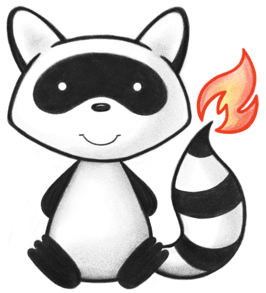
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044 045/** 046 * An association between a patient and an organization / healthcare provider(s) 047 * during which time encounters may occur. The managing organization assumes a 048 * level of responsibility for the patient during this time. 049 */ 050@ResourceDef(name = "EpisodeOfCare", profile = "http://hl7.org/fhir/StructureDefinition/EpisodeOfCare") 051public class EpisodeOfCare extends DomainResource { 052 053 public enum EpisodeOfCareStatus { 054 /** 055 * This episode of care is planned to start at the date specified in the 056 * period.start. During this status, an organization may perform assessments to 057 * determine if the patient is eligible to receive services, or be organizing to 058 * make resources available to provide care services. 059 */ 060 PLANNED, 061 /** 062 * This episode has been placed on a waitlist, pending the episode being made 063 * active (or cancelled). 064 */ 065 WAITLIST, 066 /** 067 * This episode of care is current. 068 */ 069 ACTIVE, 070 /** 071 * This episode of care is on hold; the organization has limited responsibility 072 * for the patient (such as while on respite). 073 */ 074 ONHOLD, 075 /** 076 * This episode of care is finished and the organization is not expecting to be 077 * providing further care to the patient. Can also be known as "closed", 078 * "completed" or other similar terms. 079 */ 080 FINISHED, 081 /** 082 * The episode of care was cancelled, or withdrawn from service, often selected 083 * during the planned stage as the patient may have gone elsewhere, or the 084 * circumstances have changed and the organization is unable to provide the 085 * care. It indicates that services terminated outside the planned/expected 086 * workflow. 087 */ 088 CANCELLED, 089 /** 090 * This instance should not have been part of this patient's medical record. 091 */ 092 ENTEREDINERROR, 093 /** 094 * added to help the parsers with the generic types 095 */ 096 NULL; 097 098 public static EpisodeOfCareStatus fromCode(String codeString) throws FHIRException { 099 if (codeString == null || "".equals(codeString)) 100 return null; 101 if ("planned".equals(codeString)) 102 return PLANNED; 103 if ("waitlist".equals(codeString)) 104 return WAITLIST; 105 if ("active".equals(codeString)) 106 return ACTIVE; 107 if ("onhold".equals(codeString)) 108 return ONHOLD; 109 if ("finished".equals(codeString)) 110 return FINISHED; 111 if ("cancelled".equals(codeString)) 112 return CANCELLED; 113 if ("entered-in-error".equals(codeString)) 114 return ENTEREDINERROR; 115 if (Configuration.isAcceptInvalidEnums()) 116 return null; 117 else 118 throw new FHIRException("Unknown EpisodeOfCareStatus code '" + codeString + "'"); 119 } 120 121 public String toCode() { 122 switch (this) { 123 case PLANNED: 124 return "planned"; 125 case WAITLIST: 126 return "waitlist"; 127 case ACTIVE: 128 return "active"; 129 case ONHOLD: 130 return "onhold"; 131 case FINISHED: 132 return "finished"; 133 case CANCELLED: 134 return "cancelled"; 135 case ENTEREDINERROR: 136 return "entered-in-error"; 137 case NULL: 138 return null; 139 default: 140 return "?"; 141 } 142 } 143 144 public String getSystem() { 145 switch (this) { 146 case PLANNED: 147 return "http://hl7.org/fhir/episode-of-care-status"; 148 case WAITLIST: 149 return "http://hl7.org/fhir/episode-of-care-status"; 150 case ACTIVE: 151 return "http://hl7.org/fhir/episode-of-care-status"; 152 case ONHOLD: 153 return "http://hl7.org/fhir/episode-of-care-status"; 154 case FINISHED: 155 return "http://hl7.org/fhir/episode-of-care-status"; 156 case CANCELLED: 157 return "http://hl7.org/fhir/episode-of-care-status"; 158 case ENTEREDINERROR: 159 return "http://hl7.org/fhir/episode-of-care-status"; 160 case NULL: 161 return null; 162 default: 163 return "?"; 164 } 165 } 166 167 public String getDefinition() { 168 switch (this) { 169 case PLANNED: 170 return "This episode of care is planned to start at the date specified in the period.start. During this status, an organization may perform assessments to determine if the patient is eligible to receive services, or be organizing to make resources available to provide care services."; 171 case WAITLIST: 172 return "This episode has been placed on a waitlist, pending the episode being made active (or cancelled)."; 173 case ACTIVE: 174 return "This episode of care is current."; 175 case ONHOLD: 176 return "This episode of care is on hold; the organization has limited responsibility for the patient (such as while on respite)."; 177 case FINISHED: 178 return "This episode of care is finished and the organization is not expecting to be providing further care to the patient. Can also be known as \"closed\", \"completed\" or other similar terms."; 179 case CANCELLED: 180 return "The episode of care was cancelled, or withdrawn from service, often selected during the planned stage as the patient may have gone elsewhere, or the circumstances have changed and the organization is unable to provide the care. It indicates that services terminated outside the planned/expected workflow."; 181 case ENTEREDINERROR: 182 return "This instance should not have been part of this patient's medical record."; 183 case NULL: 184 return null; 185 default: 186 return "?"; 187 } 188 } 189 190 public String getDisplay() { 191 switch (this) { 192 case PLANNED: 193 return "Planned"; 194 case WAITLIST: 195 return "Waitlist"; 196 case ACTIVE: 197 return "Active"; 198 case ONHOLD: 199 return "On Hold"; 200 case FINISHED: 201 return "Finished"; 202 case CANCELLED: 203 return "Cancelled"; 204 case ENTEREDINERROR: 205 return "Entered in Error"; 206 case NULL: 207 return null; 208 default: 209 return "?"; 210 } 211 } 212 } 213 214 public static class EpisodeOfCareStatusEnumFactory implements EnumFactory<EpisodeOfCareStatus> { 215 public EpisodeOfCareStatus fromCode(String codeString) throws IllegalArgumentException { 216 if (codeString == null || "".equals(codeString)) 217 if (codeString == null || "".equals(codeString)) 218 return null; 219 if ("planned".equals(codeString)) 220 return EpisodeOfCareStatus.PLANNED; 221 if ("waitlist".equals(codeString)) 222 return EpisodeOfCareStatus.WAITLIST; 223 if ("active".equals(codeString)) 224 return EpisodeOfCareStatus.ACTIVE; 225 if ("onhold".equals(codeString)) 226 return EpisodeOfCareStatus.ONHOLD; 227 if ("finished".equals(codeString)) 228 return EpisodeOfCareStatus.FINISHED; 229 if ("cancelled".equals(codeString)) 230 return EpisodeOfCareStatus.CANCELLED; 231 if ("entered-in-error".equals(codeString)) 232 return EpisodeOfCareStatus.ENTEREDINERROR; 233 throw new IllegalArgumentException("Unknown EpisodeOfCareStatus code '" + codeString + "'"); 234 } 235 236 public Enumeration<EpisodeOfCareStatus> fromType(PrimitiveType<?> code) throws FHIRException { 237 if (code == null) 238 return null; 239 if (code.isEmpty()) 240 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.NULL, code); 241 String codeString = code.asStringValue(); 242 if (codeString == null || "".equals(codeString)) 243 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.NULL, code); 244 if ("planned".equals(codeString)) 245 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.PLANNED, code); 246 if ("waitlist".equals(codeString)) 247 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.WAITLIST, code); 248 if ("active".equals(codeString)) 249 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.ACTIVE, code); 250 if ("onhold".equals(codeString)) 251 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.ONHOLD, code); 252 if ("finished".equals(codeString)) 253 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.FINISHED, code); 254 if ("cancelled".equals(codeString)) 255 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.CANCELLED, code); 256 if ("entered-in-error".equals(codeString)) 257 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.ENTEREDINERROR, code); 258 throw new FHIRException("Unknown EpisodeOfCareStatus code '" + codeString + "'"); 259 } 260 261 public String toCode(EpisodeOfCareStatus code) { 262 if (code == EpisodeOfCareStatus.PLANNED) 263 return "planned"; 264 if (code == EpisodeOfCareStatus.WAITLIST) 265 return "waitlist"; 266 if (code == EpisodeOfCareStatus.ACTIVE) 267 return "active"; 268 if (code == EpisodeOfCareStatus.ONHOLD) 269 return "onhold"; 270 if (code == EpisodeOfCareStatus.FINISHED) 271 return "finished"; 272 if (code == EpisodeOfCareStatus.CANCELLED) 273 return "cancelled"; 274 if (code == EpisodeOfCareStatus.ENTEREDINERROR) 275 return "entered-in-error"; 276 return "?"; 277 } 278 279 public String toSystem(EpisodeOfCareStatus code) { 280 return code.getSystem(); 281 } 282 } 283 284 @Block() 285 public static class EpisodeOfCareStatusHistoryComponent extends BackboneElement implements IBaseBackboneElement { 286 /** 287 * planned | waitlist | active | onhold | finished | cancelled. 288 */ 289 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 290 @Description(shortDefinition = "planned | waitlist | active | onhold | finished | cancelled | entered-in-error", formalDefinition = "planned | waitlist | active | onhold | finished | cancelled.") 291 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/episode-of-care-status") 292 protected Enumeration<EpisodeOfCareStatus> status; 293 294 /** 295 * The period during this EpisodeOfCare that the specific status applied. 296 */ 297 @Child(name = "period", type = { Period.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 298 @Description(shortDefinition = "Duration the EpisodeOfCare was in the specified status", formalDefinition = "The period during this EpisodeOfCare that the specific status applied.") 299 protected Period period; 300 301 private static final long serialVersionUID = -1192432864L; 302 303 /** 304 * Constructor 305 */ 306 public EpisodeOfCareStatusHistoryComponent() { 307 super(); 308 } 309 310 /** 311 * Constructor 312 */ 313 public EpisodeOfCareStatusHistoryComponent(Enumeration<EpisodeOfCareStatus> status, Period period) { 314 super(); 315 this.status = status; 316 this.period = period; 317 } 318 319 /** 320 * @return {@link #status} (planned | waitlist | active | onhold | finished | 321 * cancelled.). This is the underlying object with id, value and 322 * extensions. The accessor "getStatus" gives direct access to the value 323 */ 324 public Enumeration<EpisodeOfCareStatus> getStatusElement() { 325 if (this.status == null) 326 if (Configuration.errorOnAutoCreate()) 327 throw new Error("Attempt to auto-create EpisodeOfCareStatusHistoryComponent.status"); 328 else if (Configuration.doAutoCreate()) 329 this.status = new Enumeration<EpisodeOfCareStatus>(new EpisodeOfCareStatusEnumFactory()); // bb 330 return this.status; 331 } 332 333 public boolean hasStatusElement() { 334 return this.status != null && !this.status.isEmpty(); 335 } 336 337 public boolean hasStatus() { 338 return this.status != null && !this.status.isEmpty(); 339 } 340 341 /** 342 * @param value {@link #status} (planned | waitlist | active | onhold | finished 343 * | cancelled.). This is the underlying object with id, value and 344 * extensions. The accessor "getStatus" gives direct access to the 345 * value 346 */ 347 public EpisodeOfCareStatusHistoryComponent setStatusElement(Enumeration<EpisodeOfCareStatus> value) { 348 this.status = value; 349 return this; 350 } 351 352 /** 353 * @return planned | waitlist | active | onhold | finished | cancelled. 