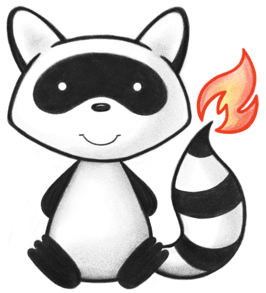
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.r4.model.Enumerations.NoteType; 042import org.hl7.fhir.r4.model.Enumerations.NoteTypeEnumFactory; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.ResourceDef; 049import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 050 051/** 052 * This resource provides: the claim details; adjudication details from the 053 * processing of a Claim; and optionally account balance information, for 054 * informing the subscriber of the benefits provided. 055 */ 056@ResourceDef(name = "ExplanationOfBenefit", profile = "http://hl7.org/fhir/StructureDefinition/ExplanationOfBenefit") 057public class ExplanationOfBenefit extends DomainResource { 058 059 public enum ExplanationOfBenefitStatus { 060 /** 061 * The resource instance is currently in-force. 062 */ 063 ACTIVE, 064 /** 065 * The resource instance is withdrawn, rescinded or reversed. 066 */ 067 CANCELLED, 068 /** 069 * A new resource instance the contents of which is not complete. 070 */ 071 DRAFT, 072 /** 073 * The resource instance was entered in error. 074 */ 075 ENTEREDINERROR, 076 /** 077 * added to help the parsers with the generic types 078 */ 079 NULL; 080 081 public static ExplanationOfBenefitStatus fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("active".equals(codeString)) 085 return ACTIVE; 086 if ("cancelled".equals(codeString)) 087 return CANCELLED; 088 if ("draft".equals(codeString)) 089 return DRAFT; 090 if ("entered-in-error".equals(codeString)) 091 return ENTEREDINERROR; 092 if (Configuration.isAcceptInvalidEnums()) 093 return null; 094 else 095 throw new FHIRException("Unknown ExplanationOfBenefitStatus code '" + codeString + "'"); 096 } 097 098 public String toCode() { 099 switch (this) { 100 case ACTIVE: 101 return "active"; 102 case CANCELLED: 103 return "cancelled"; 104 case DRAFT: 105 return "draft"; 106 case ENTEREDINERROR: 107 return "entered-in-error"; 108 case NULL: 109 return null; 110 default: 111 return "?"; 112 } 113 } 114 115 public String getSystem() { 116 switch (this) { 117 case ACTIVE: 118 return "http://hl7.org/fhir/explanationofbenefit-status"; 119 case CANCELLED: 120 return "http://hl7.org/fhir/explanationofbenefit-status"; 121 case DRAFT: 122 return "http://hl7.org/fhir/explanationofbenefit-status"; 123 case ENTEREDINERROR: 124 return "http://hl7.org/fhir/explanationofbenefit-status"; 125 case NULL: 126 return null; 127 default: 128 return "?"; 129 } 130 } 131 132 public String getDefinition() { 133 switch (this) { 134 case ACTIVE: 135 return "The resource instance is currently in-force."; 136 case CANCELLED: 137 return "The resource instance is withdrawn, rescinded or reversed."; 138 case DRAFT: 139 return "A new resource instance the contents of which is not complete."; 140 case ENTEREDINERROR: 141 return "The resource instance was entered in error."; 142 case NULL: 143 return null; 144 default: 145 return "?"; 146 } 147 } 148 149 public String getDisplay() { 150 switch (this) { 151 case ACTIVE: 152 return "Active"; 153 case CANCELLED: 154 return "Cancelled"; 155 case DRAFT: 156 return "Draft"; 157 case ENTEREDINERROR: 158 return "Entered In Error"; 159 case NULL: 160 return null; 161 default: 162 return "?"; 163 } 164 } 165 } 166 167 public static class ExplanationOfBenefitStatusEnumFactory implements EnumFactory<ExplanationOfBenefitStatus> { 168 public ExplanationOfBenefitStatus fromCode(String codeString) throws IllegalArgumentException { 169 if (codeString == null || "".equals(codeString)) 170 if (codeString == null || "".equals(codeString)) 171 return null; 172 if ("active".equals(codeString)) 173 return ExplanationOfBenefitStatus.ACTIVE; 174 if ("cancelled".equals(codeString)) 175 return ExplanationOfBenefitStatus.CANCELLED; 176 if ("draft".equals(codeString)) 177 return ExplanationOfBenefitStatus.DRAFT; 178 if ("entered-in-error".equals(codeString)) 179 return ExplanationOfBenefitStatus.ENTEREDINERROR; 180 throw new IllegalArgumentException("Unknown ExplanationOfBenefitStatus code '" + codeString + "'"); 181 } 182 183 public Enumeration<ExplanationOfBenefitStatus> fromType(PrimitiveType<?> code) throws FHIRException { 184 if (code == null) 185 return null; 186 if (code.isEmpty()) 187 return new Enumeration<ExplanationOfBenefitStatus>(this, ExplanationOfBenefitStatus.NULL, code); 188 String codeString = code.asStringValue(); 189 if (codeString == null || "".equals(codeString)) 190 return new Enumeration<ExplanationOfBenefitStatus>(this, ExplanationOfBenefitStatus.NULL, code); 191 if ("active".equals(codeString)) 192 return new Enumeration<ExplanationOfBenefitStatus>(this, ExplanationOfBenefitStatus.ACTIVE, code); 193 if ("cancelled".equals(codeString)) 194 return new Enumeration<ExplanationOfBenefitStatus>(this, ExplanationOfBenefitStatus.CANCELLED, code); 195 if ("draft".equals(codeString)) 196 return new Enumeration<ExplanationOfBenefitStatus>(this, ExplanationOfBenefitStatus.DRAFT, code); 197 if ("entered-in-error".equals(codeString)) 198 return new Enumeration<ExplanationOfBenefitStatus>(this, ExplanationOfBenefitStatus.ENTEREDINERROR, code); 199 throw new FHIRException("Unknown ExplanationOfBenefitStatus code '" + codeString + "'"); 200 } 201 202 public String toCode(ExplanationOfBenefitStatus code) { 203 if (code == ExplanationOfBenefitStatus.NULL) 204 return null; 205 if (code == ExplanationOfBenefitStatus.ACTIVE) 206 return "active"; 207 if (code == ExplanationOfBenefitStatus.CANCELLED) 208 return "cancelled"; 209 if (code == ExplanationOfBenefitStatus.DRAFT) 210 return "draft"; 211 if (code == ExplanationOfBenefitStatus.ENTEREDINERROR) 212 return "entered-in-error"; 213 return "?"; 214 } 215 216 public String toSystem(ExplanationOfBenefitStatus code) { 217 return code.getSystem(); 218 } 219 } 220 221 public enum Use { 222 /** 223 * The treatment is complete and this represents a Claim for the services. 224 */ 225 CLAIM, 226 /** 227 * The treatment is proposed and this represents a Pre-authorization for the 228 * services. 229 */ 230 PREAUTHORIZATION, 231 /** 232 * The treatment is proposed and this represents a Pre-determination for the 233 * services. 234 */ 235 PREDETERMINATION, 236 /** 237 * added to help the parsers with the generic types 238 */ 239 NULL; 240 241 public static Use fromCode(String codeString) throws FHIRException { 242 if (codeString == null || "".equals(codeString)) 243 return null; 244 if ("claim".equals(codeString)) 245 return CLAIM; 246 if ("preauthorization".equals(codeString)) 247 return PREAUTHORIZATION; 248 if ("predetermination".equals(codeString)) 249 return PREDETERMINATION; 250 if (Configuration.isAcceptInvalidEnums()) 251 return null; 252 else 253 throw new FHIRException("Unknown Use code '" + codeString + "'"); 254 } 255 256 public String toCode() { 257 switch (this) { 258 case CLAIM: 259 return "claim"; 260 case PREAUTHORIZATION: 261 return "preauthorization"; 262 case PREDETERMINATION: 263 return "predetermination"; 264 case NULL: 265 return null; 266 default: 267 return "?"; 268 } 269 } 270 271 public String getSystem() { 272 switch (this) { 273 case CLAIM: 274 return "http://hl7.org/fhir/claim-use"; 275 case PREAUTHORIZATION: 276 return "http://hl7.org/fhir/claim-use"; 277 case PREDETERMINATION: 278 return "http://hl7.org/fhir/claim-use"; 279 case NULL: 280 return null; 281 default: 282 return "?"; 283 } 284 } 285 286 public String getDefinition() { 287 switch (this) { 288 case CLAIM: 289 return "The treatment is complete and this represents a Claim for the services."; 290 case PREAUTHORIZATION: 291 return "The treatment is proposed and this represents a Pre-authorization for the services."; 292 case PREDETERMINATION: 293 return "The treatment is proposed and this represents a Pre-determination for the services."; 294 case NULL: 295 return null; 296 default: 297 return "?"; 298 } 299 } 300 301 public String getDisplay() { 302 switch (this) { 303 case CLAIM: 304 return "Claim"; 305 case PREAUTHORIZATION: 306 return "Preauthorization"; 307 case PREDETERMINATION: 308 return "Predetermination"; 309 case NULL: 310 return null; 311 default: 312 return "?"; 313 } 314 } 315 } 316 317 public static class UseEnumFactory implements EnumFactory<Use> { 318 public Use fromCode(String codeString) throws IllegalArgumentException { 319 if (codeString == null || "".equals(codeString)) 320 if (codeString == null || "".equals(codeString)) 321 return null; 322 if ("claim".equals(codeString)) 323 return Use.CLAIM; 324 if ("preauthorization".equals(codeString)) 325 return Use.PREAUTHORIZATION; 326 if ("predetermination".equals(codeString)) 327 return Use.PREDETERMINATION; 328 throw new IllegalArgumentException("Unknown Use code '" + codeString + "'"); 329 } 330 331 public Enumeration<Use> fromType(PrimitiveType<?> code) throws FHIRException { 332 if (code == null) 333 return null; 334 if (code.isEmpty()) 335 return new Enumeration<Use>(this, Use.NULL, code); 336 String codeString = code.asStringValue(); 337 if (codeString == null || "".equals(codeString)) 338 return new Enumeration<Use>(this, Use.NULL, code); 339 if ("claim".equals(codeString)) 340 return new Enumeration<Use>(this, Use.CLAIM, code); 341 if ("preauthorization".equals(codeString)) 342 return new Enumeration<Use>(this, Use.PREAUTHORIZATION, code); 343 if ("predetermination".equals(codeString)) 344 return new Enumeration<Use>(this, Use.PREDETERMINATION, code); 345 throw new FHIRException("Unknown Use code '" + codeString + "'"); 346 } 347 348 public String toCode(Use code) { 349 if (code == Use.NULL) 350 return null; 351 if (code == Use.CLAIM) 352 return "claim"; 353 if (code == Use.PREAUTHORIZATION) 354 return "preauthorization"; 355 if (code == Use.PREDETERMINATION) 356 return "predetermination"; 357 return "?"; 358 } 359 360 public String toSystem(Use code) { 361 return code.getSystem(); 362 } 363 } 364 365 public enum RemittanceOutcome { 366 /** 367 * The Claim/Pre-authorization/Pre-determination has been received but 368 * processing has not begun. 369 */ 370 QUEUED, 371 /** 372 * The processing has completed without errors 373 */ 374 COMPLETE, 375 /** 376 * One or more errors have been detected in the Claim 377 */ 378 ERROR, 379 /** 380 * No errors have been detected in the Claim and some of the adjudication has 381 * been performed. 382 */ 383 PARTIAL, 384 /** 385 * added to help the parsers with the generic types 386 */ 387 NULL; 388 389 public static RemittanceOutcome fromCode(String codeString) throws FHIRException { 390 if (codeString == null || "".equals(codeString)) 391 return null; 392 if ("queued".equals(codeString)) 393 return QUEUED; 394 if ("complete".equals(codeString)) 395 return COMPLETE; 396 if ("error".equals(codeString)) 397 return ERROR; 398 if ("partial".equals(codeString)) 399 return PARTIAL; 400 if (Configuration.isAcceptInvalidEnums()) 401 return null; 402 else 403 throw new FHIRException("Unknown RemittanceOutcome code '" + codeString + "'"); 404 } 405 406 public String toCode() { 407 switch (this) { 408 case QUEUED: 409 return "queued"; 410 case COMPLETE: 411 return "complete"; 412 case ERROR: 413 return "error"; 414 case PARTIAL: 415 return "partial"; 416 case NULL: 417 return null; 418 default: 419 return "?"; 420 } 421 } 422 423 public String getSystem() { 424 switch (this) { 425 case QUEUED: 426 return "http://hl7.org/fhir/remittance-outcome"; 427 case COMPLETE: 428 return "http://hl7.org/fhir/remittance-outcome"; 429 case ERROR: 430 return "http://hl7.org/fhir/remittance-outcome"; 431 case PARTIAL: 432 return "http://hl7.org/fhir/remittance-outcome"; 433 case NULL: 434 return null; 435 default: 436 return "?"; 437 } 438 } 439 440 public String getDefinition() { 441 switch (this) { 442 case QUEUED: 443 return "The Claim/Pre-authorization/Pre-determination has been received but processing has not begun."; 444 case COMPLETE: 445 return "The processing has completed without errors"; 446 case ERROR: 447 return "One or more errors have been detected in the Claim"; 448 case PARTIAL: 449 return "No errors have been detected in the Claim and some of the adjudication has been performed."; 450 case NULL: 451 return null; 452 default: 453 return "?"; 454 } 455 } 456 457 public String getDisplay() { 458 switch (this) { 459 case QUEUED: 460 return "Queued"; 461 case COMPLETE: 462 return "Processing Complete"; 463 case ERROR: 464 return "Error"; 465 case PARTIAL: 466 return "Partial Processing"; 467 case NULL: 468 return null; 469 default: 470 return "?"; 471 } 472 } 473 } 474 475 public static class RemittanceOutcomeEnumFactory implements EnumFactory<RemittanceOutcome> { 476 public RemittanceOutcome fromCode(String codeString) throws IllegalArgumentException { 477 if (codeString == null || "".equals(codeString)) 478 if (codeString == null || "".equals(codeString)) 479 return null; 480 if ("queued".equals(codeString)) 481 return RemittanceOutcome.QUEUED; 482 if ("complete".equals(codeString)) 483 return RemittanceOutcome.COMPLETE; 484 if ("error".equals(codeString)) 485 return RemittanceOutcome.ERROR; 486 if ("partial".equals(codeString)) 487 return RemittanceOutcome.PARTIAL; 488 throw new IllegalArgumentException("Unknown RemittanceOutcome code '" + codeString + "'"); 489 } 490 491 public Enumeration<RemittanceOutcome> fromType(PrimitiveType<?> code) throws FHIRException { 492 if (code == null) 493 return null; 494 if (code.isEmpty()) 495 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.NULL, code); 496 String codeString = code.asStringValue(); 497 if (codeString == null || "".equals(codeString)) 498 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.NULL, code); 499 if ("queued".equals(codeString)) 500 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.QUEUED, code); 501 if ("complete".equals(codeString)) 502 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.COMPLETE, code); 503 if ("error".equals(codeString)) 504 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.ERROR, code); 505 if ("partial".equals(codeString)) 506 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.PARTIAL, code); 507 throw new FHIRException("Unknown RemittanceOutcome code '" + codeString + "'"); 508 } 509 510 public String toCode(RemittanceOutcome code) { 511 if (code == RemittanceOutcome.NULL) 512 return null; 513 if (code == RemittanceOutcome.QUEUED) 514 return "queued"; 515 if (code == RemittanceOutcome.COMPLETE) 516 return "complete"; 517 if (code == RemittanceOutcome.ERROR) 518 return "error"; 519 if (code == RemittanceOutcome.PARTIAL) 520 return "partial"; 521 return "?"; 522 } 523 524 public String toSystem(RemittanceOutcome code) { 525 return code.getSystem(); 526 } 527 } 528 529 @Block() 530 public static class RelatedClaimComponent extends BackboneElement implements IBaseBackboneElement { 531 /** 532 * Reference to a related claim. 533 */ 534 @Child(name = "claim", type = { Claim.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 535 @Description(shortDefinition = "Reference to the related claim", formalDefinition = "Reference to a related claim.") 536 protected Reference claim; 537 538 /** 539 * The actual object that is the target of the reference (Reference to a related 540 * claim.) 541 */ 542 protected Claim claimTarget; 543 544 /** 545 * A code to convey how the claims are related. 546 */ 547 @Child(name = "relationship", type = { 548 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 549 @Description(shortDefinition = "How the reference claim is related", formalDefinition = "A code to convey how the claims are related.") 550 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/related-claim-relationship") 551 protected CodeableConcept relationship; 552 553 /** 554 * An alternate organizational reference to the case or file to which this 555 * particular claim pertains. 556 */ 557 @Child(name = "reference", type = { 558 Identifier.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 559 @Description(shortDefinition = "File or case reference", formalDefinition = "An alternate organizational reference to the case or file to which this particular claim pertains.") 560 protected Identifier reference; 561 562 private static final long serialVersionUID = -379338905L; 563 564 /** 565 * Constructor 566 */ 567 public RelatedClaimComponent() { 568 super(); 569 } 570 571 /** 572 * @return {@link #claim} (Reference to a related claim.) 573 */ 574 public Reference getClaim() { 575 if (this.claim == null) 576 if (Configuration.errorOnAutoCreate()) 577 throw new Error("Attempt to auto-create RelatedClaimComponent.claim"); 578 else if (Configuration.doAutoCreate()) 579 this.claim = new Reference(); // cc 580 return this.claim; 581 } 582 583 public boolean hasClaim() { 584 return this.claim != null && !this.claim.isEmpty(); 585 } 586 587 /** 588 * @param value {@link #claim} (Reference to a related claim.) 589 */ 590 public RelatedClaimComponent setClaim(Reference value) { 591 this.claim = value; 592 return this; 593 } 594 595 /** 596 * @return {@link #claim} The actual object that is the target of the reference. 597 * The reference library doesn't populate this, but you can use it to 598 * hold the resource if you resolve it. (Reference to a related claim.) 599 */ 600 public Claim getClaimTarget() { 601 if (this.claimTarget == null) 602 if (Configuration.errorOnAutoCreate()) 603 throw new Error("Attempt to auto-create RelatedClaimComponent.claim"); 604 else if (Configuration.doAutoCreate()) 605 this.claimTarget = new Claim(); // aa 606 return this.claimTarget; 607 } 608 609 /** 610 * @param value {@link #claim} The actual object that is the target of the 611 * reference. The reference library doesn't use these, but you can 612 * use it to hold the resource if you resolve it. (Reference to a 613 * related claim.) 614 */ 615 public RelatedClaimComponent setClaimTarget(Claim value) { 616 this.claimTarget = value; 617 return this; 618 } 619 620 /** 621 * @return {@link #relationship} (A code to convey how the claims are related.) 622 */ 623 public CodeableConcept getRelationship() { 624 if (this.relationship == null) 625 if (Configuration.errorOnAutoCreate()) 626 throw new Error("Attempt to auto-create RelatedClaimComponent.relationship"); 627 else if (Configuration.doAutoCreate()) 628 this.relationship = new CodeableConcept(); // cc 629 return this.relationship; 630 } 631 632 public boolean hasRelationship() { 633 return this.relationship != null && !this.relationship.isEmpty(); 634 } 635 636 /** 637 * @param value {@link #relationship} (A code to convey how the claims are 638 * related.) 639 */ 640 public RelatedClaimComponent setRelationship(CodeableConcept value) { 641 this.relationship = value; 642 return this; 643 } 644 645 /** 646 * @return {@link #reference} (An alternate organizational reference to the case 647 * or file to which this particular claim pertains.) 648 */ 649 public Identifier getReference() { 650 if (this.reference == null) 651 if (Configuration.errorOnAutoCreate()) 652 throw new Error("Attempt to auto-create RelatedClaimComponent.reference"); 653 else if (Configuration.doAutoCreate()) 654 this.reference = new Identifier(); // cc 655 return this.reference; 656 } 657 658 public boolean hasReference() { 659 return this.reference != null && !this.reference.isEmpty(); 660 } 661 662 /** 663 * @param value {@link #reference} (An alternate organizational reference to the 664 * case or file to which this particular claim pertains.) 665 */ 666 public RelatedClaimComponent setReference(Identifier value) { 667 this.reference = value; 668 return this; 669 } 670 671 protected void listChildren(List<Property> children) { 672 super.listChildren(children); 673 children.add(new Property("claim", "Reference(Claim)", "Reference to a related claim.", 0, 1, claim)); 674 children.add(new Property("relationship", "CodeableConcept", "A code to convey how the claims are related.", 0, 1, 675 relationship)); 676 children.add(new Property("reference", "Identifier", 677 "An alternate organizational reference to the case or file to which this particular claim pertains.", 0, 1, 678 reference)); 679 } 680 681 @Override 682 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 683 switch (_hash) { 684 case 94742588: 685 /* claim */ return new Property("claim", "Reference(Claim)", "Reference to a related claim.", 0, 1, claim); 686 case -261851592: 687 /* relationship */ return new Property("relationship", "CodeableConcept", 688 "A code to convey how the claims are related.", 0, 1, relationship); 689 case -925155509: 690 /* reference */ return new Property("reference", "Identifier", 691 "An alternate organizational reference to the case or file to which this particular claim pertains.", 0, 1, 692 reference); 693 default: 694 return super.getNamedProperty(_hash, _name, _checkValid); 695 } 696 697 } 698 699 @Override 700 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 701 switch (hash) { 702 case 94742588: 703 /* claim */ return this.claim == null ? new Base[0] : new Base[] { this.claim }; // Reference 704 case -261851592: 705 /* relationship */ return this.relationship == null ? new Base[0] : new Base[] { this.relationship }; // CodeableConcept 706 case -925155509: 707 /* reference */ return this.reference == null ? new Base[0] : new Base[] { this.reference }; // Identifier 708 default: 709 return super.getProperty(hash, name, checkValid); 710 } 711 712 } 713 714 @Override 715 public Base setProperty(int hash, String name, Base value) throws FHIRException { 716 switch (hash) { 717 case 94742588: // claim 718 this.claim = castToReference(value); // Reference 719 return value; 720 case -261851592: // relationship 721 this.relationship = castToCodeableConcept(value); // CodeableConcept 722 return value; 723 case -925155509: // reference 724 this.reference = castToIdentifier(value); // Identifier 725 return value; 726 default: 727 return super.setProperty(hash, name, value); 728 } 729 730 } 731 732 @Override 733 public Base setProperty(String name, Base value) throws FHIRException { 734 if (name.equals("claim")) { 735 this.claim = castToReference(value); // Reference 736 } else if (name.equals("relationship")) { 737 this.relationship = castToCodeableConcept(value); // CodeableConcept 738 } else if (name.equals("reference")) { 739 this.reference = castToIdentifier(value); // Identifier 740 } else 741 return super.setProperty(name, value); 742 return value; 743 } 744 745 @Override 746 public void removeChild(String name, Base value) throws FHIRException { 747 if (name.equals("claim")) { 748 this.claim = null; 749 } else if (name.equals("relationship")) { 750 this.relationship = null; 751 } else if (name.equals("reference")) { 752 this.reference = null; 753 } else 754 super.removeChild(name, value); 755 756 } 757 758 @Override 759 public Base makeProperty(int hash, String name) throws FHIRException { 760 switch (hash) { 761 case 94742588: 762 return getClaim(); 763 case -261851592: 764 return getRelationship(); 765 case -925155509: 766 return getReference(); 767 default: 768 return super.makeProperty(hash, name); 769 } 770 771 } 772 773 @Override 774 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 775 switch (hash) { 776 case 94742588: 777 /* claim */ return new String[] { "Reference" }; 778 case -261851592: 779 /* relationship */ return new String[] { "CodeableConcept" }; 780 case -925155509: 781 /* reference */ return new String[] { "Identifier" }; 782 default: 783 return super.getTypesForProperty(hash, name); 784 } 785 786 } 787 788 @Override 789 public Base addChild(String name) throws FHIRException { 790 if (name.equals("claim")) { 791 this.claim = new Reference(); 792 return this.claim; 793 } else if (name.equals("relationship")) { 794 this.relationship = new CodeableConcept(); 795 return this.relationship; 796 } else if (name.equals("reference")) { 797 this.reference = new Identifier(); 798 return this.reference; 799 } else 800 return super.addChild(name); 801 } 802 803 public RelatedClaimComponent copy() { 804 RelatedClaimComponent dst = new RelatedClaimComponent(); 805 copyValues(dst); 806 return dst; 807 } 808 809 public void copyValues(RelatedClaimComponent dst) { 810 super.copyValues(dst); 811 dst.claim = claim == null ? null : claim.copy(); 812 dst.relationship = relationship == null ? null : relationship.copy(); 813 dst.reference = reference == null ? null : reference.copy(); 814 } 815 816 @Override 817 public boolean equalsDeep(Base other_) { 818 if (!super.equalsDeep(other_)) 819 return false; 820 if (!(other_ instanceof RelatedClaimComponent)) 821 return false; 822 RelatedClaimComponent o = (RelatedClaimComponent) other_; 823 return compareDeep(claim, o.claim, true) && compareDeep(relationship, o.relationship, true) 824 && compareDeep(reference, o.reference, true); 825 } 826 827 @Override 828 public boolean equalsShallow(Base other_) { 829 if (!super.equalsShallow(other_)) 830 return false; 831 if (!(other_ instanceof RelatedClaimComponent)) 832 return false; 833 RelatedClaimComponent o = (RelatedClaimComponent) other_; 834 return true; 835 } 836 837 public boolean isEmpty() { 838 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(claim, relationship, reference); 839 } 840 841 public String fhirType() { 842 return "ExplanationOfBenefit.related"; 843 844 } 845 846 } 847 848 @Block() 849 public static class PayeeComponent extends BackboneElement implements IBaseBackboneElement { 850 /** 851 * Type of Party to be reimbursed: Subscriber, provider, other. 852 */ 853 @Child(name = "type", type = { 854 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 855 @Description(shortDefinition = "Category of recipient", formalDefinition = "Type of Party to be reimbursed: Subscriber, provider, other.") 856 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/payeetype") 857 protected CodeableConcept type; 858 859 /** 860 * Reference to the individual or organization to whom any payment will be made. 861 */ 862 @Child(name = "party", type = { Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, 863 RelatedPerson.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 864 @Description(shortDefinition = "Recipient reference", formalDefinition = "Reference to the individual or organization to whom any payment will be made.") 865 protected Reference party; 866 867 /** 868 * The actual object that is the target of the reference (Reference to the 869 * individual or organization to whom any payment will be made.) 870 */ 871 protected Resource partyTarget; 872 873 private static final long serialVersionUID = 1609484699L; 874 875 /** 876 * Constructor 877 */ 878 public PayeeComponent() { 879 super(); 880 } 881 882 /** 883 * @return {@link #type} (Type of Party to be reimbursed: Subscriber, provider, 884 * other.) 885 */ 886 public CodeableConcept getType() { 887 if (this.type == null) 888 if (Configuration.errorOnAutoCreate()) 889 throw new Error("Attempt to auto-create PayeeComponent.type"); 890 else if (Configuration.doAutoCreate()) 891 this.type = new CodeableConcept(); // cc 892 return this.type; 893 } 894 895 public boolean hasType() { 896 return this.type != null && !this.type.isEmpty(); 897 } 898 899 /** 900 * @param value {@link #type} (Type of Party to be reimbursed: Subscriber, 901 * provider, other.) 902 */ 903 public PayeeComponent setType(CodeableConcept value) { 904 this.type = value; 905 return this; 906 } 907 908 /** 909 * @return {@link #party} (Reference to the individual or organization to whom 910 * any payment will be made.) 911 */ 912 public Reference getParty() { 913 if (this.party == null) 914 if (Configuration.errorOnAutoCreate()) 915 throw new Error("Attempt to auto-create PayeeComponent.party"); 916 else if (Configuration.doAutoCreate()) 917 this.party = new Reference(); // cc 918 return this.party; 919 } 920 921 public boolean hasParty() { 922 return this.party != null && !this.party.isEmpty(); 923 } 924 925 /** 926 * @param value {@link #party} (Reference to the individual or organization to 927 * whom any payment will be made.) 928 */ 929 public PayeeComponent setParty(Reference value) { 930 this.party = value; 931 return this; 932 } 933 934 /** 935 * @return {@link #party} The actual object that is the target of the reference. 936 * The reference library doesn't populate this, but you can use it to 937 * hold the resource if you resolve it. (Reference to the individual or 938 * organization to whom any payment will be made.) 939 */ 940 public Resource getPartyTarget() { 941 return this.partyTarget; 942 } 943 944 /** 945 * @param value {@link #party} The actual object that is the target of the 946 * reference. The reference library doesn't use these, but you can 947 * use it to hold the resource if you resolve it. (Reference to the 948 * individual or organization to whom any payment will be made.) 949 */ 950 public PayeeComponent setPartyTarget(Resource value) { 951 this.partyTarget = value; 952 return this; 953 } 954 955 protected void listChildren(List<Property> children) { 956 super.listChildren(children); 957 children.add(new Property("type", "CodeableConcept", 958 "Type of Party to be reimbursed: Subscriber, provider, other.", 0, 1, type)); 959 children.add(new Property("party", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson)", 960 "Reference to the individual or organization to whom any payment will be made.", 0, 1, party)); 961 } 962 963 @Override 964 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 965 switch (_hash) { 966 case 3575610: 967 /* type */ return new Property("type", "CodeableConcept", 968 "Type of Party to be reimbursed: Subscriber, provider, other.", 0, 1, type); 969 case 106437350: 970 /* party */ return new Property("party", 971 "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson)", 972 "Reference to the individual or organization to whom any payment will be made.", 0, 1, party); 973 default: 974 return super.getNamedProperty(_hash, _name, _checkValid); 975 } 976 977 } 978 979 @Override 980 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 981 switch (hash) { 982 case 3575610: 983 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 984 case 106437350: 985 /* party */ return this.party == null ? new Base[0] : new Base[] { this.party }; // Reference 986 default: 987 return super.getProperty(hash, name, checkValid); 988 } 989 990 } 991 992 @Override 993 public Base setProperty(int hash, String name, Base value) throws FHIRException { 994 switch (hash) { 995 case 3575610: // type 996 this.type = castToCodeableConcept(value); // CodeableConcept 997 return value; 998 case 106437350: // party 999 this.party = castToReference(value); // Reference 1000 return value; 1001 default: 1002 return super.setProperty(hash, name, value); 1003 } 1004 1005 } 1006 1007 @Override 1008 public Base setProperty(String name, Base value) throws FHIRException { 1009 if (name.equals("type")) { 1010 this.type = castToCodeableConcept(value); // CodeableConcept 1011 } else if (name.equals("party")) { 1012 this.party = castToReference(value); // Reference 1013 } else 1014 return super.setProperty(name, value); 1015 return value; 1016 } 1017 1018 @Override 1019 public void removeChild(String name, Base value) throws FHIRException { 1020 if (name.equals("type")) { 1021 this.type = null; 1022 } else if (name.equals("party")) { 1023 this.party = null; 1024 } else 1025 super.removeChild(name, value); 1026 1027 } 1028 1029 @Override 1030 public Base makeProperty(int hash, String name) throws FHIRException { 1031 switch (hash) { 1032 case 3575610: 1033 return getType(); 1034 case 106437350: 1035 return getParty(); 1036 default: 1037 return super.makeProperty(hash, name); 1038 } 1039 1040 } 1041 1042 @Override 1043 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1044 switch (hash) { 1045 case 3575610: 1046 /* type */ return new String[] { "CodeableConcept" }; 1047 case 106437350: 1048 /* party */ return new String[] { "Reference" }; 1049 default: 1050 return super.getTypesForProperty(hash, name); 1051 } 1052 1053 } 1054 1055 @Override 1056 public Base addChild(String name) throws FHIRException { 1057 if (name.equals("type")) { 1058 this.type = new CodeableConcept(); 1059 return this.type; 1060 } else if (name.equals("party")) { 1061 this.party = new Reference(); 1062 return this.party; 1063 } else 1064 return super.addChild(name); 1065 } 1066 1067 public PayeeComponent copy() { 1068 PayeeComponent dst = new PayeeComponent(); 1069 copyValues(dst); 1070 return dst; 1071 } 1072 1073 public void copyValues(PayeeComponent dst) { 1074 super.copyValues(dst); 1075 dst.type = type == null ? null : type.copy(); 1076 dst.party = party == null ? null : party.copy(); 1077 } 1078 1079 @Override 1080 public boolean equalsDeep(Base other_) { 1081 if (!super.equalsDeep(other_)) 1082 return false; 1083 if (!(other_ instanceof PayeeComponent)) 1084 return false; 1085 PayeeComponent o = (PayeeComponent) other_; 1086 return compareDeep(type, o.type, true) && compareDeep(party, o.party, true); 1087 } 1088 1089 @Override 1090 public boolean equalsShallow(Base other_) { 1091 if (!super.equalsShallow(other_)) 1092 return false; 1093 if (!(other_ instanceof PayeeComponent)) 1094 return false; 1095 PayeeComponent o = (PayeeComponent) other_; 1096 return true; 1097 } 1098 1099 public boolean isEmpty() { 1100 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, party); 1101 } 1102 1103 public String fhirType() { 1104 return "ExplanationOfBenefit.payee"; 1105 1106 } 1107 1108 } 1109 1110 @Block() 1111 public static class CareTeamComponent extends BackboneElement implements IBaseBackboneElement { 1112 /** 1113 * A number to uniquely identify care team entries. 1114 */ 1115 @Child(name = "sequence", type = { 1116 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1117 @Description(shortDefinition = "Order of care team", formalDefinition = "A number to uniquely identify care team entries.") 1118 protected PositiveIntType sequence; 1119 1120 /** 1121 * Member of the team who provided the product or service. 1122 */ 1123 @Child(name = "provider", type = { Practitioner.class, PractitionerRole.class, 1124 Organization.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 1125 @Description(shortDefinition = "Practitioner or organization", formalDefinition = "Member of the team who provided the product or service.") 1126 protected Reference provider; 1127 1128 /** 1129 * The actual object that is the target of the reference (Member of the team who 1130 * provided the product or service.) 1131 */ 1132 protected Resource providerTarget; 1133 1134 /** 1135 * The party who is billing and/or responsible for the claimed products or 1136 * services. 1137 */ 1138 @Child(name = "responsible", type = { 1139 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1140 @Description(shortDefinition = "Indicator of the lead practitioner", formalDefinition = "The party who is billing and/or responsible for the claimed products or services.") 1141 protected BooleanType responsible; 1142 1143 /** 1144 * The lead, assisting or supervising practitioner and their discipline if a 1145 * multidisciplinary team. 1146 */ 1147 @Child(name = "role", type = { 1148 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1149 @Description(shortDefinition = "Function within the team", formalDefinition = "The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team.") 1150 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-careteamrole") 1151 protected CodeableConcept role; 1152 1153 /** 1154 * The qualification of the practitioner which is applicable for this service. 1155 */ 1156 @Child(name = "qualification", type = { 1157 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1158 @Description(shortDefinition = "Practitioner credential or specialization", formalDefinition = "The qualification of the practitioner which is applicable for this service.") 1159 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/provider-qualification") 1160 protected CodeableConcept qualification; 1161 1162 private static final long serialVersionUID = 1758966968L; 1163 1164 /** 1165 * Constructor 1166 */ 1167 public CareTeamComponent() { 1168 super(); 1169 } 1170 1171 /** 1172 * Constructor 1173 */ 1174 public CareTeamComponent(PositiveIntType sequence, Reference provider) { 1175 super(); 1176 this.sequence = sequence; 1177 this.provider = provider; 1178 } 1179 1180 /** 1181 * @return {@link #sequence} (A number to uniquely identify care team entries.). 1182 * This is the underlying object with id, value and extensions. The 1183 * accessor "getSequence" gives direct access to the value 1184 */ 1185 public PositiveIntType getSequenceElement() { 1186 if (this.sequence == null) 1187 if (Configuration.errorOnAutoCreate()) 1188 throw new Error("Attempt to auto-create CareTeamComponent.sequence"); 1189 else if (Configuration.doAutoCreate()) 1190 this.sequence = new PositiveIntType(); // bb 1191 return this.sequence; 1192 } 1193 1194 public boolean hasSequenceElement() { 1195 return this.sequence != null && !this.sequence.isEmpty(); 1196 } 1197 1198 public boolean hasSequence() { 1199 return this.sequence != null && !this.sequence.isEmpty(); 1200 } 1201 1202 /** 1203 * @param value {@link #sequence} (A number to uniquely identify care team 1204 * entries.). This is the underlying object with id, value and 1205 * extensions. The accessor "getSequence" gives direct access to 1206 * the value 1207 */ 1208 public CareTeamComponent setSequenceElement(PositiveIntType value) { 1209 this.sequence = value; 1210 return this; 1211 } 1212 1213 /** 1214 * @return A number to uniquely identify care team entries. 1215 */ 1216 public int getSequence() { 1217 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 1218 } 1219 1220 /** 1221 * @param value A number to uniquely identify care team entries. 1222 */ 1223 public CareTeamComponent setSequence(int value) { 1224 if (this.sequence == null) 1225 this.sequence = new PositiveIntType(); 1226 this.sequence.setValue(value); 1227 return this; 1228 } 1229 1230 /** 1231 * @return {@link #provider} (Member of the team who provided the product or 1232 * service.) 1233 */ 1234 public Reference getProvider() { 1235 if (this.provider == null) 1236 if (Configuration.errorOnAutoCreate()) 1237 throw new Error("Attempt to auto-create CareTeamComponent.provider"); 1238 else if (Configuration.doAutoCreate()) 1239 this.provider = new Reference(); // cc 1240 return this.provider; 1241 } 1242 1243 public boolean hasProvider() { 1244 return this.provider != null && !this.provider.isEmpty(); 1245 } 1246 1247 /** 1248 * @param value {@link #provider} (Member of the team who provided the product 1249 * or service.) 1250 */ 1251 public CareTeamComponent setProvider(Reference value) { 1252 this.provider = value; 1253 return this; 1254 } 1255 1256 /** 1257 * @return {@link #provider} The actual object that is the target of the 1258 * reference. The reference library doesn't populate this, but you can 1259 * use it to hold the resource if you resolve it. (Member of the team 1260 * who provided the product or service.) 1261 */ 1262 public Resource getProviderTarget() { 1263 return this.providerTarget; 1264 } 1265 1266 /** 1267 * @param value {@link #provider} The actual object that is the target of the 1268 * reference. The reference library doesn't use these, but you can 1269 * use it to hold the resource if you resolve it. (Member of the 1270 * team who provided the product or service.) 1271 */ 1272 public CareTeamComponent setProviderTarget(Resource value) { 1273 this.providerTarget = value; 1274 return this; 1275 } 1276 1277 /** 1278 * @return {@link #responsible} (The party who is billing and/or responsible for 1279 * the claimed products or services.). This is the underlying object 1280 * with id, value and extensions. The accessor "getResponsible" gives 1281 * direct access to the value 1282 */ 1283 public BooleanType getResponsibleElement() { 1284 if (this.responsible == null) 1285 if (Configuration.errorOnAutoCreate()) 1286 throw new Error("Attempt to auto-create CareTeamComponent.responsible"); 1287 else if (Configuration.doAutoCreate()) 1288 this.responsible = new BooleanType(); // bb 1289 return this.responsible; 1290 } 1291 1292 public boolean hasResponsibleElement() { 1293 return this.responsible != null && !this.responsible.isEmpty(); 1294 } 1295 1296 public boolean hasResponsible() { 1297 return this.responsible != null && !this.responsible.isEmpty(); 1298 } 1299 1300 /** 1301 * @param value {@link #responsible} (The party who is billing and/or 1302 * responsible for the claimed products or services.). This is the 1303 * underlying object with id, value and extensions. The accessor 1304 * "getResponsible" gives direct access to the value 1305 */ 1306 public CareTeamComponent setResponsibleElement(BooleanType value) { 1307 this.responsible = value; 1308 return this; 1309 } 1310 1311 /** 1312 * @return The party who is billing and/or responsible for the claimed products 1313 * or services. 1314 */ 1315 public boolean getResponsible() { 1316 return this.responsible == null || this.responsible.isEmpty() ? false : this.responsible.getValue(); 1317 } 1318 1319 /** 1320 * @param value The party who is billing and/or responsible for the claimed 1321 * products or services. 1322 */ 1323 public CareTeamComponent setResponsible(boolean value) { 1324 if (this.responsible == null) 1325 this.responsible = new BooleanType(); 1326 this.responsible.setValue(value); 1327 return this; 1328 } 1329 1330 /** 1331 * @return {@link #role} (The lead, assisting or supervising practitioner and 1332 * their discipline if a multidisciplinary team.) 1333 */ 1334 public CodeableConcept getRole() { 1335 if (this.role == null) 1336 if (Configuration.errorOnAutoCreate()) 1337 throw new Error("Attempt to auto-create CareTeamComponent.role"); 1338 else if (Configuration.doAutoCreate()) 1339 this.role = new CodeableConcept(); // cc 1340 return this.role; 1341 } 1342 1343 public boolean hasRole() { 1344 return this.role != null && !this.role.isEmpty(); 1345 } 1346 1347 /** 1348 * @param value {@link #role} (The lead, assisting or supervising practitioner 1349 * and their discipline if a multidisciplinary team.) 1350 */ 1351 public CareTeamComponent setRole(CodeableConcept value) { 1352 this.role = value; 1353 return this; 1354 } 1355 1356 /** 1357 * @return {@link #qualification} (The qualification of the practitioner which 1358 * is applicable for this service.) 1359 */ 1360 public CodeableConcept getQualification() { 1361 if (this.qualification == null) 1362 if (Configuration.errorOnAutoCreate()) 1363 throw new Error("Attempt to auto-create CareTeamComponent.qualification"); 1364 else if (Configuration.doAutoCreate()) 1365 this.qualification = new CodeableConcept(); // cc 1366 return this.qualification; 1367 } 1368 1369 public boolean hasQualification() { 1370 return this.qualification != null && !this.qualification.isEmpty(); 1371 } 1372 1373 /** 1374 * @param value {@link #qualification} (The qualification of the practitioner 1375 * which is applicable for this service.) 1376 */ 1377 public CareTeamComponent setQualification(CodeableConcept value) { 1378 this.qualification = value; 1379 return this; 1380 } 1381 1382 protected void listChildren(List<Property> children) { 1383 super.listChildren(children); 1384 children.add( 1385 new Property("sequence", "positiveInt", "A number to uniquely identify care team entries.", 0, 1, sequence)); 1386 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", 1387 "Member of the team who provided the product or service.", 0, 1, provider)); 1388 children.add(new Property("responsible", "boolean", 1389 "The party who is billing and/or responsible for the claimed products or services.", 0, 1, responsible)); 1390 children.add(new Property("role", "CodeableConcept", 1391 "The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team.", 0, 1, 1392 role)); 1393 children.add(new Property("qualification", "CodeableConcept", 1394 "The qualification of the practitioner which is applicable for this service.", 0, 1, qualification)); 1395 } 1396 1397 @Override 1398 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1399 switch (_hash) { 1400 case 1349547969: 1401 /* sequence */ return new Property("sequence", "positiveInt", 1402 "A number to uniquely identify care team entries.", 0, 1, sequence); 1403 case -987494927: 1404 /* provider */ return new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", 1405 "Member of the team who provided the product or service.", 0, 1, provider); 1406 case 1847674614: 1407 /* responsible */ return new Property("responsible", "boolean", 1408 "The party who is billing and/or responsible for the claimed products or services.", 0, 1, responsible); 1409 case 3506294: 1410 /* role */ return new Property("role", "CodeableConcept", 1411 "The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team.", 0, 1, 1412 role); 1413 case -631333393: 1414 /* qualification */ return new Property("qualification", "CodeableConcept", 1415 "The qualification of the practitioner which is applicable for this service.", 0, 1, qualification); 1416 default: 1417 return super.getNamedProperty(_hash, _name, _checkValid); 1418 } 1419 1420 } 1421 1422 @Override 1423 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1424 switch (hash) { 1425 case 1349547969: 1426 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 1427 case -987494927: 1428 /* provider */ return this.provider == null ? new Base[0] : new Base[] { this.provider }; // Reference 1429 case 1847674614: 1430 /* responsible */ return this.responsible == null ? new Base[0] : new Base[] { this.responsible }; // BooleanType 1431 case 3506294: 1432 /* role */ return this.role == null ? new Base[0] : new Base[] { this.role }; // CodeableConcept 1433 case -631333393: 1434 /* qualification */ return this.qualification == null ? new Base[0] : new Base[] { this.qualification }; // CodeableConcept 1435 default: 1436 return super.getProperty(hash, name, checkValid); 1437 } 1438 1439 } 1440 1441 @Override 1442 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1443 switch (hash) { 1444 case 1349547969: // sequence 1445 this.sequence = castToPositiveInt(value); // PositiveIntType 1446 return value; 1447 case -987494927: // provider 1448 this.provider = castToReference(value); // Reference 1449 return value; 1450 case 1847674614: // responsible 1451 this.responsible = castToBoolean(value); // BooleanType 1452 return value; 1453 case 3506294: // role 1454 this.role = castToCodeableConcept(value); // CodeableConcept 1455 return value; 1456 case -631333393: // qualification 1457 this.qualification = castToCodeableConcept(value); // CodeableConcept 1458 return value; 1459 default: 1460 return super.setProperty(hash, name, value); 1461 } 1462 1463 } 1464 1465 @Override 1466 public Base setProperty(String name, Base value) throws FHIRException { 1467 if (name.equals("sequence")) { 1468 this.sequence = castToPositiveInt(value); // PositiveIntType 1469 } else if (name.equals("provider")) { 1470 this.provider = castToReference(value); // Reference 1471 } else if (name.equals("responsible")) { 1472 this.responsible = castToBoolean(value); // BooleanType 1473 } else if (name.equals("role")) { 1474 this.role = castToCodeableConcept(value); // CodeableConcept 1475 } else if (name.equals("qualification")) { 1476 this.qualification = castToCodeableConcept(value); // CodeableConcept 1477 } else 1478 return super.setProperty(name, value); 1479 return value; 1480 } 1481 1482 @Override 1483 public void removeChild(String name, Base value) throws FHIRException { 1484 if (name.equals("sequence")) { 1485 this.sequence = null; 1486 } else if (name.equals("provider")) { 1487 this.provider = null; 1488 } else if (name.equals("responsible")) { 1489 this.responsible = null; 1490 } else if (name.equals("role")) { 1491 this.role = null; 1492 } else if (name.equals("qualification")) { 1493 this.qualification = null; 1494 } else 1495 super.removeChild(name, value); 1496 1497 } 1498 1499 @Override 1500 public Base makeProperty(int hash, String name) throws FHIRException { 1501 switch (hash) { 1502 case 1349547969: 1503 return getSequenceElement(); 1504 case -987494927: 1505 return getProvider(); 1506 case 1847674614: 1507 return getResponsibleElement(); 1508 case 3506294: 1509 return getRole(); 1510 case -631333393: 1511 return getQualification(); 1512 default: 1513 return super.makeProperty(hash, name); 1514 } 1515 1516 } 1517 1518 @Override 1519 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1520 switch (hash) { 1521 case 1349547969: 1522 /* sequence */ return new String[] { "positiveInt" }; 1523 case -987494927: 1524 /* provider */ return new String[] { "Reference" }; 1525 case 1847674614: 1526 /* responsible */ return new String[] { "boolean" }; 1527 case 3506294: 1528 /* role */ return new String[] { "CodeableConcept" }; 1529 case -631333393: 1530 /* qualification */ return new String[] { "CodeableConcept" }; 1531 default: 1532 return super.getTypesForProperty(hash, name); 1533 } 1534 1535 } 1536 1537 @Override 1538 public Base addChild(String name) throws FHIRException { 1539 if (name.equals("sequence")) { 1540 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.sequence"); 1541 } else if (name.equals("provider")) { 1542 this.provider = new Reference(); 1543 return this.provider; 1544 } else if (name.equals("responsible")) { 1545 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.responsible"); 1546 } else if (name.equals("role")) { 1547 this.role = new CodeableConcept(); 1548 return this.role; 1549 } else if (name.equals("qualification")) { 1550 this.qualification = new CodeableConcept(); 1551 return this.qualification; 1552 } else 1553 return super.addChild(name); 1554 } 1555 1556 public CareTeamComponent copy() { 1557 CareTeamComponent dst = new CareTeamComponent(); 1558 copyValues(dst); 1559 return dst; 1560 } 1561 1562 public void copyValues(CareTeamComponent dst) { 1563 super.copyValues(dst); 1564 dst.sequence = sequence == null ? null : sequence.copy(); 1565 dst.provider = provider == null ? null : provider.copy(); 1566 dst.responsible = responsible == null ? null : responsible.copy(); 1567 dst.role = role == null ? null : role.copy(); 1568 dst.qualification = qualification == null ? null : qualification.copy(); 1569 } 1570 1571 @Override 1572 public boolean equalsDeep(Base other_) { 1573 if (!super.equalsDeep(other_)) 1574 return false; 1575 if (!(other_ instanceof CareTeamComponent)) 1576 return false; 1577 CareTeamComponent o = (CareTeamComponent) other_; 1578 return compareDeep(sequence, o.sequence, true) && compareDeep(provider, o.provider, true) 1579 && compareDeep(responsible, o.responsible, true) && compareDeep(role, o.role, true) 1580 && compareDeep(qualification, o.qualification, true); 1581 } 1582 1583 @Override 1584 public boolean equalsShallow(Base other_) { 1585 if (!super.equalsShallow(other_)) 1586 return false; 1587 if (!(other_ instanceof CareTeamComponent)) 1588 return false; 1589 CareTeamComponent o = (CareTeamComponent) other_; 1590 return compareValues(sequence, o.sequence, true) && compareValues(responsible, o.responsible, true); 1591 } 1592 1593 public boolean isEmpty() { 1594 return super.isEmpty() 1595 && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, provider, responsible, role, qualification); 1596 } 1597 1598 public String fhirType() { 1599 return "ExplanationOfBenefit.careTeam"; 1600 1601 } 1602 1603 } 1604 1605 @Block() 1606 public static class SupportingInformationComponent extends BackboneElement implements IBaseBackboneElement { 1607 /** 1608 * A number to uniquely identify supporting information entries. 1609 */ 1610 @Child(name = "sequence", type = { 1611 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1612 @Description(shortDefinition = "Information instance identifier", formalDefinition = "A number to uniquely identify supporting information entries.") 1613 protected PositiveIntType sequence; 1614 1615 /** 1616 * The general class of the information supplied: information; exception; 1617 * accident, employment; onset, etc. 1618 */ 1619 @Child(name = "category", type = { 1620 CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 1621 @Description(shortDefinition = "Classification of the supplied information", formalDefinition = "The general class of the information supplied: information; exception; accident, employment; onset, etc.") 1622 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-informationcategory") 1623 protected CodeableConcept category; 1624 1625 /** 1626 * System and code pertaining to the specific information regarding special 1627 * conditions relating to the setting, treatment or patient for which care is 1628 * sought. 1629 */ 1630 @Child(name = "code", type = { 1631 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1632 @Description(shortDefinition = "Type of information", formalDefinition = "System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought.") 1633 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-exception") 1634 protected CodeableConcept code; 1635 1636 /** 1637 * The date when or period to which this information refers. 1638 */ 1639 @Child(name = "timing", type = { DateType.class, 1640 Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1641 @Description(shortDefinition = "When it occurred", formalDefinition = "The date when or period to which this information refers.") 1642 protected Type timing; 1643 1644 /** 1645 * Additional data or information such as resources, documents, images etc. 1646 * including references to the data or the actual inclusion of the data. 1647 */ 1648 @Child(name = "value", type = { BooleanType.class, StringType.class, Quantity.class, Attachment.class, 1649 Reference.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1650 @Description(shortDefinition = "Data to be provided", formalDefinition = "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.") 1651 protected Type value; 1652 1653 /** 1654 * Provides the reason in the situation where a reason code is required in 1655 * addition to the content. 1656 */ 1657 @Child(name = "reason", type = { Coding.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1658 @Description(shortDefinition = "Explanation for the information", formalDefinition = "Provides the reason in the situation where a reason code is required in addition to the content.") 1659 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/missing-tooth-reason") 1660 protected Coding reason; 1661 1662 private static final long serialVersionUID = -410136661L; 1663 1664 /** 1665 * Constructor 1666 */ 1667 public SupportingInformationComponent() { 1668 super(); 1669 } 1670 1671 /** 1672 * Constructor 1673 */ 1674 public SupportingInformationComponent(PositiveIntType sequence, CodeableConcept category) { 1675 super(); 1676 this.sequence = sequence; 1677 this.category = category; 1678 } 1679 1680 /** 1681 * @return {@link #sequence} (A number to uniquely identify supporting 1682 * information entries.). This is the underlying object with id, value 1683 * and extensions. The accessor "getSequence" gives direct access to the 1684 * value 1685 */ 1686 public PositiveIntType getSequenceElement() { 1687 if (this.sequence == null) 1688 if (Configuration.errorOnAutoCreate()) 1689 throw new Error("Attempt to auto-create SupportingInformationComponent.sequence"); 1690 else if (Configuration.doAutoCreate()) 1691 this.sequence = new PositiveIntType(); // bb 1692 return this.sequence; 1693 } 1694 1695 public boolean hasSequenceElement() { 1696 return this.sequence != null && !this.sequence.isEmpty(); 1697 } 1698 1699 public boolean hasSequence() { 1700 return this.sequence != null && !this.sequence.isEmpty(); 1701 } 1702 1703 /** 1704 * @param value {@link #sequence} (A number to uniquely identify supporting 1705 * information entries.). This is the underlying object with id, 1706 * value and extensions. The accessor "getSequence" gives direct 1707 * access to the value 1708 */ 1709 public SupportingInformationComponent setSequenceElement(PositiveIntType value) { 1710 this.sequence = value; 1711 return this; 1712 } 1713 1714 /** 1715 * @return A number to uniquely identify supporting information entries. 1716 */ 1717 public int getSequence() { 1718 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 1719 } 1720 1721 /** 1722 * @param value A number to uniquely identify supporting information entries. 1723 */ 1724 public SupportingInformationComponent setSequence(int value) { 1725 if (this.sequence == null) 1726 this.sequence = new PositiveIntType(); 1727 this.sequence.setValue(value); 1728 return this; 1729 } 1730 1731 /** 1732 * @return {@link #category} (The general class of the information supplied: 1733 * information; exception; accident, employment; onset, etc.) 1734 */ 1735 public CodeableConcept getCategory() { 1736 if (this.category == null) 1737 if (Configuration.errorOnAutoCreate()) 1738 throw new Error("Attempt to auto-create SupportingInformationComponent.category"); 1739 else if (Configuration.doAutoCreate()) 1740 this.category = new CodeableConcept(); // cc 1741 return this.category; 1742 } 1743 1744 public boolean hasCategory() { 1745 return this.category != null && !this.category.isEmpty(); 1746 } 1747 1748 /** 1749 * @param value {@link #category} (The general class of the information 1750 * supplied: information; exception; accident, employment; onset, 1751 * etc.) 1752 */ 1753 public SupportingInformationComponent setCategory(CodeableConcept value) { 1754 this.category = value; 1755 return this; 1756 } 1757 1758 /** 1759 * @return {@link #code} (System and code pertaining to the specific information 1760 * regarding special conditions relating to the setting, treatment or 1761 * patient for which care is sought.) 1762 */ 1763 public CodeableConcept getCode() { 1764 if (this.code == null) 1765 if (Configuration.errorOnAutoCreate()) 1766 throw new Error("Attempt to auto-create SupportingInformationComponent.code"); 1767 else if (Configuration.doAutoCreate()) 1768 this.code = new CodeableConcept(); // cc 1769 return this.code; 1770 } 1771 1772 public boolean hasCode() { 1773 return this.code != null && !this.code.isEmpty(); 1774 } 1775 1776 /** 1777 * @param value {@link #code} (System and code pertaining to the specific 1778 * information regarding special conditions relating to the 1779 * setting, treatment or patient for which care is sought.) 1780 */ 1781 public SupportingInformationComponent setCode(CodeableConcept value) { 1782 this.code = value; 1783 return this; 1784 } 1785 1786 /** 1787 * @return {@link #timing} (The date when or period to which this information 1788 * refers.) 1789 */ 1790 public Type getTiming() { 1791 return this.timing; 1792 } 1793 1794 /** 1795 * @return {@link #timing} (The date when or period to which this information 1796 * refers.) 1797 */ 1798 public DateType getTimingDateType() throws FHIRException { 1799 if (this.timing == null) 1800 this.timing = new DateType(); 1801 if (!(this.timing instanceof DateType)) 1802 throw new FHIRException("Type mismatch: the type DateType was expected, but " + this.timing.getClass().getName() 1803 + " was encountered"); 1804 return (DateType) this.timing; 1805 } 1806 1807 public boolean hasTimingDateType() { 1808 return this != null && this.timing instanceof DateType; 1809 } 1810 1811 /** 1812 * @return {@link #timing} (The date when or period to which this information 1813 * refers.) 1814 */ 1815 public Period getTimingPeriod() throws FHIRException { 1816 if (this.timing == null) 1817 this.timing = new Period(); 1818 if (!(this.timing instanceof Period)) 1819 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.timing.getClass().getName() 1820 + " was encountered"); 1821 return (Period) this.timing; 1822 } 1823 1824 public boolean hasTimingPeriod() { 1825 return this != null && this.timing instanceof Period; 1826 } 1827 1828 public boolean hasTiming() { 1829 return this.timing != null && !this.timing.isEmpty(); 1830 } 1831 1832 /** 1833 * @param value {@link #timing} (The date when or period to which this 1834 * information refers.) 1835 */ 1836 public SupportingInformationComponent setTiming(Type value) { 1837 if (value != null && !(value instanceof DateType || value instanceof Period)) 1838 throw new Error("Not the right type for ExplanationOfBenefit.supportingInfo.timing[x]: " + value.fhirType()); 1839 this.timing = value; 1840 return this; 1841 } 1842 1843 /** 1844 * @return {@link #value} (Additional data or information such as resources, 1845 * documents, images etc. including references to the data or the actual 1846 * inclusion of the data.) 1847 */ 1848 public Type getValue() { 1849 return this.value; 1850 } 1851 1852 /** 1853 * @return {@link #value} (Additional data or information such as resources, 1854 * documents, images etc. including references to the data or the actual 1855 * inclusion of the data.) 1856 */ 1857 public BooleanType getValueBooleanType() throws FHIRException { 1858 if (this.value == null) 1859 this.value = new BooleanType(); 1860 if (!(this.value instanceof BooleanType)) 1861 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 1862 + this.value.getClass().getName() + " was encountered"); 1863 return (BooleanType) this.value; 1864 } 1865 1866 public boolean hasValueBooleanType() { 1867 return this != null && this.value instanceof BooleanType; 1868 } 1869 1870 /** 1871 * @return {@link #value} (Additional data or information such as resources, 1872 * documents, images etc. including references to the data or the actual 1873 * inclusion of the data.) 1874 */ 1875 public StringType getValueStringType() throws FHIRException { 1876 if (this.value == null) 1877 this.value = new StringType(); 1878 if (!(this.value instanceof StringType)) 1879 throw new FHIRException("Type mismatch: the type StringType was expected, but " 1880 + this.value.getClass().getName() + " was encountered"); 1881 return (StringType) this.value; 1882 } 1883 1884 public boolean hasValueStringType() { 1885 return this != null && this.value instanceof StringType; 1886 } 1887 1888 /** 1889 * @return {@link #value} (Additional data or information such as resources, 1890 * documents, images etc. including references to the data or the actual 1891 * inclusion of the data.) 1892 */ 1893 public Quantity getValueQuantity() throws FHIRException { 1894 if (this.value == null) 1895 this.value = new Quantity(); 1896 if (!(this.value instanceof Quantity)) 1897 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName() 1898 + " was encountered"); 1899 return (Quantity) this.value; 1900 } 1901 1902 public boolean hasValueQuantity() { 1903 return this != null && this.value instanceof Quantity; 1904 } 1905 1906 /** 1907 * @return {@link #value} (Additional data or information such as resources, 1908 * documents, images etc. including references to the data or the actual 1909 * inclusion of the data.) 1910 */ 1911 public Attachment getValueAttachment() throws FHIRException { 1912 if (this.value == null) 1913 this.value = new Attachment(); 1914 if (!(this.value instanceof Attachment)) 1915 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 1916 + this.value.getClass().getName() + " was encountered"); 1917 return (Attachment) this.value; 1918 } 1919 1920 public boolean hasValueAttachment() { 1921 return this != null && this.value instanceof Attachment; 1922 } 1923 1924 /** 1925 * @return {@link #value} (Additional data or information such as resources, 1926 * documents, images etc. including references to the data or the actual 1927 * inclusion of the data.) 1928 */ 1929 public Reference getValueReference() throws FHIRException { 1930 if (this.value == null) 1931 this.value = new Reference(); 1932 if (!(this.value instanceof Reference)) 1933 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.value.getClass().getName() 1934 + " was encountered"); 1935 return (Reference) this.value; 1936 } 1937 1938 public boolean hasValueReference() { 1939 return this != null && this.value instanceof Reference; 1940 } 1941 1942 public boolean hasValue() { 1943 return this.value != null && !this.value.isEmpty(); 1944 } 1945 1946 /** 1947 * @param value {@link #value} (Additional data or information such as 1948 * resources, documents, images etc. including references to the 1949 * data or the actual inclusion of the data.) 1950 */ 1951 public SupportingInformationComponent setValue(Type value) { 1952 if (value != null && !(value instanceof BooleanType || value instanceof StringType || value instanceof Quantity 1953 || value instanceof Attachment || value instanceof Reference)) 1954 throw new Error("Not the right type for ExplanationOfBenefit.supportingInfo.value[x]: " + value.fhirType()); 1955 this.value = value; 1956 return this; 1957 } 1958 1959 /** 1960 * @return {@link #reason} (Provides the reason in the situation where a reason 1961 * code is required in addition to the content.) 1962 */ 1963 public Coding getReason() { 1964 if (this.reason == null) 1965 if (Configuration.errorOnAutoCreate()) 1966 throw new Error("Attempt to auto-create SupportingInformationComponent.reason"); 1967 else if (Configuration.doAutoCreate()) 1968 this.reason = new Coding(); // cc 1969 return this.reason; 1970 } 1971 1972 public boolean hasReason() { 1973 return this.reason != null && !this.reason.isEmpty(); 1974 } 1975 1976 /** 1977 * @param value {@link #reason} (Provides the reason in the situation where a 1978 * reason code is required in addition to the content.) 1979 */ 1980 public SupportingInformationComponent setReason(Coding value) { 1981 this.reason = value; 1982 return this; 1983 } 1984 1985 protected void listChildren(List<Property> children) { 1986 super.listChildren(children); 1987 children.add(new Property("sequence", "positiveInt", 1988 "A number to uniquely identify supporting information entries.", 0, 1, sequence)); 1989 children.add(new Property("category", "CodeableConcept", 1990 "The general class of the information supplied: information; exception; accident, employment; onset, etc.", 0, 1991 1, category)); 1992 children.add(new Property("code", "CodeableConcept", 1993 "System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought.", 1994 0, 1, code)); 1995 children.add(new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 1996 0, 1, timing)); 1997 children.add(new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)", 1998 "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 1999 0, 1, value)); 2000 children.add(new Property("reason", "Coding", 2001 "Provides the reason in the situation where a reason code is required in addition to the content.", 0, 1, 2002 reason)); 2003 } 2004 2005 @Override 2006 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2007 switch (_hash) { 2008 case 1349547969: 2009 /* sequence */ return new Property("sequence", "positiveInt", 2010 "A number to uniquely identify supporting information entries.", 0, 1, sequence); 2011 case 50511102: 2012 /* category */ return new Property("category", "CodeableConcept", 2013 "The general class of the information supplied: information; exception; accident, employment; onset, etc.", 2014 0, 1, category); 2015 case 3059181: 2016 /* code */ return new Property("code", "CodeableConcept", 2017 "System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought.", 2018 0, 1, code); 2019 case 164632566: 2020 /* timing[x] */ return new Property("timing[x]", "date|Period", 2021 "The date when or period to which this information refers.", 0, 1, timing); 2022 case -873664438: 2023 /* timing */ return new Property("timing[x]", "date|Period", 2024 "The date when or period to which this information refers.", 0, 1, timing); 2025 case 807935768: 2026 /* timingDate */ return new Property("timing[x]", "date|Period", 2027 "The date when or period to which this information refers.", 0, 1, timing); 2028 case -615615829: 2029 /* timingPeriod */ return new Property("timing[x]", "date|Period", 2030 "The date when or period to which this information refers.", 0, 1, timing); 2031 case -1410166417: 2032 /* value[x] */ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)", 2033 "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 2034 0, 1, value); 2035 case 111972721: 2036 /* value */ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)", 2037 "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 2038 0, 1, value); 2039 case 733421943: 2040 /* valueBoolean */ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)", 2041 "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 2042 0, 1, value); 2043 case -1424603934: 2044 /* valueString */ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)", 2045 "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 2046 0, 1, value); 2047 case -2029823716: 2048 /* valueQuantity */ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)", 2049 "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 2050 0, 1, value); 2051 case -475566732: 2052 /* valueAttachment */ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)", 2053 "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 2054 0, 1, value); 2055 case 1755241690: 2056 /* valueReference */ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)", 2057 "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 2058 0, 1, value); 2059 case -934964668: 2060 /* reason */ return new Property("reason", "Coding", 2061 "Provides the reason in the situation where a reason code is required in addition to the content.", 0, 1, 2062 reason); 2063 default: 2064 return super.getNamedProperty(_hash, _name, _checkValid); 2065 } 2066 2067 } 2068 2069 @Override 2070 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2071 switch (hash) { 2072 case 1349547969: 2073 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 2074 case 50511102: 2075 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 2076 case 3059181: 2077 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 2078 case -873664438: 2079 /* timing */ return this.timing == null ? new Base[0] : new Base[] { this.timing }; // Type 2080 case 111972721: 2081 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 2082 case -934964668: 2083 /* reason */ return this.reason == null ? new Base[0] : new Base[] { this.reason }; // Coding 2084 default: 2085 return super.getProperty(hash, name, checkValid); 2086 } 2087 2088 } 2089 2090 @Override 2091 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2092 switch (hash) { 2093 case 1349547969: // sequence 2094 this.sequence = castToPositiveInt(value); // PositiveIntType 2095 return value; 2096 case 50511102: // category 2097 this.category = castToCodeableConcept(value); // CodeableConcept 2098 return value; 2099 case 3059181: // code 2100 this.code = castToCodeableConcept(value); // CodeableConcept 2101 return value; 2102 case -873664438: // timing 2103 this.timing = castToType(value); // Type 2104 return value; 2105 case 111972721: // value 2106 this.value = castToType(value); // Type 2107 return value; 2108 case -934964668: // reason 2109 this.reason = castToCoding(value); // Coding 2110 return value; 2111 default: 2112 return super.setProperty(hash, name, value); 2113 } 2114 2115 } 2116 2117 @Override 2118 public Base setProperty(String name, Base value) throws FHIRException { 2119 if (name.equals("sequence")) { 2120 this.sequence = castToPositiveInt(value); // PositiveIntType 2121 } else if (name.equals("category")) { 2122 this.category = castToCodeableConcept(value); // CodeableConcept 2123 } else if (name.equals("code")) { 2124 this.code = castToCodeableConcept(value); // CodeableConcept 2125 } else if (name.equals("timing[x]")) { 2126 this.timing = castToType(value); // Type 2127 } else if (name.equals("value[x]")) { 2128 this.value = castToType(value); // Type 2129 } else if (name.equals("reason")) { 2130 this.reason = castToCoding(value); // Coding 2131 } else 2132 return super.setProperty(name, value); 2133 return value; 2134 } 2135 2136 @Override 2137 public void removeChild(String name, Base value) throws FHIRException { 2138 if (name.equals("sequence")) { 2139 this.sequence = null; 2140 } else if (name.equals("category")) { 2141 this.category = null; 2142 } else if (name.equals("code")) { 2143 this.code = null; 2144 } else if (name.equals("timing[x]")) { 2145 this.timing = null; 2146 } else if (name.equals("value[x]")) { 2147 this.value = null; 2148 } else if (name.equals("reason")) { 2149 this.reason = null; 2150 } else 2151 super.removeChild(name, value); 2152 2153 } 2154 2155 @Override 2156 public Base makeProperty(int hash, String name) throws FHIRException { 2157 switch (hash) { 2158 case 1349547969: 2159 return getSequenceElement(); 2160 case 50511102: 2161 return getCategory(); 2162 case 3059181: 2163 return getCode(); 2164 case 164632566: 2165 return getTiming(); 2166 case -873664438: 2167 return getTiming(); 2168 case -1410166417: 2169 return getValue(); 2170 case 111972721: 2171 return getValue(); 2172 case -934964668: 2173 return getReason(); 2174 default: 2175 return super.makeProperty(hash, name); 2176 } 2177 2178 } 2179 2180 @Override 2181 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2182 switch (hash) { 2183 case 1349547969: 2184 /* sequence */ return new String[] { "positiveInt" }; 2185 case 50511102: 2186 /* category */ return new String[] { "CodeableConcept" }; 2187 case 3059181: 2188 /* code */ return new String[] { "CodeableConcept" }; 2189 case -873664438: 2190 /* timing */ return new String[] { "date", "Period" }; 2191 case 111972721: 2192 /* value */ return new String[] { "boolean", "string", "Quantity", "Attachment", "Reference" }; 2193 case -934964668: 2194 /* reason */ return new String[] { "Coding" }; 2195 default: 2196 return super.getTypesForProperty(hash, name); 2197 } 2198 2199 } 2200 2201 @Override 2202 public Base addChild(String name) throws FHIRException { 2203 if (name.equals("sequence")) { 2204 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.sequence"); 2205 } else if (name.equals("category")) { 2206 this.category = new CodeableConcept(); 2207 return this.category; 2208 } else if (name.equals("code")) { 2209 this.code = new CodeableConcept(); 2210 return this.code; 2211 } else if (name.equals("timingDate")) { 2212 this.timing = new DateType(); 2213 return this.timing; 2214 } else if (name.equals("timingPeriod")) { 2215 this.timing = new Period(); 2216 return this.timing; 2217 } else if (name.equals("valueBoolean")) { 2218 this.value = new BooleanType(); 2219 return this.value; 2220 } else if (name.equals("valueString")) { 2221 this.value = new StringType(); 2222 return this.value; 2223 } else if (name.equals("valueQuantity")) { 2224 this.value = new Quantity(); 2225 return this.value; 2226 } else if (name.equals("valueAttachment")) { 2227 this.value = new Attachment(); 2228 return this.value; 2229 } else if (name.equals("valueReference")) { 2230 this.value = new Reference(); 2231 return this.value; 2232 } else if (name.equals("reason")) { 2233 this.reason = new Coding(); 2234 return this.reason; 2235 } else 2236 return super.addChild(name); 2237 } 2238 2239 public SupportingInformationComponent copy() { 2240 SupportingInformationComponent dst = new SupportingInformationComponent(); 2241 copyValues(dst); 2242 return dst; 2243 } 2244 2245 public void copyValues(SupportingInformationComponent dst) { 2246 super.copyValues(dst); 2247 dst.sequence = sequence == null ? null : sequence.copy(); 2248 dst.category = category == null ? null : category.copy(); 2249 dst.code = code == null ? null : code.copy(); 2250 dst.timing = timing == null ? null : timing.copy(); 2251 dst.value = value == null ? null : value.copy(); 2252 dst.reason = reason == null ? null : reason.copy(); 2253 } 2254 2255 @Override 2256 public boolean equalsDeep(Base other_) { 2257 if (!super.equalsDeep(other_)) 2258 return false; 2259 if (!(other_ instanceof SupportingInformationComponent)) 2260 return false; 2261 SupportingInformationComponent o = (SupportingInformationComponent) other_; 2262 return compareDeep(sequence, o.sequence, true) && compareDeep(category, o.category, true) 2263 && compareDeep(code, o.code, true) && compareDeep(timing, o.timing, true) && compareDeep(value, o.value, true) 2264 && compareDeep(reason, o.reason, true); 2265 } 2266 2267 @Override 2268 public boolean equalsShallow(Base other_) { 2269 if (!super.equalsShallow(other_)) 2270 return false; 2271 if (!(other_ instanceof SupportingInformationComponent)) 2272 return false; 2273 SupportingInformationComponent o = (SupportingInformationComponent) other_; 2274 return compareValues(sequence, o.sequence, true); 2275 } 2276 2277 public boolean isEmpty() { 2278 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, category, code, timing, value, reason); 2279 } 2280 2281 public String fhirType() { 2282 return "ExplanationOfBenefit.supportingInfo"; 2283 2284 } 2285 2286 } 2287 2288 @Block() 2289 public static class DiagnosisComponent extends BackboneElement implements IBaseBackboneElement { 2290 /** 2291 * A number to uniquely identify diagnosis entries. 2292 */ 2293 @Child(name = "sequence", type = { 2294 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2295 @Description(shortDefinition = "Diagnosis instance identifier", formalDefinition = "A number to uniquely identify diagnosis entries.") 2296 protected PositiveIntType sequence; 2297 2298 /** 2299 * The nature of illness or problem in a coded form or as a reference to an 2300 * external defined Condition. 2301 */ 2302 @Child(name = "diagnosis", type = { CodeableConcept.class, 2303 Condition.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 2304 @Description(shortDefinition = "Nature of illness or problem", formalDefinition = "The nature of illness or problem in a coded form or as a reference to an external defined Condition.") 2305 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/icd-10") 2306 protected Type diagnosis; 2307 2308 /** 2309 * When the condition was observed or the relative ranking. 2310 */ 2311 @Child(name = "type", type = { 2312 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2313 @Description(shortDefinition = "Timing or nature of the diagnosis", formalDefinition = "When the condition was observed or the relative ranking.") 2314 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-diagnosistype") 2315 protected List<CodeableConcept> type; 2316 2317 /** 2318 * Indication of whether the diagnosis was present on admission to a facility. 2319 */ 2320 @Child(name = "onAdmission", type = { 2321 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2322 @Description(shortDefinition = "Present on admission", formalDefinition = "Indication of whether the diagnosis was present on admission to a facility.") 2323 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-diagnosis-on-admission") 2324 protected CodeableConcept onAdmission; 2325 2326 /** 2327 * A package billing code or bundle code used to group products and services to 2328 * a particular health condition (such as heart attack) which is based on a 2329 * predetermined grouping code system. 2330 */ 2331 @Child(name = "packageCode", type = { 2332 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 2333 @Description(shortDefinition = "Package billing code", formalDefinition = "A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.") 2334 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-diagnosisrelatedgroup") 2335 protected CodeableConcept packageCode; 2336 2337 private static final long serialVersionUID = 2120593974L; 2338 2339 /** 2340 * Constructor 2341 */ 2342 public DiagnosisComponent() { 2343 super(); 2344 } 2345 2346 /** 2347 * Constructor 2348 */ 2349 public DiagnosisComponent(PositiveIntType sequence, Type diagnosis) { 2350 super(); 2351 this.sequence = sequence; 2352 this.diagnosis = diagnosis; 2353 } 2354 2355 /** 2356 * @return {@link #sequence} (A number to uniquely identify diagnosis entries.). 2357 * This is the underlying object with id, value and extensions. The 2358 * accessor "getSequence" gives direct access to the value 2359 */ 2360 public PositiveIntType getSequenceElement() { 2361 if (this.sequence == null) 2362 if (Configuration.errorOnAutoCreate()) 2363 throw new Error("Attempt to auto-create DiagnosisComponent.sequence"); 2364 else if (Configuration.doAutoCreate()) 2365 this.sequence = new PositiveIntType(); // bb 2366 return this.sequence; 2367 } 2368 2369 public boolean hasSequenceElement() { 2370 return this.sequence != null && !this.sequence.isEmpty(); 2371 } 2372 2373 public boolean hasSequence() { 2374 return this.sequence != null && !this.sequence.isEmpty(); 2375 } 2376 2377 /** 2378 * @param value {@link #sequence} (A number to uniquely identify diagnosis 2379 * entries.). This is the underlying object with id, value and 2380 * extensions. The accessor "getSequence" gives direct access to 2381 * the value 2382 */ 2383 public DiagnosisComponent setSequenceElement(PositiveIntType value) { 2384 this.sequence = value; 2385 return this; 2386 } 2387 2388 /** 2389 * @return A number to uniquely identify diagnosis entries. 2390 */ 2391 public int getSequence() { 2392 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 2393 } 2394 2395 /** 2396 * @param value A number to uniquely identify diagnosis entries. 2397 */ 2398 public DiagnosisComponent setSequence(int value) { 2399 if (this.sequence == null) 2400 this.sequence = new PositiveIntType(); 2401 this.sequence.setValue(value); 2402 return this; 2403 } 2404 2405 /** 2406 * @return {@link #diagnosis} (The nature of illness or problem in a coded form 2407 * or as a reference to an external defined Condition.) 2408 */ 2409 public Type getDiagnosis() { 2410 return this.diagnosis; 2411 } 2412 2413 /** 2414 * @return {@link #diagnosis} (The nature of illness or problem in a coded form 2415 * or as a reference to an external defined Condition.) 2416 */ 2417 public CodeableConcept getDiagnosisCodeableConcept() throws FHIRException { 2418 if (this.diagnosis == null) 2419 this.diagnosis = new CodeableConcept(); 2420 if (!(this.diagnosis instanceof CodeableConcept)) 2421 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2422 + this.diagnosis.getClass().getName() + " was encountered"); 2423 return (CodeableConcept) this.diagnosis; 2424 } 2425 2426 public boolean hasDiagnosisCodeableConcept() { 2427 return this != null && this.diagnosis instanceof CodeableConcept; 2428 } 2429 2430 /** 2431 * @return {@link #diagnosis} (The nature of illness or problem in a coded form 2432 * or as a reference to an external defined Condition.) 2433 */ 2434 public Reference getDiagnosisReference() throws FHIRException { 2435 if (this.diagnosis == null) 2436 this.diagnosis = new Reference(); 2437 if (!(this.diagnosis instanceof Reference)) 2438 throw new FHIRException("Type mismatch: the type Reference was expected, but " 2439 + this.diagnosis.getClass().getName() + " was encountered"); 2440 return (Reference) this.diagnosis; 2441 } 2442 2443 public boolean hasDiagnosisReference() { 2444 return this != null && this.diagnosis instanceof Reference; 2445 } 2446 2447 public boolean hasDiagnosis() { 2448 return this.diagnosis != null && !this.diagnosis.isEmpty(); 2449 } 2450 2451 /** 2452 * @param value {@link #diagnosis} (The nature of illness or problem in a coded 2453 * form or as a reference to an external defined Condition.) 2454 */ 2455 public DiagnosisComponent setDiagnosis(Type value) { 2456 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2457 throw new Error("Not the right type for ExplanationOfBenefit.diagnosis.diagnosis[x]: " + value.fhirType()); 2458 this.diagnosis = value; 2459 return this; 2460 } 2461 2462 /** 2463 * @return {@link #type} (When the condition was observed or the relative 2464 * ranking.) 2465 */ 2466 public List<CodeableConcept> getType() { 2467 if (this.type == null) 2468 this.type = new ArrayList<CodeableConcept>(); 2469 return this.type; 2470 } 2471 2472 /** 2473 * @return Returns a reference to <code>this</code> for easy method chaining 2474 */ 2475 public DiagnosisComponent setType(List<CodeableConcept> theType) { 2476 this.type = theType; 2477 return this; 2478 } 2479 2480 public boolean hasType() { 2481 if (this.type == null) 2482 return false; 2483 for (CodeableConcept item : this.type) 2484 if (!item.isEmpty()) 2485 return true; 2486 return false; 2487 } 2488 2489 public CodeableConcept addType() { // 3 2490 CodeableConcept t = new CodeableConcept(); 2491 if (this.type == null) 2492 this.type = new ArrayList<CodeableConcept>(); 2493 this.type.add(t); 2494 return t; 2495 } 2496 2497 public DiagnosisComponent addType(CodeableConcept t) { // 3 2498 if (t == null) 2499 return this; 2500 if (this.type == null) 2501 this.type = new ArrayList<CodeableConcept>(); 2502 this.type.add(t); 2503 return this; 2504 } 2505 2506 /** 2507 * @return The first repetition of repeating field {@link #type}, creating it if 2508 * it does not already exist 2509 */ 2510 public CodeableConcept getTypeFirstRep() { 2511 if (getType().isEmpty()) { 2512 addType(); 2513 } 2514 return getType().get(0); 2515 } 2516 2517 /** 2518 * @return {@link #onAdmission} (Indication of whether the diagnosis was present 2519 * on admission to a facility.) 2520 */ 2521 public CodeableConcept getOnAdmission() { 2522 if (this.onAdmission == null) 2523 if (Configuration.errorOnAutoCreate()) 2524 throw new Error("Attempt to auto-create DiagnosisComponent.onAdmission"); 2525 else if (Configuration.doAutoCreate()) 2526 this.onAdmission = new CodeableConcept(); // cc 2527 return this.onAdmission; 2528 } 2529 2530 public boolean hasOnAdmission() { 2531 return this.onAdmission != null && !this.onAdmission.isEmpty(); 2532 } 2533 2534 /** 2535 * @param value {@link #onAdmission} (Indication of whether the diagnosis was 2536 * present on admission to a facility.) 2537 */ 2538 public DiagnosisComponent setOnAdmission(CodeableConcept value) { 2539 this.onAdmission = value; 2540 return this; 2541 } 2542 2543 /** 2544 * @return {@link #packageCode} (A package billing code or bundle code used to 2545 * group products and services to a particular health condition (such as 2546 * heart attack) which is based on a predetermined grouping code 2547 * system.) 2548 */ 2549 public CodeableConcept getPackageCode() { 2550 if (this.packageCode == null) 2551 if (Configuration.errorOnAutoCreate()) 2552 throw new Error("Attempt to auto-create DiagnosisComponent.packageCode"); 2553 else if (Configuration.doAutoCreate()) 2554 this.packageCode = new CodeableConcept(); // cc 2555 return this.packageCode; 2556 } 2557 2558 public boolean hasPackageCode() { 2559 return this.packageCode != null && !this.packageCode.isEmpty(); 2560 } 2561 2562 /** 2563 * @param value {@link #packageCode} (A package billing code or bundle code used 2564 * to group products and services to a particular health condition 2565 * (such as heart attack) which is based on a predetermined 2566 * grouping code system.) 