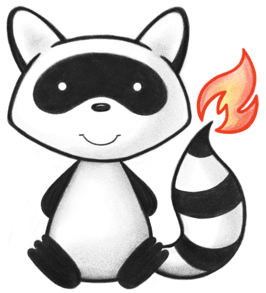
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049 050/** 051 * Example of workflow instance. 052 */ 053@ResourceDef(name = "ExampleScenario", profile = "http://hl7.org/fhir/StructureDefinition/ExampleScenario") 054@ChildOrder(names = { "url", "identifier", "version", "name", "status", "experimental", "date", "publisher", "contact", 055 "useContext", "jurisdiction", "copyright", "purpose", "actor", "instance", "process", "workflow" }) 056public class ExampleScenario extends MetadataResource { 057 058 public enum ExampleScenarioActorType { 059 /** 060 * A person. 061 */ 062 PERSON, 063 /** 064 * A system. 065 */ 066 ENTITY, 067 /** 068 * added to help the parsers with the generic types 069 */ 070 NULL; 071 072 public static ExampleScenarioActorType fromCode(String codeString) throws FHIRException { 073 if (codeString == null || "".equals(codeString)) 074 return null; 075 if ("person".equals(codeString)) 076 return PERSON; 077 if ("entity".equals(codeString)) 078 return ENTITY; 079 if (Configuration.isAcceptInvalidEnums()) 080 return null; 081 else 082 throw new FHIRException("Unknown ExampleScenarioActorType code '" + codeString + "'"); 083 } 084 085 public String toCode() { 086 switch (this) { 087 case PERSON: 088 return "person"; 089 case ENTITY: 090 return "entity"; 091 case NULL: 092 return null; 093 default: 094 return "?"; 095 } 096 } 097 098 public String getSystem() { 099 switch (this) { 100 case PERSON: 101 return "http://hl7.org/fhir/examplescenario-actor-type"; 102 case ENTITY: 103 return "http://hl7.org/fhir/examplescenario-actor-type"; 104 case NULL: 105 return null; 106 default: 107 return "?"; 108 } 109 } 110 111 public String getDefinition() { 112 switch (this) { 113 case PERSON: 114 return "A person."; 115 case ENTITY: 116 return "A system."; 117 case NULL: 118 return null; 119 default: 120 return "?"; 121 } 122 } 123 124 public String getDisplay() { 125 switch (this) { 126 case PERSON: 127 return "Person"; 128 case ENTITY: 129 return "System"; 130 case NULL: 131 return null; 132 default: 133 return "?"; 134 } 135 } 136 } 137 138 public static class ExampleScenarioActorTypeEnumFactory implements EnumFactory<ExampleScenarioActorType> { 139 public ExampleScenarioActorType fromCode(String codeString) throws IllegalArgumentException { 140 if (codeString == null || "".equals(codeString)) 141 if (codeString == null || "".equals(codeString)) 142 return null; 143 if ("person".equals(codeString)) 144 return ExampleScenarioActorType.PERSON; 145 if ("entity".equals(codeString)) 146 return ExampleScenarioActorType.ENTITY; 147 throw new IllegalArgumentException("Unknown ExampleScenarioActorType code '" + codeString + "'"); 148 } 149 150 public Enumeration<ExampleScenarioActorType> fromType(PrimitiveType<?> code) throws FHIRException { 151 if (code == null) 152 return null; 153 if (code.isEmpty()) 154 return new Enumeration<ExampleScenarioActorType>(this, ExampleScenarioActorType.NULL, code); 155 String codeString = code.asStringValue(); 156 if (codeString == null || "".equals(codeString)) 157 return new Enumeration<ExampleScenarioActorType>(this, ExampleScenarioActorType.NULL, code); 158 if ("person".equals(codeString)) 159 return new Enumeration<ExampleScenarioActorType>(this, ExampleScenarioActorType.PERSON, code); 160 if ("entity".equals(codeString)) 161 return new Enumeration<ExampleScenarioActorType>(this, ExampleScenarioActorType.ENTITY, code); 162 throw new FHIRException("Unknown ExampleScenarioActorType code '" + codeString + "'"); 163 } 164 165 public String toCode(ExampleScenarioActorType code) { 166 if (code == ExampleScenarioActorType.PERSON) 167 return "person"; 168 if (code == ExampleScenarioActorType.ENTITY) 169 return "entity"; 170 return "?"; 171 } 172 173 public String toSystem(ExampleScenarioActorType code) { 174 return code.getSystem(); 175 } 176 } 177 178 public enum FHIRResourceType { 179 /** 180 * A financial tool for tracking value accrued for a particular purpose. In the 181 * healthcare field, used to track charges for a patient, cost centers, etc. 182 */ 183 ACCOUNT, 184 /** 185 * This resource allows for the definition of some activity to be performed, 186 * independent of a particular patient, practitioner, or other performance 187 * context. 188 */ 189 ACTIVITYDEFINITION, 190 /** 191 * Actual or potential/avoided event causing unintended physical injury 192 * resulting from or contributed to by medical care, a research study or other 193 * healthcare setting factors that requires additional monitoring, treatment, or 194 * hospitalization, or that results in death. 195 */ 196 ADVERSEEVENT, 197 /** 198 * Risk of harmful or undesirable, physiological response which is unique to an 199 * individual and associated with exposure to a substance. 200 */ 201 ALLERGYINTOLERANCE, 202 /** 203 * A booking of a healthcare event among patient(s), practitioner(s), related 204 * person(s) and/or device(s) for a specific date/time. This may result in one 205 * or more Encounter(s). 206 */ 207 APPOINTMENT, 208 /** 209 * A reply to an appointment request for a patient and/or practitioner(s), such 210 * as a confirmation or rejection. 211 */ 212 APPOINTMENTRESPONSE, 213 /** 214 * A record of an event made for purposes of maintaining a security log. Typical 215 * uses include detection of intrusion attempts and monitoring for inappropriate 216 * usage. 217 */ 218 AUDITEVENT, 219 /** 220 * Basic is used for handling concepts not yet defined in FHIR, narrative-only 221 * resources that don't map to an existing resource, and custom resources not 222 * appropriate for inclusion in the FHIR specification. 223 */ 224 BASIC, 225 /** 226 * A resource that represents the data of a single raw artifact as digital 227 * content accessible in its native format. A Binary resource can contain any 228 * content, whether text, image, pdf, zip archive, etc. 229 */ 230 BINARY, 231 /** 232 * A material substance originating from a biological entity intended to be 233 * transplanted or infused into another (possibly the same) biological entity. 234 */ 235 BIOLOGICALLYDERIVEDPRODUCT, 236 /** 237 * Record details about an anatomical structure. This resource may be used when 238 * a coded concept does not provide the necessary detail needed for the use 239 * case. 240 */ 241 BODYSTRUCTURE, 242 /** 243 * A container for a collection of resources. 244 */ 245 BUNDLE, 246 /** 247 * A Capability Statement documents a set of capabilities (behaviors) of a FHIR 248 * Server for a particular version of FHIR that may be used as a statement of 249 * actual server functionality or a statement of required or desired server 250 * implementation. 251 */ 252 CAPABILITYSTATEMENT, 253 /** 254 * Describes the intention of how one or more practitioners intend to deliver 255 * care for a particular patient, group or community for a period of time, 256 * possibly limited to care for a specific condition or set of conditions. 257 */ 258 CAREPLAN, 259 /** 260 * The Care Team includes all the people and organizations who plan to 261 * participate in the coordination and delivery of care for a patient. 262 */ 263 CARETEAM, 264 /** 265 * Catalog entries are wrappers that contextualize items included in a catalog. 266 */ 267 CATALOGENTRY, 268 /** 269 * The resource ChargeItem describes the provision of healthcare provider 270 * products for a certain patient, therefore referring not only to the product, 271 * but containing in addition details of the provision, like date, time, amounts 272 * and participating organizations and persons. Main Usage of the ChargeItem is 273 * to enable the billing process and internal cost allocation. 274 */ 275 CHARGEITEM, 276 /** 277 * The ChargeItemDefinition resource provides the properties that apply to the 278 * (billing) codes necessary to calculate costs and prices. The properties may 279 * differ largely depending on type and realm, therefore this resource gives 280 * only a rough structure and requires profiling for each type of billing code 281 * system. 282 */ 283 CHARGEITEMDEFINITION, 284 /** 285 * A provider issued list of professional services and products which have been 286 * provided, or are to be provided, to a patient which is sent to an insurer for 287 * reimbursement. 288 */ 289 CLAIM, 290 /** 291 * This resource provides the adjudication details from the processing of a 292 * Claim resource. 293 */ 294 CLAIMRESPONSE, 295 /** 296 * A record of a clinical assessment performed to determine what problem(s) may 297 * affect the patient and before planning the treatments or management 298 * strategies that are best to manage a patient's condition. Assessments are 299 * often 1:1 with a clinical consultation / encounter, but this varies greatly 300 * depending on the clinical workflow. This resource is called 301 * "ClinicalImpression" rather than "ClinicalAssessment" to avoid confusion with 302 * the recording of assessment tools such as Apgar score. 303 */ 304 CLINICALIMPRESSION, 305 /** 306 * The CodeSystem resource is used to declare the existence of and describe a 307 * code system or code system supplement and its key properties, and optionally 308 * define a part or all of its content. 309 */ 310 CODESYSTEM, 311 /** 312 * An occurrence of information being transmitted; e.g. an alert that was sent 313 * to a responsible provider, a public health agency that was notified about a 314 * reportable condition. 315 */ 316 COMMUNICATION, 317 /** 318 * A request to convey information; e.g. the CDS system proposes that an alert 319 * be sent to a responsible provider, the CDS system proposes that the public 320 * health agency be notified about a reportable condition. 321 */ 322 COMMUNICATIONREQUEST, 323 /** 324 * A compartment definition that defines how resources are accessed on a server. 325 */ 326 COMPARTMENTDEFINITION, 327 /** 328 * A set of healthcare-related information that is assembled together into a 329 * single logical package that provides a single coherent statement of meaning, 330 * establishes its own context and that has clinical attestation with regard to 331 * who is making the statement. A Composition defines the structure and 332 * narrative content necessary for a document. However, a Composition alone does 333 * not constitute a document. Rather, the Composition must be the first entry in 334 * a Bundle where Bundle.type=document, and any other resources referenced from 335 * Composition must be included as subsequent entries in the Bundle (for example 336 * Patient, Practitioner, Encounter, etc.). 337 */ 338 COMPOSITION, 339 /** 340 * A statement of relationships from one set of concepts to one or more other 341 * concepts - either concepts in code systems, or data element/data element 342 * concepts, or classes in class models. 343 */ 344 CONCEPTMAP, 345 /** 346 * A clinical condition, problem, diagnosis, or other event, situation, issue, 347 * or clinical concept that has risen to a level of concern. 348 */ 349 CONDITION, 350 /** 351 * A record of a healthcare consumer?s choices, which permits or denies 352 * identified recipient(s) or recipient role(s) to perform one or more actions 353 * within a given policy context, for specific purposes and periods of time. 354 */ 355 CONSENT, 356 /** 357 * Legally enforceable, formally recorded unilateral or bilateral directive 358 * i.e., a policy or agreement. 359 */ 360 CONTRACT, 361 /** 362 * Financial instrument which may be used to reimburse or pay for health care 363 * products and services. Includes both insurance and self-payment. 364 */ 365 COVERAGE, 366 /** 367 * The CoverageEligibilityRequest provides patient and insurance coverage 368 * information to an insurer for them to respond, in the form of an 369 * CoverageEligibilityResponse, with information regarding whether the stated 370 * coverage is valid and in-force and optionally to provide the insurance 371 * details of the policy. 372 */ 373 COVERAGEELIGIBILITYREQUEST, 374 /** 375 * This resource provides eligibility and plan details from the processing of an 376 * CoverageEligibilityRequest resource. 377 */ 378 COVERAGEELIGIBILITYRESPONSE, 379 /** 380 * Indicates an actual or potential clinical issue with or between one or more 381 * active or proposed clinical actions for a patient; e.g. Drug-drug 382 * interaction, Ineffective treatment frequency, Procedure-condition conflict, 383 * etc. 384 */ 385 DETECTEDISSUE, 386 /** 387 * A type of a manufactured item that is used in the provision of healthcare 388 * without being substantially changed through that activity. The device may be 389 * a medical or non-medical device. 390 */ 391 DEVICE, 392 /** 393 * The characteristics, operational status and capabilities of a medical-related 394 * component of a medical device. 395 */ 396 DEVICEDEFINITION, 397 /** 398 * Describes a measurement, calculation or setting capability of a medical 399 * device. 400 */ 401 DEVICEMETRIC, 402 /** 403 * Represents a request for a patient to employ a medical device. The device may 404 * be an implantable device, or an external assistive device, such as a walker. 405 */ 406 DEVICEREQUEST, 407 /** 408 * A record of a device being used by a patient where the record is the result 409 * of a report from the patient or another clinician. 410 */ 411 DEVICEUSESTATEMENT, 412 /** 413 * The findings and interpretation of diagnostic tests performed on patients, 414 * groups of patients, devices, and locations, and/or specimens derived from 415 * these. The report includes clinical context such as requesting and provider 416 * information, and some mix of atomic results, images, textual and coded 417 * interpretations, and formatted representation of diagnostic reports. 418 */ 419 DIAGNOSTICREPORT, 420 /** 421 * A collection of documents compiled for a purpose together with metadata that 422 * applies to the collection. 423 */ 424 DOCUMENTMANIFEST, 425 /** 426 * A reference to a document of any kind for any purpose. Provides metadata 427 * about the document so that the document can be discovered and managed. The 428 * scope of a document is any seralized object with a mime-type, so includes 429 * formal patient centric documents (CDA), cliical notes, scanned paper, and 430 * non-patient specific documents like policy text. 431 */ 432 DOCUMENTREFERENCE, 433 /** 434 * A resource that includes narrative, extensions, and contained resources. 435 */ 436 DOMAINRESOURCE, 437 /** 438 * The EffectEvidenceSynthesis resource describes the difference in an outcome 439 * between exposures states in a population where the effect estimate is derived 440 * from a combination of research studies. 441 */ 442 EFFECTEVIDENCESYNTHESIS, 443 /** 444 * An interaction between a patient and healthcare provider(s) for the purpose 445 * of providing healthcare service(s) or assessing the health status of a 446 * patient. 447 */ 448 ENCOUNTER, 449 /** 450 * The technical details of an endpoint that can be used for electronic 451 * services, such as for web services providing XDS.b or a REST endpoint for 452 * another FHIR server. This may include any security context information. 453 */ 454 ENDPOINT, 455 /** 456 * This resource provides the insurance enrollment details to the insurer 457 * regarding a specified coverage. 458 */ 459 ENROLLMENTREQUEST, 460 /** 461 * This resource provides enrollment and plan details from the processing of an 462 * EnrollmentRequest resource. 463 */ 464 ENROLLMENTRESPONSE, 465 /** 466 * An association between a patient and an organization / healthcare provider(s) 467 * during which time encounters may occur. The managing organization assumes a 468 * level of responsibility for the patient during this time. 469 */ 470 EPISODEOFCARE, 471 /** 472 * The EventDefinition resource provides a reusable description of when a 473 * particular event can occur. 474 */ 475 EVENTDEFINITION, 476 /** 477 * The Evidence resource describes the conditional state (population and any 478 * exposures being compared within the population) and outcome (if specified) 479 * that the knowledge (evidence, assertion, recommendation) is about. 480 */ 481 EVIDENCE, 482 /** 483 * The EvidenceVariable resource describes a "PICO" element that knowledge 484 * (evidence, assertion, recommendation) is about. 485 */ 486 EVIDENCEVARIABLE, 487 /** 488 * Example of workflow instance. 489 */ 490 EXAMPLESCENARIO, 491 /** 492 * This resource provides: the claim details; adjudication details from the 493 * processing of a Claim; and optionally account balance information, for 494 * informing the subscriber of the benefits provided. 495 */ 496 EXPLANATIONOFBENEFIT, 497 /** 498 * Significant health conditions for a person related to the patient relevant in 499 * the context of care for the patient. 500 */ 501 FAMILYMEMBERHISTORY, 502 /** 503 * Prospective warnings of potential issues when providing care to the patient. 504 */ 505 FLAG, 506 /** 507 * Describes the intended objective(s) for a patient, group or organization 508 * care, for example, weight loss, restoring an activity of daily living, 509 * obtaining herd immunity via immunization, meeting a process improvement 510 * objective, etc. 511 */ 512 GOAL, 513 /** 514 * A formal computable definition of a graph of resources - that is, a coherent 515 * set of resources that form a graph by following references. The Graph 516 * Definition resource defines a set and makes rules about the set. 517 */ 518 GRAPHDEFINITION, 519 /** 520 * Represents a defined collection of entities that may be discussed or acted 521 * upon collectively but which are not expected to act collectively, and are not 522 * formally or legally recognized; i.e. a collection of entities that isn't an 523 * Organization. 524 */ 525 GROUP, 526 /** 527 * A guidance response is the formal response to a guidance request, including 528 * any output parameters returned by the evaluation, as well as the description 529 * of any proposed actions to be taken. 530 */ 531 GUIDANCERESPONSE, 532 /** 533 * The details of a healthcare service available at a location. 534 */ 535 HEALTHCARESERVICE, 536 /** 537 * Representation of the content produced in a DICOM imaging study. A study 538 * comprises a set of series, each of which includes a set of Service-Object 539 * Pair Instances (SOP Instances - images or other data) acquired or produced in 540 * a common context. A series is of only one modality (e.g. X-ray, CT, MR, 541 * ultrasound), but a study may have multiple series of different modalities. 542 */ 543 IMAGINGSTUDY, 544 /** 545 * Describes the event of a patient being administered a vaccine or a record of 546 * an immunization as reported by a patient, a clinician or another party. 547 */ 548 IMMUNIZATION, 549 /** 550 * Describes a comparison of an immunization event against published 551 * recommendations to determine if the administration is "valid" in relation to 552 * those recommendations. 553 */ 554 IMMUNIZATIONEVALUATION, 555 /** 556 * A patient's point-in-time set of recommendations (i.e. forecasting) according 557 * to a published schedule with optional supporting justification. 558 */ 559 IMMUNIZATIONRECOMMENDATION, 560 /** 561 * A set of rules of how a particular interoperability or standards problem is 562 * solved - typically through the use of FHIR resources. This resource is used 563 * to gather all the parts of an implementation guide into a logical whole and 564 * to publish a computable definition of all the parts. 565 */ 566 IMPLEMENTATIONGUIDE, 567 /** 568 * Details of a Health Insurance product/plan provided by an organization. 569 */ 570 INSURANCEPLAN, 571 /** 572 * Invoice containing collected ChargeItems from an Account with calculated 573 * individual and total price for Billing purpose. 574 */ 575 INVOICE, 576 /** 577 * The Library resource is a general-purpose container for knowledge asset 578 * definitions. It can be used to describe and expose existing knowledge assets 579 * such as logic libraries and information model descriptions, as well as to 580 * describe a collection of knowledge assets. 581 */ 582 LIBRARY, 583 /** 584 * Identifies two or more records (resource instances) that refer to the same 585 * real-world "occurrence". 586 */ 587 LINKAGE, 588 /** 589 * A list is a curated collection of resources. 590 */ 591 LIST, 592 /** 593 * Details and position information for a physical place where services are 594 * provided and resources and participants may be stored, found, contained, or 595 * accommodated. 596 */ 597 LOCATION, 598 /** 599 * The Measure resource provides the definition of a quality measure. 600 */ 601 MEASURE, 602 /** 603 * The MeasureReport resource contains the results of the calculation of a 604 * measure; and optionally a reference to the resources involved in that 605 * calculation. 606 */ 607 MEASUREREPORT, 608 /** 609 * A photo, video, or audio recording acquired or used in healthcare. The actual 610 * content may be inline or provided by direct reference. 611 */ 612 MEDIA, 613 /** 614 * This resource is primarily used for the identification and definition of a 615 * medication for the purposes of prescribing, dispensing, and administering a 616 * medication as well as for making statements about medication use. 617 */ 618 MEDICATION, 619 /** 620 * Describes the event of a patient consuming or otherwise being administered a 621 * medication. This may be as simple as swallowing a tablet or it may be a long 622 * running infusion. Related resources tie this event to the authorizing 623 * prescription, and the specific encounter between patient and health care 624 * practitioner. 625 */ 626 MEDICATIONADMINISTRATION, 627 /** 628 * Indicates that a medication product is to be or has been dispensed for a 629 * named person/patient. This includes a description of the medication product 630 * (supply) provided and the instructions for administering the medication. The 631 * medication dispense is the result of a pharmacy system responding to a 632 * medication order. 633 */ 634 MEDICATIONDISPENSE, 635 /** 636 * Information about a medication that is used to support knowledge. 637 */ 638 MEDICATIONKNOWLEDGE, 639 /** 640 * An order or request for both supply of the medication and the instructions 641 * for administration of the medication to a patient. The resource is called 642 * "MedicationRequest" rather than "MedicationPrescription" or "MedicationOrder" 643 * to generalize the use across inpatient and outpatient settings, including 644 * care plans, etc., and to harmonize with workflow patterns. 645 */ 646 MEDICATIONREQUEST, 647 /** 648 * A record of a medication that is being consumed by a patient. A 649 * MedicationStatement may indicate that the patient may be taking the 650 * medication now or has taken the medication in the past or will be taking the 651 * medication in the future. The source of this information can be the patient, 652 * significant other (such as a family member or spouse), or a clinician. A 653 * common scenario where this information is captured is during the history 654 * taking process during a patient visit or stay. The medication information may 655 * come from sources such as the patient's memory, from a prescription bottle, 656 * or from a list of medications the patient, clinician or other party 657 * maintains. 658 * 659 * The primary difference between a medication statement and a medication 660 * administration is that the medication administration has complete 661 * administration information and is based on actual administration information 662 * from the person who administered the medication. A medication statement is 663 * often, if not always, less specific. There is no required date/time when the 664 * medication was administered, in fact we only know that a source has reported 665 * the patient is taking this medication, where details such as time, quantity, 666 * or rate or even medication product may be incomplete or missing or less 667 * precise. As stated earlier, the medication statement information may come 668 * from the patient's memory, from a prescription bottle or from a list of 669 * medications the patient, clinician or other party maintains. Medication 670 * administration is more formal and is not missing detailed information. 671 */ 672 MEDICATIONSTATEMENT, 673 /** 674 * Detailed definition of a medicinal product, typically for uses other than 675 * direct patient care (e.g. regulatory use). 676 */ 677 MEDICINALPRODUCT, 678 /** 679 * The regulatory authorization of a medicinal product. 680 */ 681 MEDICINALPRODUCTAUTHORIZATION, 682 /** 683 * The clinical particulars - indications, contraindications etc. of a medicinal 684 * product, including for regulatory purposes. 685 */ 686 MEDICINALPRODUCTCONTRAINDICATION, 687 /** 688 * Indication for the Medicinal Product. 689 */ 690 MEDICINALPRODUCTINDICATION, 691 /** 692 * An ingredient of a manufactured item or pharmaceutical product. 693 */ 694 MEDICINALPRODUCTINGREDIENT, 695 /** 696 * The interactions of the medicinal product with other medicinal products, or 697 * other forms of interactions. 698 */ 699 MEDICINALPRODUCTINTERACTION, 700 /** 701 * The manufactured item as contained in the packaged medicinal product. 702 */ 703 MEDICINALPRODUCTMANUFACTURED, 704 /** 705 * A medicinal product in a container or package. 706 */ 707 MEDICINALPRODUCTPACKAGED, 708 /** 709 * A pharmaceutical product described in terms of its composition and dose form. 710 */ 711 MEDICINALPRODUCTPHARMACEUTICAL, 712 /** 713 * Describe the undesirable effects of the medicinal product. 714 */ 715 MEDICINALPRODUCTUNDESIRABLEEFFECT, 716 /** 717 * Defines the characteristics of a message that can be shared between systems, 718 * including the type of event that initiates the message, the content to be 719 * transmitted and what response(s), if any, are permitted. 720 */ 721 MESSAGEDEFINITION, 722 /** 723 * The header for a message exchange that is either requesting or responding to 724 * an action. The reference(s) that are the subject of the action as well as 725 * other information related to the action are typically transmitted in a bundle 726 * in which the MessageHeader resource instance is the first resource in the 727 * bundle. 728 */ 729 MESSAGEHEADER, 730 /** 731 * Raw data describing a biological sequence. 732 */ 733 MOLECULARSEQUENCE, 734 /** 735 * A curated namespace that issues unique symbols within that namespace for the 736 * identification of concepts, people, devices, etc. Represents a "System" used 737 * within the Identifier and Coding data types. 738 */ 739 NAMINGSYSTEM, 740 /** 741 * A request to supply a diet, formula feeding (enteral) or oral nutritional 742 * supplement to a patient/resident. 743 */ 744 NUTRITIONORDER, 745 /** 746 * Measurements and simple assertions made about a patient, device or other 747 * subject. 748 */ 749 OBSERVATION, 750 /** 751 * Set of definitional characteristics for a kind of observation or measurement 752 * produced or consumed by an orderable health care service. 753 */ 754 OBSERVATIONDEFINITION, 755 /** 756 * A formal computable definition of an operation (on the RESTful interface) or 757 * a named query (using the search interaction). 758 */ 759 OPERATIONDEFINITION, 760 /** 761 * A collection of error, warning, or information messages that result from a 762 * system action. 763 */ 764 OPERATIONOUTCOME, 765 /** 766 * A formally or informally recognized grouping of people or organizations 767 * formed for the purpose of achieving some form of collective action. Includes 768 * companies, institutions, corporations, departments, community groups, 769 * healthcare practice groups, payer/insurer, etc. 770 */ 771 ORGANIZATION, 772 /** 773 * Defines an affiliation/assotiation/relationship between 2 distinct 774 * oganizations, that is not a part-of relationship/sub-division relationship. 775 */ 776 ORGANIZATIONAFFILIATION, 777 /** 778 * This resource is a non-persisted resource used to pass information into and 779 * back from an [operation](operations.html). It has no other use, and there is 780 * no RESTful endpoint associated with it. 781 */ 782 PARAMETERS, 783 /** 784 * Demographics and other administrative information about an individual or 785 * animal receiving care or other health-related services. 786 */ 787 PATIENT, 788 /** 789 * This resource provides the status of the payment for goods and services 790 * rendered, and the request and response resource references. 791 */ 792 PAYMENTNOTICE, 793 /** 794 * This resource provides the details including amount of a payment and 795 * allocates the payment items being paid. 796 */ 797 PAYMENTRECONCILIATION, 798 /** 799 * Demographics and administrative information about a person independent of a 800 * specific health-related context. 801 */ 802 PERSON, 803 /** 804 * This resource allows for the definition of various types of plans as a 805 * sharable, consumable, and executable artifact. The resource is general enough 806 * to support the description of a broad range of clinical artifacts such as 807 * clinical decision support rules, order sets and protocols. 808 */ 809 PLANDEFINITION, 810 /** 811 * A person who is directly or indirectly involved in the provisioning of 812 * healthcare. 813 */ 814 PRACTITIONER, 815 /** 816 * A specific set of Roles/Locations/specialties/services that a practitioner 817 * may perform at an organization for a period of time. 818 */ 819 PRACTITIONERROLE, 820 /** 821 * An action that is or was performed on or for a patient. This can be a 822 * physical intervention like an operation, or less invasive like long term 823 * services, counseling, or hypnotherapy. 824 */ 825 PROCEDURE, 826 /** 827 * Provenance of a resource is a record that describes entities and processes 828 * involved in producing and delivering or otherwise influencing that resource. 829 * Provenance provides a critical foundation for assessing authenticity, 830 * enabling trust, and allowing reproducibility. Provenance assertions are a 831 * form of contextual metadata and can themselves become important records with 832 * their own provenance. Provenance statement indicates clinical significance in 833 * terms of confidence in authenticity, reliability, and trustworthiness, 834 * integrity, and stage in lifecycle (e.g. Document Completion - has the 835 * artifact been legally authenticated), all of which may impact security, 836 * privacy, and trust policies. 837 */ 838 PROVENANCE, 839 /** 840 * A structured set of questions intended to guide the collection of answers 841 * from end-users. Questionnaires provide detailed control over order, 842 * presentation, phraseology and grouping to allow coherent, consistent data 843 * collection. 844 */ 845 QUESTIONNAIRE, 846 /** 847 * A structured set of questions and their answers. The questions are ordered 848 * and grouped into coherent subsets, corresponding to the structure of the 849 * grouping of the questionnaire being responded to. 850 */ 851 QUESTIONNAIRERESPONSE, 852 /** 853 * Information about a person that is involved in the care for a patient, but 854 * who is not the target of healthcare, nor has a formal responsibility in the 855 * care process. 856 */ 857 RELATEDPERSON, 858 /** 859 * A group of related requests that can be used to capture intended activities 860 * that have inter-dependencies such as "give this medication after that one". 861 */ 862 REQUESTGROUP, 863 /** 864 * The ResearchDefinition resource describes the conditional state (population 865 * and any exposures being compared within the population) and outcome (if 866 * specified) that the knowledge (evidence, assertion, recommendation) is about. 867 */ 868 RESEARCHDEFINITION, 869 /** 870 * The ResearchElementDefinition resource describes a "PICO" element that 871 * knowledge (evidence, assertion, recommendation) is about. 872 */ 873 RESEARCHELEMENTDEFINITION, 874 /** 875 * A process where a researcher or organization plans and then executes a series 876 * of steps intended to increase the field of healthcare-related knowledge. This 877 * includes studies of safety, efficacy, comparative effectiveness and other 878 * information about medications, devices, therapies and other interventional 879 * and investigative techniques. A ResearchStudy involves the gathering of 880 * information about human or animal subjects. 881 */ 882 RESEARCHSTUDY, 883 /** 884 * A physical entity which is the primary unit of operational and/or 885 * administrative interest in a study. 886 */ 887 RESEARCHSUBJECT, 888 /** 889 * This is the base resource type for everything. 890 */ 891 RESOURCE, 892 /** 893 * An assessment of the likely outcome(s) for a patient or other subject as well 894 * as the likelihood of each outcome. 895 */ 896 RISKASSESSMENT, 897 /** 898 * The RiskEvidenceSynthesis resource describes the likelihood of an outcome in 899 * a population plus exposure state where the risk estimate is derived from a 900 * combination of research studies. 901 */ 902 RISKEVIDENCESYNTHESIS, 903 /** 904 * A container for slots of time that may be available for booking appointments. 905 */ 906 SCHEDULE, 907 /** 908 * A search parameter that defines a named search item that can be used to 909 * search/filter on a resource. 910 */ 911 SEARCHPARAMETER, 912 /** 913 * A record of a request for service such as diagnostic investigations, 914 * treatments, or operations to be performed. 915 */ 916 SERVICEREQUEST, 917 /** 918 * A slot of time on a schedule that may be available for booking appointments. 919 */ 920 SLOT, 921 /** 922 * A sample to be used for analysis. 923 */ 924 SPECIMEN, 925 /** 926 * A kind of specimen with associated set of requirements. 927 */ 928 SPECIMENDEFINITION, 929 /** 930 * A definition of a FHIR structure. This resource is used to describe the 931 * underlying resources, data types defined in FHIR, and also for describing 932 * extensions and constraints on resources and data types. 933 */ 934 STRUCTUREDEFINITION, 935 /** 936 * A Map of relationships between 2 structures that can be used to transform 937 * data. 938 */ 939 STRUCTUREMAP, 940 /** 941 * The subscription resource is used to define a push-based subscription from a 942 * server to another system. Once a subscription is registered with the server, 943 * the server checks every resource that is created or updated, and if the 944 * resource matches the given criteria, it sends a message on the defined 945 * "channel" so that another system can take an appropriate action. 946 */ 947 SUBSCRIPTION, 948 /** 949 * A homogeneous material with a definite composition. 950 */ 951 SUBSTANCE, 952 /** 953 * Nucleic acids are defined by three distinct elements: the base, sugar and 954 * linkage. Individual substance/moiety IDs will be created for each of these 955 * elements. The nucleotide sequence will be always entered in the 5?-3? 956 * direction. 957 */ 958 SUBSTANCENUCLEICACID, 959 /** 960 * Todo. 961 */ 962 SUBSTANCEPOLYMER, 963 /** 964 * A SubstanceProtein is defined as a single unit of a linear amino acid 965 * sequence, or a combination of subunits that are either covalently linked or 966 * have a defined invariant stoichiometric relationship. This includes all 967 * synthetic, recombinant and purified SubstanceProteins of defined sequence, 968 * whether the use is therapeutic or prophylactic. This set of elements will be 969 * used to describe albumins, coagulation factors, cytokines, growth factors, 970 * peptide/SubstanceProtein hormones, enzymes, toxins, toxoids, recombinant 971 * vaccines, and immunomodulators. 972 */ 973 SUBSTANCEPROTEIN, 974 /** 975 * Todo. 976 */ 977 SUBSTANCEREFERENCEINFORMATION, 978 /** 979 * Source material shall capture information on the taxonomic and anatomical 980 * origins as well as the fraction of a material that can result in or can be 981 * modified to form a substance. This set of data elements shall be used to 982 * define polymer substances isolated from biological matrices. Taxonomic and 983 * anatomical origins shall be described using a controlled vocabulary as 984 * required. This information is captured for naturally derived polymers ( . 985 * starch) and structurally diverse substances. For Organisms belonging to the 986 * Kingdom Plantae the Substance level defines the fresh material of a single 987 * species or infraspecies, the Herbal Drug and the Herbal preparation. For 988 * Herbal preparations, the fraction information will be captured at the 989 * Substance information level and additional information for herbal extracts 990 * will be captured at the Specified Substance Group 1 information level. See 991 * for further explanation the Substance Class: Structurally Diverse and the 992 * herbal annex. 993 */ 994 SUBSTANCESOURCEMATERIAL, 995 /** 996 * The detailed description of a substance, typically at a level beyond what is 997 * used for prescribing. 998 */ 999 SUBSTANCESPECIFICATION, 1000 /** 1001 * Record of delivery of what is supplied. 1002 */ 1003 SUPPLYDELIVERY, 1004 /** 1005 * A record of a request for a medication, substance or device used in the 1006 * healthcare setting. 1007 */ 1008 SUPPLYREQUEST, 1009 /** 1010 * A task to be performed. 1011 */ 1012 TASK, 1013 /** 1014 * A TerminologyCapabilities resource documents a set of capabilities 1015 * (behaviors) of a FHIR Terminology Server that may be used as a statement of 1016 * actual server functionality or a statement of required or desired server 1017 * implementation. 1018 */ 1019 TERMINOLOGYCAPABILITIES, 1020 /** 1021 * A summary of information based on the results of executing a TestScript. 1022 */ 1023 TESTREPORT, 1024 /** 1025 * A structured set of tests against a FHIR server or client implementation to 1026 * determine compliance against the FHIR specification. 1027 */ 1028 TESTSCRIPT, 1029 /** 1030 * A ValueSet resource instance specifies a set of codes drawn from one or more 1031 * code systems, intended for use in a particular context. Value sets link 1032 * between [[[CodeSystem]]] definitions and their use in [coded 1033 * elements](terminologies.html). 1034 */ 1035 VALUESET, 1036 /** 1037 * Describes validation requirements, source(s), status and dates for one or 1038 * more elements. 1039 */ 1040 VERIFICATIONRESULT, 1041 /** 1042 * An authorization for the provision of glasses and/or contact lenses to a 1043 * patient. 1044 */ 1045 VISIONPRESCRIPTION, 1046 /** 1047 * added to help the parsers with the generic types 1048 */ 1049 NULL; 1050 1051 public static FHIRResourceType fromCode(String codeString) throws FHIRException { 1052 if (codeString == null || "".equals(codeString)) 1053 return null; 1054 if ("Account".equals(codeString)) 1055 return ACCOUNT; 1056 if ("ActivityDefinition".equals(codeString)) 1057 return ACTIVITYDEFINITION; 1058 if ("AdverseEvent".equals(codeString)) 1059 return ADVERSEEVENT; 1060 if ("AllergyIntolerance".equals(codeString)) 1061 return ALLERGYINTOLERANCE; 1062 if ("Appointment".equals(codeString)) 1063 return APPOINTMENT; 1064 if ("AppointmentResponse".equals(codeString)) 1065 return APPOINTMENTRESPONSE; 1066 if ("AuditEvent".equals(codeString)) 1067 return AUDITEVENT; 1068 if ("Basic".equals(codeString)) 1069 return BASIC; 1070 if ("Binary".equals(codeString)) 1071 return BINARY; 1072 if ("BiologicallyDerivedProduct".equals(codeString)) 1073 return BIOLOGICALLYDERIVEDPRODUCT; 1074 if ("BodyStructure".equals(codeString)) 1075 return BODYSTRUCTURE; 1076 if ("Bundle".equals(codeString)) 1077 return BUNDLE; 1078 if ("CapabilityStatement".equals(codeString)) 1079 return CAPABILITYSTATEMENT; 1080 if ("CarePlan".equals(codeString)) 1081 return CAREPLAN; 1082 if ("CareTeam".equals(codeString)) 1083 return CARETEAM; 1084 if ("CatalogEntry".equals(codeString)) 1085 return CATALOGENTRY; 1086 if ("ChargeItem".equals(codeString)) 1087 return CHARGEITEM; 1088 if ("ChargeItemDefinition".equals(codeString)) 1089 return CHARGEITEMDEFINITION; 1090 if ("Claim".equals(codeString)) 1091 return CLAIM; 1092 if ("ClaimResponse".equals(codeString)) 1093 return CLAIMRESPONSE; 1094 if ("ClinicalImpression".equals(codeString)) 1095 return CLINICALIMPRESSION; 1096 if ("CodeSystem".equals(codeString)) 1097 return CODESYSTEM; 1098 if ("Communication".equals(codeString)) 1099 return COMMUNICATION; 1100 if ("CommunicationRequest".equals(codeString)) 1101 return COMMUNICATIONREQUEST; 1102 if ("CompartmentDefinition".equals(codeString)) 1103 return COMPARTMENTDEFINITION; 1104 if ("Composition".equals(codeString)) 1105 return COMPOSITION; 1106 if ("ConceptMap".equals(codeString)) 1107 return CONCEPTMAP; 1108 if ("Condition".equals(codeString)) 1109 return CONDITION; 1110 if ("Consent".equals(codeString)) 1111 return CONSENT; 1112 if ("Contract".equals(codeString)) 1113 return CONTRACT; 1114 if ("Coverage".equals(codeString)) 1115 return COVERAGE; 1116 if ("CoverageEligibilityRequest".equals(codeString)) 1117 return COVERAGEELIGIBILITYREQUEST; 1118 if ("CoverageEligibilityResponse".equals(codeString)) 1119 return COVERAGEELIGIBILITYRESPONSE; 1120 if ("DetectedIssue".equals(codeString)) 1121 return DETECTEDISSUE; 1122 if ("Device".equals(codeString)) 1123 return DEVICE; 1124 if ("DeviceDefinition".equals(codeString)) 1125 return DEVICEDEFINITION; 1126 if ("DeviceMetric".equals(codeString)) 1127 return DEVICEMETRIC; 1128 if ("DeviceRequest".equals(codeString)) 1129 return DEVICEREQUEST; 1130 if ("DeviceUseStatement".equals(codeString)) 1131 return DEVICEUSESTATEMENT; 1132 if ("DiagnosticReport".equals(codeString)) 1133 return DIAGNOSTICREPORT; 1134 if ("DocumentManifest".equals(codeString)) 1135 return DOCUMENTMANIFEST; 1136 if ("DocumentReference".equals(codeString)) 1137 return DOCUMENTREFERENCE; 1138 if ("DomainResource".equals(codeString)) 1139 return DOMAINRESOURCE; 1140 if ("EffectEvidenceSynthesis".equals(codeString)) 1141 return EFFECTEVIDENCESYNTHESIS; 1142 if ("Encounter".equals(codeString)) 1143 return ENCOUNTER; 1144 if ("Endpoint".equals(codeString)) 1145 return ENDPOINT; 1146 if ("EnrollmentRequest".equals(codeString)) 1147 return ENROLLMENTREQUEST; 1148 if ("EnrollmentResponse".equals(codeString)) 1149 return ENROLLMENTRESPONSE; 1150 if ("EpisodeOfCare".equals(codeString)) 1151 return EPISODEOFCARE; 1152 if ("EventDefinition".equals(codeString)) 1153 return EVENTDEFINITION; 1154 if ("Evidence".equals(codeString)) 1155 return EVIDENCE; 1156 if ("EvidenceVariable".equals(codeString)) 1157 return EVIDENCEVARIABLE; 1158 if ("ExampleScenario".equals(codeString)) 1159 return EXAMPLESCENARIO; 1160 if ("ExplanationOfBenefit".equals(codeString)) 1161 return EXPLANATIONOFBENEFIT; 1162 if ("FamilyMemberHistory".equals(codeString)) 1163 return FAMILYMEMBERHISTORY; 1164 if ("Flag".equals(codeString)) 1165 return FLAG; 1166 if ("Goal".equals(codeString)) 1167 return GOAL; 1168 if ("GraphDefinition".equals(codeString)) 1169 return GRAPHDEFINITION; 1170 if ("Group".equals(codeString)) 1171 return GROUP; 1172 if ("GuidanceResponse".equals(codeString)) 1173 return GUIDANCERESPONSE; 1174 if ("HealthcareService".equals(codeString)) 1175 return HEALTHCARESERVICE; 1176 if ("ImagingStudy".equals(codeString)) 1177 return IMAGINGSTUDY; 1178 if ("Immunization".equals(codeString)) 1179 return IMMUNIZATION; 1180 if ("ImmunizationEvaluation".equals(codeString)) 1181 return IMMUNIZATIONEVALUATION; 1182 if ("ImmunizationRecommendation".equals(codeString)) 1183 return IMMUNIZATIONRECOMMENDATION; 1184 if ("ImplementationGuide".equals(codeString)) 1185 return IMPLEMENTATIONGUIDE; 1186 if ("InsurancePlan".equals(codeString)) 1187 return INSURANCEPLAN; 1188 if ("Invoice".equals(codeString)) 1189 return INVOICE; 1190 if ("Library".equals(codeString)) 1191 return LIBRARY; 1192 if ("Linkage".equals(codeString)) 1193 return LINKAGE; 1194 if ("List".equals(codeString)) 1195 return LIST; 1196 if ("Location".equals(codeString)) 1197 return LOCATION; 1198 if ("Measure".equals(codeString)) 1199 return MEASURE; 1200 if ("MeasureReport".equals(codeString)) 1201 return MEASUREREPORT; 1202 if ("Media".equals(codeString)) 1203 return MEDIA; 1204 if ("Medication".equals(codeString)) 1205 return MEDICATION; 1206 if ("MedicationAdministration".equals(codeString)) 1207 return MEDICATIONADMINISTRATION; 1208 if ("MedicationDispense".equals(codeString)) 1209 return MEDICATIONDISPENSE; 1210 if ("MedicationKnowledge".equals(codeString)) 1211 return MEDICATIONKNOWLEDGE; 1212 if ("MedicationRequest".equals(codeString)) 1213 return MEDICATIONREQUEST; 1214 if ("MedicationStatement".equals(codeString)) 1215 return MEDICATIONSTATEMENT; 1216 if ("MedicinalProduct".equals(codeString)) 1217 return MEDICINALPRODUCT; 1218 if ("MedicinalProductAuthorization".equals(codeString)) 1219 return MEDICINALPRODUCTAUTHORIZATION; 1220 if ("MedicinalProductContraindication".equals(codeString)) 1221 return MEDICINALPRODUCTCONTRAINDICATION; 1222 if ("MedicinalProductIndication".equals(codeString)) 1223 return MEDICINALPRODUCTINDICATION; 1224 if ("MedicinalProductIngredient".equals(codeString)) 1225 return MEDICINALPRODUCTINGREDIENT; 1226 if ("MedicinalProductInteraction".equals(codeString)) 1227 return MEDICINALPRODUCTINTERACTION; 1228 if ("MedicinalProductManufactured".equals(codeString)) 1229 return MEDICINALPRODUCTMANUFACTURED; 1230 if ("MedicinalProductPackaged".equals(codeString)) 1231 return MEDICINALPRODUCTPACKAGED; 1232 if ("MedicinalProductPharmaceutical".equals(codeString)) 1233 return MEDICINALPRODUCTPHARMACEUTICAL; 1234 if ("MedicinalProductUndesirableEffect".equals(codeString)) 1235 return MEDICINALPRODUCTUNDESIRABLEEFFECT; 1236 if ("MessageDefinition".equals(codeString)) 1237 return MESSAGEDEFINITION; 1238 if ("MessageHeader".equals(codeString)) 1239 return MESSAGEHEADER; 1240 if ("MolecularSequence".equals(codeString)) 1241 return MOLECULARSEQUENCE; 