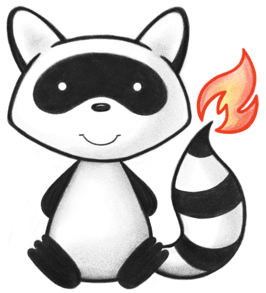
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * Describes the intended objective(s) for a patient, group or organization 049 * care, for example, weight loss, restoring an activity of daily living, 050 * obtaining herd immunity via immunization, meeting a process improvement 051 * objective, etc. 052 */ 053@ResourceDef(name = "Goal", profile = "http://hl7.org/fhir/StructureDefinition/Goal") 054public class Goal extends DomainResource { 055 056 public enum GoalLifecycleStatus { 057 /** 058 * A goal is proposed for this patient. 059 */ 060 PROPOSED, 061 /** 062 * A goal is planned for this patient. 063 */ 064 PLANNED, 065 /** 066 * A proposed goal was accepted or acknowledged. 067 */ 068 ACCEPTED, 069 /** 070 * The goal is being sought actively. 071 */ 072 ACTIVE, 073 /** 074 * The goal remains a long term objective but is no longer being actively 075 * pursued for a temporary period of time. 076 */ 077 ONHOLD, 078 /** 079 * The goal is no longer being sought. 080 */ 081 COMPLETED, 082 /** 083 * The goal has been abandoned. 084 */ 085 CANCELLED, 086 /** 087 * The goal was entered in error and voided. 088 */ 089 ENTEREDINERROR, 090 /** 091 * A proposed goal was rejected. 092 */ 093 REJECTED, 094 /** 095 * added to help the parsers with the generic types 096 */ 097 NULL; 098 099 public static GoalLifecycleStatus fromCode(String codeString) throws FHIRException { 100 if (codeString == null || "".equals(codeString)) 101 return null; 102 if ("proposed".equals(codeString)) 103 return PROPOSED; 104 if ("planned".equals(codeString)) 105 return PLANNED; 106 if ("accepted".equals(codeString)) 107 return ACCEPTED; 108 if ("active".equals(codeString)) 109 return ACTIVE; 110 if ("on-hold".equals(codeString)) 111 return ONHOLD; 112 if ("completed".equals(codeString)) 113 return COMPLETED; 114 if ("cancelled".equals(codeString)) 115 return CANCELLED; 116 if ("entered-in-error".equals(codeString)) 117 return ENTEREDINERROR; 118 if ("rejected".equals(codeString)) 119 return REJECTED; 120 if (Configuration.isAcceptInvalidEnums()) 121 return null; 122 else 123 throw new FHIRException("Unknown GoalLifecycleStatus code '" + codeString + "'"); 124 } 125 126 public String toCode() { 127 switch (this) { 128 case PROPOSED: 129 return "proposed"; 130 case PLANNED: 131 return "planned"; 132 case ACCEPTED: 133 return "accepted"; 134 case ACTIVE: 135 return "active"; 136 case ONHOLD: 137 return "on-hold"; 138 case COMPLETED: 139 return "completed"; 140 case CANCELLED: 141 return "cancelled"; 142 case ENTEREDINERROR: 143 return "entered-in-error"; 144 case REJECTED: 145 return "rejected"; 146 case NULL: 147 return null; 148 default: 149 return "?"; 150 } 151 } 152 153 public String getSystem() { 154 switch (this) { 155 case PROPOSED: 156 return "http://hl7.org/fhir/goal-status"; 157 case PLANNED: 158 return "http://hl7.org/fhir/goal-status"; 159 case ACCEPTED: 160 return "http://hl7.org/fhir/goal-status"; 161 case ACTIVE: 162 return "http://hl7.org/fhir/goal-status"; 163 case ONHOLD: 164 return "http://hl7.org/fhir/goal-status"; 165 case COMPLETED: 166 return "http://hl7.org/fhir/goal-status"; 167 case CANCELLED: 168 return "http://hl7.org/fhir/goal-status"; 169 case ENTEREDINERROR: 170 return "http://hl7.org/fhir/goal-status"; 171 case REJECTED: 172 return "http://hl7.org/fhir/goal-status"; 173 case NULL: 174 return null; 175 default: 176 return "?"; 177 } 178 } 179 180 public String getDefinition() { 181 switch (this) { 182 case PROPOSED: 183 return "A goal is proposed for this patient."; 184 case PLANNED: 185 return "A goal is planned for this patient."; 186 case ACCEPTED: 187 return "A proposed goal was accepted or acknowledged."; 188 case ACTIVE: 189 return "The goal is being sought actively."; 190 case ONHOLD: 191 return "The goal remains a long term objective but is no longer being actively pursued for a temporary period of time."; 192 case COMPLETED: 193 return "The goal is no longer being sought."; 194 case CANCELLED: 195 return "The goal has been abandoned."; 196 case ENTEREDINERROR: 197 return "The goal was entered in error and voided."; 198 case REJECTED: 199 return "A proposed goal was rejected."; 200 case NULL: 201 return null; 202 default: 203 return "?"; 204 } 205 } 206 207 public String getDisplay() { 208 switch (this) { 209 case PROPOSED: 210 return "Proposed"; 211 case PLANNED: 212 return "Planned"; 213 case ACCEPTED: 214 return "Accepted"; 215 case ACTIVE: 216 return "Active"; 217 case ONHOLD: 218 return "On Hold"; 219 case COMPLETED: 220 return "Completed"; 221 case CANCELLED: 222 return "Cancelled"; 223 case ENTEREDINERROR: 224 return "Entered in Error"; 225 case REJECTED: 226 return "Rejected"; 227 case NULL: 228 return null; 229 default: 230 return "?"; 231 } 232 } 233 } 234 235 public static class GoalLifecycleStatusEnumFactory implements EnumFactory<GoalLifecycleStatus> { 236 public GoalLifecycleStatus fromCode(String codeString) throws IllegalArgumentException { 237 if (codeString == null || "".equals(codeString)) 238 if (codeString == null || "".equals(codeString)) 239 return null; 240 if ("proposed".equals(codeString)) 241 return GoalLifecycleStatus.PROPOSED; 242 if ("planned".equals(codeString)) 243 return GoalLifecycleStatus.PLANNED; 244 if ("accepted".equals(codeString)) 245 return GoalLifecycleStatus.ACCEPTED; 246 if ("active".equals(codeString)) 247 return GoalLifecycleStatus.ACTIVE; 248 if ("on-hold".equals(codeString)) 249 return GoalLifecycleStatus.ONHOLD; 250 if ("completed".equals(codeString)) 251 return GoalLifecycleStatus.COMPLETED; 252 if ("cancelled".equals(codeString)) 253 return GoalLifecycleStatus.CANCELLED; 254 if ("entered-in-error".equals(codeString)) 255 return GoalLifecycleStatus.ENTEREDINERROR; 256 if ("rejected".equals(codeString)) 257 return GoalLifecycleStatus.REJECTED; 258 throw new IllegalArgumentException("Unknown GoalLifecycleStatus code '" + codeString + "'"); 259 } 260 261 public Enumeration<GoalLifecycleStatus> fromType(PrimitiveType<?> code) throws FHIRException { 262 if (code == null) 263 return null; 264 if (code.isEmpty()) 265 return new Enumeration<GoalLifecycleStatus>(this, GoalLifecycleStatus.NULL, code); 266 String codeString = code.asStringValue(); 267 if (codeString == null || "".equals(codeString)) 268 return new Enumeration<GoalLifecycleStatus>(this, GoalLifecycleStatus.NULL, code); 269 if ("proposed".equals(codeString)) 270 return new Enumeration<GoalLifecycleStatus>(this, GoalLifecycleStatus.PROPOSED, code); 271 if ("planned".equals(codeString)) 272 return new Enumeration<GoalLifecycleStatus>(this, GoalLifecycleStatus.PLANNED, code); 273 if ("accepted".equals(codeString)) 274 return new Enumeration<GoalLifecycleStatus>(this, GoalLifecycleStatus.ACCEPTED, code); 275 if ("active".equals(codeString)) 276 return new Enumeration<GoalLifecycleStatus>(this, GoalLifecycleStatus.ACTIVE, code); 277 if ("on-hold".equals(codeString)) 278 return new Enumeration<GoalLifecycleStatus>(this, GoalLifecycleStatus.ONHOLD, code); 279 if ("completed".equals(codeString)) 280 return new Enumeration<GoalLifecycleStatus>(this, GoalLifecycleStatus.COMPLETED, code); 281 if ("cancelled".equals(codeString)) 282 return new Enumeration<GoalLifecycleStatus>(this, GoalLifecycleStatus.CANCELLED, code); 283 if ("entered-in-error".equals(codeString)) 284 return new Enumeration<GoalLifecycleStatus>(this, GoalLifecycleStatus.ENTEREDINERROR, code); 285 if ("rejected".equals(codeString)) 286 return new Enumeration<GoalLifecycleStatus>(this, GoalLifecycleStatus.REJECTED, code); 287 throw new FHIRException("Unknown GoalLifecycleStatus code '" + codeString + "'"); 288 } 289 290 public String toCode(GoalLifecycleStatus code) { 291 if (code == GoalLifecycleStatus.NULL) 292 return null; 293 if (code == GoalLifecycleStatus.PROPOSED) 294 return "proposed"; 295 if (code == GoalLifecycleStatus.PLANNED) 296 return "planned"; 297 if (code == GoalLifecycleStatus.ACCEPTED) 298 return "accepted"; 299 if (code == GoalLifecycleStatus.ACTIVE) 300 return "active"; 301 if (code == GoalLifecycleStatus.ONHOLD) 302 return "on-hold"; 303 if (code == GoalLifecycleStatus.COMPLETED) 304 return "completed"; 305 if (code == GoalLifecycleStatus.CANCELLED) 306 return "cancelled"; 307 if (code == GoalLifecycleStatus.ENTEREDINERROR) 308 return "entered-in-error"; 309 if (code == GoalLifecycleStatus.REJECTED) 310 return "rejected"; 311 return "?"; 312 } 313 314 public String toSystem(GoalLifecycleStatus code) { 315 return code.getSystem(); 316 } 317 } 318 319 @Block() 320 public static class GoalTargetComponent extends BackboneElement implements IBaseBackboneElement { 321 /** 322 * The parameter whose value is being tracked, e.g. body weight, blood pressure, 323 * or hemoglobin A1c level. 324 */ 325 @Child(name = "measure", type = { 326 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 327 @Description(shortDefinition = "The parameter whose value is being tracked", formalDefinition = "The parameter whose value is being tracked, e.g. body weight, blood pressure, or hemoglobin A1c level.") 328 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-codes") 329 protected CodeableConcept measure; 330 331 /** 332 * The target value of the focus to be achieved to signify the fulfillment of 333 * the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the 334 * range can be specified. When a low value is missing, it indicates that the 335 * goal is achieved at any focus value at or below the high value. Similarly, if 336 * the high value is missing, it indicates that the goal is achieved at any 337 * focus value at or above the low value. 338 */ 339 @Child(name = "detail", type = { Quantity.class, Range.class, CodeableConcept.class, StringType.class, 340 BooleanType.class, IntegerType.class, 341 Ratio.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 342 @Description(shortDefinition = "The target value to be achieved", formalDefinition = "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.") 343 protected Type detail; 344 345 /** 346 * Indicates either the date or the duration after start by which the goal 347 * should be met. 348 */ 349 @Child(name = "due", type = { DateType.class, 350 Duration.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 351 @Description(shortDefinition = "Reach goal on or before", formalDefinition = "Indicates either the date or the duration after start by which the goal should be met.") 352 protected Type due; 353 354 private static final long serialVersionUID = -585108934L; 355 356 /** 357 * Constructor 358 */ 359 public GoalTargetComponent() { 360 super(); 361 } 362 363 /** 364 * @return {@link #measure} (The parameter whose value is being tracked, e.g. 365 * body weight, blood pressure, or hemoglobin A1c level.) 366 */ 367 public CodeableConcept getMeasure() { 368 if (this.measure == null) 369 if (Configuration.errorOnAutoCreate()) 370 throw new Error("Attempt to auto-create GoalTargetComponent.measure"); 371 else if (Configuration.doAutoCreate()) 372 this.measure = new CodeableConcept(); // cc 373 return this.measure; 374 } 375 376 public boolean hasMeasure() { 377 return this.measure != null && !this.measure.isEmpty(); 378 } 379 380 /** 381 * @param value {@link #measure} (The parameter whose value is being tracked, 382 * e.g. body weight, blood pressure, or hemoglobin A1c level.) 383 */ 384 public GoalTargetComponent setMeasure(CodeableConcept value) { 385 this.measure = value; 386 return this; 387 } 388 389 /** 390 * @return {@link #detail} (The target value of the focus to be achieved to 391 * signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either 392 * the high or low or both values of the range can be specified. When a 393 * low value is missing, it indicates that the goal is achieved at any 394 * focus value at or below the high value. Similarly, if the high value 395 * is missing, it indicates that the goal is achieved at any focus value 396 * at or above the low value.) 