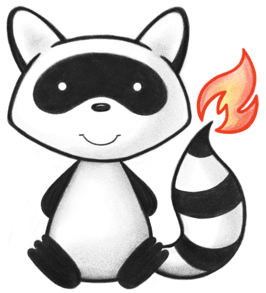
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047 048/** 049 * The EventDefinition resource provides a reusable description of when a 050 * particular event can occur. 051 */ 052@ResourceDef(name = "EventDefinition", profile = "http://hl7.org/fhir/StructureDefinition/EventDefinition") 053@ChildOrder(names = { "url", "identifier", "version", "name", "title", "subtitle", "status", "experimental", 054 "subject[x]", "date", "publisher", "contact", "description", "useContext", "jurisdiction", "purpose", "usage", 055 "copyright", "approvalDate", "lastReviewDate", "effectivePeriod", "topic", "author", "editor", "reviewer", 056 "endorser", "relatedArtifact", "trigger" }) 057public class EventDefinition extends MetadataResource { 058 059 /** 060 * A formal identifier that is used to identify this event definition when it is 061 * represented in other formats, or referenced in a specification, model, design 062 * or an instance. 063 */ 064 @Child(name = "identifier", type = { 065 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 066 @Description(shortDefinition = "Additional identifier for the event definition", formalDefinition = "A formal identifier that is used to identify this event definition when it is represented in other formats, or referenced in a specification, model, design or an instance.") 067 protected List<Identifier> identifier; 068 069 /** 070 * An explanatory or alternate title for the event definition giving additional 071 * information about its content. 072 */ 073 @Child(name = "subtitle", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 074 @Description(shortDefinition = "Subordinate title of the event definition", formalDefinition = "An explanatory or alternate title for the event definition giving additional information about its content.") 075 protected StringType subtitle; 076 077 /** 078 * A code or group definition that describes the intended subject of the event 079 * definition. 080 */ 081 @Child(name = "subject", type = { CodeableConcept.class, 082 Group.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 083 @Description(shortDefinition = "Type of individual the event definition is focused on", formalDefinition = "A code or group definition that describes the intended subject of the event definition.") 084 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/subject-type") 085 protected Type subject; 086 087 /** 088 * Explanation of why this event definition is needed and why it has been 089 * designed as it has. 090 */ 091 @Child(name = "purpose", type = { 092 MarkdownType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 093 @Description(shortDefinition = "Why this event definition is defined", formalDefinition = "Explanation of why this event definition is needed and why it has been designed as it has.") 094 protected MarkdownType purpose; 095 096 /** 097 * A detailed description of how the event definition is used from a clinical 098 * perspective. 099 */ 100 @Child(name = "usage", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 101 @Description(shortDefinition = "Describes the clinical usage of the event definition", formalDefinition = "A detailed description of how the event definition is used from a clinical perspective.") 102 protected StringType usage; 103 104 /** 105 * A copyright statement relating to the event definition and/or its contents. 106 * Copyright statements are generally legal restrictions on the use and 107 * publishing of the event definition. 108 */ 109 @Child(name = "copyright", type = { 110 MarkdownType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 111 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the event definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the event definition.") 112 protected MarkdownType copyright; 113 114 /** 115 * The date on which the resource content was approved by the publisher. 116 * Approval happens once when the content is officially approved for usage. 117 */ 118 @Child(name = "approvalDate", type = { 119 DateType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 120 @Description(shortDefinition = "When the event definition was approved by publisher", formalDefinition = "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.") 121 protected DateType approvalDate; 122 123 /** 124 * The date on which the resource content was last reviewed. Review happens 125 * periodically after approval but does not change the original approval date. 126 */ 127 @Child(name = "lastReviewDate", type = { 128 DateType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 129 @Description(shortDefinition = "When the event definition was last reviewed", formalDefinition = "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.") 130 protected DateType lastReviewDate; 131 132 /** 133 * The period during which the event definition content was or is planned to be 134 * in active use. 135 */ 136 @Child(name = "effectivePeriod", type = { 137 Period.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 138 @Description(shortDefinition = "When the event definition is expected to be used", formalDefinition = "The period during which the event definition content was or is planned to be in active use.") 139 protected Period effectivePeriod; 140 141 /** 142 * Descriptive topics related to the module. Topics provide a high-level 143 * categorization of the module that can be useful for filtering and searching. 144 */ 145 @Child(name = "topic", type = { 146 CodeableConcept.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 147 @Description(shortDefinition = "E.g. Education, Treatment, Assessment, etc.", formalDefinition = "Descriptive topics related to the module. Topics provide a high-level categorization of the module that can be useful for filtering and searching.") 148 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/definition-topic") 149 protected List<CodeableConcept> topic; 150 151 /** 152 * An individiual or organization primarily involved in the creation and 153 * maintenance of the content. 154 */ 155 @Child(name = "author", type = { 156 ContactDetail.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 157 @Description(shortDefinition = "Who authored the content", formalDefinition = "An individiual or organization primarily involved in the creation and maintenance of the content.") 158 protected List<ContactDetail> author; 159 160 /** 161 * An individual or organization primarily responsible for internal coherence of 162 * the content. 163 */ 164 @Child(name = "editor", type = { 165 ContactDetail.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 166 @Description(shortDefinition = "Who edited the content", formalDefinition = "An individual or organization primarily responsible for internal coherence of the content.") 167 protected List<ContactDetail> editor; 168 169 /** 170 * An individual or organization primarily responsible for review of some aspect 171 * of the content. 172 */ 173 @Child(name = "reviewer", type = { 174 ContactDetail.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 175 @Description(shortDefinition = "Who reviewed the content", formalDefinition = "An individual or organization primarily responsible for review of some aspect of the content.") 176 protected List<ContactDetail> reviewer; 177 178 /** 179 * An individual or organization responsible for officially endorsing the 180 * content for use in some setting. 181 */ 182 @Child(name = "endorser", type = { 183 ContactDetail.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 184 @Description(shortDefinition = "Who endorsed the content", formalDefinition = "An individual or organization responsible for officially endorsing the content for use in some setting.") 185 protected List<ContactDetail> endorser; 186 187 /** 188 * Related resources such as additional documentation, justification, or 189 * bibliographic references. 190 */ 191 @Child(name = "relatedArtifact", type = { 192 RelatedArtifact.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 193 @Description(shortDefinition = "Additional documentation, citations, etc.", formalDefinition = "Related resources such as additional documentation, justification, or bibliographic references.") 194 protected List<RelatedArtifact> relatedArtifact; 195 196 /** 197 * The trigger element defines when the event occurs. If more than one trigger 198 * condition is specified, the event fires whenever any one of the trigger 199 * conditions is met. 200 */ 201 @Child(name = "trigger", type = { 202 TriggerDefinition.class }, order = 15, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 203 @Description(shortDefinition = "\"when\" the event occurs (multiple = 'or')", formalDefinition = "The trigger element defines when the event occurs. If more than one trigger condition is specified, the event fires whenever any one of the trigger conditions is met.") 204 protected List<TriggerDefinition> trigger; 205 206 private static final long serialVersionUID = 1022506246L; 207 208 /** 209 * Constructor 210 */ 211 public EventDefinition() { 212 super(); 213 } 214 215 /** 216 * Constructor 217 */ 218 public EventDefinition(Enumeration<PublicationStatus> status) { 219 super(); 220 this.status = status; 221 } 222 223 /** 224 * @return {@link #url} (An absolute URI that is used to identify this event 225 * definition when it is referenced in a specification, model, design or 226 * an instance; also called its canonical identifier. This SHOULD be 227 * globally unique and SHOULD be a literal address at which at which an 228 * authoritative instance of this event definition is (or will be) 229 * published. This URL can be the target of a canonical reference. It 230 * SHALL remain the same when the event definition is stored on 231 * different servers.). This is the underlying object with id, value and 232 * extensions. The accessor "getUrl" gives direct access to the value 233 */ 234 public UriType getUrlElement() { 235 if (this.url == null) 236 if (Configuration.errorOnAutoCreate()) 237 throw new Error("Attempt to auto-create EventDefinition.url"); 238 else if (Configuration.doAutoCreate()) 239 this.url = new UriType(); // bb 240 return this.url; 241 } 242 243 public boolean hasUrlElement() { 244 return this.url != null && !this.url.isEmpty(); 245 } 246 247 public boolean hasUrl() { 248 return this.url != null && !this.url.isEmpty(); 249 } 250 251 /** 252 * @param value {@link #url} (An absolute URI that is used to identify this 253 * event definition when it is referenced in a specification, 254 * model, design or an instance; also called its canonical 255 * identifier. This SHOULD be globally unique and SHOULD be a 256 * literal address at which at which an authoritative instance of 257 * this event definition is (or will be) published. This URL can be 258 * the target of a canonical reference. It SHALL remain the same 259 * when the event definition is stored on different servers.). This 260 * is the underlying object with id, value and extensions. The 261 * accessor "getUrl" gives direct access to the value 262 */ 263 public EventDefinition setUrlElement(UriType value) { 264 this.url = value; 265 return this; 266 } 267 268 /** 269 * @return An absolute URI that is used to identify this event definition when 270 * it is referenced in a specification, model, design or an instance; 271 * also called its canonical identifier. This SHOULD be globally unique 272 * and SHOULD be a literal address at which at which an authoritative 273 * instance of this event definition is (or will be) published. This URL 274 * can be the target of a canonical reference. It SHALL remain the same 275 * when the event definition is stored on different servers. 276 */ 277 public String getUrl() { 278 return this.url == null ? null : this.url.getValue(); 279 } 280 281 /** 282 * @param value An absolute URI that is used to identify this event definition 283 * when it is referenced in a specification, model, design or an 284 * instance; also called its canonical identifier. This SHOULD be 285 * globally unique and SHOULD be a literal address at which at 286 * which an authoritative instance of this event definition is (or 287 * will be) published. This URL can be the target of a canonical 288 * reference. It SHALL remain the same when the event definition is 289 * stored on different servers. 290 */ 291 public EventDefinition setUrl(String value) { 292 if (Utilities.noString(value)) 293 this.url = null; 294 else { 295 if (this.url == null) 296 this.url = new UriType(); 297 this.url.setValue(value); 298 } 299 return this; 300 } 301 302 /** 303 * @return {@link #identifier} (A formal identifier that is used to identify 304 * this event definition when it is represented in other formats, or 305 * referenced in a specification, model, design or an instance.) 306 */ 307 public List<Identifier> getIdentifier() { 308 if (this.identifier == null) 309 this.identifier = new ArrayList<Identifier>(); 310 return this.identifier; 311 } 312 313 /** 314 * @return Returns a reference to <code>this</code> for easy method chaining 315 */ 316 public EventDefinition setIdentifier(List<Identifier> theIdentifier) { 317 this.identifier = theIdentifier; 318 return this; 319 } 320 321 public boolean hasIdentifier() { 322 if (this.identifier == null) 323 return false; 324 for (Identifier item : this.identifier) 325 if (!item.isEmpty()) 326 return true; 327 return false; 328 } 329 330 public Identifier addIdentifier() { // 3 331 Identifier t = new Identifier(); 332 if (this.identifier == null) 333 this.identifier = new ArrayList<Identifier>(); 334 this.identifier.add(t); 335 return t; 336 } 337 338 public EventDefinition addIdentifier(Identifier t) { // 3 339 if (t == null) 340 return this; 341 if (this.identifier == null) 342 this.identifier = new ArrayList<Identifier>(); 343 this.identifier.add(t); 344 return this; 345 } 346 347 /** 348 * @return The first repetition of repeating field {@link #identifier}, creating 349 * it if it does not already exist 350 */ 351 public Identifier getIdentifierFirstRep() { 352 if (getIdentifier().isEmpty()) { 353 addIdentifier(); 354 } 355 return getIdentifier().get(0); 356 } 357 358 /** 359 * @return {@link #version} (The identifier that is used to identify this 360 * version of the event definition when it is referenced in a 361 * specification, model, design or instance. This is an arbitrary value 362 * managed by the event definition author and is not expected to be 363 * globally unique. For example, it might be a timestamp (e.g. yyyymmdd) 364 * if a managed version is not available. There is also no expectation 365 * that versions can be placed in a lexicographical sequence.). This is 366 * the underlying object with id, value and extensions. The accessor 367 * "getVersion" gives direct access to the value 368 */ 369 public StringType getVersionElement() { 370 if (this.version == null) 371 if (Configuration.errorOnAutoCreate()) 372 throw new Error("Attempt to auto-create EventDefinition.version"); 373 else if (Configuration.doAutoCreate()) 374 this.version = new StringType(); // bb 375 return this.version; 376 } 377 378 public boolean hasVersionElement() { 379 return this.version != null && !this.version.isEmpty(); 380 } 381 382 public boolean hasVersion() { 383 return this.version != null && !this.version.isEmpty(); 384 } 385 386 /** 387 * @param value {@link #version} (The identifier that is used to identify this 388 * version of the event definition when it is referenced in a 389 * specification, model, design or instance. This is an arbitrary 390 * value managed by the event definition author and is not expected 391 * to be globally unique. For example, it might be a timestamp 392 * (e.g. yyyymmdd) if a managed version is not available. There is 393 * also no expectation that versions can be placed in a 394 * lexicographical sequence.). This is the underlying object with 395 * id, value and extensions. The accessor "getVersion" gives direct 396 * access to the value 397 */ 398 public EventDefinition setVersionElement(StringType value) { 399 this.version = value; 400 return this; 401 } 402 403 /** 404 * @return The identifier that is used to identify this version of the event 405 * definition when it is referenced in a specification, model, design or 406 * instance. This is an arbitrary value managed by the event definition 407 * author and is not expected to be globally unique. For example, it 408 * might be a timestamp (e.g. yyyymmdd) if a managed version is not 409 * available. There is also no expectation that versions can be placed 410 * in a lexicographical sequence. 