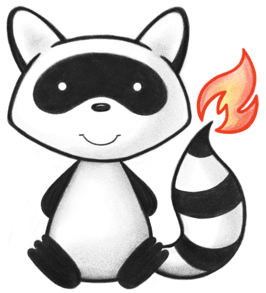
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049 050/** 051 * The EvidenceVariable resource describes a "PICO" element that knowledge 052 * (evidence, assertion, recommendation) is about. 053 */ 054@ResourceDef(name = "EvidenceVariable", profile = "http://hl7.org/fhir/StructureDefinition/EvidenceVariable") 055@ChildOrder(names = { "url", "identifier", "version", "name", "title", "shortTitle", "subtitle", "status", "date", 056 "publisher", "contact", "description", "note", "useContext", "jurisdiction", "copyright", "approvalDate", 057 "lastReviewDate", "effectivePeriod", "topic", "author", "editor", "reviewer", "endorser", "relatedArtifact", "type", 058 "characteristic" }) 059public class EvidenceVariable extends MetadataResource { 060 061 public enum EvidenceVariableType { 062 /** 063 * The variable is dichotomous, such as present or absent. 064 */ 065 DICHOTOMOUS, 066 /** 067 * The variable is a continuous result such as a quantity. 068 */ 069 CONTINUOUS, 070 /** 071 * The variable is described narratively rather than quantitatively. 072 */ 073 DESCRIPTIVE, 074 /** 075 * added to help the parsers with the generic types 076 */ 077 NULL; 078 079 public static EvidenceVariableType fromCode(String codeString) throws FHIRException { 080 if (codeString == null || "".equals(codeString)) 081 return null; 082 if ("dichotomous".equals(codeString)) 083 return DICHOTOMOUS; 084 if ("continuous".equals(codeString)) 085 return CONTINUOUS; 086 if ("descriptive".equals(codeString)) 087 return DESCRIPTIVE; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown EvidenceVariableType code '" + codeString + "'"); 092 } 093 094 public String toCode() { 095 switch (this) { 096 case DICHOTOMOUS: 097 return "dichotomous"; 098 case CONTINUOUS: 099 return "continuous"; 100 case DESCRIPTIVE: 101 return "descriptive"; 102 case NULL: 103 return null; 104 default: 105 return "?"; 106 } 107 } 108 109 public String getSystem() { 110 switch (this) { 111 case DICHOTOMOUS: 112 return "http://hl7.org/fhir/variable-type"; 113 case CONTINUOUS: 114 return "http://hl7.org/fhir/variable-type"; 115 case DESCRIPTIVE: 116 return "http://hl7.org/fhir/variable-type"; 117 case NULL: 118 return null; 119 default: 120 return "?"; 121 } 122 } 123 124 public String getDefinition() { 125 switch (this) { 126 case DICHOTOMOUS: 127 return "The variable is dichotomous, such as present or absent."; 128 case CONTINUOUS: 129 return "The variable is a continuous result such as a quantity."; 130 case DESCRIPTIVE: 131 return "The variable is described narratively rather than quantitatively."; 132 case NULL: 133 return null; 134 default: 135 return "?"; 136 } 137 } 138 139 public String getDisplay() { 140 switch (this) { 141 case DICHOTOMOUS: 142 return "Dichotomous"; 143 case CONTINUOUS: 144 return "Continuous"; 145 case DESCRIPTIVE: 146 return "Descriptive"; 147 case NULL: 148 return null; 149 default: 150 return "?"; 151 } 152 } 153 } 154 155 public static class EvidenceVariableTypeEnumFactory implements EnumFactory<EvidenceVariableType> { 156 public EvidenceVariableType fromCode(String codeString) throws IllegalArgumentException { 157 if (codeString == null || "".equals(codeString)) 158 if (codeString == null || "".equals(codeString)) 159 return null; 160 if ("dichotomous".equals(codeString)) 161 return EvidenceVariableType.DICHOTOMOUS; 162 if ("continuous".equals(codeString)) 163 return EvidenceVariableType.CONTINUOUS; 164 if ("descriptive".equals(codeString)) 165 return EvidenceVariableType.DESCRIPTIVE; 166 throw new IllegalArgumentException("Unknown EvidenceVariableType code '" + codeString + "'"); 167 } 168 169 public Enumeration<EvidenceVariableType> fromType(PrimitiveType<?> code) throws FHIRException { 170 if (code == null) 171 return null; 172 if (code.isEmpty()) 173 return new Enumeration<EvidenceVariableType>(this, EvidenceVariableType.NULL, code); 174 String codeString = code.asStringValue(); 175 if (codeString == null || "".equals(codeString)) 176 return new Enumeration<EvidenceVariableType>(this, EvidenceVariableType.NULL, code); 177 if ("dichotomous".equals(codeString)) 178 return new Enumeration<EvidenceVariableType>(this, EvidenceVariableType.DICHOTOMOUS, code); 179 if ("continuous".equals(codeString)) 180 return new Enumeration<EvidenceVariableType>(this, EvidenceVariableType.CONTINUOUS, code); 181 if ("descriptive".equals(codeString)) 182 return new Enumeration<EvidenceVariableType>(this, EvidenceVariableType.DESCRIPTIVE, code); 183 throw new FHIRException("Unknown EvidenceVariableType code '" + codeString + "'"); 184 } 185 186 public String toCode(EvidenceVariableType code) { 187 if (code == EvidenceVariableType.DICHOTOMOUS) 188 return "dichotomous"; 189 if (code == EvidenceVariableType.CONTINUOUS) 190 return "continuous"; 191 if (code == EvidenceVariableType.DESCRIPTIVE) 192 return "descriptive"; 193 return "?"; 194 } 195 196 public String toSystem(EvidenceVariableType code) { 197 return code.getSystem(); 198 } 199 } 200 201 public enum GroupMeasure { 202 /** 203 * Aggregated using Mean of participant values. 204 */ 205 MEAN, 206 /** 207 * Aggregated using Median of participant values. 208 */ 209 MEDIAN, 210 /** 211 * Aggregated using Mean of study mean values. 212 */ 213 MEANOFMEAN, 214 /** 215 * Aggregated using Mean of study median values. 216 */ 217 MEANOFMEDIAN, 218 /** 219 * Aggregated using Median of study mean values. 220 */ 221 MEDIANOFMEAN, 222 /** 223 * Aggregated using Median of study median values. 224 */ 225 MEDIANOFMEDIAN, 226 /** 227 * added to help the parsers with the generic types 228 */ 229 NULL; 230 231 public static GroupMeasure fromCode(String codeString) throws FHIRException { 232 if (codeString == null || "".equals(codeString)) 233 return null; 234 if ("mean".equals(codeString)) 235 return MEAN; 236 if ("median".equals(codeString)) 237 return MEDIAN; 238 if ("mean-of-mean".equals(codeString)) 239 return MEANOFMEAN; 240 if ("mean-of-median".equals(codeString)) 241 return MEANOFMEDIAN; 242 if ("median-of-mean".equals(codeString)) 243 return MEDIANOFMEAN; 244 if ("median-of-median".equals(codeString)) 245 return MEDIANOFMEDIAN; 246 if (Configuration.isAcceptInvalidEnums()) 247 return null; 248 else 249 throw new FHIRException("Unknown GroupMeasure code '" + codeString + "'"); 250 } 251 252 public String toCode() { 253 switch (this) { 254 case MEAN: 255 return "mean"; 256 case MEDIAN: 257 return "median"; 258 case MEANOFMEAN: 259 return "mean-of-mean"; 260 case MEANOFMEDIAN: 261 return "mean-of-median"; 262 case MEDIANOFMEAN: 263 return "median-of-mean"; 264 case MEDIANOFMEDIAN: 265 return "median-of-median"; 266 case NULL: 267 return null; 268 default: 269 return "?"; 270 } 271 } 272 273 public String getSystem() { 274 switch (this) { 275 case MEAN: 276 return "http://hl7.org/fhir/group-measure"; 277 case MEDIAN: 278 return "http://hl7.org/fhir/group-measure"; 279 case MEANOFMEAN: 280 return "http://hl7.org/fhir/group-measure"; 281 case MEANOFMEDIAN: 282 return "http://hl7.org/fhir/group-measure"; 283 case MEDIANOFMEAN: 284 return "http://hl7.org/fhir/group-measure"; 285 case MEDIANOFMEDIAN: 286 return "http://hl7.org/fhir/group-measure"; 287 case NULL: 288 return null; 289 default: 290 return "?"; 291 } 292 } 293 294 public String getDefinition() { 295 switch (this) { 296 case MEAN: 297 return "Aggregated using Mean of participant values."; 298 case MEDIAN: 299 return "Aggregated using Median of participant values."; 300 case MEANOFMEAN: 301 return "Aggregated using Mean of study mean values."; 302 case MEANOFMEDIAN: 303 return "Aggregated using Mean of study median values."; 304 case MEDIANOFMEAN: 305 return "Aggregated using Median of study mean values."; 306 case MEDIANOFMEDIAN: 307 return "Aggregated using Median of study median values."; 308 case NULL: 309 return null; 310 default: 311 return "?"; 312 } 313 } 314 315 public String getDisplay() { 316 switch (this) { 317 case MEAN: 318 return "Mean"; 319 case MEDIAN: 320 return "Median"; 321 case MEANOFMEAN: 322 return "Mean of Study Means"; 323 case MEANOFMEDIAN: 324 return "Mean of Study Medins"; 325 case MEDIANOFMEAN: 326 return "Median of Study Means"; 327 case MEDIANOFMEDIAN: 328 return "Median of Study Medians"; 329 case NULL: 330 return null; 331 default: 332 return "?"; 333 } 334 } 335 } 336 337 public static class GroupMeasureEnumFactory implements EnumFactory<GroupMeasure> { 338 public GroupMeasure fromCode(String codeString) throws IllegalArgumentException { 339 if (codeString == null || "".equals(codeString)) 340 if (codeString == null || "".equals(codeString)) 341 return null; 342 if ("mean".equals(codeString)) 343 return GroupMeasure.MEAN; 344 if ("median".equals(codeString)) 345 return GroupMeasure.MEDIAN; 346 if ("mean-of-mean".equals(codeString)) 347 return GroupMeasure.MEANOFMEAN; 348 if ("mean-of-median".equals(codeString)) 349 return GroupMeasure.MEANOFMEDIAN; 350 if ("median-of-mean".equals(codeString)) 351 return GroupMeasure.MEDIANOFMEAN; 352 if ("median-of-median".equals(codeString)) 353 return GroupMeasure.MEDIANOFMEDIAN; 354 throw new IllegalArgumentException("Unknown GroupMeasure code '" + codeString + "'"); 355 } 356 357 public Enumeration<GroupMeasure> fromType(PrimitiveType<?> code) throws FHIRException { 358 if (code == null) 359 return null; 360 if (code.isEmpty()) 361 return new Enumeration<GroupMeasure>(this, GroupMeasure.NULL, code); 362 String codeString = code.asStringValue(); 363 if (codeString == null || "".equals(codeString)) 364 return new Enumeration<GroupMeasure>(this, GroupMeasure.NULL, code); 365 if ("mean".equals(codeString)) 366 return new Enumeration<GroupMeasure>(this, GroupMeasure.MEAN, code); 367 if ("median".equals(codeString)) 368 return new Enumeration<GroupMeasure>(this, GroupMeasure.MEDIAN, code); 369 if ("mean-of-mean".equals(codeString)) 370 return new Enumeration<GroupMeasure>(this, GroupMeasure.MEANOFMEAN, code); 371 if ("mean-of-median".equals(codeString)) 372 return new Enumeration<GroupMeasure>(this, GroupMeasure.MEANOFMEDIAN, code); 373 if ("median-of-mean".equals(codeString)) 374 return new Enumeration<GroupMeasure>(this, GroupMeasure.MEDIANOFMEAN, code); 375 if ("median-of-median".equals(codeString)) 376 return new Enumeration<GroupMeasure>(this, GroupMeasure.MEDIANOFMEDIAN, code); 377 throw new FHIRException("Unknown GroupMeasure code '" + codeString + "'"); 378 } 379 380 public String toCode(GroupMeasure code) { 381 if (code == GroupMeasure.MEAN) 382 return "mean"; 383 if (code == GroupMeasure.MEDIAN) 384 return "median"; 385 if (code == GroupMeasure.MEANOFMEAN) 386 return "mean-of-mean"; 387 if (code == GroupMeasure.MEANOFMEDIAN) 388 return "mean-of-median"; 389 if (code == GroupMeasure.MEDIANOFMEAN) 390 return "median-of-mean"; 391 if (code == GroupMeasure.MEDIANOFMEDIAN) 392 return "median-of-median"; 393 return "?"; 394 } 395 396 public String toSystem(GroupMeasure code) { 397 return code.getSystem(); 398 } 399 } 400 401 @Block() 402 public static class EvidenceVariableCharacteristicComponent extends BackboneElement implements IBaseBackboneElement { 403 /** 404 * A short, natural language description of the characteristic that could be 405 * used to communicate the criteria to an end-user. 406 */ 407 @Child(name = "description", type = { 408 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 409 @Description(shortDefinition = "Natural language description of the characteristic", formalDefinition = "A short, natural language description of the characteristic that could be used to communicate the criteria to an end-user.") 410 protected StringType description; 411 412 /** 413 * Define members of the evidence element using Codes (such as condition, 414 * medication, or observation), Expressions ( using an expression language such 415 * as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in 416 * the last year). 417 */ 418 @Child(name = "definition", type = { Group.class, CanonicalType.class, CodeableConcept.class, Expression.class, 419 DataRequirement.class, TriggerDefinition.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 420 @Description(shortDefinition = "What code or expression defines members?", formalDefinition = "Define members of the evidence element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).") 421 protected Type definition; 422 423 /** 424 * Use UsageContext to define the members of the population, such as Age Ranges, 425 * Genders, Settings. 426 */ 427 @Child(name = "usageContext", type = { 428 UsageContext.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 429 @Description(shortDefinition = "What code/value pairs define members?", formalDefinition = "Use UsageContext to define the members of the population, such as Age Ranges, Genders, Settings.") 430 protected List<UsageContext> usageContext; 431 432 /** 433 * When true, members with this characteristic are excluded from the element. 434 */ 435 @Child(name = "exclude", type = { 436 BooleanType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 437 @Description(shortDefinition = "Whether the characteristic includes or excludes members", formalDefinition = "When true, members with this characteristic are excluded from the element.") 438 protected BooleanType exclude; 439 440 /** 441 * Indicates what effective period the study covers. 442 */ 443 @Child(name = "participantEffective", type = { DateTimeType.class, Period.class, Duration.class, 444 Timing.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 445 @Description(shortDefinition = "What time period do participants cover", formalDefinition = "Indicates what effective period the study covers.") 446 protected Type participantEffective; 447 448 /** 449 * Indicates duration from the participant's study entry. 450 */ 451 @Child(name = "timeFromStart", type = { 452 Duration.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 453 @Description(shortDefinition = "Observation time from study start", formalDefinition = "Indicates duration from the participant's study entry.") 454 protected Duration timeFromStart; 455 456 /** 457 * Indicates how elements are aggregated within the study effective period. 458 */ 459 @Child(name = "groupMeasure", type = { 460 CodeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 461 @Description(shortDefinition = "mean | median | mean-of-mean | mean-of-median | median-of-mean | median-of-median", formalDefinition = "Indicates how elements are aggregated within the study effective period.") 462 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/group-measure") 463 protected Enumeration<GroupMeasure> groupMeasure; 464 465 private static final long serialVersionUID = 1901961318L; 466 467 /** 468 * Constructor 469 */ 470 public EvidenceVariableCharacteristicComponent() { 471 super(); 472 } 473 474 /** 475 * Constructor 476 */ 477 public EvidenceVariableCharacteristicComponent(Type definition) { 478 super(); 479 this.definition = definition; 480 } 481 482 /** 483 * @return {@link #description} (A short, natural language description of the 484 * characteristic that could be used to communicate the criteria to an 485 * end-user.). This is the underlying object with id, value and 486 * extensions. The accessor "getDescription" gives direct access to the 487 * value 488 */ 489 public StringType getDescriptionElement() { 490 if (this.description == null) 491 if (Configuration.errorOnAutoCreate()) 492 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.description"); 493 else if (Configuration.doAutoCreate()) 494 this.description = new StringType(); // bb 495 return this.description; 496 } 497 498 public boolean hasDescriptionElement() { 499 return this.description != null && !this.description.isEmpty(); 500 } 501 502 public boolean hasDescription() { 503 return this.description != null && !this.description.isEmpty(); 504 } 505 506 /** 507 * @param value {@link #description} (A short, natural language description of 508 * the characteristic that could be used to communicate the 509 * criteria to an end-user.). This is the underlying object with 510 * id, value and extensions. The accessor "getDescription" gives 511 * direct access to the value 512 */ 513 public EvidenceVariableCharacteristicComponent setDescriptionElement(StringType value) { 514 this.description = value; 515 return this; 516 } 517 518 /** 519 * @return A short, natural language description of the characteristic that 520 * could be used to communicate the criteria to an end-user. 521 */ 522 public String getDescription() { 523 return this.description == null ? null : this.description.getValue(); 524 } 525 526 /** 527 * @param value A short, natural language description of the characteristic that 528 * could be used to communicate the criteria to an end-user. 529 */ 530 public EvidenceVariableCharacteristicComponent setDescription(String value) { 531 if (Utilities.noString(value)) 532 this.description = null; 533 else { 534 if (this.description == null) 535 this.description = new StringType(); 536 this.description.setValue(value); 537 } 538 return this; 539 } 540 541 /** 542 * @return {@link #definition} (Define members of the evidence element using 543 * Codes (such as condition, medication, or observation), Expressions ( 544 * using an expression language such as FHIRPath or CQL) or 545 * DataRequirements (such as Diabetes diagnosis onset in the last 546 * year).) 547 */ 548 public Type getDefinition() { 549 return this.definition; 550 } 551 552 /** 553 * @return {@link #definition} (Define members of the evidence element using 554 * Codes (such as condition, medication, or observation), Expressions ( 555 * using an expression language such as FHIRPath or CQL) or 556 * DataRequirements (such as Diabetes diagnosis onset in the last 557 * year).) 558 */ 559 public Reference getDefinitionReference() throws FHIRException { 560 if (this.definition == null) 561 this.definition = new Reference(); 562 if (!(this.definition instanceof Reference)) 563 throw new FHIRException("Type mismatch: the type Reference was expected, but " 564 + this.definition.getClass().getName() + " was encountered"); 565 return (Reference) this.definition; 566 } 567 568 public boolean hasDefinitionReference() { 569 return this != null && this.definition instanceof Reference; 570 } 571 572 /** 573 * @return {@link #definition} (Define members of the evidence element using 574 * Codes (such as condition, medication, or observation), Expressions ( 575 * using an expression language such as FHIRPath or CQL) or 576 * DataRequirements (such as Diabetes diagnosis onset in the last 577 * year).) 578 */ 579 public CanonicalType getDefinitionCanonicalType() throws FHIRException { 580 if (this.definition == null) 581 this.definition = new CanonicalType(); 582 if (!(this.definition instanceof CanonicalType)) 583 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but " 584 + this.definition.getClass().getName() + " was encountered"); 585 return (CanonicalType) this.definition; 586 } 587 588 public boolean hasDefinitionCanonicalType() { 589 return this != null && this.definition instanceof CanonicalType; 590 } 591 592 /** 593 * @return {@link #definition} (Define members of the evidence element using 594 * Codes (such as condition, medication, or observation), Expressions ( 595 * using an expression language such as FHIRPath or CQL) or 596 * DataRequirements (such as Diabetes diagnosis onset in the last 597 * year).) 598 */ 599 public CodeableConcept getDefinitionCodeableConcept() throws FHIRException { 600 if (this.definition == null) 601 this.definition = new CodeableConcept(); 602 if (!(this.definition instanceof CodeableConcept)) 603 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 604 + this.definition.getClass().getName() + " was encountered"); 605 return (CodeableConcept) this.definition; 606 } 607 608 public boolean hasDefinitionCodeableConcept() { 609 return this != null && this.definition instanceof CodeableConcept; 610 } 611 612 /** 613 * @return {@link #definition} (Define members of the evidence element using 614 * Codes (such as condition, medication, or observation), Expressions ( 615 * using an expression language such as FHIRPath or CQL) or 616 * DataRequirements (such as Diabetes diagnosis onset in the last 617 * year).) 618 */ 619 public Expression getDefinitionExpression() throws FHIRException { 620 if (this.definition == null) 621 this.definition = new Expression(); 622 if (!(this.definition instanceof Expression)) 623 throw new FHIRException("Type mismatch: the type Expression was expected, but " 624 + this.definition.getClass().getName() + " was encountered"); 625 return (Expression) this.definition; 626 } 627 628 public boolean hasDefinitionExpression() { 629 return this != null && this.definition instanceof Expression; 630 } 631 632 /** 633 * @return {@link #definition} (Define members of the evidence element using 634 * Codes (such as condition, medication, or observation), Expressions ( 635 * using an expression language such as FHIRPath or CQL) or 636 * DataRequirements (such as Diabetes diagnosis onset in the last 637 * year).) 