1242 if ("NamingSystem".equals(codeString)) 1243 return NAMINGSYSTEM; 1244 if ("NutritionOrder".equals(codeString)) 1245 return NUTRITIONORDER; 1246 if ("Observation".equals(codeString)) 1247 return OBSERVATION; 1248 if ("ObservationDefinition".equals(codeString)) 1249 return OBSERVATIONDEFINITION; 1250 if ("OperationDefinition".equals(codeString)) 1251 return OPERATIONDEFINITION; 1252 if ("OperationOutcome".equals(codeString)) 1253 return OPERATIONOUTCOME; 1254 if ("Organization".equals(codeString)) 1255 return ORGANIZATION; 1256 if ("OrganizationAffiliation".equals(codeString)) 1257 return ORGANIZATIONAFFILIATION; 1258 if ("Parameters".equals(codeString)) 1259 return PARAMETERS; 1260 if ("Patient".equals(codeString)) 1261 return PATIENT; 1262 if ("PaymentNotice".equals(codeString)) 1263 return PAYMENTNOTICE; 1264 if ("PaymentReconciliation".equals(codeString)) 1265 return PAYMENTRECONCILIATION; 1266 if ("Person".equals(codeString)) 1267 return PERSON; 1268 if ("PlanDefinition".equals(codeString)) 1269 return PLANDEFINITION; 1270 if ("Practitioner".equals(codeString)) 1271 return PRACTITIONER; 1272 if ("PractitionerRole".equals(codeString)) 1273 return PRACTITIONERROLE; 1274 if ("Procedure".equals(codeString)) 1275 return PROCEDURE; 1276 if ("Provenance".equals(codeString)) 1277 return PROVENANCE; 1278 if ("Questionnaire".equals(codeString)) 1279 return QUESTIONNAIRE; 1280 if ("QuestionnaireResponse".equals(codeString)) 1281 return QUESTIONNAIRERESPONSE; 1282 if ("RelatedPerson".equals(codeString)) 1283 return RELATEDPERSON; 1284 if ("RequestGroup".equals(codeString)) 1285 return REQUESTGROUP; 1286 if ("ResearchDefinition".equals(codeString)) 1287 return RESEARCHDEFINITION; 1288 if ("ResearchElementDefinition".equals(codeString)) 1289 return RESEARCHELEMENTDEFINITION; 1290 if ("ResearchStudy".equals(codeString)) 1291 return RESEARCHSTUDY; 1292 if ("ResearchSubject".equals(codeString)) 1293 return RESEARCHSUBJECT; 1294 if ("Resource".equals(codeString)) 1295 return RESOURCE; 1296 if ("RiskAssessment".equals(codeString)) 1297 return RISKASSESSMENT; 1298 if ("RiskEvidenceSynthesis".equals(codeString)) 1299 return RISKEVIDENCESYNTHESIS; 1300 if ("Schedule".equals(codeString)) 1301 return SCHEDULE; 1302 if ("SearchParameter".equals(codeString)) 1303 return SEARCHPARAMETER; 1304 if ("ServiceRequest".equals(codeString)) 1305 return SERVICEREQUEST; 1306 if ("Slot".equals(codeString)) 1307 return SLOT; 1308 if ("Specimen".equals(codeString)) 1309 return SPECIMEN; 1310 if ("SpecimenDefinition".equals(codeString)) 1311 return SPECIMENDEFINITION; 1312 if ("StructureDefinition".equals(codeString)) 1313 return STRUCTUREDEFINITION; 1314 if ("StructureMap".equals(codeString)) 1315 return STRUCTUREMAP; 1316 if ("Subscription".equals(codeString)) 1317 return SUBSCRIPTION; 1318 if ("Substance".equals(codeString)) 1319 return SUBSTANCE; 1320 if ("SubstanceNucleicAcid".equals(codeString)) 1321 return SUBSTANCENUCLEICACID; 1322 if ("SubstancePolymer".equals(codeString)) 1323 return SUBSTANCEPOLYMER; 1324 if ("SubstanceProtein".equals(codeString)) 1325 return SUBSTANCEPROTEIN; 1326 if ("SubstanceReferenceInformation".equals(codeString)) 1327 return SUBSTANCEREFERENCEINFORMATION; 1328 if ("SubstanceSourceMaterial".equals(codeString)) 1329 return SUBSTANCESOURCEMATERIAL; 1330 if ("SubstanceSpecification".equals(codeString)) 1331 return SUBSTANCESPECIFICATION; 1332 if ("SupplyDelivery".equals(codeString)) 1333 return SUPPLYDELIVERY; 1334 if ("SupplyRequest".equals(codeString)) 1335 return SUPPLYREQUEST; 1336 if ("Task".equals(codeString)) 1337 return TASK; 1338 if ("TerminologyCapabilities".equals(codeString)) 1339 return TERMINOLOGYCAPABILITIES; 1340 if ("TestReport".equals(codeString)) 1341 return TESTREPORT; 1342 if ("TestScript".equals(codeString)) 1343 return TESTSCRIPT; 1344 if ("ValueSet".equals(codeString)) 1345 return VALUESET; 1346 if ("VerificationResult".equals(codeString)) 1347 return VERIFICATIONRESULT; 1348 if ("VisionPrescription".equals(codeString)) 1349 return VISIONPRESCRIPTION; 1350 if (Configuration.isAcceptInvalidEnums()) 1351 return null; 1352 else 1353 throw new FHIRException("Unknown FHIRResourceType code '" + codeString + "'"); 1354 } 1355 1356 public String toCode() { 1357 switch (this) { 1358 case ACCOUNT: 1359 return "Account"; 1360 case ACTIVITYDEFINITION: 1361 return "ActivityDefinition"; 1362 case ADVERSEEVENT: 1363 return "AdverseEvent"; 1364 case ALLERGYINTOLERANCE: 1365 return "AllergyIntolerance"; 1366 case APPOINTMENT: 1367 return "Appointment"; 1368 case APPOINTMENTRESPONSE: 1369 return "AppointmentResponse"; 1370 case AUDITEVENT: 1371 return "AuditEvent"; 1372 case BASIC: 1373 return "Basic"; 1374 case BINARY: 1375 return "Binary"; 1376 case BIOLOGICALLYDERIVEDPRODUCT: 1377 return "BiologicallyDerivedProduct"; 1378 case BODYSTRUCTURE: 1379 return "BodyStructure"; 1380 case BUNDLE: 1381 return "Bundle"; 1382 case CAPABILITYSTATEMENT: 1383 return "CapabilityStatement"; 1384 case CAREPLAN: 1385 return "CarePlan"; 1386 case CARETEAM: 1387 return "CareTeam"; 1388 case CATALOGENTRY: 1389 return "CatalogEntry"; 1390 case CHARGEITEM: 1391 return "ChargeItem"; 1392 case CHARGEITEMDEFINITION: 1393 return "ChargeItemDefinition"; 1394 case CLAIM: 1395 return "Claim"; 1396 case CLAIMRESPONSE: 1397 return "ClaimResponse"; 1398 case CLINICALIMPRESSION: 1399 return "ClinicalImpression"; 1400 case CODESYSTEM: 1401 return "CodeSystem"; 1402 case COMMUNICATION: 1403 return "Communication"; 1404 case COMMUNICATIONREQUEST: 1405 return "CommunicationRequest"; 1406 case COMPARTMENTDEFINITION: 1407 return "CompartmentDefinition"; 1408 case COMPOSITION: 1409 return "Composition"; 1410 case CONCEPTMAP: 1411 return "ConceptMap"; 1412 case CONDITION: 1413 return "Condition"; 1414 case CONSENT: 1415 return "Consent"; 1416 case CONTRACT: 1417 return "Contract"; 1418 case COVERAGE: 1419 return "Coverage"; 1420 case COVERAGEELIGIBILITYREQUEST: 1421 return "CoverageEligibilityRequest"; 1422 case COVERAGEELIGIBILITYRESPONSE: 1423 return "CoverageEligibilityResponse"; 1424 case DETECTEDISSUE: 1425 return "DetectedIssue"; 1426 case DEVICE: 1427 return "Device"; 1428 case DEVICEDEFINITION: 1429 return "DeviceDefinition"; 1430 case DEVICEMETRIC: 1431 return "DeviceMetric"; 1432 case DEVICEREQUEST: 1433 return "DeviceRequest"; 1434 case DEVICEUSESTATEMENT: 1435 return "DeviceUseStatement"; 1436 case DIAGNOSTICREPORT: 1437 return "DiagnosticReport"; 1438 case DOCUMENTMANIFEST: 1439 return "DocumentManifest"; 1440 case DOCUMENTREFERENCE: 1441 return "DocumentReference"; 1442 case DOMAINRESOURCE: 1443 return "DomainResource"; 1444 case EFFECTEVIDENCESYNTHESIS: 1445 return "EffectEvidenceSynthesis"; 1446 case ENCOUNTER: 1447 return "Encounter"; 1448 case ENDPOINT: 1449 return "Endpoint"; 1450 case ENROLLMENTREQUEST: 1451 return "EnrollmentRequest"; 1452 case ENROLLMENTRESPONSE: 1453 return "EnrollmentResponse"; 1454 case EPISODEOFCARE: 1455 return "EpisodeOfCare"; 1456 case EVENTDEFINITION: 1457 return "EventDefinition"; 1458 case EVIDENCE: 1459 return "Evidence"; 1460 case EVIDENCEVARIABLE: 1461 return "EvidenceVariable"; 1462 case EXAMPLESCENARIO: 1463 return "ExampleScenario"; 1464 case EXPLANATIONOFBENEFIT: 1465 return "ExplanationOfBenefit"; 1466 case FAMILYMEMBERHISTORY: 1467 return "FamilyMemberHistory"; 1468 case FLAG: 1469 return "Flag"; 1470 case GOAL: 1471 return "Goal"; 1472 case GRAPHDEFINITION: 1473 return "GraphDefinition"; 1474 case GROUP: 1475 return "Group"; 1476 case GUIDANCERESPONSE: 1477 return "GuidanceResponse"; 1478 case HEALTHCARESERVICE: 1479 return "HealthcareService"; 1480 case IMAGINGSTUDY: 1481 return "ImagingStudy"; 1482 case IMMUNIZATION: 1483 return "Immunization"; 1484 case IMMUNIZATIONEVALUATION: 1485 return "ImmunizationEvaluation"; 1486 case IMMUNIZATIONRECOMMENDATION: 1487 return "ImmunizationRecommendation"; 1488 case IMPLEMENTATIONGUIDE: 1489 return "ImplementationGuide"; 1490 case INSURANCEPLAN: 1491 return "InsurancePlan"; 1492 case INVOICE: 1493 return "Invoice"; 1494 case LIBRARY: 1495 return "Library"; 1496 case LINKAGE: 1497 return "Linkage"; 1498 case LIST: 1499 return "List"; 1500 case LOCATION: 1501 return "Location"; 1502 case MEASURE: 1503 return "Measure"; 1504 case MEASUREREPORT: 1505 return "MeasureReport"; 1506 case MEDIA: 1507 return "Media"; 1508 case MEDICATION: 1509 return "Medication"; 1510 case MEDICATIONADMINISTRATION: 1511 return "MedicationAdministration"; 1512 case MEDICATIONDISPENSE: 1513 return "MedicationDispense"; 1514 case MEDICATIONKNOWLEDGE: 1515 return "MedicationKnowledge"; 1516 case MEDICATIONREQUEST: 1517 return "MedicationRequest"; 1518 case MEDICATIONSTATEMENT: 1519 return "MedicationStatement"; 1520 case MEDICINALPRODUCT: 1521 return "MedicinalProduct"; 1522 case MEDICINALPRODUCTAUTHORIZATION: 1523 return "MedicinalProductAuthorization"; 1524 case MEDICINALPRODUCTCONTRAINDICATION: 1525 return "MedicinalProductContraindication"; 1526 case MEDICINALPRODUCTINDICATION: 1527 return "MedicinalProductIndication"; 1528 case MEDICINALPRODUCTINGREDIENT: 1529 return "MedicinalProductIngredient"; 1530 case MEDICINALPRODUCTINTERACTION: 1531 return "MedicinalProductInteraction"; 1532 case MEDICINALPRODUCTMANUFACTURED: 1533 return "MedicinalProductManufactured"; 1534 case MEDICINALPRODUCTPACKAGED: 1535 return "MedicinalProductPackaged"; 1536 case MEDICINALPRODUCTPHARMACEUTICAL: 1537 return "MedicinalProductPharmaceutical"; 1538 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 1539 return "MedicinalProductUndesirableEffect"; 1540 case MESSAGEDEFINITION: 1541 return "MessageDefinition"; 1542 case MESSAGEHEADER: 1543 return "MessageHeader"; 1544 case MOLECULARSEQUENCE: 1545 return "MolecularSequence"; 1546 case NAMINGSYSTEM: 1547 return "NamingSystem"; 1548 case NUTRITIONORDER: 1549 return "NutritionOrder"; 1550 case OBSERVATION: 1551 return "Observation"; 1552 case OBSERVATIONDEFINITION: 1553 return "ObservationDefinition"; 1554 case OPERATIONDEFINITION: 1555 return "OperationDefinition"; 1556 case OPERATIONOUTCOME: 1557 return "OperationOutcome"; 1558 case ORGANIZATION: 1559 return "Organization"; 1560 case ORGANIZATIONAFFILIATION: 1561 return "OrganizationAffiliation"; 1562 case PARAMETERS: 1563 return "Parameters"; 1564 case PATIENT: 1565 return "Patient"; 1566 case PAYMENTNOTICE: 1567 return "PaymentNotice"; 1568 case PAYMENTRECONCILIATION: 1569 return "PaymentReconciliation"; 1570 case PERSON: 1571 return "Person"; 1572 case PLANDEFINITION: 1573 return "PlanDefinition"; 1574 case PRACTITIONER: 1575 return "Practitioner"; 1576 case PRACTITIONERROLE: 1577 return "PractitionerRole"; 1578 case PROCEDURE: 1579 return "Procedure"; 1580 case PROVENANCE: 1581 return "Provenance"; 1582 case QUESTIONNAIRE: 1583 return "Questionnaire"; 1584 case QUESTIONNAIRERESPONSE: 1585 return "QuestionnaireResponse"; 1586 case RELATEDPERSON: 1587 return "RelatedPerson"; 1588 case REQUESTGROUP: 1589 return "RequestGroup"; 1590 case RESEARCHDEFINITION: 1591 return "ResearchDefinition"; 1592 case RESEARCHELEMENTDEFINITION: 1593 return "ResearchElementDefinition"; 1594 case RESEARCHSTUDY: 1595 return "ResearchStudy"; 1596 case RESEARCHSUBJECT: 1597 return "ResearchSubject"; 1598 case RESOURCE: 1599 return "Resource"; 1600 case RISKASSESSMENT: 1601 return "RiskAssessment"; 1602 case RISKEVIDENCESYNTHESIS: 1603 return "RiskEvidenceSynthesis"; 1604 case SCHEDULE: 1605 return "Schedule"; 1606 case SEARCHPARAMETER: 1607 return "SearchParameter"; 1608 case SERVICEREQUEST: 1609 return "ServiceRequest"; 1610 case SLOT: 1611 return "Slot"; 1612 case SPECIMEN: 1613 return "Specimen"; 1614 case SPECIMENDEFINITION: 1615 return "SpecimenDefinition"; 1616 case STRUCTUREDEFINITION: 1617 return "StructureDefinition"; 1618 case STRUCTUREMAP: 1619 return "StructureMap"; 1620 case SUBSCRIPTION: 1621 return "Subscription"; 1622 case SUBSTANCE: 1623 return "Substance"; 1624 case SUBSTANCENUCLEICACID: 1625 return "SubstanceNucleicAcid"; 1626 case SUBSTANCEPOLYMER: 1627 return "SubstancePolymer"; 1628 case SUBSTANCEPROTEIN: 1629 return "SubstanceProtein"; 1630 case SUBSTANCEREFERENCEINFORMATION: 1631 return "SubstanceReferenceInformation"; 1632 case SUBSTANCESOURCEMATERIAL: 1633 return "SubstanceSourceMaterial"; 1634 case SUBSTANCESPECIFICATION: 1635 return "SubstanceSpecification"; 1636 case SUPPLYDELIVERY: 1637 return "SupplyDelivery"; 1638 case SUPPLYREQUEST: 1639 return "SupplyRequest"; 1640 case TASK: 1641 return "Task"; 1642 case TERMINOLOGYCAPABILITIES: 1643 return "TerminologyCapabilities"; 1644 case TESTREPORT: 1645 return "TestReport"; 1646 case TESTSCRIPT: 1647 return "TestScript"; 1648 case VALUESET: 1649 return "ValueSet"; 1650 case VERIFICATIONRESULT: 1651 return "VerificationResult"; 1652 case VISIONPRESCRIPTION: 1653 return "VisionPrescription"; 1654 case NULL: 1655 return null; 1656 default: 1657 return "?"; 1658 } 1659 } 1660 1661 public String getSystem() { 1662 switch (this) { 1663 case ACCOUNT: 1664 return "http://hl7.org/fhir/resource-types"; 1665 case ACTIVITYDEFINITION: 1666 return "http://hl7.org/fhir/resource-types"; 1667 case ADVERSEEVENT: 1668 return "http://hl7.org/fhir/resource-types"; 1669 case ALLERGYINTOLERANCE: 1670 return "http://hl7.org/fhir/resource-types"; 1671 case APPOINTMENT: 1672 return "http://hl7.org/fhir/resource-types"; 1673 case APPOINTMENTRESPONSE: 1674 return "http://hl7.org/fhir/resource-types"; 1675 case AUDITEVENT: 1676 return "http://hl7.org/fhir/resource-types"; 1677 case BASIC: 1678 return "http://hl7.org/fhir/resource-types"; 1679 case BINARY: 1680 return "http://hl7.org/fhir/resource-types"; 1681 case BIOLOGICALLYDERIVEDPRODUCT: 1682 return "http://hl7.org/fhir/resource-types"; 1683 case BODYSTRUCTURE: 1684 return "http://hl7.org/fhir/resource-types"; 1685 case BUNDLE: 1686 return "http://hl7.org/fhir/resource-types"; 1687 case CAPABILITYSTATEMENT: 1688 return "http://hl7.org/fhir/resource-types"; 1689 case CAREPLAN: 1690 return "http://hl7.org/fhir/resource-types"; 1691 case CARETEAM: 1692 return "http://hl7.org/fhir/resource-types"; 1693 case CATALOGENTRY: 1694 return "http://hl7.org/fhir/resource-types"; 1695 case CHARGEITEM: 1696 return "http://hl7.org/fhir/resource-types"; 1697 case CHARGEITEMDEFINITION: 1698 return "http://hl7.org/fhir/resource-types"; 1699 case CLAIM: 1700 return "http://hl7.org/fhir/resource-types"; 1701 case CLAIMRESPONSE: 1702 return "http://hl7.org/fhir/resource-types"; 1703 case CLINICALIMPRESSION: 1704 return "http://hl7.org/fhir/resource-types"; 1705 case CODESYSTEM: 1706 return "http://hl7.org/fhir/resource-types"; 1707 case COMMUNICATION: 1708 return "http://hl7.org/fhir/resource-types"; 1709 case COMMUNICATIONREQUEST: 1710 return "http://hl7.org/fhir/resource-types"; 1711 case COMPARTMENTDEFINITION: 1712 return "http://hl7.org/fhir/resource-types"; 1713 case COMPOSITION: 1714 return "http://hl7.org/fhir/resource-types"; 1715 case CONCEPTMAP: 1716 return "http://hl7.org/fhir/resource-types"; 1717 case CONDITION: 1718 return "http://hl7.org/fhir/resource-types"; 1719 case CONSENT: 1720 return "http://hl7.org/fhir/resource-types"; 1721 case CONTRACT: 1722 return "http://hl7.org/fhir/resource-types"; 1723 case COVERAGE: 1724 return "http://hl7.org/fhir/resource-types"; 1725 case COVERAGEELIGIBILITYREQUEST: 1726 return "http://hl7.org/fhir/resource-types"; 1727 case COVERAGEELIGIBILITYRESPONSE: 1728 return "http://hl7.org/fhir/resource-types"; 1729 case DETECTEDISSUE: 1730 return "http://hl7.org/fhir/resource-types"; 1731 case DEVICE: 1732 return "http://hl7.org/fhir/resource-types"; 1733 case DEVICEDEFINITION: 1734 return "http://hl7.org/fhir/resource-types"; 1735 case DEVICEMETRIC: 1736 return "http://hl7.org/fhir/resource-types"; 1737 case DEVICEREQUEST: 1738 return "http://hl7.org/fhir/resource-types"; 1739 case DEVICEUSESTATEMENT: 1740 return "http://hl7.org/fhir/resource-types"; 1741 case DIAGNOSTICREPORT: 1742 return "http://hl7.org/fhir/resource-types"; 1743 case DOCUMENTMANIFEST: 1744 return "http://hl7.org/fhir/resource-types"; 1745 case DOCUMENTREFERENCE: 1746 return "http://hl7.org/fhir/resource-types"; 1747 case DOMAINRESOURCE: 1748 return "http://hl7.org/fhir/resource-types"; 1749 case EFFECTEVIDENCESYNTHESIS: 1750 return "http://hl7.org/fhir/resource-types"; 1751 case ENCOUNTER: 1752 return "http://hl7.org/fhir/resource-types"; 1753 case ENDPOINT: 1754 return "http://hl7.org/fhir/resource-types"; 1755 case ENROLLMENTREQUEST: 1756 return "http://hl7.org/fhir/resource-types"; 1757 case ENROLLMENTRESPONSE: 1758 return "http://hl7.org/fhir/resource-types"; 1759 case EPISODEOFCARE: 1760 return "http://hl7.org/fhir/resource-types"; 1761 case EVENTDEFINITION: 1762 return "http://hl7.org/fhir/resource-types"; 1763 case EVIDENCE: 1764 return "http://hl7.org/fhir/resource-types"; 1765 case EVIDENCEVARIABLE: 1766 return "http://hl7.org/fhir/resource-types"; 1767 case EXAMPLESCENARIO: 1768 return "http://hl7.org/fhir/resource-types"; 1769 case EXPLANATIONOFBENEFIT: 1770 return "http://hl7.org/fhir/resource-types"; 1771 case FAMILYMEMBERHISTORY: 1772 return "http://hl7.org/fhir/resource-types"; 1773 case FLAG: 1774 return "http://hl7.org/fhir/resource-types"; 1775 case GOAL: 1776 return "http://hl7.org/fhir/resource-types"; 1777 case GRAPHDEFINITION: 1778 return "http://hl7.org/fhir/resource-types"; 1779 case GROUP: 1780 return "http://hl7.org/fhir/resource-types"; 1781 case GUIDANCERESPONSE: 1782 return "http://hl7.org/fhir/resource-types"; 1783 case HEALTHCARESERVICE: 1784 return "http://hl7.org/fhir/resource-types"; 1785 case IMAGINGSTUDY: 1786 return "http://hl7.org/fhir/resource-types"; 1787 case IMMUNIZATION: 1788 return "http://hl7.org/fhir/resource-types"; 1789 case IMMUNIZATIONEVALUATION: 1790 return "http://hl7.org/fhir/resource-types"; 1791 case IMMUNIZATIONRECOMMENDATION: 1792 return "http://hl7.org/fhir/resource-types"; 1793 case IMPLEMENTATIONGUIDE: 1794 return "http://hl7.org/fhir/resource-types"; 1795 case INSURANCEPLAN: 1796 return "http://hl7.org/fhir/resource-types"; 1797 case INVOICE: 1798 return "http://hl7.org/fhir/resource-types"; 1799 case LIBRARY: 1800 return "http://hl7.org/fhir/resource-types"; 1801 case LINKAGE: 1802 return "http://hl7.org/fhir/resource-types"; 1803 case LIST: 1804 return "http://hl7.org/fhir/resource-types"; 1805 case LOCATION: 1806 return "http://hl7.org/fhir/resource-types"; 1807 case MEASURE: 1808 return "http://hl7.org/fhir/resource-types"; 1809 case MEASUREREPORT: 1810 return "http://hl7.org/fhir/resource-types"; 1811 case MEDIA: 1812 return "http://hl7.org/fhir/resource-types"; 1813 case MEDICATION: 1814 return "http://hl7.org/fhir/resource-types"; 1815 case MEDICATIONADMINISTRATION: 1816 return "http://hl7.org/fhir/resource-types"; 1817 case MEDICATIONDISPENSE: 1818 return "http://hl7.org/fhir/resource-types"; 1819 case MEDICATIONKNOWLEDGE: 1820 return "http://hl7.org/fhir/resource-types"; 1821 case MEDICATIONREQUEST: 1822 return "http://hl7.org/fhir/resource-types"; 1823 case MEDICATIONSTATEMENT: 1824 return "http://hl7.org/fhir/resource-types"; 1825 case MEDICINALPRODUCT: 1826 return "http://hl7.org/fhir/resource-types"; 1827 case MEDICINALPRODUCTAUTHORIZATION: 1828 return "http://hl7.org/fhir/resource-types"; 1829 case MEDICINALPRODUCTCONTRAINDICATION: 1830 return "http://hl7.org/fhir/resource-types"; 1831 case MEDICINALPRODUCTINDICATION: 1832 return "http://hl7.org/fhir/resource-types"; 1833 case MEDICINALPRODUCTINGREDIENT: 1834 return "http://hl7.org/fhir/resource-types"; 1835 case MEDICINALPRODUCTINTERACTION: 1836 return "http://hl7.org/fhir/resource-types"; 1837 case MEDICINALPRODUCTMANUFACTURED: 1838 return "http://hl7.org/fhir/resource-types"; 1839 case MEDICINALPRODUCTPACKAGED: 1840 return "http://hl7.org/fhir/resource-types"; 1841 case MEDICINALPRODUCTPHARMACEUTICAL: 1842 return "http://hl7.org/fhir/resource-types"; 1843 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 1844 return "http://hl7.org/fhir/resource-types"; 1845 case MESSAGEDEFINITION: 1846 return "http://hl7.org/fhir/resource-types"; 1847 case MESSAGEHEADER: 1848 return "http://hl7.org/fhir/resource-types"; 1849 case MOLECULARSEQUENCE: 1850 return "http://hl7.org/fhir/resource-types"; 1851 case NAMINGSYSTEM: 1852 return "http://hl7.org/fhir/resource-types"; 1853 case NUTRITIONORDER: 1854 return "http://hl7.org/fhir/resource-types"; 1855 case OBSERVATION: 1856 return "http://hl7.org/fhir/resource-types"; 1857 case OBSERVATIONDEFINITION: 1858 return "http://hl7.org/fhir/resource-types"; 1859 case OPERATIONDEFINITION: 1860 return "http://hl7.org/fhir/resource-types"; 1861 case OPERATIONOUTCOME: 1862 return "http://hl7.org/fhir/resource-types"; 1863 case ORGANIZATION: 1864 return "http://hl7.org/fhir/resource-types"; 1865 case ORGANIZATIONAFFILIATION: 1866 return "http://hl7.org/fhir/resource-types"; 1867 case PARAMETERS: 1868 return "http://hl7.org/fhir/resource-types"; 1869 case PATIENT: 1870 return "http://hl7.org/fhir/resource-types"; 1871 case PAYMENTNOTICE: 1872 return "http://hl7.org/fhir/resource-types"; 1873 case PAYMENTRECONCILIATION: 1874 return "http://hl7.org/fhir/resource-types"; 1875 case PERSON: 1876 return "http://hl7.org/fhir/resource-types"; 1877 case PLANDEFINITION: 1878 return "http://hl7.org/fhir/resource-types"; 1879 case PRACTITIONER: 1880 return "http://hl7.org/fhir/resource-types"; 1881 case PRACTITIONERROLE: 1882 return "http://hl7.org/fhir/resource-types"; 1883 case PROCEDURE: 1884 return "http://hl7.org/fhir/resource-types"; 1885 case PROVENANCE: 1886 return "http://hl7.org/fhir/resource-types"; 1887 case QUESTIONNAIRE: 1888 return "http://hl7.org/fhir/resource-types"; 1889 case QUESTIONNAIRERESPONSE: 1890 return "http://hl7.org/fhir/resource-types"; 1891 case RELATEDPERSON: 1892 return "http://hl7.org/fhir/resource-types"; 1893 case REQUESTGROUP: 1894 return "http://hl7.org/fhir/resource-types"; 1895 case RESEARCHDEFINITION: 1896 return "http://hl7.org/fhir/resource-types"; 1897 case RESEARCHELEMENTDEFINITION: 1898 return "http://hl7.org/fhir/resource-types"; 1899 case RESEARCHSTUDY: 1900 return "http://hl7.org/fhir/resource-types"; 1901 case RESEARCHSUBJECT: 1902 return "http://hl7.org/fhir/resource-types"; 1903 case RESOURCE: 1904 return "http://hl7.org/fhir/resource-types"; 1905 case RISKASSESSMENT: 1906 return "http://hl7.org/fhir/resource-types"; 1907 case RISKEVIDENCESYNTHESIS: 1908 return "http://hl7.org/fhir/resource-types"; 1909 case SCHEDULE: 1910 return "http://hl7.org/fhir/resource-types"; 1911 case SEARCHPARAMETER: 1912 return "http://hl7.org/fhir/resource-types"; 1913 case SERVICEREQUEST: 1914 return "http://hl7.org/fhir/resource-types"; 1915 case SLOT: 1916 return "http://hl7.org/fhir/resource-types"; 1917 case SPECIMEN: 1918 return "http://hl7.org/fhir/resource-types"; 1919 case SPECIMENDEFINITION: 1920 return "http://hl7.org/fhir/resource-types"; 1921 case STRUCTUREDEFINITION: 1922 return "http://hl7.org/fhir/resource-types"; 1923 case STRUCTUREMAP: 1924 return "http://hl7.org/fhir/resource-types"; 1925 case SUBSCRIPTION: 1926 return "http://hl7.org/fhir/resource-types"; 1927 case SUBSTANCE: 1928 return "http://hl7.org/fhir/resource-types"; 1929 case SUBSTANCENUCLEICACID: 1930 return "http://hl7.org/fhir/resource-types"; 1931 case SUBSTANCEPOLYMER: 1932 return "http://hl7.org/fhir/resource-types"; 1933 case SUBSTANCEPROTEIN: 1934 return "http://hl7.org/fhir/resource-types"; 1935 case SUBSTANCEREFERENCEINFORMATION: 1936 return "http://hl7.org/fhir/resource-types"; 1937 case SUBSTANCESOURCEMATERIAL: 1938 return "http://hl7.org/fhir/resource-types"; 1939 case SUBSTANCESPECIFICATION: 1940 return "http://hl7.org/fhir/resource-types"; 1941 case SUPPLYDELIVERY: 1942 return "http://hl7.org/fhir/resource-types"; 1943 case SUPPLYREQUEST: 1944 return "http://hl7.org/fhir/resource-types"; 1945 case TASK: 1946 return "http://hl7.org/fhir/resource-types"; 1947 case TERMINOLOGYCAPABILITIES: 1948 return "http://hl7.org/fhir/resource-types"; 1949 case TESTREPORT: 1950 return "http://hl7.org/fhir/resource-types"; 1951 case TESTSCRIPT: 1952 return "http://hl7.org/fhir/resource-types"; 1953 case VALUESET: 1954 return "http://hl7.org/fhir/resource-types"; 1955 case VERIFICATIONRESULT: 1956 return "http://hl7.org/fhir/resource-types"; 1957 case VISIONPRESCRIPTION: 1958 return "http://hl7.org/fhir/resource-types"; 1959 case NULL: 1960 return null; 1961 default: 1962 return "?"; 1963 } 1964 } 1965 1966 public String getDefinition() { 1967 switch (this) { 1968 case ACCOUNT: 1969 return "A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centers, etc."; 1970 case ACTIVITYDEFINITION: 1971 return "This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context."; 1972 case ADVERSEEVENT: 1973 return "Actual or potential/avoided event causing unintended physical injury resulting from or contributed to by medical care, a research study or other healthcare setting factors that requires additional monitoring, treatment, or hospitalization, or that results in death."; 1974 case ALLERGYINTOLERANCE: 1975 return "Risk of harmful or undesirable, physiological response which is unique to an individual and associated with exposure to a substance."; 1976 case APPOINTMENT: 1977 return "A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s)."; 1978 case APPOINTMENTRESPONSE: 1979 return "A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection."; 1980 case AUDITEVENT: 1981 return "A record of an event made for purposes of maintaining a security log. Typical uses include detection of intrusion attempts and monitoring for inappropriate usage."; 1982 case BASIC: 1983 return "Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification."; 1984 case BINARY: 1985 return "A resource that represents the data of a single raw artifact as digital content accessible in its native format. A Binary resource can contain any content, whether text, image, pdf, zip archive, etc."; 1986 case BIOLOGICALLYDERIVEDPRODUCT: 1987 return "A material substance originating from a biological entity intended to be transplanted or infused\ninto another (possibly the same) biological entity."; 1988 case BODYSTRUCTURE: 1989 return "Record details about an anatomical structure. This resource may be used when a coded concept does not provide the necessary detail needed for the use case."; 1990 case BUNDLE: 1991 return "A container for a collection of resources."; 1992 case CAPABILITYSTATEMENT: 1993 return "A Capability Statement documents a set of capabilities (behaviors) of a FHIR Server for a particular version of FHIR that may be used as a statement of actual server functionality or a statement of required or desired server implementation."; 1994 case CAREPLAN: 1995 return "Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions."; 1996 case CARETEAM: 1997 return "The Care Team includes all the people and organizations who plan to participate in the coordination and delivery of care for a patient."; 1998 case CATALOGENTRY: 1999 return "Catalog entries are wrappers that contextualize items included in a catalog."; 2000 case CHARGEITEM: 2001 return "The resource ChargeItem describes the provision of healthcare provider products for a certain patient, therefore referring not only to the product, but containing in addition details of the provision, like date, time, amounts and participating organizations and persons. Main Usage of the ChargeItem is to enable the billing process and internal cost allocation."; 2002 case CHARGEITEMDEFINITION: 2003 return "The ChargeItemDefinition resource provides the properties that apply to the (billing) codes necessary to calculate costs and prices. The properties may differ largely depending on type and realm, therefore this resource gives only a rough structure and requires profiling for each type of billing code system."; 2004 case CLAIM: 2005 return "A provider issued list of professional services and products which have been provided, or are to be provided, to a patient which is sent to an insurer for reimbursement."; 2006 case CLAIMRESPONSE: 2007 return "This resource provides the adjudication details from the processing of a Claim resource."; 2008 case CLINICALIMPRESSION: 2009 return "A record of a clinical assessment performed to determine what problem(s) may affect the patient and before planning the treatments or management strategies that are best to manage a patient's condition. Assessments are often 1:1 with a clinical consultation / encounter, but this varies greatly depending on the clinical workflow. This resource is called \"ClinicalImpression\" rather than \"ClinicalAssessment\" to avoid confusion with the recording of assessment tools such as Apgar score."; 2010 case CODESYSTEM: 2011 return "The CodeSystem resource is used to declare the existence of and describe a code system or code system supplement and its key properties, and optionally define a part or all of its content."; 2012 case COMMUNICATION: 2013 return "An occurrence of information being transmitted; e.g. an alert that was sent to a responsible provider, a public health agency that was notified about a reportable condition."; 2014 case COMMUNICATIONREQUEST: 2015 return "A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition."; 2016 case COMPARTMENTDEFINITION: 2017 return "A compartment definition that defines how resources are accessed on a server."; 2018 case COMPOSITION: 2019 return "A set of healthcare-related information that is assembled together into a single logical package that provides a single coherent statement of meaning, establishes its own context and that has clinical attestation with regard to who is making the statement. A Composition defines the structure and narrative content necessary for a document. However, a Composition alone does not constitute a document. Rather, the Composition must be the first entry in a Bundle where Bundle.type=document, and any other resources referenced from Composition must be included as subsequent entries in the Bundle (for example Patient, Practitioner, Encounter, etc.)."; 2020 case CONCEPTMAP: 2021 return "A statement of relationships from one set of concepts to one or more other concepts - either concepts in code systems, or data element/data element concepts, or classes in class models."; 2022 case CONDITION: 2023 return "A clinical condition, problem, diagnosis, or other event, situation, issue, or clinical concept that has risen to a level of concern."; 2024 case CONSENT: 2025 return "A record of a healthcare consumer?s choices, which permits or denies identified recipient(s) or recipient role(s) to perform one or more actions within a given policy context, for specific purposes and periods of time."; 2026 case CONTRACT: 2027 return "Legally enforceable, formally recorded unilateral or bilateral directive i.e., a policy or agreement."; 2028 case COVERAGE: 2029 return "Financial instrument which may be used to reimburse or pay for health care products and services. Includes both insurance and self-payment."; 2030 case COVERAGEELIGIBILITYREQUEST: 2031 return "The CoverageEligibilityRequest provides patient and insurance coverage information to an insurer for them to respond, in the form of an CoverageEligibilityResponse, with information regarding whether the stated coverage is valid and in-force and optionally to provide the insurance details of the policy."; 2032 case COVERAGEELIGIBILITYRESPONSE: 2033 return "This resource provides eligibility and plan details from the processing of an CoverageEligibilityRequest resource."; 2034 case DETECTEDISSUE: 2035 return "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, etc."; 2036 case DEVICE: 2037 return "A type of a manufactured item that is used in the provision of healthcare without being substantially changed through that activity. The device may be a medical or non-medical device."; 2038 case DEVICEDEFINITION: 2039 return "The characteristics, operational status and capabilities of a medical-related component of a medical device."; 2040 case DEVICEMETRIC: 2041 return "Describes a measurement, calculation or setting capability of a medical device."; 2042 case DEVICEREQUEST: 2043 return "Represents a request for a patient to employ a medical device. The device may be an implantable device, or an external assistive device, such as a walker."; 2044 case DEVICEUSESTATEMENT: 2045 return "A record of a device being used by a patient where the record is the result of a report from the patient or another clinician."; 2046 case DIAGNOSTICREPORT: 2047 return "The findings and interpretation of diagnostic tests performed on patients, groups of patients, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting and provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports."; 2048 case DOCUMENTMANIFEST: 2049 return "A collection of documents compiled for a purpose together with metadata that applies to the collection."; 2050 case DOCUMENTREFERENCE: 2051 return "A reference to a document of any kind for any purpose. Provides metadata about the document so that the document can be discovered and managed. The scope of a document is any seralized object with a mime-type, so includes formal patient centric documents (CDA), cliical notes, scanned paper, and non-patient specific documents like policy text."; 2052 case DOMAINRESOURCE: 2053 return "A resource that includes narrative, extensions, and contained resources."; 2054 case EFFECTEVIDENCESYNTHESIS: 2055 return "The EffectEvidenceSynthesis resource describes the difference in an outcome between exposures states in a population where the effect estimate is derived from a combination of research studies."; 2056 case ENCOUNTER: 2057 return "An interaction between a patient and healthcare provider(s) for the purpose of providing healthcare service(s) or assessing the health status of a patient."; 2058 case ENDPOINT: 2059 return "The technical details of an endpoint that can be used for electronic services, such as for web services providing XDS.b or a REST endpoint for another FHIR server. This may include any security context information."; 2060 case ENROLLMENTREQUEST: 2061 return "This resource provides the insurance enrollment details to the insurer regarding a specified coverage."; 2062 case ENROLLMENTRESPONSE: 2063 return "This resource provides enrollment and plan details from the processing of an EnrollmentRequest resource."; 2064 case EPISODEOFCARE: 2065 return "An association between a patient and an organization / healthcare provider(s) during which time encounters may occur. The managing organization assumes a level of responsibility for the patient during this time."; 2066 case EVENTDEFINITION: 2067 return "The EventDefinition resource provides a reusable description of when a particular event can occur."; 2068 case EVIDENCE: 2069 return "The Evidence resource describes the conditional state (population and any exposures being compared within the population) and outcome (if specified) that the knowledge (evidence, assertion, recommendation) is about."; 2070 case EVIDENCEVARIABLE: 2071 return "The EvidenceVariable resource describes a \"PICO\" element that knowledge (evidence, assertion, recommendation) is about."; 2072 case EXAMPLESCENARIO: 2073 return "Example of workflow instance."; 2074 case EXPLANATIONOFBENEFIT: 2075 return "This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided."; 2076 case FAMILYMEMBERHISTORY: 2077 return "Significant health conditions for a person related to the patient relevant in the context of care for the patient."; 2078 case FLAG: 2079 return "Prospective warnings of potential issues when providing care to the patient."; 2080 case GOAL: 2081 return "Describes the intended objective(s) for a patient, group or organization care, for example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc."; 2082 case GRAPHDEFINITION: 2083 return "A formal computable definition of a graph of resources - that is, a coherent set of resources that form a graph by following references. The Graph Definition resource defines a set and makes rules about the set."; 2084 case GROUP: 2085 return "Represents a defined collection of entities that may be discussed or acted upon collectively but which are not expected to act collectively, and are not formally or legally recognized; i.e. a collection of entities that isn't an Organization."; 2086 case GUIDANCERESPONSE: 2087 return "A guidance response is the formal response to a guidance request, including any output parameters returned by the evaluation, as well as the description of any proposed actions to be taken."; 2088 case HEALTHCARESERVICE: 2089 return "The details of a healthcare service available at a location."; 2090 case IMAGINGSTUDY: 2091 return "Representation of the content produced in a DICOM imaging study. A study comprises a set of series, each of which includes a set of Service-Object Pair Instances (SOP Instances - images or other data) acquired or produced in a common context. A series is of only one modality (e.g. X-ray, CT, MR, ultrasound), but a study may have multiple series of different modalities."; 2092 case IMMUNIZATION: 2093 return "Describes the event of a patient being administered a vaccine or a record of an immunization as reported by a patient, a clinician or another party."; 2094 case IMMUNIZATIONEVALUATION: 2095 return "Describes a comparison of an immunization event against published recommendations to determine if the administration is \"valid\" in relation to those recommendations."; 2096 case IMMUNIZATIONRECOMMENDATION: 2097 return "A patient's point-in-time set of recommendations (i.e. forecasting) according to a published schedule with optional supporting justification."; 2098 case IMPLEMENTATIONGUIDE: 2099 return "A set of rules of how a particular interoperability or standards problem is solved - typically through the use of FHIR resources. This resource is used to gather all the parts of an implementation guide into a logical whole and to publish a computable definition of all the parts."; 2100 case INSURANCEPLAN: 2101 return "Details of a Health Insurance product/plan provided by an organization."; 2102 case INVOICE: 2103 return "Invoice containing collected ChargeItems from an Account with calculated individual and total price for Billing purpose."; 2104 case LIBRARY: 2105 return "The Library resource is a general-purpose container for knowledge asset definitions. It can be used to describe and expose existing knowledge assets such as logic libraries and information model descriptions, as well as to describe a collection of knowledge assets."; 2106 case LINKAGE: 2107 return "Identifies two or more records (resource instances) that refer to the same real-world \"occurrence\"."; 2108 case LIST: 2109 return "A list is a curated collection of resources."; 2110 case LOCATION: 2111 return "Details and position information for a physical place where services are provided and resources and participants may be stored, found, contained, or accommodated."; 2112 case MEASURE: 2113 return "The Measure resource provides the definition of a quality measure."; 2114 case MEASUREREPORT: 2115 return "The MeasureReport resource contains the results of the calculation of a measure; and optionally a reference to the resources involved in that calculation."; 2116 case MEDIA: 2117 return "A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference."; 2118 case MEDICATION: 2119 return "This resource is primarily used for the identification and definition of a medication for the purposes of prescribing, dispensing, and administering a medication as well as for making statements about medication use."; 2120 case MEDICATIONADMINISTRATION: 2121 return "Describes the event of a patient consuming or otherwise being administered a medication. This may be as simple as swallowing a tablet or it may be a long running infusion. Related resources tie this event to the authorizing prescription, and the specific encounter between patient and health care practitioner."; 2122 case MEDICATIONDISPENSE: 2123 return "Indicates that a medication product is to be or has been dispensed for a named person/patient. This includes a description of the medication product (supply) provided and the instructions for administering the medication. The medication dispense is the result of a pharmacy system responding to a medication order."; 2124 case MEDICATIONKNOWLEDGE: 2125 return "Information about a medication that is used to support knowledge."; 2126 case MEDICATIONREQUEST: 2127 return "An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called \"MedicationRequest\" rather than \"MedicationPrescription\" or \"MedicationOrder\" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns."; 2128 case MEDICATIONSTATEMENT: 2129 return "A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from sources such as the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains. \n\nThe primary difference between a medication statement and a medication administration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medication statement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the medication statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information."; 2130 case MEDICINALPRODUCT: 2131 return "Detailed definition of a medicinal product, typically for uses other than direct patient care (e.g. regulatory use)."; 2132 case MEDICINALPRODUCTAUTHORIZATION: 2133 return "The regulatory authorization of a medicinal product."; 2134 case MEDICINALPRODUCTCONTRAINDICATION: 2135 return "The clinical particulars - indications, contraindications etc. of a medicinal product, including for regulatory purposes."; 2136 case MEDICINALPRODUCTINDICATION: 2137 return "Indication for the Medicinal Product."; 2138 case MEDICINALPRODUCTINGREDIENT: 2139 return "An ingredient of a manufactured item or pharmaceutical product."; 2140 case MEDICINALPRODUCTINTERACTION: 2141 return "The interactions of the medicinal product with other medicinal products, or other forms of interactions."; 2142 case MEDICINALPRODUCTMANUFACTURED: 2143 return "The manufactured item as contained in the packaged medicinal product."; 2144 case MEDICINALPRODUCTPACKAGED: 2145 return "A medicinal product in a container or package."; 2146 case MEDICINALPRODUCTPHARMACEUTICAL: 2147 return "A pharmaceutical product described in terms of its composition and dose form."; 2148 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 2149 return "Describe the undesirable effects of the medicinal product."; 2150 case MESSAGEDEFINITION: 2151 return "Defines the characteristics of a message that can be shared between systems, including the type of event that initiates the message, the content to be transmitted and what response(s), if any, are permitted."; 2152 case MESSAGEHEADER: 2153 return "The header for a message exchange that is either requesting or responding to an action. The reference(s) that are the subject of the action as well as other information related to the action are typically transmitted in a bundle in which the MessageHeader resource instance is the first resource in the bundle."; 2154 case MOLECULARSEQUENCE: 2155 return "Raw data describing a biological sequence."; 2156 case NAMINGSYSTEM: 2157 return "A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a \"System\" used within the Identifier and Coding data types."; 2158 case NUTRITIONORDER: 2159 return "A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident."; 2160 case OBSERVATION: 2161 return "Measurements and simple assertions made about a patient, device or other subject."; 2162 case OBSERVATIONDEFINITION: 2163 return "Set of definitional characteristics for a kind of observation or measurement produced or consumed by an orderable health care service."; 2164 case OPERATIONDEFINITION: 2165 return "A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction)."; 2166 case OPERATIONOUTCOME: 2167 return "A collection of error, warning, or information messages that result from a system action."; 2168 case ORGANIZATION: 2169 return "A formally or informally recognized grouping of people or organizations formed for the purpose of achieving some form of collective action. Includes companies, institutions, corporations, departments, community groups, healthcare practice groups, payer/insurer, etc."; 2170 case ORGANIZATIONAFFILIATION: 2171 return "Defines an affiliation/assotiation/relationship between 2 distinct oganizations, that is not a part-of relationship/sub-division relationship."; 2172 case PARAMETERS: 2173 return "This resource is a non-persisted resource used to pass information into and back from an [operation](operations.html). It has no other use, and there is no RESTful endpoint associated with it."; 2174 case PATIENT: 2175 return "Demographics and other administrative information about an individual or animal receiving care or other health-related services."; 2176 case PAYMENTNOTICE: 2177 return "This resource provides the status of the payment for goods and services rendered, and the request and response resource references."; 2178 case PAYMENTRECONCILIATION: 2179 return "This resource provides the details including amount of a payment and allocates the payment items being paid."; 2180 case PERSON: 2181 return "Demographics and administrative information about a person independent of a specific health-related context."; 2182 case PLANDEFINITION: 2183 return "This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical artifacts such as clinical decision support rules, order sets and protocols."; 2184 case PRACTITIONER: 2185 return "A person who is directly or indirectly involved in the provisioning of healthcare."; 2186 case PRACTITIONERROLE: 2187 return "A specific set of Roles/Locations/specialties/services that a practitioner may perform at an organization for a period of time."; 2188 case PROCEDURE: 2189 return "An action that is or was performed on or for a patient. This can be a physical intervention like an operation, or less invasive like long term services, counseling, or hypnotherapy."; 2190 case PROVENANCE: 2191 return "Provenance of a resource is a record that describes entities and processes involved in producing and delivering or otherwise influencing that resource. Provenance provides a critical foundation for assessing authenticity, enabling trust, and allowing reproducibility. Provenance assertions are a form of contextual metadata and can themselves become important records with their own provenance. Provenance statement indicates clinical significance in terms of confidence in authenticity, reliability, and trustworthiness, integrity, and stage in lifecycle (e.g. Document Completion - has the artifact been legally authenticated), all of which may impact security, privacy, and trust policies."; 2192 case QUESTIONNAIRE: 2193 return "A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection."; 2194 case QUESTIONNAIRERESPONSE: 2195 return "A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the questionnaire being responded to."; 2196 case RELATEDPERSON: 2197 return "Information about a person that is involved in the care for a patient, but who is not the target of healthcare, nor has a formal responsibility in the care process."; 2198 case REQUESTGROUP: 2199 return "A group of related requests that can be used to capture intended activities that have inter-dependencies such as \"give this medication after that one\"."; 2200 case RESEARCHDEFINITION: 2201 return "The ResearchDefinition resource describes the conditional state (population and any exposures being compared within the population) and outcome (if specified) that the knowledge (evidence, assertion, recommendation) is about."; 2202 case RESEARCHELEMENTDEFINITION: 2203 return "The ResearchElementDefinition resource describes a \"PICO\" element that knowledge (evidence, assertion, recommendation) is about."; 2204 case RESEARCHSTUDY: 2205 return "A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects."; 2206 case RESEARCHSUBJECT: 2207 return "A physical entity which is the primary unit of operational and/or administrative interest in a study."; 2208 case RESOURCE: 2209 return "This is the base resource type for everything."; 2210 case RISKASSESSMENT: 2211 return "An assessment of the likely outcome(s) for a patient or other subject as well as the likelihood of each outcome."; 2212 case RISKEVIDENCESYNTHESIS: 2213 return "The RiskEvidenceSynthesis resource describes the likelihood of an outcome in a population plus exposure state where the risk estimate is derived from a combination of research studies."; 2214 case SCHEDULE: 2215 return "A container for slots of time that may be available for booking appointments."; 2216 case SEARCHPARAMETER: 2217 return "A search parameter that defines a named search item that can be used to search/filter on a resource."; 2218 case SERVICEREQUEST: 2219 return "A record of a request for service such as diagnostic investigations, treatments, or operations to be performed."; 2220 case SLOT: 2221 return "A slot of time on a schedule that may be available for booking appointments."; 2222 case SPECIMEN: 2223 return "A sample to be used for analysis."; 2224 case SPECIMENDEFINITION: 2225 return "A kind of specimen with associated set of requirements."; 2226 case STRUCTUREDEFINITION: 2227 return "A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions and constraints on resources and data types."; 2228 case STRUCTUREMAP: 2229 return "A Map of relationships between 2 structures that can be used to transform data."; 2230 case SUBSCRIPTION: 2231 return "The subscription resource is used to define a push-based subscription from a server to another system. Once a subscription is registered with the server, the server checks every resource that is created or updated, and if the resource matches the given criteria, it sends a message on the defined \"channel\" so that another system can take an appropriate action."; 2232 case SUBSTANCE: 2233 return "A homogeneous material with a definite composition."; 2234 case SUBSTANCENUCLEICACID: 2235 return "Nucleic acids are defined by three distinct elements: the base, sugar and linkage. Individual substance/moiety IDs will be created for each of these elements. The nucleotide sequence will be always entered in the 5?