411 */ 412 public String getVersion() { 413 return this.version == null ? null : this.version.getValue(); 414 } 415 416 /** 417 * @param value The identifier that is used to identify this version of the 418 * event definition when it is referenced in a specification, 419 * model, design or instance. This is an arbitrary value managed by 420 * the event definition author and is not expected to be globally 421 * unique. For example, it might be a timestamp (e.g. yyyymmdd) if 422 * a managed version is not available. There is also no expectation 423 * that versions can be placed in a lexicographical sequence. 424 */ 425 public EventDefinition setVersion(String value) { 426 if (Utilities.noString(value)) 427 this.version = null; 428 else { 429 if (this.version == null) 430 this.version = new StringType(); 431 this.version.setValue(value); 432 } 433 return this; 434 } 435 436 /** 437 * @return {@link #name} (A natural language name identifying the event 438 * definition. This name should be usable as an identifier for the 439 * module by machine processing applications such as code generation.). 440 * This is the underlying object with id, value and extensions. The 441 * accessor "getName" gives direct access to the value 442 */ 443 public StringType getNameElement() { 444 if (this.name == null) 445 if (Configuration.errorOnAutoCreate()) 446 throw new Error("Attempt to auto-create EventDefinition.name"); 447 else if (Configuration.doAutoCreate()) 448 this.name = new StringType(); // bb 449 return this.name; 450 } 451 452 public boolean hasNameElement() { 453 return this.name != null && !this.name.isEmpty(); 454 } 455 456 public boolean hasName() { 457 return this.name != null && !this.name.isEmpty(); 458 } 459 460 /** 461 * @param value {@link #name} (A natural language name identifying the event 462 * definition. This name should be usable as an identifier for the 463 * module by machine processing applications such as code 464 * generation.). This is the underlying object with id, value and 465 * extensions. The accessor "getName" gives direct access to the 466 * value 467 */ 468 public EventDefinition setNameElement(StringType value) { 469 this.name = value; 470 return this; 471 } 472 473 /** 474 * @return A natural language name identifying the event definition. This name 475 * should be usable as an identifier for the module by machine 476 * processing applications such as code generation. 477 */ 478 public String getName() { 479 return this.name == null ? null : this.name.getValue(); 480 } 481 482 /** 483 * @param value A natural language name identifying the event definition. This 484 * name should be usable as an identifier for the module by machine 485 * processing applications such as code generation. 486 */ 487 public EventDefinition setName(String value) { 488 if (Utilities.noString(value)) 489 this.name = null; 490 else { 491 if (this.name == null) 492 this.name = new StringType(); 493 this.name.setValue(value); 494 } 495 return this; 496 } 497 498 /** 499 * @return {@link #title} (A short, descriptive, user-friendly title for the 500 * event definition.). This is the underlying object with id, value and 501 * extensions. The accessor "getTitle" gives direct access to the value 502 */ 503 public StringType getTitleElement() { 504 if (this.title == null) 505 if (Configuration.errorOnAutoCreate()) 506 throw new Error("Attempt to auto-create EventDefinition.title"); 507 else if (Configuration.doAutoCreate()) 508 this.title = new StringType(); // bb 509 return this.title; 510 } 511 512 public boolean hasTitleElement() { 513 return this.title != null && !this.title.isEmpty(); 514 } 515 516 public boolean hasTitle() { 517 return this.title != null && !this.title.isEmpty(); 518 } 519 520 /** 521 * @param value {@link #title} (A short, descriptive, user-friendly title for 522 * the event definition.). This is the underlying object with id, 523 * value and extensions. The accessor "getTitle" gives direct 524 * access to the value 525 */ 526 public EventDefinition setTitleElement(StringType value) { 527 this.title = value; 528 return this; 529 } 530 531 /** 532 * @return A short, descriptive, user-friendly title for the event definition. 533 */ 534 public String getTitle() { 535 return this.title == null ? null : this.title.getValue(); 536 } 537 538 /** 539 * @param value A short, descriptive, user-friendly title for the event 540 * definition. 541 */ 542 public EventDefinition setTitle(String value) { 543 if (Utilities.noString(value)) 544 this.title = null; 545 else { 546 if (this.title == null) 547 this.title = new StringType(); 548 this.title.setValue(value); 549 } 550 return this; 551 } 552 553 /** 554 * @return {@link #subtitle} (An explanatory or alternate title for the event 555 * definition giving additional information about its content.). This is 556 * the underlying object with id, value and extensions. The accessor 557 * "getSubtitle" gives direct access to the value 558 */ 559 public StringType getSubtitleElement() { 560 if (this.subtitle == null) 561 if (Configuration.errorOnAutoCreate()) 562 throw new Error("Attempt to auto-create EventDefinition.subtitle"); 563 else if (Configuration.doAutoCreate()) 564 this.subtitle = new StringType(); // bb 565 return this.subtitle; 566 } 567 568 public boolean hasSubtitleElement() { 569 return this.subtitle != null && !this.subtitle.isEmpty(); 570 } 571 572 public boolean hasSubtitle() { 573 return this.subtitle != null && !this.subtitle.isEmpty(); 574 } 575 576 /** 577 * @param value {@link #subtitle} (An explanatory or alternate title for the 578 * event definition giving additional information about its 579 * content.). This is the underlying object with id, value and 580 * extensions. The accessor "getSubtitle" gives direct access to 581 * the value 582 */ 583 public EventDefinition setSubtitleElement(StringType value) { 584 this.subtitle = value; 585 return this; 586 } 587 588 /** 589 * @return An explanatory or alternate title for the event definition giving 590 * additional information about its content. 591 */ 592 public String getSubtitle() { 593 return this.subtitle == null ? null : this.subtitle.getValue(); 594 } 595 596 /** 597 * @param value An explanatory or alternate title for the event definition 598 * giving additional information about its content. 599 */ 600 public EventDefinition setSubtitle(String value) { 601 if (Utilities.noString(value)) 602 this.subtitle = null; 603 else { 604 if (this.subtitle == null) 605 this.subtitle = new StringType(); 606 this.subtitle.setValue(value); 607 } 608 return this; 609 } 610 611 /** 612 * @return {@link #status} (The status of this event definition. Enables 613 * tracking the life-cycle of the content.). This is the underlying 614 * object with id, value and extensions. The accessor "getStatus" gives 615 * direct access to the value 616 */ 617 public Enumeration<PublicationStatus> getStatusElement() { 618 if (this.status == null) 619 if (Configuration.errorOnAutoCreate()) 620 throw new Error("Attempt to auto-create EventDefinition.status"); 621 else if (Configuration.doAutoCreate()) 622 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 623 return this.status; 624 } 625 626 public boolean hasStatusElement() { 627 return this.status != null && !this.status.isEmpty(); 628 } 629 630 public boolean hasStatus() { 631 return this.status != null && !this.status.isEmpty(); 632 } 633 634 /** 635 * @param value {@link #status} (The status of this event definition. Enables 636 * tracking the life-cycle of the content.). This is the underlying 637 * object with id, value and extensions. The accessor "getStatus" 638 * gives direct access to the value 639 */ 640 public EventDefinition setStatusElement(Enumeration<PublicationStatus> value) { 641 this.status = value; 642 return this; 643 } 644 645 /** 646 * @return The status of this event definition. Enables tracking the life-cycle 647 * of the content. 648 */ 649 public PublicationStatus getStatus() { 650 return this.status == null ? null : this.status.getValue(); 651 } 652 653 /** 654 * @param value The status of this event definition. Enables tracking the 655 * life-cycle of the content. 656 */ 657 public EventDefinition setStatus(PublicationStatus value) { 658 if (this.status == null) 659 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 660 this.status.setValue(value); 661 return this; 662 } 663 664 /** 665 * @return {@link #experimental} (A Boolean value to indicate that this event 666 * definition is authored for testing purposes (or 667 * education/evaluation/marketing) and is not intended to be used for 668 * genuine usage.). This is the underlying object with id, value and 669 * extensions. The accessor "getExperimental" gives direct access to the 670 * value 671 */ 672 public BooleanType getExperimentalElement() { 673 if (this.experimental == null) 674 if (Configuration.errorOnAutoCreate()) 675 throw new Error("Attempt to auto-create EventDefinition.experimental"); 676 else if (Configuration.doAutoCreate()) 677 this.experimental = new BooleanType(); // bb 678 return this.experimental; 679 } 680 681 public boolean hasExperimentalElement() { 682 return this.experimental != null && !this.experimental.isEmpty(); 683 } 684 685 public boolean hasExperimental() { 686 return this.experimental != null && !this.experimental.isEmpty(); 687 } 688 689 /** 690 * @param value {@link #experimental} (A Boolean value to indicate that this 691 * event definition is authored for testing purposes (or 692 * education/evaluation/marketing) and is not intended to be used 693 * for genuine usage.). This is the underlying object with id, 694 * value and extensions. The accessor "getExperimental" gives 695 * direct access to the value 696 */ 697 public EventDefinition setExperimentalElement(BooleanType value) { 698 this.experimental = value; 699 return this; 700 } 701 702 /** 703 * @return A Boolean value to indicate that this event definition is authored 704 * for testing purposes (or education/evaluation/marketing) and is not 705 * intended to be used for genuine usage. 706 */ 707 public boolean getExperimental() { 708 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 709 } 710 711 /** 712 * @param value A Boolean value to indicate that this event definition is 713 * authored for testing purposes (or 714 * education/evaluation/marketing) and is not intended to be used 715 * for genuine usage. 716 */ 717 public EventDefinition setExperimental(boolean value) { 718 if (this.experimental == null) 719 this.experimental = new BooleanType(); 720 this.experimental.setValue(value); 721 return this; 722 } 723 724 /** 725 * @return {@link #subject} (A code or group definition that describes the 726 * intended subject of the event definition.) 727 */ 728 public Type getSubject() { 729 return this.subject; 730 } 731 732 /** 733 * @return {@link #subject} (A code or group definition that describes the 734 * intended subject of the event definition.) 735 */ 736 public CodeableConcept getSubjectCodeableConcept() throws FHIRException { 737 if (this.subject == null) 738 this.subject = new CodeableConcept(); 739 if (!(this.subject instanceof CodeableConcept)) 740 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 741 + this.subject.getClass().getName() + " was encountered"); 742 return (CodeableConcept) this.subject; 743 } 744 745 public boolean hasSubjectCodeableConcept() { 746 return this != null && this.subject instanceof CodeableConcept; 747 } 748 749 /** 750 * @return {@link #subject} (A code or group definition that describes the 751 * intended subject of the event definition.) 752 */ 753 public Reference getSubjectReference() throws FHIRException { 754 if (this.subject == null) 755 this.subject = new Reference(); 756 if (!(this.subject instanceof Reference)) 757 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.subject.getClass().getName() 758 + " was encountered"); 759 return (Reference) this.subject; 760 } 761 762 public boolean hasSubjectReference() { 763 return this != null && this.subject instanceof Reference; 764 } 765 766 public boolean hasSubject() { 767 return this.subject != null && !this.subject.isEmpty(); 768 } 769 770 /** 771 * @param value {@link #subject} (A code or group definition that describes the 772 * intended subject of the event definition.) 773 */ 774 public EventDefinition setSubject(Type value) { 775 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 776 throw new Error("Not the right type for EventDefinition.subject[x]: " + value.fhirType()); 777 this.subject = value; 778 return this; 779 } 780 781 /** 782 * @return {@link #date} (The date (and optionally time) when the event 783 * definition was published. The date must change when the business 784 * version changes and it must change if the status code changes. In 785 * addition, it should change when the substantive content of the event 786 * definition changes.). This is the underlying object with id, value 787 * and extensions. The accessor "getDate" gives direct access to the 788 * value 789 */ 790 public DateTimeType getDateElement() { 791 if (this.date == null) 792 if (Configuration.errorOnAutoCreate()) 793 throw new Error("Attempt to auto-create EventDefinition.date"); 794 else if (Configuration.doAutoCreate()) 795 this.date = new DateTimeType(); // bb 796 return this.date; 797 } 798 799 public boolean hasDateElement() { 800 return this.date != null && !this.date.isEmpty(); 801 } 802 803 public boolean hasDate() { 804 return this.date != null && !this.date.isEmpty(); 805 } 806 807 /** 808 * @param value {@link #date} (The date (and optionally time) when the event 809 * definition was published. The date must change when the business 810 * version changes and it must change if the status code changes. 