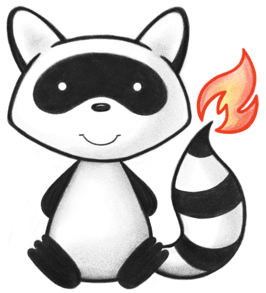
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049 050/** 051 * A formal computable definition of a graph of resources - that is, a coherent 052 * set of resources that form a graph by following references. The Graph 053 * Definition resource defines a set and makes rules about the set. 054 */ 055@ResourceDef(name = "GraphDefinition", profile = "http://hl7.org/fhir/StructureDefinition/GraphDefinition") 056@ChildOrder(names = { "url", "version", "name", "status", "experimental", "date", "publisher", "contact", "description", 057 "useContext", "jurisdiction", "purpose", "start", "profile", "link" }) 058public class GraphDefinition extends MetadataResource { 059 060 public enum GraphCompartmentUse { 061 /** 062 * This compartment rule is a condition for whether the rule applies. 063 */ 064 CONDITION, 065 /** 066 * This compartment rule is enforced on any relationships that meet the 067 * conditions. 068 */ 069 REQUIREMENT, 070 /** 071 * added to help the parsers with the generic types 072 */ 073 NULL; 074 075 public static GraphCompartmentUse fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("condition".equals(codeString)) 079 return CONDITION; 080 if ("requirement".equals(codeString)) 081 return REQUIREMENT; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown GraphCompartmentUse code '" + codeString + "'"); 086 } 087 088 public String toCode() { 089 switch (this) { 090 case CONDITION: 091 return "condition"; 092 case REQUIREMENT: 093 return "requirement"; 094 case NULL: 095 return null; 096 default: 097 return "?"; 098 } 099 } 100 101 public String getSystem() { 102 switch (this) { 103 case CONDITION: 104 return "http://hl7.org/fhir/graph-compartment-use"; 105 case REQUIREMENT: 106 return "http://hl7.org/fhir/graph-compartment-use"; 107 case NULL: 108 return null; 109 default: 110 return "?"; 111 } 112 } 113 114 public String getDefinition() { 115 switch (this) { 116 case CONDITION: 117 return "This compartment rule is a condition for whether the rule applies."; 118 case REQUIREMENT: 119 return "This compartment rule is enforced on any relationships that meet the conditions."; 120 case NULL: 121 return null; 122 default: 123 return "?"; 124 } 125 } 126 127 public String getDisplay() { 128 switch (this) { 129 case CONDITION: 130 return "Condition"; 131 case REQUIREMENT: 132 return "Requirement"; 133 case NULL: 134 return null; 135 default: 136 return "?"; 137 } 138 } 139 } 140 141 public static class GraphCompartmentUseEnumFactory implements EnumFactory<GraphCompartmentUse> { 142 public GraphCompartmentUse fromCode(String codeString) throws IllegalArgumentException { 143 if (codeString == null || "".equals(codeString)) 144 if (codeString == null || "".equals(codeString)) 145 return null; 146 if ("condition".equals(codeString)) 147 return GraphCompartmentUse.CONDITION; 148 if ("requirement".equals(codeString)) 149 return GraphCompartmentUse.REQUIREMENT; 150 throw new IllegalArgumentException("Unknown GraphCompartmentUse code '" + codeString + "'"); 151 } 152 153 public Enumeration<GraphCompartmentUse> fromType(PrimitiveType<?> code) throws FHIRException { 154 if (code == null) 155 return null; 156 if (code.isEmpty()) 157 return new Enumeration<GraphCompartmentUse>(this, GraphCompartmentUse.NULL, code); 158 String codeString = code.asStringValue(); 159 if (codeString == null || "".equals(codeString)) 160 return new Enumeration<GraphCompartmentUse>(this, GraphCompartmentUse.NULL, code); 161 if ("condition".equals(codeString)) 162 return new Enumeration<GraphCompartmentUse>(this, GraphCompartmentUse.CONDITION, code); 163 if ("requirement".equals(codeString)) 164 return new Enumeration<GraphCompartmentUse>(this, GraphCompartmentUse.REQUIREMENT, code); 165 throw new FHIRException("Unknown GraphCompartmentUse code '" + codeString + "'"); 166 } 167 168 public String toCode(GraphCompartmentUse code) { 169 if (code == GraphCompartmentUse.NULL) 170 return null; 171 if (code == GraphCompartmentUse.CONDITION) 172 return "condition"; 173 if (code == GraphCompartmentUse.REQUIREMENT) 174 return "requirement"; 175 return "?"; 176 } 177 178 public String toSystem(GraphCompartmentUse code) { 179 return code.getSystem(); 180 } 181 } 182 183 public enum CompartmentCode { 184 /** 185 * The compartment definition is for the patient compartment. 186 */ 187 PATIENT, 188 /** 189 * The compartment definition is for the encounter compartment. 190 */ 191 ENCOUNTER, 192 /** 193 * The compartment definition is for the related-person compartment. 194 */ 195 RELATEDPERSON, 196 /** 197 * The compartment definition is for the practitioner compartment. 198 */ 199 PRACTITIONER, 200 /** 201 * The compartment definition is for the device compartment. 202 */ 203 DEVICE, 204 /** 205 * added to help the parsers with the generic types 206 */ 207 NULL; 208 209 public static CompartmentCode fromCode(String codeString) throws FHIRException { 210 if (codeString == null || "".equals(codeString)) 211 return null; 212 if ("Patient".equals(codeString)) 213 return PATIENT; 214 if ("Encounter".equals(codeString)) 215 return ENCOUNTER; 216 if ("RelatedPerson".equals(codeString)) 217 return RELATEDPERSON; 218 if ("Practitioner".equals(codeString)) 219 return PRACTITIONER; 220 if ("Device".equals(codeString)) 221 return DEVICE; 222 if (Configuration.isAcceptInvalidEnums()) 223 return null; 224 else 225 throw new FHIRException("Unknown CompartmentCode code '" + codeString + "'"); 226 } 227 228 public String toCode() { 229 switch (this) { 230 case PATIENT: 231 return "Patient"; 232 case ENCOUNTER: 233 return "Encounter"; 234 case RELATEDPERSON: 235 return "RelatedPerson"; 236 case PRACTITIONER: 237 return "Practitioner"; 238 case DEVICE: 239 return "Device"; 240 case NULL: 241 return null; 242 default: 243 return "?"; 244 } 245 } 246 247 public String getSystem() { 248 switch (this) { 249 case PATIENT: 250 return "http://hl7.org/fhir/compartment-type"; 251 case ENCOUNTER: 252 return "http://hl7.org/fhir/compartment-type"; 253 case RELATEDPERSON: 254 return "http://hl7.org/fhir/compartment-type"; 255 case PRACTITIONER: 256 return "http://hl7.org/fhir/compartment-type"; 257 case DEVICE: 258 return "http://hl7.org/fhir/compartment-type"; 259 case NULL: 260 return null; 261 default: 262 return "?"; 263 } 264 } 265 266 public String getDefinition() { 267 switch (this) { 268 case PATIENT: 269 return "The compartment definition is for the patient compartment."; 270 case ENCOUNTER: 271 return "The compartment definition is for the encounter compartment."; 272 case RELATEDPERSON: 273 return "The compartment definition is for the related-person compartment."; 274 case PRACTITIONER: 275 return "The compartment definition is for the practitioner compartment."; 276 case DEVICE: 277 return "The compartment definition is for the device compartment."; 278 case NULL: 279 return null; 280 default: 281 return "?"; 282 } 283 } 284 285 public String getDisplay() { 286 switch (this) { 287 case PATIENT: 288 return "Patient"; 289 case ENCOUNTER: 290 return "Encounter"; 291 case RELATEDPERSON: 292 return "RelatedPerson"; 293 case PRACTITIONER: 294 return "Practitioner"; 295 case DEVICE: 296 return "Device"; 297 case NULL: 298 return null; 299 default: 300 return "?"; 301 } 302 } 303 } 304 305 public static class CompartmentCodeEnumFactory implements EnumFactory<CompartmentCode> { 306 public CompartmentCode fromCode(String codeString) throws IllegalArgumentException { 307 if (codeString == null || "".equals(codeString)) 308 if (codeString == null || "".equals(codeString)) 309 return null; 310 if ("Patient".equals(codeString)) 311 return CompartmentCode.PATIENT; 312 if ("Encounter".equals(codeString)) 313 return CompartmentCode.ENCOUNTER; 314 if ("RelatedPerson".equals(codeString)) 315 return CompartmentCode.RELATEDPERSON; 316 if ("Practitioner".equals(codeString)) 317 return CompartmentCode.PRACTITIONER; 318 if ("Device".equals(codeString)) 319 return CompartmentCode.DEVICE; 320 throw new IllegalArgumentException("Unknown CompartmentCode code '" + codeString + "'"); 321 } 322 323 public Enumeration<CompartmentCode> fromType(PrimitiveType<?> code) throws FHIRException { 324 if (code == null) 325 return null; 326 if (code.isEmpty()) 327 return new Enumeration<CompartmentCode>(this, CompartmentCode.NULL, code); 328 String codeString = code.asStringValue(); 329 if (codeString == null || "".equals(codeString)) 330 return new Enumeration<CompartmentCode>(this, CompartmentCode.NULL, code); 331 if ("Patient".equals(codeString)) 332 return new Enumeration<CompartmentCode>(this, CompartmentCode.PATIENT, code); 333 if ("Encounter".equals(codeString)) 334 return new Enumeration<CompartmentCode>(this, CompartmentCode.ENCOUNTER, code); 335 if ("RelatedPerson".equals(codeString)) 336 return new Enumeration<CompartmentCode>(this, CompartmentCode.RELATEDPERSON, code); 337 if ("Practitioner".equals(codeString)) 338 return new Enumeration<CompartmentCode>(this, CompartmentCode.PRACTITIONER, code); 339 if ("Device".equals(codeString)) 340 return new Enumeration<CompartmentCode>(this, CompartmentCode.DEVICE, code); 341 throw new FHIRException("Unknown CompartmentCode code '" + codeString + "'"); 342 } 343 344 public String toCode(CompartmentCode code) { 345 if (code == CompartmentCode.NULL) 346 return null; 347 if (code == CompartmentCode.PATIENT) 348 return "Patient"; 349 if (code == CompartmentCode.ENCOUNTER) 350 return "Encounter"; 351 if (code == CompartmentCode.RELATEDPERSON) 352 return "RelatedPerson"; 353 if (code == CompartmentCode.PRACTITIONER) 354 return "Practitioner"; 355 if (code == CompartmentCode.DEVICE) 356 return "Device"; 357 return "?"; 358 } 359 360 public String toSystem(CompartmentCode code) { 361 return code.getSystem(); 362 } 363 } 364 365 public enum GraphCompartmentRule { 366 /** 367 * The compartment must be identical (the same literal reference). 368 */ 369 IDENTICAL, 370 /** 371 * The compartment must be the same - the record must be about the same patient, 372 * but the reference may be different. 373 */ 374 MATCHING, 375 /** 376 * The compartment must be different. 377 */ 378 DIFFERENT, 379 /** 380 * The compartment rule is defined in the accompanying FHIRPath expression. 381 */ 382 CUSTOM, 383 /** 384 * added to help the parsers with the generic types 385 */ 386 NULL; 387 388 public static GraphCompartmentRule fromCode(String codeString) throws FHIRException { 389 if (codeString == null || "".equals(codeString)) 390 return null; 391 if ("identical".equals(codeString)) 392 return IDENTICAL; 393 if ("matching".equals(codeString)) 394 return MATCHING; 395 if ("different".equals(codeString)) 396 return DIFFERENT; 397 if ("custom".equals(codeString)) 398 return CUSTOM; 399 if (Configuration.isAcceptInvalidEnums()) 400 return null; 401 else 402 throw new FHIRException("Unknown GraphCompartmentRule code '" + codeString + "'"); 403 } 404 405 public String toCode() { 406 switch (this) { 407 case IDENTICAL: 408 return "identical"; 409 case MATCHING: 410 return "matching"; 411 case DIFFERENT: 412 return "different"; 413 case CUSTOM: 414 return "custom"; 415 case NULL: 416 return null; 417 default: 418 return "?"; 419 } 420 } 421 422 public String getSystem() { 423 switch (this) { 424 case IDENTICAL: 425 return "http://hl7.org/fhir/graph-compartment-rule"; 426 case MATCHING: 427 return "http://hl7.org/fhir/graph-compartment-rule"; 428 case DIFFERENT: 429 return "http://hl7.org/fhir/graph-compartment-rule"; 430 case CUSTOM: 431 return "http://hl7.org/fhir/graph-compartment-rule"; 432 case NULL: 433 return null; 434 default: 435 return "?"; 436 } 437 } 438 439 public String getDefinition() { 440 switch (this) { 441 case IDENTICAL: 442 return "The compartment must be identical (the same literal reference)."; 443 case MATCHING: 444 return "The compartment must be the same - the record must be about the same patient, but the reference may be different."; 445 case DIFFERENT: 446 return "The compartment must be different."; 447 case CUSTOM: 448 return "The compartment rule is defined in the accompanying FHIRPath expression."; 449 case NULL: 450 return null; 451 default: 452 return "?"; 453 } 454 } 455 456 public String getDisplay() { 457 switch (this) { 458 case IDENTICAL: 459 return "Identical"; 460 case MATCHING: 461 return "Matching"; 462 case DIFFERENT: 463 return "Different"; 464 case CUSTOM: 465 return "Custom"; 466 case NULL: 467 return null; 468 default: 469 return "?"; 470 } 471 } 472 } 473 474 public static class GraphCompartmentRuleEnumFactory implements EnumFactory<GraphCompartmentRule> { 475 public GraphCompartmentRule fromCode(String codeString) throws IllegalArgumentException { 476 if (codeString == null || "".equals(codeString)) 477 if (codeString == null || "".equals(codeString)) 478 return null; 479 if ("identical".equals(codeString)) 480 return GraphCompartmentRule.IDENTICAL; 481 if ("matching".equals(codeString)) 482 return GraphCompartmentRule.MATCHING; 483 if ("different".equals(codeString)) 484 return GraphCompartmentRule.DIFFERENT; 485 if ("custom".equals(codeString)) 486 return GraphCompartmentRule.CUSTOM; 487 throw new IllegalArgumentException("Unknown GraphCompartmentRule code '" + codeString + "'"); 488 } 489 490 public Enumeration<GraphCompartmentRule> fromType(PrimitiveType<?> code) throws FHIRException { 491 if (code == null) 492 return null; 493 if (code.isEmpty()) 494 return new Enumeration<GraphCompartmentRule>(this, GraphCompartmentRule.NULL, code); 495 String codeString = code.asStringValue(); 496 if (codeString == null || "".equals(codeString)) 497 return new Enumeration<GraphCompartmentRule>(this, GraphCompartmentRule.NULL, code); 498 if ("identical".equals(codeString)) 499 return new Enumeration<GraphCompartmentRule>(this, GraphCompartmentRule.IDENTICAL, code); 500 if ("matching".equals(codeString)) 501 return new Enumeration<GraphCompartmentRule>(this, GraphCompartmentRule.MATCHING, code); 502 if ("different".equals(codeString)) 503 return new Enumeration<GraphCompartmentRule>(this, GraphCompartmentRule.DIFFERENT, code); 504 if ("custom".equals(codeString)) 505 return new Enumeration<GraphCompartmentRule>(this, GraphCompartmentRule.CUSTOM, code); 506 throw new FHIRException("Unknown GraphCompartmentRule code '" + codeString + "'"); 507 } 508 509 public String toCode(GraphCompartmentRule code) { 510 if (code == GraphCompartmentRule.NULL) 511 return null; 512 if (code == GraphCompartmentRule.IDENTICAL) 513 return "identical"; 514 if (code == GraphCompartmentRule.MATCHING) 515 return "matching"; 516 if (code == GraphCompartmentRule.DIFFERENT) 517 return "different"; 518 if (code == GraphCompartmentRule.CUSTOM) 519 return "custom"; 520 return "?"; 521 } 522 523 public String toSystem(GraphCompartmentRule code) { 524 return code.getSystem(); 525 } 526 } 527 528 @Block() 529 public static class GraphDefinitionLinkComponent extends BackboneElement implements IBaseBackboneElement { 530 /** 531 * A FHIR expression that identifies one of FHIR References to other resources. 532 */ 533 @Child(name = "path", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 534 @Description(shortDefinition = "Path in the resource that contains the link", formalDefinition = "A FHIR expression that identifies one of FHIR References to other resources.") 535 protected StringType path; 536 537 /** 538 * Which slice (if profiled). 539 */ 540 @Child(name = "sliceName", type = { 541 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 542 @Description(shortDefinition = "Which slice (if profiled)", formalDefinition = "Which slice (if profiled).") 543 protected StringType sliceName; 544 545 /** 546 * Minimum occurrences for this link. 547 */ 548 @Child(name = "min", type = { IntegerType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 549 @Description(shortDefinition = "Minimum occurrences for this link", formalDefinition = "Minimum occurrences for this link.") 550 protected IntegerType min; 551 552 /** 553 * Maximum occurrences for this link. 554 */ 555 @Child(name = "max", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 556 @Description(shortDefinition = "Maximum occurrences for this link", formalDefinition = "Maximum occurrences for this link.") 557 protected StringType max; 558 559 /** 560 * Information about why this link is of interest in this graph definition. 561 */ 562 @Child(name = "description", type = { 563 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 564 @Description(shortDefinition = "Why this link is specified", formalDefinition = "Information about why this link is of interest in this graph definition.") 565 protected StringType description; 566 567 /** 568 * Potential target for the link. 569 */ 570 @Child(name = "target", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 571 @Description(shortDefinition = "Potential target for the link", formalDefinition = "Potential target for the link.") 572 protected List<GraphDefinitionLinkTargetComponent> target; 573 574 private static final long serialVersionUID = -593733346L; 575 576 /** 577 * Constructor 578 */ 579 public GraphDefinitionLinkComponent() { 580 super(); 581 } 582 583 /** 584 * @return {@link #path} (A FHIR expression that identifies one of FHIR 585 * References to other resources.). This is the underlying object with 586 * id, value and extensions. The accessor "getPath" gives direct access 587 * to the value 588 */ 589 public StringType getPathElement() { 590 if (this.path == null) 591 if (Configuration.errorOnAutoCreate()) 592 throw new Error("Attempt to auto-create GraphDefinitionLinkComponent.path"); 593 else if (Configuration.doAutoCreate()) 594 this.path = new StringType(); // bb 595 return this.path; 596 } 597 598 public boolean hasPathElement() { 599 return this.path != null && !this.path.isEmpty(); 600 } 601 602 public boolean hasPath() { 603 return this.path != null && !this.path.isEmpty(); 604 } 605 606 /** 607 * @param value {@link #path} (A FHIR expression that identifies one of FHIR 608 * References to other resources.). This is the underlying object 609 * with id, value and extensions. The accessor "getPath" gives 610 * direct access to the value 611 */ 612 public GraphDefinitionLinkComponent setPathElement(StringType value) { 613 this.path = value; 614 return this; 615 } 616 617 /** 618 * @return A FHIR expression that identifies one of FHIR References to other 619 * resources. 