354 */ 355 public EpisodeOfCareStatus getStatus() { 356 return this.status == null ? null : this.status.getValue(); 357 } 358 359 /** 360 * @param value planned | waitlist | active | onhold | finished | cancelled. 361 */ 362 public EpisodeOfCareStatusHistoryComponent setStatus(EpisodeOfCareStatus value) { 363 if (this.status == null) 364 this.status = new Enumeration<EpisodeOfCareStatus>(new EpisodeOfCareStatusEnumFactory()); 365 this.status.setValue(value); 366 return this; 367 } 368 369 /** 370 * @return {@link #period} (The period during this EpisodeOfCare that the 371 * specific status applied.) 372 */ 373 public Period getPeriod() { 374 if (this.period == null) 375 if (Configuration.errorOnAutoCreate()) 376 throw new Error("Attempt to auto-create EpisodeOfCareStatusHistoryComponent.period"); 377 else if (Configuration.doAutoCreate()) 378 this.period = new Period(); // cc 379 return this.period; 380 } 381 382 public boolean hasPeriod() { 383 return this.period != null && !this.period.isEmpty(); 384 } 385 386 /** 387 * @param value {@link #period} (The period during this EpisodeOfCare that the 388 * specific status applied.) 389 */ 390 public EpisodeOfCareStatusHistoryComponent setPeriod(Period value) { 391 this.period = value; 392 return this; 393 } 394 395 protected void listChildren(List<Property> children) { 396 super.listChildren(children); 397 children.add( 398 new Property("status", "code", "planned | waitlist | active | onhold | finished | cancelled.", 0, 1, status)); 399 children.add(new Property("period", "Period", 400 "The period during this EpisodeOfCare that the specific status applied.", 0, 1, period)); 401 } 402 403 @Override 404 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 405 switch (_hash) { 406 case -892481550: 407 /* status */ return new Property("status", "code", 408 "planned | waitlist | active | onhold | finished | cancelled.", 0, 1, status); 409 case -991726143: 410 /* period */ return new Property("period", "Period", 411 "The period during this EpisodeOfCare that the specific status applied.", 0, 1, period); 412 default: 413 return super.getNamedProperty(_hash, _name, _checkValid); 414 } 415 416 } 417 418 @Override 419 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 420 switch (hash) { 421 case -892481550: 422 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<EpisodeOfCareStatus> 423 case -991726143: 424 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 425 default: 426 return super.getProperty(hash, name, checkValid); 427 } 428 429 } 430 431 @Override 432 public Base setProperty(int hash, String name, Base value) throws FHIRException { 433 switch (hash) { 434 case -892481550: // status 435 value = new EpisodeOfCareStatusEnumFactory().fromType(castToCode(value)); 436 this.status = (Enumeration) value; // Enumeration<EpisodeOfCareStatus> 437 return value; 438 case -991726143: // period 439 this.period = castToPeriod(value); // Period 440 return value; 441 default: 442 return super.setProperty(hash, name, value); 443 } 444 445 } 446 447 @Override 448 public Base setProperty(String name, Base value) throws FHIRException { 449 if (name.equals("status")) { 450 value = new EpisodeOfCareStatusEnumFactory().fromType(castToCode(value)); 451 this.status = (Enumeration) value; // Enumeration<EpisodeOfCareStatus> 452 } else if (name.equals("period")) { 453 this.period = castToPeriod(value); // Period 454 } else 455 return super.setProperty(name, value); 456 return value; 457 } 458 459 @Override 460 public void removeChild(String name, Base value) throws FHIRException { 461 if (name.equals("status")) { 462 this.status = null; 463 } else if (name.equals("period")) { 464 this.period = null; 465 } else 466 super.removeChild(name, value); 467 468 } 469 470 @Override 471 public Base makeProperty(int hash, String name) throws FHIRException { 472 switch (hash) { 473 case -892481550: 474 return getStatusElement(); 475 case -991726143: 476 return getPeriod(); 477 default: 478 return super.makeProperty(hash, name); 479 } 480 481 } 482 483 @Override 484 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 485 switch (hash) { 486 case -892481550: 487 /* status */ return new String[] { "code" }; 488 case -991726143: 489 /* period */ return new String[] { "Period" }; 490 default: 491 return super.getTypesForProperty(hash, name); 492 } 493 494 } 495 496 @Override 497 public Base addChild(String name) throws FHIRException { 498 if (name.equals("status")) { 499 throw new FHIRException("Cannot call addChild on a singleton property EpisodeOfCare.status"); 500 } else if (name.equals("period")) { 501 this.period = new Period(); 502 return this.period; 503 } else 504 return super.addChild(name); 505 } 506 507 public EpisodeOfCareStatusHistoryComponent copy() { 508 EpisodeOfCareStatusHistoryComponent dst = new EpisodeOfCareStatusHistoryComponent(); 509 copyValues(dst); 510 return dst; 511 } 512 513 public void copyValues(EpisodeOfCareStatusHistoryComponent dst) { 514 super.copyValues(dst); 515 dst.status = status == null ? null : status.copy(); 516 dst.period = period == null ? null : period.copy(); 517 } 518 519 @Override 520 public boolean equalsDeep(Base other_) { 521 if (!super.equalsDeep(other_)) 522 return false; 523 if (!(other_ instanceof EpisodeOfCareStatusHistoryComponent)) 524 return false; 525 EpisodeOfCareStatusHistoryComponent o = (EpisodeOfCareStatusHistoryComponent) other_; 526 return compareDeep(status, o.status, true) && compareDeep(period, o.period, true); 527 } 528 529 @Override 530 public boolean equalsShallow(Base other_) { 531 if (!super.equalsShallow(other_)) 532 return false; 533 if (!(other_ instanceof EpisodeOfCareStatusHistoryComponent)) 534 return false; 535 EpisodeOfCareStatusHistoryComponent o = (EpisodeOfCareStatusHistoryComponent) other_; 536 return compareValues(status, o.status, true); 537 } 538 539 public boolean isEmpty() { 540 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(status, period); 541 } 542 543 public String fhirType() { 544 return "EpisodeOfCare.statusHistory"; 545 546 } 547 548 } 549 550 @Block() 551 public static class DiagnosisComponent extends BackboneElement implements IBaseBackboneElement { 552 /** 553 * A list of conditions/problems/diagnoses that this episode of care is intended 554 * to be providing care for. 555 */ 556 @Child(name = "condition", type = { 557 Condition.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 558 @Description(shortDefinition = "Conditions/problems/diagnoses this episode of care is for", formalDefinition = "A list of conditions/problems/diagnoses that this episode of care is intended to be providing care for.") 559 protected Reference condition; 560 561 /** 562 * The actual object that is the target of the reference (A list of 563 * conditions/problems/diagnoses that this episode of care is intended to be 564 * providing care for.) 565 */ 566 protected Condition conditionTarget; 567 568 /** 569 * Role that this diagnosis has within the episode of care (e.g. admission, 570 * billing, discharge ?). 571 */ 572 @Child(name = "role", type = { 573 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 574 @Description(shortDefinition = "Role that this diagnosis has within the episode of care (e.g. admission, billing, discharge ?)", formalDefinition = "Role that this diagnosis has within the episode of care (e.g. admission, billing, discharge ?).") 575 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/diagnosis-role") 576 protected CodeableConcept role; 577 578 /** 579 * Ranking of the diagnosis (for each role type). 580 */ 581 @Child(name = "rank", type = { 582 PositiveIntType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 583 @Description(shortDefinition = "Ranking of the diagnosis (for each role type)", formalDefinition = "Ranking of the diagnosis (for each role type).") 584 protected PositiveIntType rank; 585 586 private static final long serialVersionUID = 249445632L; 587 588 /** 589 * Constructor 590 */ 591 public DiagnosisComponent() { 592 super(); 593 } 594 595 /** 596 * Constructor 597 */ 598 public DiagnosisComponent(Reference condition) { 599 super(); 600 this.condition = condition; 601 } 602 603 /** 604 * @return {@link #condition} (A list of conditions/problems/diagnoses that this 605 * episode of care is intended to be providing care for.) 606 */ 607 public Reference getCondition() { 608 if (this.condition == null) 609 if (Configuration.errorOnAutoCreate()) 610 throw new Error("Attempt to auto-create DiagnosisComponent.condition"); 611 else if (Configuration.doAutoCreate()) 612 this.condition = new Reference(); // cc 613 return this.condition; 614 } 615 616 public boolean hasCondition() { 617 return this.condition != null && !this.condition.isEmpty(); 618 } 619 620 /** 621 * @param value {@link #condition} (A list of conditions/problems/diagnoses that 622 * this episode of care is intended to be providing care for.) 623 */ 624 public DiagnosisComponent setCondition(Reference value) { 625 this.condition = value; 626 return this; 627 } 628 629 /** 630 * @return {@link #condition} The actual object that is the target of the 631 * reference. The reference library doesn't populate this, but you can 632 * use it to hold the resource if you resolve it. (A list of 633 * conditions/problems/diagnoses that this episode of care is intended 634 * to be providing care for.) 635 */ 636 public Condition getConditionTarget() { 637 if (this.conditionTarget == null) 638 if (Configuration.errorOnAutoCreate()) 639 throw new Error("Attempt to auto-create DiagnosisComponent.condition"); 640 else if (Configuration.doAutoCreate()) 641 this.conditionTarget = new Condition(); // aa 642 return this.conditionTarget; 643 } 644 645 /** 646 * @param value {@link #condition} The actual object that is the target of the 647 * reference. The reference library doesn't use these, but you can 648 * use it to hold the resource if you resolve it. (A list of 649 * conditions/problems/diagnoses that this episode of care is 650 * intended to be providing care for.) 651 */ 652 public DiagnosisComponent setConditionTarget(Condition value) { 653 this.conditionTarget = value; 654 return this; 655 } 656 657 /** 658 * @return {@link #role} (Role that this diagnosis has within the episode of 659 * care (e.g. admission, billing, discharge ?).) 