397 */ 398 public Type getDetail() { 399 return this.detail; 400 } 401 402 /** 403 * @return {@link #detail} (The target value of the focus to be achieved to 404 * signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either 405 * the high or low or both values of the range can be specified. When a 406 * low value is missing, it indicates that the goal is achieved at any 407 * focus value at or below the high value. Similarly, if the high value 408 * is missing, it indicates that the goal is achieved at any focus value 409 * at or above the low value.) 410 */ 411 public Quantity getDetailQuantity() throws FHIRException { 412 if (this.detail == null) 413 this.detail = new Quantity(); 414 if (!(this.detail instanceof Quantity)) 415 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.detail.getClass().getName() 416 + " was encountered"); 417 return (Quantity) this.detail; 418 } 419 420 public boolean hasDetailQuantity() { 421 return this != null && this.detail instanceof Quantity; 422 } 423 424 /** 425 * @return {@link #detail} (The target value of the focus to be achieved to 426 * signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either 427 * the high or low or both values of the range can be specified. When a 428 * low value is missing, it indicates that the goal is achieved at any 429 * focus value at or below the high value. Similarly, if the high value 430 * is missing, it indicates that the goal is achieved at any focus value 431 * at or above the low value.) 432 */ 433 public Range getDetailRange() throws FHIRException { 434 if (this.detail == null) 435 this.detail = new Range(); 436 if (!(this.detail instanceof Range)) 437 throw new FHIRException( 438 "Type mismatch: the type Range was expected, but " + this.detail.getClass().getName() + " was encountered"); 439 return (Range) this.detail; 440 } 441 442 public boolean hasDetailRange() { 443 return this != null && this.detail instanceof Range; 444 } 445 446 /** 447 * @return {@link #detail} (The target value of the focus to be achieved to 448 * signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either 449 * the high or low or both values of the range can be specified. When a 450 * low value is missing, it indicates that the goal is achieved at any 451 * focus value at or below the high value. Similarly, if the high value 452 * is missing, it indicates that the goal is achieved at any focus value 453 * at or above the low value.) 454 */ 455 public CodeableConcept getDetailCodeableConcept() throws FHIRException { 456 if (this.detail == null) 457 this.detail = new CodeableConcept(); 458 if (!(this.detail instanceof CodeableConcept)) 459 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 460 + this.detail.getClass().getName() + " was encountered"); 461 return (CodeableConcept) this.detail; 462 } 463 464 public boolean hasDetailCodeableConcept() { 465 return this != null && this.detail instanceof CodeableConcept; 466 } 467 468 /** 469 * @return {@link #detail} (The target value of the focus to be achieved to 470 * signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either 471 * the high or low or both values of the range can be specified. When a 472 * low value is missing, it indicates that the goal is achieved at any 473 * focus value at or below the high value. Similarly, if the high value 474 * is missing, it indicates that the goal is achieved at any focus value 475 * at or above the low value.) 476 */ 477 public StringType getDetailStringType() throws FHIRException { 478 if (this.detail == null) 479 this.detail = new StringType(); 480 if (!(this.detail instanceof StringType)) 481 throw new FHIRException("Type mismatch: the type StringType was expected, but " 482 + this.detail.getClass().getName() + " was encountered"); 483 return (StringType) this.detail; 484 } 485 486 public boolean hasDetailStringType() { 487 return this != null && this.detail instanceof StringType; 488 } 489 490 /** 491 * @return {@link #detail} (The target value of the focus to be achieved to 492 * signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either 493 * the high or low or both values of the range can be specified. When a 494 * low value is missing, it indicates that the goal is achieved at any 495 * focus value at or below the high value. Similarly, if the high value 496 * is missing, it indicates that the goal is achieved at any focus value 497 * at or above the low value.) 498 */ 499 public BooleanType getDetailBooleanType() throws FHIRException { 500 if (this.detail == null) 501 this.detail = new BooleanType(); 502 if (!(this.detail instanceof BooleanType)) 503 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 504 + this.detail.getClass().getName() + " was encountered"); 505 return (BooleanType) this.detail; 506 } 507 508 public boolean hasDetailBooleanType() { 509 return this != null && this.detail instanceof BooleanType; 510 } 511 512 /** 513 * @return {@link #detail} (The target value of the focus to be achieved to 514 * signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either 515 * the high or low or both values of the range can be specified. When a 516 * low value is missing, it indicates that the goal is achieved at any 517 * focus value at or below the high value. Similarly, if the high value 518 * is missing, it indicates that the goal is achieved at any focus value 519 * at or above the low value.) 520 */ 521 public IntegerType getDetailIntegerType() throws FHIRException { 522 if (this.detail == null) 523 this.detail = new IntegerType(); 524 if (!(this.detail instanceof IntegerType)) 525 throw new FHIRException("Type mismatch: the type IntegerType was expected, but " 526 + this.detail.getClass().getName() + " was encountered"); 527 return (IntegerType) this.detail; 528 } 529 530 public boolean hasDetailIntegerType() { 531 return this != null && this.detail instanceof IntegerType; 532 } 533 534 /** 535 * @return {@link #detail} (The target value of the focus to be achieved to 536 * signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either 537 * the high or low or both values of the range can be specified. When a 538 * low value is missing, it indicates that the goal is achieved at any 539 * focus value at or below the high value. Similarly, if the high value 540 * is missing, it indicates that the goal is achieved at any focus value 541 * at or above the low value.) 542 */ 543 public Ratio getDetailRatio() throws FHIRException { 544 if (this.detail == null) 545 this.detail = new Ratio(); 546 if (!(this.detail instanceof Ratio)) 547 throw new FHIRException( 548 "Type mismatch: the type Ratio was expected, but " + this.detail.getClass().getName() + " was encountered"); 549 return (Ratio) this.detail; 550 } 551 552 public boolean hasDetailRatio() { 553 return this != null && this.detail instanceof Ratio; 554 } 555 556 public boolean hasDetail() { 557 return this.detail != null && !this.detail.isEmpty(); 558 } 559 560 /** 561 * @param value {@link #detail} (The target value of the focus to be achieved to 562 * signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. 563 * Either the high or low or both values of the range can be 564 * specified. When a low value is missing, it indicates that the 565 * goal is achieved at any focus value at or below the high value. 566 * Similarly, if the high value is missing, it indicates that the 567 * goal is achieved at any focus value at or above the low value.) 568 */ 569 public GoalTargetComponent setDetail(Type value) { 570 if (value != null && !(value instanceof Quantity || value instanceof Range || value instanceof CodeableConcept 571 || value instanceof StringType || value instanceof BooleanType || value instanceof IntegerType 572 || value instanceof Ratio)) 573 throw new Error("Not the right type for Goal.target.detail[x]: " + value.fhirType()); 574 this.detail = value; 575 return this; 576 } 577 578 /** 579 * @return {@link #due} (Indicates either the date or the duration after start 580 * by which the goal should be met.) 581 */ 582 public Type getDue() { 583 return this.due; 584 } 585 586 /** 587 * @return {@link #due} (Indicates either the date or the duration after start 588 * by which the goal should be met.) 589 */ 590 public DateType getDueDateType() throws FHIRException { 591 if (this.due == null) 592 this.due = new DateType(); 593 if (!(this.due instanceof DateType)) 594 throw new FHIRException( 595 "Type mismatch: the type DateType was expected, but " + this.due.getClass().getName() + " was encountered"); 596 return (DateType) this.due; 597 } 598 599 public boolean hasDueDateType() { 600 return this != null && this.due instanceof DateType; 601 } 602 603 /** 604 * @return {@link #due} (Indicates either the date or the duration after start 605 * by which the goal should be met.) 606 */ 607 public Duration getDueDuration() throws FHIRException { 608 if (this.due == null) 609 this.due = new Duration(); 610 if (!(this.due instanceof Duration)) 611 throw new FHIRException( 612 "Type mismatch: the type Duration was expected, but " + this.due.getClass().getName() + " was encountered"); 613 return (Duration) this.due; 614 } 615 616 public boolean hasDueDuration() { 617 return this != null && this.due instanceof Duration; 618 } 619 620 public boolean hasDue() { 621 return this.due != null && !this.due.isEmpty(); 622 } 623 624 /** 625 * @param value {@link #due} (Indicates either the date or the duration after 626 * start by which the goal should be met.) 627 */ 628 public GoalTargetComponent setDue(Type value) { 629 if (value != null && !(value instanceof DateType || value instanceof Duration)) 630 throw new Error("Not the right type for Goal.target.due[x]: " + value.fhirType()); 631 this.due = value; 632 return this; 633 } 634 635 protected void listChildren(List<Property> children) { 636 super.listChildren(children); 637 children.add(new Property("measure", "CodeableConcept", 638 "The parameter whose value is being tracked, e.g. body weight, blood pressure, or hemoglobin A1c level.", 0, 639 1, measure)); 640 children.add(new Property("detail[x]", "Quantity|Range|CodeableConcept|string|boolean|integer|Ratio", 641 "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.", 642 0, 1, detail)); 643 children.add(new Property("due[x]", "date|Duration", 644 "Indicates either the date or the duration after start by which the goal should be met.", 0, 1, due)); 645 } 646 647 @Override 648 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 649 switch (_hash) { 650 case 938321246: 651 /* measure */ return new Property("measure", "CodeableConcept", 652 "The parameter whose value is being tracked, e.g. body weight, blood pressure, or hemoglobin A1c level.", 0, 653 1, measure); 654 case -1973084529: 655 /* detail[x] */ return new Property("detail[x]", "Quantity|Range|CodeableConcept|string|boolean|integer|Ratio", 656 "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.", 657 0, 1, detail); 658 case -1335224239: 659 /* detail */ return new Property("detail[x]", "Quantity|Range|CodeableConcept|string|boolean|integer|Ratio", 660 "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.", 661 0, 1, detail); 662 case -1313079300: 663 /* detailQuantity */ return new Property("detail[x]", 664 "Quantity|Range|CodeableConcept|string|boolean|integer|Ratio", 665 "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.", 666 0, 1, detail); 667 case -2062632084: 668 /* detailRange */ return new Property("detail[x]", 669 "Quantity|Range|CodeableConcept|string|boolean|integer|Ratio", 670 "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.", 671 0, 1, detail); 672 case -175586544: 673 /* detailCodeableConcept */ return new Property("detail[x]", 674 "Quantity|Range|CodeableConcept|string|boolean|integer|Ratio", 675 "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.", 676 0, 1, detail); 677 case 529212354: 678 /* detailString */ return new Property("detail[x]", 679 "Quantity|Range|CodeableConcept|string|boolean|integer|Ratio", 680 "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.", 681 0, 1, detail); 682 case 1172184727: 683 /* detailBoolean */ return new Property("detail[x]", 684 "Quantity|Range|CodeableConcept|string|boolean|integer|Ratio", 685 "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.", 686 0, 1, detail); 687 case -1229442131: 688 /* detailInteger */ return new Property("detail[x]", 689 "Quantity|Range|CodeableConcept|string|boolean|integer|Ratio", 690 "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.", 691 0, 1, detail); 692 case -2062626246: 693 /* detailRatio */ return new Property("detail[x]", 694 "Quantity|Range|CodeableConcept|string|boolean|integer|Ratio", 695 "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.", 696 0, 1, detail); 697 case -1320900084: 698 /* due[x] */ return new Property("due[x]", "date|Duration", 699 "Indicates either the date or the duration after start by which the goal should be met.", 0, 1, due); 700 case 99828: 701 /* due */ return new Property("due[x]", "date|Duration", 702 "Indicates either the date or the duration after start by which the goal should be met.", 0, 1, due); 703 case 2001063874: 704 /* dueDate */ return new Property("due[x]", "date|Duration", 705 "Indicates either the date or the duration after start by which the goal should be met.", 0, 1, due); 706 case -620428376: 707 /* dueDuration */ return new Property("due[x]", "date|Duration", 708 "Indicates either the date or the duration after start by which the goal should be met.", 0, 1, due); 709 default: 710 return super.getNamedProperty(_hash, _name, _checkValid); 711 } 712 713 } 714 715 @Override 716 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 717 switch (hash) { 718 case 938321246: 719 /* measure */ return this.measure == null ? new Base[0] : new Base[] { this.measure }; // CodeableConcept 720 case -1335224239: 721 /* detail */ return this.detail == null ? new Base[0] : new Base[] { this.detail }; // Type 722 case 99828: 723 /* due */ return this.due == null ? new Base[0] : new Base[] { this.due }; // Type 724 default: 725 return super.getProperty(hash, name, checkValid); 726 } 727 728 } 729 730 @Override 731 public Base setProperty(int hash, String name, Base value) throws FHIRException { 732 switch (hash) { 733 case 938321246: // measure 734 this.measure = castToCodeableConcept(value); // CodeableConcept 735 return value; 736 case -1335224239: // detail 737 this.detail = castToType(value); // Type 738 return value; 739 case 99828: // due 740 this.due = castToType(value); // Type 741 return value; 742 default: 743 return super.setProperty(hash, name, value); 744 } 745 746 } 747 748 @Override 749 public Base setProperty(String name, Base value) throws FHIRException { 750 if (name.equals("measure")) { 751 this.measure = castToCodeableConcept(value); // CodeableConcept 752 } else if (name.equals("detail[x]")) { 753 this.detail = castToType(value); // Type 754 } else if (name.equals("due[x]")) { 755 this.due = castToType(value); // Type 756 } else 757 return super.setProperty(name, value); 758 return value; 759 } 760 761 @Override 762 public void removeChild(String name, Base value) throws FHIRException { 763 if (name.equals("measure")) { 764 this.measure = null; 765 } else if (name.equals("detail[x]")) { 766 this.detail = null; 767 } else if (name.equals("due[x]")) { 768 this.due = null; 769 } else 770 super.removeChild(name, value); 771 772 } 773 774 @Override 775 public Base makeProperty(int hash, String name) throws FHIRException { 776 switch (hash) { 777 case 938321246: 778 return getMeasure(); 779 case -1973084529: 780 return getDetail(); 781 case -1335224239: 782 return getDetail(); 783 case -1320900084: 784 return getDue(); 785 case 99828: 786 return getDue(); 787 default: 788 return super.makeProperty(hash, name); 789 } 790 791 } 792 793 @Override 794 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 795 switch (hash) { 796 case 938321246: 797 /* measure */ return new String[] { "CodeableConcept" }; 798 case -1335224239: 799 /* detail */ return new String[] { "Quantity", "Range", "CodeableConcept", "string", "boolean", "integer", 800 "Ratio" }; 801 case 99828: 802 /* due */ return new String[] { "date", "Duration" }; 803 default: 804 return super.getTypesForProperty(hash, name); 805 } 806 807 } 808 809 @Override 810 public Base addChild(String name) throws FHIRException { 811 if (name.equals("measure")) { 812 this.measure = new CodeableConcept(); 813 return this.measure; 814 } else if (name.equals("detailQuantity")) { 815 this.detail = new Quantity(); 816 return this.detail; 817 } else if (name.equals("detailRange")) { 818 this.detail = new Range(); 819 return this.detail; 820 } else if (name.equals("detailCodeableConcept")) { 821 this.detail = new CodeableConcept(); 822 return this.detail; 823 } else if (name.equals("detailString")) { 824 this.detail = new StringType(); 825 return this.detail; 826 } else if (name.equals("detailBoolean")) { 827 this.detail = new BooleanType(); 828 return this.detail; 829 } else if (name.equals("detailInteger")) { 830 this.detail = new IntegerType(); 831 return this.detail; 832 } else if (name.equals("detailRatio")) { 833 this.detail = new Ratio(); 834 return this.detail; 835 } else if (name.equals("dueDate")) { 836 this.due = new DateType(); 837 return this.due; 838 } else if (name.equals("dueDuration")) { 839 this.due = new Duration(); 840 return this.due; 841 } else 842 return super.addChild(name); 843 } 844 845 public GoalTargetComponent copy() { 846 GoalTargetComponent dst = new GoalTargetComponent(); 847 copyValues(dst); 848 return dst; 849 } 850 851 public void copyValues(GoalTargetComponent dst) { 852 super.copyValues(dst); 853 dst.measure = measure == null ? null : measure.copy(); 854 dst.detail = detail == null ? null : detail.copy(); 855 dst.due = due == null ? null : due.copy(); 856 } 857 858 @Override 859 public boolean equalsDeep(Base other_) { 860 if (!super.equalsDeep(other_)) 861 return false; 862 if (!(other_ instanceof GoalTargetComponent)) 863 return false; 864 GoalTargetComponent o = (GoalTargetComponent) other_; 865 return compareDeep(measure, o.measure, true) && compareDeep(detail, o.detail, true) 866 && compareDeep(due, o.due, true); 867 } 868 869 @Override 870 public boolean equalsShallow(Base other_) { 871 if (!super.equalsShallow(other_)) 872 return false; 873 if (!(other_ instanceof GoalTargetComponent)) 874 return false; 875 GoalTargetComponent o = (GoalTargetComponent) other_; 876 return true; 877 } 878 879 public boolean isEmpty() { 880 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(measure, detail, due); 881 } 882 883 public String fhirType() { 884 return "Goal.target"; 885 886 } 887 888 } 889 890 /** 891 * Business identifiers assigned to this goal by the performer or other systems 892 * which remain constant as the resource is updated and propagates from server 893 * to server. 894 */ 895 @Child(name = "identifier", type = { 896 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 897 @Description(shortDefinition = "External Ids for this goal", formalDefinition = "Business identifiers assigned to this goal by the performer or other systems which remain constant as the resource is updated and propagates from server to server.") 898 protected List<Identifier> identifier; 899 900 /** 901 * The state of the goal throughout its lifecycle. 902 */ 903 @Child(name = "lifecycleStatus", type = { 904 CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 905 @Description(shortDefinition = "proposed | planned | accepted | active | on-hold | completed | cancelled | entered-in-error | rejected", formalDefinition = "The state of the goal throughout its lifecycle.") 906 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/goal-status") 907 protected Enumeration<GoalLifecycleStatus> lifecycleStatus; 908 909 /** 910 * Describes the progression, or lack thereof, towards the goal against the 911 * target. 912 */ 913 @Child(name = "achievementStatus", type = { 914 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 915 @Description(shortDefinition = "in-progress | improving | worsening | no-change | achieved | sustaining | not-achieved | no-progress | not-attainable", formalDefinition = "Describes the progression, or lack thereof, towards the goal against the target.") 916 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/goal-achievement") 917 protected CodeableConcept achievementStatus; 918 919 /** 920 * Indicates a category the goal falls within. 921 */ 922 @Child(name = "category", type = { 923 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 924 @Description(shortDefinition = "E.g. Treatment, dietary, behavioral, etc.", formalDefinition = "Indicates a category the goal falls within.") 925 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/goal-category") 926 protected List<CodeableConcept> category; 927 928 /** 929 * Identifies the mutually agreed level of importance associated with 930 * reaching/sustaining the goal. 931 */ 932 @Child(name = "priority", type = { 933 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 934 @Description(shortDefinition = "high-priority | medium-priority | low-priority", formalDefinition = "Identifies the mutually agreed level of importance associated with reaching/sustaining the goal.") 935 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/goal-priority") 936 protected CodeableConcept priority; 937 938 /** 939 * Human-readable and/or coded description of a specific desired objective of 940 * care, such as "control blood pressure" or "negotiate an obstacle course" or 941 * "dance with child at wedding". 942 */ 943 @Child(name = "description", type = { 944 CodeableConcept.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 945 @Description(shortDefinition = "Code or text describing goal", formalDefinition = "Human-readable and/or coded description of a specific desired objective of care, such as \"control blood pressure\" or \"negotiate an obstacle course\" or \"dance with child at wedding\".") 946 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/clinical-findings") 947 protected CodeableConcept description; 948 949 /** 950 * Identifies the patient, group or organization for whom the goal is being 951 * established. 952 */ 953 @Child(name = "subject", type = { Patient.class, Group.class, 954 Organization.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 955 @Description(shortDefinition = "Who this goal is intended for", formalDefinition = "Identifies the patient, group or organization for whom the goal is being established.") 956 protected Reference subject; 957 958 /** 959 * The actual object that is the target of the reference (Identifies the 960 * patient, group or organization for whom the goal is being established.) 961 */ 962 protected Resource subjectTarget; 963 964 /** 965 * The date or event after which the goal should begin being pursued. 966 */ 967 @Child(name = "start", type = { DateType.class, 968 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 969 @Description(shortDefinition = "When goal pursuit begins", formalDefinition = "The date or event after which the goal should begin being pursued.") 970 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/goal-start-event") 971 protected Type start; 972 973 /** 974 * Indicates what should be done by when. 975 */ 976 @Child(name = "target", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 977 @Description(shortDefinition = "Target outcome for the goal", formalDefinition = "Indicates what should be done by when.") 978 protected List<GoalTargetComponent> target; 979 980 /** 981 * Identifies when the current status. I.e. When initially created, when 982 * achieved, when cancelled, etc. 983 */ 984 @Child(name = "statusDate", type = { DateType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 985 @Description(shortDefinition = "When goal status took effect", formalDefinition = "Identifies when the current status. I.e. When initially created, when achieved, when cancelled, etc.") 986 protected DateType statusDate; 987 988 /** 989 * Captures the reason for the current status. 990 */ 991 @Child(name = "statusReason", type = { 992 StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 993 @Description(shortDefinition = "Reason for current status", formalDefinition = "Captures the reason for the current status.") 