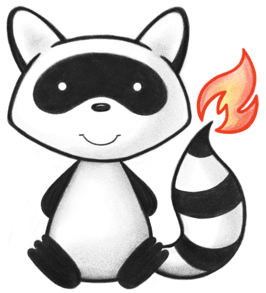
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.List; 034 035import org.hl7.fhir.exceptions.FHIRException; 036import org.hl7.fhir.instance.model.api.IBaseDatatype; 037import org.hl7.fhir.instance.model.api.IBaseExtension; 038import org.hl7.fhir.instance.model.api.IBaseHasExtensions; 039 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.DatatypeDef; 042import ca.uhn.fhir.model.api.annotation.Description; 043 044/** 045 * Optional Extension Element - found in all resources. 046 */ 047@DatatypeDef(name = "Extension") 048public class Extension extends BaseExtension implements IBaseExtension<Extension, Type>, IBaseHasExtensions { 049 050 /** 051 * Source of the definition for the extension code - a logical name or a URL. 052 */ 053 @Child(name = "url", type = { UriType.class }, order = 0, min = 1, max = 1, modifier = false, summary = false) 054 @Description(shortDefinition = "identifies the meaning of the extension", formalDefinition = "Source of the definition for the extension code - a logical name or a URL.") 055 protected UriType url; 056 057 /** 058 * Value of extension - must be one of a constrained set of the data types (see 059 * [Extensibility](extensibility.html) for a list). 060 */ 061 @Child(name = "value", type = {}, order = 1, min = 0, max = 1, modifier = false, summary = false) 062 @Description(shortDefinition = "Value of extension", formalDefinition = "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).") 063 protected org.hl7.fhir.r4.model.Type value; 064 065 private static final long serialVersionUID = 194602931L; 066 067 /** 068 * Constructor 069 */ 070 public Extension() { 071 super(); 072 } 073 074 /** 075 * Constructor 076 */ 077 public Extension(UriType url) { 078 super(); 079 this.url = url; 080 } 081 082 /** 083 * Constructor 084 */ 085 public Extension(String theUrl) { 086 setUrl(theUrl); 087 } 088 089 /** 090 * Constructor 091 */ 092 public Extension(String theUrl, IBaseDatatype theValue) { 093 setUrl(theUrl); 094 setValue(theValue); 095 } 096 097 /** 098 * @return {@link #url} (Source of the definition for the extension code - a 099 * logical name or a URL.). This is the underlying object with id, value 100 * and extensions. The accessor "getUrl" gives direct access to the 101 * value 102 */ 103 public UriType getUrlElement() { 104 if (this.url == null) 105 if (Configuration.errorOnAutoCreate()) 106 throw new Error("Attempt to auto-create Extension.url"); 107 else if (Configuration.doAutoCreate()) 108 this.url = new UriType(); // bb 109 return this.url; 110 } 111 112 public boolean hasUrlElement() { 113 return this.url != null && !this.url.isEmpty(); 114 } 115 116 public boolean hasUrl() { 117 return this.url != null && !this.url.isEmpty(); 118 } 119 120 /** 121 * @param value {@link #url} (Source of the definition for the extension code - 122 * a logical name or a URL.). This is the underlying object with 123 * id, value and extensions. The accessor "getUrl" gives direct 124 * access to the value 125 */ 126 public Extension setUrlElement(UriType value) { 127 this.url = value; 128 return this; 129 } 130 131 /** 132 * @return Source of the definition for the extension code - a logical name or a 133 * URL. 134 */ 135 public String getUrl() { 136 return this.url == null ? null : this.url.getValue(); 137 } 138 139 /** 140 * @param value Source of the definition for the extension code - a logical name 141 * or a URL. 142 */ 143 public Extension setUrl(String value) { 144 if (this.url == null) 145 this.url = new UriType(); 146 this.url.setValue(value); 147 return this; 148 } 149 150 /** 151 * @return {@link #value} (Value of extension - must be one of a constrained set 152 * of the data types (see [Extensibility](extensibility.html) for a 153 * list).) 154 */ 155 public org.hl7.fhir.r4.model.Type getValue() { 156 return this.value; 157 } 158 159 public boolean hasValue() { 160 return this.value != null && !this.value.isEmpty(); 161 } 162 163 /** 164 * @param value {@link #value} (Value of extension - must be one of a 165 * constrained set of the data types (see 166 * [Extensibility](extensibility.html) for a list).) 