-3? direction."; 2236 case SUBSTANCEPOLYMER: 2237 return "Todo."; 2238 case SUBSTANCEPROTEIN: 2239 return "A SubstanceProtein is defined as a single unit of a linear amino acid sequence, or a combination of subunits that are either covalently linked or have a defined invariant stoichiometric relationship. This includes all synthetic, recombinant and purified SubstanceProteins of defined sequence, whether the use is therapeutic or prophylactic. This set of elements will be used to describe albumins, coagulation factors, cytokines, growth factors, peptide/SubstanceProtein hormones, enzymes, toxins, toxoids, recombinant vaccines, and immunomodulators."; 2240 case SUBSTANCEREFERENCEINFORMATION: 2241 return "Todo."; 2242 case SUBSTANCESOURCEMATERIAL: 2243 return "Source material shall capture information on the taxonomic and anatomical origins as well as the fraction of a material that can result in or can be modified to form a substance. This set of data elements shall be used to define polymer substances isolated from biological matrices. Taxonomic and anatomical origins shall be described using a controlled vocabulary as required. This information is captured for naturally derived polymers ( . starch) and structurally diverse substances. For Organisms belonging to the Kingdom Plantae the Substance level defines the fresh material of a single species or infraspecies, the Herbal Drug and the Herbal preparation. For Herbal preparations, the fraction information will be captured at the Substance information level and additional information for herbal extracts will be captured at the Specified Substance Group 1 information level. See for further explanation the Substance Class: Structurally Diverse and the herbal annex."; 2244 case SUBSTANCESPECIFICATION: 2245 return "The detailed description of a substance, typically at a level beyond what is used for prescribing."; 2246 case SUPPLYDELIVERY: 2247 return "Record of delivery of what is supplied."; 2248 case SUPPLYREQUEST: 2249 return "A record of a request for a medication, substance or device used in the healthcare setting."; 2250 case TASK: 2251 return "A task to be performed."; 2252 case TERMINOLOGYCAPABILITIES: 2253 return "A TerminologyCapabilities resource documents a set of capabilities (behaviors) of a FHIR Terminology Server that may be used as a statement of actual server functionality or a statement of required or desired server implementation."; 2254 case TESTREPORT: 2255 return "A summary of information based on the results of executing a TestScript."; 2256 case TESTSCRIPT: 2257 return "A structured set of tests against a FHIR server or client implementation to determine compliance against the FHIR specification."; 2258 case VALUESET: 2259 return "A ValueSet resource instance specifies a set of codes drawn from one or more code systems, intended for use in a particular context. Value sets link between [[[CodeSystem]]] definitions and their use in [coded elements](terminologies.html)."; 2260 case VERIFICATIONRESULT: 2261 return "Describes validation requirements, source(s), status and dates for one or more elements."; 2262 case VISIONPRESCRIPTION: 2263 return "An authorization for the provision of glasses and/or contact lenses to a patient."; 2264 case NULL: 2265 return null; 2266 default: 2267 return "?"; 2268 } 2269 } 2270 2271 public String getDisplay() { 2272 switch (this) { 2273 case ACCOUNT: 2274 return "Account"; 2275 case ACTIVITYDEFINITION: 2276 return "ActivityDefinition"; 2277 case ADVERSEEVENT: 2278 return "AdverseEvent"; 2279 case ALLERGYINTOLERANCE: 2280 return "AllergyIntolerance"; 2281 case APPOINTMENT: 2282 return "Appointment"; 2283 case APPOINTMENTRESPONSE: 2284 return "AppointmentResponse"; 2285 case AUDITEVENT: 2286 return "AuditEvent"; 2287 case BASIC: 2288 return "Basic"; 2289 case BINARY: 2290 return "Binary"; 2291 case BIOLOGICALLYDERIVEDPRODUCT: 2292 return "BiologicallyDerivedProduct"; 2293 case BODYSTRUCTURE: 2294 return "BodyStructure"; 2295 case BUNDLE: 2296 return "Bundle"; 2297 case CAPABILITYSTATEMENT: 2298 return "CapabilityStatement"; 2299 case CAREPLAN: 2300 return "CarePlan"; 2301 case CARETEAM: 2302 return "CareTeam"; 2303 case CATALOGENTRY: 2304 return "CatalogEntry"; 2305 case CHARGEITEM: 2306 return "ChargeItem"; 2307 case CHARGEITEMDEFINITION: 2308 return "ChargeItemDefinition"; 2309 case CLAIM: 2310 return "Claim"; 2311 case CLAIMRESPONSE: 2312 return "ClaimResponse"; 2313 case CLINICALIMPRESSION: 2314 return "ClinicalImpression"; 2315 case CODESYSTEM: 2316 return "CodeSystem"; 2317 case COMMUNICATION: 2318 return "Communication"; 2319 case COMMUNICATIONREQUEST: 2320 return "CommunicationRequest"; 2321 case COMPARTMENTDEFINITION: 2322 return "CompartmentDefinition"; 2323 case COMPOSITION: 2324 return "Composition"; 2325 case CONCEPTMAP: 2326 return "ConceptMap"; 2327 case CONDITION: 2328 return "Condition"; 2329 case CONSENT: 2330 return "Consent"; 2331 case CONTRACT: 2332 return "Contract"; 2333 case COVERAGE: 2334 return "Coverage"; 2335 case COVERAGEELIGIBILITYREQUEST: 2336 return "CoverageEligibilityRequest"; 2337 case COVERAGEELIGIBILITYRESPONSE: 2338 return "CoverageEligibilityResponse"; 2339 case DETECTEDISSUE: 2340 return "DetectedIssue"; 2341 case DEVICE: 2342 return "Device"; 2343 case DEVICEDEFINITION: 2344 return "DeviceDefinition"; 2345 case DEVICEMETRIC: 2346 return "DeviceMetric"; 2347 case DEVICEREQUEST: 2348 return "DeviceRequest"; 2349 case DEVICEUSESTATEMENT: 2350 return "DeviceUseStatement"; 2351 case DIAGNOSTICREPORT: 2352 return "DiagnosticReport"; 2353 case DOCUMENTMANIFEST: 2354 return "DocumentManifest"; 2355 case DOCUMENTREFERENCE: 2356 return "DocumentReference"; 2357 case DOMAINRESOURCE: 2358 return "DomainResource"; 2359 case EFFECTEVIDENCESYNTHESIS: 2360 return "EffectEvidenceSynthesis"; 2361 case ENCOUNTER: 2362 return "Encounter"; 2363 case ENDPOINT: 2364 return "Endpoint"; 2365 case ENROLLMENTREQUEST: 2366 return "EnrollmentRequest"; 2367 case ENROLLMENTRESPONSE: 2368 return "EnrollmentResponse"; 2369 case EPISODEOFCARE: 2370 return "EpisodeOfCare"; 2371 case EVENTDEFINITION: 2372 return "EventDefinition"; 2373 case EVIDENCE: 2374 return "Evidence"; 2375 case EVIDENCEVARIABLE: 2376 return "EvidenceVariable"; 2377 case EXAMPLESCENARIO: 2378 return "ExampleScenario"; 2379 case EXPLANATIONOFBENEFIT: 2380 return "ExplanationOfBenefit"; 2381 case FAMILYMEMBERHISTORY: 2382 return "FamilyMemberHistory"; 2383 case FLAG: 2384 return "Flag"; 2385 case GOAL: 2386 return "Goal"; 2387 case GRAPHDEFINITION: 2388 return "GraphDefinition"; 2389 case GROUP: 2390 return "Group"; 2391 case GUIDANCERESPONSE: 2392 return "GuidanceResponse"; 2393 case HEALTHCARESERVICE: 2394 return "HealthcareService"; 2395 case IMAGINGSTUDY: 2396 return "ImagingStudy"; 2397 case IMMUNIZATION: 2398 return "Immunization"; 2399 case IMMUNIZATIONEVALUATION: 2400 return "ImmunizationEvaluation"; 2401 case IMMUNIZATIONRECOMMENDATION: 2402 return "ImmunizationRecommendation"; 2403 case IMPLEMENTATIONGUIDE: 2404 return "ImplementationGuide"; 2405 case INSURANCEPLAN: 2406 return "InsurancePlan"; 2407 case INVOICE: 2408 return "Invoice"; 2409 case LIBRARY: 2410 return "Library"; 2411 case LINKAGE: 2412 return "Linkage"; 2413 case LIST: 2414 return "List"; 2415 case LOCATION: 2416 return "Location"; 2417 case MEASURE: 2418 return "Measure"; 2419 case MEASUREREPORT: 2420 return "MeasureReport"; 2421 case MEDIA: 2422 return "Media"; 2423 case MEDICATION: 2424 return "Medication"; 2425 case MEDICATIONADMINISTRATION: 2426 return "MedicationAdministration"; 2427 case MEDICATIONDISPENSE: 2428 return "MedicationDispense"; 2429 case MEDICATIONKNOWLEDGE: 2430 return "MedicationKnowledge"; 2431 case MEDICATIONREQUEST: 2432 return "MedicationRequest"; 2433 case MEDICATIONSTATEMENT: 2434 return "MedicationStatement"; 2435 case MEDICINALPRODUCT: 2436 return "MedicinalProduct"; 2437 case MEDICINALPRODUCTAUTHORIZATION: 2438 return "MedicinalProductAuthorization"; 2439 case MEDICINALPRODUCTCONTRAINDICATION: 2440 return "MedicinalProductContraindication"; 2441 case MEDICINALPRODUCTINDICATION: 2442 return "MedicinalProductIndication"; 2443 case MEDICINALPRODUCTINGREDIENT: 2444 return "MedicinalProductIngredient"; 2445 case MEDICINALPRODUCTINTERACTION: 2446 return "MedicinalProductInteraction"; 2447 case MEDICINALPRODUCTMANUFACTURED: 2448 return "MedicinalProductManufactured"; 2449 case MEDICINALPRODUCTPACKAGED: 2450 return "MedicinalProductPackaged"; 2451 case MEDICINALPRODUCTPHARMACEUTICAL: 2452 return "MedicinalProductPharmaceutical"; 2453 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 2454 return "MedicinalProductUndesirableEffect"; 2455 case MESSAGEDEFINITION: 2456 return "MessageDefinition"; 2457 case MESSAGEHEADER: 2458 return "MessageHeader"; 2459 case MOLECULARSEQUENCE: 2460 return "MolecularSequence"; 2461 case NAMINGSYSTEM: 2462 return "NamingSystem"; 2463 case NUTRITIONORDER: 2464 return "NutritionOrder"; 2465 case OBSERVATION: 2466 return "Observation"; 2467 case OBSERVATIONDEFINITION: 2468 return "ObservationDefinition"; 2469 case OPERATIONDEFINITION: 2470 return "OperationDefinition"; 2471 case OPERATIONOUTCOME: 2472 return "OperationOutcome"; 2473 case ORGANIZATION: 2474 return "Organization"; 2475 case ORGANIZATIONAFFILIATION: 2476 return "OrganizationAffiliation"; 2477 case PARAMETERS: 2478 return "Parameters"; 2479 case PATIENT: 2480 return "Patient"; 2481 case PAYMENTNOTICE: 2482 return "PaymentNotice"; 2483 case PAYMENTRECONCILIATION: 2484 return "PaymentReconciliation"; 2485 case PERSON: 2486 return "Person"; 2487 case PLANDEFINITION: 2488 return "PlanDefinition"; 2489 case PRACTITIONER: 2490 return "Practitioner"; 2491 case PRACTITIONERROLE: 2492 return "PractitionerRole"; 2493 case PROCEDURE: 2494 return "Procedure"; 2495 case PROVENANCE: 2496 return "Provenance"; 2497 case QUESTIONNAIRE: 2498 return "Questionnaire"; 2499 case QUESTIONNAIRERESPONSE: 2500 return "QuestionnaireResponse"; 2501 case RELATEDPERSON: 2502 return "RelatedPerson"; 2503 case REQUESTGROUP: 2504 return "RequestGroup"; 2505 case RESEARCHDEFINITION: 2506 return "ResearchDefinition"; 2507 case RESEARCHELEMENTDEFINITION: 2508 return "ResearchElementDefinition"; 2509 case RESEARCHSTUDY: 2510 return "ResearchStudy"; 2511 case RESEARCHSUBJECT: 2512 return "ResearchSubject"; 2513 case RESOURCE: 2514 return "Resource"; 2515 case RISKASSESSMENT: 2516 return "RiskAssessment"; 2517 case RISKEVIDENCESYNTHESIS: 2518 return "RiskEvidenceSynthesis"; 2519 case SCHEDULE: 2520 return "Schedule"; 2521 case SEARCHPARAMETER: 2522 return "SearchParameter"; 2523 case SERVICEREQUEST: 2524 return "ServiceRequest"; 2525 case SLOT: 2526 return "Slot"; 2527 case SPECIMEN: 2528 return "Specimen"; 2529 case SPECIMENDEFINITION: 2530 return "SpecimenDefinition"; 2531 case STRUCTUREDEFINITION: 2532 return "StructureDefinition"; 2533 case STRUCTUREMAP: 2534 return "StructureMap"; 2535 case SUBSCRIPTION: 2536 return "Subscription"; 2537 case SUBSTANCE: 2538 return "Substance"; 2539 case SUBSTANCENUCLEICACID: 2540 return "SubstanceNucleicAcid"; 2541 case SUBSTANCEPOLYMER: 2542 return "SubstancePolymer"; 2543 case SUBSTANCEPROTEIN: 2544 return "SubstanceProtein"; 2545 case SUBSTANCEREFERENCEINFORMATION: 2546 return "SubstanceReferenceInformation"; 2547 case SUBSTANCESOURCEMATERIAL: 2548 return "SubstanceSourceMaterial"; 2549 case SUBSTANCESPECIFICATION: 2550 return "SubstanceSpecification"; 2551 case SUPPLYDELIVERY: 2552 return "SupplyDelivery"; 2553 case SUPPLYREQUEST: 2554 return "SupplyRequest"; 2555 case TASK: 2556 return "Task"; 2557 case TERMINOLOGYCAPABILITIES: 2558 return "TerminologyCapabilities"; 2559 case TESTREPORT: 2560 return "TestReport"; 2561 case TESTSCRIPT: 2562 return "TestScript"; 2563 case VALUESET: 2564 return "ValueSet"; 2565 case VERIFICATIONRESULT: 2566 return "VerificationResult"; 2567 case VISIONPRESCRIPTION: 2568 return "VisionPrescription"; 2569 case NULL: 2570 return null; 2571 default: 2572 return "?"; 2573 } 2574 } 2575 } 2576 2577 public static class FHIRResourceTypeEnumFactory implements EnumFactory<FHIRResourceType> { 2578 public FHIRResourceType fromCode(String codeString) throws IllegalArgumentException { 2579 if (codeString == null || "".equals(codeString)) 2580 if (codeString == null || "".equals(codeString)) 2581 return null; 2582 if ("Account".equals(codeString)) 2583 return FHIRResourceType.ACCOUNT; 2584 if ("ActivityDefinition".equals(codeString)) 2585 return FHIRResourceType.ACTIVITYDEFINITION; 2586 if ("AdverseEvent".equals(codeString)) 2587 return FHIRResourceType.ADVERSEEVENT; 2588 if ("AllergyIntolerance".equals(codeString)) 2589 return FHIRResourceType.ALLERGYINTOLERANCE; 2590 if ("Appointment".equals(codeString)) 2591 return FHIRResourceType.APPOINTMENT; 2592 if ("AppointmentResponse".equals(codeString)) 2593 return FHIRResourceType.APPOINTMENTRESPONSE; 2594 if ("AuditEvent".equals(codeString)) 2595 return FHIRResourceType.AUDITEVENT; 2596 if ("Basic".equals(codeString)) 2597 return FHIRResourceType.BASIC; 2598 if ("Binary".equals(codeString)) 2599 return FHIRResourceType.BINARY; 2600 if ("BiologicallyDerivedProduct".equals(codeString)) 2601 return FHIRResourceType.BIOLOGICALLYDERIVEDPRODUCT; 2602 if ("BodyStructure".equals(codeString)) 2603 return FHIRResourceType.BODYSTRUCTURE; 2604 if ("Bundle".equals(codeString)) 2605 return FHIRResourceType.BUNDLE; 2606 if ("CapabilityStatement".equals(codeString)) 2607 return FHIRResourceType.CAPABILITYSTATEMENT; 2608 if ("CarePlan".equals(codeString)) 2609 return FHIRResourceType.CAREPLAN; 2610 if ("CareTeam".equals(codeString)) 2611 return FHIRResourceType.CARETEAM; 2612 if ("CatalogEntry".equals(codeString)) 2613 return FHIRResourceType.CATALOGENTRY; 2614 if ("ChargeItem".equals(codeString)) 2615 return FHIRResourceType.CHARGEITEM; 2616 if ("ChargeItemDefinition".equals(codeString)) 2617 return FHIRResourceType.CHARGEITEMDEFINITION; 2618 if ("Claim".equals(codeString)) 2619 return FHIRResourceType.CLAIM; 2620 if ("ClaimResponse".equals(codeString)) 2621 return FHIRResourceType.CLAIMRESPONSE; 2622 if ("ClinicalImpression".equals(codeString)) 2623 return FHIRResourceType.CLINICALIMPRESSION; 2624 if ("CodeSystem".equals(codeString)) 2625 return FHIRResourceType.CODESYSTEM; 2626 if ("Communication".equals(codeString)) 2627 return FHIRResourceType.COMMUNICATION; 2628 if ("CommunicationRequest".equals(codeString)) 2629 return FHIRResourceType.COMMUNICATIONREQUEST; 2630 if ("CompartmentDefinition".equals(codeString)) 2631 return FHIRResourceType.COMPARTMENTDEFINITION; 2632 if ("Composition".equals(codeString)) 2633 return FHIRResourceType.COMPOSITION; 2634 if ("ConceptMap".equals(codeString)) 2635 return FHIRResourceType.CONCEPTMAP; 2636 if ("Condition".equals(codeString)) 2637 return FHIRResourceType.CONDITION; 2638 if ("Consent".equals(codeString)) 2639 return FHIRResourceType.CONSENT; 2640 if ("Contract".equals(codeString)) 2641 return FHIRResourceType.CONTRACT; 2642 if ("Coverage".equals(codeString)) 2643 return FHIRResourceType.COVERAGE; 2644 if ("CoverageEligibilityRequest".equals(codeString)) 2645 return FHIRResourceType.COVERAGEELIGIBILITYREQUEST; 2646 if ("CoverageEligibilityResponse".equals(codeString)) 2647 return FHIRResourceType.COVERAGEELIGIBILITYRESPONSE; 2648 if ("DetectedIssue".equals(codeString)) 2649 return FHIRResourceType.DETECTEDISSUE; 2650 if ("Device".equals(codeString)) 2651 return FHIRResourceType.DEVICE; 2652 if ("DeviceDefinition".equals(codeString)) 2653 return FHIRResourceType.DEVICEDEFINITION; 2654 if ("DeviceMetric".equals(codeString)) 2655 return FHIRResourceType.DEVICEMETRIC; 2656 if ("DeviceRequest".equals(codeString)) 2657 return FHIRResourceType.DEVICEREQUEST; 2658 if ("DeviceUseStatement".equals(codeString)) 2659 return FHIRResourceType.DEVICEUSESTATEMENT; 2660 if ("DiagnosticReport".equals(codeString)) 2661 return FHIRResourceType.DIAGNOSTICREPORT; 2662 if ("DocumentManifest".equals(codeString)) 2663 return FHIRResourceType.DOCUMENTMANIFEST; 2664 if ("DocumentReference".equals(codeString)) 2665 return FHIRResourceType.DOCUMENTREFERENCE; 2666 if ("DomainResource".equals(codeString)) 2667 return FHIRResourceType.DOMAINRESOURCE; 2668 if ("EffectEvidenceSynthesis".equals(codeString)) 2669 return FHIRResourceType.EFFECTEVIDENCESYNTHESIS; 2670 if ("Encounter".equals(codeString)) 2671 return FHIRResourceType.ENCOUNTER; 2672 if ("Endpoint".equals(codeString)) 2673 return FHIRResourceType.ENDPOINT; 2674 if ("EnrollmentRequest".equals(codeString)) 2675 return FHIRResourceType.ENROLLMENTREQUEST; 2676 if ("EnrollmentResponse".equals(codeString)) 2677 return FHIRResourceType.ENROLLMENTRESPONSE; 2678 if ("EpisodeOfCare".equals(codeString)) 2679 return FHIRResourceType.EPISODEOFCARE; 2680 if ("EventDefinition".equals(codeString)) 2681 return FHIRResourceType.EVENTDEFINITION; 2682 if ("Evidence".equals(codeString)) 2683 return FHIRResourceType.EVIDENCE; 2684 if ("EvidenceVariable".equals(codeString)) 2685 return FHIRResourceType.EVIDENCEVARIABLE; 2686 if ("ExampleScenario".equals(codeString)) 2687 return FHIRResourceType.EXAMPLESCENARIO; 2688 if ("ExplanationOfBenefit".equals(codeString)) 2689 return FHIRResourceType.EXPLANATIONOFBENEFIT; 2690 if ("FamilyMemberHistory".equals(codeString)) 2691 return FHIRResourceType.FAMILYMEMBERHISTORY; 2692 if ("Flag".equals(codeString)) 2693 return FHIRResourceType.FLAG; 2694 if ("Goal".equals(codeString)) 2695 return FHIRResourceType.GOAL; 2696 if ("GraphDefinition".equals(codeString)) 2697 return FHIRResourceType.GRAPHDEFINITION; 2698 if ("Group".equals(codeString)) 2699 return FHIRResourceType.GROUP; 2700 if ("GuidanceResponse".equals(codeString)) 2701 return FHIRResourceType.GUIDANCERESPONSE; 2702 if ("HealthcareService".equals(codeString)) 2703 return FHIRResourceType.HEALTHCARESERVICE; 2704 if ("ImagingStudy".equals(codeString)) 2705 return FHIRResourceType.IMAGINGSTUDY; 2706 if ("Immunization".equals(codeString)) 2707 return FHIRResourceType.IMMUNIZATION; 2708 if ("ImmunizationEvaluation".equals(codeString)) 2709 return FHIRResourceType.IMMUNIZATIONEVALUATION; 2710 if ("ImmunizationRecommendation".equals(codeString)) 2711 return FHIRResourceType.IMMUNIZATIONRECOMMENDATION; 2712 if ("ImplementationGuide".equals(codeString)) 2713 return FHIRResourceType.IMPLEMENTATIONGUIDE; 2714 if ("InsurancePlan".equals(codeString)) 2715 return FHIRResourceType.INSURANCEPLAN; 2716 if ("Invoice".equals(codeString)) 2717 return FHIRResourceType.INVOICE; 2718 if ("Library".equals(codeString)) 2719 return FHIRResourceType.LIBRARY; 2720 if ("Linkage".equals(codeString)) 2721 return FHIRResourceType.LINKAGE; 2722 if ("List".equals(codeString)) 2723 return FHIRResourceType.LIST; 2724 if ("Location".equals(codeString)) 2725 return FHIRResourceType.LOCATION; 2726 if ("Measure".equals(codeString)) 2727 return FHIRResourceType.MEASURE; 2728 if ("MeasureReport".equals(codeString)) 2729 return FHIRResourceType.MEASUREREPORT; 2730 if ("Media".equals(codeString)) 2731 return FHIRResourceType.MEDIA; 2732 if ("Medication".equals(codeString)) 2733 return FHIRResourceType.MEDICATION; 2734 if ("MedicationAdministration".equals(codeString)) 2735 return FHIRResourceType.MEDICATIONADMINISTRATION; 2736 if ("MedicationDispense".equals(codeString)) 2737 return FHIRResourceType.MEDICATIONDISPENSE; 2738 if ("MedicationKnowledge".equals(codeString)) 2739 return FHIRResourceType.MEDICATIONKNOWLEDGE; 2740 if ("MedicationRequest".equals(codeString)) 2741 return FHIRResourceType.MEDICATIONREQUEST; 2742 if ("MedicationStatement".equals(codeString)) 2743 return FHIRResourceType.MEDICATIONSTATEMENT; 2744 if ("MedicinalProduct".equals(codeString)) 2745 return FHIRResourceType.MEDICINALPRODUCT; 2746 if ("MedicinalProductAuthorization".equals(codeString)) 2747 return FHIRResourceType.MEDICINALPRODUCTAUTHORIZATION; 2748 if ("MedicinalProductContraindication".equals(codeString)) 2749 return FHIRResourceType.MEDICINALPRODUCTCONTRAINDICATION; 2750 if ("MedicinalProductIndication".equals(codeString)) 2751 return FHIRResourceType.MEDICINALPRODUCTINDICATION; 2752 if ("MedicinalProductIngredient".equals(codeString)) 2753 return FHIRResourceType.MEDICINALPRODUCTINGREDIENT; 2754 if ("MedicinalProductInteraction".equals(codeString)) 2755 return FHIRResourceType.MEDICINALPRODUCTINTERACTION; 2756 if ("MedicinalProductManufactured".equals(codeString)) 2757 return FHIRResourceType.MEDICINALPRODUCTMANUFACTURED; 2758 if ("MedicinalProductPackaged".equals(codeString)) 2759 return FHIRResourceType.MEDICINALPRODUCTPACKAGED; 2760 if ("MedicinalProductPharmaceutical".equals(codeString)) 2761 return FHIRResourceType.MEDICINALPRODUCTPHARMACEUTICAL; 2762 if ("MedicinalProductUndesirableEffect".equals(codeString)) 2763 return FHIRResourceType.MEDICINALPRODUCTUNDESIRABLEEFFECT; 2764 if ("MessageDefinition".equals(codeString)) 2765 return FHIRResourceType.MESSAGEDEFINITION; 2766 if ("MessageHeader".equals(codeString)) 2767 return FHIRResourceType.MESSAGEHEADER; 2768 if ("MolecularSequence".equals(codeString)) 2769 return FHIRResourceType.MOLECULARSEQUENCE; 2770 if ("NamingSystem".equals(codeString)) 2771 return FHIRResourceType.NAMINGSYSTEM; 2772 if ("NutritionOrder".equals(codeString)) 2773 return FHIRResourceType.NUTRITIONORDER; 2774 if ("Observation".equals(codeString)) 2775 return FHIRResourceType.OBSERVATION; 2776 if ("ObservationDefinition".equals(codeString)) 2777 return FHIRResourceType.OBSERVATIONDEFINITION; 2778 if ("OperationDefinition".equals(codeString)) 2779 return FHIRResourceType.OPERATIONDEFINITION; 2780 if ("OperationOutcome".equals(codeString)) 2781 return FHIRResourceType.OPERATIONOUTCOME; 2782 if ("Organization".equals(codeString)) 2783 return FHIRResourceType.ORGANIZATION; 2784 if ("OrganizationAffiliation".equals(codeString)) 2785 return FHIRResourceType.ORGANIZATIONAFFILIATION; 2786 if ("Parameters".equals(codeString)) 2787 return FHIRResourceType.PARAMETERS; 2788 if ("Patient".equals(codeString)) 2789 return FHIRResourceType.PATIENT; 2790 if ("PaymentNotice".equals(codeString)) 2791 return FHIRResourceType.PAYMENTNOTICE; 2792 if ("PaymentReconciliation".equals(codeString)) 2793 return FHIRResourceType.PAYMENTRECONCILIATION; 2794 if ("Person".equals(codeString)) 2795 return FHIRResourceType.PERSON; 2796 if ("PlanDefinition".equals(codeString)) 2797 return FHIRResourceType.PLANDEFINITION; 2798 if ("Practitioner".equals(codeString)) 2799 return FHIRResourceType.PRACTITIONER; 2800 if ("PractitionerRole".equals(codeString)) 2801 return FHIRResourceType.PRACTITIONERROLE; 2802 if ("Procedure".equals(codeString)) 2803 return FHIRResourceType.PROCEDURE; 2804 if ("Provenance".equals(codeString)) 2805 return FHIRResourceType.PROVENANCE; 2806 if ("Questionnaire".equals(codeString)) 2807 return FHIRResourceType.QUESTIONNAIRE; 2808 if ("QuestionnaireResponse".equals(codeString)) 2809 return FHIRResourceType.QUESTIONNAIRERESPONSE; 2810 if ("RelatedPerson".equals(codeString)) 2811 return FHIRResourceType.RELATEDPERSON; 2812 if ("RequestGroup".equals(codeString)) 2813 return FHIRResourceType.REQUESTGROUP; 2814 if ("ResearchDefinition".equals(codeString)) 2815 return FHIRResourceType.RESEARCHDEFINITION; 2816 if ("ResearchElementDefinition".equals(codeString)) 2817 return FHIRResourceType.RESEARCHELEMENTDEFINITION; 2818 if ("ResearchStudy".equals(codeString)) 2819 return FHIRResourceType.RESEARCHSTUDY; 2820 if ("ResearchSubject".equals(codeString)) 2821 return FHIRResourceType.RESEARCHSUBJECT; 2822 if ("Resource".equals(codeString)) 2823 return FHIRResourceType.RESOURCE; 2824 if ("RiskAssessment".equals(codeString)) 2825 return FHIRResourceType.RISKASSESSMENT; 2826 if ("RiskEvidenceSynthesis".equals(codeString)) 2827 return FHIRResourceType.RISKEVIDENCESYNTHESIS; 2828 if ("Schedule".equals(codeString)) 2829 return FHIRResourceType.SCHEDULE; 2830 if ("SearchParameter".equals(codeString)) 2831 return FHIRResourceType.SEARCHPARAMETER; 2832 if ("ServiceRequest".equals(codeString)) 2833 return FHIRResourceType.SERVICEREQUEST; 2834 if ("Slot".equals(codeString)) 2835 return FHIRResourceType.SLOT; 2836 if ("Specimen".equals(codeString)) 2837 return FHIRResourceType.SPECIMEN; 2838 if ("SpecimenDefinition".equals(codeString)) 2839 return FHIRResourceType.SPECIMENDEFINITION; 2840 if ("StructureDefinition".equals(codeString)) 2841 return FHIRResourceType.STRUCTUREDEFINITION; 2842 if ("StructureMap".equals(codeString)) 2843 return FHIRResourceType.STRUCTUREMAP; 2844 if ("Subscription".equals(codeString)) 2845 return FHIRResourceType.SUBSCRIPTION; 2846 if ("Substance".equals(codeString)) 2847 return FHIRResourceType.SUBSTANCE; 2848 if ("SubstanceNucleicAcid".equals(codeString)) 2849 return FHIRResourceType.SUBSTANCENUCLEICACID; 2850 if ("SubstancePolymer".equals(codeString)) 2851 return FHIRResourceType.SUBSTANCEPOLYMER; 2852 if ("SubstanceProtein".equals(codeString)) 2853 return FHIRResourceType.SUBSTANCEPROTEIN; 2854 if ("SubstanceReferenceInformation".equals(codeString)) 2855 return FHIRResourceType.SUBSTANCEREFERENCEINFORMATION; 2856 if ("SubstanceSourceMaterial".equals(codeString)) 2857 return FHIRResourceType.SUBSTANCESOURCEMATERIAL; 2858 if ("SubstanceSpecification".equals(codeString)) 2859 return FHIRResourceType.SUBSTANCESPECIFICATION; 2860 if ("SupplyDelivery".equals(codeString)) 2861 return FHIRResourceType.SUPPLYDELIVERY; 2862 if ("SupplyRequest".equals(codeString)) 2863 return FHIRResourceType.SUPPLYREQUEST; 2864 if ("Task".equals(codeString)) 2865 return FHIRResourceType.TASK; 2866 if ("TerminologyCapabilities".equals(codeString)) 2867 return FHIRResourceType.TERMINOLOGYCAPABILITIES; 2868 if ("TestReport".equals(codeString)) 2869 return FHIRResourceType.TESTREPORT; 2870 if ("TestScript".equals(codeString)) 2871 return FHIRResourceType.TESTSCRIPT; 2872 if ("ValueSet".equals(codeString)) 2873 return FHIRResourceType.VALUESET; 2874 if ("VerificationResult".equals(codeString)) 2875 return FHIRResourceType.VERIFICATIONRESULT; 2876 if ("VisionPrescription".equals(codeString)) 2877 return FHIRResourceType.VISIONPRESCRIPTION; 2878 throw new IllegalArgumentException("Unknown FHIRResourceType code '" + codeString + "'"); 2879 } 2880 2881 public Enumeration<FHIRResourceType> fromType(PrimitiveType<?> code) throws FHIRException { 2882 if (code == null) 2883 return null; 2884 if (code.isEmpty()) 2885 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.NULL, code); 2886 String codeString = code.asStringValue(); 2887 if (codeString == null || "".equals(codeString)) 2888 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.NULL, code); 2889 if ("Account".equals(codeString)) 2890 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.ACCOUNT, code); 2891 if ("ActivityDefinition".equals(codeString)) 2892 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.ACTIVITYDEFINITION, code); 2893 if ("AdverseEvent".equals(codeString)) 2894 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.ADVERSEEVENT, code); 2895 if ("AllergyIntolerance".equals(codeString)) 2896 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.ALLERGYINTOLERANCE, code); 2897 if ("Appointment".equals(codeString)) 2898 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.APPOINTMENT, code); 2899 if ("AppointmentResponse".equals(codeString)) 2900 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.APPOINTMENTRESPONSE, code); 2901 if ("AuditEvent".equals(codeString)) 2902 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.AUDITEVENT, code); 2903 if ("Basic".equals(codeString)) 2904 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.BASIC, code); 2905 if ("Binary".equals(codeString)) 2906 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.BINARY, code); 2907 if ("BiologicallyDerivedProduct".equals(codeString)) 2908 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.BIOLOGICALLYDERIVEDPRODUCT, code); 2909 if ("BodyStructure".equals(codeString)) 2910 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.BODYSTRUCTURE, code); 2911 if ("Bundle".equals(codeString)) 2912 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.BUNDLE, code); 2913 if ("CapabilityStatement".equals(codeString)) 2914 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CAPABILITYSTATEMENT, code); 2915 if ("CarePlan".equals(codeString)) 2916 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CAREPLAN, code); 2917 if ("CareTeam".equals(codeString)) 2918 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CARETEAM, code); 2919 if ("CatalogEntry".equals(codeString)) 2920 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CATALOGENTRY, code); 2921 if ("ChargeItem".equals(codeString)) 2922 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CHARGEITEM, code); 2923 if ("ChargeItemDefinition".equals(codeString)) 2924 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CHARGEITEMDEFINITION, code); 2925 if ("Claim".equals(codeString)) 2926 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CLAIM, code); 2927 if ("ClaimResponse".equals(codeString)) 2928 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CLAIMRESPONSE, code); 2929 if ("ClinicalImpression".equals(codeString)) 2930 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CLINICALIMPRESSION, code); 2931 if ("CodeSystem".equals(codeString)) 2932 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CODESYSTEM, code); 2933 if ("Communication".equals(codeString)) 2934 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.COMMUNICATION, code); 2935 if ("CommunicationRequest".equals(codeString)) 2936 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.COMMUNICATIONREQUEST, code); 2937 if ("CompartmentDefinition".equals(codeString)) 2938 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.COMPARTMENTDEFINITION, code); 2939 if ("Composition".equals(codeString)) 2940 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.COMPOSITION, code); 2941 if ("ConceptMap".equals(codeString)) 2942 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CONCEPTMAP, code); 2943 if ("Condition".equals(codeString)) 2944 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CONDITION, code); 2945 if ("Consent".equals(codeString)) 2946 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CONSENT, code); 2947 if ("Contract".equals(codeString)) 2948 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CONTRACT, code); 2949 if ("Coverage".equals(codeString)) 2950 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.COVERAGE, code); 2951 if ("CoverageEligibilityRequest".equals(codeString)) 2952 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.COVERAGEELIGIBILITYREQUEST, code); 2953 if ("CoverageEligibilityResponse".equals(codeString)) 2954 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.COVERAGEELIGIBILITYRESPONSE, code); 2955 if ("DetectedIssue".equals(codeString)) 2956 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.DETECTEDISSUE, code); 2957 if ("Device".equals(codeString)) 2958 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.DEVICE, code); 2959 if ("DeviceDefinition".equals(codeString)) 2960 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.DEVICEDEFINITION, code); 2961 if ("DeviceMetric".equals(codeString)) 2962 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.DEVICEMETRIC, code); 2963 if ("DeviceRequest".equals(codeString)) 2964 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.DEVICEREQUEST, code); 2965 if ("DeviceUseStatement".equals(codeString)) 2966 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.DEVICEUSESTATEMENT, code); 2967 if ("DiagnosticReport".equals(codeString)) 2968 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.DIAGNOSTICREPORT, code); 2969 if ("DocumentManifest".equals(codeString)) 2970 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.DOCUMENTMANIFEST, code); 2971 if ("DocumentReference".equals(codeString)) 2972 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.DOCUMENTREFERENCE, code); 2973 if ("DomainResource".equals(codeString)) 2974 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.DOMAINRESOURCE, code); 2975 if ("EffectEvidenceSynthesis".equals(codeString)) 2976 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.EFFECTEVIDENCESYNTHESIS, code); 2977 if ("Encounter".equals(codeString)) 2978 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.ENCOUNTER, code); 2979 if ("Endpoint".equals(codeString)) 2980 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.ENDPOINT, code); 2981 if ("EnrollmentRequest".equals(codeString)) 2982 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.ENROLLMENTREQUEST, code); 2983 if ("EnrollmentResponse".equals(codeString)) 2984 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.ENROLLMENTRESPONSE, code); 2985 if ("EpisodeOfCare".equals(codeString)) 2986 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.EPISODEOFCARE, code); 2987 if ("EventDefinition".equals(codeString)) 2988 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.EVENTDEFINITION, code); 2989 if ("Evidence".equals(codeString)) 2990 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.EVIDENCE, code); 2991 if ("EvidenceVariable".equals(codeString)) 2992 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.EVIDENCEVARIABLE, code); 2993 if ("ExampleScenario".equals(codeString)) 2994 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.EXAMPLESCENARIO, code); 2995 if ("ExplanationOfBenefit".equals(codeString)) 2996 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.EXPLANATIONOFBENEFIT, code); 2997 if ("FamilyMemberHistory".equals(codeString)) 2998 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.FAMILYMEMBERHISTORY, code); 2999 if ("Flag".equals(codeString)) 3000 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.FLAG, code); 3001 if ("Goal".equals(codeString)) 3002 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.GOAL, code); 3003 if ("GraphDefinition".equals(codeString)) 3004 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.GRAPHDEFINITION, code); 3005 if ("Group".equals(codeString)) 3006 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.GROUP, code); 3007 if ("GuidanceResponse".equals(codeString)) 3008 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.GUIDANCERESPONSE, code); 3009 if ("HealthcareService".equals(codeString)) 3010 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.HEALTHCARESERVICE, code); 3011 if ("ImagingStudy".equals(codeString)) 3012 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.IMAGINGSTUDY, code); 3013 if ("Immunization".equals(codeString)) 3014 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.IMMUNIZATION, code); 3015 if ("ImmunizationEvaluation".equals(codeString)) 3016 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.IMMUNIZATIONEVALUATION, code); 3017 if ("ImmunizationRecommendation".equals(codeString)) 3018 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.IMMUNIZATIONRECOMMENDATION, code); 3019 if ("ImplementationGuide".equals(codeString)) 3020 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.IMPLEMENTATIONGUIDE, code); 3021 if ("InsurancePlan".equals(codeString)) 3022 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.INSURANCEPLAN, code); 3023 if ("Invoice".equals(codeString)) 3024 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.INVOICE, code); 3025 if ("Library".equals(codeString)) 3026 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.LIBRARY, code); 3027 if ("Linkage".equals(codeString)) 3028 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.LINKAGE, code); 3029 if ("List".equals(codeString)) 3030 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.LIST, code); 3031 if ("Location".equals(codeString)) 3032 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.LOCATION, code); 3033 if ("Measure".equals(codeString)) 3034 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEASURE, code); 3035 if ("MeasureReport".equals(codeString)) 3036 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEASUREREPORT, code); 3037 if ("Media".equals(codeString)) 3038 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDIA, code); 3039 if ("Medication".equals(codeString)) 3040 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICATION, code); 3041 if ("MedicationAdministration".equals(codeString)) 3042 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICATIONADMINISTRATION, code); 3043 if ("MedicationDispense".equals(codeString)) 3044 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICATIONDISPENSE, code); 3045 if ("MedicationKnowledge".equals(codeString)) 3046 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICATIONKNOWLEDGE, code); 3047 if ("MedicationRequest".equals(codeString)) 3048 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICATIONREQUEST, code); 3049 if ("MedicationStatement".equals(codeString)) 3050 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICATIONSTATEMENT, code); 3051 if ("MedicinalProduct".equals(codeString)) 3052 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICINALPRODUCT, code); 3053 if ("MedicinalProductAuthorization".equals(codeString)) 3054 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICINALPRODUCTAUTHORIZATION, code); 3055 if ("MedicinalProductContraindication".equals(codeString)) 3056 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICINALPRODUCTCONTRAINDICATION, code); 3057 if ("MedicinalProductIndication".equals(codeString)) 3058 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICINALPRODUCTINDICATION, code); 3059 if ("MedicinalProductIngredient".equals(codeString)) 3060 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICINALPRODUCTINGREDIENT, code); 3061 if ("MedicinalProductInteraction".equals(codeString)) 3062 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICINALPRODUCTINTERACTION, code); 3063 if ("MedicinalProductManufactured".equals(codeString)) 3064 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICINALPRODUCTMANUFACTURED, code); 3065 if ("MedicinalProductPackaged".equals(codeString)) 3066 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICINALPRODUCTPACKAGED, code); 3067 if ("MedicinalProductPharmaceutical".equals(codeString)) 3068 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICINALPRODUCTPHARMACEUTICAL, code); 3069 if ("MedicinalProductUndesirableEffect".equals(codeString)) 3070 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICINALPRODUCTUNDESIRABLEEFFECT, code); 3071 if ("MessageDefinition".equals(codeString)) 3072 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MESSAGEDEFINITION, code); 3073 if ("MessageHeader".equals(codeString)) 3074 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MESSAGEHEADER, code); 3075 if ("MolecularSequence".equals(codeString)) 3076 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MOLECULARSEQUENCE, code); 3077 if ("NamingSystem".equals(codeString)) 3078 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.NAMINGSYSTEM, code); 3079 if ("NutritionOrder".equals(codeString)) 3080 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.NUTRITIONORDER, code); 3081 if ("Observation".equals(codeString)) 3082 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.OBSERVATION, code); 3083 if ("ObservationDefinition".equals(codeString)) 3084 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.OBSERVATIONDEFINITION, code); 3085 if ("OperationDefinition".equals(codeString)) 3086 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.OPERATIONDEFINITION, code); 3087 if ("OperationOutcome".equals(codeString)) 3088 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.OPERATIONOUTCOME, code); 3089 if ("Organization".equals(codeString)) 3090 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.ORGANIZATION, code); 3091 if ("OrganizationAffiliation".equals(codeString)) 3092 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.ORGANIZATIONAFFILIATION, code); 3093 if ("Parameters".equals(codeString)) 3094 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.PARAMETERS, code); 3095 if ("Patient".equals(codeString)) 3096 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.PATIENT, code); 3097 if ("PaymentNotice".equals(codeString)) 3098 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.PAYMENTNOTICE, code); 3099 if ("PaymentReconciliation".equals(codeString)) 3100 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.PAYMENTRECONCILIATION, code); 3101 if ("Person".equals(codeString)) 3102 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.PERSON, code); 3103 if ("PlanDefinition".equals(codeString)) 3104 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.PLANDEFINITION, code); 3105 if ("Practitioner".equals(codeString)) 3106 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.PRACTITIONER, code); 3107 if ("PractitionerRole".equals(codeString)) 3108 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.PRACTITIONERROLE, code); 3109 if ("Procedure".equals(codeString)) 3110 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.PROCEDURE, code); 3111 if ("Provenance".equals(codeString)) 3112 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.PROVENANCE, code); 3113 if ("Questionnaire".equals(codeString)) 3114 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.QUESTIONNAIRE, code); 3115 if ("QuestionnaireResponse".equals(codeString)) 3116 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.QUESTIONNAIRERESPONSE, code); 