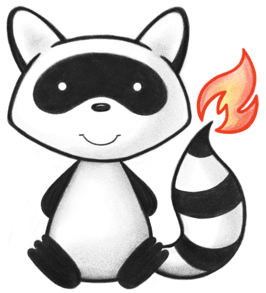
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.List; 034 035import org.hl7.fhir.exceptions.FHIRException; 036import org.hl7.fhir.instance.model.api.ICompositeType; 037import org.hl7.fhir.utilities.Utilities; 038 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.DatatypeDef; 041import ca.uhn.fhir.model.api.annotation.Description; 042 043/** 044 * A expression that is evaluated in a specified context and returns a value. 045 * The context of use of the expression must specify the context in which the 046 * expression is evaluated, and how the result of the expression is used. 047 */ 048@DatatypeDef(name = "Expression") 049public class Expression extends Type implements ICompositeType { 050 051 public enum ExpressionLanguage { 052 /** 053 * Clinical Quality Language. 054 */ 055 TEXT_CQL, 056 /** 057 * FHIRPath. 058 */ 059 TEXT_FHIRPATH, 060 /** 061 * FHIR's RESTful query syntax - typically independent of base URL. 062 */ 063 APPLICATION_XFHIRQUERY, 064 /** 065 * added to help the parsers with the generic types 066 */ 067 NULL; 068 069 public static ExpressionLanguage fromCode(String codeString) throws FHIRException { 070 if (codeString == null || "".equals(codeString)) 071 return null; 072 if ("text/cql".equals(codeString)) 073 return TEXT_CQL; 074 if ("text/fhirpath".equals(codeString)) 075 return TEXT_FHIRPATH; 076 if ("application/x-fhir-query".equals(codeString)) 077 return APPLICATION_XFHIRQUERY; 078 if (Configuration.isAcceptInvalidEnums()) 079 return null; 080 else 081 throw new FHIRException("Unknown ExpressionLanguage code '" + codeString + "'"); 082 } 083 084 public String toCode() { 085 switch (this) { 086 case TEXT_CQL: 087 return "text/cql"; 088 case TEXT_FHIRPATH: 089 return "text/fhirpath"; 090 case APPLICATION_XFHIRQUERY: 091 return "application/x-fhir-query"; 092 case NULL: 093 return null; 094 default: 095 return "?"; 096 } 097 } 098 099 public String getSystem() { 100 switch (this) { 101 case TEXT_CQL: 102 return "http://hl7.org/fhir/expression-language"; 103 case TEXT_FHIRPATH: 104 return "http://hl7.org/fhir/expression-language"; 105 case APPLICATION_XFHIRQUERY: 106 return "http://hl7.org/fhir/expression-language"; 107 case NULL: 108 return null; 109 default: 110 return "?"; 111 } 112 } 113 114 public String getDefinition() { 115 switch (this) { 116 case TEXT_CQL: 117 return "Clinical Quality Language."; 118 case TEXT_FHIRPATH: 119 return "FHIRPath."; 120 case APPLICATION_XFHIRQUERY: 121 return "FHIR's RESTful query syntax - typically independent of base URL."; 122 case NULL: 123 return null; 124 default: 125 return "?"; 126 } 127 } 128 129 public String getDisplay() { 130 switch (this) { 131 case TEXT_CQL: 132 return "CQL"; 133 case TEXT_FHIRPATH: 134 return "FHIRPath"; 135 case APPLICATION_XFHIRQUERY: 136 return "FHIR Query"; 137 case NULL: 138 return null; 139 default: 140 return "?"; 141 } 142 } 143 } 144 145 public static class ExpressionLanguageEnumFactory implements EnumFactory<ExpressionLanguage> { 146 public ExpressionLanguage fromCode(String codeString) throws IllegalArgumentException { 147 if (codeString == null || "".equals(codeString)) 148 if (codeString == null || "".equals(codeString)) 149 return null; 150 if ("text/cql".equals(codeString)) 151 return ExpressionLanguage.TEXT_CQL; 152 if ("text/fhirpath".equals(codeString)) 153 return ExpressionLanguage.TEXT_FHIRPATH; 154 if ("application/x-fhir-query".equals(codeString)) 155 return ExpressionLanguage.APPLICATION_XFHIRQUERY; 156 throw new IllegalArgumentException("Unknown ExpressionLanguage code '" + codeString + "'"); 157 } 158 159 public Enumeration<ExpressionLanguage> fromType(PrimitiveType<?