638 */ 639 public DataRequirement getDefinitionDataRequirement() throws FHIRException { 640 if (this.definition == null) 641 this.definition = new DataRequirement(); 642 if (!(this.definition instanceof DataRequirement)) 643 throw new FHIRException("Type mismatch: the type DataRequirement was expected, but " 644 + this.definition.getClass().getName() + " was encountered"); 645 return (DataRequirement) this.definition; 646 } 647 648 public boolean hasDefinitionDataRequirement() { 649 return this != null && this.definition instanceof DataRequirement; 650 } 651 652 /** 653 * @return {@link #definition} (Define members of the evidence element using 654 * Codes (such as condition, medication, or observation), Expressions ( 655 * using an expression language such as FHIRPath or CQL) or 656 * DataRequirements (such as Diabetes diagnosis onset in the last 657 * year).) 658 */ 659 public TriggerDefinition getDefinitionTriggerDefinition() throws FHIRException { 660 if (this.definition == null) 661 this.definition = new TriggerDefinition(); 662 if (!(this.definition instanceof TriggerDefinition)) 663 throw new FHIRException("Type mismatch: the type TriggerDefinition was expected, but " 664 + this.definition.getClass().getName() + " was encountered"); 665 return (TriggerDefinition) this.definition; 666 } 667 668 public boolean hasDefinitionTriggerDefinition() { 669 return this != null && this.definition instanceof TriggerDefinition; 670 } 671 672 public boolean hasDefinition() { 673 return this.definition != null && !this.definition.isEmpty(); 674 } 675 676 /** 677 * @param value {@link #definition} (Define members of the evidence element 678 * using Codes (such as condition, medication, or observation), 679 * Expressions ( using an expression language such as FHIRPath or 680 * CQL) or DataRequirements (such as Diabetes diagnosis onset in 681 * the last year).) 682 */ 683 public EvidenceVariableCharacteristicComponent setDefinition(Type value) { 684 if (value != null 685 && !(value instanceof Reference || value instanceof CanonicalType || value instanceof CodeableConcept 686 || value instanceof Expression || value instanceof DataRequirement || value instanceof TriggerDefinition)) 687 throw new Error("Not the right type for EvidenceVariable.characteristic.definition[x]: " + value.fhirType()); 688 this.definition = value; 689 return this; 690 } 691 692 /** 693 * @return {@link #usageContext} (Use UsageContext to define the members of the 694 * population, such as Age Ranges, Genders, Settings.) 695 */ 696 public List<UsageContext> getUsageContext() { 697 if (this.usageContext == null) 698 this.usageContext = new ArrayList<UsageContext>(); 699 return this.usageContext; 700 } 701 702 /** 703 * @return Returns a reference to <code>this</code> for easy method chaining 704 */ 705 public EvidenceVariableCharacteristicComponent setUsageContext(List<UsageContext> theUsageContext) { 706 this.usageContext = theUsageContext; 707 return this; 708 } 709 710 public boolean hasUsageContext() { 711 if (this.usageContext == null) 712 return false; 713 for (UsageContext item : this.usageContext) 714 if (!item.isEmpty()) 715 return true; 716 return false; 717 } 718 719 public UsageContext addUsageContext() { // 3 720 UsageContext t = new UsageContext(); 721 if (this.usageContext == null) 722 this.usageContext = new ArrayList<UsageContext>(); 723 this.usageContext.add(t); 724 return t; 725 } 726 727 public EvidenceVariableCharacteristicComponent addUsageContext(UsageContext t) { // 3 728 if (t == null) 729 return this; 730 if (this.usageContext == null) 731 this.usageContext = new ArrayList<UsageContext>(); 732 this.usageContext.add(t); 733 return this; 734 } 735 736 /** 737 * @return The first repetition of repeating field {@link #usageContext}, 738 * creating it if it does not already exist 739 */ 740 public UsageContext getUsageContextFirstRep() { 741 if (getUsageContext().isEmpty()) { 742 addUsageContext(); 743 } 744 return getUsageContext().get(0); 745 } 746 747 /** 748 * @return {@link #exclude} (When true, members with this characteristic are 749 * excluded from the element.). This is the underlying object with id, 750 * value and extensions. The accessor "getExclude" gives direct access 751 * to the value 752 */ 753 public BooleanType getExcludeElement() { 754 if (this.exclude == null) 755 if (Configuration.errorOnAutoCreate()) 756 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.exclude"); 757 else if (Configuration.doAutoCreate()) 758 this.exclude = new BooleanType(); // bb 759 return this.exclude; 760 } 761 762 public boolean hasExcludeElement() { 763 return this.exclude != null && !this.exclude.isEmpty(); 764 } 765 766 public boolean hasExclude() { 767 return this.exclude != null && !this.exclude.isEmpty(); 768 } 769 770 /** 771 * @param value {@link #exclude} (When true, members with this characteristic 772 * are excluded from the element.). This is the underlying object 773 * with id, value and extensions. The accessor "getExclude" gives 774 * direct access to the value 775 */ 776 public EvidenceVariableCharacteristicComponent setExcludeElement(BooleanType value) { 777 this.exclude = value; 778 return this; 779 } 780 781 /** 782 * @return When true, members with this characteristic are excluded from the 783 * element. 784 */ 785 public boolean getExclude() { 786 return this.exclude == null || this.exclude.isEmpty() ? false : this.exclude.getValue(); 787 } 788 789 /** 790 * @param value When true, members with this characteristic are excluded from 791 * the element. 792 */ 793 public EvidenceVariableCharacteristicComponent setExclude(boolean value) { 794 if (this.exclude == null) 795 this.exclude = new BooleanType(); 796 this.exclude.setValue(value); 797 return this; 798 } 799 800 /** 801 * @return {@link #participantEffective} (Indicates what effective period the 802 * study covers.) 803 */ 804 public Type getParticipantEffective() { 805 return this.participantEffective; 806 } 807 808 /** 809 * @return {@link #participantEffective} (Indicates what effective period the 810 * study covers.) 811 */ 812 public DateTimeType getParticipantEffectiveDateTimeType() throws FHIRException { 813 if (this.participantEffective == null) 814 this.participantEffective = new DateTimeType(); 815 if (!(this.participantEffective instanceof DateTimeType)) 816 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 817 + this.participantEffective.getClass().getName() + " was encountered"); 818 return (DateTimeType) this.participantEffective; 819 } 820 821 public boolean hasParticipantEffectiveDateTimeType() { 822 return this != null && this.participantEffective instanceof DateTimeType; 823 } 824 825 /** 826 * @return {@link #participantEffective} (Indicates what effective period the 827 * study covers.) 828 */ 829 public Period getParticipantEffectivePeriod() throws FHIRException { 830 if (this.participantEffective == null) 831 this.participantEffective = new Period(); 832 if (!(this.participantEffective instanceof Period)) 833 throw new FHIRException("Type mismatch: the type Period was expected, but " 834 + this.participantEffective.getClass().getName() + " was encountered"); 835 return (Period) this.participantEffective; 836 } 837 838 public boolean hasParticipantEffectivePeriod() { 839 return this != null && this.participantEffective instanceof Period; 840 } 841 842 /** 843 * @return {@link #participantEffective} (Indicates what effective period the 844 * study covers.) 845 */ 846 public Duration getParticipantEffectiveDuration() throws FHIRException { 847 if (this.participantEffective == null) 848 this.participantEffective = new Duration(); 849 if (!(this.participantEffective instanceof Duration)) 850 throw new FHIRException("Type mismatch: the type Duration was expected, but " 851 + this.participantEffective.getClass().getName() + " was encountered"); 852 return (Duration) this.participantEffective; 853 } 854 855 public boolean hasParticipantEffectiveDuration() { 856 return this != null && this.participantEffective instanceof Duration; 857 } 858 859 /** 860 * @return {@link #participantEffective} (Indicates what effective period the 861 * study covers.) 862 */ 863 public Timing getParticipantEffectiveTiming() throws FHIRException { 864 if (this.participantEffective == null) 865 this.participantEffective = new Timing(); 866 if (!(this.participantEffective instanceof Timing)) 867 throw new FHIRException("Type mismatch: the type Timing was expected, but " 868 + this.participantEffective.getClass().getName() + " was encountered"); 869 return (Timing) this.participantEffective; 870 } 871 872 public boolean hasParticipantEffectiveTiming() { 873 return this != null && this.participantEffective instanceof Timing; 874 } 875 876 public boolean hasParticipantEffective() { 877 return this.participantEffective != null && !this.participantEffective.isEmpty(); 878 } 879 880 /** 881 * @param value {@link #participantEffective} (Indicates what effective period 882 * the study covers.) 883 */ 884 public EvidenceVariableCharacteristicComponent setParticipantEffective(Type value) { 885 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Duration 886 || value instanceof Timing)) 887 throw new Error( 888 "Not the right type for EvidenceVariable.characteristic.participantEffective[x]: " + value.fhirType()); 889 this.participantEffective = value; 890 return this; 891 } 892 893 /** 894 * @return {@link #timeFromStart} (Indicates duration from the participant's 895 * study entry.) 896 */ 897 public Duration getTimeFromStart() { 898 if (this.timeFromStart == null) 899 if (Configuration.errorOnAutoCreate()) 900 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.timeFromStart"); 901 else if (Configuration.doAutoCreate()) 902 this.timeFromStart = new Duration(); // cc 903 return this.timeFromStart; 904 } 905 906 public boolean hasTimeFromStart() { 907 return this.timeFromStart != null && !this.timeFromStart.isEmpty(); 908 } 909 910 /** 911 * @param value {@link #timeFromStart} (Indicates duration from the 912 * participant's study entry.) 913 */ 914 public EvidenceVariableCharacteristicComponent setTimeFromStart(Duration value) { 915 this.timeFromStart = value; 916 return this; 917 } 918 919 /** 920 * @return {@link #groupMeasure} (Indicates how elements are aggregated within 921 * the study effective period.). This is the underlying object with id, 922 * value and extensions. The accessor "getGroupMeasure" gives direct 923 * access to the value 924 */ 925 public Enumeration<GroupMeasure> getGroupMeasureElement() { 926 if (this.groupMeasure == null) 927 if (Configuration.errorOnAutoCreate()) 928 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.groupMeasure"); 929 else if (Configuration.doAutoCreate()) 930 this.groupMeasure = new Enumeration<GroupMeasure>(new GroupMeasureEnumFactory()); // bb 931 return this.groupMeasure; 932 } 933 934 public boolean hasGroupMeasureElement() { 935 return this.groupMeasure != null && !this.groupMeasure.isEmpty(); 936 } 937 938 public boolean hasGroupMeasure() { 939 return this.groupMeasure != null && !this.groupMeasure.isEmpty(); 940 } 941 942 /** 943 * @param value {@link #groupMeasure} (Indicates how elements are aggregated 944 * within the study effective period.). This is the underlying 945 * object with id, value and extensions. The accessor 946 * "getGroupMeasure" gives direct access to the value 947 */ 948 public EvidenceVariableCharacteristicComponent setGroupMeasureElement(Enumeration<GroupMeasure> value) { 949 this.groupMeasure = value; 950 return this; 951 } 952 953 /** 954 * @return Indicates how elements are aggregated within the study effective 955 * period. 956 */ 957 public GroupMeasure getGroupMeasure() { 958 return this.groupMeasure == null ? null : this.groupMeasure.getValue(); 959 } 960 961 /** 962 * @param value Indicates how elements are aggregated within the study effective 963 * period. 964 */ 965 public EvidenceVariableCharacteristicComponent setGroupMeasure(GroupMeasure value) { 966 if (value == null) 967 this.groupMeasure = null; 968 else { 969 if (this.groupMeasure == null) 970 this.groupMeasure = new Enumeration<GroupMeasure>(new GroupMeasureEnumFactory()); 971 this.groupMeasure.setValue(value); 972 } 973 return this; 974 } 975 976 protected void listChildren(List<Property> children) { 977 super.listChildren(children); 978 children.add(new Property("description", "string", 979 "A short, natural language description of the characteristic that could be used to communicate the criteria to an end-user.", 980 0, 1, description)); 981 children.add(new Property("definition[x]", 982 "Reference(Group)|canonical(ActivityDefinition)|CodeableConcept|Expression|DataRequirement|TriggerDefinition", 983 "Define members of the evidence element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 984 0, 1, definition)); 985 children.add(new Property("usageContext", "UsageContext", 986 "Use UsageContext to define the members of the population, such as Age Ranges, Genders, Settings.", 0, 987 java.lang.Integer.MAX_VALUE, usageContext)); 988 children.add(new Property("exclude", "boolean", 989 "When true, members with this characteristic are excluded from the element.", 0, 1, exclude)); 990 children.add(new Property("participantEffective[x]", "dateTime|Period|Duration|Timing", 991 "Indicates what effective period the study covers.", 0, 1, participantEffective)); 992 children.add(new Property("timeFromStart", "Duration", "Indicates duration from the participant's study entry.", 993 0, 1, timeFromStart)); 994 children.add(new Property("groupMeasure", "code", 995 "Indicates how elements are aggregated within the study effective period.", 0, 1, groupMeasure)); 996 } 997 998 @Override 999 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1000 switch (_hash) { 1001 case -1724546052: 1002 /* description */ return new Property("description", "string", 1003 "A short, natural language description of the characteristic that could be used to communicate the criteria to an end-user.", 1004 0, 1, description); 1005 case -1139422643: 1006 /* definition[x] */ return new Property("definition[x]", 1007 "Reference(Group)|canonical(ActivityDefinition)|CodeableConcept|Expression|DataRequirement|TriggerDefinition", 1008 "Define members of the evidence element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1009 0, 1, definition); 1010 case -1014418093: 1011 /* definition */ return new Property("definition[x]", 1012 "Reference(Group)|canonical(ActivityDefinition)|CodeableConcept|Expression|DataRequirement|TriggerDefinition", 1013 "Define members of the evidence element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1014 0, 1, definition); 1015 case -820021448: 1016 /* definitionReference */ return new Property("definition[x]", 1017 "Reference(Group)|canonical(ActivityDefinition)|CodeableConcept|Expression|DataRequirement|TriggerDefinition", 1018 "Define members of the evidence element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1019 0, 1, definition); 1020 case 933485793: 1021 /* definitionCanonical */ return new Property("definition[x]", 1022 "Reference(Group)|canonical(ActivityDefinition)|CodeableConcept|Expression|DataRequirement|TriggerDefinition", 1023 "Define members of the evidence element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1024 0, 1, definition); 1025 case -1446002226: 1026 /* definitionCodeableConcept */ return new Property("definition[x]", 1027 "Reference(Group)|canonical(ActivityDefinition)|CodeableConcept|Expression|DataRequirement|TriggerDefinition", 1028 "Define members of the evidence element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1029 0, 1, definition); 1030 case 1463703627: 1031 /* definitionExpression */ return new Property("definition[x]", 1032 "Reference(Group)|canonical(ActivityDefinition)|CodeableConcept|Expression|DataRequirement|TriggerDefinition", 1033 "Define members of the evidence element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1034 0, 1, definition); 1035 case -660350874: 1036 /* definitionDataRequirement */ return new Property("definition[x]", 1037 "Reference(Group)|canonical(ActivityDefinition)|CodeableConcept|Expression|DataRequirement|TriggerDefinition", 1038 "Define members of the evidence element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1039 0, 1, definition); 1040 case -1130324968: 1041 /* definitionTriggerDefinition */ return new Property("definition[x]", 1042 "Reference(Group)|canonical(ActivityDefinition)|CodeableConcept|Expression|DataRequirement|TriggerDefinition", 1043 "Define members of the evidence element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1044 0, 1, definition); 1045 case 907012302: 1046 /* usageContext */ return new Property("usageContext", "UsageContext", 1047 "Use UsageContext to define the members of the population, such as Age Ranges, Genders, Settings.", 0, 1048 java.lang.Integer.MAX_VALUE, usageContext); 1049 case -1321148966: 1050 /* exclude */ return new Property("exclude", "boolean", 1051 "When true, members with this characteristic are excluded from the element.", 0, 1, exclude); 1052 case 1777308748: 1053 /* participantEffective[x] */ return new Property("participantEffective[x]", "dateTime|Period|Duration|Timing", 1054 "Indicates what effective period the study covers.", 0, 1, participantEffective); 1055 case 1376306100: 1056 /* participantEffective */ return new Property("participantEffective[x]", "dateTime|Period|Duration|Timing", 1057 "Indicates what effective period the study covers.", 0, 1, participantEffective); 1058 case -1721146513: 1059 /* participantEffectiveDateTime */ return new Property("participantEffective[x]", 1060 "dateTime|Period|Duration|Timing", "Indicates what effective period the study covers.", 0, 1, 1061 participantEffective); 1062 case -883650923: 1063 /* participantEffectivePeriod */ return new Property("participantEffective[x]", 1064 "dateTime|Period|Duration|Timing", "Indicates what effective period the study covers.", 0, 1, 1065 participantEffective); 1066 case -1210941080: 1067 /* participantEffectiveDuration */ return new Property("participantEffective[x]", 1068 "dateTime|Period|Duration|Timing", "Indicates what effective period the study covers.", 0, 1, 1069 participantEffective); 1070 case -765589218: 1071 /* participantEffectiveTiming */ return new Property("participantEffective[x]", 1072 "dateTime|Period|Duration|Timing", "Indicates what effective period the study covers.", 0, 1, 1073 participantEffective); 1074 case 2100140683: 1075 /* timeFromStart */ return new Property("timeFromStart", "Duration", 1076 "Indicates duration from the participant's study entry.", 0, 1, timeFromStart); 1077 case 588892639: 1078 /* groupMeasure */ return new Property("groupMeasure", "code", 1079 "Indicates how elements are aggregated within the study effective period.", 0, 1, groupMeasure); 1080 default: 1081 return super.getNamedProperty(_hash, _name, _checkValid); 1082 } 1083 1084 } 1085 1086 @Override 1087 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1088 switch (hash) { 1089 case -1724546052: 1090 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1091 case -1014418093: 1092 /* definition */ return this.definition == null ? new Base[0] : new Base[] { this.definition }; // Type 1093 case 907012302: 1094 /* usageContext */ return this.usageContext == null ? new Base[0] 1095 : this.usageContext.toArray(new Base[this.usageContext.size()]); // UsageContext 1096 case -1321148966: 1097 /* exclude */ return this.exclude == null ? new Base[0] : new Base[] { this.exclude }; // BooleanType 1098 case 1376306100: 1099 /* participantEffective */ return this.participantEffective == null ? new Base[0] 1100 : new Base[] { this.participantEffective }; // Type 1101 case 2100140683: 1102 /* timeFromStart */ return this.timeFromStart == null ? new Base[0] : new Base[] { this.timeFromStart }; // Duration 1103 case 588892639: 1104 /* groupMeasure */ return this.groupMeasure == null ? new Base[0] : new Base[] { this.groupMeasure }; // Enumeration<GroupMeasure> 1105 default: 1106 return super.getProperty(hash, name, checkValid); 1107 } 1108 1109 } 1110 1111 @Override 1112 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1113 switch (hash) { 1114 case -1724546052: // description 1115 this.description = castToString(value); // StringType 1116 return value; 1117 case -1014418093: // definition 1118 this.definition = castToType(value); // Type 1119 return value; 1120 case 907012302: // usageContext 1121 this.getUsageContext().add(castToUsageContext(value)); // UsageContext 1122 return value; 1123 case -1321148966: // exclude 1124 this.exclude = castToBoolean(value); // BooleanType 1125 return value; 1126 case 1376306100: // participantEffective 1127 this.participantEffective = castToType(value); // Type 1128 return value; 1129 case 2100140683: // timeFromStart 1130 this.timeFromStart = castToDuration(value); // Duration 1131 return value; 1132 case 588892639: // groupMeasure 1133 value = new GroupMeasureEnumFactory().fromType(castToCode(value)); 1134 this.groupMeasure = (Enumeration) value; // Enumeration<GroupMeasure> 1135 return value; 1136 default: 1137 return super.setProperty(hash, name, value); 1138 } 1139 1140 } 1141 1142 @Override 1143 public Base setProperty(String name, Base value) throws FHIRException { 1144 if (name.equals("description")) { 1145 this.description = castToString(value); // StringType 1146 } else if (name.equals("definition[x]")) { 1147 this.definition = castToType(value); // Type 1148 } else if (name.equals("usageContext")) { 1149 this.getUsageContext().add(castToUsageContext(value)); 1150 } else if (name.equals("exclude")) { 1151 this.exclude = castToBoolean(value); // BooleanType 1152 } else if (name.equals("participantEffective[x]")) { 1153 this.participantEffective = castToType(value); // Type 1154 } else if (name.equals("timeFromStart")) { 1155 this.timeFromStart = castToDuration(value); // Duration 1156 } else if (name.equals("groupMeasure")) { 1157 value = new GroupMeasureEnumFactory().fromType(castToCode(value)); 1158 this.groupMeasure = (Enumeration) value; // Enumeration<GroupMeasure> 1159 } else 1160 return super.setProperty(name, value); 1161 return value; 1162 } 1163 1164 @Override 1165 public void removeChild(String name, Base value) throws FHIRException { 1166 if (name.equals("description")) { 1167 this.description = null; 1168 } else if (name.equals("definition[x]")) { 1169 this.definition = null; 1170 } else if (name.equals("usageContext")) { 1171 this.getUsageContext().remove(castToUsageContext(value)); 1172 } else if (name.equals("exclude")) { 1173 this.exclude = null; 1174 } else if (name.equals("participantEffective[x]")) { 1175 this.participantEffective = null; 1176 } else if (name.equals("timeFromStart")) { 1177 this.timeFromStart = null; 1178 } else if (name.equals("groupMeasure")) { 1179 this.groupMeasure = null; 1180 } else 1181 super.removeChild(name, value); 1182 1183 } 1184 1185 @Override 1186 public Base makeProperty(int hash, String name) throws FHIRException { 1187 switch (hash) { 1188 case -1724546052: 1189 return getDescriptionElement(); 1190 case -1139422643: 1191 return getDefinition(); 1192 case -1014418093: 1193 return getDefinition(); 1194 case 907012302: 1195 return addUsageContext(); 1196 case -1321148966: 1197 return getExcludeElement(); 1198 case 1777308748: 1199 return getParticipantEffective(); 1200 case 1376306100: 1201 return getParticipantEffective(); 1202 case 2100140683: 1203 return getTimeFromStart(); 1204 case 588892639: 1205 return getGroupMeasureElement(); 1206 default: 1207 return super.makeProperty(hash, name); 1208 } 1209 1210 } 1211 1212 @Override 1213 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1214 switch (hash) { 1215 case -1724546052: 1216 /* description */ return new String[] { "string" }; 1217 case -1014418093: 1218 /* definition */ return new String[] { "Reference", "canonical", "CodeableConcept", "Expression", 1219 "DataRequirement", "TriggerDefinition" }; 1220 case 907012302: 1221 /* usageContext */ return new String[] { "UsageContext" }; 1222 case -1321148966: 1223 /* exclude */ return new String[] { "boolean" }; 1224 case 1376306100: 1225 /* participantEffective */ return new String[] { "dateTime", "Period", "Duration", "Timing" }; 1226 case 2100140683: 1227 /* timeFromStart */ return new String[] { "Duration" }; 1228 case 588892639: 1229 /* groupMeasure */ return new String[] { "code" }; 1230 default: 1231 return super.getTypesForProperty(hash, name); 1232 } 1233 1234 } 1235 1236 @Override 1237 public Base addChild(String name) throws FHIRException { 1238 if (name.equals("description")) { 1239 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.description"); 1240 } else if (name.equals("definitionReference")) { 1241 this.definition = new Reference(); 1242 return this.definition; 1243 } else if (name.equals("definitionCanonical")) { 1244 this.definition = new CanonicalType(); 1245 return this.definition; 1246 } else if (name.equals("definitionCodeableConcept")) { 1247 this.definition = new CodeableConcept(); 1248 return this.definition; 1249 } else if (name.equals("definitionExpression")) { 1250 this.definition = new Expression(); 1251 return this.definition; 1252 } else if (name.equals("definitionDataRequirement")) { 1253 this.definition = new DataRequirement(); 1254 return this.definition; 1255 } else if (name.equals("definitionTriggerDefinition")) { 1256 this.definition = new TriggerDefinition(); 1257 return this.definition; 1258 } else if (name.equals("usageContext")) { 1259 return addUsageContext(); 1260 } else if (name.equals("exclude")) { 1261 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.exclude"); 1262 } else if (name.equals("participantEffectiveDateTime")) { 1263 this.participantEffective = new DateTimeType(); 1264 return this.participantEffective; 1265 } else if (name.equals("participantEffectivePeriod")) { 1266 this.participantEffective = new Period(); 1267 return this.participantEffective; 1268 } else if (name.equals("participantEffectiveDuration")) { 1269 this.participantEffective = new Duration(); 1270 return this.participantEffective; 1271 } else if (name.equals("participantEffectiveTiming")) { 1272 this.participantEffective = new Timing(); 1273 return this.participantEffective; 1274 } else if (name.equals("timeFromStart")) { 1275 this.timeFromStart = new Duration(); 1276 return this.timeFromStart; 1277 } else if (name.equals("groupMeasure")) { 1278 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.groupMeasure"); 1279 } else 1280 return super.addChild(name); 1281 } 1282 1283 public EvidenceVariableCharacteristicComponent copy() { 1284 EvidenceVariableCharacteristicComponent dst = new EvidenceVariableCharacteristicComponent(); 1285 copyValues(dst); 1286 return dst; 1287 } 1288 1289 public void copyValues(EvidenceVariableCharacteristicComponent dst) { 1290 super.copyValues(dst); 1291 dst.description = description == null ? null : description.copy(); 1292 dst.definition = definition == null ? null : definition.copy(); 1293 if (usageContext != null) { 1294 dst.usageContext = new ArrayList<UsageContext>(); 1295 for (UsageContext i : usageContext) 1296 dst.usageContext.add(i.copy()); 1297 } 1298 ; 1299 dst.exclude = exclude == null ? null : exclude.copy(); 1300 dst.participantEffective = participantEffective == null ? null : participantEffective.copy(); 1301 dst.timeFromStart = timeFromStart == null ? null : timeFromStart.copy(); 1302 dst.groupMeasure = groupMeasure == null ? null : groupMeasure.copy(); 1303 } 1304 1305 @Override 1306 public boolean equalsDeep(Base other_) { 1307 if (!super.equalsDeep(other_)) 1308 return false; 1309 if (!(other_ instanceof EvidenceVariableCharacteristicComponent)) 1310 return false; 1311 EvidenceVariableCharacteristicComponent o = (EvidenceVariableCharacteristicComponent) other_; 1312 return compareDeep(description, o.description, true) && compareDeep(definition, o.definition, true) 1313 && compareDeep(usageContext, o.usageContext, true) && compareDeep(exclude, o.exclude, true) 1314 && compareDeep(participantEffective, o.participantEffective, true) 1315 && compareDeep(timeFromStart, o.timeFromStart, true) && compareDeep(groupMeasure, o.groupMeasure, true); 1316 } 1317 1318 @Override 1319 public boolean equalsShallow(Base other_) { 1320 if (!super.equalsShallow(other_)) 1321 return false; 1322 if (!(other_ instanceof EvidenceVariableCharacteristicComponent)) 1323 return false; 1324 EvidenceVariableCharacteristicComponent o = (EvidenceVariableCharacteristicComponent) other_; 1325 return compareValues(description, o.description, true) && compareValues(exclude, o.exclude, true) 1326 && compareValues(groupMeasure, o.groupMeasure, true); 1327 } 1328 1329 public boolean isEmpty() { 1330 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, definition, usageContext, exclude, 1331 participantEffective, timeFromStart, groupMeasure); 1332 } 1333 1334 public String fhirType() { 1335 return "EvidenceVariable.characteristic"; 1336 1337 } 1338 1339 } 1340 1341 /** 1342 * A formal identifier that is used to identify this evidence variable when it 1343 * is represented in other formats, or referenced in a specification, model, 1344 * design or an instance. 1345 */ 1346 @Child(name = "identifier", type = { 1347 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1348 @Description(shortDefinition = "Additional identifier for the evidence variable", formalDefinition = "A formal identifier that is used to identify this evidence variable when it is represented in other formats, or referenced in a specification, model, design or an instance.") 1349 protected List<Identifier> identifier; 1350 1351 /** 1352 * The short title provides an alternate title for use in informal descriptive 1353 * contexts where the full, formal title is not necessary. 1354 */ 1355 @Child(name = "shortTitle", type = { 1356 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1357 @Description(shortDefinition = "Title for use in informal contexts", formalDefinition = "The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary.") 1358 protected StringType shortTitle; 1359 1360 /** 1361 * An explanatory or alternate title for the EvidenceVariable giving additional 1362 * information about its content. 1363 */ 1364 @Child(name = "subtitle", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1365 @Description(shortDefinition = "Subordinate title of the EvidenceVariable", formalDefinition = "An explanatory or alternate title for the EvidenceVariable giving additional information about its content.") 1366 protected StringType subtitle; 1367 1368 /** 1369 * A human-readable string to clarify or explain concepts about the resource. 1370 */ 1371 @Child(name = "note", type = { 1372 Annotation.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1373 @Description(shortDefinition = "Used for footnotes or explanatory notes", formalDefinition = "A human-readable string to clarify or explain concepts about the resource.") 1374 protected List<Annotation> note; 1375 1376 /** 1377 * A copyright statement relating to the evidence variable and/or its contents. 1378 * Copyright statements are generally legal restrictions on the use and 1379 * publishing of the evidence variable. 1380 */ 1381 @Child(name = "copyright", type = { 1382 MarkdownType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1383 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the evidence variable and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the evidence variable.") 1384 protected MarkdownType copyright; 1385 1386 /** 1387 * The date on which the resource content was approved by the publisher. 1388 * Approval happens once when the content is officially approved for usage. 1389 */ 1390 @Child(name = "approvalDate", type = { 1391 DateType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1392 @Description(shortDefinition = "When the evidence variable was approved by publisher", formalDefinition = "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.") 1393 protected DateType approvalDate; 1394 1395 /** 1396 * The date on which the resource content was last reviewed. Review happens 1397 * periodically after approval but does not change the original approval date. 1398 */ 1399 @Child(name = "lastReviewDate", type = { 1400 DateType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1401 @Description(shortDefinition = "When the evidence variable was last reviewed", formalDefinition = "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.") 1402 protected DateType lastReviewDate; 1403 1404 /** 1405 * The period during which the evidence variable content was or is planned to be 1406 * in active use. 1407 */ 1408 @Child(name = "effectivePeriod", type = { 1409 Period.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1410 @Description(shortDefinition = "When the evidence variable is expected to be used", formalDefinition = "The period during which the evidence variable content was or is planned to be in active use.") 1411 protected Period effectivePeriod; 1412 1413 /** 1414 * Descriptive topics related to the content of the EvidenceVariable. Topics 1415 * provide a high-level categorization grouping types of EvidenceVariables that 1416 * can be useful for filtering and searching. 1417 */ 1418 @Child(name = "topic", type = { 1419 CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1420 @Description(shortDefinition = "The category of the EvidenceVariable, such as Education, Treatment, Assessment, etc.", formalDefinition = "Descriptive topics related to the content of the EvidenceVariable. Topics provide a high-level categorization grouping types of EvidenceVariables that can be useful for filtering and searching.") 1421 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/definition-topic") 1422 protected List<CodeableConcept> topic; 1423 1424 /** 1425 * An individiual or organization primarily involved in the creation and 1426 * maintenance of the content. 1427 */ 1428 @Child(name = "author", type = { 1429 ContactDetail.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1430 @Description(shortDefinition = "Who authored the content", formalDefinition = "An individiual or organization primarily involved in the creation and maintenance of the content.") 1431 protected List<ContactDetail> author; 1432 1433 /** 1434 * An individual or organization primarily responsible for internal coherence of 1435 * the content. 1436 */ 1437 @Child(name = "editor", type = { 1438 ContactDetail.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1439 @Description(shortDefinition = "Who edited the content", formalDefinition = "An individual or organization primarily responsible for internal coherence of the content.") 1440 protected List<ContactDetail> editor; 1441 1442 /** 1443 * An individual or organization primarily responsible for review of some aspect 1444 * of the content. 1445 */ 1446 @Child(name = "reviewer", type = { 1447 ContactDetail.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1448 @Description(shortDefinition = "Who reviewed the content", formalDefinition = "An individual or organization primarily responsible for review of some aspect of the content.") 1449 protected List<ContactDetail> reviewer; 1450 1451 /** 1452 * An individual or organization responsible for officially endorsing the 1453 * content for use in some setting. 1454 */ 1455 @Child(name = "endorser", type = { 1456 ContactDetail.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1457 @Description(shortDefinition = "Who endorsed the content", formalDefinition = "An individual or organization responsible for officially endorsing the content for use in some setting.") 1458 protected List<ContactDetail> endorser; 1459 1460 /** 1461 * Related artifacts such as additional documentation, justification, or 1462 * bibliographic references. 1463 */ 1464 @Child(name = "relatedArtifact", type = { 1465 RelatedArtifact.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1466 @Description(shortDefinition = "Additional documentation, citations, etc.", formalDefinition = "Related artifacts such as additional documentation, justification, or bibliographic references.") 1467 protected List<RelatedArtifact> relatedArtifact; 1468 1469 /** 1470 * The type of evidence element, a population, an exposure, or an outcome. 1471 */ 1472 @Child(name = "type", type = { CodeType.class }, order = 14, min = 0, max = 1, modifier = false, summary = true) 1473 @Description(shortDefinition = "dichotomous | continuous | descriptive", formalDefinition = "The type of evidence element, a population, an exposure, or an outcome.") 1474 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/variable-type") 1475 protected Enumeration<EvidenceVariableType> type; 1476 1477 /** 1478 * A characteristic that defines the members of the evidence element. Multiple 1479 * characteristics are applied with "and" semantics. 1480 */ 1481 @Child(name = "characteristic", type = {}, order = 15, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1482 @Description(shortDefinition = "What defines the members of the evidence element", formalDefinition = "A characteristic that defines the members of the evidence element. Multiple characteristics are applied with \"and\" semantics.") 1483 protected List<EvidenceVariableCharacteristicComponent> characteristic; 1484 1485 private static final long serialVersionUID = -317280154L; 1486 1487 /** 1488 * Constructor 1489 */ 1490 public EvidenceVariable() { 1491 super(); 1492 } 1493 1494 /** 1495 * Constructor 1496 */ 1497 public EvidenceVariable(Enumeration<PublicationStatus> status) { 1498 super(); 1499 this.status = status; 1500 } 1501 1502 /** 1503 * @return {@link #url} (An absolute URI that is used to identify this evidence 1504 * variable when it is referenced in a specification, model, design or 1505 * an instance; also called its canonical identifier. This SHOULD be 1506 * globally unique and SHOULD be a literal address at which at which an 1507 * authoritative instance of this evidence variable is (or will be) 1508 * published. This URL can be the target of a canonical reference. It 1509 * SHALL remain the same when the evidence variable is stored on 1510 * different servers.). This is the underlying object with id, value and 1511 * extensions. The accessor "getUrl" gives direct access to the value 1512 */ 1513 public UriType getUrlElement() { 1514 if (this.url == null) 1515 if (Configuration.errorOnAutoCreate()) 1516 throw new Error("Attempt to auto-create EvidenceVariable.url"); 1517 else if (Configuration.doAutoCreate()) 1518 this.url = new UriType(); // bb 1519 return this.url; 1520 } 1521 1522 public boolean hasUrlElement() { 1523 return this.url != null && !this.url.isEmpty(); 1524 } 1525 1526 public boolean hasUrl() { 1527 return this.url != null && !this.url.isEmpty(); 1528 } 1529 1530 /** 1531 * @param value {@link #url} (An absolute URI that is used to identify this 1532 * evidence variable when it is referenced in a specification, 1533 * model, design or an instance; also called its canonical 1534 * identifier. This SHOULD be globally unique and SHOULD be a 1535 * literal address at which at which an authoritative instance of 1536 * this evidence variable is (or will be) published. This URL can 1537 * be the target of a canonical reference. It SHALL remain the same 1538 * when the evidence variable is stored on different servers.). 1539 * This is the underlying object with id, value and extensions. The 1540 * accessor "getUrl" gives direct access to the value 1541 */ 1542 public EvidenceVariable setUrlElement(UriType value) { 1543 this.url = value; 1544 return this; 1545 } 1546 1547 /** 1548 * @return An absolute URI that is used to identify this evidence variable when 1549 * it is referenced in a specification, model, design or an instance; 1550 * also called its canonical identifier. This SHOULD be globally unique 1551 * and SHOULD be a literal address at which at which an authoritative 1552 * instance of this evidence variable is (or will be) published. This 1553 * URL can be the target of a canonical reference. It SHALL remain the 1554 * same when the evidence variable is stored on different servers. 1555 */ 1556 public String getUrl() { 1557 return this.url == null ? null : this.url.getValue(); 1558 } 1559 1560 /** 1561 * @param value An absolute URI that is used to identify this evidence variable 1562 * when it is referenced in a specification, model, design or an 1563 * instance; also called its canonical identifier. This SHOULD be 1564 * globally unique and SHOULD be a literal address at which at 1565 * which an authoritative instance of this evidence variable is (or 1566 * will be) published. This URL can be the target of a canonical 1567 * reference. It SHALL remain the same when the evidence variable 1568 * is stored on different servers. 1569 */ 1570 public EvidenceVariable setUrl(String value) { 1571 if (Utilities.noString(value)) 1572 this.url = null; 1573 else { 1574 if (this.url == null) 1575 this.url = new UriType(); 1576 this.url.setValue(value); 1577 } 1578 return this; 1579 } 1580 1581 /** 1582 * @return {@link #identifier} (A formal identifier that is used to identify 1583 * this evidence variable when it is represented in other formats, or 1584 * referenced in a specification, model, design or an instance.) 