994 protected StringType statusReason; 995 996 /** 997 * Indicates whose goal this is - patient goal, practitioner goal, etc. 998 */ 999 @Child(name = "expressedBy", type = { Patient.class, Practitioner.class, PractitionerRole.class, 1000 RelatedPerson.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 1001 @Description(shortDefinition = "Who's responsible for creating Goal?", formalDefinition = "Indicates whose goal this is - patient goal, practitioner goal, etc.") 1002 protected Reference expressedBy; 1003 1004 /** 1005 * The actual object that is the target of the reference (Indicates whose goal 1006 * this is - patient goal, practitioner goal, etc.) 1007 */ 1008 protected Resource expressedByTarget; 1009 1010 /** 1011 * The identified conditions and other health record elements that are intended 1012 * to be addressed by the goal. 1013 */ 1014 @Child(name = "addresses", type = { Condition.class, Observation.class, MedicationStatement.class, 1015 NutritionOrder.class, ServiceRequest.class, 1016 RiskAssessment.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1017 @Description(shortDefinition = "Issues addressed by this goal", formalDefinition = "The identified conditions and other health record elements that are intended to be addressed by the goal.") 1018 protected List<Reference> addresses; 1019 /** 1020 * The actual objects that are the target of the reference (The identified 1021 * conditions and other health record elements that are intended to be addressed 1022 * by the goal.) 1023 */ 1024 protected List<Resource> addressesTarget; 1025 1026 /** 1027 * Any comments related to the goal. 1028 */ 1029 @Child(name = "note", type = { 1030 Annotation.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1031 @Description(shortDefinition = "Comments about the goal", formalDefinition = "Any comments related to the goal.") 1032 protected List<Annotation> note; 1033 1034 /** 1035 * Identifies the change (or lack of change) at the point when the status of the 1036 * goal is assessed. 1037 */ 1038 @Child(name = "outcomeCode", type = { 1039 CodeableConcept.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1040 @Description(shortDefinition = "What result was achieved regarding the goal?", formalDefinition = "Identifies the change (or lack of change) at the point when the status of the goal is assessed.") 1041 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/clinical-findings") 1042 protected List<CodeableConcept> outcomeCode; 1043 1044 /** 1045 * Details of what's changed (or not changed). 1046 */ 1047 @Child(name = "outcomeReference", type = { 1048 Observation.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1049 @Description(shortDefinition = "Observation that resulted from goal", formalDefinition = "Details of what's changed (or not changed).") 1050 protected List<Reference> outcomeReference; 1051 /** 1052 * The actual objects that are the target of the reference (Details of what's 1053 * changed (or not changed).) 1054 */ 1055 protected List<Observation> outcomeReferenceTarget; 1056 1057 private static final long serialVersionUID = -1366854797L; 1058 1059 /** 1060 * Constructor 1061 */ 1062 public Goal() { 1063 super(); 1064 } 1065 1066 /** 1067 * Constructor 1068 */ 1069 public Goal(Enumeration<GoalLifecycleStatus> lifecycleStatus, CodeableConcept description, Reference subject) { 1070 super(); 1071 this.lifecycleStatus = lifecycleStatus; 1072 this.description = description; 1073 this.subject = subject; 1074 } 1075 1076 /** 1077 * @return {@link #identifier} (Business identifiers assigned to this goal by 1078 * the performer or other systems which remain constant as the resource 1079 * is updated and propagates from server to server.) 1080 */ 1081 public List<Identifier> getIdentifier() { 1082 if (this.identifier == null) 1083 this.identifier = new ArrayList<Identifier>(); 1084 return this.identifier; 1085 } 1086 1087 /** 1088 * @return Returns a reference to <code>this</code> for easy method chaining 1089 */ 1090 public Goal setIdentifier(List<Identifier> theIdentifier) { 1091 this.identifier = theIdentifier; 1092 return this; 1093 } 1094 1095 public boolean hasIdentifier() { 1096 if (this.identifier == null) 1097 return false; 1098 for (Identifier item : this.identifier) 1099 if (!item.isEmpty()) 1100 return true; 1101 return false; 1102 } 1103 1104 public Identifier addIdentifier() { // 3 1105 Identifier t = new Identifier(); 1106 if (this.identifier == null) 1107 this.identifier = new ArrayList<Identifier>(); 1108 this.identifier.add(t); 1109 return t; 1110 } 1111 1112 public Goal addIdentifier(Identifier t) { // 3 1113 if (t == null) 1114 return this; 1115 if (this.identifier == null) 1116 this.identifier = new ArrayList<Identifier>(); 1117 this.identifier.add(t); 1118 return this; 1119 } 1120 1121 /** 1122 * @return The first repetition of repeating field {@link #identifier}, creating 1123 * it if it does not already exist 1124 */ 1125 public Identifier getIdentifierFirstRep() { 1126 if (getIdentifier().isEmpty()) { 1127 addIdentifier(); 1128 } 1129 return getIdentifier().get(0); 1130 } 1131 1132 /** 1133 * @return {@link #lifecycleStatus} (The state of the goal throughout its 1134 * lifecycle.). This is the underlying object with id, value and 1135 * extensions. The accessor "getLifecycleStatus" gives direct access to 1136 * the value 1137 */ 1138 public Enumeration<GoalLifecycleStatus> getLifecycleStatusElement() { 1139 if (this.lifecycleStatus == null) 1140 if (Configuration.errorOnAutoCreate()) 1141 throw new Error("Attempt to auto-create Goal.lifecycleStatus"); 1142 else if (Configuration.doAutoCreate()) 1143 this.lifecycleStatus = new Enumeration<GoalLifecycleStatus>(new GoalLifecycleStatusEnumFactory()); // bb 1144 return this.lifecycleStatus; 1145 } 1146 1147 public boolean hasLifecycleStatusElement() { 1148 return this.lifecycleStatus != null && !this.lifecycleStatus.isEmpty(); 1149 } 1150 1151 public boolean hasLifecycleStatus() { 1152 return this.lifecycleStatus != null && !this.lifecycleStatus.isEmpty(); 1153 } 1154 1155 /** 1156 * @param value {@link #lifecycleStatus} (The state of the goal throughout its 1157 * lifecycle.). This is the underlying object with id, value and 1158 * extensions. The accessor "getLifecycleStatus" gives direct 1159 * access to the value 1160 */ 1161 public Goal setLifecycleStatusElement(Enumeration<GoalLifecycleStatus> value) { 1162 this.lifecycleStatus = value; 1163 return this; 1164 } 1165 1166 /** 1167 * @return The state of the goal throughout its lifecycle. 1168 */ 1169 public GoalLifecycleStatus getLifecycleStatus() { 1170 return this.lifecycleStatus == null ? null : this.lifecycleStatus.getValue(); 1171 } 1172 1173 /** 1174 * @param value The state of the goal throughout its lifecycle. 1175 */ 1176 public Goal setLifecycleStatus(GoalLifecycleStatus value) { 1177 if (this.lifecycleStatus == null) 1178 this.lifecycleStatus = new Enumeration<GoalLifecycleStatus>(new GoalLifecycleStatusEnumFactory()); 1179 this.lifecycleStatus.setValue(value); 1180 return this; 1181 } 1182 1183 /** 1184 * @return {@link #achievementStatus} (Describes the progression, or lack 1185 * thereof, towards the goal against the target.) 1186 */ 1187 public CodeableConcept getAchievementStatus() { 1188 if (this.achievementStatus == null) 1189 if (Configuration.errorOnAutoCreate()) 1190 throw new Error("Attempt to auto-create Goal.achievementStatus"); 1191 else if (Configuration.doAutoCreate()) 1192 this.achievementStatus = new CodeableConcept(); // cc 1193 return this.achievementStatus; 1194 } 1195 1196 public boolean hasAchievementStatus() { 1197 return this.achievementStatus != null && !this.achievementStatus.isEmpty(); 1198 } 1199 1200 /** 1201 * @param value {@link #achievementStatus} (Describes the progression, or lack 1202 * thereof, towards the goal against the target.) 1203 */ 1204 public Goal setAchievementStatus(CodeableConcept value) { 1205 this.achievementStatus = value; 1206 return this; 1207 } 1208 1209 /** 1210 * @return {@link #category} (Indicates a category the goal falls within.) 1211 */ 1212 public List<CodeableConcept> getCategory() { 1213 if (this.category == null) 1214 this.category = new ArrayList<CodeableConcept>(); 1215 return this.category; 1216 } 1217 1218 /** 1219 * @return Returns a reference to <code>this</code> for easy method chaining 1220 */ 1221 public Goal setCategory(List<CodeableConcept> theCategory) { 1222 this.category = theCategory; 1223 return this; 1224 } 1225 1226 public boolean hasCategory() { 1227 if (this.category == null) 1228 return false; 1229 for (CodeableConcept item : this.category) 1230 if (!item.isEmpty()) 1231 return true; 1232 return false; 1233 } 1234 1235 public CodeableConcept addCategory() { // 3 1236 CodeableConcept t = new CodeableConcept(); 1237 if (this.category == null) 1238 this.category = new ArrayList<CodeableConcept>(); 1239 this.category.add(t); 1240 return t; 1241 } 1242 1243 public Goal addCategory(CodeableConcept t) { // 3 1244 if (t == null) 1245 return this; 1246 if (this.category == null) 1247 this.category = new ArrayList<CodeableConcept>(); 1248 this.category.add(t); 1249 return this; 1250 } 1251 1252 /** 1253 * @return The first repetition of repeating field {@link #category}, creating 1254 * it if it does not already exist 1255 */ 1256 public CodeableConcept getCategoryFirstRep() { 1257 if (getCategory().isEmpty()) { 1258 addCategory(); 1259 } 1260 return getCategory().get(0); 1261 } 1262 1263 /** 1264 * @return {@link #priority} (Identifies the mutually agreed level of importance 1265 * associated with reaching/sustaining the goal.) 1266 */ 1267 public CodeableConcept getPriority() { 1268 if (this.priority == null) 1269 if (Configuration.errorOnAutoCreate()) 1270 throw new Error("Attempt to auto-create Goal.priority"); 1271 else if (Configuration.doAutoCreate()) 1272 this.priority = new CodeableConcept(); // cc 1273 return this.priority; 1274 } 1275 1276 public boolean hasPriority() { 1277 return this.priority != null && !this.priority.isEmpty(); 1278 } 1279 1280 /** 1281 * @param value {@link #priority} (Identifies the mutually agreed level of 1282 * importance associated with reaching/sustaining the goal.) 1283 */ 1284 public Goal setPriority(CodeableConcept value) { 1285 this.priority = value; 1286 return this; 1287 } 1288 1289 /** 1290 * @return {@link #description} (Human-readable and/or coded description of a 1291 * specific desired objective of care, such as "control blood pressure" 1292 * or "negotiate an obstacle course" or "dance with child at wedding".) 1293 */ 1294 public CodeableConcept getDescription() { 1295 if (this.description == null) 1296 if (Configuration.errorOnAutoCreate()) 1297 throw new Error("Attempt to auto-create Goal.description"); 1298 else if (Configuration.doAutoCreate()) 1299 this.description = new CodeableConcept(); // cc 1300 return this.description; 1301 } 1302 1303 public boolean hasDescription() { 1304 return this.description != null && !this.description.isEmpty(); 1305 } 1306 1307 /** 1308 * @param value {@link #description} (Human-readable and/or coded description of 1309 * a specific desired objective of care, such as "control blood 1310 * pressure" or "negotiate an obstacle course" or "dance with child 1311 * at wedding".) 1312 */ 1313 public Goal setDescription(CodeableConcept value) { 1314 this.description = value; 1315 return this; 1316 } 1317 1318 /** 1319 * @return {@link #subject} (Identifies the patient, group or organization for 1320 * whom the goal is being established.) 1321 */ 1322 public Reference getSubject() { 1323 if (this.subject == null) 1324 if (Configuration.errorOnAutoCreate()) 1325 throw new Error("Attempt to auto-create Goal.subject"); 1326 else if (Configuration.doAutoCreate()) 1327 this.subject = new Reference(); // cc 1328 return this.subject; 1329 } 1330 1331 public boolean hasSubject() { 1332 return this.subject != null && !this.subject.isEmpty(); 1333 } 1334 1335 /** 1336 * @param value {@link #subject} (Identifies the patient, group or organization 1337 * for whom the goal is being established.) 