 14458 if ("StructureDefinition".equals(codeString)) 14459 return new Enumeration<KnowledgeResourceType>(this, KnowledgeResourceType.STRUCTUREDEFINITION, code); 14460 if ("StructureMap".equals(codeString)) 14461 return new Enumeration<KnowledgeResourceType>(this, KnowledgeResourceType.STRUCTUREMAP, code); 14462 if ("ValueSet".equals(codeString)) 14463 return new Enumeration<KnowledgeResourceType>(this, KnowledgeResourceType.VALUESET, code); 14464 throw new FHIRException("Unknown KnowledgeResourceType code '" + codeString + "'"); 14465 } 14466 14467 public String toCode(KnowledgeResourceType code) { 14468 if (code == KnowledgeResourceType.NULL) 14469 return null; 14470 if (code == KnowledgeResourceType.ACTIVITYDEFINITION) 14471 return "ActivityDefinition"; 14472 if (code == KnowledgeResourceType.CODESYSTEM) 14473 return "CodeSystem"; 14474 if (code == KnowledgeResourceType.CONCEPTMAP) 14475 return "ConceptMap"; 14476 if (code == KnowledgeResourceType.LIBRARY) 14477 return "Library"; 14478 if (code == KnowledgeResourceType.MEASURE) 14479 return "Measure"; 14480 if (code == KnowledgeResourceType.PLANDEFINITION) 14481 return "PlanDefinition"; 14482 if (code == KnowledgeResourceType.STRUCTUREDEFINITION) 14483 return "StructureDefinition"; 14484 if (code == KnowledgeResourceType.STRUCTUREMAP) 14485 return "StructureMap"; 14486 if (code == KnowledgeResourceType.VALUESET) 14487 return "ValueSet"; 14488 return "?"; 14489 } 14490 14491 public String toSystem(KnowledgeResourceType code) { 14492 return code.getSystem(); 14493 } 14494 } 14495 14496 public enum MessageEvent { 14497 /** 14498 * added to help the parsers 14499 */ 14500 NULL; 14501 14502 public static MessageEvent fromCode(String codeString) throws FHIRException { 14503 if (codeString == null || "".equals(codeString)) 14504 return null; 14505 throw new FHIRException("Unknown MessageEvent code '" + codeString + "'"); 14506 } 14507 14508 public String toCode() { 14509 switch (this) { 14510 case NULL: 14511 return null; 14512 default: 14513 return "?"; 14514 } 14515 } 14516 14517 public String getSystem() { 14518 switch (this) { 14519 case NULL: 14520 return null; 14521 default: 14522 return "?"; 14523 } 14524 } 14525 14526 public String getDefinition() { 14527 switch (this) { 14528 case NULL: 14529 return null; 14530 default: 14531 return "?"; 14532 } 14533 } 14534 14535 public String getDisplay() { 14536 switch (this) { 14537 case NULL: 14538 return null; 14539 default: 14540 return "?"; 14541 } 14542 } 14543 } 14544 14545 public static class MessageEventEnumFactory implements EnumFactory<MessageEvent> { 14546 public MessageEvent fromCode(String codeString) throws IllegalArgumentException { 14547 if (codeString == null || "".equals(codeString)) 14548 if (codeString == null || "".equals(codeString)) 14549 return null; 14550 throw new IllegalArgumentException("Unknown MessageEvent code '" + codeString + "'"); 14551 } 14552 14553 public Enumeration<MessageEvent> fromType(PrimitiveType<?> code) throws FHIRException { 14554 if (code == null) 14555 return null; 14556 if (code.isEmpty()) 14557 return new Enumeration<MessageEvent>(this, MessageEvent.NULL, code); 14558 String codeString = code.asStringValue(); 14559 if (codeString == null || "".equals(codeString)) 14560 return new Enumeration<MessageEvent>(this, MessageEvent.NULL, code); 14561 throw new FHIRException("Unknown MessageEvent code '" + codeString + "'"); 14562 } 14563 14564 public String toCode(MessageEvent code) { 14565 if (code == MessageEvent.NULL) 14566 return null; 14567 return "?"; 14568 } 14569 14570 public String toSystem(MessageEvent code) { 14571 return code.getSystem(); 14572 } 14573 } 14574 14575 public enum NoteType { 14576 /** 14577 * Display the note. 14578 */ 14579 DISPLAY, 14580 /** 14581 * Print the note on the form. 14582 */ 14583 PRINT, 14584 /** 14585 * Print the note for the operator. 14586 */ 14587 PRINTOPER, 14588 /** 14589 * added to help the parsers 14590 */ 14591 NULL; 14592 14593 public static NoteType fromCode(String codeString) throws FHIRException { 14594 if (codeString == null || "".equals(codeString)) 14595 return null; 14596 if ("display".equals(codeString)) 14597 return DISPLAY; 14598 if ("print".equals(codeString)) 14599 return PRINT; 14600 if ("printoper".equals(codeString)) 14601 return PRINTOPER; 14602 throw new FHIRException("Unknown NoteType code '" + codeString + "'"); 14603 } 14604 14605 public String toCode() { 14606 switch (this) { 14607 case DISPLAY: 14608 return "display"; 14609 case PRINT: 14610 return "print"; 14611 case PRINTOPER: 14612 return "printoper"; 14613 case NULL: 14614 return null; 14615 default: 14616 return "?"; 14617 } 14618 } 14619 14620 public String getSystem() { 14621 switch (this) { 14622 case DISPLAY: 14623 return "http://hl7.org/fhir/note-type"; 14624 case PRINT: 14625 return "http://hl7.org/fhir/note-type"; 14626 case PRINTOPER: 14627 return "http://hl7.org/fhir/note-type"; 14628 case NULL: 14629 return null; 14630 default: 14631 return "?"; 14632 } 14633 } 14634 14635 public String getDefinition() { 14636 switch (this) { 14637 case DISPLAY: 14638 return "Display the note."; 14639 case PRINT: 14640 return "Print the note on the form."; 14641 case PRINTOPER: 14642 return "Print the note for the operator."; 14643 case NULL: 14644 return null; 14645 default: 14646 return "?"; 14647 } 14648 } 14649 14650 public String getDisplay() { 14651 switch (this) { 14652 case DISPLAY: 14653 return "Display"; 14654 case PRINT: 14655 return "Print (Form)"; 14656 case PRINTOPER: 14657 return "Print (Operator)"; 14658 case NULL: 14659 return null; 14660 default: 14661 return "?"; 14662 } 14663 } 14664 } 14665 14666 public static class NoteTypeEnumFactory implements EnumFactory<NoteType> { 14667 public NoteType fromCode(String codeString) throws IllegalArgumentException { 14668 if (codeString == null || "".equals(codeString)) 14669 if (codeString == null || "".equals(codeString)) 14670 return null; 14671 if ("display".equals(codeString)) 14672 return NoteType.DISPLAY; 14673 if ("print".equals(codeString)) 14674 return NoteType.PRINT; 14675 if ("printoper".equals(codeString)) 14676 return NoteType.PRINTOPER; 14677 throw new IllegalArgumentException("Unknown NoteType code '" + codeString + "'"); 14678 } 14679 14680 public Enumeration<NoteType> fromType(PrimitiveType<?> code) throws FHIRException { 14681 if (code == null) 14682 return null; 14683 if (code.isEmpty()) 14684 return new Enumeration<NoteType>(this, NoteType.NULL, code); 14685 String codeString = code.asStringValue(); 14686 if (codeString == null || "".equals(codeString)) 14687 return new Enumeration<NoteType>(this, NoteType.NULL, code); 14688 if ("display".equals(codeString)) 14689 return new Enumeration<NoteType>(this, NoteType.DISPLAY, code); 14690 if ("print".equals(codeString)) 14691 return new Enumeration<NoteType>(this, NoteType.PRINT, code); 14692 if ("printoper".equals(codeString)) 14693 return new Enumeration<NoteType>(this, NoteType.PRINTOPER, code); 14694 throw new FHIRException("Unknown NoteType code '" + codeString + "'"); 14695 } 14696 14697 public String toCode(NoteType code) { 14698 if (code == NoteType.NULL) 14699 return null; 14700 if (code == NoteType.DISPLAY) 14701 return "display"; 14702 if (code == NoteType.PRINT) 14703 return "print"; 14704 if (code == NoteType.PRINTOPER) 14705 return "printoper"; 14706 return "?"; 14707 } 14708 14709 public String toSystem(NoteType code) { 14710 return code.getSystem(); 14711 } 14712 } 14713 14714 public enum PublicationStatus { 14715 /** 14716 * This resource is still under development and is not yet considered to be 14717 * ready for normal use. 14718 */ 14719 DRAFT, 14720 /** 14721 * This resource is ready for normal use. 14722 */ 14723 ACTIVE, 14724 /** 14725 * This resource has been withdrawn or superseded and should no longer be used. 14726 */ 14727 RETIRED, 14728 /** 14729 * The authoring system does not know which of the status values currently 14730 * applies for this resource. Note: This concept is not to be used for "other" - 14731 * one of the listed statuses is presumed to apply, it's just not known which 14732 * one. 14733 */ 14734 UNKNOWN, 14735 /** 14736 * added to help the parsers 14737 */ 14738 NULL; 14739 14740 public static PublicationStatus fromCode(String codeString) throws FHIRException { 14741 if (codeString == null || "".equals(codeString)) 14742 return null; 14743 if ("draft".equals(codeString)) 14744 return DRAFT; 14745 if ("active".equals(codeString)) 14746 return ACTIVE; 14747 if ("retired".equals(codeString)) 14748 return RETIRED; 14749 if ("unknown".equals(codeString)) 14750 return UNKNOWN; 14751 throw new FHIRException("Unknown PublicationStatus code '" + codeString + "'"); 14752 } 14753 14754 public String toCode() { 14755 switch (this) { 14756 case DRAFT: 14757 return "draft"; 14758 case ACTIVE: 14759 return "active"; 14760 case RETIRED: 14761 return "retired"; 14762 case UNKNOWN: 14763 return "unknown"; 14764 case NULL: 14765 return null; 14766 default: 14767 return "?"; 14768 } 14769 } 14770 14771 public String getSystem() { 14772 switch (this) { 14773 case DRAFT: 14774 return "http://hl7.org/fhir/publication-status"; 14775 case ACTIVE: 14776 return "http://hl7.org/fhir/publication-status"; 14777 case RETIRED: 14778 return "http://hl7.org/fhir/publication-status"; 14779 case UNKNOWN: 14780 return "http://hl7.org/fhir/publication-status"; 14781 case NULL: 14782 return null; 14783 default: 14784 return "?"; 14785 } 14786 } 14787 14788 public String getDefinition() { 14789 switch (this) { 14790 case DRAFT: 14791 return "This resource is still under development and is not yet considered to be ready for normal use."; 14792 case ACTIVE: 14793 return "This resource is ready for normal use."; 14794 case RETIRED: 14795 return "This resource has been withdrawn or superseded and should no longer be used."; 14796 case UNKNOWN: 14797 return "The authoring system does not know which of the status values currently applies for this resource. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one."; 14798 case NULL: 14799 return null; 14800 default: 14801 return "?"; 14802 } 14803 } 14804 14805 public String getDisplay() { 14806 switch (this) { 14807 case DRAFT: 14808 return "Draft"; 14809 case ACTIVE: 14810 return "Active"; 14811 case RETIRED: 14812 return "Retired"; 14813 case UNKNOWN: 14814 return "Unknown"; 14815 case NULL: 14816 return null; 14817 default: 14818 return "?"; 14819 } 14820 } 14821 } 14822 14823 public static class PublicationStatusEnumFactory implements EnumFactory<PublicationStatus> { 14824 public PublicationStatus fromCode(String codeString) throws IllegalArgumentException { 14825 if (codeString == null || "".equals(codeString)) 14826 if (codeString == null || "".equals(codeString)) 14827 return null; 14828 if ("draft".equals(codeString)) 14829 return PublicationStatus.DRAFT; 14830 if ("active".equals(codeString)) 14831 return PublicationStatus.ACTIVE; 14832 if ("retired".equals(codeString)) 14833 return PublicationStatus.RETIRED; 14834 if ("unknown".equals(codeString)) 14835 return PublicationStatus.UNKNOWN; 14836 throw new IllegalArgumentException("Unknown PublicationStatus code '" + codeString + "'"); 14837 } 14838 14839 public Enumeration<PublicationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 14840 if (code == null) 14841 return null; 14842 if (code.isEmpty()) 14843 return new Enumeration<PublicationStatus>(this, PublicationStatus.NULL, code); 14844 String codeString = code.asStringValue(); 14845 if (codeString == null || "".equals(codeString)) 14846 return new Enumeration<PublicationStatus>(this, PublicationStatus.NULL, code); 14847 if ("draft".equals(codeString)) 14848 return new Enumeration<PublicationStatus>(this, PublicationStatus.DRAFT, code); 14849 if ("active".equals(codeString)) 14850 return new Enumeration<PublicationStatus>(this, PublicationStatus.ACTIVE, code); 14851 if ("retired".equals(codeString)) 14852 return new Enumeration<PublicationStatus>(this, PublicationStatus.RETIRED, code); 14853 if ("unknown".equals(codeString)) 14854 return new Enumeration<PublicationStatus>(this, PublicationStatus.UNKNOWN, code); 14855 throw new FHIRException("Unknown PublicationStatus code '" + codeString + "'"); 14856 } 14857 14858 public String toCode(PublicationStatus code) { 14859 if (code == PublicationStatus.NULL) 14860 return null; 14861 if (code == PublicationStatus.DRAFT) 14862 return "draft"; 14863 if (code == PublicationStatus.ACTIVE) 14864 return "active"; 14865 if (code == PublicationStatus.RETIRED) 14866 return "retired"; 14867 if (code == PublicationStatus.UNKNOWN) 14868 return "unknown"; 14869 return "?"; 14870 } 14871 14872 public String toSystem(PublicationStatus code) { 14873 return code.getSystem(); 14874 } 14875 } 14876 14877 public enum RemittanceOutcome { 14878 /** 14879 * The Claim/Pre-authorization/Pre-determination has been received but 14880 * processing has not begun. 14881 */ 14882 QUEUED, 14883 /** 14884 * The processing has completed without errors 14885 */ 14886 COMPLETE, 14887 /** 14888 * One or more errors have been detected in the Claim 14889 */ 14890 ERROR, 14891 /** 14892 * No errors have been detected in the Claim and some of the adjudication has 14893 * been performed. 14894 */ 14895 PARTIAL, 14896 /** 14897 * added to help the parsers 14898 */ 14899 NULL; 14900 14901 public static RemittanceOutcome fromCode(String codeString) throws FHIRException { 14902 if (codeString == null || "".equals(codeString)) 14903 return null; 14904 if ("queued".equals(codeString)) 14905 return QUEUED; 14906 if ("complete".equals(codeString)) 14907 return COMPLETE; 14908 if ("error".equals(codeString)) 14909 return ERROR; 14910 if ("partial".equals(codeString)) 14911 return PARTIAL; 14912 throw new FHIRException("Unknown RemittanceOutcome code '" + codeString + "'"); 14913 } 14914 14915 public String toCode() { 14916 switch (this) { 14917 case QUEUED: 14918 return "queued"; 14919 case COMPLETE: 14920 return "complete"; 14921 case ERROR: 14922 return "error"; 14923 case PARTIAL: 14924 return "partial"; 14925 case NULL: 14926 return null; 14927 default: 14928 return "?"; 14929 } 14930 } 14931 14932 public String getSystem() { 14933 switch (this) { 14934 case QUEUED: 14935 return "http://hl7.org/fhir/remittance-outcome"; 14936 case COMPLETE: 14937 return "http://hl7.org/fhir/remittance-outcome"; 14938 case ERROR: 14939 return "http://hl7.org/fhir/remittance-outcome"; 14940 case PARTIAL: 14941 return "http://hl7.org/fhir/remittance-outcome"; 14942 case NULL: 14943 return null; 14944 default: 14945 return "?"; 14946 } 14947 } 14948 14949 public String getDefinition() { 14950 switch (this) { 14951 case QUEUED: 14952 return "The Claim/Pre-authorization/Pre-determination has been received but processing has not begun."; 14953 case COMPLETE: 14954 return "The processing has completed without errors"; 14955 case ERROR: 14956 return "One or more errors have been detected in the Claim"; 14957 case PARTIAL: 14958 return "No errors have been detected in the Claim and some of the adjudication has been performed."; 14959 case NULL: 14960 return null; 14961 default: 14962 return "?"; 14963 } 14964 } 14965 14966 public String getDisplay() { 14967 switch (this) { 14968 case QUEUED: 14969 return "Queued"; 14970 case COMPLETE: 14971 return "Processing Complete"; 14972 case ERROR: 14973 return "Error"; 14974 case PARTIAL: 14975 return "Partial Processing"; 14976 case NULL: 14977 return null; 14978 default: 14979 return "?"; 14980 } 14981 } 14982 } 14983 14984 public static class RemittanceOutcomeEnumFactory implements EnumFactory<RemittanceOutcome> { 14985 public RemittanceOutcome fromCode(String codeString) throws IllegalArgumentException { 14986 if (codeString == null || "".equals(codeString)) 14987 if (codeString == null || "".equals(codeString)) 14988 return null; 14989 if ("queued".equals(codeString)) 14990 return RemittanceOutcome.QUEUED; 14991 if ("complete".equals(codeString)) 14992 return RemittanceOutcome.COMPLETE; 14993 if ("error".equals(codeString)) 14994 return RemittanceOutcome.ERROR; 14995 if ("partial".equals(codeString)) 14996 return RemittanceOutcome.PARTIAL; 14997 throw new IllegalArgumentException("Unknown RemittanceOutcome code '" + codeString + "'"); 14998 } 14999 15000 public Enumeration<RemittanceOutcome> fromType(PrimitiveType<?> code) throws FHIRException { 15001 if (code == null) 15002 return null; 15003 if (code.isEmpty()) 15004 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.NULL, code); 15005 String codeString = code.asStringValue(); 15006 if (codeString == null || "".equals(codeString)) 15007 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.NULL, code); 15008 if ("queued".equals(codeString)) 15009 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.QUEUED, code); 15010 if ("complete".equals(codeString)) 15011 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.COMPLETE, code); 15012 if ("error".equals(codeString)) 15013 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.ERROR, code); 15014 if ("partial".equals(codeString)) 15015 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.PARTIAL, code); 15016 throw new FHIRException("Unknown RemittanceOutcome code '" + codeString + "'"); 15017 } 15018 15019 public String toCode(RemittanceOutcome code) { 15020 if (code == RemittanceOutcome.NULL) 15021 return null; 15022 if (code == RemittanceOutcome.QUEUED) 15023 return "queued"; 15024 if (code == RemittanceOutcome.COMPLETE) 15025 return "complete"; 15026 if (code == RemittanceOutcome.ERROR) 15027 return "error"; 15028 if (code == RemittanceOutcome.PARTIAL) 15029 return "partial"; 15030 return "?"; 15031 } 15032 15033 public String toSystem(RemittanceOutcome code) { 15034 return code.getSystem(); 15035 } 15036 } 15037 15038 public enum RequestResourceType { 15039 /** 15040 * A booking of a healthcare event among patient(s), practitioner(s), related 15041 * person(s) and/or device(s) for a specific date/time. This may result in one 15042 * or more Encounter(s). 15043 */ 15044 APPOINTMENT, 15045 /** 15046 * A reply to an appointment request for a patient and/or practitioner(s), such 15047 * as a confirmation or rejection. 15048 */ 15049 APPOINTMENTRESPONSE, 15050 /** 15051 * Healthcare plan for patient or group. 15052 */ 15053 CAREPLAN, 15054 /** 15055 * Claim, Pre-determination or Pre-authorization. 15056 */ 15057 CLAIM, 15058 /** 15059 * A request for information to be sent to a receiver. 15060 */ 15061 COMMUNICATIONREQUEST, 15062 /** 15063 * Legal Agreement. 15064 */ 15065 CONTRACT, 15066 /** 15067 * Medical device request. 15068 */ 15069 DEVICEREQUEST, 15070 /** 15071 * Enrollment request. 15072 */ 15073 ENROLLMENTREQUEST, 15074 /** 15075 * Guidance or advice relating to an immunization. 15076 */ 15077 IMMUNIZATIONRECOMMENDATION, 15078 /** 15079 * Ordering of medication for patient or group. 15080 */ 15081 MEDICATIONREQUEST, 15082 /** 15083 * Diet, formula or nutritional supplement request. 15084 */ 15085 NUTRITIONORDER, 15086 /** 15087 * A record of a request for service such as diagnostic investigations, 15088 * treatments, or operations to be performed. 15089 */ 15090 SERVICEREQUEST, 15091 /** 15092 * Request for a medication, substance or device. 15093 */ 15094 SUPPLYREQUEST, 15095 /** 15096 * A task to be performed. 15097 */ 15098 TASK, 15099 /** 15100 * Prescription for vision correction products for a patient. 15101 */ 15102 VISIONPRESCRIPTION, 15103 /** 15104 * added to help the parsers 15105 */ 15106 NULL; 15107 15108 public static RequestResourceType fromCode(String codeString) throws FHIRException { 15109 if (codeString == null || "".equals(codeString)) 15110 return null; 15111 if ("Appointment".equals(codeString)) 15112 return APPOINTMENT; 15113 if ("AppointmentResponse".equals(codeString)) 15114 return APPOINTMENTRESPONSE; 15115 if ("CarePlan".equals(codeString)) 15116 return CAREPLAN; 15117 if ("Claim".equals(codeString)) 15118 return CLAIM; 15119 if ("CommunicationRequest".equals(codeString)) 15120 return COMMUNICATIONREQUEST; 15121 if ("Contract".equals(codeString)) 15122 return CONTRACT; 15123 if ("DeviceRequest".equals(codeString)) 15124 return DEVICEREQUEST; 15125 if ("EnrollmentRequest".equals(codeString)) 15126 return ENROLLMENTREQUEST; 15127 if ("ImmunizationRecommendation".equals(codeString)) 15128 return IMMUNIZATIONRECOMMENDATION; 15129 if ("MedicationRequest".equals(codeString)) 15130 return MEDICATIONREQUEST; 15131 if ("NutritionOrder".equals(codeString)) 15132 return NUTRITIONORDER; 15133 if ("ServiceRequest".equals(codeString)) 15134 return SERVICEREQUEST; 15135 if ("SupplyRequest".equals(codeString)) 15136 return SUPPLYREQUEST; 15137 if ("Task".equals(codeString)) 15138 return TASK; 15139 if ("VisionPrescription".equals(codeString)) 15140 return VISIONPRESCRIPTION; 15141 throw new FHIRException("Unknown RequestResourceType code '" + codeString + "'"); 15142 } 15143 15144 public String toCode() { 15145 switch (this) { 15146 case APPOINTMENT: 15147 return "Appointment"; 15148 case APPOINTMENTRESPONSE: 15149 return "AppointmentResponse"; 15150 case CAREPLAN: 15151 return "CarePlan"; 15152 case CLAIM: 15153 return "Claim"; 15154 case COMMUNICATIONREQUEST: 15155 return "CommunicationRequest"; 15156 case CONTRACT: 15157 return "Contract"; 15158 case DEVICEREQUEST: 15159 return "DeviceRequest"; 15160 case ENROLLMENTREQUEST: 15161 return "EnrollmentRequest"; 15162 case IMMUNIZATIONRECOMMENDATION: 15163 return "ImmunizationRecommendation"; 15164 case MEDICATIONREQUEST: 15165 return "MedicationRequest"; 15166 case NUTRITIONORDER: 15167 return "NutritionOrder"; 15168 case SERVICEREQUEST: 15169 return "ServiceRequest"; 15170 case SUPPLYREQUEST: 15171 return "SupplyRequest"; 15172 case TASK: 15173 return "Task"; 15174 case VISIONPRESCRIPTION: 15175 return "VisionPrescription"; 15176 case NULL: 15177 return null; 15178 default: 15179 return "?"; 15180 } 15181 } 15182 15183 public String getSystem() { 15184 switch (this) { 15185 case APPOINTMENT: 15186 return "http://hl7.org/fhir/request-resource-types"; 15187 case APPOINTMENTRESPONSE: 15188 return "http://hl7.org/fhir/request-resource-types"; 15189 case CAREPLAN: 15190 return "http://hl7.org/fhir/request-resource-types"; 15191 case CLAIM: 15192 return "http://hl7.org/fhir/request-resource-types"; 15193 case COMMUNICATIONREQUEST: 15194 return "http://hl7.org/fhir/request-resource-types"; 15195 case CONTRACT: 15196 return "http://hl7.org/fhir/request-resource-types"; 15197 case DEVICEREQUEST: 15198 return "http://hl7.org/fhir/request-resource-types"; 15199 case ENROLLMENTREQUEST: 15200 return "http://hl7.org/fhir/request-resource-types"; 15201 case IMMUNIZATIONRECOMMENDATION: 15202 return "http://hl7.org/fhir/request-resource-types"; 15203 case MEDICATIONREQUEST: 15204 return "http://hl7.org/fhir/request-resource-types"; 15205 case NUTRITIONORDER: 15206 return "http://hl7.org/fhir/request-resource-types"; 15207 case SERVICEREQUEST: 15208 return "http://hl7.org/fhir/request-resource-types"; 15209 case SUPPLYREQUEST: 15210 return "http://hl7.org/fhir/request-resource-types"; 15211 case TASK: 15212 return "http://hl7.org/fhir/request-resource-types"; 15213 case VISIONPRESCRIPTION: 15214 return "http://hl7.org/fhir/request-resource-types"; 15215 case NULL: 15216 return null; 15217 default: 15218 return "?"; 15219 } 15220 } 15221 15222 public String getDefinition() { 15223 switch (this) { 15224 case APPOINTMENT: 15225 return "A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s)."; 15226 case APPOINTMENTRESPONSE: 15227 return "A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection."; 15228 case CAREPLAN: 15229 return "Healthcare plan for patient or group."; 15230 case CLAIM: 15231 return "Claim, Pre-determination or Pre-authorization."; 15232 case COMMUNICATIONREQUEST: 15233 return "A request for information to be sent to a receiver."; 15234 case CONTRACT: 15235 return "Legal Agreement."; 15236 case DEVICEREQUEST: 15237 return "Medical device request."; 15238 case ENROLLMENTREQUEST: 15239 return "Enrollment request."; 15240 case IMMUNIZATIONRECOMMENDATION: 15241 return "Guidance or advice relating to an immunization."; 15242 case MEDICATIONREQUEST: 15243 return "Ordering of medication for patient or group."; 15244 case NUTRITIONORDER: 15245 return "Diet, formula or nutritional supplement request."; 15246 case SERVICEREQUEST: 15247 return "A record of a request for service such as diagnostic investigations, treatments, or operations to be performed."; 15248 case SUPPLYREQUEST: 15249 return "Request for a medication, substance or device."; 15250 case TASK: 15251 return "A task to be performed."; 15252 case VISIONPRESCRIPTION: 15253 return "Prescription for vision correction products for a patient."; 15254 case NULL: 15255 return null; 15256 default: 15257 return "?"; 15258 } 15259 } 15260 15261 public String getDisplay() { 15262 switch (this) { 15263 case APPOINTMENT: 15264 return "Appointment"; 15265 case APPOINTMENTRESPONSE: 15266 return "AppointmentResponse"; 15267 case CAREPLAN: 15268 return "CarePlan"; 15269 case CLAIM: 15270 return "Claim"; 15271 case COMMUNICATIONREQUEST: 15272 return "CommunicationRequest"; 15273 case CONTRACT: 15274 return "Contract"; 15275 case DEVICEREQUEST: 15276 return "DeviceRequest"; 15277 case ENROLLMENTREQUEST: 15278 return "EnrollmentRequest"; 15279 case IMMUNIZATIONRECOMMENDATION: 15280 return "ImmunizationRecommendation"; 15281 case MEDICATIONREQUEST: 15282 return "MedicationRequest"; 15283 case NUTRITIONORDER: 15284 return "NutritionOrder"; 15285 case SERVICEREQUEST: 15286 return "ServiceRequest"; 15287 case SUPPLYREQUEST: 15288 return "SupplyRequest"; 15289 case TASK: 15290 return "Task"; 15291 case VISIONPRESCRIPTION: 15292 return "VisionPrescription"; 15293 case NULL: 15294 return null; 15295 default: 15296 return "?"; 15297 } 15298 } 15299 } 15300 15301 public static class RequestResourceTypeEnumFactory implements EnumFactory<RequestResourceType> { 15302 public RequestResourceType fromCode(String codeString) throws IllegalArgumentException { 15303 if (codeString == null || "".equals(codeString)) 15304 if (codeString == null || "".equals(codeString)) 15305 return null; 15306 if ("Appointment".equals(codeString)) 15307 return RequestResourceType.APPOINTMENT; 15308 if ("AppointmentResponse".equals(codeString)) 15309 return RequestResourceType.APPOINTMENTRESPONSE; 15310 if ("CarePlan".equals(codeString)) 15311 return RequestResourceType.CAREPLAN; 15312 if ("Claim".equals(codeString)) 15313 return RequestResourceType.CLAIM; 15314 if ("CommunicationRequest".equals(codeString)) 15315 return RequestResourceType.COMMUNICATIONREQUEST; 15316 if ("Contract".equals(codeString)) 15317 return RequestResourceType.CONTRACT; 15318 if ("DeviceRequest".equals(codeString)) 15319 return RequestResourceType.DEVICEREQUEST; 15320 if ("EnrollmentRequest".equals(codeString)) 15321 return RequestResourceType.ENROLLMENTREQUEST; 15322 if ("ImmunizationRecommendation".equals(codeString)) 15323 return RequestResourceType.IMMUNIZATIONRECOMMENDATION; 15324 if ("MedicationRequest".equals(codeString)) 15325 return RequestResourceType.MEDICATIONREQUEST; 15326 if ("NutritionOrder".equals(codeString)) 15327 return RequestResourceType.NUTRITIONORDER; 15328 if ("ServiceRequest".equals(codeString)) 15329 return RequestResourceType.SERVICEREQUEST; 15330 if ("SupplyRequest".equals(codeString)) 15331 return RequestResourceType.SUPPLYREQUEST; 15332 if ("Task".equals(codeString)) 15333 return RequestResourceType.TASK; 15334 if ("VisionPrescription".equals(codeString)) 15335 return RequestResourceType.VISIONPRESCRIPTION; 15336 throw new IllegalArgumentException("Unknown RequestResourceType code '" + codeString + "'"); 15337 } 15338 15339 public Enumeration<RequestResourceType> fromType(PrimitiveType<?