811 * In addition, it should change when the substantive content of 812 * the event definition changes.). This is the underlying object 813 * with id, value and extensions. The accessor "getDate" gives 814 * direct access to the value 815 */ 816 public EventDefinition setDateElement(DateTimeType value) { 817 this.date = value; 818 return this; 819 } 820 821 /** 822 * @return The date (and optionally time) when the event definition was 823 * published. The date must change when the business version changes and 824 * it must change if the status code changes. In addition, it should 825 * change when the substantive content of the event definition changes. 826 */ 827 public Date getDate() { 828 return this.date == null ? null : this.date.getValue(); 829 } 830 831 /** 832 * @param value The date (and optionally time) when the event definition was 833 * published. The date must change when the business version 834 * changes and it must change if the status code changes. In 835 * addition, it should change when the substantive content of the 836 * event definition changes. 837 */ 838 public EventDefinition setDate(Date value) { 839 if (value == null) 840 this.date = null; 841 else { 842 if (this.date == null) 843 this.date = new DateTimeType(); 844 this.date.setValue(value); 845 } 846 return this; 847 } 848 849 /** 850 * @return {@link #publisher} (The name of the organization or individual that 851 * published the event definition.). This is the underlying object with 852 * id, value and extensions. The accessor "getPublisher" gives direct 853 * access to the value 854 */ 855 public StringType getPublisherElement() { 856 if (this.publisher == null) 857 if (Configuration.errorOnAutoCreate()) 858 throw new Error("Attempt to auto-create EventDefinition.publisher"); 859 else if (Configuration.doAutoCreate()) 860 this.publisher = new StringType(); // bb 861 return this.publisher; 862 } 863 864 public boolean hasPublisherElement() { 865 return this.publisher != null && !this.publisher.isEmpty(); 866 } 867 868 public boolean hasPublisher() { 869 return this.publisher != null && !this.publisher.isEmpty(); 870 } 871 872 /** 873 * @param value {@link #publisher} (The name of the organization or individual 874 * that published the event definition.). This is the underlying 875 * object with id, value and extensions. The accessor 876 * "getPublisher" gives direct access to the value 877 */ 878 public EventDefinition setPublisherElement(StringType value) { 879 this.publisher = value; 880 return this; 881 } 882 883 /** 884 * @return The name of the organization or individual that published the event 885 * definition. 886 */ 887 public String getPublisher() { 888 return this.publisher == null ? null : this.publisher.getValue(); 889 } 890 891 /** 892 * @param value The name of the organization or individual that published the 893 * event definition. 894 */ 895 public EventDefinition setPublisher(String value) { 896 if (Utilities.noString(value)) 897 this.publisher = null; 898 else { 899 if (this.publisher == null) 900 this.publisher = new StringType(); 901 this.publisher.setValue(value); 902 } 903 return this; 904 } 905 906 /** 907 * @return {@link #contact} (Contact details to assist a user in finding and 908 * communicating with the publisher.) 909 */ 910 public List<ContactDetail> getContact() { 911 if (this.contact == null) 912 this.contact = new ArrayList<ContactDetail>(); 913 return this.contact; 914 } 915 916 /** 917 * @return Returns a reference to <code>this</code> for easy method chaining 918 */ 919 public EventDefinition setContact(List<ContactDetail> theContact) { 920 this.contact = theContact; 921 return this; 922 } 923 924 public boolean hasContact() { 925 if (this.contact == null) 926 return false; 927 for (ContactDetail item : this.contact) 928 if (!item.isEmpty()) 929 return true; 930 return false; 931 } 932 933 public ContactDetail addContact() { // 3 934 ContactDetail t = new ContactDetail(); 935 if (this.contact == null) 936 this.contact = new ArrayList<ContactDetail>(); 937 this.contact.add(t); 938 return t; 939 } 940 941 public EventDefinition addContact(ContactDetail t) { // 3 942 if (t == null) 943 return this; 944 if (this.contact == null) 945 this.contact = new ArrayList<ContactDetail>(); 946 this.contact.add(t); 947 return this; 948 } 949 950 /** 951 * @return The first repetition of repeating field {@link #contact}, creating it 952 * if it does not already exist 953 */ 954 public ContactDetail getContactFirstRep() { 955 if (getContact().isEmpty()) { 956 addContact(); 957 } 958 return getContact().get(0); 959 } 960 961 /** 962 * @return {@link #description} (A free text natural language description of the 963 * event definition from a consumer's perspective.). This is the 964 * underlying object with id, value and extensions. The accessor 965 * "getDescription" gives direct access to the value 966 */ 967 public MarkdownType getDescriptionElement() { 968 if (this.description == null) 969 if (Configuration.errorOnAutoCreate()) 970 throw new Error("Attempt to auto-create EventDefinition.description"); 971 else if (Configuration.doAutoCreate()) 972 this.description = new MarkdownType(); // bb 973 return this.description; 974 } 975 976 public boolean hasDescriptionElement() { 977 return this.description != null && !this.description.isEmpty(); 978 } 979 980 public boolean hasDescription() { 981 return this.description != null && !this.description.isEmpty(); 982 } 983 984 /** 985 * @param value {@link #description} (A free text natural language description 986 * of the event definition from a consumer's perspective.). This is 987 * the underlying object with id, value and extensions. The 988 * accessor "getDescription" gives direct access to the value 989 */ 990 public EventDefinition setDescriptionElement(MarkdownType value) { 991 this.description = value; 992 return this; 993 } 994 995 /** 996 * @return A free text natural language description of the event definition from 997 * a consumer's perspective. 998 */ 999 public String getDescription() { 1000 return this.description == null ? null : this.description.getValue(); 1001 } 1002 1003 /** 1004 * @param value A free text natural language description of the event definition 1005 * from a consumer's perspective. 1006 */ 1007 public EventDefinition setDescription(String value) { 1008 if (value == null) 1009 this.description = null; 1010 else { 1011 if (this.description == null) 1012 this.description = new MarkdownType(); 1013 this.description.setValue(value); 1014 } 1015 return this; 1016 } 1017 1018 /** 1019 * @return {@link #useContext} (The content was developed with a focus and 1020 * intent of supporting the contexts that are listed. These contexts may 1021 * be general categories (gender, age, ...) or may be references to 1022 * specific programs (insurance plans, studies, ...) and may be used to 1023 * assist with indexing and searching for appropriate event definition 1024 * instances.) 1025 */ 1026 public List<UsageContext> getUseContext() { 1027 if (this.useContext == null) 1028 this.useContext = new ArrayList<UsageContext>(); 1029 return this.useContext; 1030 } 1031 1032 /** 1033 * @return Returns a reference to <code>this</code> for easy method chaining 1034 */ 1035 public EventDefinition setUseContext(List<UsageContext> theUseContext) { 1036 this.useContext = theUseContext; 1037 return this; 1038 } 1039 1040 public boolean hasUseContext() { 1041 if (this.useContext == null) 1042 return false; 1043 for (UsageContext item : this.useContext) 1044 if (!item.isEmpty()) 1045 return true; 1046 return false; 1047 } 1048 1049 public UsageContext addUseContext() { // 3 1050 UsageContext t = new UsageContext(); 1051 if (this.useContext == null) 1052 this.useContext = new ArrayList<UsageContext>(); 1053 this.useContext.add(t); 1054 return t; 1055 } 1056 1057 public EventDefinition addUseContext(UsageContext t) { // 3 1058 if (t == null) 1059 return this; 1060 if (this.useContext == null) 1061 this.useContext = new ArrayList<UsageContext>(); 1062 this.useContext.add(t); 1063 return this; 1064 } 1065 1066 /** 1067 * @return The first repetition of repeating field {@link #useContext}, creating 1068 * it if it does not already exist 1069 */ 1070 public UsageContext getUseContextFirstRep() { 1071 if (getUseContext().isEmpty()) { 1072 addUseContext(); 1073 } 1074 return getUseContext().get(0); 1075 } 1076 1077 /** 1078 * @return {@link #jurisdiction} (A legal or geographic region in which the 1079 * event definition is intended to be used.) 1080 */ 1081 public List<CodeableConcept> getJurisdiction() { 1082 if (this.jurisdiction == null) 1083 this.jurisdiction = new ArrayList<CodeableConcept>(); 1084 return this.jurisdiction; 1085 } 1086 1087 /** 1088 * @return Returns a reference to <code>this</code> for easy method chaining 1089 */ 1090 public EventDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 1091 this.jurisdiction = theJurisdiction; 1092 return this; 1093 } 1094 1095 public boolean hasJurisdiction() { 1096 if (this.jurisdiction == null) 1097 return false; 1098 for (CodeableConcept item : this.jurisdiction) 1099 if (!item.isEmpty()) 1100 return true; 1101 return false; 1102 } 1103 1104 public CodeableConcept addJurisdiction() { // 3 1105 CodeableConcept t = new CodeableConcept(); 1106 if (this.jurisdiction == null) 1107 this.jurisdiction = new ArrayList<CodeableConcept>(); 1108 this.jurisdiction.add(t); 1109 return t; 1110 } 1111 1112 public EventDefinition addJurisdiction(CodeableConcept t) { // 3 1113 if (t == null) 1114 return this; 1115 if (this.jurisdiction == null) 1116 this.jurisdiction = new ArrayList<CodeableConcept>(); 1117 this.jurisdiction.add(t); 1118 return this; 1119 } 1120 1121 /** 1122 * @return The first repetition of repeating field {@link #jurisdiction}, 1123 * creating it if it does not already exist 1124 */ 1125 public CodeableConcept getJurisdictionFirstRep() { 1126 if (getJurisdiction().isEmpty()) { 1127 addJurisdiction(); 1128 } 1129 return getJurisdiction().get(0); 1130 } 1131 1132 /** 1133 * @return {@link #purpose} (Explanation of why this event definition is needed 1134 * and why it has been designed as it has.). This is the underlying 1135 * object with id, value and extensions. The accessor "getPurpose" gives 1136 * direct access to the value 1137 */ 1138 public MarkdownType getPurposeElement() { 1139 if (this.purpose == null) 1140 if (Configuration.errorOnAutoCreate()) 1141 throw new Error("Attempt to auto-create EventDefinition.purpose"); 1142 else if (Configuration.doAutoCreate()) 1143 this.purpose = new MarkdownType(); // bb 1144 return this.purpose; 1145 } 1146 1147 public boolean hasPurposeElement() { 1148 return this.purpose != null && !this.purpose.isEmpty(); 1149 } 1150 1151 public boolean hasPurpose() { 1152 return this.purpose != null && !this.purpose.isEmpty(); 1153 } 1154 1155 /** 1156 * @param value {@link #purpose} (Explanation of why this event definition is 1157 * needed and why it has been designed as it has.). This is the 1158 * underlying object with id, value and extensions. The accessor 1159 * "getPurpose" gives direct access to the value 1160 */ 1161 public EventDefinition setPurposeElement(MarkdownType value) { 1162 this.purpose = value; 1163 return this; 1164 } 1165 1166 /** 1167 * @return Explanation of why this event definition is needed and why it has 1168 * been designed as it has. 1169 */ 1170 public String getPurpose() { 1171 return this.purpose == null ? null : this.purpose.getValue(); 1172 } 1173 1174 /** 1175 * @param value Explanation of why this event definition is needed and why it 1176 * has been designed as it has. 1177 */ 1178 public EventDefinition setPurpose(String value) { 1179 if (value == null) 1180 this.purpose = null; 1181 else { 1182 if (this.purpose == null) 1183 this.purpose = new MarkdownType(); 1184 this.purpose.setValue(value); 1185 } 1186 return this; 1187 } 1188 1189 /** 1190 * @return {@link #usage} (A detailed description of how the event definition is 1191 * used from a clinical perspective.). This is the underlying object 1192 * with id, value and extensions. The accessor "getUsage" gives direct 1193 * access to the value 1194 */ 1195 public StringType getUsageElement() { 1196 if (this.usage == null) 1197 if (Configuration.errorOnAutoCreate()) 1198 throw new Error("Attempt to auto-create EventDefinition.usage"); 1199 else if (Configuration.doAutoCreate()) 1200 this.usage = new StringType(); // bb 1201 return this.usage; 1202 } 1203 1204 public boolean hasUsageElement() { 1205 return this.usage != null && !this.usage.isEmpty(); 1206 } 1207 1208 public boolean hasUsage() { 1209 return this.usage != null && !this.usage.isEmpty(); 1210 } 1211 1212 /** 1213 * @param value {@link #usage} (A detailed description of how the event 1214 * definition is used from a clinical perspective.). This is the 1215 * underlying object with id, value and extensions. The accessor 1216 * "getUsage" gives direct access to the value 1217 */ 1218 public EventDefinition setUsageElement(StringType value) { 1219 this.usage = value; 1220 return this; 1221 } 1222 1223 /** 1224 * @return A detailed description of how the event definition is used from a 1225 * clinical perspective. 1226 */ 1227 public String getUsage() { 1228 return this.usage == null ? null : this.usage.getValue(); 1229 } 1230 1231 /** 1232 * @param value A detailed description of how the event definition is used from 1233 * a clinical perspective. 1234 */ 1235 public EventDefinition setUsage(String value) { 1236 if (Utilities.noString(value)) 1237 this.usage = null; 1238 else { 1239 if (this.usage == null) 1240 this.usage = new StringType(); 1241 this.usage.setValue(value); 1242 } 1243 return this; 1244 } 1245 1246 /** 1247 * @return {@link #copyright} (A copyright statement relating to the event 1248 * definition and/or its contents. Copyright statements are generally 1249 * legal restrictions on the use and publishing of the event 1250 * definition.). This is the underlying object with id, value and 1251 * extensions. The accessor "getCopyright" gives direct access to the 1252 * value 1253 */ 1254 public MarkdownType getCopyrightElement() { 1255 if (this.copyright == null) 1256 if (Configuration.errorOnAutoCreate()) 1257 throw new Error("Attempt to auto-create EventDefinition.copyright"); 1258 else if (Configuration.doAutoCreate()) 1259 this.copyright = new MarkdownType(); // bb 1260 return this.copyright; 1261 } 1262 1263 public boolean hasCopyrightElement() { 1264 return this.copyright != null && !this.copyright.isEmpty(); 1265 } 1266 1267 public boolean hasCopyright() { 1268 return this.copyright != null && !this.copyright.isEmpty(); 1269 } 1270 1271 /** 1272 * @param value {@link #copyright} (A copyright statement relating to the event 1273 * definition and/or its contents. Copyright statements are 1274 * generally legal restrictions on the use and publishing of the 1275 * event definition.). This is the underlying object with id, value 1276 * and extensions. The accessor "getCopyright" gives direct access 1277 * to the value 1278 */ 1279 public EventDefinition setCopyrightElement(MarkdownType value) { 1280 this.copyright = value; 1281 return this; 1282 } 1283 1284 /** 1285 * @return A copyright statement relating to the event definition and/or its 1286 * contents. Copyright statements are generally legal restrictions on 1287 * the use and publishing of the event definition. 