1338 */ 1339 public Goal setSubject(Reference value) { 1340 this.subject = value; 1341 return this; 1342 } 1343 1344 /** 1345 * @return {@link #subject} The actual object that is the target of the 1346 * reference. The reference library doesn't populate this, but you can 1347 * use it to hold the resource if you resolve it. (Identifies the 1348 * patient, group or organization for whom the goal is being 1349 * established.) 1350 */ 1351 public Resource getSubjectTarget() { 1352 return this.subjectTarget; 1353 } 1354 1355 /** 1356 * @param value {@link #subject} The actual object that is the target of the 1357 * reference. The reference library doesn't use these, but you can 1358 * use it to hold the resource if you resolve it. (Identifies the 1359 * patient, group or organization for whom the goal is being 1360 * established.) 1361 */ 1362 public Goal setSubjectTarget(Resource value) { 1363 this.subjectTarget = value; 1364 return this; 1365 } 1366 1367 /** 1368 * @return {@link #start} (The date or event after which the goal should begin 1369 * being pursued.) 1370 */ 1371 public Type getStart() { 1372 return this.start; 1373 } 1374 1375 /** 1376 * @return {@link #start} (The date or event after which the goal should begin 1377 * being pursued.) 1378 */ 1379 public DateType getStartDateType() throws FHIRException { 1380 if (this.start == null) 1381 this.start = new DateType(); 1382 if (!(this.start instanceof DateType)) 1383 throw new FHIRException( 1384 "Type mismatch: the type DateType was expected, but " + this.start.getClass().getName() + " was encountered"); 1385 return (DateType) this.start; 1386 } 1387 1388 public boolean hasStartDateType() { 1389 return this != null && this.start instanceof DateType; 1390 } 1391 1392 /** 1393 * @return {@link #start} (The date or event after which the goal should begin 1394 * being pursued.) 1395 */ 1396 public CodeableConcept getStartCodeableConcept() throws FHIRException { 1397 if (this.start == null) 1398 this.start = new CodeableConcept(); 1399 if (!(this.start instanceof CodeableConcept)) 1400 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1401 + this.start.getClass().getName() + " was encountered"); 1402 return (CodeableConcept) this.start; 1403 } 1404 1405 public boolean hasStartCodeableConcept() { 1406 return this != null && this.start instanceof CodeableConcept; 1407 } 1408 1409 public boolean hasStart() { 1410 return this.start != null && !this.start.isEmpty(); 1411 } 1412 1413 /** 1414 * @param value {@link #start} (The date or event after which the goal should 1415 * begin being pursued.) 1416 */ 1417 public Goal setStart(Type value) { 1418 if (value != null && !(value instanceof DateType || value instanceof CodeableConcept)) 1419 throw new Error("Not the right type for Goal.start[x]: " + value.fhirType()); 1420 this.start = value; 1421 return this; 1422 } 1423 1424 /** 1425 * @return {@link #target} (Indicates what should be done by when.) 1426 */ 1427 public List<GoalTargetComponent> getTarget() { 1428 if (this.target == null) 1429 this.target = new ArrayList<GoalTargetComponent>(); 1430 return this.target; 1431 } 1432 1433 /** 1434 * @return Returns a reference to <code>this</code> for easy method chaining 1435 */ 1436 public Goal setTarget(List<GoalTargetComponent> theTarget) { 1437 this.target = theTarget; 1438 return this; 1439 } 1440 1441 public boolean hasTarget() { 1442 if (this.target == null) 1443 return false; 1444 for (GoalTargetComponent item : this.target) 1445 if (!item.isEmpty()) 1446 return true; 1447 return false; 1448 } 1449 1450 public GoalTargetComponent addTarget() { // 3 1451 GoalTargetComponent t = new GoalTargetComponent(); 1452 if (this.target == null) 1453 this.target = new ArrayList<GoalTargetComponent>(); 1454 this.target.add(t); 1455 return t; 1456 } 1457 1458 public Goal addTarget(GoalTargetComponent t) { // 3 1459 if (t == null) 1460 return this; 1461 if (this.target == null) 1462 this.target = new ArrayList<GoalTargetComponent>(); 1463 this.target.add(t); 1464 return this; 1465 } 1466 1467 /** 1468 * @return The first repetition of repeating field {@link #target}, creating it 1469 * if it does not already exist 1470 */ 1471 public GoalTargetComponent getTargetFirstRep() { 1472 if (getTarget().isEmpty()) { 1473 addTarget(); 1474 } 1475 return getTarget().get(0); 1476 } 1477 1478 /** 1479 * @return {@link #statusDate} (Identifies when the current status. I.e. When 1480 * initially created, when achieved, when cancelled, etc.). This is the 1481 * underlying object with id, value and extensions. The accessor 1482 * "getStatusDate" gives direct access to the value 1483 */ 1484 public DateType getStatusDateElement() { 1485 if (this.statusDate == null) 1486 if (Configuration.errorOnAutoCreate()) 1487 throw new Error("Attempt to auto-create Goal.statusDate"); 1488 else if (Configuration.doAutoCreate()) 1489 this.statusDate = new DateType(); // bb 1490 return this.statusDate; 1491 } 1492 1493 public boolean hasStatusDateElement() { 1494 return this.statusDate != null && !this.statusDate.isEmpty(); 1495 } 1496 1497 public boolean hasStatusDate() { 1498 return this.statusDate != null && !this.statusDate.isEmpty(); 1499 } 1500 1501 /** 1502 * @param value {@link #statusDate} (Identifies when the current status. I.e. 1503 * When initially created, when achieved, when cancelled, etc.). 1504 * This is the underlying object with id, value and extensions. The 1505 * accessor "getStatusDate" gives direct access to the value 1506 */ 1507 public Goal setStatusDateElement(DateType value) { 1508 this.statusDate = value; 1509 return this; 1510 } 1511 1512 /** 1513 * @return Identifies when the current status. I.e. When initially created, when 1514 * achieved, when cancelled, etc. 1515 */ 1516 public Date getStatusDate() { 1517 return this.statusDate == null ? null : this.statusDate.getValue(); 1518 } 1519 1520 /** 1521 * @param value Identifies when the current status. I.e. When initially created, 1522 * when achieved, when cancelled, etc. 1523 */ 1524 public Goal setStatusDate(Date value) { 1525 if (value == null) 1526 this.statusDate = null; 1527 else { 1528 if (this.statusDate == null) 1529 this.statusDate = new DateType(); 1530 this.statusDate.setValue(value); 1531 } 1532 return this; 1533 } 1534 1535 /** 1536 * @return {@link #statusReason} (Captures the reason for the current status.). 1537 * This is the underlying object with id, value and extensions. The 1538 * accessor "getStatusReason" gives direct access to the value 1539 */ 1540 public StringType getStatusReasonElement() { 1541 if (this.statusReason == null) 1542 if (Configuration.errorOnAutoCreate()) 1543 throw new Error("Attempt to auto-create Goal.statusReason"); 1544 else if (Configuration.doAutoCreate()) 1545 this.statusReason = new StringType(); // bb 1546 return this.statusReason; 1547 } 1548 1549 public boolean hasStatusReasonElement() { 1550 return this.statusReason != null && !this.statusReason.isEmpty(); 1551 } 1552 1553 public boolean hasStatusReason() { 1554 return this.statusReason != null && !this.statusReason.isEmpty(); 1555 } 1556 1557 /** 1558 * @param value {@link #statusReason} (Captures the reason for the current 1559 * status.). This is the underlying object with id, value and 1560 * extensions. The accessor "getStatusReason" gives direct access 1561 * to the value 1562 */ 1563 public Goal setStatusReasonElement(StringType value) { 1564 this.statusReason = value; 1565 return this; 1566 } 1567 1568 /** 1569 * @return Captures the reason for the current status. 1570 */ 1571 public String getStatusReason() { 1572 return this.statusReason == null ? null : this.statusReason.getValue(); 1573 } 1574 1575 /** 1576 * @param value Captures the reason for the current status. 1577 */ 1578 public Goal setStatusReason(String value) { 1579 if (Utilities.noString(value)) 1580 this.statusReason = null; 1581 else { 1582 if (this.statusReason == null) 1583 this.statusReason = new StringType(); 1584 this.statusReason.setValue(value); 1585 } 1586 return this; 1587 } 1588 1589 /** 1590 * @return {@link #expressedBy} (Indicates whose goal this is - patient goal, 1591 * practitioner goal, etc.) 1592 */ 1593 public Reference getExpressedBy() { 1594 if (this.expressedBy == null) 1595 if (Configuration.errorOnAutoCreate()) 1596 throw new Error("Attempt to auto-create Goal.expressedBy"); 1597 else if (Configuration.doAutoCreate()) 1598 this.expressedBy = new Reference(); // cc 1599 return this.expressedBy; 1600 } 1601 1602 public boolean hasExpressedBy() { 1603 return this.expressedBy != null && !this.expressedBy.isEmpty(); 1604 } 1605 1606 /** 1607 * @param value {@link #expressedBy} (Indicates whose goal this is - patient 1608 * goal, practitioner goal, etc.) 1609 */ 1610 public Goal setExpressedBy(Reference value) { 1611 this.expressedBy = value; 1612 return this; 1613 } 1614 1615 /** 1616 * @return {@link #expressedBy} The actual object that is the target of the 1617 * reference. The reference library doesn't populate this, but you can 1618 * use it to hold the resource if you resolve it. (Indicates whose goal 1619 * this is - patient goal, practitioner goal, etc.) 1620 */ 1621 public Resource getExpressedByTarget() { 1622 return this.expressedByTarget; 1623 } 1624 1625 /** 1626 * @param value {@link #expressedBy} The actual object that is the target of the 1627 * reference. The reference library doesn't use these, but you can 1628 * use it to hold the resource if you resolve it. (Indicates whose 1629 * goal this is - patient goal, practitioner goal, etc.) 1630 */ 1631 public Goal setExpressedByTarget(Resource value) { 1632 this.expressedByTarget = value; 1633 return this; 1634 } 1635 1636 /** 1637 * @return {@link #addresses} (The identified conditions and other health record 1638 * elements that are intended to be addressed by the goal.) 1639 */ 1640 public List<Reference> getAddresses() { 1641 if (this.addresses == null) 1642 this.addresses = new ArrayList<Reference>(); 1643 return this.addresses; 1644 } 1645 1646 /** 1647 * @return Returns a reference to <code>this</code> for easy method chaining 1648 */ 1649 public Goal setAddresses(List<Reference> theAddresses) { 1650 this.addresses = theAddresses; 1651 return this; 1652 } 1653 1654 public boolean hasAddresses() { 1655 if (this.addresses == null) 1656 return false; 1657 for (Reference item : this.addresses) 1658 if (!item.isEmpty()) 1659 return true; 1660 return false; 1661 } 1662 1663 public Reference addAddresses() { // 3 1664 Reference t = new Reference(); 1665 if (this.addresses == null) 1666 this.addresses = new ArrayList<Reference>(); 1667 this.addresses.add(t); 1668 return t; 1669 } 1670 1671 public Goal addAddresses(Reference t) { // 3 1672 if (t == null) 1673 return this; 1674 if (this.addresses == null) 1675 this.addresses = new ArrayList<Reference>(); 1676 this.addresses.add(t); 1677 return this; 1678 } 1679 1680 /** 1681 * @return The first repetition of repeating field {@link #addresses}, creating 1682 * it if it does not already exist 1683 */ 1684 public Reference getAddressesFirstRep() { 1685 if (getAddresses().isEmpty()) { 1686 addAddresses(); 1687 } 1688 return getAddresses().get(0); 1689 } 1690 1691 /** 1692 * @deprecated Use Reference#setResource(IBaseResource) instead 1693 */ 1694 @Deprecated 1695 public List<Resource> getAddressesTarget() { 1696 if (this.addressesTarget == null) 1697 this.addressesTarget = new ArrayList<Resource>(); 1698 return this.addressesTarget; 1699 } 1700 1701 /** 1702 * @return {@link #note} (Any comments related to the goal.) 1703 */ 1704 public List<Annotation> getNote() { 1705 if (this.note == null) 1706 this.note = new ArrayList<Annotation>(); 1707 return this.note; 1708 } 1709 1710 /** 1711 * @return Returns a reference to <code>this</code> for easy method chaining 1712 */ 1713 public Goal setNote(List<Annotation> theNote) { 1714 this.note = theNote; 1715 return this; 1716 } 1717 1718 public boolean hasNote() { 1719 if (this.note == null) 1720 return false; 1721 for (Annotation item : this.note) 1722 if (!item.isEmpty()) 1723 return true; 1724 return false; 1725 } 1726 1727 public Annotation addNote() { // 3 1728 Annotation t = new Annotation(); 1729 if (this.note == null) 1730 this.note = new ArrayList<Annotation>(); 1731 this.note.add(t); 1732 return t; 1733 } 1734 1735 public Goal addNote(Annotation t) { // 3 1736 if (t == null) 1737 return this; 1738 if (this.note == null) 1739 this.note = new ArrayList<Annotation>(); 1740 this.note.add(t); 1741 return this; 1742 } 1743 1744 /** 1745 * @return The first repetition of repeating field {@link #note}, creating it if 1746 * it does not already exist 1747 */ 1748 public Annotation getNoteFirstRep() { 1749 if (getNote().isEmpty()) { 1750 addNote(); 1751 } 1752 return getNote().get(0); 1753 } 1754 1755 /** 1756 * @return {@link #outcomeCode} (Identifies the change (or lack of change) at 1757 * the point when the status of the goal is assessed.) 