> code) throws FHIRException { 160 if (code == null) 161 return null; 162 if (code.isEmpty()) 163 return new Enumeration<ExpressionLanguage>(this, ExpressionLanguage.NULL, code); 164 String codeString = code.asStringValue(); 165 if (codeString == null || "".equals(codeString)) 166 return new Enumeration<ExpressionLanguage>(this, ExpressionLanguage.NULL, code); 167 if ("text/cql".equals(codeString)) 168 return new Enumeration<ExpressionLanguage>(this, ExpressionLanguage.TEXT_CQL, code); 169 if ("text/fhirpath".equals(codeString)) 170 return new Enumeration<ExpressionLanguage>(this, ExpressionLanguage.TEXT_FHIRPATH, code); 171 if ("application/x-fhir-query".equals(codeString)) 172 return new Enumeration<ExpressionLanguage>(this, ExpressionLanguage.APPLICATION_XFHIRQUERY, code); 173 throw new FHIRException("Unknown ExpressionLanguage code '" + codeString + "'"); 174 } 175 176 public String toCode(ExpressionLanguage code) { 177 if (code == ExpressionLanguage.NULL) 178 return null; 179 if (code == ExpressionLanguage.TEXT_CQL) 180 return "text/cql"; 181 if (code == ExpressionLanguage.TEXT_FHIRPATH) 182 return "text/fhirpath"; 183 if (code == ExpressionLanguage.APPLICATION_XFHIRQUERY) 184 return "application/x-fhir-query"; 185 return "?"; 186 } 187 188 public String toSystem(ExpressionLanguage code) { 189 return code.getSystem(); 190 } 191 } 192 193 /** 194 * A brief, natural language description of the condition that effectively 195 * communicates the intended semantics. 196 */ 197 @Child(name = "description", type = { 198 StringType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 199 @Description(shortDefinition = "Natural language description of the condition", formalDefinition = "A brief, natural language description of the condition that effectively communicates the intended semantics.") 200 protected StringType description; 201 202 /** 203 * A short name assigned to the expression to allow for multiple reuse of the 204 * expression in the context where it is defined. 205 */ 206 @Child(name = "name", type = { IdType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 207 @Description(shortDefinition = "Short name assigned to expression for reuse", formalDefinition = "A short name assigned to the expression to allow for multiple reuse of the expression in the context where it is defined.") 208 protected IdType name; 209 210 /** 211 * The media type of the language for the expression. 212 */ 213 @Child(name = "language", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 214 @Description(shortDefinition = "text/cql | text/fhirpath | application/x-fhir-query | etc.", formalDefinition = "The media type of the language for the expression.") 215 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/expression-language") 216 protected CodeType language; 217 218 /** 219 * An expression in the specified language that returns a value. 220 */ 221 @Child(name = "expression", type = { 222 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 223 @Description(shortDefinition = "Expression in specified language", formalDefinition = "An expression in the specified language that returns a value.") 224 protected StringType expression; 225 226 /** 227 * A URI that defines where the expression is found. 228 */ 229 @Child(name = "reference", type = { UriType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 230 @Description(shortDefinition = "Where the expression is found", formalDefinition = "A URI that defines where the expression is found.") 231 protected UriType reference; 232 233 private static final long serialVersionUID = -941986742L; 234 235 /** 236 * Constructor 237 */ 238 public Expression() { 239 super(); 240 } 241 242 /** 243 * Constructor 244 */ 245 public Expression(CodeType language) { 246 super(); 247 this.language = language; 248 } 249 250 /** 251 * @return {@link #description} (A brief, natural language description of the 252 * condition that effectively communicates the intended semantics.). 