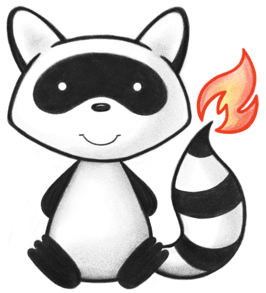
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import java.io.IOException; 033import java.net.URISyntaxException; 034import java.text.ParseException; 035import java.util.UUID; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.r4.model.ContactPoint.ContactPointSystem; 039import org.hl7.fhir.r4.model.Narrative.NarrativeStatus; 040import org.hl7.fhir.utilities.Utilities; 041import org.hl7.fhir.utilities.xhtml.XhtmlParser; 042 043/* 044Copyright (c) 2011+, HL7, Inc 045All rights reserved. 046 047Redistribution and use in source and binary forms, with or without modification, 048are permitted provided that the following conditions are met: 049 050 * Redistributions of source code must retain the above copyright notice, this 051 list of conditions and the following disclaimer. 052 * Redistributions in binary form must reproduce the above copyright notice, 053 this list of conditions and the following disclaimer in the documentation 054 and/or other materials provided with the distribution. 055 * Neither the name of HL7 nor the names of its contributors may be used to 056 endorse or promote products derived from this software without specific 057 prior written permission. 058 059THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 060ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 061WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 062IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 063INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 064NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 065PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 066WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 067ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 068POSSIBILITY OF SUCH DAMAGE. 069 070*/ 071 072public class Factory { 073 074 public static IdType newId(String value) { 075 if (value == null) 076 return null; 077 IdType res = new IdType(); 078 res.setValue(value); 079 return res; 080 } 081 082 public static StringType newString_(String value) { 083 if (value == null) 084 return null; 085 StringType res = new StringType(); 086 res.setValue(value); 087 return res; 088 } 089 090 public static UriType newUri(String value) throws URISyntaxException { 091 if (value == null) 092 return null; 093 UriType res = new UriType(); 094 res.setValue(value); 095 return res; 096 } 097 098 public static UrlType newUrl(String value) throws URISyntaxException { 099 if (value == null) 100 return null; 101 UrlType res = new UrlType(); 102 res.setValue(value); 103 return res; 104 } 105 106 public static CanonicalType newCanonical(String value) throws URISyntaxException { 107 if (value == null) 108 return null; 109 CanonicalType res = new CanonicalType(); 110 res.setValue(value); 111 return res; 112 } 113 114 public static DateTimeType newDateTime(String value) throws ParseException { 115 if (value == null) 116 return null; 117 return new DateTimeType(value); 118 } 119 120 public static DateType newDate(String value) throws ParseException { 121 if (value == null) 122 return null; 123 return new DateType(value); 124 } 125 126 public static CodeType newCode(String value) { 127 if (value == null) 128 return null; 129 CodeType res = new CodeType(); 130 res.setValue(value); 131 return res; 132 } 133 134 public static IntegerType newInteger(int value) { 135 IntegerType res = new IntegerType(); 136 res.setValue(value); 137 return res; 138 } 139 140 public static IntegerType newInteger(java.lang.Integer value) { 141 if (value == null) 142 return null; 143 IntegerType res = new IntegerType(); 144 res.setValue(value); 145 return res; 146 } 147 148 public static BooleanType newBoolean(boolean value) { 149 BooleanType res = new BooleanType(); 150 res.setValue(value); 151 return res; 152 } 153 154 public static ContactPoint newContactPoint(ContactPointSystem system, String value) { 155 ContactPoint res = new ContactPoint(); 156 res.setSystem(system); 157 res.setValue(value); 158 return res; 159 } 160 161 public static Extension newExtension(String uri, Type value, boolean evenIfNull) { 162 if (!evenIfNull && (value == null || value.isEmpty())) 163 return null; 164 Extension e = new