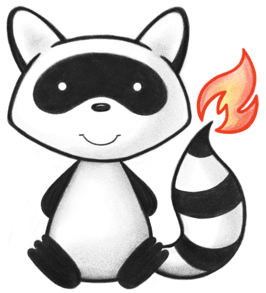
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * Represents a defined collection of entities that may be discussed or acted 048 * upon collectively but which are not expected to act collectively, and are not 049 * formally or legally recognized; i.e. a collection of entities that isn't an 050 * Organization. 051 */ 052@ResourceDef(name = "Group", profile = "http://hl7.org/fhir/StructureDefinition/Group") 053public class Group extends DomainResource { 054 055 public enum GroupType { 056 /** 057 * Group contains "person" Patient resources. 058 */ 059 PERSON, 060 /** 061 * Group contains "animal" Patient resources. 062 */ 063 ANIMAL, 064 /** 065 * Group contains healthcare practitioner resources (Practitioner or 066 * PractitionerRole). 067 */ 068 PRACTITIONER, 069 /** 070 * Group contains Device resources. 071 */ 072 DEVICE, 073 /** 074 * Group contains Medication resources. 075 */ 076 MEDICATION, 077 /** 078 * Group contains Substance resources. 079 */ 080 SUBSTANCE, 081 /** 082 * added to help the parsers with the generic types 083 */ 084 NULL; 085 086 public static GroupType fromCode(String codeString) throws FHIRException { 087 if (codeString == null || "".equals(codeString)) 088 return null; 089 if ("person".equals(codeString)) 090 return PERSON; 091 if ("animal".equals(codeString)) 092 return ANIMAL; 093 if ("practitioner".equals(codeString)) 094 return PRACTITIONER; 095 if ("device".equals(codeString)) 096 return DEVICE; 097 if ("medication".equals(codeString)) 098 return MEDICATION; 099 if ("substance".equals(codeString)) 100 return SUBSTANCE; 101 if (Configuration.isAcceptInvalidEnums()) 102 return null; 103 else 104 throw new FHIRException("Unknown GroupType code '" + codeString + "'"); 105 } 106 107 public String toCode() { 108 switch (this) { 109 case PERSON: 110 return "person"; 111 case ANIMAL: 112 return "animal"; 113 case PRACTITIONER: 114 return "practitioner"; 115 case DEVICE: 116 return "device"; 117 case MEDICATION: 118 return "medication"; 119 case SUBSTANCE: 120 return "substance"; 121 case NULL: 122 return null; 123 default: 124 return "?"; 125 } 126 } 127 128 public String getSystem() { 129 switch (this) { 130 case PERSON: 131 return "http://hl7.org/fhir/group-type"; 132 case ANIMAL: 133 return "http://hl7.org/fhir/group-type"; 134 case PRACTITIONER: 135 return "http://hl7.org/fhir/group-type"; 136 case DEVICE: 137 return "http://hl7.org/fhir/group-type"; 138 case MEDICATION: 139 return "http://hl7.org/fhir/group-type"; 140 case SUBSTANCE: 141 return "http://hl7.org/fhir/group-type"; 142 case NULL: 143 return null; 144 default: 145 return "?"; 146 } 147 } 148 149 public String getDefinition() { 150 switch (this) { 151 case PERSON: 152 return "Group contains \"person\" Patient resources."; 153 case ANIMAL: 154 return "Group contains \"animal\" Patient resources."; 155 case PRACTITIONER: 156 return "Group contains healthcare practitioner resources (Practitioner or PractitionerRole)."; 157 case DEVICE: 158 return "Group contains Device resources."; 159 case MEDICATION: 160 return "Group contains Medication resources."; 161 case SUBSTANCE: 162 return "Group contains Substance resources."; 163 case NULL: 164 return null; 165 default: 166 return "?"; 167 } 168 } 169 170 public String getDisplay() { 171 switch (this) { 172 case PERSON: 173 return "Person"; 174 case ANIMAL: 175 return "Animal"; 176 case PRACTITIONER: 177 return "Practitioner"; 178 case DEVICE: 179 return "Device"; 180 case MEDICATION: 181 return "Medication"; 182 case SUBSTANCE: 183 return "Substance"; 184 case NULL: 185 return null; 186 default: 187 return "?"; 188 } 189 } 190 } 191 192 public static class GroupTypeEnumFactory implements EnumFactory<GroupType> { 193 public GroupType fromCode(String codeString) throws IllegalArgumentException { 194 if (codeString == null || "".equals(codeString)) 195 if (codeString == null || "".equals(codeString)) 196 return null; 197 if ("person".equals(codeString)) 198 return GroupType.PERSON; 199 if ("animal".equals(codeString)) 200 return GroupType.ANIMAL; 201 if ("practitioner".equals(codeString)) 202 return GroupType.PRACTITIONER; 203 if ("device".equals(codeString)) 204 return GroupType.DEVICE; 205 if ("medication".equals(codeString)) 206 return GroupType.MEDICATION; 207 if ("substance".equals(codeString)) 208 return GroupType.SUBSTANCE; 209 throw new IllegalArgumentException("Unknown GroupType code '" + codeString + "'"); 210 } 211 212 public Enumeration<GroupType> fromType(PrimitiveType<?> code) throws FHIRException { 213 if (code == null) 214 return null; 215 if (code.isEmpty()) 216 return new Enumeration<GroupType>(this, GroupType.NULL, code); 217 String codeString = code.asStringValue(); 218 if (codeString == null || "".equals(codeString)) 219 return new Enumeration<GroupType>(this, GroupType.NULL, code); 220 if ("person".equals(codeString)) 221 return new Enumeration<GroupType>(this, GroupType.PERSON, code); 222 if ("animal".equals(codeString)) 223 return new Enumeration<GroupType>(this, GroupType.ANIMAL, code); 224 if ("practitioner".equals(codeString)) 225 return new Enumeration<GroupType>(this, GroupType.PRACTITIONER, code); 226 if ("device".equals(codeString)) 227 return new Enumeration<GroupType>(this, GroupType.DEVICE, code); 228 if ("medication".equals(codeString)) 229 return new Enumeration<GroupType>(this, GroupType.MEDICATION, code); 230 if ("substance".equals(codeString)) 231 return new Enumeration<GroupType>(this, GroupType.SUBSTANCE, code); 232 throw new FHIRException("Unknown GroupType code '" + codeString + "'"); 233 } 234 235 public String toCode(GroupType code) { 236 if (code == GroupType.PERSON) 237 return "person"; 238 if (code == GroupType.ANIMAL) 239 return "animal"; 240 if (code == GroupType.PRACTITIONER) 241 return "practitioner"; 242 if (code == GroupType.DEVICE) 243 return "device"; 244 if (code == GroupType.MEDICATION) 245 return "medication"; 246 if (code == GroupType.SUBSTANCE) 247 return "substance"; 248 return "?"; 249 } 250 251 public String toSystem(GroupType code) { 252 return code.getSystem(); 253 } 254 } 255 256 @Block() 257 public static class GroupCharacteristicComponent extends BackboneElement implements IBaseBackboneElement { 258 /** 259 * A code that identifies the kind of trait being asserted. 260 */ 261 @Child(name = "code", type = { 262 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 263 @Description(shortDefinition = "Kind of characteristic", formalDefinition = "A code that identifies the kind of trait being asserted.") 264 protected CodeableConcept code; 265 266 /** 267 * The value of the trait that holds (or does not hold - see 'exclude') for 268 * members of the group. 269 */ 270 @Child(name = "value", type = { CodeableConcept.class, BooleanType.class, Quantity.class, Range.class, 271 Reference.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 272 @Description(shortDefinition = "Value held by characteristic", formalDefinition = "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.") 273 protected Type value; 274 275 /** 276 * If true, indicates the characteristic is one that is NOT held by members of 277 * the group. 278 */ 279 @Child(name = "exclude", type = { 280 BooleanType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 281 @Description(shortDefinition = "Group includes or excludes", formalDefinition = "If true, indicates the characteristic is one that is NOT held by members of the group.") 282 protected BooleanType exclude; 283 284 /** 285 * The period over which the characteristic is tested; e.g. the patient had an 286 * operation during the month of June. 287 */ 288 @Child(name = "period", type = { Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 289 @Description(shortDefinition = "Period over which characteristic is tested", formalDefinition = "The period over which the characteristic is tested; e.g. the patient had an operation during the month of June.") 290 protected Period period; 291 292 private static final long serialVersionUID = -1000688967L; 293 294 /** 295 * Constructor 296 */ 297 public GroupCharacteristicComponent() { 298 super(); 299 } 300 301 /** 302 * Constructor 303 */ 304 public GroupCharacteristicComponent(CodeableConcept code, Type value, BooleanType exclude) { 305 super(); 306 this.code = code; 307 this.value = value; 308 this.exclude = exclude; 309 } 310 311 /** 312 * @return {@link #code} (A code that identifies the kind of trait being 313 * asserted.) 314 */ 315 public CodeableConcept getCode() { 316 if (this.code == null) 317 if (Configuration.errorOnAutoCreate()) 318 throw new Error("Attempt to auto-create GroupCharacteristicComponent.code"); 319 else if (Configuration.doAutoCreate()) 320 this.code = new CodeableConcept(); // cc 321 return this.code; 322 } 323 324 public boolean hasCode() { 325 return this.code != null && !this.code.isEmpty(); 326 } 327 328 /** 329 * @param value {@link #code} (A code that identifies the kind of trait being 330 * asserted.) 331 */ 332 public GroupCharacteristicComponent setCode(CodeableConcept value) { 333 this.code = value; 334 return this; 335 } 336 337 /** 338 * @return {@link #value} (The value of the trait that holds (or does not hold - 339 * see 'exclude') for members of the group.) 340 */ 341 public Type getValue() { 342 return this.value; 343 } 344 345 /** 346 * @return {@link #value} (The value of the trait that holds (or does not hold - 347 * see 'exclude') for members of the group.) 348 */ 349 public CodeableConcept getValueCodeableConcept() throws FHIRException { 350 if (this.value == null) 351 this.value = new CodeableConcept(); 352 if (!(this.value instanceof CodeableConcept)) 353 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 354 + this.value.getClass().getName() + " was encountered"); 355 return (CodeableConcept) this.value; 356 } 357 358 public boolean hasValueCodeableConcept() { 359 return this != null && this.value instanceof CodeableConcept; 360 } 361 362 /** 363 * @return {@link #value} (The value of the trait that holds (or does not hold - 364 * see 'exclude') for members of the group.) 