660 */ 661 public CodeableConcept getRole() { 662 if (this.role == null) 663 if (Configuration.errorOnAutoCreate()) 664 throw new Error("Attempt to auto-create DiagnosisComponent.role"); 665 else if (Configuration.doAutoCreate()) 666 this.role = new CodeableConcept(); // cc 667 return this.role; 668 } 669 670 public boolean hasRole() { 671 return this.role != null && !this.role.isEmpty(); 672 } 673 674 /** 675 * @param value {@link #role} (Role that this diagnosis has within the episode 676 * of care (e.g. admission, billing, discharge ?).) 677 */ 678 public DiagnosisComponent setRole(CodeableConcept value) { 679 this.role = value; 680 return this; 681 } 682 683 /** 684 * @return {@link #rank} (Ranking of the diagnosis (for each role type).). This 685 * is the underlying object with id, value and extensions. The accessor 686 * "getRank" gives direct access to the value 687 */ 688 public PositiveIntType getRankElement() { 689 if (this.rank == null) 690 if (Configuration.errorOnAutoCreate()) 691 throw new Error("Attempt to auto-create DiagnosisComponent.rank"); 692 else if (Configuration.doAutoCreate()) 693 this.rank = new PositiveIntType(); // bb 694 return this.rank; 695 } 696 697 public boolean hasRankElement() { 698 return this.rank != null && !this.rank.isEmpty(); 699 } 700 701 public boolean hasRank() { 702 return this.rank != null && !this.rank.isEmpty(); 703 } 704 705 /** 706 * @param value {@link #rank} (Ranking of the diagnosis (for each role type).). 707 * This is the underlying object with id, value and extensions. The 708 * accessor "getRank" gives direct access to the value 709 */ 710 public DiagnosisComponent setRankElement(PositiveIntType value) { 711 this.rank = value; 712 return this; 713 } 714 715 /** 716 * @return Ranking of the diagnosis (for each role type). 717 */ 718 public int getRank() { 719 return this.rank == null || this.rank.isEmpty() ? 0 : this.rank.getValue(); 720 } 721 722 /** 723 * @param value Ranking of the diagnosis (for each role type). 724 */ 725 public DiagnosisComponent setRank(int value) { 726 if (this.rank == null) 727 this.rank = new PositiveIntType(); 728 this.rank.setValue(value); 729 return this; 730 } 731 732 protected void listChildren(List<Property> children) { 733 super.listChildren(children); 734 children.add(new Property("condition", "Reference(Condition)", 735 "A list of conditions/problems/diagnoses that this episode of care is intended to be providing care for.", 0, 736 1, condition)); 737 children.add(new Property("role", "CodeableConcept", 738 "Role that this diagnosis has within the episode of care (e.g. admission, billing, discharge ?).", 0, 1, 739 role)); 740 children.add(new Property("rank", "positiveInt", "Ranking of the diagnosis (for each role type).", 0, 1, rank)); 741 } 742 743 @Override 744 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 745 switch (_hash) { 746 case -861311717: 747 /* condition */ return new Property("condition", "Reference(Condition)", 748 "A list of conditions/problems/diagnoses that this episode of care is intended to be providing care for.", 749 0, 1, condition); 750 case 3506294: 751 /* role */ return new Property("role", "CodeableConcept", 752 "Role that this diagnosis has within the episode of care (e.g. admission, billing, discharge ?).", 0, 1, 753 role); 754 case 3492908: 755 /* rank */ return new Property("rank", "positiveInt", "Ranking of the diagnosis (for each role type).", 0, 1, 756 rank); 757 default: 758 return super.getNamedProperty(_hash, _name, _checkValid); 759 } 760 761 } 762 763 @Override 764 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 765 switch (hash) { 766 case -861311717: 767 /* condition */ return this.condition == null ? new Base[0] : new Base[] { this.condition }; // Reference 768 case 3506294: 769 /* role */ return this.role == null ? new Base[0] : new Base[] { this.role }; // CodeableConcept 770 case 3492908: 771 /* rank */ return this.rank == null ? new Base[0] : new Base[] { this.rank }; // PositiveIntType 772 default: 773 return super.getProperty(hash, name, checkValid); 774 } 775 776 } 777 778 @Override 779 public Base setProperty(int hash, String name, Base value) throws FHIRException { 780 switch (hash) { 781 case -861311717: // condition 782 this.condition = castToReference(value); // Reference 783 return value; 784 case 3506294: // role 785 this.role = castToCodeableConcept(value); // CodeableConcept 786 return value; 787 case 3492908: // rank 788 this.rank = castToPositiveInt(value); // PositiveIntType 789 return value; 790 default: 791 return super.setProperty(hash, name, value); 792 } 793 794 } 795 796 @Override 797 public Base setProperty(String name, Base value) throws FHIRException { 798 if (name.equals("condition")) { 799 this.condition = castToReference(value); // Reference 800 } else if (name.equals("role")) { 801 this.role = castToCodeableConcept(value); // CodeableConcept 802 } else if (name.equals("rank")) { 803 this.rank = castToPositiveInt(value); // PositiveIntType 804 } else 805 return super.setProperty(name, value); 806 return value; 807 } 808 809 @Override 810 public void removeChild(String name, Base value) throws FHIRException { 811 if (name.equals("condition")) { 812 this.condition = null; 813 } else if (name.equals("role")) { 814 this.role = null; 815 } else if (name.equals("rank")) { 816 this.rank = null; 817 } else 818 super.removeChild(name, value); 819 820 } 821 822 @Override 823 public Base makeProperty(int hash, String name) throws FHIRException { 824 switch (hash) { 825 case -861311717: 826 return getCondition(); 827 case 3506294: 828 return getRole(); 829 case 3492908: 830 return getRankElement(); 831 default: 832 return super.makeProperty(hash, name); 833 } 834 835 } 836 837 @Override 838 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 839 switch (hash) { 840 case -861311717: 841 /* condition */ return new String[] { "Reference" }; 842 case 3506294: 843 /* role */ return new String[] { "CodeableConcept" }; 844 case 3492908: 845 /* rank */ return new String[] { "positiveInt" }; 846 default: 847 return super.getTypesForProperty(hash, name); 848 } 849 850 } 851 852 @Override 853 public Base addChild(String name) throws FHIRException { 854 if (name.equals("condition")) { 855 this.condition = new Reference(); 856 return this.condition; 857 } else if (name.equals("role")) { 858 this.role = new CodeableConcept(); 859 return this.role; 860 } else if (name.equals("rank")) { 861 throw new FHIRException("Cannot call addChild on a singleton property EpisodeOfCare.rank"); 862 } else 863 return super.addChild(name); 864 } 865 866 public DiagnosisComponent copy() { 867 DiagnosisComponent dst = new DiagnosisComponent(); 868 copyValues(dst); 869 return dst; 870 } 871 872 public void copyValues(DiagnosisComponent dst) { 873 super.copyValues(dst); 874 dst.condition = condition == null ? null : condition.copy(); 875 dst.role = role == null ? null : role.copy(); 876 dst.rank = rank == null ? null : rank.copy(); 877 } 878 879 @Override 880 public boolean equalsDeep(Base other_) { 881 if (!super.equalsDeep(other_)) 882 return false; 883 if (!(other_ instanceof DiagnosisComponent)) 884 return false; 885 DiagnosisComponent o = (DiagnosisComponent) other_; 886 return compareDeep(condition, o.condition, true) && compareDeep(role, o.role, true) 887 && compareDeep(rank, o.rank, true); 888 } 889 890 @Override 891 public boolean equalsShallow(Base other_) { 892 if (!super.equalsShallow(other_)) 893 return false; 894 if (!(other_ instanceof DiagnosisComponent)) 895 return false; 896 DiagnosisComponent o = (DiagnosisComponent) other_; 897 return compareValues(rank, o.rank, true); 898 } 899 900 public boolean isEmpty() { 901 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(condition, role, rank); 902 } 903 904 public String fhirType() { 905 return "EpisodeOfCare.diagnosis"; 906 907 } 908 909 } 910 911 /** 912 * The EpisodeOfCare may be known by different identifiers for different 913 * contexts of use, such as when an external agency is tracking the Episode for 914 * funding purposes. 915 */ 916 @Child(name = "identifier", type = { 917 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 918 @Description(shortDefinition = "Business Identifier(s) relevant for this EpisodeOfCare", formalDefinition = "The EpisodeOfCare may be known by different identifiers for different contexts of use, such as when an external agency is tracking the Episode for funding purposes.") 919 protected List<Identifier> identifier; 920 921 /** 922 * planned | waitlist | active | onhold | finished | cancelled. 923 */ 924 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 925 @Description(shortDefinition = "planned | waitlist | active | onhold | finished | cancelled | entered-in-error", formalDefinition = "planned | waitlist | active | onhold | finished | cancelled.") 926 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/episode-of-care-status") 927 protected Enumeration<EpisodeOfCareStatus> status; 928 929 /** 930 * The history of statuses that the EpisodeOfCare has been through (without 931 * requiring processing the history of the resource). 932 */ 933 @Child(name = "statusHistory", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 934 @Description(shortDefinition = "Past list of status codes (the current status may be included to cover the start date of the status)", formalDefinition = "The history of statuses that the EpisodeOfCare has been through (without requiring processing the history of the resource).") 935 protected List<EpisodeOfCareStatusHistoryComponent> statusHistory; 936 937 /** 938 * A classification of the type of episode of care; e.g. specialist referral, 939 * disease management, type of funded care. 940 */ 941 @Child(name = "type", type = { 942 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 943 @Description(shortDefinition = "Type/class - e.g. specialist referral, disease management", formalDefinition = "A classification of the type of episode of care; e.g. specialist referral, disease management, type of funded care.") 944 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/episodeofcare-type") 945 protected List<CodeableConcept> type; 946 947 /** 948 * The list of diagnosis relevant to this episode of care. 949 */ 950 @Child(name = "diagnosis", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 951 @Description(shortDefinition = "The list of diagnosis relevant to this episode of care", formalDefinition = "The list of diagnosis relevant to this episode of care.") 952 protected List<DiagnosisComponent> diagnosis; 953 954 /** 955 * The patient who is the focus of this episode of care. 956 */ 957 @Child(name = "patient", type = { Patient.