1288 */ 1289 public String getCopyright() { 1290 return this.copyright == null ? null : this.copyright.getValue(); 1291 } 1292 1293 /** 1294 * @param value A copyright statement relating to the event definition and/or 1295 * its contents. Copyright statements are generally legal 1296 * restrictions on the use and publishing of the event definition. 1297 */ 1298 public EventDefinition setCopyright(String value) { 1299 if (value == null) 1300 this.copyright = null; 1301 else { 1302 if (this.copyright == null) 1303 this.copyright = new MarkdownType(); 1304 this.copyright.setValue(value); 1305 } 1306 return this; 1307 } 1308 1309 /** 1310 * @return {@link #approvalDate} (The date on which the resource content was 1311 * approved by the publisher. Approval happens once when the content is 1312 * officially approved for usage.). This is the underlying object with 1313 * id, value and extensions. The accessor "getApprovalDate" gives direct 1314 * access to the value 1315 */ 1316 public DateType getApprovalDateElement() { 1317 if (this.approvalDate == null) 1318 if (Configuration.errorOnAutoCreate()) 1319 throw new Error("Attempt to auto-create EventDefinition.approvalDate"); 1320 else if (Configuration.doAutoCreate()) 1321 this.approvalDate = new DateType(); // bb 1322 return this.approvalDate; 1323 } 1324 1325 public boolean hasApprovalDateElement() { 1326 return this.approvalDate != null && !this.approvalDate.isEmpty(); 1327 } 1328 1329 public boolean hasApprovalDate() { 1330 return this.approvalDate != null && !this.approvalDate.isEmpty(); 1331 } 1332 1333 /** 1334 * @param value {@link #approvalDate} (The date on which the resource content 1335 * was approved by the publisher. Approval happens once when the 1336 * content is officially approved for usage.). This is the 1337 * underlying object with id, value and extensions. The accessor 1338 * "getApprovalDate" gives direct access to the value 1339 */ 1340 public EventDefinition setApprovalDateElement(DateType value) { 1341 this.approvalDate = value; 1342 return this; 1343 } 1344 1345 /** 1346 * @return The date on which the resource content was approved by the publisher. 1347 * Approval happens once when the content is officially approved for 1348 * usage. 1349 */ 1350 public Date getApprovalDate() { 1351 return this.approvalDate == null ? null : this.approvalDate.getValue(); 1352 } 1353 1354 /** 1355 * @param value The date on which the resource content was approved by the 1356 * publisher. Approval happens once when the content is officially 1357 * approved for usage. 1358 */ 1359 public EventDefinition setApprovalDate(Date value) { 1360 if (value == null) 1361 this.approvalDate = null; 1362 else { 1363 if (this.approvalDate == null) 1364 this.approvalDate = new DateType(); 1365 this.approvalDate.setValue(value); 1366 } 1367 return this; 1368 } 1369 1370 /** 1371 * @return {@link #lastReviewDate} (The date on which the resource content was 1372 * last reviewed. Review happens periodically after approval but does 1373 * not change the original approval date.). This is the underlying 1374 * object with id, value and extensions. The accessor 1375 * "getLastReviewDate" gives direct access to the value 1376 */ 1377 public DateType getLastReviewDateElement() { 1378 if (this.lastReviewDate == null) 1379 if (Configuration.errorOnAutoCreate()) 1380 throw new Error("Attempt to auto-create EventDefinition.lastReviewDate"); 1381 else if (Configuration.doAutoCreate()) 1382 this.lastReviewDate = new DateType(); // bb 1383 return this.lastReviewDate; 1384 } 1385 1386 public boolean hasLastReviewDateElement() { 1387 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 1388 } 1389 1390 public boolean hasLastReviewDate() { 1391 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 1392 } 1393 1394 /** 1395 * @param value {@link #lastReviewDate} (The date on which the resource content 1396 * was last reviewed. Review happens periodically after approval 1397 * but does not change the original approval date.). This is the 1398 * underlying object with id, value and extensions. The accessor 1399 * "getLastReviewDate" gives direct access to the value 1400 */ 1401 public EventDefinition setLastReviewDateElement(DateType value) { 1402 this.lastReviewDate = value; 1403 return this; 1404 } 1405 1406 /** 1407 * @return The date on which the resource content was last reviewed. Review 1408 * happens periodically after approval but does not change the original 1409 * approval date. 1410 */ 1411 public Date getLastReviewDate() { 1412 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 1413 } 1414 1415 /** 1416 * @param value The date on which the resource content was last reviewed. Review 1417 * happens periodically after approval but does not change the 1418 * original approval date. 1419 */ 1420 public EventDefinition setLastReviewDate(Date value) { 1421 if (value == null) 1422 this.lastReviewDate = null; 1423 else { 1424 if (this.lastReviewDate == null) 1425 this.lastReviewDate = new DateType(); 1426 this.lastReviewDate.setValue(value); 1427 } 1428 return this; 1429 } 1430 1431 /** 1432 * @return {@link #effectivePeriod} (The period during which the event 1433 * definition content was or is planned to be in active use.) 1434 */ 1435 public Period getEffectivePeriod() { 1436 if (this.effectivePeriod == null) 1437 if (Configuration.errorOnAutoCreate()) 1438 throw new Error("Attempt to auto-create EventDefinition.effectivePeriod"); 1439 else if (Configuration.doAutoCreate()) 1440 this.effectivePeriod = new Period(); // cc 1441 return this.effectivePeriod; 1442 } 1443 1444 public boolean hasEffectivePeriod() { 1445 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 1446 } 1447 1448 /** 1449 * @param value {@link #effectivePeriod} (The period during which the event 1450 * definition content was or is planned to be in active use.) 1451 */ 1452 public EventDefinition setEffectivePeriod(Period value) { 1453 this.effectivePeriod = value; 1454 return this; 1455 } 1456 1457 /** 1458 * @return {@link #topic} (Descriptive topics related to the module. Topics 1459 * provide a high-level categorization of the module that can be useful 1460 * for filtering and searching.) 1461 */ 1462 public List<CodeableConcept> getTopic() { 1463 if (this.topic == null) 1464 this.topic = new ArrayList<CodeableConcept>(); 1465 return this.topic; 1466 } 1467 1468 /** 1469 * @return Returns a reference to <code>this</code> for easy method chaining 1470 */ 1471 public EventDefinition setTopic(List<CodeableConcept> theTopic) { 1472 this.topic = theTopic; 1473 return this; 1474 } 1475 1476 public boolean hasTopic() { 1477 if (this.topic == null) 1478 return false; 1479 for (CodeableConcept item : this.topic) 1480 if (!item.isEmpty()) 1481 return true; 1482 return false; 1483 } 1484 1485 public CodeableConcept addTopic() { // 3 1486 CodeableConcept t = new CodeableConcept(); 1487 if (this.topic == null) 1488 this.topic = new ArrayList<CodeableConcept>(); 1489 this.topic.add(t); 1490 return t; 1491 } 1492 1493 public EventDefinition addTopic(CodeableConcept t) { // 3 1494 if (t == null) 1495 return this; 1496 if (this.topic == null) 1497 this.topic = new ArrayList<CodeableConcept>(); 1498 this.topic.add(t); 1499 return this; 1500 } 1501 1502 /** 1503 * @return The first repetition of repeating field {@link #topic}, creating it 1504 * if it does not already exist 1505 */ 1506 public CodeableConcept getTopicFirstRep() { 1507 if (getTopic().isEmpty()) { 1508 addTopic(); 1509 } 1510 return getTopic().get(0); 1511 } 1512 1513 /** 1514 * @return {@link #author} (An individiual or organization primarily involved in 1515 * the creation and maintenance of the content.) 1516 */ 1517 public List<ContactDetail> getAuthor() { 1518 if (this.author == null) 1519 this.author = new ArrayList<ContactDetail>(); 1520 return this.author; 1521 } 1522 1523 /** 1524 * @return Returns a reference to <code>this</code> for easy method chaining 1525 */ 1526 public EventDefinition setAuthor(List<ContactDetail> theAuthor) { 1527 this.author = theAuthor; 1528 return this; 1529 } 1530 1531 public boolean hasAuthor() { 1532 if (this.author == null) 1533 return false; 1534 for (ContactDetail item : this.author) 1535 if (!item.isEmpty()) 1536 return true; 1537 return false; 1538 } 1539 1540 public ContactDetail addAuthor() { // 3 1541 ContactDetail t = new ContactDetail(); 1542 if (this.author == null) 1543 this.author = new ArrayList<ContactDetail>(); 1544 this.author.add(t); 1545 return t; 1546 } 1547 1548 public EventDefinition addAuthor(ContactDetail t) { // 3 1549 if (t == null) 1550 return this; 1551 if (this.author == null) 1552 this.author = new ArrayList<ContactDetail>(); 1553 this.author.add(t); 1554 return this; 1555 } 1556 1557 /** 1558 * @return The first repetition of repeating field {@link #author}, creating it 1559 * if it does not already exist 1560 */ 1561 public ContactDetail getAuthorFirstRep() { 1562 if (getAuthor().isEmpty()) { 1563 addAuthor(); 1564 } 1565 return getAuthor().get(0); 1566 } 1567 1568 /** 1569 * @return {@link #editor} (An individual or organization primarily responsible 1570 * for internal coherence of the content.) 1571 */ 1572 public List<ContactDetail> getEditor() { 1573 if (this.editor == null) 1574 this.editor = new ArrayList<ContactDetail>(); 1575 return this.editor; 1576 } 1577 1578 /** 1579 * @return Returns a reference to <code>this</code> for easy method chaining 1580 */ 1581 public EventDefinition setEditor(List<ContactDetail> theEditor) { 1582 this.editor = theEditor; 1583 return this; 1584 } 1585 1586 public boolean hasEditor() { 1587 if (this.editor == null) 1588 return false; 1589 for (ContactDetail item : this.editor) 1590 if (!item.isEmpty()) 1591 return true; 1592 return false; 1593 } 1594 1595 public ContactDetail addEditor() { // 3 1596 ContactDetail t = new ContactDetail(); 1597 if (this.editor == null) 1598 this.editor = new ArrayList<ContactDetail>(); 1599 this.editor.add(t); 1600 return t; 1601 } 1602 1603 public EventDefinition addEditor(ContactDetail t) { // 3 1604 if (t == null) 1605 return this; 1606 if (this.editor == null) 1607 this.editor = new ArrayList<ContactDetail>(); 1608 this.editor.add(t); 1609 return this; 1610 } 1611 1612 /** 1613 * @return The first repetition of repeating field {@link #editor}, creating it 1614 * if it does not already exist 1615 */ 1616 public ContactDetail getEditorFirstRep() { 1617 if (getEditor().isEmpty()) { 1618 addEditor(); 1619 } 1620 return getEditor().get(0); 1621 } 1622 1623 /** 1624 * @return {@link #reviewer} (An individual or organization primarily 1625 * responsible for review of some aspect of the content.) 1626 */ 1627 public List<ContactDetail> getReviewer() { 1628 if (this.reviewer == null) 1629 this.reviewer = new ArrayList<ContactDetail>(); 1630 return this.reviewer; 1631 } 1632 1633 /** 1634 * @return Returns a reference to <code>this</code> for easy method chaining 1635 */ 1636 public EventDefinition setReviewer(List<ContactDetail> theReviewer) { 1637 this.reviewer = theReviewer; 1638 return this; 1639 } 1640 1641 public boolean hasReviewer() { 1642 if (this.reviewer == null) 1643 return false; 1644 for (ContactDetail item : this.reviewer) 1645 if (!item.isEmpty()) 1646 return true; 1647 return false; 1648 } 1649 1650 public ContactDetail addReviewer() { // 3 1651 ContactDetail t = new ContactDetail(); 1652 if (this.reviewer == null) 1653 this.reviewer = new ArrayList<ContactDetail>(); 1654 this.reviewer.add(t); 1655 return t; 1656 } 1657 1658 public EventDefinition addReviewer(ContactDetail t) { // 3 1659 if (t == null) 1660 return this; 1661 if (this.reviewer == null) 1662 this.reviewer = new ArrayList<ContactDetail>(); 1663 this.reviewer.add(t); 1664 return this; 1665 } 1666 1667 /** 1668 * @return The first repetition of repeating field {@link #reviewer}, creating 1669 * it if it does not already exist 1670 */ 1671 public ContactDetail getReviewerFirstRep() { 1672 if (getReviewer().isEmpty()) { 1673 addReviewer(); 1674 } 1675 return getReviewer().get(0); 1676 } 1677 1678 /** 1679 * @return {@link #endorser} (An individual or organization responsible for 1680 * officially endorsing the content for use in some setting.) 1681 */ 1682 public List<ContactDetail> getEndorser() { 1683 if (this.endorser == null) 1684 this.endorser = new ArrayList<ContactDetail>(); 1685 return this.endorser; 1686 } 1687 1688 /** 1689 * @return Returns a reference to <code>this</code> for easy method chaining 1690 */ 1691 public EventDefinition setEndorser(List<ContactDetail> theEndorser) { 1692 this.endorser = theEndorser; 1693 return this; 1694 } 1695 1696 public boolean hasEndorser() { 1697 if (this.endorser == null) 1698 return false; 1699 for (ContactDetail item : this.endorser) 1700 if (!item.isEmpty()) 1701 return true; 1702 return false; 1703 } 1704 1705 public ContactDetail addEndorser() { // 3 1706 ContactDetail t = new ContactDetail(); 1707 if (this.endorser == null) 1708 this.endorser = new ArrayList<ContactDetail>(); 1709 this.endorser.add(t); 1710 return t; 1711 } 1712 1713 public EventDefinition addEndorser(ContactDetail t) { // 3 1714 if (t == null) 1715 return this; 1716 if (this.endorser == null) 1717 this.endorser = new ArrayList<ContactDetail>(); 1718 this.endorser.add(t); 1719 return this; 1720 } 1721 1722 /** 1723 * @return The first repetition of repeating field {@link #endorser}, creating 1724 * it if it does not already exist 1725 */ 1726 public ContactDetail getEndorserFirstRep() { 1727 if (getEndorser().isEmpty()) { 1728 addEndorser(); 1729 } 1730 return getEndorser().get(0); 1731 } 1732 1733 /** 1734 * @return {@link #relatedArtifact} (Related resources such as additional 1735 * documentation, justification, or bibliographic references.) 