365 */ 366 public BooleanType getValueBooleanType() throws FHIRException { 367 if (this.value == null) 368 this.value = new BooleanType(); 369 if (!(this.value instanceof BooleanType)) 370 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 371 + this.value.getClass().getName() + " was encountered"); 372 return (BooleanType) this.value; 373 } 374 375 public boolean hasValueBooleanType() { 376 return this != null && this.value instanceof BooleanType; 377 } 378 379 /** 380 * @return {@link #value} (The value of the trait that holds (or does not hold - 381 * see 'exclude') for members of the group.) 382 */ 383 public Quantity getValueQuantity() throws FHIRException { 384 if (this.value == null) 385 this.value = new Quantity(); 386 if (!(this.value instanceof Quantity)) 387 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName() 388 + " was encountered"); 389 return (Quantity) this.value; 390 } 391 392 public boolean hasValueQuantity() { 393 return this != null && this.value instanceof Quantity; 394 } 395 396 /** 397 * @return {@link #value} (The value of the trait that holds (or does not hold - 398 * see 'exclude') for members of the group.) 399 */ 400 public Range getValueRange() throws FHIRException { 401 if (this.value == null) 402 this.value = new Range(); 403 if (!(this.value instanceof Range)) 404 throw new FHIRException( 405 "Type mismatch: the type Range was expected, but " + this.value.getClass().getName() + " was encountered"); 406 return (Range) this.value; 407 } 408 409 public boolean hasValueRange() { 410 return this != null && this.value instanceof Range; 411 } 412 413 /** 414 * @return {@link #value} (The value of the trait that holds (or does not hold - 415 * see 'exclude') for members of the group.) 416 */ 417 public Reference getValueReference() throws FHIRException { 418 if (this.value == null) 419 this.value = new Reference(); 420 if (!(this.value instanceof Reference)) 421 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.value.getClass().getName() 422 + " was encountered"); 423 return (Reference) this.value; 424 } 425 426 public boolean hasValueReference() { 427 return this != null && this.value instanceof Reference; 428 } 429 430 public boolean hasValue() { 431 return this.value != null && !this.value.isEmpty(); 432 } 433 434 /** 435 * @param value {@link #value} (The value of the trait that holds (or does not 436 * hold - see 'exclude') for members of the group.) 437 */ 438 public GroupCharacteristicComponent setValue(Type value) { 439 if (value != null && !(value instanceof CodeableConcept || value instanceof BooleanType 440 || value instanceof Quantity || value instanceof Range || value instanceof Reference)) 441 throw new Error("Not the right type for Group.characteristic.value[x]: " + value.fhirType()); 442 this.value = value; 443 return this; 444 } 445 446 /** 447 * @return {@link #exclude} (If true, indicates the characteristic is one that 448 * is NOT held by members of the group.). This is the underlying object 449 * with id, value and extensions. The accessor "getExclude" gives direct 450 * access to the value 451 */ 452 public BooleanType getExcludeElement() { 453 if (this.exclude == null) 454 if (Configuration.errorOnAutoCreate()) 455 throw new Error("Attempt to auto-create GroupCharacteristicComponent.exclude"); 456 else if (Configuration.doAutoCreate()) 457 this.exclude = new BooleanType(); // bb 458 return this.exclude; 459 } 460 461 public boolean hasExcludeElement() { 462 return this.exclude != null && !this.exclude.isEmpty(); 463 } 464 465 public boolean hasExclude() { 466 return this.exclude != null && !this.exclude.isEmpty(); 467 } 468 469 /** 470 * @param value {@link #exclude} (If true, indicates the characteristic is one 471 * that is NOT held by members of the group.). This is the 472 * underlying object with id, value and extensions. The accessor 473 * "getExclude" gives direct access to the value 474 */ 475 public GroupCharacteristicComponent setExcludeElement(BooleanType value) { 476 this.exclude = value; 477 return this; 478 } 479 480 /** 481 * @return If true, indicates the characteristic is one that is NOT held by 482 * members of the group. 483 */ 484 public boolean getExclude() { 485 return this.exclude == null || this.exclude.isEmpty() ? false : this.exclude.getValue(); 486 } 487 488 /** 489 * @param value If true, indicates the characteristic is one that is NOT held by 490 * members of the group. 491 */ 492 public GroupCharacteristicComponent setExclude(boolean value) { 493 if (this.exclude == null) 494 this.exclude = new BooleanType(); 495 this.exclude.setValue(value); 496 return this; 497 } 498 499 /** 500 * @return {@link #period} (The period over which the characteristic is tested; 501 * e.g. the patient had an operation during the month of June.) 502 */ 503 public Period getPeriod() { 504 if (this.period == null) 505 if (Configuration.errorOnAutoCreate()) 506 throw new Error("Attempt to auto-create GroupCharacteristicComponent.period"); 507 else if (Configuration.doAutoCreate()) 508 this.period = new Period(); // cc 509 return this.period; 510 } 511 512 public boolean hasPeriod() { 513 return this.period != null && !this.period.isEmpty(); 514 } 515 516 /** 517 * @param value {@link #period} (The period over which the characteristic is 518 * tested; e.g. the patient had an operation during the month of 519 * June.) 520 */ 521 public GroupCharacteristicComponent setPeriod(Period value) { 522 this.period = value; 523 return this; 524 } 525 526 protected void listChildren(List<Property> children) { 527 super.listChildren(children); 528 children.add(new Property("code", "CodeableConcept", "A code that identifies the kind of trait being asserted.", 529 0, 1, code)); 530 children.add(new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", 531 "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, 532 value)); 533 children.add(new Property("exclude", "boolean", 534 "If true, indicates the characteristic is one that is NOT held by members of the group.", 0, 1, exclude)); 535 children.add(new Property("period", "Period", 536 "The period over which the characteristic is tested; e.g. the patient had an operation during the month of June.", 537 0, 1, period)); 538 } 539 540 @Override 541 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 542 switch (_hash) { 543 case 3059181: 544 /* code */ return new Property("code", "CodeableConcept", 545 "A code that identifies the kind of trait being asserted.", 0, 1, code); 546 case -1410166417: 547 /* value[x] */ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", 548 "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, 549 value); 550 case 111972721: 551 /* value */ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", 552 "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, 553 value); 554 case 924902896: 555 /* valueCodeableConcept */ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", 556 "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, 557 value); 558 case 733421943: 559 /* valueBoolean */ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", 560 "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, 561 value); 562 case -2029823716: 563 /* valueQuantity */ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", 564 "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, 565 value); 566 case 2030761548: 567 /* valueRange */ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", 568 "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, 569 value); 570 case 1755241690: 571 /* valueReference */ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", 572 "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, 573 value); 574 case -1321148966: 575 /* exclude */ return new Property("exclude", "boolean", 576 "If true, indicates the characteristic is one that is NOT held by members of the group.", 0, 1, exclude); 577 case -991726143: 578 /* period */ return new Property("period", "Period", 579 "The period over which the characteristic is tested; e.g. the patient had an operation during the month of June.", 580 0, 1, period); 581 default: 582 return super.getNamedProperty(_hash, _name, _checkValid); 583 } 584 585 } 586 587 @Override 588 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 589 switch (hash) { 590 case 3059181: 591 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 592 case 111972721: 593 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 594 case -1321148966: 595 /* exclude */ return this.exclude == null ? new Base[0] : new Base[] { this.exclude }; // BooleanType 596 case -991726143: 597 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 598 default: 599 return super.getProperty(hash, name, checkValid); 600 } 601 602 } 603 604 @Override 605 public Base setProperty(int hash, String name, Base value) throws FHIRException { 606 switch (hash) { 607 case 3059181: // code 608 this.code = castToCodeableConcept(value); // CodeableConcept 609 return value; 610 case 111972721: // value 611 this.value = castToType(value); // Type 612 return value; 613 case -1321148966: // exclude 614 this.exclude = castToBoolean(value); // BooleanType 615 return value; 616 case -991726143: // period 617 this.period = castToPeriod(value); // Period 618 return value; 619 default: 620 return super.setProperty(hash, name, value); 621 } 622 623 } 624 625 @Override 626 public Base setProperty(String name, Base value) throws FHIRException { 627 if (name.equals("code")) { 628 this.code = castToCodeableConcept(value); // CodeableConcept 629 } else if (name.equals("value[x]")) { 630 this.value = castToType(value); // Type 631 } else if (name.equals("exclude")) { 632 this.exclude = castToBoolean(value); // BooleanType 633 } else if (name.equals("period")) { 634 this.period = castToPeriod(value); // Period 635 } else 636 return super.setProperty(name, value); 637 return value; 638 } 639 640 @Override 641 public Base makeProperty(int hash, String name) throws FHIRException { 642 switch (hash) { 643 case 3059181: 644 return getCode(); 645 case -1410166417: 646 return getValue(); 647 case 111972721: 648 return getValue(); 649 case -1321148966: 650 return getExcludeElement(); 651 case -991726143: 652 return getPeriod(); 653 default: 654 return super.makeProperty(hash, name); 655 } 656 657 } 658 659 @Override 660 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 661 switch (hash) { 662 case 3059181: 663 /* code */ return new String[] { "CodeableConcept" }; 664 case 111972721: 665 /* value */ return new String[] { "CodeableConcept", "boolean", "Quantity", "Range", "Reference" }; 666 case -1321148966: 667 /* exclude */ return new String[] { "boolean" }; 668 case -991726143: 669 /* period */ return new String[] { "Period" }; 670 default: 671 return super.getTypesForProperty(hash, name); 672 } 673 674 } 675 676 @Override 677 public Base addChild(String name) throws FHIRException { 678 if (name.equals("code")) { 679 this.code = new CodeableConcept(); 680 return this.code; 681 } else if (name.equals("valueCodeableConcept")) { 682 this.value = new CodeableConcept(); 683 return this.value; 684 } else if (name.equals("valueBoolean")) { 685 this.value = new BooleanType(); 686 return this.value; 687 } else if (name.equals("valueQuantity")) { 688 this.value = new Quantity(); 689 return this.value; 690 } else if (name.equals("valueRange")) { 691 this.value = new Range(); 692 return this.value; 693 } else if (name.equals("valueReference")) { 694 this.value = new Reference(); 695 return this.value; 696 } else if (name.equals("exclude")) { 697 throw new FHIRException("Cannot call addChild on a singleton property Group.exclude"); 698 } else if (name.equals("period")) { 699 this.period = new Period(); 700 return this.period; 701 } else 702 return super.addChild(name); 703 } 704 705 public GroupCharacteristicComponent copy() { 706 GroupCharacteristicComponent dst = new GroupCharacteristicComponent(); 707 copyValues(dst); 708 return dst; 709 } 710 711 public void copyValues(GroupCharacteristicComponent dst) { 712 super.copyValues(dst); 713 dst.code = code == null ? null : code.copy(); 714 dst.value = value == null ? null : value.copy(); 715 dst.exclude = exclude == null ? null : exclude.copy(); 716 dst.period = period == null ? null : period.copy(); 717 } 718 719 @Override 720 public boolean equalsDeep(Base other_) { 721 if (!super.equalsDeep(other_)) 722 return false; 723 if (!(other_ instanceof GroupCharacteristicComponent)) 724 return false; 725 GroupCharacteristicComponent o = (GroupCharacteristicComponent) other_; 726 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true) 727 && compareDeep(exclude, o.exclude, true) && compareDeep(period, o.period, true); 728 } 729 730 @Override 731 public boolean equalsShallow(Base other_) { 732 if (!super.equalsShallow(other_)) 733 return false; 734 if (!(other_ instanceof GroupCharacteristicComponent)) 735 return false; 736 GroupCharacteristicComponent o = (GroupCharacteristicComponent) other_; 737 return compareValues(exclude, o.exclude, true); 738 } 739 740 public boolean isEmpty() { 741 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value, exclude, period); 742 } 743 744 public String fhirType() { 745 return "Group.characteristic"; 746 747 } 748 749 } 750 751 @Block() 752 public static class GroupMemberComponent extends BackboneElement implements IBaseBackboneElement { 753 /** 754 * A reference to the entity that is a member of the group. Must be consistent 755 * with Group.type. If the entity is another group, then the type must be the 756 * same. 757 */ 758 @Child(name = "entity", type = { Patient.class, Practitioner.class, PractitionerRole.class, Device.class, 759 Medication.class, Substance.class, 760 Group.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 761 @Description(shortDefinition = "Reference to the group member", formalDefinition = "A reference to the entity that is a member of the group. Must be consistent with Group.type. If the entity is another group, then the type must be the same.") 762 protected Reference entity; 763 764 /** 765 * The actual object that is the target of the reference (A reference to the 766 * entity that is a member of the group. Must be consistent with Group.type. If 767 * the entity is another group, then the type must be the same.) 768 */ 769 protected Resource entityTarget; 770 771 /** 772 * The period that the member was in the group, if known. 773 */ 774 @Child(name = "period", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 775 @Description(shortDefinition = "Period member belonged to the group", formalDefinition = "The period that the member was in the group, if known.") 776 protected Period period; 777 778 /** 779 * A flag to indicate that the member is no longer in the group, but previously 780 * may have been a member. 781 */ 782 @Child(name = "inactive", type = { 783 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 784 @Description(shortDefinition = "If member is no longer in group", formalDefinition = "A flag to indicate that the member is no longer in the group, but previously may have been a member.") 785 protected BooleanType inactive; 786 787 private static final long serialVersionUID = -333869055L; 788 789 /** 790 * Constructor 791 */ 792 public GroupMemberComponent() { 793 super(); 794 } 795 796 /** 797 * Constructor 798 */ 799 public GroupMemberComponent(Reference entity) { 800 super(); 801 this.entity = entity; 802 } 803 804 /** 805 * @return {@link #entity} (A reference to the entity that is a member of the 806 * group. Must be consistent with Group.type. If the entity is another 807 * group, then the type must be the same.) 808 */ 809 public Reference getEntity() { 810 if (this.entity == null) 811 if (Configuration.errorOnAutoCreate()) 812 throw new Error("Attempt to auto-create GroupMemberComponent.entity"); 813 else if (Configuration.doAutoCreate()) 814 this.entity = new Reference(); // cc 815 return this.entity; 816 } 817 818 public boolean hasEntity() { 819 return this.entity != null && !this.entity.isEmpty(); 820 } 821 822 /** 823 * @param value {@link #entity} (A reference to the entity that is a member of 824 * the group. Must be consistent with Group.type. If the entity is 825 * another group, then the type must be the same.) 826 */ 827 public GroupMemberComponent setEntity(Reference value) { 828 this.entity = value; 829 return this; 830 } 831 832 /** 833 * @return {@link #entity} The actual object that is the target of the 834 * reference. The reference library doesn't populate this, but you can 835 * use it to hold the resource if you resolve it. (A reference to the 836 * entity that is a member of the group. Must be consistent with 837 * Group.type. If the entity is another group, then the type must be the 838 * same.) 839 */ 840 public Resource getEntityTarget() { 841 return this.entityTarget; 842 } 843 844 /** 845 * @param value {@link #entity} The actual object that is the target of the 846 * reference. The reference library doesn't use these, but you can 847 * use it to hold the resource if you resolve it. (A reference to 848 * the entity that is a member of the group. Must be consistent 849 * with Group.type. If the entity is another group, then the type 850 * must be the same.) 851 */ 852 public GroupMemberComponent setEntityTarget(Resource value) { 853 this.entityTarget = value; 854 return this; 855 } 856 857 /** 858 * @return {@link #period} (The period that the member was in the group, if 859 * known.) 860 */ 861 public Period getPeriod() { 862 if (this.period == null) 863 if (Configuration.errorOnAutoCreate()) 864 throw new Error("Attempt to auto-create GroupMemberComponent.period"); 865 else if (Configuration.doAutoCreate()) 866 this.period = new Period(); // cc 867 return this.period; 868 } 869 870 public boolean hasPeriod() { 871 return this.period != null && !this.period.isEmpty(); 872 } 873 874 /** 875 * @param value {@link #period} (The period that the member was in the group, if 876 * known.) 877 */ 878 public GroupMemberComponent setPeriod(Period value) { 879 this.period = value; 880 return this; 881 } 882 883 /** 884 * @return {@link #inactive} (A flag to indicate that the member is no longer in 885 * the group, but previously may have been a member.). This is the 886 * underlying object with id, value and extensions. The accessor 887 * "getInactive" gives direct access to the value 888 */ 889 public BooleanType getInactiveElement() { 890 if (this.inactive == null) 891 if (Configuration.errorOnAutoCreate()) 892 throw new Error("Attempt to auto-create GroupMemberComponent.inactive"); 893 else if (Configuration.doAutoCreate()) 894 this.inactive = new BooleanType(); // bb 895 return this.inactive; 896 } 897 898 public boolean hasInactiveElement() { 899 return this.inactive != null && !this.inactive.isEmpty(); 900 } 901 902 public boolean hasInactive() { 903 return this.inactive != null && !this.inactive.isEmpty(); 904 } 905 906 /** 907 * @param value {@link #inactive} (A flag to indicate that the member is no 908 * longer in the group, but previously may have been a member.). 909 * This is the underlying object with id, value and extensions. The 910 * accessor "getInactive" gives direct access to the value 911 */ 912 public GroupMemberComponent setInactiveElement(BooleanType value) { 913 this.inactive = value; 914 return this; 915 } 916 917 /** 918 * @return A flag to indicate that the member is no longer in the group, but 919 * previously may have been a member. 920 */ 921 public boolean getInactive() { 922 return this.inactive == null || this.inactive.isEmpty() ? false : this.inactive.getValue(); 923 } 924 925 /** 926 * @param value A flag to indicate that the member is no longer in the group, 927 * but previously may have been a member. 