167 */ 168 public Extension setValue(org.hl7.fhir.r4.model.Type value) { 169 this.value = value; 170 return this; 171 } 172 173 protected void listChildren(List<Property> children) { 174 super.listChildren(children); 175 children.add(new Property("url", "uri", 176 "Source of the definition for the extension code - a logical name or a URL.", 0, 1, url)); 177 children.add(new Property("value[x]", "*", 178 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 179 0, 1, value)); 180 } 181 182 @Override 183 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 184 switch (_hash) { 185 case 116079: 186 /* url */ return new Property("url", "uri", 187 "Source of the definition for the extension code - a logical name or a URL.", 0, 1, url); 188 case -1410166417: 189 /* value[x] */ return new Property("value[x]", "*", 190 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 191 0, 1, value); 192 case 111972721: 193 /* value */ return new Property("value[x]", "*", 194 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 195 0, 1, value); 196 case -1535024575: 197 /* valueBase64Binary */ return new Property("value[x]", "*", 198 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 199 0, 1, value); 200 case 733421943: 201 /* valueBoolean */ return new Property("value[x]", "*", 202 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 203 0, 1, value); 204 case -786218365: 205 /* valueCanonical */ return new Property("value[x]", "*", 206 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 207 0, 1, value); 208 case -766209282: 209 /* valueCode */ return new Property("value[x]", "*", 210 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 211 0, 1, value); 212 case -766192449: 213 /* valueDate */ return new Property("value[x]", "*", 214 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 215 0, 1, value); 216 case 1047929900: 217 /* valueDateTime */ return new Property("value[x]", "*", 218 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 219 0, 1, value); 220 case -2083993440: 221 /* valueDecimal */ return new Property("value[x]", "*", 222 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 223 0, 1, value); 224 case 231604844: 225 /* valueId */ return new Property("value[x]", "*", 226 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 227 0, 1, value); 228 case -1668687056: 229 /* valueInstant */ return new Property("value[x]", "*", 230 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 231 0, 1, value); 232 case -1668204915: 233 /* valueInteger */ return new Property("value[x]", "*", 234 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 235 0, 1, value); 236 case -497880704: 237 /* valueMarkdown */ return new Property("value[x]", "*", 238 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 239 0, 1, value); 240 case -1410178407: 241 /* valueOid */ return new Property("value[x]", "*", 242 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 243 0, 1, value); 244 case -1249932027: 245 /* valuePositiveInt */ return new Property("value[x]", "*", 246 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 247 0, 1, value); 248 case -1424603934: 249 /* valueString */ return new Property("value[x]", "*", 250 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 251 0, 1, value); 252 case -765708322: 253 /* valueTime */ return new Property("value[x]", "*", 254 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 255 0, 1, value); 256 case 26529417: 257 /* valueUnsignedInt */ return new Property("value[x]", "*", 258 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 259 0, 1, value); 260 case -1410172357: 261 /* valueUri */ return new Property("value[x]", "*", 262 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 263 0, 1, value); 264 case -1410172354: 265 /* valueUrl */ return new Property("value[x]", "*", 266 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 267 0, 1, value); 268 case -765667124: 269 /* valueUuid */ return new Property("value[x]", "*", 270 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 271 0, 1, value); 272 case -478981821: 273 /* valueAddress */ return new Property("value[x]", "*", 274 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 275 0, 1, value); 276 case -67108992: 277 /* valueAnnotation */ return new Property("value[x]", "*", 278 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 279 0, 1, value); 280 case -475566732: 281 /* valueAttachment */ return new Property("value[x]", "*", 282 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 283 0, 1, value); 284 case 924902896: 285 /* valueCodeableConcept */ return new Property("value[x]", "*", 286 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 287 0, 1, value); 288 case -1887705029: 289 /* valueCoding */ return new Property("value[x]", "*", 290 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 291 0, 1, value); 292 case 944904545: 293 /* valueContactPoint */ return new Property("value[x]", "*", 294 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 295 0, 1, value); 296 case -2026205465: 297 /* valueHumanName */ return new Property("value[x]", "*", 298 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 299 0, 1, value); 300 case -130498310: 301 /* valueIdentifier */ return new Property("value[x]", "*", 302 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 303 0, 1, value); 304 case -1524344174: 305 /* valuePeriod */ return new Property("value[x]", "*", 306 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 307 0, 1, value); 308 case -2029823716: 309 /* valueQuantity */ return new Property("value[x]", "*", 310 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 311 0, 1, value); 312 case 2030761548: 313 /* valueRange */ return new Property("value[x]", "*", 314 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 315 0, 1, value); 316 case 2030767386: 317 /* valueRatio */ return new Property("value[x]", "*", 318 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 319 0, 1, value); 320 case 1755241690: 321 /* valueReference */ return new Property("value[x]", "*", 322 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 323 0, 1, value); 324 case -962229101: 325 /* valueSampledData */ return new Property("value[x]", "*", 326 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 327 0, 1, value); 328 case -540985785: 329 /* valueSignature */ return new Property("value[x]", "*", 330 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 331 0, 1, value); 332 case -1406282469: 333 /* valueTiming */ return new Property("value[x]", "*", 334 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 335 0, 1, value); 336 case -1858636920: 337 /* valueDosage */ return new Property("value[x]", "*", 338 "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 339 0, 1, value); 340 default: 341 return super.getNamedProperty(_hash, _name, _checkValid); 342 } 343 344 } 345 346 @Override 347 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 348 switch (hash) { 349 case 116079: 350 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 351 case 111972721: 352 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // org.hl7.fhir.r4.model.Type 353 default: 354 return super.getProperty(hash, name, checkValid); 355 } 356 357 } 358 359 @Override 360 public Base setProperty(int hash, String name, Base value) throws FHIRException { 361 switch (hash) { 362 case 116079: // url 363 this.url = castToUri(value); // UriType 364 return value; 365 case 111972721: // value 366 this.value = castToType(value); // org.hl7.fhir.r4.model.Type 367 return value; 368 default: 369 return super.setProperty(hash, name, value); 370 } 371 372 } 373 374 @Override 375 public Base setProperty(String name, Base value) throws FHIRException { 376 if (name.equals("url")) { 377 this.url = castToUri(value); // UriType 378 } else if (name.equals("value[x]")) { 379 this.value = castToType(value); // org.hl7.fhir.r4.model.Type 380 } else 381 return super.setProperty(name, value); 382 return value; 383 } 384 385 @Override 386 public Base makeProperty(int hash, String name) throws FHIRException { 387 switch (hash) { 388 case 116079: 389 return getUrlElement(); 390 case -1410166417: 391 return getValue(); 392 case 111972721: 393 return getValue(); 394 default: 395 return super.makeProperty(hash, name); 396 } 397 398 } 399 400 @Override 401 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 402 switch (hash) { 403 case 116079: 404 /* url */ return new String[] { "uri" }; 405 case 111972721: 406 /* value */ return new String[] { "*" }; 407 default: 408 return super.getTypesForProperty(hash, name); 409 } 410 411 } 412 413 @Override 414 public Base addChild(String name) throws FHIRException { 415 if (name.equals("url")) { 416 throw new FHIRException("Cannot call addChild on a singleton property Extension.url"); 417 } else if (name.equals("valueBase64Binary")) { 418 this.value = new Base64BinaryType(); 419 return this.value; 420 } else if (name.equals("valueBoolean")) { 421 this.value = new BooleanType(); 422 return this.value; 423 } else if (name.equals("valueCanonical")) { 424 this.value = new CanonicalType(); 425 return this.value; 426 } else if (name.equals("valueCode")) { 427 this.value = new CodeType(); 428 return this.value; 429 } else if (name.equals("valueDate")) { 430 this.value = new DateType(); 431 return this.value; 432 } else if (name.equals("valueDateTime")) { 433 this.value = new DateTimeType(); 434 return this.value; 435 } else if (name.equals("valueDecimal")) { 436 this.value = new DecimalType(); 437 return this.value; 438 } else if (name.equals("valueId")) { 439 this.value = new IdType(); 440 return this.value; 441 } else if (name.equals("valueInstant")) { 442 this.value = new InstantType(); 443 return this.value; 444 } else if (name.equals("valueInteger")) { 445 this.value = new IntegerType(); 446 return this.value; 447 } else if (name.equals("valueMarkdown")) { 448 this.value = new MarkdownType(); 449 return this.value; 450 } else if (name.equals("valueOid")) { 451 this.value = new OidType(); 452 return this.value; 453 } else if (name.equals("valuePositiveInt")) { 454 this.value = new PositiveIntType(); 455 return this.value; 456 } else if (name.equals("valueString")) { 457 this.value = new StringType(); 458 return this.value; 459 } else if (name.equals("valueTime")) { 460 this.value = new TimeType(); 461 return this.value; 462 } else if (name.equals("valueUnsignedInt")) { 463 this.value = new UnsignedIntType(); 464 return this.value; 465 } else if (name.equals("valueUri")) { 466 this.value = new UriType(); 467 return this.value; 468 } else if (name.equals("valueUrl")) { 469 this.value = new UrlType(); 470 return this.value; 471 } else if (name.equals("valueUuid")) { 472 this.value = new UuidType(); 473 return this.value; 474 } else if (name.equals("valueAddress")) { 475 this.value = new Address(); 476 return this.value; 477 } else if (name.equals("valueAge")) { 478 this.value = new Age(); 479 return this.value; 480 } else if (name.equals("valueAnnotation")) { 481 this.value = new Annotation(); 482 return this.value; 483 } else if (name.equals("valueAttachment")) { 484 this.value = new Attachment(); 485 return this.value; 486 } else if (name.equals("valueCodeableConcept")) { 487 this.value = new CodeableConcept(); 488 return this.value; 489 } else if (name.equals("valueCoding")) { 490 this.value = new Coding(); 491 return this.value; 492 } else if (name.equals("valueContactPoint")) { 493 this.value = new ContactPoint(); 494 return this.value; 495 } else if (name.equals("valueCount")) { 496 this.value = new Count(); 497 return this.value; 498 } else if (name.equals("valueDistance")) { 499 this.value = new Distance(); 500 return this.value; 501 } else if (name.equals("valueDuration")) { 502 this.value = new Duration(); 503 return this.value; 504 } else if (name.equals("valueHumanName")) { 505 this.value = new HumanName(); 506 return this.value; 507 } else if (name.equals("valueIdentifier")) { 508 this.value = new Identifier(); 509 return this.value; 510 } else if (name.equals("valueMoney")) { 511 this.value = new Money(); 512 return this.value; 513 } else if (name.equals("valuePeriod")) { 514 this.value = new Period(); 515 return this.value; 516 } else if (name.equals("valueQuantity")) { 517 this.value = new Quantity(); 518 return this.value; 519 } else if (name.equals("valueRange")) { 520 this.value = new Range(); 521 return this.value; 522 } else if (name.equals("valueRatio")) { 523 this.value = new Ratio(); 524 return this.value; 525 } else if (name.equals("valueReference")) { 526 this.value = new Reference(); 527 return this.value; 528 } else if (name.equals("valueSampledData")) { 529 this.value = new SampledData(); 530 return this.value; 531 } else if (name.equals("valueSignature")) { 532 this.value = new Signature(); 533 return this.value; 534 } else if (name.equals("valueTiming")) { 535 this.value = new Timing(); 536 return this.value; 537 } else if (name.equals("valueContactDetail")) { 538 this.value = new ContactDetail(); 539 return this.value; 540 } else if (name.equals("valueContributor")) { 541 this.value = new Contributor(); 542 return this.value; 543 } else if (name.equals("valueDataRequirement")) { 544 this.value = new DataRequirement(); 545 return this.value; 546 } else if (name.equals("valueExpression")) { 547 this.value = new Expression(); 548 return this.value; 549 } else if (name.equals("valueParameterDefinition")) { 550 this.value = new ParameterDefinition(); 551 return this.value; 552 } else if (name.equals("valueRelatedArtifact")) { 553 this.value = new RelatedArtifact(); 554 return this.value; 555 } else if (name.equals("valueTriggerDefinition")) { 556 this.value = new TriggerDefinition(); 557 return this.value; 558 } else if (name.equals("valueUsageContext")) { 559 this.value = new UsageContext(); 560 return this.value; 561 } else if (name.equals("valueDosage")) { 562 this.value = new Dosage(); 563 return this.value; 564 } else if (name.equals("valueMeta")) { 565 this.value = new Meta(); 566 return this.value; 567 } else 568 return super.addChild(name); 569 } 570 571 public String fhirType() { 572 return "Extension"; 573 574 } 575 576 public Extension copy() { 577 Extension dst = new Extension(); 578 copyValues(dst); 579 return dst; 580 } 581 582 public void copyValues(Extension dst) { 583 super.copyValues(dst); 584 dst.url = url == null ? null : url.copy(); 585 dst.value = value == null ? null : value.copy(); 586 } 587 588 protected Extension typedCopy() { 589 return copy(); 590 } 591 592 @Override 593 public boolean equalsDeep(Base other_) { 594 if (!super.equalsDeep(other_)) 595 return false; 596 if (!(other_ instanceof Extension)) 597 return false; 598 Extension o = (Extension) other_; 599 return compareDeep(url, o.url, true) && compareDeep(value, o.value, true); 600 } 601 602 @Override 603 public boolean equalsShallow(Base other_) { 604 if (!super.equalsShallow(other_)) 605 return false; 606 if (!(other_ instanceof Extension)) 607 return false; 608 Extension o = (Extension) other_; 609 return compareValues(url, o.url, true); 610 } 611 612 public boolean isEmpty() { 613 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, value); 614 } 615 616}