620 */ 621 public String getPath() { 622 return this.path == null ? null : this.path.getValue(); 623 } 624 625 /** 626 * @param value A FHIR expression that identifies one of FHIR References to 627 * other resources. 628 */ 629 public GraphDefinitionLinkComponent setPath(String value) { 630 if (Utilities.noString(value)) 631 this.path = null; 632 else { 633 if (this.path == null) 634 this.path = new StringType(); 635 this.path.setValue(value); 636 } 637 return this; 638 } 639 640 /** 641 * @return {@link #sliceName} (Which slice (if profiled).). This is the 642 * underlying object with id, value and extensions. The accessor 643 * "getSliceName" gives direct access to the value 644 */ 645 public StringType getSliceNameElement() { 646 if (this.sliceName == null) 647 if (Configuration.errorOnAutoCreate()) 648 throw new Error("Attempt to auto-create GraphDefinitionLinkComponent.sliceName"); 649 else if (Configuration.doAutoCreate()) 650 this.sliceName = new StringType(); // bb 651 return this.sliceName; 652 } 653 654 public boolean hasSliceNameElement() { 655 return this.sliceName != null && !this.sliceName.isEmpty(); 656 } 657 658 public boolean hasSliceName() { 659 return this.sliceName != null && !this.sliceName.isEmpty(); 660 } 661 662 /** 663 * @param value {@link #sliceName} (Which slice (if profiled).). This is the 664 * underlying object with id, value and extensions. The accessor 665 * "getSliceName" gives direct access to the value 666 */ 667 public GraphDefinitionLinkComponent setSliceNameElement(StringType value) { 668 this.sliceName = value; 669 return this; 670 } 671 672 /** 673 * @return Which slice (if profiled). 674 */ 675 public String getSliceName() { 676 return this.sliceName == null ? null : this.sliceName.getValue(); 677 } 678 679 /** 680 * @param value Which slice (if profiled). 681 */ 682 public GraphDefinitionLinkComponent setSliceName(String value) { 683 if (Utilities.noString(value)) 684 this.sliceName = null; 685 else { 686 if (this.sliceName == null) 687 this.sliceName = new StringType(); 688 this.sliceName.setValue(value); 689 } 690 return this; 691 } 692 693 /** 694 * @return {@link #min} (Minimum occurrences for this link.). This is the 695 * underlying object with id, value and extensions. The accessor 696 * "getMin" gives direct access to the value 697 */ 698 public IntegerType getMinElement() { 699 if (this.min == null) 700 if (Configuration.errorOnAutoCreate()) 701 throw new Error("Attempt to auto-create GraphDefinitionLinkComponent.min"); 702 else if (Configuration.doAutoCreate()) 703 this.min = new IntegerType(); // bb 704 return this.min; 705 } 706 707 public boolean hasMinElement() { 708 return this.min != null && !this.min.isEmpty(); 709 } 710 711 public boolean hasMin() { 712 return this.min != null && !this.min.isEmpty(); 713 } 714 715 /** 716 * @param value {@link #min} (Minimum occurrences for this link.). This is the 717 * underlying object with id, value and extensions. The accessor 718 * "getMin" gives direct access to the value 719 */ 720 public GraphDefinitionLinkComponent setMinElement(IntegerType value) { 721 this.min = value; 722 return this; 723 } 724 725 /** 726 * @return Minimum occurrences for this link. 727 */ 728 public int getMin() { 729 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 730 } 731 732 /** 733 * @param value Minimum occurrences for this link. 734 */ 735 public GraphDefinitionLinkComponent setMin(int value) { 736 if (this.min == null) 737 this.min = new IntegerType(); 738 this.min.setValue(value); 739 return this; 740 } 741 742 /** 743 * @return {@link #max} (Maximum occurrences for this link.). This is the 744 * underlying object with id, value and extensions. The accessor 745 * "getMax" gives direct access to the value 746 */ 747 public StringType getMaxElement() { 748 if (this.max == null) 749 if (Configuration.errorOnAutoCreate()) 750 throw new Error("Attempt to auto-create GraphDefinitionLinkComponent.max"); 751 else if (Configuration.doAutoCreate()) 752 this.max = new StringType(); // bb 753 return this.max; 754 } 755 756 public boolean hasMaxElement() { 757 return this.max != null && !this.max.isEmpty(); 758 } 759 760 public boolean hasMax() { 761 return this.max != null && !this.max.isEmpty(); 762 } 763 764 /** 765 * @param value {@link #max} (Maximum occurrences for this link.). This is the 766 * underlying object with id, value and extensions. The accessor 767 * "getMax" gives direct access to the value 768 */ 769 public GraphDefinitionLinkComponent setMaxElement(StringType value) { 770 this.max = value; 771 return this; 772 } 773 774 /** 775 * @return Maximum occurrences for this link. 776 */ 777 public String getMax() { 778 return this.max == null ? null : this.max.getValue(); 779 } 780 781 /** 782 * @param value Maximum occurrences for this link. 783 */ 784 public GraphDefinitionLinkComponent setMax(String value) { 785 if (Utilities.noString(value)) 786 this.max = null; 787 else { 788 if (this.max == null) 789 this.max = new StringType(); 790 this.max.setValue(value); 791 } 792 return this; 793 } 794 795 /** 796 * @return {@link #description} (Information about why this link is of interest 797 * in this graph definition.). This is the underlying object with id, 798 * value and extensions. The accessor "getDescription" gives direct 799 * access to the value 800 */ 801 public StringType getDescriptionElement() { 802 if (this.description == null) 803 if (Configuration.errorOnAutoCreate()) 804 throw new Error("Attempt to auto-create GraphDefinitionLinkComponent.description"); 805 else if (Configuration.doAutoCreate()) 806 this.description = new StringType(); // bb 807 return this.description; 808 } 809 810 public boolean hasDescriptionElement() { 811 return this.description != null && !this.description.isEmpty(); 812 } 813 814 public boolean hasDescription() { 815 return this.description != null && !this.description.isEmpty(); 816 } 817 818 /** 819 * @param value {@link #description} (Information about why this link is of 820 * interest in this graph definition.). This is the underlying 821 * object with id, value and extensions. The accessor 822 * "getDescription" gives direct access to the value 823 */ 824 public GraphDefinitionLinkComponent setDescriptionElement(StringType value) { 825 this.description = value; 826 return this; 827 } 828 829 /** 830 * @return Information about why this link is of interest in this graph 831 * definition. 832 */ 833 public String getDescription() { 834 return this.description == null ? null : this.description.getValue(); 835 } 836 837 /** 838 * @param value Information about why this link is of interest in this graph 839 * definition. 840 */ 841 public GraphDefinitionLinkComponent setDescription(String value) { 842 if (Utilities.noString(value)) 843 this.description = null; 844 else { 845 if (this.description == null) 846 this.description = new StringType(); 847 this.description.setValue(value); 848 } 849 return this; 850 } 851 852 /** 853 * @return {@link #target} (Potential target for the link.) 854 */ 855 public List<GraphDefinitionLinkTargetComponent> getTarget() { 856 if (this.target == null) 857 this.target = new ArrayList<GraphDefinitionLinkTargetComponent>(); 858 return this.target; 859 } 860 861 /** 862 * @return Returns a reference to <code>this</code> for easy method chaining 863 */ 864 public GraphDefinitionLinkComponent setTarget(List<GraphDefinitionLinkTargetComponent> theTarget) { 865 this.target = theTarget; 866 return this; 867 } 868 869 public boolean hasTarget() { 870 if (this.target == null) 871 return false; 872 for (GraphDefinitionLinkTargetComponent item : this.target) 873 if (!item.isEmpty()) 874 return true; 875 return false; 876 } 877 878 public GraphDefinitionLinkTargetComponent addTarget() { // 3 879 GraphDefinitionLinkTargetComponent t = new GraphDefinitionLinkTargetComponent(); 880 if (this.target == null) 881 this.target = new ArrayList<GraphDefinitionLinkTargetComponent>(); 882 this.target.add(t); 883 return t; 884 } 885 886 public GraphDefinitionLinkComponent addTarget(GraphDefinitionLinkTargetComponent t) { // 3 887 if (t == null) 888 return this; 889 if (this.target == null) 890 this.target = new ArrayList<GraphDefinitionLinkTargetComponent>(); 891 this.target.add(t); 892 return this; 893 } 894 895 /** 896 * @return The first repetition of repeating field {@link #target}, creating it 897 * if it does not already exist 898 */ 899 public GraphDefinitionLinkTargetComponent getTargetFirstRep() { 900 if (getTarget().isEmpty()) { 901 addTarget(); 902 } 903 return getTarget().get(0); 904 } 905 906 protected void listChildren(List<Property> children) { 907 super.listChildren(children); 908 children.add(new Property("path", "string", 909 "A FHIR expression that identifies one of FHIR References to other resources.", 0, 1, path)); 910 children.add(new Property("sliceName", "string", "Which slice (if profiled).", 0, 1, sliceName)); 911 children.add(new Property("min", "integer", "Minimum occurrences for this link.", 0, 1, min)); 912 children.add(new Property("max", "string", "Maximum occurrences for this link.", 0, 1, max)); 913 children.add(new Property("description", "string", 914 "Information about why this link is of interest in this graph definition.", 0, 1, description)); 915 children 916 .add(new Property("target", "", "Potential target for the link.", 0, java.lang.Integer.MAX_VALUE, target)); 917 } 918 919 @Override 920 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 921 switch (_hash) { 922 case 3433509: 923 /* path */ return new Property("path", "string", 924 "A FHIR expression that identifies one of FHIR References to other resources.", 0, 1, path); 925 case -825289923: 926 /* sliceName */ return new Property("sliceName", "string", "Which slice (if profiled).", 0, 1, sliceName); 927 case 108114: 928 /* min */ return new Property("min", "integer", "Minimum occurrences for this link.", 0, 1, min); 929 case 107876: 930 /* max */ return new Property("max", "string", "Maximum occurrences for this link.", 0, 1, max); 931 case -1724546052: 932 /* description */ return new Property("description", "string", 933 "Information about why this link is of interest in this graph definition.", 0, 1, description); 934 case -880905839: 935 /* target */ return new Property("target", "", "Potential target for the link.", 0, java.lang.Integer.MAX_VALUE, 936 target); 937 default: 938 return super.getNamedProperty(_hash, _name, _checkValid); 939 } 940 941 } 942 943 @Override 944 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 945 switch (hash) { 946 case 3433509: 947 /* path */ return this.path == null ? new Base[0] : new Base[] { this.path }; // StringType 948 case -825289923: 949 /* sliceName */ return this.sliceName == null ? new Base[0] : new Base[] { this.sliceName }; // StringType 950 case 108114: 951 /* min */ return this.min == null ? new Base[0] : new Base[] { this.min }; // IntegerType 952 case 107876: 953 /* max */ return this.max == null ? new Base[0] : new Base[] { this.max }; // StringType 954 case -1724546052: 955 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 956 case -880905839: 957 /* target */ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // GraphDefinitionLinkTargetComponent 958 default: 959 return super.getProperty(hash, name, checkValid); 960 } 961 962 } 963 964 @Override 965 public Base setProperty(int hash, String name, Base value) throws FHIRException { 966 switch (hash) { 967 case 3433509: // path 968 this.path = castToString(value); // StringType 969 return value; 970 case -825289923: // sliceName 971 this.sliceName = castToString(value); // StringType 972 return value; 973 case 108114: // min 974 this.min = castToInteger(value); // IntegerType 975 return value; 976 case 107876: // max 977 this.max = castToString(value); // StringType 978 return value; 979 case -1724546052: // description 980 this.description = castToString(value); // StringType 981 return value; 982 case -880905839: // target 983 this.getTarget().add((GraphDefinitionLinkTargetComponent) value); // GraphDefinitionLinkTargetComponent 984 return value; 985 default: 986 return super.setProperty(hash, name, value); 987 } 988 989 } 990 991 @Override 992 public Base setProperty(String name, Base value) throws FHIRException { 993 if (name.equals("path")) { 994 this.path = castToString(value); // StringType 995 } else if (name.equals("sliceName")) { 996 this.sliceName = castToString(value); // StringType 997 } else if (name.equals("min")) { 998 this.min = castToInteger(value); // IntegerType 999 } else if (name.equals("max")) { 1000 this.max = castToString(value); // StringType 1001 } else if (name.equals("description")) { 1002 this.description = castToString(value); // StringType 1003 } else if (name.equals("target")) { 1004 this.getTarget().add((GraphDefinitionLinkTargetComponent) value); 1005 } else 1006 return super.setProperty(name, value); 1007 return value; 1008 } 1009 1010 @Override 1011 public void removeChild(String name, Base value) throws FHIRException { 1012 if (name.equals("path")) { 1013 this.path = null; 1014 } else if (name.equals("sliceName")) { 1015 this.sliceName = null; 1016 } else if (name.equals("min")) { 1017 this.min = null; 1018 } else if (name.equals("max")) { 1019 this.max = null; 1020 } else if (name.equals("description")) { 1021 this.description = null; 1022 } else if (name.equals("target")) { 1023 this.getTarget().remove((GraphDefinitionLinkTargetComponent) value); 1024 } else 1025 super.removeChild(name, value); 1026 1027 } 1028 1029 @Override 1030 public Base makeProperty(int hash, String name) throws FHIRException { 1031 switch (hash) { 1032 case 3433509: 1033 return getPathElement(); 1034 case -825289923: 1035 return getSliceNameElement(); 1036 case 108114: 1037 return getMinElement(); 1038 case 107876: 1039 return getMaxElement(); 1040 case -1724546052: 1041 return getDescriptionElement(); 1042 case -880905839: 1043 return addTarget(); 1044 default: 1045 return super.makeProperty(hash, name); 1046 } 1047 1048 } 1049 1050 @Override 1051 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1052 switch (hash) { 1053 case 3433509: 1054 /* path */ return new String[] { "string" }; 1055 case -825289923: 1056 /* sliceName */ return new String[] { "string" }; 1057 case 108114: 1058 /* min */ return new String[] { "integer" }; 1059 case 107876: 1060 /* max */ return new String[] { "string" }; 1061 case -1724546052: 1062 /* description */ return new String[] { "string" }; 1063 case -880905839: 1064 /* target */ return new String[] {}; 1065 default: 1066 return super.getTypesForProperty(hash, name); 1067 } 1068 1069 } 1070 1071 @Override 1072 public Base addChild(String name) throws FHIRException { 1073 if (name.equals("path")) { 1074 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.path"); 1075 } else if (name.equals("sliceName")) { 1076 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.sliceName"); 1077 } else if (name.equals("min")) { 1078 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.min"); 1079 } else if (name.equals("max")) { 1080 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.max"); 1081 } else if (name.equals("description")) { 1082 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.description"); 1083 } else if (name.equals("target")) { 1084 return addTarget(); 1085 } else 1086 return super.addChild(name); 1087 } 1088 1089 public GraphDefinitionLinkComponent copy() { 1090 GraphDefinitionLinkComponent dst = new GraphDefinitionLinkComponent(); 1091 copyValues(dst); 1092 return dst; 1093 } 1094 1095 public void copyValues(GraphDefinitionLinkComponent dst) { 1096 super.copyValues(dst); 1097 dst.path = path == null ? null : path.copy(); 1098 dst.sliceName = sliceName == null ? null : sliceName.copy(); 1099 dst.min = min == null ? null : min.copy(); 1100 dst.max = max == null ? null : max.copy(); 1101 dst.description = description == null ? null : description.copy(); 1102 if (target != null) { 1103 dst.target = new ArrayList<GraphDefinitionLinkTargetComponent>(); 1104 for (GraphDefinitionLinkTargetComponent i : target) 1105 dst.target.add(i.copy()); 1106 } 1107 ; 1108 } 1109 1110 @Override 1111 public boolean equalsDeep(Base other_) { 1112 if (!super.equalsDeep(other_)) 1113 return false; 1114 if (!(other_ instanceof GraphDefinitionLinkComponent)) 1115 return false; 1116 GraphDefinitionLinkComponent o = (GraphDefinitionLinkComponent) other_; 1117 return compareDeep(path, o.path, true) && compareDeep(sliceName, o.sliceName, true) 1118 && compareDeep(min, o.min, true) && compareDeep(max, o.max, true) 1119 && compareDeep(description, o.description, true) && compareDeep(target, o.target, true); 1120 } 1121 1122 @Override 1123 public boolean equalsShallow(Base other_) { 1124 if (!super.equalsShallow(other_)) 1125 return false; 1126 if (!