> code) throws FHIRException { 15340 if (code == null) 15341 return null; 15342 if (code.isEmpty()) 15343 return new Enumeration<RequestResourceType>(this, RequestResourceType.NULL, code); 15344 String codeString = code.asStringValue(); 15345 if (codeString == null || "".equals(codeString)) 15346 return new Enumeration<RequestResourceType>(this, RequestResourceType.NULL, code); 15347 if ("Appointment".equals(codeString)) 15348 return new Enumeration<RequestResourceType>(this, RequestResourceType.APPOINTMENT, code); 15349 if ("AppointmentResponse".equals(codeString)) 15350 return new Enumeration<RequestResourceType>(this, RequestResourceType.APPOINTMENTRESPONSE, code); 15351 if ("CarePlan".equals(codeString)) 15352 return new Enumeration<RequestResourceType>(this, RequestResourceType.CAREPLAN, code); 15353 if ("Claim".equals(codeString)) 15354 return new Enumeration<RequestResourceType>(this, RequestResourceType.CLAIM, code); 15355 if ("CommunicationRequest".equals(codeString)) 15356 return new Enumeration<RequestResourceType>(this, RequestResourceType.COMMUNICATIONREQUEST, code); 15357 if ("Contract".equals(codeString)) 15358 return new Enumeration<RequestResourceType>(this, RequestResourceType.CONTRACT, code); 15359 if ("DeviceRequest".equals(codeString)) 15360 return new Enumeration<RequestResourceType>(this, RequestResourceType.DEVICEREQUEST, code); 15361 if ("EnrollmentRequest".equals(codeString)) 15362 return new Enumeration<RequestResourceType>(this, RequestResourceType.ENROLLMENTREQUEST, code); 15363 if ("ImmunizationRecommendation".equals(codeString)) 15364 return new Enumeration<RequestResourceType>(this, RequestResourceType.IMMUNIZATIONRECOMMENDATION, code); 15365 if ("MedicationRequest".equals(codeString)) 15366 return new Enumeration<RequestResourceType>(this, RequestResourceType.MEDICATIONREQUEST, code); 15367 if ("NutritionOrder".equals(codeString)) 15368 return new Enumeration<RequestResourceType>(this, RequestResourceType.NUTRITIONORDER, code); 15369 if ("ServiceRequest".equals(codeString)) 15370 return new Enumeration<RequestResourceType>(this, RequestResourceType.SERVICEREQUEST, code); 15371 if ("SupplyRequest".equals(codeString)) 15372 return new Enumeration<RequestResourceType>(this, RequestResourceType.SUPPLYREQUEST, code); 15373 if ("Task".equals(codeString)) 15374 return new Enumeration<RequestResourceType>(this, RequestResourceType.TASK, code); 15375 if ("VisionPrescription".equals(codeString)) 15376 return new Enumeration<RequestResourceType>(this, RequestResourceType.VISIONPRESCRIPTION, code); 15377 throw new FHIRException("Unknown RequestResourceType code '" + codeString + "'"); 15378 } 15379 15380 public String toCode(RequestResourceType code) { 15381 if (code == RequestResourceType.NULL) 15382 return null; 15383 if (code == RequestResourceType.APPOINTMENT) 15384 return "Appointment"; 15385 if (code == RequestResourceType.APPOINTMENTRESPONSE) 15386 return "AppointmentResponse"; 15387 if (code == RequestResourceType.CAREPLAN) 15388 return "CarePlan"; 15389 if (code == RequestResourceType.CLAIM) 15390 return "Claim"; 15391 if (code == RequestResourceType.COMMUNICATIONREQUEST) 15392 return "CommunicationRequest"; 15393 if (code == RequestResourceType.CONTRACT) 15394 return "Contract"; 15395 if (code == RequestResourceType.DEVICEREQUEST) 15396 return "DeviceRequest"; 15397 if (code == RequestResourceType.ENROLLMENTREQUEST) 15398 return "EnrollmentRequest"; 15399 if (code == RequestResourceType.IMMUNIZATIONRECOMMENDATION) 15400 return "ImmunizationRecommendation"; 15401 if (code == RequestResourceType.MEDICATIONREQUEST) 15402 return "MedicationRequest"; 15403 if (code == RequestResourceType.NUTRITIONORDER) 15404 return "NutritionOrder"; 15405 if (code == RequestResourceType.SERVICEREQUEST) 15406 return "ServiceRequest"; 15407 if (code == RequestResourceType.SUPPLYREQUEST) 15408 return "SupplyRequest"; 15409 if (code == RequestResourceType.TASK) 15410 return "Task"; 15411 if (code == RequestResourceType.VISIONPRESCRIPTION) 15412 return "VisionPrescription"; 15413 return "?"; 15414 } 15415 15416 public String toSystem(RequestResourceType code) { 15417 return code.getSystem(); 15418 } 15419 } 15420 15421 public enum ResourceType { 15422 /** 15423 * A financial tool for tracking value accrued for a particular purpose. In the 15424 * healthcare field, used to track charges for a patient, cost centers, etc. 15425 */ 15426 ACCOUNT, 15427 /** 15428 * This resource allows for the definition of some activity to be performed, 15429 * independent of a particular patient, practitioner, or other performance 15430 * context. 15431 */ 15432 ACTIVITYDEFINITION, 15433 /** 15434 * Actual or potential/avoided event causing unintended physical injury 15435 * resulting from or contributed to by medical care, a research study or other 15436 * healthcare setting factors that requires additional monitoring, treatment, or 15437 * hospitalization, or that results in death. 15438 */ 15439 ADVERSEEVENT, 15440 /** 15441 * Risk of harmful or undesirable, physiological response which is unique to an 15442 * individual and associated with exposure to a substance. 15443 */ 15444 ALLERGYINTOLERANCE, 15445 /** 15446 * A booking of a healthcare event among patient(s), practitioner(s), related 15447 * person(s) and/or device(s) for a specific date/time. This may result in one 15448 * or more Encounter(s). 15449 */ 15450 APPOINTMENT, 15451 /** 15452 * A reply to an appointment request for a patient and/or practitioner(s), such 15453 * as a confirmation or rejection. 15454 */ 15455 APPOINTMENTRESPONSE, 15456 /** 15457 * A record of an event made for purposes of maintaining a security log. Typical 15458 * uses include detection of intrusion attempts and monitoring for inappropriate 15459 * usage. 15460 */ 15461 AUDITEVENT, 15462 /** 15463 * Basic is used for handling concepts not yet defined in FHIR, narrative-only 15464 * resources that don't map to an existing resource, and custom resources not 15465 * appropriate for inclusion in the FHIR specification. 15466 */ 15467 BASIC, 15468 /** 15469 * A resource that represents the data of a single raw artifact as digital 15470 * content accessible in its native format. A Binary resource can contain any 15471 * content, whether text, image, pdf, zip archive, etc. 15472 */ 15473 BINARY, 15474 /** 15475 * A material substance originating from a biological entity intended to be 15476 * transplanted or infused into another (possibly the same) biological entity. 15477 */ 15478 BIOLOGICALLYDERIVEDPRODUCT, 15479 /** 15480 * Record details about an anatomical structure. This resource may be used when 15481 * a coded concept does not provide the necessary detail needed for the use 15482 * case. 15483 */ 15484 BODYSTRUCTURE, 15485 /** 15486 * A container for a collection of resources. 15487 */ 15488 BUNDLE, 15489 /** 15490 * A Capability Statement documents a set of capabilities (behaviors) of a FHIR 15491 * Server for a particular version of FHIR that may be used as a statement of 15492 * actual server functionality or a statement of required or desired server 15493 * implementation. 15494 */ 15495 CAPABILITYSTATEMENT, 15496 /** 15497 * Describes the intention of how one or more practitioners intend to deliver 15498 * care for a particular patient, group or community for a period of time, 15499 * possibly limited to care for a specific condition or set of conditions. 15500 */ 15501 CAREPLAN, 15502 /** 15503 * The Care Team includes all the people and organizations who plan to 15504 * participate in the coordination and delivery of care for a patient. 15505 */ 15506 CARETEAM, 15507 /** 15508 * Catalog entries are wrappers that contextualize items included in a catalog. 15509 */ 15510 CATALOGENTRY, 15511 /** 15512 * The resource ChargeItem describes the provision of healthcare provider 15513 * products for a certain patient, therefore referring not only to the product, 15514 * but containing in addition details of the provision, like date, time, amounts 15515 * and participating organizations and persons. Main Usage of the ChargeItem is 15516 * to enable the billing process and internal cost allocation. 15517 */ 15518 CHARGEITEM, 15519 /** 15520 * The ChargeItemDefinition resource provides the properties that apply to the 15521 * (billing) codes necessary to calculate costs and prices. The properties may 15522 * differ largely depending on type and realm, therefore this resource gives 15523 * only a rough structure and requires profiling for each type of billing code 15524 * system. 15525 */ 15526 CHARGEITEMDEFINITION, 15527 /** 15528 * A provider issued list of professional services and products which have been 15529 * provided, or are to be provided, to a patient which is sent to an insurer for 15530 * reimbursement. 15531 */ 15532 CLAIM, 15533 /** 15534 * This resource provides the adjudication details from the processing of a 15535 * Claim resource. 15536 */ 15537 CLAIMRESPONSE, 15538 /** 15539 * A record of a clinical assessment performed to determine what problem(s) may 15540 * affect the patient and before planning the treatments or management 15541 * strategies that are best to manage a patient's condition. Assessments are 15542 * often 1:1 with a clinical consultation / encounter, but this varies greatly 15543 * depending on the clinical workflow. This resource is called 15544 * "ClinicalImpression" rather than "ClinicalAssessment" to avoid confusion with 15545 * the recording of assessment tools such as Apgar score. 15546 */ 15547 CLINICALIMPRESSION, 15548 /** 15549 * The CodeSystem resource is used to declare the existence of and describe a 15550 * code system or code system supplement and its key properties, and optionally 15551 * define a part or all of its content. 15552 */ 15553 CODESYSTEM, 15554 /** 15555 * An occurrence of information being transmitted; e.g. an alert that was sent 15556 * to a responsible provider, a public health agency that was notified about a 15557 * reportable condition. 15558 */ 15559 COMMUNICATION, 15560 /** 15561 * A request to convey information; e.g. the CDS system proposes that an alert 15562 * be sent to a responsible provider, the CDS system proposes that the public 15563 * health agency be notified about a reportable condition. 15564 */ 15565 COMMUNICATIONREQUEST, 15566 /** 15567 * A compartment definition that defines how resources are accessed on a server. 15568 */ 15569 COMPARTMENTDEFINITION, 15570 /** 15571 * A set of healthcare-related information that is assembled together into a 15572 * single logical package that provides a single coherent statement of meaning, 15573 * establishes its own context and that has clinical attestation with regard to 15574 * who is making the statement. A Composition defines the structure and 15575 * narrative content necessary for a document. However, a Composition alone does 15576 * not constitute a document. Rather, the Composition must be the first entry in 15577 * a Bundle where Bundle.type=document, and any other resources referenced from 15578 * Composition must be included as subsequent entries in the Bundle (for example 15579 * Patient, Practitioner, Encounter, etc.). 15580 */ 15581 COMPOSITION, 15582 /** 15583 * A statement of relationships from one set of concepts to one or more other 15584 * concepts - either concepts in code systems, or data element/data element 15585 * concepts, or classes in class models. 15586 */ 15587 CONCEPTMAP, 15588 /** 15589 * A clinical condition, problem, diagnosis, or other event, situation, issue, 15590 * or clinical concept that has risen to a level of concern. 15591 */ 15592 CONDITION, 15593 /** 15594 * A record of a healthcare consumer?s choices, which permits or denies 15595 * identified recipient(s) or recipient role(s) to perform one or more actions 15596 * within a given policy context, for specific purposes and periods of time. 15597 */ 15598 CONSENT, 15599 /** 15600 * Legally enforceable, formally recorded unilateral or bilateral directive 15601 * i.e., a policy or agreement. 15602 */ 15603 CONTRACT, 15604 /** 15605 * Financial instrument which may be used to reimburse or pay for health care 15606 * products and services. Includes both insurance and self-payment. 15607 */ 15608 COVERAGE, 15609 /** 15610 * The CoverageEligibilityRequest provides patient and insurance coverage 15611 * information to an insurer for them to respond, in the form of an 15612 * CoverageEligibilityResponse, with information regarding whether the stated 15613 * coverage is valid and in-force and optionally to provide the insurance 15614 * details of the policy. 15615 */ 15616 COVERAGEELIGIBILITYREQUEST, 15617 /** 15618 * This resource provides eligibility and plan details from the processing of an 15619 * CoverageEligibilityRequest resource. 15620 */ 15621 COVERAGEELIGIBILITYRESPONSE, 15622 /** 15623 * Indicates an actual or potential clinical issue with or between one or more 15624 * active or proposed clinical actions for a patient; e.g. Drug-drug 15625 * interaction, Ineffective treatment frequency, Procedure-condition conflict, 15626 * etc. 15627 */ 15628 DETECTEDISSUE, 15629 /** 15630 * A type of a manufactured item that is used in the provision of healthcare 15631 * without being substantially changed through that activity. The device may be 15632 * a medical or non-medical device. 15633 */ 15634 DEVICE, 15635 /** 15636 * The characteristics, operational status and capabilities of a medical-related 15637 * component of a medical device. 15638 */ 15639 DEVICEDEFINITION, 15640 /** 15641 * Describes a measurement, calculation or setting capability of a medical 15642 * device. 15643 */ 15644 DEVICEMETRIC, 15645 /** 15646 * Represents a request for a patient to employ a medical device. The device may 15647 * be an implantable device, or an external assistive device, such as a walker. 15648 */ 15649 DEVICEREQUEST, 15650 /** 15651 * A record of a device being used by a patient where the record is the result 15652 * of a report from the patient or another clinician. 15653 */ 15654 DEVICEUSESTATEMENT, 15655 /** 15656 * The findings and interpretation of diagnostic tests performed on patients, 15657 * groups of patients, devices, and locations, and/or specimens derived from 15658 * these. The report includes clinical context such as requesting and provider 15659 * information, and some mix of atomic results, images, textual and coded 15660 * interpretations, and formatted representation of diagnostic reports. 15661 */ 15662 DIAGNOSTICREPORT, 15663 /** 15664 * A collection of documents compiled for a purpose together with metadata that 15665 * applies to the collection. 15666 */ 15667 DOCUMENTMANIFEST, 15668 /** 15669 * A reference to a document of any kind for any purpose. Provides metadata 15670 * about the document so that the document can be discovered and managed. The 15671 * scope of a document is any seralized object with a mime-type, so includes 15672 * formal patient centric documents (CDA), cliical notes, scanned paper, and 15673 * non-patient specific documents like policy text. 15674 */ 15675 DOCUMENTREFERENCE, 15676 /** 15677 * A resource that includes narrative, extensions, and contained resources. 15678 */ 15679 DOMAINRESOURCE, 15680 /** 15681 * The EffectEvidenceSynthesis resource describes the difference in an outcome 15682 * between exposures states in a population where the effect estimate is derived 15683 * from a combination of research studies. 15684 */ 15685 EFFECTEVIDENCESYNTHESIS, 15686 /** 15687 * An interaction between a patient and healthcare provider(s) for the purpose 15688 * of providing healthcare service(s) or assessing the health status of a 15689 * patient. 15690 */ 15691 ENCOUNTER, 15692 /** 15693 * The technical details of an endpoint that can be used for electronic 15694 * services, such as for web services providing XDS.b or a REST endpoint for 15695 * another FHIR server. This may include any security context information. 15696 */ 15697 ENDPOINT, 15698 /** 15699 * This resource provides the insurance enrollment details to the insurer 15700 * regarding a specified coverage. 15701 */ 15702 ENROLLMENTREQUEST, 15703 /** 15704 * This resource provides enrollment and plan details from the processing of an 15705 * EnrollmentRequest resource. 15706 */ 15707 ENROLLMENTRESPONSE, 15708 /** 15709 * An association between a patient and an organization / healthcare provider(s) 15710 * during which time encounters may occur. The managing organization assumes a 15711 * level of responsibility for the patient during this time. 15712 */ 15713 EPISODEOFCARE, 15714 /** 15715 * The EventDefinition resource provides a reusable description of when a 15716 * particular event can occur. 15717 */ 15718 EVENTDEFINITION, 15719 /** 15720 * The Evidence resource describes the conditional state (population and any 15721 * exposures being compared within the population) and outcome (if specified) 15722 * that the knowledge (evidence, assertion, recommendation) is about. 15723 */ 15724 EVIDENCE, 15725 /** 15726 * The EvidenceVariable resource describes a "PICO" element that knowledge 15727 * (evidence, assertion, recommendation) is about. 15728 */ 15729 EVIDENCEVARIABLE, 15730 /** 15731 * Example of workflow instance. 15732 */ 15733 EXAMPLESCENARIO, 15734 /** 15735 * This resource provides: the claim details; adjudication details from the 15736 * processing of a Claim; and optionally account balance information, for 15737 * informing the subscriber of the benefits provided. 15738 */ 15739 EXPLANATIONOFBENEFIT, 15740 /** 15741 * Significant health conditions for a person related to the patient relevant in 15742 * the context of care for the patient. 15743 */ 15744 FAMILYMEMBERHISTORY, 15745 /** 15746 * Prospective warnings of potential issues when providing care to the patient. 15747 */ 15748 FLAG, 15749 /** 15750 * Describes the intended objective(s) for a patient, group or organization 15751 * care, for example, weight loss, restoring an activity of daily living, 15752 * obtaining herd immunity via immunization, meeting a process improvement 15753 * objective, etc. 15754 */ 15755 GOAL, 15756 /** 15757 * A formal computable definition of a graph of resources - that is, a coherent 15758 * set of resources that form a graph by following references. The Graph 15759 * Definition resource defines a set and makes rules about the set. 15760 */ 15761 GRAPHDEFINITION, 15762 /** 15763 * Represents a defined collection of entities that may be discussed or acted 15764 * upon collectively but which are not expected to act collectively, and are not 15765 * formally or legally recognized; i.e. a collection of entities that isn't an 15766 * Organization. 15767 */ 15768 GROUP, 15769 /** 15770 * A guidance response is the formal response to a guidance request, including 15771 * any output parameters returned by the evaluation, as well as the description 15772 * of any proposed actions to be taken. 15773 */ 15774 GUIDANCERESPONSE, 15775 /** 15776 * The details of a healthcare service available at a location. 15777 */ 15778 HEALTHCARESERVICE, 15779 /** 15780 * Representation of the content produced in a DICOM imaging study. A study 15781 * comprises a set of series, each of which includes a set of Service-Object 15782 * Pair Instances (SOP Instances - images or other data) acquired or produced in 15783 * a common context. A series is of only one modality (e.g. X-ray, CT, MR, 15784 * ultrasound), but a study may have multiple series of different modalities. 15785 */ 15786 IMAGINGSTUDY, 15787 /** 15788 * Describes the event of a patient being administered a vaccine or a record of 15789 * an immunization as reported by a patient, a clinician or another party. 15790 */ 15791 IMMUNIZATION, 15792 /** 15793 * Describes a comparison of an immunization event against published 15794 * recommendations to determine if the administration is "valid" in relation to 15795 * those recommendations. 15796 */ 15797 IMMUNIZATIONEVALUATION, 15798 /** 15799 * A patient's point-in-time set of recommendations (i.e. forecasting) according 15800 * to a published schedule with optional supporting justification. 15801 */ 15802 IMMUNIZATIONRECOMMENDATION, 15803 /** 15804 * A set of rules of how a particular interoperability or standards problem is 15805 * solved - typically through the use of FHIR resources. This resource is used 15806 * to gather all the parts of an implementation guide into a logical whole and 15807 * to publish a computable definition of all the parts. 15808 */ 15809 IMPLEMENTATIONGUIDE, 15810 /** 15811 * Details of a Health Insurance product/plan provided by an organization. 15812 */ 15813 INSURANCEPLAN, 15814 /** 15815 * Invoice containing collected ChargeItems from an Account with calculated 15816 * individual and total price for Billing purpose. 15817 */ 15818 INVOICE, 15819 /** 15820 * The Library resource is a general-purpose container for knowledge asset 15821 * definitions. It can be used to describe and expose existing knowledge assets 15822 * such as logic libraries and information model descriptions, as well as to 15823 * describe a collection of knowledge assets. 15824 */ 15825 LIBRARY, 15826 /** 15827 * Identifies two or more records (resource instances) that refer to the same 15828 * real-world "occurrence". 15829 */ 15830 LINKAGE, 15831 /** 15832 * A list is a curated collection of resources. 15833 */ 15834 LIST, 15835 /** 15836 * Details and position information for a physical place where services are 15837 * provided and resources and participants may be stored, found, contained, or 15838 * accommodated. 15839 */ 15840 LOCATION, 15841 /** 15842 * The Measure resource provides the definition of a quality measure. 15843 */ 15844 MEASURE, 15845 /** 15846 * The MeasureReport resource contains the results of the calculation of a 15847 * measure; and optionally a reference to the resources involved in that 15848 * calculation. 15849 */ 15850 MEASUREREPORT, 15851 /** 15852 * A photo, video, or audio recording acquired or used in healthcare. The actual 15853 * content may be inline or provided by direct reference. 