1585 */ 1586 public List<Identifier> getIdentifier() { 1587 if (this.identifier == null) 1588 this.identifier = new ArrayList<Identifier>(); 1589 return this.identifier; 1590 } 1591 1592 /** 1593 * @return Returns a reference to <code>this</code> for easy method chaining 1594 */ 1595 public EvidenceVariable setIdentifier(List<Identifier> theIdentifier) { 1596 this.identifier = theIdentifier; 1597 return this; 1598 } 1599 1600 public boolean hasIdentifier() { 1601 if (this.identifier == null) 1602 return false; 1603 for (Identifier item : this.identifier) 1604 if (!item.isEmpty()) 1605 return true; 1606 return false; 1607 } 1608 1609 public Identifier addIdentifier() { // 3 1610 Identifier t = new Identifier(); 1611 if (this.identifier == null) 1612 this.identifier = new ArrayList<Identifier>(); 1613 this.identifier.add(t); 1614 return t; 1615 } 1616 1617 public EvidenceVariable addIdentifier(Identifier t) { // 3 1618 if (t == null) 1619 return this; 1620 if (this.identifier == null) 1621 this.identifier = new ArrayList<Identifier>(); 1622 this.identifier.add(t); 1623 return this; 1624 } 1625 1626 /** 1627 * @return The first repetition of repeating field {@link #identifier}, creating 1628 * it if it does not already exist 1629 */ 1630 public Identifier getIdentifierFirstRep() { 1631 if (getIdentifier().isEmpty()) { 1632 addIdentifier(); 1633 } 1634 return getIdentifier().get(0); 1635 } 1636 1637 /** 1638 * @return {@link #version} (The identifier that is used to identify this 1639 * version of the evidence variable when it is referenced in a 1640 * specification, model, design or instance. This is an arbitrary value 1641 * managed by the evidence variable author and is not expected to be 1642 * globally unique. For example, it might be a timestamp (e.g. yyyymmdd) 1643 * if a managed version is not available. There is also no expectation 1644 * that versions can be placed in a lexicographical sequence. To provide 1645 * a version consistent with the Decision Support Service specification, 1646 * use the format Major.Minor.Revision (e.g. 1.0.0). For more 1647 * information on versioning knowledge assets, refer to the Decision 1648 * Support Service specification. Note that a version is required for 1649 * non-experimental active artifacts.). This is the underlying object 1650 * with id, value and extensions. The accessor "getVersion" gives direct 1651 * access to the value 1652 */ 1653 public StringType getVersionElement() { 1654 if (this.version == null) 1655 if (Configuration.errorOnAutoCreate()) 1656 throw new Error("Attempt to auto-create EvidenceVariable.version"); 1657 else if (Configuration.doAutoCreate()) 1658 this.version = new StringType(); // bb 1659 return this.version; 1660 } 1661 1662 public boolean hasVersionElement() { 1663 return this.version != null && !this.version.isEmpty(); 1664 } 1665 1666 public boolean hasVersion() { 1667 return this.version != null && !this.version.isEmpty(); 1668 } 1669 1670 /** 1671 * @param value {@link #version} (The identifier that is used to identify this 1672 * version of the evidence variable when it is referenced in a 1673 * specification, model, design or instance. This is an arbitrary 1674 * value managed by the evidence variable author and is not 1675 * expected to be globally unique. For example, it might be a 1676 * timestamp (e.g. yyyymmdd) if a managed version is not available. 1677 * There is also no expectation that versions can be placed in a 1678 * lexicographical sequence. To provide a version consistent with 1679 * the Decision Support Service specification, use the format 1680 * Major.Minor.Revision (e.g. 1.0.0). For more information on 1681 * versioning knowledge assets, refer to the Decision Support 1682 * Service specification. Note that a version is required for 1683 * non-experimental active artifacts.). This is the underlying 1684 * object with id, value and extensions. The accessor "getVersion" 1685 * gives direct access to the value 1686 */ 1687 public EvidenceVariable setVersionElement(StringType value) { 1688 this.version = value; 1689 return this; 1690 } 1691 1692 /** 1693 * @return The identifier that is used to identify this version of the evidence 1694 * variable when it is referenced in a specification, model, design or 1695 * instance. This is an arbitrary value managed by the evidence variable 1696 * author and is not expected to be globally unique. For example, it 1697 * might be a timestamp (e.g. yyyymmdd) if a managed version is not 1698 * available. There is also no expectation that versions can be placed 1699 * in a lexicographical sequence. To provide a version consistent with 1700 * the Decision Support Service specification, use the format 1701 * Major.Minor.Revision (e.g. 1.0.0). For more information on versioning 1702 * knowledge assets, refer to the Decision Support Service 1703 * specification. Note that a version is required for non-experimental 1704 * active artifacts. 1705 */ 1706 public String getVersion() { 1707 return this.version == null ? null : this.version.getValue(); 1708 } 1709 1710 /** 1711 * @param value The identifier that is used to identify this version of the 1712 * evidence variable when it is referenced in a specification, 1713 * model, design or instance. This is an arbitrary value managed by 1714 * the evidence variable author and is not expected to be globally 1715 * unique. For example, it might be a timestamp (e.g. yyyymmdd) if 1716 * a managed version is not available. There is also no expectation 1717 * that versions can be placed in a lexicographical sequence. To 1718 * provide a version consistent with the Decision Support Service 1719 * specification, use the format Major.Minor.Revision (e.g. 1.0.0). 1720 * For more information on versioning knowledge assets, refer to 1721 * the Decision Support Service specification. Note that a version 1722 * is required for non-experimental active artifacts. 1723 */ 1724 public EvidenceVariable setVersion(String value) { 1725 if (Utilities.noString(value)) 1726 this.version = null; 1727 else { 1728 if (this.version == null) 1729 this.version = new StringType(); 1730 this.version.setValue(value); 1731 } 1732 return this; 1733 } 1734 1735 /** 1736 * @return {@link #name} (A natural language name identifying the evidence 1737 * variable. This name should be usable as an identifier for the module 1738 * by machine processing applications such as code generation.). This is 1739 * the underlying object with id, value and extensions. The accessor 1740 * "getName" gives direct access to the value 1741 */ 1742 public StringType getNameElement() { 1743 if (this.name == null) 1744 if (Configuration.errorOnAutoCreate()) 1745 throw new Error("Attempt to auto-create EvidenceVariable.name"); 1746 else if (Configuration.doAutoCreate()) 1747 this.name = new StringType(); // bb 1748 return this.name; 1749 } 1750 1751 public boolean hasNameElement() { 1752 return this.name != null && !this.name.isEmpty(); 1753 } 1754 1755 public boolean hasName() { 1756 return this.name != null && !this.name.isEmpty(); 1757 } 1758 1759 /** 1760 * @param value {@link #name} (A natural language name identifying the evidence 1761 * variable. This name should be usable as an identifier for the 1762 * module by machine processing applications such as code 1763 * generation.). This is the underlying object with id, value and 1764 * extensions. The accessor "getName" gives direct access to the 1765 * value 1766 */ 1767 public EvidenceVariable setNameElement(StringType value) { 1768 this.name = value; 1769 return this; 1770 } 1771 1772 /** 1773 * @return A natural language name identifying the evidence variable. This name 1774 * should be usable as an identifier for the module by machine 1775 * processing applications such as code generation. 1776 */ 1777 public String getName() { 1778 return this.name == null ? null : this.name.getValue(); 1779 } 1780 1781 /** 1782 * @param value A natural language name identifying the evidence variable. This 1783 * name should be usable as an identifier for the module by machine 1784 * processing applications such as code generation. 1785 */ 1786 public EvidenceVariable setName(String value) { 1787 if (Utilities.noString(value)) 1788 this.name = null; 1789 else { 1790 if (this.name == null) 1791 this.name = new StringType(); 1792 this.name.setValue(value); 1793 } 1794 return this; 1795 } 1796 1797 /** 1798 * @return {@link #title} (A short, descriptive, user-friendly title for the 1799 * evidence variable.). This is the underlying object with id, value and 1800 * extensions. The accessor "getTitle" gives direct access to the value 1801 */ 1802 public StringType getTitleElement() { 1803 if (this.title == null) 1804 if (Configuration.errorOnAutoCreate()) 1805 throw new Error("Attempt to auto-create EvidenceVariable.title"); 1806 else if (Configuration.doAutoCreate()) 1807 this.title = new StringType(); // bb 1808 return this.title; 1809 } 1810 1811 public boolean hasTitleElement() { 1812 return this.title != null && !this.title.isEmpty(); 1813 } 1814 1815 public boolean hasTitle() { 1816 return this.title != null && !this.title.isEmpty(); 1817 } 1818 1819 /** 1820 * @param value {@link #title} (A short, descriptive, user-friendly title for 1821 * the evidence variable.). This is the underlying object with id, 1822 * value and extensions. The accessor "getTitle" gives direct 1823 * access to the value 1824 */ 1825 public EvidenceVariable setTitleElement(StringType value) { 1826 this.title = value; 1827 return this; 1828 } 1829 1830 /** 1831 * @return A short, descriptive, user-friendly title for the evidence variable. 1832 */ 1833 public String getTitle() { 1834 return this.title == null ? null : this.title.getValue(); 1835 } 1836 1837 /** 1838 * @param value A short, descriptive, user-friendly title for the evidence 1839 * variable. 1840 */ 1841 public EvidenceVariable setTitle(String value) { 1842 if (Utilities.noString(value)) 1843 this.title = null; 1844 else { 1845 if (this.title == null) 1846 this.title = new StringType(); 1847 this.title.setValue(value); 1848 } 1849 return this; 1850 } 1851 1852 /** 1853 * @return {@link #shortTitle} (The short title provides an alternate title for 1854 * use in informal descriptive contexts where the full, formal title is 1855 * not necessary.). This is the underlying object with id, value and 1856 * extensions. The accessor "getShortTitle" gives direct access to the 1857 * value 1858 */ 1859 public StringType getShortTitleElement() { 1860 if (this.shortTitle == null) 1861 if (Configuration.errorOnAutoCreate()) 1862 throw new Error("Attempt to auto-create EvidenceVariable.shortTitle"); 1863 else if (Configuration.doAutoCreate()) 1864 this.shortTitle = new StringType(); // bb 1865 return this.shortTitle; 1866 } 1867 1868 public boolean hasShortTitleElement() { 1869 return this.shortTitle != null && !this.shortTitle.isEmpty(); 1870 } 1871 1872 public boolean hasShortTitle() { 1873 return this.shortTitle != null && !this.shortTitle.isEmpty(); 1874 } 1875 1876 /** 1877 * @param value {@link #shortTitle} (The short title provides an alternate title 1878 * for use in informal descriptive contexts where the full, formal 1879 * title is not necessary.). This is the underlying object with id, 1880 * value and extensions. The accessor "getShortTitle" gives direct 1881 * access to the value 1882 */ 1883 public EvidenceVariable setShortTitleElement(StringType value) { 1884 this.shortTitle = value; 1885 return this; 1886 } 1887 1888 /** 1889 * @return The short title provides an alternate title for use in informal 1890 * descriptive contexts where the full, formal title is not necessary. 1891 */ 1892 public String getShortTitle() { 1893 return this.shortTitle == null ? null : this.shortTitle.getValue(); 1894 } 1895 1896 /** 1897 * @param value The short title provides an alternate title for use in informal 1898 * descriptive contexts where the full, formal title is not 1899 * necessary. 1900 */ 1901 public EvidenceVariable setShortTitle(String value) { 1902 if (Utilities.noString(value)) 1903 this.shortTitle = null; 1904 else { 1905 if (this.shortTitle == null) 1906 this.shortTitle = new StringType(); 1907 this.shortTitle.setValue(value); 1908 } 1909 return this; 1910 } 1911 1912 /** 1913 * @return {@link #subtitle} (An explanatory or alternate title for the 1914 * EvidenceVariable giving additional information about its content.). 1915 * This is the underlying object with id, value and extensions. The 1916 * accessor "getSubtitle" gives direct access to the value 1917 */ 1918 public StringType getSubtitleElement() { 1919 if (this.subtitle == null) 1920 if (Configuration.errorOnAutoCreate()) 1921 throw new Error("Attempt to auto-create EvidenceVariable.subtitle"); 1922 else if (Configuration.doAutoCreate()) 1923 this.subtitle = new StringType(); // bb 1924 return this.subtitle; 1925 } 1926 1927 public boolean hasSubtitleElement() { 1928 return this.subtitle != null && !this.subtitle.isEmpty(); 1929 } 1930 1931 public boolean hasSubtitle() { 1932 return this.subtitle != null && !this.subtitle.isEmpty(); 1933 } 1934 1935 /** 1936 * @param value {@link #subtitle} (An explanatory or alternate title for the 1937 * EvidenceVariable giving additional information about its 1938 * content.). This is the underlying object with id, value and 1939 * extensions. The accessor "getSubtitle" gives direct access to 1940 * the value 1941 */ 1942 public EvidenceVariable setSubtitleElement(StringType value) { 1943 this.subtitle = value; 1944 return this; 1945 } 1946 1947 /** 1948 * @return An explanatory or alternate title for the EvidenceVariable giving 1949 * additional information about its content. 1950 */ 1951 public String getSubtitle() { 1952 return this.subtitle == null ? null : this.subtitle.getValue(); 1953 } 1954 1955 /** 1956 * @param value An explanatory or alternate title for the EvidenceVariable 1957 * giving additional information about its content. 1958 */ 1959 public EvidenceVariable setSubtitle(String value) { 1960 if (Utilities.noString(value)) 1961 this.subtitle = null; 1962 else { 1963 if (this.subtitle == null) 1964 this.subtitle = new StringType(); 1965 this.subtitle.setValue(value); 1966 } 1967 return this; 1968 } 1969 1970 /** 1971 * @return {@link #status} (The status of this evidence variable. Enables 1972 * tracking the life-cycle of the content.). This is the underlying 1973 * object with id, value and extensions. The accessor "getStatus" gives 1974 * direct access to the value 1975 */ 1976 public Enumeration<PublicationStatus> getStatusElement() { 1977 if (this.status == null) 1978 if (Configuration.errorOnAutoCreate()) 1979 throw new Error("Attempt to auto-create EvidenceVariable.status"); 1980 else if (Configuration.doAutoCreate()) 1981 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1982 return this.status; 1983 } 1984 1985 public boolean hasStatusElement() { 1986 return this.status != null && !this.status.isEmpty(); 1987 } 1988 1989 public boolean hasStatus() { 1990 return this.status != null && !this.status.isEmpty(); 1991 } 1992 1993 /** 1994 * @param value {@link #status} (The status of this evidence variable. Enables 1995 * tracking the life-cycle of the content.). This is the underlying 1996 * object with id, value and extensions. The accessor "getStatus" 1997 * gives direct access to the value 1998 */ 1999 public EvidenceVariable setStatusElement(Enumeration<PublicationStatus> value) { 2000 this.status = value; 2001 return this; 2002 } 2003 2004 /** 2005 * @return The status of this evidence variable. Enables tracking the life-cycle 2006 * of the content. 2007 */ 2008 public PublicationStatus getStatus() { 2009 return this.status == null ? null : this.status.getValue(); 2010 } 2011 2012 /** 2013 * @param value The status of this evidence variable. Enables tracking the 2014 * life-cycle of the content. 2015 */ 2016 public EvidenceVariable setStatus(PublicationStatus value) { 2017 if (this.status == null) 2018 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2019 this.status.setValue(value); 2020 return this; 2021 } 2022 2023 /** 2024 * @return {@link #date} (The date (and optionally time) when the evidence 2025 * variable was published. The date must change when the business 2026 * version changes and it must change if the status code changes. In 2027 * addition, it should change when the substantive content of the 2028 * evidence variable changes.). This is the underlying object with id, 2029 * value and extensions. The accessor "getDate" gives direct access to 2030 * the value 2031 */ 2032 public DateTimeType getDateElement() { 2033 if (this.date == null) 2034 if (Configuration.errorOnAutoCreate()) 2035 throw new Error("Attempt to auto-create EvidenceVariable.date"); 2036 else if (Configuration.doAutoCreate()) 2037 this.date = new DateTimeType(); // bb 2038 return this.date; 2039 } 2040 2041 public boolean hasDateElement() { 2042 return this.date != null && !this.date.isEmpty(); 2043 } 2044 2045 public boolean hasDate() { 2046 return this.date != null && !this.date.isEmpty(); 2047 } 2048 2049 /** 2050 * @param value {@link #date} (The date (and optionally time) when the evidence 2051 * variable was published. The date must change when the business 2052 * version changes and it must change if the status code changes. 2053 * In addition, it should change when the substantive content of 2054 * the evidence variable changes.). This is the underlying object 2055 * with id, value and extensions. The accessor "getDate" gives 2056 * direct access to the value 2057 */ 2058 public EvidenceVariable setDateElement(DateTimeType value) { 2059 this.date = value; 2060 return this; 2061 } 2062 2063 /** 2064 * @return The date (and optionally time) when the evidence variable was 2065 * published. The date must change when the business version changes and 2066 * it must change if the status code changes. In addition, it should 2067 * change when the substantive content of the evidence variable changes. 2068 */ 2069 public Date getDate() { 2070 return this.date == null ? null : this.date.getValue(); 2071 } 2072 2073 /** 2074 * @param value The date (and optionally time) when the evidence variable was 2075 * published. The date must change when the business version 2076 * changes and it must change if the status code changes. In 2077 * addition, it should change when the substantive content of the 2078 * evidence variable changes. 2079 */ 2080 public EvidenceVariable setDate(Date value) { 2081 if (value == null) 2082 this.date = null; 2083 else { 2084 if (this.date == null) 2085 this.date = new DateTimeType(); 2086 this.date.setValue(value); 2087 } 2088 return this; 2089 } 2090 2091 /** 2092 * @return {@link #publisher} (The name of the organization or individual that 2093 * published the evidence variable.). This is the underlying object with 2094 * id, value and extensions. The accessor "getPublisher" gives direct 2095 * access to the value 2096 */ 2097 public StringType getPublisherElement() { 2098 if (this.publisher == null) 2099 if (Configuration.errorOnAutoCreate()) 2100 throw new Error("Attempt to auto-create EvidenceVariable.publisher"); 2101 else if (Configuration.doAutoCreate()) 2102 this.publisher = new StringType(); // bb 2103 return this.publisher; 2104 } 2105 2106 public boolean hasPublisherElement() { 2107 return this.publisher != null && !this.publisher.isEmpty(); 2108 } 2109 2110 public boolean hasPublisher() { 2111 return this.publisher != null && !this.publisher.isEmpty(); 2112 } 2113 2114 /** 2115 * @param value {@link #publisher} (The name of the organization or individual 2116 * that published the evidence variable.). This is the underlying 2117 * object with id, value and extensions. The accessor 2118 * "getPublisher" gives direct access to the value 2119 */ 2120 public EvidenceVariable setPublisherElement(StringType value) { 2121 this.publisher = value; 2122 return this; 2123 } 2124 2125 /** 2126 * @return The name of the organization or individual that published the 2127 * evidence variable. 2128 */ 2129 public String getPublisher() { 2130 return this.publisher == null ? null : this.publisher.getValue(); 2131 } 2132 2133 /** 2134 * @param value The name of the organization or individual that published the 2135 * evidence variable. 2136 */ 2137 public EvidenceVariable setPublisher(String value) { 2138 if (Utilities.noString(value)) 2139 this.publisher = null; 2140 else { 2141 if (this.publisher == null) 2142 this.publisher = new StringType(); 2143 this.publisher.setValue(value); 2144 } 2145 return this; 2146 } 2147 2148 /** 2149 * @return {@link #contact} (Contact details to assist a user in finding and 2150 * communicating with the publisher.) 