3117 if ("RelatedPerson".equals(codeString)) 3118 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.RELATEDPERSON, code); 3119 if ("RequestGroup".equals(codeString)) 3120 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.REQUESTGROUP, code); 3121 if ("ResearchDefinition".equals(codeString)) 3122 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.RESEARCHDEFINITION, code); 3123 if ("ResearchElementDefinition".equals(codeString)) 3124 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.RESEARCHELEMENTDEFINITION, code); 3125 if ("ResearchStudy".equals(codeString)) 3126 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.RESEARCHSTUDY, code); 3127 if ("ResearchSubject".equals(codeString)) 3128 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.RESEARCHSUBJECT, code); 3129 if ("Resource".equals(codeString)) 3130 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.RESOURCE, code); 3131 if ("RiskAssessment".equals(codeString)) 3132 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.RISKASSESSMENT, code); 3133 if ("RiskEvidenceSynthesis".equals(codeString)) 3134 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.RISKEVIDENCESYNTHESIS, code); 3135 if ("Schedule".equals(codeString)) 3136 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SCHEDULE, code); 3137 if ("SearchParameter".equals(codeString)) 3138 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SEARCHPARAMETER, code); 3139 if ("ServiceRequest".equals(codeString)) 3140 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SERVICEREQUEST, code); 3141 if ("Slot".equals(codeString)) 3142 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SLOT, code); 3143 if ("Specimen".equals(codeString)) 3144 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SPECIMEN, code); 3145 if ("SpecimenDefinition".equals(codeString)) 3146 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SPECIMENDEFINITION, code); 3147 if ("StructureDefinition".equals(codeString)) 3148 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.STRUCTUREDEFINITION, code); 3149 if ("StructureMap".equals(codeString)) 3150 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.STRUCTUREMAP, code); 3151 if ("Subscription".equals(codeString)) 3152 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SUBSCRIPTION, code); 3153 if ("Substance".equals(codeString)) 3154 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SUBSTANCE, code); 3155 if ("SubstanceNucleicAcid".equals(codeString)) 3156 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SUBSTANCENUCLEICACID, code); 3157 if ("SubstancePolymer".equals(codeString)) 3158 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SUBSTANCEPOLYMER, code); 3159 if ("SubstanceProtein".equals(codeString)) 3160 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SUBSTANCEPROTEIN, code); 3161 if ("SubstanceReferenceInformation".equals(codeString)) 3162 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SUBSTANCEREFERENCEINFORMATION, code); 3163 if ("SubstanceSourceMaterial".equals(codeString)) 3164 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SUBSTANCESOURCEMATERIAL, code); 3165 if ("SubstanceSpecification".equals(codeString)) 3166 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SUBSTANCESPECIFICATION, code); 3167 if ("SupplyDelivery".equals(codeString)) 3168 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SUPPLYDELIVERY, code); 3169 if ("SupplyRequest".equals(codeString)) 3170 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SUPPLYREQUEST, code); 3171 if ("Task".equals(codeString)) 3172 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.TASK, code); 3173 if ("TerminologyCapabilities".equals(codeString)) 3174 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.TERMINOLOGYCAPABILITIES, code); 3175 if ("TestReport".equals(codeString)) 3176 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.TESTREPORT, code); 3177 if ("TestScript".equals(codeString)) 3178 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.TESTSCRIPT, code); 3179 if ("ValueSet".equals(codeString)) 3180 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.VALUESET, code); 3181 if ("VerificationResult".equals(codeString)) 3182 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.VERIFICATIONRESULT, code); 3183 if ("VisionPrescription".equals(codeString)) 3184 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.VISIONPRESCRIPTION, code); 3185 throw new FHIRException("Unknown FHIRResourceType code '" + codeString + "'"); 3186 } 3187 3188 public String toCode(FHIRResourceType code) { 3189 if (code == FHIRResourceType.ACCOUNT) 3190 return "Account"; 3191 if (code == FHIRResourceType.ACTIVITYDEFINITION) 3192 return "ActivityDefinition"; 3193 if (code == FHIRResourceType.ADVERSEEVENT) 3194 return "AdverseEvent"; 3195 if (code == FHIRResourceType.ALLERGYINTOLERANCE) 3196 return "AllergyIntolerance"; 3197 if (code == FHIRResourceType.APPOINTMENT) 3198 return "Appointment"; 3199 if (code == FHIRResourceType.APPOINTMENTRESPONSE) 3200 return "AppointmentResponse"; 3201 if (code == FHIRResourceType.AUDITEVENT) 3202 return "AuditEvent"; 3203 if (code == FHIRResourceType.BASIC) 3204 return "Basic"; 3205 if (code == FHIRResourceType.BINARY) 3206 return "Binary"; 3207 if (code == FHIRResourceType.BIOLOGICALLYDERIVEDPRODUCT) 3208 return "BiologicallyDerivedProduct"; 3209 if (code == FHIRResourceType.BODYSTRUCTURE) 3210 return "BodyStructure"; 3211 if (code == FHIRResourceType.BUNDLE) 3212 return "Bundle"; 3213 if (code == FHIRResourceType.CAPABILITYSTATEMENT) 3214 return "CapabilityStatement"; 3215 if (code == FHIRResourceType.CAREPLAN) 3216 return "CarePlan"; 3217 if (code == FHIRResourceType.CARETEAM) 3218 return "CareTeam"; 3219 if (code == FHIRResourceType.CATALOGENTRY) 3220 return "CatalogEntry"; 3221 if (code == FHIRResourceType.CHARGEITEM) 3222 return "ChargeItem"; 3223 if (code == FHIRResourceType.CHARGEITEMDEFINITION) 3224 return "ChargeItemDefinition"; 3225 if (code == FHIRResourceType.CLAIM) 3226 return "Claim"; 3227 if (code == FHIRResourceType.CLAIMRESPONSE) 3228 return "ClaimResponse"; 3229 if (code == FHIRResourceType.CLINICALIMPRESSION) 3230 return "ClinicalImpression"; 3231 if (code == FHIRResourceType.CODESYSTEM) 3232 return "CodeSystem"; 3233 if (code == FHIRResourceType.COMMUNICATION) 3234 return "Communication"; 3235 if (code == FHIRResourceType.COMMUNICATIONREQUEST) 3236 return "CommunicationRequest"; 3237 if (code == FHIRResourceType.COMPARTMENTDEFINITION) 3238 return "CompartmentDefinition"; 3239 if (code == FHIRResourceType.COMPOSITION) 3240 return "Composition"; 3241 if (code == FHIRResourceType.CONCEPTMAP) 3242 return "ConceptMap"; 3243 if (code == FHIRResourceType.CONDITION) 3244 return "Condition"; 3245 if (code == FHIRResourceType.CONSENT) 3246 return "Consent"; 3247 if (code == FHIRResourceType.CONTRACT) 3248 return "Contract"; 3249 if (code == FHIRResourceType.COVERAGE) 3250 return "Coverage"; 3251 if (code == FHIRResourceType.COVERAGEELIGIBILITYREQUEST) 3252 return "CoverageEligibilityRequest"; 3253 if (code == FHIRResourceType.COVERAGEELIGIBILITYRESPONSE) 3254 return "CoverageEligibilityResponse"; 3255 if (code == FHIRResourceType.DETECTEDISSUE) 3256 return "DetectedIssue"; 3257 if (code == FHIRResourceType.DEVICE) 3258 return "Device"; 3259 if (code == FHIRResourceType.DEVICEDEFINITION) 3260 return "DeviceDefinition"; 3261 if (code == FHIRResourceType.DEVICEMETRIC) 3262 return "DeviceMetric"; 3263 if (code == FHIRResourceType.DEVICEREQUEST) 3264 return "DeviceRequest"; 3265 if (code == FHIRResourceType.DEVICEUSESTATEMENT) 3266 return "DeviceUseStatement"; 3267 if (code == FHIRResourceType.DIAGNOSTICREPORT) 3268 return "DiagnosticReport"; 3269 if (code == FHIRResourceType.DOCUMENTMANIFEST) 3270 return "DocumentManifest"; 3271 if (code == FHIRResourceType.DOCUMENTREFERENCE) 3272 return "DocumentReference"; 3273 if (code == FHIRResourceType.DOMAINRESOURCE) 3274 return "DomainResource"; 3275 if (code == FHIRResourceType.EFFECTEVIDENCESYNTHESIS) 3276 return "EffectEvidenceSynthesis"; 3277 if (code == FHIRResourceType.ENCOUNTER) 3278 return "Encounter"; 3279 if (code == FHIRResourceType.ENDPOINT) 3280 return "Endpoint"; 3281 if (code == FHIRResourceType.ENROLLMENTREQUEST) 3282 return "EnrollmentRequest"; 3283 if (code == FHIRResourceType.ENROLLMENTRESPONSE) 3284 return "EnrollmentResponse"; 3285 if (code == FHIRResourceType.EPISODEOFCARE) 3286 return "EpisodeOfCare"; 3287 if (code == FHIRResourceType.EVENTDEFINITION) 3288 return "EventDefinition"; 3289 if (code == FHIRResourceType.EVIDENCE) 3290 return "Evidence"; 3291 if (code == FHIRResourceType.EVIDENCEVARIABLE) 3292 return "EvidenceVariable"; 3293 if (code == FHIRResourceType.EXAMPLESCENARIO) 3294 return "ExampleScenario"; 3295 if (code == FHIRResourceType.EXPLANATIONOFBENEFIT) 3296 return "ExplanationOfBenefit"; 3297 if (code == FHIRResourceType.FAMILYMEMBERHISTORY) 3298 return "FamilyMemberHistory"; 3299 if (code == FHIRResourceType.FLAG) 3300 return "Flag"; 3301 if (code == FHIRResourceType.GOAL) 3302 return "Goal"; 3303 if (code == FHIRResourceType.GRAPHDEFINITION) 3304 return "GraphDefinition"; 3305 if (code == FHIRResourceType.GROUP) 3306 return "Group"; 3307 if (code == FHIRResourceType.GUIDANCERESPONSE) 3308 return "GuidanceResponse"; 3309 if (code == FHIRResourceType.HEALTHCARESERVICE) 3310 return "HealthcareService"; 3311 if (code == FHIRResourceType.IMAGINGSTUDY) 3312 return "ImagingStudy"; 3313 if (code == FHIRResourceType.IMMUNIZATION) 3314 return "Immunization"; 3315 if (code == FHIRResourceType.IMMUNIZATIONEVALUATION) 3316 return "ImmunizationEvaluation"; 3317 if (code == FHIRResourceType.IMMUNIZATIONRECOMMENDATION) 3318 return "ImmunizationRecommendation"; 3319 if (code == FHIRResourceType.IMPLEMENTATIONGUIDE) 3320 return "ImplementationGuide"; 3321 if (code == FHIRResourceType.INSURANCEPLAN) 3322 return "InsurancePlan"; 3323 if (code == FHIRResourceType.INVOICE) 3324 return "Invoice"; 3325 if (code == FHIRResourceType.LIBRARY) 3326 return "Library"; 3327 if (code == FHIRResourceType.LINKAGE) 3328 return "Linkage"; 3329 if (code == FHIRResourceType.LIST) 3330 return "List"; 3331 if (code == FHIRResourceType.LOCATION) 3332 return "Location"; 3333 if (code == FHIRResourceType.MEASURE) 3334 return "Measure"; 3335 if (code == FHIRResourceType.MEASUREREPORT) 3336 return "MeasureReport"; 3337 if (code == FHIRResourceType.MEDIA) 3338 return "Media"; 3339 if (code == FHIRResourceType.MEDICATION) 3340 return "Medication"; 3341 if (code == FHIRResourceType.MEDICATIONADMINISTRATION) 3342 return "MedicationAdministration"; 3343 if (code == FHIRResourceType.MEDICATIONDISPENSE) 3344 return "MedicationDispense"; 3345 if (code == FHIRResourceType.MEDICATIONKNOWLEDGE) 3346 return "MedicationKnowledge"; 3347 if (code == FHIRResourceType.MEDICATIONREQUEST) 3348 return "MedicationRequest"; 3349 if (code == FHIRResourceType.MEDICATIONSTATEMENT) 3350 return "MedicationStatement"; 3351 if (code == FHIRResourceType.MEDICINALPRODUCT) 3352 return "MedicinalProduct"; 3353 if (code == FHIRResourceType.MEDICINALPRODUCTAUTHORIZATION) 3354 return "MedicinalProductAuthorization"; 3355 if (code == FHIRResourceType.MEDICINALPRODUCTCONTRAINDICATION) 3356 return "MedicinalProductContraindication"; 3357 if (code == FHIRResourceType.MEDICINALPRODUCTINDICATION) 3358 return "MedicinalProductIndication"; 3359 if (code == FHIRResourceType.MEDICINALPRODUCTINGREDIENT) 3360 return "MedicinalProductIngredient"; 3361 if (code == FHIRResourceType.MEDICINALPRODUCTINTERACTION) 3362 return "MedicinalProductInteraction"; 3363 if (code == FHIRResourceType.MEDICINALPRODUCTMANUFACTURED) 3364 return "MedicinalProductManufactured"; 3365 if (code == FHIRResourceType.MEDICINALPRODUCTPACKAGED) 3366 return "MedicinalProductPackaged"; 3367 if (code == FHIRResourceType.MEDICINALPRODUCTPHARMACEUTICAL) 3368 return "MedicinalProductPharmaceutical"; 3369 if (code == FHIRResourceType.MEDICINALPRODUCTUNDESIRABLEEFFECT) 3370 return "MedicinalProductUndesirableEffect"; 3371 if (code == FHIRResourceType.MESSAGEDEFINITION) 3372 return "MessageDefinition"; 3373 if (code == FHIRResourceType.MESSAGEHEADER) 3374 return "MessageHeader"; 3375 if (code == FHIRResourceType.MOLECULARSEQUENCE) 3376 return "MolecularSequence"; 3377 if (code == FHIRResourceType.NAMINGSYSTEM) 3378 return "NamingSystem"; 3379 if (code == FHIRResourceType.NUTRITIONORDER) 3380 return "NutritionOrder"; 3381 if (code == FHIRResourceType.OBSERVATION) 3382 return "Observation"; 3383 if (code == FHIRResourceType.OBSERVATIONDEFINITION) 3384 return "ObservationDefinition"; 3385 if (code == FHIRResourceType.OPERATIONDEFINITION) 3386 return "OperationDefinition"; 3387 if (code == FHIRResourceType.OPERATIONOUTCOME) 3388 return "OperationOutcome"; 3389 if (code == FHIRResourceType.ORGANIZATION) 3390 return "Organization"; 3391 if (code == FHIRResourceType.ORGANIZATIONAFFILIATION) 3392 return "OrganizationAffiliation"; 3393 if (code == FHIRResourceType.PARAMETERS) 3394 return "Parameters"; 3395 if (code == FHIRResourceType.PATIENT) 3396 return "Patient"; 3397 if (code == FHIRResourceType.PAYMENTNOTICE) 3398 return "PaymentNotice"; 3399 if (code == FHIRResourceType.PAYMENTRECONCILIATION) 3400 return "PaymentReconciliation"; 3401 if (code == FHIRResourceType.PERSON) 3402 return "Person"; 3403 if (code == FHIRResourceType.PLANDEFINITION) 3404 return "PlanDefinition"; 3405 if (code == FHIRResourceType.PRACTITIONER) 3406 return "Practitioner"; 3407 if (code == FHIRResourceType.PRACTITIONERROLE) 3408 return "PractitionerRole"; 3409 if (code == FHIRResourceType.PROCEDURE) 3410 return "Procedure"; 3411 if (code == FHIRResourceType.PROVENANCE) 3412 return "Provenance"; 3413 if (code == FHIRResourceType.QUESTIONNAIRE) 3414 return "Questionnaire"; 3415 if (code == FHIRResourceType.QUESTIONNAIRERESPONSE) 3416 return "QuestionnaireResponse"; 3417 if (code == FHIRResourceType.RELATEDPERSON) 3418 return "RelatedPerson"; 3419 if (code == FHIRResourceType.REQUESTGROUP) 3420 return "RequestGroup"; 3421 if (code == FHIRResourceType.RESEARCHDEFINITION) 3422 return "ResearchDefinition"; 3423 if (code == FHIRResourceType.RESEARCHELEMENTDEFINITION) 3424 return "ResearchElementDefinition"; 3425 if (code == FHIRResourceType.RESEARCHSTUDY) 3426 return "ResearchStudy"; 3427 if (code == FHIRResourceType.RESEARCHSUBJECT) 3428 return "ResearchSubject"; 3429 if (code == FHIRResourceType.RESOURCE) 3430 return "Resource"; 3431 if (code == FHIRResourceType.RISKASSESSMENT) 3432 return "RiskAssessment"; 3433 if (code == FHIRResourceType.RISKEVIDENCESYNTHESIS) 3434 return "RiskEvidenceSynthesis"; 3435 if (code == FHIRResourceType.SCHEDULE) 3436 return "Schedule"; 3437 if (code == FHIRResourceType.SEARCHPARAMETER) 3438 return "SearchParameter"; 3439 if (code == FHIRResourceType.SERVICEREQUEST) 3440 return "ServiceRequest"; 3441 if (code == FHIRResourceType.SLOT) 3442 return "Slot"; 3443 if (code == FHIRResourceType.SPECIMEN) 3444 return "Specimen"; 3445 if (code == FHIRResourceType.SPECIMENDEFINITION) 3446 return "SpecimenDefinition"; 3447 if (code == FHIRResourceType.STRUCTUREDEFINITION) 3448 return "StructureDefinition"; 3449 if (code == FHIRResourceType.STRUCTUREMAP) 3450 return "StructureMap"; 3451 if (code == FHIRResourceType.SUBSCRIPTION) 3452 return "Subscription"; 3453 if (code == FHIRResourceType.SUBSTANCE) 3454 return "Substance"; 3455 if (code == FHIRResourceType.SUBSTANCENUCLEICACID) 3456 return "SubstanceNucleicAcid"; 3457 if (code == FHIRResourceType.SUBSTANCEPOLYMER) 3458 return "SubstancePolymer"; 3459 if (code == FHIRResourceType.SUBSTANCEPROTEIN) 3460 return "SubstanceProtein"; 3461 if (code == FHIRResourceType.SUBSTANCEREFERENCEINFORMATION) 3462 return "SubstanceReferenceInformation"; 3463 if (code == FHIRResourceType.SUBSTANCESOURCEMATERIAL) 3464 return "SubstanceSourceMaterial"; 3465 if (code == FHIRResourceType.SUBSTANCESPECIFICATION) 3466 return "SubstanceSpecification"; 3467 if (code == FHIRResourceType.SUPPLYDELIVERY) 3468 return "SupplyDelivery"; 3469 if (code == FHIRResourceType.SUPPLYREQUEST) 3470 return "SupplyRequest"; 3471 if (code == FHIRResourceType.TASK) 3472 return "Task"; 3473 if (code == FHIRResourceType.TERMINOLOGYCAPABILITIES) 3474 return "TerminologyCapabilities"; 3475 if (code == FHIRResourceType.TESTREPORT) 3476 return "TestReport"; 3477 if (code == FHIRResourceType.TESTSCRIPT) 3478 return "TestScript"; 3479 if (code == FHIRResourceType.VALUESET) 3480 return "ValueSet"; 3481 if (code == FHIRResourceType.VERIFICATIONRESULT) 3482 return "VerificationResult"; 3483 if (code == FHIRResourceType.VISIONPRESCRIPTION) 3484 return "VisionPrescription"; 3485 return "?"; 3486 } 3487 3488 public String toSystem(FHIRResourceType code) { 3489 return code.getSystem(); 3490 } 3491 } 3492 3493 @Block() 3494 public static class ExampleScenarioActorComponent extends BackboneElement implements IBaseBackboneElement { 3495 /** 3496 * ID or acronym of actor. 3497 */ 3498 @Child(name = "actorId", type = { 3499 StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 3500 @Description(shortDefinition = "ID or acronym of the actor", formalDefinition = "ID or acronym of actor.") 3501 protected StringType actorId; 3502 3503 /** 3504 * The type of actor - person or system. 3505 */ 3506 @Child(name = "type", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 3507 @Description(shortDefinition = "person | entity", formalDefinition = "The type of actor - person or system.") 3508 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/examplescenario-actor-type") 3509 protected Enumeration<ExampleScenarioActorType> type; 3510 3511 /** 3512 * The name of the actor as shown in the page. 3513 */ 3514 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 3515 @Description(shortDefinition = "The name of the actor as shown in the page", formalDefinition = "The name of the actor as shown in the page.") 3516 protected StringType name; 3517 3518 /** 3519 * The description of the actor. 3520 */ 3521 @Child(name = "description", type = { 3522 MarkdownType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 3523 @Description(shortDefinition = "The description of the actor", formalDefinition = "The description of the actor.") 3524 protected MarkdownType description; 3525 3526 private static final long serialVersionUID = 1348364162L; 3527 3528 /** 3529 * Constructor 3530 */ 3531 public ExampleScenarioActorComponent() { 3532 super(); 3533 } 3534 3535 /** 3536 * Constructor 3537 */ 3538 public ExampleScenarioActorComponent(StringType actorId, Enumeration<ExampleScenarioActorType> type) { 3539 super(); 3540 this.actorId = actorId; 3541 this.type = type; 3542 } 3543 3544 /** 3545 * @return {@link #actorId} (ID or acronym of actor.). This is the underlying 3546 * object with id, value and extensions. The accessor "getActorId" gives 3547 * direct access to the value 3548 */ 3549 public StringType getActorIdElement() { 3550 if (this.actorId == null) 3551 if (Configuration.errorOnAutoCreate()) 3552 throw new Error("Attempt to auto-create ExampleScenarioActorComponent.actorId"); 3553 else if (Configuration.doAutoCreate()) 3554 this.actorId = new StringType(); // bb 3555 return this.actorId; 3556 } 3557 3558 public boolean hasActorIdElement() { 3559 return this.actorId != null && !this.actorId.isEmpty(); 3560 } 3561 3562 public boolean hasActorId() { 3563 return this.actorId != null && !this.actorId.isEmpty(); 3564 } 3565 3566 /** 3567 * @param value {@link #actorId} (ID or acronym of actor.). This is the 3568 * underlying object with id, value and extensions. The accessor 3569 * "getActorId" gives direct access to the value 3570 */ 3571 public ExampleScenarioActorComponent setActorIdElement(StringType value) { 3572 this.actorId = value; 3573 return this; 3574 } 3575 3576 /** 3577 * @return ID or acronym of actor. 3578 */ 3579 public String getActorId() { 3580 return this.actorId == null ? null : this.actorId.getValue(); 3581 } 3582 3583 /** 3584 * @param value ID or acronym of actor. 3585 */ 3586 public ExampleScenarioActorComponent setActorId(String value) { 3587 if (this.actorId == null) 3588 this.actorId = new StringType(); 3589 this.actorId.setValue(value); 3590 return this; 3591 } 3592 3593 /** 3594 * @return {@link #type} (The type of actor - person or system.). This is the 3595 * underlying object with id, value and extensions. The accessor 3596 * "getType" gives direct access to the value 3597 */ 3598 public Enumeration<ExampleScenarioActorType> getTypeElement() { 3599 if (this.type == null) 3600 if (Configuration.errorOnAutoCreate()) 3601 throw new Error("Attempt to auto-create ExampleScenarioActorComponent.type"); 3602 else if (Configuration.doAutoCreate()) 3603 this.type = new Enumeration<ExampleScenarioActorType>(new ExampleScenarioActorTypeEnumFactory()); // bb 3604 return this.type; 3605 } 3606 3607 public boolean hasTypeElement() { 3608 return this.type != null && !this.type.isEmpty(); 3609 } 3610 3611 public boolean hasType() { 3612 return this.type != null && !this.type.isEmpty(); 3613 } 3614 3615 /** 3616 * @param value {@link #type} (The type of actor - person or system.). This is 3617 * the underlying object with id, value and extensions. The 3618 * accessor "getType" gives direct access to the value 3619 */ 3620 public ExampleScenarioActorComponent setTypeElement(Enumeration<ExampleScenarioActorType> value) { 3621 this.type = value; 3622 return this; 3623 } 3624 3625 /** 3626 * @return The type of actor - person or system. 3627 */ 3628 public ExampleScenarioActorType getType() { 3629 return this.type == null ? null : this.type.getValue(); 3630 } 3631 3632 /** 3633 * @param value The type of actor - person or system. 3634 */ 3635 public ExampleScenarioActorComponent setType(ExampleScenarioActorType value) { 3636 if (this.type == null) 3637 this.type = new Enumeration<ExampleScenarioActorType>(new ExampleScenarioActorTypeEnumFactory()); 3638 this.type.setValue(value); 3639 return this; 3640 } 3641 3642 /** 3643 * @return {@link #name} (The name of the actor as shown in the page.). This is 3644 * the underlying object with id, value and extensions. The accessor 3645 * "getName" gives direct access to the value 3646 */ 3647 public StringType getNameElement() { 3648 if (this.name == null) 3649 if (Configuration.errorOnAutoCreate()) 3650 throw new Error("Attempt to auto-create ExampleScenarioActorComponent.name"); 3651 else if (Configuration.doAutoCreate()) 3652 this.name = new StringType(); // bb 3653 return this.name; 3654 } 3655 3656 public boolean hasNameElement() { 3657 return this.name != null && !this.name.isEmpty(); 3658 } 3659 3660 public boolean hasName() { 3661 return this.name != null && !this.name.isEmpty(); 3662 } 3663 3664 /** 3665 * @param value {@link #name} (The name of the actor as shown in the page.). 3666 * This is the underlying object with id, value and extensions. The 3667 * accessor "getName" gives direct access to the value 3668 */ 3669 public ExampleScenarioActorComponent setNameElement(StringType value) { 3670 this.name = value; 3671 return this; 3672 } 3673 3674 /** 3675 * @return The name of the actor as shown in the page. 3676 */ 3677 public String getName() { 3678 return this.name == null ? null : this.name.getValue(); 3679 } 3680 3681 /** 3682 * @param value The name of the actor as shown in the page. 3683 */ 3684 public ExampleScenarioActorComponent setName(String value) { 3685 if (Utilities.noString(value)) 3686 this.name = null; 3687 else { 3688 if (this.name == null) 3689 this.name = new StringType(); 3690 this.name.setValue(value); 3691 } 3692 return this; 3693 } 3694 3695 /** 3696 * @return {@link #description} (The description of the actor.). This is the 3697 * underlying object with id, value and extensions. The accessor 3698 * "getDescription" gives direct access to the value 3699 */ 3700 public MarkdownType getDescriptionElement() { 3701 if (this.description == null) 3702 if (Configuration.errorOnAutoCreate()) 3703 throw new Error("Attempt to auto-create ExampleScenarioActorComponent.description"); 3704 else if (Configuration.doAutoCreate()) 3705 this.description = new MarkdownType(); // bb 3706 return this.description; 3707 } 3708 3709 public boolean hasDescriptionElement() { 3710 return this.description != null && !this.description.isEmpty(); 3711 } 3712 3713 public boolean hasDescription() { 3714 return this.description != null && !this.description.isEmpty(); 3715 } 3716 3717 /** 3718 * @param value {@link #description} (The description of the actor.). This is 3719 * the underlying object with id, value and extensions. The 3720 * accessor "getDescription" gives direct access to the value 3721 */ 3722 public ExampleScenarioActorComponent setDescriptionElement(MarkdownType value) { 3723 this.description = value; 3724 return this; 3725 } 3726 3727 /** 3728 * @return The description of the actor. 3729 */ 3730 public String getDescription() { 3731 return this.description == null ? null : this.description.getValue(); 3732 } 3733 3734 /** 3735 * @param value The description of the actor. 3736 */ 3737 public ExampleScenarioActorComponent setDescription(String value) { 3738 if (value == null) 3739 this.description = null; 3740 else { 3741 if (this.description == null) 3742 this.description = new MarkdownType(); 3743 this.description.setValue(value); 3744 } 3745 return this; 3746 } 3747 3748 protected void listChildren(List<Property> children) { 3749 super.listChildren(children); 3750 children.add(new Property("actorId", "string", "ID or acronym of actor.", 0, 1, actorId)); 3751 children.add(new Property("type", "code", "The type of actor - person or system.", 0, 1, type)); 3752 children.add(new Property("name", "string", "The name of the actor as shown in the page.", 0, 1, name)); 3753 children.add(new Property("description", "markdown", "The description of the actor.", 0, 1, description)); 3754 } 3755 3756 @Override 3757 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3758 switch (_hash) { 3759 case -1161623056: 3760 /* actorId */ return new Property("actorId", "string", "ID or acronym of actor.", 0, 1, actorId); 3761 case 3575610: 3762 /* type */ return new Property("type", "code", "The type of actor - person or system.", 0, 1, type); 3763 case 3373707: 3764 /* name */ return new Property("name", "string", "The name of the actor as shown in the page.", 0, 1, name); 3765 case -1724546052: 3766 /* description */ return new Property("description", "markdown", "The description of the actor.", 0, 1, 3767 description); 3768 default: 3769 return super.getNamedProperty(_hash, _name, _checkValid); 3770 } 3771 3772 } 3773 3774 @Override 3775 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3776 switch (hash) { 3777 case -1161623056: 3778 /* actorId */ return this.actorId == null ? new Base[0] : new Base[] { this.actorId }; // StringType 3779 case 3575610: 3780 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<ExampleScenarioActorType> 3781 case 3373707: 3782 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 3783 case -1724546052: 3784 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 3785 default: 3786 return super.getProperty(hash, name, checkValid); 3787 } 3788 3789 } 3790 3791 @Override 3792 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3793 switch (hash) { 3794 case -1161623056: // actorId 3795 this.actorId = castToString(value); // StringType 3796 return value; 3797 case 3575610: // type 3798 value = new ExampleScenarioActorTypeEnumFactory().fromType(castToCode(value)); 3799 this.type = (Enumeration) value; // Enumeration<ExampleScenarioActorType> 3800 return value; 3801 case 3373707: // name 3802 this.name = castToString(value); // StringType 3803 return value; 3804 case -1724546052: // description 3805 this.description = castToMarkdown(value); // MarkdownType 3806 return value; 3807 default: 3808 return super.setProperty(hash, name, value); 3809 } 3810 3811 } 3812 3813 @Override 3814 public Base setProperty(String name, Base value) throws FHIRException { 3815 if (name.equals("actorId")) { 3816 this.actorId = castToString(value); // StringType 3817 } else if (name.equals("type")) { 3818 value = new ExampleScenarioActorTypeEnumFactory().fromType(castToCode(value)); 3819 this.type = (Enumeration) value; // Enumeration<ExampleScenarioActorType> 3820 } else if (name.equals("name")) { 3821 this.name = castToString(value); // StringType 3822 } else if (name.equals("description")) { 3823 this.description = castToMarkdown(value); // MarkdownType 3824 } else 3825 return super.setProperty(name, value); 3826 return value; 3827 } 3828 3829 @Override 3830 public Base makeProperty(int hash, String name) throws FHIRException { 3831 switch (hash) { 3832 case -1161623056: 3833 return getActorIdElement(); 3834 case 3575610: 3835 return getTypeElement(); 3836 case 3373707: 3837 return getNameElement(); 3838 case -1724546052: 3839 return getDescriptionElement(); 3840 default: 3841 return super.makeProperty(hash, name); 3842 } 3843 3844 } 3845 3846 @Override 3847 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3848 switch (hash) { 3849 case -1161623056: 3850 /* actorId */ return new String[] { "string" }; 3851 case 3575610: 3852 /* type */ return new String[] { "code" }; 3853 case 3373707: 3854 /* name */ return new String[] { "string" }; 3855 case -1724546052: 3856 /* description */ return new String[] { "markdown" }; 3857 default: 3858 return super.getTypesForProperty(hash, name); 3859 } 3860 3861 } 3862 3863 @Override 3864 public Base addChild(String name) throws FHIRException { 3865 if (name.equals("actorId")) { 3866 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.actorId"); 3867 } else if (name.equals("type")) { 3868 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.type"); 3869 } else if (name.equals("name")) { 3870 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.name"); 3871 } else if (name.equals("description")) { 3872 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.description"); 3873 } else 3874 return super.addChild(name); 3875 } 3876 3877 public ExampleScenarioActorComponent copy() { 3878 ExampleScenarioActorComponent dst = new ExampleScenarioActorComponent(); 3879 copyValues(dst); 3880 return dst; 3881 } 3882 3883 public void copyValues(ExampleScenarioActorComponent dst) { 3884 super.copyValues(dst); 3885 dst.actorId = actorId == null ? null : actorId.copy(); 3886 dst.type = type == null ? null : type.copy(); 3887 dst.name = name == null ? null : name.copy(); 3888 dst.description = description == null ? null : description.copy(); 3889 } 3890 3891 @Override 3892 public boolean equalsDeep(Base other_) { 3893 if (!super.equalsDeep(other_)) 3894 return false; 3895 if (!(other_ instanceof ExampleScenarioActorComponent)) 3896 return false; 3897 ExampleScenarioActorComponent o = (ExampleScenarioActorComponent) other_; 3898 return compareDeep(actorId, o.actorId, true) && compareDeep(type, o.type, true) && compareDeep(name, o.name, true) 3899 && compareDeep(description, o.description, true); 3900 } 3901 3902 @Override 3903 public boolean equalsShallow(Base other_) { 3904 if (!super.equalsShallow(other_)) 3905 return false; 3906 if (!(other_ instanceof ExampleScenarioActorComponent)) 3907 return false; 3908 ExampleScenarioActorComponent o = (ExampleScenarioActorComponent) other_; 3909 return compareValues(actorId, o.actorId, true) && compareValues(type, o.type, true) 3910 && compareValues(name, o.name, true) && compareValues(description, o.description, true); 3911 } 3912 3913 public boolean isEmpty() { 3914 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(actorId, type, name, description); 3915 } 3916 3917 public String fhirType() { 3918 return "ExampleScenario.actor"; 3919 3920 } 3921 3922 } 3923 3924 @Block() 3925 public static class ExampleScenarioInstanceComponent extends BackboneElement implements IBaseBackboneElement { 3926 /** 3927 * The id of the resource for referencing. 3928 */ 3929 @Child(name = "resourceId", type = { 3930 StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 3931 @Description(shortDefinition = "The id of the resource for referencing", formalDefinition = "The id of the resource for referencing.") 3932 protected StringType resourceId; 3933 3934 /** 3935 * The type of the resource. 3936 */ 3937 @Child(name = "resourceType", type = { 3938 CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 3939 @Description(shortDefinition = "The type of the resource", formalDefinition = "The type of the resource.") 3940 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/resource-types") 3941 protected Enumeration<FHIRResourceType> resourceType; 3942 3943 /** 3944 * A short name for the resource instance. 3945 */ 3946 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 3947 @Description(shortDefinition = "A short name for the resource instance", formalDefinition = "A short name for the resource instance.") 3948 protected StringType name; 3949 3950 /** 3951 * Human-friendly description of the resource instance. 3952 */ 3953 @Child(name = "description", type = { 3954 MarkdownType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 3955 @Description(shortDefinition = "Human-friendly description of the resource instance", formalDefinition = "Human-friendly description of the resource instance.") 3956 protected MarkdownType description; 3957 3958 /** 3959 * A specific version of the resource. 3960 */ 3961 @Child(name = "version", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3962 @Description(shortDefinition = "A specific version of the resource", formalDefinition = "A specific version of the resource.") 3963 protected List<ExampleScenarioInstanceVersionComponent> version; 3964 3965 /** 3966 * Resources contained in the instance (e.g. the observations contained in a 3967 * bundle). 3968 */ 3969 @Child(name = "containedInstance", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3970 @Description(shortDefinition = "Resources contained in the instance", formalDefinition = "Resources contained in the instance (e.g. the observations contained in a bundle).") 3971 protected List<ExampleScenarioInstanceContainedInstanceComponent> containedInstance; 3972 3973 private static final long serialVersionUID = -1131860669L; 3974 3975 /** 3976 * Constructor 3977 */ 3978 public ExampleScenarioInstanceComponent() { 3979 super(); 3980 } 3981 3982 /** 3983 * Constructor 3984 */ 3985 public ExampleScenarioInstanceComponent(StringType resourceId, Enumeration<FHIRResourceType> resourceType) { 3986 super(); 3987 this.resourceId = resourceId; 3988 this.resourceType = resourceType; 3989 } 3990 3991 /** 3992 * @return {@link #resourceId} (The id of the resource for referencing.). This 3993 * is the underlying object with id, value and extensions. The accessor 3994 * "getResourceId" gives direct access to the value 3995 */ 3996 public StringType getResourceIdElement() { 3997 if (this.resourceId == null) 3998 if (Configuration.errorOnAutoCreate()) 3999 throw new Error("Attempt to auto-create ExampleScenarioInstanceComponent.resourceId"); 4000 else if (Configuration.doAutoCreate()) 4001 this.resourceId = new StringType(); // bb 4002 return this.resourceId; 4003 } 4004 4005 public boolean hasResourceIdElement() { 4006 return this.resourceId != null && !this.resourceId.isEmpty(); 4007 } 4008 4009 public boolean hasResourceId() { 4010 return this.resourceId != null && !this.resourceId.isEmpty(); 4011 } 4012 4013 /** 4014 * @param value {@link #resourceId} (The id of the resource for referencing.). 4015 * This is the underlying object with id, value and extensions. The 4016 * accessor "getResourceId" gives direct access to the value 4017 */ 4018 public ExampleScenarioInstanceComponent setResourceIdElement(StringType value) { 4019 this.resourceId = value; 4020 return this; 4021 } 4022 4023 /** 4024 * @return The id of the resource for referencing. 4025 */ 4026 public String getResourceId() { 4027 return this.resourceId == null ? null : this.resourceId.getValue(); 4028 } 4029 4030 /** 4031 * @param value The id of the resource for referencing. 4032 */ 4033 public ExampleScenarioInstanceComponent setResourceId(String value) { 4034 if (this.resourceId == null) 4035 this.resourceId = new StringType(); 4036 this.resourceId.setValue(value); 4037 return this; 4038 } 4039 4040 /** 4041 * @return {@link #resourceType} (The type of the resource.). This is the 4042 * underlying object with id, value and extensions. The accessor 4043 * "getResourceType" gives direct access to the value 4044 */ 4045 public Enumeration<FHIRResourceType> getResourceTypeElement() { 4046 if (this.resourceType == null) 4047 if (Configuration.errorOnAutoCreate()) 4048 throw new Error("Attempt to auto-create ExampleScenarioInstanceComponent.resourceType"); 4049 else if (Configuration.doAutoCreate()) 4050 this.resourceType = new Enumeration<FHIRResourceType>(new FHIRResourceTypeEnumFactory()); // bb 4051 return this.resourceType; 4052 } 4053 4054 public boolean hasResourceTypeElement() { 4055 return this.resourceType != null && !this.resourceType.isEmpty(); 4056 } 4057 4058 public boolean hasResourceType() { 4059 return this.resourceType != null && !this.resourceType.isEmpty(); 4060 } 4061 4062 /** 4063 * @param value {@link #resourceType} (The type of the resource.). This is the 4064 * underlying object with id, value and extensions. The accessor 4065 * "getResourceType" gives direct access to the value 4066 */ 4067 public ExampleScenarioInstanceComponent setResourceTypeElement(Enumeration<FHIRResourceType> value) { 4068 this.resourceType = value; 4069 return this; 4070 } 4071 4072 /** 4073 * @return The type of the resource. 4074 */ 4075 public FHIRResourceType getResourceType() { 4076 return this.resourceType == null ? null : this.resourceType.getValue(); 4077 } 4078 4079 /** 4080 * @param value The type of the resource. 4081 */ 4082 public ExampleScenarioInstanceComponent setResourceType(FHIRResourceType value) { 4083 if (this.resourceType == null) 4084 this.resourceType = new Enumeration<FHIRResourceType>(new FHIRResourceTypeEnumFactory()); 4085 this.resourceType.setValue(value); 4086 return this; 4087 } 4088 4089 /** 4090 * @return {@link #name} (A short name for the resource instance.). This is the 4091 * underlying object with id, value and extensions. The accessor 4092 * "getName" gives direct access to the value 4093 */ 4094 public StringType getNameElement() { 4095 if (this.name == null) 4096 if (Configuration.errorOnAutoCreate()) 4097 throw new Error("Attempt to auto-create ExampleScenarioInstanceComponent.name"); 4098 else if (Configuration.doAutoCreate()) 4099 this.name = new StringType(); // bb 4100 return this.name; 4101 } 4102 4103 public boolean hasNameElement() { 4104 return this.name != null && !this.name.isEmpty(); 4105 } 4106 4107 public boolean hasName() { 4108 return this.name != null && !this.name.isEmpty(); 4109 } 4110 4111 /** 4112 * @param value {@link #name} (A short name for the resource instance.). This is 4113 * the underlying object with id, value and extensions. The 4114 * accessor "getName" gives direct access to the value 4115 */ 4116 public ExampleScenarioInstanceComponent setNameElement(StringType value) { 4117 this.name = value; 4118 return this; 4119 } 4120 4121 /** 4122 * @return A short name for the resource instance. 4123 */ 4124 public String getName() { 4125 return this.name == null ? null : this.name.getValue(); 4126 } 4127 4128 /** 4129 * @param value A short name for the resource instance. 4130 */ 4131 public ExampleScenarioInstanceComponent setName(String value) { 4132 if (Utilities.noString(value)) 4133 this.name = null; 4134 else { 4135 if (this.name == null) 4136 this.name = new StringType(); 4137 this.name.setValue(value); 4138 } 4139 return this; 4140 } 4141 4142 /** 4143 * @return {@link #description} (Human-friendly description of the resource 4144 * instance.). This is the underlying object with id, value and 4145 * extensions. The accessor "getDescription" gives direct access to the 4146 * value 4147 */ 4148 public MarkdownType getDescriptionElement() { 4149 if (this.description == null) 4150 if (Configuration.errorOnAutoCreate()) 4151 throw new Error("Attempt to auto-create ExampleScenarioInstanceComponent.description"); 4152 else if (Configuration.doAutoCreate()) 4153 this.description = new MarkdownType(); // bb 4154 return this.description; 4155 } 4156 4157 public boolean hasDescriptionElement() { 4158 return this.description != null && !this.description.isEmpty(); 4159 } 4160 4161 public boolean hasDescription() { 4162 return this.description != null && !this.description.isEmpty(); 4163 } 4164 4165 /** 4166 * @param value {@link #description} (Human-friendly description of the resource 4167 * instance.). This is the underlying object with id, value and 4168 * extensions. The accessor "getDescription" gives direct access to 4169 * the value 4170 */ 4171 public ExampleScenarioInstanceComponent setDescriptionElement(MarkdownType value) { 4172 this.description = value; 4173 return this; 4174 } 4175 4176 /** 4177 * @return Human-friendly description of the resource instance. 4178 */ 4179 public String getDescription() { 4180 return this.description == null ? null : this.description.getValue(); 4181 } 4182 4183 /** 4184 * @param value Human-friendly description of the resource instance. 4185 */ 4186 public ExampleScenarioInstanceComponent setDescription(String value) { 4187 if (value == null) 4188 this.description = null; 4189 else { 4190 if (this.description == null) 4191 this.description = new MarkdownType(); 4192 this.description.setValue(value); 4193 } 4194 return this; 4195 } 4196 4197 /** 4198 * @return {@link #version} (A specific version of the resource.) 4199 */ 4200 public List<ExampleScenarioInstanceVersionComponent> getVersion() { 4201 if (this.version == null) 4202 this.version = new ArrayList<ExampleScenarioInstanceVersionComponent>(); 4203 return this.version; 4204 } 4205 4206 /** 4207 * @return Returns a reference to <code>this</code> for easy method chaining 4208 */ 4209 public ExampleScenarioInstanceComponent setVersion(List<ExampleScenarioInstanceVersionComponent> theVersion) { 4210 this.version = theVersion; 4211 return this; 4212 } 4213 4214 public boolean hasVersion() { 4215 if (this.version == null) 4216 return false; 4217 for (ExampleScenarioInstanceVersionComponent item : this.version) 4218 if (!item.isEmpty()) 4219 return true; 4220 return false; 4221 } 4222 4223 public ExampleScenarioInstanceVersionComponent addVersion() { // 3 4224 ExampleScenarioInstanceVersionComponent t = new ExampleScenarioInstanceVersionComponent(); 4225 if (this.version == null) 4226 this.version = new ArrayList<ExampleScenarioInstanceVersionComponent>(); 4227 this.version.add(t); 4228 return t; 4229 } 4230 4231 public ExampleScenarioInstanceComponent addVersion(ExampleScenarioInstanceVersionComponent t) { // 3 4232 if (t == null) 4233 return this; 4234 if (this.version == null) 4235 this.version = new ArrayList<ExampleScenarioInstanceVersionComponent>(); 4236 this.version.add(t); 4237 return this; 4238 } 4239 4240 /** 4241 * @return The first repetition of repeating field {@link #version}, creating it 4242 * if it does not already exist 4243 */ 4244 public ExampleScenarioInstanceVersionComponent getVersionFirstRep() { 4245 if (getVersion().isEmpty()) { 4246 addVersion(); 4247 } 4248 return getVersion().get(0); 4249 } 4250 4251 /** 4252 * @return {@link #containedInstance} (Resources contained in the instance (e.g. 4253 * the observations contained in a bundle).) 