2567 */ 2568 public DiagnosisComponent setPackageCode(CodeableConcept value) { 2569 this.packageCode = value; 2570 return this; 2571 } 2572 2573 protected void listChildren(List<Property> children) { 2574 super.listChildren(children); 2575 children.add( 2576 new Property("sequence", "positiveInt", "A number to uniquely identify diagnosis entries.", 0, 1, sequence)); 2577 children.add(new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", 2578 "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, 2579 diagnosis)); 2580 children.add(new Property("type", "CodeableConcept", "When the condition was observed or the relative ranking.", 2581 0, java.lang.Integer.MAX_VALUE, type)); 2582 children.add(new Property("onAdmission", "CodeableConcept", 2583 "Indication of whether the diagnosis was present on admission to a facility.", 0, 1, onAdmission)); 2584 children.add(new Property("packageCode", "CodeableConcept", 2585 "A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.", 2586 0, 1, packageCode)); 2587 } 2588 2589 @Override 2590 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2591 switch (_hash) { 2592 case 1349547969: 2593 /* sequence */ return new Property("sequence", "positiveInt", 2594 "A number to uniquely identify diagnosis entries.", 0, 1, sequence); 2595 case -1487009809: 2596 /* diagnosis[x] */ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", 2597 "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 2598 1, diagnosis); 2599 case 1196993265: 2600 /* diagnosis */ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", 2601 "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 2602 1, diagnosis); 2603 case 277781616: 2604 /* diagnosisCodeableConcept */ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", 2605 "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 2606 1, diagnosis); 2607 case 2050454362: 2608 /* diagnosisReference */ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", 2609 "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 2610 1, diagnosis); 2611 case 3575610: 2612 /* type */ return new Property("type", "CodeableConcept", 2613 "When the condition was observed or the relative ranking.", 0, java.lang.Integer.MAX_VALUE, type); 2614 case -3386134: 2615 /* onAdmission */ return new Property("onAdmission", "CodeableConcept", 2616 "Indication of whether the diagnosis was present on admission to a facility.", 0, 1, onAdmission); 2617 case 908444499: 2618 /* packageCode */ return new Property("packageCode", "CodeableConcept", 2619 "A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.", 2620 0, 1, packageCode); 2621 default: 2622 return super.getNamedProperty(_hash, _name, _checkValid); 2623 } 2624 2625 } 2626 2627 @Override 2628 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2629 switch (hash) { 2630 case 1349547969: 2631 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 2632 case 1196993265: 2633 /* diagnosis */ return this.diagnosis == null ? new Base[0] : new Base[] { this.diagnosis }; // Type 2634 case 3575610: 2635 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 2636 case -3386134: 2637 /* onAdmission */ return this.onAdmission == null ? new Base[0] : new Base[] { this.onAdmission }; // CodeableConcept 2638 case 908444499: 2639 /* packageCode */ return this.packageCode == null ? new Base[0] : new Base[] { this.packageCode }; // CodeableConcept 2640 default: 2641 return super.getProperty(hash, name, checkValid); 2642 } 2643 2644 } 2645 2646 @Override 2647 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2648 switch (hash) { 2649 case 1349547969: // sequence 2650 this.sequence = castToPositiveInt(value); // PositiveIntType 2651 return value; 2652 case 1196993265: // diagnosis 2653 this.diagnosis = castToType(value); // Type 2654 return value; 2655 case 3575610: // type 2656 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 2657 return value; 2658 case -3386134: // onAdmission 2659 this.onAdmission = castToCodeableConcept(value); // CodeableConcept 2660 return value; 2661 case 908444499: // packageCode 2662 this.packageCode = castToCodeableConcept(value); // CodeableConcept 2663 return value; 2664 default: 2665 return super.setProperty(hash, name, value); 2666 } 2667 2668 } 2669 2670 @Override 2671 public Base setProperty(String name, Base value) throws FHIRException { 2672 if (name.equals("sequence")) { 2673 this.sequence = castToPositiveInt(value); // PositiveIntType 2674 } else if (name.equals("diagnosis[x]")) { 2675 this.diagnosis = castToType(value); // Type 2676 } else if (name.equals("type")) { 2677 this.getType().add(castToCodeableConcept(value)); 2678 } else if (name.equals("onAdmission")) { 2679 this.onAdmission = castToCodeableConcept(value); // CodeableConcept 2680 } else if (name.equals("packageCode")) { 2681 this.packageCode = castToCodeableConcept(value); // CodeableConcept 2682 } else 2683 return super.setProperty(name, value); 2684 return value; 2685 } 2686 2687 @Override 2688 public void removeChild(String name, Base value) throws FHIRException { 2689 if (name.equals("sequence")) { 2690 this.sequence = null; 2691 } else if (name.equals("diagnosis[x]")) { 2692 this.diagnosis = null; 2693 } else if (name.equals("type")) { 2694 this.getType().remove(castToCodeableConcept(value)); 2695 } else if (name.equals("onAdmission")) { 2696 this.onAdmission = null; 2697 } else if (name.equals("packageCode")) { 2698 this.packageCode = null; 2699 } else 2700 super.removeChild(name, value); 2701 2702 } 2703 2704 @Override 2705 public Base makeProperty(int hash, String name) throws FHIRException { 2706 switch (hash) { 2707 case 1349547969: 2708 return getSequenceElement(); 2709 case -1487009809: 2710 return getDiagnosis(); 2711 case 1196993265: 2712 return getDiagnosis(); 2713 case 3575610: 2714 return addType(); 2715 case -3386134: 2716 return getOnAdmission(); 2717 case 908444499: 2718 return getPackageCode(); 2719 default: 2720 return super.makeProperty(hash, name); 2721 } 2722 2723 } 2724 2725 @Override 2726 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2727 switch (hash) { 2728 case 1349547969: 2729 /* sequence */ return new String[] { "positiveInt" }; 2730 case 1196993265: 2731 /* diagnosis */ return new String[] { "CodeableConcept", "Reference" }; 2732 case 3575610: 2733 /* type */ return new String[] { "CodeableConcept" }; 2734 case -3386134: 2735 /* onAdmission */ return new String[] { "CodeableConcept" }; 2736 case 908444499: 2737 /* packageCode */ return new String[] { "CodeableConcept" }; 2738 default: 2739 return super.getTypesForProperty(hash, name); 2740 } 2741 2742 } 2743 2744 @Override 2745 public Base addChild(String name) throws FHIRException { 2746 if (name.equals("sequence")) { 2747 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.sequence"); 2748 } else if (name.equals("diagnosisCodeableConcept")) { 2749 this.diagnosis = new CodeableConcept(); 2750 return this.diagnosis; 2751 } else if (name.equals("diagnosisReference")) { 2752 this.diagnosis = new Reference(); 2753 return this.diagnosis; 2754 } else if (name.equals("type")) { 2755 return addType(); 2756 } else if (name.equals("onAdmission")) { 2757 this.onAdmission = new CodeableConcept(); 2758 return this.onAdmission; 2759 } else if (name.equals("packageCode")) { 2760 this.packageCode = new CodeableConcept(); 2761 return this.packageCode; 2762 } else 2763 return super.addChild(name); 2764 } 2765 2766 public DiagnosisComponent copy() { 2767 DiagnosisComponent dst = new DiagnosisComponent(); 2768 copyValues(dst); 2769 return dst; 2770 } 2771 2772 public void copyValues(DiagnosisComponent dst) { 2773 super.copyValues(dst); 2774 dst.sequence = sequence == null ? null : sequence.copy(); 2775 dst.diagnosis = diagnosis == null ? null : diagnosis.copy(); 2776 if (type != null) { 2777 dst.type = new ArrayList<CodeableConcept>(); 2778 for (CodeableConcept i : type) 2779 dst.type.add(i.copy()); 2780 } 2781 ; 2782 dst.onAdmission = onAdmission == null ? null : onAdmission.copy(); 2783 dst.packageCode = packageCode == null ? null : packageCode.copy(); 2784 } 2785 2786 @Override 2787 public boolean equalsDeep(Base other_) { 2788 if (!super.equalsDeep(other_)) 2789 return false; 2790 if (!(other_ instanceof DiagnosisComponent)) 2791 return false; 2792 DiagnosisComponent o = (DiagnosisComponent) other_; 2793 return compareDeep(sequence, o.sequence, true) && compareDeep(diagnosis, o.diagnosis, true) 2794 && compareDeep(type, o.type, true) && compareDeep(onAdmission, o.onAdmission, true) 2795 && compareDeep(packageCode, o.packageCode, true); 2796 } 2797 2798 @Override 2799 public boolean equalsShallow(Base other_) { 2800 if (!super.equalsShallow(other_)) 2801 return false; 2802 if (!(other_ instanceof DiagnosisComponent)) 2803 return false; 2804 DiagnosisComponent o = (DiagnosisComponent) other_; 2805 return compareValues(sequence, o.sequence, true); 2806 } 2807 2808 public boolean isEmpty() { 2809 return super.isEmpty() 2810 && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, diagnosis, type, onAdmission, packageCode); 2811 } 2812 2813 public String fhirType() { 2814 return "ExplanationOfBenefit.diagnosis"; 2815 2816 } 2817 2818 } 2819 2820 @Block() 2821 public static class ProcedureComponent extends BackboneElement implements IBaseBackboneElement { 2822 /** 2823 * A number to uniquely identify procedure entries. 2824 */ 2825 @Child(name = "sequence", type = { 2826 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2827 @Description(shortDefinition = "Procedure instance identifier", formalDefinition = "A number to uniquely identify procedure entries.") 2828 protected PositiveIntType sequence; 2829 2830 /** 2831 * When the condition was observed or the relative ranking. 2832 */ 2833 @Child(name = "type", type = { 2834 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2835 @Description(shortDefinition = "Category of Procedure", formalDefinition = "When the condition was observed or the relative ranking.") 2836 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-procedure-type") 2837 protected List<CodeableConcept> type; 2838 2839 /** 2840 * Date and optionally time the procedure was performed. 2841 */ 2842 @Child(name = "date", type = { DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2843 @Description(shortDefinition = "When the procedure was performed", formalDefinition = "Date and optionally time the procedure was performed.") 2844 protected DateTimeType date; 2845 2846 /** 2847 * The code or reference to a Procedure resource which identifies the clinical 2848 * intervention performed. 2849 */ 2850 @Child(name = "procedure", type = { CodeableConcept.class, 2851 Procedure.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 2852 @Description(shortDefinition = "Specific clinical procedure", formalDefinition = "The code or reference to a Procedure resource which identifies the clinical intervention performed.") 2853 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/icd-10-procedures") 2854 protected Type procedure; 2855 2856 /** 2857 * Unique Device Identifiers associated with this line item. 2858 */ 2859 @Child(name = "udi", type = { 2860 Device.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2861 @Description(shortDefinition = "Unique device identifier", formalDefinition = "Unique Device Identifiers associated with this line item.") 2862 protected List<Reference> udi; 2863 /** 2864 * The actual objects that are the target of the reference (Unique Device 2865 * Identifiers associated with this line item.) 2866 */ 2867 protected List<Device> udiTarget; 2868 2869 private static final long serialVersionUID = 935341852L; 2870 2871 /** 2872 * Constructor 2873 */ 2874 public ProcedureComponent() { 2875 super(); 2876 } 2877 2878 /** 2879 * Constructor 2880 */ 2881 public ProcedureComponent(PositiveIntType sequence, Type procedure) { 2882 super(); 2883 this.sequence = sequence; 2884 this.procedure = procedure; 2885 } 2886 2887 /** 2888 * @return {@link #sequence} (A number to uniquely identify procedure entries.). 2889 * This is the underlying object with id, value and extensions. The 2890 * accessor "getSequence" gives direct access to the value 2891 */ 2892 public PositiveIntType getSequenceElement() { 2893 if (this.sequence == null) 2894 if (Configuration.errorOnAutoCreate()) 2895 throw new Error("Attempt to auto-create ProcedureComponent.sequence"); 2896 else if (Configuration.doAutoCreate()) 2897 this.sequence = new PositiveIntType(); // bb 2898 return this.sequence; 2899 } 2900 2901 public boolean hasSequenceElement() { 2902 return this.sequence != null && !this.sequence.isEmpty(); 2903 } 2904 2905 public boolean hasSequence() { 2906 return this.sequence != null && !this.sequence.isEmpty(); 2907 } 2908 2909 /** 2910 * @param value {@link #sequence} (A number to uniquely identify procedure 2911 * entries.). This is the underlying object with id, value and 2912 * extensions. The accessor "getSequence" gives direct access to 2913 * the value 2914 */ 2915 public ProcedureComponent setSequenceElement(PositiveIntType value) { 2916 this.sequence = value; 2917 return this; 2918 } 2919 2920 /** 2921 * @return A number to uniquely identify procedure entries. 2922 */ 2923 public int getSequence() { 2924 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 2925 } 2926 2927 /** 2928 * @param value A number to uniquely identify procedure entries. 2929 */ 2930 public ProcedureComponent setSequence(int value) { 2931 if (this.sequence == null) 2932 this.sequence = new PositiveIntType(); 2933 this.sequence.setValue(value); 2934 return this; 2935 } 2936 2937 /** 2938 * @return {@link #type} (When the condition was observed or the relative 2939 * ranking.) 2940 */ 2941 public List<CodeableConcept> getType() { 2942 if (this.type == null) 2943 this.type = new ArrayList<CodeableConcept>(); 2944 return this.type; 2945 } 2946 2947 /** 2948 * @return Returns a reference to <code>this</code> for easy method chaining 2949 */ 2950 public ProcedureComponent setType(List<CodeableConcept> theType) { 2951 this.type = theType; 2952 return this; 2953 } 2954 2955 public boolean hasType() { 2956 if (this.type == null) 2957 return false; 2958 for (CodeableConcept item : this.type) 2959 if (!item.isEmpty()) 2960 return true; 2961 return false; 2962 } 2963 2964 public CodeableConcept addType() { // 3 2965 CodeableConcept t = new CodeableConcept(); 2966 if (this.type == null) 2967 this.type = new ArrayList<CodeableConcept>(); 2968 this.type.add(t); 2969 return t; 2970 } 2971 2972 public ProcedureComponent addType(CodeableConcept t) { // 3 2973 if (t == null) 2974 return this; 2975 if (this.type == null) 2976 this.type = new ArrayList<CodeableConcept>(); 2977 this.type.add(t); 2978 return this; 2979 } 2980 2981 /** 2982 * @return The first repetition of repeating field {@link #type}, creating it if 2983 * it does not already exist 2984 */ 2985 public CodeableConcept getTypeFirstRep() { 2986 if (getType().isEmpty()) { 2987 addType(); 2988 } 2989 return getType().get(0); 2990 } 2991 2992 /** 2993 * @return {@link #date} (Date and optionally time the procedure was 2994 * performed.). This is the underlying object with id, value and 2995 * extensions. The accessor "getDate" gives direct access to the value 2996 */ 2997 public DateTimeType getDateElement() { 2998 if (this.date == null) 2999 if (Configuration.errorOnAutoCreate()) 3000 throw new Error("Attempt to auto-create ProcedureComponent.date"); 3001 else if (Configuration.doAutoCreate()) 3002 this.date = new DateTimeType(); // bb 3003 return this.date; 3004 } 3005 3006 public boolean hasDateElement() { 3007 return this.date != null && !this.date.isEmpty(); 3008 } 3009 3010 public boolean hasDate() { 3011 return this.date != null && !this.date.isEmpty(); 3012 } 3013 3014 /** 3015 * @param value {@link #date} (Date and optionally time the procedure was 3016 * performed.). This is the underlying object with id, value and 3017 * extensions. The accessor "getDate" gives direct access to the 3018 * value 3019 */ 3020 public ProcedureComponent setDateElement(DateTimeType value) { 3021 this.date = value; 3022 return this; 3023 } 3024 3025 /** 3026 * @return Date and optionally time the procedure was performed. 3027 */ 3028 public Date getDate() { 3029 return this.date == null ? null : this.date.getValue(); 3030 } 3031 3032 /** 3033 * @param value Date and optionally time the procedure was performed. 3034 */ 3035 public ProcedureComponent setDate(Date value) { 3036 if (value == null) 3037 this.date = null; 3038 else { 3039 if (this.date == null) 3040 this.date = new DateTimeType(); 3041 this.date.setValue(value); 3042 } 3043 return this; 3044 } 3045 3046 /** 3047 * @return {@link #procedure} (The code or reference to a Procedure resource 3048 * which identifies the clinical intervention performed.) 3049 */ 3050 public Type getProcedure() { 3051 return this.procedure; 3052 } 3053 3054 /** 3055 * @return {@link #procedure} (The code or reference to a Procedure resource 3056 * which identifies the clinical intervention performed.) 3057 */ 3058 public CodeableConcept getProcedureCodeableConcept() throws FHIRException { 3059 if (this.procedure == null) 3060 this.procedure = new CodeableConcept(); 3061 if (!(this.procedure instanceof CodeableConcept)) 3062 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 3063 + this.procedure.getClass().getName() + " was encountered"); 3064 return (CodeableConcept) this.procedure; 3065 } 3066 3067 public boolean hasProcedureCodeableConcept() { 3068 return this != null && this.procedure instanceof CodeableConcept; 3069 } 3070 3071 /** 3072 * @return {@link #procedure} (The code or reference to a Procedure resource 3073 * which identifies the clinical intervention performed.) 3074 */ 3075 public Reference getProcedureReference() throws FHIRException { 3076 if (this.procedure == null) 3077 this.procedure = new Reference(); 3078 if (!(this.procedure instanceof Reference)) 3079 throw new FHIRException("Type mismatch: the type Reference was expected, but " 3080 + this.procedure.getClass().getName() + " was encountered"); 3081 return (Reference) this.procedure; 3082 } 3083 3084 public boolean hasProcedureReference() { 3085 return this != null && this.procedure instanceof Reference; 3086 } 3087 3088 public boolean hasProcedure() { 3089 return this.procedure != null && !this.procedure.isEmpty(); 3090 } 3091 3092 /** 3093 * @param value {@link #procedure} (The code or reference to a Procedure 3094 * resource which identifies the clinical intervention performed.) 3095 */ 3096 public ProcedureComponent setProcedure(Type value) { 3097 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 3098 throw new Error("Not the right type for ExplanationOfBenefit.procedure.procedure[x]: " + value.fhirType()); 3099 this.procedure = value; 3100 return this; 3101 } 3102 3103 /** 3104 * @return {@link #udi} (Unique Device Identifiers associated with this line 3105 * item.) 3106 */ 3107 public List<Reference> getUdi() { 3108 if (this.udi == null) 3109 this.udi = new ArrayList<Reference>(); 3110 return this.udi; 3111 } 3112 3113 /** 3114 * @return Returns a reference to <code>this</code> for easy method chaining 3115 */ 3116 public ProcedureComponent setUdi(List<Reference> theUdi) { 3117 this.udi = theUdi; 3118 return this; 3119 } 3120 3121 public boolean hasUdi() { 3122 if (this.udi == null) 3123 return false; 3124 for (Reference item : this.udi) 3125 if (!item.isEmpty()) 3126 return true; 3127 return false; 3128 } 3129 3130 public Reference addUdi() { // 3 3131 Reference t = new Reference(); 3132 if (this.udi == null) 3133 this.udi = new ArrayList<Reference>(); 3134 this.udi.add(t); 3135 return t; 3136 } 3137 3138 public ProcedureComponent addUdi(Reference t) { // 3 3139 if (t == null) 3140 return this; 3141 if (this.udi == null) 3142 this.udi = new ArrayList<Reference>(); 3143 this.udi.add(t); 3144 return this; 3145 } 3146 3147 /** 3148 * @return The first repetition of repeating field {@link #udi}, creating it if 3149 * it does not already exist 3150 */ 3151 public Reference getUdiFirstRep() { 3152 if (getUdi().isEmpty()) { 3153 addUdi(); 3154 } 3155 return getUdi().get(0); 3156 } 3157 3158 /** 3159 * @deprecated Use Reference#setResource(IBaseResource) instead 3160 */ 3161 @Deprecated 3162 public List<Device> getUdiTarget() { 3163 if (this.udiTarget == null) 3164 this.udiTarget = new ArrayList<Device>(); 3165 return this.udiTarget; 3166 } 3167 3168 /** 3169 * @deprecated Use Reference#setResource(IBaseResource) instead 3170 */ 3171 @Deprecated 3172 public Device addUdiTarget() { 3173 Device r = new Device(); 3174 if (this.udiTarget == null) 3175 this.udiTarget = new ArrayList<Device>(); 3176 this.udiTarget.add(r); 3177 return r; 3178 } 3179 3180 protected void listChildren(List<Property> children) { 3181 super.listChildren(children); 3182 children.add( 3183 new Property("sequence", "positiveInt", "A number to uniquely identify procedure entries.", 0, 1, sequence)); 3184 children.add(new Property("type", "CodeableConcept", "When the condition was observed or the relative ranking.", 3185 0, java.lang.Integer.MAX_VALUE, type)); 3186 children 3187 .add(new Property("date", "dateTime", "Date and optionally time the procedure was performed.", 0, 1, date)); 3188 children.add(new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", 3189 "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, 3190 procedure)); 3191 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 3192 0, java.lang.Integer.MAX_VALUE, udi)); 3193 } 3194 3195 @Override 3196 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3197 switch (_hash) { 3198 case 1349547969: 3199 /* sequence */ return new Property("sequence", "positiveInt", 3200 "A number to uniquely identify procedure entries.", 0, 1, sequence); 3201 case 3575610: 3202 /* type */ return new Property("type", "CodeableConcept", 3203 "When the condition was observed or the relative ranking.", 0, java.lang.Integer.MAX_VALUE, type); 3204 case 3076014: 3205 /* date */ return new Property("date", "dateTime", "Date and optionally time the procedure was performed.", 0, 3206 1, date); 3207 case 1640074445: 3208 /* procedure[x] */ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", 3209 "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, 3210 procedure); 3211 case -1095204141: 3212 /* procedure */ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", 3213 "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, 3214 procedure); 3215 case -1284783026: 3216 /* procedureCodeableConcept */ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", 3217 "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, 3218 procedure); 3219 case 881809848: 3220 /* procedureReference */ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", 3221 "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, 3222 procedure); 3223 case 115642: 3224 /* udi */ return new Property("udi", "Reference(Device)", 3225 "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 3226 default: 3227 return super.getNamedProperty(_hash, _name, _checkValid); 3228 } 3229 3230 } 3231 3232 @Override 3233 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3234 switch (hash) { 3235 case 1349547969: 3236 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 3237 case 3575610: 3238 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 3239 case 3076014: 3240 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 3241 case -1095204141: 3242 /* procedure */ return this.procedure == null ? new Base[0] : new Base[] { this.procedure }; // Type 3243 case 115642: 3244 /* udi */ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 3245 default: 3246 return super.getProperty(hash, name, checkValid); 3247 } 3248 3249 } 3250 3251 @Override 3252 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3253 switch (hash) { 3254 case 1349547969: // sequence 3255 this.sequence = castToPositiveInt(value); // PositiveIntType 3256 return value; 3257 case 3575610: // type 3258 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 3259 return value; 3260 case 3076014: // date 3261 this.date = castToDateTime(value); // DateTimeType 3262 return value; 3263 case -1095204141: // procedure 3264 this.procedure = castToType(value); // Type 3265 return value; 3266 case 115642: // udi 3267 this.getUdi().add(castToReference(value)); // Reference 3268 return value; 3269 default: 3270 return super.setProperty(hash, name, value); 3271 } 3272 3273 } 3274 3275 @Override 3276 public Base setProperty(String name, Base value) throws FHIRException { 3277 if (name.equals("sequence")) { 3278 this.sequence = castToPositiveInt(value); // PositiveIntType 3279 } else if (name.equals("type")) { 3280 this.getType().add(castToCodeableConcept(value)); 3281 } else if (name.equals("date")) { 3282 this.date = castToDateTime(value); // DateTimeType 3283 } else if (name.equals("procedure[x]")) { 3284 this.procedure = castToType(value); // Type 3285 } else if (name.equals("udi")) { 3286 this.getUdi().add(castToReference(value)); 3287 } else 3288 return super.setProperty(name, value); 3289 return value; 3290 } 3291 3292 @Override 3293 public void removeChild(String name, Base value) throws FHIRException { 3294 if (name.equals("sequence")) { 3295 this.sequence = null; 3296 } else if (name.equals("type")) { 3297 this.getType().remove(castToCodeableConcept(value)); 3298 } else if (name.equals("date")) { 3299 this.date = null; 3300 } else if (name.equals("procedure[x]")) { 3301 this.procedure = null; 3302 } else if (name.equals("udi")) { 3303 this.getUdi().remove(castToReference(value)); 3304 } else 3305 super.removeChild(name, value); 3306 3307 } 3308 3309 @Override 3310 public Base makeProperty(int hash, String name) throws FHIRException { 3311 switch (hash) { 3312 case 1349547969: 3313 return getSequenceElement(); 3314 case 3575610: 3315 return addType(); 3316 case 3076014: 3317 return getDateElement(); 3318 case 1640074445: 3319 return getProcedure(); 3320 case -1095204141: 3321 return getProcedure(); 3322 case 115642: 3323 return addUdi(); 3324 default: 3325 return super.makeProperty(hash, name); 3326 } 3327 3328 } 3329 3330 @Override 3331 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3332 switch (hash) { 3333 case 1349547969: 3334 /* sequence */ return new String[] { "positiveInt" }; 3335 case 3575610: 3336 /* type */ return new String[] { "CodeableConcept" }; 3337 case 3076014: 3338 /* date */ return new String[] { "dateTime" }; 3339 case -1095204141: 3340 /* procedure */ return new String[] { "CodeableConcept", "Reference" }; 3341 case 115642: 3342 /* udi */ return new String[] { "Reference" }; 3343 default: 3344 return super.getTypesForProperty(hash, name); 3345 } 3346 3347 } 3348 3349 @Override 3350 public Base addChild(String name) throws FHIRException { 3351 if (name.equals("sequence")) { 3352 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.sequence"); 3353 } else if (name.equals("type")) { 3354 return addType(); 3355 } else if (name.equals("date")) { 3356 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.date"); 3357 } else if (name.equals("procedureCodeableConcept")) { 3358 this.procedure = new CodeableConcept(); 3359 return this.procedure; 3360 } else if (name.equals("procedureReference")) { 3361 this.procedure = new Reference(); 3362 return this.procedure; 3363 } else if (name.equals("udi")) { 3364 return addUdi(); 3365 } else 3366 return super.addChild(name); 3367 } 3368 3369 public ProcedureComponent copy() { 3370 ProcedureComponent dst = new ProcedureComponent(); 3371 copyValues(dst); 3372 return dst; 3373 } 3374 3375 public void copyValues(ProcedureComponent dst) { 3376 super.copyValues(dst); 3377 dst.sequence = sequence == null ? null : sequence.copy(); 3378 if (type != null) { 3379 dst.type = new ArrayList<CodeableConcept>(); 3380 for (CodeableConcept i : type) 3381 dst.type.add(i.copy()); 3382 } 3383 ; 3384 dst.date = date == null ? null : date.copy(); 3385 dst.procedure = procedure == null ? null : procedure.copy(); 3386 if (udi != null) { 3387 dst.udi = new ArrayList<Reference>(); 3388 for (Reference i : udi) 3389 dst.udi.add(i.copy()); 3390 } 3391 ; 3392 } 3393 3394 @Override 3395 public boolean equalsDeep(Base other_) { 3396 if (!super.equalsDeep(other_)) 3397 return false; 3398 if (!(other_ instanceof ProcedureComponent)) 3399 return false; 3400 ProcedureComponent o = (ProcedureComponent) other_; 3401 return compareDeep(sequence, o.sequence, true) && compareDeep(type, o.type, true) 3402 && compareDeep(date, o.date, true) && compareDeep(procedure, o.procedure, true) 3403 && compareDeep(udi, o.udi, true); 3404 } 3405 3406 @Override 3407 public boolean equalsShallow(Base other_) { 3408 if (!super.equalsShallow(other_)) 3409 return false; 3410 if (!(other_ instanceof ProcedureComponent)) 3411 return false; 3412 ProcedureComponent o = (ProcedureComponent) other_; 3413 return compareValues(sequence, o.sequence, true) && compareValues(date, o.date, true); 3414 } 3415 3416 public boolean isEmpty() { 3417 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, type, date, procedure, udi); 3418 } 3419 3420 public String fhirType() { 3421 return "ExplanationOfBenefit.procedure"; 3422 3423 } 3424 3425 } 3426 3427 @Block() 3428 public static class InsuranceComponent extends BackboneElement implements IBaseBackboneElement { 3429 /** 3430 * A flag to indicate that this Coverage is to be used for adjudication of this 3431 * claim when set to true. 3432 */ 3433 @Child(name = "focal", type = { BooleanType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 3434 @Description(shortDefinition = "Coverage to be used for adjudication", formalDefinition = "A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.") 3435 protected BooleanType focal; 3436 3437 /** 3438 * Reference to the insurance card level information contained in the Coverage 3439 * resource. The coverage issuing insurer will use these details to locate the 3440 * patient's actual coverage within the insurer's information system. 3441 */ 3442 @Child(name = "coverage", type = { Coverage.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 3443 @Description(shortDefinition = "Insurance information", formalDefinition = "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.") 3444 protected Reference coverage; 3445 3446 /** 3447 * The actual object that is the target of the reference (Reference to the 3448 * insurance card level information contained in the Coverage resource. The 3449 * coverage issuing insurer will use these details to locate the patient's 3450 * actual coverage within the insurer's information system.) 3451 */ 3452 protected Coverage coverageTarget; 3453 3454 /** 3455 * Reference numbers previously provided by the insurer to the provider to be 3456 * quoted on subsequent claims containing services or products related to the 3457 * prior authorization. 3458 */ 3459 @Child(name = "preAuthRef", type = { 3460 StringType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3461 @Description(shortDefinition = "Prior authorization reference number", formalDefinition = "Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.") 3462 protected List<StringType> preAuthRef; 3463 3464 private static final long serialVersionUID = -606383626L; 3465 3466 /** 3467 * Constructor 3468 */ 3469 public InsuranceComponent() { 3470 super(); 3471 } 3472 3473 /** 3474 * Constructor 3475 */ 3476 public InsuranceComponent(BooleanType focal, Reference coverage) { 3477 super(); 3478 this.focal = focal; 3479 this.coverage = coverage; 3480 } 3481 3482 /** 3483 * @return {@link #focal} (A flag to indicate that this Coverage is to be used 3484 * for adjudication of this claim when set to true.). This is the 3485 * underlying object with id, value and extensions. The accessor 3486 * "getFocal" gives direct access to the value 3487 */ 3488 public BooleanType getFocalElement() { 3489 if (this.focal == null) 3490 if (Configuration.errorOnAutoCreate()) 3491 throw new Error("Attempt to auto-create InsuranceComponent.focal"); 3492 else if (Configuration.doAutoCreate()) 3493 this.focal = new BooleanType(); // bb 3494 return this.focal; 3495 } 3496 3497 public boolean hasFocalElement() { 3498 return this.focal != null && !this.focal.isEmpty(); 3499 } 3500 3501 public boolean hasFocal() { 3502 return this.focal != null && !this.focal.isEmpty(); 3503 } 3504 3505 /** 3506 * @param value {@link #focal} (A flag to indicate that this Coverage is to be 3507 * used for adjudication of this claim when set to true.). This is 3508 * the underlying object with id, value and extensions. The 3509 * accessor "getFocal" gives direct access to the value 3510 */ 3511 public InsuranceComponent setFocalElement(BooleanType value) { 3512 this.focal = value; 3513 return this; 3514 } 3515 3516 /** 3517 * @return A flag to indicate that this Coverage is to be used for adjudication 3518 * of this claim when set to true. 3519 */ 3520 public boolean getFocal() { 3521 return this.focal == null || this.focal.isEmpty() ? false : this.focal.getValue(); 3522 } 3523 3524 /** 3525 * @param value A flag to indicate that this Coverage is to be used for 3526 * adjudication of this claim when set to true. 3527 */ 3528 public InsuranceComponent setFocal(boolean value) { 3529 if (this.focal == null) 3530 this.focal = new BooleanType(); 3531 this.focal.setValue(value); 3532 return this; 3533 } 3534 3535 /** 3536 * @return {@link #coverage} (Reference to the insurance card level information 3537 * contained in the Coverage resource. The coverage issuing insurer will 3538 * use these details to locate the patient's actual coverage within the 3539 * insurer's information system.) 3540 */ 3541 public Reference getCoverage() { 3542 if (this.coverage == null) 3543 if (Configuration.errorOnAutoCreate()) 3544 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 3545 else if (Configuration.doAutoCreate()) 3546 this.coverage = new Reference(); // cc 3547 return this.coverage; 3548 } 3549 3550 public boolean hasCoverage() { 3551 return this.coverage != null && !this.coverage.isEmpty(); 3552 } 3553 3554 /** 3555 * @param value {@link #coverage} (Reference to the insurance card level 3556 * information contained in the Coverage resource. The coverage 3557 * issuing insurer will use these details to locate the patient's 3558 * actual coverage within the insurer's information system.) 3559 */ 3560 public InsuranceComponent setCoverage(Reference value) { 3561 this.coverage = value; 3562 return this; 3563 } 3564 3565 /** 3566 * @return {@link #coverage} The actual object that is the target of the 3567 * reference. The reference library doesn't populate this, but you can 3568 * use it to hold the resource if you resolve it. (Reference to the 3569 * insurance card level information contained in the Coverage resource. 3570 * The coverage issuing insurer will use these details to locate the 3571 * patient's actual coverage within the insurer's information system.) 3572 */ 3573 public Coverage getCoverageTarget() { 3574 if (this.coverageTarget == null) 3575 if (Configuration.errorOnAutoCreate()) 3576 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 3577 else if (Configuration.doAutoCreate()) 3578 this.coverageTarget = new Coverage(); // aa 3579 return this.coverageTarget; 3580 } 3581 3582 /** 3583 * @param value {@link #coverage} The actual object that is the target of the 3584 * reference. The reference library doesn't use these, but you can 3585 * use it to hold the resource if you resolve it. (Reference to the 3586 * insurance card level information contained in the Coverage 3587 * resource. The coverage issuing insurer will use these details to 3588 * locate the patient's actual coverage within the insurer's 3589 * information system.) 3590 */ 3591 public InsuranceComponent setCoverageTarget(Coverage value) { 3592 this.coverageTarget = value; 3593 return this; 3594 } 3595 3596 /** 3597 * @return {@link #preAuthRef} (Reference numbers previously provided by the 3598 * insurer to the provider to be quoted on subsequent claims containing 3599 * services or products related to the prior authorization.) 3600 */ 3601 public List<StringType> getPreAuthRef() { 3602 if (this.preAuthRef == null) 3603 this.preAuthRef = new ArrayList<StringType>(); 3604 return this.preAuthRef; 3605 } 3606 3607 /** 3608 * @return Returns a reference to <code>this</code> for easy method chaining 3609 */ 3610 public InsuranceComponent setPreAuthRef(List<StringType> thePreAuthRef) { 3611 this.preAuthRef = thePreAuthRef; 3612 return this; 3613 } 3614 3615 public boolean hasPreAuthRef() { 3616 if (this.preAuthRef == null) 3617 return false; 3618 for (StringType item : this.preAuthRef) 3619 if (!item.isEmpty()) 3620 return true; 3621 return false; 3622 } 3623 3624 /** 3625 * @return {@link #preAuthRef} (Reference numbers previously provided by the 3626 * insurer to the provider to be quoted on subsequent claims containing 3627 * services or products related to the prior authorization.) 3628 */ 3629 public StringType addPreAuthRefElement() {// 2 3630 StringType t = new StringType(); 3631 if (this.preAuthRef == null) 3632 this.preAuthRef = new ArrayList<StringType>(); 3633 this.preAuthRef.add(t); 3634 return t; 3635 } 3636 3637 /** 3638 * @param value {@link #preAuthRef} (Reference numbers previously provided by 3639 * the insurer to the provider to be quoted on subsequent claims 3640 * containing services or products related to the prior 3641 * authorization.) 3642 */ 3643 public InsuranceComponent addPreAuthRef(String value) { // 1 3644 StringType t = new StringType(); 3645 t.setValue(value); 3646 if (this.preAuthRef == null) 3647 this.preAuthRef = new ArrayList<StringType>(); 3648 this.preAuthRef.add(t); 3649 return this; 3650 } 3651 3652 /** 3653 * @param value {@link #preAuthRef} (Reference numbers previously provided by 3654 * the insurer to the provider to be quoted on subsequent claims 3655 * containing services or products related to the prior 3656 * authorization.) 3657 */ 3658 public boolean hasPreAuthRef(String value) { 3659 if (this.preAuthRef == null) 3660 return false; 3661 for (StringType v : this.preAuthRef) 3662 if (v.getValue().equals(value)) // string 3663 return true; 3664 return false; 3665 } 3666 3667 protected void listChildren(List<Property> children) { 3668 super.listChildren(children); 3669 children.add(new Property("focal", "boolean", 3670 "A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.", 0, 1, 3671 focal)); 3672 children.add(new Property("coverage", "Reference(Coverage)", 3673 "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 3674 0, 1, coverage)); 3675 children.add(new Property("preAuthRef", "string", 3676 "Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.", 3677 0, java.lang.Integer.MAX_VALUE, preAuthRef)); 3678 } 3679 3680 @Override 3681 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3682 switch (_hash) { 3683 case 97604197: 3684 /* focal */ return new Property("focal", "boolean", 3685 "A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.", 0, 3686 1, focal); 3687 case -351767064: 3688 /* coverage */ return new Property("coverage", "Reference(Coverage)", 3689 "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 3690 0, 1, coverage); 3691 case 522246568: 3692 /* preAuthRef */ return new Property("preAuthRef", "string", 3693 "Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.", 3694 0, java.lang.Integer.MAX_VALUE, preAuthRef); 3695 default: 3696 return super.getNamedProperty(_hash, _name, _checkValid); 3697 } 3698 3699 } 3700 3701 @Override 3702 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3703 switch (hash) { 3704 case 97604197: 3705 /* focal */ return this.focal == null ? new Base[0] : new Base[] { this.focal }; // BooleanType 3706 case -351767064: 3707 /* coverage */ return this.coverage == null ? new Base[0] : new Base[] { this.coverage }; // Reference 3708 case 522246568: 3709 /* preAuthRef */ return this.preAuthRef == null ? new Base[0] 3710 : this.preAuthRef.toArray(new Base[this.preAuthRef.size()]); // StringType 3711 default: 3712 return super.getProperty(hash, name, checkValid); 3713 } 3714 3715 } 3716 3717 @Override 3718 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3719 switch (hash) { 3720 case 97604197: // focal 3721 this.focal = castToBoolean(value); // BooleanType 3722 return value; 3723 case -351767064: // coverage 3724 this.coverage = castToReference(value); // Reference 3725 return value; 3726 case 522246568: // preAuthRef 3727 this.getPreAuthRef().add(castToString(value)); // StringType 3728 return value; 3729 default: 3730 return super.setProperty(hash, name, value); 3731 } 3732 3733 } 3734 3735 @Override 3736 public Base setProperty(String name, Base value) throws FHIRException { 3737 if (name.equals("focal")) { 3738 this.focal = castToBoolean(value); // BooleanType 3739 } else if (name.equals("coverage")) { 3740 this.coverage = castToReference(value); // Reference 3741 } else if (name.equals("preAuthRef")) { 3742 this.getPreAuthRef().add(castToString(value)); 3743 } else 3744 return super.setProperty(name, value); 3745 return value; 3746 } 3747 3748 @Override 3749 public void removeChild(String name, Base value) throws FHIRException { 3750 if (name.equals("focal")) { 3751 this.focal = null; 3752 } else if (name.equals("coverage")) { 3753 this.coverage = null; 3754 } else if (name.equals("preAuthRef")) { 3755 this.getPreAuthRef().remove(castToString(value)); 3756 } else 3757 super.removeChild(name, value); 3758 3759 } 3760 3761 @Override 3762 public Base makeProperty(int hash, String name) throws FHIRException { 3763 switch (hash) { 3764 case 97604197: 3765 return getFocalElement(); 3766 case -351767064: 3767 return getCoverage(); 3768 case 522246568: 3769 return addPreAuthRefElement(); 3770 default: 3771 return super.makeProperty(hash, name); 3772 } 3773 3774 } 3775 3776 @Override 3777 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3778 switch (hash) { 3779 case 97604197: 3780 /* focal */ return new String[] { "boolean" }; 3781 case -351767064: 3782 /* coverage */ return new String[] { "Reference" }; 3783 case 522246568: 3784 /* preAuthRef */ return new String[] { "string" }; 3785 default: 3786 return super.getTypesForProperty(hash, name); 3787 } 3788 3789 } 3790 3791 @Override 3792 public Base addChild(String name) throws FHIRException { 3793 if (name.equals("focal")) { 3794 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.focal"); 3795 } else if (name.equals("coverage")) { 3796 this.coverage = new Reference(); 3797 return this.coverage; 3798 } else if (name.equals("preAuthRef")) { 3799 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.preAuthRef"); 3800 } else 3801 return super.addChild(name); 3802 } 3803 3804 public InsuranceComponent copy() { 3805 InsuranceComponent dst = new InsuranceComponent(); 3806 copyValues(dst); 3807 return dst; 3808 } 3809 3810 public void copyValues(InsuranceComponent dst) { 3811 super.copyValues(dst); 3812 dst.focal = focal == null ? null : focal.copy(); 3813 dst.coverage = coverage == null ? null : coverage.copy(); 3814 if (preAuthRef != null) { 3815 dst.preAuthRef = new ArrayList<StringType>(); 3816 for (StringType i : preAuthRef) 3817 dst.preAuthRef.add(i.copy()); 3818 } 3819 ; 3820 } 3821 3822 @Override 3823 public boolean equalsDeep(Base other_) { 3824 if (!super.equalsDeep(other_)) 3825 return false; 3826 if (!(other_ instanceof InsuranceComponent)) 3827 return false; 3828 InsuranceComponent o = (InsuranceComponent) other_; 3829 return compareDeep(focal, o.focal, true) && compareDeep(coverage, o.coverage, true) 3830 && compareDeep(preAuthRef, o.preAuthRef, true); 3831 } 3832 3833 @Override 3834 public boolean equalsShallow(Base other_) { 3835 if (!super.equalsShallow(other_)) 3836 return false; 3837 if (!(other_ instanceof InsuranceComponent)) 3838 return false; 3839 InsuranceComponent o = (InsuranceComponent) other_; 3840 return compareValues(focal, o.focal, true) && compareValues(preAuthRef, o.preAuthRef, true); 3841 } 3842 3843 public boolean isEmpty() { 3844 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(focal, coverage, preAuthRef); 3845 } 3846 3847 public String fhirType() { 3848 return "ExplanationOfBenefit.insurance"; 3849 3850 } 3851 3852 } 3853 3854 @Block() 3855 public static class AccidentComponent extends BackboneElement implements IBaseBackboneElement { 3856 /** 3857 * Date of an accident event related to the products and services contained in 3858 * the claim. 3859 */ 3860 @Child(name = "date", type = { DateType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 3861 @Description(shortDefinition = "When the incident occurred", formalDefinition = "Date of an accident event related to the products and services contained in the claim.") 3862 protected DateType date; 3863 3864 /** 3865 * The type or context of the accident event for the purposes of selection of 3866 * potential insurance coverages and determination of coordination between 3867 * insurers. 3868 */ 3869 @Child(name = "type", type = { 3870 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 3871 @Description(shortDefinition = "The nature of the accident", formalDefinition = "The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers.") 3872 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ActIncidentCode") 3873 protected CodeableConcept type; 3874 3875 /** 3876 * The physical location of the accident event. 3877 */ 3878 @Child(name = "location", type = { Address.class, 3879 Location.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 3880 @Description(shortDefinition = "Where the event occurred", formalDefinition = "The physical location of the accident event.") 3881 protected Type location; 3882 3883 private static final long serialVersionUID = 622904984L; 3884 3885 /** 3886 * Constructor 3887 */ 3888 public AccidentComponent() { 3889 super(); 3890 } 3891 3892 /** 3893 * @return {@link #date} (Date of an accident event related to the products and 3894 * services contained in the claim.). This is the underlying object with 3895 * id, value and extensions. The accessor "getDate" gives direct access 3896 * to the value 3897 */ 3898 public DateType getDateElement() { 3899 if (this.date == null) 3900 if (Configuration.errorOnAutoCreate()) 3901 throw new Error("Attempt to auto-create AccidentComponent.date"); 3902 else if (Configuration.doAutoCreate()) 3903 this.date = new DateType(); // bb 3904 return this.date; 3905 } 3906 3907 public boolean hasDateElement() { 3908 return this.date != null && !this.date.isEmpty(); 3909 } 3910 3911 public boolean hasDate() { 3912 return this.date != null && !this.date.isEmpty(); 3913 } 3914 3915 /** 3916 * @param value {@link #date} (Date of an accident event related to the products 3917 * and services contained in the claim.). This is the underlying 3918 * object with id, value and extensions. The accessor "getDate" 3919 * gives direct access to the value 3920 */ 3921 public AccidentComponent setDateElement(DateType value) { 3922 this.date = value; 3923 return this; 3924 } 3925 3926 /** 3927 * @return Date of an accident event related to the products and services 3928 * contained in the claim. 3929 */ 3930 public Date getDate() { 3931 return this.date == null ? null : this.date.getValue(); 3932 } 3933 3934 /** 3935 * @param value Date of an accident event related to the products and services 3936 * contained in the claim. 3937 */ 3938 public AccidentComponent setDate(Date value) { 3939 if (value == null) 3940 this.date = null; 3941 else { 3942 if (this.date == null) 3943 this.date = new DateType(); 3944 this.date.setValue(value); 3945 } 3946 return this; 3947 } 3948 3949 /** 3950 * @return {@link #type} (The type or context of the accident event for the 3951 * purposes of selection of potential insurance coverages and 3952 * determination of coordination between insurers.) 3953 */ 3954 public CodeableConcept getType() { 3955 if (this.type == null) 3956 if (Configuration.errorOnAutoCreate()) 3957 throw new Error("Attempt to auto-create AccidentComponent.type"); 3958 else if (Configuration.doAutoCreate()) 3959 this.type = new CodeableConcept(); // cc 3960 return this.type; 3961 } 3962 3963 public boolean hasType() { 3964 return this.type != null && !this.type.isEmpty(); 3965 } 3966 3967 /** 3968 * @param value {@link #type} (The type or context of the accident event for the 3969 * purposes of selection of potential insurance coverages and 3970 * determination of coordination between insurers.) 3971 */ 3972 public AccidentComponent setType(CodeableConcept value) { 3973 this.type = value; 3974 return this; 3975 } 3976 3977 /** 3978 * @return {@link #location} (The physical location of the accident event.) 3979 */ 3980 public Type getLocation() { 3981 return this.location; 3982 } 3983 3984 /** 3985 * @return {@link #location} (The physical location of the accident event.) 3986 */ 3987 public Address getLocationAddress() throws FHIRException { 3988 if (this.location == null) 3989 this.location = new Address(); 3990 if (!(this.location instanceof Address)) 3991 throw new FHIRException("Type mismatch: the type Address was expected, but " 3992 + this.location.getClass().getName() + " was encountered"); 3993 return (Address) this.location; 3994 } 3995 3996 public boolean hasLocationAddress() { 3997 return this != null && this.location instanceof Address; 3998 } 3999 4000 /** 4001 * @return {@link #location} (The physical location of the accident event.) 4002 */ 4003 public Reference getLocationReference() throws FHIRException { 4004 if (this.location == null) 4005 this.location = new Reference(); 4006 if (!(this.location instanceof Reference)) 4007 throw new FHIRException("Type mismatch: the type Reference was expected, but " 4008 + this.location.getClass().getName() + " was encountered"); 4009 return (Reference) this.location; 4010 } 4011 4012 public boolean hasLocationReference() { 4013 return this != null && this.location instanceof Reference; 4014 } 4015 4016 public boolean hasLocation() { 4017 return this.location != null && !this.location.isEmpty(); 4018 } 4019 4020 /** 4021 * @param value {@link #location} (The physical location of the accident event.) 4022 */ 4023 public AccidentComponent setLocation(Type value) { 4024 if (value != null && !(value instanceof Address || value instanceof Reference)) 4025 throw new Error("Not the right type for ExplanationOfBenefit.accident.location[x]: " + value.fhirType()); 4026 this.location = value; 4027 return this; 4028 } 4029 4030 protected void listChildren(List<Property> children) { 4031 super.listChildren(children); 4032 children.add(new Property("date", "date", 4033 "Date of an accident event related to the products and services contained in the claim.", 0, 1, date)); 4034 children.add(new Property("type", "CodeableConcept", 4035 "The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers.", 4036 0, 1, type)); 4037 children.add(new Property("location[x]", "Address|Reference(Location)", 4038 "The physical location of the accident event.", 0, 1, location)); 4039 } 4040 4041 @Override 4042 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4043 switch (_hash) { 4044 case 3076014: 4045 /* date */ return new Property("date", "date", 4046 "Date of an accident event related to the products and services contained in the claim.", 0, 1, date); 4047 case 3575610: 4048 /* type */ return new Property("type", "CodeableConcept", 4049 "The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers.", 4050 0, 1, type); 4051 case 552316075: 4052 /* location[x] */ return new Property("location[x]", "Address|Reference(Location)", 4053 "The physical location of the accident event.", 0, 1, location); 4054 case 1901043637: 4055 /* location */ return new Property("location[x]", "Address|Reference(Location)", 4056 "The physical location of the accident event.", 0, 1, location); 4057 case -1280020865: 4058 /* locationAddress */ return new Property("location[x]", "Address|Reference(Location)", 4059 "The physical location of the accident event.", 0, 1, location); 4060 case 755866390: 4061 /* locationReference */ return new Property("location[x]", "Address|Reference(Location)", 4062 "The physical location of the accident event.", 0, 1, location); 4063 default: 4064 return super.getNamedProperty(_hash, _name, _checkValid); 4065 } 4066 4067 } 4068 4069 @Override 4070 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4071 switch (hash) { 4072 case 3076014: 4073 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateType 4074 case 3575610: 4075 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 4076 case 1901043637: 4077 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Type 4078 default: 4079 return super.getProperty(hash, name, checkValid); 4080 } 4081 4082 } 4083 4084 @Override 4085 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4086 switch (hash) { 4087 case 3076014: // date 4088 this.date = castToDate(value); // DateType 4089 return value; 4090 case 3575610: // type 4091 this.type = castToCodeableConcept(value); // CodeableConcept 4092 return value; 4093 case 1901043637: // location 4094 this.location = castToType(value); // Type 4095 return value; 4096 default: 4097 return super.setProperty(hash, name, value); 4098 } 4099 4100 } 4101 4102 @Override 4103 public Base setProperty(String name, Base value) throws FHIRException { 4104 if (name.equals("date")) { 4105 this.date = castToDate(value); // DateType 4106 } else if (name.equals("type")) { 4107 this.type = castToCodeableConcept(value); // CodeableConcept 4108 } else if (name.equals("location[x]")) { 4109 this.location = castToType(value); // Type 4110 } else 4111 return super.setProperty(name, value); 4112 return value; 4113 } 4114 4115 @Override 4116 public void removeChild(String name, Base value) throws FHIRException { 4117 if (name.equals("date")) { 4118 this.date = null; 4119 } else if (name.equals("type")) { 4120 this.type = null; 4121 } else if (name.equals("location[x]")) { 4122 this.location = null; 4123 } else 4124 super.removeChild(name, value); 4125 4126 } 4127 4128 @Override 4129 public Base makeProperty(int hash, String name) throws FHIRException { 4130 switch (hash) { 4131 case 3076014: 4132 return getDateElement(); 4133 case 3575610: 4134 return getType(); 4135 case 552316075: 4136 return getLocation(); 4137 case 1901043637: 4138 return getLocation(); 4139 default: 4140 return super.makeProperty(hash, name); 4141 } 4142 4143 } 4144 4145 @Override 4146 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4147 switch (hash) { 4148 case 3076014: 4149 /* date */ return new String[] { "date" }; 4150 case 3575610: 4151 /* type */ return new String[] { "CodeableConcept" }; 4152 case 1901043637: 4153 /* location */ return new String[] { "Address", "Reference" }; 4154 default: 4155 return super.getTypesForProperty(hash, name); 4156 } 4157 4158 } 4159 4160 @Override 4161 public Base addChild(String name) throws FHIRException { 4162 if (name.equals("date")) { 4163 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.date"); 4164 } else if (name.equals("type")) { 4165 this.type = new CodeableConcept(); 4166 return this.type; 4167 } else if (name.equals("locationAddress")) { 4168 this.location = new Address(); 4169 return this.location; 4170 } else if (name.equals("locationReference")) { 4171 this.location = new Reference(); 4172 return this.location; 4173 } else 4174 return super.addChild(name); 4175 } 4176 4177 public AccidentComponent copy() { 4178 AccidentComponent dst = new AccidentComponent(); 4179 copyValues(dst); 4180 return dst; 4181 } 4182 4183 public void copyValues(AccidentComponent dst) { 4184 super.copyValues(dst); 4185 dst.date = date == null ? null : date.copy(); 4186 dst.type = type == null ? null : type.copy(); 4187 dst.location = location == null ? null : location.copy(); 4188 } 4189 4190 @Override 4191 public boolean equalsDeep(Base other_) { 4192 if (!super.equalsDeep(other_)) 4193 return false; 4194 if (!(other_ instanceof AccidentComponent)) 4195 return false; 4196 AccidentComponent o = (AccidentComponent) other_; 4197 return compareDeep(date, o.date, true) && compareDeep(type, o.type, true) 4198 && compareDeep(location, o.location, true); 4199 } 4200 4201 @Override 4202 public boolean equalsShallow(Base other_) { 4203 if (!super.equalsShallow(other_)) 4204 return false; 4205 if (!(other_ instanceof AccidentComponent)) 4206 return false; 4207 AccidentComponent o = (AccidentComponent) other_; 4208 return compareValues(date, o.date, true); 4209 } 4210 4211 public boolean isEmpty() { 4212 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(date, type, location); 4213 } 4214 4215 public String fhirType() { 4216 return "ExplanationOfBenefit.accident"; 4217 4218 } 4219 4220 } 4221 4222 @Block() 4223 public static class ItemComponent extends BackboneElement implements IBaseBackboneElement { 4224 /** 4225 * A number to uniquely identify item entries. 4226 */ 4227 @Child(name = "sequence", type = { 4228 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 4229 @Description(shortDefinition = "Item instance identifier", formalDefinition = "A number to uniquely identify item entries.") 4230 protected PositiveIntType sequence; 4231 4232 /** 4233 * Care team members related to this service or product. 4234 */ 4235 @Child(name = "careTeamSequence", type = { 4236 PositiveIntType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4237 @Description(shortDefinition = "Applicable care team members", formalDefinition = "Care team members related to this service or product.") 4238 protected List<PositiveIntType> careTeamSequence; 4239 4240 /** 4241 * Diagnoses applicable for this service or product. 4242 */ 4243 @Child(name = "diagnosisSequence", type = { 4244 PositiveIntType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4245 @Description(shortDefinition = "Applicable diagnoses", formalDefinition = "Diagnoses applicable for this service or product.") 4246 protected List<PositiveIntType> diagnosisSequence; 4247 4248 /** 4249 * Procedures applicable for this service or product. 4250 */ 4251 @Child(name = "procedureSequence", type = { 4252 PositiveIntType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4253 @Description(shortDefinition = "Applicable procedures", formalDefinition = "Procedures applicable for this service or product.") 4254 protected List<PositiveIntType> procedureSequence; 4255 4256 /** 4257 * Exceptions, special conditions and supporting information applicable for this 4258 * service or product. 4259 */ 4260 @Child(name = "informationSequence", type = { 4261 PositiveIntType.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4262 @Description(shortDefinition = "Applicable exception and supporting information", formalDefinition = "Exceptions, special conditions and supporting information applicable for this service or product.") 4263 protected List<PositiveIntType> informationSequence; 4264 4265 /** 4266 * The type of revenue or cost center providing the product and/or service. 4267 */ 4268 @Child(name = "revenue", type = { 4269 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 4270 @Description(shortDefinition = "Revenue or cost center code", formalDefinition = "The type of revenue or cost center providing the product and/or service.") 4271 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-revenue-center") 4272 protected CodeableConcept revenue; 4273 4274 /** 4275 * Code to identify the general type of benefits under which products and 4276 * services are provided. 4277 */ 4278 @Child(name = "category", type = { 4279 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 4280 @Description(shortDefinition = "Benefit classification", formalDefinition = "Code to identify the general type of benefits under which products and services are provided.") 4281 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-benefitcategory") 4282 protected CodeableConcept category; 4283 4284 /** 4285 * When the value is a group code then this item collects a set of related claim 4286 * details, otherwise this contains the product, service, drug or other billing 4287 * code for the item. 4288 */ 4289 @Child(name = "productOrService", type = { 4290 CodeableConcept.class }, order = 8, min = 1, max = 1, modifier = false, summary = false) 4291 @Description(shortDefinition = "Billing, service, product, or drug code", formalDefinition = "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.") 4292 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-uscls") 4293 protected CodeableConcept productOrService; 4294 4295 /** 4296 * Item typification or modifiers codes to convey additional context for the 4297 * product or service. 4298 */ 4299 @Child(name = "modifier", type = { 4300 CodeableConcept.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4301 @Description(shortDefinition = "Product or service billing modifiers", formalDefinition = "Item typification or modifiers codes to convey additional context for the product or service.") 4302 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-modifiers") 4303 protected List<CodeableConcept> modifier; 4304 4305 /** 4306 * Identifies the program under which this may be recovered. 4307 */ 4308 @Child(name = "programCode", type = { 4309 CodeableConcept.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4310 @Description(shortDefinition = "Program the product or service is provided under", formalDefinition = "Identifies the program under which this may be recovered.") 4311 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-program-code") 4312 protected List<CodeableConcept> programCode; 4313 4314 /** 4315 * The date or dates when the service or product was supplied, performed or 4316 * completed. 4317 */ 4318 @Child(name = "serviced", type = { DateType.class, 4319 Period.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 4320 @Description(shortDefinition = "Date or dates of service or product delivery", formalDefinition = "The date or dates when the service or product was supplied, performed or completed.") 4321 protected Type serviced; 4322 4323 /** 4324 * Where the product or service was provided. 4325 */ 4326 @Child(name = "location", type = { CodeableConcept.class, Address.class, 4327 Location.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 4328 @Description(shortDefinition = "Place of service or where product was supplied", formalDefinition = "Where the product or service was provided.") 4329 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-place") 4330 protected Type location; 4331 4332 /** 4333 * The number of repetitions of a service or product. 4334 */ 4335 @Child(name = "quantity", type = { 4336 Quantity.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 4337 @Description(shortDefinition = "Count of products or services", formalDefinition = "The number of repetitions of a service or product.") 4338 protected Quantity quantity; 4339 4340 /** 4341 * If the item is not a group then this is the fee for the product or service, 4342 * otherwise this is the total of the fees for the details of the group. 4343 */ 4344 @Child(name = "unitPrice", type = { Money.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 4345 @Description(shortDefinition = "Fee, charge or cost per item", formalDefinition = "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.") 4346 protected Money unitPrice; 4347 4348 /** 4349 * A real number that represents a multiplier used in determining the overall 4350 * value of services delivered and/or goods received. The concept of a Factor 4351 * allows for a discount or surcharge multiplier to be applied to a monetary 4352 * amount. 4353 */ 4354 @Child(name = "factor", type = { 4355 DecimalType.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 4356 @Description(shortDefinition = "Price scaling factor", formalDefinition = "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.") 4357 protected DecimalType factor; 4358 4359 /** 4360 * The quantity times the unit price for an additional service or product or 4361 * charge. 4362 */ 4363 @Child(name = "net", type = { Money.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 4364 @Description(shortDefinition = "Total item cost", formalDefinition = "The quantity times the unit price for an additional service or product or charge.") 4365 protected Money net; 4366 4367 /** 4368 * Unique Device Identifiers associated with this line item. 4369 */ 4370 @Child(name = "udi", type = { 4371 Device.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4372 @Description(shortDefinition = "Unique device identifier", formalDefinition = "Unique Device Identifiers associated with this line item.") 4373 protected List<Reference> udi; 4374 /** 4375 * The actual objects that are the target of the reference (Unique Device 4376 * Identifiers associated with this line item.) 4377 */ 4378 protected List<Device> udiTarget; 4379 4380 /** 4381 * Physical service site on the patient (limb, tooth, etc.). 4382 */ 4383 @Child(name = "bodySite", type = { 4384 CodeableConcept.class }, order = 18, min = 0, max = 1, modifier = false, summary = false) 4385 @Description(shortDefinition = "Anatomical location", formalDefinition = "Physical service site on the patient (limb, tooth, etc.).") 4386 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/tooth") 4387 protected CodeableConcept bodySite; 4388 4389 /** 4390 * A region or surface of the bodySite, e.g. limb region or tooth surface(s). 4391 */ 4392 @Child(name = "subSite", type = { 4393 CodeableConcept.class }, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4394 @Description(shortDefinition = "Anatomical sub-location", formalDefinition = "A region or surface of the bodySite, e.g. limb region or tooth surface(s).") 4395 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/surface") 4396 protected List<CodeableConcept> subSite; 4397 4398 /** 4399 * A billed item may include goods or services provided in multiple encounters. 4400 */ 4401 @Child(name = "encounter", type = { 4402 Encounter.class }, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4403 @Description(shortDefinition = "Encounters related to this billed item", formalDefinition = "A billed item may include goods or services provided in multiple encounters.") 4404 protected List<Reference> encounter; 4405 /** 4406 * The actual objects that are the target of the reference (A billed item may 4407 * include goods or services provided in multiple encounters.) 4408 */ 4409 protected List<Encounter> encounterTarget; 4410 4411 /** 4412 * The numbers associated with notes below which apply to the adjudication of 4413 * this item. 4414 */ 4415 @Child(name = "noteNumber", type = { 4416 PositiveIntType.class }, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4417 @Description(shortDefinition = "Applicable note numbers", formalDefinition = "The numbers associated with notes below which apply to the adjudication of this item.") 4418 protected List<PositiveIntType> noteNumber; 4419 4420 /** 4421 * If this item is a group then the values here are a summary of the 4422 * adjudication of the detail items. If this item is a simple product or service 4423 * then this is the result of the adjudication of this item. 4424 */ 4425 @Child(name = "adjudication", type = {}, order = 22, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4426 @Description(shortDefinition = "Adjudication details", formalDefinition = "If this item is a group then the values here are a summary of the adjudication of the detail items. If this item is a simple product or service then this is the result of the adjudication of this item.") 4427 protected List<AdjudicationComponent> adjudication; 4428 4429 /** 4430 * Second-tier of goods and services. 4431 */ 4432 @Child(name = "detail", type = {}, order = 23, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4433 @Description(shortDefinition = "Additional items", formalDefinition = "Second-tier of goods and services.") 4434 protected List<DetailComponent> detail; 4435 4436 private static final long serialVersionUID = 67419471L; 4437 4438 /** 4439 * Constructor 4440 */ 4441 public ItemComponent() { 4442 super(); 4443 } 4444 4445 /** 4446 * Constructor 4447 */ 4448 public ItemComponent(PositiveIntType sequence, CodeableConcept productOrService) { 4449 super(); 4450 this.sequence = sequence; 4451 this.productOrService = productOrService; 4452 } 4453 4454 /** 4455 * @return {@link #sequence} (A number to uniquely identify item entries.). This 4456 * is the underlying object with id, value and extensions. The accessor 4457 * "getSequence" gives direct access to the value 4458 */ 4459 public PositiveIntType getSequenceElement() { 4460 if (this.sequence == null) 4461 if (Configuration.errorOnAutoCreate()) 4462 throw new Error("Attempt to auto-create ItemComponent.sequence"); 4463 else if (Configuration.doAutoCreate()) 4464 this.sequence = new PositiveIntType(); // bb 4465 return this.sequence; 4466 } 4467 4468 public boolean hasSequenceElement() { 4469 return this.sequence != null && !this.sequence.isEmpty(); 4470 } 4471 4472 public boolean hasSequence() { 4473 return this.sequence != null && !this.sequence.isEmpty(); 4474 } 4475 4476 /** 4477 * @param value {@link #sequence} (A number to uniquely identify item entries.). 4478 * This is the underlying object with id, value and extensions. The 4479 * accessor "getSequence" gives direct access to the value 4480 */ 4481 public ItemComponent setSequenceElement(PositiveIntType value) { 4482 this.sequence = value; 4483 return this; 4484 } 4485 4486 /** 4487 * @return A number to uniquely identify item entries. 4488 */ 4489 public int getSequence() { 4490 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 4491 } 4492 4493 /** 4494 * @param value A number to uniquely identify item entries. 4495 */ 4496 public ItemComponent setSequence(int value) { 4497 if (this.sequence == null) 4498 this.sequence = new PositiveIntType(); 4499 this.sequence.setValue(value); 4500 return this; 4501 } 4502 4503 /** 4504 * @return {@link #careTeamSequence} (Care team members related to this service 4505 * or product.) 4506 */ 4507 public List<PositiveIntType> getCareTeamSequence() { 4508 if (this.careTeamSequence == null) 4509 this.careTeamSequence = new ArrayList<PositiveIntType>(); 4510 return this.careTeamSequence; 4511 } 4512 4513 /** 4514 * @return Returns a reference to <code>this</code> for easy method chaining 4515 */ 4516 public ItemComponent setCareTeamSequence(List<PositiveIntType> theCareTeamSequence) { 4517 this.careTeamSequence = theCareTeamSequence; 4518 return this; 4519 } 4520 4521 public boolean hasCareTeamSequence() { 4522 if (this.careTeamSequence == null) 4523 return false; 4524 for (PositiveIntType item : this.careTeamSequence) 4525 if (!item.isEmpty()) 4526 return true; 4527 return false; 4528 } 4529 4530 /** 4531 * @return {@link #careTeamSequence} (Care team members related to this service 4532 * or product.) 4533 */ 4534 public PositiveIntType addCareTeamSequenceElement() {// 2 4535 PositiveIntType t = new PositiveIntType(); 4536 if (this.careTeamSequence == null) 4537 this.careTeamSequence = new ArrayList<PositiveIntType>(); 4538 this.careTeamSequence.add(t); 4539 return t; 4540 } 4541 4542 /** 4543 * @param value {@link #careTeamSequence} (Care team members related to this 4544 * service or product.) 4545 */ 4546 public ItemComponent addCareTeamSequence(int value) { // 1 4547 PositiveIntType t = new PositiveIntType(); 4548 t.setValue(value); 4549 if (this.careTeamSequence == null) 4550 this.careTeamSequence = new ArrayList<PositiveIntType>(); 4551 this.careTeamSequence.add(t); 4552 return this; 4553 } 4554 4555 /** 4556 * @param value {@link #careTeamSequence} (Care team members related to this 4557 * service or product.) 4558 */ 4559 public boolean hasCareTeamSequence(int value) { 4560 if (this.careTeamSequence == null) 4561 return false; 4562 for (PositiveIntType v : this.careTeamSequence) 4563 if (v.getValue().equals(value)) // positiveInt 4564 return true; 4565 return false; 4566 } 4567 4568 /** 4569 * @return {@link #diagnosisSequence} (Diagnoses applicable for this service or 4570 * product.) 4571 */ 4572 public List<PositiveIntType> getDiagnosisSequence() { 4573 if (this.diagnosisSequence == null) 4574 this.diagnosisSequence = new ArrayList<PositiveIntType>(); 4575 return this.diagnosisSequence; 4576 } 4577 4578 /** 4579 * @return Returns a reference to <code>this</code> for easy method chaining 4580 */ 4581 public ItemComponent setDiagnosisSequence(List<PositiveIntType> theDiagnosisSequence) { 4582 this.diagnosisSequence = theDiagnosisSequence; 4583 return this; 4584 } 4585 4586 public boolean hasDiagnosisSequence() { 4587 if (this.diagnosisSequence == null) 4588 return false; 4589 for (PositiveIntType item : this.diagnosisSequence) 4590 if (!item.isEmpty()) 4591 return true; 4592 return false; 4593 } 4594 4595 /** 4596 * @return {@link #diagnosisSequence} (Diagnoses applicable for this service or 4597 * product.) 4598 */ 4599 public PositiveIntType addDiagnosisSequenceElement() {// 2 4600 PositiveIntType t = new PositiveIntType(); 4601 if (this.diagnosisSequence == null) 4602 this.diagnosisSequence = new ArrayList<PositiveIntType>(); 4603 this.diagnosisSequence.add(t); 4604 return t; 4605 } 4606 4607 /** 4608 * @param value {@link #diagnosisSequence} (Diagnoses applicable for this 4609 * service or product.) 4610 */ 4611 public ItemComponent addDiagnosisSequence(int value) { // 1 4612 PositiveIntType t = new PositiveIntType(); 4613 t.setValue(value); 4614 if (this.diagnosisSequence == null) 4615 this.diagnosisSequence = new ArrayList<PositiveIntType>(); 4616 this.diagnosisSequence.add(t); 4617 return this; 4618 } 4619 4620 /** 4621 * @param value {@link #diagnosisSequence} (Diagnoses applicable for this 4622 * service or product.) 4623 */ 4624 public boolean hasDiagnosisSequence(int value) { 4625 if (this.diagnosisSequence == null) 4626 return false; 4627 for (PositiveIntType v : this.diagnosisSequence) 4628 if (v.getValue().equals(value)) // positiveInt 4629 return true; 4630 return false; 4631 } 4632 4633 /** 4634 * @return {@link #procedureSequence} (Procedures applicable for this service or 4635 * product.) 4636 */ 4637 public List<PositiveIntType> getProcedureSequence() { 4638 if (this.procedureSequence == null) 4639 this.procedureSequence = new ArrayList<PositiveIntType>(); 4640 return this.procedureSequence; 4641 } 4642 4643 /** 4644 * @return Returns a reference to <code>this</code> for easy method chaining 4645 */ 4646 public ItemComponent setProcedureSequence(List<PositiveIntType> theProcedureSequence) { 4647 this.procedureSequence = theProcedureSequence; 4648 return this; 4649 } 4650 4651 public boolean hasProcedureSequence() { 4652 if (this.procedureSequence == null) 4653 return false; 4654 for (PositiveIntType item : this.procedureSequence) 4655 if (!item.isEmpty()) 4656 return true; 4657 return false; 4658 } 4659 4660 /** 4661 * @return {@link #procedureSequence} (Procedures applicable for this service or 4662 * product.) 4663 */ 4664 public PositiveIntType addProcedureSequenceElement() {// 2 4665 PositiveIntType t = new PositiveIntType(); 4666 if (this.procedureSequence == null) 4667 this.procedureSequence = new ArrayList<PositiveIntType>(); 4668 this.procedureSequence.add(t); 4669 return t; 4670 } 4671 4672 /** 4673 * @param value {@link #procedureSequence} (Procedures applicable for this 4674 * service or product.) 4675 */ 4676 public ItemComponent addProcedureSequence(int value) { // 1 4677 PositiveIntType t = new PositiveIntType(); 4678 t.setValue(value); 4679 if (this.procedureSequence == null) 4680 this.procedureSequence = new ArrayList<PositiveIntType>(); 4681 this.procedureSequence.add(t); 4682 return this; 4683 } 4684 4685 /** 4686 * @param value {@link #procedureSequence} (Procedures applicable for this 4687 * service or product.) 4688 */ 4689 public boolean hasProcedureSequence(int value) { 4690 if (this.procedureSequence == null) 4691 return false; 4692 for (PositiveIntType v : this.procedureSequence) 4693 if (v.getValue().equals(value)) // positiveInt 4694 return true; 4695 return false; 4696 } 4697 4698 /** 4699 * @return {@link #informationSequence} (Exceptions, special conditions and 4700 * supporting information applicable for this service or product.) 4701 */ 4702 public List<PositiveIntType> getInformationSequence() { 4703 if (this.informationSequence == null) 4704 this.informationSequence = new ArrayList<PositiveIntType>(); 4705 return this.informationSequence; 4706 } 4707 4708 /** 4709 * @return Returns a reference to <code>this</code> for easy method chaining 4710 */ 4711 public ItemComponent setInformationSequence(List<PositiveIntType> theInformationSequence) { 4712 this.informationSequence = theInformationSequence; 4713 return this; 4714 } 4715 4716 public boolean hasInformationSequence() { 4717 if (this.informationSequence == null) 4718 return false; 4719 for (PositiveIntType item : this.informationSequence) 4720 if (!item.isEmpty()) 4721 return true; 4722 return false; 4723 } 4724 4725 /** 4726 * @return {@link #informationSequence} (Exceptions, special conditions and 4727 * supporting information applicable for this service or product.) 4728 */ 4729 public PositiveIntType addInformationSequenceElement() {// 2 4730 PositiveIntType t = new PositiveIntType(); 4731 if (this.informationSequence == null) 4732 this.informationSequence = new ArrayList<PositiveIntType>(); 4733 this.informationSequence.add(t); 4734 return t; 4735 } 4736 4737 /** 4738 * @param value {@link #informationSequence} (Exceptions, special conditions and 4739 * supporting information applicable for this service or product.) 4740 */ 4741 public ItemComponent addInformationSequence(int value) { // 1 4742 PositiveIntType t = new PositiveIntType(); 4743 t.setValue(value); 4744 if (this.informationSequence == null) 4745 this.informationSequence = new ArrayList<PositiveIntType>(); 4746 this.informationSequence.add(t); 4747 return this; 4748 } 4749 4750 /** 4751 * @param value {@link #informationSequence} (Exceptions, special conditions and 4752 * supporting information applicable for this service or product.) 4753 */ 4754 public boolean hasInformationSequence(int value) { 4755 if (this.informationSequence == null) 4756 return false; 4757 for (PositiveIntType v : this.informationSequence) 4758 if (v.getValue().equals(value)) // positiveInt 4759 return true; 4760 return false; 4761 } 4762 4763 /** 4764 * @return {@link #revenue} (The type of revenue or cost center providing the 4765 * product and/or service.) 4766 */ 4767 public CodeableConcept getRevenue() { 4768 if (this.revenue == null) 4769 if (Configuration.errorOnAutoCreate()) 4770 throw new Error("Attempt to auto-create ItemComponent.revenue"); 4771 else if (Configuration.doAutoCreate()) 4772 this.revenue = new CodeableConcept(); // cc 4773 return this.revenue; 4774 } 4775 4776 public boolean hasRevenue() { 4777 return this.revenue != null && !this.revenue.isEmpty(); 4778 } 4779 4780 /** 4781 * @param value {@link #revenue} (The type of revenue or cost center providing 4782 * the product and/or service.) 4783 */ 4784 public ItemComponent setRevenue(CodeableConcept value) { 4785 this.revenue = value; 4786 return this; 4787 } 4788 4789 /** 4790 * @return {@link #category} (Code to identify the general type of benefits 4791 * under which products and services are provided.) 4792 */ 4793 public CodeableConcept getCategory() { 4794 if (this.category == null) 4795 if (Configuration.errorOnAutoCreate()) 4796 throw new Error("Attempt to auto-create ItemComponent.category"); 4797 else if (Configuration.doAutoCreate()) 4798 this.category = new CodeableConcept(); // cc 4799 return this.category; 4800 } 4801 4802 public boolean hasCategory() { 4803 return this.category != null && !this.category.isEmpty(); 4804 } 4805 4806 /** 4807 * @param value {@link #category} (Code to identify the general type of benefits 4808 * under which products and services are provided.) 4809 */ 4810 public ItemComponent setCategory(CodeableConcept value) { 4811 this.category = value; 4812 return this; 4813 } 4814 4815 /** 4816 * @return {@link #productOrService} (When the value is a group code then this 4817 * item collects a set of related claim details, otherwise this contains 4818 * the product, service, drug or other billing code for the item.) 4819 */ 4820 public CodeableConcept getProductOrService() { 4821 if (this.productOrService == null) 4822 if (Configuration.errorOnAutoCreate()) 4823 throw new Error("Attempt to auto-create ItemComponent.productOrService"); 4824 else if (Configuration.doAutoCreate()) 4825 this.productOrService = new CodeableConcept(); // cc 4826 return this.productOrService; 4827 } 4828 4829 public boolean hasProductOrService() { 4830 return this.productOrService != null && !this.productOrService.isEmpty(); 4831 } 4832 4833 /** 4834 * @param value {@link #productOrService} (When the value is a group code then 4835 * this item collects a set of related claim details, otherwise 4836 * this contains the product, service, drug or other billing code 4837 * for the item.) 4838 */ 4839 public ItemComponent setProductOrService(CodeableConcept value) { 4840 this.productOrService = value; 4841 return this; 4842 } 4843 4844 /** 4845 * @return {@link #modifier} (Item typification or modifiers codes to convey 4846 * additional context for the product or service.) 4847 */ 4848 public List<CodeableConcept> getModifier() { 4849 if (this.modifier == null) 4850 this.modifier = new ArrayList<CodeableConcept>(); 4851 return this.modifier; 4852 } 4853 4854 /** 4855 * @return Returns a reference to <code>this</code> for easy method chaining 4856 */ 4857 public ItemComponent setModifier(List<CodeableConcept> theModifier) { 4858 this.modifier = theModifier; 4859 return this; 4860 } 4861 4862 public boolean hasModifier() { 4863 if (this.modifier == null) 4864 return false; 4865 for (CodeableConcept item : this.modifier) 4866 if (!item.isEmpty()) 4867 return true; 4868 return false; 4869 } 4870 4871 public CodeableConcept addModifier() { // 3 4872 CodeableConcept t = new CodeableConcept(); 4873 if (this.modifier == null) 4874 this.modifier = new ArrayList<CodeableConcept>(); 4875 this.modifier.add(t); 4876 return t; 4877 } 4878 4879 public ItemComponent addModifier(CodeableConcept t) { // 3 4880 if (t == null) 4881 return this; 4882 if (this.modifier == null) 4883 this.modifier = new ArrayList<CodeableConcept>(); 4884 this.modifier.add(t); 4885 return this; 4886 } 4887 4888 /** 4889 * @return The first repetition of repeating field {@link #modifier}, creating 4890 * it if it does not already exist 4891 */ 4892 public CodeableConcept getModifierFirstRep() { 4893 if (getModifier().isEmpty()) { 4894 addModifier(); 4895 } 4896 return getModifier().get(0); 4897 } 4898 4899 /** 4900 * @return {@link #programCode} (Identifies the program under which this may be 4901 * recovered.) 4902 */ 4903 public List<CodeableConcept> getProgramCode() { 4904 if (this.programCode == null) 4905 this.programCode = new ArrayList<CodeableConcept>(); 4906 return this.programCode; 4907 } 4908 4909 /** 4910 * @return Returns a reference to <code>this</code> for easy method chaining 4911 */ 4912 public ItemComponent setProgramCode(List<CodeableConcept> theProgramCode) { 4913 this.programCode = theProgramCode; 4914 return this; 4915 } 4916 4917 public boolean hasProgramCode() { 4918 if (this.programCode == null) 4919 return false; 4920 for (CodeableConcept item : this.programCode) 4921 if (!item.isEmpty()) 4922 return true; 4923 return false; 4924 } 4925 4926 public CodeableConcept addProgramCode() { // 3 4927 CodeableConcept t = new CodeableConcept(); 4928 if (this.programCode == null) 4929 this.programCode = new ArrayList<CodeableConcept>(); 4930 this.programCode.add(t); 4931 return t; 4932 } 4933 4934 public ItemComponent addProgramCode(CodeableConcept t) { // 3 4935 if (t == null) 4936 return this; 4937 if (this.programCode == null) 4938 this.programCode = new ArrayList<CodeableConcept>(); 4939 this.programCode.add(t); 4940 return this; 4941 } 4942 4943 /** 4944 * @return The first repetition of repeating field {@link #programCode}, 4945 * creating it if it does not already exist 4946 */ 4947 public CodeableConcept getProgramCodeFirstRep() { 4948 if (getProgramCode().isEmpty()) { 4949 addProgramCode(); 4950 } 4951 return getProgramCode().get(0); 4952 } 4953 4954 /** 4955 * @return {@link #serviced} (The date or dates when the service or product was 4956 * supplied, performed or completed.) 4957 */ 4958 public Type getServiced() { 4959 return this.serviced; 4960 } 4961 4962 /** 4963 * @return {@link #serviced} (The date or dates when the service or product was 4964 * supplied, performed or completed.) 4965 */ 4966 public DateType getServicedDateType() throws FHIRException { 4967 if (this.serviced == null) 4968 this.serviced = new DateType(); 4969 if (!(this.serviced instanceof DateType)) 4970 throw new FHIRException("Type mismatch: the type DateType was expected, but " 4971 + this.serviced.getClass().getName() + " was encountered"); 4972 return (DateType) this.serviced; 4973 } 4974 4975 public boolean hasServicedDateType() { 4976 return this != null && this.serviced instanceof DateType; 4977 } 4978 4979 /** 4980 * @return {@link #serviced} (The date or dates when the service or product was 4981 * supplied, performed or completed.) 4982 */ 4983 public Period getServicedPeriod() throws FHIRException { 4984 if (this.serviced == null) 4985 this.serviced = new Period(); 4986 if (!(this.serviced instanceof Period)) 4987 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.serviced.getClass().getName() 4988 + " was encountered"); 4989 return (Period) this.serviced; 4990 } 4991 4992 public boolean hasServicedPeriod() { 4993 return this != null && this.serviced instanceof Period; 4994 } 4995 4996 public boolean hasServiced() { 4997 return this.serviced != null && !this.serviced.isEmpty(); 4998 } 4999 5000 /** 5001 * @param value {@link #serviced} (The date or dates when the service or product 5002 * was supplied, performed or completed.) 5003 */ 5004 public ItemComponent setServiced(Type value) { 5005 if (value != null && !(value instanceof DateType || value instanceof Period)) 5006 throw new Error("Not the right type for ExplanationOfBenefit.item.serviced[x]: " + value.fhirType()); 5007 this.serviced = value; 5008 return this; 5009 } 5010 5011 /** 5012 * @return {@link #location} (Where the product or service was provided.) 5013 */ 5014 public Type getLocation() { 5015 return this.location; 5016 } 5017 5018 /** 5019 * @return {@link #location} (Where the product or service was provided.) 5020 */ 5021 public CodeableConcept getLocationCodeableConcept() throws FHIRException { 5022 if (this.location == null) 5023 this.location = new CodeableConcept(); 5024 if (!(this.location instanceof CodeableConcept)) 5025 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 5026 + this.location.getClass().getName() + " was encountered"); 5027 return (CodeableConcept) this.location; 5028 } 5029 5030 public boolean hasLocationCodeableConcept() { 5031 return this != null && this.location instanceof CodeableConcept; 5032 } 5033 5034 /** 5035 * @return {@link #location} (Where the product or service was provided.) 5036 */ 5037 public Address getLocationAddress() throws FHIRException { 5038 if (this.location == null) 5039 this.location = new Address(); 5040 if (!(this.location instanceof Address)) 5041 throw new FHIRException("Type mismatch: the type Address was expected, but " 5042 + this.location.getClass().getName() + " was encountered"); 5043 return (Address) this.location; 5044 } 5045 5046 public boolean hasLocationAddress() { 5047 return this != null && this.location instanceof Address; 5048 } 5049 5050 /** 5051 * @return {@link #location} (Where the product or service was provided.) 5052 */ 5053 public Reference getLocationReference() throws FHIRException { 5054 if (this.location == null) 5055 this.location = new Reference(); 5056 if (!(this.location instanceof Reference)) 5057 throw new FHIRException("Type mismatch: the type Reference was expected, but " 5058 + this.location.getClass().getName() + " was encountered"); 5059 return (Reference) this.location; 5060 } 5061 5062 public boolean hasLocationReference() { 5063 return this != null && this.location instanceof Reference; 5064 } 5065 5066 public boolean hasLocation() { 5067 return this.location != null && !this.location.isEmpty(); 5068 } 5069 5070 /** 5071 * @param value {@link #location} (Where the product or service was provided.) 5072 */ 5073 public ItemComponent setLocation(Type value) { 5074 if (value != null 5075 && !(value instanceof CodeableConcept || value instanceof Address || value instanceof Reference)) 5076 throw new Error("Not the right type for ExplanationOfBenefit.item.location[x]: " + value.fhirType()); 5077 this.location = value; 5078 return this; 5079 } 5080 5081 /** 5082 * @return {@link #quantity} (The number of repetitions of a service or 5083 * product.) 5084 */ 5085 public Quantity getQuantity() { 5086 if (this.quantity == null) 5087 if (Configuration.errorOnAutoCreate()) 5088 throw new Error("Attempt to auto-create ItemComponent.quantity"); 5089 else if (Configuration.doAutoCreate()) 5090 this.quantity = new Quantity(); // cc 5091 return this.quantity; 5092 } 5093 5094 public boolean hasQuantity() { 5095 return this.quantity != null && !this.quantity.isEmpty(); 5096 } 5097 5098 /** 5099 * @param value {@link #quantity} (The number of repetitions of a service or 5100 * product.) 5101 */ 5102 public ItemComponent setQuantity(Quantity value) { 5103 this.quantity = value; 5104 return this; 5105 } 5106 5107 /** 5108 * @return {@link #unitPrice} (If the item is not a group then this is the fee 5109 * for the product or service, otherwise this is the total of the fees 5110 * for the details of the group.) 5111 */ 5112 public Money getUnitPrice() { 5113 if (this.unitPrice == null) 5114 if (Configuration.errorOnAutoCreate()) 5115 throw new Error("Attempt to auto-create ItemComponent.unitPrice"); 5116 else if (Configuration.doAutoCreate()) 5117 this.unitPrice = new Money(); // cc 5118 return this.unitPrice; 5119 } 5120 5121 public boolean hasUnitPrice() { 5122 return this.unitPrice != null && !this.unitPrice.isEmpty(); 5123 } 5124 5125 /** 5126 * @param value {@link #unitPrice} (If the item is not a group then this is the 5127 * fee for the product or service, otherwise this is the total of 5128 * the fees for the details of the group.) 5129 */ 5130 public ItemComponent setUnitPrice(Money value) { 5131 this.unitPrice = value; 5132 return this; 5133 } 5134 5135 /** 5136 * @return {@link #factor} (A real number that represents a multiplier used in 5137 * determining the overall value of services delivered and/or goods 5138 * received. The concept of a Factor allows for a discount or surcharge 5139 * multiplier to be applied to a monetary amount.). This is the 5140 * underlying object with id, value and extensions. The accessor 5141 * "getFactor" gives direct access to the value 5142 */ 5143 public DecimalType getFactorElement() { 5144 if (this.factor == null) 5145 if (Configuration.errorOnAutoCreate()) 5146 throw new Error("Attempt to auto-create ItemComponent.factor"); 5147 else if (Configuration.doAutoCreate()) 5148 this.factor = new DecimalType(); // bb 5149 return this.factor; 5150 } 5151 5152 public boolean hasFactorElement() { 5153 return this.factor != null && !this.factor.isEmpty(); 5154 } 5155 5156 public boolean hasFactor() { 5157 return this.factor != null && !this.factor.isEmpty(); 5158 } 5159 5160 /** 5161 * @param value {@link #factor} (A real number that represents a multiplier used 5162 * in determining the overall value of services delivered and/or 5163 * goods received. The concept of a Factor allows for a discount or 5164 * surcharge multiplier to be applied to a monetary amount.). This 5165 * is the underlying object with id, value and extensions. The 5166 * accessor "getFactor" gives direct access to the value 5167 */ 5168 public ItemComponent setFactorElement(DecimalType value) { 5169 this.factor = value; 5170 return this; 5171 } 5172 5173 /** 5174 * @return A real number that represents a multiplier used in determining the 5175 * overall value of services delivered and/or goods received. The 5176 * concept of a Factor allows for a discount or surcharge multiplier to 5177 * be applied to a monetary amount. 