2151 */ 2152 public List<ContactDetail> getContact() { 2153 if (this.contact == null) 2154 this.contact = new ArrayList<ContactDetail>(); 2155 return this.contact; 2156 } 2157 2158 /** 2159 * @return Returns a reference to <code>this</code> for easy method chaining 2160 */ 2161 public EvidenceVariable setContact(List<ContactDetail> theContact) { 2162 this.contact = theContact; 2163 return this; 2164 } 2165 2166 public boolean hasContact() { 2167 if (this.contact == null) 2168 return false; 2169 for (ContactDetail item : this.contact) 2170 if (!item.isEmpty()) 2171 return true; 2172 return false; 2173 } 2174 2175 public ContactDetail addContact() { // 3 2176 ContactDetail t = new ContactDetail(); 2177 if (this.contact == null) 2178 this.contact = new ArrayList<ContactDetail>(); 2179 this.contact.add(t); 2180 return t; 2181 } 2182 2183 public EvidenceVariable addContact(ContactDetail t) { // 3 2184 if (t == null) 2185 return this; 2186 if (this.contact == null) 2187 this.contact = new ArrayList<ContactDetail>(); 2188 this.contact.add(t); 2189 return this; 2190 } 2191 2192 /** 2193 * @return The first repetition of repeating field {@link #contact}, creating it 2194 * if it does not already exist 2195 */ 2196 public ContactDetail getContactFirstRep() { 2197 if (getContact().isEmpty()) { 2198 addContact(); 2199 } 2200 return getContact().get(0); 2201 } 2202 2203 /** 2204 * @return {@link #description} (A free text natural language description of the 2205 * evidence variable from a consumer's perspective.). This is the 2206 * underlying object with id, value and extensions. The accessor 2207 * "getDescription" gives direct access to the value 2208 */ 2209 public MarkdownType getDescriptionElement() { 2210 if (this.description == null) 2211 if (Configuration.errorOnAutoCreate()) 2212 throw new Error("Attempt to auto-create EvidenceVariable.description"); 2213 else if (Configuration.doAutoCreate()) 2214 this.description = new MarkdownType(); // bb 2215 return this.description; 2216 } 2217 2218 public boolean hasDescriptionElement() { 2219 return this.description != null && !this.description.isEmpty(); 2220 } 2221 2222 public boolean hasDescription() { 2223 return this.description != null && !this.description.isEmpty(); 2224 } 2225 2226 /** 2227 * @param value {@link #description} (A free text natural language description 2228 * of the evidence variable from a consumer's perspective.). This 2229 * is the underlying object with id, value and extensions. The 2230 * accessor "getDescription" gives direct access to the value 2231 */ 2232 public EvidenceVariable setDescriptionElement(MarkdownType value) { 2233 this.description = value; 2234 return this; 2235 } 2236 2237 /** 2238 * @return A free text natural language description of the evidence variable 2239 * from a consumer's perspective. 2240 */ 2241 public String getDescription() { 2242 return this.description == null ? null : this.description.getValue(); 2243 } 2244 2245 /** 2246 * @param value A free text natural language description of the evidence 2247 * variable from a consumer's perspective. 2248 */ 2249 public EvidenceVariable setDescription(String value) { 2250 if (value == null) 2251 this.description = null; 2252 else { 2253 if (this.description == null) 2254 this.description = new MarkdownType(); 2255 this.description.setValue(value); 2256 } 2257 return this; 2258 } 2259 2260 /** 2261 * @return {@link #note} (A human-readable string to clarify or explain concepts 2262 * about the resource.) 2263 */ 2264 public List<Annotation> getNote() { 2265 if (this.note == null) 2266 this.note = new ArrayList<Annotation>(); 2267 return this.note; 2268 } 2269 2270 /** 2271 * @return Returns a reference to <code>this</code> for easy method chaining 2272 */ 2273 public EvidenceVariable setNote(List<Annotation> theNote) { 2274 this.note = theNote; 2275 return this; 2276 } 2277 2278 public boolean hasNote() { 2279 if (this.note == null) 2280 return false; 2281 for (Annotation item : this.note) 2282 if (!item.isEmpty()) 2283 return true; 2284 return false; 2285 } 2286 2287 public Annotation addNote() { // 3 2288 Annotation t = new Annotation(); 2289 if (this.note == null) 2290 this.note = new ArrayList<Annotation>(); 2291 this.note.add(t); 2292 return t; 2293 } 2294 2295 public EvidenceVariable addNote(Annotation t) { // 3 2296 if (t == null) 2297 return this; 2298 if (this.note == null) 2299 this.note = new ArrayList<Annotation>(); 2300 this.note.add(t); 2301 return this; 2302 } 2303 2304 /** 2305 * @return The first repetition of repeating field {@link #note}, creating it if 2306 * it does not already exist 2307 */ 2308 public Annotation getNoteFirstRep() { 2309 if (getNote().isEmpty()) { 2310 addNote(); 2311 } 2312 return getNote().get(0); 2313 } 2314 2315 /** 2316 * @return {@link #useContext} (The content was developed with a focus and 2317 * intent of supporting the contexts that are listed. These contexts may 2318 * be general categories (gender, age, ...) or may be references to 2319 * specific programs (insurance plans, studies, ...) and may be used to 2320 * assist with indexing and searching for appropriate evidence variable 2321 * instances.) 2322 */ 2323 public List<UsageContext> getUseContext() { 2324 if (this.useContext == null) 2325 this.useContext = new ArrayList<UsageContext>(); 2326 return this.useContext; 2327 } 2328 2329 /** 2330 * @return Returns a reference to <code>this</code> for easy method chaining 2331 */ 2332 public EvidenceVariable setUseContext(List<UsageContext> theUseContext) { 2333 this.useContext = theUseContext; 2334 return this; 2335 } 2336 2337 public boolean hasUseContext() { 2338 if (this.useContext == null) 2339 return false; 2340 for (UsageContext item : this.useContext) 2341 if (!item.isEmpty()) 2342 return true; 2343 return false; 2344 } 2345 2346 public UsageContext addUseContext() { // 3 2347 UsageContext t = new UsageContext(); 2348 if (this.useContext == null) 2349 this.useContext = new ArrayList<UsageContext>(); 2350 this.useContext.add(t); 2351 return t; 2352 } 2353 2354 public EvidenceVariable addUseContext(UsageContext t) { // 3 2355 if (t == null) 2356 return this; 2357 if (this.useContext == null) 2358 this.useContext = new ArrayList<UsageContext>(); 2359 this.useContext.add(t); 2360 return this; 2361 } 2362 2363 /** 2364 * @return The first repetition of repeating field {@link #useContext}, creating 2365 * it if it does not already exist 2366 */ 2367 public UsageContext getUseContextFirstRep() { 2368 if (getUseContext().isEmpty()) { 2369 addUseContext(); 2370 } 2371 return getUseContext().get(0); 2372 } 2373 2374 /** 2375 * @return {@link #jurisdiction} (A legal or geographic region in which the 2376 * evidence variable is intended to be used.) 2377 */ 2378 public List<CodeableConcept> getJurisdiction() { 2379 if (this.jurisdiction == null) 2380 this.jurisdiction = new ArrayList<CodeableConcept>(); 2381 return this.jurisdiction; 2382 } 2383 2384 /** 2385 * @return Returns a reference to <code>this</code> for easy method chaining 2386 */ 2387 public EvidenceVariable setJurisdiction(List<CodeableConcept> theJurisdiction) { 2388 this.jurisdiction = theJurisdiction; 2389 return this; 2390 } 2391 2392 public boolean hasJurisdiction() { 2393 if (this.jurisdiction == null) 2394 return false; 2395 for (CodeableConcept item : this.jurisdiction) 2396 if (!item.isEmpty()) 2397 return true; 2398 return false; 2399 } 2400 2401 public CodeableConcept addJurisdiction() { // 3 2402 CodeableConcept t = new CodeableConcept(); 2403 if (this.jurisdiction == null) 2404 this.jurisdiction = new ArrayList<CodeableConcept>(); 2405 this.jurisdiction.add(t); 2406 return t; 2407 } 2408 2409 public EvidenceVariable addJurisdiction(CodeableConcept t) { // 3 2410 if (t == null) 2411 return this; 2412 if (this.jurisdiction == null) 2413 this.jurisdiction = new ArrayList<CodeableConcept>(); 2414 this.jurisdiction.add(t); 2415 return this; 2416 } 2417 2418 /** 2419 * @return The first repetition of repeating field {@link #jurisdiction}, 2420 * creating it if it does not already exist 2421 */ 2422 public CodeableConcept getJurisdictionFirstRep() { 2423 if (getJurisdiction().isEmpty()) { 2424 addJurisdiction(); 2425 } 2426 return getJurisdiction().get(0); 2427 } 2428 2429 /** 2430 * @return {@link #copyright} (A copyright statement relating to the evidence 2431 * variable and/or its contents. Copyright statements are generally 2432 * legal restrictions on the use and publishing of the evidence 2433 * variable.). This is the underlying object with id, value and 2434 * extensions. The accessor "getCopyright" gives direct access to the 2435 * value 2436 */ 2437 public MarkdownType getCopyrightElement() { 2438 if (this.copyright == null) 2439 if (Configuration.errorOnAutoCreate()) 2440 throw new Error("Attempt to auto-create EvidenceVariable.copyright"); 2441 else if (Configuration.doAutoCreate()) 2442 this.copyright = new MarkdownType(); // bb 2443 return this.copyright; 2444 } 2445 2446 public boolean hasCopyrightElement() { 2447 return this.copyright != null && !this.copyright.isEmpty(); 2448 } 2449 2450 public boolean hasCopyright() { 2451 return this.copyright != null && !this.copyright.isEmpty(); 2452 } 2453 2454 /** 2455 * @param value {@link #copyright} (A copyright statement relating to the 2456 * evidence variable and/or its contents. Copyright statements are 2457 * generally legal restrictions on the use and publishing of the 2458 * evidence variable.). This is the underlying object with id, 2459 * value and extensions. The accessor "getCopyright" gives direct 2460 * access to the value 2461 */ 2462 public EvidenceVariable setCopyrightElement(MarkdownType value) { 2463 this.copyright = value; 2464 return this; 2465 } 2466 2467 /** 2468 * @return A copyright statement relating to the evidence variable and/or its 2469 * contents. Copyright statements are generally legal restrictions on 2470 * the use and publishing of the evidence variable. 2471 */ 2472 public String getCopyright() { 2473 return this.copyright == null ? null : this.copyright.getValue(); 2474 } 2475 2476 /** 2477 * @param value A copyright statement relating to the evidence variable and/or 2478 * its contents. Copyright statements are generally legal 2479 * restrictions on the use and publishing of the evidence variable. 2480 */ 2481 public EvidenceVariable setCopyright(String value) { 2482 if (value == null) 2483 this.copyright = null; 2484 else { 2485 if (this.copyright == null) 2486 this.copyright = new MarkdownType(); 2487 this.copyright.setValue(value); 2488 } 2489 return this; 2490 } 2491 2492 /** 2493 * @return {@link #approvalDate} (The date on which the resource content was 2494 * approved by the publisher. Approval happens once when the content is 2495 * officially approved for usage.). This is the underlying object with 2496 * id, value and extensions. The accessor "getApprovalDate" gives direct 2497 * access to the value 2498 */ 2499 public DateType getApprovalDateElement() { 2500 if (this.approvalDate == null) 2501 if (Configuration.errorOnAutoCreate()) 2502 throw new Error("Attempt to auto-create EvidenceVariable.approvalDate"); 2503 else if (Configuration.doAutoCreate()) 2504 this.approvalDate = new DateType(); // bb 2505 return this.approvalDate; 2506 } 2507 2508 public boolean hasApprovalDateElement() { 2509 return this.approvalDate != null && !this.approvalDate.isEmpty(); 2510 } 2511 2512 public boolean hasApprovalDate() { 2513 return this.approvalDate != null && !this.approvalDate.isEmpty(); 2514 } 2515 2516 /** 2517 * @param value {@link #approvalDate} (The date on which the resource content 2518 * was approved by the publisher. Approval happens once when the 2519 * content is officially approved for usage.). This is the 2520 * underlying object with id, value and extensions. The accessor 2521 * "getApprovalDate" gives direct access to the value 2522 */ 2523 public EvidenceVariable setApprovalDateElement(DateType value) { 2524 this.approvalDate = value; 2525 return this; 2526 } 2527 2528 /** 2529 * @return The date on which the resource content was approved by the publisher. 2530 * Approval happens once when the content is officially approved for 2531 * usage. 2532 */ 2533 public Date getApprovalDate() { 2534 return this.approvalDate == null ? null : this.approvalDate.getValue(); 2535 } 2536 2537 /** 2538 * @param value The date on which the resource content was approved by the 2539 * publisher. Approval happens once when the content is officially 2540 * approved for usage. 2541 */ 2542 public EvidenceVariable setApprovalDate(Date value) { 2543 if (value == null) 2544 this.approvalDate = null; 2545 else { 2546 if (this.approvalDate == null) 2547 this.approvalDate = new DateType(); 2548 this.approvalDate.setValue(value); 2549 } 2550 return this; 2551 } 2552 2553 /** 2554 * @return {@link #lastReviewDate} (The date on which the resource content was 2555 * last reviewed. Review happens periodically after approval but does 2556 * not change the original approval date.). This is the underlying 2557 * object with id, value and extensions. The accessor 2558 * "getLastReviewDate" gives direct access to the value 2559 */ 2560 public DateType getLastReviewDateElement() { 2561 if (this.lastReviewDate == null) 2562 if (Configuration.errorOnAutoCreate()) 2563 throw new Error("Attempt to auto-create EvidenceVariable.lastReviewDate"); 2564 else if (Configuration.doAutoCreate()) 2565 this.lastReviewDate = new DateType(); // bb 2566 return this.lastReviewDate; 2567 } 2568 2569 public boolean hasLastReviewDateElement() { 2570 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 2571 } 2572 2573 public boolean hasLastReviewDate() { 2574 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 2575 } 2576 2577 /** 2578 * @param value {@link #lastReviewDate} (The date on which the resource content 2579 * was last reviewed. Review happens periodically after approval 2580 * but does not change the original approval date.). This is the 2581 * underlying object with id, value and extensions. The accessor 2582 * "getLastReviewDate" gives direct access to the value 2583 */ 2584 public EvidenceVariable setLastReviewDateElement(DateType value) { 2585 this.lastReviewDate = value; 2586 return this; 2587 } 2588 2589 /** 2590 * @return The date on which the resource content was last reviewed. Review 2591 * happens periodically after approval but does not change the original 2592 * approval date. 2593 */ 2594 public Date getLastReviewDate() { 2595 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 2596 } 2597 2598 /** 2599 * @param value The date on which the resource content was last reviewed. Review 2600 * happens periodically after approval but does not change the 2601 * original approval date. 2602 */ 2603 public EvidenceVariable setLastReviewDate(Date value) { 2604 if (value == null) 2605 this.lastReviewDate = null; 2606 else { 2607 if (this.lastReviewDate == null) 2608 this.lastReviewDate = new DateType(); 2609 this.lastReviewDate.setValue(value); 2610 } 2611 return this; 2612 } 2613 2614 /** 2615 * @return {@link #effectivePeriod} (The period during which the evidence 2616 * variable content was or is planned to be in active use.) 2617 */ 2618 public Period getEffectivePeriod() { 2619 if (this.effectivePeriod == null) 2620 if (Configuration.errorOnAutoCreate()) 2621 throw new Error("Attempt to auto-create EvidenceVariable.effectivePeriod"); 2622 else if (Configuration.doAutoCreate()) 2623 this.effectivePeriod = new Period(); // cc 2624 return this.effectivePeriod; 2625 } 2626 2627 public boolean hasEffectivePeriod() { 2628 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 2629 } 2630 2631 /** 2632 * @param value {@link #effectivePeriod} (The period during which the evidence 2633 * variable content was or is planned to be in active use.) 2634 */ 2635 public EvidenceVariable setEffectivePeriod(Period value) { 2636 this.effectivePeriod = value; 2637 return this; 2638 } 2639 2640 /** 2641 * @return {@link #topic} (Descriptive topics related to the content of the 2642 * EvidenceVariable. Topics provide a high-level categorization grouping 2643 * types of EvidenceVariables that can be useful for filtering and 2644 * searching.) 2645 */ 2646 public List<CodeableConcept> getTopic() { 2647 if (this.topic == null) 2648 this.topic = new ArrayList<CodeableConcept>(); 2649 return this.topic; 2650 } 2651 2652 /** 2653 * @return Returns a reference to <code>this</code> for easy method chaining 2654 */ 2655 public EvidenceVariable setTopic(List<CodeableConcept> theTopic) { 2656 this.topic = theTopic; 2657 return this; 2658 } 2659 2660 public boolean hasTopic() { 2661 if (this.topic == null) 2662 return false; 2663 for (CodeableConcept item : this.topic) 2664 if (!item.isEmpty()) 2665 return true; 2666 return false; 2667 } 2668 2669 public CodeableConcept addTopic() { // 3 2670 CodeableConcept t = new CodeableConcept(); 2671 if (this.topic == null) 2672 this.topic = new ArrayList<CodeableConcept>(); 2673 this.topic.add(t); 2674 return t; 2675 } 2676 2677 public EvidenceVariable addTopic(CodeableConcept t) { // 3 2678 if (t == null) 2679 return this; 2680 if (this.topic == null) 2681 this.topic = new ArrayList<CodeableConcept>(); 2682 this.topic.add(t); 2683 return this; 2684 } 2685 2686 /** 2687 * @return The first repetition of repeating field {@link #topic}, creating it 2688 * if it does not already exist 2689 */ 2690 public CodeableConcept getTopicFirstRep() { 2691 if (getTopic().isEmpty()) { 2692 addTopic(); 2693 } 2694 return getTopic().get(0); 2695 } 2696 2697 /** 2698 * @return {@link #author} (An individiual or organization primarily involved in 2699 * the creation and maintenance of the content.) 2700 */ 2701 public List<ContactDetail> getAuthor() { 2702 if (this.author == null) 2703 this.author = new ArrayList<ContactDetail>(); 2704 return this.author; 2705 } 2706 2707 /** 2708 * @return Returns a reference to <code>this</code> for easy method chaining 2709 */ 2710 public EvidenceVariable setAuthor(List<ContactDetail> theAuthor) { 2711 this.author = theAuthor; 2712 return this; 2713 } 2714 2715 public boolean hasAuthor() { 2716 if (this.author == null) 2717 return false; 2718 for (ContactDetail item : this.author) 2719 if (!item.isEmpty()) 2720 return true; 2721 return false; 2722 } 2723 2724 public ContactDetail addAuthor() { // 3 2725 ContactDetail t = new ContactDetail(); 2726 if (this.author == null) 2727 this.author = new ArrayList<ContactDetail>(); 2728 this.author.add(t); 2729 return t; 2730 } 2731 2732 public EvidenceVariable addAuthor(ContactDetail t) { // 3 2733 if (t == null) 2734 return this; 2735 if (this.author == null) 2736 this.author = new ArrayList<ContactDetail>(); 2737 this.author.add(t); 2738 return this; 2739 } 2740 2741 /** 2742 * @return The first repetition of repeating field {@link #author}, creating it 2743 * if it does not already exist 2744 */ 2745 public ContactDetail getAuthorFirstRep() { 2746 if (getAuthor().isEmpty()) { 2747 addAuthor(); 2748 } 2749 return getAuthor().get(0); 2750 } 2751 2752 /** 2753 * @return {@link #editor} (An individual or organization primarily responsible 2754 * for internal coherence of the content.) 2755 */ 2756 public List<ContactDetail> getEditor() { 2757 if (this.editor == null) 2758 this.editor = new ArrayList<ContactDetail>(); 2759 return this.editor; 2760 } 2761 2762 /** 2763 * @return Returns a reference to <code>this</code> for easy method chaining 2764 */ 2765 public EvidenceVariable setEditor(List<ContactDetail> theEditor) { 2766 this.editor = theEditor; 2767 return this; 2768 } 2769 2770 public boolean hasEditor() { 2771 if (this.editor == null) 2772 return false; 2773 for (ContactDetail item : this.editor) 2774 if (!item.isEmpty()) 2775 return true; 2776 return false; 2777 } 2778 2779 public ContactDetail addEditor() { // 3 2780 ContactDetail t = new ContactDetail(); 2781 if (this.editor == null) 2782 this.editor = new ArrayList<ContactDetail>(); 2783 this.editor.add(t); 2784 return t; 2785 } 2786 2787 public EvidenceVariable addEditor(ContactDetail t) { // 3 2788 if (t == null) 2789 return this; 2790 if (this.editor == null) 2791 this.editor = new ArrayList<ContactDetail>(); 2792 this.editor.add(t); 2793 return this; 2794 } 2795 2796 /** 2797 * @return The first repetition of repeating field {@link #editor}, creating it 2798 * if it does not already exist 2799 */ 2800 public ContactDetail getEditorFirstRep() { 2801 if (getEditor().isEmpty()) { 2802 addEditor(); 2803 } 2804 return getEditor().get(0); 2805 } 2806 2807 /** 2808 * @return {@link #reviewer} (An individual or organization primarily 2809 * responsible for review of some aspect of the content.) 2810 */ 2811 public List<ContactDetail> getReviewer() { 2812 if (this.reviewer == null) 2813 this.reviewer = new ArrayList<ContactDetail>(); 2814 return this.reviewer; 2815 } 2816 2817 /** 2818 * @return Returns a reference to <code>this</code> for easy method chaining 2819 */ 2820 public EvidenceVariable setReviewer(List<ContactDetail> theReviewer) { 2821 this.reviewer = theReviewer; 2822 return this; 2823 } 2824 2825 public boolean hasReviewer() { 2826 if (this.reviewer == null) 2827 return false; 2828 for (ContactDetail item : this.reviewer) 2829 if (!item.isEmpty()) 2830 return true; 2831 return false; 2832 } 2833 2834 public ContactDetail addReviewer() { // 3 2835 ContactDetail t = new ContactDetail(); 2836 if (this.reviewer == null) 2837 this.reviewer = new ArrayList<ContactDetail>(); 2838 this.reviewer.add(t); 2839 return t; 2840 } 2841 2842 public EvidenceVariable addReviewer(ContactDetail t) { // 3 2843 if (t == null) 2844 return this; 2845 if (this.reviewer == null) 2846 this.reviewer = new ArrayList<ContactDetail>(); 2847 this.reviewer.add(t); 2848 return this; 2849 } 2850 2851 /** 2852 * @return The first repetition of repeating field {@link #reviewer}, creating 2853 * it if it does not already exist 2854 */ 2855 public ContactDetail getReviewerFirstRep() { 2856 if (getReviewer().isEmpty()) { 2857 addReviewer(); 2858 } 2859 return getReviewer().get(0); 2860 } 2861 2862 /** 2863 * @return {@link #endorser} (An individual or organization responsible for 2864 * officially endorsing the content for use in some setting.) 