15854 */ 15855 MEDIA, 15856 /** 15857 * This resource is primarily used for the identification and definition of a 15858 * medication for the purposes of prescribing, dispensing, and administering a 15859 * medication as well as for making statements about medication use. 15860 */ 15861 MEDICATION, 15862 /** 15863 * Describes the event of a patient consuming or otherwise being administered a 15864 * medication. This may be as simple as swallowing a tablet or it may be a long 15865 * running infusion. Related resources tie this event to the authorizing 15866 * prescription, and the specific encounter between patient and health care 15867 * practitioner. 15868 */ 15869 MEDICATIONADMINISTRATION, 15870 /** 15871 * Indicates that a medication product is to be or has been dispensed for a 15872 * named person/patient. This includes a description of the medication product 15873 * (supply) provided and the instructions for administering the medication. The 15874 * medication dispense is the result of a pharmacy system responding to a 15875 * medication order. 15876 */ 15877 MEDICATIONDISPENSE, 15878 /** 15879 * Information about a medication that is used to support knowledge. 15880 */ 15881 MEDICATIONKNOWLEDGE, 15882 /** 15883 * An order or request for both supply of the medication and the instructions 15884 * for administration of the medication to a patient. The resource is called 15885 * "MedicationRequest" rather than "MedicationPrescription" or "MedicationOrder" 15886 * to generalize the use across inpatient and outpatient settings, including 15887 * care plans, etc., and to harmonize with workflow patterns. 15888 */ 15889 MEDICATIONREQUEST, 15890 /** 15891 * A record of a medication that is being consumed by a patient. A 15892 * MedicationStatement may indicate that the patient may be taking the 15893 * medication now or has taken the medication in the past or will be taking the 15894 * medication in the future. The source of this information can be the patient, 15895 * significant other (such as a family member or spouse), or a clinician. A 15896 * common scenario where this information is captured is during the history 15897 * taking process during a patient visit or stay. The medication information may 15898 * come from sources such as the patient's memory, from a prescription bottle, 15899 * or from a list of medications the patient, clinician or other party 15900 * maintains. 15901 * 15902 * The primary difference between a medication statement and a medication 15903 * administration is that the medication administration has complete 15904 * administration information and is based on actual administration information 15905 * from the person who administered the medication. A medication statement is 15906 * often, if not always, less specific. There is no required date/time when the 15907 * medication was administered, in fact we only know that a source has reported 15908 * the patient is taking this medication, where details such as time, quantity, 15909 * or rate or even medication product may be incomplete or missing or less 15910 * precise. As stated earlier, the medication statement information may come 15911 * from the patient's memory, from a prescription bottle or from a list of 15912 * medications the patient, clinician or other party maintains. Medication 15913 * administration is more formal and is not missing detailed information. 15914 */ 15915 MEDICATIONSTATEMENT, 15916 /** 15917 * Detailed definition of a medicinal product, typically for uses other than 15918 * direct patient care (e.g. regulatory use). 15919 */ 15920 MEDICINALPRODUCT, 15921 /** 15922 * The regulatory authorization of a medicinal product. 15923 */ 15924 MEDICINALPRODUCTAUTHORIZATION, 15925 /** 15926 * The clinical particulars - indications, contraindications etc. of a medicinal 15927 * product, including for regulatory purposes. 15928 */ 15929 MEDICINALPRODUCTCONTRAINDICATION, 15930 /** 15931 * Indication for the Medicinal Product. 15932 */ 15933 MEDICINALPRODUCTINDICATION, 15934 /** 15935 * An ingredient of a manufactured item or pharmaceutical product. 15936 */ 15937 MEDICINALPRODUCTINGREDIENT, 15938 /** 15939 * The interactions of the medicinal product with other medicinal products, or 15940 * other forms of interactions. 15941 */ 15942 MEDICINALPRODUCTINTERACTION, 15943 /** 15944 * The manufactured item as contained in the packaged medicinal product. 15945 */ 15946 MEDICINALPRODUCTMANUFACTURED, 15947 /** 15948 * A medicinal product in a container or package. 15949 */ 15950 MEDICINALPRODUCTPACKAGED, 15951 /** 15952 * A pharmaceutical product described in terms of its composition and dose form. 15953 */ 15954 MEDICINALPRODUCTPHARMACEUTICAL, 15955 /** 15956 * Describe the undesirable effects of the medicinal product. 15957 */ 15958 MEDICINALPRODUCTUNDESIRABLEEFFECT, 15959 /** 15960 * Defines the characteristics of a message that can be shared between systems, 15961 * including the type of event that initiates the message, the content to be 15962 * transmitted and what response(s), if any, are permitted. 15963 */ 15964 MESSAGEDEFINITION, 15965 /** 15966 * The header for a message exchange that is either requesting or responding to 15967 * an action. The reference(s) that are the subject of the action as well as 15968 * other information related to the action are typically transmitted in a bundle 15969 * in which the MessageHeader resource instance is the first resource in the 15970 * bundle. 15971 */ 15972 MESSAGEHEADER, 15973 /** 15974 * Raw data describing a biological sequence. 15975 */ 15976 MOLECULARSEQUENCE, 15977 /** 15978 * A curated namespace that issues unique symbols within that namespace for the 15979 * identification of concepts, people, devices, etc. Represents a "System" used 15980 * within the Identifier and Coding data types. 15981 */ 15982 NAMINGSYSTEM, 15983 /** 15984 * A request to supply a diet, formula feeding (enteral) or oral nutritional 15985 * supplement to a patient/resident. 15986 */ 15987 NUTRITIONORDER, 15988 /** 15989 * Measurements and simple assertions made about a patient, device or other 15990 * subject. 15991 */ 15992 OBSERVATION, 15993 /** 15994 * Set of definitional characteristics for a kind of observation or measurement 15995 * produced or consumed by an orderable health care service. 15996 */ 15997 OBSERVATIONDEFINITION, 15998 /** 15999 * A formal computable definition of an operation (on the RESTful interface) or 16000 * a named query (using the search interaction). 16001 */ 16002 OPERATIONDEFINITION, 16003 /** 16004 * A collection of error, warning, or information messages that result from a 16005 * system action. 16006 */ 16007 OPERATIONOUTCOME, 16008 /** 16009 * A formally or informally recognized grouping of people or organizations 16010 * formed for the purpose of achieving some form of collective action. Includes 16011 * companies, institutions, corporations, departments, community groups, 16012 * healthcare practice groups, payer/insurer, etc. 16013 */ 16014 ORGANIZATION, 16015 /** 16016 * Defines an affiliation/assotiation/relationship between 2 distinct 16017 * oganizations, that is not a part-of relationship/sub-division relationship. 16018 */ 16019 ORGANIZATIONAFFILIATION, 16020 /** 16021 * This resource is a non-persisted resource used to pass information into and 16022 * back from an [operation](operations.html). It has no other use, and there is 16023 * no RESTful endpoint associated with it. 16024 */ 16025 PARAMETERS, 16026 /** 16027 * Demographics and other administrative information about an individual or 16028 * animal receiving care or other health-related services. 16029 */ 16030 PATIENT, 16031 /** 16032 * This resource provides the status of the payment for goods and services 16033 * rendered, and the request and response resource references. 16034 */ 16035 PAYMENTNOTICE, 16036 /** 16037 * This resource provides the details including amount of a payment and 16038 * allocates the payment items being paid. 16039 */ 16040 PAYMENTRECONCILIATION, 16041 /** 16042 * Demographics and administrative information about a person independent of a 16043 * specific health-related context. 16044 */ 16045 PERSON, 16046 /** 16047 * This resource allows for the definition of various types of plans as a 16048 * sharable, consumable, and executable artifact. The resource is general enough 16049 * to support the description of a broad range of clinical artifacts such as 16050 * clinical decision support rules, order sets and protocols. 16051 */ 16052 PLANDEFINITION, 16053 /** 16054 * A person who is directly or indirectly involved in the provisioning of 16055 * healthcare. 16056 */ 16057 PRACTITIONER, 16058 /** 16059 * A specific set of Roles/Locations/specialties/services that a practitioner 16060 * may perform at an organization for a period of time. 16061 */ 16062 PRACTITIONERROLE, 16063 /** 16064 * An action that is or was performed on or for a patient. This can be a 16065 * physical intervention like an operation, or less invasive like long term 16066 * services, counseling, or hypnotherapy. 16067 */ 16068 PROCEDURE, 16069 /** 16070 * Provenance of a resource is a record that describes entities and processes 16071 * involved in producing and delivering or otherwise influencing that resource. 16072 * Provenance provides a critical foundation for assessing authenticity, 16073 * enabling trust, and allowing reproducibility. Provenance assertions are a 16074 * form of contextual metadata and can themselves become important records with 16075 * their own provenance. Provenance statement indicates clinical significance in 16076 * terms of confidence in authenticity, reliability, and trustworthiness, 16077 * integrity, and stage in lifecycle (e.g. Document Completion - has the 16078 * artifact been legally authenticated), all of which may impact security, 16079 * privacy, and trust policies. 16080 */ 16081 PROVENANCE, 16082 /** 16083 * A structured set of questions intended to guide the collection of answers 16084 * from end-users. Questionnaires provide detailed control over order, 16085 * presentation, phraseology and grouping to allow coherent, consistent data 16086 * collection. 16087 */ 16088 QUESTIONNAIRE, 16089 /** 16090 * A structured set of questions and their answers. The questions are ordered 16091 * and grouped into coherent subsets, corresponding to the structure of the 16092 * grouping of the questionnaire being responded to. 16093 */ 16094 QUESTIONNAIRERESPONSE, 16095 /** 16096 * Information about a person that is involved in the care for a patient, but 16097 * who is not the target of healthcare, nor has a formal responsibility in the 16098 * care process. 16099 */ 16100 RELATEDPERSON, 16101 /** 16102 * A group of related requests that can be used to capture intended activities 16103 * that have inter-dependencies such as "give this medication after that one". 16104 */ 16105 REQUESTGROUP, 16106 /** 16107 * The ResearchDefinition resource describes the conditional state (population 16108 * and any exposures being compared within the population) and outcome (if 16109 * specified) that the knowledge (evidence, assertion, recommendation) is about. 16110 */ 16111 RESEARCHDEFINITION, 16112 /** 16113 * The ResearchElementDefinition resource describes a "PICO" element that 16114 * knowledge (evidence, assertion, recommendation) is about. 16115 */ 16116 RESEARCHELEMENTDEFINITION, 16117 /** 16118 * A process where a researcher or organization plans and then executes a series 16119 * of steps intended to increase the field of healthcare-related knowledge. This 16120 * includes studies of safety, efficacy, comparative effectiveness and other 16121 * information about medications, devices, therapies and other interventional 16122 * and investigative techniques. A ResearchStudy involves the gathering of 16123 * information about human or animal subjects. 16124 */ 16125 RESEARCHSTUDY, 16126 /** 16127 * A physical entity which is the primary unit of operational and/or 16128 * administrative interest in a study. 16129 */ 16130 RESEARCHSUBJECT, 16131 /** 16132 * This is the base resource type for everything. 16133 */ 16134 RESOURCE, 16135 /** 16136 * An assessment of the likely outcome(s) for a patient or other subject as well 16137 * as the likelihood of each outcome. 16138 */ 16139 RISKASSESSMENT, 16140 /** 16141 * The RiskEvidenceSynthesis resource describes the likelihood of an outcome in 16142 * a population plus exposure state where the risk estimate is derived from a 16143 * combination of research studies. 16144 */ 16145 RISKEVIDENCESYNTHESIS, 16146 /** 16147 * A container for slots of time that may be available for booking appointments. 16148 */ 16149 SCHEDULE, 16150 /** 16151 * A search parameter that defines a named search item that can be used to 16152 * search/filter on a resource. 16153 */ 16154 SEARCHPARAMETER, 16155 /** 16156 * A record of a request for service such as diagnostic investigations, 16157 * treatments, or operations to be performed. 16158 */ 16159 SERVICEREQUEST, 16160 /** 16161 * A slot of time on a schedule that may be available for booking appointments. 16162 */ 16163 SLOT, 16164 /** 16165 * A sample to be used for analysis. 16166 */ 16167 SPECIMEN, 16168 /** 16169 * A kind of specimen with associated set of requirements. 16170 */ 16171 SPECIMENDEFINITION, 16172 /** 16173 * A definition of a FHIR structure. This resource is used to describe the 16174 * underlying resources, data types defined in FHIR, and also for describing 16175 * extensions and constraints on resources and data types. 16176 */ 16177 STRUCTUREDEFINITION, 16178 /** 16179 * A Map of relationships between 2 structures that can be used to transform 16180 * data. 16181 */ 16182 STRUCTUREMAP, 16183 /** 16184 * The subscription resource is used to define a push-based subscription from a 16185 * server to another system. Once a subscription is registered with the server, 16186 * the server checks every resource that is created or updated, and if the 16187 * resource matches the given criteria, it sends a message on the defined 16188 * "channel" so that another system can take an appropriate action. 16189 */ 16190 SUBSCRIPTION, 16191 /** 16192 * A homogeneous material with a definite composition. 16193 */ 16194 SUBSTANCE, 16195 /** 16196 * Nucleic acids are defined by three distinct elements: the base, sugar and 16197 * linkage. Individual substance/moiety IDs will be created for each of these 16198 * elements. The nucleotide sequence will be always entered in the 5?-3? 16199 * direction. 16200 */ 16201 SUBSTANCENUCLEICACID, 16202 /** 16203 * Todo. 16204 */ 16205 SUBSTANCEPOLYMER, 16206 /** 16207 * A SubstanceProtein is defined as a single unit of a linear amino acid 16208 * sequence, or a combination of subunits that are either covalently linked or 16209 * have a defined invariant stoichiometric relationship. This includes all 16210 * synthetic, recombinant and purified SubstanceProteins of defined sequence, 16211 * whether the use is therapeutic or prophylactic. This set of elements will be 16212 * used to describe albumins, coagulation factors, cytokines, growth factors, 16213 * peptide/SubstanceProtein hormones, enzymes, toxins, toxoids, recombinant 16214 * vaccines, and immunomodulators. 16215 */ 16216 SUBSTANCEPROTEIN, 16217 /** 16218 * Todo. 16219 */ 16220 SUBSTANCEREFERENCEINFORMATION, 16221 /** 16222 * Source material shall capture information on the taxonomic and anatomical 16223 * origins as well as the fraction of a material that can result in or can be 16224 * modified to form a substance. This set of data elements shall be used to 16225 * define polymer substances isolated from biological matrices. Taxonomic and 16226 * anatomical origins shall be described using a controlled vocabulary as 16227 * required. This information is captured for naturally derived polymers ( . 16228 * starch) and structurally diverse substances. For Organisms belonging to the 16229 * Kingdom Plantae the Substance level defines the fresh material of a single 16230 * species or infraspecies, the Herbal Drug and the Herbal preparation. For 16231 * Herbal preparations, the fraction information will be captured at the 16232 * Substance information level and additional information for herbal extracts 16233 * will be captured at the Specified Substance Group 1 information level. See 16234 * for further explanation the Substance Class: Structurally Diverse and the 16235 * herbal annex. 16236 */ 16237 SUBSTANCESOURCEMATERIAL, 16238 /** 16239 * The detailed description of a substance, typically at a level beyond what is 16240 * used for prescribing. 16241 */ 16242 SUBSTANCESPECIFICATION, 16243 /** 16244 * Record of delivery of what is supplied. 16245 */ 16246 SUPPLYDELIVERY, 16247 /** 16248 * A record of a request for a medication, substance or device used in the 16249 * healthcare setting. 16250 */ 16251 SUPPLYREQUEST, 16252 /** 16253 * A task to be performed. 16254 */ 16255 TASK, 16256 /** 16257 * A Terminology Capabilities documents a set of capabilities (behaviors) of a 16258 * FHIR Server that may be used as a statement of actual server functionality or 16259 * a statement of required or desired server implementation. 16260 */ 16261 TERMINOLOGYCAPABILITIES, 16262 /** 16263 * A summary of information based on the results of executing a TestScript. 16264 */ 16265 TESTREPORT, 16266 /** 16267 * A structured set of tests against a FHIR server or client implementation to 16268 * determine compliance against the FHIR specification. 16269 */ 16270 TESTSCRIPT, 16271 /** 16272 * A ValueSet resource instance specifies a set of codes drawn from one or more 16273 * code systems, intended for use in a particular context. Value sets link 16274 * between [[[CodeSystem]]] definitions and their use in [coded 16275 * elements](terminologies.html). 16276 */ 16277 VALUESET, 16278 /** 16279 * Describes validation requirements, source(s), status and dates for one or 16280 * more elements. 16281 */ 16282 VERIFICATIONRESULT, 16283 /** 16284 * An authorization for the provision of glasses and/or contact lenses to a 16285 * patient. 16286 */ 16287 VISIONPRESCRIPTION, 16288 /** 16289 * added to help the parsers 16290 */ 16291 NULL; 16292 16293 public static ResourceType fromCode(String codeString) throws FHIRException { 16294 if (codeString == null || "".equals(codeString)) 16295 return null; 16296 if ("Account".equals(codeString)) 16297 return ACCOUNT; 16298 if ("ActivityDefinition".equals(codeString)) 16299 return ACTIVITYDEFINITION; 16300 if ("AdverseEvent".equals(codeString)) 16301 return ADVERSEEVENT; 16302 if ("AllergyIntolerance".equals(codeString)) 16303 return ALLERGYINTOLERANCE; 16304 if ("Appointment".equals(codeString)) 16305 return APPOINTMENT; 16306 if ("AppointmentResponse".equals(codeString)) 16307 return APPOINTMENTRESPONSE; 16308 if ("AuditEvent".equals(codeString)) 16309 return AUDITEVENT; 16310 if ("Basic".equals(codeString)) 16311 return BASIC; 16312 if ("Binary".equals(codeString)) 16313 return BINARY; 16314 if ("BiologicallyDerivedProduct".equals(codeString)) 16315 return BIOLOGICALLYDERIVEDPRODUCT; 16316 if ("BodyStructure".equals(codeString)) 16317 return BODYSTRUCTURE; 16318 if ("Bundle".equals(codeString)) 16319 return BUNDLE; 16320 if ("CapabilityStatement".equals(codeString)) 16321 return CAPABILITYSTATEMENT; 16322 if ("CarePlan".equals(codeString)) 16323 return CAREPLAN; 16324 if ("CareTeam".equals(codeString)) 16325 return CARETEAM; 16326 if ("CatalogEntry".equals(codeString)) 16327 return CATALOGENTRY; 16328 if ("ChargeItem".equals(codeString)) 16329 return CHARGEITEM; 16330 if ("ChargeItemDefinition".equals(codeString)) 16331 return CHARGEITEMDEFINITION; 16332 if ("Claim".equals(codeString)) 16333 return CLAIM; 16334 if ("ClaimResponse".equals(codeString)) 16335 return CLAIMRESPONSE; 16336 if ("ClinicalImpression".equals(codeString)) 16337 return CLINICALIMPRESSION; 16338 if ("CodeSystem".equals(codeString)) 16339 return CODESYSTEM; 16340 if ("Communication".equals(codeString)) 16341 return COMMUNICATION; 16342 if ("CommunicationRequest".equals(codeString)) 16343 return COMMUNICATIONREQUEST; 16344 if ("CompartmentDefinition".equals(codeString)) 16345 return COMPARTMENTDEFINITION; 16346 if ("Composition".equals(codeString)) 16347 return COMPOSITION; 16348 if ("ConceptMap".equals(codeString)) 16349 return CONCEPTMAP; 16350 if ("Condition".equals(codeString)) 16351 return CONDITION; 16352 if ("Consent".equals(codeString)) 16353 return CONSENT; 16354 if ("Contract".equals(codeString)) 16355 return CONTRACT; 16356 if ("Coverage".equals(codeString)) 16357 return COVERAGE; 16358 if ("CoverageEligibilityRequest".equals(codeString)) 16359 return COVERAGEELIGIBILITYREQUEST; 16360 if ("CoverageEligibilityResponse".equals(codeString)) 16361 return COVERAGEELIGIBILITYRESPONSE; 16362 if ("DetectedIssue".equals(codeString)) 16363 return DETECTEDISSUE; 16364 if ("Device".equals(codeString)) 16365 return DEVICE; 16366 if ("DeviceDefinition".equals(codeString)) 16367 return DEVICEDEFINITION; 16368 if ("DeviceMetric".equals(codeString)) 16369 return DEVICEMETRIC; 16370 if ("DeviceRequest".equals(codeString)) 16371 return DEVICEREQUEST; 16372 if ("DeviceUseStatement".equals(codeString)) 16373 return DEVICEUSESTATEMENT; 16374 if ("DiagnosticReport".equals(codeString)) 16375 return DIAGNOSTICREPORT; 16376 if ("DocumentManifest".equals(codeString)) 16377 return DOCUMENTMANIFEST; 16378 if ("DocumentReference".equals(codeString)) 16379 return DOCUMENTREFERENCE; 16380 if ("DomainResource".equals(codeString)) 16381 return DOMAINRESOURCE; 16382 if ("EffectEvidenceSynthesis".equals(codeString)) 16383 return EFFECTEVIDENCESYNTHESIS; 16384 if ("Encounter".equals(codeString)) 16385 return ENCOUNTER; 16386 if ("Endpoint".equals(codeString)) 16387 return ENDPOINT; 16388 if ("EnrollmentRequest".equals(codeString)) 16389 return ENROLLMENTREQUEST; 16390 if ("EnrollmentResponse".equals(codeString)) 16391 return ENROLLMENTRESPONSE; 16392 if ("EpisodeOfCare".equals(codeString)) 16393 return EPISODEOFCARE; 16394 if ("EventDefinition".equals(codeString)) 16395 return EVENTDEFINITION; 16396 if ("Evidence".equals(codeString)) 16397 return EVIDENCE; 16398 if ("EvidenceVariable".equals(codeString)) 16399 return EVIDENCEVARIABLE; 16400 if ("ExampleScenario".equals(codeString)) 16401 return EXAMPLESCENARIO; 16402 if ("ExplanationOfBenefit".equals(codeString)) 16403 return EXPLANATIONOFBENEFIT; 16404 if ("FamilyMemberHistory".equals(codeString)) 16405 return FAMILYMEMBERHISTORY; 16406 if ("Flag".equals(codeString)) 16407 return FLAG; 16408 if ("Goal".equals(codeString)) 16409 return GOAL; 16410 if ("GraphDefinition".equals(codeString)) 16411 return GRAPHDEFINITION; 16412 if ("Group".equals(codeString)) 16413 return GROUP; 16414 if ("GuidanceResponse".equals(codeString)) 16415 return GUIDANCERESPONSE; 16416 if ("HealthcareService".equals(codeString)) 16417 return HEALTHCARESERVICE; 16418 if ("ImagingStudy".equals(codeString)) 16419 return IMAGINGSTUDY; 16420 if ("Immunization".equals(codeString)) 16421 return IMMUNIZATION; 16422 if ("ImmunizationEvaluation".equals(codeString)) 16423 return IMMUNIZATIONEVALUATION; 16424 if ("ImmunizationRecommendation".equals(codeString)) 16425 return IMMUNIZATIONRECOMMENDATION; 16426 if ("ImplementationGuide".equals(codeString)) 16427 return IMPLEMENTATIONGUIDE; 16428 if ("InsurancePlan".equals(codeString)) 16429 return INSURANCEPLAN; 16430 if ("Invoice".equals(codeString)) 16431 return INVOICE; 16432 if ("Library".equals(codeString)) 16433 return LIBRARY; 16434 if ("Linkage".equals(codeString)) 16435 return LINKAGE; 16436 if ("List".equals(codeString)) 16437 return LIST; 16438 if ("Location".equals(codeString)) 16439 return LOCATION; 16440 if ("Measure".equals(codeString)) 16441 return MEASURE; 16442 if ("MeasureReport".equals(codeString)) 16443 return MEASUREREPORT; 16444 if ("Media".equals(codeString)) 16445 return MEDIA; 16446 if ("Medication".equals(codeString)) 16447 return MEDICATION; 16448 if ("MedicationAdministration".equals(codeString)) 16449 return MEDICATIONADMINISTRATION; 16450 if ("MedicationDispense".equals(codeString)) 16451 return MEDICATIONDISPENSE; 16452 if ("MedicationKnowledge".equals(codeString)) 16453 return MEDICATIONKNOWLEDGE; 16454 if ("MedicationRequest".equals(codeString)) 16455 return MEDICATIONREQUEST; 16456 if ("MedicationStatement".equals(codeString)) 16457 return MEDICATIONSTATEMENT; 16458 if ("MedicinalProduct".equals(codeString)) 16459 return MEDICINALPRODUCT; 16460 if ("MedicinalProductAuthorization".equals(codeString)) 16461 return MEDICINALPRODUCTAUTHORIZATION; 16462 if ("MedicinalProductContraindication".equals(codeString)) 16463 return MEDICINALPRODUCTCONTRAINDICATION; 16464 if ("MedicinalProductIndication".equals(codeString)) 16465 return MEDICINALPRODUCTINDICATION; 16466 if ("MedicinalProductIngredient".equals(codeString)) 16467 return MEDICINALPRODUCTINGREDIENT; 16468 if ("MedicinalProductInteraction".equals(codeString)) 16469 return MEDICINALPRODUCTINTERACTION; 16470 if ("MedicinalProductManufactured".equals(codeString)) 16471 return MEDICINALPRODUCTMANUFACTURED; 16472 if ("MedicinalProductPackaged".equals(codeString)) 16473 return MEDICINALPRODUCTPACKAGED; 16474 if ("MedicinalProductPharmaceutical".equals(codeString)) 