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 958 @Description(shortDefinition = "The patient who is the focus of this episode of care", formalDefinition = "The patient who is the focus of this episode of care.") 959 protected Reference patient; 960 961 /** 962 * The actual object that is the target of the reference (The patient who is the 963 * focus of this episode of care.) 964 */ 965 protected Patient patientTarget; 966 967 /** 968 * The organization that has assumed the specific responsibilities for the 969 * specified duration. 970 */ 971 @Child(name = "managingOrganization", type = { 972 Organization.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 973 @Description(shortDefinition = "Organization that assumes care", formalDefinition = "The organization that has assumed the specific responsibilities for the specified duration.") 974 protected Reference managingOrganization; 975 976 /** 977 * The actual object that is the target of the reference (The organization that 978 * has assumed the specific responsibilities for the specified duration.) 979 */ 980 protected Organization managingOrganizationTarget; 981 982 /** 983 * The interval during which the managing organization assumes the defined 984 * responsibility. 985 */ 986 @Child(name = "period", type = { Period.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 987 @Description(shortDefinition = "Interval during responsibility is assumed", formalDefinition = "The interval during which the managing organization assumes the defined responsibility.") 988 protected Period period; 989 990 /** 991 * Referral Request(s) that are fulfilled by this EpisodeOfCare, incoming 992 * referrals. 993 */ 994 @Child(name = "referralRequest", type = { 995 ServiceRequest.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 996 @Description(shortDefinition = "Originating Referral Request(s)", formalDefinition = "Referral Request(s) that are fulfilled by this EpisodeOfCare, incoming referrals.") 997 protected List<Reference> referralRequest; 998 /** 999 * The actual objects that are the target of the reference (Referral Request(s) 1000 * that are fulfilled by this EpisodeOfCare, incoming referrals.) 1001 */ 1002 protected List<ServiceRequest> referralRequestTarget; 1003 1004 /** 1005 * The practitioner that is the care manager/care coordinator for this patient. 1006 */ 1007 @Child(name = "careManager", type = { Practitioner.class, 1008 PractitionerRole.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1009 @Description(shortDefinition = "Care manager/care coordinator for the patient", formalDefinition = "The practitioner that is the care manager/care coordinator for this patient.") 1010 protected Reference careManager; 1011 1012 /** 1013 * The actual object that is the target of the reference (The practitioner that 1014 * is the care manager/care coordinator for this patient.) 1015 */ 1016 protected Resource careManagerTarget; 1017 1018 /** 1019 * The list of practitioners that may be facilitating this episode of care for 1020 * specific purposes. 1021 */ 1022 @Child(name = "team", type = { 1023 CareTeam.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1024 @Description(shortDefinition = "Other practitioners facilitating this episode of care", formalDefinition = "The list of practitioners that may be facilitating this episode of care for specific purposes.") 1025 protected List<Reference> team; 1026 /** 1027 * The actual objects that are the target of the reference (The list of 1028 * practitioners that may be facilitating this episode of care for specific 1029 * purposes.) 1030 */ 1031 protected List<CareTeam> teamTarget; 1032 1033 /** 1034 * The set of accounts that may be used for billing for this EpisodeOfCare. 1035 */ 1036 @Child(name = "account", type = { 1037 Account.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1038 @Description(shortDefinition = "The set of accounts that may be used for billing for this EpisodeOfCare", formalDefinition = "The set of accounts that may be used for billing for this EpisodeOfCare.") 1039 protected List<Reference> account; 1040 /** 1041 * The actual objects that are the target of the reference (The set of accounts 1042 * that may be used for billing for this EpisodeOfCare.) 1043 */ 1044 protected List<Account> accountTarget; 1045 1046 private static final long serialVersionUID = 548033949L; 1047 1048 /** 1049 * Constructor 1050 */ 1051 public EpisodeOfCare() { 1052 super(); 1053 } 1054 1055 /** 1056 * Constructor 1057 */ 1058 public EpisodeOfCare(Enumeration<EpisodeOfCareStatus> status, Reference patient) { 1059 super(); 1060 this.status = status; 1061 this.patient = patient; 1062 } 1063 1064 /** 1065 * @return {@link #identifier} (The EpisodeOfCare may be known by different 1066 * identifiers for different contexts of use, such as when an external 1067 * agency is tracking the Episode for funding purposes.) 1068 */ 1069 public List<Identifier> getIdentifier() { 1070 if (this.identifier == null) 1071 this.identifier = new ArrayList<Identifier>(); 1072 return this.identifier; 1073 } 1074 1075 /** 1076 * @return Returns a reference to <code>this</code> for easy method chaining 1077 */ 1078 public EpisodeOfCare setIdentifier(List<Identifier> theIdentifier) { 1079 this.identifier = theIdentifier; 1080 return this; 1081 } 1082 1083 public boolean hasIdentifier() { 1084 if (this.identifier == null) 1085 return false; 1086 for (Identifier item : this.identifier) 1087 if (!item.isEmpty()) 1088 return true; 1089 return false; 1090 } 1091 1092 public Identifier addIdentifier() { // 3 1093 Identifier t = new Identifier(); 1094 if (this.identifier == null) 1095 this.identifier = new ArrayList<Identifier>(); 1096 this.identifier.add(t); 1097 return t; 1098 } 1099 1100 public EpisodeOfCare addIdentifier(Identifier t) { // 3 1101 if (t == null) 1102 return this; 1103 if (this.identifier == null) 1104 this.identifier = new ArrayList<Identifier>(); 1105 this.identifier.add(t); 1106 return this; 1107 } 1108 1109 /** 1110 * @return The first repetition of repeating field {@link #identifier}, creating 1111 * it if it does not already exist 1112 */ 1113 public Identifier getIdentifierFirstRep() { 1114 if (getIdentifier().isEmpty()) { 1115 addIdentifier(); 1116 } 1117 return getIdentifier().get(0); 1118 } 1119 1120 /** 1121 * @return {@link #status} (planned | waitlist | active | onhold | finished | 1122 * cancelled.). This is the underlying object with id, value and 1123 * extensions. The accessor "getStatus" gives direct access to the value 1124 */ 1125 public Enumeration<EpisodeOfCareStatus> getStatusElement() { 1126 if (this.status == null) 1127 if (Configuration.errorOnAutoCreate()) 1128 throw new Error("Attempt to auto-create EpisodeOfCare.status"); 1129 else if (Configuration.doAutoCreate()) 1130 this.status = new Enumeration<EpisodeOfCareStatus>(new EpisodeOfCareStatusEnumFactory()); // bb 1131 return this.status; 1132 } 1133 1134 public boolean hasStatusElement() { 1135 return this.status != null && !this.status.isEmpty(); 1136 } 1137 1138 public boolean hasStatus() { 1139 return this.status != null && !this.status.isEmpty(); 1140 } 1141 1142 /** 1143 * @param value {@link #status} (planned | waitlist | active | onhold | finished 1144 * | cancelled.). This is the underlying object with id, value and 1145 * extensions. The accessor "getStatus" gives direct access to the 1146 * value 1147 */ 1148 public EpisodeOfCare setStatusElement(Enumeration<EpisodeOfCareStatus> value) { 1149 this.status = value; 1150 return this; 1151 } 1152 1153 /** 1154 * @return planned | waitlist | active | onhold | finished | cancelled. 1155 */ 1156 public EpisodeOfCareStatus getStatus() { 1157 return this.status == null ? null : this.status.getValue(); 1158 } 1159 1160 /** 1161 * @param value planned | waitlist | active | onhold | finished | cancelled. 1162 */ 1163 public EpisodeOfCare setStatus(EpisodeOfCareStatus value) { 1164 if (this.status == null) 1165 this.status = new Enumeration<EpisodeOfCareStatus>(new EpisodeOfCareStatusEnumFactory()); 1166 this.status.setValue(value); 1167 return this; 1168 } 1169 1170 /** 1171 * @return {@link #statusHistory} (The history of statuses that the 1172 * EpisodeOfCare has been through (without requiring processing the 1173 * history of the resource).) 1174 */ 1175 public List<EpisodeOfCareStatusHistoryComponent> getStatusHistory() { 1176 if (this.statusHistory == null) 1177 this.statusHistory = new ArrayList<EpisodeOfCareStatusHistoryComponent>(); 1178 return this.statusHistory; 1179 } 1180 1181 /** 1182 * @return Returns a reference to <code>this</code> for easy method chaining 1183 */ 1184 public EpisodeOfCare setStatusHistory(List<EpisodeOfCareStatusHistoryComponent> theStatusHistory) { 1185 this.statusHistory = theStatusHistory; 1186 return this; 1187 } 1188 1189 public boolean hasStatusHistory() { 1190 if (this.statusHistory == null) 1191 return false; 1192 for (EpisodeOfCareStatusHistoryComponent item : this.statusHistory) 1193 if (!item.isEmpty()) 1194 return true; 1195 return false; 1196 } 1197 1198 public EpisodeOfCareStatusHistoryComponent addStatusHistory() { // 3 1199 EpisodeOfCareStatusHistoryComponent t = new EpisodeOfCareStatusHistoryComponent(); 1200 if (this.statusHistory == null) 1201 this.statusHistory = new ArrayList<EpisodeOfCareStatusHistoryComponent>(); 1202 this.statusHistory.add(t); 1203 return t; 1204 } 1205 1206 public EpisodeOfCare addStatusHistory(EpisodeOfCareStatusHistoryComponent t) { // 3 1207 if (t == null) 1208 return this; 1209 if (this.statusHistory == null) 1210 this.statusHistory = new ArrayList<EpisodeOfCareStatusHistoryComponent>(); 1211 this.statusHistory.add(t); 1212 return this; 1213 } 1214 1215 /** 1216 * @return The first repetition of repeating field {@link #statusHistory}, 1217 * creating it if it does not already exist 1218 */ 1219 public EpisodeOfCareStatusHistoryComponent getStatusHistoryFirstRep() { 1220 if (getStatusHistory().isEmpty()) { 1221 addStatusHistory(); 1222 } 1223 return getStatusHistory().get(0); 1224 } 1225 1226 /** 1227 * @return {@link #type} (A classification of the type of episode of care; e.g. 1228 * specialist referral, disease management, type of funded care.) 1229 */ 1230 public List<CodeableConcept> getType() { 1231 if (this.type == null) 1232 this.type = new ArrayList<CodeableConcept>(); 1233 return this.type; 1234 } 1235 1236 /** 1237 * @return Returns a reference to <code>this</code> for easy method chaining 1238 */ 1239 public EpisodeOfCare setType(List<CodeableConcept> theType) { 1240 this.type = theType; 1241 return this; 1242 } 1243 1244 public boolean hasType() { 1245 if (this.type == null) 1246 return false; 1247 for (CodeableConcept item : this.type) 1248 if (!item.isEmpty()) 1249 return true; 1250 return false; 1251 } 1252 1253 public CodeableConcept addType() { // 3 1254 