2865 */ 2866 public List<ContactDetail> getEndorser() { 2867 if (this.endorser == null) 2868 this.endorser = new ArrayList<ContactDetail>(); 2869 return this.endorser; 2870 } 2871 2872 /** 2873 * @return Returns a reference to <code>this</code> for easy method chaining 2874 */ 2875 public EvidenceVariable setEndorser(List<ContactDetail> theEndorser) { 2876 this.endorser = theEndorser; 2877 return this; 2878 } 2879 2880 public boolean hasEndorser() { 2881 if (this.endorser == null) 2882 return false; 2883 for (ContactDetail item : this.endorser) 2884 if (!item.isEmpty()) 2885 return true; 2886 return false; 2887 } 2888 2889 public ContactDetail addEndorser() { // 3 2890 ContactDetail t = new ContactDetail(); 2891 if (this.endorser == null) 2892 this.endorser = new ArrayList<ContactDetail>(); 2893 this.endorser.add(t); 2894 return t; 2895 } 2896 2897 public EvidenceVariable addEndorser(ContactDetail t) { // 3 2898 if (t == null) 2899 return this; 2900 if (this.endorser == null) 2901 this.endorser = new ArrayList<ContactDetail>(); 2902 this.endorser.add(t); 2903 return this; 2904 } 2905 2906 /** 2907 * @return The first repetition of repeating field {@link #endorser}, creating 2908 * it if it does not already exist 2909 */ 2910 public ContactDetail getEndorserFirstRep() { 2911 if (getEndorser().isEmpty()) { 2912 addEndorser(); 2913 } 2914 return getEndorser().get(0); 2915 } 2916 2917 /** 2918 * @return {@link #relatedArtifact} (Related artifacts such as additional 2919 * documentation, justification, or bibliographic references.) 2920 */ 2921 public List<RelatedArtifact> getRelatedArtifact() { 2922 if (this.relatedArtifact == null) 2923 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 2924 return this.relatedArtifact; 2925 } 2926 2927 /** 2928 * @return Returns a reference to <code>this</code> for easy method chaining 2929 */ 2930 public EvidenceVariable setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 2931 this.relatedArtifact = theRelatedArtifact; 2932 return this; 2933 } 2934 2935 public boolean hasRelatedArtifact() { 2936 if (this.relatedArtifact == null) 2937 return false; 2938 for (RelatedArtifact item : this.relatedArtifact) 2939 if (!item.isEmpty()) 2940 return true; 2941 return false; 2942 } 2943 2944 public RelatedArtifact addRelatedArtifact() { // 3 2945 RelatedArtifact t = new RelatedArtifact(); 2946 if (this.relatedArtifact == null) 2947 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 2948 this.relatedArtifact.add(t); 2949 return t; 2950 } 2951 2952 public EvidenceVariable addRelatedArtifact(RelatedArtifact t) { // 3 2953 if (t == null) 2954 return this; 2955 if (this.relatedArtifact == null) 2956 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 2957 this.relatedArtifact.add(t); 2958 return this; 2959 } 2960 2961 /** 2962 * @return The first repetition of repeating field {@link #relatedArtifact}, 2963 * creating it if it does not already exist 2964 */ 2965 public RelatedArtifact getRelatedArtifactFirstRep() { 2966 if (getRelatedArtifact().isEmpty()) { 2967 addRelatedArtifact(); 2968 } 2969 return getRelatedArtifact().get(0); 2970 } 2971 2972 /** 2973 * @return {@link #type} (The type of evidence element, a population, an 2974 * exposure, or an outcome.). This is the underlying object with id, 2975 * value and extensions. The accessor "getType" gives direct access to 2976 * the value 2977 */ 2978 public Enumeration<EvidenceVariableType> getTypeElement() { 2979 if (this.type == null) 2980 if (Configuration.errorOnAutoCreate()) 2981 throw new Error("Attempt to auto-create EvidenceVariable.type"); 2982 else if (Configuration.doAutoCreate()) 2983 this.type = new Enumeration<EvidenceVariableType>(new EvidenceVariableTypeEnumFactory()); // bb 2984 return this.type; 2985 } 2986 2987 public boolean hasTypeElement() { 2988 return this.type != null && !this.type.isEmpty(); 2989 } 2990 2991 public boolean hasType() { 2992 return this.type != null && !this.type.isEmpty(); 2993 } 2994 2995 /** 2996 * @param value {@link #type} (The type of evidence element, a population, an 2997 * exposure, or an outcome.). This is the underlying object with 2998 * id, value and extensions. The accessor "getType" gives direct 2999 * access to the value 3000 */ 3001 public EvidenceVariable setTypeElement(Enumeration<EvidenceVariableType> value) { 3002 this.type = value; 3003 return this; 3004 } 3005 3006 /** 3007 * @return The type of evidence element, a population, an exposure, or an 3008 * outcome. 3009 */ 3010 public EvidenceVariableType getType() { 3011 return this.type == null ? null : this.type.getValue(); 3012 } 3013 3014 /** 3015 * @param value The type of evidence element, a population, an exposure, or an 3016 * outcome. 3017 */ 3018 public EvidenceVariable setType(EvidenceVariableType value) { 3019 if (value == null) 3020 this.type = null; 3021 else { 3022 if (this.type == null) 3023 this.type = new Enumeration<EvidenceVariableType>(new EvidenceVariableTypeEnumFactory()); 3024 this.type.setValue(value); 3025 } 3026 return this; 3027 } 3028 3029 /** 3030 * @return {@link #characteristic} (A characteristic that defines the members of 3031 * the evidence element. Multiple characteristics are applied with "and" 3032 * semantics.) 3033 */ 3034 public List<EvidenceVariableCharacteristicComponent> getCharacteristic() { 3035 if (this.characteristic == null) 3036 this.characteristic = new ArrayList<EvidenceVariableCharacteristicComponent>(); 3037 return this.characteristic; 3038 } 3039 3040 /** 3041 * @return Returns a reference to <code>this</code> for easy method chaining 3042 */ 3043 public EvidenceVariable setCharacteristic(List<EvidenceVariableCharacteristicComponent> theCharacteristic) { 3044 this.characteristic = theCharacteristic; 3045 return this; 3046 } 3047 3048 public boolean hasCharacteristic() { 3049 if (this.characteristic == null) 3050 return false; 3051 for (EvidenceVariableCharacteristicComponent item : this.characteristic) 3052 if (!item.isEmpty()) 3053 return true; 3054 return false; 3055 } 3056 3057 public EvidenceVariableCharacteristicComponent addCharacteristic() { // 3 3058 EvidenceVariableCharacteristicComponent t = new EvidenceVariableCharacteristicComponent(); 3059 if (this.characteristic == null) 3060 this.characteristic = new ArrayList<EvidenceVariableCharacteristicComponent>(); 3061 this.characteristic.add(t); 3062 return t; 3063 } 3064 3065 public EvidenceVariable addCharacteristic(EvidenceVariableCharacteristicComponent t) { // 3 3066 if (t == null) 3067 return this; 3068 if (this.characteristic == null) 3069 this.characteristic = new ArrayList<EvidenceVariableCharacteristicComponent>(); 3070 this.characteristic.add(t); 3071 return this; 3072 } 3073 3074 /** 3075 * @return The first repetition of repeating field {@link #characteristic}, 3076 * creating it if it does not already exist 3077 */ 3078 public EvidenceVariableCharacteristicComponent getCharacteristicFirstRep() { 3079 if (getCharacteristic().isEmpty()) { 3080 addCharacteristic(); 3081 } 3082 return getCharacteristic().get(0); 3083 } 3084 3085 protected void listChildren(List<Property> children) { 3086 super.listChildren(children); 3087 children.add(new Property("url", "uri", 3088 "An absolute URI that is used to identify this evidence variable when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this evidence variable is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the evidence variable is stored on different servers.", 3089 0, 1, url)); 3090 children.add(new Property("identifier", "Identifier", 3091 "A formal identifier that is used to identify this evidence variable when it is represented in other formats, or referenced in a specification, model, design or an instance.", 3092 0, java.lang.Integer.MAX_VALUE, identifier)); 3093 children.add(new Property("version", "string", 3094 "The identifier that is used to identify this version of the evidence variable when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence variable author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 3095 0, 1, version)); 3096 children.add(new Property("name", "string", 3097 "A natural language name identifying the evidence variable. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 3098 0, 1, name)); 3099 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the evidence variable.", 3100 0, 1, title)); 3101 children.add(new Property("shortTitle", "string", 3102 "The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary.", 3103 0, 1, shortTitle)); 3104 children.add(new Property("subtitle", "string", 3105 "An explanatory or alternate title for the EvidenceVariable giving additional information about its content.", 3106 0, 1, subtitle)); 3107 children.add(new Property("status", "code", 3108 "The status of this evidence variable. Enables tracking the life-cycle of the content.", 0, 1, status)); 3109 children.add(new Property("date", "dateTime", 3110 "The date (and optionally time) when the evidence variable was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence variable changes.", 3111 0, 1, date)); 3112 children.add(new Property("publisher", "string", 3113 "The name of the organization or individual that published the evidence variable.", 0, 1, publisher)); 3114 children.add(new Property("contact", "ContactDetail", 3115 "Contact details to assist a user in finding and communicating with the publisher.", 0, 3116 java.lang.Integer.MAX_VALUE, contact)); 3117 children.add(new Property("description", "markdown", 3118 "A free text natural language description of the evidence variable from a consumer's perspective.", 0, 1, 3119 description)); 3120 children.add( 3121 new Property("note", "Annotation", "A human-readable string to clarify or explain concepts about the resource.", 3122 0, java.lang.Integer.MAX_VALUE, note)); 3123 children.add(new Property("useContext", "UsageContext", 3124 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence variable instances.", 3125 0, java.lang.Integer.MAX_VALUE, useContext)); 3126 children.add(new Property("jurisdiction", "CodeableConcept", 3127 "A legal or geographic region in which the evidence variable is intended to be used.", 0, 3128 java.lang.Integer.MAX_VALUE, jurisdiction)); 3129 children.add(new Property("copyright", "markdown", 3130 "A copyright statement relating to the evidence variable and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the evidence variable.", 3131 0, 1, copyright)); 3132 children.add(new Property("approvalDate", "date", 3133 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 3134 0, 1, approvalDate)); 3135 children.add(new Property("lastReviewDate", "date", 3136 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 3137 0, 1, lastReviewDate)); 3138 children.add(new Property("effectivePeriod", "Period", 3139 "The period during which the evidence variable content was or is planned to be in active use.", 0, 1, 3140 effectivePeriod)); 3141 children.add(new Property("topic", "CodeableConcept", 3142 "Descriptive topics related to the content of the EvidenceVariable. Topics provide a high-level categorization grouping types of EvidenceVariables that can be useful for filtering and searching.", 3143 0, java.lang.Integer.MAX_VALUE, topic)); 3144 children.add(new Property("author", "ContactDetail", 3145 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 3146 java.lang.Integer.MAX_VALUE, author)); 3147 children.add(new Property("editor", "ContactDetail", 3148 "An individual or organization primarily responsible for internal coherence of the content.", 0, 3149 java.lang.Integer.MAX_VALUE, editor)); 3150 children.add(new Property("reviewer", "ContactDetail", 3151 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 3152 java.lang.Integer.MAX_VALUE, reviewer)); 3153 children.add(new Property("endorser", "ContactDetail", 3154 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 3155 java.lang.Integer.MAX_VALUE, endorser)); 3156 children.add(new Property("relatedArtifact", "RelatedArtifact", 3157 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 3158 java.lang.Integer.MAX_VALUE, relatedArtifact)); 3159 children.add(new Property("type", "code", "The type of evidence element, a population, an exposure, or an outcome.", 3160 0, 1, type)); 3161 children.add(new Property("characteristic", "", 3162 "A characteristic that defines the members of the evidence element. Multiple characteristics are applied with \"and\" semantics.", 3163 0, java.lang.Integer.MAX_VALUE, characteristic)); 3164 } 3165 3166 @Override 3167 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3168 switch (_hash) { 3169 case 116079: 3170 /* url */ return new Property("url", "uri", 3171 "An absolute URI that is used to identify this evidence variable when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this evidence variable is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the evidence variable is stored on different servers.", 3172 0, 1, url); 3173 case -1618432855: 3174 /* identifier */ return new Property("identifier", "Identifier", 3175 "A formal identifier that is used to identify this evidence variable when it is represented in other formats, or referenced in a specification, model, design or an instance.", 3176 0, java.lang.Integer.MAX_VALUE, identifier); 3177 case 351608024: 3178 /* version */ return new Property("version", "string", 3179 "The identifier that is used to identify this version of the evidence variable when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence variable author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 3180 0, 1, version); 3181 case 3373707: 3182 /* name */ return new Property("name", "string", 3183 "A natural language name identifying the evidence variable. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 3184 0, 1, name); 3185 case 110371416: 3186 /* title */ return new Property("title", "string", 3187 "A short, descriptive, user-friendly title for the evidence variable.", 0, 1, title); 3188 case 1555503932: 3189 /* shortTitle */ return new Property("shortTitle", "string", 3190 "The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary.", 3191 0, 1, shortTitle); 3192 case -2060497896: 3193 /* subtitle */ return new Property("subtitle", "string", 3194 "An explanatory or alternate title for the EvidenceVariable giving additional information about its content.", 3195 0, 1, subtitle); 3196 case -892481550: 3197 /* status */ return new Property("status", "code", 3198 "The status of this evidence variable. Enables tracking the life-cycle of the content.", 0, 1, status); 3199 case 3076014: 3200 /* date */ return new Property("date", "dateTime", 3201 "The date (and optionally time) when the evidence variable was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence variable changes.", 3202 0, 1, date); 3203 case 1447404028: 3204 /* publisher */ return new Property("publisher", "string", 3205 "The name of the organization or individual that published the evidence variable.", 0, 1, publisher); 3206 case 951526432: 3207 /* contact */ return new Property("contact", "ContactDetail", 3208 "Contact details to assist a user in finding and communicating with the publisher.", 0, 3209 java.lang.Integer.MAX_VALUE, contact); 3210 case -1724546052: 3211 /* description */ return new Property("description", "markdown", 3212 "A free text natural language description of the evidence variable from a consumer's perspective.", 0, 1, 3213 description); 3214 case 3387378: 3215 /* note */ return new Property("note", "Annotation", 3216 "A human-readable string to clarify or explain concepts about the resource.", 0, java.lang.Integer.MAX_VALUE, 3217 note); 3218 case -669707736: 3219 /* useContext */ return new Property("useContext", "UsageContext", 3220 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence variable instances.", 3221 0, java.lang.Integer.MAX_VALUE, useContext); 3222 case -507075711: 3223 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 3224 "A legal or geographic region in which the evidence variable is intended to be used.", 0, 3225 java.lang.Integer.MAX_VALUE, jurisdiction); 3226 case 1522889671: 3227 /* copyright */ return new Property("copyright", "markdown", 3228 "A copyright statement relating to the evidence variable and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the evidence variable.", 3229 0, 1, copyright); 3230 case 223539345: 3231 /* approvalDate */ return new Property("approvalDate", "date", 3232 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 3233 0, 1, approvalDate); 3234 case -1687512484: 3235 /* lastReviewDate */ return new Property("lastReviewDate", "date", 3236 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 3237 0, 1, lastReviewDate); 3238 case -403934648: 3239 /* effectivePeriod */ return new Property("effectivePeriod", "Period", 3240 "The period during which the evidence variable content was or is planned to be in active use.", 0, 1, 3241 effectivePeriod); 3242 case 110546223: 3243 /* topic */ return new Property("topic", "CodeableConcept", 3244 "Descriptive topics related to the content of the EvidenceVariable. Topics provide a high-level categorization grouping types of EvidenceVariables that can be useful for filtering and searching.", 3245 0, java.lang.Integer.MAX_VALUE, topic); 3246 case -1406328437: 3247 /* author */ return new Property("author", "ContactDetail", 3248 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 3249 java.lang.Integer.MAX_VALUE, author); 3250 case -1307827859: 3251 /* editor */ return new Property("editor", "ContactDetail", 3252 "An individual or organization primarily responsible for internal coherence of the content.", 0, 3253 java.lang.Integer.MAX_VALUE, editor); 3254 case -261190139: 3255 /* reviewer */ return new Property("reviewer", "ContactDetail", 3256 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 3257 java.lang.Integer.MAX_VALUE, reviewer); 3258 case 1740277666: 3259 /* endorser */ return new Property("endorser", "ContactDetail", 3260 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 3261 java.lang.Integer.MAX_VALUE, endorser); 3262 case 666807069: 3263 /* relatedArtifact */ return new Property("relatedArtifact", "RelatedArtifact", 3264 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 3265 java.lang.Integer.MAX_VALUE, relatedArtifact); 3266 case 3575610: 3267 /* type */ return new Property("type", "code", 3268 "The type of evidence element, a population, an exposure, or an outcome.", 0, 1, type); 3269 case 366313883: 3270 /* characteristic */ return new Property("characteristic", "", 3271 "A characteristic that defines the members of the evidence element. Multiple characteristics are applied with \"and\" semantics.", 3272 0, java.lang.Integer.MAX_VALUE, characteristic); 3273 default: 3274 return super.getNamedProperty(_hash, _name, _checkValid); 3275 } 3276 3277 } 3278 3279 @Override 3280 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3281 switch (hash) { 3282 case 116079: 3283 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 3284 case -1618432855: 3285 /* identifier */ return this.identifier == null ? new Base[0] 3286 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3287 case 351608024: 3288 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 3289 case 3373707: 3290 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 3291 case 110371416: 3292 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 3293 case 1555503932: 3294 /* shortTitle */ return this.shortTitle == null ? new Base[0] : new Base[] { this.shortTitle }; // StringType 3295 case -2060497896: 3296 /* subtitle */ return this.subtitle == null ? new Base[0] : new Base[] { this.subtitle }; // StringType 3297 case -892481550: 3298 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 3299 case 3076014: 3300 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 3301 case 1447404028: 3302 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 3303 case 951526432: 3304 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 3305 case -1724546052: 3306 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 3307 case 3387378: 3308 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 3309 case -669707736: 3310 /* useContext */ return this.useContext == null ? new Base[0] 3311 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 3312 case -507075711: 3313 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 3314 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 3315 case 1522889671: 3316 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 3317 case 223539345: 3318 /* approvalDate */ return this.approvalDate == null ? new Base[0] : new Base[] { this.approvalDate }; // DateType 3319 case -1687512484: 3320 /* lastReviewDate */ return this.lastReviewDate == null ? new Base[0] : new Base[] { this.lastReviewDate }; // DateType 3321 case -403934648: 3322 /* effectivePeriod */ return this.effectivePeriod == null ? new Base[0] : new Base[] { this.effectivePeriod }; // Period 3323 case 110546223: 3324 /* topic */ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 3325 case -1406328437: 3326 /* author */ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 