4254 */ 4255 public List<ExampleScenarioInstanceContainedInstanceComponent> getContainedInstance() { 4256 if (this.containedInstance == null) 4257 this.containedInstance = new ArrayList<ExampleScenarioInstanceContainedInstanceComponent>(); 4258 return this.containedInstance; 4259 } 4260 4261 /** 4262 * @return Returns a reference to <code>this</code> for easy method chaining 4263 */ 4264 public ExampleScenarioInstanceComponent setContainedInstance( 4265 List<ExampleScenarioInstanceContainedInstanceComponent> theContainedInstance) { 4266 this.containedInstance = theContainedInstance; 4267 return this; 4268 } 4269 4270 public boolean hasContainedInstance() { 4271 if (this.containedInstance == null) 4272 return false; 4273 for (ExampleScenarioInstanceContainedInstanceComponent item : this.containedInstance) 4274 if (!item.isEmpty()) 4275 return true; 4276 return false; 4277 } 4278 4279 public ExampleScenarioInstanceContainedInstanceComponent addContainedInstance() { // 3 4280 ExampleScenarioInstanceContainedInstanceComponent t = new ExampleScenarioInstanceContainedInstanceComponent(); 4281 if (this.containedInstance == null) 4282 this.containedInstance = new ArrayList<ExampleScenarioInstanceContainedInstanceComponent>(); 4283 this.containedInstance.add(t); 4284 return t; 4285 } 4286 4287 public ExampleScenarioInstanceComponent addContainedInstance(ExampleScenarioInstanceContainedInstanceComponent t) { // 3 4288 if (t == null) 4289 return this; 4290 if (this.containedInstance == null) 4291 this.containedInstance = new ArrayList<ExampleScenarioInstanceContainedInstanceComponent>(); 4292 this.containedInstance.add(t); 4293 return this; 4294 } 4295 4296 /** 4297 * @return The first repetition of repeating field {@link #containedInstance}, 4298 * creating it if it does not already exist 4299 */ 4300 public ExampleScenarioInstanceContainedInstanceComponent getContainedInstanceFirstRep() { 4301 if (getContainedInstance().isEmpty()) { 4302 addContainedInstance(); 4303 } 4304 return getContainedInstance().get(0); 4305 } 4306 4307 protected void listChildren(List<Property> children) { 4308 super.listChildren(children); 4309 children.add(new Property("resourceId", "string", "The id of the resource for referencing.", 0, 1, resourceId)); 4310 children.add(new Property("resourceType", "code", "The type of the resource.", 0, 1, resourceType)); 4311 children.add(new Property("name", "string", "A short name for the resource instance.", 0, 1, name)); 4312 children.add(new Property("description", "markdown", "Human-friendly description of the resource instance.", 0, 1, 4313 description)); 4314 children.add( 4315 new Property("version", "", "A specific version of the resource.", 0, java.lang.Integer.MAX_VALUE, version)); 4316 children.add(new Property("containedInstance", "", 4317 "Resources contained in the instance (e.g. the observations contained in a bundle).", 0, 4318 java.lang.Integer.MAX_VALUE, containedInstance)); 4319 } 4320 4321 @Override 4322 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4323 switch (_hash) { 4324 case -1345650231: 4325 /* resourceId */ return new Property("resourceId", "string", "The id of the resource for referencing.", 0, 1, 4326 resourceId); 4327 case -384364440: 4328 /* resourceType */ return new Property("resourceType", "code", "The type of the resource.", 0, 1, resourceType); 4329 case 3373707: 4330 /* name */ return new Property("name", "string", "A short name for the resource instance.", 0, 1, name); 4331 case -1724546052: 4332 /* description */ return new Property("description", "markdown", 4333 "Human-friendly description of the resource instance.", 0, 1, description); 4334 case 351608024: 4335 /* version */ return new Property("version", "", "A specific version of the resource.", 0, 4336 java.lang.Integer.MAX_VALUE, version); 4337 case -417062360: 4338 /* containedInstance */ return new Property("containedInstance", "", 4339 "Resources contained in the instance (e.g. the observations contained in a bundle).", 0, 4340 java.lang.Integer.MAX_VALUE, containedInstance); 4341 default: 4342 return super.getNamedProperty(_hash, _name, _checkValid); 4343 } 4344 4345 } 4346 4347 @Override 4348 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4349 switch (hash) { 4350 case -1345650231: 4351 /* resourceId */ return this.resourceId == null ? new Base[0] : new Base[] { this.resourceId }; // StringType 4352 case -384364440: 4353 /* resourceType */ return this.resourceType == null ? new Base[0] : new Base[] { this.resourceType }; // Enumeration<FHIRResourceType> 4354 case 3373707: 4355 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 4356 case -1724546052: 4357 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 4358 case 351608024: 4359 /* version */ return this.version == null ? new Base[0] : this.version.toArray(new Base[this.version.size()]); // ExampleScenarioInstanceVersionComponent 4360 case -417062360: 4361 /* containedInstance */ return this.containedInstance == null ? new Base[0] 4362 : this.containedInstance.toArray(new Base[this.containedInstance.size()]); // ExampleScenarioInstanceContainedInstanceComponent 4363 default: 4364 return super.getProperty(hash, name, checkValid); 4365 } 4366 4367 } 4368 4369 @Override 4370 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4371 switch (hash) { 4372 case -1345650231: // resourceId 4373 this.resourceId = castToString(value); // StringType 4374 return value; 4375 case -384364440: // resourceType 4376 value = new FHIRResourceTypeEnumFactory().fromType(castToCode(value)); 4377 this.resourceType = (Enumeration) value; // Enumeration<FHIRResourceType> 4378 return value; 4379 case 3373707: // name 4380 this.name = castToString(value); // StringType 4381 return value; 4382 case -1724546052: // description 4383 this.description = castToMarkdown(value); // MarkdownType 4384 return value; 4385 case 351608024: // version 4386 this.getVersion().add((ExampleScenarioInstanceVersionComponent) value); // ExampleScenarioInstanceVersionComponent 4387 return value; 4388 case -417062360: // containedInstance 4389 this.getContainedInstance().add((ExampleScenarioInstanceContainedInstanceComponent) value); // ExampleScenarioInstanceContainedInstanceComponent 4390 return value; 4391 default: 4392 return super.setProperty(hash, name, value); 4393 } 4394 4395 } 4396 4397 @Override 4398 public Base setProperty(String name, Base value) throws FHIRException { 4399 if (name.equals("resourceId")) { 4400 this.resourceId = castToString(value); // StringType 4401 } else if (name.equals("resourceType")) { 4402 value = new FHIRResourceTypeEnumFactory().fromType(castToCode(value)); 4403 this.resourceType = (Enumeration) value; // Enumeration<FHIRResourceType> 4404 } else if (name.equals("name")) { 4405 this.name = castToString(value); // StringType 4406 } else if (name.equals("description")) { 4407 this.description = castToMarkdown(value); // MarkdownType 4408 } else if (name.equals("version")) { 4409 this.getVersion().add((ExampleScenarioInstanceVersionComponent) value); 4410 } else if (name.equals("containedInstance")) { 4411 this.getContainedInstance().add((ExampleScenarioInstanceContainedInstanceComponent) value); 4412 } else 4413 return super.setProperty(name, value); 4414 return value; 4415 } 4416 4417 @Override 4418 public Base makeProperty(int hash, String name) throws FHIRException { 4419 switch (hash) { 4420 case -1345650231: 4421 return getResourceIdElement(); 4422 case -384364440: 4423 return getResourceTypeElement(); 4424 case 3373707: 4425 return getNameElement(); 4426 case -1724546052: 4427 return getDescriptionElement(); 4428 case 351608024: 4429 return addVersion(); 4430 case -417062360: 4431 return addContainedInstance(); 4432 default: 4433 return super.makeProperty(hash, name); 4434 } 4435 4436 } 4437 4438 @Override 4439 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4440 switch (hash) { 4441 case -1345650231: 4442 /* resourceId */ return new String[] { "string" }; 4443 case -384364440: 4444 /* resourceType */ return new String[] { "code" }; 4445 case 3373707: 4446 /* name */ return new String[] { "string" }; 4447 case -1724546052: 4448 /* description */ return new String[] { "markdown" }; 4449 case 351608024: 4450 /* version */ return new String[] {}; 4451 case -417062360: 4452 /* containedInstance */ return new String[] {}; 4453 default: 4454 return super.getTypesForProperty(hash, name); 4455 } 4456 4457 } 4458 4459 @Override 4460 public Base addChild(String name) throws FHIRException { 4461 if (name.equals("resourceId")) { 4462 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.resourceId"); 4463 } else if (name.equals("resourceType")) { 4464 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.resourceType"); 4465 } else if (name.equals("name")) { 4466 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.name"); 4467 } else if (name.equals("description")) { 4468 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.description"); 4469 } else if (name.equals("version")) { 4470 return addVersion(); 4471 } else if (name.equals("containedInstance")) { 4472 return addContainedInstance(); 4473 } else 4474 return super.addChild(name); 4475 } 4476 4477 public ExampleScenarioInstanceComponent copy() { 4478 ExampleScenarioInstanceComponent dst = new ExampleScenarioInstanceComponent(); 4479 copyValues(dst); 4480 return dst; 4481 } 4482 4483 public void copyValues(ExampleScenarioInstanceComponent dst) { 4484 super.copyValues(dst); 4485 dst.resourceId = resourceId == null ? null : resourceId.copy(); 4486 dst.resourceType = resourceType == null ? null : resourceType.copy(); 4487 dst.name = name == null ? null : name.copy(); 4488 dst.description = description == null ? null : description.copy(); 4489 if (version != null) { 4490 dst.version = new ArrayList<ExampleScenarioInstanceVersionComponent>(); 4491 for (ExampleScenarioInstanceVersionComponent i : version) 4492 dst.version.add(i.copy()); 4493 } 4494 ; 4495 if (containedInstance != null) { 4496 dst.containedInstance = new ArrayList<ExampleScenarioInstanceContainedInstanceComponent>(); 4497 for (ExampleScenarioInstanceContainedInstanceComponent i : containedInstance) 4498 dst.containedInstance.add(i.copy()); 4499 } 4500 ; 4501 } 4502 4503 @Override 4504 public boolean equalsDeep(Base other_) { 4505 if (!super.equalsDeep(other_)) 4506 return false; 4507 if (!(other_ instanceof ExampleScenarioInstanceComponent)) 4508 return false; 4509 ExampleScenarioInstanceComponent o = (ExampleScenarioInstanceComponent) other_; 4510 return compareDeep(resourceId, o.resourceId, true) && compareDeep(resourceType, o.resourceType, true) 4511 && compareDeep(name, o.name, true) && compareDeep(description, o.description, true) 4512 && compareDeep(version, o.version, true) && compareDeep(containedInstance, o.containedInstance, true); 4513 } 4514 4515 @Override 4516 public boolean equalsShallow(Base other_) { 4517 if (!super.equalsShallow(other_)) 4518 return false; 4519 if (!(other_ instanceof ExampleScenarioInstanceComponent)) 4520 return false; 4521 ExampleScenarioInstanceComponent o = (ExampleScenarioInstanceComponent) other_; 4522 return compareValues(resourceId, o.resourceId, true) && compareValues(resourceType, o.resourceType, true) 4523 && compareValues(name, o.name, true) && compareValues(description, o.description, true); 4524 } 4525 4526 public boolean isEmpty() { 4527 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(resourceId, resourceType, name, description, 4528 version, containedInstance); 4529 } 4530 4531 public String fhirType() { 4532 return "ExampleScenario.instance"; 4533 4534 } 4535 4536 } 4537 4538 @Block() 4539 public static class ExampleScenarioInstanceVersionComponent extends BackboneElement implements IBaseBackboneElement { 4540 /** 4541 * The identifier of a specific version of a resource. 4542 */ 4543 @Child(name = "versionId", type = { 4544 StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 4545 @Description(shortDefinition = "The identifier of a specific version of a resource", formalDefinition = "The identifier of a specific version of a resource.") 4546 protected StringType versionId; 4547 4548 /** 4549 * The description of the resource version. 4550 */ 4551 @Child(name = "description", type = { 4552 MarkdownType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 4553 @Description(shortDefinition = "The description of the resource version", formalDefinition = "The description of the resource version.") 4554 protected MarkdownType description; 4555 4556 private static final long serialVersionUID = 960821913L; 4557 4558 /** 4559 * Constructor 4560 */ 4561 public ExampleScenarioInstanceVersionComponent() { 4562 super(); 4563 } 4564 4565 /** 4566 * Constructor 4567 */ 4568 public ExampleScenarioInstanceVersionComponent(StringType versionId, MarkdownType description) { 4569 super(); 4570 this.versionId = versionId; 4571 this.description = description; 4572 } 4573 4574 /** 4575 * @return {@link #versionId} (The identifier of a specific version of a 4576 * resource.). This is the underlying object with id, value and 4577 * extensions. The accessor "getVersionId" gives direct access to the 4578 * value 4579 */ 4580 public StringType getVersionIdElement() { 4581 if (this.versionId == null) 4582 if (Configuration.errorOnAutoCreate()) 4583 throw new Error("Attempt to auto-create ExampleScenarioInstanceVersionComponent.versionId"); 4584 else if (Configuration.doAutoCreate()) 4585 this.versionId = new StringType(); // bb 4586 return this.versionId; 4587 } 4588 4589 public boolean hasVersionIdElement() { 4590 return this.versionId != null && !this.versionId.isEmpty(); 4591 } 4592 4593 public boolean hasVersionId() { 4594 return this.versionId != null && !this.versionId.isEmpty(); 4595 } 4596 4597 /** 4598 * @param value {@link #versionId} (The identifier of a specific version of a 4599 * resource.). This is the underlying object with id, value and 4600 * extensions. The accessor "getVersionId" gives direct access to 4601 * the value 4602 */ 4603 public ExampleScenarioInstanceVersionComponent setVersionIdElement(StringType value) { 4604 this.versionId = value; 4605 return this; 4606 } 4607 4608 /** 4609 * @return The identifier of a specific version of a resource. 4610 */ 4611 public String getVersionId() { 4612 return this.versionId == null ? null : this.versionId.getValue(); 4613 } 4614 4615 /** 4616 * @param value The identifier of a specific version of a resource. 4617 */ 4618 public ExampleScenarioInstanceVersionComponent setVersionId(String value) { 4619 if (this.versionId == null) 4620 this.versionId = new StringType(); 4621 this.versionId.setValue(value); 4622 return this; 4623 } 4624 4625 /** 4626 * @return {@link #description} (The description of the resource version.). This 4627 * is the underlying object with id, value and extensions. The accessor 4628 * "getDescription" gives direct access to the value 4629 */ 4630 public MarkdownType getDescriptionElement() { 4631 if (this.description == null) 4632 if (Configuration.errorOnAutoCreate()) 4633 throw new Error("Attempt to auto-create ExampleScenarioInstanceVersionComponent.description"); 4634 else if (Configuration.doAutoCreate()) 4635 this.description = new MarkdownType(); // bb 4636 return this.description; 4637 } 4638 4639 public boolean hasDescriptionElement() { 4640 return this.description != null && !this.description.isEmpty(); 4641 } 4642 4643 public boolean hasDescription() { 4644 return this.description != null && !this.description.isEmpty(); 4645 } 4646 4647 /** 4648 * @param value {@link #description} (The description of the resource version.). 4649 * This is the underlying object with id, value and extensions. The 4650 * accessor "getDescription" gives direct access to the value 4651 */ 4652 public ExampleScenarioInstanceVersionComponent setDescriptionElement(MarkdownType value) { 4653 this.description = value; 4654 return this; 4655 } 4656 4657 /** 4658 * @return The description of the resource version. 4659 */ 4660 public String getDescription() { 4661 return this.description == null ? null : this.description.getValue(); 4662 } 4663 4664 /** 4665 * @param value The description of the resource version. 4666 */ 4667 public ExampleScenarioInstanceVersionComponent setDescription(String value) { 4668 if (this.description == null) 4669 this.description = new MarkdownType(); 4670 this.description.setValue(value); 4671 return this; 4672 } 4673 4674 protected void listChildren(List<Property> children) { 4675 super.listChildren(children); 4676 children.add( 4677 new Property("versionId", "string", "The identifier of a specific version of a resource.", 0, 1, versionId)); 4678 children 4679 .add(new Property("description", "markdown", "The description of the resource version.", 0, 1, description)); 4680 } 4681 4682 @Override 4683 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4684 switch (_hash) { 4685 case -1407102957: 4686 /* versionId */ return new Property("versionId", "string", 4687 "The identifier of a specific version of a resource.", 0, 1, versionId); 4688 case -1724546052: 4689 /* description */ return new Property("description", "markdown", "The description of the resource version.", 0, 4690 1, description); 4691 default: 4692 return super.getNamedProperty(_hash, _name, _checkValid); 4693 } 4694 4695 } 4696 4697 @Override 4698 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4699 switch (hash) { 4700 case -1407102957: 4701 /* versionId */ return this.versionId == null ? new Base[0] : new Base[] { this.versionId }; // StringType 4702 case -1724546052: 4703 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 4704 default: 4705 return super.getProperty(hash, name, checkValid); 4706 } 4707 4708 } 4709 4710 @Override 4711 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4712 switch (hash) { 4713 case -1407102957: // versionId 4714 this.versionId = castToString(value); // StringType 4715 return value; 4716 case -1724546052: // description 4717 this.description = castToMarkdown(value); // MarkdownType 4718 return value; 4719 default: 4720 return super.setProperty(hash, name, value); 4721 } 4722 4723 } 4724 4725 @Override 4726 public Base setProperty(String name, Base value) throws FHIRException { 4727 if (name.equals("versionId")) { 4728 this.versionId = castToString(value); // StringType 4729 } else if (name.equals("description")) { 4730 this.description = castToMarkdown(value); // MarkdownType 4731 } else 4732 return super.setProperty(name, value); 4733 return value; 4734 } 4735 4736 @Override 4737 public Base makeProperty(int hash, String name) throws FHIRException { 4738 switch (hash) { 4739 case -1407102957: 4740 return getVersionIdElement(); 4741 case -1724546052: 4742 return getDescriptionElement(); 4743 default: 4744 return super.makeProperty(hash, name); 4745 } 4746 4747 } 4748 4749 @Override 4750 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4751 switch (hash) { 4752 case -1407102957: 4753 /* versionId */ return new String[] { "string" }; 4754 case -1724546052: 4755 /* description */ return new String[] { "markdown" }; 4756 default: 4757 return super.getTypesForProperty(hash, name); 4758 } 4759 4760 } 4761 4762 @Override 4763 public Base addChild(String name) throws FHIRException { 4764 if (name.equals("versionId")) { 4765 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.versionId"); 4766 } else if (name.equals("description")) { 4767 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.description"); 4768 } else 4769 return super.addChild(name); 4770 } 4771 4772 public ExampleScenarioInstanceVersionComponent copy() { 4773 ExampleScenarioInstanceVersionComponent dst = new ExampleScenarioInstanceVersionComponent(); 4774 copyValues(dst); 4775 return dst; 4776 } 4777 4778 public void copyValues(ExampleScenarioInstanceVersionComponent dst) { 4779 super.copyValues(dst); 4780 dst.versionId = versionId == null ? null : versionId.copy(); 4781 dst.description = description == null ? null : description.copy(); 4782 } 4783 4784 @Override 4785 public boolean equalsDeep(Base other_) { 4786 if (!super.equalsDeep(other_)) 4787 return false; 4788 if (!(other_ instanceof ExampleScenarioInstanceVersionComponent)) 4789 return false; 4790 ExampleScenarioInstanceVersionComponent o = (ExampleScenarioInstanceVersionComponent) other_; 4791 return compareDeep(versionId, o.versionId, true) && compareDeep(description, o.description, true); 4792 } 4793 4794 @Override 4795 public boolean equalsShallow(Base other_) { 4796 if (!super.equalsShallow(other_)) 4797 return false; 4798 if (!(other_ instanceof ExampleScenarioInstanceVersionComponent)) 4799 return false; 4800 ExampleScenarioInstanceVersionComponent o = (ExampleScenarioInstanceVersionComponent) other_; 4801 return compareValues(versionId, o.versionId, true) && compareValues(description, o.description, true); 4802 } 4803 4804 public boolean isEmpty() { 4805 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(versionId, description); 4806 } 4807 4808 public String fhirType() { 4809 return "ExampleScenario.instance.version"; 4810 4811 } 4812 4813 } 4814 4815 @Block() 4816 public static class ExampleScenarioInstanceContainedInstanceComponent extends BackboneElement 4817 implements IBaseBackboneElement { 4818 /** 4819 * Each resource contained in the instance. 4820 */ 4821 @Child(name = "resourceId", type = { 4822 StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 4823 @Description(shortDefinition = "Each resource contained in the instance", formalDefinition = "Each resource contained in the instance.") 4824 protected StringType resourceId; 4825 4826 /** 4827 * A specific version of a resource contained in the instance. 4828 */ 4829 @Child(name = "versionId", type = { 4830 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 4831 @Description(shortDefinition = "A specific version of a resource contained in the instance", formalDefinition = "A specific version of a resource contained in the instance.") 4832 protected StringType versionId; 4833 4834 private static final long serialVersionUID = 908084124L; 4835 4836 /** 4837 * Constructor 4838 */ 4839 public ExampleScenarioInstanceContainedInstanceComponent() { 4840 super(); 4841 } 4842 4843 /** 4844 * Constructor 4845 */ 4846 public ExampleScenarioInstanceContainedInstanceComponent(StringType resourceId) { 4847 super(); 4848 this.resourceId = resourceId; 4849 } 4850 4851 /** 4852 * @return {@link #resourceId} (Each resource contained in the instance.). This 4853 * is the underlying object with id, value and extensions. The accessor 4854 * "getResourceId" gives direct access to the value 4855 */ 4856 public StringType getResourceIdElement() { 4857 if (this.resourceId == null) 4858 if (Configuration.errorOnAutoCreate()) 4859 throw new Error("Attempt to auto-create ExampleScenarioInstanceContainedInstanceComponent.resourceId"); 4860 else if (Configuration.doAutoCreate()) 4861 this.resourceId = new StringType(); // bb 4862 return this.resourceId; 4863 } 4864 4865 public boolean hasResourceIdElement() { 4866 return this.resourceId != null && !this.resourceId.isEmpty(); 4867 } 4868 4869 public boolean hasResourceId() { 4870 return this.resourceId != null && !this.resourceId.isEmpty(); 4871 } 4872 4873 /** 4874 * @param value {@link #resourceId} (Each resource contained in the instance.). 4875 * This is the underlying object with id, value and extensions. The 4876 * accessor "getResourceId" gives direct access to the value 4877 */ 4878 public ExampleScenarioInstanceContainedInstanceComponent setResourceIdElement(StringType value) { 4879 this.resourceId = value; 4880 return this; 4881 } 4882 4883 /** 4884 * @return Each resource contained in the instance. 4885 */ 4886 public String getResourceId() { 4887 return this.resourceId == null ? null : this.resourceId.getValue(); 4888 } 4889 4890 /** 4891 * @param value Each resource contained in the instance. 4892 */ 4893 public ExampleScenarioInstanceContainedInstanceComponent setResourceId(String value) { 4894 if (this.resourceId == null) 4895 this.resourceId = new StringType(); 4896 this.resourceId.setValue(value); 4897 return this; 4898 } 4899 4900 /** 4901 * @return {@link #versionId} (A specific version of a resource contained in the 4902 * instance.). This is the underlying object with id, value and 4903 * extensions. The accessor "getVersionId" gives direct access to the 4904 * value 4905 */ 4906 public StringType getVersionIdElement() { 4907 if (this.versionId == null) 4908 if (Configuration.errorOnAutoCreate()) 4909 throw new Error("Attempt to auto-create ExampleScenarioInstanceContainedInstanceComponent.versionId"); 4910 else if (Configuration.doAutoCreate()) 4911 this.versionId = new StringType(); // bb 4912 return this.versionId; 4913 } 4914 4915 public boolean hasVersionIdElement() { 4916 return this.versionId != null && !this.versionId.isEmpty(); 4917 } 4918 4919 public boolean hasVersionId() { 4920 return this.versionId != null && !this.versionId.isEmpty(); 4921 } 4922 4923 /** 4924 * @param value {@link #versionId} (A specific version of a resource contained 4925 * in the instance.). This is the underlying object with id, value 4926 * and extensions. The accessor "getVersionId" gives direct access 4927 * to the value 4928 */ 4929 public ExampleScenarioInstanceContainedInstanceComponent setVersionIdElement(StringType value) { 4930 this.versionId = value; 4931 return this; 4932 } 4933 4934 /** 4935 * @return A specific version of a resource contained in the instance. 4936 */ 4937 public String getVersionId() { 4938 return this.versionId == null ? null : this.versionId.getValue(); 4939 } 4940 4941 /** 4942 * @param value A specific version of a resource contained in the instance. 4943 */ 4944 public ExampleScenarioInstanceContainedInstanceComponent setVersionId(String value) { 4945 if (Utilities.noString(value)) 4946 this.versionId = null; 4947 else { 4948 if (this.versionId == null) 4949 this.versionId = new StringType(); 4950 this.versionId.setValue(value); 4951 } 4952 return this; 4953 } 4954 4955 protected void listChildren(List<Property> children) { 4956 super.listChildren(children); 4957 children.add(new Property("resourceId", "string", "Each resource contained in the instance.", 0, 1, resourceId)); 4958 children.add(new Property("versionId", "string", "A specific version of a resource contained in the instance.", 0, 4959 1, versionId)); 4960 } 4961 4962 @Override 4963 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4964 switch (_hash) { 4965 case -1345650231: 4966 /* resourceId */ return new Property("resourceId", "string", "Each resource contained in the instance.", 0, 1, 4967 resourceId); 4968 case -1407102957: 4969 /* versionId */ return new Property("versionId", "string", 4970 "A specific version of a resource contained in the instance.", 0, 1, versionId); 4971 default: 4972 return super.getNamedProperty(_hash, _name, _checkValid); 4973 } 4974 4975 } 4976 4977 @Override 4978 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4979 switch (hash) { 4980 case -1345650231: 4981 /* resourceId */ return this.resourceId == null ? new Base[0] : new Base[] { this.resourceId }; // StringType 4982 case -1407102957: 4983 /* versionId */ return this.versionId == null ? new Base[0] : new Base[] { this.versionId }; // StringType 4984 default: 4985 return super.getProperty(hash, name, checkValid); 4986 } 4987 4988 } 4989 4990 @Override 4991 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4992 switch (hash) { 4993 case -1345650231: // resourceId 4994 this.resourceId = castToString(value); // StringType 4995 return value; 4996 case -1407102957: // versionId 4997 this.versionId = castToString(value); // StringType 4998 return value; 4999 default: 5000 return super.setProperty(hash, name, value); 5001 } 5002 5003 } 5004 5005 @Override 5006 public Base setProperty(String name, Base value) throws FHIRException { 5007 if (name.equals("resourceId")) { 5008 this.resourceId = castToString(value); // StringType 5009 } else if (name.equals("versionId")) { 5010 this.versionId = castToString(value); // StringType 5011 } else 5012 return super.setProperty(name, value); 5013 return value; 5014 } 5015 5016 @Override 5017 public Base makeProperty(int hash, String name) throws FHIRException { 5018 switch (hash) { 5019 case -1345650231: 5020 return getResourceIdElement(); 5021 case -1407102957: 5022 return getVersionIdElement(); 5023 default: 5024 return super.makeProperty(hash, name); 5025 } 5026 5027 } 5028 5029 @Override 5030 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5031 switch (hash) { 5032 case -1345650231: 5033 /* resourceId */ return new String[] { "string" }; 5034 case -1407102957: 5035 /* versionId */ return new String[] { "string" }; 5036 default: 5037 return super.getTypesForProperty(hash, name); 5038 } 5039 5040 } 5041 5042 @Override 5043 public Base addChild(String name) throws FHIRException { 5044 if (name.equals("resourceId")) { 5045 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.resourceId"); 5046 } else if (name.equals("versionId")) { 5047 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.versionId"); 5048 } else 5049 return super.addChild(name); 5050 } 5051 5052 public ExampleScenarioInstanceContainedInstanceComponent copy() { 5053 ExampleScenarioInstanceContainedInstanceComponent dst = new ExampleScenarioInstanceContainedInstanceComponent(); 5054 copyValues(dst); 5055 return dst; 5056 } 5057 5058 public void copyValues(ExampleScenarioInstanceContainedInstanceComponent dst) { 5059 super.copyValues(dst); 5060 dst.resourceId = resourceId == null ? null : resourceId.copy(); 5061 dst.versionId = versionId == null ? null : versionId.copy(); 5062 } 5063 5064 @Override 5065 public boolean equalsDeep(Base other_) { 5066 if (!super.equalsDeep(other_)) 5067 return false; 5068 if (!(other_ instanceof ExampleScenarioInstanceContainedInstanceComponent)) 5069 return false; 5070 ExampleScenarioInstanceContainedInstanceComponent o = (ExampleScenarioInstanceContainedInstanceComponent) other_; 5071 return compareDeep(resourceId, o.resourceId, true) && compareDeep(versionId, o.versionId, true); 5072 } 5073 5074 @Override 5075 public boolean equalsShallow(Base other_) { 5076 if (!super.equalsShallow(other_)) 5077 return false; 5078 if (!(other_ instanceof ExampleScenarioInstanceContainedInstanceComponent)) 5079 return false; 5080 ExampleScenarioInstanceContainedInstanceComponent o = (ExampleScenarioInstanceContainedInstanceComponent) other_; 5081 return compareValues(resourceId, o.resourceId, true) && compareValues(versionId, o.versionId, true); 5082 } 5083 5084 public boolean isEmpty() { 5085 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(resourceId, versionId); 5086 } 5087 5088 public String fhirType() { 5089 return "ExampleScenario.instance.containedInstance"; 5090 5091 } 5092 5093 } 5094 5095 @Block() 5096 public static class ExampleScenarioProcessComponent extends BackboneElement implements IBaseBackboneElement { 5097 /** 5098 * The diagram title of the group of operations. 5099 */ 5100 @Child(name = "title", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 5101 @Description(shortDefinition = "The diagram title of the group of operations", formalDefinition = "The diagram title of the group of operations.") 5102 protected StringType title; 5103 5104 /** 5105 * A longer description of the group of operations. 5106 */ 5107 @Child(name = "description", type = { 5108 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 5109 @Description(shortDefinition = "A longer description of the group of operations", formalDefinition = "A longer description of the group of operations.") 5110 protected MarkdownType description; 5111 5112 /** 5113 * Description of initial status before the process starts. 5114 */ 5115 @Child(name = "preConditions", type = { 5116 MarkdownType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 5117 @Description(shortDefinition = "Description of initial status before the process starts", formalDefinition = "Description of initial status before the process starts.") 5118 protected MarkdownType preConditions; 5119 5120 /** 5121 * Description of final status after the process ends. 5122 */ 5123 @Child(name = "postConditions", type = { 5124 MarkdownType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 5125 @Description(shortDefinition = "Description of final status after the process ends", formalDefinition = "Description of final status after the process ends.") 5126 protected MarkdownType postConditions; 5127 5128 /** 5129 * Each step of the process. 5130 */ 5131 @Child(name = "step", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5132 @Description(shortDefinition = "Each step of the process", formalDefinition = "Each step of the process.") 5133 protected List<ExampleScenarioProcessStepComponent> step; 5134 5135 private static final long serialVersionUID = 325578043L; 5136 5137 /** 5138 * Constructor 5139 */ 5140 public ExampleScenarioProcessComponent() { 5141 super(); 5142 } 5143 5144 /** 5145 * Constructor 5146 */ 5147 public ExampleScenarioProcessComponent(StringType title) { 5148 super(); 5149 this.title = title; 5150 } 5151 5152 /** 5153 * @return {@link #title} (The diagram title of the group of operations.). This 5154 * is the underlying object with id, value and extensions. The accessor 5155 * "getTitle" gives direct access to the value 5156 */ 5157 public StringType getTitleElement() { 5158 if (this.title == null) 5159 if (Configuration.errorOnAutoCreate()) 5160 throw new Error("Attempt to auto-create ExampleScenarioProcessComponent.title"); 5161 else if (Configuration.doAutoCreate()) 5162 this.title = new StringType(); // bb 5163 return this.title; 5164 } 5165 5166 public boolean hasTitleElement() { 5167 return this.title != null && !this.title.isEmpty(); 5168 } 5169 5170 public boolean hasTitle() { 5171 return this.title != null && !this.title.isEmpty(); 5172 } 5173 5174 /** 5175 * @param value {@link #title} (The diagram title of the group of operations.). 5176 * This is the underlying object with id, value and extensions. The 5177 * accessor "getTitle" gives direct access to the value 5178 */ 5179 public ExampleScenarioProcessComponent setTitleElement(StringType value) { 5180 this.title = value; 5181 return this; 5182 } 5183 5184 /** 5185 * @return The diagram title of the group of operations. 5186 */ 5187 public String getTitle() { 5188 return this.title == null ? null : this.title.getValue(); 5189 } 5190 5191 /** 5192 * @param value The diagram title of the group of operations. 5193 */ 5194 public ExampleScenarioProcessComponent setTitle(String value) { 5195 if (this.title == null) 5196 this.title = new StringType(); 5197 this.title.setValue(value); 5198 return this; 5199 } 5200 5201 /** 5202 * @return {@link #description} (A longer description of the group of 5203 * operations.). This is the underlying object with id, value and 5204 * extensions. The accessor "getDescription" gives direct access to the 5205 * value 5206 */ 5207 public MarkdownType getDescriptionElement() { 5208 if (this.description == null) 5209 if (Configuration.errorOnAutoCreate()) 5210 throw new Error("Attempt to auto-create ExampleScenarioProcessComponent.description"); 5211 else if (Configuration.doAutoCreate()) 5212 this.description = new MarkdownType(); // bb 5213 return this.description; 5214 } 5215 5216 public boolean hasDescriptionElement() { 5217 return this.description != null && !this.description.isEmpty(); 5218 } 5219 5220 public boolean hasDescription() { 5221 return this.description != null && !this.description.isEmpty(); 5222 } 5223 5224 /** 5225 * @param value {@link #description} (A longer description of the group of 5226 * operations.). This is the underlying object with id, value and 5227 * extensions. The accessor "getDescription" gives direct access to 5228 * the value 5229 */ 5230 public ExampleScenarioProcessComponent setDescriptionElement(MarkdownType value) { 5231 this.description = value; 5232 return this; 5233 } 5234 5235 /** 5236 * @return A longer description of the group of operations. 5237 */ 5238 public String getDescription() { 5239 return this.description == null ? null : this.description.getValue(); 5240 } 5241 5242 /** 5243 * @param value A longer description of the group of operations. 5244 */ 5245 public ExampleScenarioProcessComponent setDescription(String value) { 5246 if (value == null) 5247 this.description = null; 5248 else { 5249 if (this.description == null) 5250 this.description = new MarkdownType(); 5251 this.description.setValue(value); 5252 } 5253 return this; 5254 } 5255 5256 /** 5257 * @return {@link #preConditions} (Description of initial status before the 5258 * process starts.). This is the underlying object with id, value and 5259 * extensions. The accessor "getPreConditions" gives direct access to 5260 * the value 5261 */ 5262 public MarkdownType getPreConditionsElement() { 5263 if (this.preConditions == null) 5264 if (Configuration.errorOnAutoCreate()) 5265 throw new Error("Attempt to auto-create ExampleScenarioProcessComponent.preConditions"); 5266 else if (Configuration.doAutoCreate()) 5267 this.preConditions = new MarkdownType(); // bb 5268 return this.preConditions; 5269 } 5270 5271 public boolean hasPreConditionsElement() { 5272 return this.preConditions != null && !this.preConditions.isEmpty(); 5273 } 5274 5275 public boolean hasPreConditions() { 5276 return this.preConditions != null && !this.preConditions.isEmpty(); 5277 } 5278 5279 /** 5280 * @param value {@link #preConditions} (Description of initial status before the 5281 * process starts.). This is the underlying object with id, value 5282 * and extensions. The accessor "getPreConditions" gives direct 5283 * access to the value 5284 */ 5285 public ExampleScenarioProcessComponent setPreConditionsElement(MarkdownType value) { 5286 this.preConditions = value; 5287 return this; 5288 } 5289 5290 /** 5291 * @return Description of initial status before the process starts. 5292 */ 5293 public String getPreConditions() { 5294 return this.preConditions == null ? null : this.preConditions.getValue(); 5295 } 5296 5297 /** 5298 * @param value Description of initial status before the process starts. 5299 */ 5300 public ExampleScenarioProcessComponent setPreConditions(String value) { 5301 if (value == null) 5302 this.preConditions = null; 5303 else { 5304 if (this.preConditions == null) 5305 this.preConditions = new MarkdownType(); 5306 this.preConditions.setValue(value); 5307 } 5308 return this; 5309 } 5310 5311 /** 5312 * @return {@link #postConditions} (Description of final status after the 5313 * process ends.). This is the underlying object with id, value and 5314 * extensions. The accessor "getPostConditions" gives direct access to 5315 * the value 5316 */ 5317 public MarkdownType getPostConditionsElement() { 5318 if (this.postConditions == null) 5319 if (Configuration.errorOnAutoCreate()) 5320 throw new Error("Attempt to auto-create ExampleScenarioProcessComponent.postConditions"); 5321 else if (Configuration.doAutoCreate()) 5322 this.postConditions = new MarkdownType(); // bb 5323 return this.postConditions; 5324 } 5325 5326 public boolean hasPostConditionsElement() { 5327 return this.postConditions != null && !this.postConditions.isEmpty(); 5328 } 5329 5330 public boolean hasPostConditions() { 5331 return this.postConditions != null && !this.postConditions.isEmpty(); 5332 } 5333 5334 /** 5335 * @param value {@link #postConditions} (Description of final status after the 5336 * process ends.). This is the underlying object with id, value and 5337 * extensions. The accessor "getPostConditions" gives direct access 5338 * to the value 5339 */ 5340 public ExampleScenarioProcessComponent setPostConditionsElement(MarkdownType value) { 5341 this.postConditions = value; 5342 return this; 5343 } 5344 5345 /** 5346 * @return Description of final status after the process ends. 5347 */ 5348 public String getPostConditions() { 5349 return this.postConditions == null ? null : this.postConditions.getValue(); 5350 } 5351 5352 /** 5353 * @param value Description of final status after the process ends. 5354 */ 5355 public ExampleScenarioProcessComponent setPostConditions(String value) { 5356 if (value == null) 5357 this.postConditions = null; 5358 else { 5359 if (this.postConditions == null) 5360 this.postConditions = new MarkdownType(); 5361 this.postConditions.setValue(value); 5362 } 5363 return this; 5364 } 5365 5366 /** 5367 * @return {@link #step} (Each step of the process.) 5368 */ 5369 public List<ExampleScenarioProcessStepComponent> getStep() { 5370 if (this.step == null) 5371 this.step = new ArrayList<ExampleScenarioProcessStepComponent>(); 5372 return this.step; 5373 } 5374 5375 /** 5376 * @return Returns a reference to <code>this</code> for easy method chaining 5377 */ 5378 public ExampleScenarioProcessComponent setStep(List<ExampleScenarioProcessStepComponent> theStep) { 5379 this.step = theStep; 5380 return this; 5381 } 5382 5383 public boolean hasStep() { 5384 if (this.step == null) 5385 return false; 5386 for (ExampleScenarioProcessStepComponent item : this.step) 5387 if (!item.isEmpty()) 5388 return true; 5389 return false; 5390 } 5391 5392 public ExampleScenarioProcessStepComponent addStep() { // 3 5393 ExampleScenarioProcessStepComponent t = new ExampleScenarioProcessStepComponent(); 5394 if (this.step == null) 5395 this.step = new ArrayList<ExampleScenarioProcessStepComponent>(); 5396 this.step.add(t); 5397 return t; 5398 } 5399 5400 public ExampleScenarioProcessComponent addStep(ExampleScenarioProcessStepComponent t) { // 3 5401 if (t == null) 5402 return this; 5403 if (this.step == null) 5404 this.step = new ArrayList<ExampleScenarioProcessStepComponent>(); 5405 this.step.add(t); 5406 return this; 5407 } 5408 5409 /** 5410 * @return The first repetition of repeating field {@link #step}, creating it if 5411 * it does not already exist 5412 */ 5413 public ExampleScenarioProcessStepComponent getStepFirstRep() { 5414 if (getStep().isEmpty()) { 5415 addStep(); 5416 } 5417 return getStep().get(0); 5418 } 5419 5420 protected void listChildren(List<Property> children) { 5421 super.listChildren(children); 5422 children.add(new Property("title", "string", "The diagram title of the group of operations.", 0, 1, title)); 5423 children.add(new Property("description", "markdown", "A longer description of the group of operations.", 0, 1, 5424 description)); 5425 children.add(new Property("preConditions", "markdown", "Description of initial status before the process starts.", 5426 0, 1, preConditions)); 5427 children.add(new Property("postConditions", "markdown", "Description of final status after the process ends.", 0, 5428 1, postConditions)); 5429 children.add(new Property("step", "", "Each step of the process.", 0, java.lang.Integer.MAX_VALUE, step)); 5430 } 5431 5432 @Override 5433 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5434 switch (_hash) { 5435 case 110371416: 5436 /* title */ return new Property("title", "string", "The diagram title of the group of operations.", 0, 1, 5437 title); 5438 case -1724546052: 5439 /* description */ return new Property("description", "markdown", 5440 "A longer description of the group of operations.", 0, 1, description); 5441 case -1006692933: 5442 /* preConditions */ return new Property("preConditions", "markdown", 5443 "Description of initial status before the process starts.", 0, 1, preConditions); 5444 case 1738302328: 5445 /* postConditions */ return new Property("postConditions", "markdown", 5446 "Description of final status after the process ends.", 0, 1, postConditions); 5447 case 3540684: 5448 /* step */ return new Property("step", "", "Each step of the process.", 0, java.lang.Integer.MAX_VALUE, step); 5449 default: 5450 return super.getNamedProperty(_hash, _name, _checkValid); 5451 } 5452 5453 } 5454 5455 @Override 5456 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5457 switch (hash) { 5458 case 110371416: 5459 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 5460 case -1724546052: 5461 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 5462 case -1006692933: 5463 /* preConditions */ return this.preConditions == null ? new Base[0] : new Base[] { this.preConditions }; // MarkdownType 5464 case 1738302328: 5465 /* postConditions */ return this.postConditions == null ? new Base[0] : new Base[] { this.postConditions }; // MarkdownType 5466 case 3540684: 5467 /* step */ return this.step == null ? new Base[0] : this.step.toArray(new Base[this.step.size()]); // ExampleScenarioProcessStepComponent 5468 default: 5469 return super.getProperty(hash, name, checkValid); 5470 } 5471 5472 } 5473 5474 @Override 5475 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5476 switch (hash) { 5477 case 110371416: // title 5478 this.title = castToString(value); // StringType 5479 return value; 5480 case -1724546052: // description 5481 this.description = castToMarkdown(value); // MarkdownType 5482 return value; 5483 case -1006692933: // preConditions 5484 this.preConditions = castToMarkdown(value); // MarkdownType 5485 return value; 5486 case 1738302328: // postConditions 5487 this.postConditions = castToMarkdown(value); // MarkdownType 5488 return value; 5489 case 3540684: // step 5490 this.getStep().add((ExampleScenarioProcessStepComponent) value); // ExampleScenarioProcessStepComponent 5491 return value; 5492 default: 5493 return super.setProperty(hash, name, value); 5494 } 5495 5496 } 5497 5498 @Override 5499 public Base setProperty(String name, Base value) throws FHIRException { 5500 if (name.equals("title")) { 5501 this.title = castToString(value); // StringType 5502 } else if (name.equals("description")) { 5503 this.description = castToMarkdown(value); // MarkdownType 5504 } else if (name.equals("preConditions")) { 5505 this.preConditions = castToMarkdown(value); // MarkdownType 5506 } else if (name.equals("postConditions")) { 5507 this.postConditions = castToMarkdown(value); // MarkdownType 5508 } else if (name.equals("step")) { 5509 this.getStep().add((ExampleScenarioProcessStepComponent) value); 5510 } else 5511 return super.setProperty(name, value); 5512 return value; 5513 } 5514 5515 @Override 5516 public Base makeProperty(int hash, String name) throws FHIRException { 5517 switch (hash) { 5518 case 110371416: 5519 return getTitleElement(); 5520 case -1724546052: 5521 return getDescriptionElement(); 5522 case -1006692933: 5523 return getPreConditionsElement(); 5524 case 1738302328: 5525 return getPostConditionsElement(); 5526 case 3540684: 5527 return addStep(); 5528 default: 5529 return super.makeProperty(hash, name); 5530 } 5531 5532 } 5533 5534 @Override 5535 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5536 switch (hash) { 5537 case 110371416: 5538 /* title */ return new String[] { "string" }; 5539 case -1724546052: 5540 /* description */ return new String[] { "markdown" }; 5541 case -1006692933: 5542 /* preConditions */ return new String[] { "markdown" }; 5543 case 1738302328: 5544 /* postConditions */ return new String[] { "markdown" }; 5545 case 3540684: 5546 /* step */ return new String[] {}; 5547 default: 5548 return super.getTypesForProperty(hash, name); 5549 } 5550 5551 } 5552 5553 @Override 5554 public Base addChild(String name) throws FHIRException { 5555 if (name.equals("title")) { 5556 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.title"); 5557 } else if (name.equals("description")) { 5558 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.description"); 5559 } else if (name.equals("preConditions")) { 5560 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.preConditions"); 5561 } else if (name.equals("postConditions")) { 5562 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.postConditions"); 5563 } else if (name.equals("step")) { 5564 return addStep(); 5565 } else 5566 return super.addChild(name); 5567 } 5568 5569 public ExampleScenarioProcessComponent copy() { 5570 ExampleScenarioProcessComponent dst = new ExampleScenarioProcessComponent(); 5571 copyValues(dst); 5572 return dst; 5573 } 5574 5575 public void copyValues(ExampleScenarioProcessComponent dst) { 5576 super.copyValues(dst); 5577 dst.title = title == null ? null : title.copy(); 5578 dst.description = description == null ? null : description.copy(); 5579 dst.preConditions = preConditions == null ? null : preConditions.copy(); 5580 dst.postConditions = postConditions == null ? null : postConditions.copy(); 5581 if (step != null) { 5582 dst.step = new ArrayList<ExampleScenarioProcessStepComponent>(); 5583 for (ExampleScenarioProcessStepComponent i : step) 5584 dst.step.add(i.copy()); 5585 } 5586 ; 5587 } 5588 5589 @Override 5590 public boolean equalsDeep(Base other_) { 5591 if (!super.equalsDeep(other_)) 5592 return false; 5593 if (!