5178 */ 5179 public BigDecimal getFactor() { 5180 return this.factor == null ? null : this.factor.getValue(); 5181 } 5182 5183 /** 5184 * @param value A real number that represents a multiplier used in determining 5185 * the overall value of services delivered and/or goods received. 5186 * The concept of a Factor allows for a discount or surcharge 5187 * multiplier to be applied to a monetary amount. 5188 */ 5189 public ItemComponent setFactor(BigDecimal value) { 5190 if (value == null) 5191 this.factor = null; 5192 else { 5193 if (this.factor == null) 5194 this.factor = new DecimalType(); 5195 this.factor.setValue(value); 5196 } 5197 return this; 5198 } 5199 5200 /** 5201 * @param value A real number that represents a multiplier used in determining 5202 * the overall value of services delivered and/or goods received. 5203 * The concept of a Factor allows for a discount or surcharge 5204 * multiplier to be applied to a monetary amount. 5205 */ 5206 public ItemComponent setFactor(long value) { 5207 this.factor = new DecimalType(); 5208 this.factor.setValue(value); 5209 return this; 5210 } 5211 5212 /** 5213 * @param value A real number that represents a multiplier used in determining 5214 * the overall value of services delivered and/or goods received. 5215 * The concept of a Factor allows for a discount or surcharge 5216 * multiplier to be applied to a monetary amount. 5217 */ 5218 public ItemComponent setFactor(double value) { 5219 this.factor = new DecimalType(); 5220 this.factor.setValue(value); 5221 return this; 5222 } 5223 5224 /** 5225 * @return {@link #net} (The quantity times the unit price for an additional 5226 * service or product or charge.) 5227 */ 5228 public Money getNet() { 5229 if (this.net == null) 5230 if (Configuration.errorOnAutoCreate()) 5231 throw new Error("Attempt to auto-create ItemComponent.net"); 5232 else if (Configuration.doAutoCreate()) 5233 this.net = new Money(); // cc 5234 return this.net; 5235 } 5236 5237 public boolean hasNet() { 5238 return this.net != null && !this.net.isEmpty(); 5239 } 5240 5241 /** 5242 * @param value {@link #net} (The quantity times the unit price for an 5243 * additional service or product or charge.) 5244 */ 5245 public ItemComponent setNet(Money value) { 5246 this.net = value; 5247 return this; 5248 } 5249 5250 /** 5251 * @return {@link #udi} (Unique Device Identifiers associated with this line 5252 * item.) 5253 */ 5254 public List<Reference> getUdi() { 5255 if (this.udi == null) 5256 this.udi = new ArrayList<Reference>(); 5257 return this.udi; 5258 } 5259 5260 /** 5261 * @return Returns a reference to <code>this</code> for easy method chaining 5262 */ 5263 public ItemComponent setUdi(List<Reference> theUdi) { 5264 this.udi = theUdi; 5265 return this; 5266 } 5267 5268 public boolean hasUdi() { 5269 if (this.udi == null) 5270 return false; 5271 for (Reference item : this.udi) 5272 if (!item.isEmpty()) 5273 return true; 5274 return false; 5275 } 5276 5277 public Reference addUdi() { // 3 5278 Reference t = new Reference(); 5279 if (this.udi == null) 5280 this.udi = new ArrayList<Reference>(); 5281 this.udi.add(t); 5282 return t; 5283 } 5284 5285 public ItemComponent addUdi(Reference t) { // 3 5286 if (t == null) 5287 return this; 5288 if (this.udi == null) 5289 this.udi = new ArrayList<Reference>(); 5290 this.udi.add(t); 5291 return this; 5292 } 5293 5294 /** 5295 * @return The first repetition of repeating field {@link #udi}, creating it if 5296 * it does not already exist 5297 */ 5298 public Reference getUdiFirstRep() { 5299 if (getUdi().isEmpty()) { 5300 addUdi(); 5301 } 5302 return getUdi().get(0); 5303 } 5304 5305 /** 5306 * @deprecated Use Reference#setResource(IBaseResource) instead 5307 */ 5308 @Deprecated 5309 public List<Device> getUdiTarget() { 5310 if (this.udiTarget == null) 5311 this.udiTarget = new ArrayList<Device>(); 5312 return this.udiTarget; 5313 } 5314 5315 /** 5316 * @deprecated Use Reference#setResource(IBaseResource) instead 5317 */ 5318 @Deprecated 5319 public Device addUdiTarget() { 5320 Device r = new Device(); 5321 if (this.udiTarget == null) 5322 this.udiTarget = new ArrayList<Device>(); 5323 this.udiTarget.add(r); 5324 return r; 5325 } 5326 5327 /** 5328 * @return {@link #bodySite} (Physical service site on the patient (limb, tooth, 5329 * etc.).) 5330 */ 5331 public CodeableConcept getBodySite() { 5332 if (this.bodySite == null) 5333 if (Configuration.errorOnAutoCreate()) 5334 throw new Error("Attempt to auto-create ItemComponent.bodySite"); 5335 else if (Configuration.doAutoCreate()) 5336 this.bodySite = new CodeableConcept(); // cc 5337 return this.bodySite; 5338 } 5339 5340 public boolean hasBodySite() { 5341 return this.bodySite != null && !this.bodySite.isEmpty(); 5342 } 5343 5344 /** 5345 * @param value {@link #bodySite} (Physical service site on the patient (limb, 5346 * tooth, etc.).) 5347 */ 5348 public ItemComponent setBodySite(CodeableConcept value) { 5349 this.bodySite = value; 5350 return this; 5351 } 5352 5353 /** 5354 * @return {@link #subSite} (A region or surface of the bodySite, e.g. limb 5355 * region or tooth surface(s).) 5356 */ 5357 public List<CodeableConcept> getSubSite() { 5358 if (this.subSite == null) 5359 this.subSite = new ArrayList<CodeableConcept>(); 5360 return this.subSite; 5361 } 5362 5363 /** 5364 * @return Returns a reference to <code>this</code> for easy method chaining 5365 */ 5366 public ItemComponent setSubSite(List<CodeableConcept> theSubSite) { 5367 this.subSite = theSubSite; 5368 return this; 5369 } 5370 5371 public boolean hasSubSite() { 5372 if (this.subSite == null) 5373 return false; 5374 for (CodeableConcept item : this.subSite) 5375 if (!item.isEmpty()) 5376 return true; 5377 return false; 5378 } 5379 5380 public CodeableConcept addSubSite() { // 3 5381 CodeableConcept t = new CodeableConcept(); 5382 if (this.subSite == null) 5383 this.subSite = new ArrayList<CodeableConcept>(); 5384 this.subSite.add(t); 5385 return t; 5386 } 5387 5388 public ItemComponent addSubSite(CodeableConcept t) { // 3 5389 if (t == null) 5390 return this; 5391 if (this.subSite == null) 5392 this.subSite = new ArrayList<CodeableConcept>(); 5393 this.subSite.add(t); 5394 return this; 5395 } 5396 5397 /** 5398 * @return The first repetition of repeating field {@link #subSite}, creating it 5399 * if it does not already exist 5400 */ 5401 public CodeableConcept getSubSiteFirstRep() { 5402 if (getSubSite().isEmpty()) { 5403 addSubSite(); 5404 } 5405 return getSubSite().get(0); 5406 } 5407 5408 /** 5409 * @return {@link #encounter} (A billed item may include goods or services 5410 * provided in multiple encounters.) 5411 */ 5412 public List<Reference> getEncounter() { 5413 if (this.encounter == null) 5414 this.encounter = new ArrayList<Reference>(); 5415 return this.encounter; 5416 } 5417 5418 /** 5419 * @return Returns a reference to <code>this</code> for easy method chaining 5420 */ 5421 public ItemComponent setEncounter(List<Reference> theEncounter) { 5422 this.encounter = theEncounter; 5423 return this; 5424 } 5425 5426 public boolean hasEncounter() { 5427 if (this.encounter == null) 5428 return false; 5429 for (Reference item : this.encounter) 5430 if (!item.isEmpty()) 5431 return true; 5432 return false; 5433 } 5434 5435 public Reference addEncounter() { // 3 5436 Reference t = new Reference(); 5437 if (this.encounter == null) 5438 this.encounter = new ArrayList<Reference>(); 5439 this.encounter.add(t); 5440 return t; 5441 } 5442 5443 public ItemComponent addEncounter(Reference t) { // 3 5444 if (t == null) 5445 return this; 5446 if (this.encounter == null) 5447 this.encounter = new ArrayList<Reference>(); 5448 this.encounter.add(t); 5449 return this; 5450 } 5451 5452 /** 5453 * @return The first repetition of repeating field {@link #encounter}, creating 5454 * it if it does not already exist 5455 */ 5456 public Reference getEncounterFirstRep() { 5457 if (getEncounter().isEmpty()) { 5458 addEncounter(); 5459 } 5460 return getEncounter().get(0); 5461 } 5462 5463 /** 5464 * @deprecated Use Reference#setResource(IBaseResource) instead 5465 */ 5466 @Deprecated 5467 public List<Encounter> getEncounterTarget() { 5468 if (this.encounterTarget == null) 5469 this.encounterTarget = new ArrayList<Encounter>(); 5470 return this.encounterTarget; 5471 } 5472 5473 /** 5474 * @deprecated Use Reference#setResource(IBaseResource) instead 5475 */ 5476 @Deprecated 5477 public Encounter addEncounterTarget() { 5478 Encounter r = new Encounter(); 5479 if (this.encounterTarget == null) 5480 this.encounterTarget = new ArrayList<Encounter>(); 5481 this.encounterTarget.add(r); 5482 return r; 5483 } 5484 5485 /** 5486 * @return {@link #noteNumber} (The numbers associated with notes below which 5487 * apply to the adjudication of this item.) 5488 */ 5489 public List<PositiveIntType> getNoteNumber() { 5490 if (this.noteNumber == null) 5491 this.noteNumber = new ArrayList<PositiveIntType>(); 5492 return this.noteNumber; 5493 } 5494 5495 /** 5496 * @return Returns a reference to <code>this</code> for easy method chaining 5497 */ 5498 public ItemComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 5499 this.noteNumber = theNoteNumber; 5500 return this; 5501 } 5502 5503 public boolean hasNoteNumber() { 5504 if (this.noteNumber == null) 5505 return false; 5506 for (PositiveIntType item : this.noteNumber) 5507 if (!item.isEmpty()) 5508 return true; 5509 return false; 5510 } 5511 5512 /** 5513 * @return {@link #noteNumber} (The numbers associated with notes below which 5514 * apply to the adjudication of this item.) 5515 */ 5516 public PositiveIntType addNoteNumberElement() {// 2 5517 PositiveIntType t = new PositiveIntType(); 5518 if (this.noteNumber == null) 5519 this.noteNumber = new ArrayList<PositiveIntType>(); 5520 this.noteNumber.add(t); 5521 return t; 5522 } 5523 5524 /** 5525 * @param value {@link #noteNumber} (The numbers associated with notes below 5526 * which apply to the adjudication of this item.) 5527 */ 5528 public ItemComponent addNoteNumber(int value) { // 1 5529 PositiveIntType t = new PositiveIntType(); 5530 t.setValue(value); 5531 if (this.noteNumber == null) 5532 this.noteNumber = new ArrayList<PositiveIntType>(); 5533 this.noteNumber.add(t); 5534 return this; 5535 } 5536 5537 /** 5538 * @param value {@link #noteNumber} (The numbers associated with notes below 5539 * which apply to the adjudication of this item.) 5540 */ 5541 public boolean hasNoteNumber(int value) { 5542 if (this.noteNumber == null) 5543 return false; 5544 for (PositiveIntType v : this.noteNumber) 5545 if (v.getValue().equals(value)) // positiveInt 5546 return true; 5547 return false; 5548 } 5549 5550 /** 5551 * @return {@link #adjudication} (If this item is a group then the values here 5552 * are a summary of the adjudication of the detail items. If this item 5553 * is a simple product or service then this is the result of the 5554 * adjudication of this item.) 5555 */ 5556 public List<AdjudicationComponent> getAdjudication() { 5557 if (this.adjudication == null) 5558 this.adjudication = new ArrayList<AdjudicationComponent>(); 5559 return this.adjudication; 5560 } 5561 5562 /** 5563 * @return Returns a reference to <code>this</code> for easy method chaining 5564 */ 5565 public ItemComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 5566 this.adjudication = theAdjudication; 5567 return this; 5568 } 5569 5570 public boolean hasAdjudication() { 5571 if (this.adjudication == null) 5572 return false; 5573 for (AdjudicationComponent item : this.adjudication) 5574 if (!item.isEmpty()) 5575 return true; 5576 return false; 5577 } 5578 5579 public AdjudicationComponent addAdjudication() { // 3 5580 AdjudicationComponent t = new AdjudicationComponent(); 5581 if (this.adjudication == null) 5582 this.adjudication = new ArrayList<AdjudicationComponent>(); 5583 this.adjudication.add(t); 5584 return t; 5585 } 5586 5587 public ItemComponent addAdjudication(AdjudicationComponent t) { // 3 5588 if (t == null) 5589 return this; 5590 if (this.adjudication == null) 5591 this.adjudication = new ArrayList<AdjudicationComponent>(); 5592 this.adjudication.add(t); 5593 return this; 5594 } 5595 5596 /** 5597 * @return The first repetition of repeating field {@link #adjudication}, 5598 * creating it if it does not already exist 5599 */ 5600 public AdjudicationComponent getAdjudicationFirstRep() { 5601 if (getAdjudication().isEmpty()) { 5602 addAdjudication(); 5603 } 5604 return getAdjudication().get(0); 5605 } 5606 5607 /** 5608 * @return {@link #detail} (Second-tier of goods and services.) 5609 */ 5610 public List<DetailComponent> getDetail() { 5611 if (this.detail == null) 5612 this.detail = new ArrayList<DetailComponent>(); 5613 return this.detail; 5614 } 5615 5616 /** 5617 * @return Returns a reference to <code>this</code> for easy method chaining 5618 */ 5619 public ItemComponent setDetail(List<DetailComponent> theDetail) { 5620 this.detail = theDetail; 5621 return this; 5622 } 5623 5624 public boolean hasDetail() { 5625 if (this.detail == null) 5626 return false; 5627 for (DetailComponent item : this.detail) 5628 if (!item.isEmpty()) 5629 return true; 5630 return false; 5631 } 5632 5633 public DetailComponent addDetail() { // 3 5634 DetailComponent t = new DetailComponent(); 5635 if (this.detail == null) 5636 this.detail = new ArrayList<DetailComponent>(); 5637 this.detail.add(t); 5638 return t; 5639 } 5640 5641 public ItemComponent addDetail(DetailComponent t) { // 3 5642 if (t == null) 5643 return this; 5644 if (this.detail == null) 5645 this.detail = new ArrayList<DetailComponent>(); 5646 this.detail.add(t); 5647 return this; 5648 } 5649 5650 /** 5651 * @return The first repetition of repeating field {@link #detail}, creating it 5652 * if it does not already exist 5653 */ 5654 public DetailComponent getDetailFirstRep() { 5655 if (getDetail().isEmpty()) { 5656 addDetail(); 5657 } 5658 return getDetail().get(0); 5659 } 5660 5661 protected void listChildren(List<Property> children) { 5662 super.listChildren(children); 5663 children 5664 .add(new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 1, sequence)); 5665 children.add(new Property("careTeamSequence", "positiveInt", 5666 "Care team members related to this service or product.", 0, java.lang.Integer.MAX_VALUE, careTeamSequence)); 5667 children.add(new Property("diagnosisSequence", "positiveInt", "Diagnoses applicable for this service or product.", 5668 0, java.lang.Integer.MAX_VALUE, diagnosisSequence)); 5669 children.add(new Property("procedureSequence", "positiveInt", 5670 "Procedures applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, procedureSequence)); 5671 children.add(new Property("informationSequence", "positiveInt", 5672 "Exceptions, special conditions and supporting information applicable for this service or product.", 0, 5673 java.lang.Integer.MAX_VALUE, informationSequence)); 5674 children.add(new Property("revenue", "CodeableConcept", 5675 "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 5676 children.add(new Property("category", "CodeableConcept", 5677 "Code to identify the general type of benefits under which products and services are provided.", 0, 1, 5678 category)); 5679 children.add(new Property("productOrService", "CodeableConcept", 5680 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 5681 0, 1, productOrService)); 5682 children.add(new Property("modifier", "CodeableConcept", 5683 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 5684 java.lang.Integer.MAX_VALUE, modifier)); 5685 children.add(new Property("programCode", "CodeableConcept", 5686 "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 5687 children.add(new Property("serviced[x]", "date|Period", 5688 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced)); 5689 children.add(new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 5690 "Where the product or service was provided.", 0, 1, location)); 5691 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 5692 1, quantity)); 5693 children.add(new Property("unitPrice", "Money", 5694 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 5695 0, 1, unitPrice)); 5696 children.add(new Property("factor", "decimal", 5697 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 5698 0, 1, factor)); 5699 children.add(new Property("net", "Money", 5700 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net)); 5701 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 5702 0, java.lang.Integer.MAX_VALUE, udi)); 5703 children.add(new Property("bodySite", "CodeableConcept", 5704 "Physical service site on the patient (limb, tooth, etc.).", 0, 1, bodySite)); 5705 children.add(new Property("subSite", "CodeableConcept", 5706 "A region or surface of the bodySite, e.g. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, 5707 subSite)); 5708 children.add(new Property("encounter", "Reference(Encounter)", 5709 "A billed item may include goods or services provided in multiple encounters.", 0, 5710 java.lang.Integer.MAX_VALUE, encounter)); 5711 children.add(new Property("noteNumber", "positiveInt", 5712 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 5713 java.lang.Integer.MAX_VALUE, noteNumber)); 5714 children.add(new Property("adjudication", "", 5715 "If this item is a group then the values here are a summary of the adjudication of the detail items. If this item is a simple product or service then this is the result of the adjudication of this item.", 5716 0, java.lang.Integer.MAX_VALUE, adjudication)); 5717 children.add( 5718 new Property("detail", "", "Second-tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, detail)); 5719 } 5720 5721 @Override 5722 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5723 switch (_hash) { 5724 case 1349547969: 5725 /* sequence */ return new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 5726 1, sequence); 5727 case 1070083823: 5728 /* careTeamSequence */ return new Property("careTeamSequence", "positiveInt", 5729 "Care team members related to this service or product.", 0, java.lang.Integer.MAX_VALUE, careTeamSequence); 5730 case -909769262: 5731 /* diagnosisSequence */ return new Property("diagnosisSequence", "positiveInt", 5732 "Diagnoses applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, diagnosisSequence); 5733 case -808920140: 5734 /* procedureSequence */ return new Property("procedureSequence", "positiveInt", 5735 "Procedures applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, procedureSequence); 5736 case -702585587: 5737 /* informationSequence */ return new Property("informationSequence", "positiveInt", 5738 "Exceptions, special conditions and supporting information applicable for this service or product.", 0, 5739 java.lang.Integer.MAX_VALUE, informationSequence); 5740 case 1099842588: 5741 /* revenue */ return new Property("revenue", "CodeableConcept", 5742 "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 5743 case 50511102: 5744 /* category */ return new Property("category", "CodeableConcept", 5745 "Code to identify the general type of benefits under which products and services are provided.", 0, 1, 5746 category); 5747 case 1957227299: 5748 /* productOrService */ return new Property("productOrService", "CodeableConcept", 5749 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 5750 0, 1, productOrService); 5751 case -615513385: 5752 /* modifier */ return new Property("modifier", "CodeableConcept", 5753 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 5754 java.lang.Integer.MAX_VALUE, modifier); 5755 case 1010065041: 5756 /* programCode */ return new Property("programCode", "CodeableConcept", 5757 "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 5758 case -1927922223: 5759 /* serviced[x] */ return new Property("serviced[x]", "date|Period", 5760 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 5761 case 1379209295: 5762 /* serviced */ return new Property("serviced[x]", "date|Period", 5763 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 5764 case 363246749: 5765 /* servicedDate */ return new Property("serviced[x]", "date|Period", 5766 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 5767 case 1534966512: 5768 /* servicedPeriod */ return new Property("serviced[x]", "date|Period", 5769 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 5770 case 552316075: 5771 /* location[x] */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 5772 "Where the product or service was provided.", 0, 1, location); 5773 case 1901043637: 5774 /* location */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 5775 "Where the product or service was provided.", 0, 1, location); 5776 case -1224800468: 5777 /* locationCodeableConcept */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 5778 "Where the product or service was provided.", 0, 1, location); 5779 case -1280020865: 5780 /* locationAddress */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 5781 "Where the product or service was provided.", 0, 1, location); 5782 case 755866390: 5783 /* locationReference */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 5784 "Where the product or service was provided.", 0, 1, location); 5785 case -1285004149: 5786 /* quantity */ return new Property("quantity", "SimpleQuantity", 5787 "The number of repetitions of a service or product.", 0, 1, quantity); 5788 case -486196699: 5789 /* unitPrice */ return new Property("unitPrice", "Money", 5790 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 5791 0, 1, unitPrice); 5792 case -1282148017: 5793 /* factor */ return new Property("factor", "decimal", 5794 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 5795 0, 1, factor); 5796 case 108957: 5797 /* net */ return new Property("net", "Money", 5798 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net); 5799 case 115642: 5800 /* udi */ return new Property("udi", "Reference(Device)", 5801 "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 5802 case 1702620169: 5803 /* bodySite */ return new Property("bodySite", "CodeableConcept", 5804 "Physical service site on the patient (limb, tooth, etc.).", 0, 1, bodySite); 5805 case -1868566105: 5806 /* subSite */ return new Property("subSite", "CodeableConcept", 5807 "A region or surface of the bodySite, e.g. limb region or tooth surface(s).", 0, 5808 java.lang.Integer.MAX_VALUE, subSite); 5809 case 1524132147: 5810 /* encounter */ return new Property("encounter", "Reference(Encounter)", 5811 "A billed item may include goods or services provided in multiple encounters.", 0, 5812 java.lang.Integer.MAX_VALUE, encounter); 5813 case -1110033957: 5814 /* noteNumber */ return new Property("noteNumber", "positiveInt", 5815 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 5816 java.lang.Integer.MAX_VALUE, noteNumber); 5817 case -231349275: 5818 /* adjudication */ return new Property("adjudication", "", 5819 "If this item is a group then the values here are a summary of the adjudication of the detail items. If this item is a simple product or service then this is the result of the adjudication of this item.", 5820 0, java.lang.Integer.MAX_VALUE, adjudication); 5821 case -1335224239: 5822 /* detail */ return new Property("detail", "", "Second-tier of goods and services.", 0, 5823 java.lang.Integer.MAX_VALUE, detail); 5824 default: 5825 return super.getNamedProperty(_hash, _name, _checkValid); 5826 } 5827 5828 } 5829 5830 @Override 5831 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5832 switch (hash) { 5833 case 1349547969: 5834 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 5835 case 1070083823: 5836 /* careTeamSequence */ return this.careTeamSequence == null ? new Base[0] 5837 : this.careTeamSequence.toArray(new Base[this.careTeamSequence.size()]); // PositiveIntType 5838 case -909769262: 5839 /* diagnosisSequence */ return this.diagnosisSequence == null ? new Base[0] 5840 : this.diagnosisSequence.toArray(new Base[this.diagnosisSequence.size()]); // PositiveIntType 5841 case -808920140: 5842 /* procedureSequence */ return this.procedureSequence == null ? new Base[0] 5843 : this.procedureSequence.toArray(new Base[this.procedureSequence.size()]); // PositiveIntType 5844 case -702585587: 5845 /* informationSequence */ return this.informationSequence == null ? new Base[0] 5846 : this.informationSequence.toArray(new Base[this.informationSequence.size()]); // PositiveIntType 5847 case 1099842588: 5848 /* revenue */ return this.revenue == null ? new Base[0] : new Base[] { this.revenue }; // CodeableConcept 5849 case 50511102: 5850 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 5851 case 1957227299: 5852 /* productOrService */ return this.productOrService == null ? new Base[0] 5853 : new Base[] { this.productOrService }; // CodeableConcept 5854 case -615513385: 5855 /* modifier */ return this.modifier == null ? new Base[0] 5856 : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 5857 case 1010065041: 5858 /* programCode */ return this.programCode == null ? new Base[0] 5859 : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 5860 case 1379209295: 5861 /* serviced */ return this.serviced == null ? new Base[0] : new Base[] { this.serviced }; // Type 5862 case 1901043637: 5863 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Type 5864 case -1285004149: 5865 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 5866 case -486196699: 5867 /* unitPrice */ return this.unitPrice == null ? new Base[0] : new Base[] { this.unitPrice }; // Money 5868 case -1282148017: 5869 /* factor */ return this.factor == null ? new Base[0] : new Base[] { this.factor }; // DecimalType 5870 case 108957: 5871 /* net */ return this.net == null ? new Base[0] : new Base[] { this.net }; // Money 5872 case 115642: 5873 /* udi */ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 5874 case 1702620169: 5875 /* bodySite */ return this.bodySite == null ? new Base[0] : new Base[] { this.bodySite }; // CodeableConcept 5876 case -1868566105: 5877 /* subSite */ return this.subSite == null ? new Base[0] : this.subSite.toArray(new Base[this.subSite.size()]); // CodeableConcept 5878 case 1524132147: 5879 /* encounter */ return this.encounter == null ? new Base[0] 5880 : this.encounter.toArray(new Base[this.encounter.size()]); // Reference 5881 case -1110033957: 5882 /* noteNumber */ return this.noteNumber == null ? new Base[0] 5883 : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 5884 case -231349275: 5885 /* adjudication */ return this.adjudication == null ? new Base[0] 5886 : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 5887 case -1335224239: 5888 /* detail */ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // DetailComponent 5889 default: 5890 return super.getProperty(hash, name, checkValid); 5891 } 5892 5893 } 5894 5895 @Override 5896 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5897 switch (hash) { 5898 case 1349547969: // sequence 5899 this.sequence = castToPositiveInt(value); // PositiveIntType 5900 return value; 5901 case 1070083823: // careTeamSequence 5902 this.getCareTeamSequence().add(castToPositiveInt(value)); // PositiveIntType 5903 return value; 5904 case -909769262: // diagnosisSequence 5905 this.getDiagnosisSequence().add(castToPositiveInt(value)); // PositiveIntType 5906 return value; 5907 case -808920140: // procedureSequence 5908 this.getProcedureSequence().add(castToPositiveInt(value)); // PositiveIntType 5909 return value; 5910 case -702585587: // informationSequence 5911 this.getInformationSequence().add(castToPositiveInt(value)); // PositiveIntType 5912 return value; 5913 case 1099842588: // revenue 5914 this.revenue = castToCodeableConcept(value); // CodeableConcept 5915 return value; 5916 case 50511102: // category 5917 this.category = castToCodeableConcept(value); // CodeableConcept 5918 return value; 5919 case 1957227299: // productOrService 5920 this.productOrService = castToCodeableConcept(value); // CodeableConcept 5921 return value; 5922 case -615513385: // modifier 5923 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 5924 return value; 5925 case 1010065041: // programCode 5926 this.getProgramCode().add(castToCodeableConcept(value)); // CodeableConcept 5927 return value; 5928 case 1379209295: // serviced 5929 this.serviced = castToType(value); // Type 5930 return value; 5931 case 1901043637: // location 5932 this.location = castToType(value); // Type 5933 return value; 5934 case -1285004149: // quantity 5935 this.quantity = castToQuantity(value); // Quantity 5936 return value; 5937 case -486196699: // unitPrice 5938 this.unitPrice = castToMoney(value); // Money 5939 return value; 5940 case -1282148017: // factor 5941 this.factor = castToDecimal(value); // DecimalType 5942 return value; 5943 case 108957: // net 5944 this.net = castToMoney(value); // Money 5945 return value; 5946 case 115642: // udi 5947 this.getUdi().add(castToReference(value)); // Reference 5948 return value; 5949 case 1702620169: // bodySite 5950 this.bodySite = castToCodeableConcept(value); // CodeableConcept 5951 return value; 5952 case -1868566105: // subSite 5953 this.getSubSite().add(castToCodeableConcept(value)); // CodeableConcept 5954 return value; 5955 case 1524132147: // encounter 5956 this.getEncounter().add(castToReference(value)); // Reference 5957 return value; 5958 case -1110033957: // noteNumber 5959 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 5960 return value; 5961 case -231349275: // adjudication 5962 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 5963 return value; 5964 case -1335224239: // detail 5965 this.getDetail().add((DetailComponent) value); // DetailComponent 5966 return value; 5967 default: 5968 return super.setProperty(hash, name, value); 5969 } 5970 5971 } 5972 5973 @Override 5974 public Base setProperty(String name, Base value) throws FHIRException { 5975 if (name.equals("sequence")) { 5976 this.sequence = castToPositiveInt(value); // PositiveIntType 5977 } else if (name.equals("careTeamSequence")) { 5978 this.getCareTeamSequence().add(castToPositiveInt(value)); 5979 } else if (name.equals("diagnosisSequence")) { 5980 this.getDiagnosisSequence().add(castToPositiveInt(value)); 5981 } else if (name.equals("procedureSequence")) { 5982 this.getProcedureSequence().add(castToPositiveInt(value)); 5983 } else if (name.equals("informationSequence")) { 5984 this.getInformationSequence().add(castToPositiveInt(value)); 5985 } else if (name.equals("revenue")) { 5986 this.revenue = castToCodeableConcept(value); // CodeableConcept 5987 } else if (name.equals("category")) { 5988 this.category = castToCodeableConcept(value); // CodeableConcept 5989 } else if (name.equals("productOrService")) { 5990 this.productOrService = castToCodeableConcept(value); // CodeableConcept 5991 } else if (name.equals("modifier")) { 5992 this.getModifier().add(castToCodeableConcept(value)); 5993 } else if (name.equals("programCode")) { 5994 this.getProgramCode().add(castToCodeableConcept(value)); 5995 } else if (name.equals("serviced[x]")) { 5996 this.serviced = castToType(value); // Type 5997 } else if (name.equals("location[x]")) { 5998 this.location = castToType(value); // Type 5999 } else if (name.equals("quantity")) { 6000 this.quantity = castToQuantity(value); // Quantity 6001 } else if (name.equals("unitPrice")) { 6002 this.unitPrice = castToMoney(value); // Money 6003 } else if (name.equals("factor")) { 6004 this.factor = castToDecimal(value); // DecimalType 6005 } else if (name.equals("net")) { 6006 this.net = castToMoney(value); // Money 6007 } else if (name.equals("udi")) { 6008 this.getUdi().add(castToReference(value)); 6009 } else if (name.equals("bodySite")) { 6010 this.bodySite = castToCodeableConcept(value); // CodeableConcept 6011 } else if (name.equals("subSite")) { 6012 this.getSubSite().add(castToCodeableConcept(value)); 6013 } else if (name.equals("encounter")) { 6014 this.getEncounter().add(castToReference(value)); 6015 } else if (name.equals("noteNumber")) { 6016 this.getNoteNumber().add(castToPositiveInt(value)); 6017 } else if (name.equals("adjudication")) { 6018 this.getAdjudication().add((AdjudicationComponent) value); 6019 } else if (name.equals("detail")) { 6020 this.getDetail().add((DetailComponent) value); 6021 } else 6022 return super.setProperty(name, value); 6023 return value; 6024 } 6025 6026 @Override 6027 public void removeChild(String name, Base value) throws FHIRException { 6028 if (name.equals("sequence")) { 6029 this.sequence = null; 6030 } else if (name.equals("careTeamSequence")) { 6031 this.getCareTeamSequence().remove(castToPositiveInt(value)); 6032 } else if (name.equals("diagnosisSequence")) { 6033 this.getDiagnosisSequence().remove(castToPositiveInt(value)); 6034 } else if (name.equals("procedureSequence")) { 6035 this.getProcedureSequence().remove(castToPositiveInt(value)); 6036 } else if (name.equals("informationSequence")) { 6037 this.getInformationSequence().remove(castToPositiveInt(value)); 6038 } else if (name.equals("revenue")) { 6039 this.revenue = null; 6040 } else if (name.equals("category")) { 6041 this.category = null; 6042 } else if (name.equals("productOrService")) { 6043 this.productOrService = null; 6044 } else if (name.equals("modifier")) { 6045 this.getModifier().remove(castToCodeableConcept(value)); 6046 } else if (name.equals("programCode")) { 6047 this.getProgramCode().remove(castToCodeableConcept(value)); 6048 } else if (name.equals("serviced[x]")) { 6049 this.serviced = null; 6050 } else if (name.equals("location[x]")) { 6051 this.location = null; 6052 } else if (name.equals("quantity")) { 6053 this.quantity = null; 6054 } else if (name.equals("unitPrice")) { 6055 this.unitPrice = null; 6056 } else if (name.equals("factor")) { 6057 this.factor = null; 6058 } else if (name.equals("net")) { 6059 this.net = null; 6060 } else if (name.equals("udi")) { 6061 this.getUdi().remove(castToReference(value)); 6062 } else if (name.equals("bodySite")) { 6063 this.bodySite = null; 6064 } else if (name.equals("subSite")) { 6065 this.getSubSite().remove(castToCodeableConcept(value)); 6066 } else if (name.equals("encounter")) { 6067 this.getEncounter().remove(castToReference(value)); 6068 } else if (name.equals("noteNumber")) { 6069 this.getNoteNumber().remove(castToPositiveInt(value)); 6070 } else if (name.equals("adjudication")) { 6071 this.getAdjudication().remove((AdjudicationComponent) value); 6072 } else if (name.equals("detail")) { 6073 this.getDetail().remove((DetailComponent) value); 6074 } else 6075 super.removeChild(name, value); 6076 6077 } 6078 6079 @Override 6080 public Base makeProperty(int hash, String name) throws FHIRException { 6081 switch (hash) { 6082 case 1349547969: 6083 return getSequenceElement(); 6084 case 1070083823: 6085 return addCareTeamSequenceElement(); 6086 case -909769262: 6087 return addDiagnosisSequenceElement(); 6088 case -808920140: 6089 return addProcedureSequenceElement(); 6090 case -702585587: 6091 return addInformationSequenceElement(); 6092 case 1099842588: 6093 return getRevenue(); 6094 case 50511102: 6095 return getCategory(); 6096 case 1957227299: 6097 return getProductOrService(); 6098 case -615513385: 6099 return addModifier(); 6100 case 1010065041: 6101 return addProgramCode(); 6102 case -1927922223: 6103 return getServiced(); 6104 case 1379209295: 6105 return getServiced(); 6106 case 552316075: 6107 return getLocation(); 6108 case 1901043637: 6109 return getLocation(); 6110 case -1285004149: 6111 return getQuantity(); 6112 case -486196699: 6113 return getUnitPrice(); 6114 case -1282148017: 6115 return getFactorElement(); 6116 case 108957: 6117 return getNet(); 6118 case 115642: 6119 return addUdi(); 6120 case 1702620169: 6121 return getBodySite(); 6122 case -1868566105: 6123 return addSubSite(); 6124 case 1524132147: 6125 return addEncounter(); 6126 case -1110033957: 6127 return addNoteNumberElement(); 6128 case -231349275: 6129 return addAdjudication(); 6130 case -1335224239: 6131 return addDetail(); 6132 default: 6133 return super.makeProperty(hash, name); 6134 } 6135 6136 } 6137 6138 @Override 6139 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6140 switch (hash) { 6141 case 1349547969: 6142 /* sequence */ return new String[] { "positiveInt" }; 6143 case 1070083823: 6144 /* careTeamSequence */ return new String[] { "positiveInt" }; 6145 case -909769262: 6146 /* diagnosisSequence */ return new String[] { "positiveInt" }; 6147 case -808920140: 6148 /* procedureSequence */ return new String[] { "positiveInt" }; 6149 case -702585587: 6150 /* informationSequence */ return new String[] { "positiveInt" }; 6151 case 1099842588: 6152 /* revenue */ return new String[] { "CodeableConcept" }; 6153 case 50511102: 6154 /* category */ return new String[] { "CodeableConcept" }; 6155 case 1957227299: 6156 /* productOrService */ return new String[] { "CodeableConcept" }; 6157 case -615513385: 6158 /* modifier */ return new String[] { "CodeableConcept" }; 6159 case 1010065041: 6160 /* programCode */ return new String[] { "CodeableConcept" }; 6161 case 1379209295: 6162 /* serviced */ return new String[] { "date", "Period" }; 6163 case 1901043637: 6164 /* location */ return new String[] { "CodeableConcept", "Address", "Reference" }; 6165 case -1285004149: 6166 /* quantity */ return new String[] { "SimpleQuantity" }; 6167 case -486196699: 6168 /* unitPrice */ return new String[] { "Money" }; 6169 case -1282148017: 6170 /* factor */ return new String[] { "decimal" }; 6171 case 108957: 6172 /* net */ return new String[] { "Money" }; 6173 case 115642: 6174 /* udi */ return new String[] { "Reference" }; 6175 case 1702620169: 6176 /* bodySite */ return new String[] { "CodeableConcept" }; 6177 case -1868566105: 6178 /* subSite */ return new String[] { "CodeableConcept" }; 6179 case 1524132147: 6180 /* encounter */ return new String[] { "Reference" }; 6181 case -1110033957: 6182 /* noteNumber */ return new String[] { "positiveInt" }; 6183 case -231349275: 6184 /* adjudication */ return new String[] {}; 6185 case -1335224239: 6186 /* detail */ return new String[] {}; 6187 default: 6188 return super.getTypesForProperty(hash, name); 6189 } 6190 6191 } 6192 6193 @Override 6194 public Base addChild(String name) throws FHIRException { 6195 if (name.equals("sequence")) { 6196 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.sequence"); 6197 } else if (name.equals("careTeamSequence")) { 6198 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.careTeamSequence"); 6199 } else if (name.equals("diagnosisSequence")) { 6200 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.diagnosisSequence"); 6201 } else if (name.equals("procedureSequence")) { 6202 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.procedureSequence"); 6203 } else if (name.equals("informationSequence")) { 6204 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.informationSequence"); 6205 } else if (name.equals("revenue")) { 6206 this.revenue = new CodeableConcept(); 6207 return this.revenue; 6208 } else if (name.equals("category")) { 6209 this.category = new CodeableConcept(); 6210 return this.category; 6211 } else if (name.equals("productOrService")) { 6212 this.productOrService = new CodeableConcept(); 6213 return this.productOrService; 6214 } else if (name.equals("modifier")) { 6215 return addModifier(); 6216 } else if (name.equals("programCode")) { 6217 return addProgramCode(); 6218 } else if (name.equals("servicedDate")) { 6219 this.serviced = new DateType(); 6220 return this.serviced; 6221 } else if (name.equals("servicedPeriod")) { 6222 this.serviced = new Period(); 6223 return this.serviced; 6224 } else if (name.equals("locationCodeableConcept")) { 6225 this.location = new CodeableConcept(); 6226 return this.location; 6227 } else if (name.equals("locationAddress")) { 6228 this.location = new Address(); 6229 return this.location; 6230 } else if (name.equals("locationReference")) { 6231 this.location = new Reference(); 6232 return this.location; 6233 } else if (name.equals("quantity")) { 6234 this.quantity = new Quantity(); 6235 return this.quantity; 6236 } else if (name.equals("unitPrice")) { 6237 this.unitPrice = new Money(); 6238 return this.unitPrice; 6239 } else if (name.equals("factor")) { 6240 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.factor"); 6241 } else if (name.equals("net")) { 6242 this.net = new Money(); 6243 return this.net; 6244 } else if (name.equals("udi")) { 6245 return addUdi(); 6246 } else if (name.equals("bodySite")) { 6247 this.bodySite = new CodeableConcept(); 6248 return this.bodySite; 6249 } else if (name.equals("subSite")) { 6250 return addSubSite(); 6251 } else if (name.equals("encounter")) { 6252 return addEncounter(); 6253 } else if (name.equals("noteNumber")) { 6254 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.noteNumber"); 6255 } else if (name.equals("adjudication")) { 6256 return addAdjudication(); 6257 } else if (name.equals("detail")) { 6258 return addDetail(); 6259 } else 6260 return super.addChild(name); 6261 } 6262 6263 public ItemComponent copy() { 6264 ItemComponent dst = new ItemComponent(); 6265 copyValues(dst); 6266 return dst; 6267 } 6268 6269 public void copyValues(ItemComponent dst) { 6270 super.copyValues(dst); 6271 dst.sequence = sequence == null ? null : sequence.copy(); 6272 if (careTeamSequence != null) { 6273 dst.careTeamSequence = new ArrayList<PositiveIntType>(); 6274 for (PositiveIntType i : careTeamSequence) 6275 dst.careTeamSequence.add(i.copy()); 6276 } 6277 ; 6278 if (diagnosisSequence != null) { 6279 dst.diagnosisSequence = new ArrayList<PositiveIntType>(); 6280 for (PositiveIntType i : diagnosisSequence) 6281 dst.diagnosisSequence.add(i.copy()); 6282 } 6283 ; 6284 if (procedureSequence != null) { 6285 dst.procedureSequence = new ArrayList<PositiveIntType>(); 6286 for (PositiveIntType i : procedureSequence) 6287 dst.procedureSequence.add(i.copy()); 6288 } 6289 ; 6290 if (informationSequence != null) { 6291 dst.informationSequence = new ArrayList<PositiveIntType>(); 6292 for (PositiveIntType i : informationSequence) 6293 dst.informationSequence.add(i.copy()); 6294 } 6295 ; 6296 dst.revenue = revenue == null ? null : revenue.copy(); 6297 dst.category = category == null ? null : category.copy(); 6298 dst.productOrService = productOrService == null ? null : productOrService.copy(); 6299 if (modifier != null) { 6300 dst.modifier = new ArrayList<CodeableConcept>(); 6301 for (CodeableConcept i : modifier) 6302 dst.modifier.add(i.copy()); 6303 } 6304 ; 6305 if (programCode != null) { 6306 dst.programCode = new ArrayList<CodeableConcept>(); 6307 for (CodeableConcept i : programCode) 6308 dst.programCode.add(i.copy()); 6309 } 6310 ; 6311 dst.serviced = serviced == null ? null : serviced.copy(); 6312 dst.location = location == null ? null : location.copy(); 6313 dst.quantity = quantity == null ? null : quantity.copy(); 6314 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 6315 dst.factor = factor == null ? null : factor.copy(); 6316 dst.net = net == null ? null : net.copy(); 6317 if (udi != null) { 6318 dst.udi = new ArrayList<Reference>(); 6319 for (Reference i : udi) 6320 dst.udi.add(i.copy()); 6321 } 6322 ; 6323 dst.bodySite = bodySite == null ? null : bodySite.copy(); 6324 if (subSite != null) { 6325 dst.subSite = new ArrayList<CodeableConcept>(); 6326 for (CodeableConcept i : subSite) 6327 dst.subSite.add(i.copy()); 6328 } 6329 ; 6330 if (encounter != null) { 6331 dst.encounter = new ArrayList<Reference>(); 6332 for (Reference i : encounter) 6333 dst.encounter.add(i.copy()); 6334 } 6335 ; 6336 if (noteNumber != null) { 6337 dst.noteNumber = new ArrayList<PositiveIntType>(); 6338 for (PositiveIntType i : noteNumber) 6339 dst.noteNumber.add(i.copy()); 6340 } 6341 ; 6342 if (adjudication != null) { 6343 dst.adjudication = new ArrayList<AdjudicationComponent>(); 6344 for (AdjudicationComponent i : adjudication) 6345 dst.adjudication.add(i.copy()); 6346 } 6347 ; 6348 if (detail != null) { 6349 dst.detail = new ArrayList<DetailComponent>(); 6350 for (DetailComponent i : detail) 6351 dst.detail.add(i.copy()); 6352 } 6353 ; 6354 } 6355 6356 @Override 6357 public boolean equalsDeep(Base other_) { 6358 if (!super.equalsDeep(other_)) 6359 return false; 6360 if (!(other_ instanceof ItemComponent)) 6361 return false; 6362 ItemComponent o = (ItemComponent) other_; 6363 return compareDeep(sequence, o.sequence, true) && compareDeep(careTeamSequence, o.careTeamSequence, true) 6364 && compareDeep(diagnosisSequence, o.diagnosisSequence, true) 6365 && compareDeep(procedureSequence, o.procedureSequence, true) 6366 && compareDeep(informationSequence, o.informationSequence, true) && compareDeep(revenue, o.revenue, true) 6367 && compareDeep(category, o.category, true) && compareDeep(productOrService, o.productOrService, true) 6368 && compareDeep(modifier, o.modifier, true) && compareDeep(programCode, o.programCode, true) 6369 && compareDeep(serviced, o.serviced, true) && compareDeep(location, o.location, true) 6370 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 6371 && compareDeep(factor, o.factor, true) && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) 6372 && compareDeep(bodySite, o.bodySite, true) && compareDeep(subSite, o.subSite, true) 6373 && compareDeep(encounter, o.encounter, true) && compareDeep(noteNumber, o.noteNumber, true) 6374 && compareDeep(adjudication, o.adjudication, true) && compareDeep(detail, o.detail, true); 6375 } 6376 6377 @Override 6378 public boolean equalsShallow(Base other_) { 6379 if (!super.equalsShallow(other_)) 6380 return false; 6381 if (!(other_ instanceof ItemComponent)) 6382 return false; 6383 ItemComponent o = (ItemComponent) other_; 6384 return compareValues(sequence, o.sequence, true) && compareValues(careTeamSequence, o.careTeamSequence, true) 6385 && compareValues(diagnosisSequence, o.diagnosisSequence, true) 6386 && compareValues(procedureSequence, o.procedureSequence, true) 6387 && compareValues(informationSequence, o.informationSequence, true) && compareValues(factor, o.factor, true) 6388 && compareValues(noteNumber, o.noteNumber, true); 6389 } 6390 6391 public boolean isEmpty() { 6392 return super.isEmpty() 6393 && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, careTeamSequence, diagnosisSequence, procedureSequence, 6394 informationSequence, revenue, category, productOrService, modifier, programCode, serviced, location, 6395 quantity, unitPrice, factor, net, udi, bodySite, subSite, encounter, noteNumber, adjudication, detail); 6396 } 6397 6398 public String fhirType() { 6399 return "ExplanationOfBenefit.item"; 6400 6401 } 6402 6403 } 6404 6405 @Block() 6406 public static class AdjudicationComponent extends BackboneElement implements IBaseBackboneElement { 6407 /** 6408 * A code to indicate the information type of this adjudication record. 6409 * Information types may include: the value submitted, maximum values or 6410 * percentages allowed or payable under the plan, amounts that the patient is 6411 * responsible for in-aggregate or pertaining to this item, amounts paid by 6412 * other coverages, and the benefit payable for this item. 6413 */ 6414 @Child(name = "category", type = { 6415 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 6416 @Description(shortDefinition = "Type of adjudication information", formalDefinition = "A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in-aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.") 6417 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/adjudication") 6418 protected CodeableConcept category; 6419 6420 /** 6421 * A code supporting the understanding of the adjudication result and explaining 6422 * variance from expected amount. 6423 */ 6424 @Child(name = "reason", type = { 6425 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 6426 @Description(shortDefinition = "Explanation of adjudication outcome", formalDefinition = "A code supporting the understanding of the adjudication result and explaining variance from expected amount.") 6427 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/adjudication-reason") 6428 protected CodeableConcept reason; 6429 6430 /** 6431 * Monetary amount associated with the category. 6432 */ 6433 @Child(name = "amount", type = { Money.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 6434 @Description(shortDefinition = "Monetary amount", formalDefinition = "Monetary amount associated with the category.") 6435 protected Money amount; 6436 6437 /** 6438 * A non-monetary value associated with the category. Mutually exclusive to the 6439 * amount element above. 6440 */ 6441 @Child(name = "value", type = { DecimalType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 6442 @Description(shortDefinition = "Non-monitary value", formalDefinition = "A non-monetary value associated with the category. Mutually exclusive to the amount element above.") 6443 protected DecimalType value; 6444 6445 private static final long serialVersionUID = 1559898786L; 6446 6447 /** 6448 * Constructor 6449 */ 6450 public AdjudicationComponent() { 6451 super(); 6452 } 6453 6454 /** 6455 * Constructor 6456 */ 6457 public AdjudicationComponent(CodeableConcept category) { 6458 super(); 6459 this.category = category; 6460 } 6461 6462 /** 6463 * @return {@link #category} (A code to indicate the information type of this 6464 * adjudication record. Information types may include: the value 6465 * submitted, maximum values or percentages allowed or payable under the 6466 * plan, amounts that the patient is responsible for in-aggregate or 6467 * pertaining to this item, amounts paid by other coverages, and the 6468 * benefit payable for this item.) 6469 */ 6470 public CodeableConcept getCategory() { 6471 if (this.category == null) 6472 if (Configuration.errorOnAutoCreate()) 6473 throw new Error("Attempt to auto-create AdjudicationComponent.category"); 6474 else if (Configuration.doAutoCreate()) 6475 this.category = new CodeableConcept(); // cc 6476 return this.category; 6477 } 6478 6479 public boolean hasCategory() { 6480 return this.category != null && !this.category.isEmpty(); 6481 } 6482 6483 /** 6484 * @param value {@link #category} (A code to indicate the information type of 6485 * this adjudication record. Information types may include: the 6486 * value submitted, maximum values or percentages allowed or 6487 * payable under the plan, amounts that the patient is responsible 6488 * for in-aggregate or pertaining to this item, amounts paid by 6489 * other coverages, and the benefit payable for this item.) 6490 */ 6491 public AdjudicationComponent setCategory(CodeableConcept value) { 6492 this.category = value; 6493 return this; 6494 } 6495 6496 /** 6497 * @return {@link #reason} (A code supporting the understanding of the 6498 * adjudication result and explaining variance from expected amount.) 6499 */ 6500 public CodeableConcept getReason() { 6501 if (this.reason == null) 6502 if (Configuration.errorOnAutoCreate()) 6503 throw new Error("Attempt to auto-create AdjudicationComponent.reason"); 6504 else if (Configuration.doAutoCreate()) 6505 this.reason = new CodeableConcept(); // cc 6506 return this.reason; 6507 } 6508 6509 public boolean hasReason() { 6510 return this.reason != null && !this.reason.isEmpty(); 6511 } 6512 6513 /** 6514 * @param value {@link #reason} (A code supporting the understanding of the 6515 * adjudication result and explaining variance from expected 6516 * amount.) 6517 */ 6518 public AdjudicationComponent setReason(CodeableConcept value) { 6519 this.reason = value; 6520 return this; 6521 } 6522 6523 /** 6524 * @return {@link #amount} (Monetary amount associated with the category.) 6525 */ 6526 public Money getAmount() { 6527 if (this.amount == null) 6528 if (Configuration.errorOnAutoCreate()) 6529 throw new Error("Attempt to auto-create AdjudicationComponent.amount"); 6530 else if (Configuration.doAutoCreate()) 6531 this.amount = new Money(); // cc 6532 return this.amount; 6533 } 6534 6535 public boolean hasAmount() { 6536 return this.amount != null && !this.amount.isEmpty(); 6537 } 6538 6539 /** 6540 * @param value {@link #amount} (Monetary amount associated with the category.) 6541 */ 6542 public AdjudicationComponent setAmount(Money value) { 6543 this.amount = value; 6544 return this; 6545 } 6546 6547 /** 6548 * @return {@link #value} (A non-monetary value associated with the category. 6549 * Mutually exclusive to the amount element above.). This is the 6550 * underlying object with id, value and extensions. The accessor 6551 * "getValue" gives direct access to the value 6552 */ 6553 public DecimalType getValueElement() { 6554 if (this.value == null) 6555 if (Configuration.errorOnAutoCreate()) 6556 throw new Error("Attempt to auto-create AdjudicationComponent.value"); 6557 else if (Configuration.doAutoCreate()) 6558 this.value = new DecimalType(); // bb 6559 return this.value; 6560 } 6561 6562 public boolean hasValueElement() { 6563 return this.value != null && !this.value.isEmpty(); 6564 } 6565 6566 public boolean hasValue() { 6567 return this.value != null && !this.value.isEmpty(); 6568 } 6569 6570 /** 6571 * @param value {@link #value} (A non-monetary value associated with the 6572 * category. Mutually exclusive to the amount element above.). This 6573 * is the underlying object with id, value and extensions. The 6574 * accessor "getValue" gives direct access to the value 6575 */ 6576 public AdjudicationComponent setValueElement(DecimalType value) { 6577 this.value = value; 6578 return this; 6579 } 6580 6581 /** 6582 * @return A non-monetary value associated with the category. Mutually exclusive 6583 * to the amount element above. 6584 */ 6585 public BigDecimal getValue() { 6586 return this.value == null ? null : this.value.getValue(); 6587 } 6588 6589 /** 6590 * @param value A non-monetary value associated with the category. Mutually 6591 * exclusive to the amount element above. 6592 */ 6593 public AdjudicationComponent setValue(BigDecimal value) { 6594 if (value == null) 6595 this.value = null; 6596 else { 6597 if (this.value == null) 6598 this.value = new DecimalType(); 6599 this.value.setValue(value); 6600 } 6601 return this; 6602 } 6603 6604 /** 6605 * @param value A non-monetary value associated with the category. Mutually 6606 * exclusive to the amount element above. 6607 */ 6608 public AdjudicationComponent setValue(long value) { 6609 this.value = new DecimalType(); 6610 this.value.setValue(value); 6611 return this; 6612 } 6613 6614 /** 6615 * @param value A non-monetary value associated with the category. Mutually 6616 * exclusive to the amount element above. 6617 */ 6618 public AdjudicationComponent setValue(double value) { 6619 this.value = new DecimalType(); 6620 this.value.setValue(value); 6621 return this; 6622 } 6623 6624 protected void listChildren(List<Property> children) { 6625 super.listChildren(children); 6626 children.add(new Property("category", "CodeableConcept", 6627 "A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in-aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.", 6628 0, 1, category)); 6629 children.add(new Property("reason", "CodeableConcept", 6630 "A code supporting the understanding of the adjudication result and explaining variance from expected amount.", 6631 0, 1, reason)); 6632 children.add(new Property("amount", "Money", "Monetary amount associated with the category.", 0, 1, amount)); 6633 children.add(new Property("value", "decimal", 6634 "A non-monetary value associated with the category. Mutually exclusive to the amount element above.", 0, 1, 6635 value)); 6636 } 6637 6638 @Override 6639 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6640 switch (_hash) { 6641 case 50511102: 6642 /* category */ return new Property("category", "CodeableConcept", 6643 "A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in-aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.", 6644 0, 1, category); 6645 case -934964668: 6646 /* reason */ return new Property("reason", "CodeableConcept", 6647 "A code supporting the understanding of the adjudication result and explaining variance from expected amount.", 6648 0, 1, reason); 6649 case -1413853096: 6650 /* amount */ return new Property("amount", "Money", "Monetary amount associated with the category.", 0, 1, 6651 amount); 6652 case 111972721: 6653 /* value */ return new Property("value", "decimal", 6654 "A non-monetary value associated with the category. Mutually exclusive to the amount element above.", 0, 1, 6655 value); 6656 default: 6657 return super.getNamedProperty(_hash, _name, _checkValid); 6658 } 6659 6660 } 6661 6662 @Override 6663 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6664 switch (hash) { 6665 case 50511102: 6666 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 6667 case -934964668: 6668 /* reason */ return this.reason == null ? new Base[0] : new Base[] { this.reason }; // CodeableConcept 6669 case -1413853096: 6670 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // Money 6671 case 111972721: 6672 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // DecimalType 6673 default: 6674 return super.getProperty(hash, name, checkValid); 6675 } 6676 6677 } 6678 6679 @Override 6680 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6681 switch (hash) { 6682 case 50511102: // category 6683 this.category = castToCodeableConcept(value); // CodeableConcept 6684 return value; 6685 case -934964668: // reason 6686 this.reason = castToCodeableConcept(value); // CodeableConcept 6687 return value; 6688 case -1413853096: // amount 6689 this.amount = castToMoney(value); // Money 6690 return value; 6691 case 111972721: // value 6692 this.value = castToDecimal(value); // DecimalType 6693 return value; 6694 default: 6695 return super.setProperty(hash, name, value); 6696 } 6697 6698 } 6699 6700 @Override 6701 public Base setProperty(String name, Base value) throws FHIRException { 6702 if (name.equals("category")) { 6703 this.category = castToCodeableConcept(value); // CodeableConcept 6704 } else if (name.equals("reason")) { 6705 this.reason = castToCodeableConcept(value); // CodeableConcept 6706 } else if (name.equals("amount")) { 6707 this.amount = castToMoney(value); // Money 6708 } else if (name.equals("value")) { 6709 this.value = castToDecimal(value); // DecimalType 6710 } else 6711 return super.setProperty(name, value); 6712 return value; 6713 } 6714 6715 @Override 6716 public void removeChild(String name, Base value) throws FHIRException { 6717 if (name.equals("category")) { 6718 this.category = null; 6719 } else if (name.equals("reason")) { 6720 this.reason = null; 6721 } else if (name.equals("amount")) { 6722 this.amount = null; 6723 } else if (name.equals("value")) { 6724 this.value = null; 6725 } else 6726 super.removeChild(name, value); 6727 6728 } 6729 6730 @Override 6731 public Base makeProperty(int hash, String name) throws FHIRException { 6732 switch (hash) { 6733 case 50511102: 6734 return getCategory(); 6735 case -934964668: 6736 return getReason(); 6737 case -1413853096: 6738 return getAmount(); 6739 case 111972721: 6740 return getValueElement(); 6741 default: 6742 return super.makeProperty(hash, name); 6743 } 6744 6745 } 6746 6747 @Override 6748 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6749 switch (hash) { 6750 case 50511102: 6751 /* category */ return new String[] { "CodeableConcept" }; 6752 case -934964668: 6753 /* reason */ return new String[] { "CodeableConcept" }; 6754 case -1413853096: 6755 /* amount */ return new String[] { "Money" }; 6756 case 111972721: 6757 /* value */ return new String[] { "decimal" }; 6758 default: 6759 return super.getTypesForProperty(hash, name); 6760 } 6761 6762 } 6763 6764 @Override 6765 public Base addChild(String name) throws FHIRException { 6766 if (name.equals("category")) { 6767 this.category = new CodeableConcept(); 6768 return this.category; 6769 } else if (name.equals("reason")) { 6770 this.reason = new CodeableConcept(); 6771 return this.reason; 6772 } else if (name.equals("amount")) { 6773 this.amount = new Money(); 6774 return this.amount; 6775 } else if (name.equals("value")) { 6776 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.value"); 6777 } else 6778 return super.addChild(name); 6779 } 6780 6781 public AdjudicationComponent copy() { 6782 AdjudicationComponent dst = new AdjudicationComponent(); 6783 copyValues(dst); 6784 return dst; 6785 } 6786 6787 public void copyValues(AdjudicationComponent dst) { 6788 super.copyValues(dst); 6789 dst.category = category == null ? null : category.copy(); 6790 dst.reason = reason == null ? null : reason.copy(); 6791 dst.amount = amount == null ? null : amount.copy(); 6792 dst.value = value == null ? null : value.copy(); 6793 } 6794 6795 @Override 6796 public boolean equalsDeep(Base other_) { 6797 if (!super.equalsDeep(other_)) 6798 return false; 6799 if (!(other_ instanceof AdjudicationComponent)) 6800 return false; 6801 AdjudicationComponent o = (AdjudicationComponent) other_; 6802 return compareDeep(category, o.category, true) && compareDeep(reason, o.reason, true) 6803 && compareDeep(amount, o.amount, true) && compareDeep(value, o.value, true); 6804 } 6805 6806 @Override 6807 public boolean equalsShallow(Base other_) { 6808 if (!super.equalsShallow(other_)) 6809 return false; 6810 if (!(other_ instanceof AdjudicationComponent)) 6811 return false; 6812 AdjudicationComponent o = (AdjudicationComponent) other_; 6813 return compareValues(value, o.value, true); 6814 } 6815 6816 public boolean isEmpty() { 6817 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, reason, amount, value); 6818 } 6819 6820 public String fhirType() { 6821 return "ExplanationOfBenefit.item.adjudication"; 6822 6823 } 6824 6825 } 6826 6827 @Block() 6828 public static class DetailComponent extends BackboneElement implements IBaseBackboneElement { 6829 /** 6830 * A claim detail line. Either a simple (a product or service) or a 'group' of 6831 * sub-details which are simple items. 6832 */ 6833 @Child(name = "sequence", type = { 6834 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 6835 @Description(shortDefinition = "Product or service provided", formalDefinition = "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.") 6836 protected PositiveIntType sequence; 6837 6838 /** 6839 * The type of revenue or cost center providing the product and/or service. 6840 */ 6841 @Child(name = "revenue", type = { 6842 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 6843 @Description(shortDefinition = "Revenue or cost center code", formalDefinition = "The type of revenue or cost center providing the product and/or service.") 6844 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-revenue-center") 6845 protected CodeableConcept revenue; 6846 6847 /** 6848 * Code to identify the general type of benefits under which products and 6849 * services are provided. 6850 */ 6851 @Child(name = "category", type = { 6852 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 6853 @Description(shortDefinition = "Benefit classification", formalDefinition = "Code to identify the general type of benefits under which products and services are provided.") 6854 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-benefitcategory") 6855 protected CodeableConcept category; 6856 6857 /** 6858 * When the value is a group code then this item collects a set of related claim 6859 * details, otherwise this contains the product, service, drug or other billing 6860 * code for the item. 6861 */ 6862 @Child(name = "productOrService", type = { 6863 CodeableConcept.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 6864 @Description(shortDefinition = "Billing, service, product, or drug code", formalDefinition = "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.") 6865 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-uscls") 6866 protected CodeableConcept productOrService; 6867 6868 /** 6869 * Item typification or modifiers codes to convey additional context for the 6870 * product or service. 6871 */ 6872 @Child(name = "modifier", type = { 6873 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6874 @Description(shortDefinition = "Service/Product billing modifiers", formalDefinition = "Item typification or modifiers codes to convey additional context for the product or service.") 6875 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-modifiers") 6876 protected List<CodeableConcept> modifier; 6877 6878 /** 6879 * Identifies the program under which this may be recovered. 6880 */ 6881 @Child(name = "programCode", type = { 6882 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6883 @Description(shortDefinition = "Program the product or service is provided under", formalDefinition = "Identifies the program under which this may be recovered.") 6884 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-program-code") 6885 protected List<CodeableConcept> programCode; 6886 6887 /** 6888 * The number of repetitions of a service or product. 6889 */ 6890 @Child(name = "quantity", type = { Quantity.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 6891 @Description(shortDefinition = "Count of products or services", formalDefinition = "The number of repetitions of a service or product.") 6892 protected Quantity quantity; 6893 6894 /** 6895 * If the item is not a group then this is the fee for the product or service, 6896 * otherwise this is the total of the fees for the details of the group. 6897 */ 6898 @Child(name = "unitPrice", type = { Money.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 6899 @Description(shortDefinition = "Fee, charge or cost per item", formalDefinition = "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.") 6900 protected Money unitPrice; 6901 6902 /** 6903 * A real number that represents a multiplier used in determining the overall 6904 * value of services delivered and/or goods received. The concept of a Factor 6905 * allows for a discount or surcharge multiplier to be applied to a monetary 6906 * amount. 6907 */ 6908 @Child(name = "factor", type = { 6909 DecimalType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 6910 @Description(shortDefinition = "Price scaling factor", formalDefinition = "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.") 6911 protected DecimalType factor; 6912 6913 /** 6914 * The quantity times the unit price for an additional service or product or 6915 * charge. 6916 */ 6917 @Child(name = "net", type = { Money.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 6918 @Description(shortDefinition = "Total item cost", formalDefinition = "The quantity times the unit price for an additional service or product or charge.") 6919 protected Money net; 6920 6921 /** 6922 * Unique Device Identifiers associated with this line item. 6923 */ 6924 @Child(name = "udi", type = { 6925 Device.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6926 @Description(shortDefinition = "Unique device identifier", formalDefinition = "Unique Device Identifiers associated with this line item.") 6927 protected List<Reference> udi; 6928 /** 6929 * The actual objects that are the target of the reference (Unique Device 6930 * Identifiers associated with this line item.) 6931 */ 6932 protected List<Device> udiTarget; 6933 6934 /** 6935 * The numbers associated with notes below which apply to the adjudication of 6936 * this item. 6937 */ 6938 @Child(name = "noteNumber", type = { 6939 PositiveIntType.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6940 @Description(shortDefinition = "Applicable note numbers", formalDefinition = "The numbers associated with notes below which apply to the adjudication of this item.") 6941 protected List<PositiveIntType> noteNumber; 6942 6943 /** 6944 * The adjudication results. 6945 */ 6946 @Child(name = "adjudication", type = { 6947 AdjudicationComponent.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6948 @Description(shortDefinition = "Detail level adjudication details", formalDefinition = "The adjudication results.") 6949 protected List<AdjudicationComponent> adjudication; 6950 6951 /** 6952 * Third-tier of goods and services. 6953 */ 6954 @Child(name = "subDetail", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6955 @Description(shortDefinition = "Additional items", formalDefinition = "Third-tier of goods and services.") 6956 protected List<SubDetailComponent> subDetail; 6957 6958 private static final long serialVersionUID = 225639798L; 6959 6960 /** 6961 * Constructor 6962 */ 6963 public DetailComponent() { 6964 super(); 6965 } 6966 6967 /** 6968 * Constructor 6969 */ 6970 public DetailComponent(PositiveIntType sequence, CodeableConcept productOrService) { 6971 super(); 6972 this.sequence = sequence; 6973 this.productOrService = productOrService; 6974 } 6975 6976 /** 6977 * @return {@link #sequence} (A claim detail line. Either a simple (a product or 6978 * service) or a 'group' of sub-details which are simple items.). This 6979 * is the underlying object with id, value and extensions. The accessor 6980 * "getSequence" gives direct access to the value 6981 */ 6982 public PositiveIntType getSequenceElement() { 6983 if (this.sequence == null) 6984 if (Configuration.errorOnAutoCreate()) 6985 throw new Error("Attempt to auto-create DetailComponent.sequence"); 6986 else if (Configuration.doAutoCreate()) 6987 this.sequence = new PositiveIntType(); // bb 6988 return this.sequence; 6989 } 6990 6991 public boolean hasSequenceElement() { 6992 return this.sequence != null && !this.sequence.isEmpty(); 6993 } 6994 6995 public boolean hasSequence() { 6996 return this.sequence != null && !this.sequence.isEmpty(); 6997 } 6998 6999 /** 7000 * @param value {@link #sequence} (A claim detail line. Either a simple (a 7001 * product or service) or a 'group' of sub-details which are simple 7002 * items.). This is the underlying object with id, value and 7003 * extensions. The accessor "getSequence" gives direct access to 7004 * the value 7005 */ 7006 public DetailComponent setSequenceElement(PositiveIntType value) { 7007 this.sequence = value; 7008 return this; 7009 } 7010 7011 /** 7012 * @return A claim detail line. Either a simple (a product or service) or a 7013 * 'group' of sub-details which are simple items. 7014 */ 7015 public int getSequence() { 7016 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 7017 } 7018 7019 /** 7020 * @param value A claim detail line. Either a simple (a product or service) or a 7021 * 'group' of sub-details which are simple items. 7022 */ 7023 public DetailComponent setSequence(int value) { 7024 if (this.sequence == null) 7025 this.sequence = new PositiveIntType(); 7026 this.sequence.setValue(value); 7027 return this; 7028 } 7029 7030 /** 7031 * @return {@link #revenue} (The type of revenue or cost center providing the 7032 * product and/or service.) 7033 */ 7034 public CodeableConcept getRevenue() { 7035 if (this.revenue == null) 7036 if (Configuration.errorOnAutoCreate()) 7037 throw new Error("Attempt to auto-create DetailComponent.revenue"); 7038 else if (Configuration.doAutoCreate()) 7039 this.revenue = new CodeableConcept(); // cc 7040 return this.revenue; 7041 } 7042 7043 public boolean hasRevenue() { 7044 return this.revenue != null && !this.revenue.isEmpty(); 7045 } 7046 7047 /** 7048 * @param value {@link #revenue} (The type of revenue or cost center providing 7049 * the product and/or service.) 7050 */ 7051 public DetailComponent setRevenue(CodeableConcept value) { 7052 this.revenue = value; 7053 return this; 7054 } 7055 7056 /** 7057 * @return {@link #category} (Code to identify the general type of benefits 7058 * under which products and services are provided.) 7059 */ 7060 public CodeableConcept getCategory() { 7061 if (this.category == null) 7062 if (Configuration.errorOnAutoCreate()) 7063 throw new Error("Attempt to auto-create DetailComponent.category"); 7064 else if (Configuration.doAutoCreate()) 7065 this.category = new CodeableConcept(); // cc 7066 return this.category; 7067 } 7068 7069 public boolean hasCategory() { 7070 return this.category != null && !this.category.isEmpty(); 7071 } 7072 7073 /** 7074 * @param value {@link #category} (Code to identify the general type of benefits 7075 * under which products and services are provided.) 7076 */ 7077 public DetailComponent setCategory(CodeableConcept value) { 7078 this.category = value; 7079 return this; 7080 } 7081 7082 /** 7083 * @return {@link #productOrService} (When the value is a group code then this 7084 * item collects a set of related claim details, otherwise this contains 7085 * the product, service, drug or other billing code for the item.) 7086 */ 7087 public CodeableConcept getProductOrService() { 7088 if (this.productOrService == null) 7089 if (Configuration.errorOnAutoCreate()) 7090 throw new Error("Attempt to auto-create DetailComponent.productOrService"); 7091 else if (Configuration.doAutoCreate()) 7092 this.productOrService = new CodeableConcept(); // cc 7093 return this.productOrService; 7094 } 7095 7096 public boolean hasProductOrService() { 7097 return this.productOrService != null && !this.productOrService.isEmpty(); 7098 } 7099 7100 /** 7101 * @param value {@link #productOrService} (When the value is a group code then 7102 * this item collects a set of related claim details, otherwise 7103 * this contains the product, service, drug or other billing code 7104 * for the item.) 7105 */ 7106 public DetailComponent setProductOrService(CodeableConcept value) { 7107 this.productOrService = value; 7108 return this; 7109 } 7110 7111 /** 7112 * @return {@link #modifier} (Item typification or modifiers codes to convey 7113 * additional context for the product or service.) 7114 */ 7115 public List<CodeableConcept> getModifier() { 7116 if (this.modifier == null) 7117 this.modifier = new ArrayList<CodeableConcept>(); 7118 return this.modifier; 7119 } 7120 7121 /** 7122 * @return Returns a reference to <code>this</code> for easy method chaining 7123 */ 7124 public DetailComponent setModifier(List<CodeableConcept> theModifier) { 7125 this.modifier = theModifier; 7126 return this; 7127 } 7128 7129 public boolean hasModifier() { 7130 if (this.modifier == null) 7131 return false; 7132 for (CodeableConcept item : this.modifier) 7133 if (!item.isEmpty()) 7134 return true; 7135 return false; 7136 } 7137 7138 public CodeableConcept addModifier() { // 3 7139 CodeableConcept t = new CodeableConcept(); 7140 if (this.modifier == null) 7141 this.modifier = new ArrayList<CodeableConcept>(); 7142 this.modifier.add(t); 7143 return t; 7144 } 7145 7146 public DetailComponent addModifier(CodeableConcept t) { // 3 7147 if (t == null) 7148 return this; 7149 if (this.modifier == null) 7150 this.modifier = new ArrayList<CodeableConcept>(); 7151 this.modifier.add(t); 7152 return this; 7153 } 7154 7155 /** 7156 * @return The first repetition of repeating field {@link #modifier}, creating 7157 * it if it does not already exist 7158 */ 7159 public CodeableConcept getModifierFirstRep() { 7160 if (getModifier().isEmpty()) { 7161 addModifier(); 7162 } 7163 return getModifier().get(0); 7164 } 7165 7166 /** 7167 * @return {@link #programCode} (Identifies the program under which this may be 7168 * recovered.) 7169 */ 7170 public List<CodeableConcept> getProgramCode() { 7171 if (this.programCode == null) 7172 this.programCode = new ArrayList<CodeableConcept>(); 7173 return this.programCode; 7174 } 7175 7176 /** 7177 * @return Returns a reference to <code>this</code> for easy method chaining 7178 */ 7179 public DetailComponent setProgramCode(List<CodeableConcept> theProgramCode) { 7180 this.programCode = theProgramCode; 7181 return this; 7182 } 7183 7184 public boolean hasProgramCode() { 7185 if (this.programCode == null) 7186 return false; 7187 for (CodeableConcept item : this.programCode) 7188 if (!item.isEmpty()) 7189 return true; 7190 return false; 7191 } 7192 7193 public CodeableConcept addProgramCode() { // 3 7194 CodeableConcept t = new CodeableConcept(); 7195 if (this.programCode == null) 7196 this.programCode = new ArrayList<CodeableConcept>(); 7197 this.programCode.add(t); 7198 return t; 7199 } 7200 7201 public DetailComponent addProgramCode(CodeableConcept t) { // 3 7202 if (t == null) 7203 return this; 7204 if (this.programCode == null) 7205 this.programCode = new ArrayList<CodeableConcept>(); 7206 this.programCode.add(t); 7207 return this; 7208 } 7209 7210 /** 7211 * @return The first repetition of repeating field {@link #programCode}, 7212 * creating it if it does not already exist 7213 */ 7214 public CodeableConcept getProgramCodeFirstRep() { 7215 if (getProgramCode().isEmpty()) { 7216 addProgramCode(); 7217 } 7218 return getProgramCode().get(0); 7219 } 7220 7221 /** 7222 * @return {@link #quantity} (The number of repetitions of a service or 7223 * product.) 7224 */ 7225 public Quantity getQuantity() { 7226 if (this.quantity == null) 7227 if (Configuration.errorOnAutoCreate()) 7228 throw new Error("Attempt to auto-create DetailComponent.quantity"); 7229 else if (Configuration.doAutoCreate()) 7230 this.quantity = new Quantity(); // cc 7231 return this.quantity; 7232 } 7233 7234 public boolean hasQuantity() { 7235 return this.quantity != null && !this.quantity.isEmpty(); 7236 } 7237 7238 /** 7239 * @param value {@link #quantity} (The number of repetitions of a service or 7240 * product.) 7241 */ 7242 public DetailComponent setQuantity(Quantity value) { 7243 this.quantity = value; 7244 return this; 7245 } 7246 7247 /** 7248 * @return {@link #unitPrice} (If the item is not a group then this is the fee 7249 * for the product or service, otherwise this is the total of the fees 7250 * for the details of the group.) 7251 */ 7252 public Money getUnitPrice() { 7253 if (this.unitPrice == null) 7254 if (Configuration.errorOnAutoCreate()) 7255 throw new Error("Attempt to auto-create DetailComponent.unitPrice"); 7256 else if (Configuration.doAutoCreate()) 7257 this.unitPrice = new Money(); // cc 7258 return this.unitPrice; 7259 } 7260 7261 public boolean hasUnitPrice() { 7262 return this.unitPrice != null && !this.unitPrice.isEmpty(); 7263 } 7264 7265 /** 7266 * @param value {@link #unitPrice} (If the item is not a group then this is the 7267 * fee for the product or service, otherwise this is the total of 7268 * the fees for the details of the group.) 7269 */ 7270 public DetailComponent setUnitPrice(Money value) { 7271 this.unitPrice = value; 7272 return this; 7273 } 7274 7275 /** 7276 * @return {@link #factor} (A real number that represents a multiplier used in 7277 * determining the overall value of services delivered and/or goods 7278 * received. The concept of a Factor allows for a discount or surcharge 7279 * multiplier to be applied to a monetary amount.). This is the 7280 * underlying object with id, value and extensions. The accessor 7281 * "getFactor" gives direct access to the value 7282 */ 7283 public DecimalType getFactorElement() { 7284 if (this.factor == null) 7285 if (Configuration.errorOnAutoCreate()) 7286 throw new Error("Attempt to auto-create DetailComponent.factor"); 7287 else if (Configuration.doAutoCreate()) 7288 this.factor = new DecimalType(); // bb 7289 return this.factor; 7290 } 7291 7292 public boolean hasFactorElement() { 7293 return this.factor != null && !this.factor.isEmpty(); 7294 } 7295 7296 public boolean hasFactor() { 7297 return this.factor != null && !this.factor.isEmpty(); 7298 } 7299 7300 /** 7301 * @param value {@link #factor} (A real number that represents a multiplier used 7302 * in determining the overall value of services delivered and/or 7303 * goods received. The concept of a Factor allows for a discount or 7304 * surcharge multiplier to be applied to a monetary amount.). This 7305 * is the underlying object with id, value and extensions. The 7306 * accessor "getFactor" gives direct access to the value 7307 */ 7308 public DetailComponent setFactorElement(DecimalType value) { 7309 this.factor = value; 7310 return this; 7311 } 7312 7313 /** 7314 * @return A real number that represents a multiplier used in determining the 7315 * overall value of services delivered and/or goods received. The 7316 * concept of a Factor allows for a discount or surcharge multiplier to 7317 * be applied to a monetary amount. 7318 */ 7319 public BigDecimal getFactor() { 7320 return this.factor == null ? null : this.factor.getValue(); 7321 } 7322 7323 /** 7324 * @param value A real number that represents a multiplier used in determining 7325 * the overall value of services delivered and/or goods received. 7326 * The concept of a Factor allows for a discount or surcharge 7327 * multiplier to be applied to a monetary amount. 7328 */ 7329 public DetailComponent setFactor(BigDecimal value) { 7330 if (value == null) 7331 this.factor = null; 7332 else { 7333 if (this.factor == null) 7334 this.factor = new DecimalType(); 7335 this.factor.setValue(value); 7336 } 7337 return this; 7338 } 7339 7340 /** 7341 * @param value A real number that represents a multiplier used in determining 7342 * the overall value of services delivered and/or goods received. 7343 * The concept of a Factor allows for a discount or surcharge 7344 * multiplier to be applied to a monetary amount. 7345 */ 7346 public DetailComponent setFactor(long value) { 7347 this.factor = new DecimalType(); 7348 this.factor.setValue(value); 7349 return this; 7350 } 7351 7352 /** 7353 * @param value A real number that represents a multiplier used in determining 7354 * the overall value of services delivered and/or goods received. 7355 * The concept of a Factor allows for a discount or surcharge 7356 * multiplier to be applied to a monetary amount. 7357 */ 7358 public DetailComponent setFactor(double value) { 7359 this.factor = new DecimalType(); 7360 this.factor.setValue(value); 7361 return this; 7362 } 7363 7364 /** 7365 * @return {@link #net} (The quantity times the unit price for an additional 7366 * service or product or charge.) 7367 */ 7368 public Money getNet() { 7369 if (this.net == null) 7370 if (Configuration.errorOnAutoCreate()) 7371 throw new Error("Attempt to auto-create DetailComponent.net"); 7372 else if (Configuration.doAutoCreate()) 7373 this.net = new Money(); // cc 7374 return this.net; 7375 } 7376 7377 public boolean hasNet() { 7378 return this.net != null && !this.net.isEmpty(); 7379 } 7380 7381 /** 7382 * @param value {@link #net} (The quantity times the unit price for an 7383 * additional service or product or charge.) 7384 */ 7385 public DetailComponent setNet(Money value) { 7386 this.net = value; 7387 return this; 7388 } 7389 7390 /** 7391 * @return {@link #udi} (Unique Device Identifiers associated with this line 7392 * item.) 7393 */ 7394 public List<Reference> getUdi() { 7395 if (this.udi == null) 7396 this.udi = new ArrayList<Reference>(); 7397 return this.udi; 7398 } 7399 7400 /** 7401 * @return Returns a reference to <code>this</code> for easy method chaining 7402 */ 7403 public DetailComponent setUdi(List<Reference> theUdi) { 7404 this.udi = theUdi; 7405 return this; 7406 } 7407 7408 public boolean hasUdi() { 7409 if (this.udi == null) 7410 return false; 7411 for (Reference item : this.udi) 7412 if (!item.isEmpty()) 7413 return true; 7414 return false; 7415 } 7416 7417 public Reference addUdi() { // 3 7418 Reference t = new Reference(); 7419 if (this.udi == null) 7420 this.udi = new ArrayList<Reference>(); 7421 this.udi.add(t); 7422 return t; 7423 } 7424 7425 public DetailComponent addUdi(Reference t) { // 3 7426 if (t == null) 7427 return this; 7428 if (this.udi == null) 7429 this.udi = new ArrayList<Reference>(); 7430 this.udi.add(t); 7431 return this; 7432 } 7433 7434 /** 7435 * @return The first repetition of repeating field {@link #udi}, creating it if 7436 * it does not already exist 7437 */ 7438 public Reference getUdiFirstRep() { 7439 if (getUdi().isEmpty()) { 7440 addUdi(); 7441 } 7442 return getUdi().get(0); 7443 } 7444 7445 /** 7446 * @deprecated Use Reference#setResource(IBaseResource) instead 7447 */ 7448 @Deprecated 7449 public List<Device> getUdiTarget() { 7450 if (this.udiTarget == null) 7451 this.udiTarget = new ArrayList<Device>(); 7452 return this.udiTarget; 7453 } 7454 7455 /** 7456 * @deprecated Use Reference#setResource(IBaseResource) instead 7457 */ 7458 @Deprecated 7459 public Device addUdiTarget() { 7460 Device r = new Device(); 7461 if (this.udiTarget == null) 7462 this.udiTarget = new ArrayList<Device>(); 7463 this.udiTarget.add(r); 7464 return r; 7465 } 7466 7467 /** 7468 * @return {@link #noteNumber} (The numbers associated with notes below which 7469 * apply to the adjudication of this item.) 7470 */ 7471 public List<PositiveIntType> getNoteNumber() { 7472 if (this.noteNumber == null) 7473 this.noteNumber = new ArrayList<PositiveIntType>(); 7474 return this.noteNumber; 7475 } 7476 7477 /** 7478 * @return Returns a reference to <code>this</code> for easy method chaining 7479 */ 7480 public DetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 7481 this.noteNumber = theNoteNumber; 7482 return this; 7483 } 7484 7485 public boolean hasNoteNumber() { 7486 if (this.noteNumber == null) 7487 return false; 7488 for (PositiveIntType item : this.noteNumber) 7489 if (!item.isEmpty()) 7490 return true; 7491 return false; 7492 } 7493 7494 /** 7495 * @return {@link #noteNumber} (The numbers associated with notes below which 7496 * apply to the adjudication of this item.) 7497 */ 7498 public PositiveIntType addNoteNumberElement() {// 2 7499 PositiveIntType t = new PositiveIntType(); 7500 if (this.noteNumber == null) 7501 this.noteNumber = new ArrayList<PositiveIntType>(); 7502 this.noteNumber.add(t); 7503 return t; 7504 } 7505 7506 /** 7507 * @param value {@link #noteNumber} (The numbers associated with notes below 7508 * which apply to the adjudication of this item.) 7509 */ 7510 public DetailComponent addNoteNumber(int value) { // 1 7511 PositiveIntType t = new PositiveIntType(); 7512 t.setValue(value); 7513 if (this.noteNumber == null) 7514 this.noteNumber = new ArrayList<PositiveIntType>(); 7515 this.noteNumber.add(t); 7516 return this; 7517 } 7518 7519 /** 7520 * @param value {@link #noteNumber} (The numbers associated with notes below 7521 * which apply to the adjudication of this item.) 7522 */ 7523 public boolean hasNoteNumber(int value) { 7524 if (this.noteNumber == null) 7525 return false; 7526 for (PositiveIntType v : this.noteNumber) 7527 if (v.getValue().equals(value)) // positiveInt 7528 return true; 7529 return false; 7530 } 7531 7532 /** 7533 * @return {@link #adjudication} (The adjudication results.) 7534 */ 7535 public List<AdjudicationComponent> getAdjudication() { 7536 if (this.adjudication == null) 7537 this.adjudication = new ArrayList<AdjudicationComponent>(); 7538 return this.adjudication; 7539 } 7540 7541 /** 7542 * @return Returns a reference to <code>this</code> for easy method chaining 7543 */ 7544 public DetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 7545 this.adjudication = theAdjudication; 7546 return this; 7547 } 7548 7549 public boolean hasAdjudication() { 7550 if (this.adjudication == null) 7551 return false; 7552 for (AdjudicationComponent item : this.adjudication) 7553 if (!item.isEmpty()) 7554 return true; 7555 return false; 7556 } 7557 7558 public AdjudicationComponent addAdjudication() { // 3 7559 AdjudicationComponent t = new AdjudicationComponent(); 7560 if (this.adjudication == null) 7561 this.adjudication = new ArrayList<AdjudicationComponent>(); 7562 this.adjudication.add(t); 7563 return t; 7564 } 7565 7566 public DetailComponent addAdjudication(AdjudicationComponent t) { // 3 7567 if (t == null) 7568 return this; 7569 if (this.adjudication == null) 7570 this.adjudication = new ArrayList<AdjudicationComponent>(); 7571 this.adjudication.add(t); 7572 return this; 7573 } 7574 7575 /** 7576 * @return The first repetition of repeating field {@link #adjudication}, 7577 * creating it if it does not already exist 7578 */ 7579 public AdjudicationComponent getAdjudicationFirstRep() { 7580 if (getAdjudication().isEmpty()) { 7581 addAdjudication(); 7582 } 7583 return getAdjudication().get(0); 7584 } 7585 7586 /** 7587 * @return {@link #subDetail} (Third-tier of goods and services.) 