16475 return MEDICINALPRODUCTPHARMACEUTICAL; 16476 if ("MedicinalProductUndesirableEffect".equals(codeString)) 16477 return MEDICINALPRODUCTUNDESIRABLEEFFECT; 16478 if ("MessageDefinition".equals(codeString)) 16479 return MESSAGEDEFINITION; 16480 if ("MessageHeader".equals(codeString)) 16481 return MESSAGEHEADER; 16482 if ("MolecularSequence".equals(codeString)) 16483 return MOLECULARSEQUENCE; 16484 if ("NamingSystem".equals(codeString)) 16485 return NAMINGSYSTEM; 16486 if ("NutritionOrder".equals(codeString)) 16487 return NUTRITIONORDER; 16488 if ("Observation".equals(codeString)) 16489 return OBSERVATION; 16490 if ("ObservationDefinition".equals(codeString)) 16491 return OBSERVATIONDEFINITION; 16492 if ("OperationDefinition".equals(codeString)) 16493 return OPERATIONDEFINITION; 16494 if ("OperationOutcome".equals(codeString)) 16495 return OPERATIONOUTCOME; 16496 if ("Organization".equals(codeString)) 16497 return ORGANIZATION; 16498 if ("OrganizationAffiliation".equals(codeString)) 16499 return ORGANIZATIONAFFILIATION; 16500 if ("Parameters".equals(codeString)) 16501 return PARAMETERS; 16502 if ("Patient".equals(codeString)) 16503 return PATIENT; 16504 if ("PaymentNotice".equals(codeString)) 16505 return PAYMENTNOTICE; 16506 if ("PaymentReconciliation".equals(codeString)) 16507 return PAYMENTRECONCILIATION; 16508 if ("Person".equals(codeString)) 16509 return PERSON; 16510 if ("PlanDefinition".equals(codeString)) 16511 return PLANDEFINITION; 16512 if ("Practitioner".equals(codeString)) 16513 return PRACTITIONER; 16514 if ("PractitionerRole".equals(codeString)) 16515 return PRACTITIONERROLE; 16516 if ("Procedure".equals(codeString)) 16517 return PROCEDURE; 16518 if ("Provenance".equals(codeString)) 16519 return PROVENANCE; 16520 if ("Questionnaire".equals(codeString)) 16521 return QUESTIONNAIRE; 16522 if ("QuestionnaireResponse".equals(codeString)) 16523 return QUESTIONNAIRERESPONSE; 16524 if ("RelatedPerson".equals(codeString)) 16525 return RELATEDPERSON; 16526 if ("RequestGroup".equals(codeString)) 16527 return REQUESTGROUP; 16528 if ("ResearchDefinition".equals(codeString)) 16529 return RESEARCHDEFINITION; 16530 if ("ResearchElementDefinition".equals(codeString)) 16531 return RESEARCHELEMENTDEFINITION; 16532 if ("ResearchStudy".equals(codeString)) 16533 return RESEARCHSTUDY; 16534 if ("ResearchSubject".equals(codeString)) 16535 return RESEARCHSUBJECT; 16536 if ("Resource".equals(codeString)) 16537 return RESOURCE; 16538 if ("RiskAssessment".equals(codeString)) 16539 return RISKASSESSMENT; 16540 if ("RiskEvidenceSynthesis".equals(codeString)) 16541 return RISKEVIDENCESYNTHESIS; 16542 if ("Schedule".equals(codeString)) 16543 return SCHEDULE; 16544 if ("SearchParameter".equals(codeString)) 16545 return SEARCHPARAMETER; 16546 if ("ServiceRequest".equals(codeString)) 16547 return SERVICEREQUEST; 16548 if ("Slot".equals(codeString)) 16549 return SLOT; 16550 if ("Specimen".equals(codeString)) 16551 return SPECIMEN; 16552 if ("SpecimenDefinition".equals(codeString)) 16553 return SPECIMENDEFINITION; 16554 if ("StructureDefinition".equals(codeString)) 16555 return STRUCTUREDEFINITION; 16556 if ("StructureMap".equals(codeString)) 16557 return STRUCTUREMAP; 16558 if ("Subscription".equals(codeString)) 16559 return SUBSCRIPTION; 16560 if ("Substance".equals(codeString)) 16561 return SUBSTANCE; 16562 if ("SubstanceNucleicAcid".equals(codeString)) 16563 return SUBSTANCENUCLEICACID; 16564 if ("SubstancePolymer".equals(codeString)) 16565 return SUBSTANCEPOLYMER; 16566 if ("SubstanceProtein".equals(codeString)) 16567 return SUBSTANCEPROTEIN; 16568 if ("SubstanceReferenceInformation".equals(codeString)) 16569 return SUBSTANCEREFERENCEINFORMATION; 16570 if ("SubstanceSourceMaterial".equals(codeString)) 16571 return SUBSTANCESOURCEMATERIAL; 16572 if ("SubstanceSpecification".equals(codeString)) 16573 return SUBSTANCESPECIFICATION; 16574 if ("SupplyDelivery".equals(codeString)) 16575 return SUPPLYDELIVERY; 16576 if ("SupplyRequest".equals(codeString)) 16577 return SUPPLYREQUEST; 16578 if ("Task".equals(codeString)) 16579 return TASK; 16580 if ("TerminologyCapabilities".equals(codeString)) 16581 return TERMINOLOGYCAPABILITIES; 16582 if ("TestReport".equals(codeString)) 16583 return TESTREPORT; 16584 if ("TestScript".equals(codeString)) 16585 return TESTSCRIPT; 16586 if ("ValueSet".equals(codeString)) 16587 return VALUESET; 16588 if ("VerificationResult".equals(codeString)) 16589 return VERIFICATIONRESULT; 16590 if ("VisionPrescription".equals(codeString)) 16591 return VISIONPRESCRIPTION; 16592 throw new FHIRException("Unknown ResourceType code '" + codeString + "'"); 16593 } 16594 16595 public String toCode() { 16596 switch (this) { 16597 case ACCOUNT: 16598 return "Account"; 16599 case ACTIVITYDEFINITION: 16600 return "ActivityDefinition"; 16601 case ADVERSEEVENT: 16602 return "AdverseEvent"; 16603 case ALLERGYINTOLERANCE: 16604 return "AllergyIntolerance"; 16605 case APPOINTMENT: 16606 return "Appointment"; 16607 case APPOINTMENTRESPONSE: 16608 return "AppointmentResponse"; 16609 case AUDITEVENT: 16610 return "AuditEvent"; 16611 case BASIC: 16612 return "Basic"; 16613 case BINARY: 16614 return "Binary"; 16615 case BIOLOGICALLYDERIVEDPRODUCT: 16616 return "BiologicallyDerivedProduct"; 16617 case BODYSTRUCTURE: 16618 return "BodyStructure"; 16619 case BUNDLE: 16620 return "Bundle"; 16621 case CAPABILITYSTATEMENT: 16622 return "CapabilityStatement"; 16623 case CAREPLAN: 16624 return "CarePlan"; 16625 case CARETEAM: 16626 return "CareTeam"; 16627 case CATALOGENTRY: 16628 return "CatalogEntry"; 16629 case CHARGEITEM: 16630 return "ChargeItem"; 16631 case CHARGEITEMDEFINITION: 16632 return "ChargeItemDefinition"; 16633 case CLAIM: 16634 return "Claim"; 16635 case CLAIMRESPONSE: 16636 return "ClaimResponse"; 16637 case CLINICALIMPRESSION: 16638 return "ClinicalImpression"; 16639 case CODESYSTEM: 16640 return "CodeSystem"; 16641 case COMMUNICATION: 16642 return "Communication"; 16643 case COMMUNICATIONREQUEST: 16644 return "CommunicationRequest"; 16645 case COMPARTMENTDEFINITION: 16646 return "CompartmentDefinition"; 16647 case COMPOSITION: 16648 return "Composition"; 16649 case CONCEPTMAP: 16650 return "ConceptMap"; 16651 case CONDITION: 16652 return "Condition"; 16653 case CONSENT: 16654 return "Consent"; 16655 case CONTRACT: 16656 return "Contract"; 16657 case COVERAGE: 16658 return "Coverage"; 16659 case COVERAGEELIGIBILITYREQUEST: 16660 return "CoverageEligibilityRequest"; 16661 case COVERAGEELIGIBILITYRESPONSE: 16662 return "CoverageEligibilityResponse"; 16663 case DETECTEDISSUE: 16664 return "DetectedIssue"; 16665 case DEVICE: 16666 return "Device"; 16667 case DEVICEDEFINITION: 16668 return "DeviceDefinition"; 16669 case DEVICEMETRIC: 16670 return "DeviceMetric"; 16671 case DEVICEREQUEST: 16672 return "DeviceRequest"; 16673 case DEVICEUSESTATEMENT: 16674 return "DeviceUseStatement"; 16675 case DIAGNOSTICREPORT: 16676 return "DiagnosticReport"; 16677 case DOCUMENTMANIFEST: 16678 return "DocumentManifest"; 16679 case DOCUMENTREFERENCE: 16680 return "DocumentReference"; 16681 case DOMAINRESOURCE: 16682 return "DomainResource"; 16683 case EFFECTEVIDENCESYNTHESIS: 16684 return "EffectEvidenceSynthesis"; 16685 case ENCOUNTER: 16686 return "Encounter"; 16687 case ENDPOINT: 16688 return "Endpoint"; 16689 case ENROLLMENTREQUEST: 16690 return "EnrollmentRequest"; 16691 case ENROLLMENTRESPONSE: 16692 return "EnrollmentResponse"; 16693 case EPISODEOFCARE: 16694 return "EpisodeOfCare"; 16695 case EVENTDEFINITION: 16696 return "EventDefinition"; 16697 case EVIDENCE: 16698 return "Evidence"; 16699 case EVIDENCEVARIABLE: 16700 return "EvidenceVariable"; 16701 case EXAMPLESCENARIO: 16702 return "ExampleScenario"; 16703 case EXPLANATIONOFBENEFIT: 16704 return "ExplanationOfBenefit"; 16705 case FAMILYMEMBERHISTORY: 16706 return "FamilyMemberHistory"; 16707 case FLAG: 16708 return "Flag"; 16709 case GOAL: 16710 return "Goal"; 16711 case GRAPHDEFINITION: 16712 return "GraphDefinition"; 16713 case GROUP: 16714 return "Group"; 16715 case GUIDANCERESPONSE: 16716 return "GuidanceResponse"; 16717 case HEALTHCARESERVICE: 16718 return "HealthcareService"; 16719 case IMAGINGSTUDY: 16720 return "ImagingStudy"; 16721 case IMMUNIZATION: 16722 return "Immunization"; 16723 case IMMUNIZATIONEVALUATION: 16724 return "ImmunizationEvaluation"; 16725 case IMMUNIZATIONRECOMMENDATION: 16726 return "ImmunizationRecommendation"; 16727 case IMPLEMENTATIONGUIDE: 16728 return "ImplementationGuide"; 16729 case INSURANCEPLAN: 16730 return "InsurancePlan"; 16731 case INVOICE: 16732 return "Invoice"; 16733 case LIBRARY: 16734 return "Library"; 16735 case LINKAGE: 16736 return "Linkage"; 16737 case LIST: 16738 return "List"; 16739 case LOCATION: 16740 return "Location"; 16741 case MEASURE: 16742 return "Measure"; 16743 case MEASUREREPORT: 16744 return "MeasureReport"; 16745 case MEDIA: 16746 return "Media"; 16747 case MEDICATION: 16748 return "Medication"; 16749 case MEDICATIONADMINISTRATION: 16750 return "MedicationAdministration"; 16751 case MEDICATIONDISPENSE: 16752 return "MedicationDispense"; 16753 case MEDICATIONKNOWLEDGE: 16754 return "MedicationKnowledge"; 16755 case MEDICATIONREQUEST: 16756 return "MedicationRequest"; 16757 case MEDICATIONSTATEMENT: 16758 return "MedicationStatement"; 16759 case MEDICINALPRODUCT: 16760 return "MedicinalProduct"; 16761 case MEDICINALPRODUCTAUTHORIZATION: 16762 return "MedicinalProductAuthorization"; 16763 case MEDICINALPRODUCTCONTRAINDICATION: 16764 return "MedicinalProductContraindication"; 16765 case MEDICINALPRODUCTINDICATION: 16766 return "MedicinalProductIndication"; 16767 case MEDICINALPRODUCTINGREDIENT: 16768 return "MedicinalProductIngredient"; 16769 case MEDICINALPRODUCTINTERACTION: 16770 return "MedicinalProductInteraction"; 16771 case MEDICINALPRODUCTMANUFACTURED: 16772 return "MedicinalProductManufactured"; 16773 case MEDICINALPRODUCTPACKAGED: 16774 return "MedicinalProductPackaged"; 16775 case MEDICINALPRODUCTPHARMACEUTICAL: 16776 return "MedicinalProductPharmaceutical"; 16777 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 16778 return "MedicinalProductUndesirableEffect"; 16779 case MESSAGEDEFINITION: 16780 return "MessageDefinition"; 16781 case MESSAGEHEADER: 16782 return "MessageHeader"; 16783 case MOLECULARSEQUENCE: 16784 return "MolecularSequence"; 16785 case NAMINGSYSTEM: 16786 return "NamingSystem"; 16787 case NUTRITIONORDER: 16788 return "NutritionOrder"; 16789 case OBSERVATION: 16790 return "Observation"; 16791 case OBSERVATIONDEFINITION: 16792 return "ObservationDefinition"; 16793 case OPERATIONDEFINITION: 16794 return "OperationDefinition"; 16795 case OPERATIONOUTCOME: 16796 return "OperationOutcome"; 16797 case ORGANIZATION: 16798 return "Organization"; 16799 case ORGANIZATIONAFFILIATION: 16800 return "OrganizationAffiliation"; 16801 case PARAMETERS: 16802 return "Parameters"; 16803 case PATIENT: 16804 return "Patient"; 16805 case PAYMENTNOTICE: 16806 return "PaymentNotice"; 16807 case PAYMENTRECONCILIATION: 16808 return "PaymentReconciliation"; 16809 case PERSON: 16810 return "Person"; 16811 case PLANDEFINITION: 16812 return "PlanDefinition"; 16813 case PRACTITIONER: 16814 return "Practitioner"; 16815 case PRACTITIONERROLE: 16816 return "PractitionerRole"; 16817 case PROCEDURE: 16818 return "Procedure"; 16819 case PROVENANCE: 16820 return "Provenance"; 16821 case QUESTIONNAIRE: 16822 return "Questionnaire"; 16823 case QUESTIONNAIRERESPONSE: 16824 return "QuestionnaireResponse"; 16825 case RELATEDPERSON: 16826 return "RelatedPerson"; 16827 case REQUESTGROUP: 16828 return "RequestGroup"; 16829 case RESEARCHDEFINITION: 16830 return "ResearchDefinition"; 16831 case RESEARCHELEMENTDEFINITION: 16832 return "ResearchElementDefinition"; 16833 case RESEARCHSTUDY: 16834 return "ResearchStudy"; 16835 case RESEARCHSUBJECT: 16836 return "ResearchSubject"; 16837 case RESOURCE: 16838 return "Resource"; 16839 case RISKASSESSMENT: 16840 return "RiskAssessment"; 16841 case RISKEVIDENCESYNTHESIS: 16842 return "RiskEvidenceSynthesis"; 16843 case SCHEDULE: 16844 return "Schedule"; 16845 case SEARCHPARAMETER: 16846 return "SearchParameter"; 16847 case SERVICEREQUEST: 16848 return "ServiceRequest"; 16849 case SLOT: 16850 return "Slot"; 16851 case SPECIMEN: 16852 return "Specimen"; 16853 case SPECIMENDEFINITION: 16854 return "SpecimenDefinition"; 16855 case STRUCTUREDEFINITION: 16856 return "StructureDefinition"; 16857 case STRUCTUREMAP: 16858 return "StructureMap"; 16859 case SUBSCRIPTION: 16860 return "Subscription"; 16861 case SUBSTANCE: 16862 return "Substance"; 16863 case SUBSTANCENUCLEICACID: 16864 return "SubstanceNucleicAcid"; 16865 case SUBSTANCEPOLYMER: 16866 return "SubstancePolymer"; 16867 case SUBSTANCEPROTEIN: 16868 return "SubstanceProtein"; 16869 case SUBSTANCEREFERENCEINFORMATION: 16870 return "SubstanceReferenceInformation"; 16871 case SUBSTANCESOURCEMATERIAL: 16872 return "SubstanceSourceMaterial"; 16873 case SUBSTANCESPECIFICATION: 16874 return "SubstanceSpecification"; 16875 case SUPPLYDELIVERY: 16876 return "SupplyDelivery"; 16877 case SUPPLYREQUEST: 16878 return "SupplyRequest"; 16879 case TASK: 16880 return "Task"; 16881 case TERMINOLOGYCAPABILITIES: 16882 return "TerminologyCapabilities"; 16883 case TESTREPORT: 16884 return "TestReport"; 16885 case TESTSCRIPT: 16886 return "TestScript"; 16887 case VALUESET: 16888 return "ValueSet"; 16889 case VERIFICATIONRESULT: 16890 return "VerificationResult"; 16891 case VISIONPRESCRIPTION: 16892 return "VisionPrescription"; 16893 case NULL: 16894 return null; 16895 default: 16896 return "?"; 16897 } 16898 } 16899 16900 public String getSystem() { 16901 switch (this) { 16902 case ACCOUNT: 16903 return "http://hl7.org/fhir/resource-types"; 16904 case ACTIVITYDEFINITION: 16905 return "http://hl7.org/fhir/resource-types"; 16906 case ADVERSEEVENT: 16907 return "http://hl7.org/fhir/resource-types"; 16908 case ALLERGYINTOLERANCE: 16909 return "http://hl7.org/fhir/resource-types"; 16910 case APPOINTMENT: 16911 return "http://hl7.org/fhir/resource-types"; 16912 case APPOINTMENTRESPONSE: 16913 return "http://hl7.org/fhir/resource-types"; 16914 case AUDITEVENT: 16915 return "http://hl7.org/fhir/resource-types"; 16916 case BASIC: 16917 return "http://hl7.org/fhir/resource-types"; 16918 case BINARY: 16919 return "http://hl7.org/fhir/resource-types"; 16920 case BIOLOGICALLYDERIVEDPRODUCT: 16921 return "http://hl7.org/fhir/resource-types"; 16922 case BODYSTRUCTURE: 16923 return "http://hl7.org/fhir/resource-types"; 16924 case BUNDLE: 16925 return "http://hl7.org/fhir/resource-types"; 16926 case CAPABILITYSTATEMENT: 16927 return "http://hl7.org/fhir/resource-types"; 16928 case CAREPLAN: 16929 return "http://hl7.org/fhir/resource-types"; 16930 case CARETEAM: 16931 return "http://hl7.org/fhir/resource-types"; 16932 case CATALOGENTRY: 16933 return "http://hl7.org/fhir/resource-types"; 16934 case CHARGEITEM: 16935 return "http://hl7.org/fhir/resource-types"; 16936 case CHARGEITEMDEFINITION: 16937 return "http://hl7.org/fhir/resource-types"; 16938 case CLAIM: 16939 return "http://hl7.org/fhir/resource-types"; 16940 case CLAIMRESPONSE: 16941 return "http://hl7.org/fhir/resource-types"; 16942 case CLINICALIMPRESSION: 16943 return "http://hl7.org/fhir/resource-types"; 16944 case CODESYSTEM: 16945 return "http://hl7.org/fhir/resource-types"; 16946 case COMMUNICATION: 16947 return "http://hl7.org/fhir/resource-types"; 16948 case COMMUNICATIONREQUEST: 16949 return "http://hl7.org/fhir/resource-types"; 16950 case COMPARTMENTDEFINITION: 16951 return "http://hl7.org/fhir/resource-types"; 16952 case COMPOSITION: 16953 return "http://hl7.org/fhir/resource-types"; 16954 case CONCEPTMAP: 16955 return "http://hl7.org/fhir/resource-types"; 16956 case CONDITION: 16957 return "http://hl7.org/fhir/resource-types"; 16958 case CONSENT: 16959 return "http://hl7.org/fhir/resource-types"; 16960 case CONTRACT: 16961 return "http://hl7.org/fhir/resource-types"; 16962 case COVERAGE: 16963 return "http://hl7.org/fhir/resource-types"; 16964 case COVERAGEELIGIBILITYREQUEST: 16965 return "http://hl7.org/fhir/resource-types"; 16966 case COVERAGEELIGIBILITYRESPONSE: 16967 return "http://hl7.org/fhir/resource-types"; 16968 case DETECTEDISSUE: 16969 return "http://hl7.org/fhir/resource-types"; 16970 case DEVICE: 16971 return "http://hl7.org/fhir/resource-types"; 16972 case DEVICEDEFINITION: 16973 return "http://hl7.org/fhir/resource-types"; 16974 case DEVICEMETRIC: 16975 return "http://hl7.org/fhir/resource-types"; 16976 case DEVICEREQUEST: 16977 return "http://hl7.org/fhir/resource-types"; 16978 case DEVICEUSESTATEMENT: 16979 return "http://hl7.org/fhir/resource-types"; 16980 case DIAGNOSTICREPORT: 16981 return "http://hl7.org/fhir/resource-types"; 16982 case DOCUMENTMANIFEST: 16983 return "http://hl7.org/fhir/resource-types"; 16984 case DOCUMENTREFERENCE: 16985 return "http://hl7.org/fhir/resource-types"; 16986 case DOMAINRESOURCE: 16987 return "http://hl7.org/fhir/resource-types"; 16988 case EFFECTEVIDENCESYNTHESIS: 16989 return "http://hl7.org/fhir/resource-types"; 16990 case ENCOUNTER: 16991 return "http://hl7.org/fhir/resource-types"; 16992 case ENDPOINT: 16993 return "http://hl7.org/fhir/resource-types"; 16994 case ENROLLMENTREQUEST: 16995 return "http://hl7.org/fhir/resource-types"; 16996 case ENROLLMENTRESPONSE: 16997 return "http://hl7.org/fhir/resource-types"; 16998 case EPISODEOFCARE: 16999 return "http://hl7.org/fhir/resource-types"; 17000 case EVENTDEFINITION: 17001 return "http://hl7.org/fhir/resource-types"; 17002 case EVIDENCE: 17003 return "http://hl7.org/fhir/resource-types"; 17004 case EVIDENCEVARIABLE: 17005 return "http://hl7.org/fhir/resource-types"; 17006 case EXAMPLESCENARIO: 17007 return "http://hl7.org/fhir/resource-types"; 17008 case EXPLANATIONOFBENEFIT: 17009 return "http://hl7.org/fhir/resource-types"; 17010 case FAMILYMEMBERHISTORY: 17011 return "http://hl7.org/fhir/resource-types"; 17012 case FLAG: 17013 return "http://hl7.org/fhir/resource-types"; 17014 case GOAL: 17015 return "http://hl7.org/fhir/resource-types"; 17016 case GRAPHDEFINITION: 17017 return "http://hl7.org/fhir/resource-types"; 17018 case GROUP: 17019 return "http://hl7.org/fhir/resource-types"; 17020 case GUIDANCERESPONSE: 17021 return "http://hl7.org/fhir/resource-types"; 17022 case HEALTHCARESERVICE: 17023 return "http://hl7.org/fhir/resource-types"; 17024 case IMAGINGSTUDY: 17025 return "http://hl7.org/fhir/resource-types"; 17026 case IMMUNIZATION: 17027 return "http://hl7.org/fhir/resource-types"; 17028 case IMMUNIZATIONEVALUATION: 17029 return "http://hl7.org/fhir/resource-types"; 17030 case IMMUNIZATIONRECOMMENDATION: 17031 return "http://hl7.org/fhir/resource-types"; 17032 case IMPLEMENTATIONGUIDE: 17033 return "http://hl7.org/fhir/resource-types"; 17034 case INSURANCEPLAN: 17035 return "http://hl7.org/fhir/resource-types"; 17036 case INVOICE: 17037 return "http://hl7.org/fhir/resource-types"; 17038 case LIBRARY: 17039 return "http://hl7.org/fhir/resource-types"; 17040 case LINKAGE: 17041 return "http://hl7.org/fhir/resource-types"; 17042 case LIST: 17043 return "http://hl7.org/fhir/resource-types"; 17044 case LOCATION: 17045 return "http://hl7.org/fhir/resource-types"; 17046 case MEASURE: 17047 return "http://hl7.org/fhir/resource-types"; 17048 case MEASUREREPORT: 17049 return "http://hl7.org/fhir/resource-types"; 17050 case MEDIA: 17051 return "http://hl7.org/fhir/resource-types"; 17052 case MEDICATION: 17053 return "http://hl7.org/fhir/resource-types"; 17054 case MEDICATIONADMINISTRATION: 17055 return "http://hl7.org/fhir/resource-types"; 17056 case MEDICATIONDISPENSE: 17057 return "http://hl7.org/fhir/resource-types"; 17058 case MEDICATIONKNOWLEDGE: 17059 return "http://hl7.org/fhir/resource-types"; 17060 case MEDICATIONREQUEST: 17061 return "http://hl7.org/fhir/resource-types"; 17062 case MEDICATIONSTATEMENT: 17063 return "http://hl7.org/fhir/resource-types"; 17064 case MEDICINALPRODUCT: 17065 return "http://hl7.org/fhir/resource-types"; 17066 case MEDICINALPRODUCTAUTHORIZATION: 17067 return "http://hl7.org/fhir/resource-types"; 17068 case MEDICINALPRODUCTCONTRAINDICATION: 17069 return "http://hl7.org/fhir/resource-types"; 17070 case MEDICINALPRODUCTINDICATION: 17071 return "http://hl7.org/fhir/resource-types"; 17072 case MEDICINALPRODUCTINGREDIENT: 17073 return "http://hl7.org/fhir/resource-types"; 17074 case MEDICINALPRODUCTINTERACTION: 17075 return "http://hl7.org/fhir/resource-types"; 17076 case MEDICINALPRODUCTMANUFACTURED: 17077 return "http://hl7.org/fhir/resource-types"; 17078 case MEDICINALPRODUCTPACKAGED: 17079 return "http://hl7.org/fhir/resource-types"; 17080 case MEDICINALPRODUCTPHARMACEUTICAL: 17081 return "http://hl7.org/fhir/resource-types"; 17082 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 17083 return "http://hl7.org/fhir/resource-types"; 17084 case MESSAGEDEFINITION: 17085 return "http://hl7.org/fhir/resource-types"; 17086 case MESSAGEHEADER: 17087 return "http://hl7.org/fhir/resource-types"; 17088 case MOLECULARSEQUENCE: 17089 return "http://hl7.org/fhir/resource-types"; 17090 case NAMINGSYSTEM: 17091 return "http://hl7.org/fhir/resource-types"; 17092 case NUTRITIONORDER: 17093 return "http://hl7.org/fhir/resource-types"; 17094 case OBSERVATION: 17095 return "http://hl7.org/fhir/resource-types"; 17096 case OBSERVATIONDEFINITION: 17097 return "http://hl7.org/fhir/resource-types"; 17098 case OPERATIONDEFINITION: 17099 return "http://hl7.org/fhir/resource-types"; 17100 case OPERATIONOUTCOME: 17101 return "http://hl7.org/fhir/resource-types"; 17102 case ORGANIZATION: 17103 return "http://hl7.org/fhir/resource-types"; 17104 case ORGANIZATIONAFFILIATION: 17105 return "http://hl7.org/fhir/resource-types"; 17106 case PARAMETERS: 17107 return "http://hl7.org/fhir/resource-types"; 17108 case PATIENT: 17109 return "http://hl7.org/fhir/resource-types"; 17110 case PAYMENTNOTICE: 17111 return "http://hl7.org/fhir/resource-types"; 17112 case PAYMENTRECONCILIATION: 17113 return "http://hl7.org/fhir/resource-types"; 17114 case PERSON: 17115 return "http://hl7.org/fhir/resource-types"; 17116 case PLANDEFINITION: 17117 return "http://hl7.org/fhir/resource-types"; 17118 case PRACTITIONER: 17119 return "http://hl7.org/fhir/resource-types"; 17120 case PRACTITIONERROLE: 17121 return "http://hl7.org/fhir/resource-types"; 17122 case PROCEDURE: 17123 return "http://hl7.org/fhir/resource-types"; 17124 case PROVENANCE: 17125 return "http://hl7.org/fhir/resource-types"; 17126 case QUESTIONNAIRE: 17127 return "http://hl7.org/fhir/resource-types"; 17128 case QUESTIONNAIRERESPONSE: 17129 return "http://hl7.org/fhir/resource-types"; 17130 case RELATEDPERSON: 17131 return "http://hl7.org/fhir/resource-types"; 17132 case REQUESTGROUP: 17133 return "http://hl7.org/fhir/resource-types"; 17134 case RESEARCHDEFINITION: 17135 return "http://hl7.org/fhir/resource-types"; 17136 case RESEARCHELEMENTDEFINITION: 17137 return "http://hl7.org/fhir/resource-types"; 17138 case RESEARCHSTUDY: 17139 return "http://hl7.org/fhir/resource-types"; 17140 case RESEARCHSUBJECT: 17141 return "http://hl7.org/fhir/resource-types"; 17142 case RESOURCE: 17143 return "http://hl7.org/fhir/resource-types"; 17144 case RISKASSESSMENT: 17145 return "http://hl7.org/fhir/resource-types"; 17146 case RISKEVIDENCESYNTHESIS: 17147 return "http://hl7.org/fhir/resource-types"; 17148 case SCHEDULE: 17149 return "http://hl7.org/fhir/resource-types"; 17150 case SEARCHPARAMETER: 17151 return "http://hl7.org/fhir/resource-types"; 17152 case SERVICEREQUEST: 17153 return "http://hl7.org/fhir/resource-types"; 17154 case SLOT: 17155 return "http://hl7.org/fhir/resource-types"; 17156 case SPECIMEN: 17157 return "http://hl7.org/fhir/resource-types"; 17158 case SPECIMENDEFINITION: 17159 return "http://hl7.org/fhir/resource-types"; 17160 case STRUCTUREDEFINITION: 17161 return "http://hl7.org/fhir/resource-types"; 17162 case STRUCTUREMAP: 17163 return "http://hl7.org/fhir/resource-types"; 17164 case SUBSCRIPTION: 17165 return "http://hl7.org/fhir/resource-types"; 17166 case SUBSTANCE: 17167 return "http://hl7.org/fhir/resource-types"; 17168 case SUBSTANCENUCLEICACID: 17169 return "http://hl7.org/fhir/resource-types"; 17170 case SUBSTANCEPOLYMER: 17171 return "http://hl7.org/fhir/resource-types"; 17172 case SUBSTANCEPROTEIN: 17173 return "http://hl7.org/fhir/resource-types"; 17174 case SUBSTANCEREFERENCEINFORMATION: 17175 return "http://hl7.org/fhir/resource-types"; 17176 case SUBSTANCESOURCEMATERIAL: 17177 return "http://hl7.org/fhir/resource-types"; 17178 case SUBSTANCESPECIFICATION: 17179 return "http://hl7.org/fhir/resource-types"; 17180 case SUPPLYDELIVERY: 17181 return "http://hl7.org/fhir/resource-types"; 17182 case SUPPLYREQUEST: 17183 return "http://hl7.org/fhir/resource-types"; 17184 case TASK: 17185 return "http://hl7.org/fhir/resource-types"; 17186 case TERMINOLOGYCAPABILITIES: 17187 return "http://hl7.org/fhir/resource-types"; 17188 case TESTREPORT: 17189 return "http://hl7.org/fhir/resource-types"; 17190 case TESTSCRIPT: 17191 return "http://hl7.org/fhir/resource-types"; 