(other_ instanceof GraphDefinitionLinkComponent)) 1127 return false; 1128 GraphDefinitionLinkComponent o = (GraphDefinitionLinkComponent) other_; 1129 return compareValues(path, o.path, true) && compareValues(sliceName, o.sliceName, true) 1130 && compareValues(min, o.min, true) && compareValues(max, o.max, true) 1131 && compareValues(description, o.description, true); 1132 } 1133 1134 public boolean isEmpty() { 1135 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, sliceName, min, max, description, target); 1136 } 1137 1138 public String fhirType() { 1139 return "GraphDefinition.link"; 1140 1141 } 1142 1143 } 1144 1145 @Block() 1146 public static class GraphDefinitionLinkTargetComponent extends BackboneElement implements IBaseBackboneElement { 1147 /** 1148 * Type of resource this link refers to. 1149 */ 1150 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1151 @Description(shortDefinition = "Type of resource this link refers to", formalDefinition = "Type of resource this link refers to.") 1152 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/resource-types") 1153 protected CodeType type; 1154 1155 /** 1156 * A set of parameters to look up. 1157 */ 1158 @Child(name = "params", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1159 @Description(shortDefinition = "Criteria for reverse lookup", formalDefinition = "A set of parameters to look up.") 1160 protected StringType params; 1161 1162 /** 1163 * Profile for the target resource. 1164 */ 1165 @Child(name = "profile", type = { 1166 CanonicalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1167 @Description(shortDefinition = "Profile for the target resource", formalDefinition = "Profile for the target resource.") 1168 protected CanonicalType profile; 1169 1170 /** 1171 * Compartment Consistency Rules. 1172 */ 1173 @Child(name = "compartment", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1174 @Description(shortDefinition = "Compartment Consistency Rules", formalDefinition = "Compartment Consistency Rules.") 1175 protected List<GraphDefinitionLinkTargetCompartmentComponent> compartment; 1176 1177 /** 1178 * Additional links from target resource. 1179 */ 1180 @Child(name = "link", type = { 1181 GraphDefinitionLinkComponent.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1182 @Description(shortDefinition = "Additional links from target resource", formalDefinition = "Additional links from target resource.") 1183 protected List<GraphDefinitionLinkComponent> link; 1184 1185 private static final long serialVersionUID = -35248998L; 1186 1187 /** 1188 * Constructor 1189 */ 1190 public GraphDefinitionLinkTargetComponent() { 1191 super(); 1192 } 1193 1194 /** 1195 * Constructor 1196 */ 1197 public GraphDefinitionLinkTargetComponent(CodeType type) { 1198 super(); 1199 this.type = type; 1200 } 1201 1202 /** 1203 * @return {@link #type} (Type of resource this link refers to.). This is the 1204 * underlying object with id, value and extensions. The accessor 1205 * "getType" gives direct access to the value 1206 */ 1207 public CodeType getTypeElement() { 1208 if (this.type == null) 1209 if (Configuration.errorOnAutoCreate()) 1210 throw new Error("Attempt to auto-create GraphDefinitionLinkTargetComponent.type"); 1211 else if (Configuration.doAutoCreate()) 1212 this.type = new CodeType(); // bb 1213 return this.type; 1214 } 1215 1216 public boolean hasTypeElement() { 1217 return this.type != null && !this.type.isEmpty(); 1218 } 1219 1220 public boolean hasType() { 1221 return this.type != null && !this.type.isEmpty(); 1222 } 1223 1224 /** 1225 * @param value {@link #type} (Type of resource this link refers to.). This is 1226 * the underlying object with id, value and extensions. The 1227 * accessor "getType" gives direct access to the value 1228 */ 1229 public GraphDefinitionLinkTargetComponent setTypeElement(CodeType value) { 1230 this.type = value; 1231 return this; 1232 } 1233 1234 /** 1235 * @return Type of resource this link refers to. 1236 */ 1237 public String getType() { 1238 return this.type == null ? null : this.type.getValue(); 1239 } 1240 1241 /** 1242 * @param value Type of resource this link refers to. 1243 */ 1244 public GraphDefinitionLinkTargetComponent setType(String value) { 1245 if (this.type == null) 1246 this.type = new CodeType(); 1247 this.type.setValue(value); 1248 return this; 1249 } 1250 1251 /** 1252 * @return {@link #params} (A set of parameters to look up.). This is the 1253 * underlying object with id, value and extensions. The accessor 1254 * "getParams" gives direct access to the value 1255 */ 1256 public StringType getParamsElement() { 1257 if (this.params == null) 1258 if (Configuration.errorOnAutoCreate()) 1259 throw new Error("Attempt to auto-create GraphDefinitionLinkTargetComponent.params"); 1260 else if (Configuration.doAutoCreate()) 1261 this.params = new StringType(); // bb 1262 return this.params; 1263 } 1264 1265 public boolean hasParamsElement() { 1266 return this.params != null && !this.params.isEmpty(); 1267 } 1268 1269 public boolean hasParams() { 1270 return this.params != null && !this.params.isEmpty(); 1271 } 1272 1273 /** 1274 * @param value {@link #params} (A set of parameters to look up.). This is the 1275 * underlying object with id, value and extensions. The accessor 1276 * "getParams" gives direct access to the value 1277 */ 1278 public GraphDefinitionLinkTargetComponent setParamsElement(StringType value) { 1279 this.params = value; 1280 return this; 1281 } 1282 1283 /** 1284 * @return A set of parameters to look up. 1285 */ 1286 public String getParams() { 1287 return this.params == null ? null : this.params.getValue(); 1288 } 1289 1290 /** 1291 * @param value A set of parameters to look up. 1292 */ 1293 public GraphDefinitionLinkTargetComponent setParams(String value) { 1294 if (Utilities.noString(value)) 1295 this.params = null; 1296 else { 1297 if (this.params == null) 1298 this.params = new StringType(); 1299 this.params.setValue(value); 1300 } 1301 return this; 1302 } 1303 1304 /** 1305 * @return {@link #profile} (Profile for the target resource.). This is the 1306 * underlying object with id, value and extensions. The accessor 1307 * "getProfile" gives direct access to the value 1308 */ 1309 public CanonicalType getProfileElement() { 1310 if (this.profile == null) 1311 if (Configuration.errorOnAutoCreate()) 1312 throw new Error("Attempt to auto-create GraphDefinitionLinkTargetComponent.profile"); 1313 else if (Configuration.doAutoCreate()) 1314 this.profile = new CanonicalType(); // bb 1315 return this.profile; 1316 } 1317 1318 public boolean hasProfileElement() { 1319 return this.profile != null && !this.profile.isEmpty(); 1320 } 1321 1322 public boolean hasProfile() { 1323 return this.profile != null && !this.profile.isEmpty(); 1324 } 1325 1326 /** 1327 * @param value {@link #profile} (Profile for the target resource.). This is the 1328 * underlying object with id, value and extensions. The accessor 1329 * "getProfile" gives direct access to the value 1330 */ 1331 public GraphDefinitionLinkTargetComponent setProfileElement(CanonicalType value) { 1332 this.profile = value; 1333 return this; 1334 } 1335 1336 /** 1337 * @return Profile for the target resource. 1338 */ 1339 public String getProfile() { 1340 return this.profile == null ? null : this.profile.getValue(); 1341 } 1342 1343 /** 1344 * @param value Profile for the target resource. 1345 */ 1346 public GraphDefinitionLinkTargetComponent setProfile(String value) { 1347 if (Utilities.noString(value)) 1348 this.profile = null; 1349 else { 1350 if (this.profile == null) 1351 this.profile = new CanonicalType(); 1352 this.profile.setValue(value); 1353 } 1354 return this; 1355 } 1356 1357 /** 1358 * @return {@link #compartment} (Compartment Consistency Rules.) 1359 */ 1360 public List<GraphDefinitionLinkTargetCompartmentComponent> getCompartment() { 1361 if (this.compartment == null) 1362 this.compartment = new ArrayList<GraphDefinitionLinkTargetCompartmentComponent>(); 1363 return this.compartment; 1364 } 1365 1366 /** 1367 * @return Returns a reference to <code>this</code> for easy method chaining 1368 */ 1369 public GraphDefinitionLinkTargetComponent setCompartment( 1370 List<GraphDefinitionLinkTargetCompartmentComponent> theCompartment) { 1371 this.compartment = theCompartment; 1372 return this; 1373 } 1374 1375 public boolean hasCompartment() { 1376 if (this.compartment == null) 1377 return false; 1378 for (GraphDefinitionLinkTargetCompartmentComponent item : this.compartment) 1379 if (!item.isEmpty()) 1380 return true; 1381 return false; 1382 } 1383 1384 public GraphDefinitionLinkTargetCompartmentComponent addCompartment() { // 3 1385 GraphDefinitionLinkTargetCompartmentComponent t = new GraphDefinitionLinkTargetCompartmentComponent(); 1386 if (this.compartment == null) 1387 this.compartment = new ArrayList<GraphDefinitionLinkTargetCompartmentComponent>(); 1388 this.compartment.add(t); 1389 return t; 1390 } 1391 1392 public GraphDefinitionLinkTargetComponent addCompartment(GraphDefinitionLinkTargetCompartmentComponent t) { // 3 1393 if (t == null) 1394 return this; 1395 if (this.compartment == null) 1396 this.compartment = new ArrayList<GraphDefinitionLinkTargetCompartmentComponent>(); 1397 this.compartment.add(t); 1398 return this; 1399 } 1400 1401 /** 1402 * @return The first repetition of repeating field {@link #compartment}, 1403 * creating it if it does not already exist 1404 */ 1405 public GraphDefinitionLinkTargetCompartmentComponent getCompartmentFirstRep() { 1406 if (getCompartment().isEmpty()) { 1407 addCompartment(); 1408 } 1409 return getCompartment().get(0); 1410 } 1411 1412 /** 1413 * @return {@link #link} (Additional links from target resource.) 1414 */ 1415 public List<GraphDefinitionLinkComponent> getLink() { 1416 if (this.link == null) 1417 this.link = new ArrayList<GraphDefinitionLinkComponent>(); 1418 return this.link; 1419 } 1420 1421 /** 1422 * @return Returns a reference to <code>this</code> for easy method chaining 1423 */ 1424 public GraphDefinitionLinkTargetComponent setLink(List<GraphDefinitionLinkComponent> theLink) { 1425 this.link = theLink; 1426 return this; 1427 } 1428 1429 public boolean hasLink() { 1430 if (this.link == null) 1431 return false; 1432 for (GraphDefinitionLinkComponent item : this.link) 1433 if (!item.isEmpty()) 1434 return true; 1435 return false; 1436 } 1437 1438 public GraphDefinitionLinkComponent addLink() { // 3 1439 GraphDefinitionLinkComponent t = new GraphDefinitionLinkComponent(); 1440 if (this.link == null) 1441 this.link = new ArrayList<GraphDefinitionLinkComponent>(); 1442 this.link.add(t); 1443 return t; 1444 } 1445 1446 public GraphDefinitionLinkTargetComponent addLink(GraphDefinitionLinkComponent t) { // 3 1447 if (t == null) 1448 return this; 1449 if (this.link == null) 1450 this.link = new ArrayList<GraphDefinitionLinkComponent>(); 1451 this.link.add(t); 1452 return this; 1453 } 1454 1455 /** 1456 * @return The first repetition of repeating field {@link #link}, creating it if 1457 * it does not already exist 1458 */ 1459 public GraphDefinitionLinkComponent getLinkFirstRep() { 1460 if (getLink().isEmpty()) { 1461 addLink(); 1462 } 1463 return getLink().get(0); 1464 } 1465 1466 protected void listChildren(List<Property> children) { 1467 super.listChildren(children); 1468 children.add(new Property("type", "code", "Type of resource this link refers to.", 0, 1, type)); 1469 children.add(new Property("params", "string", "A set of parameters to look up.", 0, 1, params)); 1470 children.add( 1471 new Property("profile", "canonical(StructureDefinition)", "Profile for the target resource.", 0, 1, profile)); 1472 children.add(new Property("compartment", "", "Compartment Consistency Rules.", 0, java.lang.Integer.MAX_VALUE, 1473 compartment)); 1474 children.add(new Property("link", "@GraphDefinition.link", "Additional links from target resource.", 0, 1475 java.lang.Integer.MAX_VALUE, link)); 1476 } 1477 1478 @Override 1479 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1480 switch (_hash) { 1481 case 3575610: 1482 /* type */ return new Property("type", "code", "Type of resource this link refers to.", 0, 1, type); 1483 case -995427962: 1484 /* params */ return new Property("params", "string", "A set of parameters to look up.", 0, 1, params); 1485 case -309425751: 1486 /* profile */ return new Property("profile", "canonical(StructureDefinition)", 1487 "Profile for the target resource.", 0, 1, profile); 1488 case -397756334: 1489 /* compartment */ return new Property("compartment", "", "Compartment Consistency Rules.", 0, 1490 java.lang.Integer.MAX_VALUE, compartment); 1491 case 3321850: 1492 /* link */ return new Property("link", "@GraphDefinition.link", "Additional links from target resource.", 0, 1493 java.lang.Integer.MAX_VALUE, link); 1494 default: 1495 return super.getNamedProperty(_hash, _name, _checkValid); 1496 } 1497 1498 } 1499 1500 @Override 1501 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1502 switch (hash) { 1503 case 3575610: 1504 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeType 1505 case -995427962: 1506 /* params */ return this.params == null ? new Base[0] : new Base[] { this.params }; // StringType 1507 case -309425751: 1508 /* profile */ return this.profile == null ? new Base[0] : new Base[] { this.profile }; // CanonicalType 1509 case -397756334: 1510 /* compartment */ return this.compartment == null ? new Base[0] 1511 : this.compartment.toArray(new Base[this.compartment.size()]); // GraphDefinitionLinkTargetCompartmentComponent 1512 case 3321850: 1513 /* link */ return this.link == null ? new Base[0] : this.link.toArray(new Base[this.link.size()]); // GraphDefinitionLinkComponent 1514 default: 1515 return super.getProperty(hash, name, checkValid); 1516 } 1517 1518 } 1519 1520 @Override 1521 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1522 switch (hash) { 1523 case 3575610: // type 1524 this.type = castToCode(value); // CodeType 1525 return value; 1526 case -995427962: // params 1527 this.params = castToString(value); // StringType 1528 return value; 1529 case -309425751: // profile 1530 this.profile = castToCanonical(value); // CanonicalType 1531 return value; 1532 case -397756334: // compartment 1533 this.getCompartment().add((GraphDefinitionLinkTargetCompartmentComponent) value); // GraphDefinitionLinkTargetCompartmentComponent 1534 return value; 1535 case 3321850: // link 1536 this.getLink().add((GraphDefinitionLinkComponent) value); // GraphDefinitionLinkComponent 1537 return value; 1538 default: 1539 return super.setProperty(hash, name, value); 1540 } 1541 1542 } 1543 1544 @Override 1545 public Base setProperty(String name, Base value) throws FHIRException { 1546 if (name.equals("type")) { 1547 this.type = castToCode(value); // CodeType 1548 } else if (name.equals("params")) { 1549 this.params = castToString(value); // StringType 1550 } else if (name.equals("profile")) { 1551 this.profile = castToCanonical(value); // CanonicalType 1552 } else if (name.equals("compartment")) { 1553 this.getCompartment().add((GraphDefinitionLinkTargetCompartmentComponent) value); 1554 } else if (name.equals("link")) { 1555 this.getLink().add((GraphDefinitionLinkComponent) value); 1556 } else 1557 return super.setProperty(name, value); 1558 return value; 1559 } 1560 1561 @Override 1562 public void removeChild(String name, Base value) throws FHIRException { 1563 if (name.equals("type")) { 1564 this.type = null; 1565 } else if (name.equals("params")) { 1566 this.params = null; 1567 } else if (name.equals("profile")) { 1568 this.profile = null; 1569 } else if (name.equals("compartment")) { 1570 this.getCompartment().remove((GraphDefinitionLinkTargetCompartmentComponent) value); 1571 } else if (name.equals("link")) { 1572 this.getLink().remove((GraphDefinitionLinkComponent) value); 1573 } else 1574 super.removeChild(name, value); 1575 1576 } 1577 1578 @Override 1579 public Base makeProperty(int hash, String name) throws FHIRException { 1580 switch (hash) { 1581 case 3575610: 1582 return getTypeElement(); 1583 case -995427962: 1584 return getParamsElement(); 1585 case -309425751: 1586 return getProfileElement(); 1587 case -397756334: 1588 return addCompartment(); 1589 case 3321850: 1590 return addLink(); 1591 default: 1592 return super.makeProperty(hash, name); 1593 } 1594 1595 } 1596 1597 @Override 1598 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1599 switch (hash) { 1600 case 3575610: 1601 /* type */ return new String[] { "code" }; 1602 case -995427962: 1603 /* params */ return new String[] { "string" }; 1604 case -309425751: 1605 /* profile */ return new String[] { "canonical" }; 1606 case -397756334: 1607 /* compartment */ return new String[] {}; 1608 case 3321850: 1609 /* link */ return new String[] { "@GraphDefinition.link" }; 1610 default: 1611 return super.getTypesForProperty(hash, name); 1612 } 1613 1614 } 1615 1616 @Override 1617 public Base addChild(String name) throws FHIRException { 1618 if (name.equals("type")) { 1619 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.type"); 1620 } else if (name.equals("params")) { 1621 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.params"); 1622 } else if (name.equals("profile")) { 1623 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.profile"); 1624 } else if (name.equals("compartment")) { 1625 return addCompartment(); 1626 } else if (name.equals("link")) { 1627 return addLink(); 1628 } else 1629 return super.addChild(name); 1630 } 1631 1632 public GraphDefinitionLinkTargetComponent copy() { 1633 GraphDefinitionLinkTargetComponent dst = new GraphDefinitionLinkTargetComponent(); 1634 copyValues(dst); 1635 return dst; 1636 } 1637 1638 public void copyValues(GraphDefinitionLinkTargetComponent dst) { 1639 super.copyValues(dst); 1640 dst.type = type == null ? null : type.copy(); 1641 dst.params = params == null ? null : params.copy(); 1642 dst.profile = profile == null ? null : profile.copy(); 1643 if (compartment != null) { 1644 dst.compartment = new ArrayList<GraphDefinitionLinkTargetCompartmentComponent>(); 1645 for (GraphDefinitionLinkTargetCompartmentComponent i : compartment) 1646 dst.compartment.add(i.copy()); 1647 } 1648 ; 1649 if (link != null) { 1650 dst.link = new ArrayList<GraphDefinitionLinkComponent>(); 1651 for (GraphDefinitionLinkComponent i : link) 1652 dst.link.add(i.copy()); 1653 } 1654 ; 1655 } 1656 1657 @Override 1658 public boolean equalsDeep(Base other_) { 1659 if (!super.equalsDeep(other_)) 1660 return false; 1661 if (!