7588 */ 7589 public List<SubDetailComponent> getSubDetail() { 7590 if (this.subDetail == null) 7591 this.subDetail = new ArrayList<SubDetailComponent>(); 7592 return this.subDetail; 7593 } 7594 7595 /** 7596 * @return Returns a reference to <code>this</code> for easy method chaining 7597 */ 7598 public DetailComponent setSubDetail(List<SubDetailComponent> theSubDetail) { 7599 this.subDetail = theSubDetail; 7600 return this; 7601 } 7602 7603 public boolean hasSubDetail() { 7604 if (this.subDetail == null) 7605 return false; 7606 for (SubDetailComponent item : this.subDetail) 7607 if (!item.isEmpty()) 7608 return true; 7609 return false; 7610 } 7611 7612 public SubDetailComponent addSubDetail() { // 3 7613 SubDetailComponent t = new SubDetailComponent(); 7614 if (this.subDetail == null) 7615 this.subDetail = new ArrayList<SubDetailComponent>(); 7616 this.subDetail.add(t); 7617 return t; 7618 } 7619 7620 public DetailComponent addSubDetail(SubDetailComponent t) { // 3 7621 if (t == null) 7622 return this; 7623 if (this.subDetail == null) 7624 this.subDetail = new ArrayList<SubDetailComponent>(); 7625 this.subDetail.add(t); 7626 return this; 7627 } 7628 7629 /** 7630 * @return The first repetition of repeating field {@link #subDetail}, creating 7631 * it if it does not already exist 7632 */ 7633 public SubDetailComponent getSubDetailFirstRep() { 7634 if (getSubDetail().isEmpty()) { 7635 addSubDetail(); 7636 } 7637 return getSubDetail().get(0); 7638 } 7639 7640 protected void listChildren(List<Property> children) { 7641 super.listChildren(children); 7642 children.add(new Property("sequence", "positiveInt", 7643 "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 7644 0, 1, sequence)); 7645 children.add(new Property("revenue", "CodeableConcept", 7646 "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 7647 children.add(new Property("category", "CodeableConcept", 7648 "Code to identify the general type of benefits under which products and services are provided.", 0, 1, 7649 category)); 7650 children.add(new Property("productOrService", "CodeableConcept", 7651 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 7652 0, 1, productOrService)); 7653 children.add(new Property("modifier", "CodeableConcept", 7654 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 7655 java.lang.Integer.MAX_VALUE, modifier)); 7656 children.add(new Property("programCode", "CodeableConcept", 7657 "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 7658 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 7659 1, quantity)); 7660 children.add(new Property("unitPrice", "Money", 7661 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 7662 0, 1, unitPrice)); 7663 children.add(new Property("factor", "decimal", 7664 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 7665 0, 1, factor)); 7666 children.add(new Property("net", "Money", 7667 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net)); 7668 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 7669 0, java.lang.Integer.MAX_VALUE, udi)); 7670 children.add(new Property("noteNumber", "positiveInt", 7671 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 7672 java.lang.Integer.MAX_VALUE, noteNumber)); 7673 children.add(new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results.", 7674 0, java.lang.Integer.MAX_VALUE, adjudication)); 7675 children.add(new Property("subDetail", "", "Third-tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, 7676 subDetail)); 7677 } 7678 7679 @Override 7680 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7681 switch (_hash) { 7682 case 1349547969: 7683 /* sequence */ return new Property("sequence", "positiveInt", 7684 "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 7685 0, 1, sequence); 7686 case 1099842588: 7687 /* revenue */ return new Property("revenue", "CodeableConcept", 7688 "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 7689 case 50511102: 7690 /* category */ return new Property("category", "CodeableConcept", 7691 "Code to identify the general type of benefits under which products and services are provided.", 0, 1, 7692 category); 7693 case 1957227299: 7694 /* productOrService */ return new Property("productOrService", "CodeableConcept", 7695 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 7696 0, 1, productOrService); 7697 case -615513385: 7698 /* modifier */ return new Property("modifier", "CodeableConcept", 7699 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 7700 java.lang.Integer.MAX_VALUE, modifier); 7701 case 1010065041: 7702 /* programCode */ return new Property("programCode", "CodeableConcept", 7703 "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 7704 case -1285004149: 7705 /* quantity */ return new Property("quantity", "SimpleQuantity", 7706 "The number of repetitions of a service or product.", 0, 1, quantity); 7707 case -486196699: 7708 /* unitPrice */ return new Property("unitPrice", "Money", 7709 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 7710 0, 1, unitPrice); 7711 case -1282148017: 7712 /* factor */ return new Property("factor", "decimal", 7713 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 7714 0, 1, factor); 7715 case 108957: 7716 /* net */ return new Property("net", "Money", 7717 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net); 7718 case 115642: 7719 /* udi */ return new Property("udi", "Reference(Device)", 7720 "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 7721 case -1110033957: 7722 /* noteNumber */ return new Property("noteNumber", "positiveInt", 7723 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 7724 java.lang.Integer.MAX_VALUE, noteNumber); 7725 case -231349275: 7726 /* adjudication */ return new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", 7727 "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 7728 case -828829007: 7729 /* subDetail */ return new Property("subDetail", "", "Third-tier of goods and services.", 0, 7730 java.lang.Integer.MAX_VALUE, subDetail); 7731 default: 7732 return super.getNamedProperty(_hash, _name, _checkValid); 7733 } 7734 7735 } 7736 7737 @Override 7738 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7739 switch (hash) { 7740 case 1349547969: 7741 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 7742 case 1099842588: 7743 /* revenue */ return this.revenue == null ? new Base[0] : new Base[] { this.revenue }; // CodeableConcept 7744 case 50511102: 7745 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 7746 case 1957227299: 7747 /* productOrService */ return this.productOrService == null ? new Base[0] 7748 : new Base[] { this.productOrService }; // CodeableConcept 7749 case -615513385: 7750 /* modifier */ return this.modifier == null ? new Base[0] 7751 : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 7752 case 1010065041: 7753 /* programCode */ return this.programCode == null ? new Base[0] 7754 : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 7755 case -1285004149: 7756 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 7757 case -486196699: 7758 /* unitPrice */ return this.unitPrice == null ? new Base[0] : new Base[] { this.unitPrice }; // Money 7759 case -1282148017: 7760 /* factor */ return this.factor == null ? new Base[0] : new Base[] { this.factor }; // DecimalType 7761 case 108957: 7762 /* net */ return this.net == null ? new Base[0] : new Base[] { this.net }; // Money 7763 case 115642: 7764 /* udi */ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 7765 case -1110033957: 7766 /* noteNumber */ return this.noteNumber == null ? new Base[0] 7767 : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 7768 case -231349275: 7769 /* adjudication */ return this.adjudication == null ? new Base[0] 7770 : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 7771 case -828829007: 7772 /* subDetail */ return this.subDetail == null ? new Base[0] 7773 : this.subDetail.toArray(new Base[this.subDetail.size()]); // SubDetailComponent 7774 default: 7775 return super.getProperty(hash, name, checkValid); 7776 } 7777 7778 } 7779 7780 @Override 7781 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7782 switch (hash) { 7783 case 1349547969: // sequence 7784 this.sequence = castToPositiveInt(value); // PositiveIntType 7785 return value; 7786 case 1099842588: // revenue 7787 this.revenue = castToCodeableConcept(value); // CodeableConcept 7788 return value; 7789 case 50511102: // category 7790 this.category = castToCodeableConcept(value); // CodeableConcept 7791 return value; 7792 case 1957227299: // productOrService 7793 this.productOrService = castToCodeableConcept(value); // CodeableConcept 7794 return value; 7795 case -615513385: // modifier 7796 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 7797 return value; 7798 case 1010065041: // programCode 7799 this.getProgramCode().add(castToCodeableConcept(value)); // CodeableConcept 7800 return value; 7801 case -1285004149: // quantity 7802 this.quantity = castToQuantity(value); // Quantity 7803 return value; 7804 case -486196699: // unitPrice 7805 this.unitPrice = castToMoney(value); // Money 7806 return value; 7807 case -1282148017: // factor 7808 this.factor = castToDecimal(value); // DecimalType 7809 return value; 7810 case 108957: // net 7811 this.net = castToMoney(value); // Money 7812 return value; 7813 case 115642: // udi 7814 this.getUdi().add(castToReference(value)); // Reference 7815 return value; 7816 case -1110033957: // noteNumber 7817 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 7818 return value; 7819 case -231349275: // adjudication 7820 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 7821 return value; 7822 case -828829007: // subDetail 7823 this.getSubDetail().add((SubDetailComponent) value); // SubDetailComponent 7824 return value; 7825 default: 7826 return super.setProperty(hash, name, value); 7827 } 7828 7829 } 7830 7831 @Override 7832 public Base setProperty(String name, Base value) throws FHIRException { 7833 if (name.equals("sequence")) { 7834 this.sequence = castToPositiveInt(value); // PositiveIntType 7835 } else if (name.equals("revenue")) { 7836 this.revenue = castToCodeableConcept(value); // CodeableConcept 7837 } else if (name.equals("category")) { 7838 this.category = castToCodeableConcept(value); // CodeableConcept 7839 } else if (name.equals("productOrService")) { 7840 this.productOrService = castToCodeableConcept(value); // CodeableConcept 7841 } else if (name.equals("modifier")) { 7842 this.getModifier().add(castToCodeableConcept(value)); 7843 } else if (name.equals("programCode")) { 7844 this.getProgramCode().add(castToCodeableConcept(value)); 7845 } else if (name.equals("quantity")) { 7846 this.quantity = castToQuantity(value); // Quantity 7847 } else if (name.equals("unitPrice")) { 7848 this.unitPrice = castToMoney(value); // Money 7849 } else if (name.equals("factor")) { 7850 this.factor = castToDecimal(value); // DecimalType 7851 } else if (name.equals("net")) { 7852 this.net = castToMoney(value); // Money 7853 } else if (name.equals("udi")) { 7854 this.getUdi().add(castToReference(value)); 7855 } else if (name.equals("noteNumber")) { 7856 this.getNoteNumber().add(castToPositiveInt(value)); 7857 } else if (name.equals("adjudication")) { 7858 this.getAdjudication().add((AdjudicationComponent) value); 7859 } else if (name.equals("subDetail")) { 7860 this.getSubDetail().add((SubDetailComponent) value); 7861 } else 7862 return super.setProperty(name, value); 7863 return value; 7864 } 7865 7866 @Override 7867 public void removeChild(String name, Base value) throws FHIRException { 7868 if (name.equals("sequence")) { 7869 this.sequence = null; 7870 } else if (name.equals("revenue")) { 7871 this.revenue = null; 7872 } else if (name.equals("category")) { 7873 this.category = null; 7874 } else if (name.equals("productOrService")) { 7875 this.productOrService = null; 7876 } else if (name.equals("modifier")) { 7877 this.getModifier().remove(castToCodeableConcept(value)); 7878 } else if (name.equals("programCode")) { 7879 this.getProgramCode().remove(castToCodeableConcept(value)); 7880 } else if (name.equals("quantity")) { 7881 this.quantity = null; 7882 } else if (name.equals("unitPrice")) { 7883 this.unitPrice = null; 7884 } else if (name.equals("factor")) { 7885 this.factor = null; 7886 } else if (name.equals("net")) { 7887 this.net = null; 7888 } else if (name.equals("udi")) { 7889 this.getUdi().remove(castToReference(value)); 7890 } else if (name.equals("noteNumber")) { 7891 this.getNoteNumber().remove(castToPositiveInt(value)); 7892 } else if (name.equals("adjudication")) { 7893 this.getAdjudication().remove((AdjudicationComponent) value); 7894 } else if (name.equals("subDetail")) { 7895 this.getSubDetail().remove((SubDetailComponent) value); 7896 } else 7897 super.removeChild(name, value); 7898 7899 } 7900 7901 @Override 7902 public Base makeProperty(int hash, String name) throws FHIRException { 7903 switch (hash) { 7904 case 1349547969: 7905 return getSequenceElement(); 7906 case 1099842588: 7907 return getRevenue(); 7908 case 50511102: 7909 return getCategory(); 7910 case 1957227299: 7911 return getProductOrService(); 7912 case -615513385: 7913 return addModifier(); 7914 case 1010065041: 7915 return addProgramCode(); 7916 case -1285004149: 7917 return getQuantity(); 7918 case -486196699: 7919 return getUnitPrice(); 7920 case -1282148017: 7921 return getFactorElement(); 7922 case 108957: 7923 return getNet(); 7924 case 115642: 7925 return addUdi(); 7926 case -1110033957: 7927 return addNoteNumberElement(); 7928 case -231349275: 7929 return addAdjudication(); 7930 case -828829007: 7931 return addSubDetail(); 7932 default: 7933 return super.makeProperty(hash, name); 7934 } 7935 7936 } 7937 7938 @Override 7939 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7940 switch (hash) { 7941 case 1349547969: 7942 /* sequence */ return new String[] { "positiveInt" }; 7943 case 1099842588: 7944 /* revenue */ return new String[] { "CodeableConcept" }; 7945 case 50511102: 7946 /* category */ return new String[] { "CodeableConcept" }; 7947 case 1957227299: 7948 /* productOrService */ return new String[] { "CodeableConcept" }; 7949 case -615513385: 7950 /* modifier */ return new String[] { "CodeableConcept" }; 7951 case 1010065041: 7952 /* programCode */ return new String[] { "CodeableConcept" }; 7953 case -1285004149: 7954 /* quantity */ return new String[] { "SimpleQuantity" }; 7955 case -486196699: 7956 /* unitPrice */ return new String[] { "Money" }; 7957 case -1282148017: 7958 /* factor */ return new String[] { "decimal" }; 7959 case 108957: 7960 /* net */ return new String[] { "Money" }; 7961 case 115642: 7962 /* udi */ return new String[] { "Reference" }; 7963 case -1110033957: 7964 /* noteNumber */ return new String[] { "positiveInt" }; 7965 case -231349275: 7966 /* adjudication */ return new String[] { "@ExplanationOfBenefit.item.adjudication" }; 7967 case -828829007: 7968 /* subDetail */ return new String[] {}; 7969 default: 7970 return super.getTypesForProperty(hash, name); 7971 } 7972 7973 } 7974 7975 @Override 7976 public Base addChild(String name) throws FHIRException { 7977 if (name.equals("sequence")) { 7978 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.sequence"); 7979 } else if (name.equals("revenue")) { 7980 this.revenue = new CodeableConcept(); 7981 return this.revenue; 7982 } else if (name.equals("category")) { 7983 this.category = new CodeableConcept(); 7984 return this.category; 7985 } else if (name.equals("productOrService")) { 7986 this.productOrService = new CodeableConcept(); 7987 return this.productOrService; 7988 } else if (name.equals("modifier")) { 7989 return addModifier(); 7990 } else if (name.equals("programCode")) { 7991 return addProgramCode(); 7992 } else if (name.equals("quantity")) { 7993 this.quantity = new Quantity(); 7994 return this.quantity; 7995 } else if (name.equals("unitPrice")) { 7996 this.unitPrice = new Money(); 7997 return this.unitPrice; 7998 } else if (name.equals("factor")) { 7999 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.factor"); 8000 } else if (name.equals("net")) { 8001 this.net = new Money(); 8002 return this.net; 8003 } else if (name.equals("udi")) { 8004 return addUdi(); 8005 } else if (name.equals("noteNumber")) { 8006 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.noteNumber"); 8007 } else if (name.equals("adjudication")) { 8008 return addAdjudication(); 8009 } else if (name.equals("subDetail")) { 8010 return addSubDetail(); 8011 } else 8012 return super.addChild(name); 8013 } 8014 8015 public DetailComponent copy() { 8016 DetailComponent dst = new DetailComponent(); 8017 copyValues(dst); 8018 return dst; 8019 } 8020 8021 public void copyValues(DetailComponent dst) { 8022 super.copyValues(dst); 8023 dst.sequence = sequence == null ? null : sequence.copy(); 8024 dst.revenue = revenue == null ? null : revenue.copy(); 8025 dst.category = category == null ? null : category.copy(); 8026 dst.productOrService = productOrService == null ? null : productOrService.copy(); 8027 if (modifier != null) { 8028 dst.modifier = new ArrayList<CodeableConcept>(); 8029 for (CodeableConcept i : modifier) 8030 dst.modifier.add(i.copy()); 8031 } 8032 ; 8033 if (programCode != null) { 8034 dst.programCode = new ArrayList<CodeableConcept>(); 8035 for (CodeableConcept i : programCode) 8036 dst.programCode.add(i.copy()); 8037 } 8038 ; 8039 dst.quantity = quantity == null ? null : quantity.copy(); 8040 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 8041 dst.factor = factor == null ? null : factor.copy(); 8042 dst.net = net == null ? null : net.copy(); 8043 if (udi != null) { 8044 dst.udi = new ArrayList<Reference>(); 8045 for (Reference i : udi) 8046 dst.udi.add(i.copy()); 8047 } 8048 ; 8049 if (noteNumber != null) { 8050 dst.noteNumber = new ArrayList<PositiveIntType>(); 8051 for (PositiveIntType i : noteNumber) 8052 dst.noteNumber.add(i.copy()); 8053 } 8054 ; 8055 if (adjudication != null) { 8056 dst.adjudication = new ArrayList<AdjudicationComponent>(); 8057 for (AdjudicationComponent i : adjudication) 8058 dst.adjudication.add(i.copy()); 8059 } 8060 ; 8061 if (subDetail != null) { 8062 dst.subDetail = new ArrayList<SubDetailComponent>(); 8063 for (SubDetailComponent i : subDetail) 8064 dst.subDetail.add(i.copy()); 8065 } 8066 ; 8067 } 8068 8069 @Override 8070 public boolean equalsDeep(Base other_) { 8071 if (!super.equalsDeep(other_)) 8072 return false; 8073 if (!(other_ instanceof DetailComponent)) 8074 return false; 8075 DetailComponent o = (DetailComponent) other_; 8076 return compareDeep(sequence, o.sequence, true) && compareDeep(revenue, o.revenue, true) 8077 && compareDeep(category, o.category, true) && compareDeep(productOrService, o.productOrService, true) 8078 && compareDeep(modifier, o.modifier, true) && compareDeep(programCode, o.programCode, true) 8079 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 8080 && compareDeep(factor, o.factor, true) && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) 8081 && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(adjudication, o.adjudication, true) 8082 && compareDeep(subDetail, o.subDetail, true); 8083 } 8084 8085 @Override 8086 public boolean equalsShallow(Base other_) { 8087 if (!super.equalsShallow(other_)) 8088 return false; 8089 if (!(other_ instanceof DetailComponent)) 8090 return false; 8091 DetailComponent o = (DetailComponent) other_; 8092 return compareValues(sequence, o.sequence, true) && compareValues(factor, o.factor, true) 8093 && compareValues(noteNumber, o.noteNumber, true); 8094 } 8095 8096 public boolean isEmpty() { 8097 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, revenue, category, productOrService, 8098 modifier, programCode, quantity, unitPrice, factor, net, udi, noteNumber, adjudication, subDetail); 8099 } 8100 8101 public String fhirType() { 8102 return "ExplanationOfBenefit.item.detail"; 8103 8104 } 8105 8106 } 8107 8108 @Block() 8109 public static class SubDetailComponent extends BackboneElement implements IBaseBackboneElement { 8110 /** 8111 * A claim detail line. Either a simple (a product or service) or a 'group' of 8112 * sub-details which are simple items. 8113 */ 8114 @Child(name = "sequence", type = { 8115 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 8116 @Description(shortDefinition = "Product or service provided", formalDefinition = "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.") 8117 protected PositiveIntType sequence; 8118 8119 /** 8120 * The type of revenue or cost center providing the product and/or service. 8121 */ 8122 @Child(name = "revenue", type = { 8123 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 8124 @Description(shortDefinition = "Revenue or cost center code", formalDefinition = "The type of revenue or cost center providing the product and/or service.") 8125 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-revenue-center") 8126 protected CodeableConcept revenue; 8127 8128 /** 8129 * Code to identify the general type of benefits under which products and 8130 * services are provided. 8131 */ 8132 @Child(name = "category", type = { 8133 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 8134 @Description(shortDefinition = "Benefit classification", formalDefinition = "Code to identify the general type of benefits under which products and services are provided.") 8135 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-benefitcategory") 8136 protected CodeableConcept category; 8137 8138 /** 8139 * When the value is a group code then this item collects a set of related claim 8140 * details, otherwise this contains the product, service, drug or other billing 8141 * code for the item. 8142 */ 8143 @Child(name = "productOrService", type = { 8144 CodeableConcept.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 8145 @Description(shortDefinition = "Billing, service, product, or drug code", formalDefinition = "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.") 8146 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-uscls") 8147 protected CodeableConcept productOrService; 8148 8149 /** 8150 * Item typification or modifiers codes to convey additional context for the 8151 * product or service. 8152 */ 8153 @Child(name = "modifier", type = { 8154 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8155 @Description(shortDefinition = "Service/Product billing modifiers", formalDefinition = "Item typification or modifiers codes to convey additional context for the product or service.") 8156 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-modifiers") 8157 protected List<CodeableConcept> modifier; 8158 8159 /** 8160 * Identifies the program under which this may be recovered. 8161 */ 8162 @Child(name = "programCode", type = { 8163 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8164 @Description(shortDefinition = "Program the product or service is provided under", formalDefinition = "Identifies the program under which this may be recovered.") 8165 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-program-code") 8166 protected List<CodeableConcept> programCode; 8167 8168 /** 8169 * The number of repetitions of a service or product. 8170 */ 8171 @Child(name = "quantity", type = { Quantity.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 8172 @Description(shortDefinition = "Count of products or services", formalDefinition = "The number of repetitions of a service or product.") 8173 protected Quantity quantity; 8174 8175 /** 8176 * If the item is not a group then this is the fee for the product or service, 8177 * otherwise this is the total of the fees for the details of the group. 8178 */ 8179 @Child(name = "unitPrice", type = { Money.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 8180 @Description(shortDefinition = "Fee, charge or cost per item", formalDefinition = "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.") 8181 protected Money unitPrice; 8182 8183 /** 8184 * A real number that represents a multiplier used in determining the overall 8185 * value of services delivered and/or goods received. The concept of a Factor 8186 * allows for a discount or surcharge multiplier to be applied to a monetary 8187 * amount. 8188 */ 8189 @Child(name = "factor", type = { 8190 DecimalType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 8191 @Description(shortDefinition = "Price scaling factor", formalDefinition = "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.") 8192 protected DecimalType factor; 8193 8194 /** 8195 * The quantity times the unit price for an additional service or product or 8196 * charge. 8197 */ 8198 @Child(name = "net", type = { Money.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 8199 @Description(shortDefinition = "Total item cost", formalDefinition = "The quantity times the unit price for an additional service or product or charge.") 8200 protected Money net; 8201 8202 /** 8203 * Unique Device Identifiers associated with this line item. 8204 */ 8205 @Child(name = "udi", type = { 8206 Device.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8207 @Description(shortDefinition = "Unique device identifier", formalDefinition = "Unique Device Identifiers associated with this line item.") 8208 protected List<Reference> udi; 8209 /** 8210 * The actual objects that are the target of the reference (Unique Device 8211 * Identifiers associated with this line item.) 8212 */ 8213 protected List<Device> udiTarget; 8214 8215 /** 8216 * The numbers associated with notes below which apply to the adjudication of 8217 * this item. 8218 */ 8219 @Child(name = "noteNumber", type = { 8220 PositiveIntType.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8221 @Description(shortDefinition = "Applicable note numbers", formalDefinition = "The numbers associated with notes below which apply to the adjudication of this item.") 8222 protected List<PositiveIntType> noteNumber; 8223 8224 /** 8225 * The adjudication results. 8226 */ 8227 @Child(name = "adjudication", type = { 8228 AdjudicationComponent.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8229 @Description(shortDefinition = "Subdetail level adjudication details", formalDefinition = "The adjudication results.") 8230 protected List<AdjudicationComponent> adjudication; 8231 8232 private static final long serialVersionUID = -996156853L; 8233 8234 /** 8235 * Constructor 8236 */ 8237 public SubDetailComponent() { 8238 super(); 8239 } 8240 8241 /** 8242 * Constructor 8243 */ 8244 public SubDetailComponent(PositiveIntType sequence, CodeableConcept productOrService) { 8245 super(); 8246 this.sequence = sequence; 8247 this.productOrService = productOrService; 8248 } 8249 8250 /** 8251 * @return {@link #sequence} (A claim detail line. Either a simple (a product or 8252 * service) or a 'group' of sub-details which are simple items.). This 8253 * is the underlying object with id, value and extensions. The accessor 8254 * "getSequence" gives direct access to the value 8255 */ 8256 public PositiveIntType getSequenceElement() { 8257 if (this.sequence == null) 8258 if (Configuration.errorOnAutoCreate()) 8259 throw new Error("Attempt to auto-create SubDetailComponent.sequence"); 8260 else if (Configuration.doAutoCreate()) 8261 this.sequence = new PositiveIntType(); // bb 8262 return this.sequence; 8263 } 8264 8265 public boolean hasSequenceElement() { 8266 return this.sequence != null && !this.sequence.isEmpty(); 8267 } 8268 8269 public boolean hasSequence() { 8270 return this.sequence != null && !this.sequence.isEmpty(); 8271 } 8272 8273 /** 8274 * @param value {@link #sequence} (A claim detail line. Either a simple (a 8275 * product or service) or a 'group' of sub-details which are simple 8276 * items.). This is the underlying object with id, value and 8277 * extensions. The accessor "getSequence" gives direct access to 8278 * the value 8279 */ 8280 public SubDetailComponent setSequenceElement(PositiveIntType value) { 8281 this.sequence = value; 8282 return this; 8283 } 8284 8285 /** 8286 * @return A claim detail line. Either a simple (a product or service) or a 8287 * 'group' of sub-details which are simple items. 8288 */ 8289 public int getSequence() { 8290 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 8291 } 8292 8293 /** 8294 * @param value A claim detail line. Either a simple (a product or service) or a 8295 * 'group' of sub-details which are simple items. 8296 */ 8297 public SubDetailComponent setSequence(int value) { 8298 if (this.sequence == null) 8299 this.sequence = new PositiveIntType(); 8300 this.sequence.setValue(value); 8301 return this; 8302 } 8303 8304 /** 8305 * @return {@link #revenue} (The type of revenue or cost center providing the 8306 * product and/or service.) 8307 */ 8308 public CodeableConcept getRevenue() { 8309 if (this.revenue == null) 8310 if (Configuration.errorOnAutoCreate()) 8311 throw new Error("Attempt to auto-create SubDetailComponent.revenue"); 8312 else if (Configuration.doAutoCreate()) 8313 this.revenue = new CodeableConcept(); // cc 8314 return this.revenue; 8315 } 8316 8317 public boolean hasRevenue() { 8318 return this.revenue != null && !this.revenue.isEmpty(); 8319 } 8320 8321 /** 8322 * @param value {@link #revenue} (The type of revenue or cost center providing 8323 * the product and/or service.) 8324 */ 8325 public SubDetailComponent setRevenue(CodeableConcept value) { 8326 this.revenue = value; 8327 return this; 8328 } 8329 8330 /** 8331 * @return {@link #category} (Code to identify the general type of benefits 8332 * under which products and services are provided.) 8333 */ 8334 public CodeableConcept getCategory() { 8335 if (this.category == null) 8336 if (Configuration.errorOnAutoCreate()) 8337 throw new Error("Attempt to auto-create SubDetailComponent.category"); 8338 else if (Configuration.doAutoCreate()) 8339 this.category = new CodeableConcept(); // cc 8340 return this.category; 8341 } 8342 8343 public boolean hasCategory() { 8344 return this.category != null && !this.category.isEmpty(); 8345 } 8346 8347 /** 8348 * @param value {@link #category} (Code to identify the general type of benefits 8349 * under which products and services are provided.) 8350 */ 8351 public SubDetailComponent setCategory(CodeableConcept value) { 8352 this.category = value; 8353 return this; 8354 } 8355 8356 /** 8357 * @return {@link #productOrService} (When the value is a group code then this 8358 * item collects a set of related claim details, otherwise this contains 8359 * the product, service, drug or other billing code for the item.) 8360 */ 8361 public CodeableConcept getProductOrService() { 8362 if (this.productOrService == null) 8363 if (Configuration.errorOnAutoCreate()) 8364 throw new Error("Attempt to auto-create SubDetailComponent.productOrService"); 8365 else if (Configuration.doAutoCreate()) 8366 this.productOrService = new CodeableConcept(); // cc 8367 return this.productOrService; 8368 } 8369 8370 public boolean hasProductOrService() { 8371 return this.productOrService != null && !this.productOrService.isEmpty(); 8372 } 8373 8374 /** 8375 * @param value {@link #productOrService} (When the value is a group code then 8376 * this item collects a set of related claim details, otherwise 8377 * this contains the product, service, drug or other billing code 8378 * for the item.) 8379 */ 8380 public SubDetailComponent setProductOrService(CodeableConcept value) { 8381 this.productOrService = value; 8382 return this; 8383 } 8384 8385 /** 8386 * @return {@link #modifier} (Item typification or modifiers codes to convey 8387 * additional context for the product or service.) 8388 */ 8389 public List<CodeableConcept> getModifier() { 8390 if (this.modifier == null) 8391 this.modifier = new ArrayList<CodeableConcept>(); 8392 return this.modifier; 8393 } 8394 8395 /** 8396 * @return Returns a reference to <code>this</code> for easy method chaining 8397 */ 8398 public SubDetailComponent setModifier(List<CodeableConcept> theModifier) { 8399 this.modifier = theModifier; 8400 return this; 8401 } 8402 8403 public boolean hasModifier() { 8404 if (this.modifier == null) 8405 return false; 8406 for (CodeableConcept item : this.modifier) 8407 if (!item.isEmpty()) 8408 return true; 8409 return false; 8410 } 8411 8412 public CodeableConcept addModifier() { // 3 8413 CodeableConcept t = new CodeableConcept(); 8414 if (this.modifier == null) 8415 this.modifier = new ArrayList<CodeableConcept>(); 8416 this.modifier.add(t); 8417 return t; 8418 } 8419 8420 public SubDetailComponent addModifier(CodeableConcept t) { // 3 8421 if (t == null) 8422 return this; 8423 if (this.modifier == null) 8424 this.modifier = new ArrayList<CodeableConcept>(); 8425 this.modifier.add(t); 8426 return this; 8427 } 8428 8429 /** 8430 * @return The first repetition of repeating field {@link #modifier}, creating 8431 * it if it does not already exist 8432 */ 8433 public CodeableConcept getModifierFirstRep() { 8434 if (getModifier().isEmpty()) { 8435 addModifier(); 8436 } 8437 return getModifier().get(0); 8438 } 8439 8440 /** 8441 * @return {@link #programCode} (Identifies the program under which this may be 8442 * recovered.) 8443 */ 8444 public List<CodeableConcept> getProgramCode() { 8445 if (this.programCode == null) 8446 this.programCode = new ArrayList<CodeableConcept>(); 8447 return this.programCode; 8448 } 8449 8450 /** 8451 * @return Returns a reference to <code>this</code> for easy method chaining 8452 */ 8453 public SubDetailComponent setProgramCode(List<CodeableConcept> theProgramCode) { 8454 this.programCode = theProgramCode; 8455 return this; 8456 } 8457 8458 public boolean hasProgramCode() { 8459 if (this.programCode == null) 8460 return false; 8461 for (CodeableConcept item : this.programCode) 8462 if (!item.isEmpty()) 8463 return true; 8464 return false; 8465 } 8466 8467 public CodeableConcept addProgramCode() { // 3 8468 CodeableConcept t = new CodeableConcept(); 8469 if (this.programCode == null) 8470 this.programCode = new ArrayList<CodeableConcept>(); 8471 this.programCode.add(t); 8472 return t; 8473 } 8474 8475 public SubDetailComponent addProgramCode(CodeableConcept t) { // 3 8476 if (t == null) 8477 return this; 8478 if (this.programCode == null) 8479 this.programCode = new ArrayList<CodeableConcept>(); 8480 this.programCode.add(t); 8481 return this; 8482 } 8483 8484 /** 8485 * @return The first repetition of repeating field {@link #programCode}, 8486 * creating it if it does not already exist 8487 */ 8488 public CodeableConcept getProgramCodeFirstRep() { 8489 if (getProgramCode().isEmpty()) { 8490 addProgramCode(); 8491 } 8492 return getProgramCode().get(0); 8493 } 8494 8495 /** 8496 * @return {@link #quantity} (The number of repetitions of a service or 8497 * product.) 8498 */ 8499 public Quantity getQuantity() { 8500 if (this.quantity == null) 8501 if (Configuration.errorOnAutoCreate()) 8502 throw new Error("Attempt to auto-create SubDetailComponent.quantity"); 8503 else if (Configuration.doAutoCreate()) 8504 this.quantity = new Quantity(); // cc 8505 return this.quantity; 8506 } 8507 8508 public boolean hasQuantity() { 8509 return this.quantity != null && !this.quantity.isEmpty(); 8510 } 8511 8512 /** 8513 * @param value {@link #quantity} (The number of repetitions of a service or 8514 * product.) 8515 */ 8516 public SubDetailComponent setQuantity(Quantity value) { 8517 this.quantity = value; 8518 return this; 8519 } 8520 8521 /** 8522 * @return {@link #unitPrice} (If the item is not a group then this is the fee 8523 * for the product or service, otherwise this is the total of the fees 8524 * for the details of the group.) 8525 */ 8526 public Money getUnitPrice() { 8527 if (this.unitPrice == null) 8528 if (Configuration.errorOnAutoCreate()) 8529 throw new Error("Attempt to auto-create SubDetailComponent.unitPrice"); 8530 else if (Configuration.doAutoCreate()) 8531 this.unitPrice = new Money(); // cc 8532 return this.unitPrice; 8533 } 8534 8535 public boolean hasUnitPrice() { 8536 return this.unitPrice != null && !this.unitPrice.isEmpty(); 8537 } 8538 8539 /** 8540 * @param value {@link #unitPrice} (If the item is not a group then this is the 8541 * fee for the product or service, otherwise this is the total of 8542 * the fees for the details of the group.) 8543 */ 8544 public SubDetailComponent setUnitPrice(Money value) { 8545 this.unitPrice = value; 8546 return this; 8547 } 8548 8549 /** 8550 * @return {@link #factor} (A real number that represents a multiplier used in 8551 * determining the overall value of services delivered and/or goods 8552 * received. The concept of a Factor allows for a discount or surcharge 8553 * multiplier to be applied to a monetary amount.). This is the 8554 * underlying object with id, value and extensions. The accessor 8555 * "getFactor" gives direct access to the value 8556 */ 8557 public DecimalType getFactorElement() { 8558 if (this.factor == null) 8559 if (Configuration.errorOnAutoCreate()) 8560 throw new Error("Attempt to auto-create SubDetailComponent.factor"); 8561 else if (Configuration.doAutoCreate()) 8562 this.factor = new DecimalType(); // bb 8563 return this.factor; 8564 } 8565 8566 public boolean hasFactorElement() { 8567 return this.factor != null && !this.factor.isEmpty(); 8568 } 8569 8570 public boolean hasFactor() { 8571 return this.factor != null && !this.factor.isEmpty(); 8572 } 8573 8574 /** 8575 * @param value {@link #factor} (A real number that represents a multiplier used 8576 * in determining the overall value of services delivered and/or 8577 * goods received. The concept of a Factor allows for a discount or 8578 * surcharge multiplier to be applied to a monetary amount.). This 8579 * is the underlying object with id, value and extensions. The 8580 * accessor "getFactor" gives direct access to the value 8581 */ 8582 public SubDetailComponent setFactorElement(DecimalType value) { 8583 this.factor = value; 8584 return this; 8585 } 8586 8587 /** 8588 * @return A real number that represents a multiplier used in determining the 8589 * overall value of services delivered and/or goods received. The 8590 * concept of a Factor allows for a discount or surcharge multiplier to 8591 * be applied to a monetary amount. 8592 */ 8593 public BigDecimal getFactor() { 8594 return this.factor == null ? null : this.factor.getValue(); 8595 } 8596 8597 /** 8598 * @param value A real number that represents a multiplier used in determining 8599 * the overall value of services delivered and/or goods received. 8600 * The concept of a Factor allows for a discount or surcharge 8601 * multiplier to be applied to a monetary amount. 8602 */ 8603 public SubDetailComponent setFactor(BigDecimal value) { 8604 if (value == null) 8605 this.factor = null; 8606 else { 8607 if (this.factor == null) 8608 this.factor = new DecimalType(); 8609 this.factor.setValue(value); 8610 } 8611 return this; 8612 } 8613 8614 /** 8615 * @param value A real number that represents a multiplier used in determining 8616 * the overall value of services delivered and/or goods received. 8617 * The concept of a Factor allows for a discount or surcharge 8618 * multiplier to be applied to a monetary amount. 8619 */ 8620 public SubDetailComponent setFactor(long value) { 8621 this.factor = new DecimalType(); 8622 this.factor.setValue(value); 8623 return this; 8624 } 8625 8626 /** 8627 * @param value A real number that represents a multiplier used in determining 8628 * the overall value of services delivered and/or goods received. 8629 * The concept of a Factor allows for a discount or surcharge 8630 * multiplier to be applied to a monetary amount. 8631 */ 8632 public SubDetailComponent setFactor(double value) { 8633 this.factor = new DecimalType(); 8634 this.factor.setValue(value); 8635 return this; 8636 } 8637 8638 /** 8639 * @return {@link #net} (The quantity times the unit price for an additional 8640 * service or product or charge.) 8641 */ 8642 public Money getNet() { 8643 if (this.net == null) 8644 if (Configuration.errorOnAutoCreate()) 8645 throw new Error("Attempt to auto-create SubDetailComponent.net"); 8646 else if (Configuration.doAutoCreate()) 8647 this.net = new Money(); // cc 8648 return this.net; 8649 } 8650 8651 public boolean hasNet() { 8652 return this.net != null && !this.net.isEmpty(); 8653 } 8654 8655 /** 8656 * @param value {@link #net} (The quantity times the unit price for an 8657 * additional service or product or charge.) 8658 */ 8659 public SubDetailComponent setNet(Money value) { 8660 this.net = value; 8661 return this; 8662 } 8663 8664 /** 8665 * @return {@link #udi} (Unique Device Identifiers associated with this line 8666 * item.) 8667 */ 8668 public List<Reference> getUdi() { 8669 if (this.udi == null) 8670 this.udi = new ArrayList<Reference>(); 8671 return this.udi; 8672 } 8673 8674 /** 8675 * @return Returns a reference to <code>this</code> for easy method chaining 8676 */ 8677 public SubDetailComponent setUdi(List<Reference> theUdi) { 8678 this.udi = theUdi; 8679 return this; 8680 } 8681 8682 public boolean hasUdi() { 8683 if (this.udi == null) 8684 return false; 8685 for (Reference item : this.udi) 8686 if (!item.isEmpty()) 8687 return true; 8688 return false; 8689 } 8690 8691 public Reference addUdi() { // 3 8692 Reference t = new Reference(); 8693 if (this.udi == null) 8694 this.udi = new ArrayList<Reference>(); 8695 this.udi.add(t); 8696 return t; 8697 } 8698 8699 public SubDetailComponent addUdi(Reference t) { // 3 8700 if (t == null) 8701 return this; 8702 if (this.udi == null) 8703 this.udi = new ArrayList<Reference>(); 8704 this.udi.add(t); 8705 return this; 8706 } 8707 8708 /** 8709 * @return The first repetition of repeating field {@link #udi}, creating it if 8710 * it does not already exist 8711 */ 8712 public Reference getUdiFirstRep() { 8713 if (getUdi().isEmpty()) { 8714 addUdi(); 8715 } 8716 return getUdi().get(0); 8717 } 8718 8719 /** 8720 * @deprecated Use Reference#setResource(IBaseResource) instead 8721 */ 8722 @Deprecated 8723 public List<Device> getUdiTarget() { 8724 if (this.udiTarget == null) 8725 this.udiTarget = new ArrayList<Device>(); 8726 return this.udiTarget; 8727 } 8728 8729 /** 8730 * @deprecated Use Reference#setResource(IBaseResource) instead 8731 */ 8732 @Deprecated 8733 public Device addUdiTarget() { 8734 Device r = new Device(); 8735 if (this.udiTarget == null) 8736 this.udiTarget = new ArrayList<Device>(); 8737 this.udiTarget.add(r); 8738 return r; 8739 } 8740 8741 /** 8742 * @return {@link #noteNumber} (The numbers associated with notes below which 8743 * apply to the adjudication of this item.) 8744 */ 8745 public List<PositiveIntType> getNoteNumber() { 8746 if (this.noteNumber == null) 8747 this.noteNumber = new ArrayList<PositiveIntType>(); 8748 return this.noteNumber; 8749 } 8750 8751 /** 8752 * @return Returns a reference to <code>this</code> for easy method chaining 8753 */ 8754 public SubDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 8755 this.noteNumber = theNoteNumber; 8756 return this; 8757 } 8758 8759 public boolean hasNoteNumber() { 8760 if (this.noteNumber == null) 8761 return false; 8762 for (PositiveIntType item : this.noteNumber) 8763 if (!item.isEmpty()) 8764 return true; 8765 return false; 8766 } 8767 8768 /** 8769 * @return {@link #noteNumber} (The numbers associated with notes below which 8770 * apply to the adjudication of this item.) 8771 */ 8772 public PositiveIntType addNoteNumberElement() {// 2 8773 PositiveIntType t = new PositiveIntType(); 8774 if (this.noteNumber == null) 8775 this.noteNumber = new ArrayList<PositiveIntType>(); 8776 this.noteNumber.add(t); 8777 return t; 8778 } 8779 8780 /** 8781 * @param value {@link #noteNumber} (The numbers associated with notes below 8782 * which apply to the adjudication of this item.) 8783 */ 8784 public SubDetailComponent addNoteNumber(int value) { // 1 8785 PositiveIntType t = new PositiveIntType(); 8786 t.setValue(value); 8787 if (this.noteNumber == null) 8788 this.noteNumber = new ArrayList<PositiveIntType>(); 8789 this.noteNumber.add(t); 8790 return this; 8791 } 8792 8793 /** 8794 * @param value {@link #noteNumber} (The numbers associated with notes below 8795 * which apply to the adjudication of this item.) 8796 */ 8797 public boolean hasNoteNumber(int value) { 8798 if (this.noteNumber == null) 8799 return false; 8800 for (PositiveIntType v : this.noteNumber) 8801 if (v.getValue().equals(value)) // positiveInt 8802 return true; 8803 return false; 8804 } 8805 8806 /** 8807 * @return {@link #adjudication} (The adjudication results.) 8808 */ 8809 public List<AdjudicationComponent> getAdjudication() { 8810 if (this.adjudication == null) 8811 this.adjudication = new ArrayList<AdjudicationComponent>(); 8812 return this.adjudication; 8813 } 8814 8815 /** 8816 * @return Returns a reference to <code>this</code> for easy method chaining 8817 */ 8818 public SubDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 8819 this.adjudication = theAdjudication; 8820 return this; 8821 } 8822 8823 public boolean hasAdjudication() { 8824 if (this.adjudication == null) 8825 return false; 8826 for (AdjudicationComponent item : this.adjudication) 8827 if (!item.isEmpty()) 8828 return true; 8829 return false; 8830 } 8831 8832 public AdjudicationComponent addAdjudication() { // 3 8833 AdjudicationComponent t = new AdjudicationComponent(); 8834 if (this.adjudication == null) 8835 this.adjudication = new ArrayList<AdjudicationComponent>(); 8836 this.adjudication.add(t); 8837 return t; 8838 } 8839 8840 public SubDetailComponent addAdjudication(AdjudicationComponent t) { // 3 8841 if (t == null) 8842 return this; 8843 if (this.adjudication == null) 8844 this.adjudication = new ArrayList<AdjudicationComponent>(); 8845 this.adjudication.add(t); 8846 return this; 8847 } 8848 8849 /** 8850 * @return The first repetition of repeating field {@link #adjudication}, 8851 * creating it if it does not already exist 8852 */ 8853 public AdjudicationComponent getAdjudicationFirstRep() { 8854 if (getAdjudication().isEmpty()) { 8855 addAdjudication(); 8856 } 8857 return getAdjudication().get(0); 8858 } 8859 8860 protected void listChildren(List<Property> children) { 8861 super.listChildren(children); 8862 children.add(new Property("sequence", "positiveInt", 8863 "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 8864 0, 1, sequence)); 8865 children.add(new Property("revenue", "CodeableConcept", 8866 "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 8867 children.add(new Property("category", "CodeableConcept", 8868 "Code to identify the general type of benefits under which products and services are provided.", 0, 1, 8869 category)); 8870 children.add(new Property("productOrService", "CodeableConcept", 8871 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 8872 0, 1, productOrService)); 8873 children.add(new Property("modifier", "CodeableConcept", 8874 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 8875 java.lang.Integer.MAX_VALUE, modifier)); 8876 children.add(new Property("programCode", "CodeableConcept", 8877 "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 8878 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 8879 1, quantity)); 8880 children.add(new Property("unitPrice", "Money", 8881 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 8882 0, 1, unitPrice)); 8883 children.add(new Property("factor", "decimal", 8884 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 8885 0, 1, factor)); 8886 children.add(new Property("net", "Money", 8887 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net)); 8888 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 8889 0, java.lang.Integer.MAX_VALUE, udi)); 8890 children.add(new Property("noteNumber", "positiveInt", 8891 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 8892 java.lang.Integer.MAX_VALUE, noteNumber)); 8893 children.add(new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results.", 8894 0, java.lang.Integer.MAX_VALUE, adjudication)); 8895 } 8896 8897 @Override 8898 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8899 switch (_hash) { 8900 case 1349547969: 8901 /* sequence */ return new Property("sequence", "positiveInt", 8902 "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 8903 0, 1, sequence); 8904 case 1099842588: 8905 /* revenue */ return new Property("revenue", "CodeableConcept", 8906 "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 8907 case 50511102: 8908 /* category */ return new Property("category", "CodeableConcept", 8909 "Code to identify the general type of benefits under which products and services are provided.", 0, 1, 8910 category); 8911 case 1957227299: 8912 /* productOrService */ return new Property("productOrService", "CodeableConcept", 8913 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 8914 0, 1, productOrService); 8915 case -615513385: 8916 /* modifier */ return new Property("modifier", "CodeableConcept", 8917 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 8918 java.lang.Integer.MAX_VALUE, modifier); 8919 case 1010065041: 8920 /* programCode */ return new Property("programCode", "CodeableConcept", 8921 "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 8922 case -1285004149: 8923 /* quantity */ return new Property("quantity", "SimpleQuantity", 8924 "The number of repetitions of a service or product.", 0, 1, quantity); 8925 case -486196699: 8926 /* unitPrice */ return new Property("unitPrice", "Money", 8927 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 8928 0, 1, unitPrice); 8929 case -1282148017: 8930 /* factor */ return new Property("factor", "decimal", 8931 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 8932 0, 1, factor); 8933 case 108957: 8934 /* net */ return new Property("net", "Money", 8935 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net); 8936 case 115642: 8937 /* udi */ return new Property("udi", "Reference(Device)", 8938 "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 8939 case -1110033957: 8940 /* noteNumber */ return new Property("noteNumber", "positiveInt", 8941 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 8942 java.lang.Integer.MAX_VALUE, noteNumber); 8943 case -231349275: 8944 /* adjudication */ return new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", 8945 "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 8946 default: 8947 return super.getNamedProperty(_hash, _name, _checkValid); 8948 } 8949 8950 } 8951 8952 @Override 8953 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8954 switch (hash) { 8955 case 1349547969: 8956 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 8957 case 1099842588: 8958 /* revenue */ return this.revenue == null ? new Base[0] : new Base[] { this.revenue }; // CodeableConcept 8959 case 50511102: 8960 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 8961 case 1957227299: 8962 /* productOrService */ return this.productOrService == null ? new Base[0] 8963 : new Base[] { this.productOrService }; // CodeableConcept 8964 case -615513385: 8965 /* modifier */ return this.modifier == null ? new Base[0] 8966 : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 8967 case 1010065041: 8968 /* programCode */ return this.programCode == null ? new Base[0] 8969 : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 8970 case -1285004149: 8971 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 8972 case -486196699: 8973 /* unitPrice */ return this.unitPrice == null ? new Base[0] : new Base[] { this.unitPrice }; // Money 8974 case -1282148017: 8975 /* factor */ return this.factor == null ? new Base[0] : new Base[] { this.factor }; // DecimalType 8976 case 108957: 8977 /* net */ return this.net == null ? new Base[0] : new Base[] { this.net }; // Money 8978 case 115642: 8979 /* udi */ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 8980 case -1110033957: 8981 /* noteNumber */ return this.noteNumber == null ? new Base[0] 8982 : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 8983 case -231349275: 8984 /* adjudication */ return this.adjudication == null ? new Base[0] 8985 : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 8986 default: 8987 return super.getProperty(hash, name, checkValid); 8988 } 8989 8990 } 8991 8992 @Override 8993 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8994 switch (hash) { 8995 case 1349547969: // sequence 8996 this.sequence = castToPositiveInt(value); // PositiveIntType 8997 return value; 8998 case 1099842588: // revenue 8999 this.revenue = castToCodeableConcept(value); // CodeableConcept 9000 return value; 9001 case 50511102: // category 9002 this.category = castToCodeableConcept(value); // CodeableConcept 9003 return value; 9004 case 1957227299: // productOrService 9005 this.productOrService = castToCodeableConcept(value); // CodeableConcept 9006 return value; 9007 case -615513385: // modifier 9008 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 9009 return value; 9010 case 1010065041: // programCode 9011 this.getProgramCode().add(castToCodeableConcept(value)); // CodeableConcept 9012 return value; 9013 case -1285004149: // quantity 9014 this.quantity = castToQuantity(value); // Quantity 9015 return value; 9016 case -486196699: // unitPrice 9017 this.unitPrice = castToMoney(value); // Money 9018 return value; 9019 case -1282148017: // factor 9020 this.factor = castToDecimal(value); // DecimalType 9021 return value; 9022 case 108957: // net 9023 this.net = castToMoney(value); // Money 9024 return value; 9025 case 115642: // udi 9026 this.getUdi().add(castToReference(value)); // Reference 9027 return value; 9028 case -1110033957: // noteNumber 9029 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 9030 return value; 9031 case -231349275: // adjudication 9032 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 9033 return value; 9034 default: 9035 return super.setProperty(hash, name, value); 9036 } 9037 9038 } 9039 9040 @Override 9041 public Base setProperty(String name, Base value) throws FHIRException { 9042 if (name.equals("sequence")) { 9043 this.sequence = castToPositiveInt(value); // PositiveIntType 9044 } else if (name.equals("revenue")) { 9045 this.revenue = castToCodeableConcept(value); // CodeableConcept 9046 } else if (name.equals("category")) { 9047 this.category = castToCodeableConcept(value); // CodeableConcept 9048 } else if (name.equals("productOrService")) { 9049 this.productOrService = castToCodeableConcept(value); // CodeableConcept 9050 } else if (name.equals("modifier")) { 9051 this.getModifier().add(castToCodeableConcept(value)); 9052 } else if (name.equals("programCode")) { 9053 this.getProgramCode().add(castToCodeableConcept(value)); 9054 } else if (name.equals("quantity")) { 9055 this.quantity = castToQuantity(value); // Quantity 9056 } else if (name.equals("unitPrice")) { 9057 this.unitPrice = castToMoney(value); // Money 9058 } else if (name.equals("factor")) { 9059 this.factor = castToDecimal(value); // DecimalType 9060 } else if (name.equals("net")) { 9061 this.net = castToMoney(value); // Money 9062 } else if (name.equals("udi")) { 9063 this.getUdi().add(castToReference(value)); 9064 } else if (name.equals("noteNumber")) { 9065 this.getNoteNumber().add(castToPositiveInt(value)); 9066 } else if (name.equals("adjudication")) { 9067 this.getAdjudication().add((AdjudicationComponent) value); 9068 } else 9069 return super.setProperty(name, value); 9070 return value; 9071 } 9072 9073 @Override 9074 public void removeChild(String name, Base value) throws FHIRException { 9075 if (name.equals("sequence")) { 9076 this.sequence = null; 9077 } else if (name.equals("revenue")) { 9078 this.revenue = null; 9079 } else if (name.equals("category")) { 9080 this.category = null; 9081 } else if (name.equals("productOrService")) { 9082 this.productOrService = null; 9083 } else if (name.equals("modifier")) { 9084 this.getModifier().remove(castToCodeableConcept(value)); 9085 } else if (name.equals("programCode")) { 9086 this.getProgramCode().remove(castToCodeableConcept(value)); 9087 } else if (name.equals("quantity")) { 9088 this.quantity = null; 9089 } else if (name.equals("unitPrice")) { 9090 this.unitPrice = null; 9091 } else if (name.equals("factor")) { 9092 this.factor = null; 9093 } else if (name.equals("net")) { 9094 this.net = null; 9095 } else if (name.equals("udi")) { 9096 this.getUdi().remove(castToReference(value)); 9097 } else if (name.equals("noteNumber")) { 9098 this.getNoteNumber().remove(castToPositiveInt(value)); 9099 } else if (name.equals("adjudication")) { 9100 this.getAdjudication().remove((AdjudicationComponent) value); 9101 } else 9102 super.removeChild(name, value); 9103 9104 } 9105 9106 @Override 9107 public Base makeProperty(int hash, String name) throws FHIRException { 9108 switch (hash) { 9109 case 1349547969: 9110 return getSequenceElement(); 9111 case 1099842588: 9112 return getRevenue(); 9113 case 50511102: 9114 return getCategory(); 9115 case 1957227299: 9116 return getProductOrService(); 9117 case -615513385: 9118 return addModifier(); 9119 case 1010065041: 9120 return addProgramCode(); 9121 case -1285004149: 9122 return getQuantity(); 9123 case -486196699: 9124 return getUnitPrice(); 9125 case -1282148017: 9126 return getFactorElement(); 9127 case 108957: 9128 return getNet(); 9129 case 115642: 9130 return addUdi(); 9131 case -1110033957: 9132 return addNoteNumberElement(); 9133 case -231349275: 9134 return addAdjudication(); 9135 default: 9136 return super.makeProperty(hash, name); 9137 } 9138 9139 } 9140 9141 @Override 9142 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9143 switch (hash) { 9144 case 1349547969: 9145 /* sequence */ return new String[] { "positiveInt" }; 9146 case 1099842588: 9147 /* revenue */ return new String[] { "CodeableConcept" }; 9148 case 50511102: 9149 /* category */ return new String[] { "CodeableConcept" }; 9150 case 1957227299: 9151 /* productOrService */ return new String[] { "CodeableConcept" }; 9152 case -615513385: 9153 /* modifier */ return new String[] { "CodeableConcept" }; 9154 case 1010065041: 9155 /* programCode */ return new String[] { "CodeableConcept" }; 9156 case -1285004149: 9157 /* quantity */ return new String[] { "SimpleQuantity" }; 9158 case -486196699: 9159 /* unitPrice */ return new String[] { "Money" }; 9160 case -1282148017: 9161 /* factor */ return new String[] { "decimal" }; 9162 case 108957: 9163 /* net */ return new String[] { "Money" }; 9164 case 115642: 9165 /* udi */ return new String[] { "Reference" }; 9166 case -1110033957: 9167 /* noteNumber */ return new String[] { "positiveInt" }; 9168 case -231349275: 9169 /* adjudication */ return new String[] { "@ExplanationOfBenefit.item.adjudication" }; 9170 default: 9171 return super.getTypesForProperty(hash, name); 9172 } 9173 9174 } 9175 9176 @Override 9177 public Base addChild(String name) throws FHIRException { 9178 if (name.equals("sequence")) { 9179 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.sequence"); 9180 } else if (name.equals("revenue")) { 9181 this.revenue = new CodeableConcept(); 9182 return this.revenue; 9183 } else if (name.equals("category")) { 9184 this.category = new CodeableConcept(); 9185 return this.category; 9186 } else if (name.equals("productOrService")) { 9187 this.productOrService = new CodeableConcept(); 9188 return this.productOrService; 9189 } else if (name.equals("modifier")) { 9190 return addModifier(); 9191 } else if (name.equals("programCode")) { 9192 return addProgramCode(); 9193 } else if (name.equals("quantity")) { 9194 this.quantity = new Quantity(); 9195 return this.quantity; 9196 } else if (name.equals("unitPrice")) { 9197 this.unitPrice = new Money(); 9198 return this.unitPrice; 9199 } else if (name.equals("factor")) { 9200 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.factor"); 9201 } else if (name.equals("net")) { 9202 this.net = new Money(); 9203 return this.net; 9204 } else if (name.equals("udi")) { 9205 return addUdi(); 9206 } else if (name.equals("noteNumber")) { 9207 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.noteNumber"); 9208 } else if (name.equals("adjudication")) { 9209 return addAdjudication(); 9210 } else 9211 return super.addChild(name); 9212 } 9213 9214 public SubDetailComponent copy() { 9215 SubDetailComponent dst = new SubDetailComponent(); 9216 copyValues(dst); 9217 return dst; 9218 } 9219 9220 public void copyValues(SubDetailComponent dst) { 9221 super.copyValues(dst); 9222 dst.sequence = sequence == null ? null : sequence.copy(); 9223 dst.revenue = revenue == null ? null : revenue.copy(); 9224 dst.category = category == null ? null : category.copy(); 9225 dst.productOrService = productOrService == null ? null : productOrService.copy(); 9226 if (modifier != null) { 9227 dst.modifier = new ArrayList<CodeableConcept>(); 9228 for (CodeableConcept i : modifier) 9229 dst.modifier.add(i.copy()); 9230 } 9231 ; 9232 if (programCode != null) { 9233 dst.programCode = new ArrayList<CodeableConcept>(); 9234 for (CodeableConcept i : programCode) 9235 dst.programCode.add(i.copy()); 9236 } 9237 ; 9238 dst.quantity = quantity == null ? null : quantity.copy(); 9239 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 9240 dst.factor = factor == null ? null : factor.copy(); 9241 dst.net = net == null ? null : net.copy(); 9242 if (udi != null) { 9243 dst.udi = new ArrayList<Reference>(); 9244 for (Reference i : udi) 9245 dst.udi.add(i.copy()); 9246 } 9247 ; 9248 if (noteNumber != null) { 9249 dst.noteNumber = new ArrayList<PositiveIntType>(); 9250 for (PositiveIntType i : noteNumber) 9251 dst.noteNumber.add(i.copy()); 9252 } 9253 ; 9254 if (adjudication != null) { 9255 dst.adjudication = new ArrayList<AdjudicationComponent>(); 9256 for (AdjudicationComponent i : adjudication) 9257 dst.adjudication.add(i.copy()); 9258 } 9259 ; 9260 } 9261 9262 @Override 9263 public boolean equalsDeep(Base other_) { 9264 if (!super.equalsDeep(other_)) 9265 return false; 9266 if (!(other_ instanceof SubDetailComponent)) 9267 return false; 9268 SubDetailComponent o = (SubDetailComponent) other_; 9269 return compareDeep(sequence, o.sequence, true) && compareDeep(revenue, o.revenue, true) 9270 && compareDeep(category, o.category, true) && compareDeep(productOrService, o.productOrService, true) 9271 && compareDeep(modifier, o.modifier, true) && compareDeep(programCode, o.programCode, true) 9272 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 9273 && compareDeep(factor, o.factor, true) && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) 9274 && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(adjudication, o.adjudication, true); 9275 } 9276 9277 @Override 9278 public boolean equalsShallow(Base other_) { 9279 if (!super.equalsShallow(other_)) 9280 return false; 9281 if (!(other_ instanceof SubDetailComponent)) 9282 return false; 9283 SubDetailComponent o = (SubDetailComponent) other_; 9284 return compareValues(sequence, o.sequence, true) && compareValues(factor, o.factor, true) 9285 && compareValues(noteNumber, o.noteNumber, true); 9286 } 9287 9288 public boolean isEmpty() { 9289 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, revenue, category, productOrService, 9290 modifier, programCode, quantity, unitPrice, factor, net, udi, noteNumber, adjudication); 9291 } 9292 9293 public String fhirType() { 9294 return "ExplanationOfBenefit.item.detail.subDetail"; 9295 9296 } 9297 9298 } 9299 9300 @Block() 9301 public static class AddedItemComponent extends BackboneElement implements IBaseBackboneElement { 9302 /** 9303 * Claim items which this service line is intended to replace. 9304 */ 9305 @Child(name = "itemSequence", type = { 9306 PositiveIntType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 9307 @Description(shortDefinition = "Item sequence number", formalDefinition = "Claim items which this service line is intended to replace.") 9308 protected List<PositiveIntType> itemSequence; 9309 9310 /** 9311 * The sequence number of the details within the claim item which this line is 9312 * intended to replace. 9313 */ 9314 @Child(name = "detailSequence", type = { 9315 PositiveIntType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 9316 @Description(shortDefinition = "Detail sequence number", formalDefinition = "The sequence number of the details within the claim item which this line is intended to replace.") 9317 protected List<PositiveIntType> detailSequence; 9318 9319 /** 9320 * The sequence number of the sub-details woithin the details within the claim 9321 * item which this line is intended to replace. 9322 */ 9323 @Child(name = "subDetailSequence", type = { 9324 PositiveIntType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 9325 @Description(shortDefinition = "Subdetail sequence number", formalDefinition = "The sequence number of the sub-details woithin the details within the claim item which this line is intended to replace.") 9326 protected List<PositiveIntType> subDetailSequence; 9327 9328 /** 9329 * The providers who are authorized for the services rendered to the patient. 9330 */ 9331 @Child(name = "provider", type = { Practitioner.class, PractitionerRole.class, 9332 Organization.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 9333 @Description(shortDefinition = "Authorized providers", formalDefinition = "The providers who are authorized for the services rendered to the patient.") 9334 protected List<Reference> provider; 9335 /** 9336 * The actual objects that are the target of the reference (The providers who 9337 * are authorized for the services rendered to the patient.) 9338 */ 9339 protected List<Resource> providerTarget; 9340 9341 /** 9342 * When the value is a group code then this item collects a set of related claim 9343 * details, otherwise this contains the product, service, drug or other billing 9344 * code for the item. 9345 */ 9346 @Child(name = "productOrService", type = { 9347 CodeableConcept.class }, order = 5, min = 1, max = 1, modifier = false, summary = false) 9348 @Description(shortDefinition = "Billing, service, product, or drug code", formalDefinition = "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.") 9349 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-uscls") 9350 protected CodeableConcept productOrService; 9351 9352 /** 9353 * Item typification or modifiers codes to convey additional context for the 9354 * product or service. 9355 */ 9356 @Child(name = "modifier", type = { 9357 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 9358 @Description(shortDefinition = "Service/Product billing modifiers", formalDefinition = "Item typification or modifiers codes to convey additional context for the product or service.") 9359 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-modifiers") 9360 protected List<CodeableConcept> modifier; 9361 9362 /** 9363 * Identifies the program under which this may be recovered. 9364 */ 9365 @Child(name = "programCode", type = { 9366 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 9367 @Description(shortDefinition = "Program the product or service is provided under", formalDefinition = "Identifies the program under which this may be recovered.") 9368 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-program-code") 9369 protected List<CodeableConcept> programCode; 9370 9371 /** 9372 * The date or dates when the service or product was supplied, performed or 9373 * completed. 9374 */ 9375 @Child(name = "serviced", type = { DateType.class, 9376 Period.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 9377 @Description(shortDefinition = "Date or dates of service or product delivery", formalDefinition = "The date or dates when the service or product was supplied, performed or completed.") 9378 protected Type serviced; 9379 9380 /** 9381 * Where the product or service was provided. 9382 */ 9383 @Child(name = "location", type = { CodeableConcept.class, Address.class, 9384 Location.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 9385 @Description(shortDefinition = "Place of service or where product was supplied", formalDefinition = "Where the product or service was provided.") 9386 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-place") 9387 protected Type location; 9388 9389 /** 9390 * The number of repetitions of a service or product. 9391 */ 9392 @Child(name = "quantity", type = { 9393 Quantity.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 9394 @Description(shortDefinition = "Count of products or services", formalDefinition = "The number of repetitions of a service or product.") 9395 protected Quantity quantity; 9396 9397 /** 9398 * If the item is not a group then this is the fee for the product or service, 9399 * otherwise this is the total of the fees for the details of the group. 9400 */ 9401 @Child(name = "unitPrice", type = { Money.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 9402 @Description(shortDefinition = "Fee, charge or cost per item", formalDefinition = "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.") 9403 protected Money unitPrice; 9404 9405 /** 9406 * A real number that represents a multiplier used in determining the overall 9407 * value of services delivered and/or goods received. The concept of a Factor 9408 * allows for a discount or surcharge multiplier to be applied to a monetary 9409 * amount. 9410 */ 9411 @Child(name = "factor", type = { 9412 DecimalType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 9413 @Description(shortDefinition = "Price scaling factor", formalDefinition = "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.") 9414 protected DecimalType factor; 9415 9416 /** 9417 * The quantity times the unit price for an additional service or product or 9418 * charge. 9419 */ 9420 @Child(name = "net", type = { Money.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 9421 @Description(shortDefinition = "Total item cost", formalDefinition = "The quantity times the unit price for an additional service or product or charge.") 9422 protected Money net; 9423 9424 /** 9425 * Physical service site on the patient (limb, tooth, etc.). 9426 */ 9427 @Child(name = "bodySite", type = { 9428 CodeableConcept.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 9429 @Description(shortDefinition = "Anatomical location", formalDefinition = "Physical service site on the patient (limb, tooth, etc.).") 9430 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/tooth") 9431 protected CodeableConcept bodySite; 9432 9433 /** 9434 * A region or surface of the bodySite, e.g. limb region or tooth surface(s). 9435 */ 9436 @Child(name = "subSite", type = { 9437 CodeableConcept.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 9438 @Description(shortDefinition = "Anatomical sub-location", formalDefinition = "A region or surface of the bodySite, e.g. limb region or tooth surface(s).") 9439 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/surface") 9440 protected List<CodeableConcept> subSite; 9441 9442 /** 9443 * The numbers associated with notes below which apply to the adjudication of 9444 * this item. 9445 */ 9446 @Child(name = "noteNumber", type = { 9447 PositiveIntType.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 9448 @Description(shortDefinition = "Applicable note numbers", formalDefinition = "The numbers associated with notes below which apply to the adjudication of this item.") 9449 protected List<PositiveIntType> noteNumber; 9450 9451 /** 9452 * The adjudication results. 9453 */ 9454 @Child(name = "adjudication", type = { 9455 AdjudicationComponent.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 9456 @Description(shortDefinition = "Added items adjudication", formalDefinition = "The adjudication results.") 9457 protected List<AdjudicationComponent> adjudication; 9458 9459 /** 9460 * The second-tier service adjudications for payor added services. 9461 */ 9462 @Child(name = "detail", type = {}, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 9463 @Description(shortDefinition = "Insurer added line items", formalDefinition = "The second-tier service adjudications for payor added services.") 9464 protected List<AddedItemDetailComponent> detail; 9465 9466 private static final long serialVersionUID = -206524210L; 9467 9468 /** 9469 * Constructor 9470 */ 9471 public AddedItemComponent() { 9472 super(); 9473 } 9474 9475 /** 9476 * Constructor 9477 */ 9478 public AddedItemComponent(CodeableConcept productOrService) { 9479 super(); 9480 this.productOrService = productOrService; 9481 } 9482 9483 /** 9484 * @return {@link #itemSequence} (Claim items which this service line is 9485 * intended to replace.) 9486 */ 9487 public List<PositiveIntType> getItemSequence() { 9488 if (this.itemSequence == null) 9489 this.itemSequence = new ArrayList<PositiveIntType>(); 9490 return this.itemSequence; 9491 } 9492 9493 /** 9494 * @return Returns a reference to <code>this</code> for easy method chaining 9495 */ 9496 public AddedItemComponent setItemSequence(List<PositiveIntType> theItemSequence) { 9497 this.itemSequence = theItemSequence; 9498 return this; 9499 } 9500 9501 public boolean hasItemSequence() { 9502 if (this.itemSequence == null) 9503 return false; 9504 for (PositiveIntType item : this.itemSequence) 9505 if (!item.isEmpty()) 9506 return true; 9507 return false; 9508 } 9509 9510 /** 9511 * @return {@link #itemSequence} (Claim items which this service line is 9512 * intended to replace.) 9513 */ 9514 public PositiveIntType addItemSequenceElement() {// 2 9515 PositiveIntType t = new PositiveIntType(); 9516 if (this.itemSequence == null) 9517 this.itemSequence = new ArrayList<PositiveIntType>(); 9518 this.itemSequence.add(t); 9519 return t; 9520 } 9521 9522 /** 9523 * @param value {@link #itemSequence} (Claim items which this service line is 9524 * intended to replace.) 9525 */ 9526 public AddedItemComponent addItemSequence(int value) { // 1 9527 PositiveIntType t = new PositiveIntType(); 9528 t.setValue(value); 9529 if (this.itemSequence == null) 9530 this.itemSequence = new ArrayList<PositiveIntType>(); 9531 this.itemSequence.add(t); 9532 return this; 9533 } 9534 9535 /** 9536 * @param value {@link #itemSequence} (Claim items which this service line is 9537 * intended to replace.) 9538 */ 9539 public boolean hasItemSequence(int value) { 9540 if (this.itemSequence == null) 9541 return false; 9542 for (PositiveIntType v : this.itemSequence) 9543 if (v.getValue().equals(value)) // positiveInt 9544 return true; 9545 return false; 9546 } 9547 9548 /** 9549 * @return {@link #detailSequence} (The sequence number of the details within 9550 * the claim item which this line is intended to replace.) 9551 */ 9552 public List<PositiveIntType> getDetailSequence() { 9553 if (this.detailSequence == null) 9554 this.detailSequence = new ArrayList<PositiveIntType>(); 9555 return this.detailSequence; 9556 } 9557 9558 /** 9559 * @return Returns a reference to <code>this</code> for easy method chaining 9560 */ 9561 public AddedItemComponent setDetailSequence(List<PositiveIntType> theDetailSequence) { 9562 this.detailSequence = theDetailSequence; 9563 return this; 9564 } 9565 9566 public boolean hasDetailSequence() { 9567 if (this.detailSequence == null) 9568 return false; 9569 for (PositiveIntType item : this.detailSequence) 9570 if (!item.isEmpty()) 9571 return true; 9572 return false; 9573 } 9574 9575 /** 9576 * @return {@link #detailSequence} (The sequence number of the details within 9577 * the claim item which this line is intended to replace.) 9578 */ 9579 public PositiveIntType addDetailSequenceElement() {// 2 9580 PositiveIntType t = new PositiveIntType(); 9581 if (this.detailSequence == null) 9582 this.detailSequence = new ArrayList<PositiveIntType>(); 9583 this.detailSequence.add(t); 9584 return t; 9585 } 9586 9587 /** 9588 * @param value {@link #detailSequence} (The sequence number of the details 9589 * within the claim item which this line is intended to replace.) 9590 */ 9591 public AddedItemComponent addDetailSequence(int value) { // 1 9592 PositiveIntType t = new PositiveIntType(); 9593 t.setValue(value); 9594 if (this.detailSequence == null) 9595 this.detailSequence = new ArrayList<PositiveIntType>(); 9596 this.detailSequence.add(t); 9597 return this; 9598 } 9599 9600 /** 9601 * @param value {@link #detailSequence} (The sequence number of the details 9602 * within the claim item which this line is intended to replace.) 9603 */ 9604 public boolean hasDetailSequence(int value) { 9605 if (this.detailSequence == null) 9606 return false; 9607 for (PositiveIntType v : this.detailSequence) 9608 if (v.getValue().equals(value)) // positiveInt 9609 return true; 9610 return false; 9611 } 9612 9613 /** 9614 * @return {@link #subDetailSequence} (The sequence number of the sub-details 9615 * woithin the details within the claim item which this line is intended 9616 * to replace.) 9617 */ 9618 public List<PositiveIntType> getSubDetailSequence() { 9619 if (this.subDetailSequence == null) 9620 this.subDetailSequence = new ArrayList<PositiveIntType>(); 9621 return this.subDetailSequence; 9622 } 9623 9624 /** 9625 * @return Returns a reference to <code>this</code> for easy method chaining 9626 */ 9627 public AddedItemComponent setSubDetailSequence(List<PositiveIntType> theSubDetailSequence) { 9628 this.subDetailSequence = theSubDetailSequence; 9629 return this; 9630 } 9631 9632 public boolean hasSubDetailSequence() { 9633 if (this.subDetailSequence == null) 9634 return false; 9635 for (PositiveIntType item : this.subDetailSequence) 9636 if (!item.isEmpty()) 9637 return true; 9638 return false; 9639 } 9640 9641 /** 9642 * @return {@link #subDetailSequence} (The sequence number of the sub-details 9643 * woithin the details within the claim item which this line is intended 9644 * to replace.) 9645 */ 9646 public PositiveIntType addSubDetailSequenceElement() {// 2 9647 PositiveIntType t = new PositiveIntType(); 9648 if (this.subDetailSequence == null) 9649 this.subDetailSequence = new ArrayList<PositiveIntType>(); 9650 this.subDetailSequence.add(t); 9651 return t; 9652 } 9653 9654 /** 9655 * @param value {@link #subDetailSequence} (The sequence number of the 9656 * sub-details woithin the details within the claim item which this 9657 * line is intended to replace.) 9658 */ 9659 public AddedItemComponent addSubDetailSequence(int value) { // 1 9660 PositiveIntType t = new PositiveIntType(); 9661 t.setValue(value); 9662 if (this.subDetailSequence == null) 9663 this.subDetailSequence = new ArrayList<PositiveIntType>(); 9664 this.subDetailSequence.add(t); 9665 return this; 9666 } 9667 9668 /** 9669 * @param value {@link #subDetailSequence} (The sequence number of the 9670 * sub-details woithin the details within the claim item which this 9671 * line is intended to replace.) 9672 */ 9673 public boolean hasSubDetailSequence(int value) { 9674 if (this.subDetailSequence == null) 9675 return false; 9676 for (PositiveIntType v : this.subDetailSequence) 9677 if (v.getValue().equals(value)) // positiveInt 9678 return true; 9679 return false; 9680 } 9681 9682 /** 9683 * @return {@link #provider} (The providers who are authorized for the services 9684 * rendered to the patient.) 9685 */ 9686 public List<Reference> getProvider() { 9687 if (this.provider == null) 9688 this.provider = new ArrayList<Reference>(); 9689 return this.provider; 9690 } 9691 9692 /** 9693 * @return Returns a reference to <code>this</code> for easy method chaining 9694 */ 9695 public AddedItemComponent setProvider(List<Reference> theProvider) { 9696 this.provider = theProvider; 9697 return this; 9698 } 9699 9700 public boolean hasProvider() { 9701 if (this.provider == null) 9702 return false; 9703 for (Reference item : this.provider) 9704 if (!item.isEmpty()) 9705 return true; 9706 return false; 9707 } 9708 9709 public Reference addProvider() { // 3 9710 Reference t = new Reference(); 9711 if (this.provider == null) 9712 this.provider = new ArrayList<Reference>(); 9713 this.provider.add(t); 9714 return t; 9715 } 9716 9717 public AddedItemComponent addProvider(Reference t) { // 3 9718 if (t == null) 9719 return this; 9720 if (this.provider == null) 9721 this.provider = new ArrayList<Reference>(); 9722 this.provider.add(t); 9723 return this; 9724 } 9725 9726 /** 9727 * @return The first repetition of repeating field {@link #provider}, creating 9728 * it if it does not already exist 9729 */ 9730 public Reference getProviderFirstRep() { 9731 if (getProvider().isEmpty()) { 9732 addProvider(); 9733 } 9734 return getProvider().get(0); 9735 } 9736 9737 /** 9738 * @deprecated Use Reference#setResource(IBaseResource) instead 9739 */ 9740 @Deprecated 9741 public List<Resource> getProviderTarget() { 9742 if (this.providerTarget == null) 9743 this.providerTarget = new ArrayList<Resource>(); 9744 return this.providerTarget; 9745 } 9746 9747 /** 9748 * @return {@link #productOrService} (When the value is a group code then this 9749 * item collects a set of related claim details, otherwise this contains 9750 * the product, service, drug or other billing code for the item.) 9751 */ 9752 public CodeableConcept getProductOrService() { 9753 if (this.productOrService == null) 9754 if (Configuration.errorOnAutoCreate()) 9755 throw new Error("Attempt to auto-create AddedItemComponent.productOrService"); 9756 else if (Configuration.doAutoCreate()) 9757 this.productOrService = new CodeableConcept(); // cc 9758 return this.productOrService; 9759 } 9760 9761 public boolean hasProductOrService() { 9762 return this.productOrService != null && !this.productOrService.isEmpty(); 9763 } 9764 9765 /** 9766 * @param value {@link #productOrService} (When the value is a group code then 9767 * this item collects a set of related claim details, otherwise 9768 * this contains the product, service, drug or other billing code 9769 * for the item.) 9770 */ 9771 public AddedItemComponent setProductOrService(CodeableConcept value) { 9772 this.productOrService = value; 9773 return this; 9774 } 9775 9776 /** 9777 * @return {@link #modifier} (Item typification or modifiers codes to convey 9778 * additional context for the product or service.) 9779 */ 9780 public List<CodeableConcept> getModifier() { 9781 if (this.modifier == null) 9782 this.modifier = new ArrayList<CodeableConcept>(); 9783 return this.modifier; 9784 } 9785 9786 /** 9787 * @return Returns a reference to <code>this</code> for easy method chaining 9788 */ 9789 public AddedItemComponent setModifier(List<CodeableConcept> theModifier) { 9790 this.modifier = theModifier; 9791 return this; 9792 } 9793 9794 public boolean hasModifier() { 9795 if (this.modifier == null) 9796 return false; 9797 for (CodeableConcept item : this.modifier) 9798 if (!item.isEmpty()) 9799 return true; 9800 return false; 9801 } 9802 9803 public CodeableConcept addModifier() { // 3 9804 CodeableConcept t = new CodeableConcept(); 9805 if (this.modifier == null) 9806 this.modifier = new ArrayList<CodeableConcept>(); 9807 this.modifier.add(t); 9808 return t; 9809 } 9810 9811 public AddedItemComponent addModifier(CodeableConcept t) { // 3 9812 if (t == null) 9813 return this; 9814 if (this.modifier == null) 9815 this.modifier = new ArrayList<CodeableConcept>(); 9816 this.modifier.add(t); 9817 return this; 9818 } 9819 9820 /** 9821 * @return The first repetition of repeating field {@link #modifier}, creating 9822 * it if it does not already exist 9823 */ 9824 public CodeableConcept getModifierFirstRep() { 9825 if (getModifier().isEmpty()) { 9826 addModifier(); 9827 } 9828 return getModifier().get(0); 9829 } 9830 9831 /** 9832 * @return {@link #programCode} (Identifies the program under which this may be 9833 * recovered.) 9834 */ 9835 public List<CodeableConcept> getProgramCode() { 9836 if (this.programCode == null) 9837 this.programCode = new ArrayList<CodeableConcept>(); 9838 return this.programCode; 9839 } 9840 9841 /** 9842 * @return Returns a reference to <code>this</code> for easy method chaining 9843 */ 9844 public AddedItemComponent setProgramCode(List<CodeableConcept> theProgramCode) { 9845 this.programCode = theProgramCode; 9846 return this; 9847 } 9848 9849 public boolean hasProgramCode() { 9850 if (this.programCode == null) 9851 return false; 9852 for (CodeableConcept item : this.programCode) 9853 if (!item.isEmpty()) 9854 return true; 9855 return false; 9856 } 9857 9858 public CodeableConcept addProgramCode() { // 3 9859 CodeableConcept t = new CodeableConcept(); 9860 if (this.programCode == null) 9861 this.programCode = new ArrayList<CodeableConcept>(); 9862 this.programCode.add(t); 9863 return t; 9864 } 9865 9866 public AddedItemComponent addProgramCode(CodeableConcept t) { // 3 9867 if (t == null) 9868 return this; 9869 if (this.programCode == null) 9870 this.programCode = new ArrayList<CodeableConcept>(); 9871 this.programCode.add(t); 9872 return this; 9873 } 9874 9875 /** 9876 * @return The first repetition of repeating field {@link #programCode}, 9877 * creating it if it does not already exist 9878 */ 9879 public CodeableConcept getProgramCodeFirstRep() { 9880 if (getProgramCode().isEmpty()) { 9881 addProgramCode(); 9882 } 9883 return getProgramCode().get(0); 9884 } 9885 9886 /** 9887 * @return {@link #serviced} (The date or dates when the service or product was 9888 * supplied, performed or completed.) 9889 */ 9890 public Type getServiced() { 9891 return this.serviced; 9892 } 9893 9894 /** 9895 * @return {@link #serviced} (The date or dates when the service or product was 9896 * supplied, performed or completed.) 9897 */ 9898 public DateType getServicedDateType() throws FHIRException { 9899 if (this.serviced == null) 9900 this.serviced = new DateType(); 9901 if (!(this.serviced instanceof DateType)) 9902 throw new FHIRException("Type mismatch: the type DateType was expected, but " 9903 + this.serviced.getClass().getName() + " was encountered"); 9904 return (DateType) this.serviced; 9905 } 9906 9907 public boolean hasServicedDateType() { 9908 return this != null && this.serviced instanceof DateType; 9909 } 9910 9911 /** 9912 * @return {@link #serviced} (The date or dates when the service or product was 9913 * supplied, performed or completed.) 9914 */ 9915 public Period getServicedPeriod() throws FHIRException { 9916 if (this.serviced == null) 9917 this.serviced = new Period(); 9918 if (!(this.serviced instanceof Period)) 9919 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.serviced.getClass().getName() 9920 + " was encountered"); 9921 return (Period) this.serviced; 9922 } 9923 9924 public boolean hasServicedPeriod() { 9925 return this != null && this.serviced instanceof Period; 9926 } 9927 9928 public boolean hasServiced() { 9929 return this.serviced != null && !this.serviced.isEmpty(); 9930 } 9931 9932 /** 9933 * @param value {@link #serviced} (The date or dates when the service or product 9934 * was supplied, performed or completed.) 9935 */ 9936 public AddedItemComponent setServiced(Type value) { 9937 if (value != null && !(value instanceof DateType || value instanceof Period)) 9938 throw new Error("Not the right type for ExplanationOfBenefit.addItem.serviced[x]: " + value.fhirType()); 9939 this.serviced = value; 9940 return this; 9941 } 9942 9943 /** 9944 * @return {@link #location} (Where the product or service was provided.) 9945 */ 9946 public Type getLocation() { 9947 return this.location; 9948 } 9949 9950 /** 9951 * @return {@link #location} (Where the product or service was provided.) 9952 */ 9953 public CodeableConcept getLocationCodeableConcept() throws FHIRException { 9954 if (this.location == null) 9955 this.location = new CodeableConcept(); 9956 if (!(this.location instanceof CodeableConcept)) 9957 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 9958 + this.location.getClass().getName() + " was encountered"); 9959 return (CodeableConcept) this.location; 9960 } 9961 9962 public boolean hasLocationCodeableConcept() { 9963 return this != null && this.location instanceof CodeableConcept; 9964 } 9965 9966 /** 9967 * @return {@link #location} (Where the product or service was provided.) 9968 */ 9969 public Address getLocationAddress() throws FHIRException { 9970 if (this.location == null) 9971 this.location = new Address(); 9972 if (!(this.location instanceof Address)) 9973 throw new FHIRException("Type mismatch: the type Address was expected, but " 9974 + this.location.getClass().getName() + " was encountered"); 9975 return (Address) this.location; 9976 } 9977 9978 public boolean hasLocationAddress() { 9979 return this != null && this.location instanceof Address; 9980 } 9981 9982 /** 9983 * @return {@link #location} (Where the product or service was provided.) 9984 */ 9985 public Reference getLocationReference() throws FHIRException { 9986 if (this.location == null) 9987 this.location = new Reference(); 9988 if (!(this.location instanceof Reference)) 9989 throw new FHIRException("Type mismatch: the type Reference was expected, but " 9990 + this.location.getClass().getName() + " was encountered"); 9991 return (Reference) this.location; 9992 } 9993 9994 public boolean hasLocationReference() { 9995 return this != null && this.location instanceof Reference; 9996 } 9997 9998 public boolean hasLocation() { 9999 return this.location != null && !this.location.isEmpty(); 10000 } 10001 10002 /** 10003 * @param value {@link #location} (Where the product or service was provided.) 10004 */ 10005 public AddedItemComponent setLocation(Type value) { 10006 if (value != null 10007 && !(value instanceof CodeableConcept || value instanceof Address || value instanceof Reference)) 10008 throw new Error("Not the right type for ExplanationOfBenefit.addItem.location[x]: " + value.fhirType()); 10009 this.location = value; 10010 return this; 10011 } 10012 10013 /** 10014 * @return {@link #quantity} (The number of repetitions of a service or 10015 * product.) 