17192 case VALUESET: 17193 return "http://hl7.org/fhir/resource-types"; 17194 case VERIFICATIONRESULT: 17195 return "http://hl7.org/fhir/resource-types"; 17196 case VISIONPRESCRIPTION: 17197 return "http://hl7.org/fhir/resource-types"; 17198 case NULL: 17199 return null; 17200 default: 17201 return "?"; 17202 } 17203 } 17204 17205 public String getDefinition() { 17206 switch (this) { 17207 case ACCOUNT: 17208 return "A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centers, etc."; 17209 case ACTIVITYDEFINITION: 17210 return "This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context."; 17211 case ADVERSEEVENT: 17212 return "Actual or potential/avoided event causing unintended physical injury resulting from or contributed to by medical care, a research study or other healthcare setting factors that requires additional monitoring, treatment, or hospitalization, or that results in death."; 17213 case ALLERGYINTOLERANCE: 17214 return "Risk of harmful or undesirable, physiological response which is unique to an individual and associated with exposure to a substance."; 17215 case APPOINTMENT: 17216 return "A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s)."; 17217 case APPOINTMENTRESPONSE: 17218 return "A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection."; 17219 case AUDITEVENT: 17220 return "A record of an event made for purposes of maintaining a security log. Typical uses include detection of intrusion attempts and monitoring for inappropriate usage."; 17221 case BASIC: 17222 return "Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification."; 17223 case BINARY: 17224 return "A resource that represents the data of a single raw artifact as digital content accessible in its native format. A Binary resource can contain any content, whether text, image, pdf, zip archive, etc."; 17225 case BIOLOGICALLYDERIVEDPRODUCT: 17226 return "A material substance originating from a biological entity intended to be transplanted or infused\ninto another (possibly the same) biological entity."; 17227 case BODYSTRUCTURE: 17228 return "Record details about an anatomical structure. This resource may be used when a coded concept does not provide the necessary detail needed for the use case."; 17229 case BUNDLE: 17230 return "A container for a collection of resources."; 17231 case CAPABILITYSTATEMENT: 17232 return "A Capability Statement documents a set of capabilities (behaviors) of a FHIR Server for a particular version of FHIR that may be used as a statement of actual server functionality or a statement of required or desired server implementation."; 17233 case CAREPLAN: 17234 return "Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions."; 17235 case CARETEAM: 17236 return "The Care Team includes all the people and organizations who plan to participate in the coordination and delivery of care for a patient."; 17237 case CATALOGENTRY: 17238 return "Catalog entries are wrappers that contextualize items included in a catalog."; 17239 case CHARGEITEM: 17240 return "The resource ChargeItem describes the provision of healthcare provider products for a certain patient, therefore referring not only to the product, but containing in addition details of the provision, like date, time, amounts and participating organizations and persons. Main Usage of the ChargeItem is to enable the billing process and internal cost allocation."; 17241 case CHARGEITEMDEFINITION: 17242 return "The ChargeItemDefinition resource provides the properties that apply to the (billing) codes necessary to calculate costs and prices. The properties may differ largely depending on type and realm, therefore this resource gives only a rough structure and requires profiling for each type of billing code system."; 17243 case CLAIM: 17244 return "A provider issued list of professional services and products which have been provided, or are to be provided, to a patient which is sent to an insurer for reimbursement."; 17245 case CLAIMRESPONSE: 17246 return "This resource provides the adjudication details from the processing of a Claim resource."; 17247 case CLINICALIMPRESSION: 17248 return "A record of a clinical assessment performed to determine what problem(s) may affect the patient and before planning the treatments or management strategies that are best to manage a patient's condition. Assessments are often 1:1 with a clinical consultation / encounter, but this varies greatly depending on the clinical workflow. This resource is called \"ClinicalImpression\" rather than \"ClinicalAssessment\" to avoid confusion with the recording of assessment tools such as Apgar score."; 17249 case CODESYSTEM: 17250 return "The CodeSystem resource is used to declare the existence of and describe a code system or code system supplement and its key properties, and optionally define a part or all of its content."; 17251 case COMMUNICATION: 17252 return "An occurrence of information being transmitted; e.g. an alert that was sent to a responsible provider, a public health agency that was notified about a reportable condition."; 17253 case COMMUNICATIONREQUEST: 17254 return "A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition."; 17255 case COMPARTMENTDEFINITION: 17256 return "A compartment definition that defines how resources are accessed on a server."; 17257 case COMPOSITION: 17258 return "A set of healthcare-related information that is assembled together into a single logical package that provides a single coherent statement of meaning, establishes its own context and that has clinical attestation with regard to who is making the statement. A Composition defines the structure and narrative content necessary for a document. However, a Composition alone does not constitute a document. Rather, the Composition must be the first entry in a Bundle where Bundle.type=document, and any other resources referenced from Composition must be included as subsequent entries in the Bundle (for example Patient, Practitioner, Encounter, etc.)."; 17259 case CONCEPTMAP: 17260 return "A statement of relationships from one set of concepts to one or more other concepts - either concepts in code systems, or data element/data element concepts, or classes in class models."; 17261 case CONDITION: 17262 return "A clinical condition, problem, diagnosis, or other event, situation, issue, or clinical concept that has risen to a level of concern."; 17263 case CONSENT: 17264 return "A record of a healthcare consumer?s choices, which permits or denies identified recipient(s) or recipient role(s) to perform one or more actions within a given policy context, for specific purposes and periods of time."; 17265 case CONTRACT: 17266 return "Legally enforceable, formally recorded unilateral or bilateral directive i.e., a policy or agreement."; 17267 case COVERAGE: 17268 return "Financial instrument which may be used to reimburse or pay for health care products and services. Includes both insurance and self-payment."; 17269 case COVERAGEELIGIBILITYREQUEST: 17270 return "The CoverageEligibilityRequest provides patient and insurance coverage information to an insurer for them to respond, in the form of an CoverageEligibilityResponse, with information regarding whether the stated coverage is valid and in-force and optionally to provide the insurance details of the policy."; 17271 case COVERAGEELIGIBILITYRESPONSE: 17272 return "This resource provides eligibility and plan details from the processing of an CoverageEligibilityRequest resource."; 17273 case DETECTEDISSUE: 17274 return "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, etc."; 17275 case DEVICE: 17276 return "A type of a manufactured item that is used in the provision of healthcare without being substantially changed through that activity. The device may be a medical or non-medical device."; 17277 case DEVICEDEFINITION: 17278 return "The characteristics, operational status and capabilities of a medical-related component of a medical device."; 17279 case DEVICEMETRIC: 17280 return "Describes a measurement, calculation or setting capability of a medical device."; 17281 case DEVICEREQUEST: 17282 return "Represents a request for a patient to employ a medical device. The device may be an implantable device, or an external assistive device, such as a walker."; 17283 case DEVICEUSESTATEMENT: 17284 return "A record of a device being used by a patient where the record is the result of a report from the patient or another clinician."; 17285 case DIAGNOSTICREPORT: 17286 return "The findings and interpretation of diagnostic tests performed on patients, groups of patients, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting and provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports."; 17287 case DOCUMENTMANIFEST: 17288 return "A collection of documents compiled for a purpose together with metadata that applies to the collection."; 17289 case DOCUMENTREFERENCE: 17290 return "A reference to a document of any kind for any purpose. Provides metadata about the document so that the document can be discovered and managed. The scope of a document is any seralized object with a mime-type, so includes formal patient centric documents (CDA), cliical notes, scanned paper, and non-patient specific documents like policy text."; 17291 case DOMAINRESOURCE: 17292 return "A resource that includes narrative, extensions, and contained resources."; 17293 case EFFECTEVIDENCESYNTHESIS: 17294 return "The EffectEvidenceSynthesis resource describes the difference in an outcome between exposures states in a population where the effect estimate is derived from a combination of research studies."; 17295 case ENCOUNTER: 17296 return "An interaction between a patient and healthcare provider(s) for the purpose of providing healthcare service(s) or assessing the health status of a patient."; 17297 case ENDPOINT: 17298 return "The technical details of an endpoint that can be used for electronic services, such as for web services providing XDS.b or a REST endpoint for another FHIR server. This may include any security context information."; 17299 case ENROLLMENTREQUEST: 17300 return "This resource provides the insurance enrollment details to the insurer regarding a specified coverage."; 17301 case ENROLLMENTRESPONSE: 17302 return "This resource provides enrollment and plan details from the processing of an EnrollmentRequest resource."; 17303 case EPISODEOFCARE: 17304 return "An association between a patient and an organization / healthcare provider(s) during which time encounters may occur. The managing organization assumes a level of responsibility for the patient during this time."; 17305 case EVENTDEFINITION: 17306 return "The EventDefinition resource provides a reusable description of when a particular event can occur."; 17307 case EVIDENCE: 17308 return "The Evidence resource describes the conditional state (population and any exposures being compared within the population) and outcome (if specified) that the knowledge (evidence, assertion, recommendation) is about."; 17309 case EVIDENCEVARIABLE: 17310 return "The EvidenceVariable resource describes a \"PICO\" element that knowledge (evidence, assertion, recommendation) is about."; 17311 case EXAMPLESCENARIO: 17312 return "Example of workflow instance."; 17313 case EXPLANATIONOFBENEFIT: 17314 return "This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided."; 17315 case FAMILYMEMBERHISTORY: 17316 return "Significant health conditions for a person related to the patient relevant in the context of care for the patient."; 17317 case FLAG: 17318 return "Prospective warnings of potential issues when providing care to the patient."; 17319 case GOAL: 17320 return "Describes the intended objective(s) for a patient, group or organization care, for example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc."; 17321 case GRAPHDEFINITION: 17322 return "A formal computable definition of a graph of resources - that is, a coherent set of resources that form a graph by following references. The Graph Definition resource defines a set and makes rules about the set."; 17323 case GROUP: 17324 return "Represents a defined collection of entities that may be discussed or acted upon collectively but which are not expected to act collectively, and are not formally or legally recognized; i.e. a collection of entities that isn't an Organization."; 17325 case GUIDANCERESPONSE: 17326 return "A guidance response is the formal response to a guidance request, including any output parameters returned by the evaluation, as well as the description of any proposed actions to be taken."; 17327 case HEALTHCARESERVICE: 17328 return "The details of a healthcare service available at a location."; 17329 case IMAGINGSTUDY: 17330 return "Representation of the content produced in a DICOM imaging study. A study comprises a set of series, each of which includes a set of Service-Object Pair Instances (SOP Instances - images or other data) acquired or produced in a common context. A series is of only one modality (e.g. X-ray, CT, MR, ultrasound), but a study may have multiple series of different modalities."; 17331 case IMMUNIZATION: 17332 return "Describes the event of a patient being administered a vaccine or a record of an immunization as reported by a patient, a clinician or another party."; 17333 case IMMUNIZATIONEVALUATION: 17334 return "Describes a comparison of an immunization event against published recommendations to determine if the administration is \"valid\" in relation to those recommendations."; 17335 case IMMUNIZATIONRECOMMENDATION: 17336 return "A patient's point-in-time set of recommendations (i.e. forecasting) according to a published schedule with optional supporting justification."; 17337 case IMPLEMENTATIONGUIDE: 17338 return "A set of rules of how a particular interoperability or standards problem is solved - typically through the use of FHIR resources. This resource is used to gather all the parts of an implementation guide into a logical whole and to publish a computable definition of all the parts."; 17339 case INSURANCEPLAN: 17340 return "Details of a Health Insurance product/plan provided by an organization."; 17341 case INVOICE: 17342 return "Invoice containing collected ChargeItems from an Account with calculated individual and total price for Billing purpose."; 17343 case LIBRARY: 17344 return "The Library resource is a general-purpose container for knowledge asset definitions. It can be used to describe and expose existing knowledge assets such as logic libraries and information model descriptions, as well as to describe a collection of knowledge assets."; 17345 case LINKAGE: 17346 return "Identifies two or more records (resource instances) that refer to the same real-world \"occurrence\"."; 17347 case LIST: 17348 return "A list is a curated collection of resources."; 17349 case LOCATION: 17350 return "Details and position information for a physical place where services are provided and resources and participants may be stored, found, contained, or accommodated."; 17351 case MEASURE: 17352 return "The Measure resource provides the definition of a quality measure."; 17353 case MEASUREREPORT: 17354 return "The MeasureReport resource contains the results of the calculation of a measure; and optionally a reference to the resources involved in that calculation."; 17355 case MEDIA: 17356 return "A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference."; 17357 case MEDICATION: 17358 return "This resource is primarily used for the identification and definition of a medication for the purposes of prescribing, dispensing, and administering a medication as well as for making statements about medication use."; 17359 case MEDICATIONADMINISTRATION: 17360 return "Describes the event of a patient consuming or otherwise being administered a medication. This may be as simple as swallowing a tablet or it may be a long running infusion. Related resources tie this event to the authorizing prescription, and the specific encounter between patient and health care practitioner."; 17361 case MEDICATIONDISPENSE: 17362 return "Indicates that a medication product is to be or has been dispensed for a named person/patient. This includes a description of the medication product (supply) provided and the instructions for administering the medication. The medication dispense is the result of a pharmacy system responding to a medication order."; 17363 case MEDICATIONKNOWLEDGE: 17364 return "Information about a medication that is used to support knowledge."; 17365 case MEDICATIONREQUEST: 17366 return "An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called \"MedicationRequest\" rather than \"MedicationPrescription\" or \"MedicationOrder\" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns."; 17367 case MEDICATIONSTATEMENT: 17368 return "A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from sources such as the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains. \n\nThe primary difference between a medication statement and a medication administration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medication statement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the medication statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information."; 17369 case MEDICINALPRODUCT: 17370 return "Detailed definition of a medicinal product, typically for uses other than direct patient care (e.g. regulatory use)."; 17371 case MEDICINALPRODUCTAUTHORIZATION: 17372 return "The regulatory authorization of a medicinal product."; 17373 case MEDICINALPRODUCTCONTRAINDICATION: 17374 return "The clinical particulars - indications, contraindications etc. of a medicinal product, including for regulatory purposes."; 17375 case MEDICINALPRODUCTINDICATION: 17376 return "Indication for the Medicinal Product."; 17377 case MEDICINALPRODUCTINGREDIENT: 17378 return "An ingredient of a manufactured item or pharmaceutical product."; 17379 case MEDICINALPRODUCTINTERACTION: 17380 return "The interactions of the medicinal product with other medicinal products, or other forms of interactions."; 17381 case MEDICINALPRODUCTMANUFACTURED: 17382 return "The manufactured item as contained in the packaged medicinal product."; 17383 case MEDICINALPRODUCTPACKAGED: 17384 return "A medicinal product in a container or package."; 17385 case MEDICINALPRODUCTPHARMACEUTICAL: 17386 return "A pharmaceutical product described in terms of its composition and dose form."; 17387 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 17388 return "Describe the undesirable effects of the medicinal product."; 17389 case MESSAGEDEFINITION: 17390 return "Defines the characteristics of a message that can be shared between systems, including the type of event that initiates the message, the content to be transmitted and what response(s), if any, are permitted."; 17391 case MESSAGEHEADER: 17392 return "The header for a message exchange that is either requesting or responding to an action. The reference(s) that are the subject of the action as well as other information related to the action are typically transmitted in a bundle in which the MessageHeader resource instance is the first resource in the bundle."; 17393 case MOLECULARSEQUENCE: 17394 return "Raw data describing a biological sequence."; 17395 case NAMINGSYSTEM: 17396 return "A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a \"System\" used within the Identifier and Coding data types."; 17397 case NUTRITIONORDER: 17398 return "A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident."; 17399 case OBSERVATION: 17400 return "Measurements and simple assertions made about a patient, device or other subject."; 17401 case OBSERVATIONDEFINITION: 17402 return "Set of definitional characteristics for a kind of observation or measurement produced or consumed by an orderable health care service."; 17403 case OPERATIONDEFINITION: 17404 return "A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction)."; 17405 case OPERATIONOUTCOME: 17406 return "A collection of error, warning, or information messages that result from a system action."; 17407 case ORGANIZATION: 17408 return "A formally or informally recognized grouping of people or organizations formed for the purpose of achieving some form of collective action. Includes companies, institutions, corporations, departments, community groups, healthcare practice groups, payer/insurer, etc."; 17409 case ORGANIZATIONAFFILIATION: 17410 return "Defines an affiliation/assotiation/relationship between 2 distinct oganizations, that is not a part-of relationship/sub-division relationship."; 17411 case PARAMETERS: 17412 return "This resource is a non-persisted resource used to pass information into and back from an [operation](operations.html). It has no other use, and there is no RESTful endpoint associated with it."; 17413 case PATIENT: 17414 return "Demographics and other administrative information about an individual or animal receiving care or other health-related services."; 17415 case PAYMENTNOTICE: 17416 return "This resource provides the status of the payment for goods and services rendered, and the request and response resource references."; 17417 case PAYMENTRECONCILIATION: 17418 return "This resource provides the details including amount of a payment and allocates the payment items being paid."; 17419 case PERSON: 17420 return "Demographics and administrative information about a person independent of a specific health-related context."; 17421 case PLANDEFINITION: 17422 return "This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical artifacts such as clinical decision support rules, order sets and protocols."; 17423 case PRACTITIONER: 17424 return "A person who is directly or indirectly involved in the provisioning of healthcare."; 17425 case PRACTITIONERROLE: 17426 return "A specific set of Roles/Locations/specialties/services that a practitioner may perform at an organization for a period of time."; 17427 case PROCEDURE: 17428 return "An action that is or was performed on or for a patient. This can be a physical intervention like an operation, or less invasive like long term services, counseling, or hypnotherapy."; 17429 case PROVENANCE: 17430 return "Provenance of a resource is a record that describes entities and processes involved in producing and delivering or otherwise influencing that resource. Provenance provides a critical foundation for assessing authenticity, enabling trust, and allowing reproducibility. Provenance assertions are a form of contextual metadata and can themselves become important records with their own provenance. Provenance statement indicates clinical significance in terms of confidence in authenticity, reliability, and trustworthiness, integrity, and stage in lifecycle (e.g. Document Completion - has the artifact been legally authenticated), all of which may impact security, privacy, and trust policies."; 17431 case QUESTIONNAIRE: 17432 return "A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection."; 17433 case QUESTIONNAIRERESPONSE: 17434 return "A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the questionnaire being responded to."; 17435 case RELATEDPERSON: 17436 return "Information about a person that is involved in the care for a patient, but who is not the target of healthcare, nor has a formal responsibility in the care process."; 17437 case REQUESTGROUP: 17438 return "A group of related requests that can be used to capture intended activities that have inter-dependencies such as \"give this medication after that one\"."; 17439 case RESEARCHDEFINITION: 17440 return "The ResearchDefinition resource describes the conditional state (population and any exposures being compared within the population) and outcome (if specified) that the knowledge (evidence, assertion, recommendation) is about."; 17441 case RESEARCHELEMENTDEFINITION: 17442 return "The ResearchElementDefinition resource describes a \"PICO\" element that knowledge (evidence, assertion, recommendation) is about."; 17443 case RESEARCHSTUDY: 17444 return "A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects."; 17445 case RESEARCHSUBJECT: 17446 return "A physical entity which is the primary unit of operational and/or administrative interest in a study."; 17447 case RESOURCE: 17448 return "This is the base resource type for everything."; 17449 case RISKASSESSMENT: 17450 return "An assessment of the likely outcome(s) for a patient or other subject as well as the likelihood of each outcome."; 17451 case RISKEVIDENCESYNTHESIS: 17452 return "The RiskEvidenceSynthesis resource describes the likelihood of an outcome in a population plus exposure state where the risk estimate is derived from a combination of research studies."; 17453 case SCHEDULE: 17454 return "A container for slots of time that may be available for booking appointments."; 17455 case SEARCHPARAMETER: 17456 return "A search parameter that defines a named search item that can be used to search/filter on a resource."; 17457 case SERVICEREQUEST: 17458 return "A record of a request for service such as diagnostic investigations, treatments, or operations to be performed."; 17459 case SLOT: 17460 return "A slot of time on a schedule that may be available for booking appointments."; 17461 case SPECIMEN: 17462 return "A sample to be used for analysis."; 17463 case SPECIMENDEFINITION: 17464 return "A kind of specimen with associated set of requirements."; 17465 case STRUCTUREDEFINITION: 17466 return "A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions and constraints on resources and data types."; 17467 case STRUCTUREMAP: 17468 return "A Map of relationships between 2 structures that can be used to transform data."; 17469 case SUBSCRIPTION: 17470 return "The subscription resource is used to define a push-based subscription from a server to another system. Once a subscription is registered with the server, the server checks every resource that is created or updated, and if the resource matches the given criteria, it sends a message on the defined \"channel\" so that another system can take an appropriate action."; 17471 case SUBSTANCE: 17472 return "A homogeneous material with a definite composition."; 17473 case SUBSTANCENUCLEICACID: 17474 return "Nucleic acids are defined by three distinct elements: the base, sugar and linkage. Individual substance/moiety IDs will be created for each of these elements. The nucleotide sequence will be always entered in the 5?