CodeableConcept t = new CodeableConcept(); 1255 if (this.type == null) 1256 this.type = new ArrayList<CodeableConcept>(); 1257 this.type.add(t); 1258 return t; 1259 } 1260 1261 public EpisodeOfCare addType(CodeableConcept t) { // 3 1262 if (t == null) 1263 return this; 1264 if (this.type == null) 1265 this.type = new ArrayList<CodeableConcept>(); 1266 this.type.add(t); 1267 return this; 1268 } 1269 1270 /** 1271 * @return The first repetition of repeating field {@link #type}, creating it if 1272 * it does not already exist 1273 */ 1274 public CodeableConcept getTypeFirstRep() { 1275 if (getType().isEmpty()) { 1276 addType(); 1277 } 1278 return getType().get(0); 1279 } 1280 1281 /** 1282 * @return {@link #diagnosis} (The list of diagnosis relevant to this episode of 1283 * care.) 1284 */ 1285 public List<DiagnosisComponent> getDiagnosis() { 1286 if (this.diagnosis == null) 1287 this.diagnosis = new ArrayList<DiagnosisComponent>(); 1288 return this.diagnosis; 1289 } 1290 1291 /** 1292 * @return Returns a reference to <code>this</code> for easy method chaining 1293 */ 1294 public EpisodeOfCare setDiagnosis(List<DiagnosisComponent> theDiagnosis) { 1295 this.diagnosis = theDiagnosis; 1296 return this; 1297 } 1298 1299 public boolean hasDiagnosis() { 1300 if (this.diagnosis == null) 1301 return false; 1302 for (DiagnosisComponent item : this.diagnosis) 1303 if (!item.isEmpty()) 1304 return true; 1305 return false; 1306 } 1307 1308 public DiagnosisComponent addDiagnosis() { // 3 1309 DiagnosisComponent t = new DiagnosisComponent(); 1310 if (this.diagnosis == null) 1311 this.diagnosis = new ArrayList<DiagnosisComponent>(); 1312 this.diagnosis.add(t); 1313 return t; 1314 } 1315 1316 public EpisodeOfCare addDiagnosis(DiagnosisComponent t) { // 3 1317 if (t == null) 1318 return this; 1319 if (this.diagnosis == null) 1320 this.diagnosis = new ArrayList<DiagnosisComponent>(); 1321 this.diagnosis.add(t); 1322 return this; 1323 } 1324 1325 /** 1326 * @return The first repetition of repeating field {@link #diagnosis}, creating 1327 * it if it does not already exist 1328 */ 1329 public DiagnosisComponent getDiagnosisFirstRep() { 1330 if (getDiagnosis().isEmpty()) { 1331 addDiagnosis(); 1332 } 1333 return getDiagnosis().get(0); 1334 } 1335 1336 /** 1337 * @return {@link #patient} (The patient who is the focus of this episode of 1338 * care.) 1339 */ 1340 public Reference getPatient() { 1341 if (this.patient == null) 1342 if (Configuration.errorOnAutoCreate()) 1343 throw new Error("Attempt to auto-create EpisodeOfCare.patient"); 1344 else if (Configuration.doAutoCreate()) 1345 this.patient = new Reference(); // cc 1346 return this.patient; 1347 } 1348 1349 public boolean hasPatient() { 1350 return this.patient != null && !this.patient.isEmpty(); 1351 } 1352 1353 /** 1354 * @param value {@link #patient} (The patient who is the focus of this episode 1355 * of care.) 1356 */ 1357 public EpisodeOfCare setPatient(Reference value) { 1358 this.patient = value; 1359 return this; 1360 } 1361 1362 /** 1363 * @return {@link #patient} The actual object that is the target of the 1364 * reference. The reference library doesn't populate this, but you can 1365 * use it to hold the resource if you resolve it. (The patient who is 1366 * the focus of this episode of care.) 1367 */ 1368 public Patient getPatientTarget() { 1369 if (this.patientTarget == null) 1370 if (Configuration.errorOnAutoCreate()) 1371 throw new Error("Attempt to auto-create EpisodeOfCare.patient"); 1372 else if (Configuration.doAutoCreate()) 1373 this.patientTarget = new Patient(); // aa 1374 return this.patientTarget; 1375 } 1376 1377 /** 1378 * @param value {@link #patient} The actual object that is the target of the 1379 * reference. The reference library doesn't use these, but you can 1380 * use it to hold the resource if you resolve it. (The patient who 1381 * is the focus of this episode of care.) 1382 */ 1383 public EpisodeOfCare setPatientTarget(Patient value) { 1384 this.patientTarget = value; 1385 return this; 1386 } 1387 1388 /** 1389 * @return {@link #managingOrganization} (The organization that has assumed the 1390 * specific responsibilities for the specified duration.) 1391 */ 1392 public Reference getManagingOrganization() { 1393 if (this.managingOrganization == null) 1394 if (Configuration.errorOnAutoCreate()) 1395 throw new Error("Attempt to auto-create EpisodeOfCare.managingOrganization"); 1396 else if (Configuration.doAutoCreate()) 1397 this.managingOrganization = new Reference(); // cc 1398 return this.managingOrganization; 1399 } 1400 1401 public boolean hasManagingOrganization() { 1402 return this.managingOrganization != null && !this.managingOrganization.isEmpty(); 1403 } 1404 1405 /** 1406 * @param value {@link #managingOrganization} (The organization that has assumed 1407 * the specific responsibilities for the specified duration.) 1408 */ 1409 public EpisodeOfCare setManagingOrganization(Reference value) { 1410 this.managingOrganization = value; 1411 return this; 1412 } 1413 1414 /** 1415 * @return {@link #managingOrganization} The actual object that is the target of 1416 * the reference. The reference library doesn't populate this, but you 1417 * can use it to hold the resource if you resolve it. (The organization 1418 * that has assumed the specific responsibilities for the specified 1419 * duration.) 1420 */ 1421 public Organization getManagingOrganizationTarget() { 1422 if (this.managingOrganizationTarget == null) 1423 if (Configuration.errorOnAutoCreate()) 1424 throw new Error("Attempt to auto-create EpisodeOfCare.managingOrganization"); 1425 else if (Configuration.doAutoCreate()) 1426 this.managingOrganizationTarget = new Organization(); // aa 1427 return this.managingOrganizationTarget; 1428 } 1429 1430 /** 1431 * @param value {@link #managingOrganization} The actual object that is the 1432 * target of the reference. The reference library doesn't use 1433 * these, but you can use it to hold the resource if you resolve 1434 * it. (The organization that has assumed the specific 1435 * responsibilities for the specified duration.) 1436 */ 1437 public EpisodeOfCare setManagingOrganizationTarget(Organization value) { 1438 this.managingOrganizationTarget = value; 1439 return this; 1440 } 1441 1442 /** 1443 * @return {@link #period} (The interval during which the managing organization 1444 * assumes the defined responsibility.) 1445 */ 1446 public Period getPeriod() { 1447 if (this.period == null) 1448 if (Configuration.errorOnAutoCreate()) 1449 throw new Error("Attempt to auto-create EpisodeOfCare.period"); 1450 else if (Configuration.doAutoCreate()) 1451 this.period = new Period(); // cc 1452 return this.period; 1453 } 1454 1455 public boolean hasPeriod() { 1456 return this.period != null && !this.period.isEmpty(); 1457 } 1458 1459 /** 1460 * @param value {@link #period} (The interval during which the managing 1461 * organization assumes the defined responsibility.) 1462 */ 1463 public EpisodeOfCare setPeriod(Period value) { 1464 this.period = value; 1465 return this; 1466 } 1467 1468 /** 1469 * @return {@link #referralRequest} (Referral Request(s) that are fulfilled by 1470 * this EpisodeOfCare, incoming referrals.) 1471 */ 1472 public List<Reference> getReferralRequest() { 1473 if (this.referralRequest == null) 1474 this.referralRequest = new ArrayList<Reference>(); 1475 return this.referralRequest; 1476 } 1477 1478 /** 1479 * @return Returns a reference to <code>this</code> for easy method chaining 1480 */ 1481 public EpisodeOfCare setReferralRequest(List<Reference> theReferralRequest) { 1482 this.referralRequest = theReferralRequest; 1483 return this; 1484 } 1485 1486 public boolean hasReferralRequest() { 1487 if (this.referralRequest == null) 1488 return false; 1489 for (Reference item : this.referralRequest) 1490 if (!item.isEmpty()) 1491 return true; 1492 return false; 1493 } 1494 1495 public Reference addReferralRequest() { // 3 1496 Reference t = new Reference(); 1497 if (this.referralRequest == null) 1498 this.referralRequest = new ArrayList<Reference>(); 1499 this.referralRequest.add(t); 1500 return t; 1501 } 1502 1503 public EpisodeOfCare addReferralRequest(Reference t) { // 3 1504 if (t == null) 1505 return this; 1506 if (this.referralRequest == null) 1507 this.referralRequest = new ArrayList<Reference>(); 1508 this.referralRequest.add(t); 1509 return this; 1510 } 1511 1512 /** 1513 * @return The first repetition of repeating field {@link #referralRequest}, 1514 * creating it if it does not already exist 1515 */ 1516 public Reference getReferralRequestFirstRep() { 1517 if (getReferralRequest().isEmpty()) { 1518 addReferralRequest(); 1519 } 1520 return getReferralRequest().get(0); 1521 } 1522 1523 /** 1524 * @deprecated Use Reference#setResource(IBaseResource) instead 1525 */ 1526 @Deprecated 1527 public List<ServiceRequest> getReferralRequestTarget() { 1528 if (this.referralRequestTarget == null) 1529 this.referralRequestTarget = new ArrayList<ServiceRequest>(); 1530 return this.referralRequestTarget; 1531 } 1532 1533 /** 1534 * @deprecated Use Reference#setResource(IBaseResource) instead 1535 */ 1536 @Deprecated 1537 public ServiceRequest addReferralRequestTarget() { 1538 ServiceRequest r = new ServiceRequest(); 1539 if (this.referralRequestTarget == null) 1540 this.referralRequestTarget = new ArrayList<ServiceRequest>(); 1541 this.referralRequestTarget.add(r); 1542 return r; 1543 } 1544 1545 /** 1546 * @return {@link #careManager} (The practitioner that is the care manager/care 1547 * coordinator for this patient.) 1548 */ 1549 public Reference getCareManager() { 1550 if (this.careManager == null) 1551 if (Configuration.errorOnAutoCreate()) 1552 throw new Error("Attempt to auto-create EpisodeOfCare.careManager"); 1553 else if (Configuration.doAutoCreate()) 1554 this.careManager = new Reference(); // cc 1555 return this.careManager; 1556 } 1557 1558 public boolean hasCareManager() { 1559 return this.careManager != null && !this.careManager.isEmpty(); 1560 } 1561 1562 /** 1563 * @param value {@link #careManager} (The practitioner that is the care 1564 * manager/care coordinator for this patient.) 1565 */ 1566 public EpisodeOfCare setCareManager(Reference value) { 1567 this.careManager = value; 1568 return this; 1569 } 1570 1571 /** 1572 * @return {@link #careManager} The actual object that is the target of the 1573 * reference. The reference library doesn't populate this, but you can 1574 * use it to hold the resource if you resolve it. (The practitioner that 1575 * is the care manager/care coordinator for this patient.) 1576 */ 1577 public Resource getCareManagerTarget() { 1578 return this.careManagerTarget; 1579 } 1580 1581 /** 1582 * @param value {@link #careManager} The actual object that is the target of the 1583 * reference. The reference library doesn't use these, but you can 1584 * use it to hold the resource if you resolve it. (The practitioner 1585 * that is the care manager/care coordinator for this patient.) 