(other_ instanceof ExampleScenarioProcessComponent)) 5594 return false; 5595 ExampleScenarioProcessComponent o = (ExampleScenarioProcessComponent) other_; 5596 return compareDeep(title, o.title, true) && compareDeep(description, o.description, true) 5597 && compareDeep(preConditions, o.preConditions, true) && compareDeep(postConditions, o.postConditions, true) 5598 && compareDeep(step, o.step, true); 5599 } 5600 5601 @Override 5602 public boolean equalsShallow(Base other_) { 5603 if (!super.equalsShallow(other_)) 5604 return false; 5605 if (!(other_ instanceof ExampleScenarioProcessComponent)) 5606 return false; 5607 ExampleScenarioProcessComponent o = (ExampleScenarioProcessComponent) other_; 5608 return compareValues(title, o.title, true) && compareValues(description, o.description, true) 5609 && compareValues(preConditions, o.preConditions, true) 5610 && compareValues(postConditions, o.postConditions, true); 5611 } 5612 5613 public boolean isEmpty() { 5614 return super.isEmpty() 5615 && ca.uhn.fhir.util.ElementUtil.isEmpty(title, description, preConditions, postConditions, step); 5616 } 5617 5618 public String fhirType() { 5619 return "ExampleScenario.process"; 5620 5621 } 5622 5623 } 5624 5625 @Block() 5626 public static class ExampleScenarioProcessStepComponent extends BackboneElement implements IBaseBackboneElement { 5627 /** 5628 * Nested process. 5629 */ 5630 @Child(name = "process", type = { 5631 ExampleScenarioProcessComponent.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5632 @Description(shortDefinition = "Nested process", formalDefinition = "Nested process.") 5633 protected List<ExampleScenarioProcessComponent> process; 5634 5635 /** 5636 * If there is a pause in the flow. 5637 */ 5638 @Child(name = "pause", type = { BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 5639 @Description(shortDefinition = "If there is a pause in the flow", formalDefinition = "If there is a pause in the flow.") 5640 protected BooleanType pause; 5641 5642 /** 5643 * Each interaction or action. 5644 */ 5645 @Child(name = "operation", type = {}, order = 3, min = 0, max = 1, modifier = false, summary = false) 5646 @Description(shortDefinition = "Each interaction or action", formalDefinition = "Each interaction or action.") 5647 protected ExampleScenarioProcessStepOperationComponent operation; 5648 5649 /** 5650 * Indicates an alternative step that can be taken instead of the operations on 5651 * the base step in exceptional/atypical circumstances. 5652 */ 5653 @Child(name = "alternative", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5654 @Description(shortDefinition = "Alternate non-typical step action", formalDefinition = "Indicates an alternative step that can be taken instead of the operations on the base step in exceptional/atypical circumstances.") 5655 protected List<ExampleScenarioProcessStepAlternativeComponent> alternative; 5656 5657 private static final long serialVersionUID = -894029605L; 5658 5659 /** 5660 * Constructor 5661 */ 5662 public ExampleScenarioProcessStepComponent() { 5663 super(); 5664 } 5665 5666 /** 5667 * @return {@link #process} (Nested process.) 5668 */ 5669 public List<ExampleScenarioProcessComponent> getProcess() { 5670 if (this.process == null) 5671 this.process = new ArrayList<ExampleScenarioProcessComponent>(); 5672 return this.process; 5673 } 5674 5675 /** 5676 * @return Returns a reference to <code>this</code> for easy method chaining 5677 */ 5678 public ExampleScenarioProcessStepComponent setProcess(List<ExampleScenarioProcessComponent> theProcess) { 5679 this.process = theProcess; 5680 return this; 5681 } 5682 5683 public boolean hasProcess() { 5684 if (this.process == null) 5685 return false; 5686 for (ExampleScenarioProcessComponent item : this.process) 5687 if (!item.isEmpty()) 5688 return true; 5689 return false; 5690 } 5691 5692 public ExampleScenarioProcessComponent addProcess() { // 3 5693 ExampleScenarioProcessComponent t = new ExampleScenarioProcessComponent(); 5694 if (this.process == null) 5695 this.process = new ArrayList<ExampleScenarioProcessComponent>(); 5696 this.process.add(t); 5697 return t; 5698 } 5699 5700 public ExampleScenarioProcessStepComponent addProcess(ExampleScenarioProcessComponent t) { // 3 5701 if (t == null) 5702 return this; 5703 if (this.process == null) 5704 this.process = new ArrayList<ExampleScenarioProcessComponent>(); 5705 this.process.add(t); 5706 return this; 5707 } 5708 5709 /** 5710 * @return The first repetition of repeating field {@link #process}, creating it 5711 * if it does not already exist 5712 */ 5713 public ExampleScenarioProcessComponent getProcessFirstRep() { 5714 if (getProcess().isEmpty()) { 5715 addProcess(); 5716 } 5717 return getProcess().get(0); 5718 } 5719 5720 /** 5721 * @return {@link #pause} (If there is a pause in the flow.). This is the 5722 * underlying object with id, value and extensions. The accessor 5723 * "getPause" gives direct access to the value 5724 */ 5725 public BooleanType getPauseElement() { 5726 if (this.pause == null) 5727 if (Configuration.errorOnAutoCreate()) 5728 throw new Error("Attempt to auto-create ExampleScenarioProcessStepComponent.pause"); 5729 else if (Configuration.doAutoCreate()) 5730 this.pause = new BooleanType(); // bb 5731 return this.pause; 5732 } 5733 5734 public boolean hasPauseElement() { 5735 return this.pause != null && !this.pause.isEmpty(); 5736 } 5737 5738 public boolean hasPause() { 5739 return this.pause != null && !this.pause.isEmpty(); 5740 } 5741 5742 /** 5743 * @param value {@link #pause} (If there is a pause in the flow.). This is the 5744 * underlying object with id, value and extensions. The accessor 5745 * "getPause" gives direct access to the value 5746 */ 5747 public ExampleScenarioProcessStepComponent setPauseElement(BooleanType value) { 5748 this.pause = value; 5749 return this; 5750 } 5751 5752 /** 5753 * @return If there is a pause in the flow. 5754 */ 5755 public boolean getPause() { 5756 return this.pause == null || this.pause.isEmpty() ? false : this.pause.getValue(); 5757 } 5758 5759 /** 5760 * @param value If there is a pause in the flow. 5761 */ 5762 public ExampleScenarioProcessStepComponent setPause(boolean value) { 5763 if (this.pause == null) 5764 this.pause = new BooleanType(); 5765 this.pause.setValue(value); 5766 return this; 5767 } 5768 5769 /** 5770 * @return {@link #operation} (Each interaction or action.) 5771 */ 5772 public ExampleScenarioProcessStepOperationComponent getOperation() { 5773 if (this.operation == null) 5774 if (Configuration.errorOnAutoCreate()) 5775 throw new Error("Attempt to auto-create ExampleScenarioProcessStepComponent.operation"); 5776 else if (Configuration.doAutoCreate()) 5777 this.operation = new ExampleScenarioProcessStepOperationComponent(); // cc 5778 return this.operation; 5779 } 5780 5781 public boolean hasOperation() { 5782 return this.operation != null && !this.operation.isEmpty(); 5783 } 5784 5785 /** 5786 * @param value {@link #operation} (Each interaction or action.) 5787 */ 5788 public ExampleScenarioProcessStepComponent setOperation(ExampleScenarioProcessStepOperationComponent value) { 5789 this.operation = value; 5790 return this; 5791 } 5792 5793 /** 5794 * @return {@link #alternative} (Indicates an alternative step that can be taken 5795 * instead of the operations on the base step in exceptional/atypical 5796 * circumstances.) 5797 */ 5798 public List<ExampleScenarioProcessStepAlternativeComponent> getAlternative() { 5799 if (this.alternative == null) 5800 this.alternative = new ArrayList<ExampleScenarioProcessStepAlternativeComponent>(); 5801 return this.alternative; 5802 } 5803 5804 /** 5805 * @return Returns a reference to <code>this</code> for easy method chaining 5806 */ 5807 public ExampleScenarioProcessStepComponent setAlternative( 5808 List<ExampleScenarioProcessStepAlternativeComponent> theAlternative) { 5809 this.alternative = theAlternative; 5810 return this; 5811 } 5812 5813 public boolean hasAlternative() { 5814 if (this.alternative == null) 5815 return false; 5816 for (ExampleScenarioProcessStepAlternativeComponent item : this.alternative) 5817 if (!item.isEmpty()) 5818 return true; 5819 return false; 5820 } 5821 5822 public ExampleScenarioProcessStepAlternativeComponent addAlternative() { // 3 5823 ExampleScenarioProcessStepAlternativeComponent t = new ExampleScenarioProcessStepAlternativeComponent(); 5824 if (this.alternative == null) 5825 this.alternative = new ArrayList<ExampleScenarioProcessStepAlternativeComponent>(); 5826 this.alternative.add(t); 5827 return t; 5828 } 5829 5830 public ExampleScenarioProcessStepComponent addAlternative(ExampleScenarioProcessStepAlternativeComponent t) { // 3 5831 if (t == null) 5832 return this; 5833 if (this.alternative == null) 5834 this.alternative = new ArrayList<ExampleScenarioProcessStepAlternativeComponent>(); 5835 this.alternative.add(t); 5836 return this; 5837 } 5838 5839 /** 5840 * @return The first repetition of repeating field {@link #alternative}, 5841 * creating it if it does not already exist 5842 */ 5843 public ExampleScenarioProcessStepAlternativeComponent getAlternativeFirstRep() { 5844 if (getAlternative().isEmpty()) { 5845 addAlternative(); 5846 } 5847 return getAlternative().get(0); 5848 } 5849 5850 protected void listChildren(List<Property> children) { 5851 super.listChildren(children); 5852 children.add(new Property("process", "@ExampleScenario.process", "Nested process.", 0, 5853 java.lang.Integer.MAX_VALUE, process)); 5854 children.add(new Property("pause", "boolean", "If there is a pause in the flow.", 0, 1, pause)); 5855 children.add(new Property("operation", "", "Each interaction or action.", 0, 1, operation)); 5856 children.add(new Property("alternative", "", 5857 "Indicates an alternative step that can be taken instead of the operations on the base step in exceptional/atypical circumstances.", 5858 0, java.lang.Integer.MAX_VALUE, alternative)); 5859 } 5860 5861 @Override 5862 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5863 switch (_hash) { 5864 case -309518737: 5865 /* process */ return new Property("process", "@ExampleScenario.process", "Nested process.", 0, 5866 java.lang.Integer.MAX_VALUE, process); 5867 case 106440182: 5868 /* pause */ return new Property("pause", "boolean", "If there is a pause in the flow.", 0, 1, pause); 5869 case 1662702951: 5870 /* operation */ return new Property("operation", "", "Each interaction or action.", 0, 1, operation); 5871 case -196794451: 5872 /* alternative */ return new Property("alternative", "", 5873 "Indicates an alternative step that can be taken instead of the operations on the base step in exceptional/atypical circumstances.", 5874 0, java.lang.Integer.MAX_VALUE, alternative); 5875 default: 5876 return super.getNamedProperty(_hash, _name, _checkValid); 5877 } 5878 5879 } 5880 5881 @Override 5882 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5883 switch (hash) { 5884 case -309518737: 5885 /* process */ return this.process == null ? new Base[0] : this.process.toArray(new Base[this.process.size()]); // ExampleScenarioProcessComponent 5886 case 106440182: 5887 /* pause */ return this.pause == null ? new Base[0] : new Base[] { this.pause }; // BooleanType 5888 case 1662702951: 5889 /* operation */ return this.operation == null ? new Base[0] : new Base[] { this.operation }; // ExampleScenarioProcessStepOperationComponent 5890 case -196794451: 5891 /* alternative */ return this.alternative == null ? new Base[0] 5892 : this.alternative.toArray(new Base[this.alternative.size()]); // ExampleScenarioProcessStepAlternativeComponent 5893 default: 5894 return super.getProperty(hash, name, checkValid); 5895 } 5896 5897 } 5898 5899 @Override 5900 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5901 switch (hash) { 5902 case -309518737: // process 5903 this.getProcess().add((ExampleScenarioProcessComponent) value); // ExampleScenarioProcessComponent 5904 return value; 5905 case 106440182: // pause 5906 this.pause = castToBoolean(value); // BooleanType 5907 return value; 5908 case 1662702951: // operation 5909 this.operation = (ExampleScenarioProcessStepOperationComponent) value; // ExampleScenarioProcessStepOperationComponent 5910 return value; 5911 case -196794451: // alternative 5912 this.getAlternative().add((ExampleScenarioProcessStepAlternativeComponent) value); // ExampleScenarioProcessStepAlternativeComponent 5913 return value; 5914 default: 5915 return super.setProperty(hash, name, value); 5916 } 5917 5918 } 5919 5920 @Override 5921 public Base setProperty(String name, Base value) throws FHIRException { 5922 if (name.equals("process")) { 5923 this.getProcess().add((ExampleScenarioProcessComponent) value); 5924 } else if (name.equals("pause")) { 5925 this.pause = castToBoolean(value); // BooleanType 5926 } else if (name.equals("operation")) { 5927 this.operation = (ExampleScenarioProcessStepOperationComponent) value; // ExampleScenarioProcessStepOperationComponent 5928 } else if (name.equals("alternative")) { 5929 this.getAlternative().add((ExampleScenarioProcessStepAlternativeComponent) value); 5930 } else 5931 return super.setProperty(name, value); 5932 return value; 5933 } 5934 5935 @Override 5936 public Base makeProperty(int hash, String name) throws FHIRException { 5937 switch (hash) { 5938 case -309518737: 5939 return addProcess(); 5940 case 106440182: 5941 return getPauseElement(); 5942 case 1662702951: 5943 return getOperation(); 5944 case -196794451: 5945 return addAlternative(); 5946 default: 5947 return super.makeProperty(hash, name); 5948 } 5949 5950 } 5951 5952 @Override 5953 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5954 switch (hash) { 5955 case -309518737: 5956 /* process */ return new String[] { "@ExampleScenario.process" }; 5957 case 106440182: 5958 /* pause */ return new String[] { "boolean" }; 5959 case 1662702951: 5960 /* operation */ return new String[] {}; 5961 case -196794451: 5962 /* alternative */ return new String[] {}; 5963 default: 5964 return super.getTypesForProperty(hash, name); 5965 } 5966 5967 } 5968 5969 @Override 5970 public Base addChild(String name) throws FHIRException { 5971 if (name.equals("process")) { 5972 return addProcess(); 5973 } else if (name.equals("pause")) { 5974 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.pause"); 5975 } else if (name.equals("operation")) { 5976 this.operation = new ExampleScenarioProcessStepOperationComponent(); 5977 return this.operation; 5978 } else if (name.equals("alternative")) { 5979 return addAlternative(); 5980 } else 5981 return super.addChild(name); 5982 } 5983 5984 public ExampleScenarioProcessStepComponent copy() { 5985 ExampleScenarioProcessStepComponent dst = new ExampleScenarioProcessStepComponent(); 5986 copyValues(dst); 5987 return dst; 5988 } 5989 5990 public void copyValues(ExampleScenarioProcessStepComponent dst) { 5991 super.copyValues(dst); 5992 if (process != null) { 5993 dst.process = new ArrayList<ExampleScenarioProcessComponent>(); 5994 for (ExampleScenarioProcessComponent i : process) 5995 dst.process.add(i.copy()); 5996 } 5997 ; 5998 dst.pause = pause == null ? null : pause.copy(); 5999 dst.operation = operation == null ? null : operation.copy(); 6000 if (alternative != null) { 6001 dst.alternative = new ArrayList<ExampleScenarioProcessStepAlternativeComponent>(); 6002 for (ExampleScenarioProcessStepAlternativeComponent i : alternative) 6003 dst.alternative.add(i.copy()); 6004 } 6005 ; 6006 } 6007 6008 @Override 6009 public boolean equalsDeep(Base other_) { 6010 if (!super.equalsDeep(other_)) 6011 return false; 6012 if (!(other_ instanceof ExampleScenarioProcessStepComponent)) 6013 return false; 6014 ExampleScenarioProcessStepComponent o = (ExampleScenarioProcessStepComponent) other_; 6015 return compareDeep(process, o.process, true) && compareDeep(pause, o.pause, true) 6016 && compareDeep(operation, o.operation, true) && compareDeep(alternative, o.alternative, true); 6017 } 6018 6019 @Override 6020 public boolean equalsShallow(Base other_) { 6021 if (!super.equalsShallow(other_)) 6022 return false; 6023 if (!(other_ instanceof ExampleScenarioProcessStepComponent)) 6024 return false; 6025 ExampleScenarioProcessStepComponent o = (ExampleScenarioProcessStepComponent) other_; 6026 return compareValues(pause, o.pause, true); 6027 } 6028 6029 public boolean isEmpty() { 6030 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(process, pause, operation, alternative); 6031 } 6032 6033 public String fhirType() { 6034 return "ExampleScenario.process.step"; 6035 6036 } 6037 6038 } 6039 6040 @Block() 6041 public static class ExampleScenarioProcessStepOperationComponent extends BackboneElement 6042 implements IBaseBackboneElement { 6043 /** 6044 * The sequential number of the interaction, e.g. 1.2.5. 6045 */ 6046 @Child(name = "number", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 6047 @Description(shortDefinition = "The sequential number of the interaction", formalDefinition = "The sequential number of the interaction, e.g. 1.2.5.") 6048 protected StringType number; 6049 6050 /** 6051 * The type of operation - CRUD. 6052 */ 6053 @Child(name = "type", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 6054 @Description(shortDefinition = "The type of operation - CRUD", formalDefinition = "The type of operation - CRUD.") 6055 protected StringType type; 6056 6057 /** 6058 * The human-friendly name of the interaction. 6059 */ 6060 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 6061 @Description(shortDefinition = "The human-friendly name of the interaction", formalDefinition = "The human-friendly name of the interaction.") 6062 protected StringType name; 6063 6064 /** 6065 * Who starts the transaction. 6066 */ 6067 @Child(name = "initiator", type = { 6068 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 6069 @Description(shortDefinition = "Who starts the transaction", formalDefinition = "Who starts the transaction.") 6070 protected StringType initiator; 6071 6072 /** 6073 * Who receives the transaction. 6074 */ 6075 @Child(name = "receiver", type = { 6076 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 6077 @Description(shortDefinition = "Who receives the transaction", formalDefinition = "Who receives the transaction.") 6078 protected StringType receiver; 6079 6080 /** 6081 * A comment to be inserted in the diagram. 6082 */ 6083 @Child(name = "description", type = { 6084 MarkdownType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 6085 @Description(shortDefinition = "A comment to be inserted in the diagram", formalDefinition = "A comment to be inserted in the diagram.") 6086 protected MarkdownType description; 6087 6088 /** 6089 * Whether the initiator is deactivated right after the transaction. 6090 */ 6091 @Child(name = "initiatorActive", type = { 6092 BooleanType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 6093 @Description(shortDefinition = "Whether the initiator is deactivated right after the transaction", formalDefinition = "Whether the initiator is deactivated right after the transaction.") 6094 protected BooleanType initiatorActive; 6095 6096 /** 6097 * Whether the receiver is deactivated right after the transaction. 6098 */ 6099 @Child(name = "receiverActive", type = { 6100 BooleanType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 6101 @Description(shortDefinition = "Whether the receiver is deactivated right after the transaction", formalDefinition = "Whether the receiver is deactivated right after the transaction.") 6102 protected BooleanType receiverActive; 6103 6104 /** 6105 * Each resource instance used by the initiator. 6106 */ 6107 @Child(name = "request", type = { 6108 ExampleScenarioInstanceContainedInstanceComponent.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 6109 @Description(shortDefinition = "Each resource instance used by the initiator", formalDefinition = "Each resource instance used by the initiator.") 6110 protected ExampleScenarioInstanceContainedInstanceComponent request; 6111 6112 /** 6113 * Each resource instance used by the responder. 6114 */ 6115 @Child(name = "response", type = { 6116 ExampleScenarioInstanceContainedInstanceComponent.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 6117 @Description(shortDefinition = "Each resource instance used by the responder", formalDefinition = "Each resource instance used by the responder.") 6118 protected ExampleScenarioInstanceContainedInstanceComponent response; 6119 6120 private static final long serialVersionUID = 911241906L; 6121 6122 /** 6123 * Constructor 6124 */ 6125 public ExampleScenarioProcessStepOperationComponent() { 6126 super(); 6127 } 6128 6129 /** 6130 * Constructor 6131 */ 6132 public ExampleScenarioProcessStepOperationComponent(StringType number) { 6133 super(); 6134 this.number = number; 6135 } 6136 6137 /** 6138 * @return {@link #number} (The sequential number of the interaction, e.g. 6139 * 1.2.5.). This is the underlying object with id, value and extensions. 6140 * The accessor "getNumber" gives direct access to the value 6141 */ 6142 public StringType getNumberElement() { 6143 if (this.number == null) 6144 if (Configuration.errorOnAutoCreate()) 6145 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.number"); 6146 else if (Configuration.doAutoCreate()) 6147 this.number = new StringType(); // bb 6148 return this.number; 6149 } 6150 6151 public boolean hasNumberElement() { 6152 return this.number != null && !this.number.isEmpty(); 6153 } 6154 6155 public boolean hasNumber() { 6156 return this.number != null && !this.number.isEmpty(); 6157 } 6158 6159 /** 6160 * @param value {@link #number} (The sequential number of the interaction, e.g. 6161 * 1.2.5.). This is the underlying object with id, value and 6162 * extensions. The accessor "getNumber" gives direct access to the 6163 * value 6164 */ 6165 public ExampleScenarioProcessStepOperationComponent setNumberElement(StringType value) { 6166 this.number = value; 6167 return this; 6168 } 6169 6170 /** 6171 * @return The sequential number of the interaction, e.g. 1.2.5. 6172 */ 6173 public String getNumber() { 6174 return this.number == null ? null : this.number.getValue(); 6175 } 6176 6177 /** 6178 * @param value The sequential number of the interaction, e.g. 1.2.5. 6179 */ 6180 public ExampleScenarioProcessStepOperationComponent setNumber(String value) { 6181 if (this.number == null) 6182 this.number = new StringType(); 6183 this.number.setValue(value); 6184 return this; 6185 } 6186 6187 /** 6188 * @return {@link #type} (The type of operation - CRUD.). This is the underlying 6189 * object with id, value and extensions. The accessor "getType" gives 6190 * direct access to the value 6191 */ 6192 public StringType getTypeElement() { 6193 if (this.type == null) 6194 if (Configuration.errorOnAutoCreate()) 6195 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.type"); 6196 else if (Configuration.doAutoCreate()) 6197 this.type = new StringType(); // bb 6198 return this.type; 6199 } 6200 6201 public boolean hasTypeElement() { 6202 return this.type != null && !this.type.isEmpty(); 6203 } 6204 6205 public boolean hasType() { 6206 return this.type != null && !this.type.isEmpty(); 6207 } 6208 6209 /** 6210 * @param value {@link #type} (The type of operation - CRUD.). This is the 6211 * underlying object with id, value and extensions. The accessor 6212 * "getType" gives direct access to the value 6213 */ 6214 public ExampleScenarioProcessStepOperationComponent setTypeElement(StringType value) { 6215 this.type = value; 6216 return this; 6217 } 6218 6219 /** 6220 * @return The type of operation - CRUD. 6221 */ 6222 public String getType() { 6223 return this.type == null ? null : this.type.getValue(); 6224 } 6225 6226 /** 6227 * @param value The type of operation - CRUD. 6228 */ 6229 public ExampleScenarioProcessStepOperationComponent setType(String value) { 6230 if (Utilities.noString(value)) 6231 this.type = null; 6232 else { 6233 if (this.type == null) 6234 this.type = new StringType(); 6235 this.type.setValue(value); 6236 } 6237 return this; 6238 } 6239 6240 /** 6241 * @return {@link #name} (The human-friendly name of the interaction.). This is 6242 * the underlying object with id, value and extensions. The accessor 6243 * "getName" gives direct access to the value 6244 */ 6245 public StringType getNameElement() { 6246 if (this.name == null) 6247 if (Configuration.errorOnAutoCreate()) 6248 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.name"); 6249 else if (Configuration.doAutoCreate()) 6250 this.name = new StringType(); // bb 6251 return this.name; 6252 } 6253 6254 public boolean hasNameElement() { 6255 return this.name != null && !this.name.isEmpty(); 6256 } 6257 6258 public boolean hasName() { 6259 return this.name != null && !this.name.isEmpty(); 6260 } 6261 6262 /** 6263 * @param value {@link #name} (The human-friendly name of the interaction.). 6264 * This is the underlying object with id, value and extensions. The 6265 * accessor "getName" gives direct access to the value 6266 */ 6267 public ExampleScenarioProcessStepOperationComponent setNameElement(StringType value) { 6268 this.name = value; 6269 return this; 6270 } 6271 6272 /** 6273 * @return The human-friendly name of the interaction. 6274 */ 6275 public String getName() { 6276 return this.name == null ? null : this.name.getValue(); 6277 } 6278 6279 /** 6280 * @param value The human-friendly name of the interaction. 6281 */ 6282 public ExampleScenarioProcessStepOperationComponent setName(String value) { 6283 if (Utilities.noString(value)) 6284 this.name = null; 6285 else { 6286 if (this.name == null) 6287 this.name = new StringType(); 6288 this.name.setValue(value); 6289 } 6290 return this; 6291 } 6292 6293 /** 6294 * @return {@link #initiator} (Who starts the transaction.). This is the 6295 * underlying object with id, value and extensions. The accessor 6296 * "getInitiator" gives direct access to the value 6297 */ 6298 public StringType getInitiatorElement() { 6299 if (this.initiator == null) 6300 if (Configuration.errorOnAutoCreate()) 6301 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.initiator"); 6302 else if (Configuration.doAutoCreate()) 6303 this.initiator = new StringType(); // bb 6304 return this.initiator; 6305 } 6306 6307 public boolean hasInitiatorElement() { 6308 return this.initiator != null && !this.initiator.isEmpty(); 6309 } 6310 6311 public boolean hasInitiator() { 6312 return this.initiator != null && !this.initiator.isEmpty(); 6313 } 6314 6315 /** 6316 * @param value {@link #initiator} (Who starts the transaction.). This is the 6317 * underlying object with id, value and extensions. The accessor 6318 * "getInitiator" gives direct access to the value 6319 */ 6320 public ExampleScenarioProcessStepOperationComponent setInitiatorElement(StringType value) { 6321 this.initiator = value; 6322 return this; 6323 } 6324 6325 /** 6326 * @return Who starts the transaction. 6327 */ 6328 public String getInitiator() { 6329 return this.initiator == null ? null : this.initiator.getValue(); 6330 } 6331 6332 /** 6333 * @param value Who starts the transaction. 6334 */ 6335 public ExampleScenarioProcessStepOperationComponent setInitiator(String value) { 6336 if (Utilities.noString(value)) 6337 this.initiator = null; 6338 else { 6339 if (this.initiator == null) 6340 this.initiator = new StringType(); 6341 this.initiator.setValue(value); 6342 } 6343 return this; 6344 } 6345 6346 /** 6347 * @return {@link #receiver} (Who receives the transaction.). This is the 6348 * underlying object with id, value and extensions. The accessor 6349 * "getReceiver" gives direct access to the value 6350 */ 6351 public StringType getReceiverElement() { 6352 if (this.receiver == null) 6353 if (Configuration.errorOnAutoCreate()) 6354 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.receiver"); 6355 else if (Configuration.doAutoCreate()) 6356 this.receiver = new StringType(); // bb 6357 return this.receiver; 6358 } 6359 6360 public boolean hasReceiverElement() { 6361 return this.receiver != null && !this.receiver.isEmpty(); 6362 } 6363 6364 public boolean hasReceiver() { 6365 return this.receiver != null && !this.receiver.isEmpty(); 6366 } 6367 6368 /** 6369 * @param value {@link #receiver} (Who receives the transaction.). This is the 6370 * underlying object with id, value and extensions. The accessor 6371 * "getReceiver" gives direct access to the value 6372 */ 6373 public ExampleScenarioProcessStepOperationComponent setReceiverElement(StringType value) { 6374 this.receiver = value; 6375 return this; 6376 } 6377 6378 /** 6379 * @return Who receives the transaction. 6380 */ 6381 public String getReceiver() { 6382 return this.receiver == null ? null : this.receiver.getValue(); 6383 } 6384 6385 /** 6386 * @param value Who receives the transaction. 6387 */ 6388 public ExampleScenarioProcessStepOperationComponent setReceiver(String value) { 6389 if (Utilities.noString(value)) 6390 this.receiver = null; 6391 else { 6392 if (this.receiver == null) 6393 this.receiver = new StringType(); 6394 this.receiver.setValue(value); 6395 } 6396 return this; 6397 } 6398 6399 /** 6400 * @return {@link #description} (A comment to be inserted in the diagram.). This 6401 * is the underlying object with id, value and extensions. The accessor 6402 * "getDescription" gives direct access to the value 6403 */ 6404 public MarkdownType getDescriptionElement() { 6405 if (this.description == null) 6406 if (Configuration.errorOnAutoCreate()) 6407 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.description"); 6408 else if (Configuration.doAutoCreate()) 6409 this.description = new MarkdownType(); // bb 6410 return this.description; 6411 } 6412 6413 public boolean hasDescriptionElement() { 6414 return this.description != null && !this.description.isEmpty(); 6415 } 6416 6417 public boolean hasDescription() { 6418 return this.description != null && !this.description.isEmpty(); 6419 } 6420 6421 /** 6422 * @param value {@link #description} (A comment to be inserted in the diagram.). 6423 * This is the underlying object with id, value and extensions. The 6424 * accessor "getDescription" gives direct access to the value 6425 */ 6426 public ExampleScenarioProcessStepOperationComponent setDescriptionElement(MarkdownType value) { 6427 this.description = value; 6428 return this; 6429 } 6430 6431 /** 6432 * @return A comment to be inserted in the diagram. 6433 */ 6434 public String getDescription() { 6435 return this.description == null ? null : this.description.getValue(); 6436 } 6437 6438 /** 6439 * @param value A comment to be inserted in the diagram. 6440 */ 6441 public ExampleScenarioProcessStepOperationComponent setDescription(String value) { 6442 if (value == null) 6443 this.description = null; 6444 else { 6445 if (this.description == null) 6446 this.description = new MarkdownType(); 6447 this.description.setValue(value); 6448 } 6449 return this; 6450 } 6451 6452 /** 6453 * @return {@link #initiatorActive} (Whether the initiator is deactivated right 6454 * after the transaction.). This is the underlying object with id, value 6455 * and extensions. The accessor "getInitiatorActive" gives direct access 6456 * to the value 6457 */ 6458 public BooleanType getInitiatorActiveElement() { 6459 if (this.initiatorActive == null) 6460 if (Configuration.errorOnAutoCreate()) 6461 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.initiatorActive"); 6462 else if (Configuration.doAutoCreate()) 6463 this.initiatorActive = new BooleanType(); // bb 6464 return this.initiatorActive; 6465 } 6466 6467 public boolean hasInitiatorActiveElement() { 6468 return this.initiatorActive != null && !this.initiatorActive.isEmpty(); 6469 } 6470 6471 public boolean hasInitiatorActive() { 6472 return this.initiatorActive != null && !this.initiatorActive.isEmpty(); 6473 } 6474 6475 /** 6476 * @param value {@link #initiatorActive} (Whether the initiator is deactivated 6477 * right after the transaction.). This is the underlying object 6478 * with id, value and extensions. The accessor "getInitiatorActive" 6479 * gives direct access to the value 6480 */ 6481 public ExampleScenarioProcessStepOperationComponent setInitiatorActiveElement(BooleanType value) { 6482 this.initiatorActive = value; 6483 return this; 6484 } 6485 6486 /** 6487 * @return Whether the initiator is deactivated right after the transaction. 6488 */ 6489 public boolean getInitiatorActive() { 6490 return this.initiatorActive == null || this.initiatorActive.isEmpty() ? false : this.initiatorActive.getValue(); 6491 } 6492 6493 /** 6494 * @param value Whether the initiator is deactivated right after the 6495 * transaction. 6496 */ 6497 public ExampleScenarioProcessStepOperationComponent setInitiatorActive(boolean value) { 6498 if (this.initiatorActive == null) 6499 this.initiatorActive = new BooleanType(); 6500 this.initiatorActive.setValue(value); 6501 return this; 6502 } 6503 6504 /** 6505 * @return {@link #receiverActive} (Whether the receiver is deactivated right 6506 * after the transaction.). This is the underlying object with id, value 6507 * and extensions. The accessor "getReceiverActive" gives direct access 6508 * to the value 6509 */ 6510 public BooleanType getReceiverActiveElement() { 6511 if (this.receiverActive == null) 6512 if (Configuration.errorOnAutoCreate()) 6513 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.receiverActive"); 6514 else if (Configuration.doAutoCreate()) 6515 this.receiverActive = new BooleanType(); // bb 6516 return this.receiverActive; 6517 } 6518 6519 public boolean hasReceiverActiveElement() { 6520 return this.receiverActive != null && !this.receiverActive.isEmpty(); 6521 } 6522 6523 public boolean hasReceiverActive() { 6524 return this.receiverActive != null && !this.receiverActive.isEmpty(); 6525 } 6526 6527 /** 6528 * @param value {@link #receiverActive} (Whether the receiver is deactivated 6529 * right after the transaction.). This is the underlying object 6530 * with id, value and extensions. The accessor "getReceiverActive" 6531 * gives direct access to the value 6532 */ 6533 public ExampleScenarioProcessStepOperationComponent setReceiverActiveElement(BooleanType value) { 6534 this.receiverActive = value; 6535 return this; 6536 } 6537 6538 /** 6539 * @return Whether the receiver is deactivated right after the transaction. 6540 */ 6541 public boolean getReceiverActive() { 6542 return this.receiverActive == null || this.receiverActive.isEmpty() ? false : this.receiverActive.getValue(); 6543 } 6544 6545 /** 6546 * @param value Whether the receiver is deactivated right after the transaction. 6547 */ 6548 public ExampleScenarioProcessStepOperationComponent setReceiverActive(boolean value) { 6549 if (this.receiverActive == null) 6550 this.receiverActive = new BooleanType(); 6551 this.receiverActive.setValue(value); 6552 return this; 6553 } 6554 6555 /** 6556 * @return {@link #request} (Each resource instance used by the initiator.) 6557 */ 6558 public ExampleScenarioInstanceContainedInstanceComponent getRequest() { 6559 if (this.request == null) 6560 if (Configuration.errorOnAutoCreate()) 6561 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.request"); 6562 else if (Configuration.doAutoCreate()) 6563 this.request = new ExampleScenarioInstanceContainedInstanceComponent(); // cc 6564 return this.request; 6565 } 6566 6567 public boolean hasRequest() { 6568 return this.request != null && !this.request.isEmpty(); 6569 } 6570 6571 /** 6572 * @param value {@link #request} (Each resource instance used by the initiator.) 6573 */ 6574 public ExampleScenarioProcessStepOperationComponent setRequest( 6575 ExampleScenarioInstanceContainedInstanceComponent value) { 6576 this.request = value; 6577 return this; 6578 } 6579 6580 /** 6581 * @return {@link #response} (Each resource instance used by the responder.) 6582 */ 6583 public ExampleScenarioInstanceContainedInstanceComponent getResponse() { 6584 if (this.response == null) 6585 if (Configuration.errorOnAutoCreate()) 6586 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.response"); 6587 else if (Configuration.doAutoCreate()) 6588 this.response = new ExampleScenarioInstanceContainedInstanceComponent(); // cc 6589 return this.response; 6590 } 6591 6592 public boolean hasResponse() { 6593 return this.response != null && !this.response.isEmpty(); 6594 } 6595 6596 /** 6597 * @param value {@link #response} (Each resource instance used by the 6598 * responder.) 6599 */ 6600 public ExampleScenarioProcessStepOperationComponent setResponse( 6601 ExampleScenarioInstanceContainedInstanceComponent value) { 6602 this.response = value; 6603 return this; 6604 } 6605 6606 protected void listChildren(List<Property> children) { 6607 super.listChildren(children); 6608 children 6609 .add(new Property("number", "string", "The sequential number of the interaction, e.g. 1.2.5.", 0, 1, number)); 6610 children.add(new Property("type", "string", "The type of operation - CRUD.", 0, 1, type)); 6611 children.add(new Property("name", "string", "The human-friendly name of the interaction.", 0, 1, name)); 6612 children.add(new Property("initiator", "string", "Who starts the transaction.", 0, 1, initiator)); 6613 children.add(new Property("receiver", "string", "Who receives the transaction.", 0, 1, receiver)); 6614 children 6615 .add(new Property("description", "markdown", "A comment to be inserted in the diagram.", 0, 1, description)); 6616 children.add(new Property("initiatorActive", "boolean", 6617 "Whether the initiator is deactivated right after the transaction.", 0, 1, initiatorActive)); 6618 children.add(new Property("receiverActive", "boolean", 6619 "Whether the receiver is deactivated right after the transaction.", 0, 1, receiverActive)); 6620 children.add(new Property("request", "@ExampleScenario.instance.containedInstance", 6621 "Each resource instance used by the initiator.", 0, 1, request)); 6622 children.add(new Property("response", "@ExampleScenario.instance.containedInstance", 6623 "Each resource instance used by the responder.", 0, 1, response)); 6624 } 6625 6626 @Override 6627 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6628 switch (_hash) { 6629 case -1034364087: 6630 /* number */ return new Property("number", "string", "The sequential number of the interaction, e.g. 1.2.5.", 0, 6631 1, number); 6632 case 3575610: 6633 /* type */ return new Property("type", "string", "The type of operation - CRUD.", 0, 1, type); 6634 case 3373707: 6635 /* name */ return new Property("name", "string", "The human-friendly name of the interaction.", 0, 1, name); 6636 case -248987089: 6637 /* initiator */ return new Property("initiator", "string", "Who starts the transaction.", 0, 1, initiator); 6638 case -808719889: 6639 /* receiver */ return new Property("receiver", "string", "Who receives the transaction.", 0, 1, receiver); 6640 case -1724546052: 6641 /* description */ return new Property("description", "markdown", "A comment to be inserted in the diagram.", 0, 6642 1, description); 6643 case 384339477: 6644 /* initiatorActive */ return new Property("initiatorActive", "boolean", 6645 "Whether the initiator is deactivated right after the transaction.", 0, 1, initiatorActive); 6646 case -285284907: 6647 /* receiverActive */ return new Property("receiverActive", "boolean", 6648 "Whether the receiver is deactivated right after the transaction.", 0, 1, receiverActive); 6649 case 1095692943: 6650 /* request */ return new Property("request", "@ExampleScenario.instance.containedInstance", 6651 "Each resource instance used by the initiator.", 0, 1, request); 6652 case -340323263: 6653 /* response */ return new Property("response", "@ExampleScenario.instance.containedInstance", 6654 "Each resource instance used by the responder.", 0, 1, response); 6655 default: 6656 return super.getNamedProperty(_hash, _name, _checkValid); 6657 } 6658 6659 } 6660 6661 @Override 6662 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6663 switch (hash) { 6664 case -1034364087: 6665 /* number */ return this.number == null ? new Base[0] : new Base[] { this.number }; // StringType 6666 case 3575610: 6667 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // StringType 6668 case 3373707: 6669 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 6670 case -248987089: 6671 /* initiator */ return this.initiator == null ? new Base[0] : new Base[] { this.initiator }; // StringType 6672 case -808719889: 6673 /* receiver */ return this.receiver == null ? new Base[0] : new Base[] { this.receiver }; // StringType 6674 case -1724546052: 6675 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 6676 case 384339477: 6677 /* initiatorActive */ return this.initiatorActive == null ? new Base[0] : new Base[] { this.initiatorActive }; // BooleanType 6678 case -285284907: 6679 /* receiverActive */ return this.receiverActive == null ? new Base[0] : new Base[] { this.receiverActive }; // BooleanType 6680 case 1095692943: 6681 /* request */ return this.request == null ? new Base[0] : new Base[] { this.request }; // ExampleScenarioInstanceContainedInstanceComponent 6682 case -340323263: 6683 /* response */ return this.response == null ? new Base[0] : new Base[] { this.response }; // ExampleScenarioInstanceContainedInstanceComponent 6684 default: 6685 return super.getProperty(hash, name, checkValid); 6686 } 6687 6688 } 6689 6690 @Override 6691 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6692 switch (hash) { 6693 case -1034364087: // number 6694 this.number = castToString(value); // StringType 6695 return value; 6696 case 3575610: // type 6697 this.type = castToString(value); // StringType 6698 return value; 6699 case 3373707: // name 6700 this.name = castToString(value); // StringType 6701 return value; 6702 case -248987089: // initiator 6703 this.initiator = castToString(value); // StringType 6704 return value; 6705 case -808719889: // receiver 6706 this.receiver = castToString(value); // StringType 6707 return value; 6708 case -1724546052: // description 6709 this.description = castToMarkdown(value); // MarkdownType 6710 return value; 6711 case 384339477: // initiatorActive 6712 this.initiatorActive = castToBoolean(value); // BooleanType 6713 return value; 6714 case -285284907: // receiverActive 6715 this.receiverActive = castToBoolean(value); // BooleanType 6716 return value; 6717 case 1095692943: // request 6718 this.request = (ExampleScenarioInstanceContainedInstanceComponent) value; // ExampleScenarioInstanceContainedInstanceComponent 6719 return value; 6720 case -340323263: // response 6721 this.response = (ExampleScenarioInstanceContainedInstanceComponent) value; // ExampleScenarioInstanceContainedInstanceComponent 6722 return value; 6723 default: 6724 return super.setProperty(hash, name, value); 6725 } 6726 6727 } 6728 6729 @Override 6730 public Base setProperty(String name, Base value) throws FHIRException { 6731 if (name.equals("number")) { 6732 this.number = castToString(value); // StringType 6733 } else if (name.equals("type")) { 6734 this.type = castToString(value); // StringType 6735 } else if (name.equals("name")) { 6736 this.name = castToString(value); // StringType 6737 } else if (name.equals("initiator")) { 6738 this.initiator = castToString(value); // StringType 6739 } else if (name.equals("receiver")) { 6740 this.receiver = castToString(value); // StringType 6741 } else if (name.equals("description")) { 6742 this.description = castToMarkdown(value); // MarkdownType 6743 } else if (name.equals("initiatorActive")) { 6744 this.initiatorActive = castToBoolean(value); // BooleanType 6745 } else if (name.equals("receiverActive")) { 6746 this.receiverActive = castToBoolean(value); // BooleanType 6747 } else if (name.equals("request")) { 6748 this.request = (ExampleScenarioInstanceContainedInstanceComponent) value; // ExampleScenarioInstanceContainedInstanceComponent 6749 } else if (name.equals("response")) { 6750 this.response = (ExampleScenarioInstanceContainedInstanceComponent) value; // ExampleScenarioInstanceContainedInstanceComponent 6751 } else 6752 return super.setProperty(name, value); 6753 return value; 6754 } 6755 6756 @Override 6757 public Base makeProperty(int hash, String name) throws FHIRException { 6758 switch (hash) { 6759 case -1034364087: 6760 return getNumberElement(); 6761 case 3575610: 6762 return getTypeElement(); 6763 case 3373707: 6764 return getNameElement(); 6765 case -248987089: 6766 return getInitiatorElement(); 6767 case -808719889: 6768 return getReceiverElement(); 6769 case -1724546052: 6770 return getDescriptionElement(); 6771 case 384339477: 6772 return getInitiatorActiveElement(); 6773 case -285284907: 6774 return getReceiverActiveElement(); 6775 case 1095692943: 6776 return getRequest(); 6777 case -340323263: 6778 return getResponse(); 6779 default: 6780 return super.makeProperty(hash, name); 6781 } 6782 6783 } 6784 6785 @Override 6786 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6787 switch (hash) { 6788 case -1034364087: 6789 /* number */ return new String[] { "string" }; 6790 case 3575610: 6791 /* type */ return new String[] { "string" }; 6792 case 3373707: 6793 /* name */ return new String[] { "string" }; 6794 case -248987089: 6795 /* initiator */ return new String[] { "string" }; 6796 case -808719889: 6797 /* receiver */ return new String[] { "string" }; 6798 case -1724546052: 6799 /* description */ return new String[] { "markdown" }; 6800 case 384339477: 6801 /* initiatorActive */ return new String[] { "boolean" }; 6802 case -285284907: 6803 /* receiverActive */ return new String[] { "boolean" }; 6804 case 1095692943: 6805 /* request */ return new String[] { "@ExampleScenario.instance.containedInstance" }; 6806 case -340323263: 6807 /* response */ return new String[] { "@ExampleScenario.instance.containedInstance" }; 6808 default: 6809 return super.getTypesForProperty(hash, name); 6810 } 6811 6812 } 6813 6814 @Override 6815 public Base addChild(String name) throws FHIRException { 6816 if (name.equals("number")) { 6817 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.number"); 6818 } else if (name.equals("type")) { 6819 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.type"); 6820 } else if (name.equals("name")) { 6821 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.name"); 6822 } else if (name.equals("initiator")) { 6823 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.initiator"); 6824 } else if (name.equals("receiver")) { 6825 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.receiver"); 6826 } else if (name.equals("description")) { 6827 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.description"); 6828 } else if (name.equals("initiatorActive")) { 6829 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.initiatorActive"); 6830 } else if (name.equals("receiverActive")) { 6831 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.receiverActive"); 6832 } else if (name.equals("request")) { 6833 this.request = new ExampleScenarioInstanceContainedInstanceComponent(); 6834 return this.request; 6835 } else if (name.equals("response")) { 6836 this.response = new ExampleScenarioInstanceContainedInstanceComponent(); 6837 return this.response; 6838 } else 6839 return super.addChild(name); 6840 } 6841 6842 public ExampleScenarioProcessStepOperationComponent copy() { 6843 ExampleScenarioProcessStepOperationComponent dst = new ExampleScenarioProcessStepOperationComponent(); 6844 copyValues(dst); 6845 return dst; 6846 } 6847 6848 public void copyValues(ExampleScenarioProcessStepOperationComponent dst) { 6849 super.copyValues(dst); 6850 dst.number = number == null ? null : number.copy(); 6851 dst.type = type == null ? null : type.copy(); 6852 dst.name = name == null ? null : name.copy(); 6853 dst.initiator = initiator == null ? null : initiator.copy(); 6854 dst.receiver = receiver == null ? null : receiver.copy(); 6855 dst.description = description == null ? null : description.copy(); 6856 dst.initiatorActive = initiatorActive == null ? null : initiatorActive.copy(); 6857 dst.receiverActive = receiverActive == null ? null : receiverActive.copy(); 6858 dst.request = request == null ? null : request.copy(); 6859 dst.response = response == null ? null : response.copy(); 6860 } 6861 6862 @Override 6863 public boolean equalsDeep(Base other_) { 6864 if (!super.equalsDeep(other_)) 6865 return false; 6866 if (!