-3? direction."; 17475 case SUBSTANCEPOLYMER: 17476 return "Todo."; 17477 case SUBSTANCEPROTEIN: 17478 return "A SubstanceProtein is defined as a single unit of a linear amino acid sequence, or a combination of subunits that are either covalently linked or have a defined invariant stoichiometric relationship. This includes all synthetic, recombinant and purified SubstanceProteins of defined sequence, whether the use is therapeutic or prophylactic. This set of elements will be used to describe albumins, coagulation factors, cytokines, growth factors, peptide/SubstanceProtein hormones, enzymes, toxins, toxoids, recombinant vaccines, and immunomodulators."; 17479 case SUBSTANCEREFERENCEINFORMATION: 17480 return "Todo."; 17481 case SUBSTANCESOURCEMATERIAL: 17482 return "Source material shall capture information on the taxonomic and anatomical origins as well as the fraction of a material that can result in or can be modified to form a substance. This set of data elements shall be used to define polymer substances isolated from biological matrices. Taxonomic and anatomical origins shall be described using a controlled vocabulary as required. This information is captured for naturally derived polymers ( . starch) and structurally diverse substances. For Organisms belonging to the Kingdom Plantae the Substance level defines the fresh material of a single species or infraspecies, the Herbal Drug and the Herbal preparation. For Herbal preparations, the fraction information will be captured at the Substance information level and additional information for herbal extracts will be captured at the Specified Substance Group 1 information level. See for further explanation the Substance Class: Structurally Diverse and the herbal annex."; 17483 case SUBSTANCESPECIFICATION: 17484 return "The detailed description of a substance, typically at a level beyond what is used for prescribing."; 17485 case SUPPLYDELIVERY: 17486 return "Record of delivery of what is supplied."; 17487 case SUPPLYREQUEST: 17488 return "A record of a request for a medication, substance or device used in the healthcare setting."; 17489 case TASK: 17490 return "A task to be performed."; 17491 case TERMINOLOGYCAPABILITIES: 17492 return "A Terminology Capabilities documents a set of capabilities (behaviors) of a FHIR Server that may be used as a statement of actual server functionality or a statement of required or desired server implementation."; 17493 case TESTREPORT: 17494 return "A summary of information based on the results of executing a TestScript."; 17495 case TESTSCRIPT: 17496 return "A structured set of tests against a FHIR server or client implementation to determine compliance against the FHIR specification."; 17497 case VALUESET: 17498 return "A ValueSet resource instance specifies a set of codes drawn from one or more code systems, intended for use in a particular context. Value sets link between [[[CodeSystem]]] definitions and their use in [coded elements](terminologies.html)."; 17499 case VERIFICATIONRESULT: 17500 return "Describes validation requirements, source(s), status and dates for one or more elements."; 17501 case VISIONPRESCRIPTION: 17502 return "An authorization for the provision of glasses and/or contact lenses to a patient."; 17503 case NULL: 17504 return null; 17505 default: 17506 return "?"; 17507 } 17508 } 17509 17510 public String getDisplay() { 17511 switch (this) { 17512 case ACCOUNT: 17513 return "Account"; 17514 case ACTIVITYDEFINITION: 17515 return "ActivityDefinition"; 17516 case ADVERSEEVENT: 17517 return "AdverseEvent"; 17518 case ALLERGYINTOLERANCE: 17519 return "AllergyIntolerance"; 17520 case APPOINTMENT: 17521 return "Appointment"; 17522 case APPOINTMENTRESPONSE: 17523 return "AppointmentResponse"; 17524 case AUDITEVENT: 17525 return "AuditEvent"; 17526 case BASIC: 17527 return "Basic"; 17528 case BINARY: 17529 return "Binary"; 17530 case BIOLOGICALLYDERIVEDPRODUCT: 17531 return "BiologicallyDerivedProduct"; 17532 case BODYSTRUCTURE: 17533 return "BodyStructure"; 17534 case BUNDLE: 17535 return "Bundle"; 17536 case CAPABILITYSTATEMENT: 17537 return "CapabilityStatement"; 17538 case CAREPLAN: 17539 return "CarePlan"; 17540 case CARETEAM: 17541 return "CareTeam"; 17542 case CATALOGENTRY: 17543 return "CatalogEntry"; 17544 case CHARGEITEM: 17545 return "ChargeItem"; 17546 case CHARGEITEMDEFINITION: 17547 return "ChargeItemDefinition"; 17548 case CLAIM: 17549 return "Claim"; 17550 case CLAIMRESPONSE: 17551 return "ClaimResponse"; 17552 case CLINICALIMPRESSION: 17553 return "ClinicalImpression"; 17554 case CODESYSTEM: 17555 return "CodeSystem"; 17556 case COMMUNICATION: 17557 return "Communication"; 17558 case COMMUNICATIONREQUEST: 17559 return "CommunicationRequest"; 17560 case COMPARTMENTDEFINITION: 17561 return "CompartmentDefinition"; 17562 case COMPOSITION: 17563 return "Composition"; 17564 case CONCEPTMAP: 17565 return "ConceptMap"; 17566 case CONDITION: 17567 return "Condition"; 17568 case CONSENT: 17569 return "Consent"; 17570 case CONTRACT: 17571 return "Contract"; 17572 case COVERAGE: 17573 return "Coverage"; 17574 case COVERAGEELIGIBILITYREQUEST: 17575 return "CoverageEligibilityRequest"; 17576 case COVERAGEELIGIBILITYRESPONSE: 17577 return "CoverageEligibilityResponse"; 17578 case DETECTEDISSUE: 17579 return "DetectedIssue"; 17580 case DEVICE: 17581 return "Device"; 17582 case DEVICEDEFINITION: 17583 return "DeviceDefinition"; 17584 case DEVICEMETRIC: 17585 return "DeviceMetric"; 17586 case DEVICEREQUEST: 17587 return "DeviceRequest"; 17588 case DEVICEUSESTATEMENT: 17589 return "DeviceUseStatement"; 17590 case DIAGNOSTICREPORT: 17591 return "DiagnosticReport"; 17592 case DOCUMENTMANIFEST: 17593 return "DocumentManifest"; 17594 case DOCUMENTREFERENCE: 17595 return "DocumentReference"; 17596 case DOMAINRESOURCE: 17597 return "DomainResource"; 17598 case EFFECTEVIDENCESYNTHESIS: 17599 return "EffectEvidenceSynthesis"; 17600 case ENCOUNTER: 17601 return "Encounter"; 17602 case ENDPOINT: 17603 return "Endpoint"; 17604 case ENROLLMENTREQUEST: 17605 return "EnrollmentRequest"; 17606 case ENROLLMENTRESPONSE: 17607 return "EnrollmentResponse"; 17608 case EPISODEOFCARE: 17609 return "EpisodeOfCare"; 17610 case EVENTDEFINITION: 17611 return "EventDefinition"; 17612 case EVIDENCE: 17613 return "Evidence"; 17614 case EVIDENCEVARIABLE: 17615 return "EvidenceVariable"; 17616 case EXAMPLESCENARIO: 17617 return "ExampleScenario"; 17618 case EXPLANATIONOFBENEFIT: 17619 return "ExplanationOfBenefit"; 17620 case FAMILYMEMBERHISTORY: 17621 return "FamilyMemberHistory"; 17622 case FLAG: 17623 return "Flag"; 17624 case GOAL: 17625 return "Goal"; 17626 case GRAPHDEFINITION: 17627 return "GraphDefinition"; 17628 case GROUP: 17629 return "Group"; 17630 case GUIDANCERESPONSE: 17631 return "GuidanceResponse"; 17632 case HEALTHCARESERVICE: 17633 return "HealthcareService"; 17634 case IMAGINGSTUDY: 17635 return "ImagingStudy"; 17636 case IMMUNIZATION: 17637 return "Immunization"; 17638 case IMMUNIZATIONEVALUATION: 17639 return "ImmunizationEvaluation"; 17640 case IMMUNIZATIONRECOMMENDATION: 17641 return "ImmunizationRecommendation"; 17642 case IMPLEMENTATIONGUIDE: 17643 return "ImplementationGuide"; 17644 case INSURANCEPLAN: 17645 return "InsurancePlan"; 17646 case INVOICE: 17647 return "Invoice"; 17648 case LIBRARY: 17649 return "Library"; 17650 case LINKAGE: 17651 return "Linkage"; 17652 case LIST: 17653 return "List"; 17654 case LOCATION: 17655 return "Location"; 17656 case MEASURE: 17657 return "Measure"; 17658 case MEASUREREPORT: 17659 return "MeasureReport"; 17660 case MEDIA: 17661 return "Media"; 17662 case MEDICATION: 17663 return "Medication"; 17664 case MEDICATIONADMINISTRATION: 17665 return "MedicationAdministration"; 17666 case MEDICATIONDISPENSE: 17667 return "MedicationDispense"; 17668 case MEDICATIONKNOWLEDGE: 17669 return "MedicationKnowledge"; 17670 case MEDICATIONREQUEST: 17671 return "MedicationRequest"; 17672 case MEDICATIONSTATEMENT: 17673 return "MedicationStatement"; 17674 case MEDICINALPRODUCT: 17675 return "MedicinalProduct"; 17676 case MEDICINALPRODUCTAUTHORIZATION: 17677 return "MedicinalProductAuthorization"; 17678 case MEDICINALPRODUCTCONTRAINDICATION: 17679 return "MedicinalProductContraindication"; 17680 case MEDICINALPRODUCTINDICATION: 17681 return "MedicinalProductIndication"; 17682 case MEDICINALPRODUCTINGREDIENT: 17683 return "MedicinalProductIngredient"; 17684 case MEDICINALPRODUCTINTERACTION: 17685 return "MedicinalProductInteraction"; 17686 case MEDICINALPRODUCTMANUFACTURED: 17687 return "MedicinalProductManufactured"; 17688 case MEDICINALPRODUCTPACKAGED: 17689 return "MedicinalProductPackaged"; 17690 case MEDICINALPRODUCTPHARMACEUTICAL: 17691 return "MedicinalProductPharmaceutical"; 17692 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 17693 return "MedicinalProductUndesirableEffect"; 17694 case MESSAGEDEFINITION: 17695 return "MessageDefinition"; 17696 case MESSAGEHEADER: 17697 return "MessageHeader"; 17698 case MOLECULARSEQUENCE: 17699 return "MolecularSequence"; 17700 case NAMINGSYSTEM: 17701 return "NamingSystem"; 17702 case NUTRITIONORDER: 17703 return "NutritionOrder"; 17704 case OBSERVATION: 17705 return "Observation"; 17706 case OBSERVATIONDEFINITION: 17707 return "ObservationDefinition"; 17708 case OPERATIONDEFINITION: 17709 return "OperationDefinition"; 17710 case OPERATIONOUTCOME: 17711 return "OperationOutcome"; 17712 case ORGANIZATION: 17713 return "Organization"; 17714 case ORGANIZATIONAFFILIATION: 17715 return "OrganizationAffiliation"; 17716 case PARAMETERS: 17717 return "Parameters"; 17718 case PATIENT: 17719 return "Patient"; 17720 case PAYMENTNOTICE: 17721 return "PaymentNotice"; 17722 case PAYMENTRECONCILIATION: 17723 return "PaymentReconciliation"; 17724 case PERSON: 17725 return "Person"; 17726 case PLANDEFINITION: 17727 return "PlanDefinition"; 17728 case PRACTITIONER: 17729 return "Practitioner"; 17730 case PRACTITIONERROLE: 17731 return "PractitionerRole"; 17732 case PROCEDURE: 17733 return "Procedure"; 17734 case PROVENANCE: 17735 return "Provenance"; 17736 case QUESTIONNAIRE: 17737 return "Questionnaire"; 17738 case QUESTIONNAIRERESPONSE: 17739 return "QuestionnaireResponse"; 17740 case RELATEDPERSON: 17741 return "RelatedPerson"; 17742 case REQUESTGROUP: 17743 return "RequestGroup"; 17744 case RESEARCHDEFINITION: 17745 return "ResearchDefinition"; 17746 case RESEARCHELEMENTDEFINITION: 17747 return "ResearchElementDefinition"; 17748 case RESEARCHSTUDY: 17749 return "ResearchStudy"; 17750 case RESEARCHSUBJECT: 17751 return "ResearchSubject"; 17752 case RESOURCE: 17753 return "Resource"; 17754 case RISKASSESSMENT: 17755 return "RiskAssessment"; 17756 case RISKEVIDENCESYNTHESIS: 17757 return "RiskEvidenceSynthesis"; 17758 case SCHEDULE: 17759 return "Schedule"; 17760 case SEARCHPARAMETER: 17761 return "SearchParameter"; 17762 case SERVICEREQUEST: 17763 return "ServiceRequest"; 17764 case SLOT: 17765 return "Slot"; 17766 case SPECIMEN: 17767 return "Specimen"; 17768 case SPECIMENDEFINITION: 17769 return "SpecimenDefinition"; 17770 case STRUCTUREDEFINITION: 17771 return "StructureDefinition"; 17772 case STRUCTUREMAP: 17773 return "StructureMap"; 17774 case SUBSCRIPTION: 17775 return "Subscription"; 17776 case SUBSTANCE: 17777 return "Substance"; 17778 case SUBSTANCENUCLEICACID: 17779 return "SubstanceNucleicAcid"; 17780 case SUBSTANCEPOLYMER: 17781 return "SubstancePolymer"; 17782 case SUBSTANCEPROTEIN: 17783 return "SubstanceProtein"; 17784 case SUBSTANCEREFERENCEINFORMATION: 17785 return "SubstanceReferenceInformation"; 17786 case SUBSTANCESOURCEMATERIAL: 17787 return "SubstanceSourceMaterial"; 17788 case SUBSTANCESPECIFICATION: 17789 return "SubstanceSpecification"; 17790 case SUPPLYDELIVERY: 17791 return "SupplyDelivery"; 17792 case SUPPLYREQUEST: 17793 return "SupplyRequest"; 17794 case TASK: 17795 return "Task"; 17796 case TERMINOLOGYCAPABILITIES: 17797 return "TerminologyCapabilities"; 17798 case TESTREPORT: 17799 return "TestReport"; 17800 case TESTSCRIPT: 17801 return "TestScript"; 17802 case VALUESET: 17803 return "ValueSet"; 17804 case VERIFICATIONRESULT: 17805 return "VerificationResult"; 17806 case VISIONPRESCRIPTION: 17807 return "VisionPrescription"; 17808 case NULL: 17809 return null; 17810 default: 17811 return "?"; 17812 } 17813 } 17814 } 17815 17816 public static class ResourceTypeEnumFactory implements EnumFactory<ResourceType> { 17817 public ResourceType fromCode(String codeString) throws IllegalArgumentException { 17818 if (codeString == null || "".equals(codeString)) 17819 if (codeString == null || "".equals(codeString)) 17820 return null; 17821 if ("Account".equals(codeString)) 17822 return ResourceType.ACCOUNT; 17823 if ("ActivityDefinition".equals(codeString)) 17824 return ResourceType.ACTIVITYDEFINITION; 17825 if ("AdverseEvent".equals(codeString)) 17826 return ResourceType.ADVERSEEVENT; 17827 if ("AllergyIntolerance".equals(codeString)) 17828 return ResourceType.ALLERGYINTOLERANCE; 17829 if ("Appointment".equals(codeString)) 17830 return ResourceType.APPOINTMENT; 17831 if ("AppointmentResponse".equals(codeString)) 17832 return ResourceType.APPOINTMENTRESPONSE; 17833 if ("AuditEvent".equals(codeString)) 17834 return ResourceType.AUDITEVENT; 17835 if ("Basic".equals(codeString)) 17836 return ResourceType.BASIC; 17837 if ("Binary".equals(codeString)) 17838 return ResourceType.BINARY; 17839 if ("BiologicallyDerivedProduct".equals(codeString)) 17840 return ResourceType.BIOLOGICALLYDERIVEDPRODUCT; 17841 if ("BodyStructure".equals(codeString)) 17842 return ResourceType.BODYSTRUCTURE; 17843 if ("Bundle".equals(codeString)) 17844 return ResourceType.BUNDLE; 17845 if ("CapabilityStatement".equals(codeString)) 17846 return ResourceType.CAPABILITYSTATEMENT; 17847 if ("CarePlan".equals(codeString)) 17848 return ResourceType.CAREPLAN; 17849 if ("CareTeam".equals(codeString)) 17850 return ResourceType.CARETEAM; 17851 if ("CatalogEntry".equals(codeString)) 17852 return ResourceType.CATALOGENTRY; 17853 if ("ChargeItem".equals(codeString)) 17854 return ResourceType.CHARGEITEM; 17855 if ("ChargeItemDefinition".equals(codeString)) 17856 return ResourceType.CHARGEITEMDEFINITION; 17857 if ("Claim".equals(codeString)) 17858 return ResourceType.CLAIM; 17859 if ("ClaimResponse".equals(codeString)) 17860 return ResourceType.CLAIMRESPONSE; 17861 if ("ClinicalImpression".equals(codeString)) 17862 return ResourceType.CLINICALIMPRESSION; 17863 if ("CodeSystem".equals(codeString)) 17864 return ResourceType.CODESYSTEM; 17865 if ("Communication".equals(codeString)) 17866 return ResourceType.COMMUNICATION; 17867 if ("CommunicationRequest".equals(codeString)) 17868 return ResourceType.COMMUNICATIONREQUEST; 17869 if ("CompartmentDefinition".equals(codeString)) 17870 return ResourceType.COMPARTMENTDEFINITION; 17871 if ("Composition".equals(codeString)) 17872 return ResourceType.COMPOSITION; 17873 if ("ConceptMap".equals(codeString)) 17874 return ResourceType.CONCEPTMAP; 17875 if ("Condition".equals(codeString)) 17876 return ResourceType.CONDITION; 17877 if ("Consent".equals(codeString)) 17878 return ResourceType.CONSENT; 17879 if ("Contract".equals(codeString)) 17880 return ResourceType.CONTRACT; 17881 if ("Coverage".equals(codeString)) 17882 return ResourceType.COVERAGE; 17883 if ("CoverageEligibilityRequest".equals(codeString)) 17884 return ResourceType.COVERAGEELIGIBILITYREQUEST; 17885 if ("CoverageEligibilityResponse".equals(codeString)) 17886 return ResourceType.COVERAGEELIGIBILITYRESPONSE; 17887 if ("DetectedIssue".equals(codeString)) 17888 return ResourceType.DETECTEDISSUE; 17889 if ("Device".equals(codeString)) 17890 return ResourceType.DEVICE; 17891 if ("DeviceDefinition".equals(codeString)) 17892 return ResourceType.DEVICEDEFINITION; 17893 if ("DeviceMetric".equals(codeString)) 17894 return ResourceType.DEVICEMETRIC; 17895 if ("DeviceRequest".equals(codeString)) 17896 return ResourceType.DEVICEREQUEST; 17897 if ("DeviceUseStatement".equals(codeString)) 17898 return ResourceType.DEVICEUSESTATEMENT; 17899 if ("DiagnosticReport".equals(codeString)) 17900 return ResourceType.DIAGNOSTICREPORT; 17901 if ("DocumentManifest".equals(codeString)) 17902 return ResourceType.DOCUMENTMANIFEST; 17903 if ("DocumentReference".equals(codeString)) 17904 return ResourceType.DOCUMENTREFERENCE; 17905 if ("DomainResource".equals(codeString)) 17906 return ResourceType.DOMAINRESOURCE; 17907 if ("EffectEvidenceSynthesis".equals(codeString)) 17908 return ResourceType.EFFECTEVIDENCESYNTHESIS; 17909 if ("Encounter".equals(codeString)) 17910 return ResourceType.ENCOUNTER; 17911 if ("Endpoint".equals(codeString)) 17912 return ResourceType.ENDPOINT; 17913 if ("EnrollmentRequest".equals(codeString)) 17914 return ResourceType.ENROLLMENTREQUEST; 17915 if ("EnrollmentResponse".equals(codeString)) 17916 return ResourceType.ENROLLMENTRESPONSE; 17917 if ("EpisodeOfCare".equals(codeString)) 17918 return ResourceType.EPISODEOFCARE; 17919 if ("EventDefinition".equals(codeString)) 17920 return ResourceType.EVENTDEFINITION; 17921 if ("Evidence".equals(codeString)) 17922 return ResourceType.EVIDENCE; 17923 if ("EvidenceVariable".equals(codeString)) 17924 return ResourceType.EVIDENCEVARIABLE; 17925 if ("ExampleScenario".equals(codeString)) 17926 return ResourceType.EXAMPLESCENARIO; 17927 if ("ExplanationOfBenefit".equals(codeString)) 17928 return ResourceType.EXPLANATIONOFBENEFIT; 17929 if ("FamilyMemberHistory".equals(codeString)) 17930 return ResourceType.FAMILYMEMBERHISTORY; 17931 if ("Flag".equals(codeString)) 17932 return ResourceType.FLAG; 17933 if ("Goal".equals(codeString)) 17934 return ResourceType.GOAL; 17935 if ("GraphDefinition".equals(codeString)) 17936 return ResourceType.GRAPHDEFINITION; 17937 if ("Group".equals(codeString)) 17938 return ResourceType.GROUP; 17939 if ("GuidanceResponse".equals(codeString)) 17940 return ResourceType.GUIDANCERESPONSE; 17941 if ("HealthcareService".equals(codeString)) 17942 return ResourceType.HEALTHCARESERVICE; 17943 if ("ImagingStudy".equals(codeString)) 17944 return ResourceType.IMAGINGSTUDY; 17945 if ("Immunization".equals(codeString)) 17946 return ResourceType.IMMUNIZATION; 17947 if ("ImmunizationEvaluation".equals(codeString)) 17948 return ResourceType.IMMUNIZATIONEVALUATION; 17949 if ("ImmunizationRecommendation".equals(codeString)) 17950 return ResourceType.IMMUNIZATIONRECOMMENDATION; 17951 if ("ImplementationGuide".equals(codeString)) 17952 return ResourceType.IMPLEMENTATIONGUIDE; 17953 if ("InsurancePlan".equals(codeString)) 17954 return ResourceType.INSURANCEPLAN; 17955 if ("Invoice".equals(codeString)) 17956 return ResourceType.INVOICE; 17957 if ("Library".equals(codeString)) 17958 return ResourceType.LIBRARY; 17959 if ("Linkage".equals(codeString)) 17960 return ResourceType.LINKAGE; 17961 if ("List".equals(codeString)) 17962 return ResourceType.LIST; 17963 if ("Location".equals(codeString)) 17964 return ResourceType.LOCATION; 17965 if ("Measure".equals(codeString)) 17966 return ResourceType.MEASURE; 17967 if ("MeasureReport".equals(codeString)) 17968 return ResourceType.MEASUREREPORT; 17969 if ("Media".equals(codeString)) 17970 return ResourceType.MEDIA; 17971 if ("Medication".equals(codeString)) 17972 return ResourceType.MEDICATION; 17973 if ("MedicationAdministration".equals(codeString)) 17974 return ResourceType.MEDICATIONADMINISTRATION; 17975 if ("MedicationDispense".equals(codeString)) 17976 return ResourceType.MEDICATIONDISPENSE; 17977 if ("MedicationKnowledge".equals(codeString)) 17978 return ResourceType.MEDICATIONKNOWLEDGE; 17979 if ("MedicationRequest".equals(codeString)) 17980 return ResourceType.MEDICATIONREQUEST; 17981 if ("MedicationStatement".equals(codeString)) 17982 return ResourceType.MEDICATIONSTATEMENT; 17983 if ("MedicinalProduct".equals(codeString)) 17984 return ResourceType.MEDICINALPRODUCT; 17985 if ("MedicinalProductAuthorization".equals(codeString)) 17986 return ResourceType.MEDICINALPRODUCTAUTHORIZATION; 17987 if ("MedicinalProductContraindication".equals(codeString)) 17988 return ResourceType.MEDICINALPRODUCTCONTRAINDICATION; 17989 if ("MedicinalProductIndication".equals(codeString)) 17990 return ResourceType.MEDICINALPRODUCTINDICATION; 17991 if ("MedicinalProductIngredient".equals(codeString)) 17992 return ResourceType.MEDICINALPRODUCTINGREDIENT; 17993 if ("MedicinalProductInteraction".equals(codeString)) 17994 return ResourceType.MEDICINALPRODUCTINTERACTION; 17995 if ("MedicinalProductManufactured".equals(codeString)) 17996 return ResourceType.MEDICINALPRODUCTMANUFACTURED; 17997 if ("MedicinalProductPackaged".equals(codeString)) 17998 return ResourceType.MEDICINALPRODUCTPACKAGED; 17999 if ("MedicinalProductPharmaceutical".equals(codeString)) 18000 return ResourceType.MEDICINALPRODUCTPHARMACEUTICAL; 18001 if ("MedicinalProductUndesirableEffect".equals(codeString)) 18002 return ResourceType.MEDICINALPRODUCTUNDESIRABLEEFFECT; 18003 if ("MessageDefinition".equals(codeString)) 18004 return ResourceType.MESSAGEDEFINITION; 18005 if ("MessageHeader".equals(codeString)) 18006 return ResourceType.MESSAGEHEADER; 18007 if ("MolecularSequence".equals(codeString)) 18008 return ResourceType.MOLECULARSEQUENCE; 18009 if ("NamingSystem".equals(codeString)) 18010 return ResourceType.NAMINGSYSTEM; 18011 if ("NutritionOrder".equals(codeString)) 18012 return ResourceType.NUTRITIONORDER; 18013 if ("Observation".equals(codeString)) 18014 return ResourceType.OBSERVATION; 18015 if ("ObservationDefinition".equals(codeString)) 18016 return ResourceType.OBSERVATIONDEFINITION; 18017 if ("OperationDefinition".equals(codeString)) 18018 return ResourceType.OPERATIONDEFINITION; 18019 if ("OperationOutcome".equals(codeString)) 18020 return ResourceType.OPERATIONOUTCOME; 18021 if ("Organization".equals(codeString)) 18022 return ResourceType.ORGANIZATION; 18023 if ("OrganizationAffiliation".equals(codeString)) 18024 return ResourceType.ORGANIZATIONAFFILIATION; 18025 if ("Parameters".equals(codeString)) 18026 return ResourceType.PARAMETERS; 18027 if ("Patient".equals(codeString)) 18028 return ResourceType.PATIENT; 18029 if ("PaymentNotice".equals(codeString)) 18030 return ResourceType.PAYMENTNOTICE; 18031 if ("PaymentReconciliation".equals(codeString)) 18032 return ResourceType.PAYMENTRECONCILIATION; 18033 if ("Person".equals(codeString)) 18034 return ResourceType.PERSON; 18035 if ("PlanDefinition".equals(codeString)) 18036 return ResourceType.PLANDEFINITION; 18037 if ("Practitioner".equals(codeString)) 18038 return ResourceType.PRACTITIONER; 18039 if ("PractitionerRole".equals(codeString)) 18040 return ResourceType.PRACTITIONERROLE; 18041 if ("Procedure".equals(codeString)) 18042 return ResourceType.PROCEDURE; 18043 if ("Provenance".equals(codeString)) 18044 return ResourceType.PROVENANCE; 18045 if ("Questionnaire".equals(codeString)) 18046 return ResourceType.QUESTIONNAIRE; 18047 if ("QuestionnaireResponse".equals(codeString)) 18048 return ResourceType.QUESTIONNAIRERESPONSE; 18049 if ("RelatedPerson".equals(codeString)) 18050 return ResourceType.RELATEDPERSON; 18051 if ("RequestGroup".equals(codeString)) 18052 return ResourceType.REQUESTGROUP; 18053 if ("ResearchDefinition".equals(codeString)) 18054 return ResourceType.RESEARCHDEFINITION; 18055 if ("ResearchElementDefinition".equals(codeString)) 18056 return ResourceType.RESEARCHELEMENTDEFINITION; 18057 if ("ResearchStudy".equals(codeString)) 18058 return ResourceType.RESEARCHSTUDY; 18059 if ("ResearchSubject".equals(codeString)) 18060 return ResourceType.RESEARCHSUBJECT; 18061 if ("Resource".equals(codeString)) 18062 return ResourceType.RESOURCE; 18063 if ("RiskAssessment".equals(codeString)) 18064 return ResourceType.RISKASSESSMENT; 18065 if ("RiskEvidenceSynthesis".equals(codeString)) 18066 return ResourceType.RISKEVIDENCESYNTHESIS; 18067 if ("Schedule".equals(codeString)) 18068 return ResourceType.SCHEDULE; 18069 if ("SearchParameter".equals(codeString)) 18070 return ResourceType.SEARCHPARAMETER; 18071 if ("ServiceRequest".equals(codeString)) 18072 return ResourceType.SERVICEREQUEST; 18073 if ("Slot".equals(codeString)) 18074 return ResourceType.SLOT; 18075 if ("Specimen".equals(codeString)) 18076 return ResourceType.SPECIMEN; 18077 if ("SpecimenDefinition".equals(codeString)) 18078 return ResourceType.SPECIMENDEFINITION; 18079 if ("StructureDefinition".equals(codeString)) 18080 return ResourceType.STRUCTUREDEFINITION; 18081 if ("StructureMap".equals(codeString)) 18082 return ResourceType.STRUCTUREMAP; 18083 if ("Subscription".equals(codeString)) 18084 return ResourceType.SUBSCRIPTION; 18085 if ("Substance".equals(codeString)) 18086 return ResourceType.SUBSTANCE; 18087 if ("SubstanceNucleicAcid".equals(codeString)) 18088 return ResourceType.SUBSTANCENUCLEICACID; 18089 if ("SubstancePolymer".equals(codeString)) 18090 return ResourceType.SUBSTANCEPOLYMER; 18091 if ("SubstanceProtein".equals(codeString)) 18092 return ResourceType.SUBSTANCEPROTEIN; 18093 if ("SubstanceReferenceInformation".equals(codeString)) 18094 return ResourceType.SUBSTANCEREFERENCEINFORMATION; 18095 if ("SubstanceSourceMaterial".equals(codeString)) 18096 return ResourceType.SUBSTANCESOURCEMATERIAL; 18097 if ("SubstanceSpecification".equals(codeString)) 18098 return ResourceType.SUBSTANCESPECIFICATION; 18099 if ("SupplyDelivery".equals(codeString)) 18100 return ResourceType.SUPPLYDELIVERY; 18101 if ("SupplyRequest".equals(codeString)) 18102 return ResourceType.SUPPLYREQUEST; 18103 if ("Task".equals(codeString)) 18104 return ResourceType.TASK; 18105 if ("TerminologyCapabilities".equals(codeString)) 18106 return ResourceType.TERMINOLOGYCAPABILITIES; 18107 if ("TestReport".equals(codeString)) 18108 return ResourceType.TESTREPORT; 18109 if ("TestScript".equals(codeString)) 18110 return ResourceType.TESTSCRIPT; 18111 if ("ValueSet".equals(codeString)) 18112 return ResourceType.VALUESET; 18113 if ("VerificationResult".equals(codeString)) 18114 return ResourceType.VERIFICATIONRESULT; 18115 if ("VisionPrescription".equals(codeString)) 18116 return ResourceType.VISIONPRESCRIPTION; 18117 throw new IllegalArgumentException("Unknown ResourceType code '" + codeString + "'"); 18118 } 18119 18120 public Enumeration<ResourceType> fromType(PrimitiveType<?