253 * This is the underlying object with id, value and extensions. The 254 * accessor "getDescription" gives direct access to the value 255 */ 256 public StringType getDescriptionElement() { 257 if (this.description == null) 258 if (Configuration.errorOnAutoCreate()) 259 throw new Error("Attempt to auto-create Expression.description"); 260 else if (Configuration.doAutoCreate()) 261 this.description = new StringType(); // bb 262 return this.description; 263 } 264 265 public boolean hasDescriptionElement() { 266 return this.description != null && !this.description.isEmpty(); 267 } 268 269 public boolean hasDescription() { 270 return this.description != null && !this.description.isEmpty(); 271 } 272 273 /** 274 * @param value {@link #description} (A brief, natural language description of 275 * the condition that effectively communicates the intended 276 * semantics.). This is the underlying object with id, value and 277 * extensions. The accessor "getDescription" gives direct access to 278 * the value 279 */ 280 public Expression setDescriptionElement(StringType value) { 281 this.description = value; 282 return this; 283 } 284 285 /** 286 * @return A brief, natural language description of the condition that 287 * effectively communicates the intended semantics. 288 */ 289 public String getDescription() { 290 return this.description == null ? null : this.description.getValue(); 291 } 292 293 /** 294 * @param value A brief, natural language description of the condition that 295 * effectively communicates the intended semantics. 296 */ 297 public Expression setDescription(String value) { 298 if (Utilities.noString(value)) 299 this.description = null; 300 else { 301 if (this.description == null) 302 this.description = new StringType(); 303 this.description.setValue(value); 304 } 305 return this; 306 } 307 308 /** 309 * @return {@link #name} (A short name assigned to the expression to allow for 310 * multiple reuse of the expression in the context where it is 311 * defined.). This is the underlying object with id, value and 312 * extensions. The accessor "getName" gives direct access to the value 313 */ 314 public IdType getNameElement() { 315 if (this.name == null) 316 if (Configuration.errorOnAutoCreate()) 317 throw new Error("Attempt to auto-create Expression.name"); 318 else if (Configuration.doAutoCreate()) 319 this.name = new IdType(); // bb 320 return this.name; 321 } 322 323 public boolean hasNameElement() { 324 return this.name != null && !this.name.isEmpty(); 325 } 326 327 public boolean hasName() { 328 return this.name != null && !this.name.isEmpty(); 329 } 330 331 /** 332 * @param value {@link #name} (A short name assigned to the expression to allow 333 * for multiple reuse of the expression in the context where it is 334 * defined.). This is the underlying object with id, value and 335 * extensions. The accessor "getName" gives direct access to the 336 * value 337 */ 338 public Expression setNameElement(IdType value) { 339 this.name = value; 340 return this; 341 } 342 343 /** 344 * @return A short name assigned to the expression to allow for multiple reuse 345 * of the expression in the context where it is defined. 346 */ 347 public String getName() { 348 return this.name == null ? null : this.name.getValue(); 349 } 350 351 /** 352 * @param value A short name assigned to the expression to allow for multiple 353 * reuse of the expression in the context where it is defined. 354 */ 355 public Expression setName(String value) { 356 if (Utilities.noString(value)) 357 this.name = null; 358 else { 359 if (this.name == null) 360 this.name = new IdType(); 361 this.name.setValue(value); 362 } 363 return this; 364 } 365 366 /** 367 * @return {@link #language} (The media type of the language for the 368 * expression.). This is the underlying object with id, value and 369 * extensions. The accessor "getLanguage" gives direct access to the 370 * value 371 */ 372 public CodeType getLanguageElement() { 373 if (this.language == null) 374 if (Configuration.errorOnAutoCreate()) 375 throw new Error("Attempt to auto-create Expression.language"); 376 else if (Configuration.doAutoCreate()) 377 this.language = new CodeType(); // bb 378 return this.language; 379 } 380 381 public boolean hasLanguageElement() { 382 return this.language != null && !this.language.isEmpty(); 383 } 384 385 public boolean hasLanguage() { 386 return this.language != null && !this.language.isEmpty(); 387 } 388 389 /** 390 * @param value {@link #language} (The media type of the language for the 391 * expression.). This is the underlying object with id, value and 392 * extensions. The accessor "getLanguage" gives direct access to 393 * the value 394 */ 395 public Expression setLanguageElement(CodeType value) { 396 this.language = value; 397 return this; 398 } 399 400 /** 401 * @return The media type of the language for the expression. 