1586 */ 1587 public EpisodeOfCare setCareManagerTarget(Resource value) { 1588 this.careManagerTarget = value; 1589 return this; 1590 } 1591 1592 /** 1593 * @return {@link #team} (The list of practitioners that may be facilitating 1594 * this episode of care for specific purposes.) 1595 */ 1596 public List<Reference> getTeam() { 1597 if (this.team == null) 1598 this.team = new ArrayList<Reference>(); 1599 return this.team; 1600 } 1601 1602 /** 1603 * @return Returns a reference to <code>this</code> for easy method chaining 1604 */ 1605 public EpisodeOfCare setTeam(List<Reference> theTeam) { 1606 this.team = theTeam; 1607 return this; 1608 } 1609 1610 public boolean hasTeam() { 1611 if (this.team == null) 1612 return false; 1613 for (Reference item : this.team) 1614 if (!item.isEmpty()) 1615 return true; 1616 return false; 1617 } 1618 1619 public Reference addTeam() { // 3 1620 Reference t = new Reference(); 1621 if (this.team == null) 1622 this.team = new ArrayList<Reference>(); 1623 this.team.add(t); 1624 return t; 1625 } 1626 1627 public EpisodeOfCare addTeam(Reference t) { // 3 1628 if (t == null) 1629 return this; 1630 if (this.team == null) 1631 this.team = new ArrayList<Reference>(); 1632 this.team.add(t); 1633 return this; 1634 } 1635 1636 /** 1637 * @return The first repetition of repeating field {@link #team}, creating it if 1638 * it does not already exist 1639 */ 1640 public Reference getTeamFirstRep() { 1641 if (getTeam().isEmpty()) { 1642 addTeam(); 1643 } 1644 return getTeam().get(0); 1645 } 1646 1647 /** 1648 * @deprecated Use Reference#setResource(IBaseResource) instead 1649 */ 1650 @Deprecated 1651 public List<CareTeam> getTeamTarget() { 1652 if (this.teamTarget == null) 1653 this.teamTarget = new ArrayList<CareTeam>(); 1654 return this.teamTarget; 1655 } 1656 1657 /** 1658 * @deprecated Use Reference#setResource(IBaseResource) instead 1659 */ 1660 @Deprecated 1661 public CareTeam addTeamTarget() { 1662 CareTeam r = new CareTeam(); 1663 if (this.teamTarget == null) 1664 this.teamTarget = new ArrayList<CareTeam>(); 1665 this.teamTarget.add(r); 1666 return r; 1667 } 1668 1669 /** 1670 * @return {@link #account} (The set of accounts that may be used for billing 1671 * for this EpisodeOfCare.) 1672 */ 1673 public List<Reference> getAccount() { 1674 if (this.account == null) 1675 this.account = new ArrayList<Reference>(); 1676 return this.account; 1677 } 1678 1679 /** 1680 * @return Returns a reference to <code>this</code> for easy method chaining 1681 */ 1682 public EpisodeOfCare setAccount(List<Reference> theAccount) { 1683 this.account = theAccount; 1684 return this; 1685 } 1686 1687 public boolean hasAccount() { 1688 if (this.account == null) 1689 return false; 1690 for (Reference item : this.account) 1691 if (!item.isEmpty()) 1692 return true; 1693 return false; 1694 } 1695 1696 public Reference addAccount() { // 3 1697 Reference t = new Reference(); 1698 if (this.account == null) 1699 this.account = new ArrayList<Reference>(); 1700 this.account.add(t); 1701 return t; 1702 } 1703 1704 public EpisodeOfCare addAccount(Reference t) { // 3 1705 if (t == null) 1706 return this; 1707 if (this.account == null) 1708 this.account = new ArrayList<Reference>(); 1709 this.account.add(t); 1710 return this; 1711 } 1712 1713 /** 1714 * @return The first repetition of repeating field {@link #account}, creating it 1715 * if it does not already exist 1716 */ 1717 public Reference getAccountFirstRep() { 1718 if (getAccount().isEmpty()) { 1719 addAccount(); 1720 } 1721 return getAccount().get(0); 1722 } 1723 1724 /** 1725 * @deprecated Use Reference#setResource(IBaseResource) instead 1726 */ 1727 @Deprecated 1728 public List<Account> getAccountTarget() { 1729 if (this.accountTarget == null) 1730 this.accountTarget = new ArrayList<Account>(); 1731 return this.accountTarget; 1732 } 1733 1734 /** 1735 * @deprecated Use Reference#setResource(IBaseResource) instead 1736 */ 1737 @Deprecated 1738 public Account addAccountTarget() { 1739 Account r = new Account(); 1740 if (this.accountTarget == null) 1741 this.accountTarget = new ArrayList<Account>(); 1742 this.accountTarget.add(r); 1743 return r; 1744 } 1745 1746 protected void listChildren(List<Property> children) { 1747 super.listChildren(children); 1748 children.add(new Property("identifier", "Identifier", 1749 "The EpisodeOfCare may be known by different identifiers for different contexts of use, such as when an external agency is tracking the Episode for funding purposes.", 1750 0, java.lang.Integer.MAX_VALUE, identifier)); 1751 children.add( 1752 new Property("status", "code", "planned | waitlist | active | onhold | finished | cancelled.", 0, 1, status)); 1753 children.add(new Property("statusHistory", "", 1754 "The history of statuses that the EpisodeOfCare has been through (without requiring processing the history of the resource).", 1755 0, java.lang.Integer.MAX_VALUE, statusHistory)); 1756 children.add(new Property("type", "CodeableConcept", 1757 "A classification of the type of episode of care; e.g. specialist referral, disease management, type of funded care.", 1758 0, java.lang.Integer.MAX_VALUE, type)); 1759 children.add(new Property("diagnosis", "", "The list of diagnosis relevant to this episode of care.", 0, 1760 java.lang.Integer.MAX_VALUE, diagnosis)); 1761 children.add(new Property("patient", "Reference(Patient)", "The patient who is the focus of this episode of care.", 1762 0, 1, patient)); 1763 children.add(new Property("managingOrganization", "Reference(Organization)", 1764 "The organization that has assumed the specific responsibilities for the specified duration.", 0, 1, 1765 managingOrganization)); 1766 children.add(new Property("period", "Period", 1767 "The interval during which the managing organization assumes the defined responsibility.", 0, 1, period)); 1768 children.add(new Property("referralRequest", "Reference(ServiceRequest)", 1769 "Referral Request(s) that are fulfilled by this EpisodeOfCare, incoming referrals.", 0, 1770 java.lang.Integer.MAX_VALUE, referralRequest)); 1771 children.add(new Property("careManager", "Reference(Practitioner|PractitionerRole)", 1772 "The practitioner that is the care manager/care coordinator for this patient.", 0, 1, careManager)); 1773 children.add(new Property("team", "Reference(CareTeam)", 1774 "The list of practitioners that may be facilitating this episode of care for specific purposes.", 0, 1775 java.lang.Integer.MAX_VALUE, team)); 1776 children.add(new Property("account", "Reference(Account)", 1777 "The set of accounts that may be used for billing for this EpisodeOfCare.", 0, java.lang.Integer.MAX_VALUE, 1778 account)); 1779 } 1780 1781 @Override 1782 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1783 switch (_hash) { 1784 case -1618432855: 1785 /* identifier */ return new Property("identifier", "Identifier", 1786 "The EpisodeOfCare may be known by different identifiers for different contexts of use, such as when an external agency is tracking the Episode for funding purposes.", 1787 0, java.lang.Integer.MAX_VALUE, identifier); 1788 case -892481550: 1789 /* status */ return new Property("status", "code", "planned | waitlist | active | onhold | finished | cancelled.", 1790 0, 1, status); 1791 case -986695614: 1792 /* statusHistory */ return new Property("statusHistory", "", 1793 "The history of statuses that the EpisodeOfCare has been through (without requiring processing the history of the resource).", 1794 0, java.lang.Integer.MAX_VALUE, statusHistory); 1795 case 3575610: 1796 /* type */ return new Property("type", "CodeableConcept", 1797 "A classification of the type of episode of care; e.g. specialist referral, disease management, type of funded care.", 1798 0, java.lang.Integer.MAX_VALUE, type); 1799 case 1196993265: 1800 /* diagnosis */ return new Property("diagnosis", "", "The list of diagnosis relevant to this episode of care.", 0, 1801 java.lang.Integer.MAX_VALUE, diagnosis); 1802 case -791418107: 1803 /* patient */ return new Property("patient", "Reference(Patient)", 1804 "The patient who is the focus of this episode of care.", 0, 1, patient); 1805 case -2058947787: 1806 /* managingOrganization */ return new Property("managingOrganization", "Reference(Organization)", 1807 "The organization that has assumed the specific responsibilities for the specified duration.", 0, 1, 1808 managingOrganization); 1809 case -991726143: 1810 /* period */ return new Property("period", "Period", 1811 "The interval during which the managing organization assumes the defined responsibility.", 0, 1, period); 1812 case -310299598: 1813 /* referralRequest */ return new Property("referralRequest", "Reference(ServiceRequest)", 1814 "Referral Request(s) that are fulfilled by this EpisodeOfCare, incoming referrals.", 0, 1815 java.lang.Integer.MAX_VALUE, referralRequest); 1816 case -1147746468: 1817 /* careManager */ return new Property("careManager", "Reference(Practitioner|PractitionerRole)", 1818 "The practitioner that is the care manager/care coordinator for this patient.", 0, 1, careManager); 1819 case 3555933: 1820 /* team */ return new Property("team", "Reference(CareTeam)", 1821 "The list of practitioners that may be facilitating this episode of care for specific purposes.", 0, 1822 java.lang.Integer.MAX_VALUE, team); 1823 case -1177318867: 1824 /* account */ return new Property("account", "Reference(Account)", 1825 "The set of accounts that may be used for billing for this EpisodeOfCare.", 0, java.lang.Integer.MAX_VALUE, 1826 account); 1827 default: 1828 return super.getNamedProperty(_hash, _name, _checkValid); 1829 } 1830 1831 } 1832 1833 @Override 1834 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1835 switch (hash) { 1836 case -1618432855: 1837 /* identifier */ return this.identifier == null ? new Base[0] 1838 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1839 case -892481550: 1840 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<EpisodeOfCareStatus> 1841 case -986695614: 1842 /* statusHistory */ return this.statusHistory == null ? new Base[0] 1843 : this.statusHistory.toArray(new Base[this.statusHistory.size()]); // EpisodeOfCareStatusHistoryComponent 1844 case 3575610: 1845 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 1846 case 1196993265: 1847 /* diagnosis */ return this.diagnosis == null ? new Base[0] 1848 : this.diagnosis.toArray(new Base[this.diagnosis.size()]); // DiagnosisComponent 1849 case -791418107: 1850 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 1851 case -2058947787: 1852 /* managingOrganization */ return this.managingOrganization == null ? new Base[0] 1853 : new Base[] { this.managingOrganization }; // Reference 