3327 case -1307827859: 3328 /* editor */ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 3329 case -261190139: 3330 /* reviewer */ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 3331 case 1740277666: 3332 /* endorser */ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 3333 case 666807069: 3334 /* relatedArtifact */ return this.relatedArtifact == null ? new Base[0] 3335 : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 3336 case 3575610: 3337 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<EvidenceVariableType> 3338 case 366313883: 3339 /* characteristic */ return this.characteristic == null ? new Base[0] 3340 : this.characteristic.toArray(new Base[this.characteristic.size()]); // EvidenceVariableCharacteristicComponent 3341 default: 3342 return super.getProperty(hash, name, checkValid); 3343 } 3344 3345 } 3346 3347 @Override 3348 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3349 switch (hash) { 3350 case 116079: // url 3351 this.url = castToUri(value); // UriType 3352 return value; 3353 case -1618432855: // identifier 3354 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3355 return value; 3356 case 351608024: // version 3357 this.version = castToString(value); // StringType 3358 return value; 3359 case 3373707: // name 3360 this.name = castToString(value); // StringType 3361 return value; 3362 case 110371416: // title 3363 this.title = castToString(value); // StringType 3364 return value; 3365 case 1555503932: // shortTitle 3366 this.shortTitle = castToString(value); // StringType 3367 return value; 3368 case -2060497896: // subtitle 3369 this.subtitle = castToString(value); // StringType 3370 return value; 3371 case -892481550: // status 3372 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3373 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3374 return value; 3375 case 3076014: // date 3376 this.date = castToDateTime(value); // DateTimeType 3377 return value; 3378 case 1447404028: // publisher 3379 this.publisher = castToString(value); // StringType 3380 return value; 3381 case 951526432: // contact 3382 this.getContact().add(castToContactDetail(value)); // ContactDetail 3383 return value; 3384 case -1724546052: // description 3385 this.description = castToMarkdown(value); // MarkdownType 3386 return value; 3387 case 3387378: // note 3388 this.getNote().add(castToAnnotation(value)); // Annotation 3389 return value; 3390 case -669707736: // useContext 3391 this.getUseContext().add(castToUsageContext(value)); // UsageContext 3392 return value; 3393 case -507075711: // jurisdiction 3394 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 3395 return value; 3396 case 1522889671: // copyright 3397 this.copyright = castToMarkdown(value); // MarkdownType 3398 return value; 3399 case 223539345: // approvalDate 3400 this.approvalDate = castToDate(value); // DateType 3401 return value; 3402 case -1687512484: // lastReviewDate 3403 this.lastReviewDate = castToDate(value); // DateType 3404 return value; 3405 case -403934648: // effectivePeriod 3406 this.effectivePeriod = castToPeriod(value); // Period 3407 return value; 3408 case 110546223: // topic 3409 this.getTopic().add(castToCodeableConcept(value)); // CodeableConcept 3410 return value; 3411 case -1406328437: // author 3412 this.getAuthor().add(castToContactDetail(value)); // ContactDetail 3413 return value; 3414 case -1307827859: // editor 3415 this.getEditor().add(castToContactDetail(value)); // ContactDetail 3416 return value; 3417 case -261190139: // reviewer 3418 this.getReviewer().add(castToContactDetail(value)); // ContactDetail 3419 return value; 3420 case 1740277666: // endorser 3421 this.getEndorser().add(castToContactDetail(value)); // ContactDetail 3422 return value; 3423 case 666807069: // relatedArtifact 3424 this.getRelatedArtifact().add(castToRelatedArtifact(value)); // RelatedArtifact 3425 return value; 3426 case 3575610: // type 3427 value = new EvidenceVariableTypeEnumFactory().fromType(castToCode(value)); 3428 this.type = (Enumeration) value; // Enumeration<EvidenceVariableType> 3429 return value; 3430 case 366313883: // characteristic 3431 this.getCharacteristic().add((EvidenceVariableCharacteristicComponent) value); // EvidenceVariableCharacteristicComponent 3432 return value; 3433 default: 3434 return super.setProperty(hash, name, value); 3435 } 3436 3437 } 3438 3439 @Override 3440 public Base setProperty(String name, Base value) throws FHIRException { 3441 if (name.equals("url")) { 3442 this.url = castToUri(value); // UriType 3443 } else if (name.equals("identifier")) { 3444 this.getIdentifier().add(castToIdentifier(value)); 3445 } else if (name.equals("version")) { 3446 this.version = castToString(value); // StringType 3447 } else if (name.equals("name")) { 3448 this.name = castToString(value); // StringType 3449 } else if (name.equals("title")) { 3450 this.title = castToString(value); // StringType 3451 } else if (name.equals("shortTitle")) { 3452 this.shortTitle = castToString(value); // StringType 3453 } else if (name.equals("subtitle")) { 3454 this.subtitle = castToString(value); // StringType 3455 } else if (name.equals("status")) { 3456 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3457 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3458 } else if (name.equals("date")) { 3459 this.date = castToDateTime(value); // DateTimeType 3460 } else if (name.equals("publisher")) { 3461 this.publisher = castToString(value); // StringType 3462 } else if (name.equals("contact")) { 3463 this.getContact().add(castToContactDetail(value)); 3464 } else if (name.equals("description")) { 3465 this.description = castToMarkdown(value); // MarkdownType 3466 } else if (name.equals("note")) { 3467 this.getNote().add(castToAnnotation(value)); 3468 } else if (name.equals("useContext")) { 3469 this.getUseContext().add(castToUsageContext(value)); 3470 } else if (name.equals("jurisdiction")) { 3471 this.getJurisdiction().add(castToCodeableConcept(value)); 3472 } else if (name.equals("copyright")) { 3473 this.copyright = castToMarkdown(value); // MarkdownType 3474 } else if (name.equals("approvalDate")) { 3475 this.approvalDate = castToDate(value); // DateType 3476 } else if (name.equals("lastReviewDate")) { 3477 this.lastReviewDate = castToDate(value); // DateType 3478 } else if (name.equals("effectivePeriod")) { 3479 this.effectivePeriod = castToPeriod(value); // Period 3480 } else if (name.equals("topic")) { 3481 this.getTopic().add(castToCodeableConcept(value)); 3482 } else if (name.equals("author")) { 3483 this.getAuthor().add(castToContactDetail(value)); 3484 } else if (name.equals("editor")) { 3485 this.getEditor().add(castToContactDetail(value)); 3486 } else if (name.equals("reviewer")) { 3487 this.getReviewer().add(castToContactDetail(value)); 3488 } else if (name.equals("endorser")) { 3489 this.getEndorser().add(castToContactDetail(value)); 3490 } else if (name.equals("relatedArtifact")) { 3491 this.getRelatedArtifact().add(castToRelatedArtifact(value)); 3492 } else if (name.equals("type")) { 3493 value = new EvidenceVariableTypeEnumFactory().fromType(castToCode(value)); 3494 this.type = (Enumeration) value; // Enumeration<EvidenceVariableType> 3495 } else if (name.equals("characteristic")) { 3496 this.getCharacteristic().add((EvidenceVariableCharacteristicComponent) value); 3497 } else 3498 return super.setProperty(name, value); 3499 return value; 3500 } 3501 3502 @Override 3503 public void removeChild(String name, Base value) throws FHIRException { 3504 if (name.equals("url")) { 3505 this.url = null; 3506 } else if (name.equals("identifier")) { 3507 this.getIdentifier().remove(castToIdentifier(value)); 3508 } else if (name.equals("version")) { 3509 this.version = null; 3510 } else if (name.equals("name")) { 3511 this.name = null; 3512 } else if (name.equals("title")) { 3513 this.title = null; 3514 } else if (name.equals("shortTitle")) { 3515 this.shortTitle = null; 3516 } else if (name.equals("subtitle")) { 3517 this.subtitle = null; 3518 } else if (name.equals("status")) { 3519 this.status = null; 3520 } else if (name.equals("date")) { 3521 this.date = null; 3522 } else if (name.equals("publisher")) { 3523 this.publisher = null; 3524 } else if (name.equals("contact")) { 3525 this.getContact().remove(castToContactDetail(value)); 3526 } else if (name.equals("description")) { 3527 this.description = null; 3528 } else if (name.equals("note")) { 3529 this.getNote().remove(castToAnnotation(value)); 3530 } else if (name.equals("useContext")) { 3531 this.getUseContext().remove(castToUsageContext(value)); 3532 } else if (name.equals("jurisdiction")) { 3533 this.getJurisdiction().remove(castToCodeableConcept(value)); 3534 } else if (name.equals("copyright")) { 3535 this.copyright = null; 3536 } else if (name.equals("approvalDate")) { 3537 this.approvalDate = null; 3538 } else if (name.equals("lastReviewDate")) { 3539 this.lastReviewDate = null; 3540 } else if (name.equals("effectivePeriod")) { 3541 this.effectivePeriod = null; 3542 } else if (name.equals("topic")) { 3543 this.getTopic().remove(castToCodeableConcept(value)); 3544 } else if (name.equals("author")) { 3545 this.getAuthor().remove(castToContactDetail(value)); 3546 } else if (name.equals("editor")) { 3547 this.getEditor().remove(castToContactDetail(value)); 3548 } else if (name.equals("reviewer")) { 3549 this.getReviewer().remove(castToContactDetail(value)); 3550 } else if (name.equals("endorser")) { 3551 this.getEndorser().remove(castToContactDetail(value)); 3552 } else if (name.equals("relatedArtifact")) { 3553 this.getRelatedArtifact().remove(castToRelatedArtifact(value)); 3554 } else if (name.equals("type")) { 3555 this.type = null; 3556 } else if (name.equals("characteristic")) { 3557 this.getCharacteristic().remove((EvidenceVariableCharacteristicComponent) value); 3558 } else 3559 super.removeChild(name, value); 3560 3561 } 3562 3563 @Override 3564 public Base makeProperty(int hash, String name) throws FHIRException { 3565 switch (hash) { 3566 case 116079: 3567 return getUrlElement(); 3568 case -1618432855: 3569 return addIdentifier(); 3570 case 351608024: 3571 return getVersionElement(); 3572 case 3373707: 3573 return getNameElement(); 3574 case 110371416: 3575 return getTitleElement(); 3576 case 1555503932: 3577 return getShortTitleElement(); 3578 case -2060497896: 3579 return getSubtitleElement(); 3580 case -892481550: 3581 return getStatusElement(); 3582 case 3076014: 3583 return getDateElement(); 3584 case 1447404028: 3585 return getPublisherElement(); 3586 case 951526432: 3587 return addContact(); 3588 case -1724546052: 3589 return getDescriptionElement(); 3590 case 3387378: 3591 return addNote(); 3592 case -669707736: 3593 return addUseContext(); 3594 case -507075711: 3595 return addJurisdiction(); 3596 case 1522889671: 3597 return getCopyrightElement(); 3598 case 223539345: 3599 return getApprovalDateElement(); 3600 case -1687512484: 3601 return getLastReviewDateElement(); 3602 case -403934648: 3603 return getEffectivePeriod(); 3604 case 110546223: 3605 return addTopic(); 3606 case -1406328437: 3607 return addAuthor(); 3608 case -1307827859: 3609 return addEditor(); 3610 case -261190139: 3611 return addReviewer(); 3612 case 1740277666: 3613 return addEndorser(); 3614 case 666807069: 3615 return addRelatedArtifact(); 3616 case 3575610: 3617 return getTypeElement(); 3618 case 366313883: 3619 return addCharacteristic(); 3620 default: 3621 return super.makeProperty(hash, name); 3622 } 3623 3624 } 3625 3626 @Override 3627 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3628 switch (hash) { 3629 case 116079: 3630 /* url */ return new String[] { "uri" }; 3631 case -1618432855: 3632 /* identifier */ return new String[] { "Identifier" }; 3633 case 351608024: 3634 /* version */ return new String[] { "string" }; 3635 case 3373707: 3636 /* name */ return new String[] { "string" }; 3637 case 110371416: 3638 /* title */ return new String[] { "string" }; 3639 case 1555503932: 3640 /* shortTitle */ return new String[] { "string" }; 3641 case -2060497896: 3642 /* subtitle */ return new String[] { "string" }; 3643 case -892481550: 3644 /* status */ return new String[] { "code" }; 3645 case 3076014: 3646 /* date */ return new String[] { "dateTime" }; 3647 case 1447404028: 3648 /* publisher */ return new String[] { "string" }; 3649 case 951526432: 3650 /* contact */ return new String[] { "ContactDetail" }; 3651 case -1724546052: 3652 /* description */ return new String[] { "markdown" }; 3653 case 3387378: 3654 /* note */ return new String[] { "Annotation" }; 3655 case -669707736: 3656 /* useContext */ return new String[] { "UsageContext" }; 3657 case -507075711: 3658 /* jurisdiction */ return new String[] { "CodeableConcept" }; 3659 case 1522889671: 3660 /* copyright */ return new String[] { "markdown" }; 3661 case 223539345: 3662 /* approvalDate */ return new String[] { "date" }; 3663 case -1687512484: 3664 /* lastReviewDate */ return new String[] { "date" }; 3665 case -403934648: 3666 /* effectivePeriod */ return new String[] { "Period" }; 3667 case 110546223: 3668 /* topic */ return new String[] { "CodeableConcept" }; 3669 case -1406328437: 3670 /* author */ return new String[] { "ContactDetail" }; 3671 case -1307827859: 3672 /* editor */ return new String[] { "ContactDetail" }; 3673 case -261190139: 3674 /* reviewer */ return new String[] { "ContactDetail" }; 3675 case 1740277666: 3676 /* endorser */ return new String[] { "ContactDetail" }; 3677 case 666807069: 3678 /* relatedArtifact */ return new String[] { "RelatedArtifact" }; 3679 case 3575610: 3680 /* type */ return new String[] { "code" }; 3681 case 366313883: 3682 /* characteristic */ return new String[] {}; 3683 default: 3684 return super.getTypesForProperty(hash, name); 3685 } 3686 3687 } 3688 3689 @Override 3690 public Base addChild(String name) throws FHIRException { 3691 if (name.equals("url")) { 3692 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.url"); 3693 } else if (name.equals("identifier")) { 3694 return addIdentifier(); 3695 } else if (name.equals("version")) { 3696 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.version"); 3697 } else if (name.equals("name")) { 3698 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.name"); 3699 } else if (name.equals("title")) { 3700 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.title"); 3701 } else if (name.equals("shortTitle")) { 3702 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.shortTitle"); 3703 } else if (name.equals("subtitle")) { 3704 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.subtitle"); 3705 } else if (name.equals("status")) { 3706 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.status"); 3707 } else if (name.equals("date")) { 3708 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.date"); 3709 } else if (name.equals("publisher")) { 3710 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.publisher"); 3711 } else if (name.equals("contact")) { 3712 return addContact(); 3713 } else if (name.equals("description")) { 3714 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.description"); 3715 } else if (name.equals("note")) { 3716 return addNote(); 3717 } else if (name.equals("useContext")) { 3718 return addUseContext(); 3719 } else if (name.equals("jurisdiction")) { 3720 return addJurisdiction(); 3721 } else if (name.equals("copyright")) { 3722 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.copyright"); 3723 } else if (name.equals("approvalDate")) { 3724 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.approvalDate"); 3725 } else if (name.equals("lastReviewDate")) { 3726 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.lastReviewDate"); 3727 } else if (name.equals("effectivePeriod")) { 3728 this.effectivePeriod = new Period(); 3729 return this.effectivePeriod; 3730 } else if (name.equals("topic")) { 3731 return addTopic(); 3732 } else if (name.equals("author")) { 3733 return addAuthor(); 3734 } else if (name.equals("editor")) { 3735 return addEditor(); 3736 } else if (name.equals("reviewer")) { 3737 return addReviewer(); 3738 } else if (name.equals("endorser")) { 3739 return addEndorser(); 3740 } else if (name.equals("relatedArtifact")) { 3741 return addRelatedArtifact(); 3742 } else if (name.equals("type")) { 3743 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.type"); 3744 } else if (name.equals("characteristic")) { 3745 return addCharacteristic(); 3746 } else 3747 return super.addChild(name); 3748 } 3749 3750 public String fhirType() { 3751 return "EvidenceVariable"; 3752 3753 } 3754 3755 public EvidenceVariable copy() { 3756 EvidenceVariable dst = new EvidenceVariable(); 3757 copyValues(dst); 3758 return dst; 3759 } 3760 3761 public void copyValues(EvidenceVariable dst) { 3762 super.copyValues(dst); 3763 dst.url = url == null ? null : url.copy(); 3764 if (identifier != null) { 3765 dst.identifier = new ArrayList<Identifier>(); 3766 for (Identifier i : identifier) 3767 dst.identifier.add(i.copy()); 3768 } 3769 ; 3770 dst.version = version == null ? null : version.copy(); 3771 dst.name = name == null ? null : name.copy(); 3772 dst.title = title == null ? null : title.copy(); 3773 dst.shortTitle = shortTitle == null ? null : shortTitle.copy(); 3774 dst.subtitle = subtitle == null ? null : subtitle.copy(); 3775 dst.status = status == null ? null : status.copy(); 3776 dst.date = date == null ? null : date.copy(); 3777 dst.publisher = publisher == null ? null : publisher.copy(); 3778 if (contact != null) { 3779 dst.contact = new ArrayList<ContactDetail>(); 3780 for (ContactDetail i : contact) 3781 dst.contact.add(i.copy()); 3782 } 3783 ; 3784 dst.description = description == null ? null : description.copy(); 3785 if (note != null) { 3786 dst.note = new ArrayList<Annotation>(); 3787 for (Annotation i : note) 3788 dst.note.add(i.copy()); 3789 } 3790 ; 3791 if (useContext != null) { 3792 dst.useContext = new ArrayList<UsageContext>(); 3793 for (UsageContext i : useContext) 3794 dst.useContext.add(i.copy()); 3795 } 3796 ; 3797 if (jurisdiction != null) { 3798 dst.jurisdiction = new ArrayList<CodeableConcept>(); 3799 for (CodeableConcept i : jurisdiction) 3800 dst.jurisdiction.add(i.copy()); 3801 } 3802 ; 3803 dst.copyright = copyright == null ? null : copyright.copy(); 3804 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 3805 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 3806 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 3807 if (topic != null) { 3808 dst.topic = new ArrayList<CodeableConcept>(); 3809 for (CodeableConcept i : topic) 3810 dst.topic.add(i.copy()); 3811 } 3812 ; 3813 if (author != null) { 3814 dst.author = new ArrayList<ContactDetail>(); 3815 for (ContactDetail i : author) 3816 dst.author.add(i.copy()); 3817 } 3818 ; 3819 if (editor != null) { 3820 dst.editor = new ArrayList<ContactDetail>(); 3821 for (ContactDetail i : editor) 3822 dst.editor.add(i.copy()); 3823 } 3824 ; 3825 if (reviewer != null) { 3826 dst.reviewer = new ArrayList<ContactDetail>(); 3827 for (ContactDetail i : reviewer) 3828 dst.reviewer.add(i.copy()); 3829 } 3830 ; 3831 if (endorser != null) { 3832 dst.endorser = new ArrayList<ContactDetail>(); 3833 for (ContactDetail i : endorser) 3834 dst.endorser.add(i.copy()); 3835 } 3836 ; 3837 if (relatedArtifact != null) { 3838 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 3839 for (RelatedArtifact i : relatedArtifact) 3840 dst.relatedArtifact.add(i.copy()); 3841 } 3842 ; 3843 dst.type = type == null ? null : type.copy(); 3844 if (characteristic != null) { 3845 dst.characteristic = new ArrayList<EvidenceVariableCharacteristicComponent>(); 3846 for (EvidenceVariableCharacteristicComponent i : characteristic) 3847 dst.characteristic.add(i.copy()); 3848 } 3849 ; 3850 } 3851 3852 protected EvidenceVariable typedCopy() { 3853 return copy(); 3854 } 3855 3856 @Override 3857 public boolean equalsDeep(Base other_) { 3858 if (!super.equalsDeep(other_)) 3859 return false; 3860 if (!(other_ instanceof EvidenceVariable)) 3861 return false; 3862 EvidenceVariable o = (EvidenceVariable) other_; 3863 return compareDeep(identifier, o.identifier, true) && compareDeep(shortTitle, o.shortTitle, true) 3864 && compareDeep(subtitle, o.subtitle, true) && compareDeep(note, o.note, true) 3865 && compareDeep(copyright, o.copyright, true) && compareDeep(approvalDate, o.approvalDate, true) 3866 && compareDeep(lastReviewDate, o.lastReviewDate, true) && compareDeep(effectivePeriod, o.effectivePeriod, true) 3867 && compareDeep(topic, o.topic, true) && compareDeep(author, o.author, true) 3868 && compareDeep(editor, o.editor, true) && compareDeep(reviewer, o.reviewer, true) 3869 && compareDeep(endorser, o.endorser, true) && compareDeep(relatedArtifact, o.relatedArtifact, true) 3870 && compareDeep(type, o.type, true) && compareDeep(characteristic, o.characteristic, true); 3871 } 3872 3873 @Override 3874 public boolean equalsShallow(Base other_) { 3875 if (!super.equalsShallow(other_)) 3876 return false; 3877 if (!