928 */ 929 public GroupMemberComponent setInactive(boolean value) { 930 if (this.inactive == null) 931 this.inactive = new BooleanType(); 932 this.inactive.setValue(value); 933 return this; 934 } 935 936 protected void listChildren(List<Property> children) { 937 super.listChildren(children); 938 children.add(new Property("entity", 939 "Reference(Patient|Practitioner|PractitionerRole|Device|Medication|Substance|Group)", 940 "A reference to the entity that is a member of the group. Must be consistent with Group.type. If the entity is another group, then the type must be the same.", 941 0, 1, entity)); 942 children.add( 943 new Property("period", "Period", "The period that the member was in the group, if known.", 0, 1, period)); 944 children.add(new Property("inactive", "boolean", 945 "A flag to indicate that the member is no longer in the group, but previously may have been a member.", 0, 1, 946 inactive)); 947 } 948 949 @Override 950 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 951 switch (_hash) { 952 case -1298275357: 953 /* entity */ return new Property("entity", 954 "Reference(Patient|Practitioner|PractitionerRole|Device|Medication|Substance|Group)", 955 "A reference to the entity that is a member of the group. Must be consistent with Group.type. If the entity is another group, then the type must be the same.", 956 0, 1, entity); 957 case -991726143: 958 /* period */ return new Property("period", "Period", "The period that the member was in the group, if known.", 959 0, 1, period); 960 case 24665195: 961 /* inactive */ return new Property("inactive", "boolean", 962 "A flag to indicate that the member is no longer in the group, but previously may have been a member.", 0, 963 1, inactive); 964 default: 965 return super.getNamedProperty(_hash, _name, _checkValid); 966 } 967 968 } 969 970 @Override 971 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 972 switch (hash) { 973 case -1298275357: 974 /* entity */ return this.entity == null ? new Base[0] : new Base[] { this.entity }; // Reference 975 case -991726143: 976 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 977 case 24665195: 978 /* inactive */ return this.inactive == null ? new Base[0] : new Base[] { this.inactive }; // BooleanType 979 default: 980 return super.getProperty(hash, name, checkValid); 981 } 982 983 } 984 985 @Override 986 public Base setProperty(int hash, String name, Base value) throws FHIRException { 987 switch (hash) { 988 case -1298275357: // entity 989 this.entity = castToReference(value); // Reference 990 return value; 991 case -991726143: // period 992 this.period = castToPeriod(value); // Period 993 return value; 994 case 24665195: // inactive 995 this.inactive = castToBoolean(value); // BooleanType 996 return value; 997 default: 998 return super.setProperty(hash, name, value); 999 } 1000 1001 } 1002 1003 @Override 1004 public Base setProperty(String name, Base value) throws FHIRException { 1005 if (name.equals("entity")) { 1006 this.entity = castToReference(value); // Reference 1007 } else if (name.equals("period")) { 1008 this.period = castToPeriod(value); // Period 1009 } else if (name.equals("inactive")) { 1010 this.inactive = castToBoolean(value); // BooleanType 1011 } else 1012 return super.setProperty(name, value); 1013 return value; 1014 } 1015 1016 @Override 1017 public Base makeProperty(int hash, String name) throws FHIRException { 1018 switch (hash) { 1019 case -1298275357: 1020 return getEntity(); 1021 case -991726143: 1022 return getPeriod(); 1023 case 24665195: 1024 return getInactiveElement(); 1025 default: 1026 return super.makeProperty(hash, name); 1027 } 1028 1029 } 1030 1031 @Override 1032 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1033 switch (hash) { 1034 case -1298275357: 1035 /* entity */ return new String[] { "Reference" }; 1036 case -991726143: 1037 /* period */ return new String[] { "Period" }; 1038 case 24665195: 1039 /* inactive */ return new String[] { "boolean" }; 1040 default: 1041 return super.getTypesForProperty(hash, name); 1042 } 1043 1044 } 1045 1046 @Override 1047 public Base addChild(String name) throws FHIRException { 1048 if (name.equals("entity")) { 1049 this.entity = new Reference(); 1050 return this.entity; 1051 } else if (name.equals("period")) { 1052 this.period = new Period(); 1053 return this.period; 1054 } else if (name.equals("inactive")) { 1055 throw new FHIRException("Cannot call addChild on a singleton property Group.inactive"); 1056 } else 1057 return super.addChild(name); 1058 } 1059 1060 public GroupMemberComponent copy() { 1061 GroupMemberComponent dst = new GroupMemberComponent(); 1062 copyValues(dst); 1063 return dst; 1064 } 1065 1066 public void copyValues(GroupMemberComponent dst) { 1067 super.copyValues(dst); 1068 dst.entity = entity == null ? null : entity.copy(); 1069 dst.period = period == null ? null : period.copy(); 1070 dst.inactive = inactive == null ? null : inactive.copy(); 1071 } 1072 1073 @Override 1074 public boolean equalsDeep(Base other_) { 1075 if (!super.equalsDeep(other_)) 1076 return false; 1077 if (!(other_ instanceof GroupMemberComponent)) 1078 return false; 1079 GroupMemberComponent o = (GroupMemberComponent) other_; 1080 return compareDeep(entity, o.entity, true) && compareDeep(period, o.period, true) 1081 && compareDeep(inactive, o.inactive, true); 1082 } 1083 1084 @Override 1085 public boolean equalsShallow(Base other_) { 1086 if (!super.equalsShallow(other_)) 1087 return false; 1088 if (!(other_ instanceof GroupMemberComponent)) 1089 return false; 1090 GroupMemberComponent o = (GroupMemberComponent) other_; 1091 return compareValues(inactive, o.inactive, true); 1092 } 1093 1094 public boolean isEmpty() { 1095 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(entity, period, inactive); 1096 } 1097 1098 public String fhirType() { 1099 return "Group.member"; 1100 1101 } 1102 1103 } 1104 1105 /** 1106 * A unique business identifier for this group. 1107 */ 1108 @Child(name = "identifier", type = { 1109 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1110 @Description(shortDefinition = "Unique id", formalDefinition = "A unique business identifier for this group.") 1111 protected List<Identifier> identifier; 1112 1113 /** 1114 * Indicates whether the record for the group is available for use or is merely 1115 * being retained for historical purposes. 1116 */ 1117 @Child(name = "active", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1118 @Description(shortDefinition = "Whether this group's record is in active use", formalDefinition = "Indicates whether the record for the group is available for use or is merely being retained for historical purposes.") 1119 protected BooleanType active; 1120 1121 /** 1122 * Identifies the broad classification of the kind of resources the group 1123 * includes. 1124 */ 1125 @Child(name = "type", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1126 @Description(shortDefinition = "person | animal | practitioner | device | medication | substance", formalDefinition = "Identifies the broad classification of the kind of resources the group includes.") 1127 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/group-type") 1128 protected Enumeration<GroupType> type; 1129 1130 /** 1131 * If true, indicates that the resource refers to a specific group of real 1132 * individuals. If false, the group defines a set of intended individuals. 1133 */ 1134 @Child(name = "actual", type = { BooleanType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 1135 @Description(shortDefinition = "Descriptive or actual", formalDefinition = "If true, indicates that the resource refers to a specific group of real individuals. If false, the group defines a set of intended individuals.") 1136 protected BooleanType actual; 1137 1138 /** 1139 * Provides a specific type of resource the group includes; e.g. "cow", 1140 * "syringe", etc. 1141 */ 1142 @Child(name = "code", type = { CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1143 @Description(shortDefinition = "Kind of Group members", formalDefinition = "Provides a specific type of resource the group includes; e.g. \"cow\", \"syringe\", etc.") 1144 protected CodeableConcept code; 1145 1146 /** 1147 * A label assigned to the group for human identification and communication. 1148 */ 1149 @Child(name = "name", type = { StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1150 @Description(shortDefinition = "Label for Group", formalDefinition = "A label assigned to the group for human identification and communication.") 1151 protected StringType name; 1152 1153 /** 1154 * A count of the number of resource instances that are part of the group. 1155 */ 1156 @Child(name = "quantity", type = { 1157 UnsignedIntType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1158 @Description(shortDefinition = "Number of members", formalDefinition = "A count of the number of resource instances that are part of the group.") 1159 protected UnsignedIntType quantity; 1160 1161 /** 1162 * Entity responsible for defining and maintaining Group characteristics and/or 1163 * registered members. 1164 */ 1165 @Child(name = "managingEntity", type = { Organization.class, RelatedPerson.class, Practitioner.class, 1166 PractitionerRole.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1167 @Description(shortDefinition = "Entity that is the custodian of the Group's definition", formalDefinition = "Entity responsible for defining and maintaining Group characteristics and/or registered members.") 1168 protected Reference managingEntity; 1169 1170 /** 1171 * The actual object that is the target of the reference (Entity responsible for 1172 * defining and maintaining Group characteristics and/or registered members.) 1173 */ 1174 protected Resource managingEntityTarget; 1175 1176 /** 1177 * Identifies traits whose presence r absence is shared by members of the group. 1178 */ 1179 @Child(name = "characteristic", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1180 @Description(shortDefinition = "Include / Exclude group members by Trait", formalDefinition = "Identifies traits whose presence r absence is shared by members of the group.") 1181 protected List<GroupCharacteristicComponent> characteristic; 1182 1183 /** 1184 * Identifies the resource instances that are members of the group. 