(other_ instanceof GraphDefinitionLinkTargetComponent)) 1662 return false; 1663 GraphDefinitionLinkTargetComponent o = (GraphDefinitionLinkTargetComponent) other_; 1664 return compareDeep(type, o.type, true) && compareDeep(params, o.params, true) 1665 && compareDeep(profile, o.profile, true) && compareDeep(compartment, o.compartment, true) 1666 && compareDeep(link, o.link, true); 1667 } 1668 1669 @Override 1670 public boolean equalsShallow(Base other_) { 1671 if (!super.equalsShallow(other_)) 1672 return false; 1673 if (!(other_ instanceof GraphDefinitionLinkTargetComponent)) 1674 return false; 1675 GraphDefinitionLinkTargetComponent o = (GraphDefinitionLinkTargetComponent) other_; 1676 return compareValues(type, o.type, true) && compareValues(params, o.params, true); 1677 } 1678 1679 public boolean isEmpty() { 1680 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, params, profile, compartment, link); 1681 } 1682 1683 public String fhirType() { 1684 return "GraphDefinition.link.target"; 1685 1686 } 1687 1688 } 1689 1690 @Block() 1691 public static class GraphDefinitionLinkTargetCompartmentComponent extends BackboneElement 1692 implements IBaseBackboneElement { 1693 /** 1694 * Defines how the compartment rule is used - whether it it is used to test 1695 * whether resources are subject to the rule, or whether it is a rule that must 1696 * be followed. 1697 */ 1698 @Child(name = "use", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1699 @Description(shortDefinition = "condition | requirement", formalDefinition = "Defines how the compartment rule is used - whether it it is used to test whether resources are subject to the rule, or whether it is a rule that must be followed.") 1700 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/graph-compartment-use") 1701 protected Enumeration<GraphCompartmentUse> use; 1702 1703 /** 1704 * Identifies the compartment. 1705 */ 1706 @Child(name = "code", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 1707 @Description(shortDefinition = "Patient | Encounter | RelatedPerson | Practitioner | Device", formalDefinition = "Identifies the compartment.") 1708 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/compartment-type") 1709 protected Enumeration<CompartmentCode> code; 1710 1711 /** 1712 * identical | matching | different | no-rule | custom. 1713 */ 1714 @Child(name = "rule", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 1715 @Description(shortDefinition = "identical | matching | different | custom", formalDefinition = "identical | matching | different | no-rule | custom.") 1716 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/graph-compartment-rule") 1717 protected Enumeration<GraphCompartmentRule> rule; 1718 1719 /** 1720 * Custom rule, as a FHIRPath expression. 1721 */ 1722 @Child(name = "expression", type = { 1723 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1724 @Description(shortDefinition = "Custom rule, as a FHIRPath expression", formalDefinition = "Custom rule, as a FHIRPath expression.") 1725 protected StringType expression; 1726 1727 /** 1728 * Documentation for FHIRPath expression. 1729 */ 1730 @Child(name = "description", type = { 1731 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1732 @Description(shortDefinition = "Documentation for FHIRPath expression", formalDefinition = "Documentation for FHIRPath expression.") 1733 protected StringType description; 1734 1735 private static final long serialVersionUID = 1023364175L; 1736 1737 /** 1738 * Constructor 1739 */ 1740 public GraphDefinitionLinkTargetCompartmentComponent() { 1741 super(); 1742 } 1743 1744 /** 1745 * Constructor 1746 */ 1747 public GraphDefinitionLinkTargetCompartmentComponent(Enumeration<GraphCompartmentUse> use, 1748 Enumeration<CompartmentCode> code, Enumeration<GraphCompartmentRule> rule) { 1749 super(); 1750 this.use = use; 1751 this.code = code; 1752 this.rule = rule; 1753 } 1754 1755 /** 1756 * @return {@link #use} (Defines how the compartment rule is used - whether it 1757 * it is used to test whether resources are subject to the rule, or 1758 * whether it is a rule that must be followed.). This is the underlying 1759 * object with id, value and extensions. The accessor "getUse" gives 1760 * direct access to the value 1761 */ 1762 public Enumeration<GraphCompartmentUse> getUseElement() { 1763 if (this.use == null) 1764 if (Configuration.errorOnAutoCreate()) 1765 throw new Error("Attempt to auto-create GraphDefinitionLinkTargetCompartmentComponent.use"); 1766 else if (Configuration.doAutoCreate()) 1767 this.use = new Enumeration<GraphCompartmentUse>(new GraphCompartmentUseEnumFactory()); // bb 1768 return this.use; 1769 } 1770 1771 public boolean hasUseElement() { 1772 return this.use != null && !this.use.isEmpty(); 1773 } 1774 1775 public boolean hasUse() { 1776 return this.use != null && !this.use.isEmpty(); 1777 } 1778 1779 /** 1780 * @param value {@link #use} (Defines how the compartment rule is used - whether 1781 * it it is used to test whether resources are subject to the rule, 1782 * or whether it is a rule that must be followed.). This is the 1783 * underlying object with id, value and extensions. The accessor 1784 * "getUse" gives direct access to the value 1785 */ 1786 public GraphDefinitionLinkTargetCompartmentComponent setUseElement(Enumeration<GraphCompartmentUse> value) { 1787 this.use = value; 1788 return this; 1789 } 1790 1791 /** 1792 * @return Defines how the compartment rule is used - whether it it is used to 1793 * test whether resources are subject to the rule, or whether it is a 1794 * rule that must be followed. 1795 */ 1796 public GraphCompartmentUse getUse() { 1797 return this.use == null ? null : this.use.getValue(); 1798 } 1799 1800 /** 1801 * @param value Defines how the compartment rule is used - whether it it is used 1802 * to test whether resources are subject to the rule, or whether it 1803 * is a rule that must be followed. 1804 */ 1805 public GraphDefinitionLinkTargetCompartmentComponent setUse(GraphCompartmentUse value) { 1806 if (this.use == null) 1807 this.use = new Enumeration<GraphCompartmentUse>(new GraphCompartmentUseEnumFactory()); 1808 this.use.setValue(value); 1809 return this; 1810 } 1811 1812 /** 1813 * @return {@link #code} (Identifies the compartment.). This is the underlying 1814 * object with id, value and extensions. The accessor "getCode" gives 1815 * direct access to the value 1816 */ 1817 public Enumeration<CompartmentCode> getCodeElement() { 1818 if (this.code == null) 1819 if (Configuration.errorOnAutoCreate()) 1820 throw new Error("Attempt to auto-create GraphDefinitionLinkTargetCompartmentComponent.code"); 1821 else if (Configuration.doAutoCreate()) 1822 this.code = new Enumeration<CompartmentCode>(new CompartmentCodeEnumFactory()); // bb 1823 return this.code; 1824 } 1825 1826 public boolean hasCodeElement() { 1827 return this.code != null && !this.code.isEmpty(); 1828 } 1829 1830 public boolean hasCode() { 1831 return this.code != null && !this.code.isEmpty(); 1832 } 1833 1834 /** 1835 * @param value {@link #code} (Identifies the compartment.). This is the 1836 * underlying object with id, value and extensions. The accessor 1837 * "getCode" gives direct access to the value 1838 */ 1839 public GraphDefinitionLinkTargetCompartmentComponent setCodeElement(Enumeration<CompartmentCode> value) { 1840 this.code = value; 1841 return this; 1842 } 1843 1844 /** 1845 * @return Identifies the compartment. 1846 */ 1847 public CompartmentCode getCode() { 1848 return this.code == null ? null : this.code.getValue(); 1849 } 1850 1851 /** 1852 * @param value Identifies the compartment. 1853 */ 1854 public GraphDefinitionLinkTargetCompartmentComponent setCode(CompartmentCode value) { 1855 if (this.code == null) 1856 this.code = new Enumeration<CompartmentCode>(new CompartmentCodeEnumFactory()); 1857 this.code.setValue(value); 1858 return this; 1859 } 1860 1861 /** 1862 * @return {@link #rule} (identical | matching | different | no-rule | custom.). 1863 * This is the underlying object with id, value and extensions. The 1864 * accessor "getRule" gives direct access to the value 1865 */ 1866 public Enumeration<GraphCompartmentRule> getRuleElement() { 1867 if (this.rule == null) 1868 if (Configuration.errorOnAutoCreate()) 1869 throw new Error("Attempt to auto-create GraphDefinitionLinkTargetCompartmentComponent.rule"); 1870 else if (Configuration.doAutoCreate()) 1871 this.rule = new Enumeration<GraphCompartmentRule>(new GraphCompartmentRuleEnumFactory()); // bb 1872 return this.rule; 1873 } 1874 1875 public boolean hasRuleElement() { 1876 return this.rule != null && !this.rule.isEmpty(); 1877 } 1878 1879 public boolean hasRule() { 1880 return this.rule != null && !this.rule.isEmpty(); 1881 } 1882 1883 /** 1884 * @param value {@link #rule} (identical | matching | different | no-rule | 1885 * custom.). This is the underlying object with id, value and 1886 * extensions. The accessor "getRule" gives direct access to the 1887 * value 1888 */ 1889 public GraphDefinitionLinkTargetCompartmentComponent setRuleElement(Enumeration<GraphCompartmentRule> value) { 1890 this.rule = value; 1891 return this; 1892 } 1893 1894 /** 1895 * @return identical | matching | different | no-rule | custom. 1896 */ 1897 public GraphCompartmentRule getRule() { 1898 return this.rule == null ? null : this.rule.getValue(); 1899 } 1900 1901 /** 1902 * @param value identical | matching | different | no-rule | custom. 1903 */ 1904 public GraphDefinitionLinkTargetCompartmentComponent setRule(GraphCompartmentRule value) { 1905 if (this.rule == null) 1906 this.rule = new Enumeration<GraphCompartmentRule>(new GraphCompartmentRuleEnumFactory()); 1907 this.rule.setValue(value); 1908 return this; 1909 } 1910 1911 /** 1912 * @return {@link #expression} (Custom rule, as a FHIRPath expression.). This is 1913 * the underlying object with id, value and extensions. The accessor 1914 * "getExpression" gives direct access to the value 1915 */ 1916 public StringType getExpressionElement() { 1917 if (this.expression == null) 1918 if (Configuration.errorOnAutoCreate()) 1919 throw new Error("Attempt to auto-create GraphDefinitionLinkTargetCompartmentComponent.expression"); 1920 else if (Configuration.doAutoCreate()) 1921 this.expression = new StringType(); // bb 1922 return this.expression; 1923 } 1924 1925 public boolean hasExpressionElement() { 1926 return this.expression != null && !this.expression.isEmpty(); 1927 } 1928 1929 public boolean hasExpression() { 1930 return this.expression != null && !this.expression.isEmpty(); 1931 } 1932 1933 /** 1934 * @param value {@link #expression} (Custom rule, as a FHIRPath expression.). 1935 * This is the underlying object with id, value and extensions. The 1936 * accessor "getExpression" gives direct access to the value 1937 */ 1938 public GraphDefinitionLinkTargetCompartmentComponent setExpressionElement(StringType value) { 1939 this.expression = value; 1940 return this; 1941 } 1942 1943 /** 1944 * @return Custom rule, as a FHIRPath expression. 1945 */ 1946 public String getExpression() { 1947 return this.expression == null ? null : this.expression.getValue(); 1948 } 1949 1950 /** 1951 * @param value Custom rule, as a FHIRPath expression. 1952 */ 1953 public GraphDefinitionLinkTargetCompartmentComponent setExpression(String value) { 1954 if (Utilities.noString(value)) 1955 this.expression = null; 1956 else { 1957 if (this.expression == null) 1958 this.expression = new StringType(); 1959 this.expression.setValue(value); 1960 } 1961 return this; 1962 } 1963 1964 /** 1965 * @return {@link #description} (Documentation for FHIRPath expression.). This 1966 * is the underlying object with id, value and extensions. The accessor 1967 * "getDescription" gives direct access to the value 1968 */ 1969 public StringType getDescriptionElement() { 1970 if (this.description == null) 1971 if (Configuration.errorOnAutoCreate()) 1972 throw new Error("Attempt to auto-create GraphDefinitionLinkTargetCompartmentComponent.description"); 1973 else if (Configuration.doAutoCreate()) 1974 this.description = new StringType(); // bb 1975 return this.description; 1976 } 1977 1978 public boolean hasDescriptionElement() { 1979 return this.description != null && !this.description.isEmpty(); 1980 } 1981 1982 public boolean hasDescription() { 1983 return this.description != null && !this.description.isEmpty(); 1984 } 1985 1986 /** 1987 * @param value {@link #description} (Documentation for FHIRPath expression.). 1988 * This is the underlying object with id, value and extensions. The 1989 * accessor "getDescription" gives direct access to the value 1990 */ 1991 public GraphDefinitionLinkTargetCompartmentComponent setDescriptionElement(StringType value) { 1992 this.description = value; 1993 return this; 1994 } 1995 1996 /** 1997 * @return Documentation for FHIRPath expression. 1998 */ 1999 public String getDescription() { 2000 return this.description == null ? null : this.description.getValue(); 2001 } 2002 2003 /** 2004 * @param value Documentation for FHIRPath expression. 2005 */ 2006 public GraphDefinitionLinkTargetCompartmentComponent setDescription(String value) { 2007 if (Utilities.noString(value)) 2008 this.description = null; 2009 else { 2010 if (this.description == null) 2011 this.description = new StringType(); 2012 this.description.setValue(value); 2013 } 2014 return this; 2015 } 2016 2017 protected void listChildren(List<Property> children) { 2018 super.listChildren(children); 2019 children.add(new Property("use", "code", 2020 "Defines how the compartment rule is used - whether it it is used to test whether resources are subject to the rule, or whether it is a rule that must be followed.", 2021 0, 1, use)); 2022 children.add(new Property("code", "code", "Identifies the compartment.", 0, 1, code)); 2023 children.add(new Property("rule", "code", "identical | matching | different | no-rule | custom.", 0, 1, rule)); 2024 children.add(new Property("expression", "string", "Custom rule, as a FHIRPath expression.", 0, 1, expression)); 2025 children.add(new Property("description", "string", "Documentation for FHIRPath expression.", 0, 1, description)); 2026 } 2027 2028 @Override 2029 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2030 switch (_hash) { 2031 case 116103: 2032 /* use */ return new Property("use", "code", 2033 "Defines how the compartment rule is used - whether it it is used to test whether resources are subject to the rule, or whether it is a rule that must be followed.", 2034 0, 1, use); 2035 case 3059181: 2036 /* code */ return new Property("code", "code", "Identifies the compartment.", 0, 1, code); 2037 case 3512060: 2038 /* rule */ return new Property("rule", "code", "identical | matching | different | no-rule | custom.", 0, 1, 2039 rule); 2040 case -1795452264: 2041 /* expression */ return new Property("expression", "string", "Custom rule, as a FHIRPath expression.", 0, 1, 2042 expression); 2043 case -1724546052: 2044 /* description */ return new Property("description", "string", "Documentation for FHIRPath expression.", 0, 1, 2045 description); 2046 default: 2047 return super.getNamedProperty(_hash, _name, _checkValid); 2048 } 2049 2050 } 2051 2052 @Override 2053 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2054 switch (hash) { 2055 case 116103: 2056 /* use */ return this.use == null ? new Base[0] : new Base[] { this.use }; // Enumeration<GraphCompartmentUse> 2057 case 3059181: 2058 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // Enumeration<CompartmentCode> 2059 case 3512060: 2060 /* rule */ return this.rule == null ? new Base[0] : new Base[] { this.rule }; // Enumeration<GraphCompartmentRule> 2061 case -1795452264: 2062 /* expression */ return this.expression == null ? new Base[0] : new Base[] { this.expression }; // StringType 2063 case -1724546052: 2064 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 2065 default: 2066 return super.getProperty(hash, name, checkValid); 2067 } 2068 2069 } 2070 2071 @Override 2072 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2073 switch (hash) { 2074 case 116103: // use 2075 value = new GraphCompartmentUseEnumFactory().fromType(castToCode(value)); 2076 this.use = (Enumeration) value; // Enumeration<GraphCompartmentUse> 2077 return value; 2078 case 3059181: // code 2079 value = new CompartmentCodeEnumFactory().fromType(castToCode(value)); 2080 this.code = (Enumeration) value; // Enumeration<CompartmentCode> 2081 return value; 2082 case 3512060: // rule 2083 value = new GraphCompartmentRuleEnumFactory().fromType(castToCode(value)); 2084 this.rule = (Enumeration) value; // Enumeration<GraphCompartmentRule> 2085 