10016 */ 10017 public Quantity getQuantity() { 10018 if (this.quantity == null) 10019 if (Configuration.errorOnAutoCreate()) 10020 throw new Error("Attempt to auto-create AddedItemComponent.quantity"); 10021 else if (Configuration.doAutoCreate()) 10022 this.quantity = new Quantity(); // cc 10023 return this.quantity; 10024 } 10025 10026 public boolean hasQuantity() { 10027 return this.quantity != null && !this.quantity.isEmpty(); 10028 } 10029 10030 /** 10031 * @param value {@link #quantity} (The number of repetitions of a service or 10032 * product.) 10033 */ 10034 public AddedItemComponent setQuantity(Quantity value) { 10035 this.quantity = value; 10036 return this; 10037 } 10038 10039 /** 10040 * @return {@link #unitPrice} (If the item is not a group then this is the fee 10041 * for the product or service, otherwise this is the total of the fees 10042 * for the details of the group.) 10043 */ 10044 public Money getUnitPrice() { 10045 if (this.unitPrice == null) 10046 if (Configuration.errorOnAutoCreate()) 10047 throw new Error("Attempt to auto-create AddedItemComponent.unitPrice"); 10048 else if (Configuration.doAutoCreate()) 10049 this.unitPrice = new Money(); // cc 10050 return this.unitPrice; 10051 } 10052 10053 public boolean hasUnitPrice() { 10054 return this.unitPrice != null && !this.unitPrice.isEmpty(); 10055 } 10056 10057 /** 10058 * @param value {@link #unitPrice} (If the item is not a group then this is the 10059 * fee for the product or service, otherwise this is the total of 10060 * the fees for the details of the group.) 10061 */ 10062 public AddedItemComponent setUnitPrice(Money value) { 10063 this.unitPrice = value; 10064 return this; 10065 } 10066 10067 /** 10068 * @return {@link #factor} (A real number that represents a multiplier used in 10069 * determining the overall value of services delivered and/or goods 10070 * received. The concept of a Factor allows for a discount or surcharge 10071 * multiplier to be applied to a monetary amount.). This is the 10072 * underlying object with id, value and extensions. The accessor 10073 * "getFactor" gives direct access to the value 10074 */ 10075 public DecimalType getFactorElement() { 10076 if (this.factor == null) 10077 if (Configuration.errorOnAutoCreate()) 10078 throw new Error("Attempt to auto-create AddedItemComponent.factor"); 10079 else if (Configuration.doAutoCreate()) 10080 this.factor = new DecimalType(); // bb 10081 return this.factor; 10082 } 10083 10084 public boolean hasFactorElement() { 10085 return this.factor != null && !this.factor.isEmpty(); 10086 } 10087 10088 public boolean hasFactor() { 10089 return this.factor != null && !this.factor.isEmpty(); 10090 } 10091 10092 /** 10093 * @param value {@link #factor} (A real number that represents a multiplier used 10094 * in determining the overall value of services delivered and/or 10095 * goods received. The concept of a Factor allows for a discount or 10096 * surcharge multiplier to be applied to a monetary amount.). This 10097 * is the underlying object with id, value and extensions. The 10098 * accessor "getFactor" gives direct access to the value 10099 */ 10100 public AddedItemComponent setFactorElement(DecimalType value) { 10101 this.factor = value; 10102 return this; 10103 } 10104 10105 /** 10106 * @return A real number that represents a multiplier used in determining the 10107 * overall value of services delivered and/or goods received. The 10108 * concept of a Factor allows for a discount or surcharge multiplier to 10109 * be applied to a monetary amount. 10110 */ 10111 public BigDecimal getFactor() { 10112 return this.factor == null ? null : this.factor.getValue(); 10113 } 10114 10115 /** 10116 * @param value A real number that represents a multiplier used in determining 10117 * the overall value of services delivered and/or goods received. 10118 * The concept of a Factor allows for a discount or surcharge 10119 * multiplier to be applied to a monetary amount. 10120 */ 10121 public AddedItemComponent setFactor(BigDecimal value) { 10122 if (value == null) 10123 this.factor = null; 10124 else { 10125 if (this.factor == null) 10126 this.factor = new DecimalType(); 10127 this.factor.setValue(value); 10128 } 10129 return this; 10130 } 10131 10132 /** 10133 * @param value A real number that represents a multiplier used in determining 10134 * the overall value of services delivered and/or goods received. 10135 * The concept of a Factor allows for a discount or surcharge 10136 * multiplier to be applied to a monetary amount. 10137 */ 10138 public AddedItemComponent setFactor(long value) { 10139 this.factor = new DecimalType(); 10140 this.factor.setValue(value); 10141 return this; 10142 } 10143 10144 /** 10145 * @param value A real number that represents a multiplier used in determining 10146 * the overall value of services delivered and/or goods received. 10147 * The concept of a Factor allows for a discount or surcharge 10148 * multiplier to be applied to a monetary amount. 10149 */ 10150 public AddedItemComponent setFactor(double value) { 10151 this.factor = new DecimalType(); 10152 this.factor.setValue(value); 10153 return this; 10154 } 10155 10156 /** 10157 * @return {@link #net} (The quantity times the unit price for an additional 10158 * service or product or charge.) 10159 */ 10160 public Money getNet() { 10161 if (this.net == null) 10162 if (Configuration.errorOnAutoCreate()) 10163 throw new Error("Attempt to auto-create AddedItemComponent.net"); 10164 else if (Configuration.doAutoCreate()) 10165 this.net = new Money(); // cc 10166 return this.net; 10167 } 10168 10169 public boolean hasNet() { 10170 return this.net != null && !this.net.isEmpty(); 10171 } 10172 10173 /** 10174 * @param value {@link #net} (The quantity times the unit price for an 10175 * additional service or product or charge.) 10176 */ 10177 public AddedItemComponent setNet(Money value) { 10178 this.net = value; 10179 return this; 10180 } 10181 10182 /** 10183 * @return {@link #bodySite} (Physical service site on the patient (limb, tooth, 10184 * etc.).) 10185 */ 10186 public CodeableConcept getBodySite() { 10187 if (this.bodySite == null) 10188 if (Configuration.errorOnAutoCreate()) 10189 throw new Error("Attempt to auto-create AddedItemComponent.bodySite"); 10190 else if (Configuration.doAutoCreate()) 10191 this.bodySite = new CodeableConcept(); // cc 10192 return this.bodySite; 10193 } 10194 10195 public boolean hasBodySite() { 10196 return this.bodySite != null && !this.bodySite.isEmpty(); 10197 } 10198 10199 /** 10200 * @param value {@link #bodySite} (Physical service site on the patient (limb, 10201 * tooth, etc.).) 10202 */ 10203 public AddedItemComponent setBodySite(CodeableConcept value) { 10204 this.bodySite = value; 10205 return this; 10206 } 10207 10208 /** 10209 * @return {@link #subSite} (A region or surface of the bodySite, e.g. limb 10210 * region or tooth surface(s).) 10211 */ 10212 public List<CodeableConcept> getSubSite() { 10213 if (this.subSite == null) 10214 this.subSite = new ArrayList<CodeableConcept>(); 10215 return this.subSite; 10216 } 10217 10218 /** 10219 * @return Returns a reference to <code>this</code> for easy method chaining 10220 */ 10221 public AddedItemComponent setSubSite(List<CodeableConcept> theSubSite) { 10222 this.subSite = theSubSite; 10223 return this; 10224 } 10225 10226 public boolean hasSubSite() { 10227 if (this.subSite == null) 10228 return false; 10229 for (CodeableConcept item : this.subSite) 10230 if (!item.isEmpty()) 10231 return true; 10232 return false; 10233 } 10234 10235 public CodeableConcept addSubSite() { // 3 10236 CodeableConcept t = new CodeableConcept(); 10237 if (this.subSite == null) 10238 this.subSite = new ArrayList<CodeableConcept>(); 10239 this.subSite.add(t); 10240 return t; 10241 } 10242 10243 public AddedItemComponent addSubSite(CodeableConcept t) { // 3 10244 if (t == null) 10245 return this; 10246 if (this.subSite == null) 10247 this.subSite = new ArrayList<CodeableConcept>(); 10248 this.subSite.add(t); 10249 return this; 10250 } 10251 10252 /** 10253 * @return The first repetition of repeating field {@link #subSite}, creating it 10254 * if it does not already exist 10255 */ 10256 public CodeableConcept getSubSiteFirstRep() { 10257 if (getSubSite().isEmpty()) { 10258 addSubSite(); 10259 } 10260 return getSubSite().get(0); 10261 } 10262 10263 /** 10264 * @return {@link #noteNumber} (The numbers associated with notes below which 10265 * apply to the adjudication of this item.) 10266 */ 10267 public List<PositiveIntType> getNoteNumber() { 10268 if (this.noteNumber == null) 10269 this.noteNumber = new ArrayList<PositiveIntType>(); 10270 return this.noteNumber; 10271 } 10272 10273 /** 10274 * @return Returns a reference to <code>this</code> for easy method chaining 10275 */ 10276 public AddedItemComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 10277 this.noteNumber = theNoteNumber; 10278 return this; 10279 } 10280 10281 public boolean hasNoteNumber() { 10282 if (this.noteNumber == null) 10283 return false; 10284 for (PositiveIntType item : this.noteNumber) 10285 if (!item.isEmpty()) 10286 return true; 10287 return false; 10288 } 10289 10290 /** 10291 * @return {@link #noteNumber} (The numbers associated with notes below which 10292 * apply to the adjudication of this item.) 10293 */ 10294 public PositiveIntType addNoteNumberElement() {// 2 10295 PositiveIntType t = new PositiveIntType(); 10296 if (this.noteNumber == null) 10297 this.noteNumber = new ArrayList<PositiveIntType>(); 10298 this.noteNumber.add(t); 10299 return t; 10300 } 10301 10302 /** 10303 * @param value {@link #noteNumber} (The numbers associated with notes below 10304 * which apply to the adjudication of this item.) 10305 */ 10306 public AddedItemComponent addNoteNumber(int value) { // 1 10307 PositiveIntType t = new PositiveIntType(); 10308 t.setValue(value); 10309 if (this.noteNumber == null) 10310 this.noteNumber = new ArrayList<PositiveIntType>(); 10311 this.noteNumber.add(t); 10312 return this; 10313 } 10314 10315 /** 10316 * @param value {@link #noteNumber} (The numbers associated with notes below 10317 * which apply to the adjudication of this item.) 10318 */ 10319 public boolean hasNoteNumber(int value) { 10320 if (this.noteNumber == null) 10321 return false; 10322 for (PositiveIntType v : this.noteNumber) 10323 if (v.getValue().equals(value)) // positiveInt 10324 return true; 10325 return false; 10326 } 10327 10328 /** 10329 * @return {@link #adjudication} (The adjudication results.) 10330 */ 10331 public List<AdjudicationComponent> getAdjudication() { 10332 if (this.adjudication == null) 10333 this.adjudication = new ArrayList<AdjudicationComponent>(); 10334 return this.adjudication; 10335 } 10336 10337 /** 10338 * @return Returns a reference to <code>this</code> for easy method chaining 10339 */ 10340 public AddedItemComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 10341 this.adjudication = theAdjudication; 10342 return this; 10343 } 10344 10345 public boolean hasAdjudication() { 10346 if (this.adjudication == null) 10347 return false; 10348 for (AdjudicationComponent item : this.adjudication) 10349 if (!item.isEmpty()) 10350 return true; 10351 return false; 10352 } 10353 10354 public AdjudicationComponent addAdjudication() { // 3 10355 AdjudicationComponent t = new AdjudicationComponent(); 10356 if (this.adjudication == null) 10357 this.adjudication = new ArrayList<AdjudicationComponent>(); 10358 this.adjudication.add(t); 10359 return t; 10360 } 10361 10362 public AddedItemComponent addAdjudication(AdjudicationComponent t) { // 3 10363 if (t == null) 10364 return this; 10365 if (this.adjudication == null) 10366 this.adjudication = new ArrayList<AdjudicationComponent>(); 10367 this.adjudication.add(t); 10368 return this; 10369 } 10370 10371 /** 10372 * @return The first repetition of repeating field {@link #adjudication}, 10373 * creating it if it does not already exist 10374 */ 10375 public AdjudicationComponent getAdjudicationFirstRep() { 10376 if (getAdjudication().isEmpty()) { 10377 addAdjudication(); 10378 } 10379 return getAdjudication().get(0); 10380 } 10381 10382 /** 10383 * @return {@link #detail} (The second-tier service adjudications for payor 10384 * added services.) 10385 */ 10386 public List<AddedItemDetailComponent> getDetail() { 10387 if (this.detail == null) 10388 this.detail = new ArrayList<AddedItemDetailComponent>(); 10389 return this.detail; 10390 } 10391 10392 /** 10393 * @return Returns a reference to <code>this</code> for easy method chaining 10394 */ 10395 public AddedItemComponent setDetail(List<AddedItemDetailComponent> theDetail) { 10396 this.detail = theDetail; 10397 return this; 10398 } 10399 10400 public boolean hasDetail() { 10401 if (this.detail == null) 10402 return false; 10403 for (AddedItemDetailComponent item : this.detail) 10404 if (!item.isEmpty()) 10405 return true; 10406 return false; 10407 } 10408 10409 public AddedItemDetailComponent addDetail() { // 3 10410 AddedItemDetailComponent t = new AddedItemDetailComponent(); 10411 if (this.detail == null) 10412 this.detail = new ArrayList<AddedItemDetailComponent>(); 10413 this.detail.add(t); 10414 return t; 10415 } 10416 10417 public AddedItemComponent addDetail(AddedItemDetailComponent t) { // 3 10418 if (t == null) 10419 return this; 10420 if (this.detail == null) 10421 this.detail = new ArrayList<AddedItemDetailComponent>(); 10422 this.detail.add(t); 10423 return this; 10424 } 10425 10426 /** 10427 * @return The first repetition of repeating field {@link #detail}, creating it 10428 * if it does not already exist 10429 */ 10430 public AddedItemDetailComponent getDetailFirstRep() { 10431 if (getDetail().isEmpty()) { 10432 addDetail(); 10433 } 10434 return getDetail().get(0); 10435 } 10436 10437 protected void listChildren(List<Property> children) { 10438 super.listChildren(children); 10439 children.add(new Property("itemSequence", "positiveInt", 10440 "Claim items which this service line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, itemSequence)); 10441 children.add(new Property("detailSequence", "positiveInt", 10442 "The sequence number of the details within the claim item which this line is intended to replace.", 0, 10443 java.lang.Integer.MAX_VALUE, detailSequence)); 10444 children.add(new Property("subDetailSequence", "positiveInt", 10445 "The sequence number of the sub-details woithin the details within the claim item which this line is intended to replace.", 10446 0, java.lang.Integer.MAX_VALUE, subDetailSequence)); 10447 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", 10448 "The providers who are authorized for the services rendered to the patient.", 0, java.lang.Integer.MAX_VALUE, 10449 provider)); 10450 children.add(new Property("productOrService", "CodeableConcept", 10451 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 10452 0, 1, productOrService)); 10453 children.add(new Property("modifier", "CodeableConcept", 10454 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 10455 java.lang.Integer.MAX_VALUE, modifier)); 10456 children.add(new Property("programCode", "CodeableConcept", 10457 "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 10458 children.add(new Property("serviced[x]", "date|Period", 10459 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced)); 10460 children.add(new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 10461 "Where the product or service was provided.", 0, 1, location)); 10462 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 10463 1, quantity)); 10464 children.add(new Property("unitPrice", "Money", 10465 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 10466 0, 1, unitPrice)); 10467 children.add(new Property("factor", "decimal", 10468 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 10469 0, 1, factor)); 10470 children.add(new Property("net", "Money", 10471 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net)); 10472 children.add(new Property("bodySite", "CodeableConcept", 10473 "Physical service site on the patient (limb, tooth, etc.).", 0, 1, bodySite)); 10474 children.add(new Property("subSite", "CodeableConcept", 10475 "A region or surface of the bodySite, e.g. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, 10476 subSite)); 10477 children.add(new Property("noteNumber", "positiveInt", 10478 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 10479 java.lang.Integer.MAX_VALUE, noteNumber)); 10480 children.add(new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results.", 10481 0, java.lang.Integer.MAX_VALUE, adjudication)); 10482 children.add(new Property("detail", "", "The second-tier service adjudications for payor added services.", 0, 10483 java.lang.Integer.MAX_VALUE, detail)); 10484 } 10485 10486 @Override 10487 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 10488 switch (_hash) { 10489 case 1977979892: 10490 /* itemSequence */ return new Property("itemSequence", "positiveInt", 10491 "Claim items which this service line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, 10492 itemSequence); 10493 case 1321472818: 10494 /* detailSequence */ return new Property("detailSequence", "positiveInt", 10495 "The sequence number of the details within the claim item which this line is intended to replace.", 0, 10496 java.lang.Integer.MAX_VALUE, detailSequence); 10497 case -855462510: 10498 /* subDetailSequence */ return new Property("subDetailSequence", "positiveInt", 10499 "The sequence number of the sub-details woithin the details within the claim item which this line is intended to replace.", 10500 0, java.lang.Integer.MAX_VALUE, subDetailSequence); 10501 case -987494927: 10502 /* provider */ return new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", 10503 "The providers who are authorized for the services rendered to the patient.", 0, 10504 java.lang.Integer.MAX_VALUE, provider); 10505 case 1957227299: 10506 /* productOrService */ return new Property("productOrService", "CodeableConcept", 10507 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 10508 0, 1, productOrService); 10509 case -615513385: 10510 /* modifier */ return new Property("modifier", "CodeableConcept", 10511 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 10512 java.lang.Integer.MAX_VALUE, modifier); 10513 case 1010065041: 10514 /* programCode */ return new Property("programCode", "CodeableConcept", 10515 "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 10516 case -1927922223: 10517 /* serviced[x] */ return new Property("serviced[x]", "date|Period", 10518 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 10519 case 1379209295: 10520 /* serviced */ return new Property("serviced[x]", "date|Period", 10521 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 10522 case 363246749: 10523 /* servicedDate */ return new Property("serviced[x]", "date|Period", 10524 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 10525 case 1534966512: 10526 /* servicedPeriod */ return new Property("serviced[x]", "date|Period", 10527 "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 10528 case 552316075: 10529 /* location[x] */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 10530 "Where the product or service was provided.", 0, 1, location); 10531 case 1901043637: 10532 /* location */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 10533 "Where the product or service was provided.", 0, 1, location); 10534 case -1224800468: 10535 /* locationCodeableConcept */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 10536 "Where the product or service was provided.", 0, 1, location); 10537 case -1280020865: 10538 /* locationAddress */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 10539 "Where the product or service was provided.", 0, 1, location); 10540 case 755866390: 10541 /* locationReference */ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", 10542 "Where the product or service was provided.", 0, 1, location); 10543 case -1285004149: 10544 /* quantity */ return new Property("quantity", "SimpleQuantity", 10545 "The number of repetitions of a service or product.", 0, 1, quantity); 10546 case -486196699: 10547 /* unitPrice */ return new Property("unitPrice", "Money", 10548 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 10549 0, 1, unitPrice); 10550 case -1282148017: 10551 /* factor */ return new Property("factor", "decimal", 10552 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 10553 0, 1, factor); 10554 case 108957: 10555 /* net */ return new Property("net", "Money", 10556 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net); 10557 case 1702620169: 10558 /* bodySite */ return new Property("bodySite", "CodeableConcept", 10559 "Physical service site on the patient (limb, tooth, etc.).", 0, 1, bodySite); 10560 case -1868566105: 10561 /* subSite */ return new Property("subSite", "CodeableConcept", 10562 "A region or surface of the bodySite, e.g. limb region or tooth surface(s).", 0, 10563 java.lang.Integer.MAX_VALUE, subSite); 10564 case -1110033957: 10565 /* noteNumber */ return new Property("noteNumber", "positiveInt", 10566 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 10567 java.lang.Integer.MAX_VALUE, noteNumber); 10568 case -231349275: 10569 /* adjudication */ return new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", 10570 "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 10571 case -1335224239: 10572 /* detail */ return new Property("detail", "", 10573 "The second-tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, detail); 10574 default: 10575 return super.getNamedProperty(_hash, _name, _checkValid); 10576 } 10577 10578 } 10579 10580 @Override 10581 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 10582 switch (hash) { 10583 case 1977979892: 10584 /* itemSequence */ return this.itemSequence == null ? new Base[0] 10585 : this.itemSequence.toArray(new Base[this.itemSequence.size()]); // PositiveIntType 10586 case 1321472818: 10587 /* detailSequence */ return this.detailSequence == null ? new Base[0] 10588 : this.detailSequence.toArray(new Base[this.detailSequence.size()]); // PositiveIntType 10589 case -855462510: 10590 /* subDetailSequence */ return this.subDetailSequence == null ? new Base[0] 10591 : this.subDetailSequence.toArray(new Base[this.subDetailSequence.size()]); // PositiveIntType 10592 case -987494927: 10593 /* provider */ return this.provider == null ? new Base[0] 10594 : this.provider.toArray(new Base[this.provider.size()]); // Reference 10595 case 1957227299: 10596 /* productOrService */ return this.productOrService == null ? new Base[0] 10597 : new Base[] { this.productOrService }; // CodeableConcept 10598 case -615513385: 10599 /* modifier */ return this.modifier == null ? new Base[0] 10600 : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 10601 case 1010065041: 10602 /* programCode */ return this.programCode == null ? new Base[0] 10603 : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 10604 case 1379209295: 10605 /* serviced */ return this.serviced == null ? new Base[0] : new Base[] { this.serviced }; // Type 10606 case 1901043637: 10607 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Type 10608 case -1285004149: 10609 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 10610 case -486196699: 10611 /* unitPrice */ return this.unitPrice == null ? new Base[0] : new Base[] { this.unitPrice }; // Money 10612 case -1282148017: 10613 /* factor */ return this.factor == null ? new Base[0] : new Base[] { this.factor }; // DecimalType 10614 case 108957: 10615 /* net */ return this.net == null ? new Base[0] : new Base[] { this.net }; // Money 10616 case 1702620169: 10617 /* bodySite */ return this.bodySite == null ? new Base[0] : new Base[] { this.bodySite }; // CodeableConcept 10618 case -1868566105: 10619 /* subSite */ return this.subSite == null ? new Base[0] : this.subSite.toArray(new Base[this.subSite.size()]); // CodeableConcept 10620 case -1110033957: 10621 /* noteNumber */ return this.noteNumber == null ? new Base[0] 10622 : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 10623 case -231349275: 10624 /* adjudication */ return this.adjudication == null ? new Base[0] 10625 : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 10626 case -1335224239: 10627 /* detail */ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // AddedItemDetailComponent 10628 default: 10629 return super.getProperty(hash, name, checkValid); 10630 } 10631 10632 } 10633 10634 @Override 10635 public Base setProperty(int hash, String name, Base value) throws FHIRException { 10636 switch (hash) { 10637 case 1977979892: // itemSequence 10638 this.getItemSequence().add(castToPositiveInt(value)); // PositiveIntType 10639 return value; 10640 case 1321472818: // detailSequence 10641 this.getDetailSequence().add(castToPositiveInt(value)); // PositiveIntType 10642 return value; 10643 case -855462510: // subDetailSequence 10644 this.getSubDetailSequence().add(castToPositiveInt(value)); // PositiveIntType 10645 return value; 10646 case -987494927: // provider 10647 this.getProvider().add(castToReference(value)); // Reference 10648 return value; 10649 case 1957227299: // productOrService 10650 this.productOrService = castToCodeableConcept(value); // CodeableConcept 10651 return value; 10652 case -615513385: // modifier 10653 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 10654 return value; 10655 case 1010065041: // programCode 10656 this.getProgramCode().add(castToCodeableConcept(value)); // CodeableConcept 10657 return value; 10658 case 1379209295: // serviced 10659 this.serviced = castToType(value); // Type 10660 return value; 10661 case 1901043637: // location 10662 this.location = castToType(value); // Type 10663 return value; 10664 case -1285004149: // quantity 10665 this.quantity = castToQuantity(value); // Quantity 10666 return value; 10667 case -486196699: // unitPrice 10668 this.unitPrice = castToMoney(value); // Money 10669 return value; 10670 case -1282148017: // factor 10671 this.factor = castToDecimal(value); // DecimalType 10672 return value; 10673 case 108957: // net 10674 this.net = castToMoney(value); // Money 10675 return value; 10676 case 1702620169: // bodySite 10677 this.bodySite = castToCodeableConcept(value); // CodeableConcept 10678 return value; 10679 case -1868566105: // subSite 10680 this.getSubSite().add(castToCodeableConcept(value)); // CodeableConcept 10681 return value; 10682 case -1110033957: // noteNumber 10683 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 10684 return value; 10685 case -231349275: // adjudication 10686 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 10687 return value; 10688 case -1335224239: // detail 10689 this.getDetail().add((AddedItemDetailComponent) value); // AddedItemDetailComponent 10690 return value; 10691 default: 10692 return super.setProperty(hash, name, value); 10693 } 10694 10695 } 10696 10697 @Override 10698 public Base setProperty(String name, Base value) throws FHIRException { 10699 if (name.equals("itemSequence")) { 10700 this.getItemSequence().add(castToPositiveInt(value)); 10701 } else if (name.equals("detailSequence")) { 10702 this.getDetailSequence().add(castToPositiveInt(value)); 10703 } else if (name.equals("subDetailSequence")) { 10704 this.getSubDetailSequence().add(castToPositiveInt(value)); 10705 } else if (name.equals("provider")) { 10706 this.getProvider().add(castToReference(value)); 10707 } else if (name.equals("productOrService")) { 10708 this.productOrService = castToCodeableConcept(value); // CodeableConcept 10709 } else if (name.equals("modifier")) { 10710 this.getModifier().add(castToCodeableConcept(value)); 10711 } else if (name.equals("programCode")) { 10712 this.getProgramCode().add(castToCodeableConcept(value)); 10713 } else if (name.equals("serviced[x]")) { 10714 this.serviced = castToType(value); // Type 10715 } else if (name.equals("location[x]")) { 10716 this.location = castToType(value); // Type 10717 } else if (name.equals("quantity")) { 10718 this.quantity = castToQuantity(value); // Quantity 10719 } else if (name.equals("unitPrice")) { 10720 this.unitPrice = castToMoney(value); // Money 10721 } else if (name.equals("factor")) { 10722 this.factor = castToDecimal(value); // DecimalType 10723 } else if (name.equals("net")) { 10724 this.net = castToMoney(value); // Money 10725 } else if (name.equals("bodySite")) { 10726 this.bodySite = castToCodeableConcept(value); // CodeableConcept 10727 } else if (name.equals("subSite")) { 10728 this.getSubSite().add(castToCodeableConcept(value)); 10729 } else if (name.equals("noteNumber")) { 10730 this.getNoteNumber().add(castToPositiveInt(value)); 10731 } else if (name.equals("adjudication")) { 10732 this.getAdjudication().add((AdjudicationComponent) value); 10733 } else if (name.equals("detail")) { 10734 this.getDetail().add((AddedItemDetailComponent) value); 10735 } else 10736 return super.setProperty(name, value); 10737 return value; 10738 } 10739 10740 @Override 10741 public void removeChild(String name, Base value) throws FHIRException { 10742 if (name.equals("itemSequence")) { 10743 this.getItemSequence().remove(castToPositiveInt(value)); 10744 } else if (name.equals("detailSequence")) { 10745 this.getDetailSequence().remove(castToPositiveInt(value)); 10746 } else if (name.equals("subDetailSequence")) { 10747 this.getSubDetailSequence().remove(castToPositiveInt(value)); 10748 } else if (name.equals("provider")) { 10749 this.getProvider().remove(castToReference(value)); 10750 } else if (name.equals("productOrService")) { 10751 this.productOrService = null; 10752 } else if (name.equals("modifier")) { 10753 this.getModifier().remove(castToCodeableConcept(value)); 10754 } else if (name.equals("programCode")) { 10755 this.getProgramCode().remove(castToCodeableConcept(value)); 10756 } else if (name.equals("serviced[x]")) { 10757 this.serviced = null; 10758 } else if (name.equals("location[x]")) { 10759 this.location = null; 10760 } else if (name.equals("quantity")) { 10761 this.quantity = null; 10762 } else if (name.equals("unitPrice")) { 10763 this.unitPrice = null; 10764 } else if (name.equals("factor")) { 10765 this.factor = null; 10766 } else if (name.equals("net")) { 10767 this.net = null; 10768 } else if (name.equals("bodySite")) { 10769 this.bodySite = null; 10770 } else if (name.equals("subSite")) { 10771 this.getSubSite().remove(castToCodeableConcept(value)); 10772 } else if (name.equals("noteNumber")) { 10773 this.getNoteNumber().remove(castToPositiveInt(value)); 10774 } else if (name.equals("adjudication")) { 10775 this.getAdjudication().remove((AdjudicationComponent) value); 10776 } else if (name.equals("detail")) { 10777 this.getDetail().remove((AddedItemDetailComponent) value); 10778 } else 10779 super.removeChild(name, value); 10780 10781 } 10782 10783 @Override 10784 public Base makeProperty(int hash, String name) throws FHIRException { 10785 switch (hash) { 10786 case 1977979892: 10787 return addItemSequenceElement(); 10788 case 1321472818: 10789 return addDetailSequenceElement(); 10790 case -855462510: 10791 return addSubDetailSequenceElement(); 10792 case -987494927: 10793 return addProvider(); 10794 case 1957227299: 10795 return getProductOrService(); 10796 case -615513385: 10797 return addModifier(); 10798 case 1010065041: 10799 return addProgramCode(); 10800 case -1927922223: 10801 return getServiced(); 10802 case 1379209295: 10803 return getServiced(); 10804 case 552316075: 10805 return getLocation(); 10806 case 1901043637: 10807 return getLocation(); 10808 case -1285004149: 10809 return getQuantity(); 10810 case -486196699: 10811 return getUnitPrice(); 10812 case -1282148017: 10813 return getFactorElement(); 10814 case 108957: 10815 return getNet(); 10816 case 1702620169: 10817 return getBodySite(); 10818 case -1868566105: 10819 return addSubSite(); 10820 case -1110033957: 10821 return addNoteNumberElement(); 10822 case -231349275: 10823 return addAdjudication(); 10824 case -1335224239: 10825 return addDetail(); 10826 default: 10827 return super.makeProperty(hash, name); 10828 } 10829 10830 } 10831 10832 @Override 10833 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 10834 switch (hash) { 10835 case 1977979892: 10836 /* itemSequence */ return new String[] { "positiveInt" }; 10837 case 1321472818: 10838 /* detailSequence */ return new String[] { "positiveInt" }; 10839 case -855462510: 10840 /* subDetailSequence */ return new String[] { "positiveInt" }; 10841 case -987494927: 10842 /* provider */ return new String[] { "Reference" }; 10843 case 1957227299: 10844 /* productOrService */ return new String[] { "CodeableConcept" }; 10845 case -615513385: 10846 /* modifier */ return new String[] { "CodeableConcept" }; 10847 case 1010065041: 10848 /* programCode */ return new String[] { "CodeableConcept" }; 10849 case 1379209295: 10850 /* serviced */ return new String[] { "date", "Period" }; 10851 case 1901043637: 10852 /* location */ return new String[] { "CodeableConcept", "Address", "Reference" }; 10853 case -1285004149: 10854 /* quantity */ return new String[] { "SimpleQuantity" }; 10855 case -486196699: 10856 /* unitPrice */ return new String[] { "Money" }; 10857 case -1282148017: 10858 /* factor */ return new String[] { "decimal" }; 10859 case 108957: 10860 /* net */ return new String[] { "Money" }; 10861 case 1702620169: 10862 /* bodySite */ return new String[] { "CodeableConcept" }; 10863 case -1868566105: 10864 /* subSite */ return new String[] { "CodeableConcept" }; 10865 case -1110033957: 10866 /* noteNumber */ return new String[] { "positiveInt" }; 10867 case -231349275: 10868 /* adjudication */ return new String[] { "@ExplanationOfBenefit.item.adjudication" }; 10869 case -1335224239: 10870 /* detail */ return new String[] {}; 10871 default: 10872 return super.getTypesForProperty(hash, name); 10873 } 10874 10875 } 10876 10877 @Override 10878 public Base addChild(String name) throws FHIRException { 10879 if (name.equals("itemSequence")) { 10880 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.itemSequence"); 10881 } else if (name.equals("detailSequence")) { 10882 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.detailSequence"); 10883 } else if (name.equals("subDetailSequence")) { 10884 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.subDetailSequence"); 10885 } else if (name.equals("provider")) { 10886 return addProvider(); 10887 } else if (name.equals("productOrService")) { 10888 this.productOrService = new CodeableConcept(); 10889 return this.productOrService; 10890 } else if (name.equals("modifier")) { 10891 return addModifier(); 10892 } else if (name.equals("programCode")) { 10893 return addProgramCode(); 10894 } else if (name.equals("servicedDate")) { 10895 this.serviced = new DateType(); 10896 return this.serviced; 10897 } else if (name.equals("servicedPeriod")) { 10898 this.serviced = new Period(); 10899 return this.serviced; 10900 } else if (name.equals("locationCodeableConcept")) { 10901 this.location = new CodeableConcept(); 10902 return this.location; 10903 } else if (name.equals("locationAddress")) { 10904 this.location = new Address(); 10905 return this.location; 10906 } else if (name.equals("locationReference")) { 10907 this.location = new Reference(); 10908 return this.location; 10909 } else if (name.equals("quantity")) { 10910 this.quantity = new Quantity(); 10911 return this.quantity; 10912 } else if (name.equals("unitPrice")) { 10913 this.unitPrice = new Money(); 10914 return this.unitPrice; 10915 } else if (name.equals("factor")) { 10916 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.factor"); 10917 } else if (name.equals("net")) { 10918 this.net = new Money(); 10919 return this.net; 10920 } else if (name.equals("bodySite")) { 10921 this.bodySite = new CodeableConcept(); 10922 return this.bodySite; 10923 } else if (name.equals("subSite")) { 10924 return addSubSite(); 10925 } else if (name.equals("noteNumber")) { 10926 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.noteNumber"); 10927 } else if (name.equals("adjudication")) { 10928 return addAdjudication(); 10929 } else if (name.equals("detail")) { 10930 return addDetail(); 10931 } else 10932 return super.addChild(name); 10933 } 10934 10935 public AddedItemComponent copy() { 10936 AddedItemComponent dst = new AddedItemComponent(); 10937 copyValues(dst); 10938 return dst; 10939 } 10940 10941 public void copyValues(AddedItemComponent dst) { 10942 super.copyValues(dst); 10943 if (itemSequence != null) { 10944 dst.itemSequence = new ArrayList<PositiveIntType>(); 10945 for (PositiveIntType i : itemSequence) 10946 dst.itemSequence.add(i.copy()); 10947 } 10948 ; 10949 if (detailSequence != null) { 10950 dst.detailSequence = new ArrayList<PositiveIntType>(); 10951 for (PositiveIntType i : detailSequence) 10952 dst.detailSequence.add(i.copy()); 10953 } 10954 ; 10955 if (subDetailSequence != null) { 10956 dst.subDetailSequence = new ArrayList<PositiveIntType>(); 10957 for (PositiveIntType i : subDetailSequence) 10958 dst.subDetailSequence.add(i.copy()); 10959 } 10960 ; 10961 if (provider != null) { 10962 dst.provider = new ArrayList<Reference>(); 10963 for (Reference i : provider) 10964 dst.provider.add(i.copy()); 10965 } 10966 ; 10967 dst.productOrService = productOrService == null ? null : productOrService.copy(); 10968 if (modifier != null) { 10969 dst.modifier = new ArrayList<CodeableConcept>(); 10970 for (CodeableConcept i : modifier) 10971 dst.modifier.add(i.copy()); 10972 } 10973 ; 10974 if (programCode != null) { 10975 dst.programCode = new ArrayList<CodeableConcept>(); 10976 for (CodeableConcept i : programCode) 10977 dst.programCode.add(i.copy()); 10978 } 10979 ; 10980 dst.serviced = serviced == null ? null : serviced.copy(); 10981 dst.location = location == null ? null : location.copy(); 10982 dst.quantity = quantity == null ? null : quantity.copy(); 10983 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 10984 dst.factor = factor == null ? null : factor.copy(); 10985 dst.net = net == null ? null : net.copy(); 10986 dst.bodySite = bodySite == null ? null : bodySite.copy(); 10987 if (subSite != null) { 10988 dst.subSite = new ArrayList<CodeableConcept>(); 10989 for (CodeableConcept i : subSite) 10990 dst.subSite.add(i.copy()); 10991 } 10992 ; 10993 if (noteNumber != null) { 10994 dst.noteNumber = new ArrayList<PositiveIntType>(); 10995 for (PositiveIntType i : noteNumber) 10996 dst.noteNumber.add(i.copy()); 10997 } 10998 ; 10999 if (adjudication != null) { 11000 dst.adjudication = new ArrayList<AdjudicationComponent>(); 11001 for (AdjudicationComponent i : adjudication) 11002 dst.adjudication.add(i.copy()); 11003 } 11004 ; 11005 if (detail != null) { 11006 dst.detail = new ArrayList<AddedItemDetailComponent>(); 11007 for (AddedItemDetailComponent i : detail) 11008 dst.detail.add(i.copy()); 11009 } 11010 ; 11011 } 11012 11013 @Override 11014 public boolean equalsDeep(Base other_) { 11015 if (!super.equalsDeep(other_)) 11016 return false; 11017 if (!(other_ instanceof AddedItemComponent)) 11018 return false; 11019 AddedItemComponent o = (AddedItemComponent) other_; 11020 return compareDeep(itemSequence, o.itemSequence, true) && compareDeep(detailSequence, o.detailSequence, true) 11021 && compareDeep(subDetailSequence, o.subDetailSequence, true) && compareDeep(provider, o.provider, true) 11022 && compareDeep(productOrService, o.productOrService, true) && compareDeep(modifier, o.modifier, true) 11023 && compareDeep(programCode, o.programCode, true) && compareDeep(serviced, o.serviced, true) 11024 && compareDeep(location, o.location, true) && compareDeep(quantity, o.quantity, true) 11025 && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 11026 && compareDeep(net, o.net, true) && compareDeep(bodySite, o.bodySite, true) 11027 && compareDeep(subSite, o.subSite, true) && compareDeep(noteNumber, o.noteNumber, true) 11028 && compareDeep(adjudication, o.adjudication, true) && compareDeep(detail, o.detail, true); 11029 } 11030 11031 @Override 11032 public boolean equalsShallow(Base other_) { 11033 if (!super.equalsShallow(other_)) 11034 return false; 11035 if (!(other_ instanceof AddedItemComponent)) 11036 return false; 11037 AddedItemComponent o = (AddedItemComponent) other_; 11038 return compareValues(itemSequence, o.itemSequence, true) && compareValues(detailSequence, o.detailSequence, true) 11039 && compareValues(subDetailSequence, o.subDetailSequence, true) && compareValues(factor, o.factor, true) 11040 && compareValues(noteNumber, o.noteNumber, true); 11041 } 11042 11043 public boolean isEmpty() { 11044 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(itemSequence, detailSequence, subDetailSequence, 11045 provider, productOrService, modifier, programCode, serviced, location, quantity, unitPrice, factor, net, 11046 bodySite, subSite, noteNumber, adjudication, detail); 11047 } 11048 11049 public String fhirType() { 11050 return "ExplanationOfBenefit.addItem"; 11051 11052 } 11053 11054 } 11055 11056 @Block() 11057 public static class AddedItemDetailComponent extends BackboneElement implements IBaseBackboneElement { 11058 /** 11059 * When the value is a group code then this item collects a set of related claim 11060 * details, otherwise this contains the product, service, drug or other billing 11061 * code for the item. 11062 */ 11063 @Child(name = "productOrService", type = { 11064 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 11065 @Description(shortDefinition = "Billing, service, product, or drug code", formalDefinition = "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.") 11066 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-uscls") 11067 protected CodeableConcept productOrService; 11068 11069 /** 11070 * Item typification or modifiers codes to convey additional context for the 11071 * product or service. 11072 */ 11073 @Child(name = "modifier", type = { 11074 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11075 @Description(shortDefinition = "Service/Product billing modifiers", formalDefinition = "Item typification or modifiers codes to convey additional context for the product or service.") 11076 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-modifiers") 11077 protected List<CodeableConcept> modifier; 11078 11079 /** 11080 * The number of repetitions of a service or product. 11081 */ 11082 @Child(name = "quantity", type = { Quantity.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 11083 @Description(shortDefinition = "Count of products or services", formalDefinition = "The number of repetitions of a service or product.") 11084 protected Quantity quantity; 11085 11086 /** 11087 * If the item is not a group then this is the fee for the product or service, 11088 * otherwise this is the total of the fees for the details of the group. 11089 */ 11090 @Child(name = "unitPrice", type = { Money.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 11091 @Description(shortDefinition = "Fee, charge or cost per item", formalDefinition = "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.") 11092 protected Money unitPrice; 11093 11094 /** 11095 * A real number that represents a multiplier used in determining the overall 11096 * value of services delivered and/or goods received. The concept of a Factor 11097 * allows for a discount or surcharge multiplier to be applied to a monetary 11098 * amount. 11099 */ 11100 @Child(name = "factor", type = { 11101 DecimalType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 11102 @Description(shortDefinition = "Price scaling factor", formalDefinition = "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.") 11103 protected DecimalType factor; 11104 11105 /** 11106 * The quantity times the unit price for an additional service or product or 11107 * charge. 11108 */ 11109 @Child(name = "net", type = { Money.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 11110 @Description(shortDefinition = "Total item cost", formalDefinition = "The quantity times the unit price for an additional service or product or charge.") 11111 protected Money net; 11112 11113 /** 11114 * The numbers associated with notes below which apply to the adjudication of 11115 * this item. 11116 */ 11117 @Child(name = "noteNumber", type = { 11118 PositiveIntType.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11119 @Description(shortDefinition = "Applicable note numbers", formalDefinition = "The numbers associated with notes below which apply to the adjudication of this item.") 11120 protected List<PositiveIntType> noteNumber; 11121 11122 /** 11123 * The adjudication results. 11124 */ 11125 @Child(name = "adjudication", type = { 11126 AdjudicationComponent.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11127 @Description(shortDefinition = "Added items adjudication", formalDefinition = "The adjudication results.") 11128 protected List<AdjudicationComponent> adjudication; 11129 11130 /** 11131 * The third-tier service adjudications for payor added services. 11132 */ 11133 @Child(name = "subDetail", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11134 @Description(shortDefinition = "Insurer added line items", formalDefinition = "The third-tier service adjudications for payor added services.") 11135 protected List<AddedItemDetailSubDetailComponent> subDetail; 11136 11137 private static final long serialVersionUID = 295910869L; 11138 11139 /** 11140 * Constructor 11141 */ 11142 public AddedItemDetailComponent() { 11143 super(); 11144 } 11145 11146 /** 11147 * Constructor 11148 */ 11149 public AddedItemDetailComponent(CodeableConcept productOrService) { 11150 super(); 11151 this.productOrService = productOrService; 11152 } 11153 11154 /** 11155 * @return {@link #productOrService} (When the value is a group code then this 11156 * item collects a set of related claim details, otherwise this contains 11157 * the product, service, drug or other billing code for the item.) 11158 */ 11159 public CodeableConcept getProductOrService() { 11160 if (this.productOrService == null) 11161 if (Configuration.errorOnAutoCreate()) 11162 throw new Error("Attempt to auto-create AddedItemDetailComponent.productOrService"); 11163 else if (Configuration.doAutoCreate()) 11164 this.productOrService = new CodeableConcept(); // cc 11165 return this.productOrService; 11166 } 11167 11168 public boolean hasProductOrService() { 11169 return this.productOrService != null && !this.productOrService.isEmpty(); 11170 } 11171 11172 /** 11173 * @param value {@link #productOrService} (When the value is a group code then 11174 * this item collects a set of related claim details, otherwise 11175 * this contains the product, service, drug or other billing code 11176 * for the item.) 11177 */ 11178 public AddedItemDetailComponent setProductOrService(CodeableConcept value) { 11179 this.productOrService = value; 11180 return this; 11181 } 11182 11183 /** 11184 * @return {@link #modifier} (Item typification or modifiers codes to convey 11185 * additional context for the product or service.) 11186 */ 11187 public List<CodeableConcept> getModifier() { 11188 if (this.modifier == null) 11189 this.modifier = new ArrayList<CodeableConcept>(); 11190 return this.modifier; 11191 } 11192 11193 /** 11194 * @return Returns a reference to <code>this</code> for easy method chaining 11195 */ 11196 public AddedItemDetailComponent setModifier(List<CodeableConcept> theModifier) { 11197 this.modifier = theModifier; 11198 return this; 11199 } 11200 11201 public boolean hasModifier() { 11202 if (this.modifier == null) 11203 return false; 11204 for (CodeableConcept item : this.modifier) 11205 if (!item.isEmpty()) 11206 return true; 11207 return false; 11208 } 11209 11210 public CodeableConcept addModifier() { // 3 11211 CodeableConcept t = new CodeableConcept(); 11212 if (this.modifier == null) 11213 this.modifier = new ArrayList<CodeableConcept>(); 11214 this.modifier.add(t); 11215 return t; 11216 } 11217 11218 public AddedItemDetailComponent addModifier(CodeableConcept t) { // 3 11219 if (t == null) 11220 return this; 11221 if (this.modifier == null) 11222 this.modifier = new ArrayList<CodeableConcept>(); 11223 this.modifier.add(t); 11224 return this; 11225 } 11226 11227 /** 11228 * @return The first repetition of repeating field {@link #modifier}, creating 11229 * it if it does not already exist 11230 */ 11231 public CodeableConcept getModifierFirstRep() { 11232 if (getModifier().isEmpty()) { 11233 addModifier(); 11234 } 11235 return getModifier().get(0); 11236 } 11237 11238 /** 11239 * @return {@link #quantity} (The number of repetitions of a service or 11240 * product.) 11241 */ 11242 public Quantity getQuantity() { 11243 if (this.quantity == null) 11244 if (Configuration.errorOnAutoCreate()) 11245 throw new Error("Attempt to auto-create AddedItemDetailComponent.quantity"); 11246 else if (Configuration.doAutoCreate()) 11247 this.quantity = new Quantity(); // cc 11248 return this.quantity; 11249 } 11250 11251 public boolean hasQuantity() { 11252 return this.quantity != null && !this.quantity.isEmpty(); 11253 } 11254 11255 /** 11256 * @param value {@link #quantity} (The number of repetitions of a service or 11257 * product.) 11258 */ 11259 public AddedItemDetailComponent setQuantity(Quantity value) { 11260 this.quantity = value; 11261 return this; 11262 } 11263 11264 /** 11265 * @return {@link #unitPrice} (If the item is not a group then this is the fee 11266 * for the product or service, otherwise this is the total of the fees 11267 * for the details of the group.) 11268 */ 11269 public Money getUnitPrice() { 11270 if (this.unitPrice == null) 11271 if (Configuration.errorOnAutoCreate()) 11272 throw new Error("Attempt to auto-create AddedItemDetailComponent.unitPrice"); 11273 else if (Configuration.doAutoCreate()) 11274 this.unitPrice = new Money(); // cc 11275 return this.unitPrice; 11276 } 11277 11278 public boolean hasUnitPrice() { 11279 return this.unitPrice != null && !this.unitPrice.isEmpty(); 11280 } 11281 11282 /** 11283 * @param value {@link #unitPrice} (If the item is not a group then this is the 11284 * fee for the product or service, otherwise this is the total of 11285 * the fees for the details of the group.) 11286 */ 11287 public AddedItemDetailComponent setUnitPrice(Money value) { 11288 this.unitPrice = value; 11289 return this; 11290 } 11291 11292 /** 11293 * @return {@link #factor} (A real number that represents a multiplier used in 11294 * determining the overall value of services delivered and/or goods 11295 * received. The concept of a Factor allows for a discount or surcharge 11296 * multiplier to be applied to a monetary amount.). This is the 11297 * underlying object with id, value and extensions. The accessor 11298 * "getFactor" gives direct access to the value 11299 */ 11300 public DecimalType getFactorElement() { 11301 if (this.factor == null) 11302 if (Configuration.errorOnAutoCreate()) 11303 throw new Error("Attempt to auto-create AddedItemDetailComponent.factor"); 11304 else if (Configuration.doAutoCreate()) 11305 this.factor = new DecimalType(); // bb 11306 return this.factor; 11307 } 11308 11309 public boolean hasFactorElement() { 11310 return this.factor != null && !this.factor.isEmpty(); 11311 } 11312 11313 public boolean hasFactor() { 11314 return this.factor != null && !this.factor.isEmpty(); 11315 } 11316 11317 /** 11318 * @param value {@link #factor} (A real number that represents a multiplier used 11319 * in determining the overall value of services delivered and/or 11320 * goods received. The concept of a Factor allows for a discount or 11321 * surcharge multiplier to be applied to a monetary amount.). This 11322 * is the underlying object with id, value and extensions. The 11323 * accessor "getFactor" gives direct access to the value 11324 */ 11325 public AddedItemDetailComponent setFactorElement(DecimalType value) { 11326 this.factor = value; 11327 return this; 11328 } 11329 11330 /** 11331 * @return A real number that represents a multiplier used in determining the 11332 * overall value of services delivered and/or goods received. The 11333 * concept of a Factor allows for a discount or surcharge multiplier to 11334 * be applied to a monetary amount. 11335 */ 11336 public BigDecimal getFactor() { 11337 return this.factor == null ? null : this.factor.getValue(); 11338 } 11339 11340 /** 11341 * @param value A real number that represents a multiplier used in determining 11342 * the overall value of services delivered and/or goods received. 11343 * The concept of a Factor allows for a discount or surcharge 11344 * multiplier to be applied to a monetary amount. 11345 */ 11346 public AddedItemDetailComponent setFactor(BigDecimal value) { 11347 if (value == null) 11348 this.factor = null; 11349 else { 11350 if (this.factor == null) 11351 this.factor = new DecimalType(); 11352 this.factor.setValue(value); 11353 } 11354 return this; 11355 } 11356 11357 /** 11358 * @param value A real number that represents a multiplier used in determining 11359 * the overall value of services delivered and/or goods received. 11360 * The concept of a Factor allows for a discount or surcharge 11361 * multiplier to be applied to a monetary amount. 11362 */ 11363 public AddedItemDetailComponent setFactor(long value) { 11364 this.factor = new DecimalType(); 11365 this.factor.setValue(value); 11366 return this; 11367 } 11368 11369 /** 11370 * @param value A real number that represents a multiplier used in determining 11371 * the overall value of services delivered and/or goods received. 11372 * The concept of a Factor allows for a discount or surcharge 11373 * multiplier to be applied to a monetary amount. 11374 */ 11375 public AddedItemDetailComponent setFactor(double value) { 11376 this.factor = new DecimalType(); 11377 this.factor.setValue(value); 11378 return this; 11379 } 11380 11381 /** 11382 * @return {@link #net} (The quantity times the unit price for an additional 11383 * service or product or charge.) 11384 */ 11385 public Money getNet() { 11386 if (this.net == null) 11387 if (Configuration.errorOnAutoCreate()) 11388 throw new Error("Attempt to auto-create AddedItemDetailComponent.net"); 11389 else if (Configuration.doAutoCreate()) 11390 this.net = new Money(); // cc 11391 return this.net; 11392 } 11393 11394 public boolean hasNet() { 11395 return this.net != null && !this.net.isEmpty(); 11396 } 11397 11398 /** 11399 * @param value {@link #net} (The quantity times the unit price for an 11400 * additional service or product or charge.) 11401 */ 11402 public AddedItemDetailComponent setNet(Money value) { 11403 this.net = value; 11404 return this; 11405 } 11406 11407 /** 11408 * @return {@link #noteNumber} (The numbers associated with notes below which 11409 * apply to the adjudication of this item.) 11410 */ 11411 public List<PositiveIntType> getNoteNumber() { 11412 if (this.noteNumber == null) 11413 this.noteNumber = new ArrayList<PositiveIntType>(); 11414 return this.noteNumber; 11415 } 11416 11417 /** 11418 * @return Returns a reference to <code>this</code> for easy method chaining 11419 */ 11420 public AddedItemDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 11421 this.noteNumber = theNoteNumber; 11422 return this; 11423 } 11424 11425 public boolean hasNoteNumber() { 11426 if (this.noteNumber == null) 11427 return false; 11428 for (PositiveIntType item : this.noteNumber) 11429 if (!item.isEmpty()) 11430 return true; 11431 return false; 11432 } 11433 11434 /** 11435 * @return {@link #noteNumber} (The numbers associated with notes below which 11436 * apply to the adjudication of this item.) 11437 */ 11438 public PositiveIntType addNoteNumberElement() {// 2 11439 PositiveIntType t = new PositiveIntType(); 11440 if (this.noteNumber == null) 11441 this.noteNumber = new ArrayList<PositiveIntType>(); 11442 this.noteNumber.add(t); 11443 return t; 11444 } 11445 11446 /** 11447 * @param value {@link #noteNumber} (The numbers associated with notes below 11448 * which apply to the adjudication of this item.) 11449 */ 11450 public AddedItemDetailComponent addNoteNumber(int value) { // 1 11451 PositiveIntType t = new PositiveIntType(); 11452 t.setValue(value); 11453 if (this.noteNumber == null) 11454 this.noteNumber = new ArrayList<PositiveIntType>(); 11455 this.noteNumber.add(t); 11456 return this; 11457 } 11458 11459 /** 11460 * @param value {@link #noteNumber} (The numbers associated with notes below 11461 * which apply to the adjudication of this item.) 11462 */ 11463 public boolean hasNoteNumber(int value) { 11464 if (this.noteNumber == null) 11465 return false; 11466 for (PositiveIntType v : this.noteNumber) 11467 if (v.getValue().equals(value)) // positiveInt 11468 return true; 11469 return false; 11470 } 11471 11472 /** 11473 * @return {@link #adjudication} (The adjudication results.) 11474 */ 11475 public List<AdjudicationComponent> getAdjudication() { 11476 if (this.adjudication == null) 11477 this.adjudication = new ArrayList<AdjudicationComponent>(); 11478 return this.adjudication; 11479 } 11480 11481 /** 11482 * @return Returns a reference to <code>this</code> for easy method chaining 11483 */ 11484 public AddedItemDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 11485 this.adjudication = theAdjudication; 11486 return this; 11487 } 11488 11489 public boolean hasAdjudication() { 11490 if (this.adjudication == null) 11491 return false; 11492 for (AdjudicationComponent item : this.adjudication) 11493 if (!item.isEmpty()) 11494 return true; 11495 return false; 11496 } 11497 11498 public AdjudicationComponent addAdjudication() { // 3 11499 AdjudicationComponent t = new AdjudicationComponent(); 11500 if (this.adjudication == null) 11501 this.adjudication = new ArrayList<AdjudicationComponent>(); 11502 this.adjudication.add(t); 11503 return t; 11504 } 11505 11506 public AddedItemDetailComponent addAdjudication(AdjudicationComponent t) { // 3 11507 if (t == null) 11508 return this; 11509 if (this.adjudication == null) 11510 this.adjudication = new ArrayList<AdjudicationComponent>(); 11511 this.adjudication.add(t); 11512 return this; 11513 } 11514 11515 /** 11516 * @return The first repetition of repeating field {@link #adjudication}, 11517 * creating it if it does not already exist 11518 */ 11519 public AdjudicationComponent getAdjudicationFirstRep() { 11520 if (getAdjudication().isEmpty()) { 11521 addAdjudication(); 11522 } 11523 return getAdjudication().get(0); 11524 } 11525 11526 /** 11527 * @return {@link #subDetail} (The third-tier service adjudications for payor 11528 * added services.) 11529 */ 11530 public List<AddedItemDetailSubDetailComponent> getSubDetail() { 11531 if (this.subDetail == null) 11532 this.subDetail = new ArrayList<AddedItemDetailSubDetailComponent>(); 11533 return this.subDetail; 11534 } 11535 11536 /** 11537 * @return Returns a reference to <code>this</code> for easy method chaining 11538 */ 11539 public AddedItemDetailComponent setSubDetail(List<AddedItemDetailSubDetailComponent> theSubDetail) { 11540 this.subDetail = theSubDetail; 11541 return this; 11542 } 11543 11544 public boolean hasSubDetail() { 11545 if (this.subDetail == null) 11546 return false; 11547 for (AddedItemDetailSubDetailComponent item : this.subDetail) 11548 if (!item.isEmpty()) 11549 return true; 11550 return false; 11551 } 11552 11553 public AddedItemDetailSubDetailComponent addSubDetail() { // 3 11554 AddedItemDetailSubDetailComponent t = new AddedItemDetailSubDetailComponent(); 11555 if (this.subDetail == null) 11556 this.subDetail = new ArrayList<AddedItemDetailSubDetailComponent>(); 11557 this.subDetail.add(t); 11558 return t; 11559 } 11560 11561 public AddedItemDetailComponent addSubDetail(AddedItemDetailSubDetailComponent t) { // 3 11562 if (t == null) 11563 return this; 11564 if (this.subDetail == null) 11565 this.subDetail = new ArrayList<AddedItemDetailSubDetailComponent>(); 11566 this.subDetail.add(t); 11567 return this; 11568 } 11569 11570 /** 11571 * @return The first repetition of repeating field {@link #subDetail}, creating 11572 * it if it does not already exist 11573 */ 11574 public AddedItemDetailSubDetailComponent getSubDetailFirstRep() { 11575 if (getSubDetail().isEmpty()) { 11576 addSubDetail(); 11577 } 11578 return getSubDetail().get(0); 11579 } 11580 11581 protected void listChildren(List<Property> children) { 11582 super.listChildren(children); 11583 children.add(new Property("productOrService", "CodeableConcept", 11584 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 11585 0, 1, productOrService)); 11586 children.add(new Property("modifier", "CodeableConcept", 11587 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 11588 java.lang.Integer.MAX_VALUE, modifier)); 11589 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 11590 1, quantity)); 11591 children.add(new Property("unitPrice", "Money", 11592 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 11593 0, 1, unitPrice)); 11594 children.add(new Property("factor", "decimal", 11595 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 11596 0, 1, factor)); 11597 children.add(new Property("net", "Money", 11598 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net)); 11599 children.add(new Property("noteNumber", "positiveInt", 11600 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 11601 java.lang.Integer.MAX_VALUE, noteNumber)); 11602 children.add(new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results.", 11603 0, java.lang.Integer.MAX_VALUE, adjudication)); 11604 children.add(new Property("subDetail", "", "The third-tier service adjudications for payor added services.", 0, 11605 java.lang.Integer.MAX_VALUE, subDetail)); 11606 } 11607 11608 @Override 11609 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 11610 switch (_hash) { 11611 case 1957227299: 11612 /* productOrService */ return new Property("productOrService", "CodeableConcept", 11613 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 11614 0, 1, productOrService); 11615 case -615513385: 11616 /* modifier */ return new Property("modifier", "CodeableConcept", 11617 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 11618 java.lang.Integer.MAX_VALUE, modifier); 11619 case -1285004149: 11620 /* quantity */ return new Property("quantity", "SimpleQuantity", 11621 "The number of repetitions of a service or product.", 0, 1, quantity); 11622 case -486196699: 11623 /* unitPrice */ return new Property("unitPrice", "Money", 11624 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 11625 0, 1, unitPrice); 11626 case -1282148017: 11627 /* factor */ return new Property("factor", "decimal", 11628 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 11629 0, 1, factor); 11630 case 108957: 11631 /* net */ return new Property("net", "Money", 11632 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net); 11633 case -1110033957: 11634 /* noteNumber */ return new Property("noteNumber", "positiveInt", 11635 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 11636 java.lang.Integer.MAX_VALUE, noteNumber); 11637 case -231349275: 11638 /* adjudication */ return new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", 11639 "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 11640 case -828829007: 11641 /* subDetail */ return new Property("subDetail", "", 11642 "The third-tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, 11643 subDetail); 11644 default: 11645 return super.getNamedProperty(_hash, _name, _checkValid); 11646 } 11647 11648 } 11649 11650 @Override 11651 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 11652 switch (hash) { 11653 case 1957227299: 11654 /* productOrService */ return this.productOrService == null ? new Base[0] 11655 : new Base[] { this.productOrService }; // CodeableConcept 11656 case -615513385: 11657 /* modifier */ return this.modifier == null ? new Base[0] 11658 : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 11659 case -1285004149: 11660 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 11661 case -486196699: 11662 /* unitPrice */ return this.unitPrice == null ? new Base[0] : new Base[] { this.unitPrice }; // Money 11663 case -1282148017: 11664 /* factor */ return this.factor == null ? new Base[0] : new Base[] { this.factor }; // DecimalType 11665 case 108957: 11666 /* net */ return this.net == null ? new Base[0] : new Base[] { this.net }; // Money 11667 case -1110033957: 11668 /* noteNumber */ return this.noteNumber == null ? new Base[0] 11669 : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 11670 case -231349275: 11671 /* adjudication */ return this.adjudication == null ? new Base[0] 11672 : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 11673 case -828829007: 11674 /* subDetail */ return this.subDetail == null ? new Base[0] 11675 : this.subDetail.toArray(new Base[this.subDetail.size()]); // AddedItemDetailSubDetailComponent 11676 default: 11677 return super.getProperty(hash, name, checkValid); 11678 } 11679 11680 } 11681 11682 @Override 11683 public Base setProperty(int hash, String name, Base value) throws FHIRException { 11684 switch (hash) { 11685 case 1957227299: // productOrService 11686 this.productOrService = castToCodeableConcept(value); // CodeableConcept 11687 return value; 11688 case -615513385: // modifier 11689 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 11690 return value; 11691 case -1285004149: // quantity 11692 this.quantity = castToQuantity(value); // Quantity 11693 return value; 11694 case -486196699: // unitPrice 11695 this.unitPrice = castToMoney(value); // Money 11696 return value; 11697 case -1282148017: // factor 11698 this.factor = castToDecimal(value); // DecimalType 11699 return value; 11700 case 108957: // net 11701 this.net = castToMoney(value); // Money 11702 return value; 11703 case -1110033957: // noteNumber 11704 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 11705 return value; 11706 case -231349275: // adjudication 11707 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 11708 return value; 11709 case -828829007: // subDetail 11710 this.getSubDetail().add((AddedItemDetailSubDetailComponent) value); // AddedItemDetailSubDetailComponent 11711 return value; 11712 default: 11713 return super.setProperty(hash, name, value); 11714 } 11715 11716 } 11717 11718 @Override 11719 public Base setProperty(String name, Base value) throws FHIRException { 11720 if (name.equals("productOrService")) { 11721 this.productOrService = castToCodeableConcept(value); // CodeableConcept 11722 } else if (name.equals("modifier")) { 11723 this.getModifier().add(castToCodeableConcept(value)); 11724 } else if (name.equals("quantity")) { 11725 this.quantity = castToQuantity(value); // Quantity 11726 } else if (name.equals("unitPrice")) { 11727 this.unitPrice = castToMoney(value); // Money 11728 } else if (name.equals("factor")) { 11729 this.factor = castToDecimal(value); // DecimalType 11730 } else if (name.equals("net")) { 11731 this.net = castToMoney(value); // Money 11732 } else if (name.equals("noteNumber")) { 11733 this.getNoteNumber().add(castToPositiveInt(value)); 11734 } else if (name.equals("adjudication")) { 11735 this.getAdjudication().add((AdjudicationComponent) value); 11736 } else if (name.equals("subDetail")) { 11737 this.getSubDetail().add((AddedItemDetailSubDetailComponent) value); 11738 } else 11739 return super.setProperty(name, value); 11740 return value; 11741 } 11742 11743 @Override 11744 public void removeChild(String name, Base value) throws FHIRException { 11745 if (name.equals("productOrService")) { 11746 this.productOrService = null; 11747 } else if (name.equals("modifier")) { 11748 this.getModifier().remove(castToCodeableConcept(value)); 11749 } else if (name.equals("quantity")) { 11750 this.quantity = null; 11751 } else if (name.equals("unitPrice")) { 11752 this.unitPrice = null; 11753 } else if (name.equals("factor")) { 11754 this.factor = null; 11755 } else if (name.equals("net")) { 11756 this.net = null; 11757 } else if (name.equals("noteNumber")) { 11758 this.getNoteNumber().remove(castToPositiveInt(value)); 11759 } else if (name.equals("adjudication")) { 11760 this.getAdjudication().remove((AdjudicationComponent) value); 11761 } else if (name.equals("subDetail")) { 11762 this.getSubDetail().remove((AddedItemDetailSubDetailComponent) value); 11763 } else 11764 super.removeChild(name, value); 11765 11766 } 11767 11768 @Override 11769 public Base makeProperty(int hash, String name) throws FHIRException { 11770 switch (hash) { 11771 case 1957227299: 11772 return getProductOrService(); 11773 case -615513385: 11774 return addModifier(); 11775 case -1285004149: 11776 return getQuantity(); 11777 case -486196699: 11778 return getUnitPrice(); 11779 case -1282148017: 11780 return getFactorElement(); 11781 case 108957: 11782 return getNet(); 11783 case -1110033957: 11784 return addNoteNumberElement(); 11785 case -231349275: 11786 return addAdjudication(); 11787 case -828829007: 11788 return addSubDetail(); 11789 default: 11790 return super.makeProperty(hash, name); 11791 } 11792 11793 } 11794 11795 @Override 11796 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 11797 switch (hash) { 11798 case 1957227299: 11799 /* productOrService */ return new String[] { "CodeableConcept" }; 11800 case -615513385: 11801 /* modifier */ return new String[] { "CodeableConcept" }; 11802 case -1285004149: 11803 /* quantity */ return new String[] { "SimpleQuantity" }; 11804 case -486196699: 11805 /* unitPrice */ return new String[] { "Money" }; 11806 case -1282148017: 11807 /* factor */ return new String[] { "decimal" }; 11808 case 108957: 11809 /* net */ return new String[] { "Money" }; 11810 case -1110033957: 11811 /* noteNumber */ return new String[] { "positiveInt" }; 11812 case -231349275: 11813 /* adjudication */ return new String[] { "@ExplanationOfBenefit.item.adjudication" }; 11814 case -828829007: 11815 /* subDetail */ return new String[] {}; 11816 default: 11817 return super.getTypesForProperty(hash, name); 11818 } 11819 11820 } 11821 11822 @Override 11823 public Base addChild(String name) throws FHIRException { 11824 if (name.equals("productOrService")) { 11825 this.productOrService = new CodeableConcept(); 11826 return this.productOrService; 11827 } else if (name.equals("modifier")) { 11828 return addModifier(); 11829 } else if (name.equals("quantity")) { 11830 this.quantity = new Quantity(); 11831 return this.quantity; 11832 } else if (name.equals("unitPrice")) { 11833 this.unitPrice = new Money(); 11834 return this.unitPrice; 11835 } else if (name.equals("factor")) { 11836 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.factor"); 11837 } else if (name.equals("net")) { 11838 this.net = new Money(); 11839 return this.net; 11840 } else if (name.equals("noteNumber")) { 11841 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.noteNumber"); 11842 } else if (name.equals("adjudication")) { 11843 return addAdjudication(); 11844 } else if (name.equals("subDetail")) { 11845 return addSubDetail(); 11846 } else 11847 return super.addChild(name); 11848 } 11849 11850 public AddedItemDetailComponent copy() { 11851 AddedItemDetailComponent dst = new AddedItemDetailComponent(); 11852 copyValues(dst); 11853 return dst; 11854 } 11855 11856 public void copyValues(AddedItemDetailComponent dst) { 11857 super.copyValues(dst); 11858 dst.productOrService = productOrService == null ? null : productOrService.copy(); 11859 if (modifier != null) { 11860 dst.modifier = new ArrayList<CodeableConcept>(); 11861 for (CodeableConcept i : modifier) 11862 dst.modifier.add(i.copy()); 11863 } 11864 ; 11865 dst.quantity = quantity == null ? null : quantity.copy(); 11866 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 11867 dst.factor = factor == null ? null : factor.copy(); 11868 dst.net = net == null ? null : net.copy(); 11869 if (noteNumber != null) { 11870 dst.noteNumber = new ArrayList<PositiveIntType>(); 11871 for (PositiveIntType i : noteNumber) 11872 dst.noteNumber.add(i.copy()); 11873 } 11874 ; 11875 if (adjudication != null) { 11876 dst.adjudication = new ArrayList<AdjudicationComponent>(); 11877 for (AdjudicationComponent i : adjudication) 11878 dst.adjudication.add(i.copy()); 11879 } 11880 ; 11881 if (subDetail != null) { 11882 dst.subDetail = new ArrayList<AddedItemDetailSubDetailComponent>(); 11883 for (AddedItemDetailSubDetailComponent i : subDetail) 11884 dst.subDetail.add(i.copy()); 11885 } 11886 ; 11887 } 11888 11889 @Override 11890 public boolean equalsDeep(Base other_) { 11891 if (!super.equalsDeep(other_)) 11892 return false; 11893 if (!(other_ instanceof AddedItemDetailComponent)) 11894 return false; 11895 AddedItemDetailComponent o = (AddedItemDetailComponent) other_; 11896 return compareDeep(productOrService, o.productOrService, true) && compareDeep(modifier, o.modifier, true) 11897 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 11898 && compareDeep(factor, o.factor, true) && compareDeep(net, o.net, true) 11899 && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(adjudication, o.adjudication, true) 11900 && compareDeep(subDetail, o.subDetail, true); 11901 } 11902 11903 @Override 11904 public boolean equalsShallow(Base other_) { 11905 if (!super.equalsShallow(other_)) 11906 return false; 11907 if (!(other_ instanceof AddedItemDetailComponent)) 11908 return false; 11909 AddedItemDetailComponent o = (AddedItemDetailComponent) other_; 11910 return compareValues(factor, o.factor, true) && compareValues(noteNumber, o.noteNumber, true); 11911 } 11912 11913 public boolean isEmpty() { 11914 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(productOrService, modifier, quantity, unitPrice, 11915 factor, net, noteNumber, adjudication, subDetail); 11916 } 11917 11918 public String fhirType() { 11919 return "ExplanationOfBenefit.addItem.detail"; 11920 11921 } 11922 11923 } 11924 11925 @Block() 11926 public static class AddedItemDetailSubDetailComponent extends BackboneElement implements IBaseBackboneElement { 11927 /** 11928 * When the value is a group code then this item collects a set of related claim 11929 * details, otherwise this contains the product, service, drug or other billing 11930 * code for the item. 11931 */ 11932 @Child(name = "productOrService", type = { 11933 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 11934 @Description(shortDefinition = "Billing, service, product, or drug code", formalDefinition = "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.") 11935 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-uscls") 11936 protected CodeableConcept productOrService; 11937 11938 /** 11939 * Item typification or modifiers codes to convey additional context for the 11940 * product or service. 11941 */ 11942 @Child(name = "modifier", type = { 11943 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11944 @Description(shortDefinition = "Service/Product billing modifiers", formalDefinition = "Item typification or modifiers codes to convey additional context for the product or service.") 11945 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-modifiers") 11946 protected List<CodeableConcept> modifier; 11947 11948 /** 11949 * The number of repetitions of a service or product. 11950 */ 11951 @Child(name = "quantity", type = { Quantity.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 11952 @Description(shortDefinition = "Count of products or services", formalDefinition = "The number of repetitions of a service or product.") 11953 protected Quantity quantity; 11954 11955 /** 11956 * If the item is not a group then this is the fee for the product or service, 11957 * otherwise this is the total of the fees for the details of the group. 11958 */ 11959 @Child(name = "unitPrice", type = { Money.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 11960 @Description(shortDefinition = "Fee, charge or cost per item", formalDefinition = "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.") 11961 protected Money unitPrice; 11962 11963 /** 11964 * A real number that represents a multiplier used in determining the overall 11965 * value of services delivered and/or goods received. The concept of a Factor 11966 * allows for a discount or surcharge multiplier to be applied to a monetary 11967 * amount. 11968 */ 11969 @Child(name = "factor", type = { 11970 DecimalType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 11971 @Description(shortDefinition = "Price scaling factor", formalDefinition = "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.") 11972 protected DecimalType factor; 11973 11974 /** 11975 * The quantity times the unit price for an additional service or product or 11976 * charge. 11977 */ 11978 @Child(name = "net", type = { Money.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 11979 @Description(shortDefinition = "Total item cost", formalDefinition = "The quantity times the unit price for an additional service or product or charge.") 11980 protected Money net; 11981 11982 /** 11983 * The numbers associated with notes below which apply to the adjudication of 11984 * this item. 11985 */ 11986 @Child(name = "noteNumber", type = { 11987 PositiveIntType.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11988 @Description(shortDefinition = "Applicable note numbers", formalDefinition = "The numbers associated with notes below which apply to the adjudication of this item.") 11989 protected List<PositiveIntType> noteNumber; 11990 11991 /** 11992 * The adjudication results. 11993 */ 11994 @Child(name = "adjudication", type = { 11995 AdjudicationComponent.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11996 @Description(shortDefinition = "Added items adjudication", formalDefinition = "The adjudication results.") 11997 protected List<AdjudicationComponent> adjudication; 11998 11999 private static final long serialVersionUID = 1301363592L; 12000 12001 /** 12002 * Constructor 12003 */ 12004 public AddedItemDetailSubDetailComponent() { 12005 super(); 12006 } 12007 12008 /** 12009 * Constructor 12010 */ 12011 public AddedItemDetailSubDetailComponent(CodeableConcept productOrService) { 12012 super(); 12013 this.productOrService = productOrService; 12014 } 12015 12016 /** 12017 * @return {@link #productOrService} (When the value is a group code then this 12018 * item collects a set of related claim details, otherwise this contains 12019 * the product, service, drug or other billing code for the item.) 12020 */ 12021 public CodeableConcept getProductOrService() { 12022 if (this.productOrService == null) 12023 if (Configuration.errorOnAutoCreate()) 12024 throw new Error("Attempt to auto-create AddedItemDetailSubDetailComponent.productOrService"); 12025 else if (Configuration.doAutoCreate()) 12026 this.productOrService = new CodeableConcept(); // cc 12027 return this.productOrService; 12028 } 12029 12030 public boolean hasProductOrService() { 12031 return this.productOrService != null && !this.productOrService.isEmpty(); 12032 } 12033 12034 /** 12035 * @param value {@link #productOrService} (When the value is a group code then 12036 * this item collects a set of related claim details, otherwise 12037 * this contains the product, service, drug or other billing code 12038 * for the item.) 12039 */ 12040 public AddedItemDetailSubDetailComponent setProductOrService(CodeableConcept value) { 12041 this.productOrService = value; 12042 return this; 12043 } 12044 12045 /** 12046 * @return {@link #modifier} (Item typification or modifiers codes to convey 12047 * additional context for the product or service.) 12048 */ 12049 public List<CodeableConcept> getModifier() { 12050 if (this.modifier == null) 12051 this.modifier = new ArrayList<CodeableConcept>(); 12052 return this.modifier; 12053 } 12054 12055 /** 12056 * @return Returns a reference to <code>this</code> for easy method chaining 12057 */ 12058 public AddedItemDetailSubDetailComponent setModifier(List<CodeableConcept> theModifier) { 12059 this.modifier = theModifier; 12060 return this; 12061 } 12062 12063 public boolean hasModifier() { 12064 if (this.modifier == null) 12065 return false; 12066 for (CodeableConcept item : this.modifier) 12067 if (!item.isEmpty()) 12068 return true; 12069 return false; 12070 } 12071 12072 public CodeableConcept addModifier() { // 3 12073 CodeableConcept t = new CodeableConcept(); 12074 if (this.modifier == null) 12075 this.modifier = new ArrayList<CodeableConcept>(); 12076 this.modifier.add(t); 12077 return t; 12078 } 12079 12080 public AddedItemDetailSubDetailComponent addModifier(CodeableConcept t) { // 3 12081 if (t == null) 12082 return this; 12083 if (this.modifier == null) 12084 this.modifier = new ArrayList<CodeableConcept>(); 12085 this.modifier.add(t); 12086 return this; 12087 } 12088 12089 /** 12090 * @return The first repetition of repeating field {@link #modifier}, creating 12091 * it if it does not already exist 12092 */ 12093 public CodeableConcept getModifierFirstRep() { 12094 if (getModifier().isEmpty()) { 12095 addModifier(); 12096 } 12097 return getModifier().get(0); 12098 } 12099 12100 /** 12101 * @return {@link #quantity} (The number of repetitions of a service or 12102 * product.) 12103 */ 12104 public Quantity getQuantity() { 12105 if (this.quantity == null) 12106 if (Configuration.errorOnAutoCreate()) 12107 throw new Error("Attempt to auto-create AddedItemDetailSubDetailComponent.quantity"); 12108 else if (Configuration.doAutoCreate()) 12109 this.quantity = new Quantity(); // cc 12110 return this.quantity; 12111 } 12112 12113 public boolean hasQuantity() { 12114 return this.quantity != null && !this.quantity.isEmpty(); 12115 } 12116 12117 /** 12118 * @param value {@link #quantity} (The number of repetitions of a service or 12119 * product.) 12120 */ 12121 public AddedItemDetailSubDetailComponent setQuantity(Quantity value) { 12122 this.quantity = value; 12123 return this; 12124 } 12125 12126 /** 12127 * @return {@link #unitPrice} (If the item is not a group then this is the fee 12128 * for the product or service, otherwise this is the total of the fees 12129 * for the details of the group.) 12130 */ 12131 public Money getUnitPrice() { 12132 if (this.unitPrice == null) 12133 if (Configuration.errorOnAutoCreate()) 12134 throw new Error("Attempt to auto-create AddedItemDetailSubDetailComponent.unitPrice"); 12135 else if (Configuration.doAutoCreate()) 12136 this.unitPrice = new Money(); // cc 12137 return this.unitPrice; 12138 } 12139 12140 public boolean hasUnitPrice() { 12141 return this.unitPrice != null && !this.unitPrice.isEmpty(); 12142 } 12143 12144 /** 12145 * @param value {@link #unitPrice} (If the item is not a group then this is the 12146 * fee for the product or service, otherwise this is the total of 12147 * the fees for the details of the group.) 12148 */ 12149 public AddedItemDetailSubDetailComponent setUnitPrice(Money value) { 12150 this.unitPrice = value; 12151 return this; 12152 } 12153 12154 /** 12155 * @return {@link #factor} (A real number that represents a multiplier used in 12156 * determining the overall value of services delivered and/or goods 12157 * received. The concept of a Factor allows for a discount or surcharge 12158 * multiplier to be applied to a monetary amount.). This is the 12159 * underlying object with id, value and extensions. The accessor 12160 * "getFactor" gives direct access to the value 12161 */ 12162 public DecimalType getFactorElement() { 12163 if (this.factor == null) 12164 if (Configuration.errorOnAutoCreate()) 12165 throw new Error("Attempt to auto-create AddedItemDetailSubDetailComponent.factor"); 12166 else if (Configuration.doAutoCreate()) 12167 this.factor = new DecimalType(); // bb 12168 return this.factor; 12169 } 12170 12171 public boolean hasFactorElement() { 12172 return this.factor != null && !this.factor.isEmpty(); 12173 } 12174 12175 public boolean hasFactor() { 12176 return this.factor != null && !this.factor.isEmpty(); 12177 } 12178 12179 /** 12180 * @param value {@link #factor} (A real number that represents a multiplier used 12181 * in determining the overall value of services delivered and/or 12182 * goods received. The concept of a Factor allows for a discount or 12183 * surcharge multiplier to be applied to a monetary amount.). This 12184 * is the underlying object with id, value and extensions. The 12185 * accessor "getFactor" gives direct access to the value 12186 */ 12187 public AddedItemDetailSubDetailComponent setFactorElement(DecimalType value) { 12188 this.factor = value; 12189 return this; 12190 } 12191 12192 /** 12193 * @return A real number that represents a multiplier used in determining the 12194 * overall value of services delivered and/or goods received. The 12195 * concept of a Factor allows for a discount or surcharge multiplier to 12196 * be applied to a monetary amount. 12197 */ 12198 public BigDecimal getFactor() { 12199 return this.factor == null ? null : this.factor.getValue(); 12200 } 12201 12202 /** 12203 * @param value A real number that represents a multiplier used in determining 12204 * the overall value of services delivered and/or goods received. 12205 * The concept of a Factor allows for a discount or surcharge 12206 * multiplier to be applied to a monetary amount. 12207 */ 12208 public AddedItemDetailSubDetailComponent setFactor(BigDecimal value) { 12209 if (value == null) 12210 this.factor = null; 12211 else { 12212 if (this.factor == null) 12213 this.factor = new DecimalType(); 12214 this.factor.setValue(value); 12215 } 12216 return this; 12217 } 12218 12219 /** 12220 * @param value A real number that represents a multiplier used in determining 12221 * the overall value of services delivered and/or goods received. 12222 * The concept of a Factor allows for a discount or surcharge 12223 * multiplier to be applied to a monetary amount. 12224 */ 12225 public AddedItemDetailSubDetailComponent setFactor(long value) { 12226 this.factor = new DecimalType(); 12227 this.factor.setValue(value); 12228 return this; 12229 } 12230 12231 /** 12232 * @param value A real number that represents a multiplier used in determining 12233 * the overall value of services delivered and/or goods received. 12234 * The concept of a Factor allows for a discount or surcharge 12235 * multiplier to be applied to a monetary amount. 12236 */ 12237 public AddedItemDetailSubDetailComponent setFactor(double value) { 12238 this.factor = new DecimalType(); 12239 this.factor.setValue(value); 12240 return this; 12241 } 12242 12243 /** 12244 * @return {@link #net} (The quantity times the unit price for an additional 12245 * service or product or charge.) 12246 */ 12247 public Money getNet() { 12248 if (this.net == null) 12249 if (Configuration.errorOnAutoCreate()) 12250 throw new Error("Attempt to auto-create AddedItemDetailSubDetailComponent.net"); 12251 else if (Configuration.doAutoCreate()) 12252 this.net = new Money(); // cc 12253 return this.net; 12254 } 12255 12256 public boolean hasNet() { 12257 return this.net != null && !this.net.isEmpty(); 12258 } 12259 12260 /** 12261 * @param value {@link #net} (The quantity times the unit price for an 12262 * additional service or product or charge.) 12263 */ 12264 public AddedItemDetailSubDetailComponent setNet(Money value) { 12265 this.net = value; 12266 return this; 12267 } 12268 12269 /** 12270 * @return {@link #noteNumber} (The numbers associated with notes below which 12271 * apply to the adjudication of this item.) 12272 */ 12273 public List<PositiveIntType> getNoteNumber() { 12274 if (this.noteNumber == null) 12275 this.noteNumber = new ArrayList<PositiveIntType>(); 12276 return this.noteNumber; 12277 } 12278 12279 /** 12280 * @return Returns a reference to <code>this</code> for easy method chaining 12281 */ 12282 public AddedItemDetailSubDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 12283 this.noteNumber = theNoteNumber; 12284 return this; 12285 } 12286 12287 public boolean hasNoteNumber() { 12288 if (this.noteNumber == null) 12289 return false; 12290 for (PositiveIntType item : this.noteNumber) 12291 if (!item.isEmpty()) 12292 return true; 12293 return false; 12294 } 12295 12296 /** 12297 * @return {@link #noteNumber} (The numbers associated with notes below which 12298 * apply to the adjudication of this item.) 12299 */ 12300 public PositiveIntType addNoteNumberElement() {// 2 12301 PositiveIntType t = new PositiveIntType(); 12302 if (this.noteNumber == null) 12303 this.noteNumber = new ArrayList<PositiveIntType>(); 12304 this.noteNumber.add(t); 12305 return t; 12306 } 12307 12308 /** 12309 * @param value {@link #noteNumber} (The numbers associated with notes below 12310 * which apply to the adjudication of this item.) 12311 */ 12312 public AddedItemDetailSubDetailComponent addNoteNumber(int value) { // 1 12313 PositiveIntType t = new PositiveIntType(); 12314 t.setValue(value); 12315 if (this.noteNumber == null) 12316 this.noteNumber = new ArrayList<PositiveIntType>(); 12317 this.noteNumber.add(t); 12318 return this; 12319 } 12320 12321 /** 12322 * @param value {@link #noteNumber} (The numbers associated with notes below 12323 * which apply to the adjudication of this item.) 12324 */ 12325 public boolean hasNoteNumber(int value) { 12326 if (this.noteNumber == null) 12327 return false; 12328 for (PositiveIntType v : this.noteNumber) 12329 if (v.getValue().equals(value)) // positiveInt 12330 return true; 12331 return false; 12332 } 12333 12334 /** 12335 * @return {@link #adjudication} (The adjudication results.) 