1185 */ 1186 @Child(name = "member", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1187 @Description(shortDefinition = "Who or what is in group", formalDefinition = "Identifies the resource instances that are members of the group.") 1188 protected List<GroupMemberComponent> member; 1189 1190 private static final long serialVersionUID = -550945963L; 1191 1192 /** 1193 * Constructor 1194 */ 1195 public Group() { 1196 super(); 1197 } 1198 1199 /** 1200 * Constructor 1201 */ 1202 public Group(Enumeration<GroupType> type, BooleanType actual) { 1203 super(); 1204 this.type = type; 1205 this.actual = actual; 1206 } 1207 1208 /** 1209 * @return {@link #identifier} (A unique business identifier for this group.) 1210 */ 1211 public List<Identifier> getIdentifier() { 1212 if (this.identifier == null) 1213 this.identifier = new ArrayList<Identifier>(); 1214 return this.identifier; 1215 } 1216 1217 /** 1218 * @return Returns a reference to <code>this</code> for easy method chaining 1219 */ 1220 public Group setIdentifier(List<Identifier> theIdentifier) { 1221 this.identifier = theIdentifier; 1222 return this; 1223 } 1224 1225 public boolean hasIdentifier() { 1226 if (this.identifier == null) 1227 return false; 1228 for (Identifier item : this.identifier) 1229 if (!item.isEmpty()) 1230 return true; 1231 return false; 1232 } 1233 1234 public Identifier addIdentifier() { // 3 1235 Identifier t = new Identifier(); 1236 if (this.identifier == null) 1237 this.identifier = new ArrayList<Identifier>(); 1238 this.identifier.add(t); 1239 return t; 1240 } 1241 1242 public Group addIdentifier(Identifier t) { // 3 1243 if (t == null) 1244 return this; 1245 if (this.identifier == null) 1246 this.identifier = new ArrayList<Identifier>(); 1247 this.identifier.add(t); 1248 return this; 1249 } 1250 1251 /** 1252 * @return The first repetition of repeating field {@link #identifier}, creating 1253 * it if it does not already exist 1254 */ 1255 public Identifier getIdentifierFirstRep() { 1256 if (getIdentifier().isEmpty()) { 1257 addIdentifier(); 1258 } 1259 return getIdentifier().get(0); 1260 } 1261 1262 /** 1263 * @return {@link #active} (Indicates whether the record for the group is 1264 * available for use or is merely being retained for historical 1265 * purposes.). This is the underlying object with id, value and 1266 * extensions. The accessor "getActive" gives direct access to the value 1267 */ 1268 public BooleanType getActiveElement() { 1269 if (this.active == null) 1270 if (Configuration.errorOnAutoCreate()) 1271 throw new Error("Attempt to auto-create Group.active"); 1272 else if (Configuration.doAutoCreate()) 1273 this.active = new BooleanType(); // bb 1274 return this.active; 1275 } 1276 1277 public boolean hasActiveElement() { 1278 return this.active != null && !this.active.isEmpty(); 1279 } 1280 1281 public boolean hasActive() { 1282 return this.active != null && !this.active.isEmpty(); 1283 } 1284 1285 /** 1286 * @param value {@link #active} (Indicates whether the record for the group is 1287 * available for use or is merely being retained for historical 1288 * purposes.). This is the underlying object with id, value and 1289 * extensions. The accessor "getActive" gives direct access to the 1290 * value 1291 */ 1292 public Group setActiveElement(BooleanType value) { 1293 this.active = value; 1294 return this; 1295 } 1296 1297 /** 1298 * @return Indicates whether the record for the group is available for use or is 1299 * merely being retained for historical purposes. 1300 */ 1301 public boolean getActive() { 1302 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 1303 } 1304 1305 /** 1306 * @param value Indicates whether the record for the group is available for use 1307 * or is merely being retained for historical purposes. 1308 */ 1309 public Group setActive(boolean value) { 1310 if (this.active == null) 1311 this.active = new BooleanType(); 1312 this.active.setValue(value); 1313 return this; 1314 } 1315 1316 /** 1317 * @return {@link #type} (Identifies the broad classification of the kind of 1318 * resources the group includes.). This is the underlying object with 1319 * id, value and extensions. The accessor "getType" gives direct access 1320 * to the value 1321 */ 1322 public Enumeration<GroupType> getTypeElement() { 1323 if (this.type == null) 1324 if (Configuration.errorOnAutoCreate()) 1325 throw new Error("Attempt to auto-create Group.type"); 1326 else if (Configuration.doAutoCreate()) 1327 this.type = new Enumeration<GroupType>(new GroupTypeEnumFactory()); // bb 1328 return this.type; 1329 } 1330 1331 public boolean hasTypeElement() { 1332 return this.type != null && !this.type.isEmpty(); 1333 } 1334 1335 public boolean hasType() { 1336 return this.type != null && !this.type.isEmpty(); 1337 } 1338 1339 /** 1340 * @param value {@link #type} (Identifies the broad classification of the kind 1341 * of resources the group includes.). This is the underlying object 1342 * with id, value and extensions. The accessor "getType" gives 1343 * direct access to the value 1344 */ 1345 public Group setTypeElement(Enumeration<GroupType> value) { 1346 this.type = value; 1347 return this; 1348 } 1349 1350 /** 1351 * @return Identifies the broad classification of the kind of resources the 1352 * group includes. 1353 */ 1354 public GroupType getType() { 1355 return this.type == null ? null : this.type.getValue(); 1356 } 1357 1358 /** 1359 * @param value Identifies the broad classification of the kind of resources the 1360 * group includes. 1361 */ 1362 public Group setType(GroupType value) { 1363 if (this.type == null) 1364 this.type = new Enumeration<GroupType>(new GroupTypeEnumFactory()); 1365 this.type.setValue(value); 1366 return this; 1367 } 1368 1369 /** 1370 * @return {@link #actual} (If true, indicates that the resource refers to a 1371 * specific group of real individuals. If false, the group defines a set 1372 * of intended individuals.). This is the underlying object with id, 1373 * value and extensions. The accessor "getActual" gives direct access to 1374 * the value 1375 */ 1376 public BooleanType getActualElement() { 1377 if (this.actual == null) 1378 if (Configuration.errorOnAutoCreate()) 1379 throw new Error("Attempt to auto-create Group.actual"); 1380 else if (Configuration.doAutoCreate()) 1381 this.actual = new BooleanType(); // bb 1382 return this.actual; 1383 } 1384 1385 public boolean hasActualElement() { 1386 return this.actual != null && !this.actual.isEmpty(); 1387 } 1388 1389 public boolean hasActual() { 1390 return this.actual != null && !this.actual.isEmpty(); 1391 } 1392 1393 /** 1394 * @param value {@link #actual} (If true, indicates that the resource refers to 1395 * a specific group of real individuals. If false, the group 1396 * defines a set of intended individuals.). This is the underlying 1397 * object with id, value and extensions. The accessor "getActual" 1398 * gives direct access to the value 1399 */ 1400 public Group setActualElement(BooleanType value) { 1401 this.actual = value; 1402 return this; 1403 } 1404 1405 /** 1406 * @return If true, indicates that the resource refers to a specific group of 1407 * real individuals. If false, the group defines a set of intended 1408 * individuals. 1409 */ 1410 public boolean getActual() { 1411 return this.actual == null || this.actual.isEmpty() ? false : this.actual.getValue(); 1412 } 1413 1414 /** 1415 * @param value If true, indicates that the resource refers to a specific group 1416 * of real individuals. If false, the group defines a set of 1417 * intended individuals. 1418 */ 1419 public Group setActual(boolean value) { 1420 if (this.actual == null) 1421 this.actual = new BooleanType(); 1422 this.actual.setValue(value); 1423 return this; 1424 } 1425 1426 /** 1427 * @return {@link #code} (Provides a specific type of resource the group 1428 * includes; e.g. "cow", "syringe", etc.) 1429 */ 1430 public CodeableConcept getCode() { 1431 if (this.code == null) 1432 if (Configuration.errorOnAutoCreate()) 1433 throw new Error("Attempt to auto-create Group.code"); 1434 else if (Configuration.doAutoCreate()) 1435 this.code = new CodeableConcept(); // cc 1436 return this.code; 1437 } 1438 1439 public boolean hasCode() { 1440 return this.code != null && !this.code.isEmpty(); 1441 } 1442 1443 /** 1444 * @param value {@link #code} (Provides a specific type of resource the group 1445 * includes; e.g. "cow", "syringe", etc.) 1446 */ 1447 public Group setCode(CodeableConcept value) { 1448 this.code = value; 1449 return this; 1450 } 1451 1452 /** 1453 * @return {@link #name} (A label assigned to the group for human identification 1454 * and communication.). This is the underlying object with id, value and 1455 * extensions. The accessor "getName" gives direct access to the value 1456 */ 1457 public StringType getNameElement() { 1458 if (this.name == null) 1459 if (Configuration.errorOnAutoCreate()) 1460 throw new Error("Attempt to auto-create Group.name"); 1461 else if (Configuration.doAutoCreate()) 1462 this.name = new StringType(); // bb 1463 return this.name; 1464 } 1465 1466 public boolean hasNameElement() { 1467 return this.name != null && !this.name.isEmpty(); 1468 } 1469 1470 public boolean hasName() { 1471 return this.name != null && !this.name.isEmpty(); 1472 } 1473 1474 /** 1475 * @param value {@link #name} (A label assigned to the group for human 1476 * identification and communication.). This is the underlying 1477 * object with id, value and extensions. The accessor "getName" 1478 * gives direct access to the value 1479 */ 1480 public Group setNameElement(StringType value) { 1481 this.name = value; 1482 return this; 1483 } 1484 1485 /** 1486 * @return A label assigned to the group for human identification and 1487 * communication. 1488 */ 1489 public String getName() { 1490 return this.name == null ? null : this.name.getValue(); 1491 } 1492 1493 /** 1494 * @param value A label assigned to the group for human identification and 1495 * communication. 