Extension(); 165 e.setUrl(uri); 166 e.setValue(value); 167 return e; 168 } 169 170 public static CodeableConcept newCodeableConcept(String code, String system, String display) { 171 CodeableConcept cc = new CodeableConcept(); 172 Coding c = new Coding(); 173 c.setCode(code); 174 c.setSystem(system); 175 c.setDisplay(display); 176 cc.getCoding().add(c); 177 return cc; 178 } 179 180 public static Reference makeReference(String url) { 181 Reference rr = new Reference(); 182 rr.setReference(url); 183 return rr; 184 } 185 186 public static Narrative newNarrative(NarrativeStatus status, String html) throws IOException, FHIRException { 187 Narrative n = new Narrative(); 188 n.setStatus(status); 189 try { 190 n.setDiv(new XhtmlParser().parseFragment("<div>" + Utilities.escapeXml(html) + "</div>")); 191 } catch (org.hl7.fhir.exceptions.FHIRException e) { 192 throw new FHIRException(e.getMessage(), e); 193 } 194 return n; 195 } 196 197 public static Coding makeCoding(String code) throws FHIRException { 198 String[] parts = code.split("\\|"); 199 Coding c = new Coding(); 200 if (parts.length == 2) { 201 c.setSystem(parts[0]); 202 c.setCode(parts[1]); 203 } else if (parts.length == 3) { 204 c.setSystem(parts[0]); 205 c.setCode(parts[1]); 206 c.setDisplay(parts[2]); 207 } else 208 throw new FHIRException("Unable to understand the code '" + code + "'. Use the format system|code(|display)"); 209 return c; 210 } 211 212 public static Reference makeReference(String url, String text) { 213 Reference rr = new Reference(); 214 rr.setReference(url); 215 if (!Utilities.noString(text)) 216 rr.setDisplay(text); 217 return rr; 218 } 219 220 public static String createUUID() { 221 return "urn:uuid:" + UUID.randomUUID().toString().toLowerCase(); 222 } 223 224 public Type create(String name) throws FHIRException { 225 if (name.equals("boolean")) 226 return new BooleanType(); 227 else if (name.equals("integer")) 228 return new IntegerType(); 229 else if (name.equals("decimal")) 230 return new DecimalType(); 231 else if (name.equals("base64Binary")) 232 return new Base64BinaryType(); 233 else if (name.equals("instant")) 234 return new InstantType(); 235 else if (name.equals("string")) 236 return new StringType(); 237 else if (name.equals("uri")) 238 return new UriType(); 239 else if (name.equals("url")) 240 return new UrlType(); 241 else if (name.equals("canonical")) 242 return new CanonicalType(); 243 else if (name.equals("date")) 244 return new DateType(); 245 else if (name.equals("dateTime")) 246 return new DateTimeType(); 247 else if (name.equals("time")) 248 return new TimeType(); 249 else if (name.equals("code")) 250 return new CodeType(); 251 else if (name.equals("oid")) 252 return new OidType(); 253 else if (name.equals("id")) 254 return new IdType(); 255 else if (name.equals("unsignedInt")) 256 return new UnsignedIntType(); 257 else if (name.equals("positiveInt")) 258 return new PositiveIntType(); 259 else if (name.equals("markdown")) 260 return new MarkdownType(); 261 else if (name.equals("Annotation")) 262 return new Annotation(); 263 else if (name.equals("Attachment")) 264 return new Attachment(); 265 else if (name.equals("Identifier")) 266 return new Identifier(); 267 else if (name.equals("CodeableConcept")) 268 return new CodeableConcept(); 269 else if (name.equals("Coding")) 270 return new Coding(); 271 else if (name.equals("Quantity")) 272 return new Quantity(); 273 else if (name.equals("Range")) 274 return new Range(); 275 else if (name.equals("Period")) 276 return new Period(); 277 else if (name.equals("Ratio")) 278 return new Ratio(); 279 else if (name.equals("SampledData")) 280 return new SampledData(); 281 else if (name.equals("Signature")) 282 return new Signature(); 283 else if (name.equals("HumanName")) 284 return new HumanName(); 285 else if (name.equals("Address")) 286 return new Address(); 287 else if (name.equals("ContactPoint")) 288 return new ContactPoint(); 289 else if (name.equals("Timing")) 290 return new Timing(); 291 else if (name.equals("Reference")) 292 return new Reference(); 293 else if (name.equals("Meta")) 294 return new Meta(); 295 else 296 throw new FHIRException("Unknown data type name " + name); 297 } 298}