1758 */ 1759 public List<CodeableConcept> getOutcomeCode() { 1760 if (this.outcomeCode == null) 1761 this.outcomeCode = new ArrayList<CodeableConcept>(); 1762 return this.outcomeCode; 1763 } 1764 1765 /** 1766 * @return Returns a reference to <code>this</code> for easy method chaining 1767 */ 1768 public Goal setOutcomeCode(List<CodeableConcept> theOutcomeCode) { 1769 this.outcomeCode = theOutcomeCode; 1770 return this; 1771 } 1772 1773 public boolean hasOutcomeCode() { 1774 if (this.outcomeCode == null) 1775 return false; 1776 for (CodeableConcept item : this.outcomeCode) 1777 if (!item.isEmpty()) 1778 return true; 1779 return false; 1780 } 1781 1782 public CodeableConcept addOutcomeCode() { // 3 1783 CodeableConcept t = new CodeableConcept(); 1784 if (this.outcomeCode == null) 1785 this.outcomeCode = new ArrayList<CodeableConcept>(); 1786 this.outcomeCode.add(t); 1787 return t; 1788 } 1789 1790 public Goal addOutcomeCode(CodeableConcept t) { // 3 1791 if (t == null) 1792 return this; 1793 if (this.outcomeCode == null) 1794 this.outcomeCode = new ArrayList<CodeableConcept>(); 1795 this.outcomeCode.add(t); 1796 return this; 1797 } 1798 1799 /** 1800 * @return The first repetition of repeating field {@link #outcomeCode}, 1801 * creating it if it does not already exist 1802 */ 1803 public CodeableConcept getOutcomeCodeFirstRep() { 1804 if (getOutcomeCode().isEmpty()) { 1805 addOutcomeCode(); 1806 } 1807 return getOutcomeCode().get(0); 1808 } 1809 1810 /** 1811 * @return {@link #outcomeReference} (Details of what's changed (or not 1812 * changed).) 1813 */ 1814 public List<Reference> getOutcomeReference() { 1815 if (this.outcomeReference == null) 1816 this.outcomeReference = new ArrayList<Reference>(); 1817 return this.outcomeReference; 1818 } 1819 1820 /** 1821 * @return Returns a reference to <code>this</code> for easy method chaining 1822 */ 1823 public Goal setOutcomeReference(List<Reference> theOutcomeReference) { 1824 this.outcomeReference = theOutcomeReference; 1825 return this; 1826 } 1827 1828 public boolean hasOutcomeReference() { 1829 if (this.outcomeReference == null) 1830 return false; 1831 for (Reference item : this.outcomeReference) 1832 if (!item.isEmpty()) 1833 return true; 1834 return false; 1835 } 1836 1837 public Reference addOutcomeReference() { // 3 1838 Reference t = new Reference(); 1839 if (this.outcomeReference == null) 1840 this.outcomeReference = new ArrayList<Reference>(); 1841 this.outcomeReference.add(t); 1842 return t; 1843 } 1844 1845 public Goal addOutcomeReference(Reference t) { // 3 1846 if (t == null) 1847 return this; 1848 if (this.outcomeReference == null) 1849 this.outcomeReference = new ArrayList<Reference>(); 1850 this.outcomeReference.add(t); 1851 return this; 1852 } 1853 1854 /** 1855 * @return The first repetition of repeating field {@link #outcomeReference}, 1856 * creating it if it does not already exist 1857 */ 1858 public Reference getOutcomeReferenceFirstRep() { 1859 if (getOutcomeReference().isEmpty()) { 1860 addOutcomeReference(); 1861 } 1862 return getOutcomeReference().get(0); 1863 } 1864 1865 /** 1866 * @deprecated Use Reference#setResource(IBaseResource) instead 1867 */ 1868 @Deprecated 1869 public List<Observation> getOutcomeReferenceTarget() { 1870 if (this.outcomeReferenceTarget == null) 1871 this.outcomeReferenceTarget = new ArrayList<Observation>(); 1872 return this.outcomeReferenceTarget; 1873 } 1874 1875 /** 1876 * @deprecated Use Reference#setResource(IBaseResource) instead 1877 */ 1878 @Deprecated 1879 public Observation addOutcomeReferenceTarget() { 1880 Observation r = new Observation(); 1881 if (this.outcomeReferenceTarget == null) 1882 this.outcomeReferenceTarget = new ArrayList<Observation>(); 1883 this.outcomeReferenceTarget.add(r); 1884 return r; 1885 } 1886 1887 protected void listChildren(List<Property> children) { 1888 super.listChildren(children); 1889 children.add(new Property("identifier", "Identifier", 1890 "Business identifiers assigned to this goal by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 1891 0, java.lang.Integer.MAX_VALUE, identifier)); 1892 children.add(new Property("lifecycleStatus", "code", "The state of the goal throughout its lifecycle.", 0, 1, 1893 lifecycleStatus)); 1894 children.add(new Property("achievementStatus", "CodeableConcept", 1895 "Describes the progression, or lack thereof, towards the goal against the target.", 0, 1, achievementStatus)); 1896 children.add(new Property("category", "CodeableConcept", "Indicates a category the goal falls within.", 0, 1897 java.lang.Integer.MAX_VALUE, category)); 1898 children.add(new Property("priority", "CodeableConcept", 1899 "Identifies the mutually agreed level of importance associated with reaching/sustaining the goal.", 0, 1, 1900 priority)); 1901 children.add(new Property("description", "CodeableConcept", 1902 "Human-readable and/or coded description of a specific desired objective of care, such as \"control blood pressure\" or \"negotiate an obstacle course\" or \"dance with child at wedding\".", 1903 0, 1, description)); 1904 children.add(new Property("subject", "Reference(Patient|Group|Organization)", 1905 "Identifies the patient, group or organization for whom the goal is being established.", 0, 1, subject)); 1906 children.add(new Property("start[x]", "date|CodeableConcept", 1907 "The date or event after which the goal should begin being pursued.", 0, 1, start)); 1908 children.add( 1909 new Property("target", "", "Indicates what should be done by when.", 0, java.lang.Integer.MAX_VALUE, target)); 1910 children.add(new Property("statusDate", "date", 1911 "Identifies when the current status. I.e. When initially created, when achieved, when cancelled, etc.", 0, 1, 1912 statusDate)); 1913 children 1914 .add(new Property("statusReason", "string", "Captures the reason for the current status.", 0, 1, statusReason)); 1915 children.add(new Property("expressedBy", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson)", 1916 "Indicates whose goal this is - patient goal, practitioner goal, etc.", 0, 1, expressedBy)); 1917 children.add(new Property("addresses", 1918 "Reference(Condition|Observation|MedicationStatement|NutritionOrder|ServiceRequest|RiskAssessment)", 1919 "The identified conditions and other health record elements that are intended to be addressed by the goal.", 0, 1920 java.lang.Integer.MAX_VALUE, addresses)); 1921 children.add( 1922 new Property("note", "Annotation", "Any comments related to the goal.", 0, java.lang.Integer.MAX_VALUE, note)); 1923 children.add(new Property("outcomeCode", "CodeableConcept", 1924 "Identifies the change (or lack of change) at the point when the status of the goal is assessed.", 0, 1925 java.lang.Integer.MAX_VALUE, outcomeCode)); 1926 children.add(new Property("outcomeReference", "Reference(Observation)", 1927 "Details of what's changed (or not changed).", 0, java.lang.Integer.MAX_VALUE, outcomeReference)); 1928 } 1929 1930 @Override 1931 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1932 switch (_hash) { 1933 case -1618432855: 1934 /* identifier */ return new Property("identifier", "Identifier", 1935 "Business identifiers assigned to this goal by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 1936 0, java.lang.Integer.MAX_VALUE, identifier); 1937 case 1165552636: 1938 /* lifecycleStatus */ return new Property("lifecycleStatus", "code", 1939 "The state of the goal throughout its lifecycle.", 0, 1, lifecycleStatus); 1940 case 104524801: 1941 /* achievementStatus */ return new Property("achievementStatus", "CodeableConcept", 1942 "Describes the progression, or lack thereof, towards the goal against the target.", 0, 1, achievementStatus); 1943 case 50511102: 1944 /* category */ return new Property("category", "CodeableConcept", "Indicates a category the goal falls within.", 1945 0, java.lang.Integer.MAX_VALUE, category); 1946 case -1165461084: 1947 /* priority */ return new Property("priority", "CodeableConcept", 1948 "Identifies the mutually agreed level of importance associated with reaching/sustaining the goal.", 0, 1, 1949 priority); 1950 case -1724546052: 1951 /* description */ return new Property("description", "CodeableConcept", 1952 "Human-readable and/or coded description of a specific desired objective of care, such as \"control blood pressure\" or \"negotiate an obstacle course\" or \"dance with child at wedding\".", 1953 0, 1, description); 1954 case -1867885268: 1955 /* subject */ return new Property("subject", "Reference(Patient|Group|Organization)", 1956 "Identifies the patient, group or organization for whom the goal is being established.", 0, 1, subject); 1957 case 1316793566: 1958 /* start[x] */ return new Property("start[x]", "date|CodeableConcept", 1959 "The date or event after which the goal should begin being pursued.", 0, 1, start); 1960 case 109757538: 1961 /* start */ return new Property("start[x]", "date|CodeableConcept", 1962 "The date or event after which the goal should begin being pursued.", 0, 1, start); 1963 case -2129778896: 1964 /* startDate */ return new Property("start[x]", "date|CodeableConcept", 1965 "The date or event after which the goal should begin being pursued.", 0, 1, start); 1966 case -1758833953: 1967 /* startCodeableConcept */ return new Property("start[x]", "date|CodeableConcept", 1968 "The date or event after which the goal should begin being pursued.", 0, 1, start); 1969 case -880905839: 1970 /* target */ return new Property("target", "", "Indicates what should be done by when.", 0, 1971 java.lang.Integer.MAX_VALUE, target); 1972 case 247524032: 1973 /* statusDate */ return new Property("statusDate", "date", 1974 "Identifies when the current status. I.e. When initially created, when achieved, when cancelled, etc.", 0, 1, 1975 statusDate); 1976 case 2051346646: 1977 /* statusReason */ return new Property("statusReason", "string", "Captures the reason for the current status.", 0, 1978 1, statusReason); 1979 case 175423686: 1980 /* expressedBy */ return new Property("expressedBy", 1981 "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson)", 1982 "Indicates whose goal this is - patient goal, practitioner goal, etc.", 0, 1, expressedBy); 1983 case 874544034: 1984 /* addresses */ return new Property("addresses", 1985 "Reference(Condition|Observation|MedicationStatement|NutritionOrder|ServiceRequest|RiskAssessment)", 1986 "The identified conditions and other health record elements that are intended to be addressed by the goal.", 1987 0, java.lang.Integer.MAX_VALUE, addresses); 1988 case 3387378: 1989 /* note */ return new Property("note", "Annotation", "Any comments related to the goal.", 0, 1990 java.lang.Integer.MAX_VALUE, note); 1991 case 1062482015: 1992 /* outcomeCode */ return new Property("outcomeCode", "CodeableConcept", 1993 "Identifies the change (or lack of change) at the point when the status of the goal is assessed.", 0, 1994 java.lang.Integer.MAX_VALUE, outcomeCode); 1995 case -782273511: 1996 /* outcomeReference */ return new Property("outcomeReference", "Reference(Observation)", 1997 "Details of what's changed (or not changed).", 0, java.lang.Integer.MAX_VALUE, outcomeReference); 1998 default: 1999 return super.getNamedProperty(_hash, _name, _checkValid); 2000 } 2001 2002 } 2003 2004 @Override 2005 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2006 switch (hash) { 2007 case -1618432855: 2008 /* identifier */ return this.identifier == null ? new Base[0] 2009 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2010 case 1165552636: 2011 /* lifecycleStatus */ return this.lifecycleStatus == null ? new Base[0] : new Base[] { this.lifecycleStatus }; // Enumeration<GoalLifecycleStatus> 2012 case 104524801: 2013 /* achievementStatus */ return this.achievementStatus == null ? new Base[0] 2014 : new Base[] { this.achievementStatus }; // CodeableConcept 2015 case 50511102: 2016 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2017 case -1165461084: 2018 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // CodeableConcept 2019 case -1724546052: 2020 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // CodeableConcept 2021 case -1867885268: 2022 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2023 case 109757538: 2024 /* start */ return this.start == null ? new Base[0] : new Base[] { this.start }; // Type 2025 case -880905839: 2026 /* target */ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // GoalTargetComponent 2027 case 247524032: 2028 /* statusDate */ return this.statusDate == null ? new Base[0] : new Base[] { this.statusDate }; // DateType 2029 case 2051346646: 2030 /* statusReason */ return this.statusReason == null ? new Base[0] : new Base[] { this.statusReason }; // StringType 2031 case 175423686: 2032 /* expressedBy */ return this.expressedBy == null ? new Base[0] : new Base[] { this.expressedBy }; // Reference 2033 case 874544034: 2034 /* addresses */ return this.addresses == null ? new Base[0] 2035 : this.addresses.toArray(new Base[this.addresses.size()]); // Reference 2036 case 3387378: 2037 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2038 case 1062482015: 2039 /* outcomeCode */ return this.outcomeCode == null ? new Base[0] 2040 : this.outcomeCode.toArray(new Base[this.outcomeCode.size()]); // CodeableConcept 2041 case -782273511: 2042 /* outcomeReference */ return this.outcomeReference == null ? new Base[0] 2043 : this.outcomeReference.toArray(new Base[this.outcomeReference.size()]); // Reference 2044 default: 2045 return super.getProperty(hash, name, checkValid); 2046 } 2047 2048 } 2049 2050 @Override 2051 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2052 switch (hash) { 2053 case -1618432855: // identifier 2054 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2055 return value; 2056 case 1165552636: // lifecycleStatus 2057 value = new GoalLifecycleStatusEnumFactory().fromType(castToCode(value)); 2058 this.lifecycleStatus = (Enumeration) value; // Enumeration<GoalLifecycleStatus> 2059 return value; 2060 case 104524801: // achievementStatus 2061 this.achievementStatus = castToCodeableConcept(value); // CodeableConcept 2062 return value; 2063 case 50511102: // category 2064 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 2065 return value; 2066 case -1165461084: // priority 2067 this.priority = castToCodeableConcept(value); // CodeableConcept 2068 return value; 2069 case -1724546052: // description 2070 this.description = castToCodeableConcept(value); // CodeableConcept 2071 return value; 2072 case -1867885268: // subject 2073 this.subject = castToReference(value); // Reference 2074 return value; 2075 case 109757538: // start 2076 this.start = castToType(value); // Type 2077 return value; 2078 case -880905839: // target 2079 this.getTarget().add((GoalTargetComponent) value); // GoalTargetComponent 2080 return value; 2081 case 247524032: // statusDate 2082 this.statusDate = castToDate(value); // DateType 2083 return value; 2084 case 2051346646: // statusReason 2085 this.statusReason = castToString(value); // StringType 2086 return value; 2087 case 175423686: // expressedBy 2088 this.expressedBy = castToReference(value); // Reference 2089 return value; 2090 case 874544034: // addresses 2091 this.getAddresses().add(castToReference(value)); // Reference 2092 return value; 2093 case 3387378: // note 2094 this.getNote().add(castToAnnotation(value)); // Annotation 2095 return value; 2096 case 1062482015: // outcomeCode 2097 this.getOutcomeCode().add(castToCodeableConcept(value)); // CodeableConcept 2098 return value; 2099 case -782273511: // outcomeReference 2100 this.getOutcomeReference().add(castToReference(value)); // Reference 2101 return value; 2102 default: 2103 return super.setProperty(hash, name, value); 2104 } 2105 2106 } 2107 2108 @Override 2109 public Base setProperty(String name, Base value) throws FHIRException { 2110 if (name.equals("identifier")) { 2111 this.getIdentifier().add(castToIdentifier(value)); 2112 } else if (name.equals("lifecycleStatus")) { 2113 value = new GoalLifecycleStatusEnumFactory().fromType(castToCode(value)); 2114 this.lifecycleStatus = (Enumeration) value; // Enumeration<GoalLifecycleStatus> 2115 } else if (name.equals("achievementStatus")) { 2116 this.achievementStatus = castToCodeableConcept(value); // CodeableConcept 2117 } else if (name.equals("category")) { 2118 this.getCategory().add(castToCodeableConcept(value)); 2119 } else if (name.equals("priority")) { 2120 this.priority = castToCodeableConcept(value); // CodeableConcept 2121 } else if (name.equals("description")) { 2122 this.description = castToCodeableConcept(value); // CodeableConcept 2123 } else if (name.equals("subject")) { 2124 this.subject = castToReference(value); // Reference 2125 } else if (name.equals("start[x]")) { 2126 this.start = castToType(value); // Type 2127 } else if (name.equals("target")) { 2128 this.getTarget().add((GoalTargetComponent) value); 2129 } else if (name.equals("statusDate")) { 2130 this.statusDate = castToDate(value); // DateType 2131 } else if (name.equals("statusReason")) { 2132 this.statusReason = castToString(value); // StringType 2133 } else if (name.equals("expressedBy")) { 2134 this.expressedBy = castToReference(value); // Reference 2135 } else if (name.equals("addresses")) { 2136 this.getAddresses().add(castToReference(value)); 2137 } else if (name.equals("note")) { 2138 this.getNote().add(castToAnnotation(value)); 2139 } else if (name.equals("outcomeCode")) { 2140 this.getOutcomeCode().add(castToCodeableConcept(value)); 2141 } else if (name.equals("outcomeReference")) { 2142 this.getOutcomeReference().add(castToReference(value)); 2143 } else 2144 return super.setProperty(name, value); 2145 return value; 2146 } 2147 2148 @Override 2149 public void removeChild(String name, Base value) throws FHIRException { 2150 if (name.equals("identifier")) { 2151 this.getIdentifier().remove(castToIdentifier(value)); 2152 } else if (name.equals("lifecycleStatus")) { 2153 this.lifecycleStatus = null; 2154 } else if (name.equals("achievementStatus")) { 2155 this.achievementStatus = null; 2156 } else if (name.equals("category")) { 2157 this.getCategory().remove(castToCodeableConcept(value)); 2158 } else if (name.equals("priority")) { 2159 this.priority = null; 2160 } else if (name.equals("description")) { 2161 this.description = null; 2162 } else if (name.equals("subject")) { 2163 this.subject = null; 2164 } else if (name.equals("start[x]")) { 2165 this.start = null; 2166 } else if (name.equals("target")) { 2167 this.getTarget().remove((GoalTargetComponent) value); 2168 } else if (name.equals("statusDate")) { 2169 this.statusDate = null; 2170 } else if (name.equals("statusReason")) { 2171 this.statusReason = null; 2172 } else if (name.equals("expressedBy")) { 2173 this.expressedBy = null; 2174 } else if (name.equals("addresses")) { 2175 this.getAddresses().remove(castToReference(value)); 2176 } else if (name.equals("note")) { 2177 this.getNote().remove(castToAnnotation(value)); 2178 } else if (name.equals("outcomeCode")) { 2179 this.getOutcomeCode().remove(castToCodeableConcept(value)); 2180 } else if (name.equals("outcomeReference")) { 2181 this.getOutcomeReference().remove(castToReference(value)); 2182 } else 2183 super.removeChild(name, value); 2184 2185 } 2186 2187 @Override 2188 public Base makeProperty(int hash, String name) throws FHIRException { 2189 switch (hash) { 2190 case -1618432855: 2191 return addIdentifier(); 2192 case 1165552636: 2193 return getLifecycleStatusElement(); 2194 case 104524801: 2195 return getAchievementStatus(); 2196 case 50511102: 2197 return addCategory(); 2198 case -1165461084: 2199 return getPriority(); 2200 case -1724546052: 2201 return getDescription(); 2202 case -1867885268: 2203 return getSubject(); 2204 case 1316793566: 2205 return getStart(); 2206 case 109757538: 2207 return getStart(); 2208 case -880905839: 2209 return addTarget(); 2210 case 247524032: 2211 return getStatusDateElement(); 2212 case 2051346646: 2213 return getStatusReasonElement(); 2214 case 175423686: 2215 return getExpressedBy(); 2216 case 874544034: 2217 return addAddresses(); 2218 case 3387378: 2219 return addNote(); 2220 case 1062482015: 2221 return addOutcomeCode(); 2222 case -782273511: 2223 return addOutcomeReference(); 2224 default: 2225 return super.makeProperty(hash, name); 2226 } 2227 2228 } 2229 2230 @Override 2231 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2232 switch (hash) { 2233 case -1618432855: 2234 /* identifier */ return new String[] { "Identifier" }; 2235 case 1165552636: 2236 /* lifecycleStatus */ return new String[] { "code" }; 2237 case 104524801: 2238 /* achievementStatus */ return new String[] { "CodeableConcept" }; 2239 case 50511102: 2240 /* category */ return new String[] { "CodeableConcept" }; 2241 case -1165461084: 2242 /* priority */ return new String[] { "CodeableConcept" }; 2243 case -1724546052: 2244 /* description */ return new String[] { "CodeableConcept" }; 2245 case -1867885268: 2246 /* subject */ return new String[] { "Reference" }; 2247 case 109757538: 2248 /* start */ return new String[] { "date", "CodeableConcept" }; 2249 case -880905839: 2250 /* target */ return new String[] {}; 2251 case 247524032: 2252 /* statusDate */ return new String[] { "date" }; 2253 case 2051346646: 2254 /* statusReason */ return new String[] { "string" }; 2255 case 175423686: 2256 /* expressedBy */ return new String[] { "Reference" }; 2257 case 874544034: 2258 /* addresses */ return new String[] { "Reference" }; 2259 case 3387378: 2260 /* note */ return new String[] { "Annotation" }; 2261 case 1062482015: 2262 /* outcomeCode */ return new String[] { "CodeableConcept" }; 2263 case -782273511: 2264 /* outcomeReference */ return new String[] { "Reference" }; 2265 default: 2266 return super.getTypesForProperty(hash, name); 2267 } 2268 2269 } 2270 2271 @Override 2272 public Base addChild(String name) throws FHIRException { 2273 if (name.equals("identifier")) { 2274 return addIdentifier(); 2275 } else if (name.equals("lifecycleStatus")) { 2276 throw new FHIRException("Cannot call addChild on a singleton property Goal.lifecycleStatus"); 2277 } else if (name.equals("achievementStatus")) { 2278 this.achievementStatus = new CodeableConcept(); 2279 return this.achievementStatus; 2280 } else if (name.equals("category")) { 2281 return addCategory(); 2282 } else if (name.equals("priority")) { 2283 this.priority = new CodeableConcept(); 2284 return this.priority; 2285 } else if (name.equals("description")) { 2286 this.description = new CodeableConcept(); 2287 return this.description; 2288 } else if (name.equals("subject")) { 2289 this.subject = new Reference(); 2290 return this.subject; 2291 } else if (name.equals("startDate")) { 2292 this.start = new DateType(); 2293 return this.start; 2294 } else if (name.equals("startCodeableConcept")) { 2295 this.start = new CodeableConcept(); 2296 return this.start; 2297 } else if (name.equals("target")) { 2298 return addTarget(); 2299 } else if (name.equals("statusDate")) { 2300 throw new FHIRException("Cannot