(other_ instanceof ExampleScenarioProcessStepOperationComponent)) 6867 return false; 6868 ExampleScenarioProcessStepOperationComponent o = (ExampleScenarioProcessStepOperationComponent) other_; 6869 return compareDeep(number, o.number, true) && compareDeep(type, o.type, true) && compareDeep(name, o.name, true) 6870 && compareDeep(initiator, o.initiator, true) && compareDeep(receiver, o.receiver, true) 6871 && compareDeep(description, o.description, true) && compareDeep(initiatorActive, o.initiatorActive, true) 6872 && compareDeep(receiverActive, o.receiverActive, true) && compareDeep(request, o.request, true) 6873 && compareDeep(response, o.response, true); 6874 } 6875 6876 @Override 6877 public boolean equalsShallow(Base other_) { 6878 if (!super.equalsShallow(other_)) 6879 return false; 6880 if (!(other_ instanceof ExampleScenarioProcessStepOperationComponent)) 6881 return false; 6882 ExampleScenarioProcessStepOperationComponent o = (ExampleScenarioProcessStepOperationComponent) other_; 6883 return compareValues(number, o.number, true) && compareValues(type, o.type, true) 6884 && compareValues(name, o.name, true) && compareValues(initiator, o.initiator, true) 6885 && compareValues(receiver, o.receiver, true) && compareValues(description, o.description, true) 6886 && compareValues(initiatorActive, o.initiatorActive, true) 6887 && compareValues(receiverActive, o.receiverActive, true); 6888 } 6889 6890 public boolean isEmpty() { 6891 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(number, type, name, initiator, receiver, 6892 description, initiatorActive, receiverActive, request, response); 6893 } 6894 6895 public String fhirType() { 6896 return "ExampleScenario.process.step.operation"; 6897 6898 } 6899 6900 } 6901 6902 @Block() 6903 public static class ExampleScenarioProcessStepAlternativeComponent extends BackboneElement 6904 implements IBaseBackboneElement { 6905 /** 6906 * The label to display for the alternative that gives a sense of the 6907 * circumstance in which the alternative should be invoked. 6908 */ 6909 @Child(name = "title", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 6910 @Description(shortDefinition = "Label for alternative", formalDefinition = "The label to display for the alternative that gives a sense of the circumstance in which the alternative should be invoked.") 6911 protected StringType title; 6912 6913 /** 6914 * A human-readable description of the alternative explaining when the 6915 * alternative should occur rather than the base step. 6916 */ 6917 @Child(name = "description", type = { 6918 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 6919 @Description(shortDefinition = "A human-readable description of each option", formalDefinition = "A human-readable description of the alternative explaining when the alternative should occur rather than the base step.") 6920 protected MarkdownType description; 6921 6922 /** 6923 * What happens in each alternative option. 6924 */ 6925 @Child(name = "step", type = { 6926 ExampleScenarioProcessStepComponent.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6927 @Description(shortDefinition = "What happens in each alternative option", formalDefinition = "What happens in each alternative option.") 6928 protected List<ExampleScenarioProcessStepComponent> step; 6929 6930 private static final long serialVersionUID = -254687460L; 6931 6932 /** 6933 * Constructor 6934 */ 6935 public ExampleScenarioProcessStepAlternativeComponent() { 6936 super(); 6937 } 6938 6939 /** 6940 * Constructor 6941 */ 6942 public ExampleScenarioProcessStepAlternativeComponent(StringType title) { 6943 super(); 6944 this.title = title; 6945 } 6946 6947 /** 6948 * @return {@link #title} (The label to display for the alternative that gives a 6949 * sense of the circumstance in which the alternative should be 6950 * invoked.). This is the underlying object with id, value and 6951 * extensions. The accessor "getTitle" gives direct access to the value 6952 */ 6953 public StringType getTitleElement() { 6954 if (this.title == null) 6955 if (Configuration.errorOnAutoCreate()) 6956 throw new Error("Attempt to auto-create ExampleScenarioProcessStepAlternativeComponent.title"); 6957 else if (Configuration.doAutoCreate()) 6958 this.title = new StringType(); // bb 6959 return this.title; 6960 } 6961 6962 public boolean hasTitleElement() { 6963 return this.title != null && !this.title.isEmpty(); 6964 } 6965 6966 public boolean hasTitle() { 6967 return this.title != null && !this.title.isEmpty(); 6968 } 6969 6970 /** 6971 * @param value {@link #title} (The label to display for the alternative that 6972 * gives a sense of the circumstance in which the alternative 6973 * should be invoked.). This is the underlying object with id, 6974 * value and extensions. The accessor "getTitle" gives direct 6975 * access to the value 6976 */ 6977 public ExampleScenarioProcessStepAlternativeComponent setTitleElement(StringType value) { 6978 this.title = value; 6979 return this; 6980 } 6981 6982 /** 6983 * @return The label to display for the alternative that gives a sense of the 6984 * circumstance in which the alternative should be invoked. 6985 */ 6986 public String getTitle() { 6987 return this.title == null ? null : this.title.getValue(); 6988 } 6989 6990 /** 6991 * @param value The label to display for the alternative that gives a sense of 6992 * the circumstance in which the alternative should be invoked. 6993 */ 6994 public ExampleScenarioProcessStepAlternativeComponent setTitle(String value) { 6995 if (this.title == null) 6996 this.title = new StringType(); 6997 this.title.setValue(value); 6998 return this; 6999 } 7000 7001 /** 7002 * @return {@link #description} (A human-readable description of the alternative 7003 * explaining when the alternative should occur rather than the base 7004 * step.). This is the underlying object with id, value and extensions. 7005 * The accessor "getDescription" gives direct access to the value 7006 */ 7007 public MarkdownType getDescriptionElement() { 7008 if (this.description == null) 7009 if (Configuration.errorOnAutoCreate()) 7010 throw new Error("Attempt to auto-create ExampleScenarioProcessStepAlternativeComponent.description"); 7011 else if (Configuration.doAutoCreate()) 7012 this.description = new MarkdownType(); // bb 7013 return this.description; 7014 } 7015 7016 public boolean hasDescriptionElement() { 7017 return this.description != null && !this.description.isEmpty(); 7018 } 7019 7020 public boolean hasDescription() { 7021 return this.description != null && !this.description.isEmpty(); 7022 } 7023 7024 /** 7025 * @param value {@link #description} (A human-readable description of the 7026 * alternative explaining when the alternative should occur rather 7027 * than the base step.). This is the underlying object with id, 7028 * value and extensions. The accessor "getDescription" gives direct 7029 * access to the value 7030 */ 7031 public ExampleScenarioProcessStepAlternativeComponent setDescriptionElement(MarkdownType value) { 7032 this.description = value; 7033 return this; 7034 } 7035 7036 /** 7037 * @return A human-readable description of the alternative explaining when the 7038 * alternative should occur rather than the base step. 7039 */ 7040 public String getDescription() { 7041 return this.description == null ? null : this.description.getValue(); 7042 } 7043 7044 /** 7045 * @param value A human-readable description of the alternative explaining when 7046 * the alternative should occur rather than the base step. 7047 */ 7048 public ExampleScenarioProcessStepAlternativeComponent setDescription(String value) { 7049 if (value == null) 7050 this.description = null; 7051 else { 7052 if (this.description == null) 7053 this.description = new MarkdownType(); 7054 this.description.setValue(value); 7055 } 7056 return this; 7057 } 7058 7059 /** 7060 * @return {@link #step} (What happens in each alternative option.) 7061 */ 7062 public List<ExampleScenarioProcessStepComponent> getStep() { 7063 if (this.step == null) 7064 this.step = new ArrayList<ExampleScenarioProcessStepComponent>(); 7065 return this.step; 7066 } 7067 7068 /** 7069 * @return Returns a reference to <code>this</code> for easy method chaining 7070 */ 7071 public ExampleScenarioProcessStepAlternativeComponent setStep(List<ExampleScenarioProcessStepComponent> theStep) { 7072 this.step = theStep; 7073 return this; 7074 } 7075 7076 public boolean hasStep() { 7077 if (this.step == null) 7078 return false; 7079 for (ExampleScenarioProcessStepComponent item : this.step) 7080 if (!item.isEmpty()) 7081 return true; 7082 return false; 7083 } 7084 7085 public ExampleScenarioProcessStepComponent addStep() { // 3 7086 ExampleScenarioProcessStepComponent t = new ExampleScenarioProcessStepComponent(); 7087 if (this.step == null) 7088 this.step = new ArrayList<ExampleScenarioProcessStepComponent>(); 7089 this.step.add(t); 7090 return t; 7091 } 7092 7093 public ExampleScenarioProcessStepAlternativeComponent addStep(ExampleScenarioProcessStepComponent t) { // 3 7094 if (t == null) 7095 return this; 7096 if (this.step == null) 7097 this.step = new ArrayList<ExampleScenarioProcessStepComponent>(); 7098 this.step.add(t); 7099 return this; 7100 } 7101 7102 /** 7103 * @return The first repetition of repeating field {@link #step}, creating it if 7104 * it does not already exist 7105 */ 7106 public ExampleScenarioProcessStepComponent getStepFirstRep() { 7107 if (getStep().isEmpty()) { 7108 addStep(); 7109 } 7110 return getStep().get(0); 7111 } 7112 7113 protected void listChildren(List<Property> children) { 7114 super.listChildren(children); 7115 children.add(new Property("title", "string", 7116 "The label to display for the alternative that gives a sense of the circumstance in which the alternative should be invoked.", 7117 0, 1, title)); 7118 children.add(new Property("description", "markdown", 7119 "A human-readable description of the alternative explaining when the alternative should occur rather than the base step.", 7120 0, 1, description)); 7121 children.add(new Property("step", "@ExampleScenario.process.step", "What happens in each alternative option.", 0, 7122 java.lang.Integer.MAX_VALUE, step)); 7123 } 7124 7125 @Override 7126 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7127 switch (_hash) { 7128 case 110371416: 7129 /* title */ return new Property("title", "string", 7130 "The label to display for the alternative that gives a sense of the circumstance in which the alternative should be invoked.", 7131 0, 1, title); 7132 case -1724546052: 7133 /* description */ return new Property("description", "markdown", 7134 "A human-readable description of the alternative explaining when the alternative should occur rather than the base step.", 7135 0, 1, description); 7136 case 3540684: 7137 /* step */ return new Property("step", "@ExampleScenario.process.step", 7138 "What happens in each alternative option.", 0, java.lang.Integer.MAX_VALUE, step); 7139 default: 7140 return super.getNamedProperty(_hash, _name, _checkValid); 7141 } 7142 7143 } 7144 7145 @Override 7146 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7147 switch (hash) { 7148 case 110371416: 7149 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 7150 case -1724546052: 7151 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 7152 case 3540684: 7153 /* step */ return this.step == null ? new Base[0] : this.step.toArray(new Base[this.step.size()]); // ExampleScenarioProcessStepComponent 7154 default: 7155 return super.getProperty(hash, name, checkValid); 7156 } 7157 7158 } 7159 7160 @Override 7161 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7162 switch (hash) { 7163 case 110371416: // title 7164 this.title = castToString(value); // StringType 7165 return value; 7166 case -1724546052: // description 7167 this.description = castToMarkdown(value); // MarkdownType 7168 return value; 7169 case 3540684: // step 7170 this.getStep().add((ExampleScenarioProcessStepComponent) value); // ExampleScenarioProcessStepComponent 7171 return value; 7172 default: 7173 return super.setProperty(hash, name, value); 7174 } 7175 7176 } 7177 7178 @Override 7179 public Base setProperty(String name, Base value) throws FHIRException { 7180 if (name.equals("title")) { 7181 this.title = castToString(value); // StringType 7182 } else if (name.equals("description")) { 7183 this.description = castToMarkdown(value); // MarkdownType 7184 } else if (name.equals("step")) { 7185 this.getStep().add((ExampleScenarioProcessStepComponent) value); 7186 } else 7187 return super.setProperty(name, value); 7188 return value; 7189 } 7190 7191 @Override 7192 public Base makeProperty(int hash, String name) throws FHIRException { 7193 switch (hash) { 7194 case 110371416: 7195 return getTitleElement(); 7196 case -1724546052: 7197 return getDescriptionElement(); 7198 case 3540684: 7199 return addStep(); 7200 default: 7201 return super.makeProperty(hash, name); 7202 } 7203 7204 } 7205 7206 @Override 7207 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7208 switch (hash) { 7209 case 110371416: 7210 /* title */ return new String[] { "string" }; 7211 case -1724546052: 7212 /* description */ return new String[] { "markdown" }; 7213 case 3540684: 7214 /* step */ return new String[] { "@ExampleScenario.process.step" }; 7215 default: 7216 return super.getTypesForProperty(hash, name); 7217 } 7218 7219 } 7220 7221 @Override 7222 public Base addChild(String name) throws FHIRException { 7223 if (name.equals("title")) { 7224 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.title"); 7225 } else if (name.equals("description")) { 7226 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.description"); 7227 } else if (name.equals("step")) { 7228 return addStep(); 7229 } else 7230 return super.addChild(name); 7231 } 7232 7233 public ExampleScenarioProcessStepAlternativeComponent copy() { 7234 ExampleScenarioProcessStepAlternativeComponent dst = new ExampleScenarioProcessStepAlternativeComponent(); 7235 copyValues(dst); 7236 return dst; 7237 } 7238 7239 public void copyValues(ExampleScenarioProcessStepAlternativeComponent dst) { 7240 super.copyValues(dst); 7241 dst.title = title == null ? null : title.copy(); 7242 dst.description = description == null ? null : description.copy(); 7243 if (step != null) { 7244 dst.step = new ArrayList<ExampleScenarioProcessStepComponent>(); 7245 for (ExampleScenarioProcessStepComponent i : step) 7246 dst.step.add(i.copy()); 7247 } 7248 ; 7249 } 7250 7251 @Override 7252 public boolean equalsDeep(Base other_) { 7253 if (!super.equalsDeep(other_)) 7254 return false; 7255 if (!(other_ instanceof ExampleScenarioProcessStepAlternativeComponent)) 7256 return false; 7257 ExampleScenarioProcessStepAlternativeComponent o = (ExampleScenarioProcessStepAlternativeComponent) other_; 7258 return compareDeep(title, o.title, true) && compareDeep(description, o.description, true) 7259 && compareDeep(step, o.step, true); 7260 } 7261 7262 @Override 7263 public boolean equalsShallow(Base other_) { 7264 if (!super.equalsShallow(other_)) 7265 return false; 7266 if (!(other_ instanceof ExampleScenarioProcessStepAlternativeComponent)) 7267 return false; 7268 ExampleScenarioProcessStepAlternativeComponent o = (ExampleScenarioProcessStepAlternativeComponent) other_; 7269 return compareValues(title, o.title, true) && compareValues(description, o.description, true); 7270 } 7271 7272 public boolean isEmpty() { 7273 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(title, description, step); 7274 } 7275 7276 public String fhirType() { 7277 return "ExampleScenario.process.step.alternative"; 7278 7279 } 7280 7281 } 7282 7283 /** 7284 * A formal identifier that is used to identify this example scenario when it is 7285 * represented in other formats, or referenced in a specification, model, design 7286 * or an instance. 7287 */ 7288 @Child(name = "identifier", type = { 7289 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 7290 @Description(shortDefinition = "Additional identifier for the example scenario", formalDefinition = "A formal identifier that is used to identify this example scenario when it is represented in other formats, or referenced in a specification, model, design or an instance.") 7291 protected List<Identifier> identifier; 7292 7293 /** 7294 * A copyright statement relating to the example scenario and/or its contents. 7295 * Copyright statements are generally legal restrictions on the use and 7296 * publishing of the example scenario. 7297 */ 7298 @Child(name = "copyright", type = { 7299 MarkdownType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 7300 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the example scenario and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the example scenario.") 7301 protected MarkdownType copyright; 7302 7303 /** 7304 * What the example scenario resource is created for. This should not be used to 7305 * show the business purpose of the scenario itself, but the purpose of 7306 * documenting a scenario. 7307 */ 7308 @Child(name = "purpose", type = { 7309 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 7310 @Description(shortDefinition = "The purpose of the example, e.g. to illustrate a scenario", formalDefinition = "What the example scenario resource is created for. This should not be used to show the business purpose of the scenario itself, but the purpose of documenting a scenario.") 7311 protected MarkdownType purpose; 7312 7313 /** 7314 * Actor participating in the resource. 7315 */ 7316 @Child(name = "actor", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 7317 @Description(shortDefinition = "Actor participating in the resource", formalDefinition = "Actor participating in the resource.") 7318 protected List<ExampleScenarioActorComponent> actor; 7319 7320 /** 7321 * Each resource and each version that is present in the workflow. 7322 */ 7323 @Child(name = "instance", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 7324 @Description(shortDefinition = "Each resource and each version that is present in the workflow", formalDefinition = "Each resource and each version that is present in the workflow.") 7325 protected List<ExampleScenarioInstanceComponent> instance; 7326 7327 /** 7328 * Each major process - a group of operations. 7329 */ 7330 @Child(name = "process", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 7331 @Description(shortDefinition = "Each major process - a group of operations", formalDefinition = "Each major process - a group of operations.") 7332 protected List<ExampleScenarioProcessComponent> process; 7333 7334 /** 7335 * Another nested workflow. 7336 */ 7337 @Child(name = "workflow", type = { 7338 CanonicalType.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 7339 @Description(shortDefinition = "Another nested workflow", formalDefinition = "Another nested workflow.") 7340 protected List<CanonicalType> workflow; 7341 7342 private static final long serialVersionUID = 758248907L; 7343 7344 /** 7345 * Constructor 7346 */ 7347 public ExampleScenario() { 7348 super(); 7349 } 7350 7351 /** 7352 * Constructor 7353 */ 7354 public ExampleScenario(Enumeration<PublicationStatus> status) { 7355 super(); 7356 this.status = status; 7357 } 7358 7359 /** 7360 * @return {@link #url} (An absolute URI that is used to identify this example 7361 * scenario when it is referenced in a specification, model, design or 7362 * an instance; also called its canonical identifier. This SHOULD be 7363 * globally unique and SHOULD be a literal address at which at which an 7364 * authoritative instance of this example scenario is (or will be) 7365 * published. This URL can be the target of a canonical reference. It 7366 * SHALL remain the same when the example scenario is stored on 7367 * different servers.). This is the underlying object with id, value and 7368 * extensions. The accessor "getUrl" gives direct access to the value 7369 */ 7370 public UriType getUrlElement() { 7371 if (this.url == null) 7372 if (Configuration.errorOnAutoCreate()) 7373 throw new Error("Attempt to auto-create ExampleScenario.url"); 7374 else if (Configuration.doAutoCreate()) 7375 this.url = new UriType(); // bb 7376 return this.url; 7377 } 7378 7379 public boolean hasUrlElement() { 7380 return this.url != null && !this.url.isEmpty(); 7381 } 7382 7383 public boolean hasUrl() { 7384 return this.url != null && !this.url.isEmpty(); 7385 } 7386 7387 /** 7388 * @param value {@link #url} (An absolute URI that is used to identify this 7389 * example scenario when it is referenced in a specification, 7390 * model, design or an instance; also called its canonical 7391 * identifier. This SHOULD be globally unique and SHOULD be a 7392 * literal address at which at which an authoritative instance of 7393 * this example scenario is (or will be) published. This URL can be 7394 * the target of a canonical reference. It SHALL remain the same 7395 * when the example scenario is stored on different servers.). This 7396 * is the underlying object with id, value and extensions. The 7397 * accessor "getUrl" gives direct access to the value 7398 */ 7399 public ExampleScenario setUrlElement(UriType value) { 7400 this.url = value; 7401 return this; 7402 } 7403 7404 /** 7405 * @return An absolute URI that is used to identify this example scenario when 7406 * it is referenced in a specification, model, design or an instance; 7407 * also called its canonical identifier. This SHOULD be globally unique 7408 * and SHOULD be a literal address at which at which an authoritative 7409 * instance of this example scenario is (or will be) published. This URL 7410 * can be the target of a canonical reference. It SHALL remain the same 7411 * when the example scenario is stored on different servers. 7412 */ 7413 public String getUrl() { 7414 return this.url == null ? null : this.url.getValue(); 7415 } 7416 7417 /** 7418 * @param value An absolute URI that is used to identify this example scenario 7419 * when it is referenced in a specification, model, design or an 7420 * instance; also called its canonical identifier. This SHOULD be 7421 * globally unique and SHOULD be a literal address at which at 7422 * which an authoritative instance of this example scenario is (or 7423 * will be) published. This URL can be the target of a canonical 7424 * reference. It SHALL remain the same when the example scenario is 7425 * stored on different servers. 7426 */ 7427 public ExampleScenario setUrl(String value) { 7428 if (Utilities.noString(value)) 7429 this.url = null; 7430 else { 7431 if (this.url == null) 7432 this.url = new UriType(); 7433 this.url.setValue(value); 7434 } 7435 return this; 7436 } 7437 7438 /** 7439 * @return {@link #identifier} (A formal identifier that is used to identify 7440 * this example scenario when it is represented in other formats, or 7441 * referenced in a specification, model, design or an instance.) 7442 */ 7443 public List<Identifier> getIdentifier() { 7444 if (this.identifier == null) 7445 this.identifier = new ArrayList<Identifier>(); 7446 return this.identifier; 7447 } 7448 7449 /** 7450 * @return Returns a reference to <code>this</code> for easy method chaining 7451 */ 7452 public ExampleScenario setIdentifier(List<Identifier> theIdentifier) { 7453 this.identifier = theIdentifier; 7454 return this; 7455 } 7456 7457 public boolean hasIdentifier() { 7458 if (this.identifier == null) 7459 return false; 7460 for (Identifier item : this.identifier) 7461 if (!item.isEmpty()) 7462 return true; 7463 return false; 7464 } 7465 7466 public Identifier addIdentifier() { // 3 7467 Identifier t = new Identifier(); 7468 if (this.identifier == null) 7469 this.identifier = new ArrayList<Identifier>(); 7470 this.identifier.add(t); 7471 return t; 7472 } 7473 7474 public ExampleScenario addIdentifier(Identifier t) { // 3 7475 if (t == null) 7476 return this; 7477 if (this.identifier == null) 7478 this.identifier = new ArrayList<Identifier>(); 7479 this.identifier.add(t); 7480 return this; 7481 } 7482 7483 /** 7484 * @return The first repetition of repeating field {@link #identifier}, creating 7485 * it if it does not already exist 7486 */ 7487 public Identifier getIdentifierFirstRep() { 7488 if (getIdentifier().isEmpty()) { 7489 addIdentifier(); 7490 } 7491 return getIdentifier().get(0); 7492 } 7493 7494 /** 7495 * @return {@link #version} (The identifier that is used to identify this 7496 * version of the example scenario when it is referenced in a 7497 * specification, model, design or instance. This is an arbitrary value 7498 * managed by the example scenario author and is not expected to be 7499 * globally unique. For example, it might be a timestamp (e.g. yyyymmdd) 7500 * if a managed version is not available. There is also no expectation 7501 * that versions can be placed in a lexicographical sequence.). This is 7502 * the underlying object with id, value and extensions. The accessor 7503 * "getVersion" gives direct access to the value 7504 */ 7505 public StringType getVersionElement() { 7506 if (this.version == null) 7507 if (Configuration.errorOnAutoCreate()) 7508 throw new Error("Attempt to auto-create ExampleScenario.version"); 7509 else if (Configuration.doAutoCreate()) 7510 this.version = new StringType(); // bb 7511 return this.version; 7512 } 7513 7514 public boolean hasVersionElement() { 7515 return this.version != null && !this.version.isEmpty(); 7516 } 7517 7518 public boolean hasVersion() { 7519 return this.version != null && !this.version.isEmpty(); 7520 } 7521 7522 /** 7523 * @param value {@link #version} (The identifier that is used to identify this 7524 * version of the example scenario when it is referenced in a 7525 * specification, model, design or instance. This is an arbitrary 7526 * value managed by the example scenario author and is not expected 7527 * to be globally unique. For example, it might be a timestamp 7528 * (e.g. yyyymmdd) if a managed version is not available. There is 7529 * also no expectation that versions can be placed in a 7530 * lexicographical sequence.). This is the underlying object with 7531 * id, value and extensions. The accessor "getVersion" gives direct 7532 * access to the value 7533 */ 7534 public ExampleScenario setVersionElement(StringType value) { 7535 this.version = value; 7536 return this; 7537 } 7538 7539 /** 7540 * @return The identifier that is used to identify this version of the example 7541 * scenario when it is referenced in a specification, model, design or 7542 * instance. This is an arbitrary value managed by the example scenario 7543 * author and is not expected to be globally unique. For example, it 7544 * might be a timestamp (e.g. yyyymmdd) if a managed version is not 7545 * available. There is also no expectation that versions can be placed 7546 * in a lexicographical sequence. 7547 */ 7548 public String getVersion() { 7549 return this.version == null ? null : this.version.getValue(); 7550 } 7551 7552 /** 7553 * @param value The identifier that is used to identify this version of the 7554 * example scenario when it is referenced in a specification, 7555 * model, design or instance. This is an arbitrary value managed by 7556 * the example scenario author and is not expected to be globally 7557 * unique. For example, it might be a timestamp (e.g. yyyymmdd) if 7558 * a managed version is not available. There is also no expectation 7559 * that versions can be placed in a lexicographical sequence. 7560 */ 7561 public ExampleScenario setVersion(String value) { 7562 if (Utilities.noString(value)) 7563 this.version = null; 7564 else { 7565 if (this.version == null) 7566 this.version = new StringType(); 7567 this.version.setValue(value); 7568 } 7569 return this; 7570 } 7571 7572 /** 7573 * @return {@link #name} (A natural language name identifying the example 7574 * scenario. This name should be usable as an identifier for the module 7575 * by machine processing applications such as code generation.). This is 7576 * the underlying object with id, value and extensions. The accessor 7577 * "getName" gives direct access to the value 7578 */ 7579 public StringType getNameElement() { 7580 if (this.name == null) 7581 if (Configuration.errorOnAutoCreate()) 7582 throw new Error("Attempt to auto-create ExampleScenario.name"); 7583 else if (Configuration.doAutoCreate()) 7584 this.name = new StringType(); // bb 7585 return this.name; 7586 } 7587 7588 public boolean hasNameElement() { 7589 return this.name != null && !this.name.isEmpty(); 7590 } 7591 7592 public boolean hasName() { 7593 return this.name != null && !this.name.isEmpty(); 7594 } 7595 7596 /** 7597 * @param value {@link #name} (A natural language name identifying the example 7598 * scenario. This name should be usable as an identifier for the 7599 * module by machine processing applications such as code 7600 * generation.). This is the underlying object with id, value and 7601 * extensions. The accessor "getName" gives direct access to the 7602 * value 7603 */ 7604 public ExampleScenario setNameElement(StringType value) { 7605 this.name = value; 7606 return this; 7607 } 7608 7609 /** 7610 * @return A natural language name identifying the example scenario. This name 7611 * should be usable as an identifier for the module by machine 7612 * processing applications such as code generation. 7613 */ 7614 public String getName() { 7615 return this.name == null ? null : this.name.getValue(); 7616 } 7617 7618 /** 7619 * @param value A natural language name identifying the example scenario. This 7620 * name should be usable as an identifier for the module by machine 7621 * processing applications such as code generation. 7622 */ 7623 public ExampleScenario setName(String value) { 7624 if (Utilities.noString(value)) 7625 this.name = null; 7626 else { 7627 if (this.name == null) 7628 this.name = new StringType(); 7629 this.name.setValue(value); 7630 } 7631 return this; 7632 } 7633 7634 /** 7635 * @return {@link #status} (The status of this example scenario. Enables 7636 * tracking the life-cycle of the content.). This is the underlying 7637 * object with id, value and extensions. The accessor "getStatus" gives 7638 * direct access to the value 7639 */ 7640 public Enumeration<PublicationStatus> getStatusElement() { 7641 if (this.status == null) 7642 if (Configuration.errorOnAutoCreate()) 7643 throw new Error("Attempt to auto-create ExampleScenario.status"); 7644 else if (Configuration.doAutoCreate()) 7645 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 7646 return this.status; 7647 } 7648 7649 public boolean hasStatusElement() { 7650 return this.status != null && !this.status.isEmpty(); 7651 } 7652 7653 public boolean hasStatus() { 7654 return this.status != null && !this.status.isEmpty(); 7655 } 7656 7657 /** 7658 * @param value {@link #status} (The status of this example scenario. Enables 7659 * tracking the life-cycle of the content.). This is the underlying 7660 * object with id, value and extensions. The accessor "getStatus" 7661 * gives direct access to the value 7662 */ 7663 public ExampleScenario setStatusElement(Enumeration<PublicationStatus> value) { 7664 this.status = value; 7665 return this; 7666 } 7667 7668 /** 7669 * @return The status of this example scenario. Enables tracking the life-cycle 7670 * of the content. 7671 */ 7672 public PublicationStatus getStatus() { 7673 return this.status == null ? null : this.status.getValue(); 7674 } 7675 7676 /** 7677 * @param value The status of this example scenario. Enables tracking the 7678 * life-cycle of the content. 7679 */ 7680 public ExampleScenario setStatus(PublicationStatus value) { 7681 if (this.status == null) 7682 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 7683 this.status.setValue(value); 7684 return this; 7685 } 7686 7687 /** 7688 * @return {@link #experimental} (A Boolean value to indicate that this example 7689 * scenario is authored for testing purposes (or 7690 * education/evaluation/marketing) and is not intended to be used for 7691 * genuine usage.). This is the underlying object with id, value and 7692 * extensions. The accessor "getExperimental" gives direct access to the 7693 * value 7694 */ 7695 public BooleanType getExperimentalElement() { 7696 if (this.experimental == null) 7697 if (Configuration.errorOnAutoCreate()) 7698 throw new Error("Attempt to auto-create ExampleScenario.experimental"); 7699 else if (Configuration.doAutoCreate()) 7700 this.experimental = new BooleanType(); // bb 7701 return this.experimental; 7702 } 7703 7704 public boolean hasExperimentalElement() { 7705 return this.experimental != null && !this.experimental.isEmpty(); 7706 } 7707 7708 public boolean hasExperimental() { 7709 return this.experimental != null && !this.experimental.isEmpty(); 7710 } 7711 7712 /** 7713 * @param value {@link #experimental} (A Boolean value to indicate that this 7714 * example scenario is authored for testing purposes (or 7715 * education/evaluation/marketing) and is not intended to be used 7716 * for genuine usage.). This is the underlying object with id, 7717 * value and extensions. The accessor "getExperimental" gives 7718 * direct access to the value 7719 */ 7720 public ExampleScenario setExperimentalElement(BooleanType value) { 7721 this.experimental = value; 7722 return this; 7723 } 7724 7725 /** 7726 * @return A Boolean value to indicate that this example scenario is authored 7727 * for testing purposes (or education/evaluation/marketing) and is not 7728 * intended to be used for genuine usage. 7729 */ 7730 public boolean getExperimental() { 7731 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 7732 } 7733 7734 /** 7735 * @param value A Boolean value to indicate that this example scenario is 7736 * authored for testing purposes (or 7737 * education/evaluation/marketing) and is not intended to be used 7738 * for genuine usage. 7739 */ 7740 public ExampleScenario setExperimental(boolean value) { 7741 if (this.experimental == null) 7742 this.experimental = new BooleanType(); 7743 this.experimental.setValue(value); 7744 return this; 7745 } 7746 7747 /** 7748 * @return {@link #date} (The date (and optionally time) when the example 7749 * scenario was published. The date must change when the business 7750 * version changes and it must change if the status code changes. In 7751 * addition, it should change when the substantive content of the 7752 * example scenario changes. (e.g. the 'content logical definition').). 7753 * This is the underlying object with id, value and extensions. The 7754 * accessor "getDate" gives direct access to the value 7755 */ 7756 public DateTimeType getDateElement() { 7757 if (this.date == null) 7758 if (Configuration.errorOnAutoCreate()) 7759 throw new Error("Attempt to auto-create ExampleScenario.date"); 7760 else if (Configuration.doAutoCreate()) 7761 this.date = new DateTimeType(); // bb 7762 return this.date; 7763 } 7764 7765 public boolean hasDateElement() { 7766 return this.date != null && !this.date.isEmpty(); 7767 } 7768 7769 public boolean hasDate() { 7770 return this.date != null && !this.date.isEmpty(); 7771 } 7772 7773 /** 7774 * @param value {@link #date} (The date (and optionally time) when the example 7775 * scenario was published. The date must change when the business 7776 * version changes and it must change if the status code changes. 7777 * In addition, it should change when the substantive content of 7778 * the example scenario changes. (e.g. the 'content logical 7779 * definition').). This is the underlying object with id, value and 7780 * extensions. The accessor "getDate" gives direct access to the 7781 * value 7782 */ 7783 public ExampleScenario setDateElement(DateTimeType value) { 7784 this.date = value; 7785 return this; 7786 } 7787 7788 /** 7789 * @return The date (and optionally time) when the example scenario was 7790 * published. The date must change when the business version changes and 7791 * it must change if the status code changes. In addition, it should 7792 * change when the substantive content of the example scenario changes. 7793 * (e.g. the 'content logical definition'). 7794 */ 7795 public Date getDate() { 7796 return this.date == null ? null : this.date.getValue(); 7797 } 7798 7799 /** 7800 * @param value The date (and optionally time) when the example scenario was 7801 * published. The date must change when the business version 7802 * changes and it must change if the status code changes. In 7803 * addition, it should change when the substantive content of the 7804 * example scenario changes. (e.g. the 'content logical 7805 * definition'). 7806 */ 7807 public ExampleScenario setDate(Date value) { 7808 if (value == null) 7809 this.date = null; 7810 else { 7811 if (this.date == null) 7812 this.date = new DateTimeType(); 7813 this.date.setValue(value); 7814 } 7815 return this; 7816 } 7817 7818 /** 7819 * @return {@link #publisher} (The name of the organization or individual that 7820 * published the example scenario.). This is the underlying object with 7821 * id, value and extensions. The accessor "getPublisher" gives direct 7822 * access to the value 7823 */ 7824 public StringType getPublisherElement() { 7825 if (this.publisher == null) 7826 if (Configuration.errorOnAutoCreate()) 7827 throw new Error("Attempt to auto-create ExampleScenario.publisher"); 7828 else if (Configuration.doAutoCreate()) 7829 this.publisher = new StringType(); // bb 7830 return this.publisher; 7831 } 7832 7833 public boolean hasPublisherElement() { 7834 return this.publisher != null && !this.publisher.isEmpty(); 7835 } 7836 7837 public boolean hasPublisher() { 7838 return this.publisher != null && !this.publisher.isEmpty(); 7839 } 7840 7841 /** 7842 * @param value {@link #publisher} (The name of the organization or individual 7843 * that published the example scenario.). This is the underlying 7844 * object with id, value and extensions. The accessor 7845 * "getPublisher" gives direct access to the value 7846 */ 7847 public ExampleScenario setPublisherElement(StringType value) { 7848 this.publisher = value; 7849 return this; 7850 } 7851 7852 /** 7853 * @return The name of the organization or individual that published the example 7854 * scenario. 7855 */ 7856 public String getPublisher() { 7857 return this.publisher == null ? null : this.publisher.getValue(); 7858 } 7859 7860 /** 7861 * @param value The name of the organization or individual that published the 7862 * example scenario. 7863 */ 7864 public ExampleScenario setPublisher(String value) { 7865 if (Utilities.noString(value)) 7866 this.publisher = null; 7867 else { 7868 if (this.publisher == null) 7869 this.publisher = new StringType(); 7870 this.publisher.setValue(value); 7871 } 7872 return this; 7873 } 7874 7875 /** 7876 * @return {@link #contact} (Contact details to assist a user in finding and 7877 * communicating with the publisher.) 7878 */ 7879 public List<ContactDetail> getContact() { 7880 if (this.contact == null) 7881 this.contact = new ArrayList<ContactDetail>(); 7882 return this.contact; 7883 } 7884 7885 /** 7886 * @return Returns a reference to <code>this</code> for easy method chaining 7887 */ 7888 public ExampleScenario setContact(List<ContactDetail> theContact) { 7889 this.contact = theContact; 7890 return this; 7891 } 7892 7893 public boolean hasContact() { 7894 if (this.contact == null) 7895 return false; 7896 for (ContactDetail item : this.contact) 7897 if (!item.isEmpty()) 7898 return true; 7899 return false; 7900 } 7901 7902 public ContactDetail addContact() { // 3 7903 ContactDetail t = new ContactDetail(); 7904 if (this.contact == null) 7905 this.contact = new ArrayList<ContactDetail>(); 7906 this.contact.add(t); 7907 return t; 7908 } 7909 7910 public ExampleScenario addContact(ContactDetail t) { // 3 7911 if (t == null) 7912 return this; 7913 if (this.contact == null) 7914 this.contact = new ArrayList<ContactDetail>(); 7915 this.contact.add(t); 7916 return this; 7917 } 7918 7919 /** 7920 * @return The first repetition of repeating field {@link #contact}, creating it 7921 * if it does not already exist 7922 */ 7923 public ContactDetail getContactFirstRep() { 7924 if (getContact().isEmpty()) { 7925 addContact(); 7926 } 7927 return getContact().get(0); 7928 } 7929 7930 /** 7931 * @return {@link #useContext} (The content was developed with a focus and 7932 * intent of supporting the contexts that are listed. These contexts may 7933 * be general categories (gender, age, ...) or may be references to 7934 * specific programs (insurance plans, studies, ...) and may be used to 7935 * assist with indexing and searching for appropriate example scenario 7936 * instances.) 