return value; 2086 case -1795452264: // expression 2087 this.expression = castToString(value); // StringType 2088 return value; 2089 case -1724546052: // description 2090 this.description = castToString(value); // StringType 2091 return value; 2092 default: 2093 return super.setProperty(hash, name, value); 2094 } 2095 2096 } 2097 2098 @Override 2099 public Base setProperty(String name, Base value) throws FHIRException { 2100 if (name.equals("use")) { 2101 value = new GraphCompartmentUseEnumFactory().fromType(castToCode(value)); 2102 this.use = (Enumeration) value; // Enumeration<GraphCompartmentUse> 2103 } else if (name.equals("code")) { 2104 value = new CompartmentCodeEnumFactory().fromType(castToCode(value)); 2105 this.code = (Enumeration) value; // Enumeration<CompartmentCode> 2106 } else if (name.equals("rule")) { 2107 value = new GraphCompartmentRuleEnumFactory().fromType(castToCode(value)); 2108 this.rule = (Enumeration) value; // Enumeration<GraphCompartmentRule> 2109 } else if (name.equals("expression")) { 2110 this.expression = castToString(value); // StringType 2111 } else if (name.equals("description")) { 2112 this.description = castToString(value); // StringType 2113 } else 2114 return super.setProperty(name, value); 2115 return value; 2116 } 2117 2118 @Override 2119 public void removeChild(String name, Base value) throws FHIRException { 2120 if (name.equals("use")) { 2121 this.use = null; 2122 } else if (name.equals("code")) { 2123 this.code = null; 2124 } else if (name.equals("rule")) { 2125 this.rule = null; 2126 } else if (name.equals("expression")) { 2127 this.expression = null; 2128 } else if (name.equals("description")) { 2129 this.description = null; 2130 } else 2131 super.removeChild(name, value); 2132 2133 } 2134 2135 @Override 2136 public Base makeProperty(int hash, String name) throws FHIRException { 2137 switch (hash) { 2138 case 116103: 2139 return getUseElement(); 2140 case 3059181: 2141 return getCodeElement(); 2142 case 3512060: 2143 return getRuleElement(); 2144 case -1795452264: 2145 return getExpressionElement(); 2146 case -1724546052: 2147 return getDescriptionElement(); 2148 default: 2149 return super.makeProperty(hash, name); 2150 } 2151 2152 } 2153 2154 @Override 2155 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2156 switch (hash) { 2157 case 116103: 2158 /* use */ return new String[] { "code" }; 2159 case 3059181: 2160 /* code */ return new String[] { "code" }; 2161 case 3512060: 2162 /* rule */ return new String[] { "code" }; 2163 case -1795452264: 2164 /* expression */ return new String[] { "string" }; 2165 case -1724546052: 2166 /* description */ return new String[] { "string" }; 2167 default: 2168 return super.getTypesForProperty(hash, name); 2169 } 2170 2171 } 2172 2173 @Override 2174 public Base addChild(String name) throws FHIRException { 2175 if (name.equals("use")) { 2176 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.use"); 2177 } else if (name.equals("code")) { 2178 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.code"); 2179 } else if (name.equals("rule")) { 2180 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.rule"); 2181 } else if (name.equals("expression")) { 2182 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.expression"); 2183 } else if (name.equals("description")) { 2184 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.description"); 2185 } else 2186 return super.addChild(name); 2187 } 2188 2189 public GraphDefinitionLinkTargetCompartmentComponent copy() { 2190 GraphDefinitionLinkTargetCompartmentComponent dst = new GraphDefinitionLinkTargetCompartmentComponent(); 2191 copyValues(dst); 2192 return dst; 2193 } 2194 2195 public void copyValues(GraphDefinitionLinkTargetCompartmentComponent dst) { 2196 super.copyValues(dst); 2197 dst.use = use == null ? null : use.copy(); 2198 dst.code = code == null ? null : code.copy(); 2199 dst.rule = rule == null ? null : rule.copy(); 2200 dst.expression = expression == null ? null : expression.copy(); 2201 dst.description = description == null ? null : description.copy(); 2202 } 2203 2204 @Override 2205 public boolean equalsDeep(Base other_) { 2206 if (!super.equalsDeep(other_)) 2207 return false; 2208 if (!(other_ instanceof GraphDefinitionLinkTargetCompartmentComponent)) 2209 return false; 2210 GraphDefinitionLinkTargetCompartmentComponent o = (GraphDefinitionLinkTargetCompartmentComponent) other_; 2211 return compareDeep(use, o.use, true) && compareDeep(code, o.code, true) && compareDeep(rule, o.rule, true) 2212 && compareDeep(expression, o.expression, true) && compareDeep(description, o.description, true); 2213 } 2214 2215 @Override 2216 public boolean equalsShallow(Base other_) { 2217 if (!super.equalsShallow(other_)) 2218 return false; 2219 if (!(other_ instanceof GraphDefinitionLinkTargetCompartmentComponent)) 2220 return false; 2221 GraphDefinitionLinkTargetCompartmentComponent o = (GraphDefinitionLinkTargetCompartmentComponent) other_; 2222 return compareValues(use, o.use, true) && compareValues(code, o.code, true) && compareValues(rule, o.rule, true) 2223 && compareValues(expression, o.expression, true) && compareValues(description, o.description, true); 2224 } 2225 2226 public boolean isEmpty() { 2227 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(use, code, rule, expression, description); 2228 } 2229 2230 public String fhirType() { 2231 return "GraphDefinition.link.target.compartment"; 2232 2233 } 2234 2235 } 2236 2237 /** 2238 * Explanation of why this graph definition is needed and why it has been 2239 * designed as it has. 2240 */ 2241 @Child(name = "purpose", type = { 2242 MarkdownType.class }, order = 0, min = 0, max = 1, modifier = false, summary = false) 2243 @Description(shortDefinition = "Why this graph definition is defined", formalDefinition = "Explanation of why this graph definition is needed and why it has been designed as it has.") 2244 protected MarkdownType purpose; 2245 2246 /** 2247 * The type of FHIR resource at which instances of this graph start. 2248 */ 2249 @Child(name = "start", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2250 @Description(shortDefinition = "Type of resource at which the graph starts", formalDefinition = "The type of FHIR resource at which instances of this graph start.") 2251 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/resource-types") 2252 protected CodeType start; 2253 2254 /** 2255 * The profile that describes the use of the base resource. 2256 */ 2257 @Child(name = "profile", type = { 2258 CanonicalType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2259 @Description(shortDefinition = "Profile on base resource", formalDefinition = "The profile that describes the use of the base resource.") 2260 protected CanonicalType profile; 2261 2262 /** 2263 * Links this graph makes rules about. 2264 */ 2265 @Child(name = "link", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2266 @Description(shortDefinition = "Links this graph makes rules about", formalDefinition = "Links this graph makes rules about.") 2267 protected List<GraphDefinitionLinkComponent> link; 2268 2269 private static final long serialVersionUID = 1529157887L; 2270 2271 /** 2272 * Constructor 2273 */ 2274 public GraphDefinition() { 2275 super(); 2276 } 2277 2278 /** 2279 * Constructor 2280 */ 2281 public GraphDefinition(StringType name, Enumeration<PublicationStatus> status, CodeType start) { 2282 super(); 2283 this.name = name; 2284 this.status = status; 2285 this.start = start; 2286 } 2287 2288 /** 2289 * @return {@link #url} (An absolute URI that is used to identify this graph 2290 * definition when it is referenced in a specification, model, design or 2291 * an instance; also called its canonical identifier. This SHOULD be 2292 * globally unique and SHOULD be a literal address at which at which an 2293 * authoritative instance of this graph definition is (or will be) 2294 * published. This URL can be the target of a canonical reference. It 2295 * SHALL remain the same when the graph definition is stored on 2296 * different servers.). This is the underlying object with id, value and 2297 * extensions. The accessor "getUrl" gives direct access to the value 2298 */ 2299 public UriType getUrlElement() { 2300 if (this.url == null) 2301 if (Configuration.errorOnAutoCreate()) 2302 throw new Error("Attempt to auto-create GraphDefinition.url"); 2303 else if (Configuration.doAutoCreate()) 2304 this.url = new UriType(); // bb 2305 return this.url; 2306 } 2307 2308 public boolean hasUrlElement() { 2309 return this.url != null && !this.url.isEmpty(); 2310 } 2311 2312 public boolean hasUrl() { 2313 return this.url != null && !this.url.isEmpty(); 2314 } 2315 2316 /** 2317 * @param value {@link #url} (An absolute URI that is used to identify this 2318 * graph definition when it is referenced in a specification, 2319 * model, design or an instance; also called its canonical 2320 * identifier. This SHOULD be globally unique and SHOULD be a 2321 * literal address at which at which an authoritative instance of 2322 * this graph definition is (or will be) published. This URL can be 2323 * the target of a canonical reference. It SHALL remain the same 2324 * when the graph definition is stored on different servers.). This 2325 * is the underlying object with id, value and extensions. The 2326 * accessor "getUrl" gives direct access to the value 2327 */ 2328 public GraphDefinition setUrlElement(UriType value) { 2329 this.url = value; 2330 return this; 2331 } 2332 2333 /** 2334 * @return An absolute URI that is used to identify this graph definition when 2335 * it is referenced in a specification, model, design or an instance; 2336 * also called its canonical identifier. This SHOULD be globally unique 2337 * and SHOULD be a literal address at which at which an authoritative 2338 * instance of this graph definition is (or will be) published. This URL 2339 * can be the target of a canonical reference. It SHALL remain the same 2340 * when the graph definition is stored on different servers. 2341 */ 2342 public String getUrl() { 2343 return this.url == null ? null : this.url.getValue(); 2344 } 2345 2346 /** 2347 * @param value An absolute URI that is used to identify this graph definition 2348 * when it is referenced in a specification, model, design or an 2349 * instance; also called its canonical identifier. This SHOULD be 2350 * globally unique and SHOULD be a literal address at which at 2351 * which an authoritative instance of this graph definition is (or 2352 * will be) published. This URL can be the target of a canonical 2353 * reference. It SHALL remain the same when the graph definition is 2354 * stored on different servers. 2355 */ 2356 public GraphDefinition setUrl(String value) { 2357 if (Utilities.noString(value)) 2358 this.url = null; 2359 else { 2360 if (this.url == null) 2361 this.url = new UriType(); 2362 this.url.setValue(value); 2363 } 2364 return this; 2365 } 2366 2367 /** 2368 * @return {@link #version} (The identifier that is used to identify this 2369 * version of the graph definition when it is referenced in a 2370 * specification, model, design or instance. This is an arbitrary value 2371 * managed by the graph definition author and is not expected to be 2372 * globally unique. For example, it might be a timestamp (e.g. yyyymmdd) 2373 * if a managed version is not available. There is also no expectation 2374 * that versions can be placed in a lexicographical sequence.). This is 2375 * the underlying object with id, value and extensions. The accessor 2376 * "getVersion" gives direct access to the value 2377 */ 2378 public StringType getVersionElement() { 2379 if (this.version == null) 2380 if (Configuration.errorOnAutoCreate()) 2381 throw new Error("Attempt to auto-create GraphDefinition.version"); 2382 else if (Configuration.doAutoCreate()) 2383 this.version = new StringType(); // bb 2384 return this.version; 2385 } 2386 2387 public boolean hasVersionElement() { 2388 return this.version != null && !this.version.isEmpty(); 2389 } 2390 2391 public boolean hasVersion() { 2392 return this.version != null && !this.version.isEmpty(); 2393 } 2394 2395 /** 2396 * @param value {@link #version} (The identifier that is used to identify this 2397 * version of the graph definition when it is referenced in a 2398 * specification, model, design or instance. This is an arbitrary 2399 * value managed by the graph definition author and is not expected 2400 * to be globally unique. For example, it might be a timestamp 2401 * (e.g. yyyymmdd) if a managed version is not available. There is 2402 * also no expectation that versions can be placed in a 2403 * lexicographical sequence.). This is the underlying object with 2404 * id, value and extensions. The accessor "getVersion" gives direct 2405 * access to the value 2406 */ 2407 public GraphDefinition setVersionElement(StringType value) { 2408 this.version = value; 2409 return this; 2410 } 2411 2412 /** 2413 * @return The identifier that is used to identify this version of the graph 2414 * definition when it is referenced in a specification, model, design or 2415 * instance. This is an arbitrary value managed by the graph definition 2416 * author and is not expected to be globally unique. For example, it 2417 * might be a timestamp (e.g. yyyymmdd) if a managed version is not 2418 * available. There is also no expectation that versions can be placed 2419 * in a lexicographical sequence. 2420 */ 2421 public String getVersion() { 2422 return this.version == null ? null : this.version.getValue(); 2423 } 2424 2425 /** 2426 * @param value The identifier that is used to identify this version of the 2427 * graph definition when it is referenced in a specification, 2428 * model, design or instance. This is an arbitrary value managed by 2429 * the graph definition author and is not expected to be globally 2430 * unique. For example, it might be a timestamp (e.g. yyyymmdd) if 2431 * a managed version is not available. There is also no expectation 2432 * that versions can be placed in a lexicographical sequence. 2433 */ 2434 public GraphDefinition setVersion(String value) { 2435 if (Utilities.noString(value)) 2436 this.version = null; 2437 else { 2438 if (this.version == null) 2439 this.version = new StringType(); 2440 this.version.setValue(value); 2441 } 2442 return this; 2443 } 2444 2445 /** 2446 * @return {@link #name} (A natural language name identifying the graph 2447 * definition. This name should be usable as an identifier for the 2448 * module by machine processing applications such as code generation.). 2449 * This is the underlying object with id, value and extensions. The 2450 * accessor "getName" gives direct access to the value 2451 */ 2452 public StringType getNameElement() { 2453 if (this.name == null) 2454 if (Configuration.errorOnAutoCreate()) 2455 throw new Error("Attempt to auto-create GraphDefinition.name"); 2456 else if (Configuration.doAutoCreate()) 2457 this.name = new StringType(); // bb 2458 return this.name; 2459 } 2460 2461 public boolean hasNameElement() { 2462 return this.name != null && !this.name.isEmpty(); 2463 } 2464 2465 public boolean hasName() { 2466 return this.name != null && !this.name.isEmpty(); 2467 } 2468 2469 /** 2470 * @param value {@link #name} (A natural language name identifying the graph 2471 * definition. This name should be usable as an identifier for the 2472 * module by machine processing applications such as code 2473 * generation.). This is the underlying object with id, value and 2474 * extensions. The accessor "getName" gives direct access to the 2475 * value 2476 */ 2477 public GraphDefinition setNameElement(StringType value) { 2478 this.name = value; 2479 return this; 2480 } 2481 2482 /** 2483 * @return A natural language name identifying the graph definition. This name 2484 * should be usable as an identifier for the module by machine 2485 * processing applications such as code generation. 2486 */ 2487 public String getName() { 2488 return this.name == null ? null : this.name.getValue(); 2489 } 2490 2491 /** 2492 * @param value A natural language name identifying the graph definition. This 2493 * name should be usable as an identifier for the module by machine 2494 * processing applications such as code generation. 2495 */ 2496 public GraphDefinition setName(String value) { 2497 if (this.name == null) 2498 this.name = new StringType(); 2499 this.name.setValue(value); 2500 return this; 2501 } 2502 2503 /** 2504 * @return {@link #status} (The status of this graph definition. Enables 2505 * tracking the life-cycle of the content.). This is the underlying 2506 * object with id, value and extensions. The accessor "getStatus" gives 2507 * direct access to the value 2508 */ 2509 public Enumeration<PublicationStatus> getStatusElement() { 2510 if (this.status == null) 2511 if (Configuration.errorOnAutoCreate()) 2512 throw new Error("Attempt to auto-create GraphDefinition.status"); 2513 else if (Configuration.doAutoCreate()) 2514 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2515 return this.status; 2516 } 2517 2518 public boolean hasStatusElement() { 2519 return this.status != null && !this.status.isEmpty(); 2520 } 2521 2522 public boolean hasStatus() { 2523 return this.status != null && !this.status.isEmpty(); 2524 } 2525 2526 /** 2527 * @param value {@link #status} (The status of this graph definition. Enables 2528 * tracking the life-cycle of the content.). This is the underlying 2529 * object with id, value and extensions. The accessor "getStatus" 2530 * gives direct access to the value 2531 */ 2532 public GraphDefinition setStatusElement(Enumeration<PublicationStatus> value) { 2533 this.status = value; 2534 return this; 2535 } 2536 2537 /** 2538 * @return The status of this graph definition. Enables tracking the life-cycle 2539 * of the content. 