call addChild on a singleton property Goal.statusDate"); 2301 } else if (name.equals("statusReason")) { 2302 throw new FHIRException("Cannot call addChild on a singleton property Goal.statusReason"); 2303 } else if (name.equals("expressedBy")) { 2304 this.expressedBy = new Reference(); 2305 return this.expressedBy; 2306 } else if (name.equals("addresses")) { 2307 return addAddresses(); 2308 } else if (name.equals("note")) { 2309 return addNote(); 2310 } else if (name.equals("outcomeCode")) { 2311 return addOutcomeCode(); 2312 } else if (name.equals("outcomeReference")) { 2313 return addOutcomeReference(); 2314 } else 2315 return super.addChild(name); 2316 } 2317 2318 public String fhirType() { 2319 return "Goal"; 2320 2321 } 2322 2323 public Goal copy() { 2324 Goal dst = new Goal(); 2325 copyValues(dst); 2326 return dst; 2327 } 2328 2329 public void copyValues(Goal dst) { 2330 super.copyValues(dst); 2331 if (identifier != null) { 2332 dst.identifier = new ArrayList<Identifier>(); 2333 for (Identifier i : identifier) 2334 dst.identifier.add(i.copy()); 2335 } 2336 ; 2337 dst.lifecycleStatus = lifecycleStatus == null ? null : lifecycleStatus.copy(); 2338 dst.achievementStatus = achievementStatus == null ? null : achievementStatus.copy(); 2339 if (category != null) { 2340 dst.category = new ArrayList<CodeableConcept>(); 2341 for (CodeableConcept i : category) 2342 dst.category.add(i.copy()); 2343 } 2344 ; 2345 dst.priority = priority == null ? null : priority.copy(); 2346 dst.description = description == null ? null : description.copy(); 2347 dst.subject = subject == null ? null : subject.copy(); 2348 dst.start = start == null ? null : start.copy(); 2349 if (target != null) { 2350 dst.target = new ArrayList<GoalTargetComponent>(); 2351 for (GoalTargetComponent i : target) 2352 dst.target.add(i.copy()); 2353 } 2354 ; 2355 dst.statusDate = statusDate == null ? null : statusDate.copy(); 2356 dst.statusReason = statusReason == null ? null : statusReason.copy(); 2357 dst.expressedBy = expressedBy == null ? null : expressedBy.copy(); 2358 if (addresses != null) { 2359 dst.addresses = new ArrayList<Reference>(); 2360 for (Reference i : addresses) 2361 dst.addresses.add(i.copy()); 2362 } 2363 ; 2364 if (note != null) { 2365 dst.note = new ArrayList<Annotation>(); 2366 for (Annotation i : note) 2367 dst.note.add(i.copy()); 2368 } 2369 ; 2370 if (outcomeCode != null) { 2371 dst.outcomeCode = new ArrayList<CodeableConcept>(); 2372 for (CodeableConcept i : outcomeCode) 2373 dst.outcomeCode.add(i.copy()); 2374 } 2375 ; 2376 if (outcomeReference != null) { 2377 dst.outcomeReference = new ArrayList<Reference>(); 2378 for (Reference i : outcomeReference) 2379 dst.outcomeReference.add(i.copy()); 2380 } 2381 ; 2382 } 2383 2384 protected Goal typedCopy() { 2385 return copy(); 2386 } 2387 2388 @Override 2389 public boolean equalsDeep(Base other_) { 2390 if (!super.equalsDeep(other_)) 2391 return false; 2392 if (!(other_ instanceof Goal)) 2393 return false; 2394 Goal o = (Goal) other_; 2395 return compareDeep(identifier, o.identifier, true) && compareDeep(lifecycleStatus, o.lifecycleStatus, true) 2396 && compareDeep(achievementStatus, o.achievementStatus, true) && compareDeep(category, o.category, true) 2397 && compareDeep(priority, o.priority, true) && compareDeep(description, o.description, true) 2398 && compareDeep(subject, o.subject, true) && compareDeep(start, o.start, true) 2399 && compareDeep(target, o.target, true) && compareDeep(statusDate, o.statusDate, true) 2400 && compareDeep(statusReason, o.statusReason, true) && compareDeep(expressedBy, o.expressedBy, true) 2401 && compareDeep(addresses, o.addresses, true) && compareDeep(note, o.note, true) 2402 && compareDeep(outcomeCode, o.outcomeCode, true) && compareDeep(outcomeReference, o.outcomeReference, true); 2403 } 2404 2405 @Override 2406 public boolean equalsShallow(Base other_) { 2407 if (!super.equalsShallow(other_)) 2408 return false; 2409 if (!(other_ instanceof Goal)) 2410 return false; 2411 Goal o = (Goal) other_; 2412 return compareValues(lifecycleStatus, o.lifecycleStatus, true) && compareValues(statusDate, o.statusDate, true) 2413 && compareValues(statusReason, o.statusReason, true); 2414 } 2415 2416 public boolean isEmpty() { 2417 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, lifecycleStatus, achievementStatus, 2418 category, priority, description, subject, start, target, statusDate, statusReason, expressedBy, addresses, note, 2419 outcomeCode, outcomeReference); 2420 } 2421 2422 @Override 2423 public ResourceType getResourceType() { 2424 return ResourceType.Goal; 2425 } 2426 2427 /** 2428 * Search parameter: <b>identifier</b> 2429 * <p> 2430 * Description: <b>External Ids for this goal</b><br> 2431 * Type: <b>token</b><br> 2432 * Path: <b>Goal.identifier</b><br> 2433 * </p> 2434 */ 2435 @SearchParamDefinition(name = "identifier", path = "Goal.identifier", description = "External Ids for this goal", type = "token") 2436 public static final String SP_IDENTIFIER = "identifier"; 2437 /** 2438 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2439 * <p> 2440 * Description: <b>External Ids for this goal</b><br> 2441 * Type: <b>token</b><br> 2442 * Path: <b>Goal.identifier</b><br> 2443 * </p> 2444 */ 2445 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2446 SP_IDENTIFIER); 2447 2448 /** 2449 * Search parameter: <b>lifecycle-status</b> 2450 * <p> 2451 * Description: <b>proposed | planned | accepted | active | on-hold | completed 2452 * | cancelled | entered-in-error | rejected</b><br> 2453 * Type: <b>token</b><br> 2454 * Path: <b>Goal.lifecycleStatus</b><br> 2455 * </p> 2456 */ 2457 @SearchParamDefinition(name = "lifecycle-status", path = "Goal.lifecycleStatus", description = "proposed | planned | accepted | active | on-hold | completed | cancelled | entered-in-error | rejected", type = "token") 2458 public static final String SP_LIFECYCLE_STATUS = "lifecycle-status"; 2459 /** 2460 * <b>Fluent Client</b> search parameter constant for <b>lifecycle-status</b> 2461 * <p> 2462 * Description: <b>proposed | planned | accepted | active | on-hold | completed 2463 * | cancelled | entered-in-error | rejected</b><br> 2464 * Type: <b>token</b><br> 2465 * Path: <b>Goal.lifecycleStatus</b><br> 2466 * </p> 2467 */ 2468 public static final ca.uhn.fhir.rest.gclient.TokenClientParam LIFECYCLE_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2469 SP_LIFECYCLE_STATUS); 2470 2471 /** 2472 * Search parameter: <b>achievement-status</b> 2473 * <p> 2474 * Description: <b>in-progress | improving | worsening | no-change | achieved | 2475 * sustaining | not-achieved | no-progress | not-attainable</b><br> 2476 * Type: <b>token</b><br> 2477 * Path: <b>Goal.achievementStatus</b><br> 2478 * </p> 2479 */ 2480 @SearchParamDefinition(name = "achievement-status", path = "Goal.achievementStatus", description = "in-progress | improving | worsening | no-change | achieved | sustaining | not-achieved | no-progress | not-attainable", type = "token") 2481 public static final String SP_ACHIEVEMENT_STATUS = "achievement-status"; 2482 /** 2483 * <b>Fluent Client</b> search parameter constant for <b>achievement-status</b> 2484 * <p> 2485 * Description: <b>in-progress | improving | worsening | no-change | achieved | 2486 * sustaining | not-achieved | no-progress | not-attainable</b><br> 2487 * Type: <b>token</b><br> 2488 * Path: <b>Goal.achievementStatus</b><br> 2489 * </p> 2490 */ 2491 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACHIEVEMENT_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2492 SP_ACHIEVEMENT_STATUS); 2493 2494 /** 2495 * Search parameter: <b>patient</b> 2496 * <p> 2497 * Description: <b>Who this goal is intended for</b><br> 2498 * Type: <b>reference</b><br> 2499 * Path: <b>Goal.subject</b><br> 2500 * </p> 2501 */ 2502 @SearchParamDefinition(name = "patient", path = "Goal.subject.where(resolve() is Patient)", description = "Who this goal is intended for", type = "reference", providesMembershipIn = { 2503 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 2504 public static final String SP_PATIENT = "patient"; 2505 /** 2506 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2507 * <p> 2508 * Description: <b>Who this goal is intended for</b><br> 2509 * Type: <b>reference</b><br> 2510 * Path: <b>Goal.subject</b><br> 2511 * </p> 2512 */ 2513 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2514 SP_PATIENT); 2515 2516 /** 2517 * Constant for fluent queries to be used to add include statements. Specifies 2518 * the path value of "<b>Goal:patient</b>". 2519 */ 2520 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Goal:patient") 2521 .toLocked(); 2522 2523 /** 2524 * Search parameter: <b>subject</b> 2525 * <p> 2526 * Description: <b>Who this goal is intended for</b><br> 2527 * Type: <b>reference</b><br> 2528 * Path: <b>Goal.subject</b><br> 2529 * </p> 2530 */ 2531 @SearchParamDefinition(name = "subject", path = "Goal.subject", description = "Who this goal is intended for", type = "reference", target = { 2532 Group.class, Organization.class, Patient.class }) 2533 public static final String SP_SUBJECT = "subject"; 2534 /** 2535 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2536 * <p> 2537 * Description: <b>Who this goal is intended for</b><br> 2538 * Type: <b>reference</b><br> 2539 * Path: <b>Goal.subject</b><br> 2540 * </p> 2541 */ 2542 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2543 SP_SUBJECT); 2544 2545 /** 2546 * Constant for fluent queries to be used to add include statements. Specifies 2547 * the path value of "<b>Goal:subject</b>". 2548 */ 2549 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Goal:subject") 2550 .toLocked(); 2551 2552 /** 2553 * Search parameter: <b>start-date</b> 2554 * <p> 2555 * Description: <b>When goal pursuit begins</b><br> 2556 * Type: <b>date</b><br> 2557 * Path: <b>Goal.startDate</b><br> 2558 * </p> 2559 */ 2560 @SearchParamDefinition(name = "start-date", path = "(Goal.start as date)", description = "When goal pursuit begins", type = "date") 2561 public static final String SP_START_DATE = "start-date"; 2562 /** 2563 * <b>Fluent Client</b> search parameter constant for <b>start-date</b> 2564 * <p> 2565 * Description: <b>When goal pursuit begins</b><br> 2566 * Type: <b>date</b><br> 2567 * Path: <b>Goal.startDate</b><br> 2568 * </p> 2569 */ 2570 public static final ca.uhn.fhir.rest.gclient.DateClientParam START_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2571 SP_START_DATE); 2572 2573 /** 2574 * Search parameter: <b>category</b> 2575 * <p> 2576 * Description: <b>E.g. Treatment, dietary, behavioral, etc.</b><br> 2577 * Type: <b>token</b><br> 2578 * Path: <b>Goal.category</b><br> 2579 * </p> 2580 */ 2581 @SearchParamDefinition(name = "category", path = "Goal.category", description = "E.g. Treatment, dietary, behavioral, etc.", type = "token") 2582 public static final String SP_CATEGORY = "category"; 2583 /** 2584 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2585 * <p> 2586 * Description: <b>E.g. Treatment, dietary, behavioral, etc.</b><br> 2587 * Type: <b>token</b><br> 2588 * Path: <b>Goal.category</b><br> 2589 * </p> 2590 */ 2591 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2592 SP_CATEGORY); 2593 2594 /** 2595 * Search parameter: <b>target-date</b> 2596 * <p> 2597 * Description: <b>Reach goal on or before</b><br> 2598 * Type: <b>date</b><br> 2599 * Path: <b>Goal.target.dueDate</b><br> 2600 * </p> 2601 */ 2602 @SearchParamDefinition(name = "target-date", path = "(Goal.target.due as date)", description = "Reach goal on or before", type = "date") 2603 public static final String SP_TARGET_DATE = "target-date"; 2604 /** 2605 * <b>Fluent Client</b> search parameter constant for <b>target-date</b> 2606 * <p> 2607 * Description: <b>Reach goal on or before</b><br> 2608 * Type: <b>date</b><br> 2609 * Path: <b>Goal.target.dueDate</b><br> 2610 * </p> 2611 */ 2612 public static final ca.uhn.fhir.rest.gclient.DateClientParam TARGET_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2613 SP_TARGET_DATE); 2614 2615}