7937 */ 7938 public List<UsageContext> getUseContext() { 7939 if (this.useContext == null) 7940 this.useContext = new ArrayList<UsageContext>(); 7941 return this.useContext; 7942 } 7943 7944 /** 7945 * @return Returns a reference to <code>this</code> for easy method chaining 7946 */ 7947 public ExampleScenario setUseContext(List<UsageContext> theUseContext) { 7948 this.useContext = theUseContext; 7949 return this; 7950 } 7951 7952 public boolean hasUseContext() { 7953 if (this.useContext == null) 7954 return false; 7955 for (UsageContext item : this.useContext) 7956 if (!item.isEmpty()) 7957 return true; 7958 return false; 7959 } 7960 7961 public UsageContext addUseContext() { // 3 7962 UsageContext t = new UsageContext(); 7963 if (this.useContext == null) 7964 this.useContext = new ArrayList<UsageContext>(); 7965 this.useContext.add(t); 7966 return t; 7967 } 7968 7969 public ExampleScenario addUseContext(UsageContext t) { // 3 7970 if (t == null) 7971 return this; 7972 if (this.useContext == null) 7973 this.useContext = new ArrayList<UsageContext>(); 7974 this.useContext.add(t); 7975 return this; 7976 } 7977 7978 /** 7979 * @return The first repetition of repeating field {@link #useContext}, creating 7980 * it if it does not already exist 7981 */ 7982 public UsageContext getUseContextFirstRep() { 7983 if (getUseContext().isEmpty()) { 7984 addUseContext(); 7985 } 7986 return getUseContext().get(0); 7987 } 7988 7989 /** 7990 * @return {@link #jurisdiction} (A legal or geographic region in which the 7991 * example scenario is intended to be used.) 7992 */ 7993 public List<CodeableConcept> getJurisdiction() { 7994 if (this.jurisdiction == null) 7995 this.jurisdiction = new ArrayList<CodeableConcept>(); 7996 return this.jurisdiction; 7997 } 7998 7999 /** 8000 * @return Returns a reference to <code>this</code> for easy method chaining 8001 */ 8002 public ExampleScenario setJurisdiction(List<CodeableConcept> theJurisdiction) { 8003 this.jurisdiction = theJurisdiction; 8004 return this; 8005 } 8006 8007 public boolean hasJurisdiction() { 8008 if (this.jurisdiction == null) 8009 return false; 8010 for (CodeableConcept item : this.jurisdiction) 8011 if (!item.isEmpty()) 8012 return true; 8013 return false; 8014 } 8015 8016 public CodeableConcept addJurisdiction() { // 3 8017 CodeableConcept t = new CodeableConcept(); 8018 if (this.jurisdiction == null) 8019 this.jurisdiction = new ArrayList<CodeableConcept>(); 8020 this.jurisdiction.add(t); 8021 return t; 8022 } 8023 8024 public ExampleScenario addJurisdiction(CodeableConcept t) { // 3 8025 if (t == null) 8026 return this; 8027 if (this.jurisdiction == null) 8028 this.jurisdiction = new ArrayList<CodeableConcept>(); 8029 this.jurisdiction.add(t); 8030 return this; 8031 } 8032 8033 /** 8034 * @return The first repetition of repeating field {@link #jurisdiction}, 8035 * creating it if it does not already exist 8036 */ 8037 public CodeableConcept getJurisdictionFirstRep() { 8038 if (getJurisdiction().isEmpty()) { 8039 addJurisdiction(); 8040 } 8041 return getJurisdiction().get(0); 8042 } 8043 8044 /** 8045 * @return {@link #copyright} (A copyright statement relating to the example 8046 * scenario and/or its contents. Copyright statements are generally 8047 * legal restrictions on the use and publishing of the example 8048 * scenario.). This is the underlying object with id, value and 8049 * extensions. The accessor "getCopyright" gives direct access to the 8050 * value 8051 */ 8052 public MarkdownType getCopyrightElement() { 8053 if (this.copyright == null) 8054 if (Configuration.errorOnAutoCreate()) 8055 throw new Error("Attempt to auto-create ExampleScenario.copyright"); 8056 else if (Configuration.doAutoCreate()) 8057 this.copyright = new MarkdownType(); // bb 8058 return this.copyright; 8059 } 8060 8061 public boolean hasCopyrightElement() { 8062 return this.copyright != null && !this.copyright.isEmpty(); 8063 } 8064 8065 public boolean hasCopyright() { 8066 return this.copyright != null && !this.copyright.isEmpty(); 8067 } 8068 8069 /** 8070 * @param value {@link #copyright} (A copyright statement relating to the 8071 * example scenario and/or its contents. Copyright statements are 8072 * generally legal restrictions on the use and publishing of the 8073 * example scenario.). This is the underlying object with id, value 8074 * and extensions. The accessor "getCopyright" gives direct access 8075 * to the value 8076 */ 8077 public ExampleScenario setCopyrightElement(MarkdownType value) { 8078 this.copyright = value; 8079 return this; 8080 } 8081 8082 /** 8083 * @return A copyright statement relating to the example scenario and/or its 8084 * contents. Copyright statements are generally legal restrictions on 8085 * the use and publishing of the example scenario. 8086 */ 8087 public String getCopyright() { 8088 return this.copyright == null ? null : this.copyright.getValue(); 8089 } 8090 8091 /** 8092 * @param value A copyright statement relating to the example scenario and/or 8093 * its contents. Copyright statements are generally legal 8094 * restrictions on the use and publishing of the example scenario. 8095 */ 8096 public ExampleScenario setCopyright(String value) { 8097 if (value == null) 8098 this.copyright = null; 8099 else { 8100 if (this.copyright == null) 8101 this.copyright = new MarkdownType(); 8102 this.copyright.setValue(value); 8103 } 8104 return this; 8105 } 8106 8107 /** 8108 * @return {@link #purpose} (What the example scenario resource is created for. 8109 * This should not be used to show the business purpose of the scenario 8110 * itself, but the purpose of documenting a scenario.). This is the 8111 * underlying object with id, value and extensions. The accessor 8112 * "getPurpose" gives direct access to the value 8113 */ 8114 public MarkdownType getPurposeElement() { 8115 if (this.purpose == null) 8116 if (Configuration.errorOnAutoCreate()) 8117 throw new Error("Attempt to auto-create ExampleScenario.purpose"); 8118 else if (Configuration.doAutoCreate()) 8119 this.purpose = new MarkdownType(); // bb 8120 return this.purpose; 8121 } 8122 8123 public boolean hasPurposeElement() { 8124 return this.purpose != null && !this.purpose.isEmpty(); 8125 } 8126 8127 public boolean hasPurpose() { 8128 return this.purpose != null && !this.purpose.isEmpty(); 8129 } 8130 8131 /** 8132 * @param value {@link #purpose} (What the example scenario resource is created 8133 * for. This should not be used to show the business purpose of the 8134 * scenario itself, but the purpose of documenting a scenario.). 8135 * This is the underlying object with id, value and extensions. The 8136 * accessor "getPurpose" gives direct access to the value 8137 */ 8138 public ExampleScenario setPurposeElement(MarkdownType value) { 8139 this.purpose = value; 8140 return this; 8141 } 8142 8143 /** 8144 * @return What the example scenario resource is created for. This should not be 8145 * used to show the business purpose of the scenario itself, but the 8146 * purpose of documenting a scenario. 8147 */ 8148 public String getPurpose() { 8149 return this.purpose == null ? null : this.purpose.getValue(); 8150 } 8151 8152 /** 8153 * @param value What the example scenario resource is created for. This should 8154 * not be used to show the business purpose of the scenario itself, 8155 * but the purpose of documenting a scenario. 8156 */ 8157 public ExampleScenario setPurpose(String value) { 8158 if (value == null) 8159 this.purpose = null; 8160 else { 8161 if (this.purpose == null) 8162 this.purpose = new MarkdownType(); 8163 this.purpose.setValue(value); 8164 } 8165 return this; 8166 } 8167 8168 /** 8169 * @return {@link #actor} (Actor participating in the resource.) 8170 */ 8171 public List<ExampleScenarioActorComponent> getActor() { 8172 if (this.actor == null) 8173 this.actor = new ArrayList<ExampleScenarioActorComponent>(); 8174 return this.actor; 8175 } 8176 8177 /** 8178 * @return Returns a reference to <code>this</code> for easy method chaining 8179 */ 8180 public ExampleScenario setActor(List<ExampleScenarioActorComponent> theActor) { 8181 this.actor = theActor; 8182 return this; 8183 } 8184 8185 public boolean hasActor() { 8186 if (this.actor == null) 8187 return false; 8188 for (ExampleScenarioActorComponent item : this.actor) 8189 if (!item.isEmpty()) 8190 return true; 8191 return false; 8192 } 8193 8194 public ExampleScenarioActorComponent addActor() { // 3 8195 ExampleScenarioActorComponent t = new ExampleScenarioActorComponent(); 8196 if (this.actor == null) 8197 this.actor = new ArrayList<ExampleScenarioActorComponent>(); 8198 this.actor.add(t); 8199 return t; 8200 } 8201 8202 public ExampleScenario addActor(ExampleScenarioActorComponent t) { // 3 8203 if (t == null) 8204 return this; 8205 if (this.actor == null) 8206 this.actor = new ArrayList<ExampleScenarioActorComponent>(); 8207 this.actor.add(t); 8208 return this; 8209 } 8210 8211 /** 8212 * @return The first repetition of repeating field {@link #actor}, creating it 8213 * if it does not already exist 8214 */ 8215 public ExampleScenarioActorComponent getActorFirstRep() { 8216 if (getActor().isEmpty()) { 8217 addActor(); 8218 } 8219 return getActor().get(0); 8220 } 8221 8222 /** 8223 * @return {@link #instance} (Each resource and each version that is present in 8224 * the workflow.) 8225 */ 8226 public List<ExampleScenarioInstanceComponent> getInstance() { 8227 if (this.instance == null) 8228 this.instance = new ArrayList<ExampleScenarioInstanceComponent>(); 8229 return this.instance; 8230 } 8231 8232 /** 8233 * @return Returns a reference to <code>this</code> for easy method chaining 8234 */ 8235 public ExampleScenario setInstance(List<ExampleScenarioInstanceComponent> theInstance) { 8236 this.instance = theInstance; 8237 return this; 8238 } 8239 8240 public boolean hasInstance() { 8241 if (this.instance == null) 8242 return false; 8243 for (ExampleScenarioInstanceComponent item : this.instance) 8244 if (!item.isEmpty()) 8245 return true; 8246 return false; 8247 } 8248 8249 public ExampleScenarioInstanceComponent addInstance() { // 3 8250 ExampleScenarioInstanceComponent t = new ExampleScenarioInstanceComponent(); 8251 if (this.instance == null) 8252 this.instance = new ArrayList<ExampleScenarioInstanceComponent>(); 8253 this.instance.add(t); 8254 return t; 8255 } 8256 8257 public ExampleScenario addInstance(ExampleScenarioInstanceComponent t) { // 3 8258 if (t == null) 8259 return this; 8260 if (this.instance == null) 8261 this.instance = new ArrayList<ExampleScenarioInstanceComponent>(); 8262 this.instance.add(t); 8263 return this; 8264 } 8265 8266 /** 8267 * @return The first repetition of repeating field {@link #instance}, creating 8268 * it if it does not already exist 8269 */ 8270 public ExampleScenarioInstanceComponent getInstanceFirstRep() { 8271 if (getInstance().isEmpty()) { 8272 addInstance(); 8273 } 8274 return getInstance().get(0); 8275 } 8276 8277 /** 8278 * @return {@link #process} (Each major process - a group of operations.) 8279 */ 8280 public List<ExampleScenarioProcessComponent> getProcess() { 8281 if (this.process == null) 8282 this.process = new ArrayList<ExampleScenarioProcessComponent>(); 8283 return this.process; 8284 } 8285 8286 /** 8287 * @return Returns a reference to <code>this</code> for easy method chaining 8288 */ 8289 public ExampleScenario setProcess(List<ExampleScenarioProcessComponent> theProcess) { 8290 this.process = theProcess; 8291 return this; 8292 } 8293 8294 public boolean hasProcess() { 8295 if (this.process == null) 8296 return false; 8297 for (ExampleScenarioProcessComponent item : this.process) 8298 if (!item.isEmpty()) 8299 return true; 8300 return false; 8301 } 8302 8303 public ExampleScenarioProcessComponent addProcess() { // 3 8304 ExampleScenarioProcessComponent t = new ExampleScenarioProcessComponent(); 8305 if (this.process == null) 8306 this.process = new ArrayList<ExampleScenarioProcessComponent>(); 8307 this.process.add(t); 8308 return t; 8309 } 8310 8311 public ExampleScenario addProcess(ExampleScenarioProcessComponent t) { // 3 8312 if (t == null) 8313 return this; 8314 if (this.process == null) 8315 this.process = new ArrayList<ExampleScenarioProcessComponent>(); 8316 this.process.add(t); 8317 return this; 8318 } 8319 8320 /** 8321 * @return The first repetition of repeating field {@link #process}, creating it 8322 * if it does not already exist 8323 */ 8324 public ExampleScenarioProcessComponent getProcessFirstRep() { 8325 if (getProcess().isEmpty()) { 8326 addProcess(); 8327 } 8328 return getProcess().get(0); 8329 } 8330 8331 /** 8332 * @return {@link #workflow} (Another nested workflow.) 8333 */ 8334 public List<CanonicalType> getWorkflow() { 8335 if (this.workflow == null) 8336 this.workflow = new ArrayList<CanonicalType>(); 8337 return this.workflow; 8338 } 8339 8340 /** 8341 * @return Returns a reference to <code>this</code> for easy method chaining 8342 */ 8343 public ExampleScenario setWorkflow(List<CanonicalType> theWorkflow) { 8344 this.workflow = theWorkflow; 8345 return this; 8346 } 8347 8348 public boolean hasWorkflow() { 8349 if (this.workflow == null) 8350 return false; 8351 for (CanonicalType item : this.workflow) 8352 if (!item.isEmpty()) 8353 return true; 8354 return false; 8355 } 8356 8357 /** 8358 * @return {@link #workflow} (Another nested workflow.) 8359 */ 8360 public CanonicalType addWorkflowElement() {// 2 8361 CanonicalType t = new CanonicalType(); 8362 if (this.workflow == null) 8363 this.workflow = new ArrayList<CanonicalType>(); 8364 this.workflow.add(t); 8365 return t; 8366 } 8367 8368 /** 8369 * @param value {@link #workflow} (Another nested workflow.) 8370 */ 8371 public ExampleScenario addWorkflow(String value) { // 1 8372 CanonicalType t = new CanonicalType(); 8373 t.setValue(value); 8374 if (this.workflow == null) 8375 this.workflow = new ArrayList<CanonicalType>(); 8376 this.workflow.add(t); 8377 return this; 8378 } 8379 8380 /** 8381 * @param value {@link #workflow} (Another nested workflow.) 8382 */ 8383 public boolean hasWorkflow(String value) { 8384 if (this.workflow == null) 8385 return false; 8386 for (CanonicalType v : this.workflow) 8387 if (v.getValue().equals(value)) // canonical(ExampleScenario) 8388 return true; 8389 return false; 8390 } 8391 8392 protected void listChildren(List<Property> children) { 8393 super.listChildren(children); 8394 children.add(new Property("url", "uri", 8395 "An absolute URI that is used to identify this example scenario when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this example scenario is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the example scenario is stored on different servers.", 8396 0, 1, url)); 8397 children.add(new Property("identifier", "Identifier", 8398 "A formal identifier that is used to identify this example scenario when it is represented in other formats, or referenced in a specification, model, design or an instance.", 8399 0, java.lang.Integer.MAX_VALUE, identifier)); 8400 children.add(new Property("version", "string", 8401 "The identifier that is used to identify this version of the example scenario when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the example scenario author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 8402 0, 1, version)); 8403 children.add(new Property("name", "string", 8404 "A natural language name identifying the example scenario. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 8405 0, 1, name)); 8406 children.add(new Property("status", "code", 8407 "The status of this example scenario. Enables tracking the life-cycle of the content.", 0, 1, status)); 8408 children.add(new Property("experimental", "boolean", 8409 "A Boolean value to indicate that this example scenario is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 8410 0, 1, experimental)); 8411 children.add(new Property("date", "dateTime", 8412 "The date (and optionally time) when the example scenario was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the example scenario changes. (e.g. the 'content logical definition').", 8413 0, 1, date)); 8414 children.add(new Property("publisher", "string", 8415 "The name of the organization or individual that published the example scenario.", 0, 1, publisher)); 8416 children.add(new Property("contact", "ContactDetail", 8417 "Contact details to assist a user in finding and communicating with the publisher.", 0, 8418 java.lang.Integer.MAX_VALUE, contact)); 8419 children.add(new Property("useContext", "UsageContext", 8420 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate example scenario instances.", 8421 0, java.lang.Integer.MAX_VALUE, useContext)); 8422 children.add(new Property("jurisdiction", "CodeableConcept", 8423 "A legal or geographic region in which the example scenario is intended to be used.", 0, 8424 java.lang.Integer.MAX_VALUE, jurisdiction)); 8425 children.add(new Property("copyright", "markdown", 8426 "A copyright statement relating to the example scenario and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the example scenario.", 8427 0, 1, copyright)); 8428 children.add(new Property("purpose", "markdown", 8429 "What the example scenario resource is created for. This should not be used to show the business purpose of the scenario itself, but the purpose of documenting a scenario.", 8430 0, 1, purpose)); 8431 children 8432 .add(new Property("actor", "", "Actor participating in the resource.", 0, java.lang.Integer.MAX_VALUE, actor)); 8433 children.add(new Property("instance", "", "Each resource and each version that is present in the workflow.", 0, 8434 java.lang.Integer.MAX_VALUE, instance)); 8435 children.add(new Property("process", "", "Each major process - a group of operations.", 0, 8436 java.lang.Integer.MAX_VALUE, process)); 8437 children.add(new Property("workflow", "canonical(ExampleScenario)", "Another nested workflow.", 0, 8438 java.lang.Integer.MAX_VALUE, workflow)); 8439 } 8440 8441 @Override 8442 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8443 switch (_hash) { 8444 case 116079: 8445 /* url */ return new Property("url", "uri", 8446 "An absolute URI that is used to identify this example scenario when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this example scenario is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the example scenario is stored on different servers.", 8447 0, 1, url); 8448 case -1618432855: 8449 /* identifier */ return new Property("identifier", "Identifier", 8450 "A formal identifier that is used to identify this example scenario when it is represented in other formats, or referenced in a specification, model, design or an instance.", 8451 0, java.lang.Integer.MAX_VALUE, identifier); 8452 case 351608024: 8453 /* version */ return new Property("version", "string", 8454 "The identifier that is used to identify this version of the example scenario when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the example scenario author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 8455 0, 1, version); 8456 case 3373707: 8457 /* name */ return new Property("name", "string", 8458 "A natural language name identifying the example scenario. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 8459 0, 1, name); 8460 case -892481550: 8461 /* status */ return new Property("status", "code", 8462 "The status of this example scenario. Enables tracking the life-cycle of the content.", 0, 1, status); 8463 case -404562712: 8464 /* experimental */ return new Property("experimental", "boolean", 8465 "A Boolean value to indicate that this example scenario is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 8466 0, 1, experimental); 8467 case 3076014: 8468 /* date */ return new Property("date", "dateTime", 8469 "The date (and optionally time) when the example scenario was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the example scenario changes. (e.g. the 'content logical definition').", 8470 0, 1, date); 8471 case 1447404028: 8472 /* publisher */ return new Property("publisher", "string", 8473 "The name of the organization or individual that published the example scenario.", 0, 1, publisher); 8474 case 951526432: 8475 /* contact */ return new Property("contact", "ContactDetail", 8476 "Contact details to assist a user in finding and communicating with the publisher.", 0, 8477 java.lang.Integer.MAX_VALUE, contact); 8478 case -669707736: 8479 /* useContext */ return new Property("useContext", "UsageContext", 8480 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate example scenario instances.", 8481 0, java.lang.Integer.MAX_VALUE, useContext); 8482 case -507075711: 8483 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 8484 "A legal or geographic region in which the example scenario is intended to be used.", 0, 8485 java.lang.Integer.MAX_VALUE, jurisdiction); 8486 case 1522889671: 8487 /* copyright */ return new Property("copyright", "markdown", 8488 "A copyright statement relating to the example scenario and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the example scenario.", 8489 0, 1, copyright); 8490 case -220463842: 8491 /* purpose */ return new Property("purpose", "markdown", 8492 "What the example scenario resource is created for. This should not be used to show the business purpose of the scenario itself, but the purpose of documenting a scenario.", 8493 0, 1, purpose); 8494 case 92645877: 8495 /* actor */ return new Property("actor", "", "Actor participating in the resource.", 0, 8496 java.lang.Integer.MAX_VALUE, actor); 8497 case 555127957: 8498 /* instance */ return new Property("instance", "", 8499 "Each resource and each version that is present in the workflow.", 0, java.lang.Integer.MAX_VALUE, instance); 8500 case -309518737: 8501 /* process */ return new Property("process", "", "Each major process - a group of operations.", 0, 8502 java.lang.Integer.MAX_VALUE, process); 8503 case 35379135: 8504 /* workflow */ return new Property("workflow", "canonical(ExampleScenario)", "Another nested workflow.", 0, 8505 java.lang.Integer.MAX_VALUE, workflow); 8506 default: 8507 return super.getNamedProperty(_hash, _name, _checkValid); 8508 } 8509 8510 } 8511 8512 @Override 8513 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8514 switch (hash) { 8515 case 116079: 8516 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 8517 case -1618432855: 8518 /* identifier */ return this.identifier == null ? new Base[0] 8519 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 8520 case 351608024: 8521 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 8522 case 3373707: 8523 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 8524 case -892481550: 8525 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 8526 case -404562712: 8527 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 8528 case 3076014: 8529 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 8530 case 1447404028: 8531 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 8532 case 951526432: 8533 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 8534 case -669707736: 8535 /* useContext */ return this.useContext == null ? new Base[0] 8536 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 8537 case -507075711: 8538 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 8539 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 8540 case 1522889671: 8541 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 8542 case -220463842: 8543 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 8544 case 92645877: 8545 /* actor */ return this.actor == null ? new Base[0] : this.actor.toArray(new Base[this.actor.size()]); // ExampleScenarioActorComponent 8546 case 555127957: 8547 /* instance */ return this.instance == null ? new Base[0] : this.instance.toArray(new Base[this.instance.size()]); // ExampleScenarioInstanceComponent 8548 case -309518737: 8549 /* process */ return this.process == null ? new Base[0] : this.process.toArray(new Base[this.process.size()]); // ExampleScenarioProcessComponent 8550 case 35379135: 8551 /* workflow */ return this.workflow == null ? new Base[0] : this.workflow.toArray(new Base[this.workflow.size()]); // CanonicalType 8552 default: 8553 return super.getProperty(hash, name, checkValid); 8554 } 8555 8556 } 8557 8558 @Override 8559 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8560 switch (hash) { 8561 case 116079: // url 8562 this.url = castToUri(value); // UriType 8563 return value; 8564 case -1618432855: // identifier 8565 this.getIdentifier().add(castToIdentifier(value)); // Identifier 8566 return value; 8567 case 351608024: // version 8568 this.version = castToString(value); // StringType 8569 return value; 8570 case 3373707: // name 8571 this.name = castToString(value); // StringType 8572 return value; 8573 case -892481550: // status 8574 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 8575 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 8576 return value; 8577 case -404562712: // experimental 8578 this.experimental = castToBoolean(value); // BooleanType 8579 return value; 8580 case 3076014: // date 8581 this.date = castToDateTime(value); // DateTimeType 8582 return value; 8583 case 1447404028: // publisher 8584 this.publisher = castToString(value); // StringType 8585 return value; 8586 case 951526432: // contact 8587 this.getContact().add(castToContactDetail(value)); // ContactDetail 8588 return value; 8589 case -669707736: // useContext 8590 this.getUseContext().add(castToUsageContext(value)); // UsageContext 8591 return value; 8592 case -507075711: // jurisdiction 8593 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 8594 return value; 8595 case 1522889671: // copyright 8596 this.copyright = castToMarkdown(value); // MarkdownType 8597 return value; 8598 case -220463842: // purpose 8599 this.purpose = castToMarkdown(value); // MarkdownType 8600 return value; 8601 case 92645877: // actor 8602 this.getActor().add((ExampleScenarioActorComponent) value); // ExampleScenarioActorComponent 8603 return value; 8604 case 555127957: // instance 8605 this.getInstance().add((ExampleScenarioInstanceComponent) value); // ExampleScenarioInstanceComponent 8606 return value; 8607 case -309518737: // process 8608 this.getProcess().add((ExampleScenarioProcessComponent) value); // ExampleScenarioProcessComponent 8609 return value; 8610 case 35379135: // workflow 8611 this.getWorkflow().add(castToCanonical(value)); // CanonicalType 8612 return value; 8613 default: 8614 return super.setProperty(hash, name, value); 8615 } 8616 8617 } 8618 8619 @Override 8620 public Base setProperty(String name, Base value) throws FHIRException { 8621 if (name.equals("url")) { 8622 this.url = castToUri(value); // UriType 8623 } else if (name.equals("identifier")) { 8624 this.getIdentifier().add(castToIdentifier(value)); 8625 } else if (name.equals("version")) { 8626 this.version = castToString(value); // StringType 8627 } else if (name.equals("name")) { 8628 this.name = castToString(value); // StringType 8629 } else if (name.equals("status")) { 8630 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 8631 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 8632 } else if (name.equals("experimental")) { 8633 this.experimental = castToBoolean(value); // BooleanType 8634 } else if (name.equals("date")) { 8635 this.date = castToDateTime(value); // DateTimeType 8636 } else if (name.equals("publisher")) { 8637 this.publisher = castToString(value); // StringType 8638 } else if (name.equals("contact")) { 8639 this.getContact().add(castToContactDetail(value)); 8640 } else if (name.equals("useContext")) { 8641 this.getUseContext().add(castToUsageContext(value)); 8642 } else if (name.equals("jurisdiction")) { 8643 this.getJurisdiction().add(castToCodeableConcept(value)); 8644 } else if (name.equals("copyright")) { 8645 this.copyright = castToMarkdown(value); // MarkdownType 8646 } else if (name.equals("purpose")) { 8647 this.purpose = castToMarkdown(value); // MarkdownType 8648 } else if (name.equals("actor")) { 8649 this.getActor().add((ExampleScenarioActorComponent) value); 8650 } else if (name.equals("instance")) { 8651 this.getInstance().add((ExampleScenarioInstanceComponent) value); 8652 } else if (name.equals("process")) { 8653 this.getProcess().add((ExampleScenarioProcessComponent) value); 8654 } else if (name.equals("workflow")) { 8655 this.getWorkflow().add(castToCanonical(value)); 8656 } else 8657 return super.setProperty(name, value); 8658 return value; 8659 } 8660 8661 @Override 8662 public Base makeProperty(int hash, String name) throws FHIRException { 8663 switch (hash) { 8664 case 116079: 8665 return getUrlElement(); 8666 case -1618432855: 8667 return addIdentifier(); 8668 case 351608024: 8669 return getVersionElement(); 8670 case 3373707: 8671 return getNameElement(); 8672 case -892481550: 8673 return getStatusElement(); 8674 case -404562712: 8675 return getExperimentalElement(); 8676 case 3076014: 8677 return getDateElement(); 8678 case 1447404028: 8679 return getPublisherElement(); 8680 case 951526432: 8681 return addContact(); 8682 case -669707736: 8683 return addUseContext(); 8684 case -507075711: 8685 return addJurisdiction(); 8686 case 1522889671: 8687 return getCopyrightElement(); 8688 case -220463842: 8689 return getPurposeElement(); 8690 case 92645877: 8691 return addActor(); 8692 case 555127957: 8693 return addInstance(); 8694 case -309518737: 8695 return addProcess(); 8696 case 35379135: 8697 return addWorkflowElement(); 8698 default: 8699 return super.makeProperty(hash, name); 8700 } 8701 8702 } 8703 8704 @Override 8705 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8706 switch (hash) { 8707 case 116079: 8708 /* url */ return new String[] { "uri" }; 8709 case -1618432855: 8710 /* identifier */ return new String[] { "Identifier" }; 8711 case 351608024: 8712 /* version */ return new String[] { "string" }; 8713 case 3373707: 8714 /* name */ return new String[] { "string" }; 8715 case -892481550: 8716 /* status */ return new String[] { "code" }; 8717 case -404562712: 8718 /* experimental */ return new String[] { "boolean" }; 8719 case 3076014: 8720 /* date */ return new String[] { "dateTime" }; 8721 case 1447404028: 8722 /* publisher */ return new String[] { "string" }; 8723 case 951526432: 8724 /* contact */ return new String[] { "ContactDetail" }; 8725 case -669707736: 8726 /* useContext */ return new String[] { "UsageContext" }; 8727 case -507075711: 8728 /* jurisdiction */ return new String[] { "CodeableConcept" }; 8729 case 1522889671: 8730 /* copyright */ return new String[] { "markdown" }; 8731 case -220463842: 8732 /* purpose */ return new String[] { "markdown" }; 8733 case 92645877: 8734 /* actor */ return new String[] {}; 8735 case 555127957: 8736 /* instance */ return new String[] {}; 8737 case -309518737: 8738 /* process */ return new String[] {}; 8739 case 35379135: 8740 /* workflow */ return new String[] { "canonical" }; 8741 default: 8742 return super.getTypesForProperty(hash, name); 8743 } 8744 8745 } 8746 8747 @Override 8748 public Base addChild(String name) throws FHIRException { 8749 if (name.equals("url")) { 8750 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.url"); 8751 } else if (name.equals("identifier")) { 8752 return addIdentifier(); 8753 } else if (name.equals("version")) { 8754 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.version"); 8755 } else if (name.equals("name")) { 8756 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.name"); 8757 } else if (name.equals("status")) { 8758 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.status"); 8759 } else if (name.equals("experimental")) { 8760 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.experimental"); 8761 } else if (name.equals("date")) { 8762 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.date"); 8763 } else if (name.equals("publisher")) { 8764 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.publisher"); 8765 } else if (name.equals("contact")) { 8766 return addContact(); 8767 } else if (name.equals("useContext")) { 8768 return addUseContext(); 8769 } else if (name.equals("jurisdiction")) { 8770 return addJurisdiction(); 8771 } else if (name.equals("copyright")) { 8772 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.copyright"); 8773 } else if (name.equals("purpose")) { 8774 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.purpose"); 8775 } else if (name.equals("actor")) { 8776 return addActor(); 8777 } else if (name.equals("instance")) { 8778 return addInstance(); 8779 } else if (name.equals("process")) { 8780 return addProcess(); 8781 } else if (name.equals("workflow")) { 8782 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.workflow"); 8783 } else 8784 return super.addChild(name); 8785 } 8786 8787 public String fhirType() { 8788 return "ExampleScenario"; 8789 8790 } 8791 8792 public ExampleScenario copy() { 8793 ExampleScenario dst = new ExampleScenario(); 8794 copyValues(dst); 8795 return dst; 8796 } 8797 8798 public void copyValues(ExampleScenario dst) { 8799 super.copyValues(dst); 8800 dst.url = url == null ? null : url.copy(); 8801 if (identifier != null) { 8802 dst.identifier = new ArrayList<Identifier>(); 8803 for (Identifier i : identifier) 8804 dst.identifier.add(i.copy()); 8805 } 8806 ; 8807 dst.version = version == null ? null : version.copy(); 8808 dst.name = name == null ? null : name.copy(); 8809 dst.status = status == null ? null : status.copy(); 8810 dst.experimental = experimental == null ? null : experimental.copy(); 8811 dst.date = date == null ? null : date.copy(); 8812 dst.publisher = publisher == null ? null : publisher.copy(); 8813 if (contact != null) { 8814 dst.contact = new ArrayList<ContactDetail>(); 8815 for (ContactDetail i : contact) 8816 dst.contact.add(i.copy()); 8817 } 8818 ; 8819 if (useContext != null) { 8820 dst.useContext = new ArrayList<UsageContext>(); 8821 for (UsageContext i : useContext) 8822 dst.useContext.add(i.copy()); 8823 } 8824 ; 8825 if (jurisdiction != null) { 8826 dst.jurisdiction = new ArrayList<CodeableConcept>(); 8827 for (CodeableConcept i : jurisdiction) 8828 dst.jurisdiction.add(i.copy()); 8829 } 8830 ; 8831 dst.copyright = copyright == null ? null : copyright.copy(); 8832 dst.purpose = purpose == null ? null : purpose.copy(); 8833 if (actor != null) { 8834 dst.actor = new ArrayList<ExampleScenarioActorComponent>(); 8835 for (ExampleScenarioActorComponent i : actor) 8836 dst.actor.add(i.copy()); 8837 } 8838 ; 8839 if (instance != null) { 8840 dst.instance = new ArrayList<ExampleScenarioInstanceComponent>(); 8841 for (ExampleScenarioInstanceComponent i : instance) 8842 dst.instance.add(i.copy()); 8843 } 8844 ; 8845 if (process != null) { 8846 dst.process = new ArrayList<ExampleScenarioProcessComponent>(); 8847 for (ExampleScenarioProcessComponent i : process) 8848 dst.process.add(i.copy()); 8849 } 8850 ; 8851 if (workflow != null) { 8852 dst.workflow = new ArrayList<CanonicalType>(); 8853 for (CanonicalType i : workflow) 8854 dst.workflow.add(i.copy()); 8855 } 8856 ; 8857 } 8858 8859 protected ExampleScenario typedCopy() { 8860 return copy(); 8861 } 8862 8863 @Override 8864 public boolean equalsDeep(Base other_) { 8865 if (!super.equalsDeep(other_)) 8866 return false; 8867 if (!(other_ instanceof ExampleScenario)) 8868 return false; 8869 ExampleScenario o = (ExampleScenario) other_; 8870 return compareDeep(identifier, o.identifier, true) && compareDeep(copyright, o.copyright, true) 8871 && compareDeep(purpose, o.purpose, true) && compareDeep(actor, o.actor, true) 8872 && compareDeep(instance, o.instance, true) && compareDeep(process, o.process, true) 8873 && compareDeep(workflow, o.workflow, true); 8874 } 8875 8876 @Override 8877 public boolean equalsShallow(Base other_) { 8878 if (!super.equalsShallow(other_)) 8879 return false; 8880 if (!(other_ instanceof ExampleScenario)) 8881 return false; 8882 ExampleScenario o = (ExampleScenario) other_; 8883 return compareValues(copyright, o.copyright, true) && compareValues(purpose, o.purpose, true); 8884 } 8885 8886 public boolean isEmpty() { 8887 return super.isEmpty() 8888 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, copyright, purpose, actor, instance, process, workflow); 8889 } 8890 8891 @Override 8892 public ResourceType getResourceType() { 8893 return ResourceType.ExampleScenario; 8894 } 8895 8896 /** 8897 * Search parameter: <b>date</b> 8898 * <p> 8899 * Description: <b>The example scenario publication date</b><br> 8900 * Type: <b>date</b><br> 8901 * Path: <b>ExampleScenario.date</b><br> 8902 * </p> 8903 */ 8904 @SearchParamDefinition(name = "date", path = "ExampleScenario.date", description = "The example scenario publication date", type = "date") 8905 public static final String SP_DATE = "date"; 8906 /** 8907 * <b>Fluent Client</b> search parameter constant for <b>date</b> 8908 * <p> 8909 * Description: <b>The example scenario publication date</b><br> 8910 * Type: <b>date</b><br> 8911 * Path: <b>ExampleScenario.date</b><br> 8912 * </p> 8913 */ 8914 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 8915 SP_DATE); 8916 8917 /** 8918 * Search parameter: <b>identifier</b> 8919 * <p> 8920 * Description: <b>External identifier for the example scenario</b><br> 8921 * Type: <b>token</b><br> 8922 * Path: <b>ExampleScenario.identifier</b><br> 8923 * </p> 8924 */ 8925 @SearchParamDefinition(name = "identifier", path = "ExampleScenario.identifier", description = "External identifier for the example scenario", type = "token") 8926 public static final String SP_IDENTIFIER = "identifier"; 8927 /** 8928 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 8929 * <p> 8930 * Description: <b>External identifier for the example scenario</b><br> 8931 * Type: <b>token</b><br> 8932 * Path: <b>ExampleScenario.identifier</b><br> 8933 * </p> 8934 */ 8935 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 8936 SP_IDENTIFIER); 8937 8938 /** 8939 * Search parameter: <b>context-type-value</b> 8940 * <p> 8941 * Description: <b>A use context type and value assigned to the example 8942 * scenario</b><br> 8943 * Type: <b>composite</b><br> 8944 * Path: <b></b><br> 8945 * </p> 8946 */ 8947 @SearchParamDefinition(name = "context-type-value", path = "ExampleScenario.useContext", description = "A use context type and value assigned to the example scenario", type = "composite", compositeOf = { 8948 "context-type", "context" }) 8949 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 8950 /** 8951 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 8952 * <p> 8953 * Description: <b>A use context type and value assigned to the example 8954 * scenario</b><br> 8955 * Type: <b>composite</b><br> 8956 * Path: <b></b><br> 8957 * </p> 8958 */ 8959 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 8960 SP_CONTEXT_TYPE_VALUE); 8961 8962 /** 8963 * Search parameter: <b>jurisdiction</b> 8964 * <p> 8965 * Description: <b>Intended jurisdiction for the example scenario</b><br> 8966 * Type: <b>token</b><br> 8967 * Path: <b>ExampleScenario.jurisdiction</b><br> 8968 * </p> 8969 */ 8970 @SearchParamDefinition(name = "jurisdiction", path = "ExampleScenario.jurisdiction", description = "Intended jurisdiction for the example scenario", type = "token") 8971 public static final String SP_JURISDICTION = "jurisdiction"; 8972 /** 8973 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 8974 * <p> 8975 * Description: <b>Intended jurisdiction for the example scenario</b><br> 8976 * Type: <b>token</b><br> 8977 * Path: <b>ExampleScenario.jurisdiction</b><br> 8978 * </p> 8979 */ 8980 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 8981 SP_JURISDICTION); 8982 8983 /** 8984 * Search parameter: <b>context-type</b> 8985 * <p> 8986 * Description: <b>A type of use context assigned to the example 8987 * scenario</b><br> 8988 * Type: <b>token</b><br> 8989 * Path: <b>ExampleScenario.useContext.code</b><br> 8990 * </p> 8991 */ 8992 @SearchParamDefinition(name = "context-type", path = "ExampleScenario.useContext.code", description = "A type of use context assigned to the example scenario", type = "token") 8993 public static final String SP_CONTEXT_TYPE = "context-type"; 8994 /** 8995 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 8996 * <p> 8997 * Description: <b>A type of use context assigned to the example 8998 * scenario</b><br> 8999 * Type: <b>token</b><br> 9000 * Path: <b>ExampleScenario.useContext.code</b><br> 9001 * </p> 9002 */ 9003 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 9004 SP_CONTEXT_TYPE); 9005 9006 /** 9007 * Search parameter: <b>version</b> 9008 * <p> 9009 * Description: <b>The business version of the example scenario</b><br> 9010 * Type: <b>token</b><br> 9011 * Path: <b>ExampleScenario.version</b><br> 9012 * </p> 9013 */ 9014 @SearchParamDefinition(name = "version", path = "ExampleScenario.version", description = "The business version of the example scenario", type = "token") 9015 public static final String SP_VERSION = "version"; 9016 /** 9017 * <b>Fluent Client</b> search parameter constant for <b>version</b> 9018 * <p> 9019 * Description: <b>The business version of the example scenario</b><br> 9020 * Type: <b>token</b><br> 9021 * Path: <b>ExampleScenario.version</b><br> 9022 * </p> 9023 */ 9024 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 9025 SP_VERSION); 9026 9027 /** 9028 * Search parameter: <b>url</b> 9029 * <p> 9030 * Description: <b>The uri that identifies the example scenario</b><br> 9031 * Type: <b>uri</b><br> 9032 * Path: <b>ExampleScenario.url</b><br> 9033 * </p> 9034 */ 9035 @SearchParamDefinition(name = "url", path = "ExampleScenario.url", description = "The uri that identifies the example scenario", type = "uri") 9036 public static final String SP_URL = "url"; 9037 /** 9038 * <b>Fluent Client</b> search parameter constant for <b>url</b> 9039 * <p> 9040 * Description: <b>The uri that identifies the example scenario</b><br> 9041 * Type: <b>uri</b><br> 9042 * Path: <b>ExampleScenario.url</b><br> 9043 * </p> 9044 */ 9045 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 9046 9047 /** 9048 * Search parameter: <b>context-quantity</b> 9049 * <p> 9050 * Description: <b>A quantity- or range-valued use context assigned to the 9051 * example scenario</b><br> 9052 * Type: <b>quantity</b><br> 9053 * Path: <b>ExampleScenario.useContext.valueQuantity, 9054 * ExampleScenario.useContext.valueRange</b><br> 9055 * </p> 9056 */ 9057 @SearchParamDefinition(name = "context-quantity", path = "(ExampleScenario.useContext.value as Quantity) | (ExampleScenario.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the example scenario", type = "quantity") 9058 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 9059 /** 9060 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 9061 * <p> 9062 * Description: <b>A quantity- or range-valued use context assigned to the 9063 * example scenario</b><br> 9064 * Type: <b>quantity</b><br> 9065 * Path: <b>ExampleScenario.useContext.valueQuantity, 9066 * ExampleScenario.useContext.valueRange</b><br> 9067 * </p> 9068 */ 9069 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 9070 SP_CONTEXT_QUANTITY); 9071 9072 /** 9073 * Search parameter: <b>name</b> 9074 * <p> 9075 * Description: <b>Computationally friendly name of the example scenario</b><br> 9076 * Type: <b>string</b><br> 9077 * Path: <b>ExampleScenario.name</b><br> 9078 * </p> 9079 */ 9080 @SearchParamDefinition(name = "name", path = "ExampleScenario.name", description = "Computationally friendly name of the example scenario", type = "string") 9081 public static final String SP_NAME = "name"; 9082 /** 9083 * <b>Fluent Client</b> search parameter constant for <b>name</b> 9084 * <p> 9085 * Description: <b>Computationally friendly name of the example scenario</b><br> 9086 * Type: <b>string</b><br> 9087 * Path: <b>ExampleScenario.name</b><br> 9088 * </p> 9089 */ 9090 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 9091 SP_NAME); 9092 9093 /** 9094 * Search parameter: <b>context</b> 9095 * <p> 9096 * Description: <b>A use context assigned to the example scenario</b><br> 9097 * Type: <b>token</b><br> 9098 * Path: <b>ExampleScenario.useContext.valueCodeableConcept</b><br> 9099 * </p> 9100 */ 9101 @SearchParamDefinition(name = "context", path = "(ExampleScenario.useContext.value as CodeableConcept)", description = "A use context assigned to the example scenario", type = "token") 9102 public static final String SP_CONTEXT = "context"; 9103 /** 9104 * <b>Fluent Client</b> search parameter constant for <b>context</b> 9105 * <p> 9106 * Description: <b>A use context assigned to the example scenario</b><br> 9107 * Type: <b>token</b><br> 9108 * Path: <b>ExampleScenario.useContext.valueCodeableConcept</b><br> 9109 * </p> 9110 */ 9111 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 9112 SP_CONTEXT); 9113 9114 /** 9115 * Search parameter: <b>publisher</b> 9116 * <p> 9117 * Description: <b>Name of the publisher of the example scenario</b><br> 9118 * Type: <b>string</b><br> 9119 * Path: <b>ExampleScenario.publisher</b><br> 9120 * </p> 9121 */ 9122 @SearchParamDefinition(name = "publisher", path = "ExampleScenario.publisher", description = "Name of the publisher of the example scenario", type = "string") 9123 public static final String SP_PUBLISHER = "publisher"; 9124 /** 9125 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 9126 * <p> 9127 * Description: <b>Name of the publisher of the example scenario</b><br> 9128 * Type: <b>string</b><br> 9129 * Path: <b>ExampleScenario.publisher</b><br> 9130 * </p> 9131 */ 9132 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 9133 SP_PUBLISHER); 9134 9135 /** 9136 * Search parameter: <b>context-type-quantity</b> 9137 * <p> 9138 * Description: <b>A use context type and quantity- or range-based value 9139 * assigned to the example scenario</b><br> 9140 * Type: <b>composite</b><br> 9141 * Path: <b></b><br> 9142 * </p> 9143 */ 9144 @SearchParamDefinition(name = "context-type-quantity", path = "ExampleScenario.useContext", description = "A use context type and quantity- or range-based value assigned to the example scenario", type = "composite", compositeOf = { 9145 "context-type", "context-quantity" }) 9146 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 9147 /** 9148 * <b>Fluent Client</b> search parameter constant for 9149 * <b>context-type-quantity</b> 9150 * <p> 9151 * Description: <b>A use context type and quantity- or range-based value 9152 * assigned to the example scenario</b><br> 9153 * Type: <b>composite</b><br> 9154 * Path: <b></b><br> 9155 * </p> 9156 */ 9157 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 9158 SP_CONTEXT_TYPE_QUANTITY); 9159 9160 /** 9161 * Search parameter: <b>status</b> 9162 * <p> 9163 * Description: <b>The current status of the example scenario</b><br> 9164 * Type: <b>token</b><br> 9165 * Path: <b>ExampleScenario.status</b><br> 9166 * </p> 9167 */ 9168 @SearchParamDefinition(name = "status", path = "ExampleScenario.status", description = "The current status of the example scenario", type = "token") 9169 public static final String SP_STATUS = "status"; 9170 /** 9171 * <b>Fluent Client</b> search parameter constant for <b>status</b> 9172 * <p> 9173 * Description: <b>The current status of the example scenario</b><br> 9174 * Type: <b>token</b><br> 9175 * Path: <b>ExampleScenario.status</b><br> 9176 * </p> 9177 */ 9178 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 9179 SP_STATUS); 9180 9181}