12336 */ 12337 public List<AdjudicationComponent> getAdjudication() { 12338 if (this.adjudication == null) 12339 this.adjudication = new ArrayList<AdjudicationComponent>(); 12340 return this.adjudication; 12341 } 12342 12343 /** 12344 * @return Returns a reference to <code>this</code> for easy method chaining 12345 */ 12346 public AddedItemDetailSubDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 12347 this.adjudication = theAdjudication; 12348 return this; 12349 } 12350 12351 public boolean hasAdjudication() { 12352 if (this.adjudication == null) 12353 return false; 12354 for (AdjudicationComponent item : this.adjudication) 12355 if (!item.isEmpty()) 12356 return true; 12357 return false; 12358 } 12359 12360 public AdjudicationComponent addAdjudication() { // 3 12361 AdjudicationComponent t = new AdjudicationComponent(); 12362 if (this.adjudication == null) 12363 this.adjudication = new ArrayList<AdjudicationComponent>(); 12364 this.adjudication.add(t); 12365 return t; 12366 } 12367 12368 public AddedItemDetailSubDetailComponent addAdjudication(AdjudicationComponent t) { // 3 12369 if (t == null) 12370 return this; 12371 if (this.adjudication == null) 12372 this.adjudication = new ArrayList<AdjudicationComponent>(); 12373 this.adjudication.add(t); 12374 return this; 12375 } 12376 12377 /** 12378 * @return The first repetition of repeating field {@link #adjudication}, 12379 * creating it if it does not already exist 12380 */ 12381 public AdjudicationComponent getAdjudicationFirstRep() { 12382 if (getAdjudication().isEmpty()) { 12383 addAdjudication(); 12384 } 12385 return getAdjudication().get(0); 12386 } 12387 12388 protected void listChildren(List<Property> children) { 12389 super.listChildren(children); 12390 children.add(new Property("productOrService", "CodeableConcept", 12391 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 12392 0, 1, productOrService)); 12393 children.add(new Property("modifier", "CodeableConcept", 12394 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 12395 java.lang.Integer.MAX_VALUE, modifier)); 12396 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 12397 1, quantity)); 12398 children.add(new Property("unitPrice", "Money", 12399 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 12400 0, 1, unitPrice)); 12401 children.add(new Property("factor", "decimal", 12402 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 12403 0, 1, factor)); 12404 children.add(new Property("net", "Money", 12405 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net)); 12406 children.add(new Property("noteNumber", "positiveInt", 12407 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 12408 java.lang.Integer.MAX_VALUE, noteNumber)); 12409 children.add(new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results.", 12410 0, java.lang.Integer.MAX_VALUE, adjudication)); 12411 } 12412 12413 @Override 12414 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 12415 switch (_hash) { 12416 case 1957227299: 12417 /* productOrService */ return new Property("productOrService", "CodeableConcept", 12418 "When the value is a group code then this item collects a set of related claim details, otherwise this contains the product, service, drug or other billing code for the item.", 12419 0, 1, productOrService); 12420 case -615513385: 12421 /* modifier */ return new Property("modifier", "CodeableConcept", 12422 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 12423 java.lang.Integer.MAX_VALUE, modifier); 12424 case -1285004149: 12425 /* quantity */ return new Property("quantity", "SimpleQuantity", 12426 "The number of repetitions of a service or product.", 0, 1, quantity); 12427 case -486196699: 12428 /* unitPrice */ return new Property("unitPrice", "Money", 12429 "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 12430 0, 1, unitPrice); 12431 case -1282148017: 12432 /* factor */ return new Property("factor", "decimal", 12433 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 12434 0, 1, factor); 12435 case 108957: 12436 /* net */ return new Property("net", "Money", 12437 "The quantity times the unit price for an additional service or product or charge.", 0, 1, net); 12438 case -1110033957: 12439 /* noteNumber */ return new Property("noteNumber", "positiveInt", 12440 "The numbers associated with notes below which apply to the adjudication of this item.", 0, 12441 java.lang.Integer.MAX_VALUE, noteNumber); 12442 case -231349275: 12443 /* adjudication */ return new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", 12444 "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 12445 default: 12446 return super.getNamedProperty(_hash, _name, _checkValid); 12447 } 12448 12449 } 12450 12451 @Override 12452 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 12453 switch (hash) { 12454 case 1957227299: 12455 /* productOrService */ return this.productOrService == null ? new Base[0] 12456 : new Base[] { this.productOrService }; // CodeableConcept 12457 case -615513385: 12458 /* modifier */ return this.modifier == null ? new Base[0] 12459 : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 12460 case -1285004149: 12461 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 12462 case -486196699: 12463 /* unitPrice */ return this.unitPrice == null ? new Base[0] : new Base[] { this.unitPrice }; // Money 12464 case -1282148017: 12465 /* factor */ return this.factor == null ? new Base[0] : new Base[] { this.factor }; // DecimalType 12466 case 108957: 12467 /* net */ return this.net == null ? new Base[0] : new Base[] { this.net }; // Money 12468 case -1110033957: 12469 /* noteNumber */ return this.noteNumber == null ? new Base[0] 12470 : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 12471 case -231349275: 12472 /* adjudication */ return this.adjudication == null ? new Base[0] 12473 : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 12474 default: 12475 return super.getProperty(hash, name, checkValid); 12476 } 12477 12478 } 12479 12480 @Override 12481 public Base setProperty(int hash, String name, Base value) throws FHIRException { 12482 switch (hash) { 12483 case 1957227299: // productOrService 12484 this.productOrService = castToCodeableConcept(value); // CodeableConcept 12485 return value; 12486 case -615513385: // modifier 12487 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 12488 return value; 12489 case -1285004149: // quantity 12490 this.quantity = castToQuantity(value); // Quantity 12491 return value; 12492 case -486196699: // unitPrice 12493 this.unitPrice = castToMoney(value); // Money 12494 return value; 12495 case -1282148017: // factor 12496 this.factor = castToDecimal(value); // DecimalType 12497 return value; 12498 case 108957: // net 12499 this.net = castToMoney(value); // Money 12500 return value; 12501 case -1110033957: // noteNumber 12502 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 12503 return value; 12504 case -231349275: // adjudication 12505 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 12506 return value; 12507 default: 12508 return super.setProperty(hash, name, value); 12509 } 12510 12511 } 12512 12513 @Override 12514 public Base setProperty(String name, Base value) throws FHIRException { 12515 if (name.equals("productOrService")) { 12516 this.productOrService = castToCodeableConcept(value); // CodeableConcept 12517 } else if (name.equals("modifier")) { 12518 this.getModifier().add(castToCodeableConcept(value)); 12519 } else if (name.equals("quantity")) { 12520 this.quantity = castToQuantity(value); // Quantity 12521 } else if (name.equals("unitPrice")) { 12522 this.unitPrice = castToMoney(value); // Money 12523 } else if (name.equals("factor")) { 12524 this.factor = castToDecimal(value); // DecimalType 12525 } else if (name.equals("net")) { 12526 this.net = castToMoney(value); // Money 12527 } else if (name.equals("noteNumber")) { 12528 this.getNoteNumber().add(castToPositiveInt(value)); 12529 } else if (name.equals("adjudication")) { 12530 this.getAdjudication().add((AdjudicationComponent) value); 12531 } else 12532 return super.setProperty(name, value); 12533 return value; 12534 } 12535 12536 @Override 12537 public void removeChild(String name, Base value) throws FHIRException { 12538 if (name.equals("productOrService")) { 12539 this.productOrService = null; 12540 } else if (name.equals("modifier")) { 12541 this.getModifier().remove(castToCodeableConcept(value)); 12542 } else if (name.equals("quantity")) { 12543 this.quantity = null; 12544 } else if (name.equals("unitPrice")) { 12545 this.unitPrice = null; 12546 } else if (name.equals("factor")) { 12547 this.factor = null; 12548 } else if (name.equals("net")) { 12549 this.net = null; 12550 } else if (name.equals("noteNumber")) { 12551 this.getNoteNumber().remove(castToPositiveInt(value)); 12552 } else if (name.equals("adjudication")) { 12553 this.getAdjudication().remove((AdjudicationComponent) value); 12554 } else 12555 super.removeChild(name, value); 12556 12557 } 12558 12559 @Override 12560 public Base makeProperty(int hash, String name) throws FHIRException { 12561 switch (hash) { 12562 case 1957227299: 12563 return getProductOrService(); 12564 case -615513385: 12565 return addModifier(); 12566 case -1285004149: 12567 return getQuantity(); 12568 case -486196699: 12569 return getUnitPrice(); 12570 case -1282148017: 12571 return getFactorElement(); 12572 case 108957: 12573 return getNet(); 12574 case -1110033957: 12575 return addNoteNumberElement(); 12576 case -231349275: 12577 return addAdjudication(); 12578 default: 12579 return super.makeProperty(hash, name); 12580 } 12581 12582 } 12583 12584 @Override 12585 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 12586 switch (hash) { 12587 case 1957227299: 12588 /* productOrService */ return new String[] { "CodeableConcept" }; 12589 case -615513385: 12590 /* modifier */ return new String[] { "CodeableConcept" }; 12591 case -1285004149: 12592 /* quantity */ return new String[] { "SimpleQuantity" }; 12593 case -486196699: 12594 /* unitPrice */ return new String[] { "Money" }; 12595 case -1282148017: 12596 /* factor */ return new String[] { "decimal" }; 12597 case 108957: 12598 /* net */ return new String[] { "Money" }; 12599 case -1110033957: 12600 /* noteNumber */ return new String[] { "positiveInt" }; 12601 case -231349275: 12602 /* adjudication */ return new String[] { "@ExplanationOfBenefit.item.adjudication" }; 12603 default: 12604 return super.getTypesForProperty(hash, name); 12605 } 12606 12607 } 12608 12609 @Override 12610 public Base addChild(String name) throws FHIRException { 12611 if (name.equals("productOrService")) { 12612 this.productOrService = new CodeableConcept(); 12613 return this.productOrService; 12614 } else if (name.equals("modifier")) { 12615 return addModifier(); 12616 } else if (name.equals("quantity")) { 12617 this.quantity = new Quantity(); 12618 return this.quantity; 12619 } else if (name.equals("unitPrice")) { 12620 this.unitPrice = new Money(); 12621 return this.unitPrice; 12622 } else if (name.equals("factor")) { 12623 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.factor"); 12624 } else if (name.equals("net")) { 12625 this.net = new Money(); 12626 return this.net; 12627 } else if (name.equals("noteNumber")) { 12628 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.noteNumber"); 12629 } else if (name.equals("adjudication")) { 12630 return addAdjudication(); 12631 } else 12632 return super.addChild(name); 12633 } 12634 12635 public AddedItemDetailSubDetailComponent copy() { 12636 AddedItemDetailSubDetailComponent dst = new AddedItemDetailSubDetailComponent(); 12637 copyValues(dst); 12638 return dst; 12639 } 12640 12641 public void copyValues(AddedItemDetailSubDetailComponent dst) { 12642 super.copyValues(dst); 12643 dst.productOrService = productOrService == null ? null : productOrService.copy(); 12644 if (modifier != null) { 12645 dst.modifier = new ArrayList<CodeableConcept>(); 12646 for (CodeableConcept i : modifier) 12647 dst.modifier.add(i.copy()); 12648 } 12649 ; 12650 dst.quantity = quantity == null ? null : quantity.copy(); 12651 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 12652 dst.factor = factor == null ? null : factor.copy(); 12653 dst.net = net == null ? null : net.copy(); 12654 if (noteNumber != null) { 12655 dst.noteNumber = new ArrayList<PositiveIntType>(); 12656 for (PositiveIntType i : noteNumber) 12657 dst.noteNumber.add(i.copy()); 12658 } 12659 ; 12660 if (adjudication != null) { 12661 dst.adjudication = new ArrayList<AdjudicationComponent>(); 12662 for (AdjudicationComponent i : adjudication) 12663 dst.adjudication.add(i.copy()); 12664 } 12665 ; 12666 } 12667 12668 @Override 12669 public boolean equalsDeep(Base other_) { 12670 if (!super.equalsDeep(other_)) 12671 return false; 12672 if (!(other_ instanceof AddedItemDetailSubDetailComponent)) 12673 return false; 12674 AddedItemDetailSubDetailComponent o = (AddedItemDetailSubDetailComponent) other_; 12675 return compareDeep(productOrService, o.productOrService, true) && compareDeep(modifier, o.modifier, true) 12676 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 12677 && compareDeep(factor, o.factor, true) && compareDeep(net, o.net, true) 12678 && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(adjudication, o.adjudication, true); 12679 } 12680 12681 @Override 12682 public boolean equalsShallow(Base other_) { 12683 if (!super.equalsShallow(other_)) 12684 return false; 12685 if (!(other_ instanceof AddedItemDetailSubDetailComponent)) 12686 return false; 12687 AddedItemDetailSubDetailComponent o = (AddedItemDetailSubDetailComponent) other_; 12688 return compareValues(factor, o.factor, true) && compareValues(noteNumber, o.noteNumber, true); 12689 } 12690 12691 public boolean isEmpty() { 12692 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(productOrService, modifier, quantity, unitPrice, 12693 factor, net, noteNumber, adjudication); 12694 } 12695 12696 public String fhirType() { 12697 return "ExplanationOfBenefit.addItem.detail.subDetail"; 12698 12699 } 12700 12701 } 12702 12703 @Block() 12704 public static class TotalComponent extends BackboneElement implements IBaseBackboneElement { 12705 /** 12706 * A code to indicate the information type of this adjudication record. 12707 * Information types may include: the value submitted, maximum values or 12708 * percentages allowed or payable under the plan, amounts that the patient is 12709 * responsible for in aggregate or pertaining to this item, amounts paid by 12710 * other coverages, and the benefit payable for this item. 12711 */ 12712 @Child(name = "category", type = { 12713 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 12714 @Description(shortDefinition = "Type of adjudication information", formalDefinition = "A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.") 12715 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/adjudication") 12716 protected CodeableConcept category; 12717 12718 /** 12719 * Monetary total amount associated with the category. 12720 */ 12721 @Child(name = "amount", type = { Money.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 12722 @Description(shortDefinition = "Financial total for the category", formalDefinition = "Monetary total amount associated with the category.") 12723 protected Money amount; 12724 12725 private static final long serialVersionUID = 2012310309L; 12726 12727 /** 12728 * Constructor 12729 */ 12730 public TotalComponent() { 12731 super(); 12732 } 12733 12734 /** 12735 * Constructor 12736 */ 12737 public TotalComponent(CodeableConcept category, Money amount) { 12738 super(); 12739 this.category = category; 12740 this.amount = amount; 12741 } 12742 12743 /** 12744 * @return {@link #category} (A code to indicate the information type of this 12745 * adjudication record. Information types may include: the value 12746 * submitted, maximum values or percentages allowed or payable under the 12747 * plan, amounts that the patient is responsible for in aggregate or 12748 * pertaining to this item, amounts paid by other coverages, and the 12749 * benefit payable for this item.) 12750 */ 12751 public CodeableConcept getCategory() { 12752 if (this.category == null) 12753 if (Configuration.errorOnAutoCreate()) 12754 throw new Error("Attempt to auto-create TotalComponent.category"); 12755 else if (Configuration.doAutoCreate()) 12756 this.category = new CodeableConcept(); // cc 12757 return this.category; 12758 } 12759 12760 public boolean hasCategory() { 12761 return this.category != null && !this.category.isEmpty(); 12762 } 12763 12764 /** 12765 * @param value {@link #category} (A code to indicate the information type of 12766 * this adjudication record. Information types may include: the 12767 * value submitted, maximum values or percentages allowed or 12768 * payable under the plan, amounts that the patient is responsible 12769 * for in aggregate or pertaining to this item, amounts paid by 12770 * other coverages, and the benefit payable for this item.) 12771 */ 12772 public TotalComponent setCategory(CodeableConcept value) { 12773 this.category = value; 12774 return this; 12775 } 12776 12777 /** 12778 * @return {@link #amount} (Monetary total amount associated with the category.) 12779 */ 12780 public Money getAmount() { 12781 if (this.amount == null) 12782 if (Configuration.errorOnAutoCreate()) 12783 throw new Error("Attempt to auto-create TotalComponent.amount"); 12784 else if (Configuration.doAutoCreate()) 12785 this.amount = new Money(); // cc 12786 return this.amount; 12787 } 12788 12789 public boolean hasAmount() { 12790 return this.amount != null && !this.amount.isEmpty(); 12791 } 12792 12793 /** 12794 * @param value {@link #amount} (Monetary total amount associated with the 12795 * category.) 12796 */ 12797 public TotalComponent setAmount(Money value) { 12798 this.amount = value; 12799 return this; 12800 } 12801 12802 protected void listChildren(List<Property> children) { 12803 super.listChildren(children); 12804 children.add(new Property("category", "CodeableConcept", 12805 "A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.", 12806 0, 1, category)); 12807 children 12808 .add(new Property("amount", "Money", "Monetary total amount associated with the category.", 0, 1, amount)); 12809 } 12810 12811 @Override 12812 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 12813 switch (_hash) { 12814 case 50511102: 12815 /* category */ return new Property("category", "CodeableConcept", 12816 "A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.", 12817 0, 1, category); 12818 case -1413853096: 12819 /* amount */ return new Property("amount", "Money", "Monetary total amount associated with the category.", 0, 1, 12820 amount); 12821 default: 12822 return super.getNamedProperty(_hash, _name, _checkValid); 12823 } 12824 12825 } 12826 12827 @Override 12828 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 12829 switch (hash) { 12830 case 50511102: 12831 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 12832 case -1413853096: 12833 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // Money 12834 default: 12835 return super.getProperty(hash, name, checkValid); 12836 } 12837 12838 } 12839 12840 @Override 12841 public Base setProperty(int hash, String name, Base value) throws FHIRException { 12842 switch (hash) { 12843 case 50511102: // category 12844 this.category = castToCodeableConcept(value); // CodeableConcept 12845 return value; 12846 case -1413853096: // amount 12847 this.amount = castToMoney(value); // Money 12848 return value; 12849 default: 12850 return super.setProperty(hash, name, value); 12851 } 12852 12853 } 12854 12855 @Override 12856 public Base setProperty(String name, Base value) throws FHIRException { 12857 if (name.equals("category")) { 12858 this.category = castToCodeableConcept(value); // CodeableConcept 12859 } else if (name.equals("amount")) { 12860 this.amount = castToMoney(value); // Money 12861 } else 12862 return super.setProperty(name, value); 12863 return value; 12864 } 12865 12866 @Override 12867 public void removeChild(String name, Base value) throws FHIRException { 12868 if (name.equals("category")) { 12869 this.category = null; 12870 } else if (name.equals("amount")) { 12871 this.amount = null; 12872 } else 12873 super.removeChild(name, value); 12874 12875 } 12876 12877 @Override 12878 public Base makeProperty(int hash, String name) throws FHIRException { 12879 switch (hash) { 12880 case 50511102: 12881 return getCategory(); 12882 case -1413853096: 12883 return getAmount(); 12884 default: 12885 return super.makeProperty(hash, name); 12886 } 12887 12888 } 12889 12890 @Override 12891 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 12892 switch (hash) { 12893 case 50511102: 12894 /* category */ return new String[] { "CodeableConcept" }; 12895 case -1413853096: 12896 /* amount */ return new String[] { "Money" }; 12897 default: 12898 return super.getTypesForProperty(hash, name); 12899 } 12900 12901 } 12902 12903 @Override 12904 public Base addChild(String name) throws FHIRException { 12905 if (name.equals("category")) { 12906 this.category = new CodeableConcept(); 12907 return this.category; 12908 } else if (name.equals("amount")) { 12909 this.amount = new Money(); 12910 return this.amount; 12911 } else 12912 return super.addChild(name); 12913 } 12914 12915 public TotalComponent copy() { 12916 TotalComponent dst = new TotalComponent(); 12917 copyValues(dst); 12918 return dst; 12919 } 12920 12921 public void copyValues(TotalComponent dst) { 12922 super.copyValues(dst); 12923 dst.category = category == null ? null : category.copy(); 12924 dst.amount = amount == null ? null : amount.copy(); 12925 } 12926 12927 @Override 12928 public boolean equalsDeep(Base other_) { 12929 if (!super.equalsDeep(other_)) 12930 return false; 12931 if (!(other_ instanceof TotalComponent)) 12932 return false; 12933 TotalComponent o = (TotalComponent) other_; 12934 return compareDeep(category, o.category, true) && compareDeep(amount, o.amount, true); 12935 } 12936 12937 @Override 12938 public boolean equalsShallow(Base other_) { 12939 if (!super.equalsShallow(other_)) 12940 return false; 12941 if (!(other_ instanceof TotalComponent)) 12942 return false; 12943 TotalComponent o = (TotalComponent) other_; 12944 return true; 12945 } 12946 12947 public boolean isEmpty() { 12948 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, amount); 12949 } 12950 12951 public String fhirType() { 12952 return "ExplanationOfBenefit.total"; 12953 12954 } 12955 12956 } 12957 12958 @Block() 12959 public static class PaymentComponent extends BackboneElement implements IBaseBackboneElement { 12960 /** 12961 * Whether this represents partial or complete payment of the benefits payable. 12962 */ 12963 @Child(name = "type", type = { 12964 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 12965 @Description(shortDefinition = "Partial or complete payment", formalDefinition = "Whether this represents partial or complete payment of the benefits payable.") 12966 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-paymenttype") 12967 protected CodeableConcept type; 12968 12969 /** 12970 * Total amount of all adjustments to this payment included in this transaction 12971 * which are not related to this claim's adjudication. 12972 */ 12973 @Child(name = "adjustment", type = { Money.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 12974 @Description(shortDefinition = "Payment adjustment for non-claim issues", formalDefinition = "Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication.") 12975 protected Money adjustment; 12976 12977 /** 12978 * Reason for the payment adjustment. 12979 */ 12980 @Child(name = "adjustmentReason", type = { 12981 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 12982 @Description(shortDefinition = "Explanation for the variance", formalDefinition = "Reason for the payment adjustment.") 12983 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/payment-adjustment-reason") 12984 protected CodeableConcept adjustmentReason; 12985 12986 /** 12987 * Estimated date the payment will be issued or the actual issue date of 12988 * payment. 12989 */ 12990 @Child(name = "date", type = { DateType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 12991 @Description(shortDefinition = "Expected date of payment", formalDefinition = "Estimated date the payment will be issued or the actual issue date of payment.") 12992 protected DateType date; 12993 12994 /** 12995 * Benefits payable less any payment adjustment. 12996 */ 12997 @Child(name = "amount", type = { Money.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 12998 @Description(shortDefinition = "Payable amount after adjustment", formalDefinition = "Benefits payable less any payment adjustment.") 12999 protected Money amount; 13000 13001 /** 13002 * Issuer's unique identifier for the payment instrument. 13003 */ 13004 @Child(name = "identifier", type = { 13005 Identifier.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 13006 @Description(shortDefinition = "Business identifier for the payment", formalDefinition = "Issuer's unique identifier for the payment instrument.") 13007 protected Identifier identifier; 13008 13009 private static final long serialVersionUID = 1539906026L; 13010 13011 /** 13012 * Constructor 13013 */ 13014 public PaymentComponent() { 13015 super(); 13016 } 13017 13018 /** 13019 * @return {@link #type} (Whether this represents partial or complete payment of 13020 * the benefits payable.) 13021 */ 13022 public CodeableConcept getType() { 13023 if (this.type == null) 13024 if (Configuration.errorOnAutoCreate()) 13025 throw new Error("Attempt to auto-create PaymentComponent.type"); 13026 else if (Configuration.doAutoCreate()) 13027 this.type = new CodeableConcept(); // cc 13028 return this.type; 13029 } 13030 13031 public boolean hasType() { 13032 return this.type != null && !this.type.isEmpty(); 13033 } 13034 13035 /** 13036 * @param value {@link #type} (Whether this represents partial or complete 13037 * payment of the benefits payable.) 13038 */ 13039 public PaymentComponent setType(CodeableConcept value) { 13040 this.type = value; 13041 return this; 13042 } 13043 13044 /** 13045 * @return {@link #adjustment} (Total amount of all adjustments to this payment 13046 * included in this transaction which are not related to this claim's 13047 * adjudication.) 13048 */ 13049 public Money getAdjustment() { 13050 if (this.adjustment == null) 13051 if (Configuration.errorOnAutoCreate()) 13052 throw new Error("Attempt to auto-create PaymentComponent.adjustment"); 13053 else if (Configuration.doAutoCreate()) 13054 this.adjustment = new Money(); // cc 13055 return this.adjustment; 13056 } 13057 13058 public boolean hasAdjustment() { 13059 return this.adjustment != null && !this.adjustment.isEmpty(); 13060 } 13061 13062 /** 13063 * @param value {@link #adjustment} (Total amount of all adjustments to this 13064 * payment included in this transaction which are not related to 13065 * this claim's adjudication.) 13066 */ 13067 public PaymentComponent setAdjustment(Money value) { 13068 this.adjustment = value; 13069 return this; 13070 } 13071 13072 /** 13073 * @return {@link #adjustmentReason} (Reason for the payment adjustment.) 13074 */ 13075 public CodeableConcept getAdjustmentReason() { 13076 if (this.adjustmentReason == null) 13077 if (Configuration.errorOnAutoCreate()) 13078 throw new Error("Attempt to auto-create PaymentComponent.adjustmentReason"); 13079 else if (Configuration.doAutoCreate()) 13080 this.adjustmentReason = new CodeableConcept(); // cc 13081 return this.adjustmentReason; 13082 } 13083 13084 public boolean hasAdjustmentReason() { 13085 return this.adjustmentReason != null && !this.adjustmentReason.isEmpty(); 13086 } 13087 13088 /** 13089 * @param value {@link #adjustmentReason} (Reason for the payment adjustment.) 13090 */ 13091 public PaymentComponent setAdjustmentReason(CodeableConcept value) { 13092 this.adjustmentReason = value; 13093 return this; 13094 } 13095 13096 /** 13097 * @return {@link #date} (Estimated date the payment will be issued or the 13098 * actual issue date of payment.). This is the underlying object with 13099 * id, value and extensions. The accessor "getDate" gives direct access 13100 * to the value 13101 */ 13102 public DateType getDateElement() { 13103 if (this.date == null) 13104 if (Configuration.errorOnAutoCreate()) 13105 throw new Error("Attempt to auto-create PaymentComponent.date"); 13106 else if (Configuration.doAutoCreate()) 13107 this.date = new DateType(); // bb 13108 return this.date; 13109 } 13110 13111 public boolean hasDateElement() { 13112 return this.date != null && !this.date.isEmpty(); 13113 } 13114 13115 public boolean hasDate() { 13116 return this.date != null && !this.date.isEmpty(); 13117 } 13118 13119 /** 13120 * @param value {@link #date} (Estimated date the payment will be issued or the 13121 * actual issue date of payment.). This is the underlying object 13122 * with id, value and extensions. The accessor "getDate" gives 13123 * direct access to the value 13124 */ 13125 public PaymentComponent setDateElement(DateType value) { 13126 this.date = value; 13127 return this; 13128 } 13129 13130 /** 13131 * @return Estimated date the payment will be issued or the actual issue date of 13132 * payment. 13133 */ 13134 public Date getDate() { 13135 return this.date == null ? null : this.date.getValue(); 13136 } 13137 13138 /** 13139 * @param value Estimated date the payment will be issued or the actual issue 13140 * date of payment. 13141 */ 13142 public PaymentComponent setDate(Date value) { 13143 if (value == null) 13144 this.date = null; 13145 else { 13146 if (this.date == null) 13147 this.date = new DateType(); 13148 this.date.setValue(value); 13149 } 13150 return this; 13151 } 13152 13153 /** 13154 * @return {@link #amount} (Benefits payable less any payment adjustment.) 13155 */ 13156 public Money getAmount() { 13157 if (this.amount == null) 13158 if (Configuration.errorOnAutoCreate()) 13159 throw new Error("Attempt to auto-create PaymentComponent.amount"); 13160 else if (Configuration.doAutoCreate()) 13161 this.amount = new Money(); // cc 13162 return this.amount; 13163 } 13164 13165 public boolean hasAmount() { 13166 return this.amount != null && !this.amount.isEmpty(); 13167 } 13168 13169 /** 13170 * @param value {@link #amount} (Benefits payable less any payment adjustment.) 13171 */ 13172 public PaymentComponent setAmount(Money value) { 13173 this.amount = value; 13174 return this; 13175 } 13176 13177 /** 13178 * @return {@link #identifier} (Issuer's unique identifier for the payment 13179 * instrument.) 13180 */ 13181 public Identifier getIdentifier() { 13182 if (this.identifier == null) 13183 if (Configuration.errorOnAutoCreate()) 13184 throw new Error("Attempt to auto-create PaymentComponent.identifier"); 13185 else if (Configuration.doAutoCreate()) 13186 this.identifier = new Identifier(); // cc 13187 return this.identifier; 13188 } 13189 13190 public boolean hasIdentifier() { 13191 return this.identifier != null && !this.identifier.isEmpty(); 13192 } 13193 13194 /** 13195 * @param value {@link #identifier} (Issuer's unique identifier for the payment 13196 * instrument.) 13197 */ 13198 public PaymentComponent setIdentifier(Identifier value) { 13199 this.identifier = value; 13200 return this; 13201 } 13202 13203 protected void listChildren(List<Property> children) { 13204 super.listChildren(children); 13205 children.add(new Property("type", "CodeableConcept", 13206 "Whether this represents partial or complete payment of the benefits payable.", 0, 1, type)); 13207 children.add(new Property("adjustment", "Money", 13208 "Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication.", 13209 0, 1, adjustment)); 13210 children.add(new Property("adjustmentReason", "CodeableConcept", "Reason for the payment adjustment.", 0, 1, 13211 adjustmentReason)); 13212 children.add(new Property("date", "date", 13213 "Estimated date the payment will be issued or the actual issue date of payment.", 0, 1, date)); 13214 children.add(new Property("amount", "Money", "Benefits payable less any payment adjustment.", 0, 1, amount)); 13215 children.add(new Property("identifier", "Identifier", "Issuer's unique identifier for the payment instrument.", 0, 13216 1, identifier)); 13217 } 13218 13219 @Override 13220 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 13221 switch (_hash) { 13222 case 3575610: 13223 /* type */ return new Property("type", "CodeableConcept", 13224 "Whether this represents partial or complete payment of the benefits payable.", 0, 1, type); 13225 case 1977085293: 13226 /* adjustment */ return new Property("adjustment", "Money", 13227 "Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication.", 13228 0, 1, adjustment); 13229 case -1255938543: 13230 /* adjustmentReason */ return new Property("adjustmentReason", "CodeableConcept", 13231 "Reason for the payment adjustment.", 0, 1, adjustmentReason); 13232 case 3076014: 13233 /* date */ return new Property("date", "date", 13234 "Estimated date the payment will be issued or the actual issue date of payment.", 0, 1, date); 13235 case -1413853096: 13236 /* amount */ return new Property("amount", "Money", "Benefits payable less any payment adjustment.", 0, 1, 13237 amount); 13238 case -1618432855: 13239 /* identifier */ return new Property("identifier", "Identifier", 13240 "Issuer's unique identifier for the payment instrument.", 0, 1, identifier); 13241 default: 13242 return super.getNamedProperty(_hash, _name, _checkValid); 13243 } 13244 13245 } 13246 13247 @Override 13248 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 13249 switch (hash) { 13250 case 3575610: 13251 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 13252 case 1977085293: 13253 /* adjustment */ return this.adjustment == null ? new Base[0] : new Base[] { this.adjustment }; // Money 13254 case -1255938543: 13255 /* adjustmentReason */ return this.adjustmentReason == null ? new Base[0] 13256 : new Base[] { this.adjustmentReason }; // CodeableConcept 13257 case 3076014: 13258 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateType 13259 case -1413853096: 13260 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // Money 13261 case -1618432855: 13262 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 13263 default: 13264 return super.getProperty(hash, name, checkValid); 13265 } 13266 13267 } 13268 13269 @Override 13270 public Base setProperty(int hash, String name, Base value) throws FHIRException { 13271 switch (hash) { 13272 case 3575610: // type 13273 this.type = castToCodeableConcept(value); // CodeableConcept 13274 return value; 13275 case 1977085293: // adjustment 13276 this.adjustment = castToMoney(value); // Money 13277 return value; 13278 case -1255938543: // adjustmentReason 13279 this.adjustmentReason = castToCodeableConcept(value); // CodeableConcept 13280 return value; 13281 case 3076014: // date 13282 this.date = castToDate(value); // DateType 13283 return value; 13284 case -1413853096: // amount 13285 this.amount = castToMoney(value); // Money 13286 return value; 13287 case -1618432855: // identifier 13288 this.identifier = castToIdentifier(value); // Identifier 13289 return value; 13290 default: 13291 return super.setProperty(hash, name, value); 13292 } 13293 13294 } 13295 13296 @Override 13297 public Base setProperty(String name, Base value) throws FHIRException { 13298 if (name.equals("type")) { 13299 this.type = castToCodeableConcept(value); // CodeableConcept 13300 } else if (name.equals("adjustment")) { 13301 this.adjustment = castToMoney(value); // Money 13302 } else if (name.equals("adjustmentReason")) { 13303 this.adjustmentReason = castToCodeableConcept(value); // CodeableConcept 13304 } else if (name.equals("date")) { 13305 this.date = castToDate(value); // DateType 13306 } else if (name.equals("amount")) { 13307 this.amount = castToMoney(value); // Money 13308 } else if (name.equals("identifier")) { 13309 this.identifier = castToIdentifier(value); // Identifier 13310 } else 13311 return super.setProperty(name, value); 13312 return value; 13313 } 13314 13315 @Override 13316 public void removeChild(String name, Base value) throws FHIRException { 13317 if (name.equals("type")) { 13318 this.type = null; 13319 } else if (name.equals("adjustment")) { 13320 this.adjustment = null; 13321 } else if (name.equals("adjustmentReason")) { 13322 this.adjustmentReason = null; 13323 } else if (name.equals("date")) { 13324 this.date = null; 13325 } else if (name.equals("amount")) { 13326 this.amount = null; 13327 } else if (name.equals("identifier")) { 13328 this.identifier = null; 13329 } else 13330 super.removeChild(name, value); 13331 13332 } 13333 13334 @Override 13335 public Base makeProperty(int hash, String name) throws FHIRException { 13336 switch (hash) { 13337 case 3575610: 13338 return getType(); 13339 case 1977085293: 13340 return getAdjustment(); 13341 case -1255938543: 13342 return getAdjustmentReason(); 13343 case 3076014: 13344 return getDateElement(); 13345 case -1413853096: 13346 return getAmount(); 13347 case -1618432855: 13348 return getIdentifier(); 13349 default: 13350 return super.makeProperty(hash, name); 13351 } 13352 13353 } 13354 13355 @Override 13356 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 13357 switch (hash) { 13358 case 3575610: 13359 /* type */ return new String[] { "CodeableConcept" }; 13360 case 1977085293: 13361 /* adjustment */ return new String[] { "Money" }; 13362 case -1255938543: 13363 /* adjustmentReason */ return new String[] { "CodeableConcept" }; 13364 case 3076014: 13365 /* date */ return new String[] { "date" }; 13366 case -1413853096: 13367 /* amount */ return new String[] { "Money" }; 13368 case -1618432855: 13369 /* identifier */ return new String[] { "Identifier" }; 13370 default: 13371 return super.getTypesForProperty(hash, name); 13372 } 13373 13374 } 13375 13376 @Override 13377 public Base addChild(String name) throws FHIRException { 13378 if (name.equals("type")) { 13379 this.type = new CodeableConcept(); 13380 return this.type; 13381 } else if (name.equals("adjustment")) { 13382 this.adjustment = new Money(); 13383 return this.adjustment; 13384 } else if (name.equals("adjustmentReason")) { 13385 this.adjustmentReason = new CodeableConcept(); 13386 return this.adjustmentReason; 13387 } else if (name.equals("date")) { 13388 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.date"); 13389 } else if (name.equals("amount")) { 13390 this.amount = new Money(); 13391 return this.amount; 13392 } else if (name.equals("identifier")) { 13393 this.identifier = new Identifier(); 13394 return this.identifier; 13395 } else 13396 return super.addChild(name); 13397 } 13398 13399 public PaymentComponent copy() { 13400 PaymentComponent dst = new PaymentComponent(); 13401 copyValues(dst); 13402 return dst; 13403 } 13404 13405 public void copyValues(PaymentComponent dst) { 13406 super.copyValues(dst); 13407 dst.type = type == null ? null : type.copy(); 13408 dst.adjustment = adjustment == null ? null : adjustment.copy(); 13409 dst.adjustmentReason = adjustmentReason == null ? null : adjustmentReason.copy(); 13410 dst.date = date == null ? null : date.copy(); 13411 dst.amount = amount == null ? null : amount.copy(); 13412 dst.identifier = identifier == null ? null : identifier.copy(); 13413 } 13414 13415 @Override 13416 public boolean equalsDeep(Base other_) { 13417 if (!super.equalsDeep(other_)) 13418 return false; 13419 if (!(other_ instanceof PaymentComponent)) 13420 return false; 13421 PaymentComponent o = (PaymentComponent) other_; 13422 return compareDeep(type, o.type, true) && compareDeep(adjustment, o.adjustment, true) 13423 && compareDeep(adjustmentReason, o.adjustmentReason, true) && compareDeep(date, o.date, true) 13424 && compareDeep(amount, o.amount, true) && compareDeep(identifier, o.identifier, true); 13425 } 13426 13427 @Override 13428 public boolean equalsShallow(Base other_) { 13429 if (!super.equalsShallow(other_)) 13430 return false; 13431 if (!(other_ instanceof PaymentComponent)) 13432 return false; 13433 PaymentComponent o = (PaymentComponent) other_; 13434 return compareValues(date, o.date, true); 13435 } 13436 13437 public boolean isEmpty() { 13438 return super.isEmpty() 13439 && ca.uhn.fhir.util.ElementUtil.isEmpty(type, adjustment, adjustmentReason, date, amount, identifier); 13440 } 13441 13442 public String fhirType() { 13443 return "ExplanationOfBenefit.payment"; 13444 13445 } 13446 13447 } 13448 13449 @Block() 13450 public static class NoteComponent extends BackboneElement implements IBaseBackboneElement { 13451 /** 13452 * A number to uniquely identify a note entry. 13453 */ 13454 @Child(name = "number", type = { 13455 PositiveIntType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 13456 @Description(shortDefinition = "Note instance identifier", formalDefinition = "A number to uniquely identify a note entry.") 13457 protected PositiveIntType number; 13458 13459 /** 13460 * The business purpose of the note text. 13461 */ 13462 @Child(name = "type", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 13463 @Description(shortDefinition = "display | print | printoper", formalDefinition = "The business purpose of the note text.") 13464 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/note-type") 13465 protected Enumeration<NoteType> type; 13466 13467 /** 13468 * The explanation or description associated with the processing. 13469 */ 13470 @Child(name = "text", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 13471 @Description(shortDefinition = "Note explanatory text", formalDefinition = "The explanation or description associated with the processing.") 13472 protected StringType text; 13473 13474 /** 13475 * A code to define the language used in the text of the note. 13476 */ 13477 @Child(name = "language", type = { 13478 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 13479 @Description(shortDefinition = "Language of the text", formalDefinition = "A code to define the language used in the text of the note.") 13480 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/languages") 13481 protected CodeableConcept language; 13482 13483 private static final long serialVersionUID = -385184277L; 13484 13485 /** 13486 * Constructor 13487 */ 13488 public NoteComponent() { 13489 super(); 13490 } 13491 13492 /** 13493 * @return {@link #number} (A number to uniquely identify a note entry.). This 13494 * is the underlying object with id, value and extensions. The accessor 13495 * "getNumber" gives direct access to the value 13496 */ 13497 public PositiveIntType getNumberElement() { 13498 if (this.number == null) 13499 if (Configuration.errorOnAutoCreate()) 13500 throw new Error("Attempt to auto-create NoteComponent.number"); 13501 else if (Configuration.doAutoCreate()) 13502 this.number = new PositiveIntType(); // bb 13503 return this.number; 13504 } 13505 13506 public boolean hasNumberElement() { 13507 return this.number != null && !this.number.isEmpty(); 13508 } 13509 13510 public boolean hasNumber() { 13511 return this.number != null && !this.number.isEmpty(); 13512 } 13513 13514 /** 13515 * @param value {@link #number} (A number to uniquely identify a note entry.). 13516 * This is the underlying object with id, value and extensions. The 13517 * accessor "getNumber" gives direct access to the value 13518 */ 13519 public NoteComponent setNumberElement(PositiveIntType value) { 13520 this.number = value; 13521 return this; 13522 } 13523 13524 /** 13525 * @return A number to uniquely identify a note entry. 13526 */ 13527 public int getNumber() { 13528 return this.number == null || this.number.isEmpty() ? 0 : this.number.getValue(); 13529 } 13530 13531 /** 13532 * @param value A number to uniquely identify a note entry. 13533 */ 13534 public NoteComponent setNumber(int value) { 13535 if (this.number == null) 13536 this.number = new PositiveIntType(); 13537 this.number.setValue(value); 13538 return this; 13539 } 13540 13541 /** 13542 * @return {@link #type} (The business purpose of the note text.). This is the 13543 * underlying object with id, value and extensions. The accessor 13544 * "getType" gives direct access to the value 13545 */ 13546 public Enumeration<NoteType> getTypeElement() { 13547 if (this.type == null) 13548 if (Configuration.errorOnAutoCreate()) 13549 throw new Error("Attempt to auto-create NoteComponent.type"); 13550 else if (Configuration.doAutoCreate()) 13551 this.type = new Enumeration<NoteType>(new NoteTypeEnumFactory()); // bb 13552 return this.type; 13553 } 13554 13555 public boolean hasTypeElement() { 13556 return this.type != null && !this.type.isEmpty(); 13557 } 13558 13559 public boolean hasType() { 13560 return this.type != null && !this.type.isEmpty(); 13561 } 13562 13563 /** 13564 * @param value {@link #type} (The business purpose of the note text.). This is 13565 * the underlying object with id, value and extensions. The 13566 * accessor "getType" gives direct access to the value 13567 */ 13568 public NoteComponent setTypeElement(Enumeration<NoteType> value) { 13569 this.type = value; 13570 return this; 13571 } 13572 13573 /** 13574 * @return The business purpose of the note text. 13575 */ 13576 public NoteType getType() { 13577 return this.type == null ? null : this.type.getValue(); 13578 } 13579 13580 /** 13581 * @param value The business purpose of the note text. 13582 */ 13583 public NoteComponent setType(NoteType value) { 13584 if (value == null) 13585 this.type = null; 13586 else { 13587 if (this.type == null) 13588 this.type = new Enumeration<NoteType>(new NoteTypeEnumFactory()); 13589 this.type.setValue(value); 13590 } 13591 return this; 13592 } 13593 13594 /** 13595 * @return {@link #text} (The explanation or description associated with the 13596 * processing.). This is the underlying object with id, value and 13597 * extensions. The accessor "getText" gives direct access to the value 13598 */ 13599 public StringType getTextElement() { 13600 if (this.text == null) 13601 if (Configuration.errorOnAutoCreate()) 13602 throw new Error("Attempt to auto-create NoteComponent.text"); 13603 else if (Configuration.doAutoCreate()) 13604 this.text = new StringType(); // bb 13605 return this.text; 13606 } 13607 13608 public boolean hasTextElement() { 13609 return this.text != null && !this.text.isEmpty(); 13610 } 13611 13612 public boolean hasText() { 13613 return this.text != null && !this.text.isEmpty(); 13614 } 13615 13616 /** 13617 * @param value {@link #text} (The explanation or description associated with 13618 * the processing.). This is the underlying object with id, value 13619 * and extensions. The accessor "getText" gives direct access to 13620 * the value 13621 */ 13622 public NoteComponent setTextElement(StringType value) { 13623 this.text = value; 13624 return this; 13625 } 13626 13627 /** 13628 * @return The explanation or description associated with the processing. 13629 */ 13630 public String getText() { 13631 return this.text == null ? null : this.text.getValue(); 13632 } 13633 13634 /** 13635 * @param value The explanation or description associated with the processing. 13636 */ 13637 public NoteComponent setText(String value) { 13638 if (Utilities.noString(value)) 13639 this.text = null; 13640 else { 13641 if (this.text == null) 13642 this.text = new StringType(); 13643 this.text.setValue(value); 13644 } 13645 return this; 13646 } 13647 13648 /** 13649 * @return {@link #language} (A code to define the language used in the text of 13650 * the note.) 13651 */ 13652 public CodeableConcept getLanguage() { 13653 if (this.language == null) 13654 if (Configuration.errorOnAutoCreate()) 13655 throw new Error("Attempt to auto-create NoteComponent.language"); 13656 else if (Configuration.doAutoCreate()) 13657 this.language = new CodeableConcept(); // cc 13658 return this.language; 13659 } 13660 13661 public boolean hasLanguage() { 13662 return this.language != null && !this.language.isEmpty(); 13663 } 13664 13665 /** 13666 * @param value {@link #language} (A code to define the language used in the 13667 * text of the note.) 13668 */ 13669 public NoteComponent setLanguage(CodeableConcept value) { 13670 this.language = value; 13671 return this; 13672 } 13673 13674 protected void listChildren(List<Property> children) { 13675 super.listChildren(children); 13676 children.add(new Property("number", "positiveInt", "A number to uniquely identify a note entry.", 0, 1, number)); 13677 children.add(new Property("type", "code", "The business purpose of the note text.", 0, 1, type)); 13678 children.add( 13679 new Property("text", "string", "The explanation or description associated with the processing.", 0, 1, text)); 13680 children.add(new Property("language", "CodeableConcept", 13681 "A code to define the language used in the text of the note.", 0, 1, language)); 13682 } 13683 13684 @Override 13685 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 13686 switch (_hash) { 13687 case -1034364087: 13688 /* number */ return new Property("number", "positiveInt", "A number to uniquely identify a note entry.", 0, 1, 13689 number); 13690 case 3575610: 13691 /* type */ return new Property("type", "code", "The business purpose of the note text.", 0, 1, type); 13692 case 3556653: 13693 /* text */ return new Property("text", "string", 13694 "The explanation or description associated with the processing.", 0, 1, text); 13695 case -1613589672: 13696 /* language */ return new Property("language", "CodeableConcept", 13697 "A code to define the language used in the text of the note.", 0, 1, language); 13698 default: 13699 return super.getNamedProperty(_hash, _name, _checkValid); 13700 } 13701 13702 } 13703 13704 @Override 13705 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 13706 switch (hash) { 13707 case -1034364087: 13708 /* number */ return this.number == null ? new Base[0] : new Base[] { this.number }; // PositiveIntType 13709 case 3575610: 13710 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<NoteType> 13711 case 3556653: 13712 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType 13713 case -1613589672: 13714 /* language */ return this.language == null ? new Base[0] : new Base[] { this.language }; // CodeableConcept 13715 default: 13716 return super.getProperty(hash, name, checkValid); 13717 } 13718 13719 } 13720 13721 @Override 13722 public Base setProperty(int hash, String name, Base value) throws FHIRException { 13723 switch (hash) { 13724 case -1034364087: // number 13725 this.number = castToPositiveInt(value); // PositiveIntType 13726 return value; 13727 case 3575610: // type 13728 value = new NoteTypeEnumFactory().fromType(castToCode(value)); 13729 this.type = (Enumeration) value; // Enumeration<NoteType> 13730 return value; 13731 case 3556653: // text 13732 this.text = castToString(value); // StringType 13733 return value; 13734 case -1613589672: // language 13735 this.language = castToCodeableConcept(value); // CodeableConcept 13736 return value; 13737 default: 13738 return super.setProperty(hash, name, value); 13739 } 13740 13741 } 13742 13743 @Override 13744 public Base setProperty(String name, Base value) throws FHIRException { 13745 if (name.equals("number")) { 13746 this.number = castToPositiveInt(value); // PositiveIntType 13747 } else if (name.equals("type")) { 13748 value = new NoteTypeEnumFactory().fromType(castToCode(value)); 13749 this.type = (Enumeration) value; // Enumeration<NoteType> 13750 } else if (name.equals("text")) { 13751 this.text = castToString(value); // StringType 13752 } else if (name.equals("language")) { 13753 this.language = castToCodeableConcept(value); // CodeableConcept 13754 } else 13755 return super.setProperty(name, value); 13756 return value; 13757 } 13758 13759 @Override 13760 public void removeChild(String name, Base value) throws FHIRException { 13761 if (name.equals("number")) { 13762 this.number = null; 13763 } else if (name.equals("type")) { 13764 this.type = null; 13765 } else if (name.equals("text")) { 13766 this.text = null; 13767 } else if (name.equals("language")) { 13768 this.language = null; 13769 } else 13770 super.removeChild(name, value); 13771 13772 } 13773 13774 @Override 13775 public Base makeProperty(int hash, String name) throws FHIRException { 13776 switch (hash) { 13777 case -1034364087: 13778 return getNumberElement(); 13779 case 3575610: 13780 return getTypeElement(); 13781 case 3556653: 13782 return getTextElement(); 13783 case -1613589672: 13784 return getLanguage(); 13785 default: 13786 return super.makeProperty(hash, name); 13787 } 13788 13789 } 13790 13791 @Override 13792 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 13793 switch (hash) { 13794 case -1034364087: 13795 /* number */ return new String[] { "positiveInt" }; 13796 case 3575610: 13797 /* type */ return new String[] { "code" }; 13798 case 3556653: 13799 /* text */ return new String[] { "string" }; 13800 case -1613589672: 13801 /* language */ return new String[] { "CodeableConcept" }; 13802 default: 13803 return super.getTypesForProperty(hash, name); 13804 } 13805 13806 } 13807 13808 @Override 13809 public Base addChild(String name) throws FHIRException { 13810 if (name.equals("number")) { 13811 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.number"); 13812 } else if (name.equals("type")) { 13813 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.type"); 13814 } else if (name.equals("text")) { 13815 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.text"); 13816 } else if (name.equals("language")) { 13817 this.language = new CodeableConcept(); 13818 return this.language; 13819 } else 13820 return super.addChild(name); 13821 } 13822 13823 public NoteComponent copy() { 13824 NoteComponent dst = new NoteComponent(); 13825 copyValues(dst); 13826 return dst; 13827 } 13828 13829 public void copyValues(NoteComponent dst) { 13830 super.copyValues(dst); 13831 dst.number = number == null ? null : number.copy(); 13832 dst.type = type == null ? null : type.copy(); 13833 dst.text = text == null ? null : text.copy(); 13834 dst.language = language == null ? null : language.copy(); 13835 } 13836 13837 @Override 13838 public boolean equalsDeep(Base other_) { 13839 if (!super.equalsDeep(other_)) 13840 return false; 13841 if (!(other_ instanceof NoteComponent)) 13842 return false; 13843 NoteComponent o = (NoteComponent) other_; 13844 return compareDeep(number, o.number, true) && compareDeep(type, o.type, true) && compareDeep(text, o.text, true) 13845 && compareDeep(language, o.language, true); 13846 } 13847 13848 @Override 13849 public boolean equalsShallow(Base other_) { 13850 if (!super.equalsShallow(other_)) 13851 return false; 13852 if (!(other_ instanceof NoteComponent)) 13853 return false; 13854 NoteComponent o = (NoteComponent) other_; 13855 return compareValues(number, o.number, true) && compareValues(type, o.type, true) 13856 && compareValues(text, o.text, true); 13857 } 13858 13859 public boolean isEmpty() { 13860 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(number, type, text, language); 13861 } 13862 13863 public String fhirType() { 13864 return "ExplanationOfBenefit.processNote"; 13865 13866 } 13867 13868 } 13869 13870 @Block() 13871 public static class BenefitBalanceComponent extends BackboneElement implements IBaseBackboneElement { 13872 /** 13873 * Code to identify the general type of benefits under which products and 13874 * services are provided. 13875 */ 13876 @Child(name = "category", type = { 13877 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 13878 @Description(shortDefinition = "Benefit classification", formalDefinition = "Code to identify the general type of benefits under which products and services are provided.") 13879 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-benefitcategory") 13880 protected CodeableConcept category; 13881 13882 /** 13883 * True if the indicated class of service is excluded from the plan, missing or 13884 * False indicates the product or service is included in the coverage. 13885 */ 13886 @Child(name = "excluded", type = { 13887 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 13888 @Description(shortDefinition = "Excluded from the plan", formalDefinition = "True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage.") 13889 protected BooleanType excluded; 13890 13891 /** 13892 * A short name or tag for the benefit. 13893 */ 13894 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 13895 @Description(shortDefinition = "Short name for the benefit", formalDefinition = "A short name or tag for the benefit.") 13896 protected StringType name; 13897 13898 /** 13899 * A richer description of the benefit or services covered. 13900 */ 13901 @Child(name = "description", type = { 13902 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 13903 @Description(shortDefinition = "Description of the benefit or services covered", formalDefinition = "A richer description of the benefit or services covered.") 13904 protected StringType description; 13905 13906 /** 13907 * Is a flag to indicate whether the benefits refer to in-network providers or 13908 * out-of-network providers. 13909 */ 13910 @Child(name = "network", type = { 13911 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 13912 @Description(shortDefinition = "In or out of network", formalDefinition = "Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers.") 13913 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/benefit-network") 13914 protected CodeableConcept network; 13915 13916 /** 13917 * Indicates if the benefits apply to an individual or to the family. 13918 */ 13919 @Child(name = "unit", type = { 13920 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 13921 @Description(shortDefinition = "Individual or family", formalDefinition = "Indicates if the benefits apply to an individual or to the family.") 13922 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/benefit-unit") 13923 protected CodeableConcept unit; 13924 13925 /** 13926 * The term or period of the values such as 'maximum lifetime benefit' or 13927 * 'maximum annual visits'. 13928 */ 13929 @Child(name = "term", type = { 13930 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 13931 @Description(shortDefinition = "Annual or lifetime", formalDefinition = "The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'.") 13932 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/benefit-term") 13933 protected CodeableConcept term; 13934 13935 /** 13936 * Benefits Used to date. 13937 */ 13938 @Child(name = "financial", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 13939 @Description(shortDefinition = "Benefit Summary", formalDefinition = "Benefits Used to date.") 13940 protected List<BenefitComponent> financial; 13941 13942 private static final long serialVersionUID = -1889655824L; 13943 13944 /** 13945 * Constructor 13946 */ 13947 public BenefitBalanceComponent() { 13948 super(); 13949 } 13950 13951 /** 13952 * Constructor 13953 */ 13954 public BenefitBalanceComponent(CodeableConcept category) { 13955 super(); 13956 this.category = category; 13957 } 13958 13959 /** 13960 * @return {@link #category} (Code to identify the general type of benefits 13961 * under which products and services are provided.) 13962 */ 13963 public CodeableConcept getCategory() { 13964 if (this.category == null) 13965 if (Configuration.errorOnAutoCreate()) 13966 throw new Error("Attempt to auto-create BenefitBalanceComponent.category"); 13967 else if (Configuration.doAutoCreate()) 13968 this.category = new CodeableConcept(); // cc 13969 return this.category; 13970 } 13971 13972 public boolean hasCategory() { 13973 return this.category != null && !this.category.isEmpty(); 13974 } 13975 13976 /** 13977 * @param value {@link #category} (Code to identify the general type of benefits 13978 * under which products and services are provided.) 13979 */ 13980 public BenefitBalanceComponent setCategory(CodeableConcept value) { 13981 this.category = value; 13982 return this; 13983 } 13984 13985 /** 13986 * @return {@link #excluded} (True if the indicated class of service is excluded 13987 * from the plan, missing or False indicates the product or service is 13988 * included in the coverage.). This is the underlying object with id, 13989 * value and extensions. The accessor "getExcluded" gives direct access 13990 * to the value 13991 */ 13992 public BooleanType getExcludedElement() { 13993 if (this.excluded == null) 13994 if (Configuration.errorOnAutoCreate()) 13995 throw new Error("Attempt to auto-create BenefitBalanceComponent.excluded"); 13996 else if (Configuration.doAutoCreate()) 13997 this.excluded = new BooleanType(); // bb 13998 return this.excluded; 13999 } 14000 14001 public boolean hasExcludedElement() { 14002 return this.excluded != null && !this.excluded.isEmpty(); 14003 } 14004 14005 public boolean hasExcluded() { 14006 return this.excluded != null && !this.excluded.isEmpty(); 14007 } 14008 14009 /** 14010 * @param value {@link #excluded} (True if the indicated class of service is 14011 * excluded from the plan, missing or False indicates the product 14012 * or service is included in the coverage.). This is the underlying 14013 * object with id, value and extensions. The accessor "getExcluded" 14014 * gives direct access to the value 14015 */ 14016 public BenefitBalanceComponent setExcludedElement(BooleanType value) { 14017 this.excluded = value; 14018 return this; 14019 } 14020 14021 /** 14022 * @return True if the indicated class of service is excluded from the plan, 14023 * missing or False indicates the product or service is included in the 14024 * coverage. 14025 */ 14026 public boolean getExcluded() { 14027 return this.excluded == null || this.excluded.isEmpty() ? false : this.excluded.getValue(); 14028 } 14029 14030 /** 14031 * @param value True if the indicated class of service is excluded from the 14032 * plan, missing or False indicates the product or service is 14033 * included in the coverage. 14034 */ 14035 public BenefitBalanceComponent setExcluded(boolean value) { 14036 if (this.excluded == null) 14037 this.excluded = new BooleanType(); 14038 this.excluded.setValue(value); 14039 return this; 14040 } 14041 14042 /** 14043 * @return {@link #name} (A short name or tag for the benefit.). This is the 14044 * underlying object with id, value and extensions. The accessor 14045 * "getName" gives direct access to the value 14046 */ 14047 public StringType getNameElement() { 14048 if (this.name == null) 14049 if (Configuration.errorOnAutoCreate()) 14050 throw new Error("Attempt to auto-create BenefitBalanceComponent.name"); 14051 else if (Configuration.doAutoCreate()) 14052 this.name = new StringType(); // bb 14053 return this.name; 14054 } 14055 14056 public boolean hasNameElement() { 14057 return this.name != null && !this.name.isEmpty(); 14058 } 14059 14060 public boolean hasName() { 14061 return this.name != null && !this.name.isEmpty(); 14062 } 14063 14064 /** 14065 * @param value {@link #name} (A short name or tag for the benefit.). This is 14066 * the underlying object with id, value and extensions. The 14067 * accessor "getName" gives direct access to the value 14068 */ 14069 public BenefitBalanceComponent setNameElement(StringType value) { 14070 this.name = value; 14071 return this; 14072 } 14073 14074 /** 14075 * @return A short name or tag for the benefit. 14076 */ 14077 public String getName() { 14078 return this.name == null ? null : this.name.getValue(); 14079 } 14080 14081 /** 14082 * @param value A short name or tag for the benefit. 14083 */ 14084 public BenefitBalanceComponent setName(String value) { 14085 if (Utilities.noString(value)) 14086 this.name = null; 14087 else { 14088 if (this.name == null) 14089 this.name = new StringType(); 14090 this.name.setValue(value); 14091 } 14092 return this; 14093 } 14094 14095 /** 14096 * @return {@link #description} (A richer description of the benefit or services 14097 * covered.). This is the underlying object with id, value and 14098 * extensions. The accessor "getDescription" gives direct access to the 14099 * value 14100 */ 14101 public StringType getDescriptionElement() { 14102 if (this.description == null) 14103 if (Configuration.errorOnAutoCreate()) 14104 throw new Error("Attempt to auto-create BenefitBalanceComponent.description"); 14105 else if (Configuration.doAutoCreate()) 14106 this.description = new StringType(); // bb 14107 return this.description; 14108 } 14109 14110 public boolean hasDescriptionElement() { 14111 return this.description != null && !this.description.isEmpty(); 14112 } 14113 14114 public boolean hasDescription() { 14115 return this.description != null && !this.description.isEmpty(); 14116 } 14117 14118 /** 14119 * @param value {@link #description} (A richer description of the benefit or 14120 * services covered.). This is the underlying object with id, value 14121 * and extensions. The accessor "getDescription" gives direct 14122 * access to the value 14123 */ 14124 public BenefitBalanceComponent setDescriptionElement(StringType value) { 14125 this.description = value; 14126 return this; 14127 } 14128 14129 /** 14130 * @return A richer description of the benefit or services covered. 14131 */ 14132 public String getDescription() { 14133 return this.description == null ? null : this.description.getValue(); 14134 } 14135 14136 /** 14137 * @param value A richer description of the benefit or services covered. 14138 */ 14139 public BenefitBalanceComponent setDescription(String value) { 14140 if (Utilities.noString(value)) 14141 this.description = null; 14142 else { 14143 if (this.description == null) 14144 this.description = new StringType(); 14145 this.description.setValue(value); 14146 } 14147 return this; 14148 } 14149 14150 /** 14151 * @return {@link #network} (Is a flag to indicate whether the benefits refer to 14152 * in-network providers or out-of-network providers.) 14153 */ 14154 public CodeableConcept getNetwork() { 14155 if (this.network == null) 14156 if (Configuration.errorOnAutoCreate()) 14157 throw new Error("Attempt to auto-create BenefitBalanceComponent.network"); 14158 else if (Configuration.doAutoCreate()) 14159 this.network = new CodeableConcept(); // cc 14160 return this.network; 14161 } 14162 14163 public boolean hasNetwork() { 14164 return this.network != null && !this.network.isEmpty(); 14165 } 14166 14167 /** 14168 * @param value {@link #network} (Is a flag to indicate whether the benefits 14169 * refer to in-network providers or out-of-network providers.) 14170 */ 14171 public BenefitBalanceComponent setNetwork(CodeableConcept value) { 14172 this.network = value; 14173 return this; 14174 } 14175 14176 /** 14177 * @return {@link #unit} (Indicates if the benefits apply to an individual or to 14178 * the family.) 14179 */ 14180 public CodeableConcept getUnit() { 14181 if (this.unit == null) 14182 if (Configuration.errorOnAutoCreate()) 14183 throw new Error("Attempt to auto-create BenefitBalanceComponent.unit"); 14184 else if (Configuration.doAutoCreate()) 14185 this.unit = new CodeableConcept(); // cc 14186 return this.unit; 14187 } 14188 14189 public boolean hasUnit() { 14190 return this.unit != null && !this.unit.isEmpty(); 14191 } 14192 14193 /** 14194 * @param value {@link #unit} (Indicates if the benefits apply to an individual 14195 * or to the family.) 14196 */ 14197 public BenefitBalanceComponent setUnit(CodeableConcept value) { 14198 this.unit = value; 14199 return this; 14200 } 14201 14202 /** 14203 * @return {@link #term} (The term or period of the values such as 'maximum 14204 * lifetime benefit' or 'maximum annual visits'.) 14205 */ 14206 public CodeableConcept getTerm() { 14207 if (this.term == null) 14208 if (Configuration.errorOnAutoCreate()) 14209 throw new Error("Attempt to auto-create BenefitBalanceComponent.term"); 14210 else if (Configuration.doAutoCreate()) 14211 this.term = new CodeableConcept(); // cc 14212 return this.term; 14213 } 14214 14215 public boolean hasTerm() { 14216 return this.term != null && !this.term.isEmpty(); 14217 } 14218 14219 /** 14220 * @param value {@link #term} (The term or period of the values such as 'maximum 14221 * lifetime benefit' or 'maximum annual visits'.) 14222 */ 14223 public BenefitBalanceComponent setTerm(CodeableConcept value) { 14224 this.term = value; 14225 return this; 14226 } 14227 14228 /** 14229 * @return {@link #financial} (Benefits Used to date.) 14230 */ 14231 public List<BenefitComponent> getFinancial() { 14232 if (this.financial == null) 14233 this.financial = new ArrayList<BenefitComponent>(); 14234 return this.financial; 14235 } 14236 14237 /** 14238 * @return Returns a reference to <code>this</code> for easy method chaining 14239 */ 14240 public BenefitBalanceComponent setFinancial(List<BenefitComponent> theFinancial) { 14241 this.financial = theFinancial; 14242 return this; 14243 } 14244 14245 public boolean hasFinancial() { 14246 if (this.financial == null) 14247 return false; 14248 for (BenefitComponent item : this.financial) 14249 if (!item.isEmpty()) 14250 return true; 14251 return false; 14252 } 14253 14254 public BenefitComponent addFinancial() { // 3 14255 BenefitComponent t = new BenefitComponent(); 14256 if (this.financial == null) 14257 this.financial = new ArrayList<BenefitComponent>(); 14258 this.financial.add(t); 14259 return t; 14260 } 14261 14262 public BenefitBalanceComponent addFinancial(BenefitComponent t) { // 3 14263 if (t == null) 14264 return this; 14265 if (this.financial == null) 14266 this.financial = new ArrayList<BenefitComponent>(); 14267 this.financial.add(t); 14268 return this; 14269 } 14270 14271 /** 14272 * @return The first repetition of repeating field {@link #financial}, creating 14273 * it if it does not already exist 14274 */ 14275 public BenefitComponent getFinancialFirstRep() { 14276 if (getFinancial().isEmpty()) { 14277 addFinancial(); 14278 } 14279 return getFinancial().get(0); 14280 } 14281 14282 protected void listChildren(List<Property> children) { 14283 super.listChildren(children); 14284 children.add(new Property("category", "CodeableConcept", 14285 "Code to identify the general type of benefits under which products and services are provided.", 0, 1, 14286 category)); 14287 children.add(new Property("excluded", "boolean", 14288 "True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage.", 14289 0, 1, excluded)); 14290 children.add(new Property("name", "string", "A short name or tag for the benefit.", 0, 1, name)); 14291 children.add(new Property("description", "string", "A richer description of the benefit or services covered.", 0, 14292 1, description)); 14293 children.add(new Property("network", "CodeableConcept", 14294 "Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers.", 0, 1, 14295 network)); 14296 children.add(new Property("unit", "CodeableConcept", 14297 "Indicates if the benefits apply to an individual or to the family.", 0, 1, unit)); 14298 children.add(new Property("term", "CodeableConcept", 14299 "The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'.", 0, 1, 14300 term)); 14301 children.add(new Property("financial", "", "Benefits Used to date.", 0, java.lang.Integer.MAX_VALUE, financial)); 14302 } 14303 14304 @Override 14305 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 14306 switch (_hash) { 14307 case 50511102: 14308 /* category */ return new Property("category", "CodeableConcept", 14309 "Code to identify the general type of benefits under which products and services are provided.", 0, 1, 14310 category); 14311 case 1994055114: 14312 /* excluded */ return new Property("excluded", "boolean", 14313 "True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage.", 14314 0, 1, excluded); 14315 case 3373707: 14316 /* name */ return new Property("name", "string", "A short name or tag for the benefit.", 0, 1, name); 14317 case -1724546052: 14318 /* description */ return new Property("description", "string", 14319 "A richer description of the benefit or services covered.", 0, 1, description); 14320 case 1843485230: 14321 /* network */ return new Property("network", "CodeableConcept", 14322 "Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers.", 0, 14323 1, network); 14324 case 3594628: 14325 /* unit */ return new Property("unit", "CodeableConcept", 14326 "Indicates if the benefits apply to an individual or to the family.", 0, 1, unit); 14327 case 3556460: 14328 /* term */ return new Property("term", "CodeableConcept", 14329 "The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'.", 0, 1, 14330 term); 14331 case 357555337: 14332 /* financial */ return new Property("financial", "", "Benefits Used to date.", 0, java.lang.Integer.MAX_VALUE, 14333 financial); 14334 default: 14335 return super.getNamedProperty(_hash, _name, _checkValid); 14336 } 14337 14338 } 14339 14340 @Override 14341 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 14342 switch (hash) { 14343 case 50511102: 14344 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 14345 case 1994055114: 14346 /* excluded */ return this.excluded == null ? new Base[0] : new Base[] { this.excluded }; // BooleanType 14347 case 3373707: 14348 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 14349 case -1724546052: 14350 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 14351 case 1843485230: 14352 /* network */ return this.network == null ? new Base[0] : new Base[] { this.network }; // CodeableConcept 14353 case 3594628: 14354 /* unit */ return this.unit == null ? new Base[0] : new Base[] { this.unit }; // CodeableConcept 14355 case 3556460: 14356 /* term */ return this.term == null ? new Base[0] : new Base[] { this.term }; // CodeableConcept 14357 case 357555337: 14358 /* financial */ return this.financial == null ? new Base[0] 14359 : this.financial.toArray(new Base[this.financial.size()]); // BenefitComponent 14360 default: 14361 return super.getProperty(hash, name, checkValid); 14362 } 14363 14364 } 14365 14366 @Override 14367 public Base setProperty(int hash, String name, Base value) throws FHIRException { 14368 switch (hash) { 14369 case 50511102: // category 14370 this.category = castToCodeableConcept(value); // CodeableConcept 14371 return value; 14372 case 1994055114: // excluded 14373 this.excluded = castToBoolean(value); // BooleanType 14374 return value; 14375 case 3373707: // name 14376 this.name = castToString(value); // StringType 14377 return value; 14378 case -1724546052: // description 14379 this.description = castToString(value); // StringType 14380 return value; 14381 case 1843485230: // network 14382 this.network = castToCodeableConcept(value); // CodeableConcept 14383 return value; 14384 case 3594628: // unit 14385 this.unit = castToCodeableConcept(value); // CodeableConcept 14386 return value; 14387 case 3556460: // term 14388 this.term = castToCodeableConcept(value); // CodeableConcept 14389 return value; 14390 case 357555337: // financial 14391 this.getFinancial().add((BenefitComponent) value); // BenefitComponent 14392 return value; 14393 default: 14394 return super.setProperty(hash, name, value); 14395 } 14396 14397 } 14398 14399 @Override 14400 public Base setProperty(String name, Base value) throws FHIRException { 14401 if (name.equals("category")) { 14402 this.category = castToCodeableConcept(value); // CodeableConcept 14403 } else if (name.equals("excluded")) { 14404 this.excluded = castToBoolean(value); // BooleanType 14405 } else if (name.equals("name")) { 14406 this.name = castToString(value); // StringType 14407 } else if (name.equals("description")) { 14408 this.description = castToString(value); // StringType 14409 } else if (name.equals("network")) { 14410 this.network = castToCodeableConcept(value); // CodeableConcept 14411 } else if (name.equals("unit")) { 14412 this.unit = castToCodeableConcept(value); // CodeableConcept 14413 } else if (name.equals("term")) { 14414 this.term = castToCodeableConcept(value); // CodeableConcept 14415 } else if (name.equals("financial")) { 14416 this.getFinancial().add((BenefitComponent) value); 14417 } else 14418 return super.setProperty(name, value); 14419 return value; 14420 } 14421 14422 @Override 14423 public void removeChild(String name, Base value) throws FHIRException { 14424 if (name.equals("category")) { 14425 this.category = null; 14426 } else if (name.equals("excluded")) { 14427 this.excluded = null; 14428 } else if (name.equals("name")) { 14429 this.name = null; 14430 } else if (name.equals("description")) { 14431 this.description = null; 14432 } else if (name.equals("network")) { 14433 this.network = null; 14434 } else if (name.equals("unit")) { 14435 this.unit = null; 14436 } else if (name.equals("term")) { 14437 this.term = null; 14438 } else if (name.equals("financial")) { 14439 this.getFinancial().remove((BenefitComponent) value); 14440 } else 14441 super.removeChild(name, value); 14442 14443 } 14444 14445 @Override 14446 public Base makeProperty(int hash, String name) throws FHIRException { 14447 switch (hash) { 14448 case 50511102: 14449 return getCategory(); 14450 case 1994055114: 14451 return getExcludedElement(); 14452 case 3373707: 14453 return getNameElement(); 14454 case -1724546052: 14455 return getDescriptionElement(); 14456 case 1843485230: 14457 return getNetwork(); 14458 case 3594628: 14459 return getUnit(); 14460 case 3556460: 14461 return getTerm(); 14462 case 357555337: 14463 return addFinancial(); 14464 default: 14465 return super.makeProperty(hash, name); 14466 } 14467 14468 } 14469 14470 @Override 14471 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 14472 switch (hash) { 14473 case 50511102: 14474 /* category */ return new String[] { "CodeableConcept" }; 14475 case 1994055114: 14476 /* excluded */ return new String[] { "boolean" }; 14477 case 3373707: 14478 /* name */ return new String[] { "string" }; 14479 case -1724546052: 14480 /* description */ return new String[] { "string" }; 14481 case 1843485230: 14482 /* network */ return new String[] { "CodeableConcept" }; 14483 case 3594628: 14484 /* unit */ return new String[] { "CodeableConcept" }; 14485 case 3556460: 14486 /* term */ return new String[] { "CodeableConcept" }; 14487 case 357555337: 14488 /* financial */ return new String[] {}; 14489 default: 14490 return super.getTypesForProperty(hash, name); 14491 } 14492 14493 } 14494 14495 @Override 14496 public Base addChild(String name) throws FHIRException { 14497 if (name.equals("category")) { 14498 this.category = new CodeableConcept(); 14499 return this.category; 14500 } else if (name.equals("excluded")) { 14501 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.excluded"); 14502 } else if (name.equals("name")) { 14503 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.name"); 14504 } else if (name.equals("description")) { 14505 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.description"); 14506 } else if (name.equals("network")) { 14507 this.network = new CodeableConcept(); 14508 return this.network; 14509 } else if (name.equals("unit")) { 14510 this.unit = new CodeableConcept(); 14511 return this.unit; 14512 } else if (name.equals("term")) { 14513 this.term = new CodeableConcept(); 14514 return this.term; 14515 } else if (name.equals("financial")) { 14516 return addFinancial(); 14517 } else 14518 return super.addChild(name); 14519 } 14520 14521 public BenefitBalanceComponent copy() { 14522 BenefitBalanceComponent dst = new BenefitBalanceComponent(); 14523 copyValues(dst); 14524 return dst; 14525 } 14526 14527 public void copyValues(BenefitBalanceComponent dst) { 14528 super.copyValues(dst); 14529 dst.category = category == null ? null : category.copy(); 14530 dst.excluded = excluded == null ? null : excluded.copy(); 14531 dst.name = name == null ? null : name.copy(); 14532 dst.description = description == null ? null : description.copy(); 14533 dst.network = network == null ? null : network.copy(); 14534 dst.unit = unit == null ? null : unit.copy(); 14535 dst.term = term == null ? null : term.copy(); 14536 if (financial != null) { 14537 dst.financial = new ArrayList<BenefitComponent>(); 14538 for (BenefitComponent i : financial) 14539 dst.financial.add(i.copy()); 14540 } 14541 ; 14542 } 14543 14544 @Override 14545 public boolean equalsDeep(Base other_) { 14546 if (!super.equalsDeep(other_)) 14547 return false; 14548 if (!(other_ instanceof BenefitBalanceComponent)) 14549 return false; 14550 BenefitBalanceComponent o = (BenefitBalanceComponent) other_; 14551 return compareDeep(category, o.category, true) && compareDeep(excluded, o.excluded, true) 14552 && compareDeep(name, o.name, true) && compareDeep(description, o.description, true) 14553 && compareDeep(network, o.network, true) && compareDeep(unit, o.unit, true) && compareDeep(term, o.term, true) 14554 && compareDeep(financial, o.financial, true); 14555 } 14556 14557 @Override 14558 public boolean equalsShallow(Base other_) { 14559 if (!super.equalsShallow(other_)) 14560 return false; 14561 if (!(other_ instanceof BenefitBalanceComponent)) 14562 return false; 14563 BenefitBalanceComponent o = (BenefitBalanceComponent) other_; 14564 return compareValues(excluded, o.excluded, true) && compareValues(name, o.name, true) 14565 && compareValues(description, o.description, true); 14566 } 14567 14568 public boolean isEmpty() { 14569 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, excluded, name, description, network, 14570 unit, term, financial); 14571 } 14572 14573 public String fhirType() { 14574 return "ExplanationOfBenefit.benefitBalance"; 14575 14576 } 14577 14578 } 14579 14580 @Block() 14581 public static class BenefitComponent extends BackboneElement implements IBaseBackboneElement { 14582 /** 14583 * Classification of benefit being provided. 14584 */ 14585 @Child(name = "type", type = { 14586 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 14587 @Description(shortDefinition = "Benefit classification", formalDefinition = "Classification of benefit being provided.") 14588 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/benefit-type") 14589 protected CodeableConcept type; 14590 14591 /** 14592 * The quantity of the benefit which is permitted under the coverage. 14593 */ 14594 @Child(name = "allowed", type = { UnsignedIntType.class, StringType.class, 14595 Money.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 14596 @Description(shortDefinition = "Benefits allowed", formalDefinition = "The quantity of the benefit which is permitted under the coverage.") 14597 protected Type allowed; 14598 14599 /** 14600 * The quantity of the benefit which have been consumed to date. 14601 */ 14602 @Child(name = "used", type = { UnsignedIntType.class, 14603 Money.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 14604 @Description(shortDefinition = "Benefits used", formalDefinition = "The quantity of the benefit which have been consumed to date.") 14605 protected Type used; 14606 14607 private static final long serialVersionUID = -1506285314L; 14608 14609 /** 14610 * Constructor 14611 */ 14612 public BenefitComponent() { 14613 super(); 14614 } 14615 14616 /** 14617 * Constructor 14618 */ 14619 public BenefitComponent(CodeableConcept type) { 14620 super(); 14621 this.type = type; 14622 } 14623 14624 /** 14625 * @return {@link #type} (Classification of benefit being provided.) 14626 */ 14627 public CodeableConcept getType() { 14628 if (this.type == null) 14629 if (Configuration.errorOnAutoCreate()) 14630 throw new Error("Attempt to auto-create BenefitComponent.type"); 14631 else if (Configuration.doAutoCreate()) 14632 this.type = new CodeableConcept(); // cc 14633 return this.type; 14634 } 14635 14636 public boolean hasType() { 14637 return this.type != null && !this.type.isEmpty(); 14638 } 14639 14640 /** 14641 * @param value {@link #type} (Classification of benefit being provided.) 14642 */ 14643 public BenefitComponent setType(CodeableConcept value) { 14644 this.type = value; 14645 return this; 14646 } 14647 14648 /** 14649 * @return {@link #allowed} (The quantity of the benefit which is permitted 14650 * under the coverage.) 14651 */ 14652 public Type getAllowed() { 14653 return this.allowed; 14654 } 14655 14656 /** 14657 * @return {@link #allowed} (The quantity of the benefit which is permitted 14658 * under the coverage.) 14659 */ 14660 public UnsignedIntType getAllowedUnsignedIntType() throws FHIRException { 14661 if (this.allowed == null) 14662 this.allowed = new UnsignedIntType(); 14663 if (!(this.allowed instanceof UnsignedIntType)) 14664 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but " 14665 + this.allowed.getClass().getName() + " was encountered"); 14666 return (UnsignedIntType) this.allowed; 14667 } 14668 14669 public boolean hasAllowedUnsignedIntType() { 14670 return this != null && this.allowed instanceof UnsignedIntType; 14671 } 14672 14673 /** 14674 * @return {@link #allowed} (The quantity of the benefit which is permitted 14675 * under the coverage.) 14676 */ 14677 public StringType getAllowedStringType() throws FHIRException { 14678 if (this.allowed == null) 14679 this.allowed = new StringType(); 14680 if (!(this.allowed instanceof StringType)) 14681 throw new FHIRException("Type mismatch: the type StringType was expected, but " 14682 + this.allowed.getClass().getName() + " was encountered"); 14683 return (StringType) this.allowed; 14684 } 14685 14686 public boolean hasAllowedStringType() { 14687 return this != null && this.allowed instanceof StringType; 14688 } 14689 14690 /** 14691 * @return {@link #allowed} (The quantity of the benefit which is permitted 14692 * under the coverage.) 14693 */ 14694 public Money getAllowedMoney() throws FHIRException { 14695 if (this.allowed == null) 14696 this.allowed = new Money(); 14697 if (!(this.allowed instanceof Money)) 14698 throw new FHIRException("Type mismatch: the type Money was expected, but " + this.allowed.getClass().getName() 14699 + " was encountered"); 14700 return (Money) this.allowed; 14701 } 14702 14703 public boolean hasAllowedMoney() { 14704 return this != null && this.allowed instanceof Money; 14705 } 14706 14707 public boolean hasAllowed() { 14708 return this.allowed != null && !this.allowed.isEmpty(); 14709 } 14710 14711 /** 14712 * @param value {@link #allowed} (The quantity of the benefit which is permitted 14713 * under the coverage.) 14714 */ 14715 public BenefitComponent setAllowed(Type value) { 14716 if (value != null && !(value instanceof UnsignedIntType || value instanceof StringType || value instanceof Money)) 14717 throw new Error( 14718 "Not the right type for ExplanationOfBenefit.benefitBalance.financial.allowed[x]: " + value.fhirType()); 14719 this.allowed = value; 14720 return this; 14721 } 14722 14723 /** 14724 * @return {@link #used} (The quantity of the benefit which have been consumed 14725 * to date.) 14726 */ 14727 public Type getUsed() { 14728 return this.used; 14729 } 14730 14731 /** 14732 * @return {@link #used} (The quantity of the benefit which have been consumed 14733 * to date.) 14734 */ 14735 public UnsignedIntType getUsedUnsignedIntType() throws FHIRException { 14736 if (this.used == null) 14737 this.used = new UnsignedIntType(); 14738 if (!(this.used instanceof UnsignedIntType)) 14739 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but " 14740 + this.used.getClass().getName() + " was encountered"); 14741 return (UnsignedIntType) this.used; 14742 } 14743 14744 public boolean hasUsedUnsignedIntType() { 14745 return this != null && this.used instanceof UnsignedIntType; 14746 } 14747 14748 /** 14749 * @return {@link #used} (The quantity of the benefit which have been consumed 14750 * to date.) 14751 */ 14752 public Money getUsedMoney() throws FHIRException { 14753 if (this.used == null) 14754 this.used = new Money(); 14755 if (!(this.used instanceof Money)) 14756 throw new FHIRException( 14757 "Type mismatch: the type Money was expected, but " + this.used.getClass().getName() + " was encountered"); 14758 return (Money) this.used; 14759 } 14760 14761 public boolean hasUsedMoney() { 14762 return this != null && this.used instanceof Money; 14763 } 14764 14765 public boolean hasUsed() { 14766 return this.used != null && !this.used.isEmpty(); 14767 } 14768 14769 /** 14770 * @param value {@link #used} (The quantity of the benefit which have been 14771 * consumed to date.) 14772 */ 14773 public BenefitComponent setUsed(Type value) { 14774 if (value != null && !