1496 */ 1497 public Group setName(String value) { 1498 if (Utilities.noString(value)) 1499 this.name = null; 1500 else { 1501 if (this.name == null) 1502 this.name = new StringType(); 1503 this.name.setValue(value); 1504 } 1505 return this; 1506 } 1507 1508 /** 1509 * @return {@link #quantity} (A count of the number of resource instances that 1510 * are part of the group.). This is the underlying object with id, value 1511 * and extensions. The accessor "getQuantity" gives direct access to the 1512 * value 1513 */ 1514 public UnsignedIntType getQuantityElement() { 1515 if (this.quantity == null) 1516 if (Configuration.errorOnAutoCreate()) 1517 throw new Error("Attempt to auto-create Group.quantity"); 1518 else if (Configuration.doAutoCreate()) 1519 this.quantity = new UnsignedIntType(); // bb 1520 return this.quantity; 1521 } 1522 1523 public boolean hasQuantityElement() { 1524 return this.quantity != null && !this.quantity.isEmpty(); 1525 } 1526 1527 public boolean hasQuantity() { 1528 return this.quantity != null && !this.quantity.isEmpty(); 1529 } 1530 1531 /** 1532 * @param value {@link #quantity} (A count of the number of resource instances 1533 * that are part of the group.). This is the underlying object with 1534 * id, value and extensions. The accessor "getQuantity" gives 1535 * direct access to the value 1536 */ 1537 public Group setQuantityElement(UnsignedIntType value) { 1538 this.quantity = value; 1539 return this; 1540 } 1541 1542 /** 1543 * @return A count of the number of resource instances that are part of the 1544 * group. 1545 */ 1546 public int getQuantity() { 1547 return this.quantity == null || this.quantity.isEmpty() ? 0 : this.quantity.getValue(); 1548 } 1549 1550 /** 1551 * @param value A count of the number of resource instances that are part of the 1552 * group. 1553 */ 1554 public Group setQuantity(int value) { 1555 if (this.quantity == null) 1556 this.quantity = new UnsignedIntType(); 1557 this.quantity.setValue(value); 1558 return this; 1559 } 1560 1561 /** 1562 * @return {@link #managingEntity} (Entity responsible for defining and 1563 * maintaining Group characteristics and/or registered members.) 1564 */ 1565 public Reference getManagingEntity() { 1566 if (this.managingEntity == null) 1567 if (Configuration.errorOnAutoCreate()) 1568 throw new Error("Attempt to auto-create Group.managingEntity"); 1569 else if (Configuration.doAutoCreate()) 1570 this.managingEntity = new Reference(); // cc 1571 return this.managingEntity; 1572 } 1573 1574 public boolean hasManagingEntity() { 1575 return this.managingEntity != null && !this.managingEntity.isEmpty(); 1576 } 1577 1578 /** 1579 * @param value {@link #managingEntity} (Entity responsible for defining and 1580 * maintaining Group characteristics and/or registered members.) 1581 */ 1582 public Group setManagingEntity(Reference value) { 1583 this.managingEntity = value; 1584 return this; 1585 } 1586 1587 /** 1588 * @return {@link #managingEntity} The actual object that is the target of the 1589 * reference. The reference library doesn't populate this, but you can 1590 * use it to hold the resource if you resolve it. (Entity responsible 1591 * for defining and maintaining Group characteristics and/or registered 1592 * members.) 1593 */ 1594 public Resource getManagingEntityTarget() { 1595 return this.managingEntityTarget; 1596 } 1597 1598 /** 1599 * @param value {@link #managingEntity} The actual object that is the target of 1600 * the reference. The reference library doesn't use these, but you 1601 * can use it to hold the resource if you resolve it. (Entity 1602 * responsible for defining and maintaining Group characteristics 1603 * and/or registered members.) 1604 */ 1605 public Group setManagingEntityTarget(Resource value) { 1606 this.managingEntityTarget = value; 1607 return this; 1608 } 1609 1610 /** 1611 * @return {@link #characteristic} (Identifies traits whose presence r absence 1612 * is shared by members of the group.) 1613 */ 1614 public List<GroupCharacteristicComponent> getCharacteristic() { 1615 if (this.characteristic == null) 1616 this.characteristic = new ArrayList<GroupCharacteristicComponent>(); 1617 return this.characteristic; 1618 } 1619 1620 /** 1621 * @return Returns a reference to <code>this</code> for easy method chaining 1622 */ 1623 public Group setCharacteristic(List<GroupCharacteristicComponent> theCharacteristic) { 1624 this.characteristic = theCharacteristic; 1625 return this; 1626 } 1627 1628 public boolean hasCharacteristic() { 1629 if (this.characteristic == null) 1630 return false; 1631 for (GroupCharacteristicComponent item : this.characteristic) 1632 if (!item.isEmpty()) 1633 return true; 1634 return false; 1635 } 1636 1637 public GroupCharacteristicComponent addCharacteristic() { // 3 1638 GroupCharacteristicComponent t = new GroupCharacteristicComponent(); 1639 if (this.characteristic == null) 1640 this.characteristic = new ArrayList<GroupCharacteristicComponent>(); 1641 this.characteristic.add(t); 1642 return t; 1643 } 1644 1645 public Group addCharacteristic(GroupCharacteristicComponent t) { // 3 1646 if (t == null) 1647 return this; 1648 if (this.characteristic == null) 1649 this.characteristic = new ArrayList<GroupCharacteristicComponent>(); 1650 this.characteristic.add(t); 1651 return this; 1652 } 1653 1654 /** 1655 * @return The first repetition of repeating field {@link #characteristic}, 1656 * creating it if it does not already exist 1657 */ 1658 public GroupCharacteristicComponent getCharacteristicFirstRep() { 1659 if (getCharacteristic().isEmpty()) { 1660 addCharacteristic(); 1661 } 1662 return getCharacteristic().get(0); 1663 } 1664 1665 /** 1666 * @return {@link #member} (Identifies the resource instances that are members 1667 * of the group.) 1668 */ 1669 public List<GroupMemberComponent> getMember() { 1670 if (this.member == null) 1671 this.member = new ArrayList<GroupMemberComponent>(); 1672 return this.member; 1673 } 1674 1675 /** 1676 * @return Returns a reference to <code>this</code> for easy method chaining 1677 */ 1678 public Group setMember(List<GroupMemberComponent> theMember) { 1679 this.member = theMember; 1680 return this; 1681 } 1682 1683 public boolean hasMember() { 1684 if (this.member == null) 1685 return false; 1686 for (GroupMemberComponent item : this.member) 1687 if (!item.isEmpty()) 1688 return true; 1689 return false; 1690 } 1691 1692 public GroupMemberComponent addMember() { // 3 1693 GroupMemberComponent t = new GroupMemberComponent(); 1694 if (this.member == null) 1695 this.member = new ArrayList<GroupMemberComponent>(); 1696 this.member.add(t); 1697 return t; 1698 } 1699 1700 public Group addMember(GroupMemberComponent t) { // 3 1701 if (t == null) 1702 return this; 1703 if (this.member == null) 1704 this.member = new ArrayList<GroupMemberComponent>(); 1705 this.member.add(t); 1706 return this; 1707 } 1708 1709 /** 1710 * @return The first repetition of repeating field {@link #member}, creating it 1711 * if it does not already exist 1712 */ 1713 public GroupMemberComponent getMemberFirstRep() { 1714 if (getMember().isEmpty()) { 1715 addMember(); 1716 } 1717 return getMember().get(0); 1718 } 1719 1720 protected void listChildren(List<Property> children) { 1721 super.listChildren(children); 1722 children.add(new Property("identifier", "Identifier", "A unique business identifier for this group.", 0, 1723 java.lang.Integer.MAX_VALUE, identifier)); 1724 children.add(new Property("active", "boolean", 1725 "Indicates whether the record for the group is available for use or is merely being retained for historical purposes.", 1726 0, 1, active)); 1727 children.add(new Property("type", "code", 1728 "Identifies the broad classification of the kind of resources the group includes.", 0, 1, type)); 1729 children.add(new Property("actual", "boolean", 1730 "If true, indicates that the resource refers to a specific group of real individuals. If false, the group defines a set of intended individuals.", 1731 0, 1, actual)); 1732 children.add(new Property("code", "CodeableConcept", 1733 "Provides a specific type of resource the group includes; e.g. \"cow\", \"syringe\", etc.", 0, 1, code)); 1734 children.add(new Property("name", "string", 1735 "A label assigned to the group for human identification and communication.", 0, 1, name)); 1736 children.add(new Property("quantity", "unsignedInt", 1737 "A count of the number of resource instances that are part of the group.", 0, 1, quantity)); 1738 children.add(new Property("managingEntity", "Reference(Organization|RelatedPerson|Practitioner|PractitionerRole)", 1739 "Entity responsible for defining and maintaining Group characteristics and/or registered members.", 0, 1, 1740 managingEntity)); 1741 children.add(new Property("characteristic", "", 1742 "Identifies traits whose presence r absence is shared by members of the group.", 0, java.lang.Integer.MAX_VALUE, 1743 characteristic)); 1744 children.add(new Property("member", "", "Identifies the resource instances that are members of the group.", 0, 1745 java.lang.Integer.MAX_VALUE, member)); 1746 } 1747 1748 @Override 1749 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1750 switch (_hash) { 1751 case -1618432855: 1752 /* identifier */ return new Property("identifier", "Identifier", "A unique business identifier for this group.", 1753 0, java.lang.Integer.MAX_VALUE, identifier); 1754 case -1422950650: 1755 /* active */ return new Property("active", "boolean", 1756 "Indicates whether the record for the group is available for use or is merely being retained for historical purposes.", 1757 0, 1, active); 1758 case 3575610: 1759 /* type */ return new Property("type", "code", 1760 "Identifies the broad classification of the kind of resources the group includes.", 0, 1, type); 1761 case -1422939762: 1762 /* actual */ return new Property("actual", "boolean", 1763 "If true, indicates that the resource refers to a specific group of real individuals. If false, the group defines a set of intended individuals.", 1764 0, 1, actual); 1765 case 3059181: 1766 /* code */ return new Property("code", "CodeableConcept", 