1854 case -991726143: 1855 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 1856 case -310299598: 1857 /* referralRequest */ return this.referralRequest == null ? new Base[0] 1858 : this.referralRequest.toArray(new Base[this.referralRequest.size()]); // Reference 1859 case -1147746468: 1860 /* careManager */ return this.careManager == null ? new Base[0] : new Base[] { this.careManager }; // Reference 1861 case 3555933: 1862 /* team */ return this.team == null ? new Base[0] : this.team.toArray(new Base[this.team.size()]); // Reference 1863 case -1177318867: 1864 /* account */ return this.account == null ? new Base[0] : this.account.toArray(new Base[this.account.size()]); // Reference 1865 default: 1866 return super.getProperty(hash, name, checkValid); 1867 } 1868 1869 } 1870 1871 @Override 1872 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1873 switch (hash) { 1874 case -1618432855: // identifier 1875 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1876 return value; 1877 case -892481550: // status 1878 value = new EpisodeOfCareStatusEnumFactory().fromType(castToCode(value)); 1879 this.status = (Enumeration) value; // Enumeration<EpisodeOfCareStatus> 1880 return value; 1881 case -986695614: // statusHistory 1882 this.getStatusHistory().add((EpisodeOfCareStatusHistoryComponent) value); // EpisodeOfCareStatusHistoryComponent 1883 return value; 1884 case 3575610: // type 1885 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 1886 return value; 1887 case 1196993265: // diagnosis 1888 this.getDiagnosis().add((DiagnosisComponent) value); // DiagnosisComponent 1889 return value; 1890 case -791418107: // patient 1891 this.patient = castToReference(value); // Reference 1892 return value; 1893 case -2058947787: // managingOrganization 1894 this.managingOrganization = castToReference(value); // Reference 1895 return value; 1896 case -991726143: // period 1897 this.period = castToPeriod(value); // Period 1898 return value; 1899 case -310299598: // referralRequest 1900 this.getReferralRequest().add(castToReference(value)); // Reference 1901 return value; 1902 case -1147746468: // careManager 1903 this.careManager = castToReference(value); // Reference 1904 return value; 1905 case 3555933: // team 1906 this.getTeam().add(castToReference(value)); // Reference 1907 return value; 1908 case -1177318867: // account 1909 this.getAccount().add(castToReference(value)); // Reference 1910 return value; 1911 default: 1912 return super.setProperty(hash, name, value); 1913 } 1914 1915 } 1916 1917 @Override 1918 public Base setProperty(String name, Base value) throws FHIRException { 1919 if (name.equals("identifier")) { 1920 this.getIdentifier().add(castToIdentifier(value)); 1921 } else if (name.equals("status")) { 1922 value = new EpisodeOfCareStatusEnumFactory().fromType(castToCode(value)); 1923 this.status = (Enumeration) value; // Enumeration<EpisodeOfCareStatus> 1924 } else if (name.equals("statusHistory")) { 1925 this.getStatusHistory().add((EpisodeOfCareStatusHistoryComponent) value); 1926 } else if (name.equals("type")) { 1927 this.getType().add(castToCodeableConcept(value)); 1928 } else if (name.equals("diagnosis")) { 1929 this.getDiagnosis().add((DiagnosisComponent) value); 1930 } else if (name.equals("patient")) { 1931 this.patient = castToReference(value); // Reference 1932 } else if (name.equals("managingOrganization")) { 1933 this.managingOrganization = castToReference(value); // Reference 1934 } else if (name.equals("period")) { 1935 this.period = castToPeriod(value); // Period 1936 } else if (name.equals("referralRequest")) { 1937 this.getReferralRequest().add(castToReference(value)); 1938 } else if (name.equals("careManager")) { 1939 this.careManager = castToReference(value); // Reference 1940 } else if (name.equals("team")) { 1941 this.getTeam().add(castToReference(value)); 1942 } else if (name.equals("account")) { 1943 this.getAccount().add(castToReference(value)); 1944 } else 1945 return super.setProperty(name, value); 1946 return value; 1947 } 1948 1949 @Override 1950 public void removeChild(String name, Base value) throws FHIRException { 1951 if (name.equals("identifier")) { 1952 this.getIdentifier().remove(castToIdentifier(value)); 1953 } else if (name.equals("status")) { 1954 this.status = null; 1955 } else if (name.equals("statusHistory")) { 1956 this.getStatusHistory().remove((EpisodeOfCareStatusHistoryComponent) value); 1957 } else if (name.equals("type")) { 1958 this.getType().remove(castToCodeableConcept(value)); 1959 } else if (name.equals("diagnosis")) { 1960 this.getDiagnosis().remove((DiagnosisComponent) value); 1961 } else if (name.equals("patient")) { 1962 this.patient = null; 1963 } else if (name.equals("managingOrganization")) { 1964 this.managingOrganization = null; 1965 } else if (name.equals("period")) { 1966 this.period = null; 1967 } else if (name.equals("referralRequest")) { 1968 this.getReferralRequest().remove(castToReference(value)); 1969 } else if (name.equals("careManager")) { 1970 this.careManager = null; 1971 } else if (name.equals("team")) { 1972 this.getTeam().remove(castToReference(value)); 1973 } else if (name.equals("account")) { 1974 this.getAccount().remove(castToReference(value)); 1975 } else 1976 super.removeChild(name, value); 1977 1978 } 1979 1980 @Override 1981 public Base makeProperty(int hash, String name) throws FHIRException { 1982 switch (hash) { 1983 case -1618432855: 1984 return addIdentifier(); 1985 case -892481550: 1986 return getStatusElement(); 1987 case -986695614: 1988 return addStatusHistory(); 1989 case 3575610: 1990 return addType(); 1991 case 1196993265: 1992 return addDiagnosis(); 1993 case -791418107: 1994 return getPatient(); 1995 case -2058947787: 1996 return getManagingOrganization(); 1997 case -991726143: 1998 return getPeriod(); 1999 case -310299598: 2000 return addReferralRequest(); 2001 case -1147746468: 2002 return getCareManager(); 2003 case 3555933: 2004 return addTeam(); 2005 case -1177318867: 2006 return addAccount(); 2007 default: 2008 return super.makeProperty(hash, name); 2009 } 2010 2011 } 2012 2013 @Override 2014 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2015 switch (hash) { 2016 case -1618432855: 2017 /* identifier */ return new String[] { "Identifier" }; 2018 case -892481550: 2019 /* status */ return new String[] { "code" }; 2020 case -986695614: 2021 /* statusHistory */ return new String[] {}; 2022 case 3575610: 2023 /* type */ return new String[] { "CodeableConcept" }; 2024 case 1196993265: 2025 /* diagnosis */ return new String[] {}; 2026 case -791418107: 2027 /* patient */ return new String[] { "Reference" }; 2028 case -2058947787: 2029 /* managingOrganization */ return new String[] { "Reference" }; 2030 case -991726143: 2031 /* period */ return new String[] { "Period" }; 2032 case -310299598: 2033 /* referralRequest */ return new String[] { "Reference" }; 2034 case -1147746468: 2035 /* careManager */ return new String[] { "Reference" }; 2036 case 3555933: 2037 /* team */ return new String[] { "Reference" }; 2038 case -1177318867: 2039 /* account */ return new String[] { "Reference" }; 2040 default: 2041 return super.getTypesForProperty(hash, name); 2042 } 2043 2044 } 2045 2046 @Override 2047 public Base addChild(String name) throws FHIRException { 2048 if (name.equals("identifier")) { 2049 return addIdentifier(); 2050 } else if (name.equals("status")) { 2051 throw new FHIRException("Cannot call addChild on a singleton property EpisodeOfCare.status"); 2052 } else if (name.equals("statusHistory")) { 2053 return addStatusHistory(); 2054 } else if (name.equals("type")) { 2055 return addType(); 2056 } else if (name.equals("diagnosis")) { 2057 return addDiagnosis(); 2058 } else if (name.equals("patient")) { 2059 this.patient = new Reference(); 2060 return this.patient; 2061 } else if (name.equals("managingOrganization")) { 2062 this.managingOrganization = new Reference(); 2063 return this.managingOrganization; 2064 } else if (name.equals("period")) { 2065 this.period = new Period(); 2066 return this.period; 2067 } else if (name.equals("referralRequest")) { 2068 return addReferralRequest(); 2069 } else if (name.equals("careManager")) { 2070 this.careManager = new Reference(); 2071 return this.careManager; 2072 } else if (name.equals("team")) { 2073 return addTeam(); 2074 } else if (name.equals("account")) { 2075 return addAccount(); 2076 } else 2077 return super.addChild(name); 2078 } 2079 2080 public String fhirType() { 2081 return "EpisodeOfCare"; 2082 2083 } 2084 2085 public EpisodeOfCare copy() { 2086 EpisodeOfCare dst = new EpisodeOfCare(); 2087 copyValues(dst); 2088 return dst; 2089 } 2090 2091 public void copyValues(EpisodeOfCare dst) { 2092 super.copyValues(dst); 2093 if (identifier != null) { 2094 dst.identifier = new ArrayList<Identifier>(); 2095 for (Identifier i : identifier) 2096 dst.identifier.add(i.copy()); 2097 } 2098 ; 2099 dst.status = status == null ? null : status.copy(); 2100 if (statusHistory != null) { 2101 dst.statusHistory = new ArrayList<EpisodeOfCareStatusHistoryComponent>(); 2102 for (EpisodeOfCareStatusHistoryComponent i : statusHistory) 2103 dst.statusHistory.add(i.copy()); 2104 } 2105 ; 2106 if (type != null) { 2107 dst.type = new ArrayList<CodeableConcept>(); 2108 for (CodeableConcept i : type) 2109 dst.type.add(i.copy()); 2110 } 2111 ; 2112 if (diagnosis != null) { 2113 dst.diagnosis = new ArrayList<DiagnosisComponent>(); 2114 for (DiagnosisComponent i : diagnosis) 2115 dst.diagnosis.add(i.copy()); 2116 } 2117 ; 2118 dst.patient = patient == null ? null : patient.copy(); 2119 dst.managingOrganization = managingOrganization == null ? null : managingOrganization.copy(); 2120 dst.period = period == null ? null : period.copy(); 2121 if (referralRequest != null) { 2122 dst.referralRequest = new ArrayList<Reference>(); 2123 for (Reference i : referralRequest) 2124 dst.referralRequest.add(i.copy()); 2125 } 2126 ; 2127 dst.careManager = careManager == null ? null : careManager.copy(); 2128 if (team != null) { 2129 dst.team = new ArrayList<Reference>(); 2130 for (Reference i : team) 2131 dst.team.add(i.copy()); 2132 } 2133 ; 2134 if (account != null) { 2135 dst.account = new ArrayList<Reference>(); 2136 for (Reference i : account) 2137 dst.account.add(i.copy()); 2138 } 2139 ; 2140 } 2141 2142 protected EpisodeOfCare typedCopy() { 2143 return copy(); 2144 } 2145 2146 @Override 2147 public boolean equalsDeep(Base other_) { 2148 if (!super.equalsDeep(other_)) 2149 return false; 2150 if (!