(value instanceof UnsignedIntType || value instanceof Money)) 14775 throw new Error( 14776 "Not the right type for ExplanationOfBenefit.benefitBalance.financial.used[x]: " + value.fhirType()); 14777 this.used = value; 14778 return this; 14779 } 14780 14781 protected void listChildren(List<Property> children) { 14782 super.listChildren(children); 14783 children.add(new Property("type", "CodeableConcept", "Classification of benefit being provided.", 0, 1, type)); 14784 children.add(new Property("allowed[x]", "unsignedInt|string|Money", 14785 "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed)); 14786 children.add(new Property("used[x]", "unsignedInt|Money", 14787 "The quantity of the benefit which have been consumed to date.", 0, 1, used)); 14788 } 14789 14790 @Override 14791 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 14792 switch (_hash) { 14793 case 3575610: 14794 /* type */ return new Property("type", "CodeableConcept", "Classification of benefit being provided.", 0, 1, 14795 type); 14796 case -1336663592: 14797 /* allowed[x] */ return new Property("allowed[x]", "unsignedInt|string|Money", 14798 "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed); 14799 case -911343192: 14800 /* allowed */ return new Property("allowed[x]", "unsignedInt|string|Money", 14801 "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed); 14802 case 1668802034: 14803 /* allowedUnsignedInt */ return new Property("allowed[x]", "unsignedInt|string|Money", 14804 "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed); 14805 case -2135265319: 14806 /* allowedString */ return new Property("allowed[x]", "unsignedInt|string|Money", 14807 "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed); 14808 case -351668232: 14809 /* allowedMoney */ return new Property("allowed[x]", "unsignedInt|string|Money", 14810 "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed); 14811 case -147553373: 14812 /* used[x] */ return new Property("used[x]", "unsignedInt|Money", 14813 "The quantity of the benefit which have been consumed to date.", 0, 1, used); 14814 case 3599293: 14815 /* used */ return new Property("used[x]", "unsignedInt|Money", 14816 "The quantity of the benefit which have been consumed to date.", 0, 1, used); 14817 case 1252740285: 14818 /* usedUnsignedInt */ return new Property("used[x]", "unsignedInt|Money", 14819 "The quantity of the benefit which have been consumed to date.", 0, 1, used); 14820 case -78048509: 14821 /* usedMoney */ return new Property("used[x]", "unsignedInt|Money", 14822 "The quantity of the benefit which have been consumed to date.", 0, 1, used); 14823 default: 14824 return super.getNamedProperty(_hash, _name, _checkValid); 14825 } 14826 14827 } 14828 14829 @Override 14830 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 14831 switch (hash) { 14832 case 3575610: 14833 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 14834 case -911343192: 14835 /* allowed */ return this.allowed == null ? new Base[0] : new Base[] { this.allowed }; // Type 14836 case 3599293: 14837 /* used */ return this.used == null ? new Base[0] : new Base[] { this.used }; // Type 14838 default: 14839 return super.getProperty(hash, name, checkValid); 14840 } 14841 14842 } 14843 14844 @Override 14845 public Base setProperty(int hash, String name, Base value) throws FHIRException { 14846 switch (hash) { 14847 case 3575610: // type 14848 this.type = castToCodeableConcept(value); // CodeableConcept 14849 return value; 14850 case -911343192: // allowed 14851 this.allowed = castToType(value); // Type 14852 return value; 14853 case 3599293: // used 14854 this.used = castToType(value); // Type 14855 return value; 14856 default: 14857 return super.setProperty(hash, name, value); 14858 } 14859 14860 } 14861 14862 @Override 14863 public Base setProperty(String name, Base value) throws FHIRException { 14864 if (name.equals("type")) { 14865 this.type = castToCodeableConcept(value); // CodeableConcept 14866 } else if (name.equals("allowed[x]")) { 14867 this.allowed = castToType(value); // Type 14868 } else if (name.equals("used[x]")) { 14869 this.used = castToType(value); // Type 14870 } else 14871 return super.setProperty(name, value); 14872 return value; 14873 } 14874 14875 @Override 14876 public void removeChild(String name, Base value) throws FHIRException { 14877 if (name.equals("type")) { 14878 this.type = null; 14879 } else if (name.equals("allowed[x]")) { 14880 this.allowed = null; 14881 } else if (name.equals("used[x]")) { 14882 this.used = null; 14883 } else 14884 super.removeChild(name, value); 14885 14886 } 14887 14888 @Override 14889 public Base makeProperty(int hash, String name) throws FHIRException { 14890 switch (hash) { 14891 case 3575610: 14892 return getType(); 14893 case -1336663592: 14894 return getAllowed(); 14895 case -911343192: 14896 return getAllowed(); 14897 case -147553373: 14898 return getUsed(); 14899 case 3599293: 14900 return getUsed(); 14901 default: 14902 return super.makeProperty(hash, name); 14903 } 14904 14905 } 14906 14907 @Override 14908 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 14909 switch (hash) { 14910 case 3575610: 14911 /* type */ return new String[] { "CodeableConcept" }; 14912 case -911343192: 14913 /* allowed */ return new String[] { "unsignedInt", "string", "Money" }; 14914 case 3599293: 14915 /* used */ return new String[] { "unsignedInt", "Money" }; 14916 default: 14917 return super.getTypesForProperty(hash, name); 14918 } 14919 14920 } 14921 14922 @Override 14923 public Base addChild(String name) throws FHIRException { 14924 if (name.equals("type")) { 14925 this.type = new CodeableConcept(); 14926 return this.type; 14927 } else if (name.equals("allowedUnsignedInt")) { 14928 this.allowed = new UnsignedIntType(); 14929 return this.allowed; 14930 } else if (name.equals("allowedString")) { 14931 this.allowed = new StringType(); 14932 return this.allowed; 14933 } else if (name.equals("allowedMoney")) { 14934 this.allowed = new Money(); 14935 return this.allowed; 14936 } else if (name.equals("usedUnsignedInt")) { 14937 this.used = new UnsignedIntType(); 14938 return this.used; 14939 } else if (name.equals("usedMoney")) { 14940 this.used = new Money(); 14941 return this.used; 14942 } else 14943 return super.addChild(name); 14944 } 14945 14946 public BenefitComponent copy() { 14947 BenefitComponent dst = new BenefitComponent(); 14948 copyValues(dst); 14949 return dst; 14950 } 14951 14952 public void copyValues(BenefitComponent dst) { 14953 super.copyValues(dst); 14954 dst.type = type == null ? null : type.copy(); 14955 dst.allowed = allowed == null ? null : allowed.copy(); 14956 dst.used = used == null ? null : used.copy(); 14957 } 14958 14959 @Override 14960 public boolean equalsDeep(Base other_) { 14961 if (!super.equalsDeep(other_)) 14962 return false; 14963 if (!(other_ instanceof BenefitComponent)) 14964 return false; 14965 BenefitComponent o = (BenefitComponent) other_; 14966 return compareDeep(type, o.type, true) && compareDeep(allowed, o.allowed, true) 14967 && compareDeep(used, o.used, true); 14968 } 14969 14970 @Override 14971 public boolean equalsShallow(Base other_) { 14972 if (!super.equalsShallow(other_)) 14973 return false; 14974 if (!(other_ instanceof BenefitComponent)) 14975 return false; 14976 BenefitComponent o = (BenefitComponent) other_; 14977 return true; 14978 } 14979 14980 public boolean isEmpty() { 14981 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, allowed, used); 14982 } 14983 14984 public String fhirType() { 14985 return "ExplanationOfBenefit.benefitBalance.financial"; 14986 14987 } 14988 14989 } 14990 14991 /** 14992 * A unique identifier assigned to this explanation of benefit. 14993 */ 14994 @Child(name = "identifier", type = { 14995 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 14996 @Description(shortDefinition = "Business Identifier for the resource", formalDefinition = "A unique identifier assigned to this explanation of benefit.") 14997 protected List<Identifier> identifier; 14998 14999 /** 15000 * The status of the resource instance. 15001 */ 15002 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 15003 @Description(shortDefinition = "active | cancelled | draft | entered-in-error", formalDefinition = "The status of the resource instance.") 15004 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/explanationofbenefit-status") 15005 protected Enumeration<ExplanationOfBenefitStatus> status; 15006 15007 /** 15008 * The category of claim, e.g. oral, pharmacy, vision, institutional, 15009 * professional. 15010 */ 15011 @Child(name = "type", type = { CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 15012 @Description(shortDefinition = "Category or discipline", formalDefinition = "The category of claim, e.g. oral, pharmacy, vision, institutional, professional.") 15013 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-type") 15014 protected CodeableConcept type; 15015 15016 /** 15017 * A finer grained suite of claim type codes which may convey additional 15018 * information such as Inpatient vs Outpatient and/or a specialty service. 15019 */ 15020 @Child(name = "subType", type = { 15021 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 15022 @Description(shortDefinition = "More granular claim type", formalDefinition = "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.") 15023 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-subtype") 15024 protected CodeableConcept subType; 15025 15026 /** 15027 * A code to indicate whether the nature of the request is: to request 15028 * adjudication of products and services previously rendered; or requesting 15029 * authorization and adjudication for provision in the future; or requesting the 15030 * non-binding adjudication of the listed products and services which could be 15031 * provided in the future. 15032 */ 15033 @Child(name = "use", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 15034 @Description(shortDefinition = "claim | preauthorization | predetermination", formalDefinition = "A code to indicate whether the nature of the request is: to request adjudication of products and services previously rendered; or requesting authorization and adjudication for provision in the future; or requesting the non-binding adjudication of the listed products and services which could be provided in the future.") 15035 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-use") 15036 protected Enumeration<Use> use; 15037 15038 /** 15039 * The party to whom the professional services and/or products have been 15040 * supplied or are being considered and for whom actual for forecast 15041 * reimbursement is sought. 15042 */ 15043 @Child(name = "patient", type = { Patient.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 15044 @Description(shortDefinition = "The recipient of the products and services", formalDefinition = "The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for forecast reimbursement is sought.") 15045 protected Reference patient; 15046 15047 /** 15048 * The actual object that is the target of the reference (The party to whom the 15049 * professional services and/or products have been supplied or are being 15050 * considered and for whom actual for forecast reimbursement is sought.) 15051 */ 15052 protected Patient patientTarget; 15053 15054 /** 15055 * The period for which charges are being submitted. 15056 */ 15057 @Child(name = "billablePeriod", type = { 15058 Period.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 15059 @Description(shortDefinition = "Relevant time frame for the claim", formalDefinition = "The period for which charges are being submitted.") 15060 protected Period billablePeriod; 15061 15062 /** 15063 * The date this resource was created. 15064 */ 15065 @Child(name = "created", type = { DateTimeType.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 15066 @Description(shortDefinition = "Response creation date", formalDefinition = "The date this resource was created.") 15067 protected DateTimeType created; 15068 15069 /** 15070 * Individual who created the claim, predetermination or preauthorization. 15071 */ 15072 @Child(name = "enterer", type = { Practitioner.class, 15073 PractitionerRole.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 15074 @Description(shortDefinition = "Author of the claim", formalDefinition = "Individual who created the claim, predetermination or preauthorization.") 15075 protected Reference enterer; 15076 15077 /** 15078 * The actual object that is the target of the reference (Individual who created 15079 * the claim, predetermination or preauthorization.) 15080 */ 15081 protected Resource entererTarget; 15082 15083 /** 15084 * The party responsible for authorization, adjudication and reimbursement. 15085 */ 15086 @Child(name = "insurer", type = { Organization.class }, order = 9, min = 1, max = 1, modifier = false, summary = true) 15087 @Description(shortDefinition = "Party responsible for reimbursement", formalDefinition = "The party responsible for authorization, adjudication and reimbursement.") 15088 protected Reference insurer; 15089 15090 /** 15091 * The actual object that is the target of the reference (The party responsible 15092 * for authorization, adjudication and reimbursement.) 15093 */ 15094 protected Organization insurerTarget; 15095 15096 /** 15097 * The provider which is responsible for the claim, predetermination or 15098 * preauthorization. 15099 */ 15100 @Child(name = "provider", type = { Practitioner.class, PractitionerRole.class, 15101 Organization.class }, order = 10, min = 1, max = 1, modifier = false, summary = true) 15102 @Description(shortDefinition = "Party responsible for the claim", formalDefinition = "The provider which is responsible for the claim, predetermination or preauthorization.") 15103 protected Reference provider; 15104 15105 /** 15106 * The actual object that is the target of the reference (The provider which is 15107 * responsible for the claim, predetermination or preauthorization.) 15108 */ 15109 protected Resource providerTarget; 15110 15111 /** 15112 * The provider-required urgency of processing the request. Typical values 15113 * include: stat, routine deferred. 15114 */ 15115 @Child(name = "priority", type = { 15116 CodeableConcept.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 15117 @Description(shortDefinition = "Desired processing urgency", formalDefinition = "The provider-required urgency of processing the request. Typical values include: stat, routine deferred.") 15118 protected CodeableConcept priority; 15119 15120 /** 15121 * A code to indicate whether and for whom funds are to be reserved for future 15122 * claims. 15123 */ 15124 @Child(name = "fundsReserveRequested", type = { 15125 CodeableConcept.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 15126 @Description(shortDefinition = "For whom to reserve funds", formalDefinition = "A code to indicate whether and for whom funds are to be reserved for future claims.") 15127 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/fundsreserve") 15128 protected CodeableConcept fundsReserveRequested; 15129 15130 /** 15131 * A code, used only on a response to a preauthorization, to indicate whether 15132 * the benefits payable have been reserved and for whom. 15133 */ 15134 @Child(name = "fundsReserve", type = { 15135 CodeableConcept.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 15136 @Description(shortDefinition = "Funds reserved status", formalDefinition = "A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom.") 15137 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/fundsreserve") 15138 protected CodeableConcept fundsReserve; 15139 15140 /** 15141 * Other claims which are related to this claim such as prior submissions or 15142 * claims for related services or for the same event. 15143 */ 15144 @Child(name = "related", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 15145 @Description(shortDefinition = "Prior or corollary claims", formalDefinition = "Other claims which are related to this claim such as prior submissions or claims for related services or for the same event.") 15146 protected List<RelatedClaimComponent> related; 15147 15148 /** 15149 * Prescription to support the dispensing of pharmacy, device or vision 15150 * products. 15151 */ 15152 @Child(name = "prescription", type = { MedicationRequest.class, 15153 VisionPrescription.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 15154 @Description(shortDefinition = "Prescription authorizing services or products", formalDefinition = "Prescription to support the dispensing of pharmacy, device or vision products.") 15155 protected Reference prescription; 15156 15157 /** 15158 * The actual object that is the target of the reference (Prescription to 15159 * support the dispensing of pharmacy, device or vision products.) 15160 */ 15161 protected Resource prescriptionTarget; 15162 15163 /** 15164 * Original prescription which has been superseded by this prescription to 15165 * support the dispensing of pharmacy services, medications or products. 15166 */ 15167 @Child(name = "originalPrescription", type = { 15168 MedicationRequest.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 15169 @Description(shortDefinition = "Original prescription if superceded by fulfiller", formalDefinition = "Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products.") 15170 protected Reference originalPrescription; 15171 15172 /** 15173 * The actual object that is the target of the reference (Original prescription 15174 * which has been superseded by this prescription to support the dispensing of 15175 * pharmacy services, medications or products.) 15176 */ 15177 protected MedicationRequest originalPrescriptionTarget; 15178 15179 /** 15180 * The party to be reimbursed for cost of the products and services according to 15181 * the terms of the policy. 15182 */ 15183 @Child(name = "payee", type = {}, order = 17, min = 0, max = 1, modifier = false, summary = false) 15184 @Description(shortDefinition = "Recipient of benefits payable", formalDefinition = "The party to be reimbursed for cost of the products and services according to the terms of the policy.") 15185 protected PayeeComponent payee; 15186 15187 /** 15188 * A reference to a referral resource. 15189 */ 15190 @Child(name = "referral", type = { 15191 ServiceRequest.class }, order = 18, min = 0, max = 1, modifier = false, summary = false) 15192 @Description(shortDefinition = "Treatment Referral", formalDefinition = "A reference to a referral resource.") 15193 protected Reference referral; 15194 15195 /** 15196 * The actual object that is the target of the reference (A reference to a 15197 * referral resource.) 15198 */ 15199 protected ServiceRequest referralTarget; 15200 15201 /** 15202 * Facility where the services were provided. 15203 */ 15204 @Child(name = "facility", type = { Location.class }, order = 19, min = 0, max = 1, modifier = false, summary = false) 15205 @Description(shortDefinition = "Servicing Facility", formalDefinition = "Facility where the services were provided.") 15206 protected Reference facility; 15207 15208 /** 15209 * The actual object that is the target of the reference (Facility where the 15210 * services were provided.) 15211 */ 15212 protected Location facilityTarget; 15213 15214 /** 15215 * The business identifier for the instance of the adjudication request: claim 15216 * predetermination or preauthorization. 15217 */ 15218 @Child(name = "claim", type = { Claim.class }, order = 20, min = 0, max = 1, modifier = false, summary = false) 15219 @Description(shortDefinition = "Claim reference", formalDefinition = "The business identifier for the instance of the adjudication request: claim predetermination or preauthorization.") 15220 protected Reference claim; 15221 15222 /** 15223 * The actual object that is the target of the reference (The business 15224 * identifier for the instance of the adjudication request: claim 15225 * predetermination or preauthorization.) 15226 */ 15227 protected Claim claimTarget; 15228 15229 /** 15230 * The business identifier for the instance of the adjudication response: claim, 15231 * predetermination or preauthorization response. 15232 */ 15233 @Child(name = "claimResponse", type = { 15234 ClaimResponse.class }, order = 21, min = 0, max = 1, modifier = false, summary = false) 15235 @Description(shortDefinition = "Claim response reference", formalDefinition = "The business identifier for the instance of the adjudication response: claim, predetermination or preauthorization response.") 15236 protected Reference claimResponse; 15237 15238 /** 15239 * The actual object that is the target of the reference (The business 15240 * identifier for the instance of the adjudication response: claim, 15241 * predetermination or preauthorization response.) 15242 */ 15243 protected ClaimResponse claimResponseTarget; 15244 15245 /** 15246 * The outcome of the claim, predetermination, or preauthorization processing. 15247 */ 15248 @Child(name = "outcome", type = { CodeType.class }, order = 22, min = 1, max = 1, modifier = false, summary = true) 15249 @Description(shortDefinition = "queued | complete | error | partial", formalDefinition = "The outcome of the claim, predetermination, or preauthorization processing.") 15250 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/remittance-outcome") 15251 protected Enumeration<RemittanceOutcome> outcome; 15252 15253 /** 15254 * A human readable description of the status of the adjudication. 15255 */ 15256 @Child(name = "disposition", type = { 15257 StringType.class }, order = 23, min = 0, max = 1, modifier = false, summary = false) 15258 @Description(shortDefinition = "Disposition Message", formalDefinition = "A human readable description of the status of the adjudication.") 15259 protected StringType disposition; 15260 15261 /** 15262 * Reference from the Insurer which is used in later communications which refers 15263 * to this adjudication. 15264 */ 15265 @Child(name = "preAuthRef", type = { 15266 StringType.class }, order = 24, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 15267 @Description(shortDefinition = "Preauthorization reference", formalDefinition = "Reference from the Insurer which is used in later communications which refers to this adjudication.") 15268 protected List<StringType> preAuthRef; 15269 15270 /** 15271 * The timeframe during which the supplied preauthorization reference may be 15272 * quoted on claims to obtain the adjudication as provided. 15273 */ 15274 @Child(name = "preAuthRefPeriod", type = { 15275 Period.class }, order = 25, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 15276 @Description(shortDefinition = "Preauthorization in-effect period", formalDefinition = "The timeframe during which the supplied preauthorization reference may be quoted on claims to obtain the adjudication as provided.") 15277 protected List<Period> preAuthRefPeriod; 15278 15279 /** 15280 * The members of the team who provided the products and services. 15281 */ 15282 @Child(name = "careTeam", type = {}, order = 26, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 15283 @Description(shortDefinition = "Care Team members", formalDefinition = "The members of the team who provided the products and services.") 15284 protected List<CareTeamComponent> careTeam; 15285 15286 /** 15287 * Additional information codes regarding exceptions, special considerations, 15288 * the condition, situation, prior or concurrent issues. 15289 */ 15290 @Child(name = "supportingInfo", type = {}, order = 27, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 15291 @Description(shortDefinition = "Supporting information", formalDefinition = "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.") 15292 protected List<SupportingInformationComponent> supportingInfo; 15293 15294 /** 15295 * Information about diagnoses relevant to the claim items. 15296 */ 15297 @Child(name = "diagnosis", type = {}, order = 28, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 15298 @Description(shortDefinition = "Pertinent diagnosis information", formalDefinition = "Information about diagnoses relevant to the claim items.") 15299 protected List<DiagnosisComponent> diagnosis; 15300 15301 /** 15302 * Procedures performed on the patient relevant to the billing items with the 15303 * claim. 15304 */ 15305 @Child(name = "procedure", type = {}, order = 29, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 15306 @Description(shortDefinition = "Clinical procedures performed", formalDefinition = "Procedures performed on the patient relevant to the billing items with the claim.") 15307 protected List<ProcedureComponent> procedure; 15308 15309 /** 15310 * This indicates the relative order of a series of EOBs related to different 15311 * coverages for the same suite of services. 15312 */ 15313 @Child(name = "precedence", type = { 15314 PositiveIntType.class }, order = 30, min = 0, max = 1, modifier = false, summary = false) 15315 @Description(shortDefinition = "Precedence (primary, secondary, etc.)", formalDefinition = "This indicates the relative order of a series of EOBs related to different coverages for the same suite of services.") 15316 protected PositiveIntType precedence; 15317 15318 /** 15319 * Financial instruments for reimbursement for the health care products and 15320 * services specified on the claim. 15321 */ 15322 @Child(name = "insurance", type = {}, order = 31, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 15323 @Description(shortDefinition = "Patient insurance information", formalDefinition = "Financial instruments for reimbursement for the health care products and services specified on the claim.") 15324 protected List<InsuranceComponent> insurance; 15325 15326 /** 15327 * Details of a accident which resulted in injuries which required the products 15328 * and services listed in the claim. 15329 */ 15330 @Child(name = "accident", type = {}, order = 32, min = 0, max = 1, modifier = false, summary = false) 15331 @Description(shortDefinition = "Details of the event", formalDefinition = "Details of a accident which resulted in injuries which required the products and services listed in the claim.") 15332 protected AccidentComponent accident; 15333 15334 /** 15335 * A claim line. Either a simple (a product or service) or a 'group' of details 15336 * which can also be a simple items or groups of sub-details. 15337 */ 15338 @Child(name = "item", type = {}, order = 33, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 15339 @Description(shortDefinition = "Product or service provided", formalDefinition = "A claim line. Either a simple (a product or service) or a 'group' of details which can also be a simple items or groups of sub-details.") 15340 protected List<ItemComponent> item; 15341 15342 /** 15343 * The first-tier service adjudications for payor added product or service 15344 * lines. 15345 */ 15346 @Child(name = "addItem", type = {}, order = 34, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 15347 @Description(shortDefinition = "Insurer added line items", formalDefinition = "The first-tier service adjudications for payor added product or service lines.") 15348 protected List<AddedItemComponent> addItem; 15349 15350 /** 15351 * The adjudication results which are presented at the header level rather than 15352 * at the line-item or add-item levels. 15353 */ 15354 @Child(name = "adjudication", type = { 15355 AdjudicationComponent.class }, order = 35, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 15356 @Description(shortDefinition = "Header-level adjudication", formalDefinition = "The adjudication results which are presented at the header level rather than at the line-item or add-item levels.") 15357 protected List<AdjudicationComponent> adjudication; 15358 15359 /** 15360 * Categorized monetary totals for the adjudication. 15361 */ 15362 @Child(name = "total", type = {}, order = 36, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 15363 @Description(shortDefinition = "Adjudication totals", formalDefinition = "Categorized monetary totals for the adjudication.") 15364 protected List<TotalComponent> total; 15365 15366 /** 15367 * Payment details for the adjudication of the claim. 15368 */ 15369 @Child(name = "payment", type = {}, order = 37, min = 0, max = 1, modifier = false, summary = false) 15370 @Description(shortDefinition = "Payment Details", formalDefinition = "Payment details for the adjudication of the claim.") 15371 protected PaymentComponent payment; 15372 15373 /** 15374 * A code for the form to be used for printing the content. 15375 */ 15376 @Child(name = "formCode", type = { 15377 CodeableConcept.class }, order = 38, min = 0, max = 1, modifier = false, summary = false) 15378 @Description(shortDefinition = "Printed form identifier", formalDefinition = "A code for the form to be used for printing the content.") 15379 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/forms") 15380 protected CodeableConcept formCode; 15381 15382 /** 15383 * The actual form, by reference or inclusion, for printing the content or an 15384 * EOB. 15385 */ 15386 @Child(name = "form", type = { Attachment.class }, order = 39, min = 0, max = 1, modifier = false, summary = false) 15387 @Description(shortDefinition = "Printed reference or actual form", formalDefinition = "The actual form, by reference or inclusion, for printing the content or an EOB.") 15388 protected Attachment form; 15389 15390 /** 15391 * A note that describes or explains adjudication results in a human readable 15392 * form. 15393 */ 15394 @Child(name = "processNote", type = {}, order = 40, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 15395 @Description(shortDefinition = "Note concerning adjudication", formalDefinition = "A note that describes or explains adjudication results in a human readable form.") 15396 protected List<NoteComponent> processNote; 15397 15398 /** 15399 * The term of the benefits documented in this response. 15400 */ 15401 @Child(name = "benefitPeriod", type = { 15402 Period.class }, order = 41, min = 0, max = 1, modifier = false, summary = false) 15403 @Description(shortDefinition = "When the benefits are applicable", formalDefinition = "The term of the benefits documented in this response.") 15404 protected Period benefitPeriod; 15405 15406 /** 15407 * Balance by Benefit Category. 15408 */ 15409 @Child(name = "benefitBalance", type = {}, order = 42, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 15410 @Description(shortDefinition = "Balance by Benefit Category", formalDefinition = "Balance by Benefit Category.") 15411 protected List<BenefitBalanceComponent> benefitBalance; 15412 15413 private static final long serialVersionUID = -1515422099L; 15414 15415 /** 15416 * Constructor 15417 */ 15418 public ExplanationOfBenefit() { 15419 super(); 15420 } 15421 15422 /** 15423 * Constructor 15424 */ 15425 public ExplanationOfBenefit(Enumeration<ExplanationOfBenefitStatus> status, CodeableConcept type, 15426 Enumeration<Use> use, Reference patient, DateTimeType created, Reference insurer, Reference provider, 15427 Enumeration<RemittanceOutcome> outcome) { 15428 super(); 15429 this.status = status; 15430 this.type = type; 15431 this.use = use; 15432 this.patient = patient; 15433 this.created = created; 15434 this.insurer = insurer; 15435 this.provider = provider; 15436 this.outcome = outcome; 15437 } 15438 15439 /** 15440 * @return {@link #identifier} (A unique identifier assigned to this explanation 15441 * of benefit.) 15442 */ 15443 public List<Identifier> getIdentifier() { 15444 if (this.identifier == null) 15445 this.identifier = new ArrayList<Identifier>(); 15446 return this.identifier; 15447 } 15448 15449 /** 15450 * @return Returns a reference to <code>this</code> for easy method chaining 15451 */ 15452 public ExplanationOfBenefit setIdentifier(List<Identifier> theIdentifier) { 15453 this.identifier = theIdentifier; 15454 return this; 15455 } 15456 15457 public boolean hasIdentifier() { 15458 if (this.identifier == null) 15459 return false; 15460 for (Identifier item : this.identifier) 15461 if (!item.isEmpty()) 15462 return true; 15463 return false; 15464 } 15465 15466 public Identifier addIdentifier() { // 3 15467 Identifier t = new Identifier(); 15468 if (this.identifier == null) 15469 this.identifier = new ArrayList<Identifier>(); 15470 this.identifier.add(t); 15471 return t; 15472 } 15473 15474 public ExplanationOfBenefit addIdentifier(Identifier t) { // 3 15475 if (t == null) 15476 return this; 15477 if (this.identifier == null) 15478 this.identifier = new ArrayList<Identifier>(); 15479 this.identifier.add(t); 15480 return this; 15481 } 15482 15483 /** 15484 * @return The first repetition of repeating field {@link #identifier}, creating 15485 * it if it does not already exist 15486 */ 15487 public Identifier getIdentifierFirstRep() { 15488 if (getIdentifier().isEmpty()) { 15489 addIdentifier(); 15490 } 15491 return getIdentifier().get(0); 15492 } 15493 15494 /** 15495 * @return {@link #status} (The status of the resource instance.). This is the 15496 * underlying object with id, value and extensions. The accessor 15497 * "getStatus" gives direct access to the value 15498 */ 15499 public Enumeration<ExplanationOfBenefitStatus> getStatusElement() { 15500 if (this.status == null) 15501 if (Configuration.errorOnAutoCreate()) 15502 throw new Error("Attempt to auto-create ExplanationOfBenefit.status"); 15503 else if (Configuration.doAutoCreate()) 15504 this.status = new Enumeration<ExplanationOfBenefitStatus>(new ExplanationOfBenefitStatusEnumFactory()); // bb 15505 return this.status; 15506 } 15507 15508 public boolean hasStatusElement() { 15509 return this.status != null && !this.status.isEmpty(); 15510 } 15511 15512 public boolean hasStatus() { 15513 return this.status != null && !this.status.isEmpty(); 15514 } 15515 15516 /** 15517 * @param value {@link #status} (The status of the resource instance.). This is 15518 * the underlying object with id, value and extensions. The 15519 * accessor "getStatus" gives direct access to the value 15520 */ 15521 public ExplanationOfBenefit setStatusElement(Enumeration<ExplanationOfBenefitStatus> value) { 15522 this.status = value; 15523 return this; 15524 } 15525 15526 /** 15527 * @return The status of the resource instance. 15528 */ 15529 public ExplanationOfBenefitStatus getStatus() { 15530 return this.status == null ? null : this.status.getValue(); 15531 } 15532 15533 /** 15534 * @param value The status of the resource instance. 15535 */ 15536 public ExplanationOfBenefit setStatus(ExplanationOfBenefitStatus value) { 15537 if (this.status == null) 15538 this.status = new Enumeration<ExplanationOfBenefitStatus>(new ExplanationOfBenefitStatusEnumFactory()); 15539 this.status.setValue(value); 15540 return this; 15541 } 15542 15543 /** 15544 * @return {@link #type} (The category of claim, e.g. oral, pharmacy, vision, 15545 * institutional, professional.) 15546 */ 15547 public CodeableConcept getType() { 15548 if (this.type == null) 15549 if (Configuration.errorOnAutoCreate()) 15550 throw new Error("Attempt to auto-create ExplanationOfBenefit.type"); 15551 else if (Configuration.doAutoCreate()) 15552 this.type = new CodeableConcept(); // cc 15553 return this.type; 15554 } 15555 15556 public boolean hasType() { 15557 return this.type != null && !this.type.isEmpty(); 15558 } 15559 15560 /** 15561 * @param value {@link #type} (The category of claim, e.g. oral, pharmacy, 15562 * vision, institutional, professional.) 15563 */ 15564 public ExplanationOfBenefit setType(CodeableConcept value) { 15565 this.type = value; 15566 return this; 15567 } 15568 15569 /** 15570 * @return {@link #subType} (A finer grained suite of claim type codes which may 15571 * convey additional information such as Inpatient vs Outpatient and/or 15572 * a specialty service.) 15573 */ 15574 public CodeableConcept getSubType() { 15575 if (this.subType == null) 15576 if (Configuration.errorOnAutoCreate()) 15577 throw new Error("Attempt to auto-create ExplanationOfBenefit.subType"); 15578 else if (Configuration.doAutoCreate()) 15579 this.subType = new CodeableConcept(); // cc 15580 return this.subType; 15581 } 15582 15583 public boolean hasSubType() { 15584 return this.subType != null && !this.subType.isEmpty(); 15585 } 15586 15587 /** 15588 * @param value {@link #subType} (A finer grained suite of claim type codes 15589 * which may convey additional information such as Inpatient vs 15590 * Outpatient and/or a specialty service.) 15591 */ 15592 public ExplanationOfBenefit setSubType(CodeableConcept value) { 15593 this.subType = value; 15594 return this; 15595 } 15596 15597 /** 15598 * @return {@link #use} (A code to indicate whether the nature of the request 15599 * is: to request adjudication of products and services previously 15600 * rendered; or requesting authorization and adjudication for provision 15601 * in the future; or requesting the non-binding adjudication of the 15602 * listed products and services which could be provided in the future.). 15603 * This is the underlying object with id, value and extensions. The 15604 * accessor "getUse" gives direct access to the value 15605 */ 15606 public Enumeration<Use> getUseElement() { 15607 if (this.use == null) 15608 if (Configuration.errorOnAutoCreate()) 15609 throw new Error("Attempt to auto-create ExplanationOfBenefit.use"); 15610 else if (Configuration.doAutoCreate()) 15611 this.use = new Enumeration<Use>(new UseEnumFactory()); // bb 15612 return this.use; 15613 } 15614 15615 public boolean hasUseElement() { 15616 return this.use != null && !this.use.isEmpty(); 15617 } 15618 15619 public boolean hasUse() { 15620 return this.use != null && !this.use.isEmpty(); 15621 } 15622 15623 /** 15624 * @param value {@link #use} (A code to indicate whether the nature of the 15625 * request is: to request adjudication of products and services 15626 * previously rendered; or requesting authorization and 15627 * adjudication for provision in the future; or requesting the 15628 * non-binding adjudication of the listed products and services 15629 * which could be provided in the future.). This is the underlying 15630 * object with id, value and extensions. The accessor "getUse" 15631 * gives direct access to the value 15632 */ 15633 public ExplanationOfBenefit setUseElement(Enumeration<Use> value) { 15634 this.use = value; 15635 return this; 15636 } 15637 15638 /** 15639 * @return A code to indicate whether the nature of the request is: to request 15640 * adjudication of products and services previously rendered; or 15641 * requesting authorization and adjudication for provision in the 15642 * future; or requesting the non-binding adjudication of the listed 15643 * products and services which could be provided in the future. 15644 */ 15645 public Use getUse() { 15646 return this.use == null ? null : this.use.getValue(); 15647 } 15648 15649 /** 15650 * @param value A code to indicate whether the nature of the request is: to 15651 * request adjudication of products and services previously 15652 * rendered; or requesting authorization and adjudication for 15653 * provision in the future; or requesting the non-binding 15654 * adjudication of the listed products and services which could be 15655 * provided in the future. 15656 */ 15657 public ExplanationOfBenefit setUse(Use value) { 15658 if (this.use == null) 15659 this.use = new Enumeration<Use>(new UseEnumFactory()); 15660 this.use.setValue(value); 15661 return this; 15662 } 15663 15664 /** 15665 * @return {@link #patient} (The party to whom the professional services and/or 15666 * products have been supplied or are being considered and for whom 15667 * actual for forecast reimbursement is sought.) 15668 */ 15669 public Reference getPatient() { 15670 if (this.patient == null) 15671 if (Configuration.errorOnAutoCreate()) 15672 throw new Error("Attempt to auto-create ExplanationOfBenefit.patient"); 15673 else if (Configuration.doAutoCreate()) 15674 this.patient = new Reference(); // cc 15675 return this.patient; 15676 } 15677 15678 public boolean hasPatient() { 15679 return this.patient != null && !this.patient.isEmpty(); 15680 } 15681 15682 /** 15683 * @param value {@link #patient} (The party to whom the professional services 15684 * and/or products have been supplied or are being considered and 15685 * for whom actual for forecast reimbursement is sought.) 15686 */ 15687 public ExplanationOfBenefit setPatient(Reference value) { 15688 this.patient = value; 15689 return this; 15690 } 15691 15692 /** 15693 * @return {@link #patient} The actual object that is the target of the 15694 * reference. The reference library doesn't populate this, but you can 15695 * use it to hold the resource if you resolve it. (The party to whom the 15696 * professional services and/or products have been supplied or are being 15697 * considered and for whom actual for forecast reimbursement is sought.) 15698 */ 15699 public Patient getPatientTarget() { 15700 if (this.patientTarget == null) 15701 if (Configuration.errorOnAutoCreate()) 15702 throw new Error("Attempt to auto-create ExplanationOfBenefit.patient"); 15703 else if (Configuration.doAutoCreate()) 15704 this.patientTarget = new Patient(); // aa 15705 return this.patientTarget; 15706 } 15707 15708 /** 15709 * @param value {@link #patient} The actual object that is the target of the 15710 * reference. The reference library doesn't use these, but you can 15711 * use it to hold the resource if you resolve it. (The party to 15712 * whom the professional services and/or products have been 15713 * supplied or are being considered and for whom actual for 15714 * forecast reimbursement is sought.) 15715 */ 15716 public ExplanationOfBenefit setPatientTarget(Patient value) { 15717 this.patientTarget = value; 15718 return this; 15719 } 15720 15721 /** 15722 * @return {@link #billablePeriod} (The period for which charges are being 15723 * submitted.) 15724 */ 15725 public Period getBillablePeriod() { 15726 if (this.billablePeriod == null) 15727 if (Configuration.errorOnAutoCreate()) 15728 throw new Error("Attempt to auto-create ExplanationOfBenefit.billablePeriod"); 15729 else if (Configuration.doAutoCreate()) 15730 this.billablePeriod = new Period(); // cc 15731 return this.billablePeriod; 15732 } 15733 15734 public boolean hasBillablePeriod() { 15735 return this.billablePeriod != null && !this.billablePeriod.isEmpty(); 15736 } 15737 15738 /** 15739 * @param value {@link #billablePeriod} (The period for which charges are being 15740 * submitted.) 15741 */ 15742 public ExplanationOfBenefit setBillablePeriod(Period value) { 15743 this.billablePeriod = value; 15744 return this; 15745 } 15746 15747 /** 15748 * @return {@link #created} (The date this resource was created.). This is the 15749 * underlying object with id, value and extensions. The accessor 15750 * "getCreated" gives direct access to the value 15751 */ 15752 public DateTimeType getCreatedElement() { 15753 if (this.created == null) 15754 if (Configuration.errorOnAutoCreate()) 15755 throw new Error("Attempt to auto-create ExplanationOfBenefit.created"); 15756 else if (Configuration.doAutoCreate()) 15757 this.created = new DateTimeType(); // bb 15758 return this.created; 15759 } 15760 15761 public boolean hasCreatedElement() { 15762 return this.created != null && !this.created.isEmpty(); 15763 } 15764 15765 public boolean hasCreated() { 15766 return this.created != null && !this.created.isEmpty(); 15767 } 15768 15769 /** 15770 * @param value {@link #created} (The date this resource was created.). This is 15771 * the underlying object with id, value and extensions. The 15772 * accessor "getCreated" gives direct access to the value 15773 */ 15774 public ExplanationOfBenefit setCreatedElement(DateTimeType value) { 15775 this.created = value; 15776 return this; 15777 } 15778 15779 /** 15780 * @return The date this resource was created. 15781 */ 15782 public Date getCreated() { 15783 return this.created == null ? null : this.created.getValue(); 15784 } 15785 15786 /** 15787 * @param value The date this resource was created. 15788 */ 15789 public ExplanationOfBenefit setCreated(Date value) { 15790 if (this.created == null) 15791 this.created = new DateTimeType(); 15792 this.created.setValue(value); 15793 return this; 15794 } 15795 15796 /** 15797 * @return {@link #enterer} (Individual who created the claim, predetermination 15798 * or preauthorization.) 15799 */ 15800 public Reference getEnterer() { 15801 if (this.enterer == null) 15802 if (Configuration.errorOnAutoCreate()) 15803 throw new Error("Attempt to auto-create ExplanationOfBenefit.enterer"); 15804 else if (Configuration.doAutoCreate()) 15805 this.enterer = new Reference(); // cc 15806 return this.enterer; 15807 } 15808 15809 public boolean hasEnterer() { 15810 return this.enterer != null && !this.enterer.isEmpty(); 15811 } 15812 15813 /** 15814 * @param value {@link #enterer} (Individual who created the claim, 15815 * predetermination or preauthorization.) 15816 */ 15817 public ExplanationOfBenefit setEnterer(Reference value) { 15818 this.enterer = value; 15819 return this; 15820 } 15821 15822 /** 15823 * @return {@link #enterer} The actual object that is the target of the 15824 * reference. The reference library doesn't populate this, but you can 15825 * use it to hold the resource if you resolve it. (Individual who 15826 * created the claim, predetermination or preauthorization.) 15827 */ 15828 public Resource getEntererTarget() { 15829 return this.entererTarget; 15830 } 15831 15832 /** 15833 * @param value {@link #enterer} The actual object that is the target of the 15834 * reference. The reference library doesn't use these, but you can 15835 * use it to hold the resource if you resolve it. (Individual who 15836 * created the claim, predetermination or preauthorization.) 15837 */ 15838 public ExplanationOfBenefit setEntererTarget(Resource value) { 15839 this.entererTarget = value; 15840 return this; 15841 } 15842 15843 /** 15844 * @return {@link #insurer} (The party responsible for authorization, 15845 * adjudication and reimbursement.) 15846 */ 15847 public Reference getInsurer() { 15848 if (this.insurer == null) 15849 if (Configuration.errorOnAutoCreate()) 15850 throw new Error("Attempt to auto-create ExplanationOfBenefit.insurer"); 15851 else if (Configuration.doAutoCreate()) 15852 this.insurer = new Reference(); // cc 15853 return this.insurer; 15854 } 15855 15856 public boolean hasInsurer() { 15857 return this.insurer != null && !this.insurer.isEmpty(); 15858 } 15859 15860 /** 15861 * @param value {@link #insurer} (The party responsible for authorization, 15862 * adjudication and reimbursement.) 15863 */ 15864 public ExplanationOfBenefit setInsurer(Reference value) { 15865 this.insurer = value; 15866 return this; 15867 } 15868 15869 /** 15870 * @return {@link #insurer} The actual object that is the target of the 15871 * reference. The reference library doesn't populate this, but you can 15872 * use it to hold the resource if you resolve it. (The party responsible 15873 * for authorization, adjudication and reimbursement.) 15874 */ 15875 public Organization getInsurerTarget() { 15876 if (this.insurerTarget == null) 15877 if (Configuration.errorOnAutoCreate()) 15878 throw new Error("Attempt to auto-create ExplanationOfBenefit.insurer"); 15879 else if (Configuration.doAutoCreate()) 15880 this.insurerTarget = new Organization(); // aa 15881 return this.insurerTarget; 15882 } 15883 15884 /** 15885 * @param value {@link #insurer} The actual object that is the target of the 15886 * reference. The reference library doesn't use these, but you can 15887 * use it to hold the resource if you resolve it. (The party 15888 * responsible for authorization, adjudication and reimbursement.) 15889 */ 15890 public ExplanationOfBenefit setInsurerTarget(Organization value) { 15891 this.insurerTarget = value; 15892 return this; 15893 } 15894 15895 /** 15896 * @return {@link #provider} (The provider which is responsible for the claim, 15897 * predetermination or preauthorization.) 15898 */ 15899 public Reference getProvider() { 15900 if (this.provider == null) 15901 if (Configuration.errorOnAutoCreate()) 15902 throw new Error("Attempt to auto-create ExplanationOfBenefit.provider"); 15903 else if (Configuration.doAutoCreate()) 15904 this.provider = new Reference(); // cc 15905 return this.provider; 15906 } 15907 15908 public boolean hasProvider() { 15909 return this.provider != null && !this.provider.isEmpty(); 15910 } 15911 15912 /** 15913 * @param value {@link #provider} (The provider which is responsible for the 15914 * claim, predetermination or preauthorization.) 15915 */ 15916 public ExplanationOfBenefit setProvider(Reference value) { 15917 this.provider = value; 15918 return this; 15919 } 15920 15921 /** 15922 * @return {@link #provider} The actual object that is the target of the 15923 * reference. The reference library doesn't populate this, but you can 15924 * use it to hold the resource if you resolve it. (The provider which is 15925 * responsible for the claim, predetermination or preauthorization.) 15926 */ 15927 public Resource getProviderTarget() { 15928 return this.providerTarget; 15929 } 15930 15931 /** 15932 * @param value {@link #provider} The actual object that is the target of the 15933 * reference. The reference library doesn't use these, but you can 15934 * use it to hold the resource if you resolve it. (The provider 15935 * which is responsible for the claim, predetermination or 15936 * preauthorization.) 15937 */ 15938 public ExplanationOfBenefit setProviderTarget(Resource value) { 15939 this.providerTarget = value; 15940 return this; 15941 } 15942 15943 /** 15944 * @return {@link #priority} (The provider-required urgency of processing the 15945 * request. Typical values include: stat, routine deferred.) 15946 */ 15947 public CodeableConcept getPriority() { 15948 if (this.priority == null) 15949 if (Configuration.errorOnAutoCreate()) 15950 throw new Error("Attempt to auto-create ExplanationOfBenefit.priority"); 15951 else if (Configuration.doAutoCreate()) 15952 this.priority = new CodeableConcept(); // cc 15953 return this.priority; 15954 } 15955 15956 public boolean hasPriority() { 15957 return this.priority != null && !this.priority.isEmpty(); 15958 } 15959 15960 /** 15961 * @param value {@link #priority} (The provider-required urgency of processing 15962 * the request. Typical values include: stat, routine deferred.) 15963 */ 15964 public ExplanationOfBenefit setPriority(CodeableConcept value) { 15965 this.priority = value; 15966 return this; 15967 } 15968 15969 /** 15970 * @return {@link #fundsReserveRequested} (A code to indicate whether and for 15971 * whom funds are to be reserved for future claims.) 15972 */ 15973 public CodeableConcept getFundsReserveRequested() { 15974 if (this.fundsReserveRequested == null) 15975 if (Configuration.errorOnAutoCreate()) 15976 throw new Error("Attempt to auto-create ExplanationOfBenefit.fundsReserveRequested"); 15977 else if (Configuration.doAutoCreate()) 15978 this.fundsReserveRequested = new CodeableConcept(); // cc 15979 return this.fundsReserveRequested; 15980 } 15981 15982 public boolean hasFundsReserveRequested() { 15983 return this.fundsReserveRequested != null && !this.fundsReserveRequested.isEmpty(); 15984 } 15985 15986 /** 15987 * @param value {@link #fundsReserveRequested} (A code to indicate whether and 15988 * for whom funds are to be reserved for future claims.) 15989 */ 15990 public ExplanationOfBenefit setFundsReserveRequested(CodeableConcept value) { 15991 this.fundsReserveRequested = value; 15992 return this; 15993 } 15994 15995 /** 15996 * @return {@link #fundsReserve} (A code, used only on a response to a 15997 * preauthorization, to indicate whether the benefits payable have been 15998 * reserved and for whom.) 15999 */ 16000 public CodeableConcept getFundsReserve() { 16001 if (this.fundsReserve == null) 16002 if (Configuration.errorOnAutoCreate()) 16003 throw new Error("Attempt to auto-create ExplanationOfBenefit.fundsReserve"); 16004 else if (Configuration.doAutoCreate()) 16005 this.fundsReserve = new CodeableConcept(); // cc 16006 return this.fundsReserve; 16007 } 16008 16009 public boolean hasFundsReserve() { 16010 return this.fundsReserve != null && !this.fundsReserve.isEmpty(); 16011 } 16012 16013 /** 16014 * @param value {@link #fundsReserve} (A code, used only on a response to a 16015 * preauthorization, to indicate whether the benefits payable have 16016 * been reserved and for whom.) 16017 */ 16018 public ExplanationOfBenefit setFundsReserve(CodeableConcept value) { 16019 this.fundsReserve = value; 16020 return this; 16021 } 16022 16023 /** 16024 * @return {@link #related} (Other claims which are related to this claim such 16025 * as prior submissions or claims for related services or for the same 16026 * event.) 16027 */ 16028 public List<RelatedClaimComponent> getRelated() { 16029 if (this.related == null) 16030 this.related = new ArrayList<RelatedClaimComponent>(); 16031 return this.related; 16032 } 16033 16034 /** 16035 * @return Returns a reference to <code>this</code> for easy method chaining 16036 */ 16037 public ExplanationOfBenefit setRelated(List<RelatedClaimComponent> theRelated) { 16038 this.related = theRelated; 16039 return this; 16040 } 16041 16042 public boolean hasRelated() { 16043 if (this.related == null) 16044 return false; 16045 for (RelatedClaimComponent item : this.related) 16046 if (!item.isEmpty()) 16047 return true; 16048 return false; 16049 } 16050 16051 public RelatedClaimComponent addRelated() { // 3 16052 RelatedClaimComponent t = new RelatedClaimComponent(); 16053 if (this.related == null) 16054 this.related = new ArrayList<RelatedClaimComponent>(); 16055 this.related.add(t); 16056 return t; 16057 } 16058 16059 public ExplanationOfBenefit addRelated(RelatedClaimComponent t) { // 3 16060 if (t == null) 16061 return this; 16062 if (this.related == null) 16063 this.related = new ArrayList<RelatedClaimComponent>(); 16064 this.related.add(t); 16065 return this; 16066 } 16067 16068 /** 16069 * @return The first repetition of repeating field {@link #related}, creating it 16070 * if it does not already exist 16071 */ 16072 public RelatedClaimComponent getRelatedFirstRep() { 16073 if (getRelated().isEmpty()) { 16074 addRelated(); 16075 } 16076 return getRelated().get(0); 16077 } 16078 16079 /** 16080 * @return {@link #prescription} (Prescription to support the dispensing of 16081 * pharmacy, device or vision products.) 16082 */ 16083 public Reference getPrescription() { 16084 if (this.prescription == null) 16085 if (Configuration.errorOnAutoCreate()) 16086 throw new Error("Attempt to auto-create ExplanationOfBenefit.prescription"); 16087 else if (Configuration.doAutoCreate()) 16088 this.prescription = new Reference(); // cc 16089 return this.prescription; 16090 } 16091 16092 public boolean hasPrescription() { 16093 return this.prescription != null && !this.prescription.isEmpty(); 16094 } 16095 16096 /** 16097 * @param value {@link #prescription} (Prescription to support the dispensing of 16098 * pharmacy, device or vision products.) 16099 */ 16100 public ExplanationOfBenefit setPrescription(Reference value) { 16101 this.prescription = value; 16102 return this; 16103 } 16104 16105 /** 16106 * @return {@link #prescription} The actual object that is the target of the 16107 * reference. The reference library doesn't populate this, but you can 16108 * use it to hold the resource if you resolve it. (Prescription to 16109 * support the dispensing of pharmacy, device or vision products.) 16110 */ 16111 public Resource getPrescriptionTarget() { 16112 return this.prescriptionTarget; 16113 } 16114 16115 /** 16116 * @param value {@link #prescription} The actual object that is the target of 16117 * the reference. The reference library doesn't use these, but you 16118 * can use it to hold the resource if you resolve it. (Prescription 16119 * to support the dispensing of pharmacy, device or vision 16120 * products.) 16121 */ 16122 public ExplanationOfBenefit setPrescriptionTarget(Resource value) { 16123 this.prescriptionTarget = value; 16124 return this; 16125 } 16126 16127 /** 16128 * @return {@link #originalPrescription} (Original prescription which has been 16129 * superseded by this prescription to support the dispensing of pharmacy 16130 * services, medications or products.) 16131 */ 16132 public Reference getOriginalPrescription() { 16133 if (this.originalPrescription == null) 16134 if (Configuration.errorOnAutoCreate()) 16135 throw new Error("Attempt to auto-create ExplanationOfBenefit.originalPrescription"); 16136 else if (Configuration.doAutoCreate()) 16137 this.originalPrescription = new Reference(); // cc 16138 return this.originalPrescription; 16139 } 16140 16141 public boolean hasOriginalPrescription() { 16142 return this.originalPrescription != null && !this.originalPrescription.isEmpty(); 16143 } 16144 16145 /** 16146 * @param value {@link #originalPrescription} (Original prescription which has 16147 * been superseded by this prescription to support the dispensing 16148 * of pharmacy services, medications or products.) 16149 */ 16150 public ExplanationOfBenefit setOriginalPrescription(Reference value) { 16151 this.originalPrescription = value; 16152 return this; 16153 } 16154 16155 /** 16156 * @return {@link #originalPrescription} The actual object that is the target of 16157 * the reference. The reference library doesn't populate this, but you 16158 * can use it to hold the resource if you resolve it. (Original 16159 * prescription which has been superseded by this prescription to 16160 * support the dispensing of pharmacy services, medications or 16161 * products.) 16162 */ 16163 public MedicationRequest getOriginalPrescriptionTarget() { 16164 if (this.originalPrescriptionTarget == null) 16165 if (Configuration.errorOnAutoCreate()) 16166 throw new Error("Attempt to auto-create ExplanationOfBenefit.originalPrescription"); 16167 else if (Configuration.doAutoCreate()) 16168 this.originalPrescriptionTarget = new MedicationRequest(); // aa 16169 return this.originalPrescriptionTarget; 16170 } 16171 16172 /** 16173 * @param value {@link #originalPrescription} The actual object that is the 16174 * target of the reference. The reference library doesn't use 16175 * these, but you can use it to hold the resource if you resolve 16176 * it. (Original prescription which has been superseded by this 16177 * prescription to support the dispensing of pharmacy services, 16178 * medications or products.) 16179 */ 16180 public ExplanationOfBenefit setOriginalPrescriptionTarget(MedicationRequest value) { 16181 this.originalPrescriptionTarget = value; 16182 return this; 16183 } 16184 16185 /** 16186 * @return {@link #payee} (The party to be reimbursed for cost of the products 16187 * and services according to the terms of the policy.) 16188 */ 16189 public PayeeComponent getPayee() { 16190 if (this.payee == null) 16191 if (Configuration.errorOnAutoCreate()) 16192 throw new Error("Attempt to auto-create ExplanationOfBenefit.payee"); 16193 else if (Configuration.doAutoCreate()) 16194 this.payee = new PayeeComponent(); // cc 16195 return this.payee; 16196 } 16197 16198 public boolean hasPayee() { 16199 return this.payee != null && !this.payee.isEmpty(); 16200 } 16201 16202 /** 16203 * @param value {@link #payee} (The party to be reimbursed for cost of the 16204 * products and services according to the terms of the policy.) 16205 */ 16206 public ExplanationOfBenefit setPayee(PayeeComponent value) { 16207 this.payee = value; 16208 return this; 16209 } 16210 16211 /** 16212 * @return {@link #referral} (A reference to a referral resource.) 16213 */ 16214 public Reference getReferral() { 16215 if (this.referral == null) 16216 if (Configuration.errorOnAutoCreate()) 16217 throw new Error("Attempt to auto-create ExplanationOfBenefit.referral"); 16218 else if (Configuration.doAutoCreate()) 16219 this.referral = new Reference(); // cc 16220 return this.referral; 16221 } 16222 16223 public boolean hasReferral() { 16224 return this.referral != null && !this.referral.isEmpty(); 16225 } 16226 16227 /** 16228 * @param value {@link #referral} (A reference to a referral resource.) 16229 */ 16230 public ExplanationOfBenefit setReferral(Reference value) { 16231 this.referral = value; 16232 return this; 16233 } 16234 16235 /** 16236 * @return {@link #referral} The actual object that is the target of the 16237 * reference. The reference library doesn't populate this, but you can 16238 * use it to hold the resource if you resolve it. (A reference to a 16239 * referral resource.) 16240 */ 16241 public ServiceRequest getReferralTarget() { 16242 if (this.referralTarget == null) 16243 if (Configuration.errorOnAutoCreate()) 16244 throw new Error("Attempt to auto-create ExplanationOfBenefit.referral"); 16245 else if (Configuration.doAutoCreate()) 16246 this.referralTarget = new ServiceRequest(); // aa 16247 return this.referralTarget; 16248 } 16249 16250 /** 16251 * @param value {@link #referral} The actual object that is the target of the 16252 * reference. The reference library doesn't use these, but you can 16253 * use it to hold the resource if you resolve it. (A reference to a 16254 * referral resource.) 16255 */ 16256 public ExplanationOfBenefit setReferralTarget(ServiceRequest value) { 16257 this.referralTarget = value; 16258 return this; 16259 } 16260 16261 /** 16262 * @return {@link #facility} (Facility where the services were provided.) 16263 */ 16264 public Reference getFacility() { 16265 if (this.facility == null) 16266 if (Configuration.errorOnAutoCreate()) 16267 throw new Error("Attempt to auto-create ExplanationOfBenefit.facility"); 16268 else if (Configuration.doAutoCreate()) 16269 this.facility = new Reference(); // cc 16270 return this.facility; 16271 } 16272 16273 public boolean hasFacility() { 16274 return this.facility != null && !this.facility.isEmpty(); 16275 } 16276 16277 /** 16278 * @param value {@link #facility} (Facility where the services were provided.) 16279 */ 16280 public ExplanationOfBenefit setFacility(Reference value) { 16281 this.facility = value; 16282 return this; 16283 } 16284 16285 /** 16286 * @return {@link #facility} The actual object that is the target of the 16287 * reference. The reference library doesn't populate this, but you can 16288 * use it to hold the resource if you resolve it. (Facility where the 16289 * services were provided.) 16290 */ 16291 public Location getFacilityTarget() { 16292 if (this.facilityTarget == null) 16293 if (Configuration.errorOnAutoCreate()) 16294 throw new Error("Attempt to auto-create ExplanationOfBenefit.facility"); 16295 else if (Configuration.doAutoCreate()) 16296 this.facilityTarget = new Location(); // aa 16297 return this.facilityTarget; 16298 } 16299 16300 /** 16301 * @param value {@link #facility} The actual object that is the target of the 16302 * reference. The reference library doesn't use these, but you can 16303 * use it to hold the resource if you resolve it. (Facility where 16304 * the services were provided.) 16305 */ 16306 public ExplanationOfBenefit setFacilityTarget(Location value) { 16307 this.facilityTarget = value; 16308 return this; 16309 } 16310 16311 /** 16312 * @return {@link #claim} (The business identifier for the instance of the 16313 * adjudication request: claim predetermination or preauthorization.) 16314 */ 16315 public Reference getClaim() { 16316 if (this.claim == null) 16317 if (Configuration.errorOnAutoCreate()) 16318 throw new Error("Attempt to auto-create ExplanationOfBenefit.claim"); 16319 else if (Configuration.doAutoCreate()) 16320 this.claim = new Reference(); // cc 16321 return this.claim; 16322 } 16323 16324 public boolean hasClaim() { 16325 return this.claim != null && !this.claim.isEmpty(); 16326 } 16327 16328 /** 16329 * @param value {@link #claim} (The business identifier for the instance of the 16330 * adjudication request: claim predetermination or 16331 * preauthorization.) 16332 */ 16333 public ExplanationOfBenefit setClaim(Reference value) { 16334 this.claim = value; 16335 return this; 16336 } 16337 16338 /** 16339 * @return {@link #claim} The actual object that is the target of the reference. 16340 * The reference library doesn't populate this, but you can use it to 16341 * hold the resource if you resolve it. (The business identifier for the 16342 * instance of the adjudication request: claim predetermination or 16343 * preauthorization.) 16344 */ 16345 public Claim getClaimTarget() { 16346 if (this.claimTarget == null) 16347 if (Configuration.errorOnAutoCreate()) 16348 throw new Error("Attempt to auto-create ExplanationOfBenefit.claim"); 16349 else if (Configuration.doAutoCreate()) 16350 this.claimTarget = new Claim(); // aa 16351 return this.claimTarget; 16352 } 16353 16354 /** 16355 * @param value {@link #claim} The actual object that is the target of the 16356 * reference. The reference library doesn't use these, but you can 16357 * use it to hold the resource if you resolve it. (The business 16358 * identifier for the instance of the adjudication request: claim 16359 * predetermination or preauthorization.) 16360 */ 16361 public ExplanationOfBenefit setClaimTarget(Claim value) { 16362 this.claimTarget = value; 16363 return this; 16364 } 16365 16366 /** 16367 * @return {@link #claimResponse} (The business identifier for the instance of 16368 * the adjudication response: claim, predetermination or 16369 * preauthorization response.) 16370 */ 16371 public Reference getClaimResponse() { 16372 if (this.claimResponse == null) 16373 if (Configuration.errorOnAutoCreate()) 16374 throw new Error("Attempt to auto-create ExplanationOfBenefit.claimResponse"); 16375 else if (Configuration.doAutoCreate()) 16376 this.claimResponse = new Reference(); // cc 16377 return this.claimResponse; 16378 } 16379 16380 public boolean hasClaimResponse() { 16381 return this.claimResponse != null && !this.claimResponse.isEmpty(); 16382 } 16383 16384 /** 16385 * @param value {@link #claimResponse} (The business identifier for the instance 16386 * of the adjudication response: claim, predetermination or 16387 * preauthorization response.) 16388 */ 16389 public ExplanationOfBenefit setClaimResponse(Reference value) { 16390 this.claimResponse = value; 16391 return this; 16392 } 16393 16394 /** 16395 * @return {@link #claimResponse} The actual object that is the target of the 16396 * reference. The reference library doesn't populate this, but you can 16397 * use it to hold the resource if you resolve it. (The business 16398 * identifier for the instance of the adjudication response: claim, 16399 * predetermination or preauthorization response.) 16400 */ 16401 public ClaimResponse getClaimResponseTarget() { 16402 if (this.claimResponseTarget == null) 16403 if (Configuration.errorOnAutoCreate()) 16404 throw new Error("Attempt to auto-create ExplanationOfBenefit.claimResponse"); 16405 else if (Configuration.doAutoCreate()) 16406 this.claimResponseTarget = new ClaimResponse(); // aa 16407 return this.claimResponseTarget; 16408 } 16409 16410 /** 16411 * @param value {@link #claimResponse} The actual object that is the target of 16412 * the reference. The reference library doesn't use these, but you 16413 * can use it to hold the resource if you resolve it. (The business 16414 * identifier for the instance of the adjudication response: claim, 16415 * predetermination or preauthorization response.) 16416 */ 16417 public ExplanationOfBenefit setClaimResponseTarget(ClaimResponse value) { 16418 this.claimResponseTarget = value; 16419 return this; 16420 } 16421 16422 /** 16423 * @return {@link #outcome} (The outcome of the claim, predetermination, or 16424 * preauthorization processing.). This is the underlying object with id, 16425 * value and extensions. The accessor "getOutcome" gives direct access 16426 * to the value 16427 */ 16428 public Enumeration<RemittanceOutcome> getOutcomeElement() { 16429 if (this.outcome == null) 16430 if (Configuration.errorOnAutoCreate()) 16431 throw new Error("Attempt to auto-create ExplanationOfBenefit.outcome"); 16432 else if (Configuration.doAutoCreate()) 16433 this.outcome = new Enumeration<RemittanceOutcome>(new RemittanceOutcomeEnumFactory()); // bb 16434 return this.outcome; 16435 } 16436 16437 public boolean hasOutcomeElement() { 16438 return this.outcome != null && !this.outcome.isEmpty(); 16439 } 16440 16441 public boolean hasOutcome() { 16442 return this.outcome != null && !this.outcome.isEmpty(); 16443 } 16444 16445 /** 16446 * @param value {@link #outcome} (The outcome of the claim, predetermination, or 16447 * preauthorization processing.). This is the underlying object 16448 * with id, value and extensions. The accessor "getOutcome" gives 16449 * direct access to the value 16450 */ 16451 public ExplanationOfBenefit setOutcomeElement(Enumeration<RemittanceOutcome> value) { 16452 this.outcome = value; 16453 return this; 16454 } 16455 16456 /** 16457 * @return The outcome of the claim, predetermination, or preauthorization 16458 * processing. 16459 */ 16460 public RemittanceOutcome getOutcome() { 16461 return this.outcome == null ? null : this.outcome.getValue(); 16462 } 16463 16464 /** 16465 * @param value The outcome of the claim, predetermination, or preauthorization 16466 * processing. 16467 */ 16468 public ExplanationOfBenefit setOutcome(RemittanceOutcome value) { 16469 if (this.outcome == null) 16470 this.outcome = new Enumeration<RemittanceOutcome>(new RemittanceOutcomeEnumFactory()); 16471 this.outcome.setValue(value); 16472 return this; 16473 } 16474 16475 /** 16476 * @return {@link #disposition} (A human readable description of the status of 16477 * the adjudication.). This is the underlying object with id, value and 16478 * extensions. The accessor "getDisposition" gives direct access to the 16479 * value 16480 */ 16481 public StringType getDispositionElement() { 16482 if (this.disposition == null) 16483 if (Configuration.errorOnAutoCreate()) 16484 throw new Error("Attempt to auto-create ExplanationOfBenefit.disposition"); 16485 else if (Configuration.doAutoCreate()) 16486 this.disposition = new StringType(); // bb 16487 return this.disposition; 16488 } 16489 16490 public boolean hasDispositionElement() { 16491 return this.disposition != null && !this.disposition.isEmpty(); 16492 } 16493 16494 public boolean hasDisposition() { 16495 return this.disposition != null && !this.disposition.isEmpty(); 16496 } 16497 16498 /** 16499 * @param value {@link #disposition} (A human readable description of the status 16500 * of the adjudication.). This is the underlying object with id, 16501 * value and extensions. The accessor "getDisposition" gives direct 16502 * access to the value 16503 */ 16504 public ExplanationOfBenefit setDispositionElement(StringType value) { 16505 this.disposition = value; 16506 return this; 16507 } 16508 16509 /** 16510 * @return A human readable description of the status of the adjudication. 16511 */ 16512 public String getDisposition() { 16513 return this.disposition == null ? null : this.disposition.getValue(); 16514 } 16515 16516 /** 16517 * @param value A human readable description of the status of the adjudication. 16518 */ 16519 public ExplanationOfBenefit setDisposition(String value) { 16520 if (Utilities.noString(value)) 16521 this.disposition = null; 16522 else { 16523 if (this.disposition == null) 16524 this.disposition = new StringType(); 16525 this.disposition.setValue(value); 16526 } 16527 return this; 16528 } 16529 16530 /** 16531 * @return {@link #preAuthRef} (Reference from the Insurer which is used in 16532 * later communications which refers to this adjudication.) 16533 */ 16534 public List<StringType> getPreAuthRef() { 16535 if (this.preAuthRef == null) 16536 this.preAuthRef = new ArrayList<StringType>(); 16537 return this.preAuthRef; 16538 } 16539 16540 /** 16541 * @return Returns a reference to <code>this</code> for easy method chaining 16542 */ 16543 public ExplanationOfBenefit setPreAuthRef(List<StringType> thePreAuthRef) { 16544 this.preAuthRef = thePreAuthRef; 16545 return this; 16546 } 16547 16548 public boolean hasPreAuthRef() { 16549 if (this.preAuthRef == null) 16550 return false; 16551 for (StringType item : this.preAuthRef) 16552 if (!item.isEmpty()) 16553 return true; 16554 return false; 16555 } 16556 16557 /** 16558 * @return {@link #preAuthRef} (Reference from the Insurer which is used in 16559 * later communications which refers to this adjudication.) 16560 */ 16561 public StringType addPreAuthRefElement() {// 2 16562 StringType t = new StringType(); 16563 if (this.preAuthRef == null) 16564 this.preAuthRef = new ArrayList<StringType>(); 16565 this.preAuthRef.add(t); 16566 return t; 16567 } 16568 16569 /** 16570 * @param value {@link #preAuthRef} (Reference from the Insurer which is used in 16571 * later communications which refers to this adjudication.) 16572 */ 16573 public ExplanationOfBenefit addPreAuthRef(String value) { // 1 16574 StringType t = new StringType(); 16575 t.setValue(value); 16576 if (this.preAuthRef == null) 16577 this.preAuthRef = new ArrayList<StringType>(); 16578 this.preAuthRef.add(t); 16579 return this; 16580 } 16581 16582 /** 16583 * @param value {@link #preAuthRef} (Reference from the Insurer which is used in 16584 * later communications which refers to this adjudication.) 16585 */ 16586 public boolean hasPreAuthRef(String value) { 16587 if (this.preAuthRef == null) 16588 return false; 16589 for (StringType v : this.preAuthRef) 16590 if (v.getValue().equals(value)) // string 16591 return true; 16592 return false; 16593 } 16594 16595 /** 16596 * @return {@link #preAuthRefPeriod} (The timeframe during which the supplied 16597 * preauthorization reference may be quoted on claims to obtain the 16598 * adjudication as provided.) 16599 */ 16600 public List<Period> getPreAuthRefPeriod() { 16601 if (this.preAuthRefPeriod == null) 16602 this.preAuthRefPeriod = new ArrayList<Period>(); 16603 return this.preAuthRefPeriod; 16604 } 16605 16606 /** 16607 * @return Returns a reference to <code>this</code> for easy method chaining 16608 */ 16609 public ExplanationOfBenefit setPreAuthRefPeriod(List<Period> thePreAuthRefPeriod) { 16610 this.preAuthRefPeriod = thePreAuthRefPeriod; 16611 return this; 16612 } 16613 16614 public boolean hasPreAuthRefPeriod() { 16615 if (this.preAuthRefPeriod == null) 16616 return false; 16617 for (Period item : this.preAuthRefPeriod) 16618 if (!item.isEmpty()) 16619 return true; 16620 return false; 16621 } 16622 16623 public Period addPreAuthRefPeriod() { // 3 16624 Period t = new Period(); 16625 if (this.preAuthRefPeriod == null) 16626 this.preAuthRefPeriod = new ArrayList<Period>(); 16627 this.preAuthRefPeriod.add(t); 16628 return t; 16629 } 16630 16631 public ExplanationOfBenefit addPreAuthRefPeriod(Period t) { // 3 16632 if (t == null) 16633 return this; 16634 if (this.preAuthRefPeriod == null) 16635 this.preAuthRefPeriod = new ArrayList<Period>(); 16636 this.preAuthRefPeriod.add(t); 16637 return this; 16638 } 16639 16640 /** 16641 * @return The first repetition of repeating field {@link #preAuthRefPeriod}, 16642 * creating it if it does not already exist 16643 */ 16644 public Period getPreAuthRefPeriodFirstRep() { 16645 if (getPreAuthRefPeriod().isEmpty()) { 16646 addPreAuthRefPeriod(); 16647 } 16648 return getPreAuthRefPeriod().get(0); 16649 } 16650 16651 /** 16652 * @return {@link #careTeam} (The members of the team who provided the products 16653 * and services.) 16654 */ 16655 public List<CareTeamComponent> getCareTeam() { 16656 if (this.careTeam == null) 16657 this.careTeam = new ArrayList<CareTeamComponent>(); 16658 return this.careTeam; 16659 } 16660 16661 /** 16662 * @return Returns a reference to <code>this</code> for easy method chaining 16663 */ 16664 public ExplanationOfBenefit setCareTeam(List<CareTeamComponent> theCareTeam) { 16665 this.careTeam = theCareTeam; 16666 return this; 16667 } 16668 16669 public boolean hasCareTeam() { 16670 if (this.careTeam == null) 16671 return false; 16672 for (CareTeamComponent item : this.careTeam) 16673 if (!item.isEmpty()) 16674 return true; 16675 return false; 16676 } 16677 16678 public CareTeamComponent addCareTeam() { // 3 16679 CareTeamComponent t = new CareTeamComponent(); 16680 if (this.careTeam == null) 16681 this.careTeam = new ArrayList<CareTeamComponent>(); 16682 this.careTeam.add(t); 16683 return t; 16684 } 16685 16686 public ExplanationOfBenefit addCareTeam(CareTeamComponent t) { // 3 16687 if (t == null) 16688 return this; 16689 if (this.careTeam == null) 16690 this.careTeam = new ArrayList<CareTeamComponent>(); 16691 this.careTeam.add(t); 16692 return this; 16693 } 16694 16695 /** 16696 * @return The first repetition of repeating field {@link #careTeam}, creating 16697 * it if it does not already exist 16698 */ 16699 public CareTeamComponent getCareTeamFirstRep() { 16700 if (getCareTeam().isEmpty()) { 16701 addCareTeam(); 16702 } 16703 return getCareTeam().get(0); 16704 } 16705 16706 /** 16707 * @return {@link #supportingInfo} (Additional information codes regarding 16708 * exceptions, special considerations, the condition, situation, prior 16709 * or concurrent issues.) 16710 */ 16711 public List<SupportingInformationComponent> getSupportingInfo() { 16712 if (this.supportingInfo == null) 16713 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 16714 return this.supportingInfo; 16715 } 16716 16717 /** 16718 * @return Returns a reference to <code>this</code> for easy method chaining 16719 */ 16720 public ExplanationOfBenefit setSupportingInfo(List<SupportingInformationComponent> theSupportingInfo) { 16721 this.supportingInfo = theSupportingInfo; 16722 return this; 16723 } 16724 16725 public boolean hasSupportingInfo() { 16726 if (this.supportingInfo == null) 16727 return false; 16728 for (SupportingInformationComponent item : this.supportingInfo) 16729 if (!item.isEmpty()) 16730 return true; 16731 return false; 16732 } 16733 16734 public SupportingInformationComponent addSupportingInfo() { // 3 16735 SupportingInformationComponent t = new SupportingInformationComponent(); 16736 if (this.supportingInfo == null) 16737 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 16738 this.supportingInfo.add(t); 16739 return t; 16740 } 16741 16742 public ExplanationOfBenefit addSupportingInfo(SupportingInformationComponent t) { // 3 16743 if (t == null) 16744 return this; 16745 if (this.supportingInfo == null) 16746 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 16747 this.supportingInfo.add(t); 16748 return this; 16749 } 16750 16751 /** 16752 * @return The first repetition of repeating field {@link #supportingInfo}, 16753 * creating it if it does not already exist 16754 */ 16755 public SupportingInformationComponent getSupportingInfoFirstRep() { 16756 if (getSupportingInfo().isEmpty()) { 16757 addSupportingInfo(); 16758 } 16759 return getSupportingInfo().get(0); 16760 } 16761 16762 /** 16763 * @return {@link #diagnosis} (Information about diagnoses relevant to the claim 16764 * items.) 16765 */ 16766 public List<DiagnosisComponent> getDiagnosis() { 16767 if (this.diagnosis == null) 16768 this.diagnosis = new ArrayList<DiagnosisComponent>(); 16769 return this.diagnosis; 16770 } 16771 16772 /** 16773 * @return Returns a reference to <code>this</code> for easy method chaining 16774 */ 16775 public ExplanationOfBenefit setDiagnosis(List<DiagnosisComponent> theDiagnosis) { 16776 this.diagnosis = theDiagnosis; 16777 return this; 16778 } 16779 16780 public boolean hasDiagnosis() { 16781 if (this.diagnosis == null) 16782 return false; 16783 for (DiagnosisComponent item : this.diagnosis) 16784 if (!item.isEmpty()) 16785 return true; 16786 return false; 16787 } 16788 16789 public DiagnosisComponent addDiagnosis() { // 3 16790 DiagnosisComponent t = new DiagnosisComponent(); 16791 if (this.diagnosis == null) 16792 this.diagnosis = new ArrayList<DiagnosisComponent>(); 16793 this.diagnosis.add(t); 16794 return t; 16795 } 16796 16797 public ExplanationOfBenefit addDiagnosis(DiagnosisComponent t) { // 3 16798 if (t == null) 16799 return this; 16800 if (this.diagnosis == null) 16801 this.diagnosis = new ArrayList<DiagnosisComponent>(); 16802 this.diagnosis.add(t); 16803 return this; 16804 } 16805 16806 /** 16807 * @return The first repetition of repeating field {@link #diagnosis}, creating 16808 * it if it does not already exist 16809 */ 16810 public DiagnosisComponent getDiagnosisFirstRep() { 16811 if (getDiagnosis().isEmpty()) { 16812 addDiagnosis(); 16813 } 16814 return getDiagnosis().get(0); 16815 } 16816 16817 /** 16818 * @return {@link #procedure} (Procedures performed on the patient relevant to 16819 * the billing items with the claim.) 16820 */ 16821 public List<ProcedureComponent> getProcedure() { 16822 if (this.procedure == null) 16823 this.procedure = new ArrayList<ProcedureComponent>(); 16824 return this.procedure; 16825 } 16826 16827 /** 16828 * @return Returns a reference to <code>this</code> for easy method chaining 16829 */ 16830 public ExplanationOfBenefit setProcedure(List<ProcedureComponent> theProcedure) { 16831 this.procedure = theProcedure; 16832 return this; 16833 } 16834 16835 public boolean hasProcedure() { 16836 if (this.procedure == null) 16837 return false; 16838 for (ProcedureComponent item : this.procedure) 16839 if (!item.isEmpty()) 16840 return true; 16841 return false; 16842 } 16843 16844 public ProcedureComponent addProcedure() { // 3 16845 ProcedureComponent t = new ProcedureComponent(); 16846 if (this.procedure == null) 16847 this.procedure = new ArrayList<ProcedureComponent>(); 16848 this.procedure.add(t); 16849 return t; 16850 } 16851 16852 public ExplanationOfBenefit addProcedure(ProcedureComponent t) { // 3 16853 if (t == null) 16854 return this; 16855 if (this.procedure == null) 16856 this.procedure = new ArrayList<ProcedureComponent>(); 16857 this.procedure.add(t); 16858 return this; 16859 } 16860 16861 /** 16862 * @return The first repetition of repeating field {@link #procedure}, creating 16863 * it if it does not already exist 16864 */ 16865 public ProcedureComponent getProcedureFirstRep() { 16866 if (getProcedure().isEmpty()) { 16867 addProcedure(); 16868 } 16869 return getProcedure().get(0); 16870 } 16871 16872 /** 16873 * @return {@link #precedence} (This indicates the relative order of a series of 16874 * EOBs related to different coverages for the same suite of services.). 16875 * This is the underlying object with id, value and extensions. The 16876 * accessor "getPrecedence" gives direct access to the value 16877 */ 16878 public PositiveIntType getPrecedenceElement() { 16879 if (this.precedence == null) 16880 if (Configuration.errorOnAutoCreate()) 16881 throw new Error("Attempt to auto-create ExplanationOfBenefit.precedence"); 16882 else if (Configuration.doAutoCreate()) 16883 this.precedence = new PositiveIntType(); // bb 16884 return this.precedence; 16885 } 16886 16887 public boolean hasPrecedenceElement() { 16888 return this.precedence != null && !this.precedence.isEmpty(); 16889 } 16890 16891 public boolean hasPrecedence() { 16892 return this.precedence != null && !this.precedence.isEmpty(); 16893 } 16894 16895 /** 16896 * @param value {@link #precedence} (This indicates the relative order of a 16897 * series of EOBs related to different coverages for the same suite 16898 * of services.). This is the underlying object with id, value and 16899 * extensions. The accessor "getPrecedence" gives direct access to 16900 * the value 16901 */ 16902 public ExplanationOfBenefit setPrecedenceElement(PositiveIntType value) { 16903 this.precedence = value; 16904 return this; 16905 } 16906 16907 /** 16908 * @return This indicates the relative order of a series of EOBs related to 16909 * different coverages for the same suite of services. 16910 */ 16911 public int getPrecedence() { 16912 return this.precedence == null || this.precedence.isEmpty() ? 0 : this.precedence.getValue(); 16913 } 16914 16915 /** 16916 * @param value This indicates the relative order of a series of EOBs related to 16917 * different coverages for the same suite of services. 16918 */ 16919 public ExplanationOfBenefit setPrecedence(int value) { 16920 if (this.precedence == null) 16921 this.precedence = new PositiveIntType(); 16922 this.precedence.setValue(value); 16923 return this; 16924 } 16925 16926 /** 16927 * @return {@link #insurance} (Financial instruments for reimbursement for the 16928 * health care products and services specified on the claim.) 16929 */ 16930 public List<InsuranceComponent> getInsurance() { 16931 if (this.insurance == null) 16932 this.insurance = new ArrayList<InsuranceComponent>(); 16933 return this.insurance; 16934 } 16935 16936 /** 16937 * @return Returns a reference to <code>this</code> for easy method chaining 16938 */ 16939 public ExplanationOfBenefit setInsurance(List<InsuranceComponent> theInsurance) { 16940 this.insurance = theInsurance; 16941 return this; 16942 } 16943 16944 public boolean hasInsurance() { 16945 if (this.insurance == null) 16946 return false; 16947 for (InsuranceComponent item : this.insurance) 16948 if (!item.isEmpty()) 16949 return true; 16950 return false; 16951 } 16952 16953 public InsuranceComponent addInsurance() { // 3 16954 InsuranceComponent t = new InsuranceComponent(); 16955 if (this.insurance == null) 16956 this.insurance = new ArrayList<InsuranceComponent>(); 16957 this.insurance.add(t); 16958 return t; 16959 } 16960 16961 public ExplanationOfBenefit addInsurance(InsuranceComponent t) { // 3 16962 if (t == null) 16963 return this; 16964 if (this.insurance == null) 16965 this.insurance = new ArrayList<InsuranceComponent>(); 16966 this.insurance.add(t); 16967 return this; 16968 } 16969 16970 /** 16971 * @return The first repetition of repeating field {@link #insurance}, creating 16972 * it if it does not already exist 16973 */ 16974 public InsuranceComponent getInsuranceFirstRep() { 16975 if (getInsurance().isEmpty()) { 16976 addInsurance(); 16977 } 16978 return getInsurance().get(0); 16979 } 16980 16981 /** 16982 * @return {@link #accident} (Details of a accident which resulted in injuries 16983 * which required the products and services listed in the claim.) 16984 */ 16985 public AccidentComponent getAccident() { 16986 if (this.accident == null) 16987 if (Configuration.errorOnAutoCreate()) 16988 throw new Error("Attempt to auto-create ExplanationOfBenefit.accident"); 16989 else if (Configuration.doAutoCreate()) 16990 this.accident = new AccidentComponent(); // cc 16991 return this.accident; 16992 } 16993 16994 public boolean hasAccident() { 16995 return this.accident != null && !this.accident.isEmpty(); 16996 } 16997 16998 /** 16999 * @param value {@link #accident} (Details of a accident which resulted in 17000 * injuries which required the products and services listed in the 17001 * claim.) 17002 */ 17003 public ExplanationOfBenefit setAccident(AccidentComponent value) { 17004 this.accident = value; 17005 return this; 17006 } 17007 17008 /** 17009 * @return {@link #item} (A claim line. Either a simple (a product or service) 17010 * or a 'group' of details which can also be a simple items or groups of 17011 * sub-details.) 17012 */ 17013 public List<ItemComponent> getItem() { 17014 if (this.item == null) 17015 this.item = new ArrayList<ItemComponent>(); 17016 return this.item; 17017 } 17018 17019 /** 17020 * @return Returns a reference to <code>this</code> for easy method chaining 17021 */ 17022 public ExplanationOfBenefit setItem(List<ItemComponent> theItem) { 17023 this.item = theItem; 17024 return this; 17025 } 17026 17027 public boolean hasItem() { 17028 if (this.item == null) 17029 return false; 17030 for (ItemComponent item : this.item) 17031 if (!item.isEmpty()) 17032 return true; 17033 return false; 17034 } 17035 17036 public ItemComponent addItem() { // 3 17037 ItemComponent t = new ItemComponent(); 17038 if (this.item == null) 17039 this.item = new ArrayList<ItemComponent>(); 17040 this.item.add(t); 17041 return t; 17042 } 17043 17044 public ExplanationOfBenefit addItem(ItemComponent t) { // 3 17045 if (t == null) 17046 return this; 17047 if (this.item == null) 17048 this.item = new ArrayList<ItemComponent>(); 17049 this.item.add(t); 17050 return this; 17051 } 17052 17053 /** 17054 * @return The first repetition of repeating field {@link #item}, creating it if 17055 * it does not already exist 17056 */ 17057 public ItemComponent getItemFirstRep() { 17058 if (getItem().isEmpty()) { 17059 addItem(); 17060 } 17061 return getItem().get(0); 17062 } 17063 17064 /** 17065 * @return {@link #addItem} (The first-tier service adjudications for payor 17066 * added product or service lines.) 17067 */ 17068 public List<AddedItemComponent> getAddItem() { 17069 if (this.addItem == null) 17070 this.addItem = new ArrayList<AddedItemComponent>(); 17071 return this.addItem; 17072 } 17073 17074 /** 17075 * @return Returns a reference to <code>this</code> for easy method chaining 17076 */ 17077 public ExplanationOfBenefit setAddItem(List<AddedItemComponent> theAddItem) { 17078 this.addItem = theAddItem; 17079 return this; 17080 } 17081 17082 public boolean hasAddItem() { 17083 if (this.addItem == null) 17084 return false; 17085 for (AddedItemComponent item : this.addItem) 17086 if (!item.isEmpty()) 17087 return true; 17088 return false; 17089 } 17090 17091 public AddedItemComponent addAddItem() { // 3 17092 AddedItemComponent t = new AddedItemComponent(); 17093 if (this.addItem == null) 17094 this.addItem = new ArrayList<AddedItemComponent>(); 17095 this.addItem.add(t); 17096 return t; 17097 } 17098 17099 public ExplanationOfBenefit addAddItem(AddedItemComponent t) { // 3 17100 if (t == null) 17101 return this; 17102 if (this.addItem == null) 17103 this.addItem = new ArrayList<AddedItemComponent>(); 17104 this.addItem.add(t); 17105 return this; 17106 } 17107 17108 /** 17109 * @return The first repetition of repeating field {@link #addItem}, creating it 17110 * if it does not already exist 17111 */ 17112 public AddedItemComponent getAddItemFirstRep() { 17113 if (getAddItem().isEmpty()) { 17114 addAddItem(); 17115 } 17116 return getAddItem().get(0); 17117 } 17118 17119 /** 17120 * @return {@link #adjudication} (The adjudication results which are presented 17121 * at the header level rather than at the line-item or add-item levels.) 