1767 "Provides a specific type of resource the group includes; e.g. \"cow\", \"syringe\", etc.", 0, 1, code); 1768 case 3373707: 1769 /* name */ return new Property("name", "string", 1770 "A label assigned to the group for human identification and communication.", 0, 1, name); 1771 case -1285004149: 1772 /* quantity */ return new Property("quantity", "unsignedInt", 1773 "A count of the number of resource instances that are part of the group.", 0, 1, quantity); 1774 case -988474523: 1775 /* managingEntity */ return new Property("managingEntity", 1776 "Reference(Organization|RelatedPerson|Practitioner|PractitionerRole)", 1777 "Entity responsible for defining and maintaining Group characteristics and/or registered members.", 0, 1, 1778 managingEntity); 1779 case 366313883: 1780 /* characteristic */ return new Property("characteristic", "", 1781 "Identifies traits whose presence r absence is shared by members of the group.", 0, 1782 java.lang.Integer.MAX_VALUE, characteristic); 1783 case -1077769574: 1784 /* member */ return new Property("member", "", "Identifies the resource instances that are members of the group.", 1785 0, java.lang.Integer.MAX_VALUE, member); 1786 default: 1787 return super.getNamedProperty(_hash, _name, _checkValid); 1788 } 1789 1790 } 1791 1792 @Override 1793 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1794 switch (hash) { 1795 case -1618432855: 1796 /* identifier */ return this.identifier == null ? new Base[0] 1797 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1798 case -1422950650: 1799 /* active */ return this.active == null ? new Base[0] : new Base[] { this.active }; // BooleanType 1800 case 3575610: 1801 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<GroupType> 1802 case -1422939762: 1803 /* actual */ return this.actual == null ? new Base[0] : new Base[] { this.actual }; // BooleanType 1804 case 3059181: 1805 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 1806 case 3373707: 1807 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 1808 case -1285004149: 1809 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // UnsignedIntType 1810 case -988474523: 1811 /* managingEntity */ return this.managingEntity == null ? new Base[0] : new Base[] { this.managingEntity }; // Reference 1812 case 366313883: 1813 /* characteristic */ return this.characteristic == null ? new Base[0] 1814 : this.characteristic.toArray(new Base[this.characteristic.size()]); // GroupCharacteristicComponent 1815 case -1077769574: 1816 /* member */ return this.member == null ? new Base[0] : this.member.toArray(new Base[this.member.size()]); // GroupMemberComponent 1817 default: 1818 return super.getProperty(hash, name, checkValid); 1819 } 1820 1821 } 1822 1823 @Override 1824 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1825 switch (hash) { 1826 case -1618432855: // identifier 1827 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1828 return value; 1829 case -1422950650: // active 1830 this.active = castToBoolean(value); // BooleanType 1831 return value; 1832 case 3575610: // type 1833 value = new GroupTypeEnumFactory().fromType(castToCode(value)); 1834 this.type = (Enumeration) value; // Enumeration<GroupType> 1835 return value; 1836 case -1422939762: // actual 1837 this.actual = castToBoolean(value); // BooleanType 1838 return value; 1839 case 3059181: // code 1840 this.code = castToCodeableConcept(value); // CodeableConcept 1841 return value; 1842 case 3373707: // name 1843 this.name = castToString(value); // StringType 1844 return value; 1845 case -1285004149: // quantity 1846 this.quantity = castToUnsignedInt(value); // UnsignedIntType 1847 return value; 1848 case -988474523: // managingEntity 1849 this.managingEntity = castToReference(value); // Reference 1850 return value; 1851 case 366313883: // characteristic 1852 this.getCharacteristic().add((GroupCharacteristicComponent) value); // GroupCharacteristicComponent 1853 return value; 1854 case -1077769574: // member 1855 this.getMember().add((GroupMemberComponent) value); // GroupMemberComponent 1856 return value; 1857 default: 1858 return super.setProperty(hash, name, value); 1859 } 1860 1861 } 1862 1863 @Override 1864 public Base setProperty(String name, Base value) throws FHIRException { 1865 if (name.equals("identifier")) { 1866 this.getIdentifier().add(castToIdentifier(value)); 1867 } else if (name.equals("active")) { 1868 this.active = castToBoolean(value); // BooleanType 1869 } else if (name.equals("type")) { 1870 value = new GroupTypeEnumFactory().fromType(castToCode(value)); 1871 this.type = (Enumeration) value; // Enumeration<GroupType> 1872 } else if (name.equals("actual")) { 1873 this.actual = castToBoolean(value); // BooleanType 1874 } else if (name.equals("code")) { 1875 this.code = castToCodeableConcept(value); // CodeableConcept 1876 } else if (name.equals("name")) { 1877 this.name = castToString(value); // StringType 1878 } else if (name.equals("quantity")) { 1879 this.quantity = castToUnsignedInt(value); // UnsignedIntType 1880 } else if (name.equals("managingEntity")) { 1881 this.managingEntity = castToReference(value); // Reference 1882 } else if (name.equals("characteristic")) { 1883 this.getCharacteristic().add((GroupCharacteristicComponent) value); 1884 } else if (name.equals("member")) { 1885 this.getMember().add((GroupMemberComponent) value); 1886 } else 1887 return super.setProperty(name, value); 1888 return value; 1889 } 1890 1891 @Override 1892 public Base makeProperty(int hash, String name) throws FHIRException { 1893 switch (hash) { 1894 case -1618432855: 1895 return addIdentifier(); 1896 case -1422950650: 1897 return getActiveElement(); 1898 case 3575610: 1899 return getTypeElement(); 1900 case -1422939762: 1901 return getActualElement(); 1902 case 3059181: 1903 return getCode(); 1904 case 3373707: 1905 return getNameElement(); 1906 case -1285004149: 1907 return getQuantityElement(); 1908 case -988474523: 1909 return getManagingEntity(); 1910 case 366313883: 1911 return addCharacteristic(); 1912 case -1077769574: 1913 return addMember(); 1914 default: 1915 return super.makeProperty(hash, name); 1916 } 1917 1918 } 1919 1920 @Override 1921 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1922 switch (hash) { 1923 case -1618432855: 1924 /* identifier */ return new String[] { "Identifier" }; 1925 case -1422950650: 1926 /* active */ return new String[] { "boolean" }; 1927 case 3575610: 1928 /* type */ return new String[] { "code" }; 1929 case -1422939762: 1930 /* actual */ return new String[] { "boolean" }; 1931 case 3059181: 1932 /* code */ return new String[] { "CodeableConcept" }; 1933 case 3373707: 1934 /* name */ return new String[] { "string" }; 1935 case -1285004149: 1936 /* quantity */ return new String[] { "unsignedInt" }; 1937 case -988474523: 1938 /* managingEntity */ return new String[] { "Reference" }; 1939 case 366313883: 1940 /* characteristic */ return new String[] {}; 1941 case -1077769574: 1942 /* member */ return new String[] {}; 1943 default: 1944 return super.getTypesForProperty(hash, name); 1945 } 1946 1947 } 1948 1949 @Override 1950 public Base addChild(String name) throws FHIRException { 1951 if (name.equals("identifier")) { 1952 return addIdentifier(); 1953 } else if (name.equals("active")) { 1954 throw new FHIRException("Cannot call addChild on a singleton property Group.active"); 1955 } else if (name.equals("type")) { 1956 throw new FHIRException("Cannot call addChild on a singleton property Group.type"); 1957 } else if (name.equals("actual")) { 1958 throw new FHIRException("Cannot call addChild on a singleton property Group.actual"); 1959 } else if (name.equals("code")) { 1960 this.code = new CodeableConcept(); 1961 return this.code; 1962 } else if (name.equals("name")) { 1963 throw new FHIRException("Cannot call addChild on a singleton property Group.name"); 1964 } else if (name.equals("quantity")) { 1965 throw new FHIRException("Cannot call addChild on a singleton property Group.quantity"); 1966 } else if (name.equals("managingEntity")) { 1967 this.managingEntity = new Reference(); 1968 return this.managingEntity; 1969 } else if (name.equals("characteristic")) { 1970 return addCharacteristic(); 1971 } else if (name.equals("member")) { 1972 return addMember(); 1973 } else 1974 return super.addChild(name); 1975 } 1976 1977 public String fhirType() { 1978 return "Group"; 1979 1980 } 1981 1982 public Group copy() { 1983 Group dst = new Group(); 1984 copyValues(dst); 1985 return dst; 1986 } 1987 1988 public void copyValues(Group dst) { 1989 super.copyValues(dst); 1990 if (identifier != null) { 1991 dst.identifier = new ArrayList<Identifier>(); 1992 for (Identifier i : identifier) 1993 dst.identifier.add(i.copy()); 1994 } 1995 ; 1996 dst.active = active == null ? null : active.copy(); 1997 dst.type = type == null ? null : type.copy(); 1998 dst.actual = actual == null ? null : actual.copy(); 1999 dst.code = code == null ? null : code.copy(); 2000 dst.name = name == null ? null : name.copy(); 2001 dst.quantity = quantity == null ? null : quantity.copy(); 2002 dst.managingEntity = managingEntity == null ? null : managingEntity.copy(); 2003 if (characteristic != null) { 2004 dst.characteristic = new ArrayList<GroupCharacteristicComponent>(); 2005 for (GroupCharacteristicComponent i : characteristic) 2006 dst.characteristic.add(i.copy()); 2007 } 2008 ; 2009 if (member != null) { 2010 dst.member = new ArrayList<GroupMemberComponent>(); 2011 for (GroupMemberComponent i : member) 2012 dst.member.add(i.copy()); 2013 } 2014 ; 2015 } 2016 2017 protected Group typedCopy() { 2018 return copy(); 2019 } 2020 2021 @Override 2022 public boolean equalsDeep(Base other_) { 2023 if (!super.equalsDeep(other_)) 2024 return false; 2025 if (!