402 */ 403 public String getLanguage() { 404 return this.language == null ? null : this.language.getValue(); 405 } 406 407 /** 408 * @param value The media type of the language for the expression. 409 */ 410 public Expression setLanguage(String value) { 411 if (this.language == null) 412 this.language = new CodeType(); 413 this.language.setValue(value); 414 return this; 415 } 416 417 /** 418 * @return {@link #expression} (An expression in the specified language that 419 * returns a value.). This is the underlying object with id, value and 420 * extensions. The accessor "getExpression" gives direct access to the 421 * value 422 */ 423 public StringType getExpressionElement() { 424 if (this.expression == null) 425 if (Configuration.errorOnAutoCreate()) 426 throw new Error("Attempt to auto-create Expression.expression"); 427 else if (Configuration.doAutoCreate()) 428 this.expression = new StringType(); // bb 429 return this.expression; 430 } 431 432 public boolean hasExpressionElement() { 433 return this.expression != null && !this.expression.isEmpty(); 434 } 435 436 public boolean hasExpression() { 437 return this.expression != null && !this.expression.isEmpty(); 438 } 439 440 /** 441 * @param value {@link #expression} (An expression in the specified language 442 * that returns a value.). This is the underlying object with id, 443 * value and extensions. The accessor "getExpression" gives direct 444 * access to the value 445 */ 446 public Expression setExpressionElement(StringType value) { 447 this.expression = value; 448 return this; 449 } 450 451 /** 452 * @return An expression in the specified language that returns a value. 453 */ 454 public String getExpression() { 455 return this.expression == null ? null : this.expression.getValue(); 456 } 457 458 /** 459 * @param value An expression in the specified language that returns a value. 460 */ 461 public Expression setExpression(String value) { 462 if (Utilities.noString(value)) 463 this.expression = null; 464 else { 465 if (this.expression == null) 466 this.expression = new StringType(); 467 this.expression.setValue(value); 468 } 469 return this; 470 } 471 472 /** 473 * @return {@link #reference} (A URI that defines where the expression is 474 * found.). This is the underlying object with id, value and extensions. 475 * The accessor "getReference" gives direct access to the value 476 */ 477 public UriType getReferenceElement() { 478 if (this.reference == null) 479 if (Configuration.errorOnAutoCreate()) 480 throw new Error("Attempt to auto-create Expression.reference"); 481 else if (Configuration.doAutoCreate()) 482 this.reference = new UriType(); // bb 483 return this.reference; 484 } 485 486 public boolean hasReferenceElement() { 487 return this.reference != null && !this.reference.isEmpty(); 488 } 489 490 public boolean hasReference() { 491 return this.reference != null && !this.reference.isEmpty(); 492 } 493 494 /** 495 * @param value {@link #reference} (A URI that defines where the expression is 496 * found.). This is the underlying object with id, value and 497 * extensions. The accessor "getReference" gives direct access to 498 * the value 499 */ 500 public Expression setReferenceElement(UriType value) { 501 this.reference = value; 502 return this; 503 } 504 505 /** 506 * @return A URI that defines where the expression is found. 507 */ 508 public String getReference() { 509 return this.reference == null ? null : this.reference.getValue(); 510 } 511 512 /** 513 * @param value A URI that defines where the expression is found. 