2540 */ 2541 public PublicationStatus getStatus() { 2542 return this.status == null ? null : this.status.getValue(); 2543 } 2544 2545 /** 2546 * @param value The status of this graph definition. Enables tracking the 2547 * life-cycle of the content. 2548 */ 2549 public GraphDefinition setStatus(PublicationStatus value) { 2550 if (this.status == null) 2551 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2552 this.status.setValue(value); 2553 return this; 2554 } 2555 2556 /** 2557 * @return {@link #experimental} (A Boolean value to indicate that this graph 2558 * definition is authored for testing purposes (or 2559 * education/evaluation/marketing) and is not intended to be used for 2560 * genuine usage.). This is the underlying object with id, value and 2561 * extensions. The accessor "getExperimental" gives direct access to the 2562 * value 2563 */ 2564 public BooleanType getExperimentalElement() { 2565 if (this.experimental == null) 2566 if (Configuration.errorOnAutoCreate()) 2567 throw new Error("Attempt to auto-create GraphDefinition.experimental"); 2568 else if (Configuration.doAutoCreate()) 2569 this.experimental = new BooleanType(); // bb 2570 return this.experimental; 2571 } 2572 2573 public boolean hasExperimentalElement() { 2574 return this.experimental != null && !this.experimental.isEmpty(); 2575 } 2576 2577 public boolean hasExperimental() { 2578 return this.experimental != null && !this.experimental.isEmpty(); 2579 } 2580 2581 /** 2582 * @param value {@link #experimental} (A Boolean value to indicate that this 2583 * graph definition is authored for testing purposes (or 2584 * education/evaluation/marketing) and is not intended to be used 2585 * for genuine usage.). This is the underlying object with id, 2586 * value and extensions. The accessor "getExperimental" gives 2587 * direct access to the value 2588 */ 2589 public GraphDefinition setExperimentalElement(BooleanType value) { 2590 this.experimental = value; 2591 return this; 2592 } 2593 2594 /** 2595 * @return A Boolean value to indicate that this graph definition is authored 2596 * for testing purposes (or education/evaluation/marketing) and is not 2597 * intended to be used for genuine usage. 2598 */ 2599 public boolean getExperimental() { 2600 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 2601 } 2602 2603 /** 2604 * @param value A Boolean value to indicate that this graph definition is 2605 * authored for testing purposes (or 2606 * education/evaluation/marketing) and is not intended to be used 2607 * for genuine usage. 2608 */ 2609 public GraphDefinition setExperimental(boolean value) { 2610 if (this.experimental == null) 2611 this.experimental = new BooleanType(); 2612 this.experimental.setValue(value); 2613 return this; 2614 } 2615 2616 /** 2617 * @return {@link #date} (The date (and optionally time) when the graph 2618 * definition was published. The date must change when the business 2619 * version changes and it must change if the status code changes. In 2620 * addition, it should change when the substantive content of the graph 2621 * definition changes.). This is the underlying object with id, value 2622 * and extensions. The accessor "getDate" gives direct access to the 2623 * value 2624 */ 2625 public DateTimeType getDateElement() { 2626 if (this.date == null) 2627 if (Configuration.errorOnAutoCreate()) 2628 throw new Error("Attempt to auto-create GraphDefinition.date"); 2629 else if (Configuration.doAutoCreate()) 2630 this.date = new DateTimeType(); // bb 2631 return this.date; 2632 } 2633 2634 public boolean hasDateElement() { 2635 return this.date != null && !this.date.isEmpty(); 2636 } 2637 2638 public boolean hasDate() { 2639 return this.date != null && !this.date.isEmpty(); 2640 } 2641 2642 /** 2643 * @param value {@link #date} (The date (and optionally time) when the graph 2644 * definition was published. The date must change when the business 2645 * version changes and it must change if the status code changes. 2646 * In addition, it should change when the substantive content of 2647 * the graph definition changes.). This is the underlying object 2648 * with id, value and extensions. The accessor "getDate" gives 2649 * direct access to the value 2650 */ 2651 public GraphDefinition setDateElement(DateTimeType value) { 2652 this.date = value; 2653 return this; 2654 } 2655 2656 /** 2657 * @return The date (and optionally time) when the graph definition was 2658 * published. The date must change when the business version changes and 2659 * it must change if the status code changes. In addition, it should 2660 * change when the substantive content of the graph definition changes. 2661 */ 2662 public Date getDate() { 2663 return this.date == null ? null : this.date.getValue(); 2664 } 2665 2666 /** 2667 * @param value The date (and optionally time) when the graph definition was 2668 * published. The date must change when the business version 2669 * changes and it must change if the status code changes. In 2670 * addition, it should change when the substantive content of the 2671 * graph definition changes. 2672 */ 2673 public GraphDefinition setDate(Date value) { 2674 if (value == null) 2675 this.date = null; 2676 else { 2677 if (this.date == null) 2678 this.date = new DateTimeType(); 2679 this.date.setValue(value); 2680 } 2681 return this; 2682 } 2683 2684 /** 2685 * @return {@link #publisher} (The name of the organization or individual that 2686 * published the graph definition.). This is the underlying object with 2687 * id, value and extensions. The accessor "getPublisher" gives direct 2688 * access to the value 2689 */ 2690 public StringType getPublisherElement() { 2691 if (this.publisher == null) 2692 if (Configuration.errorOnAutoCreate()) 2693 throw new Error("Attempt to auto-create GraphDefinition.publisher"); 2694 else if (Configuration.doAutoCreate()) 2695 this.publisher = new StringType(); // bb 2696 return this.publisher; 2697 } 2698 2699 public boolean hasPublisherElement() { 2700 return this.publisher != null && !this.publisher.isEmpty(); 2701 } 2702 2703 public boolean hasPublisher() { 2704 return this.publisher != null && !this.publisher.isEmpty(); 2705 } 2706 2707 /** 2708 * @param value {@link #publisher} (The name of the organization or individual 2709 * that published the graph definition.). This is the underlying 2710 * object with id, value and extensions. The accessor 2711 * "getPublisher" gives direct access to the value 2712 */ 2713 public GraphDefinition setPublisherElement(StringType value) { 2714 this.publisher = value; 2715 return this; 2716 } 2717 2718 /** 2719 * @return The name of the organization or individual that published the graph 2720 * definition. 2721 */ 2722 public String getPublisher() { 2723 return this.publisher == null ? null : this.publisher.getValue(); 2724 } 2725 2726 /** 2727 * @param value The name of the organization or individual that published the 2728 * graph definition. 2729 */ 2730 public GraphDefinition setPublisher(String value) { 2731 if (Utilities.noString(value)) 2732 this.publisher = null; 2733 else { 2734 if (this.publisher == null) 2735 this.publisher = new StringType(); 2736 this.publisher.setValue(value); 2737 } 2738 return this; 2739 } 2740 2741 /** 2742 * @return {@link #contact} (Contact details to assist a user in finding and 2743 * communicating with the publisher.) 2744 */ 2745 public List<ContactDetail> getContact() { 2746 if (this.contact == null) 2747 this.contact = new ArrayList<ContactDetail>(); 2748 return this.contact; 2749 } 2750 2751 /** 2752 * @return Returns a reference to <code>this</code> for easy method chaining 2753 */ 2754 public GraphDefinition setContact(List<ContactDetail> theContact) { 2755 this.contact = theContact; 2756 return this; 2757 } 2758 2759 public boolean hasContact() { 2760 if (this.contact == null) 2761 return false; 2762 for (ContactDetail item : this.contact) 2763 if (!item.isEmpty()) 2764 return true; 2765 return false; 2766 } 2767 2768 public ContactDetail addContact() { // 3 2769 ContactDetail t = new ContactDetail(); 2770 if (this.contact == null) 2771 this.contact = new ArrayList<ContactDetail>(); 2772 this.contact.add(t); 2773 return t; 2774 } 2775 2776 public GraphDefinition addContact(ContactDetail t) { // 3 2777 if (t == null) 2778 return this; 2779 if (this.contact == null) 2780 this.contact = new ArrayList<ContactDetail>(); 2781 this.contact.add(t); 2782 return this; 2783 } 2784 2785 /** 2786 * @return The first repetition of repeating field {@link #contact}, creating it 2787 * if it does not already exist 2788 */ 2789 public ContactDetail getContactFirstRep() { 2790 if (getContact().isEmpty()) { 2791 addContact(); 2792 } 2793 return getContact().get(0); 2794 } 2795 2796 /** 2797 * @return {@link #description} (A free text natural language description of the 2798 * graph definition from a consumer's perspective.). This is the 2799 * underlying object with id, value and extensions. The accessor 2800 * "getDescription" gives direct access to the value 2801 */ 2802 public MarkdownType getDescriptionElement() { 2803 if (this.description == null) 2804 if (Configuration.errorOnAutoCreate()) 2805 throw new Error("Attempt to auto-create GraphDefinition.description"); 2806 else if (Configuration.doAutoCreate()) 2807 this.description = new MarkdownType(); // bb 2808 return this.description; 2809 } 2810 2811 public boolean hasDescriptionElement() { 2812 return this.description != null && !this.description.isEmpty(); 2813 } 2814 2815 public boolean hasDescription() { 2816 return this.description != null && !this.description.isEmpty(); 2817 } 2818 2819 /** 2820 * @param value {@link #description} (A free text natural language description 2821 * of the graph definition from a consumer's perspective.). This is 2822 * the underlying object with id, value and extensions. The 2823 * accessor "getDescription" gives direct access to the value 2824 */ 2825 public GraphDefinition setDescriptionElement(MarkdownType value) { 2826 this.description = value; 2827 return this; 2828 } 2829 2830 /** 2831 * @return A free text natural language description of the graph definition from 2832 * a consumer's perspective. 2833 */ 2834 public String getDescription() { 2835 return this.description == null ? null : this.description.getValue(); 2836 } 2837 2838 /** 2839 * @param value A free text natural language description of the graph definition 2840 * from a consumer's perspective. 2841 */ 2842 public GraphDefinition setDescription(String value) { 2843 if (value == null) 2844 this.description = null; 2845 else { 2846 if (this.description == null) 2847 this.description = new MarkdownType(); 2848 this.description.setValue(value); 2849 } 2850 return this; 2851 } 2852 2853 /** 2854 * @return {@link #useContext} (The content was developed with a focus and 2855 * intent of supporting the contexts that are listed. These contexts may 2856 * be general categories (gender, age, ...) or may be references to 2857 * specific programs (insurance plans, studies, ...) and may be used to 2858 * assist with indexing and searching for appropriate graph definition 2859 * instances.) 2860 */ 2861 public List<UsageContext> getUseContext() { 2862 if (this.useContext == null) 2863 this.useContext = new ArrayList<UsageContext>(); 2864 return this.useContext; 2865 } 2866 2867 /** 2868 * @return Returns a reference to <code>this</code> for easy method chaining 2869 */ 2870 public GraphDefinition setUseContext(List<UsageContext> theUseContext) { 2871 this.useContext = theUseContext; 2872 return this; 2873 } 2874 2875 public boolean hasUseContext() { 2876 if (this.useContext == null) 2877 return false; 2878 for (UsageContext item : this.useContext) 2879 if (!item.isEmpty()) 2880 return true; 2881 return false; 2882 } 2883 2884 public UsageContext addUseContext() { // 3 2885 UsageContext t = new UsageContext(); 2886 if (this.useContext == null) 2887 this.useContext = new ArrayList<UsageContext>(); 2888 this.useContext.add(t); 2889 return t; 2890 } 2891 2892 public GraphDefinition addUseContext(UsageContext t) { // 3 2893 if (t == null) 2894 return this; 2895 if (this.useContext == null) 2896 this.useContext = new ArrayList<UsageContext>(); 2897 this.useContext.add(t); 2898 return this; 2899 } 2900 2901 /** 2902 * @return The first repetition of repeating field {@link #useContext}, creating 2903 * it if it does not already exist 2904 */ 2905 public UsageContext getUseContextFirstRep() { 2906 if (getUseContext().isEmpty()) { 2907 addUseContext(); 2908 } 2909 return getUseContext().get(0); 2910 } 2911 2912 /** 2913 * @return {@link #jurisdiction} (A legal or geographic region in which the 2914 * graph definition is intended to be used.) 2915 */ 2916 public List<CodeableConcept> getJurisdiction() { 2917 if (this.jurisdiction == null) 2918 this.jurisdiction = new ArrayList<CodeableConcept>(); 2919 return this.jurisdiction; 2920 } 2921 2922 /** 2923 * @return Returns a reference to <code>this</code> for easy method chaining 2924 */ 2925 public GraphDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 2926 this.jurisdiction = theJurisdiction; 2927 return this; 2928 } 2929 2930 public boolean hasJurisdiction() { 2931 if (this.jurisdiction == null) 2932 return false; 2933 for (CodeableConcept item : this.jurisdiction) 2934 if (!item.isEmpty()) 2935 return true; 2936 return false; 2937 } 2938 2939 public CodeableConcept addJurisdiction() { // 3 2940 CodeableConcept t = new CodeableConcept(); 2941 if (this.jurisdiction == null) 2942 this.jurisdiction = new ArrayList<CodeableConcept>(); 2943 this.jurisdiction.add(t); 2944 return t; 2945 } 2946 2947 public GraphDefinition addJurisdiction(CodeableConcept t) { // 3 2948 if (t == null) 2949 return this; 2950 if (this.jurisdiction == null) 2951 this.jurisdiction = new ArrayList<CodeableConcept>(); 2952 this.jurisdiction.add(t); 2953 return this; 2954 } 2955 2956 /** 2957 * @return The first repetition of repeating field {@link #jurisdiction}, 2958 * creating it if it does not already exist 2959 */ 2960 public CodeableConcept getJurisdictionFirstRep() { 2961 if (getJurisdiction().isEmpty()) { 2962 addJurisdiction(); 2963 } 2964 return getJurisdiction().get(0); 2965 } 2966 2967 /** 2968 * @return {@link #purpose} (Explanation of why this graph definition is needed 2969 * and why it has been designed as it has.). This is the underlying 2970 * object with id, value and extensions. The accessor "getPurpose" gives 2971 * direct access to the value 2972 */ 2973 public MarkdownType getPurposeElement() { 2974 if (this.purpose == null) 2975 if (Configuration.errorOnAutoCreate()) 2976 throw new Error("Attempt to auto-create GraphDefinition.purpose"); 2977 else if (Configuration.doAutoCreate()) 2978 this.purpose = new MarkdownType(); // bb 2979 return this.purpose; 2980 } 2981 2982 public boolean hasPurposeElement() { 2983 return this.purpose != null && !this.purpose.isEmpty(); 2984 } 2985 2986 public boolean hasPurpose() { 2987 return this.purpose != null && !this.purpose.isEmpty(); 2988 } 2989 2990 /** 2991 * @param value {@link #purpose} (Explanation of why this graph definition is 2992 * needed and why it has been designed as it has.). This is the 2993 * underlying object with id, value and extensions. The accessor 2994 * "getPurpose" gives direct access to the value 2995 */ 2996 public GraphDefinition setPurposeElement(MarkdownType value) { 2997 this.purpose = value; 2998 return this; 2999 } 3000 3001 /** 3002 * @return Explanation of why this graph definition is needed and why it has 3003 * been designed as it has. 3004 */ 3005 public String getPurpose() { 3006 return this.purpose == null ? null : this.purpose.getValue(); 3007 } 3008 3009 /** 3010 * @param value Explanation of why this graph definition is needed and why it 3011 * has been designed as it has. 3012 */ 3013 public GraphDefinition setPurpose(String value) { 3014 if (value == null) 3015 this.purpose = null; 3016 else { 3017 if (this.purpose == null) 3018 this.purpose = new MarkdownType(); 3019 this.purpose.setValue(value); 3020 } 3021 return this; 3022 } 3023 3024 /** 3025 * @return {@link #start} (The type of FHIR resource at which instances of this 3026 * graph start.). This is the underlying object with id, value and 3027 * extensions. The accessor "getStart" gives direct access to the value 3028 */ 3029 public CodeType getStartElement() { 3030 if (this.start == null) 3031 if (Configuration.errorOnAutoCreate()) 3032 throw new Error("Attempt to auto-create GraphDefinition.start"); 3033 else if (Configuration.doAutoCreate()) 3034 this.start = new CodeType(); // bb 3035 return this.start; 3036 } 3037 3038 public boolean hasStartElement() { 3039 return this.start != null && !this.start.isEmpty(); 3040 } 3041 3042 public boolean hasStart() { 3043 return this.start != null && !this.start.isEmpty(); 3044 } 3045 3046 /** 3047 * @param value {@link #start} (The type of FHIR resource at which instances of 3048 * this graph start.). This is the underlying object with id, value 3049 * and extensions. The accessor "getStart" gives direct access to 3050 * the value 3051 */ 3052 public GraphDefinition setStartElement(CodeType value) { 3053 this.start = value; 3054 return this; 3055 } 3056 3057 /** 3058 * @return The type of FHIR resource at which instances of this graph start. 