1736 */ 1737 public List<RelatedArtifact> getRelatedArtifact() { 1738 if (this.relatedArtifact == null) 1739 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1740 return this.relatedArtifact; 1741 } 1742 1743 /** 1744 * @return Returns a reference to <code>this</code> for easy method chaining 1745 */ 1746 public EventDefinition setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 1747 this.relatedArtifact = theRelatedArtifact; 1748 return this; 1749 } 1750 1751 public boolean hasRelatedArtifact() { 1752 if (this.relatedArtifact == null) 1753 return false; 1754 for (RelatedArtifact item : this.relatedArtifact) 1755 if (!item.isEmpty()) 1756 return true; 1757 return false; 1758 } 1759 1760 public RelatedArtifact addRelatedArtifact() { // 3 1761 RelatedArtifact t = new RelatedArtifact(); 1762 if (this.relatedArtifact == null) 1763 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1764 this.relatedArtifact.add(t); 1765 return t; 1766 } 1767 1768 public EventDefinition addRelatedArtifact(RelatedArtifact t) { // 3 1769 if (t == null) 1770 return this; 1771 if (this.relatedArtifact == null) 1772 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1773 this.relatedArtifact.add(t); 1774 return this; 1775 } 1776 1777 /** 1778 * @return The first repetition of repeating field {@link #relatedArtifact}, 1779 * creating it if it does not already exist 1780 */ 1781 public RelatedArtifact getRelatedArtifactFirstRep() { 1782 if (getRelatedArtifact().isEmpty()) { 1783 addRelatedArtifact(); 1784 } 1785 return getRelatedArtifact().get(0); 1786 } 1787 1788 /** 1789 * @return {@link #trigger} (The trigger element defines when the event occurs. 1790 * If more than one trigger condition is specified, the event fires 1791 * whenever any one of the trigger conditions is met.) 1792 */ 1793 public List<TriggerDefinition> getTrigger() { 1794 if (this.trigger == null) 1795 this.trigger = new ArrayList<TriggerDefinition>(); 1796 return this.trigger; 1797 } 1798 1799 /** 1800 * @return Returns a reference to <code>this</code> for easy method chaining 1801 */ 1802 public EventDefinition setTrigger(List<TriggerDefinition> theTrigger) { 1803 this.trigger = theTrigger; 1804 return this; 1805 } 1806 1807 public boolean hasTrigger() { 1808 if (this.trigger == null) 1809 return false; 1810 for (TriggerDefinition item : this.trigger) 1811 if (!item.isEmpty()) 1812 return true; 1813 return false; 1814 } 1815 1816 public TriggerDefinition addTrigger() { // 3 1817 TriggerDefinition t = new TriggerDefinition(); 1818 if (this.trigger == null) 1819 this.trigger = new ArrayList<TriggerDefinition>(); 1820 this.trigger.add(t); 1821 return t; 1822 } 1823 1824 public EventDefinition addTrigger(TriggerDefinition t) { // 3 1825 if (t == null) 1826 return this; 1827 if (this.trigger == null) 1828 this.trigger = new ArrayList<TriggerDefinition>(); 1829 this.trigger.add(t); 1830 return this; 1831 } 1832 1833 /** 1834 * @return The first repetition of repeating field {@link #trigger}, creating it 1835 * if it does not already exist 1836 */ 1837 public TriggerDefinition getTriggerFirstRep() { 1838 if (getTrigger().isEmpty()) { 1839 addTrigger(); 1840 } 1841 return getTrigger().get(0); 1842 } 1843 1844 protected void listChildren(List<Property> children) { 1845 super.listChildren(children); 1846 children.add(new Property("url", "uri", 1847 "An absolute URI that is used to identify this event definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this event definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the event definition is stored on different servers.", 1848 0, 1, url)); 1849 children.add(new Property("identifier", "Identifier", 1850 "A formal identifier that is used to identify this event definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 1851 0, java.lang.Integer.MAX_VALUE, identifier)); 1852 children.add(new Property("version", "string", 1853 "The identifier that is used to identify this version of the event definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the event definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 1854 0, 1, version)); 1855 children.add(new Property("name", "string", 1856 "A natural language name identifying the event definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 1857 0, 1, name)); 1858 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the event definition.", 1859 0, 1, title)); 1860 children.add(new Property("subtitle", "string", 1861 "An explanatory or alternate title for the event definition giving additional information about its content.", 1862 0, 1, subtitle)); 1863 children.add(new Property("status", "code", 1864 "The status of this event definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 1865 children.add(new Property("experimental", "boolean", 1866 "A Boolean value to indicate that this event definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 1867 0, 1, experimental)); 1868 children.add(new Property("subject[x]", "CodeableConcept|Reference(Group)", 1869 "A code or group definition that describes the intended subject of the event definition.", 0, 1, subject)); 1870 children.add(new Property("date", "dateTime", 1871 "The date (and optionally time) when the event definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the event definition changes.", 1872 0, 1, date)); 1873 children.add(new Property("publisher", "string", 1874 "The name of the organization or individual that published the event definition.", 0, 1, publisher)); 1875 children.add(new Property("contact", "ContactDetail", 1876 "Contact details to assist a user in finding and communicating with the publisher.", 0, 1877 java.lang.Integer.MAX_VALUE, contact)); 1878 children.add(new Property("description", "markdown", 1879 "A free text natural language description of the event definition from a consumer's perspective.", 0, 1, 1880 description)); 1881 children.add(new Property("useContext", "UsageContext", 1882 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate event definition instances.", 1883 0, java.lang.Integer.MAX_VALUE, useContext)); 1884 children.add(new Property("jurisdiction", "CodeableConcept", 1885 "A legal or geographic region in which the event definition is intended to be used.", 0, 1886 java.lang.Integer.MAX_VALUE, jurisdiction)); 1887 children.add(new Property("purpose", "markdown", 1888 "Explanation of why this event definition is needed and why it has been designed as it has.", 0, 1, purpose)); 1889 children.add(new Property("usage", "string", 1890 "A detailed description of how the event definition is used from a clinical perspective.", 0, 1, usage)); 1891 children.add(new Property("copyright", "markdown", 1892 "A copyright statement relating to the event definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the event definition.", 1893 0, 1, copyright)); 1894 children.add(new Property("approvalDate", "date", 1895 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 1896 0, 1, approvalDate)); 1897 children.add(new Property("lastReviewDate", "date", 1898 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 1899 0, 1, lastReviewDate)); 1900 children.add(new Property("effectivePeriod", "Period", 1901 "The period during which the event definition content was or is planned to be in active use.", 0, 1, 1902 effectivePeriod)); 1903 children.add(new Property("topic", "CodeableConcept", 1904 "Descriptive topics related to the module. Topics provide a high-level categorization of the module that can be useful for filtering and searching.", 1905 0, java.lang.Integer.MAX_VALUE, topic)); 1906 children.add(new Property("author", "ContactDetail", 1907 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 1908 java.lang.Integer.MAX_VALUE, author)); 1909 children.add(new Property("editor", "ContactDetail", 1910 "An individual or organization primarily responsible for internal coherence of the content.", 0, 1911 java.lang.Integer.MAX_VALUE, editor)); 1912 children.add(new Property("reviewer", "ContactDetail", 1913 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 1914 java.lang.Integer.MAX_VALUE, reviewer)); 1915 children.add(new Property("endorser", "ContactDetail", 1916 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 1917 java.lang.Integer.MAX_VALUE, endorser)); 1918 children.add(new Property("relatedArtifact", "RelatedArtifact", 1919 "Related resources such as additional documentation, justification, or bibliographic references.", 0, 1920 java.lang.Integer.MAX_VALUE, relatedArtifact)); 1921 children.add(new Property("trigger", "TriggerDefinition", 1922 "The trigger element defines when the event occurs. If more than one trigger condition is specified, the event fires whenever any one of the trigger conditions is met.", 1923 0, java.lang.Integer.MAX_VALUE, trigger)); 1924 } 1925 1926 @Override 1927 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1928 switch (_hash) { 1929 case 116079: 1930 /* url */ return new Property("url", "uri", 1931 "An absolute URI that is used to identify this event definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this event definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the event definition is stored on different servers.", 1932 0, 1, url); 1933 case -1618432855: 1934 /* identifier */ return new Property("identifier", "Identifier", 1935 "A formal identifier that is used to identify this event definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 1936 0, java.lang.Integer.MAX_VALUE, identifier); 1937 case 351608024: 1938 /* version */ return new Property("version", "string", 1939 "The identifier that is used to identify this version of the event definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the event definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 1940 0, 1, version); 1941 case 3373707: 1942 /* name */ return new Property("name", "string", 1943 "A natural language name identifying the event definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 1944 0, 1, name); 1945 case 110371416: 1946 /* title */ return new Property("title", "string", 1947 "A short, descriptive, user-friendly title for the event definition.", 0, 1, title); 1948 case -2060497896: 1949 /* subtitle */ return new Property("subtitle", "string", 1950 "An explanatory or alternate title for the event definition giving additional information about its content.", 1951 0, 1, subtitle); 1952 case -892481550: 1953 /* status */ return new Property("status", "code", 1954 "The status of this event definition. Enables tracking the life-cycle of the content.", 0, 1, status); 1955 case -404562712: 1956 /* experimental */ return new Property("experimental", "boolean", 1957 "A Boolean value to indicate that this event definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 1958 0, 1, experimental); 1959 case -573640748: 1960 /* subject[x] */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 1961 "A code or group definition that describes the intended subject of the event definition.", 0, 1, subject); 1962 case -1867885268: 1963 /* subject */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 1964 "A code or group definition that describes the intended subject of the event definition.", 0, 1, subject); 1965 case -1257122603: 1966 /* subjectCodeableConcept */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 1967 "A code or group definition that describes the intended subject of the event definition.", 0, 1, subject); 1968 case 772938623: 1969 /* subjectReference */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 1970 "A code or group definition that describes the intended subject of the event definition.", 0, 1, subject); 1971 case 3076014: 1972 /* date */ return new Property("date", "dateTime", 1973 "The date (and optionally time) when the event definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the event definition changes.", 1974 0, 1, date); 1975 case 1447404028: 1976 /* publisher */ return new Property("publisher", "string", 1977 "The name of the organization or individual that published the event definition.", 0, 1, publisher); 1978 case 951526432: 1979 /* contact */ return new Property("contact", "ContactDetail", 1980 "Contact details to assist a user in finding and communicating with the publisher.", 0, 1981 java.lang.Integer.MAX_VALUE, contact); 1982 case -1724546052: 1983 /* description */ return new Property("description", "markdown", 1984 "A free text natural language description of the event definition from a consumer's perspective.", 0, 1, 1985 description); 1986 case -669707736: 1987 /* useContext */ return new Property("useContext", "UsageContext", 1988 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate event definition instances.", 1989 0, java.lang.Integer.MAX_VALUE, useContext); 1990 case -507075711: 1991 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 1992 "A legal or geographic region in which the event definition is intended to be used.", 0, 1993 java.lang.Integer.MAX_VALUE, jurisdiction); 1994 case -220463842: 1995 /* purpose */ return new Property("purpose", "markdown", 1996 "Explanation of why this event definition is needed and why it has been designed as it has.", 0, 1, purpose); 1997 case 111574433: 1998 /* usage */ return new Property("usage", "string", 1999 "A detailed description of how the event definition is used from a clinical perspective.", 0, 1, usage); 2000 case 1522889671: 2001 /* copyright */ return new Property("copyright", "markdown", 2002 "A copyright statement relating to the event definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the event definition.", 2003 0, 1, copyright); 2004 case 223539345: 2005 /* approvalDate */ return new Property("approvalDate", "date", 2006 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 2007 0, 1, approvalDate); 2008 case -1687512484: 2009 /* lastReviewDate */ return new Property("lastReviewDate", "date", 2010 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 2011 0, 1, lastReviewDate); 2012 case -403934648: 2013 /* effectivePeriod */ return new Property("effectivePeriod", "Period", 2014 "The period during which the event definition content was or is planned to be in active use.", 0, 1, 2015 effectivePeriod); 2016 case 110546223: 2017 /* topic */ return new Property("topic", "CodeableConcept", 2018 "Descriptive topics related to the module. Topics provide a high-level categorization of the module that can be useful for filtering and searching.", 2019 0, java.lang.Integer.MAX_VALUE, topic); 2020 case -1406328437: 2021 /* author */ return new Property("author", "ContactDetail", 2022 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 2023 java.lang.Integer.MAX_VALUE, author); 2024 case -1307827859: 2025 /* editor */ return new Property("editor", "ContactDetail", 2026 "An individual or organization primarily responsible for internal coherence of the content.", 0, 2027 java.lang.Integer.MAX_VALUE, editor); 2028 case -261190139: 2029 /* reviewer */ return new Property("reviewer", "ContactDetail", 2030 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 2031 java.lang.Integer.MAX_VALUE, reviewer); 2032 case 1740277666: 2033 /* endorser */ return new Property("endorser", "ContactDetail", 2034 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 2035 java.lang.Integer.MAX_VALUE, endorser); 2036 case 666807069: 2037 /* relatedArtifact */ return new Property("relatedArtifact", "RelatedArtifact", 2038 "Related resources such as additional documentation, justification, or bibliographic references.", 