17122 */ 17123 public List<AdjudicationComponent> getAdjudication() { 17124 if (this.adjudication == null) 17125 this.adjudication = new ArrayList<AdjudicationComponent>(); 17126 return this.adjudication; 17127 } 17128 17129 /** 17130 * @return Returns a reference to <code>this</code> for easy method chaining 17131 */ 17132 public ExplanationOfBenefit setAdjudication(List<AdjudicationComponent> theAdjudication) { 17133 this.adjudication = theAdjudication; 17134 return this; 17135 } 17136 17137 public boolean hasAdjudication() { 17138 if (this.adjudication == null) 17139 return false; 17140 for (AdjudicationComponent item : this.adjudication) 17141 if (!item.isEmpty()) 17142 return true; 17143 return false; 17144 } 17145 17146 public AdjudicationComponent addAdjudication() { // 3 17147 AdjudicationComponent t = new AdjudicationComponent(); 17148 if (this.adjudication == null) 17149 this.adjudication = new ArrayList<AdjudicationComponent>(); 17150 this.adjudication.add(t); 17151 return t; 17152 } 17153 17154 public ExplanationOfBenefit addAdjudication(AdjudicationComponent t) { // 3 17155 if (t == null) 17156 return this; 17157 if (this.adjudication == null) 17158 this.adjudication = new ArrayList<AdjudicationComponent>(); 17159 this.adjudication.add(t); 17160 return this; 17161 } 17162 17163 /** 17164 * @return The first repetition of repeating field {@link #adjudication}, 17165 * creating it if it does not already exist 17166 */ 17167 public AdjudicationComponent getAdjudicationFirstRep() { 17168 if (getAdjudication().isEmpty()) { 17169 addAdjudication(); 17170 } 17171 return getAdjudication().get(0); 17172 } 17173 17174 /** 17175 * @return {@link #total} (Categorized monetary totals for the adjudication.) 17176 */ 17177 public List<TotalComponent> getTotal() { 17178 if (this.total == null) 17179 this.total = new ArrayList<TotalComponent>(); 17180 return this.total; 17181 } 17182 17183 /** 17184 * @return Returns a reference to <code>this</code> for easy method chaining 17185 */ 17186 public ExplanationOfBenefit setTotal(List<TotalComponent> theTotal) { 17187 this.total = theTotal; 17188 return this; 17189 } 17190 17191 public boolean hasTotal() { 17192 if (this.total == null) 17193 return false; 17194 for (TotalComponent item : this.total) 17195 if (!item.isEmpty()) 17196 return true; 17197 return false; 17198 } 17199 17200 public TotalComponent addTotal() { // 3 17201 TotalComponent t = new TotalComponent(); 17202 if (this.total == null) 17203 this.total = new ArrayList<TotalComponent>(); 17204 this.total.add(t); 17205 return t; 17206 } 17207 17208 public ExplanationOfBenefit addTotal(TotalComponent t) { // 3 17209 if (t == null) 17210 return this; 17211 if (this.total == null) 17212 this.total = new ArrayList<TotalComponent>(); 17213 this.total.add(t); 17214 return this; 17215 } 17216 17217 /** 17218 * @return The first repetition of repeating field {@link #total}, creating it 17219 * if it does not already exist 17220 */ 17221 public TotalComponent getTotalFirstRep() { 17222 if (getTotal().isEmpty()) { 17223 addTotal(); 17224 } 17225 return getTotal().get(0); 17226 } 17227 17228 /** 17229 * @return {@link #payment} (Payment details for the adjudication of the claim.) 17230 */ 17231 public PaymentComponent getPayment() { 17232 if (this.payment == null) 17233 if (Configuration.errorOnAutoCreate()) 17234 throw new Error("Attempt to auto-create ExplanationOfBenefit.payment"); 17235 else if (Configuration.doAutoCreate()) 17236 this.payment = new PaymentComponent(); // cc 17237 return this.payment; 17238 } 17239 17240 public boolean hasPayment() { 17241 return this.payment != null && !this.payment.isEmpty(); 17242 } 17243 17244 /** 17245 * @param value {@link #payment} (Payment details for the adjudication of the 17246 * claim.) 17247 */ 17248 public ExplanationOfBenefit setPayment(PaymentComponent value) { 17249 this.payment = value; 17250 return this; 17251 } 17252 17253 /** 17254 * @return {@link #formCode} (A code for the form to be used for printing the 17255 * content.) 17256 */ 17257 public CodeableConcept getFormCode() { 17258 if (this.formCode == null) 17259 if (Configuration.errorOnAutoCreate()) 17260 throw new Error("Attempt to auto-create ExplanationOfBenefit.formCode"); 17261 else if (Configuration.doAutoCreate()) 17262 this.formCode = new CodeableConcept(); // cc 17263 return this.formCode; 17264 } 17265 17266 public boolean hasFormCode() { 17267 return this.formCode != null && !this.formCode.isEmpty(); 17268 } 17269 17270 /** 17271 * @param value {@link #formCode} (A code for the form to be used for printing 17272 * the content.) 17273 */ 17274 public ExplanationOfBenefit setFormCode(CodeableConcept value) { 17275 this.formCode = value; 17276 return this; 17277 } 17278 17279 /** 17280 * @return {@link #form} (The actual form, by reference or inclusion, for 17281 * printing the content or an EOB.) 17282 */ 17283 public Attachment getForm() { 17284 if (this.form == null) 17285 if (Configuration.errorOnAutoCreate()) 17286 throw new Error("Attempt to auto-create ExplanationOfBenefit.form"); 17287 else if (Configuration.doAutoCreate()) 17288 this.form = new Attachment(); // cc 17289 return this.form; 17290 } 17291 17292 public boolean hasForm() { 17293 return this.form != null && !this.form.isEmpty(); 17294 } 17295 17296 /** 17297 * @param value {@link #form} (The actual form, by reference or inclusion, for 17298 * printing the content or an EOB.) 17299 */ 17300 public ExplanationOfBenefit setForm(Attachment value) { 17301 this.form = value; 17302 return this; 17303 } 17304 17305 /** 17306 * @return {@link #processNote} (A note that describes or explains adjudication 17307 * results in a human readable form.) 17308 */ 17309 public List<NoteComponent> getProcessNote() { 17310 if (this.processNote == null) 17311 this.processNote = new ArrayList<NoteComponent>(); 17312 return this.processNote; 17313 } 17314 17315 /** 17316 * @return Returns a reference to <code>this</code> for easy method chaining 17317 */ 17318 public ExplanationOfBenefit setProcessNote(List<NoteComponent> theProcessNote) { 17319 this.processNote = theProcessNote; 17320 return this; 17321 } 17322 17323 public boolean hasProcessNote() { 17324 if (this.processNote == null) 17325 return false; 17326 for (NoteComponent item : this.processNote) 17327 if (!item.isEmpty()) 17328 return true; 17329 return false; 17330 } 17331 17332 public NoteComponent addProcessNote() { // 3 17333 NoteComponent t = new NoteComponent(); 17334 if (this.processNote == null) 17335 this.processNote = new ArrayList<NoteComponent>(); 17336 this.processNote.add(t); 17337 return t; 17338 } 17339 17340 public ExplanationOfBenefit addProcessNote(NoteComponent t) { // 3 17341 if (t == null) 17342 return this; 17343 if (this.processNote == null) 17344 this.processNote = new ArrayList<NoteComponent>(); 17345 this.processNote.add(t); 17346 return this; 17347 } 17348 17349 /** 17350 * @return The first repetition of repeating field {@link #processNote}, 17351 * creating it if it does not already exist 17352 */ 17353 public NoteComponent getProcessNoteFirstRep() { 17354 if (getProcessNote().isEmpty()) { 17355 addProcessNote(); 17356 } 17357 return getProcessNote().get(0); 17358 } 17359 17360 /** 17361 * @return {@link #benefitPeriod} (The term of the benefits documented in this 17362 * response.) 17363 */ 17364 public Period getBenefitPeriod() { 17365 if (this.benefitPeriod == null) 17366 if (Configuration.errorOnAutoCreate()) 17367 throw new Error("Attempt to auto-create ExplanationOfBenefit.benefitPeriod"); 17368 else if (Configuration.doAutoCreate()) 17369 this.benefitPeriod = new Period(); // cc 17370 return this.benefitPeriod; 17371 } 17372 17373 public boolean hasBenefitPeriod() { 17374 return this.benefitPeriod != null && !this.benefitPeriod.isEmpty(); 17375 } 17376 17377 /** 17378 * @param value {@link #benefitPeriod} (The term of the benefits documented in 17379 * this response.) 17380 */ 17381 public ExplanationOfBenefit setBenefitPeriod(Period value) { 17382 this.benefitPeriod = value; 17383 return this; 17384 } 17385 17386 /** 17387 * @return {@link #benefitBalance} (Balance by Benefit Category.) 17388 */ 17389 public List<BenefitBalanceComponent> getBenefitBalance() { 17390 if (this.benefitBalance == null) 17391 this.benefitBalance = new ArrayList<BenefitBalanceComponent>(); 17392 return this.benefitBalance; 17393 } 17394 17395 /** 17396 * @return Returns a reference to <code>this</code> for easy method chaining 17397 */ 17398 public ExplanationOfBenefit setBenefitBalance(List<BenefitBalanceComponent> theBenefitBalance) { 17399 this.benefitBalance = theBenefitBalance; 17400 return this; 17401 } 17402 17403 public boolean hasBenefitBalance() { 17404 if (this.benefitBalance == null) 17405 return false; 17406 for (BenefitBalanceComponent item : this.benefitBalance) 17407 if (!item.isEmpty()) 17408 return true; 17409 return false; 17410 } 17411 17412 public BenefitBalanceComponent addBenefitBalance() { // 3 17413 BenefitBalanceComponent t = new BenefitBalanceComponent(); 17414 if (this.benefitBalance == null) 17415 this.benefitBalance = new ArrayList<BenefitBalanceComponent>(); 17416 this.benefitBalance.add(t); 17417 return t; 17418 } 17419 17420 public ExplanationOfBenefit addBenefitBalance(BenefitBalanceComponent t) { // 3 17421 if (t == null) 17422 return this; 17423 if (this.benefitBalance == null) 17424 this.benefitBalance = new ArrayList<BenefitBalanceComponent>(); 17425 this.benefitBalance.add(t); 17426 return this; 17427 } 17428 17429 /** 17430 * @return The first repetition of repeating field {@link #benefitBalance}, 17431 * creating it if it does not already exist 17432 */ 17433 public BenefitBalanceComponent getBenefitBalanceFirstRep() { 17434 if (getBenefitBalance().isEmpty()) { 17435 addBenefitBalance(); 17436 } 17437 return getBenefitBalance().get(0); 17438 } 17439 17440 protected void listChildren(List<Property> children) { 17441 super.listChildren(children); 17442 children.add(new Property("identifier", "Identifier", 17443 "A unique identifier assigned to this explanation of benefit.", 0, java.lang.Integer.MAX_VALUE, identifier)); 17444 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 17445 children.add(new Property("type", "CodeableConcept", 17446 "The category of claim, e.g. oral, pharmacy, vision, institutional, professional.", 0, 1, type)); 17447 children.add(new Property("subType", "CodeableConcept", 17448 "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.", 17449 0, 1, subType)); 17450 children.add(new Property("use", "code", 17451 "A code to indicate whether the nature of the request is: to request adjudication of products and services previously rendered; or requesting authorization and adjudication for provision in the future; or requesting the non-binding adjudication of the listed products and services which could be provided in the future.", 17452 0, 1, use)); 17453 children.add(new Property("patient", "Reference(Patient)", 17454 "The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for forecast reimbursement is sought.", 17455 0, 1, patient)); 17456 children.add(new Property("billablePeriod", "Period", "The period for which charges are being submitted.", 0, 1, 17457 billablePeriod)); 17458 children.add(new Property("created", "dateTime", "The date this resource was created.", 0, 1, created)); 17459 children.add(new Property("enterer", "Reference(Practitioner|PractitionerRole)", 17460 "Individual who created the claim, predetermination or preauthorization.", 0, 1, enterer)); 17461 children.add(new Property("insurer", "Reference(Organization)", 17462 "The party responsible for authorization, adjudication and reimbursement.", 0, 1, insurer)); 17463 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", 17464 "The provider which is responsible for the claim, predetermination or preauthorization.", 0, 1, provider)); 17465 children.add(new Property("priority", "CodeableConcept", 17466 "The provider-required urgency of processing the request. Typical values include: stat, routine deferred.", 0, 17467 1, priority)); 17468 children.add(new Property("fundsReserveRequested", "CodeableConcept", 17469 "A code to indicate whether and for whom funds are to be reserved for future claims.", 0, 1, 17470 fundsReserveRequested)); 17471 children.add(new Property("fundsReserve", "CodeableConcept", 17472 "A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom.", 17473 0, 1, fundsReserve)); 17474 children.add(new Property("related", "", 17475 "Other claims which are related to this claim such as prior submissions or claims for related services or for the same event.", 17476 0, java.lang.Integer.MAX_VALUE, related)); 17477 children.add(new Property("prescription", "Reference(MedicationRequest|VisionPrescription)", 17478 "Prescription to support the dispensing of pharmacy, device or vision products.", 0, 1, prescription)); 17479 children.add(new Property("originalPrescription", "Reference(MedicationRequest)", 17480 "Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products.", 17481 0, 1, originalPrescription)); 17482 children.add(new Property("payee", "", 17483 "The party to be reimbursed for cost of the products and services according to the terms of the policy.", 0, 1, 17484 payee)); 17485 children.add( 17486 new Property("referral", "Reference(ServiceRequest)", "A reference to a referral resource.", 0, 1, referral)); 17487 children.add( 17488 new Property("facility", "Reference(Location)", "Facility where the services were provided.", 0, 1, facility)); 17489 children.add(new Property("claim", "Reference(Claim)", 17490 "The business identifier for the instance of the adjudication request: claim predetermination or preauthorization.", 17491 0, 1, claim)); 17492 children.add(new Property("claimResponse", "Reference(ClaimResponse)", 17493 "The business identifier for the instance of the adjudication response: claim, predetermination or preauthorization response.", 17494 0, 1, claimResponse)); 17495 children.add(new Property("outcome", "code", 17496 "The outcome of the claim, predetermination, or preauthorization processing.", 0, 1, outcome)); 17497 children.add(new Property("disposition", "string", 17498 "A human readable description of the status of the adjudication.", 0, 1, disposition)); 17499 children.add(new Property("preAuthRef", "string", 17500 "Reference from the Insurer which is used in later communications which refers to this adjudication.", 0, 17501 java.lang.Integer.MAX_VALUE, preAuthRef)); 17502 children.add(new Property("preAuthRefPeriod", "Period", 17503 "The timeframe during which the supplied preauthorization reference may be quoted on claims to obtain the adjudication as provided.", 17504 0, java.lang.Integer.MAX_VALUE, preAuthRefPeriod)); 17505 children.add(new Property("careTeam", "", "The members of the team who provided the products and services.", 0, 17506 java.lang.Integer.MAX_VALUE, careTeam)); 17507 children.add(new Property("supportingInfo", "", 17508 "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.", 17509 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 17510 children.add(new Property("diagnosis", "", "Information about diagnoses relevant to the claim items.", 0, 17511 java.lang.Integer.MAX_VALUE, diagnosis)); 17512 children.add(new Property("procedure", "", 17513 "Procedures performed on the patient relevant to the billing items with the claim.", 0, 17514 java.lang.Integer.MAX_VALUE, procedure)); 17515 children.add(new Property("precedence", "positiveInt", 17516 "This indicates the relative order of a series of EOBs related to different coverages for the same suite of services.", 17517 0, 1, precedence)); 17518 children.add(new Property("insurance", "", 17519 "Financial instruments for reimbursement for the health care products and services specified on the claim.", 0, 17520 java.lang.Integer.MAX_VALUE, insurance)); 17521 children.add(new Property("accident", "", 17522 "Details of a accident which resulted in injuries which required the products and services listed in the claim.", 17523 0, 1, accident)); 17524 children.add(new Property("item", "", 17525 "A claim line. Either a simple (a product or service) or a 'group' of details which can also be a simple items or groups of sub-details.", 17526 0, java.lang.Integer.MAX_VALUE, item)); 17527 children.add( 17528 new Property("addItem", "", "The first-tier service adjudications for payor added product or service lines.", 0, 17529 java.lang.Integer.MAX_VALUE, addItem)); 17530 children.add(new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", 17531 "The adjudication results which are presented at the header level rather than at the line-item or add-item levels.", 17532 0, java.lang.Integer.MAX_VALUE, adjudication)); 17533 children.add(new Property("total", "", "Categorized monetary totals for the adjudication.", 0, 17534 java.lang.Integer.MAX_VALUE, total)); 17535 children.add(new Property("payment", "", "Payment details for the adjudication of the claim.", 0, 1, payment)); 17536 children.add(new Property("formCode", "CodeableConcept", "A code for the form to be used for printing the content.", 17537 0, 1, formCode)); 17538 children.add(new Property("form", "Attachment", 17539 "The actual form, by reference or inclusion, for printing the content or an EOB.", 0, 1, form)); 17540 children.add(new Property("processNote", "", 17541 "A note that describes or explains adjudication results in a human readable form.", 0, 17542 java.lang.Integer.MAX_VALUE, processNote)); 17543 children.add(new Property("benefitPeriod", "Period", "The term of the benefits documented in this response.", 0, 1, 17544 benefitPeriod)); 17545 children.add(new Property("benefitBalance", "", "Balance by Benefit Category.", 0, java.lang.Integer.MAX_VALUE, 17546 benefitBalance)); 17547 } 17548 17549 @Override 17550 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 17551 switch (_hash) { 17552 case -1618432855: 17553 /* identifier */ return new Property("identifier", "Identifier", 17554 "A unique identifier assigned to this explanation of benefit.", 0, java.lang.Integer.MAX_VALUE, identifier); 17555 case -892481550: 17556 /* status */ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 17557 case 3575610: 17558 /* type */ return new Property("type", "CodeableConcept", 17559 "The category of claim, e.g. oral, pharmacy, vision, institutional, professional.", 0, 1, type); 17560 case -1868521062: 17561 /* subType */ return new Property("subType", "CodeableConcept", 17562 "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.", 17563 0, 1, subType); 17564 case 116103: 17565 /* use */ return new Property("use", "code", 17566 "A code to indicate whether the nature of the request is: to request adjudication of products and services previously rendered; or requesting authorization and adjudication for provision in the future; or requesting the non-binding adjudication of the listed products and services which could be provided in the future.", 17567 0, 1, use); 17568 case -791418107: 17569 /* patient */ return new Property("patient", "Reference(Patient)", 17570 "The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for forecast reimbursement is sought.", 17571 0, 1, patient); 17572 case -332066046: 17573 /* billablePeriod */ return new Property("billablePeriod", "Period", 17574 "The period for which charges are being submitted.", 0, 1, billablePeriod); 17575 case 1028554472: 17576 /* created */ return new Property("created", "dateTime", "The date this resource was created.", 0, 1, created); 17577 case -1591951995: 17578 /* enterer */ return new Property("enterer", "Reference(Practitioner|PractitionerRole)", 17579 "Individual who created the claim, predetermination or preauthorization.", 0, 1, enterer); 17580 case 1957615864: 17581 /* insurer */ return new Property("insurer", "Reference(Organization)", 17582 "The party responsible for authorization, adjudication and reimbursement.", 0, 1, insurer); 17583 case -987494927: 17584 /* provider */ return new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", 17585 "The provider which is responsible for the claim, predetermination or preauthorization.", 0, 1, provider); 17586 case -1165461084: 17587 /* priority */ return new Property("priority", "CodeableConcept", 17588 "The provider-required urgency of processing the request. Typical values include: stat, routine deferred.", 0, 17589 1, priority); 17590 case -1688904576: 17591 /* fundsReserveRequested */ return new Property("fundsReserveRequested", "CodeableConcept", 17592 "A code to indicate whether and for whom funds are to be reserved for future claims.", 0, 1, 17593 fundsReserveRequested); 17594 case 1314609806: 17595 /* fundsReserve */ return new Property("fundsReserve", "CodeableConcept", 17596 "A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom.", 17597 0, 1, fundsReserve); 17598 case 1090493483: 17599 /* related */ return new Property("related", "", 17600 "Other claims which are related to this claim such as prior submissions or claims for related services or for the same event.", 17601 0, java.lang.Integer.MAX_VALUE, related); 17602 case 460301338: 17603 /* prescription */ return new Property("prescription", "Reference(MedicationRequest|VisionPrescription)", 17604 "Prescription to support the dispensing of pharmacy, device or vision products.", 0, 1, prescription); 17605 case -1814015861: 17606 /* originalPrescription */ return new Property("originalPrescription", "Reference(MedicationRequest)", 17607 "Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products.", 17608 0, 1, originalPrescription); 17609 case 106443592: 17610 /* payee */ return new Property("payee", "", 17611 "The party to be reimbursed for cost of the products and services according to the terms of the policy.", 0, 17612 1, payee); 17613 case -722568291: 17614 /* referral */ return new Property("referral", "Reference(ServiceRequest)", "A reference to a referral resource.", 17615 0, 1, referral); 17616 case 501116579: 17617 /* facility */ return new Property("facility", "Reference(Location)", 17618 "Facility where the services were provided.", 0, 1, facility); 17619 case 94742588: 17620 /* claim */ return new Property("claim", "Reference(Claim)", 17621 "The business identifier for the instance of the adjudication request: claim predetermination or preauthorization.", 17622 0, 1, claim); 17623 case 689513629: 17624 /* claimResponse */ return new Property("claimResponse", "Reference(ClaimResponse)", 17625 "The business identifier for the instance of the adjudication response: claim, predetermination or preauthorization response.", 17626 0, 1, claimResponse); 17627 case -1106507950: 17628 /* outcome */ return new Property("outcome", "code", 17629 "The outcome of the claim, predetermination, or preauthorization processing.", 0, 1, outcome); 17630 case 583380919: 17631 /* disposition */ return new Property("disposition", "string", 17632 "A human readable description of the status of the adjudication.", 0, 1, disposition); 17633 case 522246568: 17634 /* preAuthRef */ return new Property("preAuthRef", "string", 17635 "Reference from the Insurer which is used in later communications which refers to this adjudication.", 0, 17636 java.lang.Integer.MAX_VALUE, preAuthRef); 17637 case -1262920311: 17638 /* preAuthRefPeriod */ return new Property("preAuthRefPeriod", "Period", 17639 "The timeframe during which the supplied preauthorization reference may be quoted on claims to obtain the adjudication as provided.", 17640 0, java.lang.Integer.MAX_VALUE, preAuthRefPeriod); 17641 case -7323378: 17642 /* careTeam */ return new Property("careTeam", "", 17643 "The members of the team who provided the products and services.", 0, java.lang.Integer.MAX_VALUE, careTeam); 17644 case 1922406657: 17645 /* supportingInfo */ return new Property("supportingInfo", "", 17646 "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.", 17647 0, java.lang.Integer.MAX_VALUE, supportingInfo); 17648 case 1196993265: 17649 /* diagnosis */ return new Property("diagnosis", "", "Information about diagnoses relevant to the claim items.", 17650 0, java.lang.Integer.MAX_VALUE, diagnosis); 17651 case -1095204141: 17652 /* procedure */ return new Property("procedure", "", 17653 "Procedures performed on the patient relevant to the billing items with the claim.", 0, 17654 java.lang.Integer.MAX_VALUE, procedure); 17655 case 159695370: 17656 /* precedence */ return new Property("precedence", "positiveInt", 17657 "This indicates the relative order of a series of EOBs related to different coverages for the same suite of services.", 17658 0, 1, precedence); 17659 case 73049818: 17660 /* insurance */ return new Property("insurance", "", 17661 "Financial instruments for reimbursement for the health care products and services specified on the claim.", 17662 0, java.lang.Integer.MAX_VALUE, insurance); 17663 case -2143202801: 17664 /* accident */ return new Property("accident", "", 17665 "Details of a accident which resulted in injuries which required the products and services listed in the claim.", 17666 0, 1, accident); 17667 case 3242771: 17668 /* item */ return new Property("item", "", 17669 "A claim line. Either a simple (a product or service) or a 'group' of details which can also be a simple items or groups of sub-details.", 17670 0, java.lang.Integer.MAX_VALUE, item); 17671 case -1148899500: 17672 /* addItem */ return new Property("addItem", "", 17673 "The first-tier service adjudications for payor added product or service lines.", 0, 17674 java.lang.Integer.MAX_VALUE, addItem); 17675 case -231349275: 17676 /* adjudication */ return new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", 17677 "The adjudication results which are presented at the header level rather than at the line-item or add-item levels.", 17678 0, java.lang.Integer.MAX_VALUE, adjudication); 17679 case 110549828: 17680 /* total */ return new Property("total", "", "Categorized monetary totals for the adjudication.", 0, 17681 java.lang.Integer.MAX_VALUE, total); 17682 case -786681338: 17683 /* payment */ return new Property("payment", "", "Payment details for the adjudication of the claim.", 0, 1, 17684 payment); 17685 case 473181393: 17686 /* formCode */ return new Property("formCode", "CodeableConcept", 17687 "A code for the form to be used for printing the content.", 0, 1, formCode); 17688 case 3148996: 17689 /* form */ return new Property("form", "Attachment", 17690 "The actual form, by reference or inclusion, for printing the content or an EOB.", 0, 1, form); 17691 case 202339073: 17692 /* processNote */ return new Property("processNote", "", 17693 "A note that describes or explains adjudication results in a human readable form.", 0, 17694 java.lang.Integer.MAX_VALUE, processNote); 17695 case -407369416: 17696 /* benefitPeriod */ return new Property("benefitPeriod", "Period", 17697 "The term of the benefits documented in this response.", 0, 1, benefitPeriod); 17698 case 596003397: 17699 /* benefitBalance */ return new Property("benefitBalance", "", "Balance by Benefit Category.", 0, 17700 java.lang.Integer.MAX_VALUE, benefitBalance); 17701 default: 17702 return super.getNamedProperty(_hash, _name, _checkValid); 17703 } 17704 17705 } 17706 17707 @Override 17708 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 17709 switch (hash) { 17710 case -1618432855: 17711 /* identifier */ return this.identifier == null ? new Base[0] 17712 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 17713 case -892481550: 17714 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<ExplanationOfBenefitStatus> 17715 case 3575610: 17716 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 17717 case -1868521062: 17718 /* subType */ return this.subType == null ? new Base[0] : new Base[] { this.subType }; // CodeableConcept 17719 case 116103: 17720 /* use */ return this.use == null ? new Base[0] : new Base[] { this.use }; // Enumeration<Use> 17721 case -791418107: 17722 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 17723 case -332066046: 17724 /* billablePeriod */ return this.billablePeriod == null ? new Base[0] : new Base[] { this.billablePeriod }; // Period 17725 case 1028554472: 17726 /* created */ return this.created == null ? new Base[0] : new Base[] { this.created }; // DateTimeType 17727 case -1591951995: 17728 /* enterer */ return this.enterer == null ? new Base[0] : new Base[] { this.enterer }; // Reference 17729 case 1957615864: 17730 /* insurer */ return this.insurer == null ? new Base[0] : new Base[] { this.insurer }; // Reference 17731 case -987494927: 17732 /* provider */ return this.provider == null ? new Base[0] : new Base[] { this.provider }; // Reference 17733 case -1165461084: 17734 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // CodeableConcept 17735 case -1688904576: 17736 /* fundsReserveRequested */ return this.fundsReserveRequested == null ? new Base[0] 17737 : new Base[] { this.fundsReserveRequested }; // CodeableConcept 17738 case 1314609806: 17739 /* fundsReserve */ return this.fundsReserve == null ? new Base[0] : new Base[] { this.fundsReserve }; // CodeableConcept 17740 case 1090493483: 17741 /* related */ return this.related == null ? new Base[0] : this.related.toArray(new Base[this.related.size()]); // RelatedClaimComponent 17742 case 460301338: 17743 /* prescription */ return this.prescription == null ? new Base[0] : new Base[] { this.prescription }; // Reference 17744 case -1814015861: 17745 /* originalPrescription */ return this.originalPrescription == null ? new Base[0] 17746 : new Base[] { this.originalPrescription }; // Reference 17747 case 106443592: 17748 /* payee */ return this.payee == null ? new Base[0] : new Base[] { this.payee }; // PayeeComponent 17749 case -722568291: 17750 /* referral */ return this.referral == null ? new Base[0] : new Base[] { this.referral }; // Reference 17751 case 501116579: 17752 /* facility */ return this.facility == null ? new Base[0] : new Base[] { this.facility }; // Reference 17753 case 94742588: 17754 /* claim */ return this.claim == null ? new Base[0] : new Base[] { this.claim }; // Reference 17755 case 689513629: 17756 /* claimResponse */ return this.claimResponse == null ? new Base[0] : new Base[] { this.claimResponse }; // Reference 17757 case -1106507950: 17758 /* outcome */ return this.outcome == null ? new Base[0] : new Base[] { this.outcome }; // Enumeration<RemittanceOutcome> 17759 case 583380919: 17760 /* disposition */ return this.disposition == null ? new Base[0] : new Base[] { this.disposition }; // StringType 17761 case 522246568: 17762 /* preAuthRef */ return this.preAuthRef == null ? new Base[0] 17763 : this.preAuthRef.toArray(new Base[this.preAuthRef.size()]); // StringType 17764 case -1262920311: 17765 /* preAuthRefPeriod */ return this.preAuthRefPeriod == null ? new Base[0] 17766 : this.preAuthRefPeriod.toArray(new Base[this.preAuthRefPeriod.size()]); // Period 17767 case -7323378: 17768 /* careTeam */ return this.careTeam == null ? new Base[0] : this.careTeam.toArray(new Base[this.careTeam.size()]); // CareTeamComponent 17769 case 1922406657: 17770 /* supportingInfo */ return this.supportingInfo == null ? new Base[0] 17771 : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // SupportingInformationComponent 17772 case 1196993265: 17773 /* diagnosis */ return this.diagnosis == null ? new Base[0] 17774 : this.diagnosis.toArray(new Base[this.diagnosis.size()]); // DiagnosisComponent 17775 case -1095204141: 17776 /* procedure */ return this.procedure == null ? new Base[0] 17777 : this.procedure.toArray(new Base[this.procedure.size()]); // ProcedureComponent 17778 case 159695370: 17779 /* precedence */ return this.precedence == null ? new Base[0] : new Base[] { this.precedence }; // PositiveIntType 17780 case 73049818: 17781 /* insurance */ return this.insurance == null ? new Base[0] 17782 : this.insurance.toArray(new Base[this.insurance.size()]); // InsuranceComponent 17783 case -2143202801: 17784 /* accident */ return this.accident == null ? new Base[0] : new Base[] { this.accident }; // AccidentComponent 17785 case 3242771: 17786 /* item */ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // ItemComponent 17787 case -1148899500: 17788 /* addItem */ return this.addItem == null ? new Base[0] : this.addItem.toArray(new Base[this.addItem.size()]); // AddedItemComponent 17789 case -231349275: 17790 /* adjudication */ return this.adjudication == null ? new Base[0] 17791 : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 17792 case 110549828: 17793 /* total */ return this.total == null ? new Base[0] : this.total.toArray(new Base[this.total.size()]); // TotalComponent 17794 case -786681338: 17795 /* payment */ return this.payment == null ? new Base[0] : new Base[] { this.payment }; // PaymentComponent 17796 case 473181393: 17797 /* formCode */ return this.formCode == null ? new Base[0] : new Base[] { this.formCode }; // CodeableConcept 17798 case 3148996: 17799 /* form */ return this.form == null ? new Base[0] : new Base[] { this.form }; // Attachment 17800 case 202339073: 17801 /* processNote */ return this.processNote == null ? new Base[0] 17802 : this.processNote.toArray(new Base[this.processNote.size()]); // NoteComponent 17803 case -407369416: 17804 /* benefitPeriod */ return this.benefitPeriod == null ? new Base[0] : new Base[] { this.benefitPeriod }; // Period 17805 case 596003397: 17806 /* benefitBalance */ return this.benefitBalance == null ? new Base[0] 17807 : this.benefitBalance.toArray(new Base[this.benefitBalance.size()]); // BenefitBalanceComponent 17808 default: 17809 return super.getProperty(hash, name, checkValid); 17810 } 17811 17812 } 17813 17814 @Override 17815 public Base setProperty(int hash, String name, Base value) throws FHIRException { 17816 switch (hash) { 17817 case -1618432855: // identifier 17818 this.getIdentifier().add(castToIdentifier(value)); // Identifier 17819 return value; 17820 case -892481550: // status 17821 value = new ExplanationOfBenefitStatusEnumFactory().fromType(castToCode(value)); 17822 this.status = (Enumeration) value; // Enumeration<ExplanationOfBenefitStatus> 17823 return value; 17824 case 3575610: // type 17825 this.type = castToCodeableConcept(value); // CodeableConcept 17826 return value; 17827 case -1868521062: // subType 17828 this.subType = castToCodeableConcept(value); // CodeableConcept 17829 return value; 17830 case 116103: // use 17831 value = new UseEnumFactory().fromType(castToCode(value)); 17832 this.use = (Enumeration) value; // Enumeration<Use> 17833 return value; 17834 case -791418107: // patient 17835 this.patient = castToReference(value); // Reference 17836 return value; 17837 case -332066046: // billablePeriod 17838 this.billablePeriod = castToPeriod(value); // Period 17839 return value; 17840 case 1028554472: // created 17841 this.created = castToDateTime(value); // DateTimeType 17842 return value; 17843 case -1591951995: // enterer 17844 this.enterer = castToReference(value); // Reference 17845 return value; 17846 case 1957615864: // insurer 17847 this.insurer = castToReference(value); // Reference 17848 return value; 17849 case -987494927: // provider 17850 this.provider = castToReference(value); // Reference 17851 return value; 17852 case -1165461084: // priority 17853 this.priority = castToCodeableConcept(value); // CodeableConcept 17854 return value; 17855 case -1688904576: // fundsReserveRequested 17856 this.fundsReserveRequested = castToCodeableConcept(value); // CodeableConcept 17857 return value; 17858 case 1314609806: // fundsReserve 17859 this.fundsReserve = castToCodeableConcept(value); // CodeableConcept 17860 return value; 17861 case 1090493483: // related 17862 this.getRelated().add((RelatedClaimComponent) value); // RelatedClaimComponent 17863 return value; 17864 case 460301338: // prescription 17865 this.prescription = castToReference(value); // Reference 17866 return value; 17867 case -1814015861: // originalPrescription 17868 this.originalPrescription = castToReference(value); // Reference 17869 return value; 17870 case 106443592: // payee 17871 this.payee = (PayeeComponent) value; // PayeeComponent 17872 return value; 17873 case -722568291: // referral 17874 this.referral = castToReference(value); // Reference 17875 return value; 17876 case 501116579: // facility 17877 this.facility = castToReference(value); // Reference 17878 return value; 17879 case 94742588: // claim 17880 this.claim = castToReference(value); // Reference 17881 return value; 17882 case 689513629: // claimResponse 17883 this.claimResponse = castToReference(value); // Reference 17884 return value; 17885 case -1106507950: // outcome 17886 value = new RemittanceOutcomeEnumFactory().fromType(castToCode(value)); 17887 this.outcome = (Enumeration) value; // Enumeration<RemittanceOutcome> 17888 return value; 17889 case 583380919: // disposition 17890 this.disposition = castToString(value); // StringType 17891 return value; 17892 case 522246568: // preAuthRef 17893 this.getPreAuthRef().add(castToString(value)); // StringType 17894 return value; 17895 case -1262920311: // preAuthRefPeriod 17896 this.getPreAuthRefPeriod().add(castToPeriod(value)); // Period 17897 return value; 17898 case -7323378: // careTeam 17899 this.getCareTeam().add((CareTeamComponent) value); // CareTeamComponent 17900 return value; 17901 case 1922406657: // supportingInfo 17902 this.getSupportingInfo().add((SupportingInformationComponent) value); // SupportingInformationComponent 17903 return value; 17904 case 1196993265: // diagnosis 17905 this.getDiagnosis().add((DiagnosisComponent) value); // DiagnosisComponent 17906 return value; 17907 case -1095204141: // procedure 17908 this.getProcedure().add((ProcedureComponent) value); // ProcedureComponent 17909 return value; 17910 case 159695370: // precedence 17911 this.precedence = castToPositiveInt(value); // PositiveIntType 17912 return value; 17913 case 73049818: // insurance 17914 this.getInsurance().add((InsuranceComponent) value); // InsuranceComponent 17915 return value; 17916 case -2143202801: // accident 17917 this.accident = (AccidentComponent) value; // AccidentComponent 17918 return value; 17919 case 3242771: // item 17920 this.getItem().add((ItemComponent) value); // ItemComponent 17921 return value; 17922 case -1148899500: // addItem 17923 this.getAddItem().add((AddedItemComponent) value); // AddedItemComponent 17924 return value; 17925 case -231349275: // adjudication 17926 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 17927 return value; 17928 case 110549828: // total 17929 this.getTotal().add((TotalComponent) value); // TotalComponent 17930 return value; 17931 case -786681338: // payment 17932 this.payment = (PaymentComponent) value; // PaymentComponent 17933 return value; 17934 case 473181393: // formCode 17935 this.formCode = castToCodeableConcept(value); // CodeableConcept 17936 return value; 17937 case 3148996: // form 17938 this.form = castToAttachment(value); // Attachment 17939 return value; 17940 case 202339073: // processNote 17941 this.getProcessNote().add((NoteComponent) value); // NoteComponent 17942 return value; 17943 case -407369416: // benefitPeriod 17944 this.benefitPeriod = castToPeriod(value); // Period 17945 return value; 17946 case 596003397: // benefitBalance 17947 this.getBenefitBalance().add((BenefitBalanceComponent) value); // BenefitBalanceComponent 17948 return value; 17949 default: 17950 return super.setProperty(hash, name, value); 17951 } 17952 17953 } 17954 17955 @Override 17956 public Base setProperty(String name, Base value) throws FHIRException { 17957 if (name.equals("identifier")) { 17958 this.getIdentifier().add(castToIdentifier(value)); 17959 } else if (name.equals("status")) { 17960 value = new ExplanationOfBenefitStatusEnumFactory().fromType(castToCode(value)); 17961 this.status = (Enumeration) value; // Enumeration<ExplanationOfBenefitStatus> 17962 } else if (name.equals("type")) { 17963 this.type = castToCodeableConcept(value); // CodeableConcept 17964 } else if (name.equals("subType")) { 17965 this.subType = castToCodeableConcept(value); // CodeableConcept 17966 } else if (name.equals("use")) { 17967 value = new UseEnumFactory().fromType(castToCode(value)); 17968 this.use = (Enumeration) value; // Enumeration<Use> 17969 } else if (name.equals("patient")) { 17970 this.patient = castToReference(value); // Reference 17971 } else if (name.equals("billablePeriod")) { 17972 this.billablePeriod = castToPeriod(value); // Period 17973 } else if (name.equals("created")) { 17974 this.created = castToDateTime(value); // DateTimeType 17975 } else if (name.equals("enterer")) { 17976 this.enterer = castToReference(value); // Reference 17977 } else if (name.equals("insurer")) { 17978 this.insurer = castToReference(value); // Reference 17979 } else if (name.equals("provider")) { 17980 this.provider = castToReference(value); // Reference 17981 } else if (name.equals("priority")) { 17982 this.priority = castToCodeableConcept(value); // CodeableConcept 17983 } else if (name.equals("fundsReserveRequested")) { 17984 this.fundsReserveRequested = castToCodeableConcept(value); // CodeableConcept 17985 } else if (name.equals("fundsReserve")) { 17986 this.fundsReserve = castToCodeableConcept(value); // CodeableConcept 17987 } else if (name.equals("related")) { 17988 this.getRelated().add((RelatedClaimComponent) value); 17989 } else if (name.equals("prescription")) { 17990 this.prescription = castToReference(value); // Reference 17991 } else if (name.equals("originalPrescription")) { 17992 this.originalPrescription = castToReference(value); // Reference 17993 } else if (name.equals("payee")) { 17994 this.payee = (PayeeComponent) value; // PayeeComponent 17995 } else if (name.equals("referral")) { 17996 this.referral = castToReference(value); // Reference 17997 } else if (name.equals("facility")) { 17998 this.facility = castToReference(value); // Reference 17999 } else if (name.equals("claim")) { 18000 this.claim = castToReference(value); // Reference 18001 } else if (name.equals("claimResponse")) { 18002 this.claimResponse = castToReference(value); // Reference 18003 } else if (name.equals("outcome")) { 18004 value = new RemittanceOutcomeEnumFactory().fromType(castToCode(value)); 18005 this.outcome = (Enumeration) value; // Enumeration<RemittanceOutcome> 18006 } else if (name.equals("disposition")) { 18007 this.disposition = castToString(value); // StringType 18008 } else if (name.equals("preAuthRef")) { 18009 this.getPreAuthRef().add(castToString(value)); 18010 } else if (name.equals("preAuthRefPeriod")) { 18011 this.getPreAuthRefPeriod().add(castToPeriod(value)); 18012 } else if (name.equals("careTeam")) { 18013 this.getCareTeam().add((CareTeamComponent) value); 18014 } else if (name.equals("supportingInfo")) { 18015 this.getSupportingInfo().add((SupportingInformationComponent) value); 18016 } else if (name.equals("diagnosis")) { 18017 this.getDiagnosis().add((DiagnosisComponent) value); 18018 } else if (name.equals("procedure")) { 18019 this.getProcedure().add((ProcedureComponent) value); 18020 } else if (name.equals("precedence")) { 18021 this.precedence = castToPositiveInt(value); // PositiveIntType 18022 } else if (name.equals("insurance")) { 18023 this.getInsurance().add((InsuranceComponent) value); 18024 } else if (name.equals("accident")) { 18025 this.accident = (AccidentComponent) value; // AccidentComponent 18026 } else if (name.equals("item")) { 18027 this.getItem().add((ItemComponent) value); 18028 } else if (name.equals("addItem")) { 18029 this.getAddItem().add((AddedItemComponent) value); 18030 } else if (name.equals("adjudication")) { 18031 this.getAdjudication().add((AdjudicationComponent) value); 18032 } else if (name.equals("total")) { 18033 this.getTotal().add((TotalComponent) value); 18034 } else if (name.equals("payment")) { 18035 this.payment = (PaymentComponent) value; // PaymentComponent 18036 } else if (name.equals("formCode")) { 18037 this.formCode = castToCodeableConcept(value); // CodeableConcept 18038 } else if (name.equals("form")) { 18039 this.form = castToAttachment(value); // Attachment 18040 } else if (name.equals("processNote")) { 18041 this.getProcessNote().add((NoteComponent) value); 18042 } else if (name.equals("benefitPeriod")) { 18043 this.benefitPeriod = castToPeriod(value); // Period 18044 } else if (name.equals("benefitBalance")) { 18045 this.getBenefitBalance().add((BenefitBalanceComponent) value); 18046 } else 18047 return super.setProperty(name, value); 18048 return value; 18049 } 18050 18051 @Override 18052 public void removeChild(String name, Base value) throws FHIRException { 18053 if (name.equals("identifier")) { 18054 this.getIdentifier().remove(castToIdentifier(value)); 18055 } else if (name.equals("status")) { 18056 this.status = null; 18057 } else if (name.equals("type")) { 18058 this.type = null; 18059 } else if (name.equals("subType")) { 18060 this.subType = null; 18061 } else if (name.equals("use")) { 18062 this.use = null; 18063 } else if (name.equals("patient")) { 18064 this.patient = null; 18065 } else if (name.equals("billablePeriod")) { 18066 this.billablePeriod = null; 18067 } else if (name.equals("created")) { 18068 this.created = null; 18069 } else if (name.equals("enterer")) { 18070 this.enterer = null; 18071 } else if (name.equals("insurer")) { 18072 this.insurer = null; 18073 } else if (name.equals("provider")) { 18074 this.provider = null; 18075 } else if (name.equals("priority")) { 18076 this.priority = null; 18077 } else if (name.equals("fundsReserveRequested")) { 18078 this.fundsReserveRequested = null; 18079 } else if (name.equals("fundsReserve")) { 18080 this.fundsReserve = null; 18081 } else if (name.equals("related")) { 18082 this.getRelated().remove((RelatedClaimComponent) value); 18083 } else if (name.equals("prescription")) { 18084 this.prescription = null; 18085 } else if (name.equals("originalPrescription")) { 18086 this.originalPrescription = null; 18087 } else if (name.equals("payee")) { 18088 this.payee = (PayeeComponent) value; // PayeeComponent 18089 } else if (name.equals("referral")) { 18090 this.referral = null; 18091 } else if (name.equals("facility")) { 18092 this.facility = null; 18093 } else if (name.equals("claim")) { 18094 this.claim = null; 18095 } else if (name.equals("claimResponse")) { 18096 this.claimResponse = null; 18097 } else if (name.equals("outcome")) { 18098 this.outcome = null; 18099 } else if (name.equals("disposition")) { 18100 this.disposition = null; 18101 } else if (name.equals("preAuthRef")) { 18102 this.getPreAuthRef().remove(castToString(value)); 18103 } else if (name.equals("preAuthRefPeriod")) { 18104 this.getPreAuthRefPeriod().remove(castToPeriod(value)); 18105 } else if (name.equals("careTeam")) { 18106 this.getCareTeam().remove((CareTeamComponent) value); 18107 } else if (name.equals("supportingInfo")) { 18108 this.getSupportingInfo().remove((SupportingInformationComponent) value); 18109 } else if (name.equals("diagnosis")) { 18110 this.getDiagnosis().remove((DiagnosisComponent) value); 18111 } else if (name.equals("procedure")) { 18112 this.getProcedure().remove((ProcedureComponent) value); 18113 } else if (name.equals("precedence")) { 18114 this.precedence = null; 18115 } else if (name.equals("insurance")) { 18116 this.getInsurance().remove((InsuranceComponent) value); 18117 } else if (name.equals("accident")) { 18118 this.accident = (AccidentComponent) value; // AccidentComponent 18119 } else if (name.equals("item")) { 18120 this.getItem().remove((ItemComponent) value); 18121 } else if (name.equals("addItem")) { 18122 this.getAddItem().remove((AddedItemComponent) value); 18123 } else if (name.equals("adjudication")) { 18124 this.getAdjudication().remove((AdjudicationComponent) value); 18125 } else if (name.equals("total")) { 18126 this.getTotal().remove((TotalComponent) value); 18127 } else if (name.equals("payment")) { 18128 this.payment = (PaymentComponent) value; // PaymentComponent 18129 } else if (name.equals("formCode")) { 18130 this.formCode = null; 18131 } else if (name.equals("form")) { 18132 this.form = null; 18133 } else if (name.equals("processNote")) { 18134 this.getProcessNote().remove((NoteComponent) value); 18135 } else if (name.equals("benefitPeriod")) { 18136 this.benefitPeriod = null; 18137 } else if (name.equals("benefitBalance")) { 18138 this.getBenefitBalance().remove((BenefitBalanceComponent) value); 18139 } else 18140 super.removeChild(name, value); 18141 18142 } 18143 18144 @Override 18145 public Base makeProperty(int hash, String name) throws FHIRException { 18146 switch (hash) { 18147 case -1618432855: 18148 return addIdentifier(); 18149 case -892481550: 18150 return getStatusElement(); 18151 case 3575610: 18152 return getType(); 18153 case -1868521062: 18154 return getSubType(); 18155 case 116103: 18156 return getUseElement(); 18157 case -791418107: 18158 return getPatient(); 18159 case -332066046: 18160 return getBillablePeriod(); 18161 case 1028554472: 18162 return getCreatedElement(); 18163 case -1591951995: 18164 return getEnterer(); 18165 case 1957615864: 18166 return getInsurer(); 18167 case -987494927: 18168 return getProvider(); 18169 case -1165461084: 18170 return getPriority(); 18171 case -1688904576: 18172 return getFundsReserveRequested(); 18173 case 1314609806: 18174 return getFundsReserve(); 18175 case 1090493483: 18176 return addRelated(); 18177 case 460301338: 18178 return getPrescription(); 18179 case -1814015861: 18180 return getOriginalPrescription(); 18181 case 106443592: 18182 return getPayee(); 18183 case -722568291: 18184 return getReferral(); 18185 case 501116579: 18186 return getFacility(); 18187 case 94742588: 18188 return getClaim(); 18189 case 689513629: 18190 return getClaimResponse(); 18191 case -1106507950: 18192 return getOutcomeElement(); 18193 case 583380919: 18194 return getDispositionElement(); 18195 case 522246568: 18196 return addPreAuthRefElement(); 18197 case -1262920311: 18198 return addPreAuthRefPeriod(); 18199 case -7323378: 18200 return addCareTeam(); 18201 case 1922406657: 18202 return addSupportingInfo(); 18203 case 1196993265: 18204 return addDiagnosis(); 18205 case -1095204141: 18206 return addProcedure(); 18207 case 159695370: 18208 return getPrecedenceElement(); 18209 case 73049818: 18210 return addInsurance(); 18211 case -2143202801: 18212 return getAccident(); 18213 case 3242771: 18214 return addItem(); 18215 case -1148899500: 18216 return addAddItem(); 18217 case -231349275: 18218 return addAdjudication(); 18219 case 110549828: 18220 return addTotal(); 18221 case -786681338: 18222 return getPayment(); 18223 case 473181393: 18224 return getFormCode(); 18225 case 3148996: 18226 return getForm(); 18227 case 202339073: 18228 return addProcessNote(); 18229 case -407369416: 18230 return getBenefitPeriod(); 18231 case 596003397: 18232 return addBenefitBalance(); 18233 default: 18234 return super.makeProperty(hash, name); 18235 } 18236 18237 } 18238 18239 @Override 18240 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 18241 switch (hash) { 18242 case -1618432855: 18243 /* identifier */ return new String[] { "Identifier" }; 18244 case -892481550: 18245 /* status */ return new String[] { "code" }; 18246 case 3575610: 18247 /* type */ return new String[] { "CodeableConcept" }; 18248 case -1868521062: 18249 /* subType */ return new String[] { "CodeableConcept" }; 18250 case 116103: 18251 /* use */ return new String[] { "code" }; 18252 case -791418107: 18253 /* patient */ return new String[] { "Reference" }; 18254 case -332066046: 18255 /* billablePeriod */ return new String[] { "Period" }; 18256 case 1028554472: 18257 /* created */ return new String[] { "dateTime" }; 18258 case -1591951995: 18259 /* enterer */ return new String[] { "Reference" }; 18260 case 1957615864: 18261 /* insurer */ return new String[] { "Reference" }; 18262 case -987494927: 18263 /* provider */ return new String[] { "Reference" }; 18264 case -1165461084: 18265 /* priority */ return new String[] { "CodeableConcept" }; 18266 case -1688904576: 18267 /* fundsReserveRequested */ return new String[] { "CodeableConcept" }; 18268 case 1314609806: 18269 /* fundsReserve */ return new String[] { "CodeableConcept" }; 18270 case 1090493483: 18271 /* related */ return new String[] {}; 18272 case 460301338: 18273 /* prescription */ return new String[] { "Reference" }; 18274 case -1814015861: 18275 /* originalPrescription */ return new String[] { "Reference" }; 18276 case 106443592: 18277 /* payee */ return new String[] {}; 18278 case -722568291: 18279 /* referral */ return new String[] { "Reference" }; 18280 case 501116579: 18281 /* facility */ return new String[] { "Reference" }; 18282 case 94742588: 18283 /* claim */ return new String[] { "Reference" }; 18284 case 689513629: 18285 /* claimResponse */ return new String[] { "Reference" }; 18286 case -1106507950: 18287 /* outcome */ return new String[] { "code" }; 18288 case 583380919: 18289 /* disposition */ return new String[] { "string" }; 18290 case 522246568: 18291 /* preAuthRef */ return new String[] { "string" }; 18292 case -1262920311: 18293 /* preAuthRefPeriod */ return new String[] { "Period" }; 18294 case -7323378: 18295 /* careTeam */ return new String[] {}; 18296 case 1922406657: 18297 /* supportingInfo */ return new String[] {}; 18298 case 1196993265: 18299 /* diagnosis */ return new String[] {}; 18300 case -1095204141: 18301 /* procedure */ return new String[] {}; 18302 case 159695370: 18303 /* precedence */ return new String[] { "positiveInt" }; 18304 case 73049818: 18305 /* insurance */ return new String[] {}; 18306 case -2143202801: 18307 /* accident */ return new String[] {}; 18308 case 3242771: 18309 /* item */ return new String[] {}; 18310 case -1148899500: 18311 /* addItem */ return new String[] {}; 18312 case -231349275: 18313 /* adjudication */ return new String[] { "@ExplanationOfBenefit.item.adjudication" }; 18314 case 110549828: 18315 /* total */ return new String[] {}; 18316 case -786681338: 18317 /* payment */ return new String[] {}; 18318 case 473181393: 18319 /* formCode */ return new String[] { "CodeableConcept" }; 18320 case 3148996: 18321 /* form */ return new String[] { "Attachment" }; 18322 case 202339073: 18323 /* processNote */ return new String[] {}; 18324 case -407369416: 18325 /* benefitPeriod */ return new String[] { "Period" }; 18326 case 596003397: 18327 /* benefitBalance */ return new String[] {}; 18328 default: 18329 return super.getTypesForProperty(hash, name); 18330 } 18331 18332 } 18333 18334 @Override 18335 public Base addChild(String name) throws FHIRException { 18336 if (name.equals("identifier")) { 18337 return addIdentifier(); 18338 } else if (name.equals("status")) { 18339 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.status"); 18340 } else if (name.equals("type")) { 18341 this.type = new CodeableConcept(); 18342 return this.type; 18343 } else if (name.equals("subType")) { 18344 this.subType = new CodeableConcept(); 18345 return this.subType; 18346 } else if (name.equals("use")) { 18347 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.use"); 18348 } else if (name.equals("patient")) { 18349 this.patient = new Reference(); 18350 return this.patient; 18351 } else if (name.equals("billablePeriod")) { 18352 this.billablePeriod = new Period(); 18353 return this.billablePeriod; 18354 } else if (name.equals("created")) { 18355 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.created"); 18356 } else if (name.equals("enterer")) { 18357 this.enterer = new Reference(); 18358 return this.enterer; 18359 } else if (name.equals("insurer")) { 18360 this.insurer = new Reference(); 18361 return this.insurer; 18362 } else if (name.equals("provider")) { 18363 this.provider = new Reference(); 18364 return this.provider; 18365 } else if (name.equals("priority")) { 18366 this.priority = new CodeableConcept(); 18367 return this.priority; 18368 } else if (name.equals("fundsReserveRequested")) { 18369 this.fundsReserveRequested = new CodeableConcept(); 18370 return this.fundsReserveRequested; 18371 } else if (name.equals("fundsReserve")) { 18372 this.fundsReserve = new CodeableConcept(); 18373 return this.fundsReserve; 18374 } else if (name.equals("related")) { 18375 return addRelated(); 18376 } else if (name.equals("prescription")) { 18377 this.prescription = new Reference(); 18378 return this.prescription; 18379 } else if (name.equals("originalPrescription")) { 18380 this.originalPrescription = new Reference(); 18381 return this.originalPrescription; 18382 } else if (name.equals("payee")) { 18383 this.payee = new PayeeComponent(); 18384 return this.payee; 18385 } else if (name.equals("referral")) { 18386 this.referral = new Reference(); 18387 return this.referral; 18388 } else if (name.equals("facility")) { 18389 this.facility = new Reference(); 18390 return this.facility; 18391 } else if (name.equals("claim")) { 18392 this.claim = new Reference(); 18393 return this.claim; 18394 } else if (name.equals("claimResponse")) { 18395 this.claimResponse = new Reference(); 18396 return this.claimResponse; 18397 } else if (name.equals("outcome")) { 18398 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.outcome"); 18399 } else if (name.equals("disposition")) { 18400 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.disposition"); 18401 } else if (name.equals("preAuthRef")) { 18402 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.preAuthRef"); 18403 } else if (name.equals("preAuthRefPeriod")) { 18404 return addPreAuthRefPeriod(); 18405 } else if (name.equals("careTeam")) { 18406 return addCareTeam(); 18407 } else if (name.equals("supportingInfo")) { 18408 return addSupportingInfo(); 18409 } else if (name.equals("diagnosis")) { 18410 return addDiagnosis(); 18411 } else if (name.equals("procedure")) { 18412 return addProcedure(); 18413 } else if (name.equals("precedence")) { 18414 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.precedence"); 18415 } else if (name.equals("insurance")) { 18416 return addInsurance(); 18417 } else if (name.equals("accident")) { 18418 this.accident = new AccidentComponent(); 18419 return this.accident; 18420 } else if (name.equals("item")) { 18421 return addItem(); 18422 } else if (name.equals("addItem")) { 18423 return addAddItem(); 18424 } else if (name.equals("adjudication")) { 18425 return addAdjudication(); 18426 } else if (name.equals("total")) { 18427 return addTotal(); 18428 } else if (name.equals("payment")) { 18429 this.payment = new PaymentComponent(); 18430 return this.payment; 18431 } else if (name.equals("formCode")) { 18432 this.formCode = new CodeableConcept(); 18433 return this.formCode; 18434 } else if (name.equals("form")) { 18435 this.form = new Attachment(); 18436 return this.form; 18437 } else if (name.equals("processNote")) { 18438 return addProcessNote(); 18439 } else if (name.equals("benefitPeriod")) { 18440 this.benefitPeriod = new Period(); 18441 return this.benefitPeriod; 18442 } else if (name.equals("benefitBalance")) { 18443 return addBenefitBalance(); 18444 } else 18445 return super.addChild(name); 18446 } 18447 18448 public String fhirType() { 18449 return "ExplanationOfBenefit"; 18450 18451 } 18452 18453 public ExplanationOfBenefit copy() { 18454 ExplanationOfBenefit dst = new ExplanationOfBenefit(); 18455 copyValues(dst); 18456 return dst; 18457 } 18458 18459 public void copyValues(ExplanationOfBenefit dst) { 18460 super.copyValues(dst); 18461 if (identifier != null) { 18462 dst.identifier = new ArrayList<Identifier>(); 18463 for (Identifier i : identifier) 18464 dst.identifier.add(i.copy()); 18465 } 18466 ; 18467 dst.status = status == null ? null : status.copy(); 18468 dst.type = type == null ? null : type.copy(); 18469 dst.subType = subType == null ? null : subType.copy(); 18470 dst.use = use == null ? null : use.copy(); 18471 dst.patient = patient == null ? null : patient.copy(); 18472 dst.billablePeriod = billablePeriod == null ? null : billablePeriod.copy(); 18473 dst.created = created == null ? null : created.copy(); 18474 dst.enterer = enterer == null ? null : enterer.copy(); 18475 dst.insurer = insurer == null ? null : insurer.copy(); 18476 dst.provider = provider == null ? null : provider.copy(); 18477 dst.priority = priority == null ? null : priority.copy(); 18478 dst.fundsReserveRequested = fundsReserveRequested == null ? null : fundsReserveRequested.copy(); 18479 dst.fundsReserve = fundsReserve == null ? null : fundsReserve.copy(); 18480 if (related != null) { 18481 dst.related = new ArrayList<RelatedClaimComponent>(); 18482 for (RelatedClaimComponent i : related) 18483 dst.related.add(i.copy()); 18484 } 18485 ; 18486 dst.prescription = prescription == null ? null : prescription.copy(); 18487 dst.originalPrescription = originalPrescription == null ? null : originalPrescription.copy(); 18488 dst.payee = payee == null ? null : payee.copy(); 18489 dst.referral = referral == null ? null : referral.copy(); 18490 dst.facility = facility == null ? null : facility.copy(); 18491 dst.claim = claim == null ? null : claim.copy(); 18492 dst.claimResponse = claimResponse == null ? null : claimResponse.copy(); 18493 dst.outcome = outcome == null ? null : outcome.copy(); 18494 dst.disposition = disposition == null ? null : disposition.copy(); 18495 if (preAuthRef != null) { 18496 dst.preAuthRef = new ArrayList<StringType>(); 18497 for (StringType i : preAuthRef) 18498 dst.preAuthRef.add(i.copy()); 18499 } 18500 ; 18501 if (preAuthRefPeriod != null) { 18502 dst.preAuthRefPeriod = new ArrayList<Period>(); 18503 for (Period i : preAuthRefPeriod) 18504 dst.preAuthRefPeriod.add(i.copy()); 18505 } 18506 ; 18507 if (careTeam != null) { 18508 dst.careTeam = new ArrayList<CareTeamComponent>(); 18509 for (CareTeamComponent i : careTeam) 18510 dst.careTeam.add(i.copy()); 18511 } 18512 ; 18513 if (supportingInfo != null) { 18514 dst.supportingInfo = new ArrayList<SupportingInformationComponent>(); 18515 for (SupportingInformationComponent i : supportingInfo) 18516 dst.supportingInfo.add(i.copy()); 18517 } 18518 ; 18519 if (diagnosis != null) { 18520 dst.diagnosis = new ArrayList<DiagnosisComponent>(); 18521 for (DiagnosisComponent i : diagnosis) 18522 dst.diagnosis.add(i.copy()); 18523 } 18524 ; 18525 if (procedure != null) { 18526 dst.procedure = new ArrayList<ProcedureComponent>(); 18527 for (ProcedureComponent i : procedure) 18528 dst.procedure.add(i.copy()); 18529 } 18530 ; 18531 dst.precedence = precedence == null ? null : precedence.copy(); 18532 if (insurance != null) { 18533 dst.insurance = new ArrayList<InsuranceComponent>(); 18534 for (InsuranceComponent i : insurance) 18535 dst.insurance.add(i.copy()); 18536 } 18537 ; 18538 dst.accident = accident == null ? null : accident.copy(); 18539 if (item != null) { 18540 dst.item = new ArrayList<ItemComponent>(); 18541 for (ItemComponent i : item) 18542 dst.item.add(i.copy()); 18543 } 18544 ; 18545 if (addItem != null) { 18546 dst.addItem = new ArrayList<AddedItemComponent>(); 18547 for (AddedItemComponent i : addItem) 18548 dst.addItem.add(i.copy()); 18549 } 18550 ; 18551 if (adjudication != null) { 18552 dst.adjudication = new ArrayList<AdjudicationComponent>(); 18553 for (AdjudicationComponent i : adjudication) 18554 dst.adjudication.add(i.copy()); 18555 } 18556 ; 18557 if (total != null) { 18558 dst.total = new ArrayList<TotalComponent>(); 18559 for (TotalComponent i : total) 18560 dst.total.add(i.copy()); 18561 } 18562 ; 18563 dst.payment = payment == null ? null : payment.copy(); 18564 dst.formCode = formCode == null ? null : formCode.copy(); 18565 dst.form = form == null ? null : form.copy(); 18566 if (processNote != null) { 18567 dst.processNote = new ArrayList<NoteComponent>(); 18568 for (NoteComponent i : processNote) 18569 dst.processNote.add(i.copy()); 18570 } 18571 ; 18572 dst.benefitPeriod = benefitPeriod == null ? null : benefitPeriod.copy(); 18573 if (benefitBalance != null) { 18574 dst.benefitBalance = new ArrayList<BenefitBalanceComponent>(); 18575 for (BenefitBalanceComponent i : benefitBalance) 18576 dst.benefitBalance.add(i.copy()); 18577 } 18578 ; 18579 } 18580 18581 protected ExplanationOfBenefit typedCopy() { 18582 return copy(); 18583 } 18584 18585 @Override 18586 public boolean equalsDeep(Base other_) { 18587 if (!super.equalsDeep(other_)) 18588 return false; 18589 if (!(other_ instanceof ExplanationOfBenefit)) 18590 return false; 18591 ExplanationOfBenefit o = (ExplanationOfBenefit) other_; 18592 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 18593 && compareDeep(type, o.type, true) && compareDeep(subType, o.subType, true) && compareDeep(use, o.use, true) 18594 && compareDeep(patient, o.patient, true) && compareDeep(billablePeriod, o.billablePeriod, true) 18595 && compareDeep(created, o.created, true) && compareDeep(enterer, o.enterer, true) 18596 && compareDeep(insurer, o.insurer, true) && compareDeep(provider, o.provider, true) 18597 && compareDeep(priority, o.priority, true) && compareDeep(fundsReserveRequested, o.fundsReserveRequested, true) 18598 && compareDeep(fundsReserve, o.fundsReserve, true) && compareDeep(related, o.related, true) 18599 && compareDeep(prescription, o.prescription, true) 18600 && compareDeep(originalPrescription, o.originalPrescription, true) && compareDeep(payee, o.payee, true) 18601 && compareDeep(referral, o.referral, true) && compareDeep(facility, o.facility, true) 18602 && compareDeep(claim, o.claim, true) && compareDeep(claimResponse, o.claimResponse, true) 18603 && compareDeep(outcome, o.outcome, true) && compareDeep(disposition, o.disposition, true) 18604 && compareDeep(preAuthRef, o.preAuthRef, true) && compareDeep(preAuthRefPeriod, o.preAuthRefPeriod, true) 18605 && compareDeep(careTeam, o.careTeam, true) && compareDeep(supportingInfo, o.supportingInfo, true) 18606 && compareDeep(diagnosis, o.diagnosis, true) && compareDeep(procedure, o.procedure, true) 18607 && compareDeep(precedence, o.precedence, true) && compareDeep(insurance, o.insurance, true) 18608 && compareDeep(accident, o.accident, true) && compareDeep(item, o.item, true) 18609 && compareDeep(addItem, o.addItem, true) && compareDeep(adjudication, o.adjudication, true) 18610 && compareDeep(total, o.total, true) && compareDeep(payment, o.payment, true) 18611 && compareDeep(formCode, o.formCode, true) && compareDeep(form, o.form, true) 18612 && compareDeep(processNote, o.processNote, true) && compareDeep(benefitPeriod, o.benefitPeriod, true) 18613 && compareDeep(benefitBalance, o.benefitBalance, true); 18614 } 18615 18616 @Override 18617 public boolean equalsShallow(Base other_) { 18618 if (!super.equalsShallow(other_)) 18619 return false; 18620 if (!(other_ instanceof ExplanationOfBenefit)) 18621 return false; 18622 ExplanationOfBenefit o = (ExplanationOfBenefit) other_; 18623 return compareValues(status, o.status, true) && compareValues(use, o.use, true) 18624 && compareValues(created, o.created, true) && compareValues(outcome, o.outcome, true) 18625 && compareValues(disposition, o.disposition, true) && compareValues(preAuthRef, o.preAuthRef, true) 18626 && compareValues(precedence, o.precedence, true); 18627 } 18628 18629 public boolean isEmpty() { 18630 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, type, subType, use, patient, 18631 billablePeriod, created, enterer, insurer, provider, priority, fundsReserveRequested, fundsReserve, related, 18632 prescription, originalPrescription, payee, referral, facility, claim, claimResponse, outcome, disposition, 18633 preAuthRef, preAuthRefPeriod, careTeam, supportingInfo, diagnosis, procedure, precedence, insurance, accident, 18634 item, addItem, adjudication, total, payment, formCode, form, processNote, benefitPeriod, benefitBalance); 18635 } 18636 18637 @Override 18638 public ResourceType getResourceType() { 18639 return ResourceType.ExplanationOfBenefit; 18640 } 18641 18642 /** 18643 * Search parameter: <b>coverage</b> 18644 * <p> 18645 * Description: <b>The plan under which the claim was adjudicated</b><br> 18646 * Type: <b>reference</b><br> 18647 * Path: <b>ExplanationOfBenefit.insurance.coverage</b><br> 18648 * </p> 18649 */ 18650 @SearchParamDefinition(name = "coverage", path = "ExplanationOfBenefit.insurance.coverage", description = "The plan under which the claim was adjudicated", type = "reference", target = { 18651 Coverage.class }) 18652 public static final String SP_COVERAGE = "coverage"; 18653 /** 18654 * <b>Fluent Client</b> search parameter constant for <b>coverage</b> 18655 * <p> 18656 * Description: <b>The plan under which the claim was adjudicated</b><br> 18657 * Type: <b>reference</b><br> 18658 * Path: <b>ExplanationOfBenefit.insurance.coverage</b><br> 18659 * </p> 18660 */ 18661 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COVERAGE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 18662 SP_COVERAGE); 18663 18664 /** 18665 * Constant for fluent queries to be used to add include statements. Specifies 18666 * the path value of "<b>ExplanationOfBenefit:coverage</b>". 18667 */ 18668 public static final ca.uhn.fhir.model.api.Include INCLUDE_COVERAGE = new ca.uhn.fhir.model.api.Include( 18669 "ExplanationOfBenefit:coverage").toLocked(); 18670 18671 /** 18672 * Search parameter: <b>care-team</b> 18673 * <p> 18674 * Description: <b>Member of the CareTeam</b><br> 18675 * Type: <b>reference</b><br> 18676 * Path: <b>ExplanationOfBenefit.careTeam.provider</b><br> 18677 * </p> 18678 */ 18679 @SearchParamDefinition(name = "care-team", path = "ExplanationOfBenefit.careTeam.provider", description = "Member of the CareTeam", type = "reference", providesMembershipIn = { 18680 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 18681 Practitioner.class, PractitionerRole.class }) 18682 public static final String SP_CARE_TEAM = "care-team"; 18683 /** 18684 * <b>Fluent Client</b> search parameter constant for <b>care-team</b> 18685 * <p> 18686 * Description: <b>Member of the CareTeam</b><br> 18687 * Type: <b>reference</b><br> 18688 * Path: <b>ExplanationOfBenefit.careTeam.provider</b><br> 18689 * </p> 18690 */ 18691 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CARE_TEAM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 18692 SP_CARE_TEAM); 18693 18694 /** 18695 * Constant for fluent queries to be used to add include statements. Specifies 18696 * the path value of "<b>ExplanationOfBenefit:care-team</b>". 18697 */ 18698 public static final ca.uhn.fhir.model.api.Include INCLUDE_CARE_TEAM = new ca.uhn.fhir.model.api.Include( 18699 "ExplanationOfBenefit:care-team").toLocked(); 18700 18701 /** 18702 * Search parameter: <b>identifier</b> 18703 * <p> 18704 * Description: <b>The business identifier of the Explanation of Benefit</b><br> 18705 * Type: <b>token</b><br> 18706 * Path: <b>ExplanationOfBenefit.identifier</b><br> 18707 * </p> 18708 */ 18709 @SearchParamDefinition(name = "identifier", path = "ExplanationOfBenefit.identifier", description = "The business identifier of the Explanation of Benefit", type = "token") 18710 public static final String SP_IDENTIFIER = "identifier"; 18711 /** 18712 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 18713 * <p> 18714 * Description: <b>The business identifier of the Explanation of Benefit</b><br> 18715 * Type: <b>token</b><br> 18716 * Path: <b>ExplanationOfBenefit.identifier</b><br> 18717 * </p> 18718 */ 18719 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 18720 SP_IDENTIFIER); 18721 18722 /** 18723 * Search parameter: <b>created</b> 18724 * <p> 18725 * Description: <b>The creation date for the EOB</b><br> 18726 * Type: <b>date</b><br> 18727 * Path: <b>ExplanationOfBenefit.created</b><br> 18728 * </p> 18729 */ 18730 @SearchParamDefinition(name = "created", path = "ExplanationOfBenefit.created", description = "The creation date for the EOB", type = "date") 18731 public static final String SP_CREATED = "created"; 18732 /** 18733 * <b>Fluent Client</b> search parameter constant for <b>created</b> 18734 * <p> 18735 * Description: <b>The creation date for the EOB</b><br> 18736 * Type: <b>date</b><br> 18737 * Path: <b>ExplanationOfBenefit.created</b><br> 18738 * </p> 18739 */ 18740 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam( 18741 SP_CREATED); 18742 18743 /** 18744 * Search parameter: <b>encounter</b> 18745 * <p> 18746 * Description: <b>Encounters associated with a billed line item</b><br> 18747 * Type: <b>reference</b><br> 18748 * Path: <b>ExplanationOfBenefit.item.encounter</b><br> 18749 * </p> 18750 */ 18751 @SearchParamDefinition(name = "encounter", path = "ExplanationOfBenefit.item.encounter", description = "Encounters associated with a billed line item", type = "reference", providesMembershipIn = { 18752 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 18753 public static final String SP_ENCOUNTER = "encounter"; 18754 /** 18755 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 18756 * <p> 18757 * Description: <b>Encounters associated with a billed line item</b><br> 18758 * Type: <b>reference</b><br> 18759 * Path: <b>ExplanationOfBenefit.item.encounter</b><br> 18760 * </p> 18761 */ 18762 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 18763 SP_ENCOUNTER); 18764 18765 /** 18766 * Constant for fluent queries to be used to add include statements. Specifies 18767 * the path value of "<b>ExplanationOfBenefit:encounter</b>". 18768 */ 18769 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 18770 "ExplanationOfBenefit:encounter").toLocked(); 18771 18772 /** 18773 * Search parameter: <b>payee</b> 18774 * <p> 18775 * Description: <b>The party receiving any payment for the Claim</b><br> 18776 * Type: <b>reference</b><br> 18777 * Path: <b>ExplanationOfBenefit.payee.party</b><br> 18778 * </p> 18779 */ 18780 @SearchParamDefinition(name = "payee", path = "ExplanationOfBenefit.payee.party", description = "The party receiving any payment for the Claim", type = "reference", providesMembershipIn = { 18781 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 18782 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 18783 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Organization.class, 18784 Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 18785 public static final String SP_PAYEE = "payee"; 18786 /** 18787 * <b>Fluent Client</b> search parameter constant for <b>payee</b> 18788 * <p> 18789 * Description: <b>The party receiving any payment for the Claim</b><br> 18790 * Type: <b>reference</b><br> 18791 * Path: <b>ExplanationOfBenefit.payee.party</b><br> 18792 * </p> 18793 */ 18794 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PAYEE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 18795 SP_PAYEE); 18796 18797 /** 18798 * Constant for fluent queries to be used to add include statements. Specifies 18799 * the path value of "<b>ExplanationOfBenefit:payee</b>". 18800 */ 18801 public static final ca.uhn.fhir.model.api.Include INCLUDE_PAYEE = new ca.uhn.fhir.model.api.Include( 18802 "ExplanationOfBenefit:payee").toLocked(); 18803 18804 /** 18805 * Search parameter: <b>disposition</b> 18806 * <p> 18807 * Description: <b>The contents of the disposition message</b><br> 18808 * Type: <b>string</b><br> 18809 * Path: <b>ExplanationOfBenefit.disposition</b><br> 18810 * </p> 18811 */ 18812 @SearchParamDefinition(name = "disposition", path = "ExplanationOfBenefit.disposition", description = "The contents of the disposition message", type = "string") 18813 public static final String SP_DISPOSITION = "disposition"; 18814 /** 18815 * <b>Fluent Client</b> search parameter constant for <b>disposition</b> 18816 * <p> 18817 * Description: <b>The contents of the disposition message</b><br> 18818 * Type: <b>string</b><br> 18819 * Path: <b>ExplanationOfBenefit.disposition</b><br> 18820 * </p> 18821 */ 18822 public static final ca.uhn.fhir.rest.gclient.StringClientParam DISPOSITION = new ca.uhn.fhir.rest.gclient.StringClientParam( 18823 SP_DISPOSITION); 18824 18825 /** 18826 * Search parameter: <b>provider</b> 18827 * <p> 18828 * Description: <b>The reference to the provider</b><br> 18829 * Type: <b>reference</b><br> 18830 * Path: <b>ExplanationOfBenefit.provider</b><br> 18831 * </p> 18832 */ 18833 @SearchParamDefinition(name = "provider", path = "ExplanationOfBenefit.provider", description = "The reference to the provider", type = "reference", providesMembershipIn = { 18834 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 18835 Practitioner.class, PractitionerRole.class }) 18836 public static final String SP_PROVIDER = "provider"; 18837 /** 18838 * <b>Fluent Client</b> search parameter constant for <b>provider</b> 18839 * <p> 18840 * Description: <b>The reference to the provider</b><br> 18841 * Type: <b>reference</b><br> 18842 * Path: <b>ExplanationOfBenefit.provider</b><br> 18843 * </p> 18844 */ 18845 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 18846 SP_PROVIDER); 18847 18848 /** 18849 * Constant for fluent queries to be used to add include statements. Specifies 18850 * the path value of "<b>ExplanationOfBenefit:provider</b>". 18851 */ 18852 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROVIDER = new ca.uhn.fhir.model.api.Include( 18853 "ExplanationOfBenefit:provider").toLocked(); 18854 18855 /** 18856 * Search parameter: <b>patient</b> 18857 * <p> 18858 * Description: <b>The reference to the patient</b><br> 18859 * Type: <b>reference</b><br> 18860 * Path: <b>ExplanationOfBenefit.patient</b><br> 18861 * </p> 18862 */ 18863 @SearchParamDefinition(name = "patient", path = "ExplanationOfBenefit.patient", description = "The reference to the patient", type = "reference", providesMembershipIn = { 18864 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 18865 public static final String SP_PATIENT = "patient"; 18866 /** 18867 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 18868 * <p> 18869 * Description: <b>The reference to the patient</b><br> 18870 * Type: <b>reference</b><br> 18871 * Path: <b>ExplanationOfBenefit.patient</b><br> 18872 * </p> 18873 */ 18874 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 18875 SP_PATIENT); 18876 18877 /** 18878 * Constant for fluent queries to be used to add include statements. Specifies 18879 * the path value of "<b>ExplanationOfBenefit:patient</b>". 18880 */ 18881 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 18882 "ExplanationOfBenefit:patient").toLocked(); 18883 18884 /** 18885 * Search parameter: <b>detail-udi</b> 18886 * <p> 18887 * Description: <b>UDI associated with a line item detail product or 18888 * service</b><br> 18889 * Type: <b>reference</b><br> 18890 * Path: <b>ExplanationOfBenefit.item.detail.udi</b><br> 18891 * </p> 18892 */ 18893 @SearchParamDefinition(name = "detail-udi", path = "ExplanationOfBenefit.item.detail.udi", description = "UDI associated with a line item detail product or service", type = "reference", providesMembershipIn = { 18894 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device") }, target = { Device.class }) 18895 public static final String SP_DETAIL_UDI = "detail-udi"; 18896 /** 18897 * <b>Fluent Client</b> search parameter constant for <b>detail-udi</b> 18898 * <p> 18899 * Description: <b>UDI associated with a line item detail product or 18900 * service</b><br> 18901 * Type: <b>reference</b><br> 18902 * Path: <b>ExplanationOfBenefit.item.detail.udi</b><br> 18903 * </p> 18904 */ 18905 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DETAIL_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 18906 SP_DETAIL_UDI); 18907 18908 /** 18909 * Constant for fluent queries to be used to add include statements. Specifies 18910 * the path value of "<b>ExplanationOfBenefit:detail-udi</b>". 18911 */ 18912 public static final ca.uhn.fhir.model.api.Include INCLUDE_DETAIL_UDI = new ca.uhn.fhir.model.api.Include( 18913 "ExplanationOfBenefit:detail-udi").toLocked(); 18914 18915 /** 18916 * Search parameter: <b>claim</b> 18917 * <p> 18918 * Description: <b>The reference to the claim</b><br> 18919 * Type: <b>reference</b><br> 18920 * Path: <b>ExplanationOfBenefit.claim</b><br> 18921 * </p> 18922 */ 18923 @SearchParamDefinition(name = "claim", path = "ExplanationOfBenefit.claim", description = "The reference to the claim", type = "reference", target = { 18924 Claim.class }) 18925 public static final String SP_CLAIM = "claim"; 18926 /** 18927 * <b>Fluent Client</b> search parameter constant for <b>claim</b> 18928 * <p> 18929 * Description: <b>The reference to the claim</b><br> 18930 * Type: <b>reference</b><br> 18931 * Path: <b>ExplanationOfBenefit.claim</b><br> 18932 * </p> 18933 */ 18934 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CLAIM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 18935 SP_CLAIM); 18936 18937 /** 18938 * Constant for fluent queries to be used to add include statements. Specifies 18939 * the path value of "<b>ExplanationOfBenefit:claim</b>". 18940 */ 18941 public static final ca.uhn.fhir.model.api.Include INCLUDE_CLAIM = new ca.uhn.fhir.model.api.Include( 18942 "ExplanationOfBenefit:claim").toLocked(); 18943 18944 /** 18945 * Search parameter: <b>enterer</b> 18946 * <p> 18947 * Description: <b>The party responsible for the entry of the Claim</b><br> 18948 * Type: <b>reference</b><br> 18949 * Path: <b>ExplanationOfBenefit.enterer</b><br> 18950 * </p> 18951 */ 18952 @SearchParamDefinition(name = "enterer", path = "ExplanationOfBenefit.enterer", description = "The party responsible for the entry of the Claim", type = "reference", providesMembershipIn = { 18953 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Practitioner.class, 18954 PractitionerRole.class }) 18955 public static final String SP_ENTERER = "enterer"; 18956 /** 18957 * <b>Fluent Client</b> search parameter constant for <b>enterer</b> 18958 * <p> 18959 * Description: <b>The party responsible for the entry of the Claim</b><br> 18960 * Type: <b>reference</b><br> 18961 * Path: <b>ExplanationOfBenefit.enterer</b><br> 18962 * </p> 18963 */ 18964 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTERER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 18965 SP_ENTERER); 18966 18967 /** 18968 * Constant for fluent queries to be used to add include statements. Specifies 18969 * the path value of "<b>ExplanationOfBenefit:enterer</b>". 18970 */ 18971 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTERER = new ca.uhn.fhir.model.api.Include( 18972 "ExplanationOfBenefit:enterer").toLocked(); 18973 18974 /** 18975 * Search parameter: <b>procedure-udi</b> 18976 * <p> 18977 * Description: <b>UDI associated with a procedure</b><br> 18978 * Type: <b>reference</b><br> 18979 * Path: <b>ExplanationOfBenefit.procedure.udi</b><br> 18980 * </p> 18981 */ 18982 @SearchParamDefinition(name = "procedure-udi", path = "ExplanationOfBenefit.procedure.udi", description = "UDI associated with a procedure", type = "reference", providesMembershipIn = { 18983 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device") }, target = { Device.class }) 18984 public static final String SP_PROCEDURE_UDI = "procedure-udi"; 18985 /** 18986 * <b>Fluent Client</b> search parameter constant for <b>procedure-udi</b> 18987 * <p> 18988 * Description: <b>UDI associated with a procedure</b><br> 18989 * Type: <b>reference</b><br> 18990 * Path: <b>ExplanationOfBenefit.procedure.udi</b><br> 18991 * </p> 18992 */ 18993 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROCEDURE_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 18994 SP_PROCEDURE_UDI); 18995 18996 /** 18997 * Constant for fluent queries to be used to add include statements. Specifies 18998 * the path value of "<b>ExplanationOfBenefit:procedure-udi</b>". 18999 */ 19000 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROCEDURE_UDI = new ca.uhn.fhir.model.api.Include( 19001 "ExplanationOfBenefit:procedure-udi").toLocked(); 19002 19003 /** 19004 * Search parameter: <b>subdetail-udi</b> 19005 * <p> 19006 * Description: <b>UDI associated with a line item detail subdetail product or 19007 * service</b><br> 19008 * Type: <b>reference</b><br> 19009 * Path: <b>ExplanationOfBenefit.item.detail.subDetail.udi</b><br> 19010 * </p> 19011 */ 19012 @SearchParamDefinition(name = "subdetail-udi", path = "ExplanationOfBenefit.item.detail.subDetail.udi", description = "UDI associated with a line item detail subdetail product or service", type = "reference", providesMembershipIn = { 19013 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device") }, target = { Device.class }) 19014 public static final String SP_SUBDETAIL_UDI = "subdetail-udi"; 19015 /** 19016 * <b>Fluent Client</b> search parameter constant for <b>subdetail-udi</b> 19017 * <p> 19018 * Description: <b>UDI associated with a line item detail subdetail product or 19019 * service</b><br> 19020 * Type: <b>reference</b><br> 19021 * Path: <b>ExplanationOfBenefit.item.detail.subDetail.udi</b><br> 19022 * </p> 19023 */ 19024 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBDETAIL_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 19025 SP_SUBDETAIL_UDI); 19026 19027 /** 19028 * Constant for fluent queries to be used to add include statements. Specifies 19029 * the path value of "<b>ExplanationOfBenefit:subdetail-udi</b>". 19030 */ 19031 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBDETAIL_UDI = new ca.uhn.fhir.model.api.Include( 19032 "ExplanationOfBenefit:subdetail-udi").toLocked(); 19033 19034 /** 19035 * Search parameter: <b>facility</b> 19036 * <p> 19037 * Description: <b>Facility responsible for the goods and services</b><br> 19038 * Type: <b>reference</b><br> 19039 * Path: <b>ExplanationOfBenefit.facility</b><br> 19040 * </p> 19041 */ 19042 @SearchParamDefinition(name = "facility", path = "ExplanationOfBenefit.facility", description = "Facility responsible for the goods and services", type = "reference", target = { 19043 Location.class }) 19044 public static final String SP_FACILITY = "facility"; 19045 /** 19046 * <b>Fluent Client</b> search parameter constant for <b>facility</b> 19047 * <p> 19048 * Description: <b>Facility responsible for the goods and services</b><br> 19049 * Type: <b>reference</b><br> 19050 * Path: <b>ExplanationOfBenefit.facility</b><br> 19051 * </p> 19052 */ 19053 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FACILITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 19054 SP_FACILITY); 19055 19056 /** 19057 * Constant for fluent queries to be used to add include statements. Specifies 19058 * the path value of "<b>ExplanationOfBenefit:facility</b>". 19059 */ 19060 public static final ca.uhn.fhir.model.api.Include INCLUDE_FACILITY = new ca.uhn.fhir.model.api.Include( 19061 "ExplanationOfBenefit:facility").toLocked(); 19062 19063 /** 19064 * Search parameter: <b>item-udi</b> 19065 * <p> 19066 * Description: <b>UDI associated with a line item product or service</b><br> 19067 * Type: <b>reference</b><br> 19068 * Path: <b>ExplanationOfBenefit.item.udi</b><br> 19069 * </p> 19070 */ 19071 @SearchParamDefinition(name = "item-udi", path = "ExplanationOfBenefit.item.udi", description = "UDI associated with a line item product or service", type = "reference", providesMembershipIn = { 19072 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device") }, target = { Device.class }) 19073 public static final String SP_ITEM_UDI = "item-udi"; 19074 /** 19075 * <b>Fluent Client</b> search parameter constant for <b>item-udi</b> 19076 * <p> 19077 * Description: <b>UDI associated with a line item product or service</b><br> 19078 * Type: <b>reference</b><br> 19079 * Path: <b>ExplanationOfBenefit.item.udi</b><br> 19080 * </p> 19081 */ 19082 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ITEM_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 19083 SP_ITEM_UDI); 19084 19085 /** 19086 * Constant for fluent queries to be used to add include statements. Specifies 19087 * the path value of "<b>ExplanationOfBenefit:item-udi</b>". 19088 */ 19089 public static final ca.uhn.fhir.model.api.Include INCLUDE_ITEM_UDI = new ca.uhn.fhir.model.api.Include( 19090 "ExplanationOfBenefit:item-udi").toLocked(); 19091 19092 /** 19093 * Search parameter: <b>status</b> 19094 * <p> 19095 * Description: <b>Status of the instance</b><br> 19096 * Type: <b>token</b><br> 19097 * Path: <b>ExplanationOfBenefit.status</b><br> 19098 * </p> 19099 */ 19100 @SearchParamDefinition(name = "status", path = "ExplanationOfBenefit.status", description = "Status of the instance", type = "token") 19101 public static final String SP_STATUS = "status"; 19102 /** 19103 * <b>Fluent Client</b> search parameter constant for <b>status</b> 19104 * <p> 19105 * Description: <b>Status of the instance</b><br> 19106 * Type: <b>token</b><br> 19107 * Path: <b>ExplanationOfBenefit.status</b><br> 19108 * </p> 19109 */ 19110 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 19111 SP_STATUS); 19112 19113}