(other_ instanceof EvidenceVariable)) 3878 return false; 3879 EvidenceVariable o = (EvidenceVariable) other_; 3880 return compareValues(shortTitle, o.shortTitle, true) && compareValues(subtitle, o.subtitle, true) 3881 && compareValues(copyright, o.copyright, true) && compareValues(approvalDate, o.approvalDate, true) 3882 && compareValues(lastReviewDate, o.lastReviewDate, true) && compareValues(type, o.type, true); 3883 } 3884 3885 public boolean isEmpty() { 3886 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, shortTitle, subtitle, note, copyright, 3887 approvalDate, lastReviewDate, effectivePeriod, topic, author, editor, reviewer, endorser, relatedArtifact, type, 3888 characteristic); 3889 } 3890 3891 @Override 3892 public ResourceType getResourceType() { 3893 return ResourceType.EvidenceVariable; 3894 } 3895 3896 /** 3897 * Search parameter: <b>date</b> 3898 * <p> 3899 * Description: <b>The evidence variable publication date</b><br> 3900 * Type: <b>date</b><br> 3901 * Path: <b>EvidenceVariable.date</b><br> 3902 * </p> 3903 */ 3904 @SearchParamDefinition(name = "date", path = "EvidenceVariable.date", description = "The evidence variable publication date", type = "date") 3905 public static final String SP_DATE = "date"; 3906 /** 3907 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3908 * <p> 3909 * Description: <b>The evidence variable publication date</b><br> 3910 * Type: <b>date</b><br> 3911 * Path: <b>EvidenceVariable.date</b><br> 3912 * </p> 3913 */ 3914 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3915 SP_DATE); 3916 3917 /** 3918 * Search parameter: <b>identifier</b> 3919 * <p> 3920 * Description: <b>External identifier for the evidence variable</b><br> 3921 * Type: <b>token</b><br> 3922 * Path: <b>EvidenceVariable.identifier</b><br> 3923 * </p> 3924 */ 3925 @SearchParamDefinition(name = "identifier", path = "EvidenceVariable.identifier", description = "External identifier for the evidence variable", type = "token") 3926 public static final String SP_IDENTIFIER = "identifier"; 3927 /** 3928 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3929 * <p> 3930 * Description: <b>External identifier for the evidence variable</b><br> 3931 * Type: <b>token</b><br> 3932 * Path: <b>EvidenceVariable.identifier</b><br> 3933 * </p> 3934 */ 3935 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3936 SP_IDENTIFIER); 3937 3938 /** 3939 * Search parameter: <b>successor</b> 3940 * <p> 3941 * Description: <b>What resource is being referenced</b><br> 3942 * Type: <b>reference</b><br> 3943 * Path: <b>EvidenceVariable.relatedArtifact.resource</b><br> 3944 * </p> 3945 */ 3946 @SearchParamDefinition(name = "successor", path = "EvidenceVariable.relatedArtifact.where(type='successor').resource", description = "What resource is being referenced", type = "reference") 3947 public static final String SP_SUCCESSOR = "successor"; 3948 /** 3949 * <b>Fluent Client</b> search parameter constant for <b>successor</b> 3950 * <p> 3951 * Description: <b>What resource is being referenced</b><br> 3952 * Type: <b>reference</b><br> 3953 * Path: <b>EvidenceVariable.relatedArtifact.resource</b><br> 3954 * </p> 3955 */ 3956 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUCCESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3957 SP_SUCCESSOR); 3958 3959 /** 3960 * Constant for fluent queries to be used to add include statements. Specifies 3961 * the path value of "<b>EvidenceVariable:successor</b>". 3962 */ 3963 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUCCESSOR = new ca.uhn.fhir.model.api.Include( 3964 "EvidenceVariable:successor").toLocked(); 3965 3966 /** 3967 * Search parameter: <b>context-type-value</b> 3968 * <p> 3969 * Description: <b>A use context type and value assigned to the evidence 3970 * variable</b><br> 3971 * Type: <b>composite</b><br> 3972 * Path: <b></b><br> 3973 * </p> 3974 */ 3975 @SearchParamDefinition(name = "context-type-value", path = "EvidenceVariable.useContext", description = "A use context type and value assigned to the evidence variable", type = "composite", compositeOf = { 3976 "context-type", "context" }) 3977 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 3978 /** 3979 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 3980 * <p> 3981 * Description: <b>A use context type and value assigned to the evidence 3982 * variable</b><br> 3983 * Type: <b>composite</b><br> 3984 * Path: <b></b><br> 3985 * </p> 3986 */ 3987 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 3988 SP_CONTEXT_TYPE_VALUE); 3989 3990 /** 3991 * Search parameter: <b>jurisdiction</b> 3992 * <p> 3993 * Description: <b>Intended jurisdiction for the evidence variable</b><br> 3994 * Type: <b>token</b><br> 3995 * Path: <b>EvidenceVariable.jurisdiction</b><br> 3996 * </p> 3997 */ 3998 @SearchParamDefinition(name = "jurisdiction", path = "EvidenceVariable.jurisdiction", description = "Intended jurisdiction for the evidence variable", type = "token") 3999 public static final String SP_JURISDICTION = "jurisdiction"; 4000 /** 4001 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 4002 * <p> 4003 * Description: <b>Intended jurisdiction for the evidence variable</b><br> 4004 * Type: <b>token</b><br> 4005 * Path: <b>EvidenceVariable.jurisdiction</b><br> 4006 * </p> 4007 */ 4008 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4009 SP_JURISDICTION); 4010 4011 /** 4012 * Search parameter: <b>description</b> 4013 * <p> 4014 * Description: <b>The description of the evidence variable</b><br> 4015 * Type: <b>string</b><br> 4016 * Path: <b>EvidenceVariable.description</b><br> 4017 * </p> 4018 */ 4019 @SearchParamDefinition(name = "description", path = "EvidenceVariable.description", description = "The description of the evidence variable", type = "string") 4020 public static final String SP_DESCRIPTION = "description"; 4021 /** 4022 * <b>Fluent Client</b> search parameter constant for <b>description</b> 4023 * <p> 4024 * Description: <b>The description of the evidence variable</b><br> 4025 * Type: <b>string</b><br> 4026 * Path: <b>EvidenceVariable.description</b><br> 4027 * </p> 4028 */ 4029 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 4030 SP_DESCRIPTION); 4031 4032 /** 4033 * Search parameter: <b>derived-from</b> 4034 * <p> 4035 * Description: <b>What resource is being referenced</b><br> 4036 * Type: <b>reference</b><br> 4037 * Path: <b>EvidenceVariable.relatedArtifact.resource</b><br> 4038 * </p> 4039 */ 4040 @SearchParamDefinition(name = "derived-from", path = "EvidenceVariable.relatedArtifact.where(type='derived-from').resource", description = "What resource is being referenced", type = "reference") 4041 public static final String SP_DERIVED_FROM = "derived-from"; 4042 /** 4043 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 4044 * <p> 4045 * Description: <b>What resource is being referenced</b><br> 4046 * Type: <b>reference</b><br> 4047 * Path: <b>EvidenceVariable.relatedArtifact.resource</b><br> 4048 * </p> 4049 */ 4050 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4051 SP_DERIVED_FROM); 4052 4053 /** 4054 * Constant for fluent queries to be used to add include statements. Specifies 4055 * the path value of "<b>EvidenceVariable:derived-from</b>". 4056 */ 4057 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include( 4058 "EvidenceVariable:derived-from").toLocked(); 4059 4060 /** 4061 * Search parameter: <b>context-type</b> 4062 * <p> 4063 * Description: <b>A type of use context assigned to the evidence 4064 * variable</b><br> 4065 * Type: <b>token</b><br> 4066 * Path: <b>EvidenceVariable.useContext.code</b><br> 4067 * </p> 4068 */ 4069 @SearchParamDefinition(name = "context-type", path = "EvidenceVariable.useContext.code", description = "A type of use context assigned to the evidence variable", type = "token") 4070 public static final String SP_CONTEXT_TYPE = "context-type"; 4071 /** 4072 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 4073 * <p> 4074 * Description: <b>A type of use context assigned to the evidence 4075 * variable</b><br> 4076 * Type: <b>token</b><br> 4077 * Path: <b>EvidenceVariable.useContext.code</b><br> 4078 * </p> 4079 */ 4080 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4081 SP_CONTEXT_TYPE); 4082 4083 /** 4084 * Search parameter: <b>predecessor</b> 4085 * <p> 4086 * Description: <b>What resource is being referenced</b><br> 4087 * Type: <b>reference</b><br> 4088 * Path: <b>EvidenceVariable.relatedArtifact.resource</b><br> 4089 * </p> 4090 */ 4091 @SearchParamDefinition(name = "predecessor", path = "EvidenceVariable.relatedArtifact.where(type='predecessor').resource", description = "What resource is being referenced", type = "reference") 4092 public static final String SP_PREDECESSOR = "predecessor"; 4093 /** 4094 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 4095 * <p> 4096 * Description: <b>What resource is being referenced</b><br> 4097 * Type: <b>reference</b><br> 4098 * Path: <b>EvidenceVariable.relatedArtifact.resource</b><br> 4099 * </p> 4100 */ 4101 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4102 SP_PREDECESSOR); 4103 4104 /** 4105 * Constant for fluent queries to be used to add include statements. Specifies 4106 * the path value of "<b>EvidenceVariable:predecessor</b>". 4107 */ 4108 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include( 4109 "EvidenceVariable:predecessor").toLocked(); 4110 4111 /** 4112 * Search parameter: <b>title</b> 4113 * <p> 4114 * Description: <b>The human-friendly name of the evidence variable</b><br> 4115 * Type: <b>string</b><br> 4116 * Path: <b>EvidenceVariable.title</b><br> 4117 * </p> 4118 */ 4119 @SearchParamDefinition(name = "title", path = "EvidenceVariable.title", description = "The human-friendly name of the evidence variable", type = "string") 4120 public static final String SP_TITLE = "title"; 4121 /** 4122 * <b>Fluent Client</b> search parameter constant for <b>title</b> 4123 * <p> 4124 * Description: <b>The human-friendly name of the evidence variable</b><br> 4125 * Type: <b>string</b><br> 4126 * Path: <b>EvidenceVariable.title</b><br> 4127 * </p> 4128 */ 4129 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 4130 SP_TITLE); 4131 4132 /** 4133 * Search parameter: <b>composed-of</b> 4134 * <p> 4135 * Description: <b>What resource is being referenced</b><br> 4136 * Type: <b>reference</b><br> 4137 * Path: <b>EvidenceVariable.relatedArtifact.resource</b><br> 4138 * </p> 4139 */ 4140 @SearchParamDefinition(name = "composed-of", path = "EvidenceVariable.relatedArtifact.where(type='composed-of').resource", description = "What resource is being referenced", type = "reference") 4141 public static final String SP_COMPOSED_OF = "composed-of"; 4142 /** 4143 * <b>Fluent Client</b> search parameter constant for <b>composed-of</b> 4144 * <p> 4145 * Description: <b>What resource is being referenced</b><br> 4146 * Type: <b>reference</b><br> 4147 * Path: <b>EvidenceVariable.relatedArtifact.resource</b><br> 4148 * </p> 4149 */ 4150 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSED_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4151 SP_COMPOSED_OF); 4152 4153 /** 4154 * Constant for fluent queries to be used to add include statements. Specifies 4155 * the path value of "<b>EvidenceVariable:composed-of</b>". 4156 */ 4157 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSED_OF = new ca.uhn.fhir.model.api.Include( 4158 "EvidenceVariable:composed-of").toLocked(); 4159 4160 /** 4161 * Search parameter: <b>version</b> 4162 * <p> 4163 * Description: <b>The business version of the evidence variable</b><br> 4164 * Type: <b>token</b><br> 4165 * Path: <b>EvidenceVariable.version</b><br> 4166 * </p> 4167 */ 4168 @SearchParamDefinition(name = "version", path = "EvidenceVariable.version", description = "The business version of the evidence variable", type = "token") 4169 public static final String SP_VERSION = "version"; 4170 /** 4171 * <b>Fluent Client</b> search parameter constant for <b>version</b> 4172 * <p> 4173 * Description: <b>The business version of the evidence variable</b><br> 4174 * Type: <b>token</b><br> 4175 * Path: <b>EvidenceVariable.version</b><br> 4176 * </p> 4177 */ 4178 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4179 SP_VERSION); 4180 4181 /** 4182 * Search parameter: <b>url</b> 4183 * <p> 4184 * Description: <b>The uri that identifies the evidence variable</b><br> 4185 * Type: <b>uri</b><br> 4186 * Path: <b>EvidenceVariable.url</b><br> 4187 * </p> 4188 */ 4189 @SearchParamDefinition(name = "url", path = "EvidenceVariable.url", description = "The uri that identifies the evidence variable", type = "uri") 4190 public static final String SP_URL = "url"; 4191 /** 4192 * <b>Fluent Client</b> search parameter constant for <b>url</b> 4193 * <p> 4194 * Description: <b>The uri that identifies the evidence variable</b><br> 4195 * Type: <b>uri</b><br> 4196 * Path: <b>EvidenceVariable.url</b><br> 4197 * </p> 4198 */ 4199 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 4200 4201 /** 4202 * Search parameter: <b>context-quantity</b> 4203 * <p> 4204 * Description: <b>A quantity- or range-valued use context assigned to the 4205 * evidence variable</b><br> 4206 * Type: <b>quantity</b><br> 4207 * Path: <b>EvidenceVariable.useContext.valueQuantity, 4208 * EvidenceVariable.useContext.valueRange</b><br> 4209 * </p> 4210 */ 4211 @SearchParamDefinition(name = "context-quantity", path = "(EvidenceVariable.useContext.value as Quantity) | (EvidenceVariable.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the evidence variable", type = "quantity") 4212 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 4213 /** 4214 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 4215 * <p> 4216 * Description: <b>A quantity- or range-valued use context assigned to the 4217 * evidence variable</b><br> 4218 * Type: <b>quantity</b><br> 4219 * Path: <b>EvidenceVariable.useContext.valueQuantity, 4220 * EvidenceVariable.useContext.valueRange</b><br> 4221 * </p> 4222 */ 4223 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 4224 SP_CONTEXT_QUANTITY); 4225 4226 /** 4227 * Search parameter: <b>effective</b> 4228 * <p> 4229 * Description: <b>The time during which the evidence variable is intended to be 4230 * in use</b><br> 4231 * Type: <b>date</b><br> 4232 * Path: <b>EvidenceVariable.effectivePeriod</b><br> 4233 * </p> 4234 */ 4235 @SearchParamDefinition(name = "effective", path = "EvidenceVariable.effectivePeriod", description = "The time during which the evidence variable is intended to be in use", type = "date") 4236 public static final String SP_EFFECTIVE = "effective"; 4237 /** 4238 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 4239 * <p> 4240 * Description: <b>The time during which the evidence variable is intended to be 4241 * in use</b><br> 4242 * Type: <b>date</b><br> 4243 * Path: <b>EvidenceVariable.effectivePeriod</b><br> 4244 * </p> 4245 */ 4246 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam( 4247 SP_EFFECTIVE); 4248 4249 /** 4250 * Search parameter: <b>depends-on</b> 4251 * <p> 4252 * Description: <b>What resource is being referenced</b><br> 4253 * Type: <b>reference</b><br> 4254 * Path: <b>EvidenceVariable.relatedArtifact.resource</b><br> 4255 * </p> 4256 */ 4257 @SearchParamDefinition(name = "depends-on", path = "EvidenceVariable.relatedArtifact.where(type='depends-on').resource", description = "What resource is being referenced", type = "reference") 4258 public static final String SP_DEPENDS_ON = "depends-on"; 4259 /** 4260 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 4261 * <p> 4262 * Description: <b>What resource is being referenced</b><br> 4263 * Type: <b>reference</b><br> 4264 * Path: <b>EvidenceVariable.relatedArtifact.resource</b><br> 4265 * </p> 4266 */ 4267 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4268 SP_DEPENDS_ON); 4269 4270 /** 4271 * Constant for fluent queries to be used to add include statements. Specifies 4272 * the path value of "<b>EvidenceVariable:depends-on</b>". 4273 */ 4274 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include( 4275 "EvidenceVariable:depends-on").toLocked(); 4276 4277 /** 4278 * Search parameter: <b>name</b> 4279 * <p> 4280 * Description: <b>Computationally friendly name of the evidence 4281 * variable</b><br> 4282 * Type: <b>string</b><br> 4283 * Path: <b>EvidenceVariable.name</b><br> 4284 * </p> 4285 */ 4286 @SearchParamDefinition(name = "name", path = "EvidenceVariable.name", description = "Computationally friendly name of the evidence variable", type = "string") 4287 public static final String SP_NAME = "name"; 4288 /** 4289 * <b>Fluent Client</b> search parameter constant for <b>name</b> 4290 * <p> 4291 * Description: <b>Computationally friendly name of the evidence 4292 * variable</b><br> 4293 * Type: <b>string</b><br> 4294 * Path: <b>EvidenceVariable.name</b><br> 4295 * </p> 4296 */ 4297 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 4298 SP_NAME); 4299 4300 /** 4301 * Search parameter: <b>context</b> 4302 * <p> 4303 * Description: <b>A use context assigned to the evidence variable</b><br> 4304 * Type: <b>token</b><br> 4305 * Path: <b>EvidenceVariable.useContext.valueCodeableConcept</b><br> 4306 * </p> 4307 */ 4308 @SearchParamDefinition(name = "context", path = "(EvidenceVariable.useContext.value as CodeableConcept)", description = "A use context assigned to the evidence variable", type = "token") 4309 public static final String SP_CONTEXT = "context"; 4310 /** 4311 * <b>Fluent Client</b> search parameter constant for <b>context</b> 4312 * <p> 4313 * Description: <b>A use context assigned to the evidence variable</b><br> 4314 * Type: <b>token</b><br> 4315 * Path: <b>EvidenceVariable.useContext.valueCodeableConcept</b><br> 4316 * </p> 4317 */ 4318 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4319 SP_CONTEXT); 4320 4321 /** 4322 * Search parameter: <b>publisher</b> 4323 * <p> 4324 * Description: <b>Name of the publisher of the evidence variable</b><br> 4325 * Type: <b>string</b><br> 4326 * Path: <b>EvidenceVariable.publisher</b><br> 4327 * </p> 4328 */ 4329 @SearchParamDefinition(name = "publisher", path = "EvidenceVariable.publisher", description = "Name of the publisher of the evidence variable", type = "string") 4330 public static final String SP_PUBLISHER = "publisher"; 4331 /** 4332 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 4333 * <p> 4334 * Description: <b>Name of the publisher of the evidence variable</b><br> 4335 * Type: <b>string</b><br> 4336 * Path: <b>EvidenceVariable.publisher</b><br> 4337 * </p> 4338 */ 4339 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 4340 SP_PUBLISHER); 4341 4342 /** 4343 * Search parameter: <b>topic</b> 4344 * <p> 4345 * Description: <b>Topics associated with the EvidenceVariable</b><br> 4346 * Type: <b>token</b><br> 4347 * Path: <b>EvidenceVariable.topic</b><br> 4348 * </p> 4349 */ 4350 @SearchParamDefinition(name = "topic", path = "EvidenceVariable.topic", description = "Topics associated with the EvidenceVariable", type = "token") 4351 public static final String SP_TOPIC = "topic"; 4352 /** 4353 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 4354 * <p> 4355 * Description: <b>Topics associated with the EvidenceVariable</b><br> 4356 * Type: <b>token</b><br> 4357 * Path: <b>EvidenceVariable.topic</b><br> 4358 * </p> 4359 */ 4360 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4361 SP_TOPIC); 4362 4363 /** 4364 * Search parameter: <b>context-type-quantity</b> 4365 * <p> 4366 * Description: <b>A use context type and quantity- or range-based value 4367 * assigned to the evidence variable</b><br> 4368 * Type: <b>composite</b><br> 4369 * Path: <b></b><br> 4370 * </p> 4371 */ 4372 @SearchParamDefinition(name = "context-type-quantity", path = "EvidenceVariable.useContext", description = "A use context type and quantity- or range-based value assigned to the evidence variable", type = "composite", compositeOf = { 4373 "context-type", "context-quantity" }) 4374 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 4375 /** 4376 * <b>Fluent Client</b> search parameter constant for 4377 * <b>context-type-quantity</b> 4378 * <p> 4379 * Description: <b>A use context type and quantity- or range-based value 4380 * assigned to the evidence variable</b><br> 4381 * Type: <b>composite</b><br> 4382 * Path: <b></b><br> 4383 * </p> 4384 */ 4385 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 4386 SP_CONTEXT_TYPE_QUANTITY); 4387 4388 /** 4389 * Search parameter: <b>status</b> 4390 * <p> 4391 * Description: <b>The current status of the evidence variable</b><br> 4392 * Type: <b>token</b><br> 4393 * Path: <b>EvidenceVariable.status</b><br> 4394 * </p> 4395 */ 4396 @SearchParamDefinition(name = "status", path = "EvidenceVariable.status", description = "The current status of the evidence variable", type = "token") 4397 public static final String SP_STATUS = "status"; 4398 /** 4399 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4400 * <p> 4401 * Description: <b>The current status of the evidence variable</b><br> 4402 * Type: <b>token</b><br> 4403 * Path: <b>EvidenceVariable.status</b><br> 4404 * </p> 4405 */ 4406 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4407 SP_STATUS); 4408 4409}