0, 2039 java.lang.Integer.MAX_VALUE, relatedArtifact); 2040 case -1059891784: 2041 /* trigger */ return new Property("trigger", "TriggerDefinition", 2042 "The trigger element defines when the event occurs. If more than one trigger condition is specified, the event fires whenever any one of the trigger conditions is met.", 2043 0, java.lang.Integer.MAX_VALUE, trigger); 2044 default: 2045 return super.getNamedProperty(_hash, _name, _checkValid); 2046 } 2047 2048 } 2049 2050 @Override 2051 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2052 switch (hash) { 2053 case 116079: 2054 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 2055 case -1618432855: 2056 /* identifier */ return this.identifier == null ? new Base[0] 2057 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2058 case 351608024: 2059 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 2060 case 3373707: 2061 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 2062 case 110371416: 2063 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 2064 case -2060497896: 2065 /* subtitle */ return this.subtitle == null ? new Base[0] : new Base[] { this.subtitle }; // StringType 2066 case -892481550: 2067 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 2068 case -404562712: 2069 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 2070 case -1867885268: 2071 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Type 2072 case 3076014: 2073 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 2074 case 1447404028: 2075 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 2076 case 951526432: 2077 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 2078 case -1724546052: 2079 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 2080 case -669707736: 2081 /* useContext */ return this.useContext == null ? new Base[0] 2082 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 2083 case -507075711: 2084 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 2085 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 2086 case -220463842: 2087 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 2088 case 111574433: 2089 /* usage */ return this.usage == null ? new Base[0] : new Base[] { this.usage }; // StringType 2090 case 1522889671: 2091 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 2092 case 223539345: 2093 /* approvalDate */ return this.approvalDate == null ? new Base[0] : new Base[] { this.approvalDate }; // DateType 2094 case -1687512484: 2095 /* lastReviewDate */ return this.lastReviewDate == null ? new Base[0] : new Base[] { this.lastReviewDate }; // DateType 2096 case -403934648: 2097 /* effectivePeriod */ return this.effectivePeriod == null ? new Base[0] : new Base[] { this.effectivePeriod }; // Period 2098 case 110546223: 2099 /* topic */ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 2100 case -1406328437: 2101 /* author */ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 2102 case -1307827859: 2103 /* editor */ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 2104 case -261190139: 2105 /* reviewer */ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 2106 case 1740277666: 2107 /* endorser */ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 2108 case 666807069: 2109 /* relatedArtifact */ return this.relatedArtifact == null ? new Base[0] 2110 : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 2111 case -1059891784: 2112 /* trigger */ return this.trigger == null ? new Base[0] : this.trigger.toArray(new Base[this.trigger.size()]); // TriggerDefinition 2113 default: 2114 return super.getProperty(hash, name, checkValid); 2115 } 2116 2117 } 2118 2119 @Override 2120 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2121 switch (hash) { 2122 case 116079: // url 2123 this.url = castToUri(value); // UriType 2124 return value; 2125 case -1618432855: // identifier 2126 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2127 return value; 2128 case 351608024: // version 2129 this.version = castToString(value); // StringType 2130 return value; 2131 case 3373707: // name 2132 this.name = castToString(value); // StringType 2133 return value; 2134 case 110371416: // title 2135 this.title = castToString(value); // StringType 2136 return value; 2137 case -2060497896: // subtitle 2138 this.subtitle = castToString(value); // StringType 2139 return value; 2140 case -892481550: // status 2141 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 2142 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2143 return value; 2144 case -404562712: // experimental 2145 this.experimental = castToBoolean(value); // BooleanType 2146 return value; 2147 case -1867885268: // subject 2148 this.subject = castToType(value); // Type 2149 return value; 2150 case 3076014: // date 2151 this.date = castToDateTime(value); // DateTimeType 2152 return value; 2153 case 1447404028: // publisher 2154 this.publisher = castToString(value); // StringType 2155 return value; 2156 case 951526432: // contact 2157 this.getContact().add(castToContactDetail(value)); // ContactDetail 2158 return value; 2159 case -1724546052: // description 2160 this.description = castToMarkdown(value); // MarkdownType 2161 return value; 2162 case -669707736: // useContext 2163 this.getUseContext().add(castToUsageContext(value)); // UsageContext 2164 return value; 2165 case -507075711: // jurisdiction 2166 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 2167 return value; 2168 case -220463842: // purpose 2169 this.purpose = castToMarkdown(value); // MarkdownType 2170 return value; 2171 case 111574433: // usage 2172 this.usage = castToString(value); // StringType 2173 return value; 2174 case 1522889671: // copyright 2175 this.copyright = castToMarkdown(value); // MarkdownType 2176 return value; 2177 case 223539345: // approvalDate 2178 this.approvalDate = castToDate(value); // DateType 2179 return value; 2180 case -1687512484: // lastReviewDate 2181 this.lastReviewDate = castToDate(value); // DateType 2182 return value; 2183 case -403934648: // effectivePeriod 2184 this.effectivePeriod = castToPeriod(value); // Period 2185 return value; 2186 case 110546223: // topic 2187 this.getTopic().add(castToCodeableConcept(value)); // CodeableConcept 2188 return value; 2189 case -1406328437: // author 2190 this.getAuthor().add(castToContactDetail(value)); // ContactDetail 2191 return value; 2192 case -1307827859: // editor 2193 this.getEditor().add(castToContactDetail(value)); // ContactDetail 2194 return value; 2195 case -261190139: // reviewer 2196 this.getReviewer().add(castToContactDetail(value)); // ContactDetail 2197 return value; 2198 case 1740277666: // endorser 2199 this.getEndorser().add(castToContactDetail(value)); // ContactDetail 2200 return value; 2201 case 666807069: // relatedArtifact 2202 this.getRelatedArtifact().add(castToRelatedArtifact(value)); // RelatedArtifact 2203 return value; 2204 case -1059891784: // trigger 2205 this.getTrigger().add(castToTriggerDefinition(value)); // TriggerDefinition 2206 return value; 2207 default: 2208 return super.setProperty(hash, name, value); 2209 } 2210 2211 } 2212 2213 @Override 2214 public Base setProperty(String name, Base value) throws FHIRException { 2215 if (name.equals("url")) { 2216 this.url = castToUri(value); // UriType 2217 } else if (name.equals("identifier")) { 2218 this.getIdentifier().add(castToIdentifier(value)); 2219 } else if (name.equals("version")) { 2220 this.version = castToString(value); // StringType 2221 } else if (name.equals("name")) { 2222 this.name = castToString(value); // StringType 2223 } else if (name.equals("title")) { 2224 this.title = castToString(value); // StringType 2225 } else if (name.equals("subtitle")) { 2226 this.subtitle = castToString(value); // StringType 2227 } else if (name.equals("status")) { 2228 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 2229 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2230 } else if (name.equals("experimental")) { 2231 this.experimental = castToBoolean(value); // BooleanType 2232 } else if (name.equals("subject[x]")) { 2233 this.subject = castToType(value); // Type 2234 } else if (name.equals("date")) { 2235 this.date = castToDateTime(value); // DateTimeType 2236 } else if (name.equals("publisher")) { 2237 this.publisher = castToString(value); // StringType 2238 } else if (name.equals("contact")) { 2239 this.getContact().add(castToContactDetail(value)); 2240 } else if (name.equals("description")) { 2241 this.description = castToMarkdown(value); // MarkdownType 2242 } else if (name.equals("useContext")) { 2243 this.getUseContext().add(castToUsageContext(value)); 2244 } else if (name.equals("jurisdiction")) { 2245 this.getJurisdiction().add(castToCodeableConcept(value)); 2246 } else if (name.equals("purpose")) { 2247 this.purpose = castToMarkdown(value); // MarkdownType 2248 } else if (name.equals("usage")) { 2249 this.usage = castToString(value); // StringType 2250 } else if (name.equals("copyright")) { 2251 this.copyright = castToMarkdown(value); // MarkdownType 2252 } else if (name.equals("approvalDate")) { 2253 this.approvalDate = castToDate(value); // DateType 2254 } else if (name.equals("lastReviewDate")) { 2255 this.lastReviewDate = castToDate(value); // DateType 2256 } else if (name.equals("effectivePeriod")) { 2257 this.effectivePeriod = castToPeriod(value); // Period 2258 } else if (name.equals("topic")) { 2259 this.getTopic().add(castToCodeableConcept(value)); 2260 } else if (name.equals("author")) { 2261 this.getAuthor().add(castToContactDetail(value)); 2262 } else if (name.equals("editor")) { 2263 this.getEditor().add(castToContactDetail(value)); 2264 } else if (name.equals("reviewer")) { 2265 this.getReviewer().add(castToContactDetail(value)); 2266 } else if (name.equals("endorser")) { 2267 this.getEndorser().add(castToContactDetail(value)); 2268 } else if (name.equals("relatedArtifact")) { 2269 this.getRelatedArtifact().add(castToRelatedArtifact(value)); 2270 } else if (name.equals("trigger")) { 2271 this.getTrigger().add(castToTriggerDefinition(value)); 2272 } else 2273 return super.setProperty(name, value); 2274 return value; 2275 } 2276 2277 @Override 2278 public void removeChild(String name, Base value) throws FHIRException { 2279 if (name.equals("url")) { 2280 this.url = null; 2281 } else if (name.equals("identifier")) { 2282 this.getIdentifier().remove(castToIdentifier(value)); 2283 } else if (name.equals("version")) { 2284 this.version = null; 2285 } else if (name.equals("name")) { 2286 this.name = null; 2287 } else if (name.equals("title")) { 2288 this.title = null; 2289 } else if (name.equals("subtitle")) { 2290 this.subtitle = null; 2291 } else if (name.equals("status")) { 2292 this.status = null; 2293 } else if (name.equals("experimental")) { 2294 this.experimental = null; 2295 } else if (name.equals("subject[x]")) { 2296 this.subject = null; 2297 } else if (name.equals("date")) { 2298 this.date = null; 2299 } else if (name.equals("publisher")) { 2300 this.publisher = null; 2301 } else if (name.equals("contact")) { 2302 this.getContact().remove(castToContactDetail(value)); 2303 } else if (name.equals("description")) { 2304 this.description = null; 2305 } else if (name.equals("useContext")) { 2306 this.getUseContext().remove(castToUsageContext(value)); 2307 } else if (name.equals("jurisdiction")) { 2308 this.getJurisdiction().remove(castToCodeableConcept(value)); 2309 } else if (name.equals("purpose")) { 2310 this.purpose = null; 2311 } else if (name.equals("usage")) { 2312 this.usage = null; 2313 } else if (name.equals("copyright")) { 2314 this.copyright = null; 2315 } else if (name.equals("approvalDate")) { 2316 this.approvalDate = null; 2317 } else if (name.equals("lastReviewDate")) { 2318 this.lastReviewDate = null; 2319 } else if (name.equals("effectivePeriod")) { 2320 this.effectivePeriod = null; 2321 } else if (name.equals("topic")) { 2322 this.getTopic().remove(castToCodeableConcept(value)); 2323 } else if (name.equals("author")) { 2324 this.getAuthor().remove(castToContactDetail(value)); 2325 } else if (name.equals("editor")) { 2326 this.getEditor().remove(castToContactDetail(value)); 2327 } else if (name.equals("reviewer")) { 2328 this.getReviewer().remove(castToContactDetail(value)); 2329 } else if (name.equals("endorser")) { 2330 this.getEndorser().remove(castToContactDetail(value)); 2331 } else if (name.equals("relatedArtifact")) { 2332 this.getRelatedArtifact().remove(castToRelatedArtifact(value)); 2333 } else if (name.equals("trigger")) { 2334 this.getTrigger().remove(castToTriggerDefinition(value)); 2335 } else 2336 super.removeChild(name, value); 2337 2338 } 2339 2340 @Override 2341 public Base makeProperty(int hash, String name) throws FHIRException { 2342 switch (hash) { 2343 case 116079: 2344 return getUrlElement(); 2345 case -1618432855: 2346 return addIdentifier(); 2347 case 351608024: 2348 return getVersionElement(); 2349 case 3373707: 2350 return getNameElement(); 2351 case 110371416: 2352 return getTitleElement(); 2353 case -2060497896: 2354 return getSubtitleElement(); 2355 case -892481550: 2356 return getStatusElement(); 2357 case -404562712: 2358 return getExperimentalElement(); 2359 case -573640748: 2360 return getSubject(); 2361 case -1867885268: 2362 return getSubject(); 2363 case 3076014: 2364 return getDateElement(); 2365 case 1447404028: 2366 return getPublisherElement(); 2367 case 951526432: 2368 return addContact(); 2369 case -1724546052: 2370 return getDescriptionElement(); 2371 case -669707736: 2372 return addUseContext(); 2373 case -507075711: 2374 return addJurisdiction(); 2375 case -220463842: 2376 return getPurposeElement(); 2377 case 111574433: 2378 return getUsageElement(); 2379 case 1522889671: 2380 return getCopyrightElement(); 2381 case 223539345: 2382 return getApprovalDateElement(); 2383 case -1687512484: 2384 return getLastReviewDateElement(); 2385 case -403934648: 2386 return getEffectivePeriod(); 2387 case 110546223: 2388 return addTopic(); 2389 case -1406328437: 2390 return addAuthor(); 2391 case -1307827859: 2392 return addEditor(); 2393 case -261190139: 2394 return addReviewer(); 2395 case 1740277666: 2396 return addEndorser(); 2397 case 666807069: 2398 return addRelatedArtifact(); 2399 case -1059891784: 2400 return addTrigger(); 2401 default: 2402 return super.makeProperty(hash, name); 2403 } 2404 2405 } 2406 2407 @Override 2408 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2409 switch (hash) { 2410 case 116079: 2411 /* url */ return new String[] { "uri" }; 2412 case -1618432855: 2413 /* identifier */ return new String[] { "Identifier" }; 2414 case 351608024: 2415 /* version */ return new String[] { "string" }; 2416 case 3373707: 2417 /* name */ return new String[] { "string" }; 2418 case 110371416: 2419 /* title */ return new String[] { "string" }; 2420 case -2060497896: 2421 /* subtitle */ return new String[] { "string" }; 2422 case -892481550: 2423 /* status */ return new String[] { "code" }; 2424 case -404562712: 2425 /* experimental */ return new String[] { "boolean" }; 