3059 */ 3060 public String getStart() { 3061 return this.start == null ? null : this.start.getValue(); 3062 } 3063 3064 /** 3065 * @param value The type of FHIR resource at which instances of this graph 3066 * start. 3067 */ 3068 public GraphDefinition setStart(String value) { 3069 if (this.start == null) 3070 this.start = new CodeType(); 3071 this.start.setValue(value); 3072 return this; 3073 } 3074 3075 /** 3076 * @return {@link #profile} (The profile that describes the use of the base 3077 * resource.). This is the underlying object with id, value and 3078 * extensions. The accessor "getProfile" gives direct access to the 3079 * value 3080 */ 3081 public CanonicalType getProfileElement() { 3082 if (this.profile == null) 3083 if (Configuration.errorOnAutoCreate()) 3084 throw new Error("Attempt to auto-create GraphDefinition.profile"); 3085 else if (Configuration.doAutoCreate()) 3086 this.profile = new CanonicalType(); // bb 3087 return this.profile; 3088 } 3089 3090 public boolean hasProfileElement() { 3091 return this.profile != null && !this.profile.isEmpty(); 3092 } 3093 3094 public boolean hasProfile() { 3095 return this.profile != null && !this.profile.isEmpty(); 3096 } 3097 3098 /** 3099 * @param value {@link #profile} (The profile that describes the use of the base 3100 * resource.). This is the underlying object with id, value and 3101 * extensions. The accessor "getProfile" gives direct access to the 3102 * value 3103 */ 3104 public GraphDefinition setProfileElement(CanonicalType value) { 3105 this.profile = value; 3106 return this; 3107 } 3108 3109 /** 3110 * @return The profile that describes the use of the base resource. 3111 */ 3112 public String getProfile() { 3113 return this.profile == null ? null : this.profile.getValue(); 3114 } 3115 3116 /** 3117 * @param value The profile that describes the use of the base resource. 3118 */ 3119 public GraphDefinition setProfile(String value) { 3120 if (Utilities.noString(value)) 3121 this.profile = null; 3122 else { 3123 if (this.profile == null) 3124 this.profile = new CanonicalType(); 3125 this.profile.setValue(value); 3126 } 3127 return this; 3128 } 3129 3130 /** 3131 * @return {@link #link} (Links this graph makes rules about.) 3132 */ 3133 public List<GraphDefinitionLinkComponent> getLink() { 3134 if (this.link == null) 3135 this.link = new ArrayList<GraphDefinitionLinkComponent>(); 3136 return this.link; 3137 } 3138 3139 /** 3140 * @return Returns a reference to <code>this</code> for easy method chaining 3141 */ 3142 public GraphDefinition setLink(List<GraphDefinitionLinkComponent> theLink) { 3143 this.link = theLink; 3144 return this; 3145 } 3146 3147 public boolean hasLink() { 3148 if (this.link == null) 3149 return false; 3150 for (GraphDefinitionLinkComponent item : this.link) 3151 if (!item.isEmpty()) 3152 return true; 3153 return false; 3154 } 3155 3156 public GraphDefinitionLinkComponent addLink() { // 3 3157 GraphDefinitionLinkComponent t = new GraphDefinitionLinkComponent(); 3158 if (this.link == null) 3159 this.link = new ArrayList<GraphDefinitionLinkComponent>(); 3160 this.link.add(t); 3161 return t; 3162 } 3163 3164 public GraphDefinition addLink(GraphDefinitionLinkComponent t) { // 3 3165 if (t == null) 3166 return this; 3167 if (this.link == null) 3168 this.link = new ArrayList<GraphDefinitionLinkComponent>(); 3169 this.link.add(t); 3170 return this; 3171 } 3172 3173 /** 3174 * @return The first repetition of repeating field {@link #link}, creating it if 3175 * it does not already exist 3176 */ 3177 public GraphDefinitionLinkComponent getLinkFirstRep() { 3178 if (getLink().isEmpty()) { 3179 addLink(); 3180 } 3181 return getLink().get(0); 3182 } 3183 3184 protected void listChildren(List<Property> children) { 3185 super.listChildren(children); 3186 children.add(new Property("url", "uri", 3187 "An absolute URI that is used to identify this graph definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this graph definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the graph definition is stored on different servers.", 3188 0, 1, url)); 3189 children.add(new Property("version", "string", 3190 "The identifier that is used to identify this version of the graph definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the graph definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 3191 0, 1, version)); 3192 children.add(new Property("name", "string", 3193 "A natural language name identifying the graph definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 3194 0, 1, name)); 3195 children.add(new Property("status", "code", 3196 "The status of this graph definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 3197 children.add(new Property("experimental", "boolean", 3198 "A Boolean value to indicate that this graph definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 3199 0, 1, experimental)); 3200 children.add(new Property("date", "dateTime", 3201 "The date (and optionally time) when the graph definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the graph definition changes.", 3202 0, 1, date)); 3203 children.add(new Property("publisher", "string", 3204 "The name of the organization or individual that published the graph definition.", 0, 1, publisher)); 3205 children.add(new Property("contact", "ContactDetail", 3206 "Contact details to assist a user in finding and communicating with the publisher.", 0, 3207 java.lang.Integer.MAX_VALUE, contact)); 3208 children.add(new Property("description", "markdown", 3209 "A free text natural language description of the graph definition from a consumer's perspective.", 0, 1, 3210 description)); 3211 children.add(new Property("useContext", "UsageContext", 3212 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate graph definition instances.", 3213 0, java.lang.Integer.MAX_VALUE, useContext)); 3214 children.add(new Property("jurisdiction", "CodeableConcept", 3215 "A legal or geographic region in which the graph definition is intended to be used.", 0, 3216 java.lang.Integer.MAX_VALUE, jurisdiction)); 3217 children.add(new Property("purpose", "markdown", 3218 "Explanation of why this graph definition is needed and why it has been designed as it has.", 0, 1, purpose)); 3219 children.add(new Property("start", "code", "The type of FHIR resource at which instances of this graph start.", 0, 3220 1, start)); 3221 children.add(new Property("profile", "canonical(StructureDefinition)", 3222 "The profile that describes the use of the base resource.", 0, 1, profile)); 3223 children.add(new Property("link", "", "Links this graph makes rules about.", 0, java.lang.Integer.MAX_VALUE, link)); 3224 } 3225 3226 @Override 3227 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3228 switch (_hash) { 3229 case 116079: 3230 /* url */ return new Property("url", "uri", 3231 "An absolute URI that is used to identify this graph definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this graph definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the graph definition is stored on different servers.", 3232 0, 1, url); 3233 case 351608024: 3234 /* version */ return new Property("version", "string", 3235 "The identifier that is used to identify this version of the graph definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the graph definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 3236 0, 1, version); 3237 case 3373707: 3238 /* name */ return new Property("name", "string", 3239 "A natural language name identifying the graph definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 3240 0, 1, name); 3241 case -892481550: 3242 /* status */ return new Property("status", "code", 3243 "The status of this graph definition. Enables tracking the life-cycle of the content.", 0, 1, status); 3244 case -404562712: 3245 /* experimental */ return new Property("experimental", "boolean", 3246 "A Boolean value to indicate that this graph definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 3247 0, 1, experimental); 3248 case 3076014: 3249 /* date */ return new Property("date", "dateTime", 3250 "The date (and optionally time) when the graph definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the graph definition changes.", 3251 0, 1, date); 3252 case 1447404028: 3253 /* publisher */ return new Property("publisher", "string", 3254 "The name of the organization or individual that published the graph definition.", 0, 1, publisher); 3255 case 951526432: 3256 /* contact */ return new Property("contact", "ContactDetail", 3257 "Contact details to assist a user in finding and communicating with the publisher.", 0, 3258 java.lang.Integer.MAX_VALUE, contact); 3259 case -1724546052: 3260 /* description */ return new Property("description", "markdown", 3261 "A free text natural language description of the graph definition from a consumer's perspective.", 0, 1, 3262 description); 3263 case -669707736: 3264 /* useContext */ return new Property("useContext", "UsageContext", 3265 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate graph definition instances.", 3266 0, java.lang.Integer.MAX_VALUE, useContext); 3267 case -507075711: 3268 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 3269 "A legal or geographic region in which the graph definition is intended to be used.", 0, 3270 java.lang.Integer.MAX_VALUE, jurisdiction); 3271 case -220463842: 3272 /* purpose */ return new Property("purpose", "markdown", 3273 "Explanation of why this graph definition is needed and why it has been designed as it has.", 0, 1, purpose); 3274 case 109757538: 3275 /* start */ return new Property("start", "code", 3276 "The type of FHIR resource at which instances of this graph start.", 0, 1, start); 3277 case -309425751: 3278 /* profile */ return new Property("profile", "canonical(StructureDefinition)", 3279 "The profile that describes the use of the base resource.", 0, 1, profile); 3280 case 3321850: 3281 /* link */ return new Property("link", "", "Links this graph makes rules about.", 0, java.lang.Integer.MAX_VALUE, 3282 link); 3283 default: 3284 return super.getNamedProperty(_hash, _name, _checkValid); 3285 } 3286 3287 } 3288 3289 @Override 3290 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3291 switch (hash) { 3292 case 116079: 3293 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 3294 case 351608024: 3295 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 3296 case 3373707: 3297 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 3298 case -892481550: 3299 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 3300 case -404562712: 3301 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 3302 case 3076014: 3303 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 3304 case 1447404028: 3305 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 3306 case 951526432: 3307 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 3308 case -1724546052: 3309 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 3310 case -669707736: 3311 /* useContext */ return this.useContext == null ? new Base[0] 3312 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 3313 case -507075711: 3314 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 3315 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 3316 case -220463842: 3317 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 3318 case 109757538: 3319 /* start */ return this.start == null ? new Base[0] : new Base[] { this.start }; // CodeType 3320 case -309425751: 3321 /* profile */ return this.profile == null ? new Base[0] : new Base[] { this.profile }; // CanonicalType 3322 case 3321850: 3323 /* link */ return this.link == null ? new Base[0] : this.link.toArray(new Base[this.link.size()]); // GraphDefinitionLinkComponent 3324 default: 3325 return super.getProperty(hash, name, checkValid); 3326 } 3327 3328 } 3329 3330 @Override 3331 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3332 switch (hash) { 3333 case 116079: // url 3334 this.url = castToUri(value); // UriType 3335 return value; 3336 case 351608024: // version 3337 this.version = castToString(value); // StringType 3338 return value; 3339 case 3373707: // name 3340 this.name = castToString(value); // StringType 3341 return value; 3342 case -892481550: // status 3343 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3344 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3345 return value; 3346 case -404562712: // experimental 3347 this.experimental = castToBoolean(value); // BooleanType 3348 return value; 3349 case 3076014: // date 3350 this.date = castToDateTime(value); // DateTimeType 3351 return value; 3352 case 1447404028: // publisher 3353 this.publisher = castToString(value); // StringType 3354 return value; 3355 case 951526432: // contact 3356 this.getContact().add(castToContactDetail(value)); // ContactDetail 3357 return value; 3358 case -1724546052: // description 3359 this.description = castToMarkdown(value); // MarkdownType 3360 return value; 3361 case -669707736: // useContext 3362 this.getUseContext().add(castToUsageContext(value)); // UsageContext 3363 return value; 3364 case -507075711: // jurisdiction 3365 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 3366 return value; 3367 case -220463842: // purpose 3368 this.purpose = castToMarkdown(value); // MarkdownType 3369 return value; 3370 case 109757538: // start 3371 this.start = castToCode(value); // CodeType 3372 return value; 3373 case -309425751: // profile 3374 this.profile = castToCanonical(value); // CanonicalType 3375 return value; 3376 case 3321850: // link 3377 this.getLink().add((GraphDefinitionLinkComponent) value); // GraphDefinitionLinkComponent 3378 return value; 3379 default: 3380 return super.setProperty(hash, name, value); 3381 } 3382 3383 } 3384 3385 @Override 3386 public Base setProperty(String name, Base value) throws FHIRException { 3387 if (name.equals("url")) { 3388 this.url = castToUri(value); // UriType 3389 } else if (name.equals("version")) { 3390 this.version = castToString(value); // StringType 3391 } else if (name.equals("name")) { 3392 this.name = castToString(value); // StringType 3393 } else if (name.equals("status")) { 3394 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3395 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3396 } else if (name.equals("experimental")) { 3397 this.experimental = castToBoolean(value); // BooleanType 3398 } else if (name.equals("date")) { 3399 this.date = castToDateTime(value); // DateTimeType 3400 } else if (name.equals("publisher")) { 3401 this.publisher = castToString(value); // StringType 3402 } else if (name.equals("contact")) { 3403 this.getContact().add(castToContactDetail(value)); 3404 } else if (name.equals("description")) { 3405 this.description = castToMarkdown(value); // MarkdownType 3406 } else if (name.equals("useContext")) { 3407 this.getUseContext().add(castToUsageContext(value)); 3408 } else if (name.equals("jurisdiction")) { 3409 this.getJurisdiction().add(castToCodeableConcept(value)); 3410 } else if (name.equals("purpose")) { 3411 this.purpose = castToMarkdown(value); // MarkdownType 3412 } else if (name.equals("start")) { 3413 this.start = castToCode(value); // CodeType 3414 } else if (name.equals("profile")) { 3415 this.profile = castToCanonical(value); // CanonicalType 3416 } else if (name.equals("link")) { 3417 this.getLink().add((GraphDefinitionLinkComponent) value); 3418 } else 3419 return super.setProperty(name, value); 3420 return value; 3421 } 3422 3423 @Override 3424 public void removeChild(String name, Base value) throws FHIRException { 3425 if (name.equals("url")) { 3426 this.url = null; 3427 } else if (name.equals("version")) { 3428 this.version = null; 3429 } else if (name.equals("name")) { 3430 this.name = null; 3431 } else if (name.equals("status")) { 3432 this.status = null; 3433 } else if (name.equals("experimental")) { 3434 this.experimental = null; 3435 } else if (name.equals("date")) { 3436 this.date = null; 3437 } else if (name.equals("publisher")) { 3438 this.publisher = null; 3439 } else if (name.equals("contact")) { 3440 this.getContact().remove(castToContactDetail(value)); 3441 } else if (name.equals("description")) { 3442 this.description = null; 3443 } else if (name.equals("useContext")) { 3444 this.getUseContext().remove(castToUsageContext(value)); 3445 } else if (name.equals("jurisdiction")) { 3446 this.getJurisdiction().remove(castToCodeableConcept(value)); 3447 } else if (name.equals("purpose")) { 3448 this.purpose = null; 3449 } else if (name.equals("start")) { 3450 this.start = null; 3451 } else if (name.equals("profile")) { 3452 this.profile = null; 3453 } else if (name.equals("link")) { 3454 this.getLink().remove((GraphDefinitionLinkComponent) value); 3455 } else 3456 super.removeChild(name, value); 3457 3458 } 3459 3460 @Override 3461 public Base makeProperty(int hash, String name) throws FHIRException { 3462 switch (hash) { 3463 case 116079: 3464 return getUrlElement(); 3465 case 351608024: 3466 return getVersionElement(); 3467 case 3373707: 3468 return getNameElement(); 3469 case -892481550: 3470 return getStatusElement(); 3471 case -404562712: 3472 return getExperimentalElement(); 3473 case 3076014: 3474 return getDateElement(); 3475 case 1447404028: 3476 return getPublisherElement(); 3477 case 951526432: 3478 return addContact(); 3479 case -1724546052: 3480 return getDescriptionElement(); 3481 case -669707736: 3482 return addUseContext(); 3483 case -507075711: 3484 return addJurisdiction(); 3485 case -220463842: 3486 return getPurposeElement(); 3487 case 109757538: 3488 return getStartElement(); 3489 case -309425751: 3490 return getProfileElement(); 3491 case 3321850: 3492 return addLink(); 3493 default: 