514 */ 515 public Expression setReference(String value) { 516 if (Utilities.noString(value)) 517 this.reference = null; 518 else { 519 if (this.reference == null) 520 this.reference = new UriType(); 521 this.reference.setValue(value); 522 } 523 return this; 524 } 525 526 protected void listChildren(List<Property> children) { 527 super.listChildren(children); 528 children.add(new Property("description", "string", 529 "A brief, natural language description of the condition that effectively communicates the intended semantics.", 530 0, 1, description)); 531 children.add(new Property("name", "id", 532 "A short name assigned to the expression to allow for multiple reuse of the expression in the context where it is defined.", 533 0, 1, name)); 534 children 535 .add(new Property("language", "code", "The media type of the language for the expression.", 0, 1, language)); 536 children.add(new Property("expression", "string", "An expression in the specified language that returns a value.", 537 0, 1, expression)); 538 children 539 .add(new Property("reference", "uri", "A URI that defines where the expression is found.", 0, 1, reference)); 540 } 541 542 @Override 543 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 544 switch (_hash) { 545 case -1724546052: 546 /* description */ return new Property("description", "string", 547 "A brief, natural language description of the condition that effectively communicates the intended semantics.", 548 0, 1, description); 549 case 3373707: 550 /* name */ return new Property("name", "id", 551 "A short name assigned to the expression to allow for multiple reuse of the expression in the context where it is defined.", 552 0, 1, name); 553 case -1613589672: 554 /* language */ return new Property("language", "code", "The media type of the language for the expression.", 0, 1, 555 language); 556 case -1795452264: 557 /* expression */ return new Property("expression", "string", 558 "An expression in the specified language that returns a value.", 0, 1, expression); 559 case -925155509: 560 /* reference */ return new Property("reference", "uri", "A URI that defines where the expression is found.", 0, 1, 561 reference); 562 default: 563 return super.getNamedProperty(_hash, _name, _checkValid); 564 } 565 566 } 567 568 @Override 569 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 570 switch (hash) { 571 case -1724546052: 572 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 573 case 3373707: 574 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // IdType 575 case -1613589672: 576 /* language */ return this.language == null ? new Base[0] : new Base[] { this.language }; // Enumeration<ExpressionLanguage> 577 case -1795452264: 578 /* expression */ return this.expression == null ? new Base[0] : new Base[] { this.expression }; // StringType 579 case -925155509: 580 /* reference */ return this.reference == null ? new Base[0] : new Base[] { this.reference }; // UriType 581 default: 582 return super.getProperty(hash, name, checkValid); 583 } 584 585 } 586 587 @Override 588 public Base setProperty(int hash, String name, Base value) throws FHIRException { 589 switch (hash) { 590 case -1724546052: // description 591 this.description = castToString(value); // StringType 592 return value; 593 case 3373707: // name 594 this.name = castToId(value); // IdType 595 return value; 596 case -1613589672: // language 597 this.language = castToCode(value); // Enumeration<ExpressionLanguage> 598 return value; 599 case -1795452264: // expression 600 this.expression = castToString(value); // StringType 601 return value; 602 case -925155509: // reference 603 this.reference = castToUri(value); // UriType 604 return value; 605 default: 606 return super.setProperty(hash, name, value); 607 } 608 609 } 610 611 @Override 612 public Base setProperty(String name, Base value) throws FHIRException { 613 if (name.equals("description")) { 614 this.description = castToString(value); // StringType 615 } else if (name.equals("name")) { 616 this.name = castToId(value); // IdType 617 } else if (name.equals("language")) { 618 this.language = castToCode(value); // Enumeration<ExpressionLanguage> 619 } else if (name.equals("expression")) { 620 this.expression = castToString(value); // StringType 621 } else if (name.equals("reference")) { 622 this.reference = castToUri(value); // UriType 623 } else 624 return