> code) throws FHIRException { 18121 if (code == null) 18122 return null; 18123 if (code.isEmpty()) 18124 return new Enumeration<ResourceType>(this, ResourceType.NULL, code); 18125 String codeString = code.asStringValue(); 18126 if (codeString == null || "".equals(codeString)) 18127 return new Enumeration<ResourceType>(this, ResourceType.NULL, code); 18128 if ("Account".equals(codeString)) 18129 return new Enumeration<ResourceType>(this, ResourceType.ACCOUNT, code); 18130 if ("ActivityDefinition".equals(codeString)) 18131 return new Enumeration<ResourceType>(this, ResourceType.ACTIVITYDEFINITION, code); 18132 if ("AdverseEvent".equals(codeString)) 18133 return new Enumeration<ResourceType>(this, ResourceType.ADVERSEEVENT, code); 18134 if ("AllergyIntolerance".equals(codeString)) 18135 return new Enumeration<ResourceType>(this, ResourceType.ALLERGYINTOLERANCE, code); 18136 if ("Appointment".equals(codeString)) 18137 return new Enumeration<ResourceType>(this, ResourceType.APPOINTMENT, code); 18138 if ("AppointmentResponse".equals(codeString)) 18139 return new Enumeration<ResourceType>(this, ResourceType.APPOINTMENTRESPONSE, code); 18140 if ("AuditEvent".equals(codeString)) 18141 return new Enumeration<ResourceType>(this, ResourceType.AUDITEVENT, code); 18142 if ("Basic".equals(codeString)) 18143 return new Enumeration<ResourceType>(this, ResourceType.BASIC, code); 18144 if ("Binary".equals(codeString)) 18145 return new Enumeration<ResourceType>(this, ResourceType.BINARY, code); 18146 if ("BiologicallyDerivedProduct".equals(codeString)) 18147 return new Enumeration<ResourceType>(this, ResourceType.BIOLOGICALLYDERIVEDPRODUCT, code); 18148 if ("BodyStructure".equals(codeString)) 18149 return new Enumeration<ResourceType>(this, ResourceType.BODYSTRUCTURE, code); 18150 if ("Bundle".equals(codeString)) 18151 return new Enumeration<ResourceType>(this, ResourceType.BUNDLE, code); 18152 if ("CapabilityStatement".equals(codeString)) 18153 return new Enumeration<ResourceType>(this, ResourceType.CAPABILITYSTATEMENT, code); 18154 if ("CarePlan".equals(codeString)) 18155 return new Enumeration<ResourceType>(this, ResourceType.CAREPLAN, code); 18156 if ("CareTeam".equals(codeString)) 18157 return new Enumeration<ResourceType>(this, ResourceType.CARETEAM, code); 18158 if ("CatalogEntry".equals(codeString)) 18159 return new Enumeration<ResourceType>(this, ResourceType.CATALOGENTRY, code); 18160 if ("ChargeItem".equals(codeString)) 18161 return new Enumeration<ResourceType>(this, ResourceType.CHARGEITEM, code); 18162 if ("ChargeItemDefinition".equals(codeString)) 18163 return new Enumeration<ResourceType>(this, ResourceType.CHARGEITEMDEFINITION, code); 18164 if ("Claim".equals(codeString)) 18165 return new Enumeration<ResourceType>(this, ResourceType.CLAIM, code); 18166 if ("ClaimResponse".equals(codeString)) 18167 return new Enumeration<ResourceType>(this, ResourceType.CLAIMRESPONSE, code); 18168 if ("ClinicalImpression".equals(codeString)) 18169 return new Enumeration<ResourceType>(this, ResourceType.CLINICALIMPRESSION, code); 18170 if ("CodeSystem".equals(codeString)) 18171 return new Enumeration<ResourceType>(this, ResourceType.CODESYSTEM, code); 18172 if ("Communication".equals(codeString)) 18173 return new Enumeration<ResourceType>(this, ResourceType.COMMUNICATION, code); 18174 if ("CommunicationRequest".equals(codeString)) 18175 return new Enumeration<ResourceType>(this, ResourceType.COMMUNICATIONREQUEST, code); 18176 if ("CompartmentDefinition".equals(codeString)) 18177 return new Enumeration<ResourceType>(this, ResourceType.COMPARTMENTDEFINITION, code); 18178 if ("Composition".equals(codeString)) 18179 return new Enumeration<ResourceType>(this, ResourceType.COMPOSITION, code); 18180 if ("ConceptMap".equals(codeString)) 18181 return new Enumeration<ResourceType>(this, ResourceType.CONCEPTMAP, code); 18182 if ("Condition".equals(codeString)) 18183 return new Enumeration<ResourceType>(this, ResourceType.CONDITION, code); 18184 if ("Consent".equals(codeString)) 18185 return new Enumeration<ResourceType>(this, ResourceType.CONSENT, code); 18186 if ("Contract".equals(codeString)) 18187 return new Enumeration<ResourceType>(this, ResourceType.CONTRACT, code); 18188 if ("Coverage".equals(codeString)) 18189 return new Enumeration<ResourceType>(this, ResourceType.COVERAGE, code); 18190 if ("CoverageEligibilityRequest".equals(codeString)) 18191 return new Enumeration<ResourceType>(this, ResourceType.COVERAGEELIGIBILITYREQUEST, code); 18192 if ("CoverageEligibilityResponse".equals(codeString)) 18193 return new Enumeration<ResourceType>(this, ResourceType.COVERAGEELIGIBILITYRESPONSE, code); 18194 if ("DetectedIssue".equals(codeString)) 18195 return new Enumeration<ResourceType>(this, ResourceType.DETECTEDISSUE, code); 18196 if ("Device".equals(codeString)) 18197 return new Enumeration<ResourceType>(this, ResourceType.DEVICE, code); 18198 if ("DeviceDefinition".equals(codeString)) 18199 return new Enumeration<ResourceType>(this, ResourceType.DEVICEDEFINITION, code); 18200 if ("DeviceMetric".equals(codeString)) 18201 return new Enumeration<ResourceType>(this, ResourceType.DEVICEMETRIC, code); 18202 if ("DeviceRequest".equals(codeString)) 18203 return new Enumeration<ResourceType>(this, ResourceType.DEVICEREQUEST, code); 18204 if ("DeviceUseStatement".equals(codeString)) 18205 return new Enumeration<ResourceType>(this, ResourceType.DEVICEUSESTATEMENT, code); 18206 if ("DiagnosticReport".equals(codeString)) 18207 return new Enumeration<ResourceType>(this, ResourceType.DIAGNOSTICREPORT, code); 18208 if ("DocumentManifest".equals(codeString)) 18209 return new Enumeration<ResourceType>(this, ResourceType.DOCUMENTMANIFEST, code); 18210 if ("DocumentReference".equals(codeString)) 18211 return new Enumeration<ResourceType>(this, ResourceType.DOCUMENTREFERENCE, code); 18212 if ("DomainResource".equals(codeString)) 18213 return new Enumeration<ResourceType>(this, ResourceType.DOMAINRESOURCE, code); 18214 if ("EffectEvidenceSynthesis".equals(codeString)) 18215 return new Enumeration<ResourceType>(this, ResourceType.EFFECTEVIDENCESYNTHESIS, code); 18216 if ("Encounter".equals(codeString)) 18217 return new Enumeration<ResourceType>(this, ResourceType.ENCOUNTER, code); 18218 if ("Endpoint".equals(codeString)) 18219 return new Enumeration<ResourceType>(this, ResourceType.ENDPOINT, code); 18220 if ("EnrollmentRequest".equals(codeString)) 18221 return new Enumeration<ResourceType>(this, ResourceType.ENROLLMENTREQUEST, code); 18222 if ("EnrollmentResponse".equals(codeString)) 18223 return new Enumeration<ResourceType>(this, ResourceType.ENROLLMENTRESPONSE, code); 18224 if ("EpisodeOfCare".equals(codeString)) 18225 return new Enumeration<ResourceType>(this, ResourceType.EPISODEOFCARE, code); 18226 if ("EventDefinition".equals(codeString)) 18227 return new Enumeration<ResourceType>(this, ResourceType.EVENTDEFINITION, code); 18228 if ("Evidence".equals(codeString)) 18229 return new Enumeration<ResourceType>(this, ResourceType.EVIDENCE, code); 18230 if ("EvidenceVariable".equals(codeString)) 18231 return new Enumeration<ResourceType>(this, ResourceType.EVIDENCEVARIABLE, code); 18232 if ("ExampleScenario".equals(codeString)) 18233 return new Enumeration<ResourceType>(this, ResourceType.EXAMPLESCENARIO, code); 18234 if ("ExplanationOfBenefit".equals(codeString)) 18235 return new Enumeration<ResourceType>(this, ResourceType.EXPLANATIONOFBENEFIT, code); 18236 if ("FamilyMemberHistory".equals(codeString)) 18237 return new Enumeration<ResourceType>(this, ResourceType.FAMILYMEMBERHISTORY, code); 18238 if ("Flag".equals(codeString)) 18239 return new Enumeration<ResourceType>(this, ResourceType.FLAG, code); 18240 if ("Goal".equals(codeString)) 18241 return new Enumeration<ResourceType>(this, ResourceType.GOAL, code); 18242 if ("GraphDefinition".equals(codeString)) 18243 return new Enumeration<ResourceType>(this, ResourceType.GRAPHDEFINITION, code); 18244 if ("Group".equals(codeString)) 18245 return new Enumeration<ResourceType>(this, ResourceType.GROUP, code); 18246 if ("GuidanceResponse".equals(codeString)) 18247 return new Enumeration<ResourceType>(this, ResourceType.GUIDANCERESPONSE, code); 18248 if ("HealthcareService".equals(codeString)) 18249 return new Enumeration<ResourceType>(this, ResourceType.HEALTHCARESERVICE, code); 18250 if ("ImagingStudy".equals(codeString)) 18251 return new Enumeration<ResourceType>(this, ResourceType.IMAGINGSTUDY, code); 18252 if ("Immunization".equals(codeString)) 18253 return new Enumeration<ResourceType>(this, ResourceType.IMMUNIZATION, code); 18254 if ("ImmunizationEvaluation".equals(codeString)) 18255 return new Enumeration<ResourceType>(this, ResourceType.IMMUNIZATIONEVALUATION, code); 18256 if ("ImmunizationRecommendation".equals(codeString)) 18257 return new Enumeration<ResourceType>(this, ResourceType.IMMUNIZATIONRECOMMENDATION, code); 18258 if ("ImplementationGuide".equals(codeString)) 18259 return new Enumeration<ResourceType>(this, ResourceType.IMPLEMENTATIONGUIDE, code); 18260 if ("InsurancePlan".equals(codeString)) 18261 return new Enumeration<ResourceType>(this, ResourceType.INSURANCEPLAN, code); 18262 if ("Invoice".equals(codeString)) 18263 return new Enumeration<ResourceType>(this, ResourceType.INVOICE, code); 18264 if ("Library".equals(codeString)) 18265 return new Enumeration<ResourceType>(this, ResourceType.LIBRARY, code); 18266 if ("Linkage".equals(codeString)) 18267 return new Enumeration<ResourceType>(this, ResourceType.LINKAGE, code); 18268 if ("List".equals(codeString)) 18269 return new Enumeration<ResourceType>(this, ResourceType.LIST, code); 18270 if ("Location".equals(codeString)) 18271 return new Enumeration<ResourceType>(this, ResourceType.LOCATION, code); 18272 if ("Measure".equals(codeString)) 18273 return new Enumeration<ResourceType>(this, ResourceType.MEASURE, code); 18274 if ("MeasureReport".equals(codeString)) 18275 return new Enumeration<ResourceType>(this, ResourceType.MEASUREREPORT, code); 18276 if ("Media".equals(codeString)) 18277 return new Enumeration<ResourceType>(this, ResourceType.MEDIA, code); 18278 if ("Medication".equals(codeString)) 18279 return new Enumeration<ResourceType>(this, ResourceType.MEDICATION, code); 18280 if ("MedicationAdministration".equals(codeString)) 18281 return new Enumeration<ResourceType>(this, ResourceType.MEDICATIONADMINISTRATION, code); 18282 if ("MedicationDispense".equals(codeString)) 18283 return new Enumeration<ResourceType>(this, ResourceType.MEDICATIONDISPENSE, code); 18284 if ("MedicationKnowledge".equals(codeString)) 18285 return new Enumeration<ResourceType>(this, ResourceType.MEDICATIONKNOWLEDGE, code); 18286 if ("MedicationRequest".equals(codeString)) 18287 return new Enumeration<ResourceType>(this, ResourceType.MEDICATIONREQUEST, code); 18288 if ("MedicationStatement".equals(codeString)) 18289 return new Enumeration<ResourceType>(this, ResourceType.MEDICATIONSTATEMENT, code); 18290 if ("MedicinalProduct".equals(codeString)) 18291 return new Enumeration<ResourceType>(this, ResourceType.MEDICINALPRODUCT, code); 18292 if ("MedicinalProductAuthorization".equals(codeString)) 18293 return new Enumeration<ResourceType>(this, ResourceType.MEDICINALPRODUCTAUTHORIZATION, code); 18294 if ("MedicinalProductContraindication".equals(codeString)) 18295 return new Enumeration<ResourceType>(this, ResourceType.MEDICINALPRODUCTCONTRAINDICATION, code); 18296 if ("MedicinalProductIndication".equals(codeString)) 18297 return new Enumeration<ResourceType>(this, ResourceType.MEDICINALPRODUCTINDICATION, code); 18298 if ("MedicinalProductIngredient".equals(codeString)) 18299 return new Enumeration<ResourceType>(this, ResourceType.MEDICINALPRODUCTINGREDIENT, code); 18300 if ("MedicinalProductInteraction".equals(codeString)) 18301 return new Enumeration<ResourceType>(this, ResourceType.MEDICINALPRODUCTINTERACTION, code); 18302 if ("MedicinalProductManufactured".equals(codeString)) 18303 return new Enumeration<ResourceType>(this, ResourceType.MEDICINALPRODUCTMANUFACTURED, code); 18304 if ("MedicinalProductPackaged".equals(codeString)) 18305 return new Enumeration<ResourceType>(this, ResourceType.MEDICINALPRODUCTPACKAGED, code); 18306 if ("MedicinalProductPharmaceutical".equals(codeString)) 18307 return new Enumeration<ResourceType>(this, ResourceType.MEDICINALPRODUCTPHARMACEUTICAL, code); 18308 if ("MedicinalProductUndesirableEffect".equals(codeString)) 18309 return new Enumeration<ResourceType>(this, ResourceType.MEDICINALPRODUCTUNDESIRABLEEFFECT, code); 18310 if ("MessageDefinition".equals(codeString)) 18311 return new Enumeration<ResourceType>(this, ResourceType.MESSAGEDEFINITION, code); 18312 if ("MessageHeader".equals(codeString)) 18313 return new Enumeration<ResourceType>(this, ResourceType.MESSAGEHEADER, code); 18314 if ("MolecularSequence".equals(codeString)) 18315 return new Enumeration<ResourceType>(this, ResourceType.MOLECULARSEQUENCE, code); 18316 if ("NamingSystem".equals(codeString)) 18317 return new Enumeration<ResourceType>(this, ResourceType.NAMINGSYSTEM, code); 18318 if ("NutritionOrder".equals(codeString)) 18319 return new Enumeration<ResourceType>(this, ResourceType.NUTRITIONORDER, code); 18320 if ("Observation".equals(codeString)) 18321 return new Enumeration<ResourceType>(this, ResourceType.OBSERVATION, code); 18322 if ("ObservationDefinition".equals(codeString)) 18323 return new Enumeration<ResourceType>(this, ResourceType.OBSERVATIONDEFINITION, code); 18324 if ("OperationDefinition".equals(codeString)) 18325 return new Enumeration<ResourceType>(this, ResourceType.OPERATIONDEFINITION, code); 18326 if ("OperationOutcome".equals(codeString)) 18327 return new Enumeration<ResourceType>(this, ResourceType.OPERATIONOUTCOME, code); 18328 if ("Organization".equals(codeString)) 18329 return new Enumeration<ResourceType>(this, ResourceType.ORGANIZATION, code); 18330 if ("OrganizationAffiliation".equals(codeString)) 18331 return new Enumeration<ResourceType>(this, ResourceType.ORGANIZATIONAFFILIATION, code); 18332 if ("Parameters".equals(codeString)) 18333 return new Enumeration<ResourceType>(this, ResourceType.PARAMETERS, code); 18334 if ("Patient".equals(codeString)) 18335 return new Enumeration<ResourceType>(this, ResourceType.PATIENT, code); 18336 if ("PaymentNotice".equals(codeString)) 18337 return new Enumeration<ResourceType>(this, ResourceType.PAYMENTNOTICE, code); 18338 if ("PaymentReconciliation".equals(codeString)) 18339 return new Enumeration<ResourceType>(this, ResourceType.PAYMENTRECONCILIATION, code); 18340 if ("Person".equals(codeString)) 18341 return new Enumeration<ResourceType>(this, ResourceType.PERSON, code); 18342 if ("PlanDefinition".equals(codeString)) 18343 return new Enumeration<ResourceType>(this, ResourceType.PLANDEFINITION, code); 18344 if ("Practitioner".equals(codeString)) 18345 return new Enumeration<ResourceType>(this, ResourceType.PRACTITIONER, code); 18346 if ("PractitionerRole".equals(codeString)) 18347 return new Enumeration<ResourceType>(this, ResourceType.PRACTITIONERROLE, code); 18348 if ("Procedure".equals(codeString)) 18349 return new Enumeration<ResourceType>(this, ResourceType.PROCEDURE, code); 18350 if ("Provenance".equals(codeString)) 18351 return new Enumeration<ResourceType>(this, ResourceType.PROVENANCE, code); 18352 if ("Questionnaire".equals(codeString)) 18353 return new Enumeration<ResourceType>(this, ResourceType.QUESTIONNAIRE, code); 18354 if ("QuestionnaireResponse".equals(codeString)) 18355 return new Enumeration<ResourceType>(this, ResourceType.QUESTIONNAIRERESPONSE, code); 18356 if ("RelatedPerson".equals(codeString)) 18357 return new Enumeration<ResourceType>(this, ResourceType.RELATEDPERSON, code); 18358 if ("RequestGroup".equals(codeString)) 18359 return new Enumeration<ResourceType>(this, ResourceType.REQUESTGROUP, code); 18360 if ("ResearchDefinition".equals(codeString)) 18361 return new Enumeration<ResourceType>(this, ResourceType.RESEARCHDEFINITION, code); 18362 if ("ResearchElementDefinition".equals(codeString)) 18363 return new Enumeration<ResourceType>(this, ResourceType.RESEARCHELEMENTDEFINITION, code); 18364 if ("ResearchStudy".equals(codeString)) 18365 return new Enumeration<ResourceType>(this, ResourceType.RESEARCHSTUDY, code); 18366 if ("ResearchSubject".equals(codeString)) 18367 return new Enumeration<ResourceType>(this, ResourceType.RESEARCHSUBJECT, code); 18368 if ("Resource".equals(codeString)) 18369 return new Enumeration<ResourceType>(this, ResourceType.RESOURCE, code); 18370 if ("RiskAssessment".equals(codeString)) 18371 return new Enumeration<ResourceType>(this, ResourceType.RISKASSESSMENT, code); 18372 if ("RiskEvidenceSynthesis".equals(codeString)) 18373 return new Enumeration<ResourceType>(this, ResourceType.RISKEVIDENCESYNTHESIS, code); 18374 if ("Schedule".equals(codeString)) 18375 return new Enumeration<ResourceType>(this, ResourceType.SCHEDULE, code); 18376 if ("SearchParameter".equals(codeString)) 18377 return new Enumeration<ResourceType>(this, ResourceType.SEARCHPARAMETER, code); 18378 if ("ServiceRequest".equals(codeString)) 18379 return new Enumeration<ResourceType>(this, ResourceType.SERVICEREQUEST, code); 18380 if ("Slot".equals(codeString)) 18381 return new Enumeration<ResourceType>(this, ResourceType.SLOT, code); 18382 if ("Specimen".equals(codeString)) 18383 return new Enumeration<ResourceType>(this, ResourceType.SPECIMEN, code); 18384 if ("SpecimenDefinition".equals(codeString)) 18385 return new Enumeration<ResourceType>(this, ResourceType.SPECIMENDEFINITION, code); 18386 if ("StructureDefinition".equals(codeString)) 18387 return new Enumeration<ResourceType>(this, ResourceType.STRUCTUREDEFINITION, code); 18388 if ("StructureMap".equals(codeString)) 18389 return new Enumeration<ResourceType>(this, ResourceType.STRUCTUREMAP, code); 18390 if ("Subscription".equals(codeString)) 18391 return new Enumeration<ResourceType>(this, ResourceType.SUBSCRIPTION, code); 18392 if ("Substance".equals(codeString)) 18393 return new Enumeration<ResourceType>(this, ResourceType.SUBSTANCE, code); 18394 if ("SubstanceNucleicAcid".equals(codeString)) 18395 return new Enumeration<ResourceType>(this, ResourceType.SUBSTANCENUCLEICACID, code); 18396 if ("SubstancePolymer".equals(codeString)) 18397 return new Enumeration<ResourceType>(this, ResourceType.SUBSTANCEPOLYMER, code); 18398 if ("SubstanceProtein".equals(codeString)) 18399 return new Enumeration<ResourceType>(this, ResourceType.SUBSTANCEPROTEIN, code); 18400 if ("SubstanceReferenceInformation".equals(codeString)) 18401 return new Enumeration<ResourceType>(this, ResourceType.SUBSTANCEREFERENCEINFORMATION, code); 18402 if ("SubstanceSourceMaterial".equals(codeString)) 18403 return new Enumeration<ResourceType>(this, ResourceType.SUBSTANCESOURCEMATERIAL, code); 18404 if ("SubstanceSpecification".equals(codeString)) 18405 return new Enumeration<ResourceType>(this, ResourceType.SUBSTANCESPECIFICATION, code); 18406 if ("SupplyDelivery".equals(codeString)) 18407 return new Enumeration<ResourceType>(this, ResourceType.SUPPLYDELIVERY, code); 18408 if ("SupplyRequest".equals(codeString)) 18409 return new Enumeration<ResourceType>(this, ResourceType.SUPPLYREQUEST, code); 18410 if ("Task".equals(codeString)) 18411 return new Enumeration<ResourceType>(this, ResourceType.TASK, code); 18412 if ("TerminologyCapabilities".equals(codeString)) 18413 return new Enumeration<ResourceType>(this, ResourceType.TERMINOLOGYCAPABILITIES, code); 18414 if ("TestReport".equals(codeString)) 18415 return new Enumeration<ResourceType>(this, ResourceType.TESTREPORT, code); 18416 if ("TestScript".equals(codeString)) 18417 return new Enumeration<ResourceType>(this, ResourceType.TESTSCRIPT, code); 18418 if ("ValueSet".equals(codeString)) 18419 return new Enumeration<ResourceType>(this, ResourceType.VALUESET, code); 18420 if ("VerificationResult".equals(codeString)) 18421 return new Enumeration<ResourceType>(this, ResourceType.VERIFICATIONRESULT, code); 18422 if ("VisionPrescription".equals(codeString)) 18423 return new Enumeration<ResourceType>(this, ResourceType.VISIONPRESCRIPTION, code); 18424 throw new FHIRException("Unknown ResourceType code '" + codeString + "'"); 18425 } 18426 18427 public String toCode(ResourceType code) { 18428 if (code == ResourceType.NULL) 18429 return null; 18430 if (code == ResourceType.ACCOUNT) 18431 return "Account"; 18432 if (code == ResourceType.ACTIVITYDEFINITION) 18433 return "ActivityDefinition"; 18434 if (code == ResourceType.ADVERSEEVENT) 18435 return "AdverseEvent"; 18436 if (code == ResourceType.ALLERGYINTOLERANCE) 18437 return "AllergyIntolerance"; 18438 if (code == ResourceType.APPOINTMENT) 18439 return "Appointment"; 18440 if (code == ResourceType.APPOINTMENTRESPONSE) 18441 return "AppointmentResponse"; 18442 if (code == ResourceType.AUDITEVENT) 18443 return "AuditEvent"; 18444 if (code == ResourceType.BASIC) 18445 return "Basic"; 18446 if (code == ResourceType.BINARY) 18447 return "Binary"; 18448 if (code == ResourceType.BIOLOGICALLYDERIVEDPRODUCT) 18449 return "BiologicallyDerivedProduct"; 18450 if (code == ResourceType.BODYSTRUCTURE) 18451 return "BodyStructure"; 18452 if (code == ResourceType.BUNDLE) 18453 return "Bundle"; 18454 if (code == ResourceType.CAPABILITYSTATEMENT) 18455 return "CapabilityStatement"; 18456 if (code == ResourceType.CAREPLAN) 18457 return "CarePlan"; 18458 if (code == ResourceType.CARETEAM) 18459 return "CareTeam"; 18460 if (code == ResourceType.CATALOGENTRY) 18461 return "CatalogEntry"; 18462 if (code == ResourceType.CHARGEITEM) 18463 return "ChargeItem"; 18464 if (code == ResourceType.CHARGEITEMDEFINITION) 18465 return "ChargeItemDefinition"; 18466 if (code == ResourceType.CLAIM) 18467 return "Claim"; 18468 if (code == ResourceType.CLAIMRESPONSE) 18469 return "ClaimResponse"; 18470 if (code == ResourceType.CLINICALIMPRESSION) 18471 return "ClinicalImpression"; 18472 if (code == ResourceType.CODESYSTEM) 18473 return "CodeSystem"; 18474 if (code == ResourceType.COMMUNICATION) 18475 return "Communication"; 18476 if (code == ResourceType.COMMUNICATIONREQUEST) 18477 return "CommunicationRequest"; 18478 if (code == ResourceType.COMPARTMENTDEFINITION) 18479 return "CompartmentDefinition"; 18480 if (code == ResourceType.COMPOSITION) 18481 return "Composition"; 18482 if (code == ResourceType.CONCEPTMAP) 18483 return "ConceptMap"; 18484 if (code == ResourceType.CONDITION) 18485 return "Condition"; 18486 if (code == ResourceType.CONSENT) 18487 return "Consent"; 18488 if (code == ResourceType.CONTRACT) 18489 return "Contract"; 18490 if (code == ResourceType.COVERAGE) 18491 return "Coverage"; 