(other_ instanceof Group)) 2026 return false; 2027 Group o = (Group) other_; 2028 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) 2029 && compareDeep(type, o.type, true) && compareDeep(actual, o.actual, true) && compareDeep(code, o.code, true) 2030 && compareDeep(name, o.name, true) && compareDeep(quantity, o.quantity, true) 2031 && compareDeep(managingEntity, o.managingEntity, true) && compareDeep(characteristic, o.characteristic, true) 2032 && compareDeep(member, o.member, true); 2033 } 2034 2035 @Override 2036 public boolean equalsShallow(Base other_) { 2037 if (!super.equalsShallow(other_)) 2038 return false; 2039 if (!(other_ instanceof Group)) 2040 return false; 2041 Group o = (Group) other_; 2042 return compareValues(active, o.active, true) && compareValues(type, o.type, true) 2043 && compareValues(actual, o.actual, true) && compareValues(name, o.name, true) 2044 && compareValues(quantity, o.quantity, true); 2045 } 2046 2047 public boolean isEmpty() { 2048 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, type, actual, code, name, 2049 quantity, managingEntity, characteristic, member); 2050 } 2051 2052 @Override 2053 public ResourceType getResourceType() { 2054 return ResourceType.Group; 2055 } 2056 2057 /** 2058 * Search parameter: <b>actual</b> 2059 * <p> 2060 * Description: <b>Descriptive or actual</b><br> 2061 * Type: <b>token</b><br> 2062 * Path: <b>Group.actual</b><br> 2063 * </p> 2064 */ 2065 @SearchParamDefinition(name = "actual", path = "Group.actual", description = "Descriptive or actual", type = "token") 2066 public static final String SP_ACTUAL = "actual"; 2067 /** 2068 * <b>Fluent Client</b> search parameter constant for <b>actual</b> 2069 * <p> 2070 * Description: <b>Descriptive or actual</b><br> 2071 * Type: <b>token</b><br> 2072 * Path: <b>Group.actual</b><br> 2073 * </p> 2074 */ 2075 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTUAL = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2076 SP_ACTUAL); 2077 2078 /** 2079 * Search parameter: <b>identifier</b> 2080 * <p> 2081 * Description: <b>Unique id</b><br> 2082 * Type: <b>token</b><br> 2083 * Path: <b>Group.identifier</b><br> 2084 * </p> 2085 */ 2086 @SearchParamDefinition(name = "identifier", path = "Group.identifier", description = "Unique id", type = "token") 2087 public static final String SP_IDENTIFIER = "identifier"; 2088 /** 2089 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2090 * <p> 2091 * Description: <b>Unique id</b><br> 2092 * Type: <b>token</b><br> 2093 * Path: <b>Group.identifier</b><br> 2094 * </p> 2095 */ 2096 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2097 SP_IDENTIFIER); 2098 2099 /** 2100 * Search parameter: <b>characteristic-value</b> 2101 * <p> 2102 * Description: <b>A composite of both characteristic and value</b><br> 2103 * Type: <b>composite</b><br> 2104 * Path: <b></b><br> 2105 * </p> 2106 */ 2107 @SearchParamDefinition(name = "characteristic-value", path = "Group.characteristic", description = "A composite of both characteristic and value", type = "composite", compositeOf = { 2108 "characteristic", "value" }) 2109 public static final String SP_CHARACTERISTIC_VALUE = "characteristic-value"; 2110 /** 2111 * <b>Fluent Client</b> search parameter constant for 2112 * <b>characteristic-value</b> 2113 * <p> 2114 * Description: <b>A composite of both characteristic and value</b><br> 2115 * Type: <b>composite</b><br> 2116 * Path: <b></b><br> 2117 * </p> 2118 */ 2119 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CHARACTERISTIC_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 2120 SP_CHARACTERISTIC_VALUE); 2121 2122 /** 2123 * Search parameter: <b>managing-entity</b> 2124 * <p> 2125 * Description: <b>Entity that is the custodian of the Group's 2126 * definition</b><br> 2127 * Type: <b>reference</b><br> 2128 * Path: <b>Group.managingEntity</b><br> 2129 * </p> 2130 */ 2131 @SearchParamDefinition(name = "managing-entity", path = "Group.managingEntity", description = "Entity that is the custodian of the Group's definition", type = "reference", target = { 2132 Organization.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 2133 public static final String SP_MANAGING_ENTITY = "managing-entity"; 2134 /** 2135 * <b>Fluent Client</b> search parameter constant for <b>managing-entity</b> 2136 * <p> 2137 * Description: <b>Entity that is the custodian of the Group's 2138 * definition</b><br> 2139 * Type: <b>reference</b><br> 2140 * Path: <b>Group.managingEntity</b><br> 2141 * </p> 2142 */ 2143 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MANAGING_ENTITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2144 SP_MANAGING_ENTITY); 2145 2146 /** 2147 * Constant for fluent queries to be used to add include statements. Specifies 2148 * the path value of "<b>Group:managing-entity</b>". 2149 */ 2150 public static final ca.uhn.fhir.model.api.Include INCLUDE_MANAGING_ENTITY = new ca.uhn.fhir.model.api.Include( 2151 "Group:managing-entity").toLocked(); 2152 2153 /** 2154 * Search parameter: <b>code</b> 2155 * <p> 2156 * Description: <b>The kind of resources contained</b><br> 2157 * Type: <b>token</b><br> 2158 * Path: <b>Group.code</b><br> 2159 * </p> 2160 */ 2161 @SearchParamDefinition(name = "code", path = "Group.code", description = "The kind of resources contained", type = "token") 2162 public static final String SP_CODE = "code"; 2163 /** 2164 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2165 * <p> 2166 * Description: <b>The kind of resources contained</b><br> 2167 * Type: <b>token</b><br> 2168 * Path: <b>Group.code</b><br> 2169 * </p> 2170 */ 2171 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2172 SP_CODE); 2173 2174 /** 2175 * Search parameter: <b>member</b> 2176 * <p> 2177 * Description: <b>Reference to the group member</b><br> 2178 * Type: <b>reference</b><br> 2179 * Path: <b>Group.member.entity</b><br> 2180 * </p> 2181 */ 2182 @SearchParamDefinition(name = "member", path = "Group.member.entity", description = "Reference to the group member", type = "reference", providesMembershipIn = { 2183 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 2184 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 2185 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Device.class, Group.class, 2186 Medication.class, Patient.class, Practitioner.class, PractitionerRole.class, Substance.class }) 2187 public static final String SP_MEMBER = "member"; 2188 /** 2189 * <b>Fluent Client</b> search parameter constant for <b>member</b> 2190 * <p> 2191 * Description: <b>Reference to the group member</b><br> 2192 * Type: <b>reference</b><br> 2193 * Path: <b>Group.member.entity</b><br> 2194 * </p> 2195 */ 2196 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEMBER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2197 SP_MEMBER); 2198 2199 /** 2200 * Constant for fluent queries to be used to add include statements. Specifies 2201 * the path value of "<b>Group:member</b>". 2202 */ 2203 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEMBER = new ca.uhn.fhir.model.api.Include("Group:member") 2204 .toLocked(); 2205 2206 /** 2207 * Search parameter: <b>exclude</b> 2208 * <p> 2209 * Description: <b>Group includes or excludes</b><br> 2210 * Type: <b>token</b><br> 2211 * Path: <b>Group.characteristic.exclude</b><br> 2212 * </p> 2213 */ 2214 @SearchParamDefinition(name = "exclude", path = "Group.characteristic.exclude", description = "Group includes or excludes", type = "token") 2215 public static final String SP_EXCLUDE = "exclude"; 2216 /** 2217 * <b>Fluent Client</b> search parameter constant for <b>exclude</b> 2218 * <p> 2219 * Description: <b>Group includes or excludes</b><br> 2220 * Type: <b>token</b><br> 2221 * Path: <b>Group.characteristic.exclude</b><br> 2222 * </p> 2223 */ 2224 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EXCLUDE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2225 SP_EXCLUDE); 2226 2227 /** 2228 * Search parameter: <b>type</b> 2229 * <p> 2230 * Description: <b>The type of resources the group contains</b><br> 2231 * Type: <b>token</b><br> 2232 * Path: <b>Group.type</b><br> 2233 * </p> 2234 */ 2235 @SearchParamDefinition(name = "type", path = "Group.type", description = "The type of resources the group contains", type = "token") 2236 public static final String SP_TYPE = "type"; 2237 /** 2238 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2239 * <p> 2240 * Description: <b>The type of resources the group contains</b><br> 2241 * Type: <b>token</b><br> 2242 * Path: <b>Group.type</b><br> 2243 * </p> 2244 */ 2245 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2246 SP_TYPE); 2247 2248 /** 2249 * Search parameter: <b>value</b> 2250 * <p> 2251 * Description: <b>Value held by characteristic</b><br> 2252 * Type: <b>token</b><br> 2253 * Path: <b>Group.characteristic.value[x]</b><br> 2254 * </p> 2255 */ 2256 @SearchParamDefinition(name = "value", path = "(Group.characteristic.value as CodeableConcept) | (Group.characteristic.value as boolean)", description = "Value held by characteristic", type = "token") 2257 public static final String SP_VALUE = "value"; 2258 /** 2259 * <b>Fluent Client</b> search parameter constant for <b>value</b> 2260 * <p> 2261 * Description: <b>Value held by characteristic</b><br> 2262 * Type: <b>token</b><br> 2263 * Path: <b>Group.characteristic.value[x]</b><br> 2264 * </p> 2265 */ 2266 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VALUE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2267 SP_VALUE); 2268 2269 /** 2270 * Search parameter: <b>characteristic</b> 2271 * <p> 2272 * Description: <b>Kind of characteristic</b><br> 2273 * Type: <b>token</b><br> 2274 * Path: <b>Group.characteristic.code</b><br> 2275 * </p> 2276 */ 2277 @SearchParamDefinition(name = "characteristic", path = "Group.characteristic.code", description = "Kind of characteristic", type = "token") 2278 public static final String SP_CHARACTERISTIC = "characteristic"; 2279 /** 2280 * <b>Fluent Client</b> search parameter constant for <b>characteristic</b> 2281 * <p> 2282 * Description: <b>Kind of characteristic</b><br> 2283 * Type: <b>token</b><br> 2284 * Path: <b>Group.characteristic.code</b><br> 2285 * </p> 2286 */ 2287 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CHARACTERISTIC = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2288 SP_CHARACTERISTIC); 2289 2290}