super.setProperty(name, value); 625 return value; 626 } 627 628 @Override 629 public void removeChild(String name, Base value) throws FHIRException { 630 if (name.equals("description")) { 631 this.description = null; 632 } else if (name.equals("name")) { 633 this.name = null; 634 } else if (name.equals("language")) { 635 this.language = null; 636 } else if (name.equals("expression")) { 637 this.expression = null; 638 } else if (name.equals("reference")) { 639 this.reference = null; 640 } else 641 super.removeChild(name, value); 642 643 } 644 645 @Override 646 public Base makeProperty(int hash, String name) throws FHIRException { 647 switch (hash) { 648 case -1724546052: 649 return getDescriptionElement(); 650 case 3373707: 651 return getNameElement(); 652 case -1613589672: 653 return getLanguageElement(); 654 case -1795452264: 655 return getExpressionElement(); 656 case -925155509: 657 return getReferenceElement(); 658 default: 659 return super.makeProperty(hash, name); 660 } 661 662 } 663 664 @Override 665 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 666 switch (hash) { 667 case -1724546052: 668 /* description */ return new String[] { "string" }; 669 case 3373707: 670 /* name */ return new String[] { "id" }; 671 case -1613589672: 672 /* language */ return new String[] { "code" }; 673 case -1795452264: 674 /* expression */ return new String[] { "string" }; 675 case -925155509: 676 /* reference */ return new String[] { "uri" }; 677 default: 678 return super.getTypesForProperty(hash, name); 679 } 680 681 } 682 683 @Override 684 public Base addChild(String name) throws FHIRException { 685 if (name.equals("description")) { 686 throw new FHIRException("Cannot call addChild on a singleton property Expression.description"); 687 } else if (name.equals("name")) { 688 throw new FHIRException("Cannot call addChild on a singleton property Expression.name"); 689 } else if (name.equals("language")) { 690 throw new FHIRException("Cannot call addChild on a singleton property Expression.language"); 691 } else if (name.equals("expression")) { 692 throw new FHIRException("Cannot call addChild on a singleton property Expression.expression"); 693 } else if (name.equals("reference")) { 694 throw new FHIRException("Cannot call addChild on a singleton property Expression.reference"); 695 } else 696 return super.addChild(name); 697 } 698 699 public String fhirType() { 700 return "Expression"; 701 702 } 703 704 public Expression copy() { 705 Expression dst = new Expression(); 706 copyValues(dst); 707 return dst; 708 } 709 710 public void copyValues(Expression dst) { 711 super.copyValues(dst); 712 dst.description = description == null ? null : description.copy(); 713 dst.name = name == null ? null : name.copy(); 714 dst.language = language == null ? null : language.copy(); 715 dst.expression = expression == null ? null : expression.copy(); 716 dst.reference = reference == null ? null : reference.copy(); 717 } 718 719 protected Expression typedCopy() { 720 return copy(); 721 } 722 723 @Override 724 public boolean equalsDeep(Base other_) { 725 if (!super.equalsDeep(other_)) 726 return false; 727 if (!(other_ instanceof Expression)) 728 return false; 729 Expression o = (Expression) other_; 730 return compareDeep(description, o.description, true) && compareDeep(name, o.name, true) 731 && compareDeep(language, o.language, true) && compareDeep(expression, o.expression, true) 732 && compareDeep(reference, o.reference, true); 733 } 734 735 @Override 736 public boolean equalsShallow(Base other_) { 737 if (!super.equalsShallow(other_)) 738 return false; 739 if (!(other_ instanceof Expression)) 740 return false; 741 Expression o = (Expression) other_; 742 return compareValues(description, o.description, true) && compareValues(name, o.name, true) 743 && compareValues(language, o.language, true) && compareValues(expression, o.expression, true) 744 && compareValues(reference, o.reference, true); 745 } 746 747 public boolean isEmpty() { 748 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, name, language, expression, reference); 749 } 750 751}