3494 return super.makeProperty(hash, name); 3495 } 3496 3497 } 3498 3499 @Override 3500 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3501 switch (hash) { 3502 case 116079: 3503 /* url */ return new String[] { "uri" }; 3504 case 351608024: 3505 /* version */ return new String[] { "string" }; 3506 case 3373707: 3507 /* name */ return new String[] { "string" }; 3508 case -892481550: 3509 /* status */ return new String[] { "code" }; 3510 case -404562712: 3511 /* experimental */ return new String[] { "boolean" }; 3512 case 3076014: 3513 /* date */ return new String[] { "dateTime" }; 3514 case 1447404028: 3515 /* publisher */ return new String[] { "string" }; 3516 case 951526432: 3517 /* contact */ return new String[] { "ContactDetail" }; 3518 case -1724546052: 3519 /* description */ return new String[] { "markdown" }; 3520 case -669707736: 3521 /* useContext */ return new String[] { "UsageContext" }; 3522 case -507075711: 3523 /* jurisdiction */ return new String[] { "CodeableConcept" }; 3524 case -220463842: 3525 /* purpose */ return new String[] { "markdown" }; 3526 case 109757538: 3527 /* start */ return new String[] { "code" }; 3528 case -309425751: 3529 /* profile */ return new String[] { "canonical" }; 3530 case 3321850: 3531 /* link */ return new String[] {}; 3532 default: 3533 return super.getTypesForProperty(hash, name); 3534 } 3535 3536 } 3537 3538 @Override 3539 public Base addChild(String name) throws FHIRException { 3540 if (name.equals("url")) { 3541 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.url"); 3542 } else if (name.equals("version")) { 3543 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.version"); 3544 } else if (name.equals("name")) { 3545 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.name"); 3546 } else if (name.equals("status")) { 3547 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.status"); 3548 } else if (name.equals("experimental")) { 3549 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.experimental"); 3550 } else if (name.equals("date")) { 3551 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.date"); 3552 } else if (name.equals("publisher")) { 3553 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.publisher"); 3554 } else if (name.equals("contact")) { 3555 return addContact(); 3556 } else if (name.equals("description")) { 3557 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.description"); 3558 } else if (name.equals("useContext")) { 3559 return addUseContext(); 3560 } else if (name.equals("jurisdiction")) { 3561 return addJurisdiction(); 3562 } else if (name.equals("purpose")) { 3563 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.purpose"); 3564 } else if (name.equals("start")) { 3565 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.start"); 3566 } else if (name.equals("profile")) { 3567 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.profile"); 3568 } else if (name.equals("link")) { 3569 return addLink(); 3570 } else 3571 return super.addChild(name); 3572 } 3573 3574 public String fhirType() { 3575 return "GraphDefinition"; 3576 3577 } 3578 3579 public GraphDefinition copy() { 3580 GraphDefinition dst = new GraphDefinition(); 3581 copyValues(dst); 3582 return dst; 3583 } 3584 3585 public void copyValues(GraphDefinition dst) { 3586 super.copyValues(dst); 3587 dst.url = url == null ? null : url.copy(); 3588 dst.version = version == null ? null : version.copy(); 3589 dst.name = name == null ? null : name.copy(); 3590 dst.status = status == null ? null : status.copy(); 3591 dst.experimental = experimental == null ? null : experimental.copy(); 3592 dst.date = date == null ? null : date.copy(); 3593 dst.publisher = publisher == null ? null : publisher.copy(); 3594 if (contact != null) { 3595 dst.contact = new ArrayList<ContactDetail>(); 3596 for (ContactDetail i : contact) 3597 dst.contact.add(i.copy()); 3598 } 3599 ; 3600 dst.description = description == null ? null : description.copy(); 3601 if (useContext != null) { 3602 dst.useContext = new ArrayList<UsageContext>(); 3603 for (UsageContext i : useContext) 3604 dst.useContext.add(i.copy()); 3605 } 3606 ; 3607 if (jurisdiction != null) { 3608 dst.jurisdiction = new ArrayList<CodeableConcept>(); 3609 for (CodeableConcept i : jurisdiction) 3610 dst.jurisdiction.add(i.copy()); 3611 } 3612 ; 3613 dst.purpose = purpose == null ? null : purpose.copy(); 3614 dst.start = start == null ? null : start.copy(); 3615 dst.profile = profile == null ? null : profile.copy(); 3616 if (link != null) { 3617 dst.link = new ArrayList<GraphDefinitionLinkComponent>(); 3618 for (GraphDefinitionLinkComponent i : link) 3619 dst.link.add(i.copy()); 3620 } 3621 ; 3622 } 3623 3624 protected GraphDefinition typedCopy() { 3625 return copy(); 3626 } 3627 3628 @Override 3629 public boolean equalsDeep(Base other_) { 3630 if (!super.equalsDeep(other_)) 3631 return false; 3632 if (!(other_ instanceof GraphDefinition)) 3633 return false; 3634 GraphDefinition o = (GraphDefinition) other_; 3635 return compareDeep(purpose, o.purpose, true) && compareDeep(start, o.start, true) 3636 && compareDeep(profile, o.profile, true) && compareDeep(link, o.link, true); 3637 } 3638 3639 @Override 3640 public boolean equalsShallow(Base other_) { 3641 if (!super.equalsShallow(other_)) 3642 return false; 3643 if (!(other_ instanceof GraphDefinition)) 3644 return false; 3645 GraphDefinition o = (GraphDefinition) other_; 3646 return compareValues(purpose, o.purpose, true) && compareValues(start, o.start, true); 3647 } 3648 3649 public boolean isEmpty() { 3650 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(purpose, start, profile, link); 3651 } 3652 3653 @Override 3654 public ResourceType getResourceType() { 3655 return ResourceType.GraphDefinition; 3656 } 3657 3658 /** 3659 * Search parameter: <b>date</b> 3660 * <p> 3661 * Description: <b>The graph definition publication date</b><br> 3662 * Type: <b>date</b><br> 3663 * Path: <b>GraphDefinition.date</b><br> 3664 * </p> 3665 */ 3666 @SearchParamDefinition(name = "date", path = "GraphDefinition.date", description = "The graph definition publication date", type = "date") 3667 public static final String SP_DATE = "date"; 3668 /** 3669 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3670 * <p> 3671 * Description: <b>The graph definition publication date</b><br> 3672 * Type: <b>date</b><br> 3673 * Path: <b>GraphDefinition.date</b><br> 3674 * </p> 3675 */ 3676 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3677 SP_DATE); 3678 3679 /** 3680 * Search parameter: <b>context-type-value</b> 3681 * <p> 3682 * Description: <b>A use context type and value assigned to the graph 3683 * definition</b><br> 3684 * Type: <b>composite</b><br> 3685 * Path: <b></b><br> 3686 * </p> 3687 */ 3688 @SearchParamDefinition(name = "context-type-value", path = "GraphDefinition.useContext", description = "A use context type and value assigned to the graph definition", type = "composite", compositeOf = { 3689 "context-type", "context" }) 3690 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 3691 /** 3692 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 3693 * <p> 3694 * Description: <b>A use context type and value assigned to the graph 3695 * definition</b><br> 3696 * Type: <b>composite</b><br> 3697 * Path: <b></b><br> 3698 * </p> 3699 */ 3700 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 3701 SP_CONTEXT_TYPE_VALUE); 3702 3703 /** 3704 * Search parameter: <b>jurisdiction</b> 3705 * <p> 3706 * Description: <b>Intended jurisdiction for the graph definition</b><br> 3707 * Type: <b>token</b><br> 3708 * Path: <b>GraphDefinition.jurisdiction</b><br> 3709 * </p> 3710 */ 3711 @SearchParamDefinition(name = "jurisdiction", path = "GraphDefinition.jurisdiction", description = "Intended jurisdiction for the graph definition", type = "token") 3712 public static final String SP_JURISDICTION = "jurisdiction"; 3713 /** 3714 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 3715 * <p> 3716 * Description: <b>Intended jurisdiction for the graph definition</b><br> 3717 * Type: <b>token</b><br> 3718 * Path: <b>GraphDefinition.jurisdiction</b><br> 3719 * </p> 3720 */ 3721 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3722 SP_JURISDICTION); 3723 3724 /** 3725 * Search parameter: <b>start</b> 3726 * <p> 3727 * Description: <b>Type of resource at which the graph starts</b><br> 3728 * Type: <b>token</b><br> 3729 * Path: <b>GraphDefinition.start</b><br> 3730 * </p> 3731 */ 3732 @SearchParamDefinition(name = "start", path = "GraphDefinition.start", description = "Type of resource at which the graph starts", type = "token") 3733 public static final String SP_START = "start"; 3734 /** 3735 * <b>Fluent Client</b> search parameter constant for <b>start</b> 3736 * <p> 3737 * Description: <b>Type of resource at which the graph starts</b><br> 3738 * Type: <b>token</b><br> 3739 * Path: <b>GraphDefinition.start</b><br> 3740 * </p> 3741 */ 3742 public static final ca.uhn.fhir.rest.gclient.TokenClientParam START = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3743 SP_START); 3744 3745 /** 3746 * Search parameter: <b>description</b> 3747 * <p> 3748 * Description: <b>The description of the graph definition</b><br> 3749 * Type: <b>string</b><br> 3750 * Path: <b>GraphDefinition.description</b><br> 3751 * </p> 3752 */ 3753 @SearchParamDefinition(name = "description", path = "GraphDefinition.description", description = "The description of the graph definition", type = "string") 3754 public static final String SP_DESCRIPTION = "description"; 3755 /** 3756 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3757 * <p> 3758 * Description: <b>The description of the graph definition</b><br> 3759 * Type: <b>string</b><br> 3760 * Path: <b>GraphDefinition.description</b><br> 3761 * </p> 3762 */ 3763 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 3764 SP_DESCRIPTION); 3765 3766 /** 3767 * Search parameter: <b>context-type</b> 3768 * <p> 3769 * Description: <b>A type of use context assigned to the graph 3770 * definition</b><br> 3771 * Type: <b>token</b><br> 3772 * Path: <b>GraphDefinition.useContext.code</b><br> 3773 * </p> 3774 */ 3775 @SearchParamDefinition(name = "context-type", path = "GraphDefinition.useContext.code", description = "A type of use context assigned to the graph definition", type = "token") 3776 public static final String SP_CONTEXT_TYPE = "context-type"; 3777 /** 3778 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 3779 * <p> 3780 * Description: <b>A type of use context assigned to the graph 3781 * definition</b><br> 3782 * Type: <b>token</b><br> 3783 * Path: <b>GraphDefinition.useContext.code</b><br> 3784 * </p> 3785 */ 3786 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3787 SP_CONTEXT_TYPE); 3788 3789 /** 3790 * Search parameter: <b>version</b> 3791 * <p> 3792 * Description: <b>The business version of the graph definition</b><br> 3793 * Type: <b>token</b><br> 3794 * Path: <b>GraphDefinition.version</b><br> 3795 * </p> 3796 */ 3797 @SearchParamDefinition(name = "version", path = "GraphDefinition.version", description = "The business version of the graph definition", type = "token") 3798 public static final String SP_VERSION = "version"; 3799 /** 3800 * <b>Fluent Client</b> search parameter constant for <b>version</b> 3801 * <p> 3802 * Description: <b>The business version of the graph definition</b><br> 3803 * Type: <b>token</b><br> 3804 * Path: <b>GraphDefinition.version</b><br> 3805 * </p> 3806 */ 3807 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3808 SP_VERSION); 3809 3810 /** 3811 * Search parameter: <b>url</b> 3812 * <p> 3813 * Description: <b>The uri that identifies the graph definition</b><br> 3814 * Type: <b>uri</b><br> 3815 * Path: <b>GraphDefinition.url</b><br> 3816 * </p> 3817 */ 3818 @SearchParamDefinition(name = "url", path = "GraphDefinition.url", description = "The uri that identifies the graph definition", type = "uri") 3819 public static final String SP_URL = "url"; 3820 /** 3821 * <b>Fluent Client</b> search parameter constant for <b>url</b> 3822 * <p> 3823 * Description: <b>The uri that identifies the graph definition</b><br> 3824 * Type: <b>uri</b><br> 3825 * Path: <b>GraphDefinition.url</b><br> 3826 * </p> 3827 */ 3828 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 3829 3830 /** 3831 * Search parameter: <b>context-quantity</b> 3832 * <p> 3833 * Description: <b>A quantity- or range-valued use context assigned to the graph 3834 * definition</b><br> 3835 * Type: <b>quantity</b><br> 3836 * Path: <b>GraphDefinition.useContext.valueQuantity, 3837 * GraphDefinition.useContext.valueRange</b><br> 3838 * </p> 3839 */ 3840 @SearchParamDefinition(name = "context-quantity", path = "(GraphDefinition.useContext.value as Quantity) | (GraphDefinition.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the graph definition", type = "quantity") 3841 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 3842 /** 3843 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 3844 * <p> 3845 * Description: <b>A quantity- or range-valued use context assigned to the graph 3846 * definition</b><br> 3847 * Type: <b>quantity</b><br> 3848 * Path: <b>GraphDefinition.useContext.valueQuantity, 3849 * GraphDefinition.useContext.valueRange</b><br> 3850 * </p> 3851 */ 3852 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 3853 SP_CONTEXT_QUANTITY); 3854 3855 /** 3856 * Search parameter: <b>name</b> 3857 * <p> 3858 * Description: <b>Computationally friendly name of the graph definition</b><br> 3859 * Type: <b>string</b><br> 3860 * Path: <b>GraphDefinition.name</b><br> 3861 * </p> 3862 */ 3863 @SearchParamDefinition(name = "name", path = "GraphDefinition.name", description = "Computationally friendly name of the graph definition", type = "string") 3864 public static final String SP_NAME = "name"; 3865 /** 3866 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3867 * <p> 3868 * Description: <b>Computationally friendly name of the graph definition</b><br> 3869 * Type: <b>string</b><br> 3870 * Path: <b>GraphDefinition.name</b><br> 3871 * </p> 3872 */ 3873 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 3874 SP_NAME); 3875 3876 /** 3877 * Search parameter: <b>context</b> 3878 * <p> 3879 * Description: <b>A use context assigned to the graph definition</b><br> 3880 * Type: <b>token</b><br> 3881 * Path: <b>GraphDefinition.useContext.valueCodeableConcept</b><br> 3882 * </p> 3883 */ 3884 @SearchParamDefinition(name = "context", path = "(GraphDefinition.useContext.value as CodeableConcept)", description = "A use context assigned to the graph definition", type = "token") 3885 public static final String SP_CONTEXT = "context"; 3886 /** 3887 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3888 * <p> 3889 * Description: <b>A use context assigned to the graph definition</b><br> 3890 * Type: <b>token</b><br> 3891 * Path: <b>GraphDefinition.useContext.valueCodeableConcept</b><br> 3892 * </p> 3893 */ 3894 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3895 SP_CONTEXT); 3896 3897 /** 3898 * Search parameter: <b>publisher</b> 3899 * <p> 3900 * Description: <b>Name of the publisher of the graph definition</b><br> 3901 * Type: <b>string</b><br> 3902 * Path: <b>GraphDefinition.publisher</b><br> 3903 * </p> 3904 */ 3905 @SearchParamDefinition(name = "publisher", path = "GraphDefinition.publisher", description = "Name of the publisher of the graph definition", type = "string") 3906 public static final String SP_PUBLISHER = "publisher"; 3907 /** 3908 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 3909 * <p> 3910 * Description: <b>Name of the publisher of the graph definition</b><br> 3911 * Type: <b>string</b><br> 3912 * Path: <b>GraphDefinition.publisher</b><br> 3913 * </p> 3914 */ 3915 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 3916 SP_PUBLISHER); 3917 3918 /** 3919 * Search parameter: <b>context-type-quantity</b> 3920 * <p> 3921 * Description: <b>A use context type and quantity- or range-based value 3922 * assigned to the graph definition</b><br> 3923 * Type: <b>composite</b><br> 3924 * Path: <b></b><br> 3925 * </p> 3926 */ 3927 @SearchParamDefinition(name = "context-type-quantity", path = "GraphDefinition.useContext", description = "A use context type and quantity- or range-based value assigned to the graph definition", type = "composite", compositeOf = { 3928 "context-type", "context-quantity" }) 3929 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 3930 /** 3931 * <b>Fluent Client</b> search parameter constant for 3932 * <b>context-type-quantity</b> 3933 * <p> 3934 * Description: <b>A use context type and quantity- or range-based value 3935 * assigned to the graph definition</b><br> 3936 * Type: <b>composite</b><br> 3937 * Path: <b></b><br> 3938 * </p> 3939 */ 3940 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 3941 SP_CONTEXT_TYPE_QUANTITY); 3942 3943 /** 3944 * Search parameter: <b>status</b> 3945 * <p> 3946 * Description: <b>The current status of the graph definition</b><br> 3947 * Type: <b>token</b><br> 3948 * Path: <b>GraphDefinition.status</b><br> 3949 * </p> 3950 */ 3951 @SearchParamDefinition(name = "status", path = "GraphDefinition.status", description = "The current status of the graph definition", type = "token") 3952 public static final String SP_STATUS = "status"; 3953 /** 3954 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3955 * <p> 3956 * Description: <b>The current status of the graph definition</b><br> 3957 * Type: <b>token</b><br> 3958 * Path: <b>GraphDefinition.status</b><br> 3959 * </p> 3960 */ 3961 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3962 SP_STATUS); 3963 3964}