2426 case -1867885268: 2427 /* subject */ return new String[] { "CodeableConcept", "Reference" }; 2428 case 3076014: 2429 /* date */ return new String[] { "dateTime" }; 2430 case 1447404028: 2431 /* publisher */ return new String[] { "string" }; 2432 case 951526432: 2433 /* contact */ return new String[] { "ContactDetail" }; 2434 case -1724546052: 2435 /* description */ return new String[] { "markdown" }; 2436 case -669707736: 2437 /* useContext */ return new String[] { "UsageContext" }; 2438 case -507075711: 2439 /* jurisdiction */ return new String[] { "CodeableConcept" }; 2440 case -220463842: 2441 /* purpose */ return new String[] { "markdown" }; 2442 case 111574433: 2443 /* usage */ return new String[] { "string" }; 2444 case 1522889671: 2445 /* copyright */ return new String[] { "markdown" }; 2446 case 223539345: 2447 /* approvalDate */ return new String[] { "date" }; 2448 case -1687512484: 2449 /* lastReviewDate */ return new String[] { "date" }; 2450 case -403934648: 2451 /* effectivePeriod */ return new String[] { "Period" }; 2452 case 110546223: 2453 /* topic */ return new String[] { "CodeableConcept" }; 2454 case -1406328437: 2455 /* author */ return new String[] { "ContactDetail" }; 2456 case -1307827859: 2457 /* editor */ return new String[] { "ContactDetail" }; 2458 case -261190139: 2459 /* reviewer */ return new String[] { "ContactDetail" }; 2460 case 1740277666: 2461 /* endorser */ return new String[] { "ContactDetail" }; 2462 case 666807069: 2463 /* relatedArtifact */ return new String[] { "RelatedArtifact" }; 2464 case -1059891784: 2465 /* trigger */ return new String[] { "TriggerDefinition" }; 2466 default: 2467 return super.getTypesForProperty(hash, name); 2468 } 2469 2470 } 2471 2472 @Override 2473 public Base addChild(String name) throws FHIRException { 2474 if (name.equals("url")) { 2475 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.url"); 2476 } else if (name.equals("identifier")) { 2477 return addIdentifier(); 2478 } else if (name.equals("version")) { 2479 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.version"); 2480 } else if (name.equals("name")) { 2481 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.name"); 2482 } else if (name.equals("title")) { 2483 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.title"); 2484 } else if (name.equals("subtitle")) { 2485 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.subtitle"); 2486 } else if (name.equals("status")) { 2487 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.status"); 2488 } else if (name.equals("experimental")) { 2489 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.experimental"); 2490 } else if (name.equals("subjectCodeableConcept")) { 2491 this.subject = new CodeableConcept(); 2492 return this.subject; 2493 } else if (name.equals("subjectReference")) { 2494 this.subject = new Reference(); 2495 return this.subject; 2496 } else if (name.equals("date")) { 2497 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.date"); 2498 } else if (name.equals("publisher")) { 2499 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.publisher"); 2500 } else if (name.equals("contact")) { 2501 return addContact(); 2502 } else if (name.equals("description")) { 2503 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.description"); 2504 } else if (name.equals("useContext")) { 2505 return addUseContext(); 2506 } else if (name.equals("jurisdiction")) { 2507 return addJurisdiction(); 2508 } else if (name.equals("purpose")) { 2509 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.purpose"); 2510 } else if (name.equals("usage")) { 2511 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.usage"); 2512 } else if (name.equals("copyright")) { 2513 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.copyright"); 2514 } else if (name.equals("approvalDate")) { 2515 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.approvalDate"); 2516 } else if (name.equals("lastReviewDate")) { 2517 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.lastReviewDate"); 2518 } else if (name.equals("effectivePeriod")) { 2519 this.effectivePeriod = new Period(); 2520 return this.effectivePeriod; 2521 } else if (name.equals("topic")) { 2522 return addTopic(); 2523 } else if (name.equals("author")) { 2524 return addAuthor(); 2525 } else if (name.equals("editor")) { 2526 return addEditor(); 2527 } else if (name.equals("reviewer")) { 2528 return addReviewer(); 2529 } else if (name.equals("endorser")) { 2530 return addEndorser(); 2531 } else if (name.equals("relatedArtifact")) { 2532 return addRelatedArtifact(); 2533 } else if (name.equals("trigger")) { 2534 return addTrigger(); 2535 } else 2536 return super.addChild(name); 2537 } 2538 2539 public String fhirType() { 2540 return "EventDefinition"; 2541 2542 } 2543 2544 public EventDefinition copy() { 2545 EventDefinition dst = new EventDefinition(); 2546 copyValues(dst); 2547 return dst; 2548 } 2549 2550 public void copyValues(EventDefinition dst) { 2551 super.copyValues(dst); 2552 dst.url = url == null ? null : url.copy(); 2553 if (identifier != null) { 2554 dst.identifier = new ArrayList<Identifier>(); 2555 for (Identifier i : identifier) 2556 dst.identifier.add(i.copy()); 2557 } 2558 ; 2559 dst.version = version == null ? null : version.copy(); 2560 dst.name = name == null ? null : name.copy(); 2561 dst.title = title == null ? null : title.copy(); 2562 dst.subtitle = subtitle == null ? null : subtitle.copy(); 2563 dst.status = status == null ? null : status.copy(); 2564 dst.experimental = experimental == null ? null : experimental.copy(); 2565 dst.subject = subject == null ? null : subject.copy(); 2566 dst.date = date == null ? null : date.copy(); 2567 dst.publisher = publisher == null ? null : publisher.copy(); 2568 if (contact != null) { 2569 dst.contact = new ArrayList<ContactDetail>(); 2570 for (ContactDetail i : contact) 2571 dst.contact.add(i.copy()); 2572 } 2573 ; 2574 dst.description = description == null ? null : description.copy(); 2575 if (useContext != null) { 2576 dst.useContext = new ArrayList<UsageContext>(); 2577 for (UsageContext i : useContext) 2578 dst.useContext.add(i.copy()); 2579 } 2580 ; 2581 if (jurisdiction != null) { 2582 dst.jurisdiction = new ArrayList<CodeableConcept>(); 2583 for (CodeableConcept i : jurisdiction) 2584 dst.jurisdiction.add(i.copy()); 2585 } 2586 ; 2587 dst.purpose = purpose == null ? null : purpose.copy(); 2588 dst.usage = usage == null ? null : usage.copy(); 2589 dst.copyright = copyright == null ? null : copyright.copy(); 2590 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 2591 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 2592 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 2593 if (topic != null) { 2594 dst.topic = new ArrayList<CodeableConcept>(); 2595 for (CodeableConcept i : topic) 2596 dst.topic.add(i.copy()); 2597 } 2598 ; 2599 if (author != null) { 2600 dst.author = new ArrayList<ContactDetail>(); 2601 for (ContactDetail i : author) 2602 dst.author.add(i.copy()); 2603 } 2604 ; 2605 if (editor != null) { 2606 dst.editor = new ArrayList<ContactDetail>(); 2607 for (ContactDetail i : editor) 2608 dst.editor.add(i.copy()); 2609 } 2610 ; 2611 if (reviewer != null) { 2612 dst.reviewer = new ArrayList<ContactDetail>(); 2613 for (ContactDetail i : reviewer) 2614 dst.reviewer.add(i.copy()); 2615 } 2616 ; 2617 if (endorser != null) { 2618 dst.endorser = new ArrayList<ContactDetail>(); 2619 for (ContactDetail i : endorser) 2620 dst.endorser.add(i.copy()); 2621 } 2622 ; 2623 if (relatedArtifact != null) { 2624 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 2625 for (RelatedArtifact i : relatedArtifact) 2626 dst.relatedArtifact.add(i.copy()); 2627 } 2628 ; 2629 if (trigger != null) { 2630 dst.trigger = new ArrayList<TriggerDefinition>(); 2631 for (TriggerDefinition i : trigger) 2632 dst.trigger.add(i.copy()); 2633 } 2634 ; 2635 } 2636 2637 protected EventDefinition typedCopy() { 2638 return copy(); 2639 } 2640 2641 @Override 2642 public boolean equalsDeep(Base other_) { 2643 if (!super.equalsDeep(other_)) 2644 return false; 2645 if (!(other_ instanceof EventDefinition)) 2646 return false; 2647 EventDefinition o = (EventDefinition) other_; 2648 return compareDeep(identifier, o.identifier, true) && compareDeep(subtitle, o.subtitle, true) 2649 && compareDeep(subject, o.subject, true) && compareDeep(purpose, o.purpose, true) 2650 && compareDeep(usage, o.usage, true) && compareDeep(copyright, o.copyright, true) 2651 && compareDeep(approvalDate, o.approvalDate, true) && compareDeep(lastReviewDate, o.lastReviewDate, true) 2652 && compareDeep(effectivePeriod, o.effectivePeriod, true) && compareDeep(topic, o.topic, true) 2653 && compareDeep(author, o.author, true) && compareDeep(editor, o.editor, true) 2654 && compareDeep(reviewer, o.reviewer, true) && compareDeep(endorser, o.endorser, true) 2655 && compareDeep(relatedArtifact, o.relatedArtifact, true) && compareDeep(trigger, o.trigger, true); 2656 } 2657 2658 @Override 2659 public boolean equalsShallow(Base other_) { 2660 if (!super.equalsShallow(other_)) 2661 return false; 2662 if (!(other_ instanceof EventDefinition)) 2663 return false; 2664 EventDefinition o = (EventDefinition) other_; 2665 return compareValues(subtitle, o.subtitle, true) && compareValues(purpose, o.purpose, true) 2666 && compareValues(usage, o.usage, true) && compareValues(copyright, o.copyright, true) 2667 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true); 2668 } 2669 2670 public boolean isEmpty() { 2671 return super.isEmpty() 2672 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, subtitle, subject, purpose, usage, copyright, approvalDate, 2673 lastReviewDate, effectivePeriod, topic, author, editor, reviewer, endorser, relatedArtifact, trigger); 2674 } 2675 2676 @Override 2677 public ResourceType getResourceType() { 2678 return ResourceType.EventDefinition; 2679 } 2680 2681 /** 2682 * Search parameter: <b>date</b> 2683 * <p> 2684 * Description: <b>The event definition publication date</b><br> 2685 * Type: <b>date</b><br> 2686 * Path: <b>EventDefinition.date</b><br> 2687 * </p> 2688 */ 2689 @SearchParamDefinition(name = "date", path = "EventDefinition.date", description = "The event definition publication date", type = "date") 2690 public static final String SP_DATE = "date"; 2691 /** 2692 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2693 * <p> 2694 * Description: <b>The event definition publication date</b><br> 2695 * Type: <b>date</b><br> 2696 * Path: <b>EventDefinition.date</b><br> 2697 * </p> 2698 */ 2699 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2700 SP_DATE); 2701 2702 /** 2703 * Search parameter: <b>identifier</b> 2704 * <p> 2705 * Description: <b>External identifier for the event definition</b><br> 2706 * Type: <b>token</b><br> 2707 * Path: <b>EventDefinition.identifier</b><br> 2708 * </p> 2709 */ 2710 @SearchParamDefinition(name = "identifier", path = "EventDefinition.identifier", description = "External identifier for the event definition", type = "token") 2711 public static final String SP_IDENTIFIER = "identifier"; 2712 /** 2713 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2714 * <p> 2715 * Description: <b>External identifier for the event definition</b><br> 2716 * Type: <b>token</b><br> 2717 * Path: <b>EventDefinition.identifier</b><br> 2718 * </p> 2719 */ 2720 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2721 SP_IDENTIFIER); 2722 2723 /** 2724 * Search parameter: <b>successor</b> 2725 * <p> 2726 * Description: <b>What resource is being referenced</b><br> 2727 * Type: <b>reference</b><br> 2728 * Path: <b>EventDefinition.relatedArtifact.resource</b><br> 2729 * </p> 2730 */ 2731 @SearchParamDefinition(name = "successor", path = "EventDefinition.relatedArtifact.where(type='successor').resource", description = "What resource is being referenced", type = "reference") 2732 public static final String SP_SUCCESSOR = "successor"; 2733 /** 2734 * <b>Fluent Client</b> search parameter constant for <b>successor</b> 2735 * <p> 2736 * Description: <b>What resource is being referenced</b><br> 2737 * Type: <b>reference</b><br> 2738 * Path: <b>EventDefinition.relatedArtifact.resource</b><br> 2739 * </p> 2740 */ 2741 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUCCESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2742 SP_SUCCESSOR); 2743 2744 /** 2745 * Constant for fluent queries to be used to add include statements. Specifies 2746 * the path value of "<b>EventDefinition:successor</b>". 2747 */ 2748 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUCCESSOR = new ca.uhn.fhir.model.api.Include( 2749 "EventDefinition:successor").toLocked(); 2750 2751 /** 2752 * Search parameter: <b>context-type-value</b> 2753 * <p> 2754 * Description: <b>A use context type and value assigned to the event 2755 * definition</b><br> 2756 * Type: <b>composite</b><br> 2757 * Path: <b></b><br> 2758 * </p> 2759 */ 2760 @SearchParamDefinition(name = "context-type-value", path = "EventDefinition.useContext", description = "A use context type and value assigned to the event definition", type = "composite", compositeOf = { 2761 "context-type", "context" }) 2762 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 2763 /** 2764 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 2765 * <p> 2766 * Description: <b>A use context type and value assigned to the event 2767 * definition</b><br> 2768 * Type: <b>composite</b><br> 2769 * Path: <b></b><br> 2770 * </p> 2771 */ 2772 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 2773 SP_CONTEXT_TYPE_VALUE); 2774 2775 /** 2776 * Search parameter: <b>jurisdiction</b> 2777 * <p> 2778 * Description: <b>Intended jurisdiction for the event definition</b><br> 2779 * Type: <b>token</b><br> 2780 * Path: <b>EventDefinition.jurisdiction</b><br> 2781 * </p> 2782 */ 2783 @SearchParamDefinition(name = "jurisdiction", path = "EventDefinition.jurisdiction", description = "Intended jurisdiction for the event definition", type = "token") 2784 public static final String SP_JURISDICTION = "jurisdiction"; 2785 /** 2786 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 2787 * <p> 2788 * Description: <b>Intended jurisdiction for the event definition</b><br> 2789 * Type: <b>token</b><br> 2790 * Path: <b>EventDefinition.jurisdiction</b><br> 2791 * </p> 2792 */ 2793 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2794 SP_JURISDICTION); 2795 2796 /** 2797 * Search parameter: <b>description</b> 2798 * <p> 2799 * Description: <b>The description of the event definition</b><br> 2800 * Type: <b>string</b><br> 2801 * Path: <b>EventDefinition.description</b><br> 2802 * </p> 2803 */ 2804 @SearchParamDefinition(name = "description", path = "EventDefinition.description", description = "The description of the event definition", type = "string") 2805 public static final String SP_DESCRIPTION = "description"; 2806 /** 2807 * <b>Fluent Client</b> search parameter constant for <b>description</b> 2808 * <p> 2809 * Description: <b>The description of the event definition</b><br> 2810 * Type: <b>string</b><br> 2811 * Path: <b>EventDefinition.description</b><br> 2812 * </p> 2813 */ 2814 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 2815 SP_DESCRIPTION); 2816 2817 /** 2818 * Search parameter: <b>derived-from</b> 2819 * <p> 2820 * Description: <b>What resource is being referenced</b><br> 2821 * Type: <b>reference</b><br> 2822 * Path: <b>EventDefinition.relatedArtifact.resource</b><br> 2823 * </p> 2824 */ 2825 @SearchParamDefinition(name = "derived-from", path = "EventDefinition.relatedArtifact.where(type='derived-from').resource", description = "What resource is being referenced", type = "reference") 2826 public static final String SP_DERIVED_FROM = "derived-from"; 2827 /** 2828 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 2829 * <p> 2830 * Description: <b>What resource is being referenced</b><br> 2831 * Type: <b>reference</b><br> 2832 * Path: <b>EventDefinition.relatedArtifact.resource</b><br> 2833 * </p> 2834 */ 2835 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2836 SP_DERIVED_FROM); 2837 2838 /** 2839 * Constant for fluent queries to be used to add include statements. Specifies 2840 * the path value of "<b>EventDefinition:derived-from</b>". 