18492 if (code == ResourceType.COVERAGEELIGIBILITYREQUEST) 18493 return "CoverageEligibilityRequest"; 18494 if (code == ResourceType.COVERAGEELIGIBILITYRESPONSE) 18495 return "CoverageEligibilityResponse"; 18496 if (code == ResourceType.DETECTEDISSUE) 18497 return "DetectedIssue"; 18498 if (code == ResourceType.DEVICE) 18499 return "Device"; 18500 if (code == ResourceType.DEVICEDEFINITION) 18501 return "DeviceDefinition"; 18502 if (code == ResourceType.DEVICEMETRIC) 18503 return "DeviceMetric"; 18504 if (code == ResourceType.DEVICEREQUEST) 18505 return "DeviceRequest"; 18506 if (code == ResourceType.DEVICEUSESTATEMENT) 18507 return "DeviceUseStatement"; 18508 if (code == ResourceType.DIAGNOSTICREPORT) 18509 return "DiagnosticReport"; 18510 if (code == ResourceType.DOCUMENTMANIFEST) 18511 return "DocumentManifest"; 18512 if (code == ResourceType.DOCUMENTREFERENCE) 18513 return "DocumentReference"; 18514 if (code == ResourceType.DOMAINRESOURCE) 18515 return "DomainResource"; 18516 if (code == ResourceType.EFFECTEVIDENCESYNTHESIS) 18517 return "EffectEvidenceSynthesis"; 18518 if (code == ResourceType.ENCOUNTER) 18519 return "Encounter"; 18520 if (code == ResourceType.ENDPOINT) 18521 return "Endpoint"; 18522 if (code == ResourceType.ENROLLMENTREQUEST) 18523 return "EnrollmentRequest"; 18524 if (code == ResourceType.ENROLLMENTRESPONSE) 18525 return "EnrollmentResponse"; 18526 if (code == ResourceType.EPISODEOFCARE) 18527 return "EpisodeOfCare"; 18528 if (code == ResourceType.EVENTDEFINITION) 18529 return "EventDefinition"; 18530 if (code == ResourceType.EVIDENCE) 18531 return "Evidence"; 18532 if (code == ResourceType.EVIDENCEVARIABLE) 18533 return "EvidenceVariable"; 18534 if (code == ResourceType.EXAMPLESCENARIO) 18535 return "ExampleScenario"; 18536 if (code == ResourceType.EXPLANATIONOFBENEFIT) 18537 return "ExplanationOfBenefit"; 18538 if (code == ResourceType.FAMILYMEMBERHISTORY) 18539 return "FamilyMemberHistory"; 18540 if (code == ResourceType.FLAG) 18541 return "Flag"; 18542 if (code == ResourceType.GOAL) 18543 return "Goal"; 18544 if (code == ResourceType.GRAPHDEFINITION) 18545 return "GraphDefinition"; 18546 if (code == ResourceType.GROUP) 18547 return "Group"; 18548 if (code == ResourceType.GUIDANCERESPONSE) 18549 return "GuidanceResponse"; 18550 if (code == ResourceType.HEALTHCARESERVICE) 18551 return "HealthcareService"; 18552 if (code == ResourceType.IMAGINGSTUDY) 18553 return "ImagingStudy"; 18554 if (code == ResourceType.IMMUNIZATION) 18555 return "Immunization"; 18556 if (code == ResourceType.IMMUNIZATIONEVALUATION) 18557 return "ImmunizationEvaluation"; 18558 if (code == ResourceType.IMMUNIZATIONRECOMMENDATION) 18559 return "ImmunizationRecommendation"; 18560 if (code == ResourceType.IMPLEMENTATIONGUIDE) 18561 return "ImplementationGuide"; 18562 if (code == ResourceType.INSURANCEPLAN) 18563 return "InsurancePlan"; 18564 if (code == ResourceType.INVOICE) 18565 return "Invoice"; 18566 if (code == ResourceType.LIBRARY) 18567 return "Library"; 18568 if (code == ResourceType.LINKAGE) 18569 return "Linkage"; 18570 if (code == ResourceType.LIST) 18571 return "List"; 18572 if (code == ResourceType.LOCATION) 18573 return "Location"; 18574 if (code == ResourceType.MEASURE) 18575 return "Measure"; 18576 if (code == ResourceType.MEASUREREPORT) 18577 return "MeasureReport"; 18578 if (code == ResourceType.MEDIA) 18579 return "Media"; 18580 if (code == ResourceType.MEDICATION) 18581 return "Medication"; 18582 if (code == ResourceType.MEDICATIONADMINISTRATION) 18583 return "MedicationAdministration"; 18584 if (code == ResourceType.MEDICATIONDISPENSE) 18585 return "MedicationDispense"; 18586 if (code == ResourceType.MEDICATIONKNOWLEDGE) 18587 return "MedicationKnowledge"; 18588 if (code == ResourceType.MEDICATIONREQUEST) 18589 return "MedicationRequest"; 18590 if (code == ResourceType.MEDICATIONSTATEMENT) 18591 return "MedicationStatement"; 18592 if (code == ResourceType.MEDICINALPRODUCT) 18593 return "MedicinalProduct"; 18594 if (code == ResourceType.MEDICINALPRODUCTAUTHORIZATION) 18595 return "MedicinalProductAuthorization"; 18596 if (code == ResourceType.MEDICINALPRODUCTCONTRAINDICATION) 18597 return "MedicinalProductContraindication"; 18598 if (code == ResourceType.MEDICINALPRODUCTINDICATION) 18599 return "MedicinalProductIndication"; 18600 if (code == ResourceType.MEDICINALPRODUCTINGREDIENT) 18601 return "MedicinalProductIngredient"; 18602 if (code == ResourceType.MEDICINALPRODUCTINTERACTION) 18603 return "MedicinalProductInteraction"; 18604 if (code == ResourceType.MEDICINALPRODUCTMANUFACTURED) 18605 return "MedicinalProductManufactured"; 18606 if (code == ResourceType.MEDICINALPRODUCTPACKAGED) 18607 return "MedicinalProductPackaged"; 18608 if (code == ResourceType.MEDICINALPRODUCTPHARMACEUTICAL) 18609 return "MedicinalProductPharmaceutical"; 18610 if (code == ResourceType.MEDICINALPRODUCTUNDESIRABLEEFFECT) 18611 return "MedicinalProductUndesirableEffect"; 18612 if (code == ResourceType.MESSAGEDEFINITION) 18613 return "MessageDefinition"; 18614 if (code == ResourceType.MESSAGEHEADER) 18615 return "MessageHeader"; 18616 if (code == ResourceType.MOLECULARSEQUENCE) 18617 return "MolecularSequence"; 18618 if (code == ResourceType.NAMINGSYSTEM) 18619 return "NamingSystem"; 18620 if (code == ResourceType.NUTRITIONORDER) 18621 return "NutritionOrder"; 18622 if (code == ResourceType.OBSERVATION) 18623 return "Observation"; 18624 if (code == ResourceType.OBSERVATIONDEFINITION) 18625 return "ObservationDefinition"; 18626 if (code == ResourceType.OPERATIONDEFINITION) 18627 return "OperationDefinition"; 18628 if (code == ResourceType.OPERATIONOUTCOME) 18629 return "OperationOutcome"; 18630 if (code == ResourceType.ORGANIZATION) 18631 return "Organization"; 18632 if (code == ResourceType.ORGANIZATIONAFFILIATION) 18633 return "OrganizationAffiliation"; 18634 if (code == ResourceType.PARAMETERS) 18635 return "Parameters"; 18636 if (code == ResourceType.PATIENT) 18637 return "Patient"; 18638 if (code == ResourceType.PAYMENTNOTICE) 18639 return "PaymentNotice"; 18640 if (code == ResourceType.PAYMENTRECONCILIATION) 18641 return "PaymentReconciliation"; 18642 if (code == ResourceType.PERSON) 18643 return "Person"; 18644 if (code == ResourceType.PLANDEFINITION) 18645 return "PlanDefinition"; 18646 if (code == ResourceType.PRACTITIONER) 18647 return "Practitioner"; 18648 if (code == ResourceType.PRACTITIONERROLE) 18649 return "PractitionerRole"; 18650 if (code == ResourceType.PROCEDURE) 18651 return "Procedure"; 18652 if (code == ResourceType.PROVENANCE) 18653 return "Provenance"; 18654 if (code == ResourceType.QUESTIONNAIRE) 18655 return "Questionnaire"; 18656 if (code == ResourceType.QUESTIONNAIRERESPONSE) 18657 return "QuestionnaireResponse"; 18658 if (code == ResourceType.RELATEDPERSON) 18659 return "RelatedPerson"; 18660 if (code == ResourceType.REQUESTGROUP) 18661 return "RequestGroup"; 18662 if (code == ResourceType.RESEARCHDEFINITION) 18663 return "ResearchDefinition"; 18664 if (code == ResourceType.RESEARCHELEMENTDEFINITION) 18665 return "ResearchElementDefinition"; 18666 if (code == ResourceType.RESEARCHSTUDY) 18667 return "ResearchStudy"; 18668 if (code == ResourceType.RESEARCHSUBJECT) 18669 return "ResearchSubject"; 18670 if (code == ResourceType.RESOURCE) 18671 return "Resource"; 18672 if (code == ResourceType.RISKASSESSMENT) 18673 return "RiskAssessment"; 18674 if (code == ResourceType.RISKEVIDENCESYNTHESIS) 18675 return "RiskEvidenceSynthesis"; 18676 if (code == ResourceType.SCHEDULE) 18677 return "Schedule"; 18678 if (code == ResourceType.SEARCHPARAMETER) 18679 return "SearchParameter"; 18680 if (code == ResourceType.SERVICEREQUEST) 18681 return "ServiceRequest"; 18682 if (code == ResourceType.SLOT) 18683 return "Slot"; 18684 if (code == ResourceType.SPECIMEN) 18685 return "Specimen"; 18686 if (code == ResourceType.SPECIMENDEFINITION) 18687 return "SpecimenDefinition"; 18688 if (code == ResourceType.STRUCTUREDEFINITION) 18689 return "StructureDefinition"; 18690 if (code == ResourceType.STRUCTUREMAP) 18691 return "StructureMap"; 18692 if (code == ResourceType.SUBSCRIPTION) 18693 return "Subscription"; 18694 if (code == ResourceType.SUBSTANCE) 18695 return "Substance"; 18696 if (code == ResourceType.SUBSTANCENUCLEICACID) 18697 return "SubstanceNucleicAcid"; 18698 if (code == ResourceType.SUBSTANCEPOLYMER) 18699 return "SubstancePolymer"; 18700 if (code == ResourceType.SUBSTANCEPROTEIN) 18701 return "SubstanceProtein"; 18702 if (code == ResourceType.SUBSTANCEREFERENCEINFORMATION) 18703 return "SubstanceReferenceInformation"; 18704 if (code == ResourceType.SUBSTANCESOURCEMATERIAL) 18705 return "SubstanceSourceMaterial"; 18706 if (code == ResourceType.SUBSTANCESPECIFICATION) 18707 return "SubstanceSpecification"; 18708 if (code == ResourceType.SUPPLYDELIVERY) 18709 return "SupplyDelivery"; 18710 if (code == ResourceType.SUPPLYREQUEST) 18711 return "SupplyRequest"; 18712 if (code == ResourceType.TASK) 18713 return "Task"; 18714 if (code == ResourceType.TERMINOLOGYCAPABILITIES) 18715 return "TerminologyCapabilities"; 18716 if (code == ResourceType.TESTREPORT) 18717 return "TestReport"; 18718 if (code == ResourceType.TESTSCRIPT) 18719 return "TestScript"; 18720 if (code == ResourceType.VALUESET) 18721 return "ValueSet"; 18722 if (code == ResourceType.VERIFICATIONRESULT) 18723 return "VerificationResult"; 18724 if (code == ResourceType.VISIONPRESCRIPTION) 18725 return "VisionPrescription"; 18726 return "?"; 18727 } 18728 18729 public String toSystem(ResourceType code) { 18730 return code.getSystem(); 18731 } 18732 } 18733 18734 public enum SearchParamType { 18735 /** 18736 * Search parameter SHALL be a number (a whole number, or a decimal). 18737 */ 18738 NUMBER, 18739 /** 18740 * Search parameter is on a date/time. The date format is the standard XML 18741 * format, though other formats may be supported. 18742 */ 18743 DATE, 18744 /** 18745 * Search parameter is a simple string, like a name part. Search is 18746 * case-insensitive and accent-insensitive. May match just the start of a 18747 * string. String parameters may contain spaces. 18748 */ 18749 STRING, 18750 /** 18751 * Search parameter on a coded element or identifier. May be used to search 18752 * through the text, display, code and code/codesystem (for codes) and label, 18753 * system and key (for identifier). Its value is either a string or a pair of 18754 * namespace and value, separated by a "|", depending on the modifier used. 18755 */ 18756 TOKEN, 18757 /** 18758 * A reference to another resource (Reference or canonical). 18759 */ 18760 REFERENCE, 18761 /** 18762 * A composite search parameter that combines a search on two values together. 18763 */ 18764 COMPOSITE, 18765 /** 18766 * A search parameter that searches on a quantity. 18767 */ 18768 QUANTITY, 18769 /** 18770 * A search parameter that searches on a URI (RFC 3986). 18771 */ 18772 URI, 18773 /** 18774 * Special logic applies to this parameter per the description of the search 18775 * parameter. 18776 */ 18777 SPECIAL, 18778 /** 18779 * added to help the parsers 18780 */ 18781 NULL; 18782 18783 public static SearchParamType fromCode(String codeString) throws FHIRException { 18784 if (codeString == null || "".equals(codeString)) 18785 return null; 18786 if ("number".equals(codeString)) 18787 return NUMBER; 18788 if ("date".equals(codeString)) 18789 return DATE; 18790 if ("string".equals(codeString)) 18791 return STRING; 18792 if ("token".equals(codeString)) 18793 return TOKEN; 18794 if ("reference".equals(codeString)) 18795 return REFERENCE; 18796 if ("composite".equals(codeString)) 18797 return COMPOSITE; 18798 if ("quantity".equals(codeString)) 18799 return QUANTITY; 18800 if ("uri".equals(codeString)) 18801 return URI; 18802 if ("special".equals(codeString)) 18803 return SPECIAL; 18804 throw new FHIRException("Unknown SearchParamType code '" + codeString + "'"); 18805 } 18806 18807 public String toCode() { 18808 switch (this) { 18809 case NUMBER: 18810 return "number"; 18811 case DATE: 18812 return "date"; 18813 case STRING: 18814 return "string"; 18815 case TOKEN: 18816 return "token"; 18817 case REFERENCE: 18818 return "reference"; 18819 case COMPOSITE: 18820 return "composite"; 18821 case QUANTITY: 18822 return "quantity"; 18823 case URI: 18824 return "uri"; 18825 case SPECIAL: 18826 return "special"; 18827 case NULL: 18828 return null; 18829 default: 18830 return "?"; 18831 } 18832 } 18833 18834 public String getSystem() { 18835 switch (this) { 18836 case NUMBER: 18837 return "http://hl7.org/fhir/search-param-type"; 18838 case DATE: 18839 return "http://hl7.org/fhir/search-param-type"; 18840 case STRING: 18841 return "http://hl7.org/fhir/search-param-type"; 18842 case TOKEN: 18843 return "http://hl7.org/fhir/search-param-type"; 18844 case REFERENCE: 18845 return "http://hl7.org/fhir/search-param-type"; 18846 case COMPOSITE: 18847 return "http://hl7.org/fhir/search-param-type"; 18848 case QUANTITY: 18849 return "http://hl7.org/fhir/search-param-type"; 18850 case URI: 18851 return "http://hl7.org/fhir/search-param-type"; 18852 case SPECIAL: 18853 return "http://hl7.org/fhir/search-param-type"; 18854 case NULL: 18855 return null; 18856 default: 18857 return "?"; 18858 } 18859 } 18860 18861 public String getDefinition() { 18862 switch (this) { 18863 case NUMBER: 18864 return "Search parameter SHALL be a number (a whole number, or a decimal)."; 18865 case DATE: 18866 return "Search parameter is on a date/time. The date format is the standard XML format, though other formats may be supported."; 18867 case STRING: 18868 return "Search parameter is a simple string, like a name part. Search is case-insensitive and accent-insensitive. May match just the start of a string. String parameters may contain spaces."; 18869 case TOKEN: 18870 return "Search parameter on a coded element or identifier. May be used to search through the text, display, code and code/codesystem (for codes) and label, system and key (for identifier). Its value is either a string or a pair of namespace and value, separated by a \"|\", depending on the modifier used."; 18871 case REFERENCE: 18872 return "A reference to another resource (Reference or canonical)."; 18873 case COMPOSITE: 18874 return "A composite search parameter that combines a search on two values together."; 18875 case QUANTITY: 18876 return "A search parameter that searches on a quantity."; 18877 case URI: 18878 return "A search parameter that searches on a URI (RFC 3986)."; 18879 case SPECIAL: 18880 return "Special logic applies to this parameter per the description of the search parameter."; 18881 case NULL: 18882 return null; 18883 default: 18884 return "?"; 18885 } 18886 } 18887 18888 public String getDisplay() { 18889 switch (this) { 18890 case NUMBER: 18891 return "Number"; 18892 case DATE: 18893 return "Date/DateTime"; 18894 case STRING: 18895 return "String"; 18896 case TOKEN: 18897 return "Token"; 18898 case REFERENCE: 18899 return "Reference"; 18900 case COMPOSITE: 18901 return "Composite"; 18902 case QUANTITY: 18903 return "Quantity"; 18904 case URI: 18905 return "URI"; 18906 case SPECIAL: 18907 return "Special"; 18908 case NULL: 18909 return null; 18910 default: 18911 return "?"; 18912 } 18913 } 18914 } 18915 18916 public static class SearchParamTypeEnumFactory implements EnumFactory<SearchParamType> { 18917 public SearchParamType fromCode(String codeString) throws IllegalArgumentException { 18918 if (codeString == null || "".equals(codeString)) 18919 if (codeString == null || "".equals(codeString)) 18920 return null; 18921 if ("number".equals(codeString)) 18922 return SearchParamType.NUMBER; 18923 if ("date".equals(codeString)) 18924 return SearchParamType.DATE; 18925 if ("string".equals(codeString)) 18926 return SearchParamType.STRING; 18927 if ("token".equals(codeString)) 18928 return SearchParamType.TOKEN; 18929 if ("reference".equals(codeString)) 18930 return SearchParamType.REFERENCE; 18931 if ("composite".equals(codeString)) 18932 return SearchParamType.COMPOSITE; 18933 if ("quantity".equals(codeString)) 18934 return SearchParamType.QUANTITY; 18935 if ("uri".equals(codeString)) 18936 return SearchParamType.URI; 18937 if ("special".equals(codeString)) 18938 return SearchParamType.SPECIAL; 18939 throw new IllegalArgumentException("Unknown SearchParamType code '" + codeString + "'"); 18940 } 18941 18942 public Enumeration<SearchParamType> fromType(PrimitiveType<?> code) throws FHIRException { 18943 if (code == null) 18944 return null; 18945 if (code.isEmpty()) 18946 return new Enumeration<SearchParamType>(this, SearchParamType.NULL, code); 18947 String codeString = code.asStringValue(); 18948 if (codeString == null || "".equals(codeString)) 18949 return new Enumeration<SearchParamType>(this, SearchParamType.NULL, code); 18950 if ("number".equals(codeString)) 18951 return new Enumeration<SearchParamType>(this, SearchParamType.NUMBER, code); 18952 if ("date".equals(codeString)) 18953 return new Enumeration<SearchParamType>(this, SearchParamType.DATE, code); 18954 if ("string".equals(codeString)) 18955 return new Enumeration<SearchParamType>(this, SearchParamType.STRING, code); 18956 if ("token".equals(codeString)) 18957 return new Enumeration<SearchParamType>(this, SearchParamType.TOKEN, code); 18958 if ("reference".equals(codeString)) 18959 return new Enumeration<SearchParamType>(this, SearchParamType.REFERENCE, code); 18960 if ("composite".equals(codeString)) 18961 return new Enumeration<SearchParamType>(this, SearchParamType.COMPOSITE, code); 18962 if ("quantity".equals(codeString)) 18963 return new Enumeration<SearchParamType>(this, SearchParamType.QUANTITY, code); 18964 if ("uri".equals(codeString)) 18965 return new Enumeration<SearchParamType>(this, SearchParamType.URI, code); 18966 if ("special".equals(codeString)) 18967 return new Enumeration<SearchParamType>(this, SearchParamType.SPECIAL, code); 18968 throw new FHIRException("Unknown SearchParamType code '" + codeString + "'"); 18969 } 18970 18971 public String toCode(SearchParamType code) { 18972 if (code == SearchParamType.NULL) 18973 return null; 18974 if (code == SearchParamType.NUMBER) 18975 return "number"; 18976 if (code == SearchParamType.DATE) 18977 return "date"; 18978 if (code == SearchParamType.STRING) 18979 return "string"; 18980 if (code == SearchParamType.TOKEN) 18981 return "token"; 18982 if (code == SearchParamType.REFERENCE) 18983 return "reference"; 18984 if (code == SearchParamType.COMPOSITE) 18985 return "composite"; 18986 if (code == SearchParamType.QUANTITY) 18987 return "quantity"; 18988 if (code == SearchParamType.URI) 18989 return "uri"; 18990 if (code == SearchParamType.SPECIAL) 18991 return "special"; 18992 return "?"; 18993 } 18994 18995 public String toSystem(SearchParamType code) { 18996 return code.getSystem(); 18997 } 18998 } 18999 19000 public enum SpecialValues { 19001 /** 19002 * Boolean true. 19003 */ 19004 TRUE, 19005 /** 19006 * Boolean false. 19007 */ 19008 FALSE, 19009 /** 19010 * The content is greater than zero, but too small to be quantified. 19011 */ 19012 TRACE, 19013 /** 19014 * The specific quantity is not known, but is known to be non-zero and is not 19015 * specified because it makes up the bulk of the material. 19016 */ 19017 SUFFICIENT, 19018 /** 19019 * The value is no longer available. 19020 */ 19021 WITHDRAWN, 19022 /** 19023 * The are no known applicable values in this context. 19024 */ 19025 NILKNOWN, 19026 /** 19027 * added to help the parsers 19028 */ 19029 NULL; 19030 19031 public static SpecialValues fromCode(String codeString) throws FHIRException { 19032 if (codeString == null || "".equals(codeString)) 19033 return null; 19034 if ("true".equals(codeString)) 19035 return TRUE; 19036 if ("false".equals(codeString)) 19037 return FALSE; 19038 if ("trace".equals(codeString)) 19039 return TRACE; 19040 if ("sufficient".equals(codeString)) 19041 return SUFFICIENT; 19042 if ("withdrawn".equals(codeString)) 19043 return WITHDRAWN; 19044 if ("nil-known".equals(codeString)) 19045 return NILKNOWN; 19046 throw new FHIRException("Unknown SpecialValues code '" + codeString + "'"); 19047 } 19048 19049 public String toCode() { 19050 switch (this) { 19051 case TRUE: 19052 return "true"; 19053 case FALSE: 19054 return "false"; 19055 case TRACE: 19056 return "trace"; 19057 case SUFFICIENT: 19058 return "sufficient"; 19059 case WITHDRAWN: 19060 return "withdrawn"; 19061 case NILKNOWN: 19062 return "nil-known"; 19063 case NULL: 19064 return null; 19065 default: 19066 return "?"; 19067 } 19068 } 19069 19070 public String getSystem() { 19071 switch (this) { 19072 case TRUE: 19073 return "http://terminology.hl7.org/CodeSystem/special-values"; 19074 case FALSE: 19075 return "http://terminology.hl7.org/CodeSystem/special-values"; 19076 case TRACE: 19077 return "http://terminology.hl7.org/CodeSystem/special-values"; 19078 case SUFFICIENT: 19079 return "http://terminology.hl7.org/CodeSystem/special-values"; 19080 case WITHDRAWN: 19081 return "http://terminology.hl7.org/CodeSystem/special-values"; 19082 case NILKNOWN: 19083 return "http://terminology.hl7.org/CodeSystem/special-values"; 19084 case NULL: 19085 return null; 19086 default: 19087 return "?"; 19088 } 19089 } 19090 19091 public String getDefinition() { 19092 switch (this) { 19093 case TRUE: 19094 return "Boolean true."; 19095 case FALSE: 19096 return "Boolean false."; 19097 case TRACE: 19098 return "The content is greater than zero, but too small to be quantified."; 19099 case SUFFICIENT: 19100 return "The specific quantity is not known, but is known to be non-zero and is not specified because it makes up the bulk of the material."; 19101 case WITHDRAWN: 19102 return "The value is no longer available."; 19103 case NILKNOWN: 19104 return "The are no known applicable values in this context."; 19105 case NULL: 19106 return null; 19107 default: 19108 return "?"; 19109 } 19110 } 19111 19112 public String getDisplay() { 19113 switch (this) { 19114 case TRUE: 19115 return "true"; 19116 case FALSE: 19117 return "false"; 19118 case TRACE: 19119 return "Trace Amount Detected"; 19120 case SUFFICIENT: 19121 return "Sufficient Quantity"; 19122 case WITHDRAWN: 19123 return "Value Withdrawn"; 19124 case NILKNOWN: 19125 return "Nil Known"; 19126 case NULL: 19127 return null; 19128 default: 19129 return "?"; 19130 } 19131 } 19132 } 19133 19134 public static class SpecialValuesEnumFactory implements EnumFactory<SpecialValues> { 19135 public SpecialValues fromCode(String codeString) throws IllegalArgumentException { 19136 if (codeString == null || "".equals(codeString)) 19137 if (codeString == null || "".equals(codeString)) 19138 return null; 19139 if ("true".equals(codeString)) 19140 return SpecialValues.TRUE; 19141 if ("false".equals(codeString)) 19142 return SpecialValues.FALSE; 19143 if ("trace".equals(codeString)) 19144 return SpecialValues.TRACE; 19145 if ("sufficient".equals(codeString)) 19146 return SpecialValues.SUFFICIENT; 19147 if ("withdrawn".equals(codeString)) 19148 return SpecialValues.WITHDRAWN; 19149 if ("nil-known".equals(codeString)) 19150 return SpecialValues.NILKNOWN; 19151 throw new IllegalArgumentException("Unknown SpecialValues code '" + codeString + "'"); 19152 } 19153 19154 public Enumeration<SpecialValues> fromType(PrimitiveType<?> code) throws FHIRException { 19155 if (code == null) 19156 return null; 19157 if (code.isEmpty()) 19158 return new Enumeration<SpecialValues>(this, SpecialValues.NULL, code); 19159 String codeString = code.asStringValue(); 19160 if (codeString == null || "".equals(codeString)) 19161 return new Enumeration<SpecialValues>(this, SpecialValues.NULL, code); 19162 if ("true".equals(codeString)) 19163 return new Enumeration<SpecialValues>(this, SpecialValues.TRUE, code); 19164 if ("false".equals(codeString)) 19165 return new Enumeration<SpecialValues>(this, SpecialValues.FALSE, code); 19166 if ("trace".equals(codeString)) 19167 return new Enumeration<SpecialValues>(this, SpecialValues.TRACE, code); 19168 if ("sufficient".equals(codeString)) 19169 return new Enumeration<SpecialValues>(this, SpecialValues.SUFFICIENT, code); 19170 if ("withdrawn".equals(codeString)) 19171 return new Enumeration<SpecialValues>(this, SpecialValues.WITHDRAWN, code); 19172 if ("nil-known".equals(codeString)) 19173 return new Enumeration<SpecialValues>(this, SpecialValues.NILKNOWN, code); 19174 throw new FHIRException("Unknown SpecialValues code '" + codeString + "'"); 19175 } 19176 19177 public String toCode(SpecialValues code) { 19178 if (code == SpecialValues.NULL) 19179 return null; 19180 if (code == SpecialValues.TRUE) 19181 return "true"; 19182 if (code == SpecialValues.FALSE) 19183 return "false"; 19184 if (code == SpecialValues.TRACE) 19185 return "trace"; 19186 if (code == SpecialValues.SUFFICIENT) 19187 return "sufficient"; 19188 if (code == SpecialValues.WITHDRAWN) 19189 return "withdrawn"; 19190 if (code == SpecialValues.NILKNOWN) 19191 return "nil-known"; 19192 return "?"; 19193 } 19194 19195 public String toSystem(SpecialValues code) { 19196 return code.getSystem(); 19197 } 19198 } 19199 19200}