(other_ instanceof EpisodeOfCare)) 2151 return false; 2152 EpisodeOfCare o = (EpisodeOfCare) other_; 2153 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 2154 && compareDeep(statusHistory, o.statusHistory, true) && compareDeep(type, o.type, true) 2155 && compareDeep(diagnosis, o.diagnosis, true) && compareDeep(patient, o.patient, true) 2156 && compareDeep(managingOrganization, o.managingOrganization, true) && compareDeep(period, o.period, true) 2157 && compareDeep(referralRequest, o.referralRequest, true) && compareDeep(careManager, o.careManager, true) 2158 && compareDeep(team, o.team, true) && compareDeep(account, o.account, true); 2159 } 2160 2161 @Override 2162 public boolean equalsShallow(Base other_) { 2163 if (!super.equalsShallow(other_)) 2164 return false; 2165 if (!(other_ instanceof EpisodeOfCare)) 2166 return false; 2167 EpisodeOfCare o = (EpisodeOfCare) other_; 2168 return compareValues(status, o.status, true); 2169 } 2170 2171 public boolean isEmpty() { 2172 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, statusHistory, type, diagnosis, 2173 patient, managingOrganization, period, referralRequest, careManager, team, account); 2174 } 2175 2176 @Override 2177 public ResourceType getResourceType() { 2178 return ResourceType.EpisodeOfCare; 2179 } 2180 2181 /** 2182 * Search parameter: <b>date</b> 2183 * <p> 2184 * Description: <b>The provided date search value falls within the episode of 2185 * care's period</b><br> 2186 * Type: <b>date</b><br> 2187 * Path: <b>EpisodeOfCare.period</b><br> 2188 * </p> 2189 */ 2190 @SearchParamDefinition(name = "date", path = "EpisodeOfCare.period", description = "The provided date search value falls within the episode of care's period", type = "date") 2191 public static final String SP_DATE = "date"; 2192 /** 2193 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2194 * <p> 2195 * Description: <b>The provided date search value falls within the episode of 2196 * care's period</b><br> 2197 * Type: <b>date</b><br> 2198 * Path: <b>EpisodeOfCare.period</b><br> 2199 * </p> 2200 */ 2201 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2202 SP_DATE); 2203 2204 /** 2205 * Search parameter: <b>identifier</b> 2206 * <p> 2207 * Description: <b>Business Identifier(s) relevant for this 2208 * EpisodeOfCare</b><br> 2209 * Type: <b>token</b><br> 2210 * Path: <b>EpisodeOfCare.identifier</b><br> 2211 * </p> 2212 */ 2213 @SearchParamDefinition(name = "identifier", path = "EpisodeOfCare.identifier", description = "Business Identifier(s) relevant for this EpisodeOfCare", type = "token") 2214 public static final String SP_IDENTIFIER = "identifier"; 2215 /** 2216 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2217 * <p> 2218 * Description: <b>Business Identifier(s) relevant for this 2219 * EpisodeOfCare</b><br> 2220 * Type: <b>token</b><br> 2221 * Path: <b>EpisodeOfCare.identifier</b><br> 2222 * </p> 2223 */ 2224 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2225 SP_IDENTIFIER); 2226 2227 /** 2228 * Search parameter: <b>condition</b> 2229 * <p> 2230 * Description: <b>Conditions/problems/diagnoses this episode of care is 2231 * for</b><br> 2232 * Type: <b>reference</b><br> 2233 * Path: <b>EpisodeOfCare.diagnosis.condition</b><br> 2234 * </p> 2235 */ 2236 @SearchParamDefinition(name = "condition", path = "EpisodeOfCare.diagnosis.condition", description = "Conditions/problems/diagnoses this episode of care is for", type = "reference", target = { 2237 Condition.class }) 2238 public static final String SP_CONDITION = "condition"; 2239 /** 2240 * <b>Fluent Client</b> search parameter constant for <b>condition</b> 2241 * <p> 2242 * Description: <b>Conditions/problems/diagnoses this episode of care is 2243 * for</b><br> 2244 * Type: <b>reference</b><br> 2245 * Path: <b>EpisodeOfCare.diagnosis.condition</b><br> 2246 * </p> 2247 */ 2248 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONDITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2249 SP_CONDITION); 2250 2251 /** 2252 * Constant for fluent queries to be used to add include statements. Specifies 2253 * the path value of "<b>EpisodeOfCare:condition</b>". 2254 */ 2255 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONDITION = new ca.uhn.fhir.model.api.Include( 2256 "EpisodeOfCare:condition").toLocked(); 2257 2258 /** 2259 * Search parameter: <b>patient</b> 2260 * <p> 2261 * Description: <b>The patient who is the focus of this episode of care</b><br> 2262 * Type: <b>reference</b><br> 2263 * Path: <b>EpisodeOfCare.patient</b><br> 2264 * </p> 2265 */ 2266 @SearchParamDefinition(name = "patient", path = "EpisodeOfCare.patient", description = "The patient who is the focus of this episode of care", type = "reference", providesMembershipIn = { 2267 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 2268 public static final String SP_PATIENT = "patient"; 2269 /** 2270 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2271 * <p> 2272 * Description: <b>The patient who is the focus of this episode of care</b><br> 2273 * Type: <b>reference</b><br> 2274 * Path: <b>EpisodeOfCare.patient</b><br> 2275 * </p> 2276 */ 2277 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2278 SP_PATIENT); 2279 2280 /** 2281 * Constant for fluent queries to be used to add include statements. Specifies 2282 * the path value of "<b>EpisodeOfCare:patient</b>". 2283 */ 2284 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 2285 "EpisodeOfCare:patient").toLocked(); 2286 2287 /** 2288 * Search parameter: <b>organization</b> 2289 * <p> 2290 * Description: <b>The organization that has assumed the specific 2291 * responsibilities of this EpisodeOfCare</b><br> 2292 * Type: <b>reference</b><br> 2293 * Path: <b>EpisodeOfCare.managingOrganization</b><br> 2294 * </p> 2295 */ 2296 @SearchParamDefinition(name = "organization", path = "EpisodeOfCare.managingOrganization", description = "The organization that has assumed the specific responsibilities of this EpisodeOfCare", type = "reference", target = { 2297 Organization.class }) 2298 public static final String SP_ORGANIZATION = "organization"; 2299 /** 2300 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 2301 * <p> 2302 * Description: <b>The organization that has assumed the specific 2303 * responsibilities of this EpisodeOfCare</b><br> 2304 * Type: <b>reference</b><br> 2305 * Path: <b>EpisodeOfCare.managingOrganization</b><br> 2306 * </p> 2307 */ 2308 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2309 SP_ORGANIZATION); 2310 2311 /** 2312 * Constant for fluent queries to be used to add include statements. Specifies 2313 * the path value of "<b>EpisodeOfCare:organization</b>". 2314 */ 2315 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include( 2316 "EpisodeOfCare:organization").toLocked(); 2317 2318 /** 2319 * Search parameter: <b>type</b> 2320 * <p> 2321 * Description: <b>Type/class - e.g. specialist referral, disease 2322 * management</b><br> 2323 * Type: <b>token</b><br> 2324 * Path: <b>EpisodeOfCare.type</b><br> 2325 * </p> 2326 */ 2327 @SearchParamDefinition(name = "type", path = "EpisodeOfCare.type", description = "Type/class - e.g. specialist referral, disease management", type = "token") 2328 public static final String SP_TYPE = "type"; 2329 /** 2330 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2331 * <p> 2332 * Description: <b>Type/class - e.g. specialist referral, disease 2333 * management</b><br> 2334 * Type: <b>token</b><br> 2335 * Path: <b>EpisodeOfCare.type</b><br> 2336 * </p> 2337 */ 2338 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2339 SP_TYPE); 2340 2341 /** 2342 * Search parameter: <b>care-manager</b> 2343 * <p> 2344 * Description: <b>Care manager/care coordinator for the patient</b><br> 2345 * Type: <b>reference</b><br> 2346 * Path: <b>EpisodeOfCare.careManager</b><br> 2347 * </p> 2348 */ 2349 @SearchParamDefinition(name = "care-manager", path = "EpisodeOfCare.careManager.where(resolve() is Practitioner)", description = "Care manager/care coordinator for the patient", type = "reference", providesMembershipIn = { 2350 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Practitioner.class }) 2351 public static final String SP_CARE_MANAGER = "care-manager"; 2352 /** 2353 * <b>Fluent Client</b> search parameter constant for <b>care-manager</b> 2354 * <p> 2355 * Description: <b>Care manager/care coordinator for the patient</b><br> 2356 * Type: <b>reference</b><br> 2357 * Path: <b>EpisodeOfCare.careManager</b><br> 2358 * </p> 2359 */ 2360 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CARE_MANAGER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2361 SP_CARE_MANAGER); 2362 2363 /** 2364 * Constant for fluent queries to be used to add include statements. Specifies 2365 * the path value of "<b>EpisodeOfCare:care-manager</b>". 2366 */ 2367 public static final ca.uhn.fhir.model.api.Include INCLUDE_CARE_MANAGER = new ca.uhn.fhir.model.api.Include( 2368 "EpisodeOfCare:care-manager").toLocked(); 2369 2370 /** 2371 * Search parameter: <b>status</b> 2372 * <p> 2373 * Description: <b>The current status of the Episode of Care as provided (does 2374 * not check the status history collection)</b><br> 2375 * Type: <b>token</b><br> 2376 * Path: <b>EpisodeOfCare.status</b><br> 2377 * </p> 2378 */ 2379 @SearchParamDefinition(name = "status", path = "EpisodeOfCare.status", description = "The current status of the Episode of Care as provided (does not check the status history collection)", type = "token") 2380 public static final String SP_STATUS = "status"; 2381 /** 2382 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2383 * <p> 2384 * Description: <b>The current status of the Episode of Care as provided (does 2385 * not check the status history collection)</b><br> 2386 * Type: <b>token</b><br> 2387 * Path: <b>EpisodeOfCare.status</b><br> 2388 * </p> 2389 */ 2390 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2391 SP_STATUS); 2392 2393 /** 2394 * Search parameter: <b>incoming-referral</b> 2395 * <p> 2396 * Description: <b>Incoming Referral Request</b><br> 2397 * Type: <b>reference</b><br> 2398 * Path: <b>EpisodeOfCare.referralRequest</b><br> 2399 * </p> 2400 */ 2401 @SearchParamDefinition(name = "incoming-referral", path = "EpisodeOfCare.referralRequest", description = "Incoming Referral Request", type = "reference", target = { 2402 ServiceRequest.class }) 2403 public static final String SP_INCOMING_REFERRAL = "incoming-referral"; 2404 /** 2405 * <b>Fluent Client</b> search parameter constant for <b>incoming-referral</b> 2406 * <p> 2407 * Description: <b>Incoming Referral Request</b><br> 2408 * Type: <b>reference</b><br> 2409 * Path: <b>EpisodeOfCare.referralRequest</b><br> 2410 * </p> 2411 */ 2412 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INCOMING_REFERRAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2413 SP_INCOMING_REFERRAL); 2414 2415 /** 2416 * Constant for fluent queries to be used to add include statements. Specifies 2417 * the path value of "<b>EpisodeOfCare:incoming-referral</b>". 2418 */ 2419 public static final ca.uhn.fhir.model.api.Include INCLUDE_INCOMING_REFERRAL = new ca.uhn.fhir.model.api.Include( 2420 "EpisodeOfCare:incoming-referral").toLocked(); 2421 2422}