2841 */ 2842 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include( 2843 "EventDefinition:derived-from").toLocked(); 2844 2845 /** 2846 * Search parameter: <b>context-type</b> 2847 * <p> 2848 * Description: <b>A type of use context assigned to the event 2849 * definition</b><br> 2850 * Type: <b>token</b><br> 2851 * Path: <b>EventDefinition.useContext.code</b><br> 2852 * </p> 2853 */ 2854 @SearchParamDefinition(name = "context-type", path = "EventDefinition.useContext.code", description = "A type of use context assigned to the event definition", type = "token") 2855 public static final String SP_CONTEXT_TYPE = "context-type"; 2856 /** 2857 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 2858 * <p> 2859 * Description: <b>A type of use context assigned to the event 2860 * definition</b><br> 2861 * Type: <b>token</b><br> 2862 * Path: <b>EventDefinition.useContext.code</b><br> 2863 * </p> 2864 */ 2865 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2866 SP_CONTEXT_TYPE); 2867 2868 /** 2869 * Search parameter: <b>predecessor</b> 2870 * <p> 2871 * Description: <b>What resource is being referenced</b><br> 2872 * Type: <b>reference</b><br> 2873 * Path: <b>EventDefinition.relatedArtifact.resource</b><br> 2874 * </p> 2875 */ 2876 @SearchParamDefinition(name = "predecessor", path = "EventDefinition.relatedArtifact.where(type='predecessor').resource", description = "What resource is being referenced", type = "reference") 2877 public static final String SP_PREDECESSOR = "predecessor"; 2878 /** 2879 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 2880 * <p> 2881 * Description: <b>What resource is being referenced</b><br> 2882 * Type: <b>reference</b><br> 2883 * Path: <b>EventDefinition.relatedArtifact.resource</b><br> 2884 * </p> 2885 */ 2886 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2887 SP_PREDECESSOR); 2888 2889 /** 2890 * Constant for fluent queries to be used to add include statements. Specifies 2891 * the path value of "<b>EventDefinition:predecessor</b>". 2892 */ 2893 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include( 2894 "EventDefinition:predecessor").toLocked(); 2895 2896 /** 2897 * Search parameter: <b>title</b> 2898 * <p> 2899 * Description: <b>The human-friendly name of the event definition</b><br> 2900 * Type: <b>string</b><br> 2901 * Path: <b>EventDefinition.title</b><br> 2902 * </p> 2903 */ 2904 @SearchParamDefinition(name = "title", path = "EventDefinition.title", description = "The human-friendly name of the event definition", type = "string") 2905 public static final String SP_TITLE = "title"; 2906 /** 2907 * <b>Fluent Client</b> search parameter constant for <b>title</b> 2908 * <p> 2909 * Description: <b>The human-friendly name of the event definition</b><br> 2910 * Type: <b>string</b><br> 2911 * Path: <b>EventDefinition.title</b><br> 2912 * </p> 2913 */ 2914 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 2915 SP_TITLE); 2916 2917 /** 2918 * Search parameter: <b>composed-of</b> 2919 * <p> 2920 * Description: <b>What resource is being referenced</b><br> 2921 * Type: <b>reference</b><br> 2922 * Path: <b>EventDefinition.relatedArtifact.resource</b><br> 2923 * </p> 2924 */ 2925 @SearchParamDefinition(name = "composed-of", path = "EventDefinition.relatedArtifact.where(type='composed-of').resource", description = "What resource is being referenced", type = "reference") 2926 public static final String SP_COMPOSED_OF = "composed-of"; 2927 /** 2928 * <b>Fluent Client</b> search parameter constant for <b>composed-of</b> 2929 * <p> 2930 * Description: <b>What resource is being referenced</b><br> 2931 * Type: <b>reference</b><br> 2932 * Path: <b>EventDefinition.relatedArtifact.resource</b><br> 2933 * </p> 2934 */ 2935 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSED_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2936 SP_COMPOSED_OF); 2937 2938 /** 2939 * Constant for fluent queries to be used to add include statements. Specifies 2940 * the path value of "<b>EventDefinition:composed-of</b>". 2941 */ 2942 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSED_OF = new ca.uhn.fhir.model.api.Include( 2943 "EventDefinition:composed-of").toLocked(); 2944 2945 /** 2946 * Search parameter: <b>version</b> 2947 * <p> 2948 * Description: <b>The business version of the event definition</b><br> 2949 * Type: <b>token</b><br> 2950 * Path: <b>EventDefinition.version</b><br> 2951 * </p> 2952 */ 2953 @SearchParamDefinition(name = "version", path = "EventDefinition.version", description = "The business version of the event definition", type = "token") 2954 public static final String SP_VERSION = "version"; 2955 /** 2956 * <b>Fluent Client</b> search parameter constant for <b>version</b> 2957 * <p> 2958 * Description: <b>The business version of the event definition</b><br> 2959 * Type: <b>token</b><br> 2960 * Path: <b>EventDefinition.version</b><br> 2961 * </p> 2962 */ 2963 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2964 SP_VERSION); 2965 2966 /** 2967 * Search parameter: <b>url</b> 2968 * <p> 2969 * Description: <b>The uri that identifies the event definition</b><br> 2970 * Type: <b>uri</b><br> 2971 * Path: <b>EventDefinition.url</b><br> 2972 * </p> 2973 */ 2974 @SearchParamDefinition(name = "url", path = "EventDefinition.url", description = "The uri that identifies the event definition", type = "uri") 2975 public static final String SP_URL = "url"; 2976 /** 2977 * <b>Fluent Client</b> search parameter constant for <b>url</b> 2978 * <p> 2979 * Description: <b>The uri that identifies the event definition</b><br> 2980 * Type: <b>uri</b><br> 2981 * Path: <b>EventDefinition.url</b><br> 2982 * </p> 2983 */ 2984 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 2985 2986 /** 2987 * Search parameter: <b>context-quantity</b> 2988 * <p> 2989 * Description: <b>A quantity- or range-valued use context assigned to the event 2990 * definition</b><br> 2991 * Type: <b>quantity</b><br> 2992 * Path: <b>EventDefinition.useContext.valueQuantity, 2993 * EventDefinition.useContext.valueRange</b><br> 2994 * </p> 2995 */ 2996 @SearchParamDefinition(name = "context-quantity", path = "(EventDefinition.useContext.value as Quantity) | (EventDefinition.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the event definition", type = "quantity") 2997 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 2998 /** 2999 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 3000 * <p> 3001 * Description: <b>A quantity- or range-valued use context assigned to the event 3002 * definition</b><br> 3003 * Type: <b>quantity</b><br> 3004 * Path: <b>EventDefinition.useContext.valueQuantity, 3005 * EventDefinition.useContext.valueRange</b><br> 3006 * </p> 3007 */ 3008 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 3009 SP_CONTEXT_QUANTITY); 3010 3011 /** 3012 * Search parameter: <b>effective</b> 3013 * <p> 3014 * Description: <b>The time during which the event definition is intended to be 3015 * in use</b><br> 3016 * Type: <b>date</b><br> 3017 * Path: <b>EventDefinition.effectivePeriod</b><br> 3018 * </p> 3019 */ 3020 @SearchParamDefinition(name = "effective", path = "EventDefinition.effectivePeriod", description = "The time during which the event definition is intended to be in use", type = "date") 3021 public static final String SP_EFFECTIVE = "effective"; 3022 /** 3023 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 3024 * <p> 3025 * Description: <b>The time during which the event definition is intended to be 3026 * in use</b><br> 3027 * Type: <b>date</b><br> 3028 * Path: <b>EventDefinition.effectivePeriod</b><br> 3029 * </p> 3030 */ 3031 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3032 SP_EFFECTIVE); 3033 3034 /** 3035 * Search parameter: <b>depends-on</b> 3036 * <p> 3037 * Description: <b>What resource is being referenced</b><br> 3038 * Type: <b>reference</b><br> 3039 * Path: <b>EventDefinition.relatedArtifact.resource</b><br> 3040 * </p> 3041 */ 3042 @SearchParamDefinition(name = "depends-on", path = "EventDefinition.relatedArtifact.where(type='depends-on').resource", description = "What resource is being referenced", type = "reference") 3043 public static final String SP_DEPENDS_ON = "depends-on"; 3044 /** 3045 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 3046 * <p> 3047 * Description: <b>What resource is being referenced</b><br> 3048 * Type: <b>reference</b><br> 3049 * Path: <b>EventDefinition.relatedArtifact.resource</b><br> 3050 * </p> 3051 */ 3052 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3053 SP_DEPENDS_ON); 3054 3055 /** 3056 * Constant for fluent queries to be used to add include statements. Specifies 3057 * the path value of "<b>EventDefinition:depends-on</b>". 3058 */ 3059 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include( 3060 "EventDefinition:depends-on").toLocked(); 3061 3062 /** 3063 * Search parameter: <b>name</b> 3064 * <p> 3065 * Description: <b>Computationally friendly name of the event definition</b><br> 3066 * Type: <b>string</b><br> 3067 * Path: <b>EventDefinition.name</b><br> 3068 * </p> 3069 */ 3070 @SearchParamDefinition(name = "name", path = "EventDefinition.name", description = "Computationally friendly name of the event definition", type = "string") 3071 public static final String SP_NAME = "name"; 3072 /** 3073 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3074 * <p> 3075 * Description: <b>Computationally friendly name of the event definition</b><br> 3076 * Type: <b>string</b><br> 3077 * Path: <b>EventDefinition.name</b><br> 3078 * </p> 3079 */ 3080 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 3081 SP_NAME); 3082 3083 /** 3084 * Search parameter: <b>context</b> 3085 * <p> 3086 * Description: <b>A use context assigned to the event definition</b><br> 3087 * Type: <b>token</b><br> 3088 * Path: <b>EventDefinition.useContext.valueCodeableConcept</b><br> 3089 * </p> 3090 */ 3091 @SearchParamDefinition(name = "context", path = "(EventDefinition.useContext.value as CodeableConcept)", description = "A use context assigned to the event definition", type = "token") 3092 public static final String SP_CONTEXT = "context"; 3093 /** 3094 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3095 * <p> 3096 * Description: <b>A use context assigned to the event definition</b><br> 3097 * Type: <b>token</b><br> 3098 * Path: <b>EventDefinition.useContext.valueCodeableConcept</b><br> 3099 * </p> 3100 */ 3101 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3102 SP_CONTEXT); 3103 3104 /** 3105 * Search parameter: <b>publisher</b> 3106 * <p> 3107 * Description: <b>Name of the publisher of the event definition</b><br> 3108 * Type: <b>string</b><br> 3109 * Path: <b>EventDefinition.publisher</b><br> 3110 * </p> 3111 */ 3112 @SearchParamDefinition(name = "publisher", path = "EventDefinition.publisher", description = "Name of the publisher of the event definition", type = "string") 3113 public static final String SP_PUBLISHER = "publisher"; 3114 /** 3115 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 3116 * <p> 3117 * Description: <b>Name of the publisher of the event definition</b><br> 3118 * Type: <b>string</b><br> 3119 * Path: <b>EventDefinition.publisher</b><br> 3120 * </p> 3121 */ 3122 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 3123 SP_PUBLISHER); 3124 3125 /** 3126 * Search parameter: <b>topic</b> 3127 * <p> 3128 * Description: <b>Topics associated with the module</b><br> 3129 * Type: <b>token</b><br> 3130 * Path: <b>EventDefinition.topic</b><br> 3131 * </p> 3132 */ 3133 @SearchParamDefinition(name = "topic", path = "EventDefinition.topic", description = "Topics associated with the module", type = "token") 3134 public static final String SP_TOPIC = "topic"; 3135 /** 3136 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 3137 * <p> 3138 * Description: <b>Topics associated with the module</b><br> 3139 * Type: <b>token</b><br> 3140 * Path: <b>EventDefinition.topic</b><br> 3141 * </p> 3142 */ 3143 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3144 SP_TOPIC); 3145 3146 /** 3147 * Search parameter: <b>context-type-quantity</b> 3148 * <p> 3149 * Description: <b>A use context type and quantity- or range-based value 3150 * assigned to the event definition</b><br> 3151 * Type: <b>composite</b><br> 3152 * Path: <b></b><br> 3153 * </p> 3154 */ 3155 @SearchParamDefinition(name = "context-type-quantity", path = "EventDefinition.useContext", description = "A use context type and quantity- or range-based value assigned to the event definition", type = "composite", compositeOf = { 3156 "context-type", "context-quantity" }) 3157 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 3158 /** 3159 * <b>Fluent Client</b> search parameter constant for 3160 * <b>context-type-quantity</b> 3161 * <p> 3162 * Description: <b>A use context type and quantity- or range-based value 3163 * assigned to the event definition</b><br> 3164 * Type: <b>composite</b><br> 3165 * Path: <b></b><br> 3166 * </p> 3167 */ 3168 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 3169 SP_CONTEXT_TYPE_QUANTITY); 3170 3171 /** 3172 * Search parameter: <b>status</b> 3173 * <p> 3174 * Description: <b>The current status of the event definition</b><br> 3175 * Type: <b>token</b><br> 3176 * Path: <b>EventDefinition.status</b><br> 3177 * </p> 3178 */ 3179 @SearchParamDefinition(name = "status", path = "EventDefinition.status", description = "The current status of the event definition", type = "token") 3180 public static final String SP_STATUS = "status"; 3181 /** 3182 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3183 * <p> 3184 * Description: <b>The current status of the event definition</b><br> 3185 * Type: <b>token</b><br> 3186 * Path: <b>EventDefinition.status</b><br> 3187 * </p> 3188 */ 3189 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3190 SP_STATUS); 3191 3192}